Class Class Class this pointer Constructors Destructor Copy
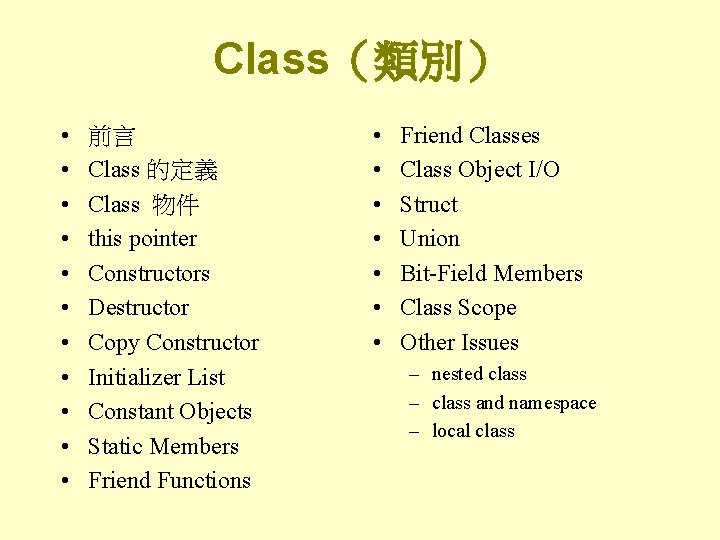
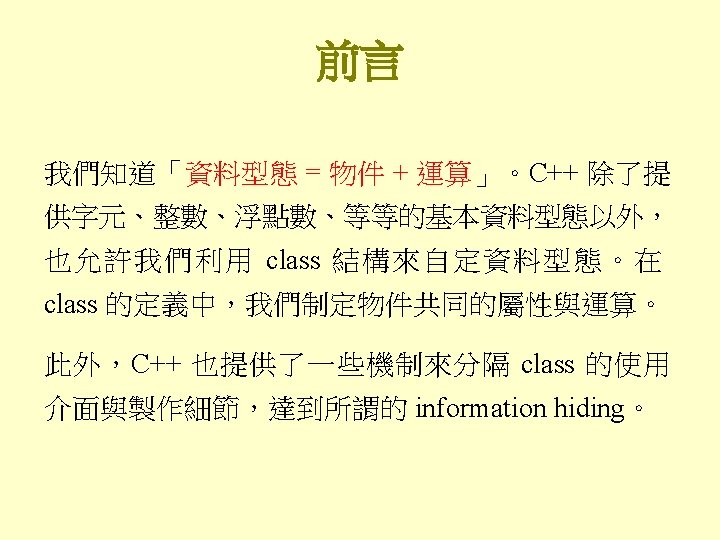
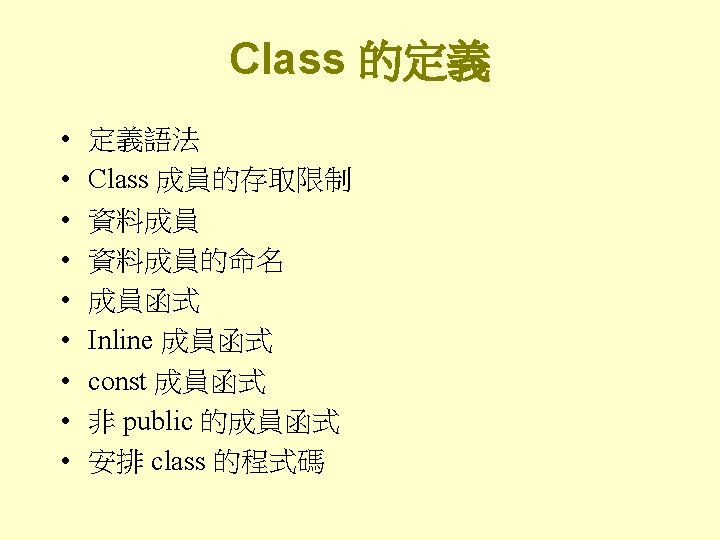
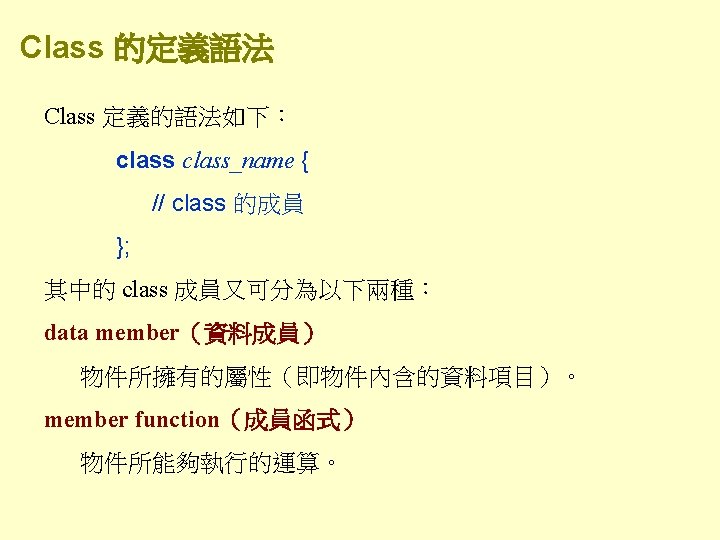
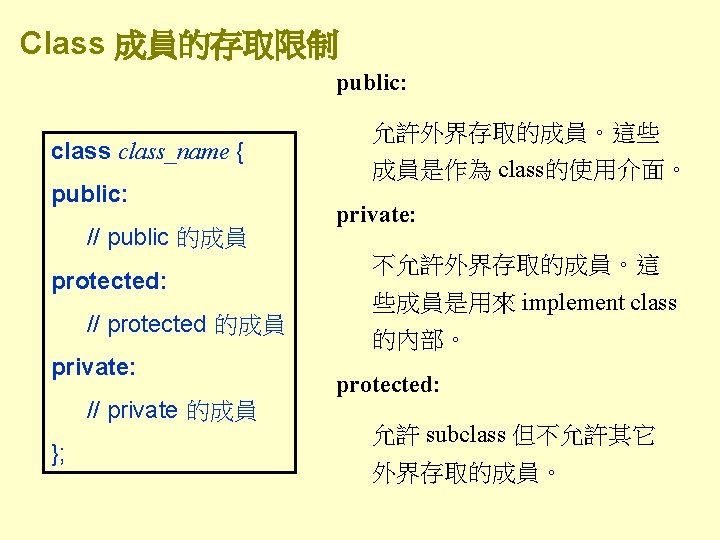
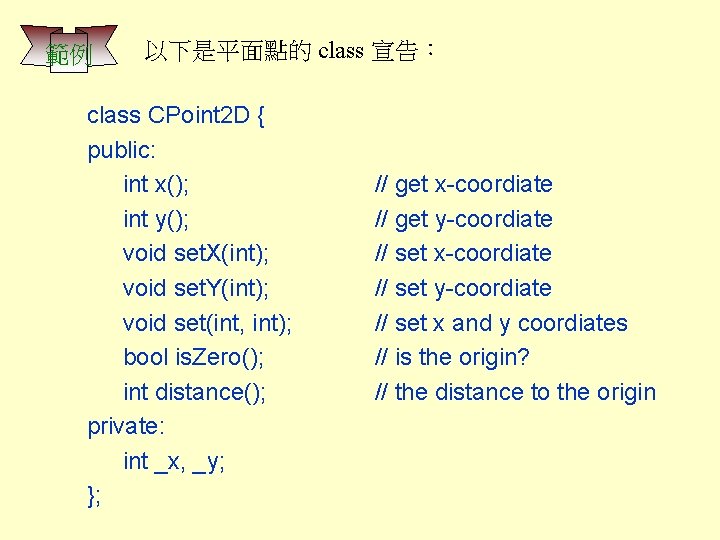
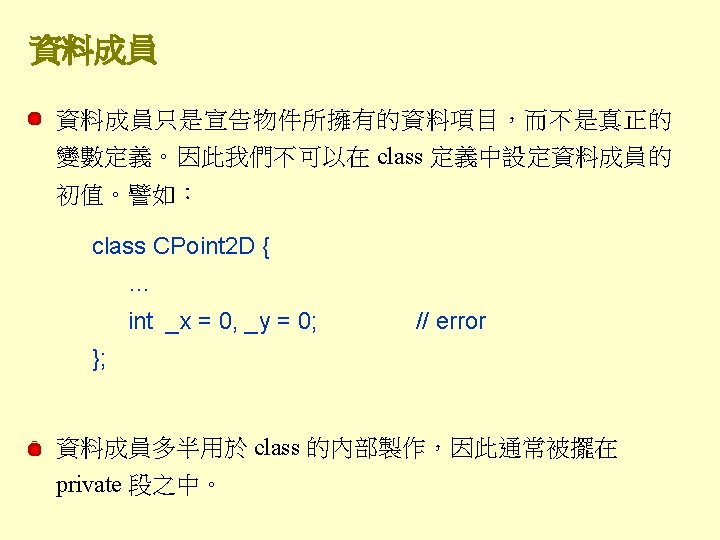
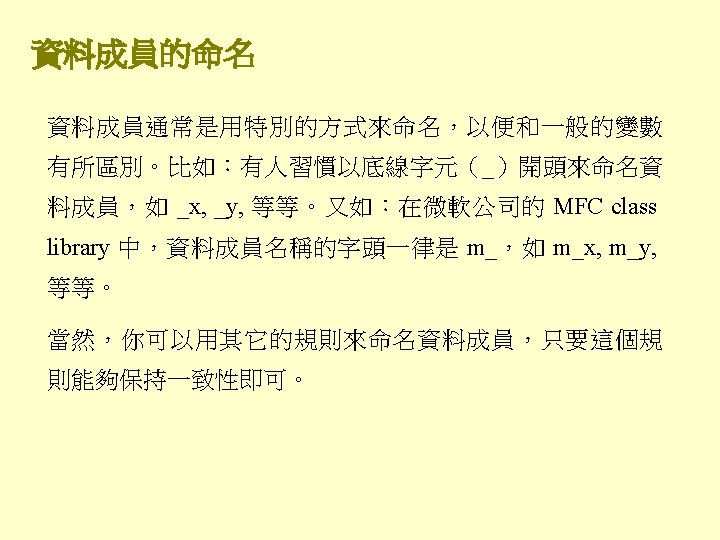
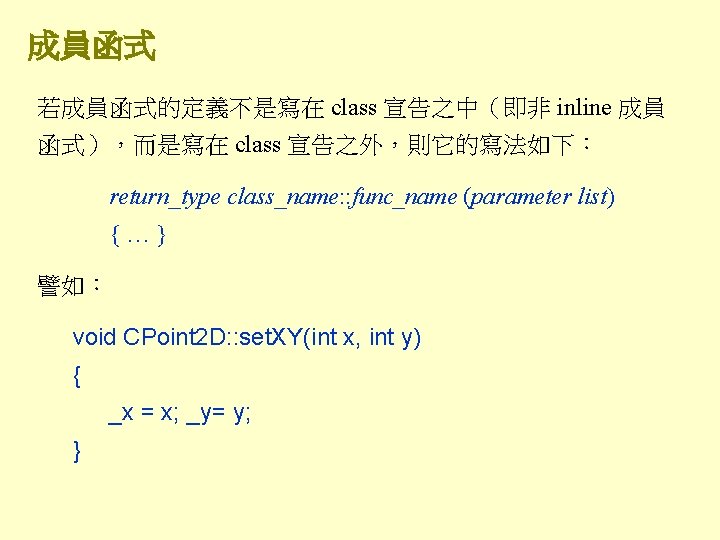
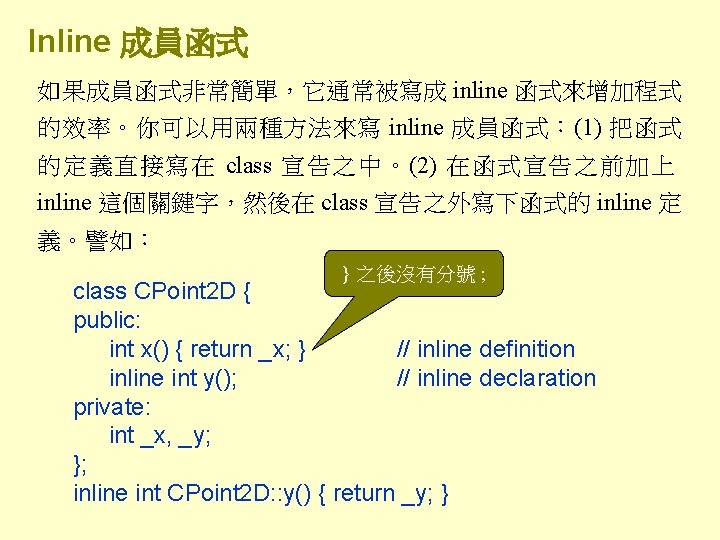
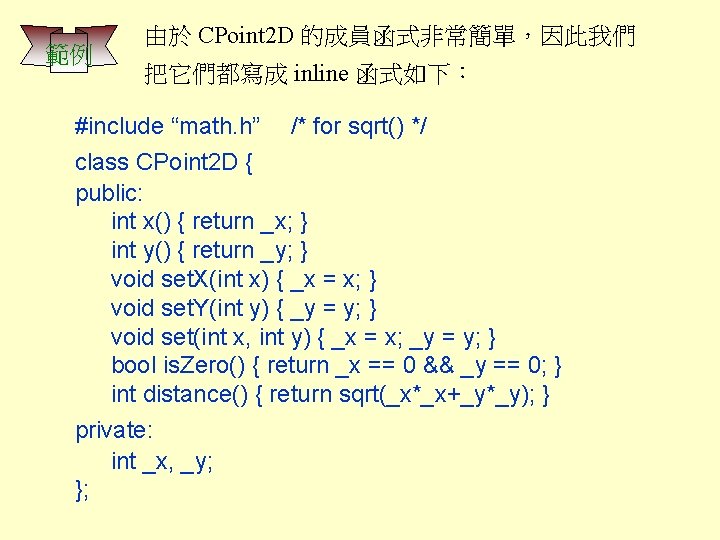
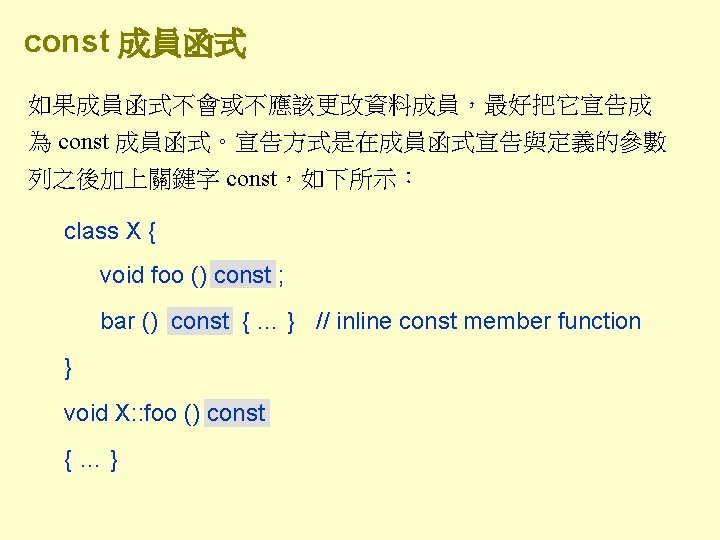
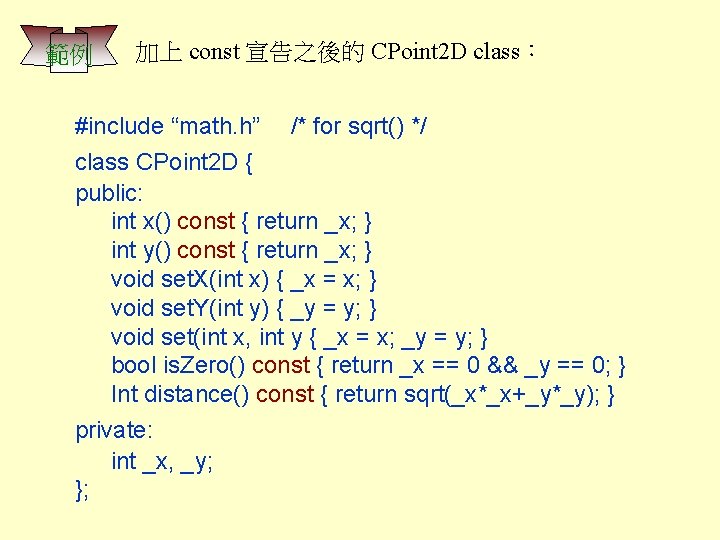
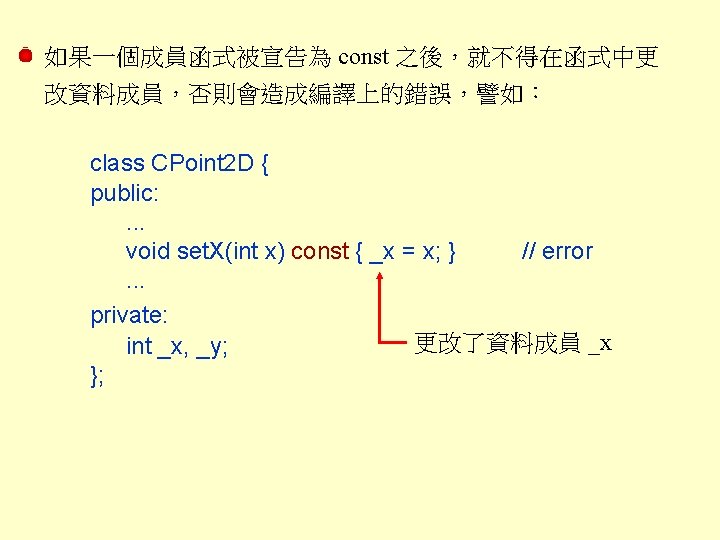
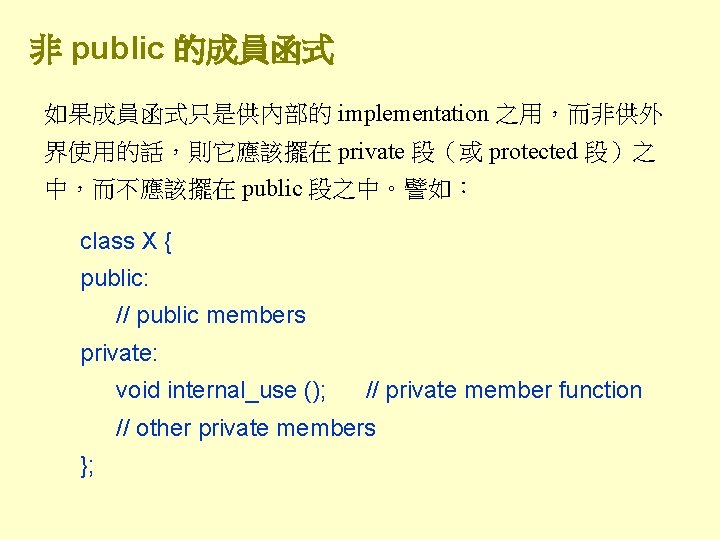
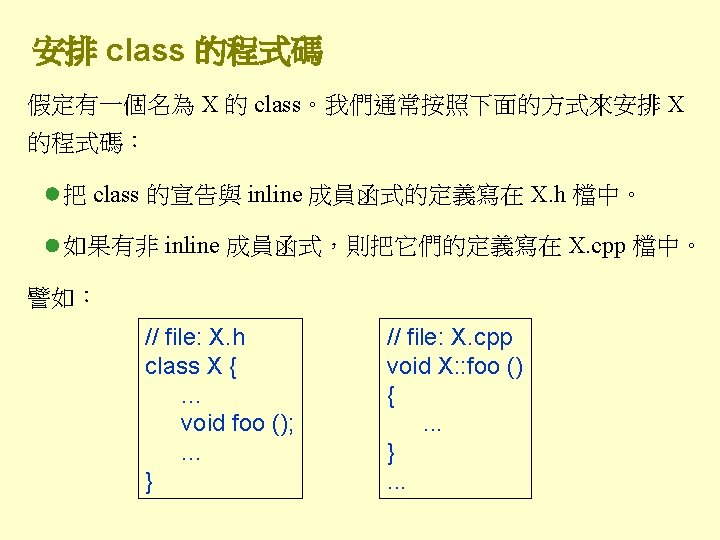
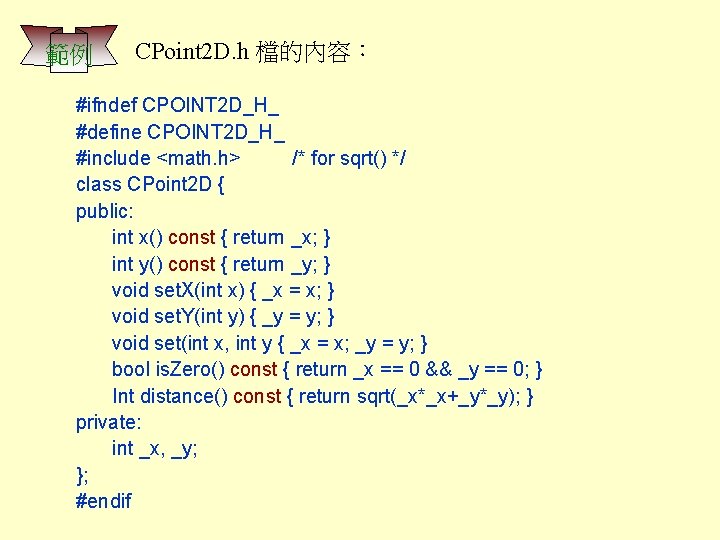
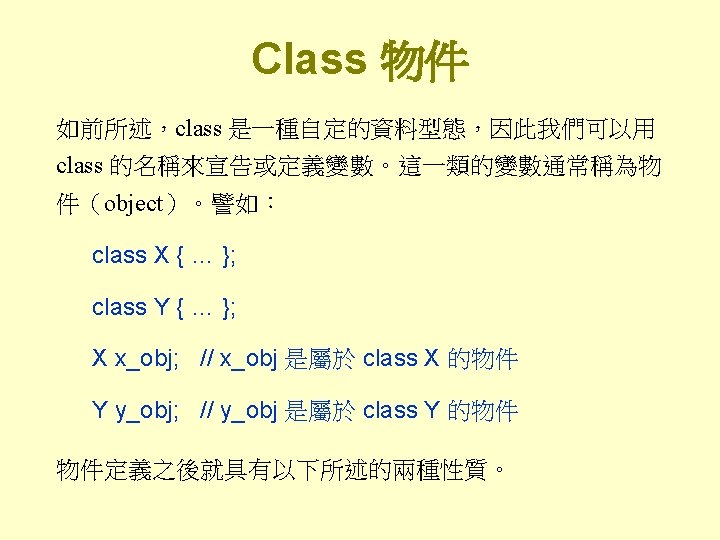
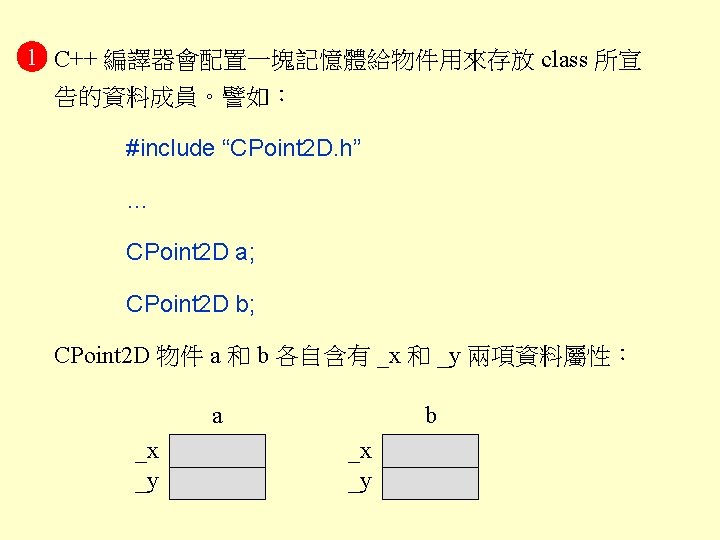
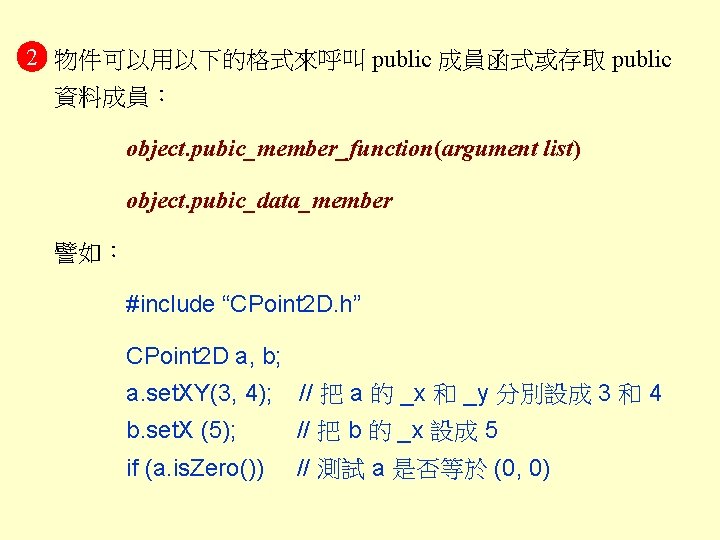
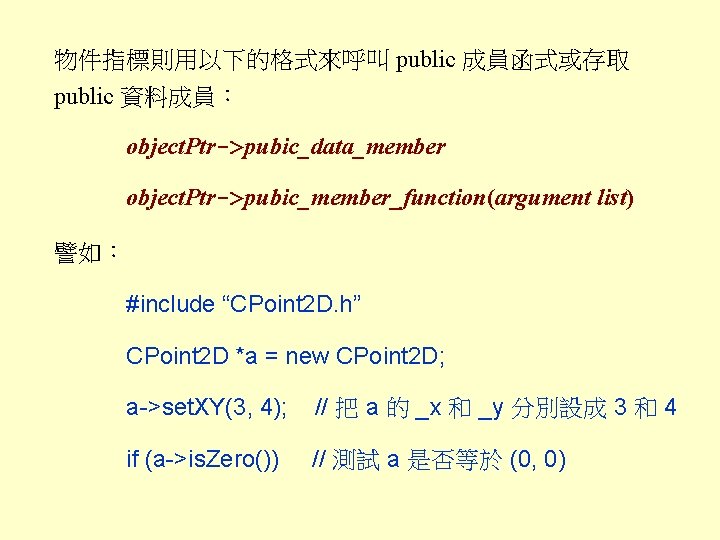
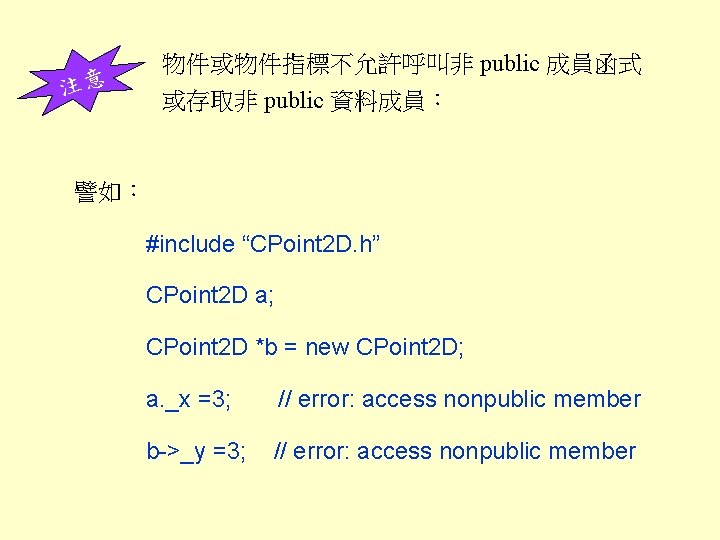
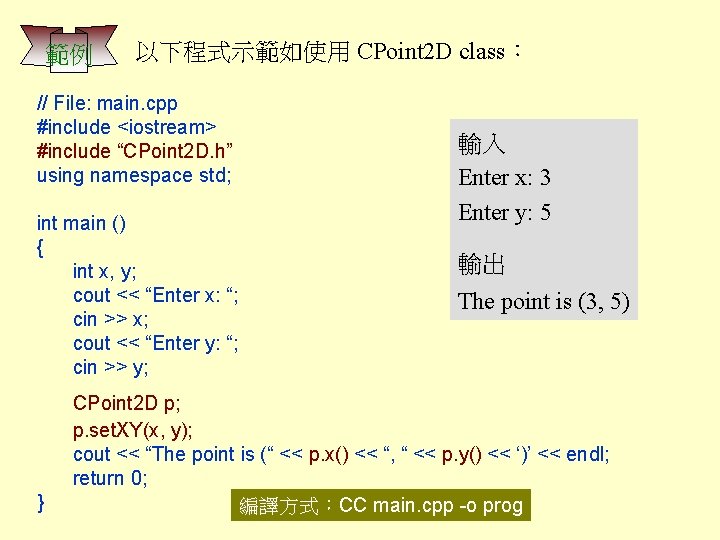
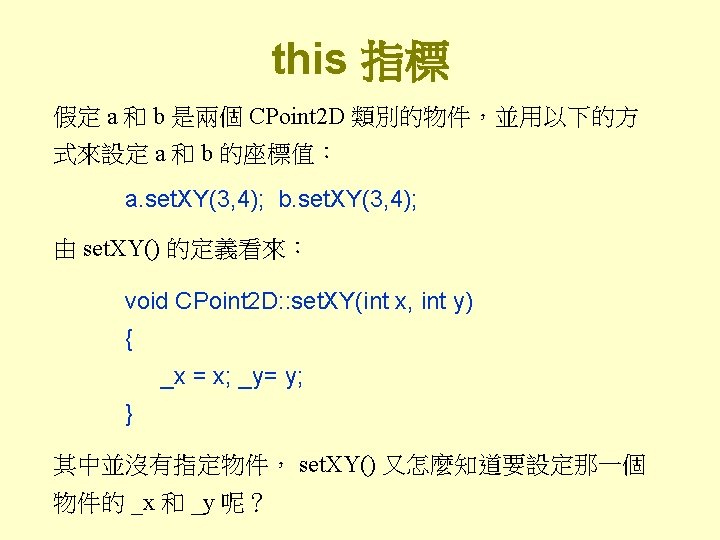
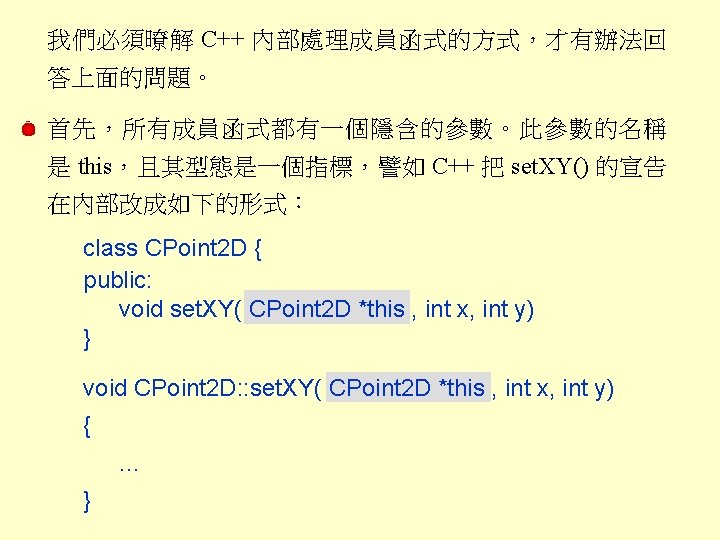
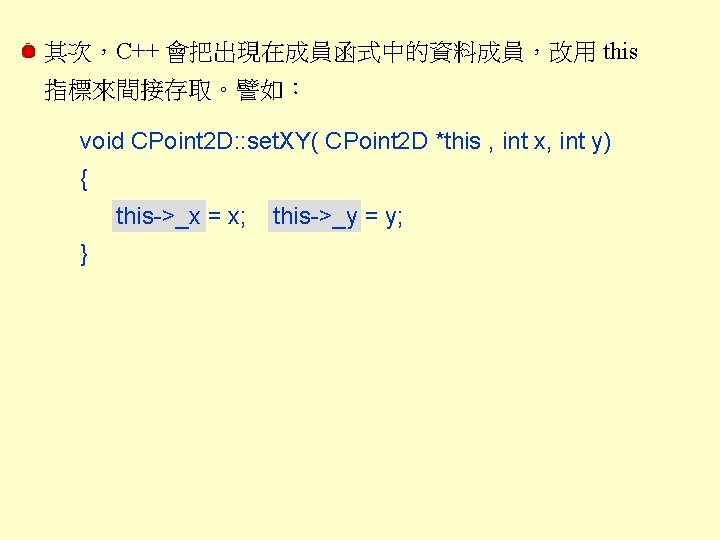
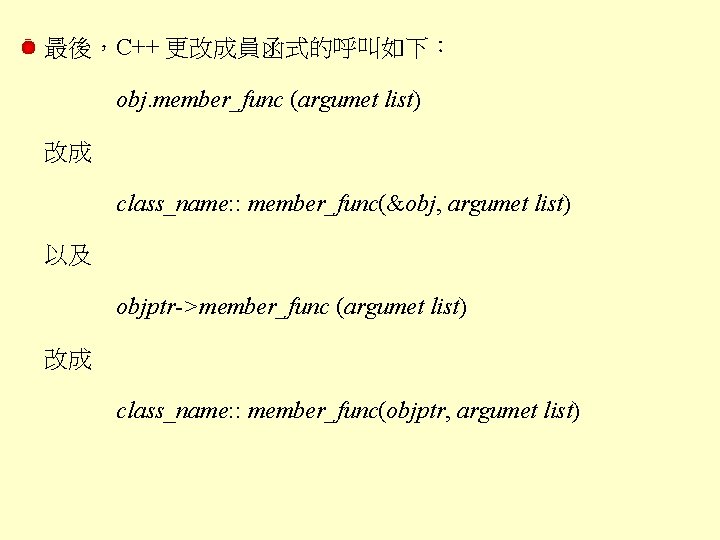
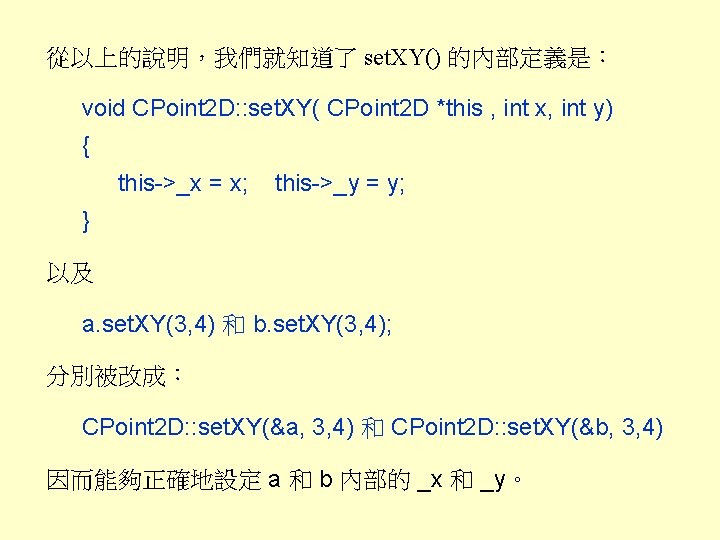
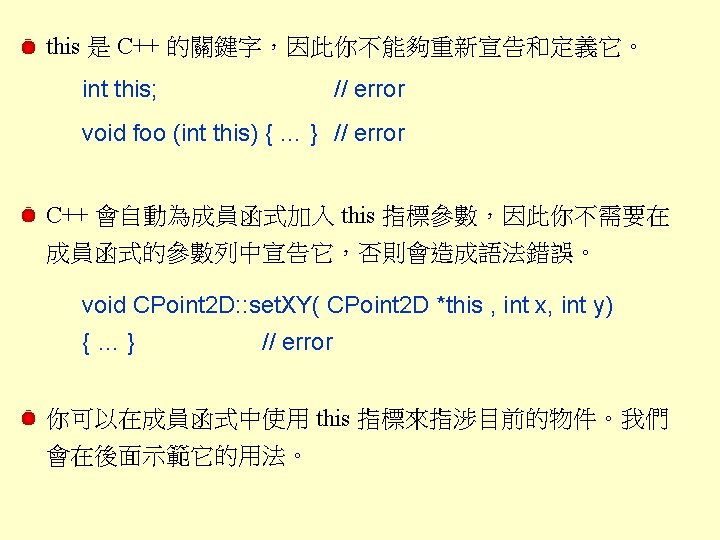
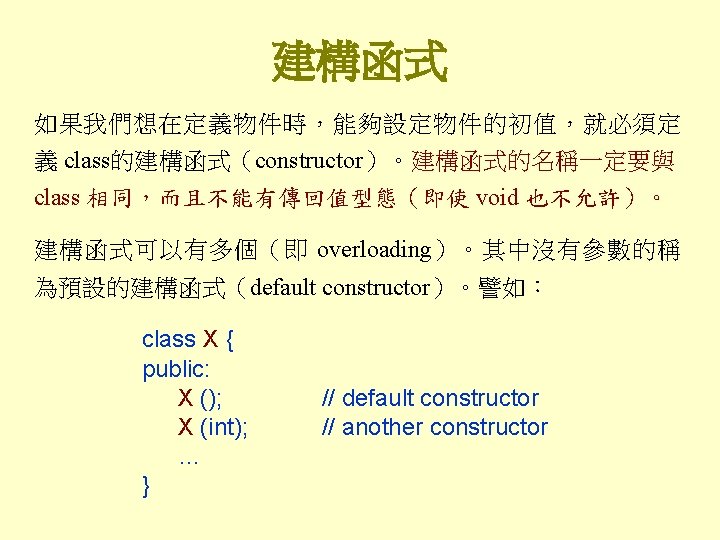
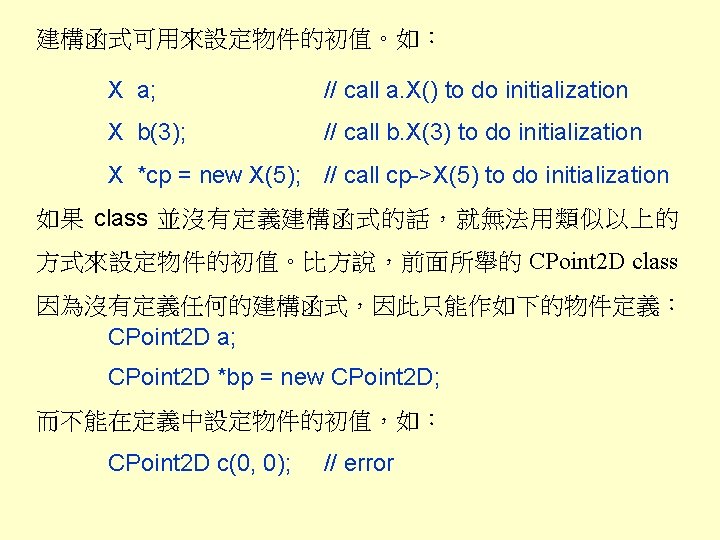
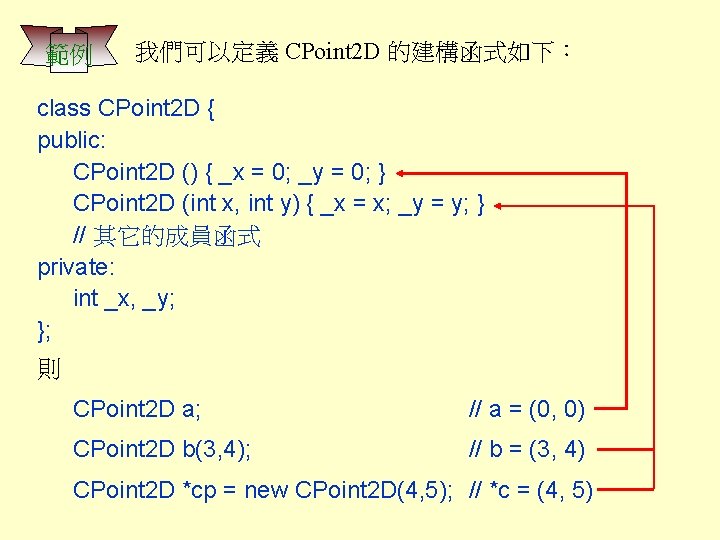
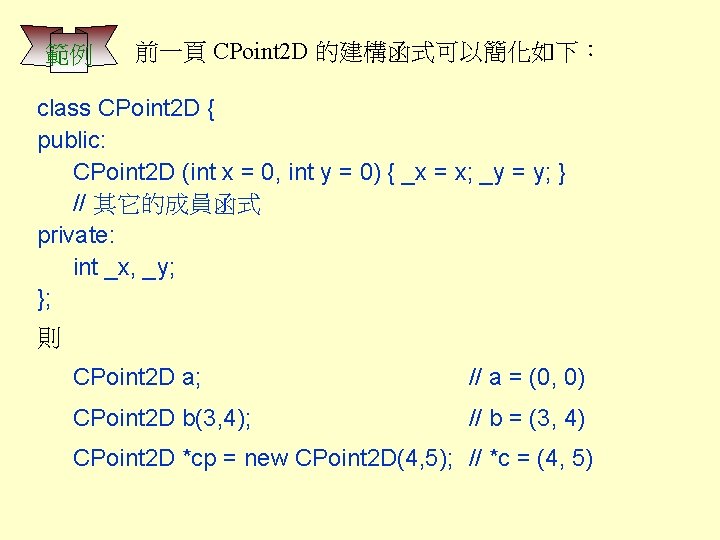
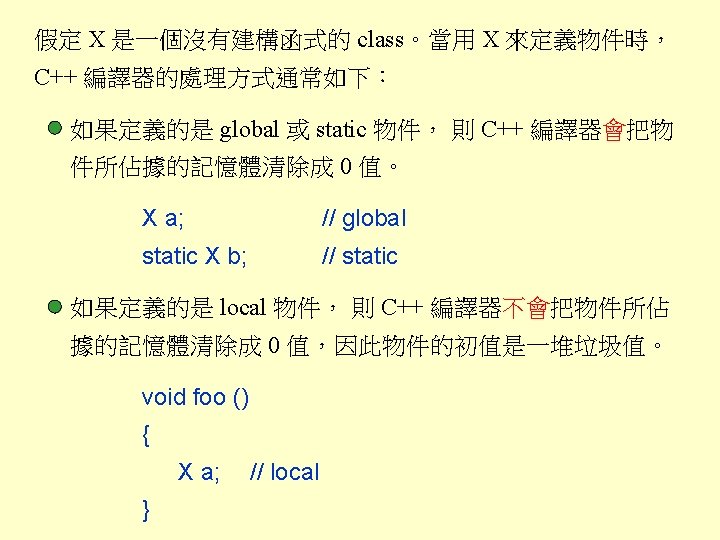
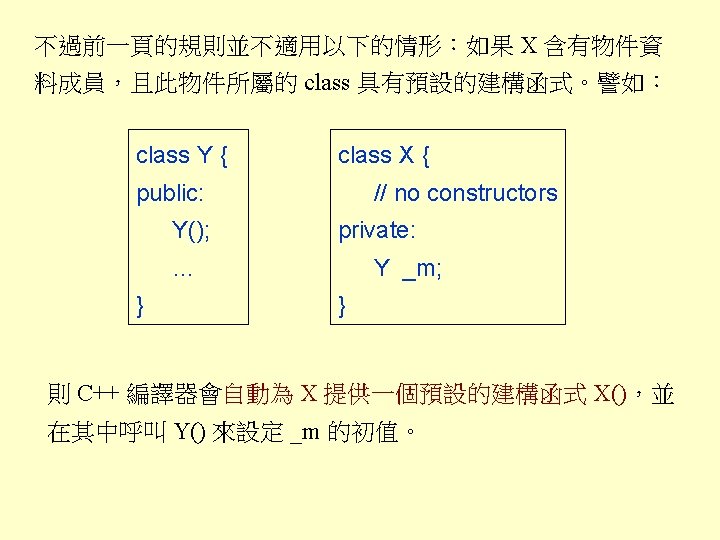
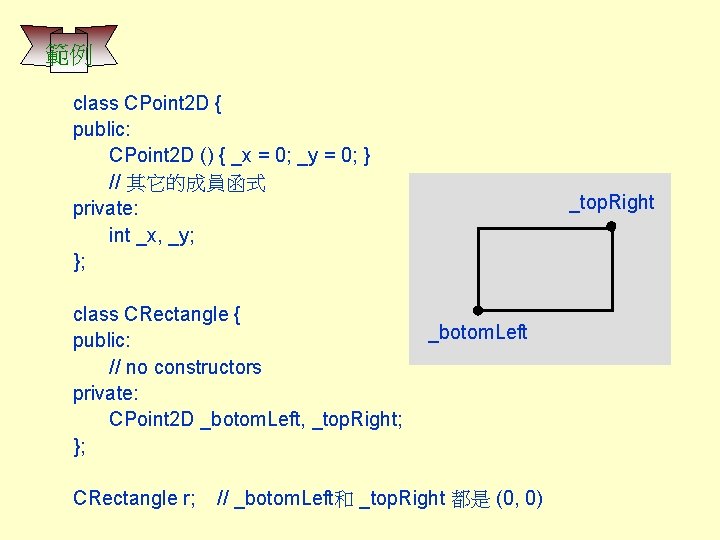
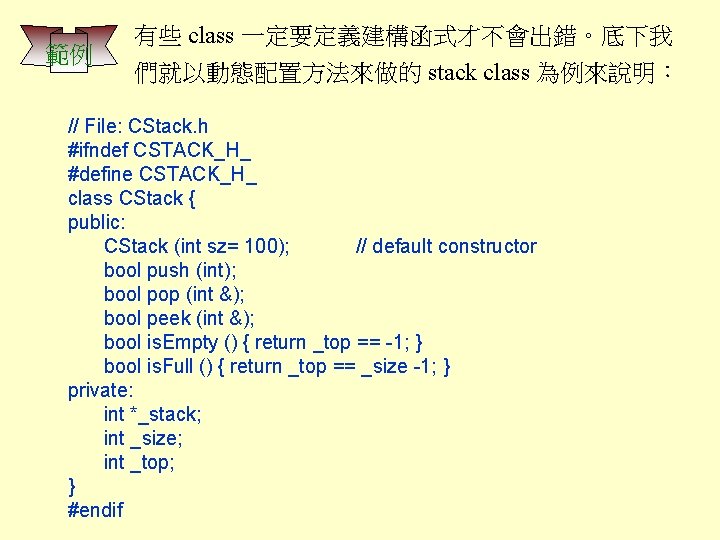
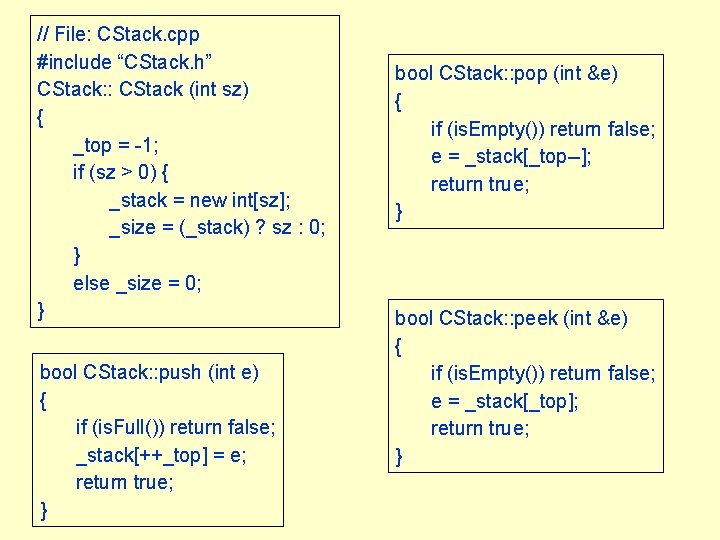
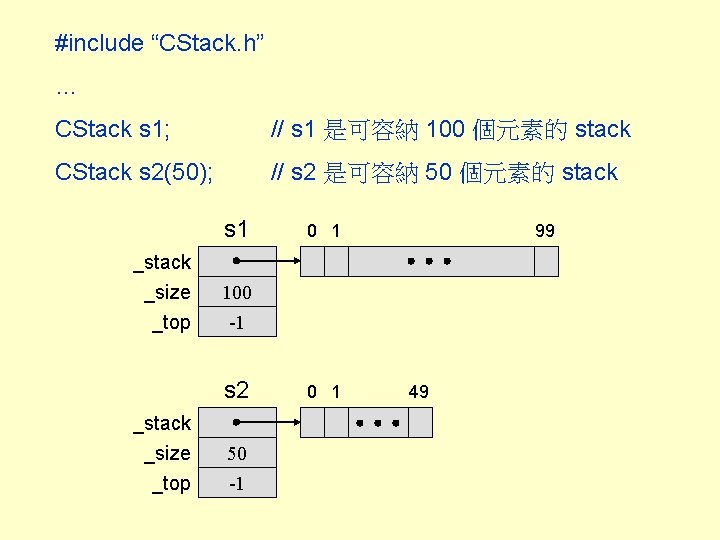
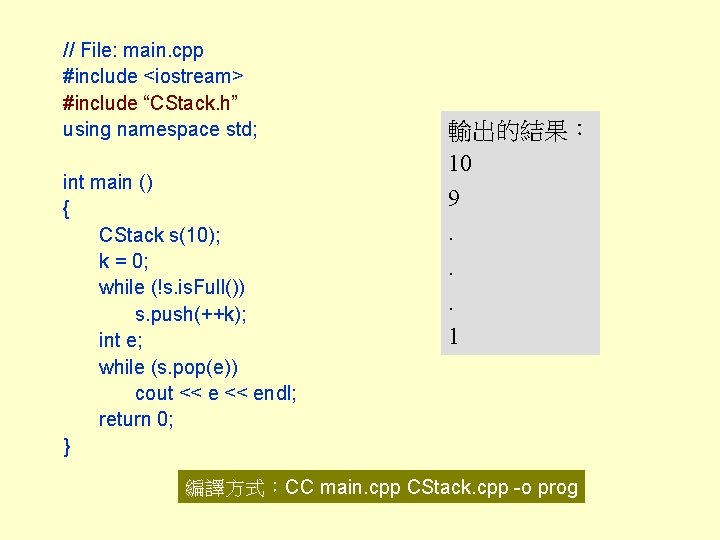
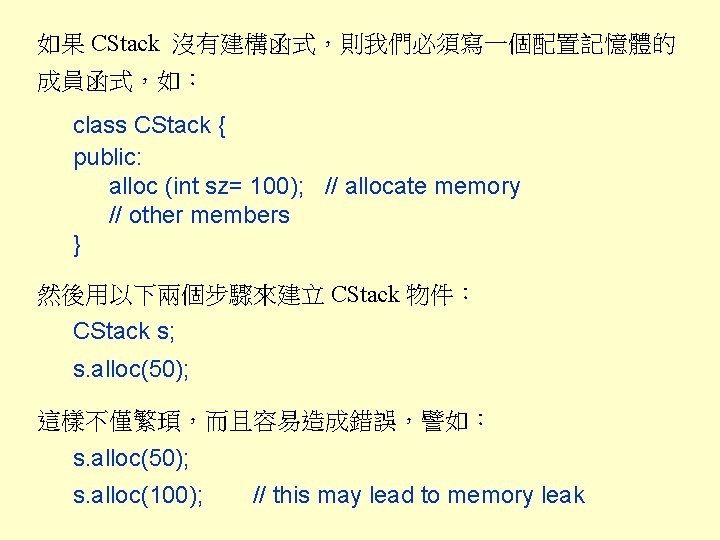
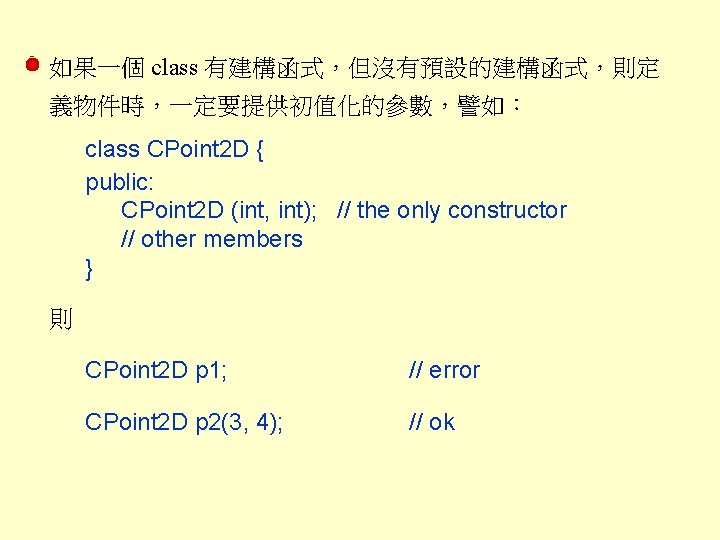
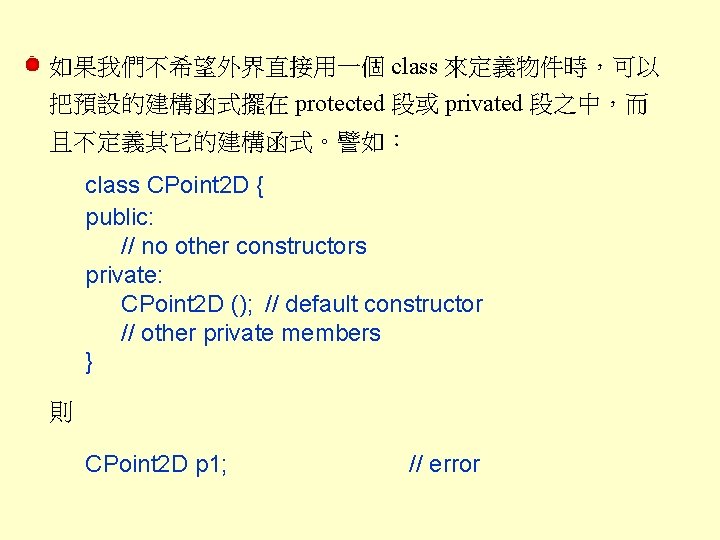
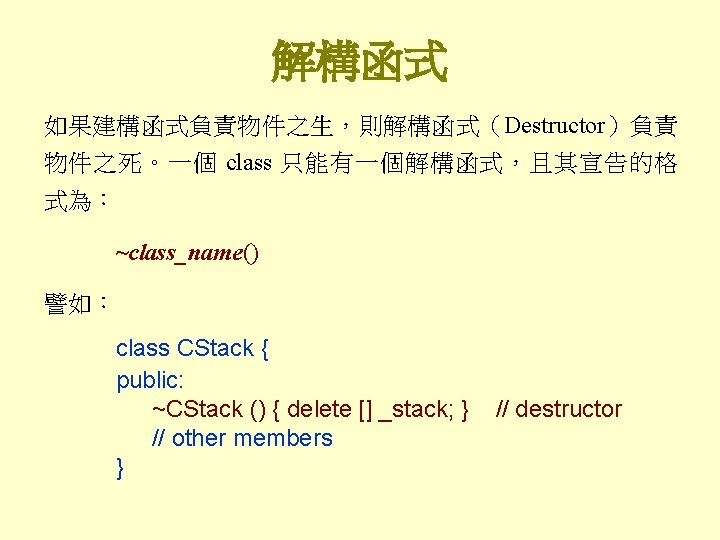
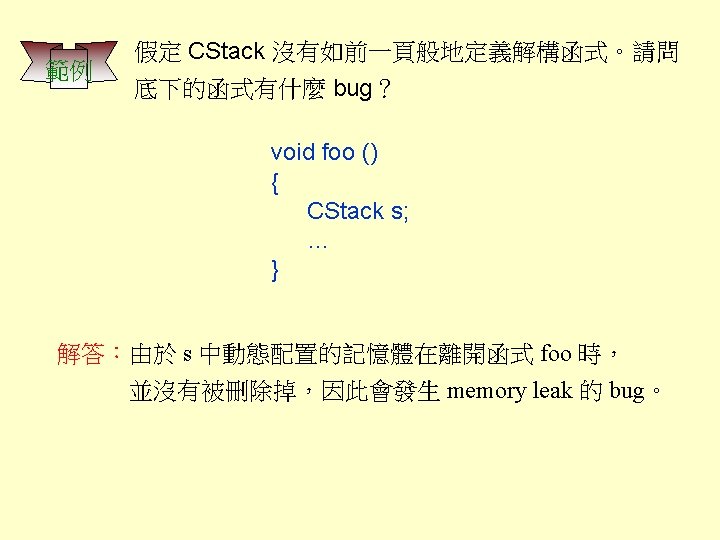
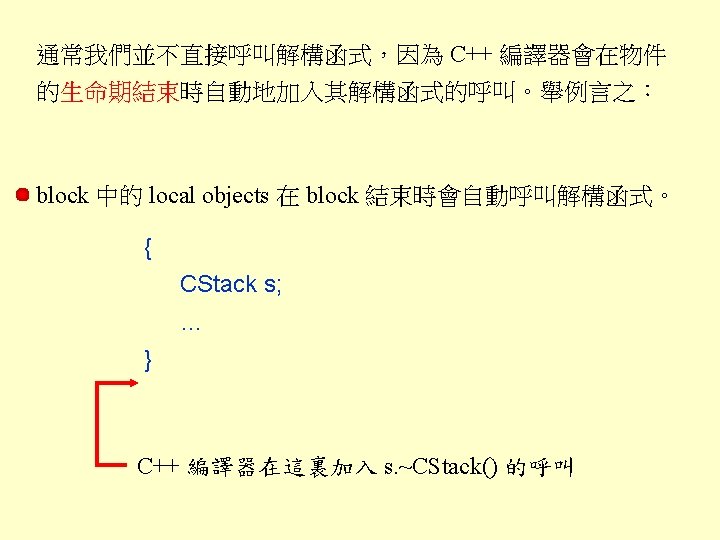
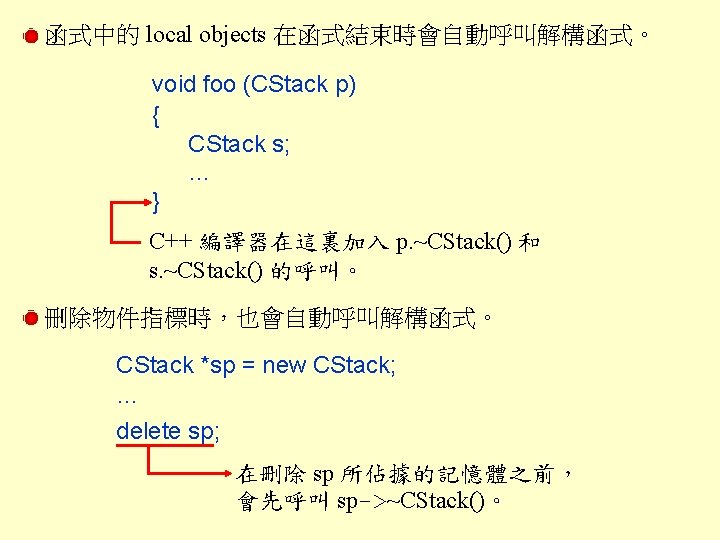
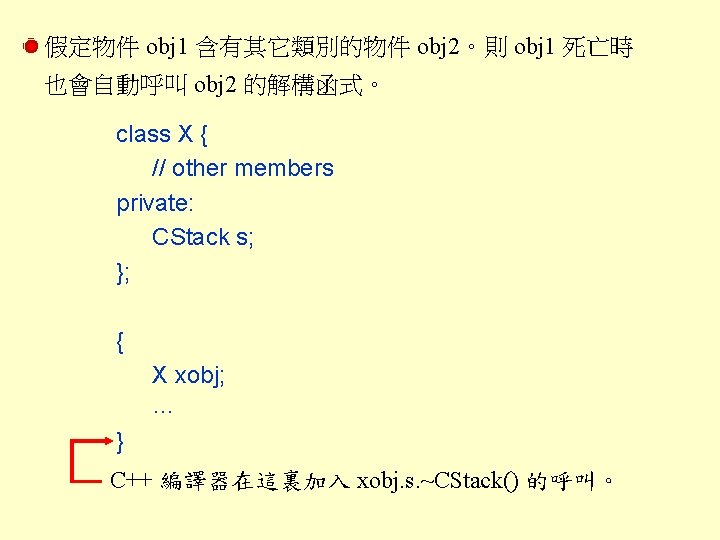
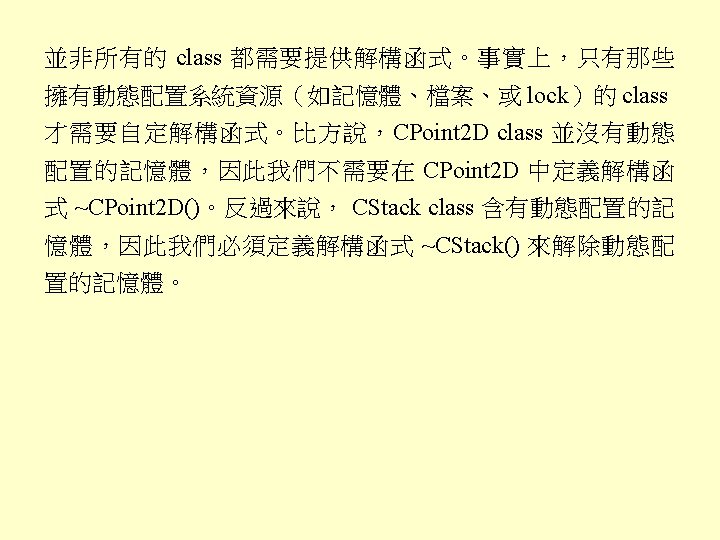
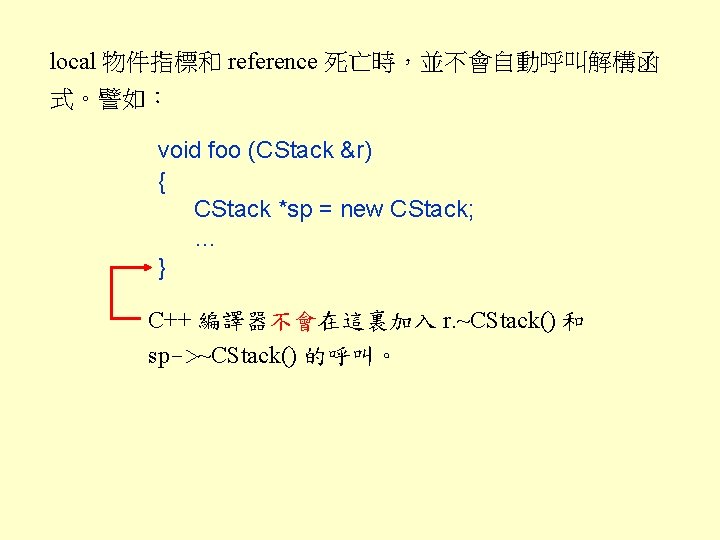
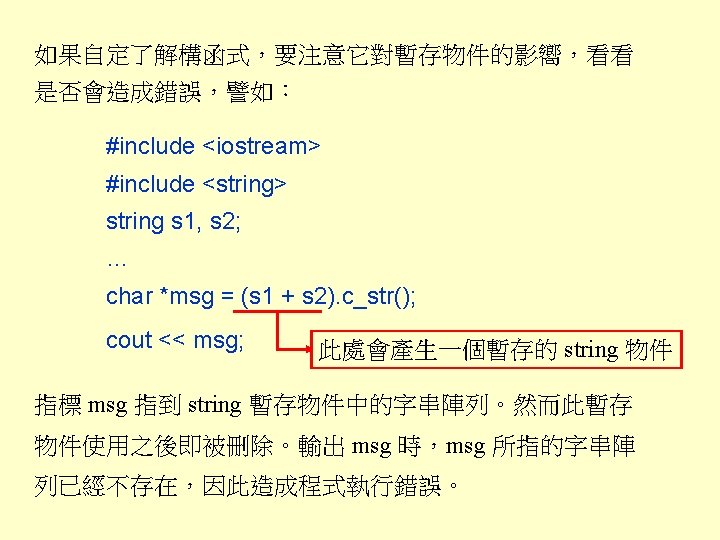
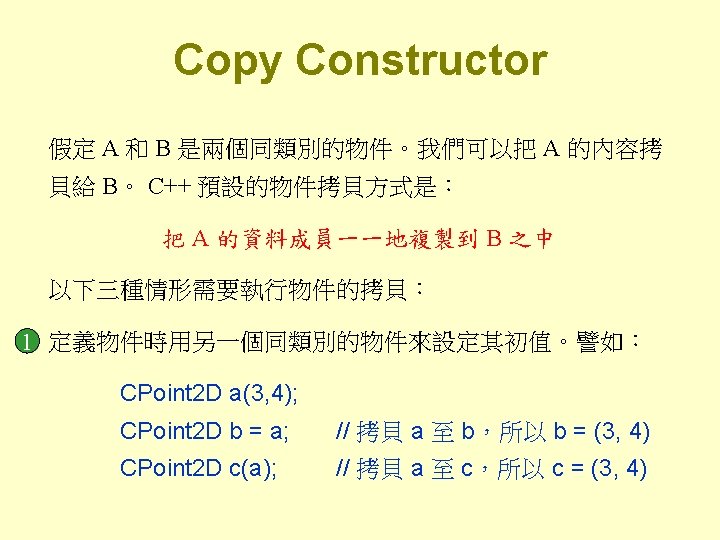
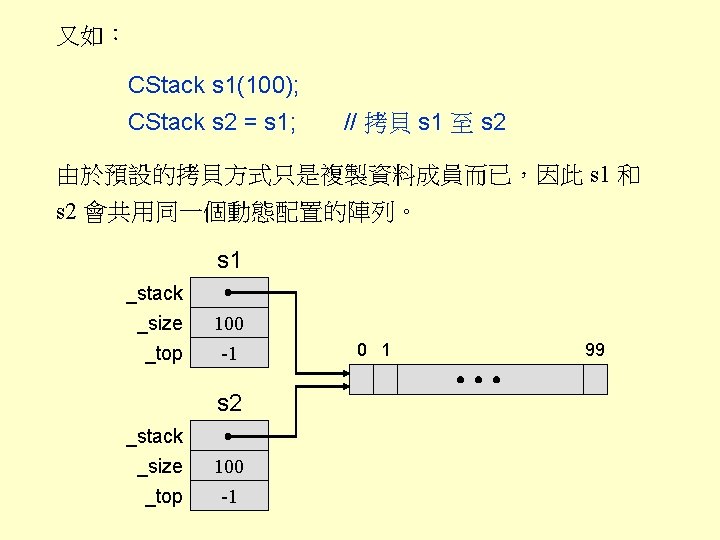
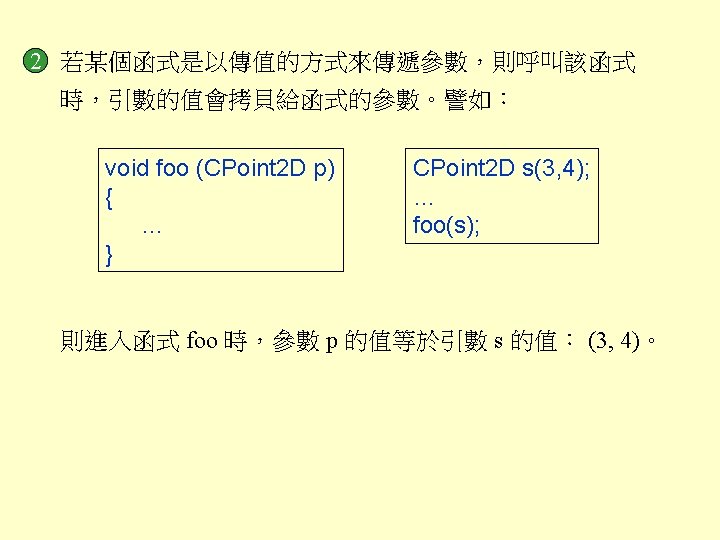
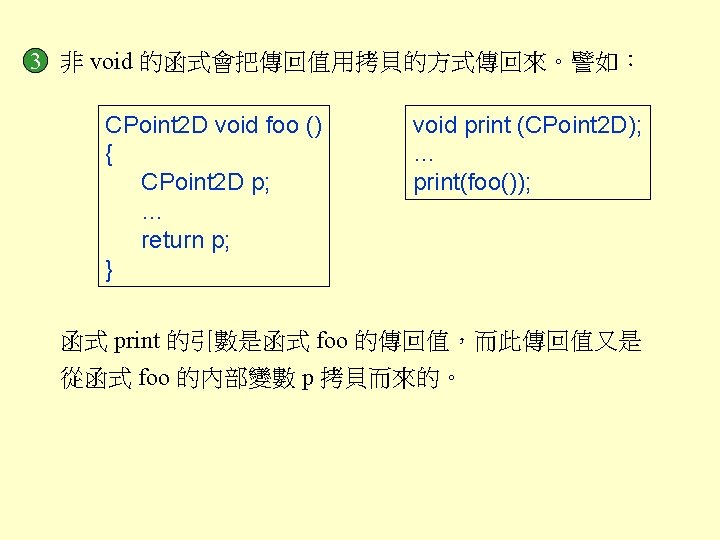
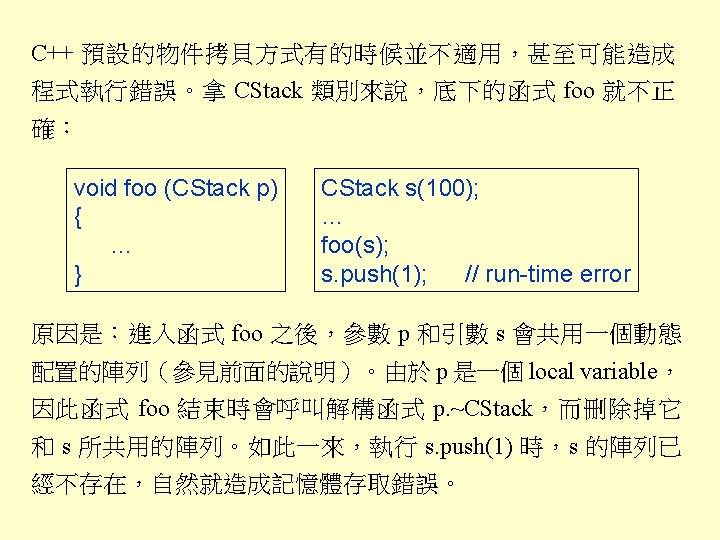
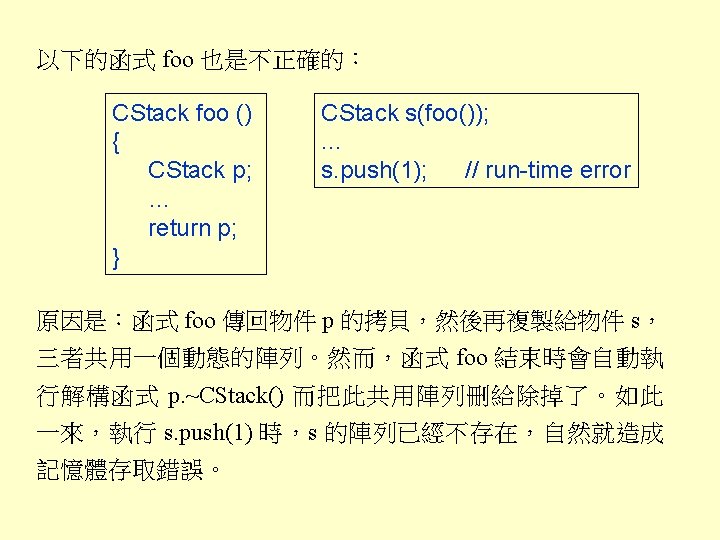
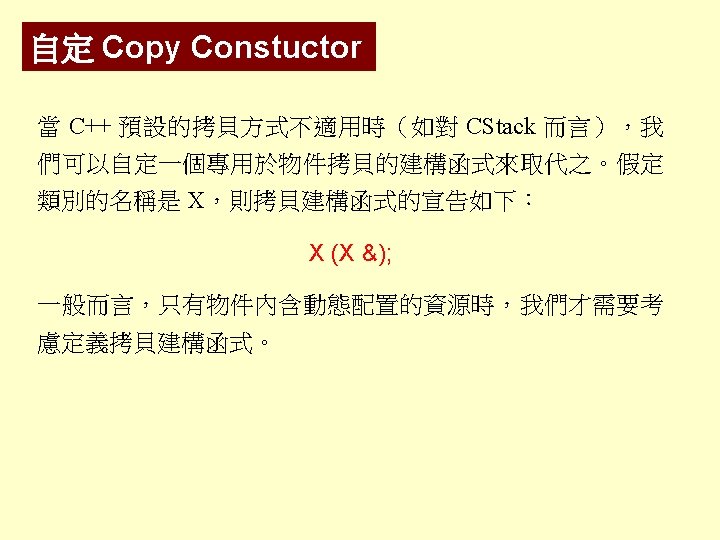
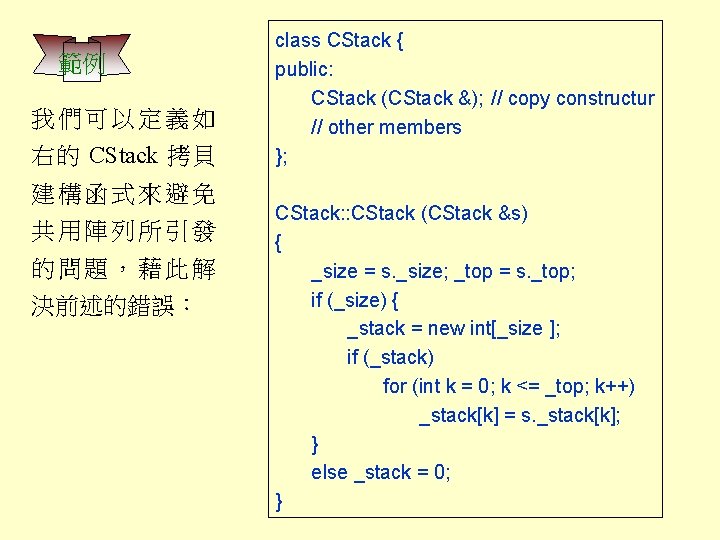
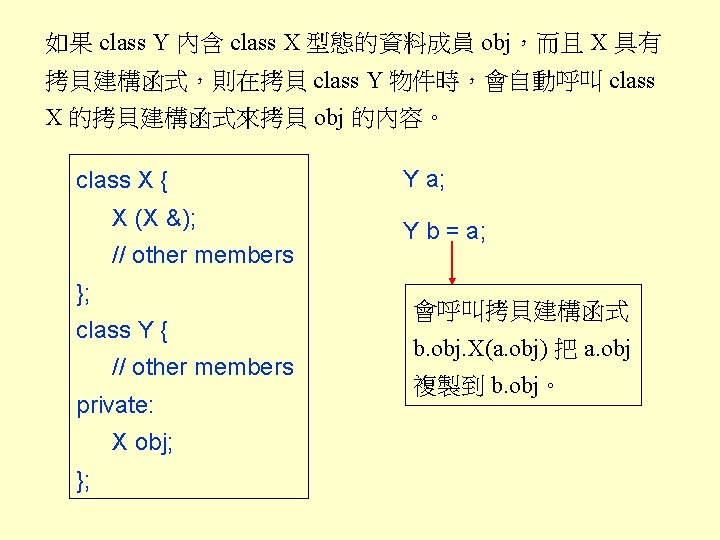
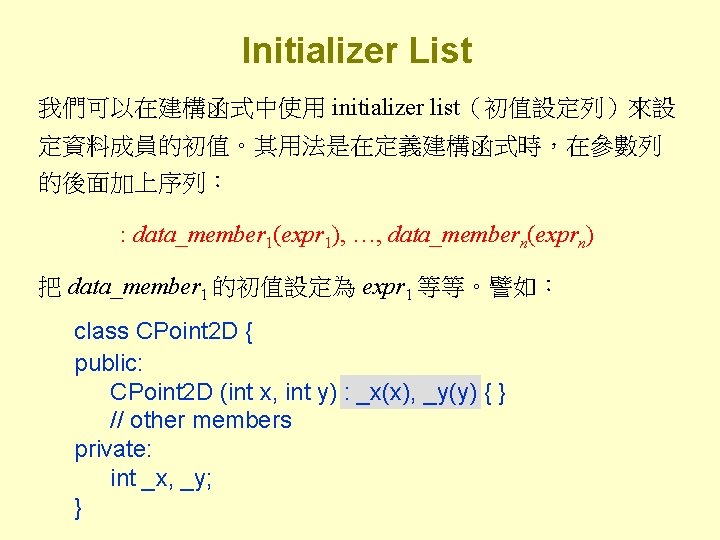
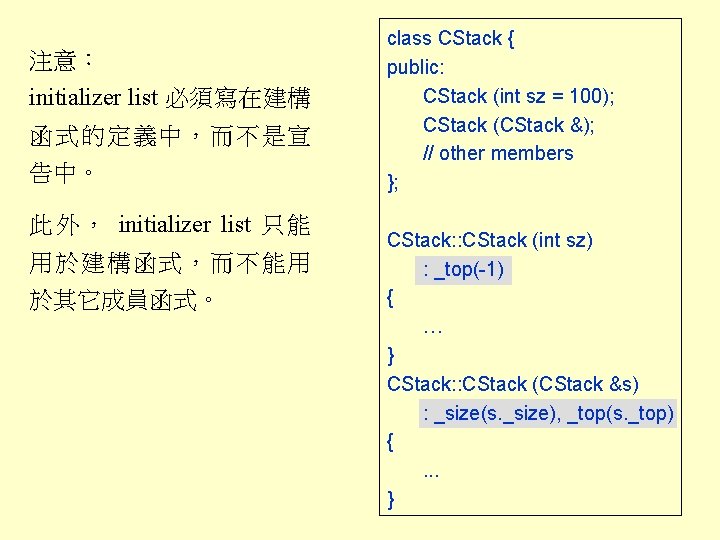
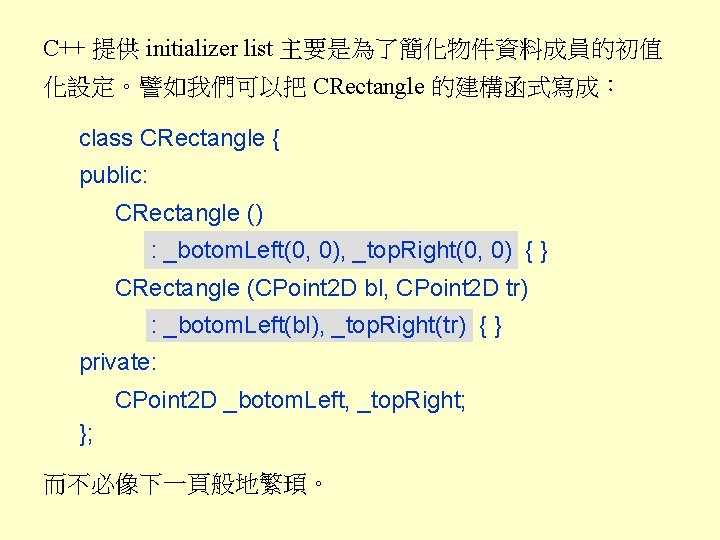
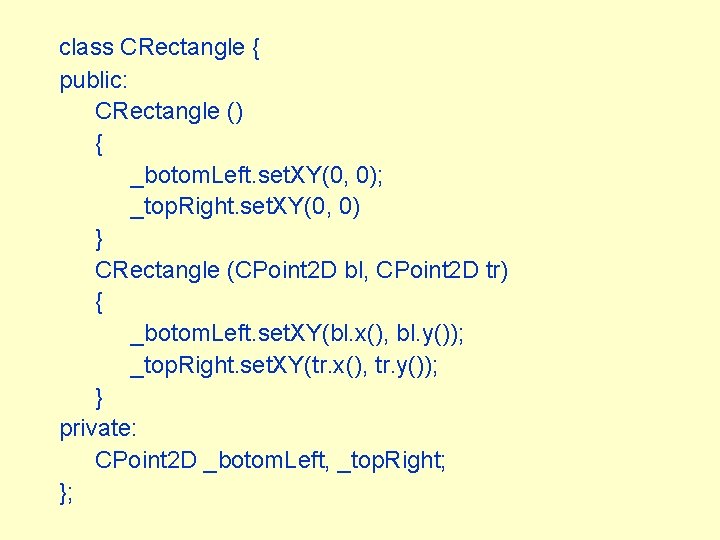
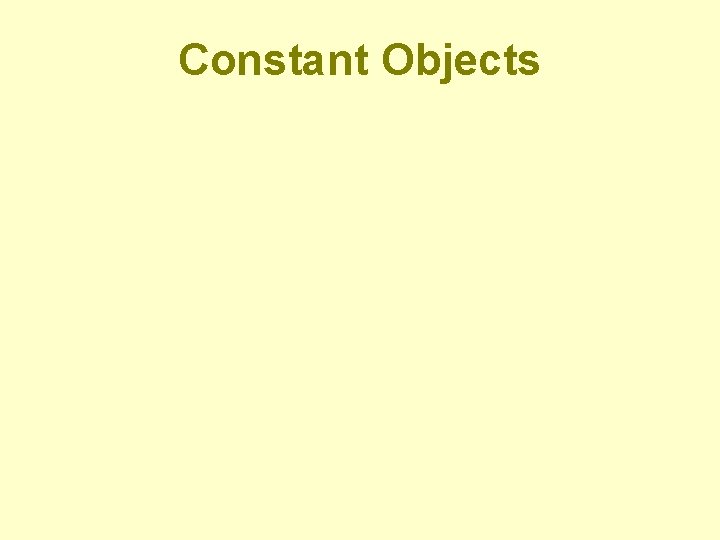
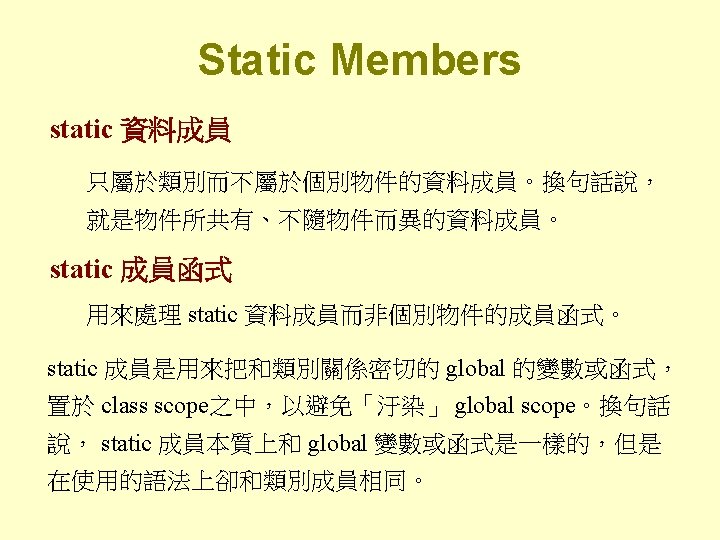
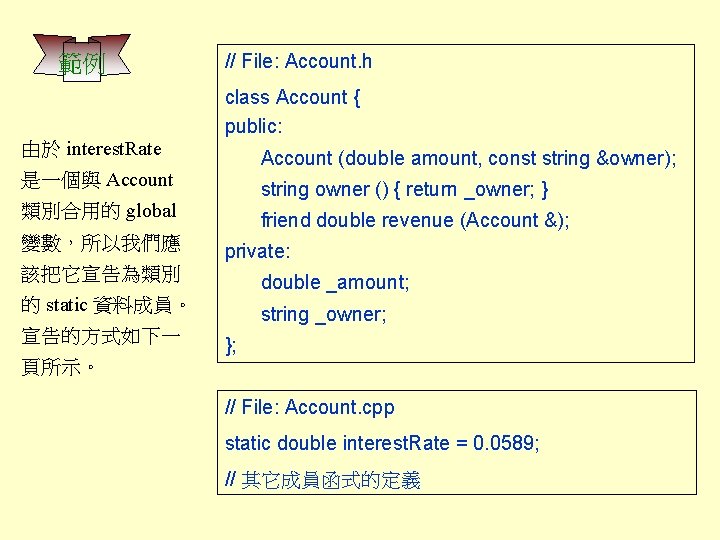
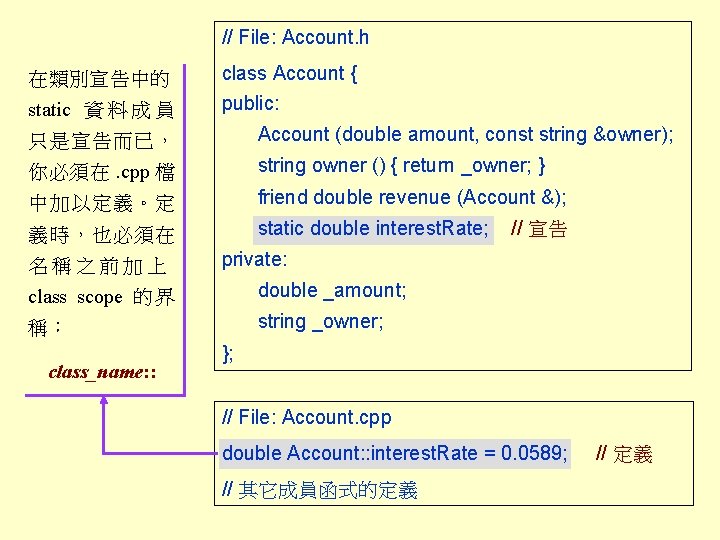
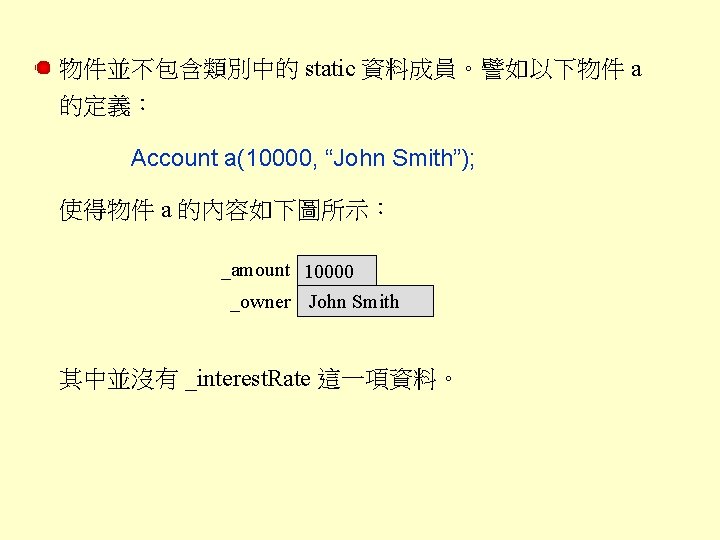
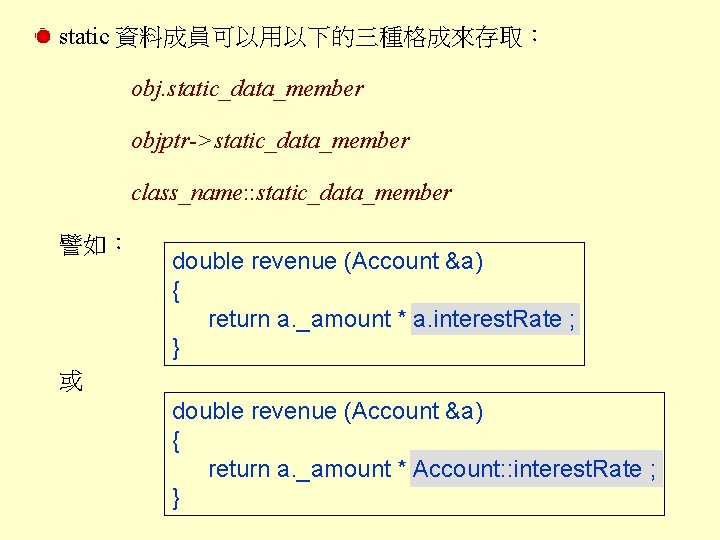
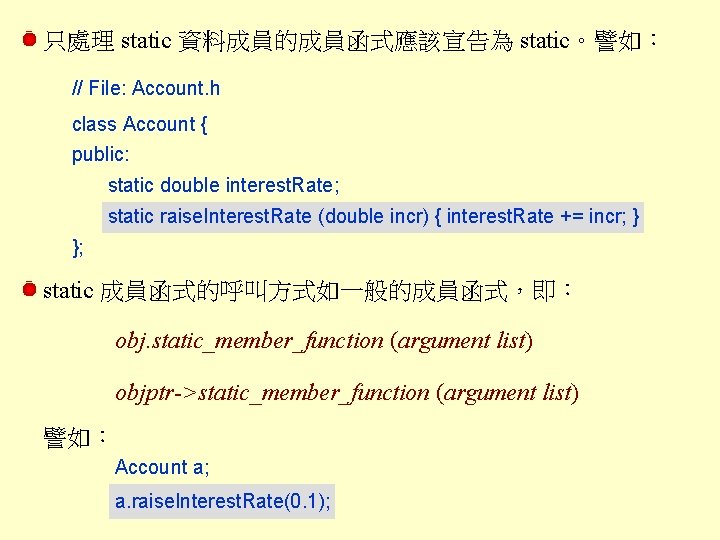
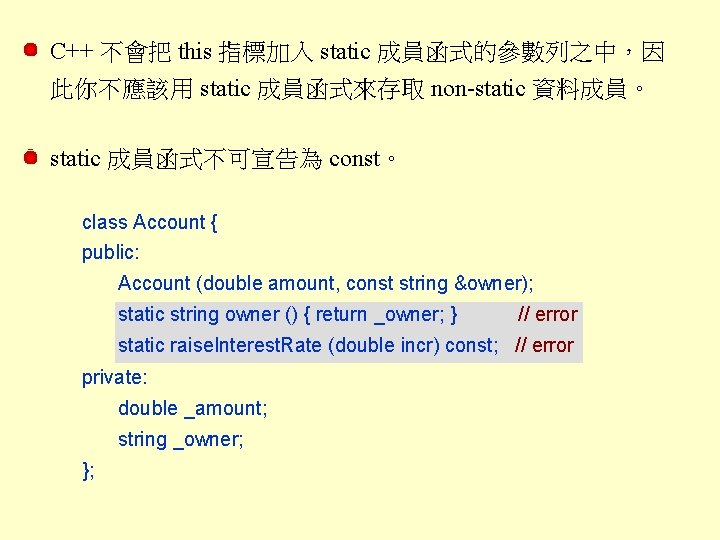
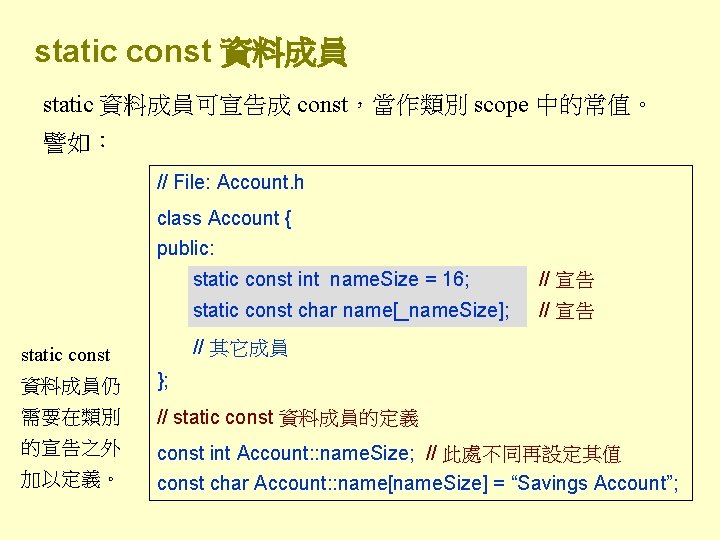
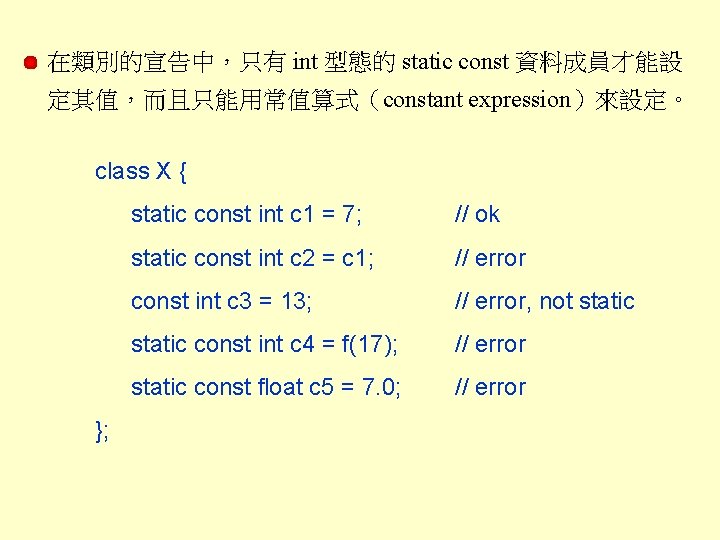
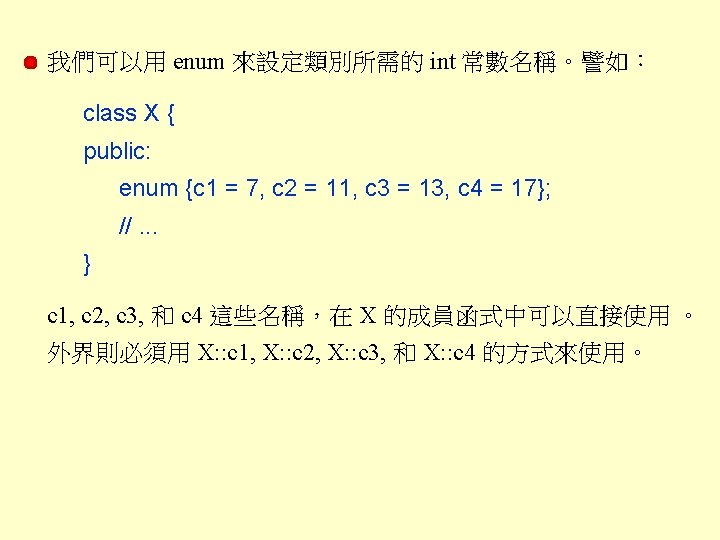
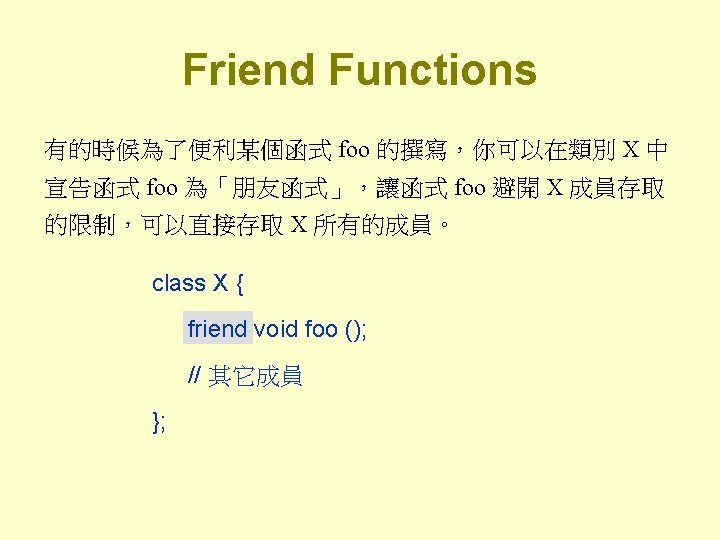
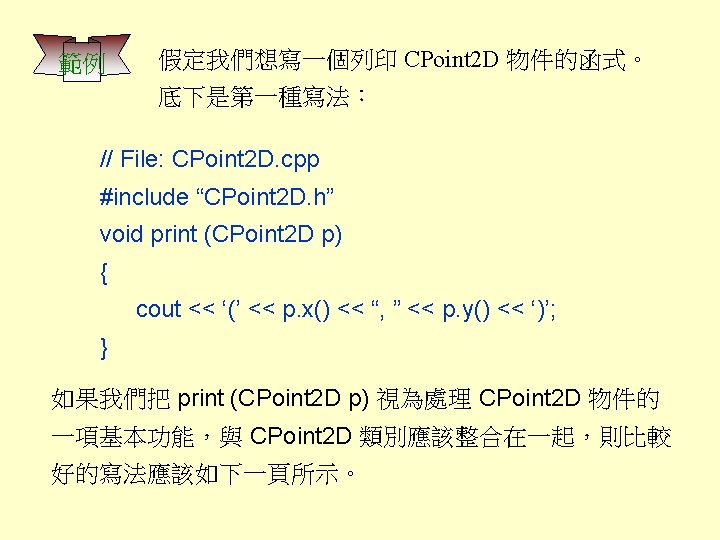
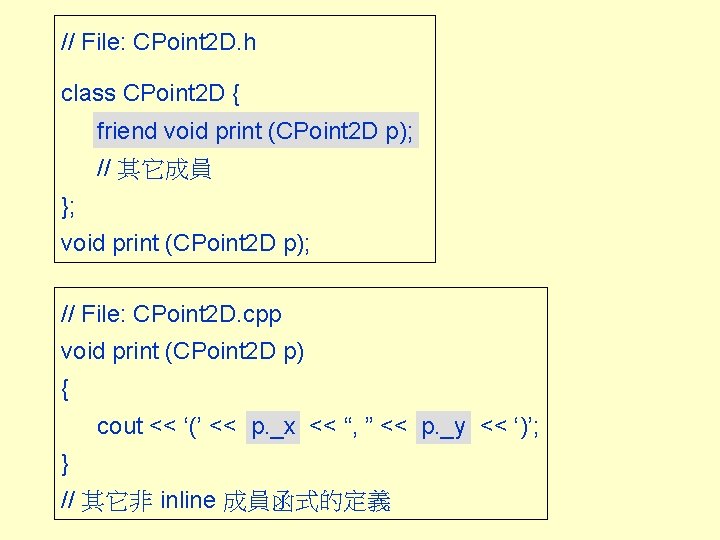
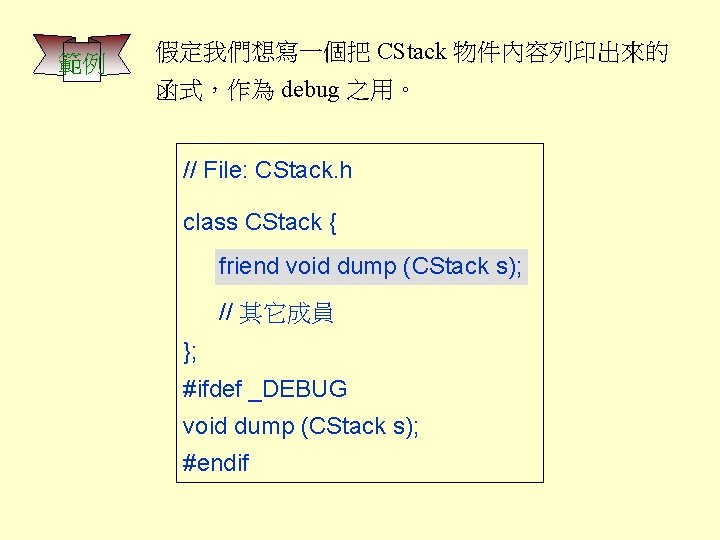
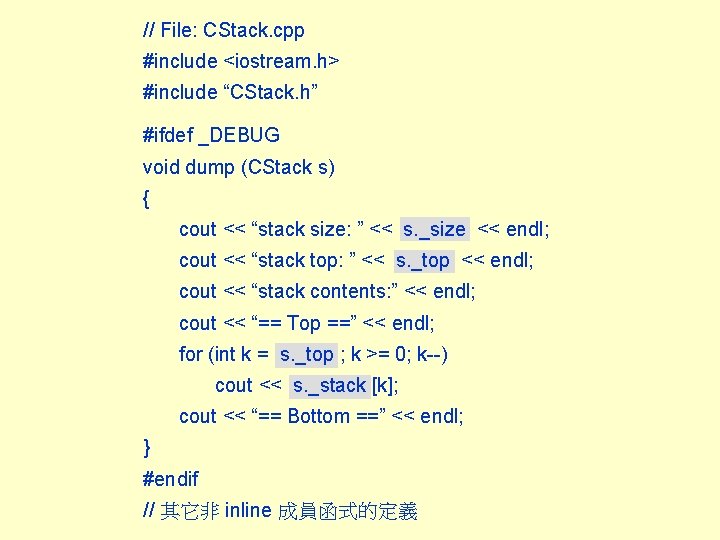
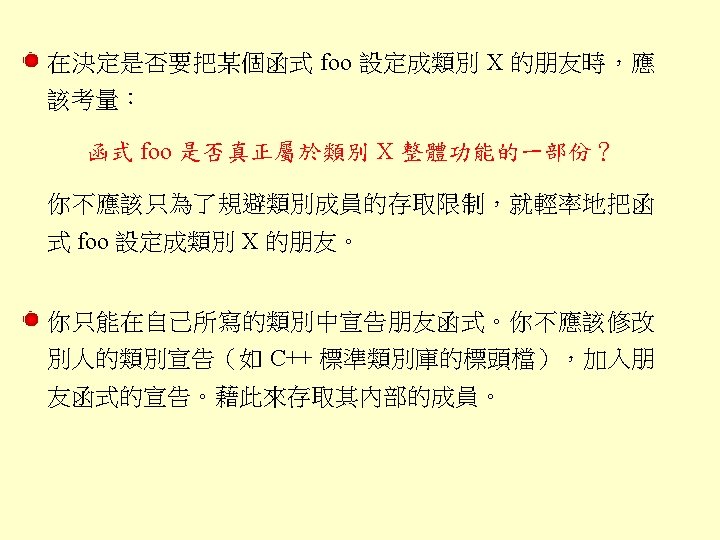
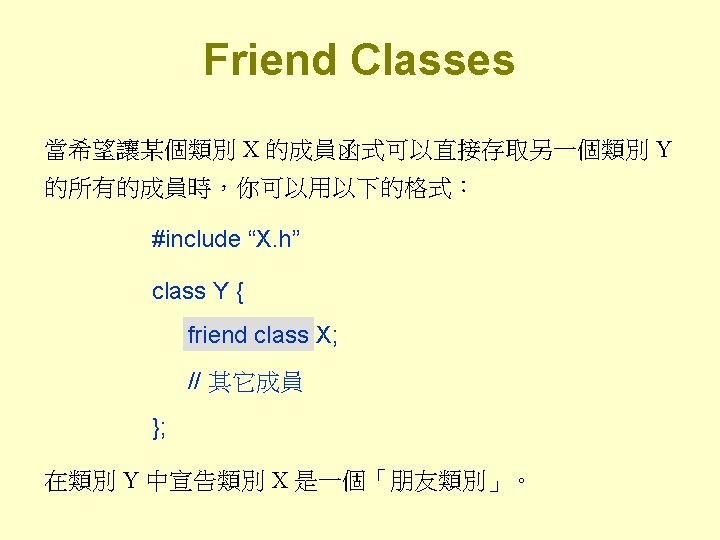
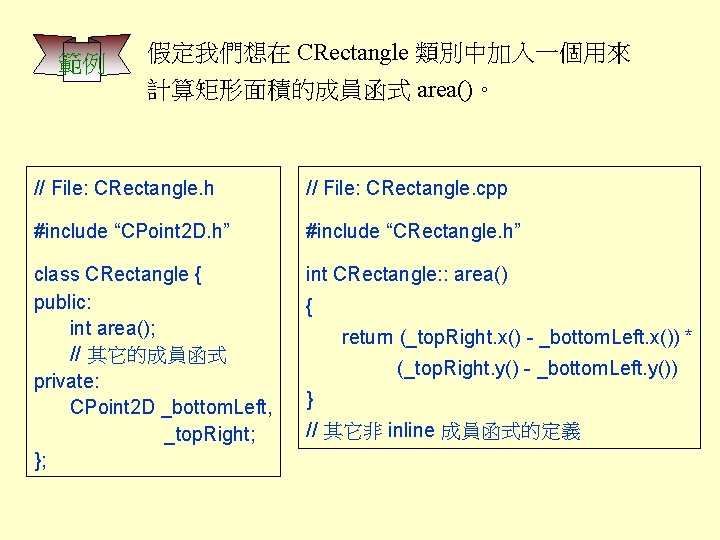
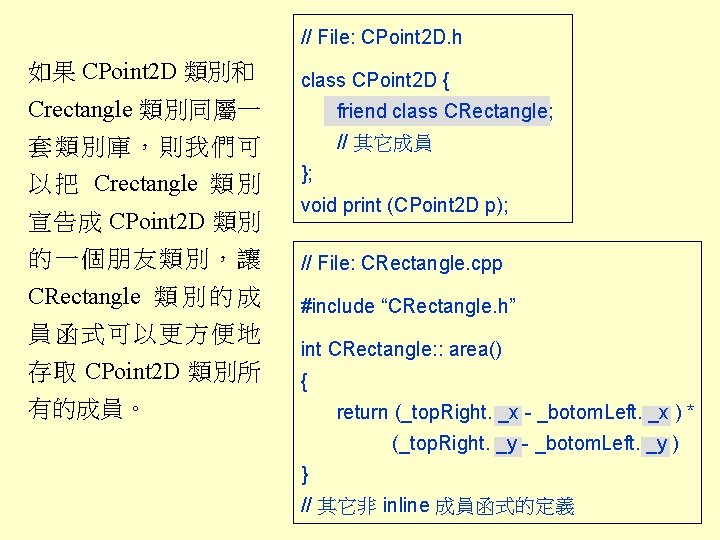
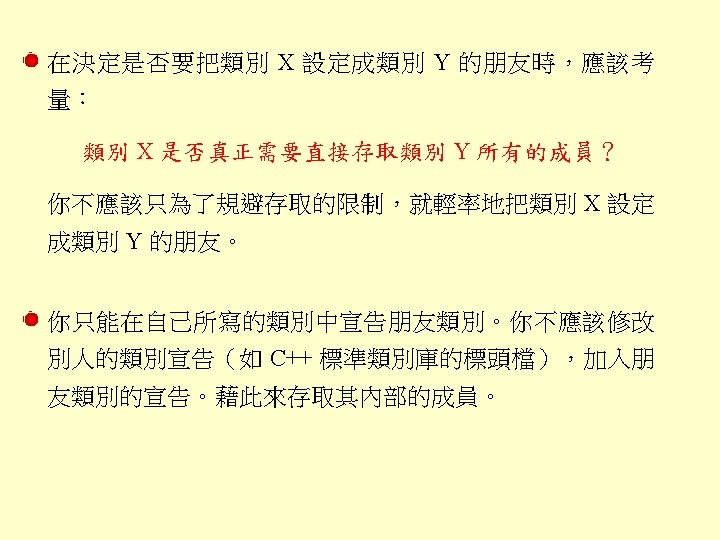
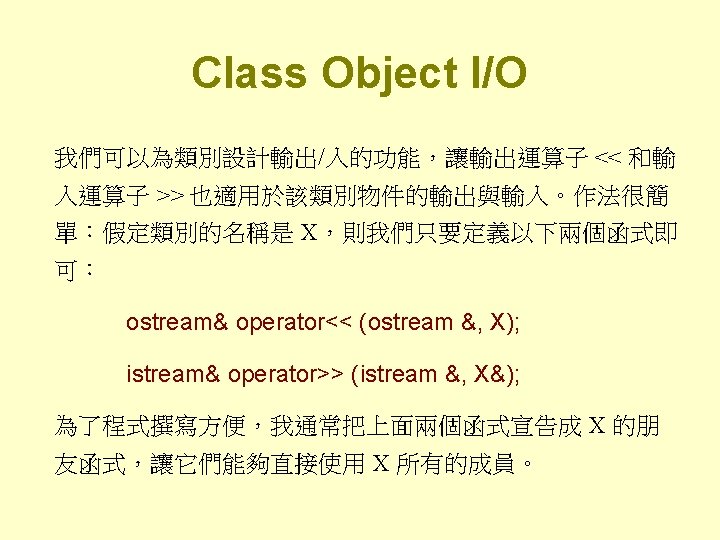
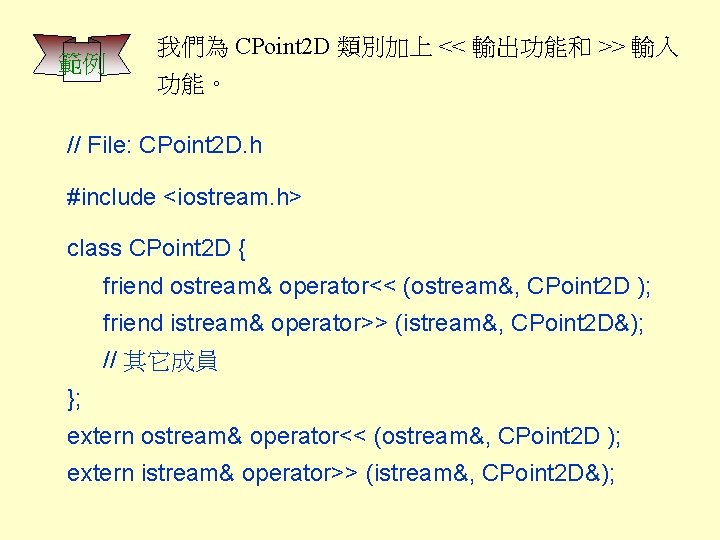
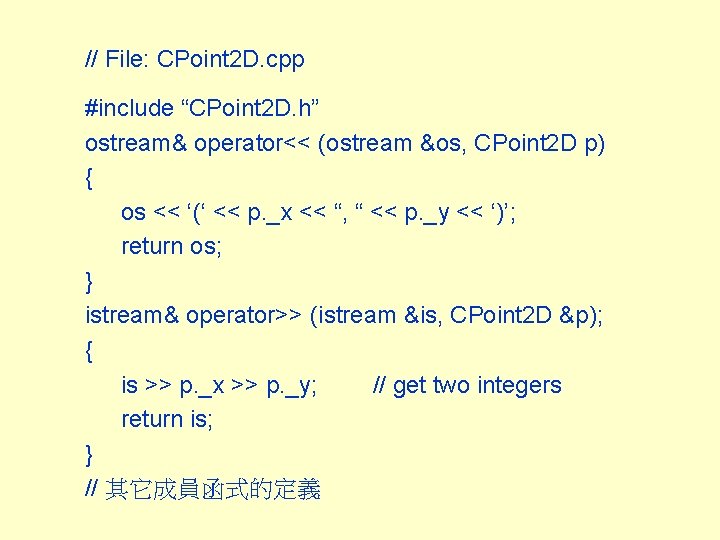
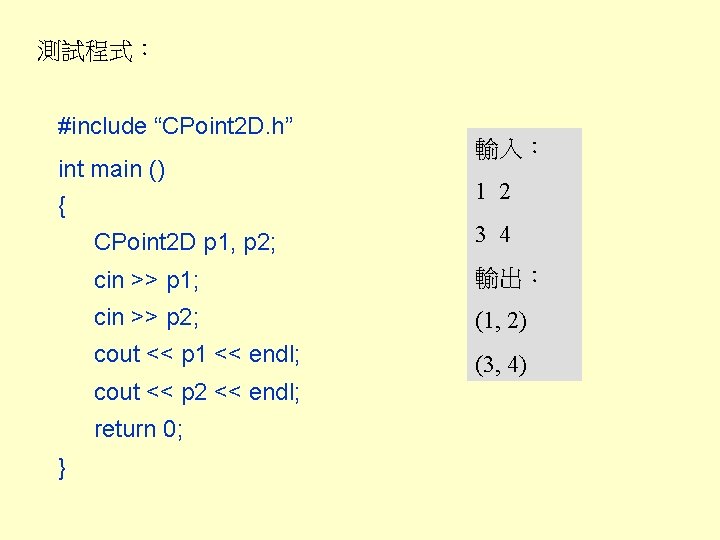
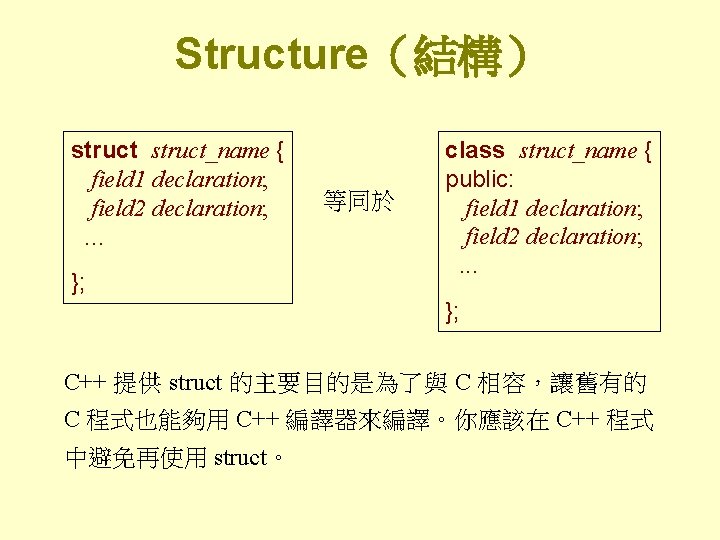
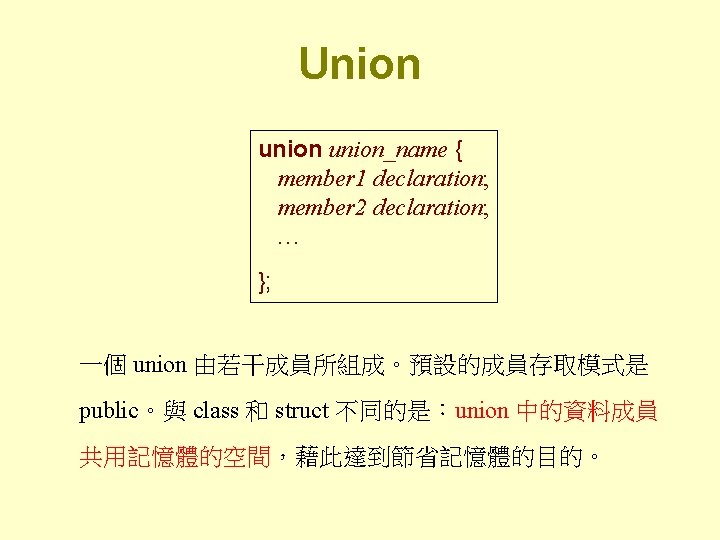
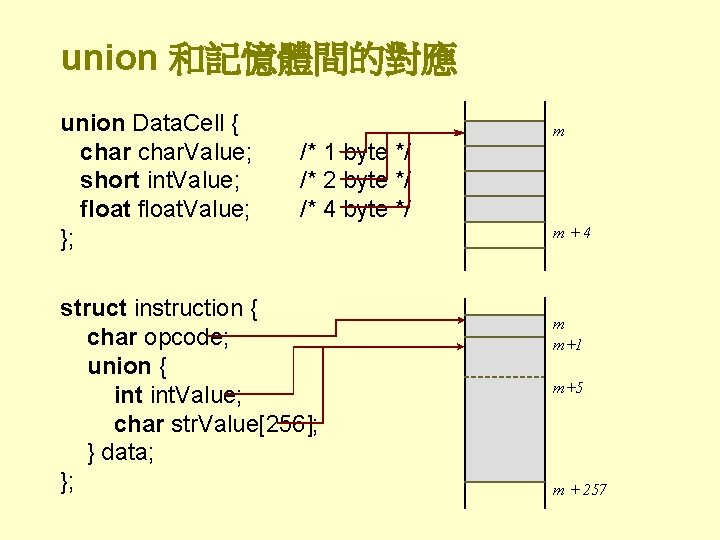
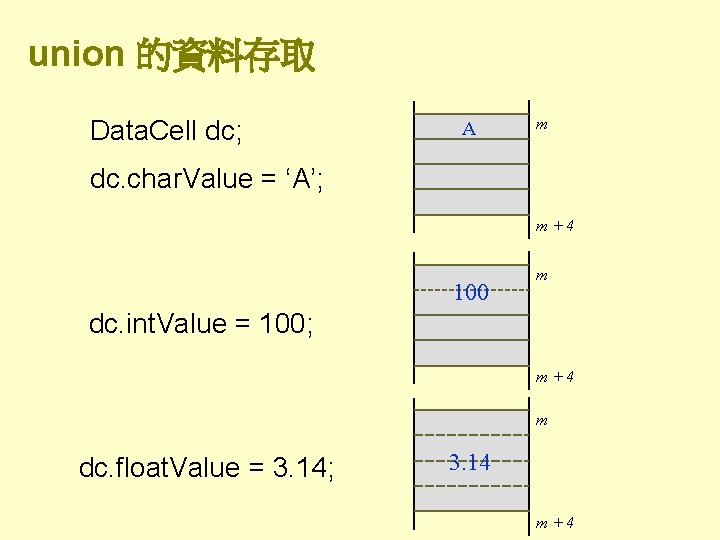
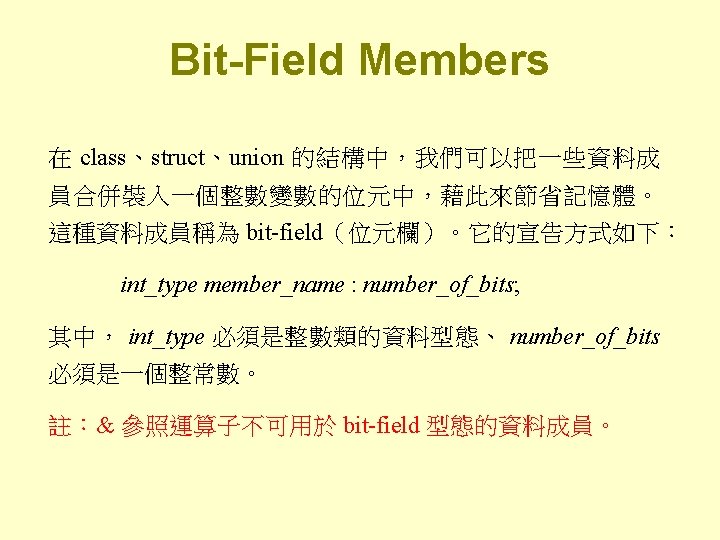
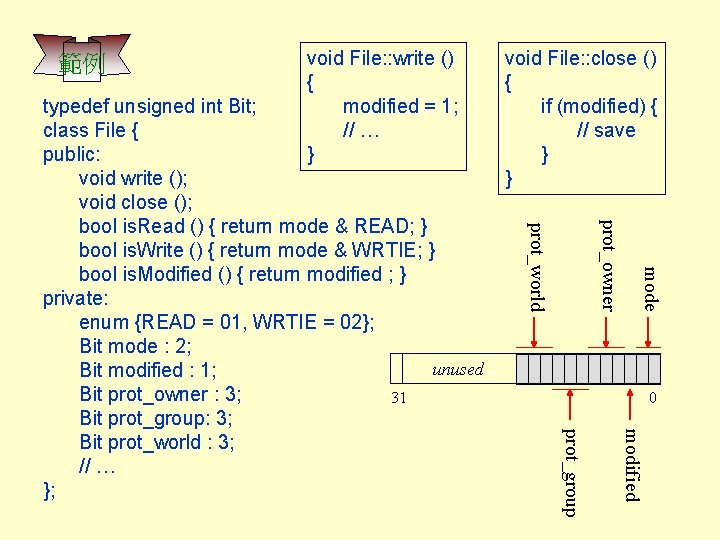
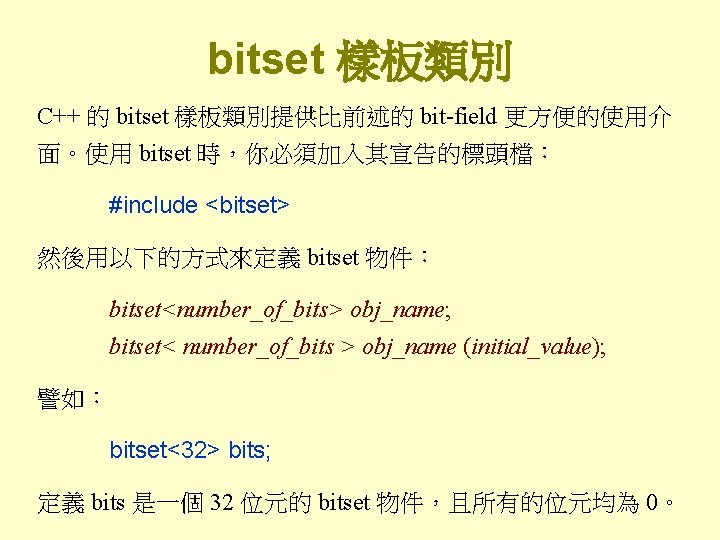
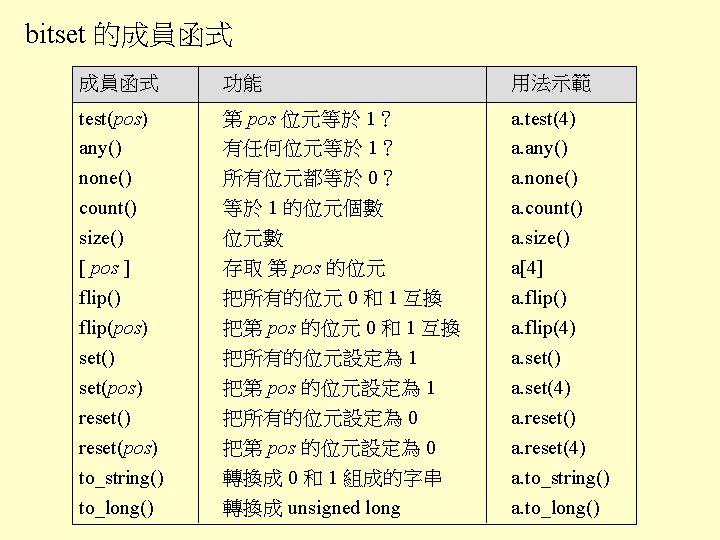
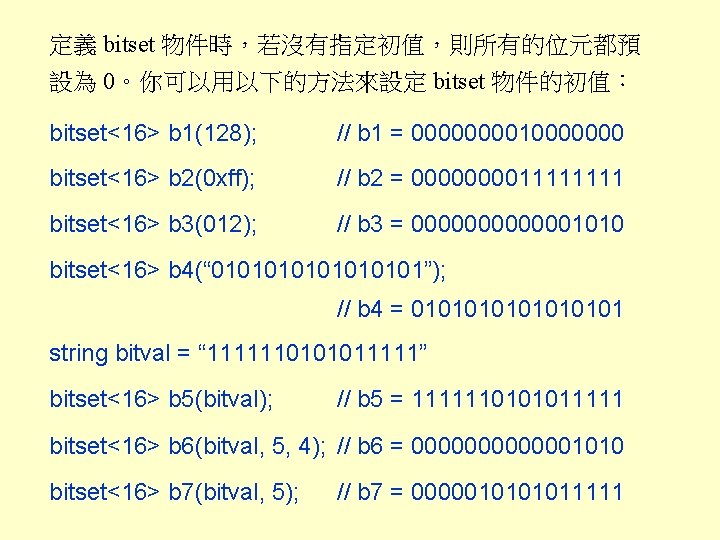
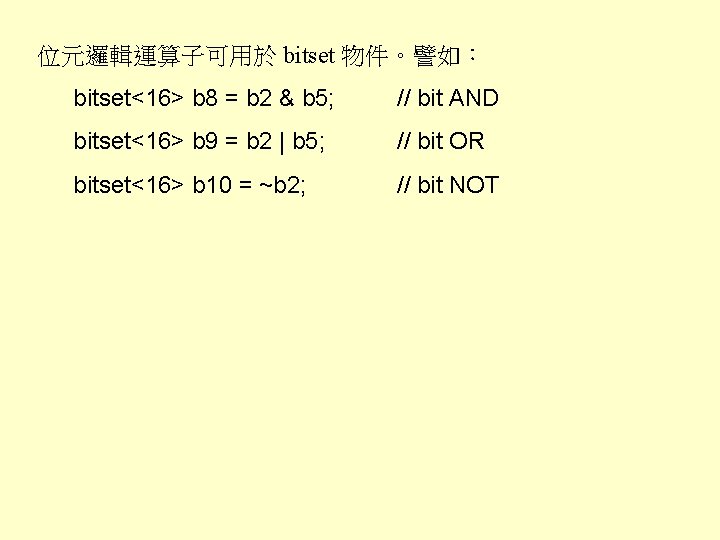
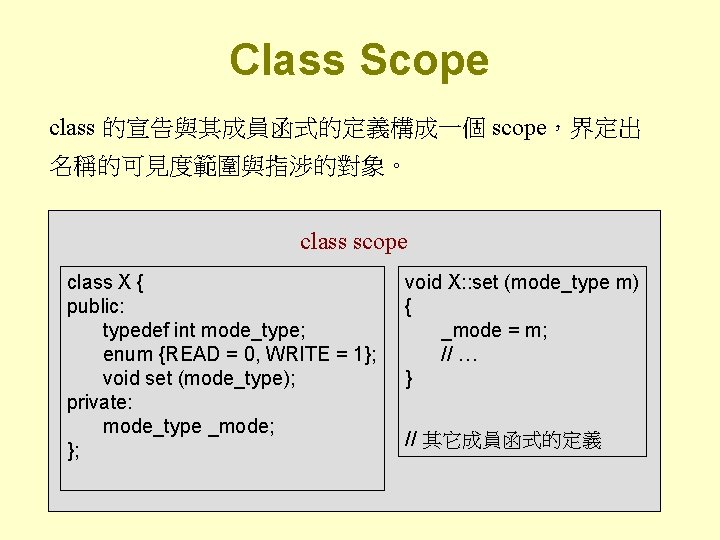
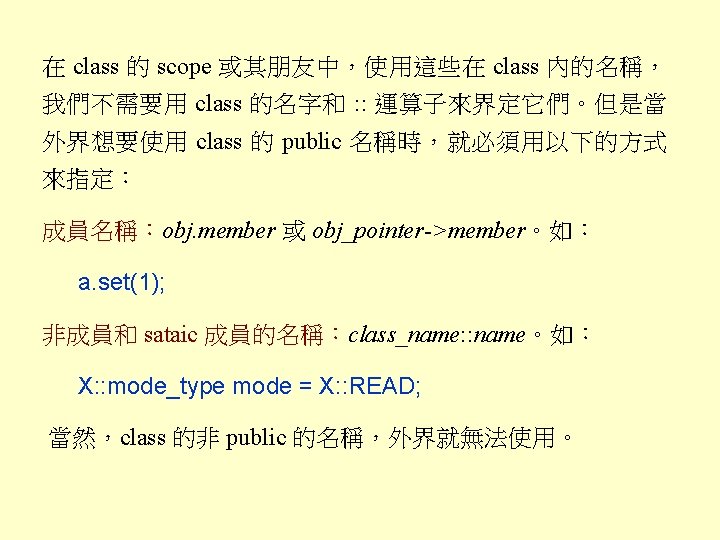
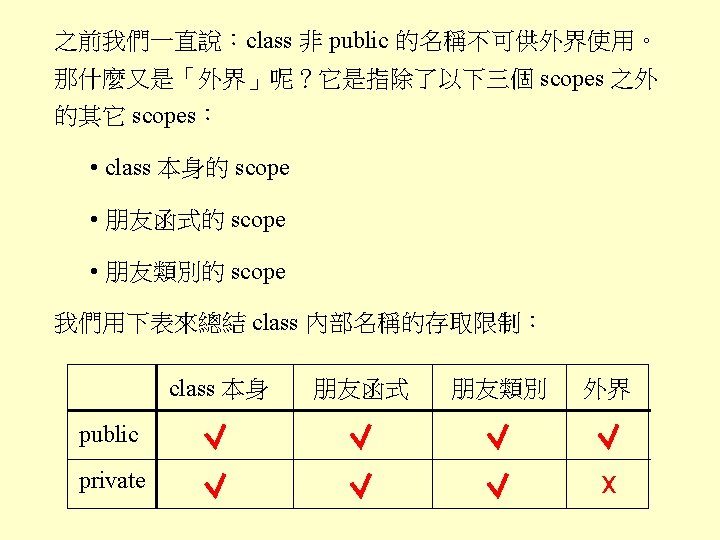
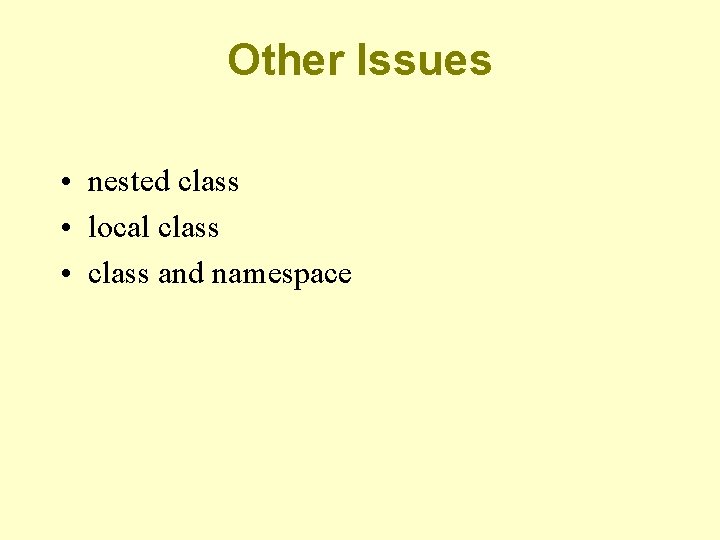
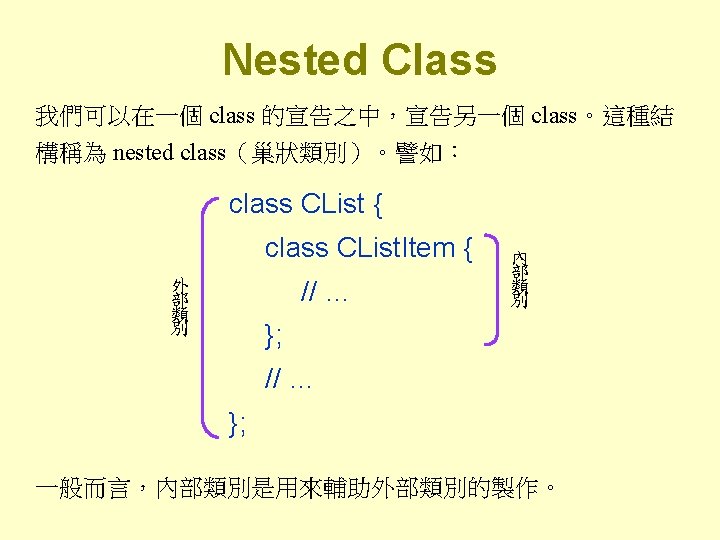
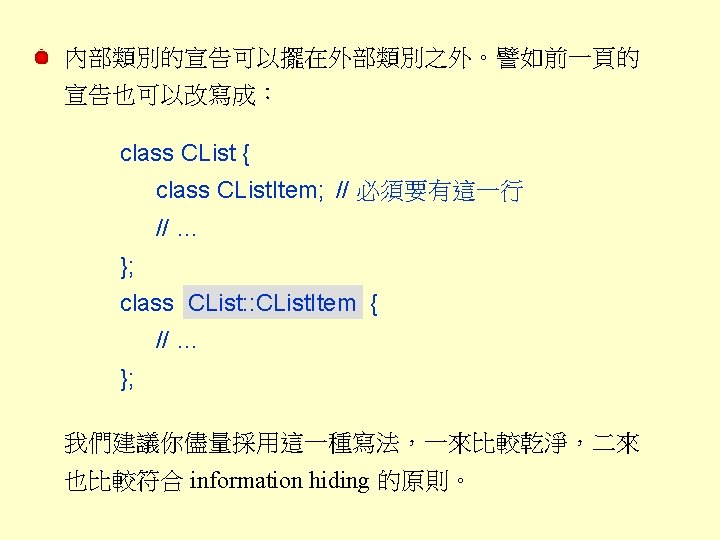
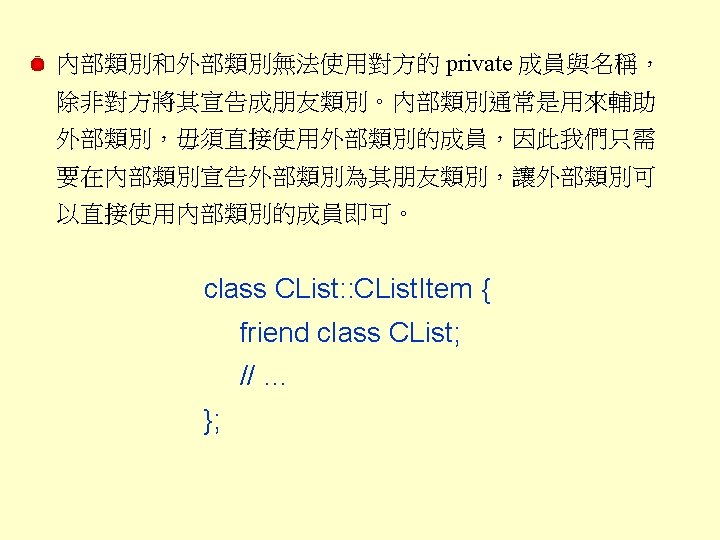
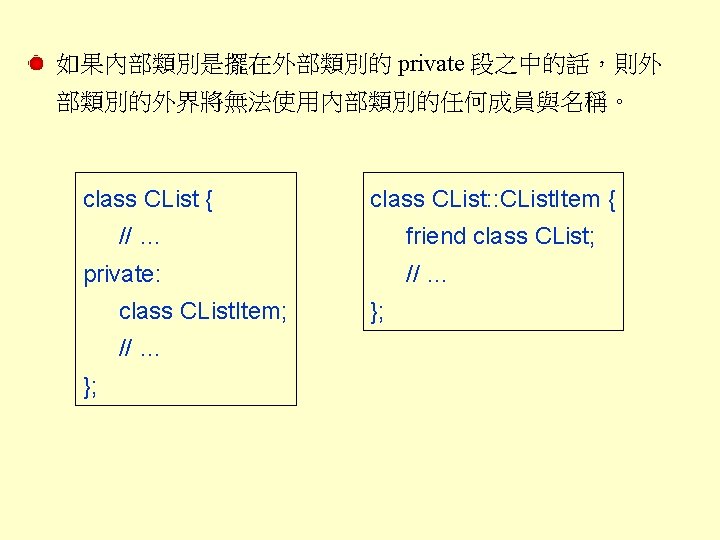
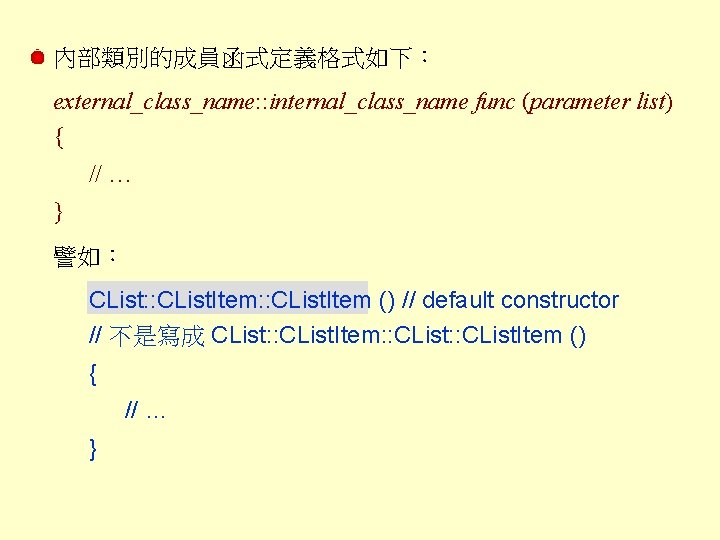
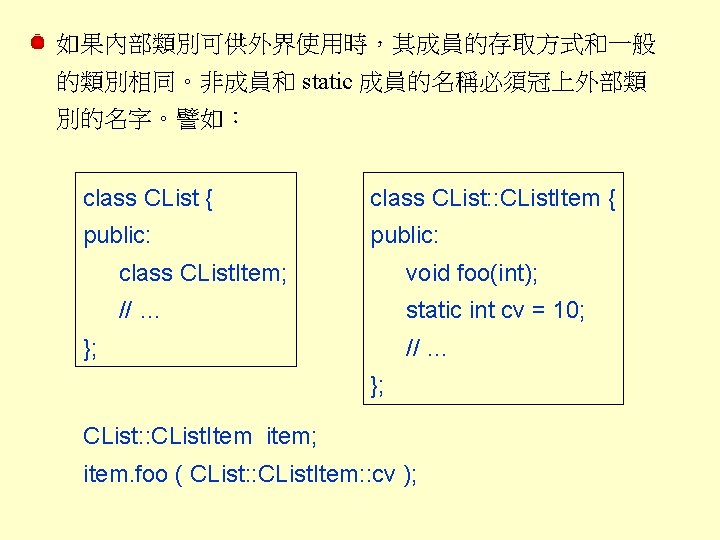
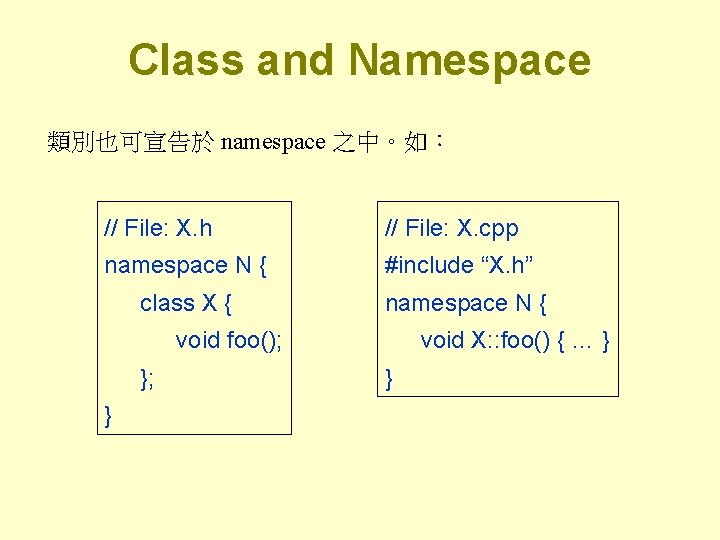
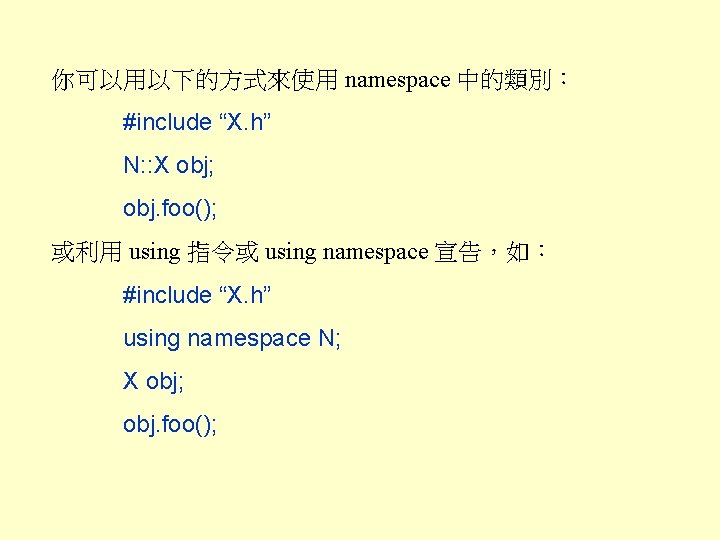
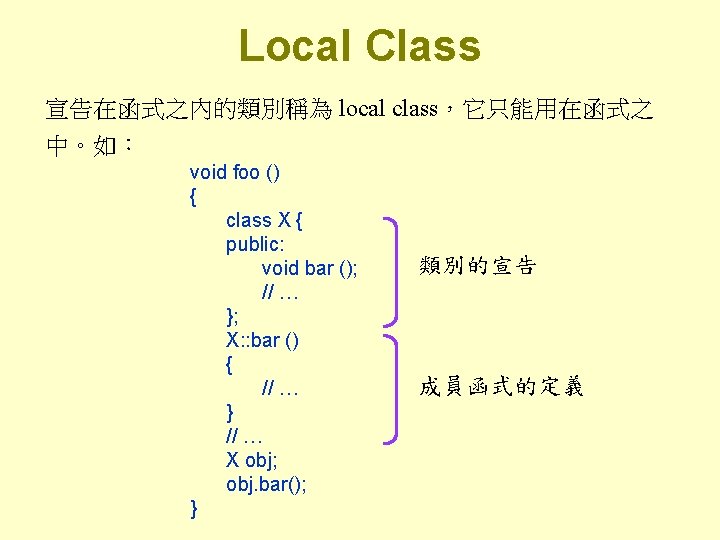
- Slides: 112
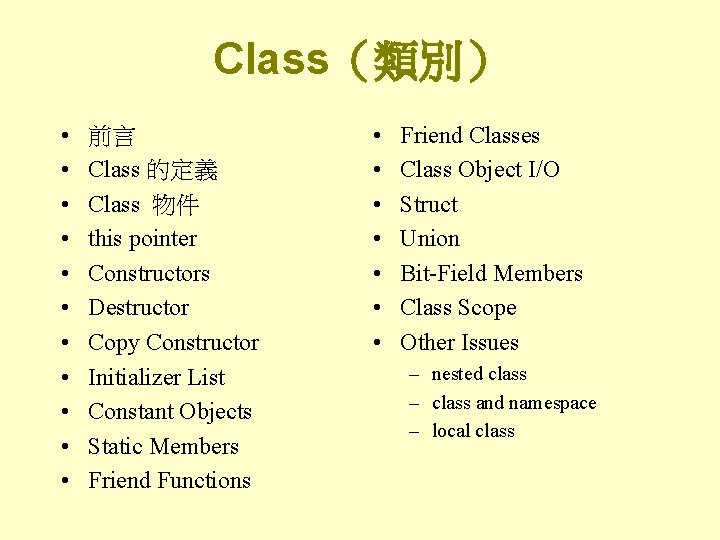
Class(類別) • • • 前言 Class 的定義 Class 物件 this pointer Constructors Destructor Copy Constructor Initializer List Constant Objects Static Members Friend Functions • • Friend Classes Class Object I/O Struct Union Bit-Field Members Class Scope Other Issues – nested class – class and namespace – local class
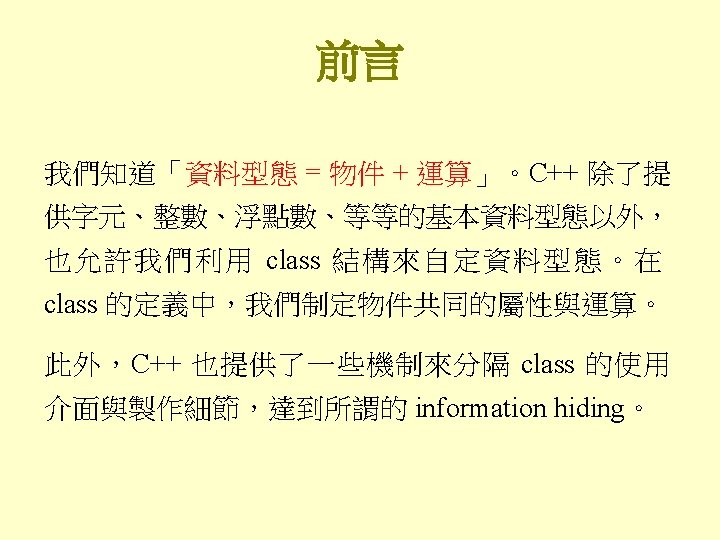
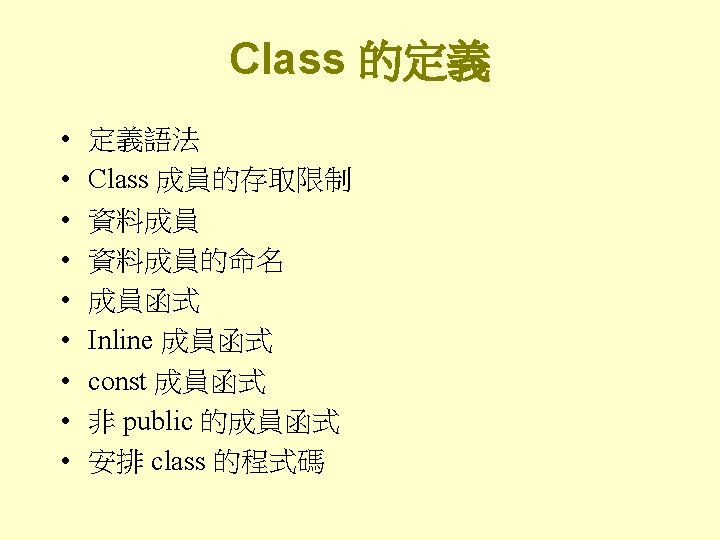
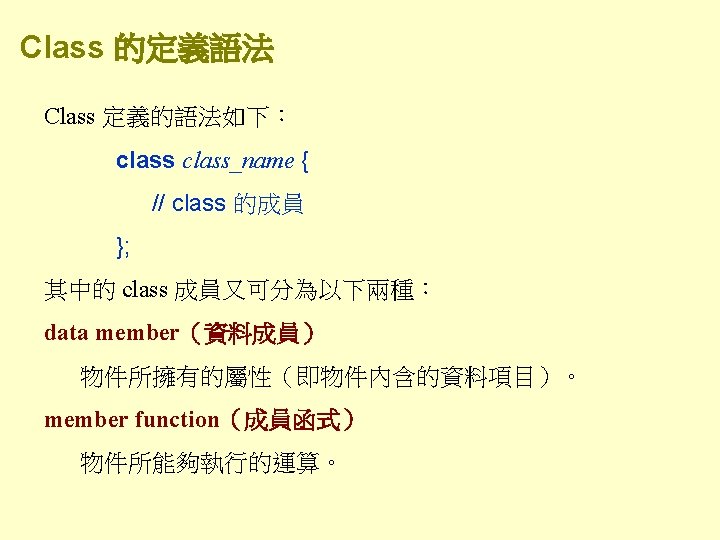
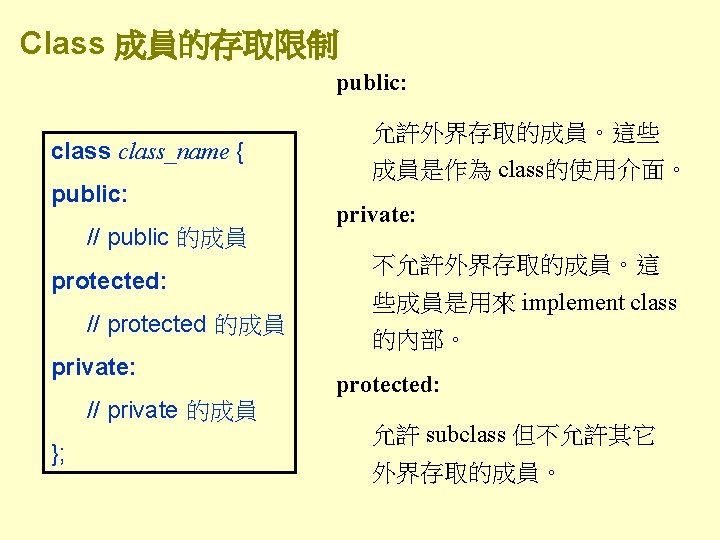
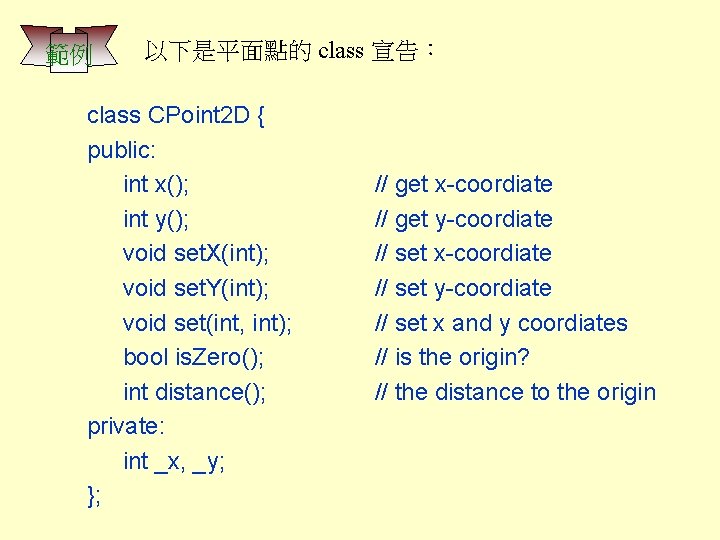
範例 以下是平面點的 class 宣告: class CPoint 2 D { public: int x(); int y(); void set. X(int); void set. Y(int); void set(int, int); bool is. Zero(); int distance(); private: int _x, _y; }; // get x-coordiate // get y-coordiate // set x-coordiate // set y-coordiate // set x and y coordiates // is the origin? // the distance to the origin
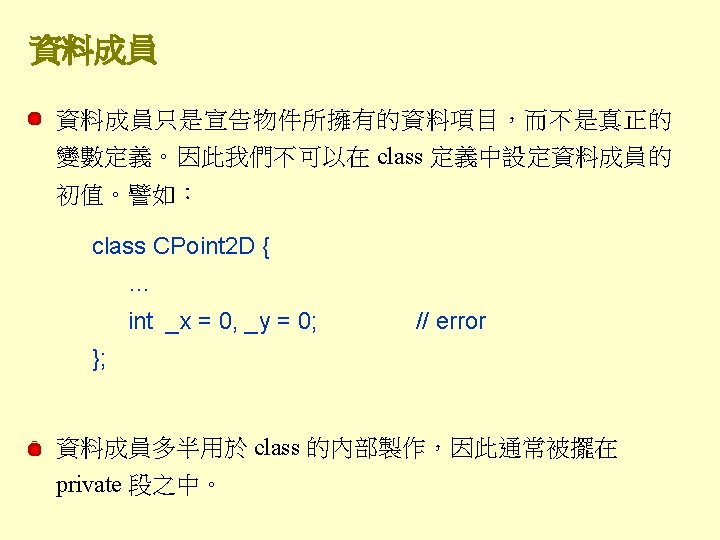
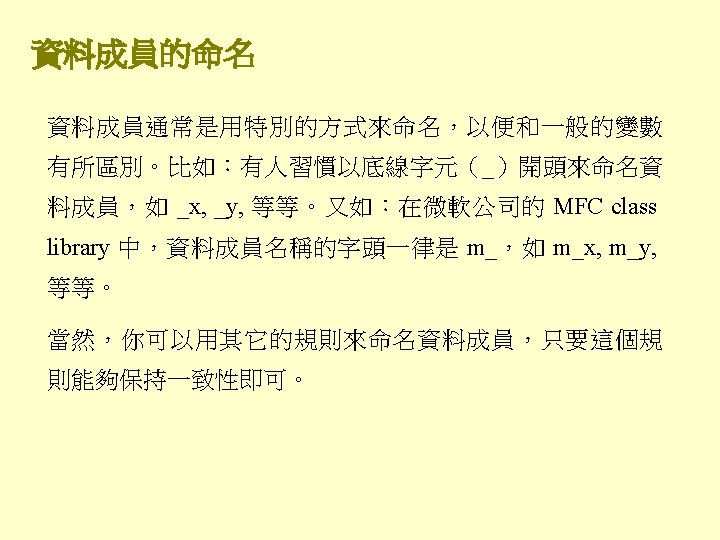
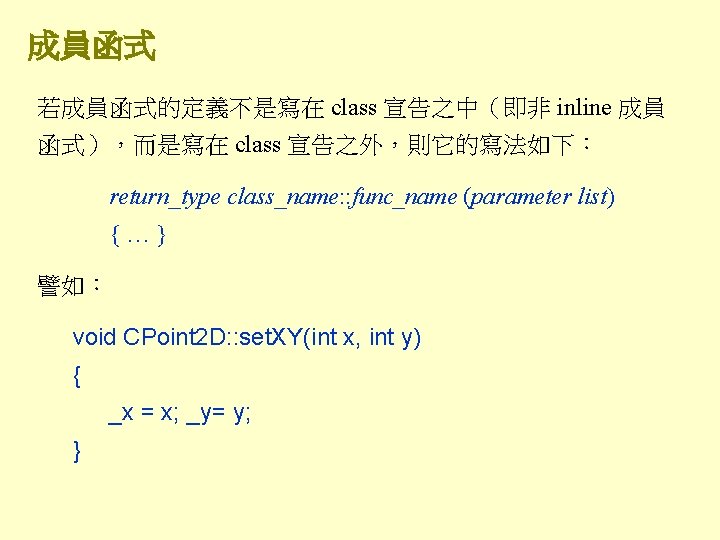
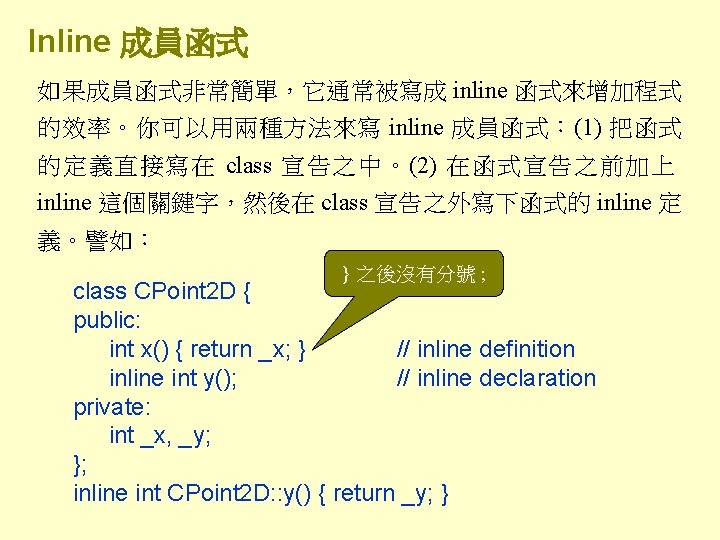
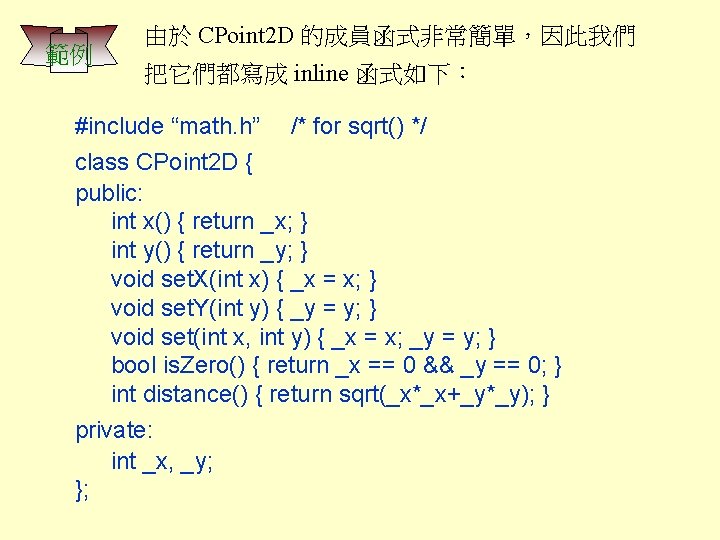
範例 由於 CPoint 2 D 的成員函式非常簡單,因此我們 把它們都寫成 inline 函式如下: #include “math. h” /* for sqrt() */ class CPoint 2 D { public: int x() { return _x; } int y() { return _y; } void set. X(int x) { _x = x; } void set. Y(int y) { _y = y; } void set(int x, int y) { _x = x; _y = y; } bool is. Zero() { return _x == 0 && _y == 0; } int distance() { return sqrt(_x*_x+_y*_y); } private: int _x, _y; };
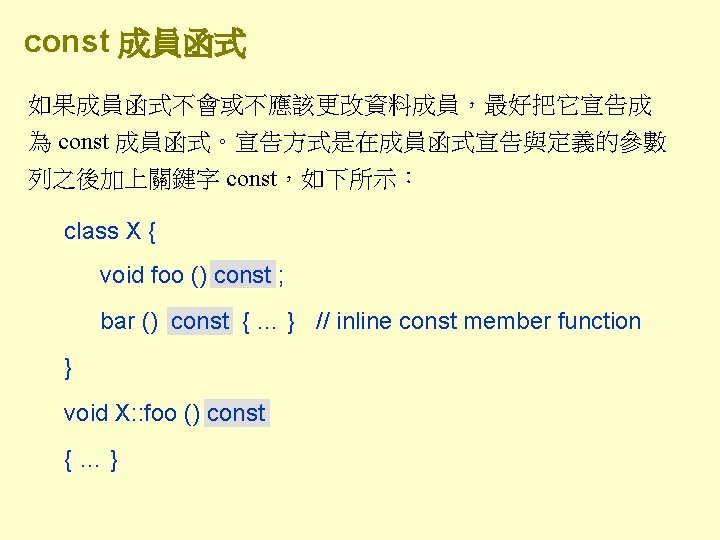
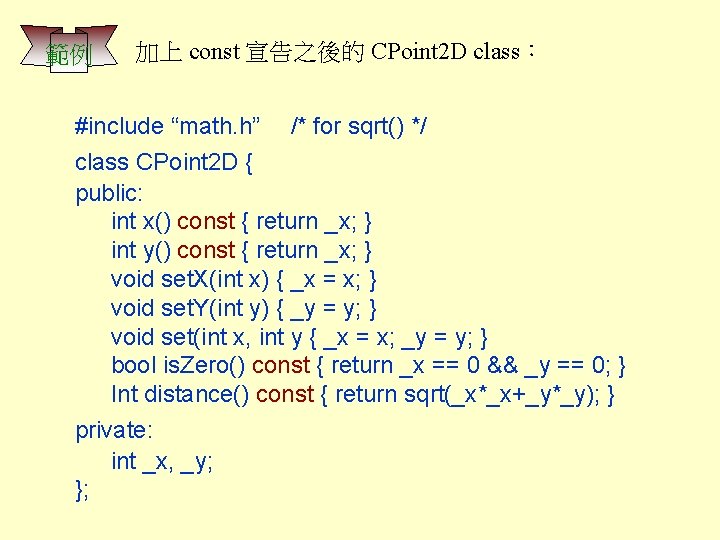
範例 加上 const 宣告之後的 CPoint 2 D class: #include “math. h” /* for sqrt() */ class CPoint 2 D { public: int x() const { return _x; } int y() const { return _x; } void set. X(int x) { _x = x; } void set. Y(int y) { _y = y; } void set(int x, int y { _x = x; _y = y; } bool is. Zero() const { return _x == 0 && _y == 0; } Int distance() const { return sqrt(_x*_x+_y*_y); } private: int _x, _y; };
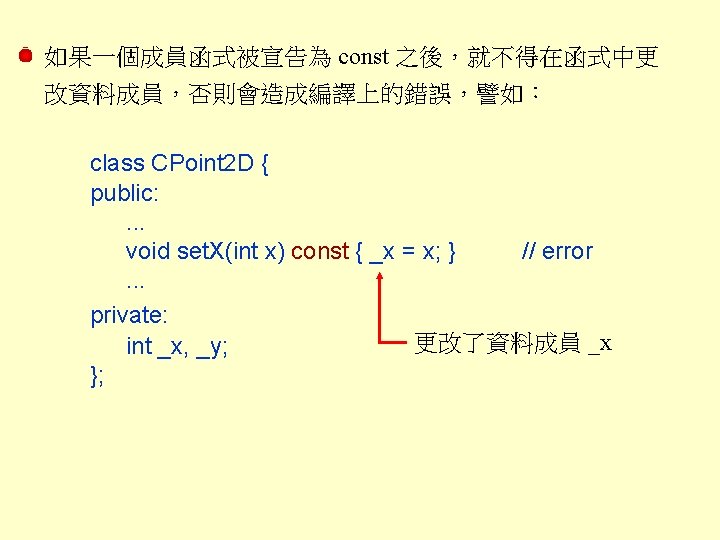
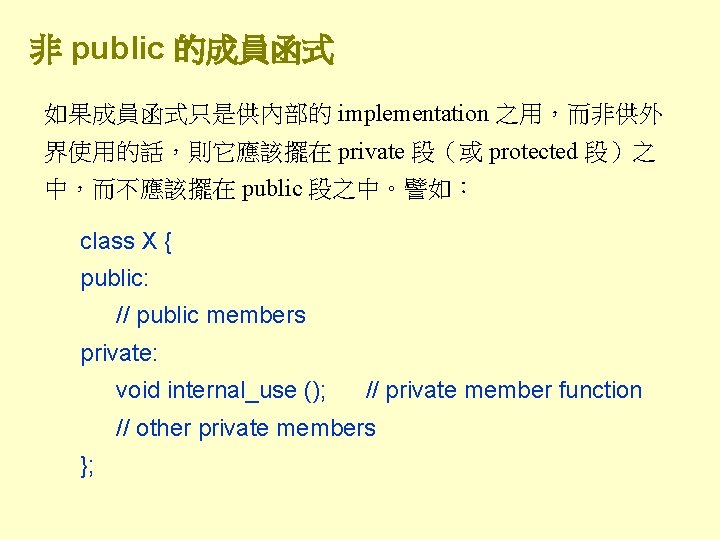
非 public 的成員函式 如果成員函式只是供內部的 implementation 之用,而非供外 界使用的話,則它應該擺在 private 段(或 protected 段)之 中,而不應該擺在 public 段之中。譬如: class X { public: // public members private: void internal_use (); // private member function // other private members };
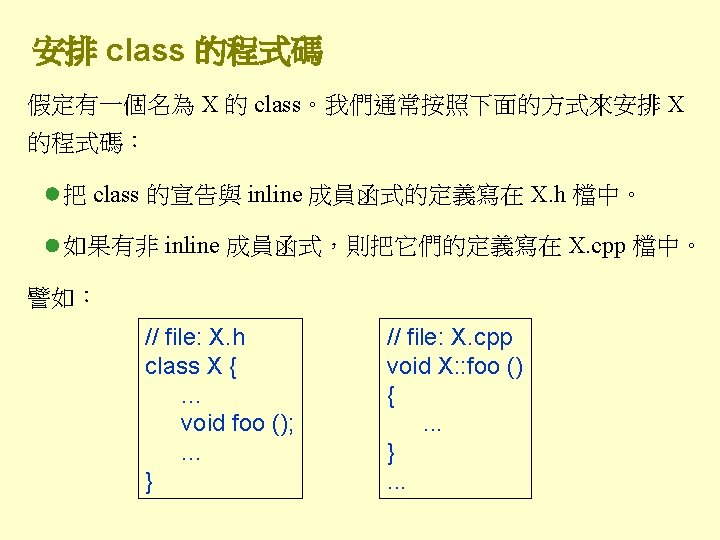
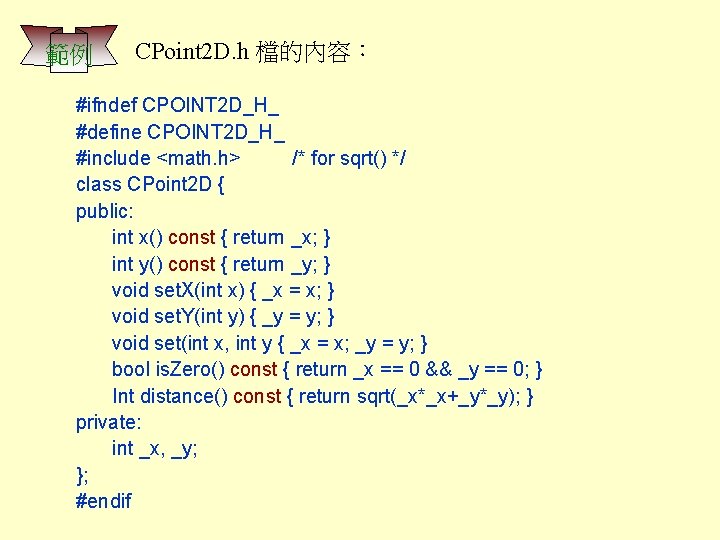
範例 CPoint 2 D. h 檔的內容: #ifndef CPOINT 2 D_H_ #define CPOINT 2 D_H_ #include <math. h> /* for sqrt() */ class CPoint 2 D { public: int x() const { return _x; } int y() const { return _y; } void set. X(int x) { _x = x; } void set. Y(int y) { _y = y; } void set(int x, int y { _x = x; _y = y; } bool is. Zero() const { return _x == 0 && _y == 0; } Int distance() const { return sqrt(_x*_x+_y*_y); } private: int _x, _y; }; #endif
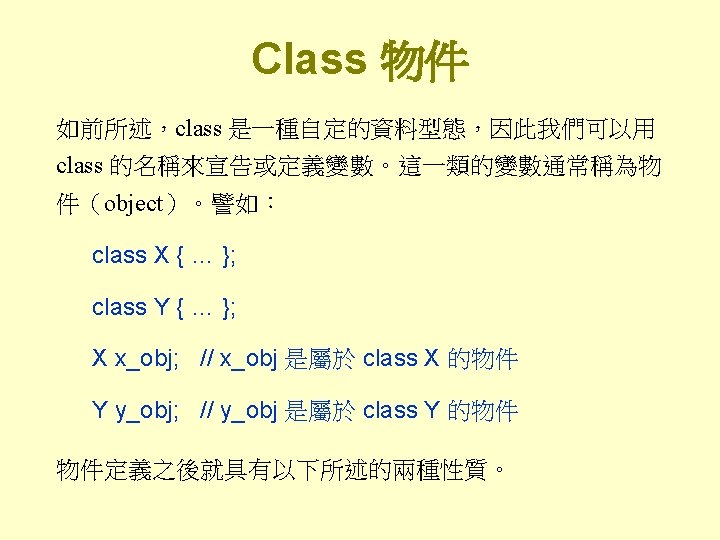
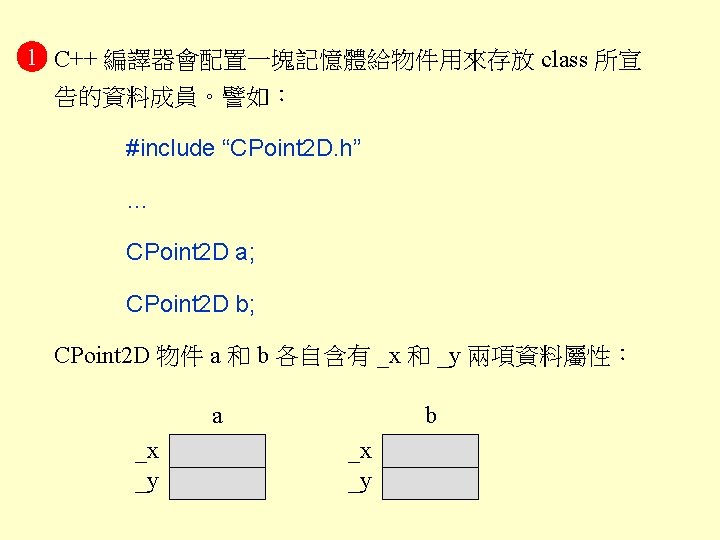
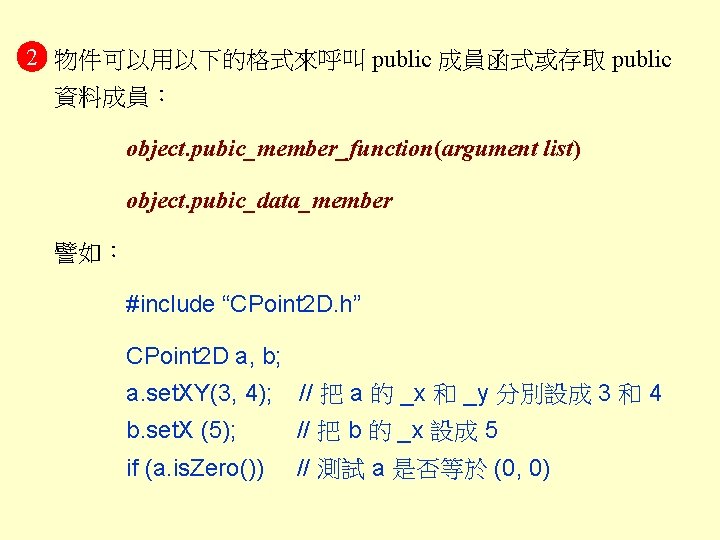
2 物件可以用以下的格式來呼叫 public 成員函式或存取 public 資料成員: object. pubic_member_function(argument list) object. pubic_data_member 譬如: #include “CPoint 2 D. h” CPoint 2 D a, b; a. set. XY(3, 4); // 把 a 的 _x 和 _y 分別設成 3 和 4 b. set. X (5); // 把 b 的 _x 設成 5 if (a. is. Zero()) // 測試 a 是否等於 (0, 0)
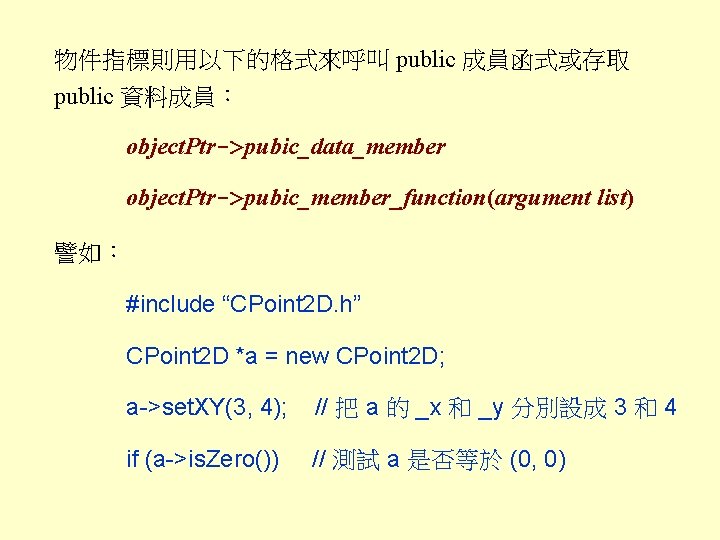
物件指標則用以下的格式來呼叫 public 成員函式或存取 public 資料成員: object. Ptr->pubic_data_member object. Ptr->pubic_member_function(argument list) 譬如: #include “CPoint 2 D. h” CPoint 2 D *a = new CPoint 2 D; a->set. XY(3, 4); // 把 a 的 _x 和 _y 分別設成 3 和 4 if (a->is. Zero()) // 測試 a 是否等於 (0, 0)
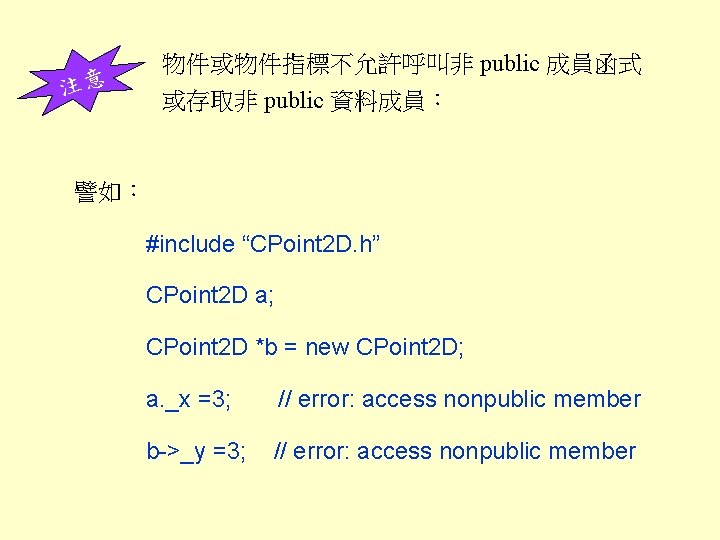
注意 物件或物件指標不允許呼叫非 public 成員函式 或存取非 public 資料成員: 譬如: #include “CPoint 2 D. h” CPoint 2 D a; CPoint 2 D *b = new CPoint 2 D; a. _x =3; // error: access nonpublic member b->_y =3; // error: access nonpublic member
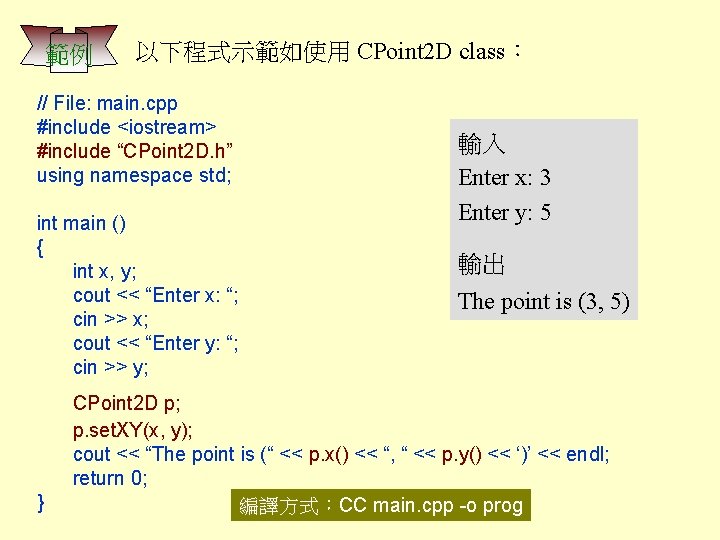
範例 以下程式示範如使用 CPoint 2 D class: // File: main. cpp #include <iostream> #include “CPoint 2 D. h” using namespace std; int main () { int x, y; cout << “Enter x: “; cin >> x; cout << “Enter y: “; cin >> y; } 輸入 Enter x: 3 Enter y: 5 輸出 The point is (3, 5) CPoint 2 D p; p. set. XY(x, y); cout << “The point is (“ << p. x() << “, “ << p. y() << ‘)’ << endl; return 0; 編譯方式:CC main. cpp -o prog
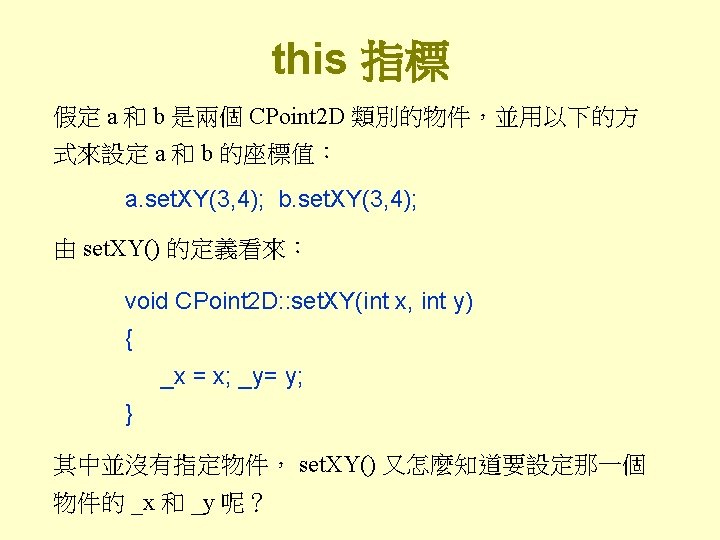
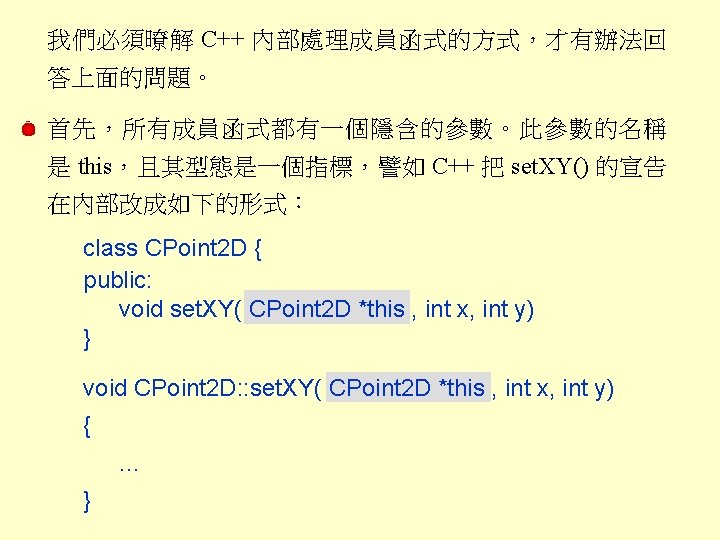
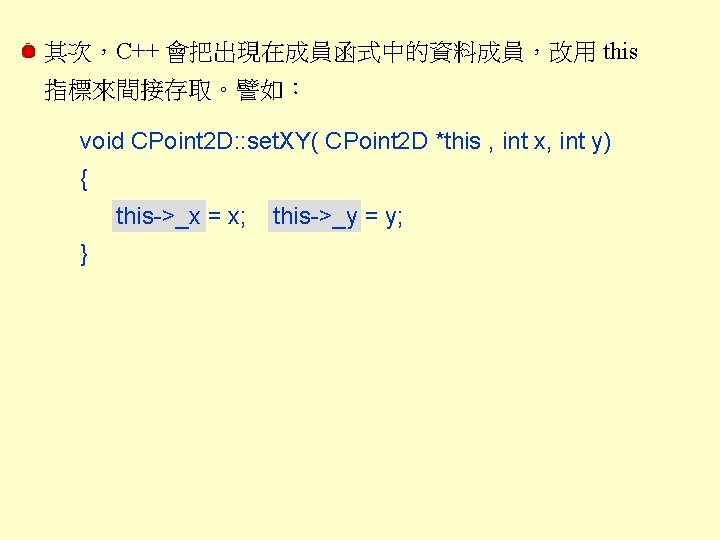
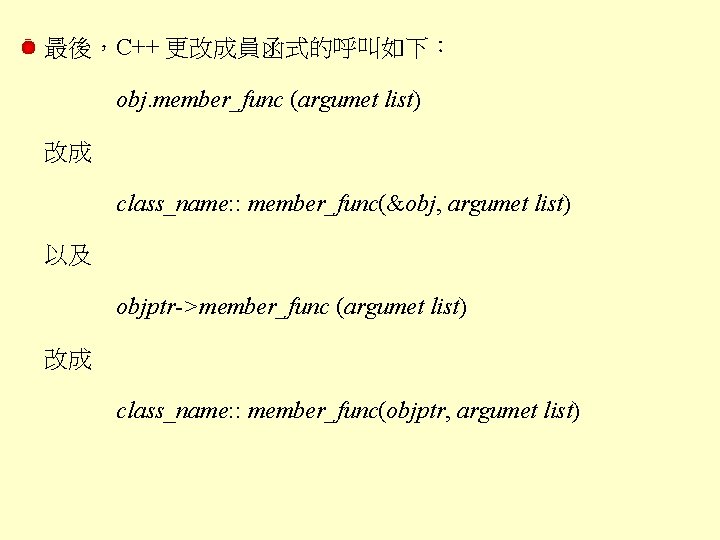
最後,C++ 更改成員函式的呼叫如下: obj. member_func (argumet list) 改成 class_name: : member_func(&obj, argumet list) 以及 objptr->member_func (argumet list) 改成 class_name: : member_func(objptr, argumet list)
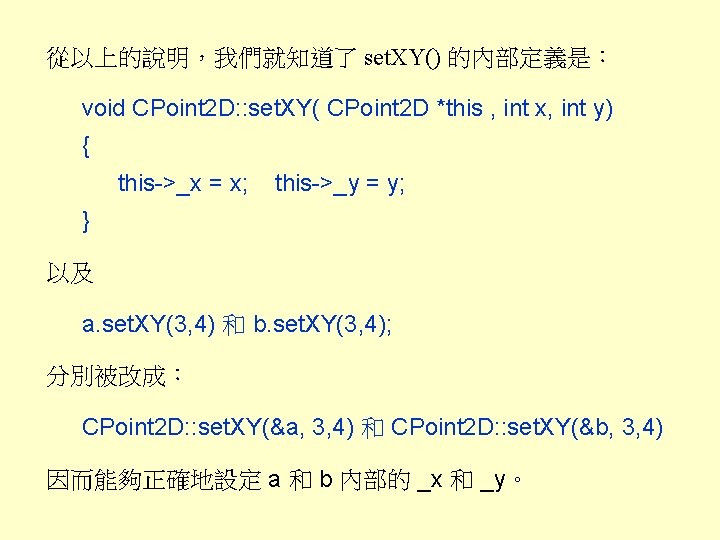
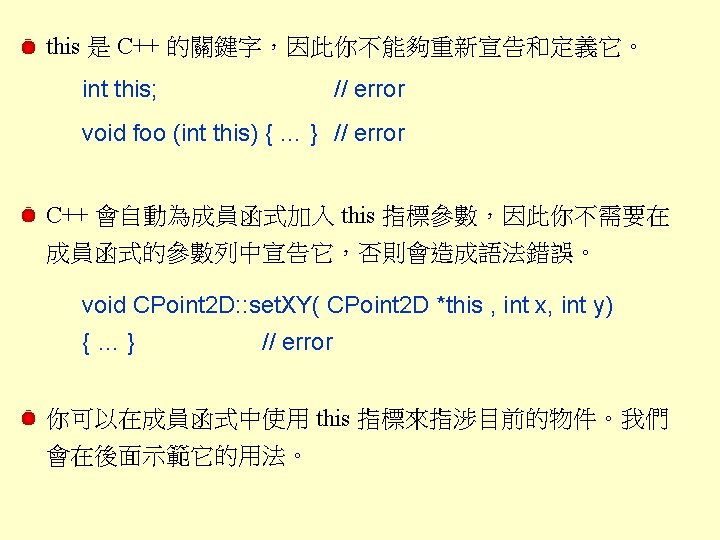
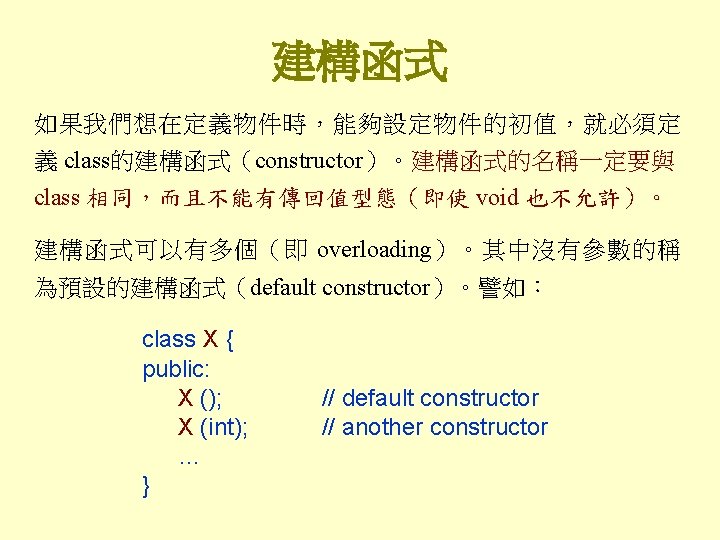
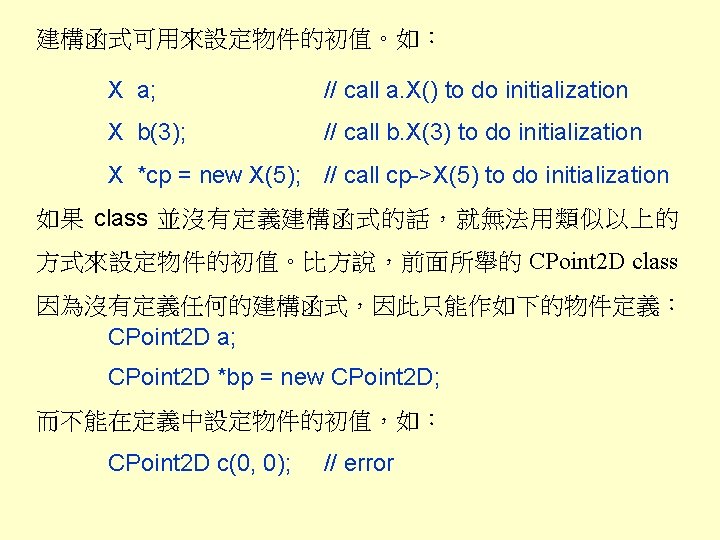
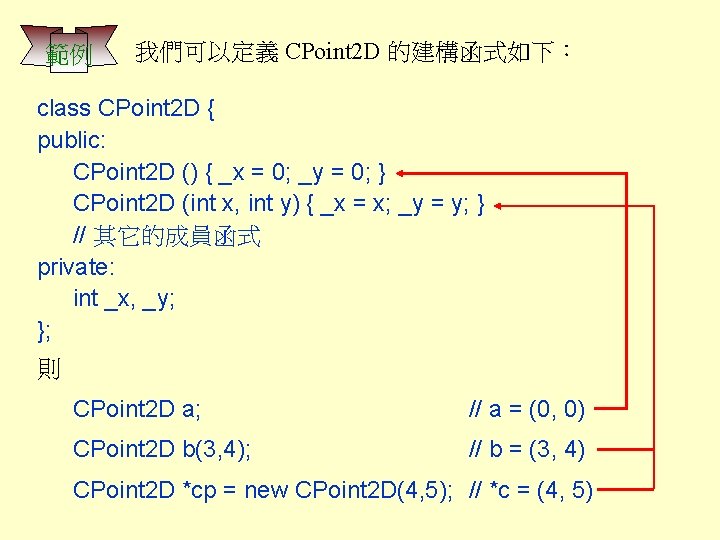
範例 我們可以定義 CPoint 2 D 的建構函式如下: class CPoint 2 D { public: CPoint 2 D () { _x = 0; _y = 0; } CPoint 2 D (int x, int y) { _x = x; _y = y; } // 其它的成員函式 private: int _x, _y; }; 則 CPoint 2 D a; // a = (0, 0) CPoint 2 D b(3, 4); // b = (3, 4) CPoint 2 D *cp = new CPoint 2 D(4, 5); // *c = (4, 5)
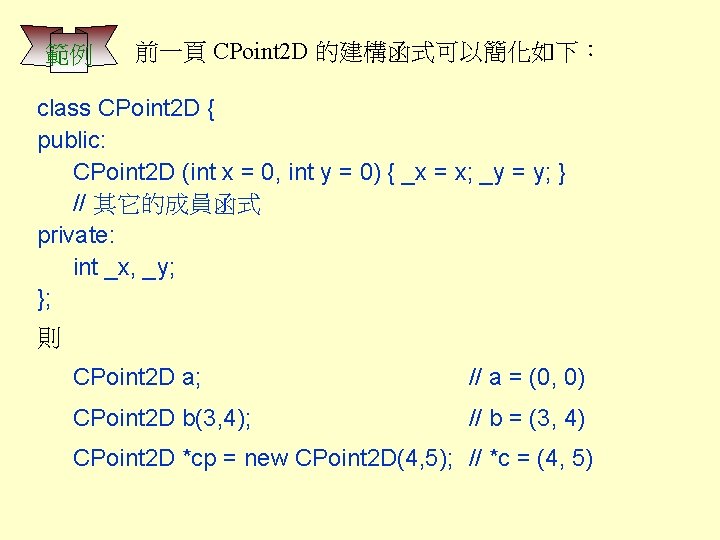
範例 前一頁 CPoint 2 D 的建構函式可以簡化如下: class CPoint 2 D { public: CPoint 2 D (int x = 0, int y = 0) { _x = x; _y = y; } // 其它的成員函式 private: int _x, _y; }; 則 CPoint 2 D a; // a = (0, 0) CPoint 2 D b(3, 4); // b = (3, 4) CPoint 2 D *cp = new CPoint 2 D(4, 5); // *c = (4, 5)
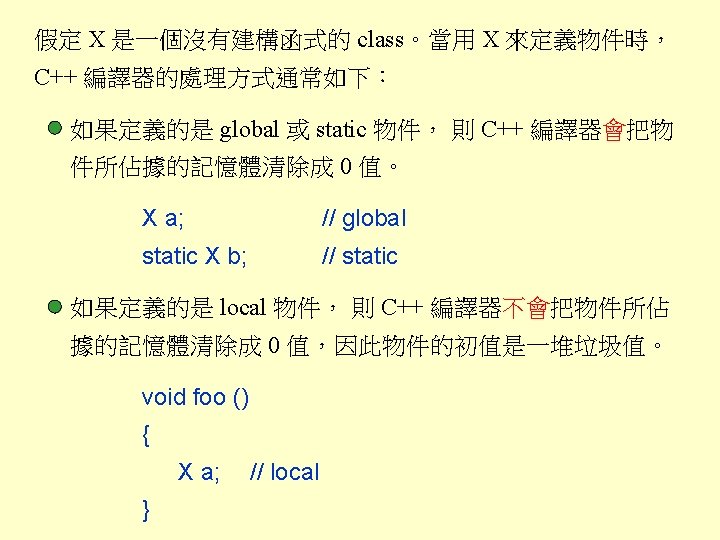
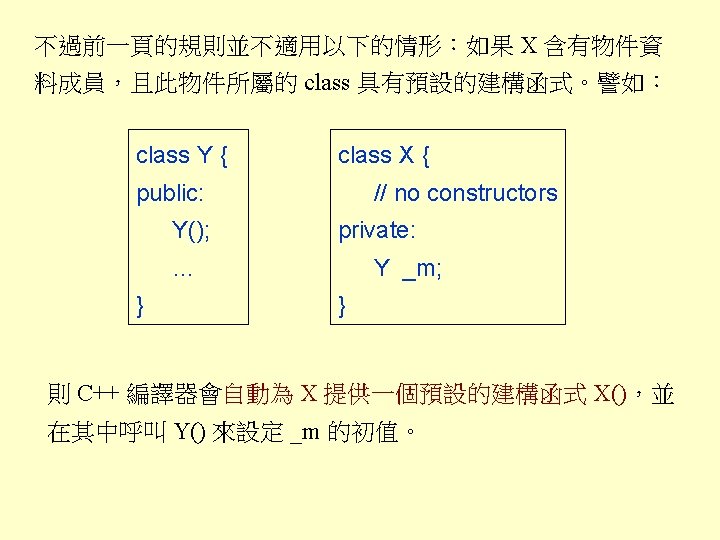
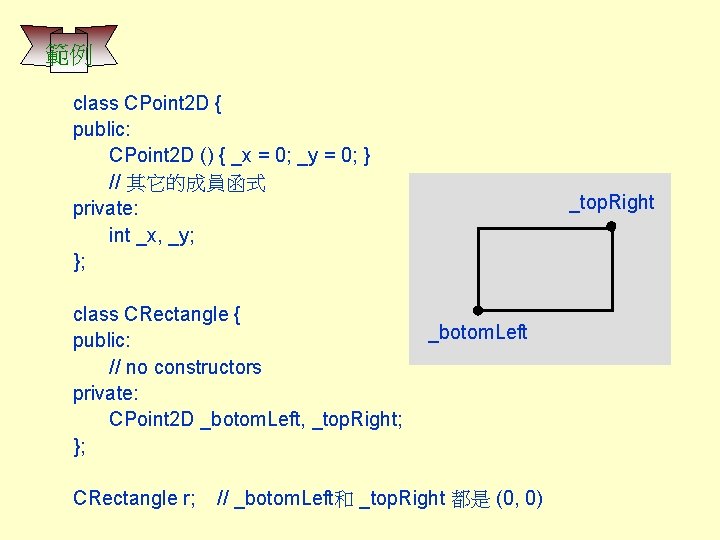
範例 class CPoint 2 D { public: CPoint 2 D () { _x = 0; _y = 0; } // 其它的成員函式 private: int _x, _y; }; class CRectangle { public: // no constructors private: CPoint 2 D _botom. Left, _top. Right; }; CRectangle r; _top. Right _botom. Left // _botom. Left和 _top. Right 都是 (0, 0)
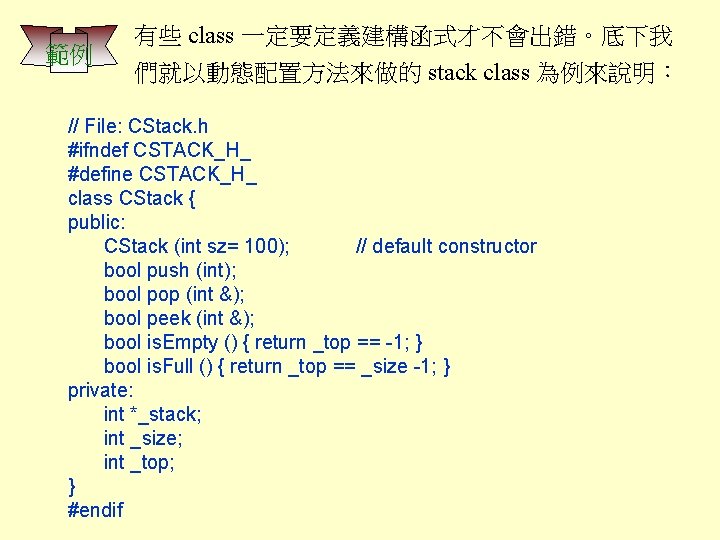
範例 有些 class 一定要定義建構函式才不會出錯。底下我 們就以動態配置方法來做的 stack class 為例來說明: // File: CStack. h #ifndef CSTACK_H_ #define CSTACK_H_ class CStack { public: CStack (int sz= 100); // default constructor bool push (int); bool pop (int &); bool peek (int &); bool is. Empty () { return _top == -1; } bool is. Full () { return _top == _size -1; } private: int *_stack; int _size; int _top; } #endif
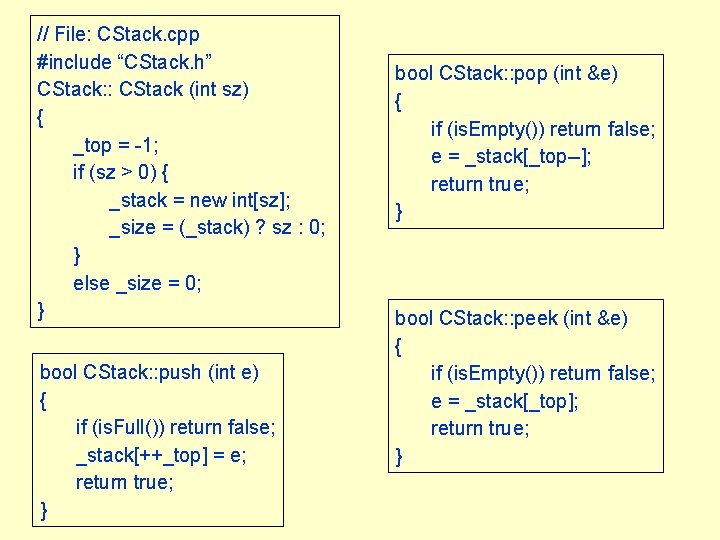
// File: CStack. cpp #include “CStack. h” CStack: : CStack (int sz) { _top = -1; if (sz > 0) { _stack = new int[sz]; _size = (_stack) ? sz : 0; } else _size = 0; } bool CStack: : push (int e) { if (is. Full()) return false; _stack[++_top] = e; return true; } bool CStack: : pop (int &e) { if (is. Empty()) return false; e = _stack[_top--]; return true; } bool CStack: : peek (int &e) { if (is. Empty()) return false; e = _stack[_top]; return true; }
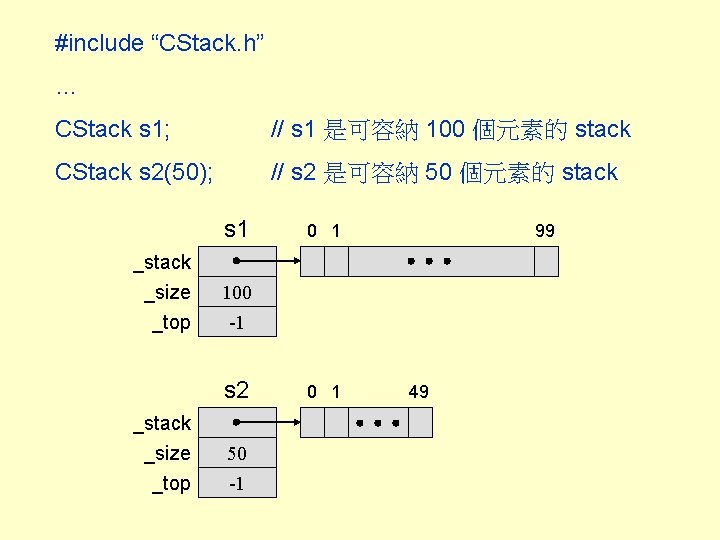
#include “CStack. h” … CStack s 1; // s 1 是可容納 100 個元素的 stack CStack s 2(50); // s 2 是可容納 50 個元素的 stack s 1 _stack _size _top 99 100 -1 s 2 _stack _size _top 0 1 50 -1 0 1 49
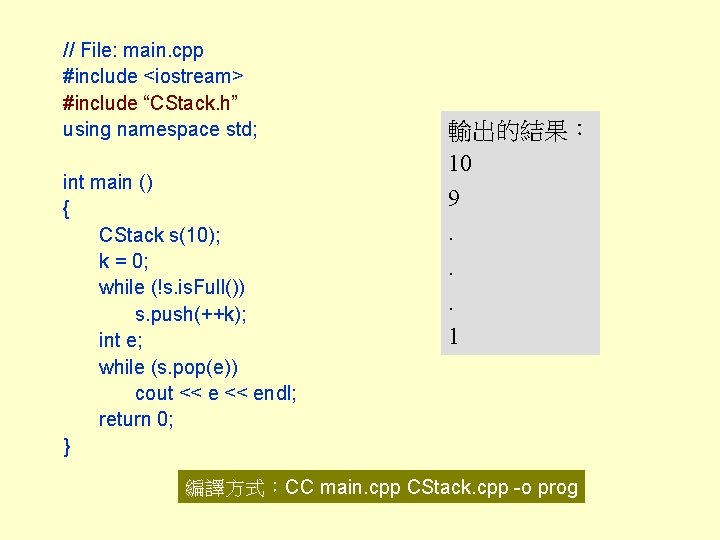
// File: main. cpp #include <iostream> #include “CStack. h” using namespace std; int main () { CStack s(10); k = 0; while (!s. is. Full()) s. push(++k); int e; while (s. pop(e)) cout << endl; return 0; } 輸出的結果: 10 9. . . 1 編譯方式:CC main. cpp CStack. cpp -o prog
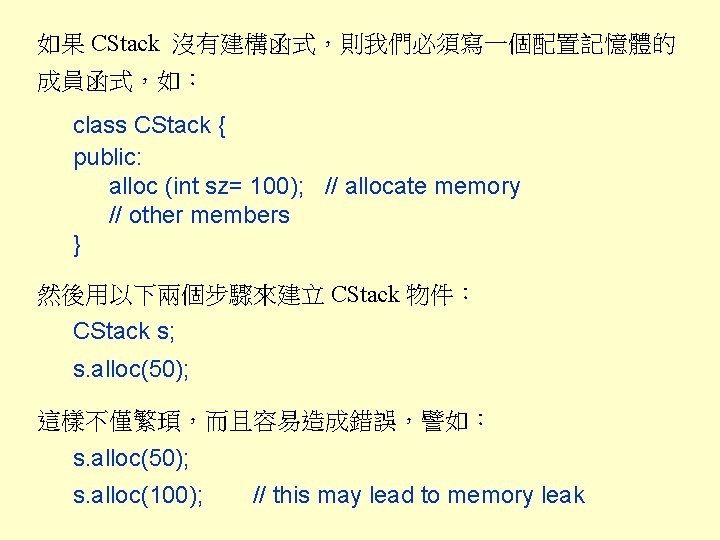
如果 CStack 沒有建構函式,則我們必須寫一個配置記憶體的 成員函式,如: class CStack { public: alloc (int sz= 100); // allocate memory // other members } 然後用以下兩個步驟來建立 CStack 物件: CStack s; s. alloc(50); 這樣不僅繁頊,而且容易造成錯誤,譬如: s. alloc(50); s. alloc(100); // this may lead to memory leak
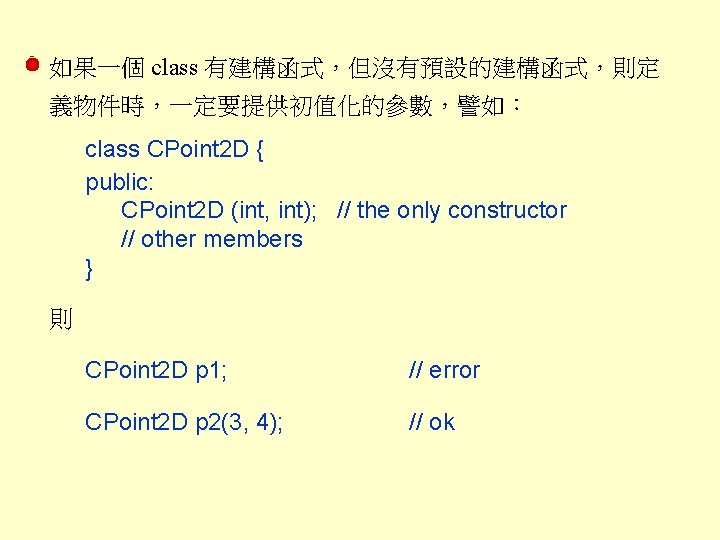
如果一個 class 有建構函式,但沒有預設的建構函式,則定 義物件時,一定要提供初值化的參數,譬如: class CPoint 2 D { public: CPoint 2 D (int, int); // the only constructor // other members } 則 CPoint 2 D p 1; // error CPoint 2 D p 2(3, 4); // ok
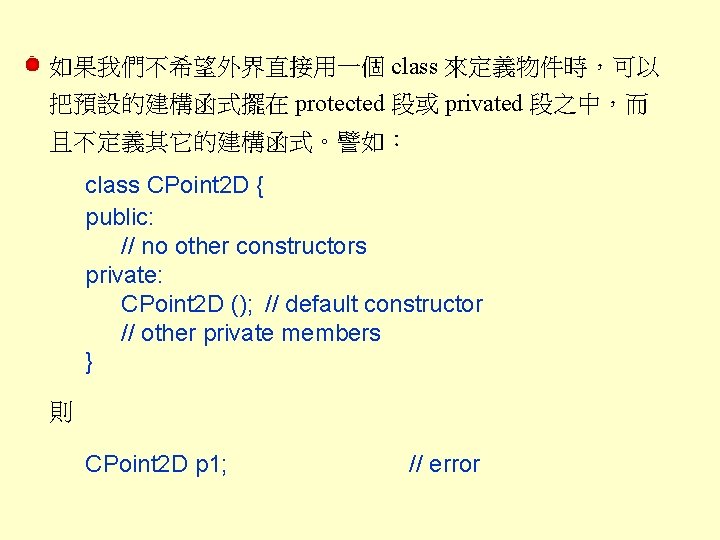
如果我們不希望外界直接用一個 class 來定義物件時,可以 把預設的建構函式擺在 protected 段或 privated 段之中,而 且不定義其它的建構函式。譬如: class CPoint 2 D { public: // no other constructors private: CPoint 2 D (); // default constructor // other private members } 則 CPoint 2 D p 1; // error
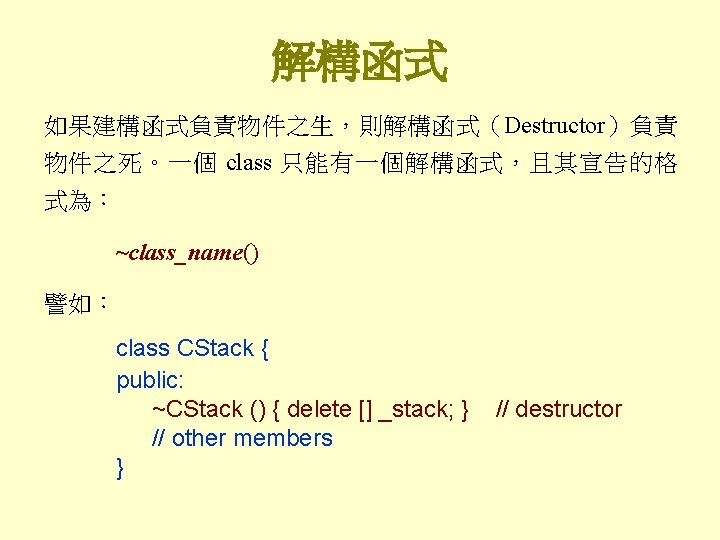
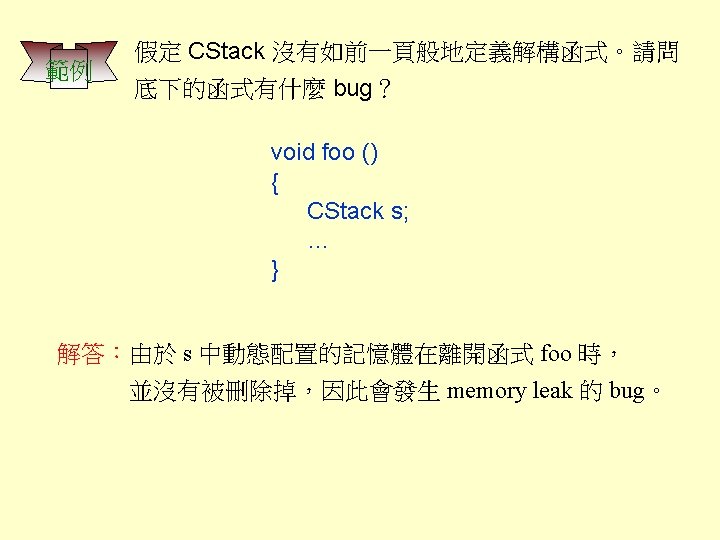
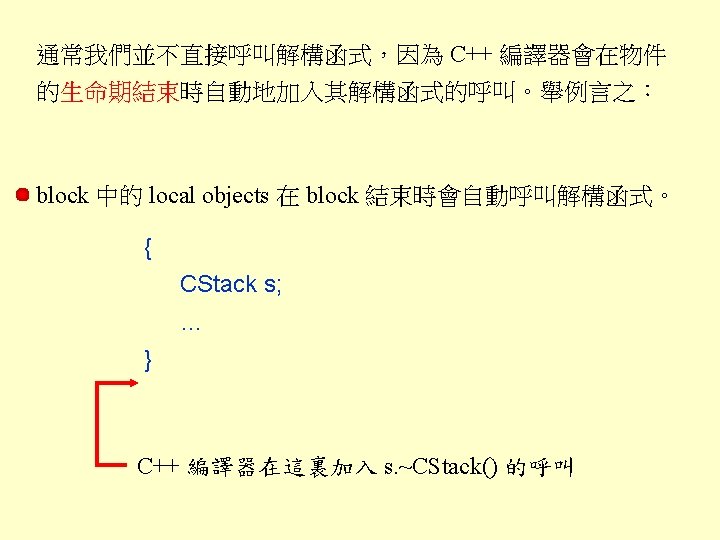
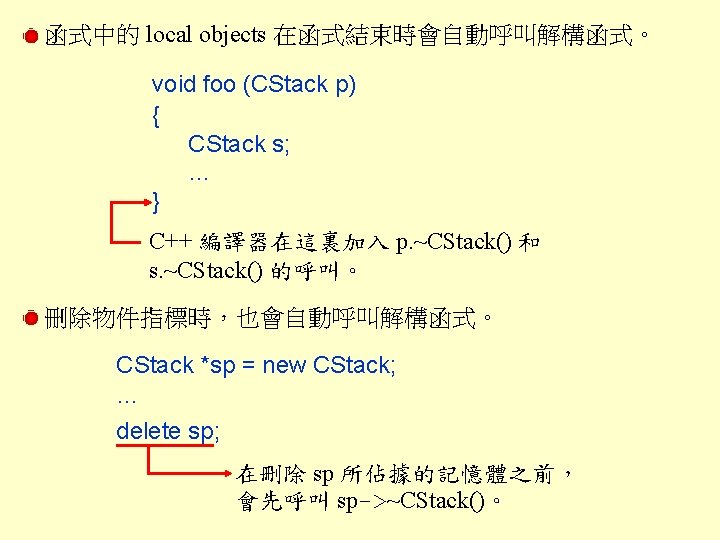
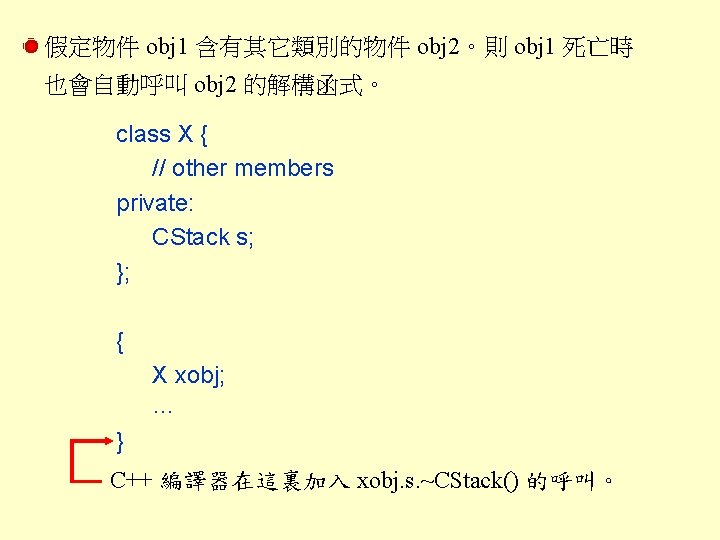
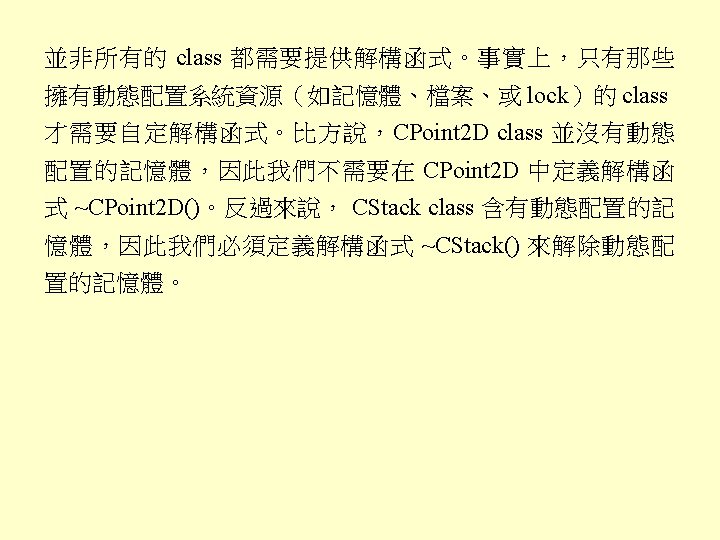
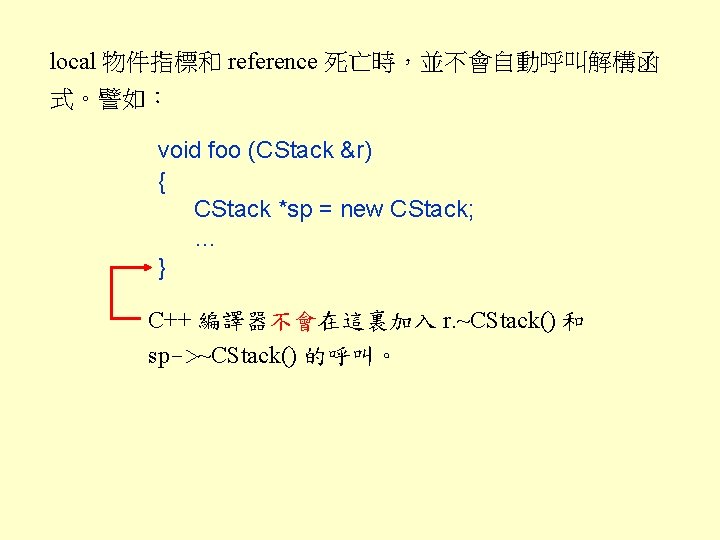
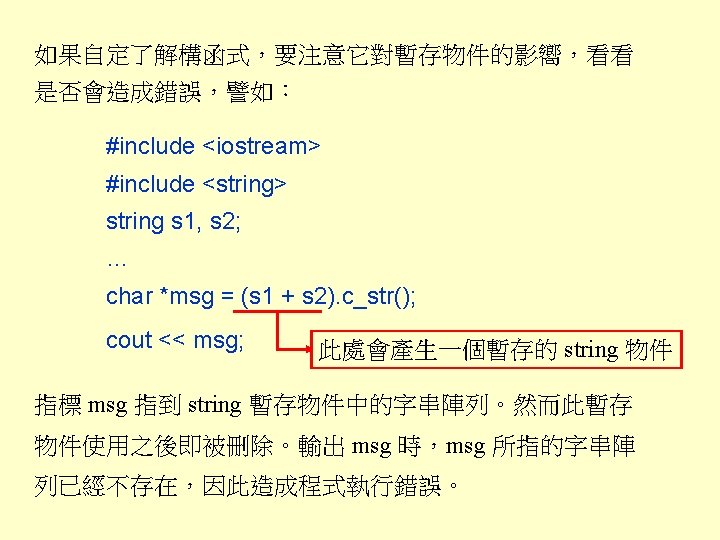
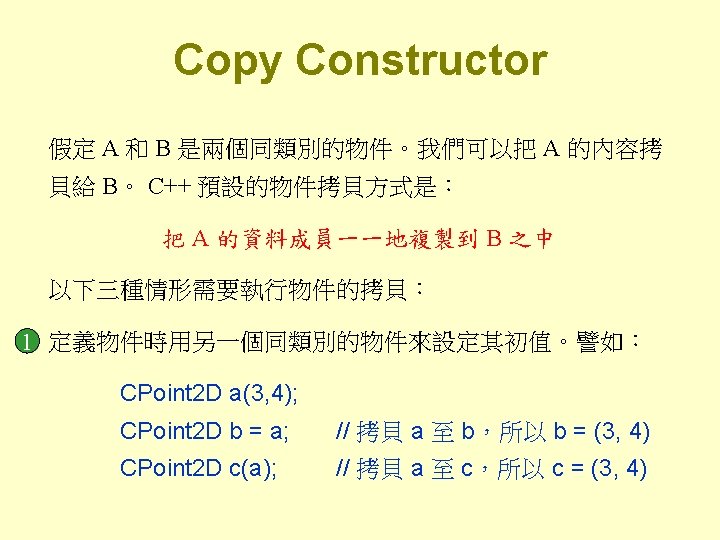
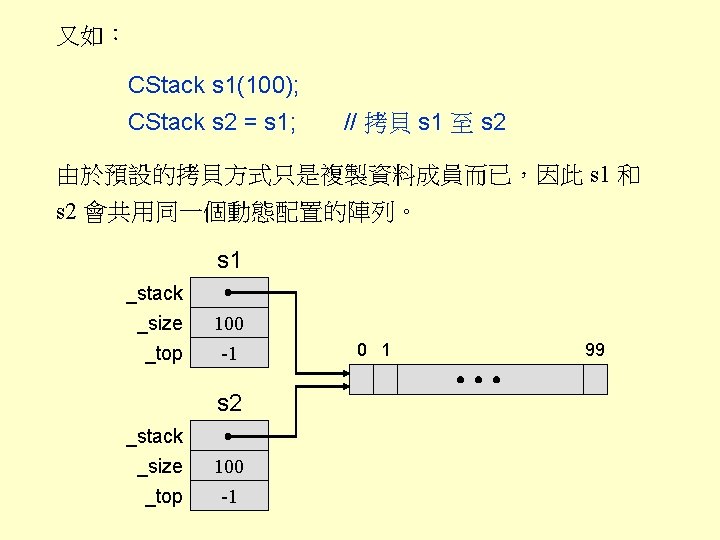
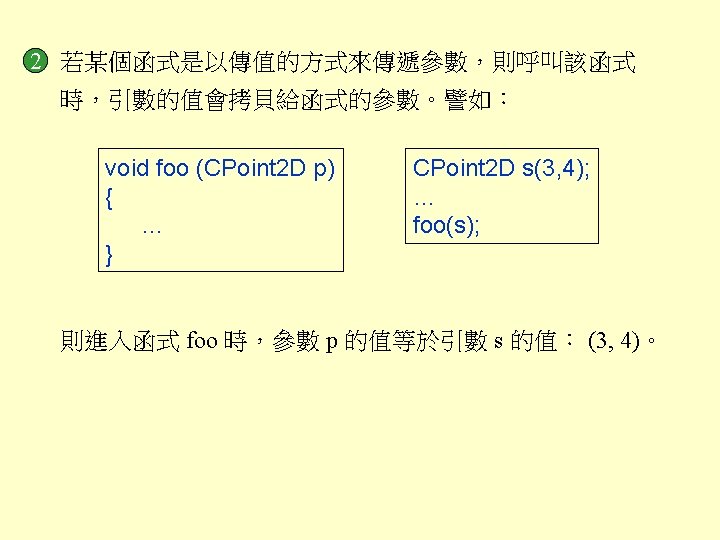
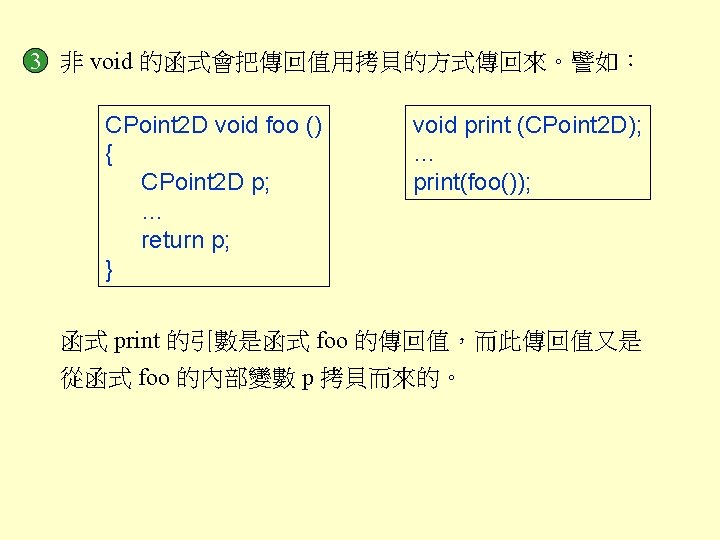
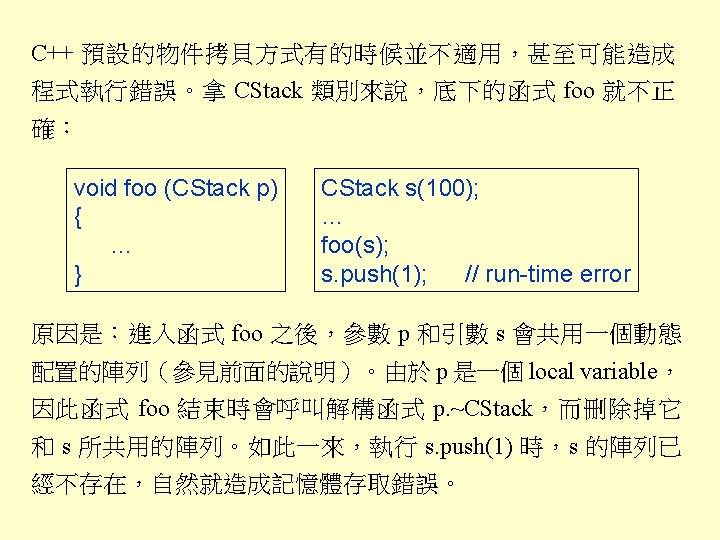
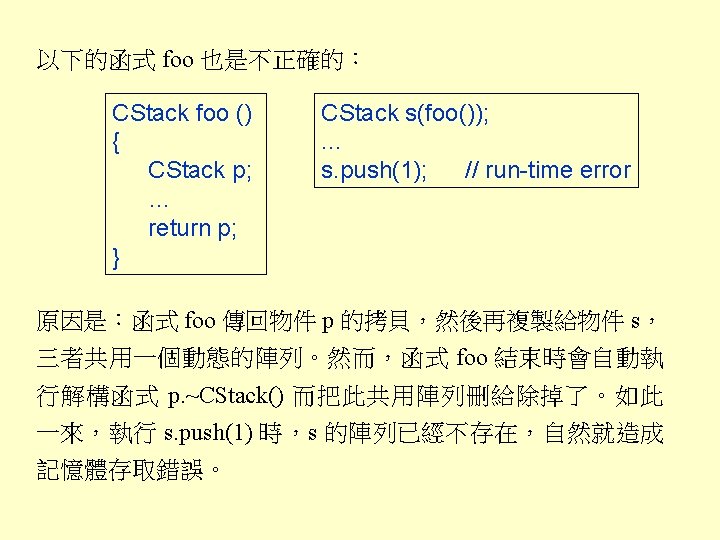
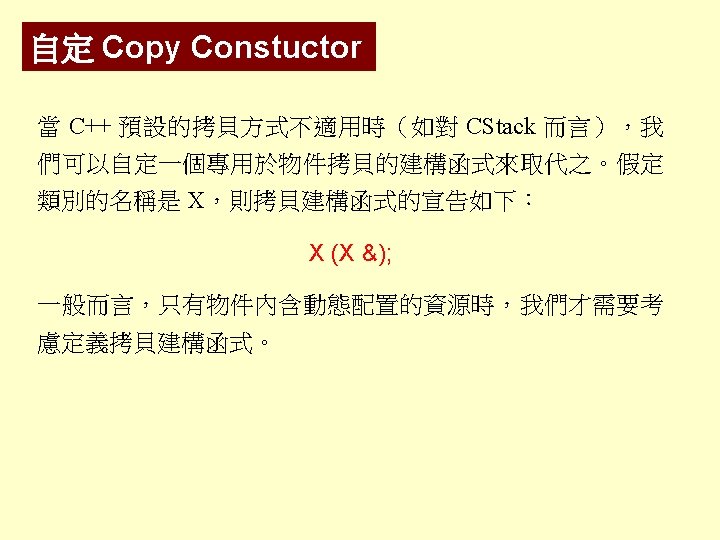
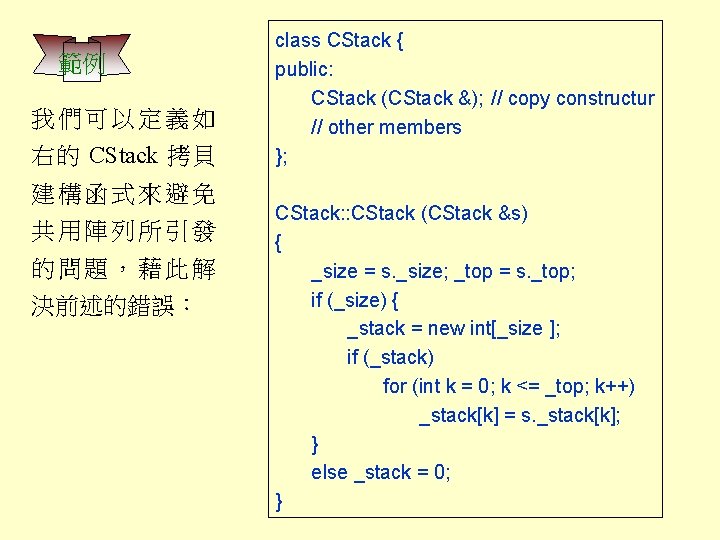
範例 我們可以定義如 右的 CStack 拷貝 建構函式來避免 共用陣列所引發 的問題,藉此解 決前述的錯誤: class CStack { public: CStack (CStack &); // copy constructur // other members }; CStack: : CStack (CStack &s) { _size = s. _size; _top = s. _top; if (_size) { _stack = new int[_size ]; if (_stack) for (int k = 0; k <= _top; k++) _stack[k] = s. _stack[k]; } else _stack = 0; }
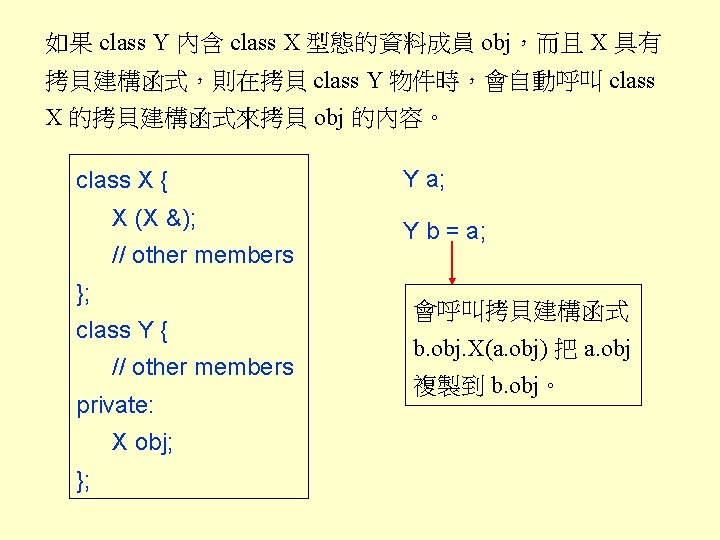
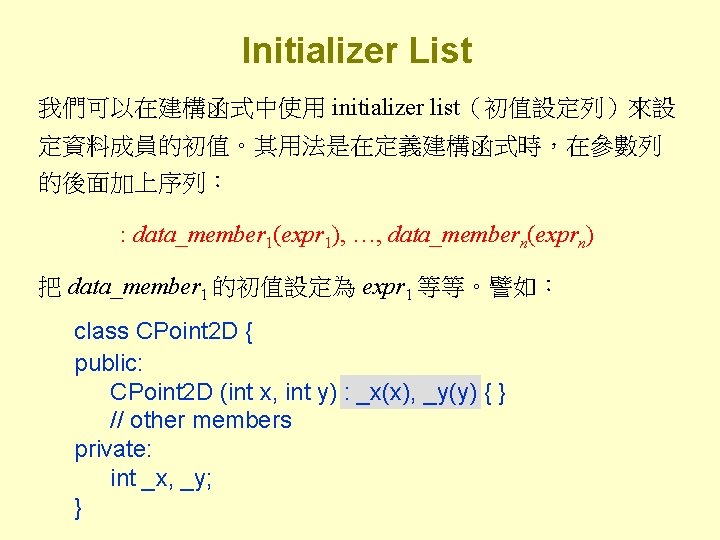
Initializer List 我們可以在建構函式中使用 initializer list(初值設定列)來設 定資料成員的初值。其用法是在定義建構函式時,在參數列 的後面加上序列: : data_member 1(expr 1), …, data_membern(exprn) 把 data_member 1 的初值設定為 expr 1 等等。譬如: class CPoint 2 D { public: CPoint 2 D (int x, int y) : _x(x), _y(y) { } // other members private: int _x, _y; }
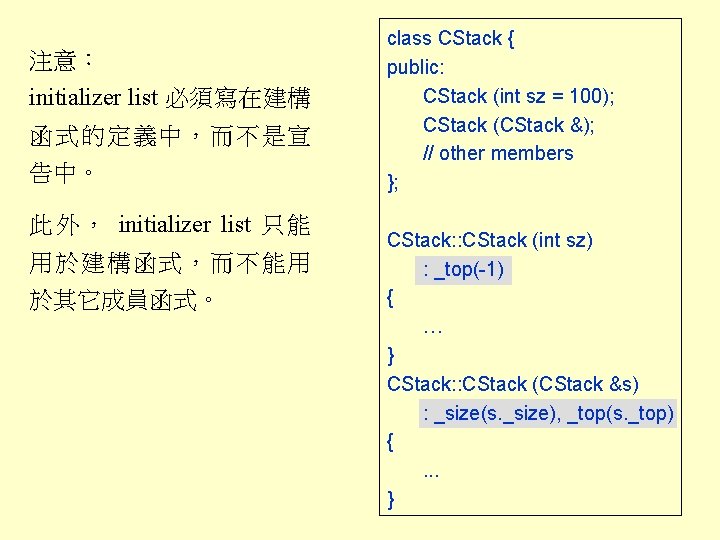
注意: initializer list 必須寫在建構 函式的定義中,而不是宣 告中。 此外, initializer list 只能 用於建構函式,而不能用 於其它成員函式。 class CStack { public: CStack (int sz = 100); CStack (CStack &); // other members }; CStack: : CStack (int sz) : _top(-1) { … } CStack: : CStack (CStack &s) : _size(s. _size), _top(s. _top) {. . . }
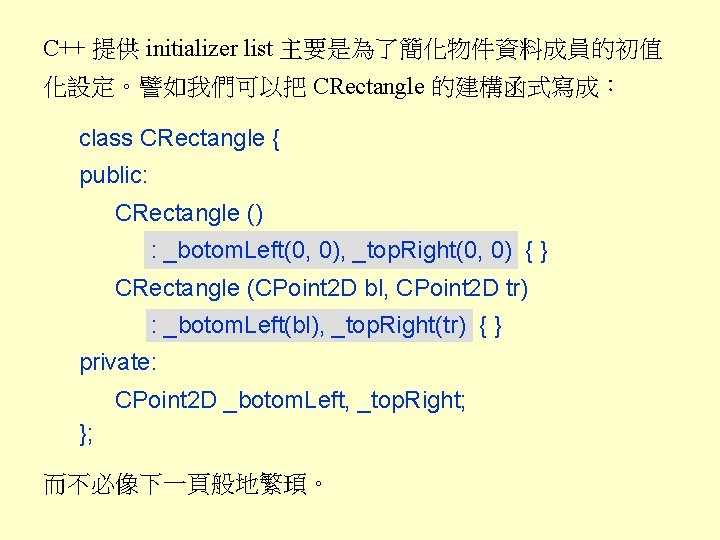
C++ 提供 initializer list 主要是為了簡化物件資料成員的初值 化設定。譬如我們可以把 CRectangle 的建構函式寫成: class CRectangle { public: CRectangle () : _botom. Left(0, 0), _top. Right(0, 0) { } CRectangle (CPoint 2 D bl, CPoint 2 D tr) : _botom. Left(bl), _top. Right(tr) { } private: CPoint 2 D _botom. Left, _top. Right; }; 而不必像下一頁般地繁頊。
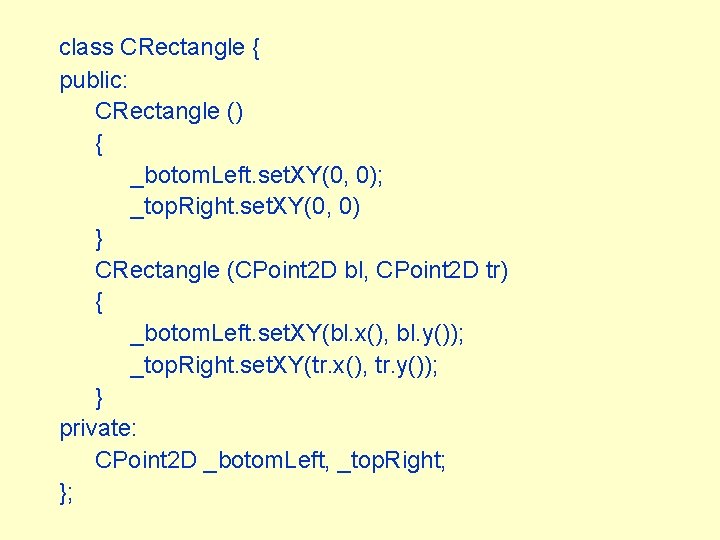
class CRectangle { public: CRectangle () { _botom. Left. set. XY(0, 0); _top. Right. set. XY(0, 0) } CRectangle (CPoint 2 D bl, CPoint 2 D tr) { _botom. Left. set. XY(bl. x(), bl. y()); _top. Right. set. XY(tr. x(), tr. y()); } private: CPoint 2 D _botom. Left, _top. Right; };
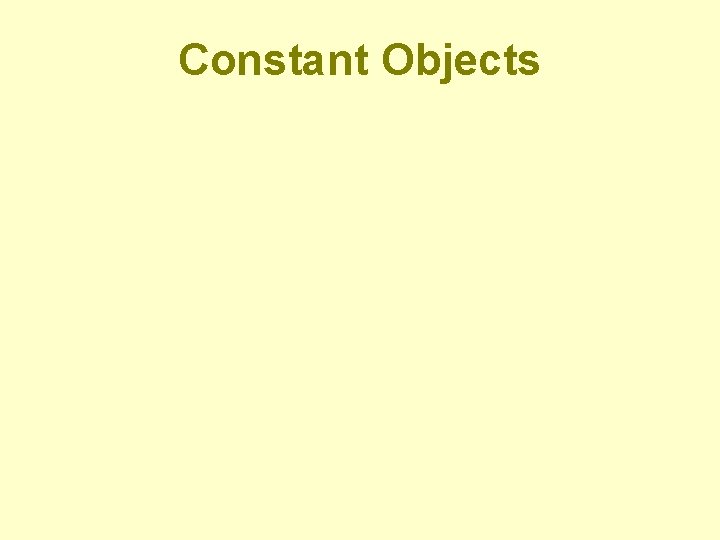
Constant Objects
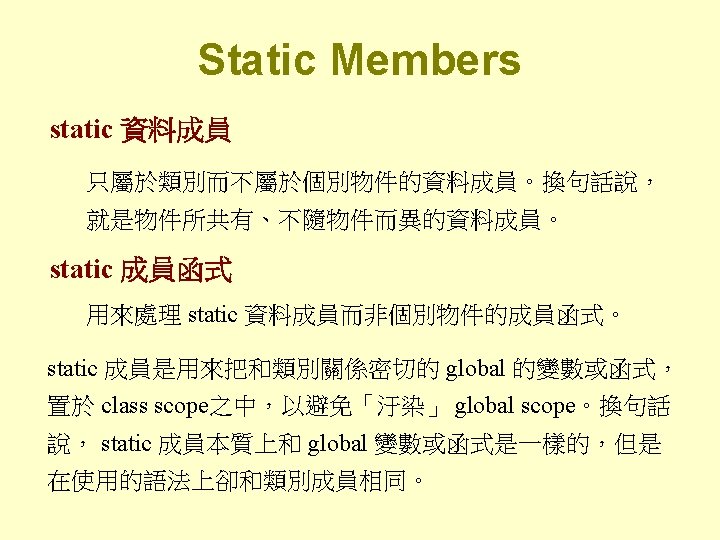
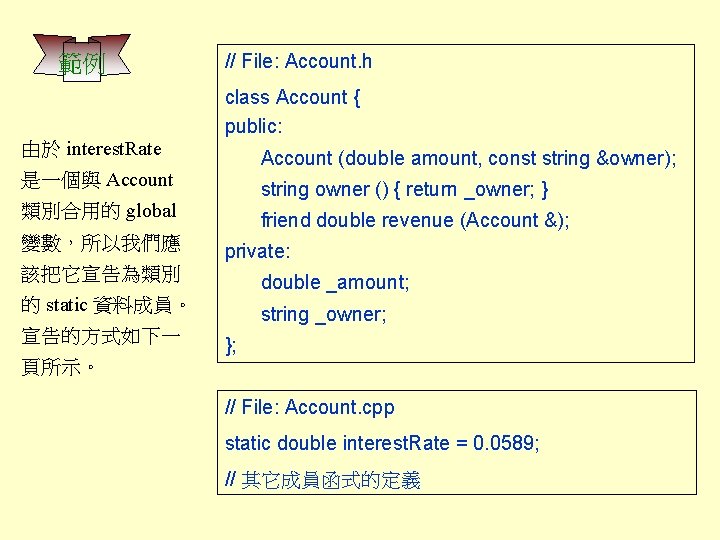
範例 由於 interest. Rate // File: Account. h class Account { public: Account (double amount, const string &owner); 是一個與 Account string owner () { return _owner; } 類別合用的 global 變數,所以我們應 friend double revenue (Account &); private: 該把它宣告為類別 double _amount; 的 static 資料成員。 宣告的方式如下一 string _owner; }; 頁所示。 // File: Account. cpp static double interest. Rate = 0. 0589; // 其它成員函式的定義
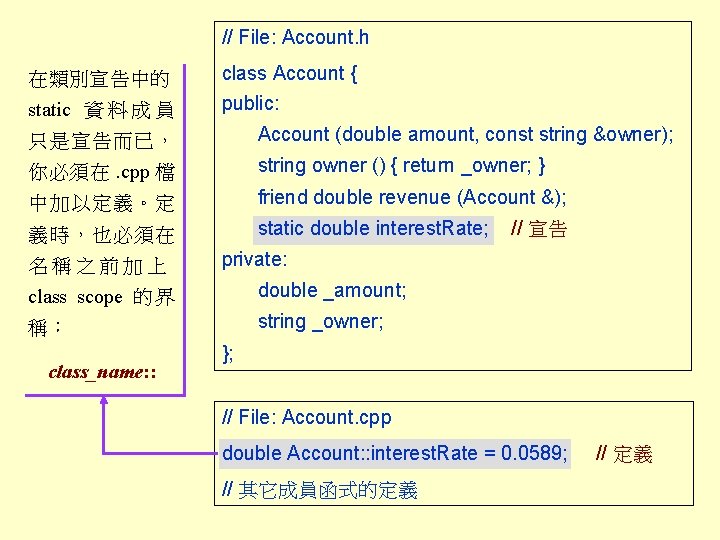
// File: Account. h 在類別宣告中的 static 資 料 成 員 class Account { public: 只是宣告而已, Account (double amount, const string &owner); 你必須在. cpp 檔 string owner () { return _owner; } 中加以定義。定 friend double revenue (Account &); 義時,也必須在 static double interest. Rate; 名稱之前加上 private: class scope 的 界 double _amount; 稱: string _owner; class_name: : // 宣告 }; // File: Account. cpp double Account: : interest. Rate = 0. 0589; // 其它成員函式的定義 // 定義
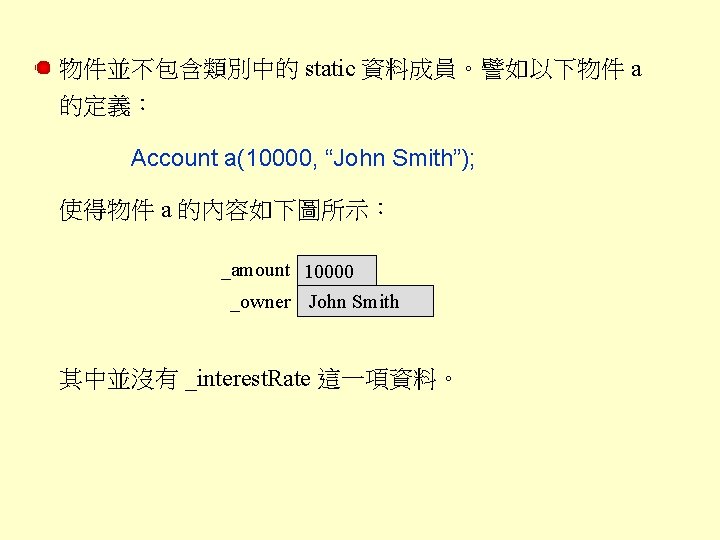
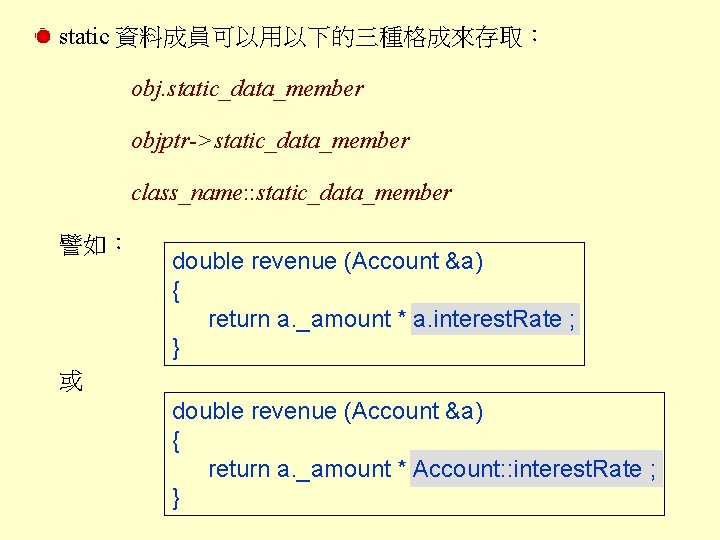
static 資料成員可以用以下的三種格成來存取: obj. static_data_member objptr->static_data_member class_name: : static_data_member 譬如: double revenue (Account &a) { return a. _amount * a. interest. Rate ; } 或 double revenue (Account &a) { return a. _amount * Account: : interest. Rate ; }
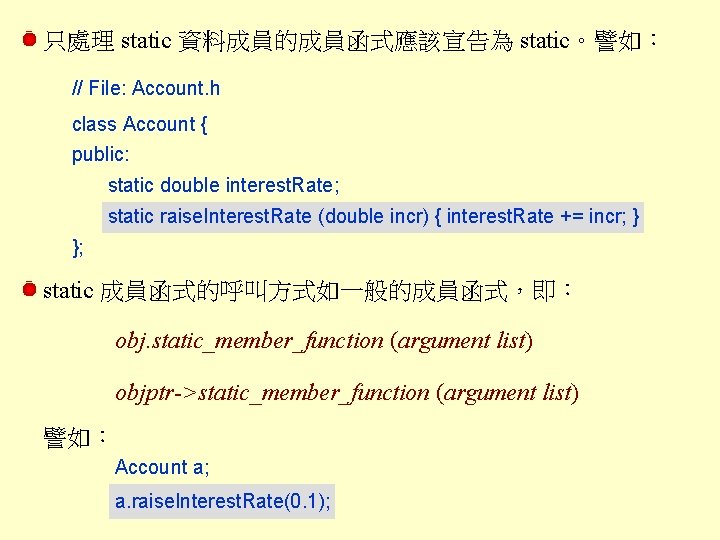
只處理 static 資料成員的成員函式應該宣告為 static。譬如: // File: Account. h class Account { public: static double interest. Rate; static raise. Interest. Rate (double incr) { interest. Rate += incr; } }; static 成員函式的呼叫方式如一般的成員函式,即: obj. static_member_function (argument list) objptr->static_member_function (argument list) 譬如: Account a; a. raise. Interest. Rate(0. 1);
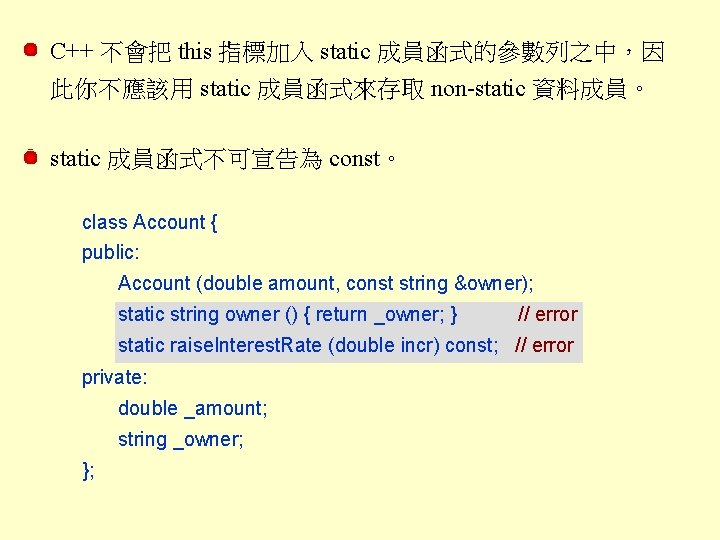
C++ 不會把 this 指標加入 static 成員函式的參數列之中,因 此你不應該用 static 成員函式來存取 non-static 資料成員。 static 成員函式不可宣告為 const。 class Account { public: Account (double amount, const string &owner); static string owner () { return _owner; } // error static raise. Interest. Rate (double incr) const; // error private: double _amount; string _owner; };
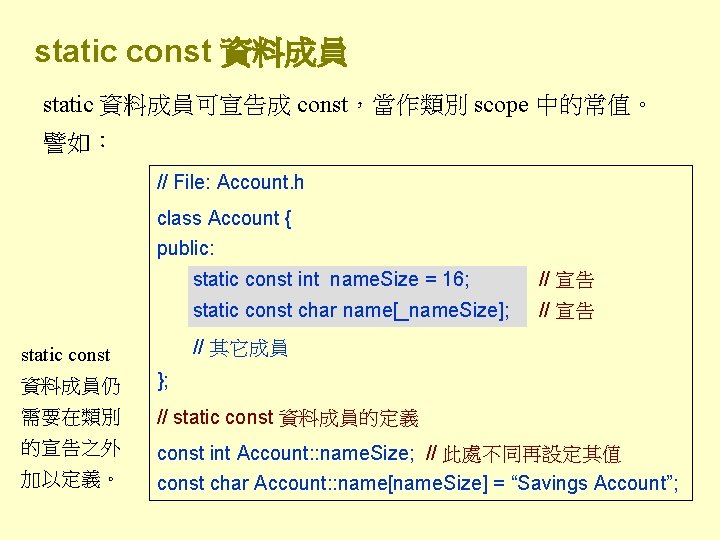
static const 資料成員 static 資料成員可宣告成 const,當作類別 scope 中的常值。 譬如: // File: Account. h class Account { public: static const int name. Size = 16; // 宣告 static const char name[_name. Size]; // 宣告 // 其它成員 static const 資料成員仍 }; 需要在類別 // static const 資料成員的定義 的宣告之外 const int Account: : name. Size; // 此處不同再設定其值 加以定義。 const char Account: : name[name. Size] = “Savings Account”;
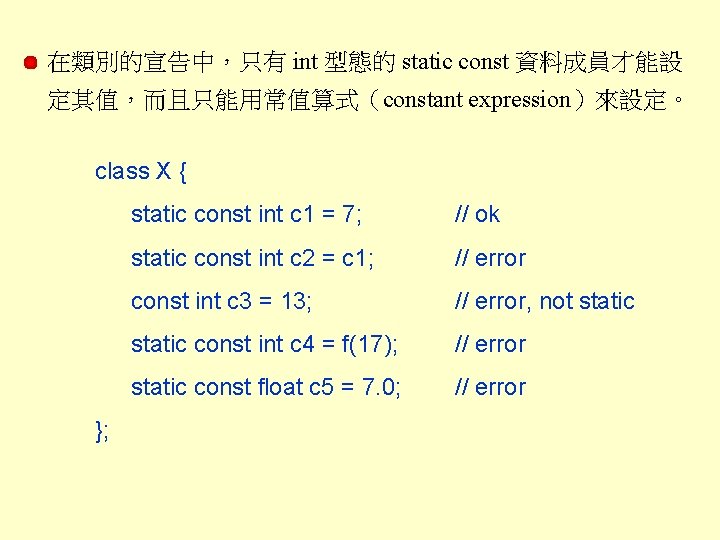
在類別的宣告中,只有 int 型態的 static const 資料成員才能設 定其值,而且只能用常值算式(constant expression)來設定。 class X { }; static const int c 1 = 7; // ok static const int c 2 = c 1; // error const int c 3 = 13; // error, not static const int c 4 = f(17); // error static const float c 5 = 7. 0; // error
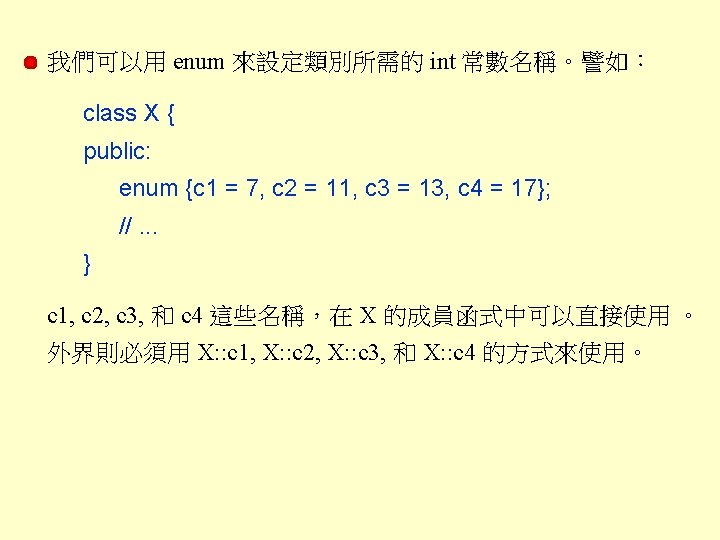
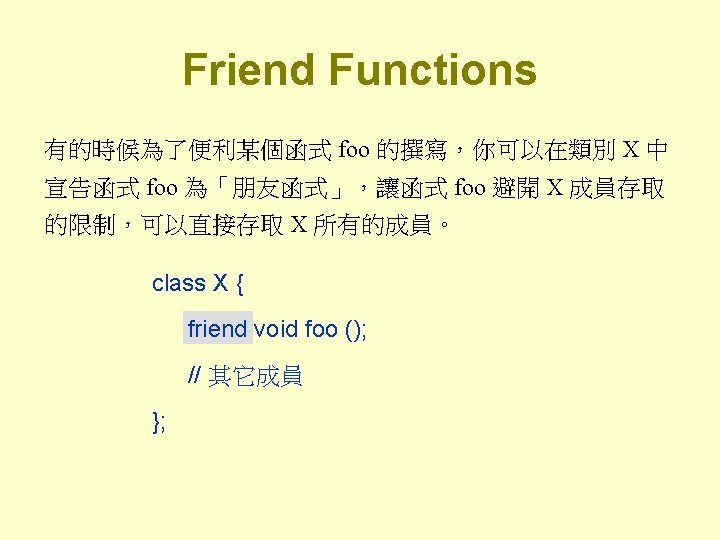
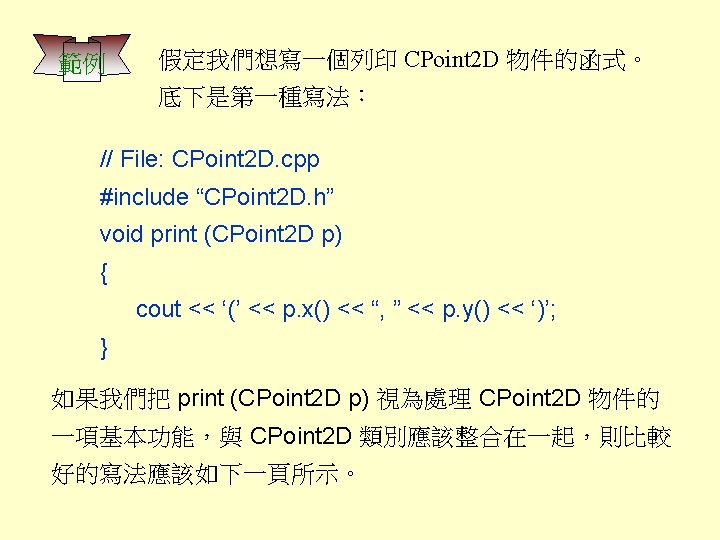
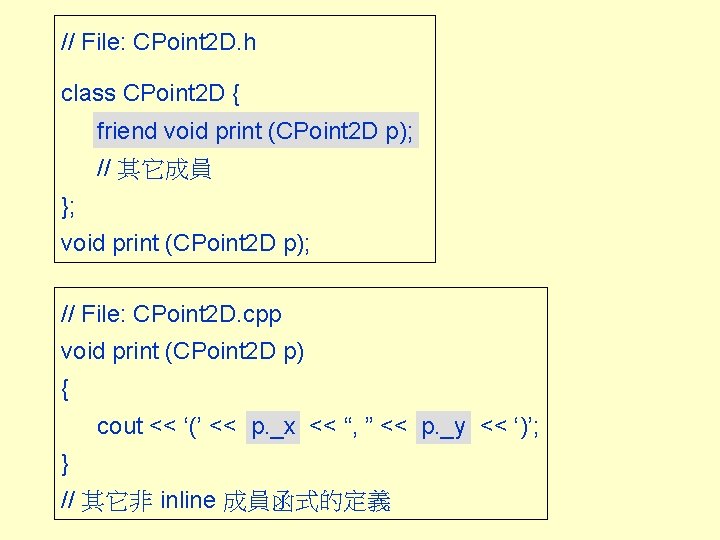
// File: CPoint 2 D. h class CPoint 2 D { friend void print (CPoint 2 D p); // 其它成員 }; void print (CPoint 2 D p); // File: CPoint 2 D. cpp void print (CPoint 2 D p) { cout << ‘(’ << p. _x << “, ” << p. _y << ‘)’; } // 其它非 inline 成員函式的定義
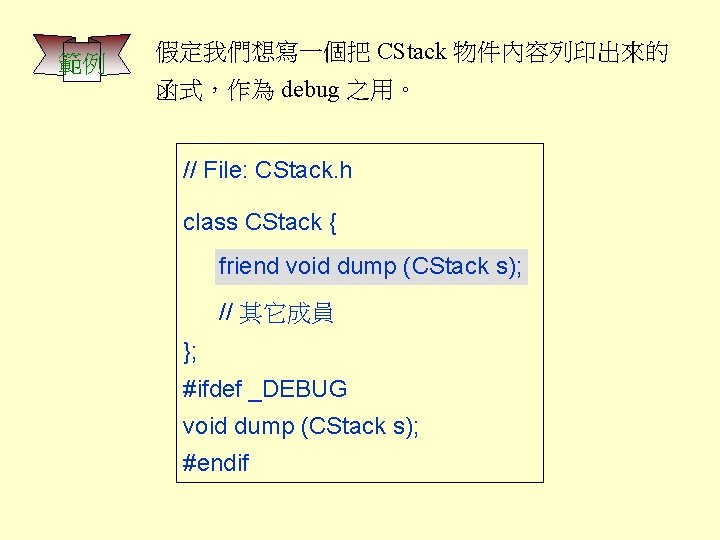
範例 假定我們想寫一個把 CStack 物件內容列印出來的 函式,作為 debug 之用。 // File: CStack. h class CStack { friend void dump (CStack s); // 其它成員 }; #ifdef _DEBUG void dump (CStack s); #endif
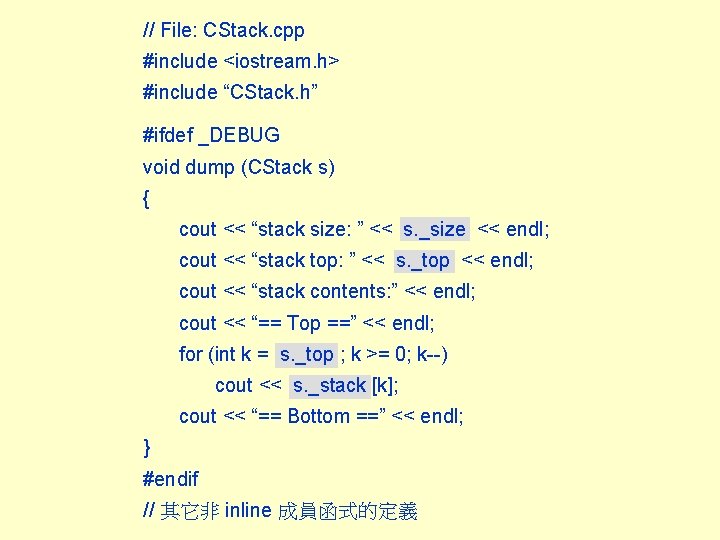
// File: CStack. cpp #include <iostream. h> #include “CStack. h” #ifdef _DEBUG void dump (CStack s) { cout << “stack size: ” << s. _size << endl; cout << “stack top: ” << s. _top << endl; cout << “stack contents: ” << endl; cout << “== Top ==” << endl; for (int k = s. _top ; k >= 0; k--) cout << s. _stack [k]; cout << “== Bottom ==” << endl; } #endif // 其它非 inline 成員函式的定義
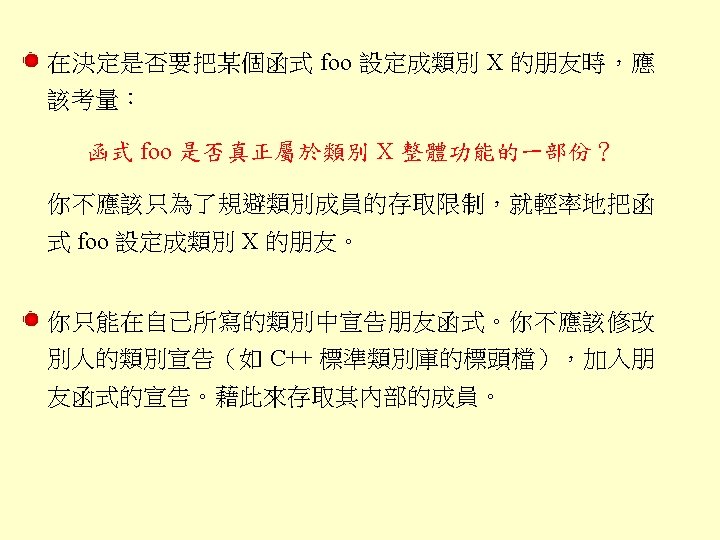
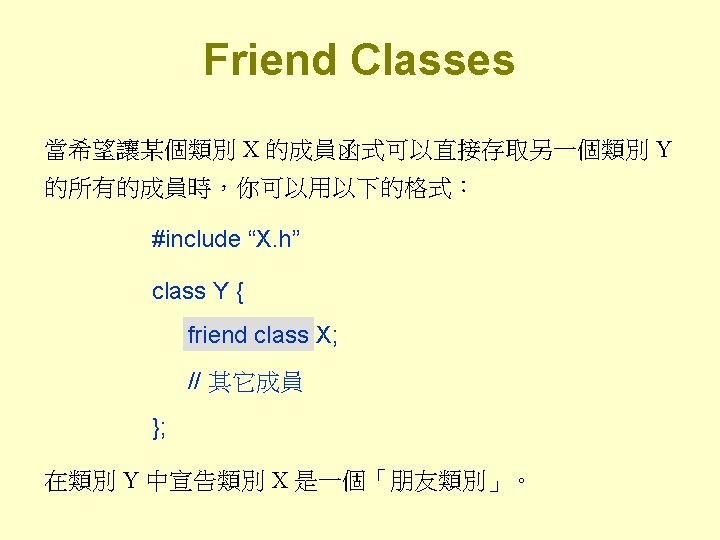
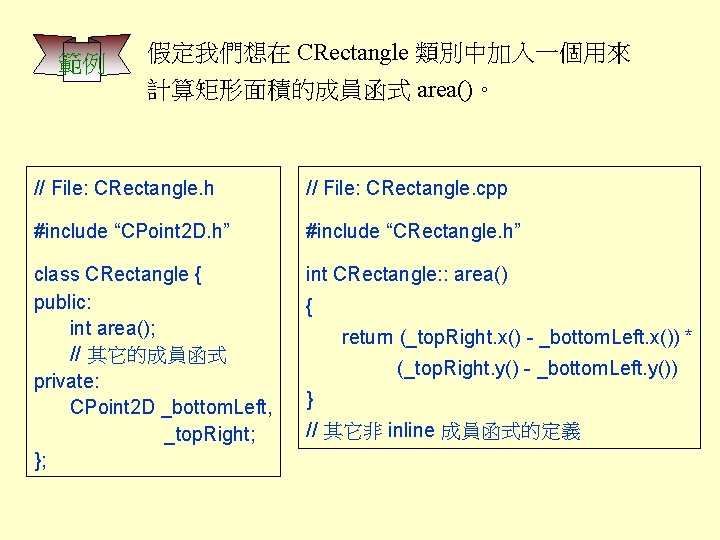
範例 假定我們想在 CRectangle 類別中加入一個用來 計算矩形面積的成員函式 area()。 // File: CRectangle. h // File: CRectangle. cpp #include “CPoint 2 D. h” #include “CRectangle. h” class CRectangle { public: int area(); // 其它的成員函式 private: CPoint 2 D _bottom. Left, _top. Right; }; int CRectangle: : area() { return (_top. Right. x() - _bottom. Left. x()) * (_top. Right. y() - _bottom. Left. y()) } // 其它非 inline 成員函式的定義
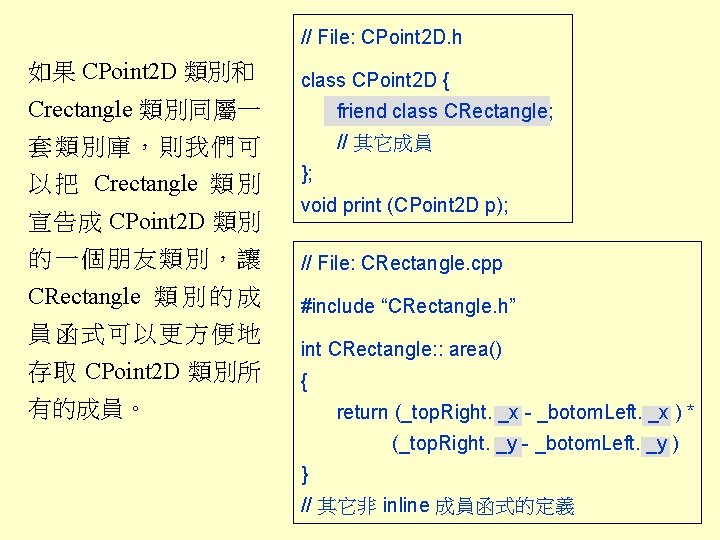
// File: CPoint 2 D. h 如果 CPoint 2 D 類別和 class CPoint 2 D { Crectangle 類別同屬一 friend class CRectangle; 套類別庫,則我們可 // 其它成員 以 把 Crectangle 類 別 宣告成 CPoint 2 D 類別 }; void print (CPoint 2 D p); 的一個朋友類別,讓 // File: CRectangle. cpp CRectangle 類 別 的 成 #include “CRectangle. h” 員函式可以更方便地 存取 CPoint 2 D 類別所 int CRectangle: : area() { 有的成員。 return (_top. Right. _x - _botom. Left. _x ) * (_top. Right. _y - _botom. Left. _y ) } // 其它非 inline 成員函式的定義
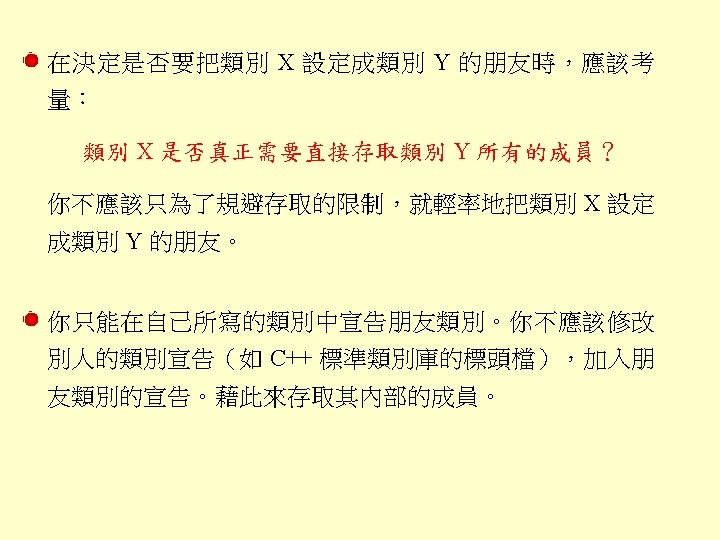
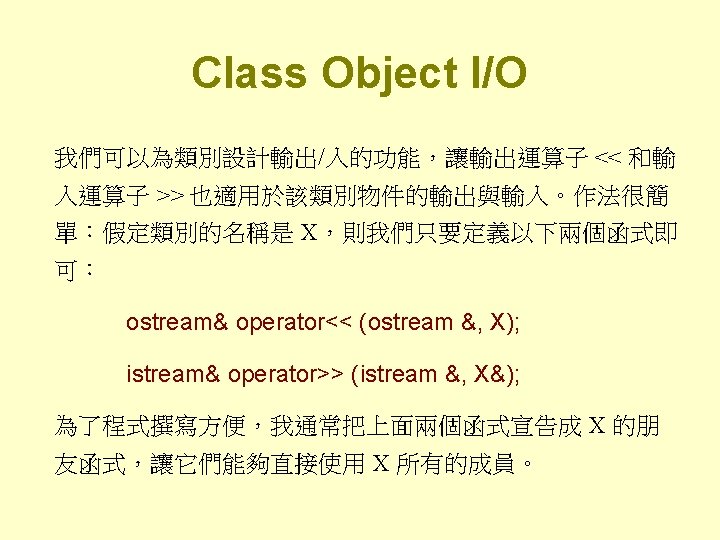
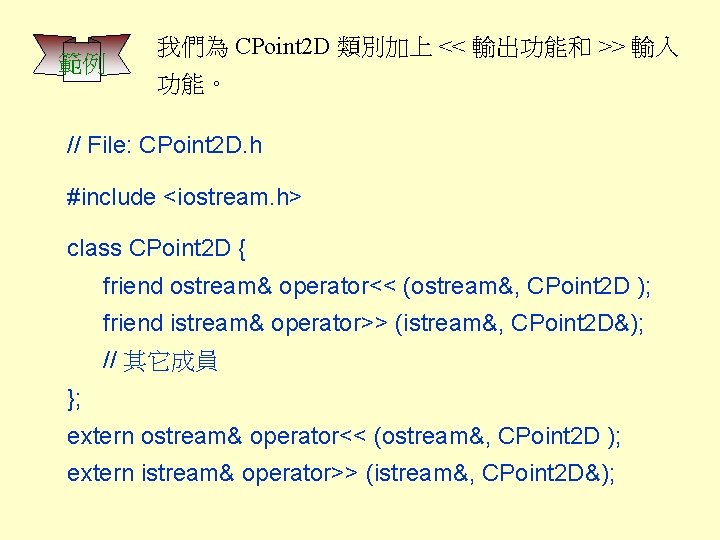
範例 我們為 CPoint 2 D 類別加上 << 輸出功能和 >> 輸入 功能。 // File: CPoint 2 D. h #include <iostream. h> class CPoint 2 D { friend ostream& operator<< (ostream&, CPoint 2 D ); friend istream& operator>> (istream&, CPoint 2 D&); // 其它成員 }; extern ostream& operator<< (ostream&, CPoint 2 D ); extern istream& operator>> (istream&, CPoint 2 D&);
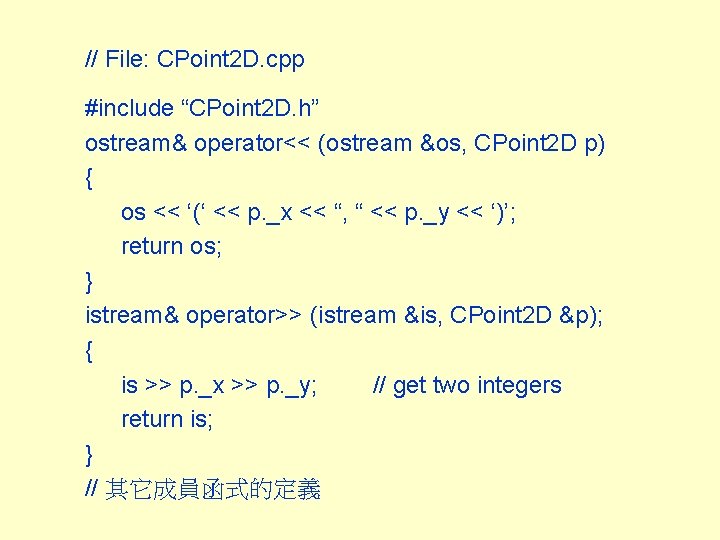
// File: CPoint 2 D. cpp #include “CPoint 2 D. h” ostream& operator<< (ostream &os, CPoint 2 D p) { os << ‘(‘ << p. _x << “, “ << p. _y << ‘)’; return os; } istream& operator>> (istream &is, CPoint 2 D &p); { is >> p. _x >> p. _y; // get two integers return is; } // 其它成員函式的定義
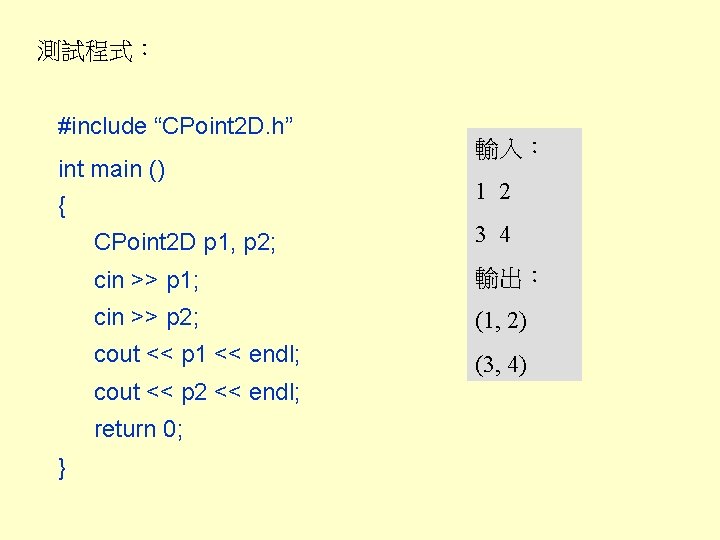
測試程式: #include “CPoint 2 D. h” int main () { 1 2 CPoint 2 D p 1, p 2; 3 4 cin >> p 1; 輸出: cin >> p 2; (1, 2) cout << p 1 << endl; (3, 4) cout << p 2 << endl; return 0; } 輸入:
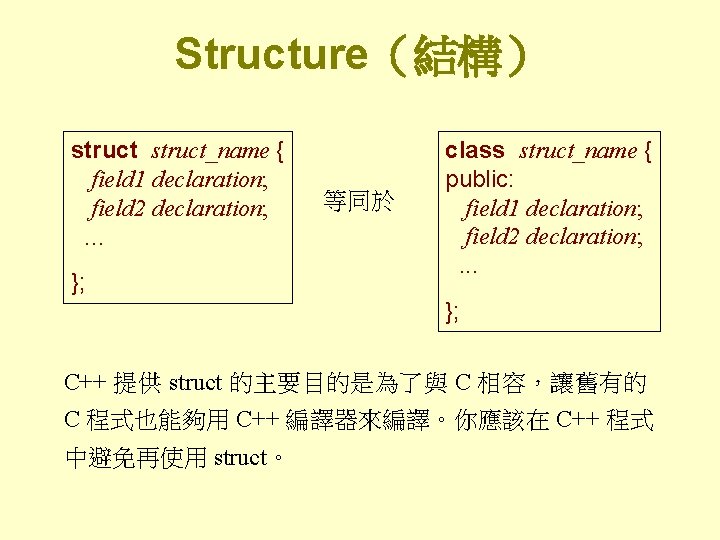
Structure(結構) struct_name { field 1 declaration; field 2 declaration; . . . }; 等同於 class struct_name { public: field 1 declaration; field 2 declaration; . . . }; C++ 提供 struct 的主要目的是為了與 C 相容,讓舊有的 C 程式也能夠用 C++ 編譯器來編譯。你應該在 C++ 程式 中避免再使用 struct。
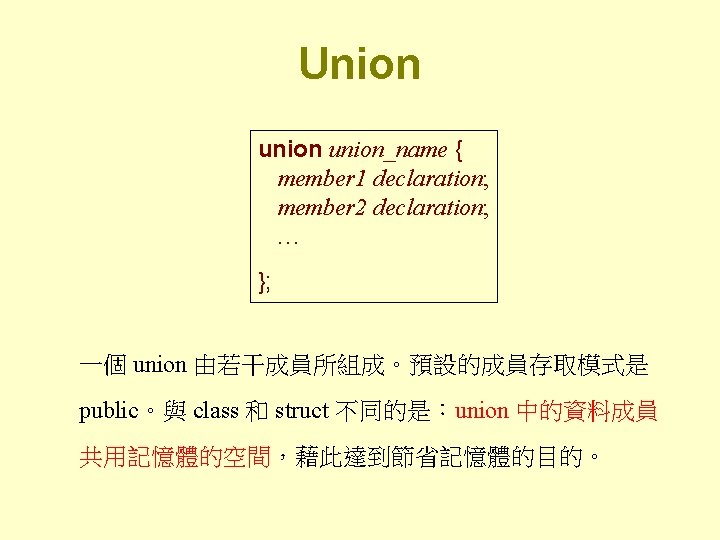
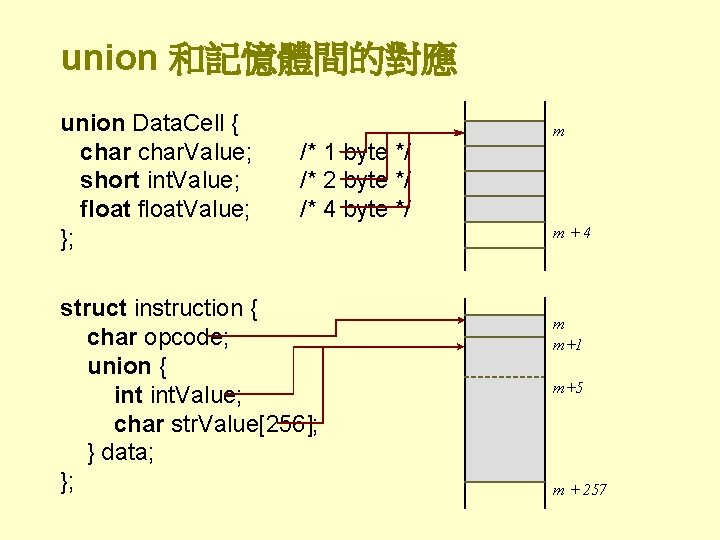
union 和記憶體間的對應 union Data. Cell { char. Value; short int. Value; float. Value; }; /* 1 byte */ /* 2 byte */ /* 4 byte */ struct instruction { char opcode; union { int. Value; char str. Value[256]; } data; }; m m+4 m m+1 m+5 m + 257
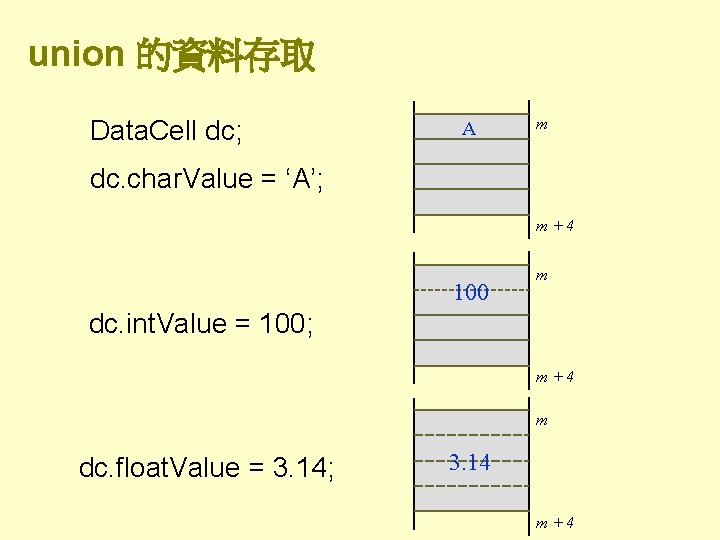
union 的資料存取 Data. Cell dc; A m dc. char. Value = ‘A’; m+4 100 m dc. int. Value = 100; m+4 m dc. float. Value = 3. 14; 3. 14 m+4
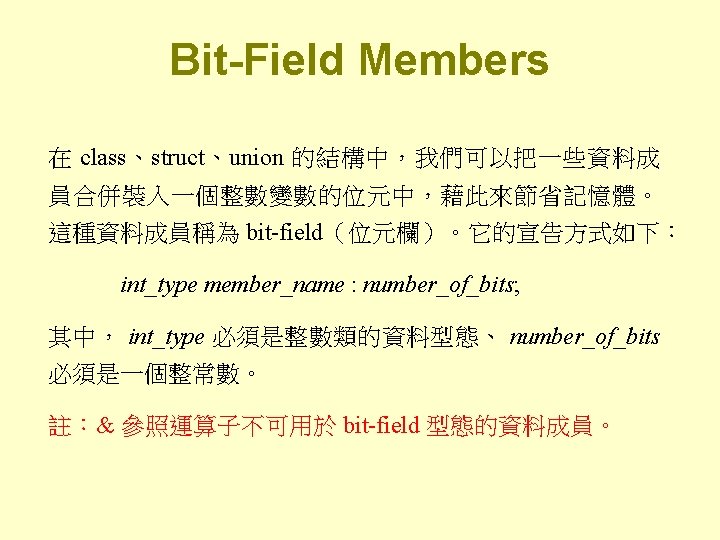
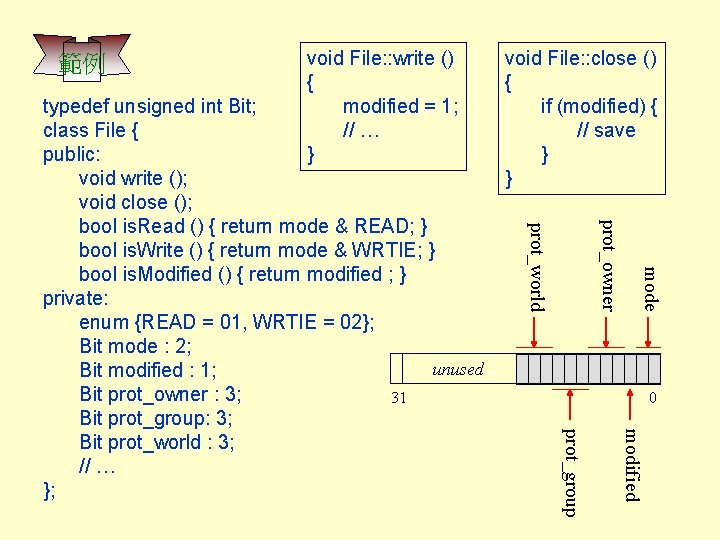
範例 void File: : write () { modified = 1; // … } mode prot_owner prot_world 0 modified prot_group typedef unsigned int Bit; class File { public: void write (); void close (); bool is. Read () { return mode & READ; } bool is. Write () { return mode & WRTIE; } bool is. Modified () { return modified ; } private: enum {READ = 01, WRTIE = 02}; Bit mode : 2; unused Bit modified : 1; Bit prot_owner : 3; 31 Bit prot_group: 3; Bit prot_world : 3; // … }; void File: : close () { if (modified) { // save } }
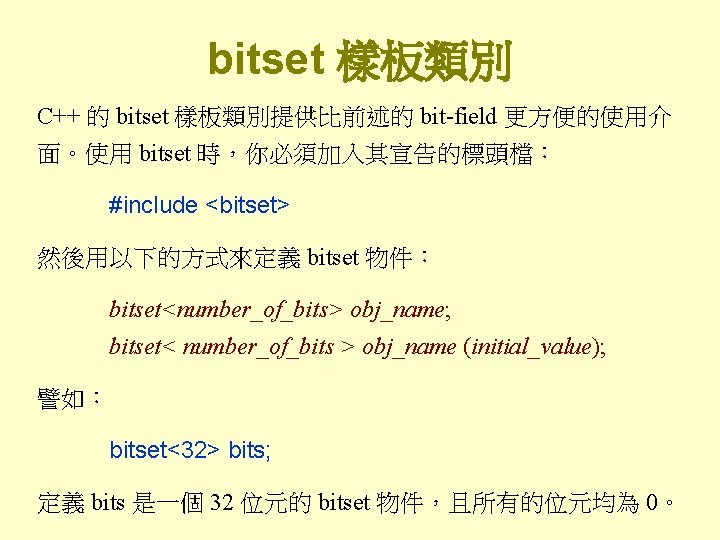
bitset 樣板類別 C++ 的 bitset 樣板類別提供比前述的 bit-field 更方便的使用介 面。使用 bitset 時,你必須加入其宣告的標頭檔: #include <bitset> 然後用以下的方式來定義 bitset 物件: bitset<number_of_bits> obj_name; bitset< number_of_bits > obj_name (initial_value); 譬如: bitset<32> bits; 定義 bits 是一個 32 位元的 bitset 物件,且所有的位元均為 0。
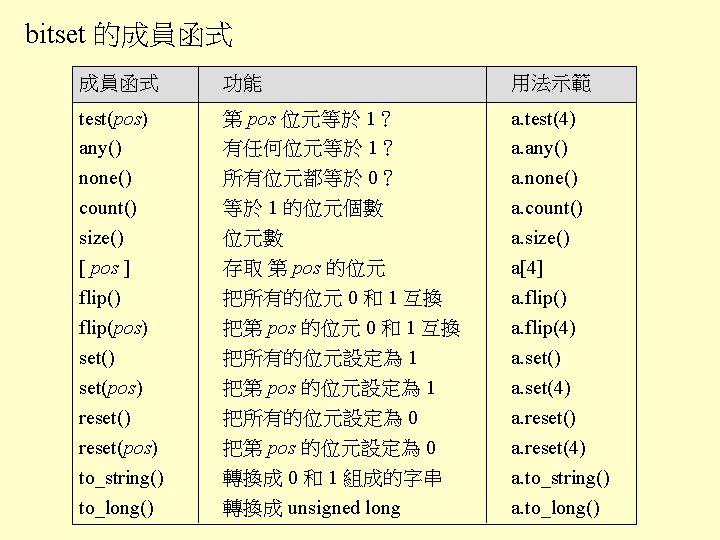
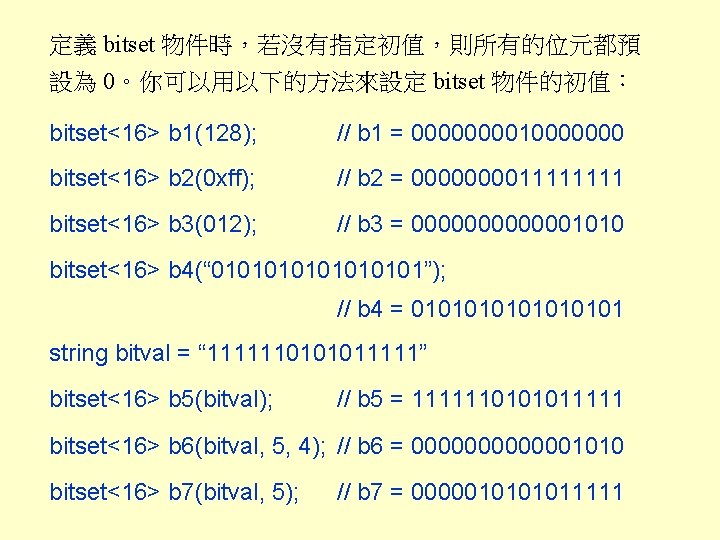
定義 bitset 物件時,若沒有指定初值,則所有的位元都預 設為 0。你可以用以下的方法來設定 bitset 物件的初值: bitset<16> b 1(128); // b 1 = 000010000000 bitset<16> b 2(0 xff); // b 2 = 00001111 bitset<16> b 3(012); // b 3 = 0000001010 bitset<16> b 4(“ 01010101”); // b 4 = 01010101 string bitval = “ 1111110101011111” bitset<16> b 5(bitval); // b 5 = 1111110101011111 bitset<16> b 6(bitval, 5, 4); // b 6 = 0000001010 bitset<16> b 7(bitval, 5); // b 7 = 000001011111
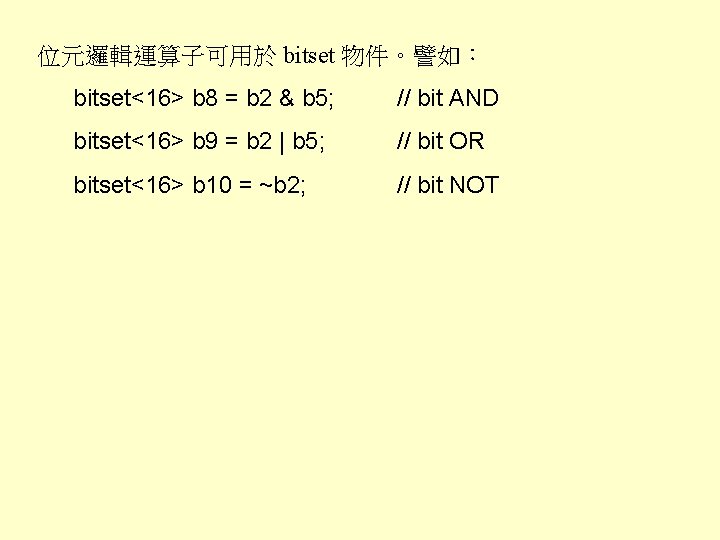
位元邏輯運算子可用於 bitset 物件。譬如: bitset<16> b 8 = b 2 & b 5; // bit AND bitset<16> b 9 = b 2 | b 5; // bit OR bitset<16> b 10 = ~b 2; // bit NOT
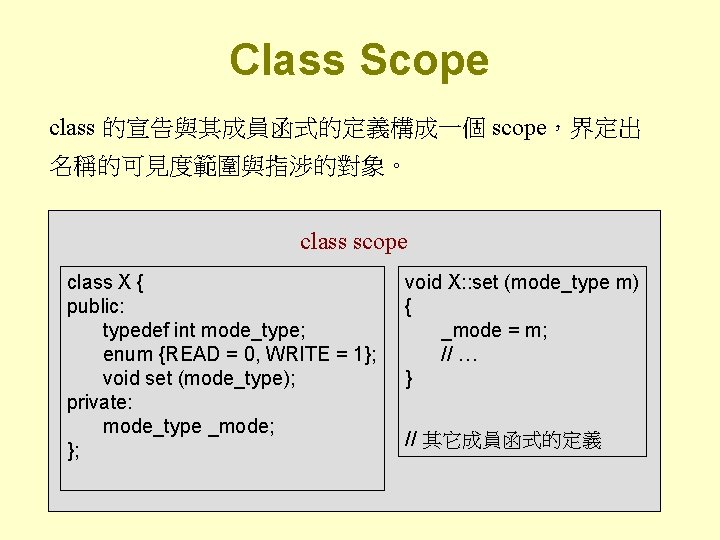
Class Scope class 的宣告與其成員函式的定義構成一個 scope,界定出 名稱的可見度範圍與指涉的對象。 class scope class X { public: typedef int mode_type; enum {READ = 0, WRITE = 1}; void set (mode_type); private: mode_type _mode; }; void X: : set (mode_type m) { _mode = m; // … } // 其它成員函式的定義
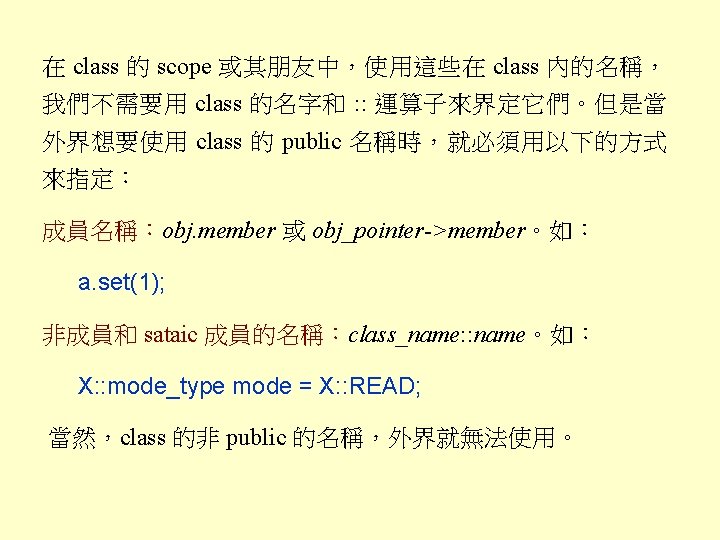
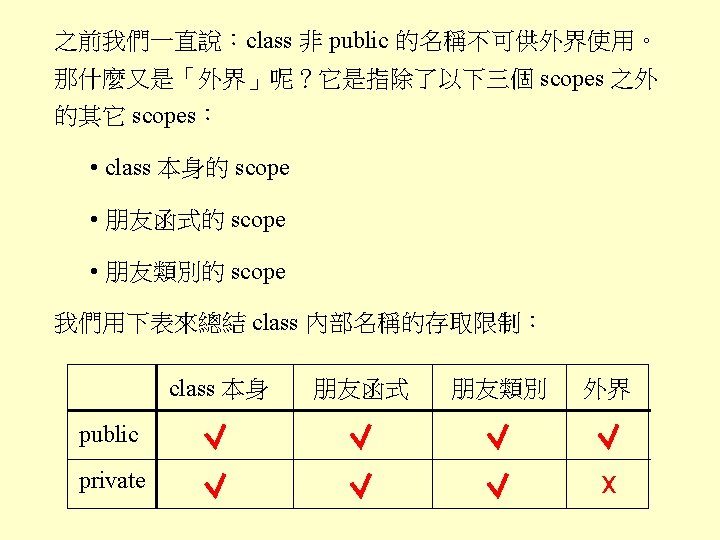
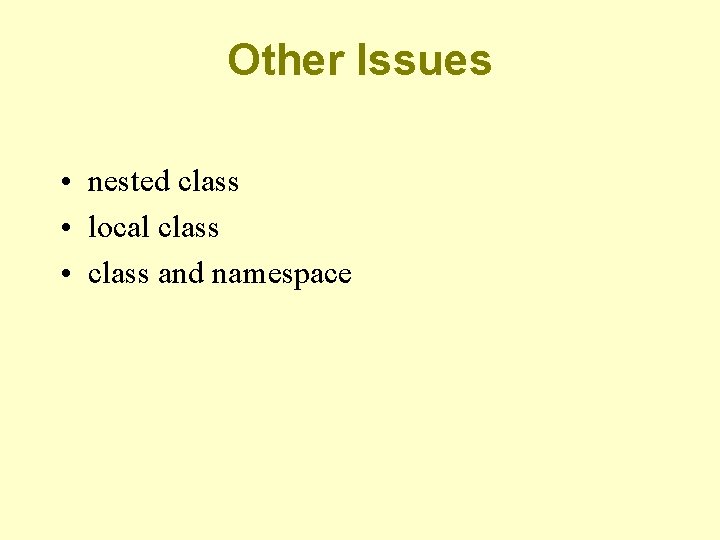
Other Issues • nested class • local class • class and namespace
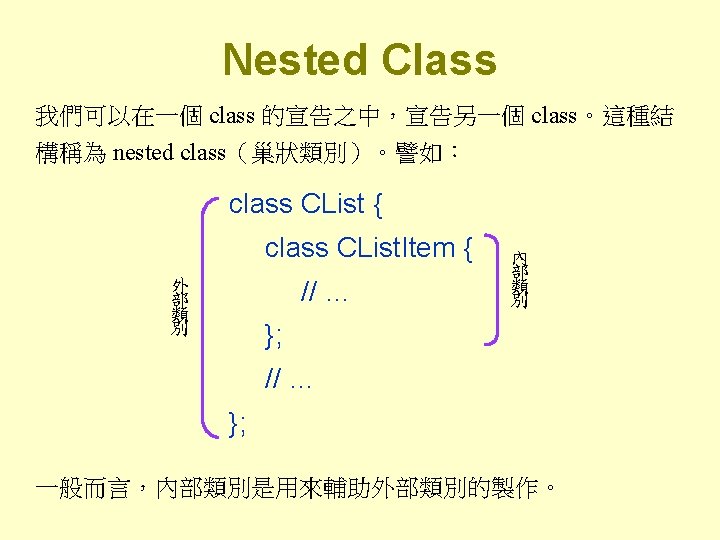
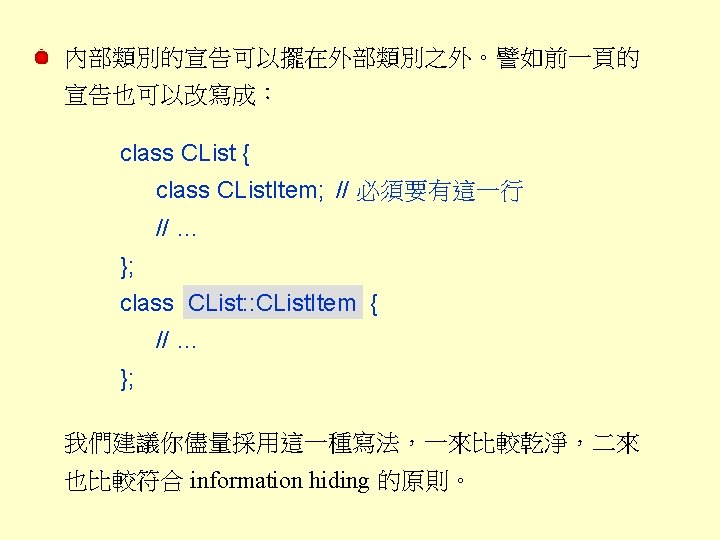
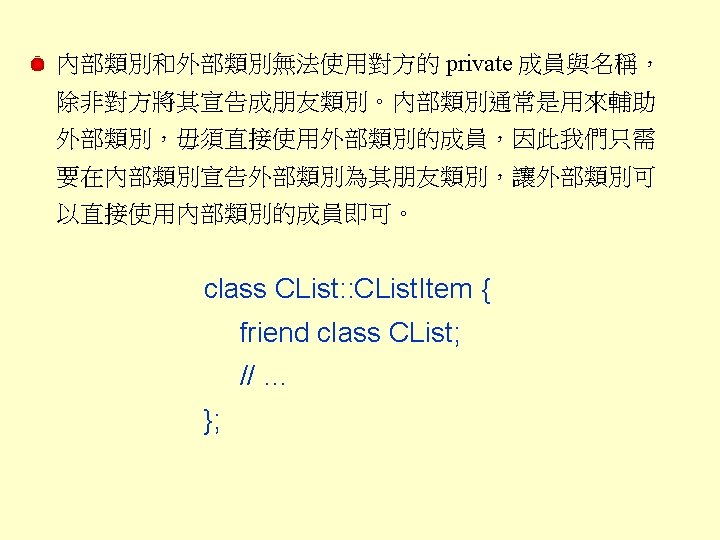
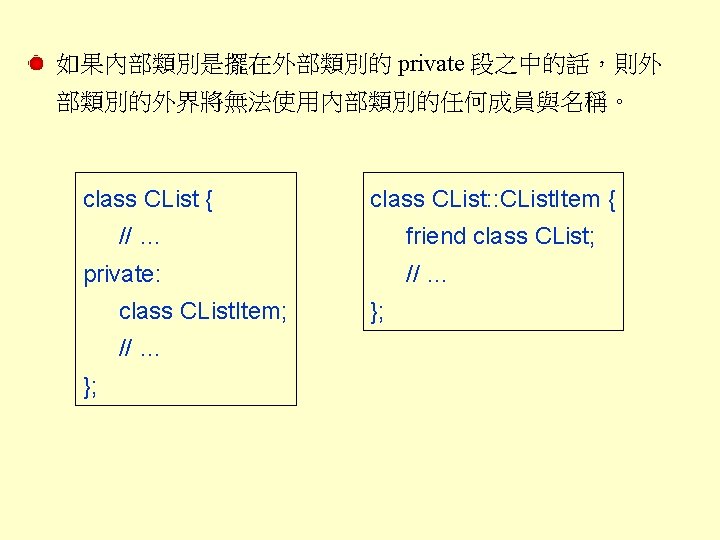
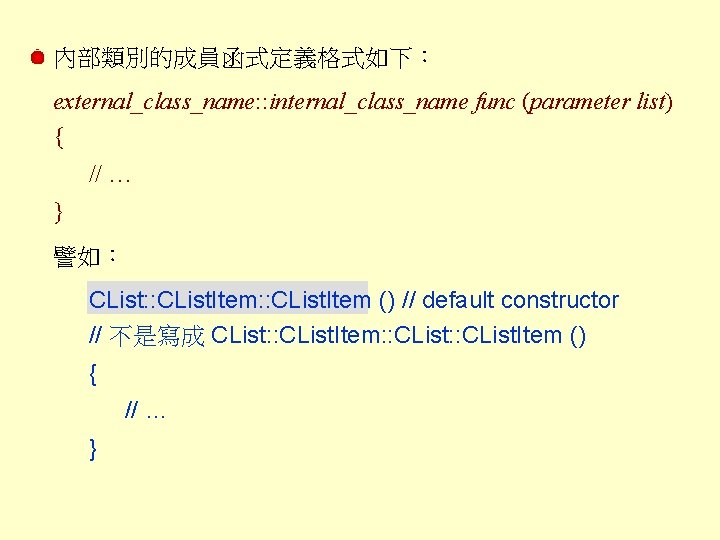
內部類別的成員函式定義格式如下: external_class_name: : internal_class_name func (parameter list) { // … } 譬如: CList: : CList. Item () // default constructor // 不是寫成 CList: : CList. Item: : CList. Item () { // … }
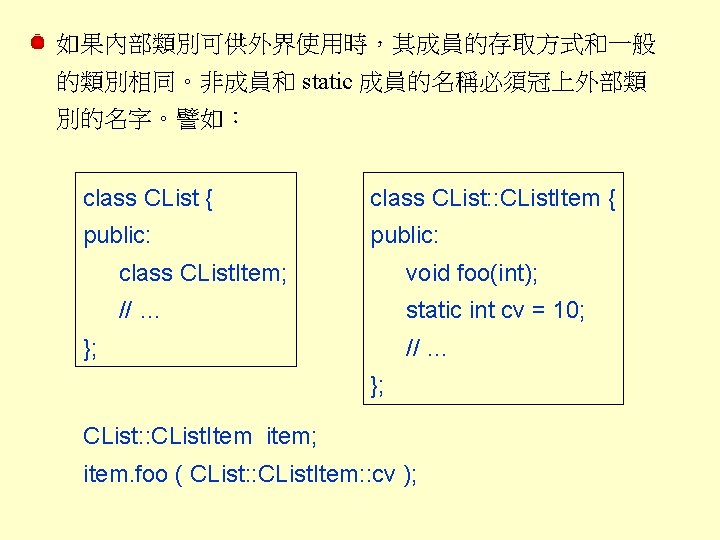
如果內部類別可供外界使用時,其成員的存取方式和一般 的類別相同。非成員和 static 成員的名稱必須冠上外部類 別的名字。譬如: class CList { class CList: : CList. Item { public: class CList. Item; void foo(int); // … static int cv = 10; }; // … }; CList: : CList. Item item; item. foo ( CList: : CList. Item: : cv );
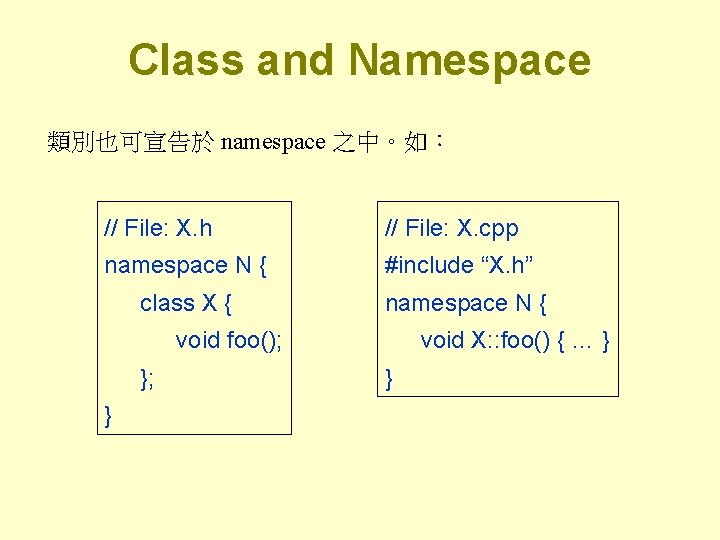
Class and Namespace 類別也可宣告於 namespace 之中。如: // File: X. h // File: X. cpp namespace N { #include “X. h” class X { namespace N { void foo(); }; } void X: : foo() { … } }
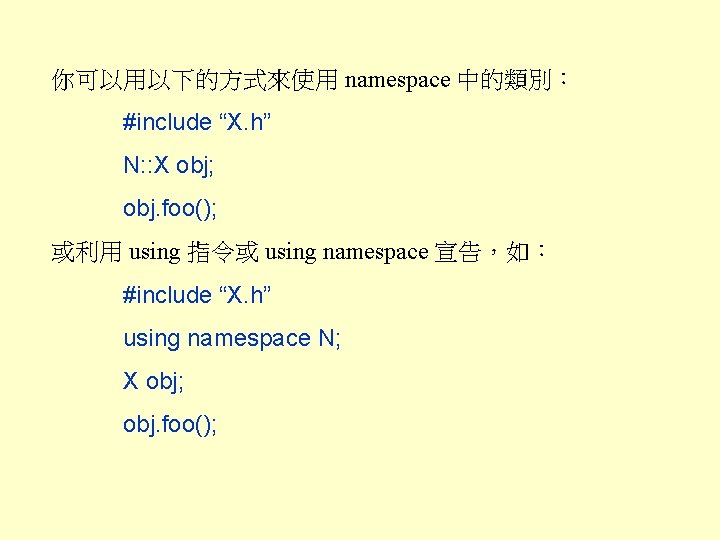
你可以用以下的方式來使用 namespace 中的類別: #include “X. h” N: : X obj; obj. foo(); 或利用 using 指令或 using namespace 宣告,如: #include “X. h” using namespace N; X obj; obj. foo();
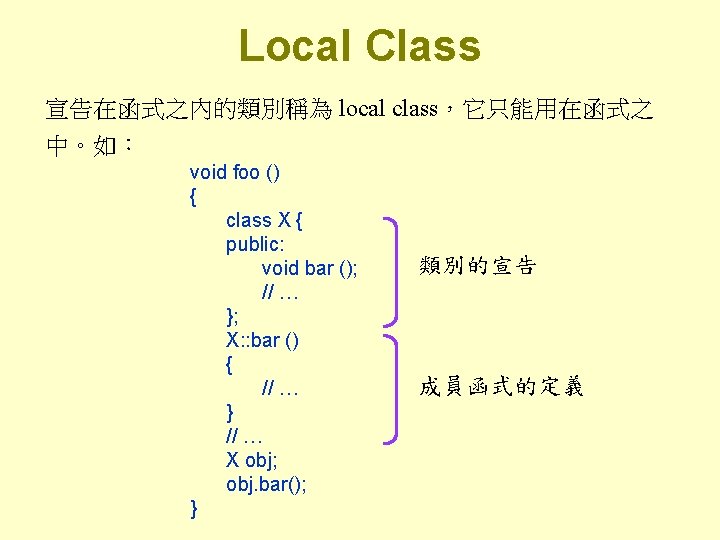
Pointer pointer
Constant pointer and pointer to constant
Constant pointer and pointer to constant
What is pointer to pointer in c
Pointer expressions
Constant pointer and pointer to constant
Pointer pointer
Constant to pointer in c
Copy class
Constructors and destructors in c
Method signature consists of
Considerate constructors scoring matrix
What is constructor in java
Considerate constructors best practice hub
What is a virtual function c++
Has virtual functions and accessible non-virtual destructor
Aspidiotus destructor
Destructor blas de lezo
Aspidiotus destructor
C# struct vs class
Malik jahan khan
A pointer is
C++ pointer vs reference
A pointer is
Dangling pointer in c
Pointer rapat
Table index
Stack pointer nedir
Lederteam daginstitutionsområdet
What is pointer
Teknik pointer
Impica
Tipe data user defined
Pointer generator
Pointer programming
Pointer subterfuge
One full revolution of the pointer on the dial equals
Teknik pointer
A pointer is
What is pointer
Se pointer comme une fleur
Variabel yang nilainya berupa alamat adalah
Explicit pointers
In the statement "int *arr[4];",arr is
Skip pointers in information retrieval
Please move your pointer
Mast cell tumor german shorthaired pointer
#include "stdafx.h"
If the head pointer points to nullptr, this indicates
Teknik pointer
In the statement int* p, q; p and q are pointer variables.
Pointer notation
Pass by pointer
Stevie pointer
Petanque technique pour pointer
Xp1024xp
C pointer basics
Importance of pointers in c
Daniel pointer
Are there pointers in java
Hip pointer moi
Pengertian pointer
Pointer c++
Boris laser pointer
Inter pointer
For skip pointer, more skip leads to
6 pointer kyc
Gruppefordeling
Laser pointer
Swizzling glsl
Pointer struktur data
Urgent pointer
Pointer politeknik
Pseudocode with functions
A pointer is
What is file handling in c
Pointer illustration
Xp1024xp
Pointer in java
Konsep dasar pointer
Pointer string array in c
Tragal pointer
Sarah pointer
Contoh string
Flowchart pointer c++
Npointer
Pointer in memory
Cointer pointer
Functor vs function pointer
Define stack pointer
Pointer subterfuge
Hip pointer injury pictures
Define a pointer
Dangling pointer definition
Jesus copy paste
Hard copy encyclopedia
Elements of advertisement
Four parts of a print ad
Lines to copy paste
Copy these sentences
Perbezaan copy and paste dan cut and paste
Capital letter after question mark
Disadvantages of garbage collection
Title copy and paste
Foto copy ktp
Food web yellowstone
Gold copy in test data management
Copy this in your notebook
Nrxn duplication
How to write a yearbook story
Make a copy
Deep copy constructor c++
E copy example