Pointers The significance of pointers in C is
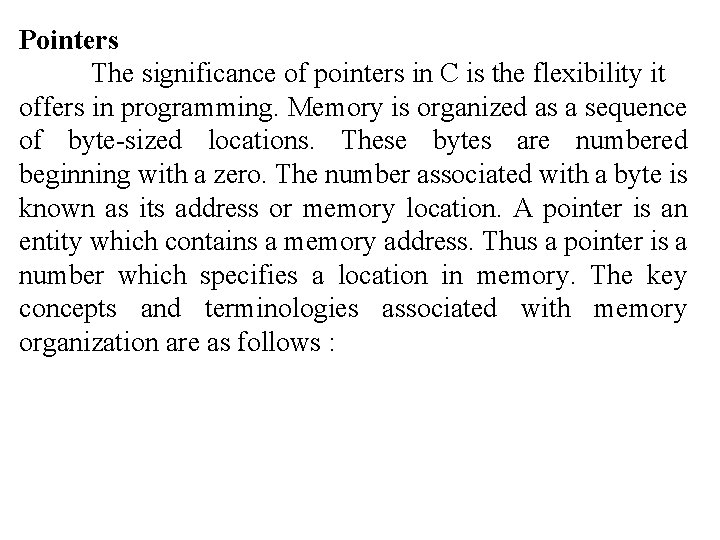
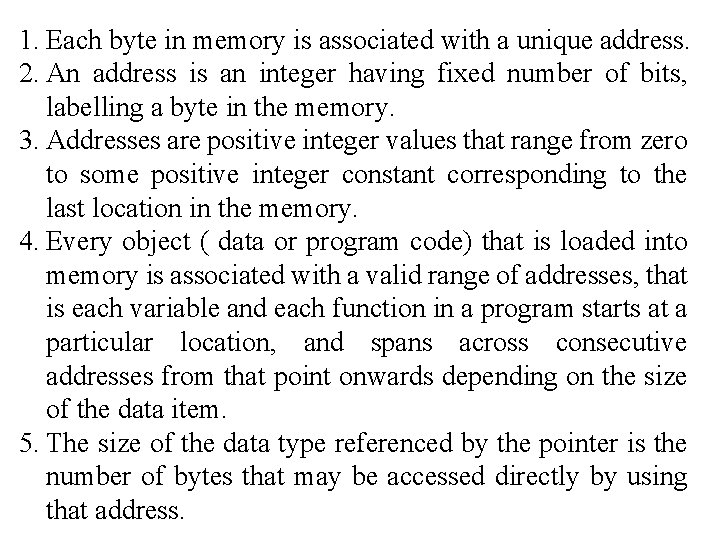
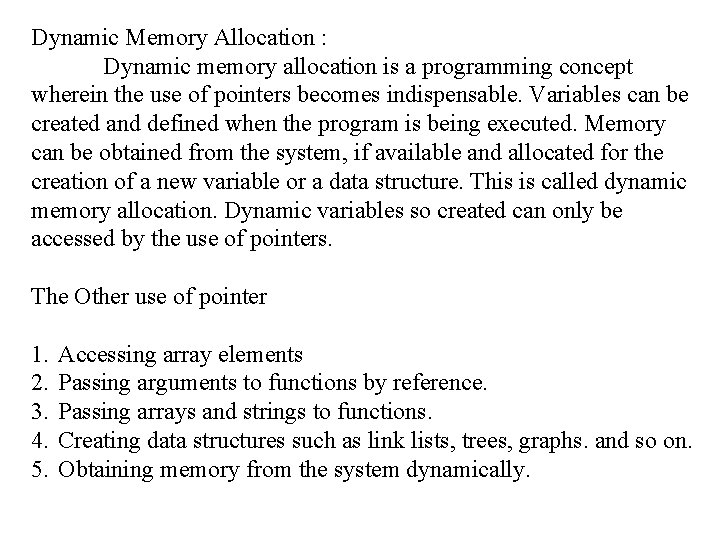
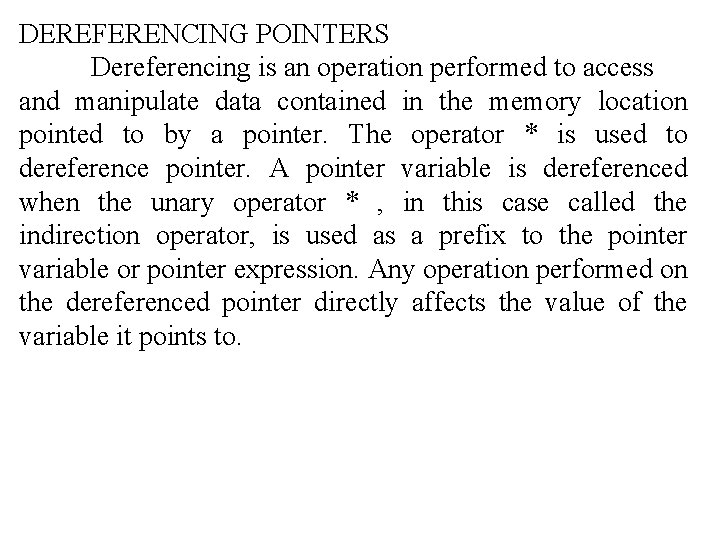
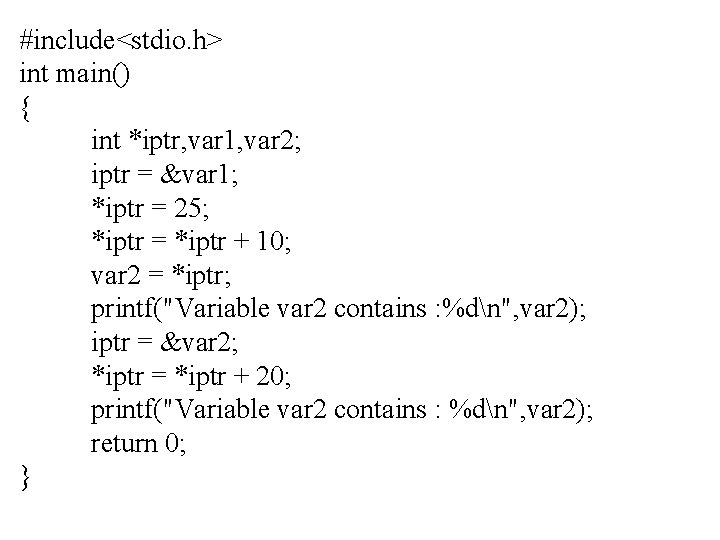
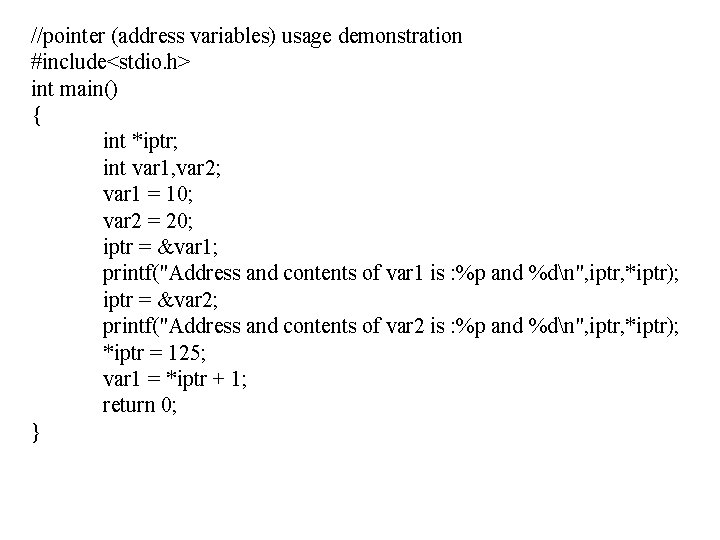
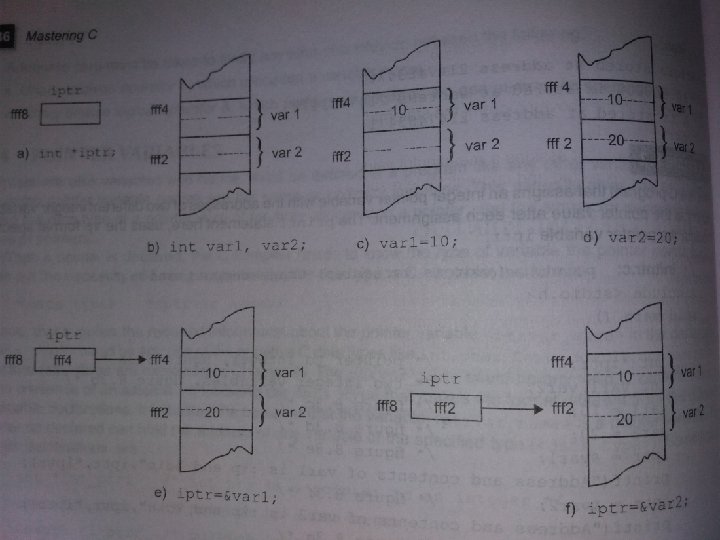
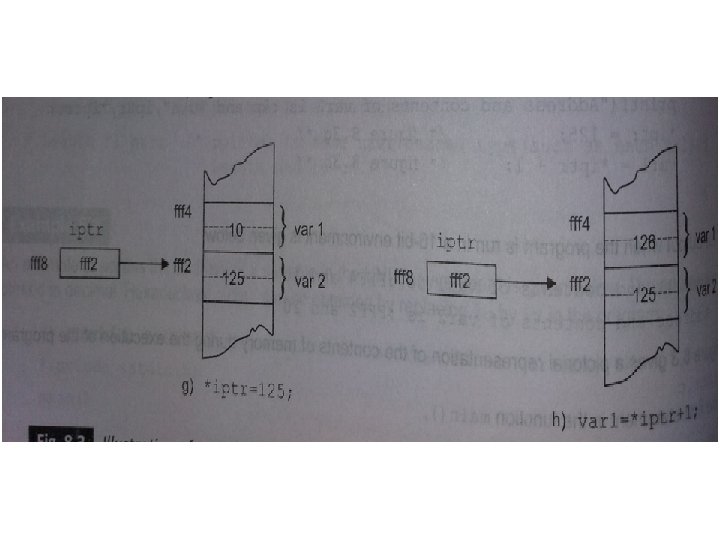
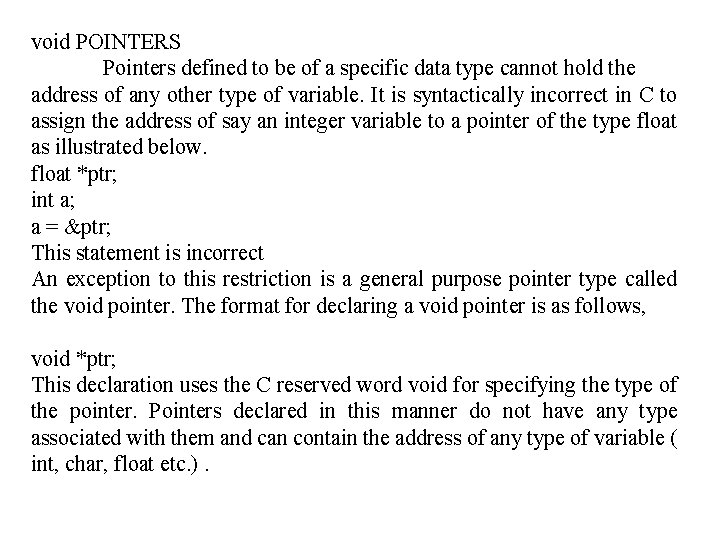
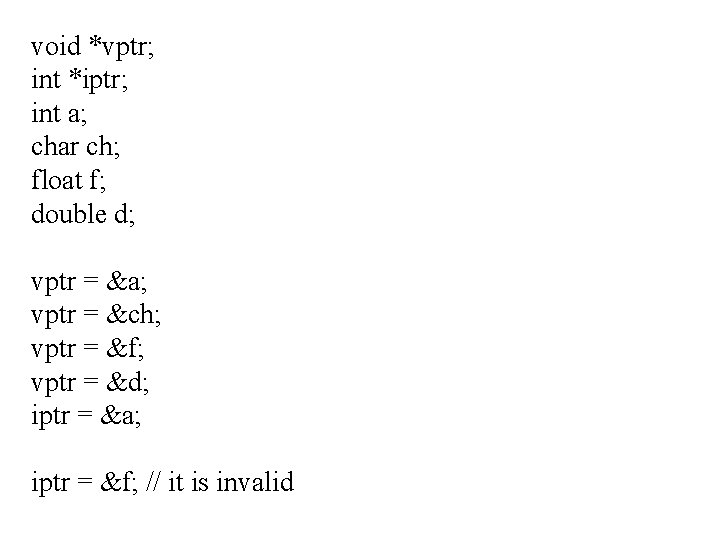
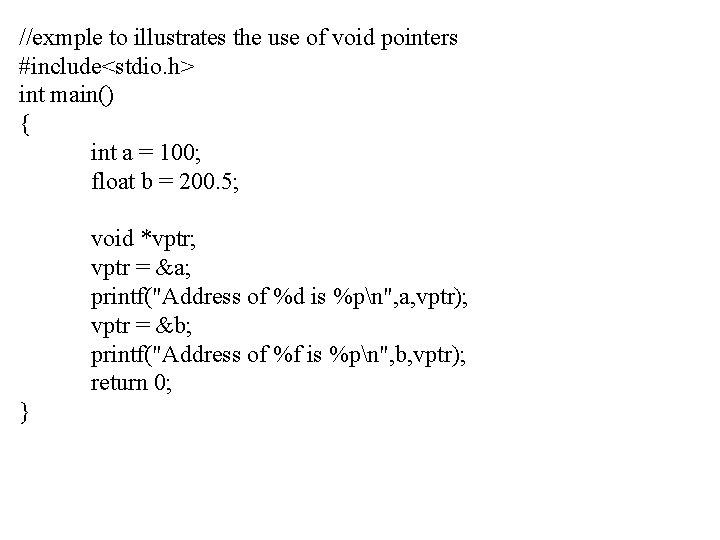
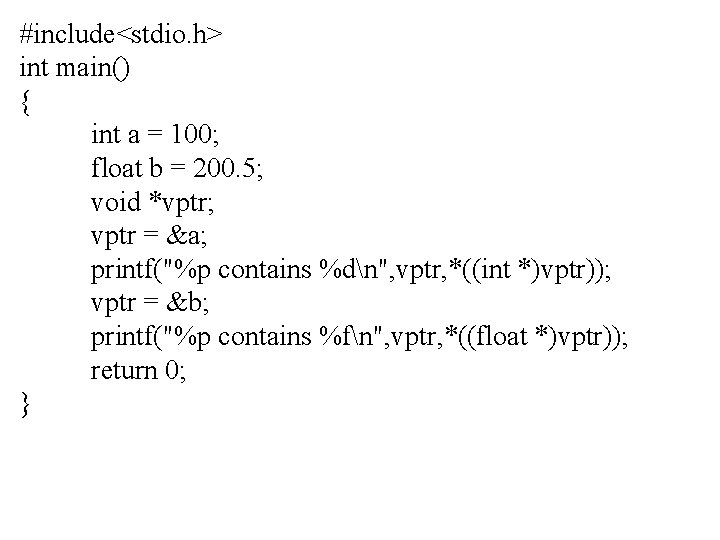
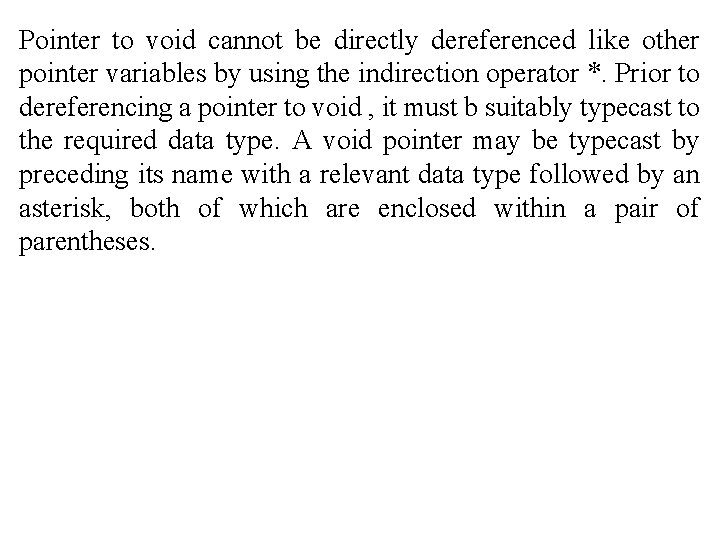
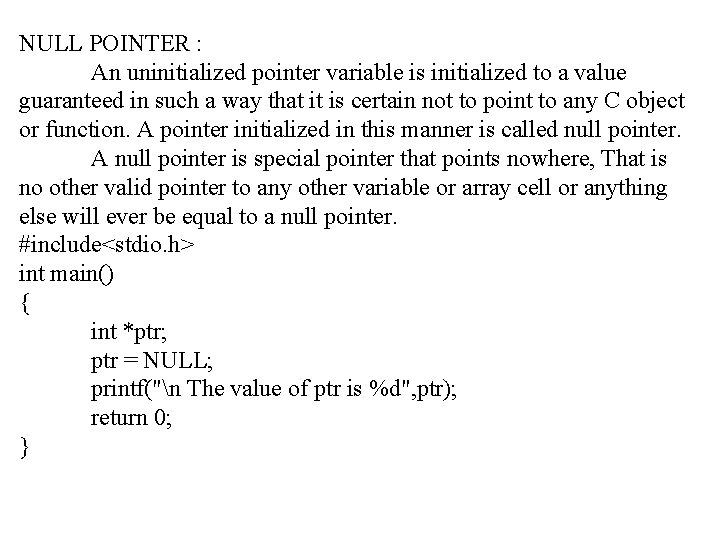
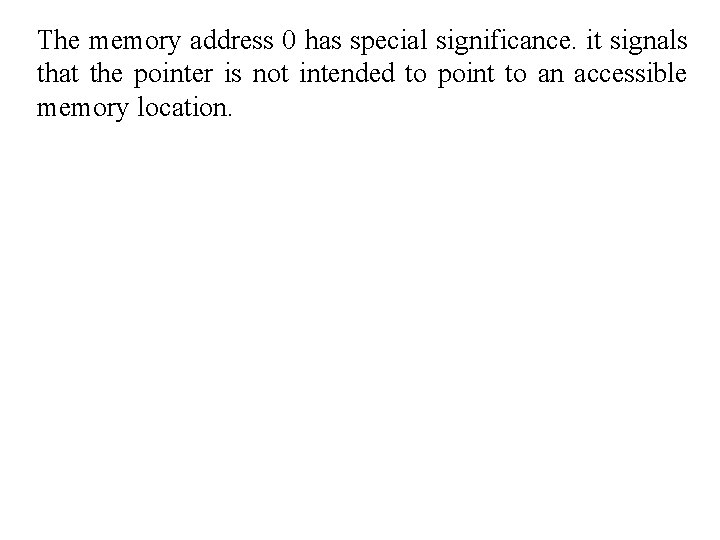
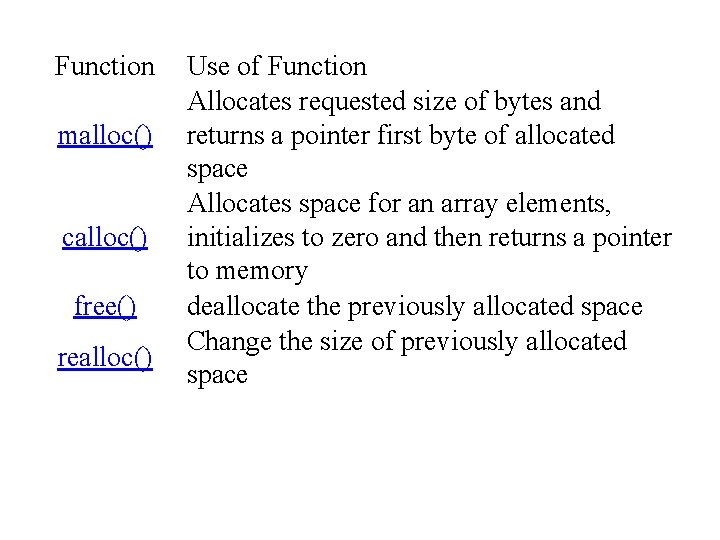
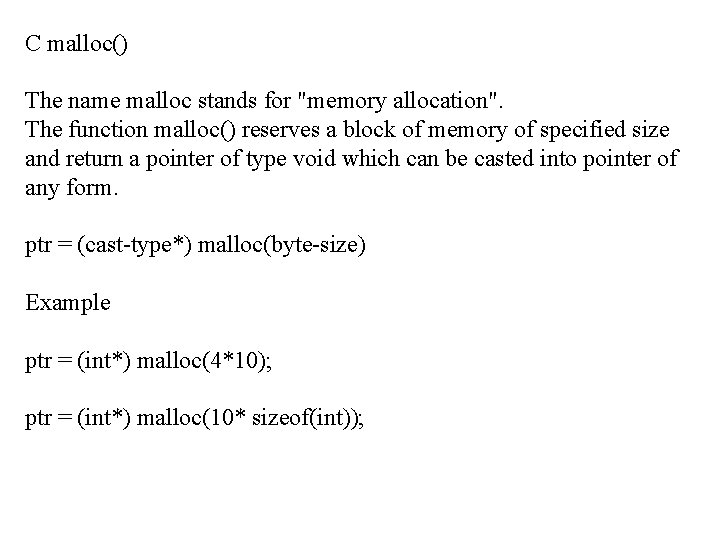
- Slides: 17
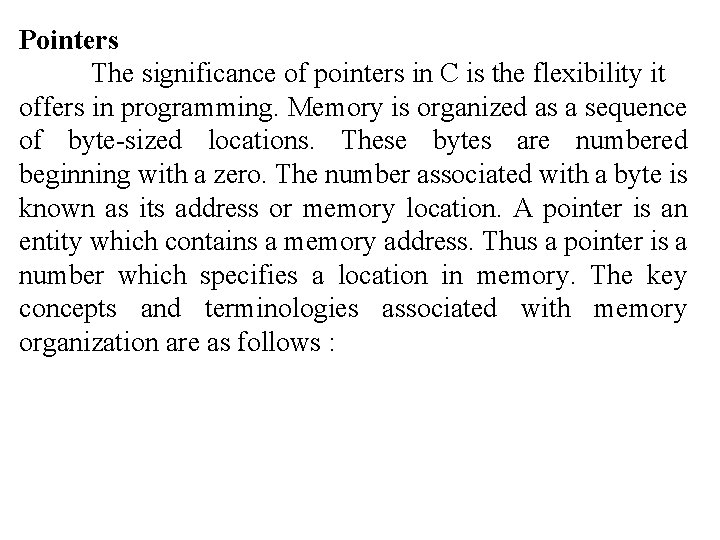
Pointers The significance of pointers in C is the flexibility it offers in programming. Memory is organized as a sequence of byte-sized locations. These bytes are numbered beginning with a zero. The number associated with a byte is known as its address or memory location. A pointer is an entity which contains a memory address. Thus a pointer is a number which specifies a location in memory. The key concepts and terminologies associated with memory organization are as follows :
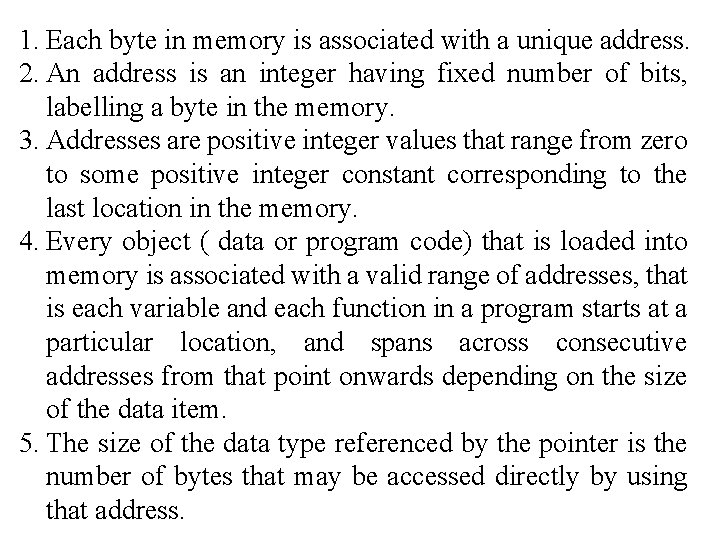
1. Each byte in memory is associated with a unique address. 2. An address is an integer having fixed number of bits, labelling a byte in the memory. 3. Addresses are positive integer values that range from zero to some positive integer constant corresponding to the last location in the memory. 4. Every object ( data or program code) that is loaded into memory is associated with a valid range of addresses, that is each variable and each function in a program starts at a particular location, and spans across consecutive addresses from that point onwards depending on the size of the data item. 5. The size of the data type referenced by the pointer is the number of bytes that may be accessed directly by using that address.
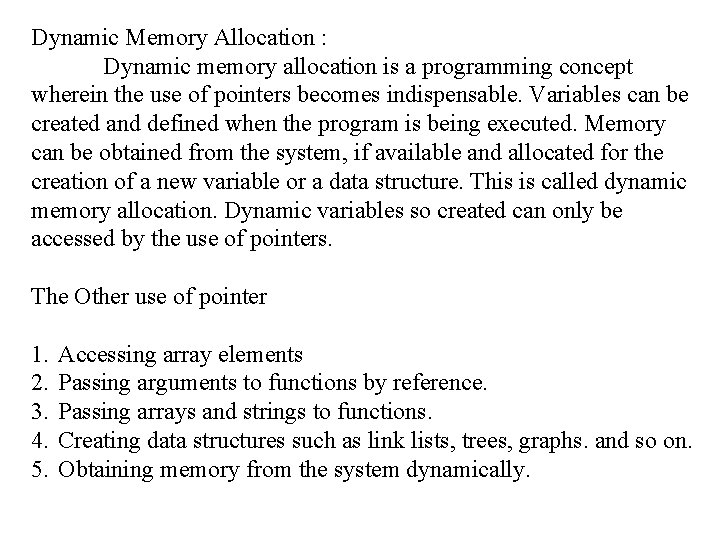
Dynamic Memory Allocation : Dynamic memory allocation is a programming concept wherein the use of pointers becomes indispensable. Variables can be created and defined when the program is being executed. Memory can be obtained from the system, if available and allocated for the creation of a new variable or a data structure. This is called dynamic memory allocation. Dynamic variables so created can only be accessed by the use of pointers. The Other use of pointer 1. 2. 3. 4. 5. Accessing array elements Passing arguments to functions by reference. Passing arrays and strings to functions. Creating data structures such as link lists, trees, graphs. and so on. Obtaining memory from the system dynamically.
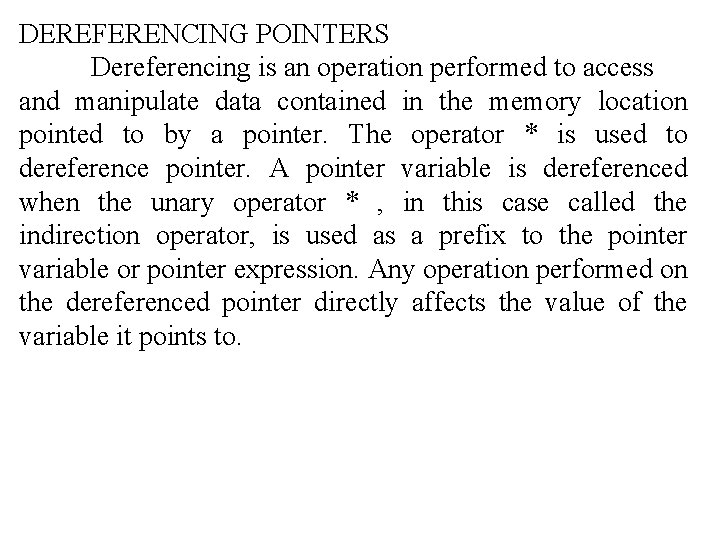
DEREFERENCING POINTERS Dereferencing is an operation performed to access and manipulate data contained in the memory location pointed to by a pointer. The operator * is used to dereference pointer. A pointer variable is dereferenced when the unary operator * , in this case called the indirection operator, is used as a prefix to the pointer variable or pointer expression. Any operation performed on the dereferenced pointer directly affects the value of the variable it points to.
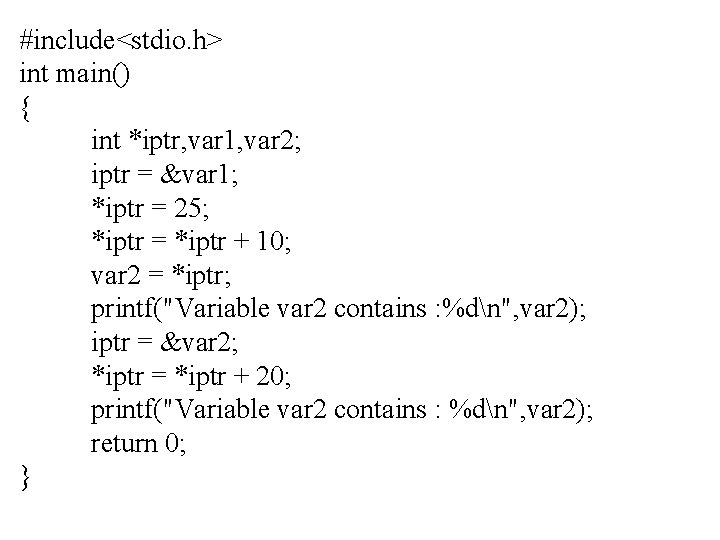
#include<stdio. h> int main() { int *iptr, var 1, var 2; iptr = &var 1; *iptr = 25; *iptr = *iptr + 10; var 2 = *iptr; printf("Variable var 2 contains : %dn", var 2); iptr = &var 2; *iptr = *iptr + 20; printf("Variable var 2 contains : %dn", var 2); return 0; }
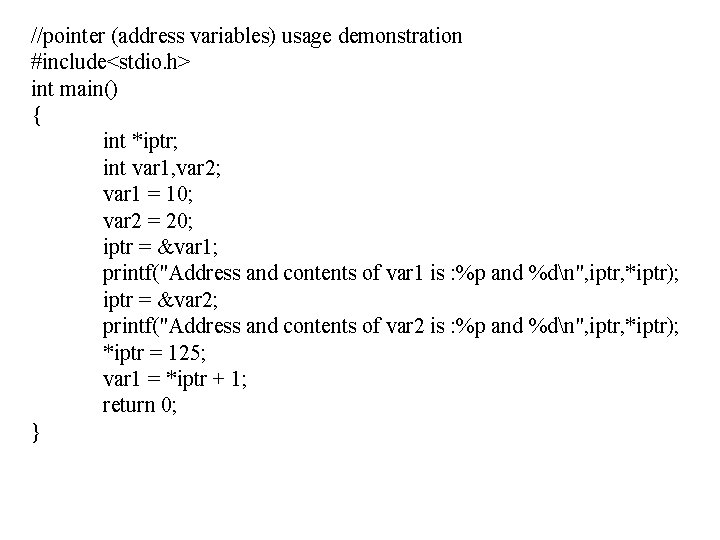
//pointer (address variables) usage demonstration #include<stdio. h> int main() { int *iptr; int var 1, var 2; var 1 = 10; var 2 = 20; iptr = &var 1; printf("Address and contents of var 1 is : %p and %dn", iptr, *iptr); iptr = &var 2; printf("Address and contents of var 2 is : %p and %dn", iptr, *iptr); *iptr = 125; var 1 = *iptr + 1; return 0; }
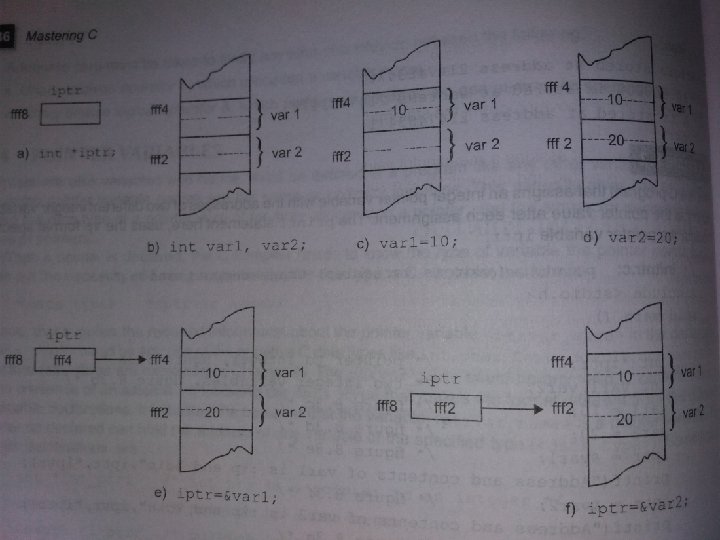
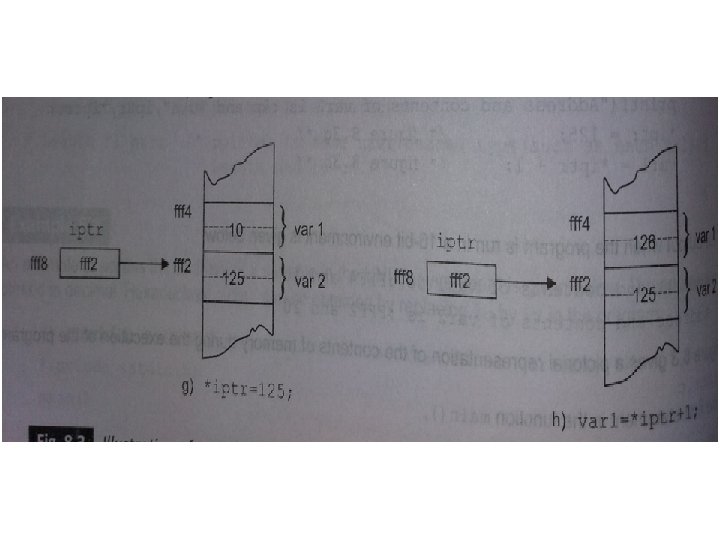
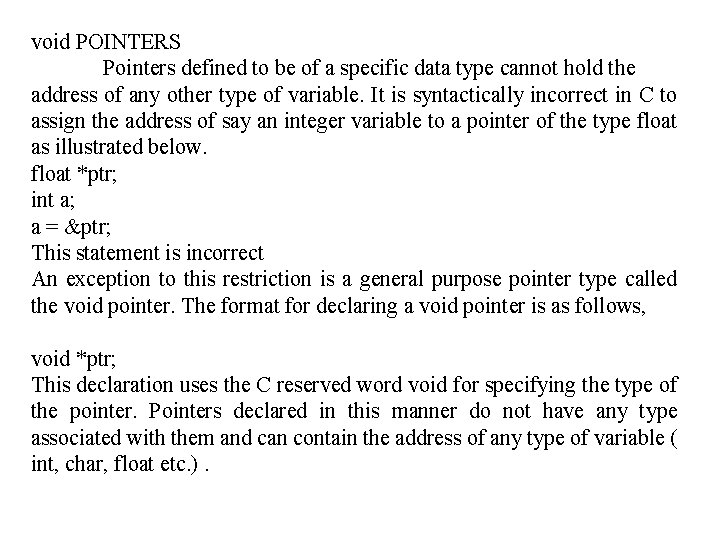
void POINTERS Pointers defined to be of a specific data type cannot hold the address of any other type of variable. It is syntactically incorrect in C to assign the address of say an integer variable to a pointer of the type float as illustrated below. float *ptr; int a; a = &ptr; This statement is incorrect An exception to this restriction is a general purpose pointer type called the void pointer. The format for declaring a void pointer is as follows, void *ptr; This declaration uses the C reserved word void for specifying the type of the pointer. Pointers declared in this manner do not have any type associated with them and can contain the address of any type of variable ( int, char, float etc. ).
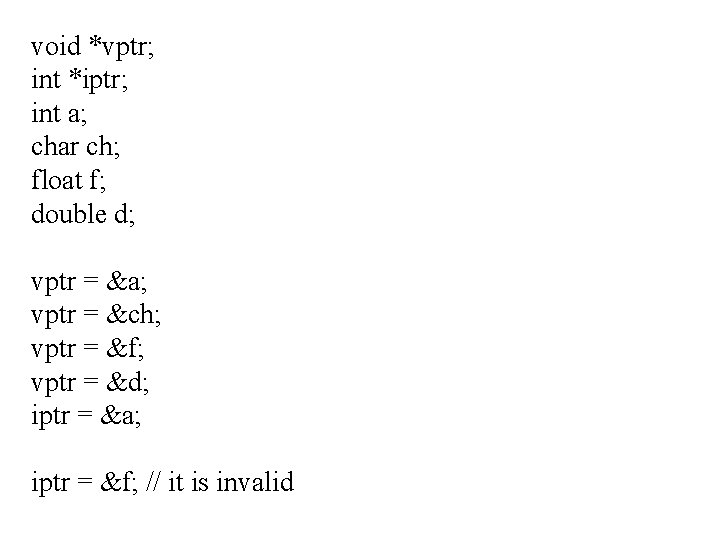
void *vptr; int *iptr; int a; char ch; float f; double d; vptr = &a; vptr = &ch; vptr = &f; vptr = &d; iptr = &a; iptr = &f; // it is invalid
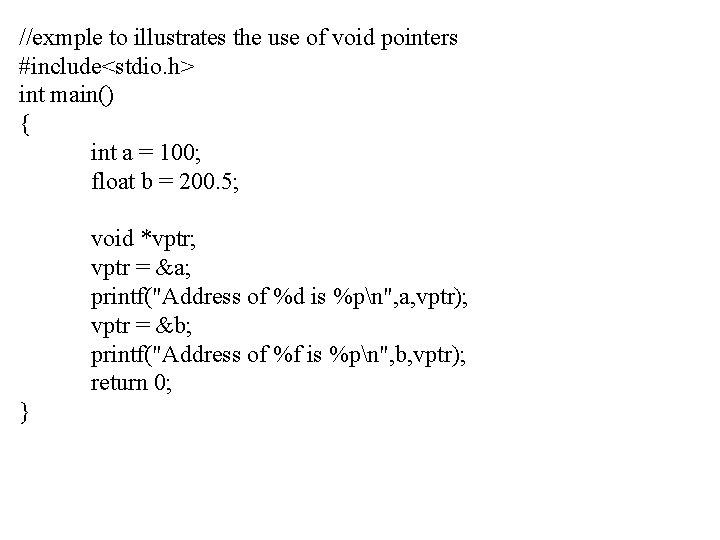
//exmple to illustrates the use of void pointers #include<stdio. h> int main() { int a = 100; float b = 200. 5; void *vptr; vptr = &a; printf("Address of %d is %pn", a, vptr); vptr = &b; printf("Address of %f is %pn", b, vptr); return 0; }
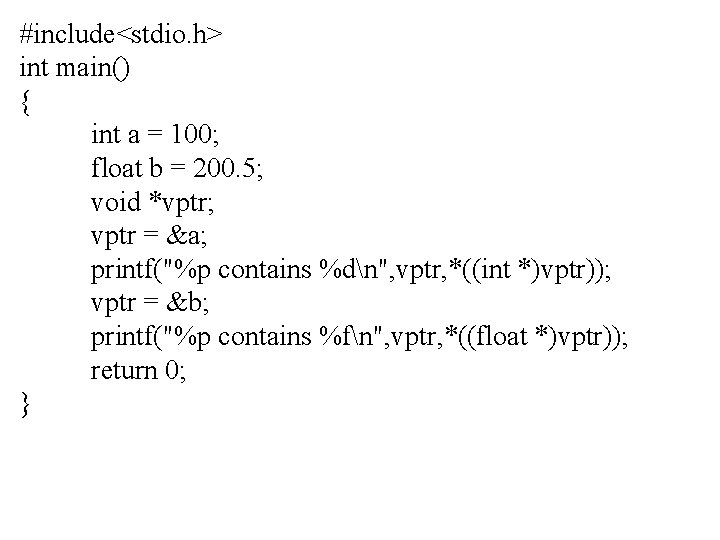
#include<stdio. h> int main() { int a = 100; float b = 200. 5; void *vptr; vptr = &a; printf("%p contains %dn", vptr, *((int *)vptr)); vptr = &b; printf("%p contains %fn", vptr, *((float *)vptr)); return 0; }
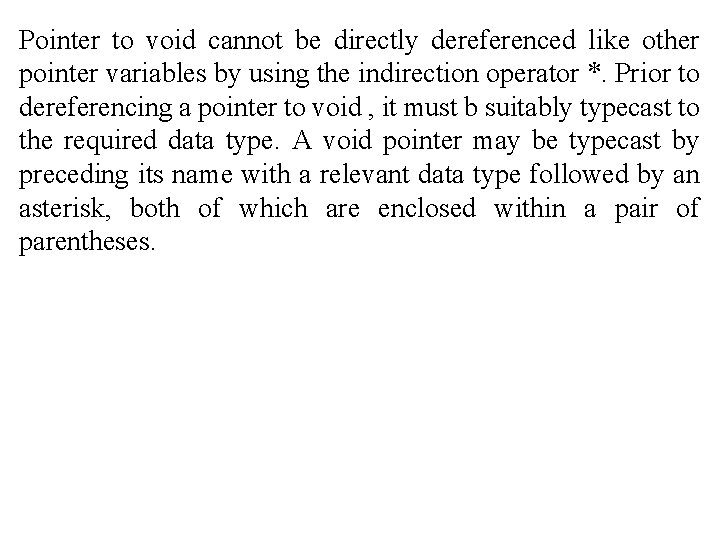
Pointer to void cannot be directly dereferenced like other pointer variables by using the indirection operator *. Prior to dereferencing a pointer to void , it must b suitably typecast to the required data type. A void pointer may be typecast by preceding its name with a relevant data type followed by an asterisk, both of which are enclosed within a pair of parentheses.
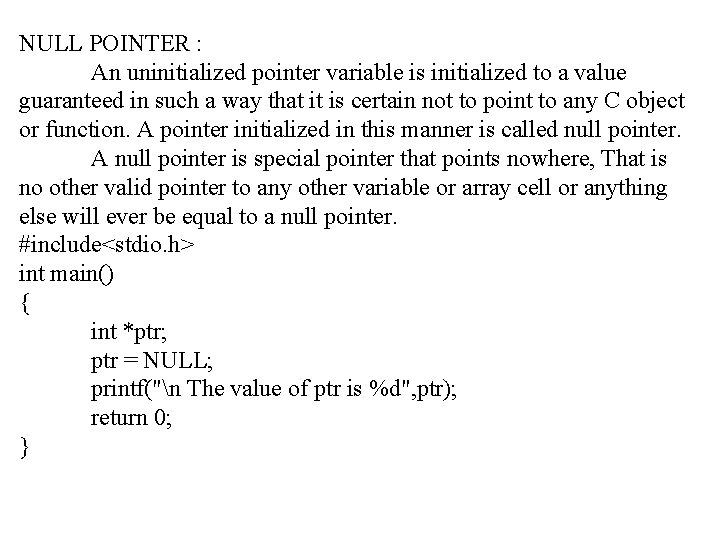
NULL POINTER : An uninitialized pointer variable is initialized to a value guaranteed in such a way that it is certain not to point to any C object or function. A pointer initialized in this manner is called null pointer. A null pointer is special pointer that points nowhere, That is no other valid pointer to any other variable or array cell or anything else will ever be equal to a null pointer. #include<stdio. h> int main() { int *ptr; ptr = NULL; printf("n The value of ptr is %d", ptr); return 0; }
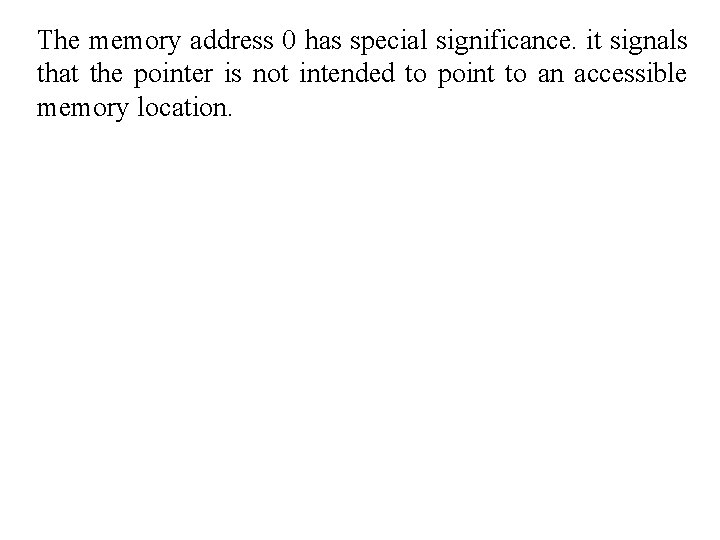
The memory address 0 has special significance. it signals that the pointer is not intended to point to an accessible memory location.
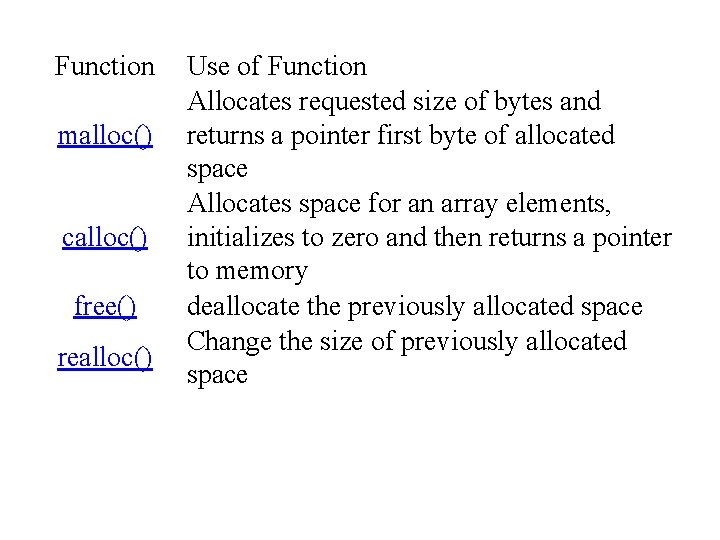
Function malloc() calloc() free() realloc() Use of Function Allocates requested size of bytes and returns a pointer first byte of allocated space Allocates space for an array elements, initializes to zero and then returns a pointer to memory deallocate the previously allocated space Change the size of previously allocated space
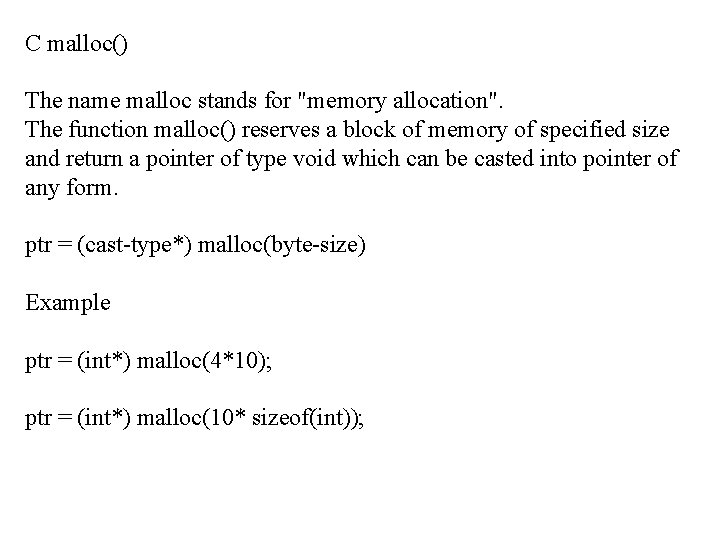
C malloc() The name malloc stands for "memory allocation". The function malloc() reserves a block of memory of specified size and return a pointer of type void which can be casted into pointer of any form. ptr = (cast-type*) malloc(byte-size) Example ptr = (int*) malloc(4*10); ptr = (int*) malloc(10* sizeof(int));