Chapter 10 Pointers Starting Out with C Early
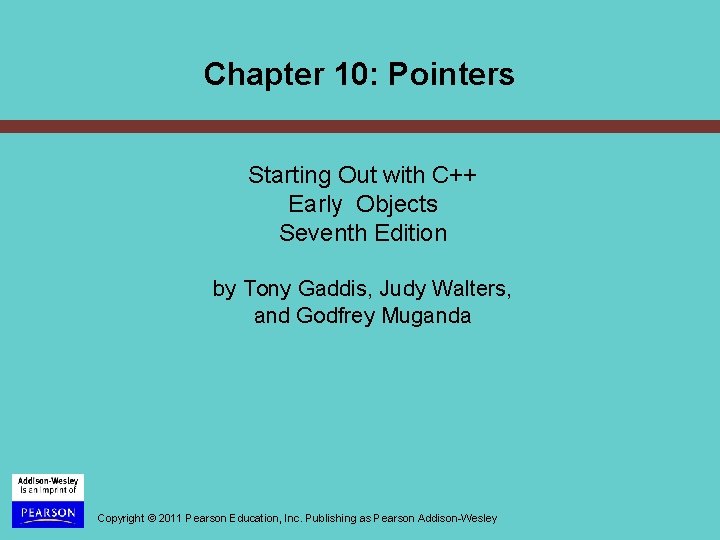
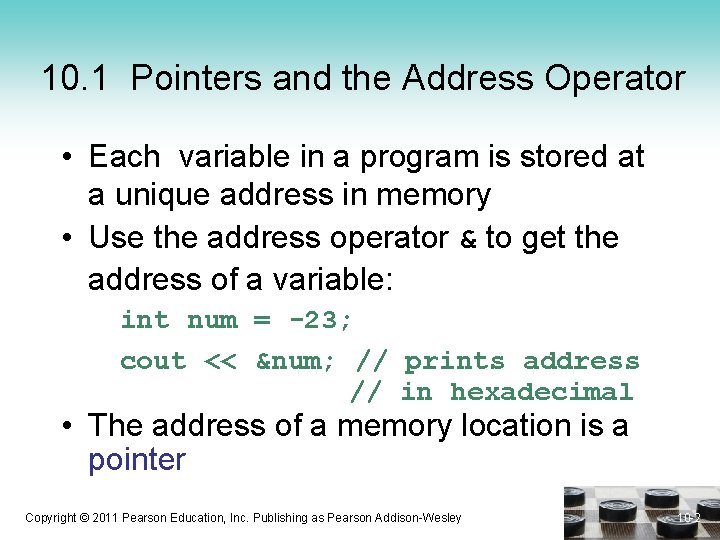
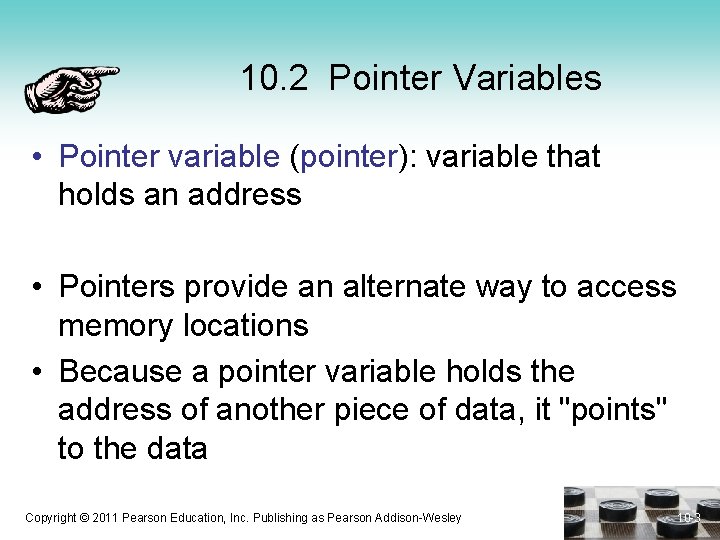
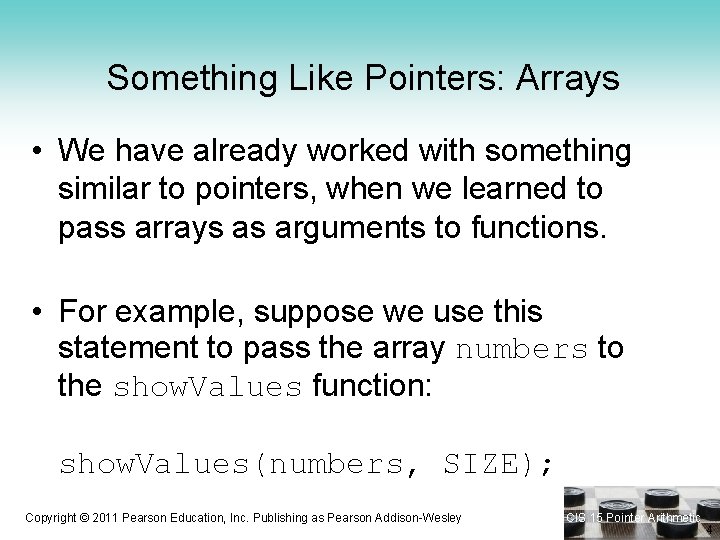
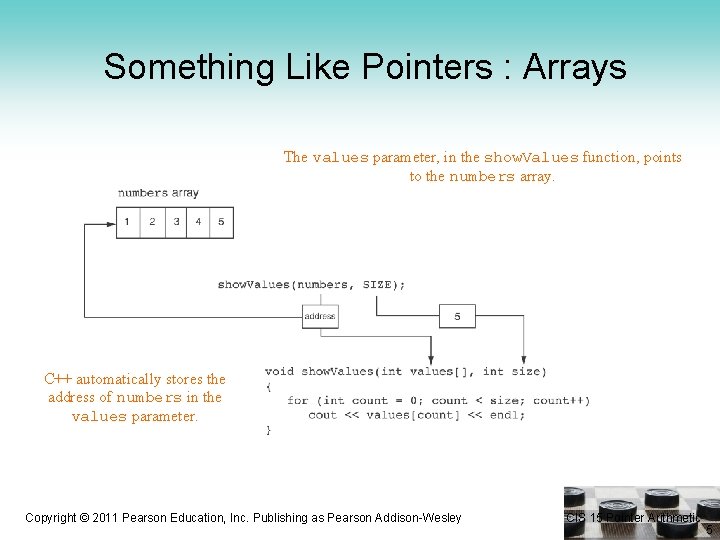
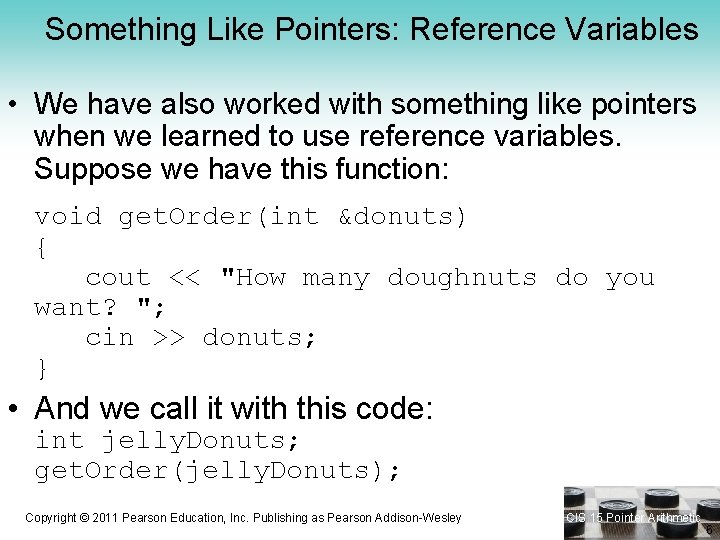
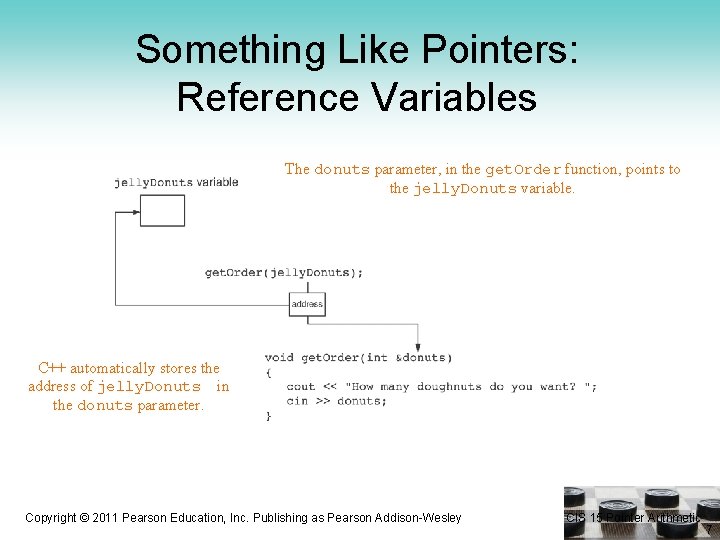
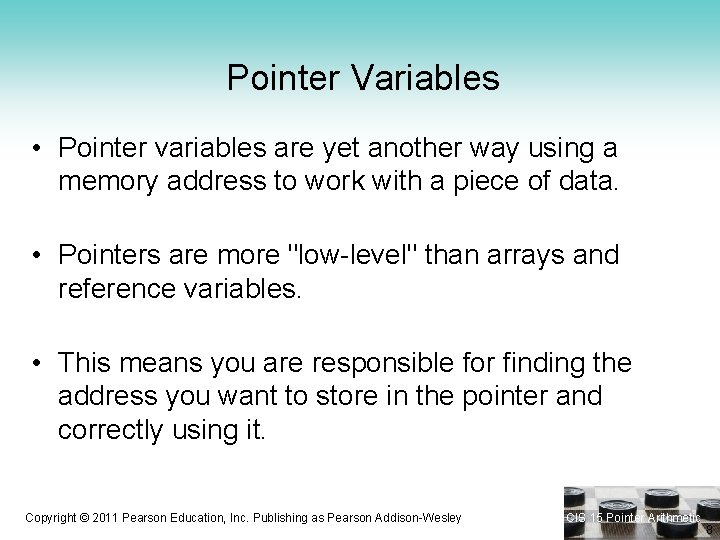
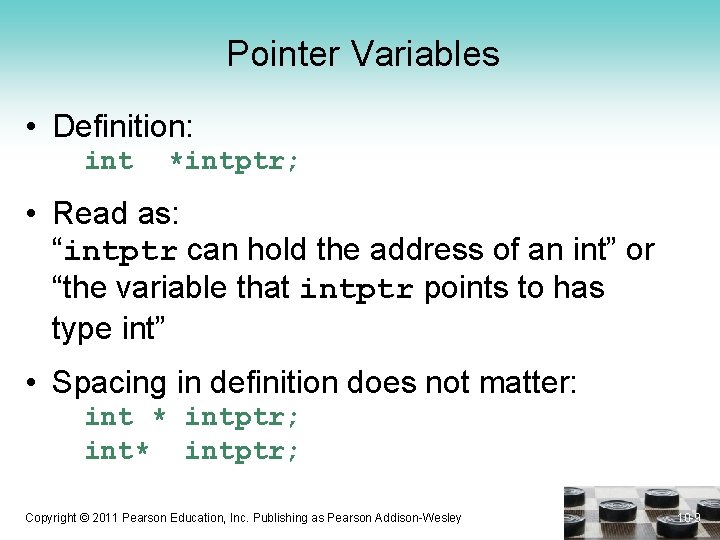
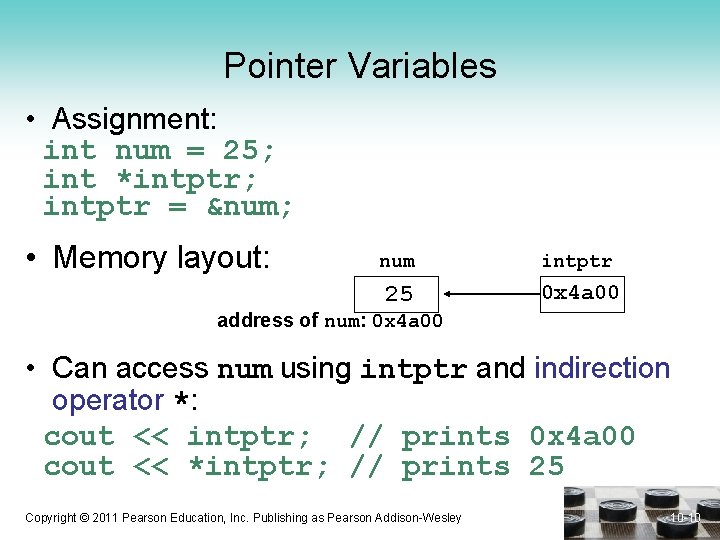
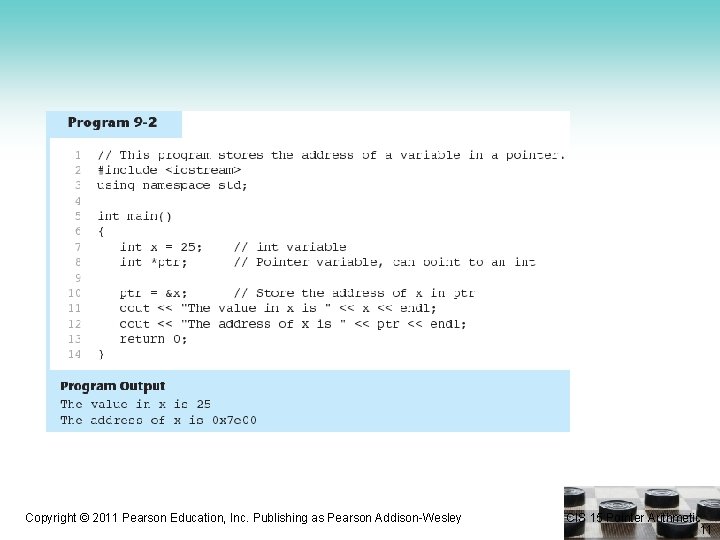
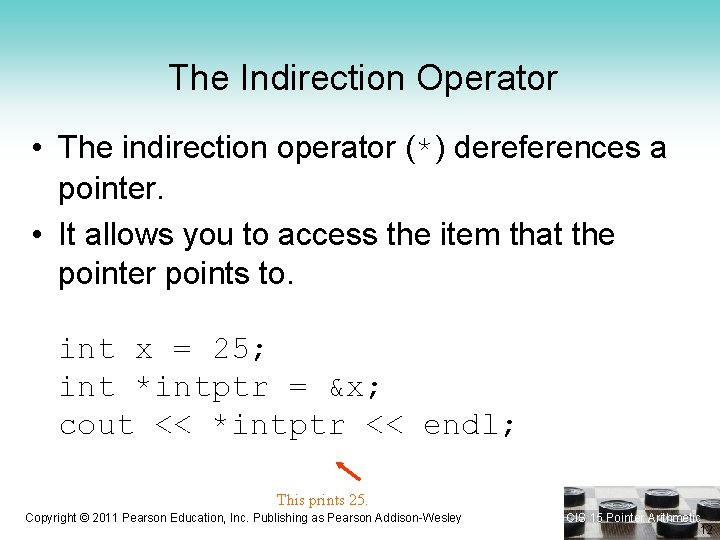
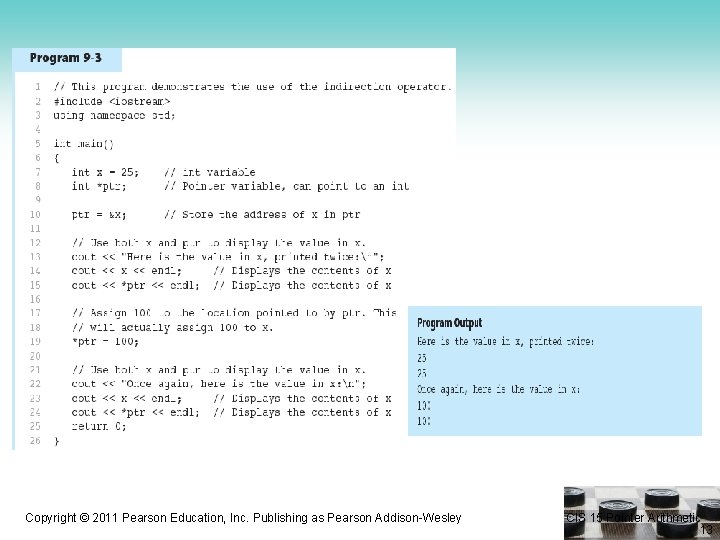
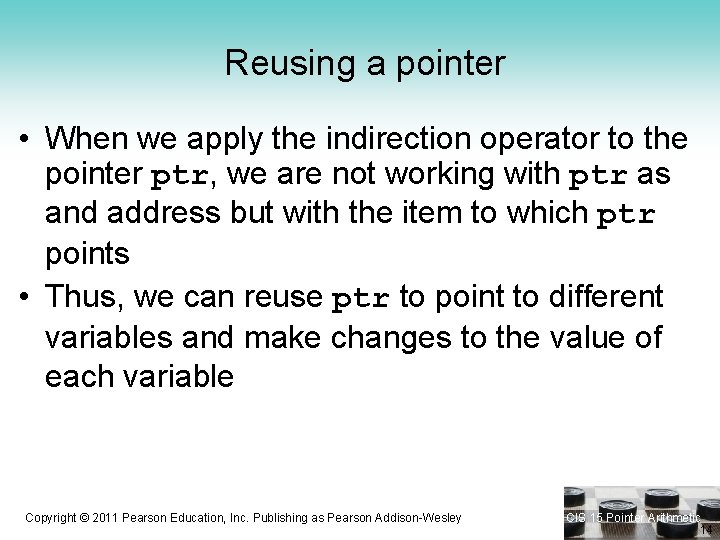
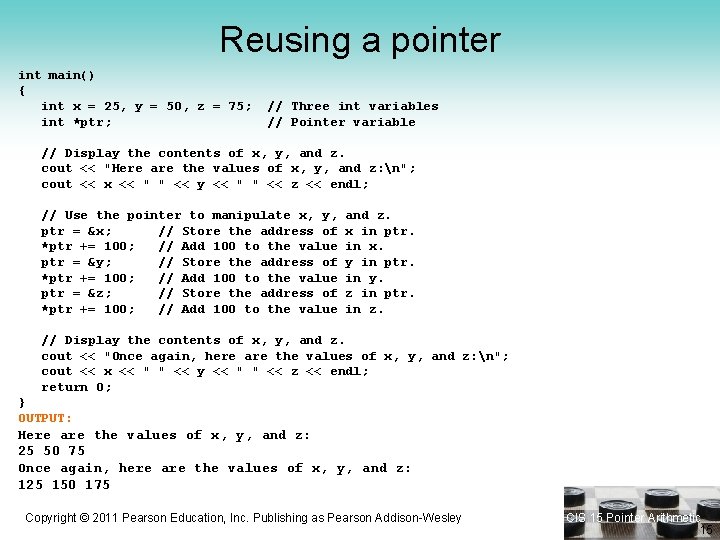
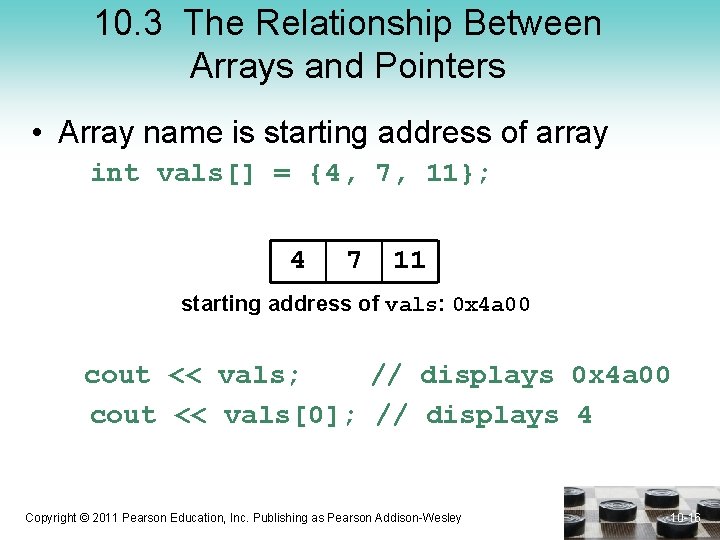
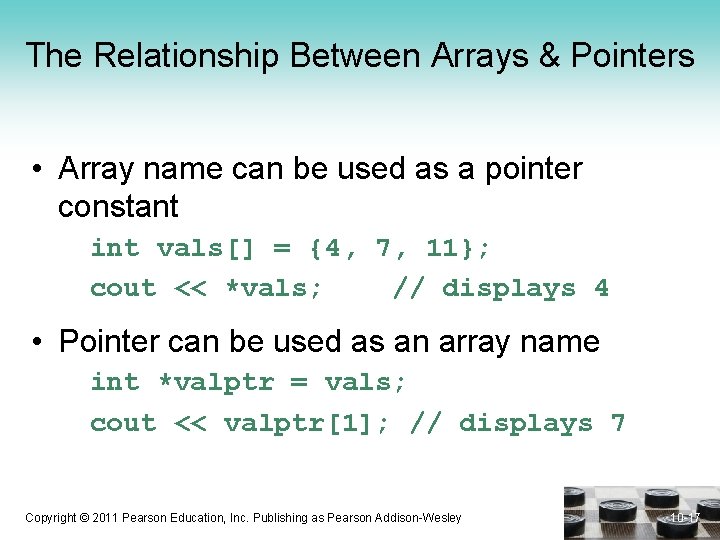
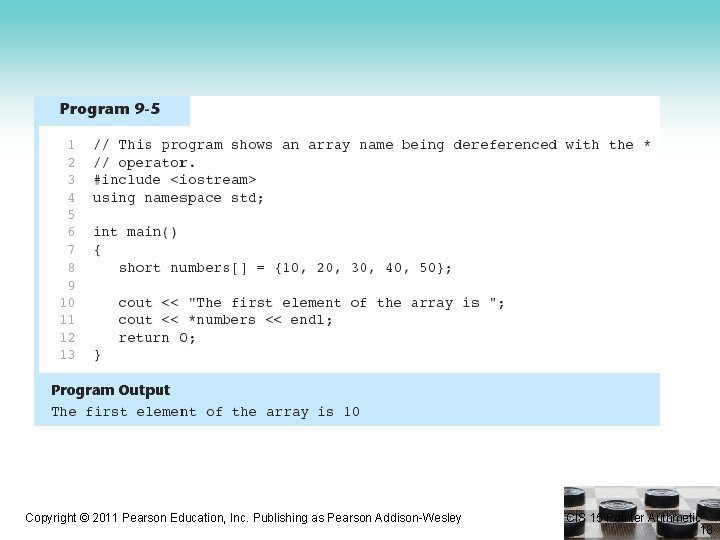
![Pointers in Expressions • Given: int vals[]={4, 7, 11}; int *valptr = vals; • Pointers in Expressions • Given: int vals[]={4, 7, 11}; int *valptr = vals; •](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-19.jpg)
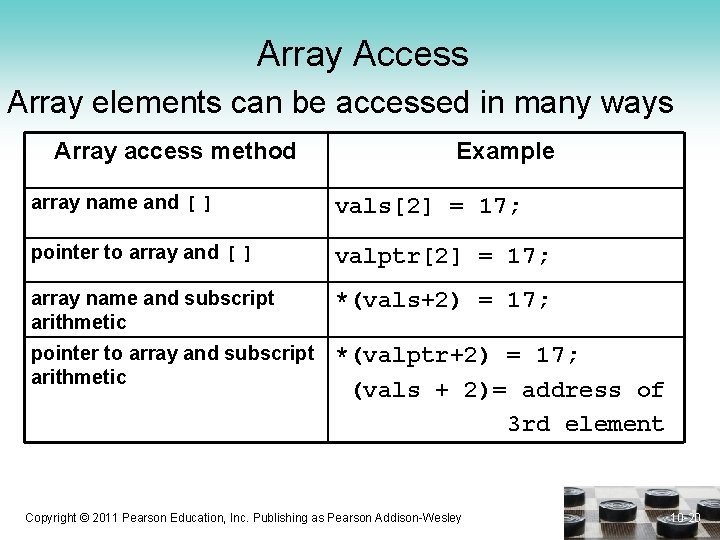
![Array Access • Array notation vals[i] is equivalent to the pointer notation *(vals + Array Access • Array notation vals[i] is equivalent to the pointer notation *(vals +](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-21.jpg)
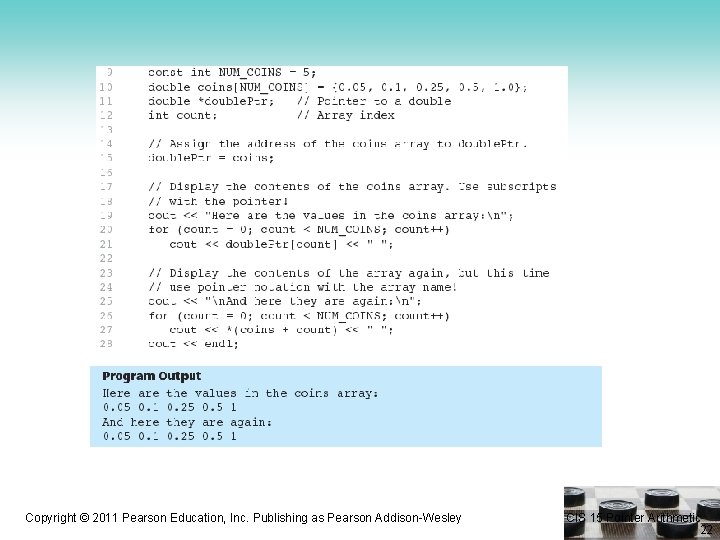
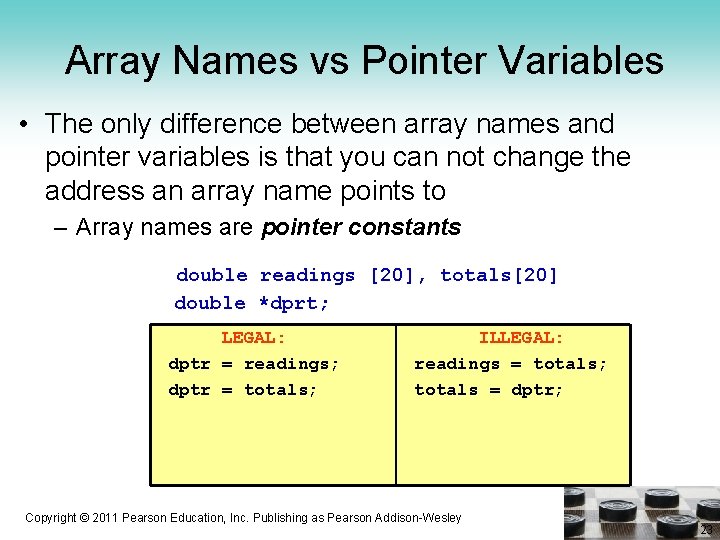
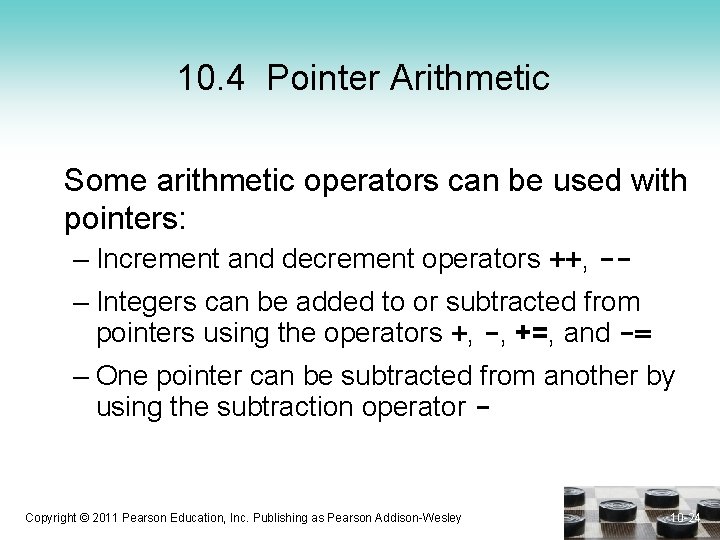
![Pointer Arithmetic Assume the variable definitions int vals[]={4, 7, 11}; int *valptr = vals; Pointer Arithmetic Assume the variable definitions int vals[]={4, 7, 11}; int *valptr = vals;](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-25.jpg)
![More on Pointer Arithmetic Assume the variable definitions: int vals[]={4, 7, 11}; int *valptr More on Pointer Arithmetic Assume the variable definitions: int vals[]={4, 7, 11}; int *valptr](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-26.jpg)
![More on Pointer Arithmetic Assume the variable definitions: int vals[]={4, 7, 11}; int *valptr More on Pointer Arithmetic Assume the variable definitions: int vals[]={4, 7, 11}; int *valptr](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-27.jpg)
![More on Pointer Arithmetic Assume the variable definitions int vals[] = {4, 7, 11}; More on Pointer Arithmetic Assume the variable definitions int vals[] = {4, 7, 11};](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-28.jpg)
![Pointer Arithmetic int vals[]={4, 7, 11}; int *valptr = vals; cout<<"nvalptr++: "<<valptr++; cout<<" *valptr: Pointer Arithmetic int vals[]={4, 7, 11}; int *valptr = vals; cout<<"nvalptr++: "<<valptr++; cout<<" *valptr:](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-29.jpg)
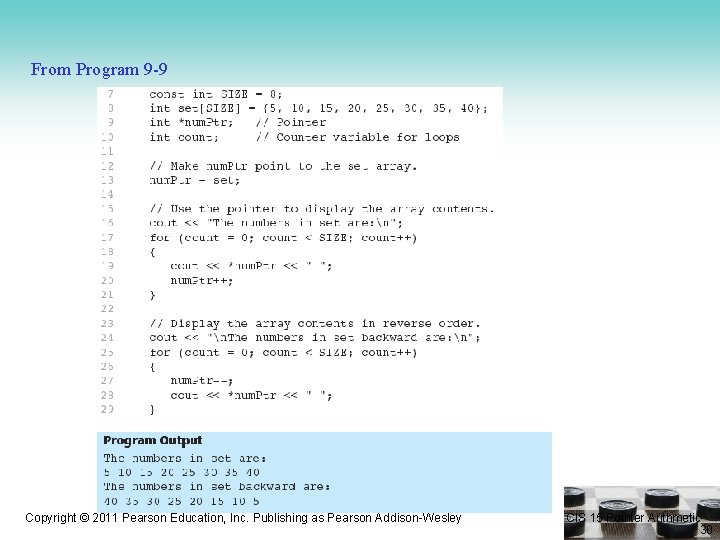
![Pointer Arithmetic - Summary Operation Example ++, -- int vals[]={4, 7, 11}; int *valptr Pointer Arithmetic - Summary Operation Example ++, -- int vals[]={4, 7, 11}; int *valptr](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-31.jpg)
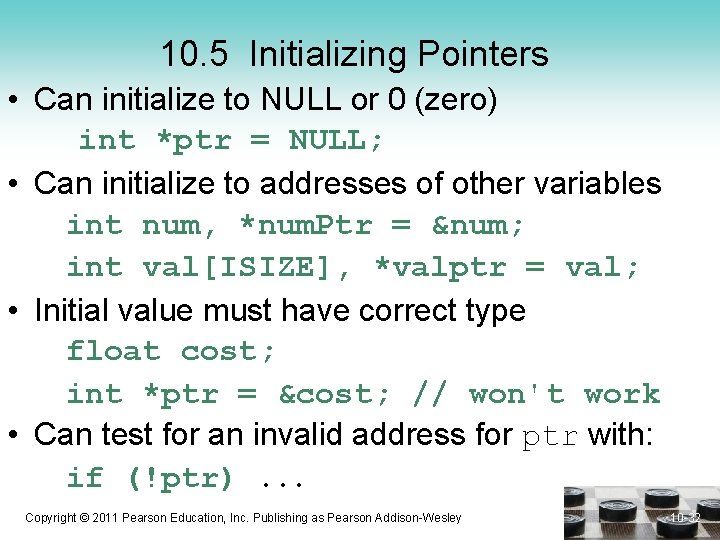
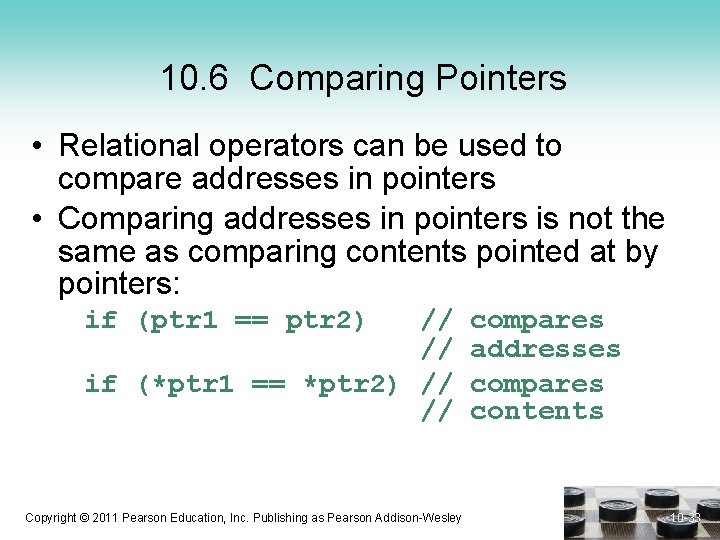
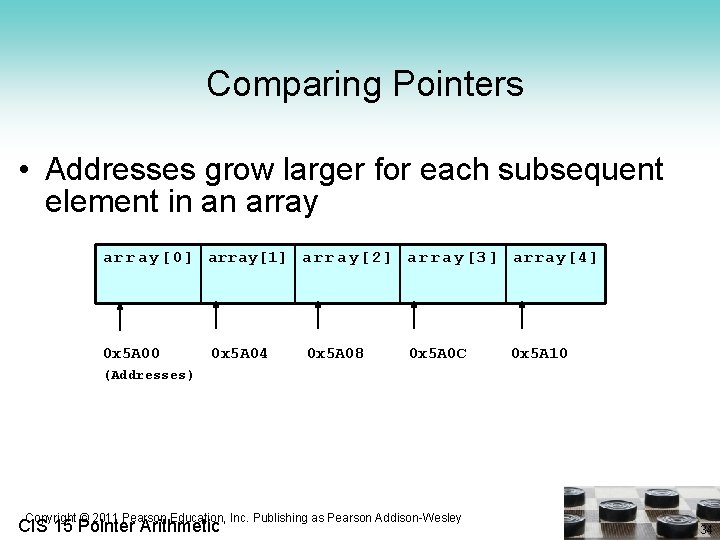
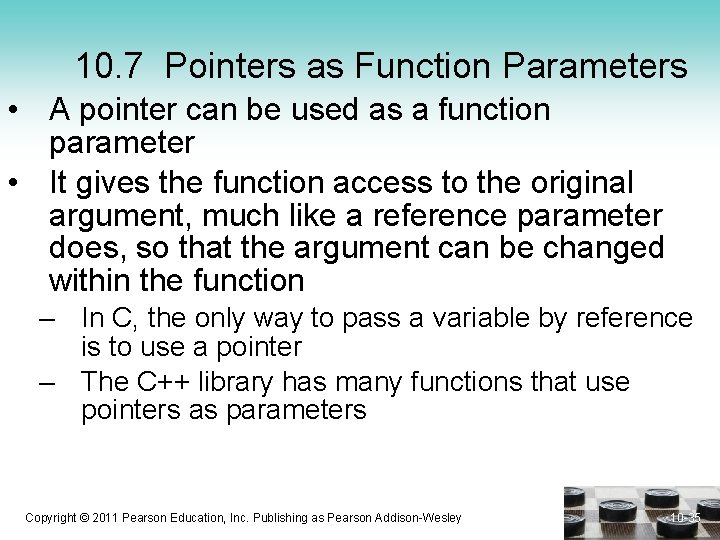
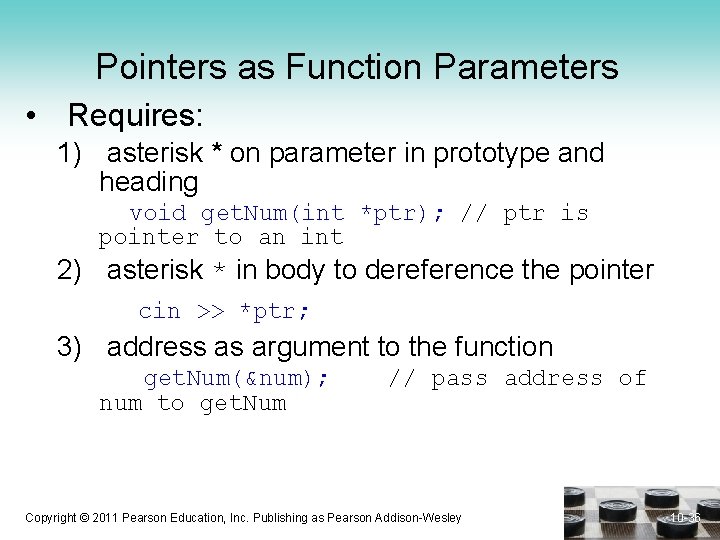
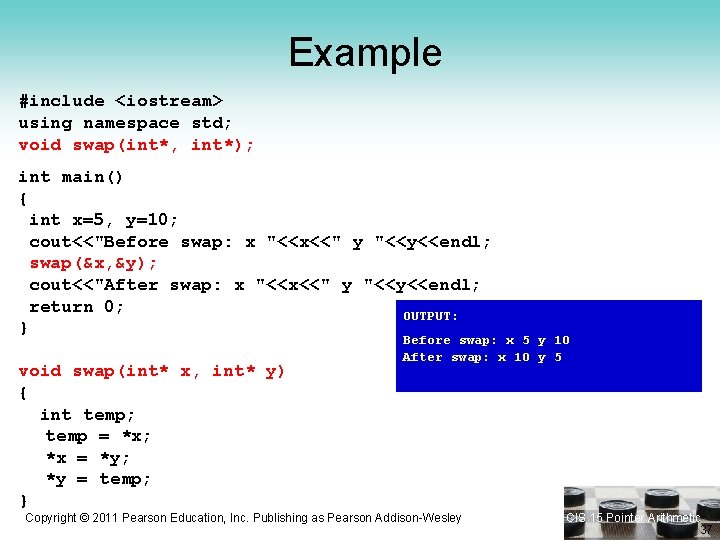
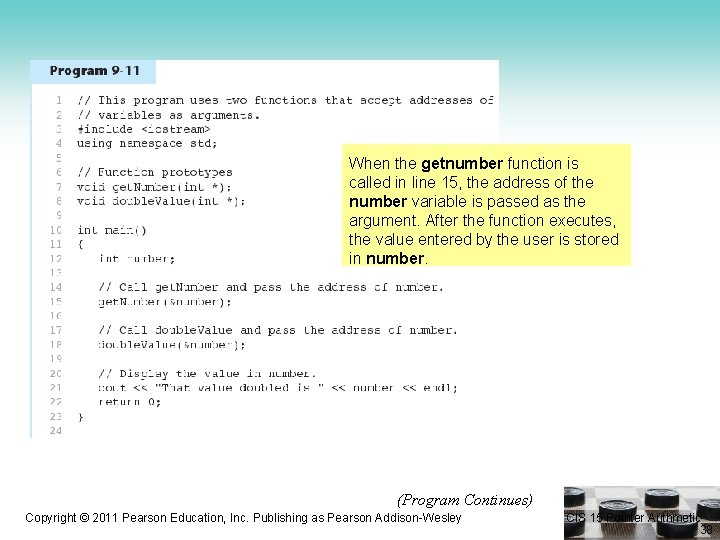
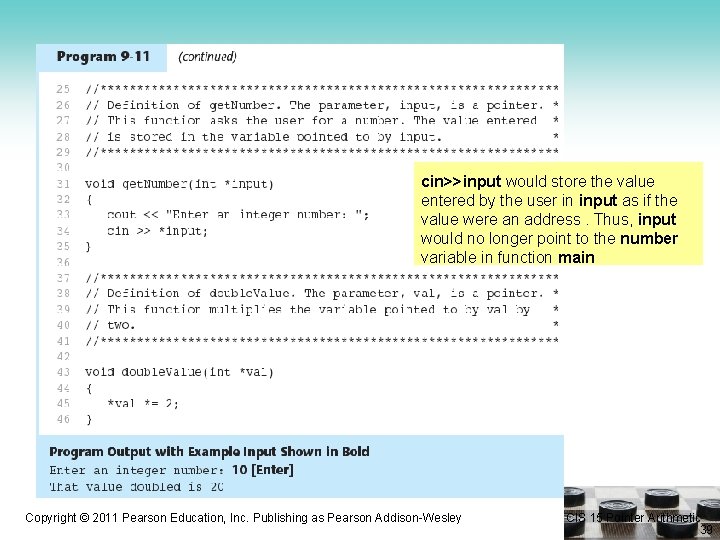
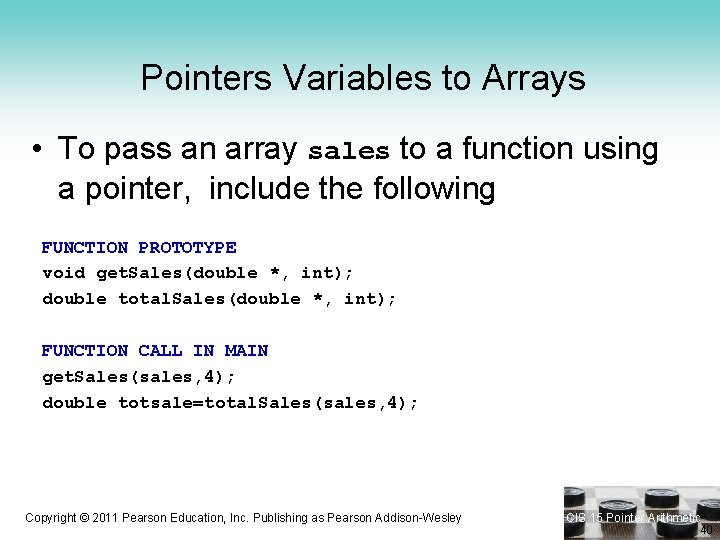
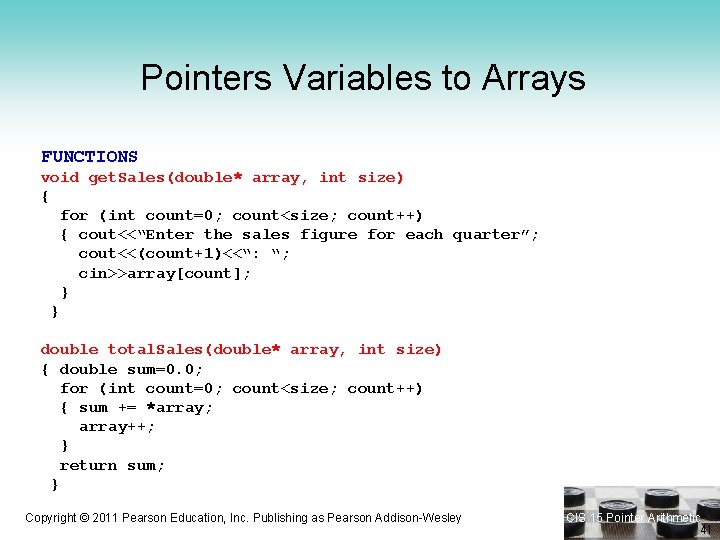
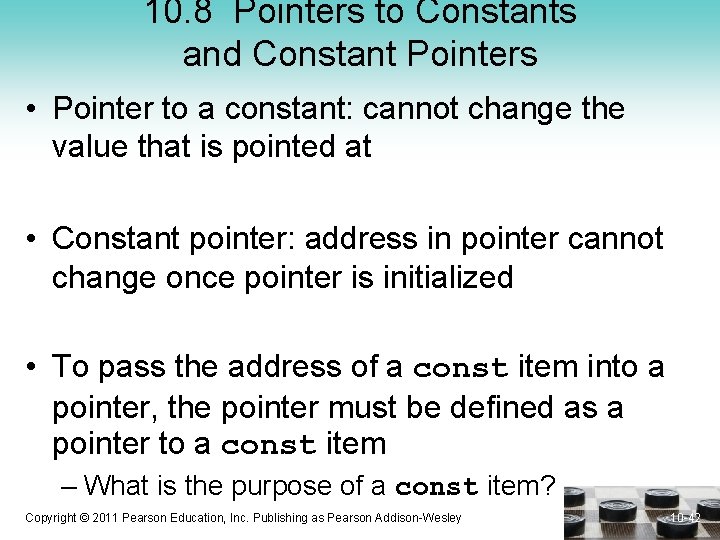
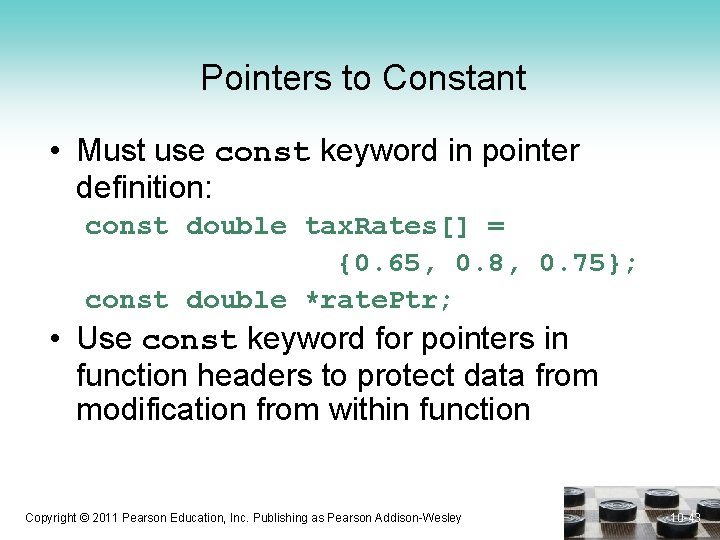
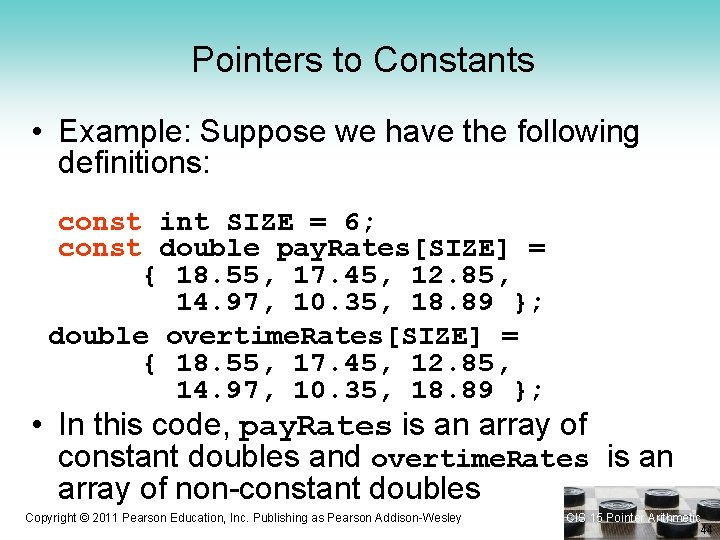
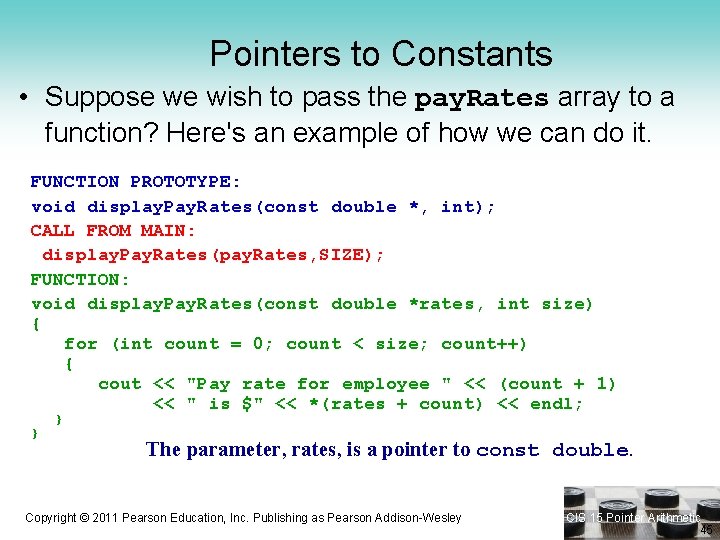
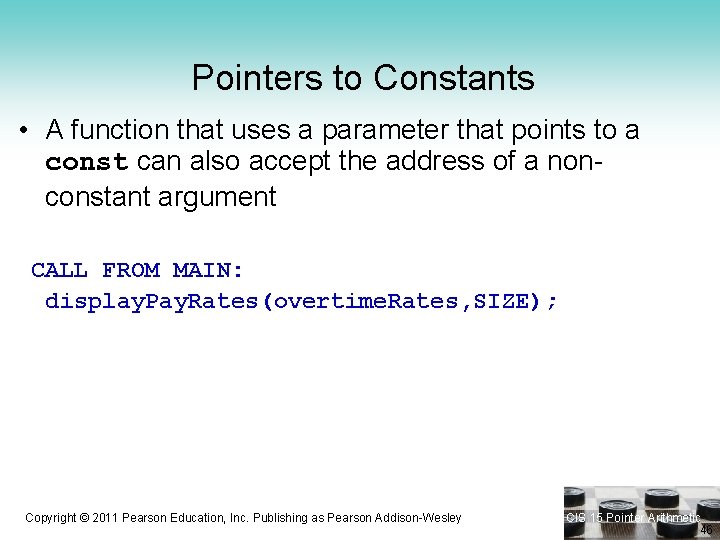
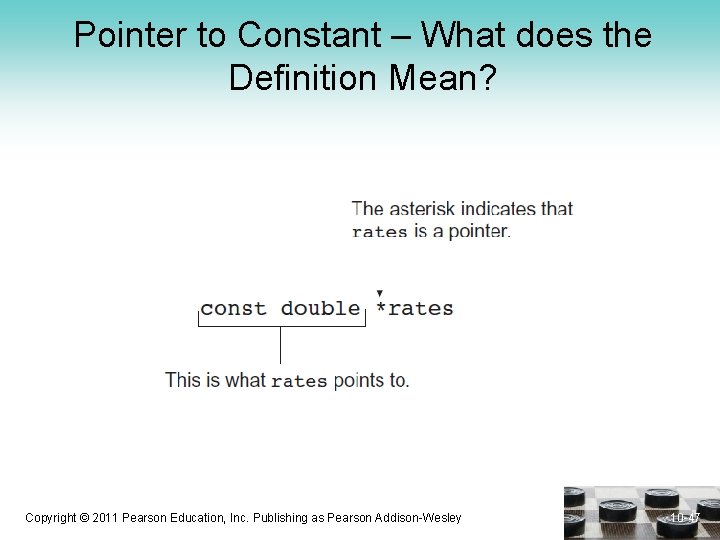
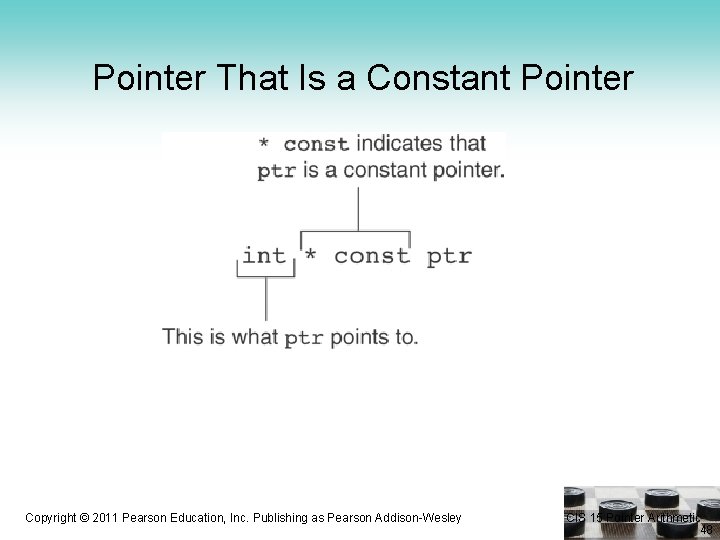
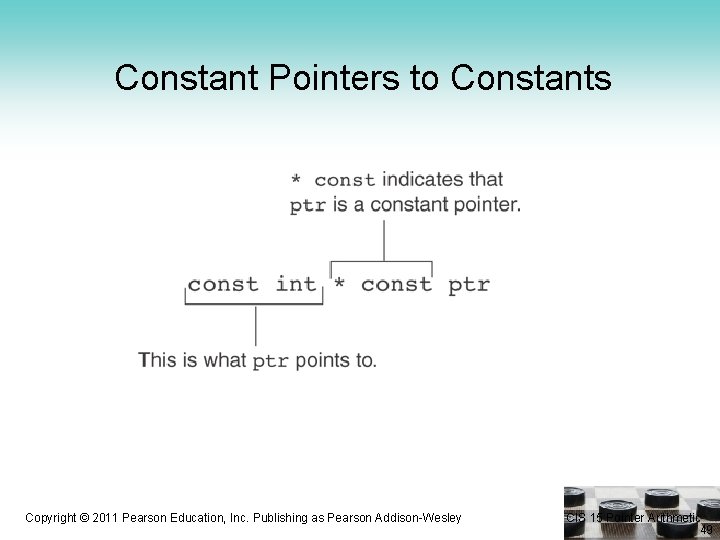
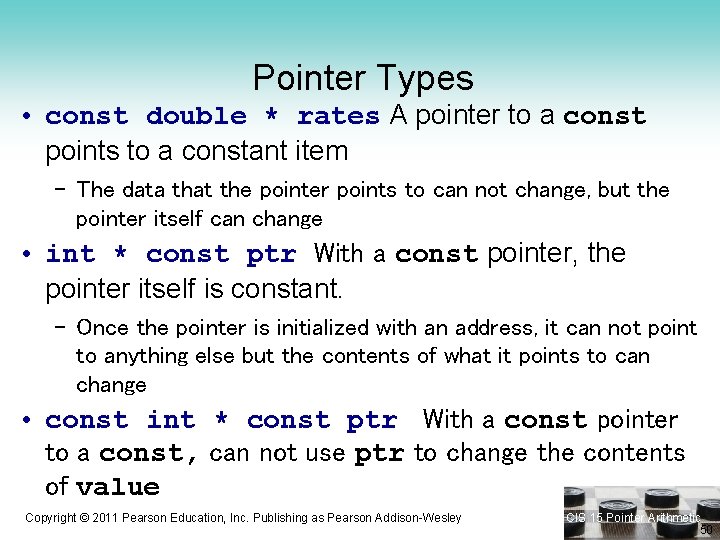
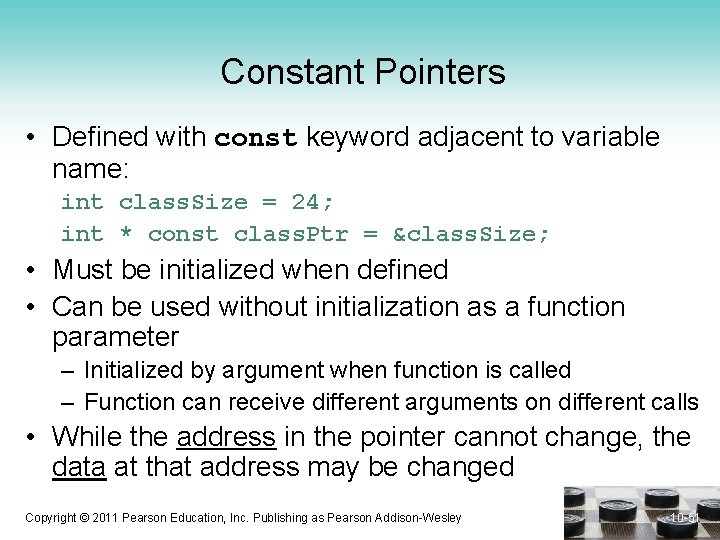
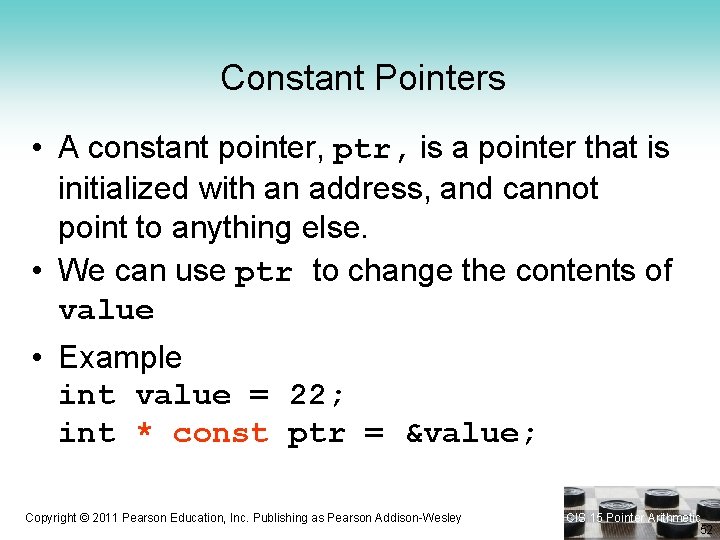
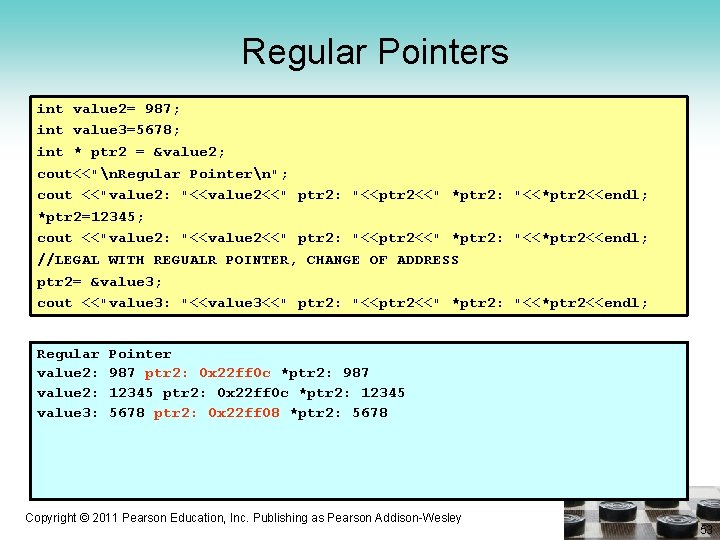
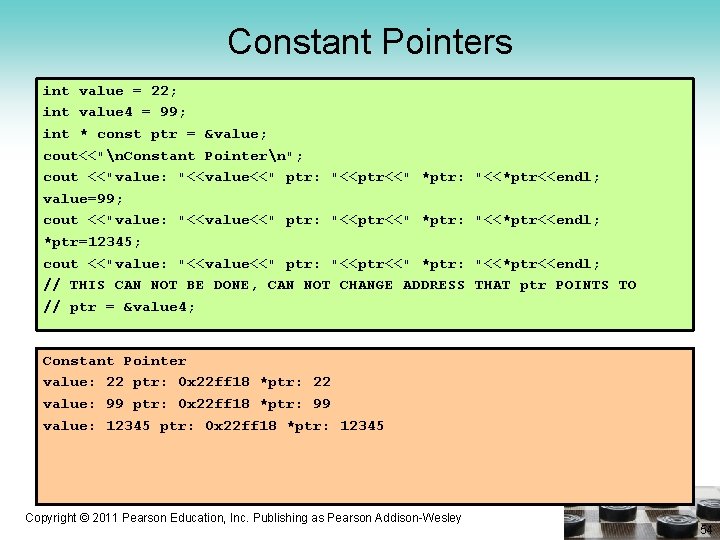
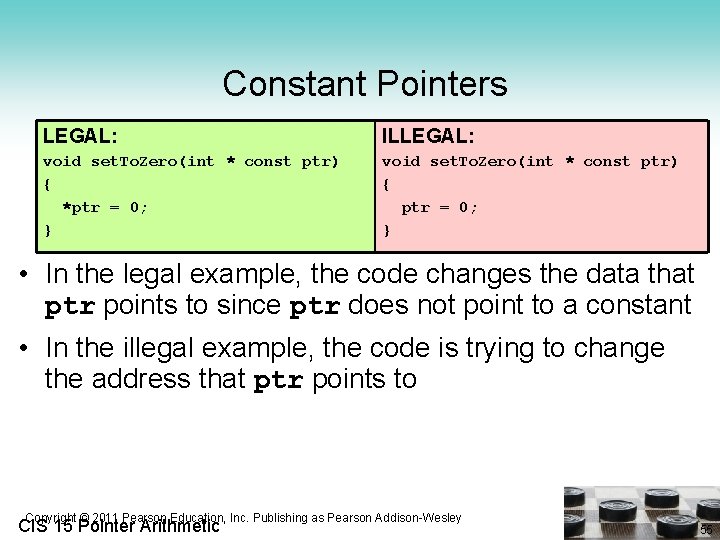
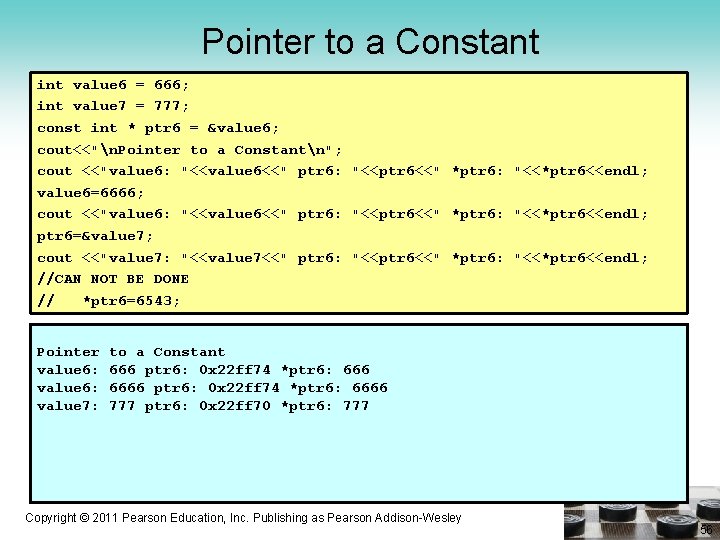
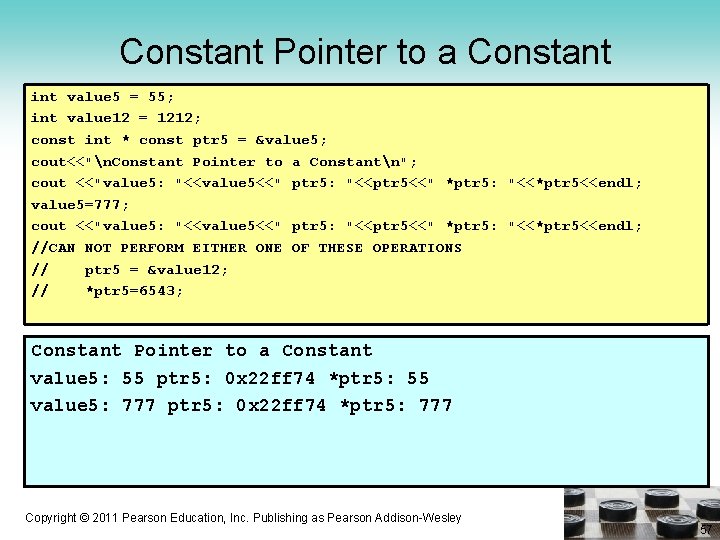
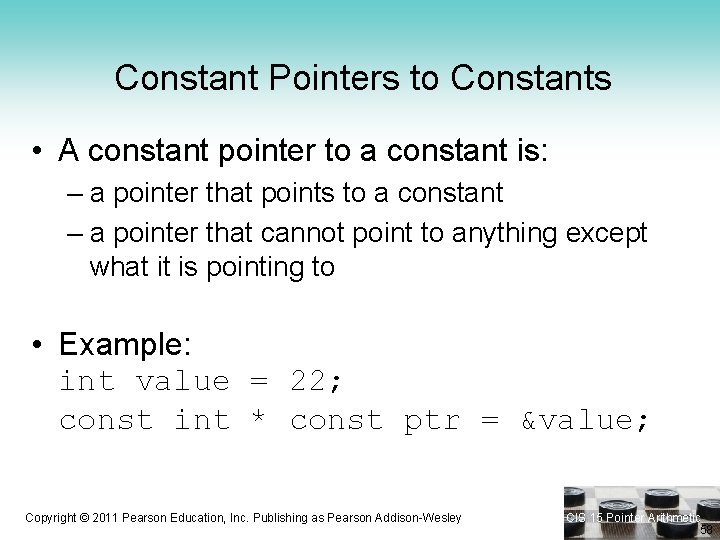
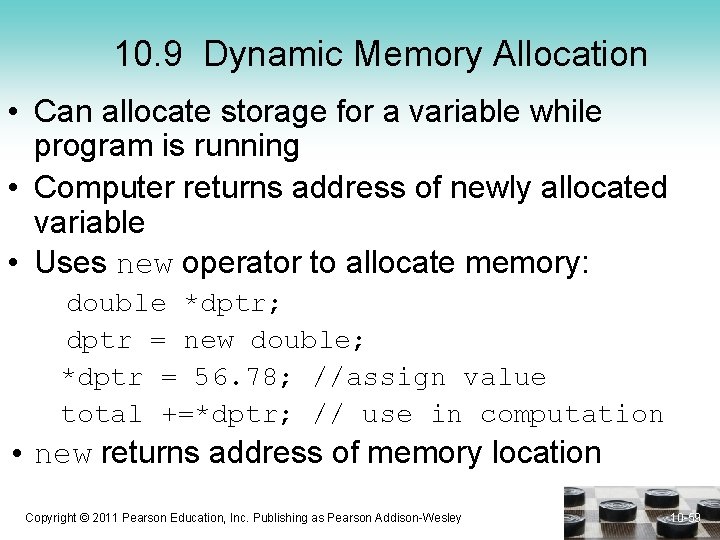
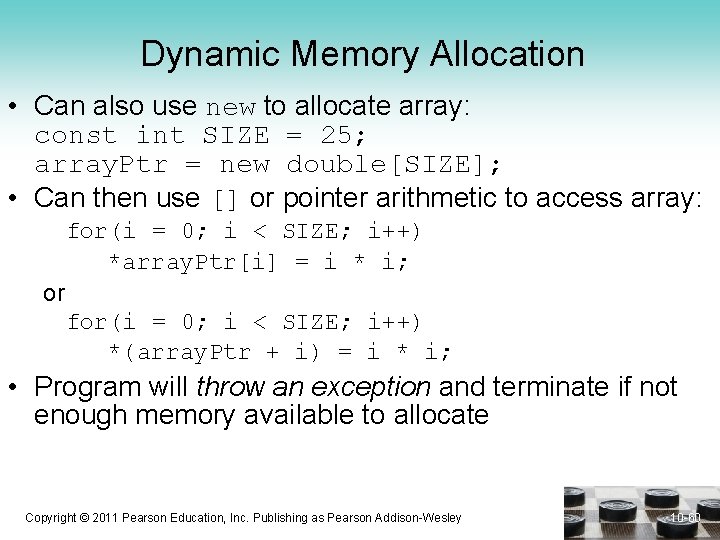
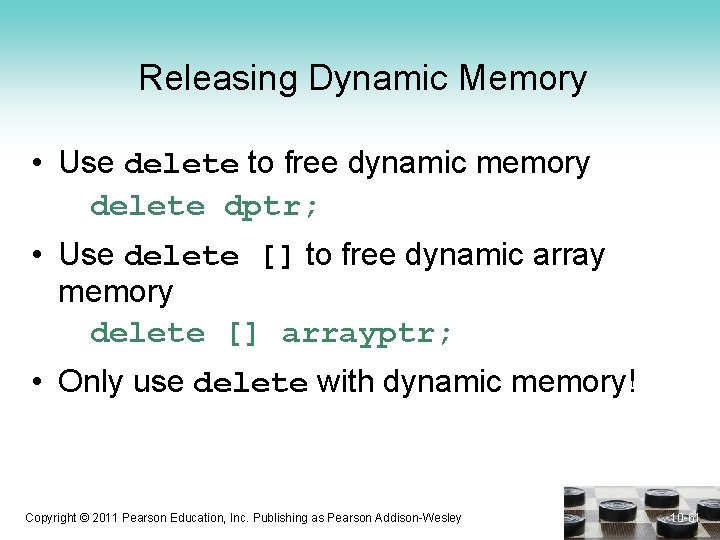
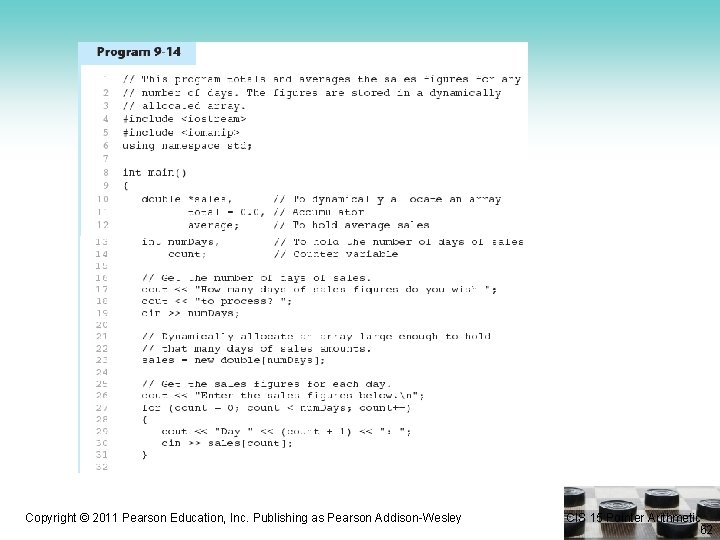
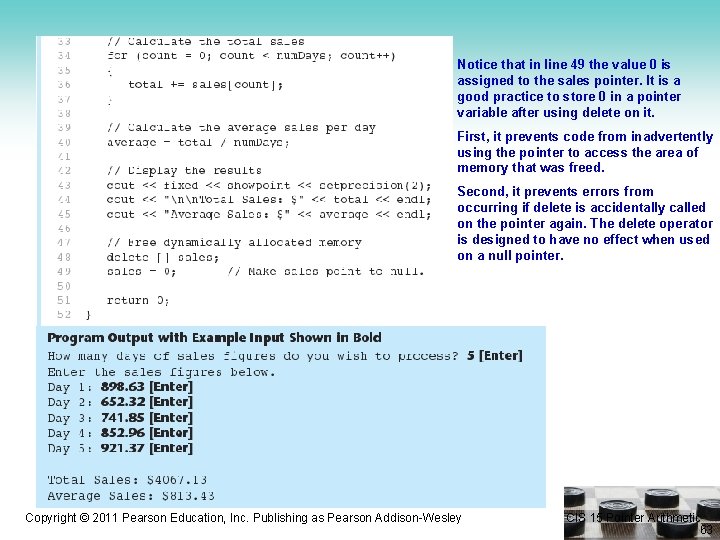
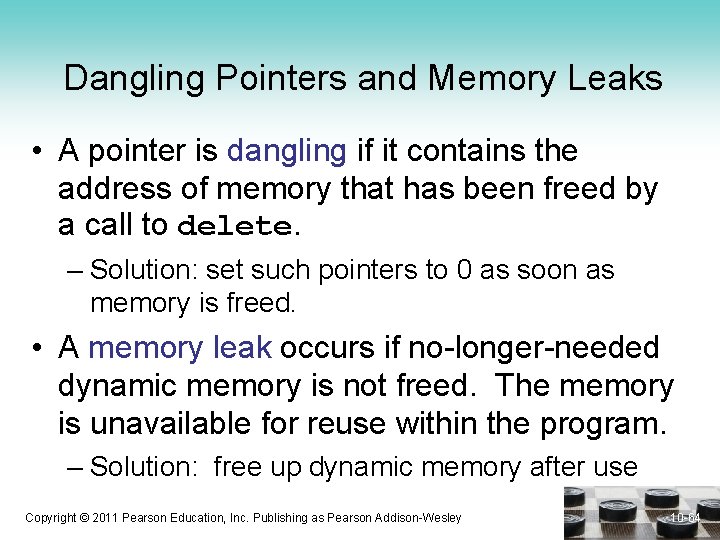
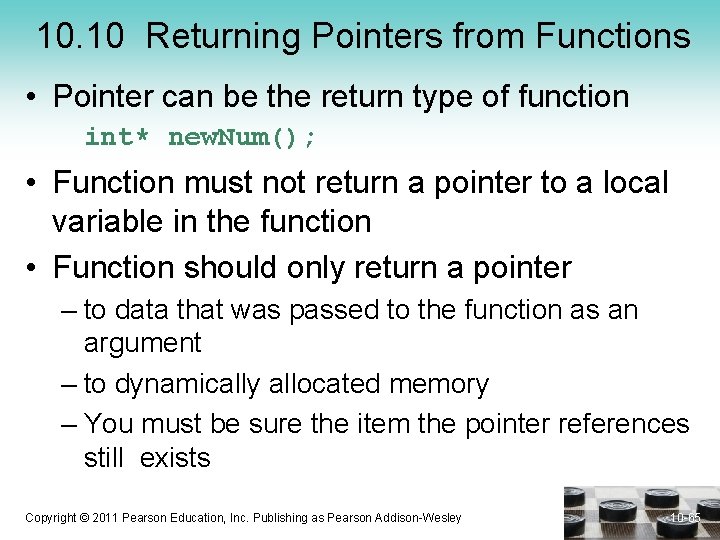
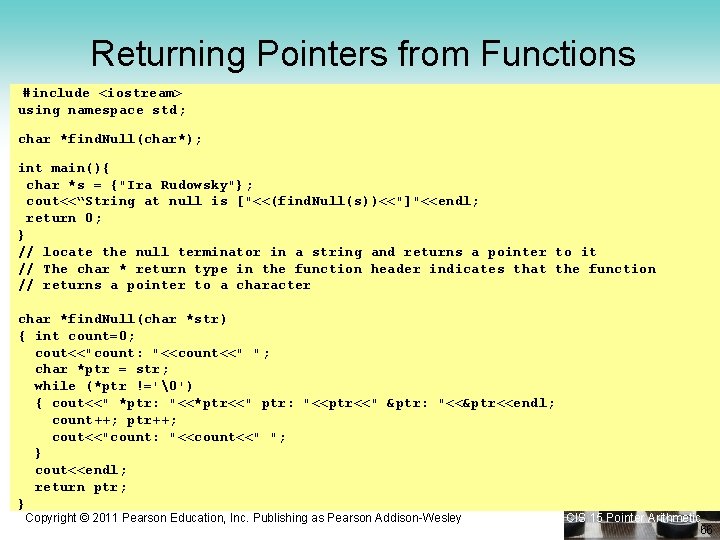
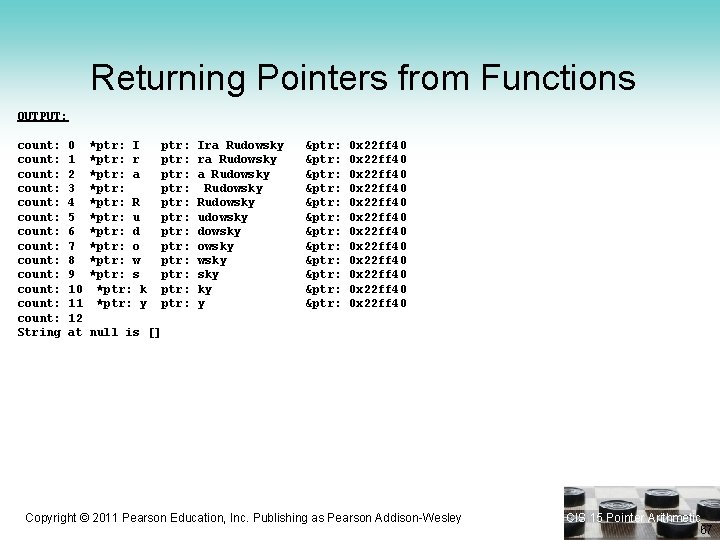
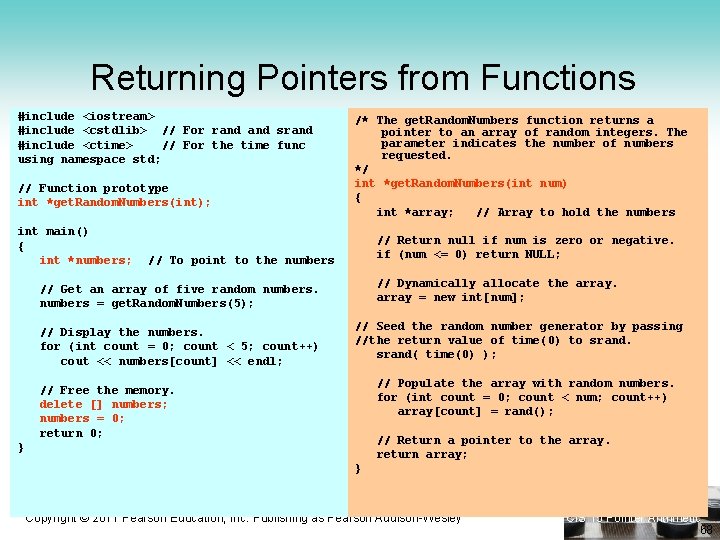
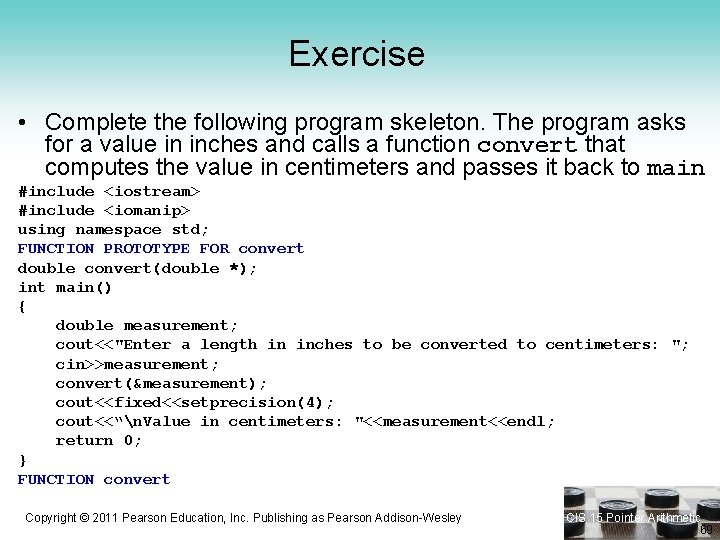
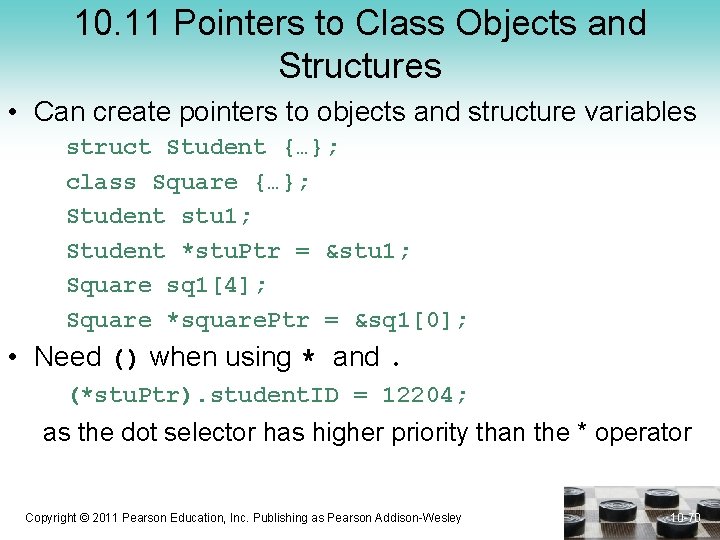
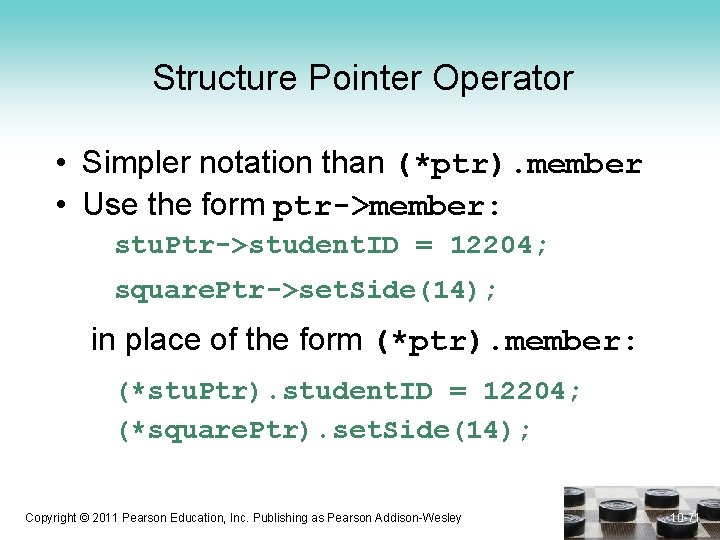
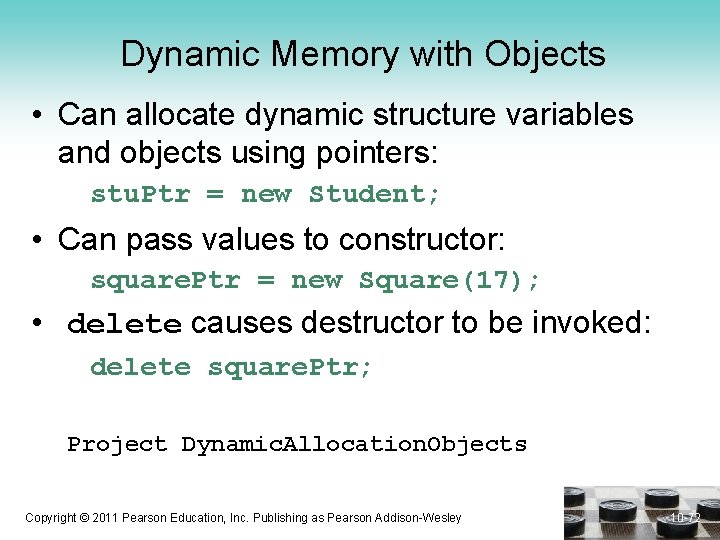
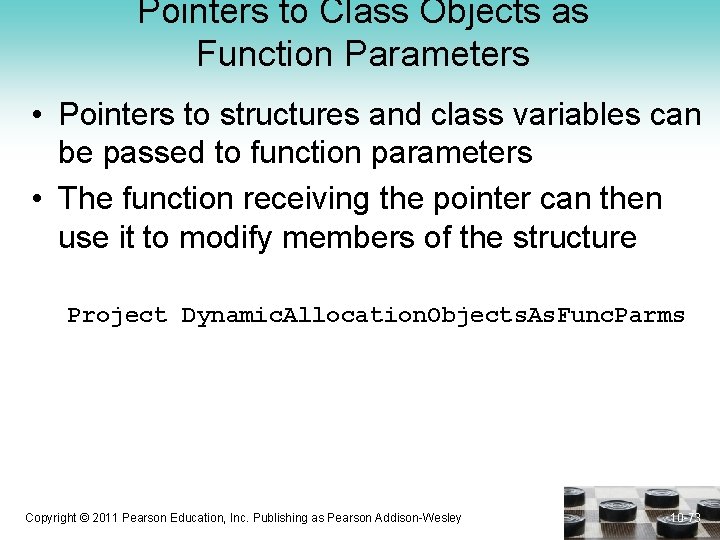
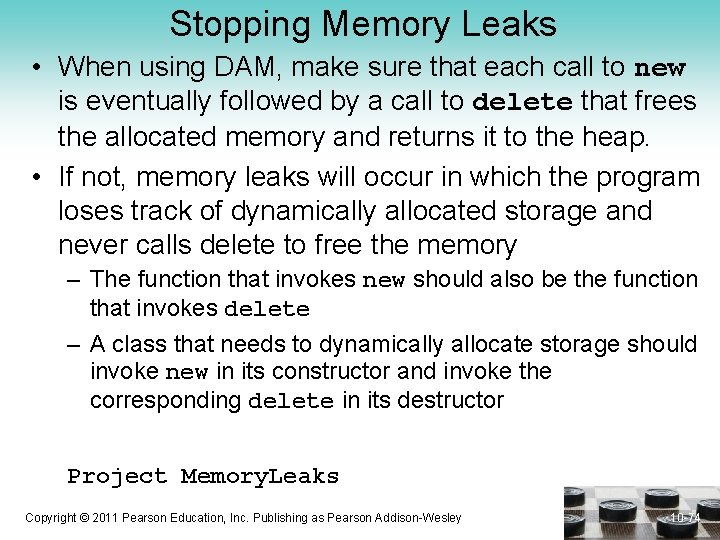
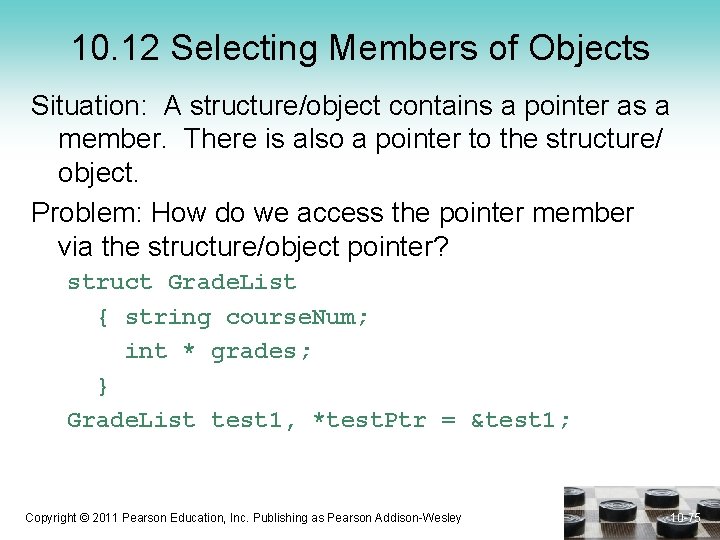
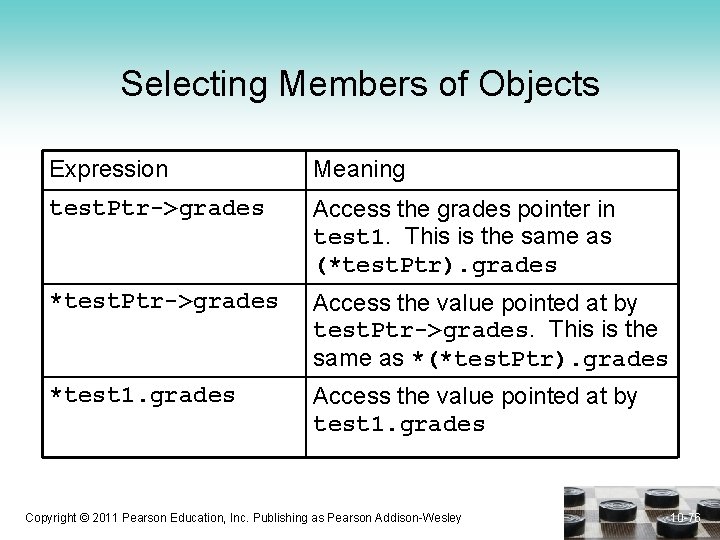
![Selecting Members of Objects struct Grade. Info { char name[25]; int *test. Scores; double Selecting Members of Objects struct Grade. Info { char name[25]; int *test. Scores; double](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-77.jpg)
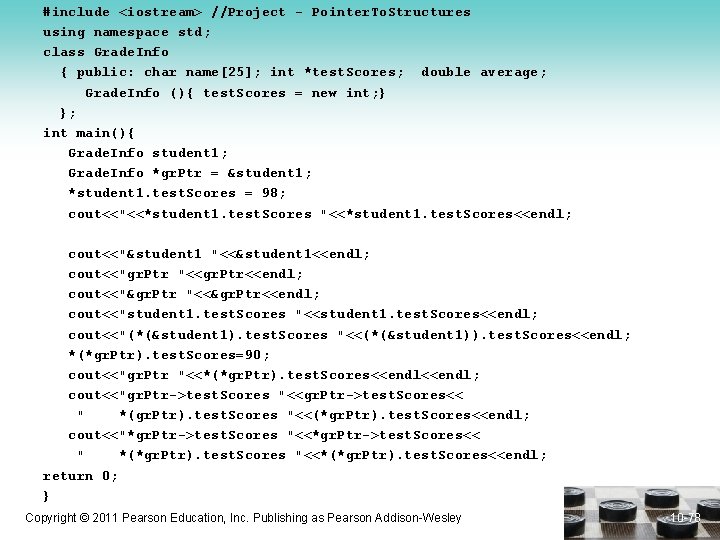
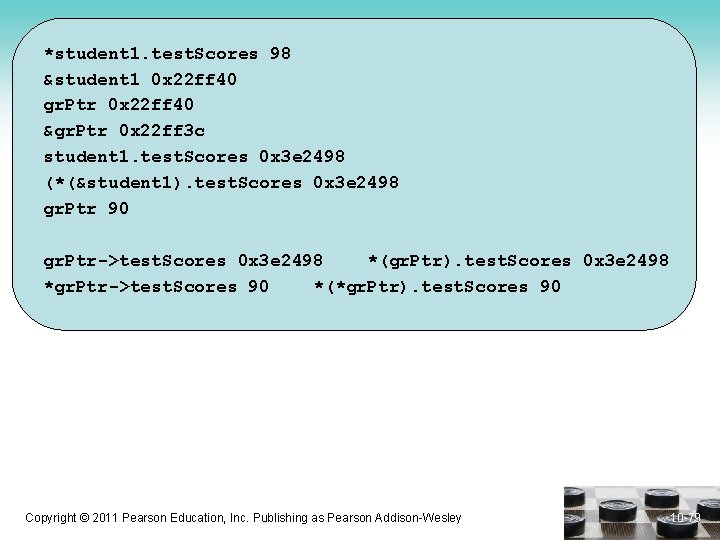
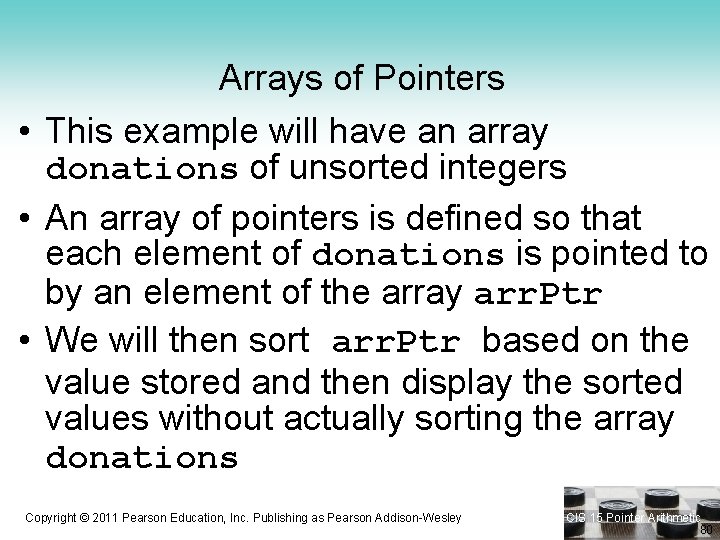
![#include <iostream> using namespace std; Arrays of Pointers void arr. Select. Sort(int *[], int); #include <iostream> using namespace std; Arrays of Pointers void arr. Select. Sort(int *[], int);](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-81.jpg)
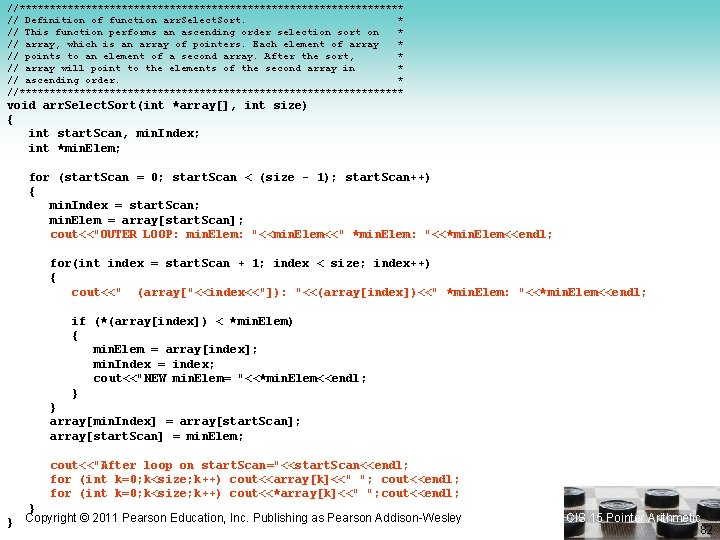
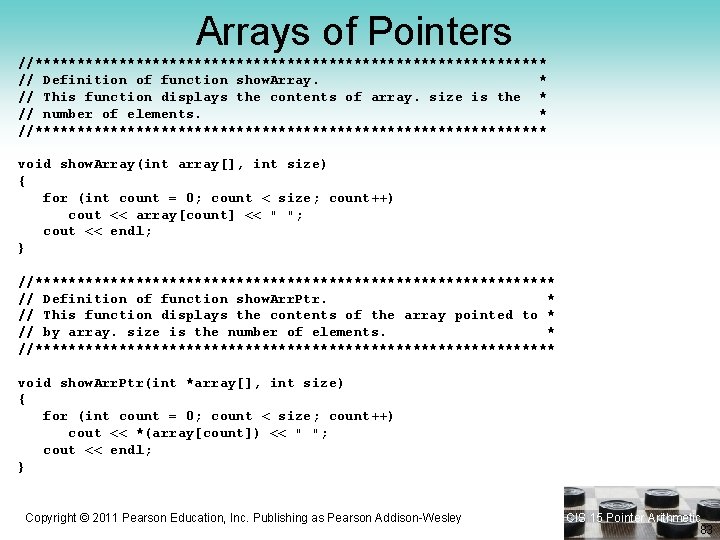
![Arrays of Pointers arr. Ptr[0]: 0 x 22 ff 50 *arr. Ptr[0]: 10 &donations[0]: Arrays of Pointers arr. Ptr[0]: 0 x 22 ff 50 *arr. Ptr[0]: 10 &donations[0]:](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-84.jpg)
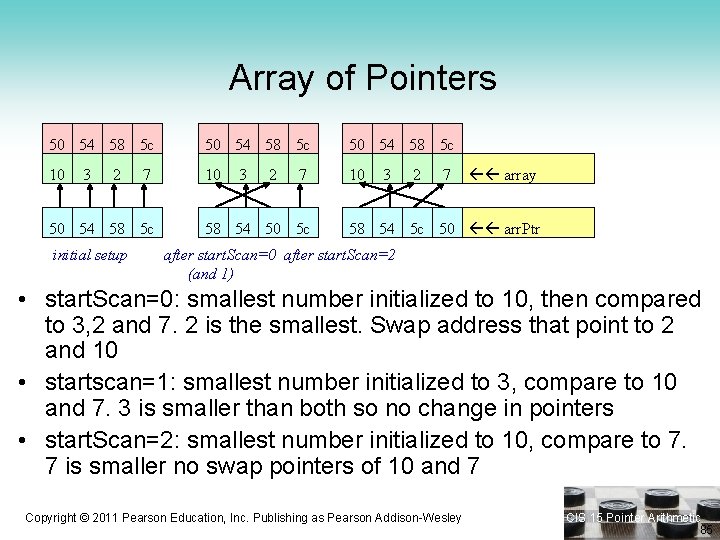
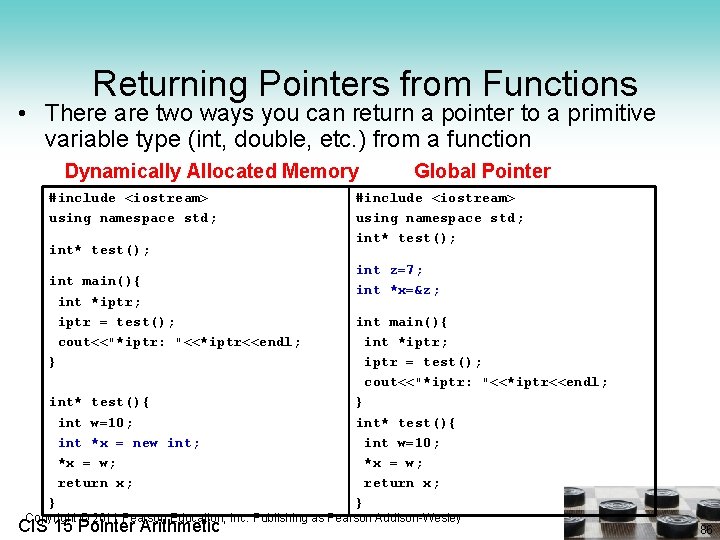
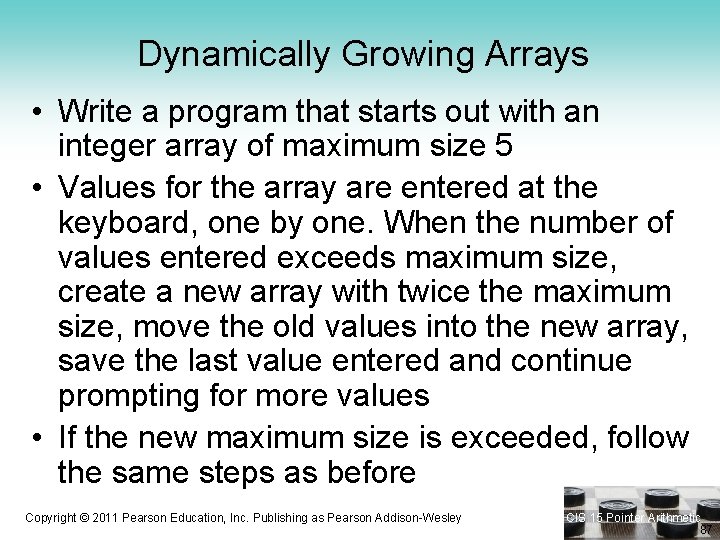
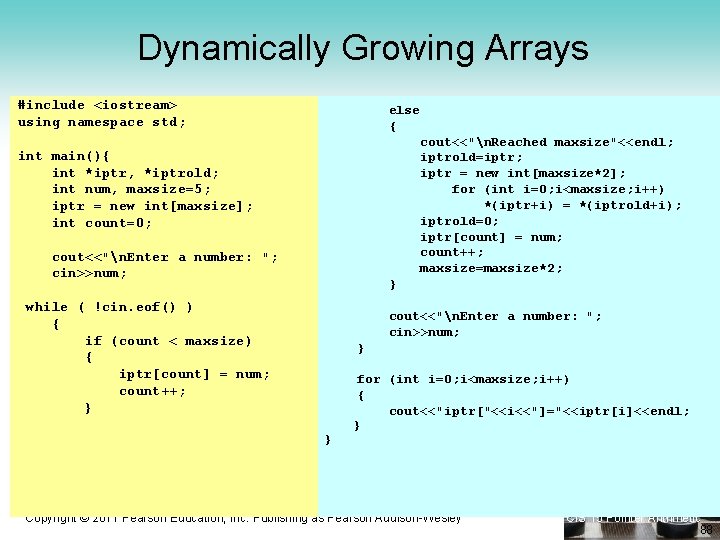
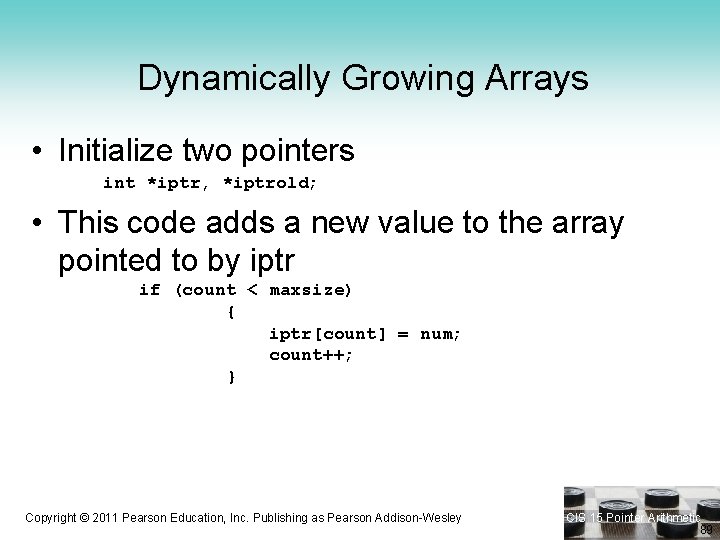
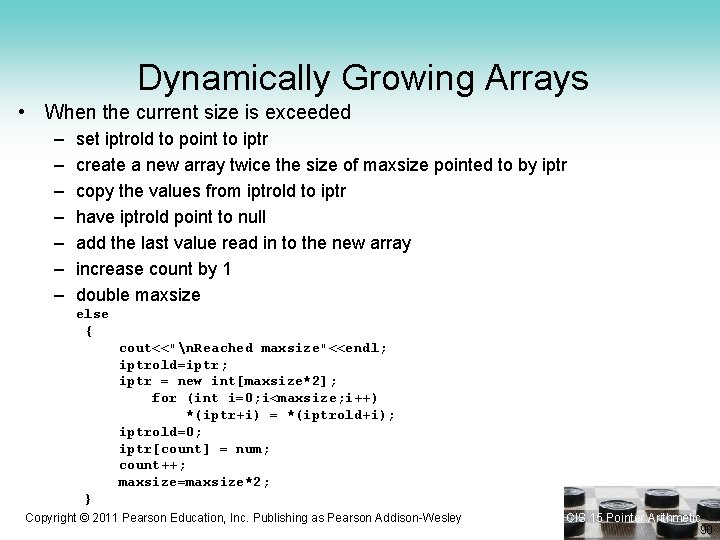
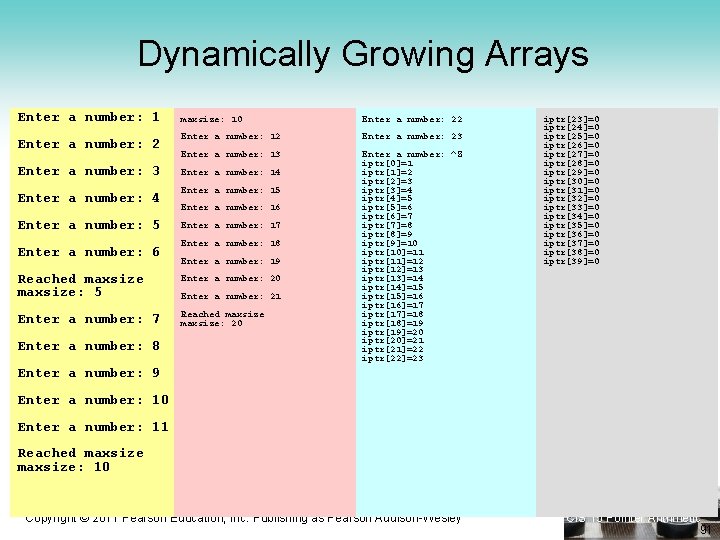
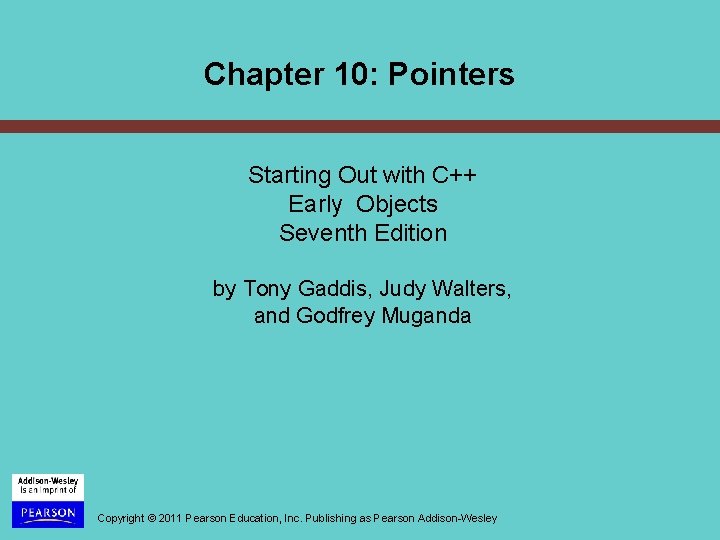
- Slides: 92
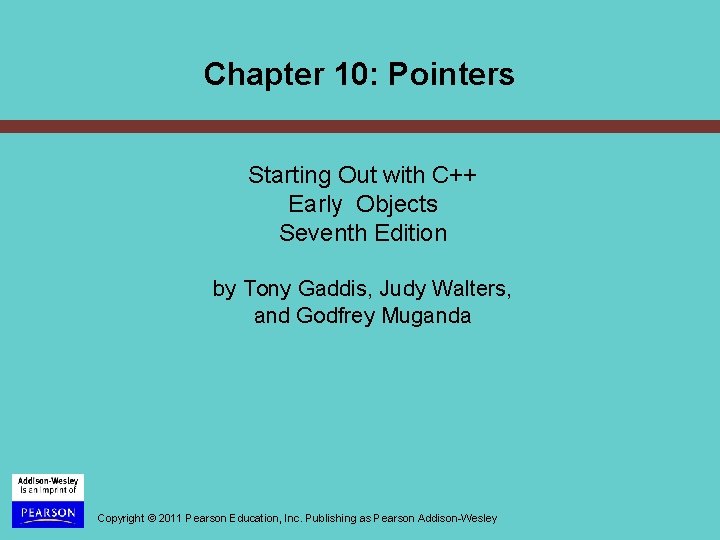
Chapter 10: Pointers Starting Out with C++ Early Objects Seventh Edition by Tony Gaddis, Judy Walters, and Godfrey Muganda Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley
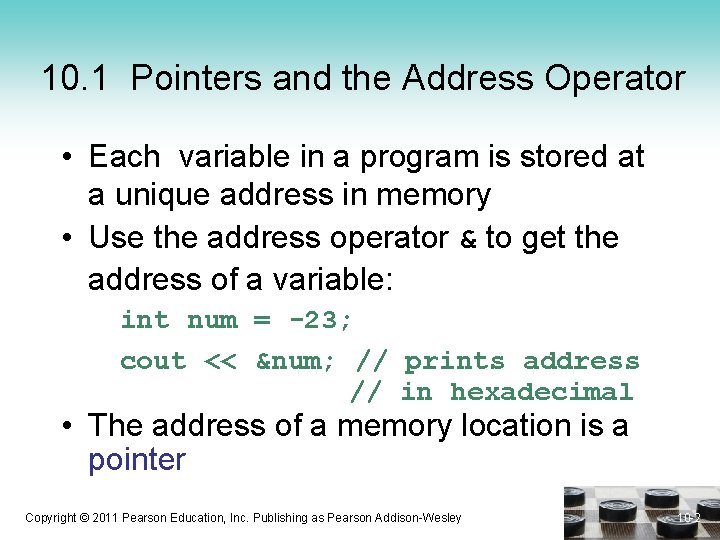
10. 1 Pointers and the Address Operator • Each variable in a program is stored at a unique address in memory • Use the address operator & to get the address of a variable: int num = -23; cout << # // prints address // in hexadecimal • The address of a memory location is a pointer Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -2
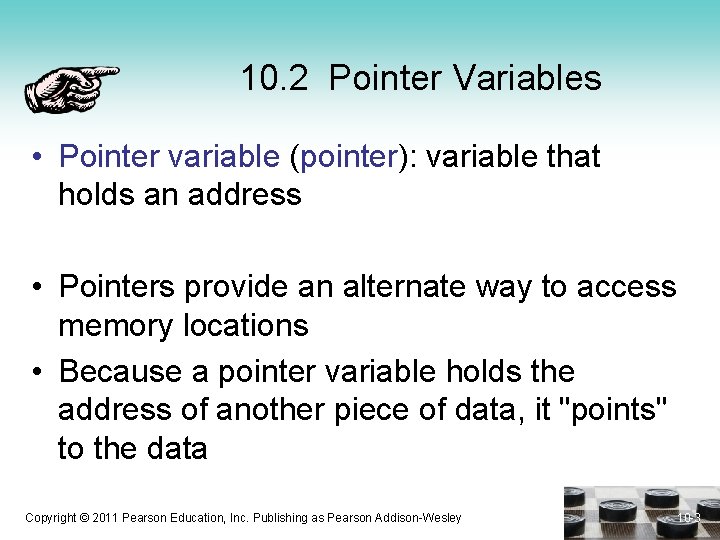
10. 2 Pointer Variables • Pointer variable (pointer): variable that holds an address • Pointers provide an alternate way to access memory locations • Because a pointer variable holds the address of another piece of data, it "points" to the data Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -3
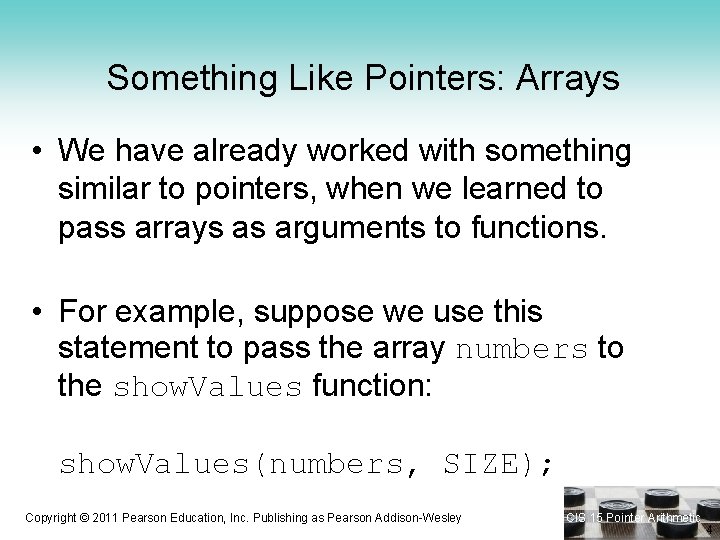
Something Like Pointers: Arrays • We have already worked with something similar to pointers, when we learned to pass arrays as arguments to functions. • For example, suppose we use this statement to pass the array numbers to the show. Values function: show. Values(numbers, SIZE); Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 4
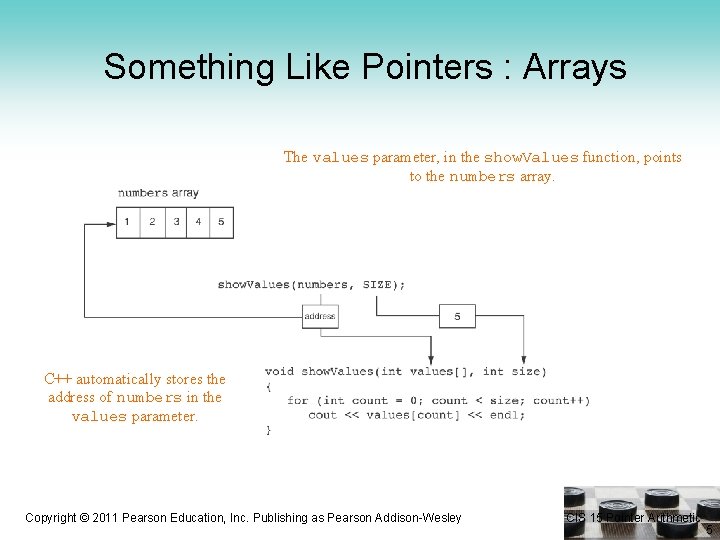
Something Like Pointers : Arrays The values parameter, in the show. Values function, points to the numbers array. C++ automatically stores the address of numbers in the values parameter. Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 5
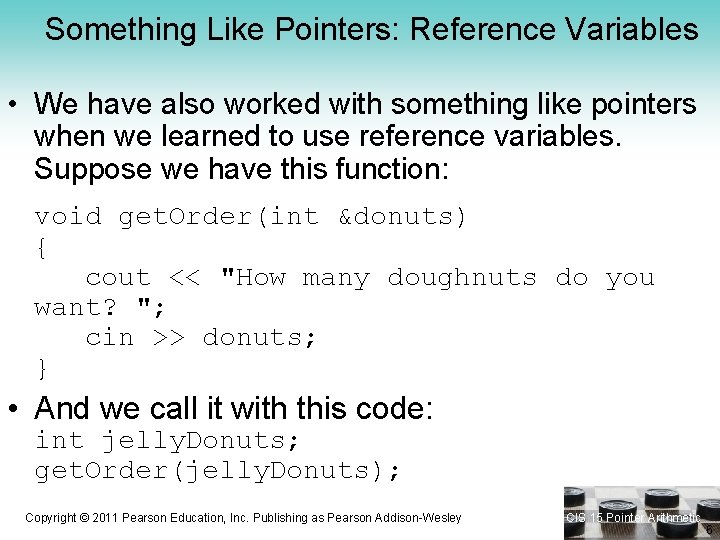
Something Like Pointers: Reference Variables • We have also worked with something like pointers when we learned to use reference variables. Suppose we have this function: void get. Order(int &donuts) { cout << "How many doughnuts do you want? "; cin >> donuts; } • And we call it with this code: int jelly. Donuts; get. Order(jelly. Donuts); Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 6
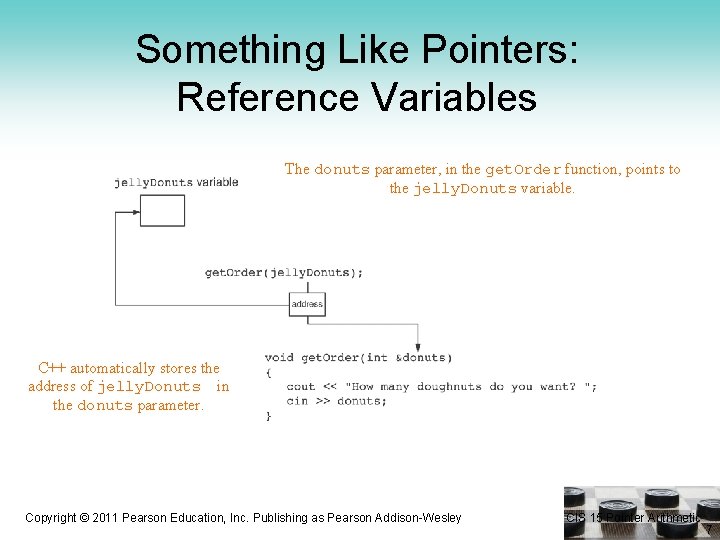
Something Like Pointers: Reference Variables The donuts parameter, in the get. Order function, points to the jelly. Donuts variable. C++ automatically stores the address of jelly. Donuts in the donuts parameter. Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 7
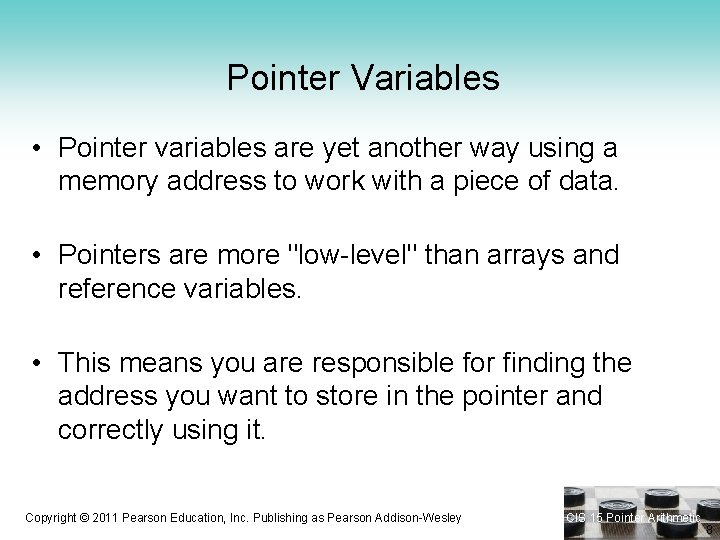
Pointer Variables • Pointer variables are yet another way using a memory address to work with a piece of data. • Pointers are more "low-level" than arrays and reference variables. • This means you are responsible for finding the address you want to store in the pointer and correctly using it. Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 8
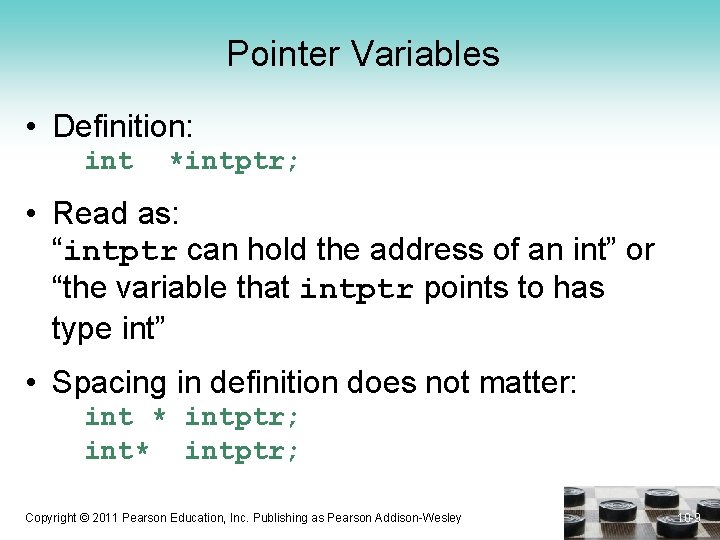
Pointer Variables • Definition: int *intptr; • Read as: “intptr can hold the address of an int” or “the variable that intptr points to has type int” • Spacing in definition does not matter: int * intptr; int* intptr; Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -9
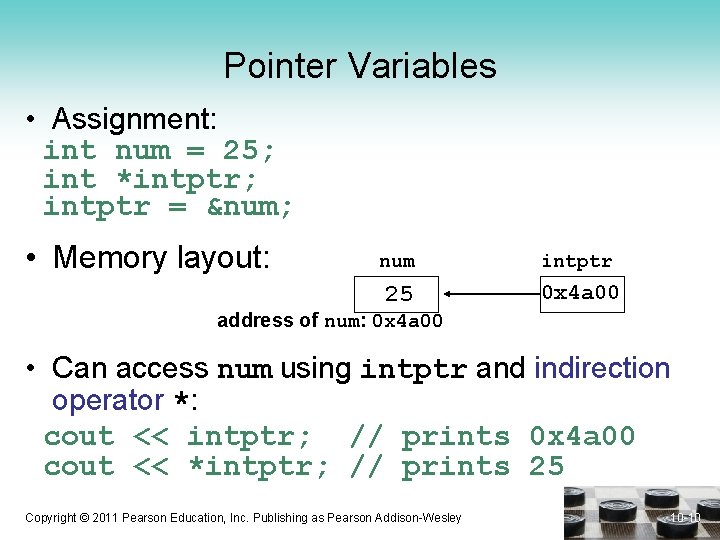
Pointer Variables • Assignment: int num = 25; int *intptr; intptr = # • Memory layout: num intptr 25 0 x 4 a 00 address of num: 0 x 4 a 00 • Can access num using intptr and indirection operator *: cout << intptr; // prints 0 x 4 a 00 cout << *intptr; // prints 25 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -10
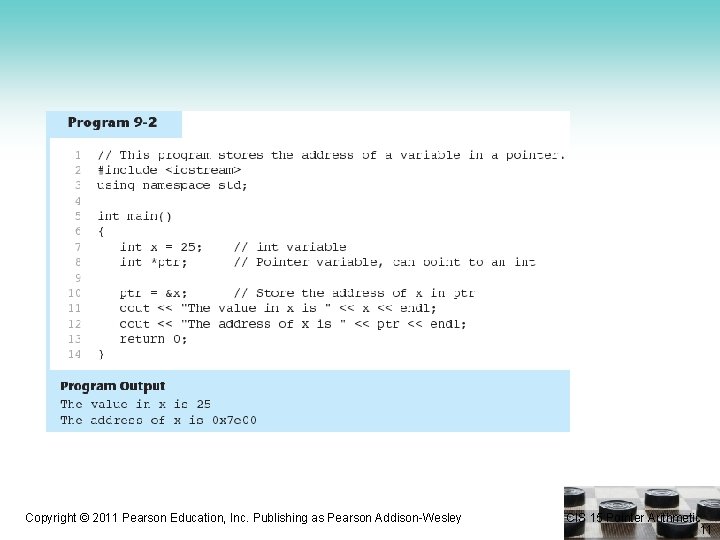
Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 11
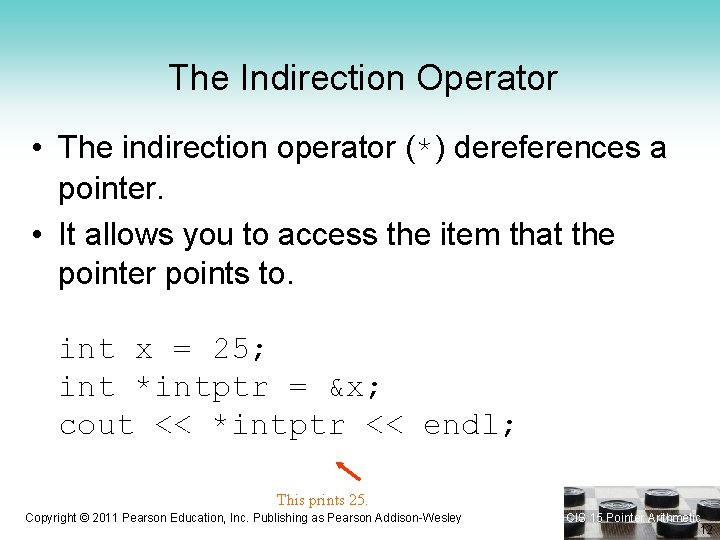
The Indirection Operator • The indirection operator (*) dereferences a pointer. • It allows you to access the item that the pointer points to. int x = 25; int *intptr = &x; cout << *intptr << endl; This prints 25. Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 12
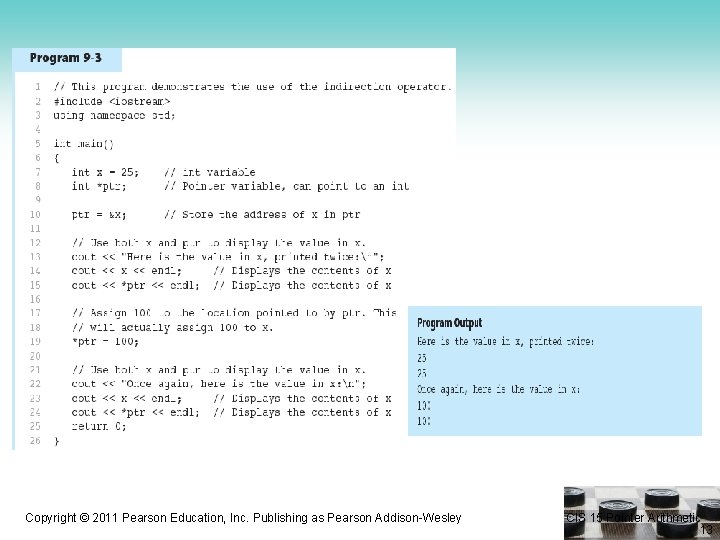
Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 13
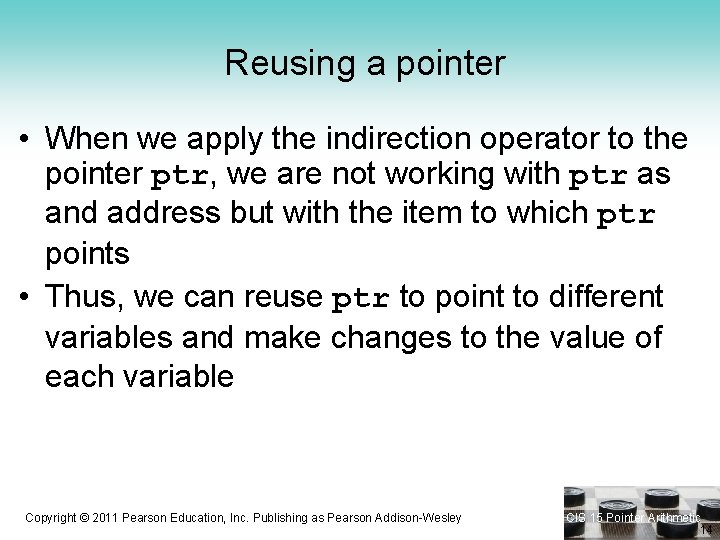
Reusing a pointer • When we apply the indirection operator to the pointer ptr, we are not working with ptr as and address but with the item to which ptr points • Thus, we can reuse ptr to point to different variables and make changes to the value of each variable Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 14
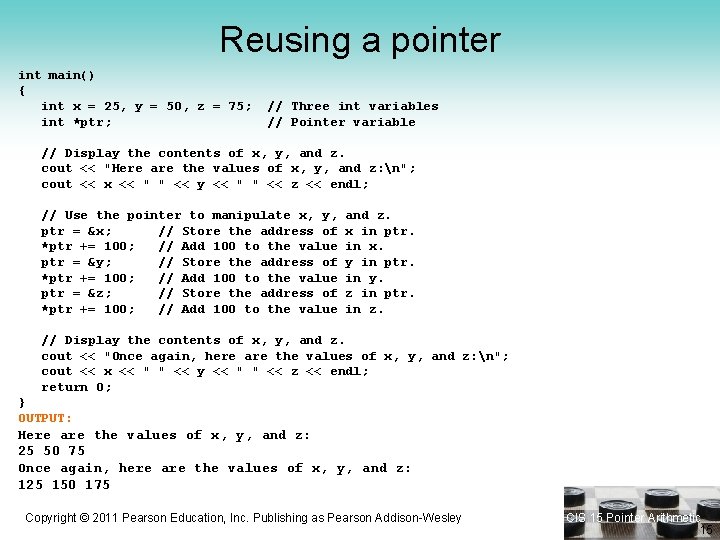
Reusing a pointer int main() { int x = 25, y = 50, z = 75; int *ptr; // Three int variables // Pointer variable // Display the contents of x, y, and z. cout << "Here are the values of x, y, and z: n"; cout << x << " " << y << " " << z << endl; // Use the pointer to manipulate x, y, ptr = &x; // Store the address of *ptr += 100; // Add 100 to the value ptr = &y; // Store the address of *ptr += 100; // Add 100 to the value ptr = &z; // Store the address of *ptr += 100; // Add 100 to the value and z. x in ptr. in x. y in ptr. in y. z in ptr. in z. // Display the contents of x, y, and z. cout << "Once again, here are the values of x, y, and z: n"; cout << x << " " << y << " " << z << endl; return 0; } OUTPUT: Here are the values of x, y, and z: 25 50 75 Once again, here are the values of x, y, and z: 125 150 175 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 15
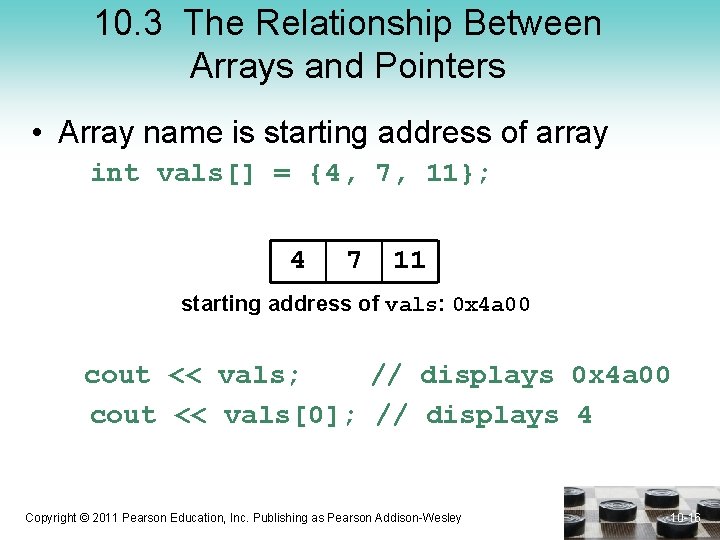
10. 3 The Relationship Between Arrays and Pointers • Array name is starting address of array int vals[] = {4, 7, 11}; 4 7 11 starting address of vals: 0 x 4 a 00 cout << vals; // displays 0 x 4 a 00 cout << vals[0]; // displays 4 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -16
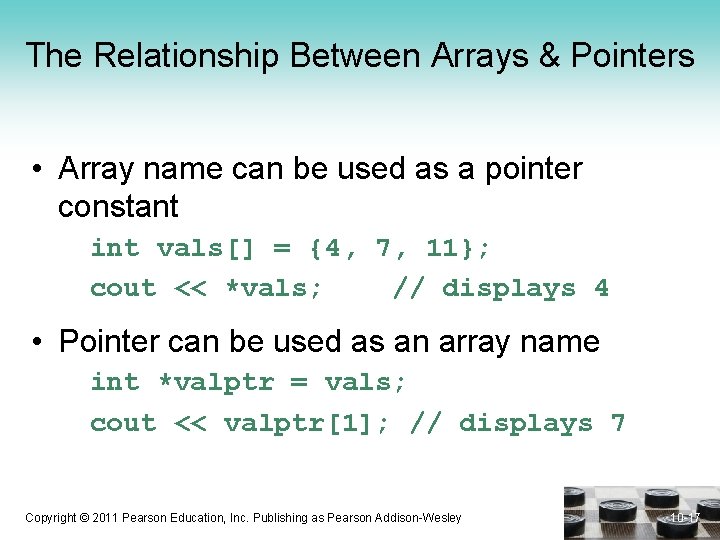
The Relationship Between Arrays & Pointers • Array name can be used as a pointer constant int vals[] = {4, 7, 11}; cout << *vals; // displays 4 • Pointer can be used as an array name int *valptr = vals; cout << valptr[1]; // displays 7 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -17
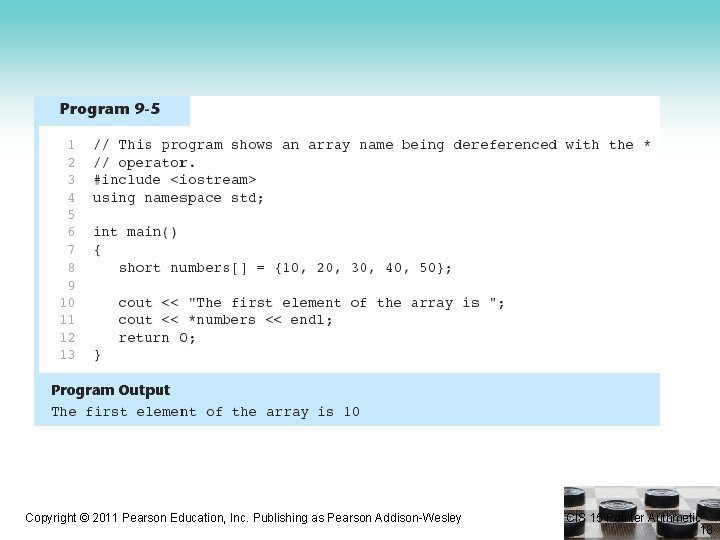
Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 18
![Pointers in Expressions Given int vals4 7 11 int valptr vals Pointers in Expressions • Given: int vals[]={4, 7, 11}; int *valptr = vals; •](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-19.jpg)
Pointers in Expressions • Given: int vals[]={4, 7, 11}; int *valptr = vals; • What is valptr + 1? • It means (address in valptr) + (1 * size of an int) cout << *(valptr+1); // displays 7 cout << *(valptr+2); // displays 11 • Must use ( ) in expression Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -19
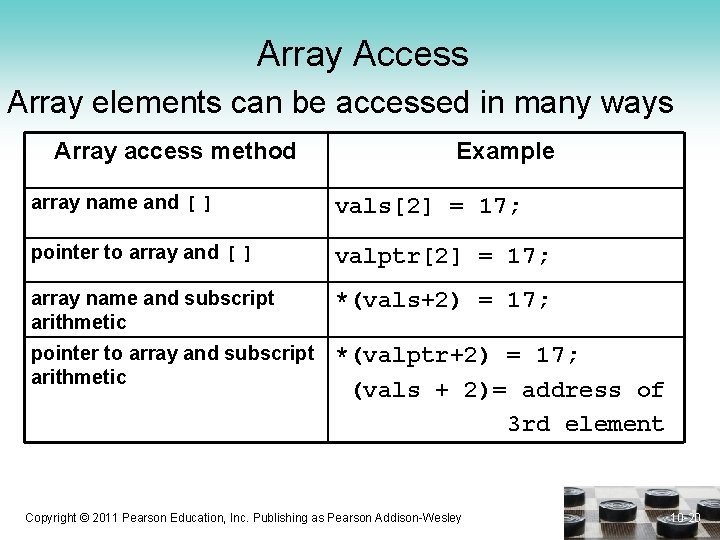
Array Access Array elements can be accessed in many ways Array access method Example array name and [ ] vals[2] = 17; pointer to array and [ ] valptr[2] = 17; array name and subscript arithmetic *(vals+2) = 17; pointer to array and subscript arithmetic *(valptr+2) = 17; (vals + 2)= address of 3 rd element Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -20
![Array Access Array notation valsi is equivalent to the pointer notation vals Array Access • Array notation vals[i] is equivalent to the pointer notation *(vals +](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-21.jpg)
Array Access • Array notation vals[i] is equivalent to the pointer notation *(vals + i) • No bounds checking performed on array access Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -21
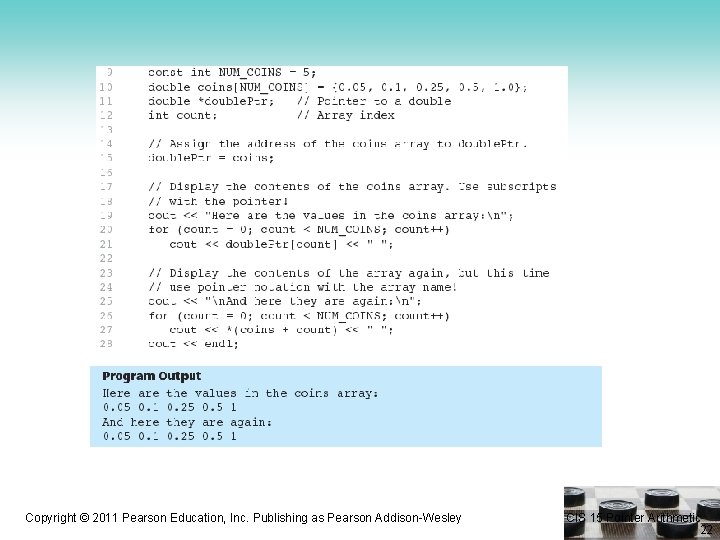
Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 22
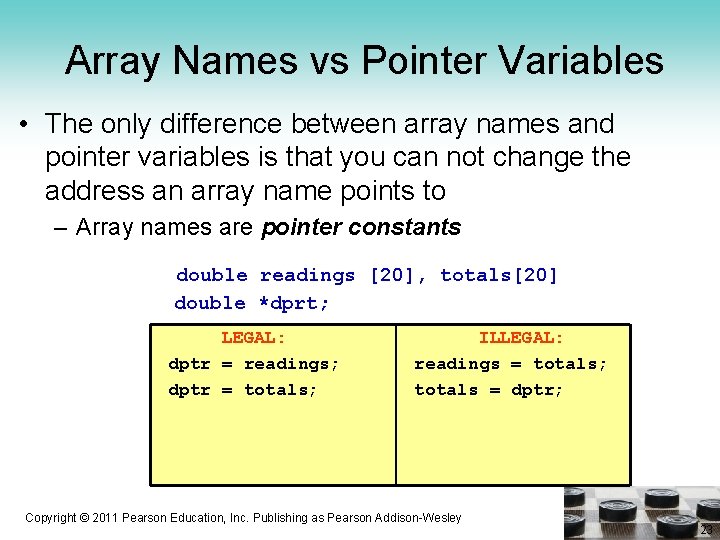
Array Names vs Pointer Variables • The only difference between array names and pointer variables is that you can not change the address an array name points to – Array names are pointer constants double readings [20], totals[20] double *dprt; LEGAL: dptr = readings; dptr = totals; ILLEGAL: readings = totals; totals = dptr; Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 23
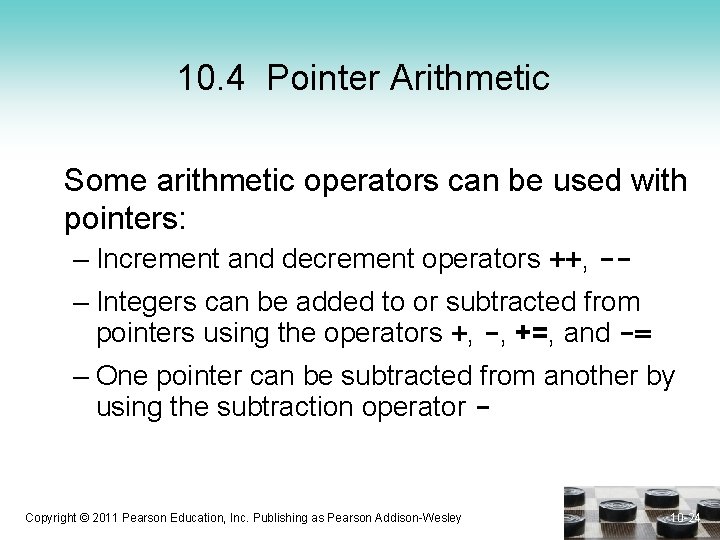
10. 4 Pointer Arithmetic Some arithmetic operators can be used with pointers: – Increment and decrement operators ++, -– Integers can be added to or subtracted from pointers using the operators +, -, +=, and -= – One pointer can be subtracted from another by using the subtraction operator - Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -24
![Pointer Arithmetic Assume the variable definitions int vals4 7 11 int valptr vals Pointer Arithmetic Assume the variable definitions int vals[]={4, 7, 11}; int *valptr = vals;](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-25.jpg)
Pointer Arithmetic Assume the variable definitions int vals[]={4, 7, 11}; int *valptr = vals; Examples of use of ++ and -valptr++; // points at 7 valptr--; // now points at 4 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -25
![More on Pointer Arithmetic Assume the variable definitions int vals4 7 11 int valptr More on Pointer Arithmetic Assume the variable definitions: int vals[]={4, 7, 11}; int *valptr](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-26.jpg)
More on Pointer Arithmetic Assume the variable definitions: int vals[]={4, 7, 11}; int *valptr = vals; Example of the use of + to add an int to a pointer: cout << *(valptr + 2) This statement will print 11 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -26
![More on Pointer Arithmetic Assume the variable definitions int vals4 7 11 int valptr More on Pointer Arithmetic Assume the variable definitions: int vals[]={4, 7, 11}; int *valptr](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-27.jpg)
More on Pointer Arithmetic Assume the variable definitions: int vals[]={4, 7, 11}; int *valptr = vals; Example of use of +=: valptr = vals; // points at 4 valptr += 2; // points at 11 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -27
![More on Pointer Arithmetic Assume the variable definitions int vals 4 7 11 More on Pointer Arithmetic Assume the variable definitions int vals[] = {4, 7, 11};](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-28.jpg)
More on Pointer Arithmetic Assume the variable definitions int vals[] = {4, 7, 11}; int *valptr = vals; Example of pointer subtraction valptr += 2; cout << valptr - vals; This statement prints 2: the number of ints between valptr and vals Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -28
![Pointer Arithmetic int vals4 7 11 int valptr vals coutnvalptr valptr cout valptr Pointer Arithmetic int vals[]={4, 7, 11}; int *valptr = vals; cout<<"nvalptr++: "<<valptr++; cout<<" *valptr:](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-29.jpg)
Pointer Arithmetic int vals[]={4, 7, 11}; int *valptr = vals; cout<<"nvalptr++: "<<valptr++; cout<<" *valptr: "<<*valptr<<endl; valptr++: 0 x 22 ff 20 *valptr: 7 cout<<"valptr--: "<<valptr--; cout<<" *valptr: "<<*valptr<<endl; valptr--: 0 x 22 ff 24 *valptr: 4 *(valptr+2): 11 cout <<"*(valptr+2): "<< *(valptr + 2)<<endl; valptr = vals; valptr+=2; cout<<"valptr+=2: "<<valptr<<endl; int y = valptr-vals; cout<<"valptr: "<<valptr<<" vals: "<<vals; cout<<" *valptr: "<<*valptr<<" *vals: "<<*vals<<endl; cout<<" valptr minus vals: "<<y<<endl; valptr+=2: 0 x 22 ff 28 valptr: 0 x 22 ff 28 vals: 0 x 22 ff 20 *valptr: 11 *vals: 4 valptr minus vals: 2 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 29
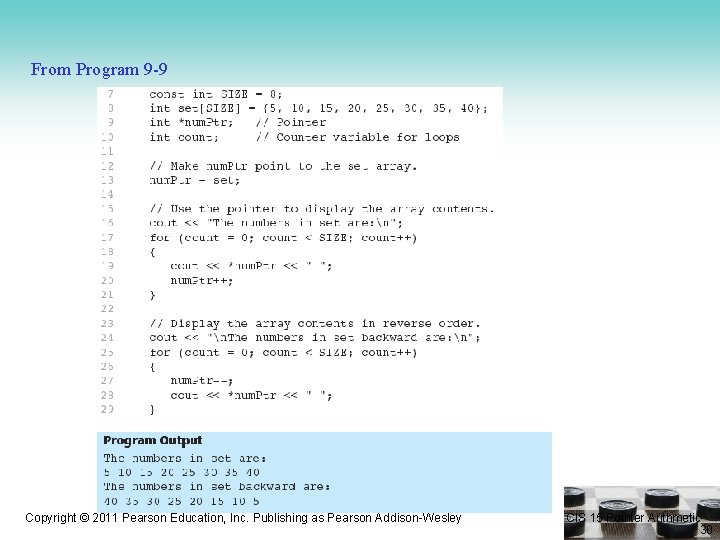
From Program 9 -9 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 30
![Pointer Arithmetic Summary Operation Example int vals4 7 11 int valptr Pointer Arithmetic - Summary Operation Example ++, -- int vals[]={4, 7, 11}; int *valptr](https://slidetodoc.com/presentation_image_h/94606bee7162c4c5331e1d9cdd3b3c3a/image-31.jpg)
Pointer Arithmetic - Summary Operation Example ++, -- int vals[]={4, 7, 11}; int *valptr = vals; valptr++; // points at 7 valptr--; // now points at 4 cout << *(valptr + 2); // 11 +, - (pointer and int) +=, -= (pointer and int) - (pointer from pointer) valptr = vals; // points at 4 valptr += 2; // points at 11 cout << valptr–val; // difference //(number of ints) between valptr // and val Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 31
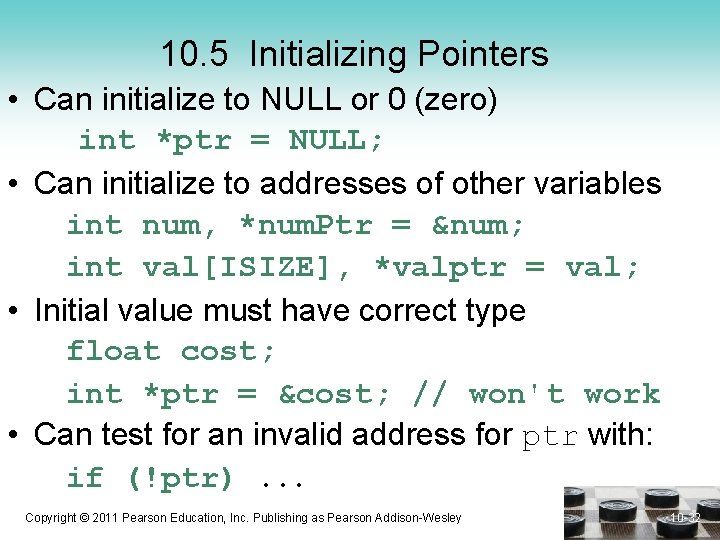
10. 5 Initializing Pointers • Can initialize to NULL or 0 (zero) int *ptr = NULL; • Can initialize to addresses of other variables int num, *num. Ptr = # int val[ISIZE], *valptr = val; • Initial value must have correct type float cost; int *ptr = &cost; // won't work • Can test for an invalid address for ptr with: if (!ptr). . . Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -32
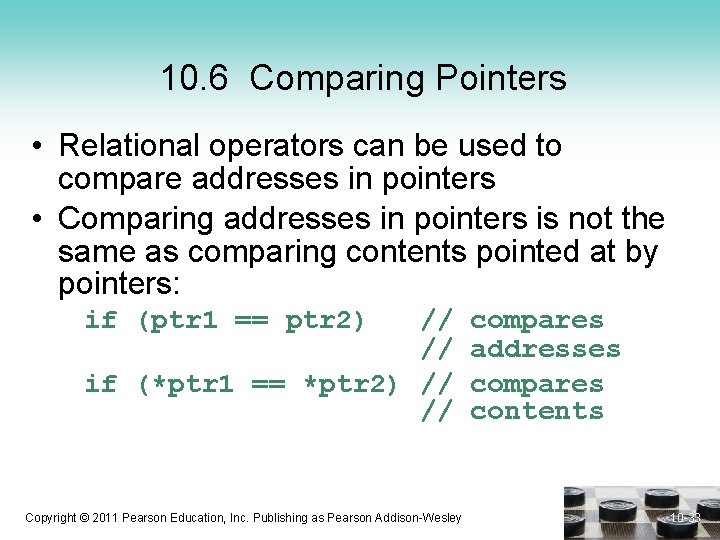
10. 6 Comparing Pointers • Relational operators can be used to compare addresses in pointers • Comparing addresses in pointers is not the same as comparing contents pointed at by pointers: if (ptr 1 == ptr 2) // // if (*ptr 1 == *ptr 2) // // Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley compares addresses compares contents 10 -33
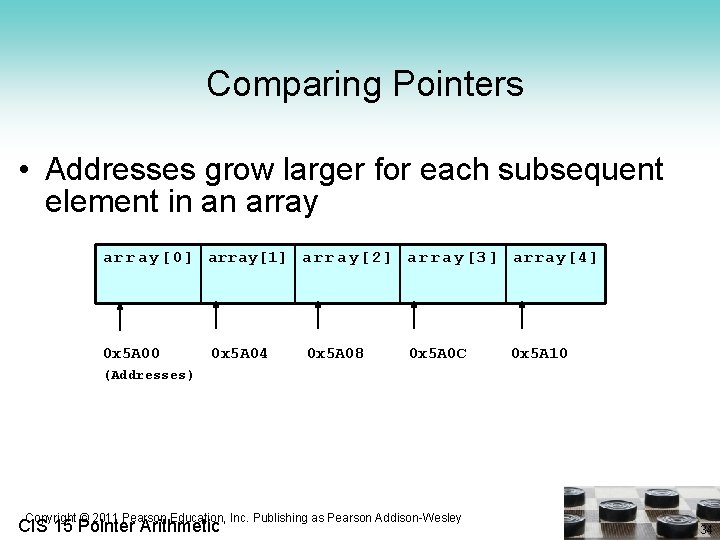
Comparing Pointers • Addresses grow larger for each subsequent element in an array a r r a y [ 0 ] array[1] a r r a y [ 2 ] a r r a y [ 3 ] array[4] 0 x 5 A 00 0 x 5 A 04 0 x 5 A 08 0 x 5 A 0 C 0 x 5 A 10 (Addresses) Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 34
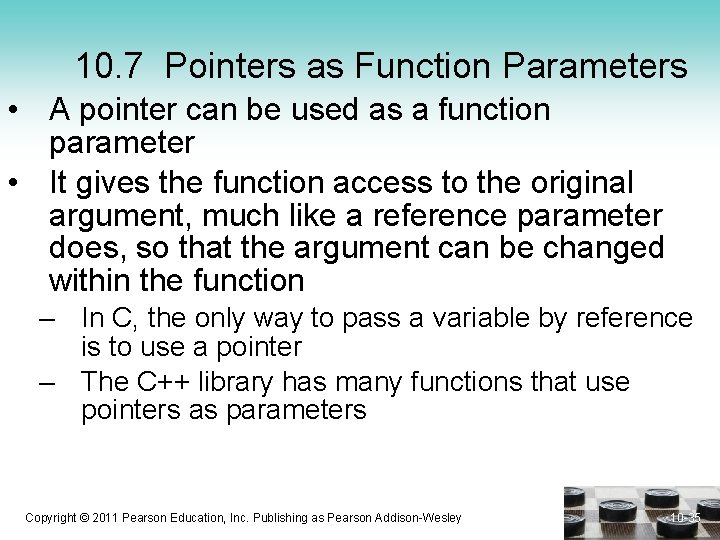
10. 7 Pointers as Function Parameters • A pointer can be used as a function parameter • It gives the function access to the original argument, much like a reference parameter does, so that the argument can be changed within the function – In C, the only way to pass a variable by reference is to use a pointer – The C++ library has many functions that use pointers as parameters Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -35
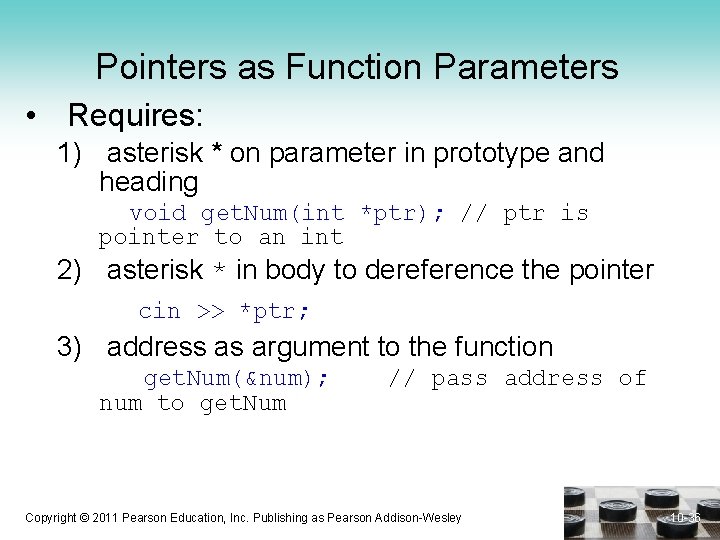
Pointers as Function Parameters • Requires: 1) asterisk * on parameter in prototype and heading void get. Num(int *ptr); // ptr is pointer to an int 2) asterisk * in body to dereference the pointer cin >> *ptr; 3) address as argument to the function get. Num(&num); num to get. Num // pass address of Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -36
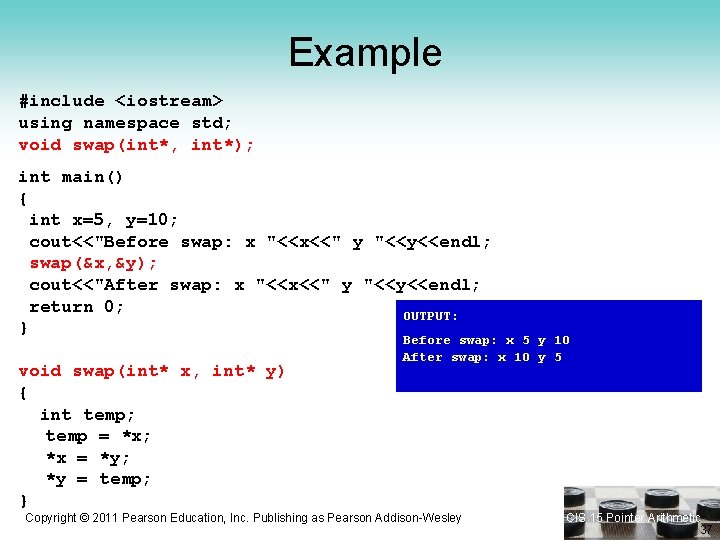
Example #include <iostream> using namespace std; void swap(int*, int*); int main() { int x=5, y=10; cout<<"Before swap: x "<<x<<" y "<<y<<endl; swap(&x, &y); cout<<"After swap: x "<<x<<" y "<<y<<endl; return 0; OUTPUT: } void swap(int* x, int* y) { int temp; temp = *x; *x = *y; *y = temp; } Before swap: x 5 y 10 After swap: x 10 y 5 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 37
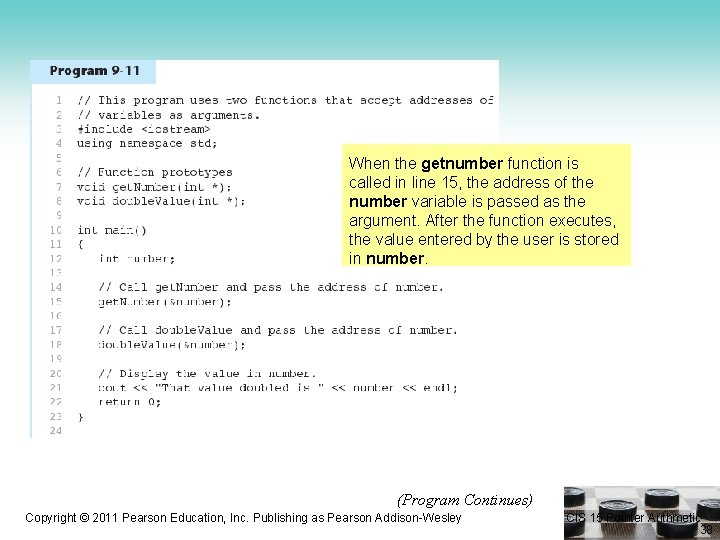
When the getnumber function is called in line 15, the address of the number variable is passed as the argument. After the function executes, the value entered by the user is stored in number. (Program Continues) Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 38
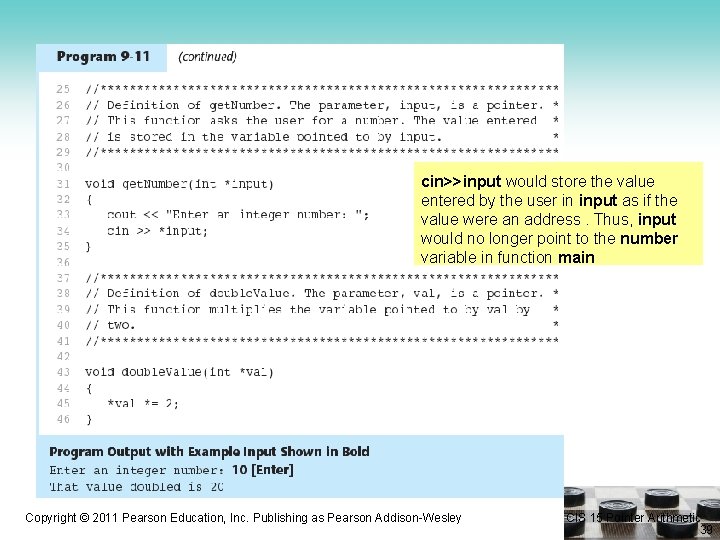
cin>>input would store the value entered by the user in input as if the value were an address. Thus, input would no longer point to the number variable in function main Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 39
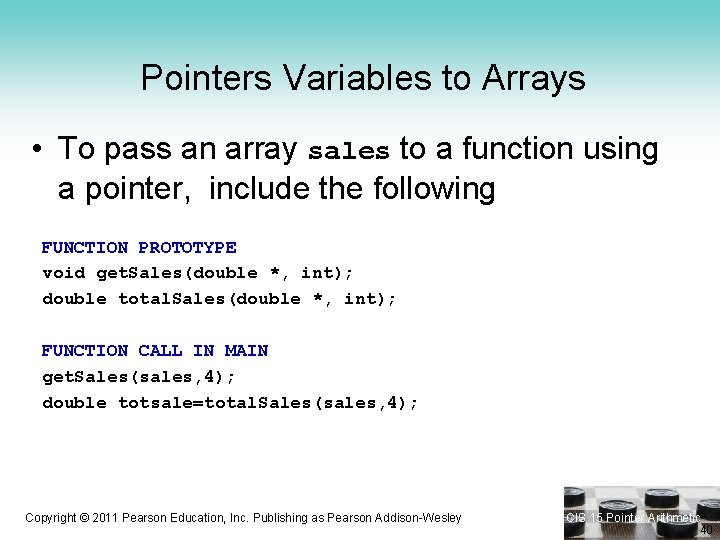
Pointers Variables to Arrays • To pass an array sales to a function using a pointer, include the following FUNCTION PROTOTYPE void get. Sales(double *, int); double total. Sales(double *, int); FUNCTION CALL IN MAIN get. Sales(sales, 4); double totsale=total. Sales(sales, 4); Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 40
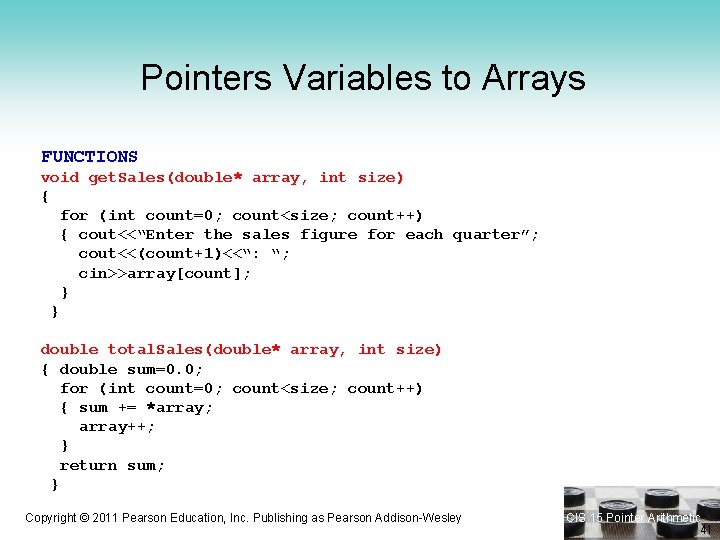
Pointers Variables to Arrays FUNCTIONS void get. Sales(double* array, int size) { for (int count=0; count<size; count++) { cout<<“Enter the sales figure for each quarter”; cout<<(count+1)<<“: “; cin>>array[count]; } } double total. Sales(double* array, int size) { double sum=0. 0; for (int count=0; count<size; count++) { sum += *array; array++; } return sum; } Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 41
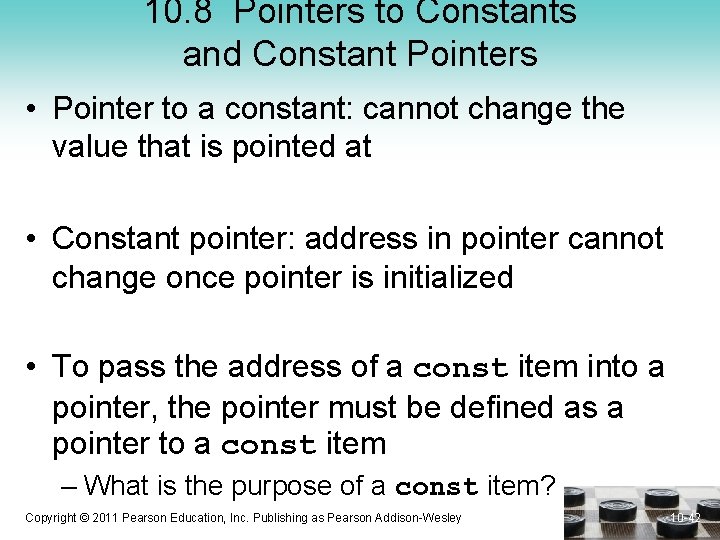
10. 8 Pointers to Constants and Constant Pointers • Pointer to a constant: cannot change the value that is pointed at • Constant pointer: address in pointer cannot change once pointer is initialized • To pass the address of a const item into a pointer, the pointer must be defined as a pointer to a const item – What is the purpose of a const item? Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -42
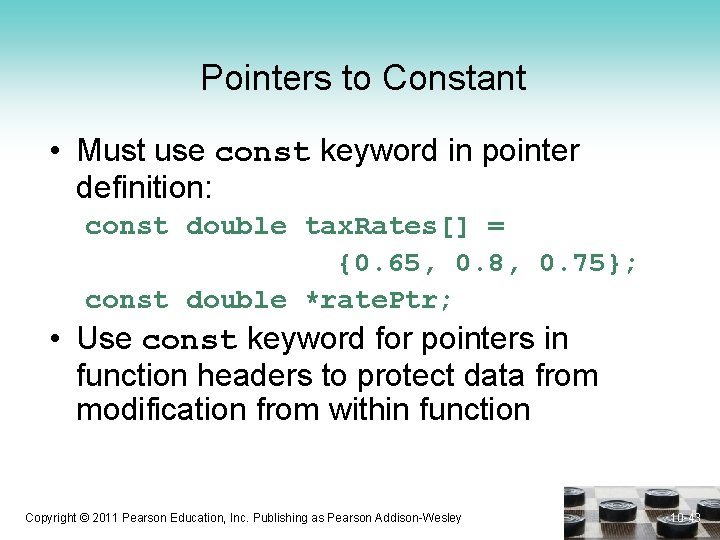
Pointers to Constant • Must use const keyword in pointer definition: const double tax. Rates[] = {0. 65, 0. 8, 0. 75}; const double *rate. Ptr; • Use const keyword for pointers in function headers to protect data from modification from within function Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -43
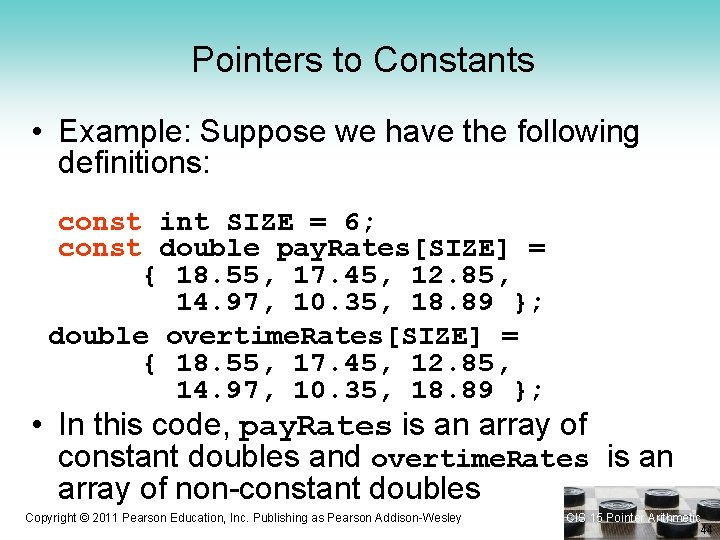
Pointers to Constants • Example: Suppose we have the following definitions: const int SIZE = 6; const double pay. Rates[SIZE] = { 18. 55, 17. 45, 12. 85, 14. 97, 10. 35, 18. 89 }; double overtime. Rates[SIZE] = { 18. 55, 17. 45, 12. 85, 14. 97, 10. 35, 18. 89 }; • In this code, pay. Rates is an array of constant doubles and overtime. Rates is an array of non-constant doubles Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 44
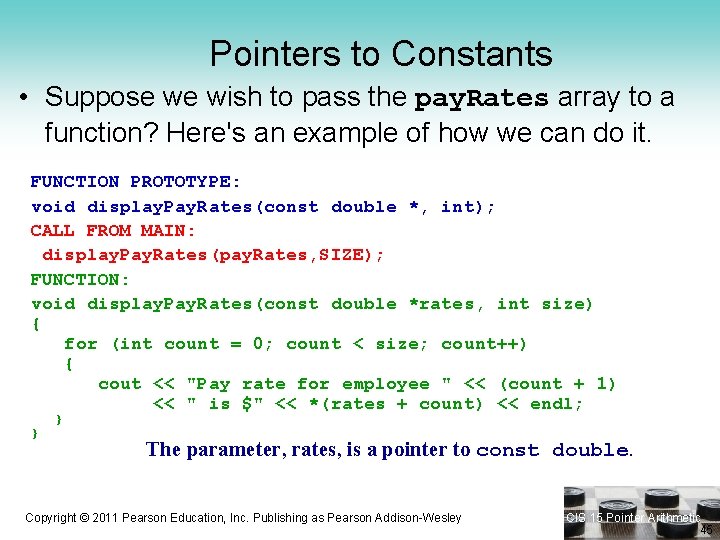
Pointers to Constants • Suppose we wish to pass the pay. Rates array to a function? Here's an example of how we can do it. FUNCTION PROTOTYPE: void display. Pay. Rates(const double *, int); CALL FROM MAIN: display. Pay. Rates(pay. Rates, SIZE); FUNCTION: void display. Pay. Rates(const double *rates, int size) { for (int count = 0; count < size; count++) { cout << "Pay rate for employee " << (count + 1) << " is $" << *(rates + count) << endl; } } The parameter, rates, is a pointer to const double. Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 45
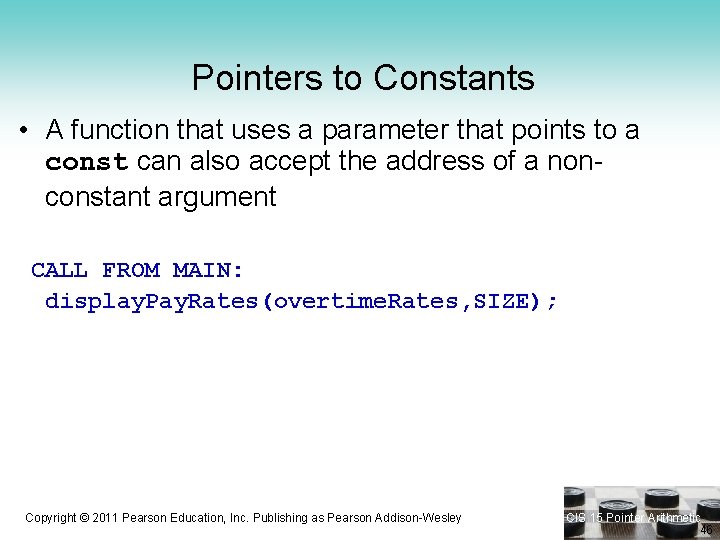
Pointers to Constants • A function that uses a parameter that points to a const can also accept the address of a nonconstant argument CALL FROM MAIN: display. Pay. Rates(overtime. Rates, SIZE); Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 46
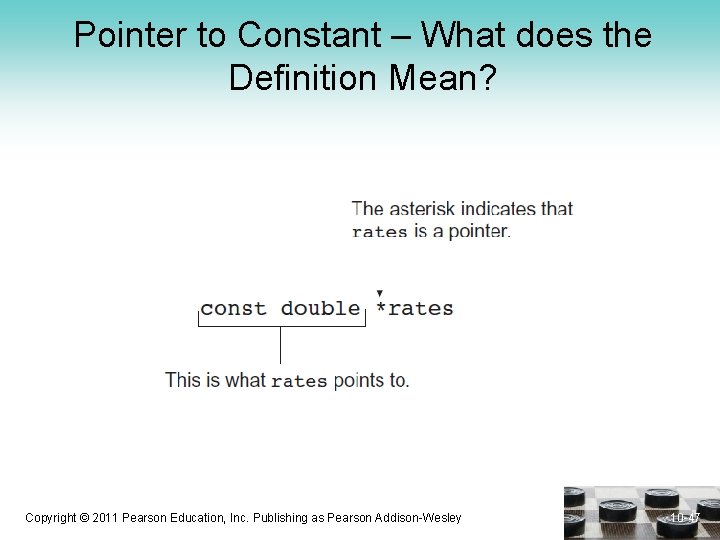
Pointer to Constant – What does the Definition Mean? Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -47
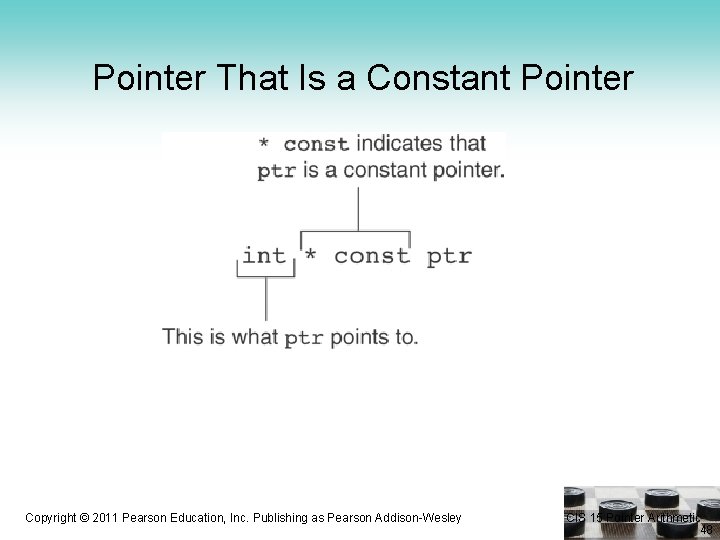
Pointer That Is a Constant Pointer Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 48
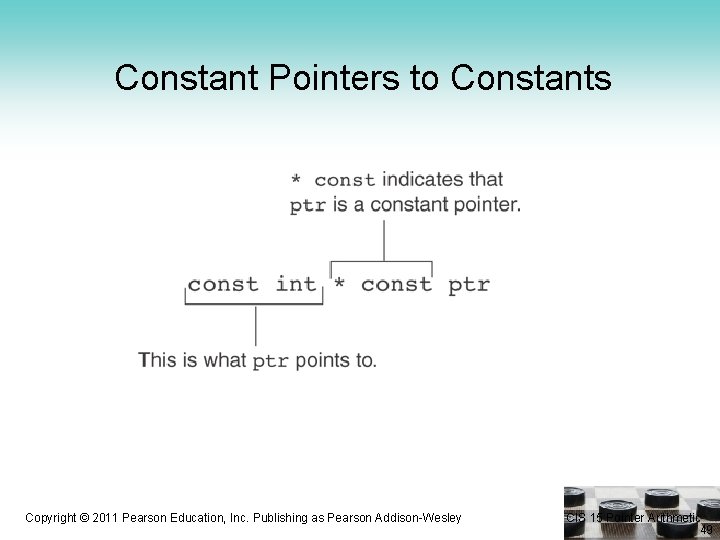
Constant Pointers to Constants Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 49
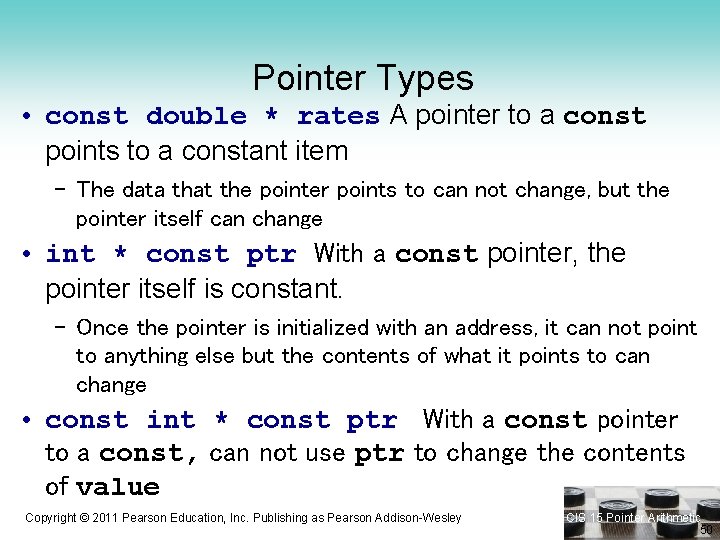
Pointer Types • const double * rates A pointer to a const points to a constant item – The data that the pointer points to can not change, but the pointer itself can change • int * const ptr With a const pointer, the pointer itself is constant. – Once the pointer is initialized with an address, it can not point to anything else but the contents of what it points to can change • const int * const ptr With a const pointer to a const, can not use ptr to change the contents of value Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 50
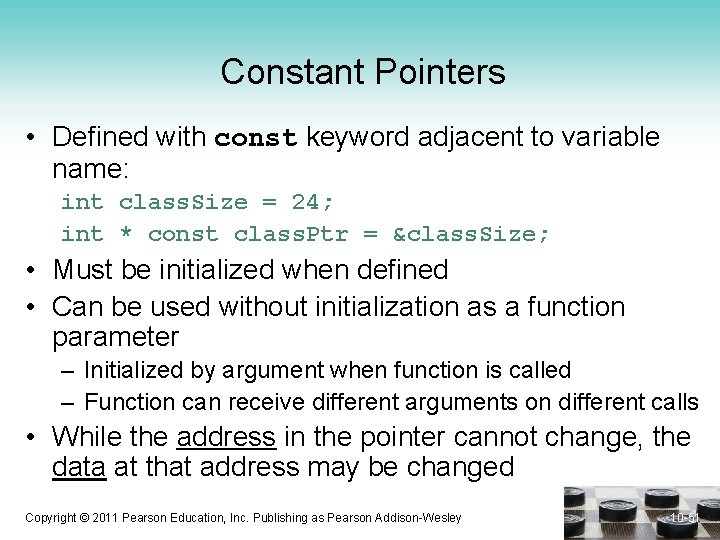
Constant Pointers • Defined with const keyword adjacent to variable name: int class. Size = 24; int * const class. Ptr = &class. Size; • Must be initialized when defined • Can be used without initialization as a function parameter – Initialized by argument when function is called – Function can receive different arguments on different calls • While the address in the pointer cannot change, the data at that address may be changed Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -51
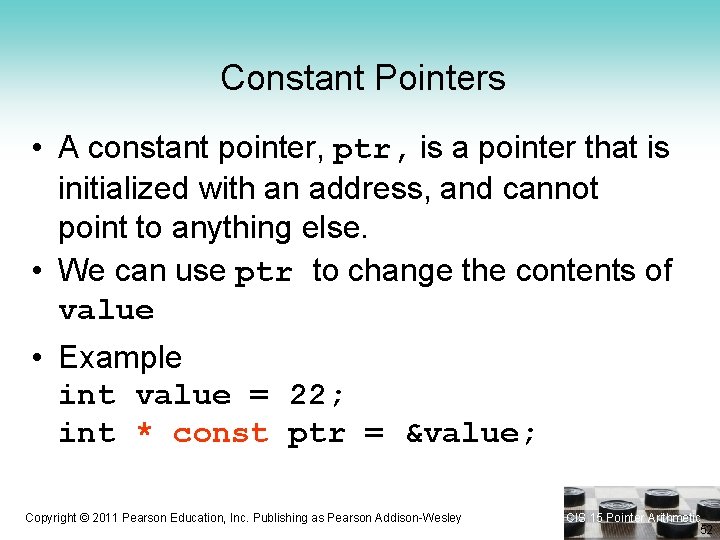
Constant Pointers • A constant pointer, ptr, is a pointer that is initialized with an address, and cannot point to anything else. • We can use ptr to change the contents of value • Example int value = 22; int * const ptr = &value; Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 52
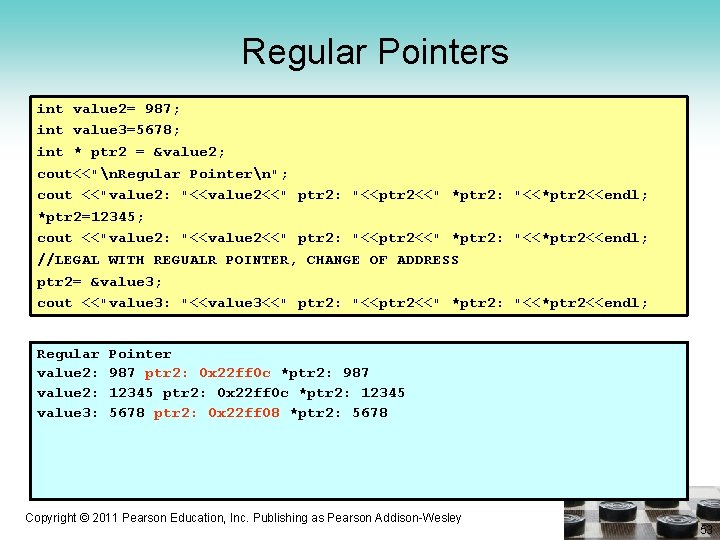
Regular Pointers int value 2= 987; int value 3=5678; int * ptr 2 = &value 2; cout<<"n. Regular Pointern"; cout <<"value 2: "<<value 2<<" ptr 2: "<<ptr 2<<" *ptr 2: "<<*ptr 2<<endl; *ptr 2=12345; cout <<"value 2: "<<value 2<<" ptr 2: "<<ptr 2<<" *ptr 2: "<<*ptr 2<<endl; //LEGAL WITH REGUALR POINTER, CHANGE OF ADDRESS ptr 2= &value 3; cout <<"value 3: "<<value 3<<" ptr 2: "<<ptr 2<<" *ptr 2: "<<*ptr 2<<endl; Regular value 2: value 3: Pointer 987 ptr 2: 0 x 22 ff 0 c *ptr 2: 987 12345 ptr 2: 0 x 22 ff 0 c *ptr 2: 12345 5678 ptr 2: 0 x 22 ff 08 *ptr 2: 5678 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 53
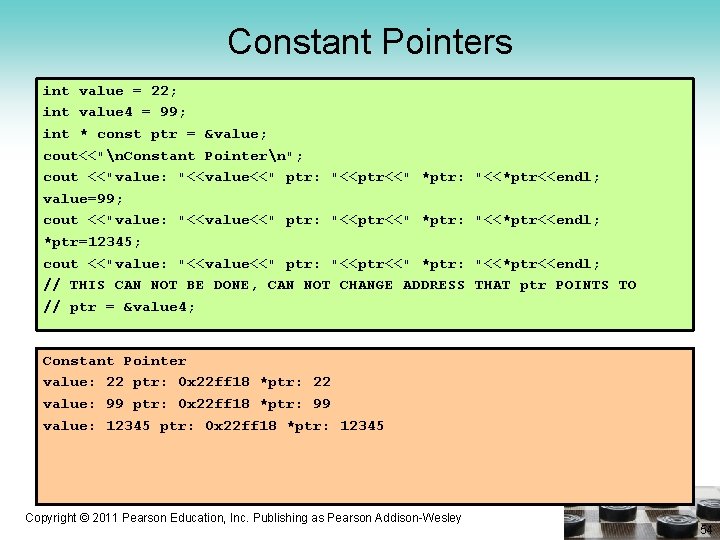
Constant Pointers int value = 22; int value 4 = 99; int * const ptr = &value; cout<<"n. Constant Pointern"; cout <<"value: "<<value<<" ptr: "<<ptr<<" *ptr: value=99; cout <<"value: "<<value<<" ptr: "<<ptr<<" *ptr: *ptr=12345; cout <<"value: "<<value<<" ptr: "<<ptr<<" *ptr: // THIS CAN NOT BE DONE, CAN NOT CHANGE ADDRESS // ptr = &value 4; "<<*ptr<<endl; THAT ptr POINTS TO Constant Pointer value: 22 ptr: 0 x 22 ff 18 *ptr: 22 value: 99 ptr: 0 x 22 ff 18 *ptr: 99 value: 12345 ptr: 0 x 22 ff 18 *ptr: 12345 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 54
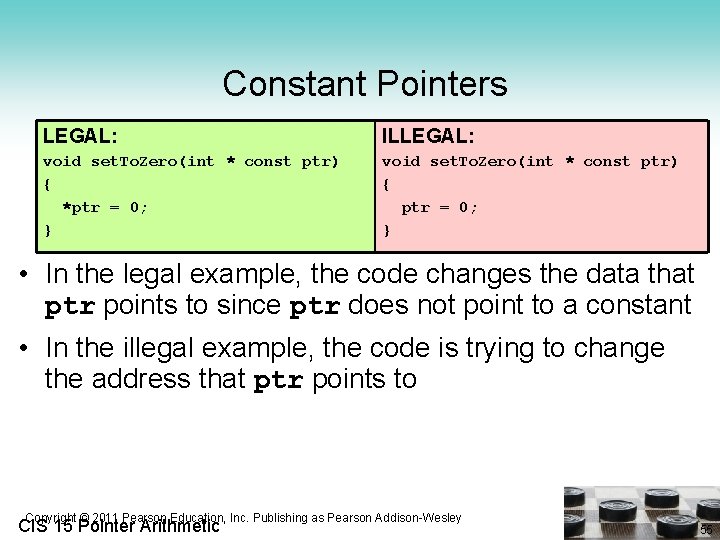
Constant Pointers LEGAL: ILLEGAL: void set. To. Zero(int * const ptr) { *ptr = 0; } void set. To. Zero(int * const ptr) { ptr = 0; } • In the legal example, the code changes the data that ptr points to since ptr does not point to a constant • In the illegal example, the code is trying to change the address that ptr points to Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 55
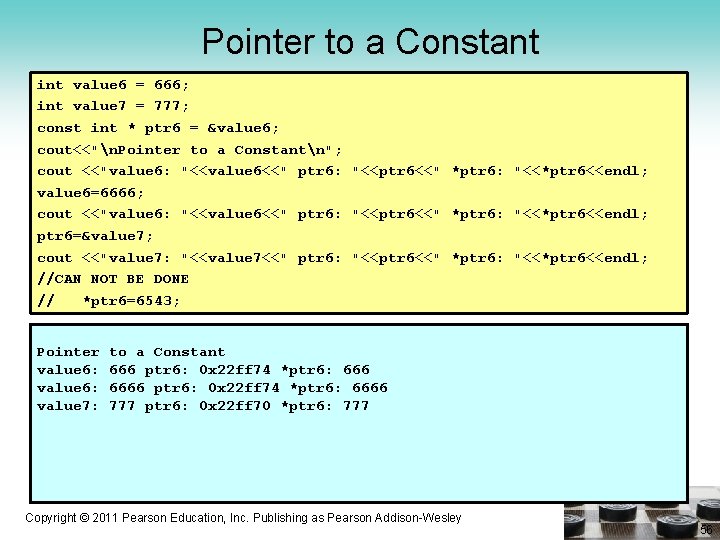
Pointer to a Constant int value 6 = 666; int value 7 = 777; const int * ptr 6 = &value 6; cout<<"n. Pointer to a Constantn"; cout <<"value 6: "<<value 6<<" ptr 6: "<<ptr 6<<" *ptr 6: "<<*ptr 6<<endl; value 6=6666; cout <<"value 6: "<<value 6<<" ptr 6: "<<ptr 6<<" *ptr 6: "<<*ptr 6<<endl; ptr 6=&value 7; cout <<"value 7: "<<value 7<<" ptr 6: "<<ptr 6<<" *ptr 6: "<<*ptr 6<<endl; //CAN NOT BE DONE // *ptr 6=6543; Pointer value 6: value 7: to a Constant 666 ptr 6: 0 x 22 ff 74 *ptr 6: 6666 777 ptr 6: 0 x 22 ff 70 *ptr 6: 777 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 56
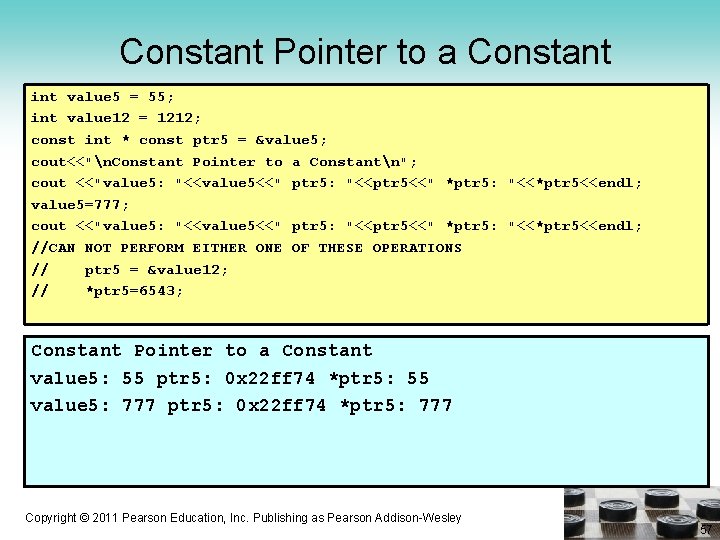
Constant Pointer to a Constant int value 5 = 55; int value 12 = 1212; const int * const ptr 5 = &value 5; cout<<"n. Constant Pointer to a Constantn"; cout <<"value 5: "<<value 5<<" ptr 5: "<<ptr 5<<" *ptr 5: "<<*ptr 5<<endl; value 5=777; cout <<"value 5: "<<value 5<<" ptr 5: "<<ptr 5<<" *ptr 5: "<<*ptr 5<<endl; //CAN NOT PERFORM EITHER ONE OF THESE OPERATIONS // ptr 5 = &value 12; // *ptr 5=6543; Constant Pointer to a Constant value 5: 55 ptr 5: 0 x 22 ff 74 *ptr 5: 55 value 5: 777 ptr 5: 0 x 22 ff 74 *ptr 5: 777 Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 57
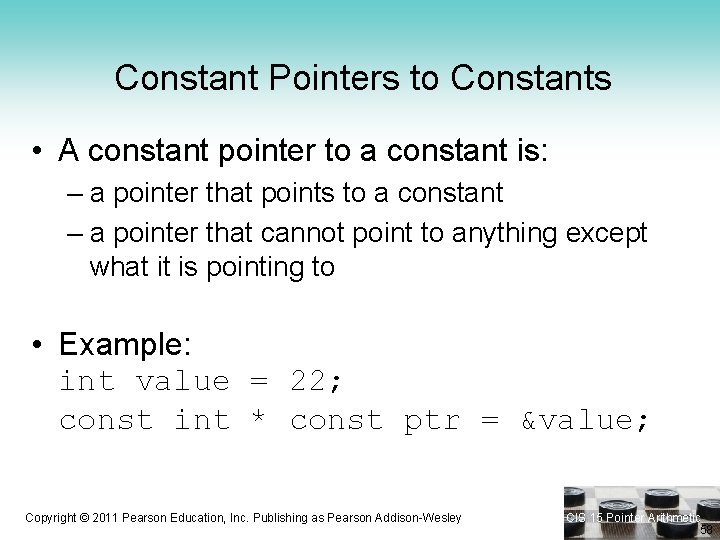
Constant Pointers to Constants • A constant pointer to a constant is: – a pointer that points to a constant – a pointer that cannot point to anything except what it is pointing to • Example: int value = 22; const int * const ptr = &value; Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 58
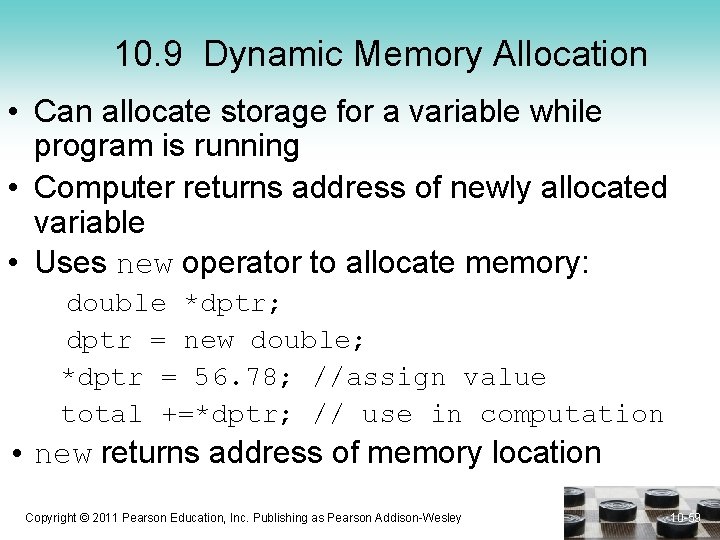
10. 9 Dynamic Memory Allocation • Can allocate storage for a variable while program is running • Computer returns address of newly allocated variable • Uses new operator to allocate memory: double *dptr; dptr = new double; *dptr = 56. 78; //assign value total +=*dptr; // use in computation • new returns address of memory location Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -59
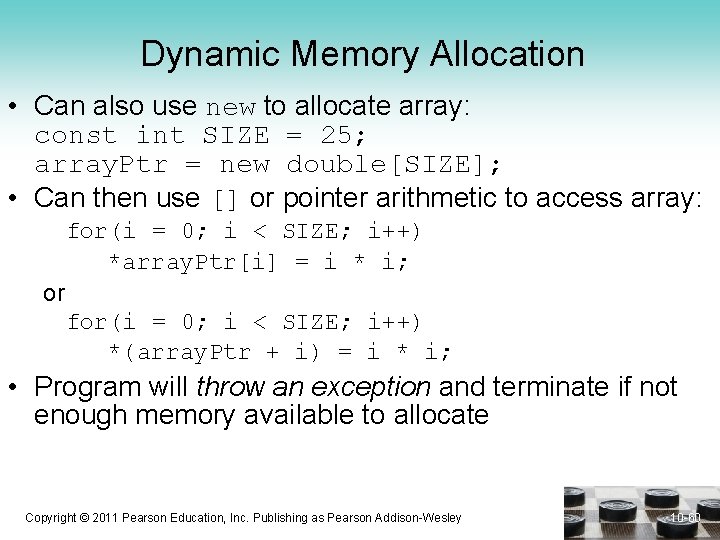
Dynamic Memory Allocation • Can also use new to allocate array: const int SIZE = 25; array. Ptr = new double[SIZE]; • Can then use [] or pointer arithmetic to access array: for(i = 0; i < SIZE; i++) *array. Ptr[i] = i * i; or for(i = 0; i < SIZE; i++) *(array. Ptr + i) = i * i; • Program will throw an exception and terminate if not enough memory available to allocate Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -60
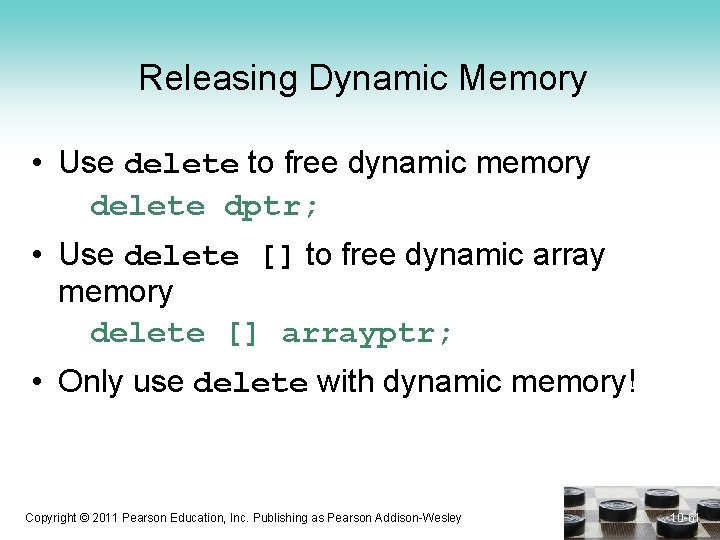
Releasing Dynamic Memory • Use delete to free dynamic memory delete dptr; • Use delete [] to free dynamic array memory delete [] arrayptr; • Only use delete with dynamic memory! Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -61
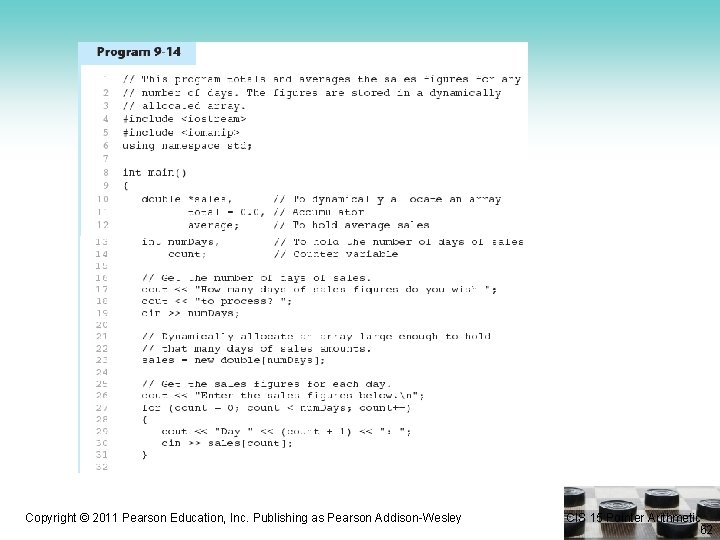
Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 62
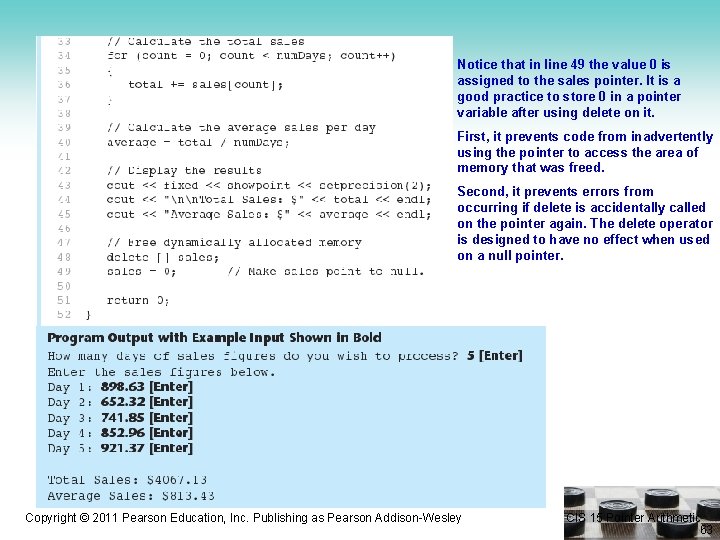
Notice that in line 49 the value 0 is assigned to the sales pointer. It is a good practice to store 0 in a pointer variable after using delete on it. First, it prevents code from inadvertently using the pointer to access the area of memory that was freed. Second, it prevents errors from occurring if delete is accidentally called on the pointer again. The delete operator is designed to have no effect when used on a null pointer. Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley CIS 15 Pointer Arithmetic 63
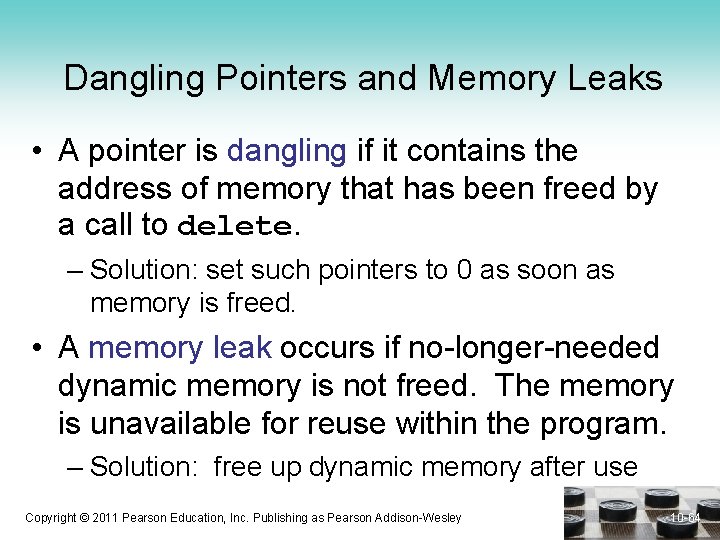
Dangling Pointers and Memory Leaks • A pointer is dangling if it contains the address of memory that has been freed by a call to delete. – Solution: set such pointers to 0 as soon as memory is freed. • A memory leak occurs if no-longer-needed dynamic memory is not freed. The memory is unavailable for reuse within the program. – Solution: free up dynamic memory after use Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -64
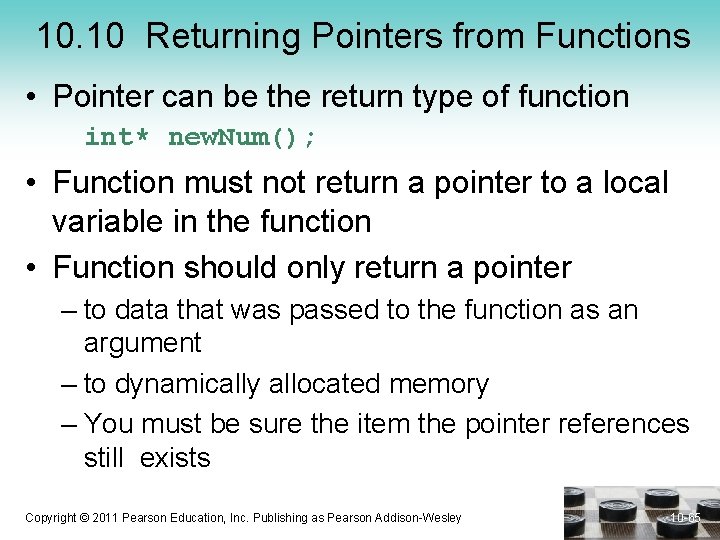
10. 10 Returning Pointers from Functions • Pointer can be the return type of function int* new. Num(); • Function must not return a pointer to a local variable in the function • Function should only return a pointer – to data that was passed to the function as an argument – to dynamically allocated memory – You must be sure the item the pointer references still exists Copyright © 2011 Pearson Education, Inc. Publishing as Pearson Addison-Wesley 10 -65
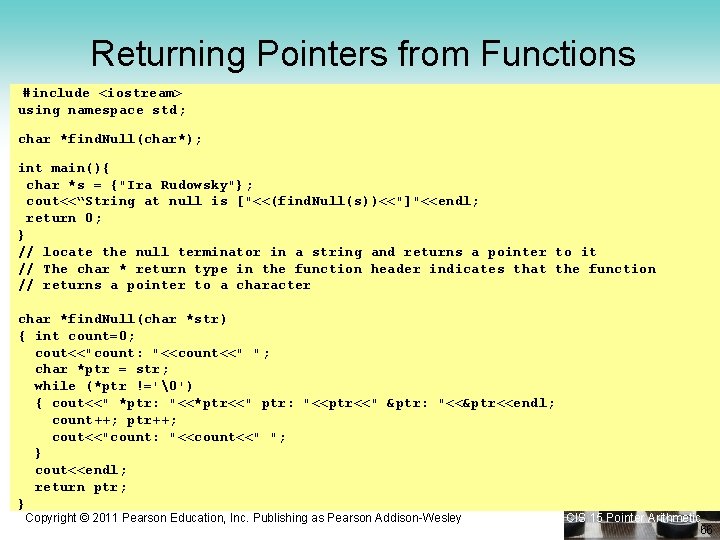
Returning Pointers from Functions #include <iostream> using namespace std; char *find. Null(char*); int main(){ char *s = {"Ira Rudowsky"}; cout<<“String at null is ["<<(find. Null(s))<<"]"<<endl; return 0; } // locate the null terminator in a string and returns a pointer to it // The char * return type in the function header indicates that the function // returns a pointer to a character char *find. Null(char *str) { int count=0; cout<<"count: "<<count<<" "; char *ptr = str; while (*ptr !='