Servlet Session Tracking Persistent information n A server
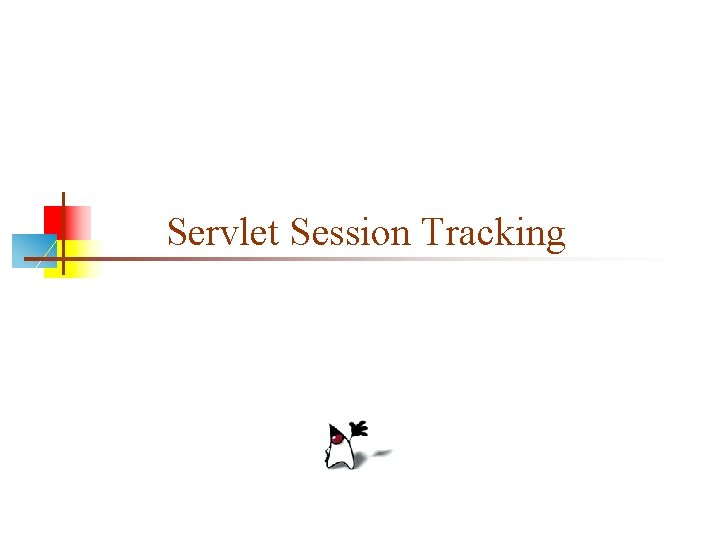
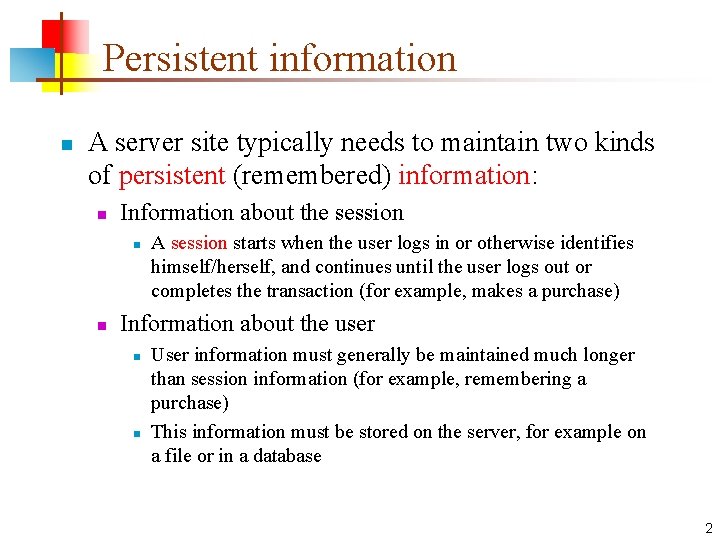
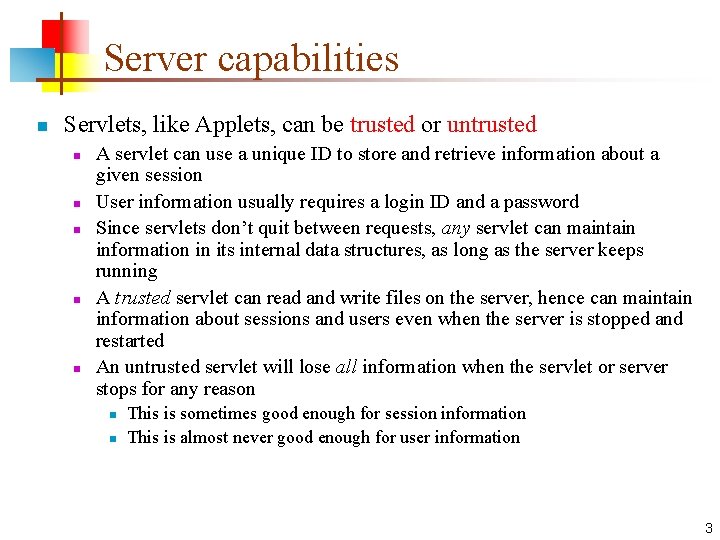
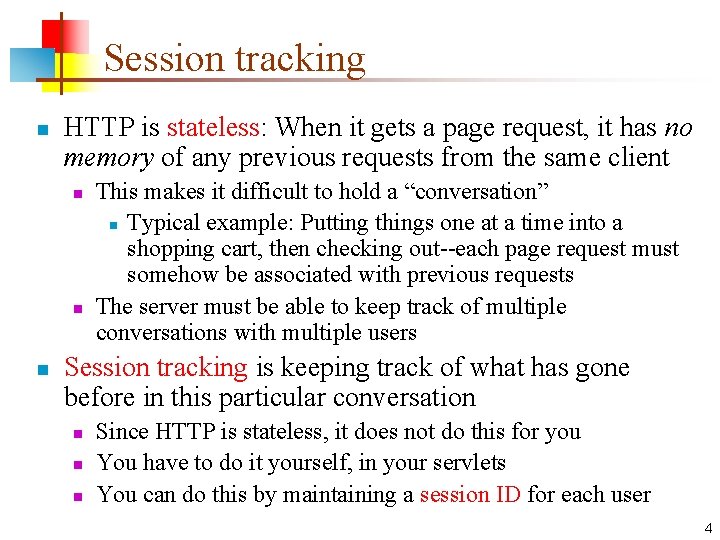
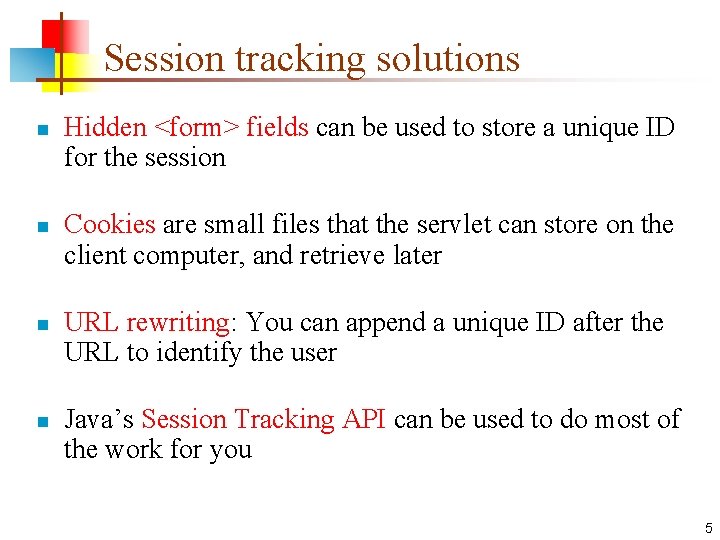
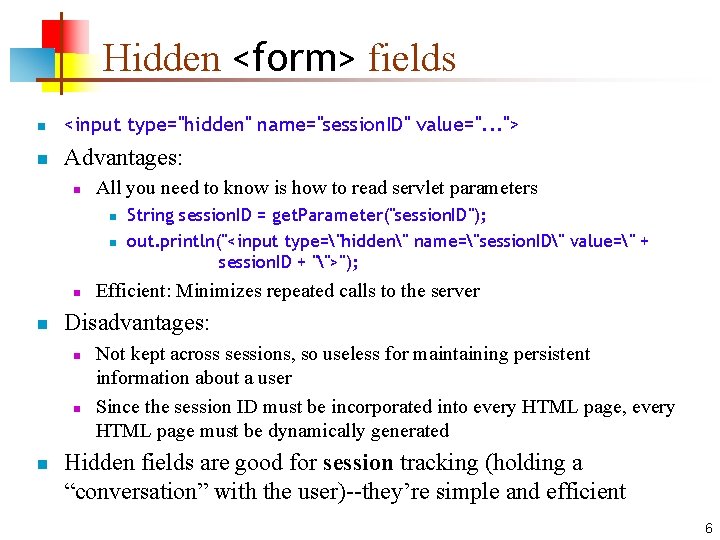
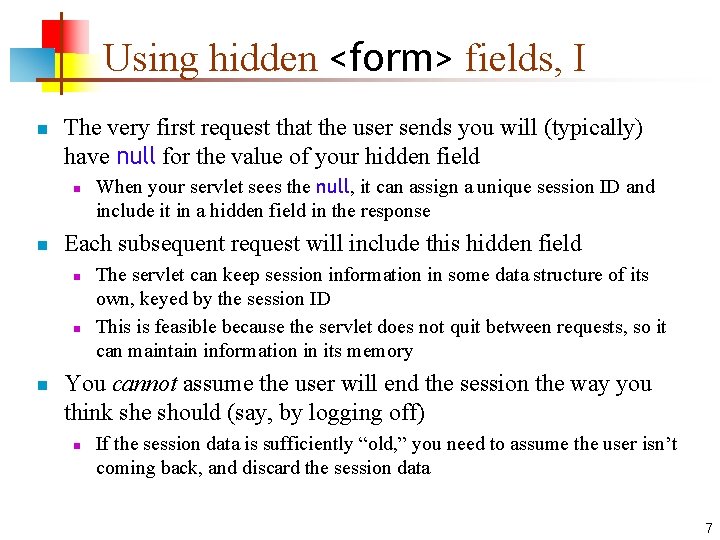
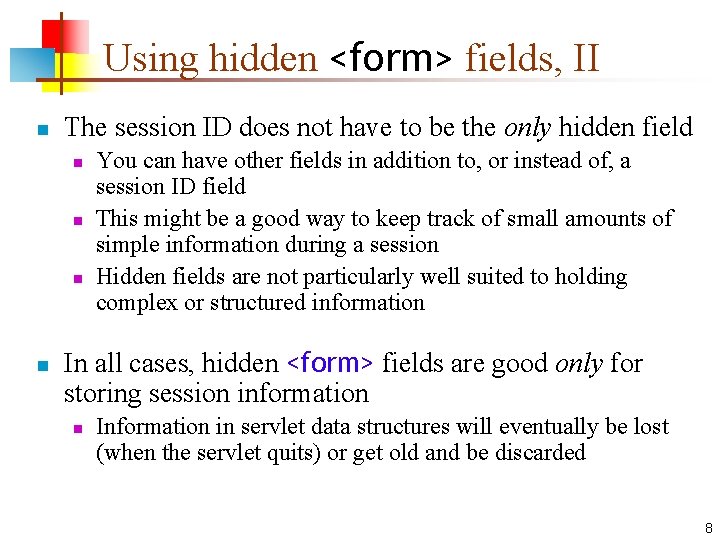
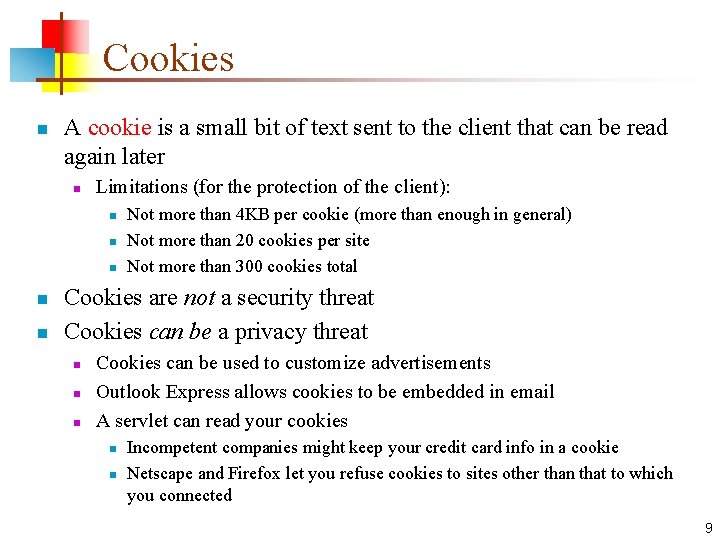
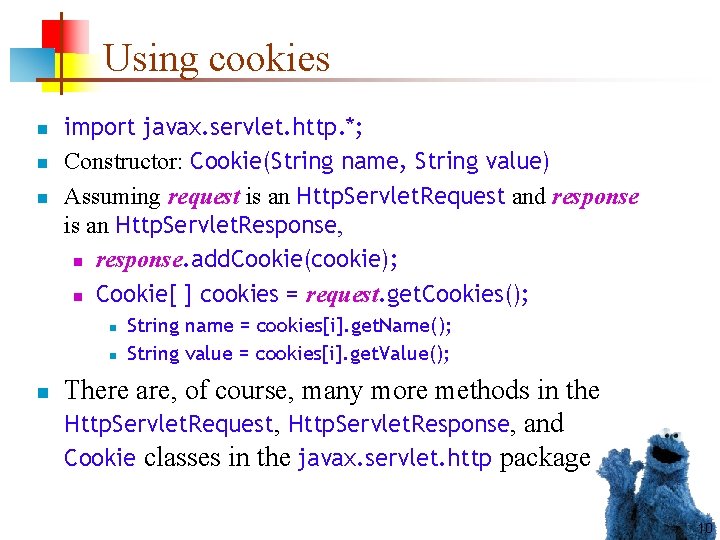
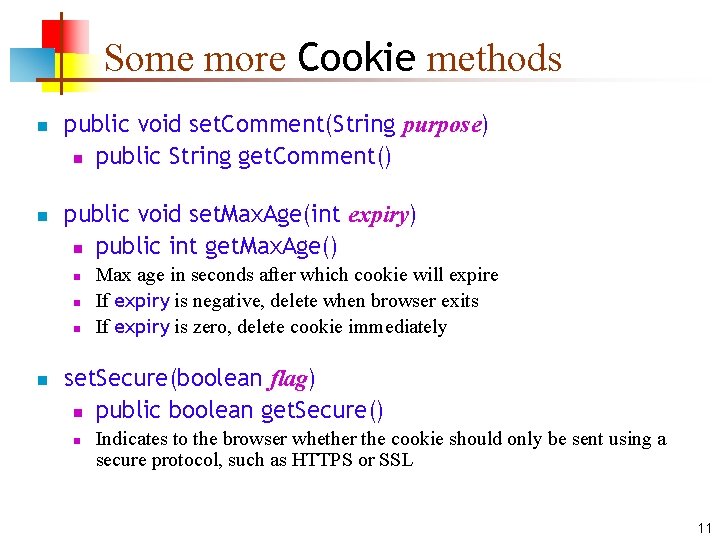
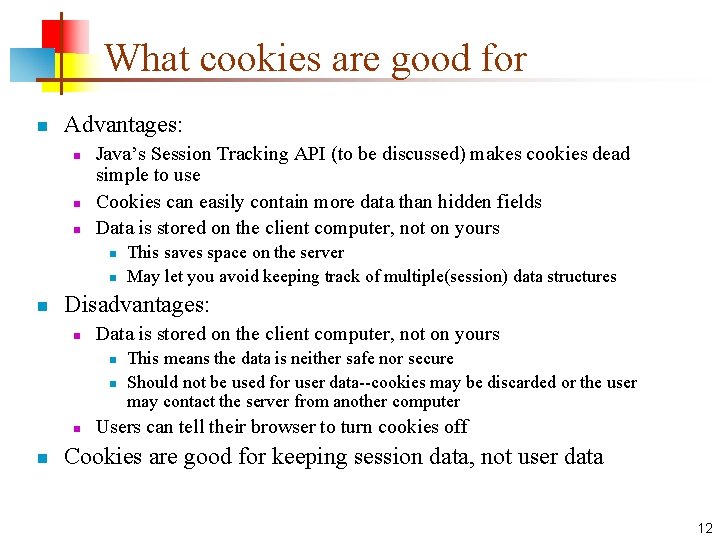
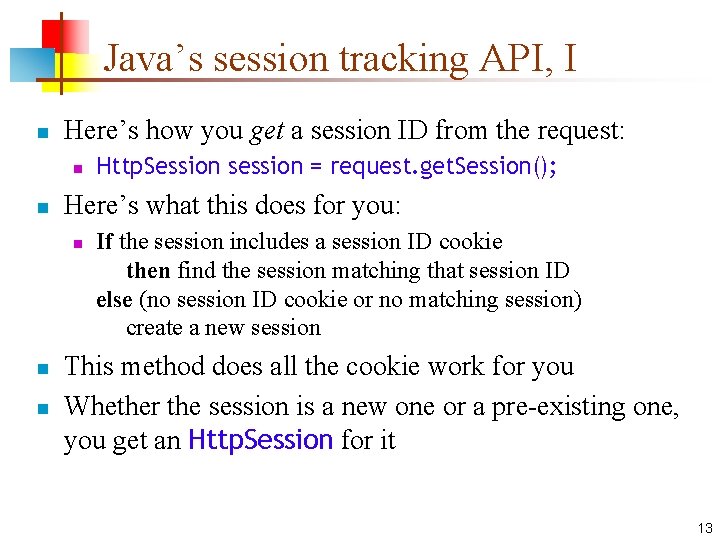
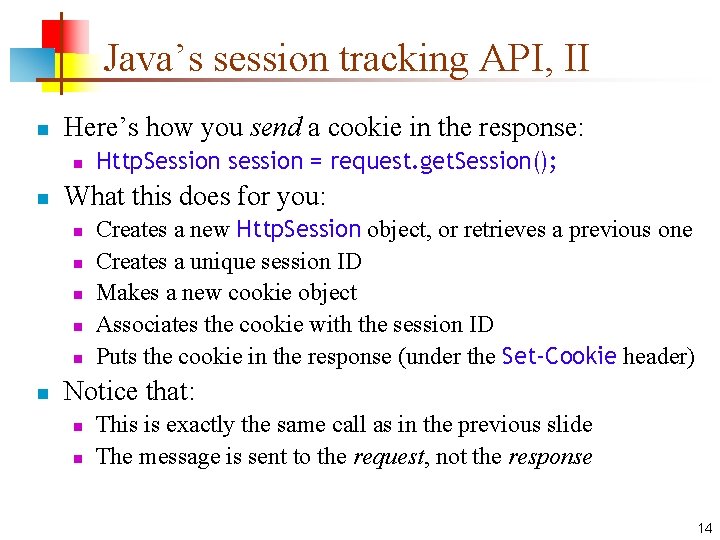
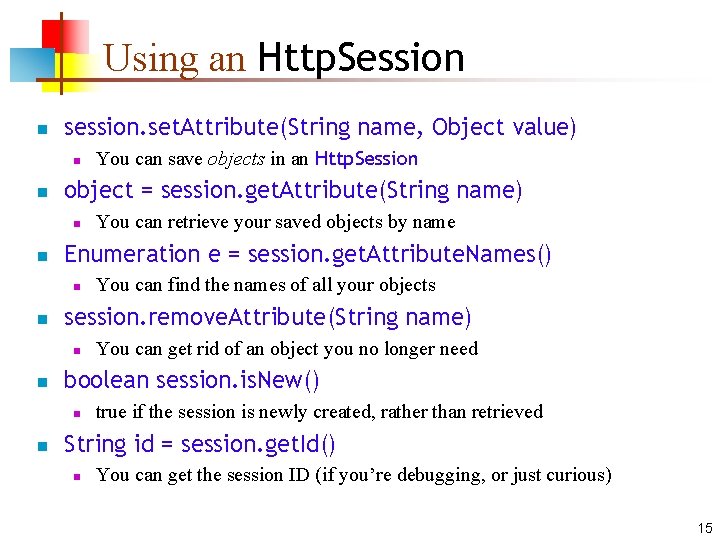
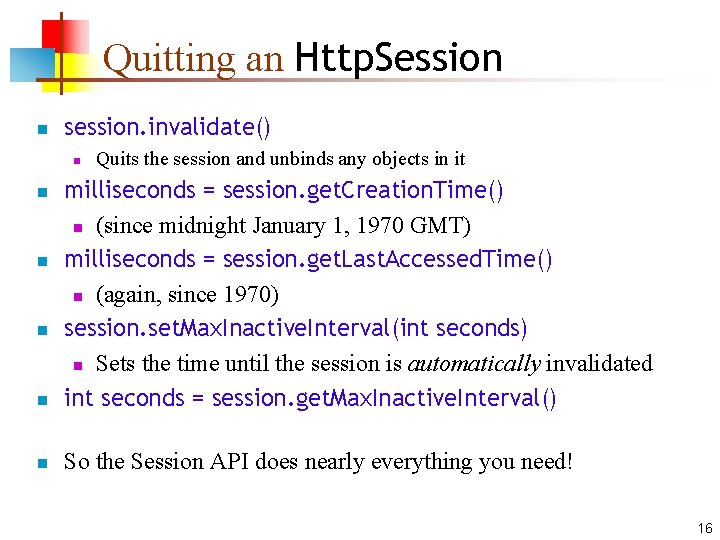
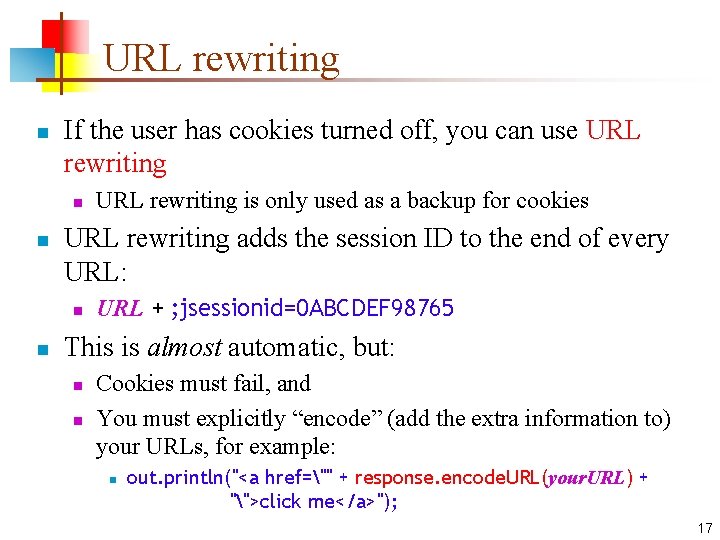
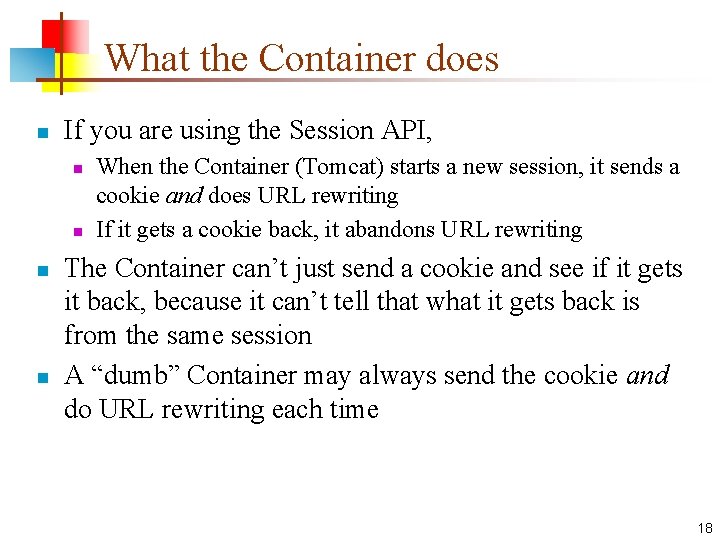
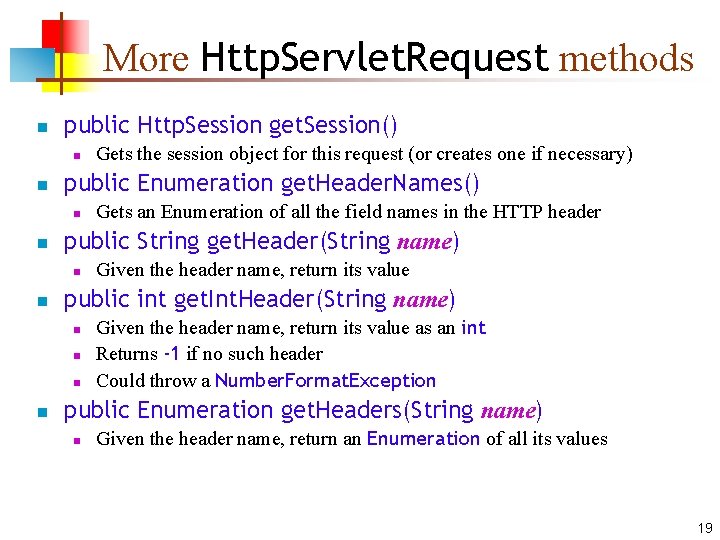
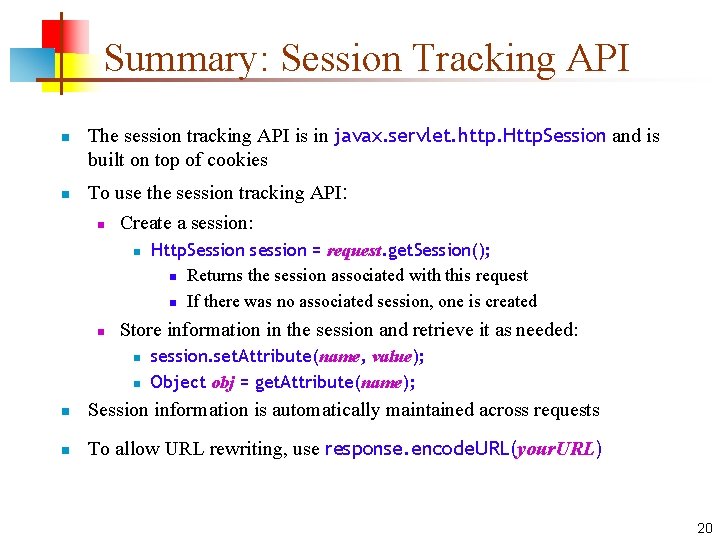
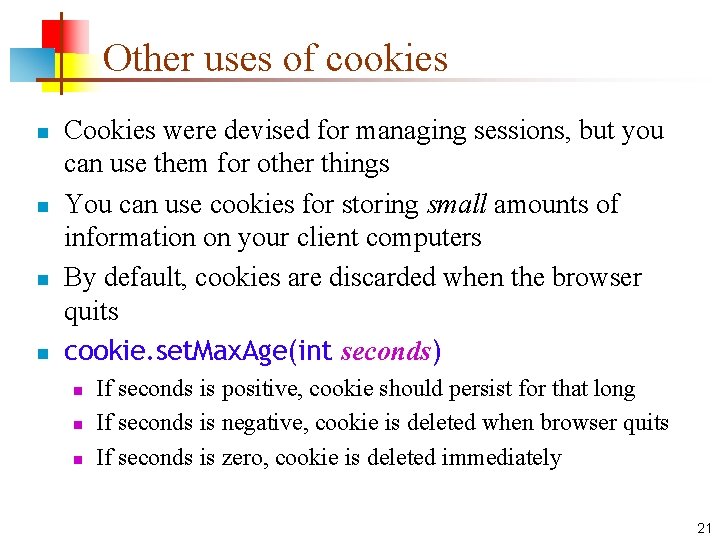
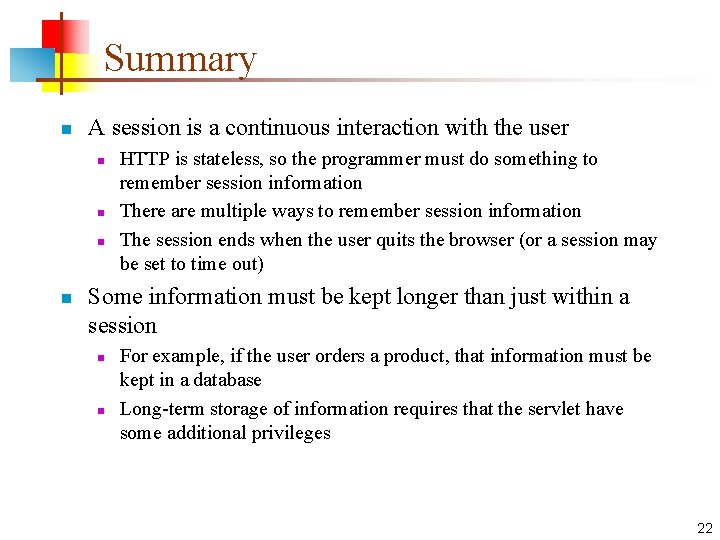
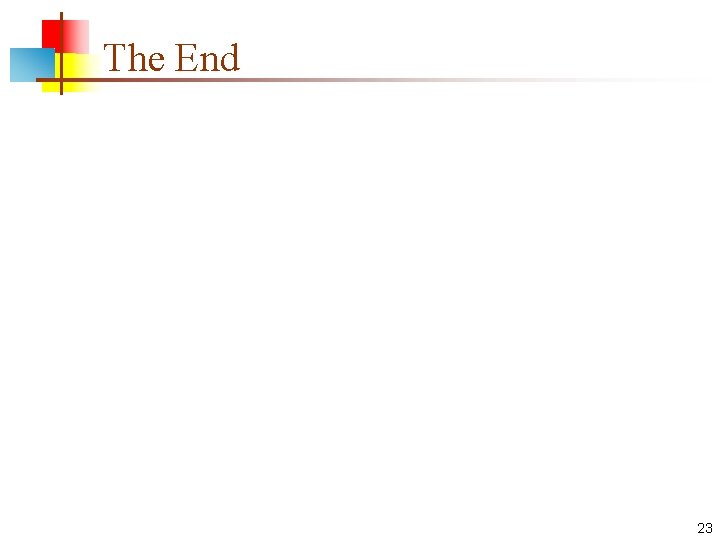
- Slides: 23
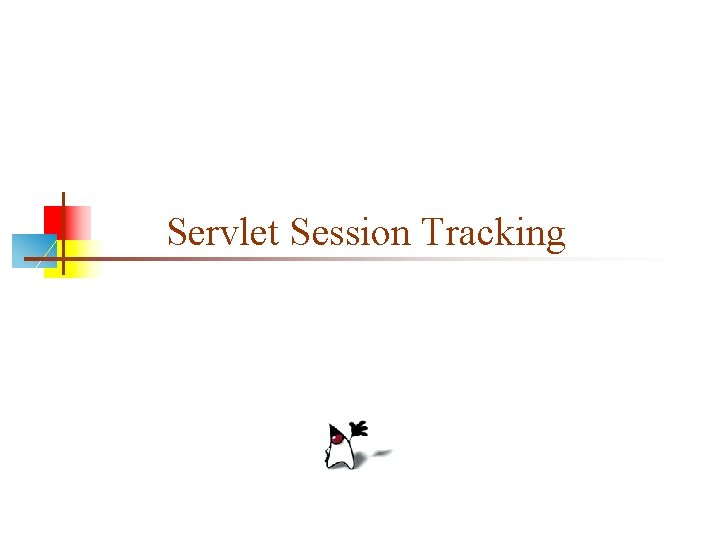
Servlet Session Tracking
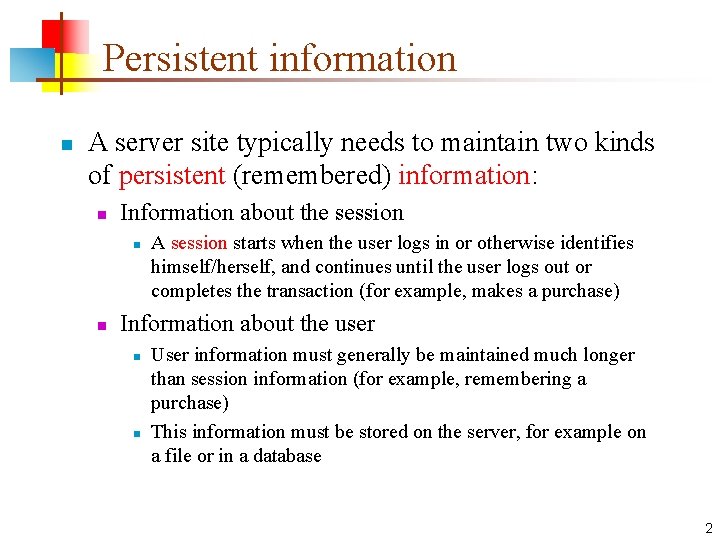
Persistent information n A server site typically needs to maintain two kinds of persistent (remembered) information: n Information about the session n n A session starts when the user logs in or otherwise identifies himself/herself, and continues until the user logs out or completes the transaction (for example, makes a purchase) Information about the user n n User information must generally be maintained much longer than session information (for example, remembering a purchase) This information must be stored on the server, for example on a file or in a database 2
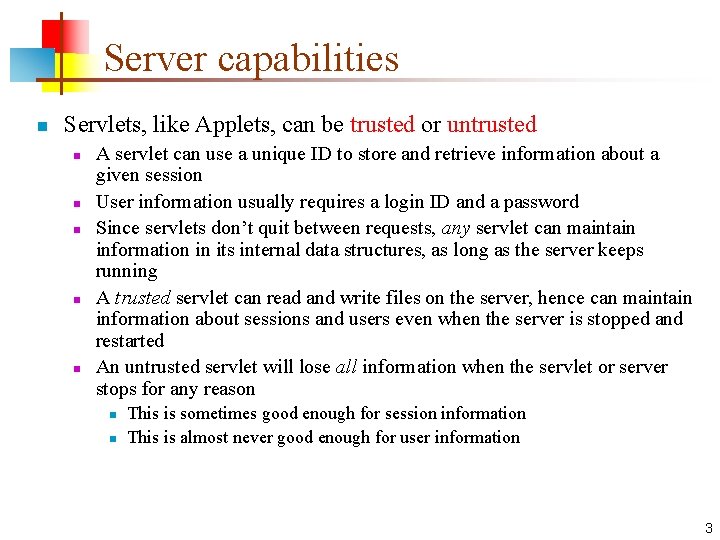
Server capabilities n Servlets, like Applets, can be trusted or untrusted n n n A servlet can use a unique ID to store and retrieve information about a given session User information usually requires a login ID and a password Since servlets don’t quit between requests, any servlet can maintain information in its internal data structures, as long as the server keeps running A trusted servlet can read and write files on the server, hence can maintain information about sessions and users even when the server is stopped and restarted An untrusted servlet will lose all information when the servlet or server stops for any reason n n This is sometimes good enough for session information This is almost never good enough for user information 3
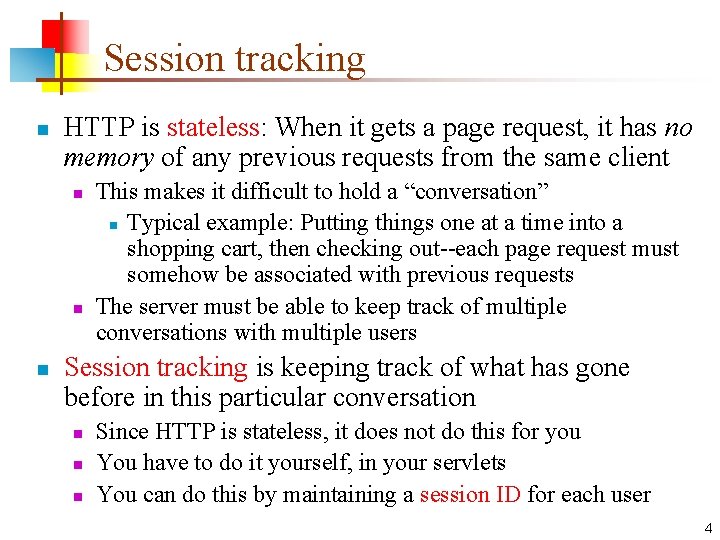
Session tracking n HTTP is stateless: When it gets a page request, it has no memory of any previous requests from the same client n n n This makes it difficult to hold a “conversation” n Typical example: Putting things one at a time into a shopping cart, then checking out--each page request must somehow be associated with previous requests The server must be able to keep track of multiple conversations with multiple users Session tracking is keeping track of what has gone before in this particular conversation n Since HTTP is stateless, it does not do this for you You have to do it yourself, in your servlets You can do this by maintaining a session ID for each user 4
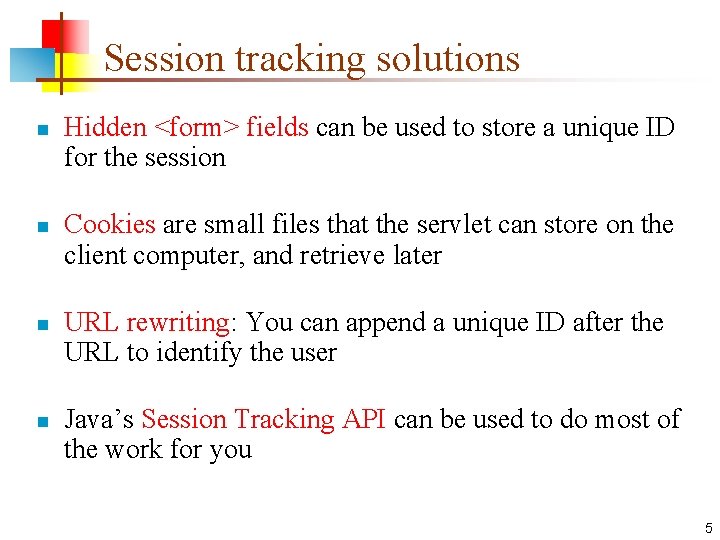
Session tracking solutions n n Hidden <form> fields can be used to store a unique ID for the session Cookies are small files that the servlet can store on the client computer, and retrieve later URL rewriting: You can append a unique ID after the URL to identify the user Java’s Session Tracking API can be used to do most of the work for you 5
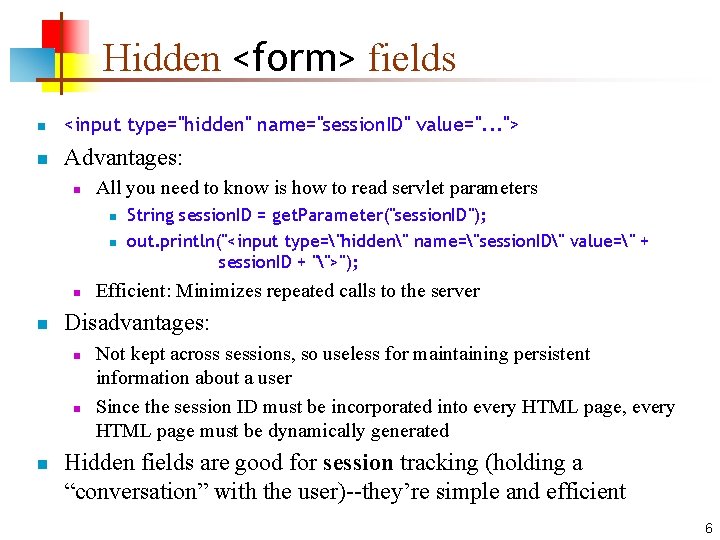
Hidden <form> fields n <input type="hidden" name="session. ID" value=". . . "> n Advantages: n All you need to know is how to read servlet parameters n n Efficient: Minimizes repeated calls to the server Disadvantages: n n n String session. ID = get. Parameter("session. ID"); out. println("<input type="hidden" name="session. ID" value=" + session. ID + "">"); Not kept across sessions, so useless for maintaining persistent information about a user Since the session ID must be incorporated into every HTML page, every HTML page must be dynamically generated Hidden fields are good for session tracking (holding a “conversation” with the user)--they’re simple and efficient 6
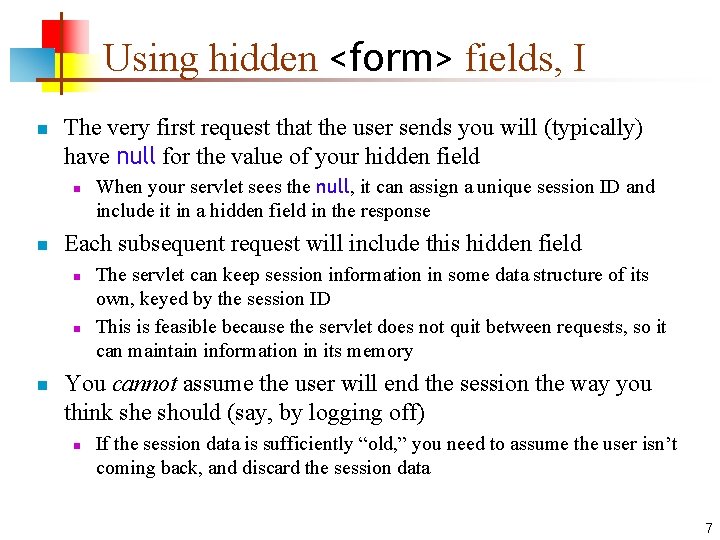
Using hidden <form> fields, I n The very first request that the user sends you will (typically) have null for the value of your hidden field n n Each subsequent request will include this hidden field n n n When your servlet sees the null, it can assign a unique session ID and include it in a hidden field in the response The servlet can keep session information in some data structure of its own, keyed by the session ID This is feasible because the servlet does not quit between requests, so it can maintain information in its memory You cannot assume the user will end the session the way you think she should (say, by logging off) n If the session data is sufficiently “old, ” you need to assume the user isn’t coming back, and discard the session data 7
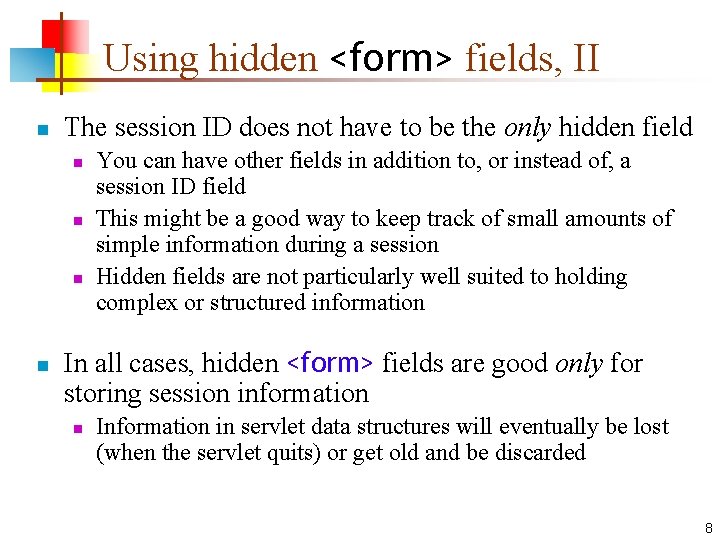
Using hidden <form> fields, II n The session ID does not have to be the only hidden field n n You can have other fields in addition to, or instead of, a session ID field This might be a good way to keep track of small amounts of simple information during a session Hidden fields are not particularly well suited to holding complex or structured information In all cases, hidden <form> fields are good only for storing session information n Information in servlet data structures will eventually be lost (when the servlet quits) or get old and be discarded 8
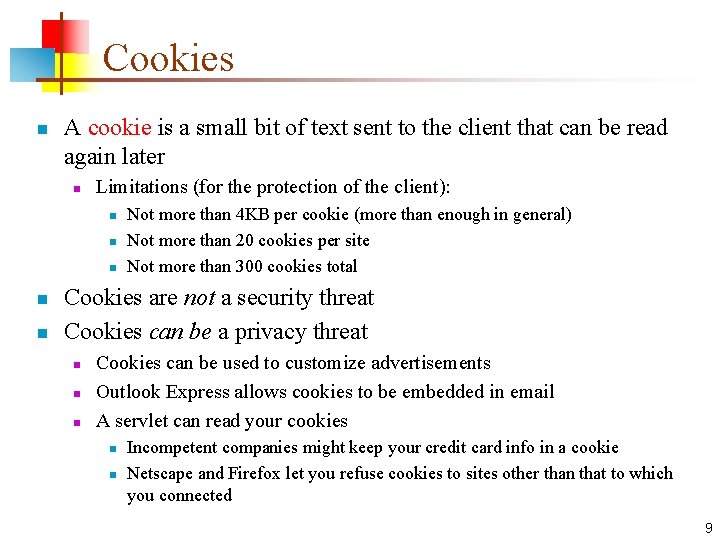
Cookies n A cookie is a small bit of text sent to the client that can be read again later n Limitations (for the protection of the client): n n n Not more than 4 KB per cookie (more than enough in general) Not more than 20 cookies per site Not more than 300 cookies total Cookies are not a security threat Cookies can be a privacy threat n n n Cookies can be used to customize advertisements Outlook Express allows cookies to be embedded in email A servlet can read your cookies n n Incompetent companies might keep your credit card info in a cookie Netscape and Firefox let you refuse cookies to sites other than that to which you connected 9
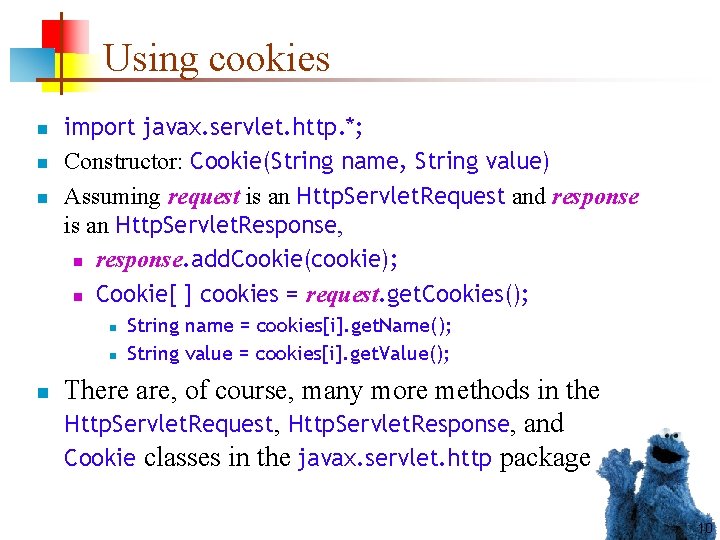
Using cookies n n n import javax. servlet. http. *; Constructor: Cookie(String name, String value) Assuming request is an Http. Servlet. Request and response is an Http. Servlet. Response, n response. add. Cookie(cookie); n Cookie[ ] cookies = request. get. Cookies(); n n n String name = cookies[i]. get. Name(); String value = cookies[i]. get. Value(); There are, of course, many more methods in the Http. Servlet. Request, Http. Servlet. Response, and Cookie classes in the javax. servlet. http package 10
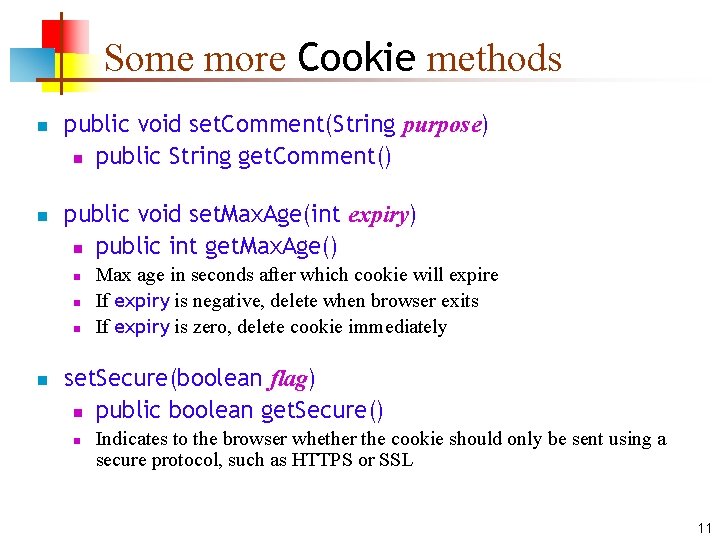
Some more Cookie methods n n public void set. Comment(String purpose) n public String get. Comment() public void set. Max. Age(int expiry) n public int get. Max. Age() n n Max age in seconds after which cookie will expire If expiry is negative, delete when browser exits If expiry is zero, delete cookie immediately set. Secure(boolean flag) n public boolean get. Secure() n Indicates to the browser whether the cookie should only be sent using a secure protocol, such as HTTPS or SSL 11
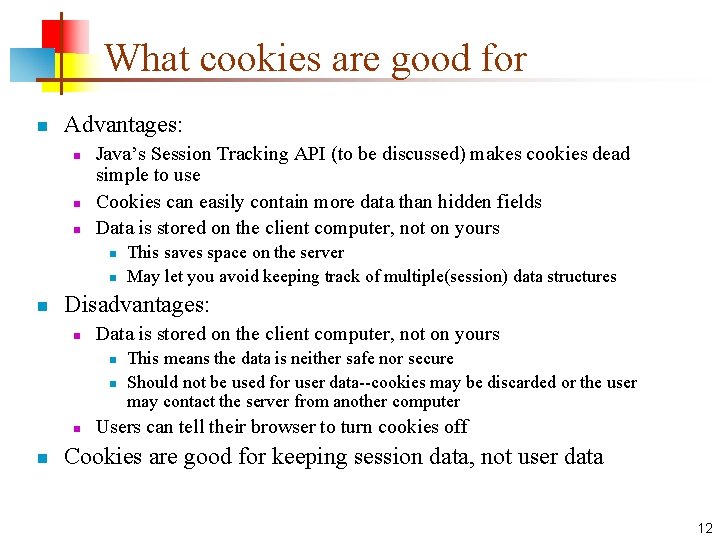
What cookies are good for n Advantages: n n n Java’s Session Tracking API (to be discussed) makes cookies dead simple to use Cookies can easily contain more data than hidden fields Data is stored on the client computer, not on yours n n n Disadvantages: n Data is stored on the client computer, not on yours n n This saves space on the server May let you avoid keeping track of multiple(session) data structures This means the data is neither safe nor secure Should not be used for user data--cookies may be discarded or the user may contact the server from another computer Users can tell their browser to turn cookies off Cookies are good for keeping session data, not user data 12
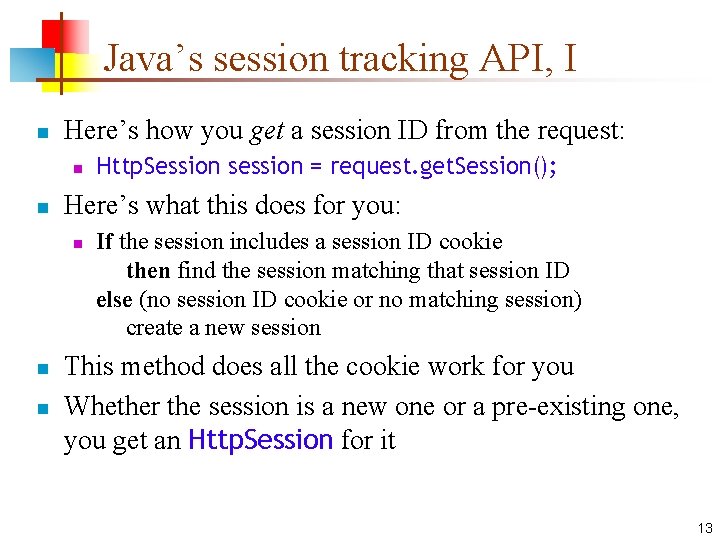
Java’s session tracking API, I n Here’s how you get a session ID from the request: n n Here’s what this does for you: n n n Http. Session session = request. get. Session(); If the session includes a session ID cookie then find the session matching that session ID else (no session ID cookie or no matching session) create a new session This method does all the cookie work for you Whether the session is a new one or a pre-existing one, you get an Http. Session for it 13
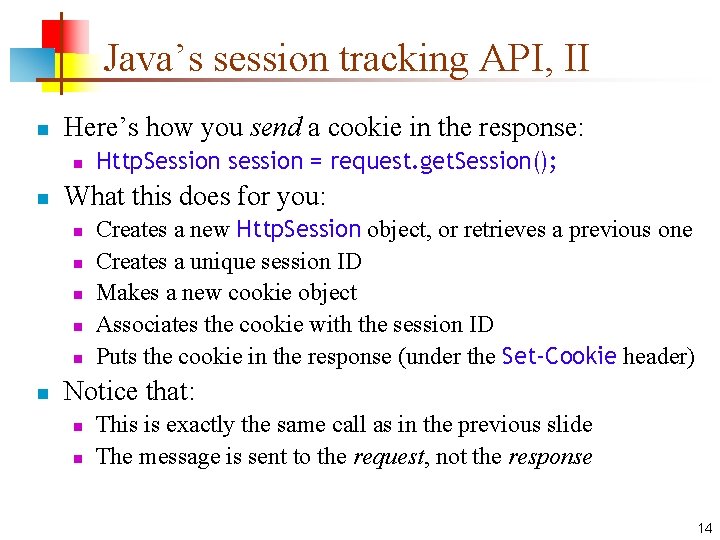
Java’s session tracking API, II n Here’s how you send a cookie in the response: n n What this does for you: n n n Http. Session session = request. get. Session(); Creates a new Http. Session object, or retrieves a previous one Creates a unique session ID Makes a new cookie object Associates the cookie with the session ID Puts the cookie in the response (under the Set-Cookie header) Notice that: n n This is exactly the same call as in the previous slide The message is sent to the request, not the response 14
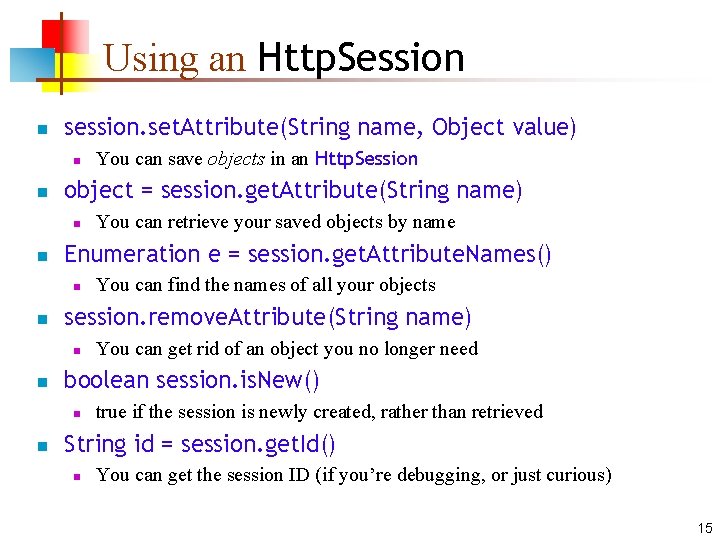
Using an Http. Session n session. set. Attribute(String name, Object value) n n object = session. get. Attribute(String name) n n You can get rid of an object you no longer need boolean session. is. New() n n You can find the names of all your objects session. remove. Attribute(String name) n n You can retrieve your saved objects by name Enumeration e = session. get. Attribute. Names() n n You can save objects in an Http. Session true if the session is newly created, rather than retrieved String id = session. get. Id() n You can get the session ID (if you’re debugging, or just curious) 15
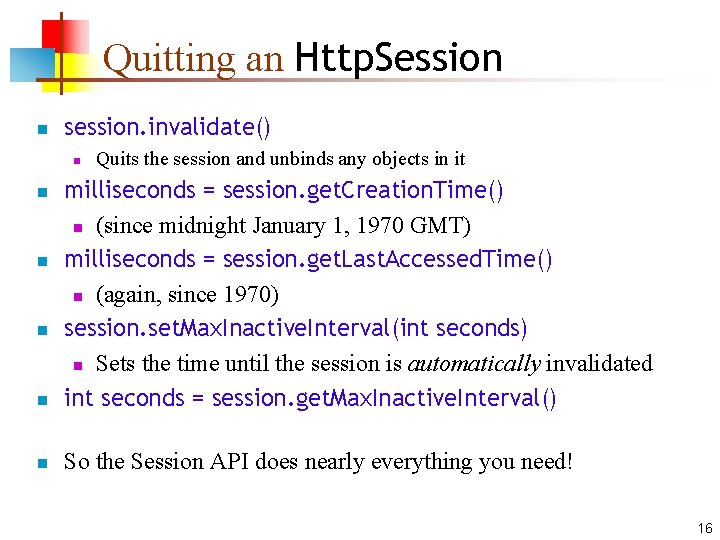
Quitting an Http. Session n session. invalidate() n Quits the session and unbinds any objects in it n milliseconds = session. get. Creation. Time() n (since midnight January 1, 1970 GMT) milliseconds = session. get. Last. Accessed. Time() n (again, since 1970) session. set. Max. Inactive. Interval(int seconds) n Sets the time until the session is automatically invalidated int seconds = session. get. Max. Inactive. Interval() n So the Session API does nearly everything you need! n n n 16
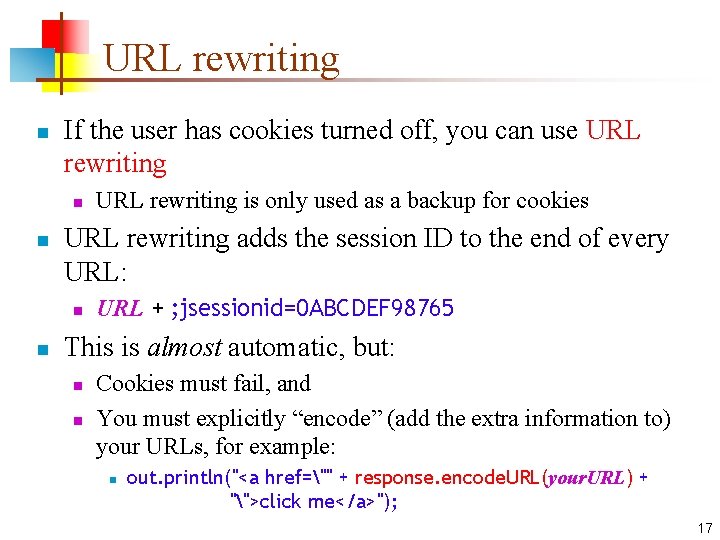
URL rewriting n If the user has cookies turned off, you can use URL rewriting n n URL rewriting adds the session ID to the end of every URL: n n URL rewriting is only used as a backup for cookies URL + ; jsessionid=0 ABCDEF 98765 This is almost automatic, but: n n Cookies must fail, and You must explicitly “encode” (add the extra information to) your URLs, for example: n out. println("<a href="" + response. encode. URL(your. URL) + "">click me</a>"); 17
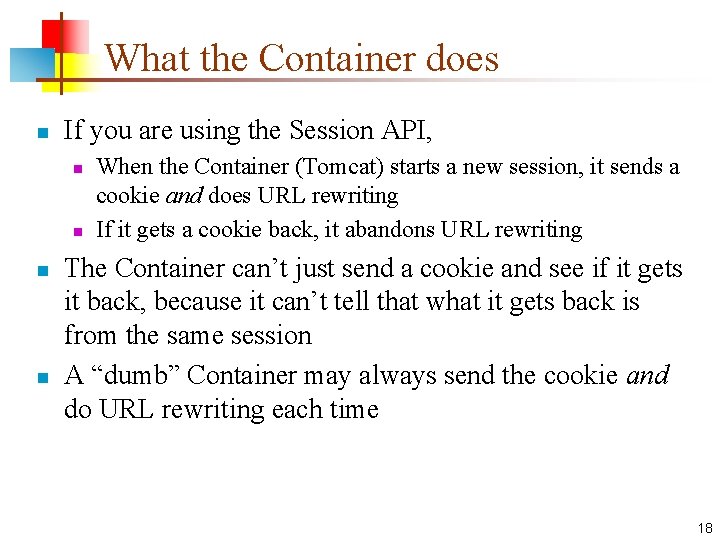
What the Container does n If you are using the Session API, n n When the Container (Tomcat) starts a new session, it sends a cookie and does URL rewriting If it gets a cookie back, it abandons URL rewriting The Container can’t just send a cookie and see if it gets it back, because it can’t tell that what it gets back is from the same session A “dumb” Container may always send the cookie and do URL rewriting each time 18
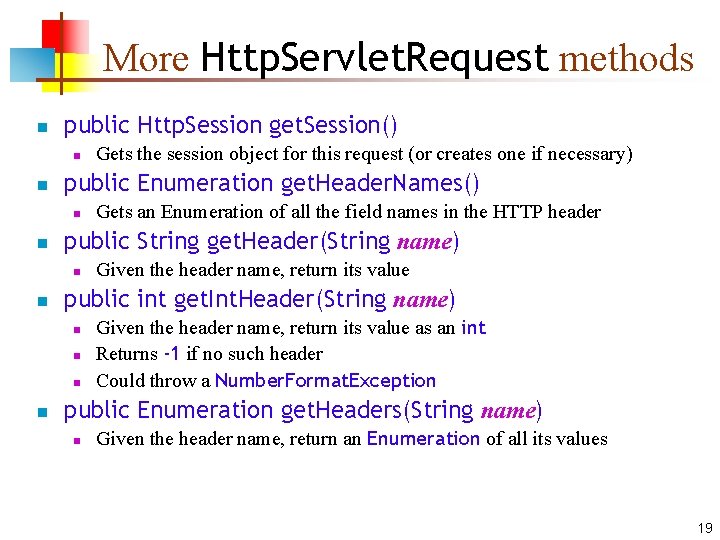
More Http. Servlet. Request methods n public Http. Session get. Session() n n public Enumeration get. Header. Names() n n Given the header name, return its value public int get. Int. Header(String name) n n Gets an Enumeration of all the field names in the HTTP header public String get. Header(String name) n n Gets the session object for this request (or creates one if necessary) Given the header name, return its value as an int Returns -1 if no such header Could throw a Number. Format. Exception public Enumeration get. Headers(String name) n Given the header name, return an Enumeration of all its values 19
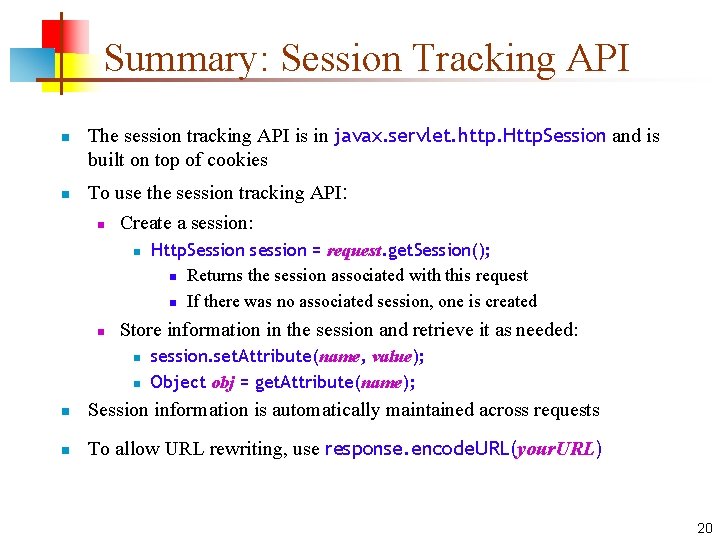
Summary: Session Tracking API n n The session tracking API is in javax. servlet. http. Http. Session and is built on top of cookies To use the session tracking API: n Create a session: n n Http. Session session = request. get. Session(); n Returns the session associated with this request n If there was no associated session, one is created Store information in the session and retrieve it as needed: n n session. set. Attribute(name, value); Object obj = get. Attribute(name); n Session information is automatically maintained across requests n To allow URL rewriting, use response. encode. URL(your. URL) 20
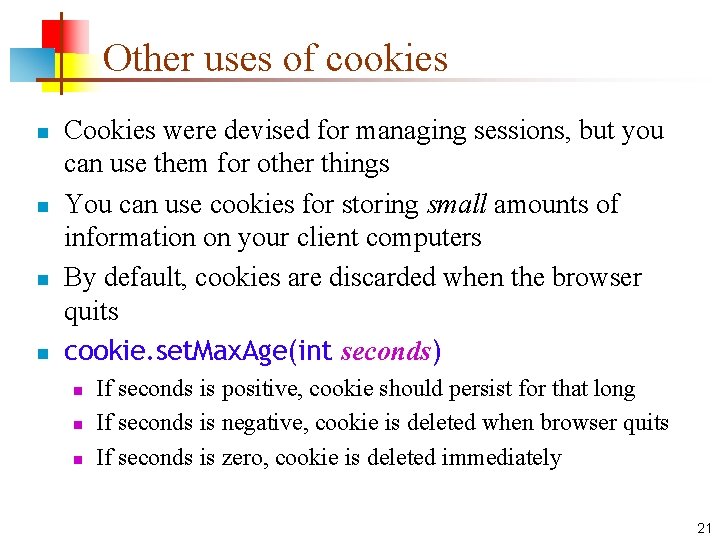
Other uses of cookies n n Cookies were devised for managing sessions, but you can use them for other things You can use cookies for storing small amounts of information on your client computers By default, cookies are discarded when the browser quits cookie. set. Max. Age(int seconds) n n n If seconds is positive, cookie should persist for that long If seconds is negative, cookie is deleted when browser quits If seconds is zero, cookie is deleted immediately 21
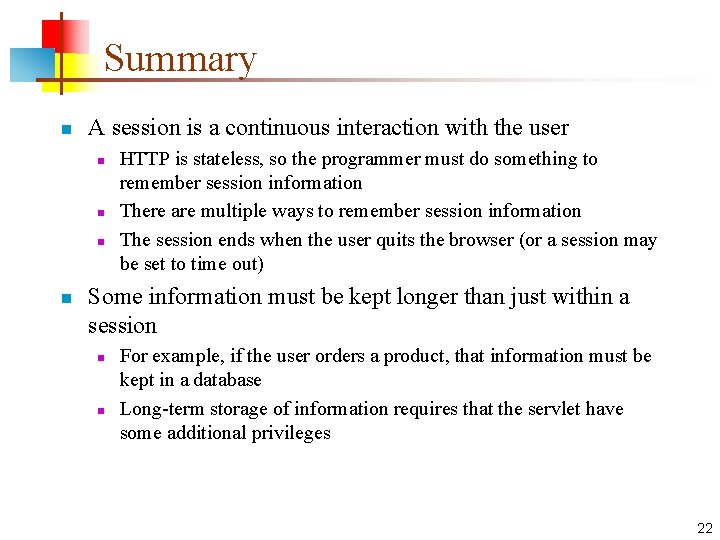
Summary n A session is a continuous interaction with the user n n HTTP is stateless, so the programmer must do something to remember session information There are multiple ways to remember session information The session ends when the user quits the browser (or a session may be set to time out) Some information must be kept longer than just within a session n n For example, if the user orders a product, that information must be kept in a database Long-term storage of information requires that the servlet have some additional privileges 22
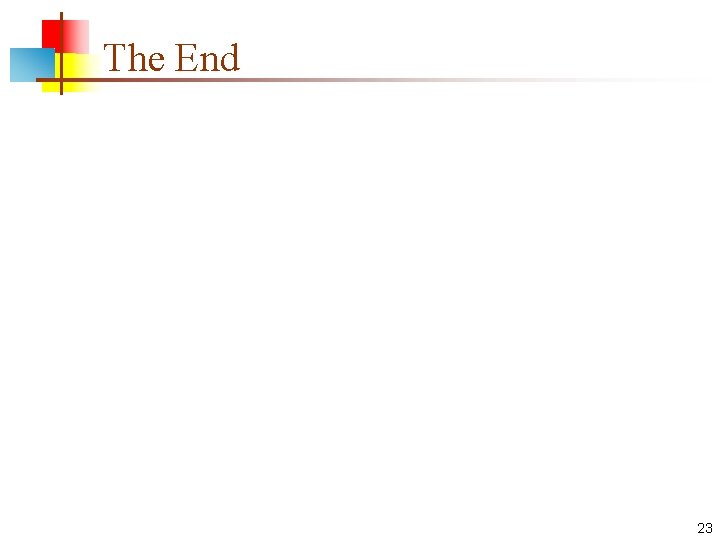
The End 23
Session tracking in servlet
Server.servlet.session.persistent
Servlet maintain session in mcq
Persistent vs non persistent http
Asp.net session management
Aka graduate information session
Absn seattle
Plt information session
Wemba wharton
Parent information session
Java jsp servlet tutorial
Tomcat servlet container
Servlet
Request 69
Java server pages
Jsp vs servlet
Servlet filter dispatcher
Hello
Import javax.servlet
Servlet chaining
Redirect and forward
Jsp jdbc
Javax.servlet
Servlet 4