More on Constructors Parameterized Constructor with Default Value
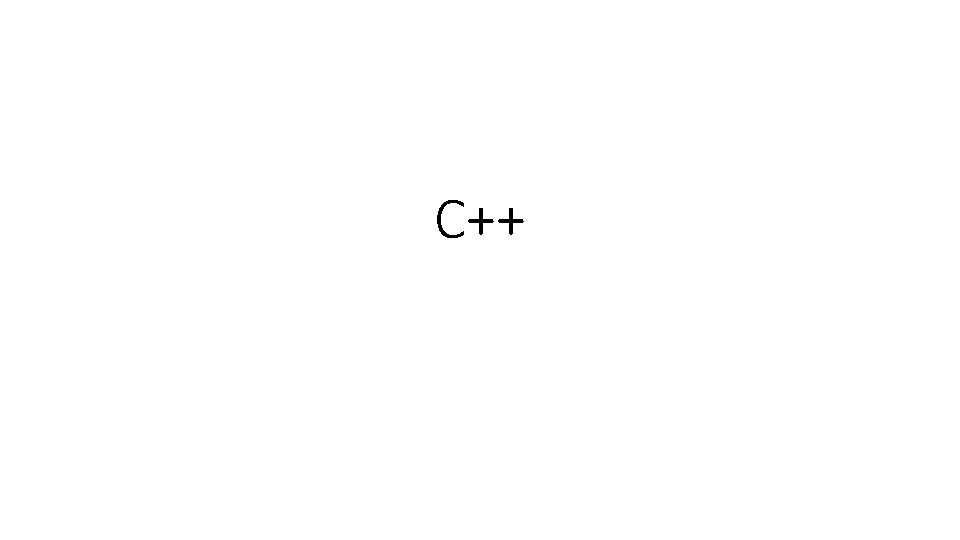
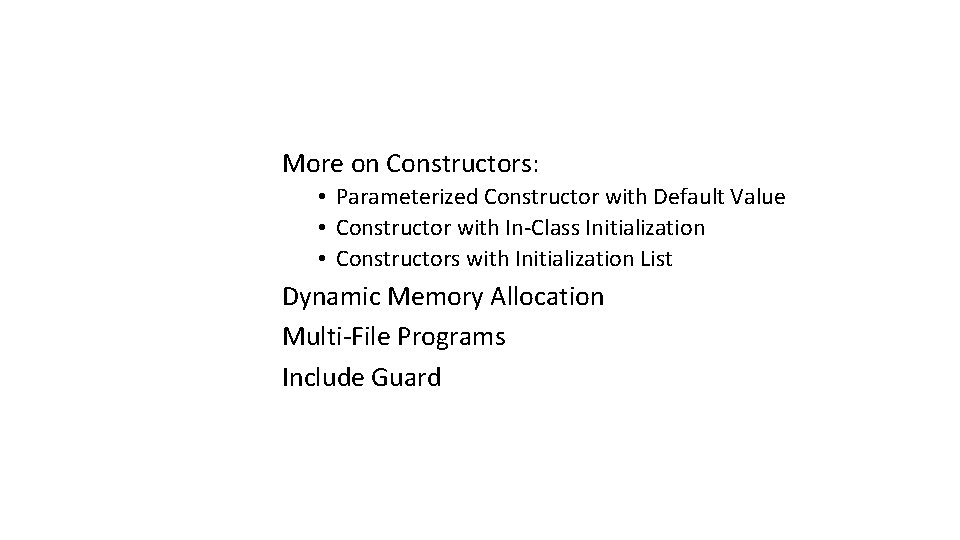
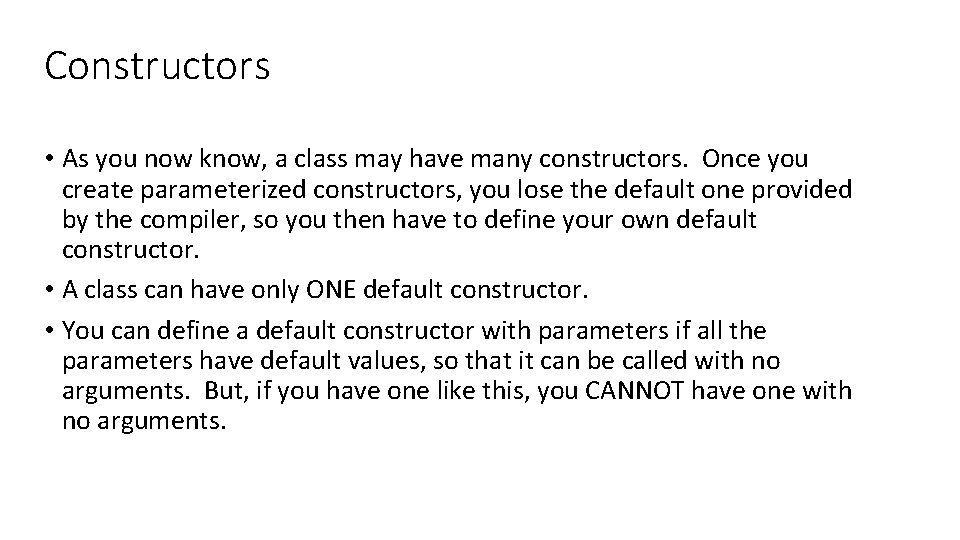
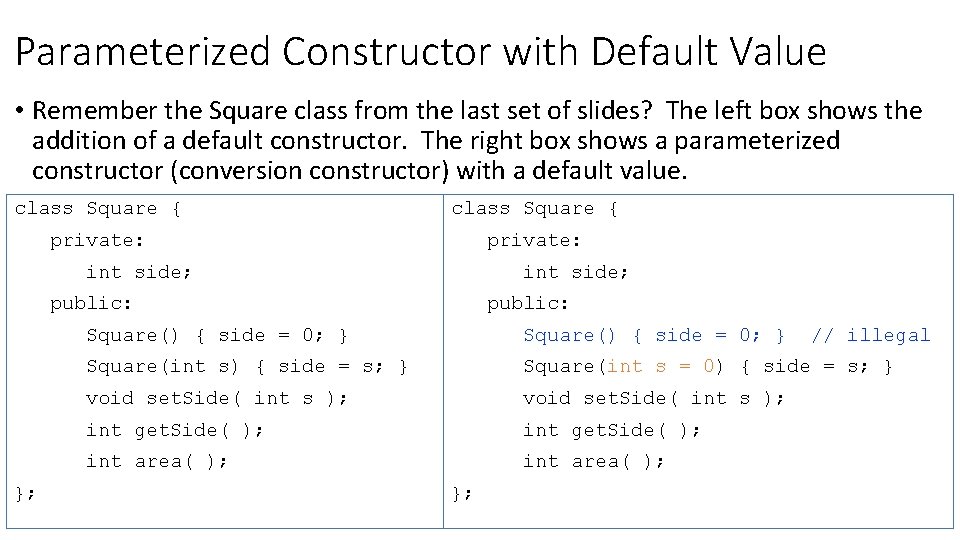
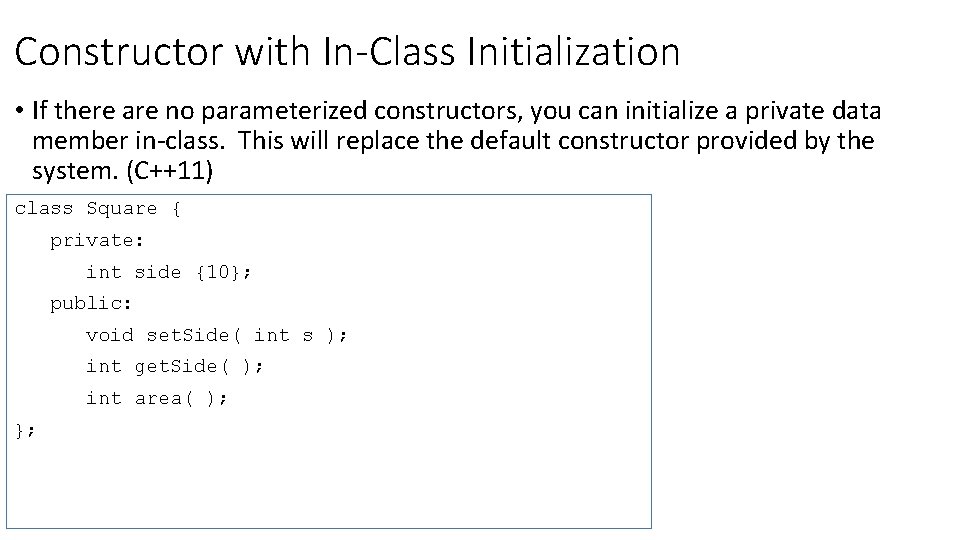
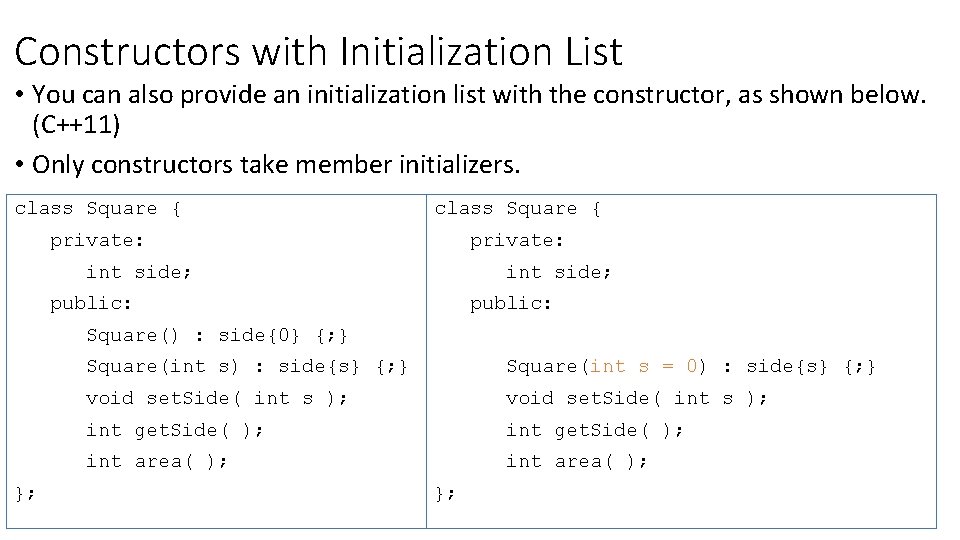
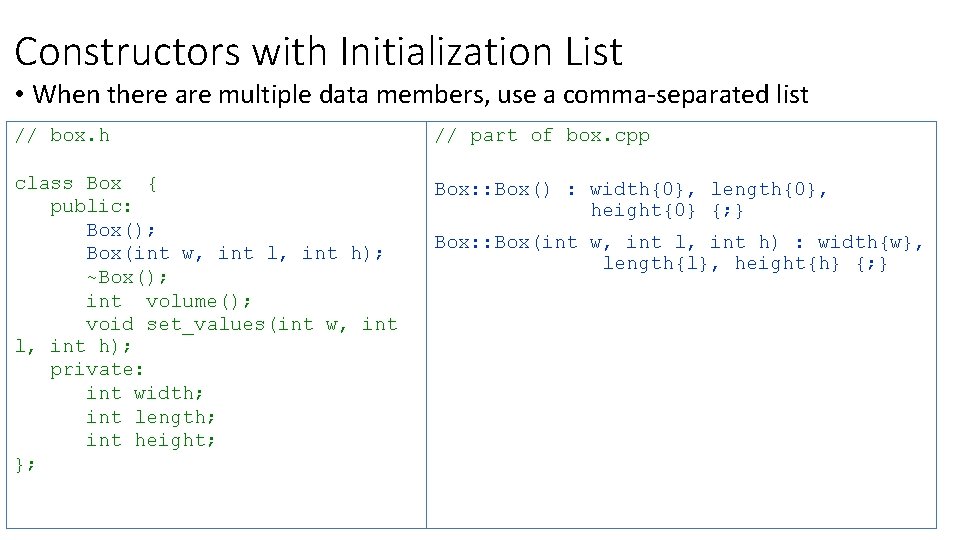
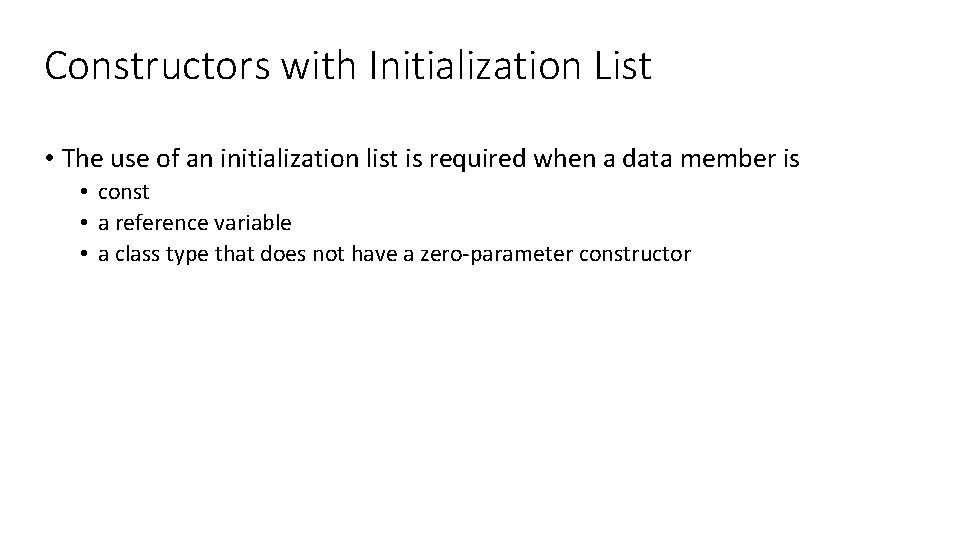
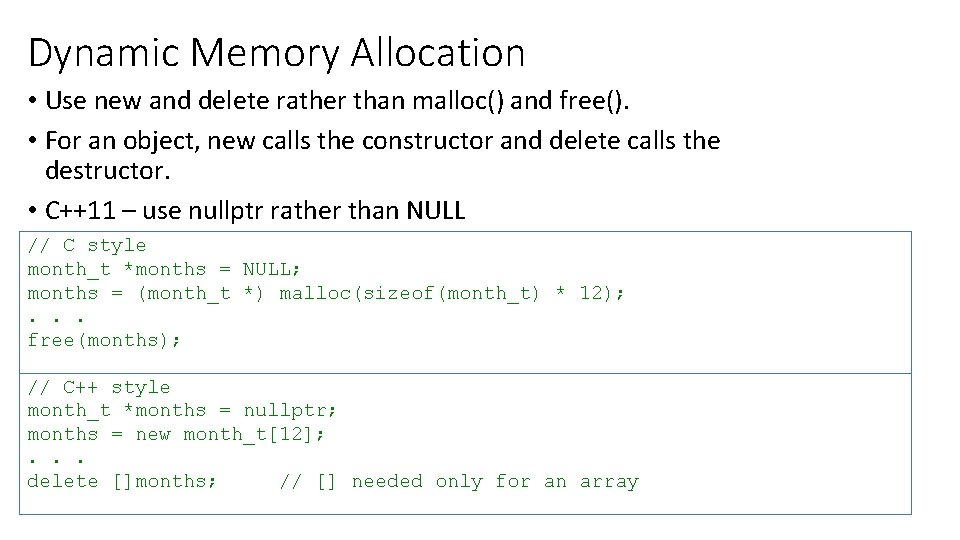
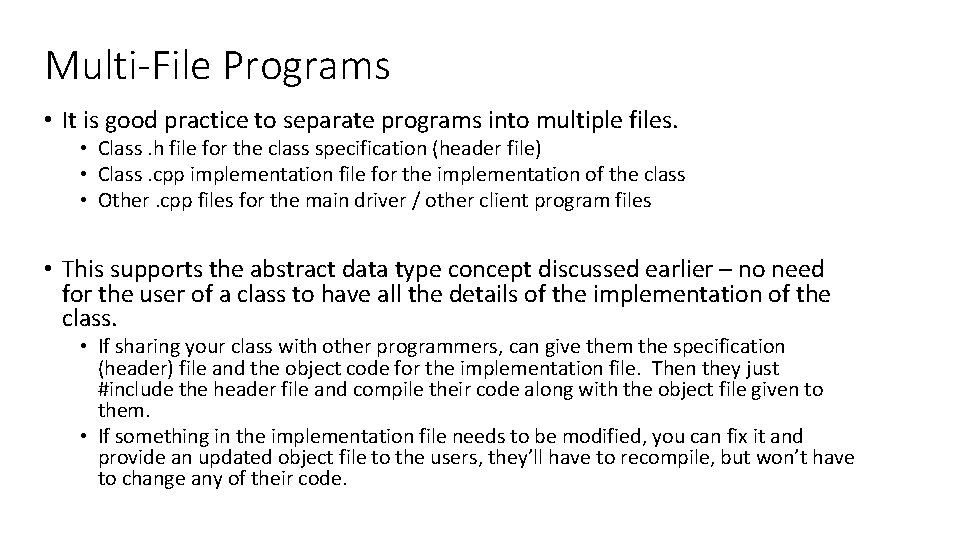
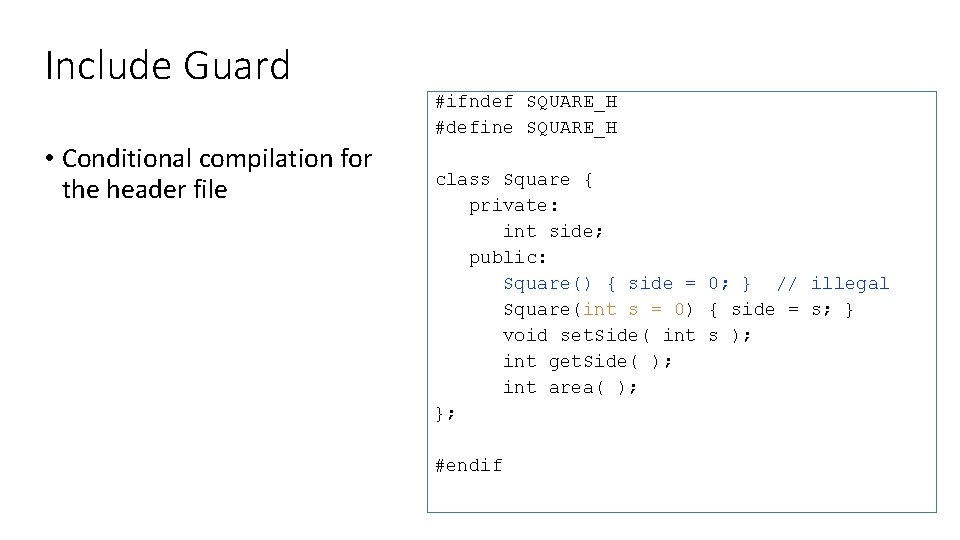
- Slides: 11
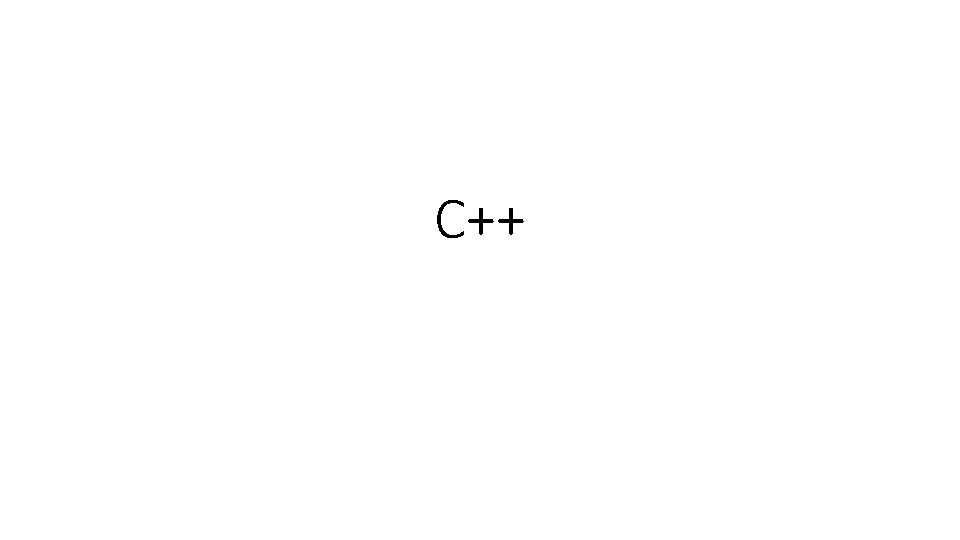
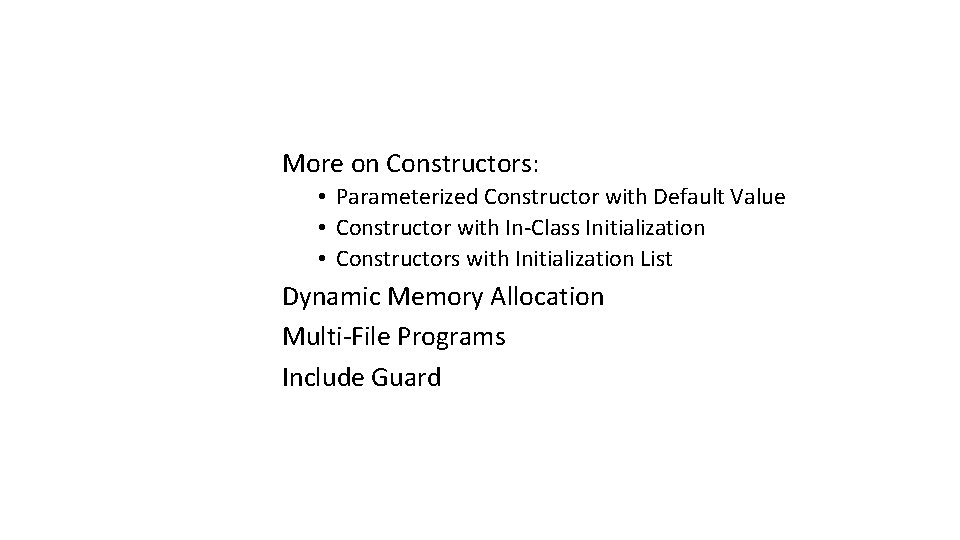
More on Constructors: • Parameterized Constructor with Default Value • Constructor with In-Class Initialization • Constructors with Initialization List Dynamic Memory Allocation Multi-File Programs Include Guard
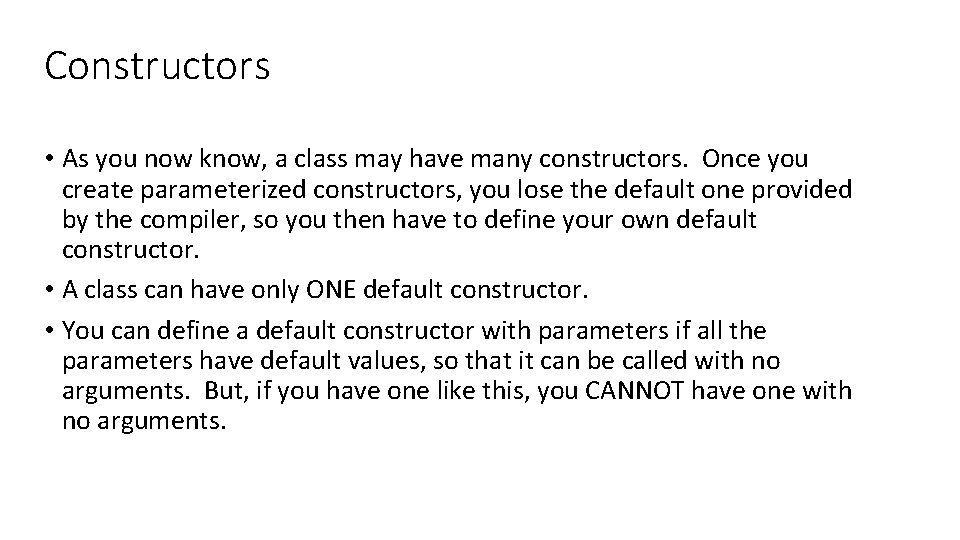
Constructors • As you now know, a class may have many constructors. Once you create parameterized constructors, you lose the default one provided by the compiler, so you then have to define your own default constructor. • A class can have only ONE default constructor. • You can define a default constructor with parameters if all the parameters have default values, so that it can be called with no arguments. But, if you have one like this, you CANNOT have one with no arguments.
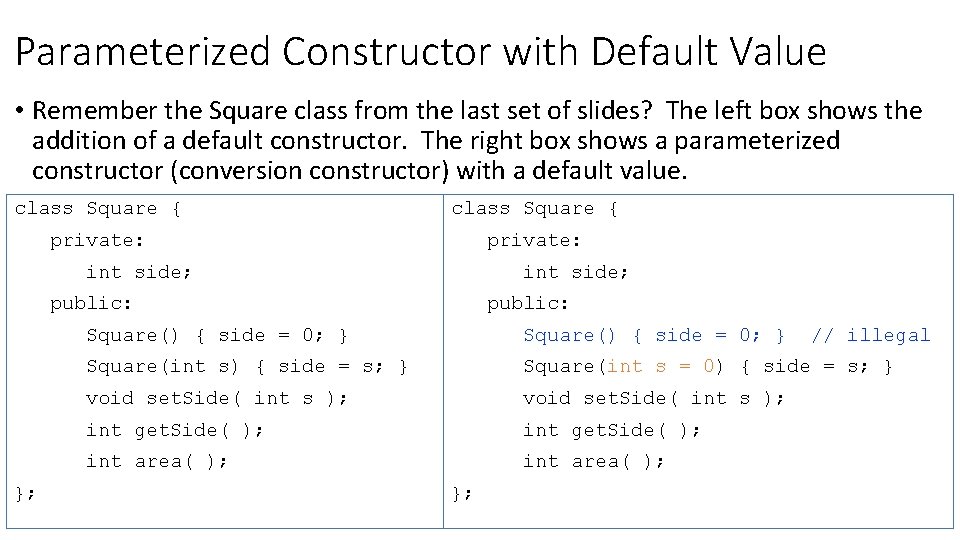
Parameterized Constructor with Default Value • Remember the Square class from the last set of slides? The left box shows the addition of a default constructor. The right box shows a parameterized constructor (conversion constructor) with a default value. class Square { private: int side; public: }; public: Square() { side = 0; } Square(int s) { side = s; } Square(int s = 0) { side = s; } void set. Side( int s ); int get. Side( ); int area( ); }; // illegal
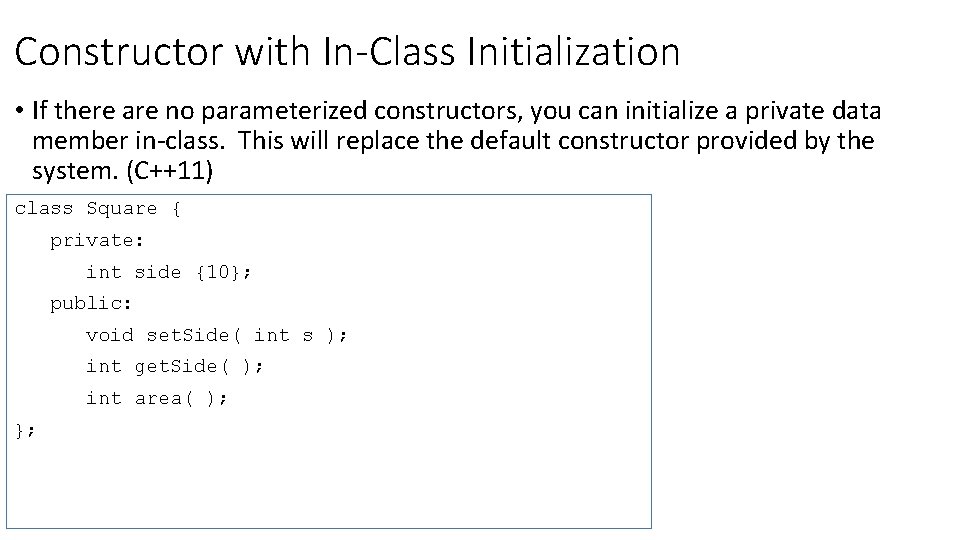
Constructor with In-Class Initialization • If there are no parameterized constructors, you can initialize a private data member in-class. This will replace the default constructor provided by the system. (C++11) class Square { private: int side {10}; public: void set. Side( int s ); int get. Side( ); int area( ); };
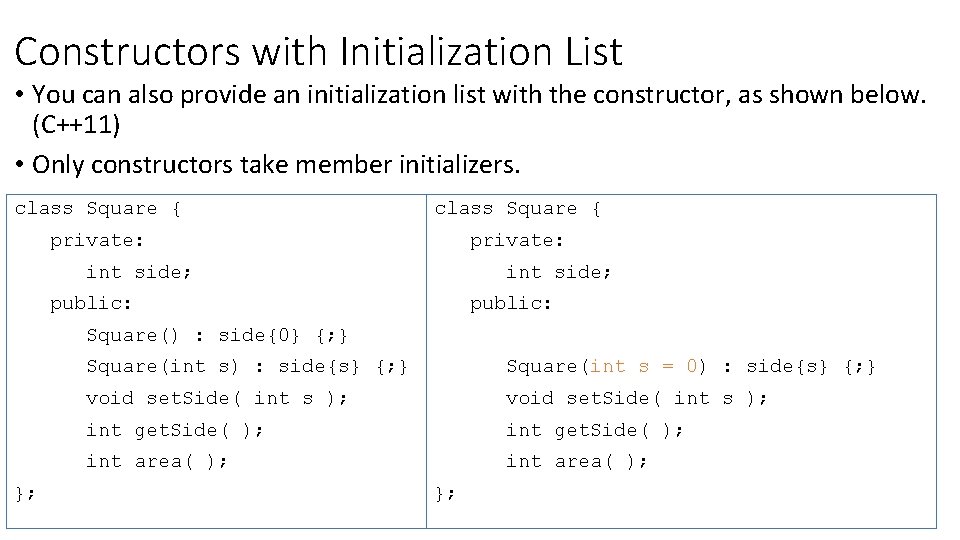
Constructors with Initialization List • You can also provide an initialization list with the constructor, as shown below. (C++11) • Only constructors take member initializers. class Square { private: int side; public: Square() : side{0} {; } }; Square(int s) : side{s} {; } Square(int s = 0) : side{s} {; } void set. Side( int s ); int get. Side( ); int area( ); };
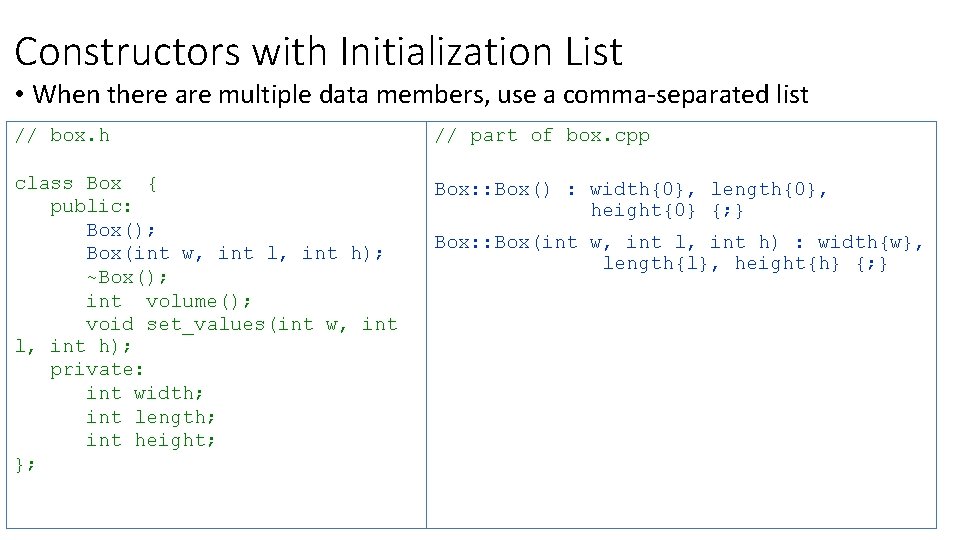
Constructors with Initialization List • When there are multiple data members, use a comma-separated list // box. h // part of box. cpp class Box { public: Box(); Box(int w, int l, int h); ~Box(); int volume(); void set_values(int w, int l, int h); private: int width; int length; int height; }; Box: : Box() : width{0}, length{0}, height{0} {; } Box: : Box(int w, int l, int h) : width{w}, length{l}, height{h} {; }
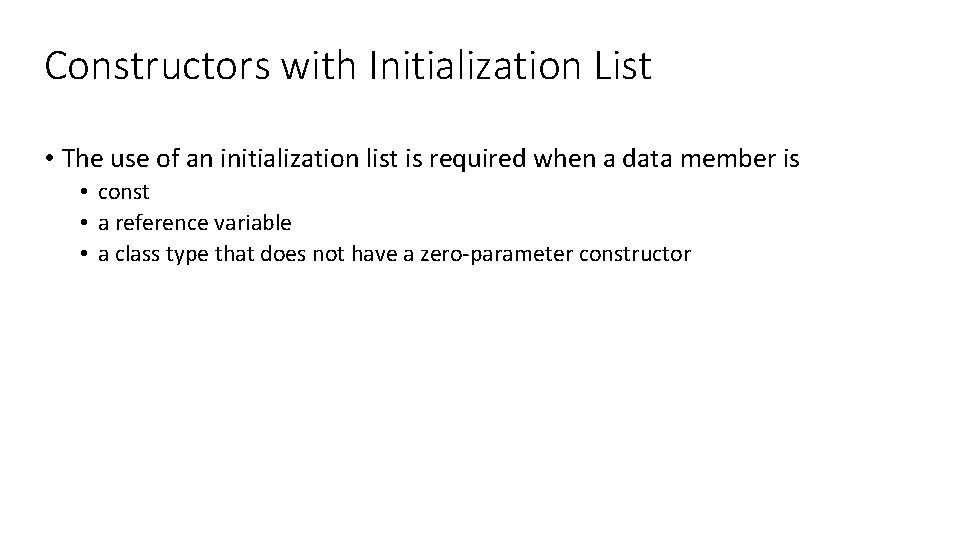
Constructors with Initialization List • The use of an initialization list is required when a data member is • const • a reference variable • a class type that does not have a zero-parameter constructor
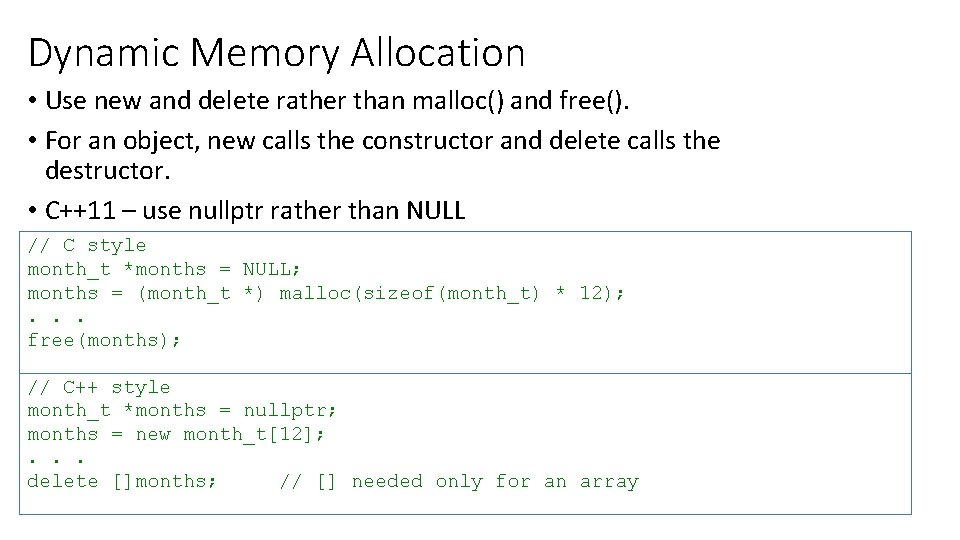
Dynamic Memory Allocation • Use new and delete rather than malloc() and free(). • For an object, new calls the constructor and delete calls the destructor. • C++11 – use nullptr rather than NULL // C style month_t *months = NULL; months = (month_t *) malloc(sizeof(month_t) * 12); . . . free(months); // C++ style month_t *months = nullptr; months = new month_t[12]; . . . delete []months; // [] needed only for an array
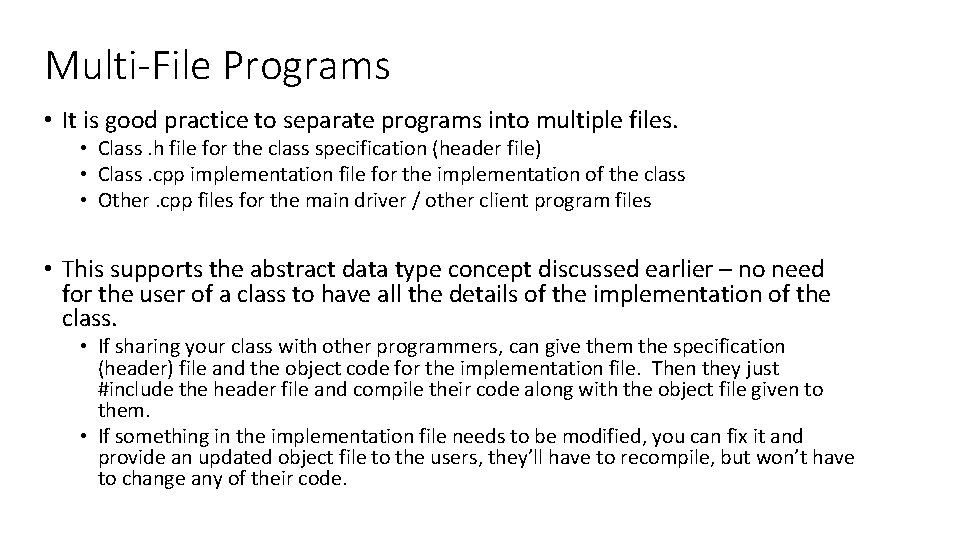
Multi-File Programs • It is good practice to separate programs into multiple files. • Class. h file for the class specification (header file) • Class. cpp implementation file for the implementation of the class • Other. cpp files for the main driver / other client program files • This supports the abstract data type concept discussed earlier – no need for the user of a class to have all the details of the implementation of the class. • If sharing your class with other programmers, can give them the specification (header) file and the object code for the implementation file. Then they just #include the header file and compile their code along with the object file given to them. • If something in the implementation file needs to be modified, you can fix it and provide an updated object file to the users, they’ll have to recompile, but won’t have to change any of their code.
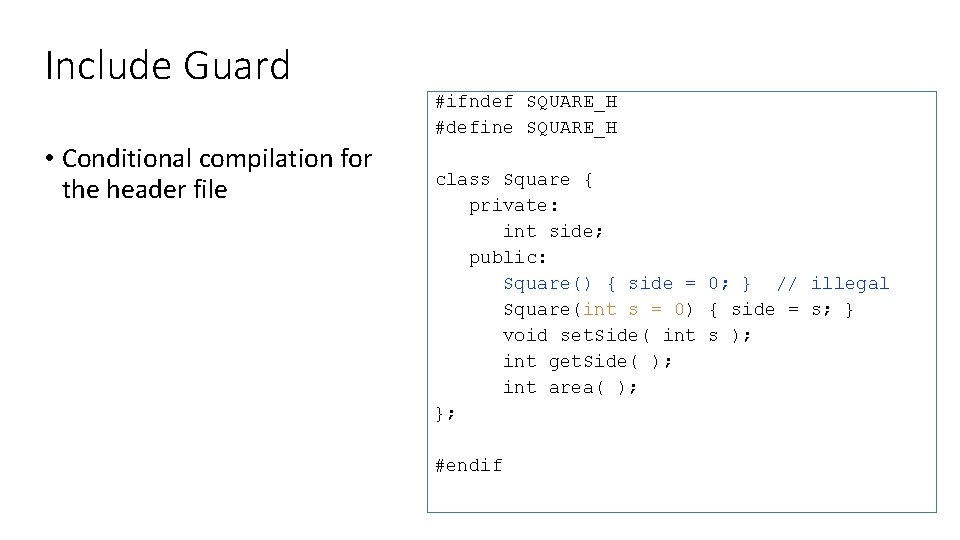
Include Guard #ifndef SQUARE_H #define SQUARE_H • Conditional compilation for the header file class Square { private: int side; public: Square() { side = 0; } // illegal Square(int s = 0) { side = s; } void set. Side( int s ); int get. Side( ); int area( ); }; #endif