Exercise 6 Stack 1 Stack is a data
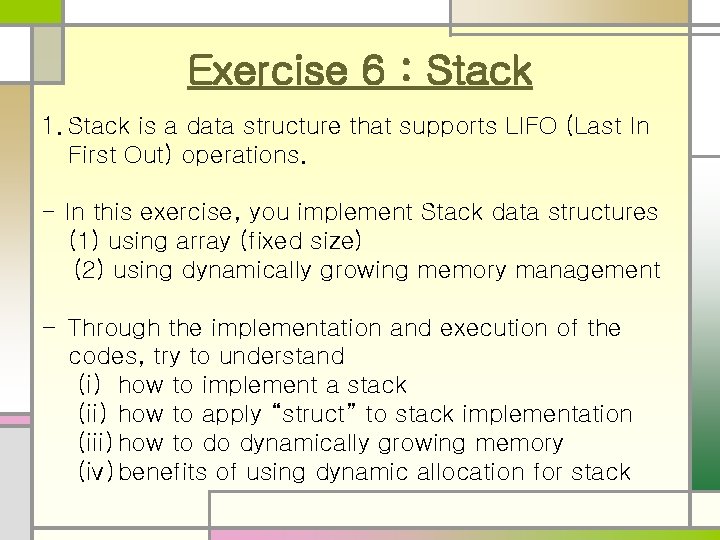
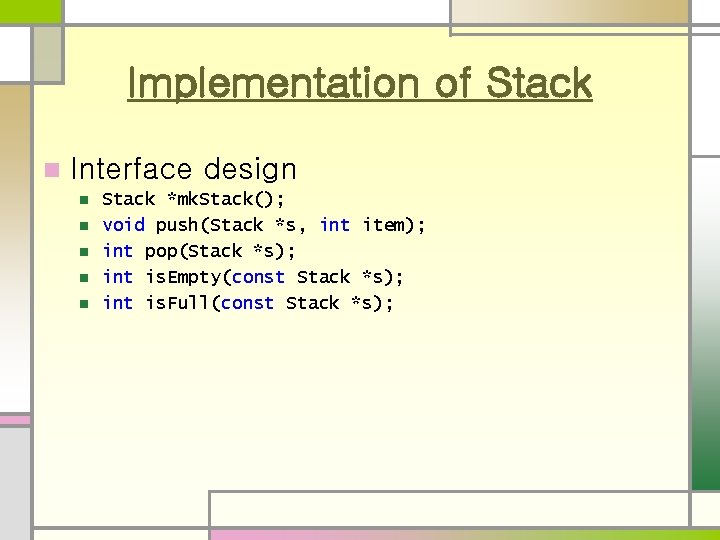
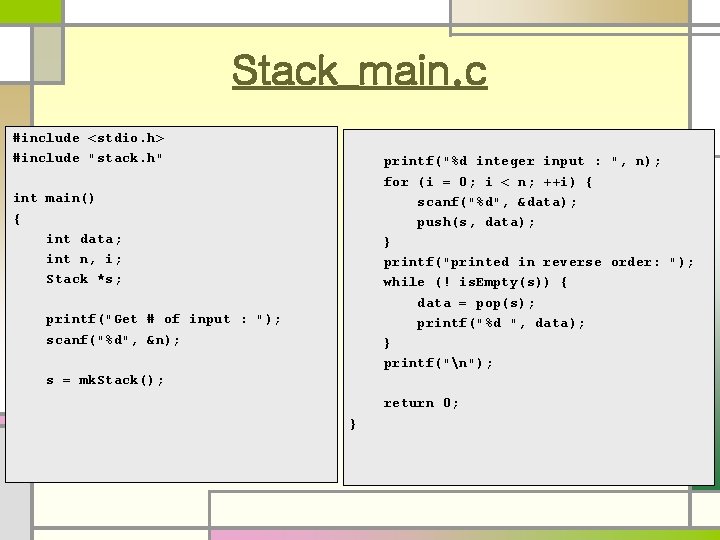
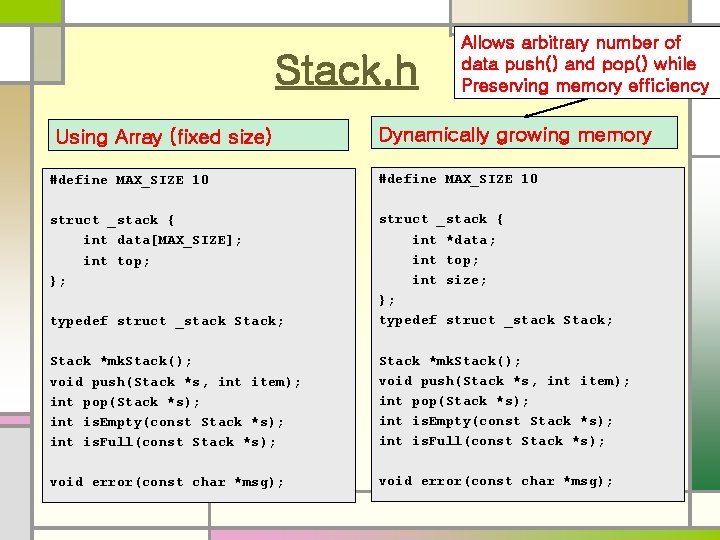
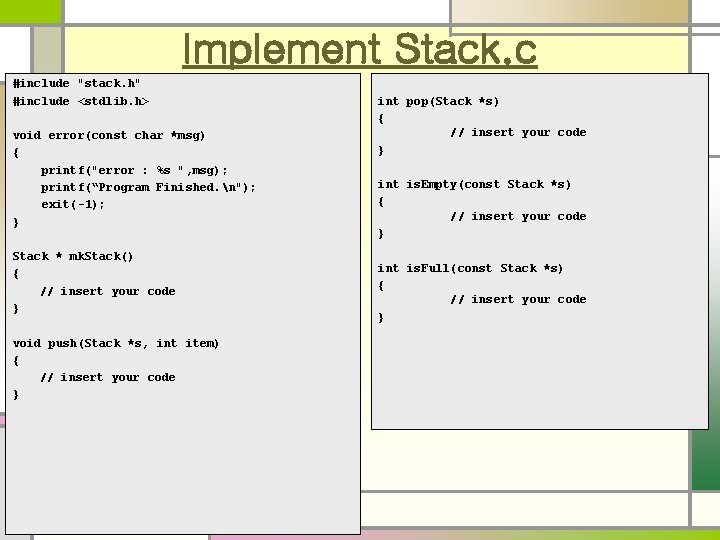
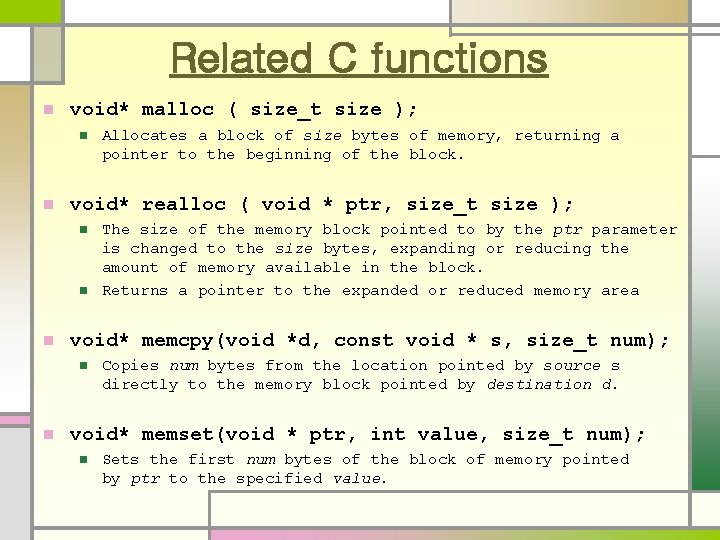
- Slides: 6
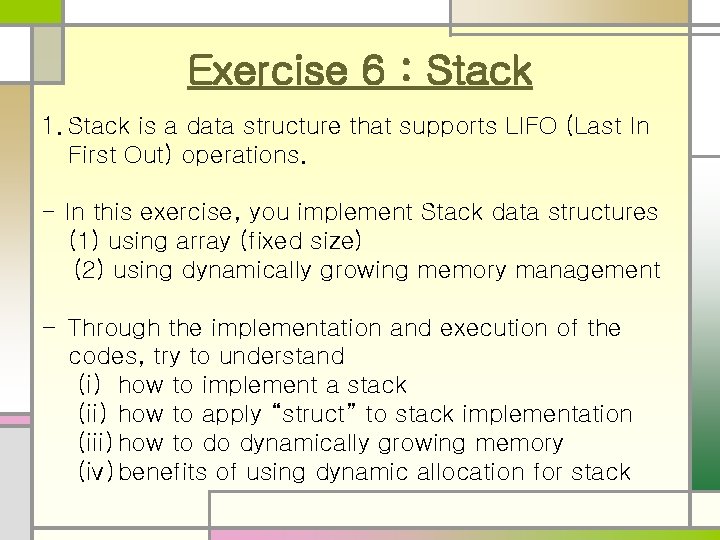
Exercise 6 : Stack 1. Stack is a data structure that supports LIFO (Last In First Out) operations. - In this exercise, you implement Stack data structures (1) using array (fixed size) (2) using dynamically growing memory management - Through the implementation and execution of the codes, try to understand (i) how to implement a stack (ii) how to apply “struct” to stack implementation (iii) how to do dynamically growing memory (iv) benefits of using dynamic allocation for stack
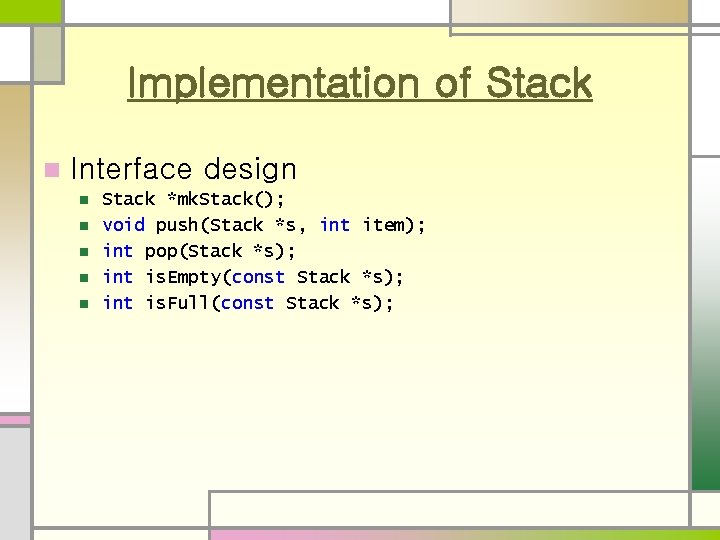
Implementation of Stack n Interface design n n Stack *mk. Stack(); void push(Stack *s, int item); int pop(Stack *s); int is. Empty(const Stack *s); int is. Full(const Stack *s);
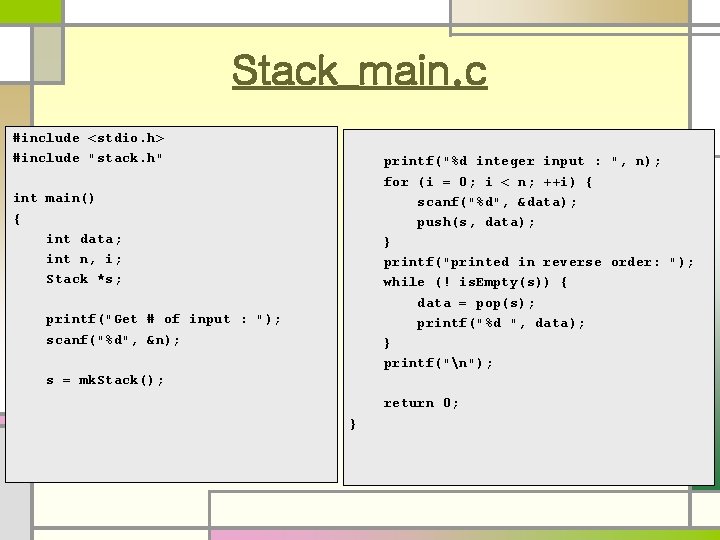
Stack_main. c #include <stdio. h> #include "stack. h" printf("%d integer input : ", n); for (i = 0; i < n; ++i) { scanf("%d", &data); push(s, data); } printf("printed in reverse order: "); while (! is. Empty(s)) { data = pop(s); printf("%d ", data); } printf("n"); int main() { int data; int n, i; Stack *s; printf("Get # of input : "); scanf("%d", &n); s = mk. Stack(); return 0; }
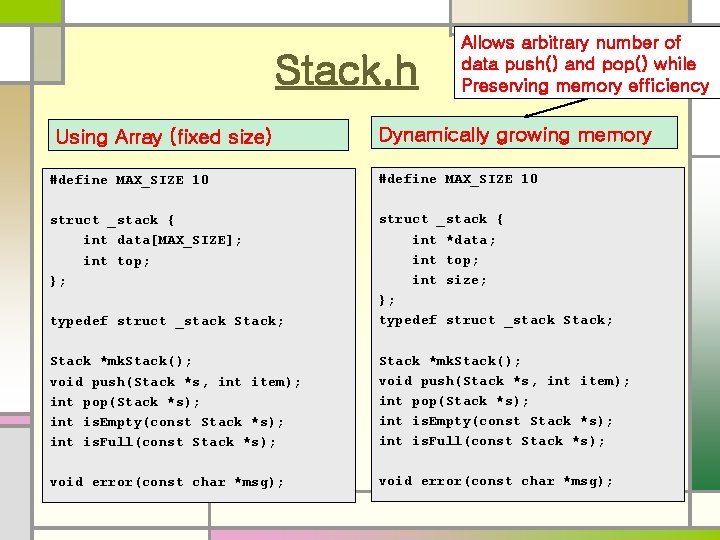
Stack. h Using Array (fixed size) Allows arbitrary number of data push() and pop() while Preserving memory efficiency Dynamically growing memory #define MAX_SIZE 10 struct _stack { int data[MAX_SIZE]; int top; }; typedef struct _stack Stack; struct _stack { int *data; int top; int size; }; typedef struct _stack Stack; Stack *mk. Stack(); void push(Stack *s, int item); int pop(Stack *s); int is. Empty(const Stack *s); int is. Full(const Stack *s); void error(const char *msg);
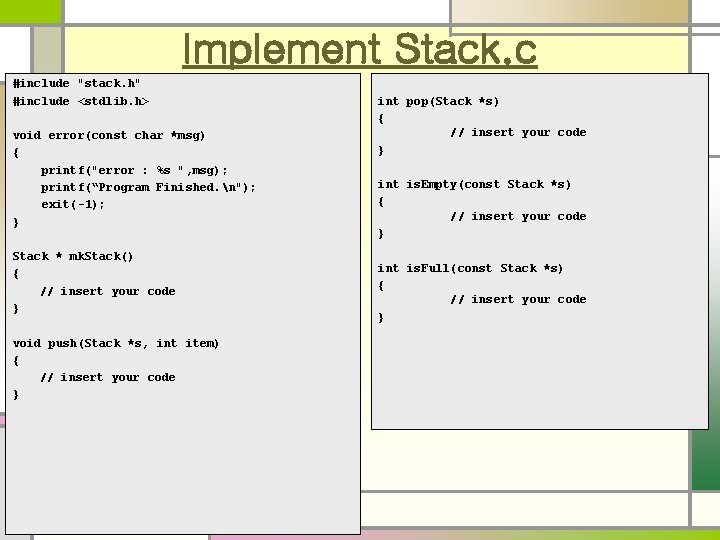
Implement Stack. c #include "stack. h" #include <stdlib. h> void error(const char *msg) { printf("error : %s ", msg); printf(“Program Finished. n"); exit(-1); } Stack * mk. Stack() { // insert your code } void push(Stack *s, int item) { // insert your code } int pop(Stack *s) { // insert your code } int is. Empty(const Stack *s) { // insert your code } int is. Full(const Stack *s) { // insert your code }
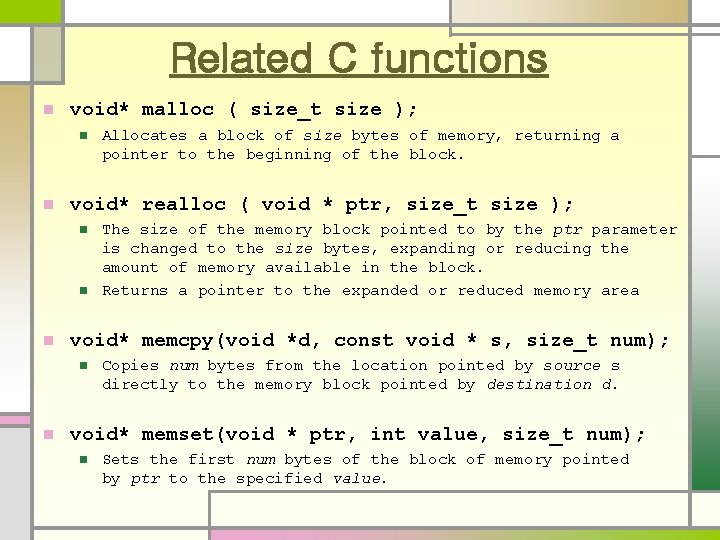
Related C functions n void* malloc ( size_t size ); n n void* realloc ( void * ptr, size_t size ); n n n The size of the memory block pointed to by the ptr parameter is changed to the size bytes, expanding or reducing the amount of memory available in the block. Returns a pointer to the expanded or reduced memory area void* memcpy(void *d, const void * s, size_t num); n n Allocates a block of size bytes of memory, returning a pointer to the beginning of the block. Copies num bytes from the location pointed by source s directly to the memory block pointed by destination d. void* memset(void * ptr, int value, size_t num); n Sets the first num bytes of the block of memory pointed by ptr to the specified value.