CHAPTER 7 USERDEFINED FUNCTIONS II In this chapter
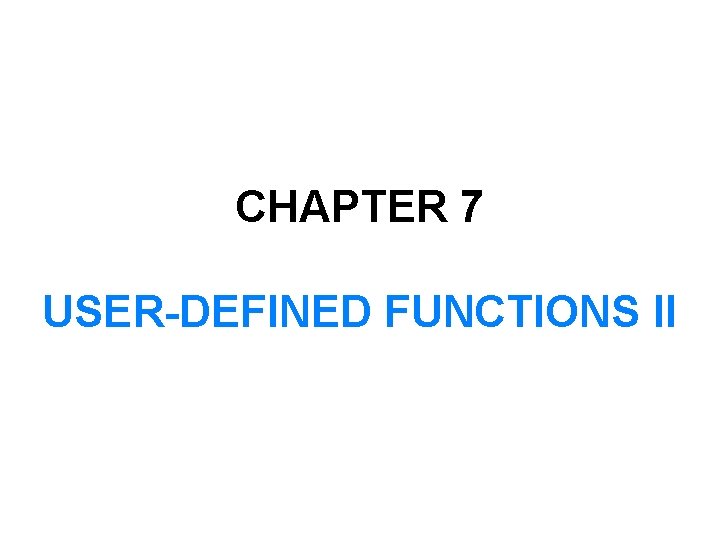
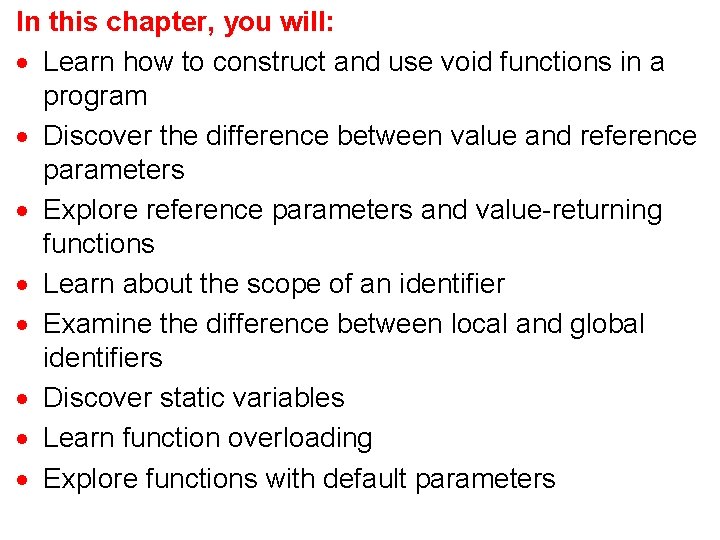
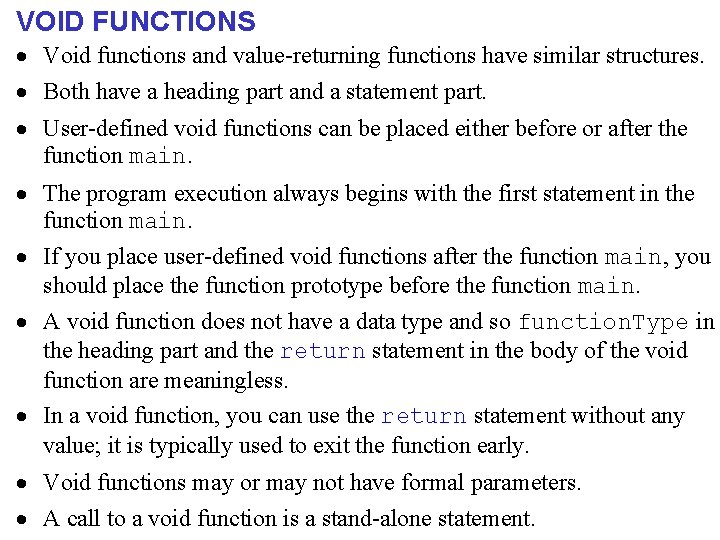
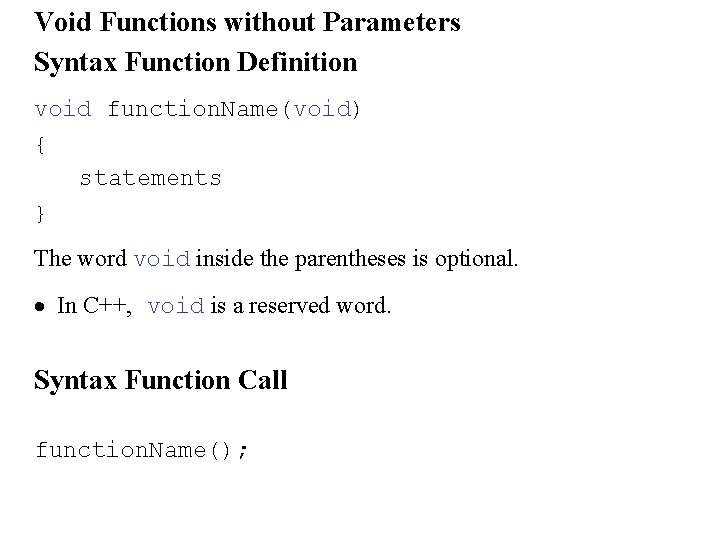
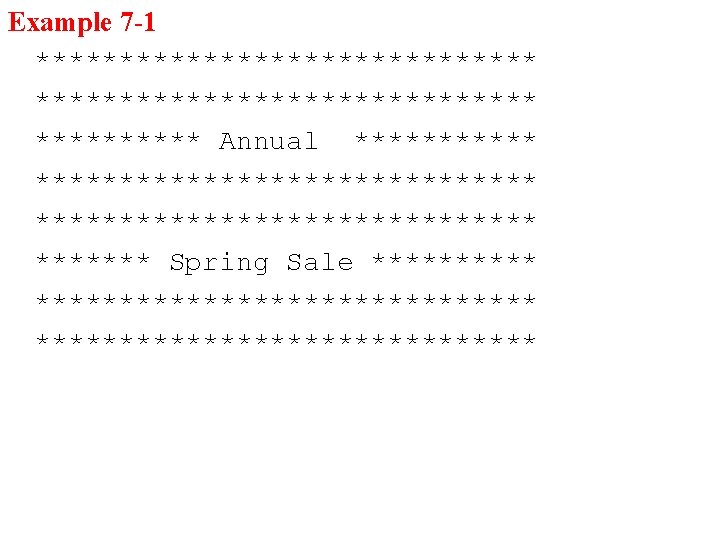
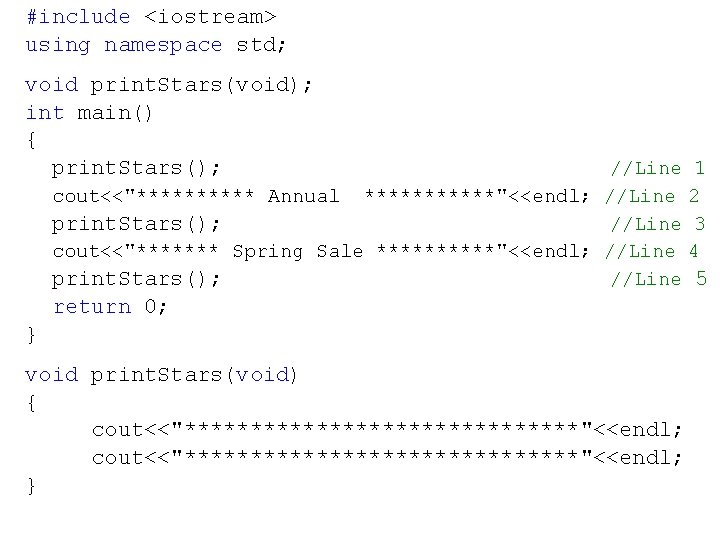
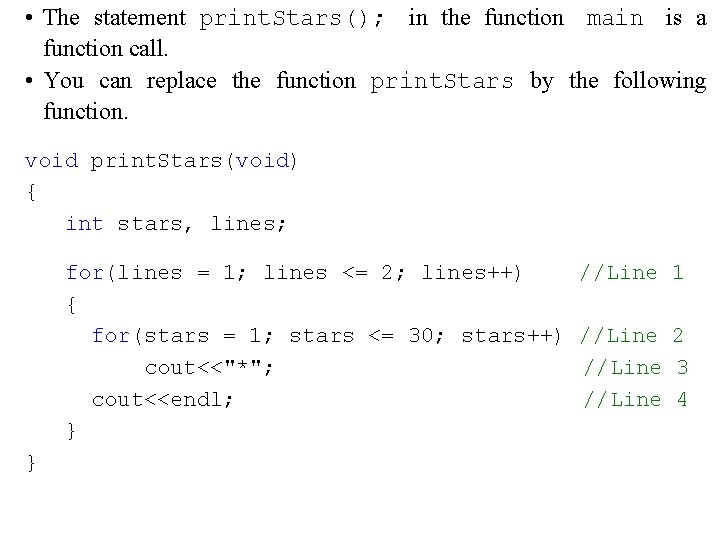
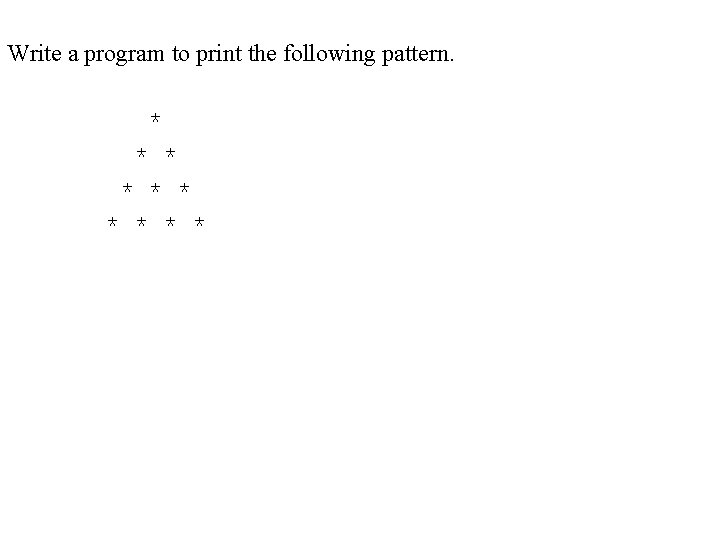
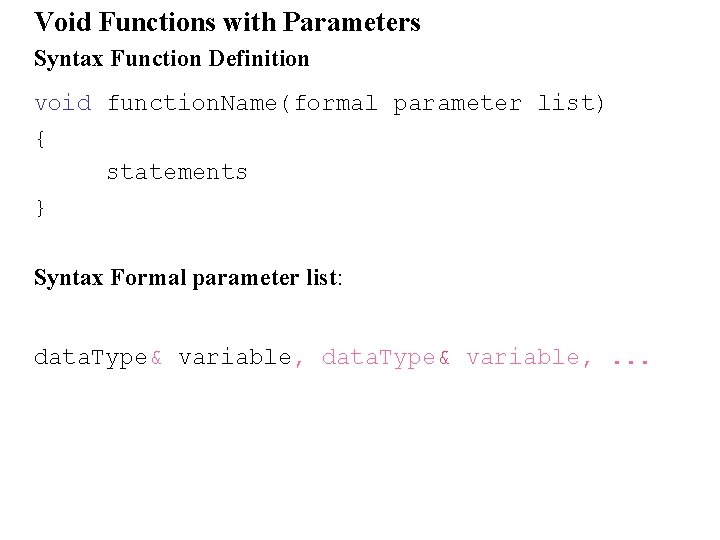
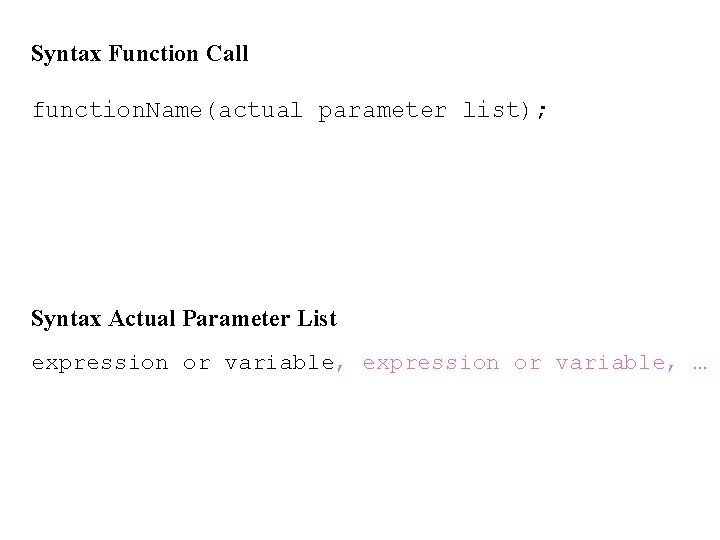
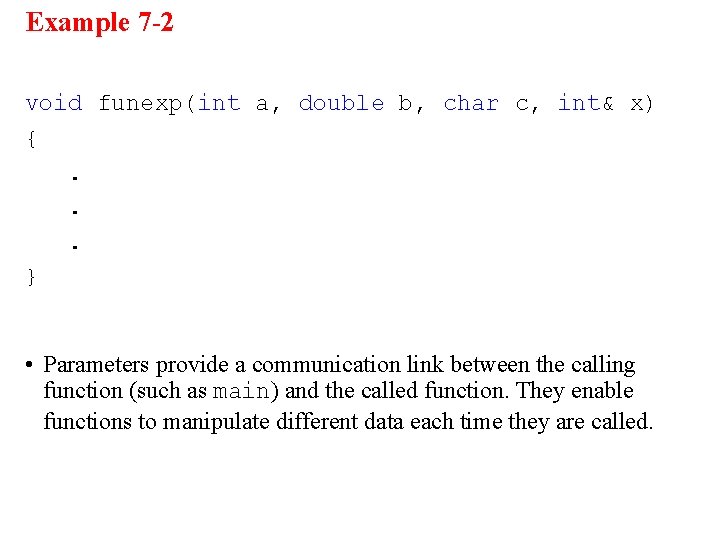
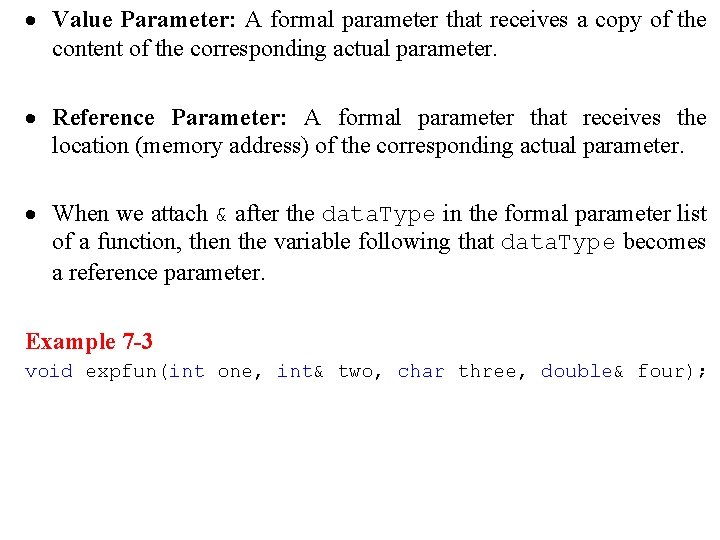
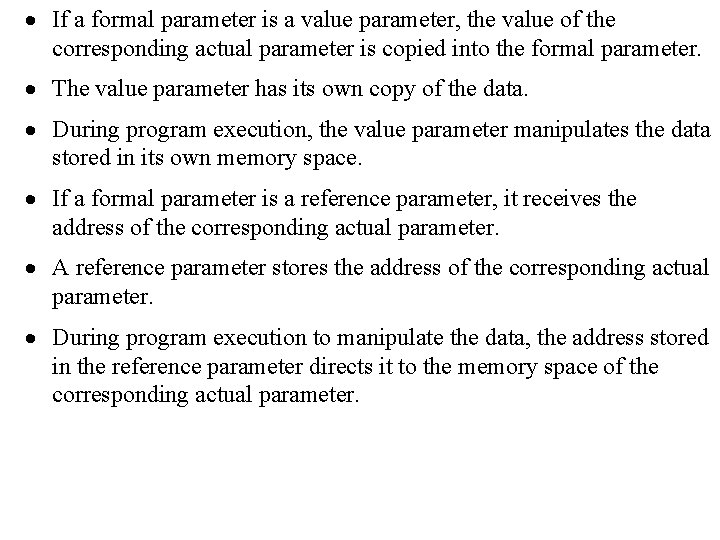
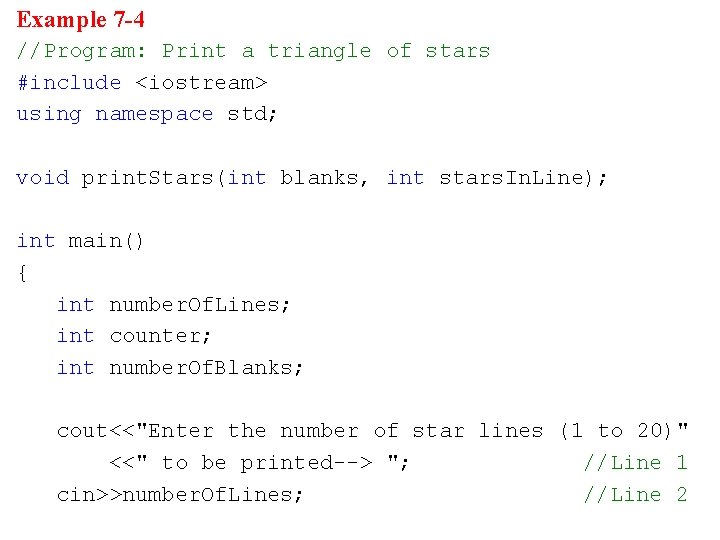
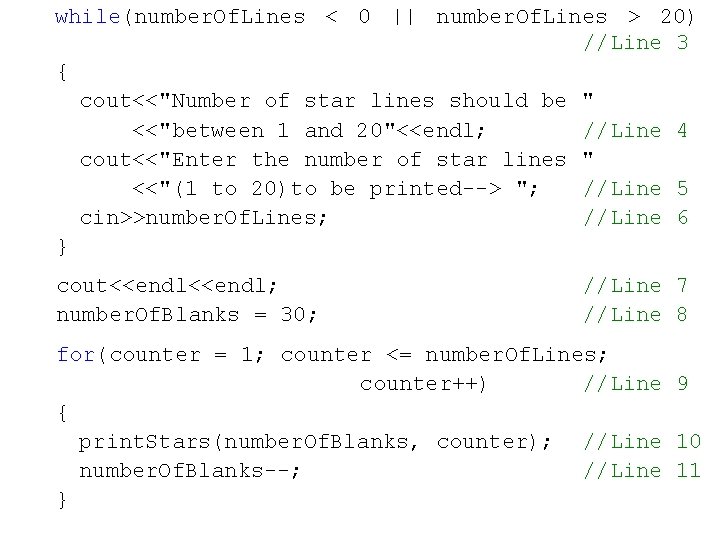
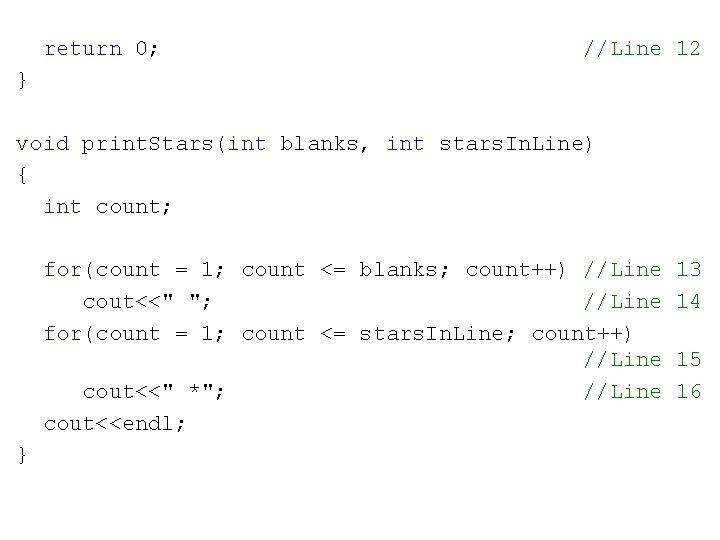
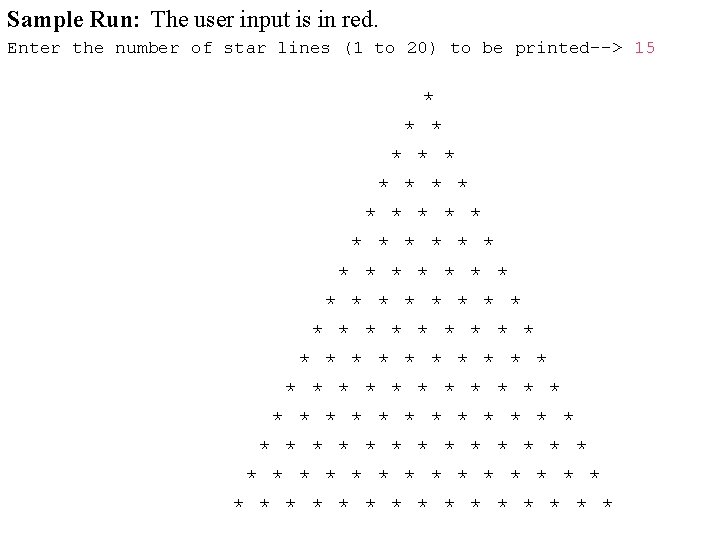
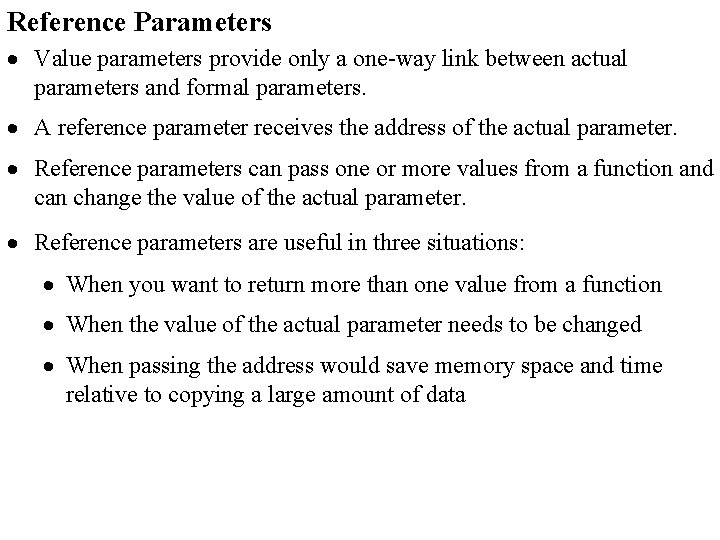
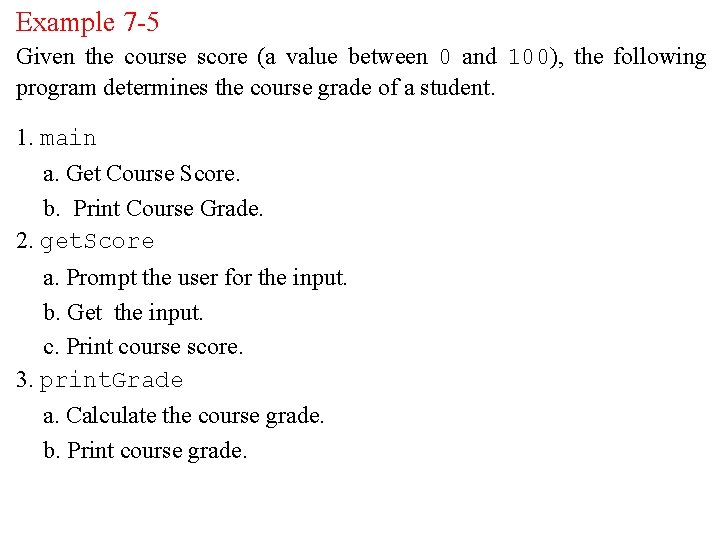
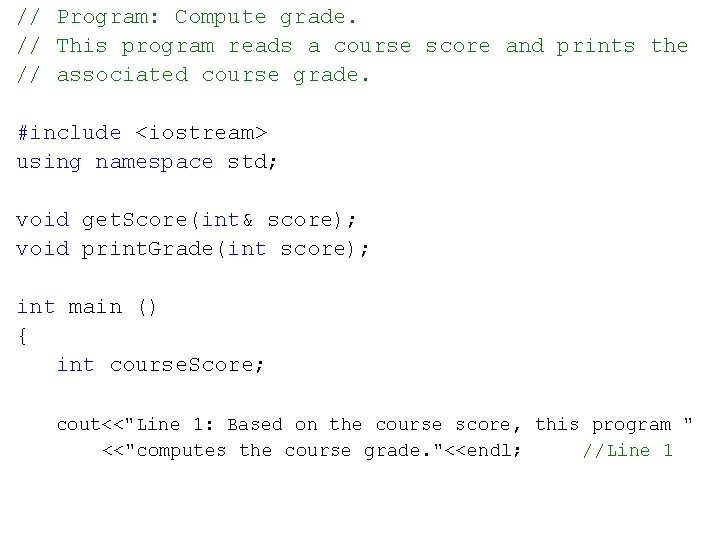
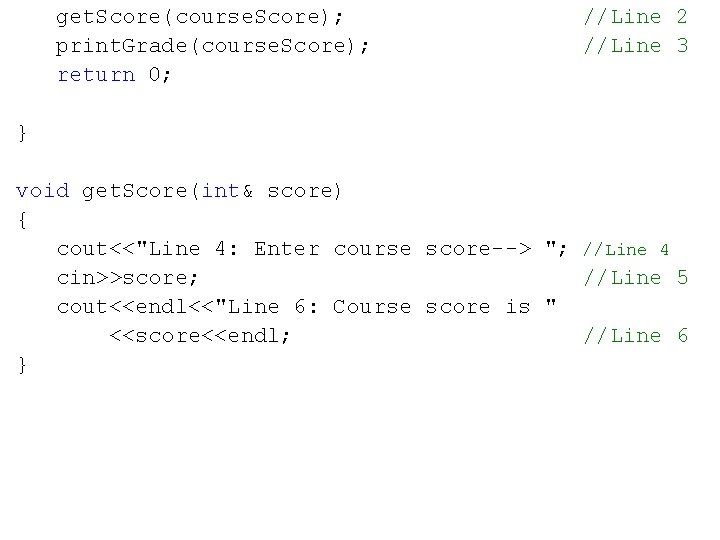
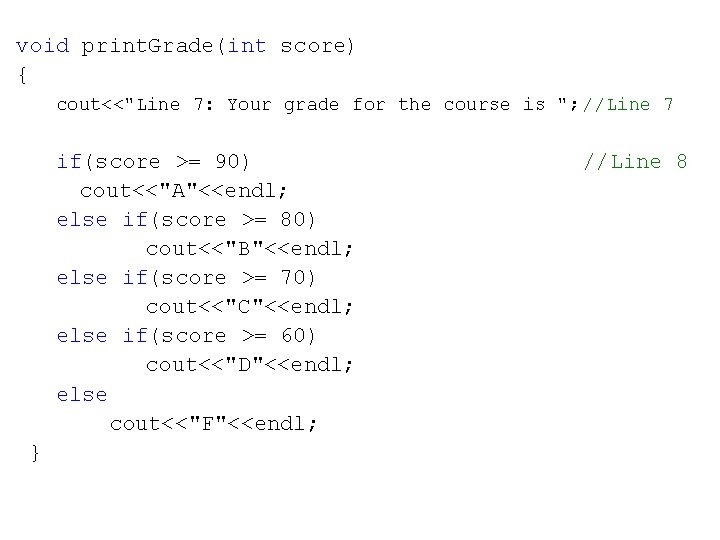
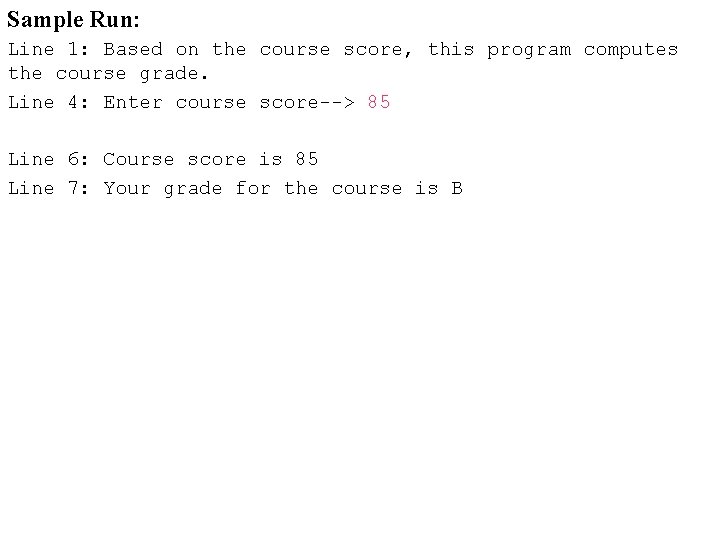
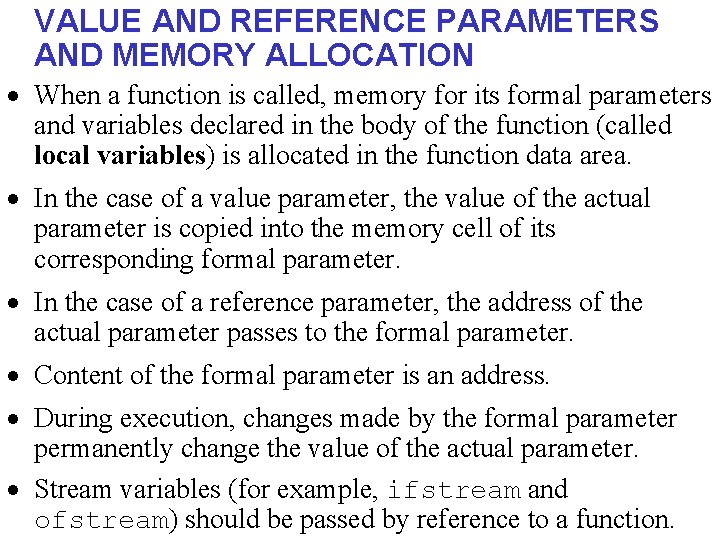
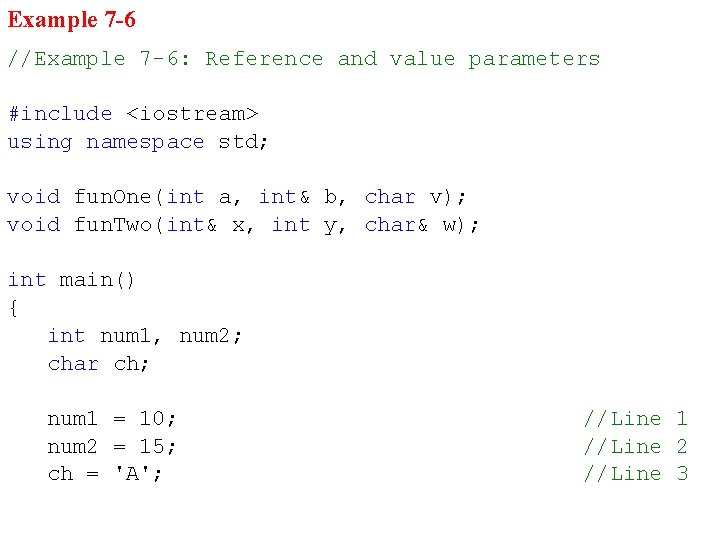
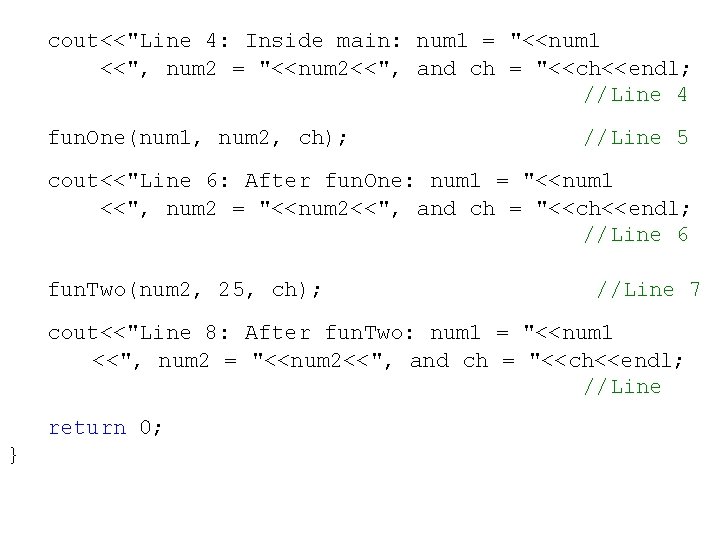
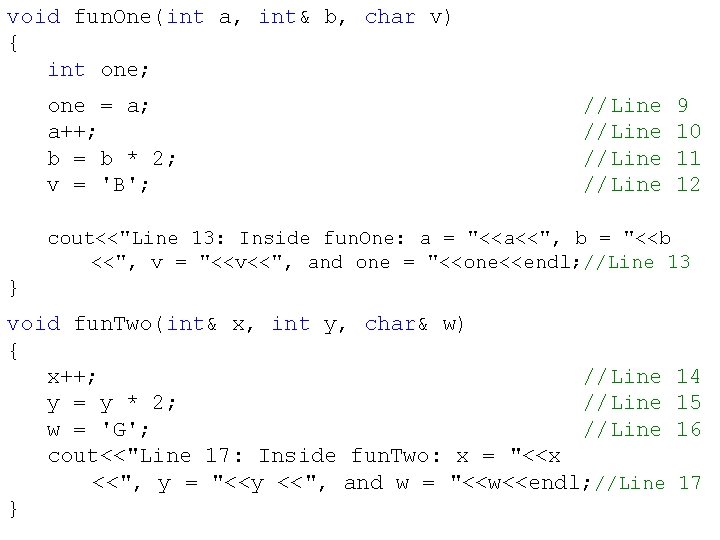
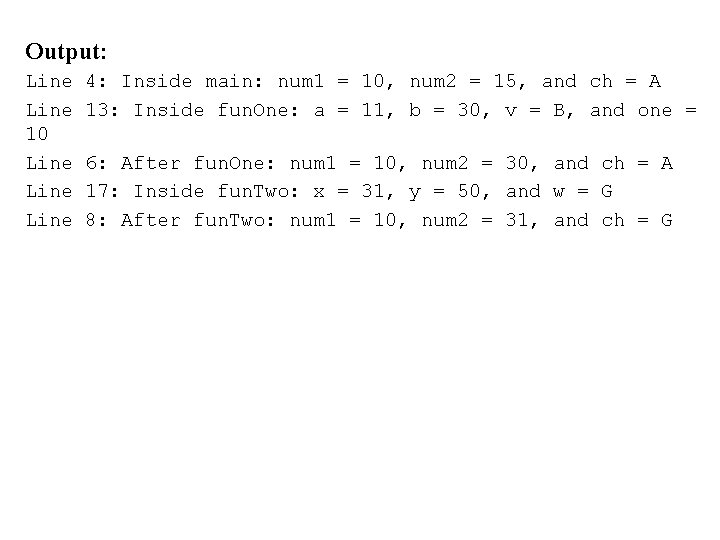
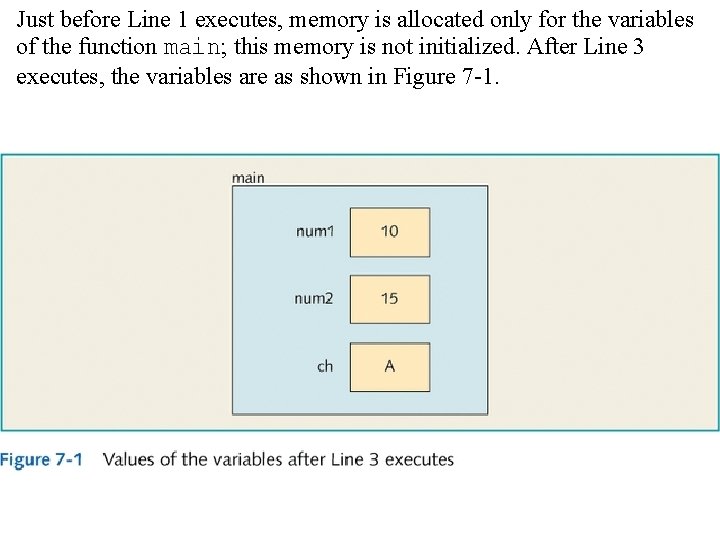
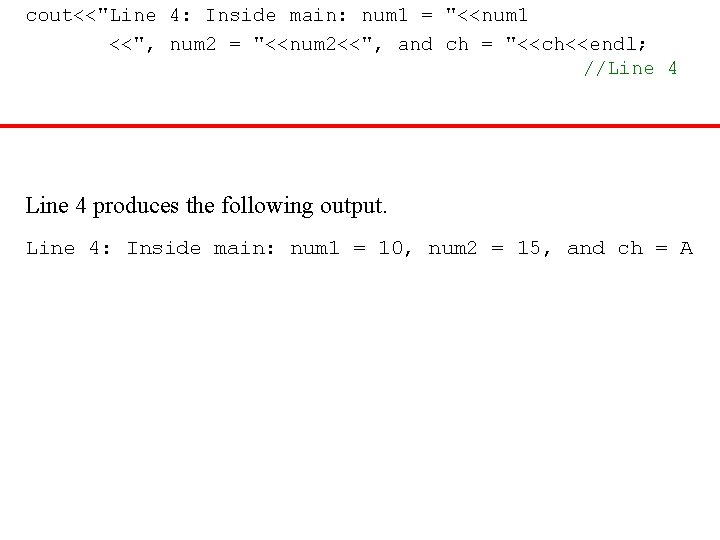
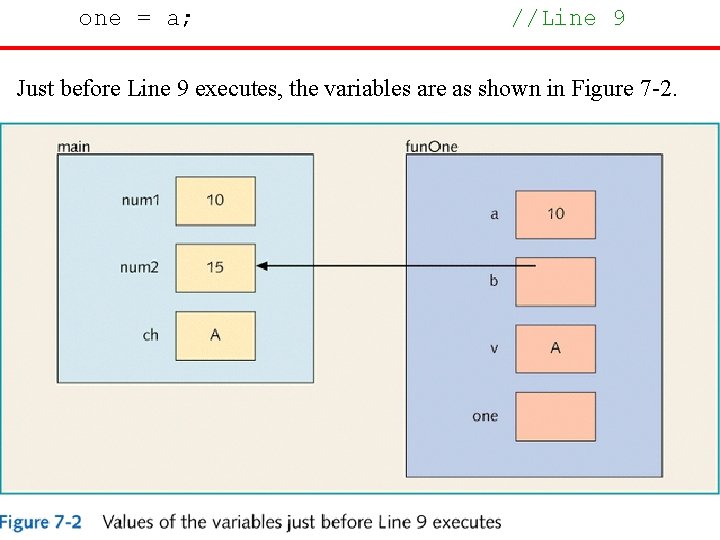
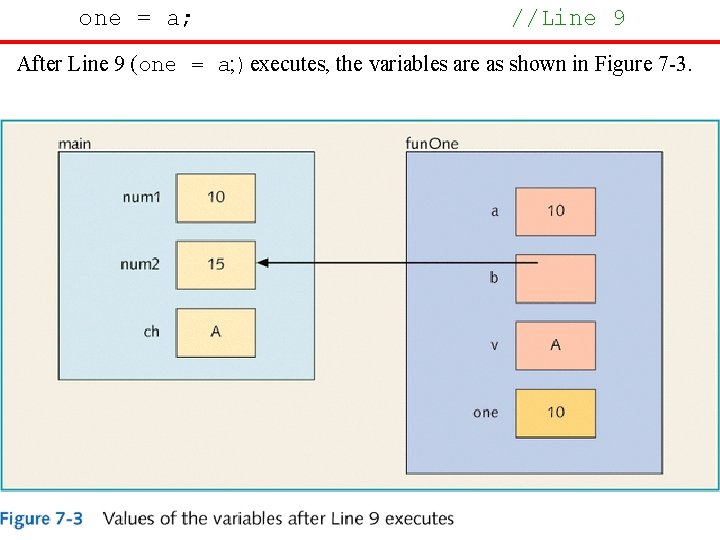
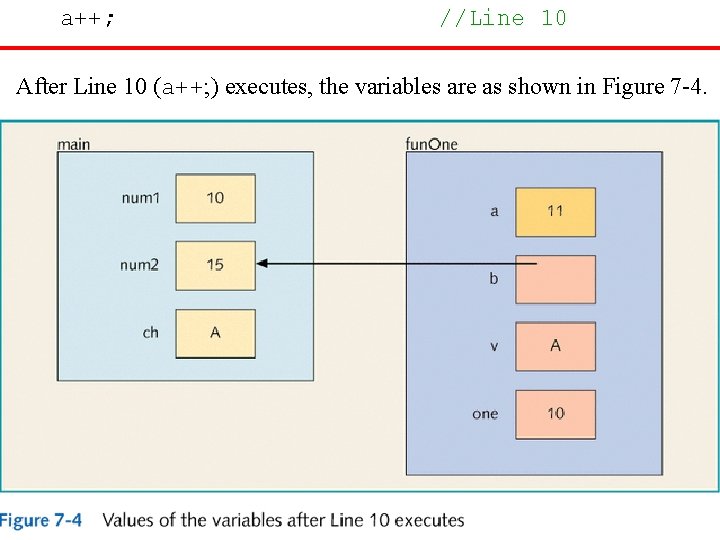
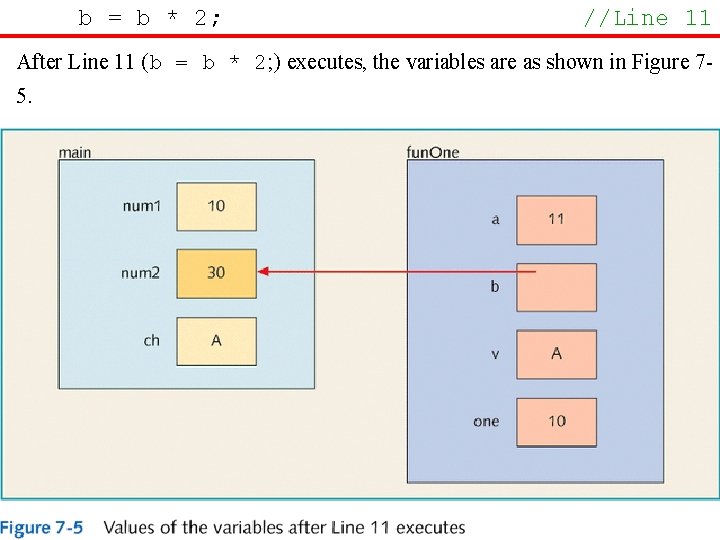
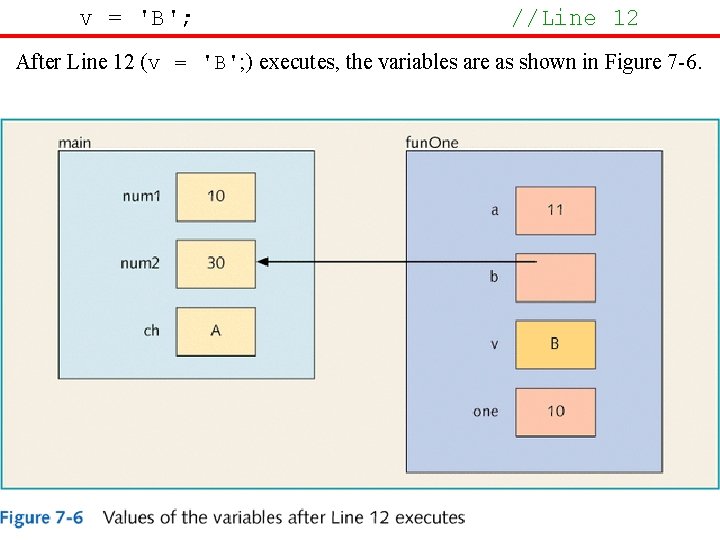
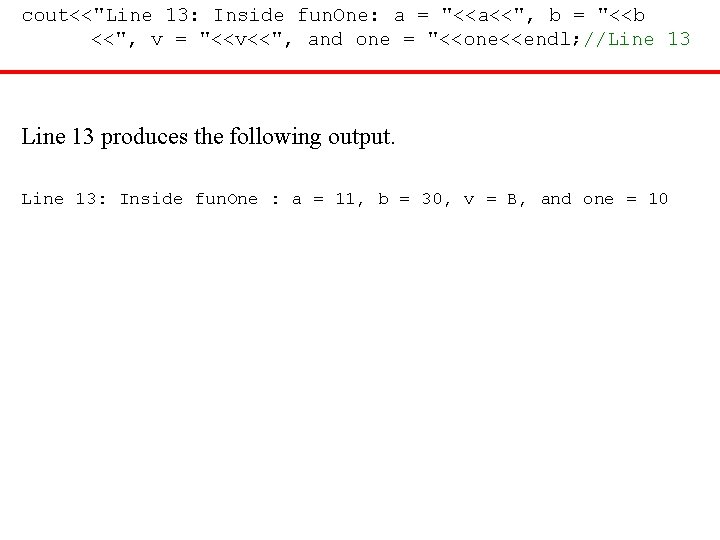
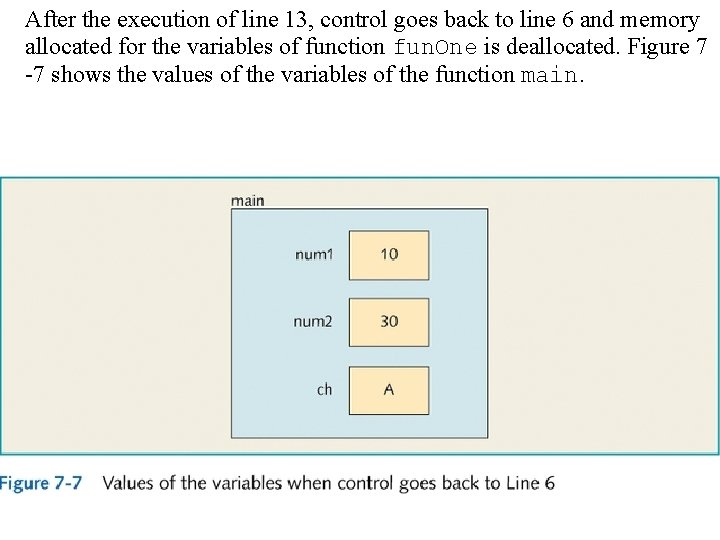
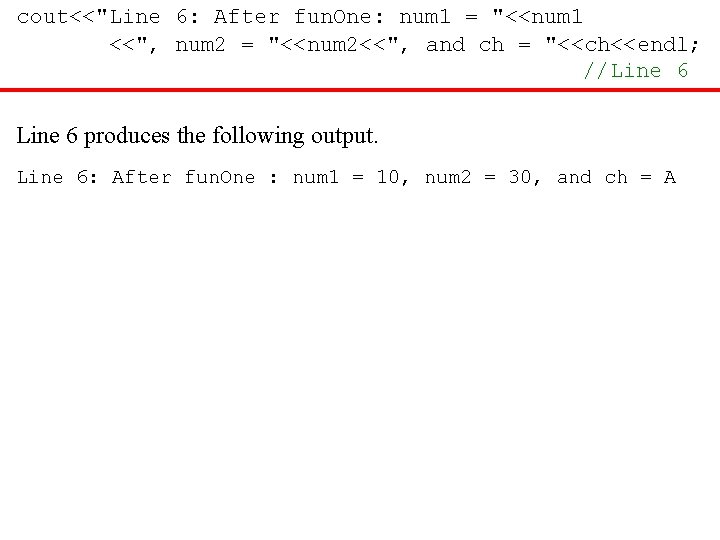
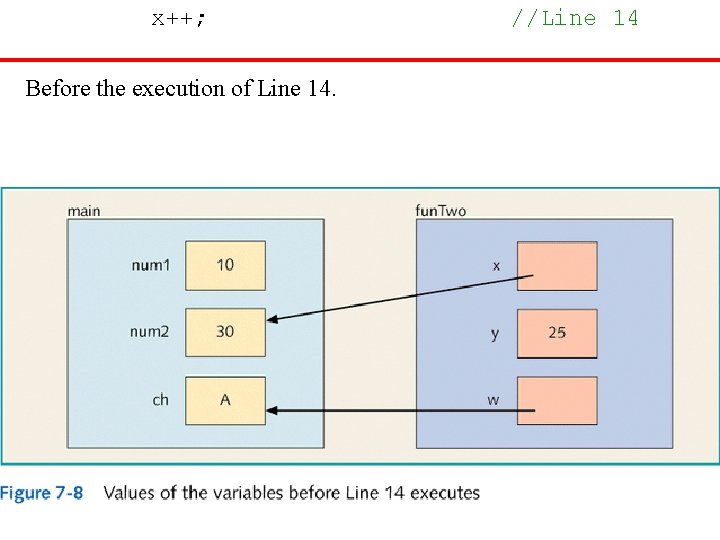
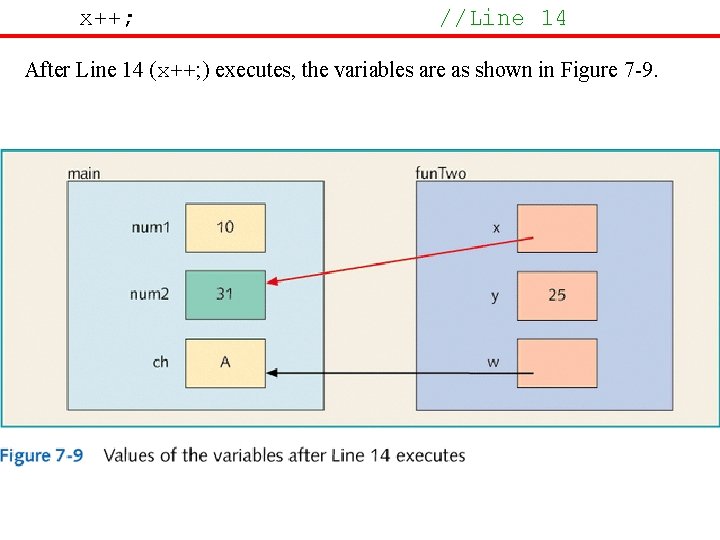
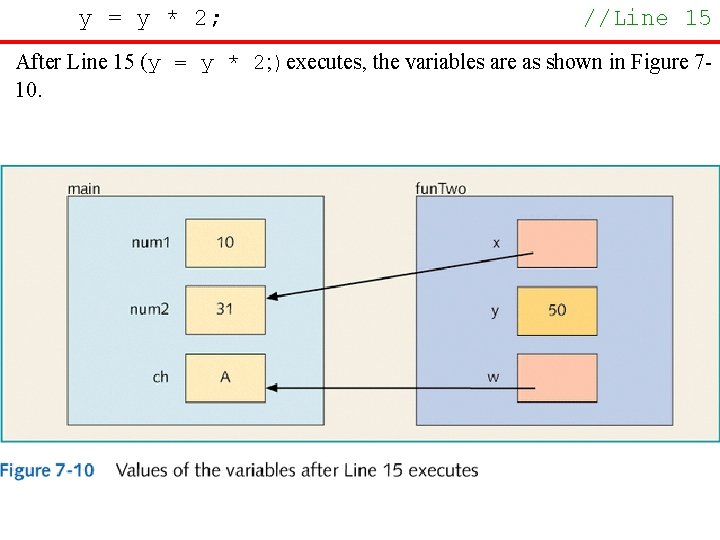
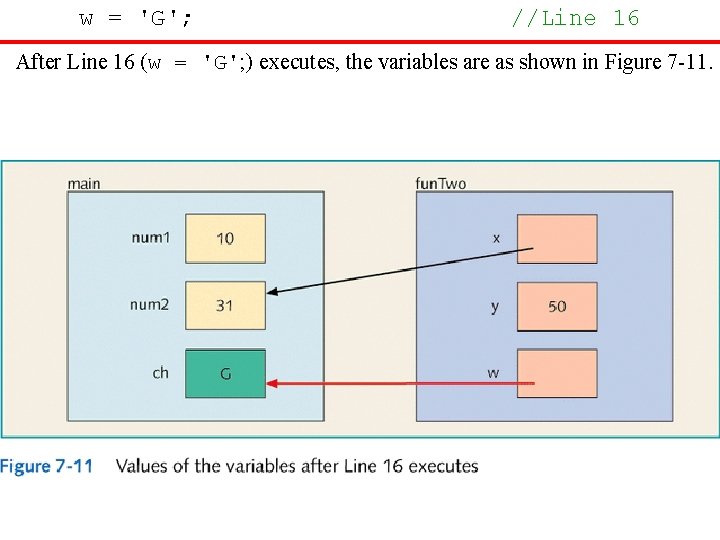
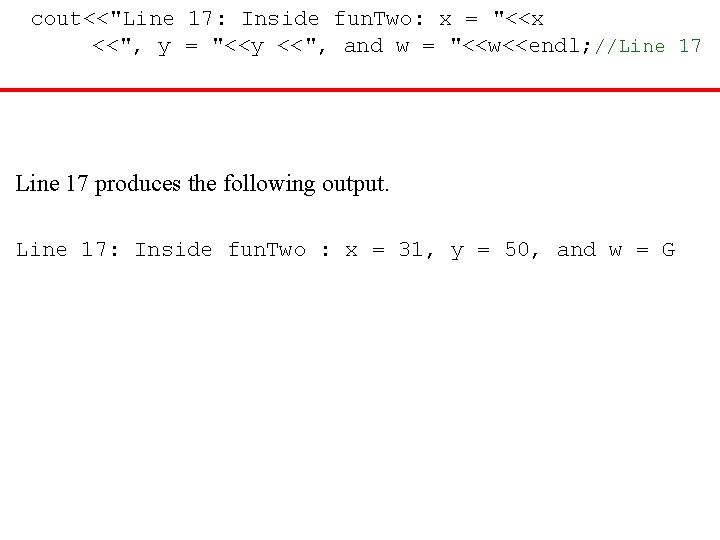
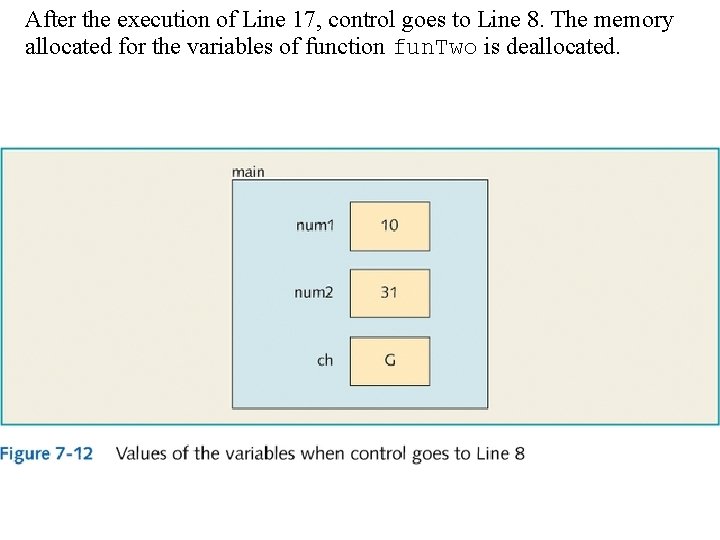
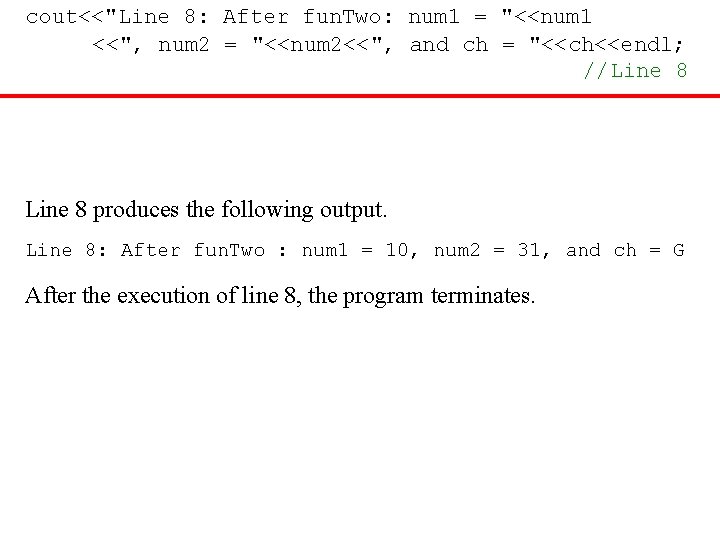
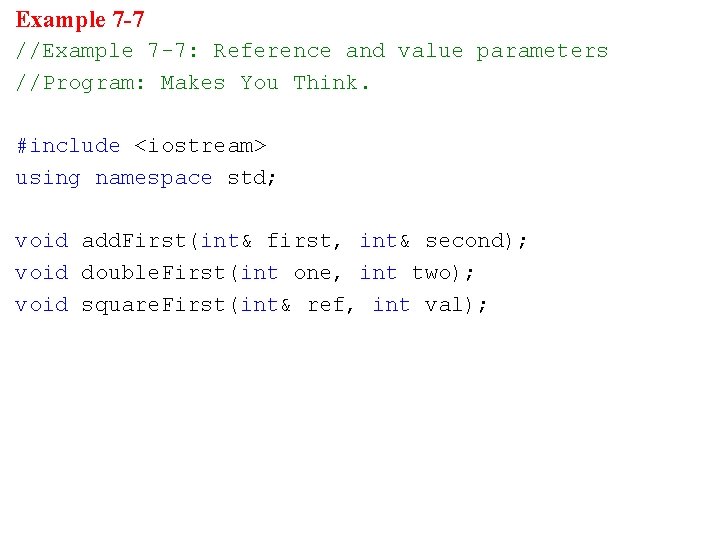
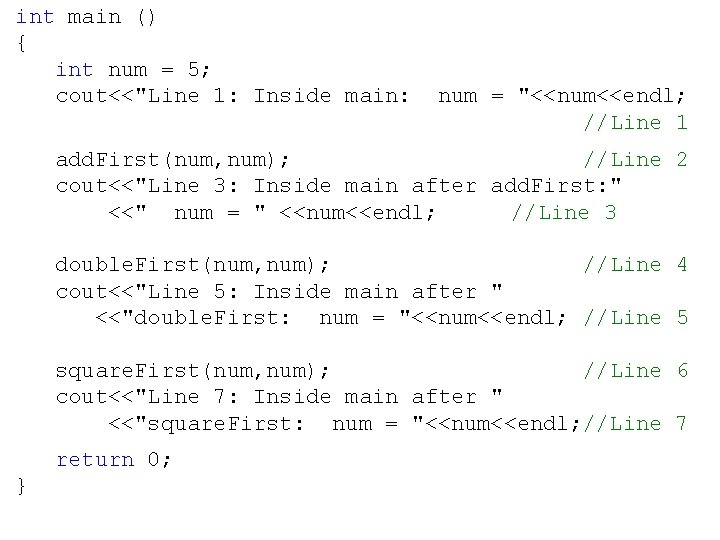
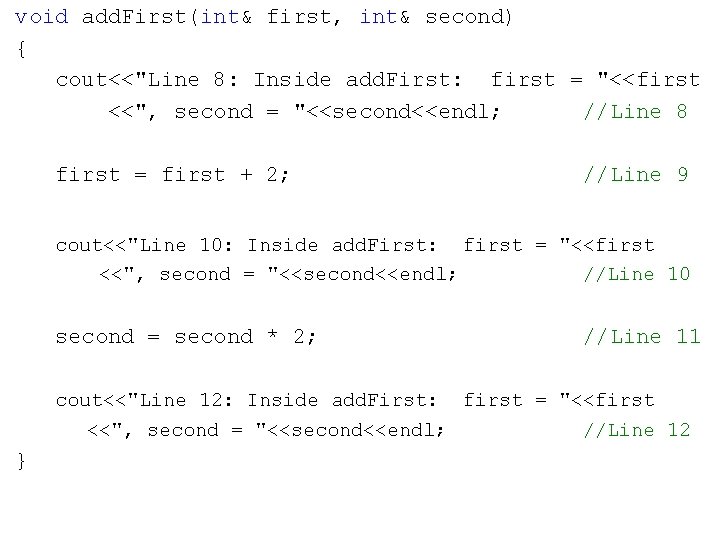
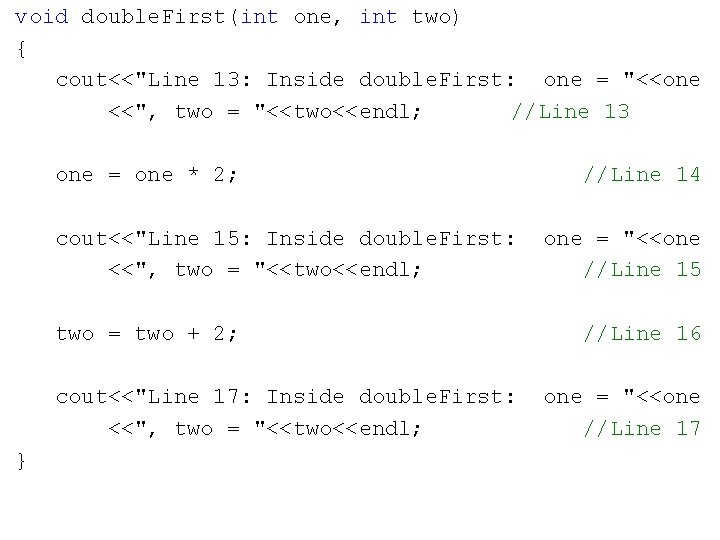
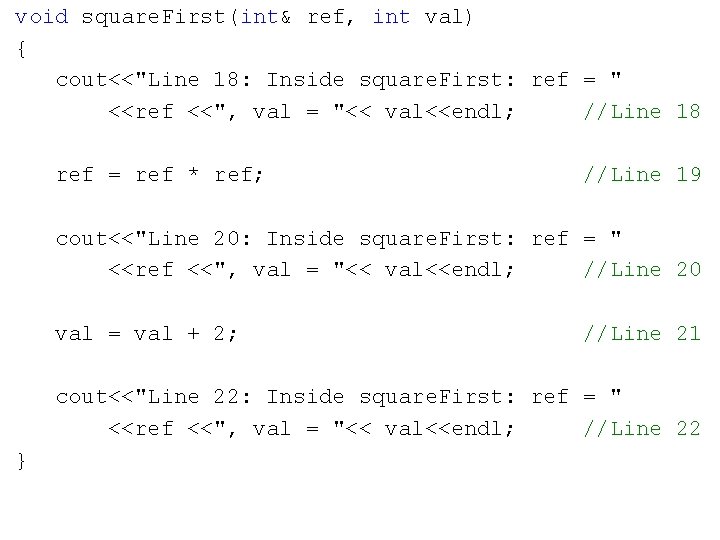
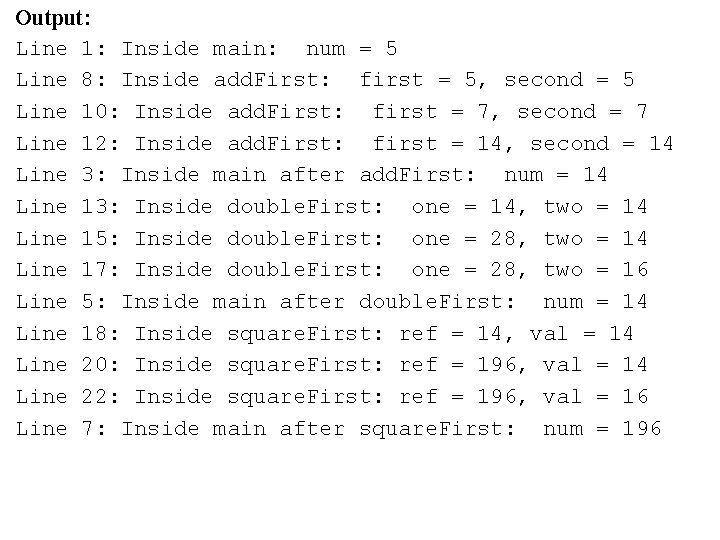
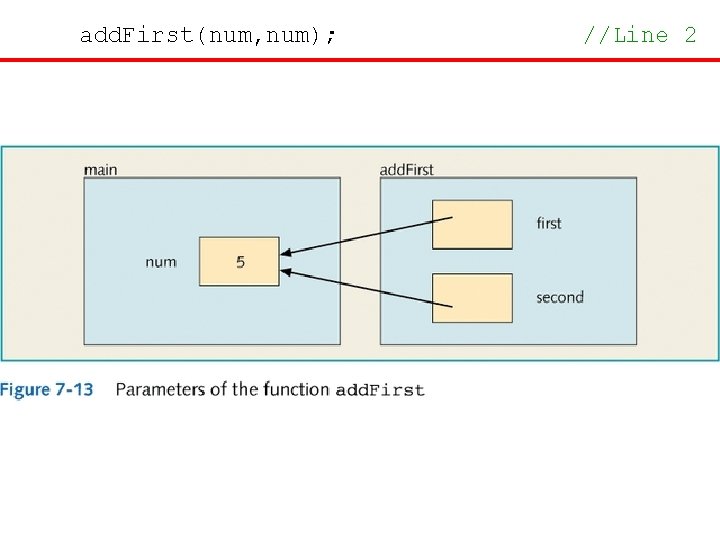
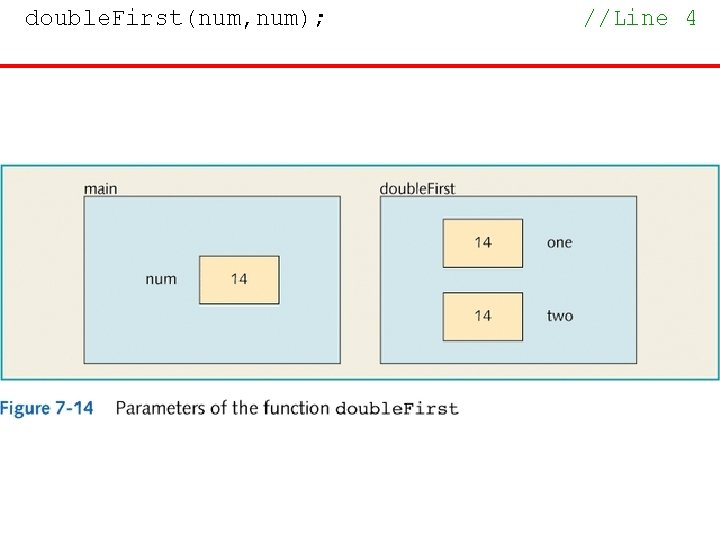
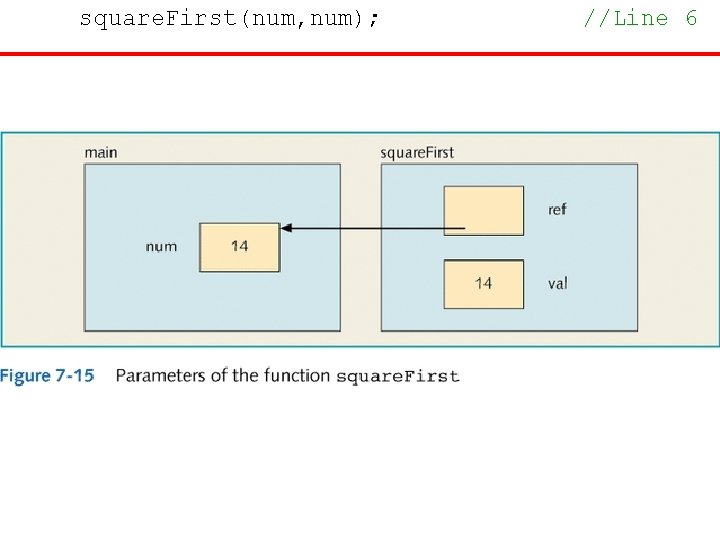
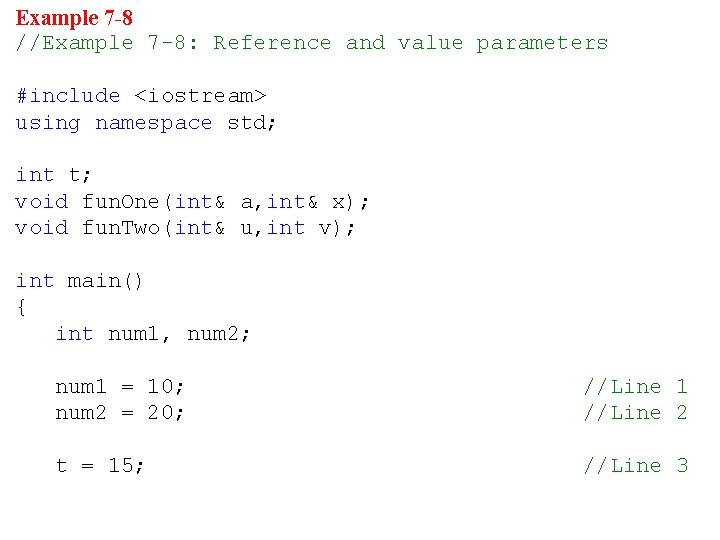
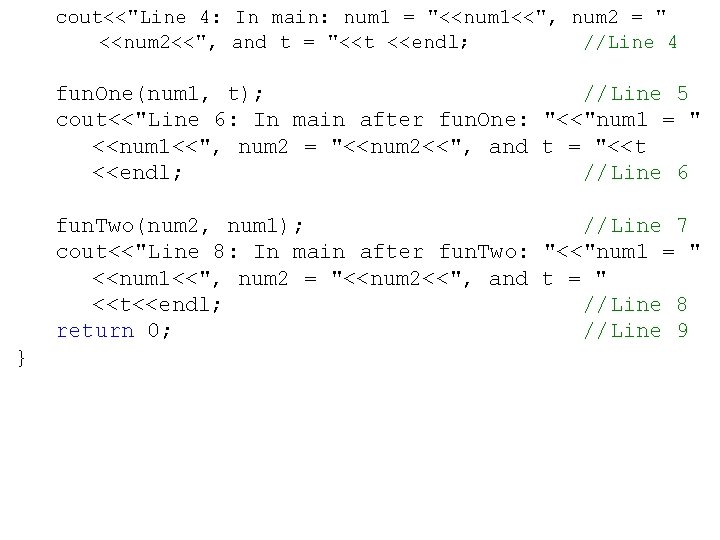
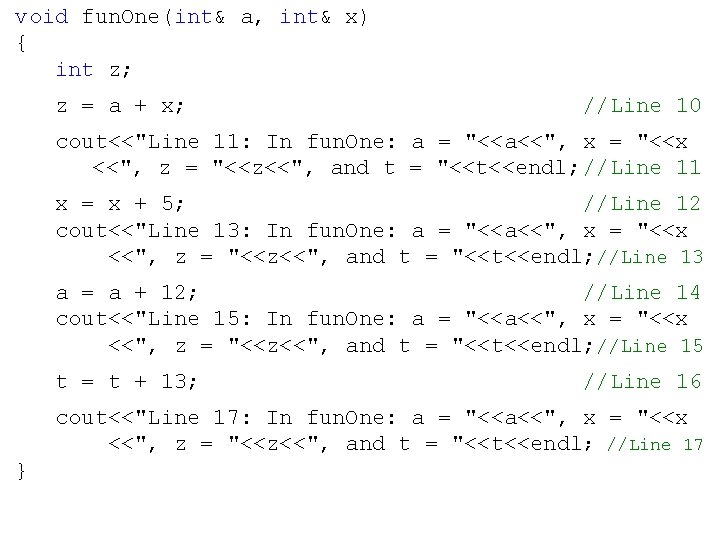
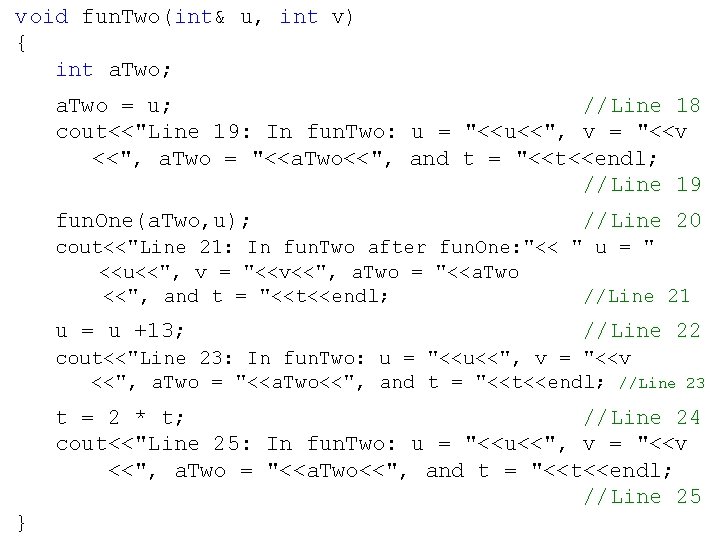
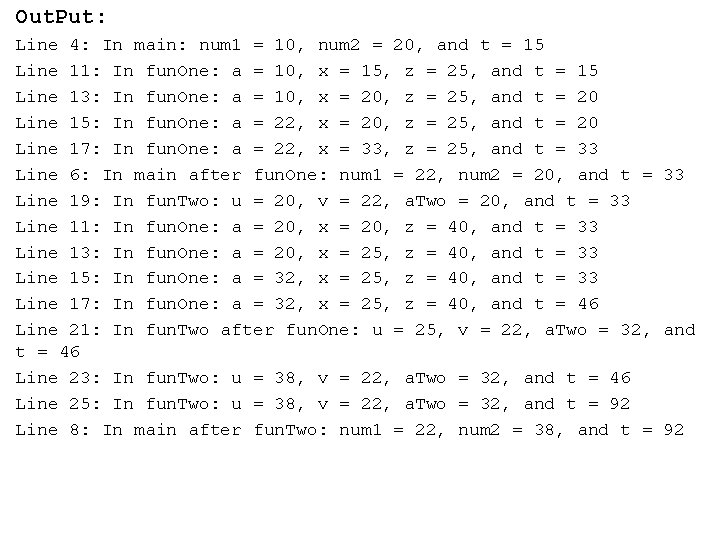
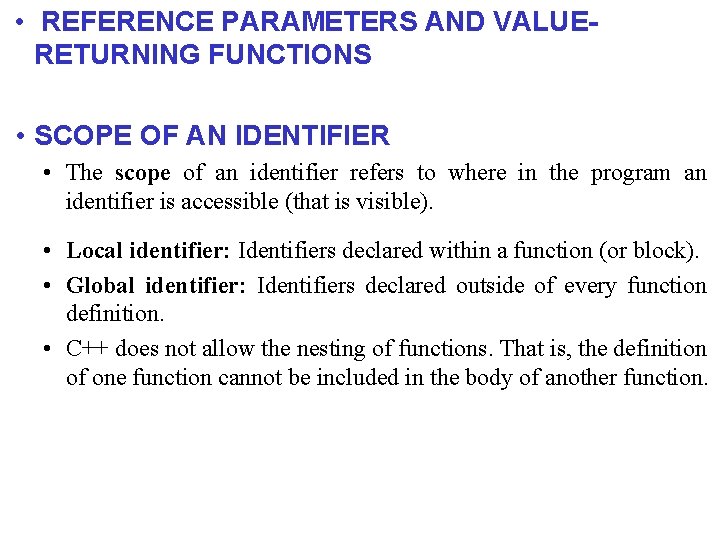
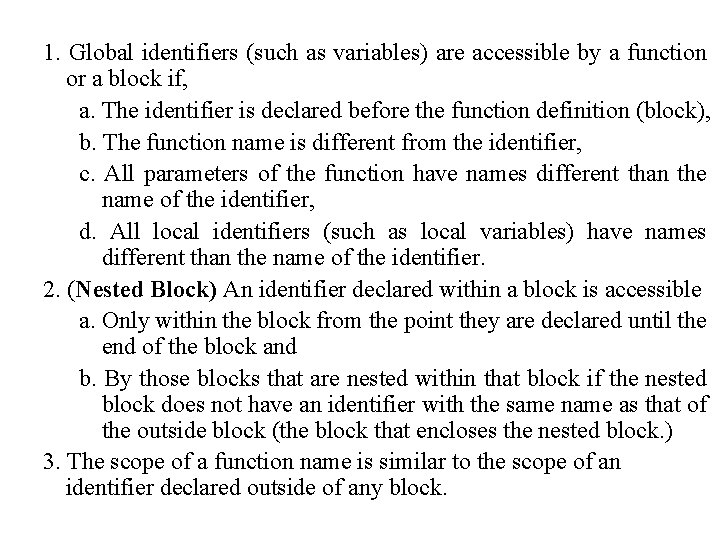
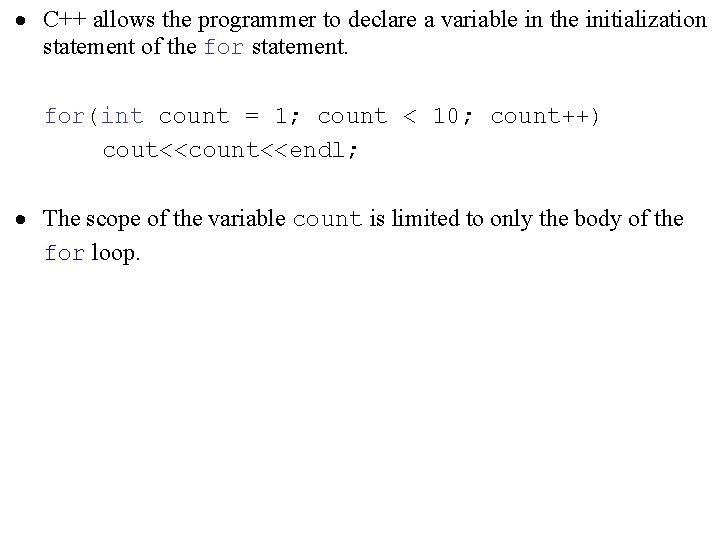
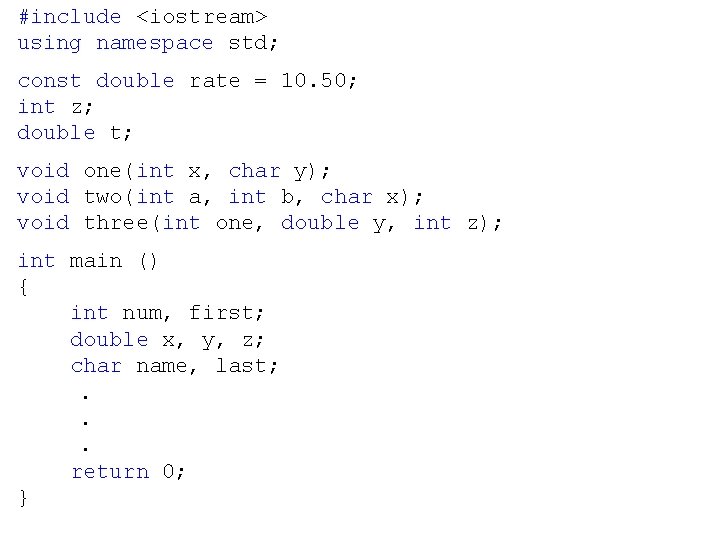
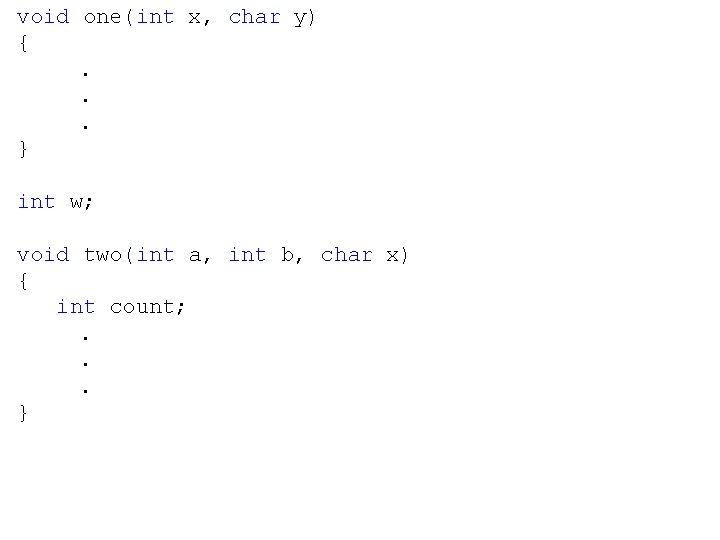
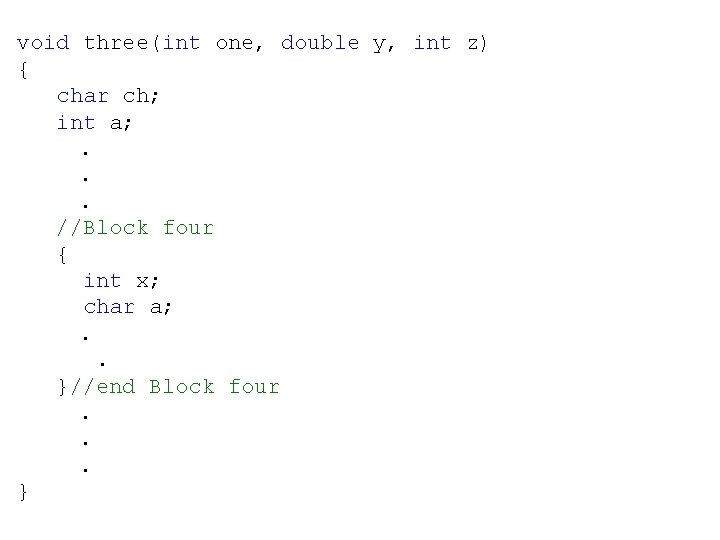
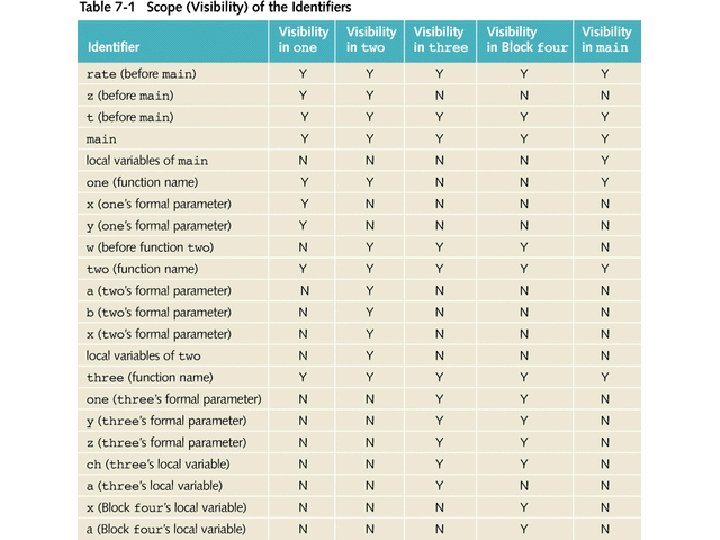
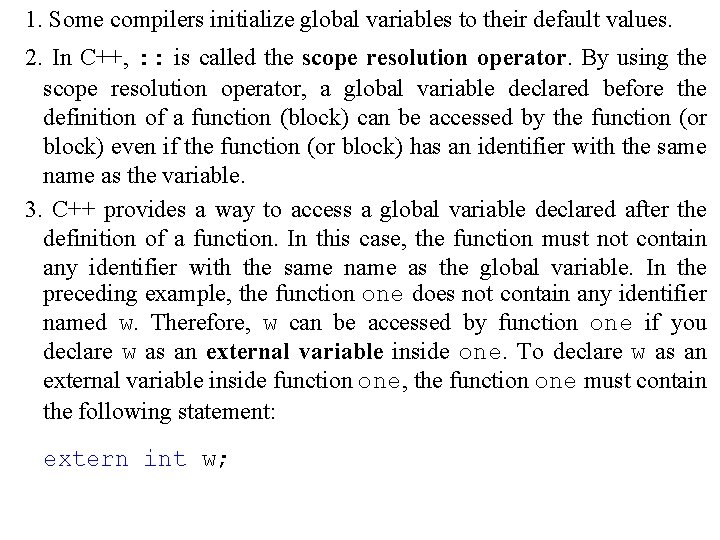
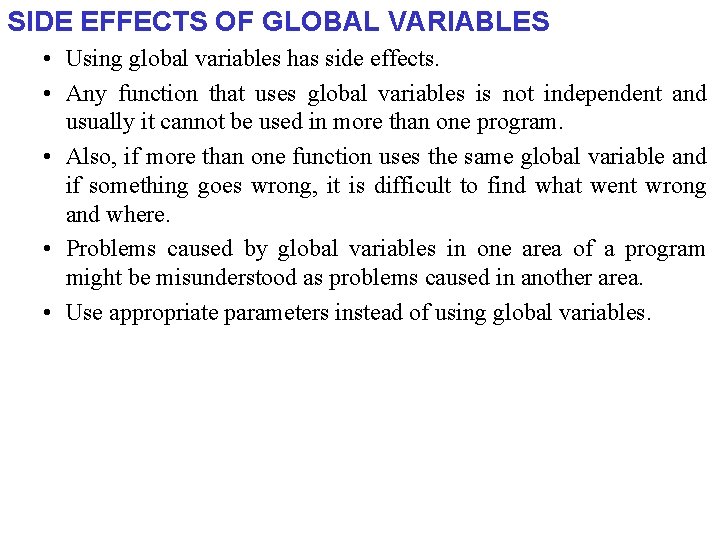
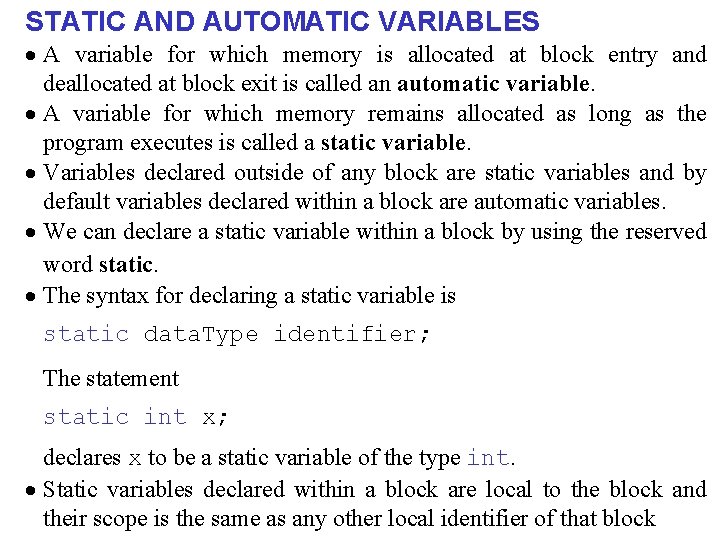
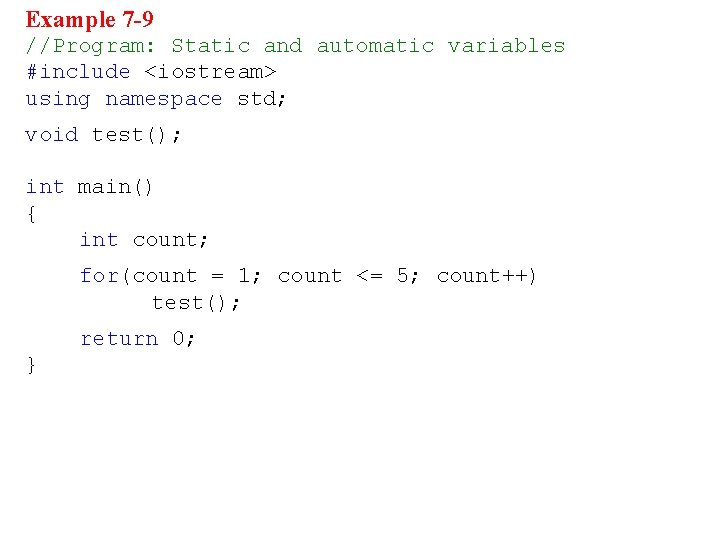
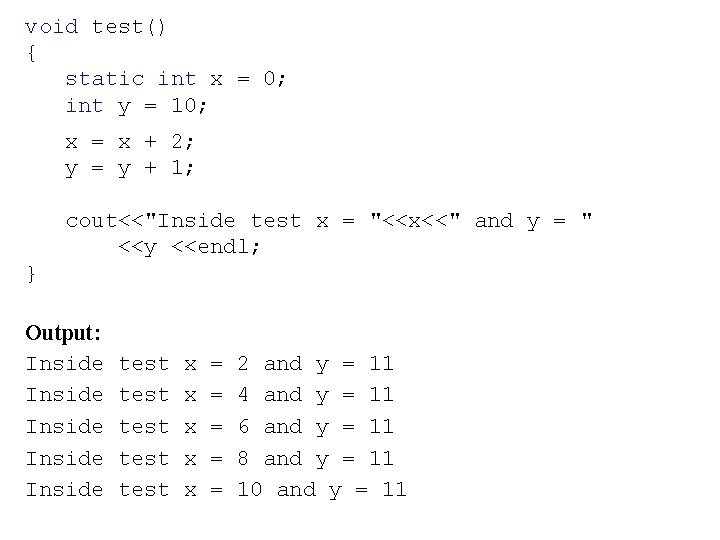
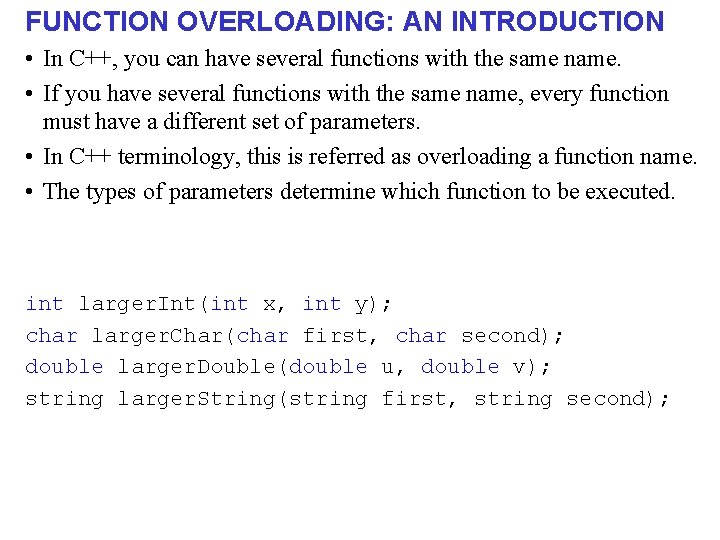
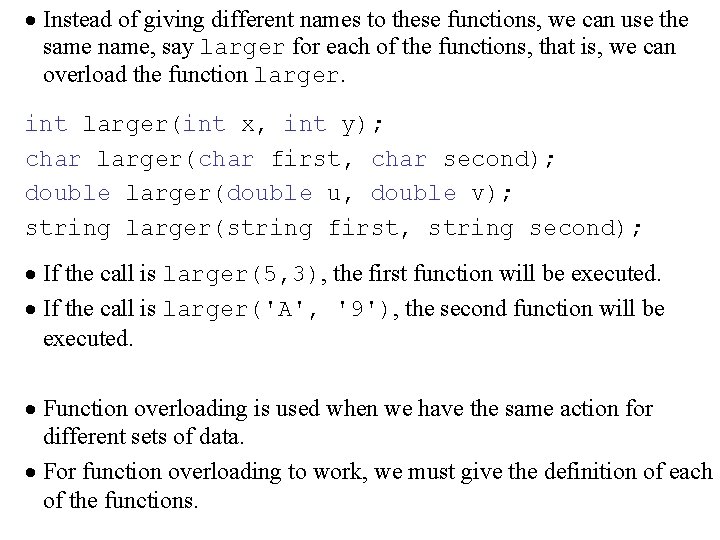
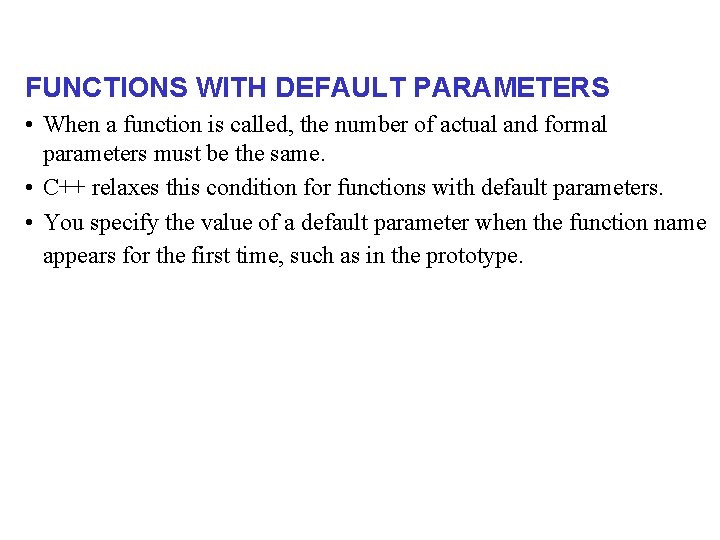
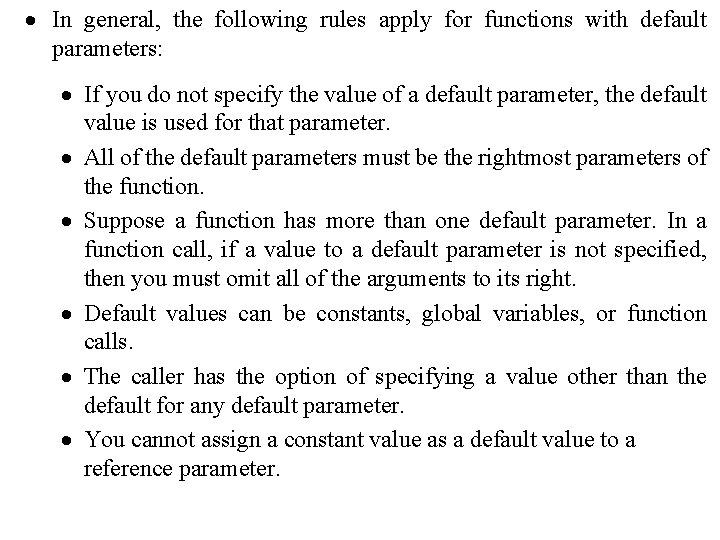
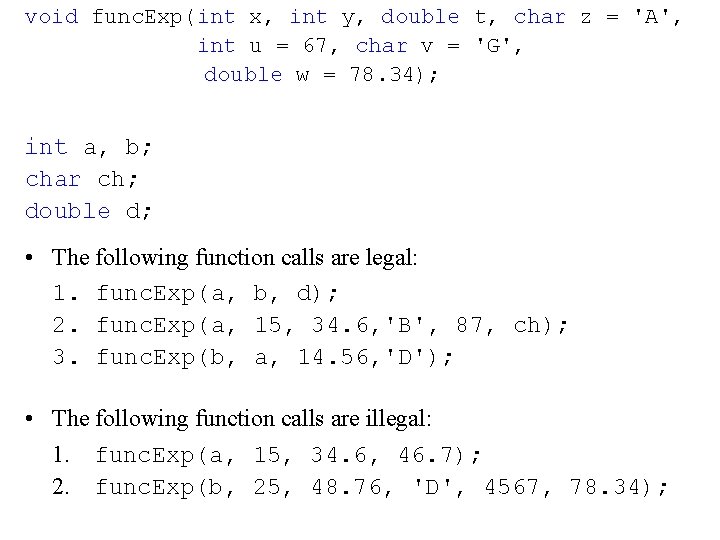
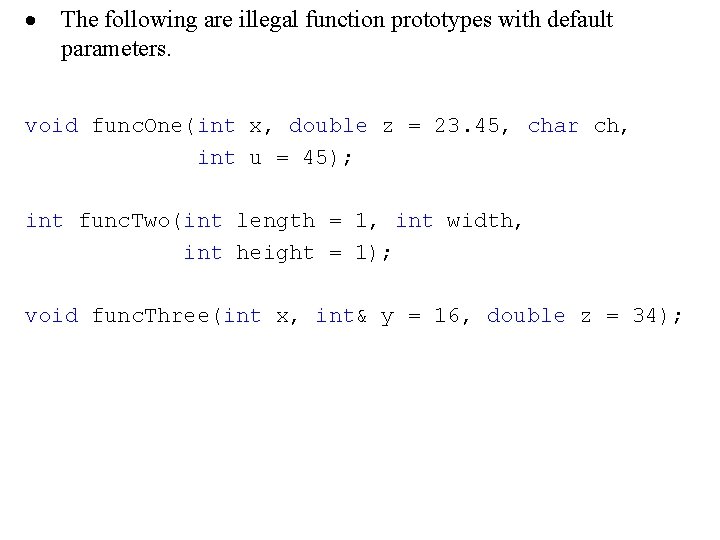
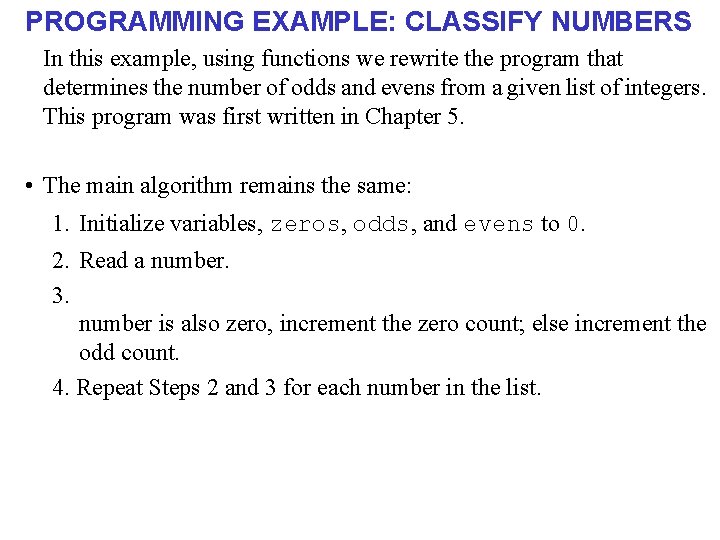
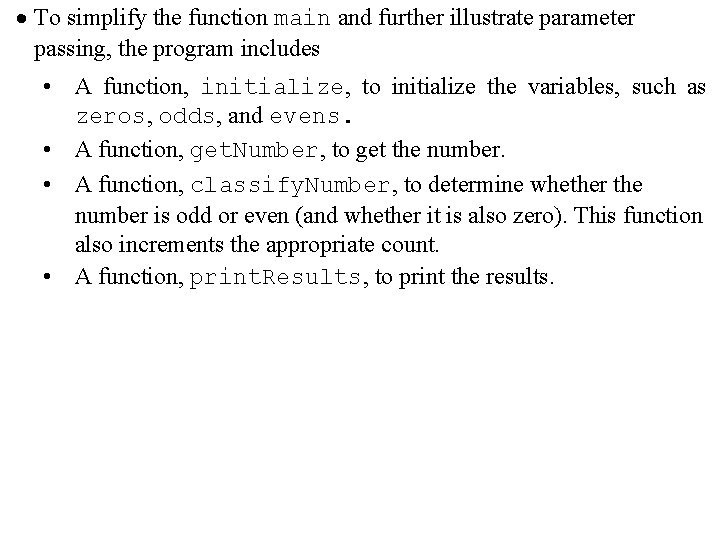
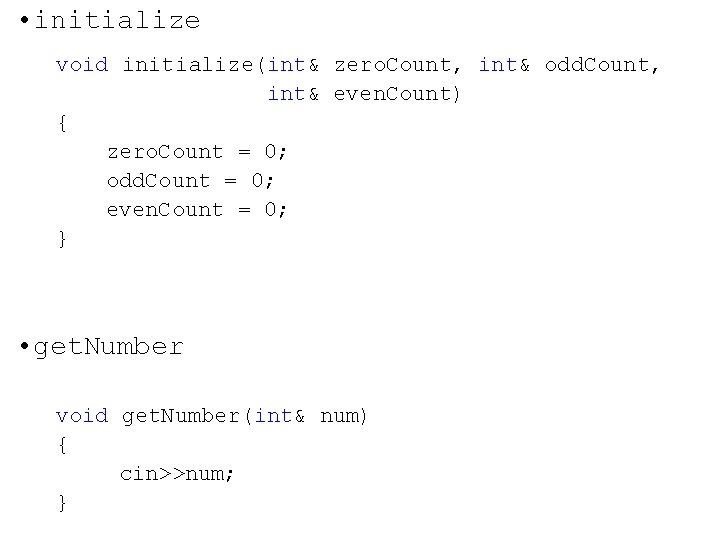
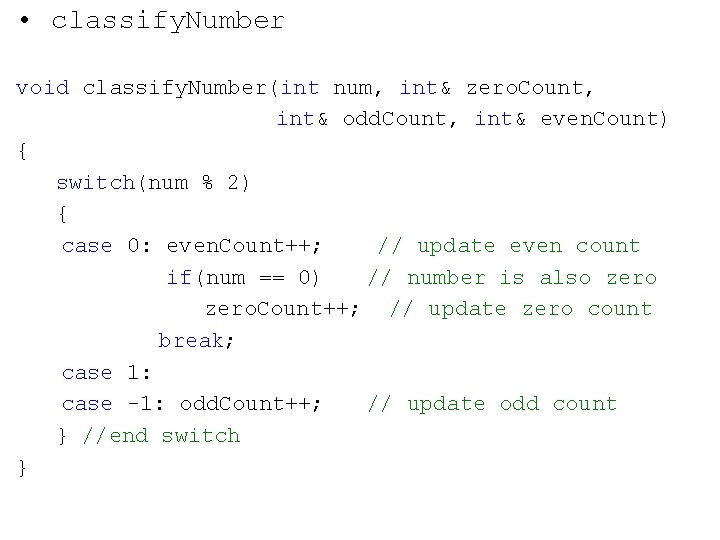
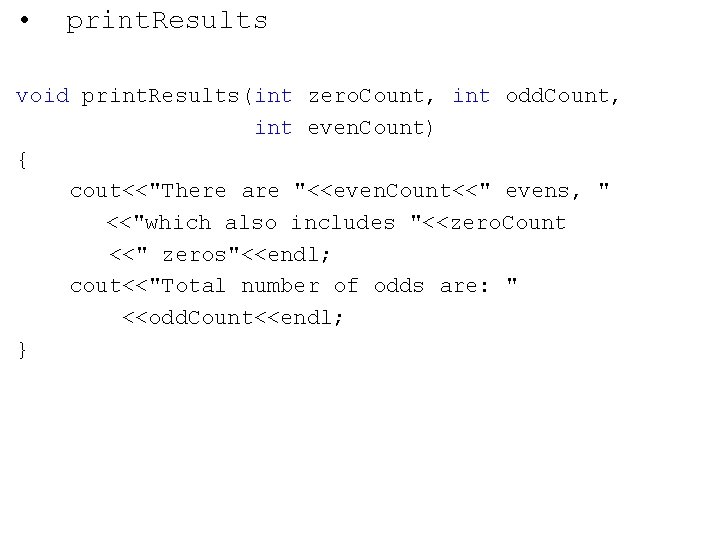
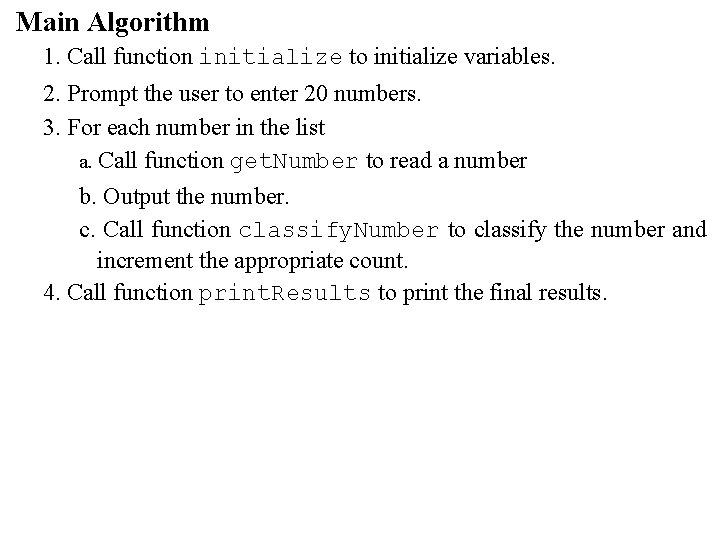
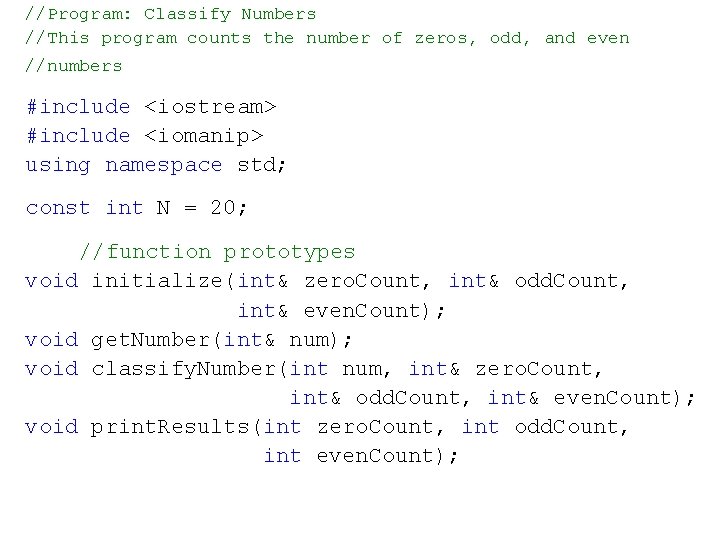
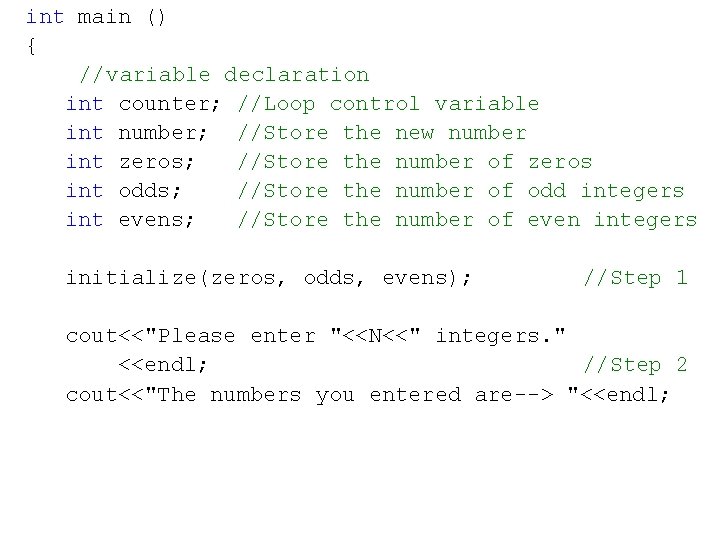
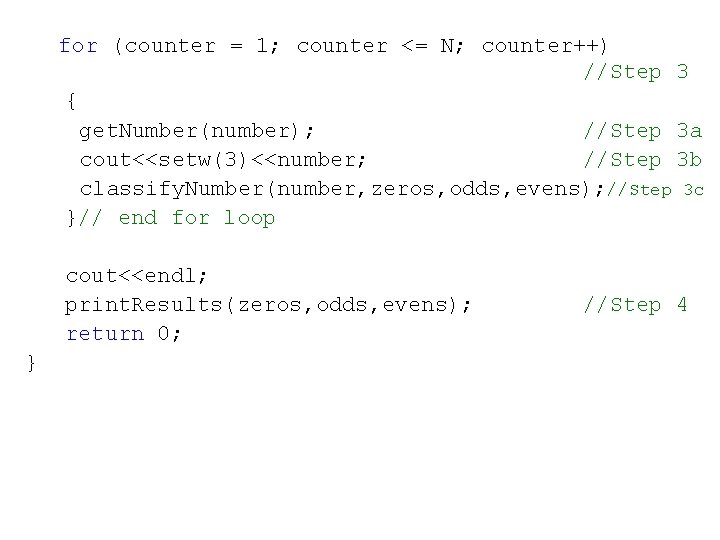
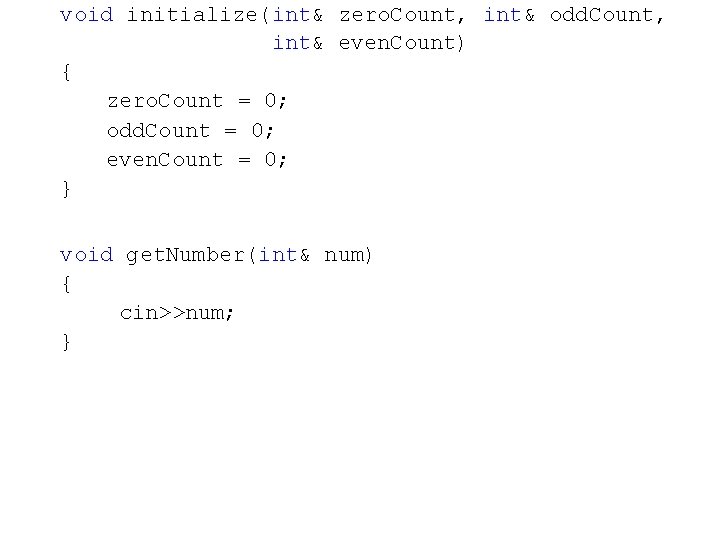
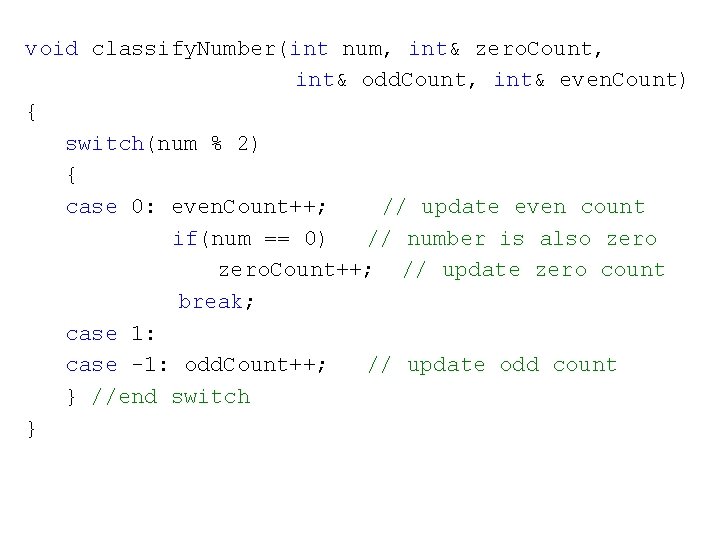
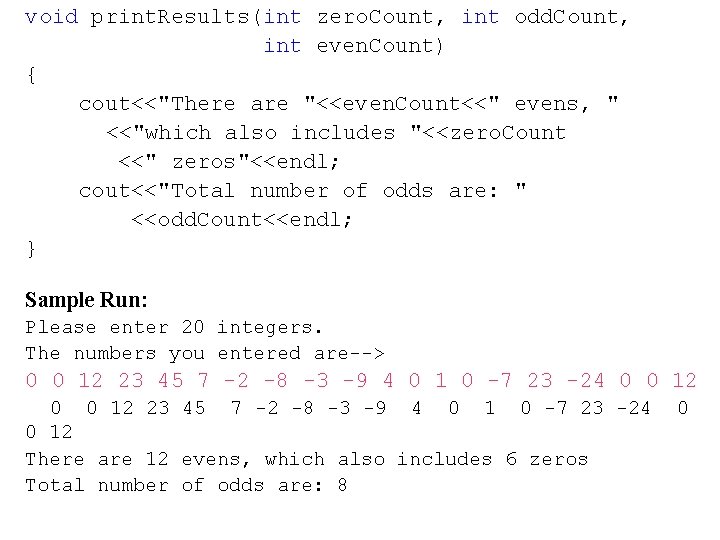
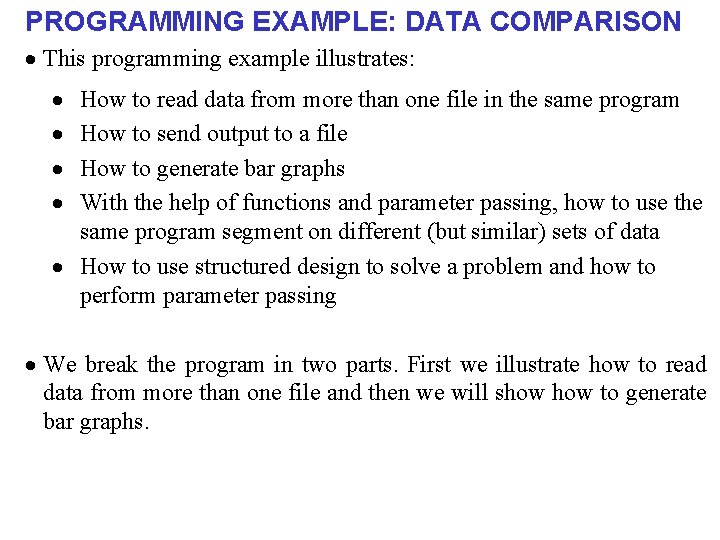
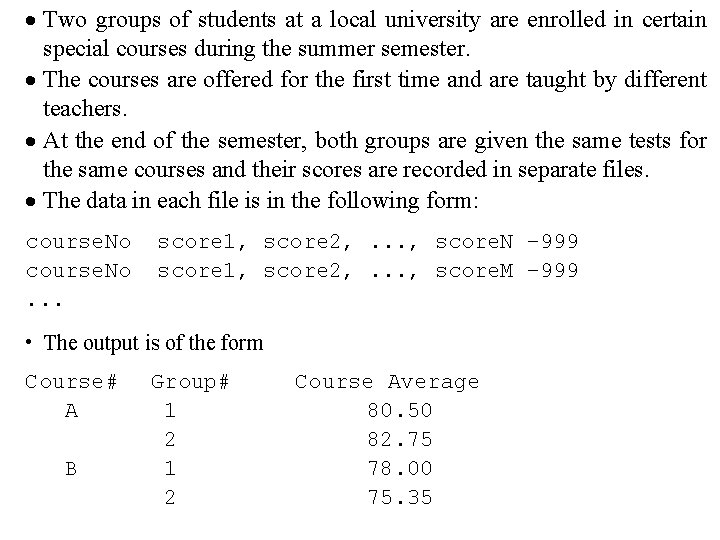
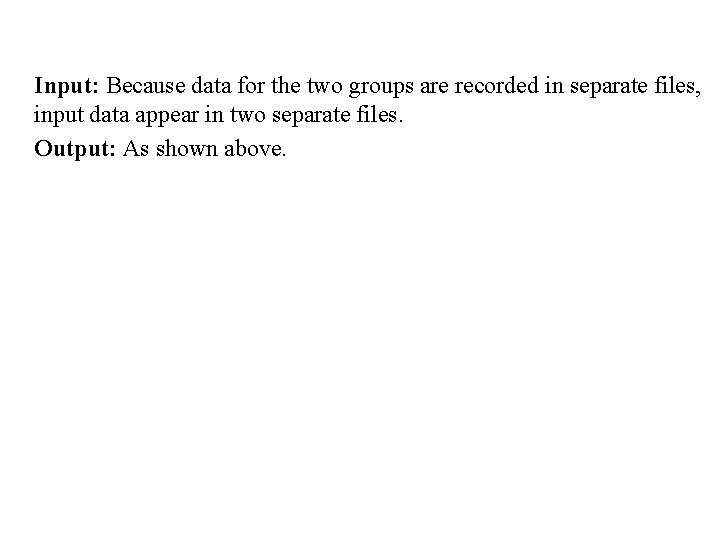
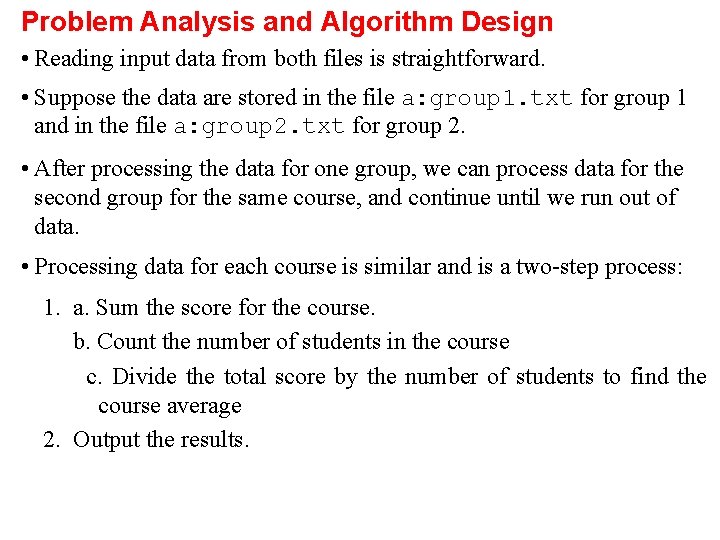
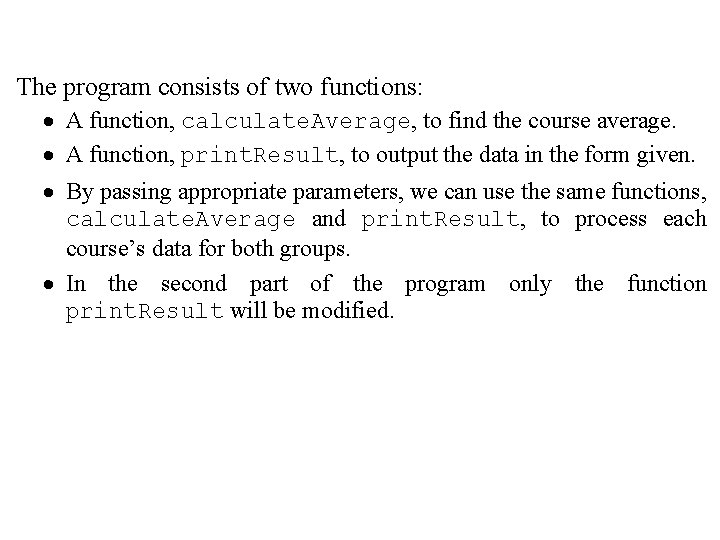
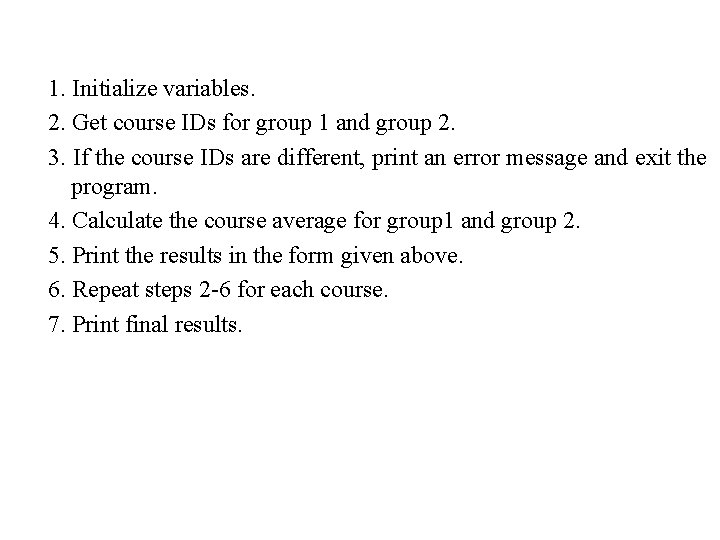
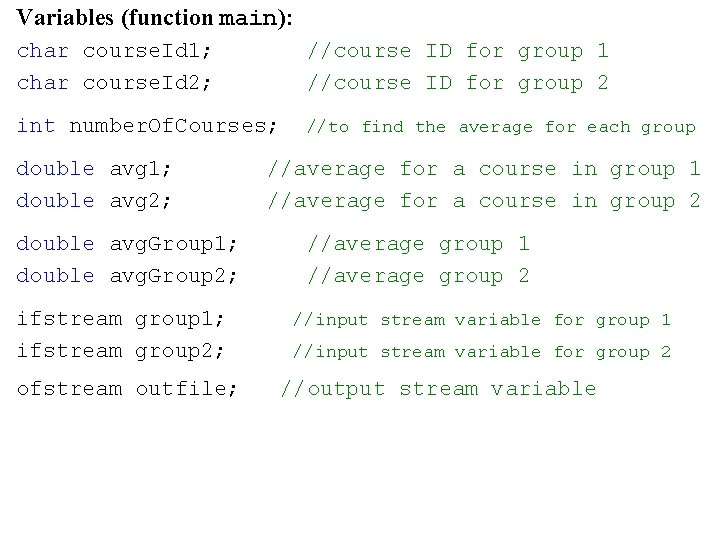
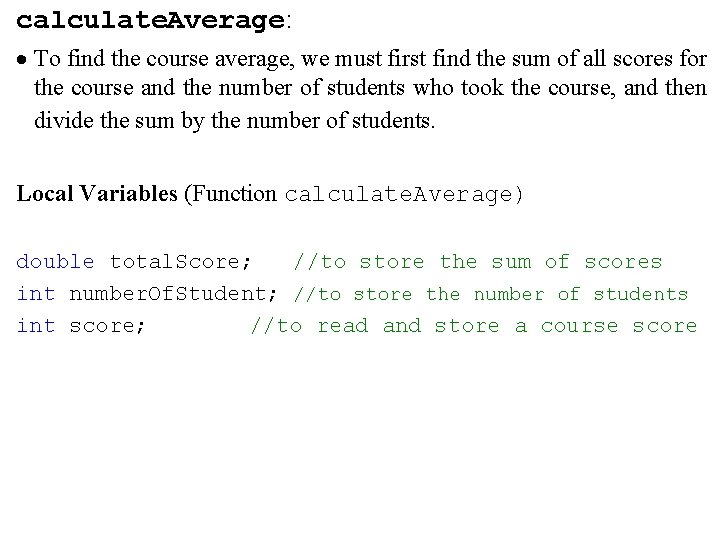
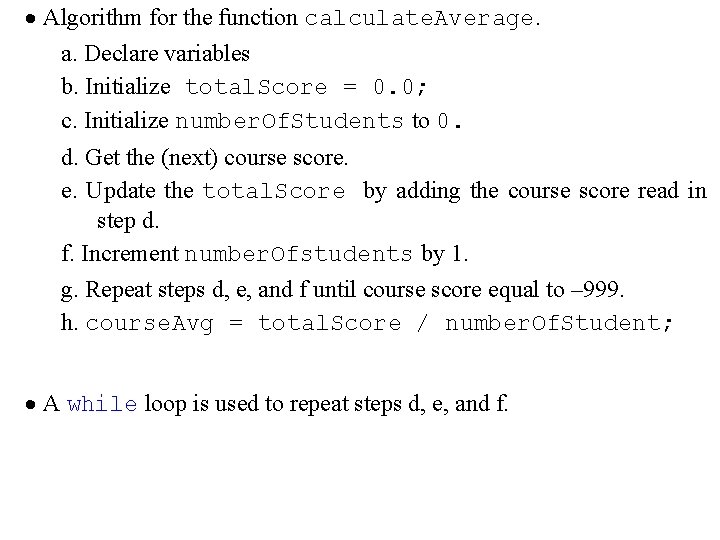
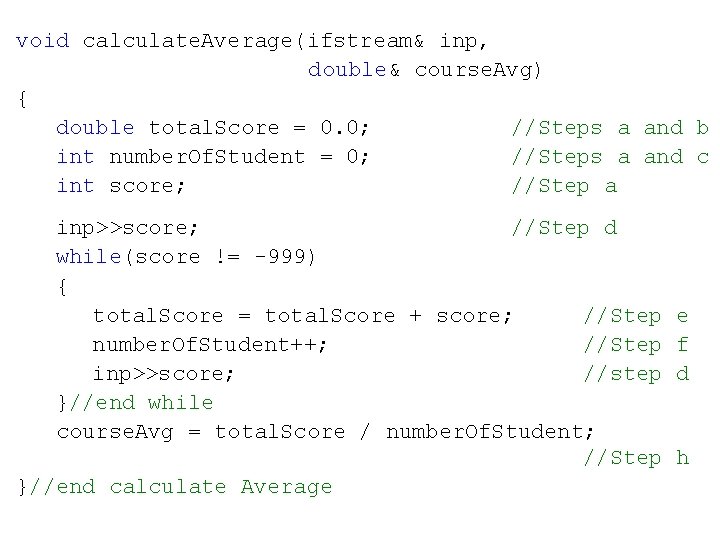
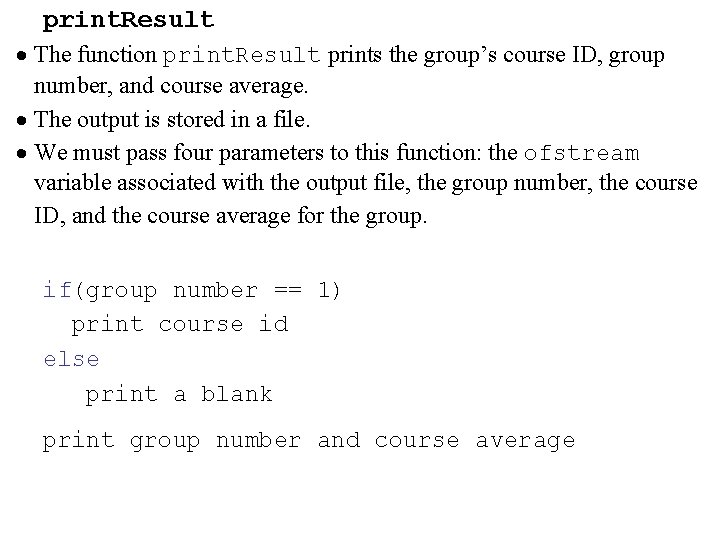
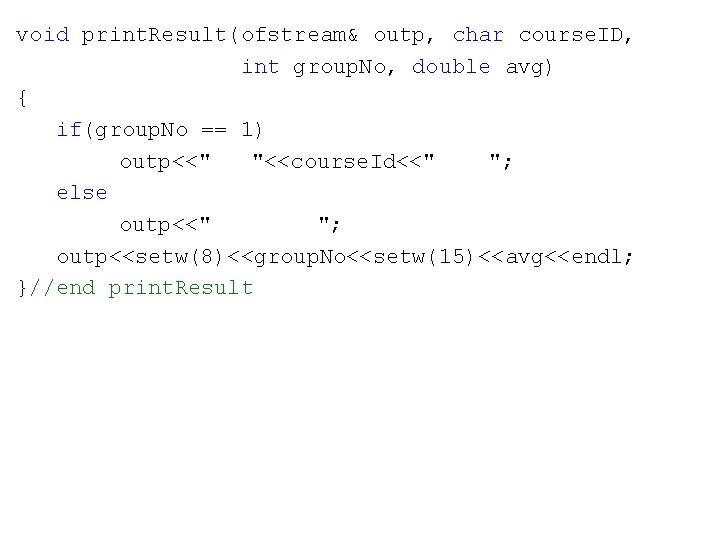
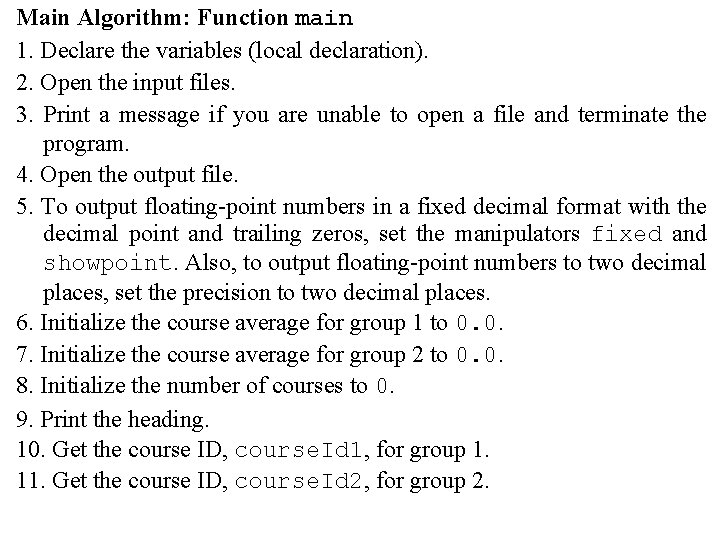
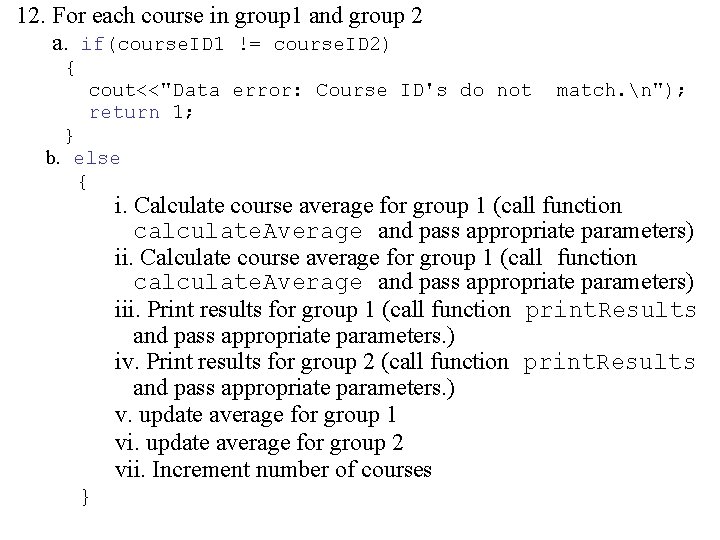
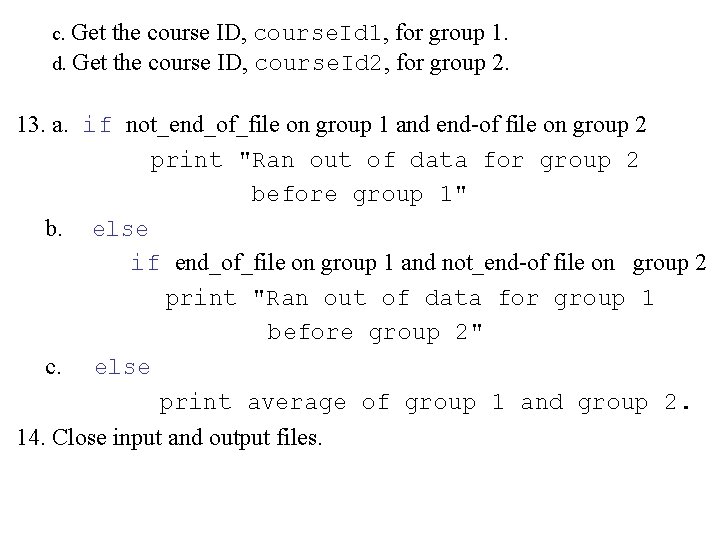
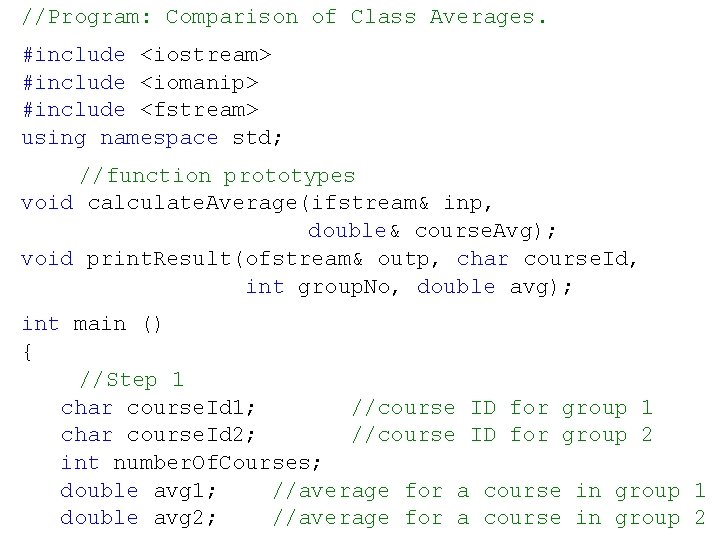
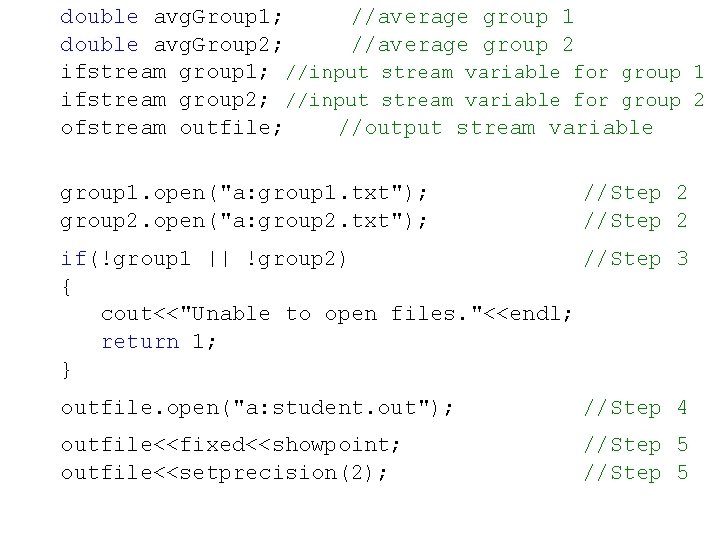
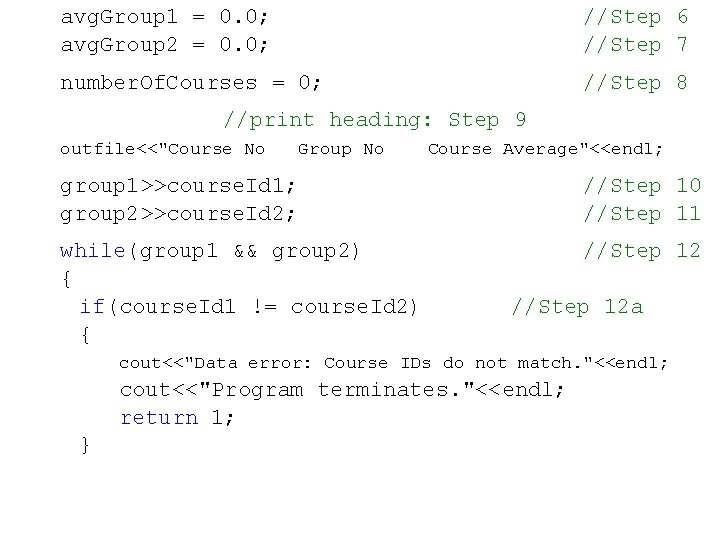
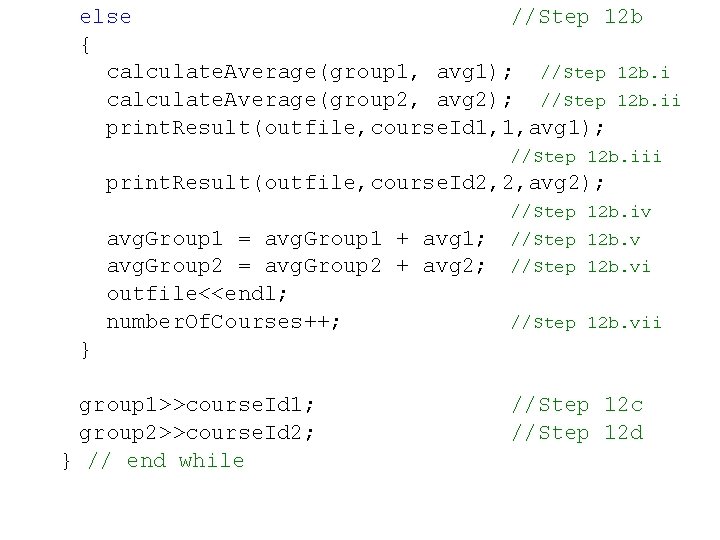
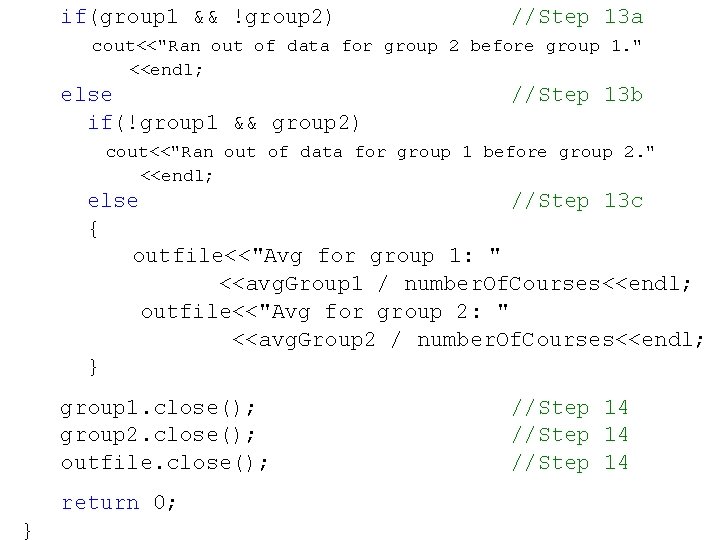
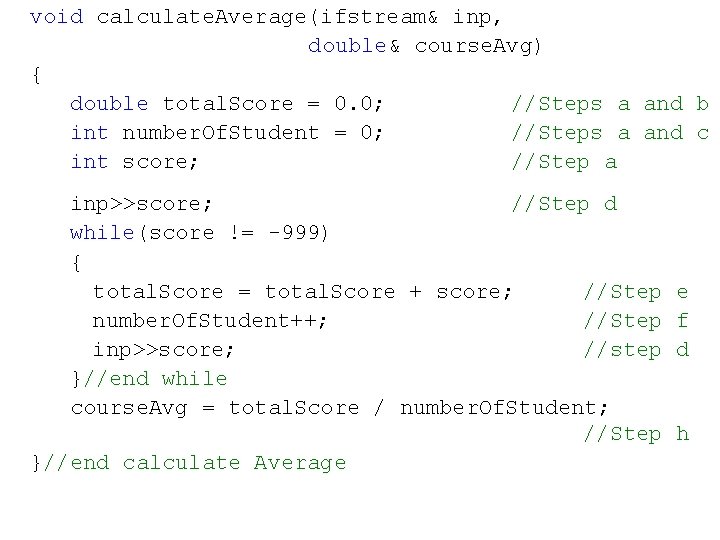
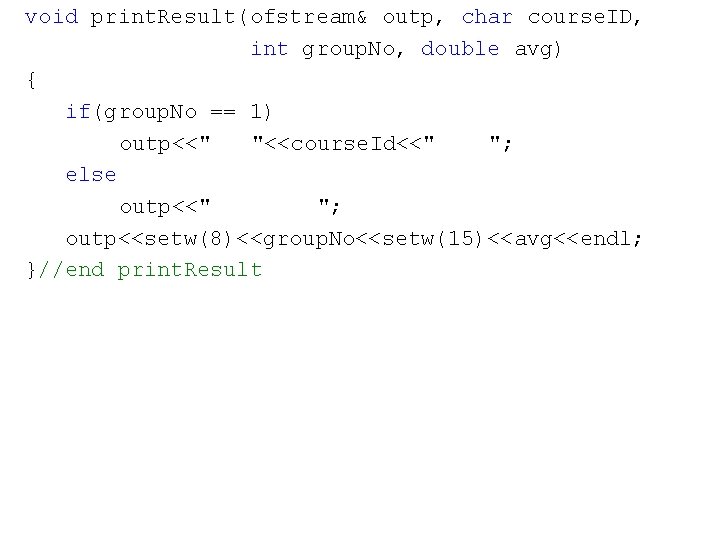
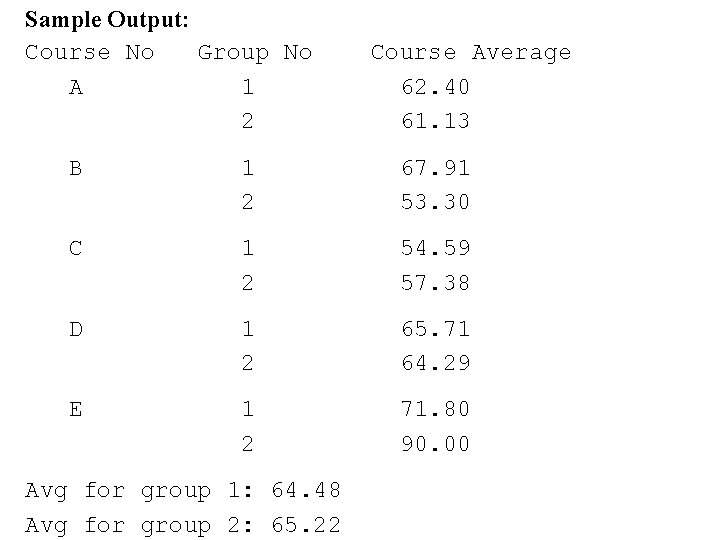
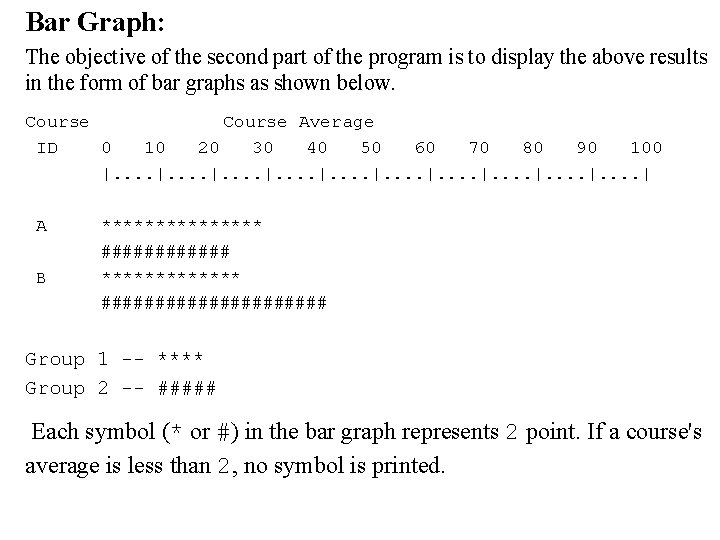
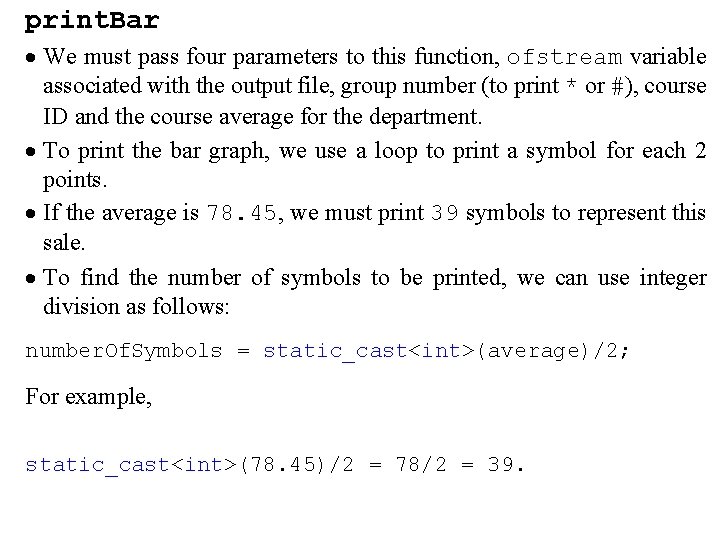
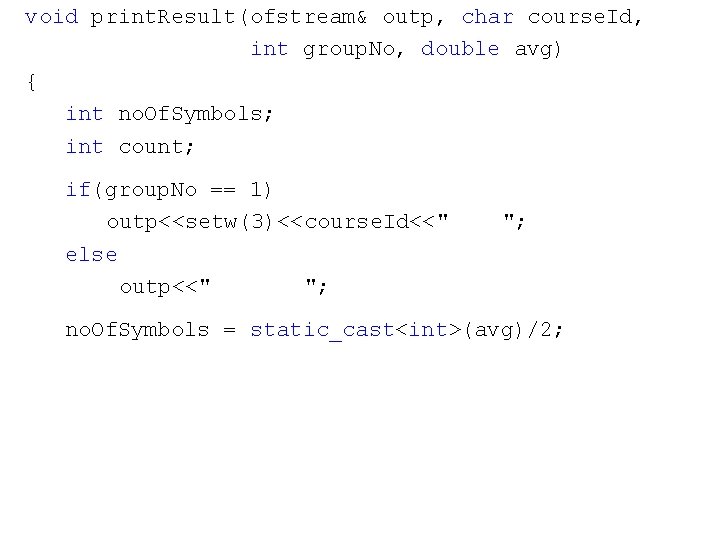
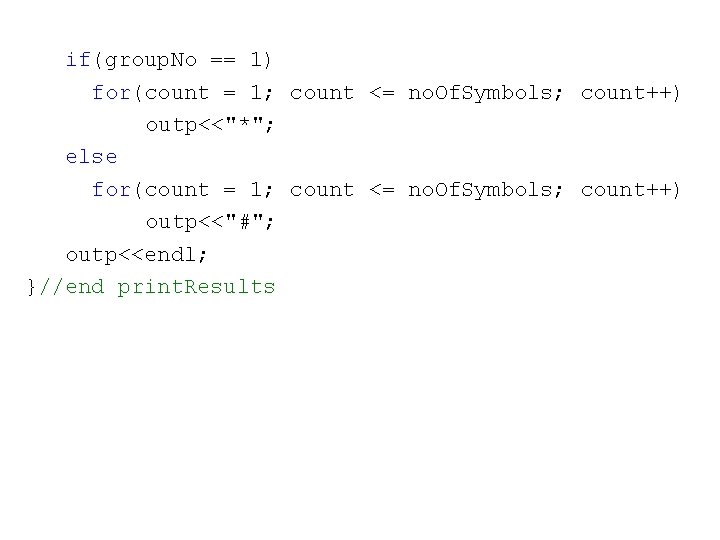
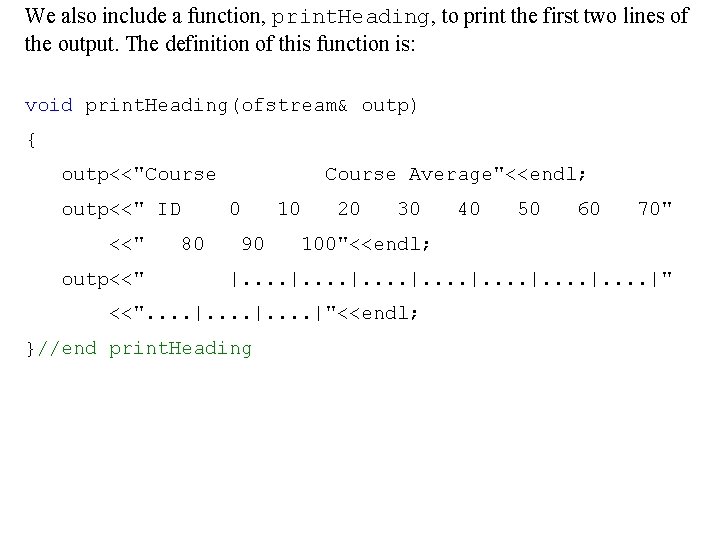
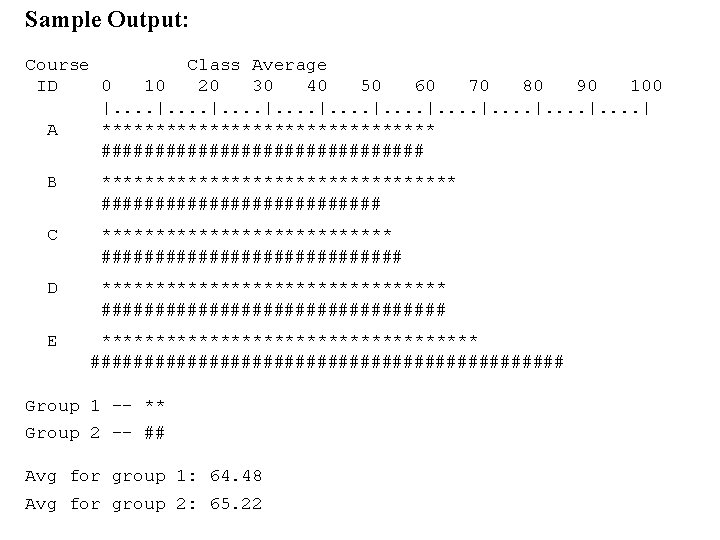
- Slides: 118
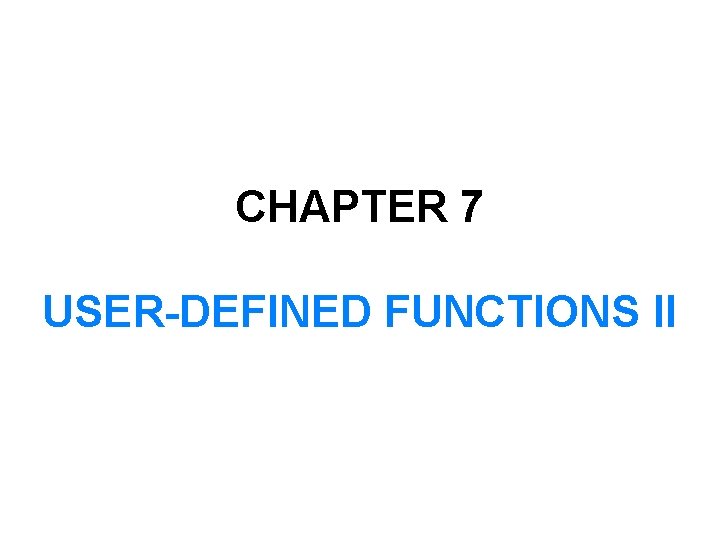
CHAPTER 7 USER-DEFINED FUNCTIONS II
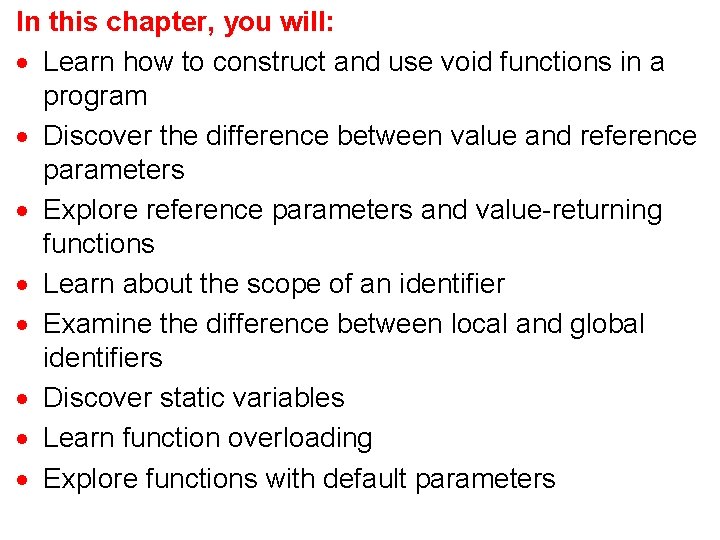
In this chapter, you will: · Learn how to construct and use void functions in a program · Discover the difference between value and reference parameters · Explore reference parameters and value-returning functions · Learn about the scope of an identifier · Examine the difference between local and global identifiers · Discover static variables · Learn function overloading · Explore functions with default parameters
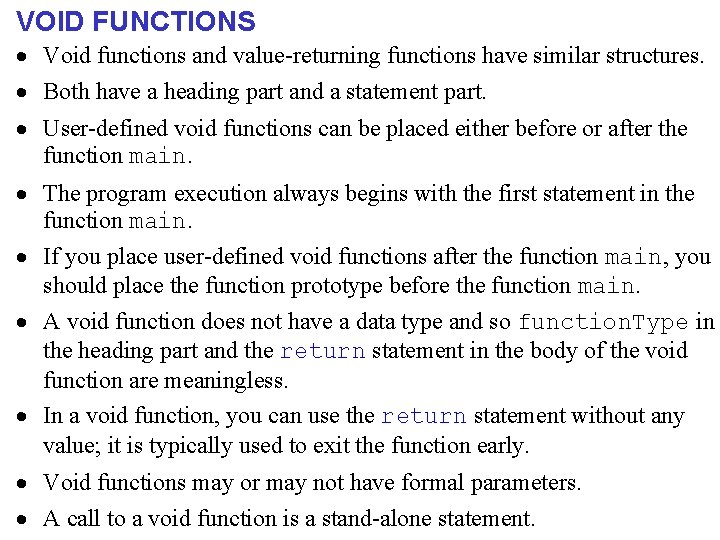
VOID FUNCTIONS · Void functions and value-returning functions have similar structures. · Both have a heading part and a statement part. · User-defined void functions can be placed either before or after the function main. · The program execution always begins with the first statement in the function main. · If you place user-defined void functions after the function main, you should place the function prototype before the function main. · A void function does not have a data type and so function. Type in the heading part and the return statement in the body of the void function are meaningless. · In a void function, you can use the return statement without any value; it is typically used to exit the function early. · Void functions may or may not have formal parameters. · A call to a void function is a stand-alone statement.
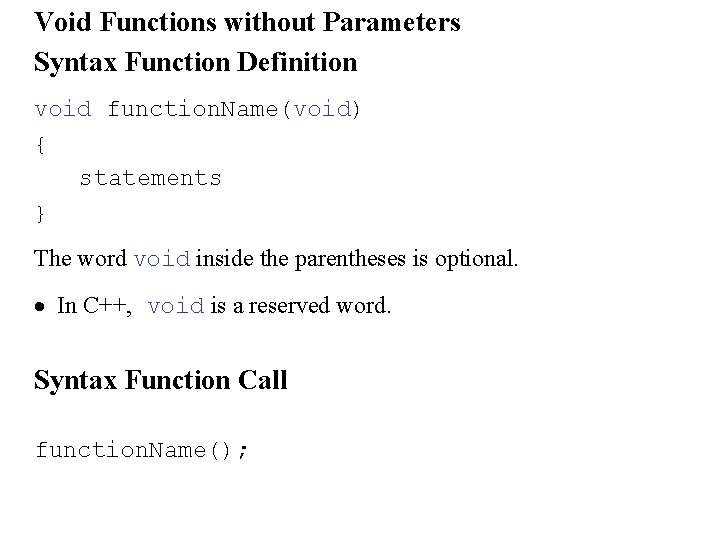
Void Functions without Parameters Syntax Function Definition void function. Name(void) { statements } The word void inside the parentheses is optional. · In C++, void is a reserved word. Syntax Function Call function. Name();
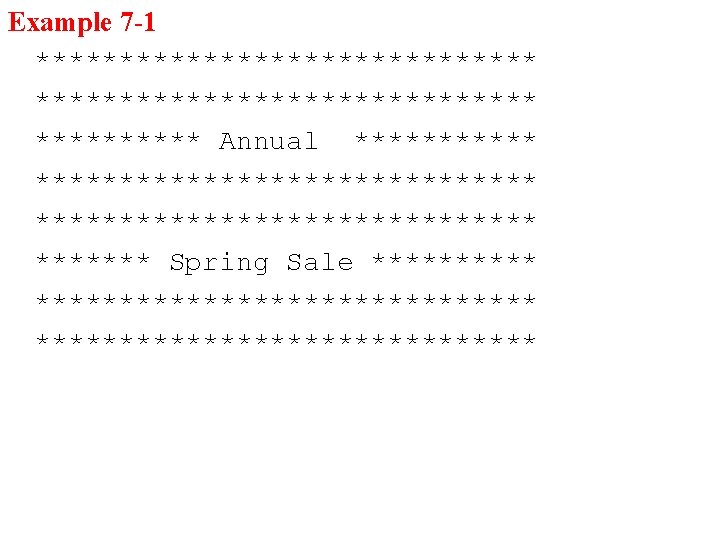
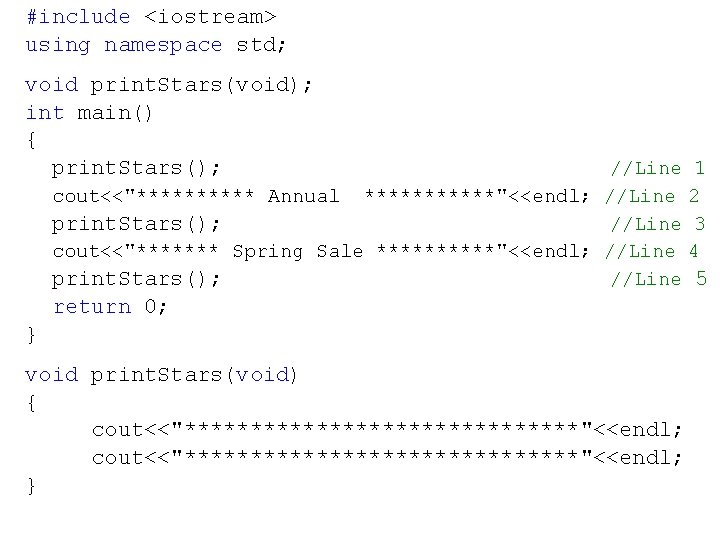
#include <iostream> using namespace std; void print. Stars(void); int main() { print. Stars(); //Line cout<<"***** Annual ******"<<endl; //Line print. Stars(); //Line cout<<"******* Spring Sale *****"<<endl; //Line print. Stars(); //Line return 0; } void print. Stars(void) { cout<<"******************************"<<endl; } 1 2 3 4 5
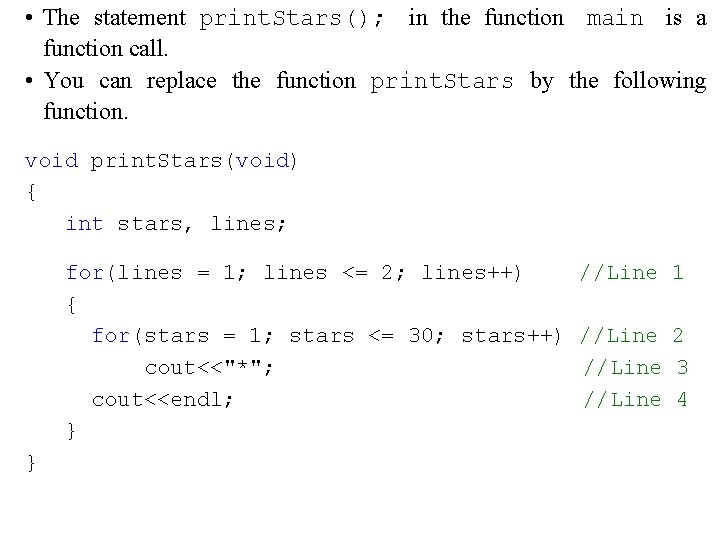
• The statement print. Stars(); in the function main is a function call. • You can replace the function print. Stars by the following function. void print. Stars(void) { int stars, lines; for(lines = 1; lines <= 2; lines++) { for(stars = 1; stars <= 30; stars++) cout<<"*"; cout<<endl; } } //Line 1 //Line 2 //Line 3 //Line 4
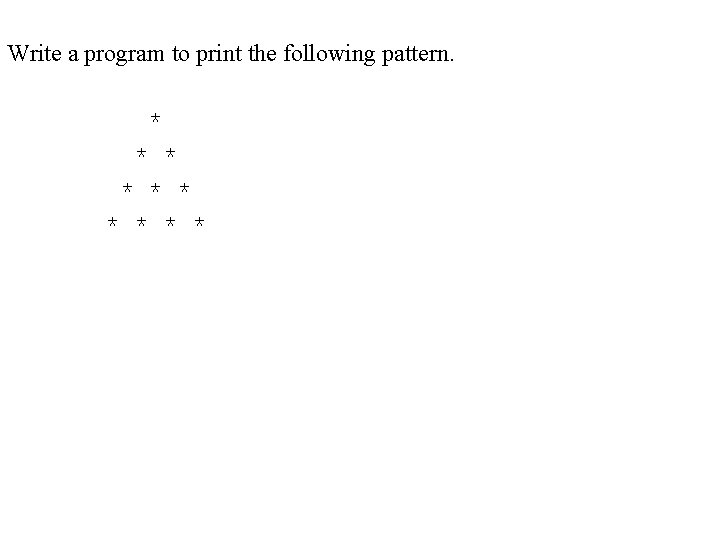
Write a program to print the following pattern. * * * * *
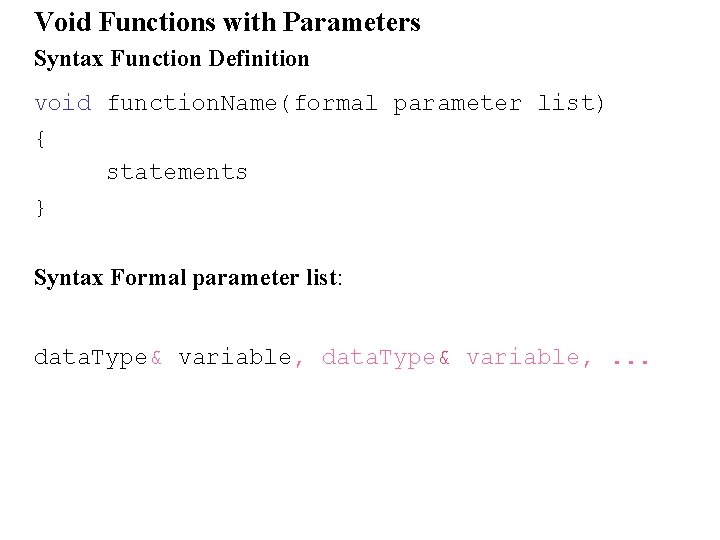
Void Functions with Parameters Syntax Function Definition void function. Name(formal parameter list) { statements } Syntax Formal parameter list: data. Type& variable, . . .
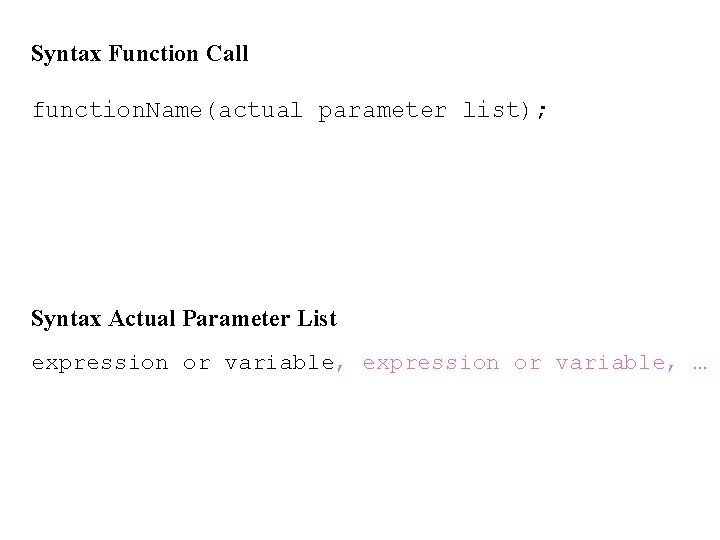
Syntax Function Call function. Name(actual parameter list); Syntax Actual Parameter List expression or variable, …
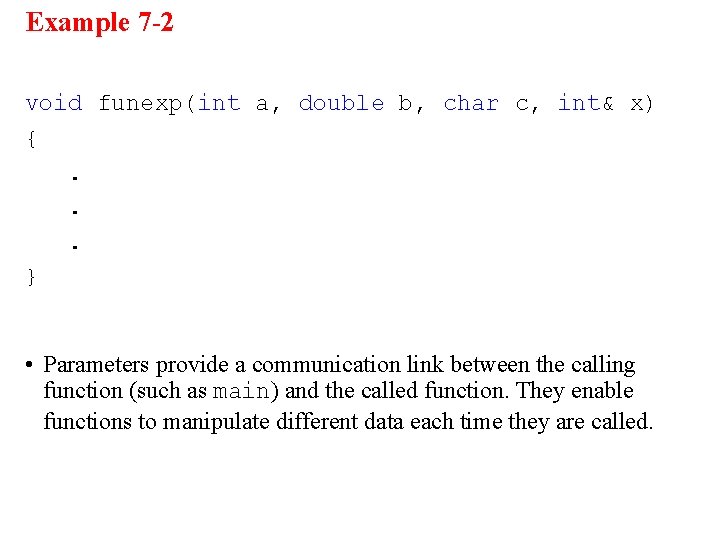
Example 7 -2 void funexp(int a, double b, char c, int& x) {. . . } • Parameters provide a communication link between the calling function (such as main) and the called function. They enable functions to manipulate different data each time they are called.
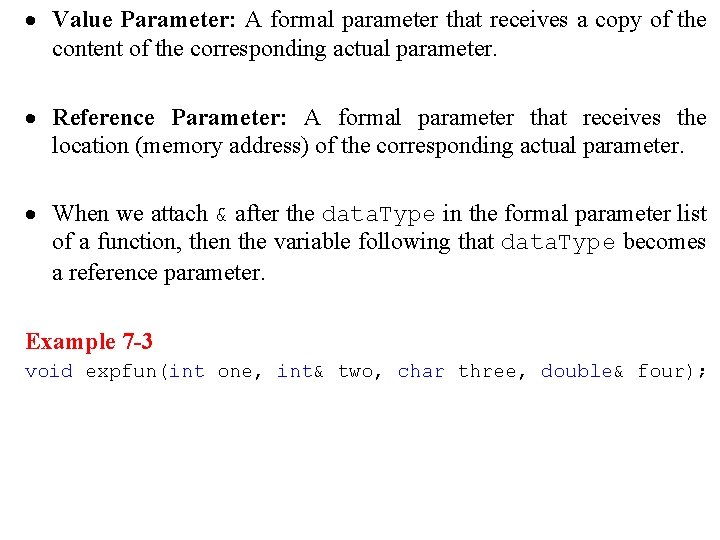
· Value Parameter: A formal parameter that receives a copy of the content of the corresponding actual parameter. · Reference Parameter: A formal parameter that receives the location (memory address) of the corresponding actual parameter. · When we attach & after the data. Type in the formal parameter list of a function, then the variable following that data. Type becomes a reference parameter. Example 7 -3 void expfun(int one, int& two, char three, double& four);
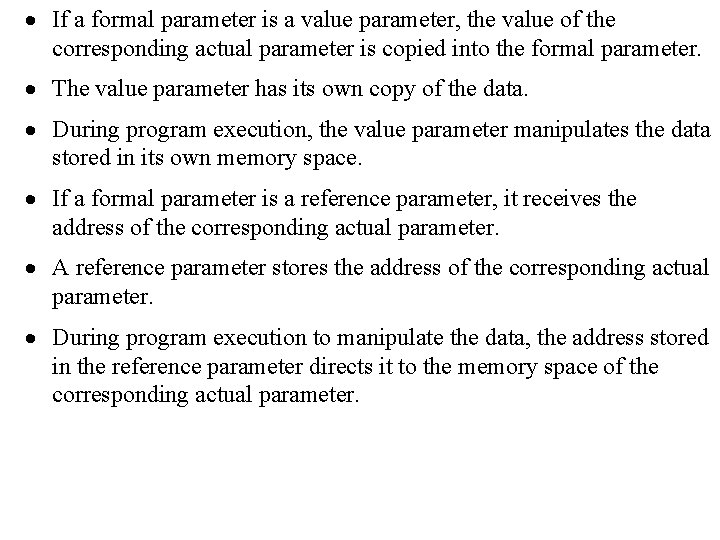
· If a formal parameter is a value parameter, the value of the corresponding actual parameter is copied into the formal parameter. · The value parameter has its own copy of the data. · During program execution, the value parameter manipulates the data stored in its own memory space. · If a formal parameter is a reference parameter, it receives the address of the corresponding actual parameter. · A reference parameter stores the address of the corresponding actual parameter. · During program execution to manipulate the data, the address stored in the reference parameter directs it to the memory space of the corresponding actual parameter.
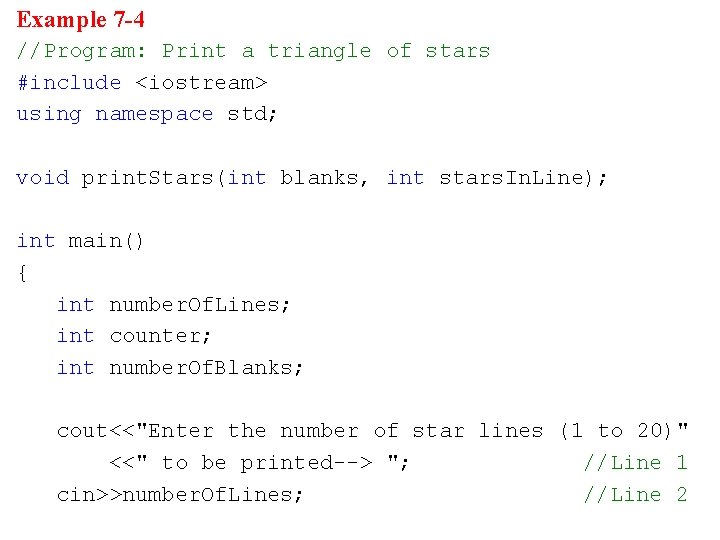
Example 7 -4 //Program: Print a triangle of stars #include <iostream> using namespace std; void print. Stars(int blanks, int stars. In. Line); int main() { int number. Of. Lines; int counter; int number. Of. Blanks; cout<<"Enter the number of star lines (1 to 20)" <<" to be printed--> "; //Line 1 cin>>number. Of. Lines; //Line 2
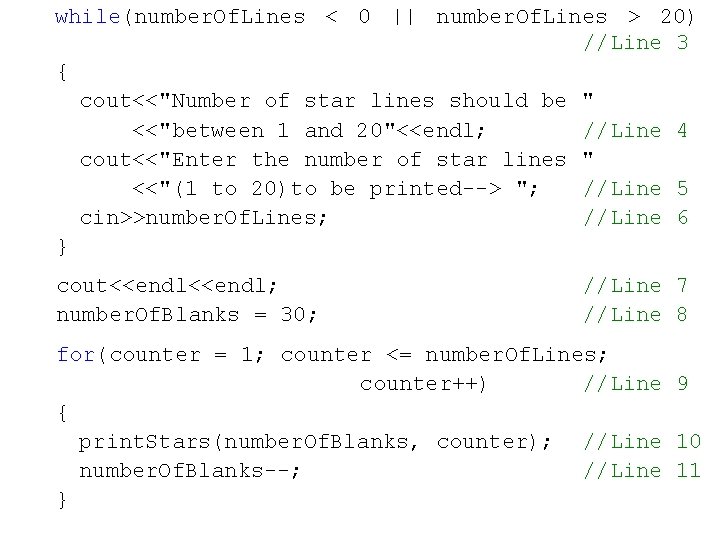
while(number. Of. Lines < 0 || number. Of. Lines > 20) //Line 3 { cout<<"Number of star lines should be " <<"between 1 and 20"<<endl; //Line 4 cout<<"Enter the number of star lines " <<"(1 to 20)to be printed--> "; //Line 5 cin>>number. Of. Lines; //Line 6 } cout<<endl; number. Of. Blanks = 30; //Line 7 //Line 8 for(counter = 1; counter <= number. Of. Lines; counter++) //Line 9 { print. Stars(number. Of. Blanks, counter); //Line 10 number. Of. Blanks--; //Line 11 }
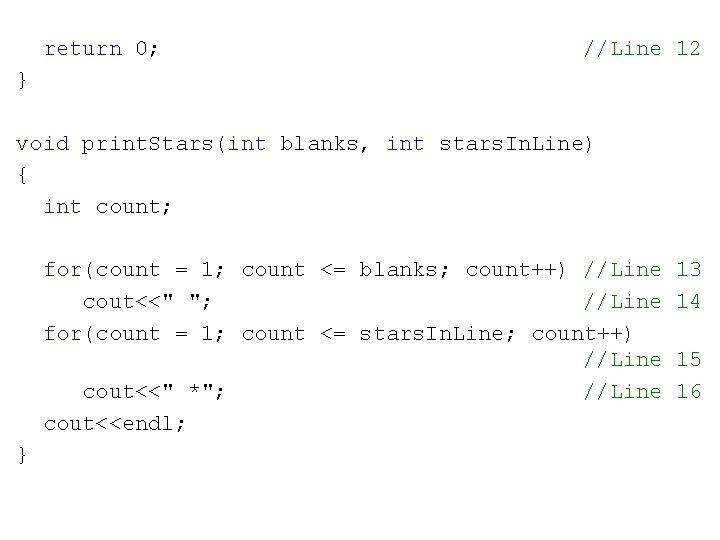
return 0; //Line 12 } void print. Stars(int blanks, int stars. In. Line) { int count; for(count = 1; count <= blanks; count++) //Line cout<<" "; //Line for(count = 1; count <= stars. In. Line; count++) //Line cout<<" *"; //Line cout<<endl; } 13 14 15 16
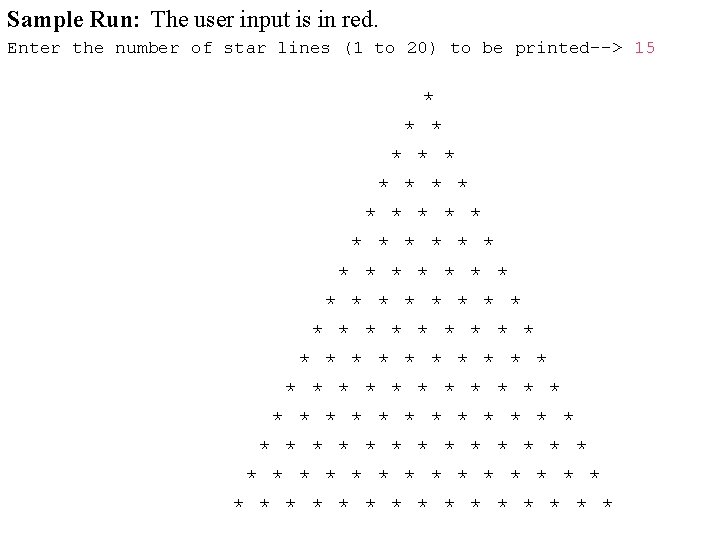
Sample Run: The user input is in red. Enter the number of star lines (1 to 20) to be printed--> 15 * * * * * * * * * * * * * * * * * * * * * * * * * * * * * *
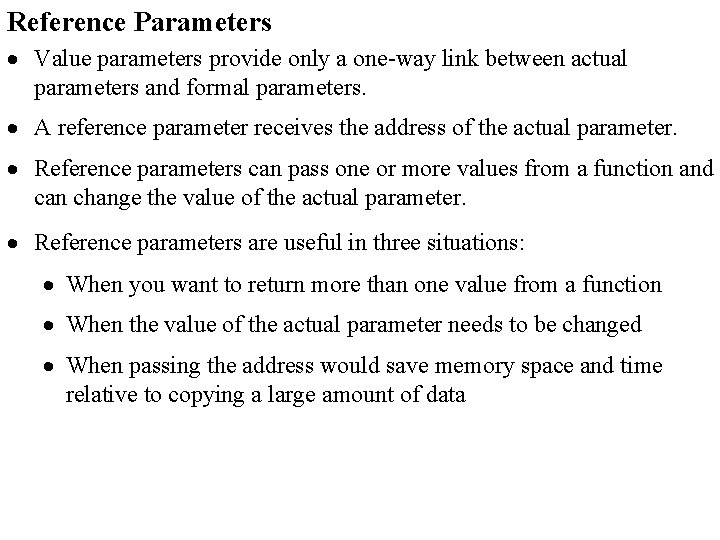
Reference Parameters · Value parameters provide only a one-way link between actual parameters and formal parameters. · A reference parameter receives the address of the actual parameter. · Reference parameters can pass one or more values from a function and can change the value of the actual parameter. · Reference parameters are useful in three situations: · When you want to return more than one value from a function · When the value of the actual parameter needs to be changed · When passing the address would save memory space and time relative to copying a large amount of data
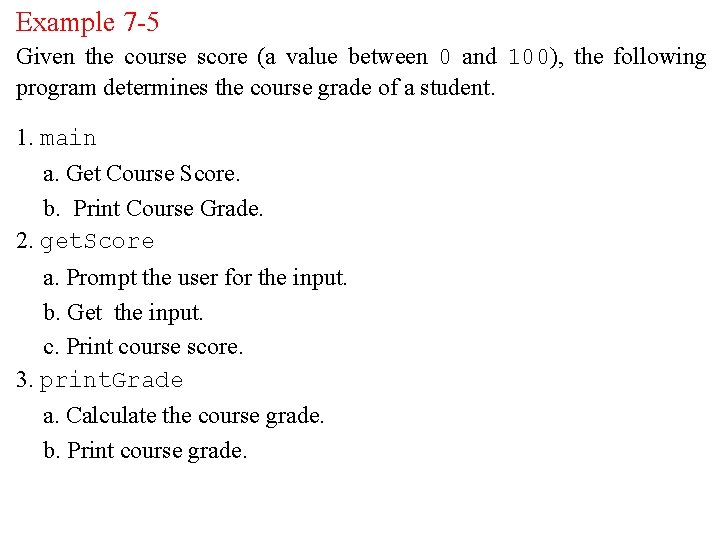
Example 7 -5 Given the course score (a value between 0 and 100), the following program determines the course grade of a student. 1. main a. Get Course Score. b. Print Course Grade. 2. get. Score a. Prompt the user for the input. b. Get the input. c. Print course score. 3. print. Grade a. Calculate the course grade. b. Print course grade.
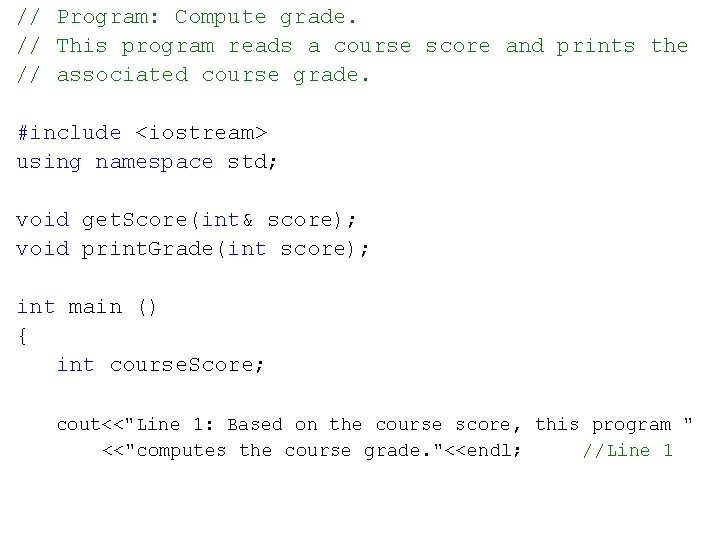
// Program: Compute grade. // This program reads a course score and prints the // associated course grade. #include <iostream> using namespace std; void get. Score(int& score); void print. Grade(int score); int main () { int course. Score; cout<<"Line 1: Based on the course score, this program " <<"computes the course grade. "<<endl; //Line 1
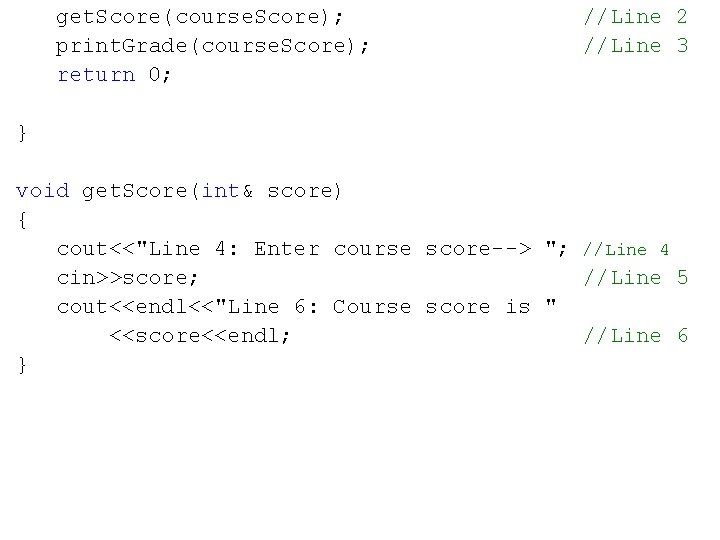
get. Score(course. Score); print. Grade(course. Score); return 0; //Line 2 //Line 3 } void get. Score(int& score) { cout<<"Line 4: Enter course score--> "; //Line 4 cin>>score; //Line 5 cout<<endl<<"Line 6: Course score is " <<score<<endl; //Line 6 }
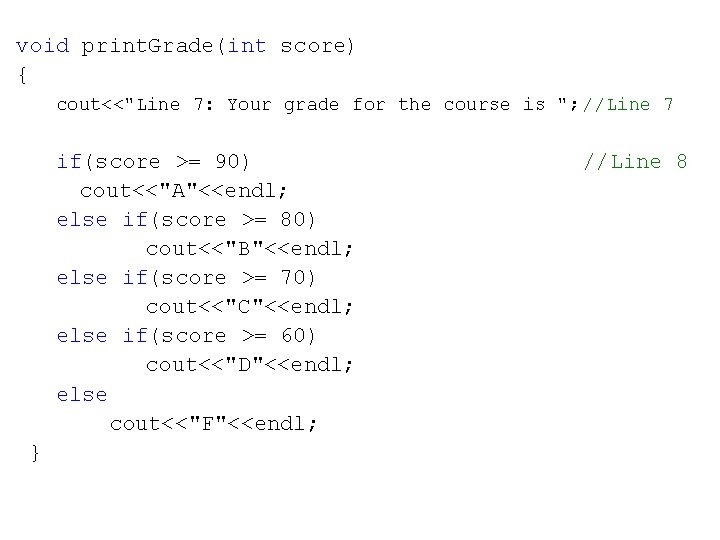
void print. Grade(int score) { cout<<"Line 7: Your grade for the course is "; //Line 7 if(score >= 90) cout<<"A"<<endl; else if(score >= 80) cout<<"B"<<endl; else if(score >= 70) cout<<"C"<<endl; else if(score >= 60) cout<<"D"<<endl; else cout<<"F"<<endl; } //Line 8
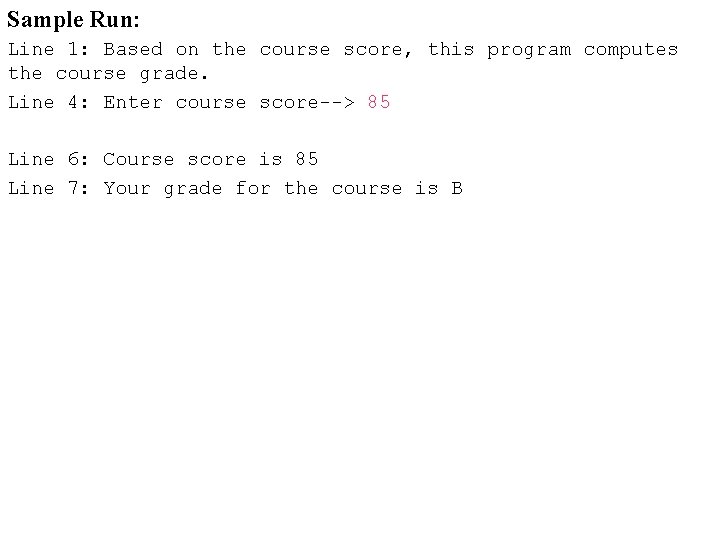
Sample Run: Line 1: Based on the course score, this program computes the course grade. Line 4: Enter course score--> 85 Line 6: Course score is 85 Line 7: Your grade for the course is B
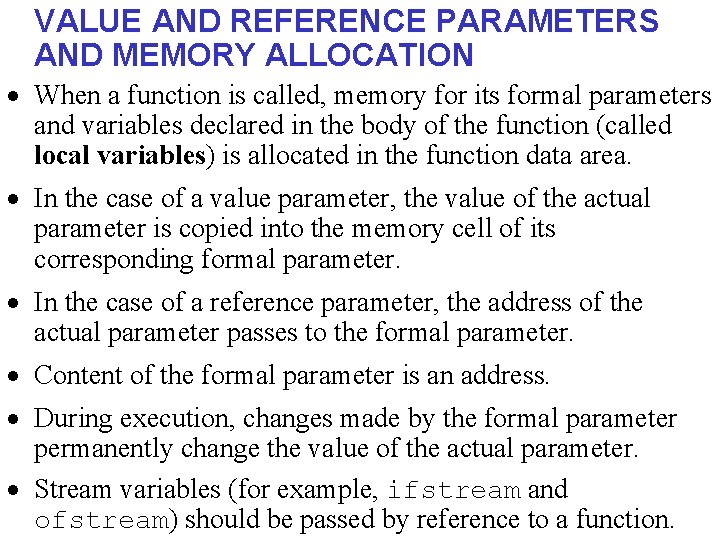
VALUE AND REFERENCE PARAMETERS AND MEMORY ALLOCATION · When a function is called, memory for its formal parameters and variables declared in the body of the function (called local variables) is allocated in the function data area. · In the case of a value parameter, the value of the actual parameter is copied into the memory cell of its corresponding formal parameter. · In the case of a reference parameter, the address of the actual parameter passes to the formal parameter. · Content of the formal parameter is an address. · During execution, changes made by the formal parameter permanently change the value of the actual parameter. · Stream variables (for example, ifstream and ofstream) should be passed by reference to a function.
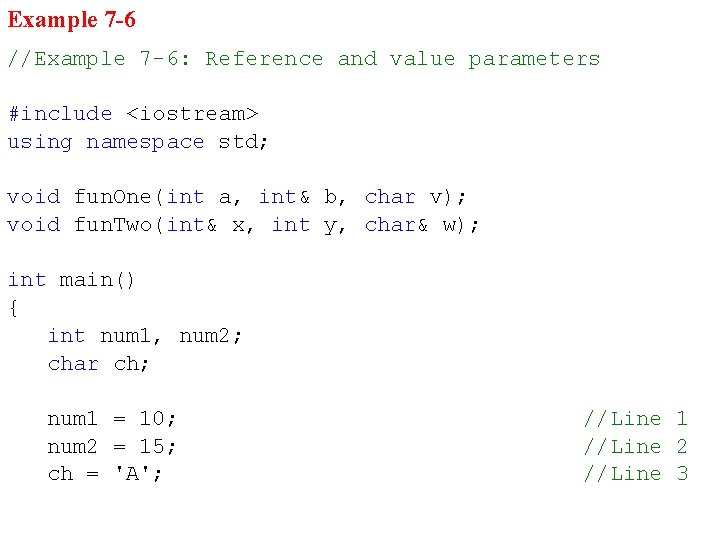
Example 7 -6 //Example 7 -6: Reference and value parameters #include <iostream> using namespace std; void fun. One(int a, int& b, char v); void fun. Two(int& x, int y, char& w); int main() { int num 1, num 2; char ch; num 1 = 10; num 2 = 15; ch = 'A'; //Line 1 //Line 2 //Line 3
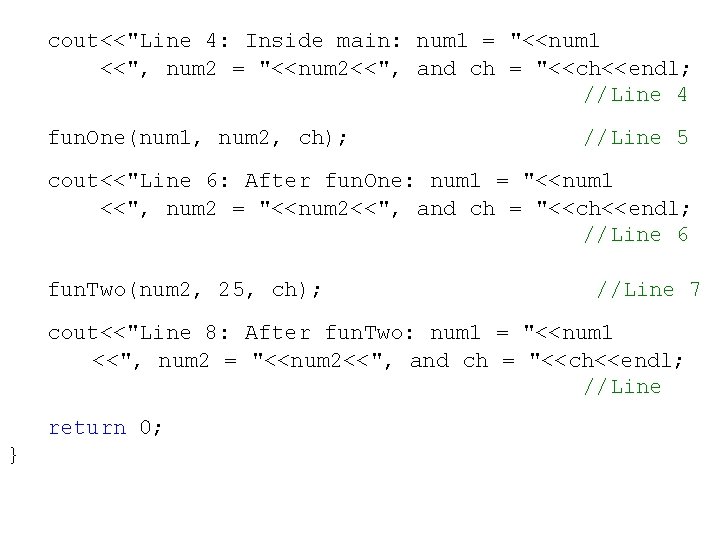
cout<<"Line 4: Inside main: num 1 = "<<num 1 <<", num 2 = "<<num 2<<", and ch = "<<ch<<endl; //Line 4 fun. One(num 1, num 2, ch); //Line 5 cout<<"Line 6: After fun. One: num 1 = "<<num 1 <<", num 2 = "<<num 2<<", and ch = "<<ch<<endl; //Line 6 fun. Two(num 2, 25, ch); //Line 7 cout<<"Line 8: After fun. Two: num 1 = "<<num 1 <<", num 2 = "<<num 2<<", and ch = "<<ch<<endl; //Line return 0; }
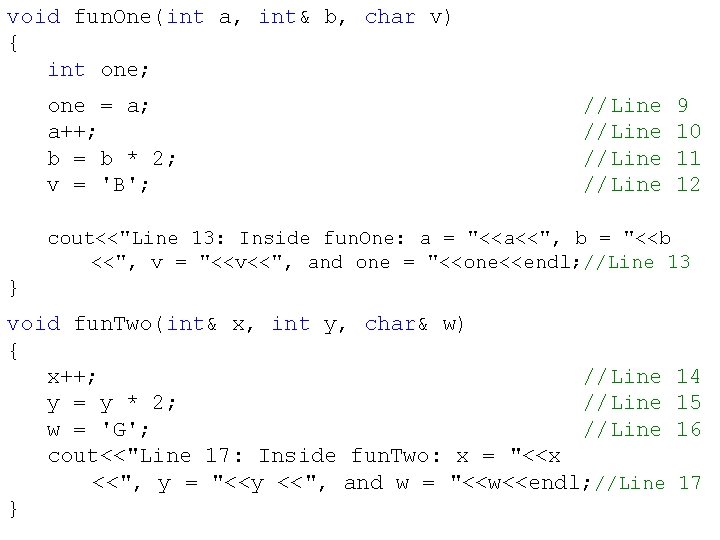
void fun. One(int a, int& b, char v) { int one; one = a; a++; b = b * 2; v = 'B'; //Line 9 10 11 12 cout<<"Line 13: Inside fun. One: a = "<<a<<", b = "<<b <<", v = "<<v<<", and one = "<<one<<endl; //Line 13 } void fun. Two(int& x, int y, char& w) { x++; //Line y = y * 2; //Line w = 'G'; //Line cout<<"Line 17: Inside fun. Two: x = "<<x <<", y = "<<y <<", and w = "<<w<<endl; //Line } 14 15 16 17
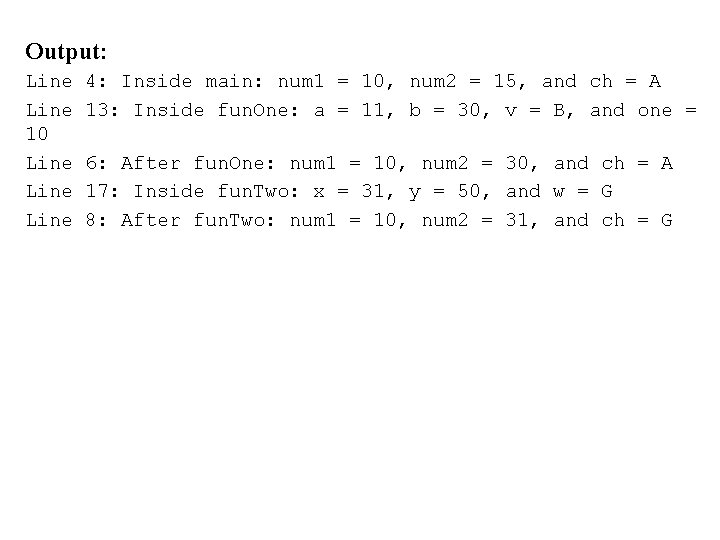
Output: Line 10 Line 4: Inside main: num 1 = 10, num 2 = 15, and ch = A 13: Inside fun. One: a = 11, b = 30, v = B, and one = 6: After fun. One: num 1 = 10, num 2 = 30, and ch = A 17: Inside fun. Two: x = 31, y = 50, and w = G 8: After fun. Two: num 1 = 10, num 2 = 31, and ch = G
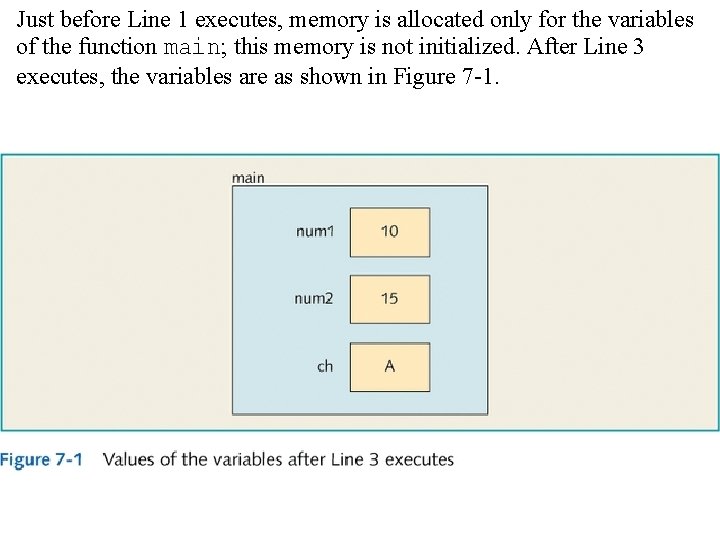
Just before Line 1 executes, memory is allocated only for the variables of the function main; this memory is not initialized. After Line 3 executes, the variables are as shown in Figure 7 -1.
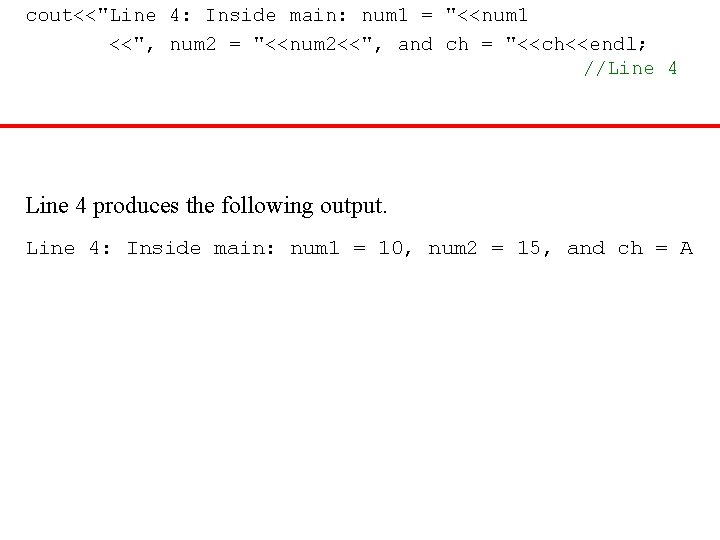
cout<<"Line 4: Inside main: num 1 = "<<num 1 <<", num 2 = "<<num 2<<", and ch = "<<ch<<endl; //Line 4 produces the following output. Line 4: Inside main: num 1 = 10, num 2 = 15, and ch = A
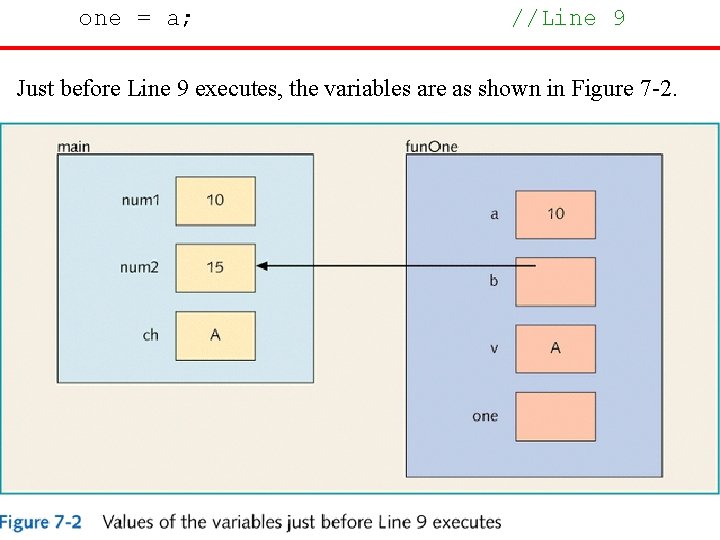
one = a; //Line 9 Just before Line 9 executes, the variables are as shown in Figure 7 -2.
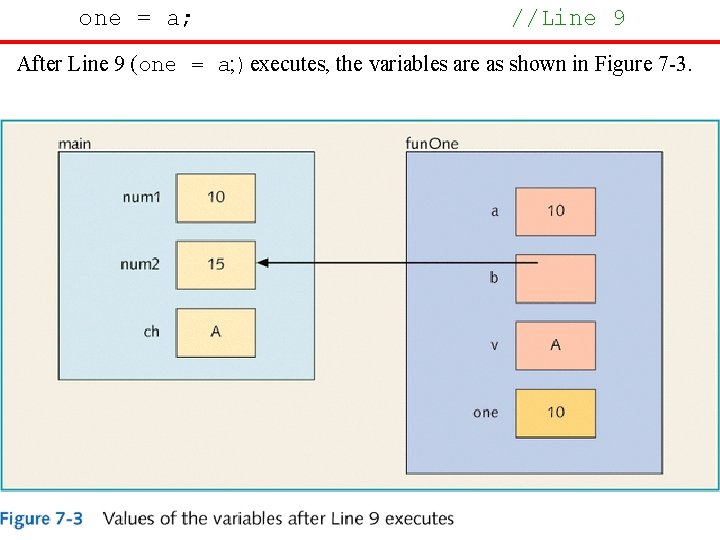
one = a; //Line 9 After Line 9 (one = a; )executes, the variables are as shown in Figure 7 -3.
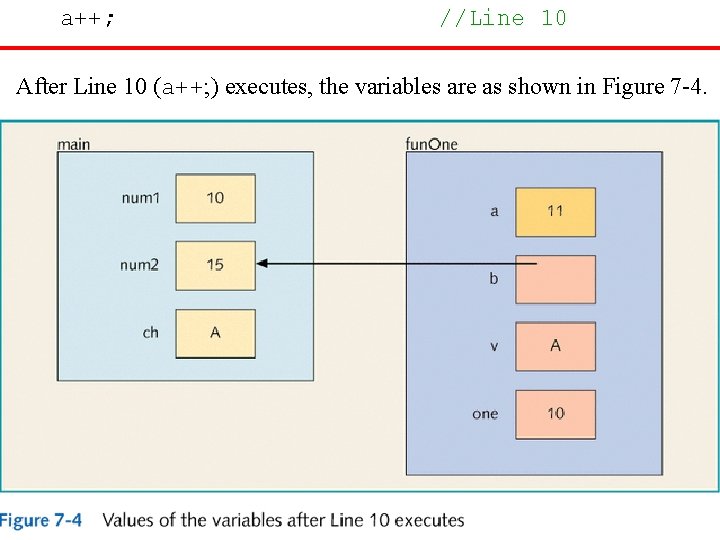
a++; //Line 10 After Line 10 (a++; ) executes, the variables are as shown in Figure 7 -4.
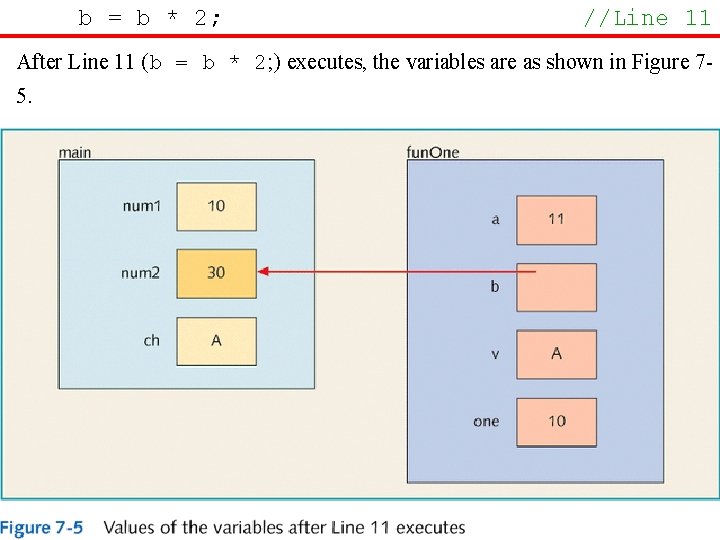
b = b * 2; //Line 11 After Line 11 (b = b * 2; ) executes, the variables are as shown in Figure 75.
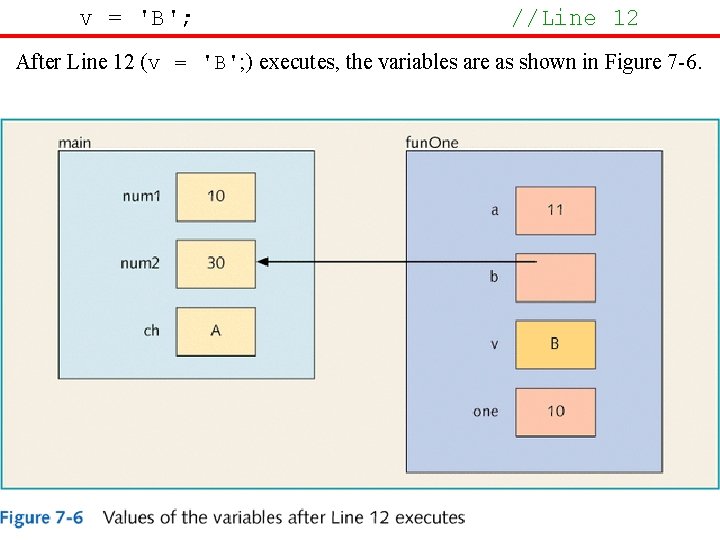
v = 'B'; //Line 12 After Line 12 (v = 'B'; ) executes, the variables are as shown in Figure 7 -6.
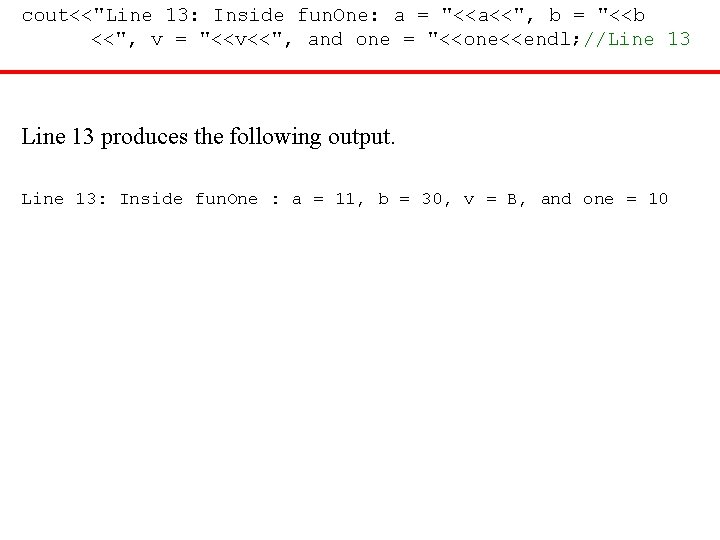
cout<<"Line 13: Inside fun. One: a = "<<a<<", b = "<<b <<", v = "<<v<<", and one = "<<one<<endl; //Line 13 produces the following output. Line 13: Inside fun. One : a = 11, b = 30, v = B, and one = 10
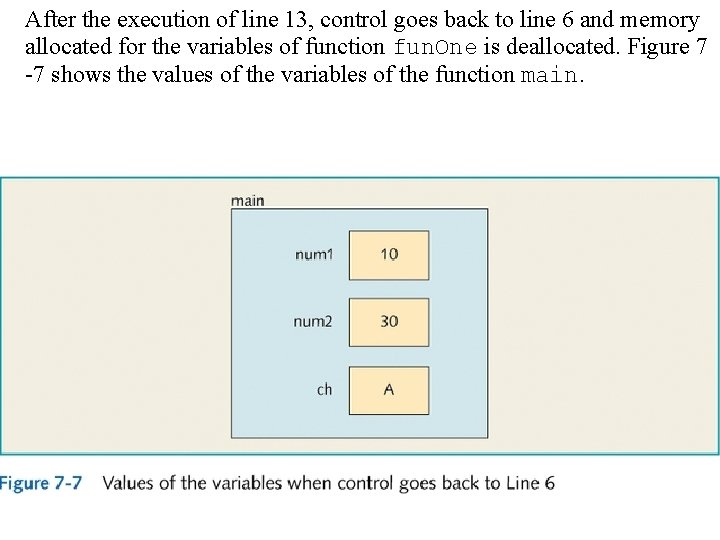
After the execution of line 13, control goes back to line 6 and memory allocated for the variables of function fun. One is deallocated. Figure 7 -7 shows the values of the variables of the function main.
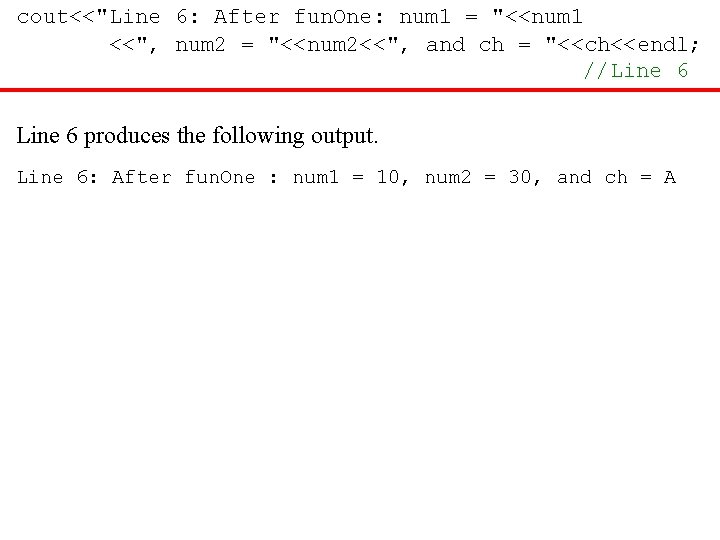
cout<<"Line 6: After fun. One: num 1 = "<<num 1 <<", num 2 = "<<num 2<<", and ch = "<<ch<<endl; //Line 6 produces the following output. Line 6: After fun. One : num 1 = 10, num 2 = 30, and ch = A
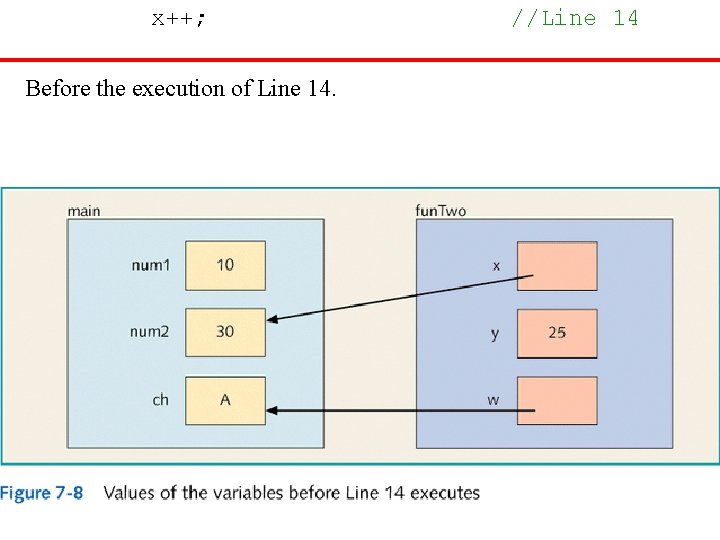
x++; Before the execution of Line 14. //Line 14
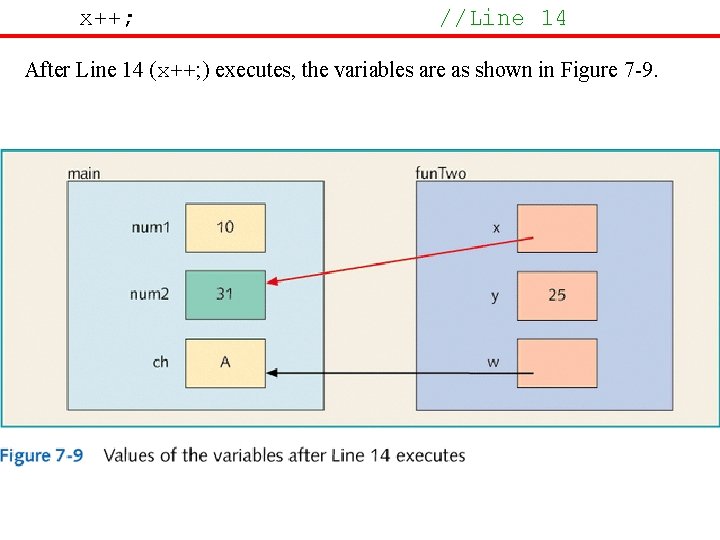
x++; //Line 14 After Line 14 (x++; ) executes, the variables are as shown in Figure 7 -9.
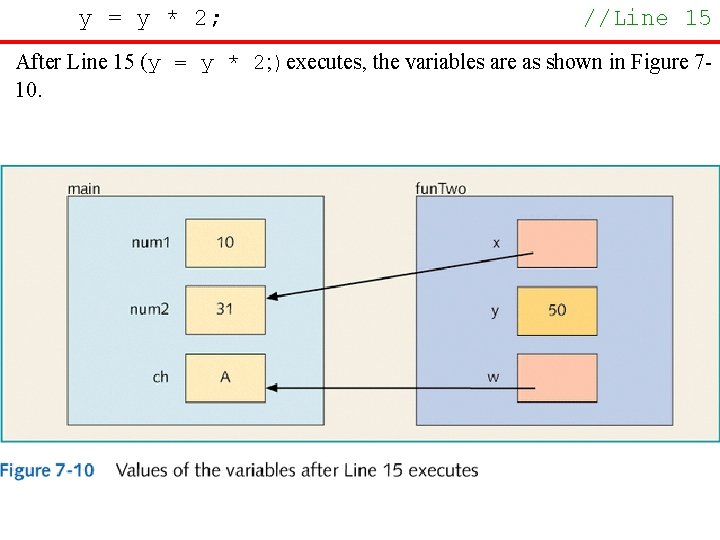
y = y * 2; //Line 15 After Line 15 (y = y * 2; )executes, the variables are as shown in Figure 710.
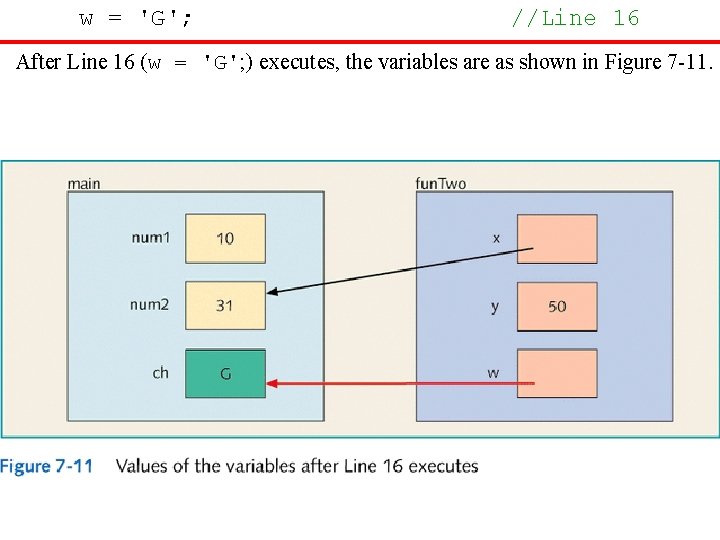
w = 'G'; //Line 16 After Line 16 (w = 'G'; ) executes, the variables are as shown in Figure 7 -11.
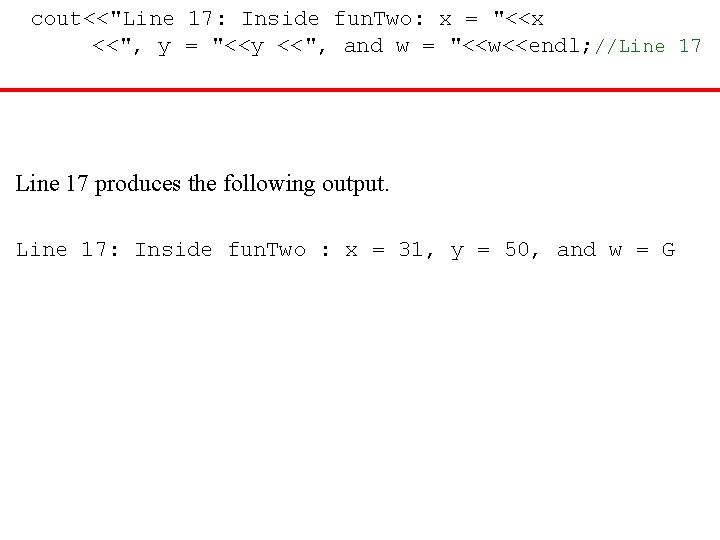
cout<<"Line 17: Inside fun. Two: x = "<<x <<", y = "<<y <<", and w = "<<w<<endl; //Line 17 produces the following output. Line 17: Inside fun. Two : x = 31, y = 50, and w = G
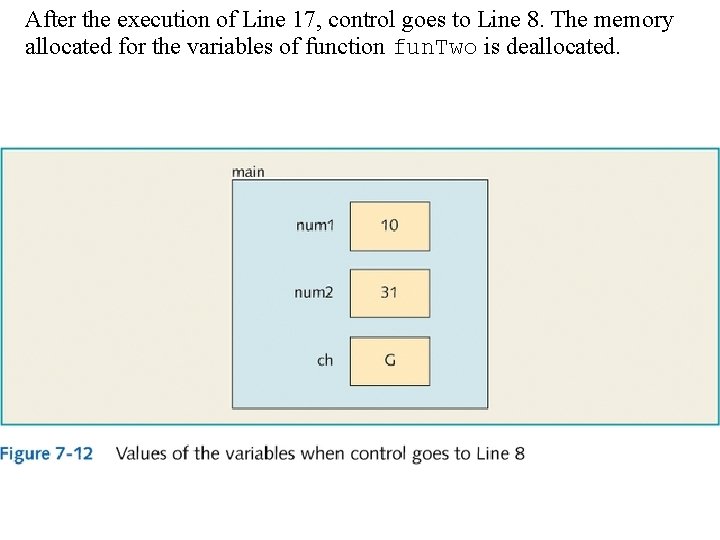
After the execution of Line 17, control goes to Line 8. The memory allocated for the variables of function fun. Two is deallocated.
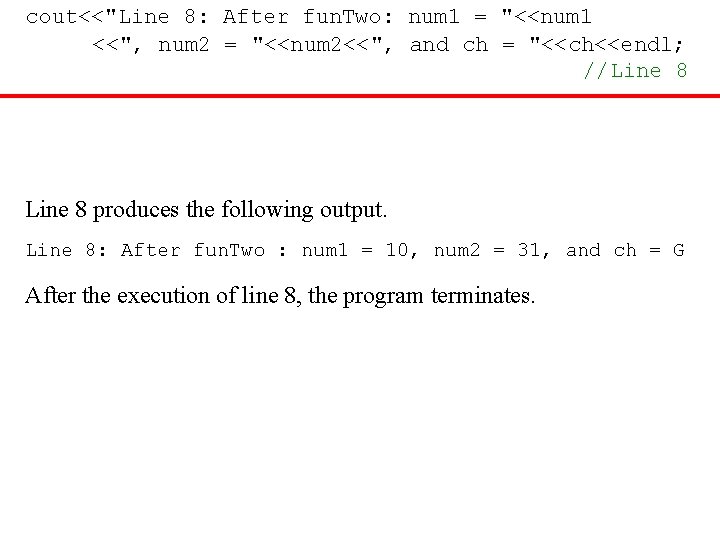
cout<<"Line 8: After fun. Two: num 1 = "<<num 1 <<", num 2 = "<<num 2<<", and ch = "<<ch<<endl; //Line 8 produces the following output. Line 8: After fun. Two : num 1 = 10, num 2 = 31, and ch = G After the execution of line 8, the program terminates.
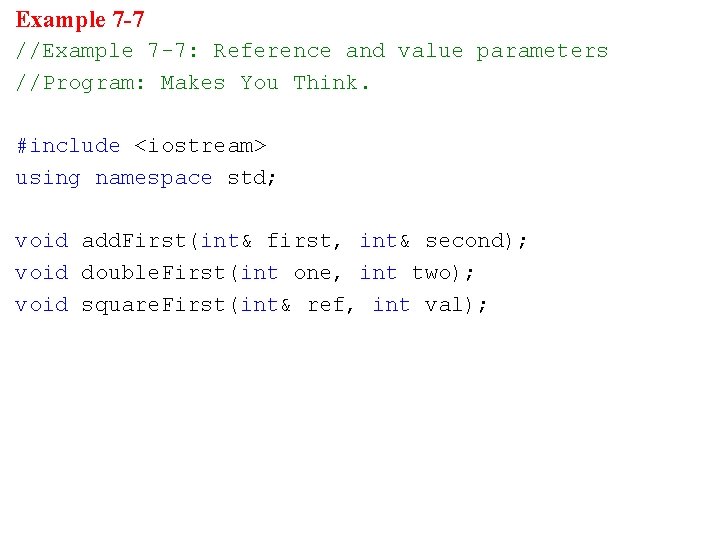
Example 7 -7 //Example 7 -7: Reference and value parameters //Program: Makes You Think. #include <iostream> using namespace std; void add. First(int& first, int& second); void double. First(int one, int two); void square. First(int& ref, int val);
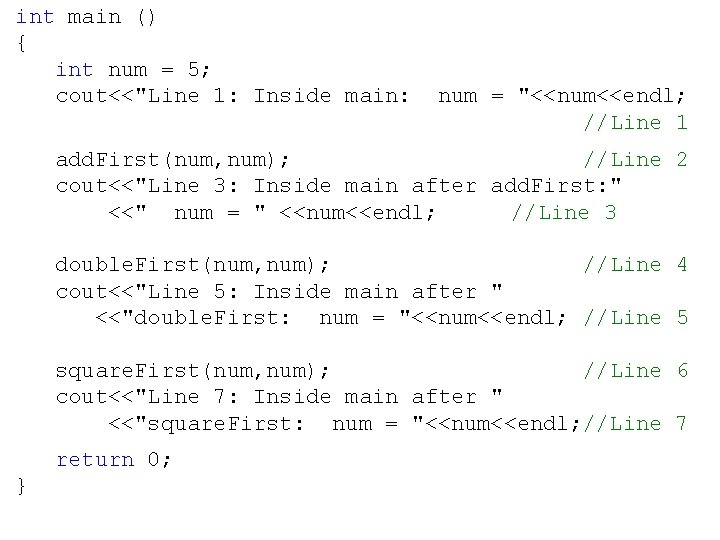
int main () { int num = 5; cout<<"Line 1: Inside main: num = "<<num<<endl; //Line 1 add. First(num, num); //Line 2 cout<<"Line 3: Inside main after add. First: " <<" num = " <<num<<endl; //Line 3 double. First(num, num); //Line 4 cout<<"Line 5: Inside main after " <<"double. First: num = "<<num<<endl; //Line 5 square. First(num, num); //Line 6 cout<<"Line 7: Inside main after " <<"square. First: num = "<<num<<endl; //Line 7 return 0; }
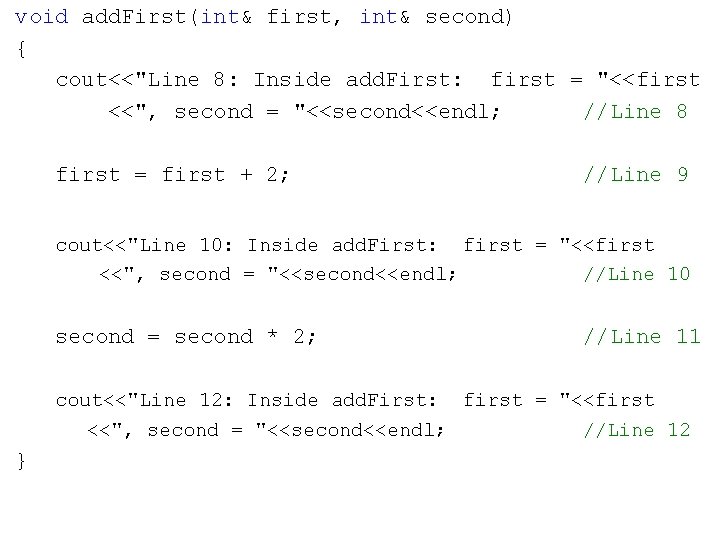
void add. First(int& first, int& second) { cout<<"Line 8: Inside add. First: first = "<<first <<", second = "<<second<<endl; //Line 8 first = first + 2; //Line 9 cout<<"Line 10: Inside add. First: first = "<<first <<", second = "<<second<<endl; //Line 10 second = second * 2; //Line 11 cout<<"Line 12: Inside add. First: first = "<<first <<", second = "<<second<<endl; //Line 12 }
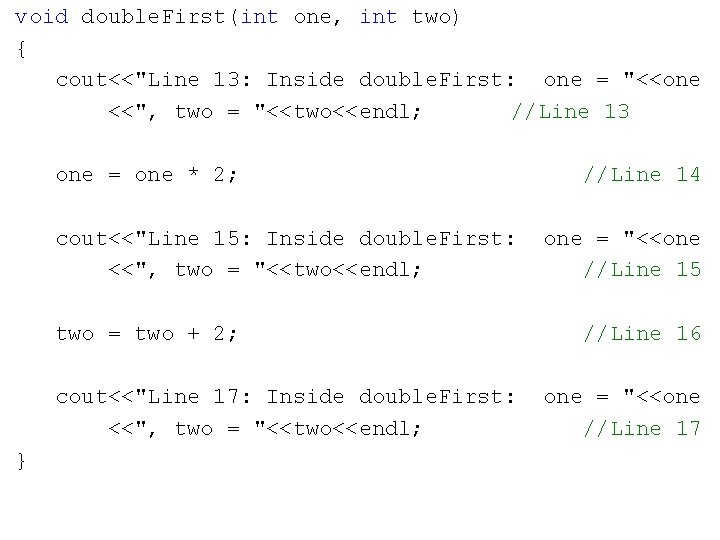
void double. First(int one, int two) { cout<<"Line 13: Inside double. First: one = "<<one <<", two = "<<two<<endl; //Line 13 one = one * 2; cout<<"Line 15: Inside double. First: <<", two = "<<two<<endl; two = two + 2; cout<<"Line 17: Inside double. First: <<", two = "<<two<<endl; } //Line 14 one = "<<one //Line 15 //Line 16 one = "<<one //Line 17
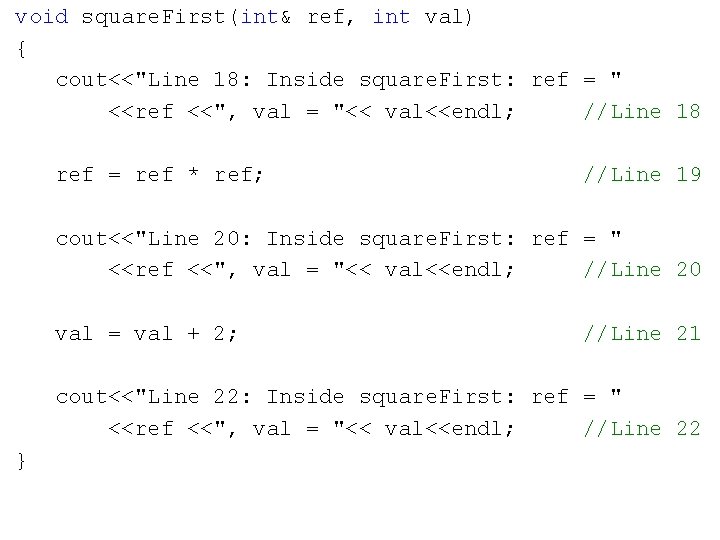
void square. First(int& ref, int val) { cout<<"Line 18: Inside square. First: ref = " <<ref <<", val = "<< val<<endl; //Line 18 ref = ref * ref; //Line 19 cout<<"Line 20: Inside square. First: ref = " <<ref <<", val = "<< val<<endl; //Line 20 val = val + 2; //Line 21 cout<<"Line 22: Inside square. First: ref = " <<ref <<", val = "<< val<<endl; //Line 22 }
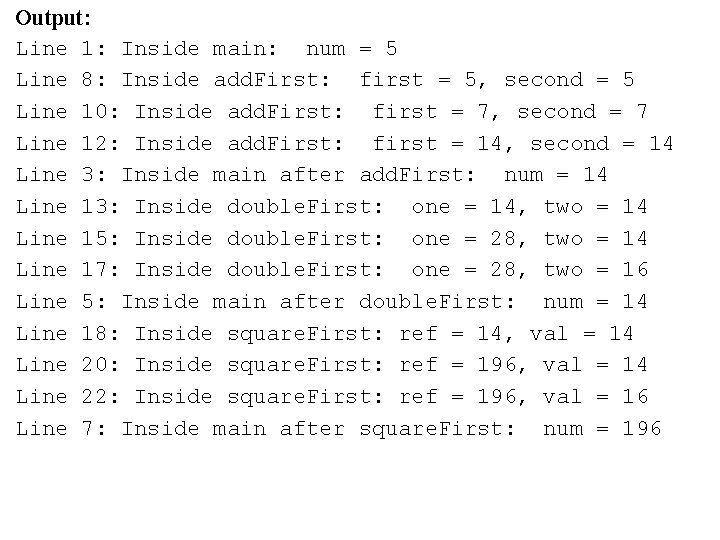
Output: Line 1: Inside main: num = 5 Line 8: Inside add. First: first = 5, second = 5 Line 10: Inside add. First: first = 7, second = 7 Line 12: Inside add. First: first = 14, second = 14 Line 3: Inside main after add. First: num = 14 Line 13: Inside double. First: one = 14, two = 14 Line 15: Inside double. First: one = 28, two = 14 Line 17: Inside double. First: one = 28, two = 16 Line 5: Inside main after double. First: num = 14 Line 18: Inside square. First: ref = 14, val = 14 Line 20: Inside square. First: ref = 196, val = 14 Line 22: Inside square. First: ref = 196, val = 16 Line 7: Inside main after square. First: num = 196
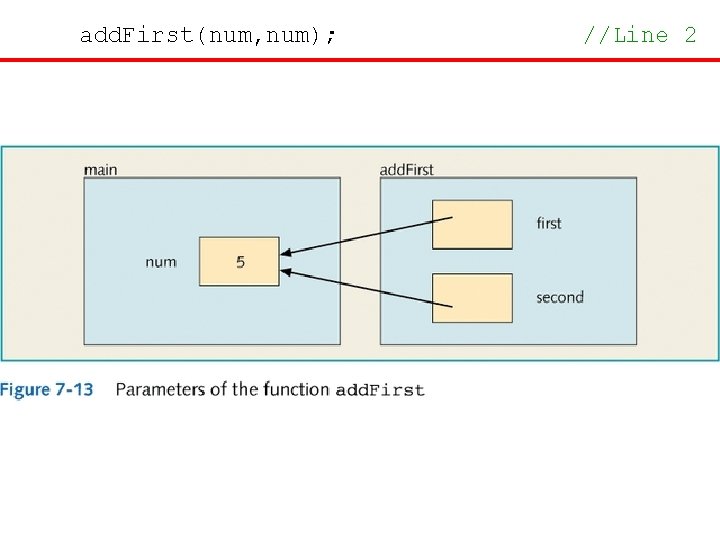
add. First(num, num); //Line 2
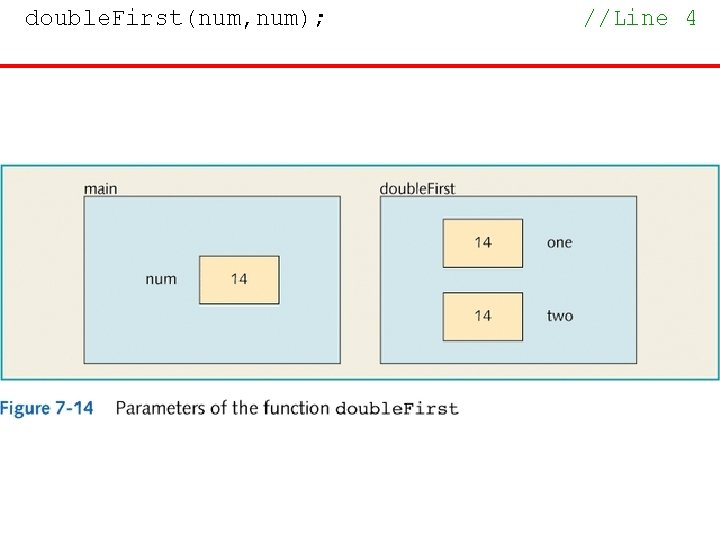
double. First(num, num); //Line 4
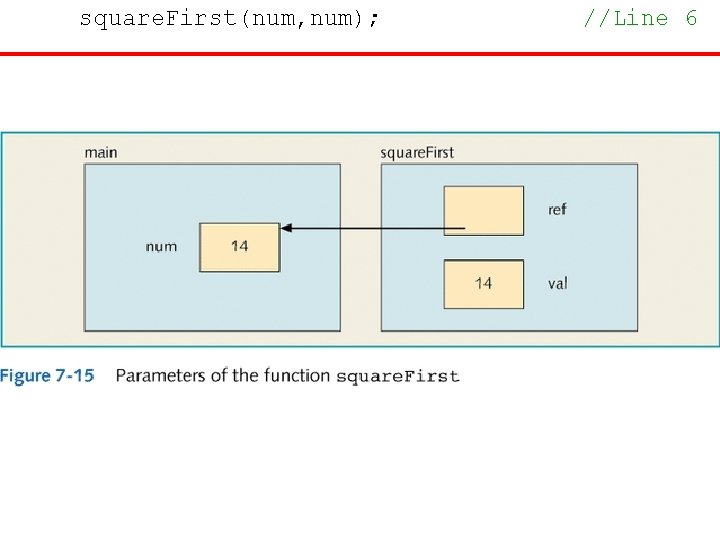
square. First(num, num); //Line 6
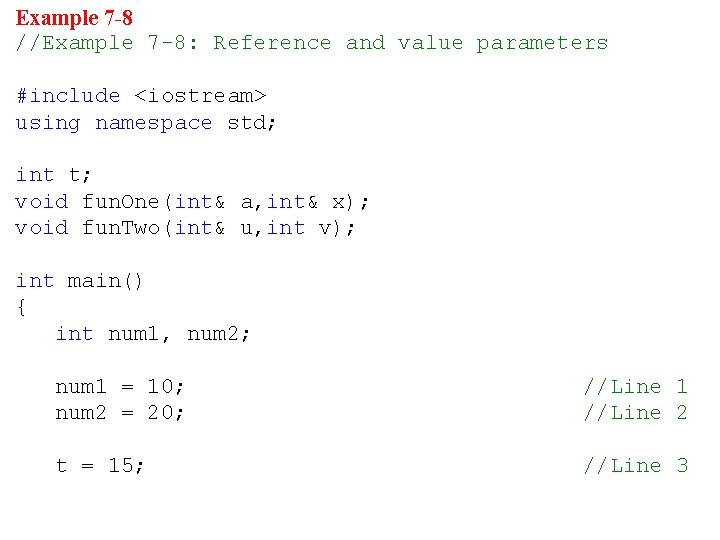
Example 7 -8 //Example 7 -8: Reference and value parameters #include <iostream> using namespace std; int t; void fun. One(int& a, int& x); void fun. Two(int& u, int v); int main() { int num 1, num 2; num 1 = 10; num 2 = 20; //Line 1 //Line 2 t = 15; //Line 3
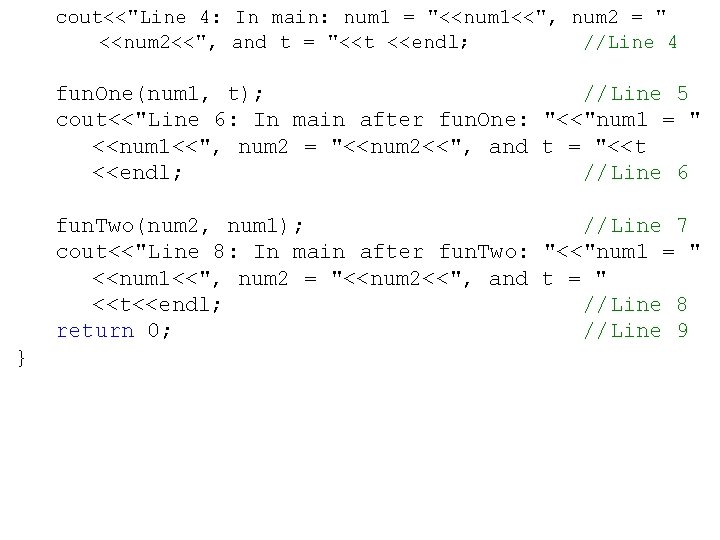
cout<<"Line 4: In main: num 1 = "<<num 1<<", num 2 = " <<num 2<<", and t = "<<t <<endl; //Line 4 fun. One(num 1, t); //Line 5 cout<<"Line 6: In main after fun. One: "<<"num 1 = " <<num 1<<", num 2 = "<<num 2<<", and t = "<<t <<endl; //Line 6 fun. Two(num 2, num 1); //Line 7 cout<<"Line 8: In main after fun. Two: "<<"num 1 = " <<num 1<<", num 2 = "<<num 2<<", and t = " <<t<<endl; //Line 8 return 0; //Line 9 }
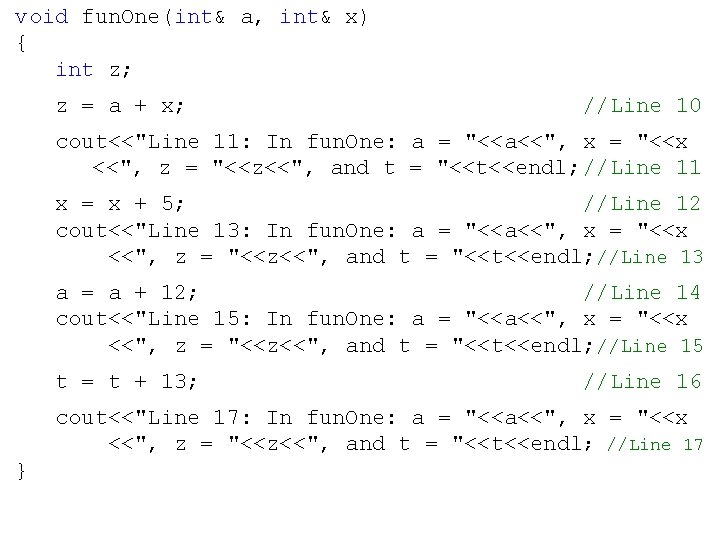
void fun. One(int& a, int& x) { int z; z = a + x; //Line 10 cout<<"Line 11: In fun. One: a = "<<a<<", x = "<<x <<", z = "<<z<<", and t = "<<t<<endl; //Line 11 x = x + 5; //Line 12 cout<<"Line 13: In fun. One: a = "<<a<<", x = "<<x <<", z = "<<z<<", and t = "<<t<<endl; //Line 13 a = a + 12; //Line 14 cout<<"Line 15: In fun. One: a = "<<a<<", x = "<<x <<", z = "<<z<<", and t = "<<t<<endl; //Line 15 t = t + 13; //Line 16 cout<<"Line 17: In fun. One: a = "<<a<<", x = "<<x <<", z = "<<z<<", and t = "<<t<<endl; //Line 17 }
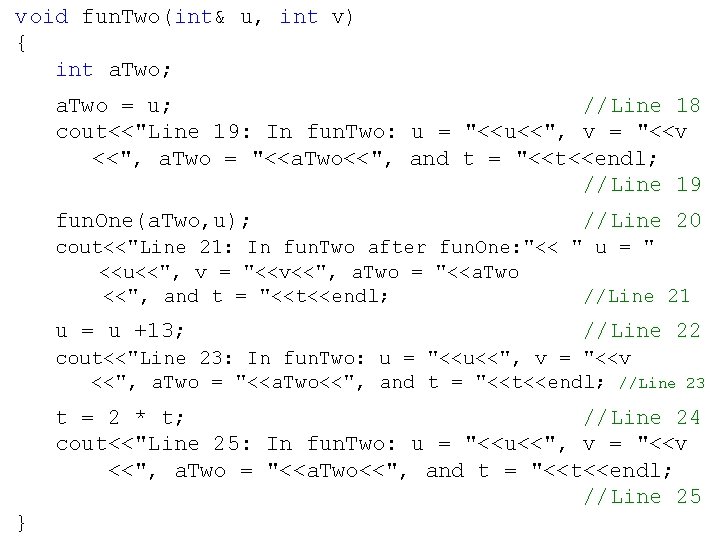
void fun. Two(int& u, int v) { int a. Two; a. Two = u; //Line 18 cout<<"Line 19: In fun. Two: u = "<<u<<", v = "<<v <<", a. Two = "<<a. Two<<", and t = "<<t<<endl; //Line 19 fun. One(a. Two, u); //Line 20 cout<<"Line 21: In fun. Two after fun. One: "<< " u = " <<u<<", v = "<<v<<", a. Two = "<<a. Two <<", and t = "<<t<<endl; //Line 21 u = u +13; //Line 22 cout<<"Line 23: In fun. Two: u = "<<u<<", v = "<<v <<", a. Two = "<<a. Two<<", and t = "<<t<<endl; //Line 23 t = 2 * t; //Line 24 cout<<"Line 25: In fun. Two: u = "<<u<<", v = "<<v <<", a. Two = "<<a. Two<<", and t = "<<t<<endl; //Line 25 }
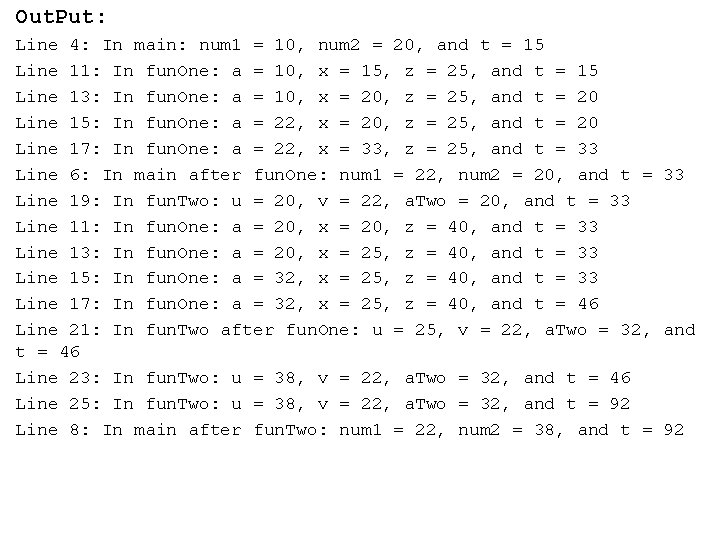
Out. Put: Line 4: In main: num 1 = 10, num 2 = 20, and t = 15 Line 11: In fun. One: a = 10, x = 15, z = 25, and t = 15 Line 13: In fun. One: a = 10, x = 20, z = 25, and t = 20 Line 15: In fun. One: a = 22, x = 20, z = 25, and t = 20 Line 17: In fun. One: a = 22, x = 33, z = 25, and t = 33 Line 6: In main after fun. One: num 1 = 22, num 2 = 20, and t = 33 Line 19: In fun. Two: u = 20, v = 22, a. Two = 20, and t = 33 Line 11: In fun. One: a = 20, x = 20, z = 40, and t = 33 Line 13: In fun. One: a = 20, x = 25, z = 40, and t = 33 Line 15: In fun. One: a = 32, x = 25, z = 40, and t = 33 Line 17: In fun. One: a = 32, x = 25, z = 40, and t = 46 Line 21: In fun. Two after fun. One: u = 25, v = 22, a. Two = 32, and t = 46 Line 23: In fun. Two: u = 38, v = 22, a. Two = 32, and t = 46 Line 25: In fun. Two: u = 38, v = 22, a. Two = 32, and t = 92 Line 8: In main after fun. Two: num 1 = 22, num 2 = 38, and t = 92
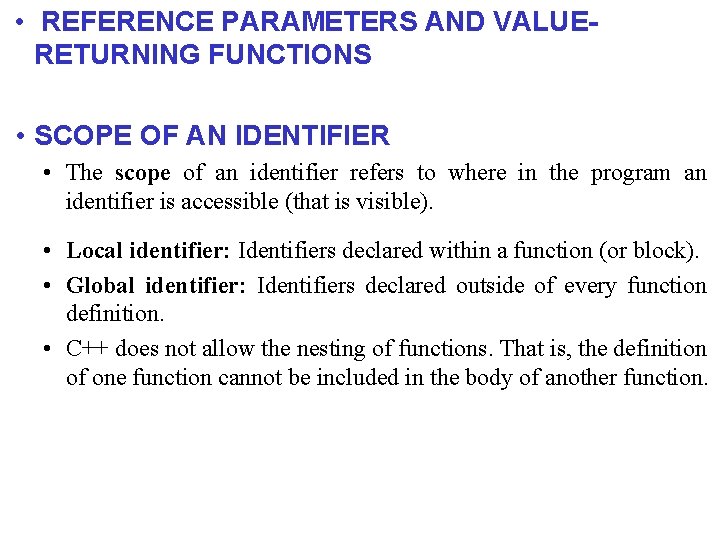
• REFERENCE PARAMETERS AND VALUERETURNING FUNCTIONS • SCOPE OF AN IDENTIFIER • The scope of an identifier refers to where in the program an identifier is accessible (that is visible). • Local identifier: Identifiers declared within a function (or block). • Global identifier: Identifiers declared outside of every function definition. • C++ does not allow the nesting of functions. That is, the definition of one function cannot be included in the body of another function.
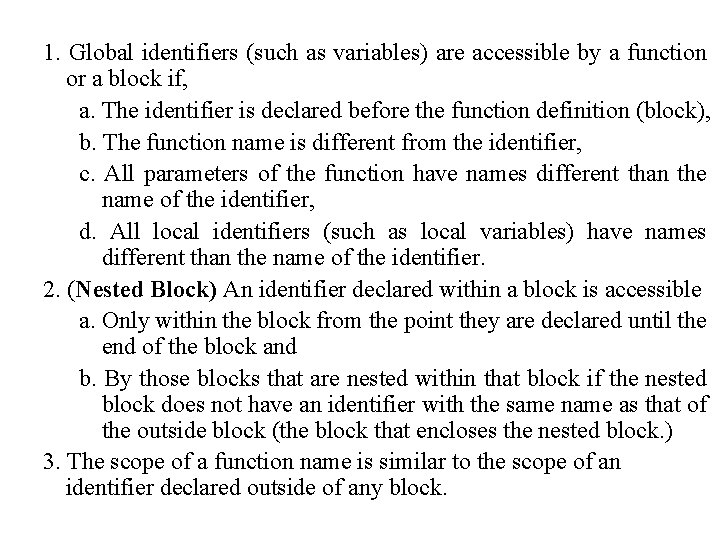
1. Global identifiers (such as variables) are accessible by a function or a block if, a. The identifier is declared before the function definition (block), b. The function name is different from the identifier, c. All parameters of the function have names different than the name of the identifier, d. All local identifiers (such as local variables) have names different than the name of the identifier. 2. (Nested Block) An identifier declared within a block is accessible a. Only within the block from the point they are declared until the end of the block and b. By those blocks that are nested within that block if the nested block does not have an identifier with the same name as that of the outside block (the block that encloses the nested block. ) 3. The scope of a function name is similar to the scope of an identifier declared outside of any block.
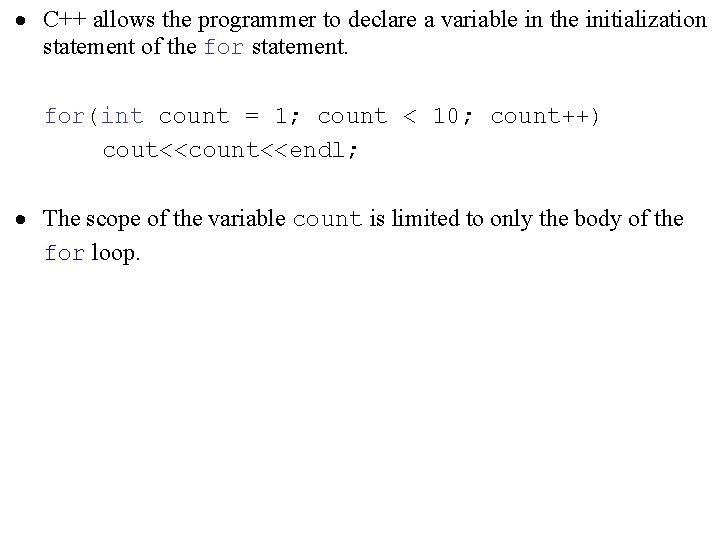
· C++ allows the programmer to declare a variable in the initialization statement of the for statement. for(int count = 1; count < 10; count++) cout<<count<<endl; · The scope of the variable count is limited to only the body of the for loop.
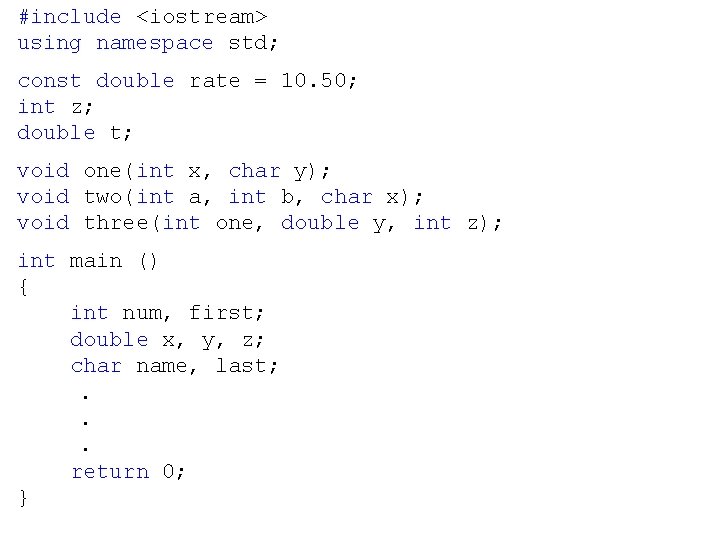
#include <iostream> using namespace std; const double rate = 10. 50; int z; double t; void one(int x, char y); void two(int a, int b, char x); void three(int one, double y, int z); int main () { int num, first; double x, y, z; char name, last; . . . return 0; }
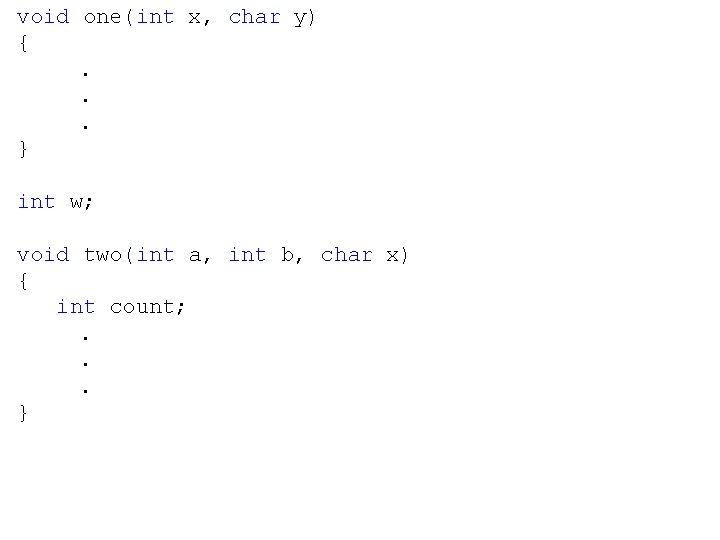
void one(int x, char y) {. . . } int w; void two(int a, int b, char x) { int count; . . . }
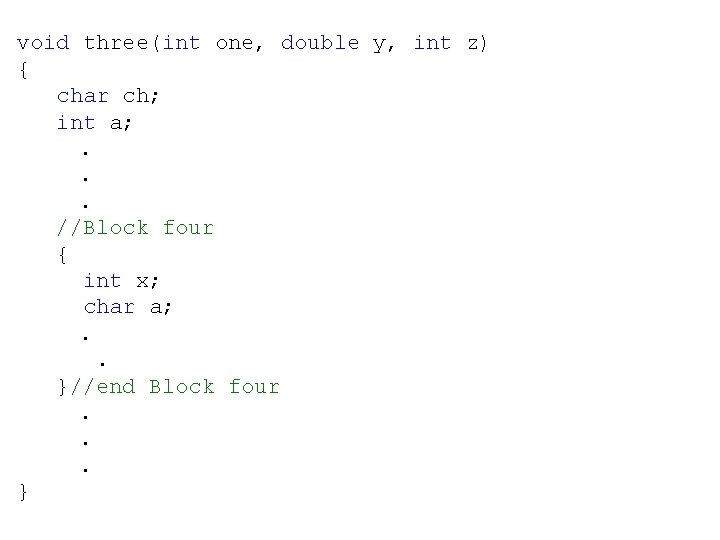
void three(int one, double y, int z) { char ch; int a; . . . //Block four { int x; char a; . . }//end Block four. . . }
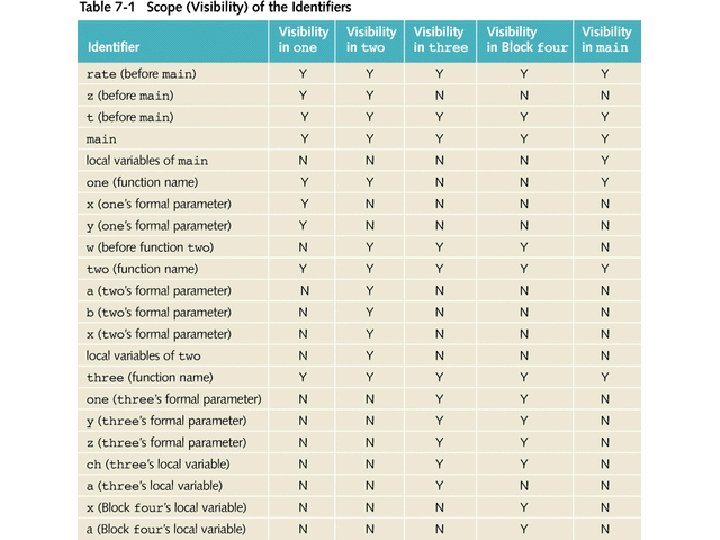
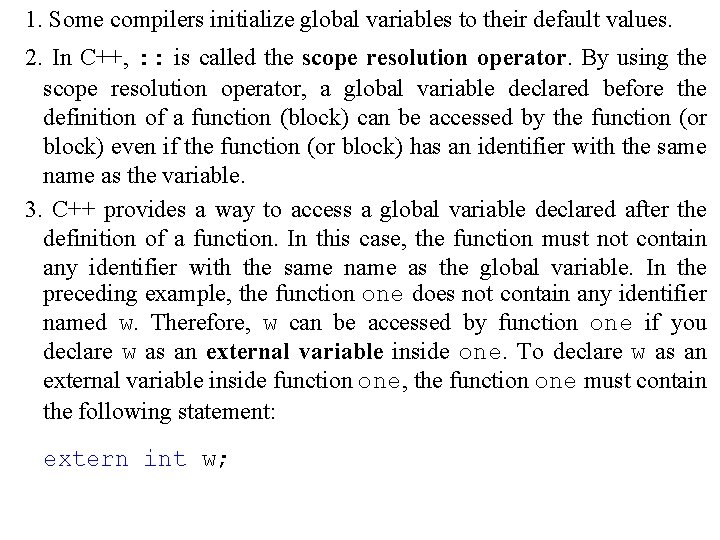
1. Some compilers initialize global variables to their default values. 2. In C++, : : is called the scope resolution operator. By using the scope resolution operator, a global variable declared before the definition of a function (block) can be accessed by the function (or block) even if the function (or block) has an identifier with the same name as the variable. 3. C++ provides a way to access a global variable declared after the definition of a function. In this case, the function must not contain any identifier with the same name as the global variable. In the preceding example, the function one does not contain any identifier named w. Therefore, w can be accessed by function one if you declare w as an external variable inside one. To declare w as an external variable inside function one, the function one must contain the following statement: extern int w;
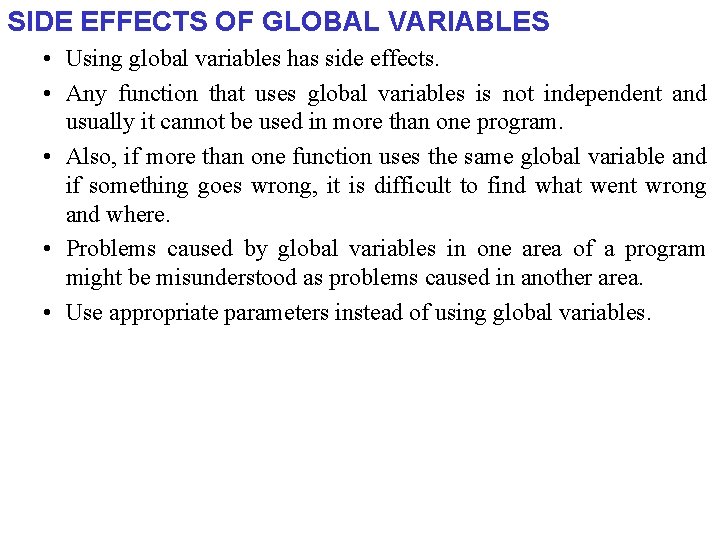
SIDE EFFECTS OF GLOBAL VARIABLES • Using global variables has side effects. • Any function that uses global variables is not independent and usually it cannot be used in more than one program. • Also, if more than one function uses the same global variable and if something goes wrong, it is difficult to find what went wrong and where. • Problems caused by global variables in one area of a program might be misunderstood as problems caused in another area. • Use appropriate parameters instead of using global variables.
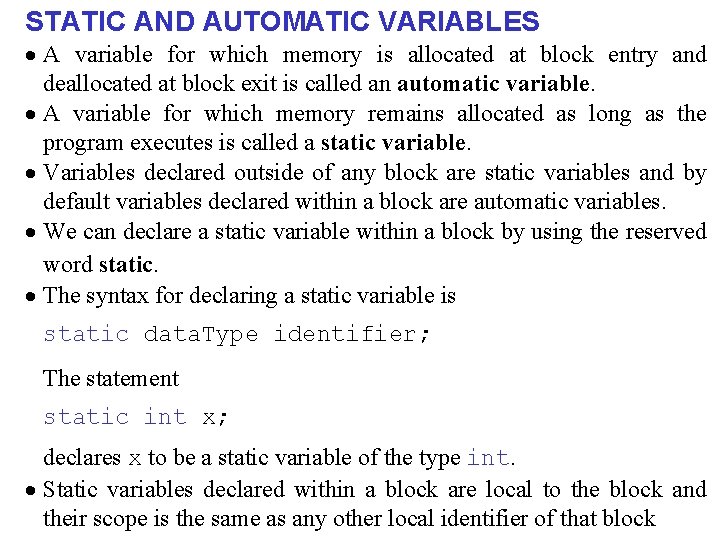
STATIC AND AUTOMATIC VARIABLES · A variable for which memory is allocated at block entry and deallocated at block exit is called an automatic variable. · A variable for which memory remains allocated as long as the program executes is called a static variable. · Variables declared outside of any block are static variables and by default variables declared within a block are automatic variables. · We can declare a static variable within a block by using the reserved word static. · The syntax for declaring a static variable is static data. Type identifier; The statement static int x; declares x to be a static variable of the type int. · Static variables declared within a block are local to the block and their scope is the same as any other local identifier of that block
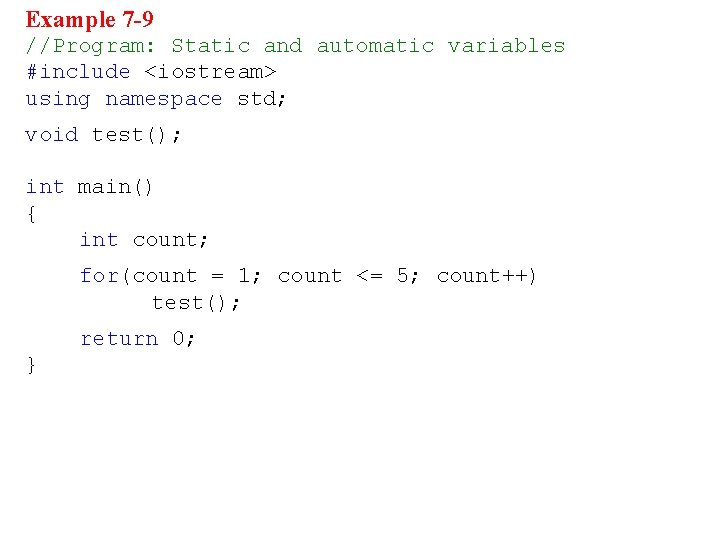
Example 7 -9 //Program: Static and automatic variables #include <iostream> using namespace std; void test(); int main() { int count; for(count = 1; count <= 5; count++) test(); return 0; }
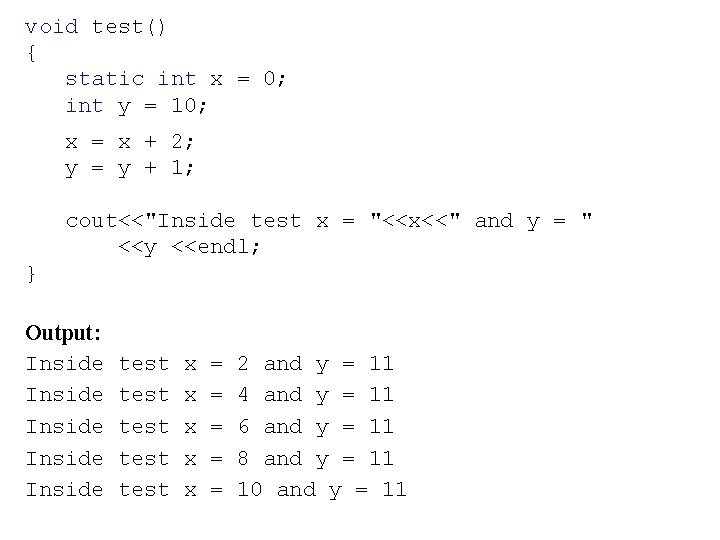
void test() { static int x = 0; int y = 10; x = x + 2; y = y + 1; cout<<"Inside test x = "<<x<<" and y = " <<y <<endl; } Output: Inside Inside test test x x x = = = 2 and y = 11 4 and y = 11 6 and y = 11 8 and y = 11 10 and y = 11
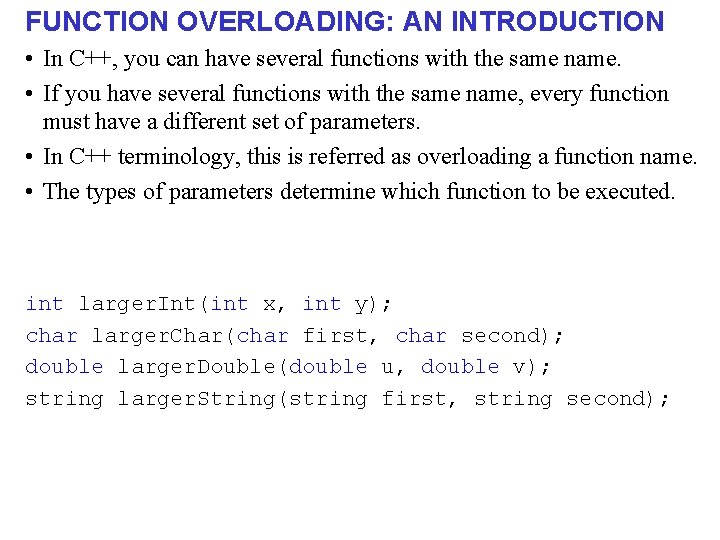
FUNCTION OVERLOADING: AN INTRODUCTION • In C++, you can have several functions with the same name. • If you have several functions with the same name, every function must have a different set of parameters. • In C++ terminology, this is referred as overloading a function name. • The types of parameters determine which function to be executed. int larger. Int(int x, int y); char larger. Char(char first, char second); double larger. Double(double u, double v); string larger. String(string first, string second);
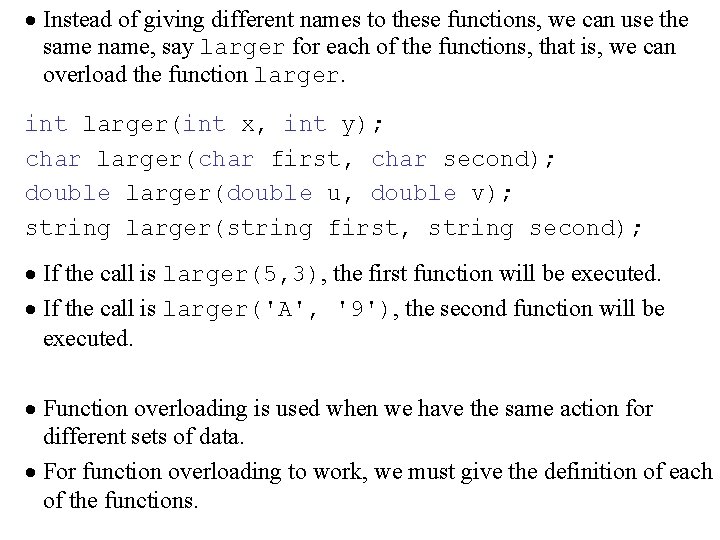
· Instead of giving different names to these functions, we can use the same name, say larger for each of the functions, that is, we can overload the function larger. int larger(int x, int y); char larger(char first, char second); double larger(double u, double v); string larger(string first, string second); · If the call is larger(5, 3), the first function will be executed. · If the call is larger('A', '9'), the second function will be executed. · Function overloading is used when we have the same action for different sets of data. · For function overloading to work, we must give the definition of each of the functions.
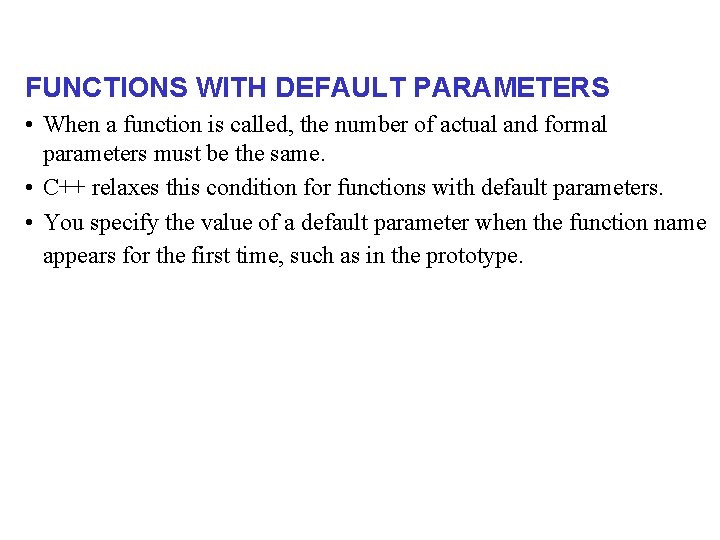
FUNCTIONS WITH DEFAULT PARAMETERS • When a function is called, the number of actual and formal parameters must be the same. • C++ relaxes this condition for functions with default parameters. • You specify the value of a default parameter when the function name appears for the first time, such as in the prototype.
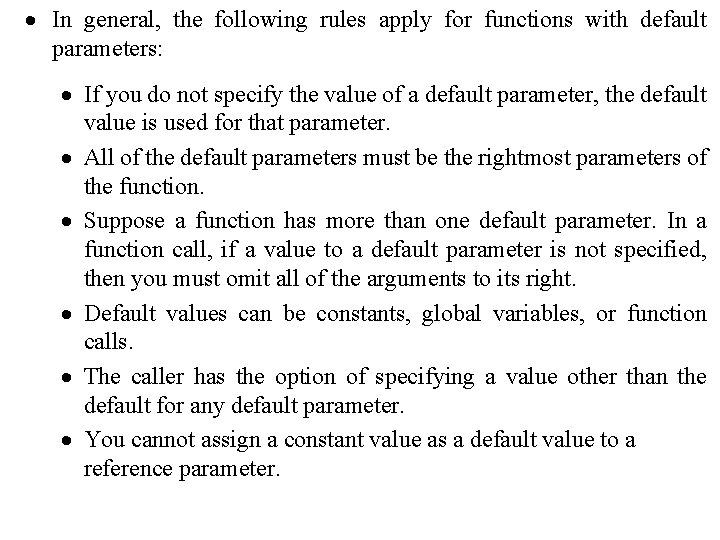
· In general, the following rules apply for functions with default parameters: · If you do not specify the value of a default parameter, the default value is used for that parameter. · All of the default parameters must be the rightmost parameters of the function. · Suppose a function has more than one default parameter. In a function call, if a value to a default parameter is not specified, then you must omit all of the arguments to its right. · Default values can be constants, global variables, or function calls. · The caller has the option of specifying a value other than the default for any default parameter. · You cannot assign a constant value as a default value to a reference parameter.
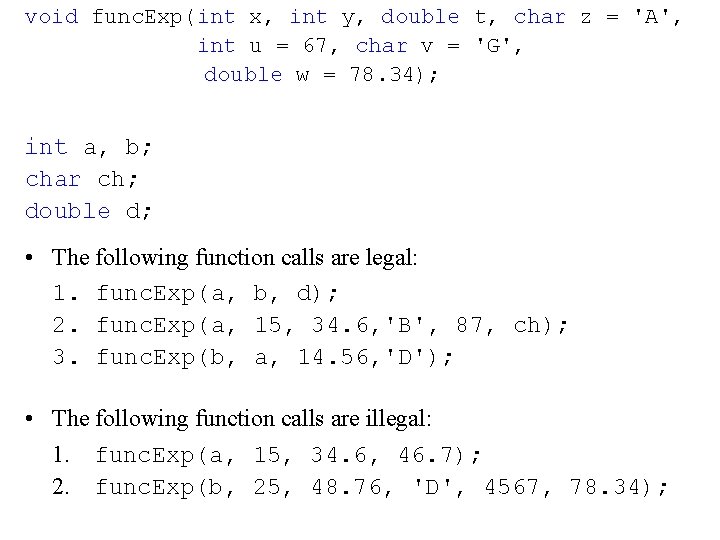
void func. Exp(int x, int y, double t, char z = 'A', int u = 67, char v = 'G', double w = 78. 34); int a, b; char ch; double d; • The following function calls are legal: 1. func. Exp(a, b, d); 2. func. Exp(a, 15, 34. 6, 'B', 87, ch); 3. func. Exp(b, a, 14. 56, 'D'); • The following function calls are illegal: 1. func. Exp(a, 15, 34. 6, 46. 7); 2. func. Exp(b, 25, 48. 76, 'D', 4567, 78. 34);
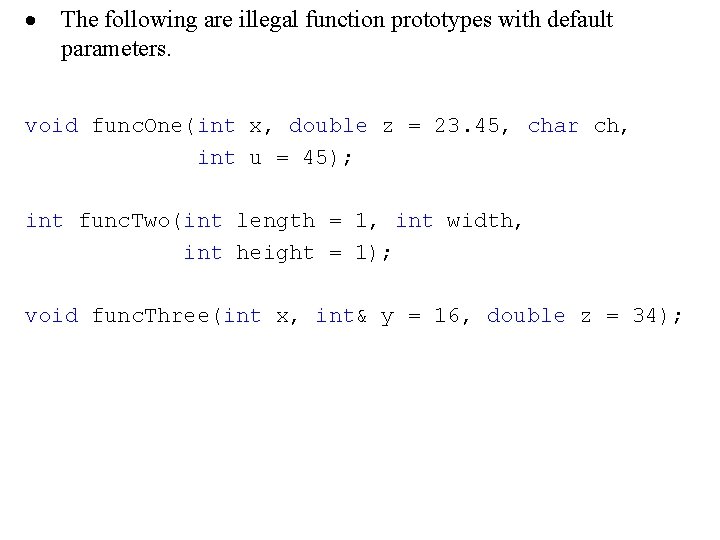
· The following are illegal function prototypes with default parameters. void func. One(int x, double z = 23. 45, char ch, int u = 45); int func. Two(int length = 1, int width, int height = 1); void func. Three(int x, int& y = 16, double z = 34);
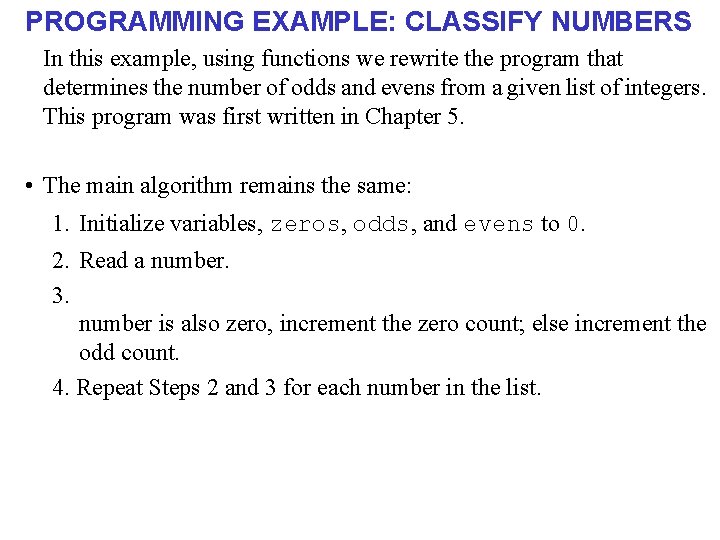
PROGRAMMING EXAMPLE: CLASSIFY NUMBERS In this example, using functions we rewrite the program that determines the number of odds and evens from a given list of integers. This program was first written in Chapter 5. • The main algorithm remains the same: 1. Initialize variables, zeros, odds, and evens to 0. 2. Read a number. 3. number is also zero, increment the zero count; else increment the odd count. 4. Repeat Steps 2 and 3 for each number in the list.
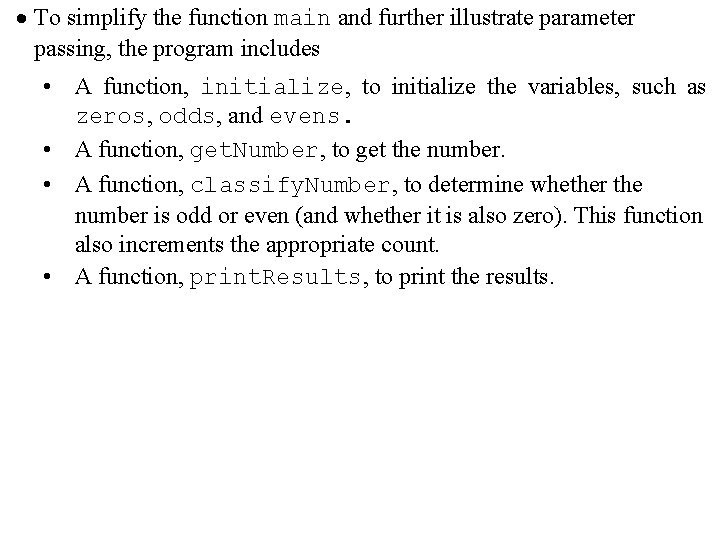
· To simplify the function main and further illustrate parameter passing, the program includes • A function, initialize, to initialize the variables, such as zeros, odds, and evens. • A function, get. Number, to get the number. • A function, classify. Number, to determine whether the number is odd or even (and whether it is also zero). This function also increments the appropriate count. • A function, print. Results, to print the results.
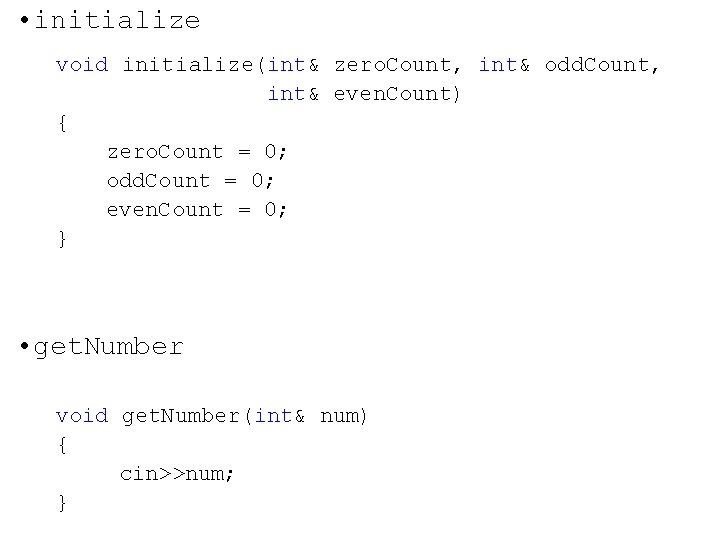
• initialize void initialize(int& zero. Count, int& odd. Count, int& even. Count) { zero. Count = 0; odd. Count = 0; even. Count = 0; } • get. Number void get. Number(int& num) { cin>>num; }
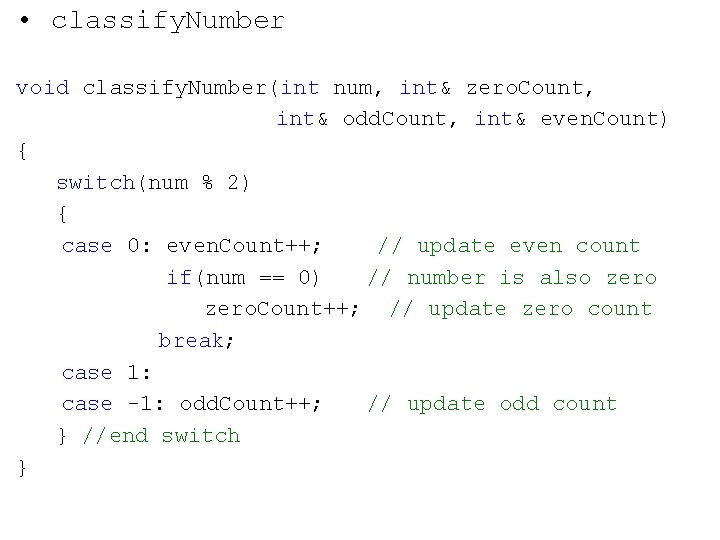
• classify. Number void classify. Number(int num, int& zero. Count, int& odd. Count, int& even. Count) { switch(num % 2) { case 0: even. Count++; // update even count if(num == 0) // number is also zero. Count++; // update zero count break; case 1: case -1: odd. Count++; // update odd count } //end switch }
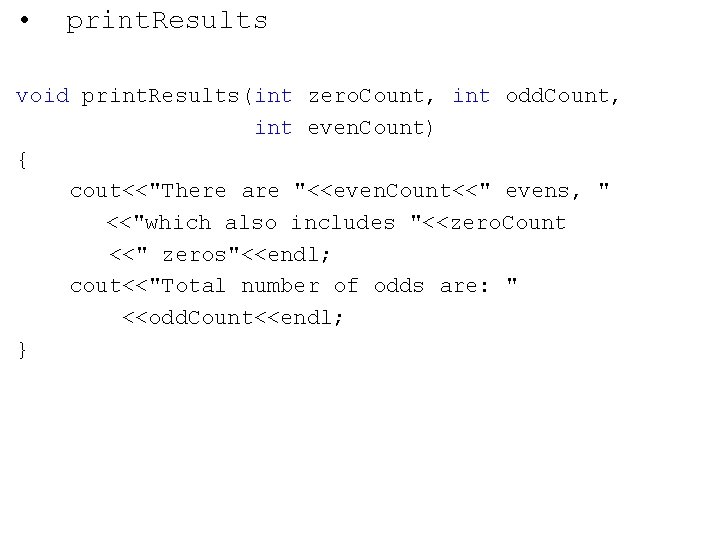
• print. Results void print. Results(int zero. Count, int odd. Count, int even. Count) { cout<<"There are "<<even. Count<<" evens, " <<"which also includes "<<zero. Count <<" zeros"<<endl; cout<<"Total number of odds are: " <<odd. Count<<endl; }
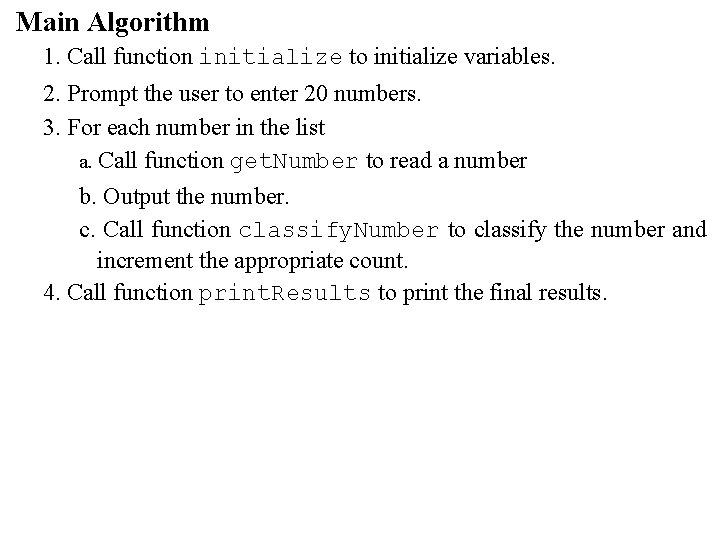
Main Algorithm 1. Call function initialize to initialize variables. 2. Prompt the user to enter 20 numbers. 3. For each number in the list a. Call function get. Number to read a number b. Output the number. c. Call function classify. Number to classify the number and increment the appropriate count. 4. Call function print. Results to print the final results.
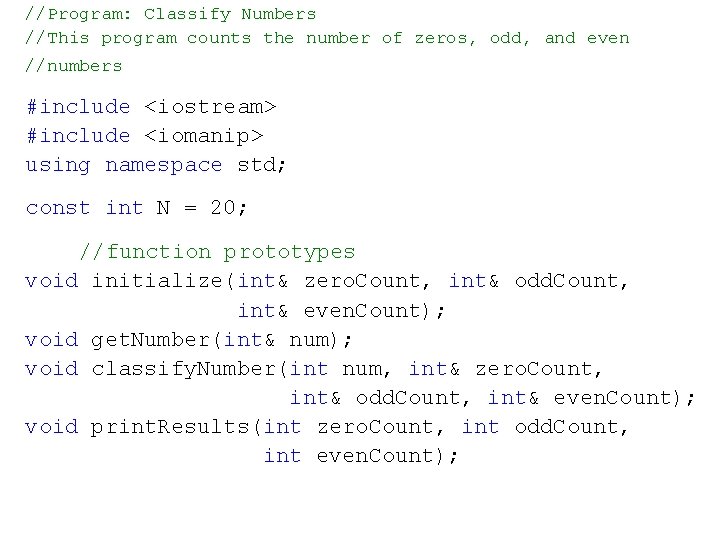
//Program: Classify Numbers //This program counts the number of zeros, odd, and even //numbers #include <iostream> #include <iomanip> using namespace std; const int N = 20; //function prototypes void initialize(int& zero. Count, int& odd. Count, int& even. Count); void get. Number(int& num); void classify. Number(int num, int& zero. Count, int& odd. Count, int& even. Count); void print. Results(int zero. Count, int odd. Count, int even. Count);
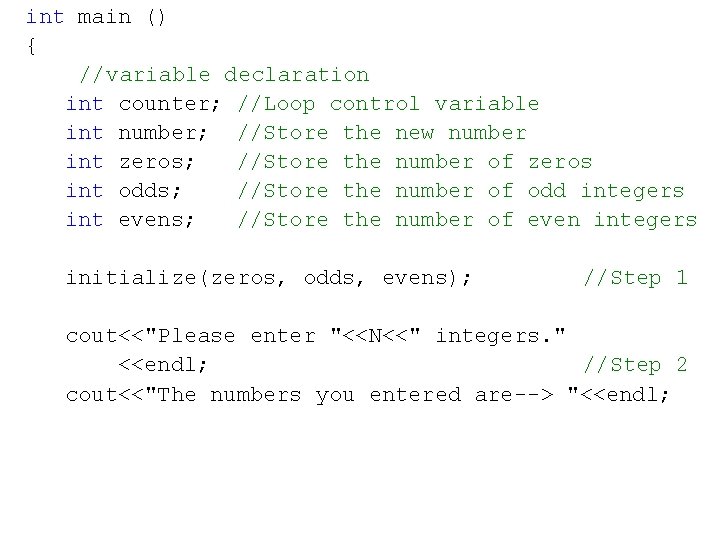
int main () { //variable declaration int counter; //Loop control variable int number; //Store the new number int zeros; //Store the number of zeros int odds; //Store the number of odd integers int evens; //Store the number of even integers initialize(zeros, odds, evens); //Step 1 cout<<"Please enter "<<N<<" integers. " <<endl; //Step 2 cout<<"The numbers you entered are--> "<<endl;
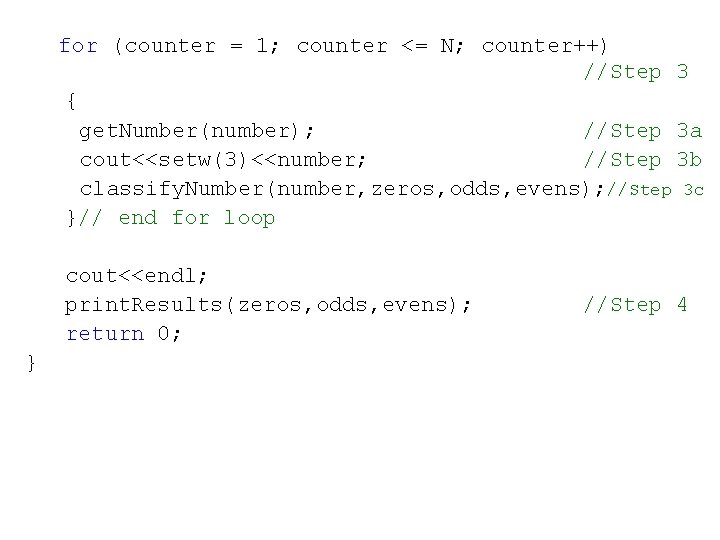
for (counter = 1; counter <= N; counter++) //Step 3 { get. Number(number); //Step 3 a cout<<setw(3)<<number; //Step 3 b classify. Number(number, zeros, odds, evens); //Step 3 c }// end for loop cout<<endl; print. Results(zeros, odds, evens); return 0; } //Step 4
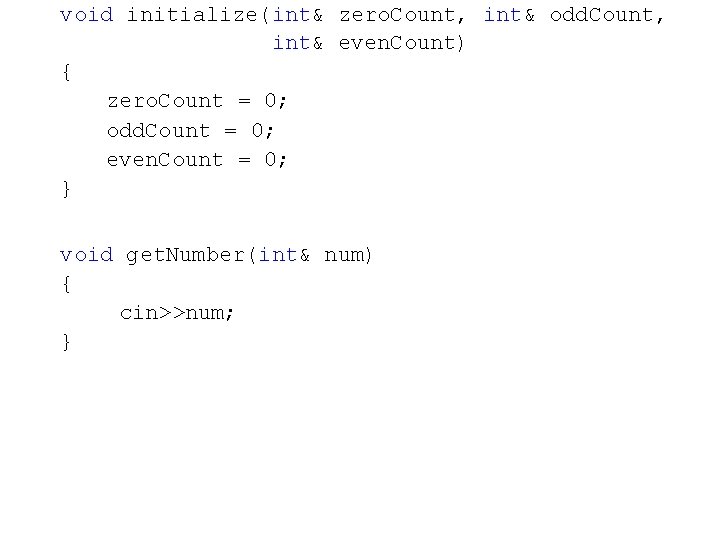
void initialize(int& zero. Count, int& odd. Count, int& even. Count) { zero. Count = 0; odd. Count = 0; even. Count = 0; } void get. Number(int& num) { cin>>num; }
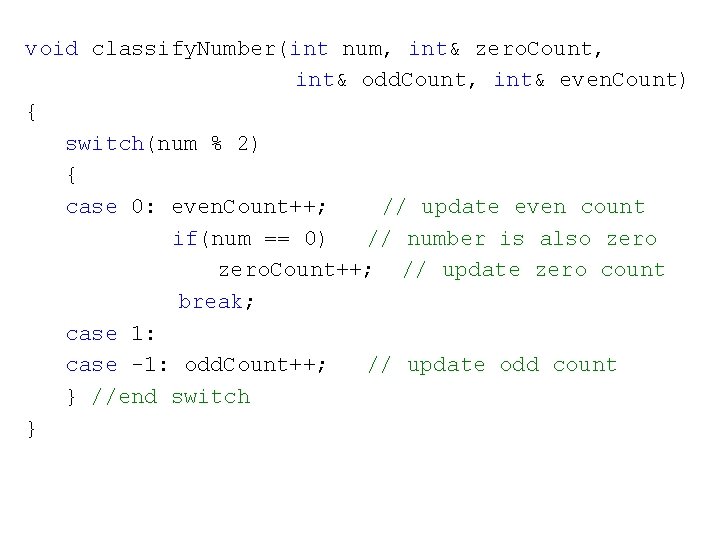
void classify. Number(int num, int& zero. Count, int& odd. Count, int& even. Count) { switch(num % 2) { case 0: even. Count++; // update even count if(num == 0) // number is also zero. Count++; // update zero count break; case 1: case -1: odd. Count++; // update odd count } //end switch }
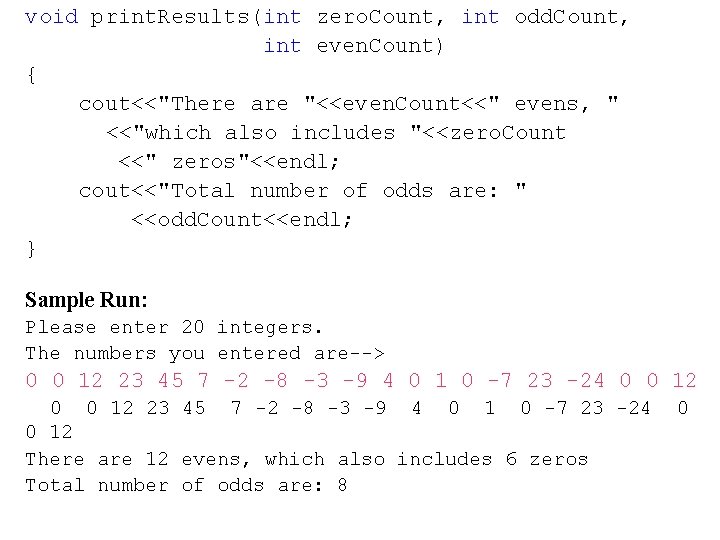
void print. Results(int zero. Count, int odd. Count, int even. Count) { cout<<"There are "<<even. Count<<" evens, " <<"which also includes "<<zero. Count <<" zeros"<<endl; cout<<"Total number of odds are: " <<odd. Count<<endl; } Sample Run: Please enter 20 integers. The numbers you entered are--> 0 0 12 23 45 7 -2 -8 -3 -9 4 0 1 0 -7 23 -24 0 12 There are 12 evens, which also includes 6 zeros Total number of odds are: 8 0
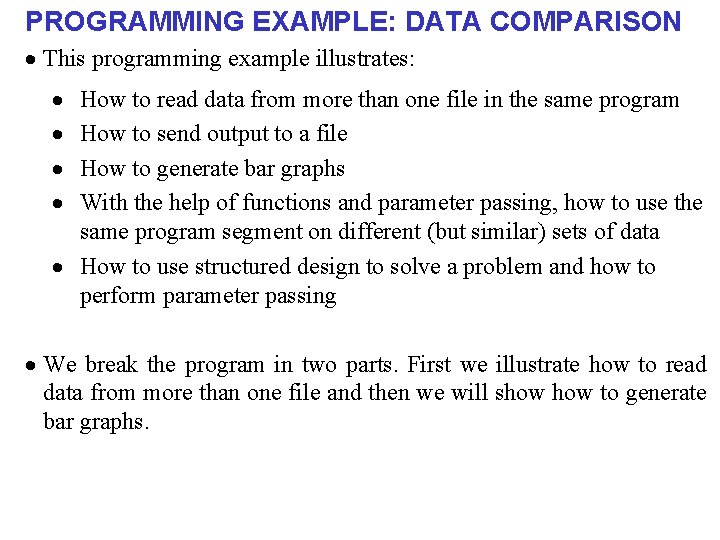
PROGRAMMING EXAMPLE: DATA COMPARISON · This programming example illustrates: · · How to read data from more than one file in the same program How to send output to a file How to generate bar graphs With the help of functions and parameter passing, how to use the same program segment on different (but similar) sets of data · How to use structured design to solve a problem and how to perform parameter passing · We break the program in two parts. First we illustrate how to read data from more than one file and then we will show to generate bar graphs.
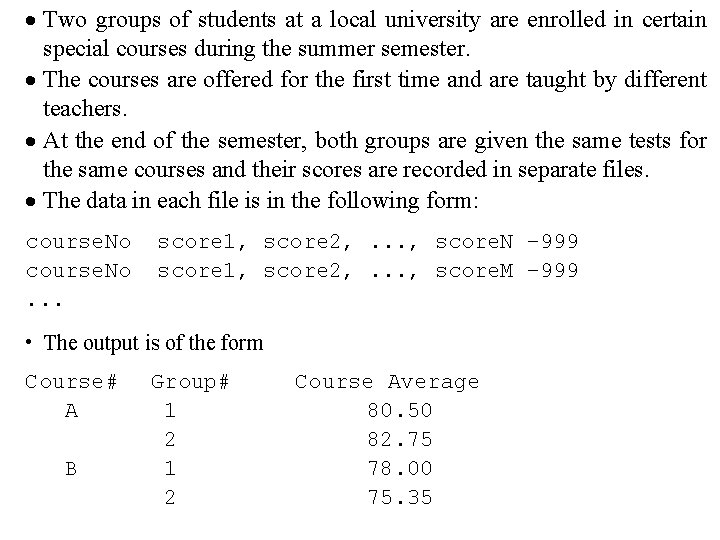
· Two groups of students at a local university are enrolled in certain special courses during the summer semester. · The courses are offered for the first time and are taught by different teachers. · At the end of the semester, both groups are given the same tests for the same courses and their scores are recorded in separate files. · The data in each file is in the following form: course. No. . . score 1, score 2, . . . , score. N – 999 score 1, score 2, . . . , score. M – 999 • The output is of the form Course# A B Group# 1 2 Course Average 80. 50 82. 75 78. 00 75. 35
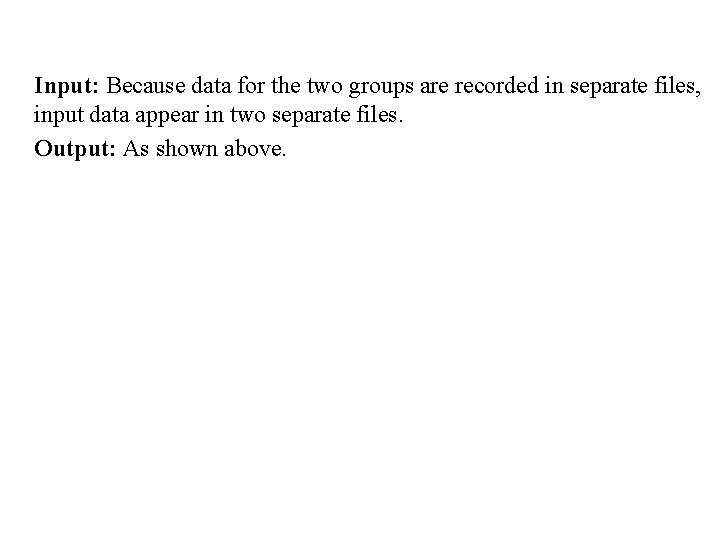
Input: Because data for the two groups are recorded in separate files, input data appear in two separate files. Output: As shown above.
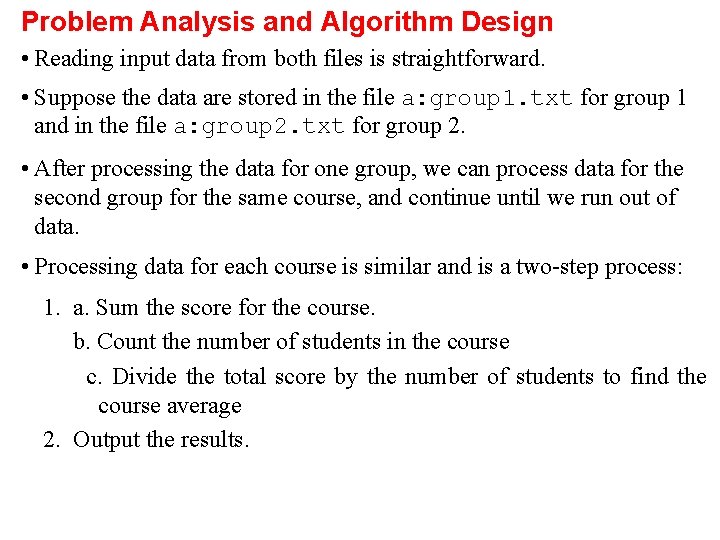
Problem Analysis and Algorithm Design • Reading input data from both files is straightforward. • Suppose the data are stored in the file a: group 1. txt for group 1 and in the file a: group 2. txt for group 2. • After processing the data for one group, we can process data for the second group for the same course, and continue until we run out of data. • Processing data for each course is similar and is a two-step process: 1. a. Sum the score for the course. b. Count the number of students in the course c. Divide the total score by the number of students to find the course average 2. Output the results.
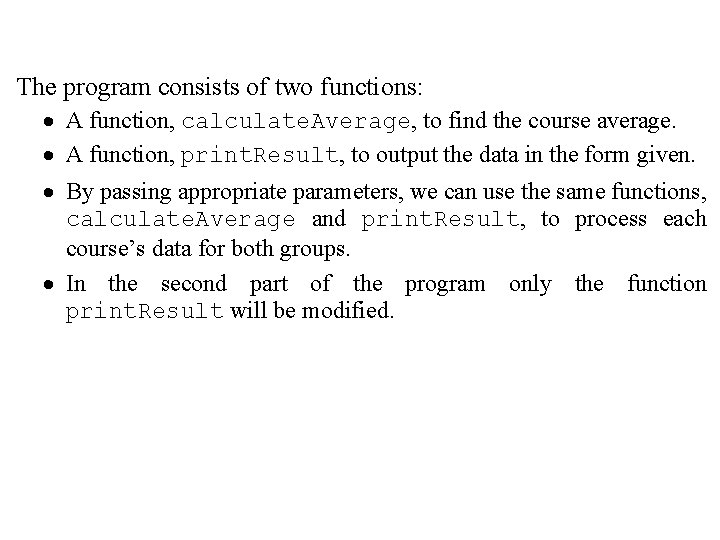
The program consists of two functions: · A function, calculate. Average, to find the course average. · A function, print. Result, to output the data in the form given. · By passing appropriate parameters, we can use the same functions, calculate. Average and print. Result, to process each course’s data for both groups. · In the second part of the program only the function print. Result will be modified.
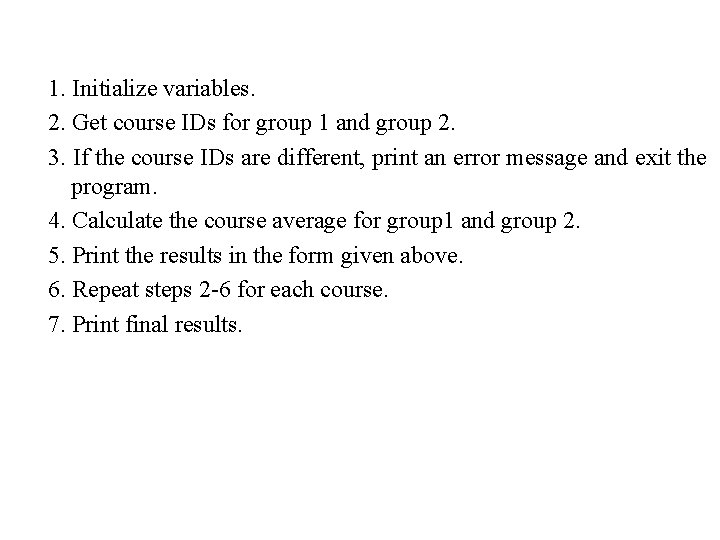
1. Initialize variables. 2. Get course IDs for group 1 and group 2. 3. If the course IDs are different, print an error message and exit the program. 4. Calculate the course average for group 1 and group 2. 5. Print the results in the form given above. 6. Repeat steps 2 -6 for each course. 7. Print final results.
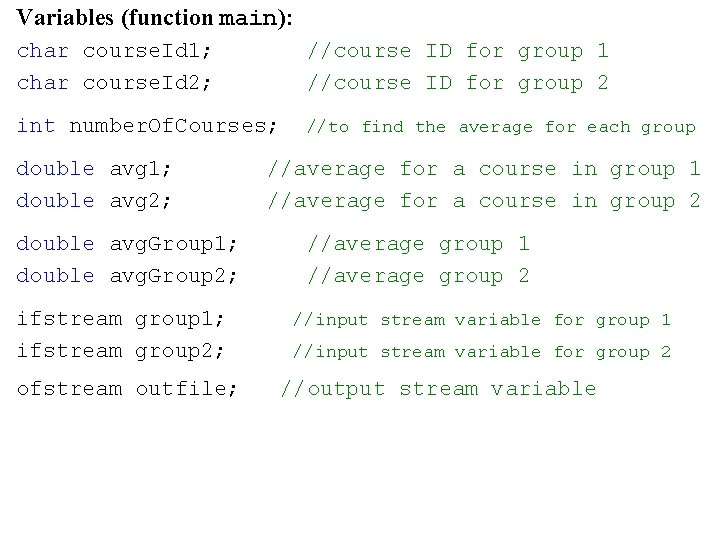
Variables (function main): char course. Id 1; char course. Id 2; //course ID for group 1 //course ID for group 2 int number. Of. Courses; //to find the average for each group double avg 1; double avg 2; double avg. Group 1; double avg. Group 2; ifstream group 1; ifstream group 2; ofstream outfile; //average for a course in group 1 //average for a course in group 2 //average group 1 //average group 2 //input stream variable for group 1 //input stream variable for group 2 //output stream variable
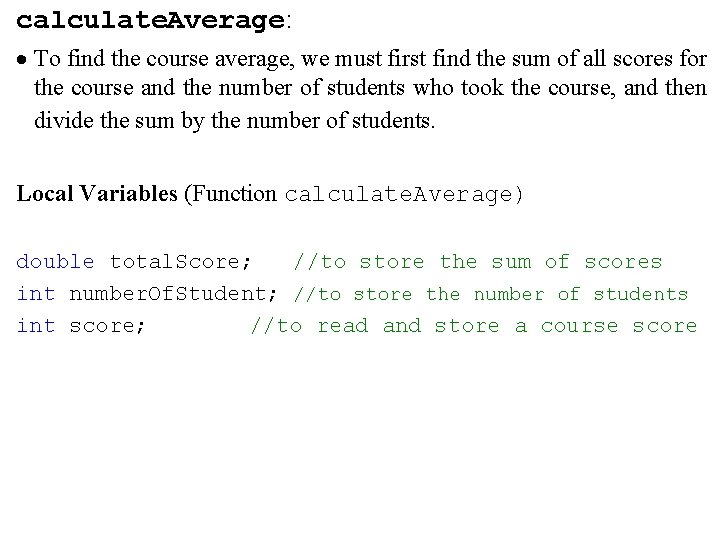
calculate. Average: · To find the course average, we must first find the sum of all scores for the course and the number of students who took the course, and then divide the sum by the number of students. Local Variables (Function calculate. Average) double total. Score; //to store the sum of scores int number. Of. Student; //to store the number of students int score; //to read and store a course score
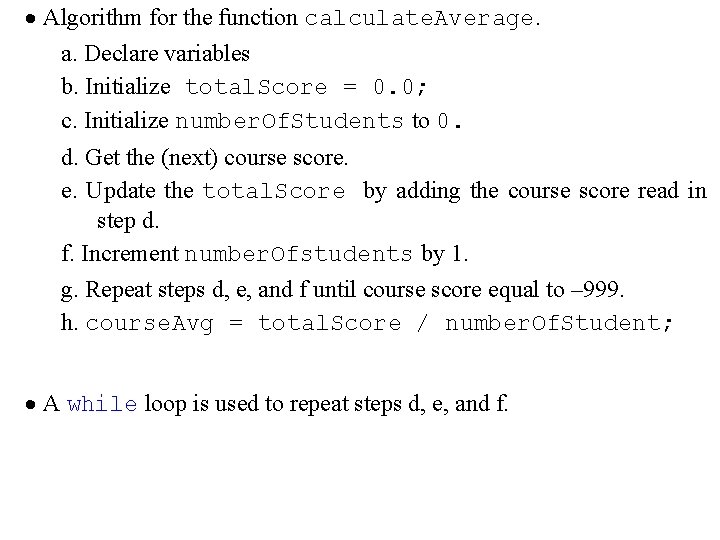
· Algorithm for the function calculate. Average. a. Declare variables b. Initialize total. Score = 0. 0; c. Initialize number. Of. Students to 0. d. Get the (next) course score. e. Update the total. Score by adding the course score read in step d. f. Increment number. Ofstudents by 1. g. Repeat steps d, e, and f until course score equal to – 999. h. course. Avg = total. Score / number. Of. Student; · A while loop is used to repeat steps d, e, and f.
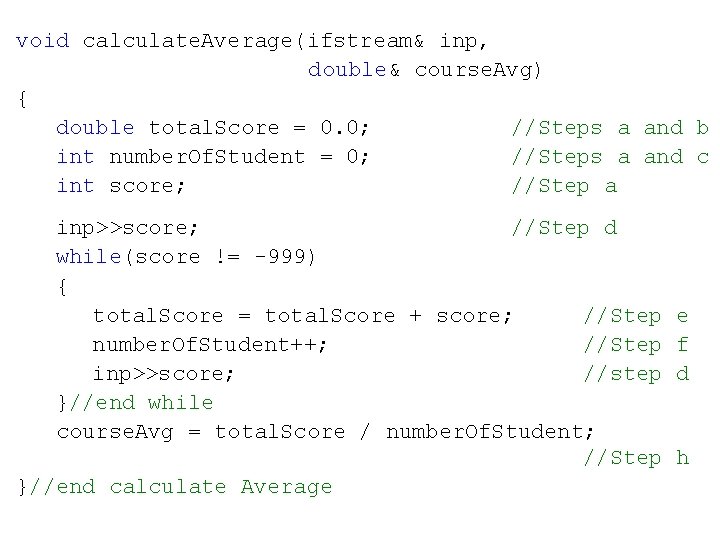
void calculate. Average(ifstream& inp, double& course. Avg) { double total. Score = 0. 0; //Steps a and b int number. Of. Student = 0; //Steps a and c int score; //Step a inp>>score; //Step d while(score != -999) { total. Score = total. Score + score; //Step number. Of. Student++; //Step inp>>score; //step }//end while course. Avg = total. Score / number. Of. Student; //Step }//end calculate Average e f d h
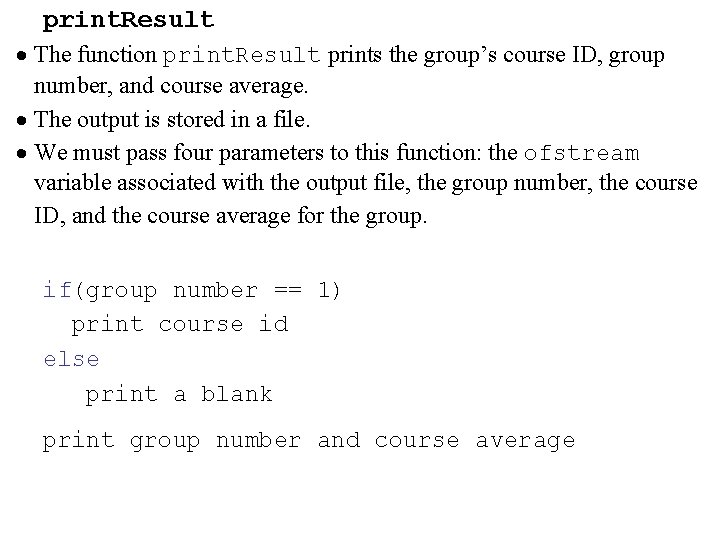
print. Result · The function print. Result prints the group’s course ID, group number, and course average. · The output is stored in a file. · We must pass four parameters to this function: the ofstream variable associated with the output file, the group number, the course ID, and the course average for the group. if(group number == 1) print course id else print a blank print group number and course average
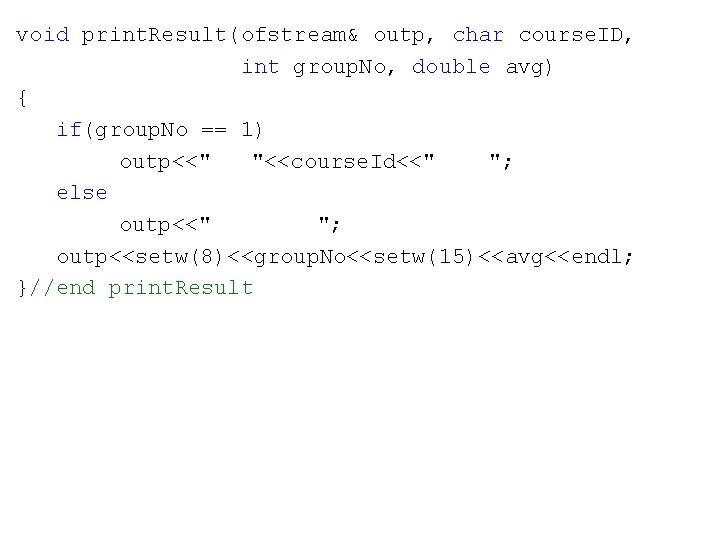
void print. Result(ofstream& outp, char course. ID, int group. No, double avg) { if(group. No == 1) outp<<" "<<course. Id<<" "; else outp<<" "; outp<<setw(8)<<group. No<<setw(15)<<avg<<endl; }//end print. Result
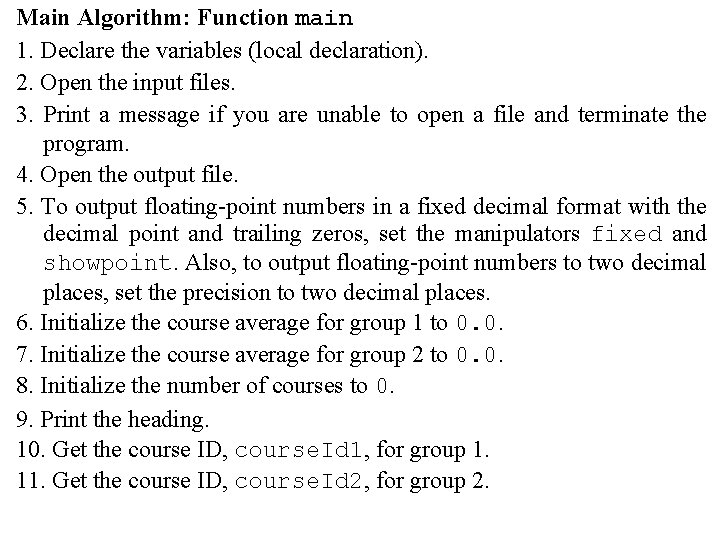
Main Algorithm: Function main 1. Declare the variables (local declaration). 2. Open the input files. 3. Print a message if you are unable to open a file and terminate the program. 4. Open the output file. 5. To output floating-point numbers in a fixed decimal format with the decimal point and trailing zeros, set the manipulators fixed and showpoint. Also, to output floating-point numbers to two decimal places, set the precision to two decimal places. 6. Initialize the course average for group 1 to 0. 0. 7. Initialize the course average for group 2 to 0. 0. 8. Initialize the number of courses to 0. 9. Print the heading. 10. Get the course ID, course. Id 1, for group 1. 11. Get the course ID, course. Id 2, for group 2.
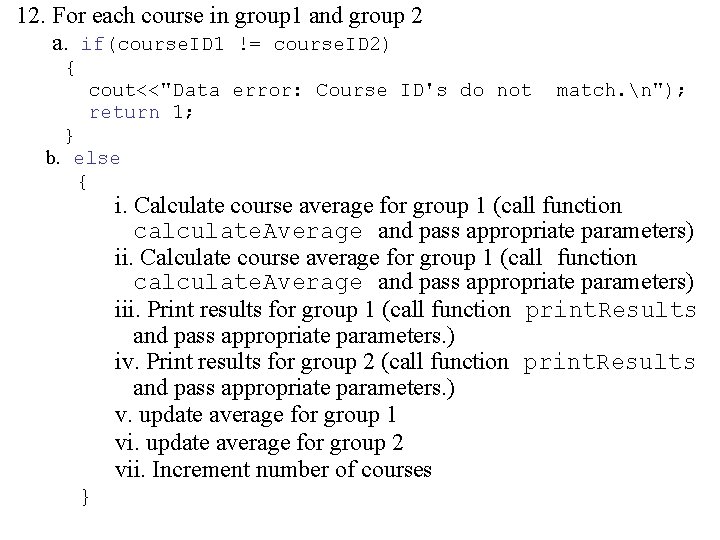
12. For each course in group 1 and group 2 a. if(course. ID 1 != course. ID 2) { cout<<"Data error: Course ID's do not return 1; } b. else { match. n"); i. Calculate course average for group 1 (call function calculate. Average and pass appropriate parameters) iii. Print results for group 1 (call function print. Results and pass appropriate parameters. ) iv. Print results for group 2 (call function print. Results and pass appropriate parameters. ) v. update average for group 1 vi. update average for group 2 vii. Increment number of courses }
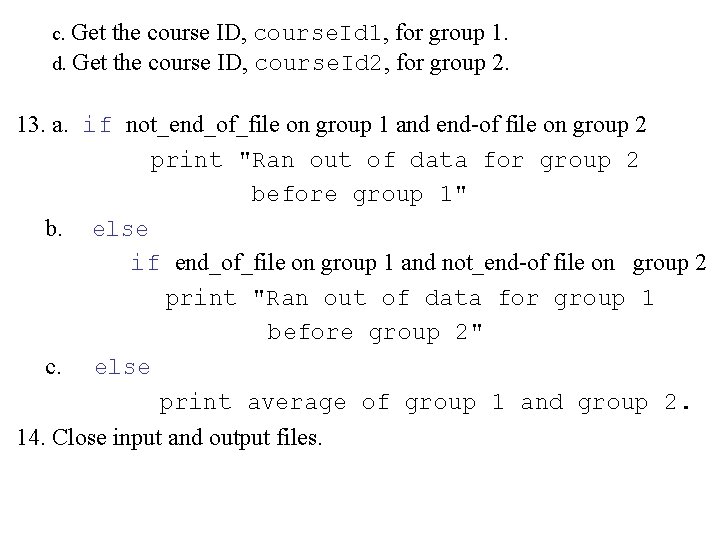
c. Get the course ID, course. Id 1, for group 1. d. Get the course ID, course. Id 2, for group 2. 13. a. if not_end_of_file on group 1 and end-of file on group 2 print "Ran out of data for group 2 before group 1" b. else if end_of_file on group 1 and not_end-of file on group 2 print "Ran out of data for group 1 before group 2" c. else print average of group 1 and group 2. 14. Close input and output files.
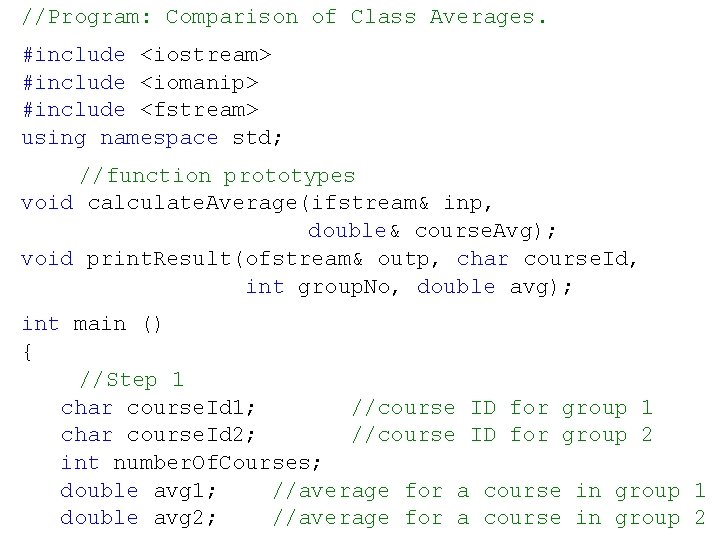
//Program: Comparison of Class Averages. #include <iostream> #include <iomanip> #include <fstream> using namespace std; //function prototypes void calculate. Average(ifstream& inp, double& course. Avg); void print. Result(ofstream& outp, char course. Id, int group. No, double avg); int main () { //Step 1 char course. Id 1; //course ID for group 1 char course. Id 2; //course ID for group 2 int number. Of. Courses; double avg 1; //average for a course in group 1 double avg 2; //average for a course in group 2
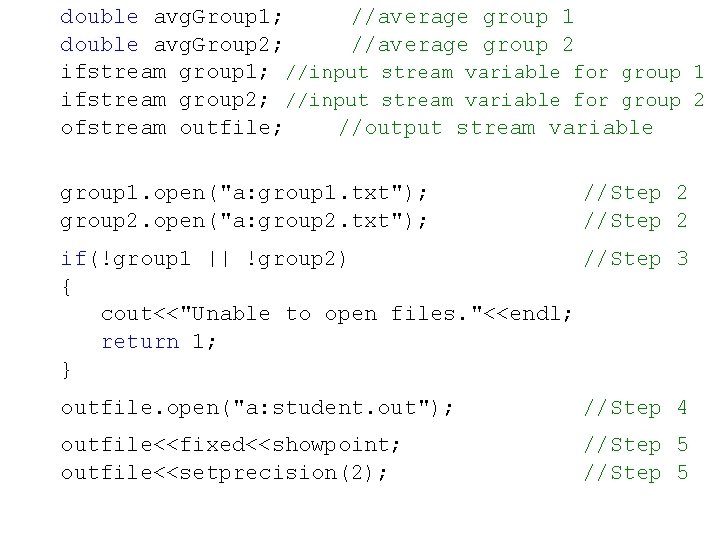
double avg. Group 1; //average group 1 double avg. Group 2; //average group 2 ifstream group 1; //input stream variable for group 1 ifstream group 2; //input stream variable for group 2 ofstream outfile; //output stream variable group 1. open("a: group 1. txt"); group 2. open("a: group 2. txt"); //Step 2 if(!group 1 || !group 2) //Step 3 { cout<<"Unable to open files. "<<endl; return 1; } outfile. open("a: student. out"); //Step 4 outfile<<fixed<<showpoint; outfile<<setprecision(2); //Step 5
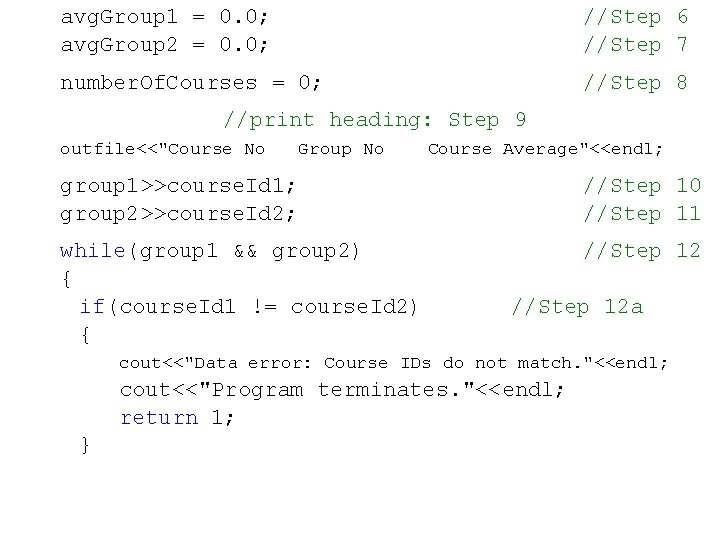
avg. Group 1 = 0. 0; avg. Group 2 = 0. 0; //Step 6 //Step 7 number. Of. Courses = 0; //Step 8 //print heading: Step 9 outfile<<"Course No Group No Course Average"<<endl; group 1>>course. Id 1; group 2>>course. Id 2; //Step 10 //Step 11 while(group 1 && group 2) { if(course. Id 1 != course. Id 2) { //Step 12 a cout<<"Data error: Course IDs do not match. "<<endl; cout<<"Program terminates. "<<endl; return 1; }
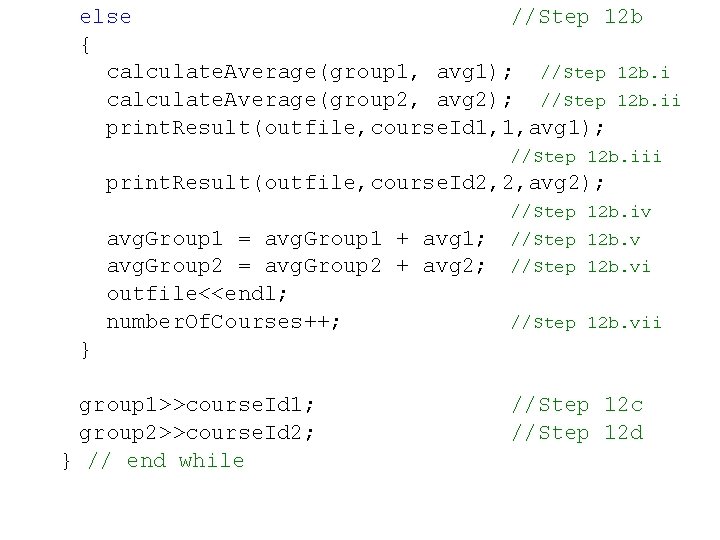
else //Step 12 b { calculate. Average(group 1, avg 1); //Step 12 b. i calculate. Average(group 2, avg 2); //Step 12 b. ii print. Result(outfile, course. Id 1, 1, avg 1); //Step 12 b. iii print. Result(outfile, course. Id 2, 2, avg 2); //Step 12 b. iv avg. Group 1 = avg. Group 1 + avg 1; avg. Group 2 = avg. Group 2 + avg 2; outfile<<endl; number. Of. Courses++; //Step 12 b. vii } group 1>>course. Id 1; group 2>>course. Id 2; } // end while //Step 12 c //Step 12 d
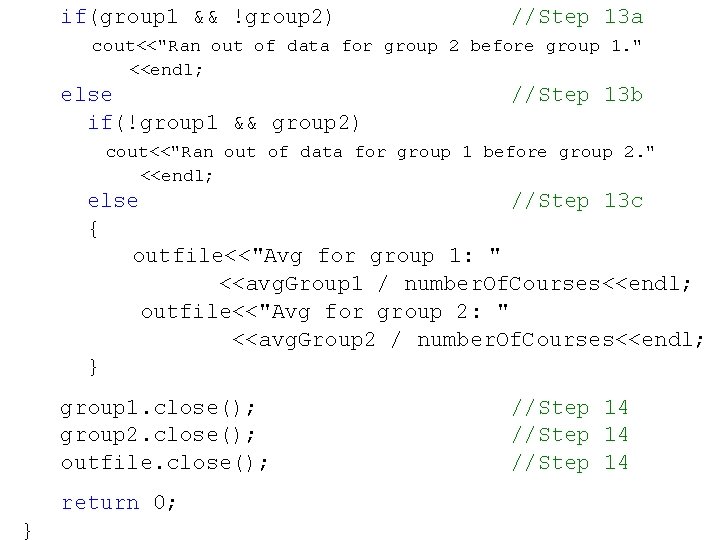
if(group 1 && !group 2) //Step 13 a cout<<"Ran out of data for group 2 before group 1. " <<endl; else if(!group 1 && group 2) //Step 13 b cout<<"Ran out of data for group 1 before group 2. " <<endl; else //Step 13 c { outfile<<"Avg for group 1: " <<avg. Group 1 / number. Of. Courses<<endl; outfile<<"Avg for group 2: " <<avg. Group 2 / number. Of. Courses<<endl; } group 1. close(); group 2. close(); outfile. close(); return 0; } //Step 14
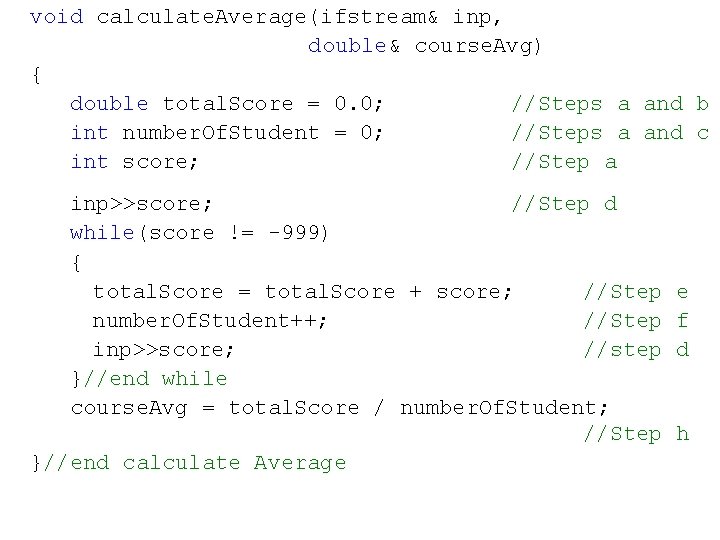
void calculate. Average(ifstream& inp, double& course. Avg) { double total. Score = 0. 0; //Steps a and b int number. Of. Student = 0; //Steps a and c int score; //Step a inp>>score; //Step d while(score != -999) { total. Score = total. Score + score; //Step number. Of. Student++; //Step inp>>score; //step }//end while course. Avg = total. Score / number. Of. Student; //Step }//end calculate Average e f d h
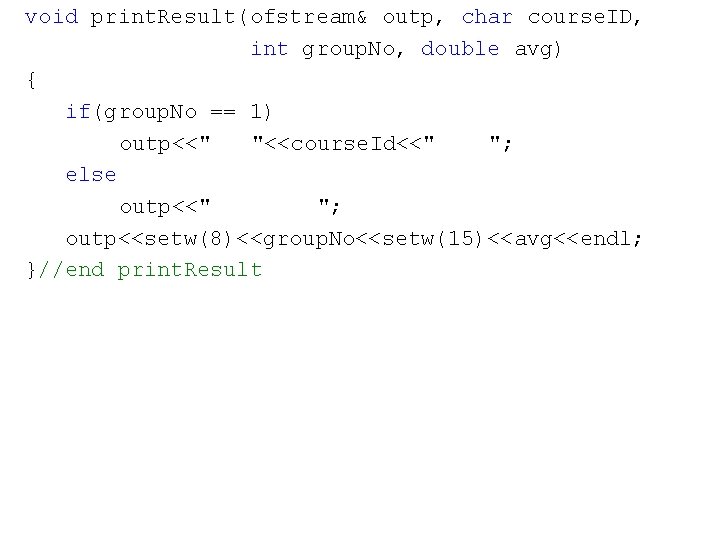
void print. Result(ofstream& outp, char course. ID, int group. No, double avg) { if(group. No == 1) outp<<" "<<course. Id<<" "; else outp<<" "; outp<<setw(8)<<group. No<<setw(15)<<avg<<endl; }//end print. Result
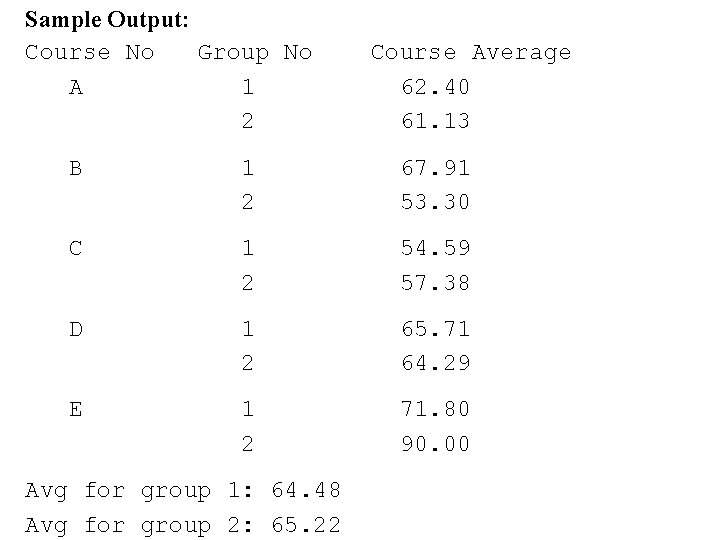
Sample Output: Course No Group No A 1 2 Course Average 62. 40 61. 13 B 1 2 67. 91 53. 30 C 1 2 54. 59 57. 38 D 1 2 65. 71 64. 29 E 1 2 71. 80 90. 00 Avg for group 1: 64. 48 Avg for group 2: 65. 22
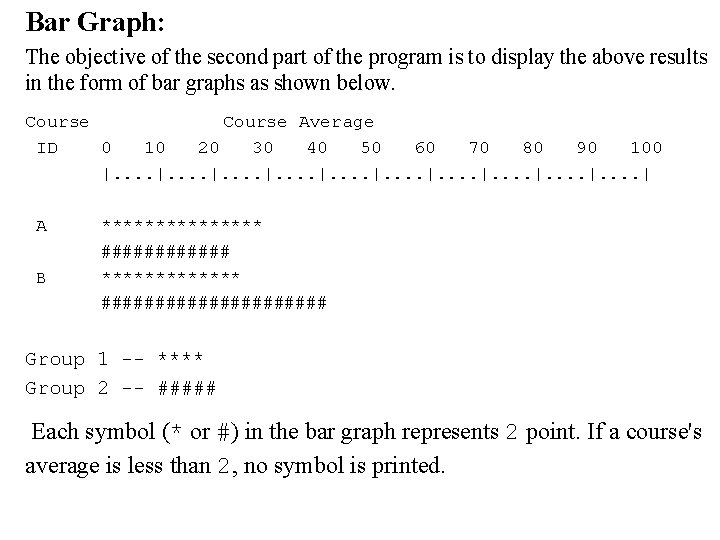
Bar Graph: The objective of the second part of the program is to display the above results in the form of bar graphs as shown below. Course Average ID 0 10 20 30 40 50 60 70 80 90 100 |. . . . | A B ******** ########### Group 1 -- **** Group 2 -- ##### Each symbol (* or #) in the bar graph represents 2 point. If a course's average is less than 2, no symbol is printed.
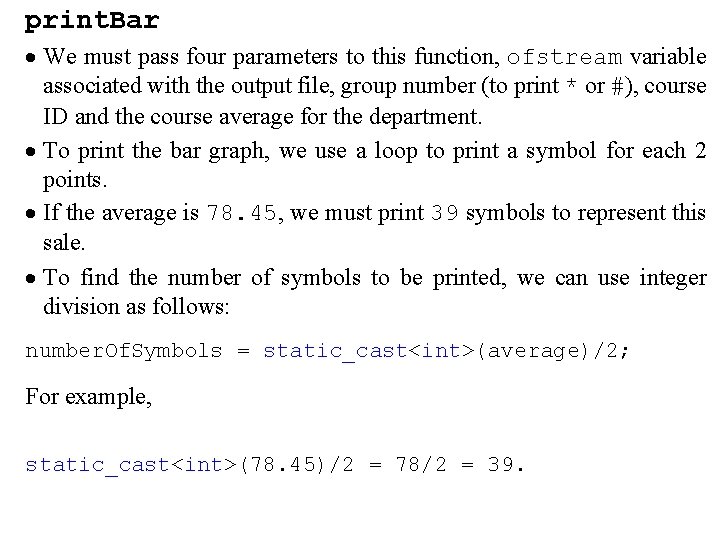
print. Bar · We must pass four parameters to this function, ofstream variable associated with the output file, group number (to print * or #), course ID and the course average for the department. · To print the bar graph, we use a loop to print a symbol for each 2 points. · If the average is 78. 45, we must print 39 symbols to represent this sale. · To find the number of symbols to be printed, we can use integer division as follows: number. Of. Symbols = static_cast<int>(average)/2; For example, static_cast<int>(78. 45)/2 = 78/2 = 39.
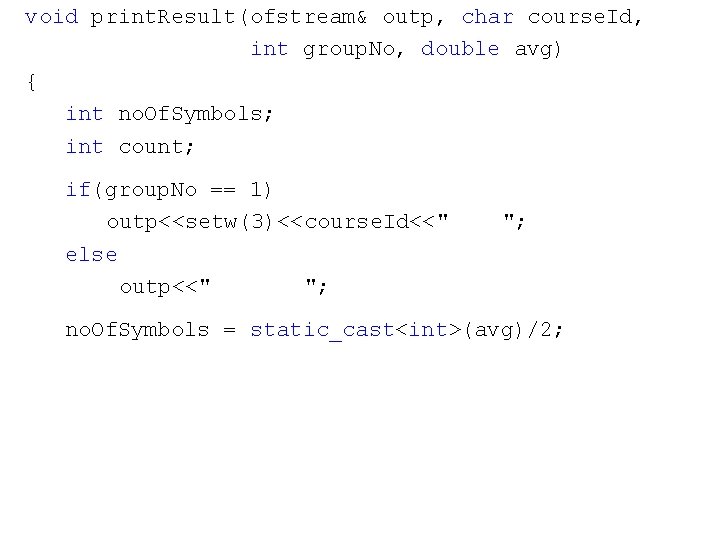
void print. Result(ofstream& outp, char course. Id, int group. No, double avg) { int no. Of. Symbols; int count; if(group. No == 1) outp<<setw(3)<<course. Id<<" else outp<<" "; no. Of. Symbols = static_cast<int>(avg)/2;
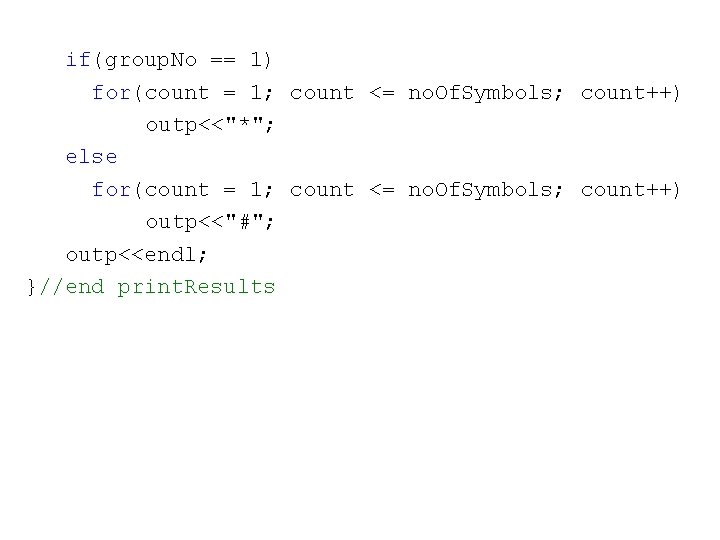
if(group. No == 1) for(count = 1; count <= no. Of. Symbols; count++) outp<<"*"; else for(count = 1; count <= no. Of. Symbols; count++) outp<<"#"; outp<<endl; }//end print. Results
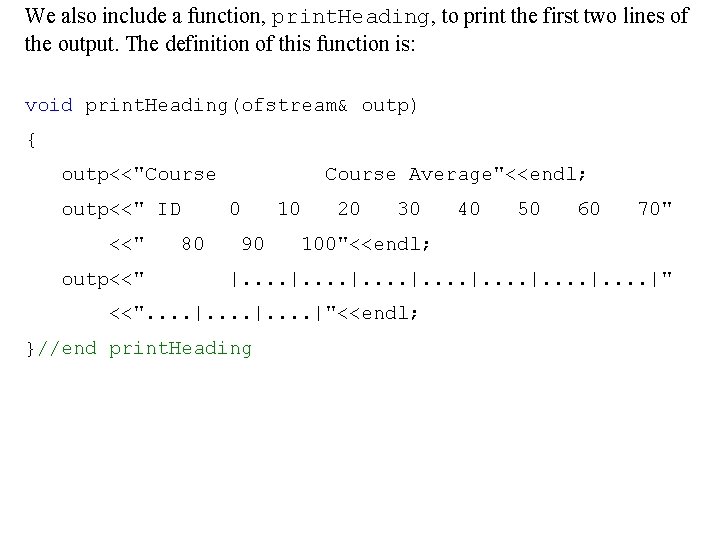
We also include a function, print. Heading, to print the first two lines of the output. The definition of this function is: void print. Heading(ofstream& outp) { outp<<"Course outp<<" ID <<" outp<<" Course Average"<<endl; 0 80 10 90 20 30 40 50 60 70" 100"<<endl; |. . . . |" <<". . . . |"<<endl; }//end print. Heading
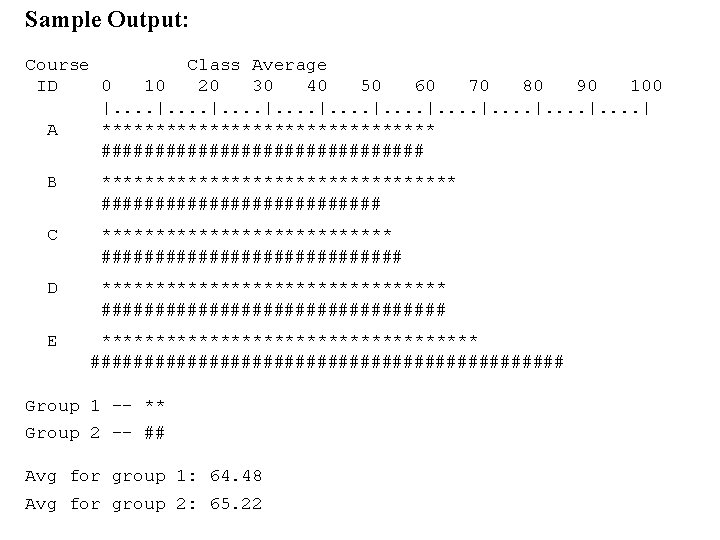
Piecewise function absolute value
Evaluating functions
Evaluating functions and operations on functions
Chapter 9 quadratic equations and functions
Chapter 8 quadratic functions and equations answer key
Chapter 6 exponential and logarithmic functions answers
Chapter 4 polynomial and rational functions
Chapter 4 exponential and logarithmic functions
Chapter 4 exponential and logarithmic functions
Chapter 3 polynomial and rational functions
Structure and functions of the executive branch
Exponential function form
Property of equality for exponential functions
Lesson 8 extra practice quadratic functions
Chapter 6 trigonometry
Factoring patterns
Exploring quadratic graphs
Chapter 4 trigonometric functions
Chapter 1 functions and their graphs
Six trigonometric functions of special angles
Lesson 5-2
Logarithmic rules
Chapter 3 linear and quadratic functions
Chapter 2 functions and graphs
Chapter 11 school policies and their functions
Chapter 1 quadratic functions and factoring
Chapter 9 exponential and logarithmic functions answer key
Chapter 9 exponential and logarithmic functions answer key
Chapter 5 trigonometric functions
Chapter 5 exponential and logarithmic functions answer key
Chapter 4 trigonometric functions
Chapter 3 graphing linear functions answer key
Chapter 2 functions and their graphs answers
Chapter 1 graphs functions and models answers
Chapter 13 trigonometric functions answers
Excel chapter 2 working with formulas and functions
Chapter 5 exponential and logarithmic functions
Chapter 3 polynomial and rational functions
Chapter 3 exponential and logarithmic functions
Chapter 2 functions and graphs
Chapter 11 structure and functions of the executive branch
What are the three categories of yeast breads
Real world piecewise functions
Constant ratio exponential functions
Parton distribution functions
Functions of wood
What is a polynomial coefficient
Vitamin c biochemical function
Minerals and their functions sources and deficiency chart
Functions of vitamin e
Functions of vitamin e
Visual basic string functions
Arti kale
Vestibular apparatus
Vertical y horizontal
Vertex form of a quadratic
3 ways to classify vegetables
Vector functions and space curves
Rational functions parent function
Using transformations to graph quadratic functions
Translating quadratic functions
Mathematical functions in java
Using recursion in models and decision making sheet 3
Absolute value graph transformations
Adh function
Microscopic structure of ureter
Association operator in pl sql example
Unit 8 rational functions homework 1
Unit 8 exponential and logarithmic functions
All real numbers on graph
Cho sha cao
Parent function for rational functions
Rational expressions and functions
Unit 6 test study guide radical functions answer key
Unit 6 radical functions homework 3
Logarithmic functions rules
Unit 5 polynomial functions homework 7
Unit 5 polynomial functions
Unit 5 exponential and logarithmic functions answers
Accountancy of lawyers
Log function
Function of a connecting rod
Function of finance department
Reciprocal trig functions
Trigonometry cofunction
What are the 6 trig functions
Three basic trigonometric functions
As91575
Horizontal answer key
Transforming linear functions algebra 2
Transformations of sine and cosine functions
Sine period
Vertical stretch or shrink
Telophase diagram
What are the two basic functions of a tire?
Pericardiu
Functions of brics
Wto functions
The usa
Palatine bone
The skin performs all of the following except
5 main functions of the skeleton
What are the three roles of marketing research?
Hr functions chart
Fuctions of ovary
Direct variation as a power
Neuron anatomy
How do muscles work in pairs
Muscular system
2 function of muscular system
Math class java
Test for gilbert syndrome
3 main functions heart
3 main functions heart
Jakobson's functions of language
Social function examples
Functions of music in film
Sinc to rect
The five functions of management