Dynamic Memory Allocation Alan L Cox alcrice edu
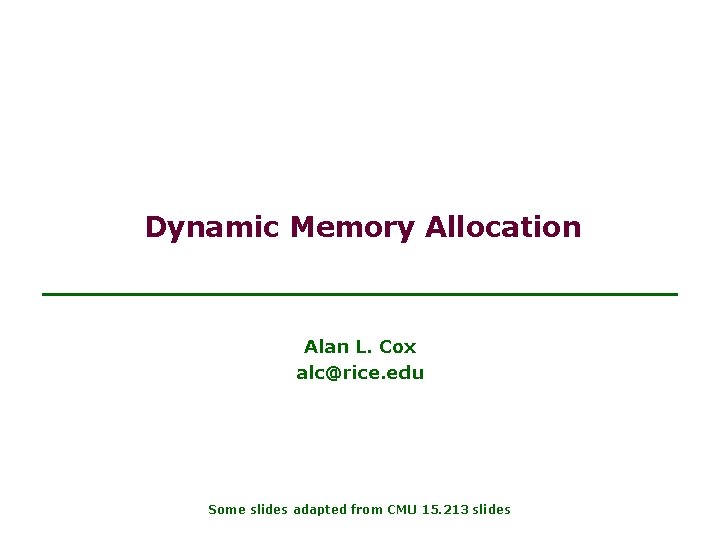
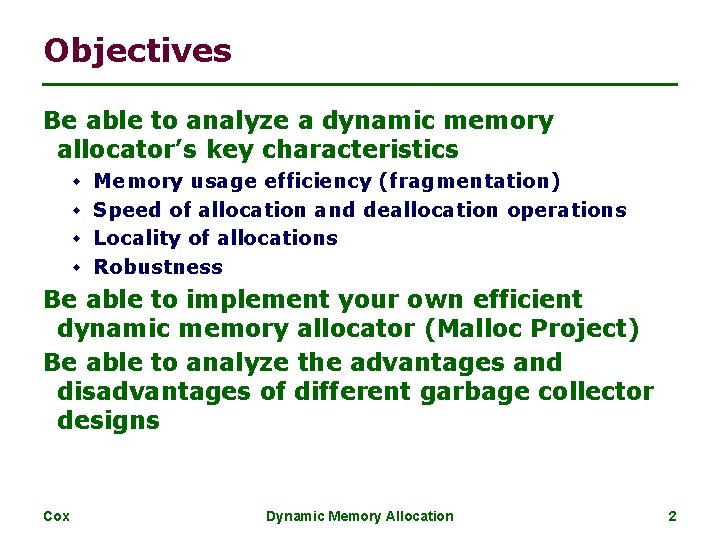
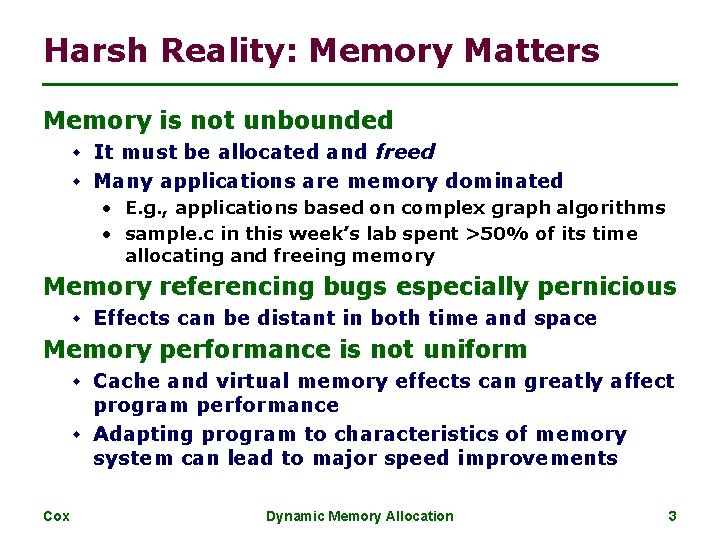
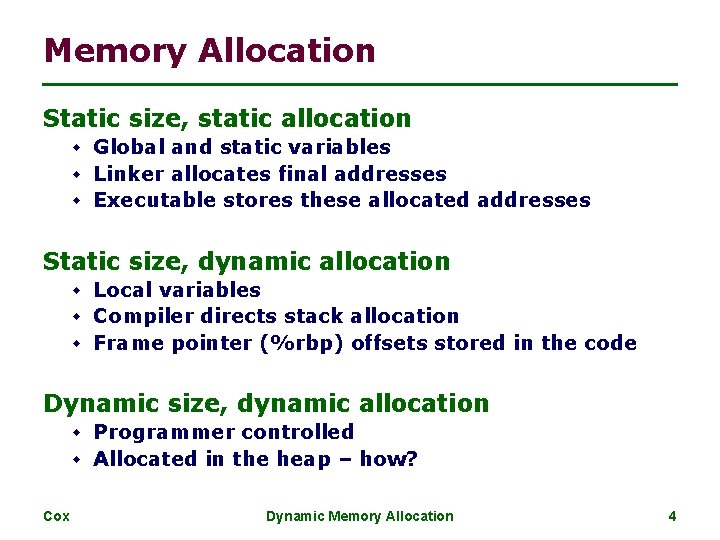
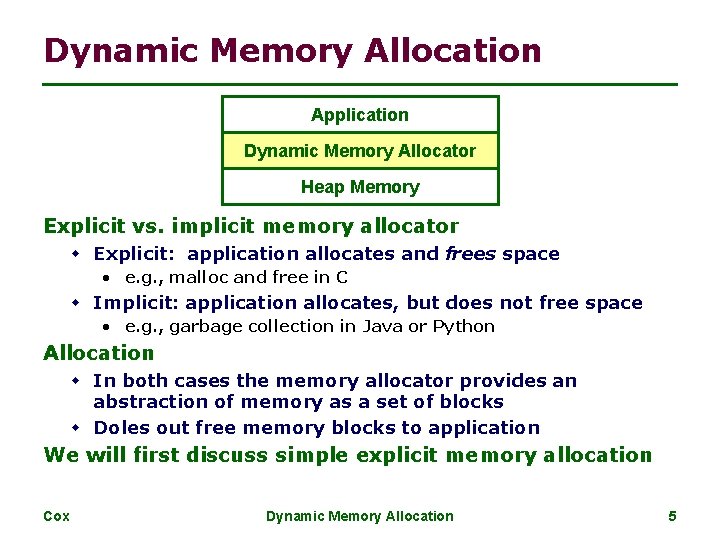
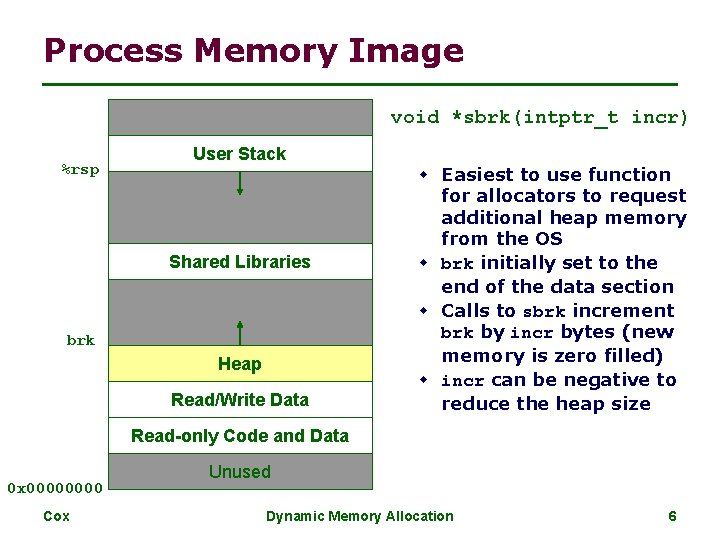
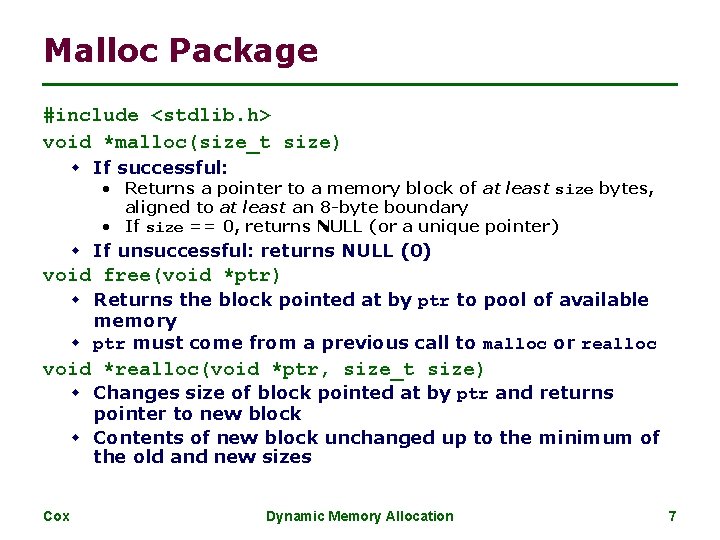
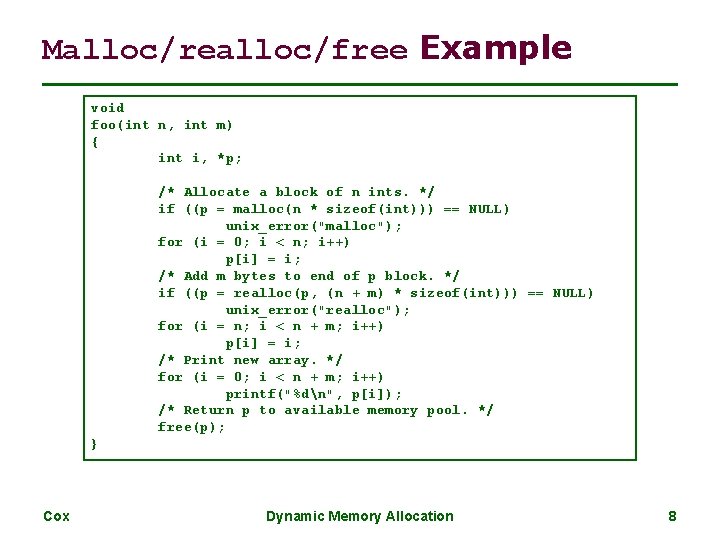
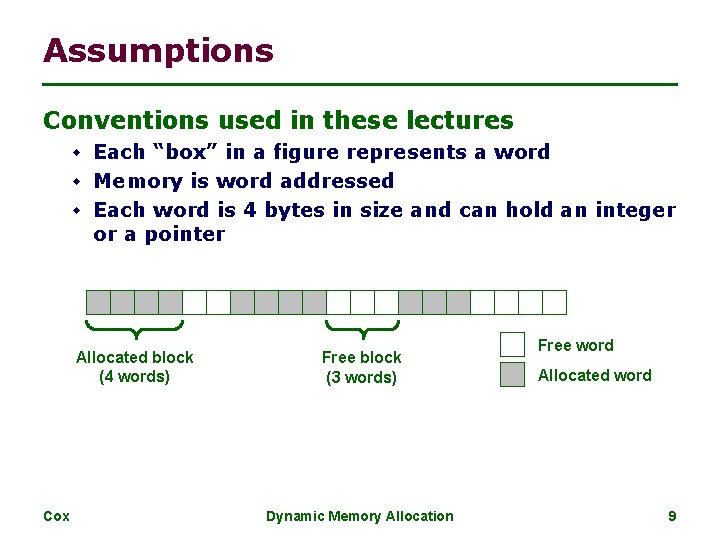
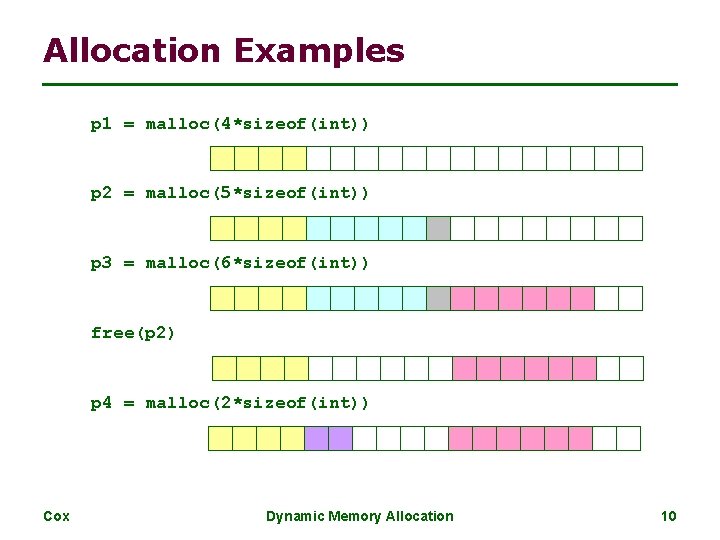
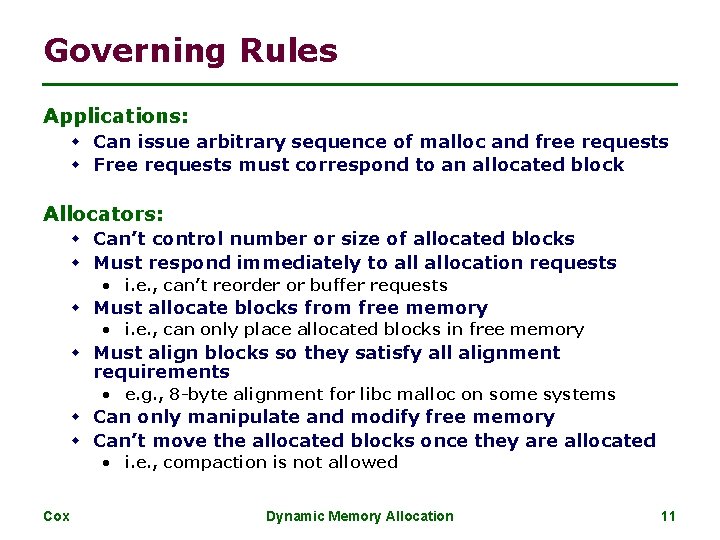
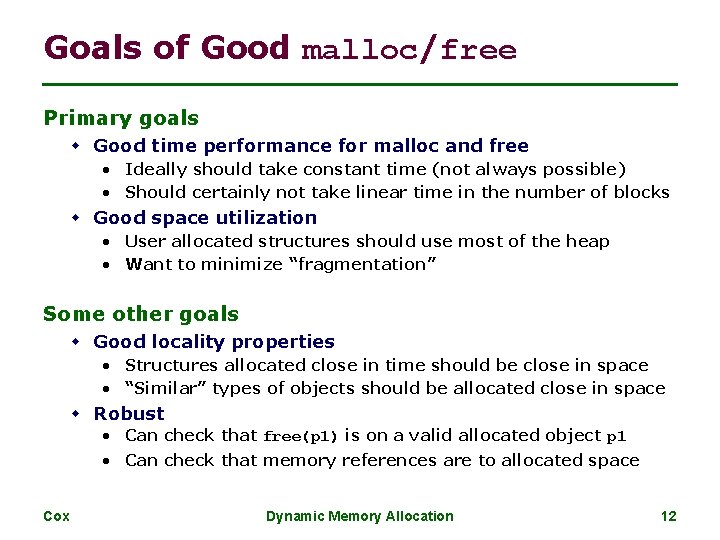
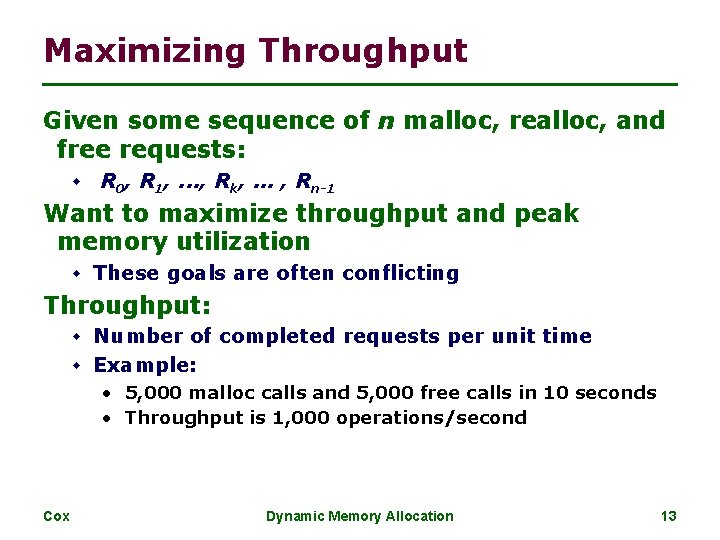
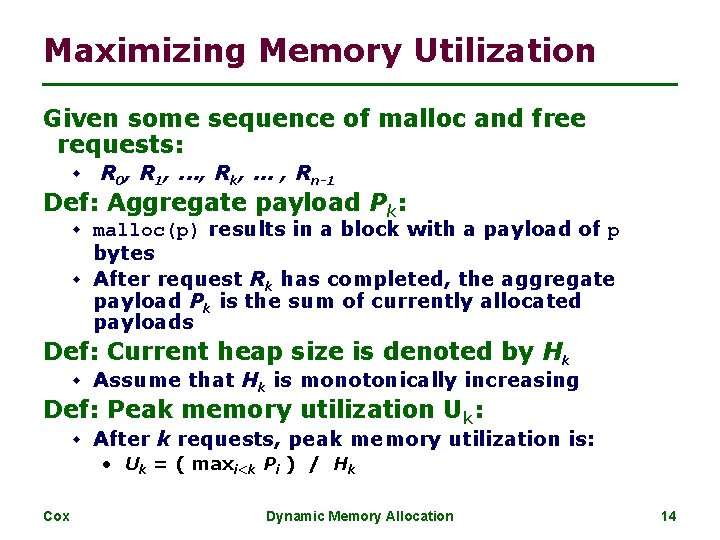
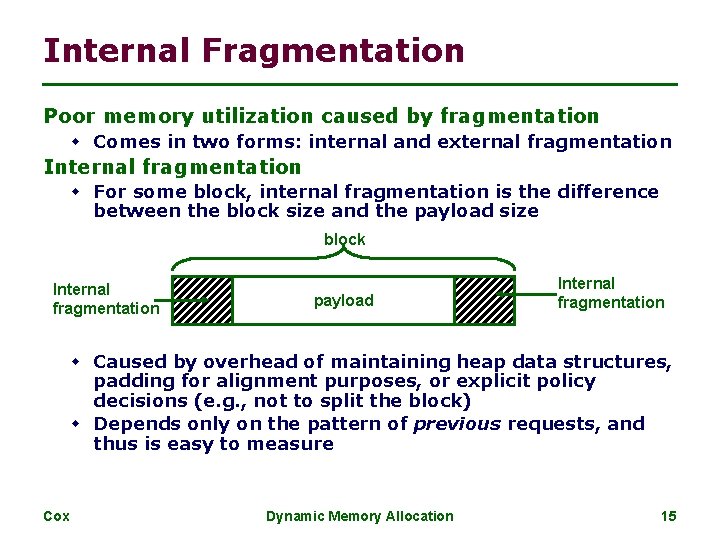
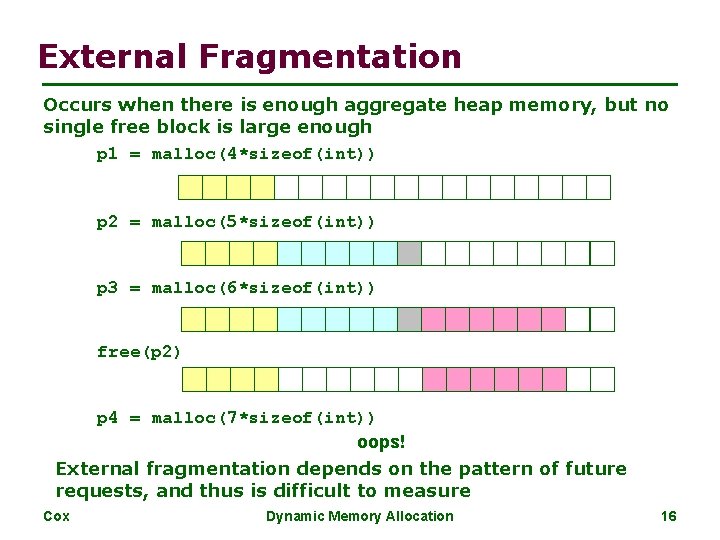
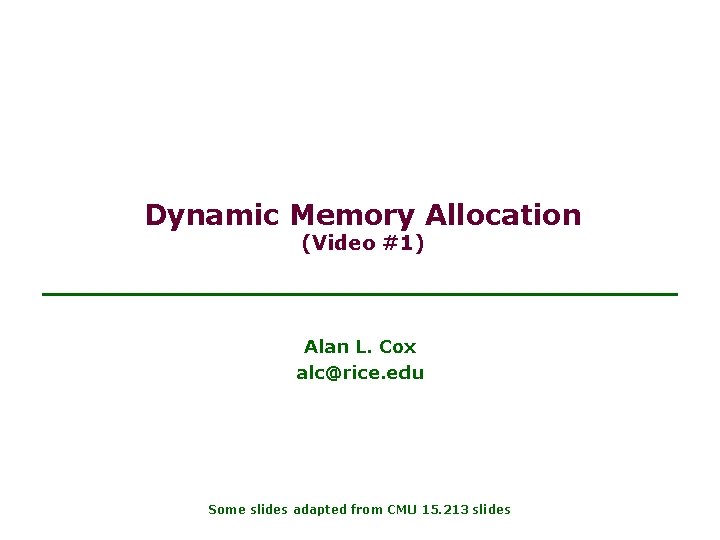
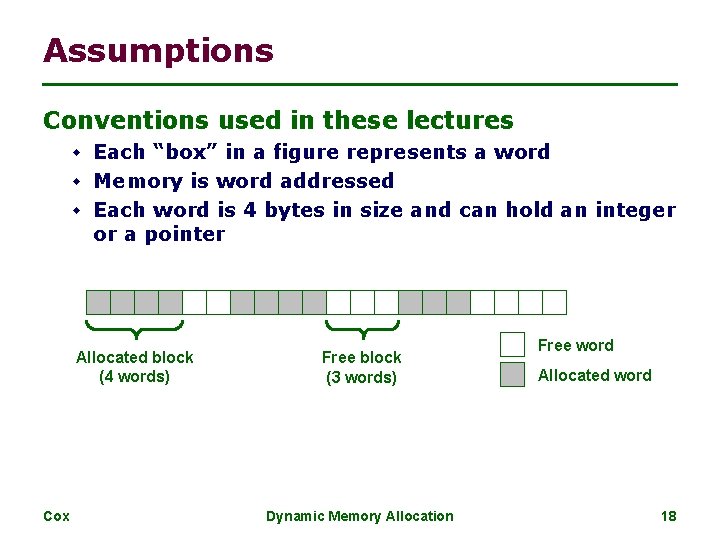
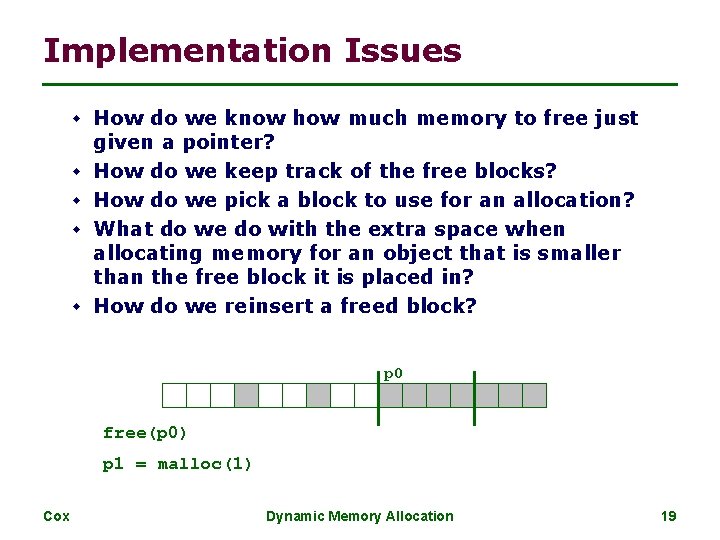
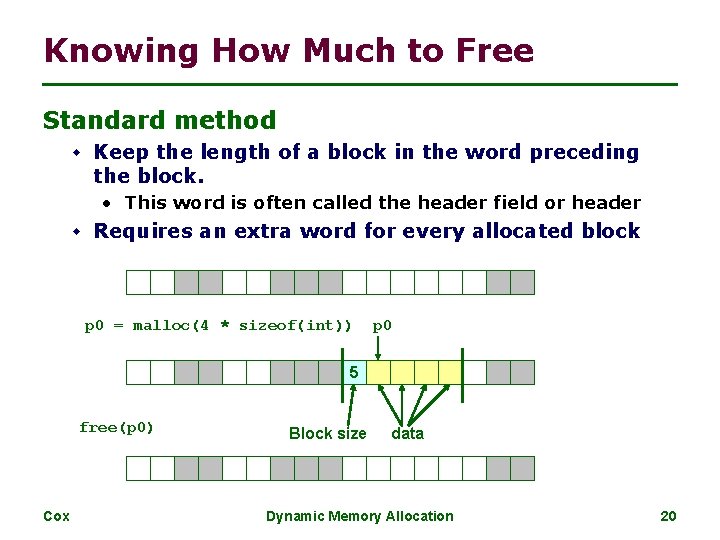
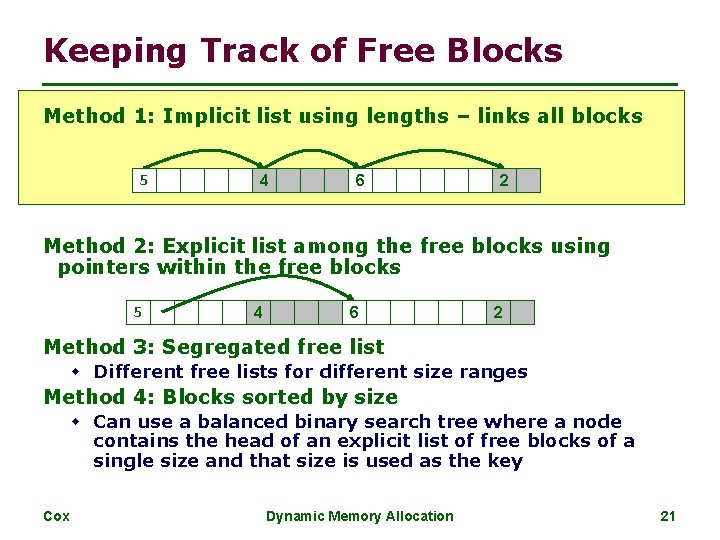
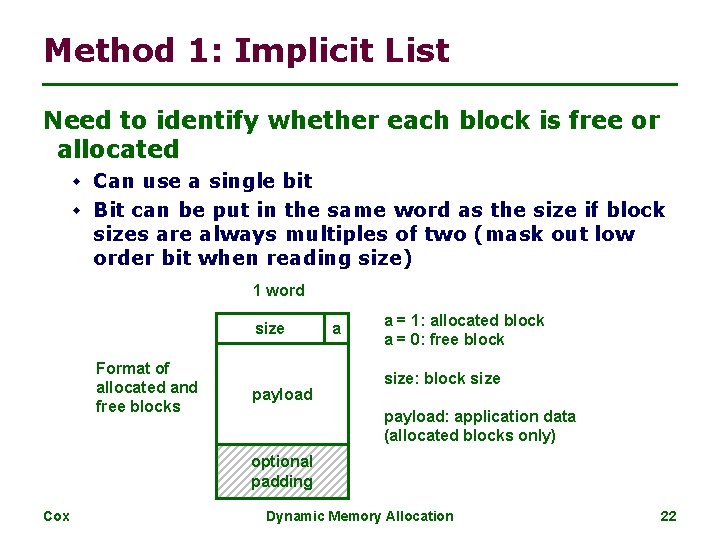
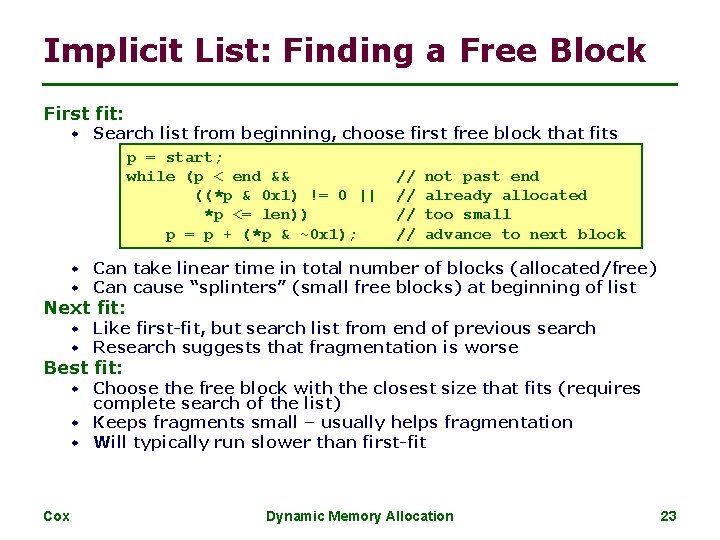
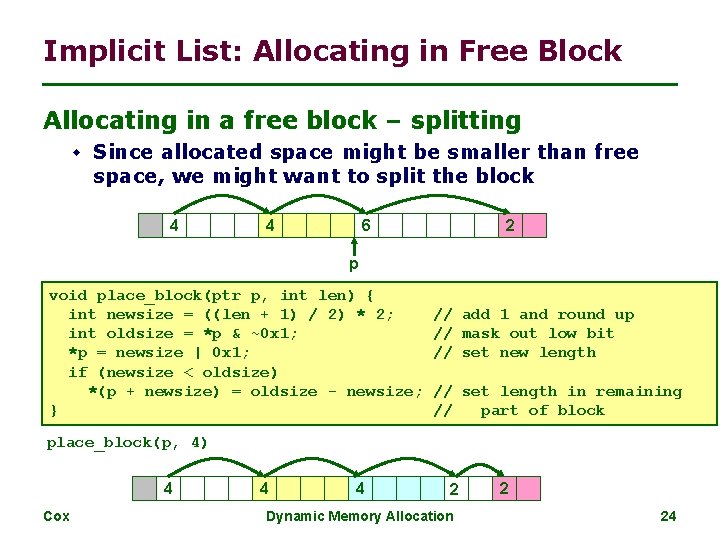
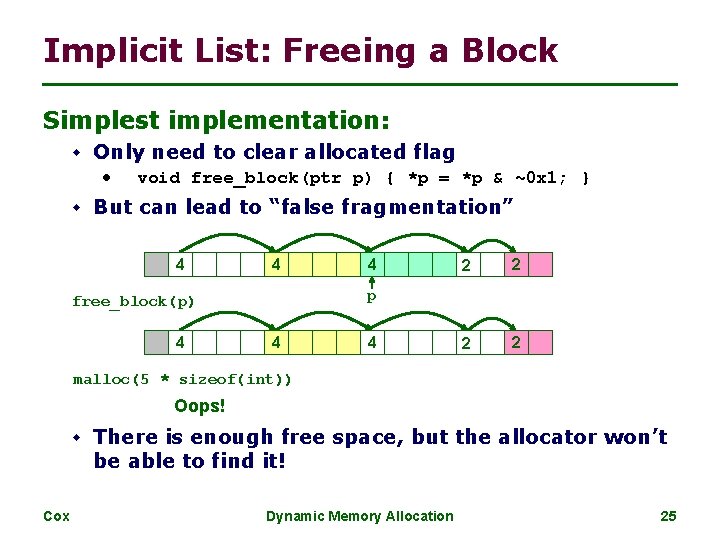
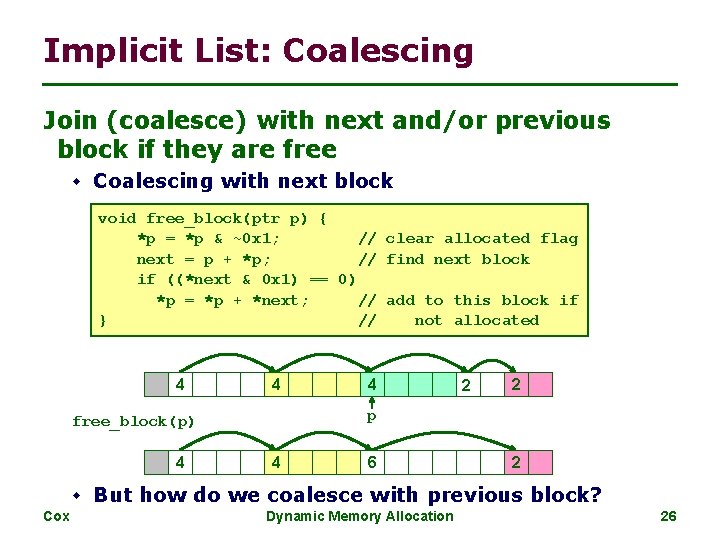
![Implicit List: Bidirectional Coalescing Boundary tags [Knuth 73] w Replicate header word at end Implicit List: Bidirectional Coalescing Boundary tags [Knuth 73] w Replicate header word at end](https://slidetodoc.com/presentation_image_h/eb36a141b805fda6ea68ddf029af9b2b/image-27.jpg)
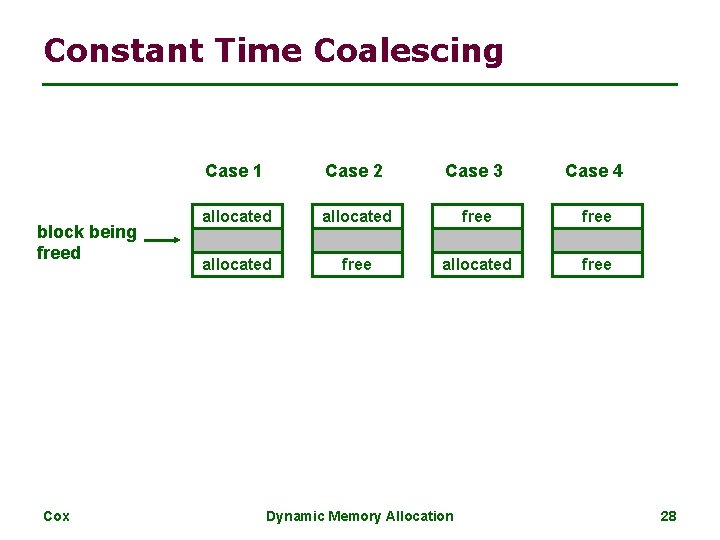
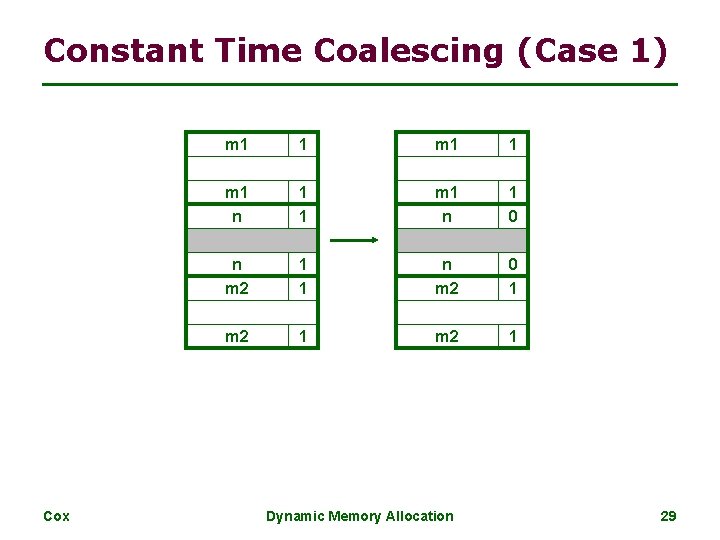
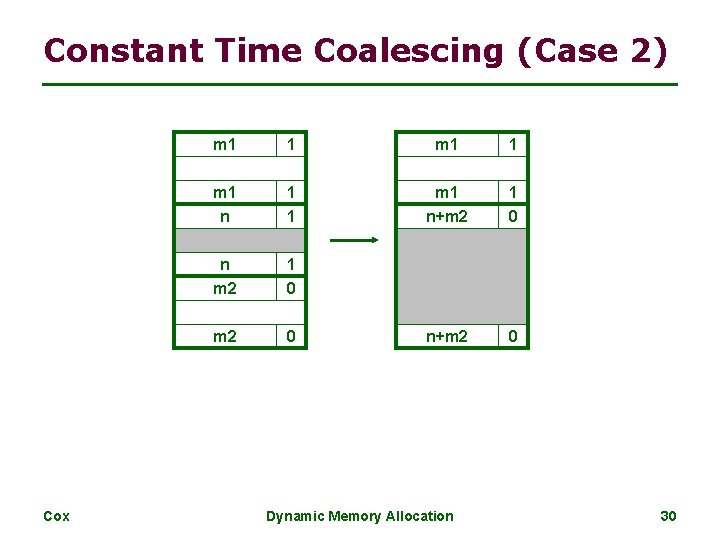
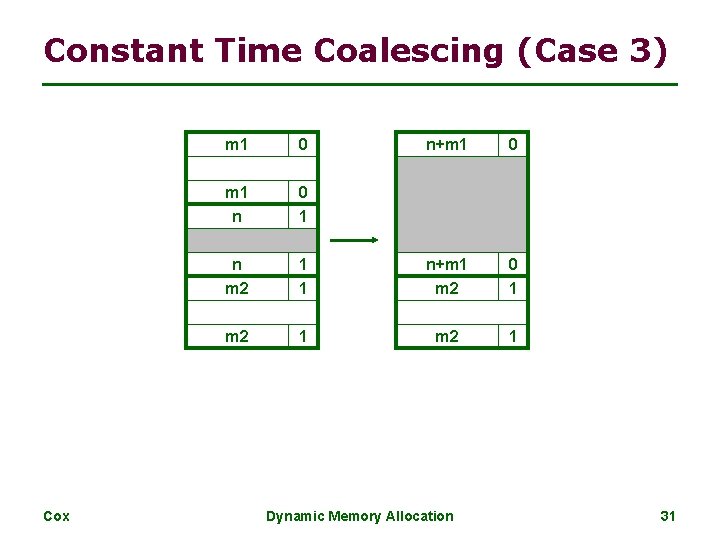
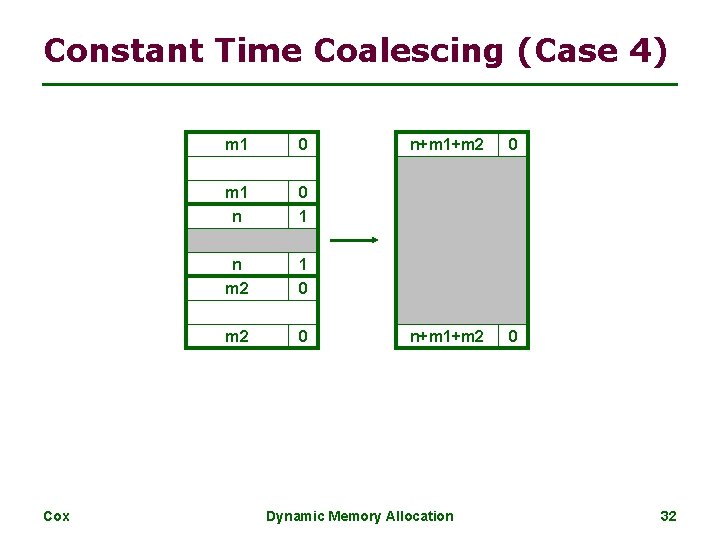
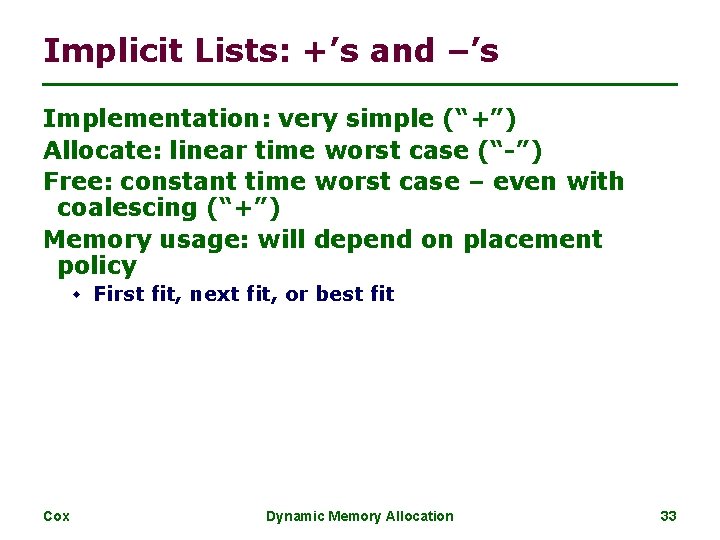
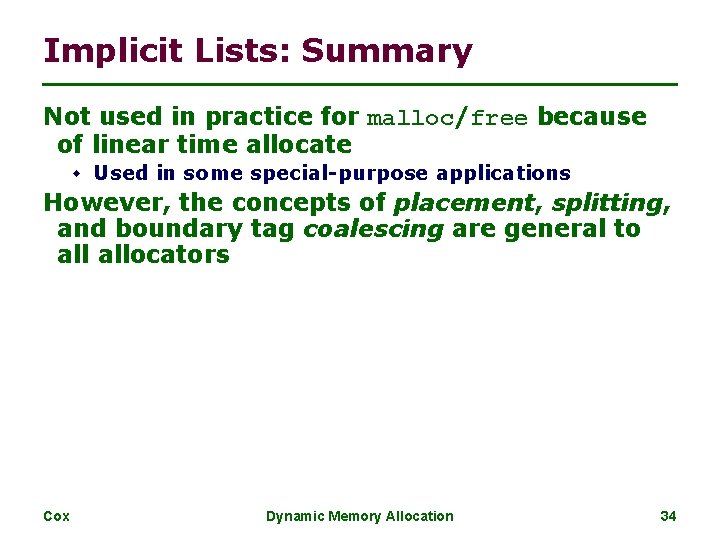
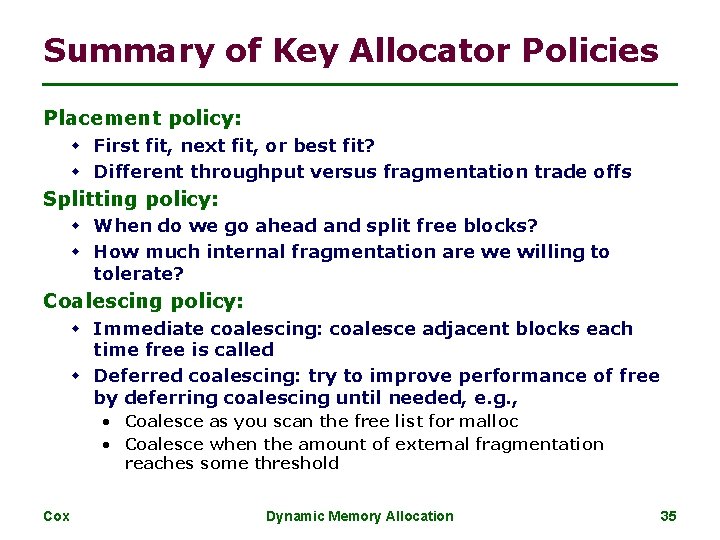
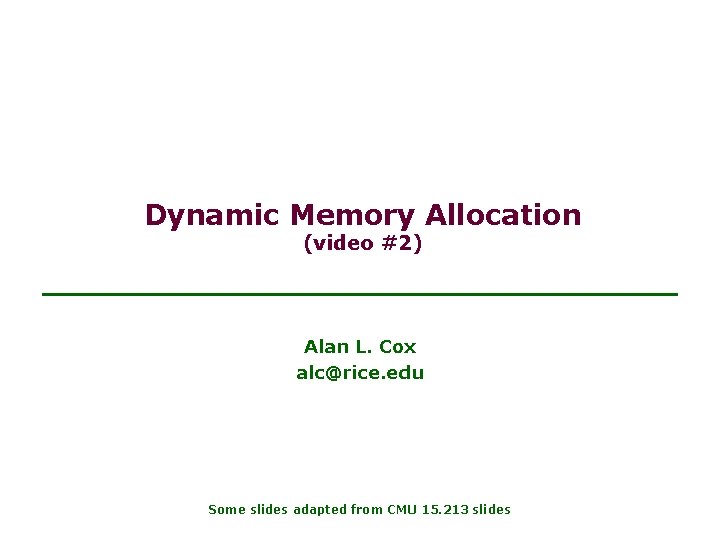
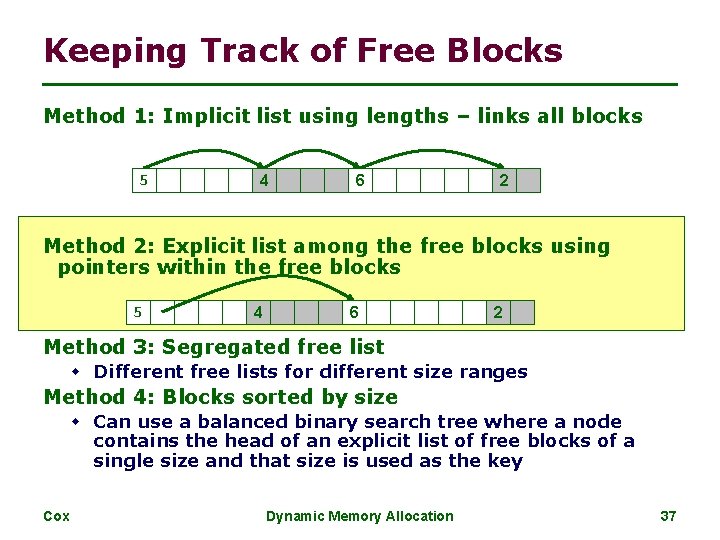
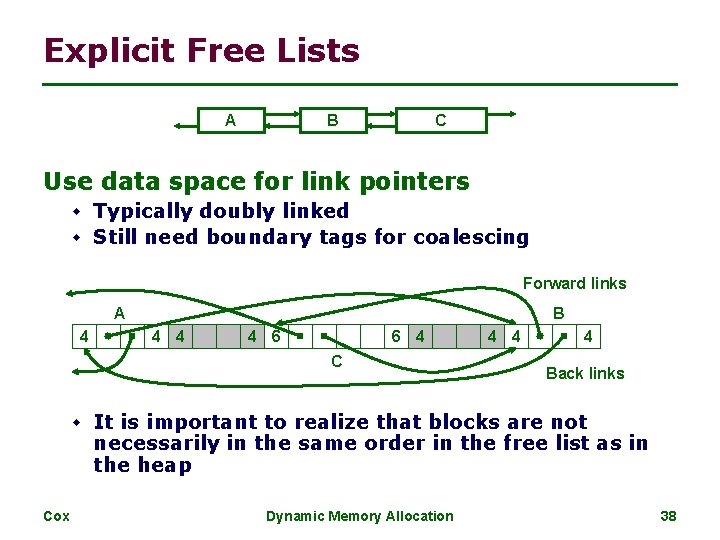
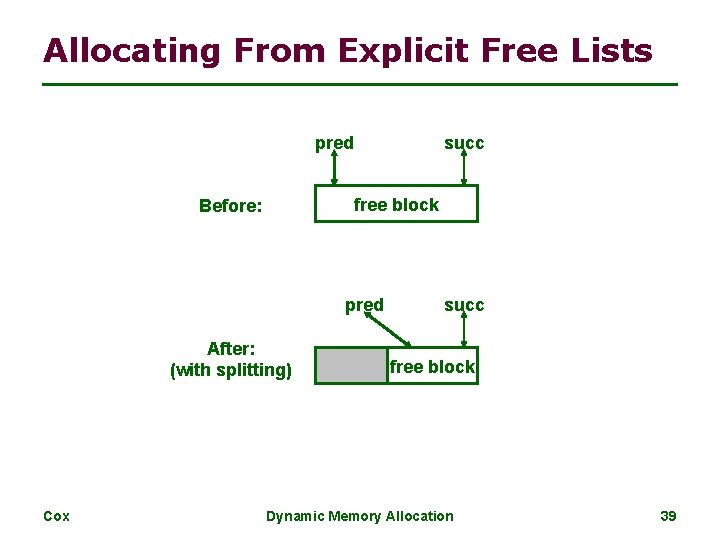
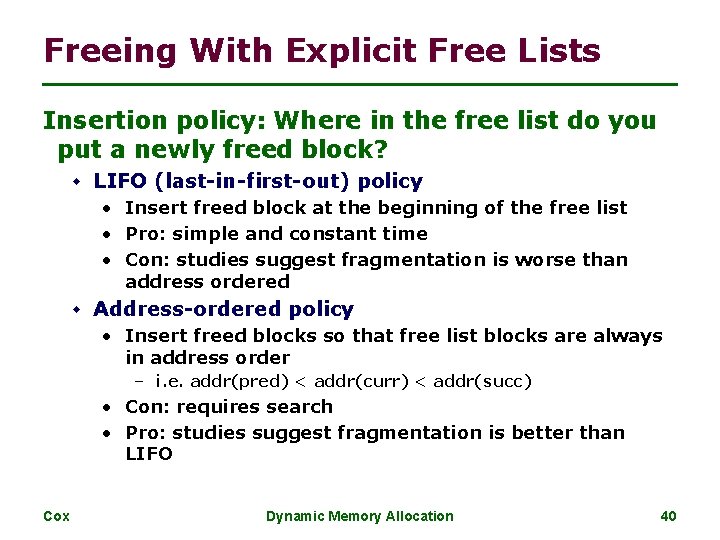
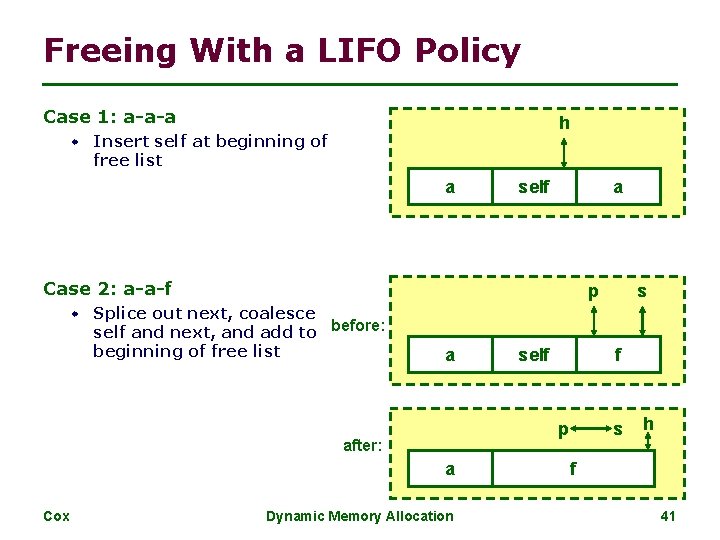
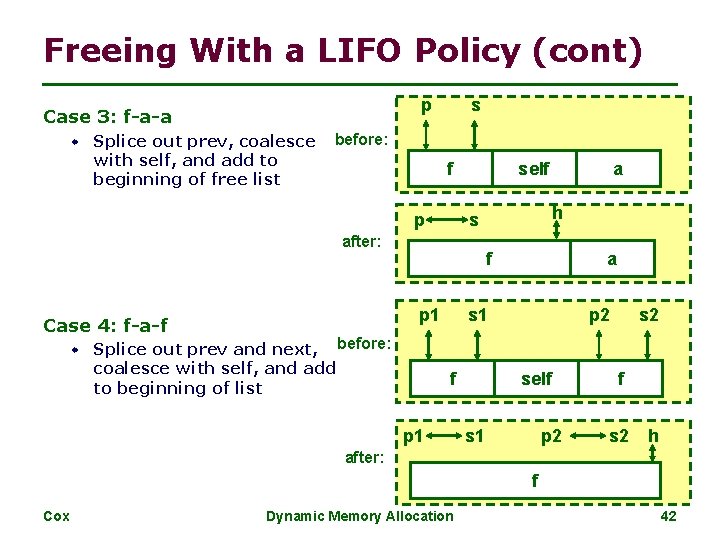
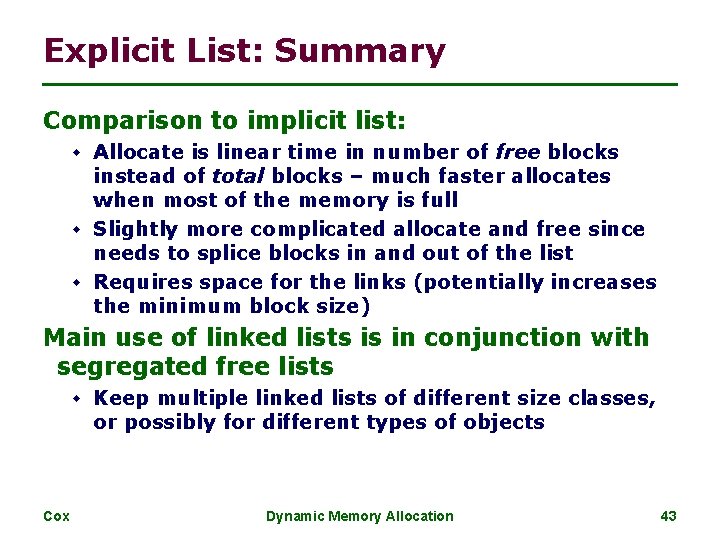
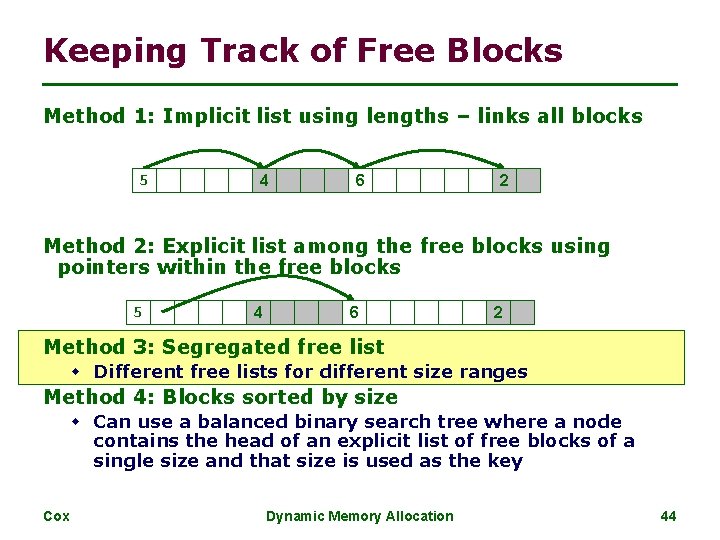
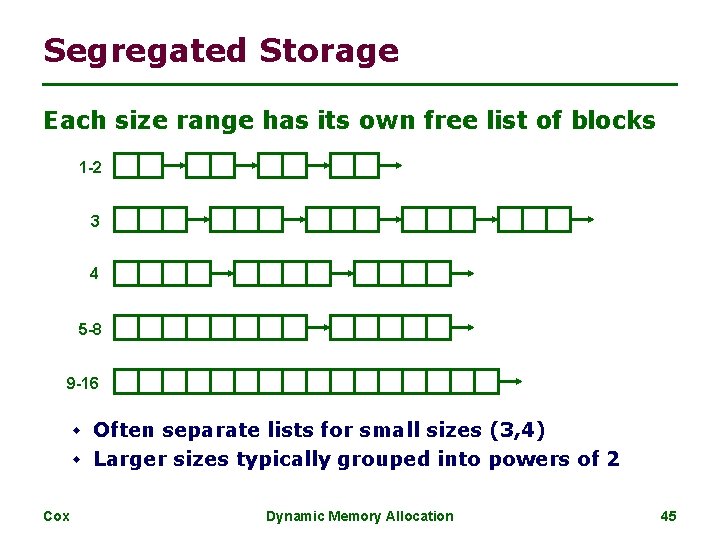
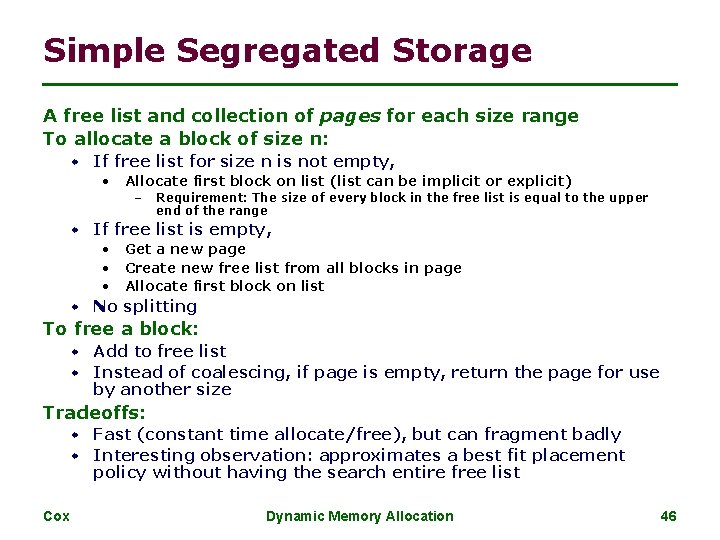
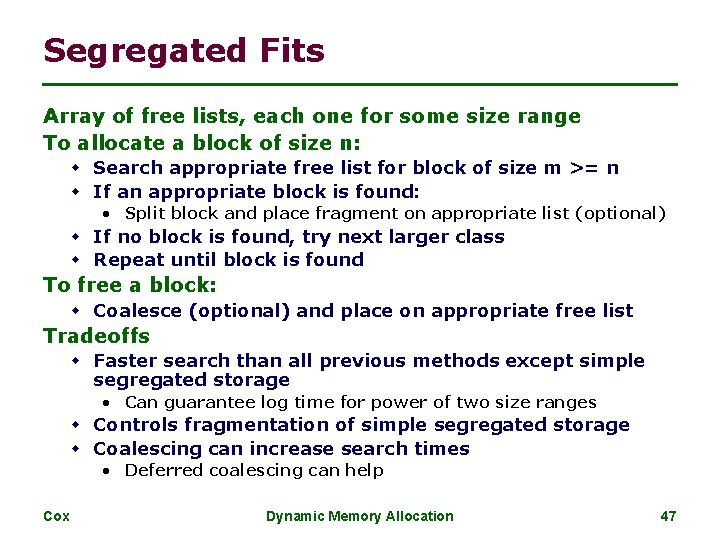
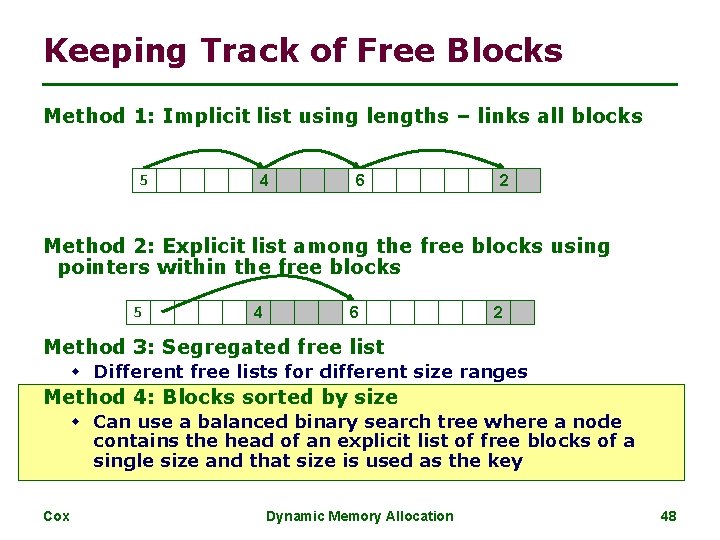
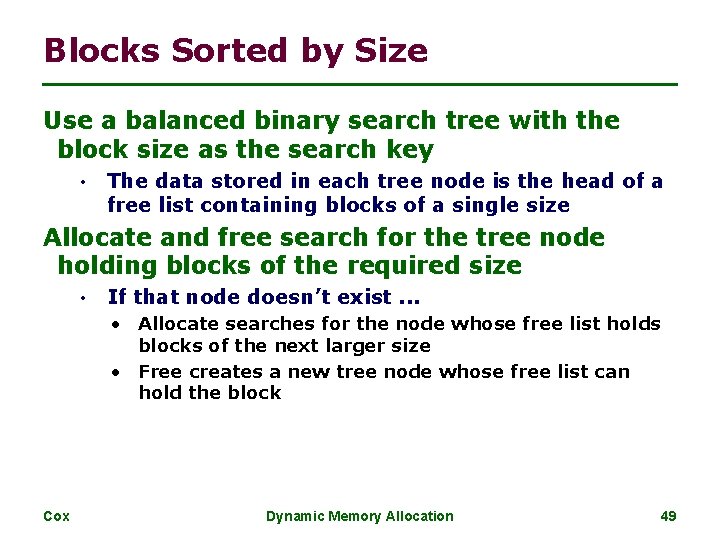
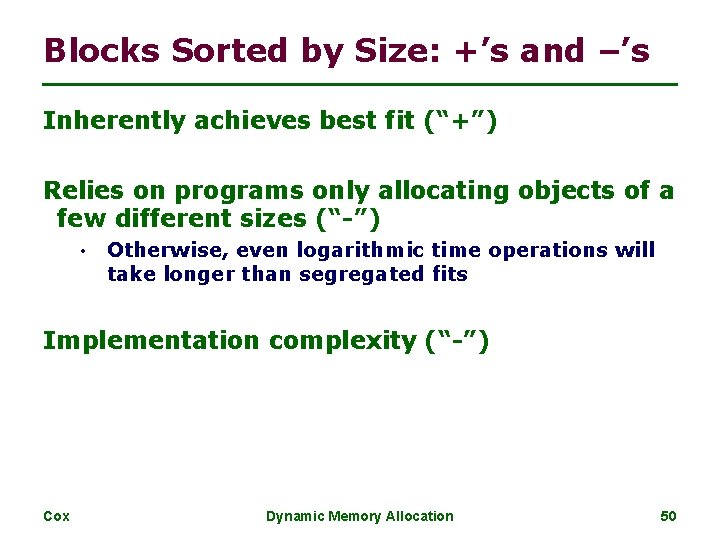
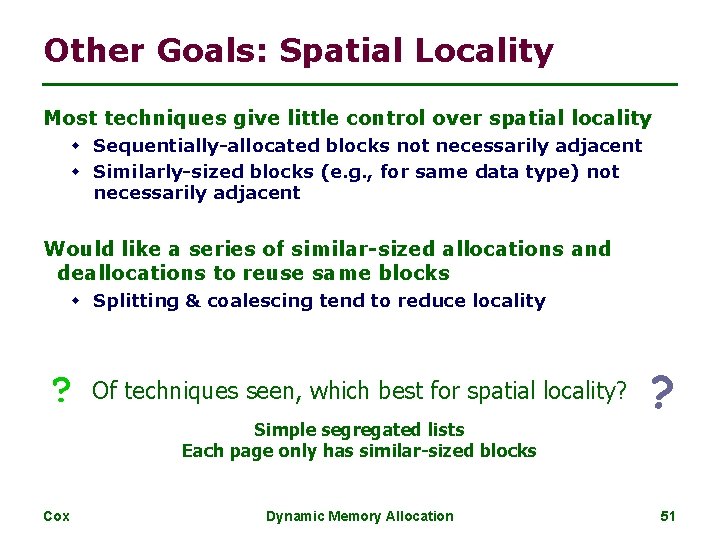
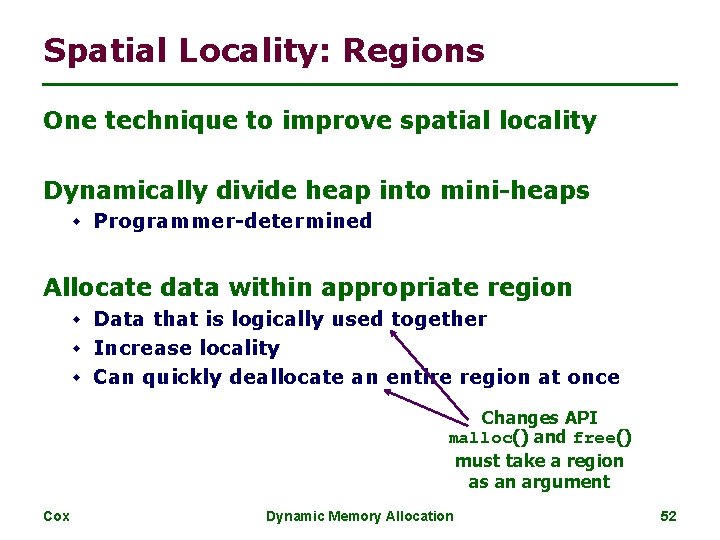
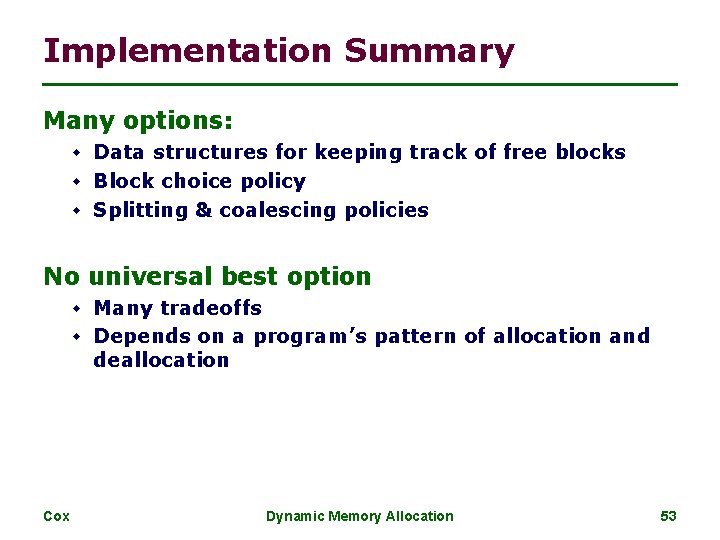
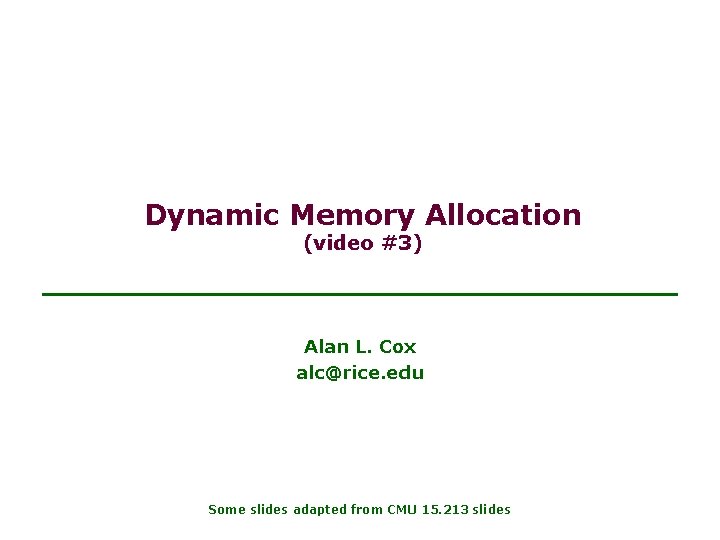
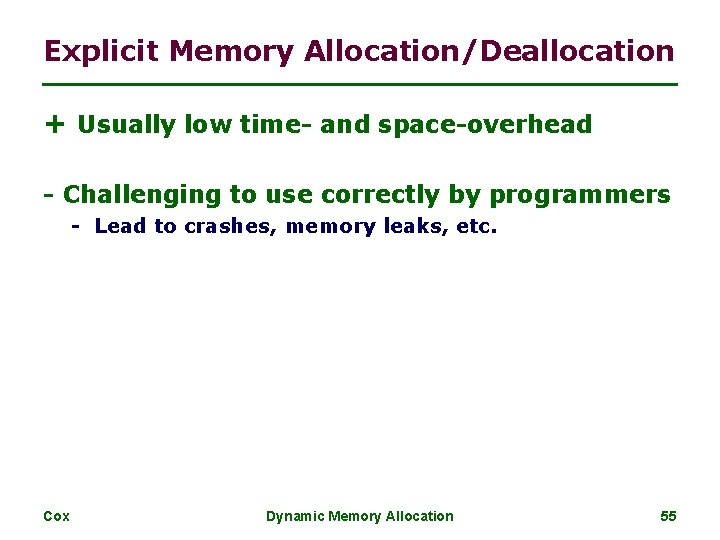
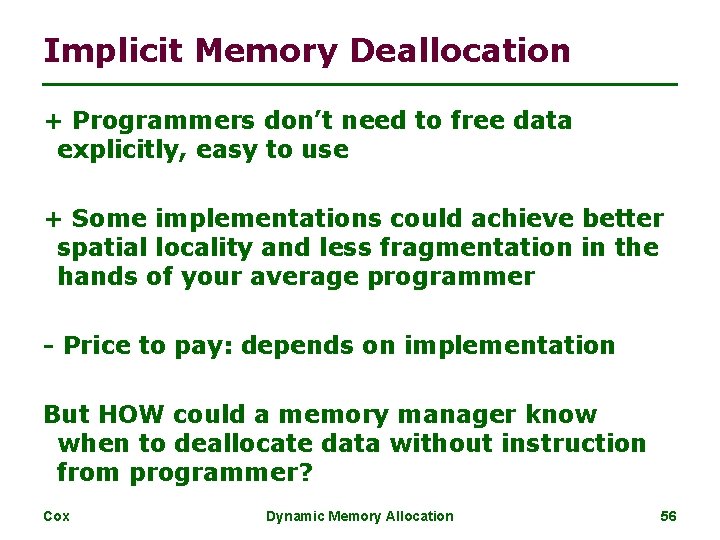
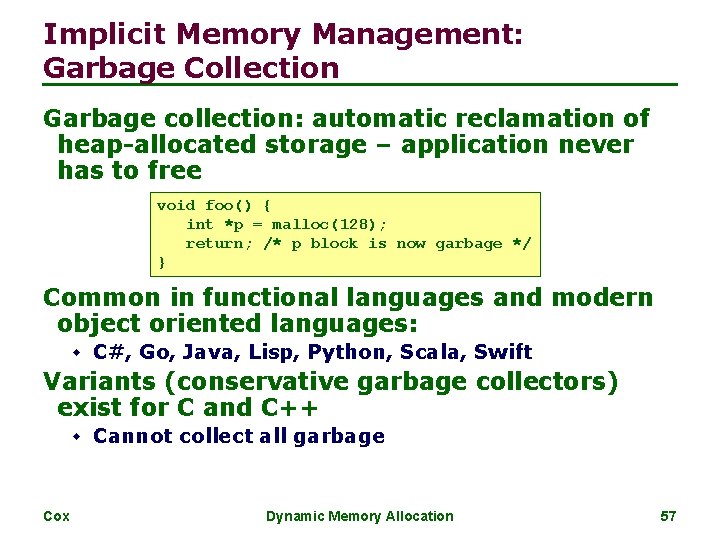
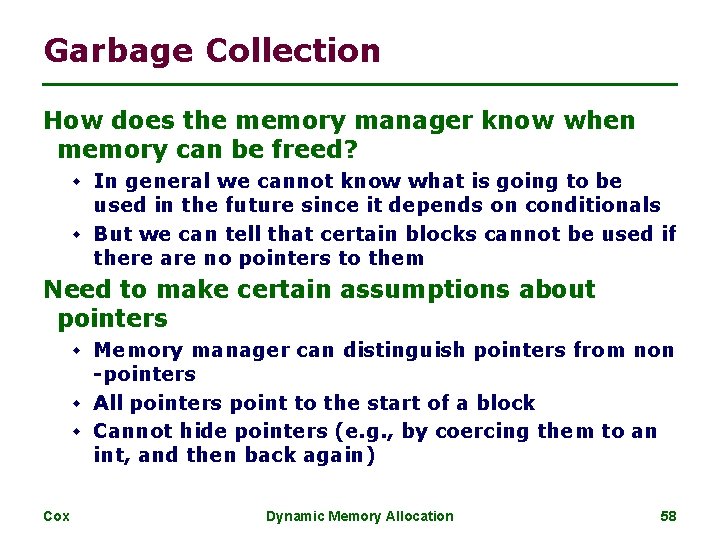
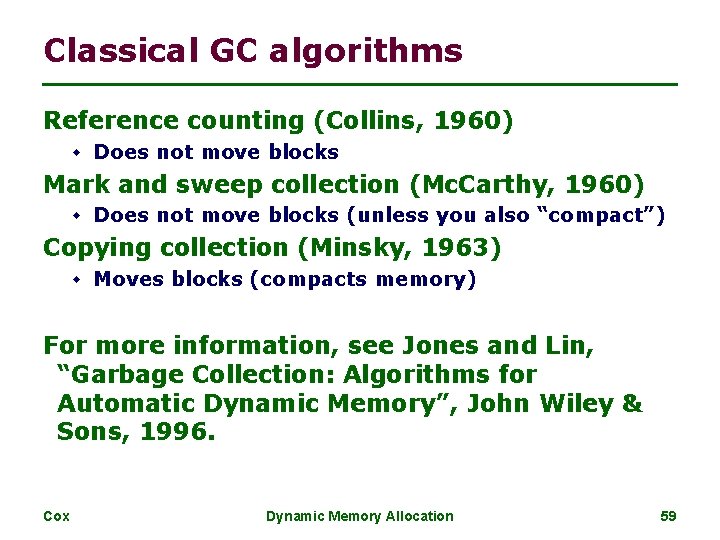
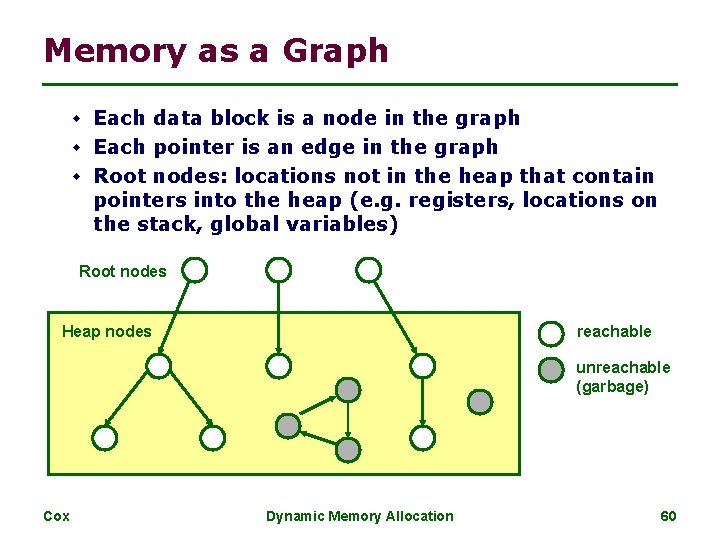
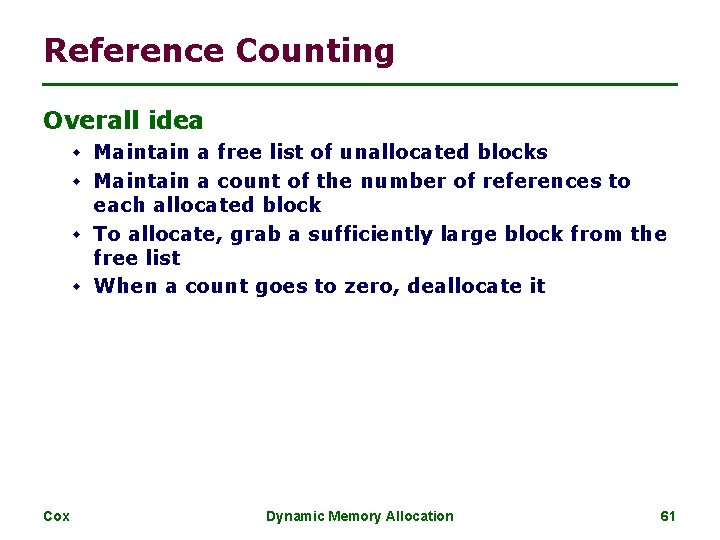
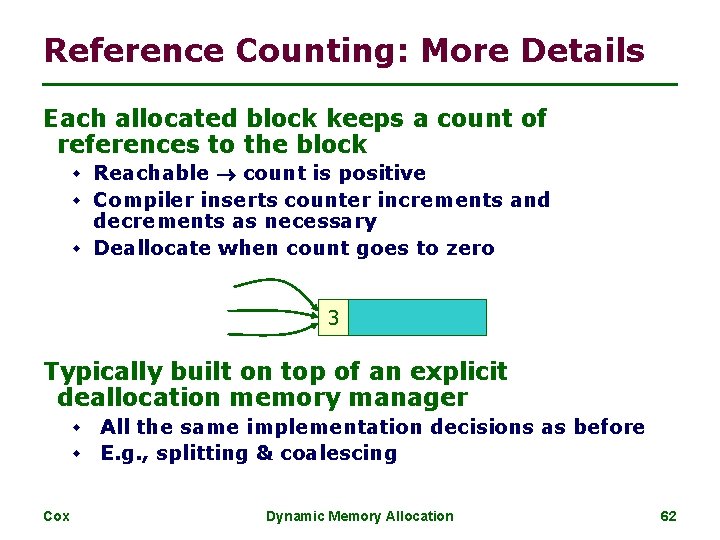
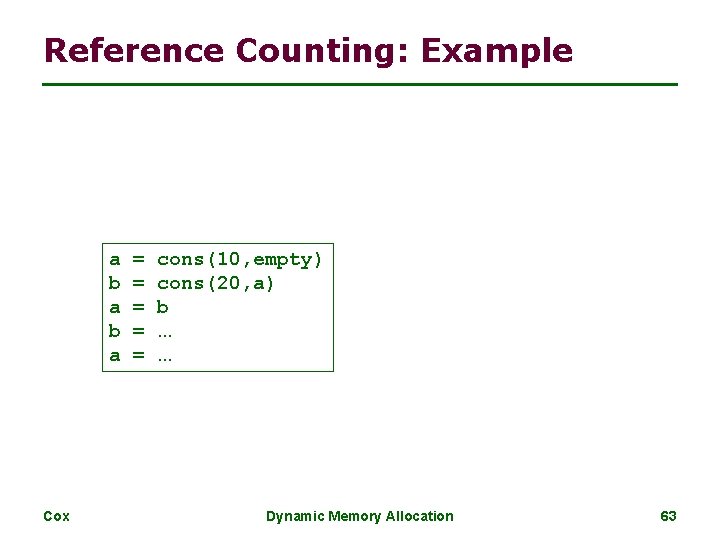
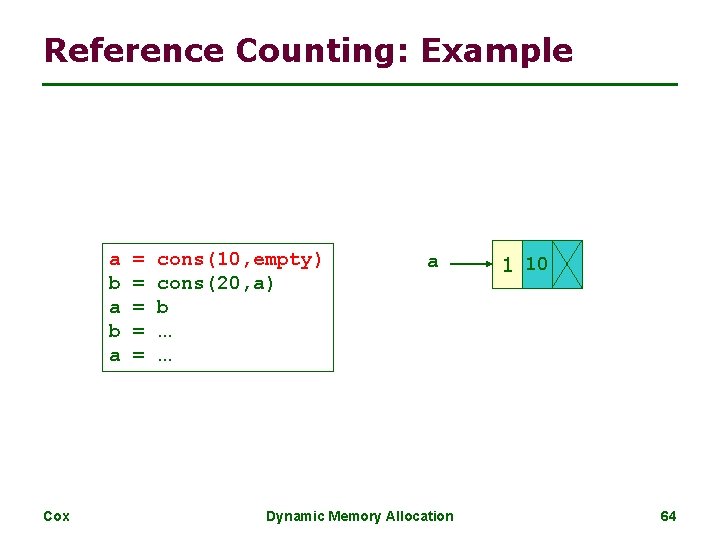
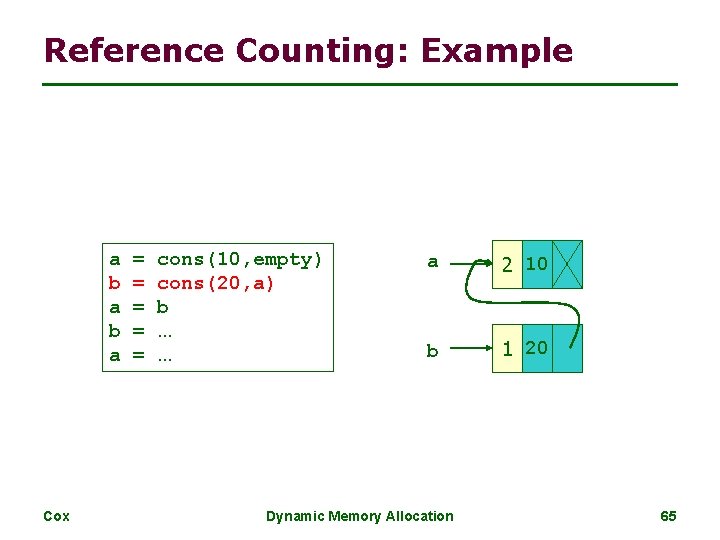
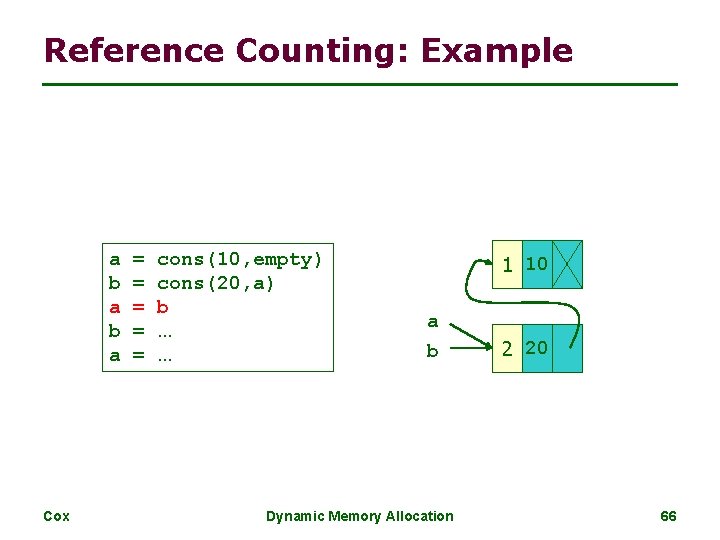
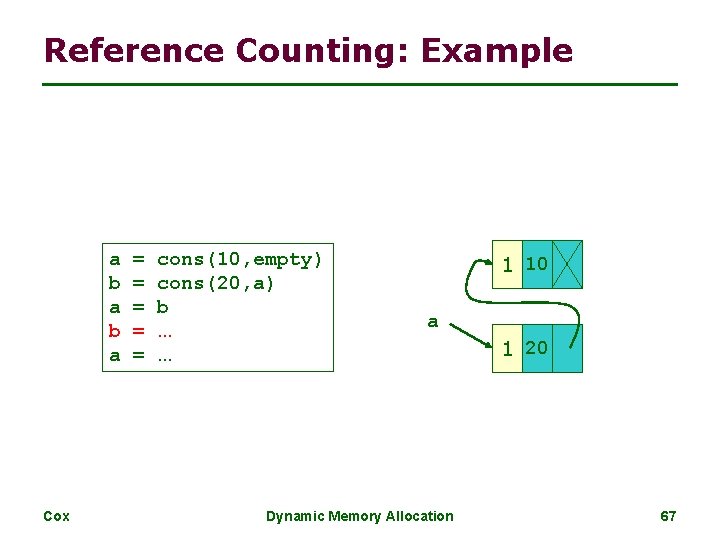
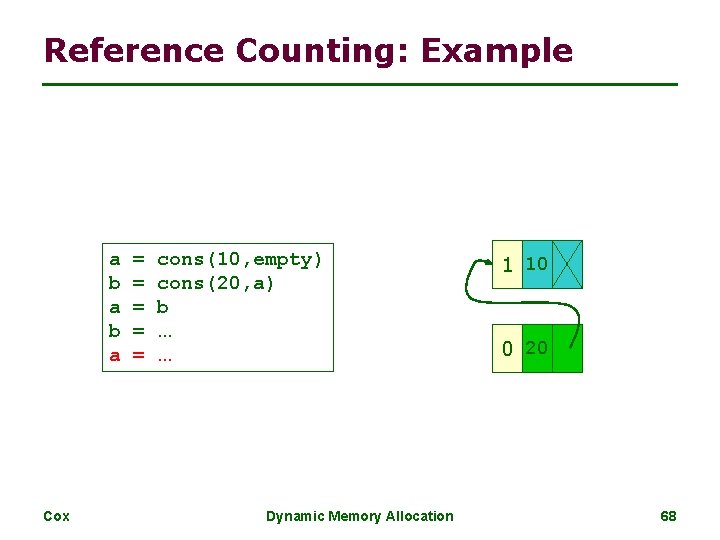
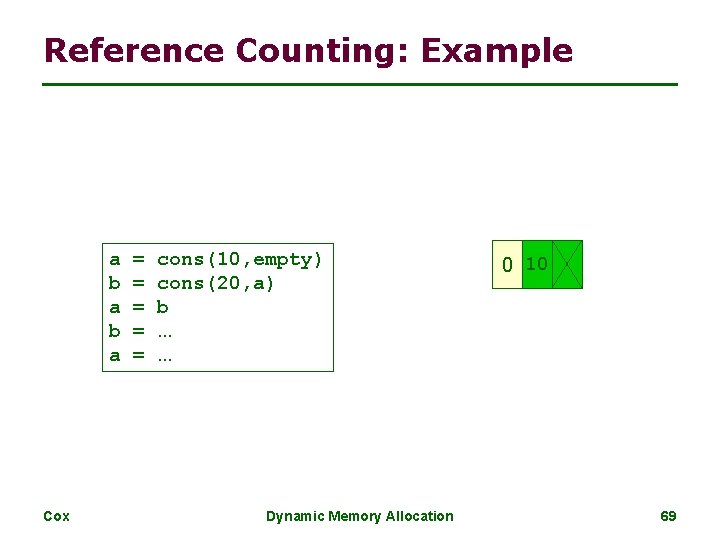
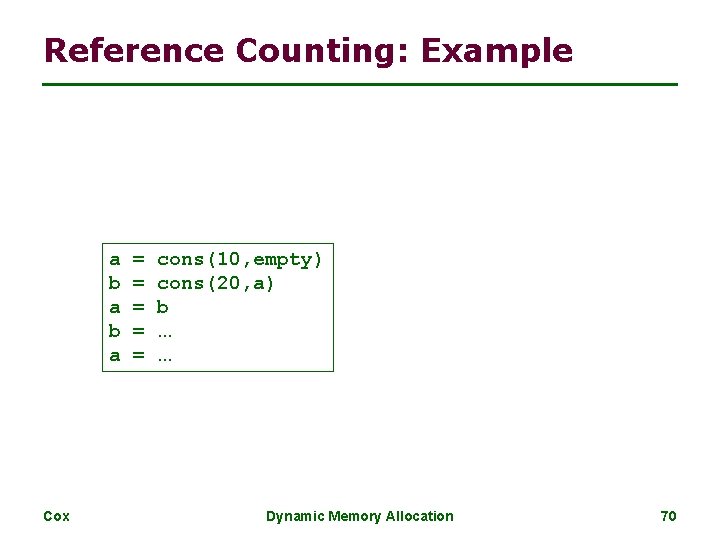
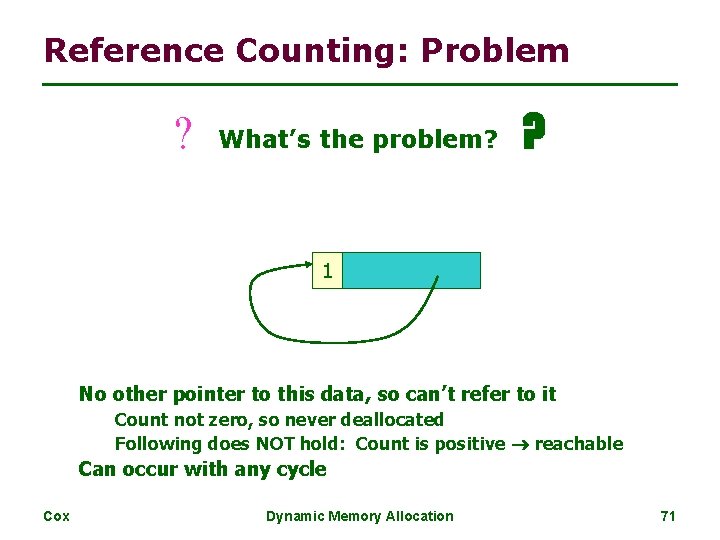
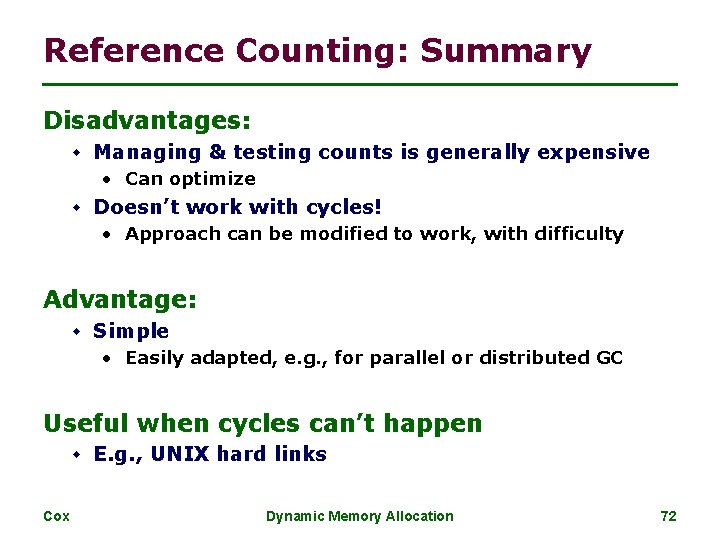
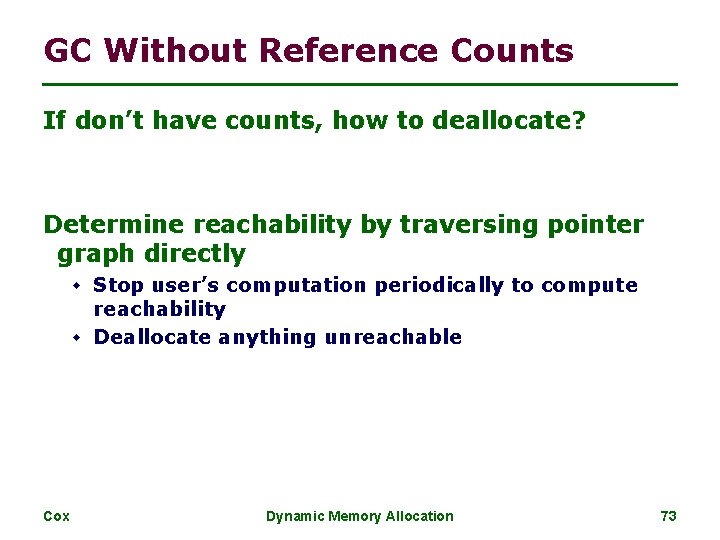
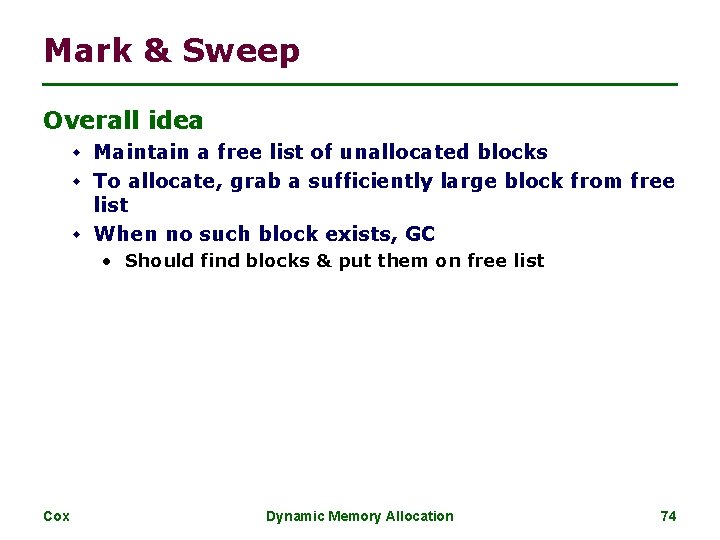
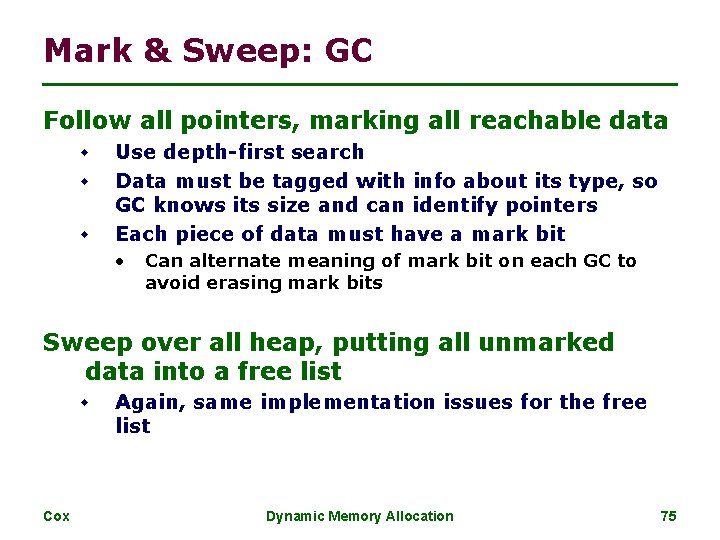
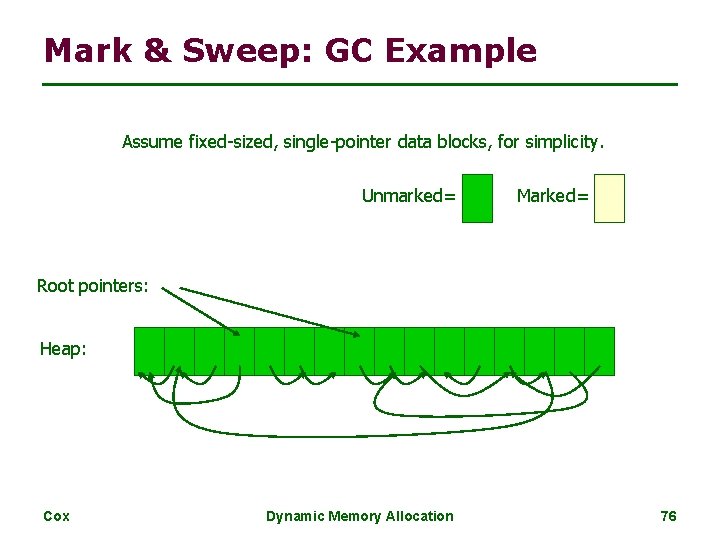
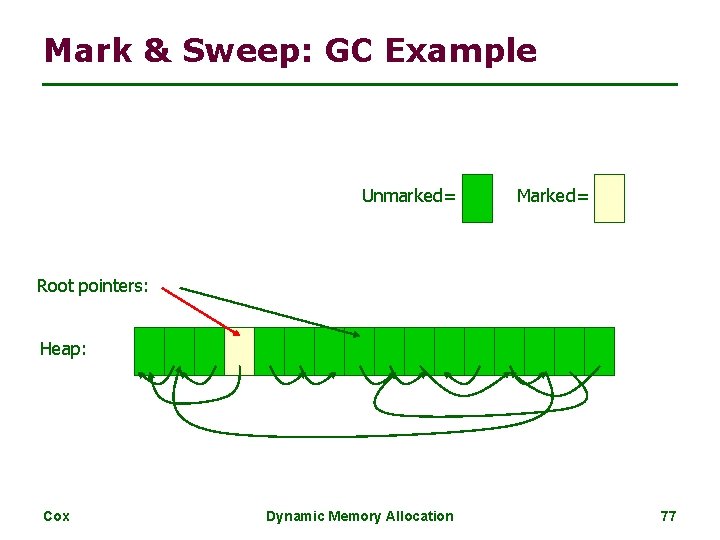
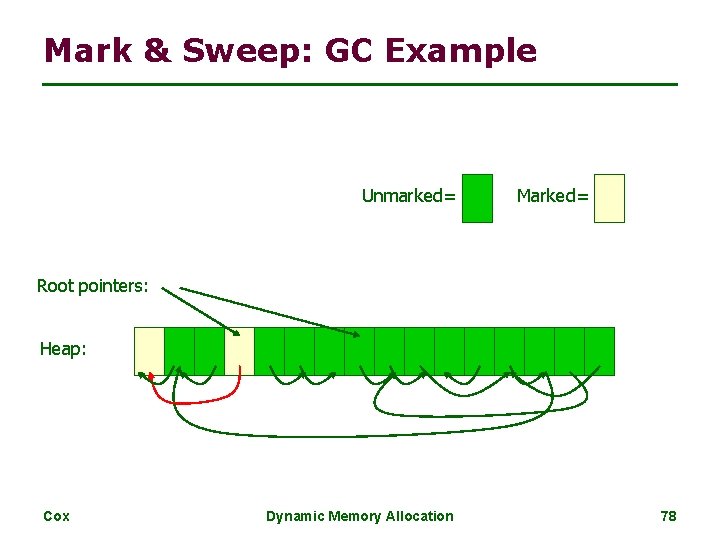
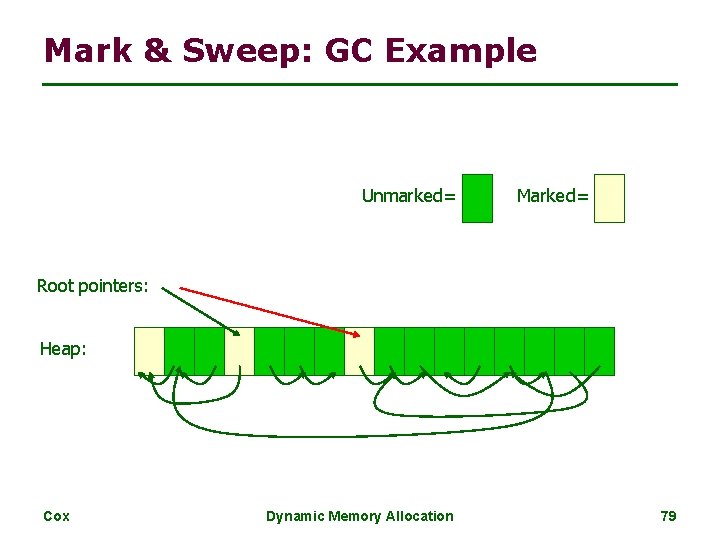
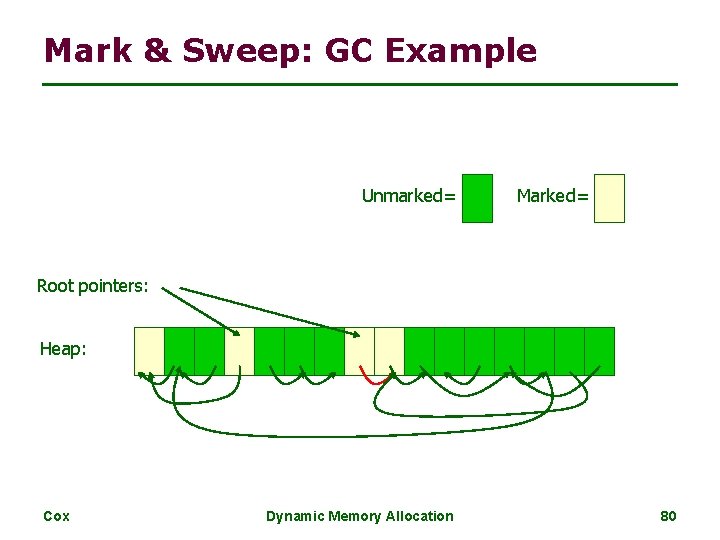
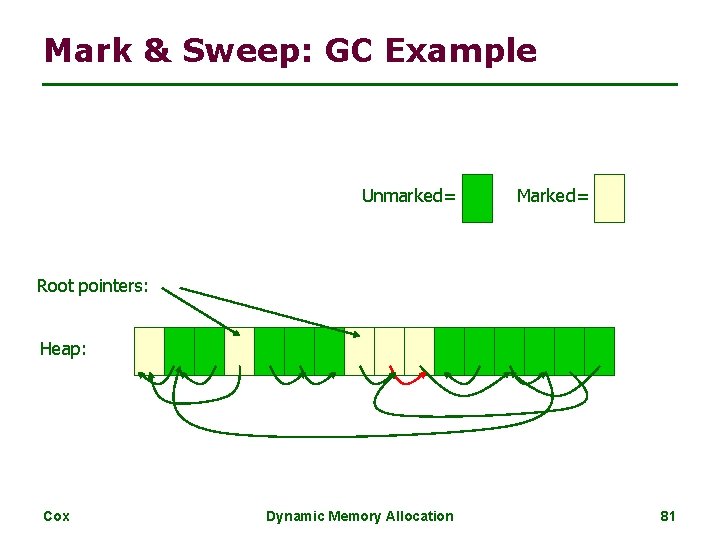
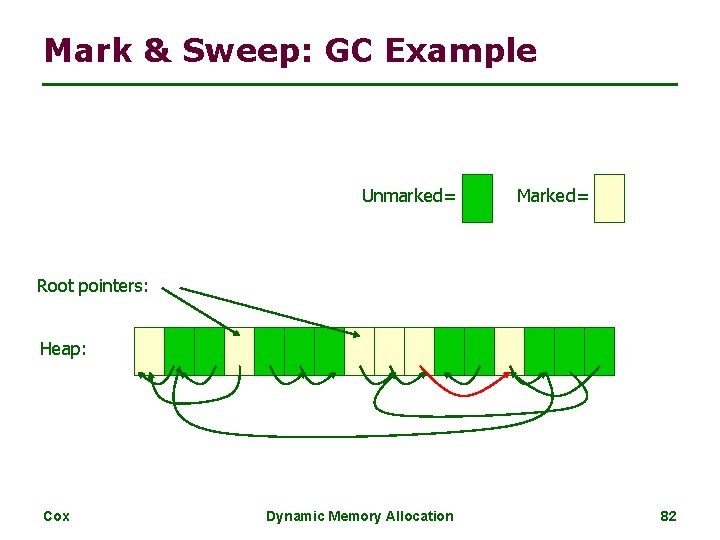
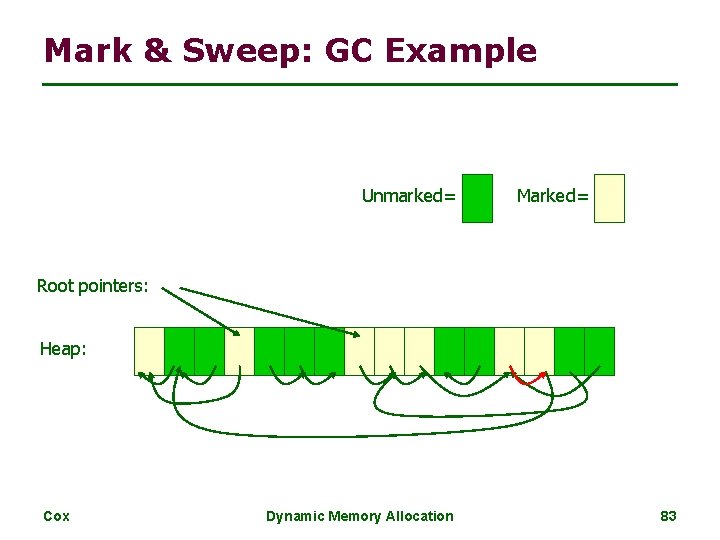
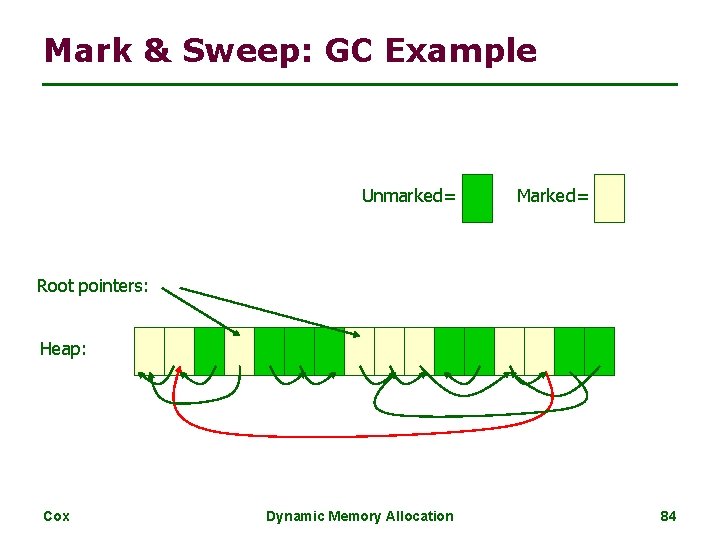
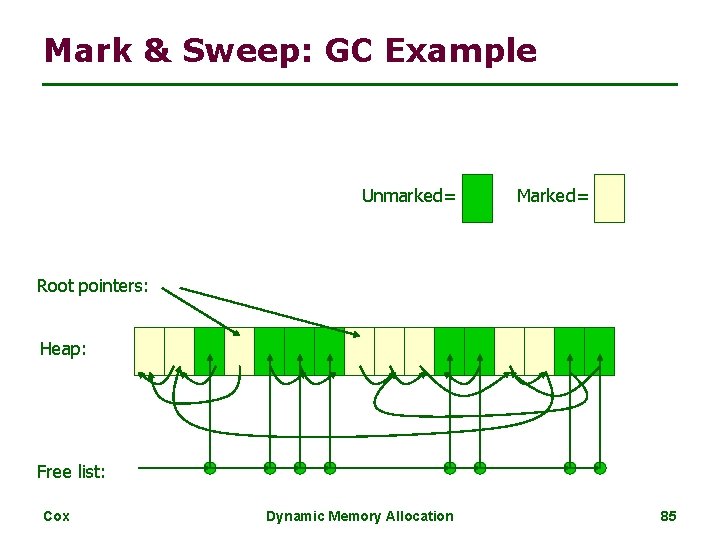
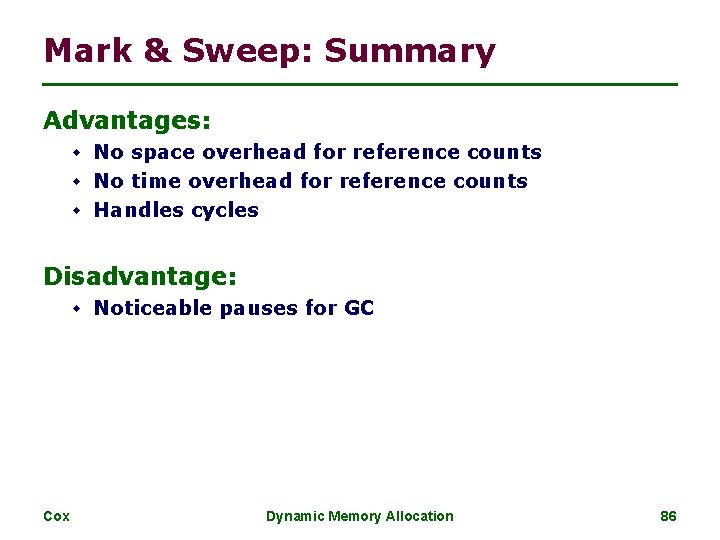
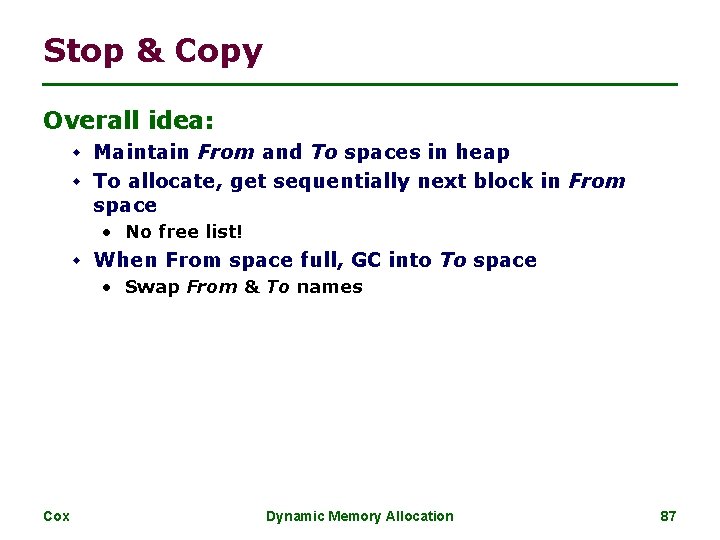
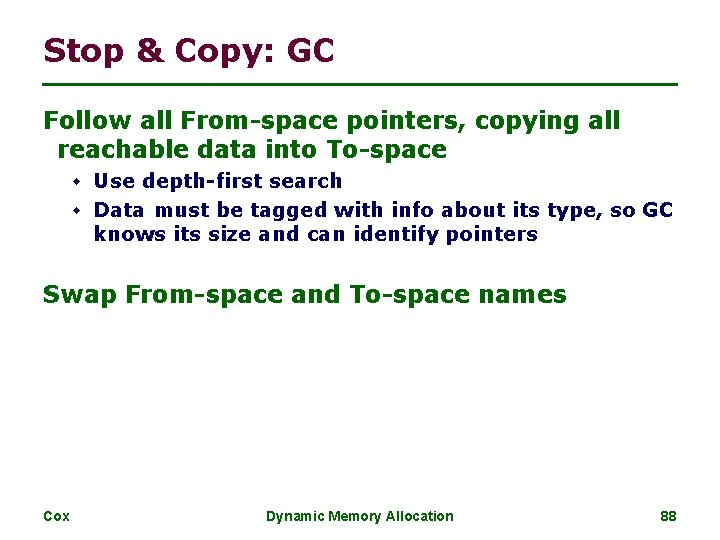
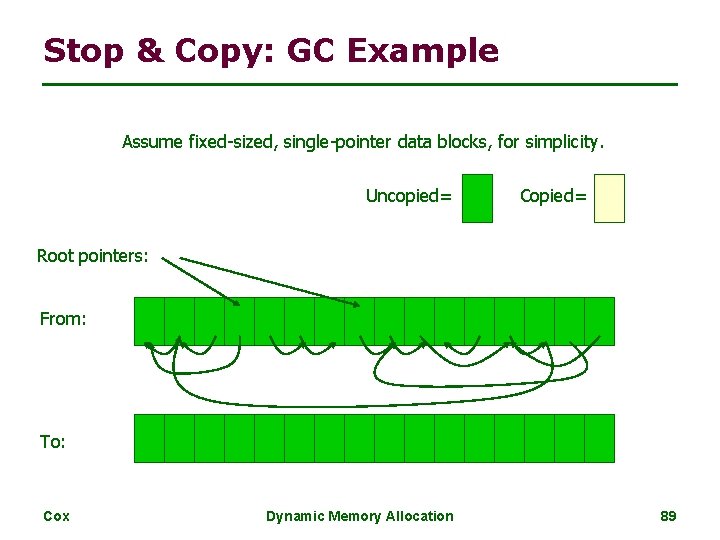
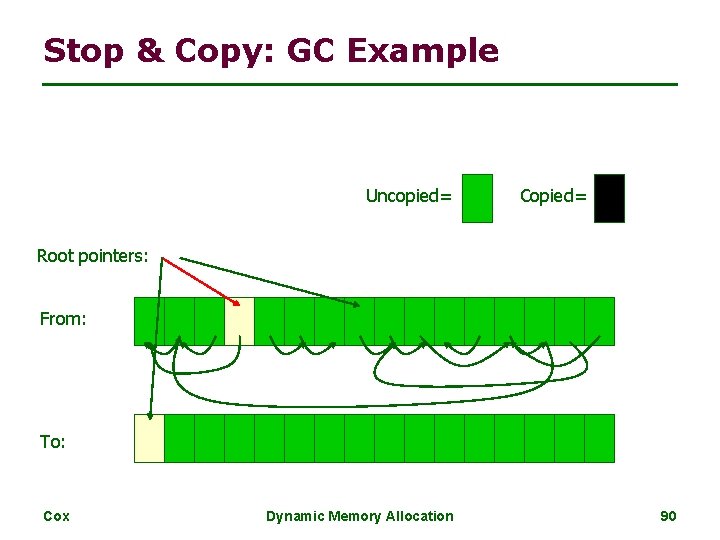
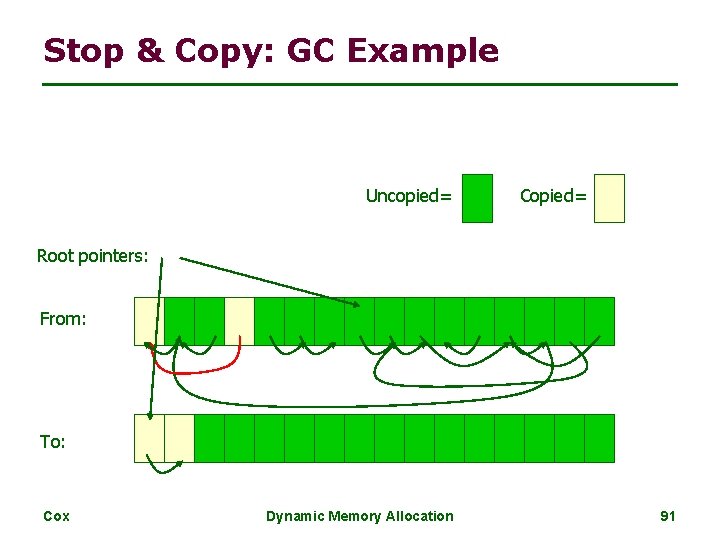
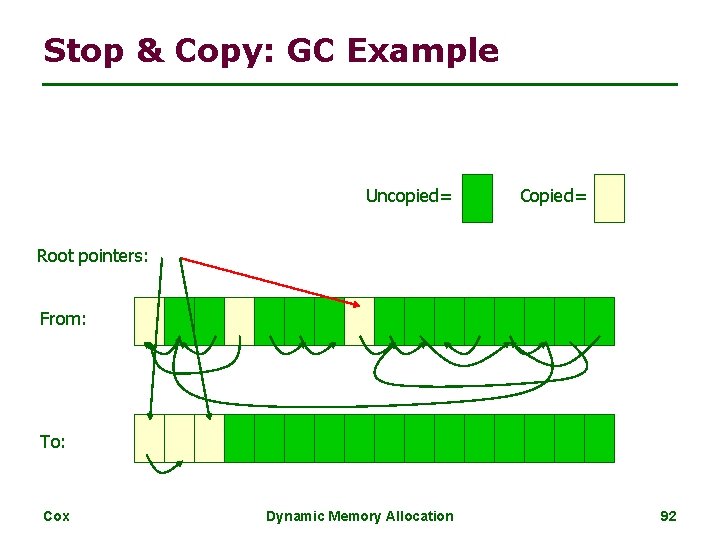
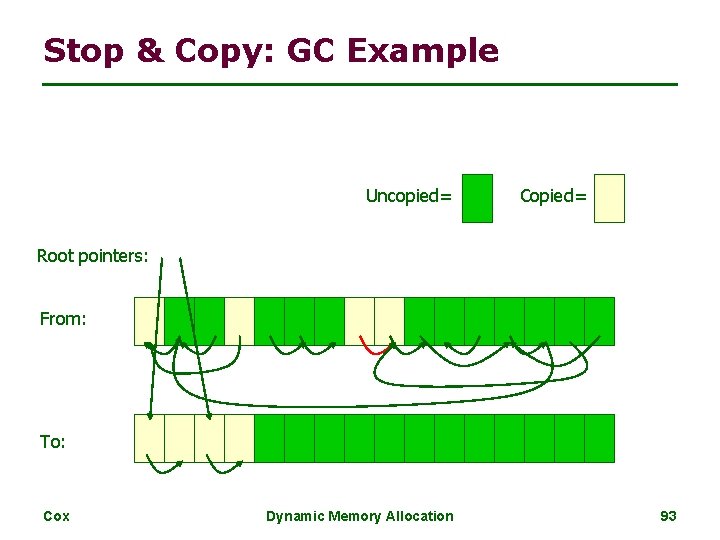
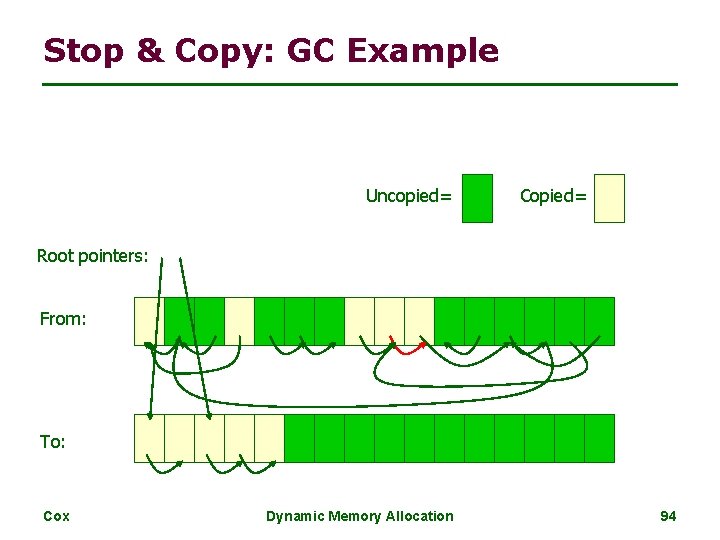
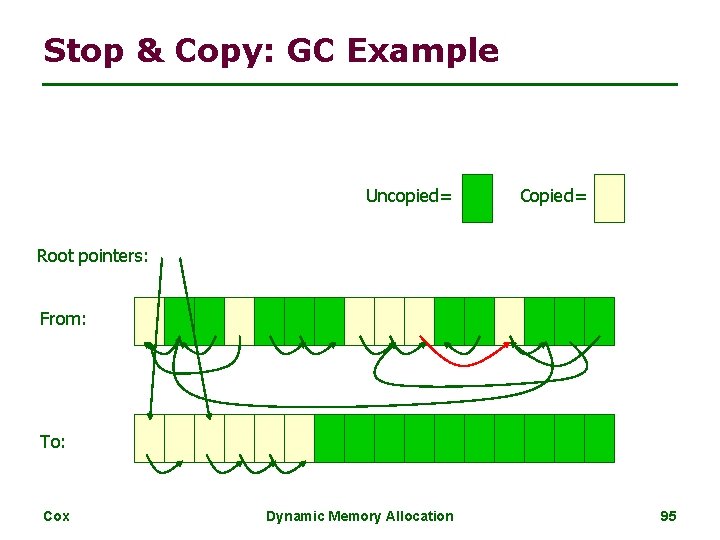
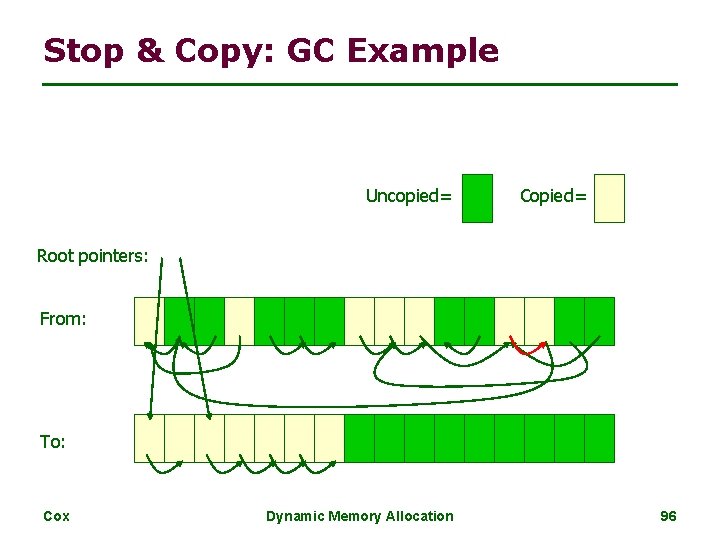
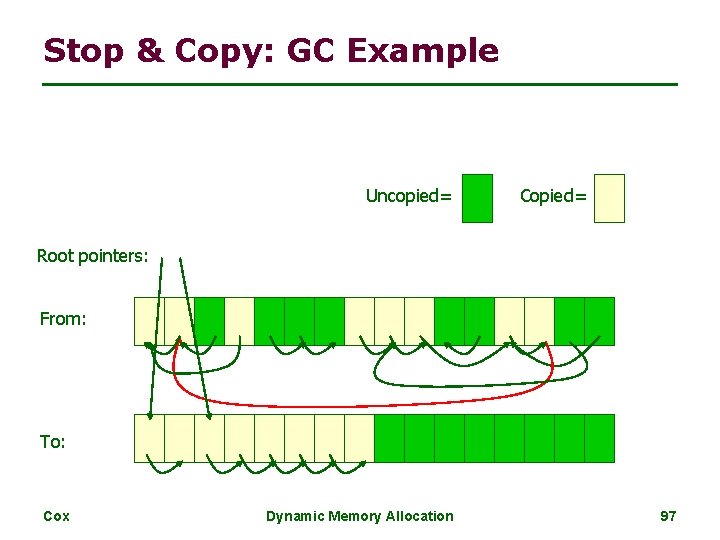
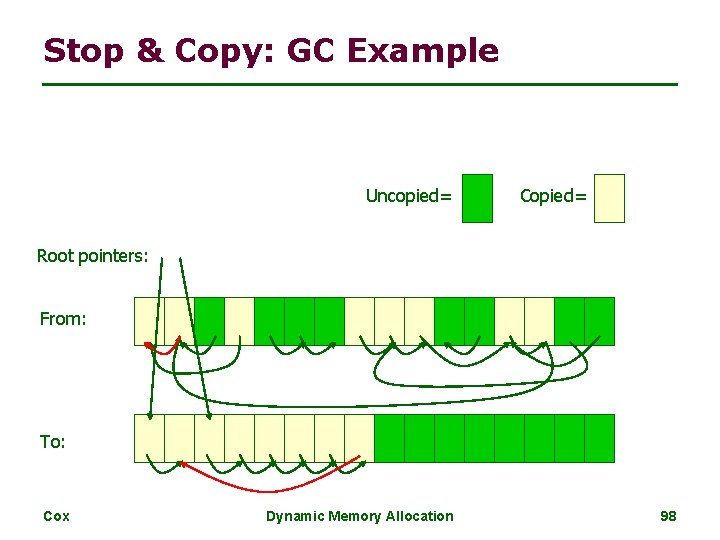
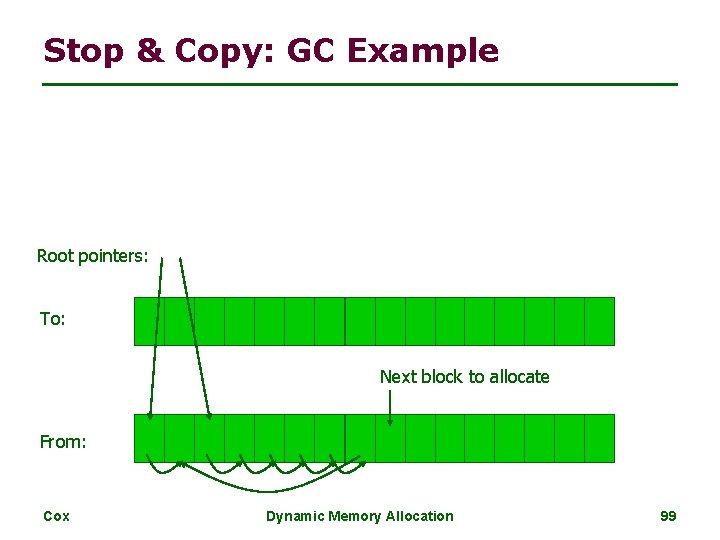
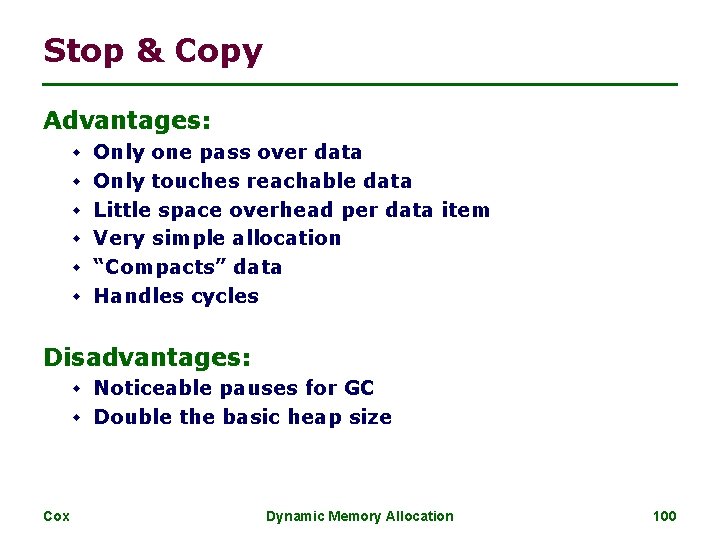
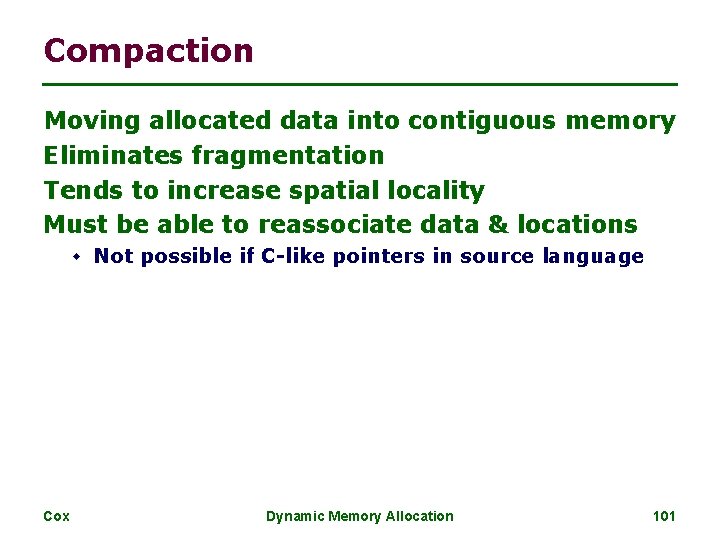
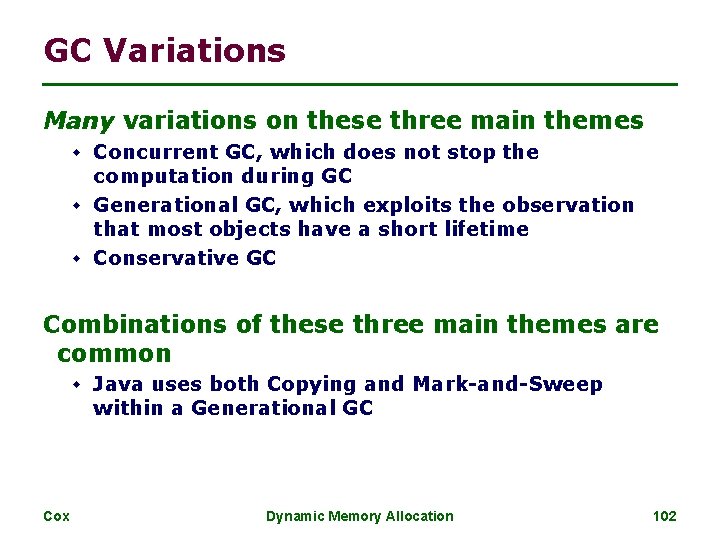
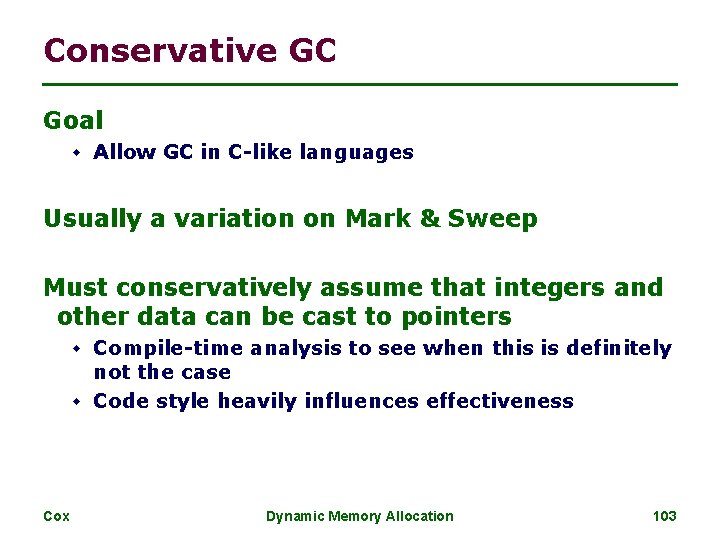
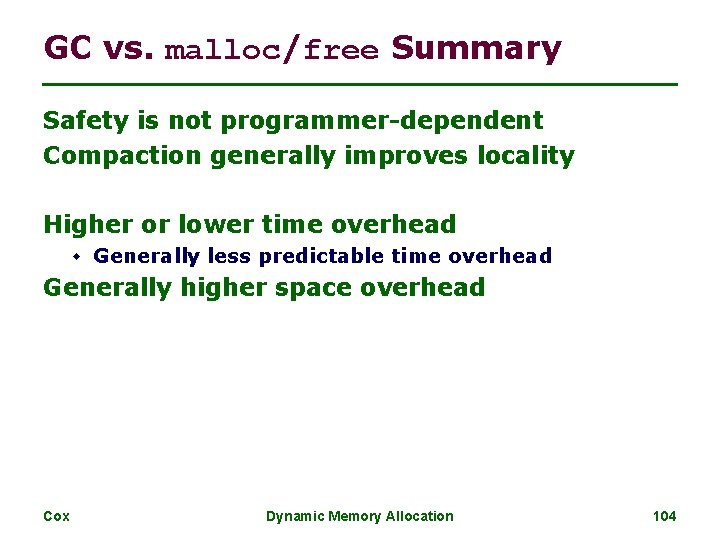
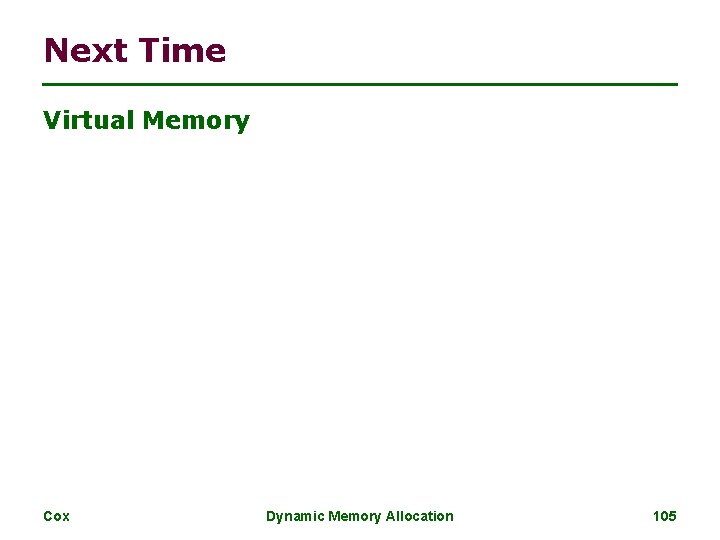
- Slides: 105
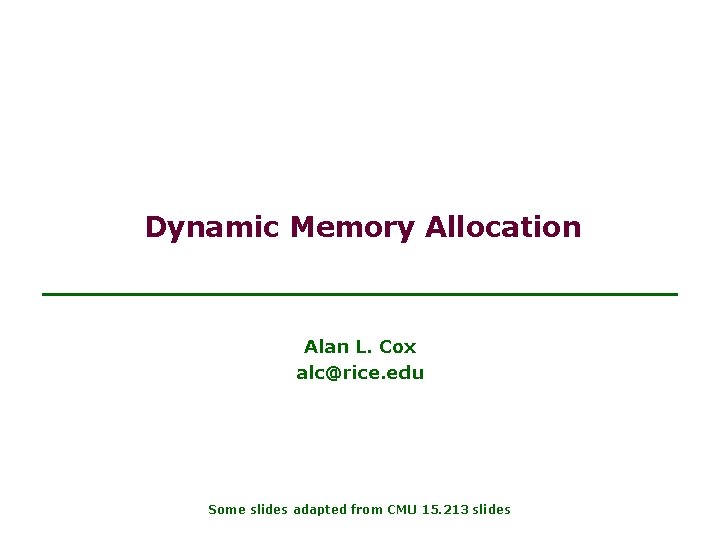
Dynamic Memory Allocation Alan L. Cox alc@rice. edu Some slides adapted from CMU 15. 213 slides
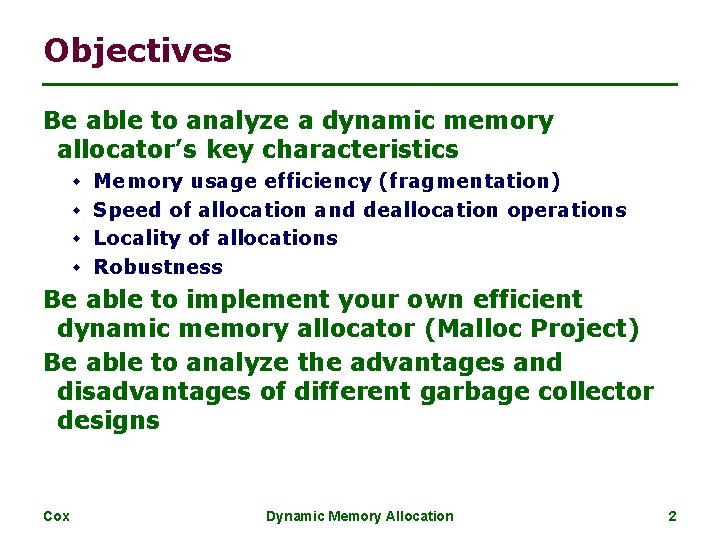
Objectives Be able to analyze a dynamic memory allocator’s key characteristics w Memory usage efficiency (fragmentation) w Speed of allocation and deallocation operations w Locality of allocations w Robustness Be able to implement your own efficient dynamic memory allocator (Malloc Project) Be able to analyze the advantages and disadvantages of different garbage collector designs Cox Dynamic Memory Allocation 2
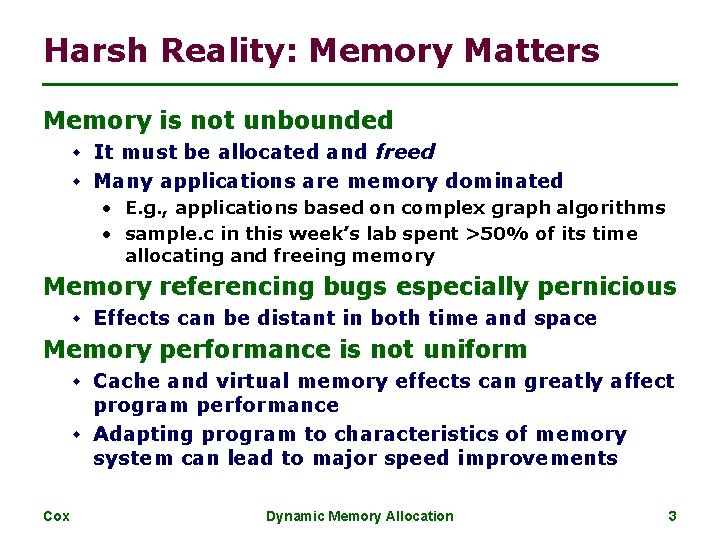
Harsh Reality: Memory Matters Memory is not unbounded w It must be allocated and freed w Many applications are memory dominated • E. g. , applications based on complex graph algorithms • sample. c in this week’s lab spent >50% of its time allocating and freeing memory Memory referencing bugs especially pernicious w Effects can be distant in both time and space Memory performance is not uniform w Cache and virtual memory effects can greatly affect program performance w Adapting program to characteristics of memory system can lead to major speed improvements Cox Dynamic Memory Allocation 3
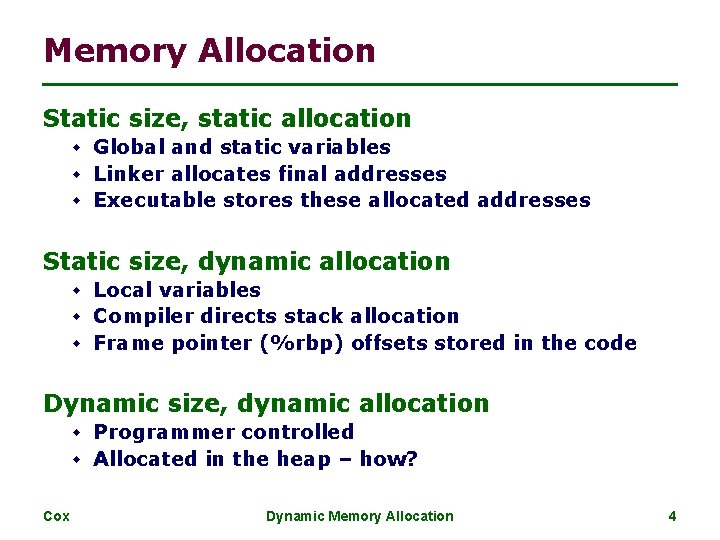
Memory Allocation Static size, static allocation w Global and static variables w Linker allocates final addresses w Executable stores these allocated addresses Static size, dynamic allocation w Local variables w Compiler directs stack allocation w Frame pointer (%rbp) offsets stored in the code Dynamic size, dynamic allocation w Programmer controlled w Allocated in the heap – how? Cox Dynamic Memory Allocation 4
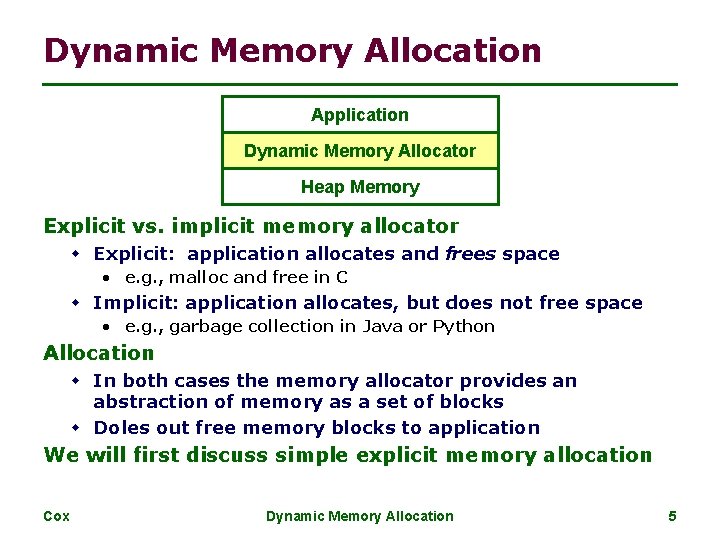
Dynamic Memory Allocation Application Dynamic Memory Allocator Heap Memory Explicit vs. implicit memory allocator w Explicit: application allocates and frees space • e. g. , malloc and free in C w Implicit: application allocates, but does not free space • e. g. , garbage collection in Java or Python Allocation w In both cases the memory allocator provides an abstraction of memory as a set of blocks w Doles out free memory blocks to application We will first discuss simple explicit memory allocation Cox Dynamic Memory Allocation 5
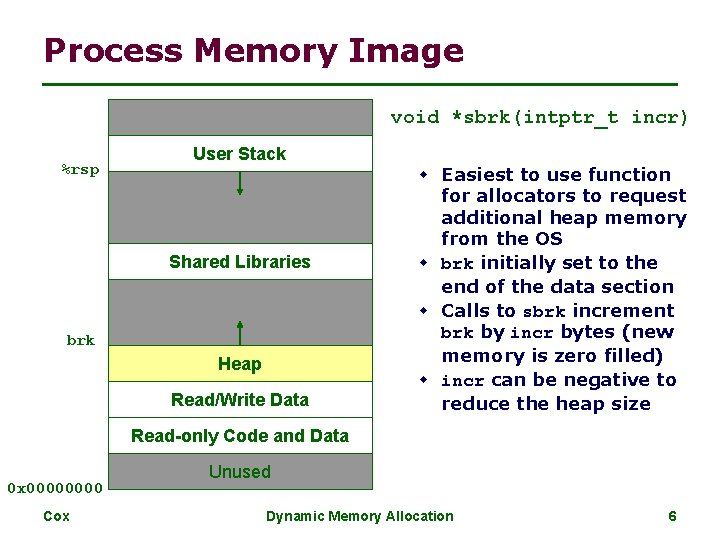
Process Memory Image void *sbrk(intptr_t incr) %rsp User Stack Shared Libraries brk Heap Read/Write Data w Easiest to use function for allocators to request additional heap memory from the OS w brk initially set to the end of the data section w Calls to sbrk increment brk by incr bytes (new memory is zero filled) w incr can be negative to reduce the heap size Read-only Code and Data 0 x 0000 Cox Unused Dynamic Memory Allocation 6
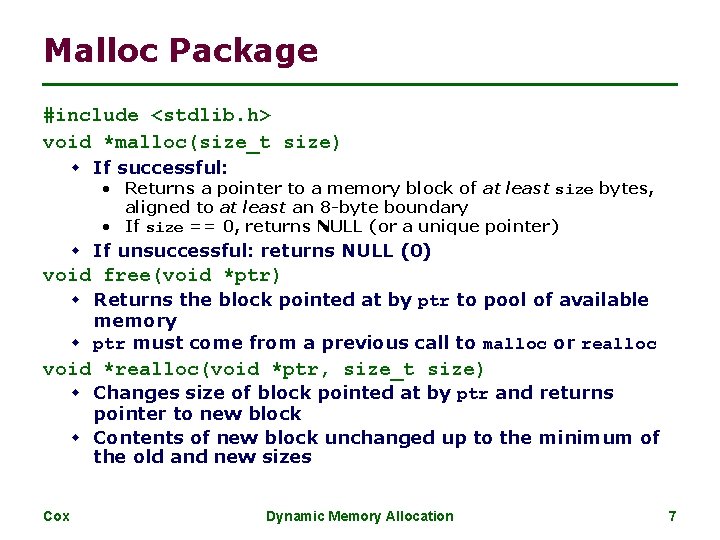
Malloc Package #include <stdlib. h> void *malloc(size_t size) w If successful: • Returns a pointer to a memory block of at least size bytes, aligned to at least an 8 -byte boundary • If size == 0, returns NULL (or a unique pointer) w If unsuccessful: returns NULL (0) void free(void *ptr) w Returns the block pointed at by ptr to pool of available memory w ptr must come from a previous call to malloc or realloc void *realloc(void *ptr, size_t size) w Changes size of block pointed at by ptr and returns pointer to new block w Contents of new block unchanged up to the minimum of the old and new sizes Cox Dynamic Memory Allocation 7
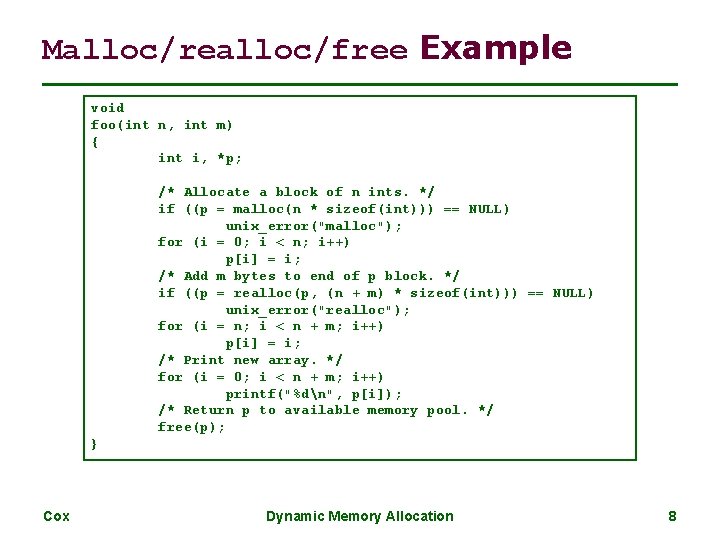
Malloc/realloc/free Example void foo(int n, int m) { int i, *p; /* Allocate a block of n ints. */ if ((p = malloc(n * sizeof(int))) == NULL) unix_error("malloc"); for (i = 0; i < n; i++) p[i] = i; /* Add m bytes to end of p block. */ if ((p = realloc(p, (n + m) * sizeof(int))) == NULL) unix_error("realloc"); for (i = n; i < n + m; i++) p[i] = i; /* Print new array. */ for (i = 0; i < n + m; i++) printf("%dn", p[i]); /* Return p to available memory pool. */ free(p); } Cox Dynamic Memory Allocation 8
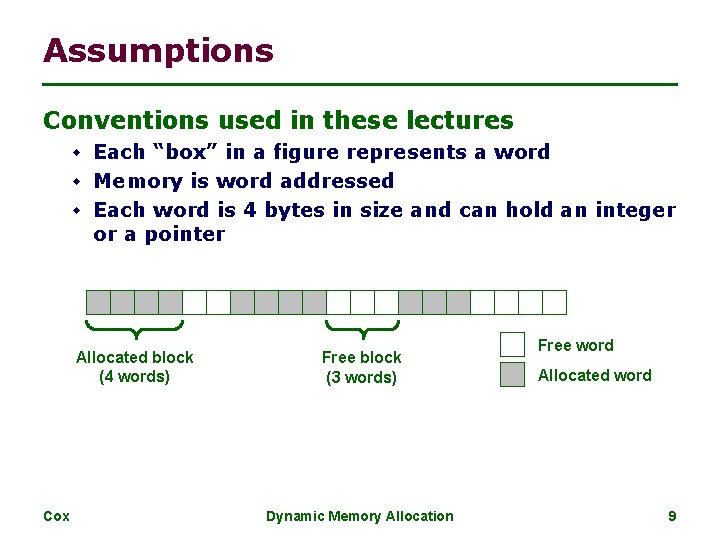
Assumptions Conventions used in these lectures w Each “box” in a figure represents a word w Memory is word addressed w Each word is 4 bytes in size and can hold an integer or a pointer Allocated block (4 words) Cox Free block (3 words) Dynamic Memory Allocation Free word Allocated word 9
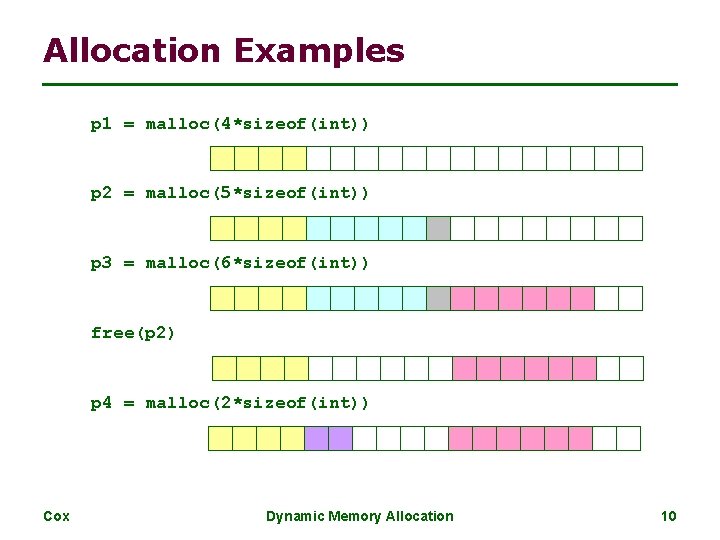
Allocation Examples p 1 = malloc(4*sizeof(int)) p 2 = malloc(5*sizeof(int)) p 3 = malloc(6*sizeof(int)) free(p 2) p 4 = malloc(2*sizeof(int)) Cox Dynamic Memory Allocation 10
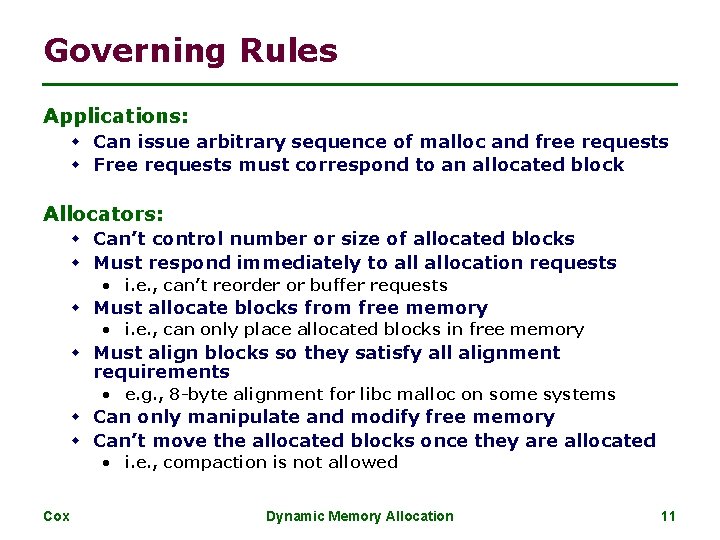
Governing Rules Applications: w Can issue arbitrary sequence of malloc and free requests w Free requests must correspond to an allocated block Allocators: w Can’t control number or size of allocated blocks w Must respond immediately to allocation requests • i. e. , can’t reorder or buffer requests w Must allocate blocks from free memory • i. e. , can only place allocated blocks in free memory w Must align blocks so they satisfy all alignment requirements • e. g. , 8 -byte alignment for libc malloc on some systems w Can only manipulate and modify free memory w Can’t move the allocated blocks once they are allocated • i. e. , compaction is not allowed Cox Dynamic Memory Allocation 11
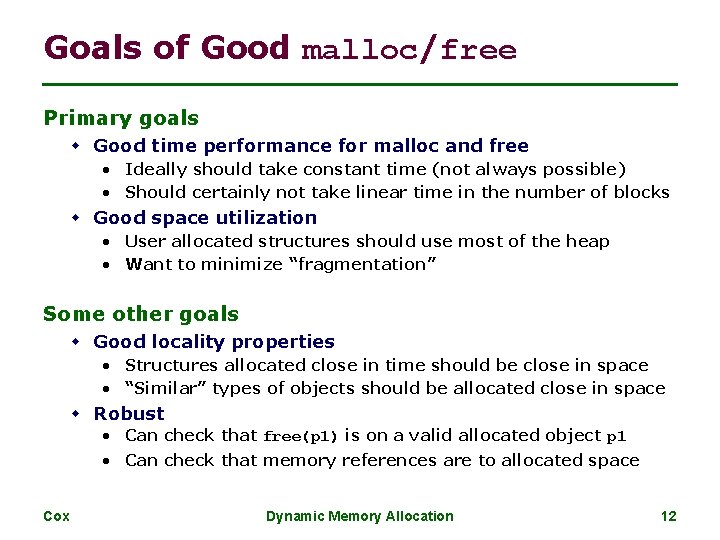
Goals of Good malloc/free Primary goals w Good time performance for malloc and free • Ideally should take constant time (not always possible) • Should certainly not take linear time in the number of blocks w Good space utilization • User allocated structures should use most of the heap • Want to minimize “fragmentation” Some other goals w Good locality properties • Structures allocated close in time should be close in space • “Similar” types of objects should be allocated close in space w Robust • Can check that free(p 1) is on a valid allocated object p 1 • Can check that memory references are to allocated space Cox Dynamic Memory Allocation 12
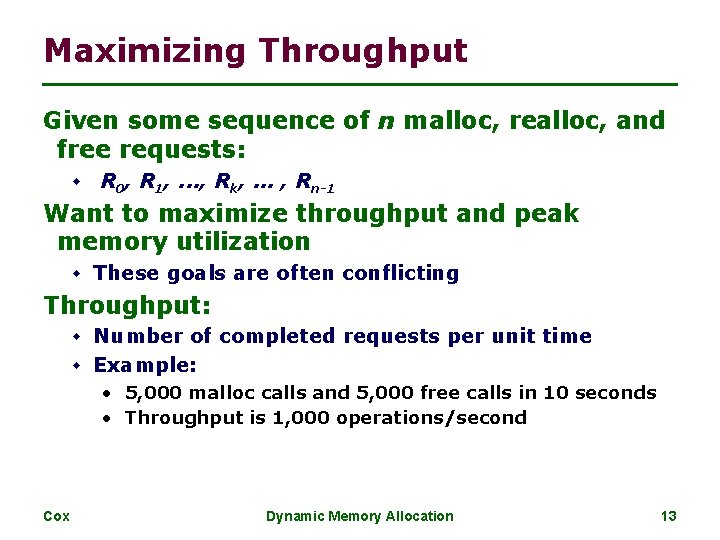
Maximizing Throughput Given some sequence of n malloc, realloc, and free requests: w R 0, R 1, . . . , Rk, . . . , Rn-1 Want to maximize throughput and peak memory utilization w These goals are often conflicting Throughput: w Number of completed requests per unit time w Example: • 5, 000 malloc calls and 5, 000 free calls in 10 seconds • Throughput is 1, 000 operations/second Cox Dynamic Memory Allocation 13
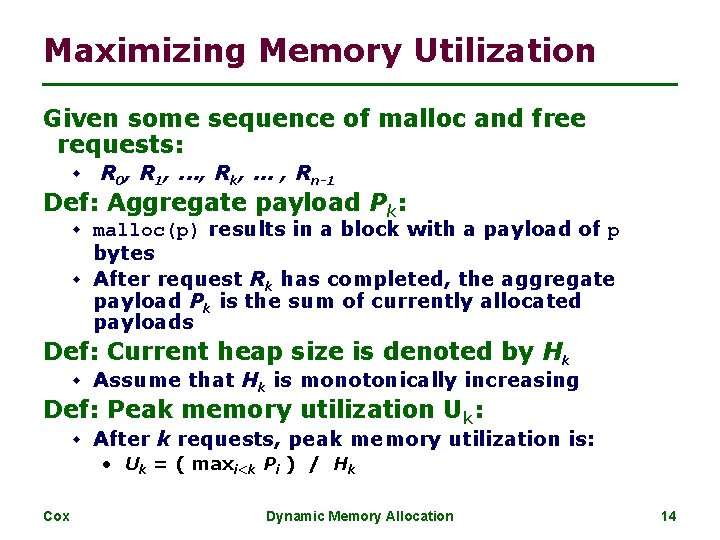
Maximizing Memory Utilization Given some sequence of malloc and free requests: w R 0, R 1, . . . , Rk, . . . , Rn-1 Def: Aggregate payload Pk: w malloc(p) results in a block with a payload of p bytes w After request Rk has completed, the aggregate payload Pk is the sum of currently allocated payloads Def: Current heap size is denoted by Hk w Assume that Hk is monotonically increasing Def: Peak memory utilization Uk: w After k requests, peak memory utilization is: • Uk = ( maxi<k Pi ) / Hk Cox Dynamic Memory Allocation 14
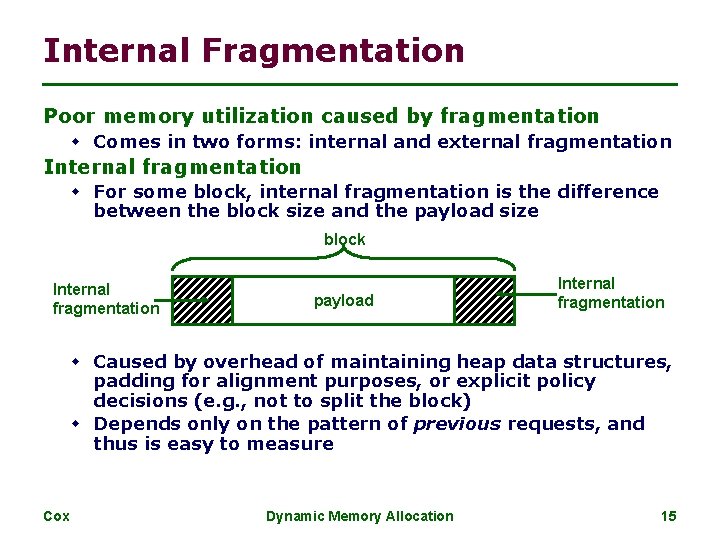
Internal Fragmentation Poor memory utilization caused by fragmentation w Comes in two forms: internal and external fragmentation Internal fragmentation w For some block, internal fragmentation is the difference between the block size and the payload size block Internal fragmentation payload Internal fragmentation w Caused by overhead of maintaining heap data structures, padding for alignment purposes, or explicit policy decisions (e. g. , not to split the block) w Depends only on the pattern of previous requests, and thus is easy to measure Cox Dynamic Memory Allocation 15
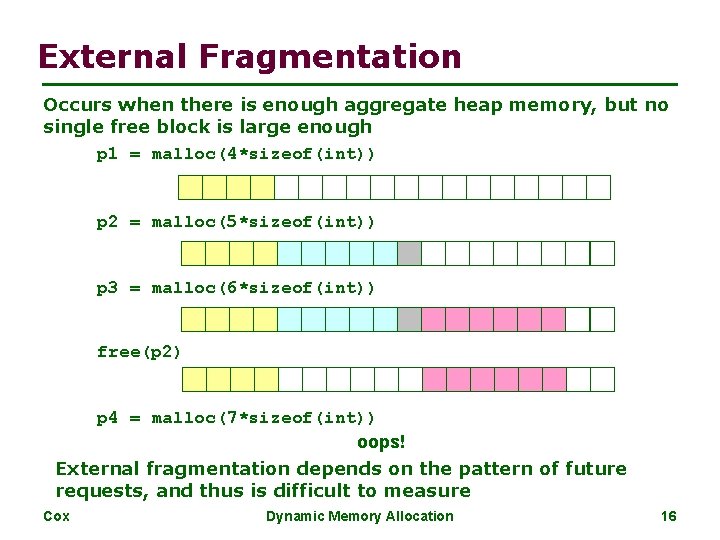
External Fragmentation Occurs when there is enough aggregate heap memory, but no single free block is large enough p 1 = malloc(4*sizeof(int)) p 2 = malloc(5*sizeof(int)) p 3 = malloc(6*sizeof(int)) free(p 2) p 4 = malloc(7*sizeof(int)) oops! External fragmentation depends on the pattern of future requests, and thus is difficult to measure Cox Dynamic Memory Allocation 16
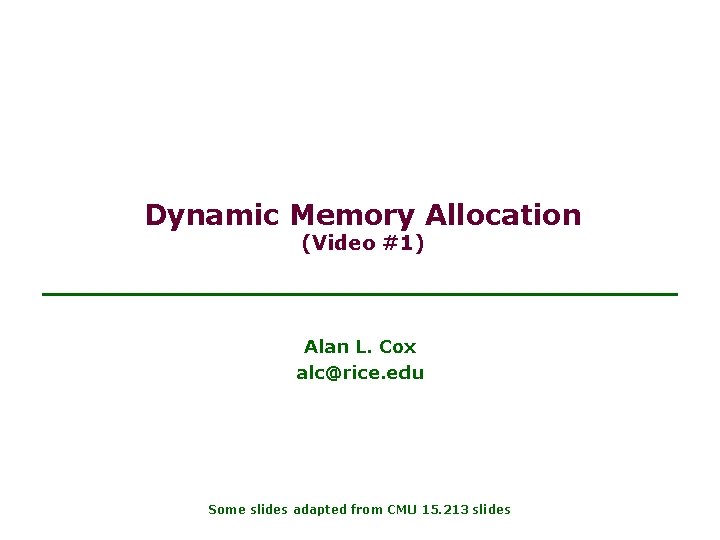
Dynamic Memory Allocation (Video #1) Alan L. Cox alc@rice. edu Some slides adapted from CMU 15. 213 slides
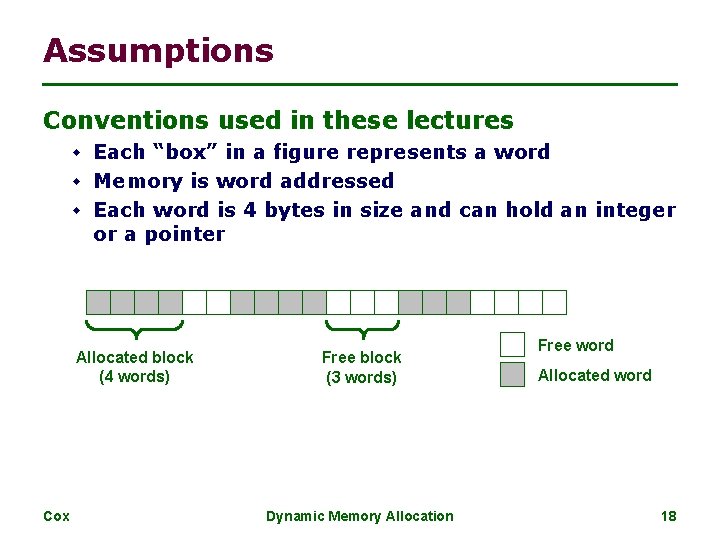
Assumptions Conventions used in these lectures w Each “box” in a figure represents a word w Memory is word addressed w Each word is 4 bytes in size and can hold an integer or a pointer Allocated block (4 words) Cox Free block (3 words) Dynamic Memory Allocation Free word Allocated word 18
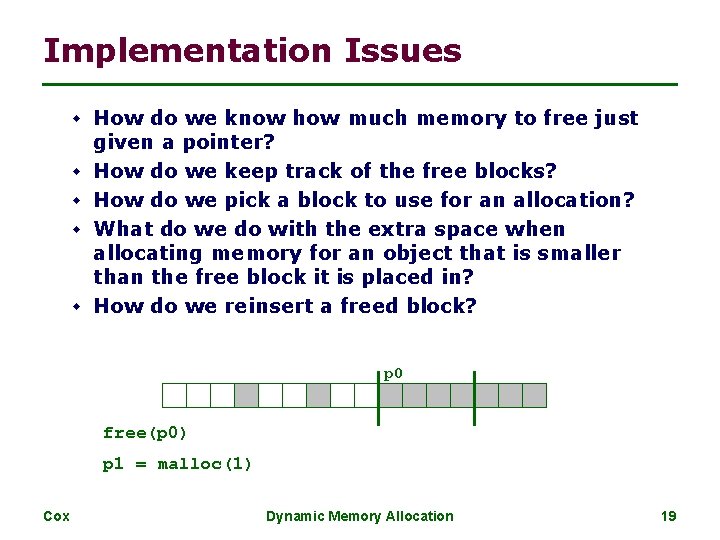
Implementation Issues w How do we know how much memory to free just w w given a pointer? How do we keep track of the free blocks? How do we pick a block to use for an allocation? What do we do with the extra space when allocating memory for an object that is smaller than the free block it is placed in? How do we reinsert a freed block? p 0 free(p 0) p 1 = malloc(1) Cox Dynamic Memory Allocation 19
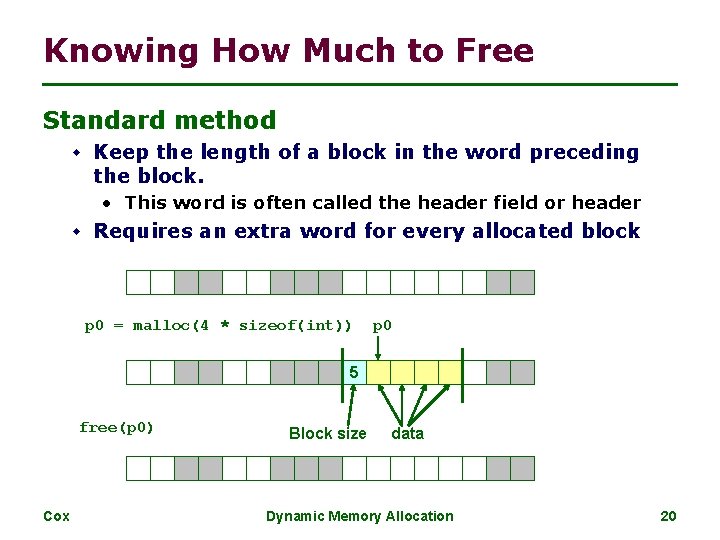
Knowing How Much to Free Standard method w Keep the length of a block in the word preceding the block. • This word is often called the header field or header w Requires an extra word for every allocated block p 0 = malloc(4 * sizeof(int)) p 0 5 free(p 0) Cox Block size data Dynamic Memory Allocation 20
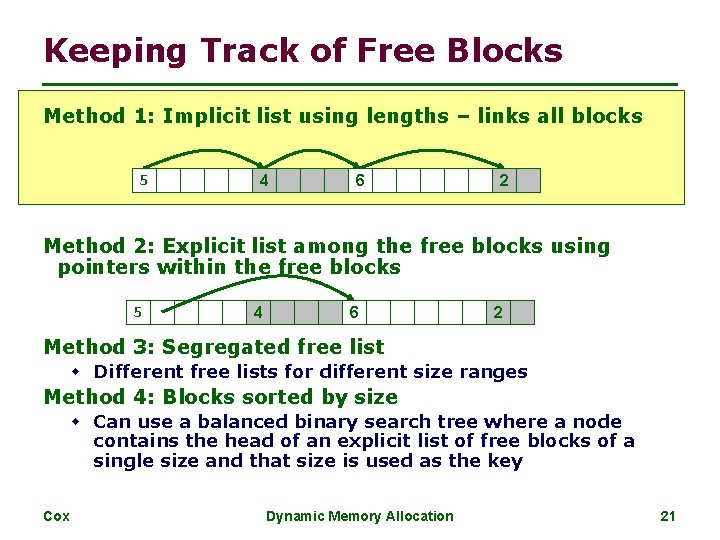
Keeping Track of Free Blocks Method 1: Implicit list using lengths – links all blocks 5 4 6 2 Method 2: Explicit list among the free blocks using pointers within the free blocks 5 4 6 2 Method 3: Segregated free list w Different free lists for different size ranges Method 4: Blocks sorted by size w Can use a balanced binary search tree where a node contains the head of an explicit list of free blocks of a single size and that size is used as the key Cox Dynamic Memory Allocation 21
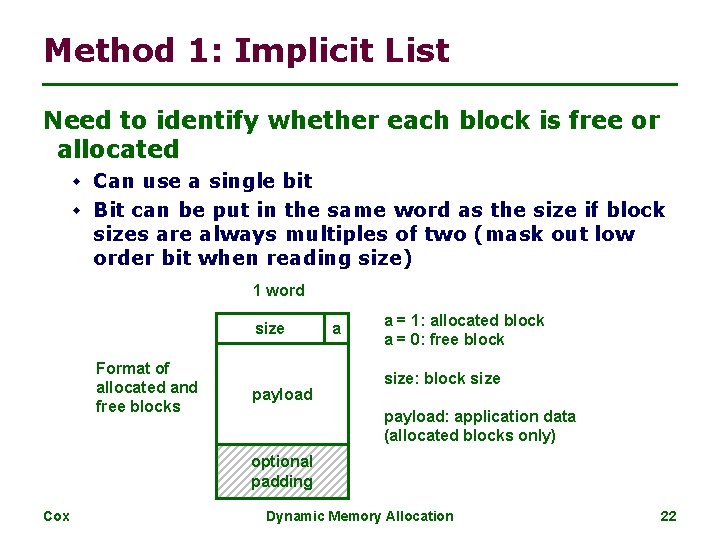
Method 1: Implicit List Need to identify whether each block is free or allocated w Can use a single bit w Bit can be put in the same word as the size if block sizes are always multiples of two (mask out low order bit when reading size) 1 word size Format of allocated and free blocks payload a a = 1: allocated block a = 0: free block size: block size payload: application data (allocated blocks only) optional padding Cox Dynamic Memory Allocation 22
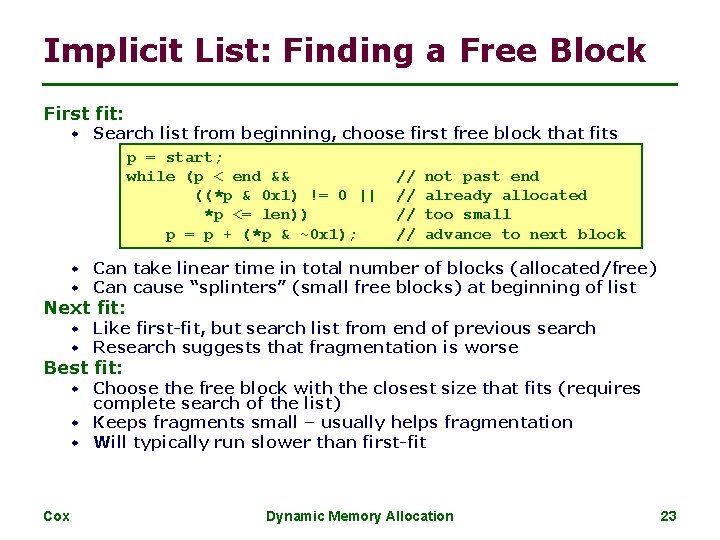
Implicit List: Finding a Free Block First fit: w Search list from beginning, choose first free block that fits p = start; while (p < end && ((*p & 0 x 1) != 0 || *p <= len)) p = p + (*p & ~0 x 1); // // not past end already allocated too small advance to next block w Can take linear time in total number of blocks (allocated/free) w Can cause “splinters” (small free blocks) at beginning of list Next fit: w Like first-fit, but search list from end of previous search w Research suggests that fragmentation is worse Best fit: w Choose the free block with the closest size that fits (requires complete search of the list) w Keeps fragments small – usually helps fragmentation w Will typically run slower than first-fit Cox Dynamic Memory Allocation 23
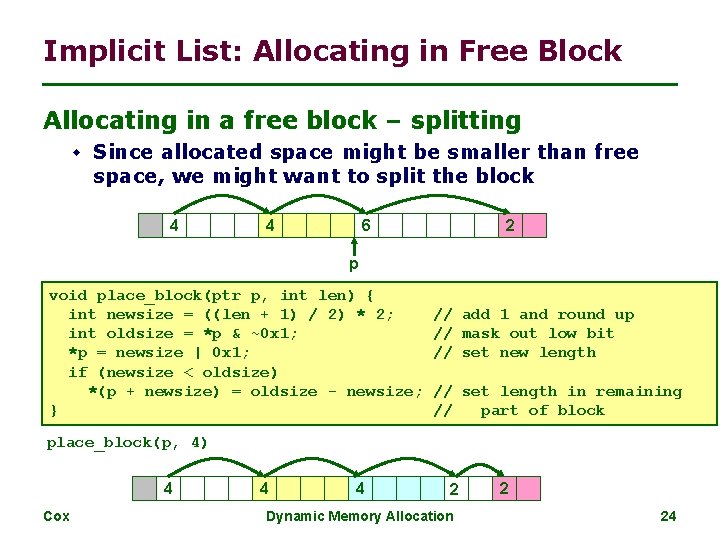
Implicit List: Allocating in Free Block Allocating in a free block – splitting w Since allocated space might be smaller than free space, we might want to split the block 4 4 6 2 p void place_block(ptr p, int len) { int newsize = ((len + 1) / 2) * 2; int oldsize = *p & ~0 x 1; *p = newsize | 0 x 1; if (newsize < oldsize) *(p + newsize) = oldsize - newsize; } // add 1 and round up // mask out low bit // set new length // set length in remaining // part of block place_block(p, 4) 4 Cox 4 4 2 Dynamic Memory Allocation 2 24
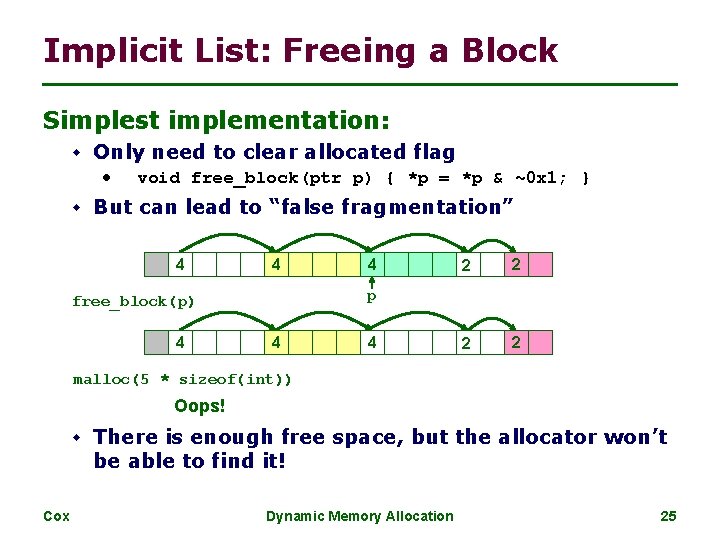
Implicit List: Freeing a Block Simplest implementation: w Only need to clear allocated flag • void free_block(ptr p) { *p = *p & ~0 x 1; } w But can lead to “false fragmentation” 4 4 2 2 p free_block(p) 4 4 malloc(5 * sizeof(int)) Oops! w There is enough free space, but the allocator won’t be able to find it! Cox Dynamic Memory Allocation 25
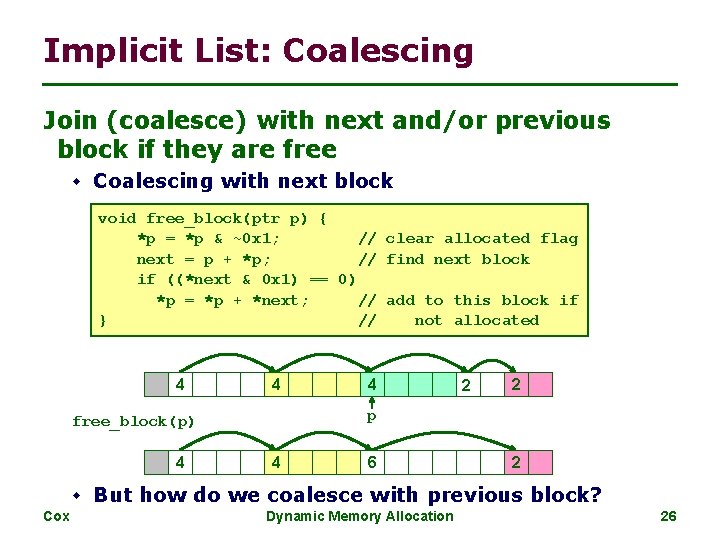
Implicit List: Coalescing Join (coalesce) with next and/or previous block if they are free w Coalescing with next block • void free_block(ptr p) { } *p = *p & ~0 x 1; // clear allocated flag next = p + *p; // find next block if ((*next & 0 x 1) == 0) *p = *p + *next; // add to this block if // not allocated 4 4 2 2 p free_block(p) 4 4 4 6 2 w But how do we coalesce with previous block? Cox Dynamic Memory Allocation 26
![Implicit List Bidirectional Coalescing Boundary tags Knuth 73 w Replicate header word at end Implicit List: Bidirectional Coalescing Boundary tags [Knuth 73] w Replicate header word at end](https://slidetodoc.com/presentation_image_h/eb36a141b805fda6ea68ddf029af9b2b/image-27.jpg)
Implicit List: Bidirectional Coalescing Boundary tags [Knuth 73] w Replicate header word at end of block w Allows us to traverse the “list” backwards, but requires extra space w Important and general technique! Header Format of allocated and free blocks Cox a payload and padding Boundary tag (footer) 4 size 4 6 a = 1: allocated block a = 0: free block size: total block size a payload: application data (allocated blocks only) 6 4 Dynamic Memory Allocation 4 27
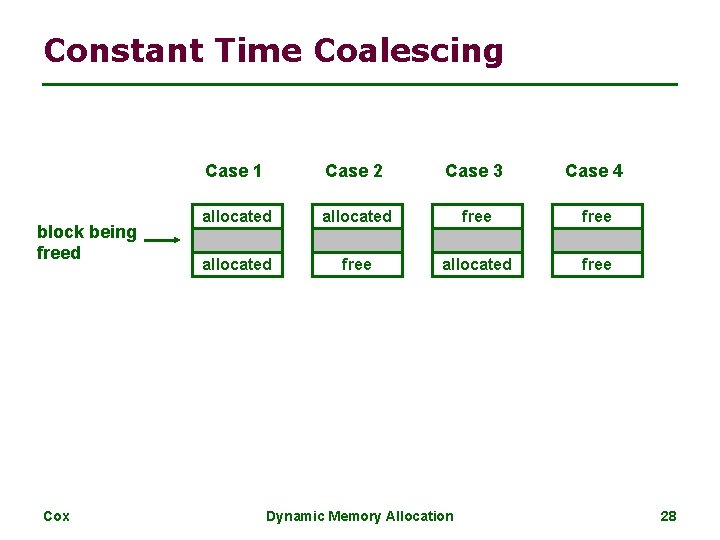
Constant Time Coalescing block being freed Cox Case 1 Case 2 Case 3 Case 4 allocated free Dynamic Memory Allocation 28
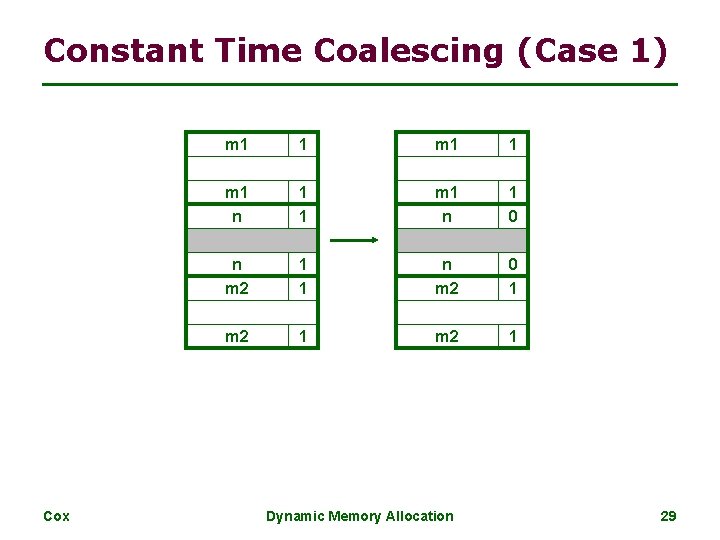
Constant Time Coalescing (Case 1) Cox m 1 1 m 1 n 1 0 n m 2 1 1 n m 2 0 1 m 2 1 Dynamic Memory Allocation 29
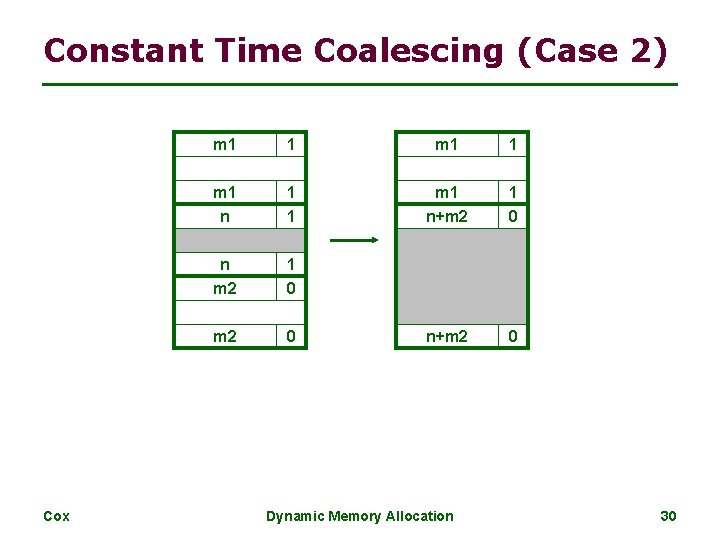
Constant Time Coalescing (Case 2) Cox m 1 1 m 1 n+m 2 1 0 n m 2 1 0 m 2 0 n+m 2 0 Dynamic Memory Allocation 30
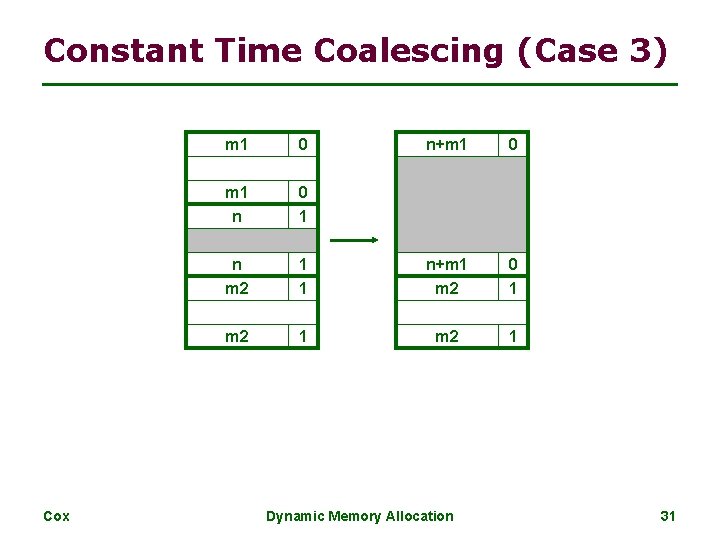
Constant Time Coalescing (Case 3) Cox m 1 0 n+m 1 0 m 1 n 0 1 n m 2 1 1 n+m 1 m 2 0 1 m 2 1 Dynamic Memory Allocation 31
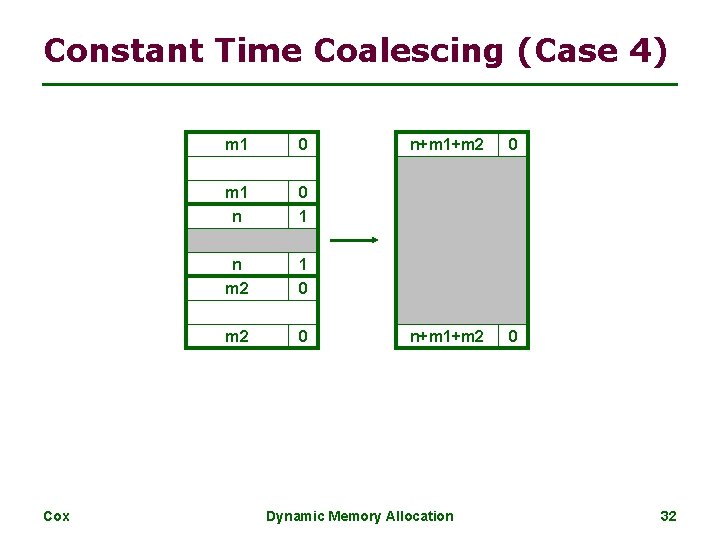
Constant Time Coalescing (Case 4) Cox m 1 0 m 1 n 0 1 n m 2 1 0 m 2 0 n+m 1+m 2 0 Dynamic Memory Allocation 32
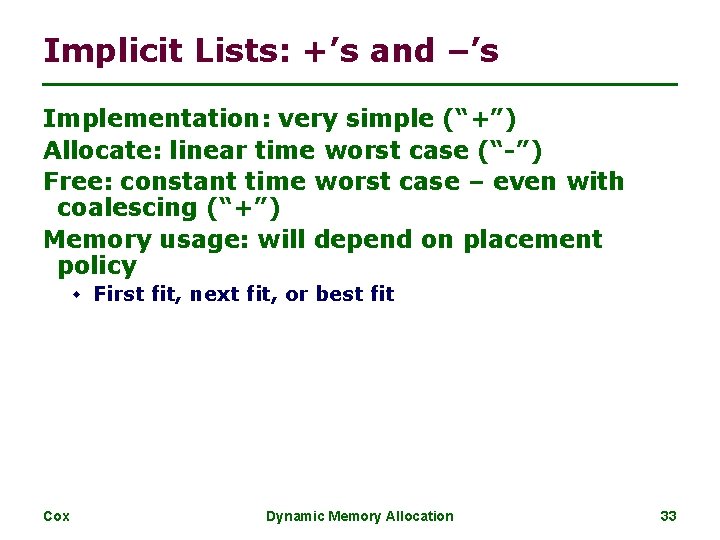
Implicit Lists: +’s and –’s Implementation: very simple (“+”) Allocate: linear time worst case (“-”) Free: constant time worst case – even with coalescing (“+”) Memory usage: will depend on placement policy w First fit, next fit, or best fit Cox Dynamic Memory Allocation 33
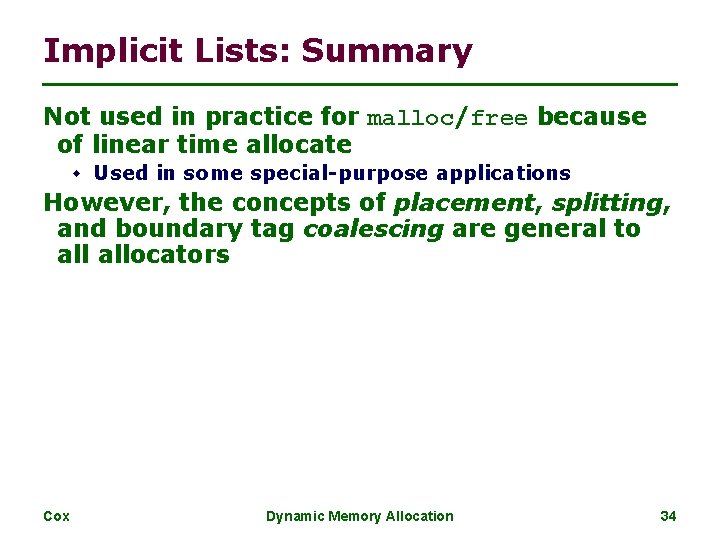
Implicit Lists: Summary Not used in practice for malloc/free because of linear time allocate w Used in some special-purpose applications However, the concepts of placement, splitting, and boundary tag coalescing are general to allocators Cox Dynamic Memory Allocation 34
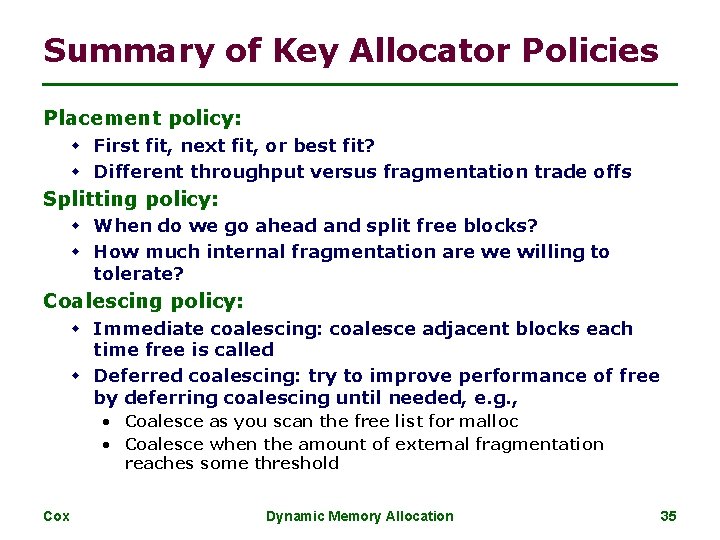
Summary of Key Allocator Policies Placement policy: w First fit, next fit, or best fit? w Different throughput versus fragmentation trade offs Splitting policy: w When do we go ahead and split free blocks? w How much internal fragmentation are we willing to tolerate? Coalescing policy: w Immediate coalescing: coalesce adjacent blocks each time free is called w Deferred coalescing: try to improve performance of free by deferring coalescing until needed, e. g. , • Coalesce as you scan the free list for malloc • Coalesce when the amount of external fragmentation reaches some threshold Cox Dynamic Memory Allocation 35
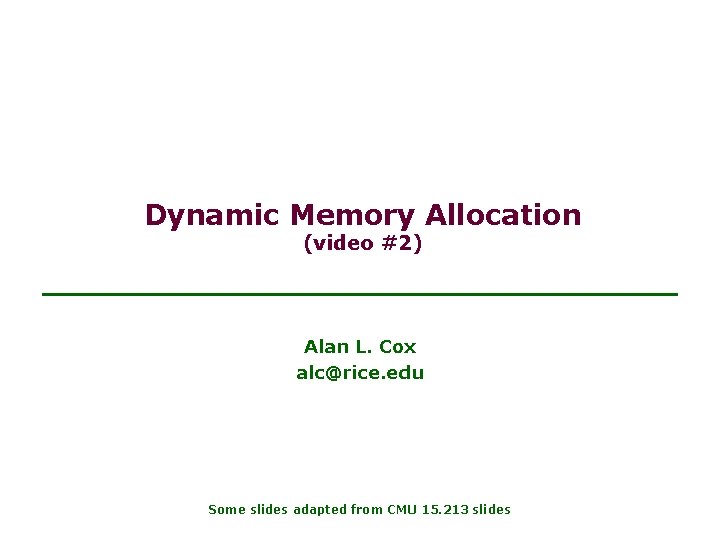
Dynamic Memory Allocation (video #2) Alan L. Cox alc@rice. edu Some slides adapted from CMU 15. 213 slides
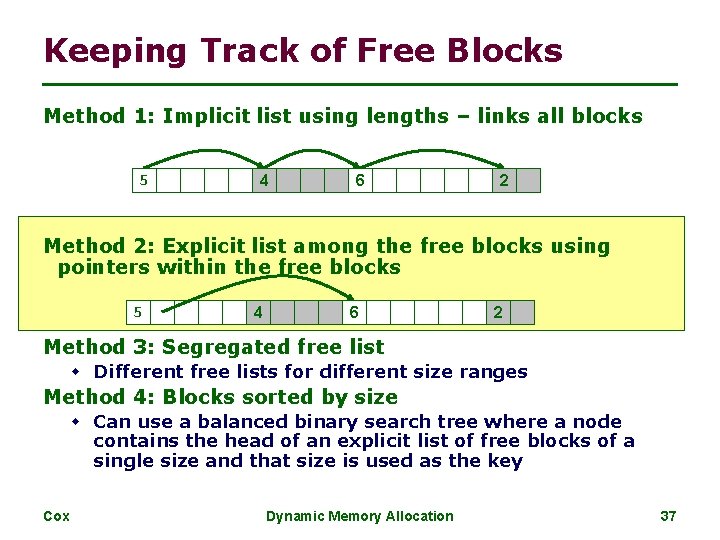
Keeping Track of Free Blocks Method 1: Implicit list using lengths – links all blocks 5 4 6 2 Method 2: Explicit list among the free blocks using pointers within the free blocks 5 4 6 2 Method 3: Segregated free list w Different free lists for different size ranges Method 4: Blocks sorted by size w Can use a balanced binary search tree where a node contains the head of an explicit list of free blocks of a single size and that size is used as the key Cox Dynamic Memory Allocation 37
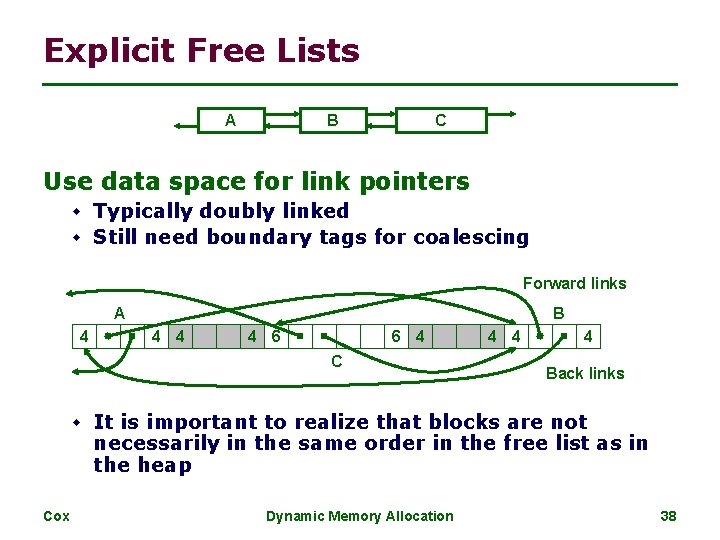
Explicit Free Lists A B C Use data space for link pointers w Typically doubly linked w Still need boundary tags for coalescing Forward links A 4 B 4 4 4 6 6 4 C 4 4 4 Back links w It is important to realize that blocks are not necessarily in the same order in the free list as in the heap Cox Dynamic Memory Allocation 38
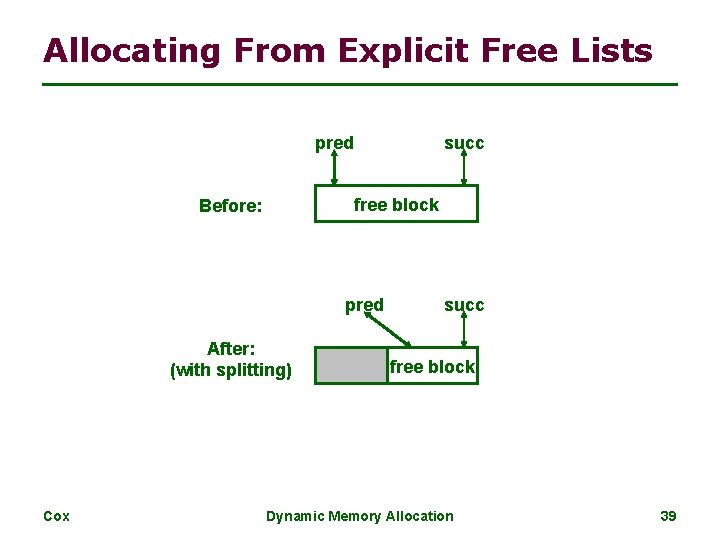
Allocating From Explicit Free Lists pred succ free block Before: pred After: (with splitting) Cox succ free block Dynamic Memory Allocation 39
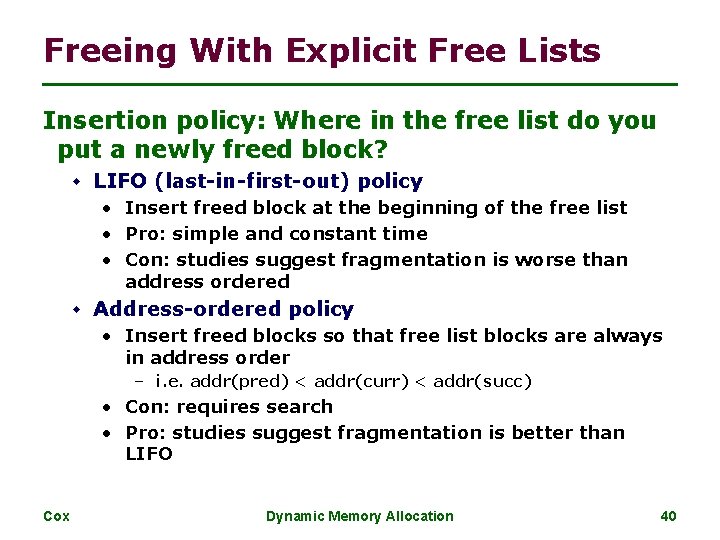
Freeing With Explicit Free Lists Insertion policy: Where in the free list do you put a newly freed block? w LIFO (last-in-first-out) policy • Insert freed block at the beginning of the free list • Pro: simple and constant time • Con: studies suggest fragmentation is worse than address ordered w Address-ordered policy • Insert freed blocks so that free list blocks are always in address order – i. e. addr(pred) < addr(curr) < addr(succ) • Con: requires search • Pro: studies suggest fragmentation is better than LIFO Cox Dynamic Memory Allocation 40
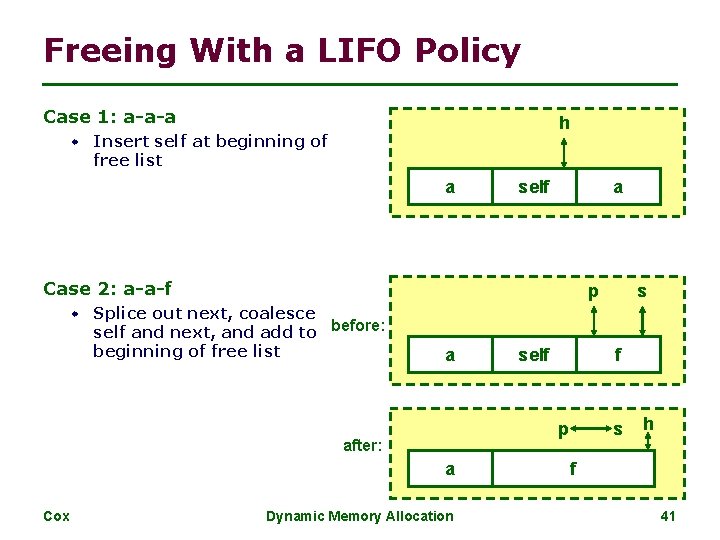
Freeing With a LIFO Policy Case 1: a-a-a h w Insert self at beginning of free list a self a Case 2: a-a-f p s w Splice out next, coalesce self and next, and add to before: beginning of free list a f p after: a Cox self Dynamic Memory Allocation s h f 41
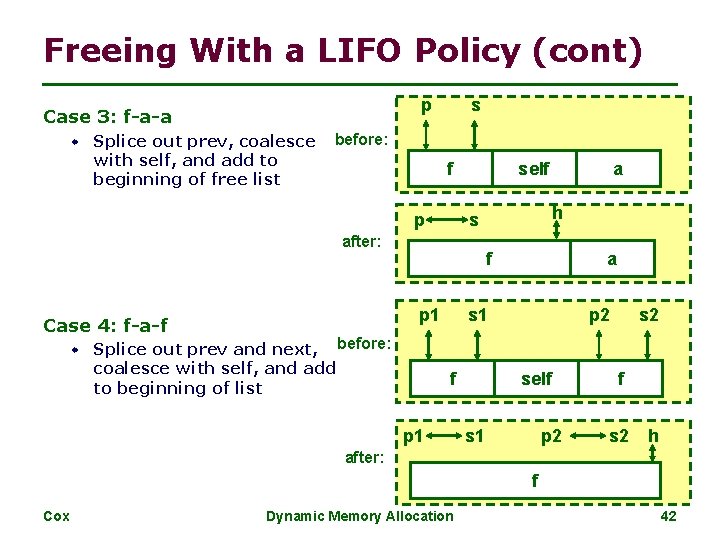
Freeing With a LIFO Policy (cont) p Case 3: f-a-a w Splice out prev, coalesce s before: with self, and add to beginning of free list f p self f p 1 Case 4: f-a-f w Splice out prev and next, h s after: a a s 1 p 2 s 2 before: coalesce with self, and add to beginning of list f p 1 self s 1 p 2 f s 2 h after: f Cox Dynamic Memory Allocation 42
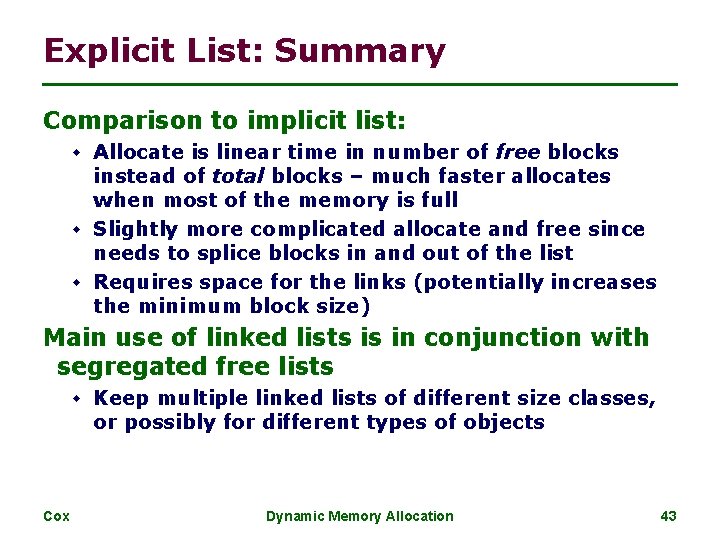
Explicit List: Summary Comparison to implicit list: w Allocate is linear time in number of free blocks instead of total blocks – much faster allocates when most of the memory is full w Slightly more complicated allocate and free since needs to splice blocks in and out of the list w Requires space for the links (potentially increases the minimum block size) Main use of linked lists is in conjunction with segregated free lists w Keep multiple linked lists of different size classes, or possibly for different types of objects Cox Dynamic Memory Allocation 43
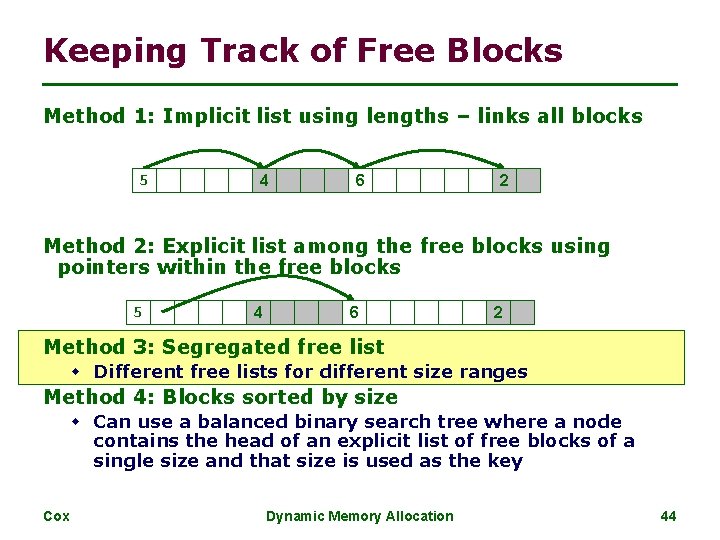
Keeping Track of Free Blocks Method 1: Implicit list using lengths – links all blocks 5 4 6 2 Method 2: Explicit list among the free blocks using pointers within the free blocks 5 4 6 2 Method 3: Segregated free list w Different free lists for different size ranges Method 4: Blocks sorted by size w Can use a balanced binary search tree where a node contains the head of an explicit list of free blocks of a single size and that size is used as the key Cox Dynamic Memory Allocation 44
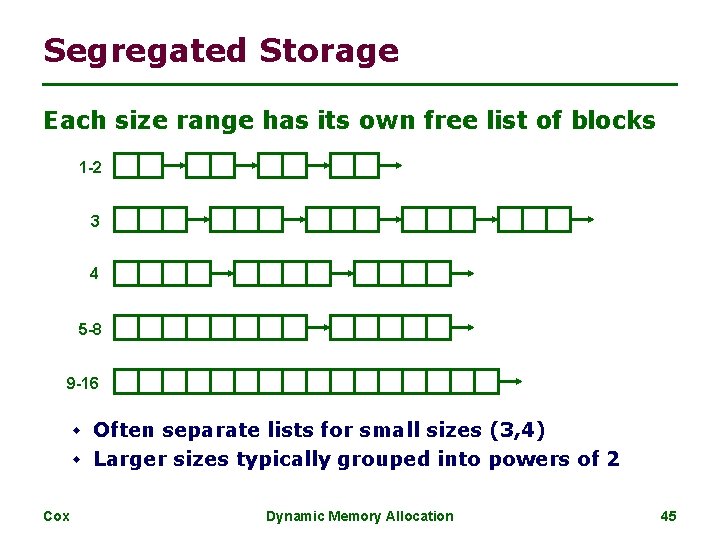
Segregated Storage Each size range has its own free list of blocks 1 -2 3 4 5 -8 9 -16 w Often separate lists for small sizes (3, 4) w Larger sizes typically grouped into powers of 2 Cox Dynamic Memory Allocation 45
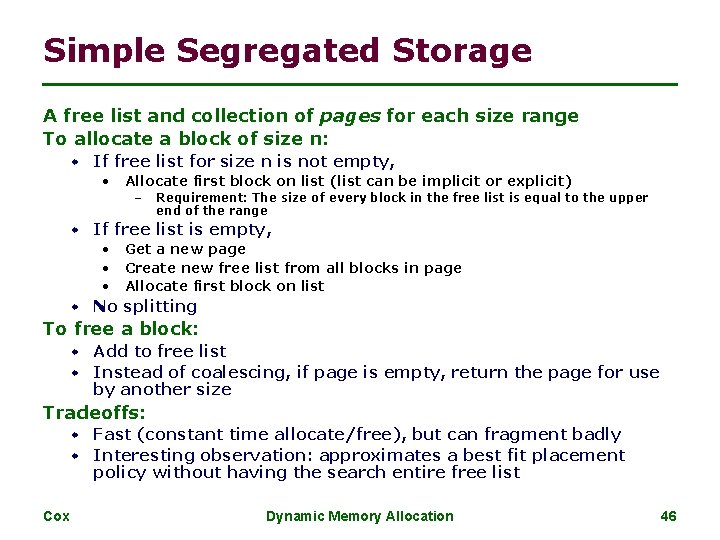
Simple Segregated Storage A free list and collection of pages for each size range To allocate a block of size n: w If free list for size n is not empty, • Allocate first block on list (list can be implicit or explicit) – Requirement: The size of every block in the free list is equal to the upper end of the range w If free list is empty, • Get a new page • Create new free list from all blocks in page • Allocate first block on list w No splitting To free a block: w Add to free list w Instead of coalescing, if page is empty, return the page for use by another size Tradeoffs: w Fast (constant time allocate/free), but can fragment badly w Interesting observation: approximates a best fit placement policy without having the search entire free list Cox Dynamic Memory Allocation 46
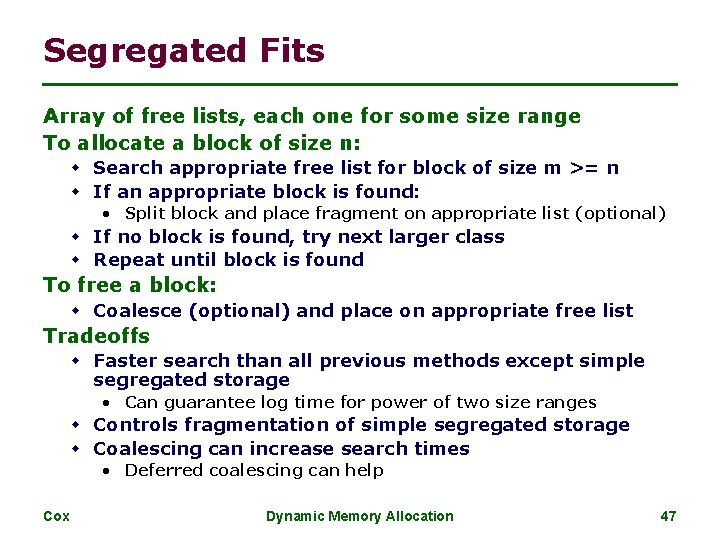
Segregated Fits Array of free lists, each one for some size range To allocate a block of size n: w Search appropriate free list for block of size m >= n w If an appropriate block is found: • Split block and place fragment on appropriate list (optional) w If no block is found, try next larger class w Repeat until block is found To free a block: w Coalesce (optional) and place on appropriate free list Tradeoffs w Faster search than all previous methods except simple segregated storage • Can guarantee log time for power of two size ranges w Controls fragmentation of simple segregated storage w Coalescing can increase search times • Deferred coalescing can help Cox Dynamic Memory Allocation 47
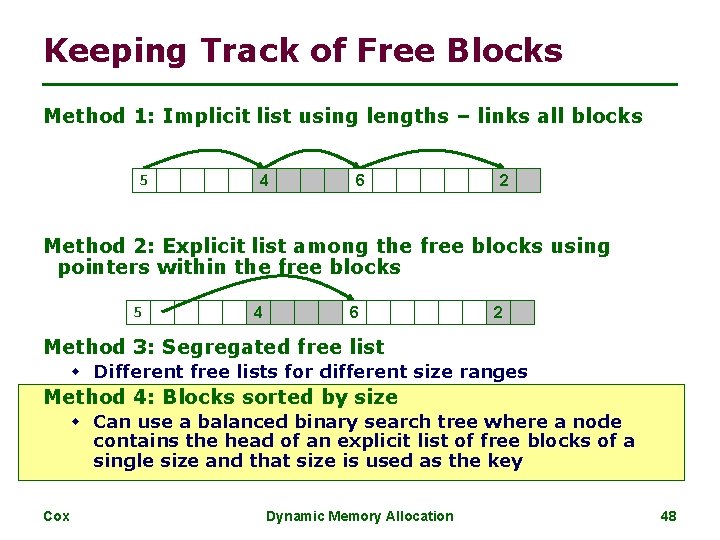
Keeping Track of Free Blocks Method 1: Implicit list using lengths – links all blocks 5 4 6 2 Method 2: Explicit list among the free blocks using pointers within the free blocks 5 4 6 2 Method 3: Segregated free list w Different free lists for different size ranges Method 4: Blocks sorted by size w Can use a balanced binary search tree where a node contains the head of an explicit list of free blocks of a single size and that size is used as the key Cox Dynamic Memory Allocation 48
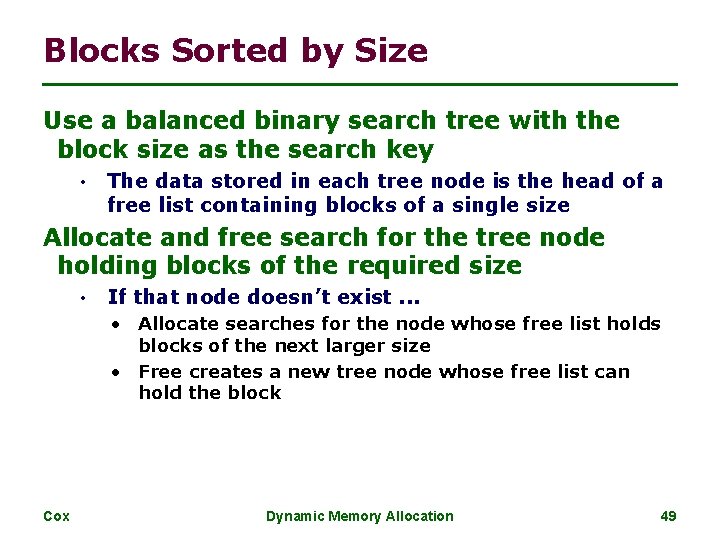
Blocks Sorted by Size Use a balanced binary search tree with the block size as the search key • The data stored in each tree node is the head of a free list containing blocks of a single size Allocate and free search for the tree node holding blocks of the required size • If that node doesn’t exist. . . • Allocate searches for the node whose free list holds blocks of the next larger size • Free creates a new tree node whose free list can hold the block Cox Dynamic Memory Allocation 49
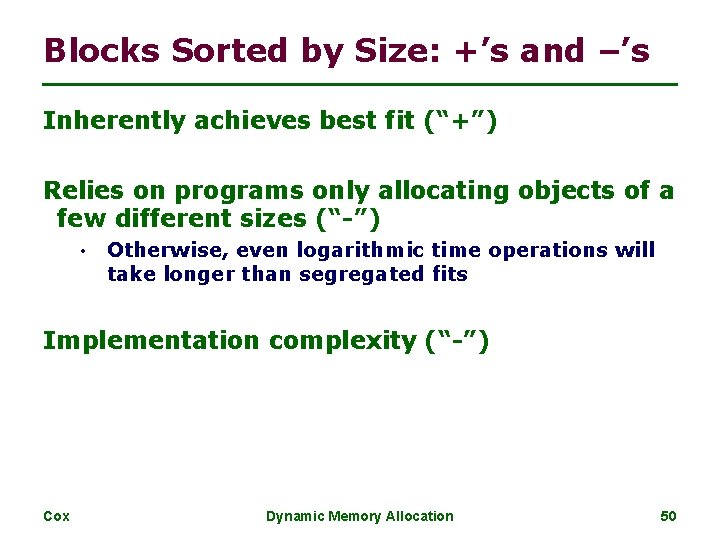
Blocks Sorted by Size: +’s and –’s Inherently achieves best fit (“+”) Relies on programs only allocating objects of a few different sizes (“-”) • Otherwise, even logarithmic time operations will take longer than segregated fits Implementation complexity (“-”) Cox Dynamic Memory Allocation 50
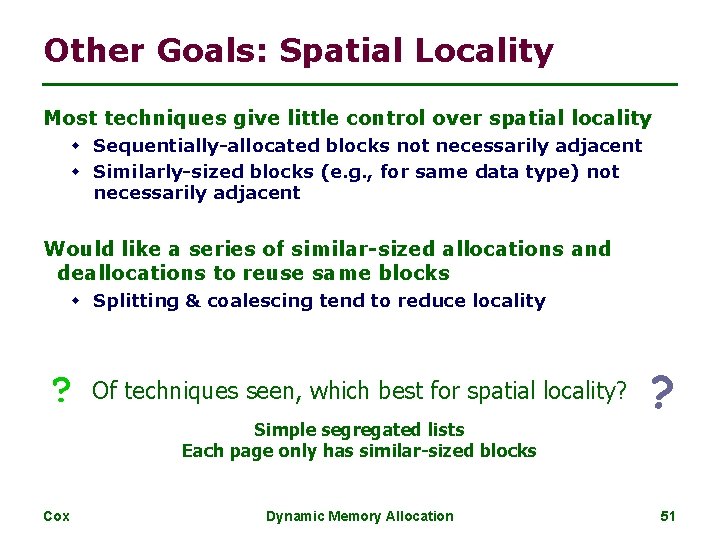
Other Goals: Spatial Locality Most techniques give little control over spatial locality w Sequentially-allocated blocks not necessarily adjacent w Similarly-sized blocks (e. g. , for same data type) not necessarily adjacent Would like a series of similar-sized allocations and deallocations to reuse same blocks w Splitting & coalescing tend to reduce locality ? Of techniques seen, which best for spatial locality? Simple segregated lists Each page only has similar-sized blocks Cox Dynamic Memory Allocation ? 51
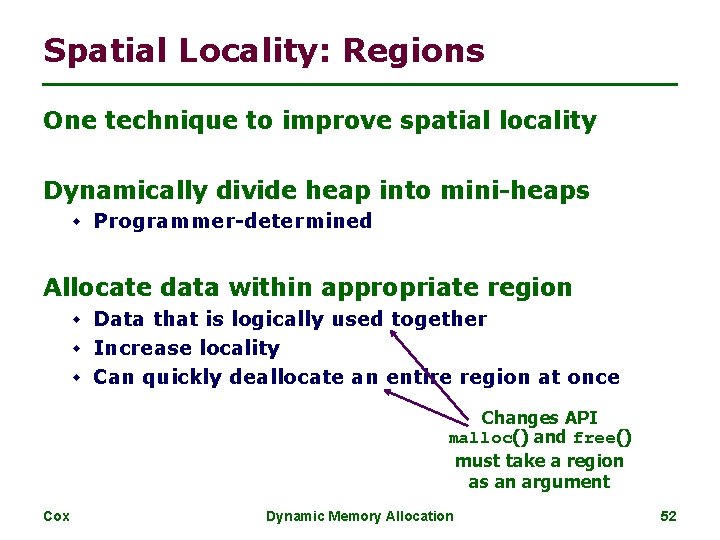
Spatial Locality: Regions One technique to improve spatial locality Dynamically divide heap into mini-heaps w Programmer-determined Allocate data within appropriate region w Data that is logically used together w Increase locality w Can quickly deallocate an entire region at once Changes API malloc() and free() must take a region as an argument Cox Dynamic Memory Allocation 52
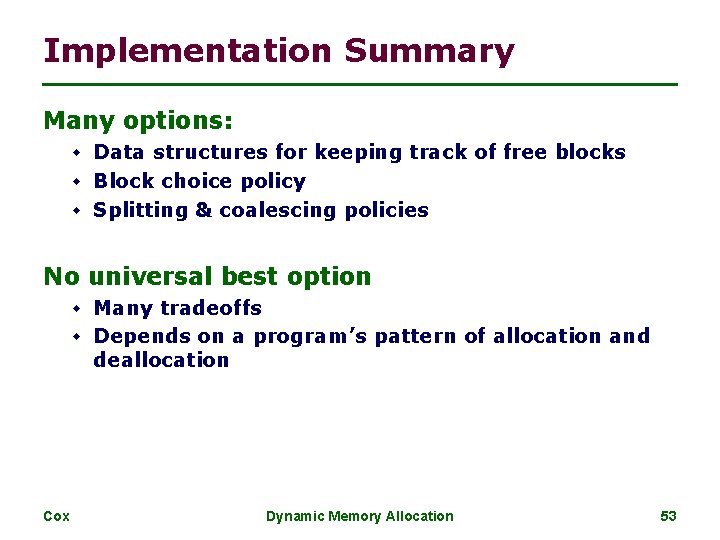
Implementation Summary Many options: w Data structures for keeping track of free blocks w Block choice policy w Splitting & coalescing policies No universal best option w Many tradeoffs w Depends on a program’s pattern of allocation and deallocation Cox Dynamic Memory Allocation 53
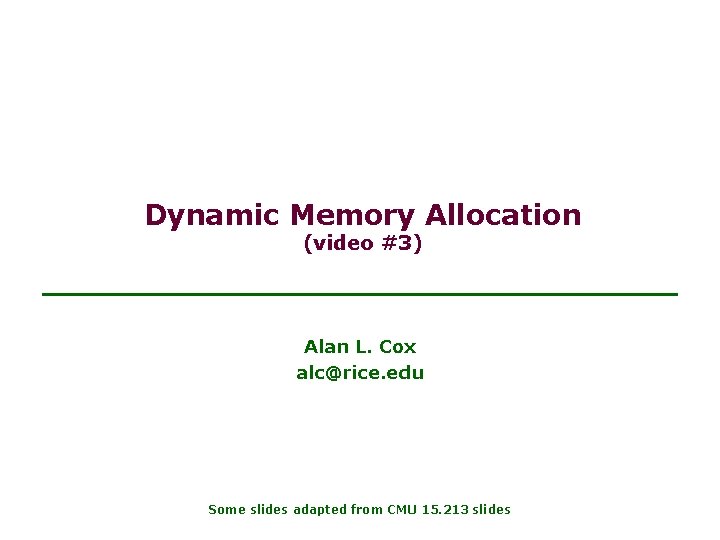
Dynamic Memory Allocation (video #3) Alan L. Cox alc@rice. edu Some slides adapted from CMU 15. 213 slides
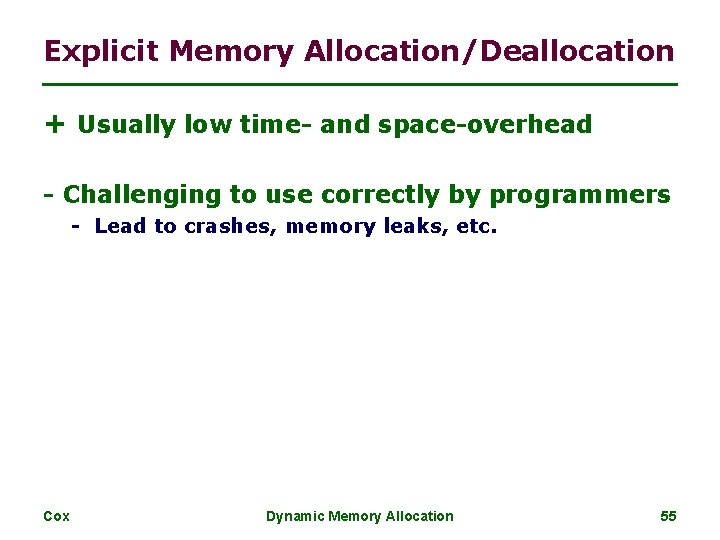
Explicit Memory Allocation/Deallocation + Usually low time- and space-overhead - Challenging to use correctly by programmers - Lead to crashes, memory leaks, etc. Cox Dynamic Memory Allocation 55
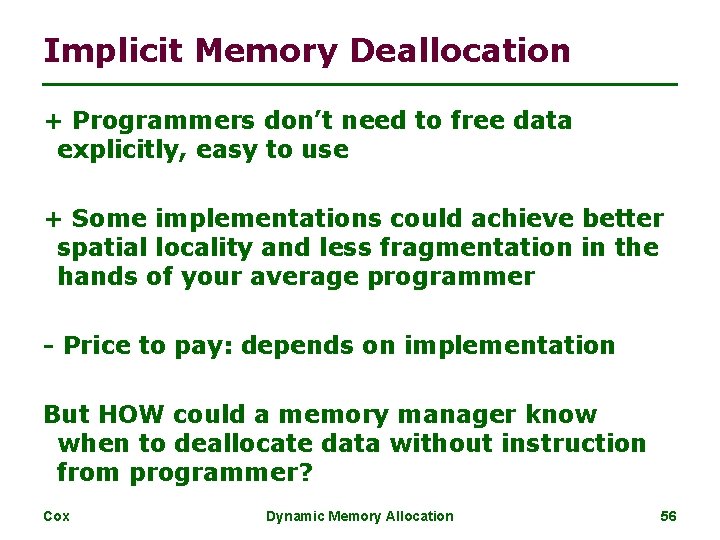
Implicit Memory Deallocation + Programmers don’t need to free data explicitly, easy to use + Some implementations could achieve better spatial locality and less fragmentation in the hands of your average programmer - Price to pay: depends on implementation But HOW could a memory manager know when to deallocate data without instruction from programmer? Cox Dynamic Memory Allocation 56
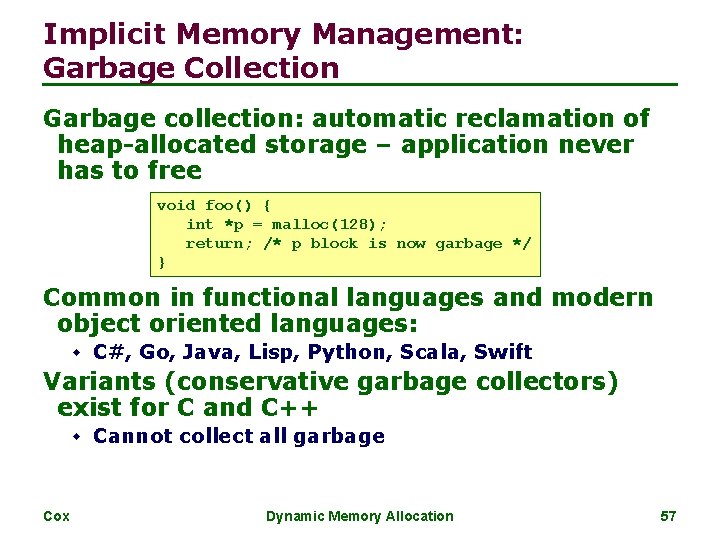
Implicit Memory Management: Garbage Collection Garbage collection: automatic reclamation of heap-allocated storage – application never has to free void foo() { int *p = malloc(128); return; /* p block is now garbage */ } Common in functional languages and modern object oriented languages: w C#, Go, Java, Lisp, Python, Scala, Swift Variants (conservative garbage collectors) exist for C and C++ w Cannot collect all garbage Cox Dynamic Memory Allocation 57
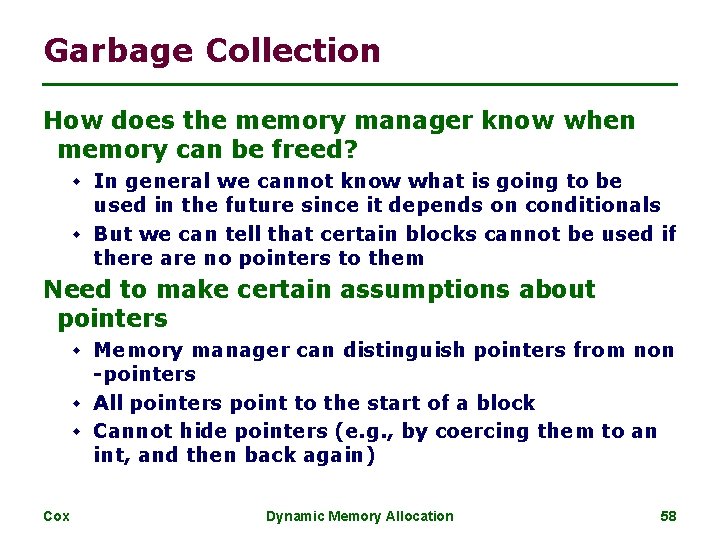
Garbage Collection How does the memory manager know when memory can be freed? w In general we cannot know what is going to be used in the future since it depends on conditionals w But we can tell that certain blocks cannot be used if there are no pointers to them Need to make certain assumptions about pointers w Memory manager can distinguish pointers from non -pointers w All pointers point to the start of a block w Cannot hide pointers (e. g. , by coercing them to an int, and then back again) Cox Dynamic Memory Allocation 58
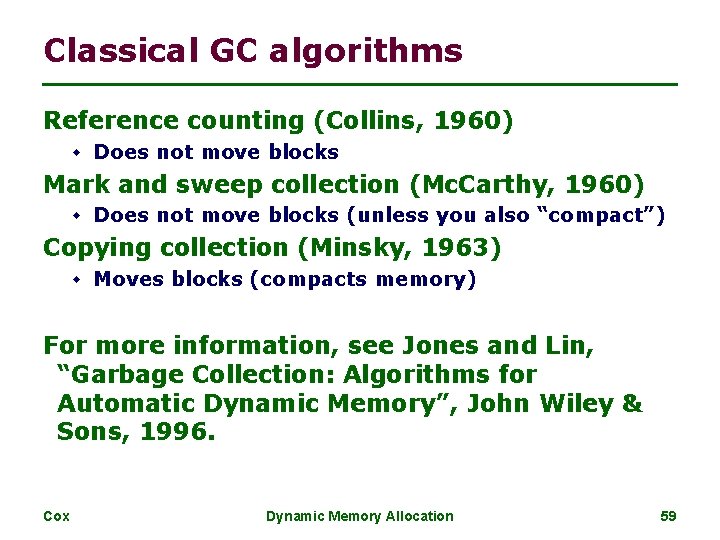
Classical GC algorithms Reference counting (Collins, 1960) w Does not move blocks Mark and sweep collection (Mc. Carthy, 1960) w Does not move blocks (unless you also “compact”) Copying collection (Minsky, 1963) w Moves blocks (compacts memory) For more information, see Jones and Lin, “Garbage Collection: Algorithms for Automatic Dynamic Memory”, John Wiley & Sons, 1996. Cox Dynamic Memory Allocation 59
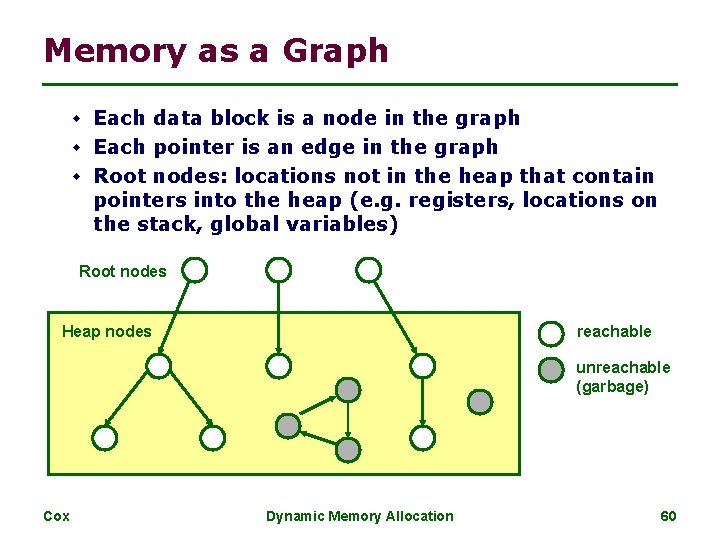
Memory as a Graph w Each data block is a node in the graph w Each pointer is an edge in the graph w Root nodes: locations not in the heap that contain pointers into the heap (e. g. registers, locations on the stack, global variables) Root nodes Heap nodes reachable unreachable (garbage) Cox Dynamic Memory Allocation 60
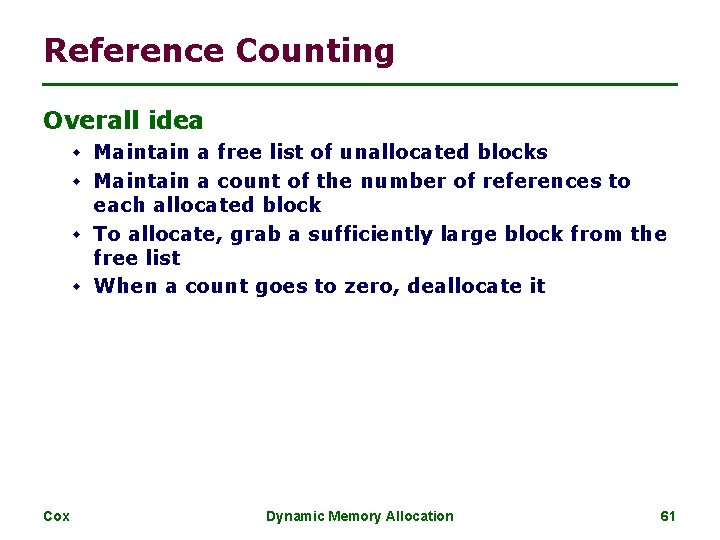
Reference Counting Overall idea w Maintain a free list of unallocated blocks w Maintain a count of the number of references to each allocated block w To allocate, grab a sufficiently large block from the free list w When a count goes to zero, deallocate it Cox Dynamic Memory Allocation 61
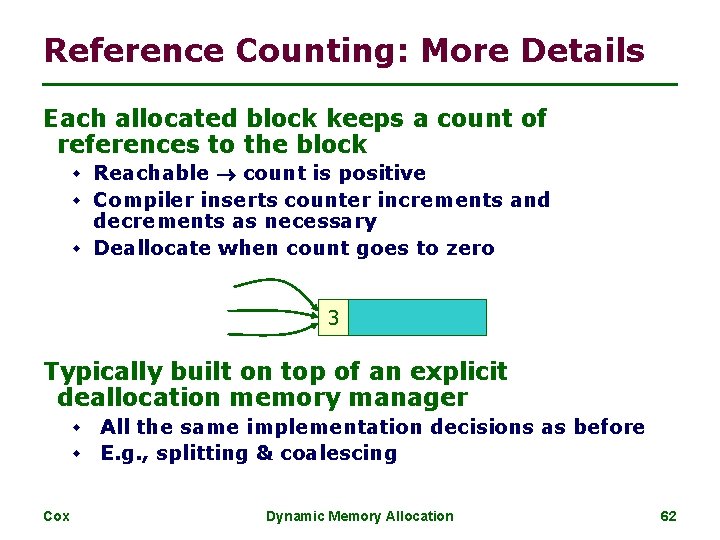
Reference Counting: More Details Each allocated block keeps a count of references to the block w Reachable count is positive w Compiler inserts counter increments and decrements as necessary w Deallocate when count goes to zero 3 Typically built on top of an explicit deallocation memory manager w w Cox All the same implementation decisions as before E. g. , splitting & coalescing Dynamic Memory Allocation 62
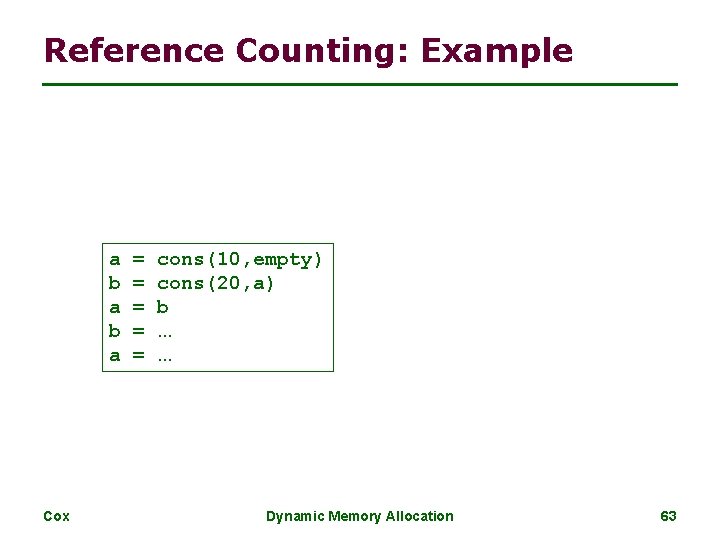
Reference Counting: Example a b a Cox = = = cons(10, empty) cons(20, a) b … … Dynamic Memory Allocation 63
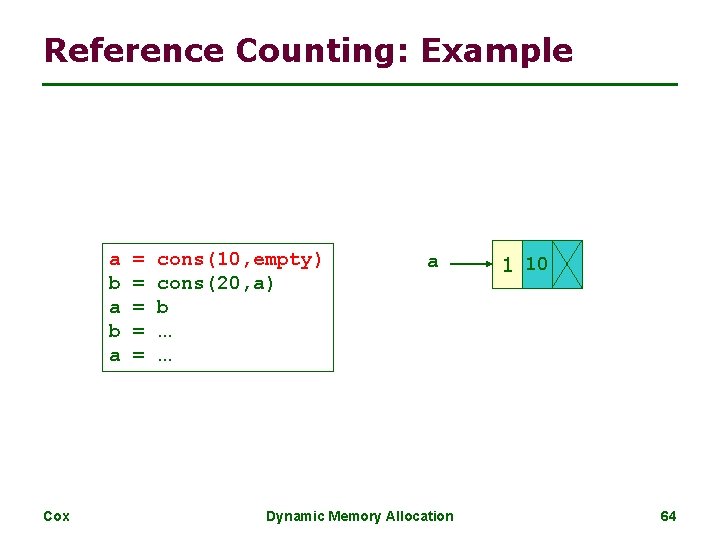
Reference Counting: Example a b a Cox = = = cons(10, empty) cons(20, a) b … … a Dynamic Memory Allocation 1 10 64
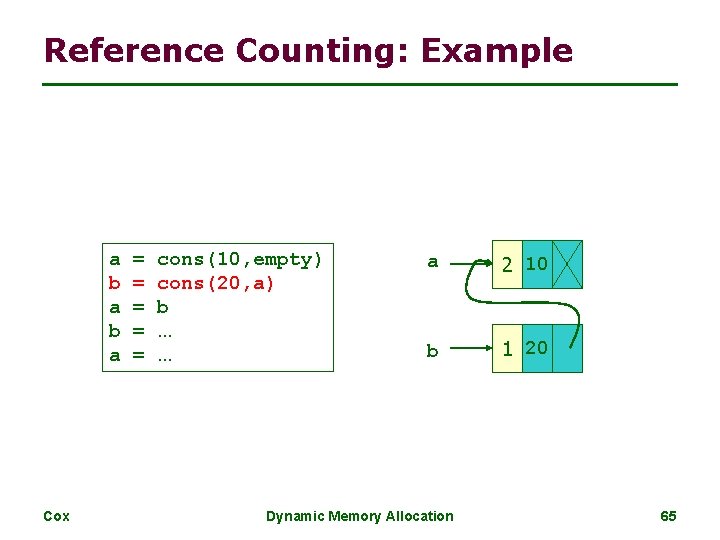
Reference Counting: Example a b a Cox = = = cons(10, empty) cons(20, a) b … … a 2 10 b 1 20 Dynamic Memory Allocation 65
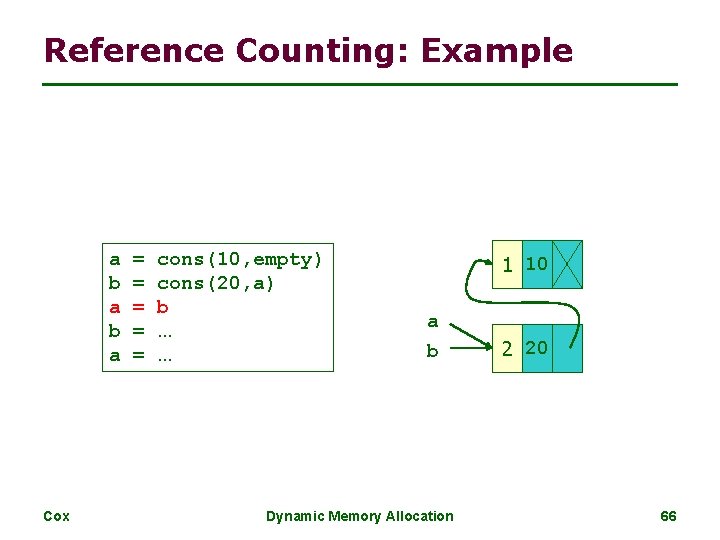
Reference Counting: Example a b a Cox = = = cons(10, empty) cons(20, a) b … … 1 10 a b Dynamic Memory Allocation 2 20 66
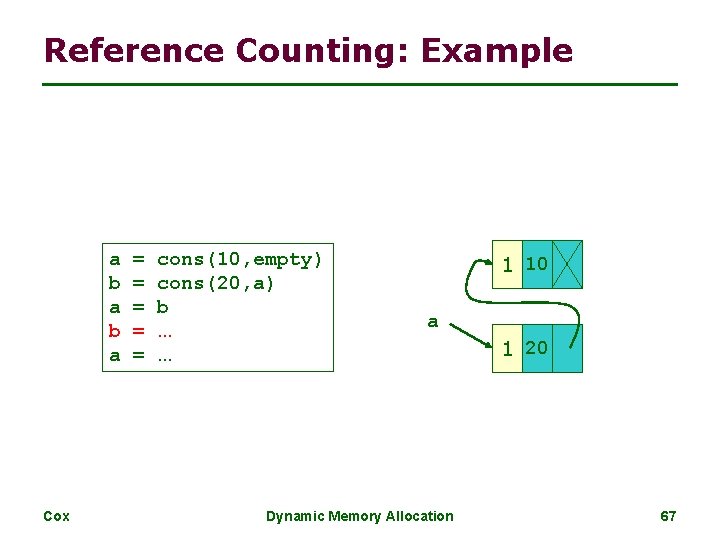
Reference Counting: Example a b a Cox = = = cons(10, empty) cons(20, a) b … … 1 10 a Dynamic Memory Allocation 1 20 67
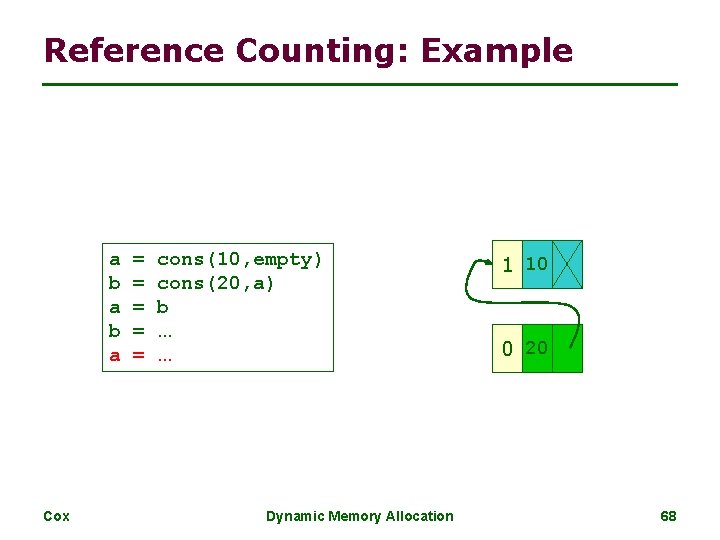
Reference Counting: Example a b a Cox = = = cons(10, empty) cons(20, a) b … … Dynamic Memory Allocation 1 10 0 20 68
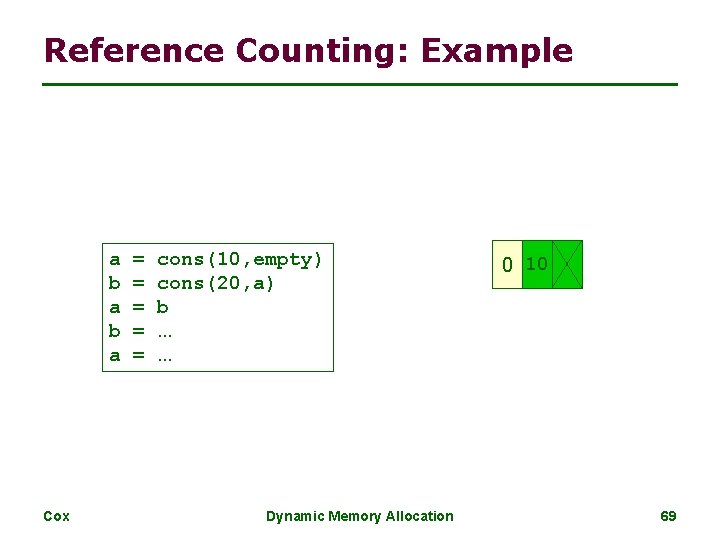
Reference Counting: Example a b a Cox = = = cons(10, empty) cons(20, a) b … … Dynamic Memory Allocation 0 10 69
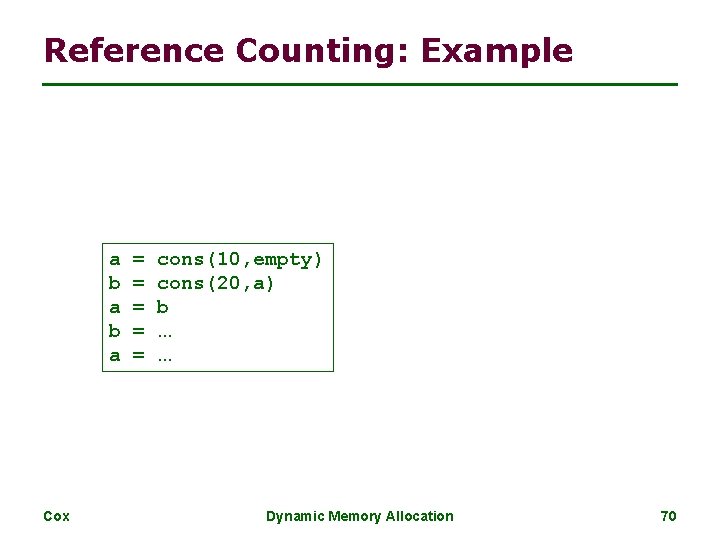
Reference Counting: Example a b a Cox = = = cons(10, empty) cons(20, a) b … … Dynamic Memory Allocation 70
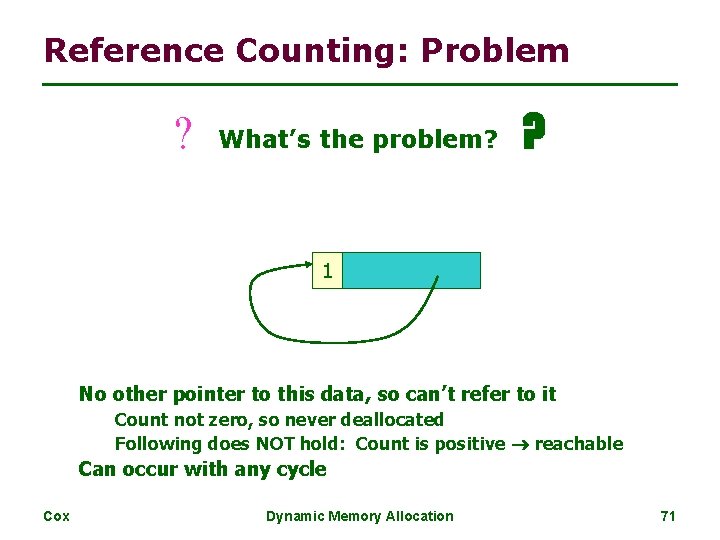
Reference Counting: Problem ? What’s the problem? ? 1 No other pointer to this data, so can’t refer to it Count not zero, so never deallocated Following does NOT hold: Count is positive reachable Can occur with any cycle Cox Dynamic Memory Allocation 71
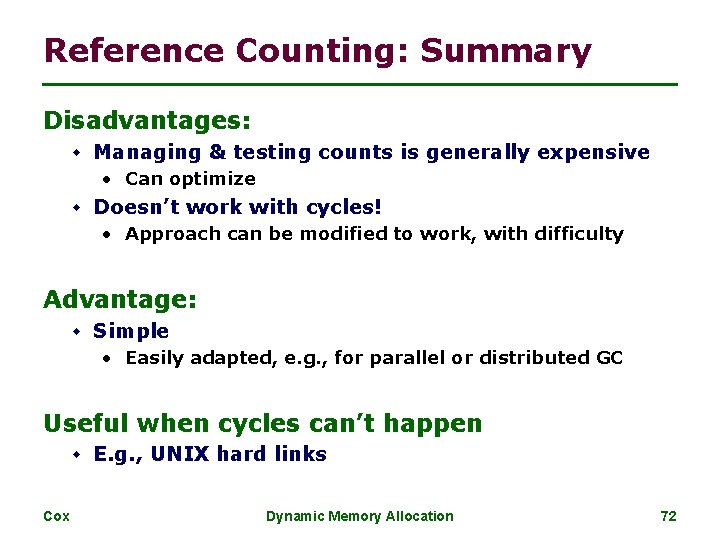
Reference Counting: Summary Disadvantages: w Managing & testing counts is generally expensive • Can optimize w Doesn’t work with cycles! • Approach can be modified to work, with difficulty Advantage: w Simple • Easily adapted, e. g. , for parallel or distributed GC Useful when cycles can’t happen w E. g. , UNIX hard links Cox Dynamic Memory Allocation 72
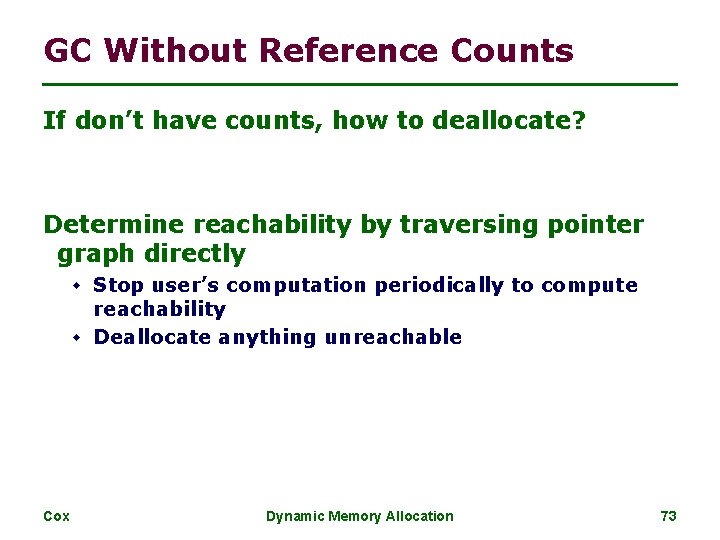
GC Without Reference Counts If don’t have counts, how to deallocate? Determine reachability by traversing pointer graph directly w Stop user’s computation periodically to compute reachability w Deallocate anything unreachable Cox Dynamic Memory Allocation 73
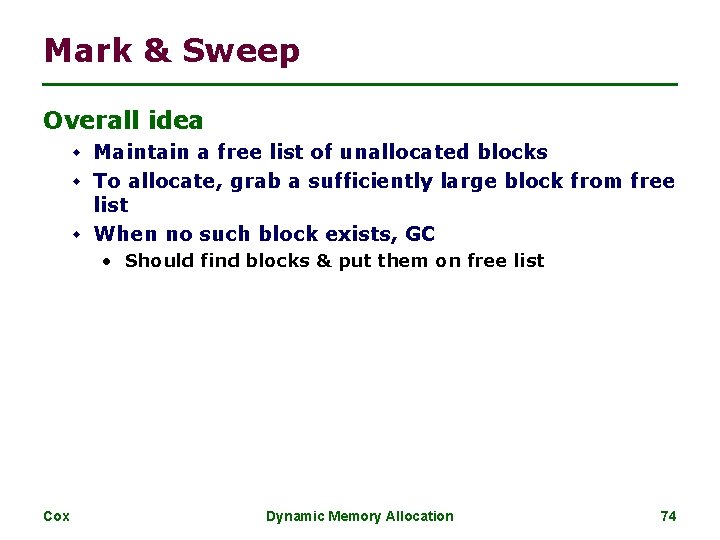
Mark & Sweep Overall idea w Maintain a free list of unallocated blocks w To allocate, grab a sufficiently large block from free list w When no such block exists, GC • Should find blocks & put them on free list Cox Dynamic Memory Allocation 74
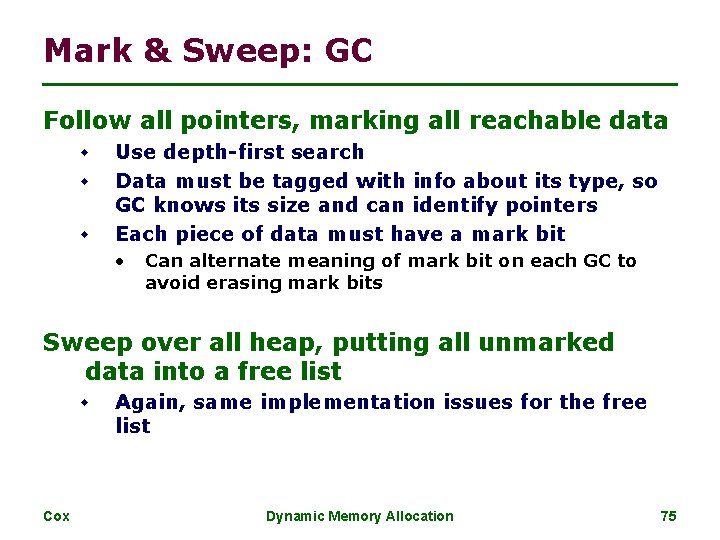
Mark & Sweep: GC Follow all pointers, marking all reachable data w w w Use depth-first search Data must be tagged with info about its type, so GC knows its size and can identify pointers Each piece of data must have a mark bit • Can alternate meaning of mark bit on each GC to avoid erasing mark bits Sweep over all heap, putting all unmarked data into a free list w Cox Again, same implementation issues for the free list Dynamic Memory Allocation 75
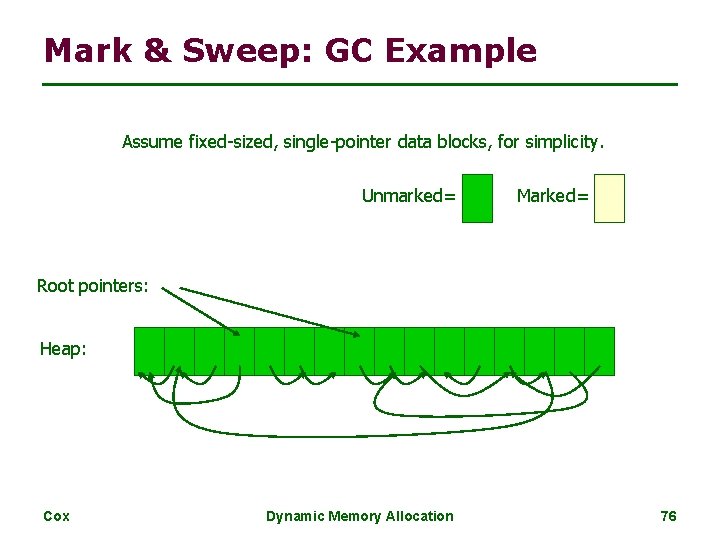
Mark & Sweep: GC Example Assume fixed-sized, single-pointer data blocks, for simplicity. Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 76
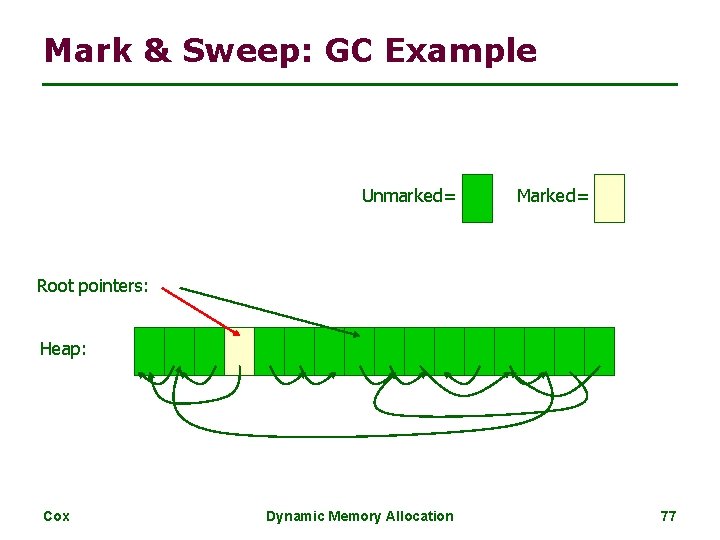
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 77
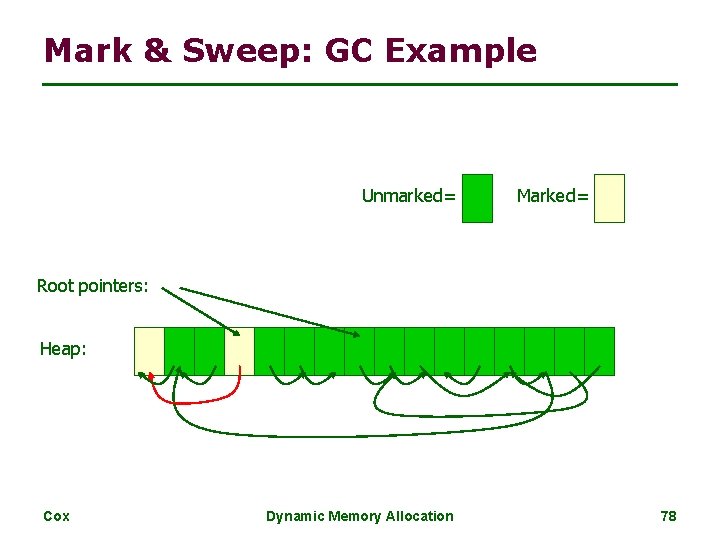
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 78
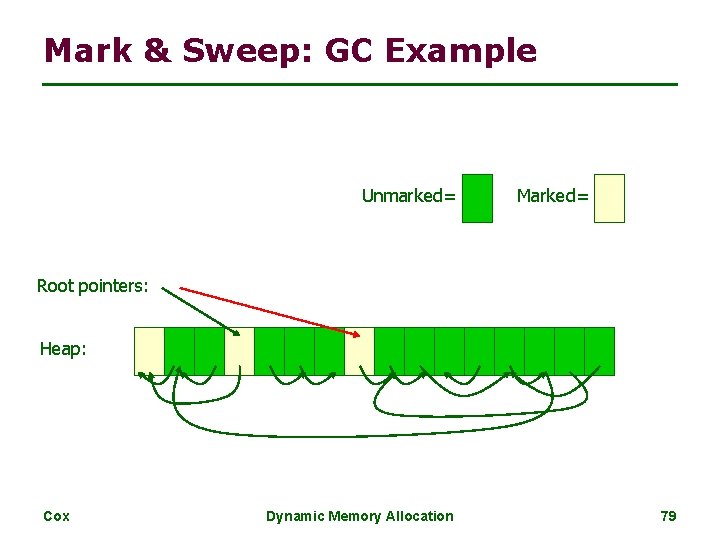
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 79
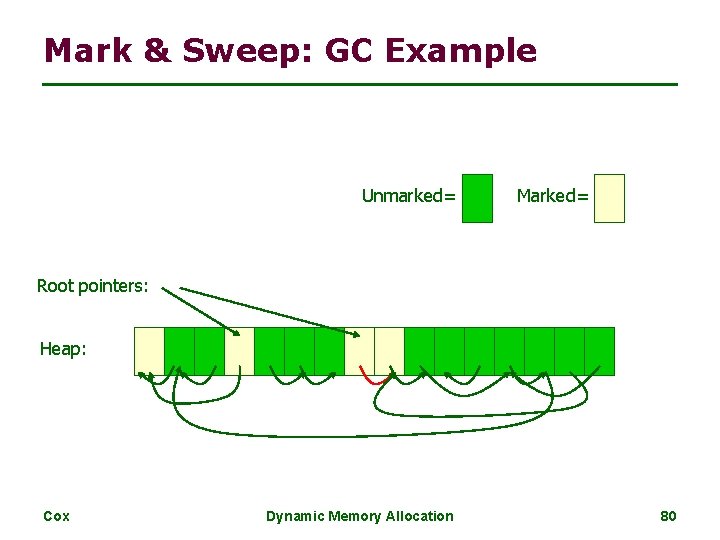
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 80
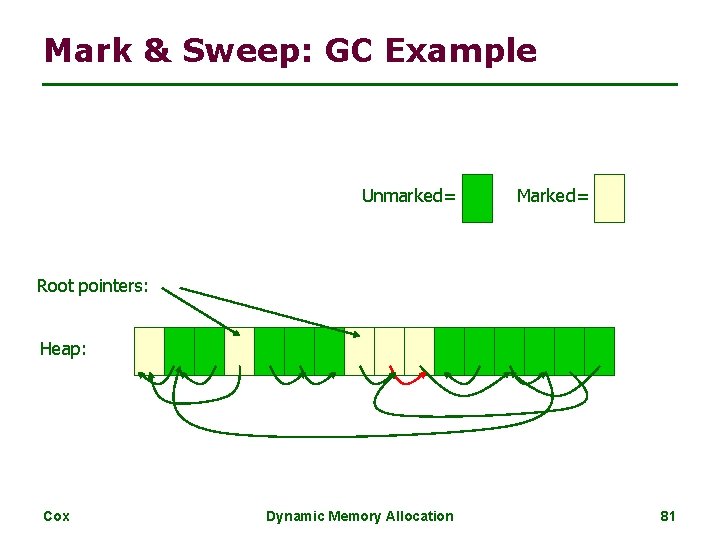
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 81
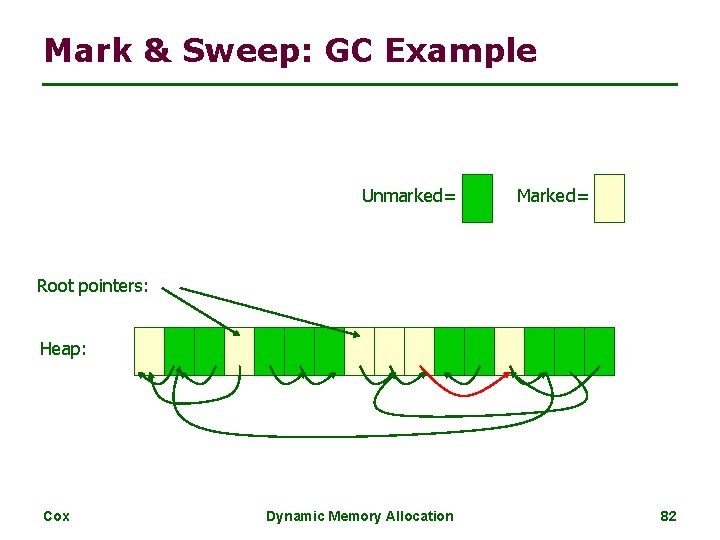
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 82
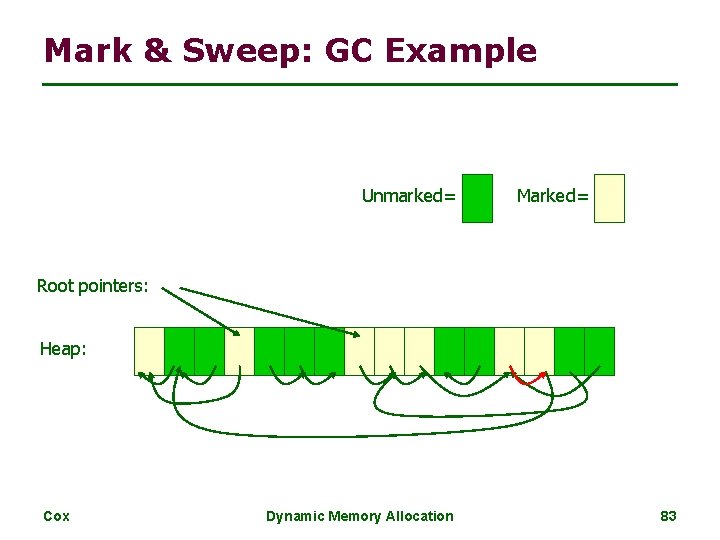
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 83
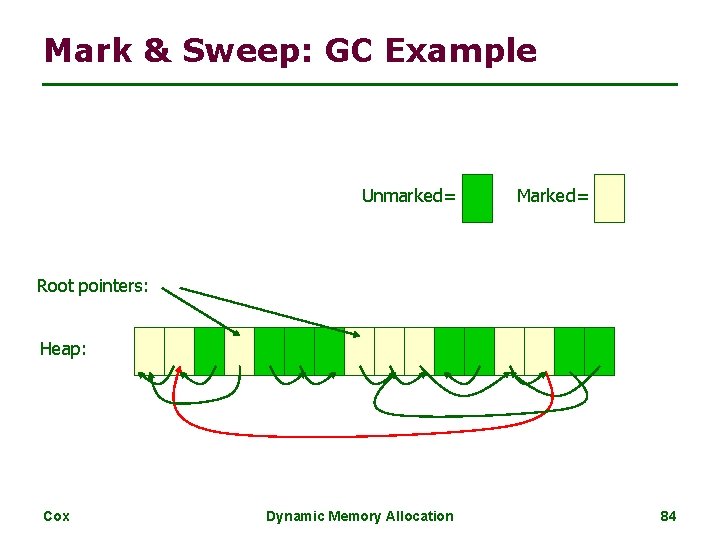
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Cox Dynamic Memory Allocation 84
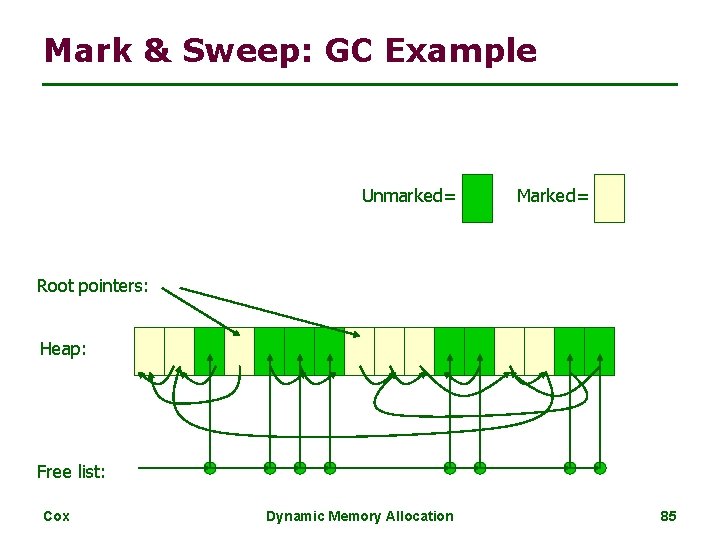
Mark & Sweep: GC Example Unmarked= Marked= Root pointers: Heap: Free list: Cox Dynamic Memory Allocation 85
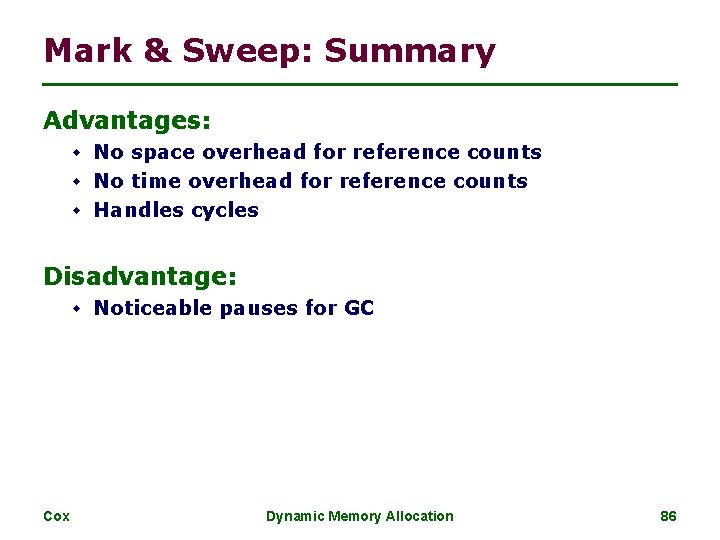
Mark & Sweep: Summary Advantages: w No space overhead for reference counts w No time overhead for reference counts w Handles cycles Disadvantage: w Noticeable pauses for GC Cox Dynamic Memory Allocation 86
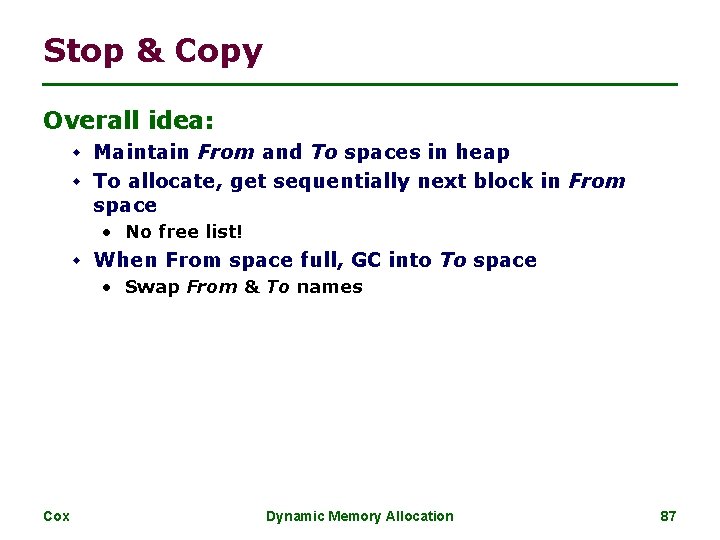
Stop & Copy Overall idea: w Maintain From and To spaces in heap w To allocate, get sequentially next block in From space • No free list! w When From space full, GC into To space • Swap From & To names Cox Dynamic Memory Allocation 87
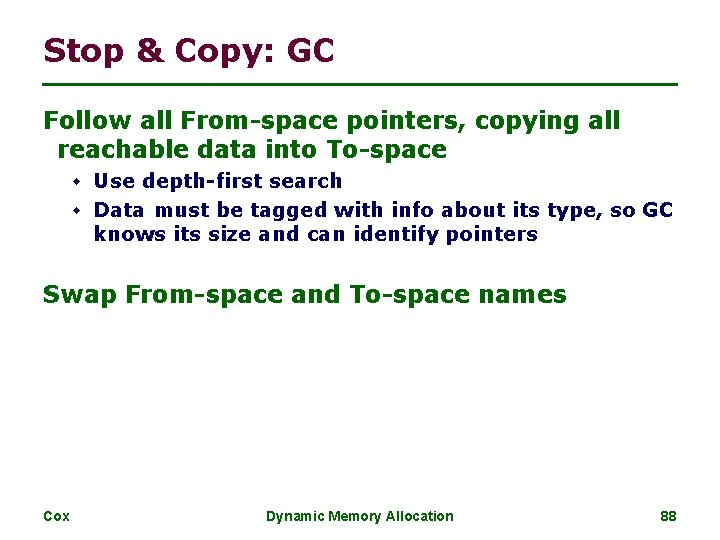
Stop & Copy: GC Follow all From-space pointers, copying all reachable data into To-space w Use depth-first search w Data must be tagged with info about its type, so GC knows its size and can identify pointers Swap From-space and To-space names Cox Dynamic Memory Allocation 88
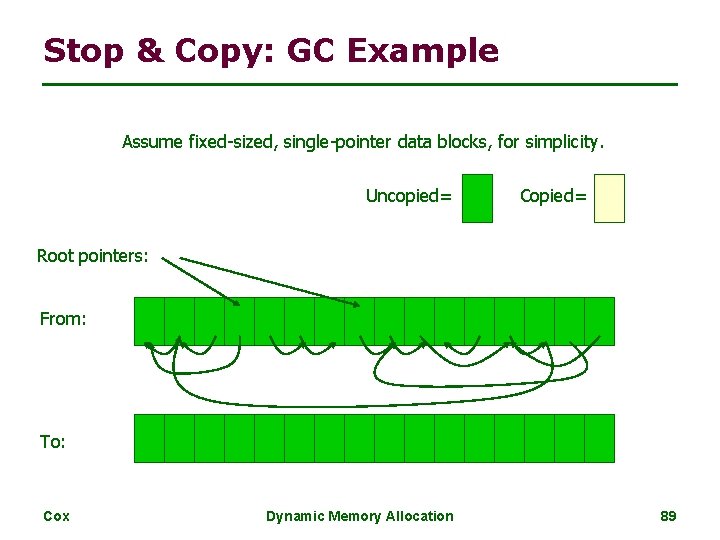
Stop & Copy: GC Example Assume fixed-sized, single-pointer data blocks, for simplicity. Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 89
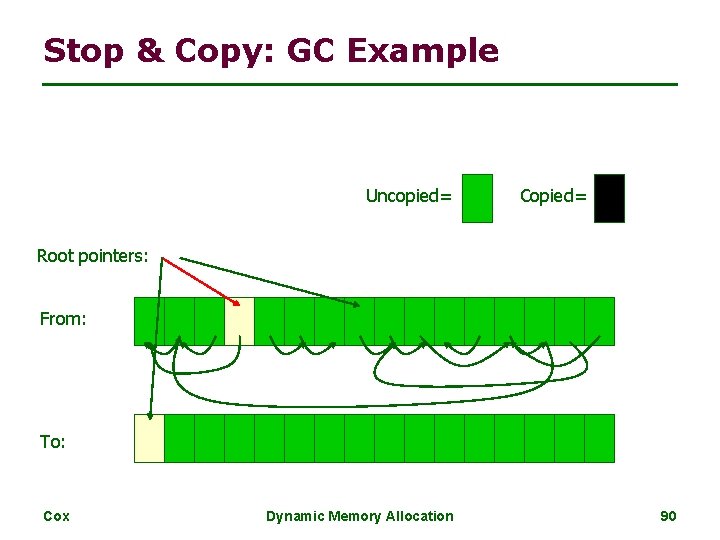
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 90
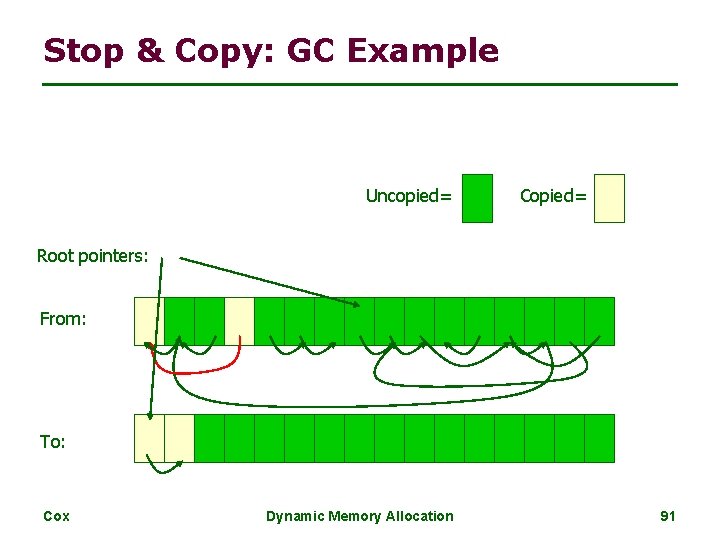
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 91
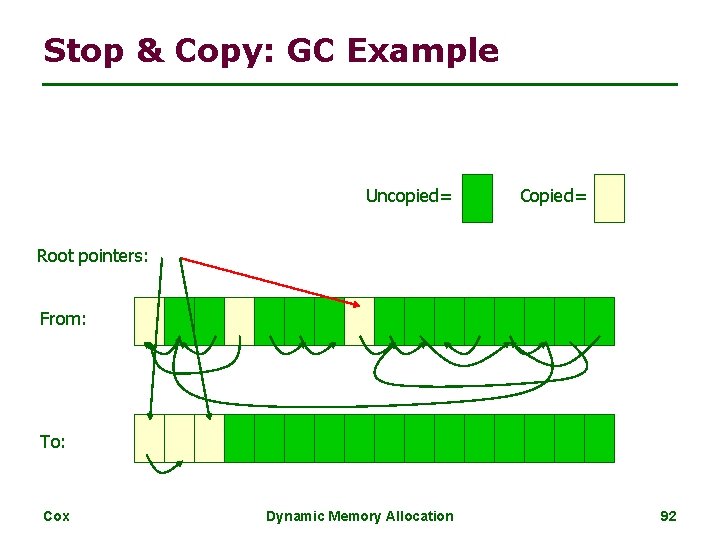
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 92
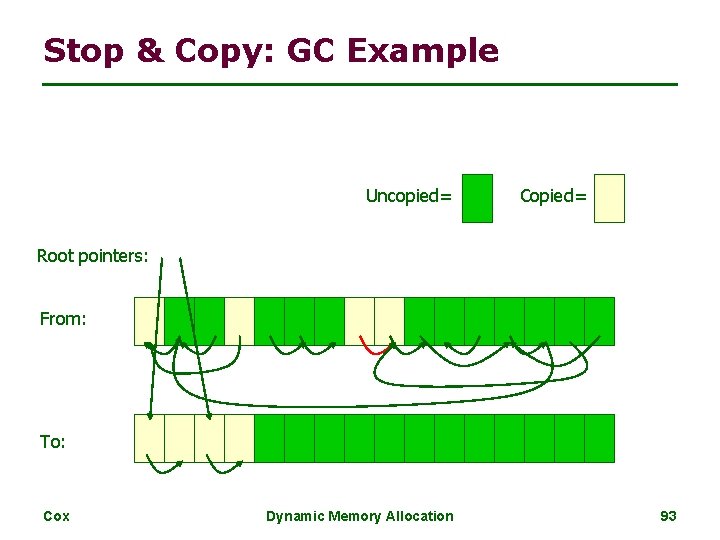
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 93
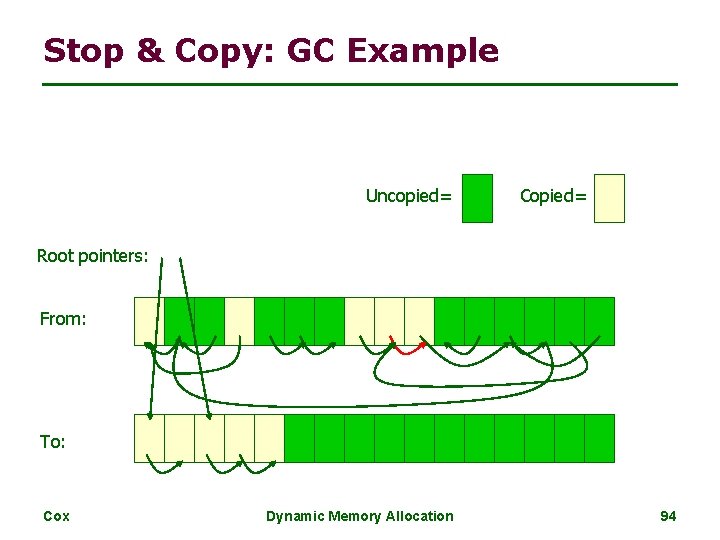
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 94
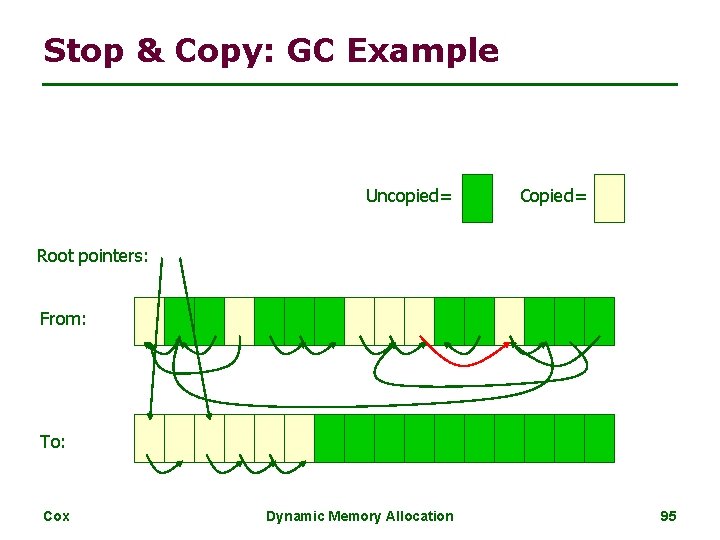
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 95
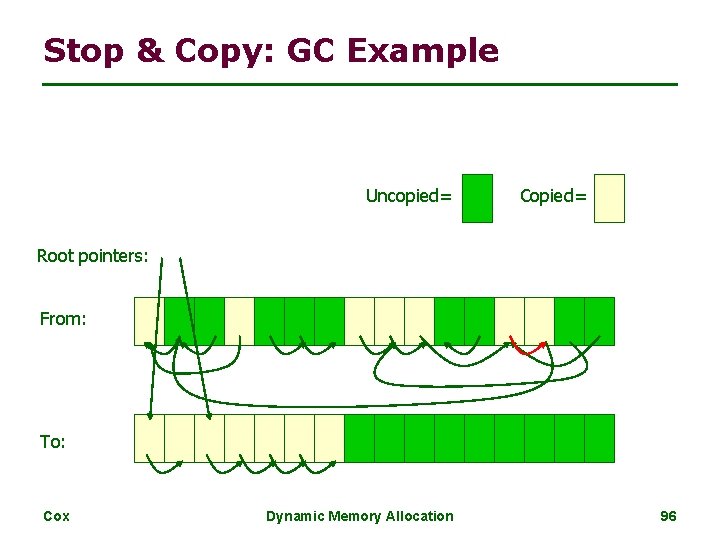
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 96
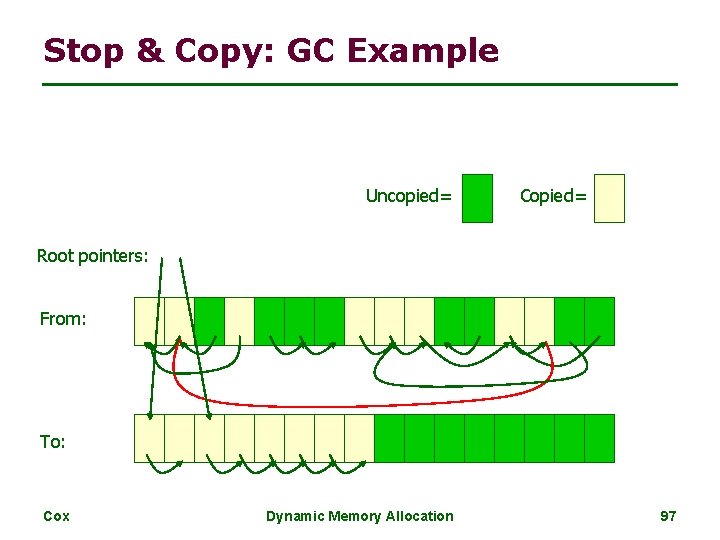
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 97
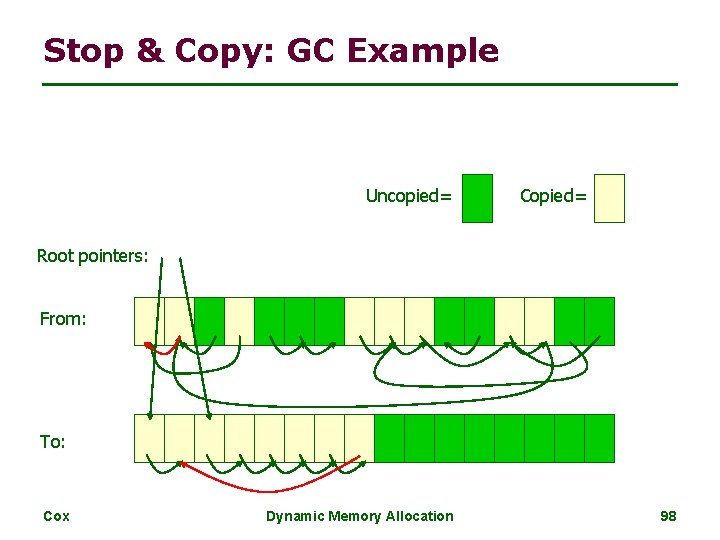
Stop & Copy: GC Example Uncopied= Copied= Root pointers: From: To: Cox Dynamic Memory Allocation 98
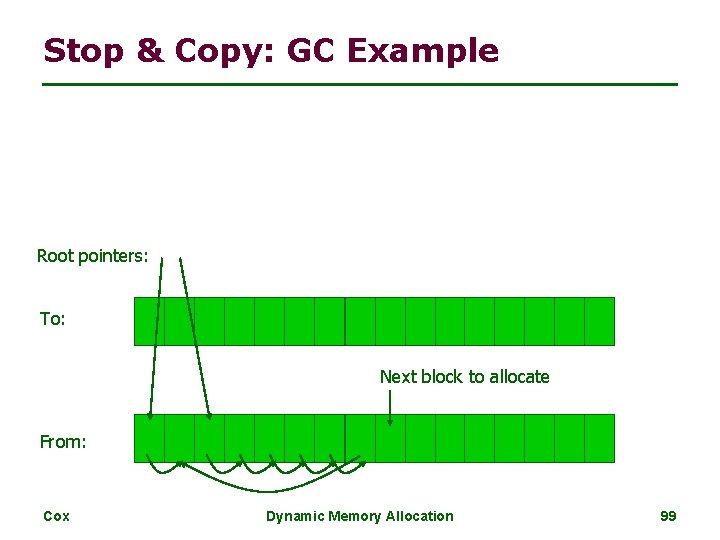
Stop & Copy: GC Example Root pointers: To: Next block to allocate From: Cox Dynamic Memory Allocation 99
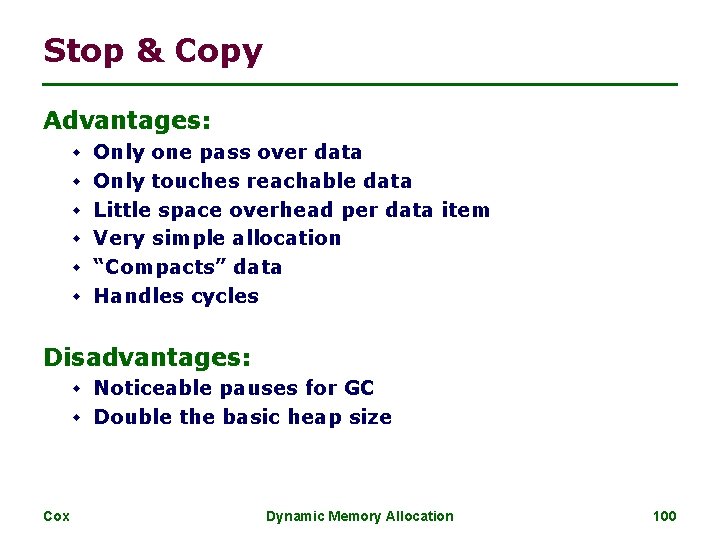
Stop & Copy Advantages: w Only one pass over data w Only touches reachable data w Little space overhead per data item w Very simple allocation w “Compacts” data w Handles cycles Disadvantages: w Noticeable pauses for GC w Double the basic heap size Cox Dynamic Memory Allocation 100
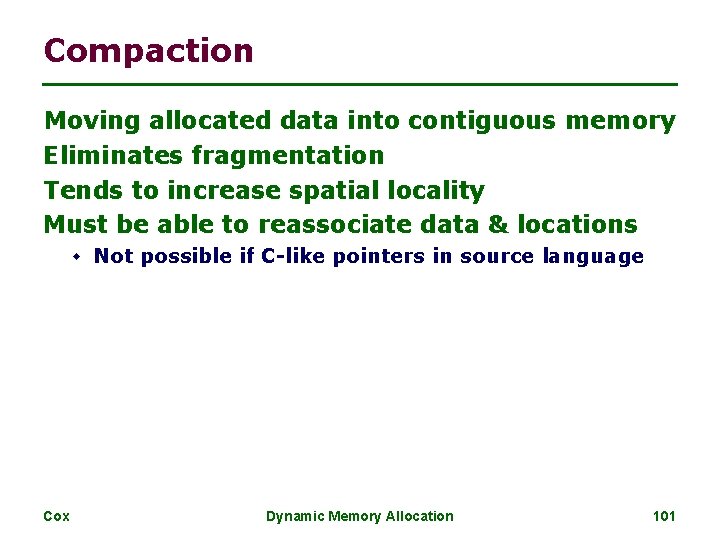
Compaction Moving allocated data into contiguous memory Eliminates fragmentation Tends to increase spatial locality Must be able to reassociate data & locations w Not possible if C-like pointers in source language Cox Dynamic Memory Allocation 101
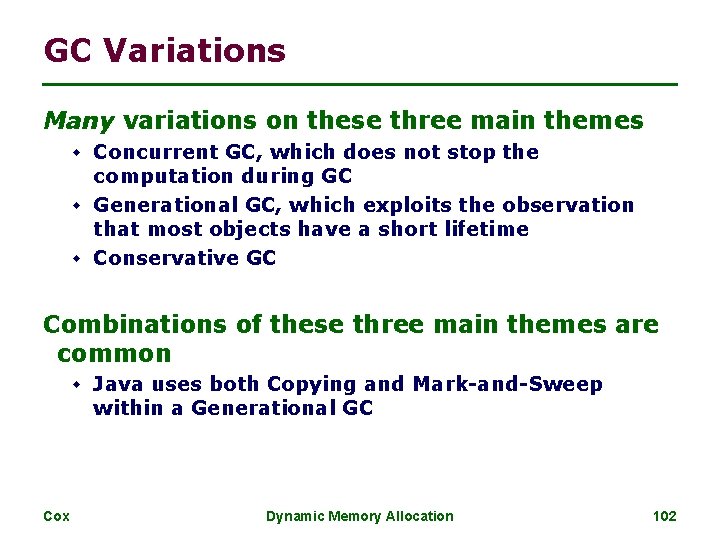
GC Variations Many variations on these three main themes w Concurrent GC, which does not stop the computation during GC w Generational GC, which exploits the observation that most objects have a short lifetime w Conservative GC Combinations of these three main themes are common w Java uses both Copying and Mark-and-Sweep within a Generational GC Cox Dynamic Memory Allocation 102
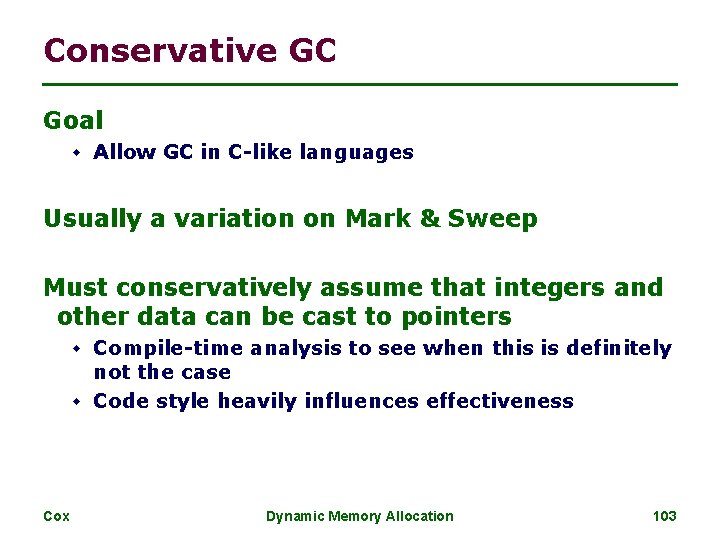
Conservative GC Goal w Allow GC in C-like languages Usually a variation on Mark & Sweep Must conservatively assume that integers and other data can be cast to pointers w Compile-time analysis to see when this is definitely not the case w Code style heavily influences effectiveness Cox Dynamic Memory Allocation 103
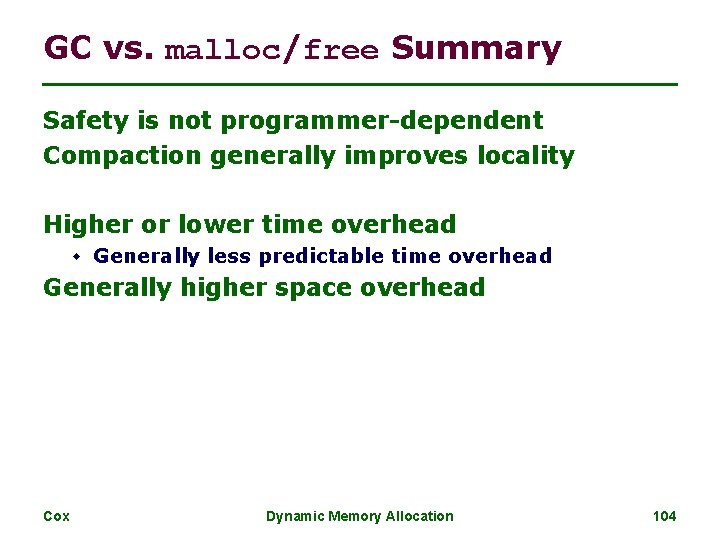
GC vs. malloc/free Summary Safety is not programmer-dependent Compaction generally improves locality Higher or lower time overhead w Generally less predictable time overhead Generally higher space overhead Cox Dynamic Memory Allocation 104
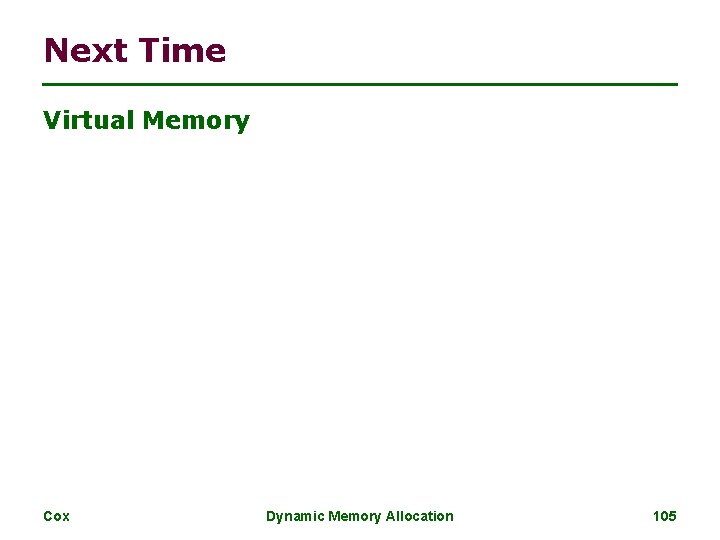
Next Time Virtual Memory Cox Dynamic Memory Allocation 105
Example of dynamic memory allocation
Knuth’s boundary tags
Disadvantages of dynamic programming
Tcmalloc
Example of dynamic memory allocation
Dynamic memory allocation in data structure
Dynamic memory allocation in data structure
Dma dynamic memory allocation
Cara kerja memori dinamis c++
Dynamic memory allocation in java
Dynamic memory allocation
Alan cox rice
Alan cox rice
Contiguous allocation vs linked allocation
Dynamic strategies for asset allocation
Dynamic storage allocation problem
Polymorphism dynamic allocation
Assumptions for dynamic channel allocation
Dynamic storage allocation
Media access control
Buddy memory allocation implementation
Single user contiguous scheme
Paged segmentation
First fit allocation
Reduce external fragmentation
Paged memory management
What are two goals of multitasking memory allocation
Contiguous memory
Non contiguous memory allocation
Jihad
Non contiguous memory allocation
Bitmap and linked list in os
Memory allocation
Zig memory allocation
Next fit memory allocation
Buddy system memory allocation
Edu.sharif.edu
Transferered
Faculty washington edu chudler
Rdram vs sdram
The allocation map
Dynamic memory management
Dynamic memory management
Semantic features definition
Explicit and implicit memory
Long term memory vs short term memory
Internal memory and external memory
Primary memory and secondary memory
Logical address
Which memory is the actual working memory?
Page fault
Virtual memory in memory hierarchy consists of
Eidetic memory vs iconic memory
Symmetric shared memory architecture
Inhibidores selectivos de la cox-2
Mpeg to jpeg
Cox regression
Cox 2 inibitori
Cox hosting
Black and cox model
Alicia cox ph
Raymond cox qc
Jessica cox biografia
Wfo cox
Minitab box cox
Biography on kobe bryant
Metoda coxa
Efpractice
Cox.net
Cox 2 inhibitors
Penny cox uf
Cox=eox/tox
Cox
Brian cox dunedin
An introduction to rheology
Cox emil
Cox mill elementary school
Tom cox intermediate
Cox urban furniture
Nelson and cox
Satistics
Nelson and cox
Nele cox
Nelson and cox
Box-cox dönüşümü
Wash sector cox's bazar
Logistinen regressioanalyysi
Ponto isoelétrico
Cox spam blocker
Cox investor relations
Paul howat
Carrie cox
Klovenmodel
Purchase price allocation pwc
Overhead allocation
Turn construction
Cloud cost allocation
Capital allocation line
What is proportional allocation
Spectrum allocation
Resource allocation in software project management
Capital market line
Resources allocation and mobilization plan (ramp)
Allocation concealment
Allocation wells
Single partition allocation