Dynamic Memory Allocation Application Dynamic Memory Allocator Heap
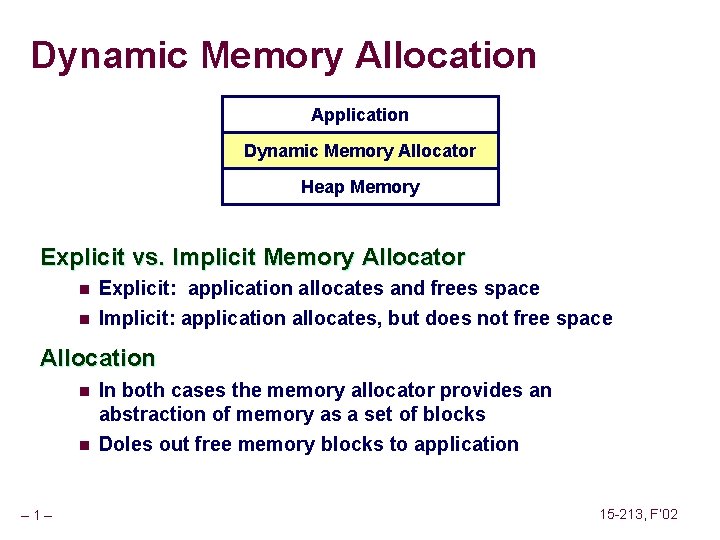
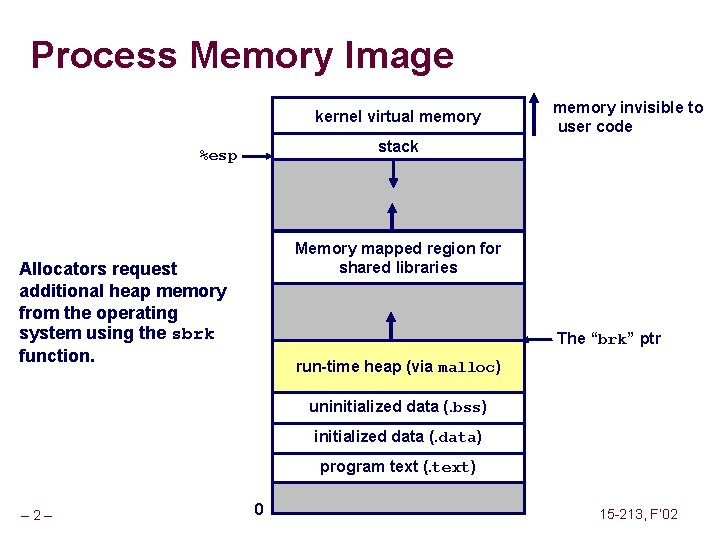
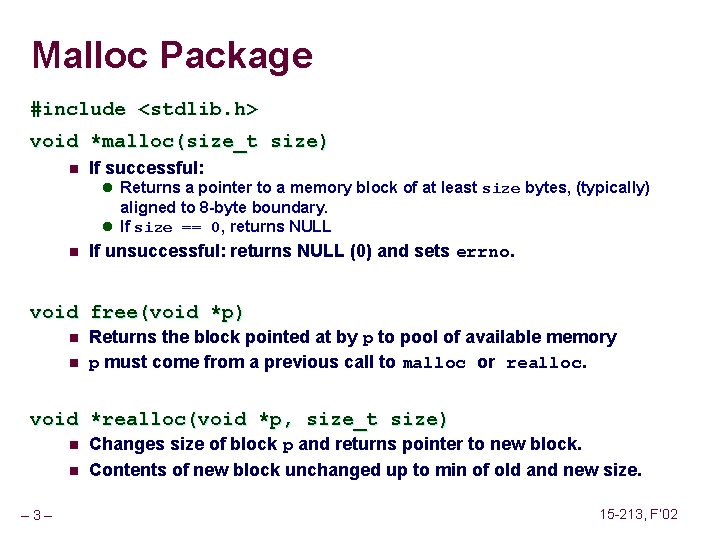
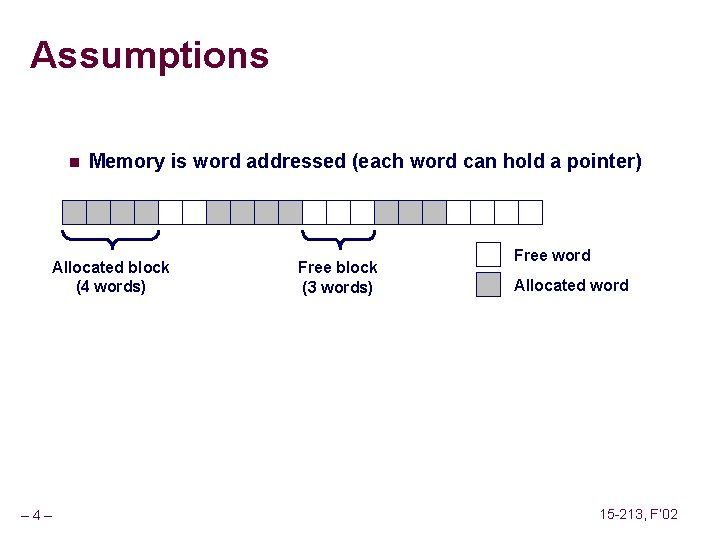
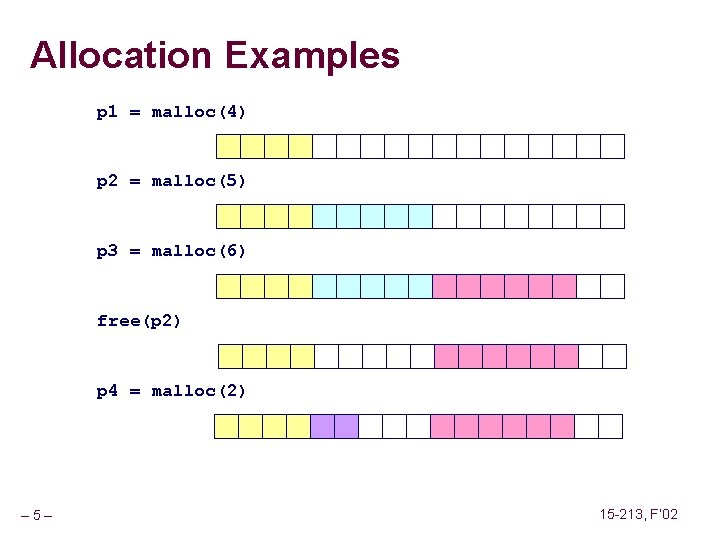
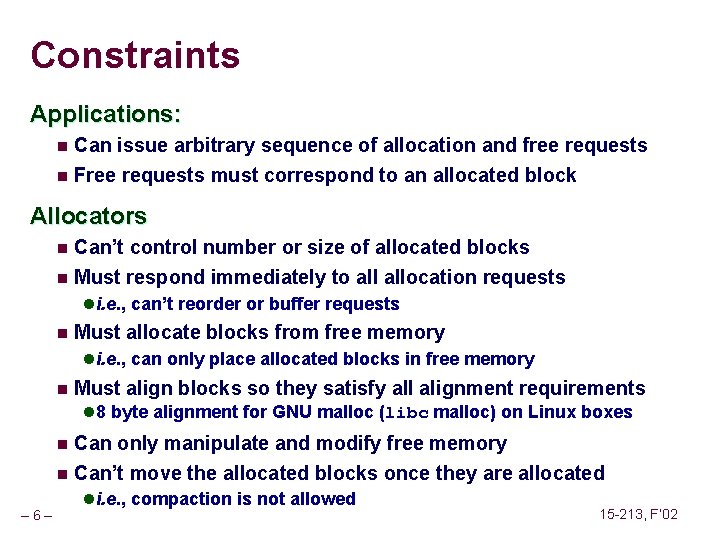
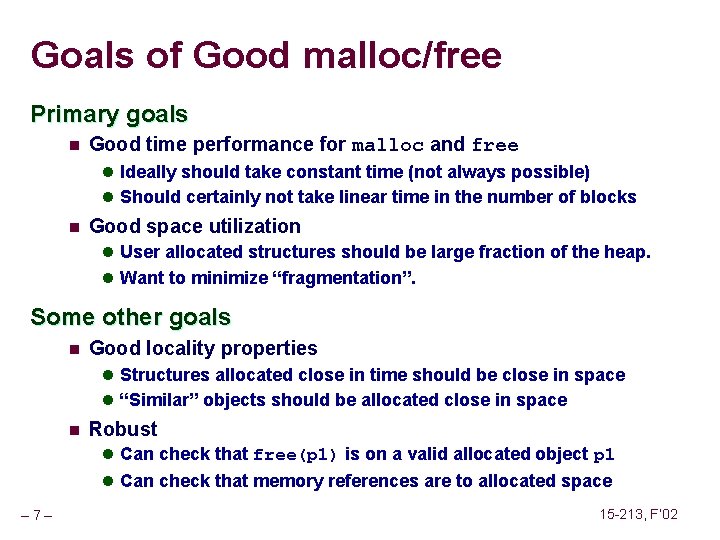
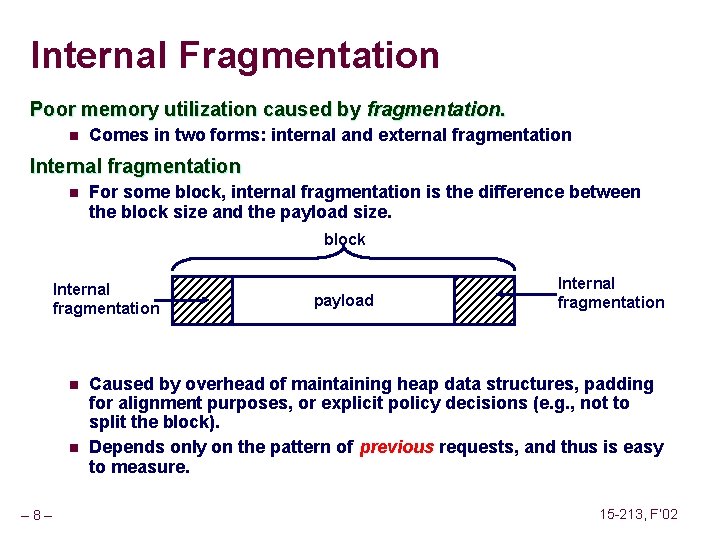
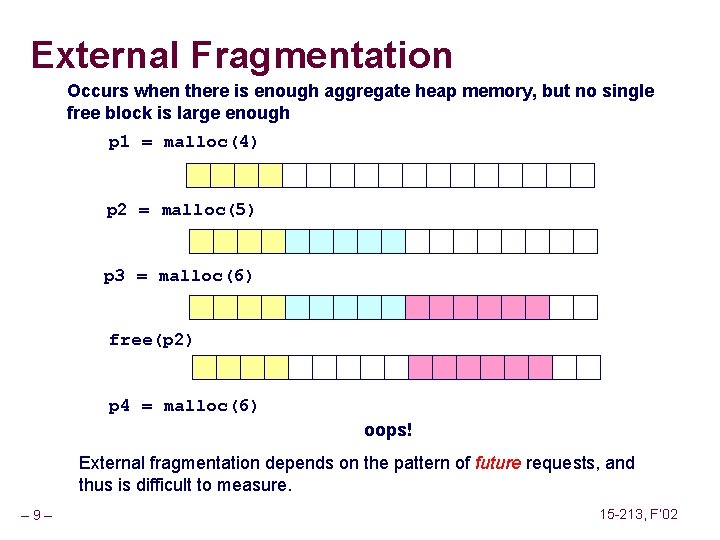
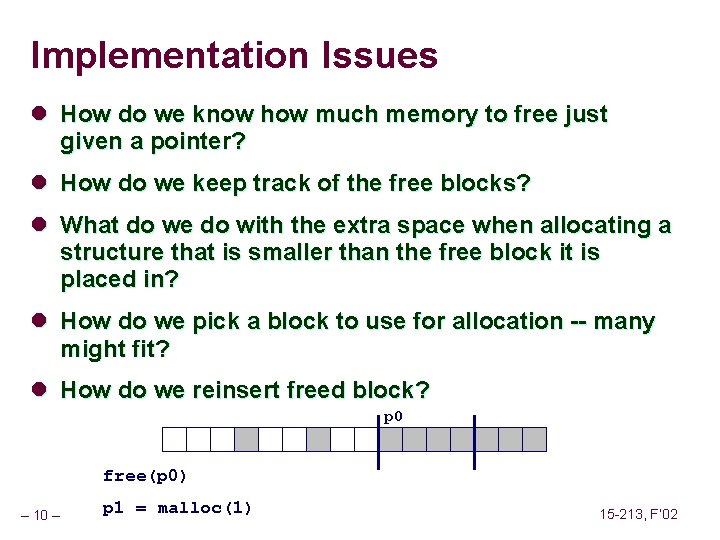
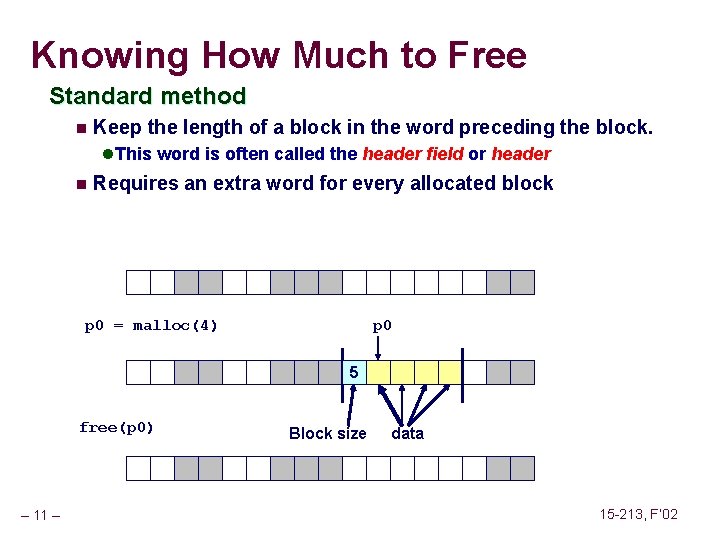
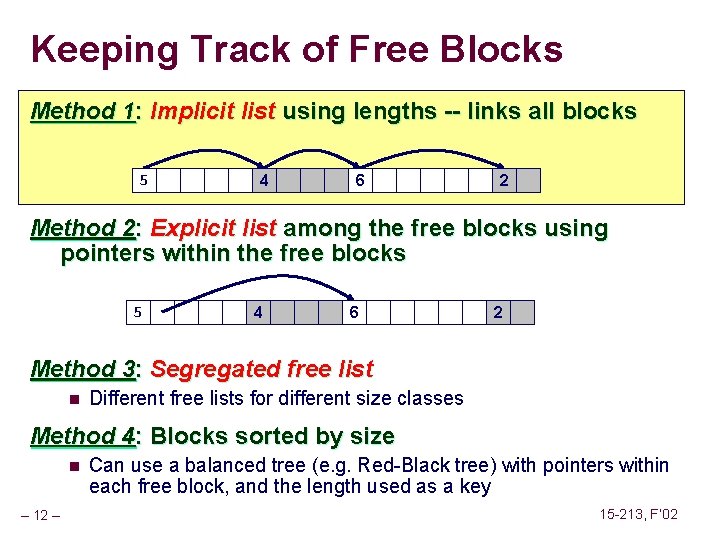
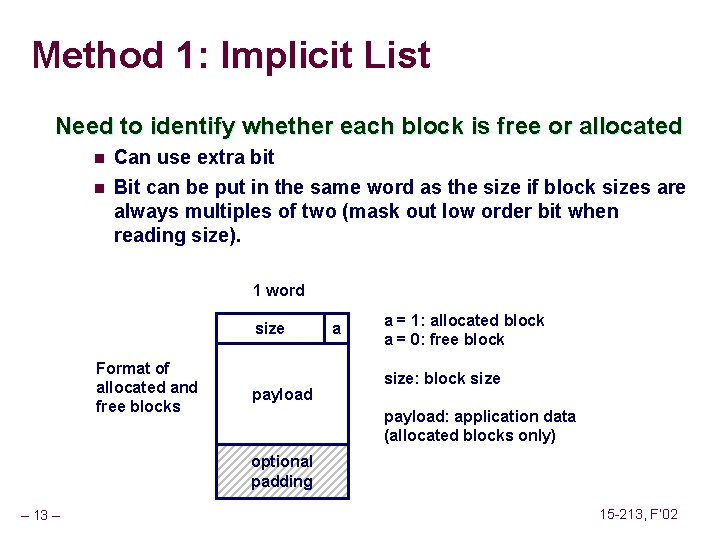
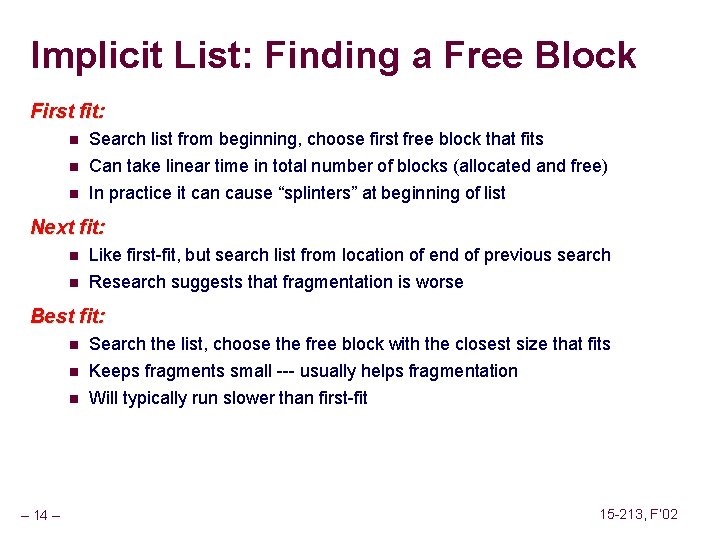
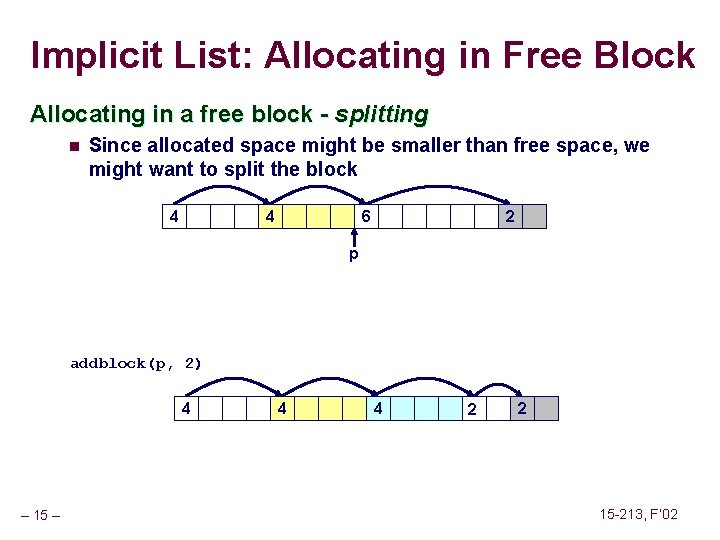
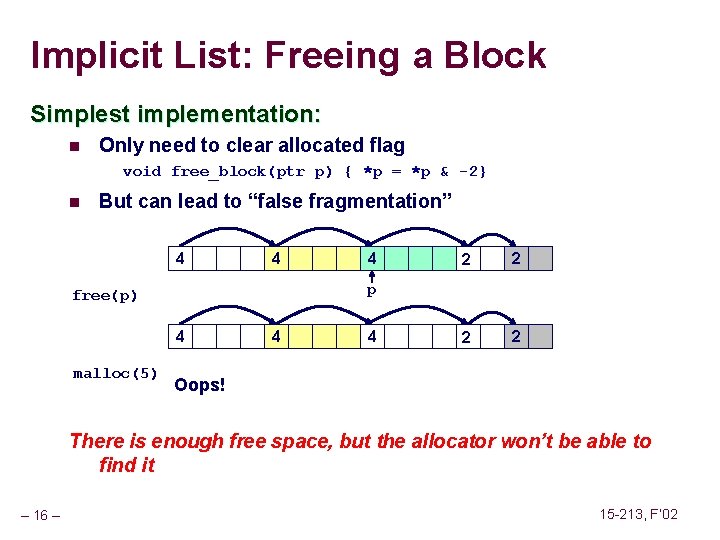
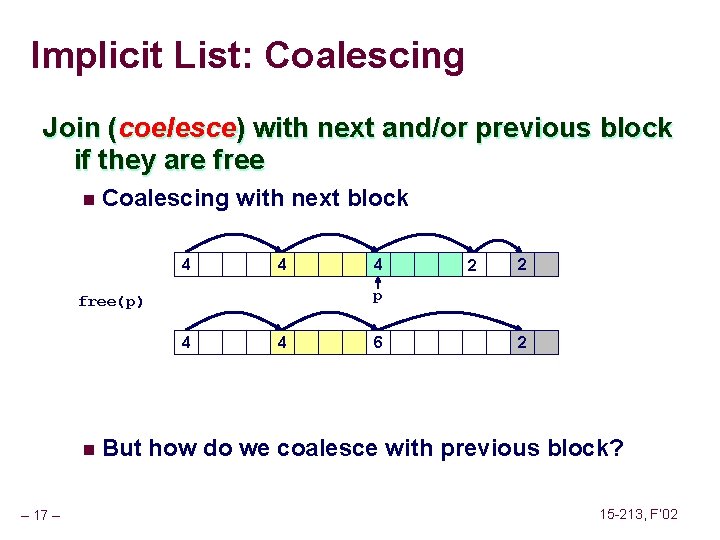
![Implicit List: Bidirectional Coalescing Boundary tags [Knuth 73] n n n Replicate size/allocated word Implicit List: Bidirectional Coalescing Boundary tags [Knuth 73] n n n Replicate size/allocated word](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-18.jpg)
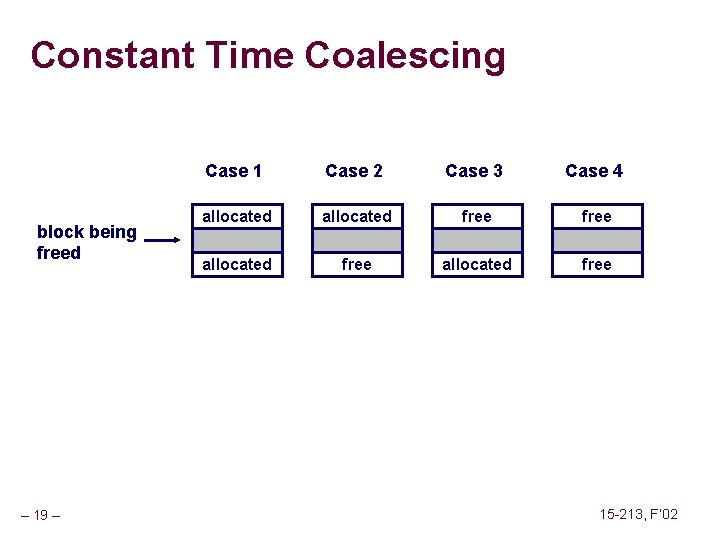
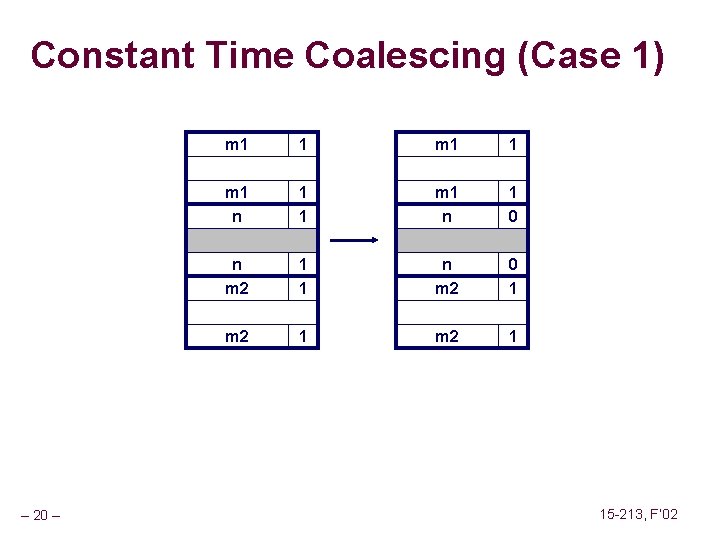
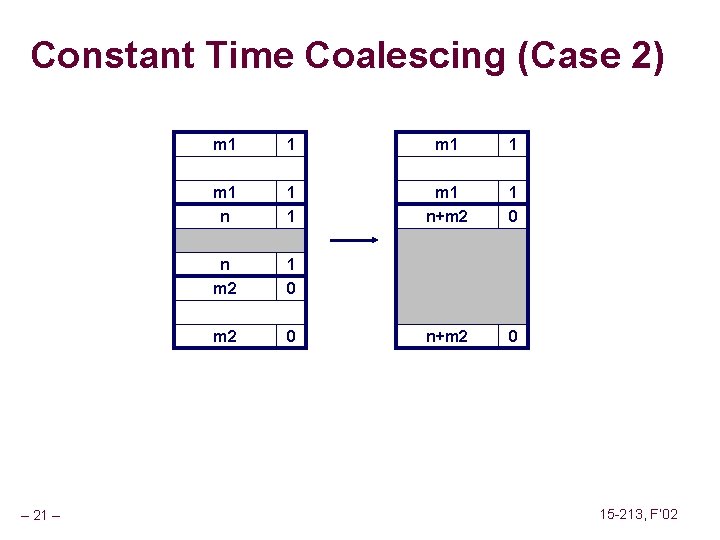
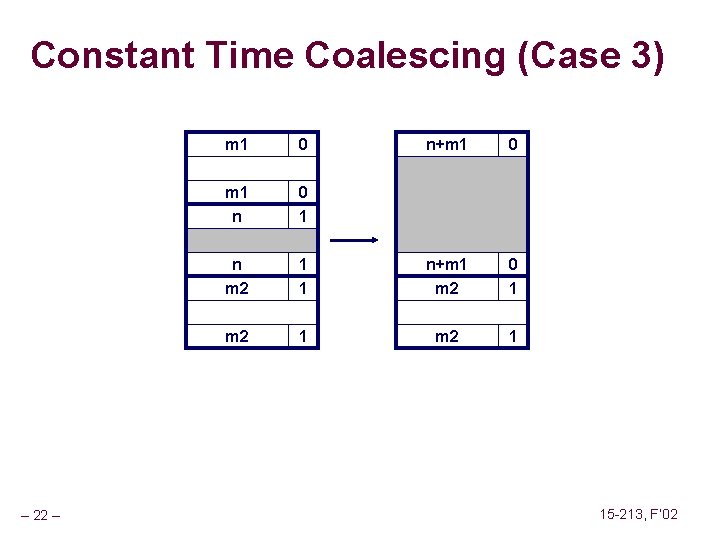
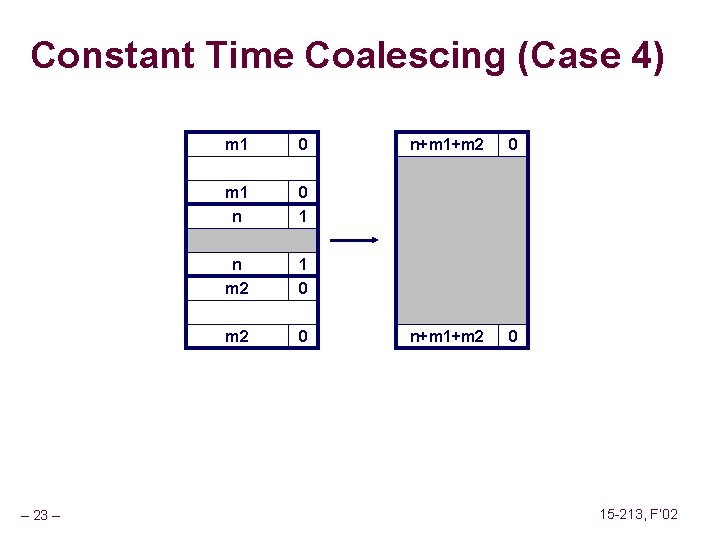
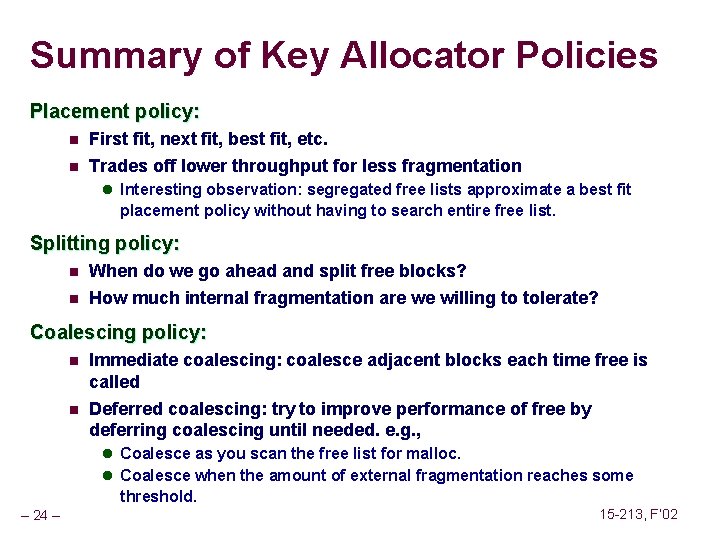
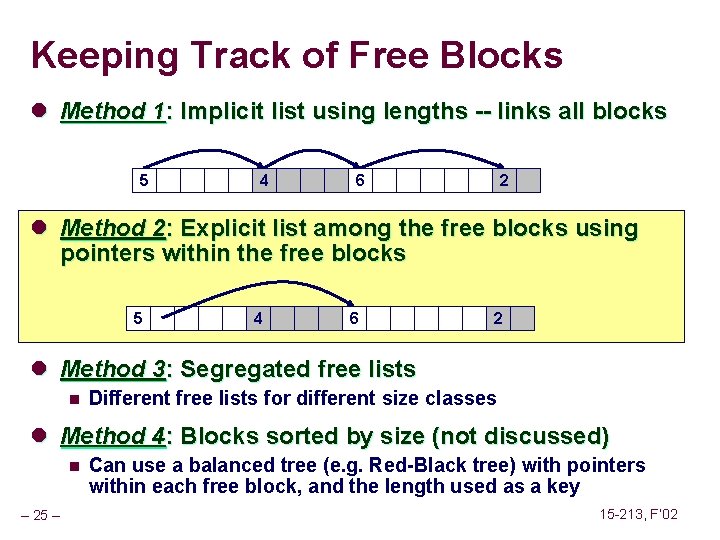
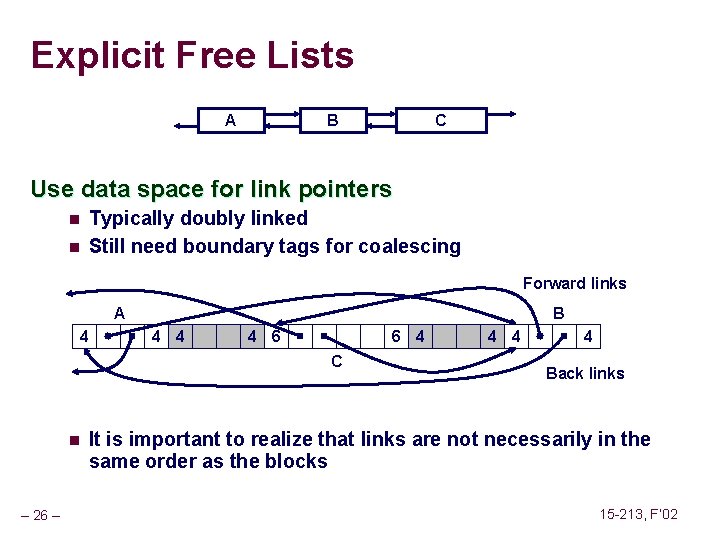
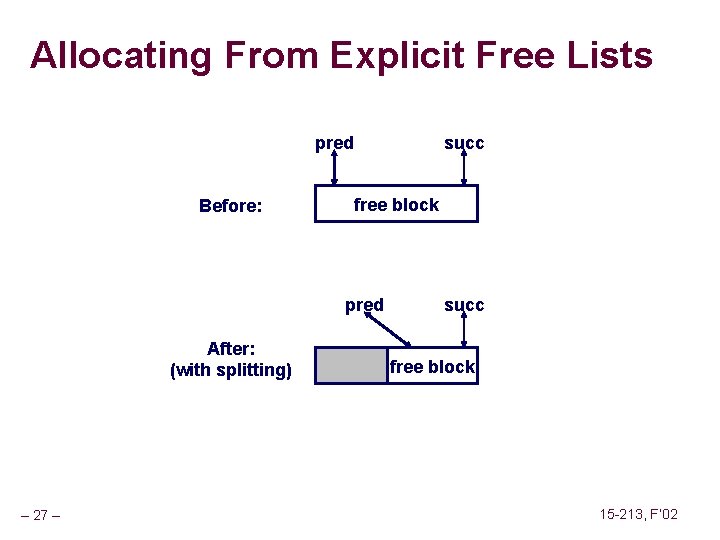
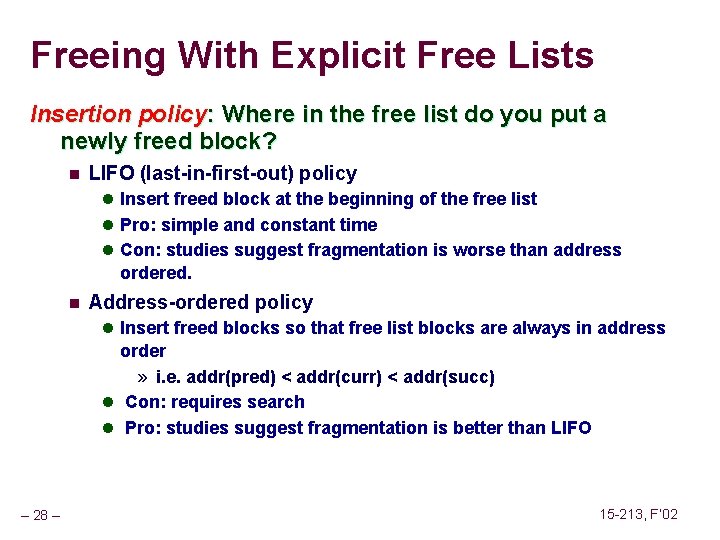
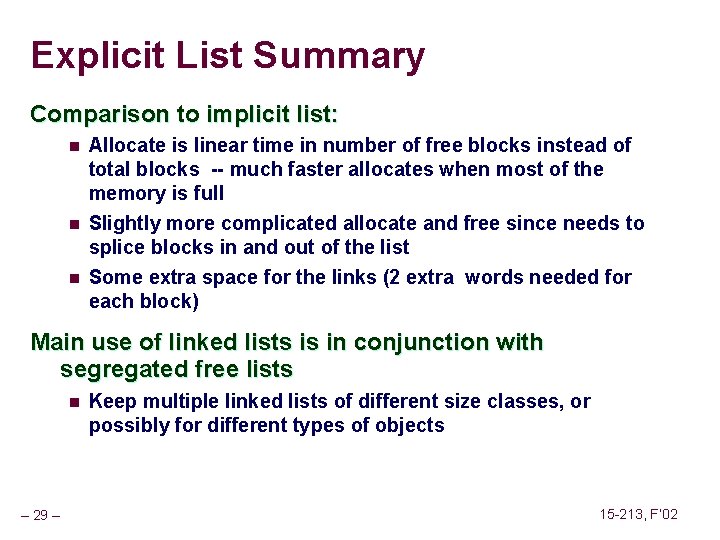
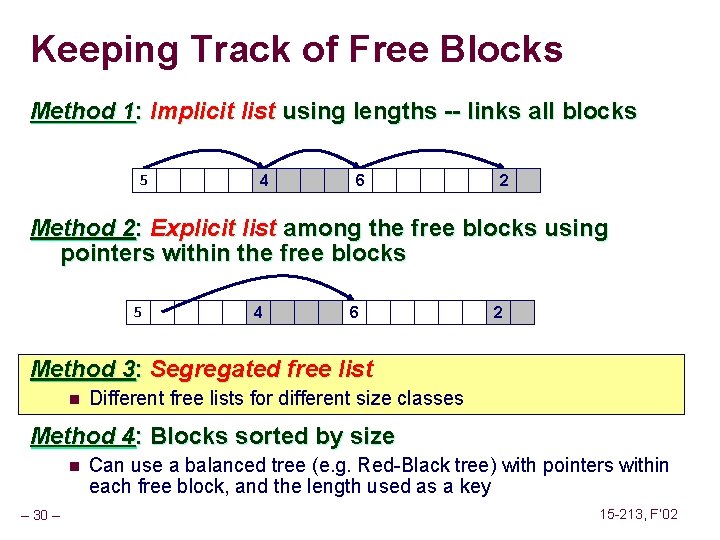
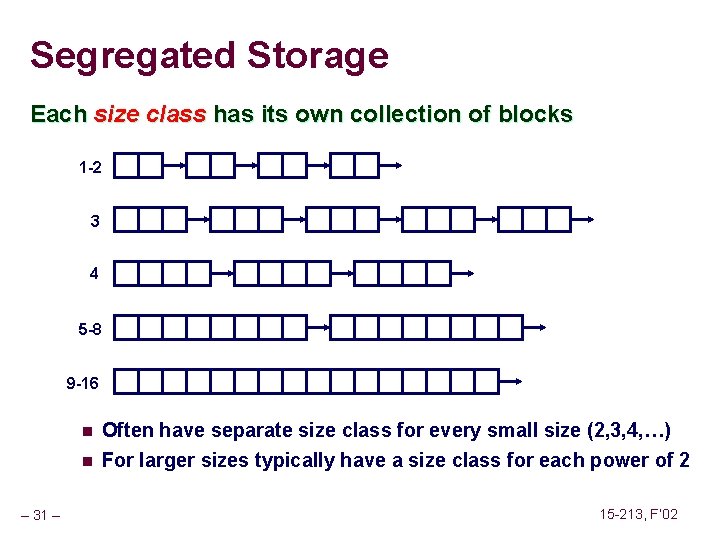
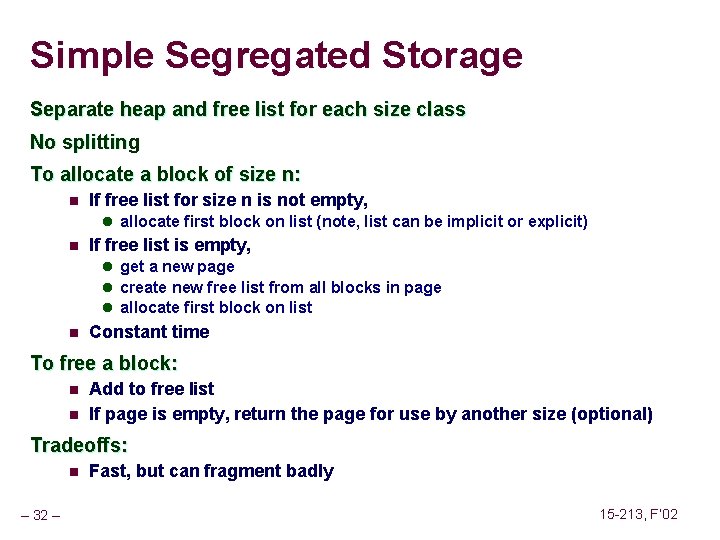
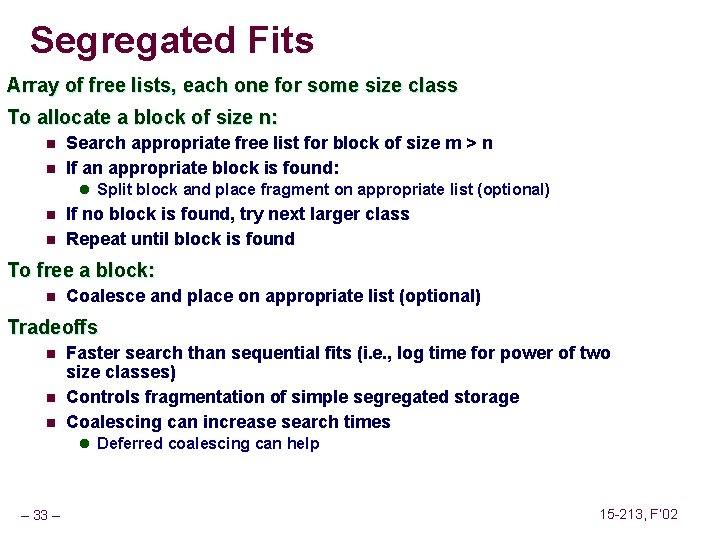
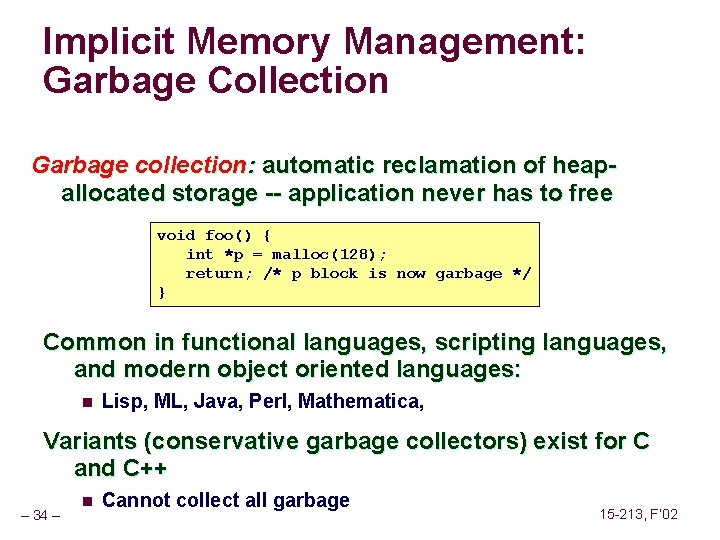
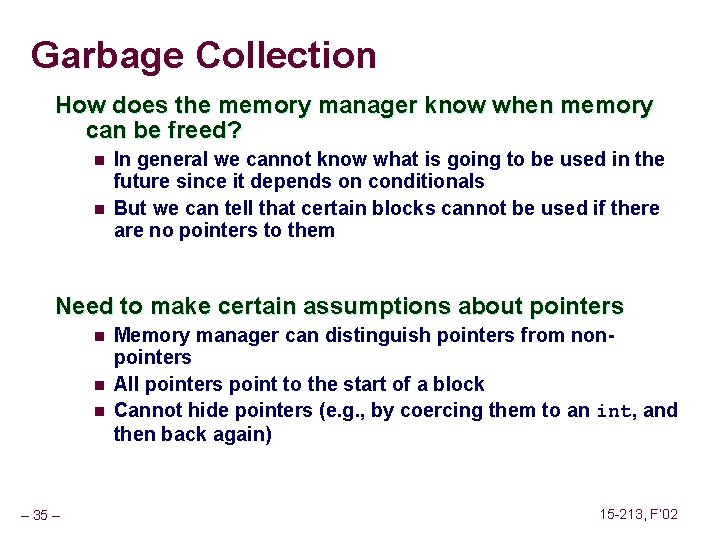
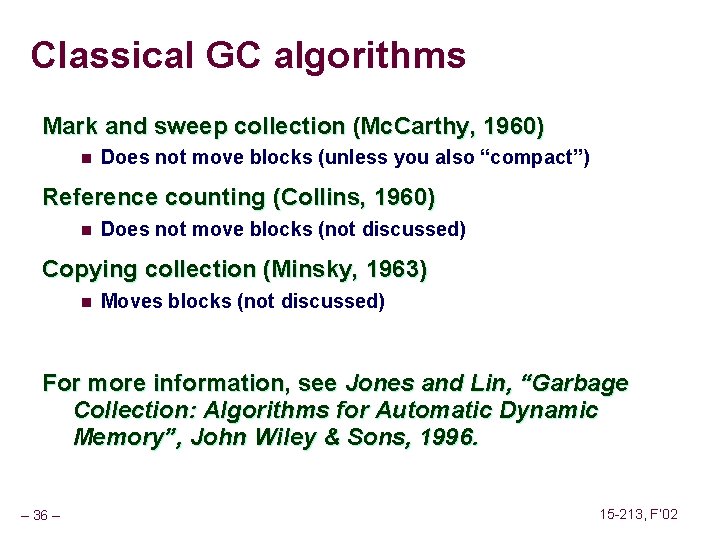
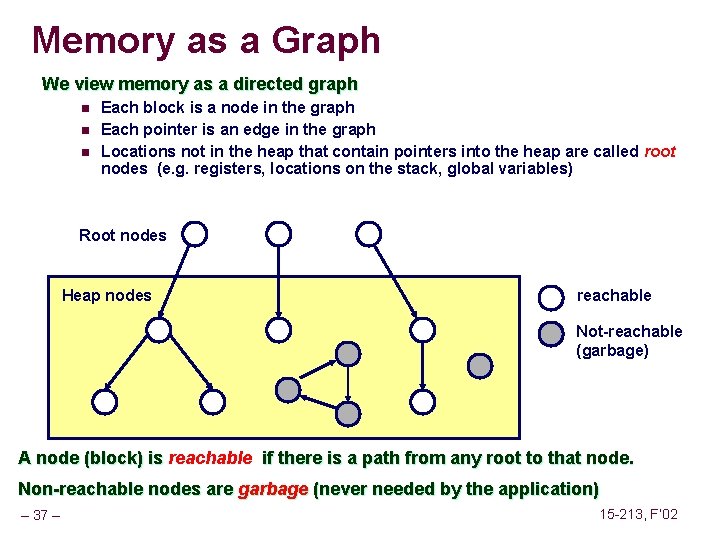
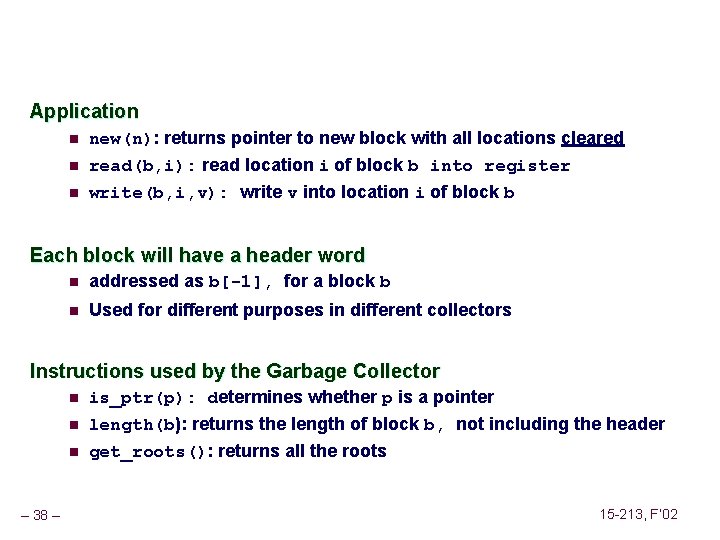
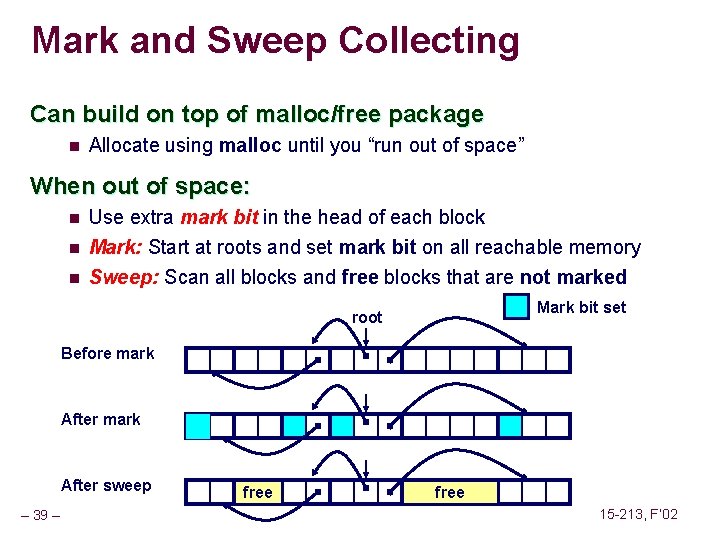
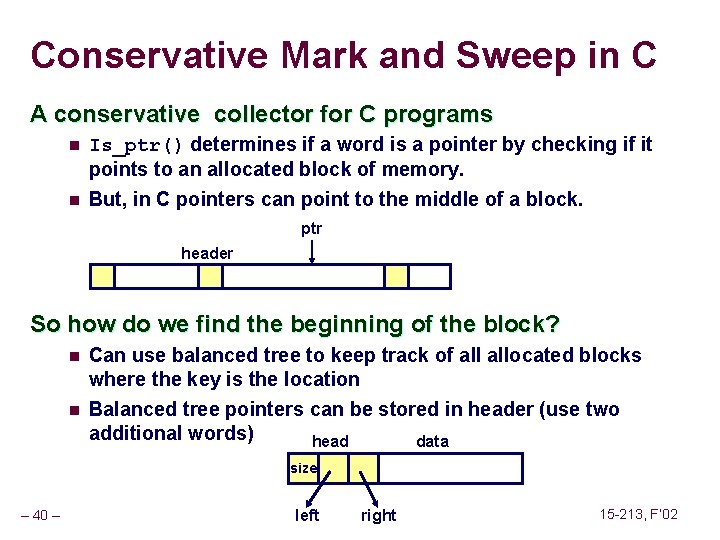
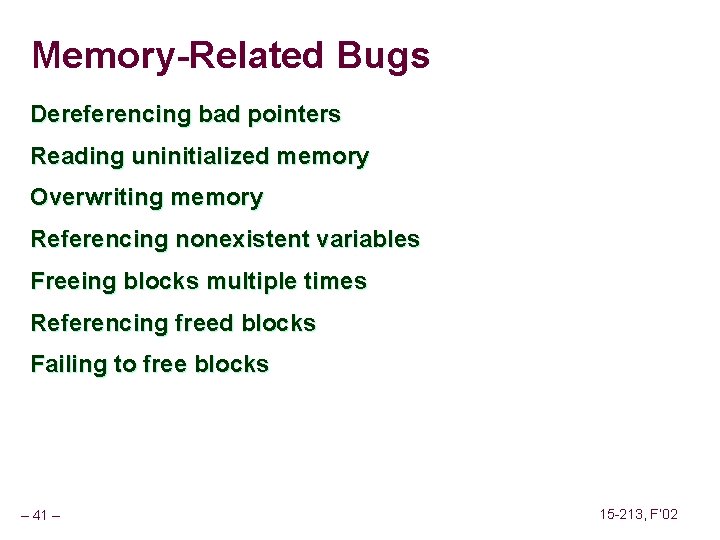
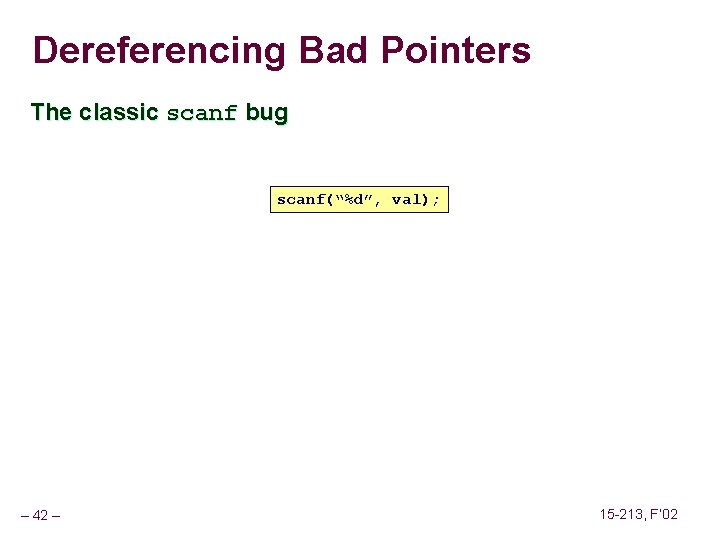
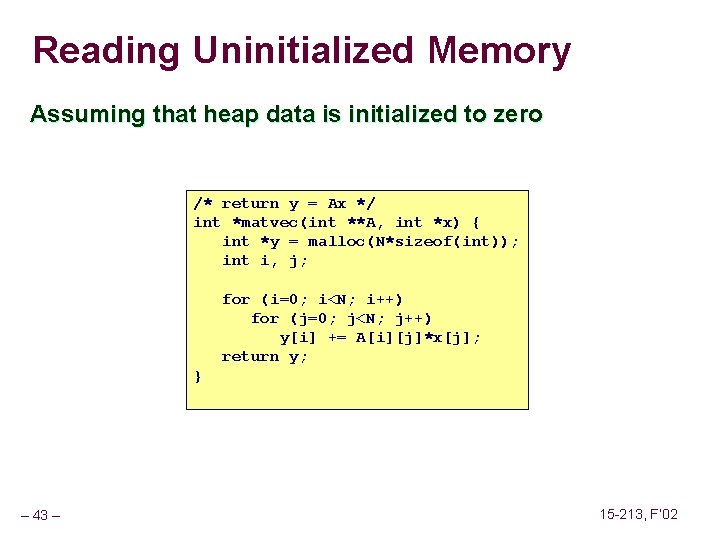
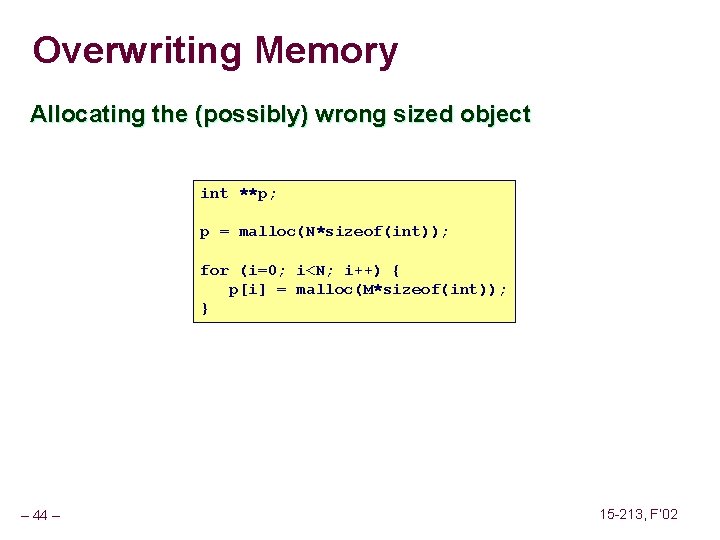
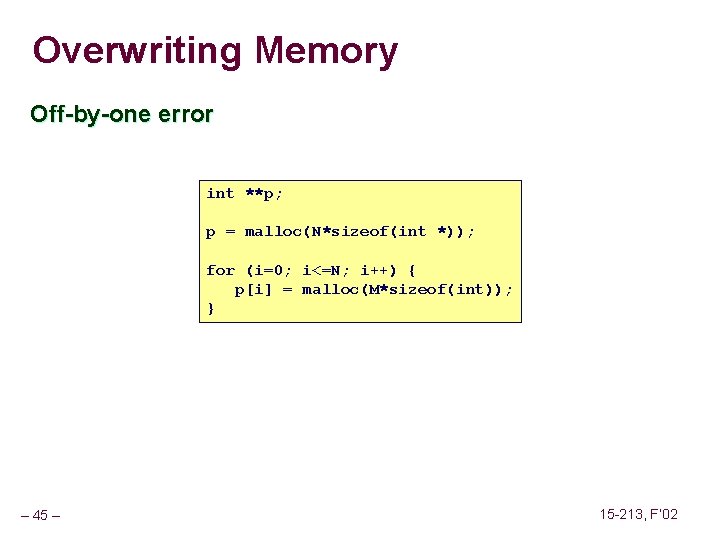
![Overwriting Memory Not checking the max string size char s[8]; int i; gets(s); /* Overwriting Memory Not checking the max string size char s[8]; int i; gets(s); /*](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-46.jpg)
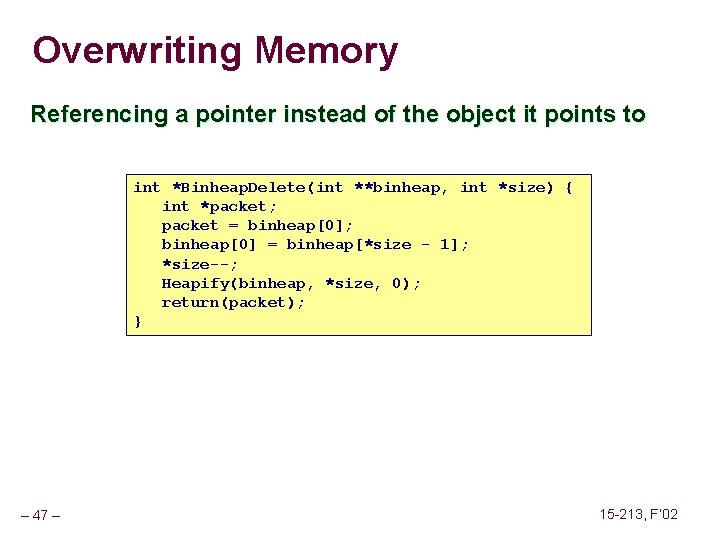
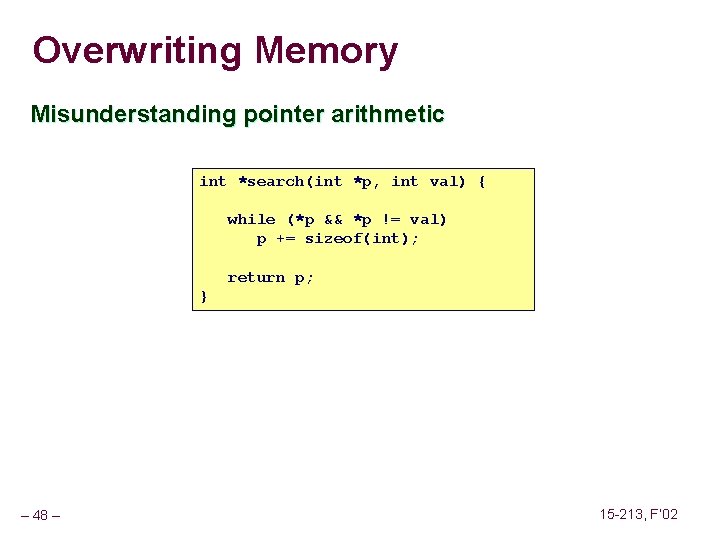
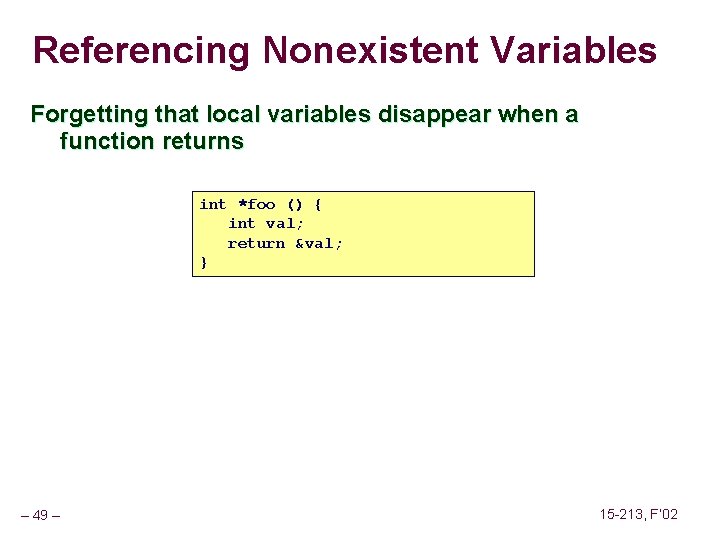
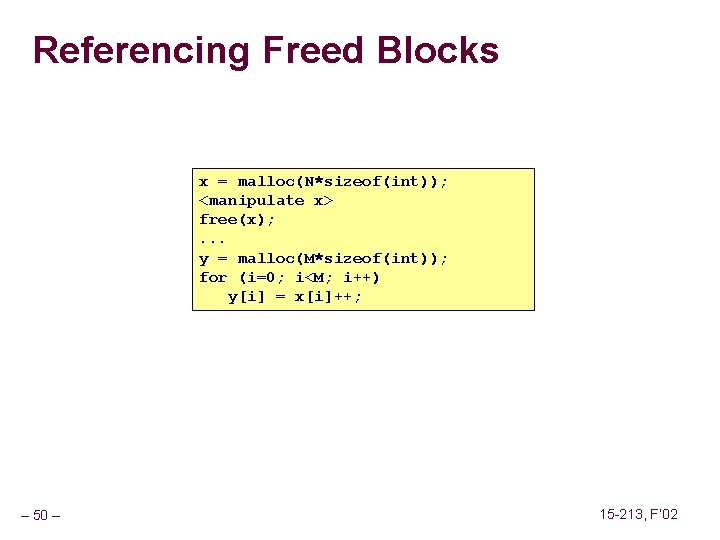
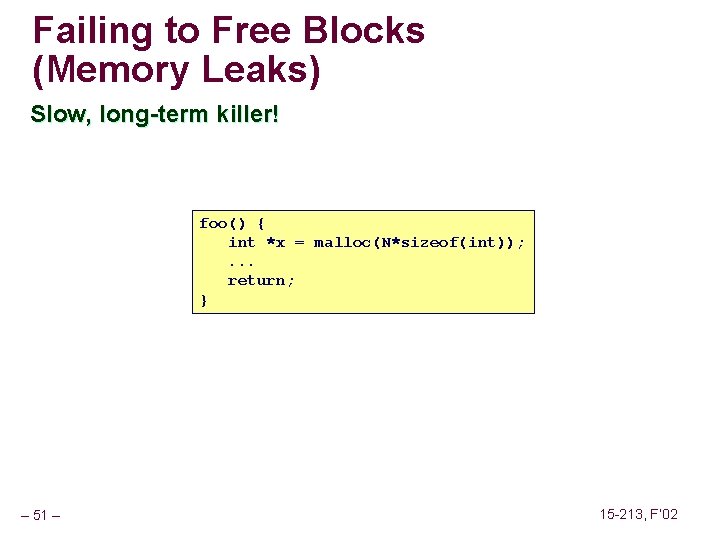
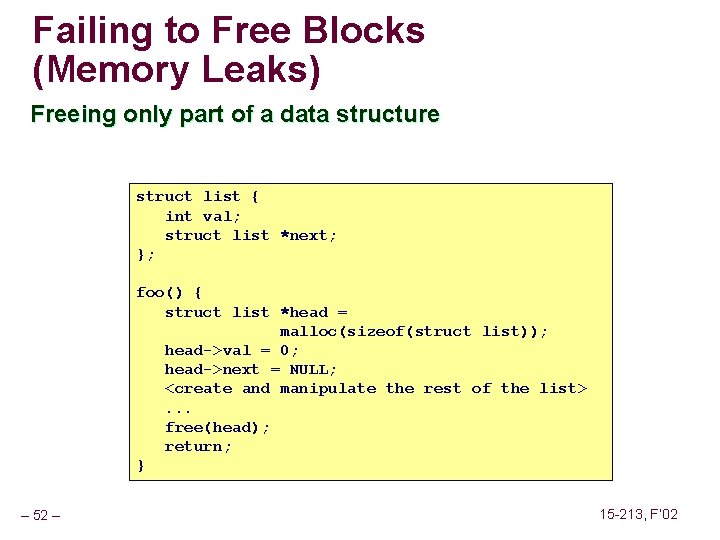
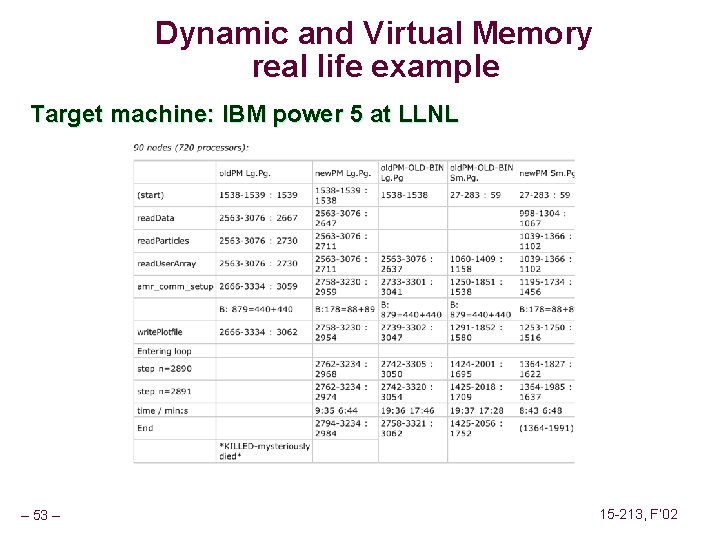
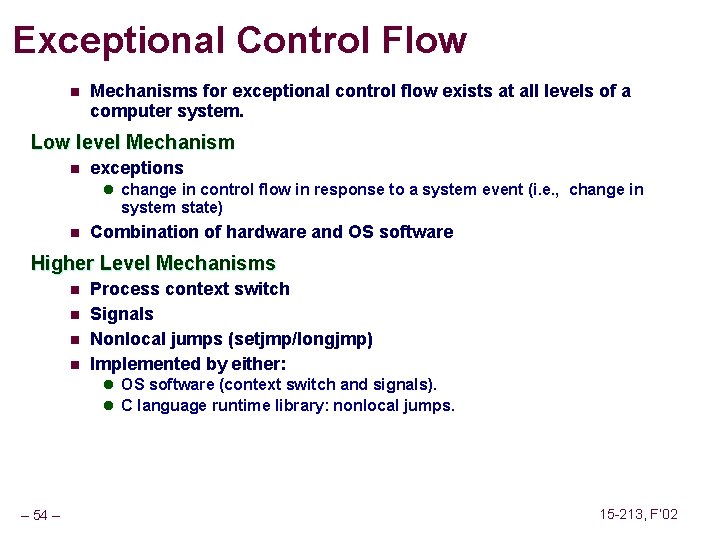
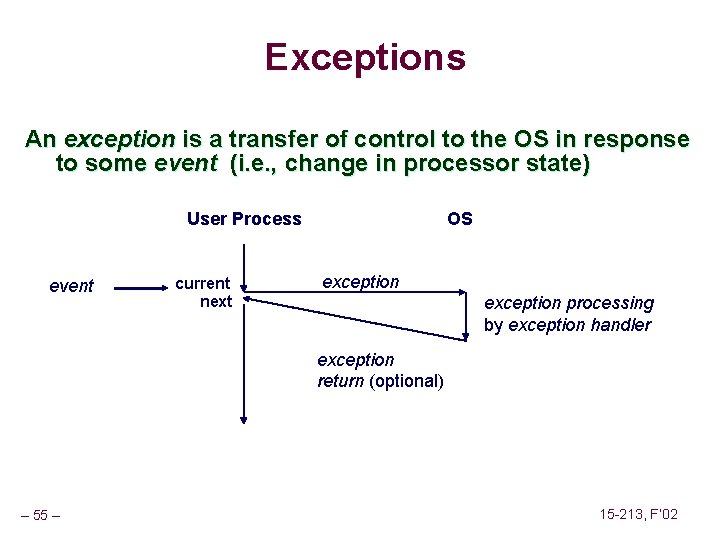
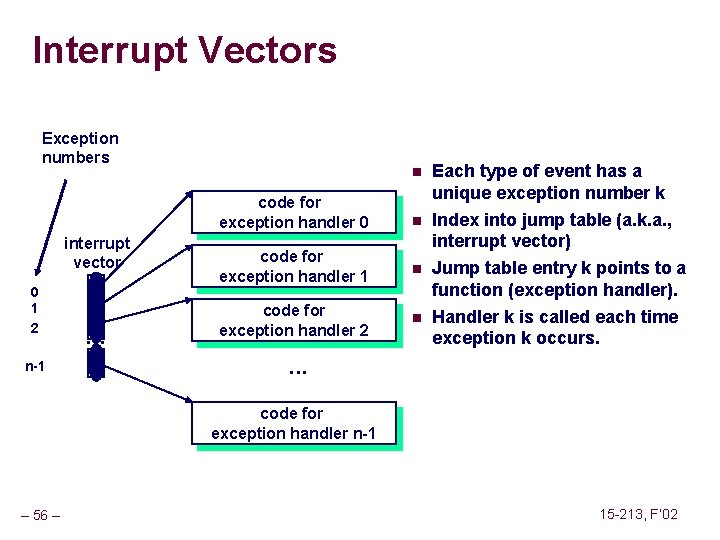
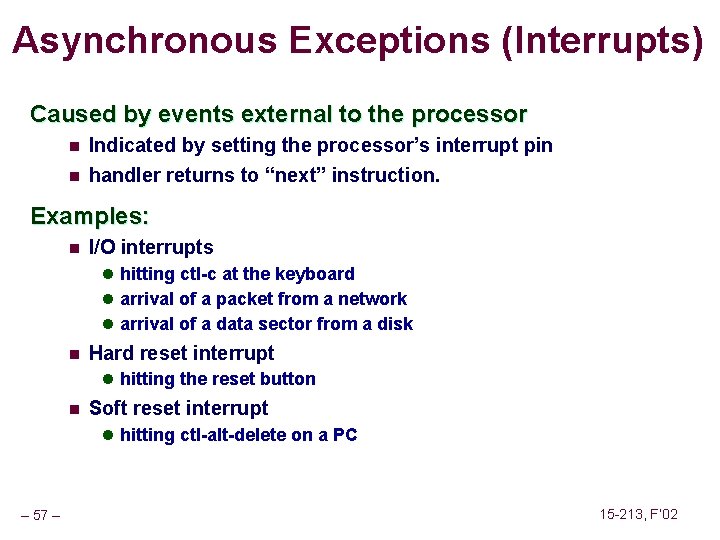
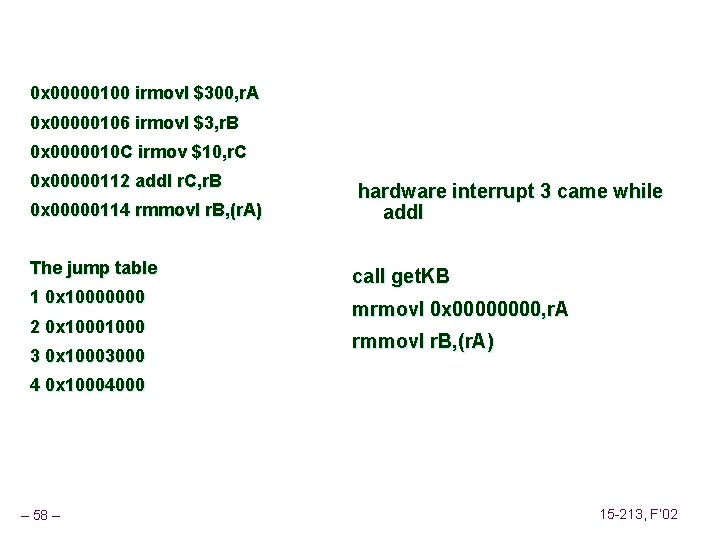
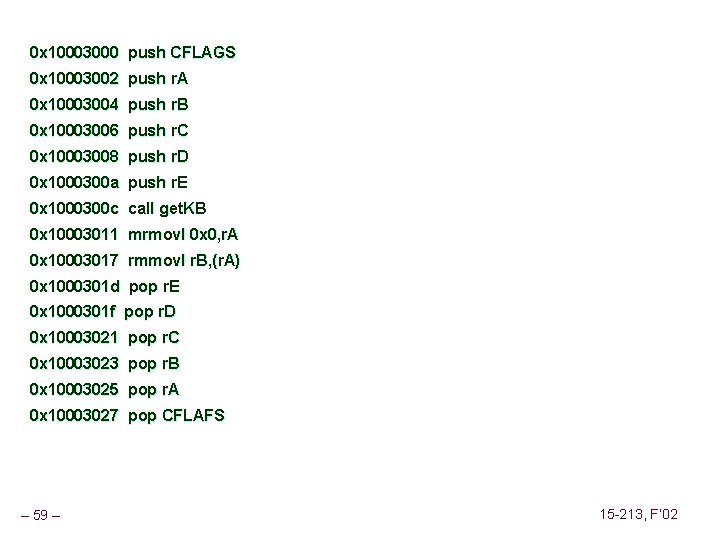
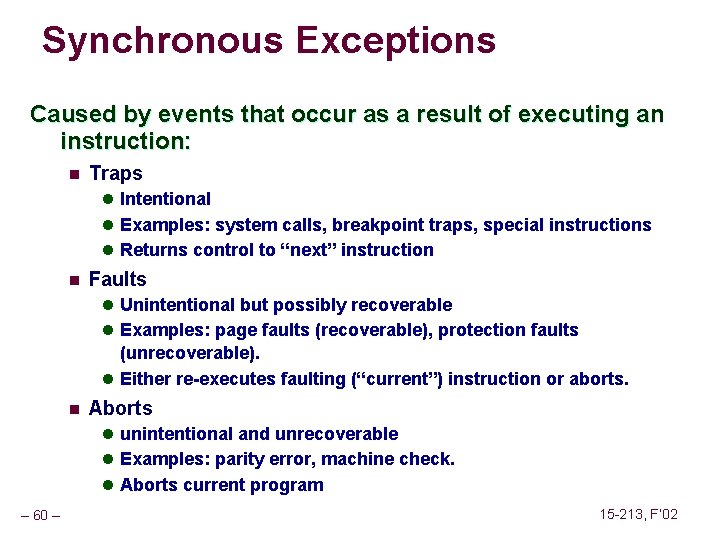
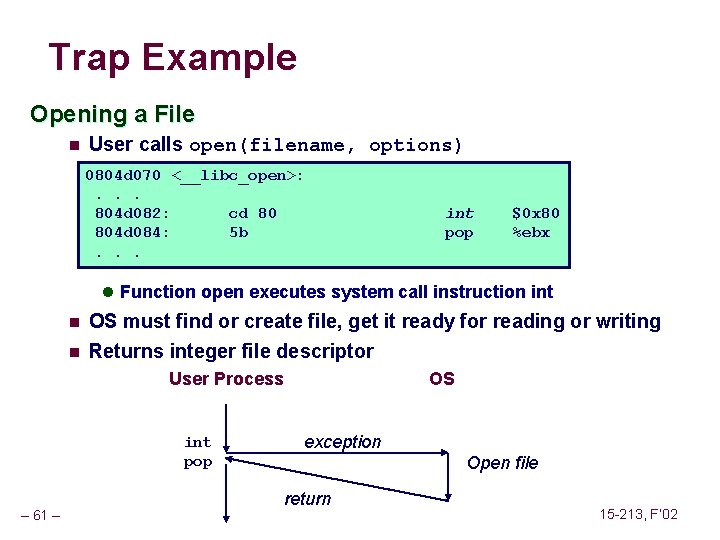
![Fault Example #1 int a[1000]; main () { a[500] = 13; } Memory Reference Fault Example #1 int a[1000]; main () { a[500] = 13; } Memory Reference](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-62.jpg)
![Fault Example #2 int a[1000]; main () { a[5000] = 13; } Memory Reference Fault Example #2 int a[1000]; main () { a[5000] = 13; } Memory Reference](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-63.jpg)
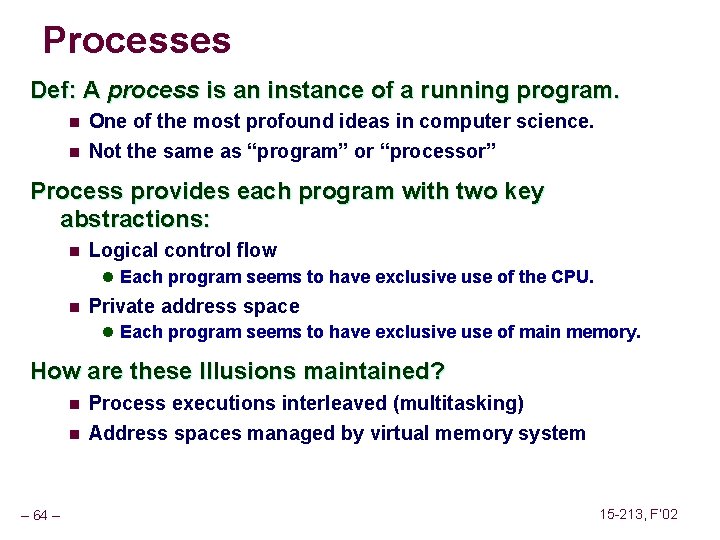
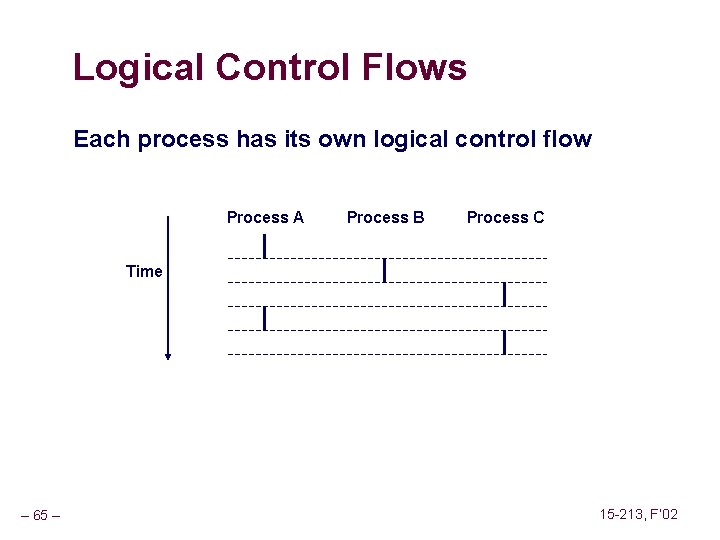
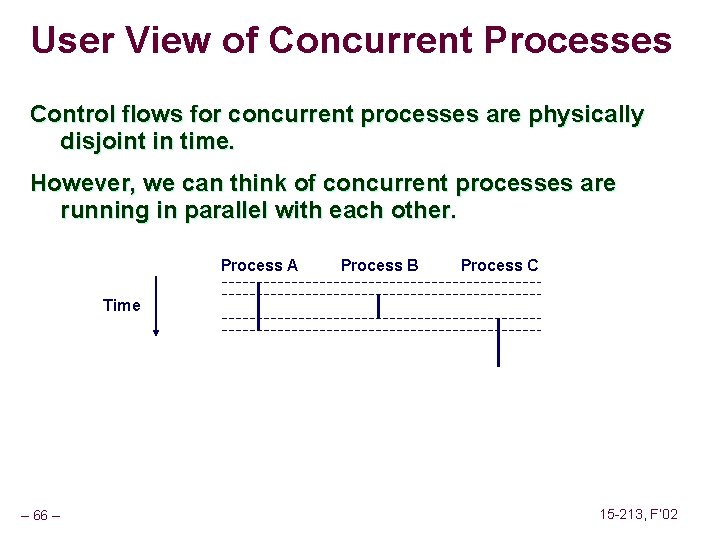
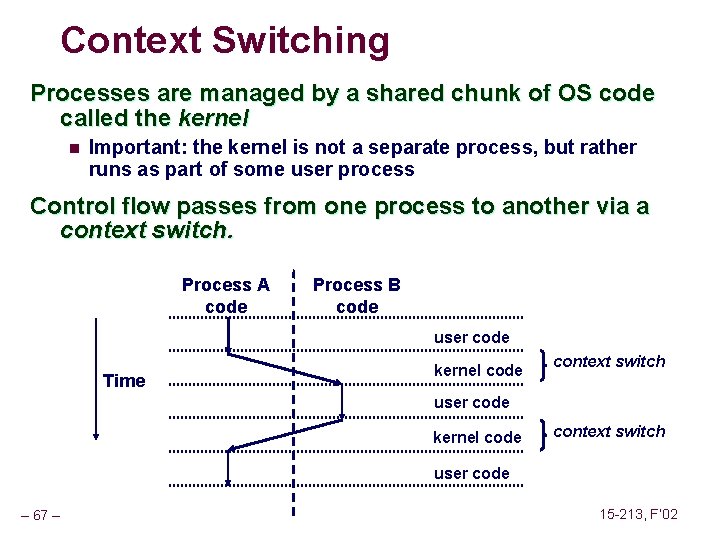
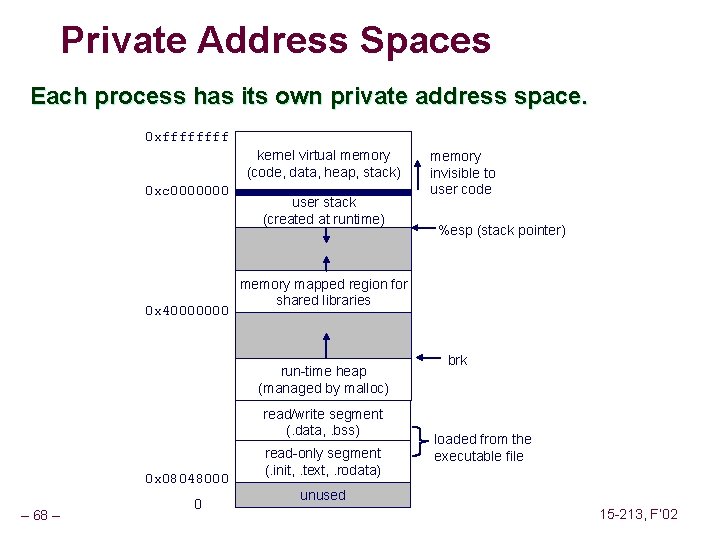
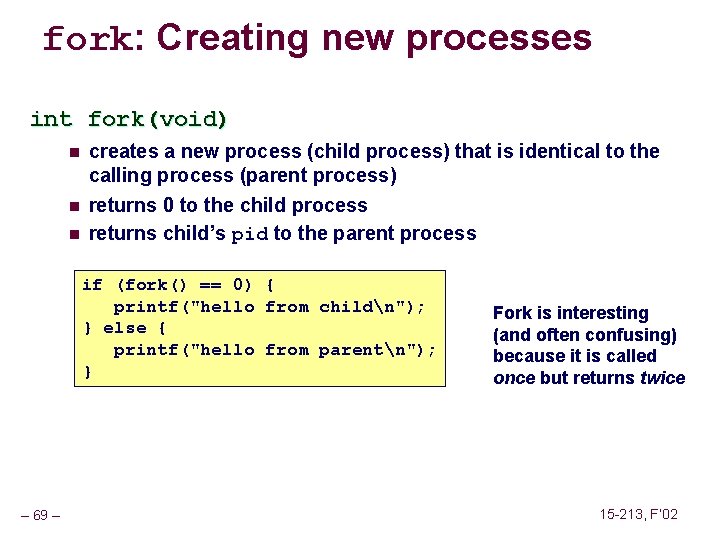
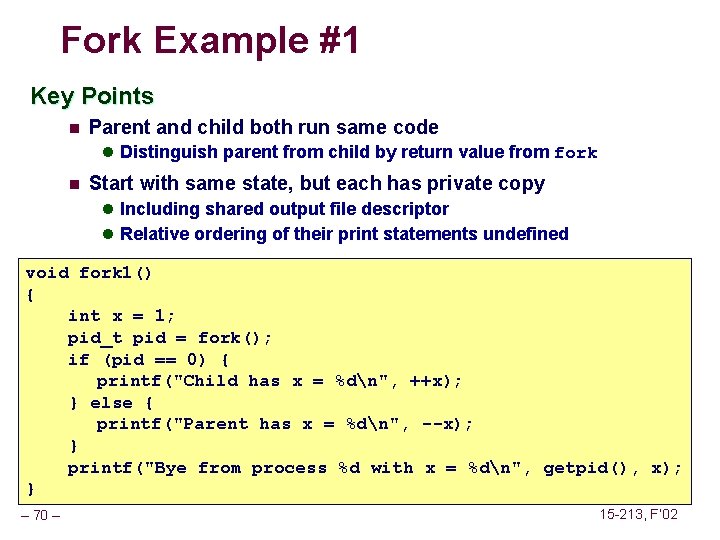
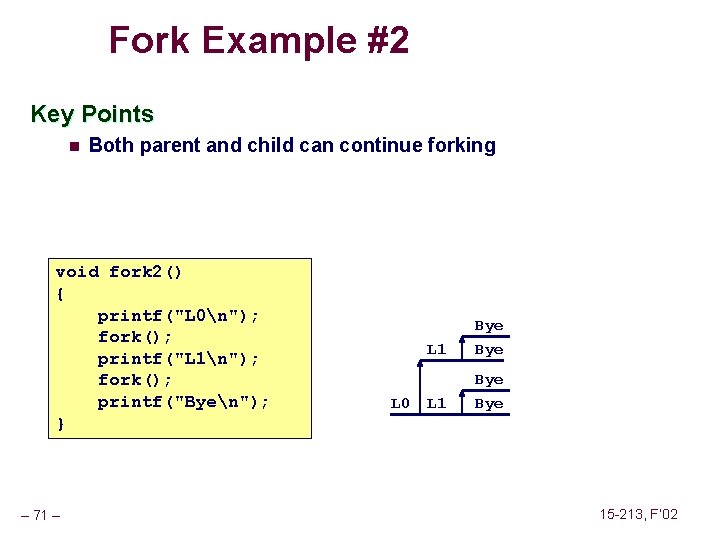
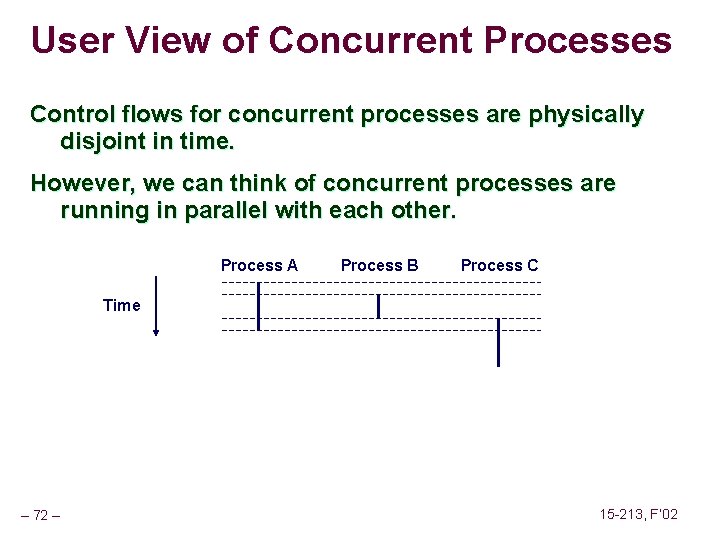
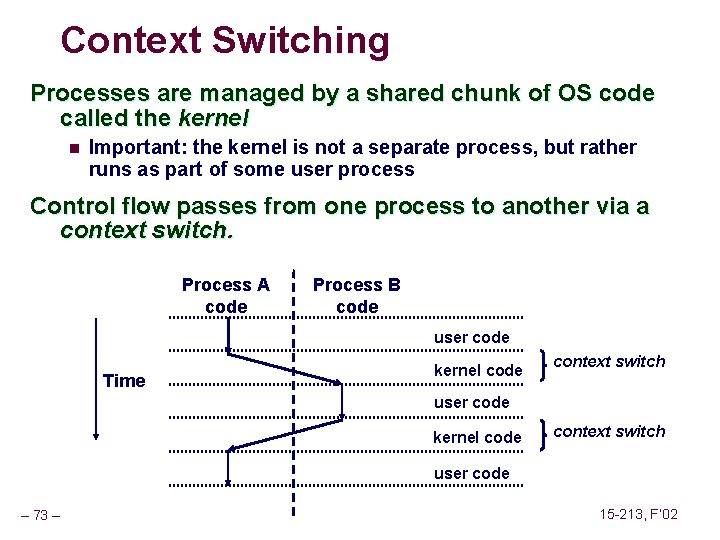
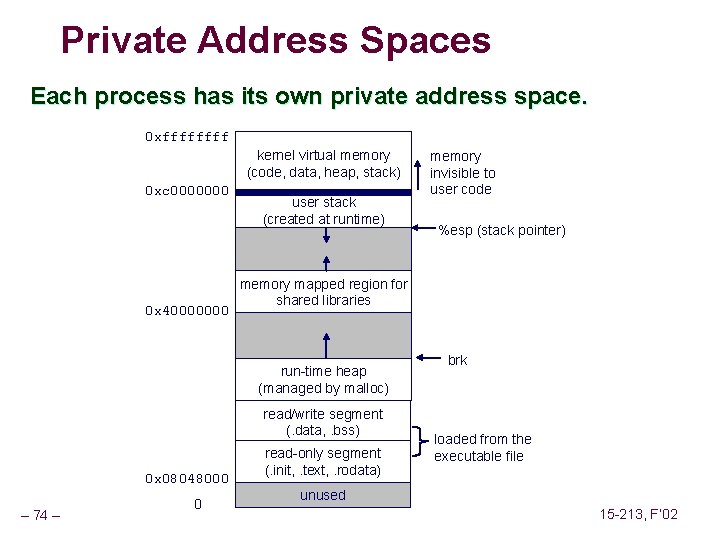
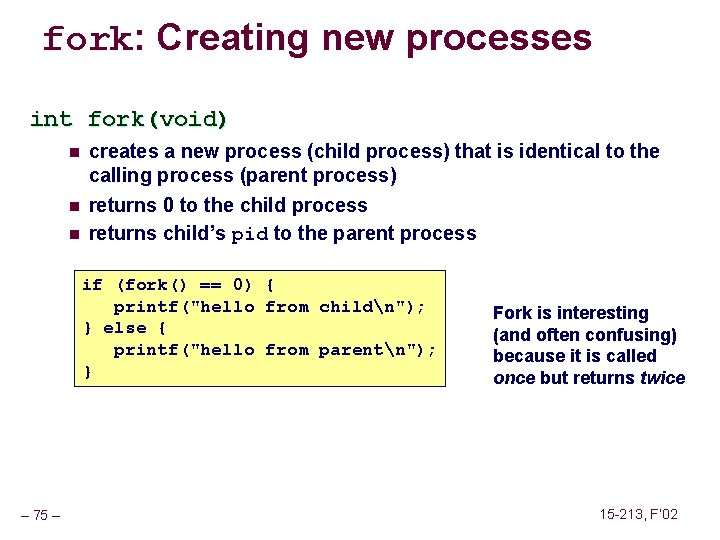
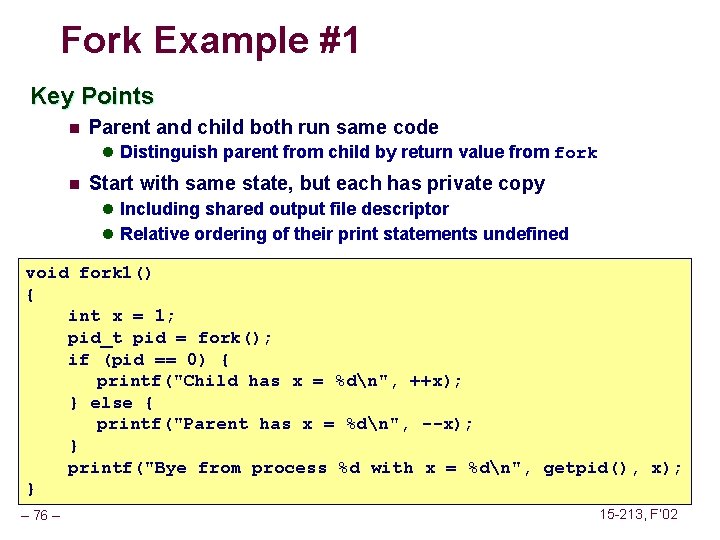
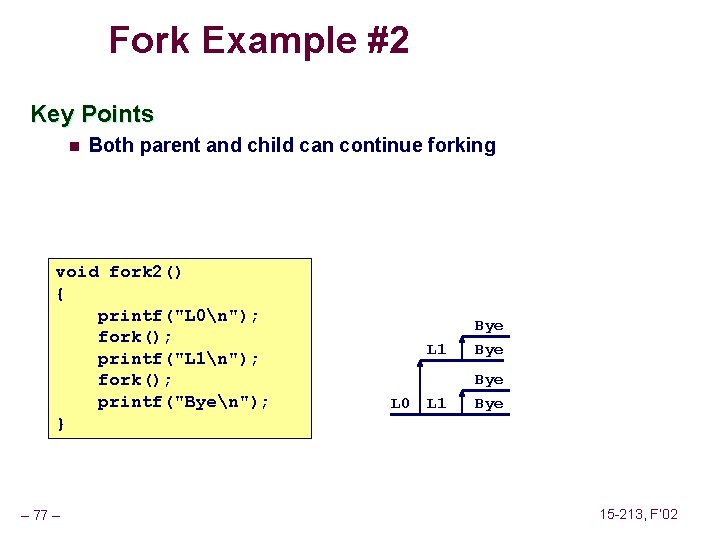
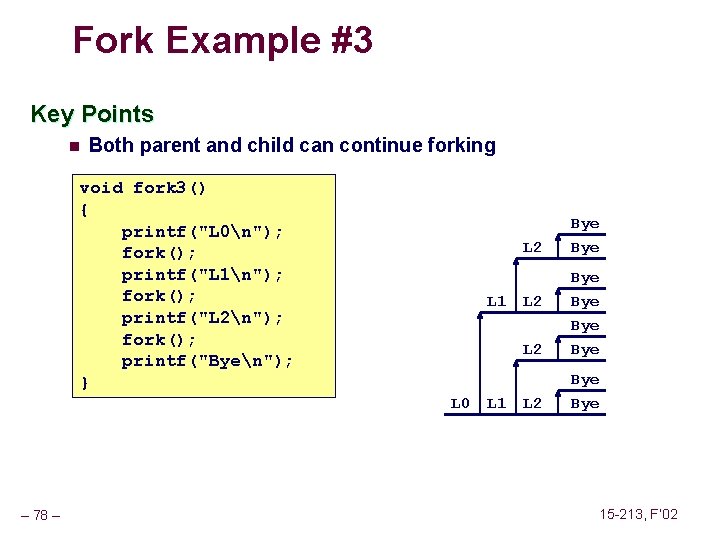
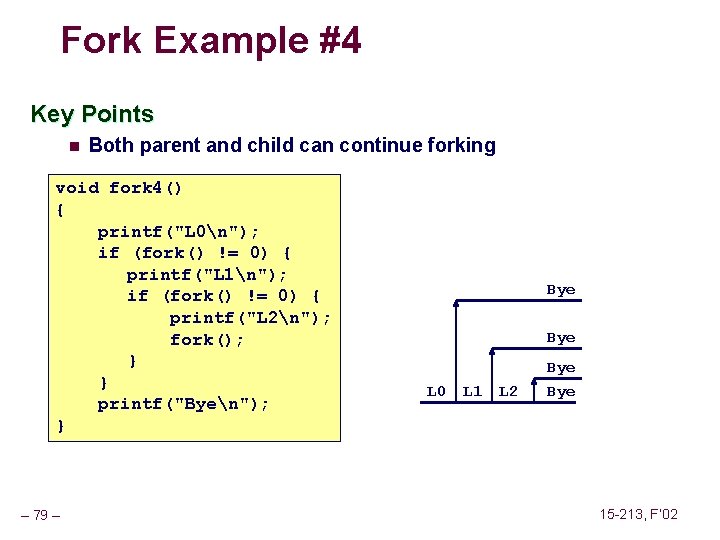
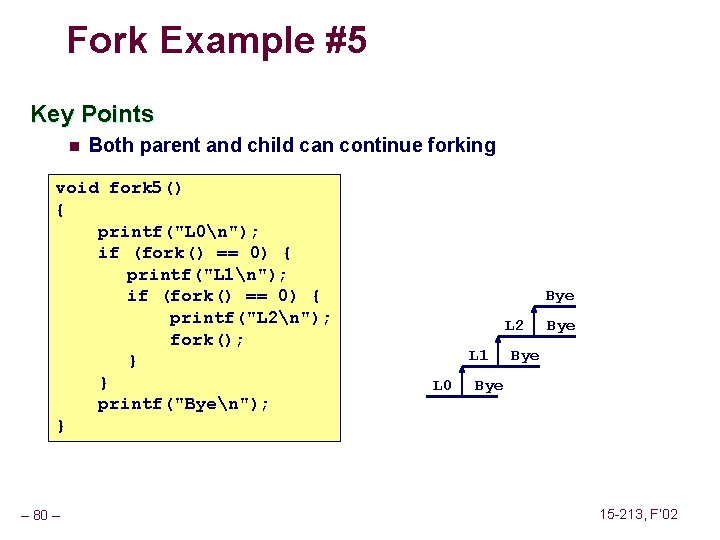
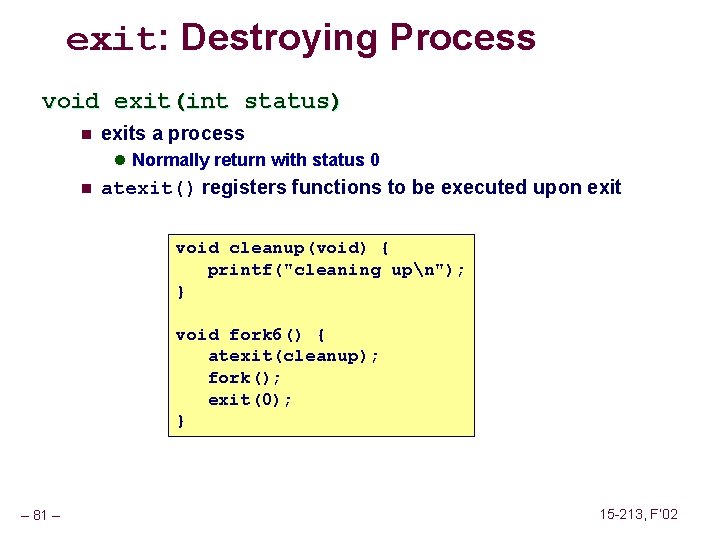
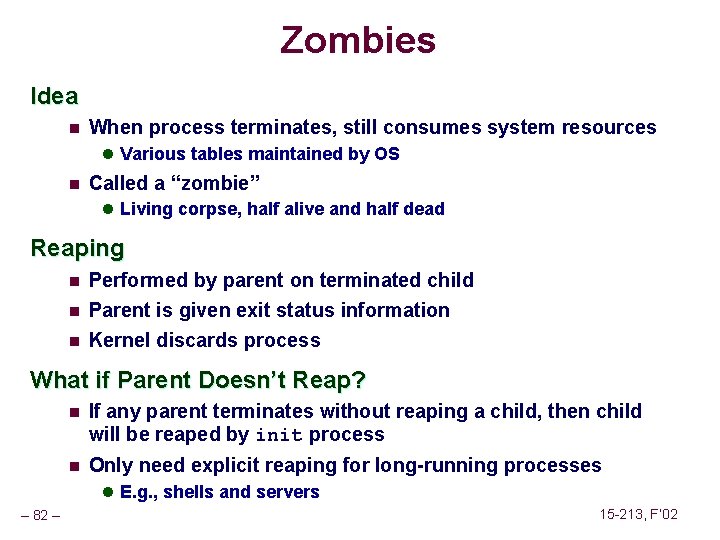
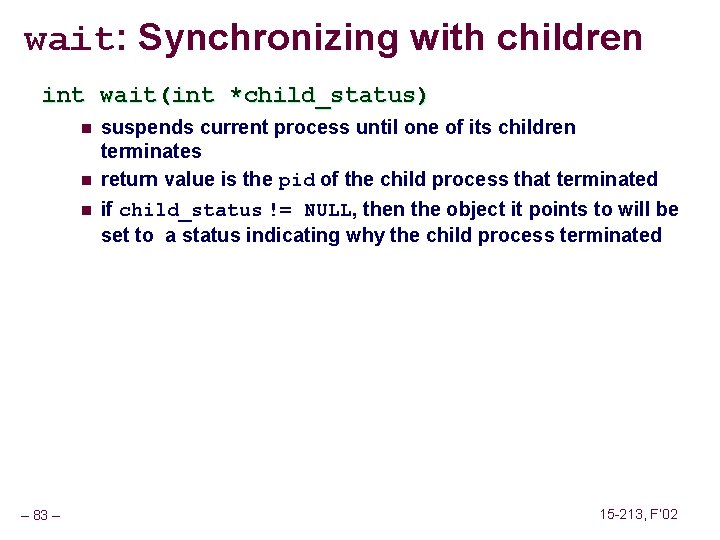
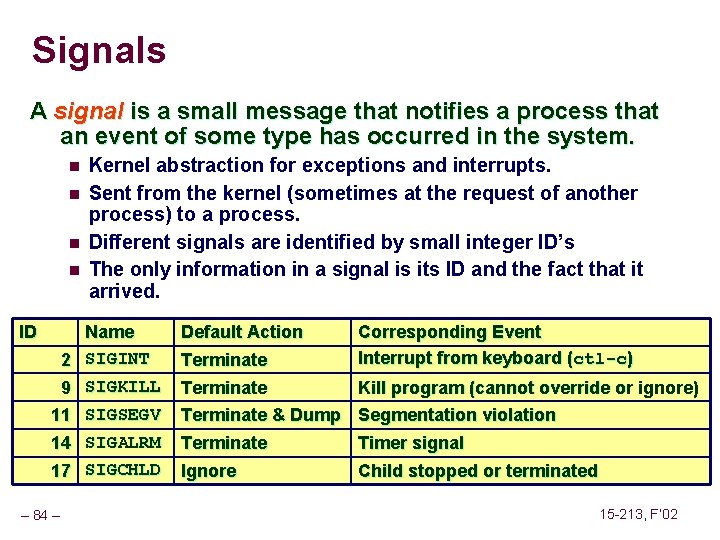
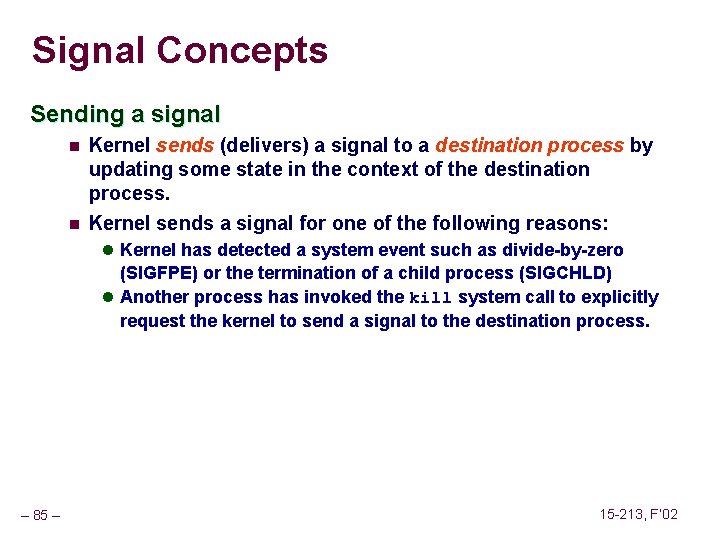
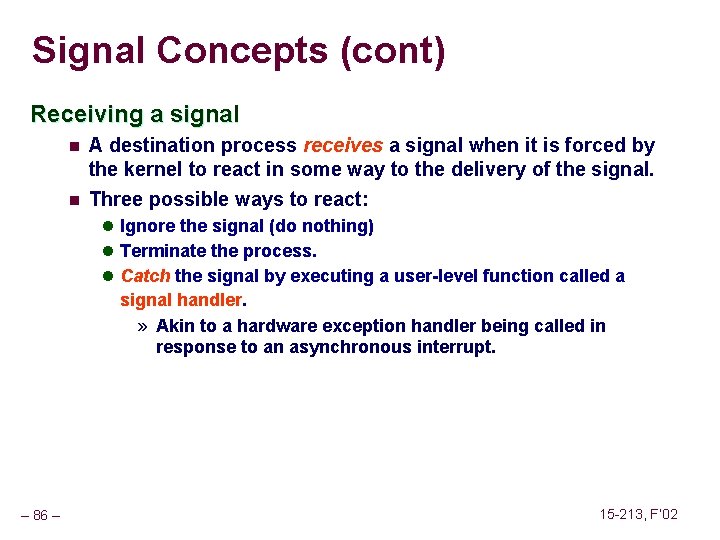
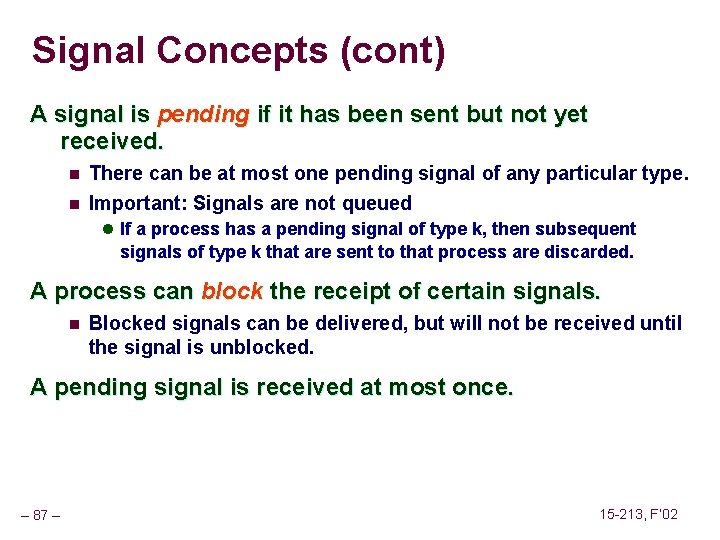
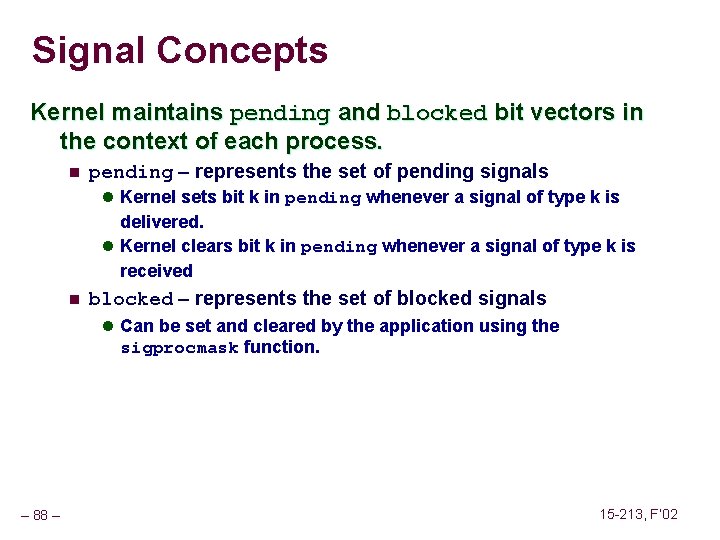
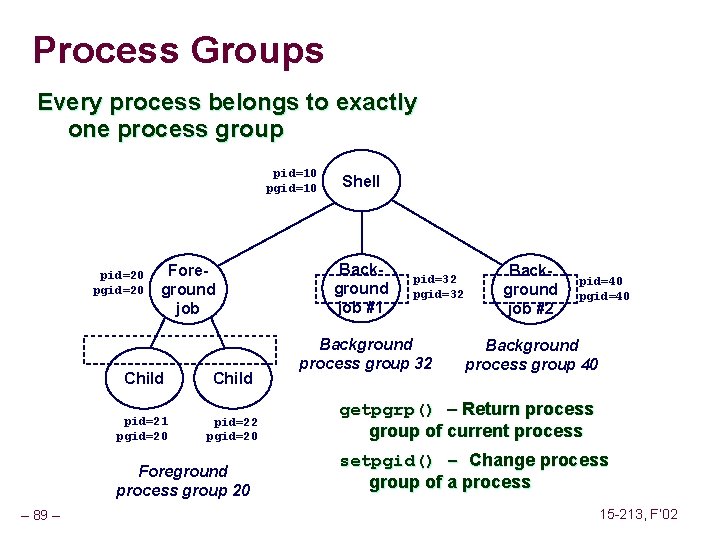
![Sending Signals with kill Function void fork 12() { pid_t pid[N]; int i, child_status; Sending Signals with kill Function void fork 12() { pid_t pid[N]; int i, child_status;](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-90.jpg)
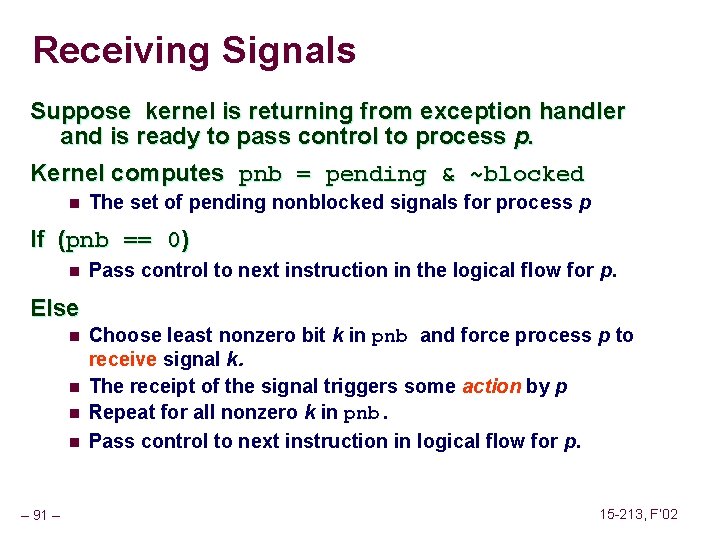
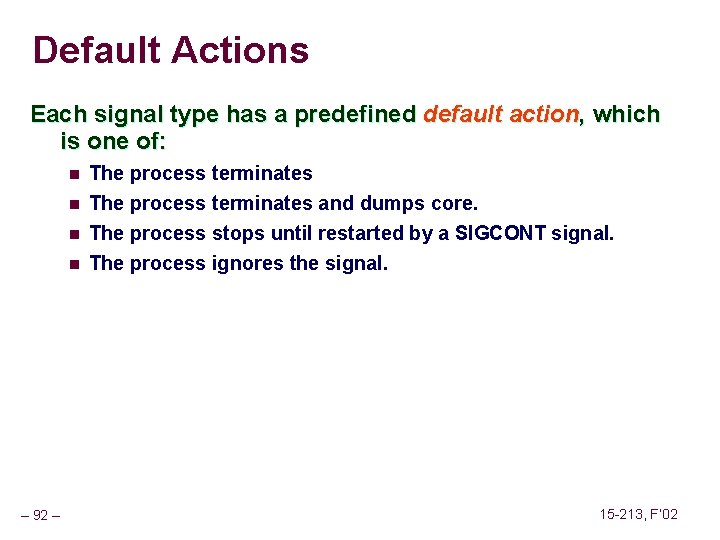
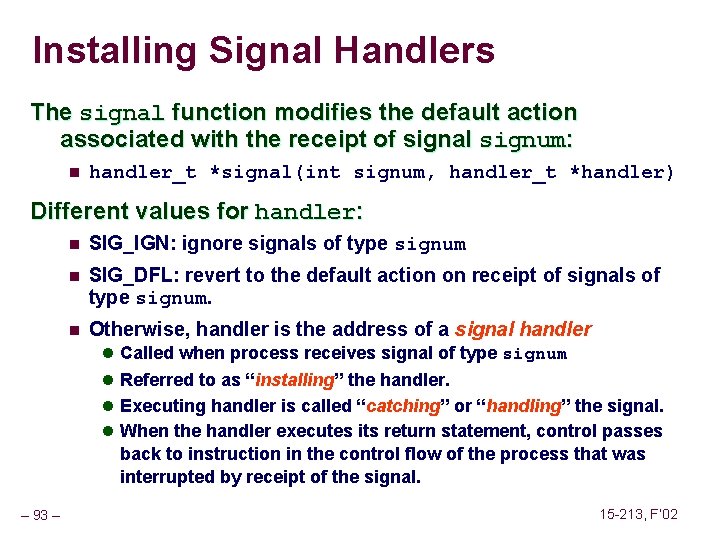
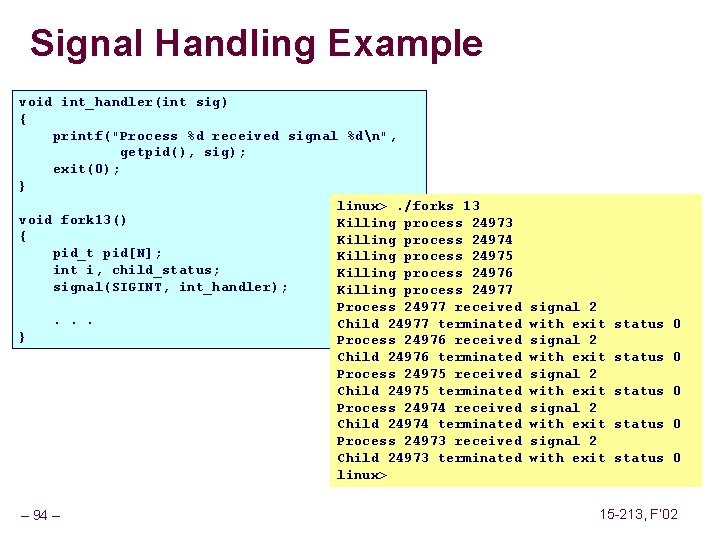
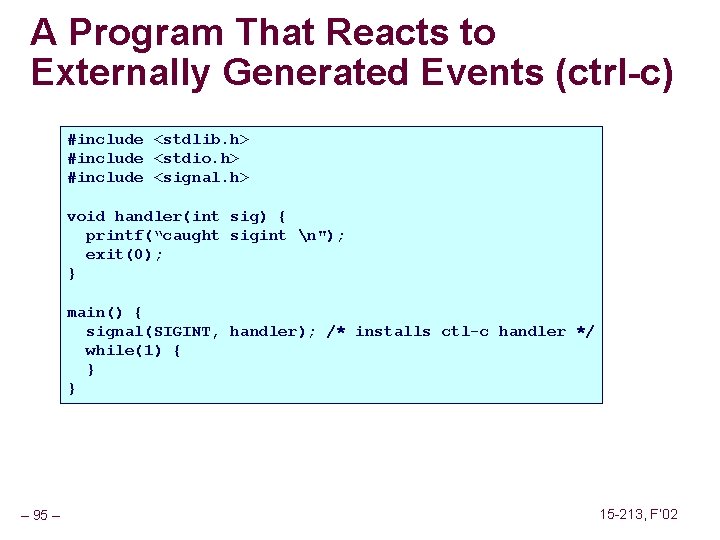
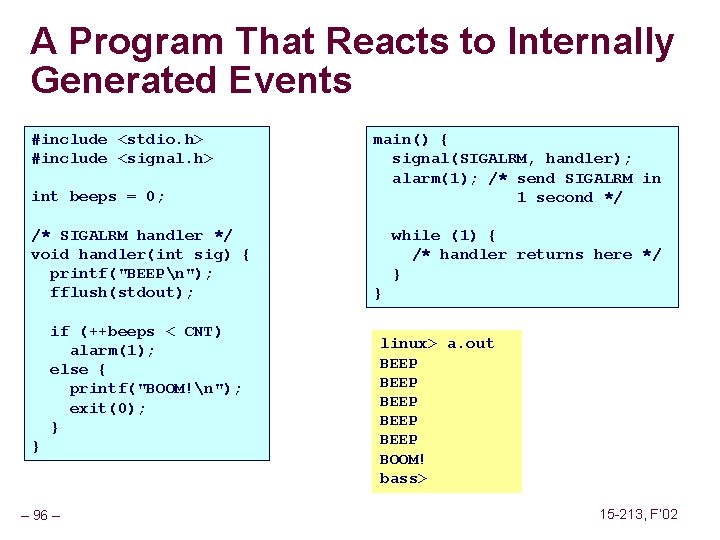
- Slides: 96
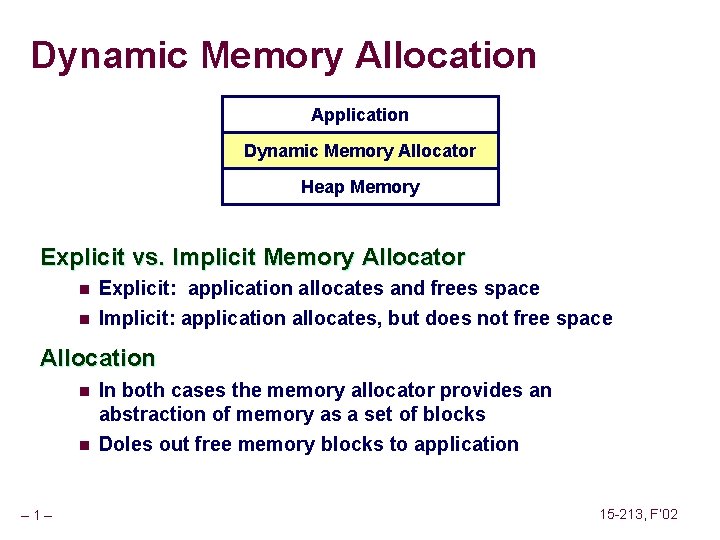
Dynamic Memory Allocation Application Dynamic Memory Allocator Heap Memory Explicit vs. Implicit Memory Allocator n Explicit: application allocates and frees space n Implicit: application allocates, but does not free space Allocation n n – 1– In both cases the memory allocator provides an abstraction of memory as a set of blocks Doles out free memory blocks to application 15 -213, F’ 02
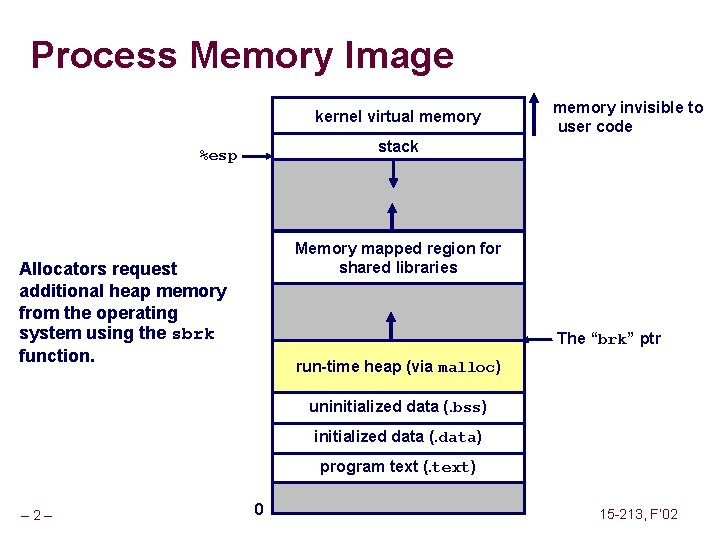
Process Memory Image kernel virtual memory invisible to user code stack %esp Memory mapped region for shared libraries Allocators request additional heap memory from the operating system using the sbrk function. The “brk” ptr run-time heap (via malloc) uninitialized data (. bss) initialized data (. data) program text (. text) – 2– 0 15 -213, F’ 02
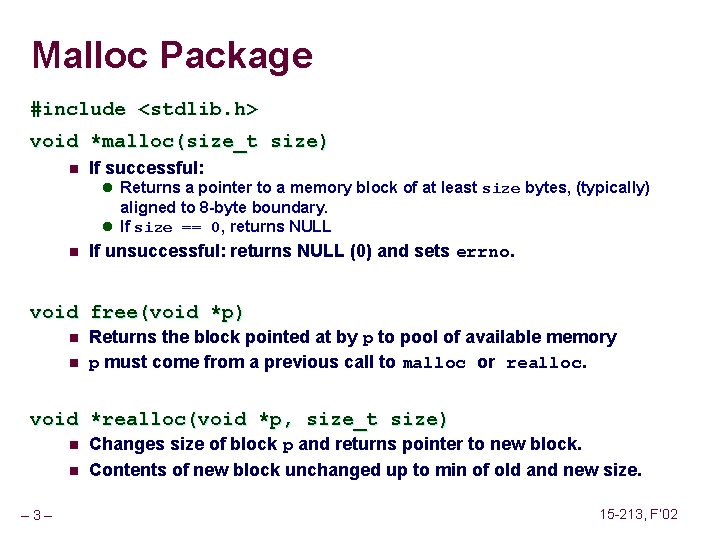
Malloc Package #include <stdlib. h> void *malloc(size_t size) n If successful: l Returns a pointer to a memory block of at least size bytes, (typically) aligned to 8 -byte boundary. l If size == 0, returns NULL n If unsuccessful: returns NULL (0) and sets errno. void free(void *p) n n Returns the block pointed at by p to pool of available memory p must come from a previous call to malloc or realloc. void *realloc(void *p, size_t size) n n – 3– Changes size of block p and returns pointer to new block. Contents of new block unchanged up to min of old and new size. 15 -213, F’ 02
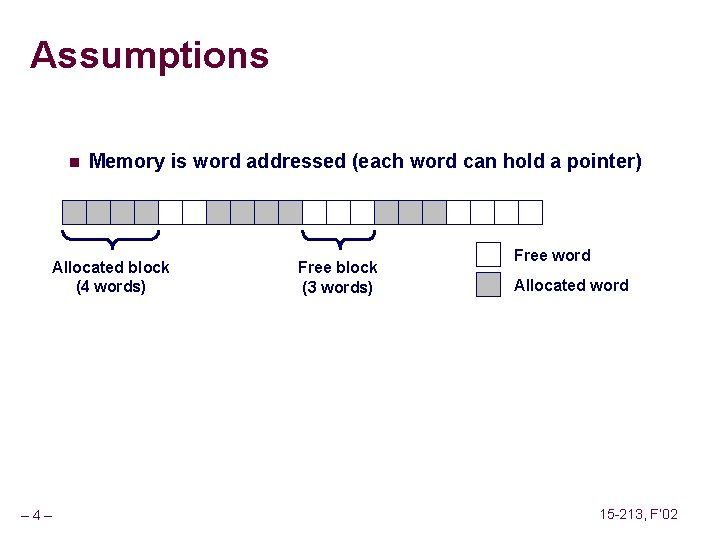
Assumptions n Memory is word addressed (each word can hold a pointer) Allocated block (4 words) – 4– Free block (3 words) Free word Allocated word 15 -213, F’ 02
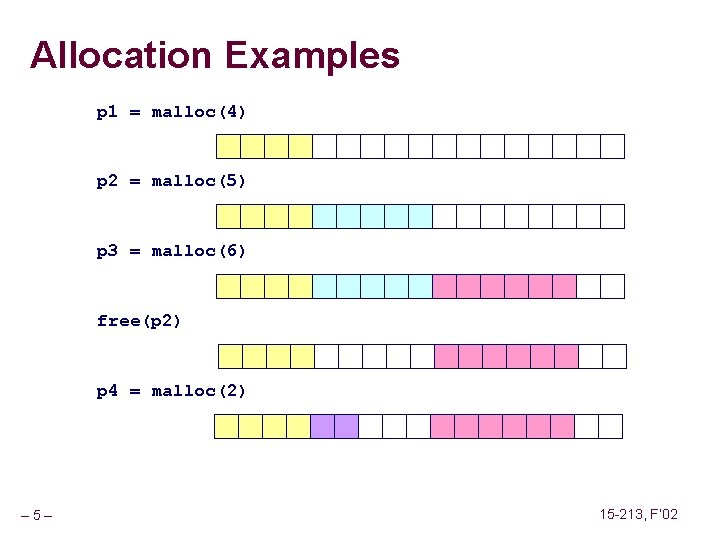
Allocation Examples p 1 = malloc(4) p 2 = malloc(5) p 3 = malloc(6) free(p 2) p 4 = malloc(2) – 5– 15 -213, F’ 02
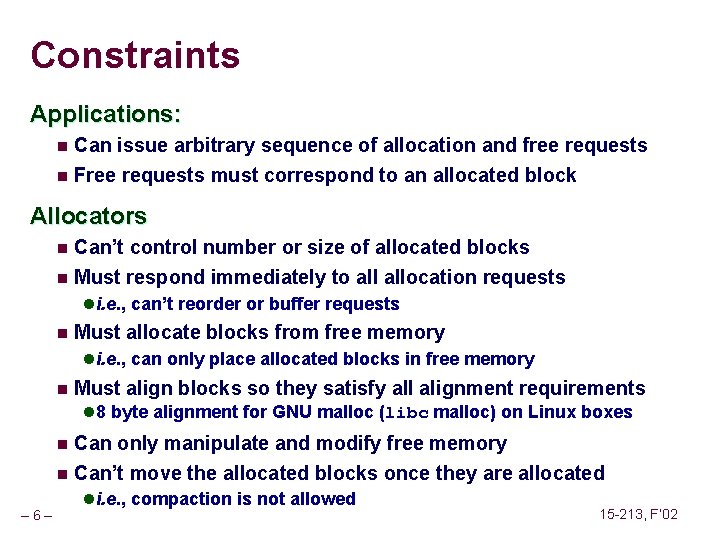
Constraints Applications: n Can issue arbitrary sequence of allocation and free requests n Free requests must correspond to an allocated block Allocators Can’t control number or size of allocated blocks n Must respond immediately to allocation requests n l i. e. , can’t reorder or buffer requests n Must allocate blocks from free memory l i. e. , can only place allocated blocks in free memory n Must align blocks so they satisfy all alignment requirements l 8 byte alignment for GNU malloc (libc malloc) on Linux boxes Can only manipulate and modify free memory n Can’t move the allocated blocks once they are allocated n – 6– l i. e. , compaction is not allowed 15 -213, F’ 02
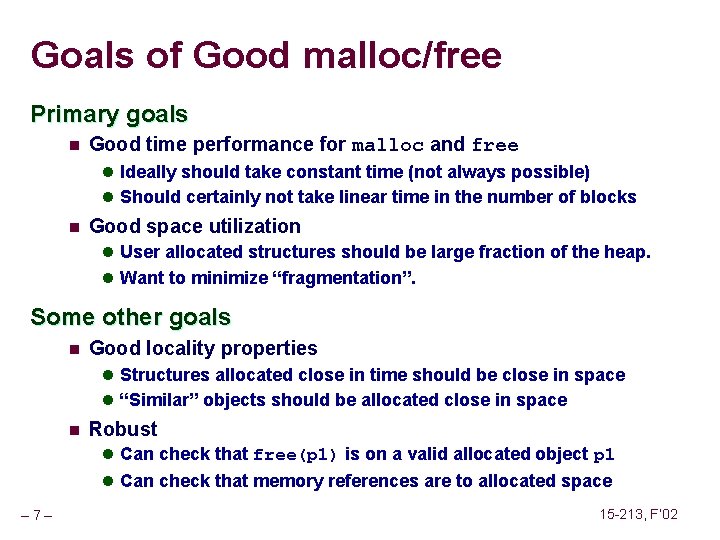
Goals of Good malloc/free Primary goals n Good time performance for malloc and free l Ideally should take constant time (not always possible) l Should certainly not take linear time in the number of blocks n Good space utilization l User allocated structures should be large fraction of the heap. l Want to minimize “fragmentation”. Some other goals n Good locality properties l Structures allocated close in time should be close in space l “Similar” objects should be allocated close in space n Robust l Can check that free(p 1) is on a valid allocated object p 1 l Can check that memory references are to allocated space – 7– 15 -213, F’ 02
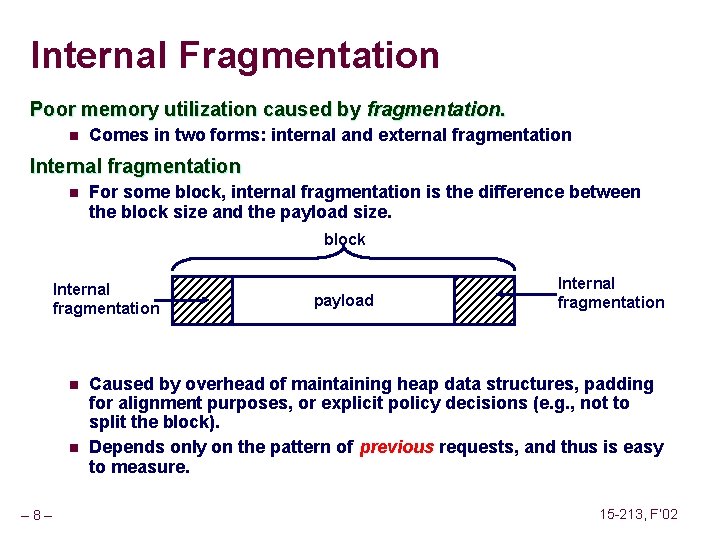
Internal Fragmentation Poor memory utilization caused by fragmentation. n Comes in two forms: internal and external fragmentation Internal fragmentation n For some block, internal fragmentation is the difference between the block size and the payload size. block Internal fragmentation n n – 8– payload Internal fragmentation Caused by overhead of maintaining heap data structures, padding for alignment purposes, or explicit policy decisions (e. g. , not to split the block). Depends only on the pattern of previous requests, and thus is easy to measure. 15 -213, F’ 02
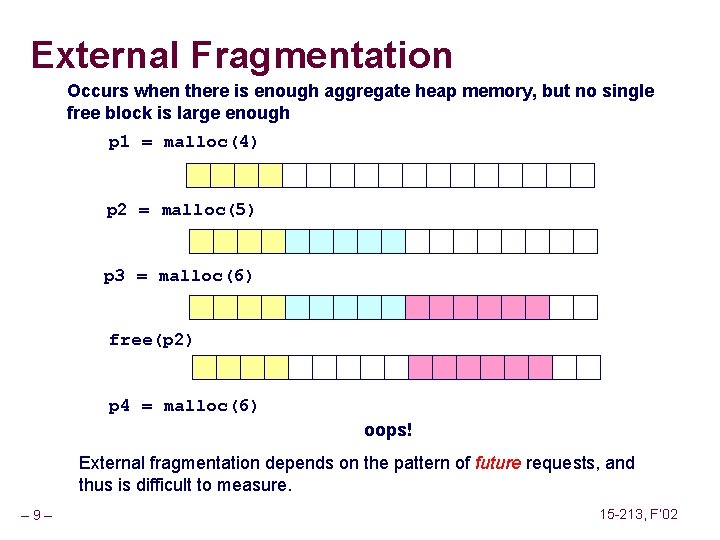
External Fragmentation Occurs when there is enough aggregate heap memory, but no single free block is large enough p 1 = malloc(4) p 2 = malloc(5) p 3 = malloc(6) free(p 2) p 4 = malloc(6) oops! External fragmentation depends on the pattern of future requests, and thus is difficult to measure. – 9– 15 -213, F’ 02
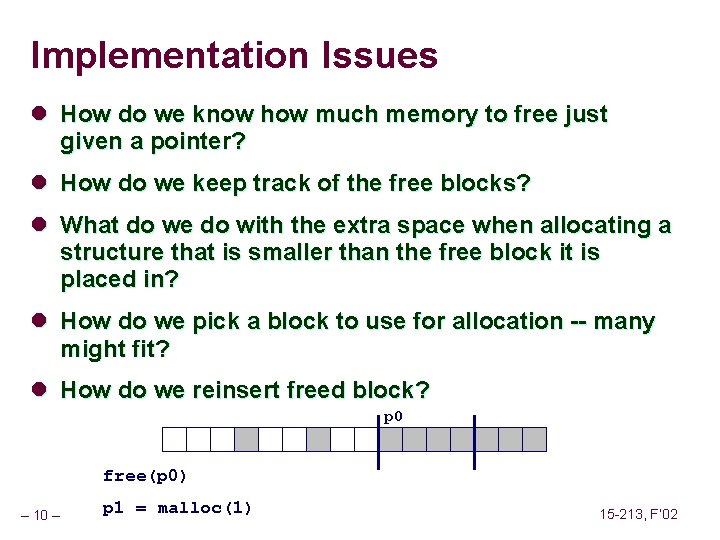
Implementation Issues l How do we know how much memory to free just given a pointer? l How do we keep track of the free blocks? l What do we do with the extra space when allocating a structure that is smaller than the free block it is placed in? l How do we pick a block to use for allocation -- many might fit? l How do we reinsert freed block? p 0 free(p 0) – 10 – p 1 = malloc(1) 15 -213, F’ 02
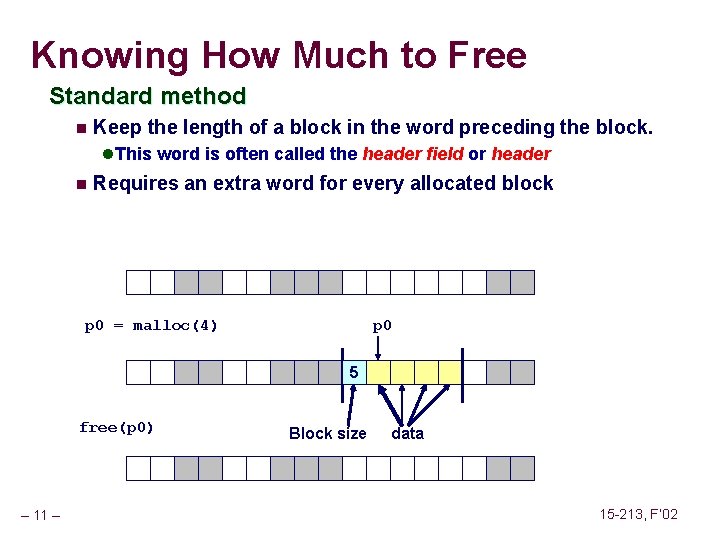
Knowing How Much to Free Standard method n Keep the length of a block in the word preceding the block. l This word is often called the header field or header n Requires an extra word for every allocated block p 0 = malloc(4) p 0 5 free(p 0) – 11 – Block size data 15 -213, F’ 02
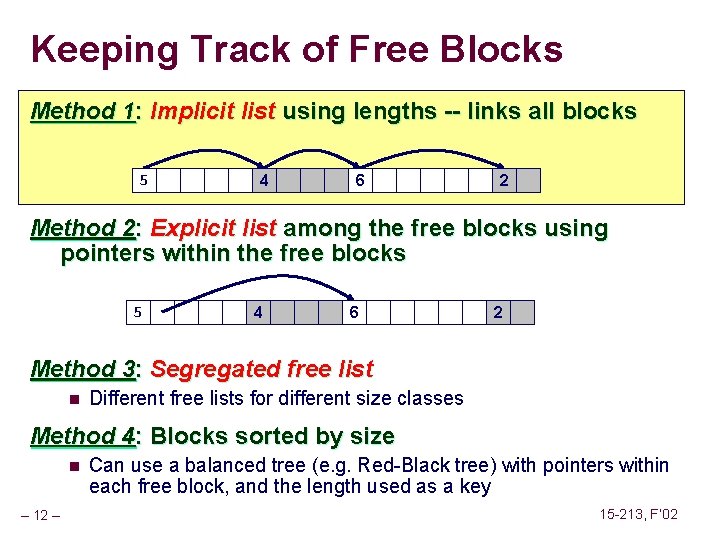
Keeping Track of Free Blocks Method 1: Implicit list using lengths -- links all blocks 5 4 6 2 Method 2: Explicit list among the free blocks using pointers within the free blocks 5 4 6 2 Method 3: Segregated free list n Different free lists for different size classes Method 4: Blocks sorted by size n – 12 – Can use a balanced tree (e. g. Red-Black tree) with pointers within each free block, and the length used as a key 15 -213, F’ 02
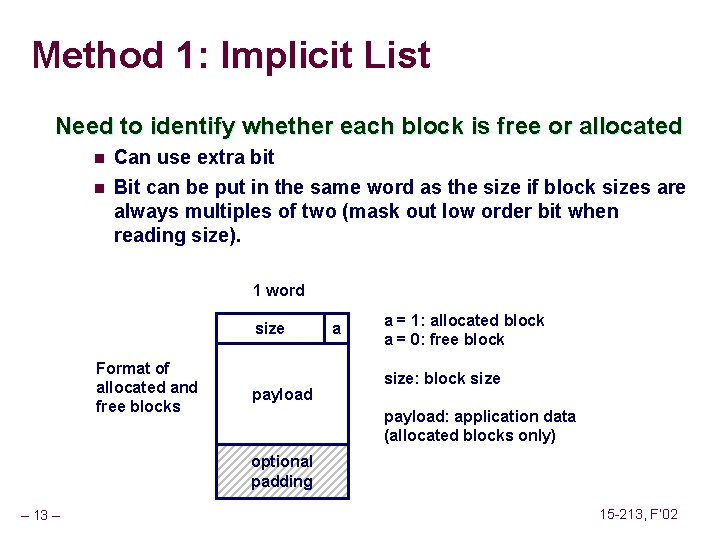
Method 1: Implicit List Need to identify whether each block is free or allocated n Can use extra bit n Bit can be put in the same word as the size if block sizes are always multiples of two (mask out low order bit when reading size). 1 word size Format of allocated and free blocks payload a a = 1: allocated block a = 0: free block size: block size payload: application data (allocated blocks only) optional padding – 13 – 15 -213, F’ 02
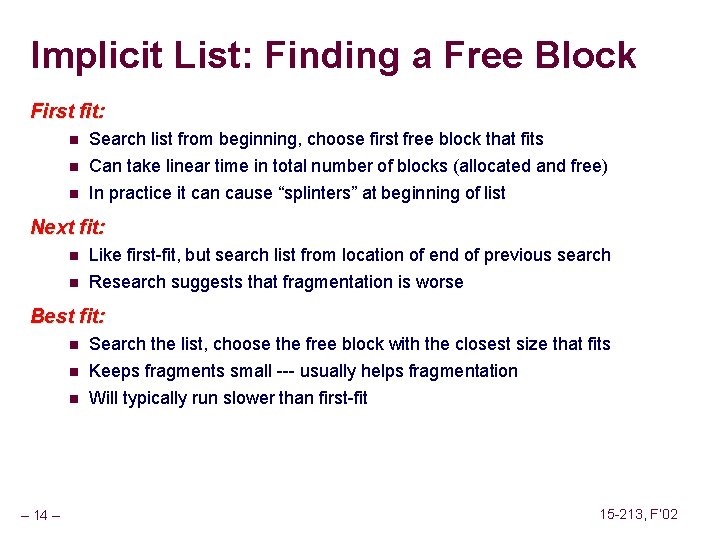
Implicit List: Finding a Free Block First fit: n Search list from beginning, choose first free block that fits Can take linear time in total number of blocks (allocated and free) n In practice it can cause “splinters” at beginning of list n Next fit: n n Like first-fit, but search list from location of end of previous search Research suggests that fragmentation is worse Best fit: n Search the list, choose the free block with the closest size that fits n Keeps fragments small --- usually helps fragmentation Will typically run slower than first-fit n – 14 – 15 -213, F’ 02
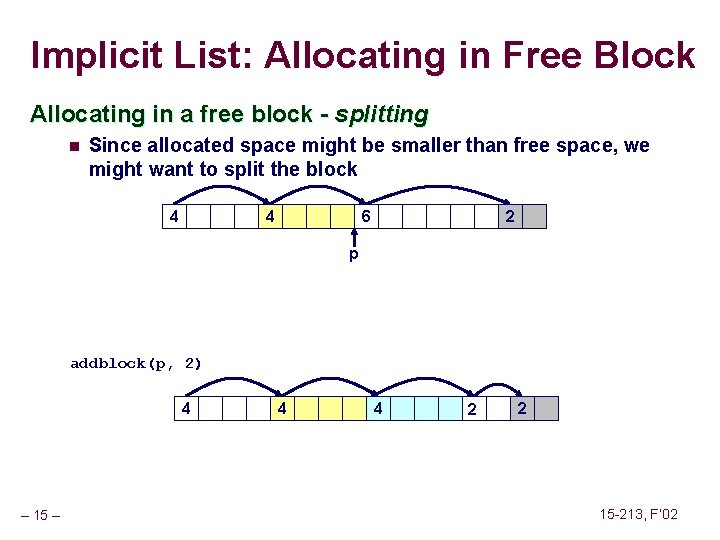
Implicit List: Allocating in Free Block Allocating in a free block - splitting n Since allocated space might be smaller than free space, we might want to split the block 4 4 6 2 p addblock(p, 2) 4 – 15 – 4 4 2 2 15 -213, F’ 02
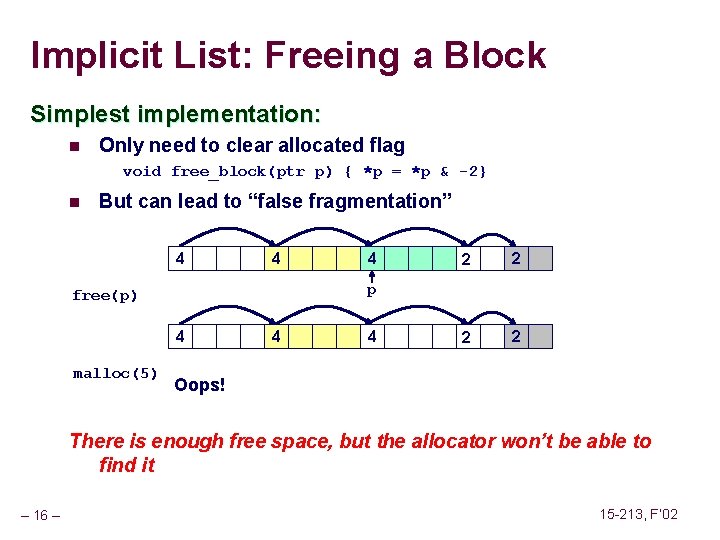
Implicit List: Freeing a Block Simplest implementation: n Only need to clear allocated flag void free_block(ptr p) { *p = *p & -2} n But can lead to “false fragmentation” 4 4 2 2 p free(p) 4 malloc(5) 4 4 4 Oops! There is enough free space, but the allocator won’t be able to find it – 16 – 15 -213, F’ 02
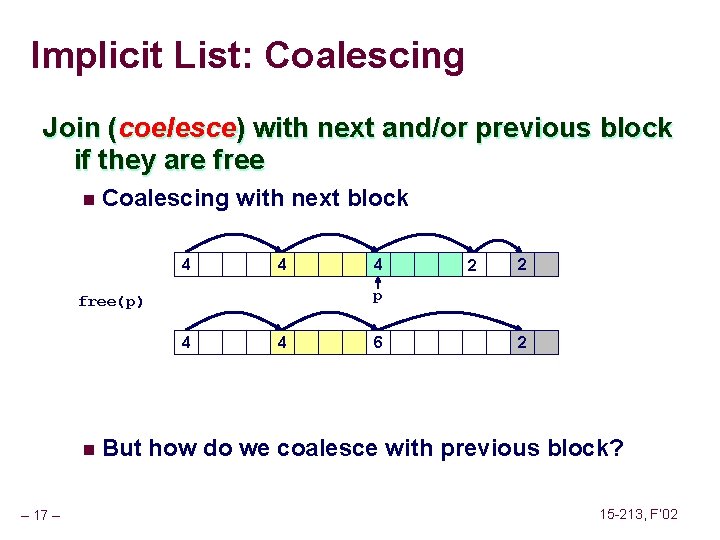
Implicit List: Coalescing Join (coelesce) with next and/or previous block if they are free n Coalescing with next block 4 4 4 – 17 – 2 2 p free(p) n 4 4 6 2 But how do we coalesce with previous block? 15 -213, F’ 02
![Implicit List Bidirectional Coalescing Boundary tags Knuth 73 n n n Replicate sizeallocated word Implicit List: Bidirectional Coalescing Boundary tags [Knuth 73] n n n Replicate size/allocated word](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-18.jpg)
Implicit List: Bidirectional Coalescing Boundary tags [Knuth 73] n n n Replicate size/allocated word at bottom of free blocks Allows us to traverse the “list” backwards, but requires extra space Important and general technique! 1 word Header Format of allocated and free blocks – 18 – a payload and padding Boundary tag (footer) 4 size 4 6 a = 1: allocated block a = 0: free block size: total block size a payload: application data (allocated blocks only) 6 4 4 15 -213, F’ 02
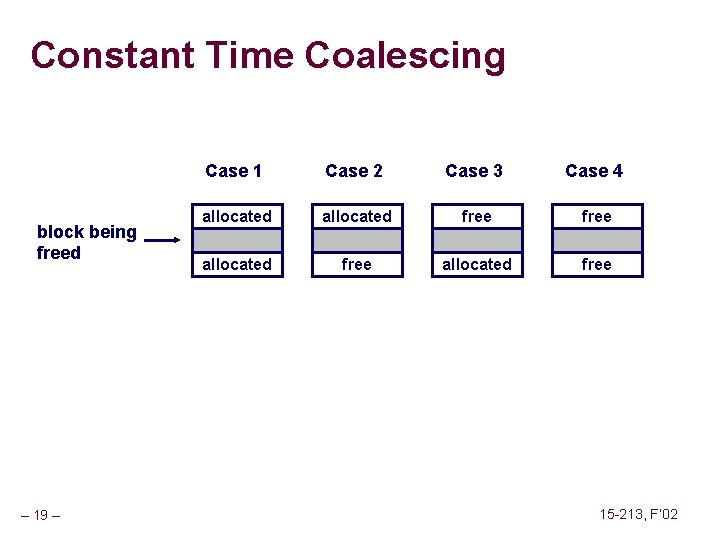
Constant Time Coalescing block being freed – 19 – Case 1 Case 2 Case 3 Case 4 allocated free 15 -213, F’ 02
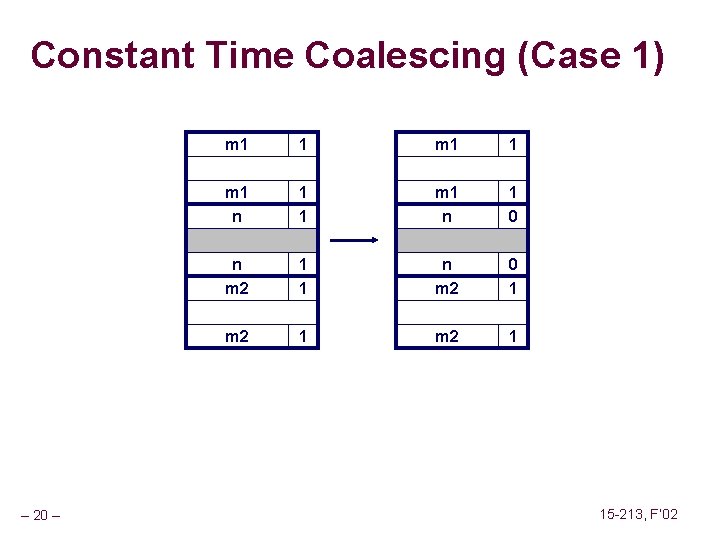
Constant Time Coalescing (Case 1) – 20 – m 1 1 m 1 n 1 0 n m 2 1 1 n m 2 0 1 m 2 1 15 -213, F’ 02
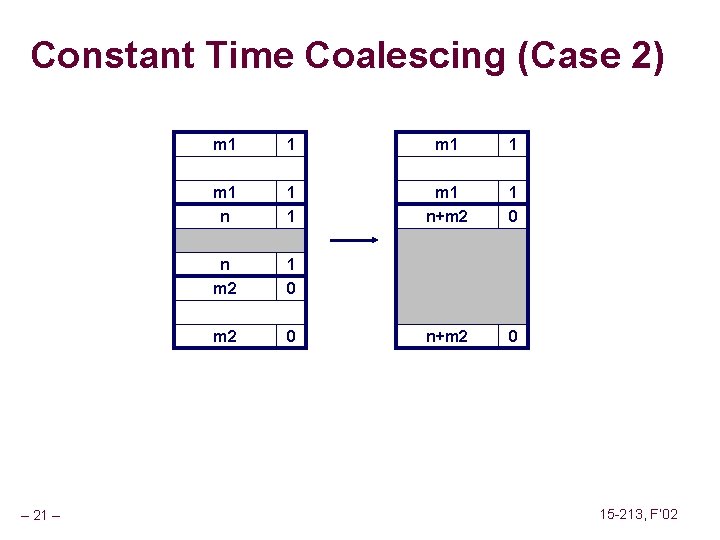
Constant Time Coalescing (Case 2) – 21 – m 1 1 m 1 n+m 2 1 0 n m 2 1 0 m 2 0 n+m 2 0 15 -213, F’ 02
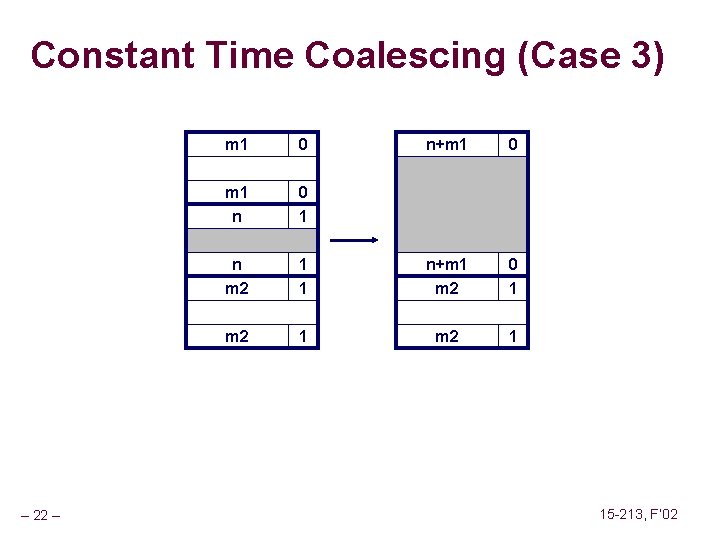
Constant Time Coalescing (Case 3) – 22 – m 1 0 n+m 1 0 m 1 n 0 1 n m 2 1 1 n+m 1 m 2 0 1 m 2 1 15 -213, F’ 02
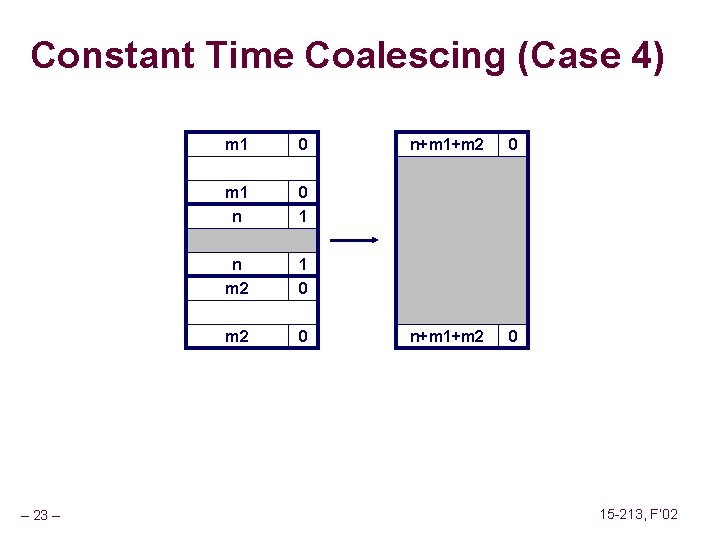
Constant Time Coalescing (Case 4) – 23 – m 1 0 m 1 n 0 1 n m 2 1 0 m 2 0 n+m 1+m 2 0 15 -213, F’ 02
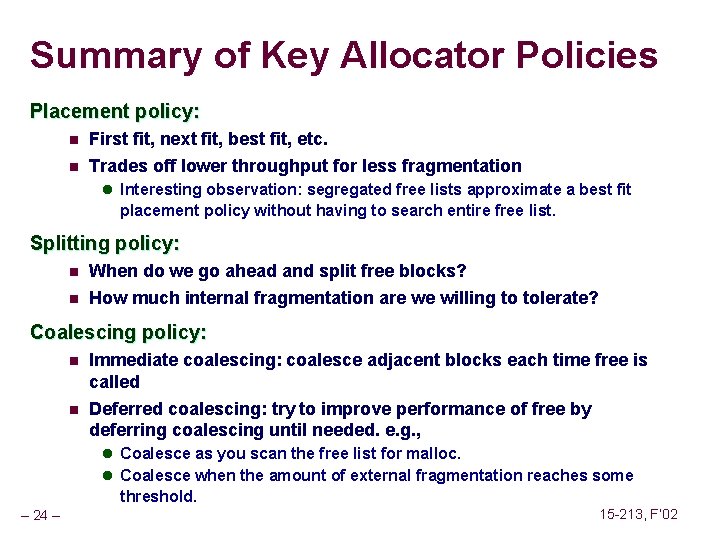
Summary of Key Allocator Policies Placement policy: n n First fit, next fit, best fit, etc. Trades off lower throughput for less fragmentation l Interesting observation: segregated free lists approximate a best fit placement policy without having to search entire free list. Splitting policy: n n When do we go ahead and split free blocks? How much internal fragmentation are we willing to tolerate? Coalescing policy: n n Immediate coalescing: coalesce adjacent blocks each time free is called Deferred coalescing: try to improve performance of free by deferring coalescing until needed. e. g. , l Coalesce as you scan the free list for malloc. l Coalesce when the amount of external fragmentation reaches some threshold. – 24 – 15 -213, F’ 02
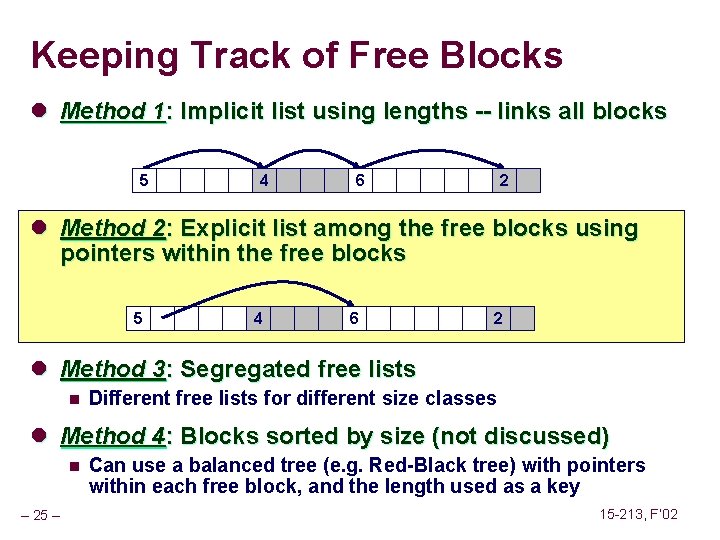
Keeping Track of Free Blocks l Method 1: Implicit list using lengths -- links all blocks 5 4 6 2 l Method 2: Explicit list among the free blocks using pointers within the free blocks 5 4 6 2 l Method 3: Segregated free lists n Different free lists for different size classes l Method 4: Blocks sorted by size (not discussed) n – 25 – Can use a balanced tree (e. g. Red-Black tree) with pointers within each free block, and the length used as a key 15 -213, F’ 02
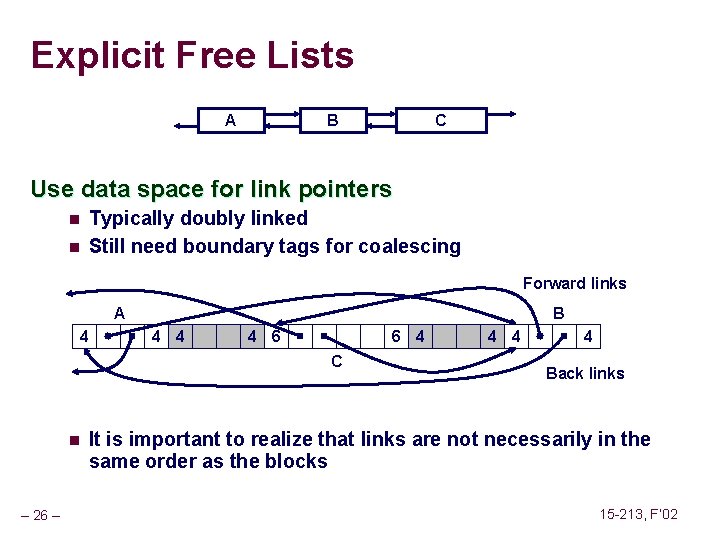
Explicit Free Lists A B C Use data space for link pointers n n Typically doubly linked Still need boundary tags for coalescing Forward links A 4 B 4 4 4 6 6 4 C n – 26 – 4 4 4 Back links It is important to realize that links are not necessarily in the same order as the blocks 15 -213, F’ 02
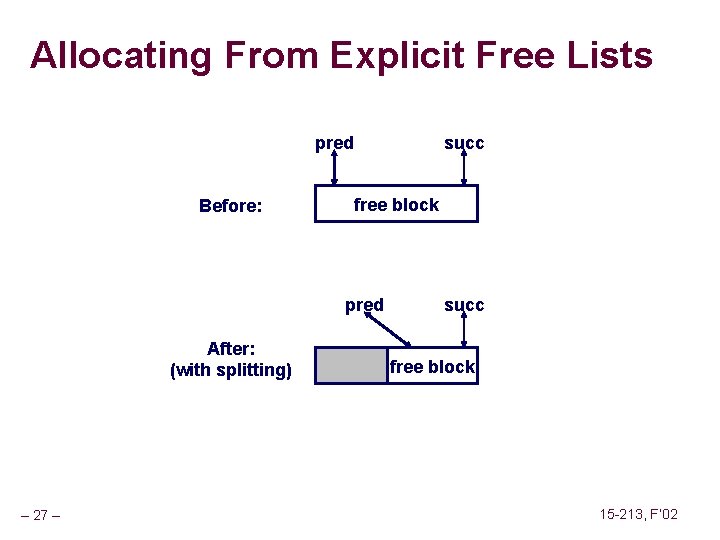
Allocating From Explicit Free Lists pred Before: succ free block pred After: (with splitting) – 27 – succ free block 15 -213, F’ 02
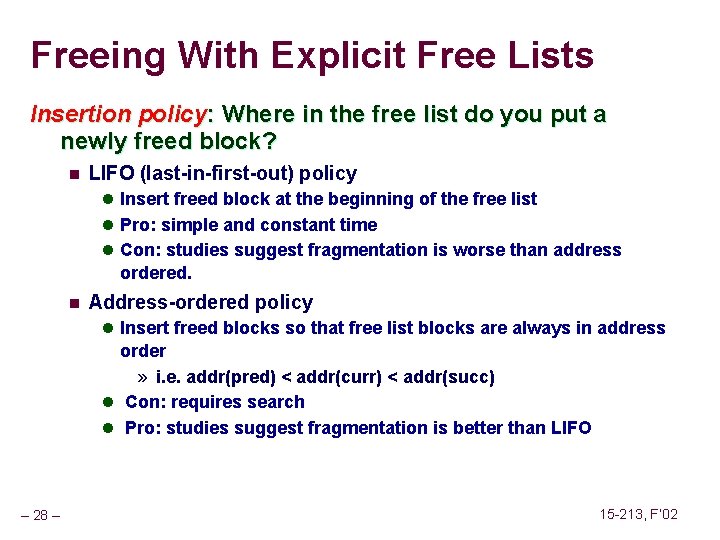
Freeing With Explicit Free Lists Insertion policy: Where in the free list do you put a newly freed block? n LIFO (last-in-first-out) policy l Insert freed block at the beginning of the free list l Pro: simple and constant time l Con: studies suggest fragmentation is worse than address ordered. n Address-ordered policy l Insert freed blocks so that free list blocks are always in address order » i. e. addr(pred) < addr(curr) < addr(succ) l Con: requires search l Pro: studies suggest fragmentation is better than LIFO – 28 – 15 -213, F’ 02
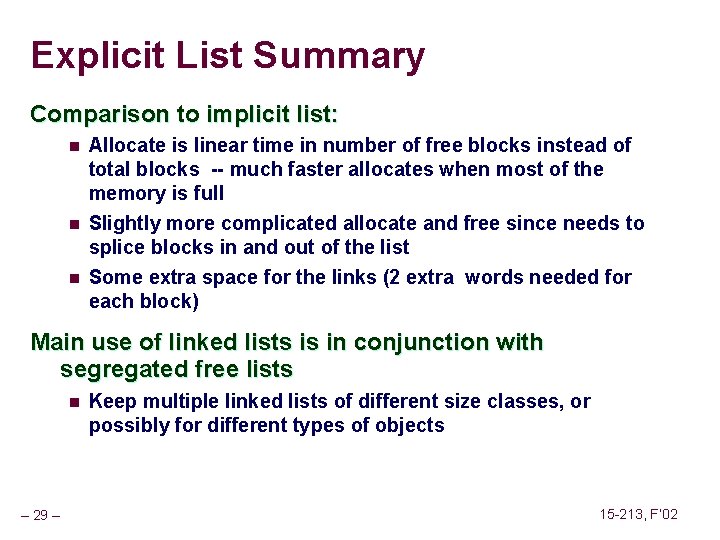
Explicit List Summary Comparison to implicit list: n n n Allocate is linear time in number of free blocks instead of total blocks -- much faster allocates when most of the memory is full Slightly more complicated allocate and free since needs to splice blocks in and out of the list Some extra space for the links (2 extra words needed for each block) Main use of linked lists is in conjunction with segregated free lists n – 29 – Keep multiple linked lists of different size classes, or possibly for different types of objects 15 -213, F’ 02
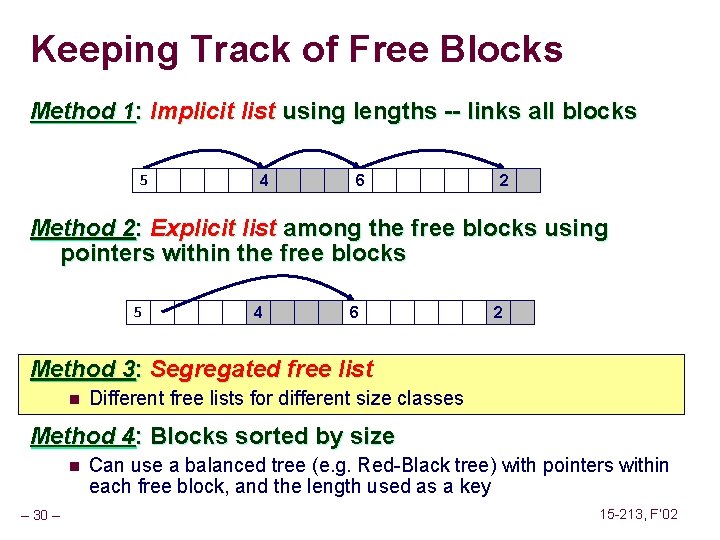
Keeping Track of Free Blocks Method 1: Implicit list using lengths -- links all blocks 5 4 6 2 Method 2: Explicit list among the free blocks using pointers within the free blocks 5 4 6 2 Method 3: Segregated free list n Different free lists for different size classes Method 4: Blocks sorted by size n – 30 – Can use a balanced tree (e. g. Red-Black tree) with pointers within each free block, and the length used as a key 15 -213, F’ 02
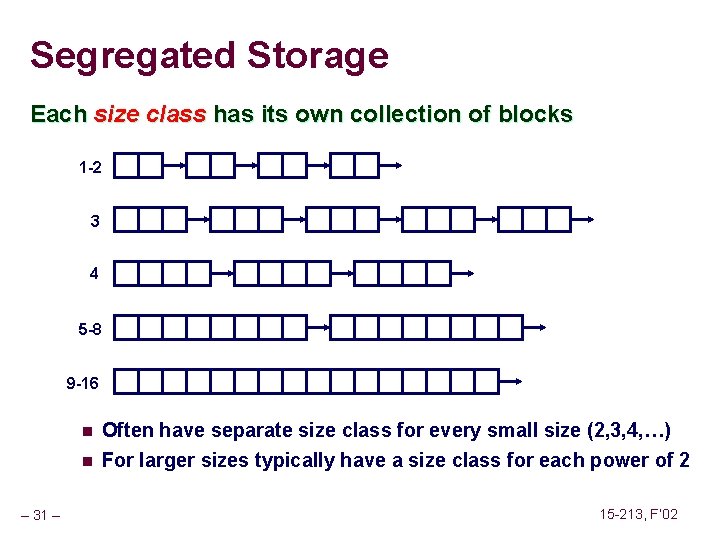
Segregated Storage Each size class has its own collection of blocks 1 -2 3 4 5 -8 9 -16 n n – 31 – Often have separate size class for every small size (2, 3, 4, …) For larger sizes typically have a size class for each power of 2 15 -213, F’ 02
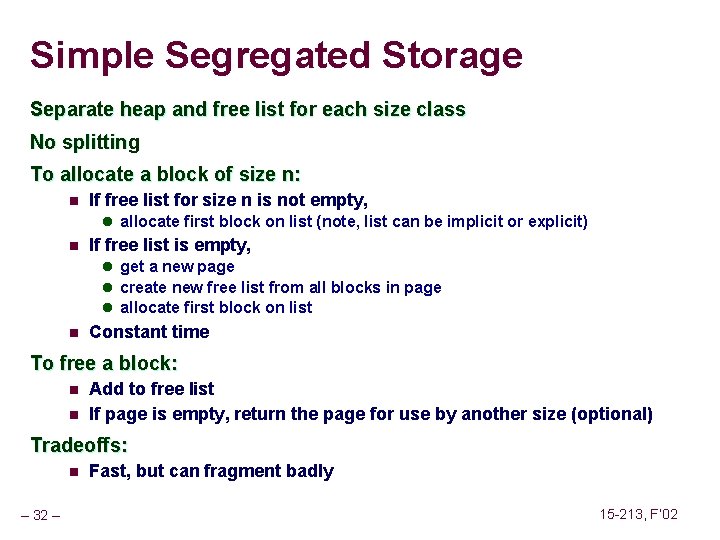
Simple Segregated Storage Separate heap and free list for each size class No splitting To allocate a block of size n: n If free list for size n is not empty, l allocate first block on list (note, list can be implicit or explicit) n If free list is empty, l get a new page l create new free list from all blocks in page l allocate first block on list n Constant time To free a block: n n Add to free list If page is empty, return the page for use by another size (optional) Tradeoffs: n – 32 – Fast, but can fragment badly 15 -213, F’ 02
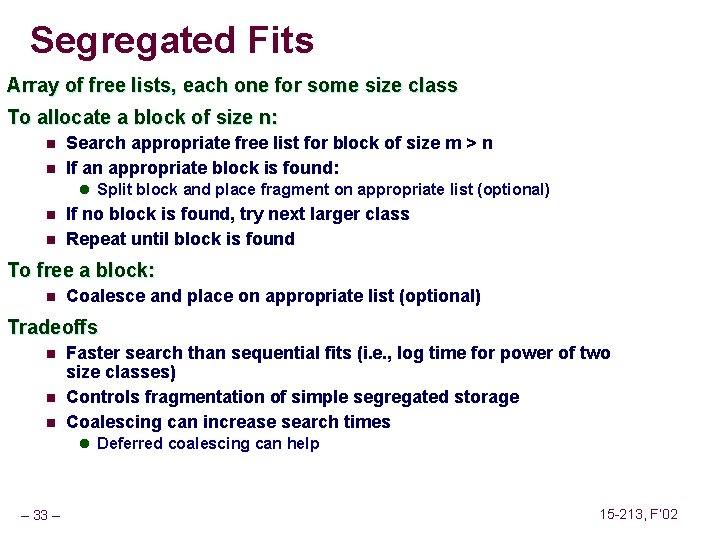
Segregated Fits Array of free lists, each one for some size class To allocate a block of size n: n n Search appropriate free list for block of size m > n If an appropriate block is found: l Split block and place fragment on appropriate list (optional) n n If no block is found, try next larger class Repeat until block is found To free a block: n Coalesce and place on appropriate list (optional) Tradeoffs n n n Faster search than sequential fits (i. e. , log time for power of two size classes) Controls fragmentation of simple segregated storage Coalescing can increase search times l Deferred coalescing can help – 33 – 15 -213, F’ 02
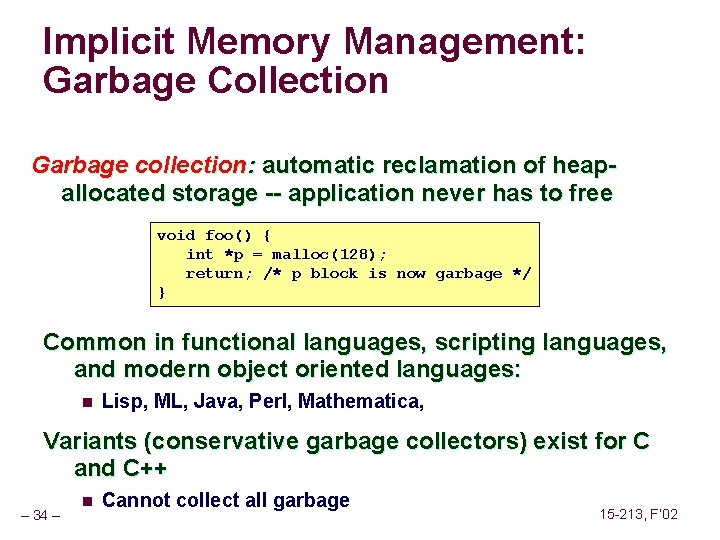
Implicit Memory Management: Garbage Collection Garbage collection: automatic reclamation of heapallocated storage -- application never has to free void foo() { int *p = malloc(128); return; /* p block is now garbage */ } Common in functional languages, scripting languages, and modern object oriented languages: n Lisp, ML, Java, Perl, Mathematica, Variants (conservative garbage collectors) exist for C and C++ – 34 – n Cannot collect all garbage 15 -213, F’ 02
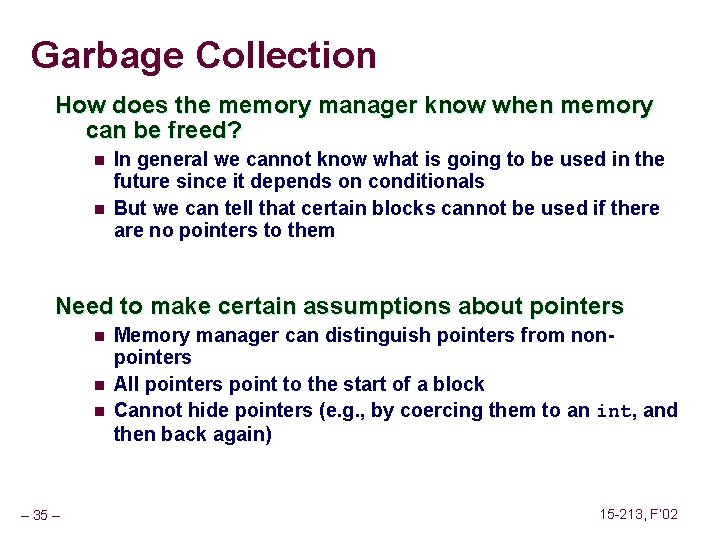
Garbage Collection How does the memory manager know when memory can be freed? n n In general we cannot know what is going to be used in the future since it depends on conditionals But we can tell that certain blocks cannot be used if there are no pointers to them Need to make certain assumptions about pointers n n n – 35 – Memory manager can distinguish pointers from nonpointers All pointers point to the start of a block Cannot hide pointers (e. g. , by coercing them to an int, and then back again) 15 -213, F’ 02
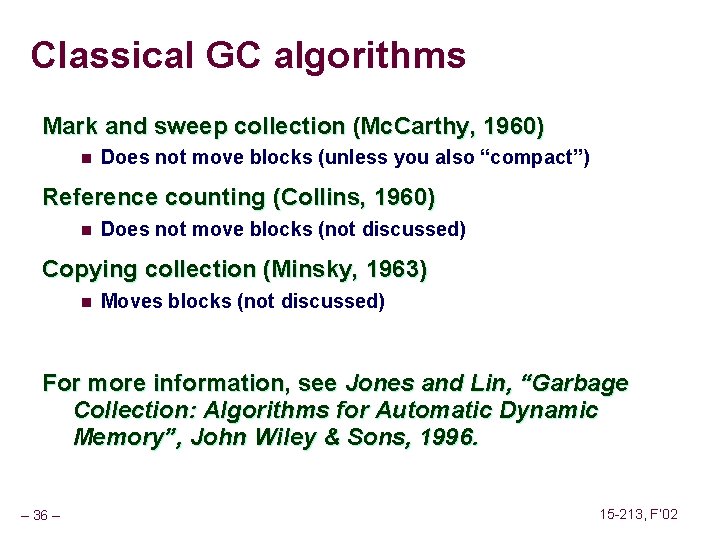
Classical GC algorithms Mark and sweep collection (Mc. Carthy, 1960) n Does not move blocks (unless you also “compact”) Reference counting (Collins, 1960) n Does not move blocks (not discussed) Copying collection (Minsky, 1963) n Moves blocks (not discussed) For more information, see Jones and Lin, “Garbage Collection: Algorithms for Automatic Dynamic Memory”, John Wiley & Sons, 1996. – 36 – 15 -213, F’ 02
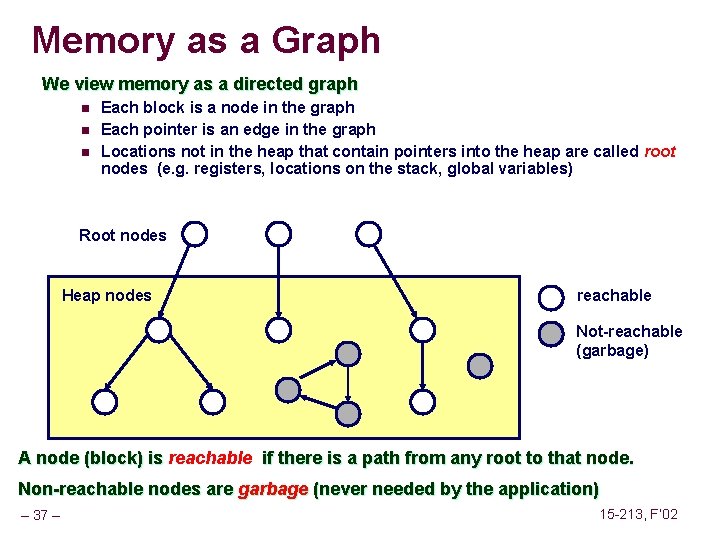
Memory as a Graph We view memory as a directed graph n n n Each block is a node in the graph Each pointer is an edge in the graph Locations not in the heap that contain pointers into the heap are called root nodes (e. g. registers, locations on the stack, global variables) Root nodes Heap nodes reachable Not-reachable (garbage) A node (block) is reachable if there is a path from any root to that node. Non-reachable nodes are garbage (never needed by the application) – 37 – 15 -213, F’ 02
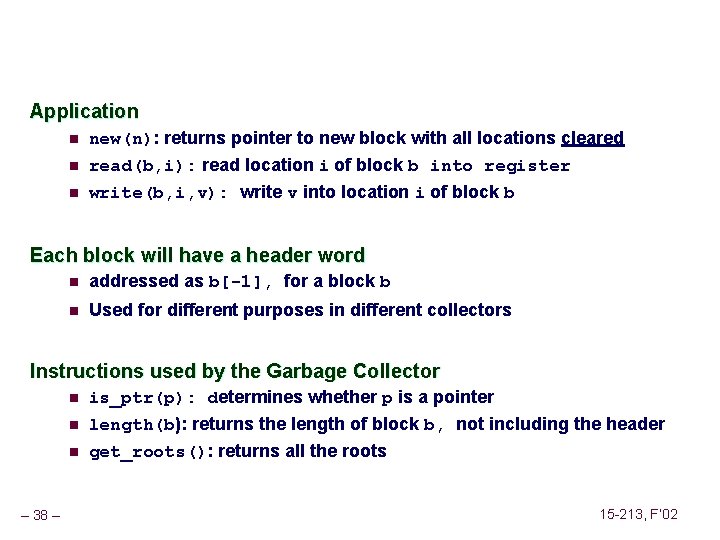
Application n new(n): returns pointer to new block with all locations cleared read(b, i): read location i of block b into register write(b, i, v): write v into location i of block b Each block will have a header word n addressed as b[-1], for a block b n Used for different purposes in different collectors Instructions used by the Garbage Collector n n n – 38 – is_ptr(p): determines whether p is a pointer length(b): returns the length of block b, not including the header get_roots(): returns all the roots 15 -213, F’ 02
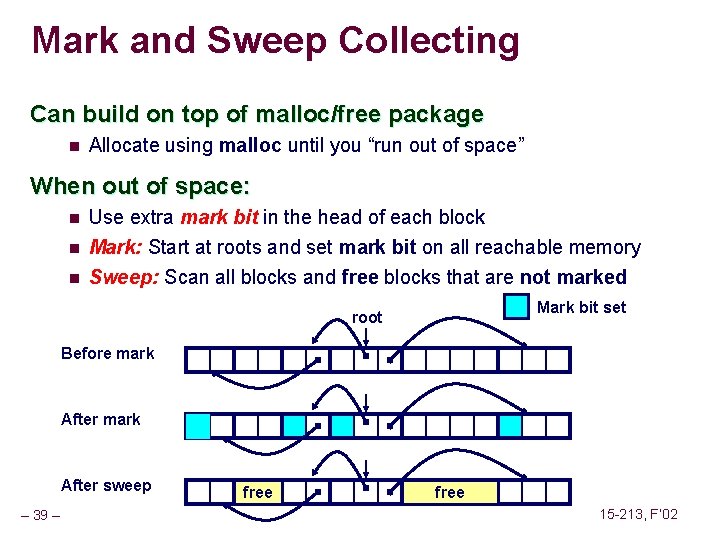
Mark and Sweep Collecting Can build on top of malloc/free package n Allocate using malloc until you “run out of space” When out of space: n n n Use extra mark bit in the head of each block Mark: Start at roots and set mark bit on all reachable memory Sweep: Scan all blocks and free blocks that are not marked Mark bit set root Before mark After sweep – 39 – free 15 -213, F’ 02
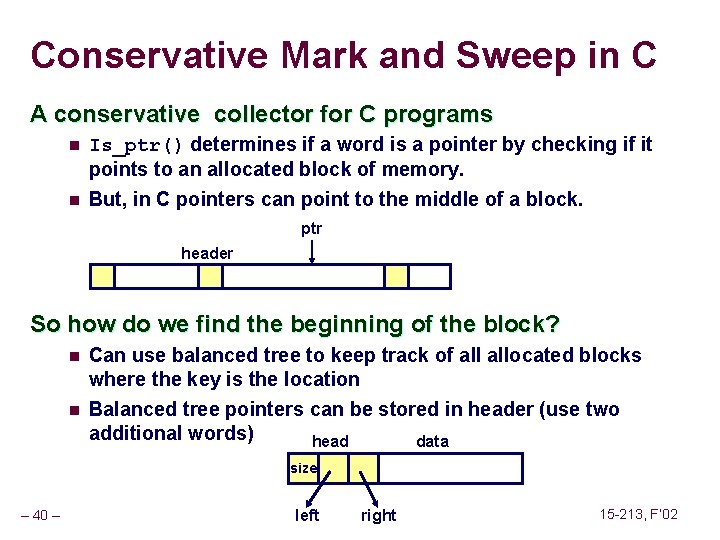
Conservative Mark and Sweep in C A conservative collector for C programs n n Is_ptr() determines if a word is a pointer by checking if it points to an allocated block of memory. But, in C pointers can point to the middle of a block. ptr header So how do we find the beginning of the block? n n Can use balanced tree to keep track of allocated blocks where the key is the location Balanced tree pointers can be stored in header (use two additional words) head data size – 40 – left right 15 -213, F’ 02
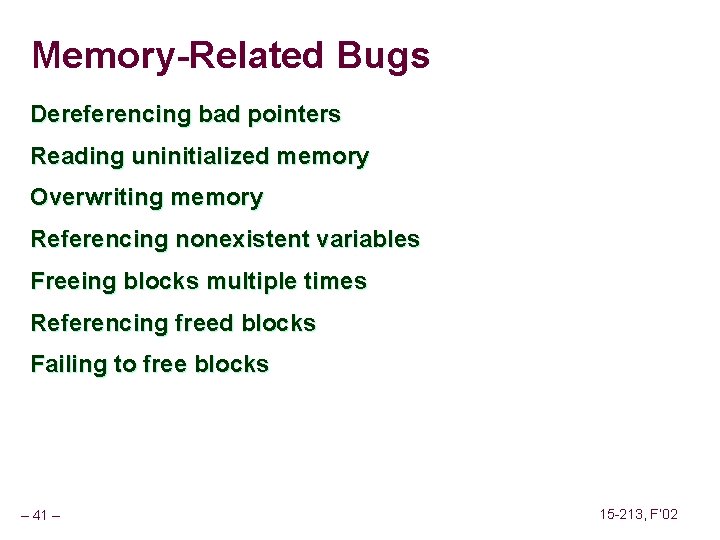
Memory-Related Bugs Dereferencing bad pointers Reading uninitialized memory Overwriting memory Referencing nonexistent variables Freeing blocks multiple times Referencing freed blocks Failing to free blocks – 41 – 15 -213, F’ 02
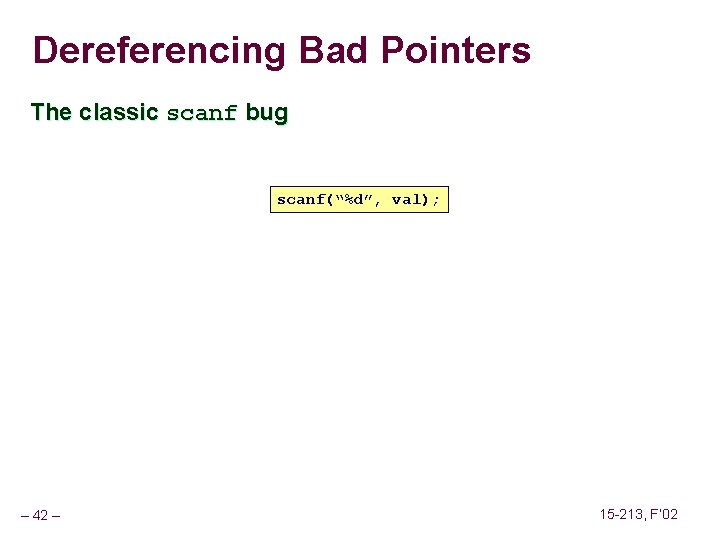
Dereferencing Bad Pointers The classic scanf bug scanf(“%d”, val); – 42 – 15 -213, F’ 02
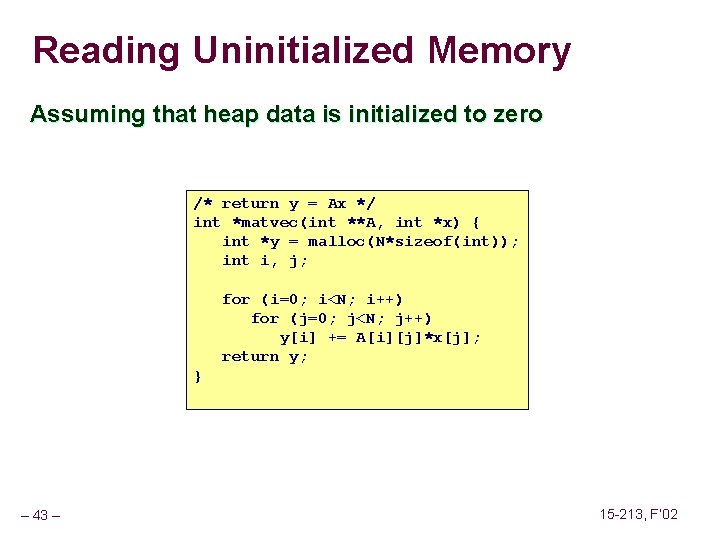
Reading Uninitialized Memory Assuming that heap data is initialized to zero /* return y = Ax */ int *matvec(int **A, int *x) { int *y = malloc(N*sizeof(int)); int i, j; for (i=0; i<N; i++) for (j=0; j<N; j++) y[i] += A[i][j]*x[j]; return y; } – 43 – 15 -213, F’ 02
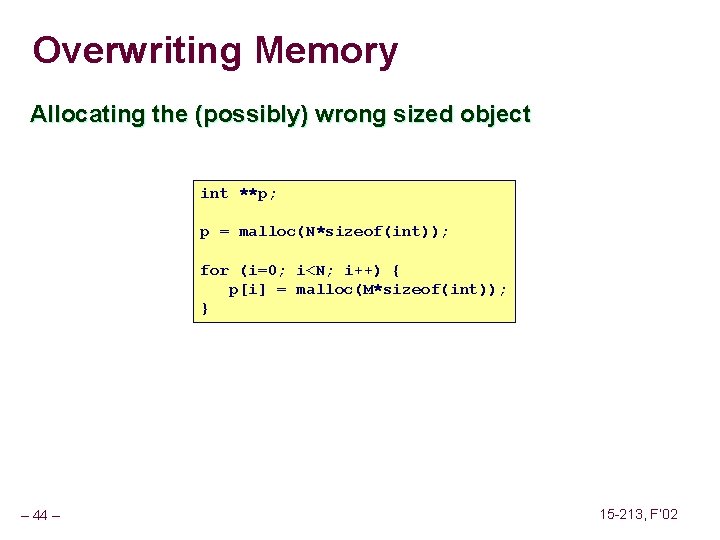
Overwriting Memory Allocating the (possibly) wrong sized object int **p; p = malloc(N*sizeof(int)); for (i=0; i<N; i++) { p[i] = malloc(M*sizeof(int)); } – 44 – 15 -213, F’ 02
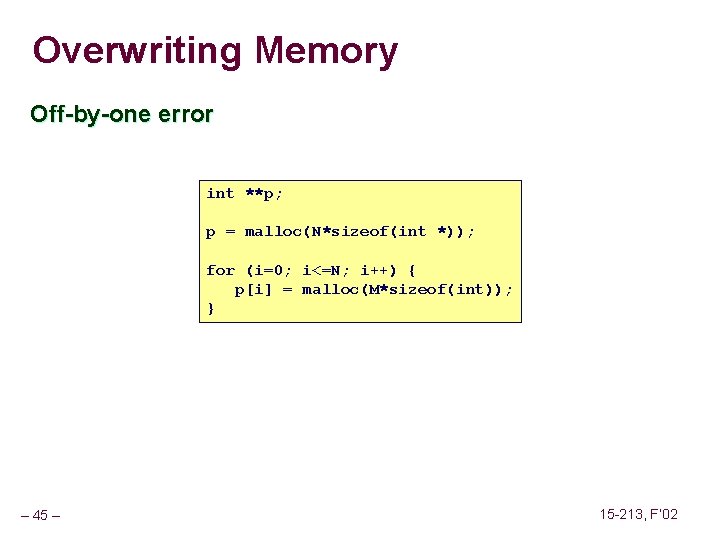
Overwriting Memory Off-by-one error int **p; p = malloc(N*sizeof(int *)); for (i=0; i<=N; i++) { p[i] = malloc(M*sizeof(int)); } – 45 – 15 -213, F’ 02
![Overwriting Memory Not checking the max string size char s8 int i getss Overwriting Memory Not checking the max string size char s[8]; int i; gets(s); /*](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-46.jpg)
Overwriting Memory Not checking the max string size char s[8]; int i; gets(s); /* reads “ 123456789” from stdin */ Basis for classic buffer overflow attacks n n – 46 – 1988 Internet worm Modern attacks on Web servers 15 -213, F’ 02
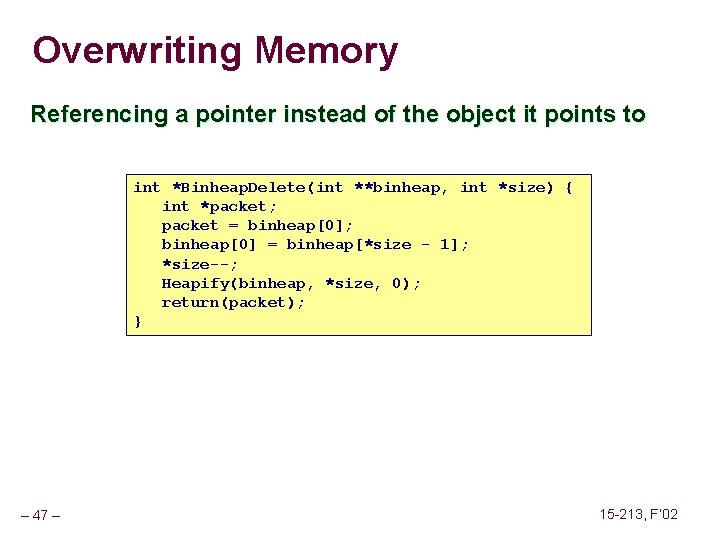
Overwriting Memory Referencing a pointer instead of the object it points to int *Binheap. Delete(int **binheap, int *size) { int *packet; packet = binheap[0]; binheap[0] = binheap[*size - 1]; *size--; Heapify(binheap, *size, 0); return(packet); } – 47 – 15 -213, F’ 02
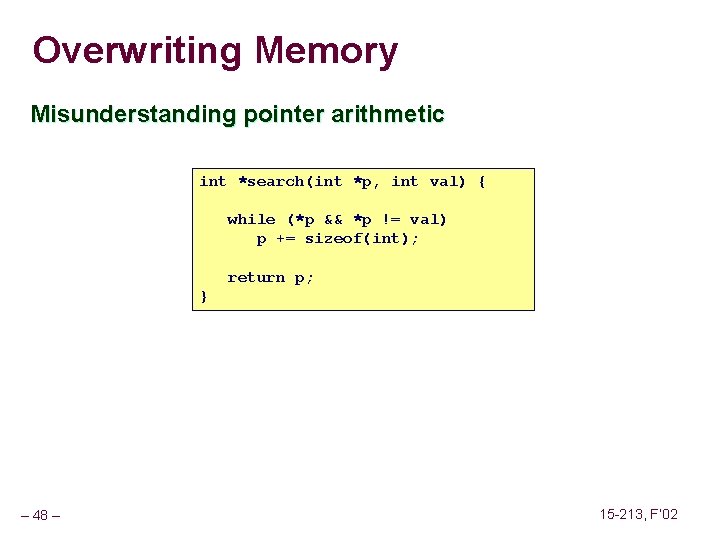
Overwriting Memory Misunderstanding pointer arithmetic int *search(int *p, int val) { while (*p && *p != val) p += sizeof(int); return p; } – 48 – 15 -213, F’ 02
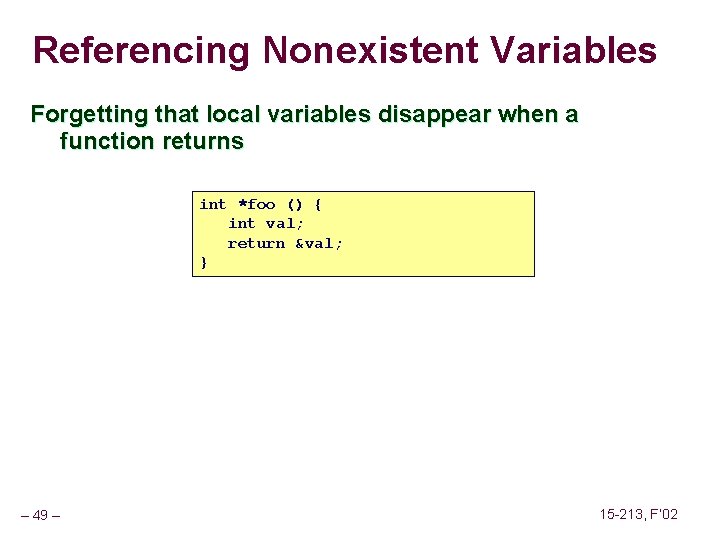
Referencing Nonexistent Variables Forgetting that local variables disappear when a function returns int *foo () { int val; return &val; } – 49 – 15 -213, F’ 02
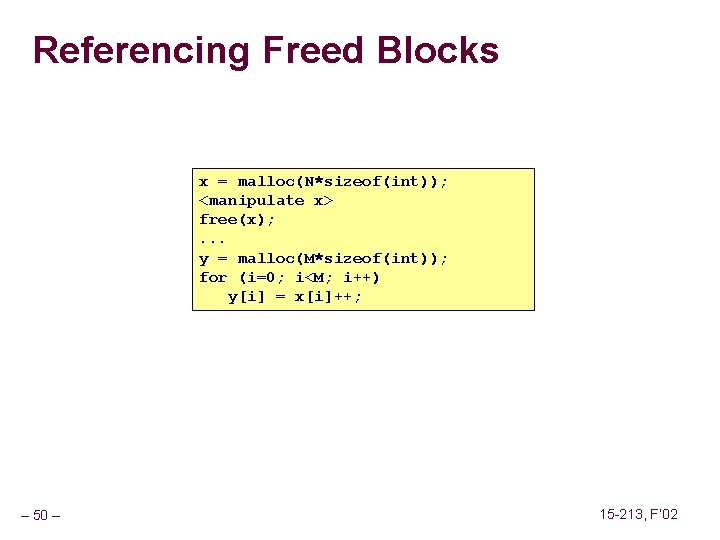
Referencing Freed Blocks x = malloc(N*sizeof(int)); <manipulate x> free(x); . . . y = malloc(M*sizeof(int)); for (i=0; i<M; i++) y[i] = x[i]++; – 50 – 15 -213, F’ 02
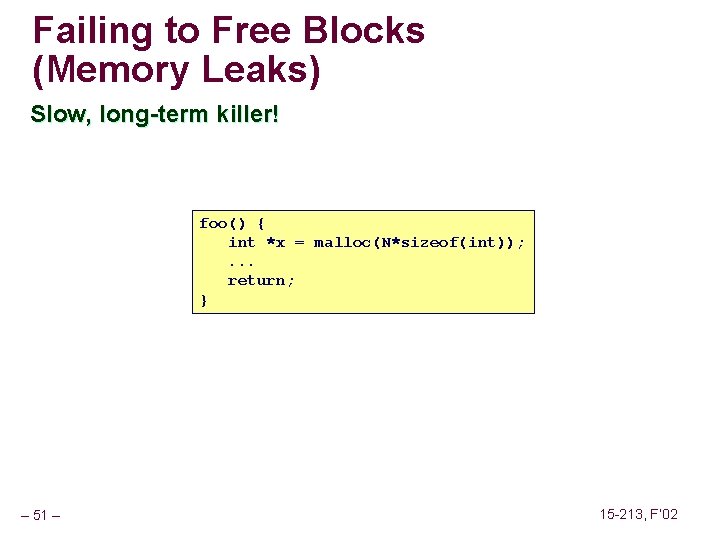
Failing to Free Blocks (Memory Leaks) Slow, long-term killer! foo() { int *x = malloc(N*sizeof(int)); . . . return; } – 51 – 15 -213, F’ 02
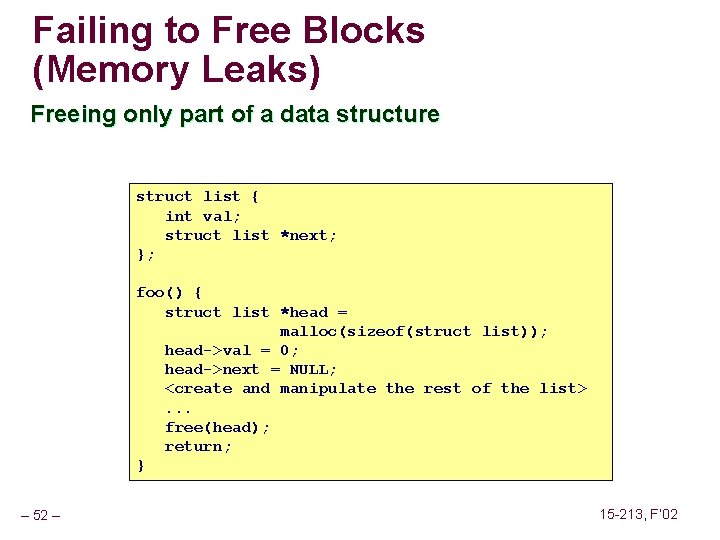
Failing to Free Blocks (Memory Leaks) Freeing only part of a data structure struct list { int val; struct list *next; }; foo() { struct list *head = malloc(sizeof(struct list)); head->val = 0; head->next = NULL; <create and manipulate the rest of the list>. . . free(head); return; } – 52 – 15 -213, F’ 02
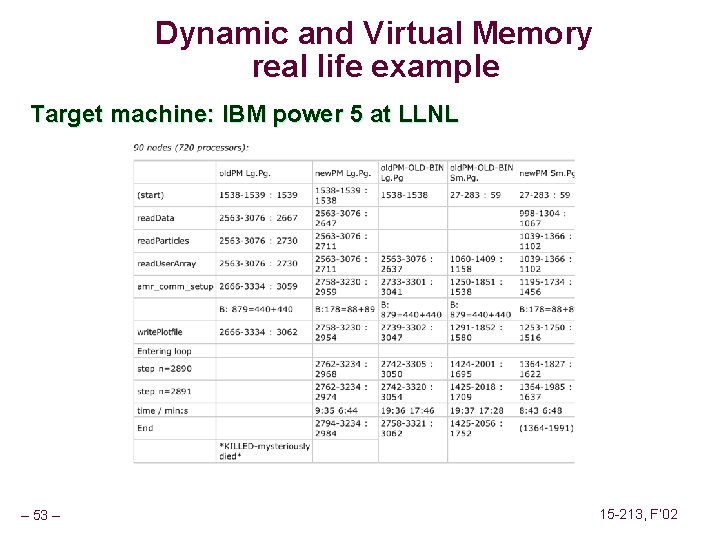
Dynamic and Virtual Memory real life example Target machine: IBM power 5 at LLNL – 53 – 15 -213, F’ 02
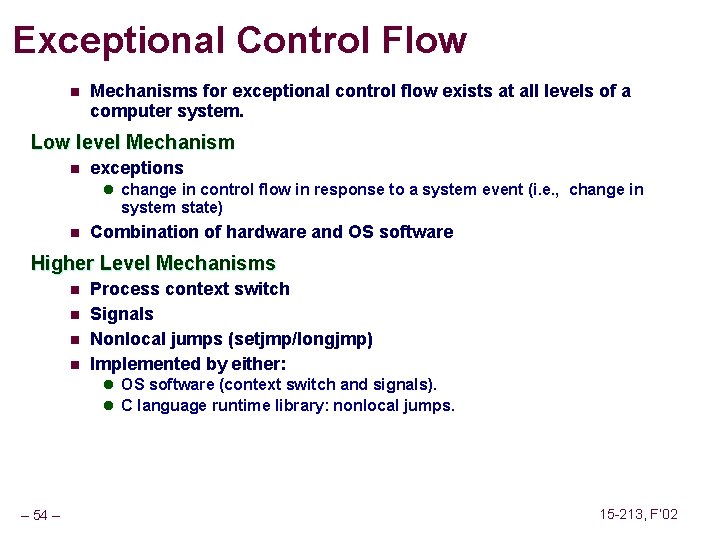
Exceptional Control Flow n Mechanisms for exceptional control flow exists at all levels of a computer system. Low level Mechanism n exceptions l change in control flow in response to a system event (i. e. , change in system state) n Combination of hardware and OS software Higher Level Mechanisms n n Process context switch Signals Nonlocal jumps (setjmp/longjmp) Implemented by either: l OS software (context switch and signals). l C language runtime library: nonlocal jumps. – 54 – 15 -213, F’ 02
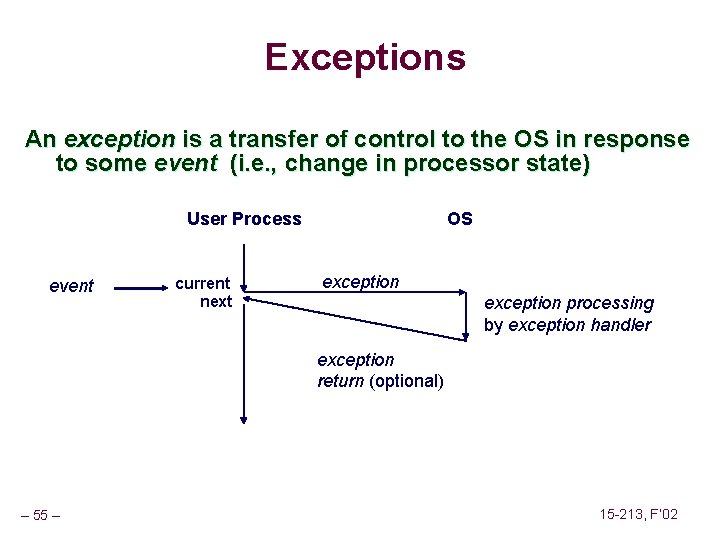
Exceptions An exception is a transfer of control to the OS in response to some event (i. e. , change in processor state) User Process event current next OS exception processing by exception handler exception return (optional) – 55 – 15 -213, F’ 02
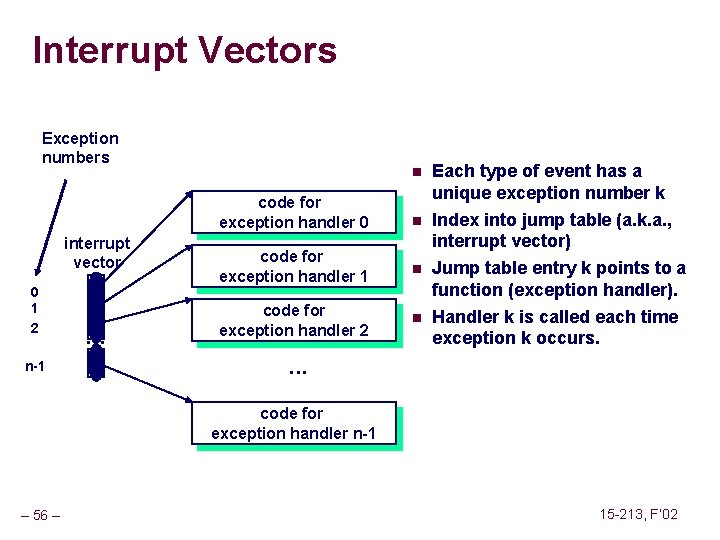
Interrupt Vectors Exception numbers n code for exception handler 0 interrupt vector 0 1 2 n-1 . . . n code for exception handler 1 n code for exception handler 2 n Each type of event has a unique exception number k Index into jump table (a. k. a. , interrupt vector) Jump table entry k points to a function (exception handler). Handler k is called each time exception k occurs. . code for exception handler n-1 – 56 – 15 -213, F’ 02
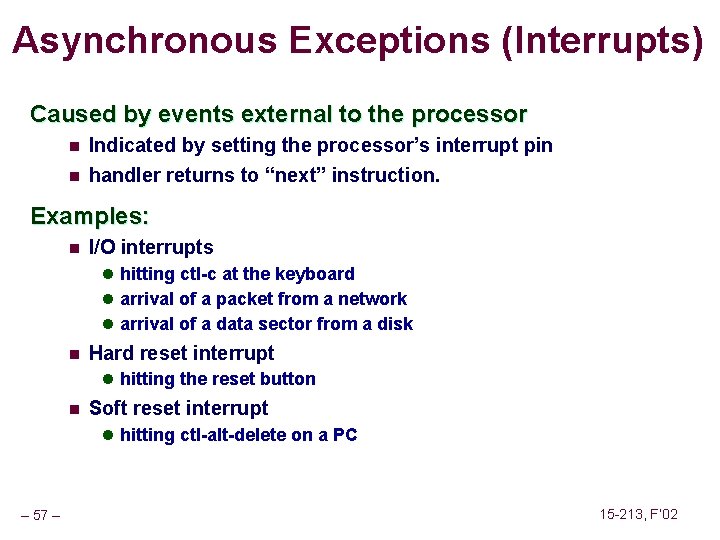
Asynchronous Exceptions (Interrupts) Caused by events external to the processor n Indicated by setting the processor’s interrupt pin n handler returns to “next” instruction. Examples: n I/O interrupts l hitting ctl-c at the keyboard l arrival of a packet from a network l arrival of a data sector from a disk n Hard reset interrupt l hitting the reset button n Soft reset interrupt l hitting ctl-alt-delete on a PC – 57 – 15 -213, F’ 02
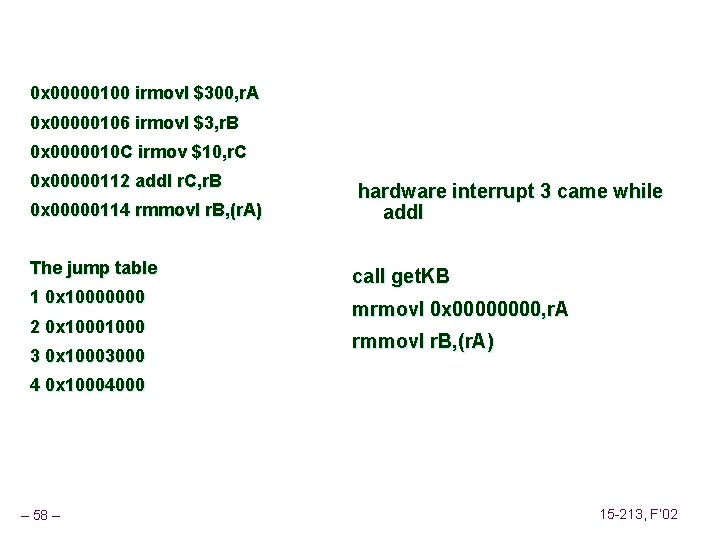
0 x 00000100 irmovl $300, r. A 0 x 00000106 irmovl $3, r. B 0 x 0000010 C irmov $10, r. C 0 x 00000112 addl r. C, r. B 0 x 00000114 rmmovl r. B, (r. A) The jump table 1 0 x 10000000 2 0 x 1000 3 0 x 10003000 hardware interrupt 3 came while addl call get. KB mrmovl 0 x 0000, r. A rmmovl r. B, (r. A) 4 0 x 10004000 – 58 – 15 -213, F’ 02
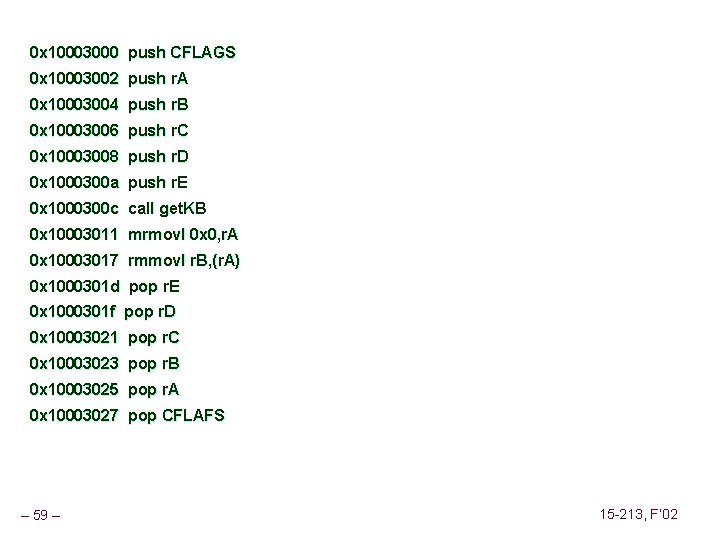
0 x 10003000 push CFLAGS 0 x 10003002 push r. A 0 x 10003004 push r. B 0 x 10003006 push r. C 0 x 10003008 push r. D 0 x 1000300 a push r. E 0 x 1000300 c call get. KB 0 x 10003011 mrmovl 0 x 0, r. A 0 x 10003017 rmmovl r. B, (r. A) 0 x 1000301 d pop r. E 0 x 1000301 f pop r. D 0 x 10003021 pop r. C 0 x 10003023 pop r. B 0 x 10003025 pop r. A 0 x 10003027 pop CFLAFS – 59 – 15 -213, F’ 02
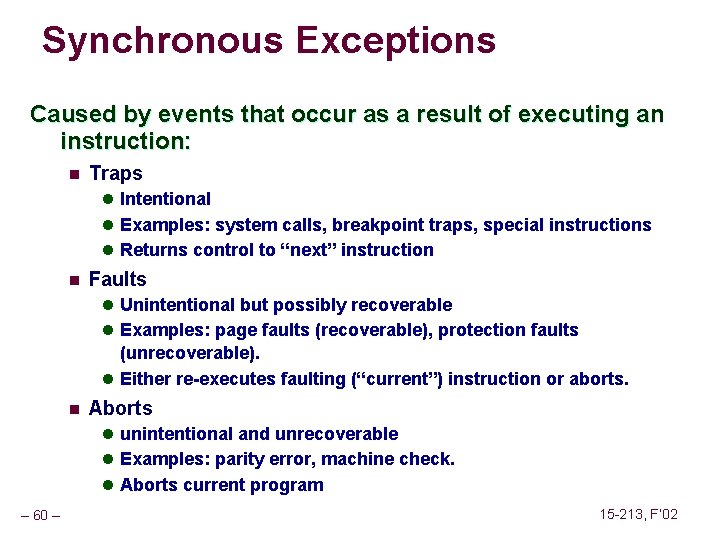
Synchronous Exceptions Caused by events that occur as a result of executing an instruction: n Traps l Intentional l Examples: system calls, breakpoint traps, special instructions l Returns control to “next” instruction n Faults l Unintentional but possibly recoverable l Examples: page faults (recoverable), protection faults (unrecoverable). l Either re-executes faulting (“current”) instruction or aborts. n Aborts l unintentional and unrecoverable l Examples: parity error, machine check. l Aborts current program – 60 – 15 -213, F’ 02
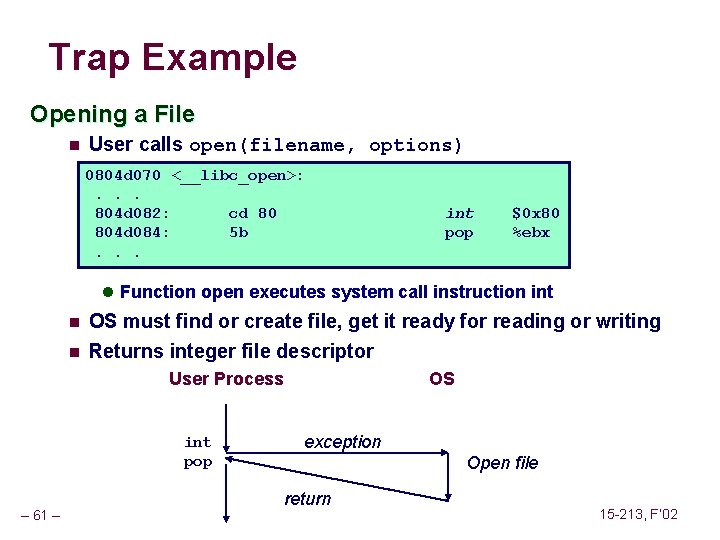
Trap Example Opening a File n User calls open(filename, options) 0804 d 070 <__libc_open>: . . . 804 d 082: cd 80 804 d 084: 5 b. . . int pop $0 x 80 %ebx l Function open executes system call instruction int n OS must find or create file, get it ready for reading or writing n Returns integer file descriptor User Process int pop – 61 – OS exception Open file return 15 -213, F’ 02
![Fault Example 1 int a1000 main a500 13 Memory Reference Fault Example #1 int a[1000]; main () { a[500] = 13; } Memory Reference](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-62.jpg)
Fault Example #1 int a[1000]; main () { a[500] = 13; } Memory Reference n n User writes to memory location That portion (page) of user’s memory is currently on disk 80483 b 7: c 7 05 10 9 d 04 08 0 d movl n Page handler must load page into physical memory n Returns to faulting instruction n Successful on second try User Process event movl OS page fault return – 62 – $0 xd, 0 x 8049 d 10 Create page and load into memory 15 -213, F’ 02
![Fault Example 2 int a1000 main a5000 13 Memory Reference Fault Example #2 int a[1000]; main () { a[5000] = 13; } Memory Reference](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-63.jpg)
Fault Example #2 int a[1000]; main () { a[5000] = 13; } Memory Reference n n User writes to memory location Address is not valid 80483 b 7: c 7 05 60 e 3 04 08 0 d movl $0 xd, 0 x 804 e 360 n Page handler detects invalid address n Sends SIGSEG signal to user process n User process exits with “segmentation fault” User Process event movl OS page fault Detect invalid address Signal process – 63 – 15 -213, F’ 02
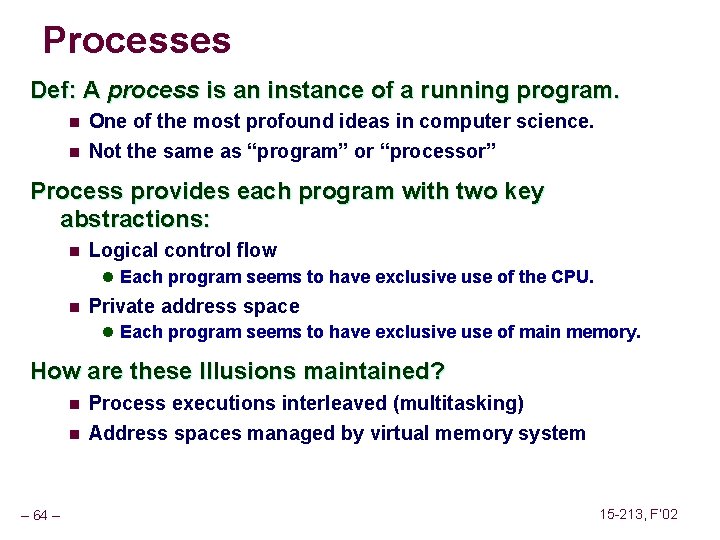
Processes Def: A process is an instance of a running program. n One of the most profound ideas in computer science. n Not the same as “program” or “processor” Process provides each program with two key abstractions: n Logical control flow l Each program seems to have exclusive use of the CPU. n Private address space l Each program seems to have exclusive use of main memory. How are these Illusions maintained? n n – 64 – Process executions interleaved (multitasking) Address spaces managed by virtual memory system 15 -213, F’ 02
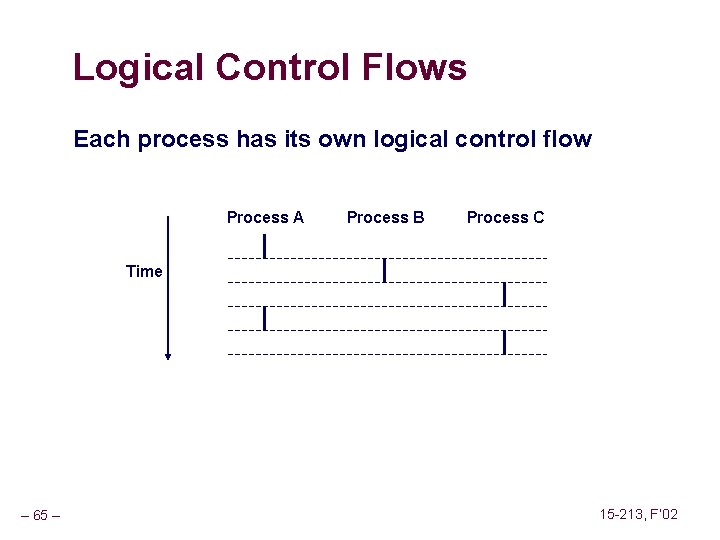
Logical Control Flows Each process has its own logical control flow Process A Process B Process C Time – 65 – 15 -213, F’ 02
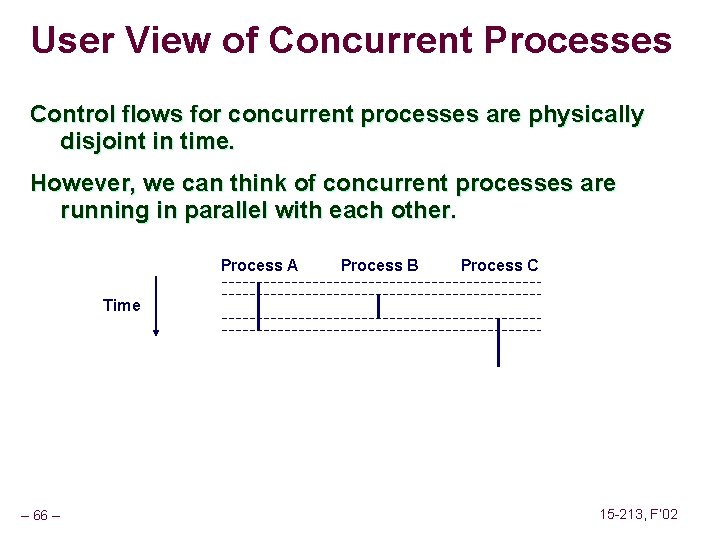
User View of Concurrent Processes Control flows for concurrent processes are physically disjoint in time. However, we can think of concurrent processes are running in parallel with each other. Process A Process B Process C Time – 66 – 15 -213, F’ 02
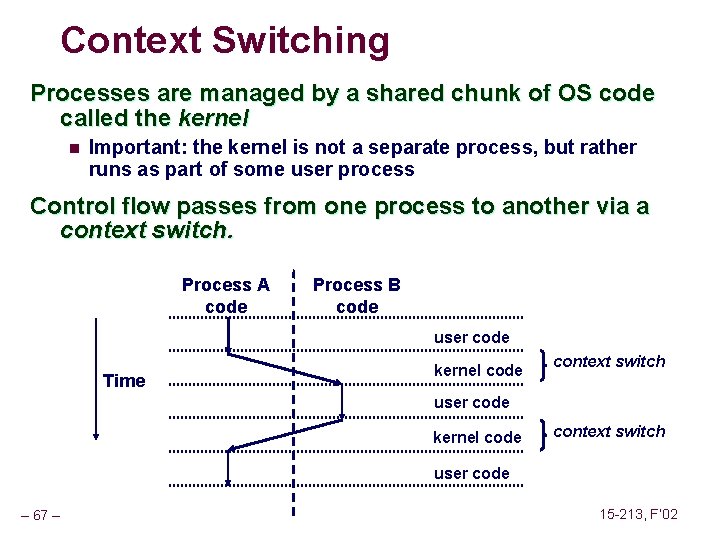
Context Switching Processes are managed by a shared chunk of OS code called the kernel n Important: the kernel is not a separate process, but rather runs as part of some user process Control flow passes from one process to another via a context switch. Process A code Process B code user code Time kernel code context switch user code – 67 – 15 -213, F’ 02
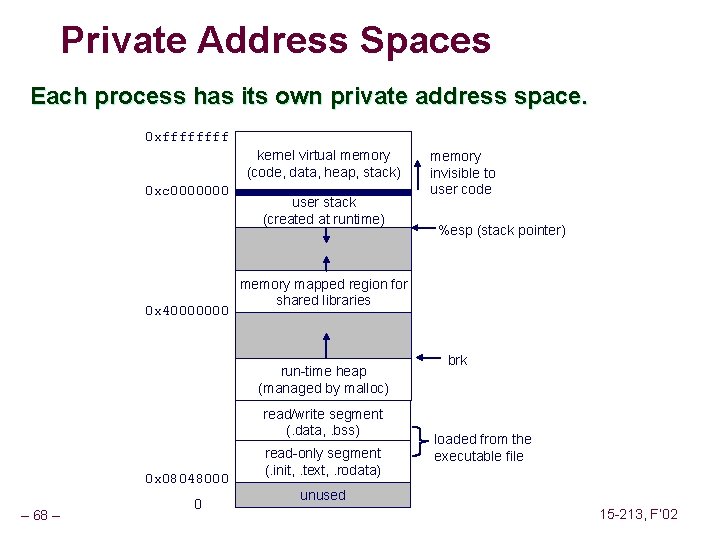
Private Address Spaces Each process has its own private address space. 0 xffff kernel virtual memory (code, data, heap, stack) 0 xc 0000000 0 x 40000000 user stack (created at runtime) read/write segment (. data, . bss) – 68 – 0 %esp (stack pointer) memory mapped region for shared libraries run-time heap (managed by malloc) 0 x 08048000 memory invisible to user code read-only segment (. init, . text, . rodata) brk loaded from the executable file unused 15 -213, F’ 02
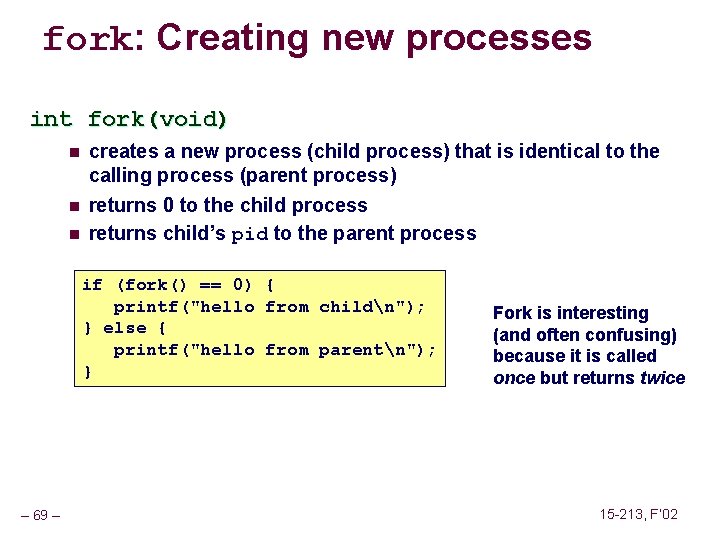
fork: Creating new processes int fork(void) n n n creates a new process (child process) that is identical to the calling process (parent process) returns 0 to the child process returns child’s pid to the parent process if (fork() == 0) { printf("hello from childn"); } else { printf("hello from parentn"); } – 69 – Fork is interesting (and often confusing) because it is called once but returns twice 15 -213, F’ 02
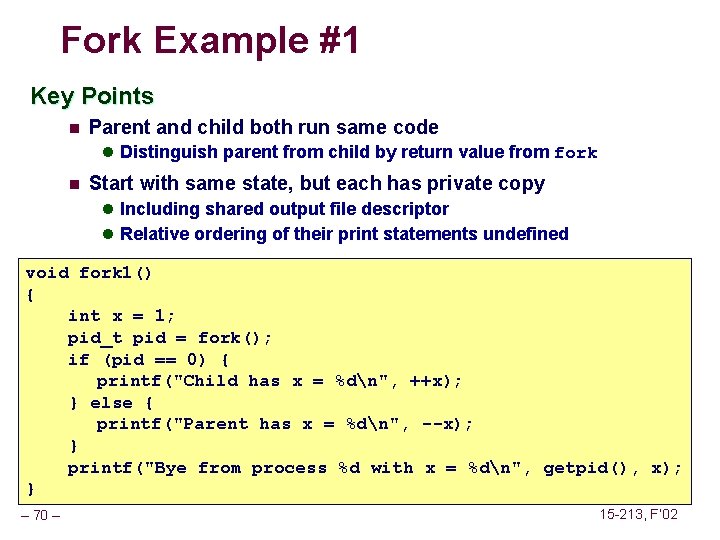
Fork Example #1 Key Points n Parent and child both run same code l Distinguish parent from child by return value from fork n Start with same state, but each has private copy l Including shared output file descriptor l Relative ordering of their print statements undefined void fork 1() { int x = 1; pid_t pid = fork(); if (pid == 0) { printf("Child has x = %dn", ++x); } else { printf("Parent has x = %dn", --x); } printf("Bye from process %d with x = %dn", getpid(), x); } – 70 – 15 -213, F’ 02
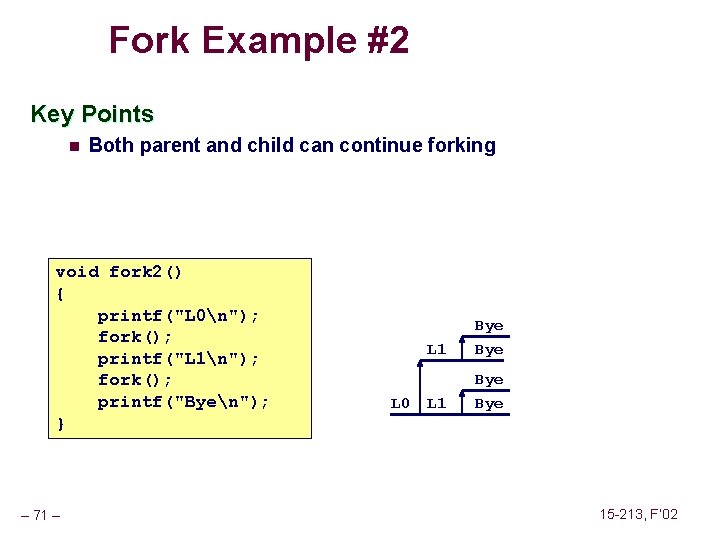
Fork Example #2 Key Points n Both parent and child can continue forking void fork 2() { printf("L 0n"); fork(); printf("L 1n"); fork(); printf("Byen"); } – 71 – L 0 L 1 Bye Bye 15 -213, F’ 02
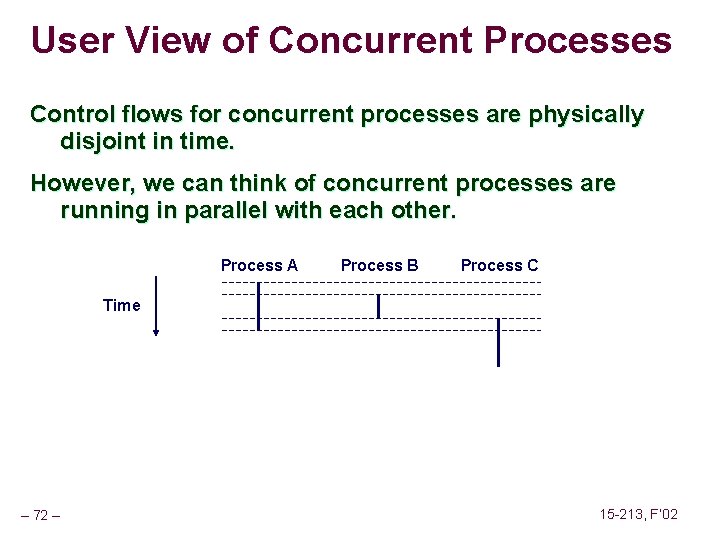
User View of Concurrent Processes Control flows for concurrent processes are physically disjoint in time. However, we can think of concurrent processes are running in parallel with each other. Process A Process B Process C Time – 72 – 15 -213, F’ 02
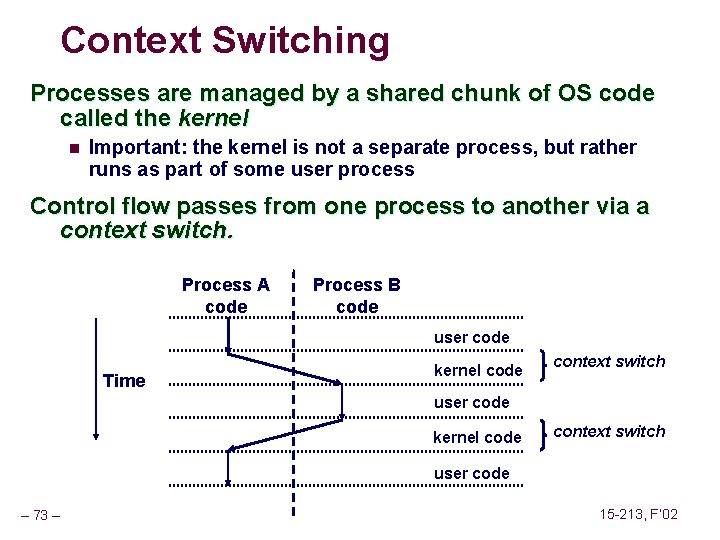
Context Switching Processes are managed by a shared chunk of OS code called the kernel n Important: the kernel is not a separate process, but rather runs as part of some user process Control flow passes from one process to another via a context switch. Process A code Process B code user code Time kernel code context switch user code – 73 – 15 -213, F’ 02
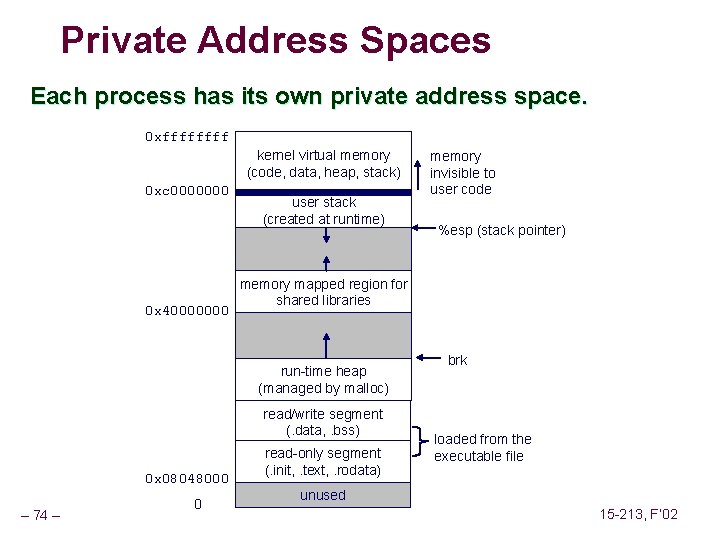
Private Address Spaces Each process has its own private address space. 0 xffff kernel virtual memory (code, data, heap, stack) 0 xc 0000000 0 x 40000000 user stack (created at runtime) read/write segment (. data, . bss) – 74 – 0 %esp (stack pointer) memory mapped region for shared libraries run-time heap (managed by malloc) 0 x 08048000 memory invisible to user code read-only segment (. init, . text, . rodata) brk loaded from the executable file unused 15 -213, F’ 02
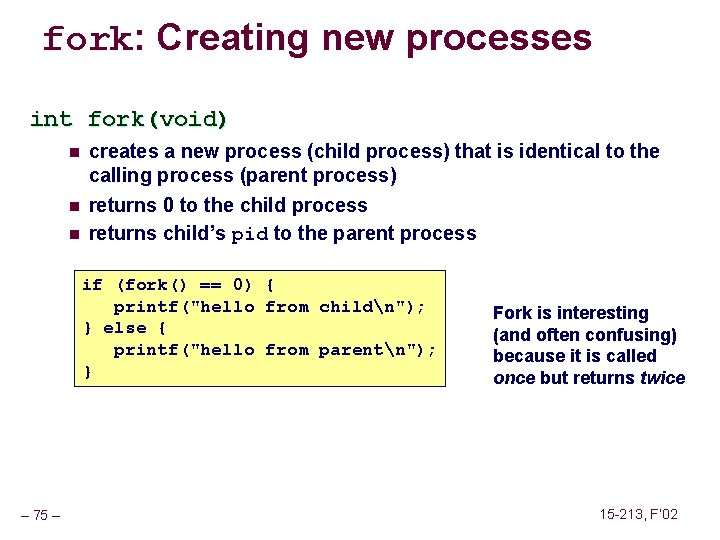
fork: Creating new processes int fork(void) n n n creates a new process (child process) that is identical to the calling process (parent process) returns 0 to the child process returns child’s pid to the parent process if (fork() == 0) { printf("hello from childn"); } else { printf("hello from parentn"); } – 75 – Fork is interesting (and often confusing) because it is called once but returns twice 15 -213, F’ 02
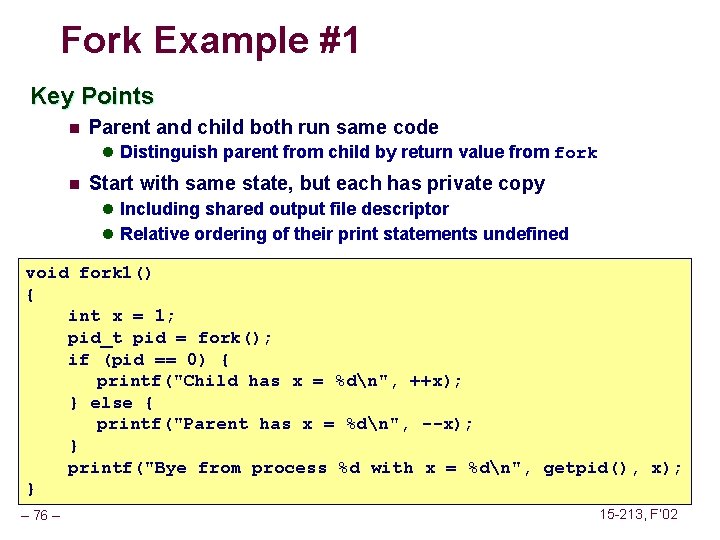
Fork Example #1 Key Points n Parent and child both run same code l Distinguish parent from child by return value from fork n Start with same state, but each has private copy l Including shared output file descriptor l Relative ordering of their print statements undefined void fork 1() { int x = 1; pid_t pid = fork(); if (pid == 0) { printf("Child has x = %dn", ++x); } else { printf("Parent has x = %dn", --x); } printf("Bye from process %d with x = %dn", getpid(), x); } – 76 – 15 -213, F’ 02
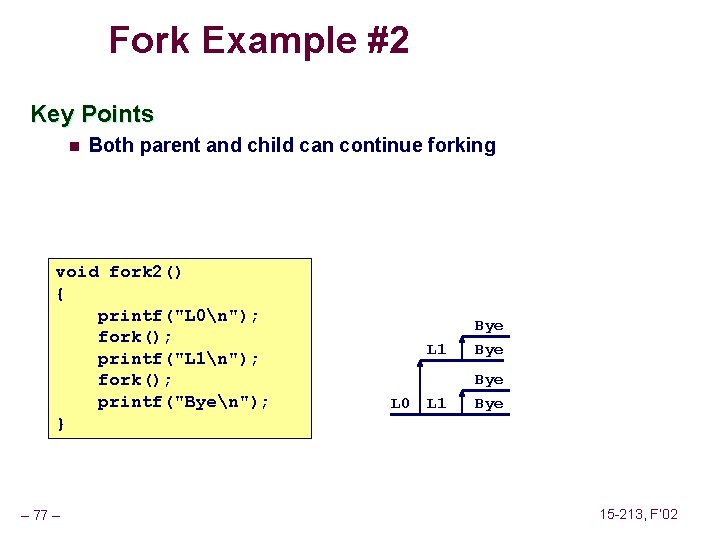
Fork Example #2 Key Points n Both parent and child can continue forking void fork 2() { printf("L 0n"); fork(); printf("L 1n"); fork(); printf("Byen"); } – 77 – L 0 L 1 Bye Bye 15 -213, F’ 02
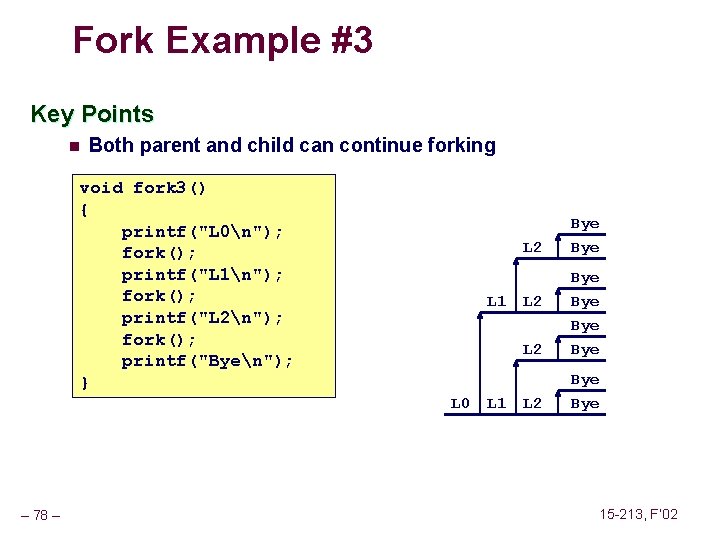
Fork Example #3 Key Points n Both parent and child can continue forking void fork 3() { printf("L 0n"); fork(); printf("L 1n"); fork(); printf("L 2n"); fork(); printf("Byen"); } L 1 L 0 – 78 – L 1 L 2 Bye Bye 15 -213, F’ 02
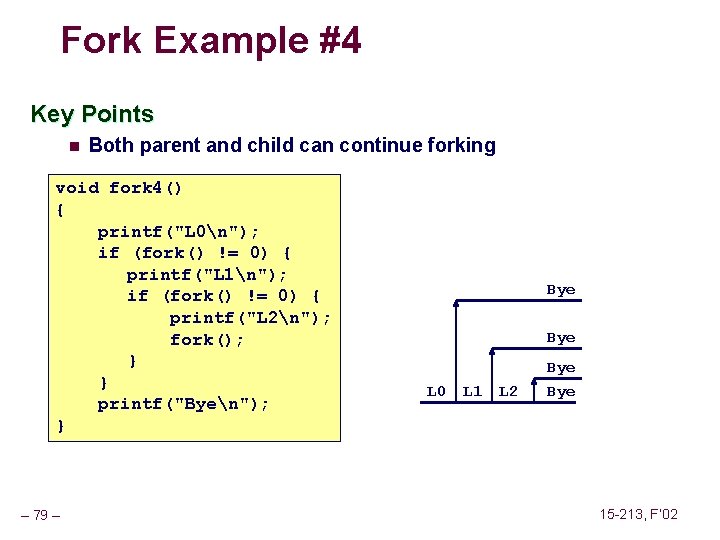
Fork Example #4 Key Points n Both parent and child can continue forking void fork 4() { printf("L 0n"); if (fork() != 0) { printf("L 1n"); if (fork() != 0) { printf("L 2n"); fork(); } } printf("Byen"); } – 79 – Bye L 0 L 1 L 2 Bye 15 -213, F’ 02
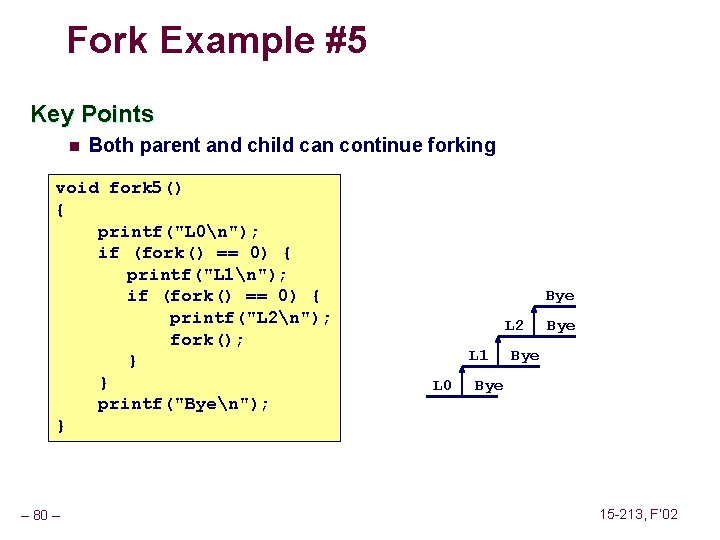
Fork Example #5 Key Points n Both parent and child can continue forking void fork 5() { printf("L 0n"); if (fork() == 0) { printf("L 1n"); if (fork() == 0) { printf("L 2n"); fork(); } } printf("Byen"); } – 80 – Bye L 2 L 1 L 0 Bye Bye 15 -213, F’ 02
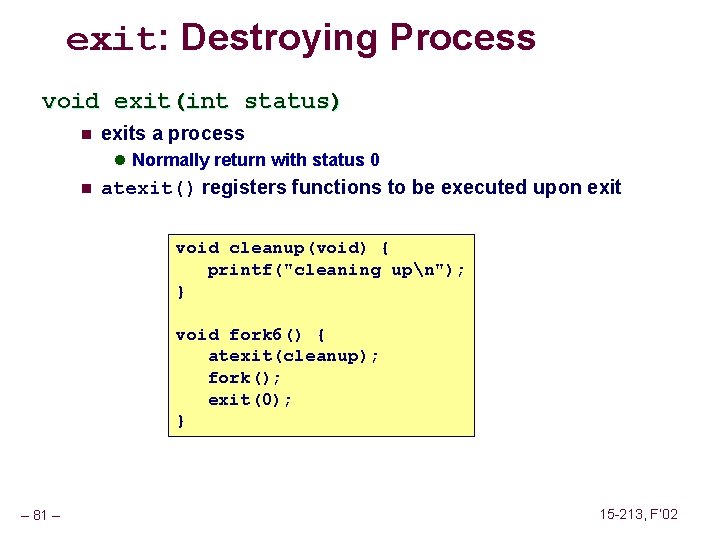
exit: Destroying Process void exit(int status) n exits a process l Normally return with status 0 n atexit() registers functions to be executed upon exit void cleanup(void) { printf("cleaning upn"); } void fork 6() { atexit(cleanup); fork(); exit(0); } – 81 – 15 -213, F’ 02
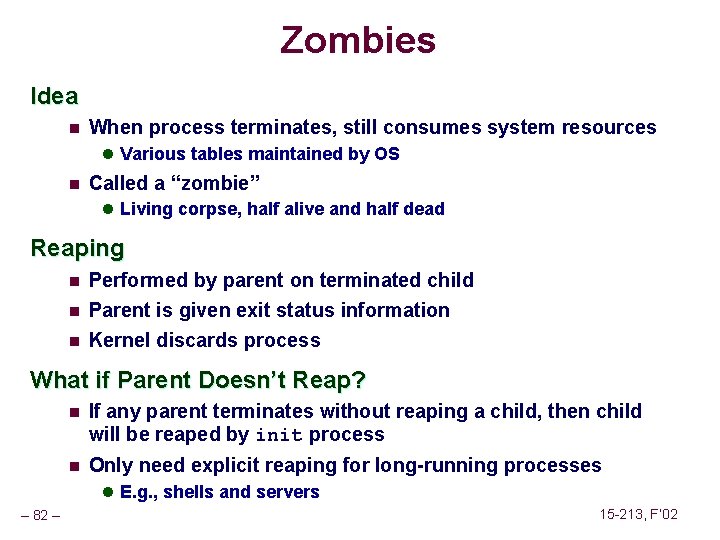
Zombies Idea n When process terminates, still consumes system resources l Various tables maintained by OS n Called a “zombie” l Living corpse, half alive and half dead Reaping n n n Performed by parent on terminated child Parent is given exit status information Kernel discards process What if Parent Doesn’t Reap? n If any parent terminates without reaping a child, then child will be reaped by init process n Only need explicit reaping for long-running processes l E. g. , shells and servers – 82 – 15 -213, F’ 02
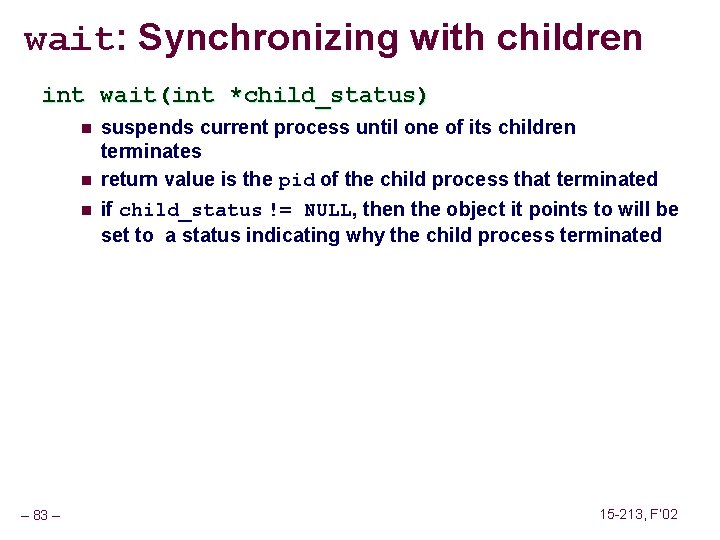
wait: Synchronizing with children int wait(int *child_status) n n n – 83 – suspends current process until one of its children terminates return value is the pid of the child process that terminated if child_status != NULL, then the object it points to will be set to a status indicating why the child process terminated 15 -213, F’ 02
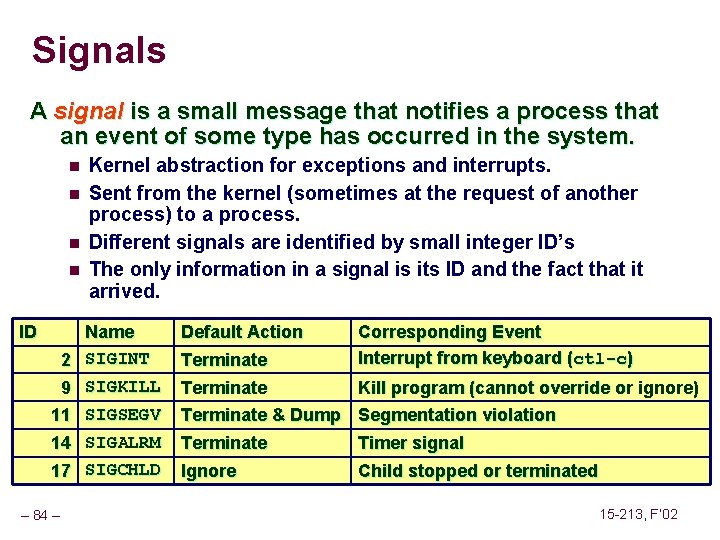
Signals A signal is a small message that notifies a process that an event of some type has occurred in the system. n n ID Kernel abstraction for exceptions and interrupts. Sent from the kernel (sometimes at the request of another process) to a process. Different signals are identified by small integer ID’s The only information in a signal is its ID and the fact that it arrived. Name 2 SIGINT 9 SIGKILL 11 SIGSEGV 14 SIGALRM Default Action Terminate 17 SIGCHLD Ignore – 84 – Corresponding Event Interrupt from keyboard (ctl-c) Terminate Kill program (cannot override or ignore) Terminate & Dump Segmentation violation Terminate Timer signal Child stopped or terminated 15 -213, F’ 02
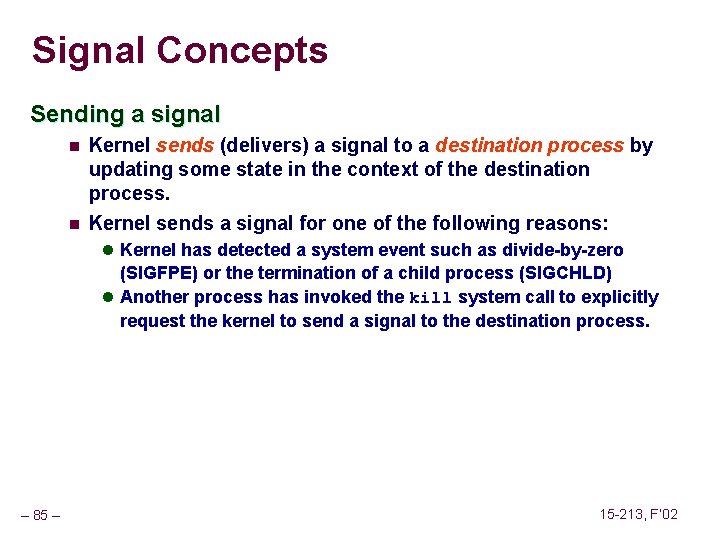
Signal Concepts Sending a signal n n Kernel sends (delivers) a signal to a destination process by updating some state in the context of the destination process. Kernel sends a signal for one of the following reasons: l Kernel has detected a system event such as divide-by-zero (SIGFPE) or the termination of a child process (SIGCHLD) l Another process has invoked the kill system call to explicitly request the kernel to send a signal to the destination process. – 85 – 15 -213, F’ 02
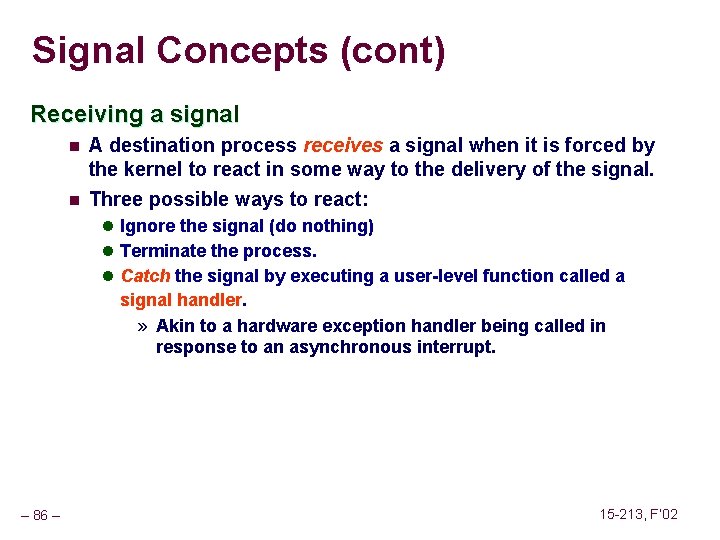
Signal Concepts (cont) Receiving a signal n n A destination process receives a signal when it is forced by the kernel to react in some way to the delivery of the signal. Three possible ways to react: l Ignore the signal (do nothing) l Terminate the process. l Catch the signal by executing a user-level function called a signal handler. » Akin to a hardware exception handler being called in response to an asynchronous interrupt. – 86 – 15 -213, F’ 02
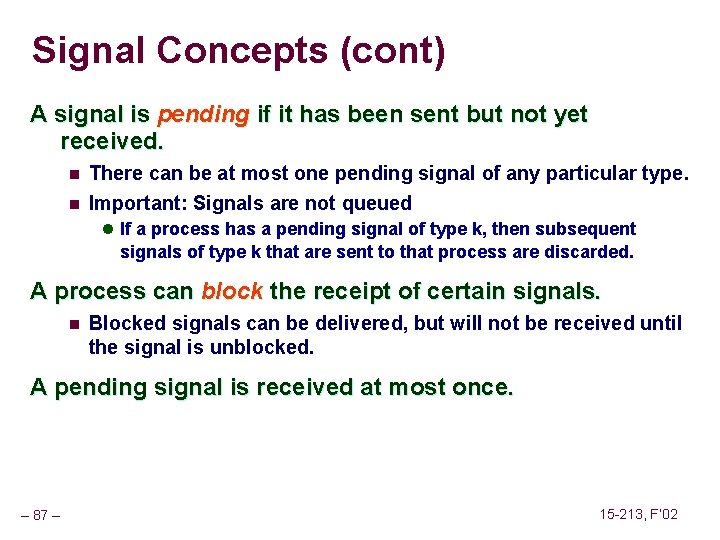
Signal Concepts (cont) A signal is pending if it has been sent but not yet received. n n There can be at most one pending signal of any particular type. Important: Signals are not queued l If a process has a pending signal of type k, then subsequent signals of type k that are sent to that process are discarded. A process can block the receipt of certain signals. n Blocked signals can be delivered, but will not be received until the signal is unblocked. A pending signal is received at most once. – 87 – 15 -213, F’ 02
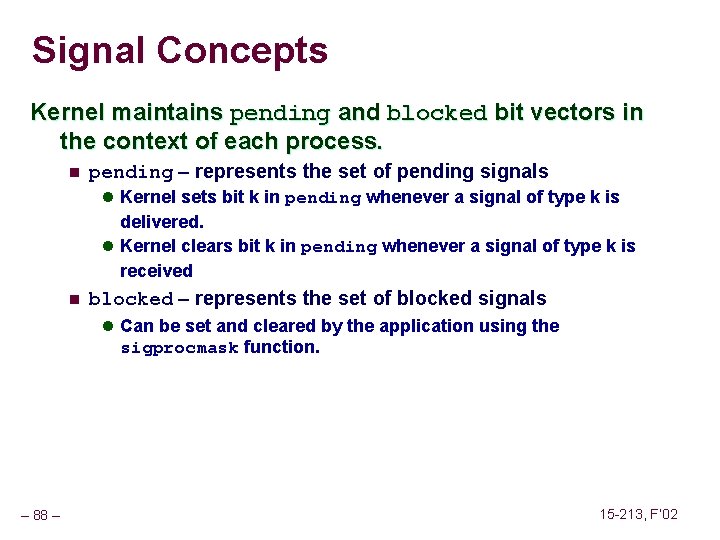
Signal Concepts Kernel maintains pending and blocked bit vectors in the context of each process. n pending – represents the set of pending signals l Kernel sets bit k in pending whenever a signal of type k is delivered. l Kernel clears bit k in pending whenever a signal of type k is received n blocked – represents the set of blocked signals l Can be set and cleared by the application using the sigprocmask function. – 88 – 15 -213, F’ 02
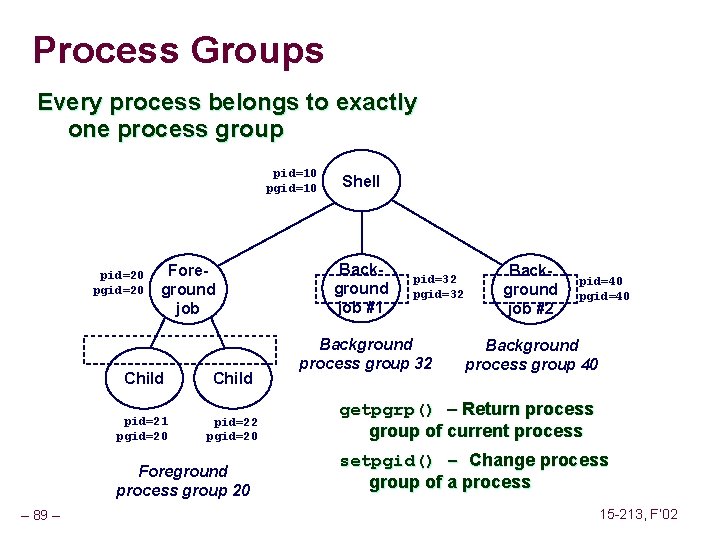
Process Groups Every process belongs to exactly one process group pid=10 pgid=10 pid=20 pgid=20 Foreground job Child pid=21 pgid=20 pid=22 pgid=20 Foreground process group 20 – 89 – Shell Background job #1 pid=32 pgid=32 Background process group 32 Background job #2 pid=40 pgid=40 Background process group 40 getpgrp() – Return process group of current process setpgid() – Change process group of a process 15 -213, F’ 02
![Sending Signals with kill Function void fork 12 pidt pidN int i childstatus Sending Signals with kill Function void fork 12() { pid_t pid[N]; int i, child_status;](https://slidetodoc.com/presentation_image_h/2487793147ef8fbe8fce23805bc1a408/image-90.jpg)
Sending Signals with kill Function void fork 12() { pid_t pid[N]; int i, child_status; for (i = 0; i < N; i++) if ((pid[i] = fork()) == 0) while(1); /* Child infinite loop */ /* Parent terminates the child processes */ for (i = 0; i < N; i++) { printf("Killing process %dn", pid[i]); kill(pid[i], SIGINT); } /* Parent reaps terminated children */ for (i = 0; i < N; i++) { pid_t wpid = wait(&child_status); if (WIFEXITED(child_status)) printf("Child %d terminated with exit status %dn", wpid, WEXITSTATUS(child_status)); else printf("Child %d terminated abnormallyn", wpid); } } – 90 – 15 -213, F’ 02
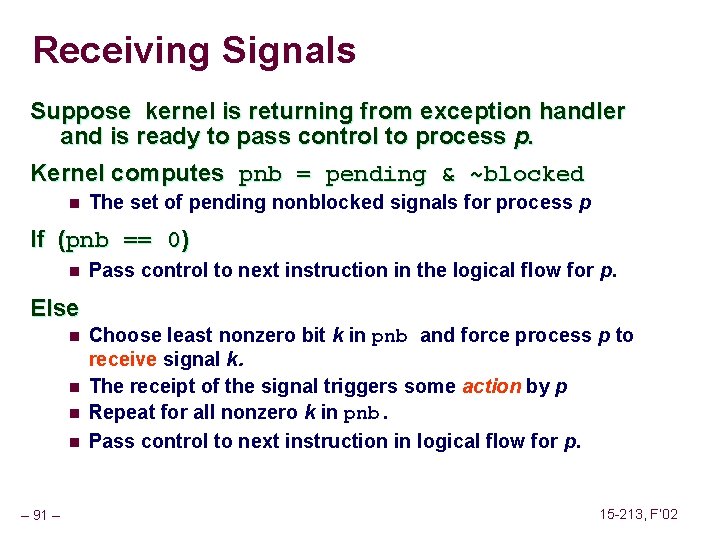
Receiving Signals Suppose kernel is returning from exception handler and is ready to pass control to process p. Kernel computes pnb = pending & ~blocked n The set of pending nonblocked signals for process p If (pnb == 0) n Pass control to next instruction in the logical flow for p. Else n n – 91 – Choose least nonzero bit k in pnb and force process p to receive signal k. The receipt of the signal triggers some action by p Repeat for all nonzero k in pnb. Pass control to next instruction in logical flow for p. 15 -213, F’ 02
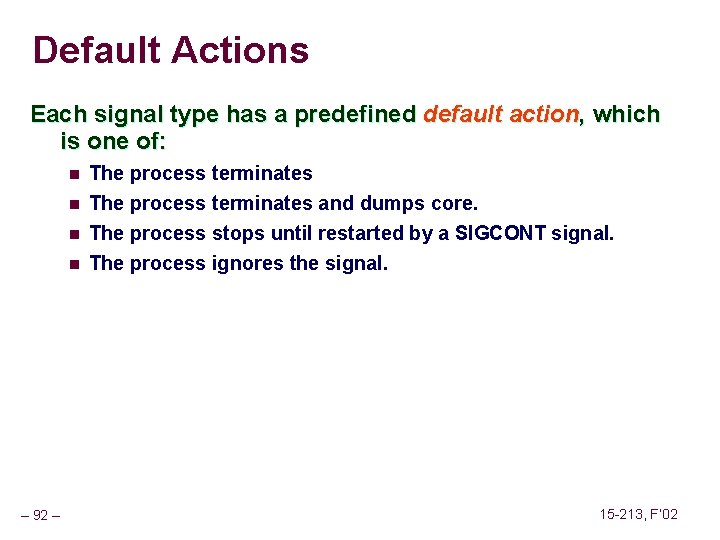
Default Actions Each signal type has a predefined default action, which is one of: n n – 92 – The process terminates and dumps core. The process stops until restarted by a SIGCONT signal. The process ignores the signal. 15 -213, F’ 02
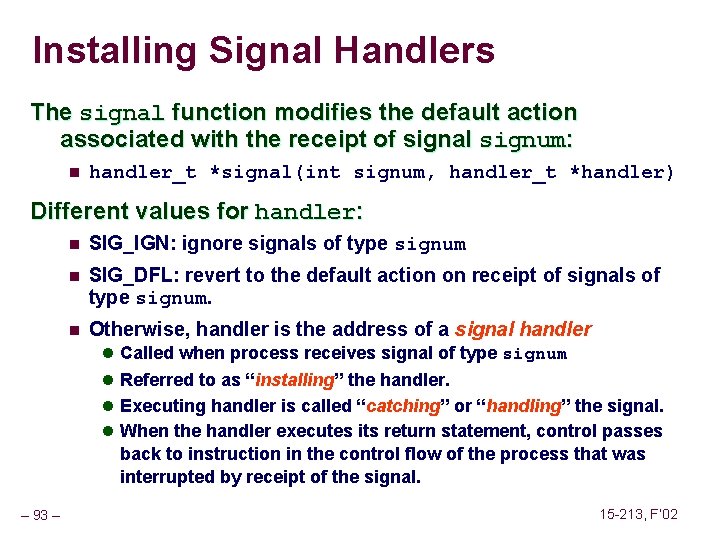
Installing Signal Handlers The signal function modifies the default action associated with the receipt of signal signum: n handler_t *signal(int signum, handler_t *handler) Different values for handler: n SIG_IGN: ignore signals of type signum n SIG_DFL: revert to the default action on receipt of signals of type signum. n Otherwise, handler is the address of a signal handler l Called when process receives signal of type signum l Referred to as “installing” the handler. l Executing handler is called “catching” or “handling” the signal. l When the handler executes its return statement, control passes back to instruction in the control flow of the process that was interrupted by receipt of the signal. – 93 – 15 -213, F’ 02
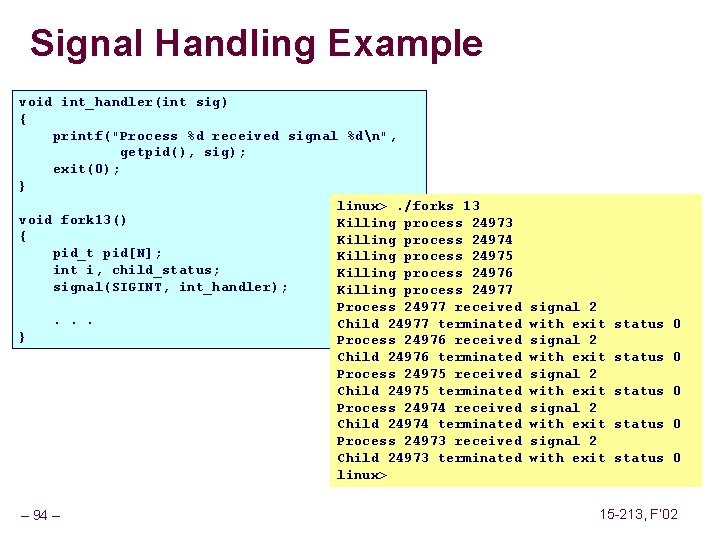
Signal Handling Example void int_handler(int sig) { printf("Process %d received signal %dn", getpid(), sig); exit(0); } linux>. /forks 13 void fork 13() Killing process 24973 { Killing process 24974 pid_t pid[N]; Killing process 24975 int i, child_status; Killing process 24976 signal(SIGINT, int_handler); Killing process 24977 Process 24977 received. . . Child 24977 terminated } Process 24976 received Child 24976 terminated Process 24975 received Child 24975 terminated Process 24974 received Child 24974 terminated Process 24973 received Child 24973 terminated linux> – 94 – signal 2 with exit signal 2 with exit status 0 status 0 15 -213, F’ 02
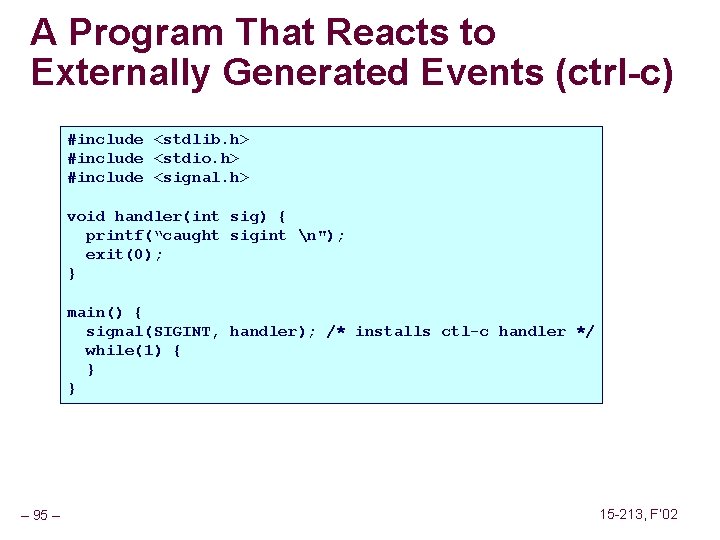
A Program That Reacts to Externally Generated Events (ctrl-c) #include <stdlib. h> #include <stdio. h> #include <signal. h> void handler(int sig) { printf(“caught sigint n"); exit(0); } main() { signal(SIGINT, handler); /* installs ctl-c handler */ while(1) { } } – 95 – 15 -213, F’ 02
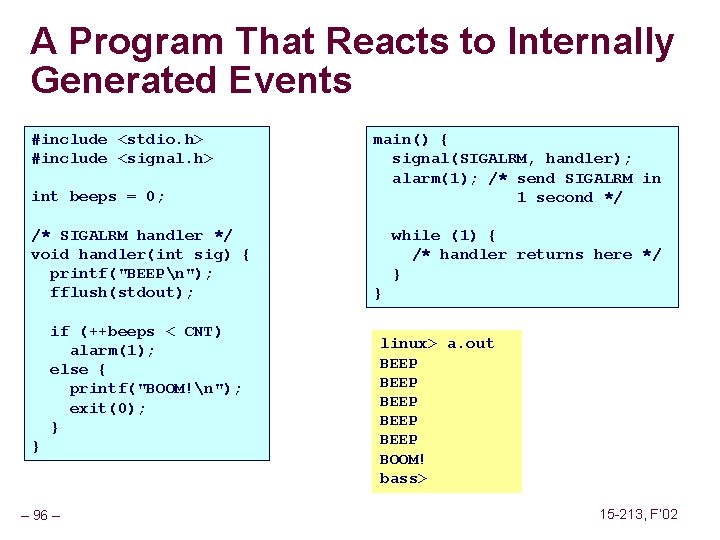
A Program That Reacts to Internally Generated Events #include <stdio. h> #include <signal. h> int beeps = 0; /* SIGALRM handler */ void handler(int sig) { printf("BEEPn"); fflush(stdout); if (++beeps < CNT) alarm(1); else { printf("BOOM!n"); exit(0); } } – 96 – main() { signal(SIGALRM, handler); alarm(1); /* send SIGALRM in 1 second */ while (1) { /* handler returns here */ } } linux> a. out BEEP BEEP BOOM! bass> 15 -213, F’ 02
Linux memory allocator
Doug lea memory allocator
Example of dynamic memory allocation
Explicit memory allocation
Disadvantages of dynamic programming
Tcmalloc
Example of dynamic memory allocation
Dynamic data structure
Dynamic memory allocation in data structure
Dynamic c programming
Types of memory allocation in c
Memory allocation in java
Malloc calloc free
Heap vs binary heap
Heap allocation
Alan cox rice
Linked allocation
The resource allocator role
Vuw my allocator
Seglist allocator
Binary buddy allocator
Fibonacci heap application
Implicit heap dynamic variables
Stack and heap memory
Dynamic strategies for asset allocation
Dynamic storage-allocation problem
Polymorphism dynamic allocation
Assumptions for dynamic channel allocation
Dynamic storage allocation
0 0005
Buddy memory allocation implementation
Single contiguous memory management
Demand paged memory allocation
Memory allocation policy
In contiguous memory allocation has no cure
Segmented/demand paged memory allocation
What are two goals of multitasking memory allocation
Contiguous memory
Non contiguous memory allocation
Q concepts
Non contiguous memory allocation
Quick fit memory allocation
Memory allocation
Zig memory allocation
Memory allocation policy
Buddy system memory allocation
Transferered
Rdram vs sdram
A free map determines which blocks are free, allocated.
Dynamic memory management
Dynamic memory management
Episodic vs semantic memory
Implicit and explicit memory
Long term memory vs short term memory
Internal memory and external memory
Primary memory and secondary memory
Logical memory is broken into
Which memory is the actual working memory?
Virtual memory
Virtual memory in memory hierarchy consists of
Eidetic memory vs iconic memory
Symmetric shared memory architecture
Windows heap exploitation
Binomial heap calculator
What are heap-organized tables
Heap sort adalah
Percolate down heap java
Priority queue using heap
Soal distribusi binomial doc
Magnetic field of two magnets
Hash table visualization
Fibonacci tree visualization
Heap sort animation
Heap sort running time
Caseitu
Agrega
Heap fibonacci
Select sort
Fibonacci heap
Symmetric min max heap
Ternary heap
Double ended priority queue
Shallow heap
Stratus cumulus nimbus
Heap increase key
Who invented selection sort
Collective noun of stamps
Fib heap
Binomial heap with example
The heap operation sift-up is used when.
Fibonacci heap amortized analysis
Min heap insertion time complexity
Double ended heap
Heap files in dbms
Westchester senior services
Pairing heap
Pairing heap