Dynamic Memory Allocation Agenda Process Layout Memory Allocation
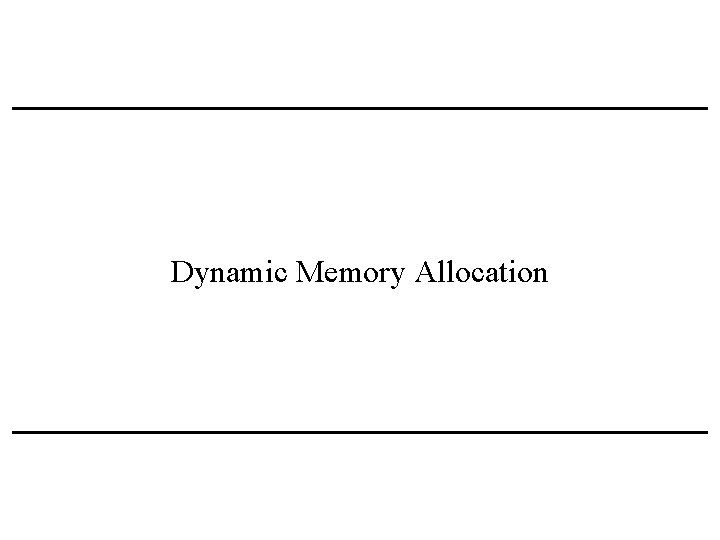
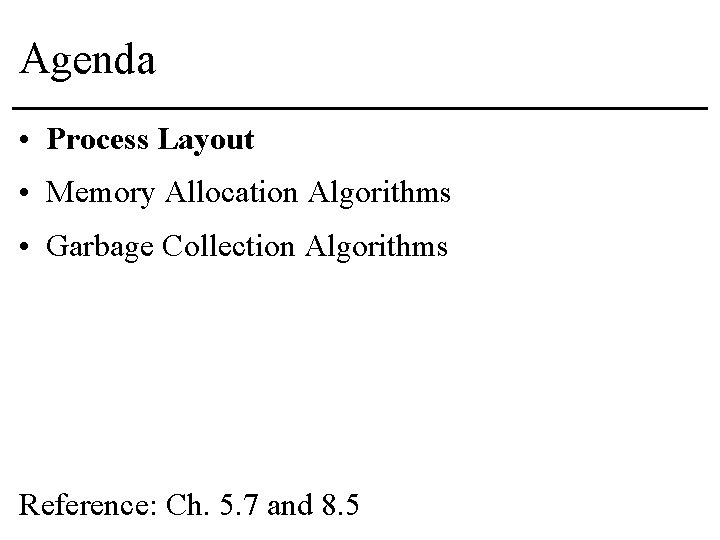
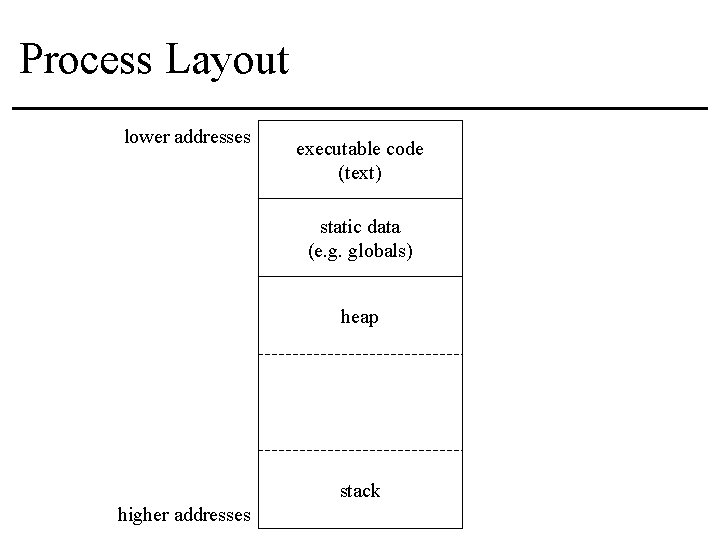
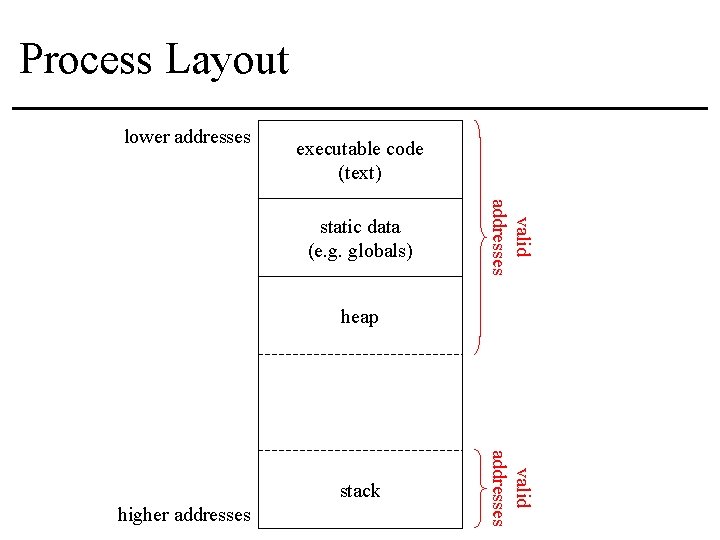
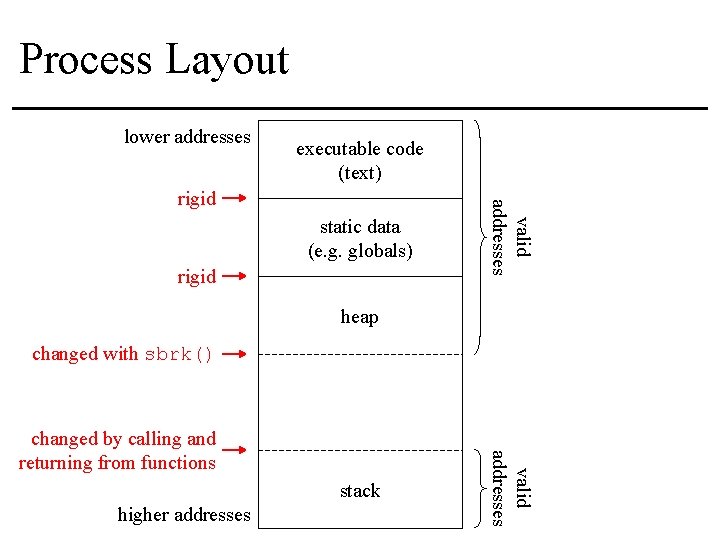
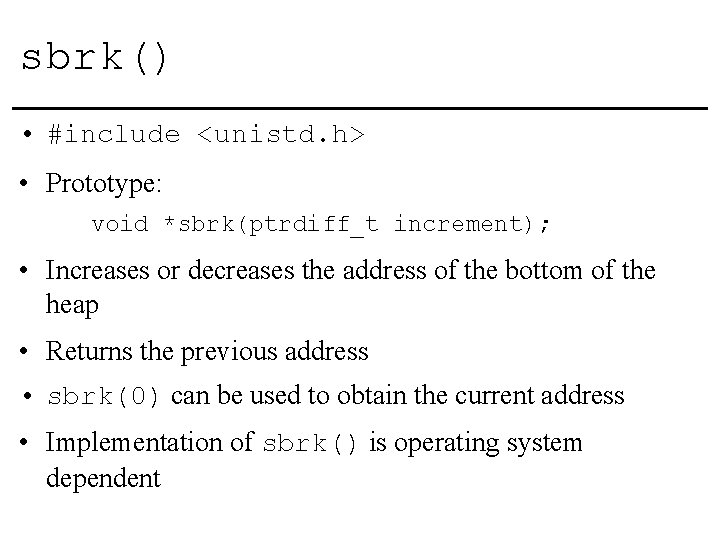
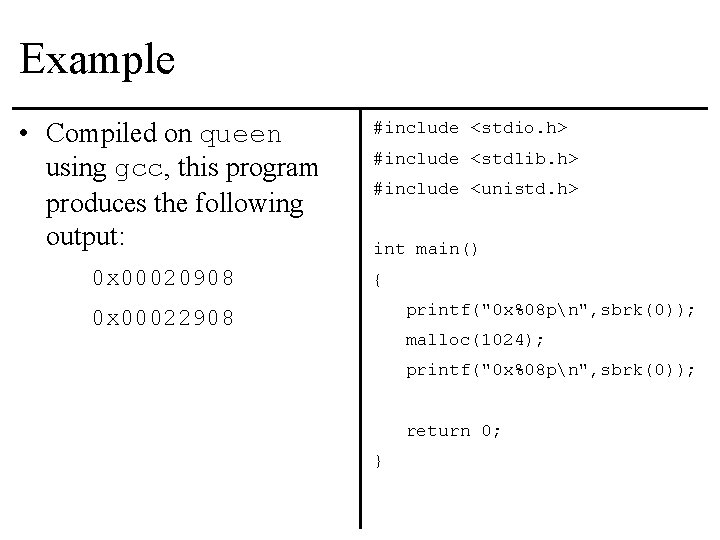
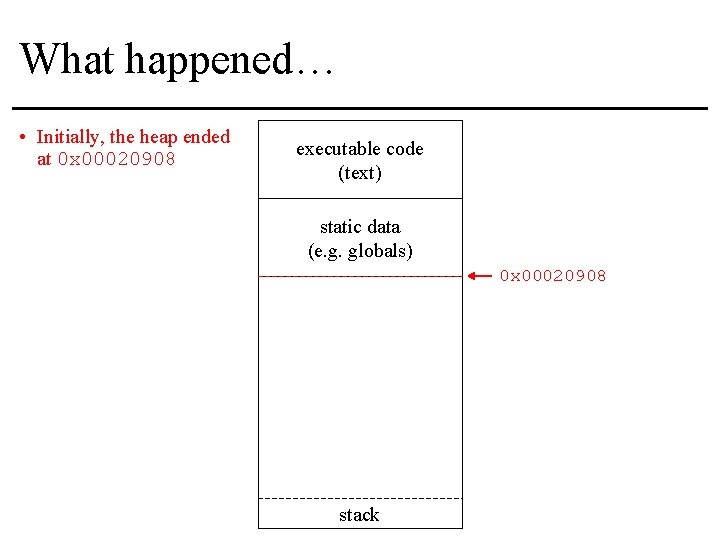
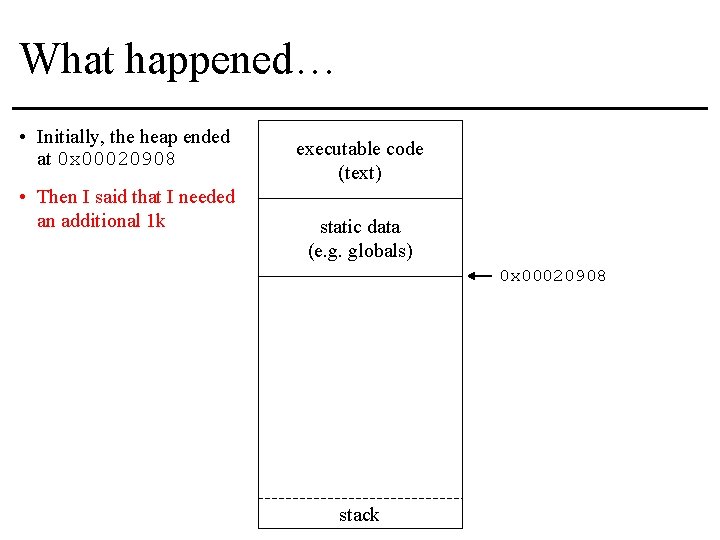
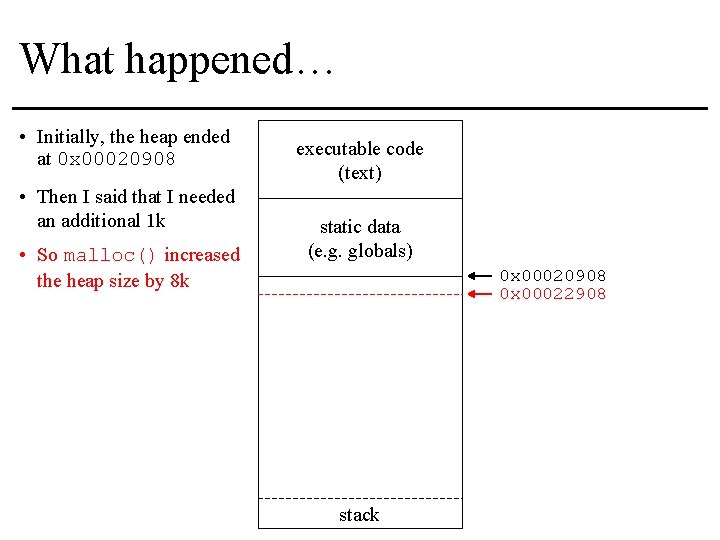
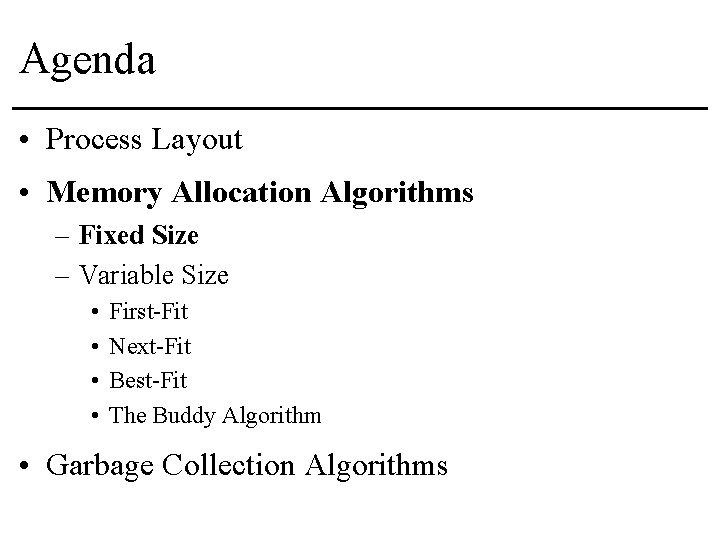
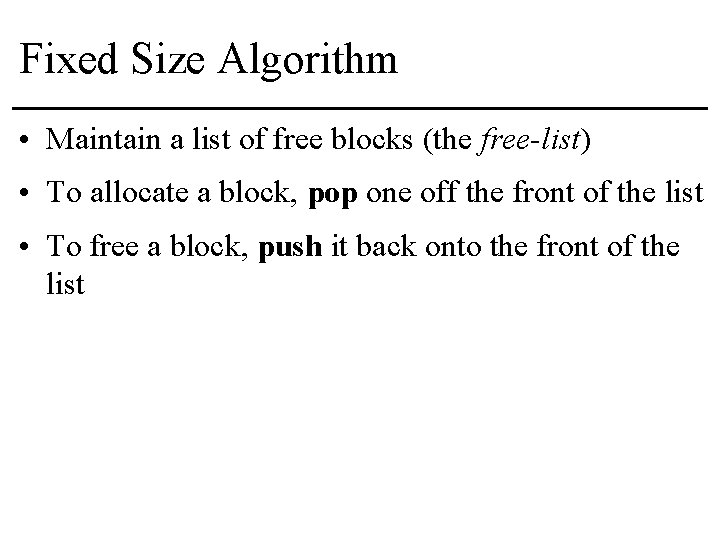
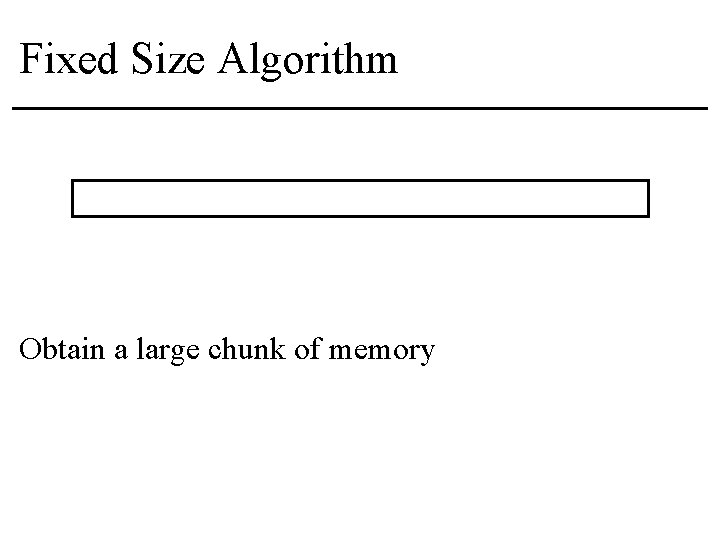
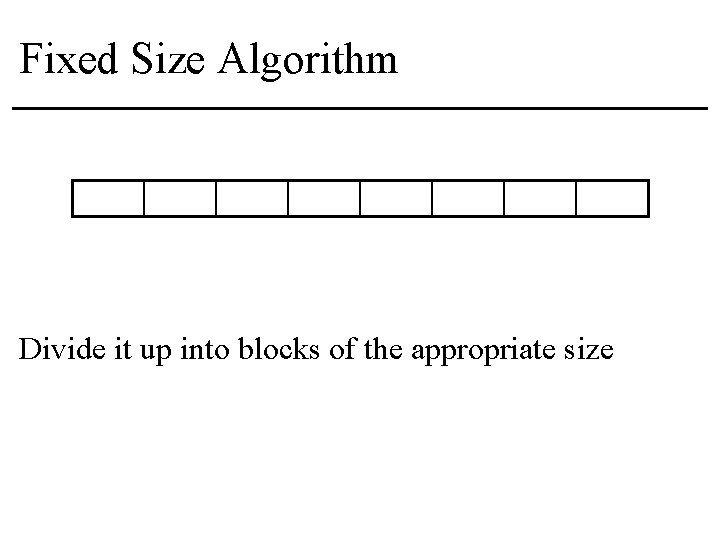
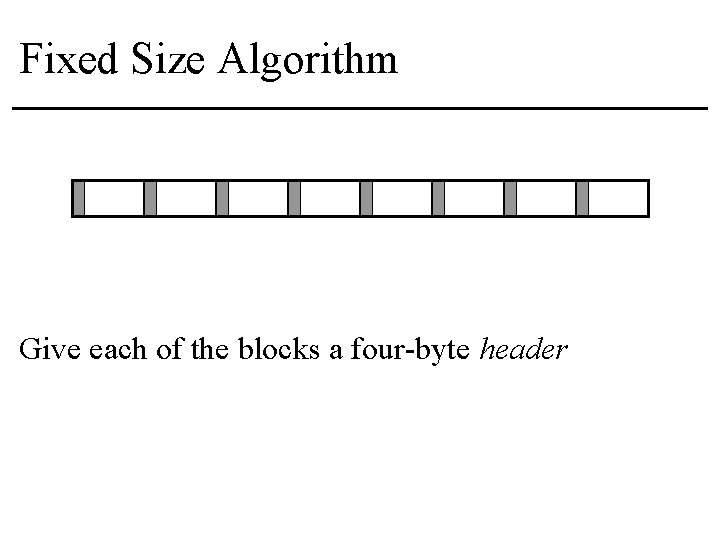
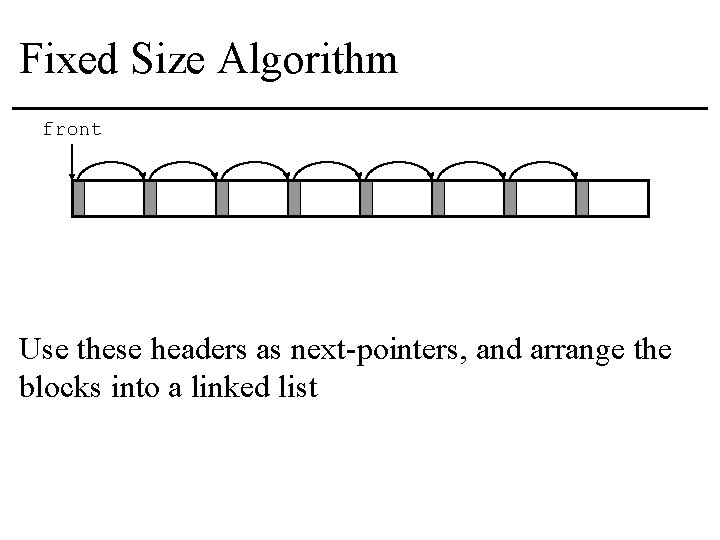
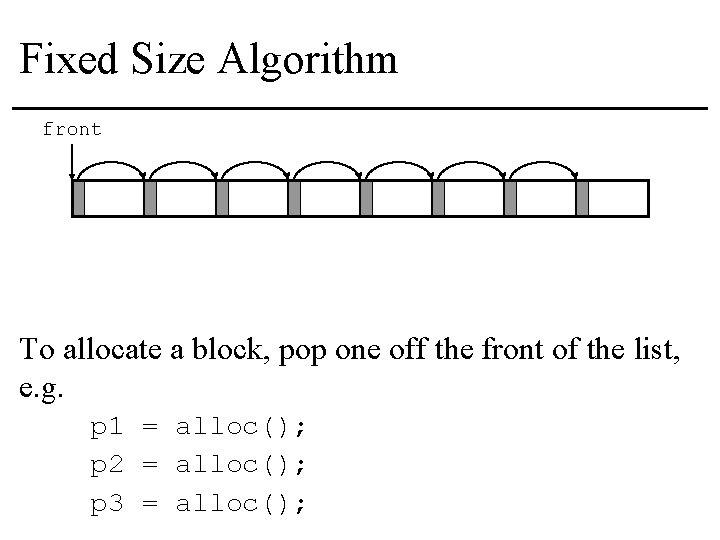
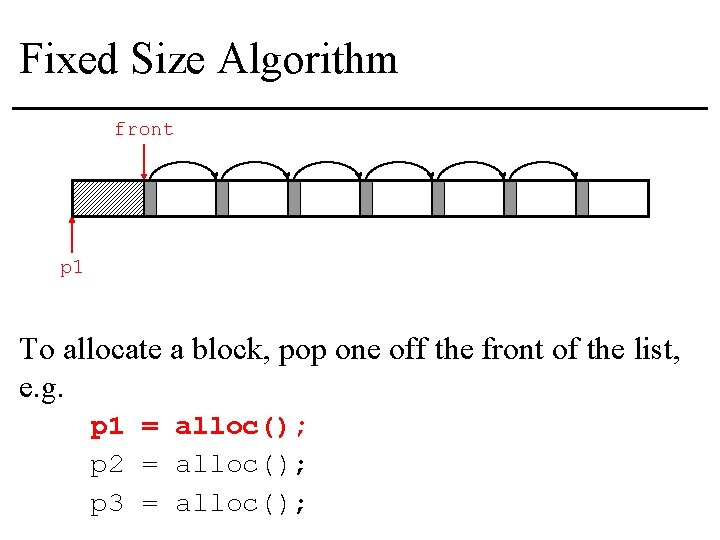
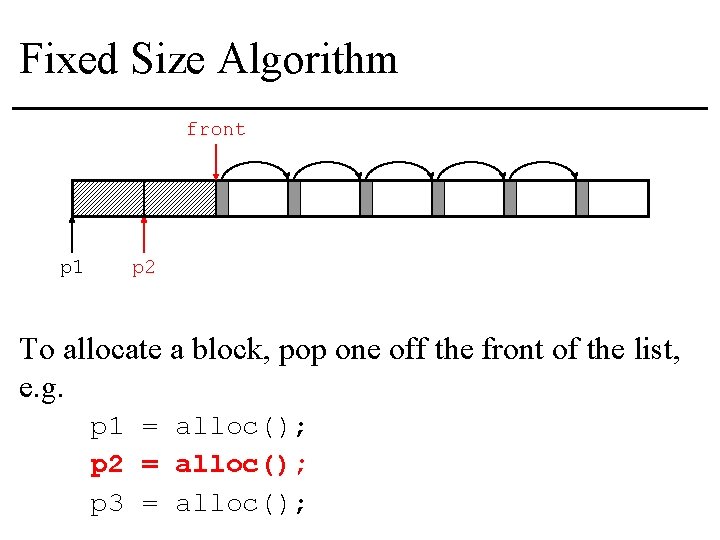
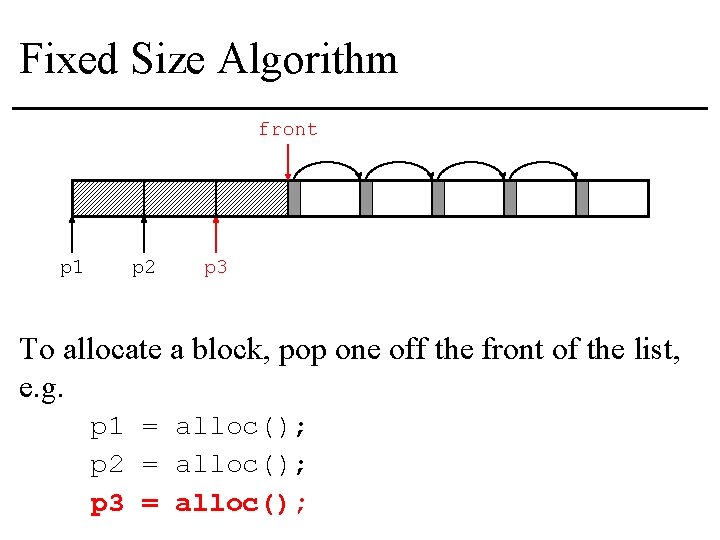
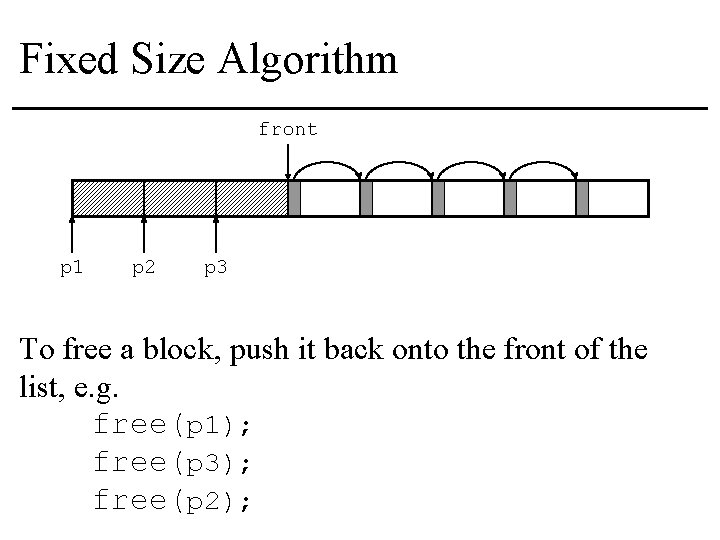
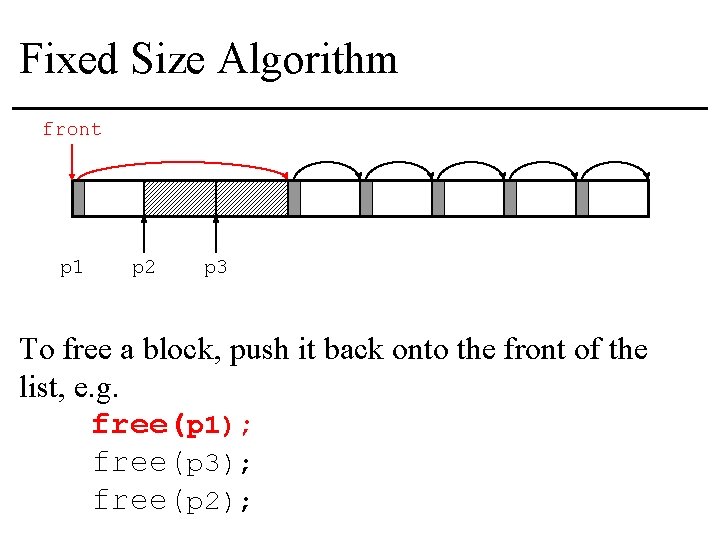
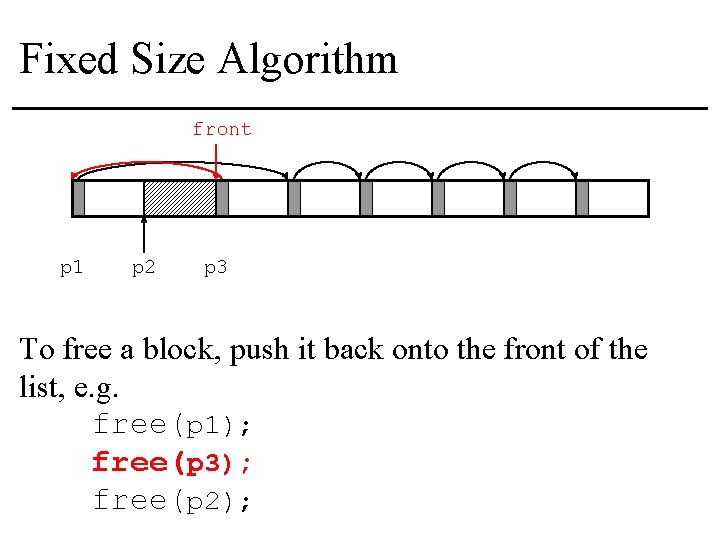
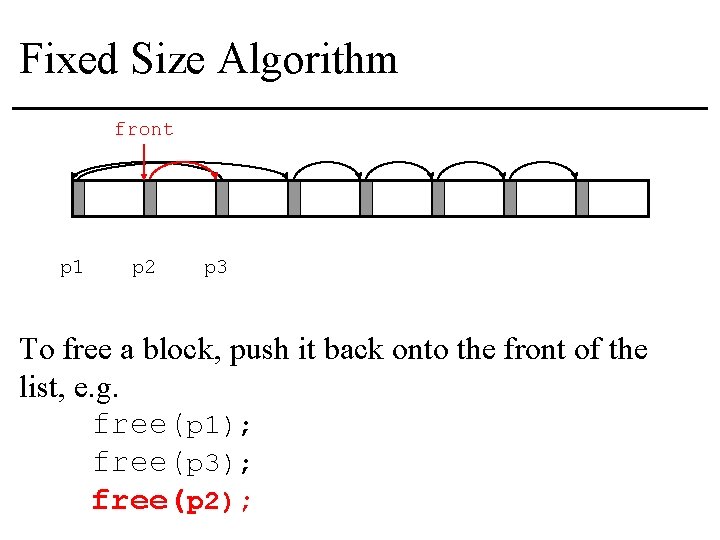
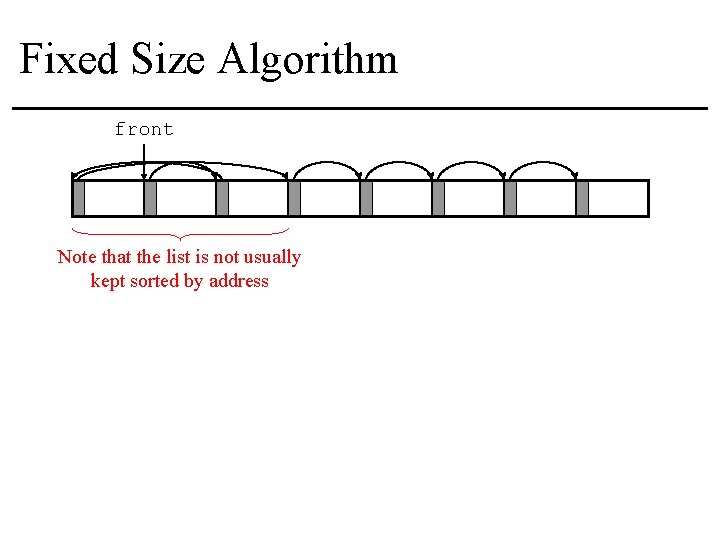
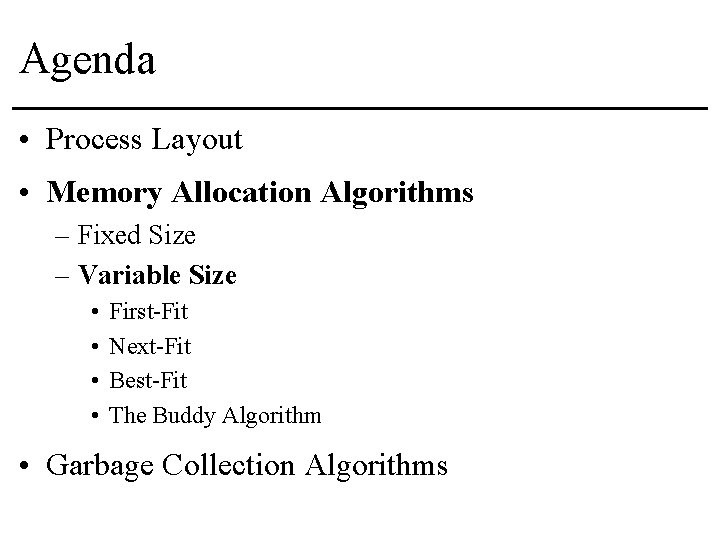
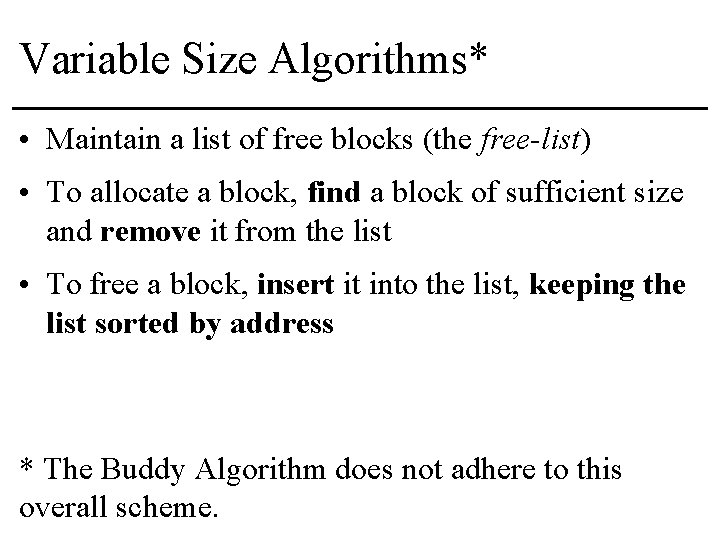
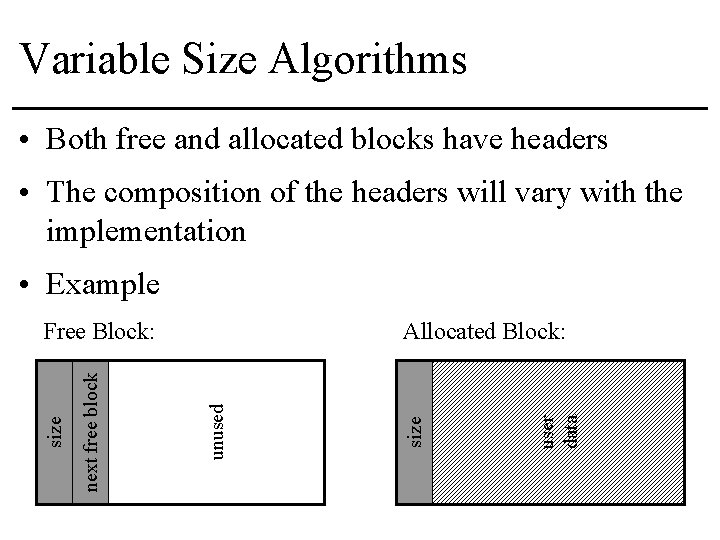
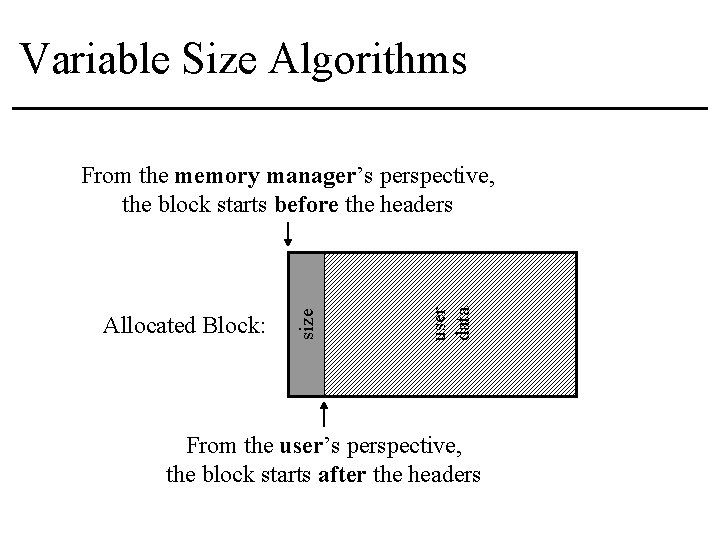
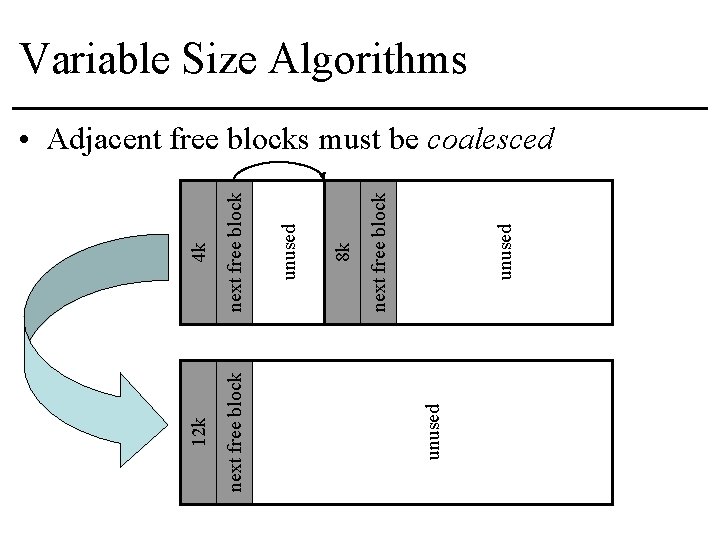
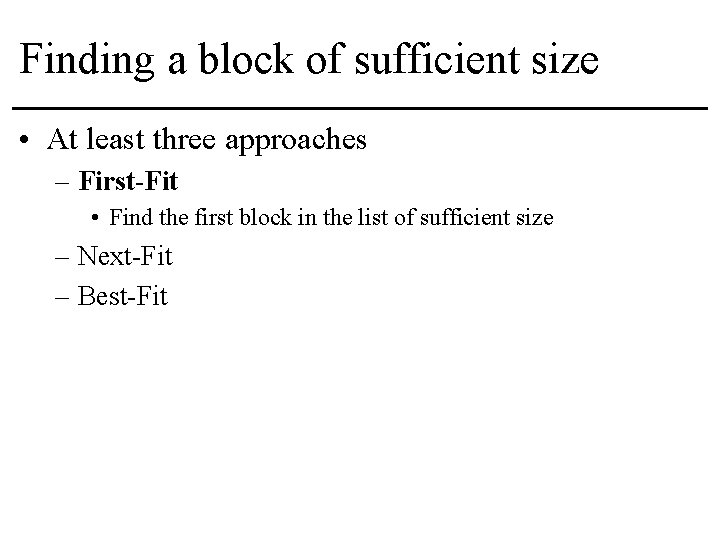
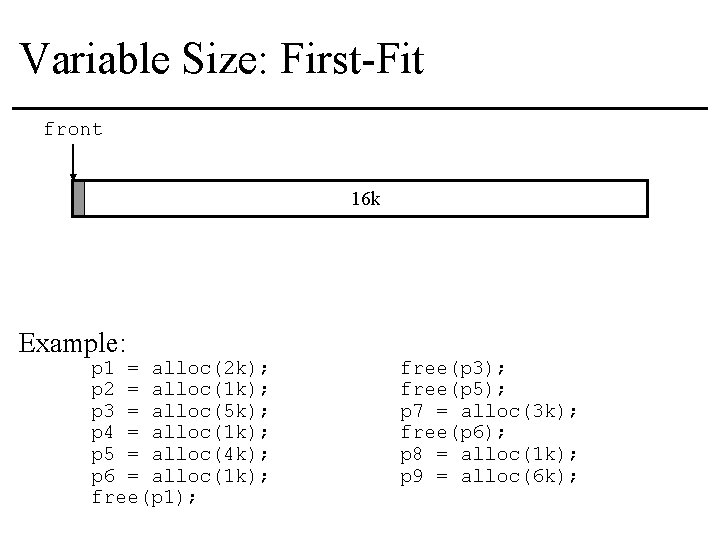
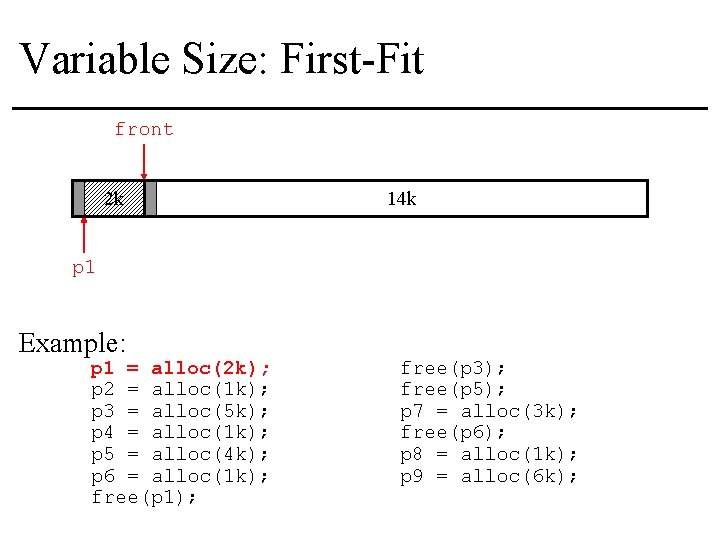
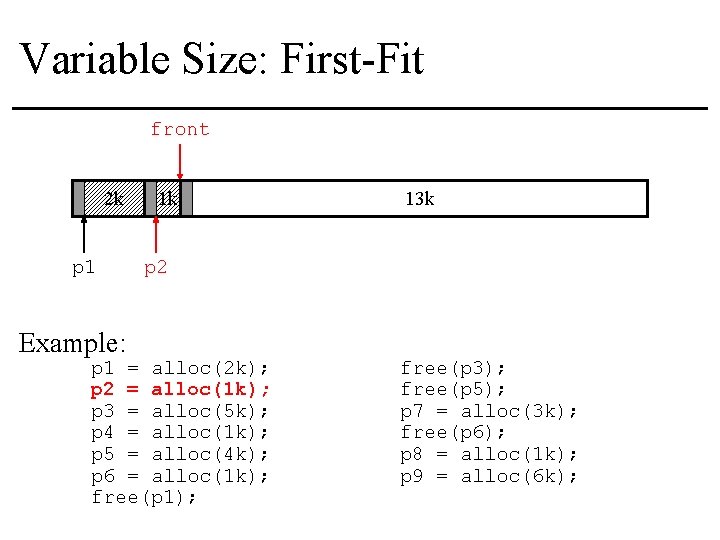
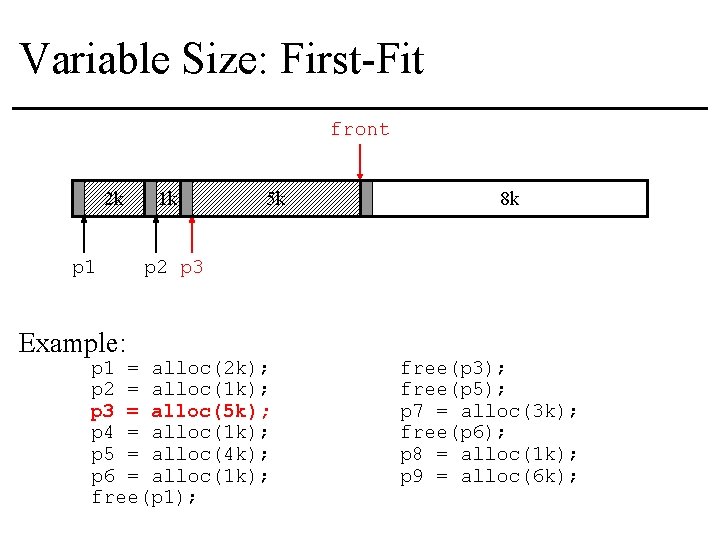
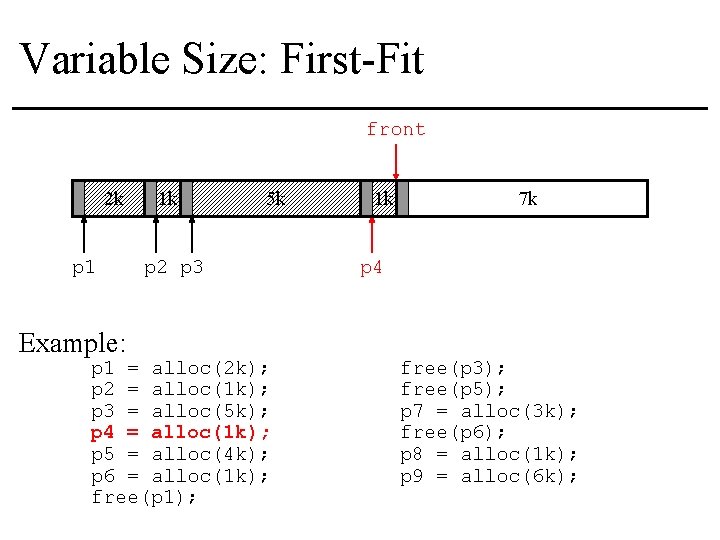
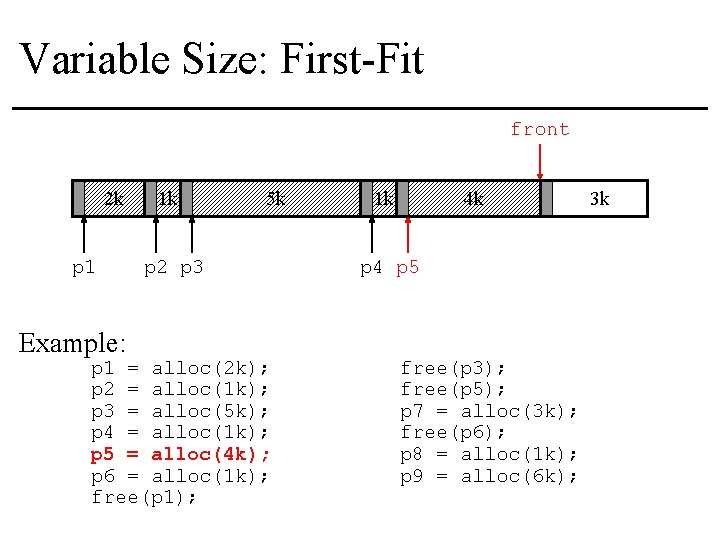
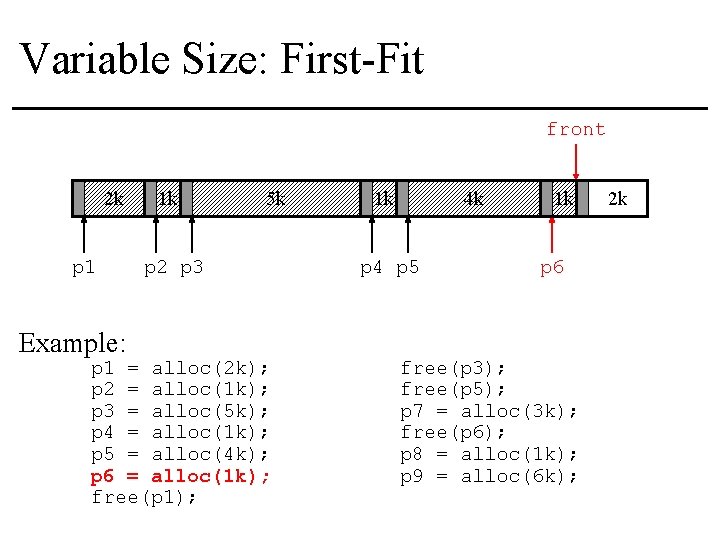
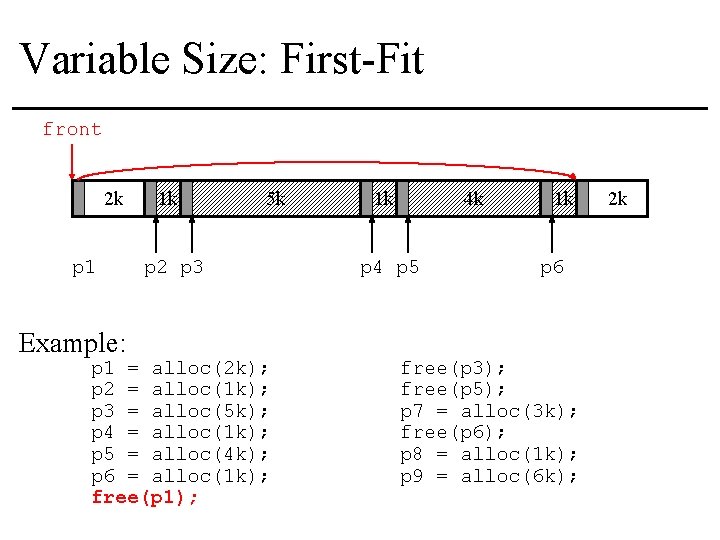
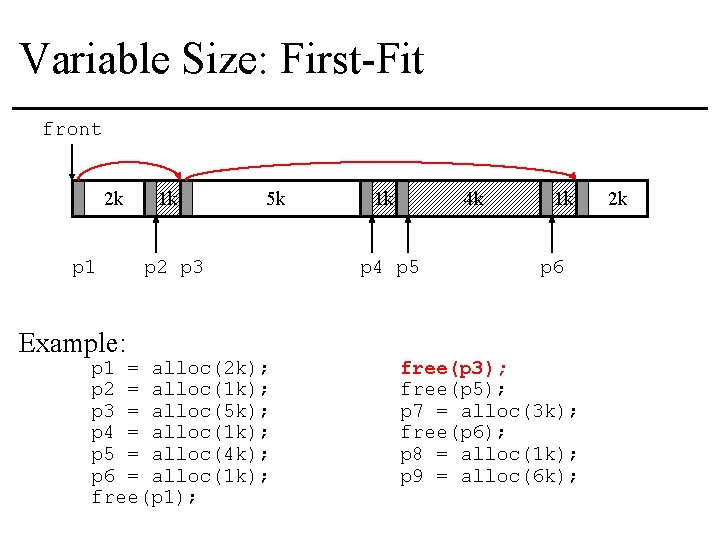
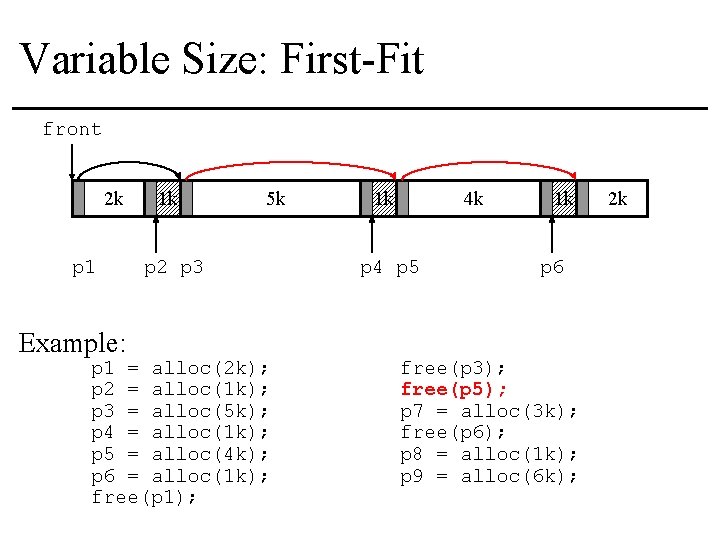
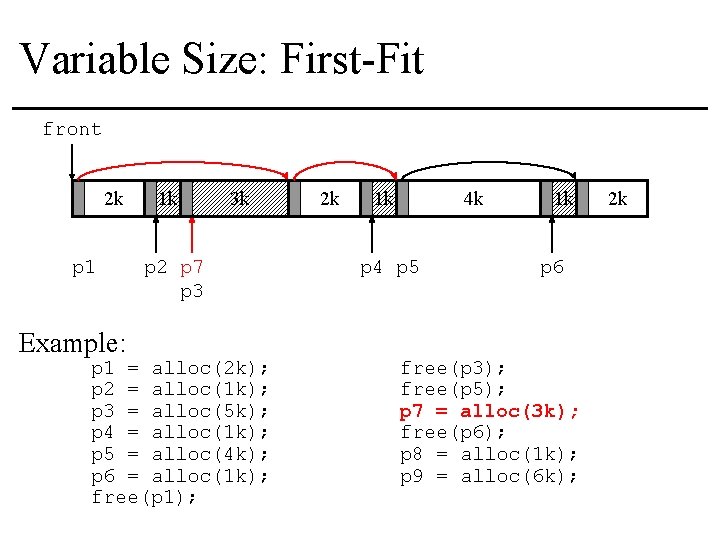
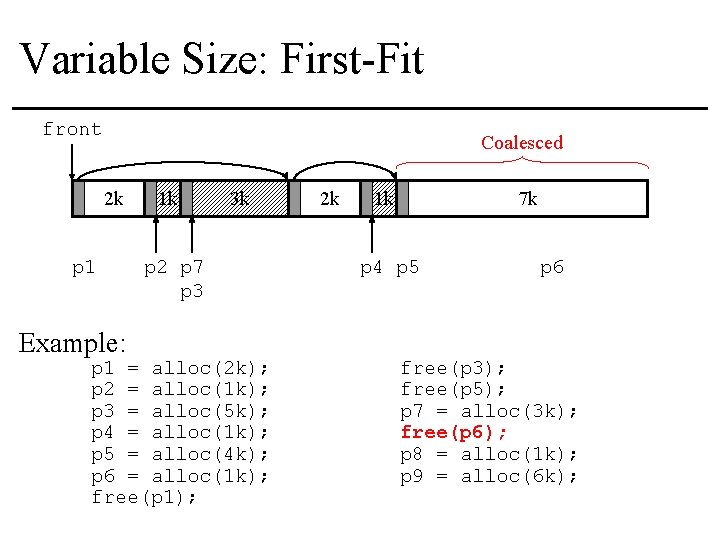
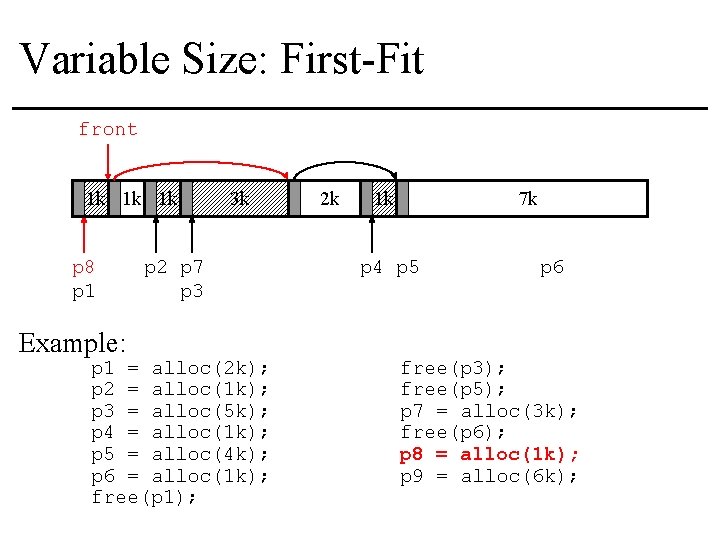
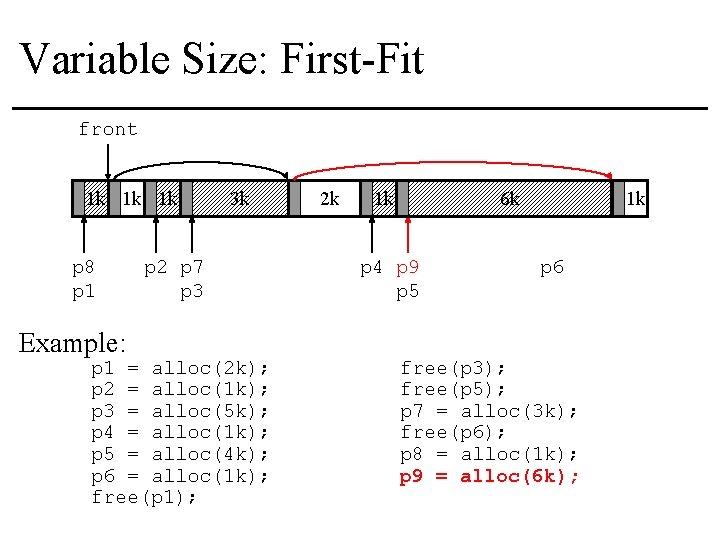
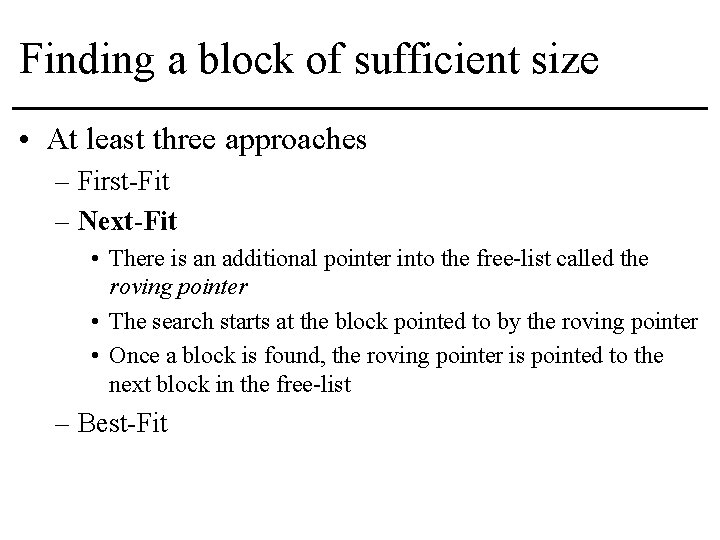
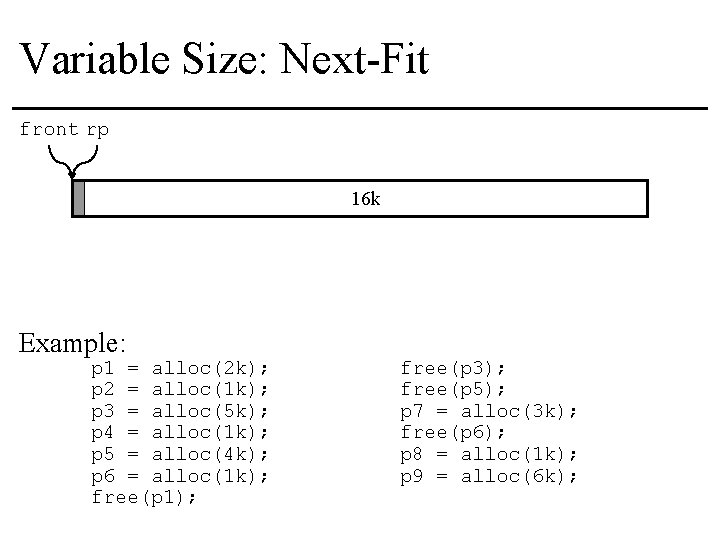
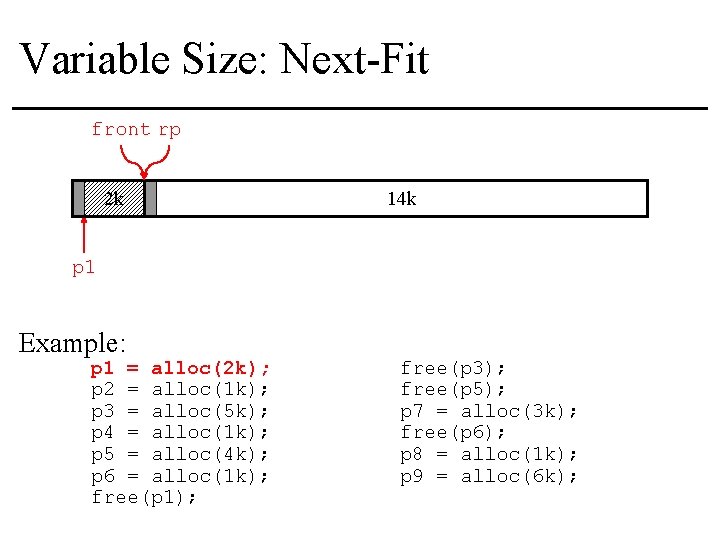
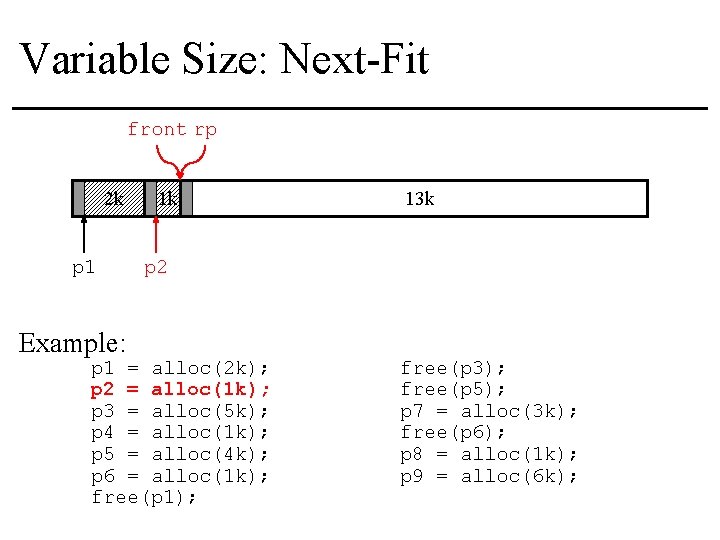
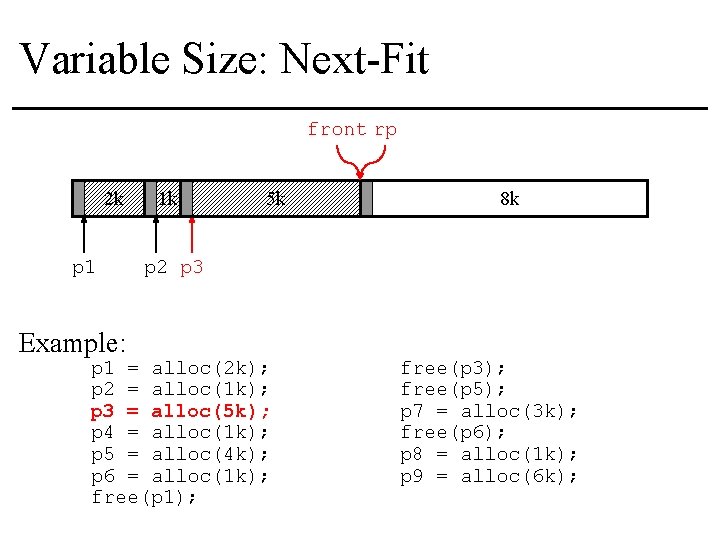
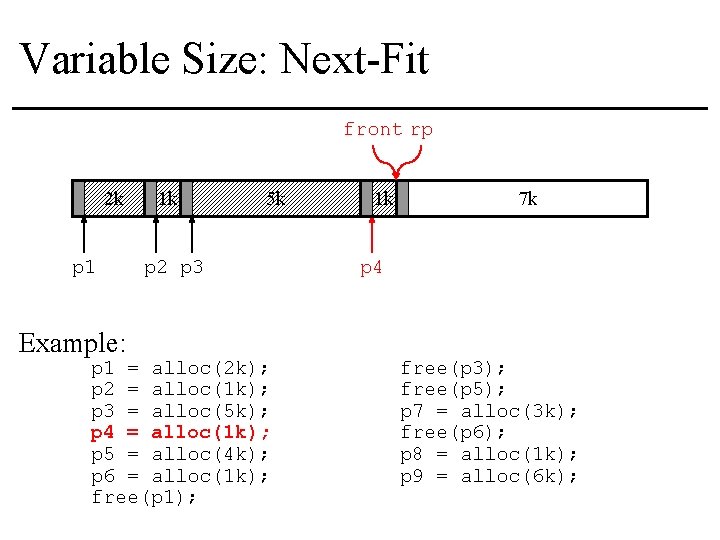
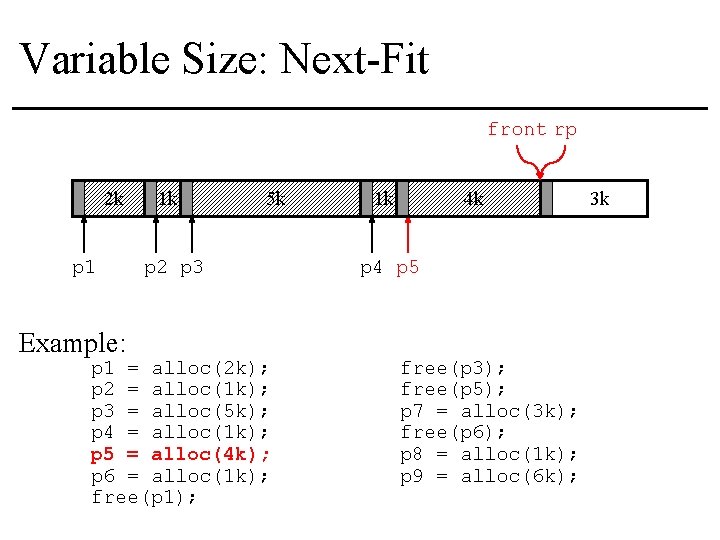
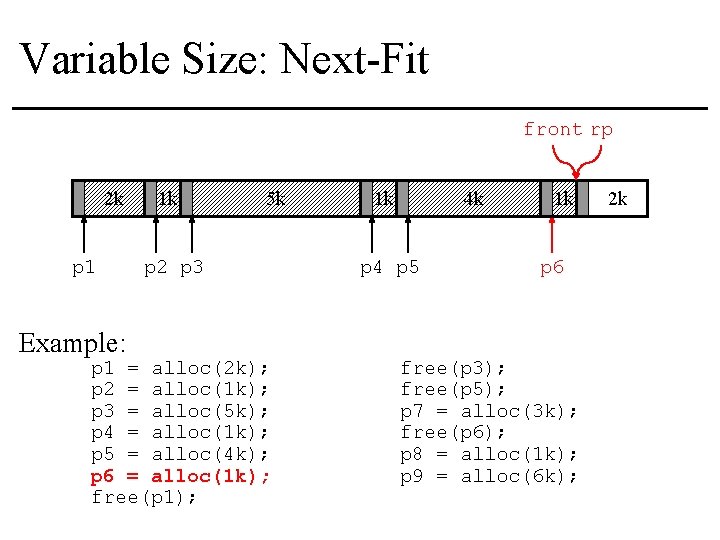
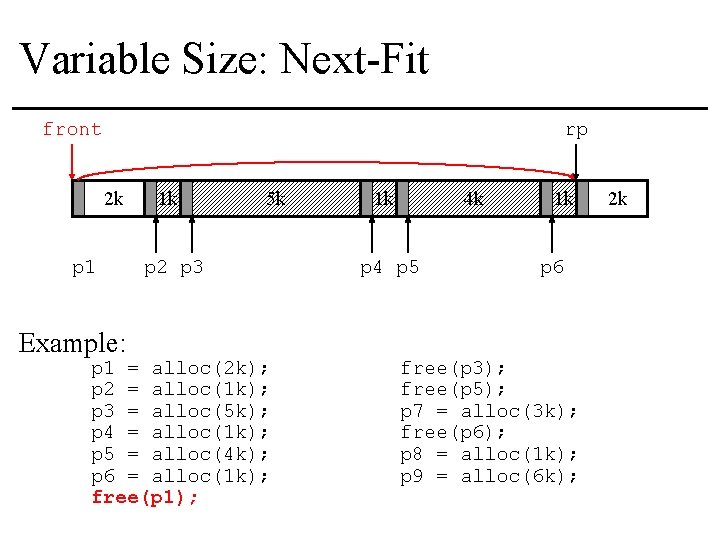
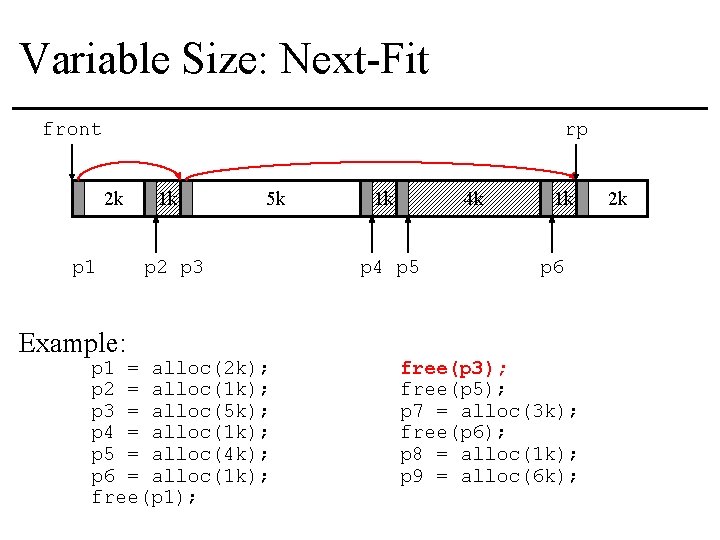
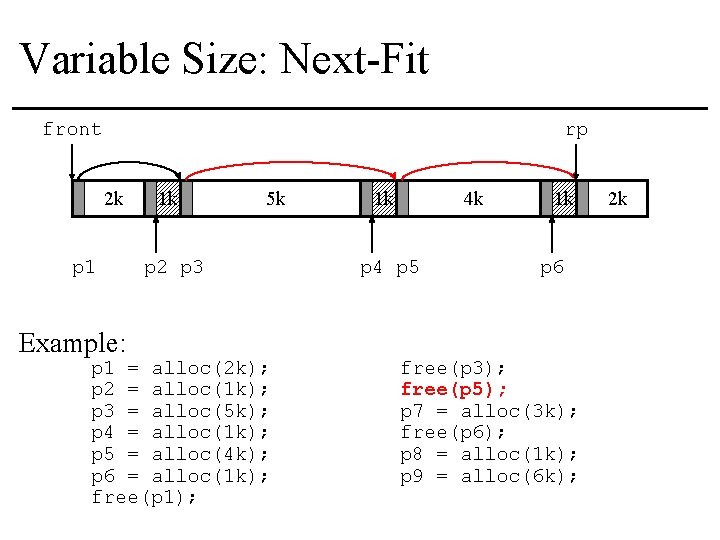
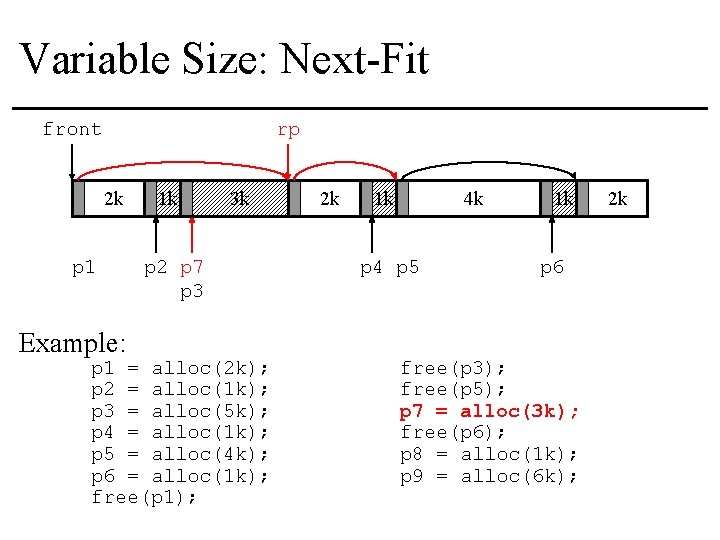
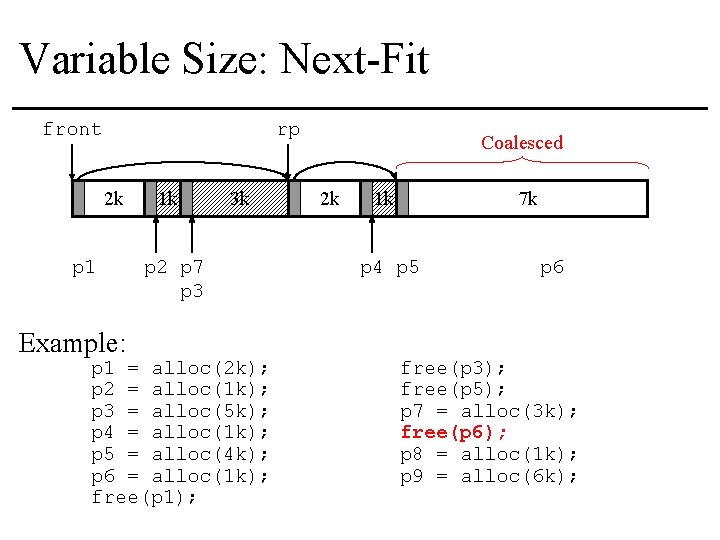
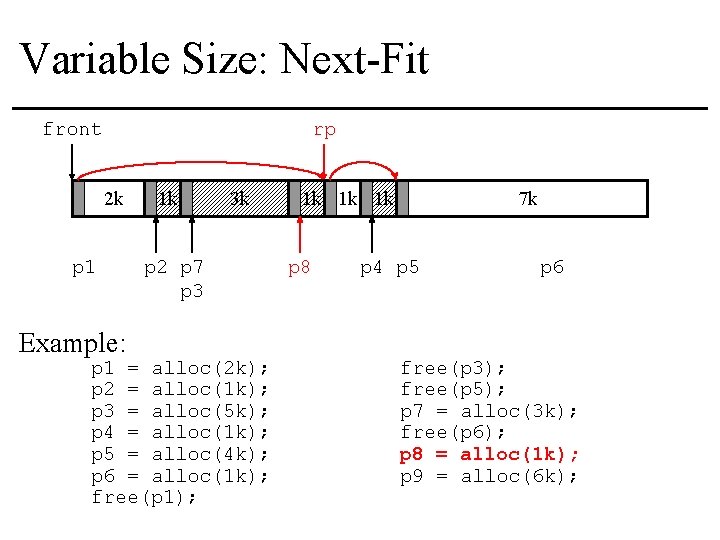
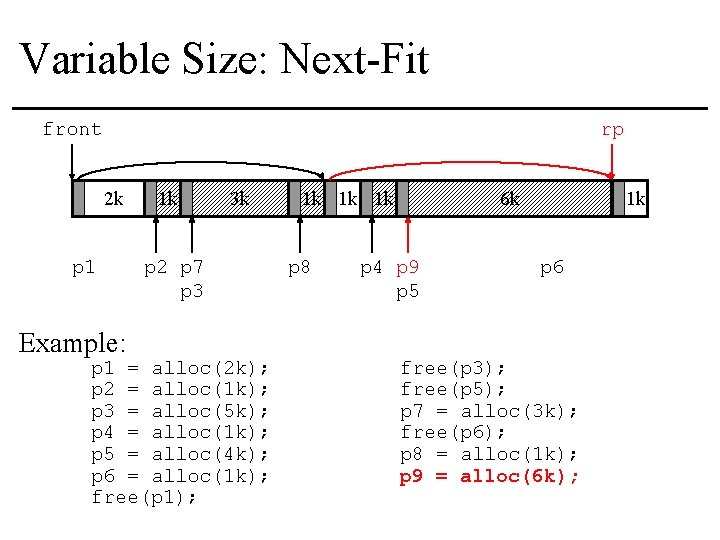
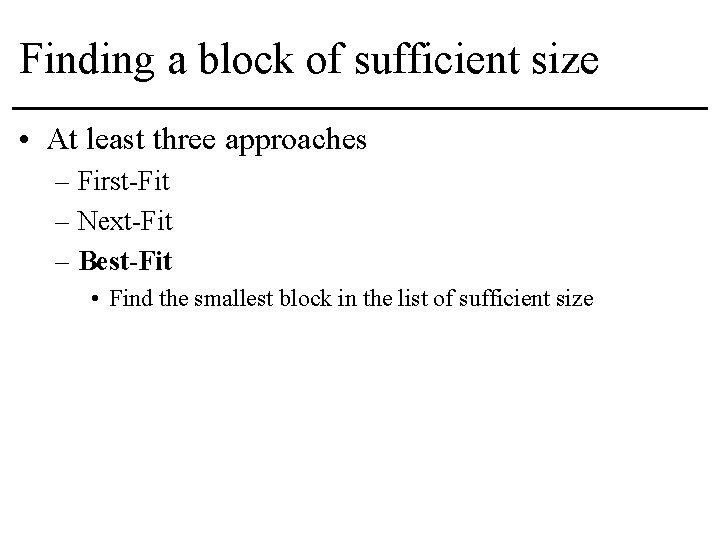
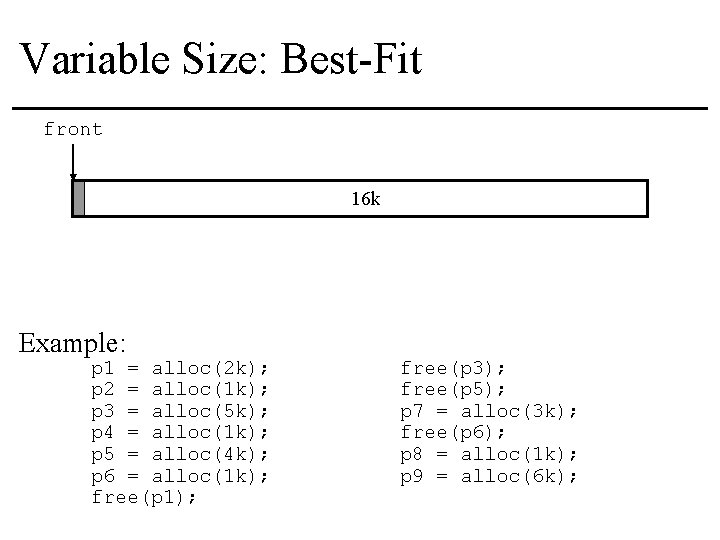
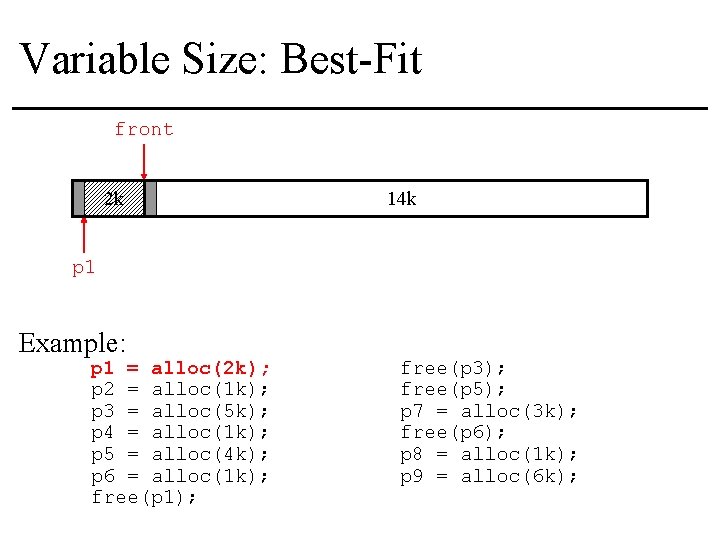
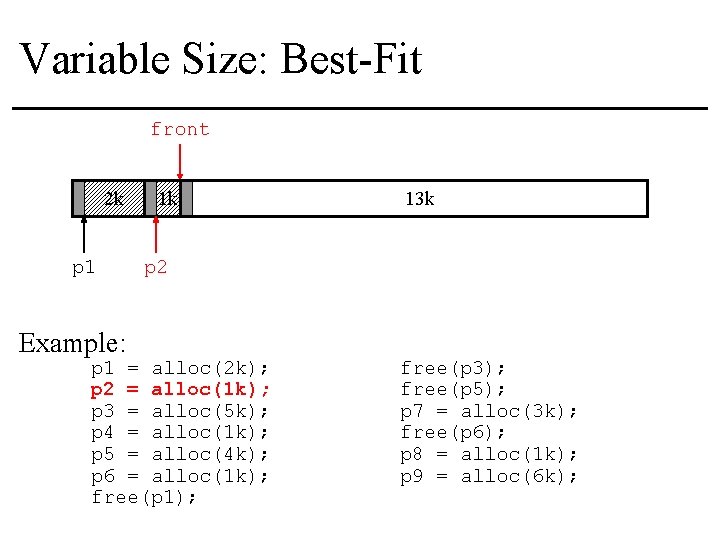
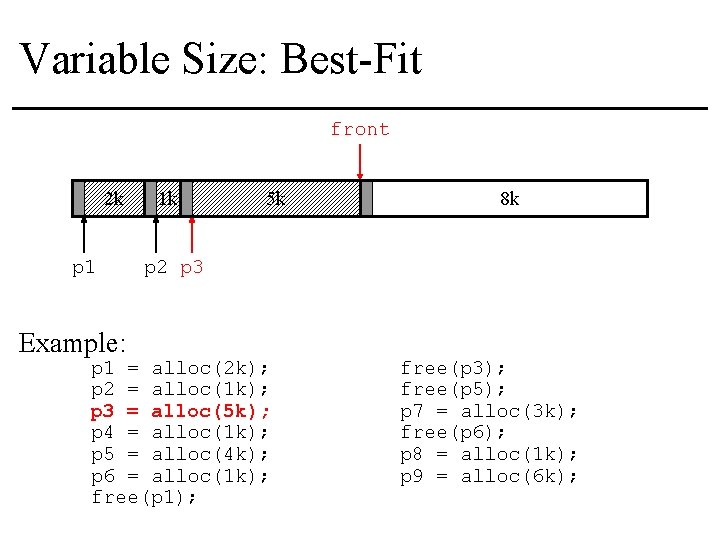
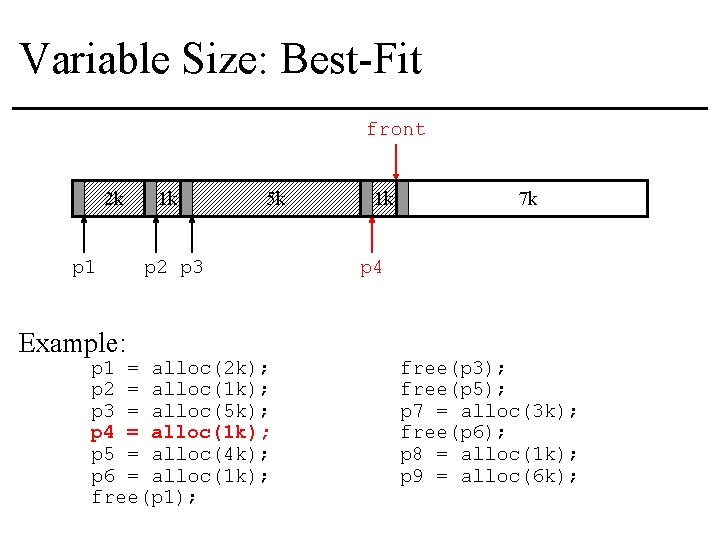
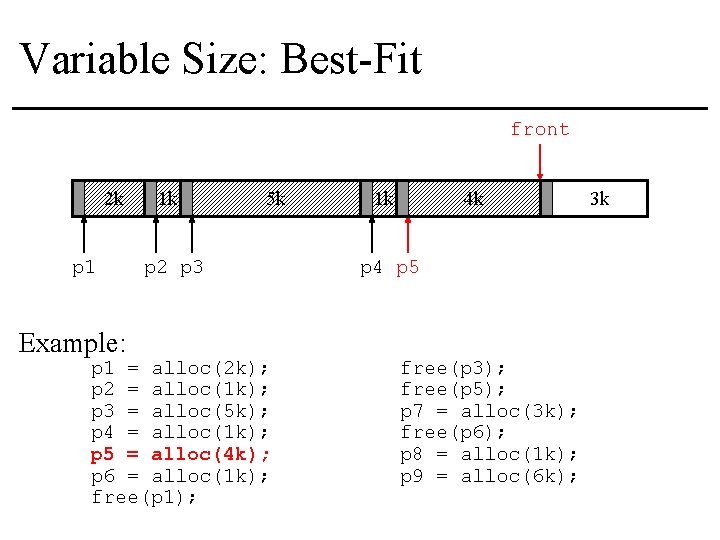
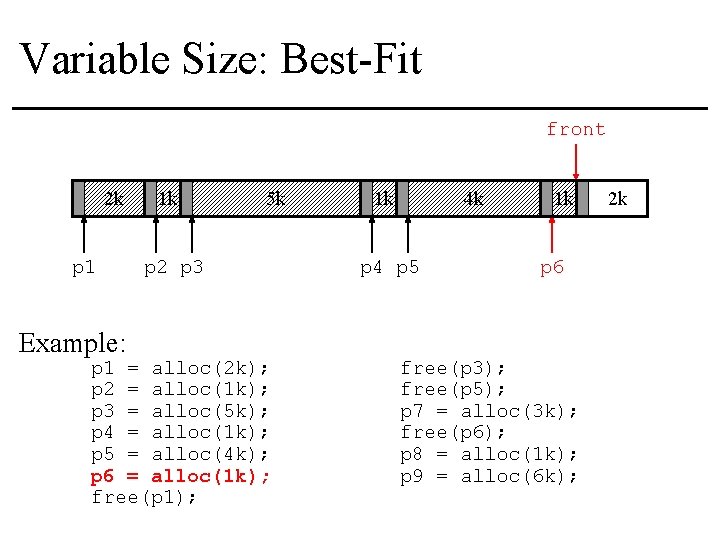
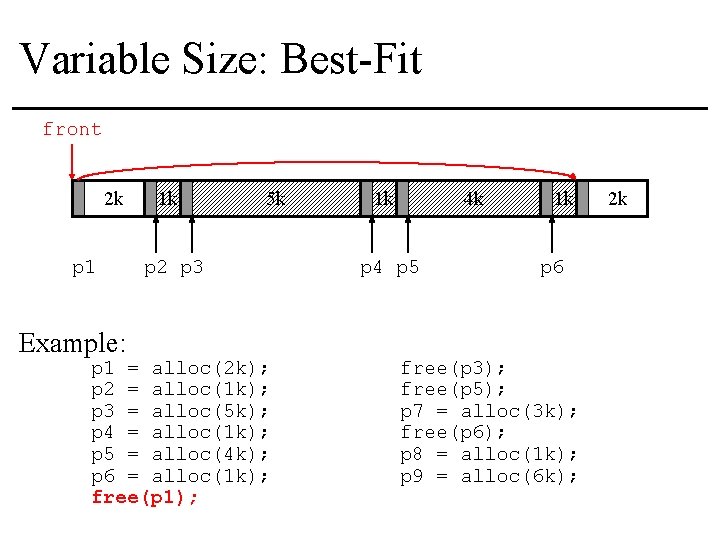
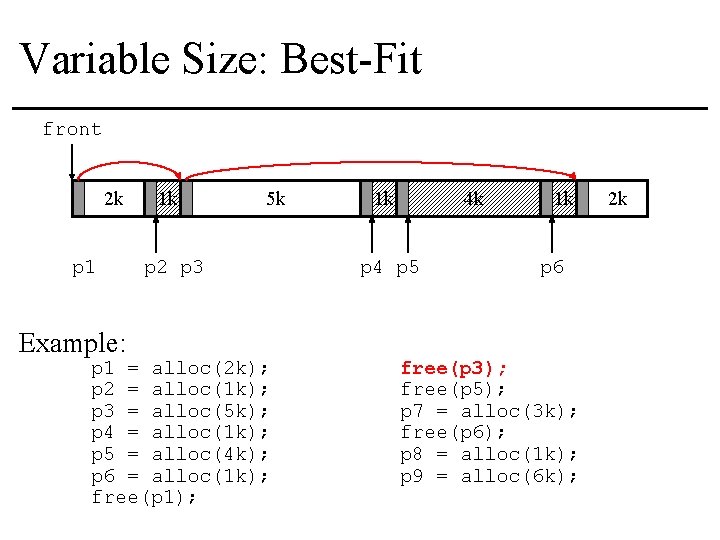
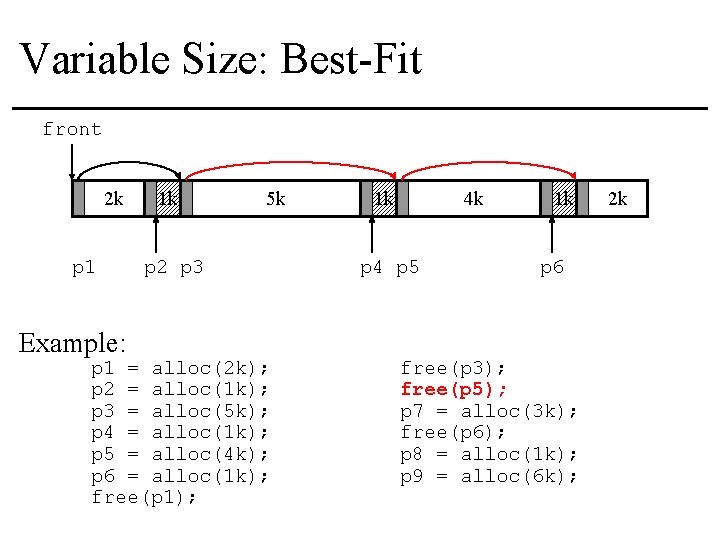
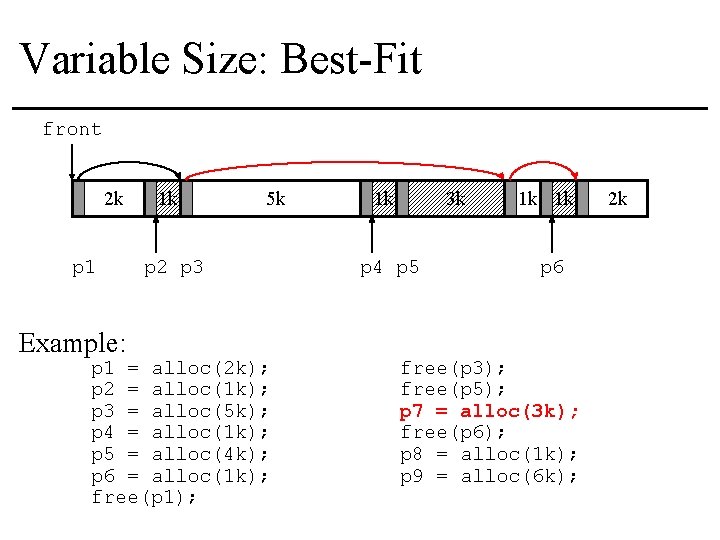
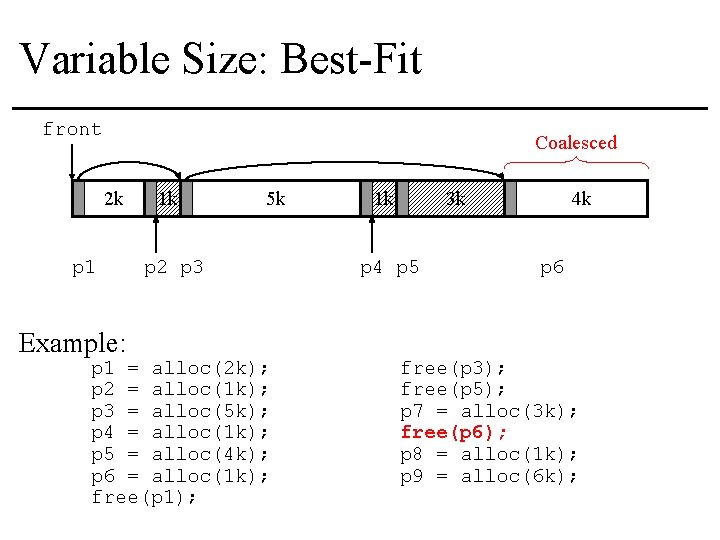
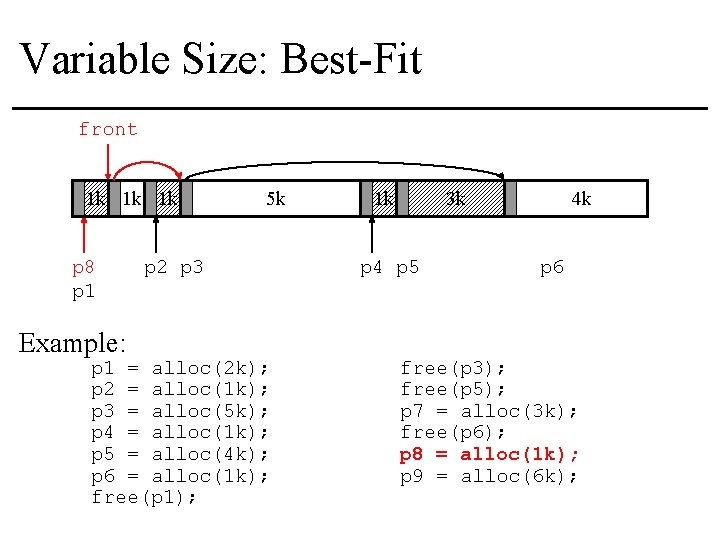
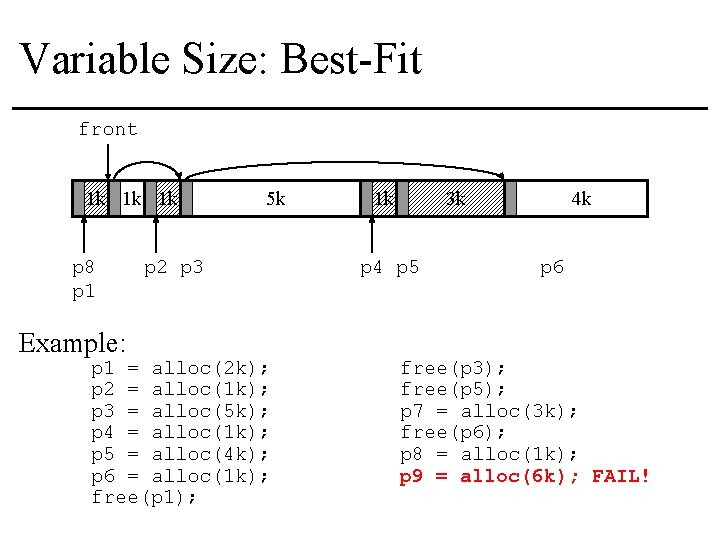
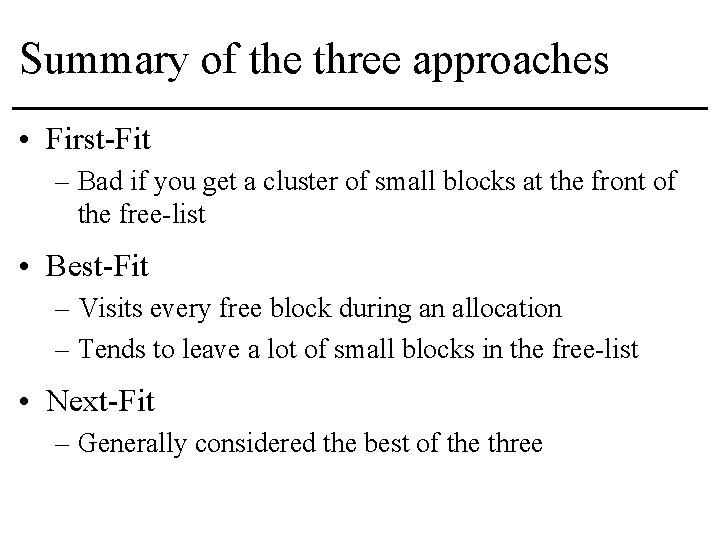
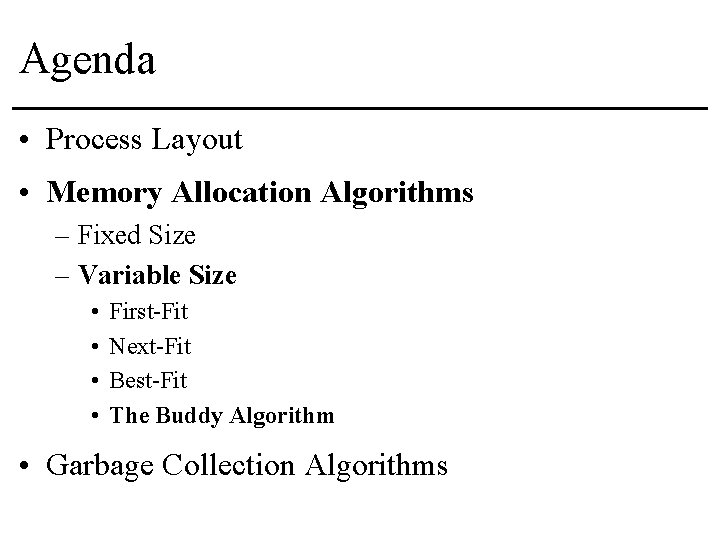
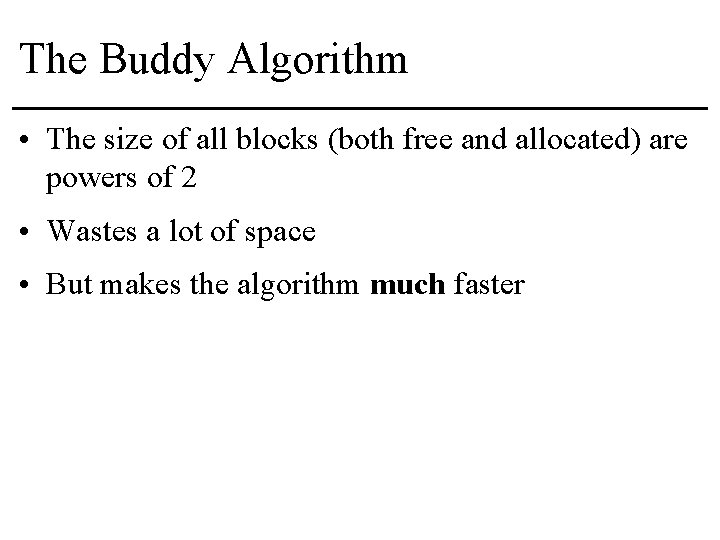
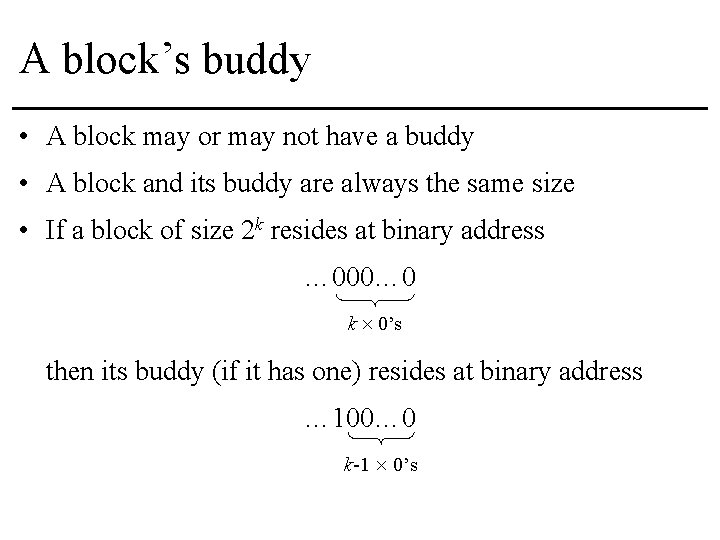
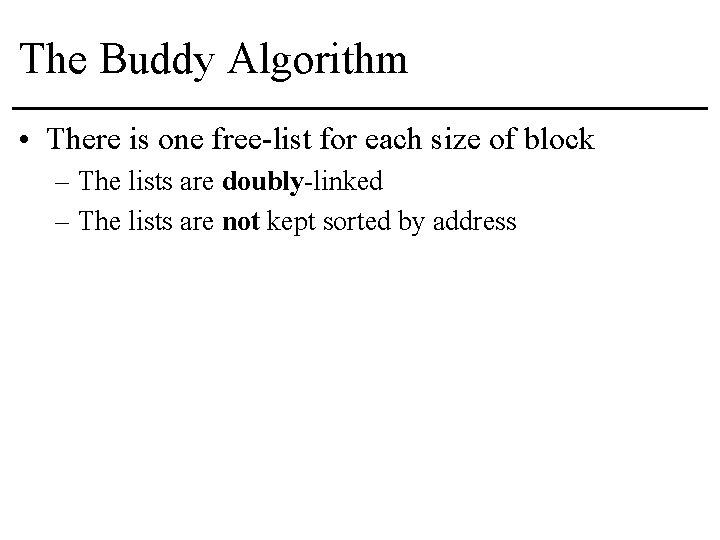
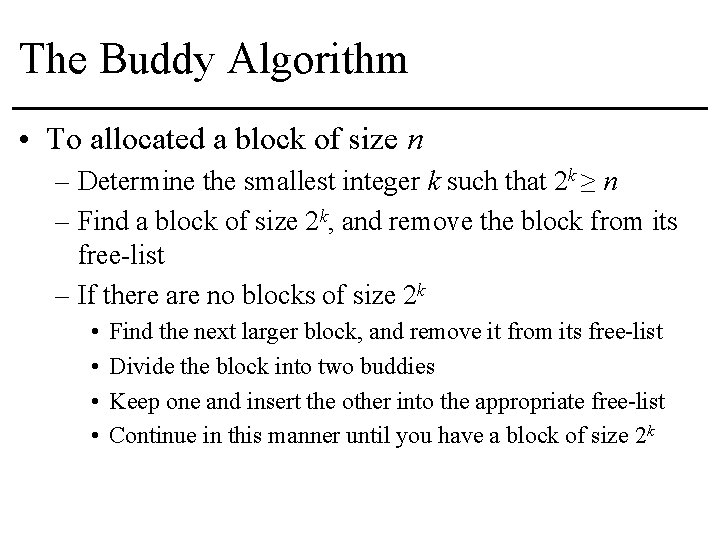
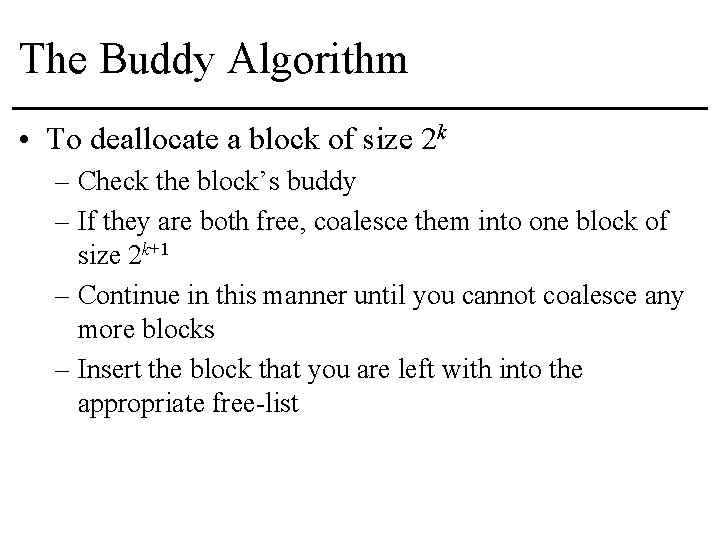
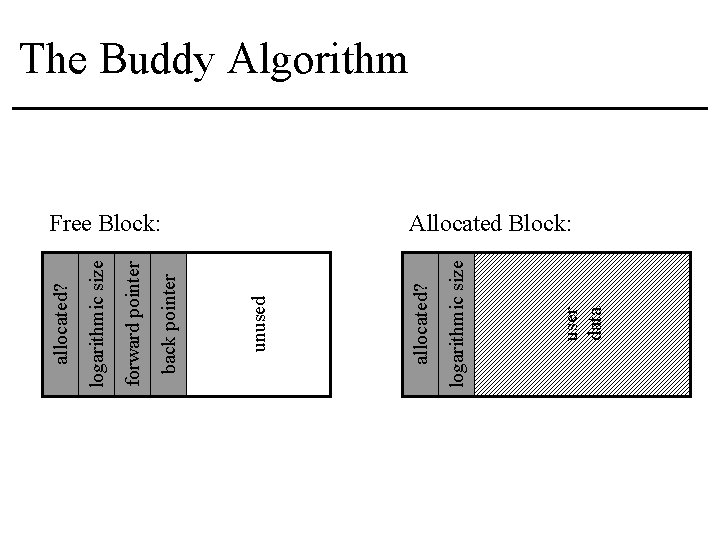
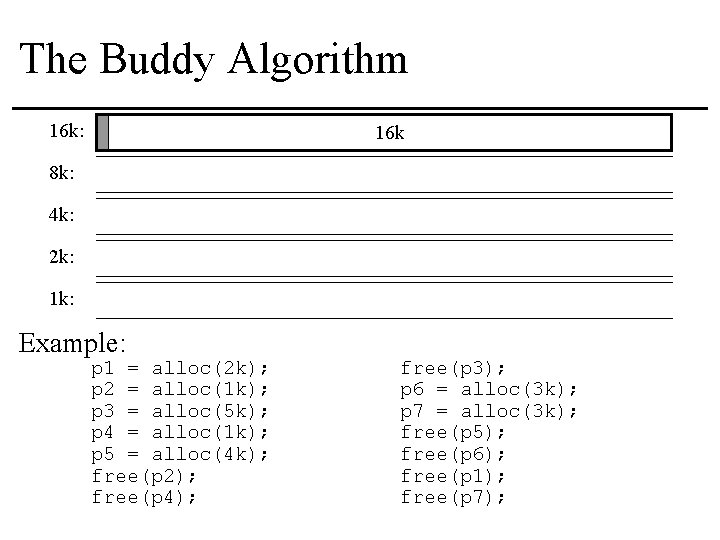
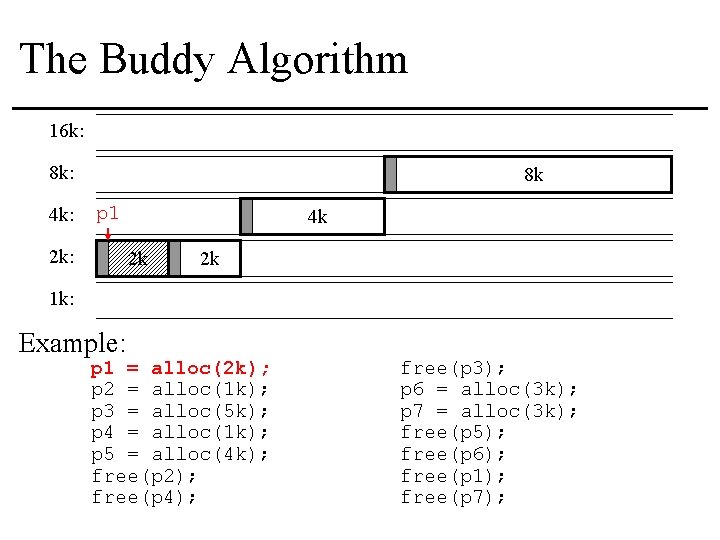
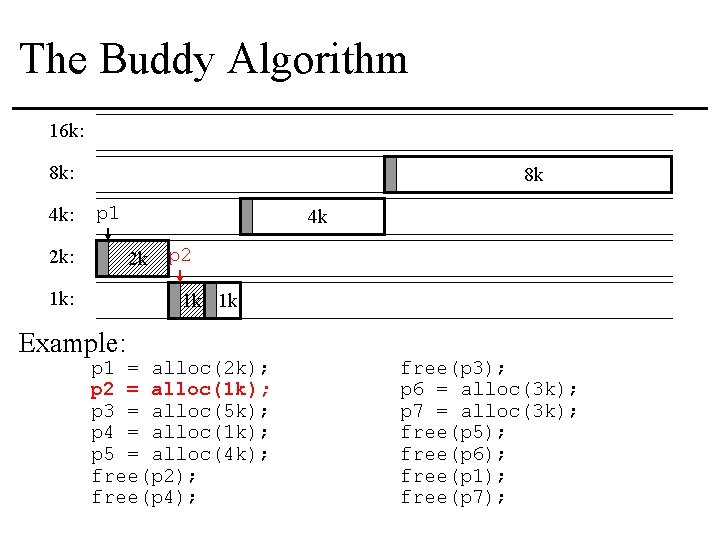
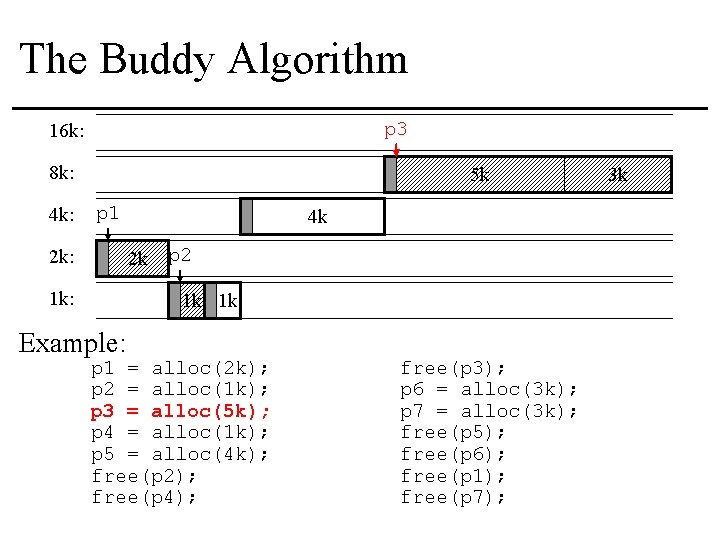
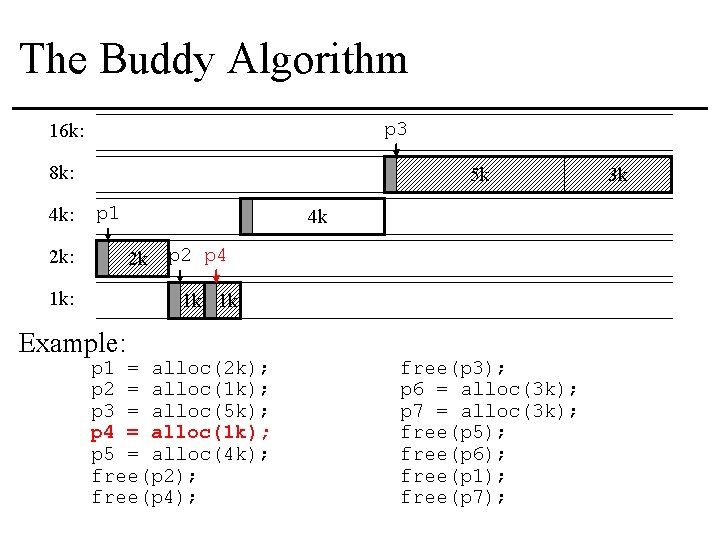
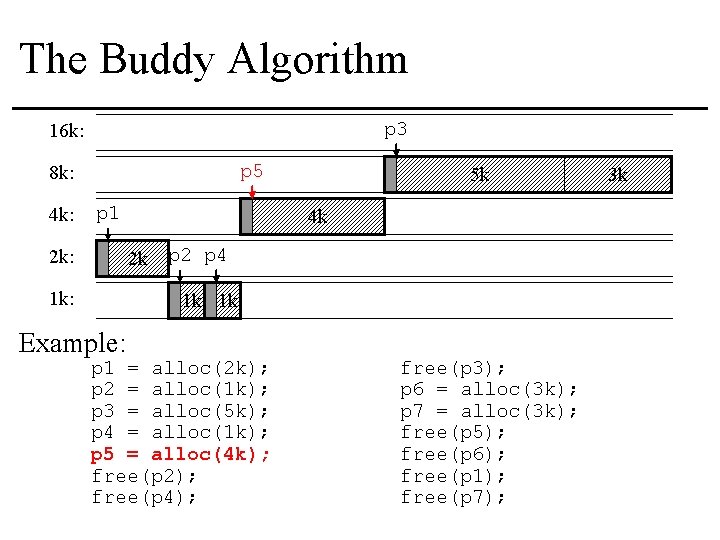
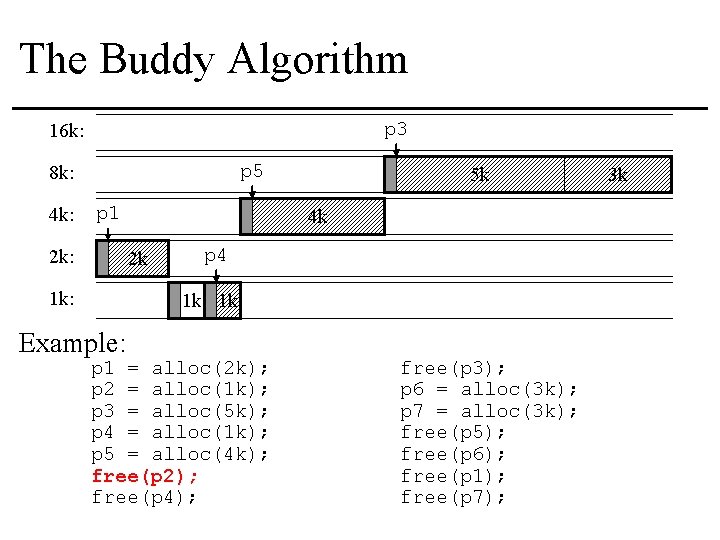
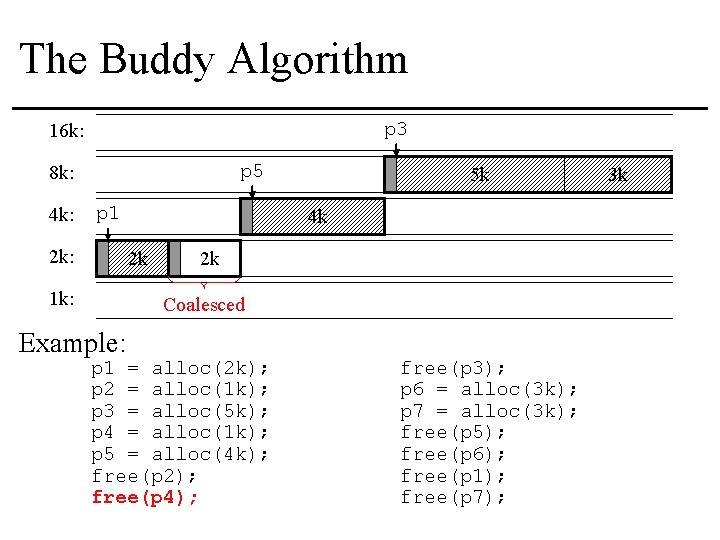
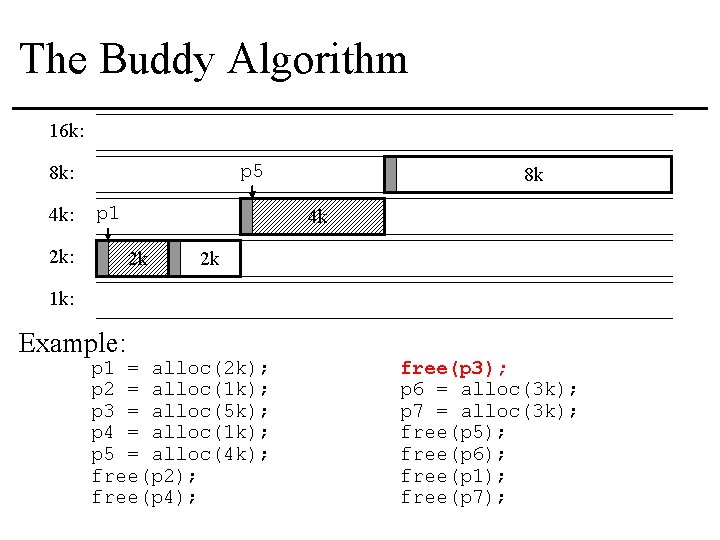
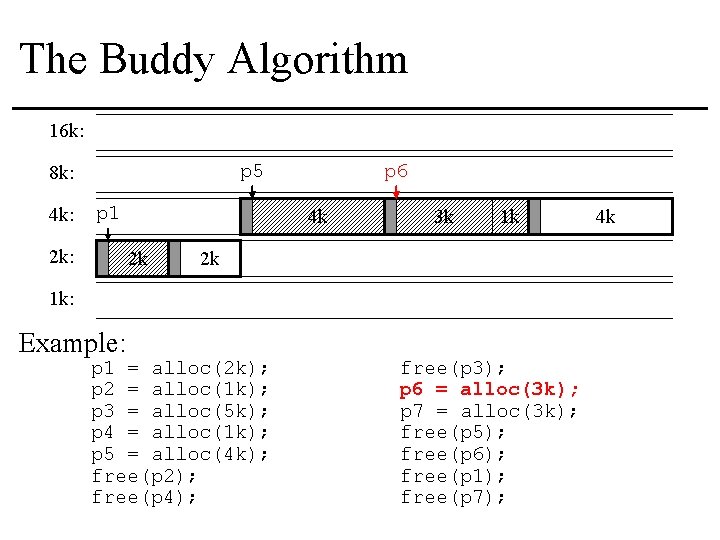
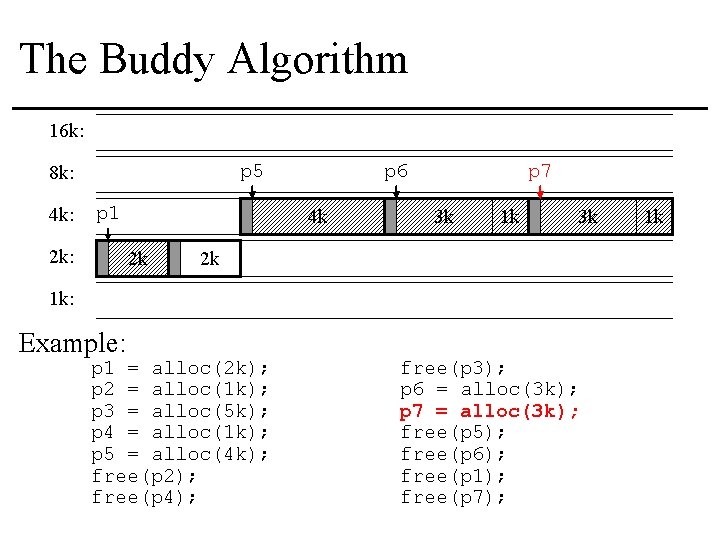
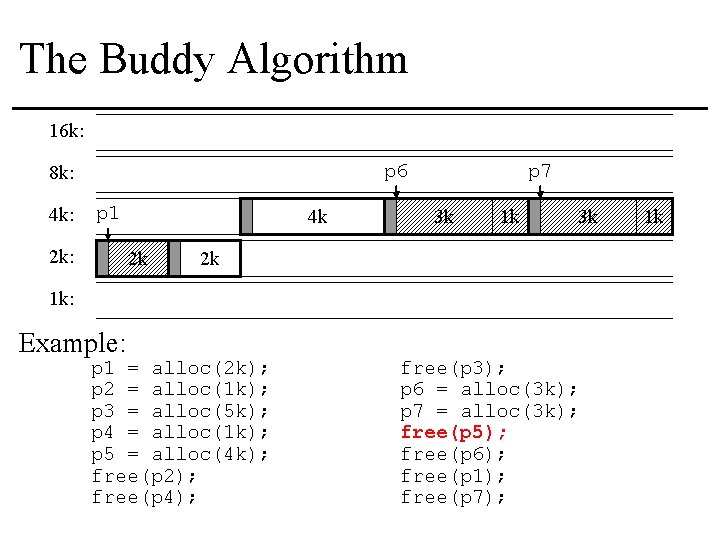
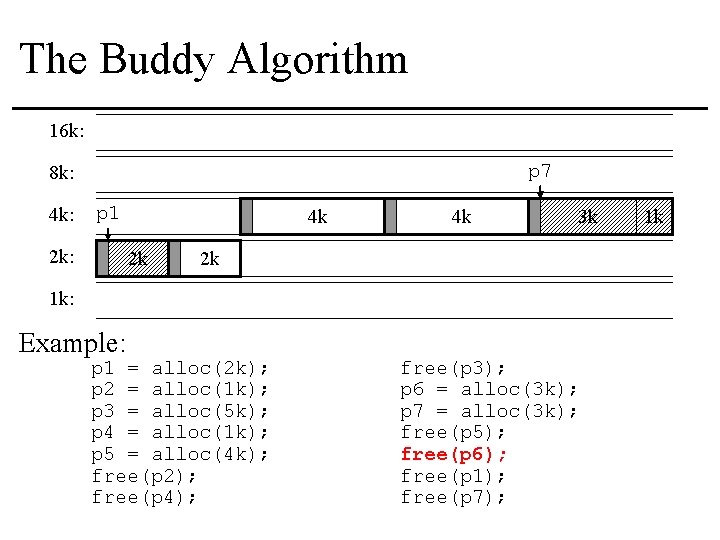
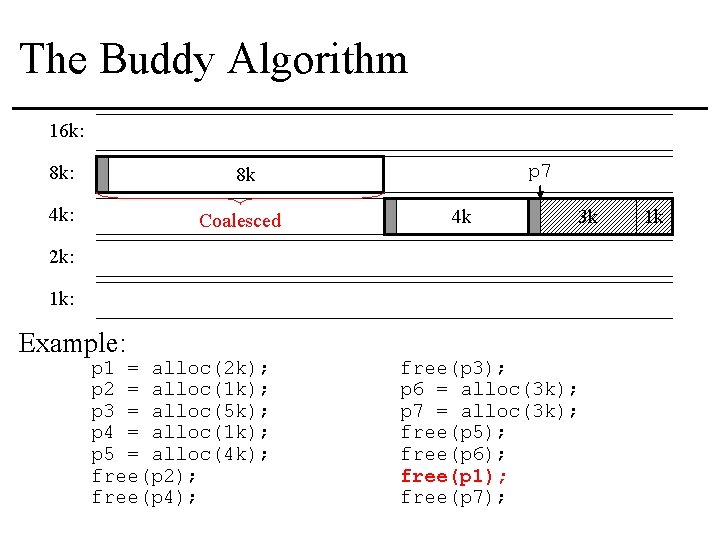
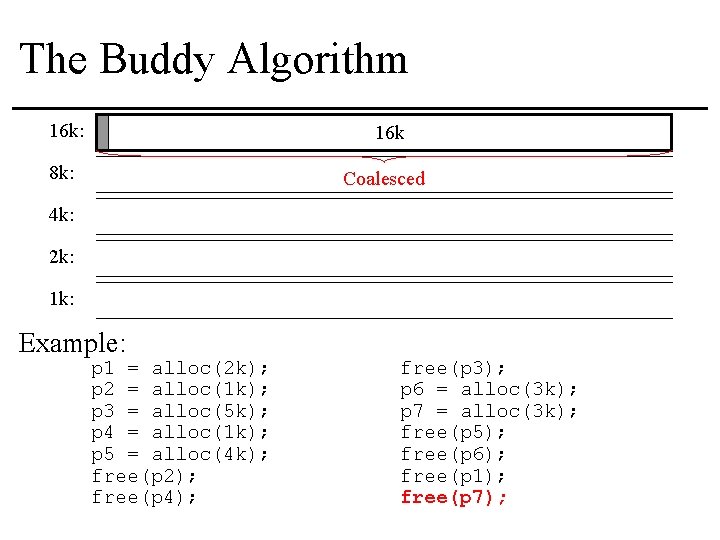
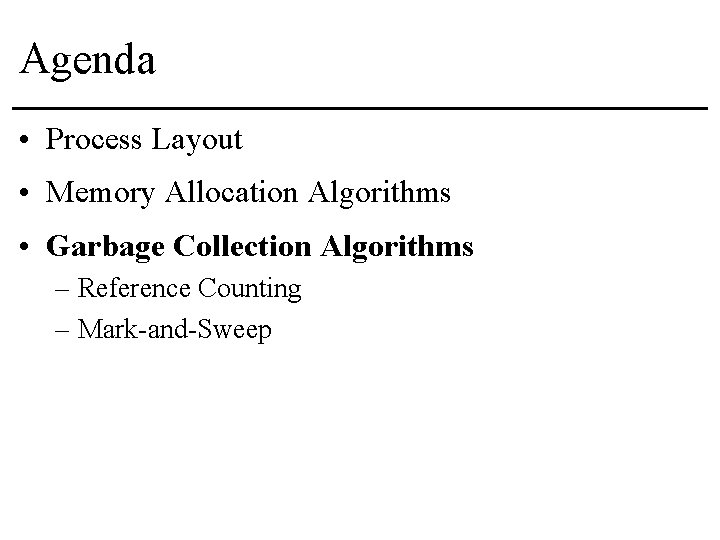
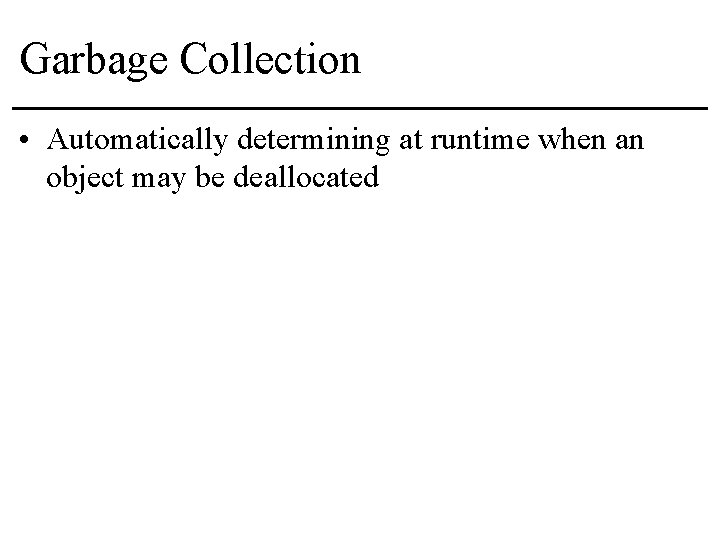
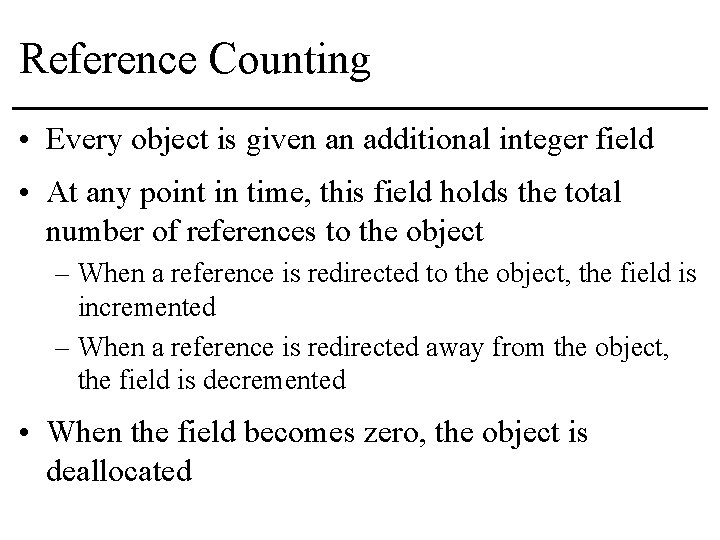
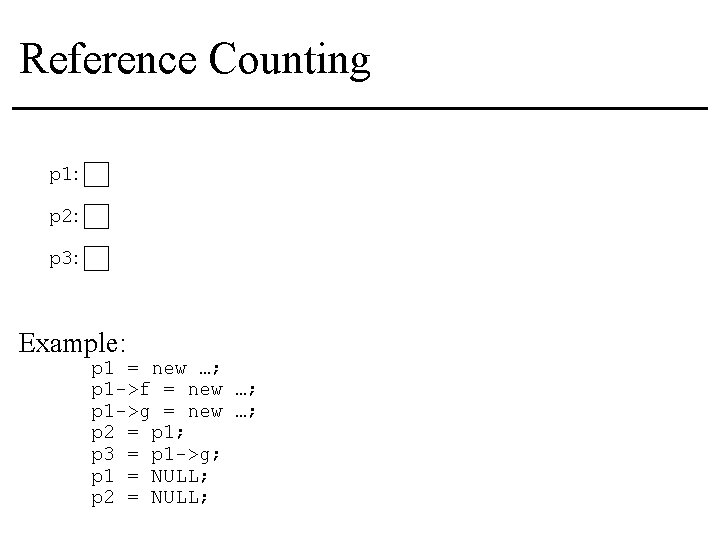
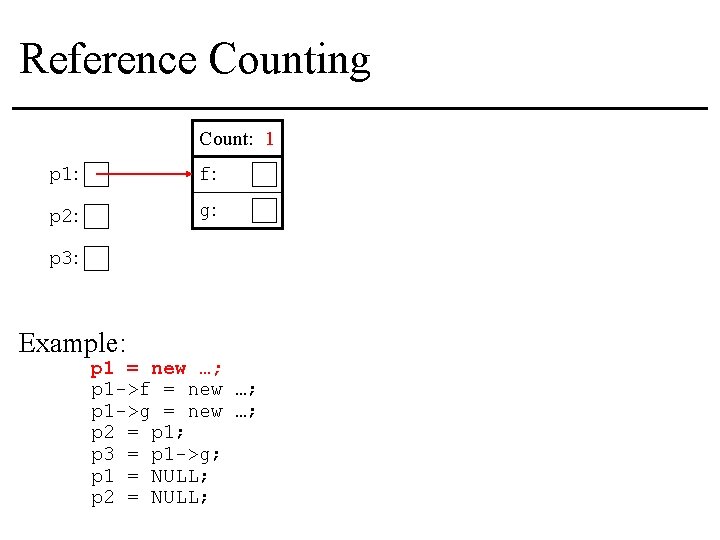
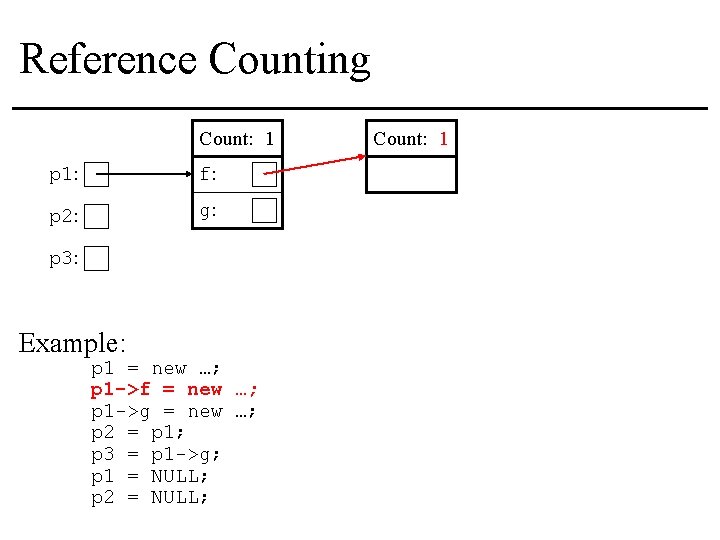
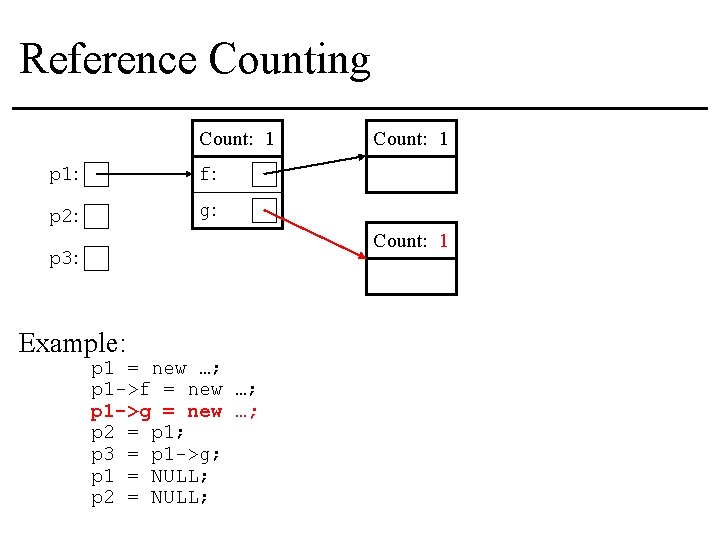
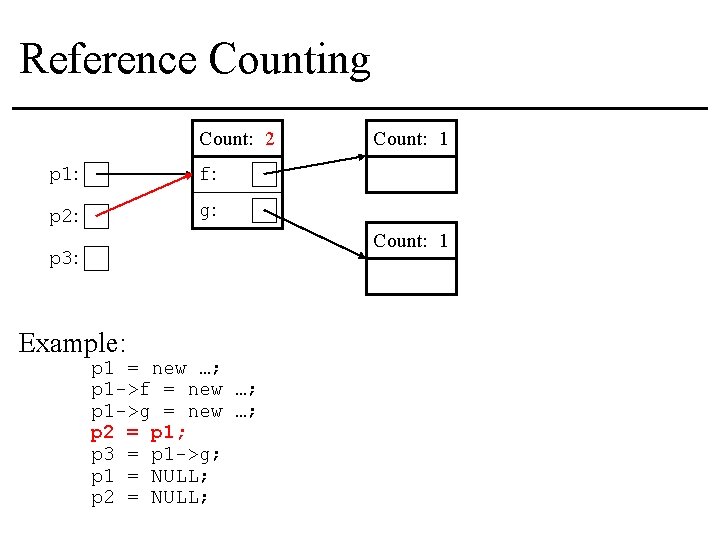
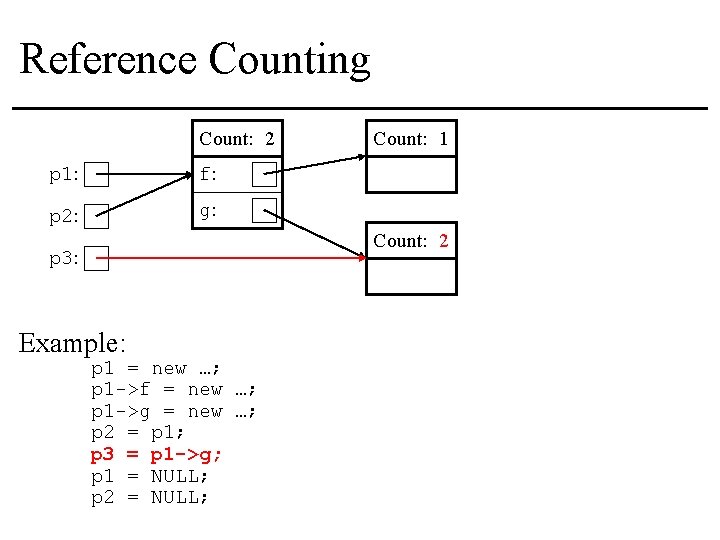
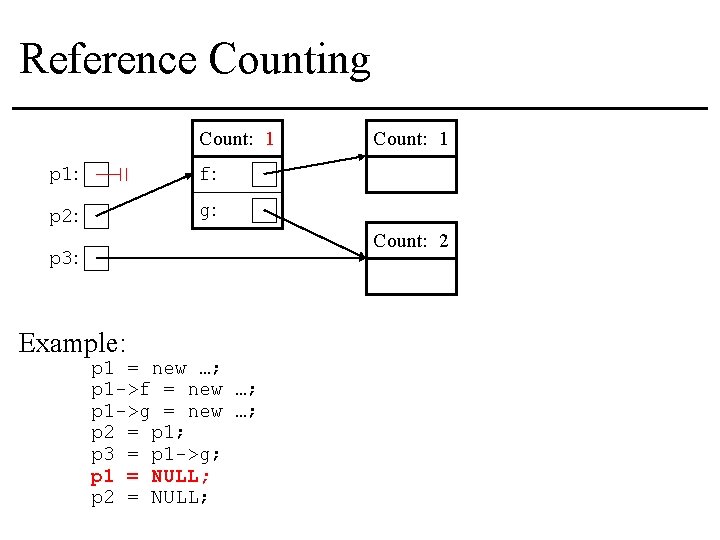
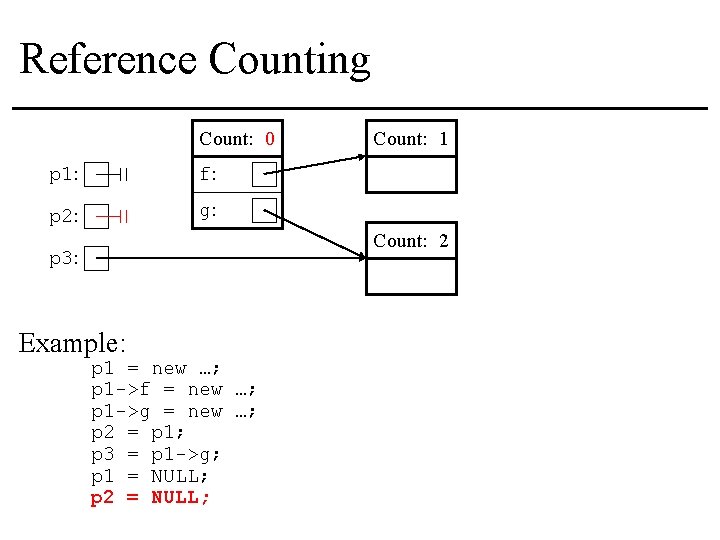
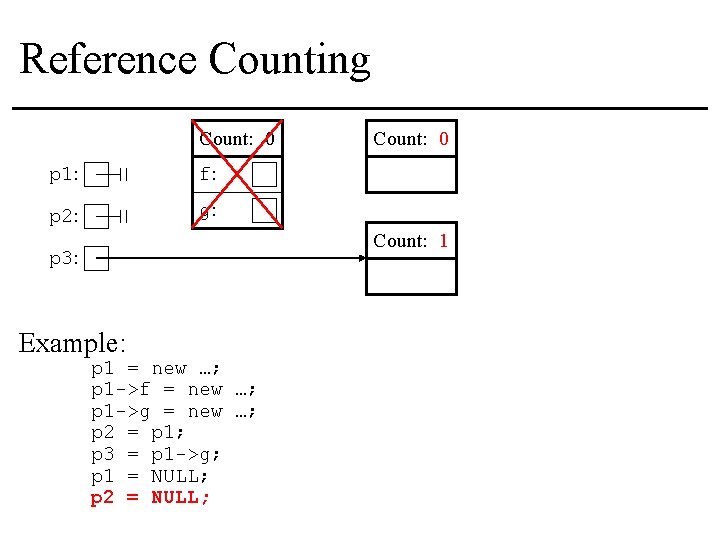
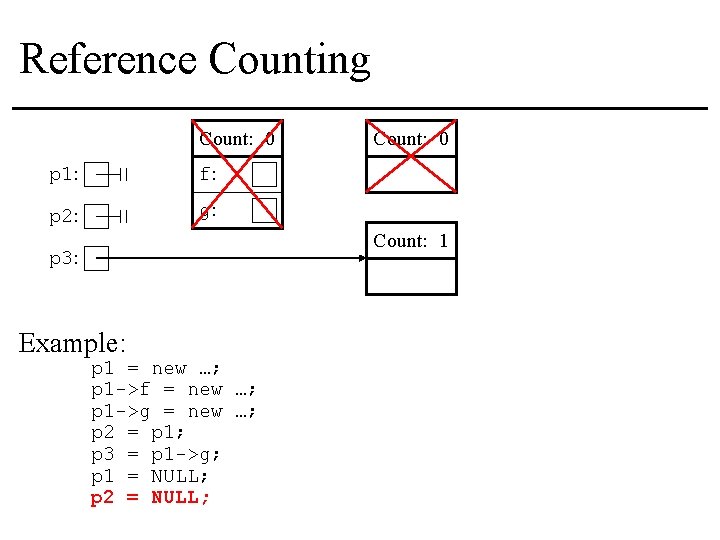
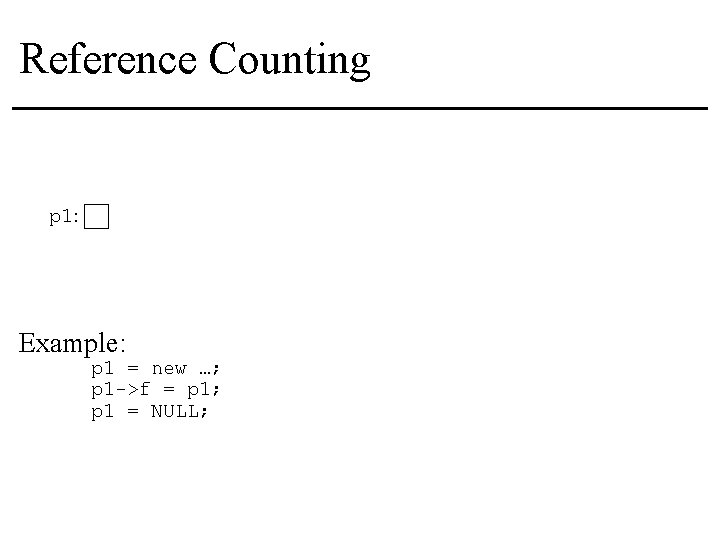
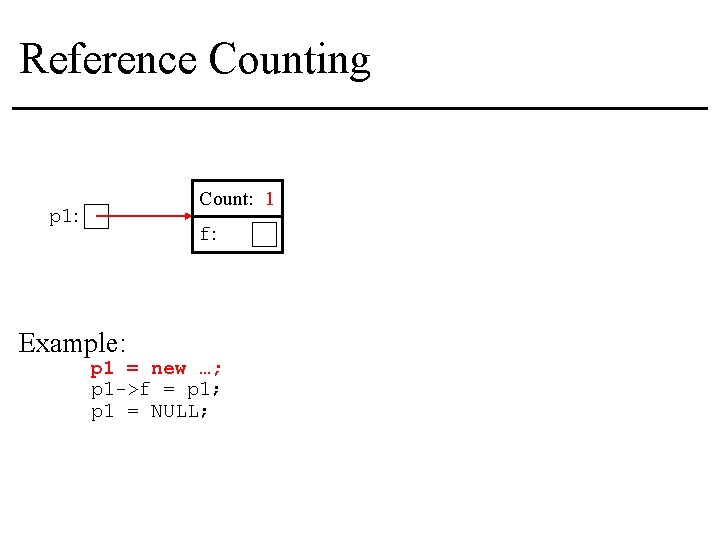
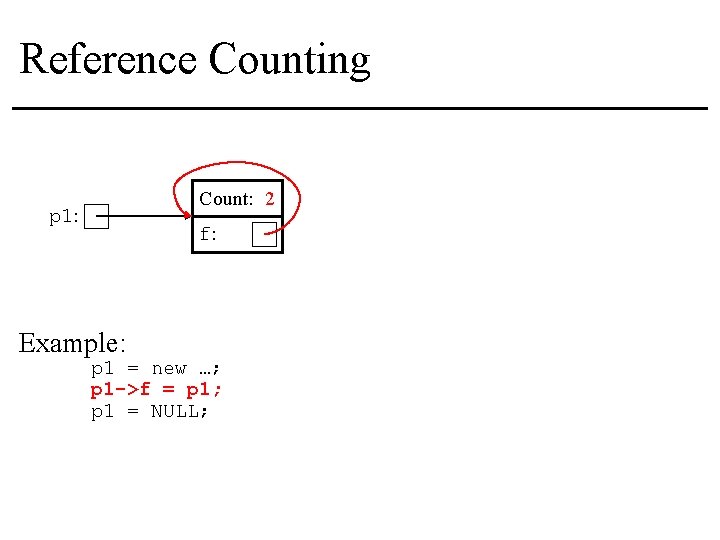
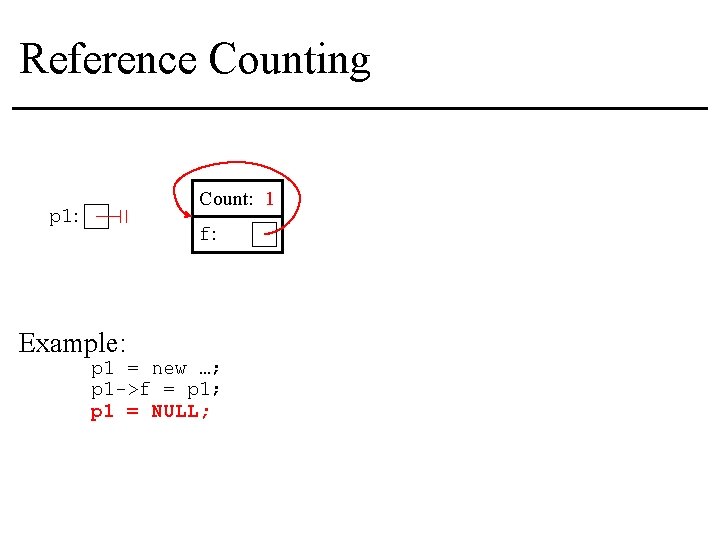
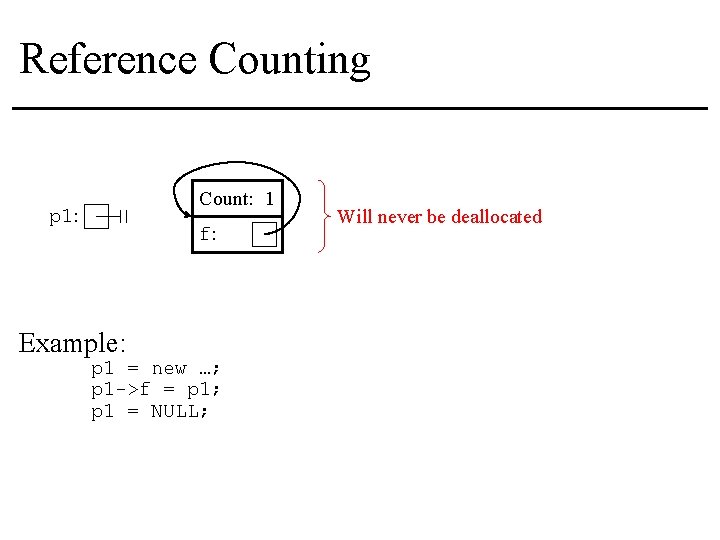
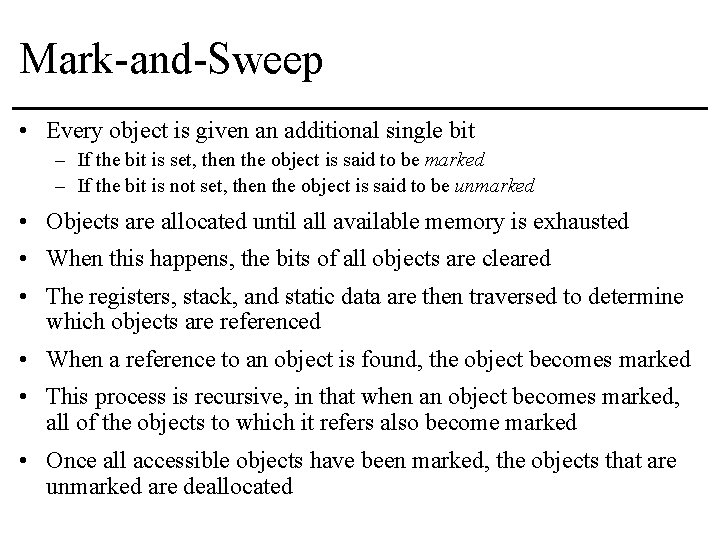
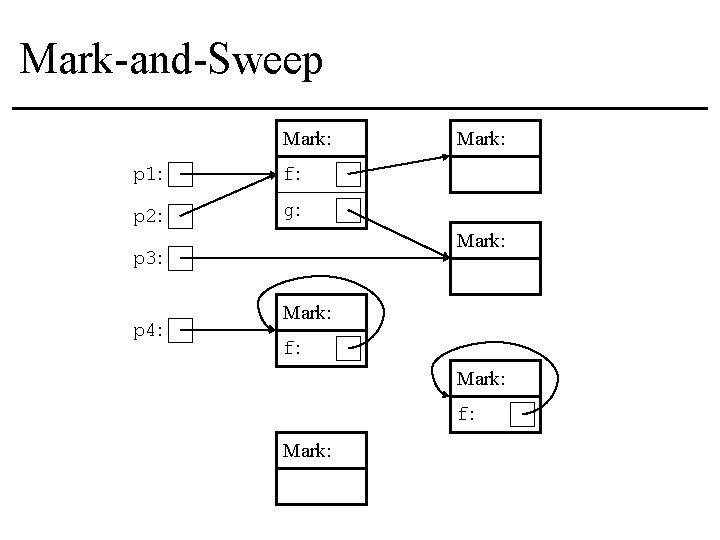
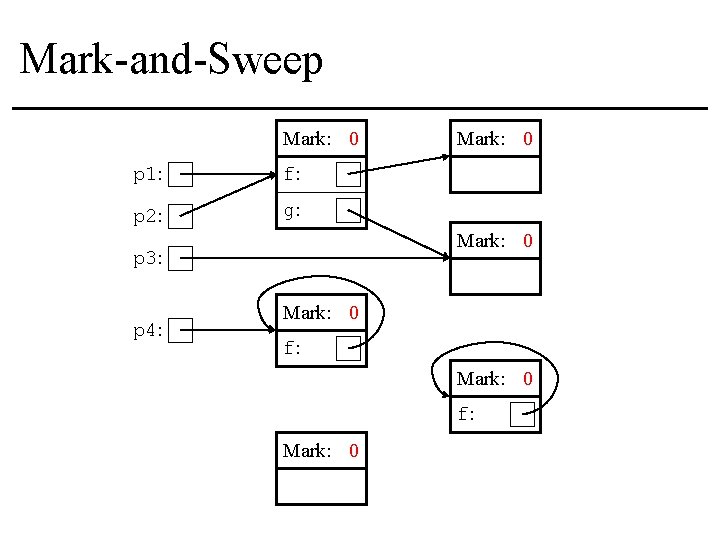
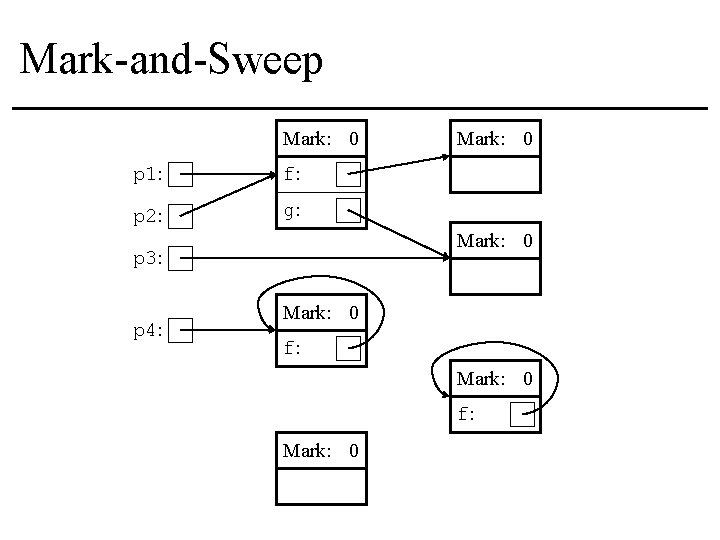
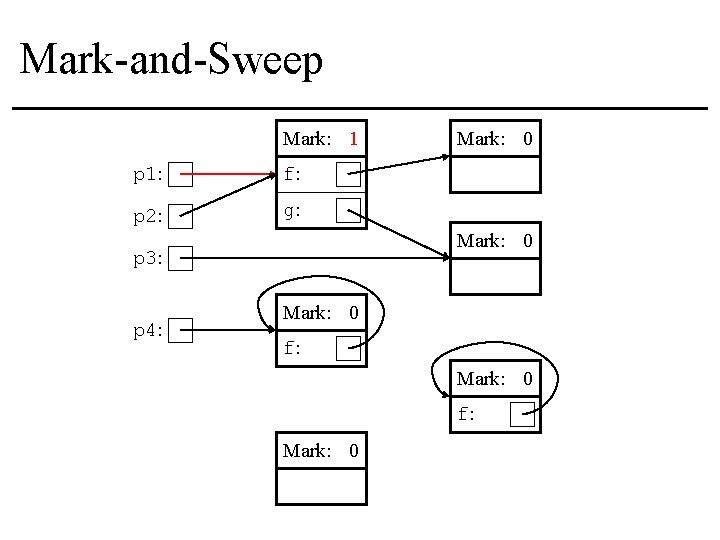
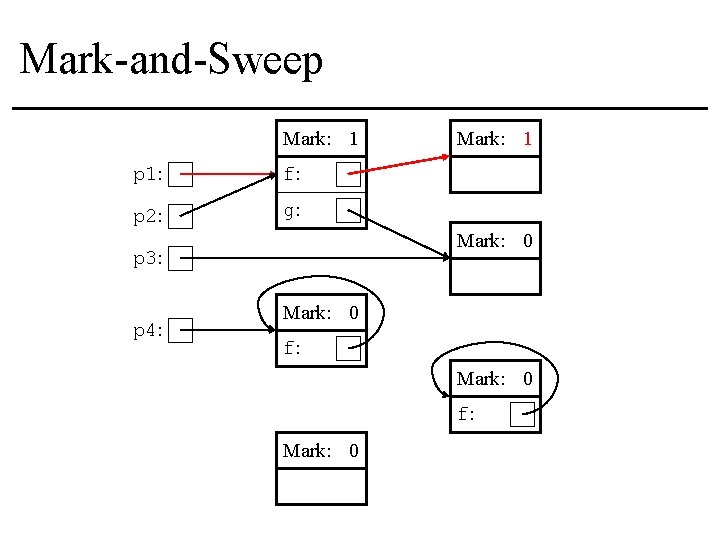
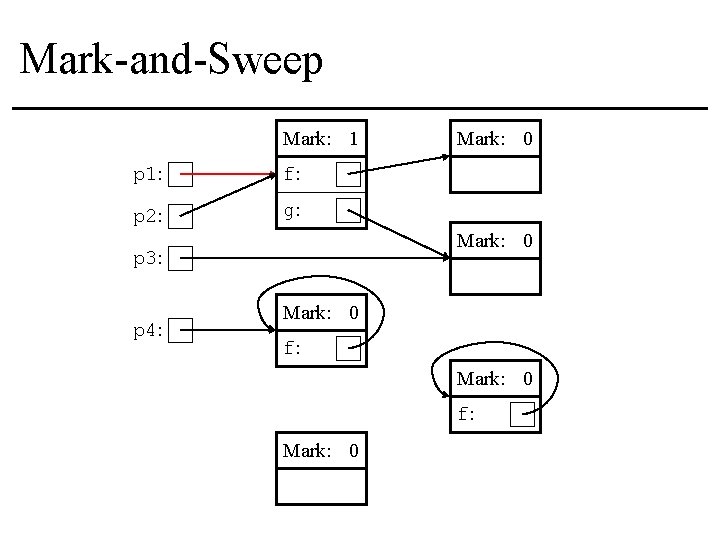
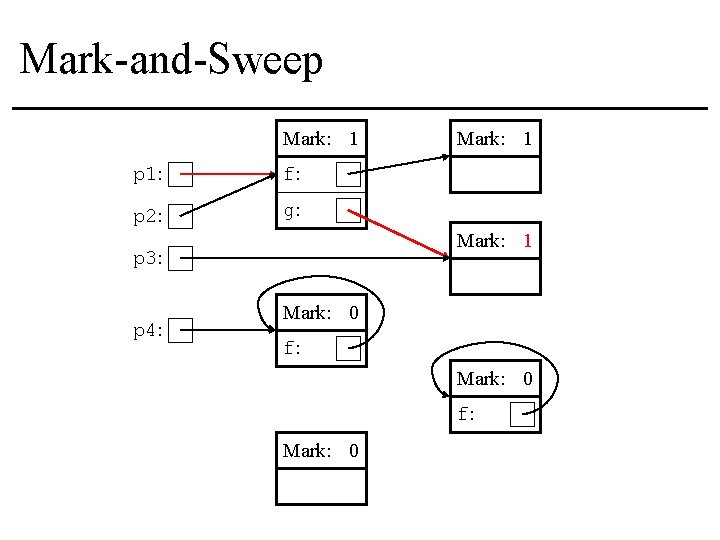
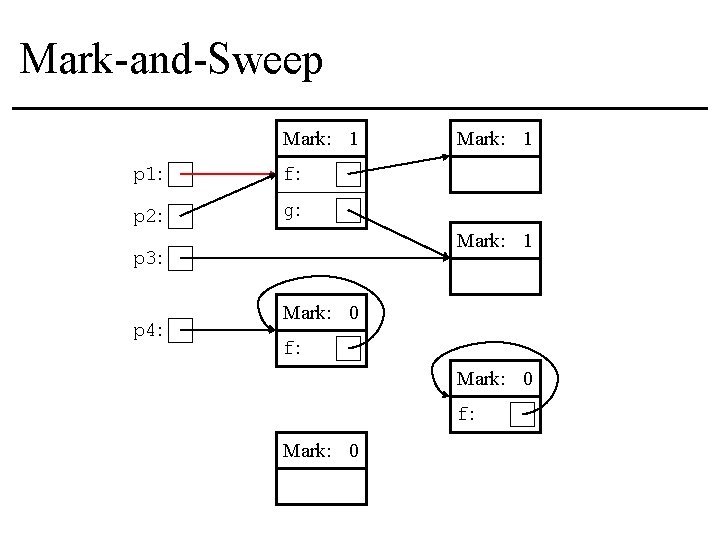
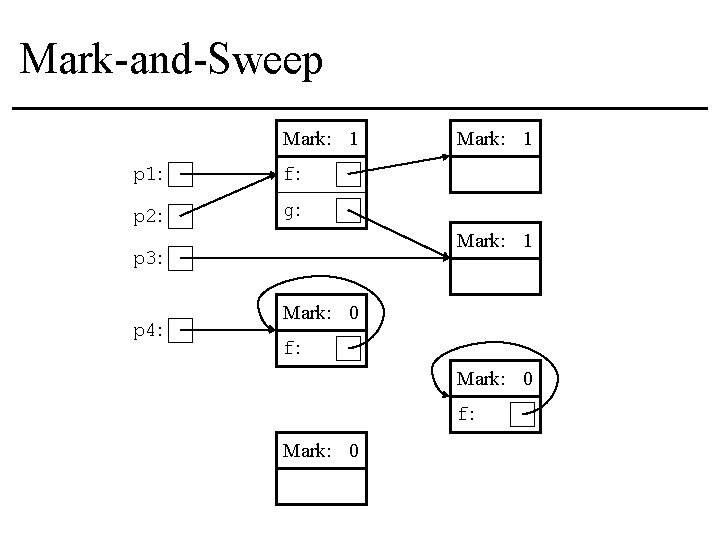
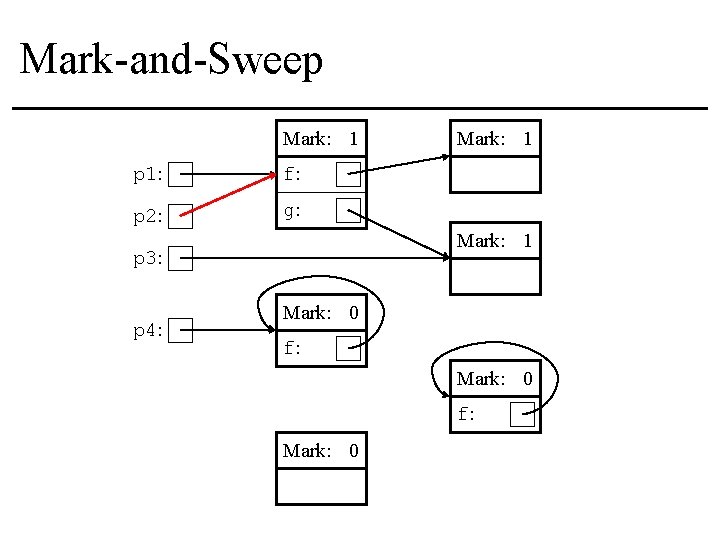
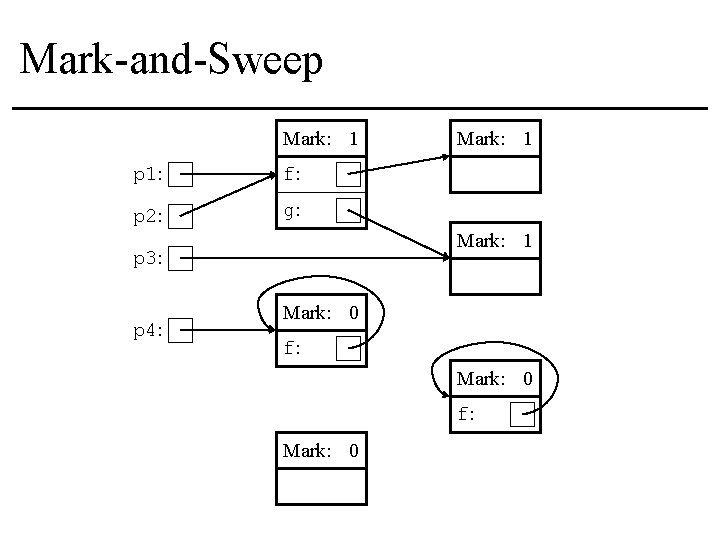
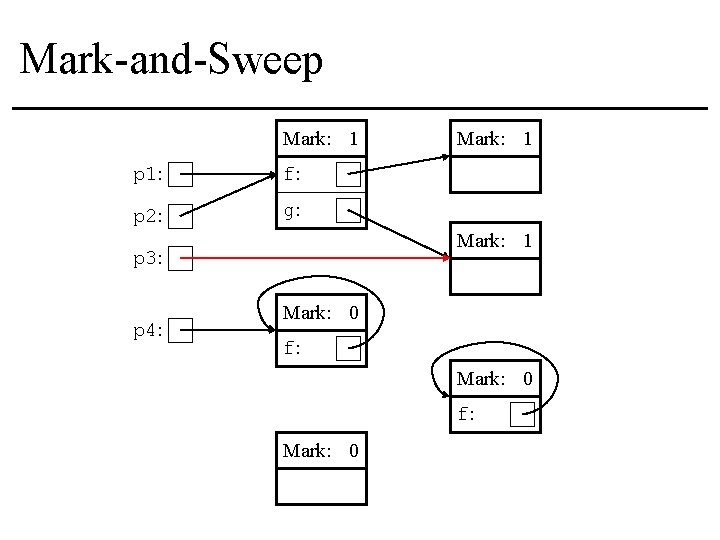
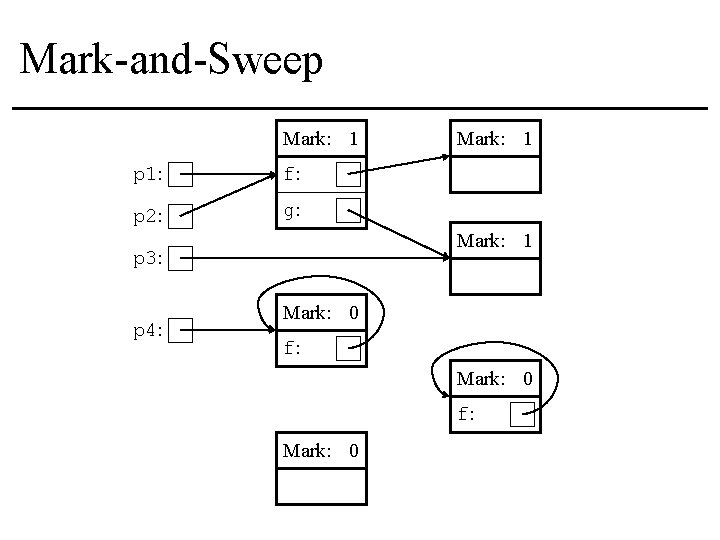
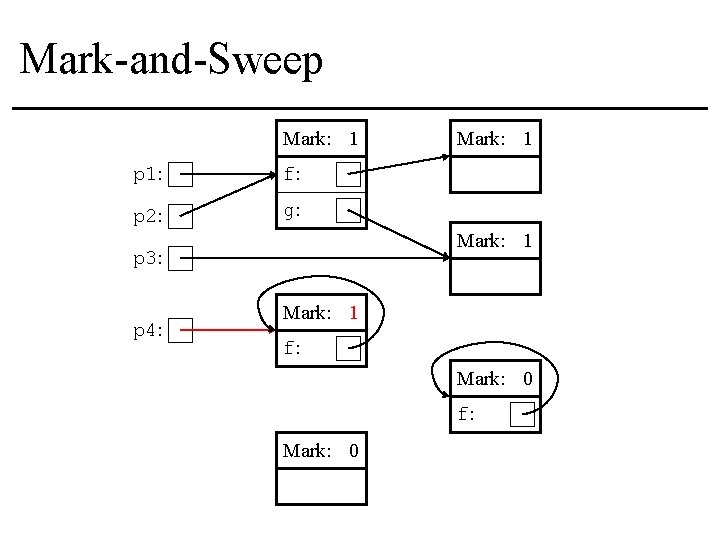
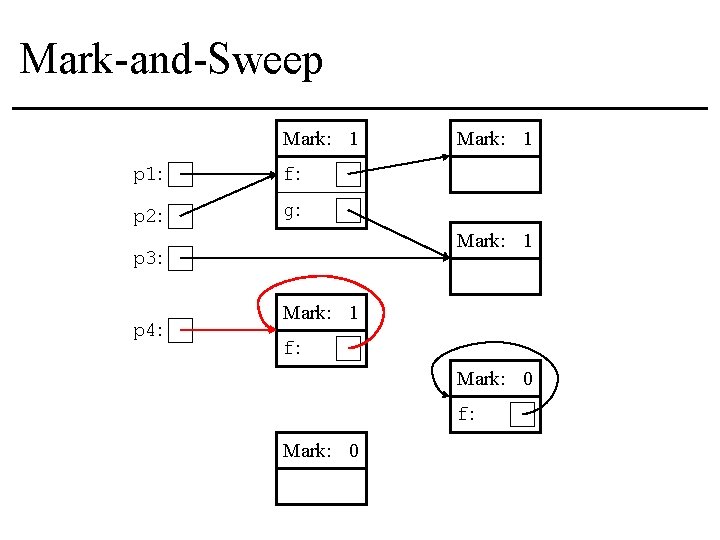
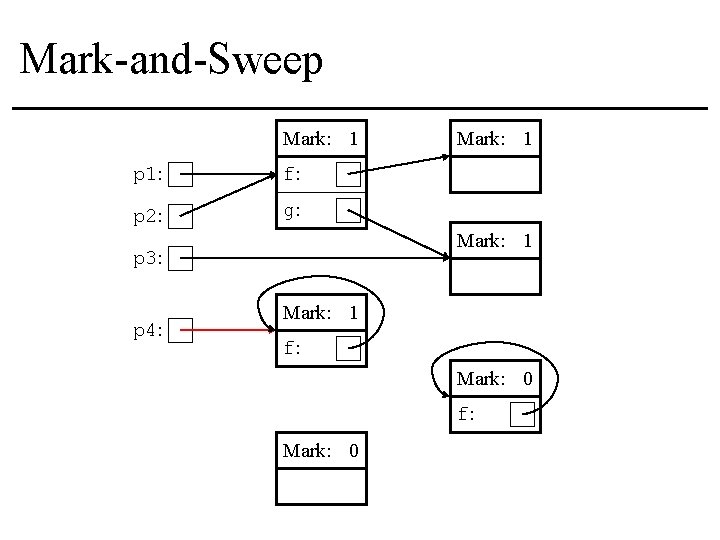
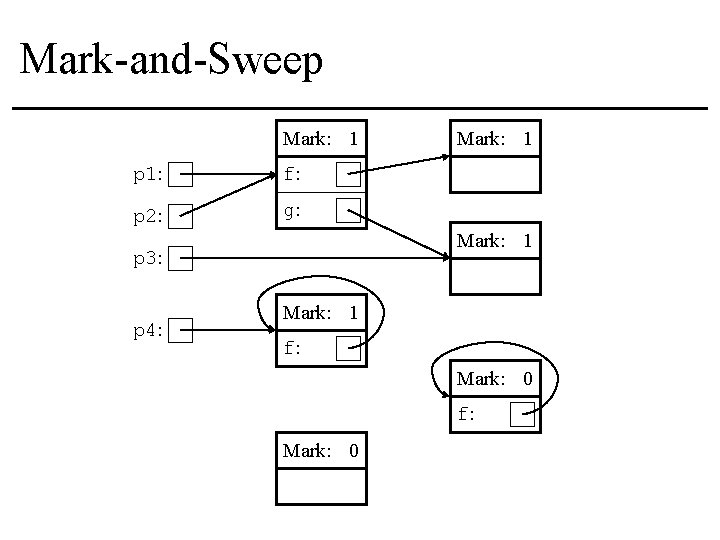
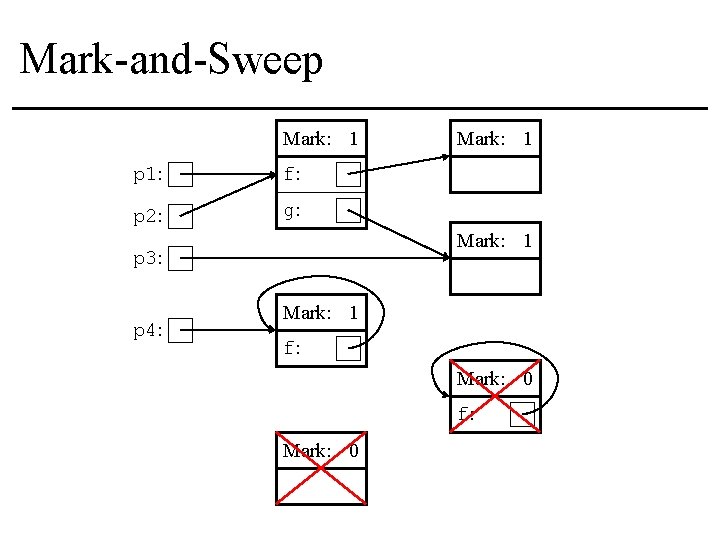
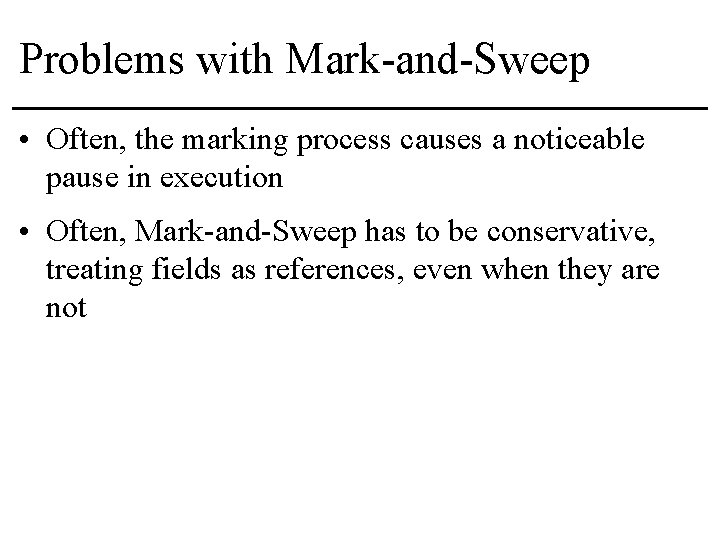
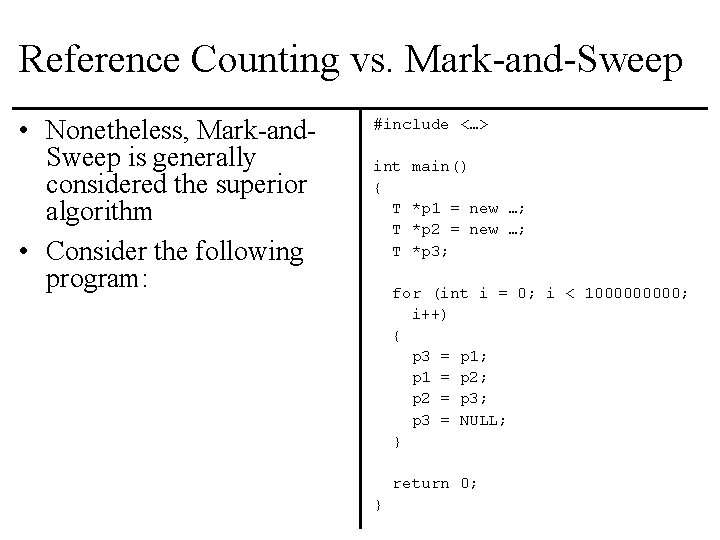
- Slides: 137
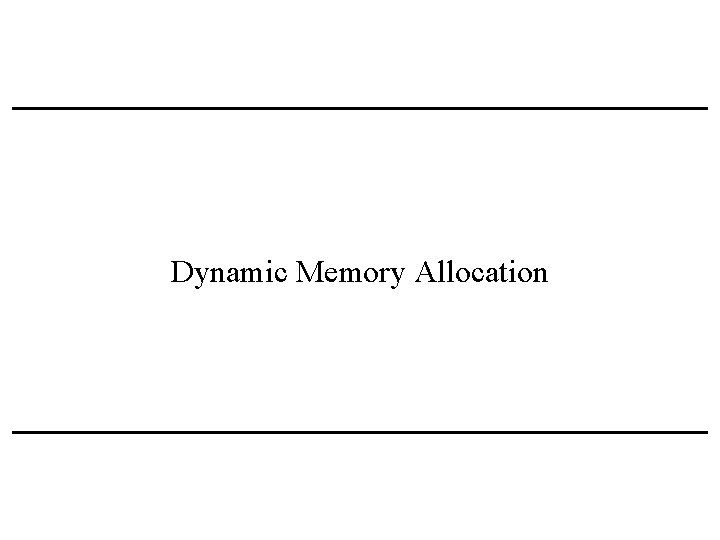
Dynamic Memory Allocation
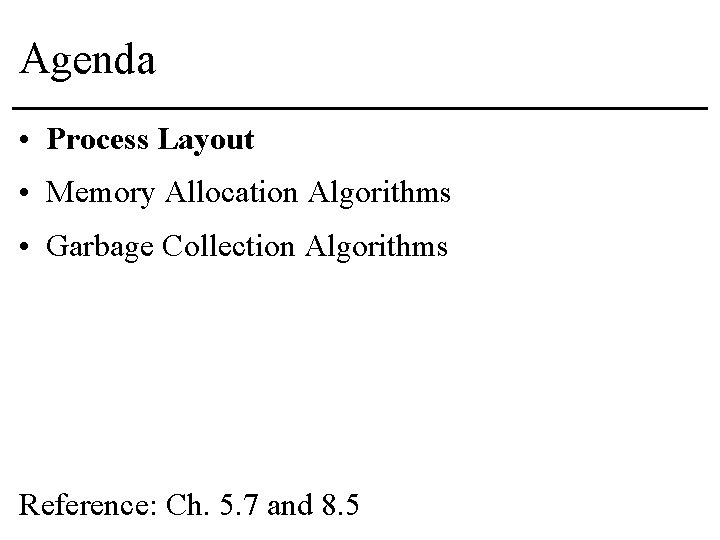
Agenda • Process Layout • Memory Allocation Algorithms • Garbage Collection Algorithms Reference: Ch. 5. 7 and 8. 5
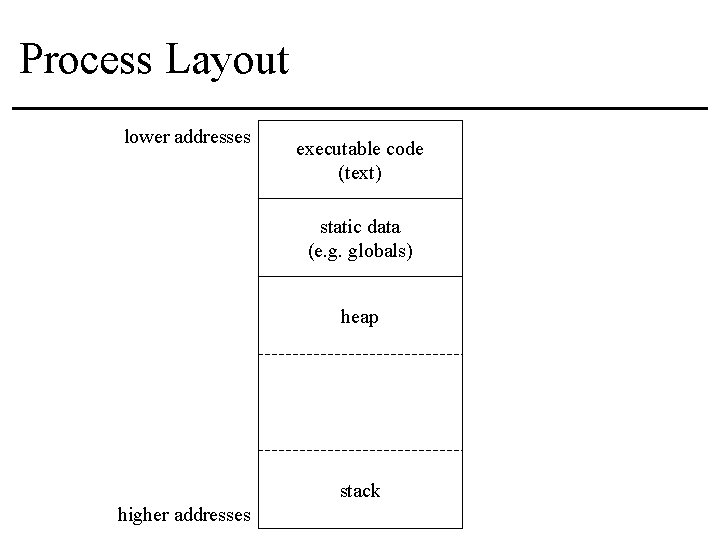
Process Layout lower addresses executable code (text) static data (e. g. globals) heap stack higher addresses
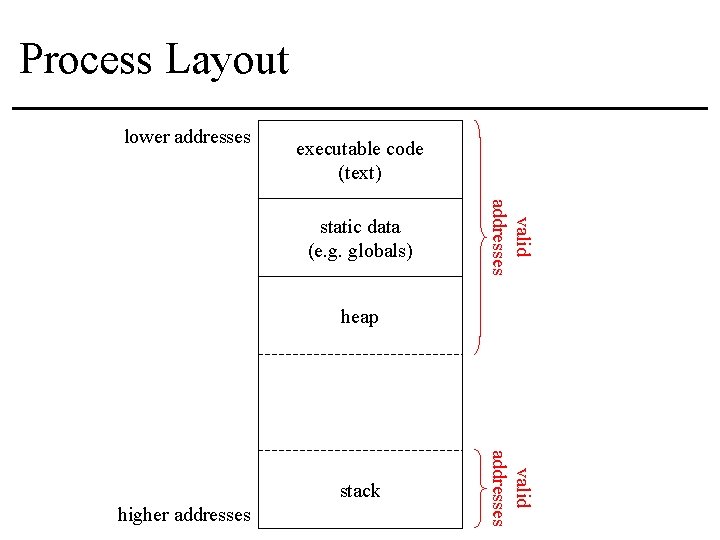
Process Layout lower addresses executable code (text) valid addresses static data (e. g. globals) heap higher addresses valid addresses stack
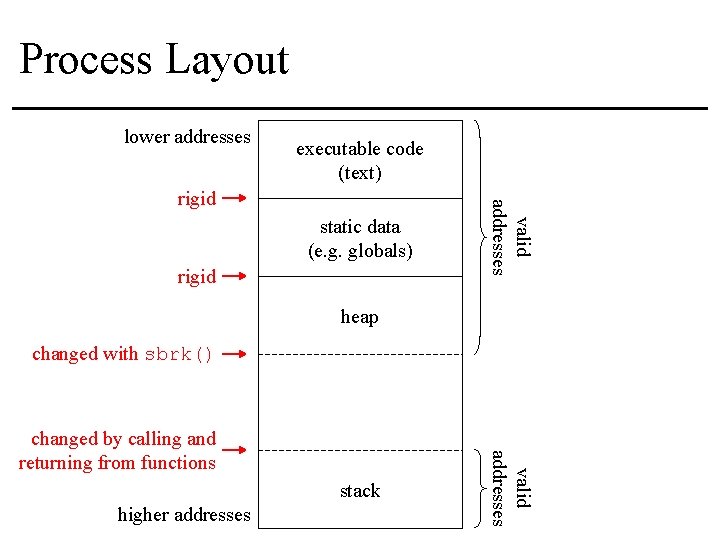
Process Layout lower addresses executable code (text) static data (e. g. globals) rigid valid addresses rigid heap changed with sbrk() stack higher addresses valid addresses changed by calling and returning from functions
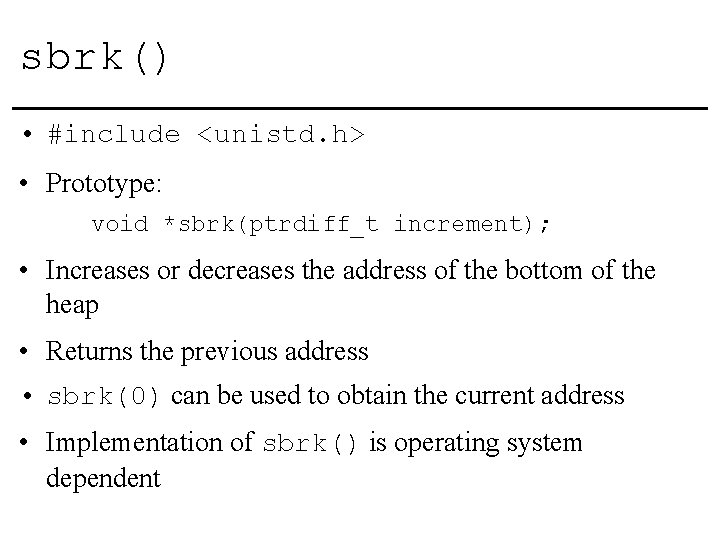
sbrk() • #include <unistd. h> • Prototype: void *sbrk(ptrdiff_t increment); • Increases or decreases the address of the bottom of the heap • Returns the previous address • sbrk(0) can be used to obtain the current address • Implementation of sbrk() is operating system dependent
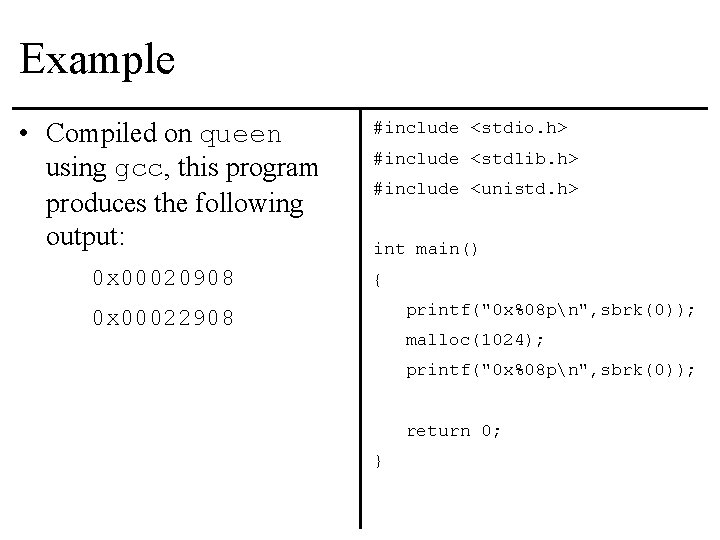
Example • Compiled on queen using gcc, this program produces the following output: 0 x 00020908 #include <stdio. h> #include <stdlib. h> #include <unistd. h> int main() { printf("0 x%08 pn", sbrk(0)); 0 x 00022908 malloc(1024); printf("0 x%08 pn", sbrk(0)); return 0; }
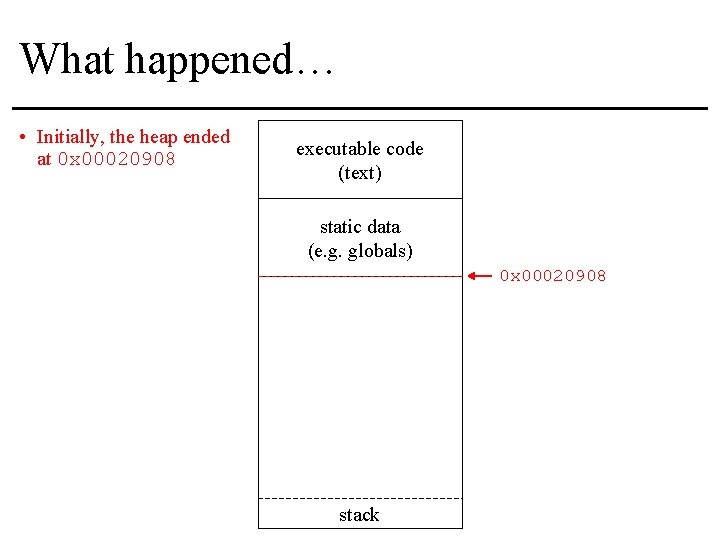
What happened… • Initially, the heap ended at 0 x 00020908 executable code (text) static data (e. g. globals) 0 x 00020908 stack
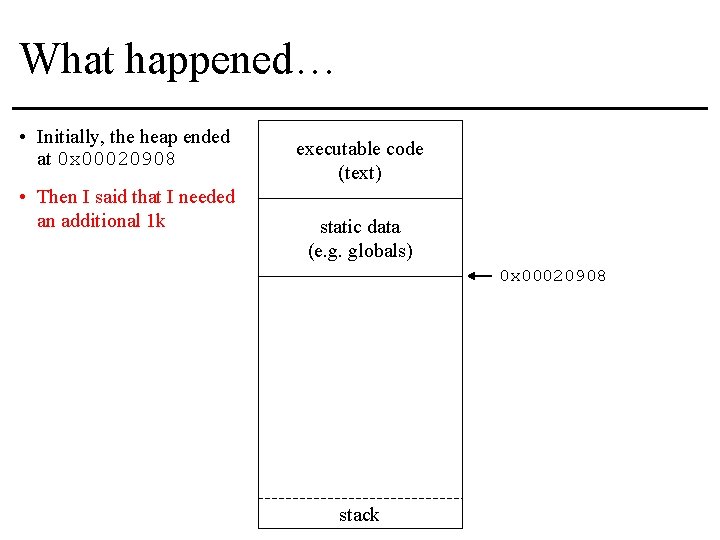
What happened… • Initially, the heap ended at 0 x 00020908 • Then I said that I needed an additional 1 k executable code (text) static data (e. g. globals) 0 x 00020908 stack
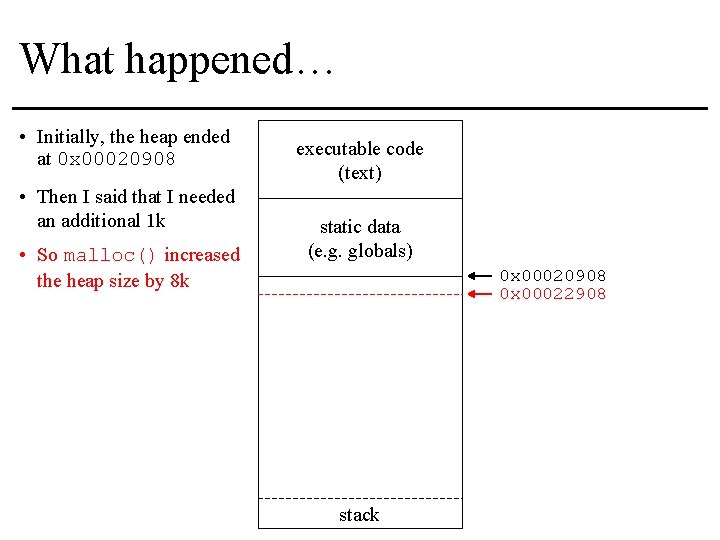
What happened… • Initially, the heap ended at 0 x 00020908 • Then I said that I needed an additional 1 k • So malloc() increased the heap size by 8 k executable code (text) static data (e. g. globals) 0 x 00020908 0 x 00022908 stack
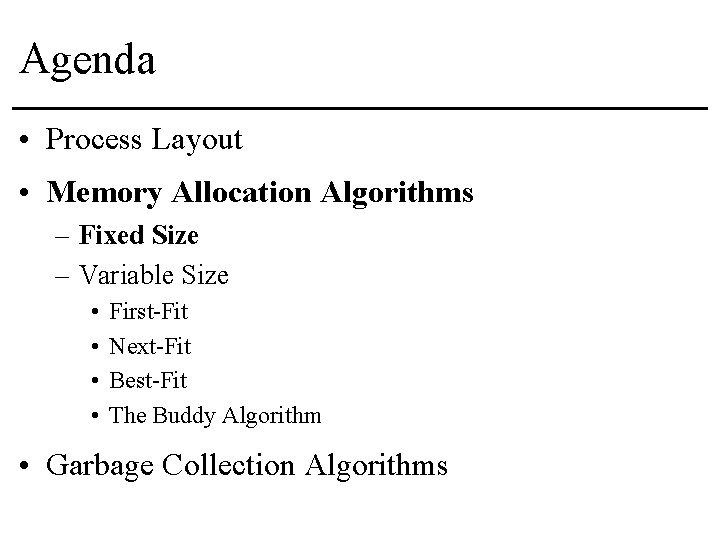
Agenda • Process Layout • Memory Allocation Algorithms – Fixed Size – Variable Size • • First-Fit Next-Fit Best-Fit The Buddy Algorithm • Garbage Collection Algorithms
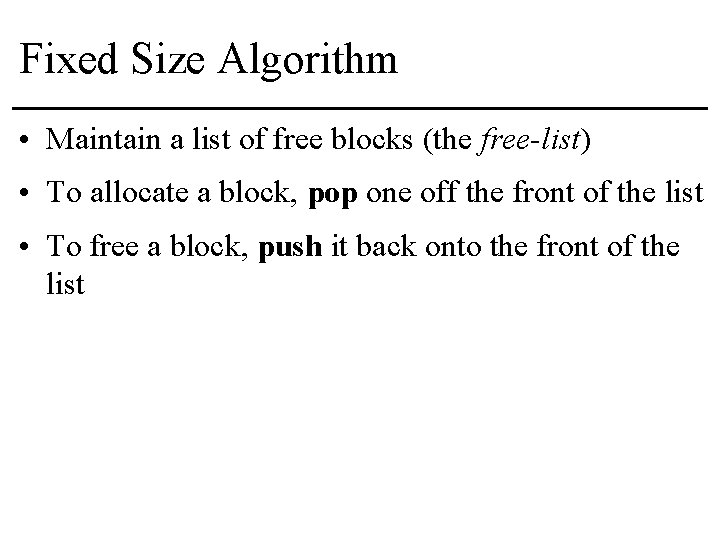
Fixed Size Algorithm • Maintain a list of free blocks (the free-list) • To allocate a block, pop one off the front of the list • To free a block, push it back onto the front of the list
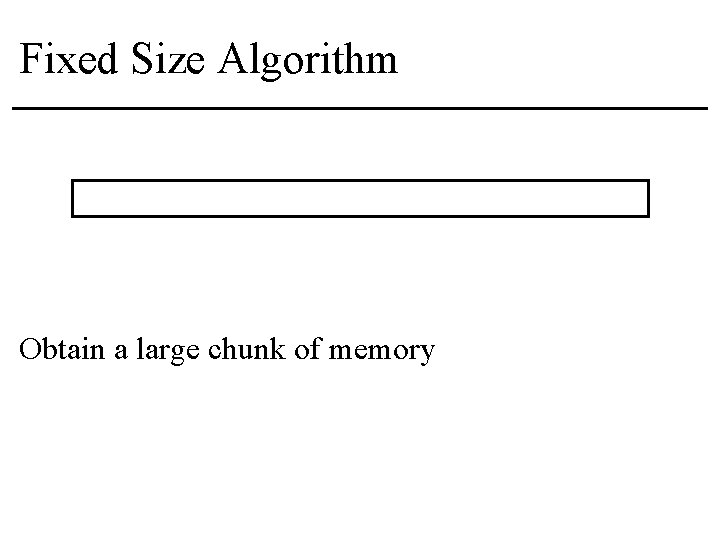
Fixed Size Algorithm Obtain a large chunk of memory
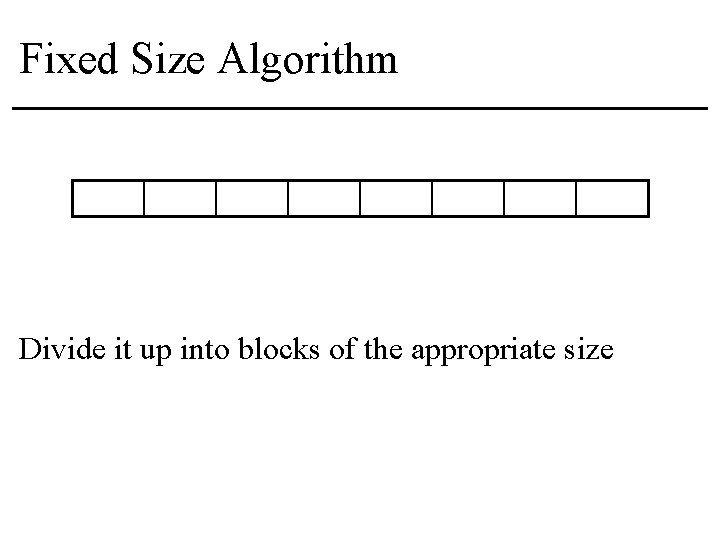
Fixed Size Algorithm Divide it up into blocks of the appropriate size
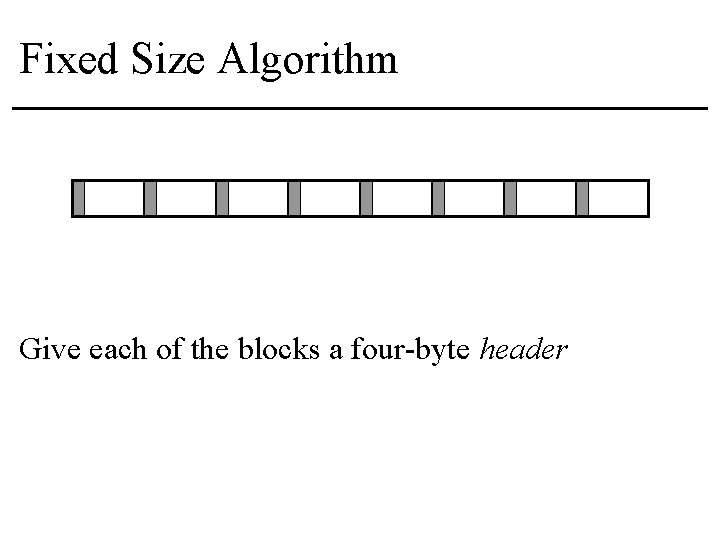
Fixed Size Algorithm Give each of the blocks a four-byte header
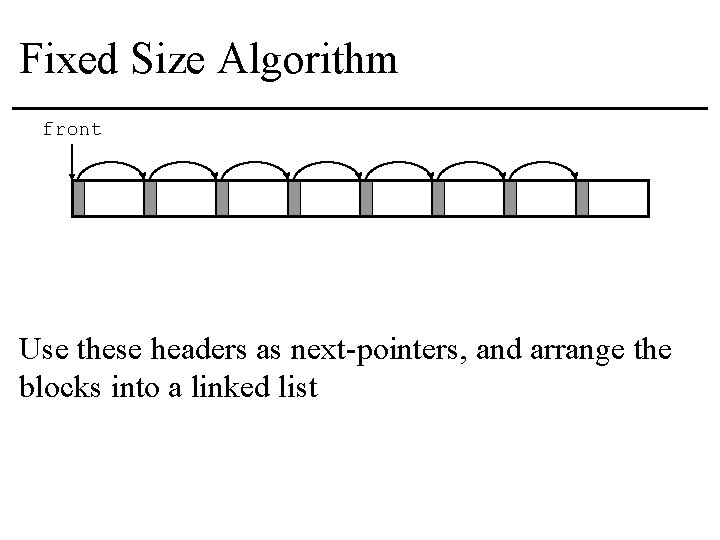
Fixed Size Algorithm front Use these headers as next-pointers, and arrange the blocks into a linked list
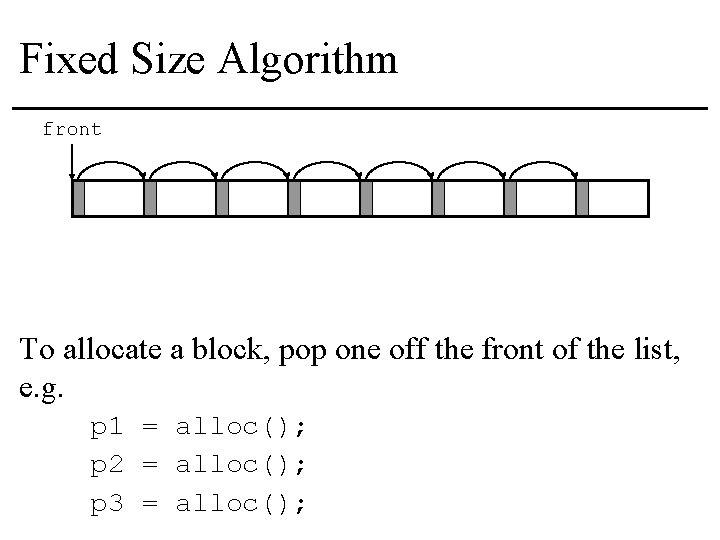
Fixed Size Algorithm front To allocate a block, pop one off the front of the list, e. g. p 1 = alloc(); p 2 = alloc(); p 3 = alloc();
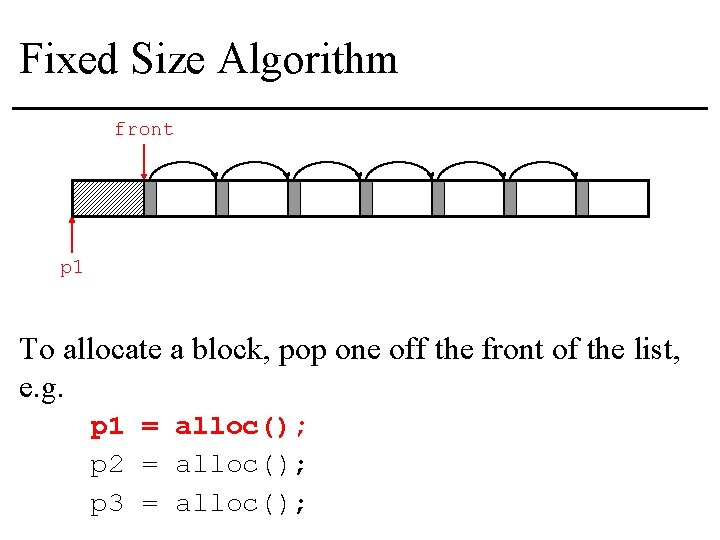
Fixed Size Algorithm front p 1 To allocate a block, pop one off the front of the list, e. g. p 1 = alloc(); p 2 = alloc(); p 3 = alloc();
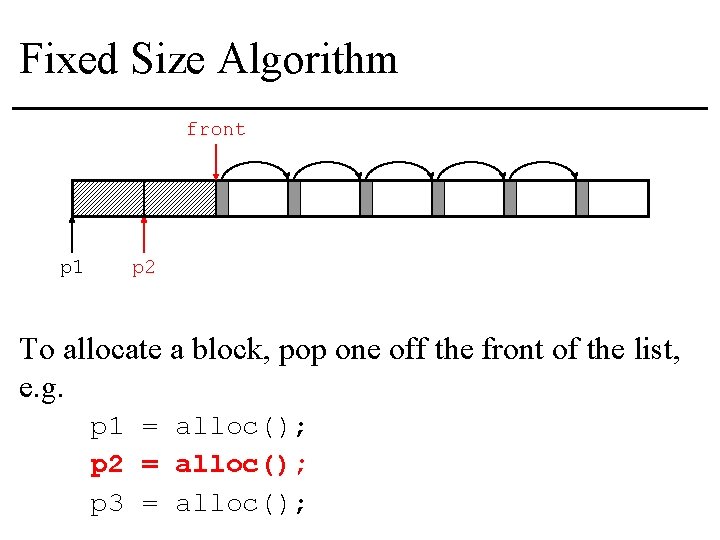
Fixed Size Algorithm front p 1 p 2 To allocate a block, pop one off the front of the list, e. g. p 1 = alloc(); p 2 = alloc(); p 3 = alloc();
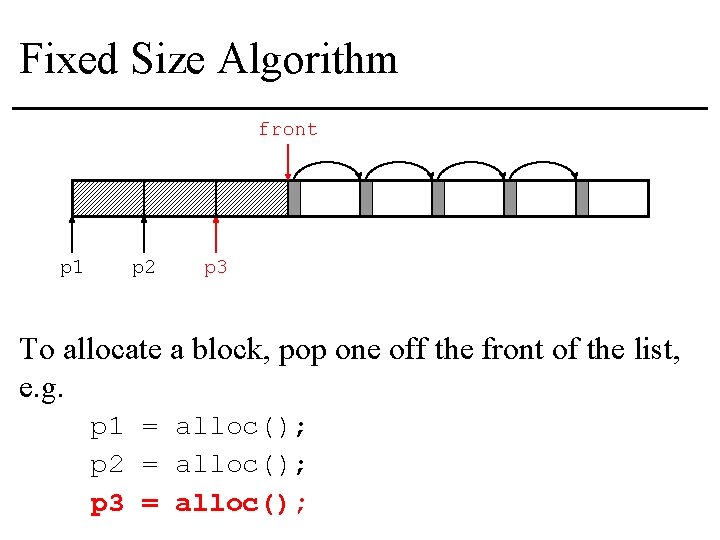
Fixed Size Algorithm front p 1 p 2 p 3 To allocate a block, pop one off the front of the list, e. g. p 1 = alloc(); p 2 = alloc(); p 3 = alloc();
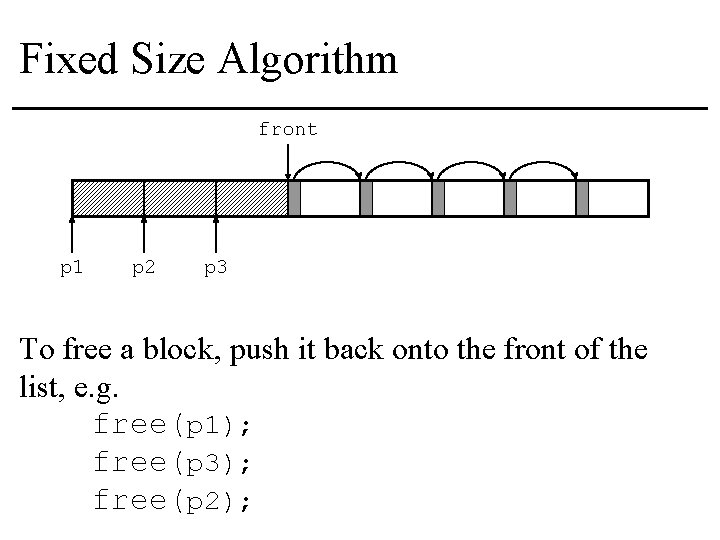
Fixed Size Algorithm front p 1 p 2 p 3 To free a block, push it back onto the front of the list, e. g. free(p 1); free(p 3); free(p 2);
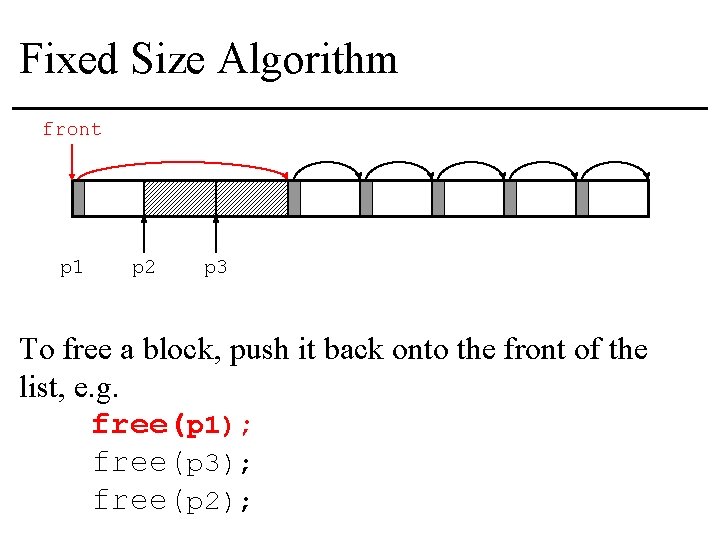
Fixed Size Algorithm front p 1 p 2 p 3 To free a block, push it back onto the front of the list, e. g. free(p 1); free(p 3); free(p 2);
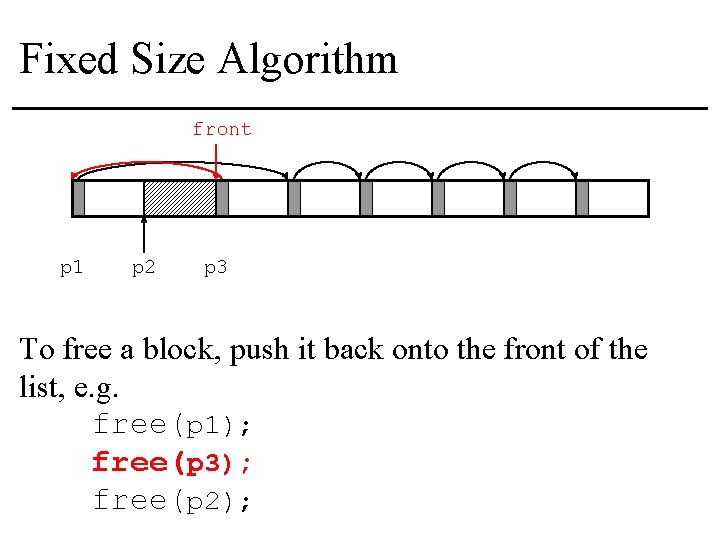
Fixed Size Algorithm front p 1 p 2 p 3 To free a block, push it back onto the front of the list, e. g. free(p 1); free(p 3); free(p 2);
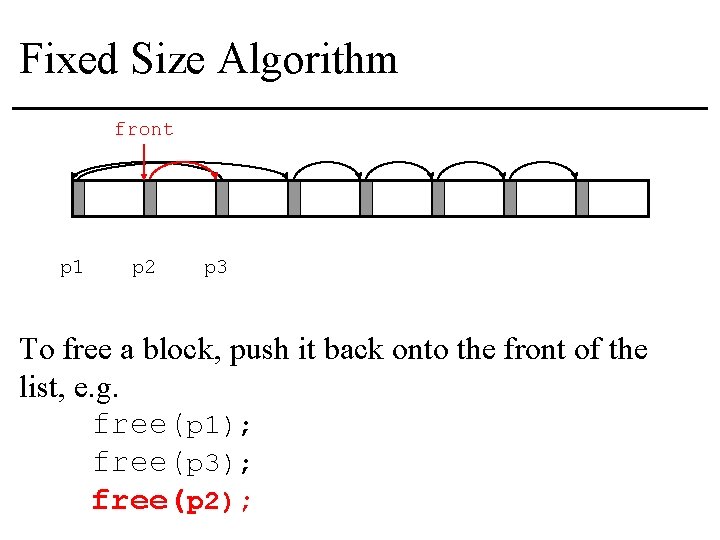
Fixed Size Algorithm front p 1 p 2 p 3 To free a block, push it back onto the front of the list, e. g. free(p 1); free(p 3); free(p 2);
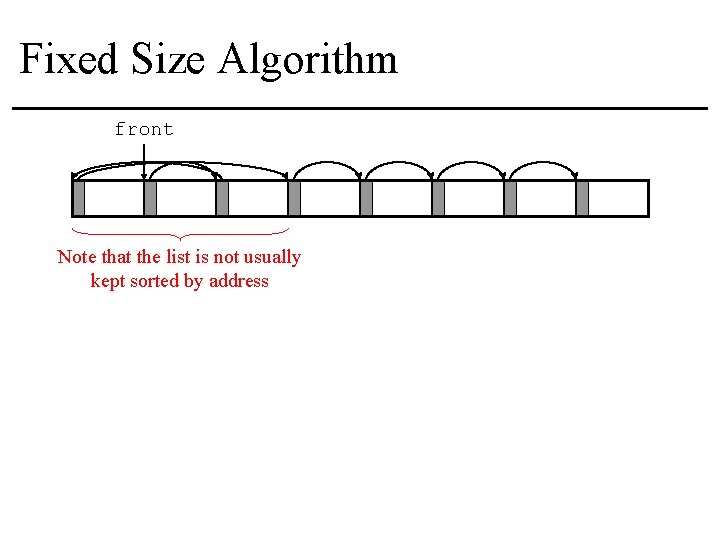
Fixed Size Algorithm front Note that the list is not usually kept sorted by address
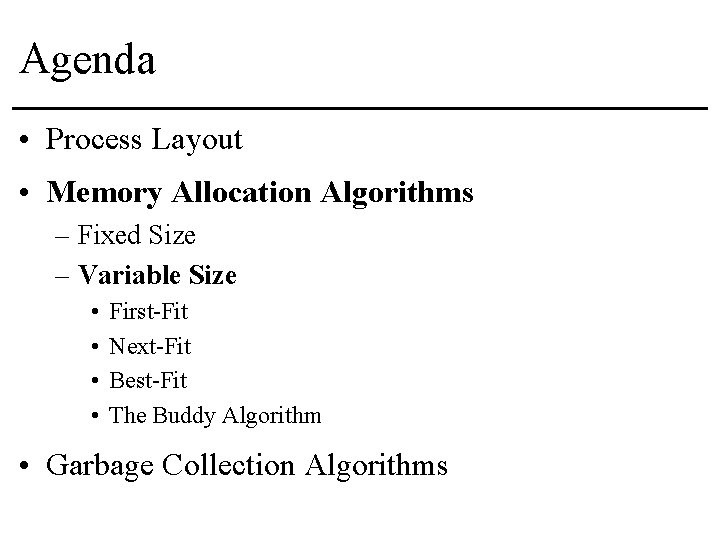
Agenda • Process Layout • Memory Allocation Algorithms – Fixed Size – Variable Size • • First-Fit Next-Fit Best-Fit The Buddy Algorithm • Garbage Collection Algorithms
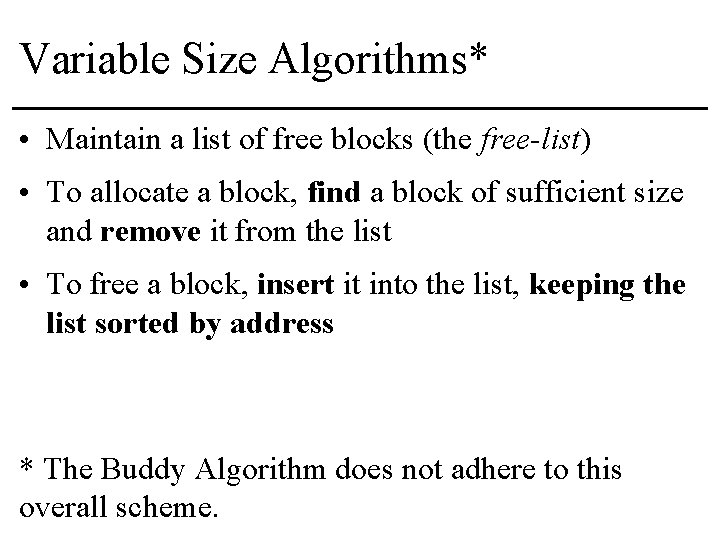
Variable Size Algorithms* • Maintain a list of free blocks (the free-list) • To allocate a block, find a block of sufficient size and remove it from the list • To free a block, insert it into the list, keeping the list sorted by address * The Buddy Algorithm does not adhere to this overall scheme.
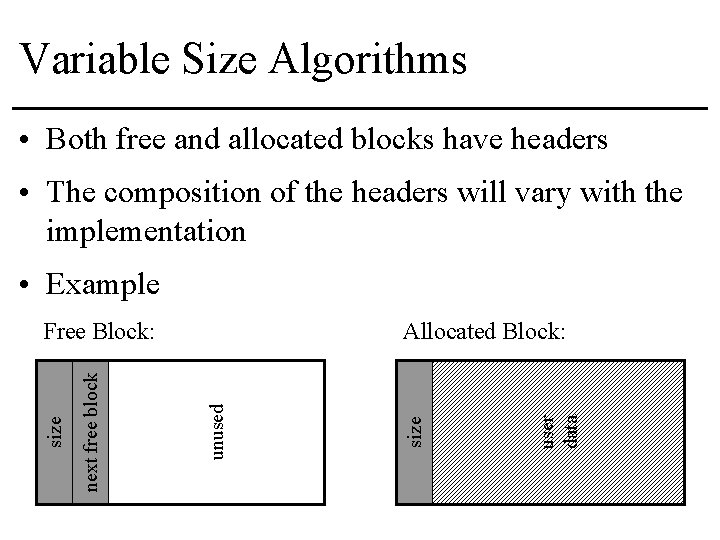
Variable Size Algorithms • Both free and allocated blocks have headers • The composition of the headers will vary with the implementation • Example user data size Allocated Block: unused next free block size Free Block:
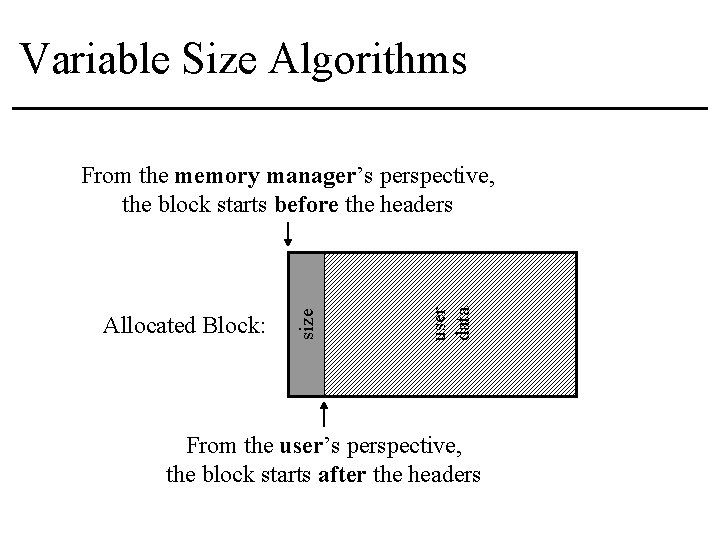
Variable Size Algorithms user data Allocated Block: size From the memory manager’s perspective, the block starts before the headers From the user’s perspective, the block starts after the headers
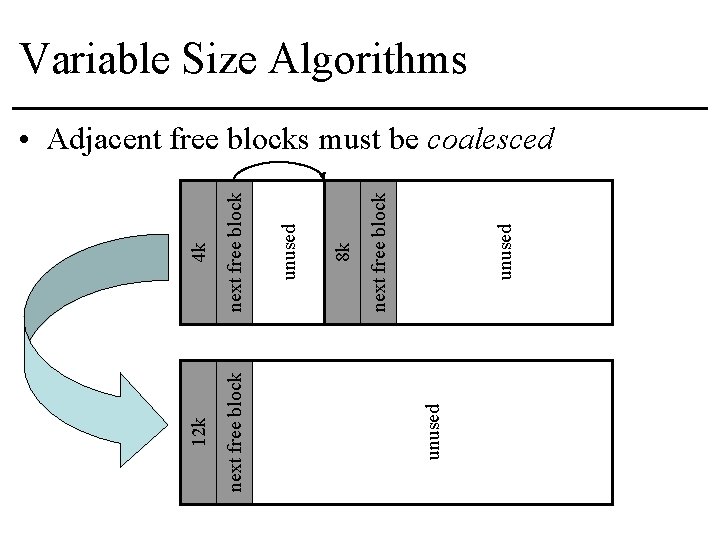
unused next free block 8 k unused 4 k 12 k Variable Size Algorithms • Adjacent free blocks must be coalesced
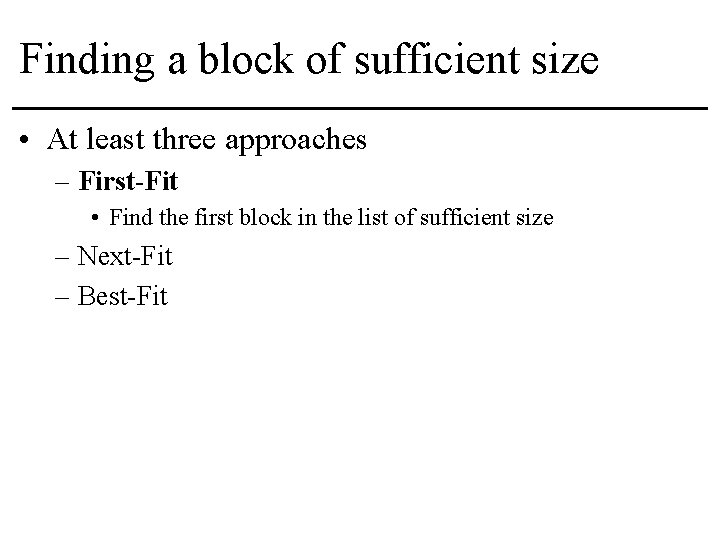
Finding a block of sufficient size • At least three approaches – First-Fit • Find the first block in the list of sufficient size – Next-Fit – Best-Fit
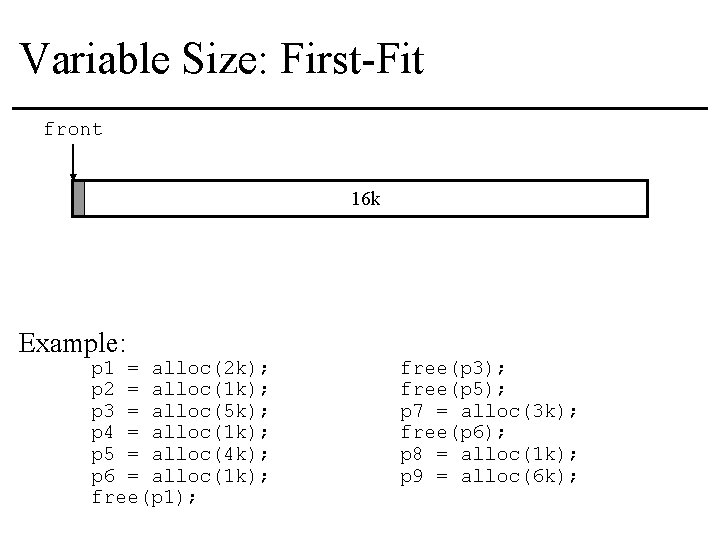
Variable Size: First-Fit front 16 k Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
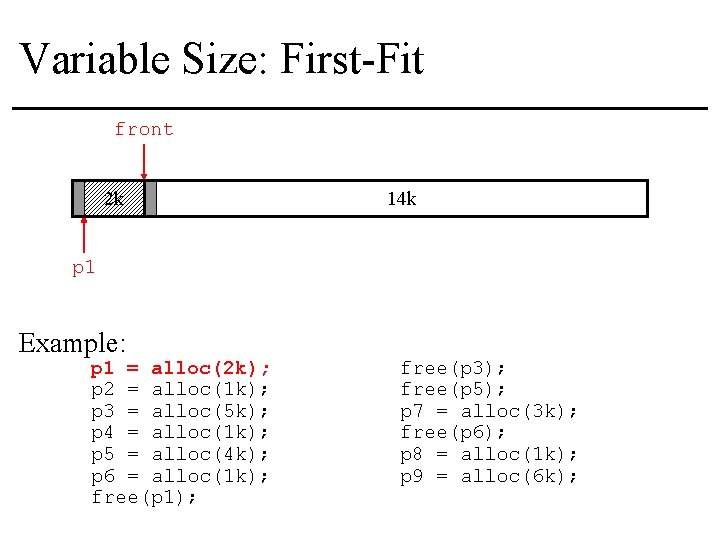
Variable Size: First-Fit front 2 k 14 k p 1 Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
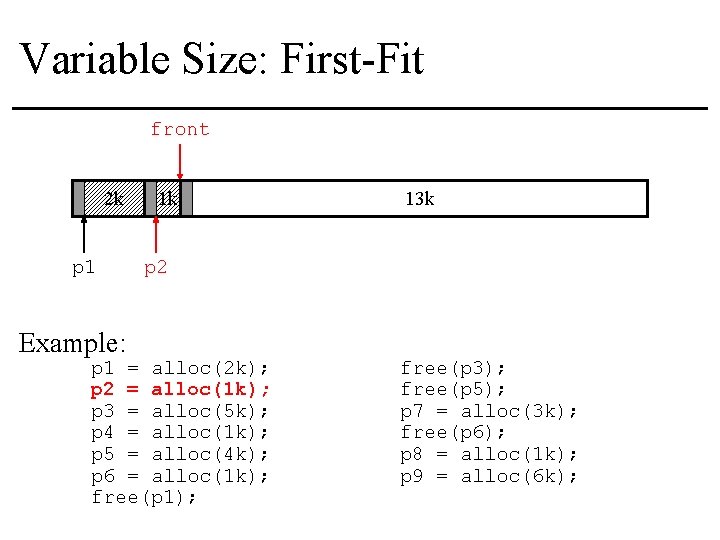
Variable Size: First-Fit front 2 k p 1 Example: 1 k 13 k p 2 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
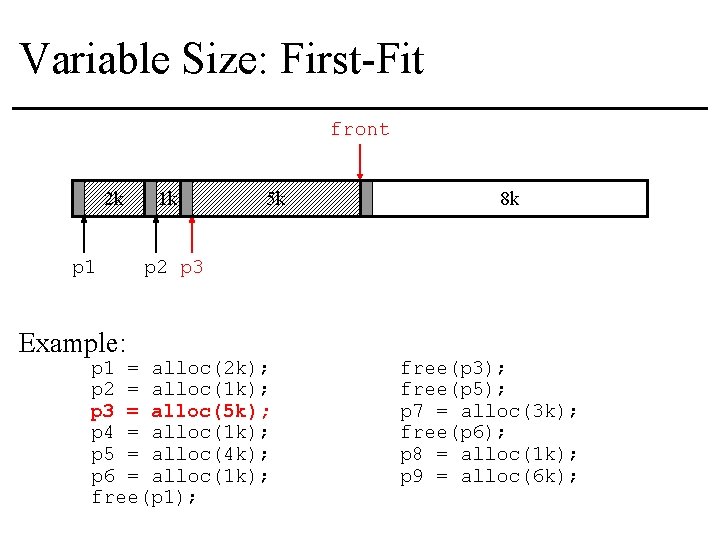
Variable Size: First-Fit front 2 k p 1 Example: 1 k 5 k 8 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
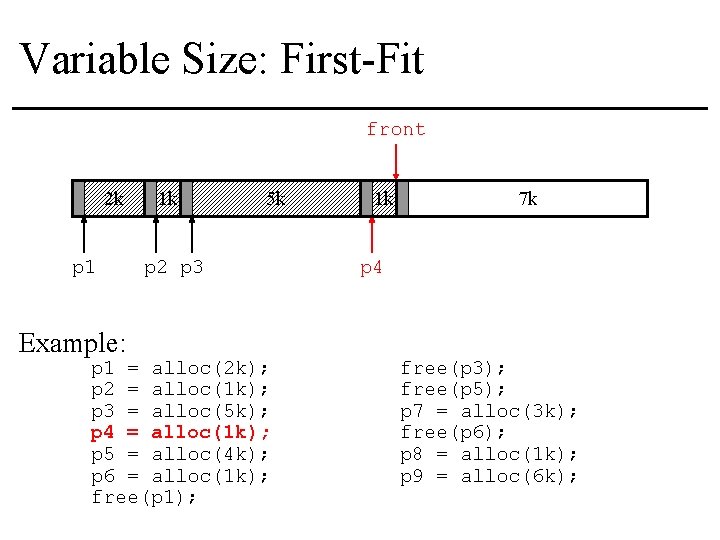
Variable Size: First-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 7 k p 4 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
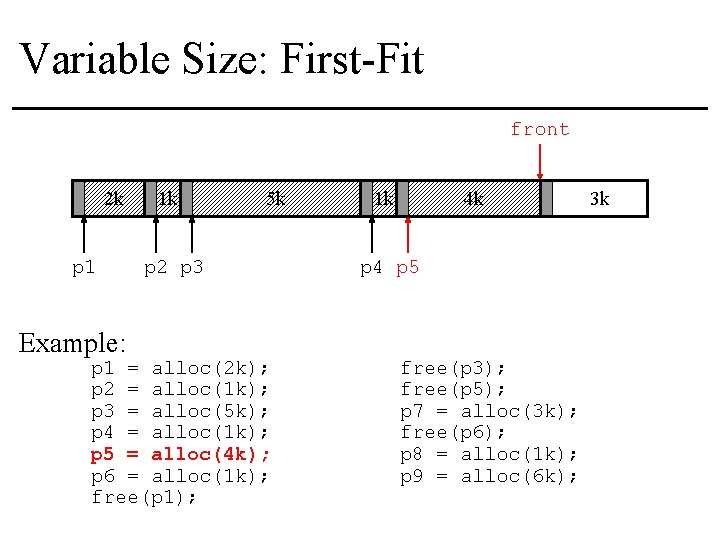
Variable Size: First-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 3 k
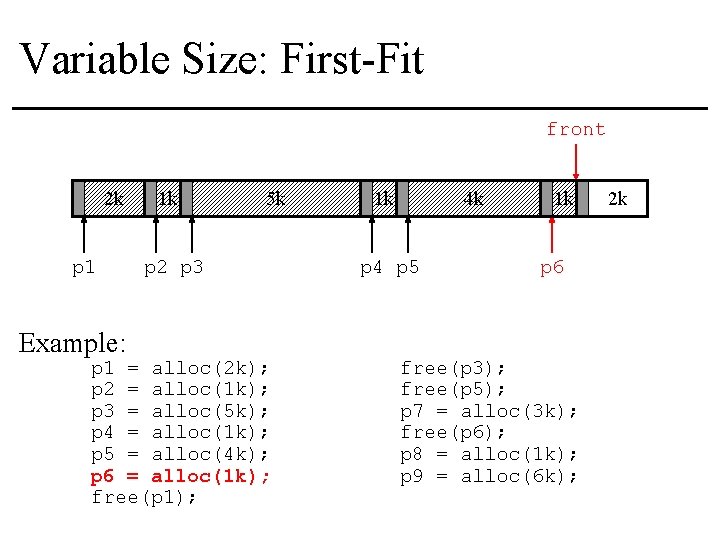
Variable Size: First-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
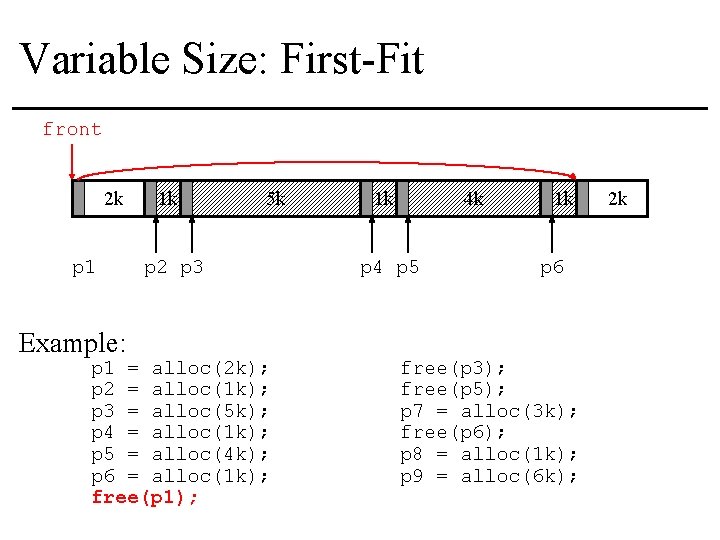
Variable Size: First-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
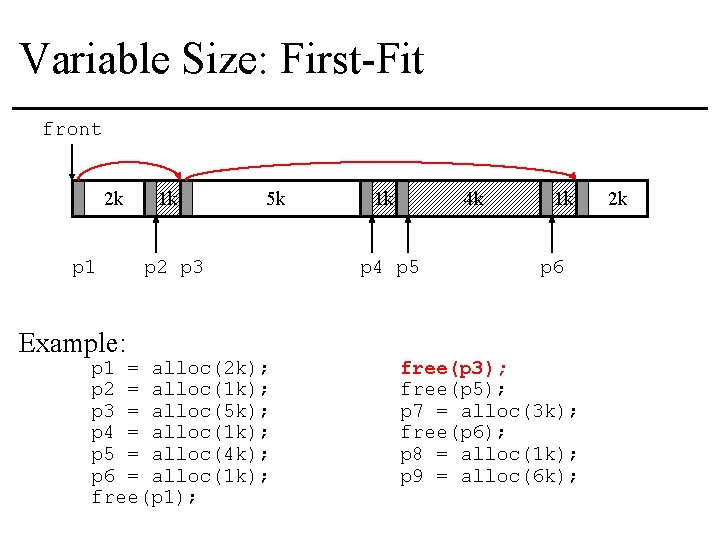
Variable Size: First-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
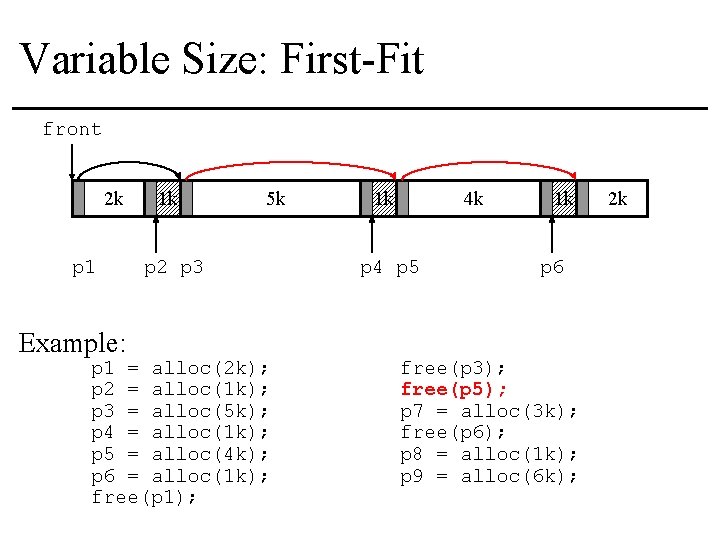
Variable Size: First-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
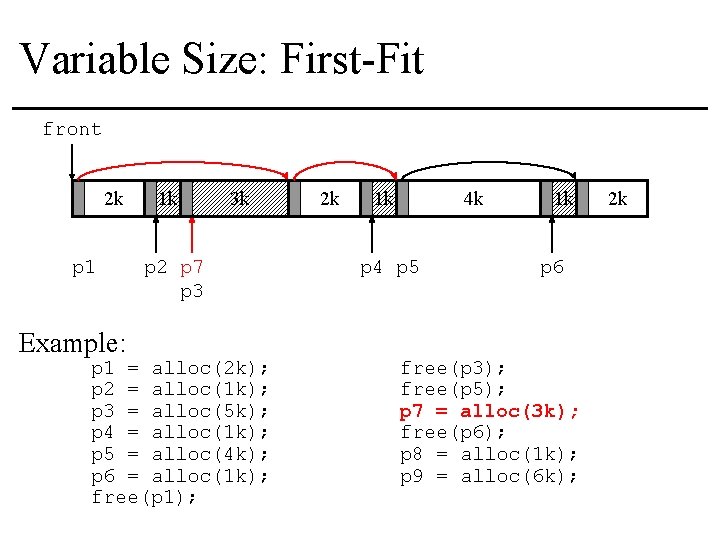
Variable Size: First-Fit front 2 k p 1 Example: 1 k 3 k p 2 p 7 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 2 k 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
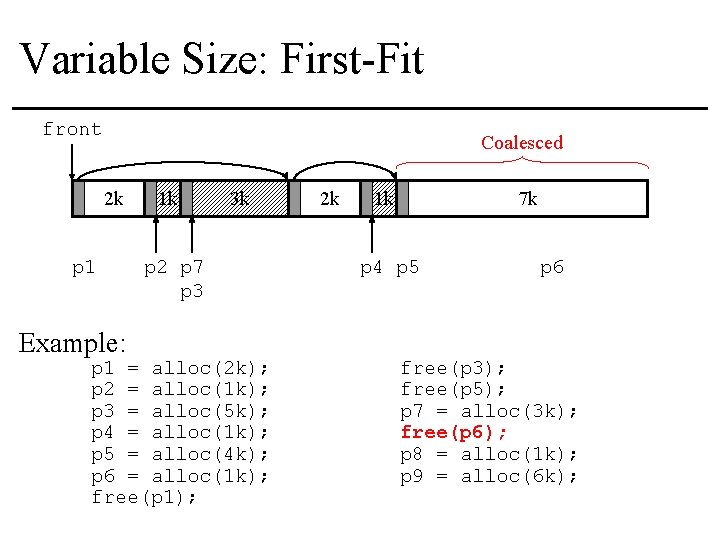
Variable Size: First-Fit front Coalesced 2 k p 1 Example: 1 k 3 k p 2 p 7 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 2 k 1 k 7 k p 4 p 5 p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
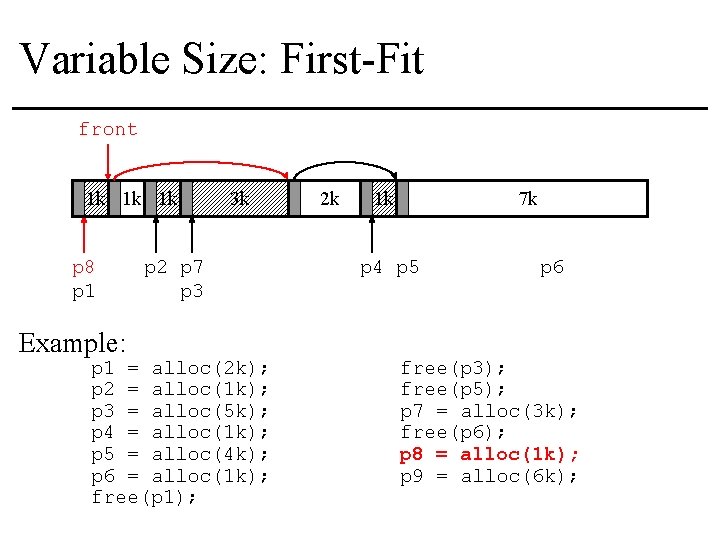
Variable Size: First-Fit front 1 k 1 k 1 k p 8 p 1 Example: 3 k p 2 p 7 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 2 k 1 k 7 k p 4 p 5 p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
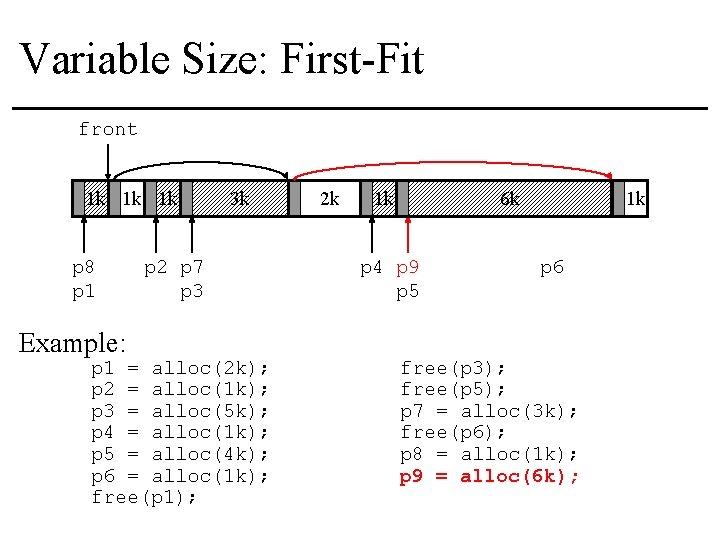
Variable Size: First-Fit front 1 k 1 k 1 k p 8 p 1 Example: 3 k p 2 p 7 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 2 k 1 k 6 k p 4 p 9 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
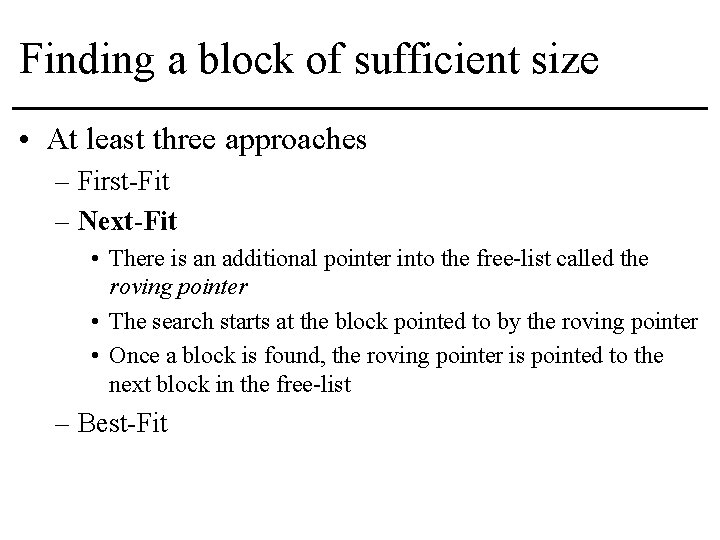
Finding a block of sufficient size • At least three approaches – First-Fit – Next-Fit • There is an additional pointer into the free-list called the roving pointer • The search starts at the block pointed to by the roving pointer • Once a block is found, the roving pointer is pointed to the next block in the free-list – Best-Fit
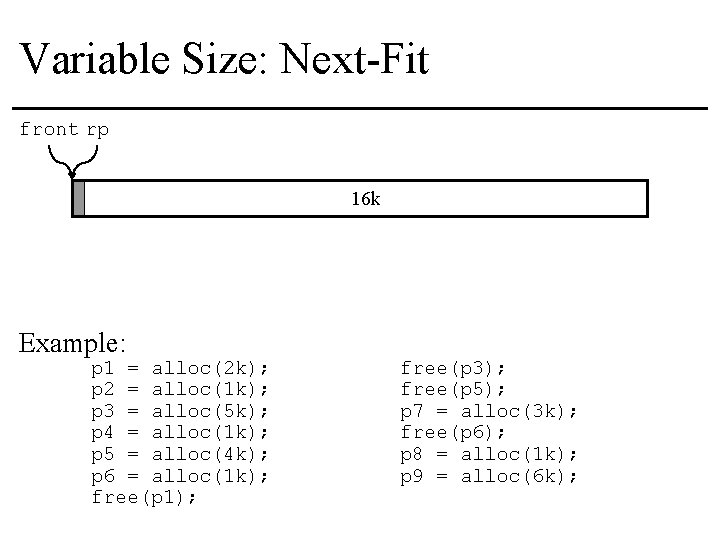
Variable Size: Next-Fit front rp 16 k Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
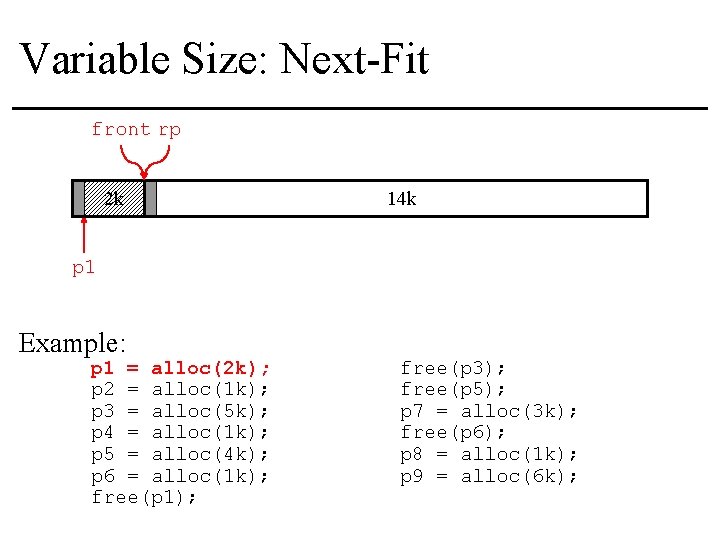
Variable Size: Next-Fit front rp 2 k 14 k p 1 Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
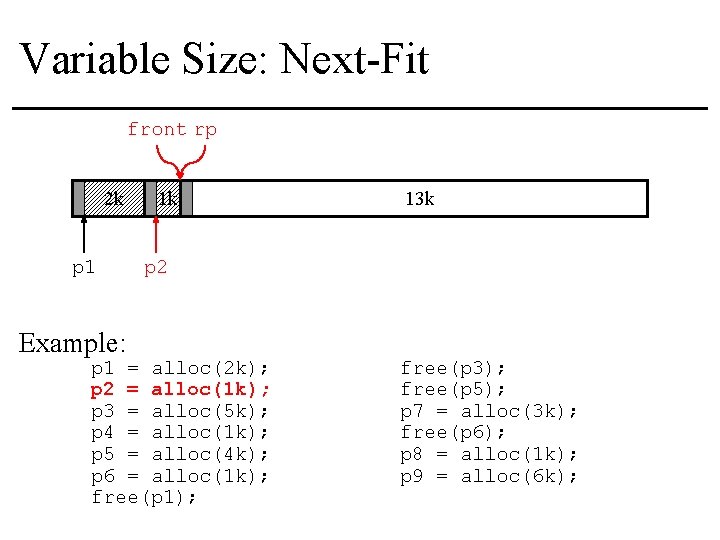
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 13 k p 2 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
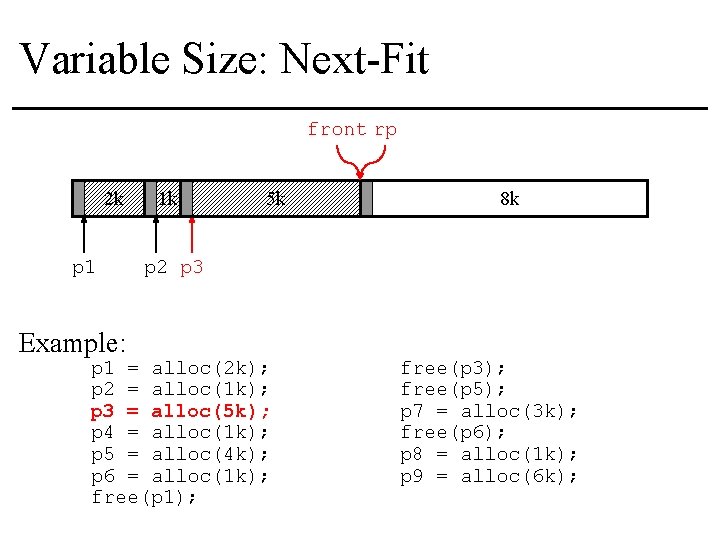
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 5 k 8 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
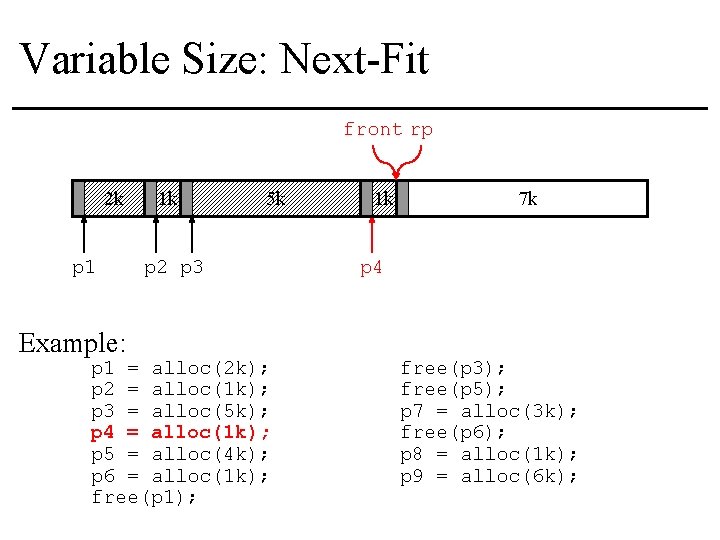
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 7 k p 4 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
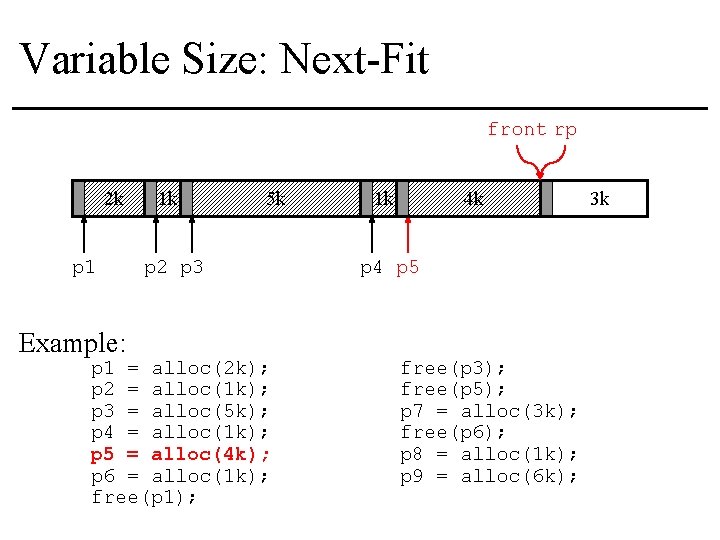
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 3 k
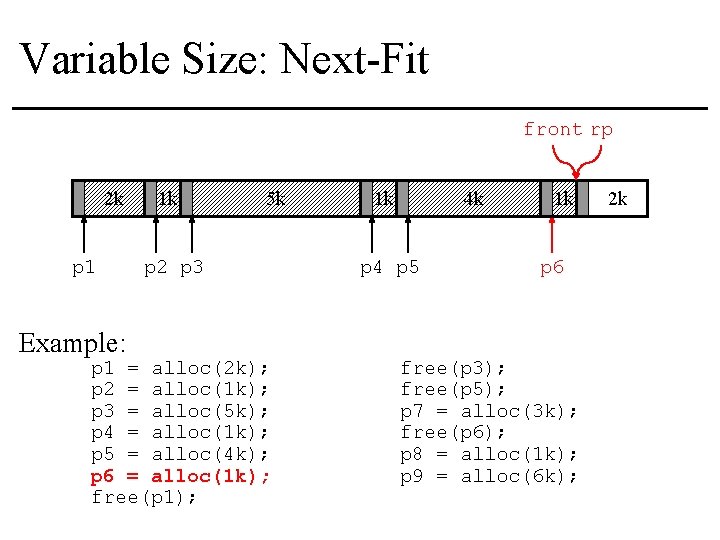
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
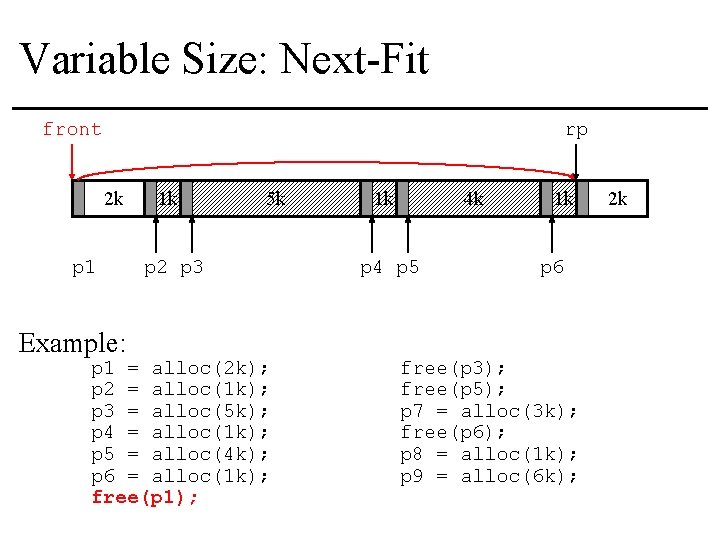
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
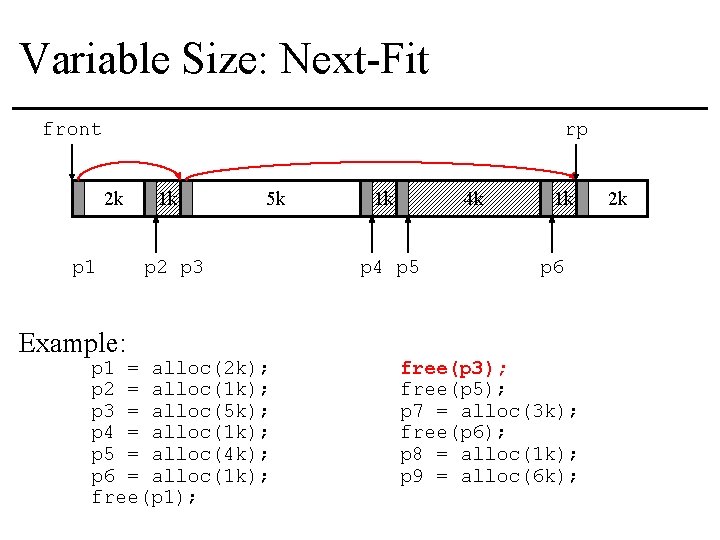
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
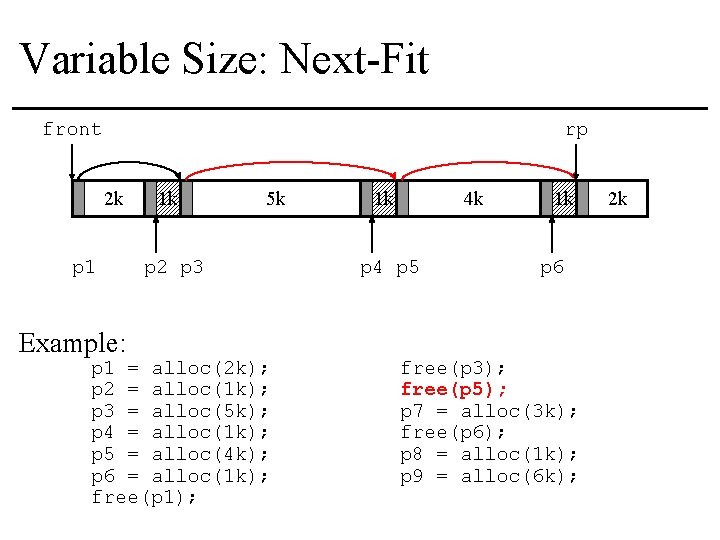
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
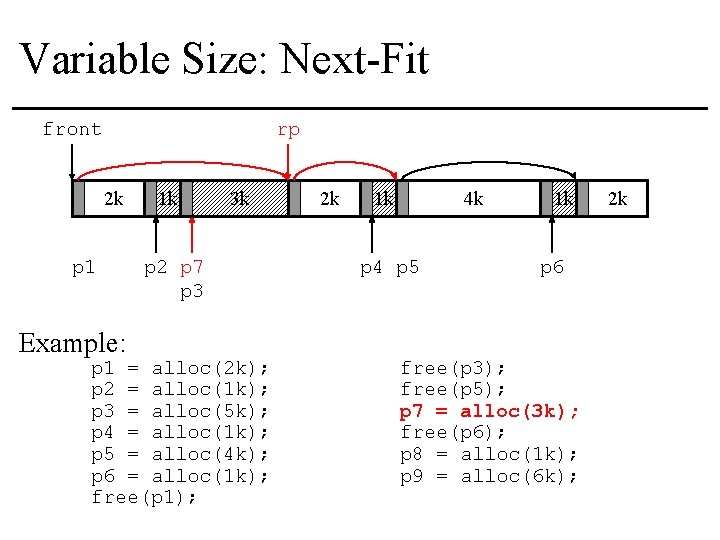
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 3 k p 2 p 7 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 2 k 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
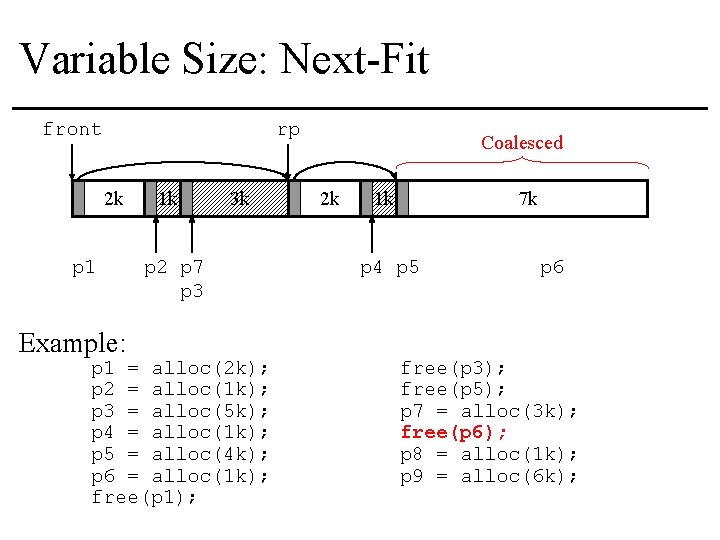
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 3 k p 2 p 7 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); Coalesced 2 k 1 k 7 k p 4 p 5 p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
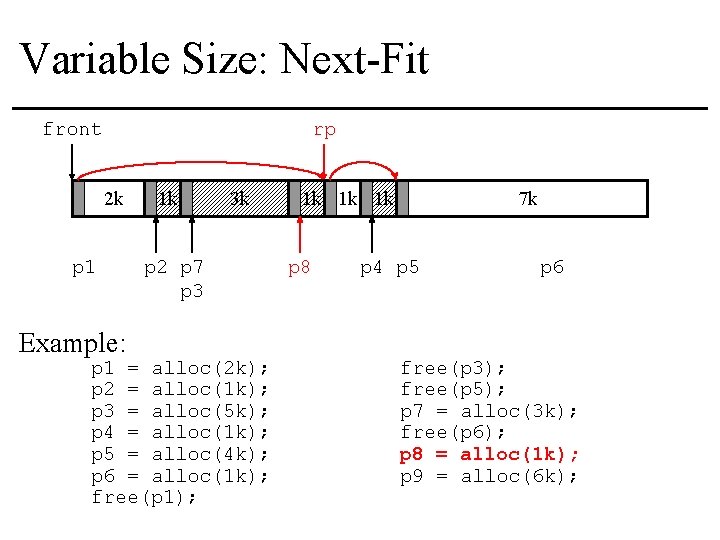
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 3 k p 2 p 7 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 1 k 1 k p 8 7 k p 4 p 5 p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
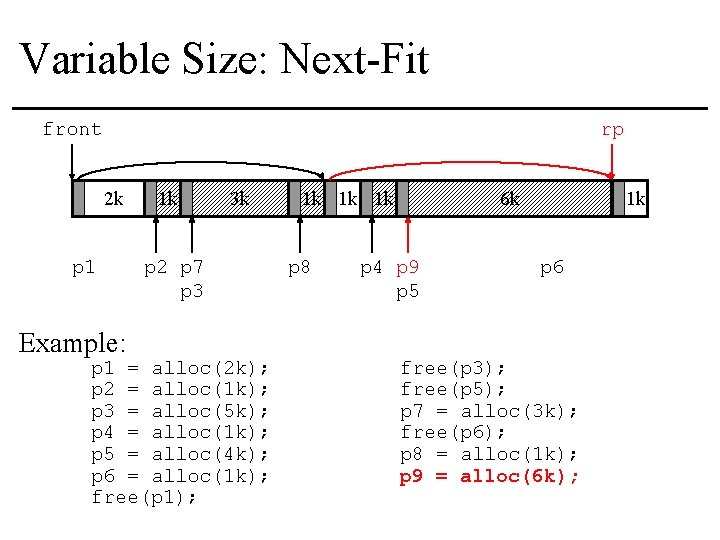
Variable Size: Next-Fit front rp 2 k p 1 Example: 1 k 3 k p 2 p 7 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 1 k 1 k p 8 6 k p 4 p 9 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
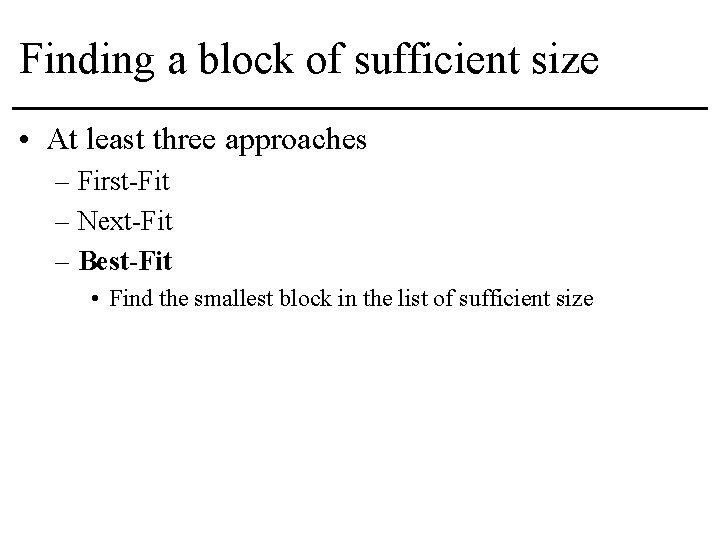
Finding a block of sufficient size • At least three approaches – First-Fit – Next-Fit – Best-Fit • Find the smallest block in the list of sufficient size
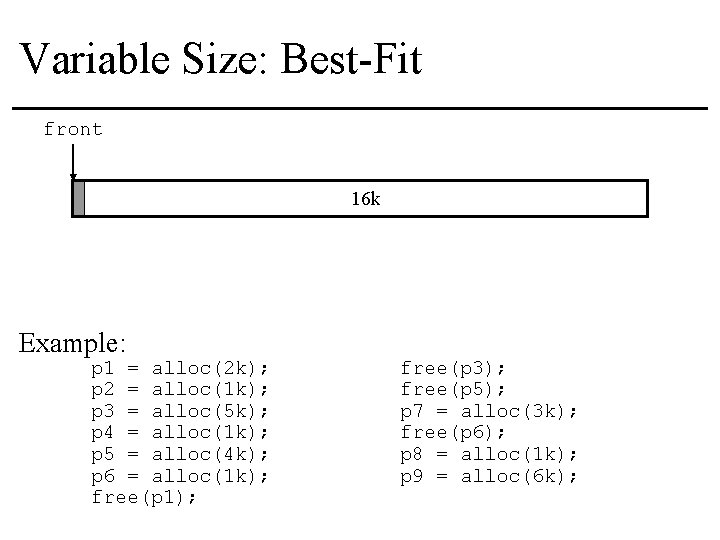
Variable Size: Best-Fit front 16 k Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
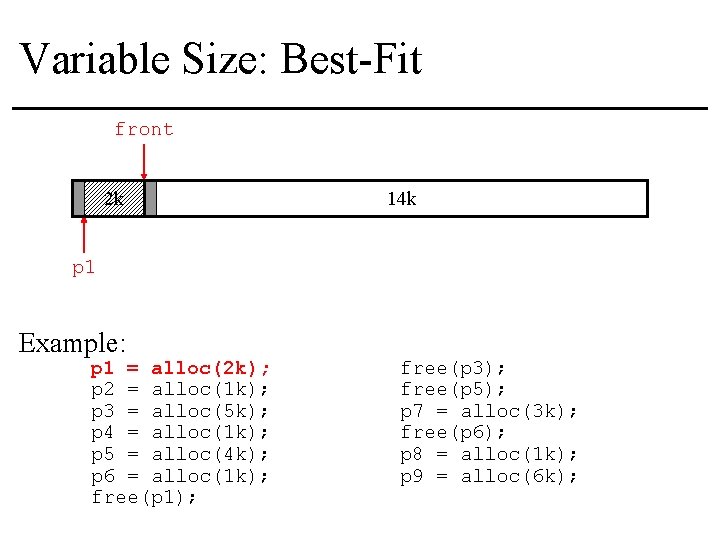
Variable Size: Best-Fit front 2 k 14 k p 1 Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
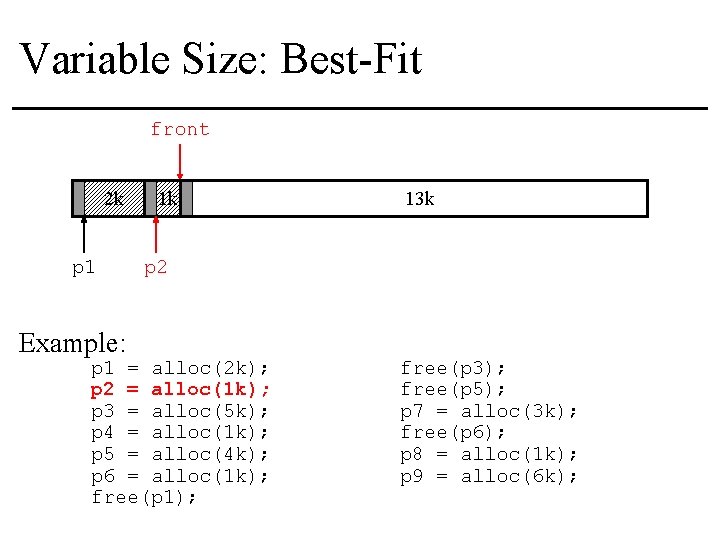
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 13 k p 2 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
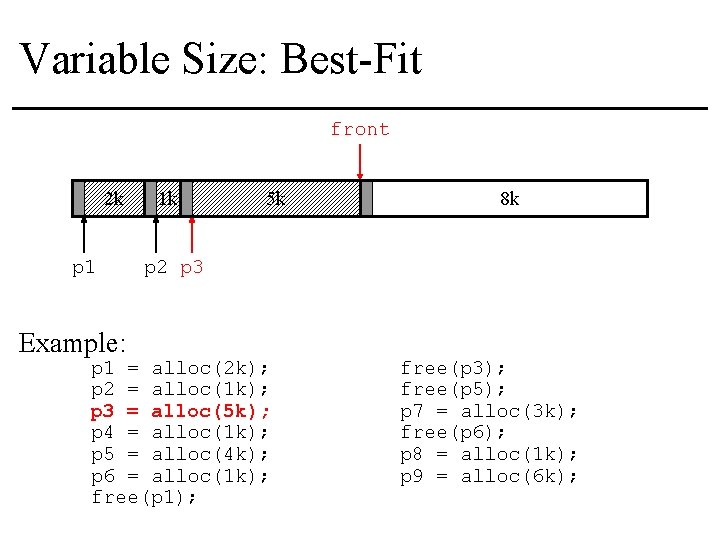
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 5 k 8 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
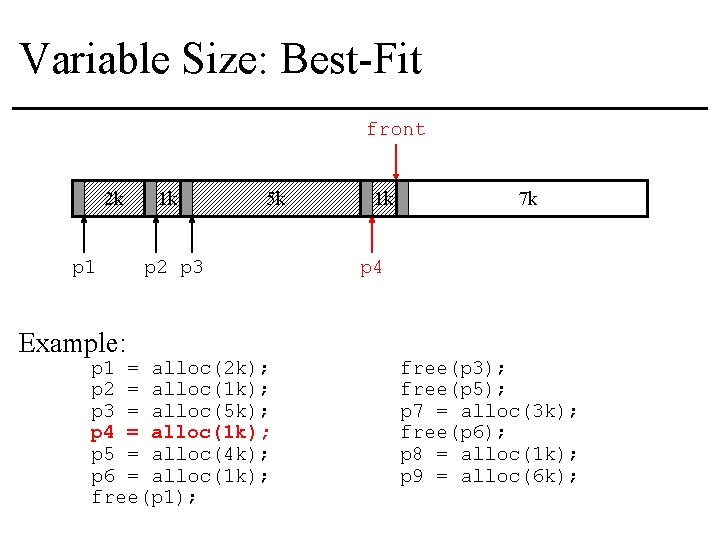
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 7 k p 4 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
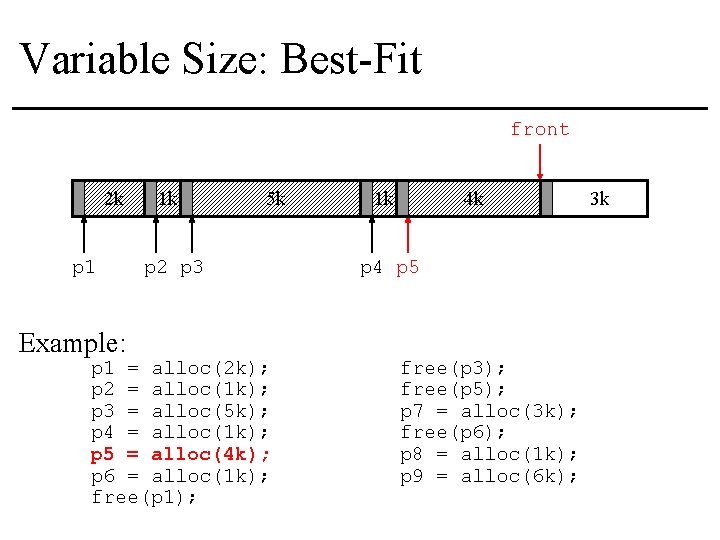
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 3 k
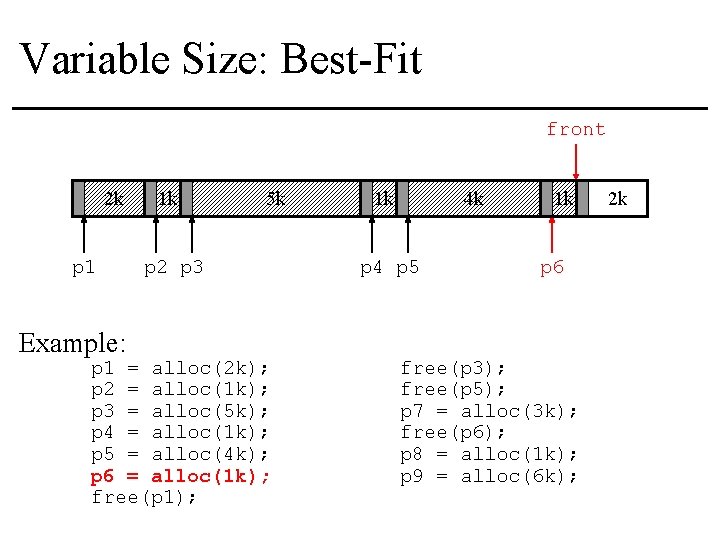
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
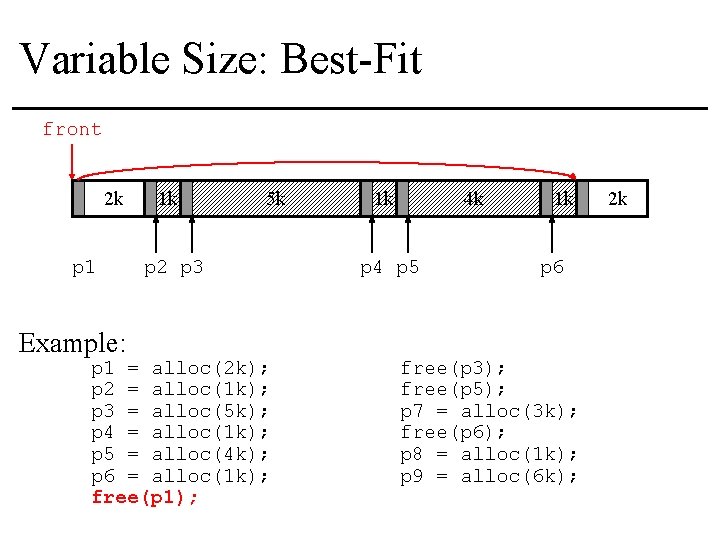
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
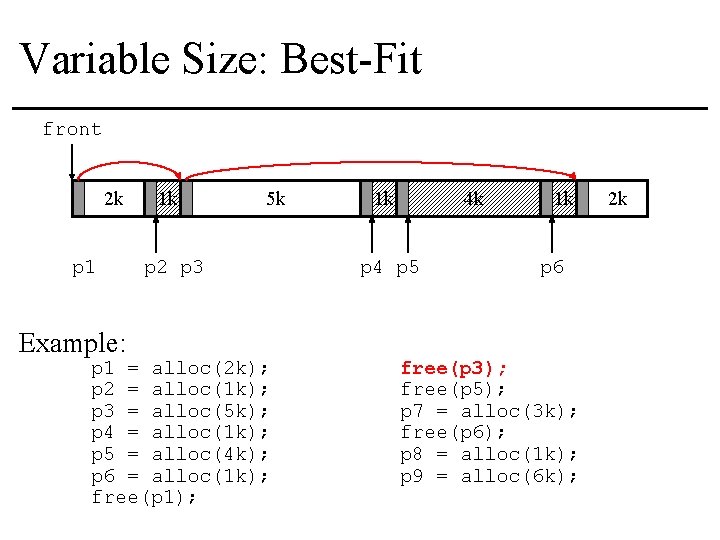
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
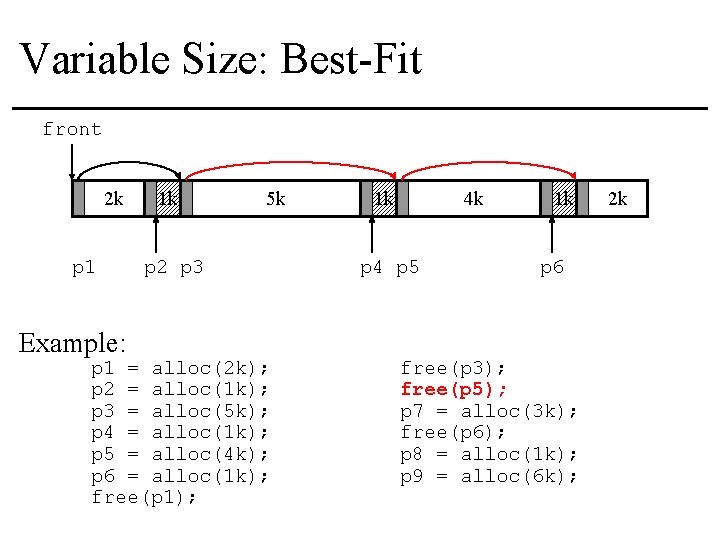
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 4 k p 4 p 5 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
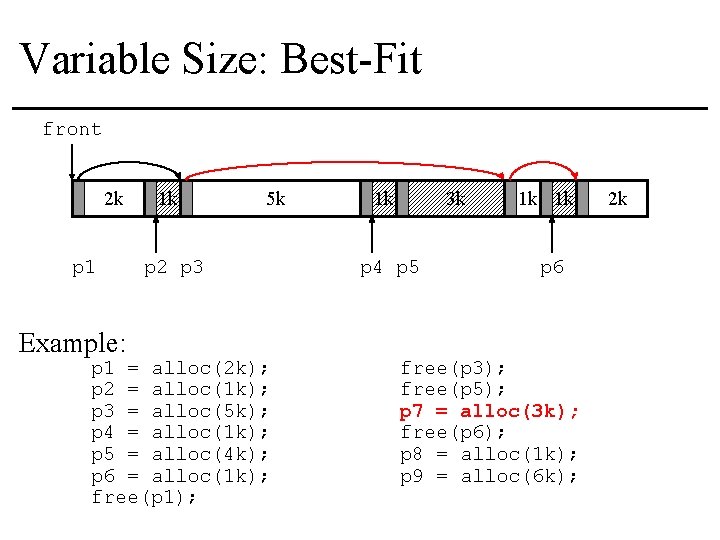
Variable Size: Best-Fit front 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 3 k p 4 p 5 1 k 1 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); 2 k
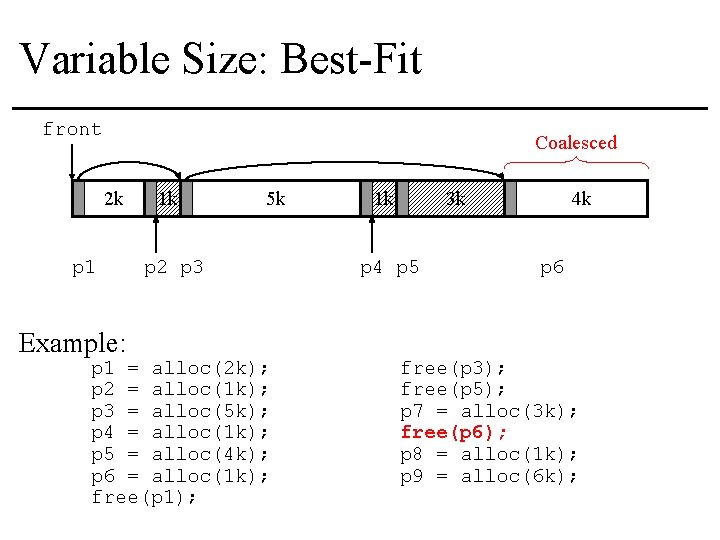
Variable Size: Best-Fit front Coalesced 2 k p 1 Example: 1 k 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 3 k p 4 p 5 4 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
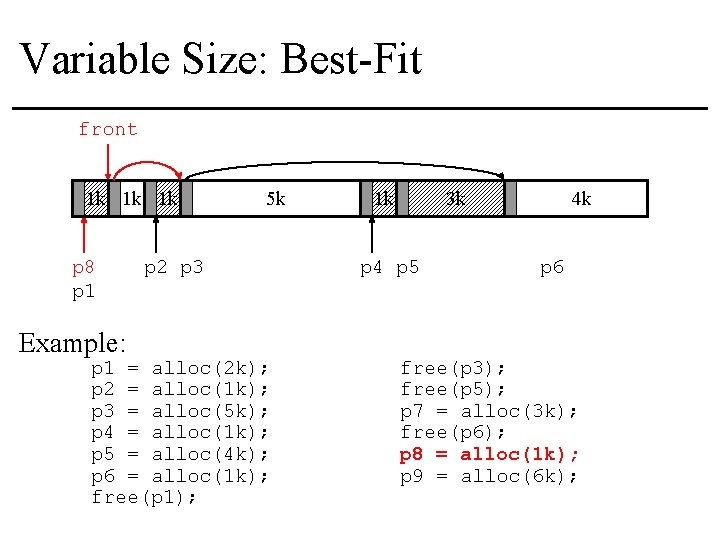
Variable Size: Best-Fit front 1 k 1 k 1 k p 8 p 1 Example: 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 3 k p 4 p 5 4 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k);
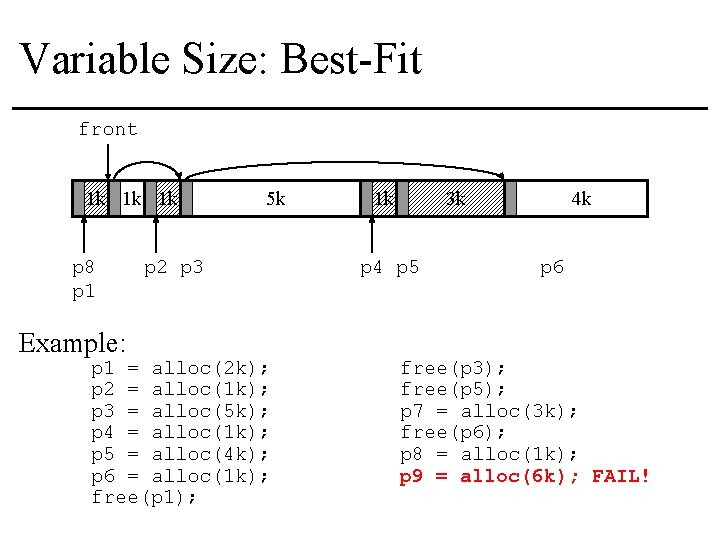
Variable Size: Best-Fit front 1 k 1 k 1 k p 8 p 1 Example: 5 k p 2 p 3 p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); p 6 = alloc(1 k); free(p 1); 1 k 3 k p 4 p 5 4 k p 6 free(p 3); free(p 5); p 7 = alloc(3 k); free(p 6); p 8 = alloc(1 k); p 9 = alloc(6 k); FAIL!
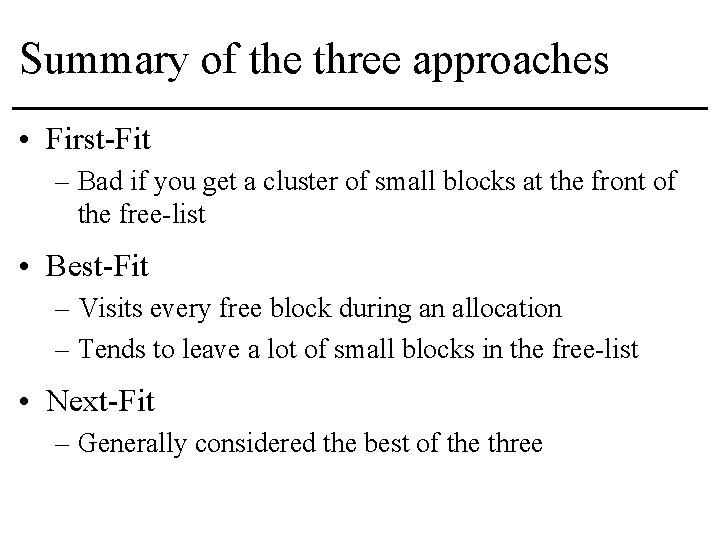
Summary of the three approaches • First-Fit – Bad if you get a cluster of small blocks at the front of the free-list • Best-Fit – Visits every free block during an allocation – Tends to leave a lot of small blocks in the free-list • Next-Fit – Generally considered the best of the three
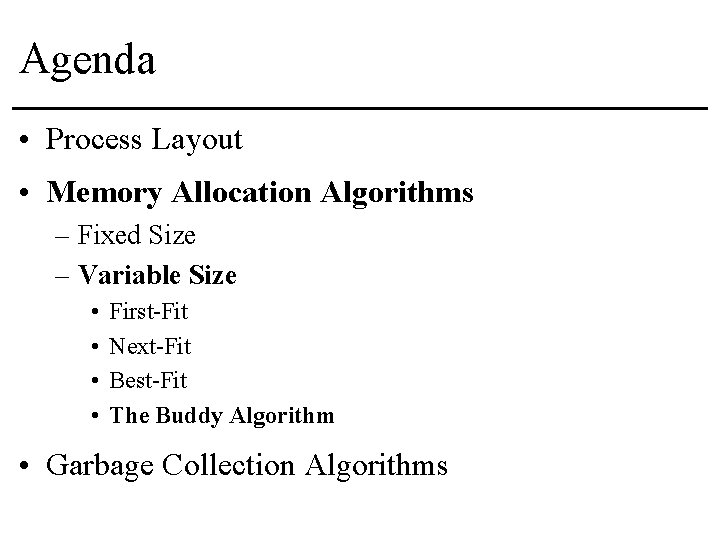
Agenda • Process Layout • Memory Allocation Algorithms – Fixed Size – Variable Size • • First-Fit Next-Fit Best-Fit The Buddy Algorithm • Garbage Collection Algorithms
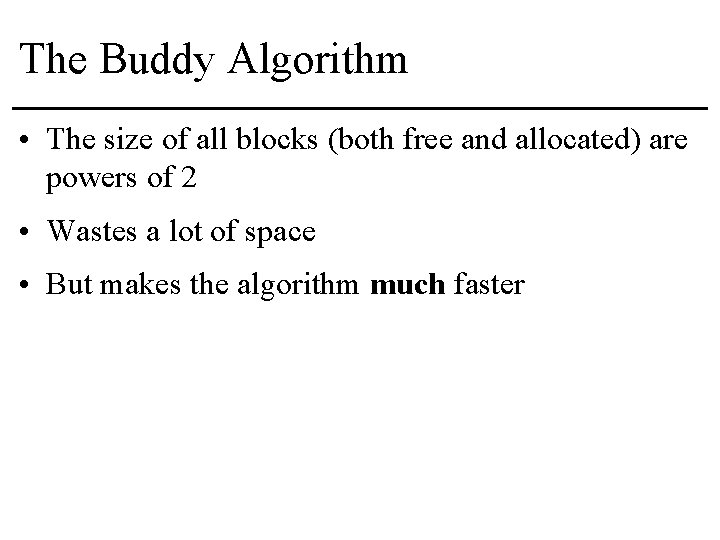
The Buddy Algorithm • The size of all blocks (both free and allocated) are powers of 2 • Wastes a lot of space • But makes the algorithm much faster
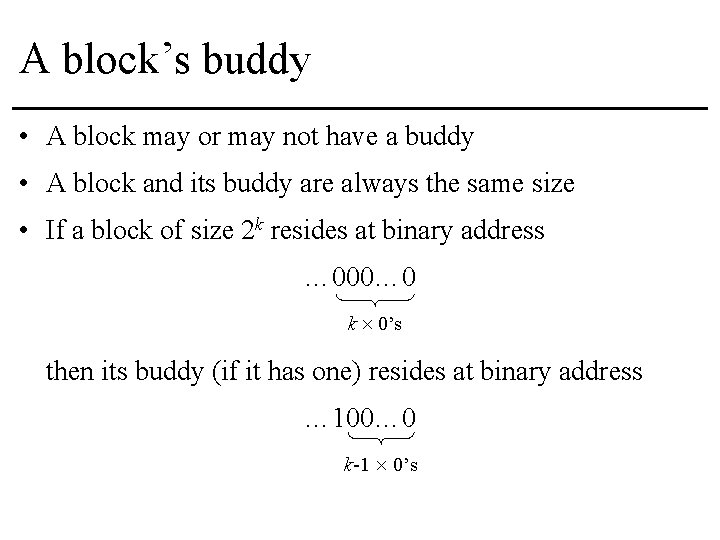
A block’s buddy • A block may or may not have a buddy • A block and its buddy are always the same size • If a block of size 2 k resides at binary address … 000… 0 k 0’s then its buddy (if it has one) resides at binary address … 100… 0 k-1 0’s
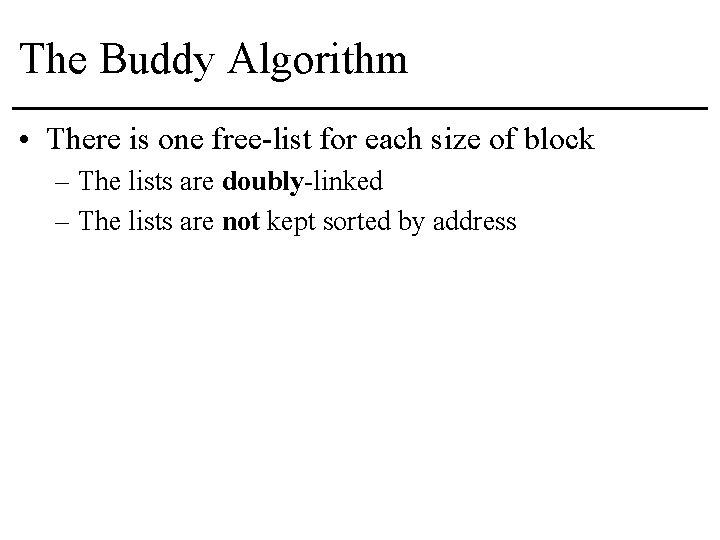
The Buddy Algorithm • There is one free-list for each size of block – The lists are doubly-linked – The lists are not kept sorted by address
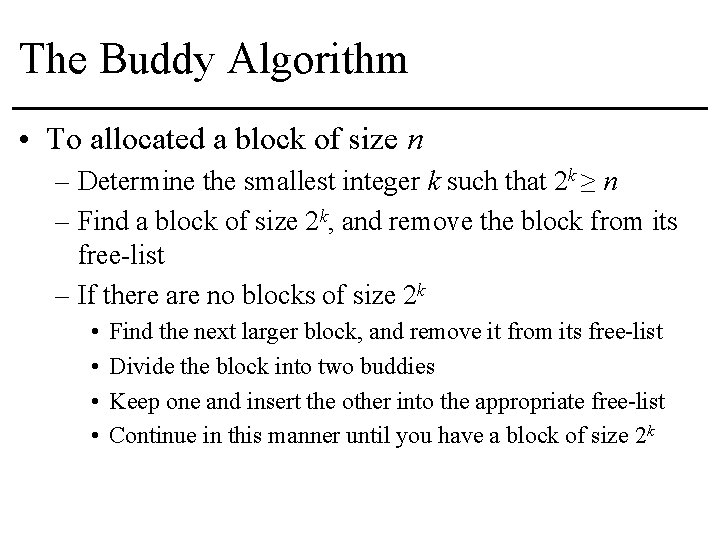
The Buddy Algorithm • To allocated a block of size n – Determine the smallest integer k such that 2 k ≥ n – Find a block of size 2 k, and remove the block from its free-list – If there are no blocks of size 2 k • • Find the next larger block, and remove it from its free-list Divide the block into two buddies Keep one and insert the other into the appropriate free-list Continue in this manner until you have a block of size 2 k
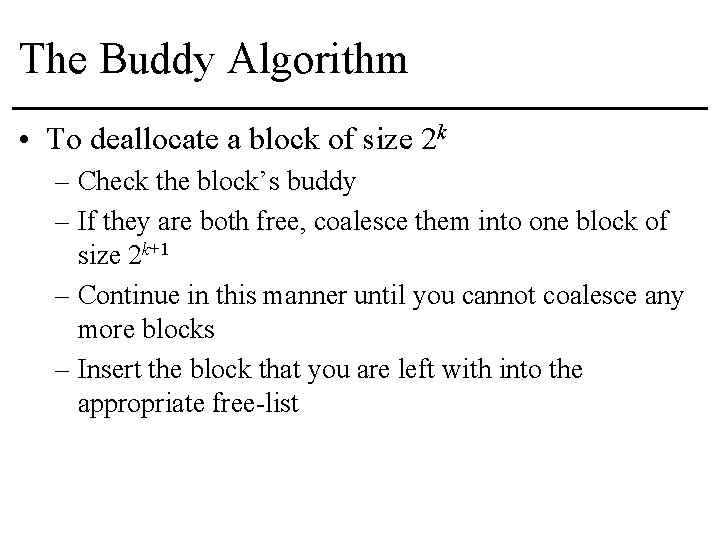
The Buddy Algorithm • To deallocate a block of size 2 k – Check the block’s buddy – If they are both free, coalesce them into one block of size 2 k+1 – Continue in this manner until you cannot coalesce any more blocks – Insert the block that you are left with into the appropriate free-list
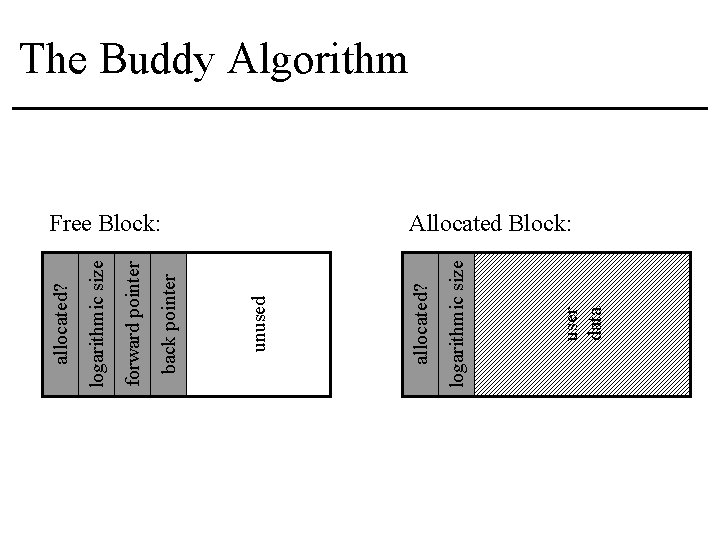
user data logarithmic size Free Block: allocated? unused back pointer forward pointer logarithmic size allocated? The Buddy Algorithm Allocated Block:
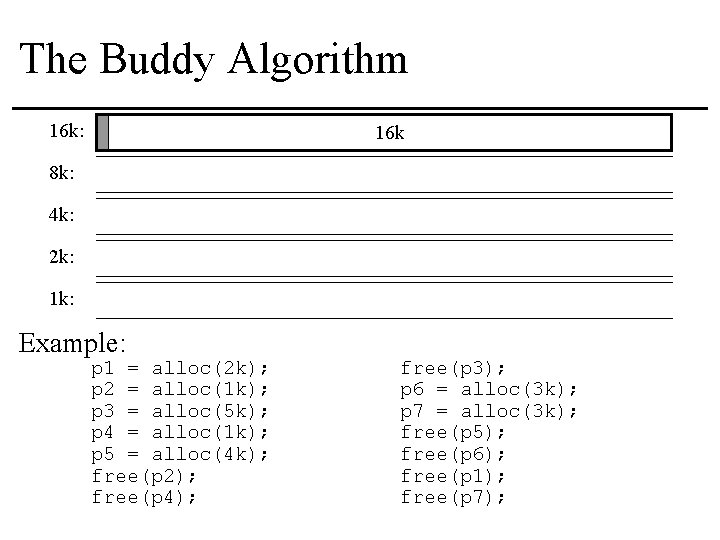
The Buddy Algorithm 16 k: 16 k 8 k: 4 k: 2 k: 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7);
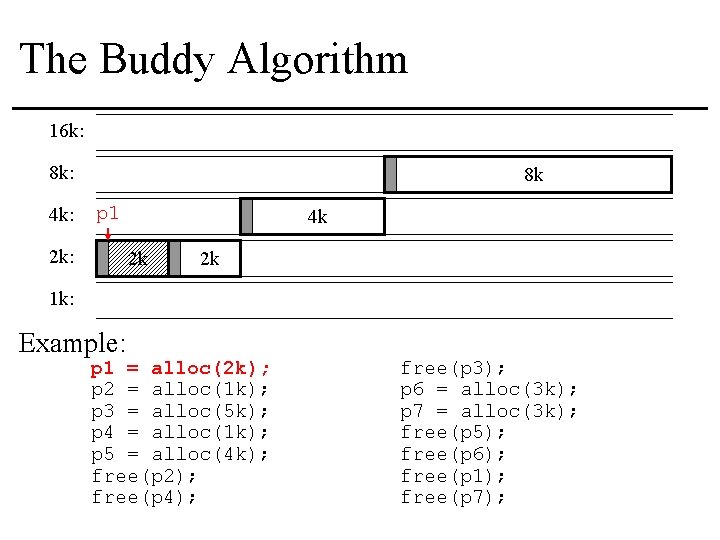
The Buddy Algorithm 16 k: 8 k: 4 k: 8 k p 1 2 k: 4 k 2 k 2 k 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7);
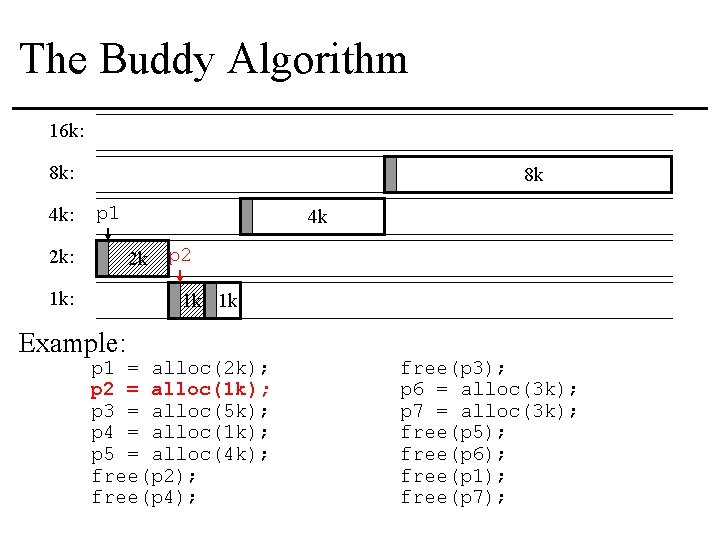
The Buddy Algorithm 16 k: 8 k: 4 k: 8 k p 1 4 k 2 k p 2 2 k: 1 k: 1 k 1 k Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7);
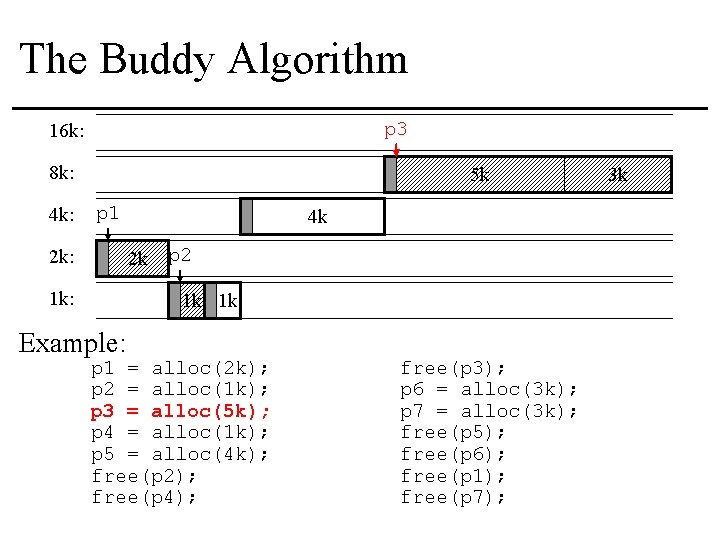
The Buddy Algorithm p 3 16 k: 8 k: 4 k: 5 k p 1 4 k 2 k p 2 2 k: 1 k: 1 k 1 k Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 3 k
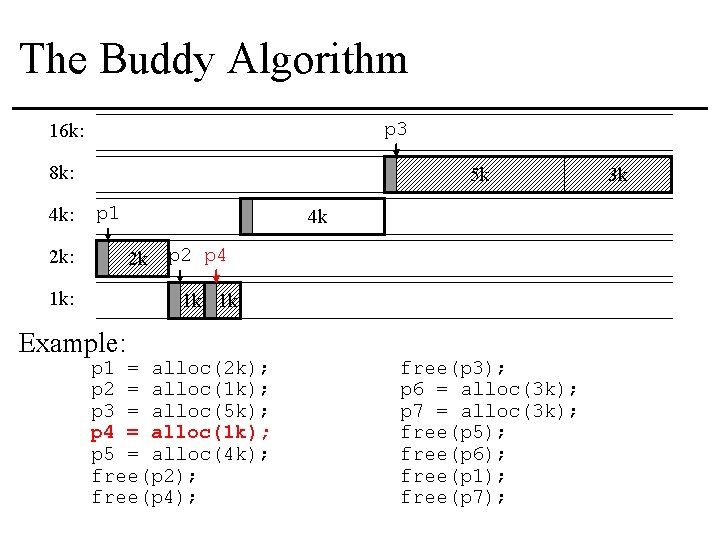
The Buddy Algorithm p 3 16 k: 8 k: 4 k: 5 k p 1 4 k 2 k p 2 p 4 2 k: 1 k: 1 k 1 k Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 3 k
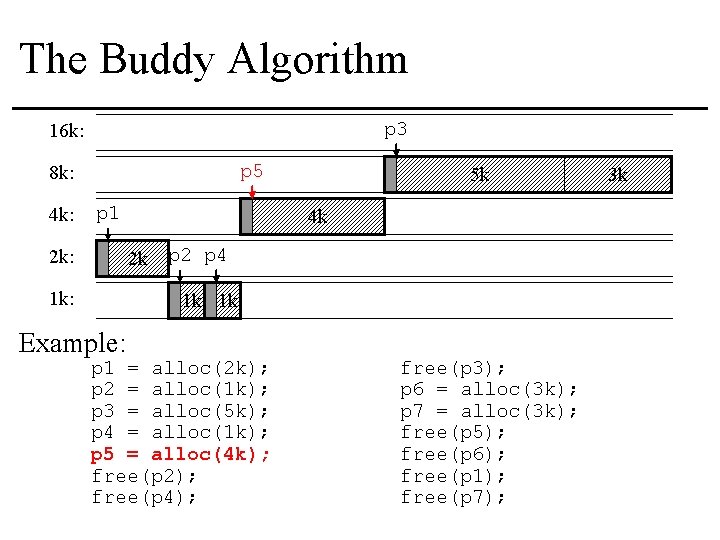
The Buddy Algorithm p 3 16 k: p 5 8 k: 4 k: p 1 5 k 4 k 2 k p 2 p 4 2 k: 1 k: 1 k 1 k Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 3 k
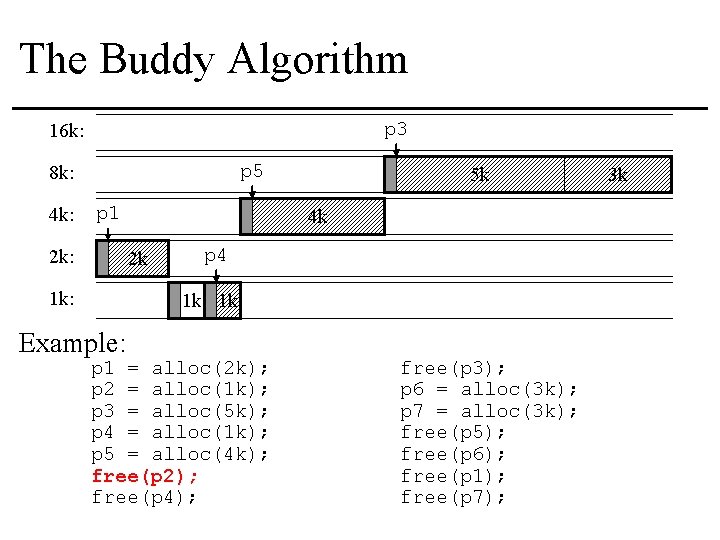
The Buddy Algorithm p 3 16 k: p 5 8 k: 4 k: p 1 2 k: 4 k 2 k 1 k: 5 k p 4 1 k 1 k Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 3 k
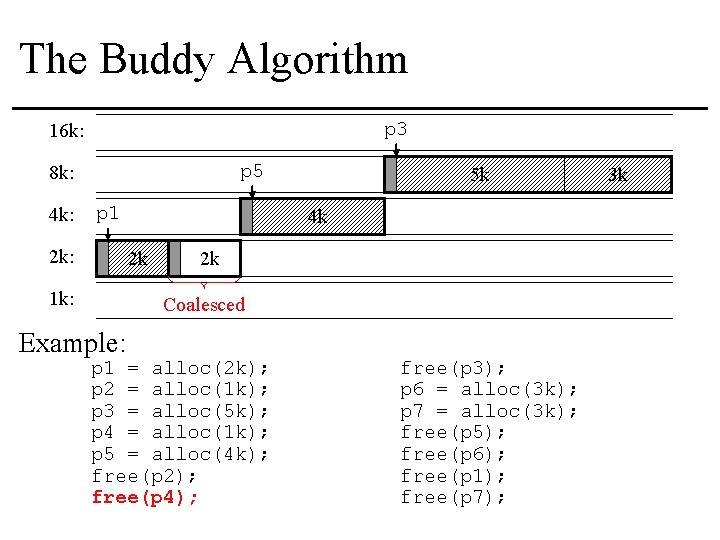
The Buddy Algorithm p 3 16 k: p 5 8 k: 4 k: p 1 2 k: 4 k 2 k 1 k: 5 k 2 k Coalesced Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 3 k
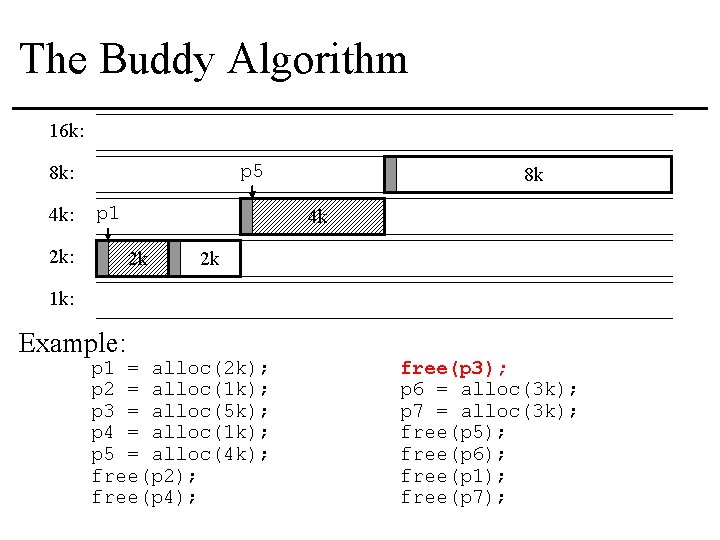
The Buddy Algorithm 16 k: p 5 8 k: 4 k: p 1 2 k: 8 k 4 k 2 k 2 k 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7);
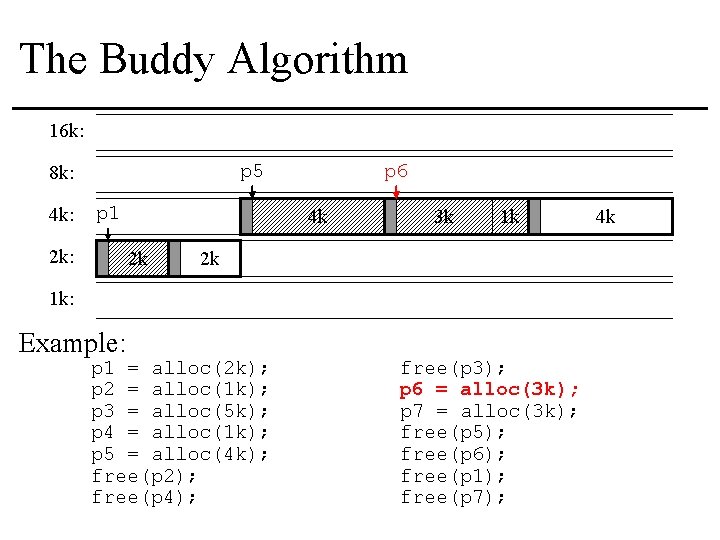
The Buddy Algorithm 16 k: p 5 8 k: 4 k: p 1 2 k: p 6 4 k 2 k 3 k 1 k 2 k 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 4 k
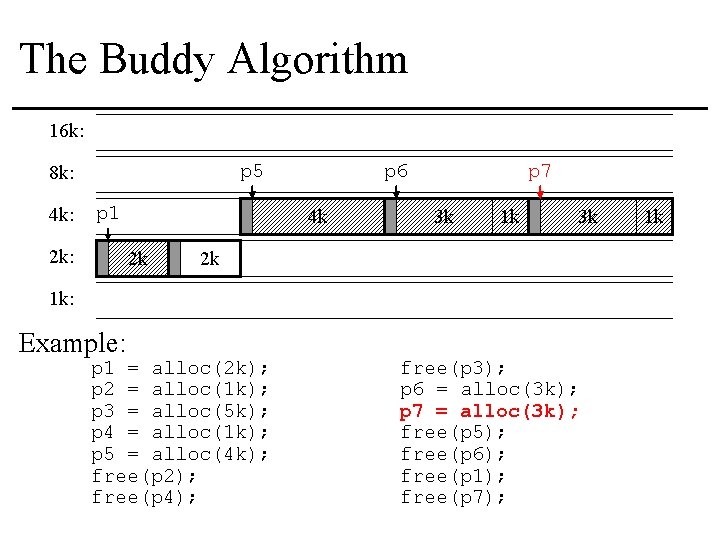
The Buddy Algorithm 16 k: p 5 8 k: 4 k: p 1 2 k: p 6 4 k 2 k p 7 3 k 1 k 3 k 2 k 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 1 k
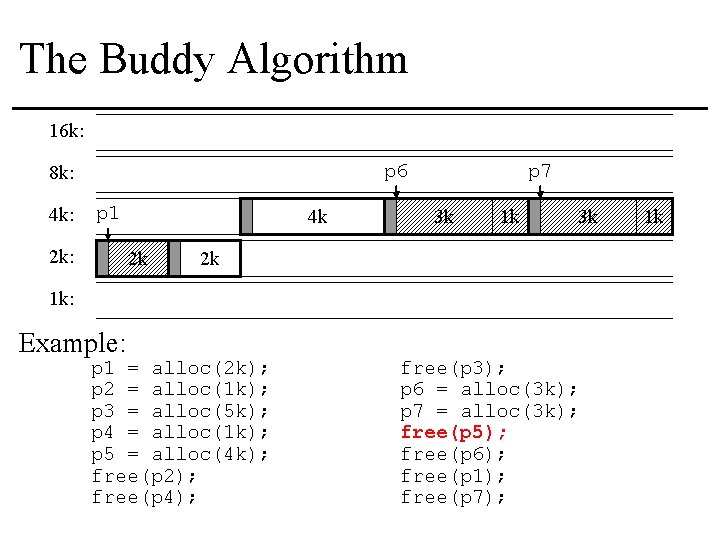
The Buddy Algorithm 16 k: p 6 8 k: 4 k: p 1 2 k: 4 k 2 k p 7 3 k 1 k 3 k 2 k 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 1 k
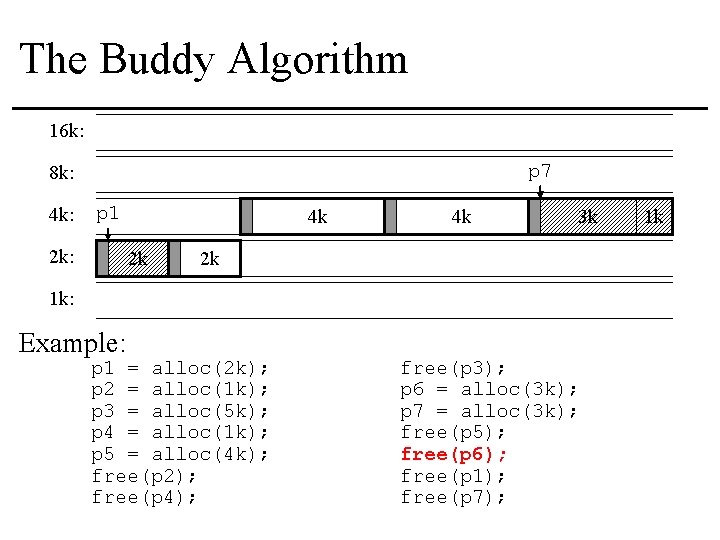
The Buddy Algorithm 16 k: p 7 8 k: 4 k: p 1 2 k: 4 k 2 k 4 k 3 k 2 k 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 1 k
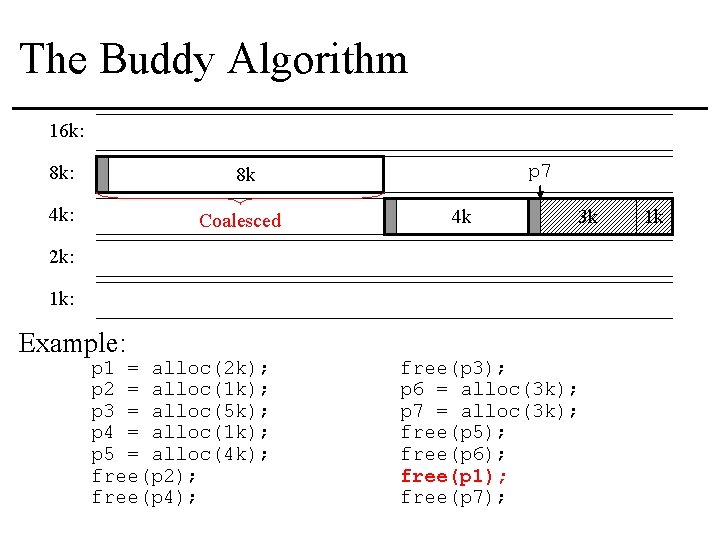
The Buddy Algorithm 16 k: 8 k: p 7 8 k 4 k: Coalesced 4 k 3 k 2 k: 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7); 1 k
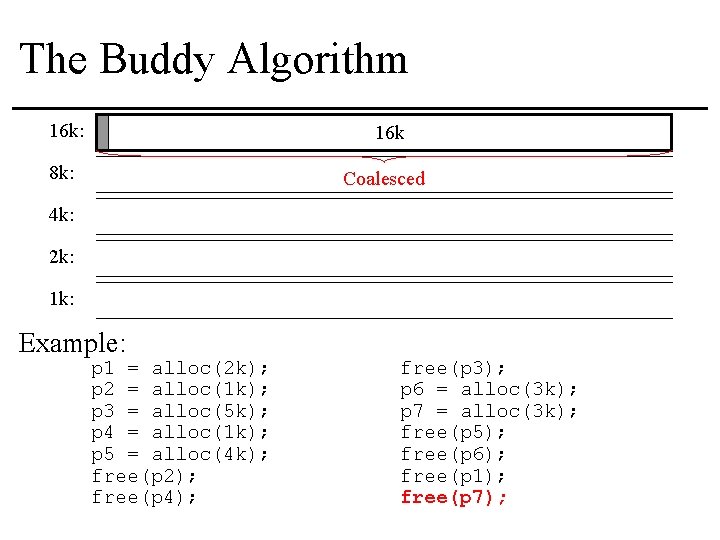
The Buddy Algorithm 16 k: 16 k 8 k: Coalesced 4 k: 2 k: 1 k: Example: p 1 = alloc(2 k); p 2 = alloc(1 k); p 3 = alloc(5 k); p 4 = alloc(1 k); p 5 = alloc(4 k); free(p 2); free(p 4); free(p 3); p 6 = alloc(3 k); p 7 = alloc(3 k); free(p 5); free(p 6); free(p 1); free(p 7);
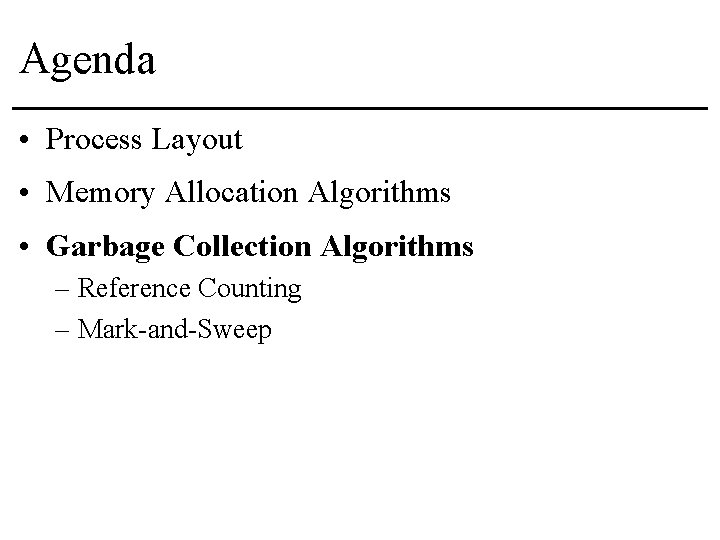
Agenda • Process Layout • Memory Allocation Algorithms • Garbage Collection Algorithms – Reference Counting – Mark-and-Sweep
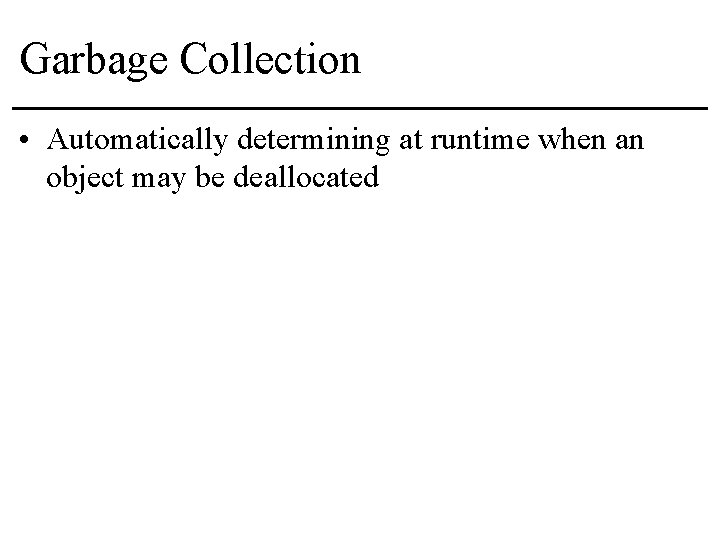
Garbage Collection • Automatically determining at runtime when an object may be deallocated
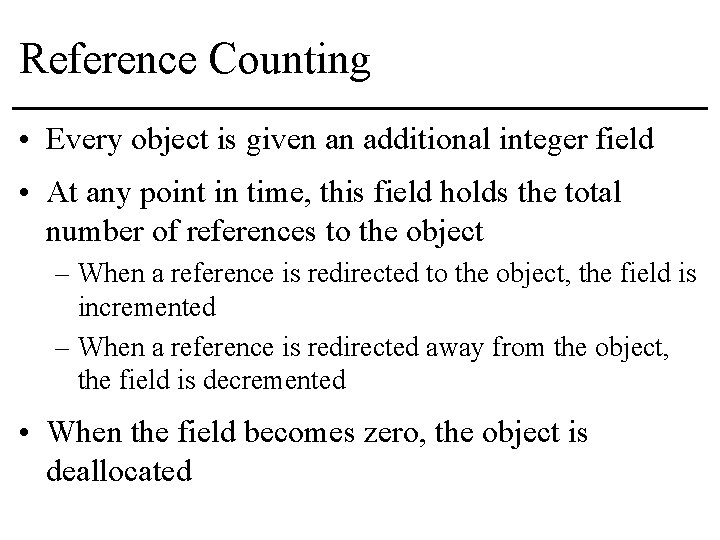
Reference Counting • Every object is given an additional integer field • At any point in time, this field holds the total number of references to the object – When a reference is redirected to the object, the field is incremented – When a reference is redirected away from the object, the field is decremented • When the field becomes zero, the object is deallocated
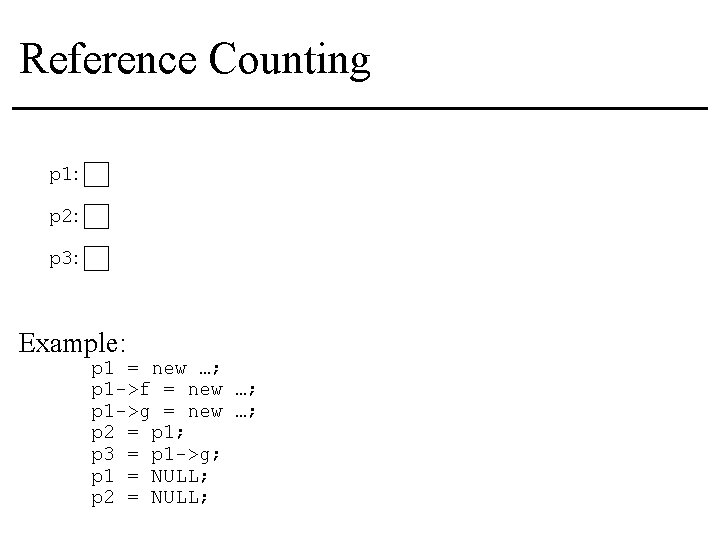
Reference Counting p 1: p 2: p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
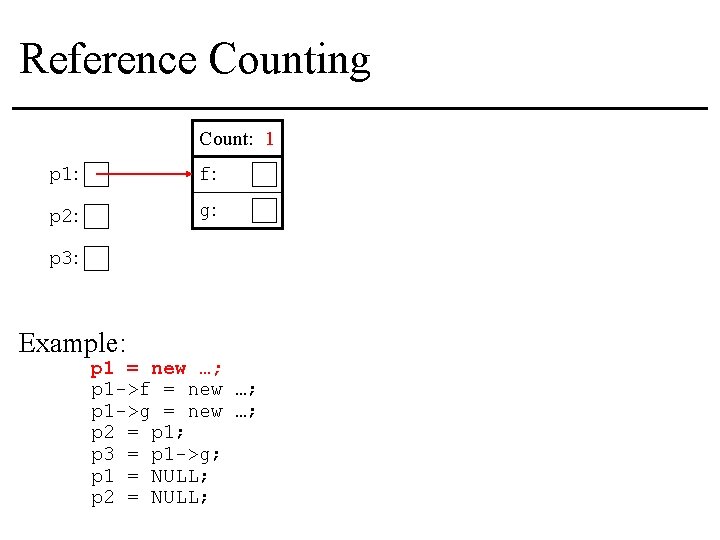
Reference Counting Count: 1 p 1: f: p 2: g: p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
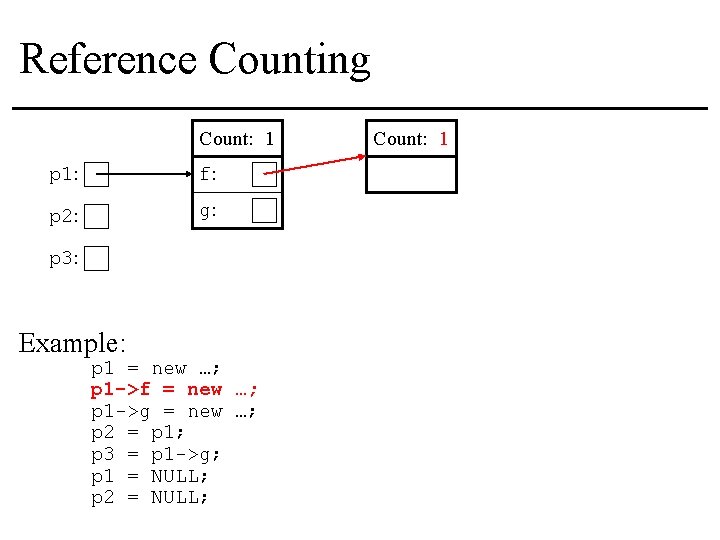
Reference Counting Count: 1 p 1: f: p 2: g: p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL; Count: 1
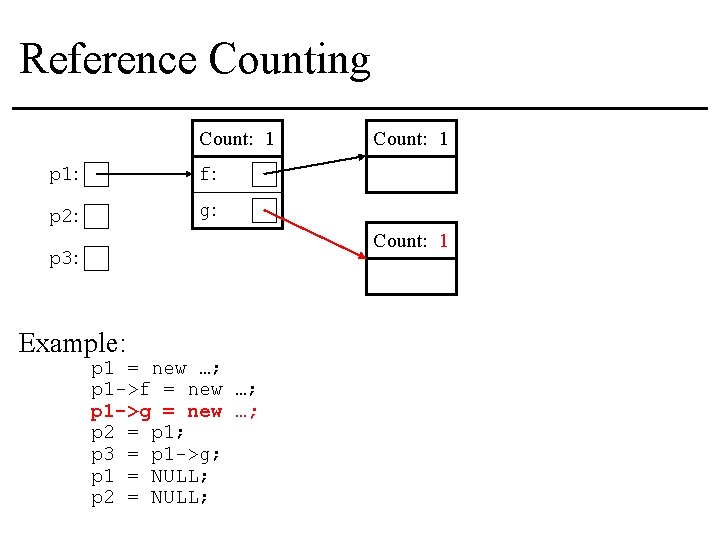
Reference Counting Count: 1 p 1: f: p 2: g: Count: 1 p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
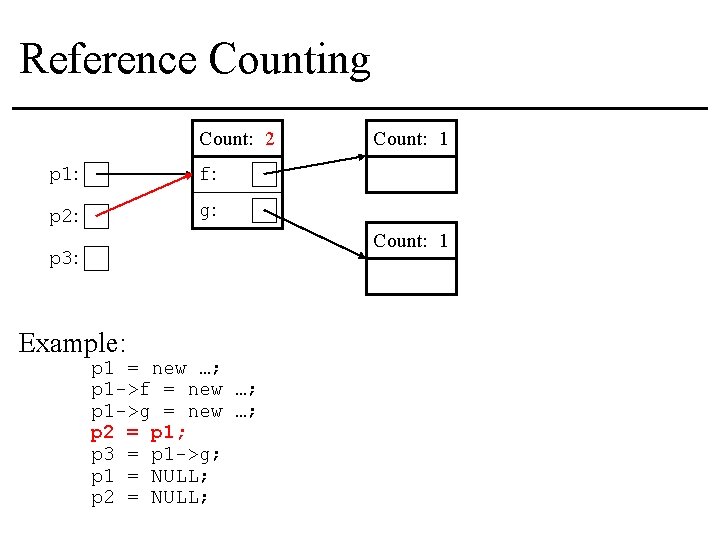
Reference Counting Count: 2 p 1: f: p 2: g: Count: 1 p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
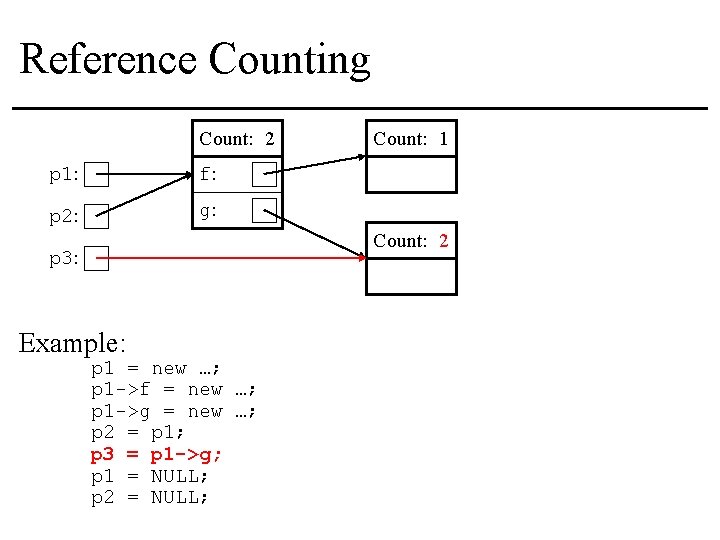
Reference Counting Count: 2 p 1: f: p 2: g: Count: 1 Count: 2 p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
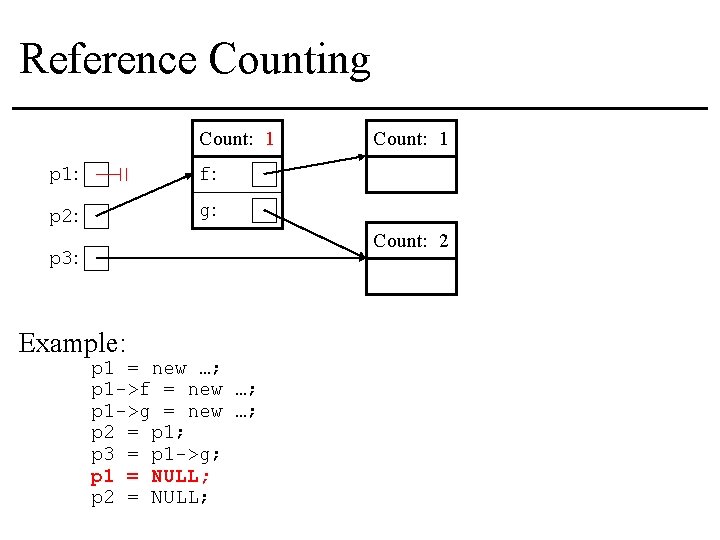
Reference Counting Count: 1 p 1: f: p 2: g: Count: 1 Count: 2 p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
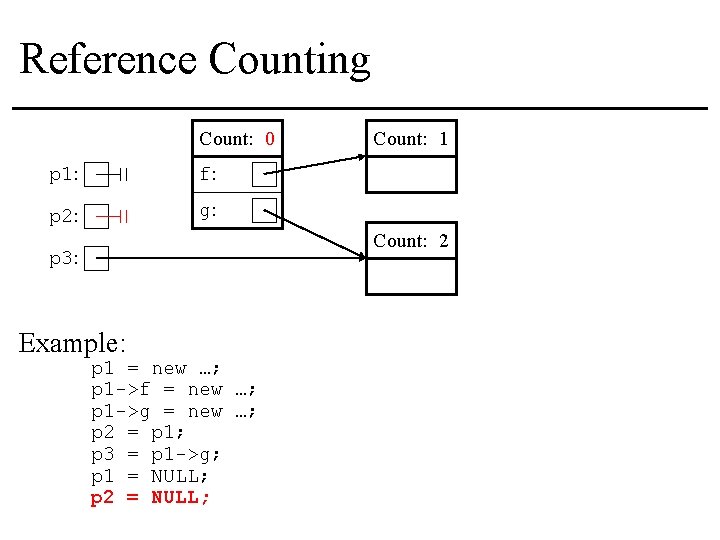
Reference Counting Count: 0 p 1: f: p 2: g: Count: 1 Count: 2 p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
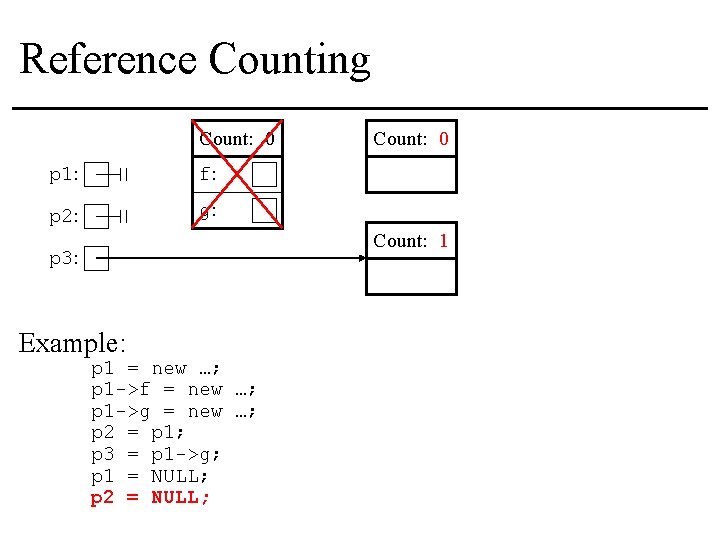
Reference Counting Count: 0 p 1: f: p 2: g: Count: 0 Count: 1 p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
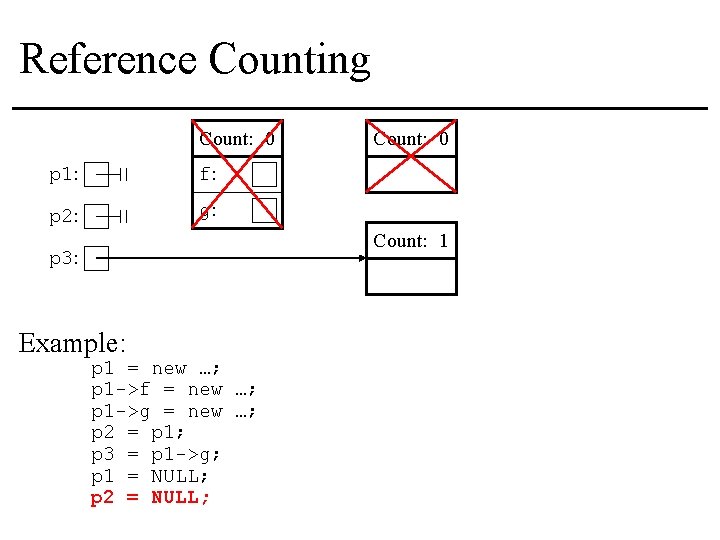
Reference Counting Count: 0 p 1: f: p 2: g: Count: 0 Count: 1 p 3: Example: p 1 = new …; p 1 ->f = new …; p 1 ->g = new …; p 2 = p 1; p 3 = p 1 ->g; p 1 = NULL; p 2 = NULL;
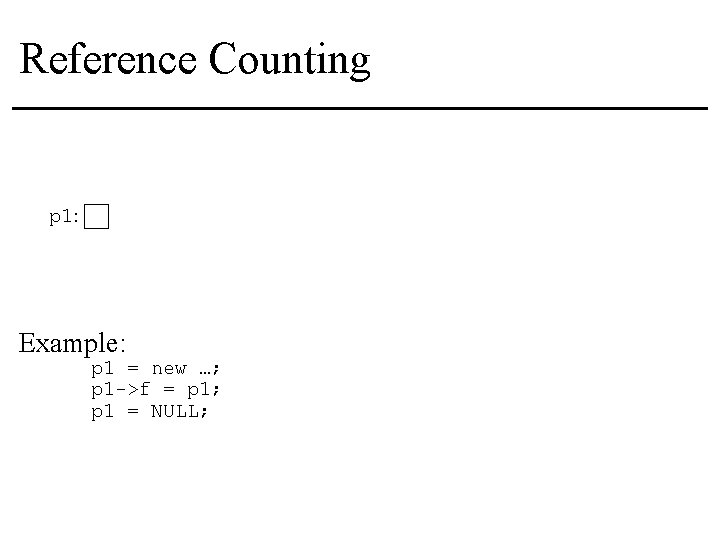
Reference Counting p 1: Example: p 1 = new …; p 1 ->f = p 1; p 1 = NULL;
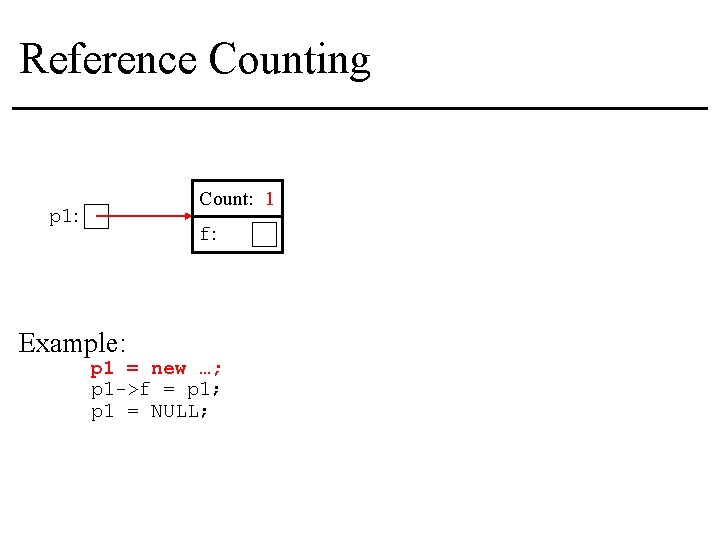
Reference Counting Count: 1 p 1: f: Example: p 1 = new …; p 1 ->f = p 1; p 1 = NULL;
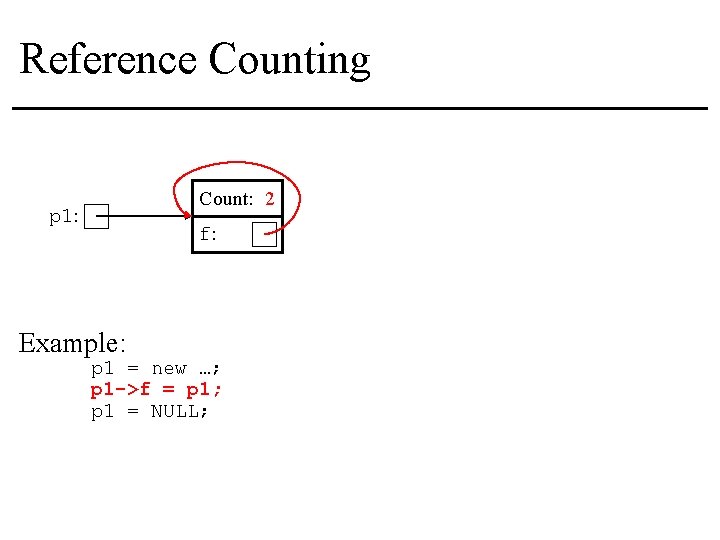
Reference Counting Count: 2 p 1: f: Example: p 1 = new …; p 1 ->f = p 1; p 1 = NULL;
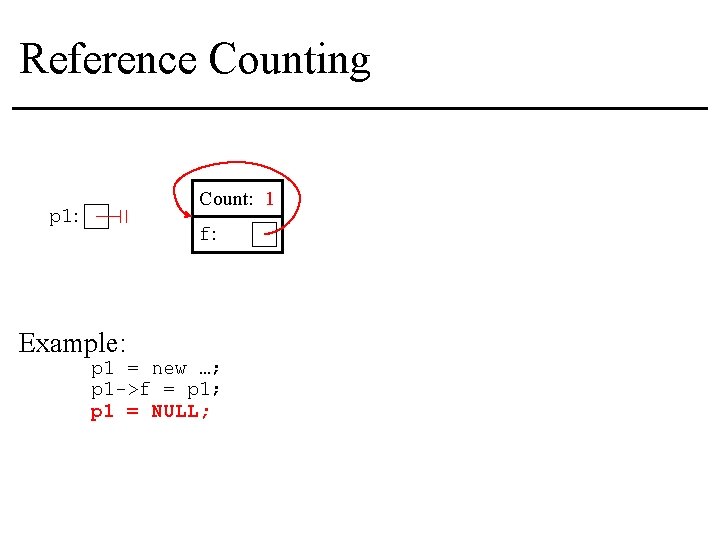
Reference Counting Count: 1 p 1: f: Example: p 1 = new …; p 1 ->f = p 1; p 1 = NULL;
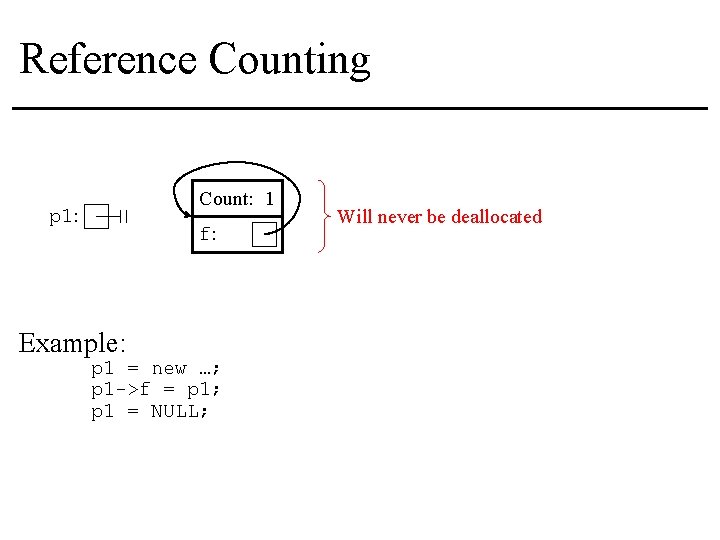
Reference Counting Count: 1 p 1: f: Example: p 1 = new …; p 1 ->f = p 1; p 1 = NULL; Will never be deallocated
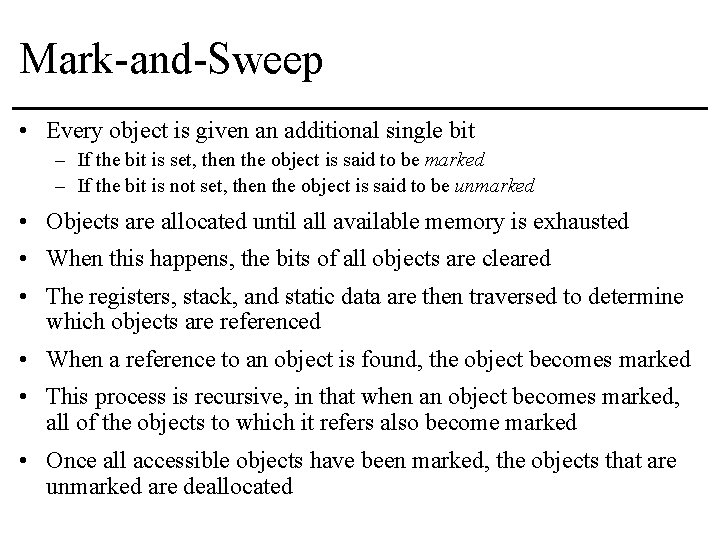
Mark-and-Sweep • Every object is given an additional single bit – If the bit is set, then the object is said to be marked – If the bit is not set, then the object is said to be unmarked • Objects are allocated until all available memory is exhausted • When this happens, the bits of all objects are cleared • The registers, stack, and static data are then traversed to determine which objects are referenced • When a reference to an object is found, the object becomes marked • This process is recursive, in that when an object becomes marked, all of the objects to which it refers also become marked • Once all accessible objects have been marked, the objects that are unmarked are deallocated
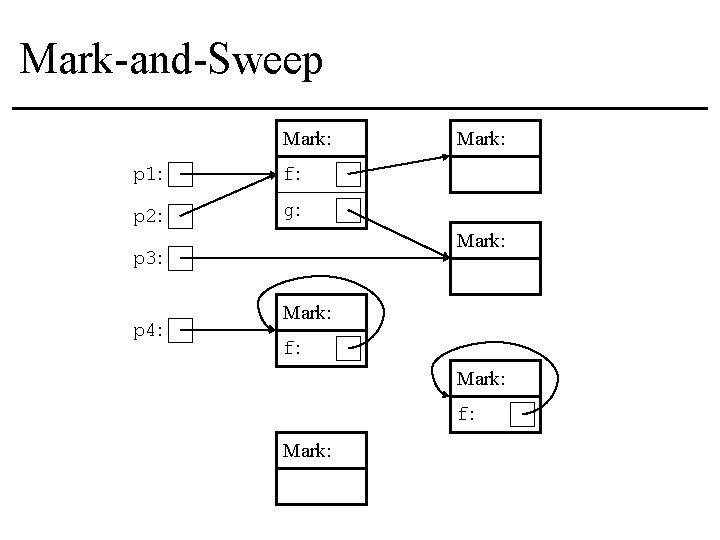
Mark-and-Sweep Mark: p 1: f: p 2: g: Mark: p 3: p 4: Mark: f: Mark:
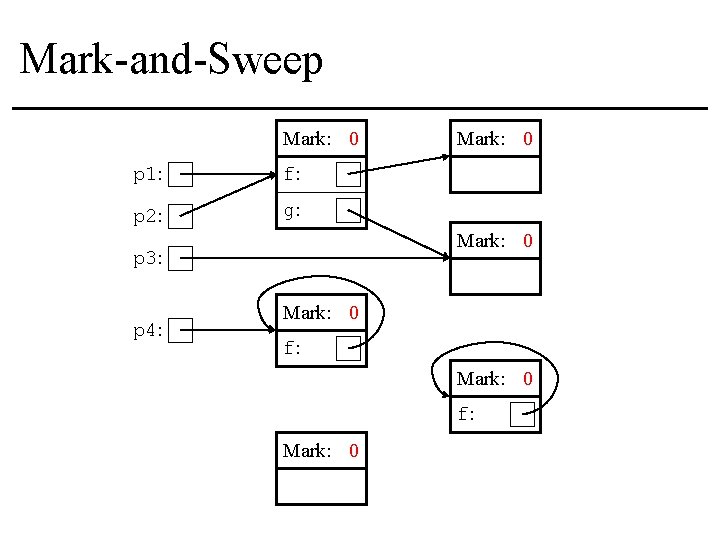
Mark-and-Sweep Mark: 0 p 1: f: p 2: g: Mark: 0 p 3: p 4: Mark: 0 f: Mark: 0
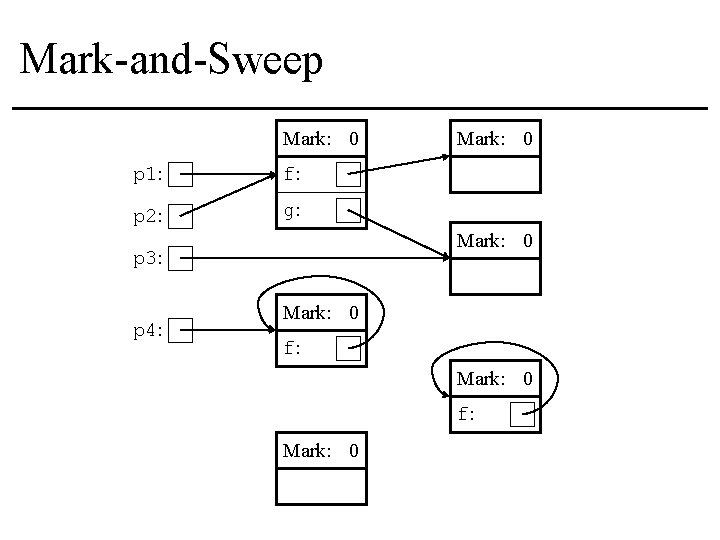
Mark-and-Sweep Mark: 0 p 1: f: p 2: g: Mark: 0 p 3: p 4: Mark: 0 f: Mark: 0
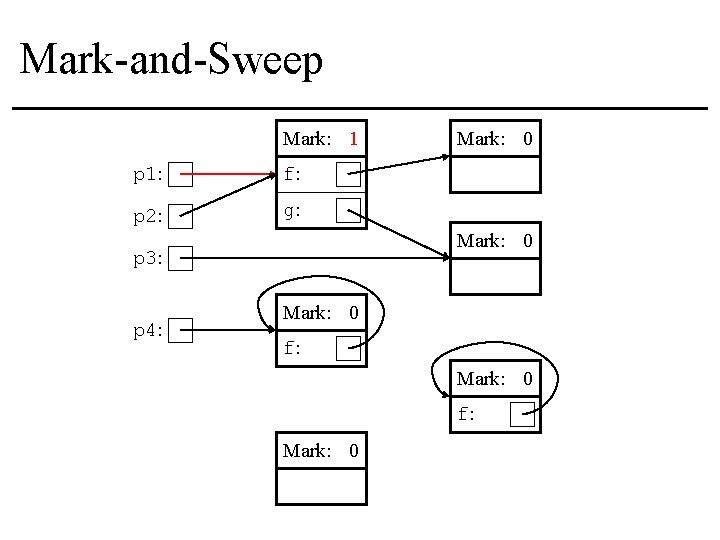
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 0 p 3: p 4: Mark: 0 f: Mark: 0
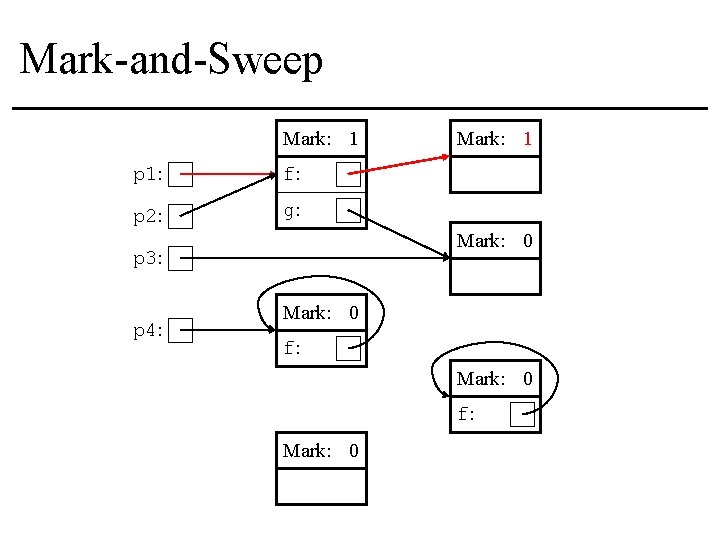
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 0 p 3: p 4: Mark: 1 Mark: 0 f: Mark: 0
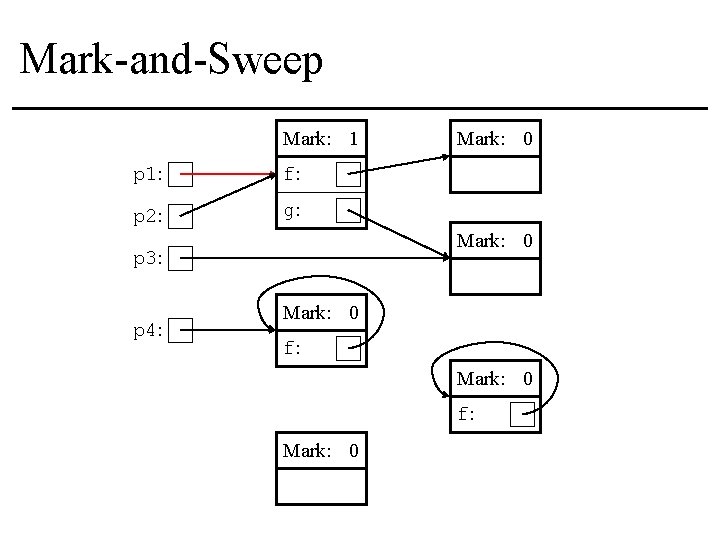
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 0 p 3: p 4: Mark: 0 f: Mark: 0
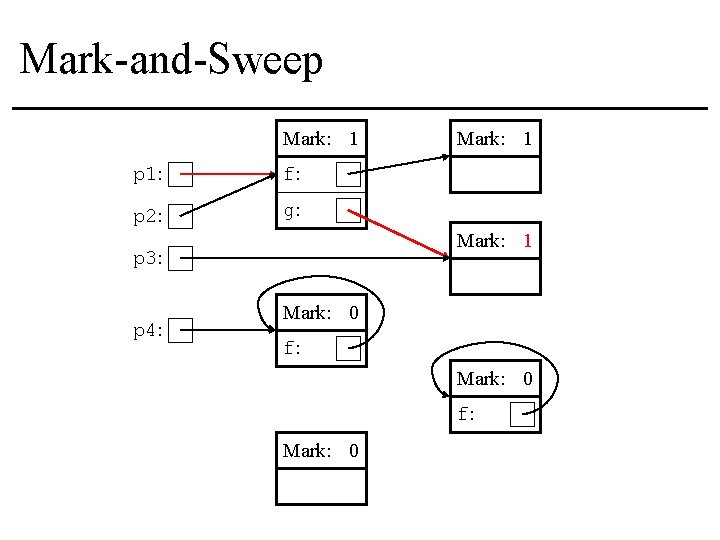
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 Mark: 0 f: Mark: 0
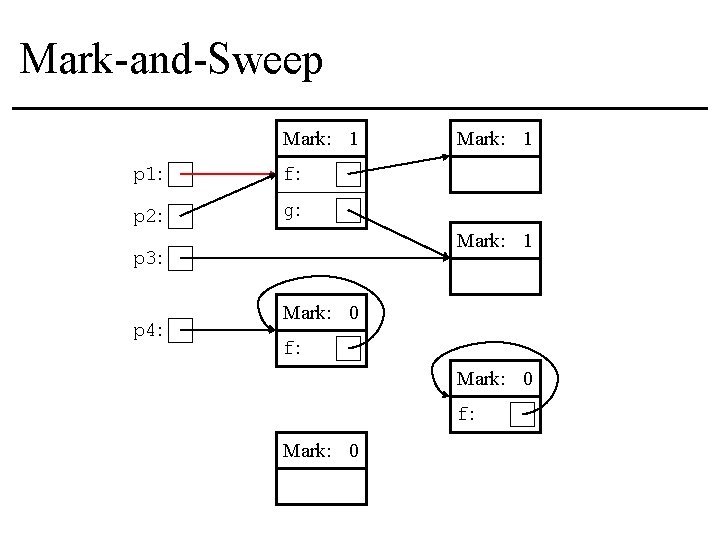
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 Mark: 0 f: Mark: 0
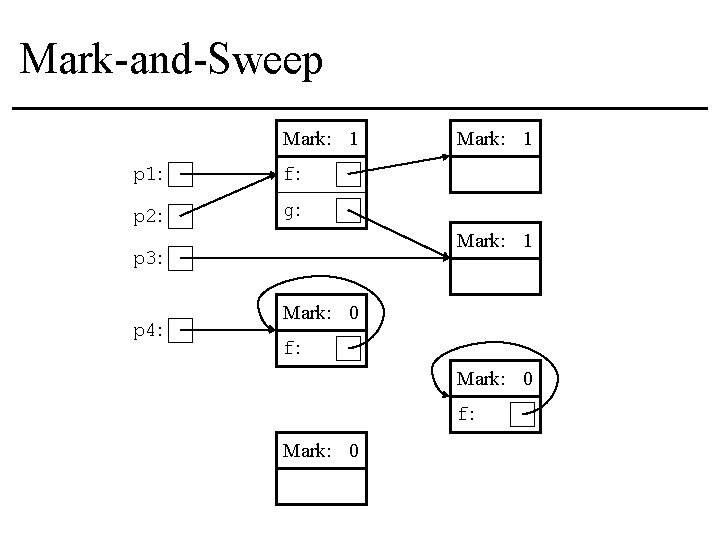
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 Mark: 0 f: Mark: 0
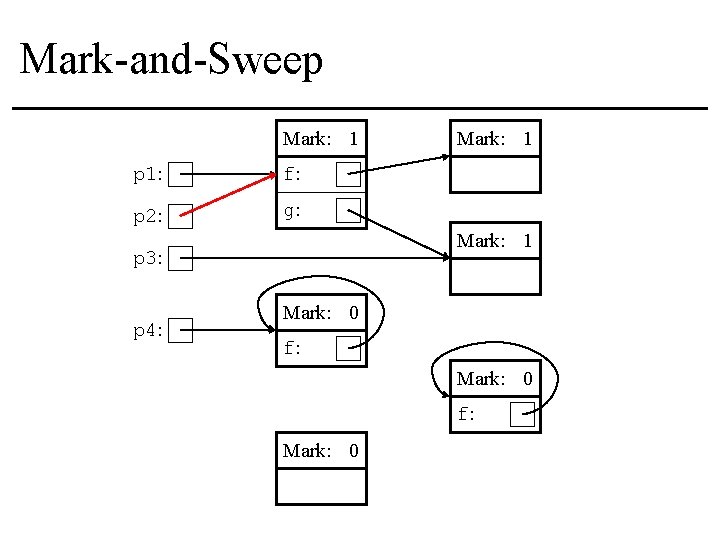
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 Mark: 0 f: Mark: 0
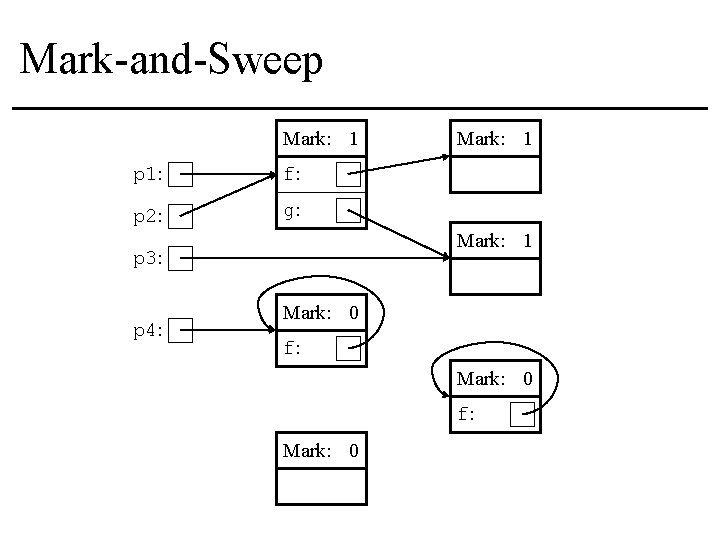
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 Mark: 0 f: Mark: 0
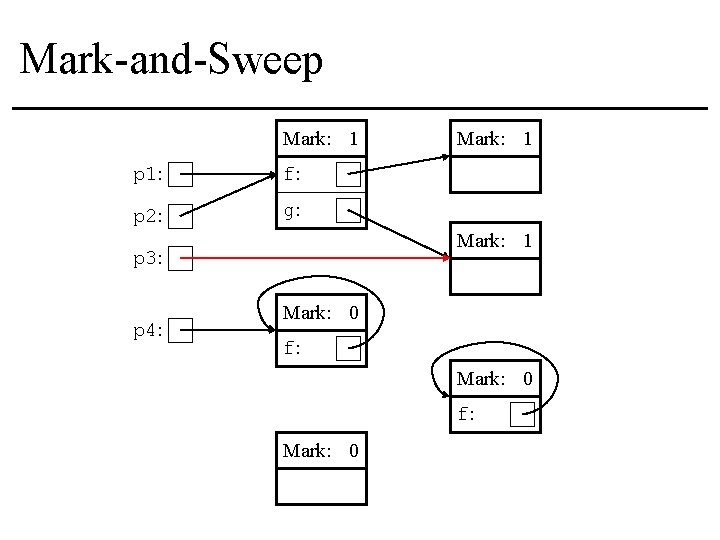
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 Mark: 0 f: Mark: 0
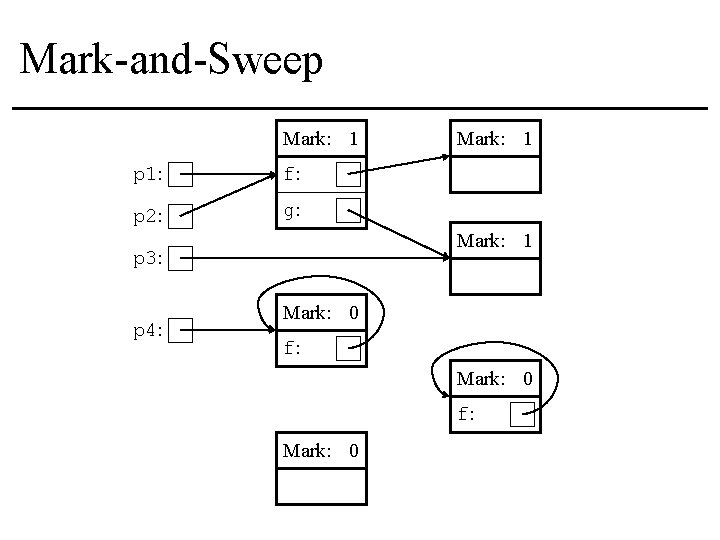
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 Mark: 0 f: Mark: 0
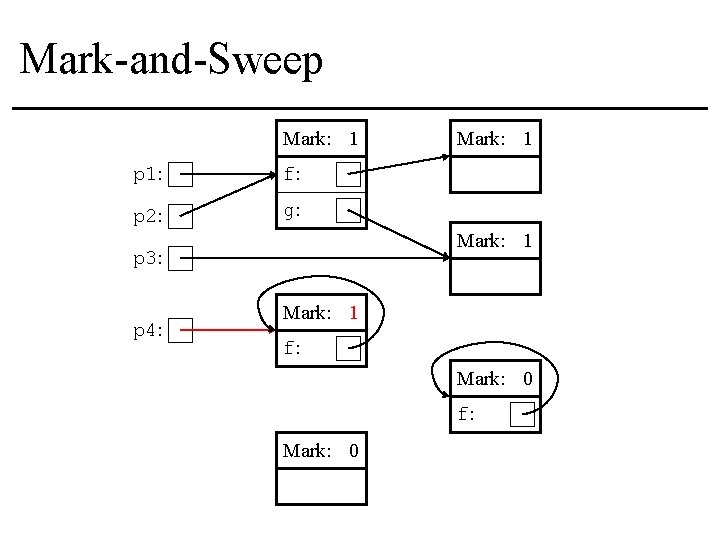
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 f: Mark: 0
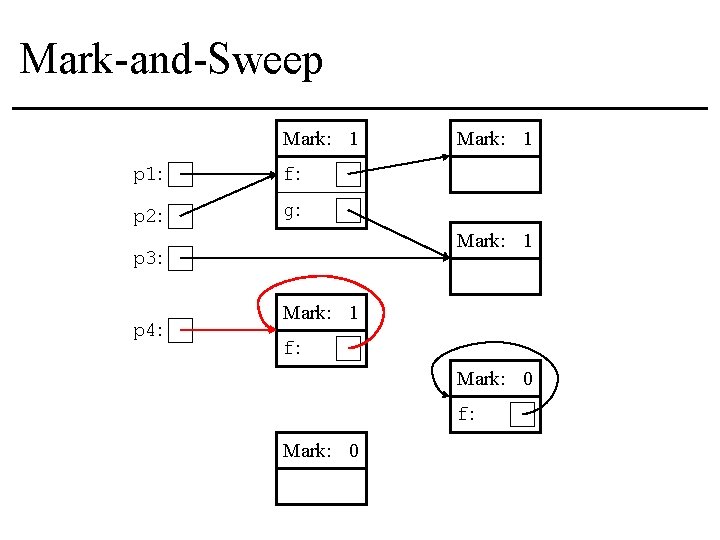
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 f: Mark: 0
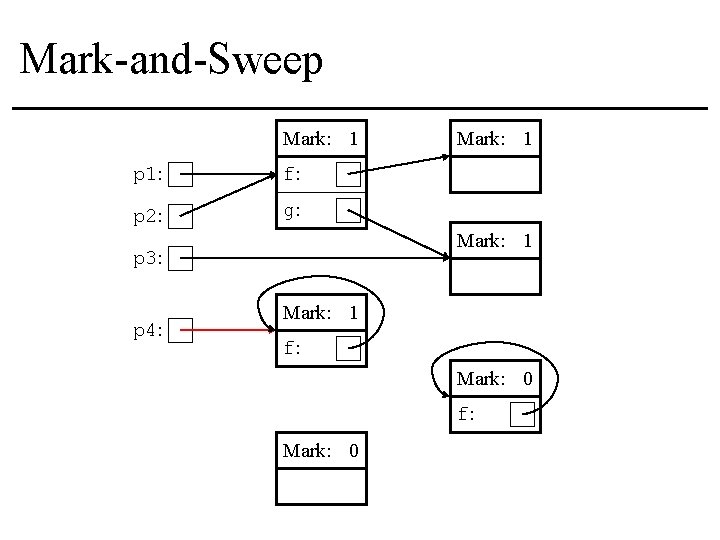
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 f: Mark: 0
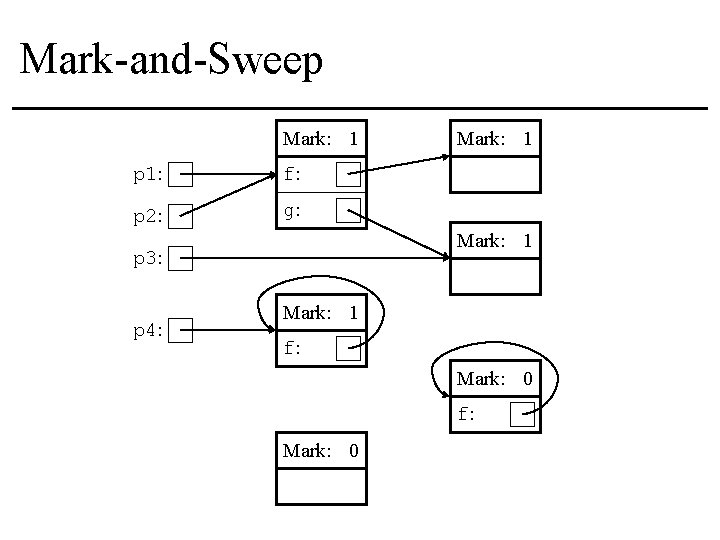
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 f: Mark: 0
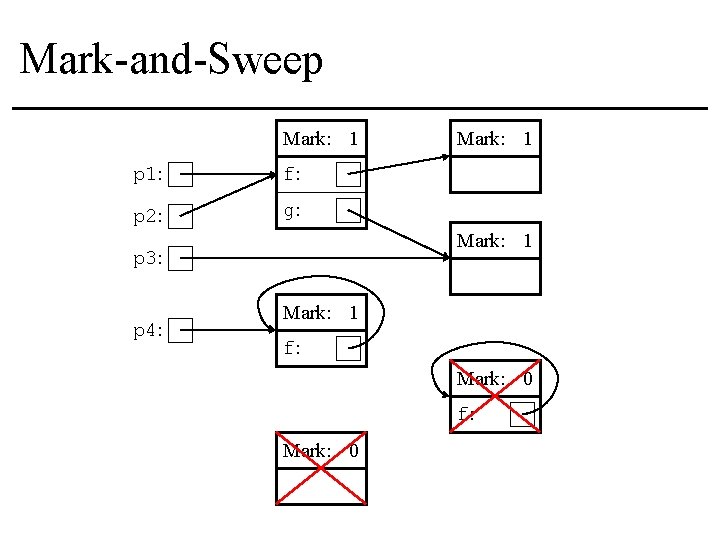
Mark-and-Sweep Mark: 1 p 1: f: p 2: g: Mark: 1 p 3: p 4: Mark: 1 f: Mark: 0
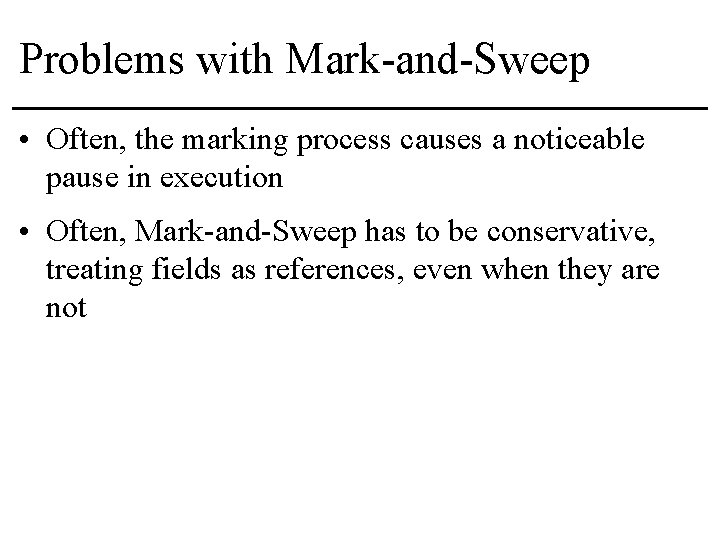
Problems with Mark-and-Sweep • Often, the marking process causes a noticeable pause in execution • Often, Mark-and-Sweep has to be conservative, treating fields as references, even when they are not
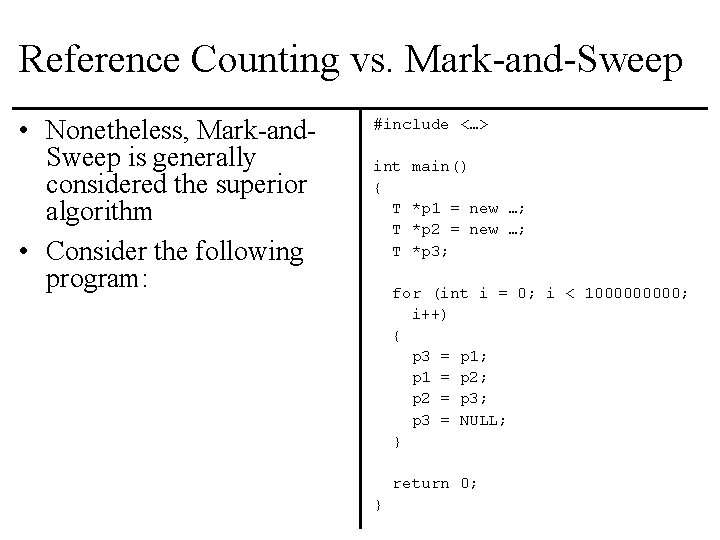
Reference Counting vs. Mark-and-Sweep • Nonetheless, Mark-and. Sweep is generally considered the superior algorithm • Consider the following program: #include <…> int { T T T main() *p 1 = new …; *p 2 = new …; *p 3; for (int i = 0; i < 100000; i++) { p 3 = p 1; p 1 = p 2; p 2 = p 3; p 3 = NULL; } return 0; }
Example of dynamic memory allocation
Explicit memory allocation
Advantages of dynamic memory allocation
Tcmalloc
Example of dynamic memory allocation
Dynamic data structure
Dynamic memory allocation in data structure
Dma dynamic memory allocation
Dynamic memory c++
Dynamic memory allocation in java
Calloc free
Linked allocation
Process memory layout
Agenda sistemica y agenda institucional
Dynamic strategies for asset allocation
Dynamic storage-allocation problem
Polymorphism dynamic allocation
Assumptions for dynamic channel allocation
Dynamic storage allocation
Dynamic bandwidth allocation in gpon
Buddy memory allocation implementation
Single user contiguous
Paged segmentation
First fit best fit worst fit testing
In contiguous memory allocation has no cure
Paged memory management
What are two goals of multitasking memory allocation
Non contiguous memory allocation
Non contiguous memory allocation
Paging in non contiguous memory allocation
Non contiguous memory allocation
Quick fit memory allocation
Memory allocation
Zig memory allocation
First fit next fit best fit worst fit
Cs 537
Transferered
Denominator layout
Smil head layout root-layout
Cddat
Fixed vs fluid layout
Asset allocation process
Double data rate synchronous dynamic random access memory
Allocating kernel memory in os
Dynamic memory management
Dynamic memory management
Stack memory layout
Hoon programming language
Virtual memory layout
Rpiw process
Episodic memory vs semantic memory
Implicit explicit memory
Long term memory vs short term memory
Internal memory and external memory
Primary memory and secondary memory
Physical address vs logical address
Which memory is the actual working memory?
Virtual memory and cache memory
Virtual memory in memory hierarchy consists of
Eidetic memory vs iconic memory
Shared memory vs distributed memory
The view that criminality is a dynamic process
Dynamic process chemistry
Importance of process selection and facility layout
Process selection and facility layout
The objective of layout strategy is to
Retail layout operations management
Process oriented layout example
Interdepartmental flow graph
Objectives of a good plant layout
Product vs process layout
Facility layout objectives
Process selection and facility layout
Chapter 6 process selection and facility layout
A hybrid layout combines
Fixed-position layouts
Process selection and facility layout
Process selection and facility layout
Contoh process oriented layout
Product-oriented layout example
Layout flow shop
Adm1848w
Process of memory encoding
Process of memory encoding
Purchase price allocation pwc
Variable overhead efficiency variance
Turn constructional unit
Cloud cost allocation
Capital allocation line
Variance of population proportion
Spectrum allocation
Software project management resource allocation
Capital allocation line
Resources allocation and mobilization plan (ramp)
Paul montgomery cork
Allocation wells in texas
Memory management in os
Heap allocation
Assembly heap allocation
Industrial estate types
Capital allocation line
Predetermined overhead allocation rate
Two objectives of industrial estate
Flexible asset allocation
Role allocation davis and moore
In departmental accounts
Purchase price allocation example
Executive order 12919
Allocation concealment
Spectrum allocation
Resource allocation vs resource leveling
First stage allocation
Incremental revenue allocation method
Predetermined overhead allocation rate
Capital allocation between risky and risk-free assets
Dynamics 365 lead allocation
Allocation concealment
Response allocation definition
Support department adalah
Module viewtype
Time allocation example
Allocation pacea gj
Channel allocation lan adalah
Purchase price allocation esempio
What is file allocation table (fat)
Register allocation
File allocation table example
Buddy system allocation
Sex allocation
Relocatable partitioned allocation
Revenue allocation methods
Cost allocation and profitability analysis
Cache allocation
Territory analysis and account classification
Gef star allocation
Algoritmo dello struzzo
Resource histogram
Wait-for graph