Dynamic Memory Allocation 1 Dynamic Memory Allocation n
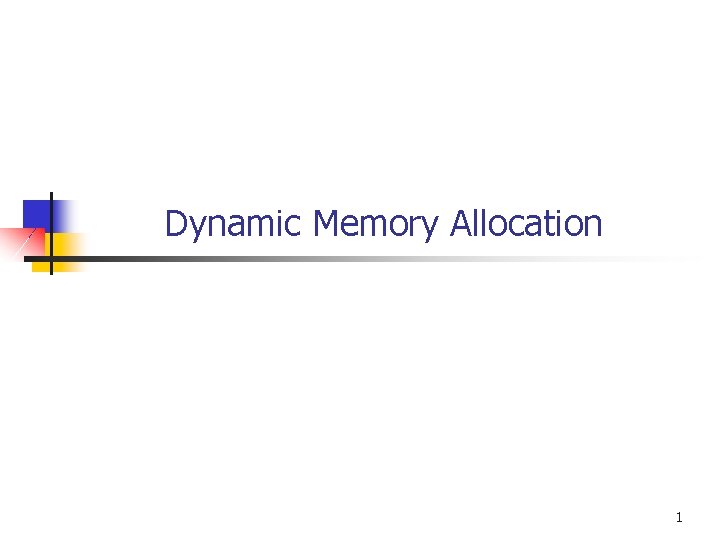
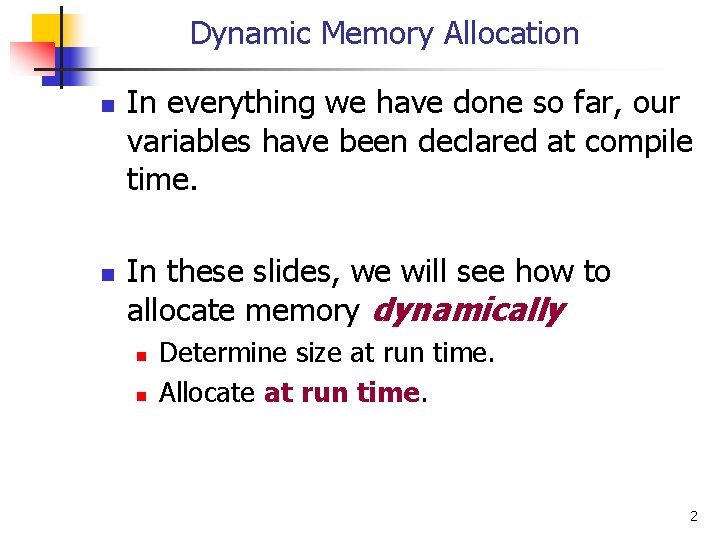
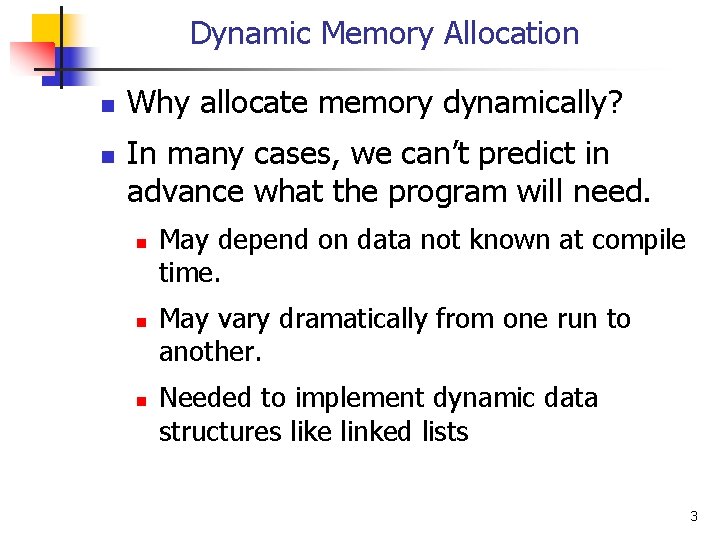
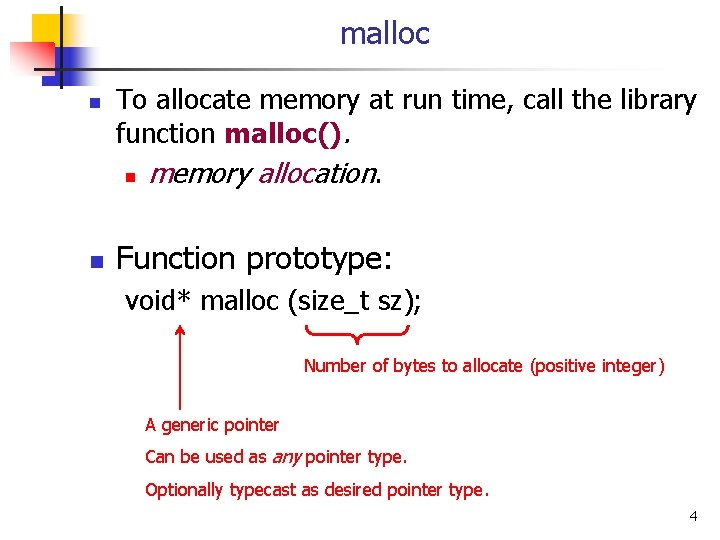
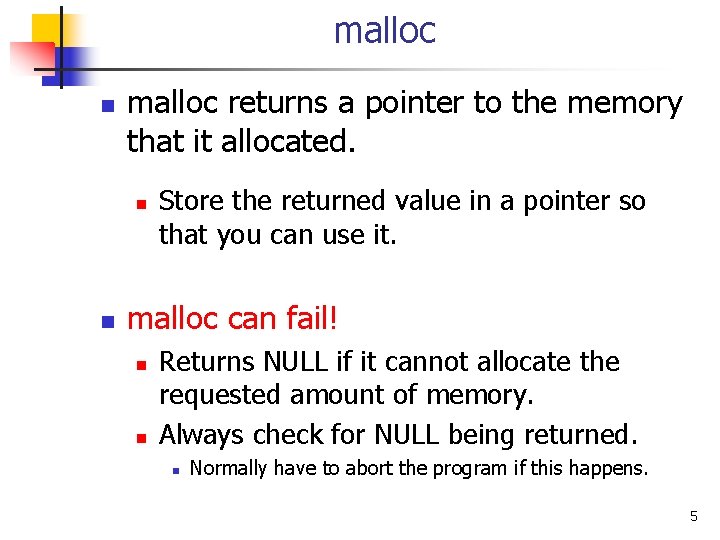
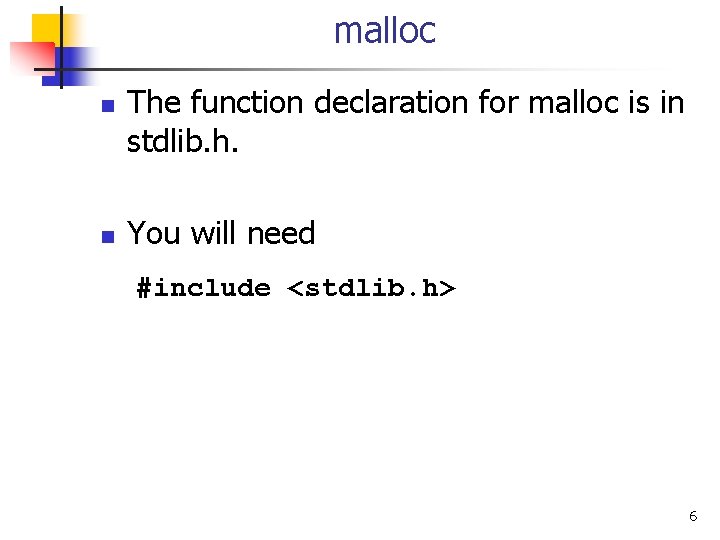
![Pointers and Arrays n n n When an array declaration like int A[10]is executed, Pointers and Arrays n n n When an array declaration like int A[10]is executed,](https://slidetodoc.com/presentation_image_h/0516d5602c754948d9e552a3372fc451/image-7.jpg)
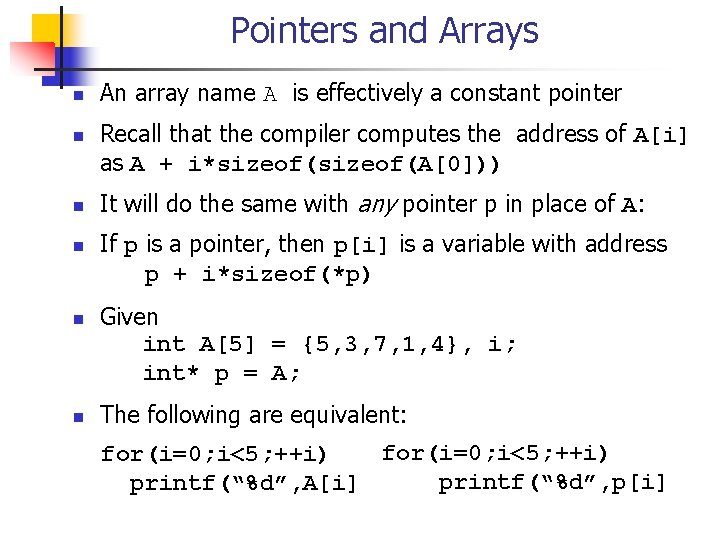
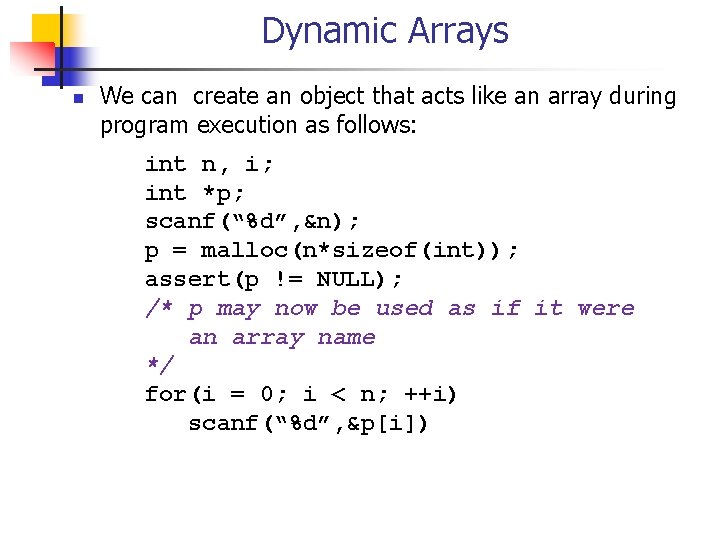
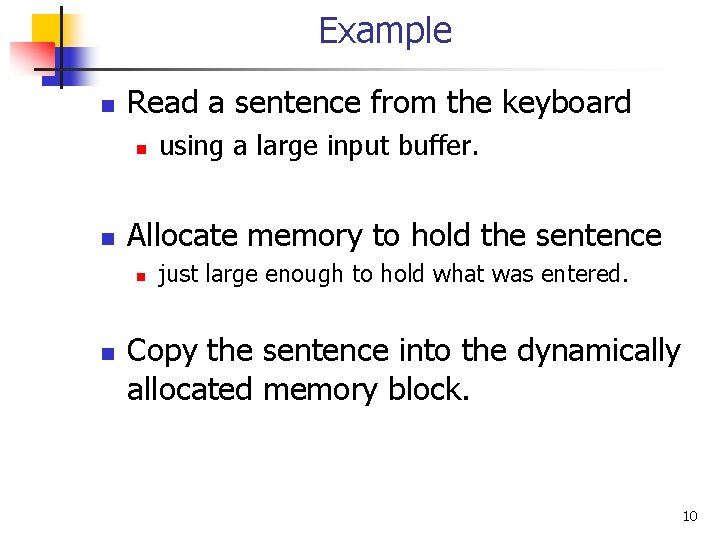
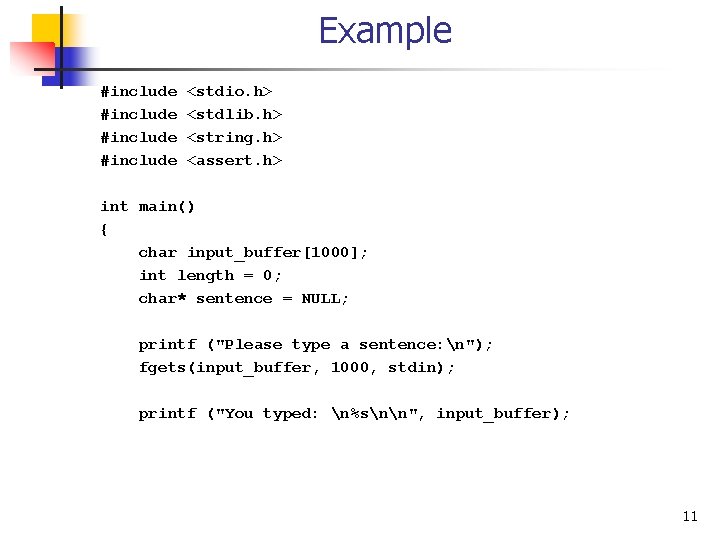
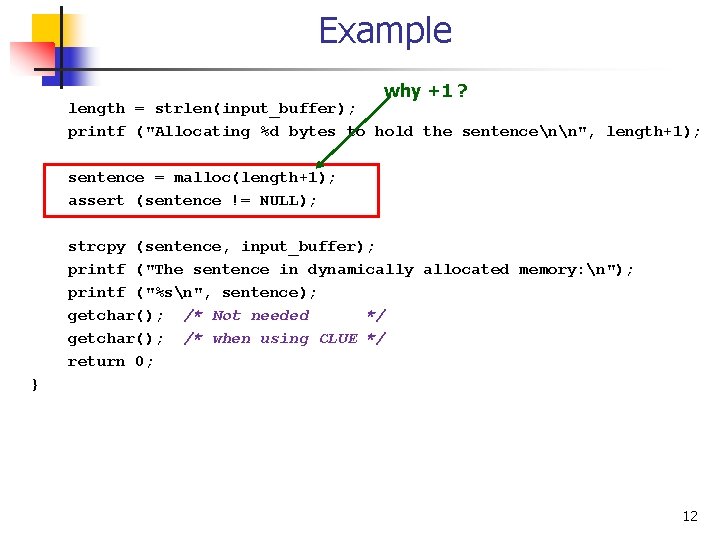
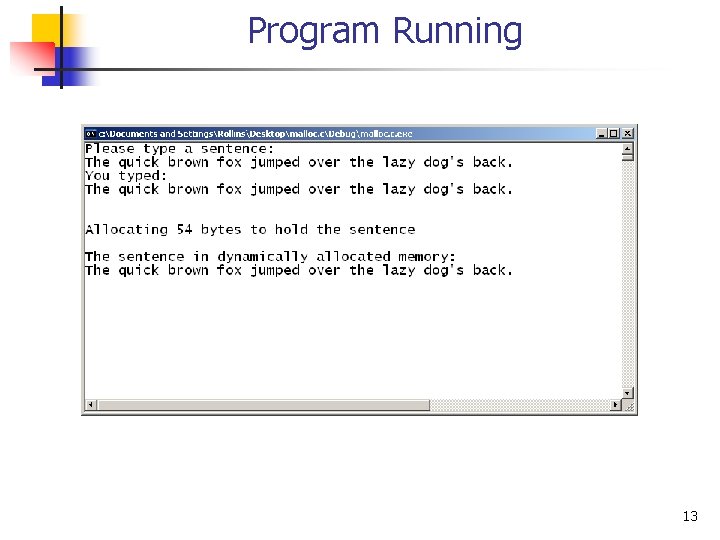
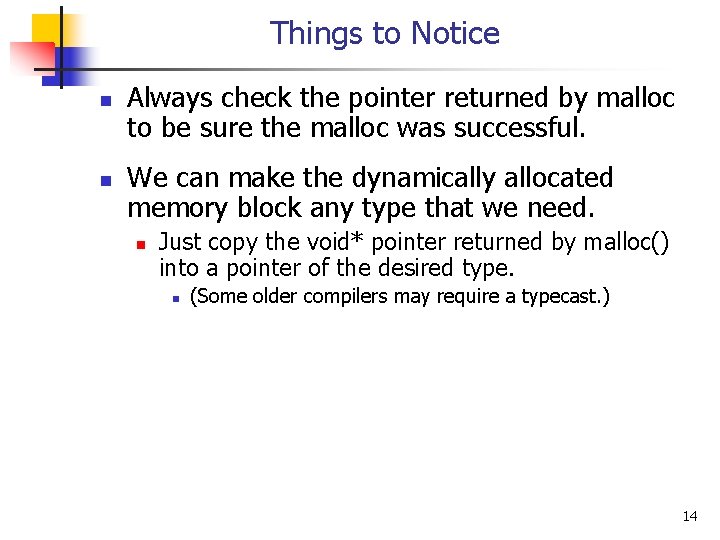
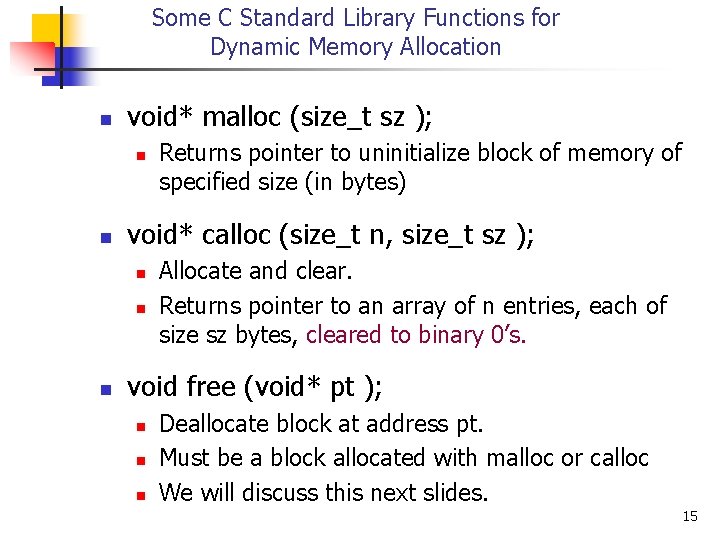
- Slides: 15
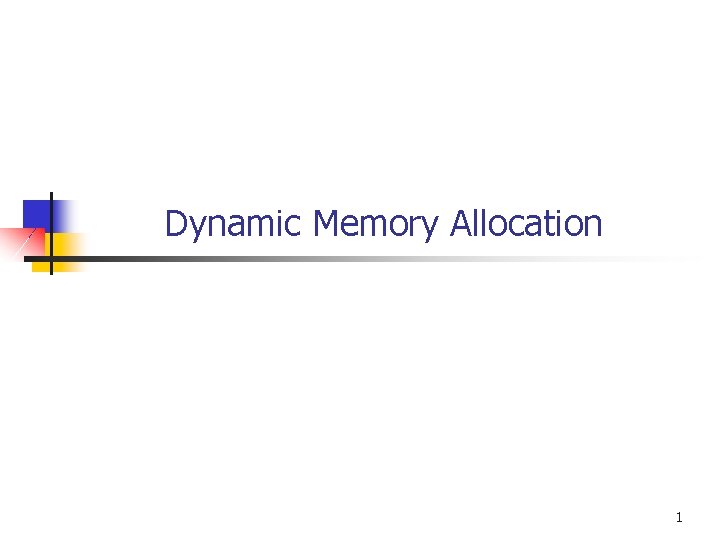
Dynamic Memory Allocation 1
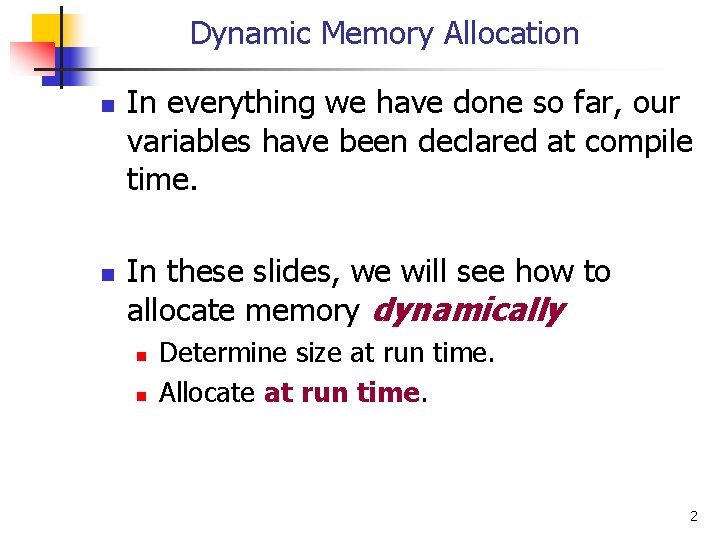
Dynamic Memory Allocation n n In everything we have done so far, our variables have been declared at compile time. In these slides, we will see how to allocate memory dynamically n n Determine size at run time. Allocate at run time. 2
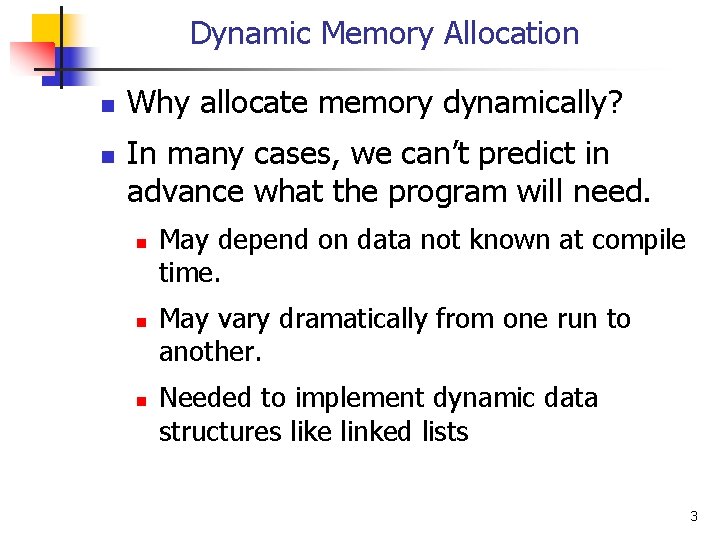
Dynamic Memory Allocation n n Why allocate memory dynamically? In many cases, we can’t predict in advance what the program will need. n n n May depend on data not known at compile time. May vary dramatically from one run to another. Needed to implement dynamic data structures like linked lists 3
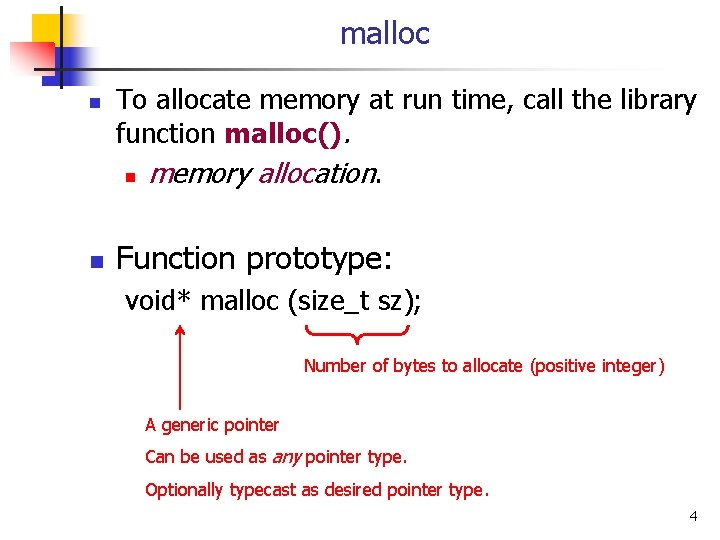
malloc n n To allocate memory at run time, call the library function malloc(). n memory allocation. Function prototype: void* malloc (size_t sz); Number of bytes to allocate (positive integer) A generic pointer Can be used as any pointer type. Optionally typecast as desired pointer type. 4
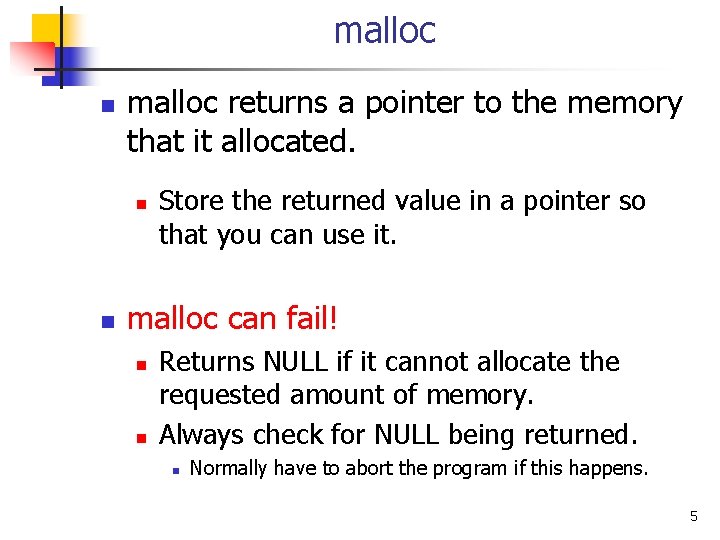
malloc n malloc returns a pointer to the memory that it allocated. n n Store the returned value in a pointer so that you can use it. malloc can fail! n n Returns NULL if it cannot allocate the requested amount of memory. Always check for NULL being returned. n Normally have to abort the program if this happens. 5
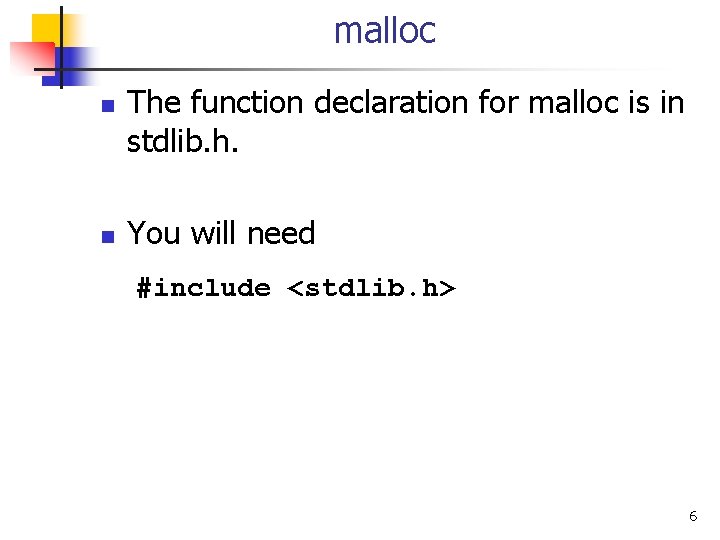
malloc n n The function declaration for malloc is in stdlib. h. You will need #include <stdlib. h> 6
![Pointers and Arrays n n n When an array declaration like int A10is executed Pointers and Arrays n n n When an array declaration like int A[10]is executed,](https://slidetodoc.com/presentation_image_h/0516d5602c754948d9e552a3372fc451/image-7.jpg)
Pointers and Arrays n n n When an array declaration like int A[10]is executed, the compiler allocates a block of 10*sizeof(int) bytes; then sets A equal to the address of the first byte of that block Thus the value of A is just &A[0]. Recall that an array variable may not be the left-hand side of an assignment statement Thus, A is effectively a constant pointer 7
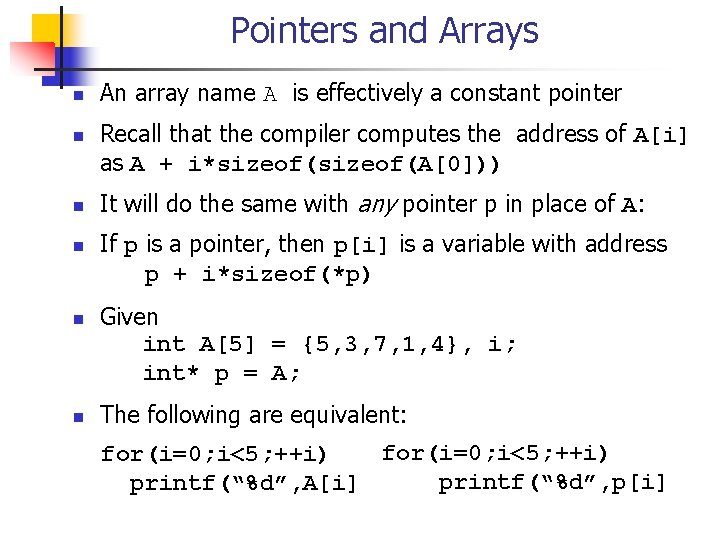
Pointers and Arrays n n n An array name A is effectively a constant pointer Recall that the compiler computes the address of A[i] as A + i*sizeof(A[0])) It will do the same with any pointer p in place of A: If p is a pointer, then p[i] is a variable with address p + i*sizeof(*p) Given int A[5] = {5, 3, 7, 1, 4}, i; int* p = A; The following are equivalent: for(i=0; i<5; ++i) printf(“%d”, p[i] printf(“%d”, A[i]
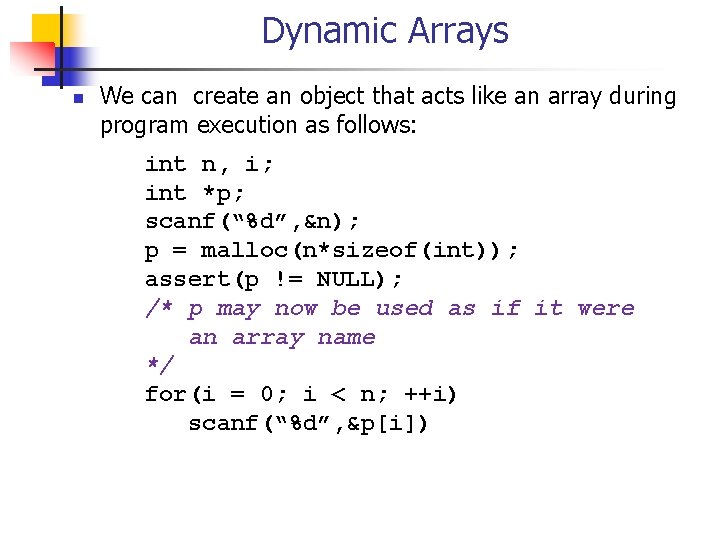
Dynamic Arrays n We can create an object that acts like an array during program execution as follows: int n, i; int *p; scanf(“%d”, &n); p = malloc(n*sizeof(int)); assert(p != NULL); /* p may now be used as if it were an array name */ for(i = 0; i < n; ++i) scanf(“%d”, &p[i])
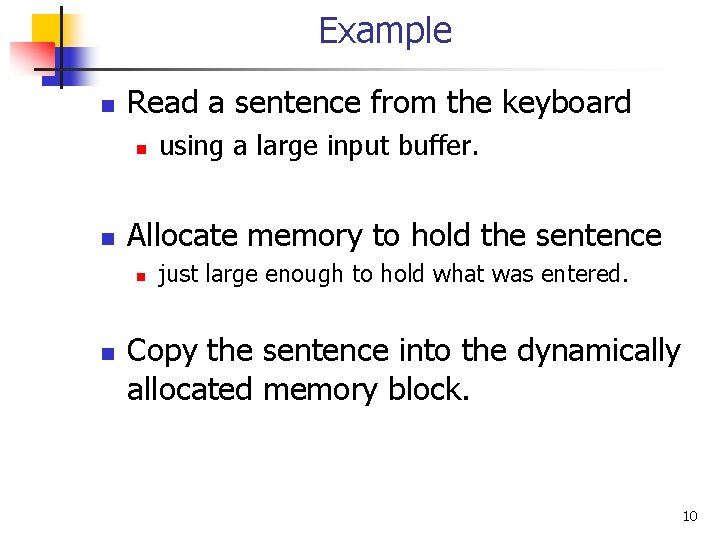
Example n Read a sentence from the keyboard n n Allocate memory to hold the sentence n n using a large input buffer. just large enough to hold what was entered. Copy the sentence into the dynamically allocated memory block. 10
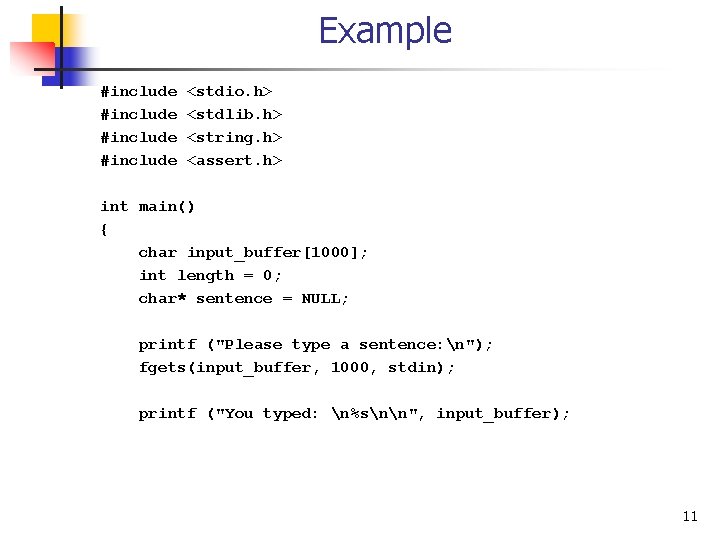
Example #include <stdio. h> <stdlib. h> <string. h> <assert. h> int main() { char input_buffer[1000]; int length = 0; char* sentence = NULL; printf ("Please type a sentence: n"); fgets(input_buffer, 1000, stdin); printf ("You typed: n%snn", input_buffer); 11
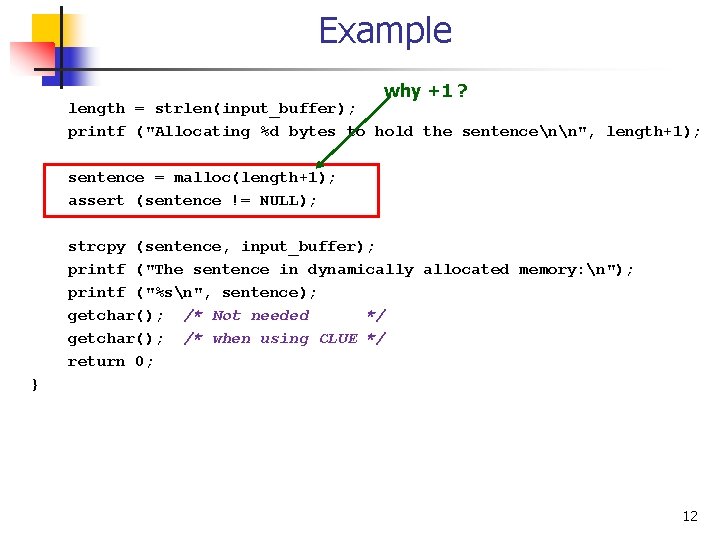
Example why +1 ? length = strlen(input_buffer); printf ("Allocating %d bytes to hold the sentencenn", length+1); sentence = malloc(length+1); assert (sentence != NULL); strcpy (sentence, input_buffer); printf ("The sentence in dynamically allocated memory: n"); printf ("%sn", sentence); getchar(); /* Not needed */ getchar(); /* when using CLUE */ return 0; } 12
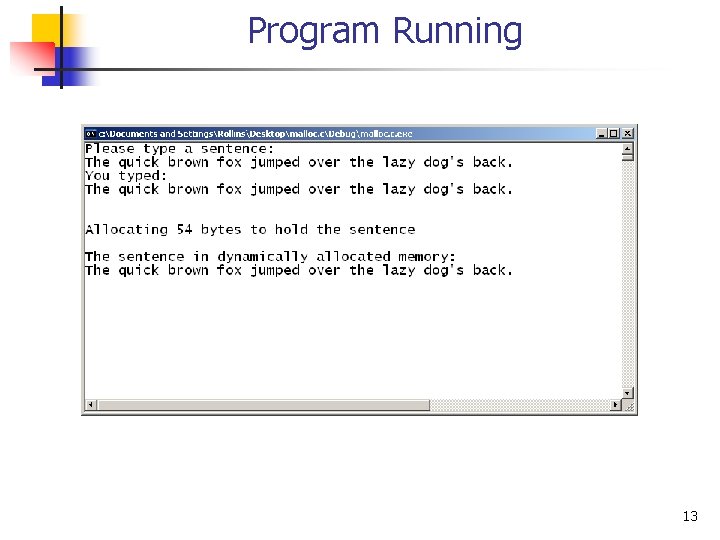
Program Running 13
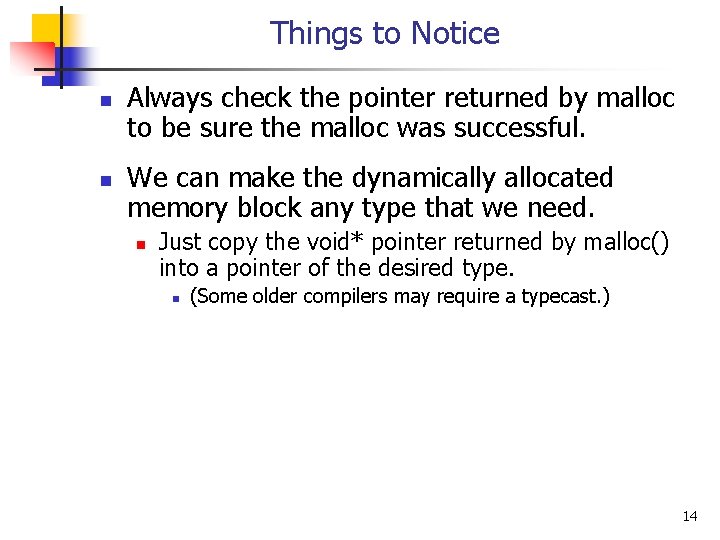
Things to Notice n n Always check the pointer returned by malloc to be sure the malloc was successful. We can make the dynamically allocated memory block any type that we need. n Just copy the void* pointer returned by malloc() into a pointer of the desired type. n (Some older compilers may require a typecast. ) 14
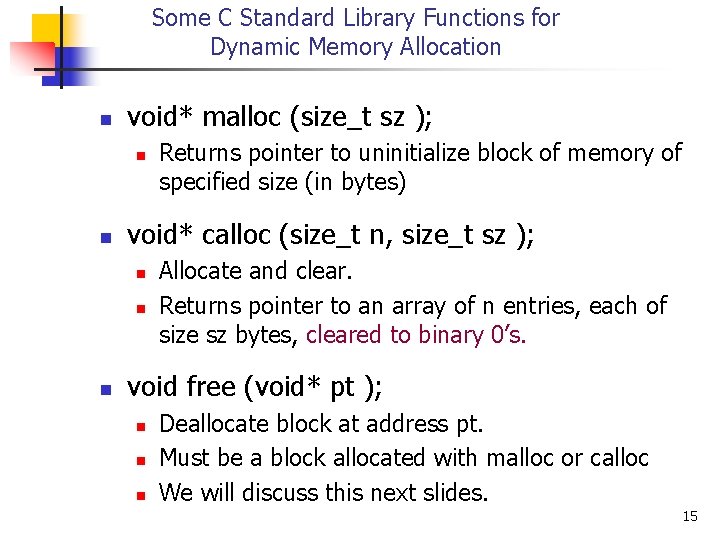
Some C Standard Library Functions for Dynamic Memory Allocation n void* malloc (size_t sz ); n n void* calloc (size_t n, size_t sz ); n n n Returns pointer to uninitialize block of memory of specified size (in bytes) Allocate and clear. Returns pointer to an array of n entries, each of size sz bytes, cleared to binary 0’s. void free (void* pt ); n n n Deallocate block at address pt. Must be a block allocated with malloc or calloc We will discuss this next slides. 15
Malloc calloc free
Dynamic memory allocation
Dynamic c programming
Example of dynamic memory allocation
Example of dynamic memory allocation
Memory allocation in c++
Knuth's boundary tags
Dynamic memory allocation in data structure
Memory allocation in java
Malloc function
Dynamic memory allocation in data structure
Contiguous allocation vs linked allocation
Assumptions for dynamic channel allocation
Polymorphism dynamic allocation
Dynamic storage allocation
Dynamic strategies for asset allocation