Dynamic Memory Allocation malloc calloc free Program Memory
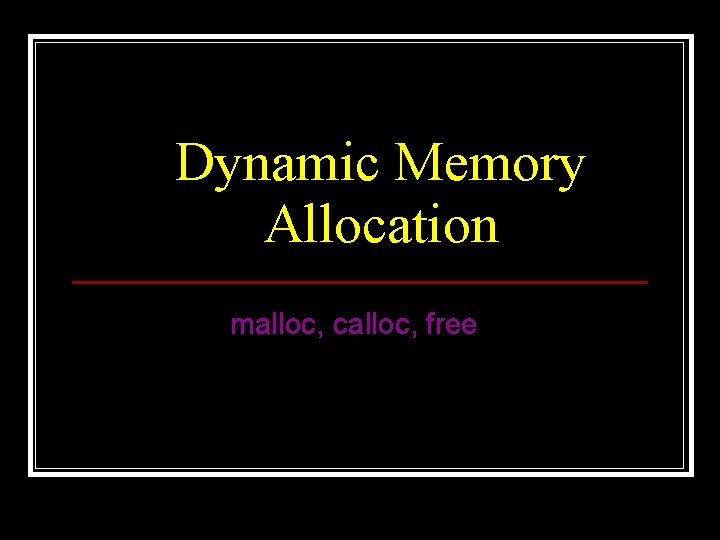
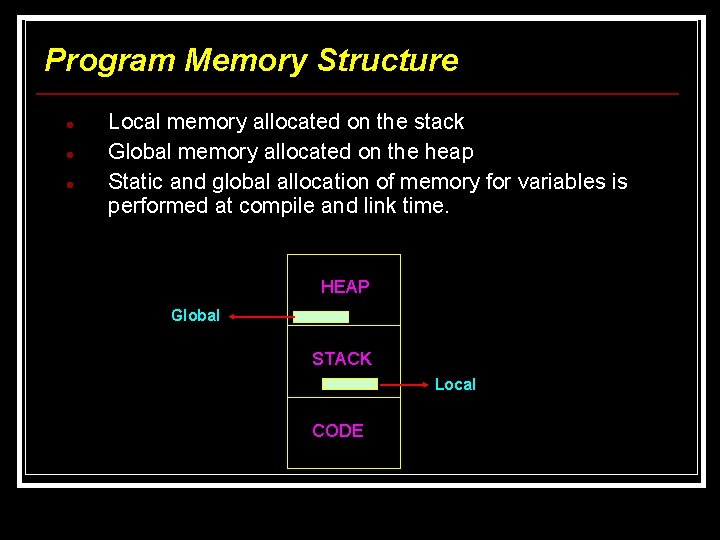
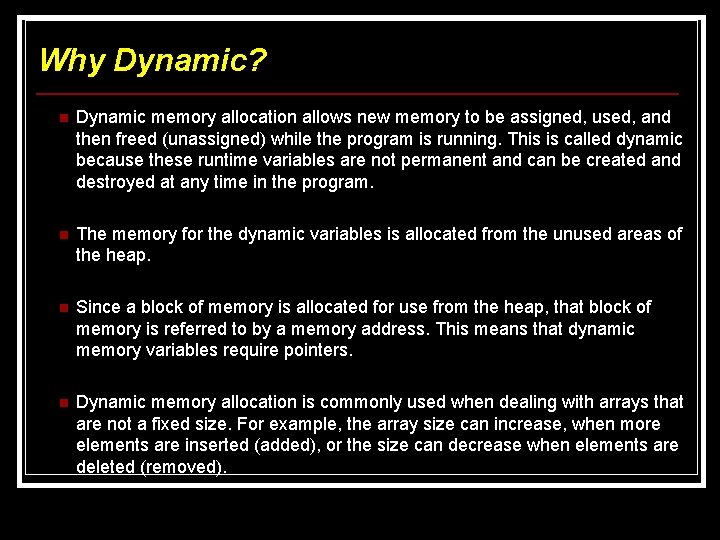
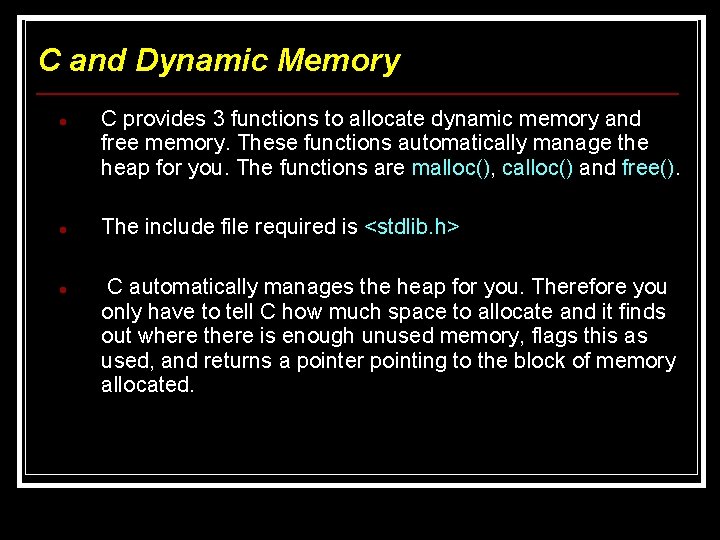
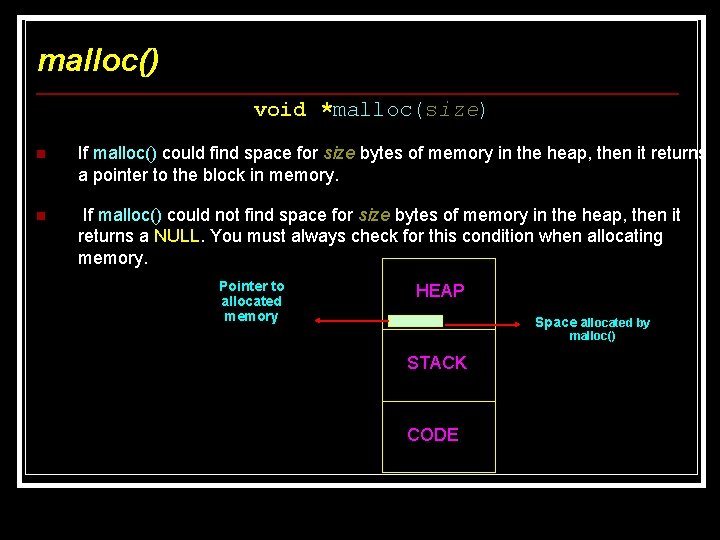
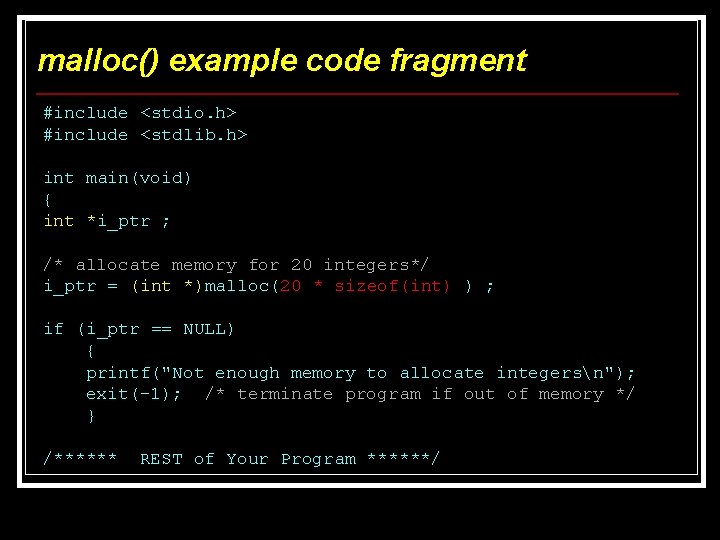
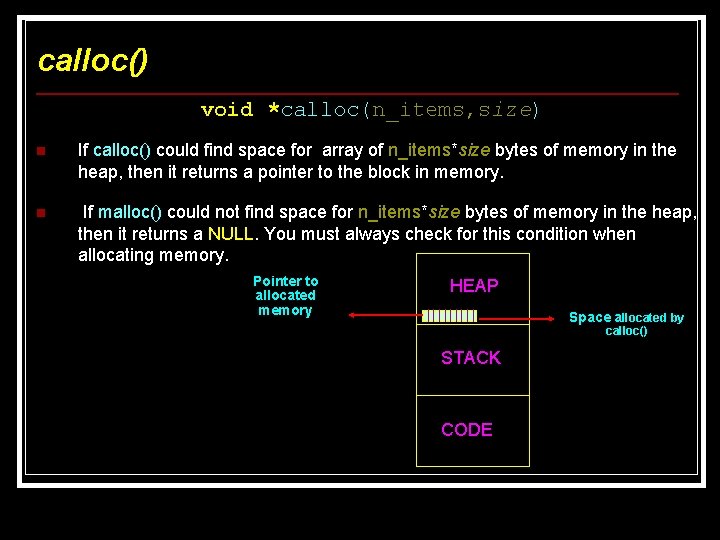
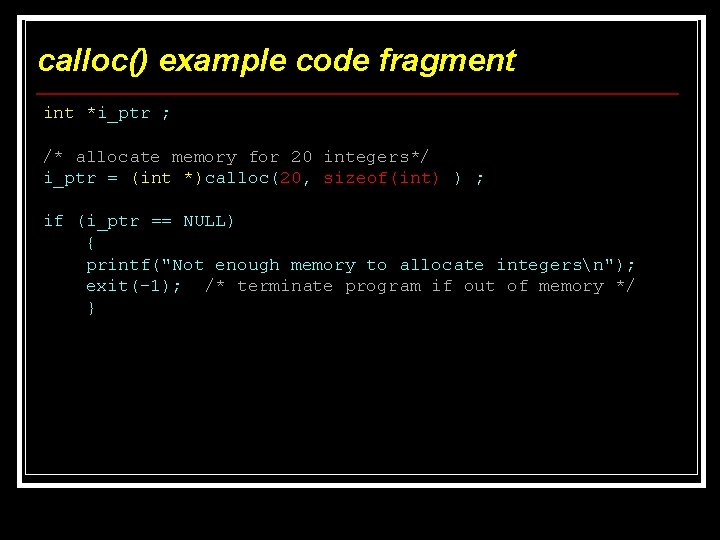
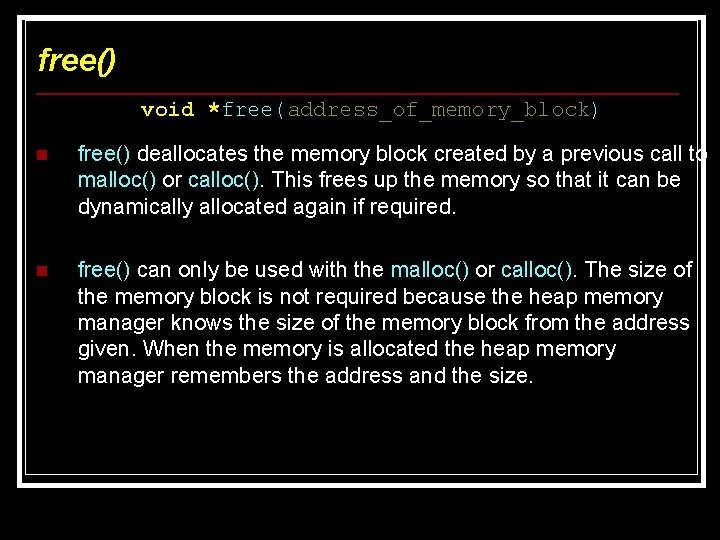
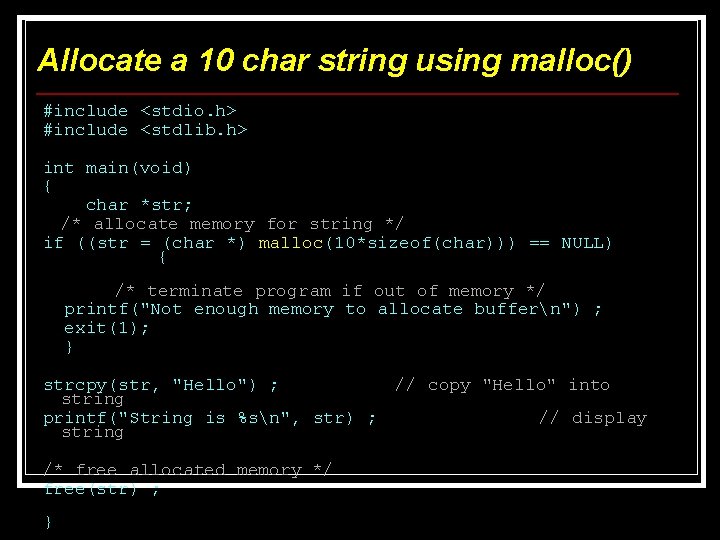
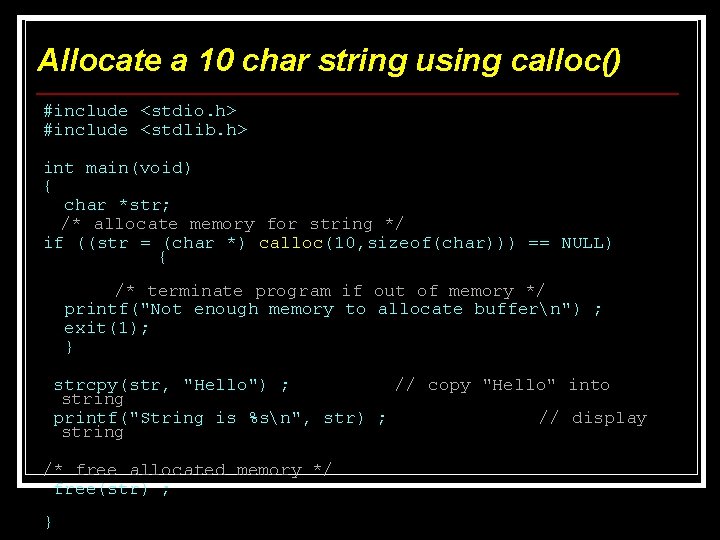
- Slides: 11
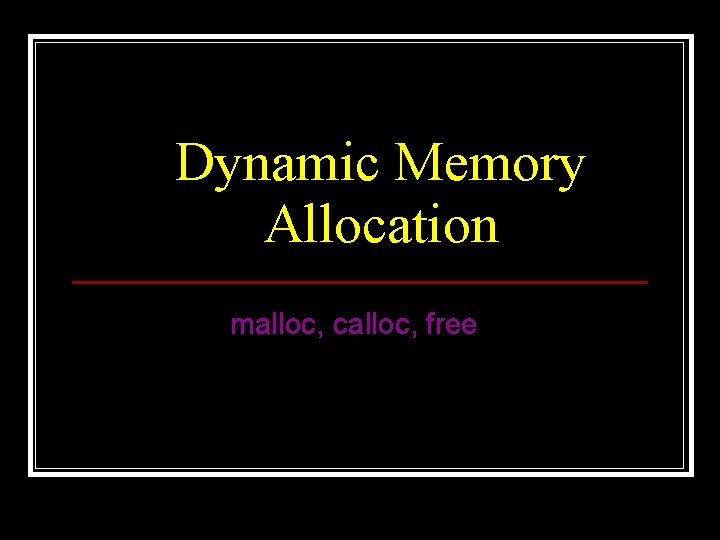
Dynamic Memory Allocation malloc, calloc, free
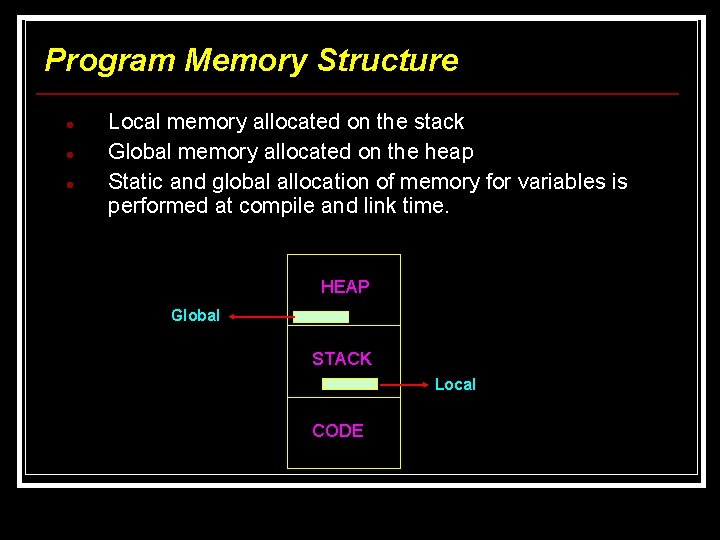
Program Memory Structure Local memory allocated on the stack Global memory allocated on the heap Static and global allocation of memory for variables is performed at compile and link time. HEAP Global STACK Local CODE
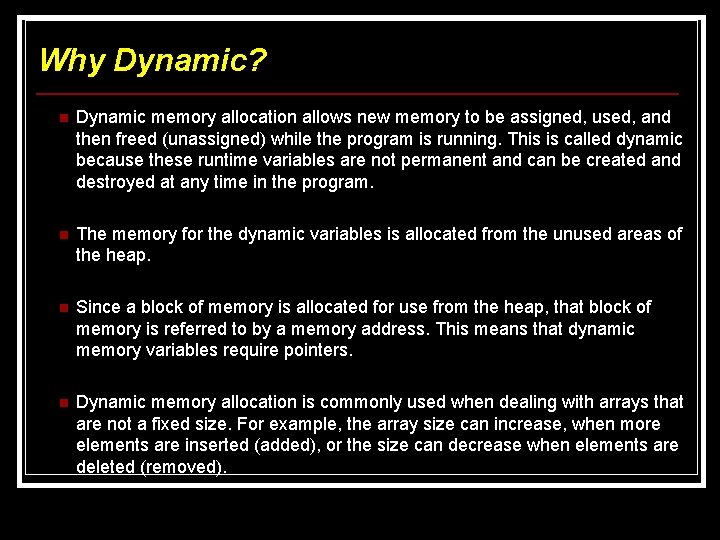
Why Dynamic? n Dynamic memory allocation allows new memory to be assigned, used, and then freed (unassigned) while the program is running. This is called dynamic because these runtime variables are not permanent and can be created and destroyed at any time in the program. n The memory for the dynamic variables is allocated from the unused areas of the heap. n Since a block of memory is allocated for use from the heap, that block of memory is referred to by a memory address. This means that dynamic memory variables require pointers. n Dynamic memory allocation is commonly used when dealing with arrays that are not a fixed size. For example, the array size can increase, when more elements are inserted (added), or the size can decrease when elements are deleted (removed).
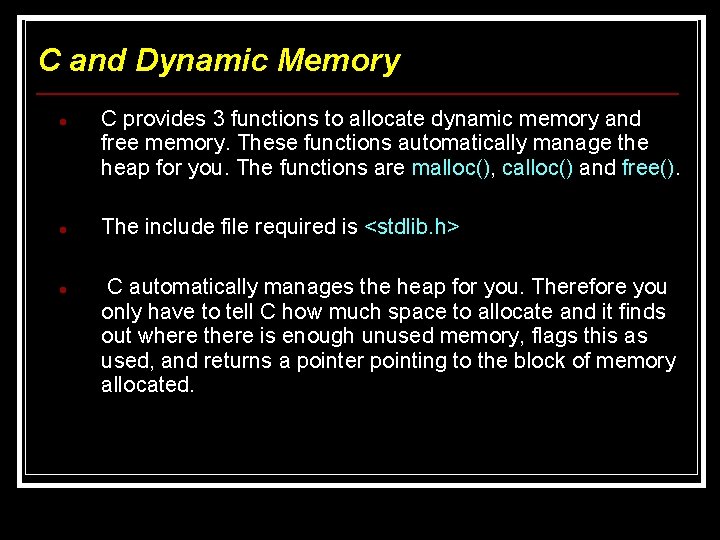
C and Dynamic Memory C provides 3 functions to allocate dynamic memory and free memory. These functions automatically manage the heap for you. The functions are malloc(), calloc() and free(). The include file required is <stdlib. h> C automatically manages the heap for you. Therefore you only have to tell C how much space to allocate and it finds out where there is enough unused memory, flags this as used, and returns a pointer pointing to the block of memory allocated.
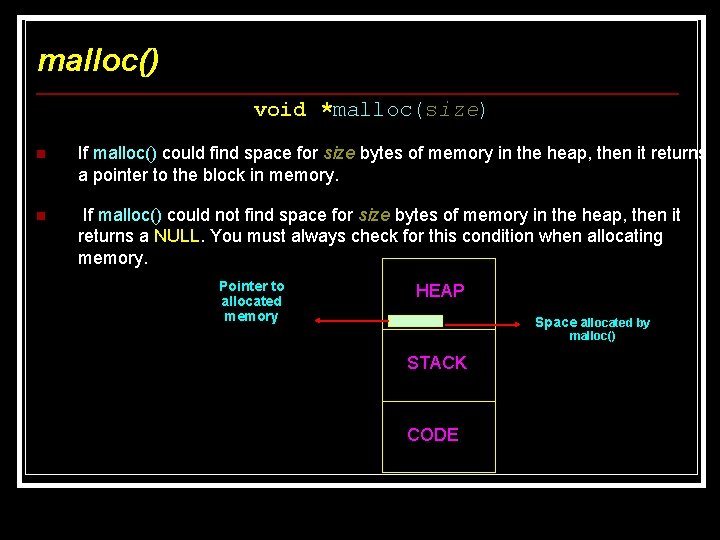
malloc() void *malloc(size) n If malloc() could find space for size bytes of memory in the heap, then it returns a pointer to the block in memory. n If malloc() could not find space for size bytes of memory in the heap, then it returns a NULL. You must always check for this condition when allocating memory. Pointer to allocated memory HEAP Space allocated by malloc() STACK CODE
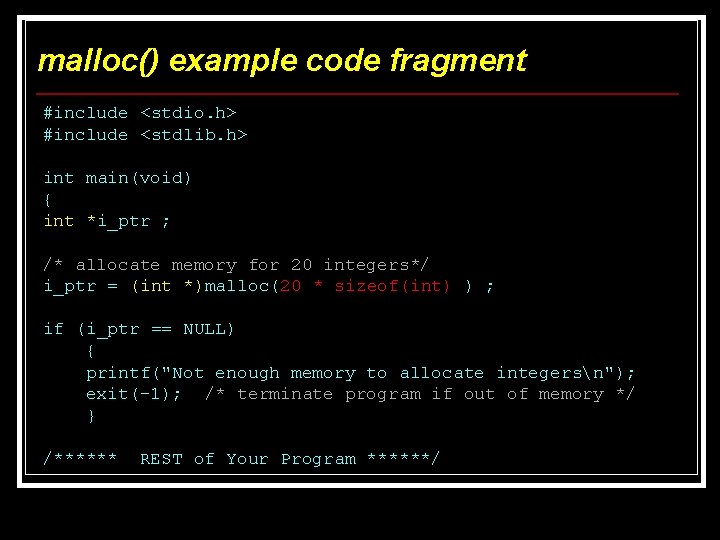
malloc() example code fragment #include <stdio. h> #include <stdlib. h> int main(void) { int *i_ptr ; /* allocate memory for 20 integers*/ i_ptr = (int *)malloc(20 * sizeof(int) ) ; if (i_ptr == NULL) { printf("Not enough memory to allocate integersn"); exit(-1); /* terminate program if out of memory */ } /****** REST of Your Program ******/
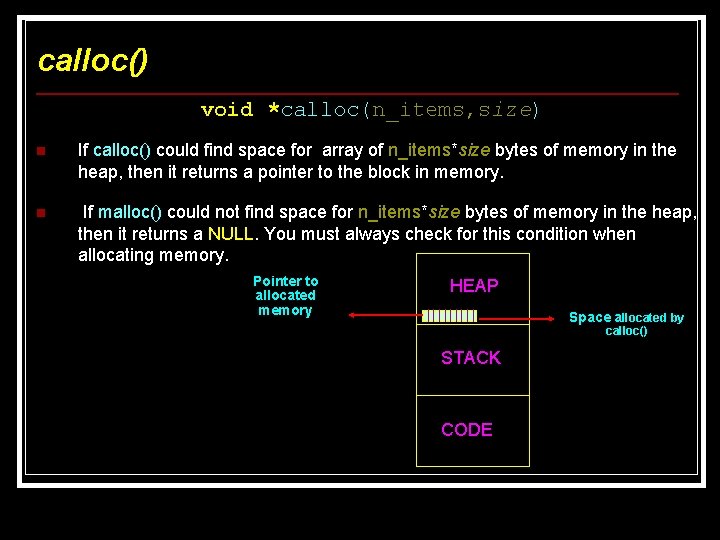
calloc() void *calloc(n_items, size) n If calloc() could find space for array of n_items*size bytes of memory in the heap, then it returns a pointer to the block in memory. n If malloc() could not find space for n_items*size bytes of memory in the heap, then it returns a NULL. You must always check for this condition when allocating memory. Pointer to allocated memory HEAP Space allocated by calloc() STACK CODE
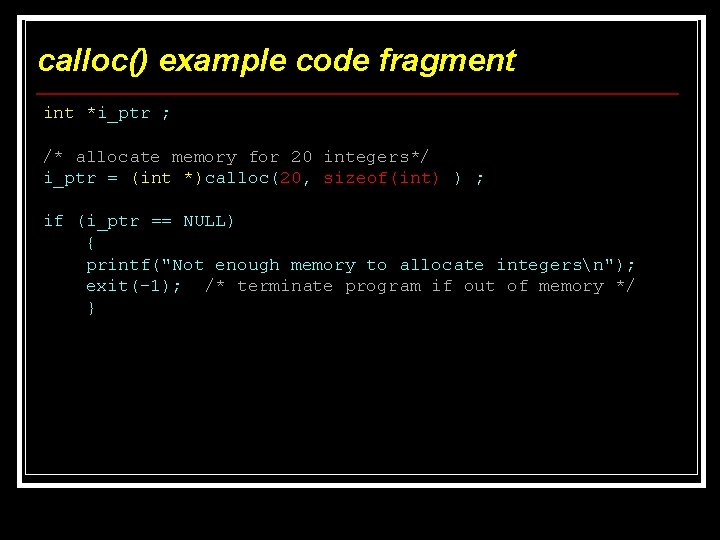
calloc() example code fragment int *i_ptr ; /* allocate memory for 20 integers*/ i_ptr = (int *)calloc(20, sizeof(int) ) ; if (i_ptr == NULL) { printf("Not enough memory to allocate integersn"); exit(-1); /* terminate program if out of memory */ }
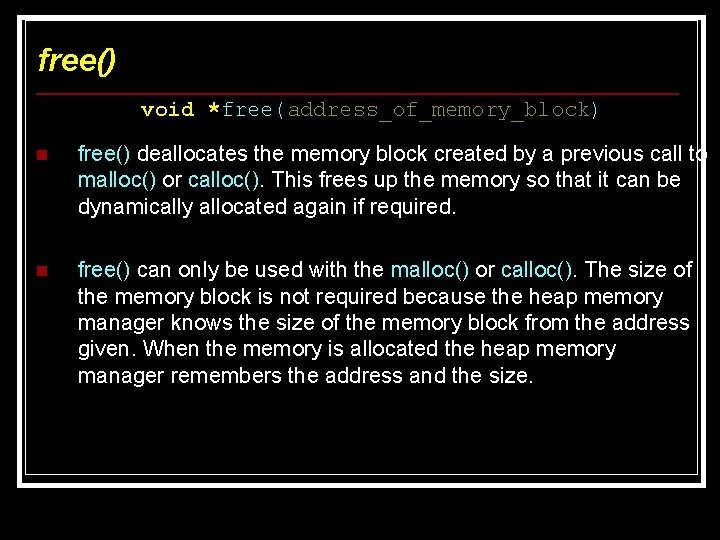
free() void *free(address_of_memory_block) n free() deallocates the memory block created by a previous call to malloc() or calloc(). This frees up the memory so that it can be dynamically allocated again if required. n free() can only be used with the malloc() or calloc(). The size of the memory block is not required because the heap memory manager knows the size of the memory block from the address given. When the memory is allocated the heap memory manager remembers the address and the size.
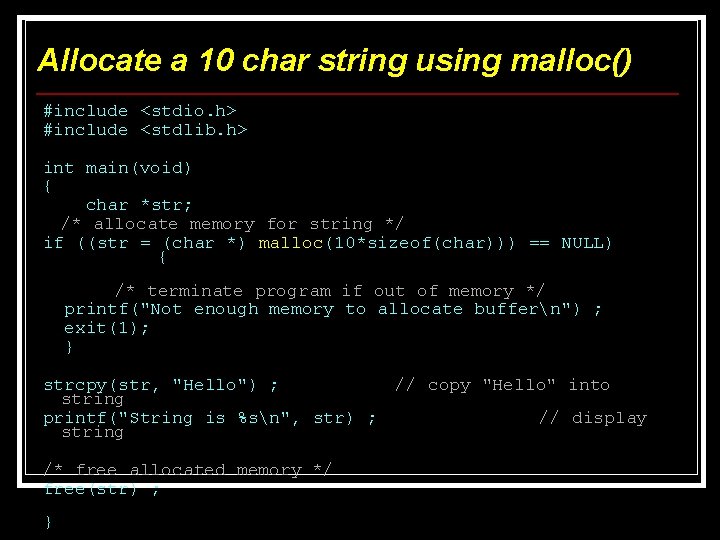
Allocate a 10 char string using malloc() #include <stdio. h> #include <stdlib. h> int main(void) { char *str; /* allocate memory for string */ if ((str = (char *) malloc(10*sizeof(char))) == NULL) { /* terminate program if out of memory */ printf("Not enough memory to allocate buffern") ; exit(1); } strcpy(str, "Hello") ; // copy "Hello" into string printf("String is %sn", str) ; // display string /* free allocated memory */ free(str) ; }
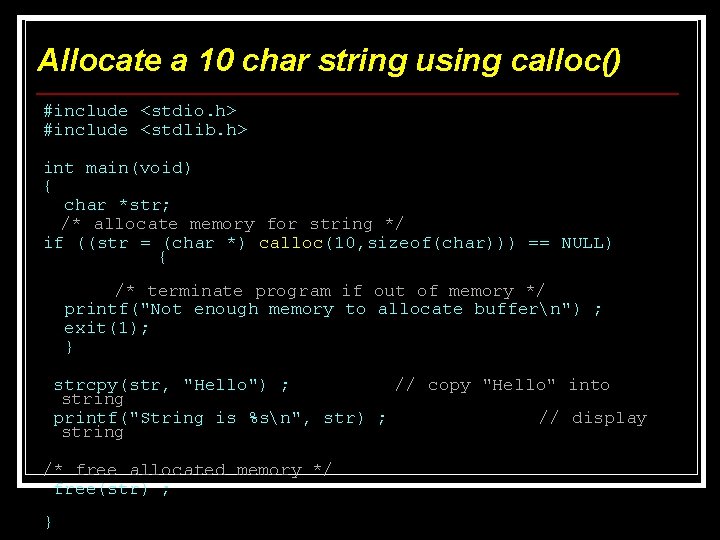
Allocate a 10 char string using calloc() #include <stdio. h> #include <stdlib. h> int main(void) { char *str; /* allocate memory for string */ if ((str = (char *) calloc(10, sizeof(char))) == NULL) { /* terminate program if out of memory */ printf("Not enough memory to allocate buffern") ; exit(1); } strcpy(str, "Hello") ; // copy "Hello" into string printf("String is %sn", str) ; // display string /* free allocated memory */ free(str) ; }
Calloc example
What is malloc and calloc in c
Example of dynamic memory allocation
Dynamic memory allocation in data structure
Disadvantages of dynamic memory allocation in c
Knuth's boundary tags
Example of dynamic memory allocation
Dynamic data structure
Dynamic memory allocation in data structure
Dynamic c programming
In which segment dynamic memory allocated
Dynamic memory allocation in java