Python Revision Damian Gordon Python Structured Programming Damian
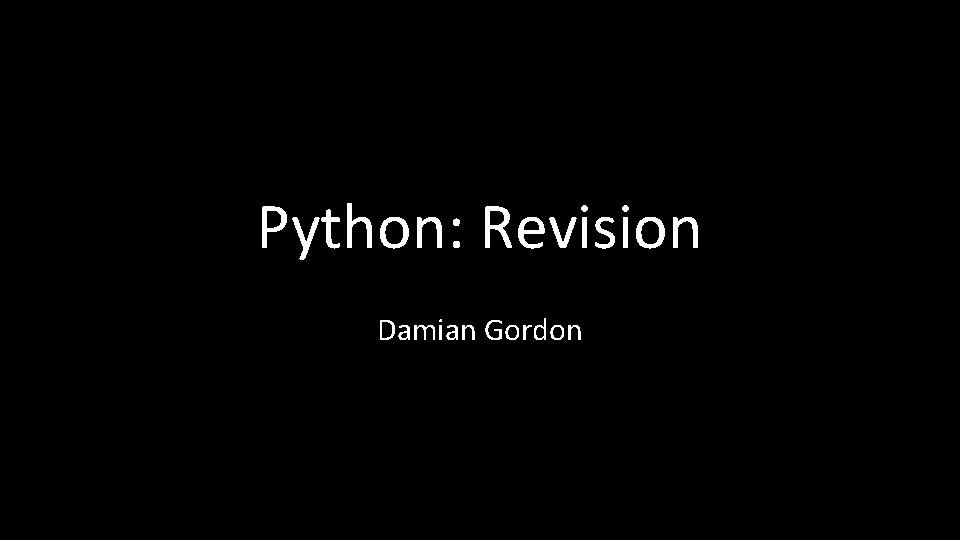
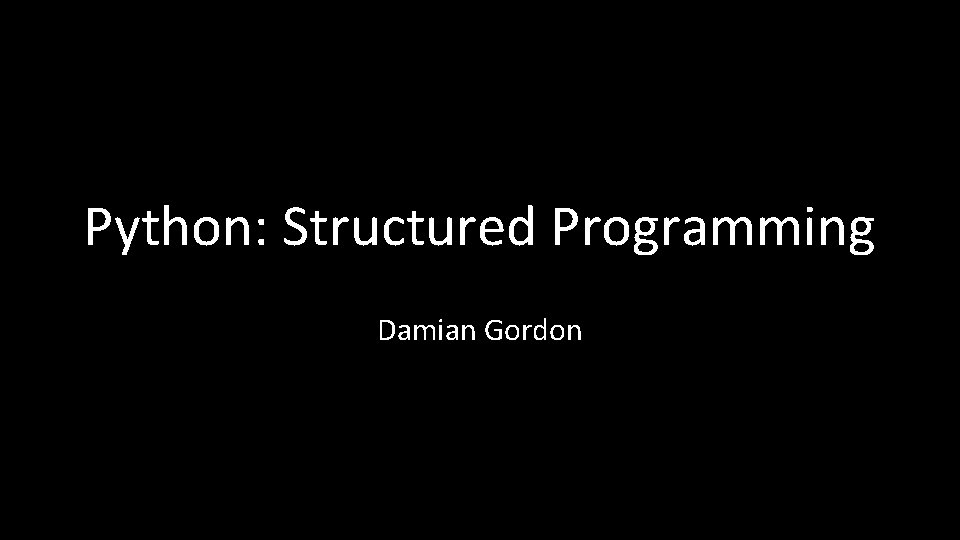
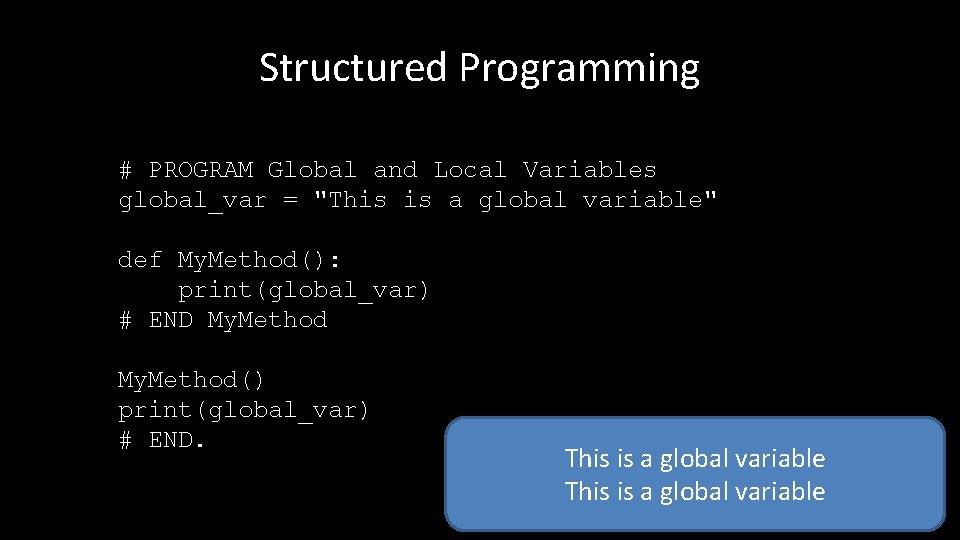
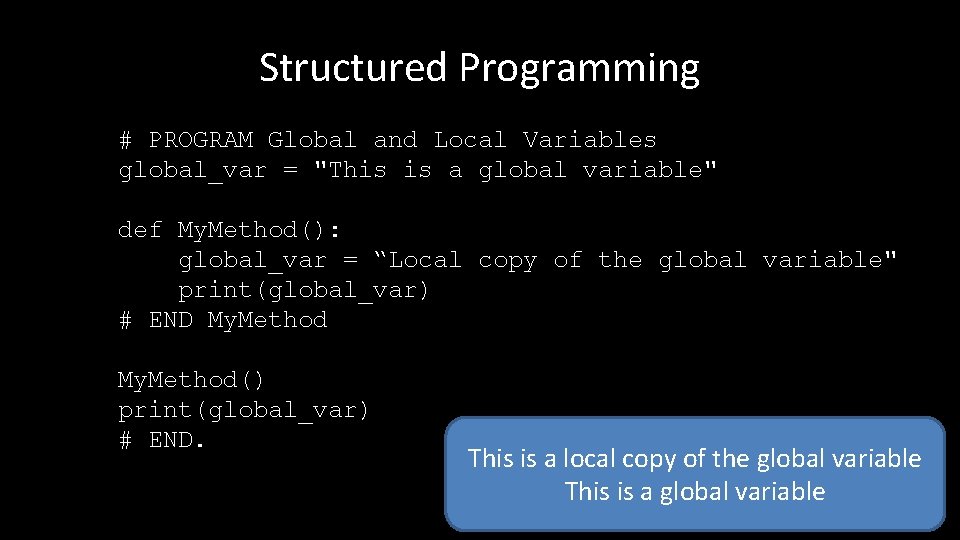
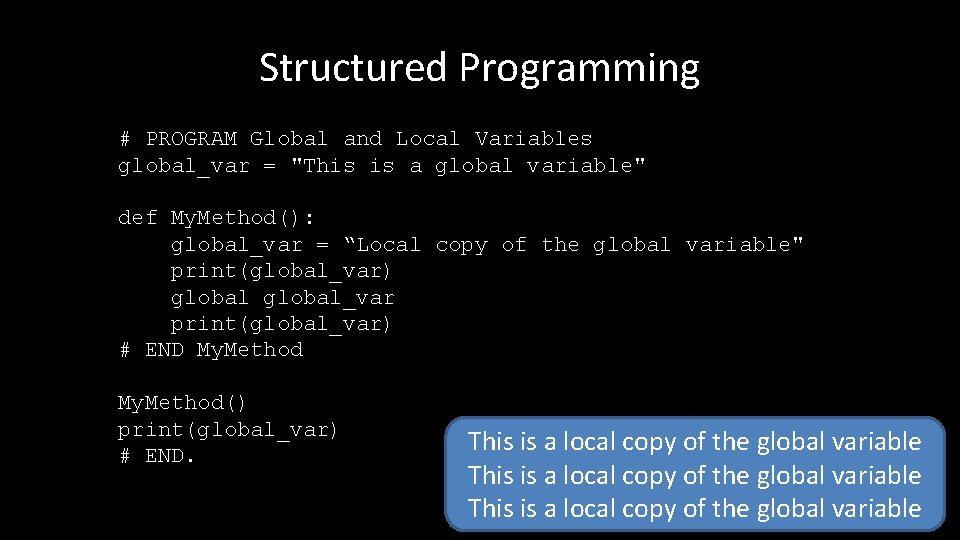
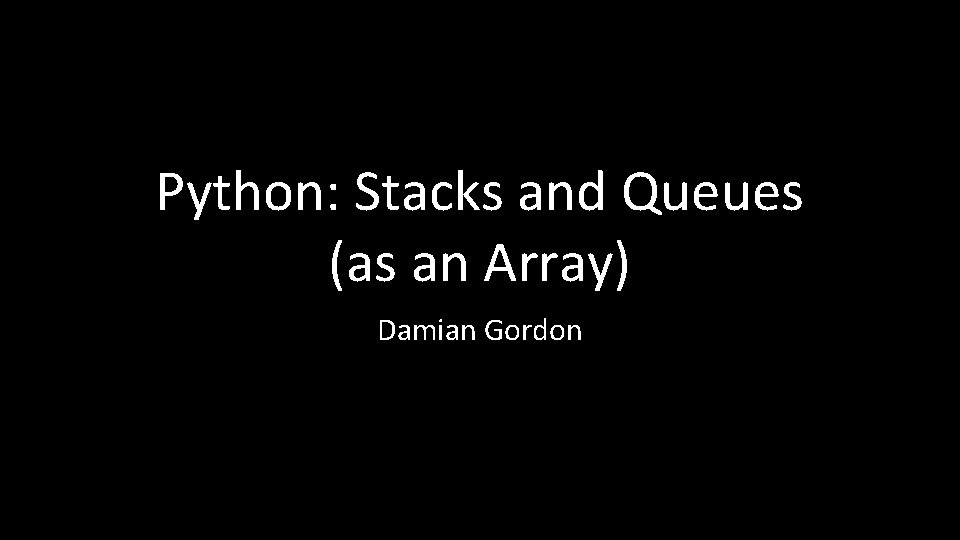
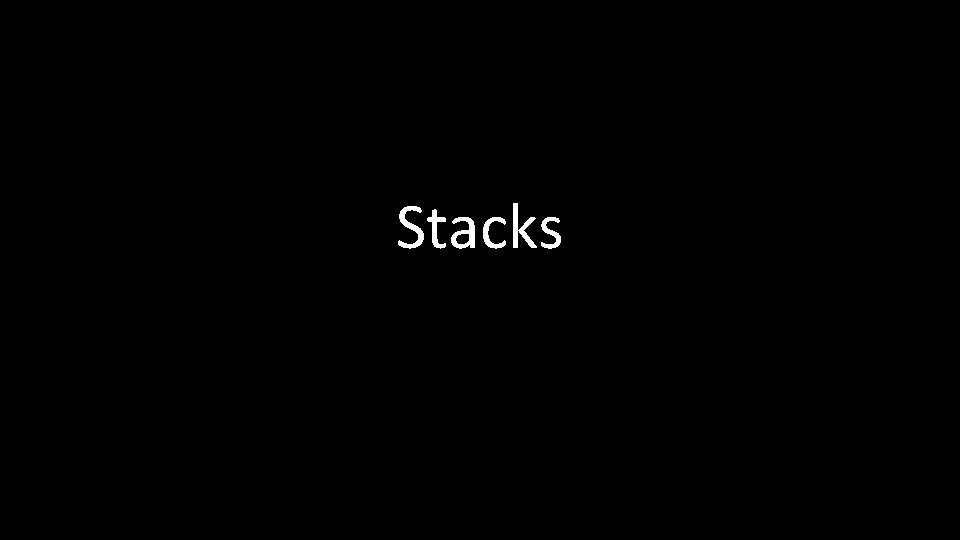
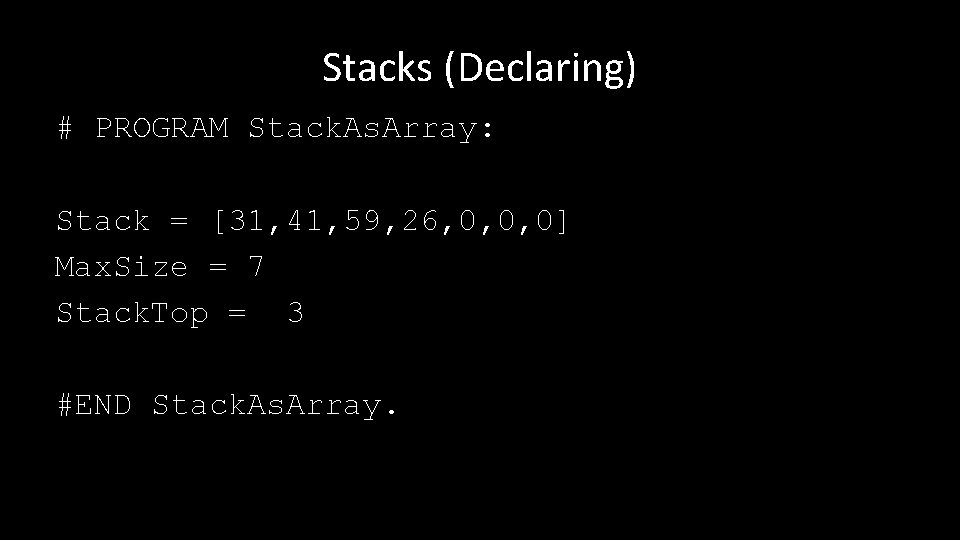
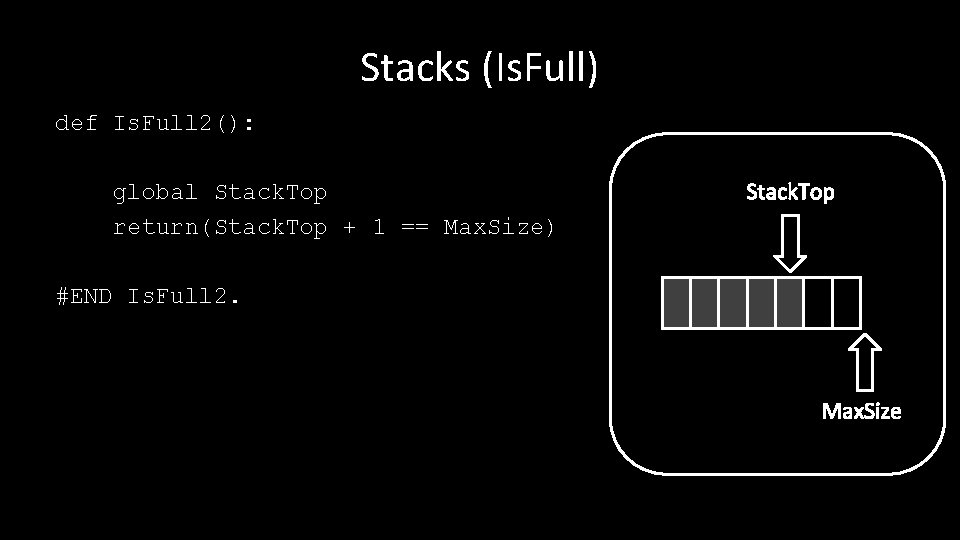
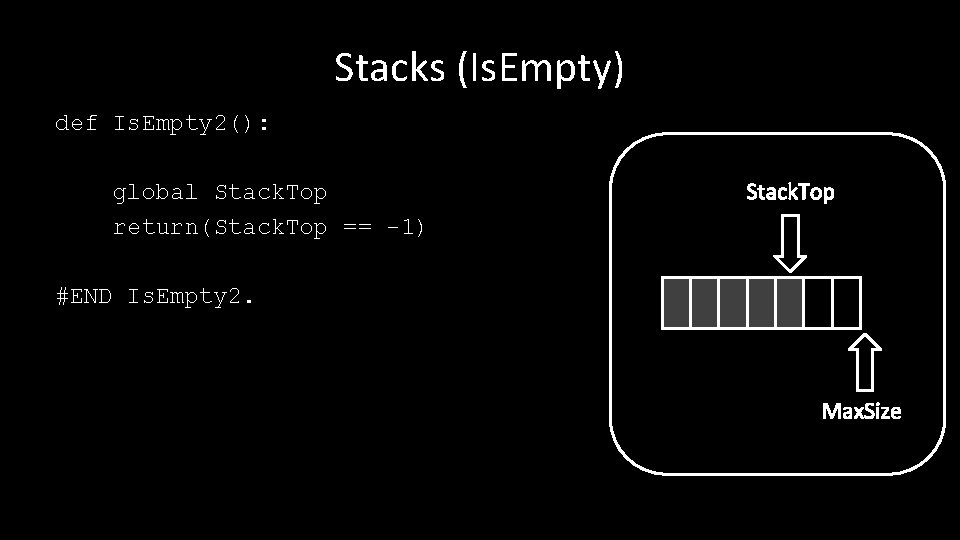
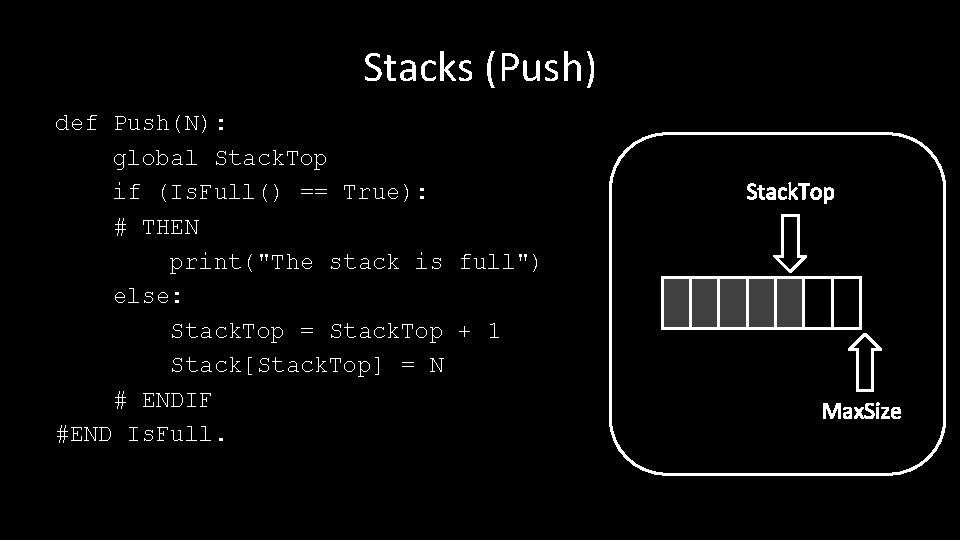
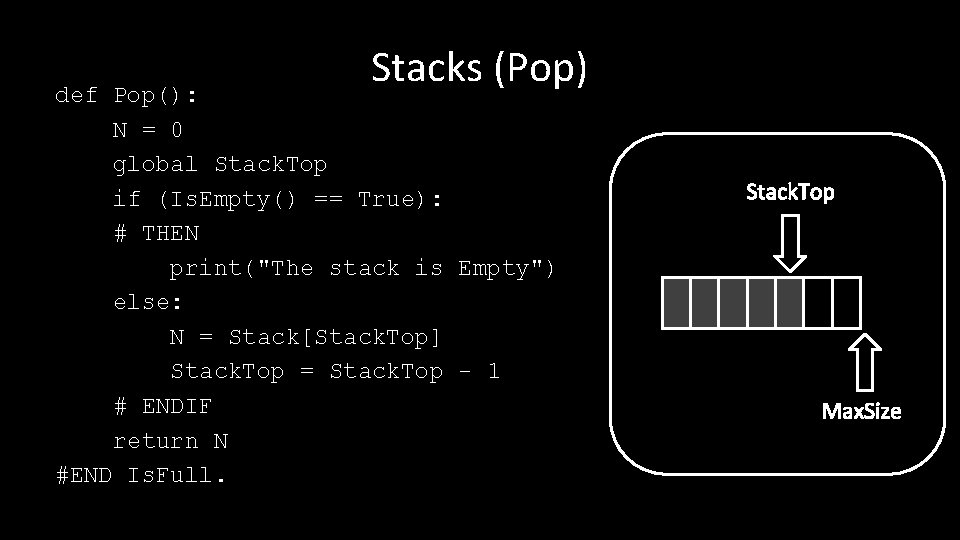
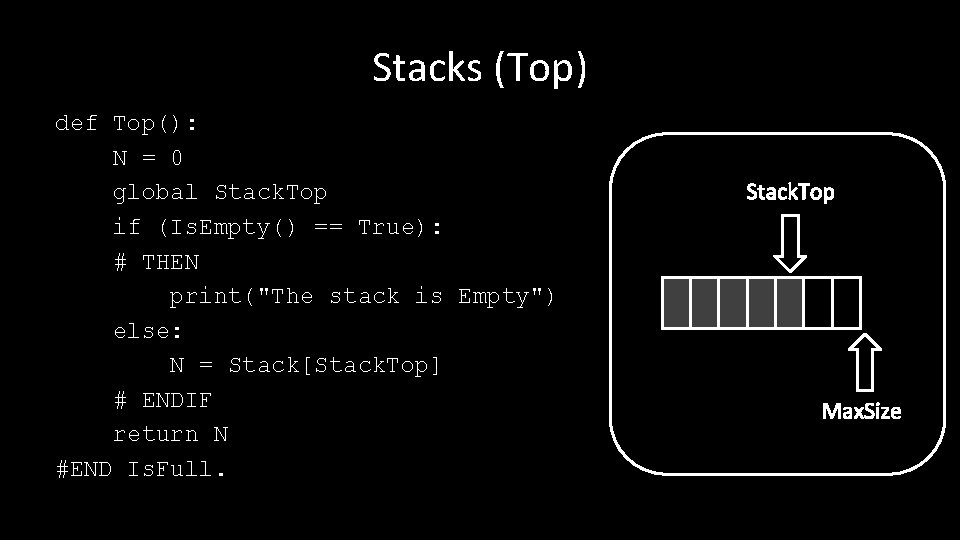
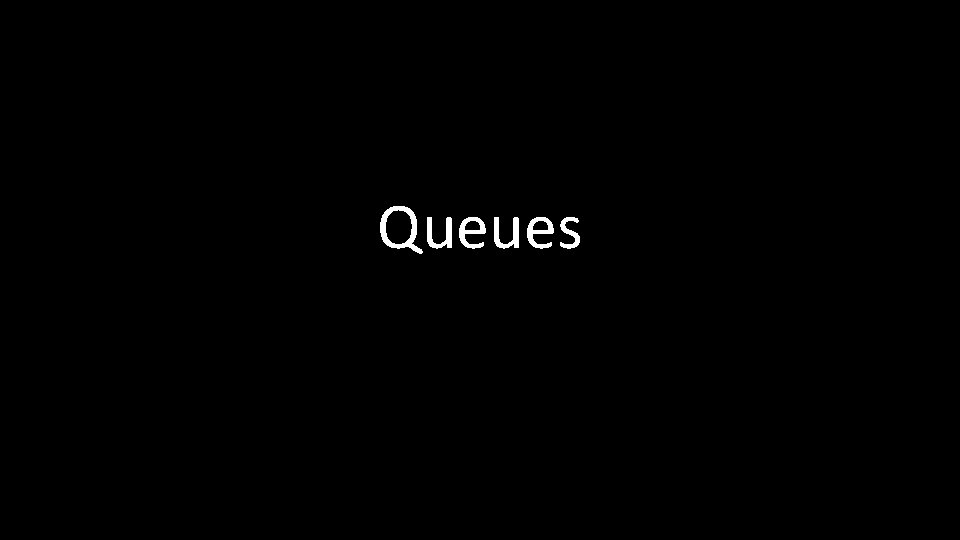
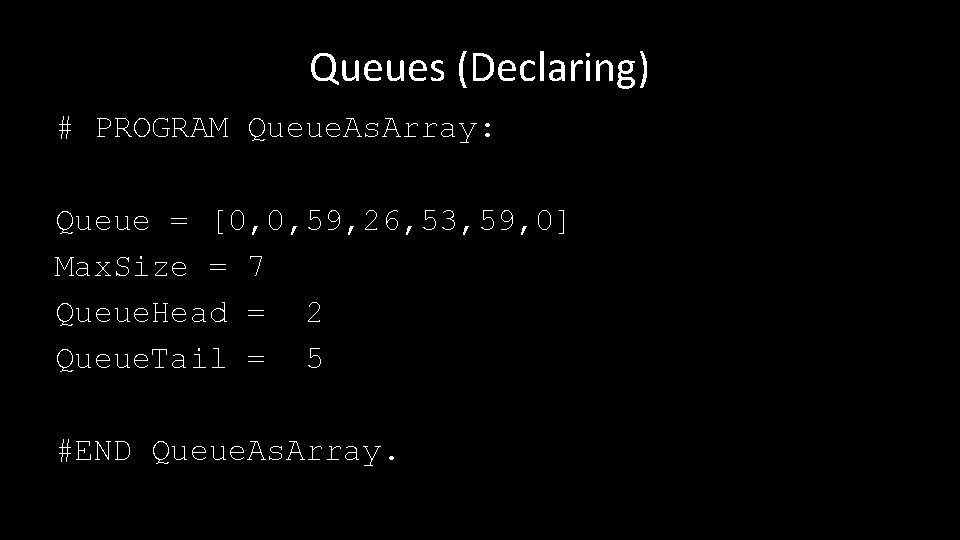
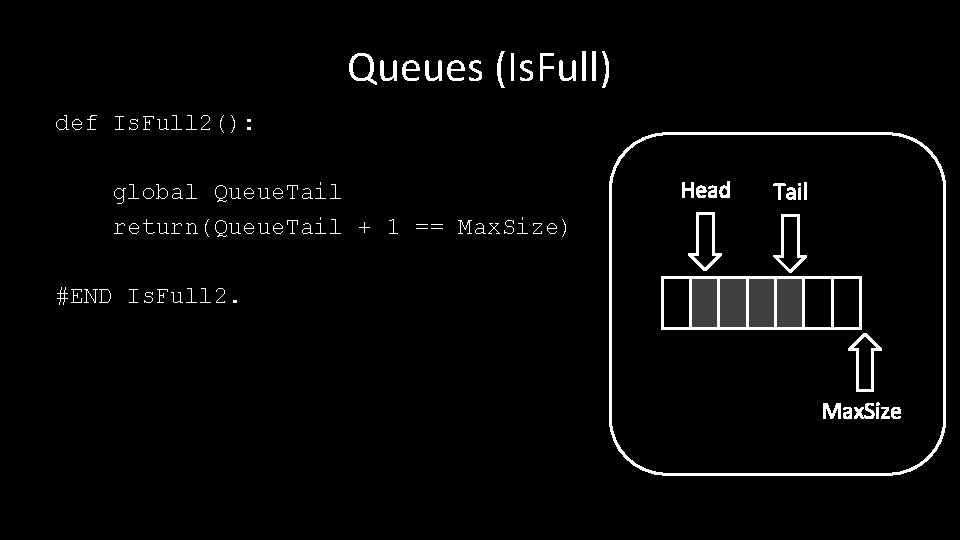
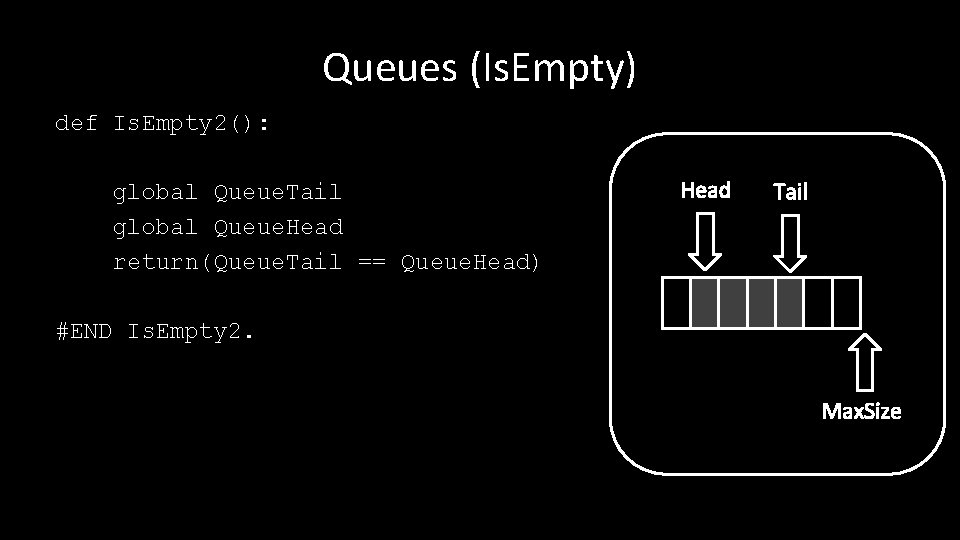
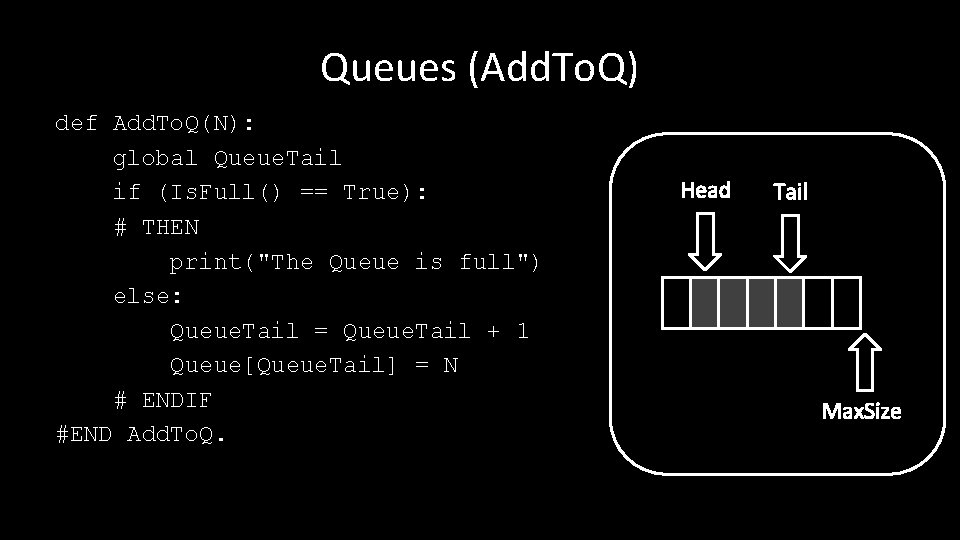
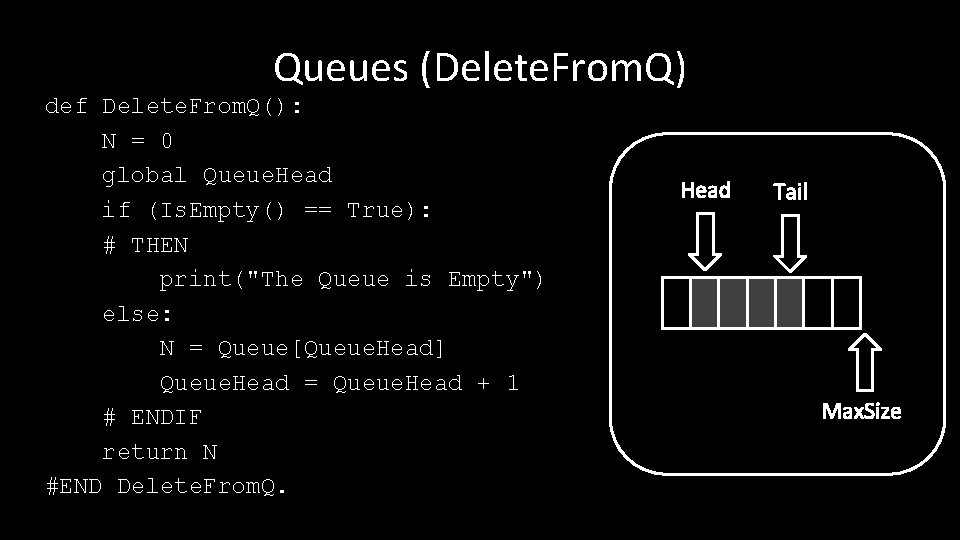
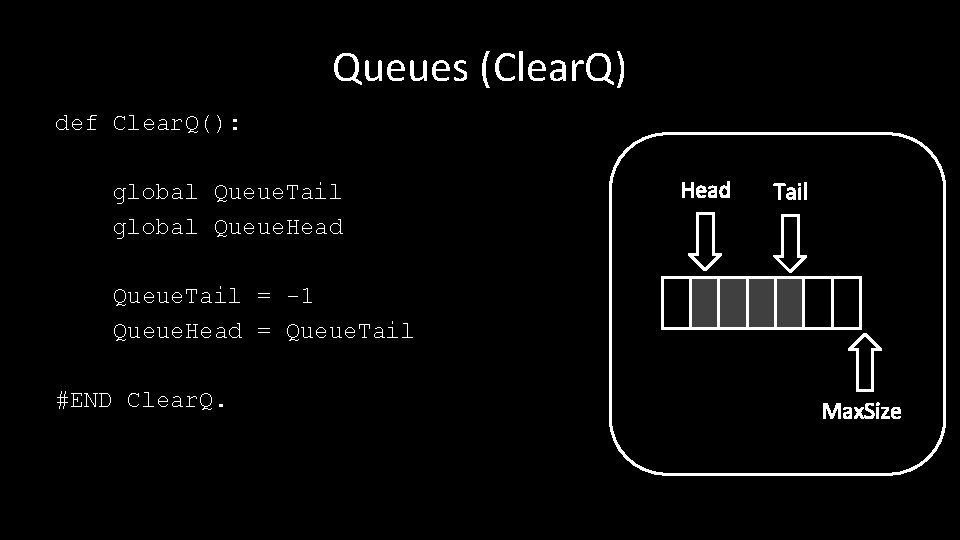
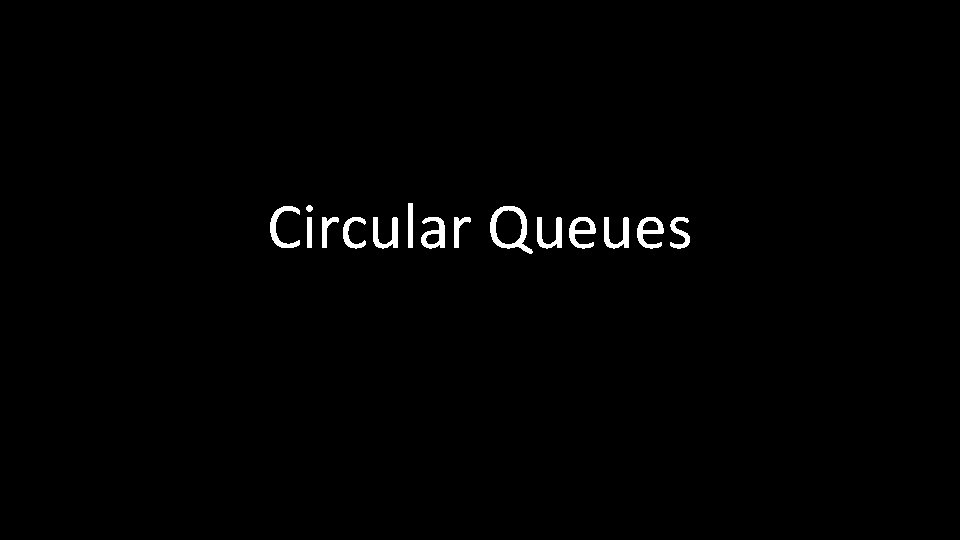
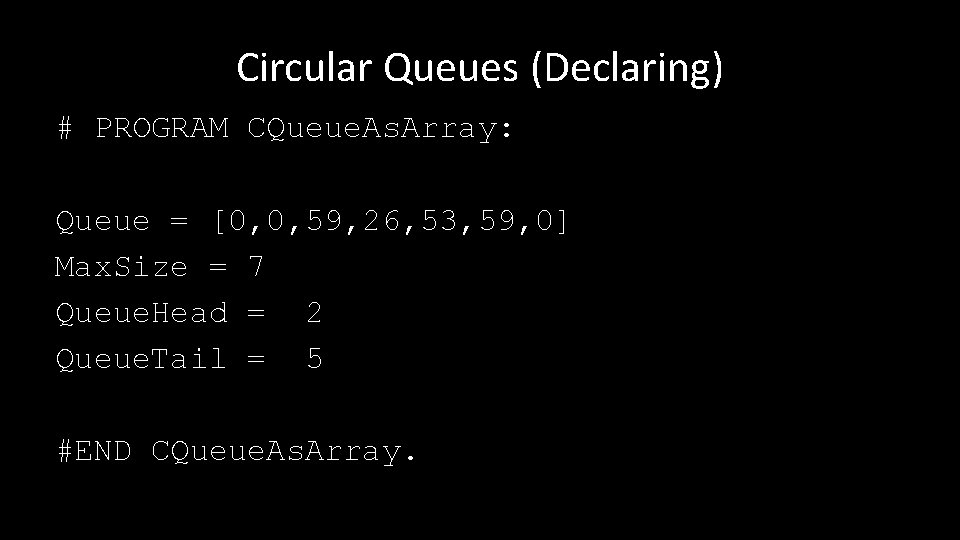
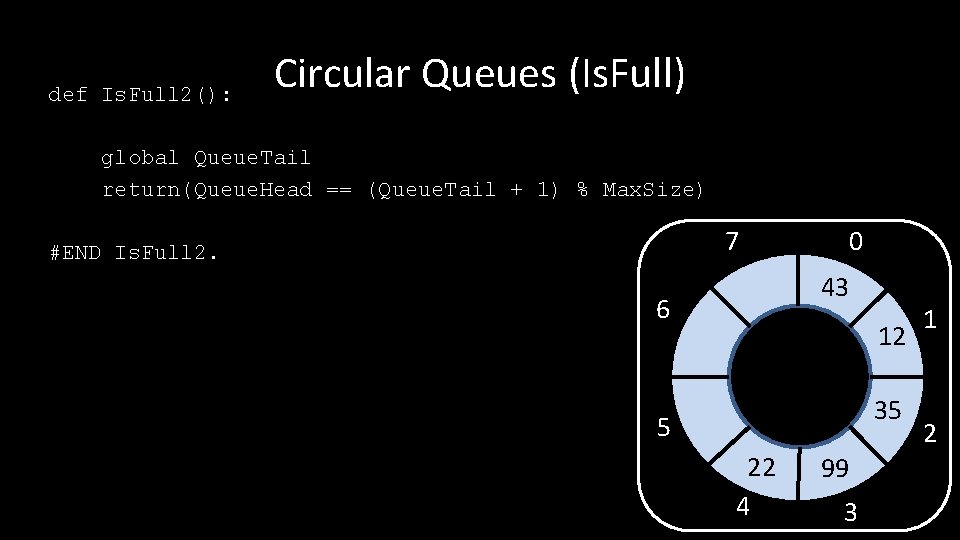
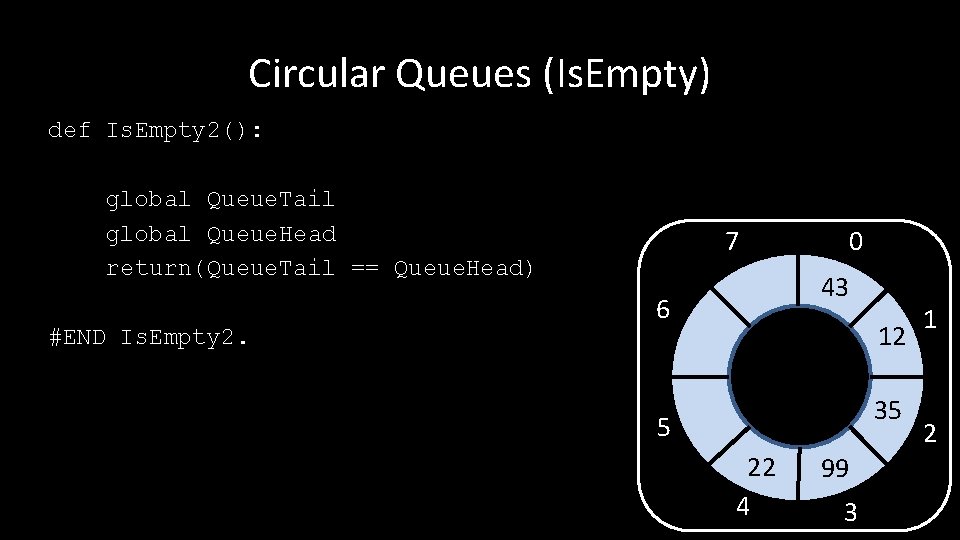
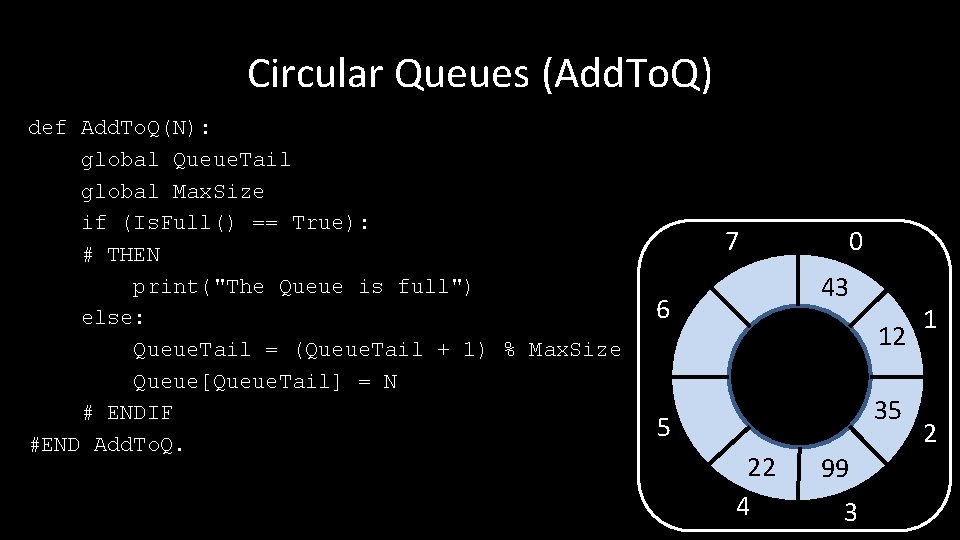
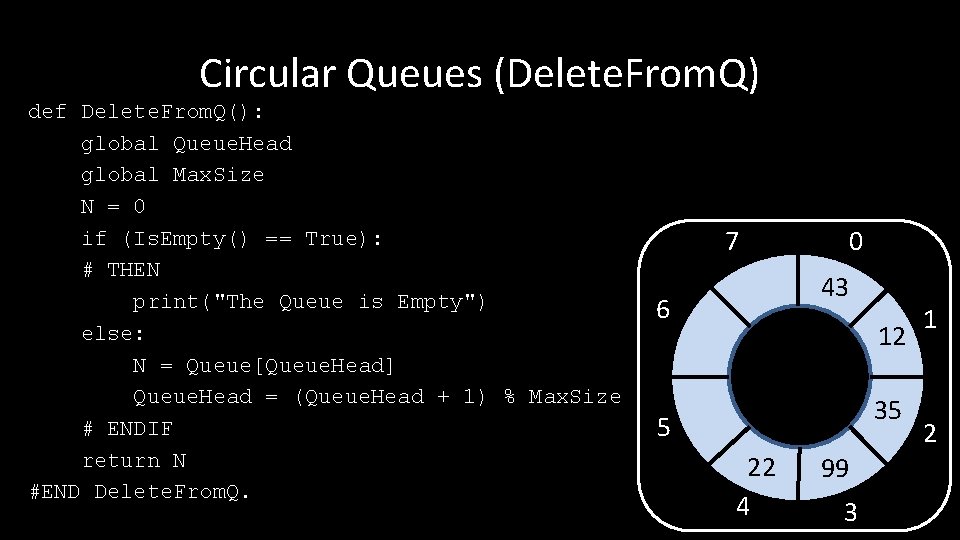
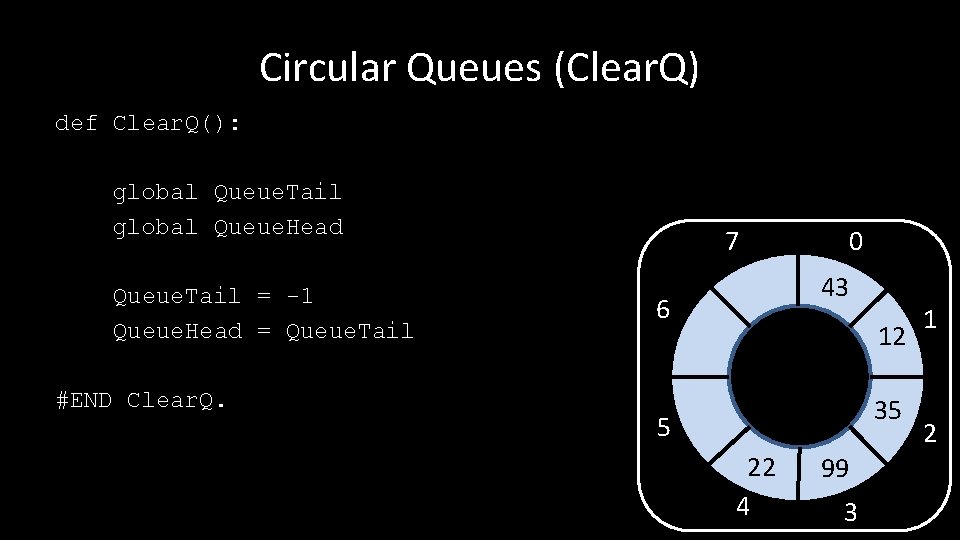
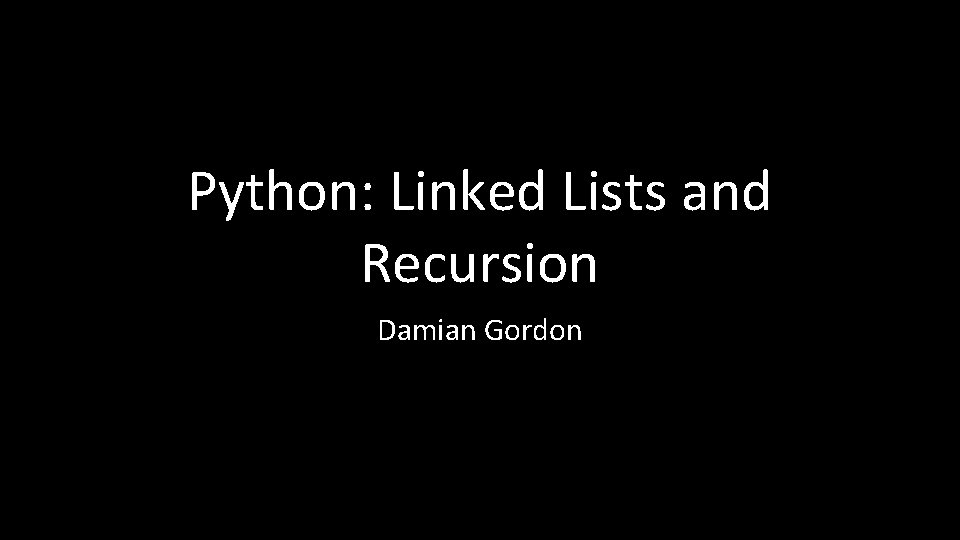
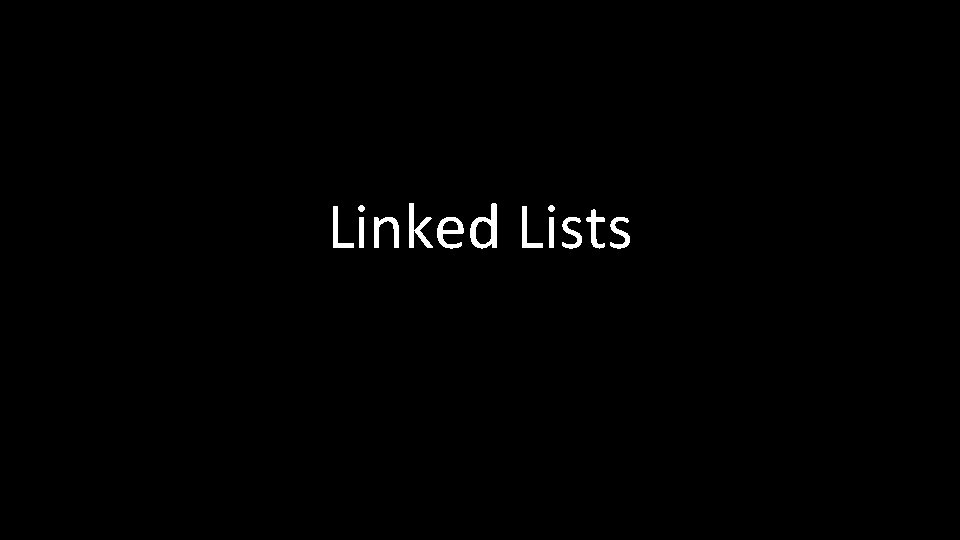
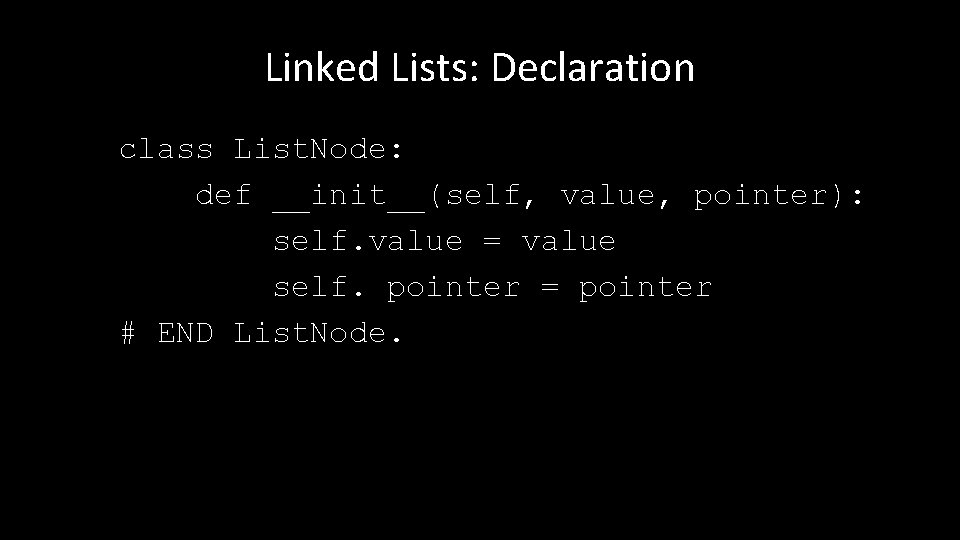
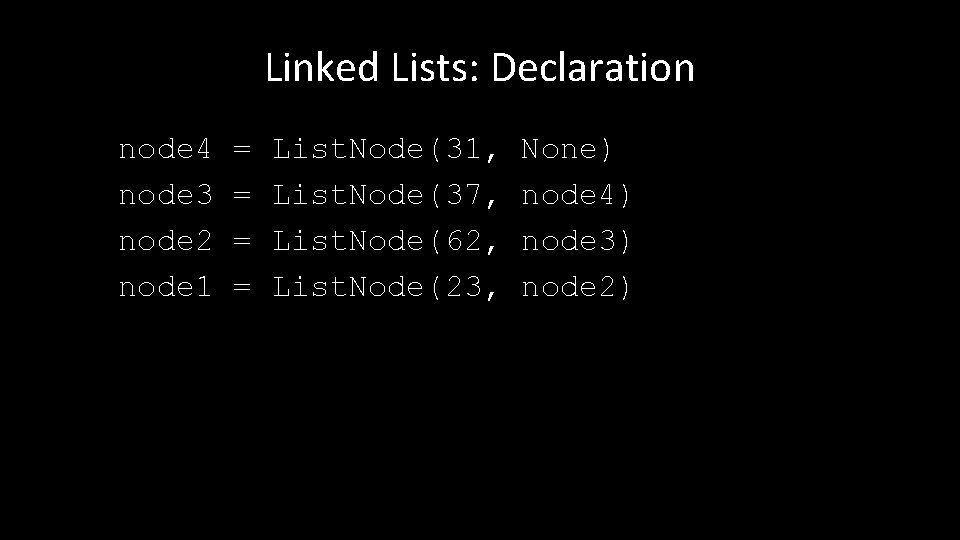
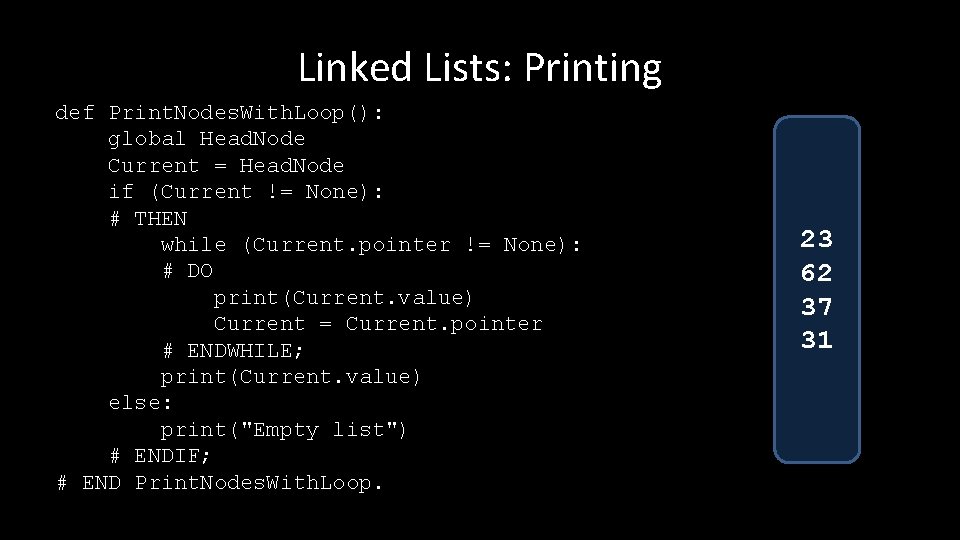
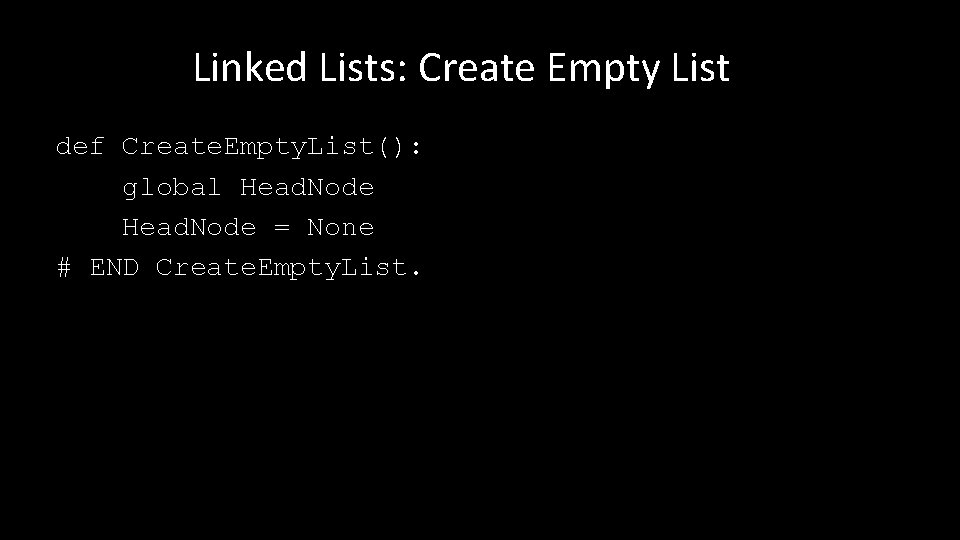
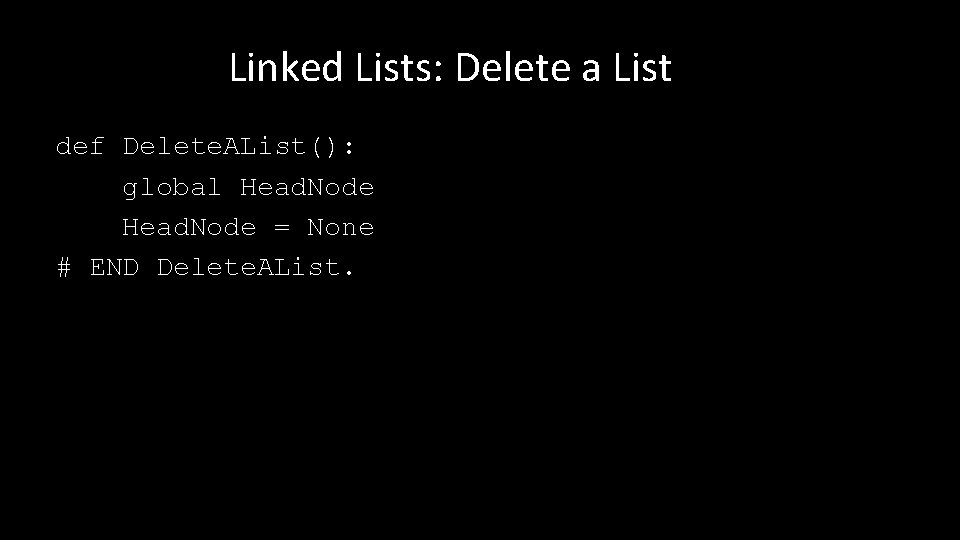
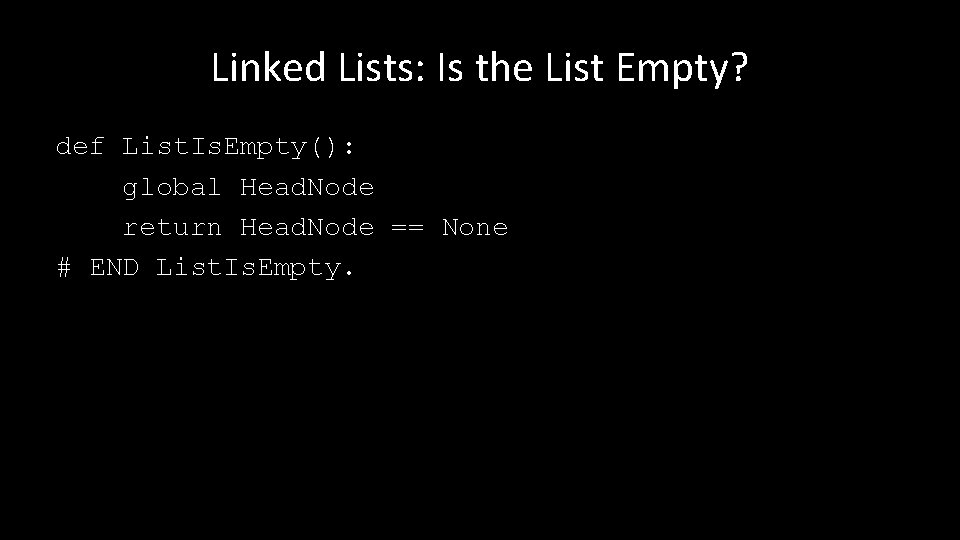
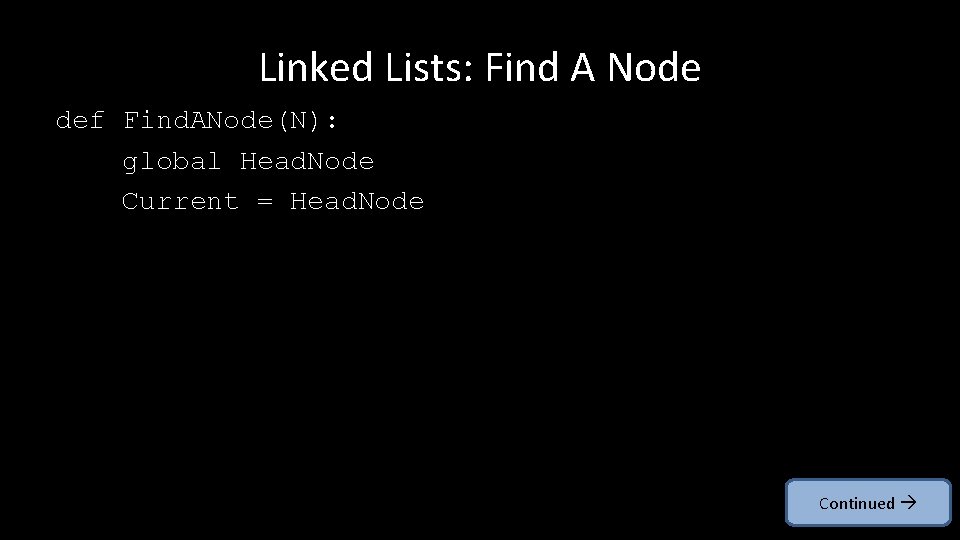
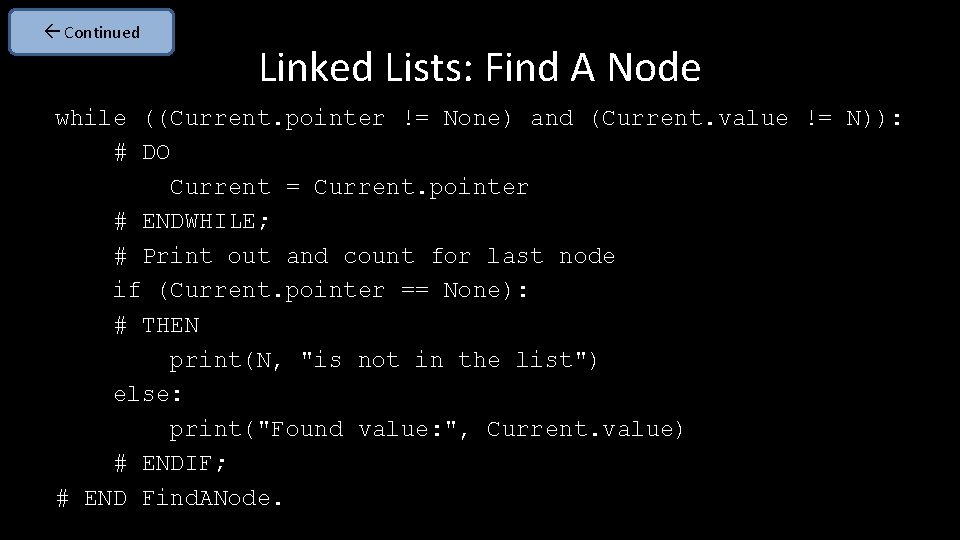
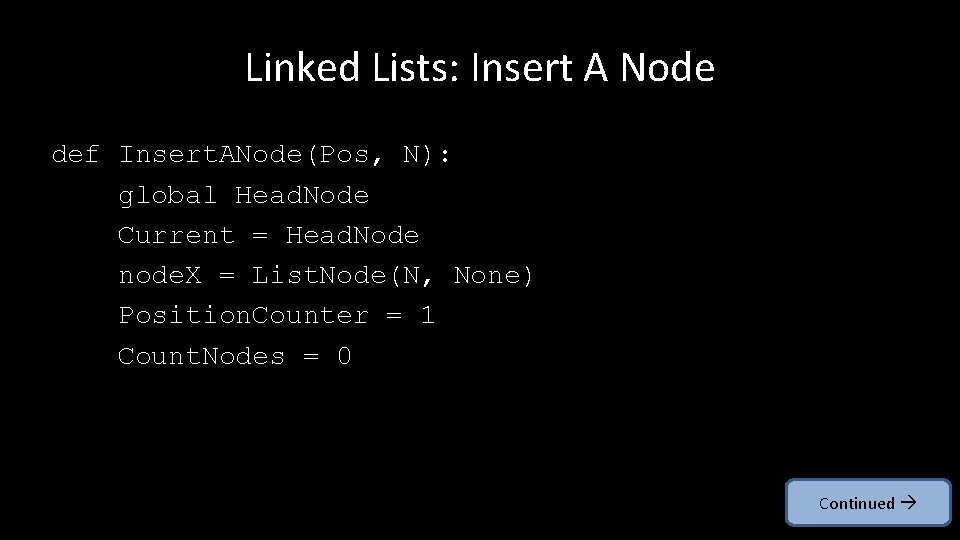
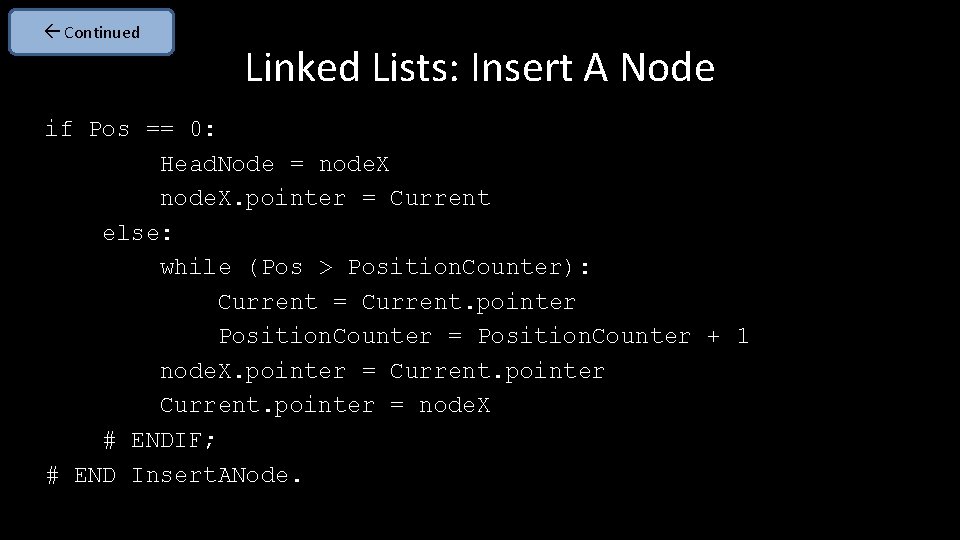
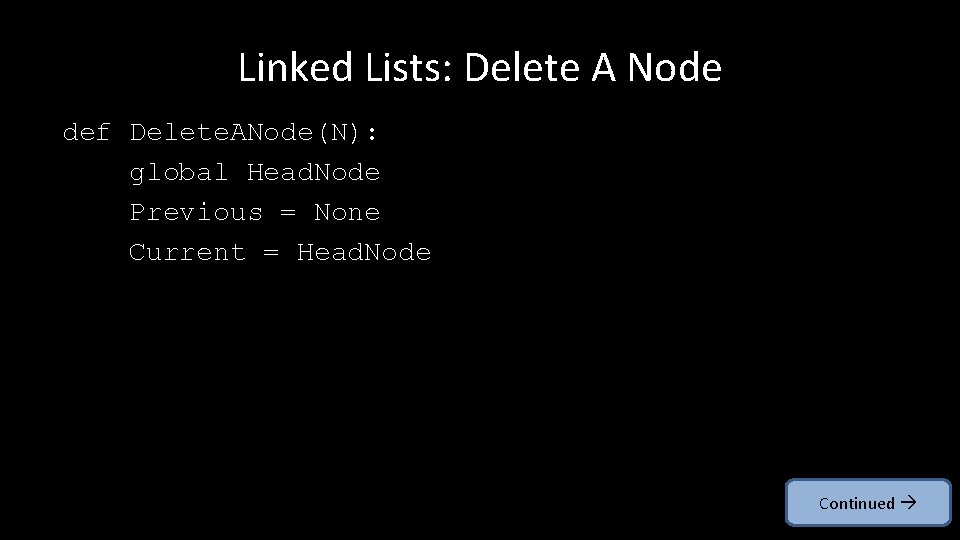
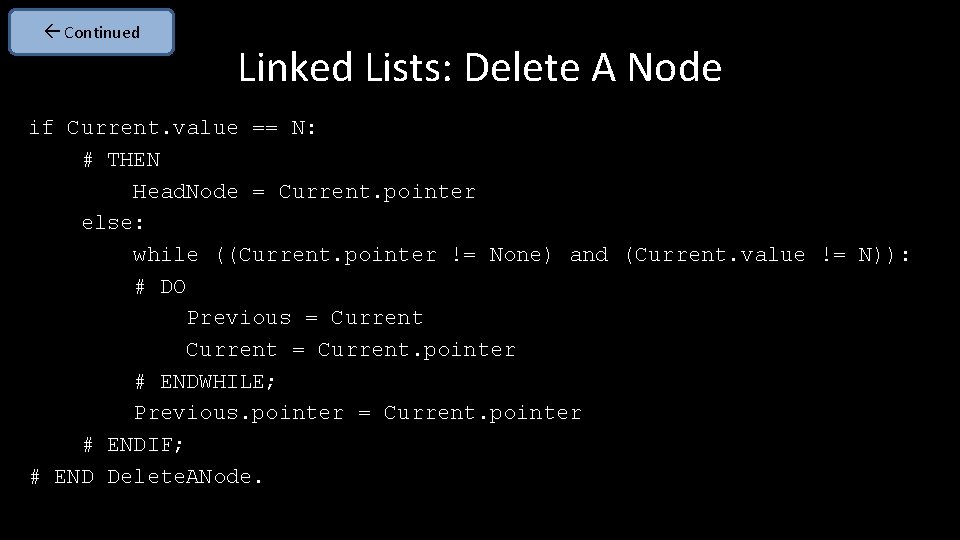
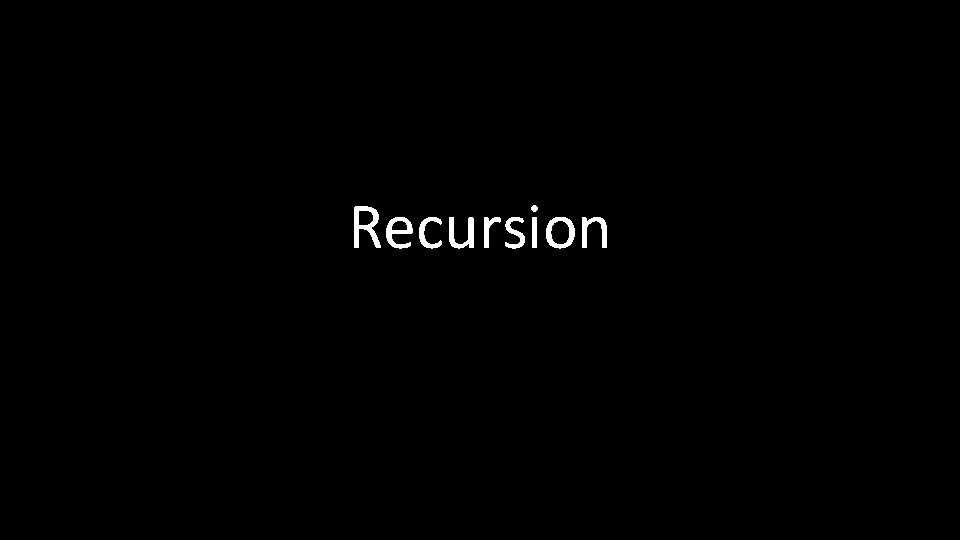
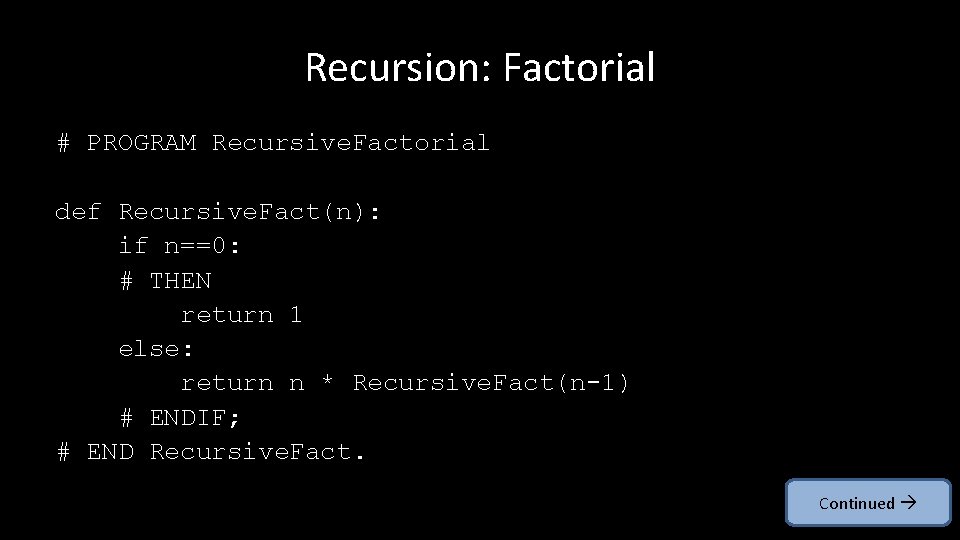
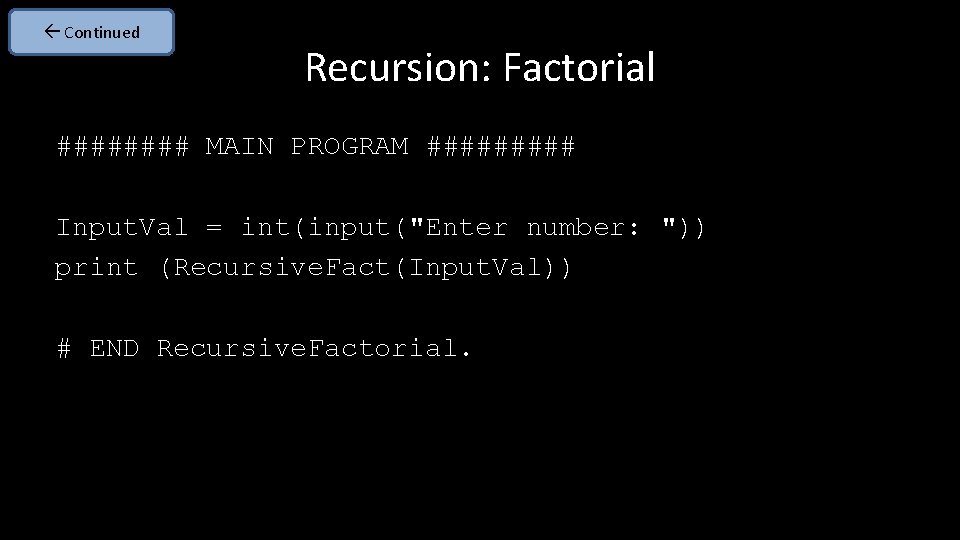
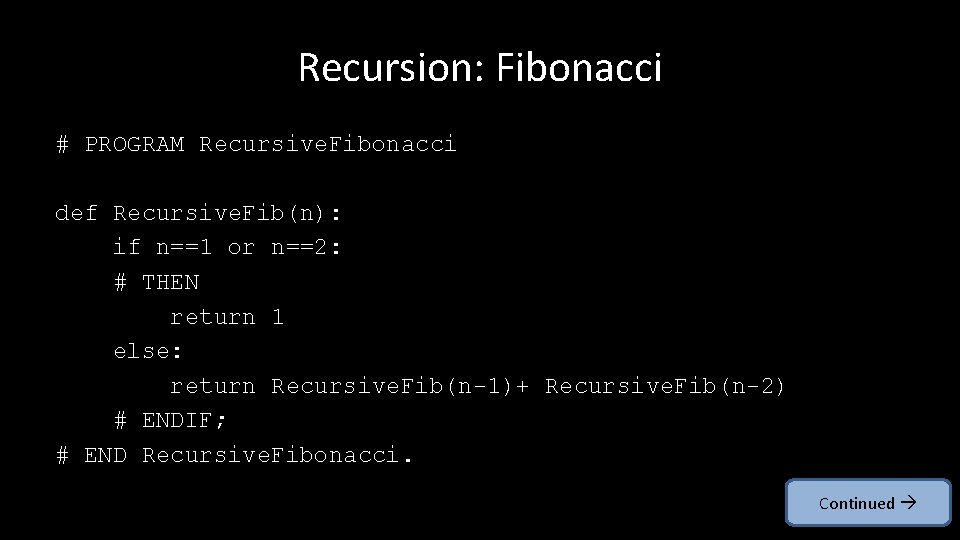
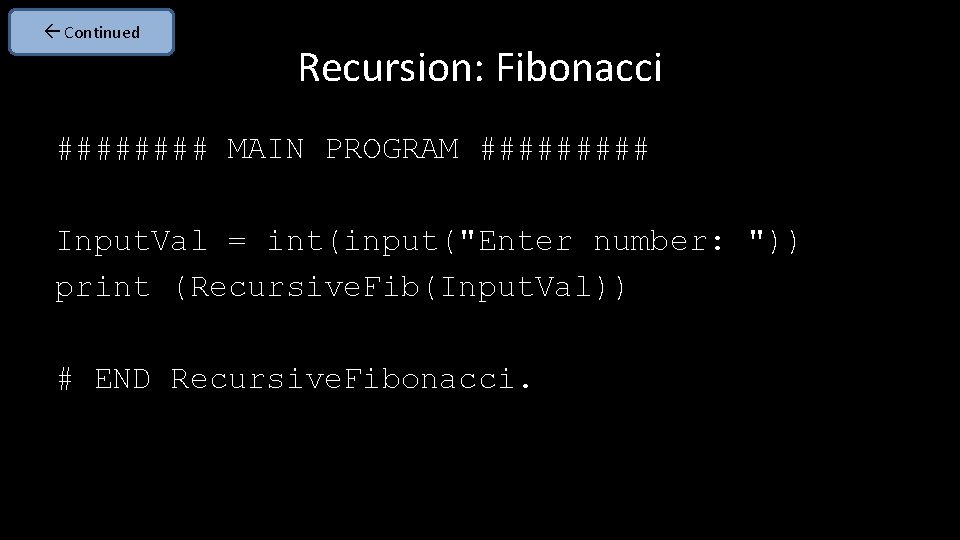
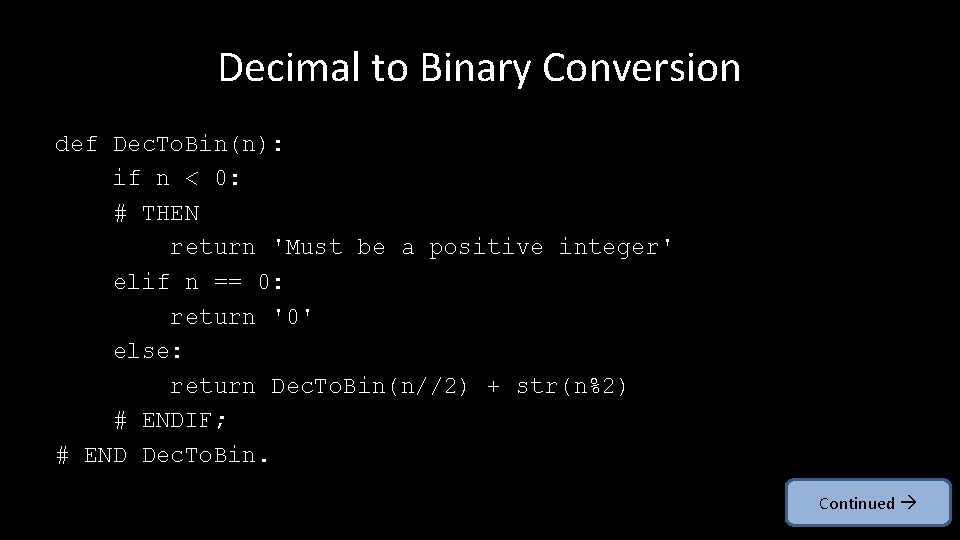
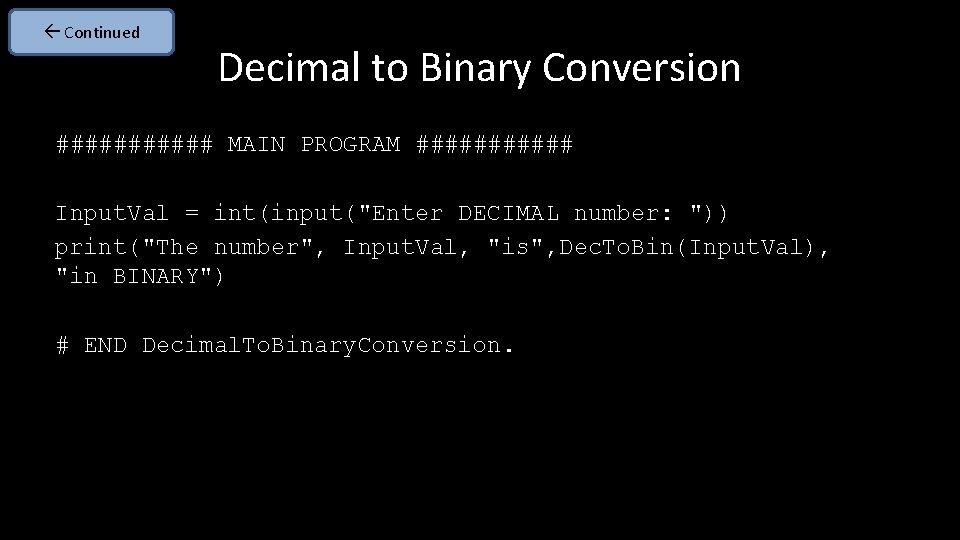
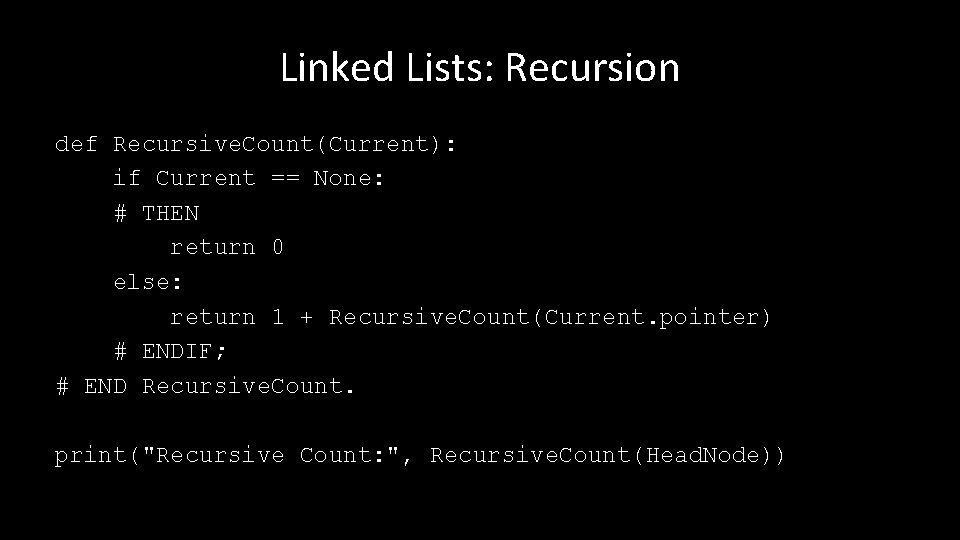
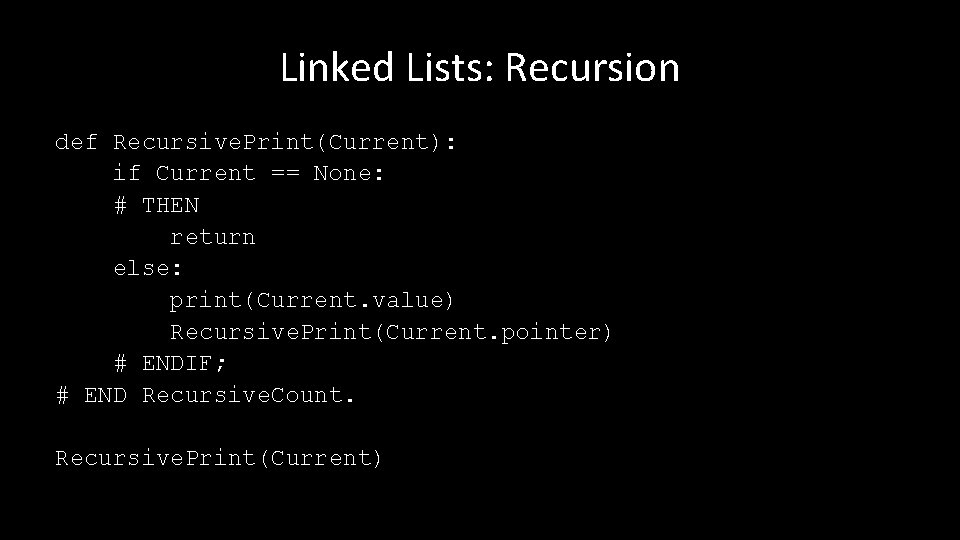
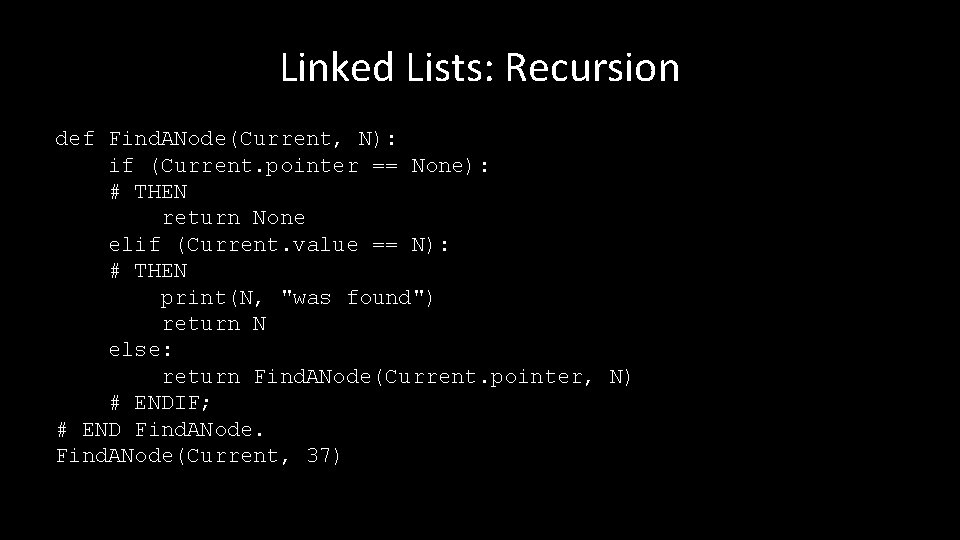
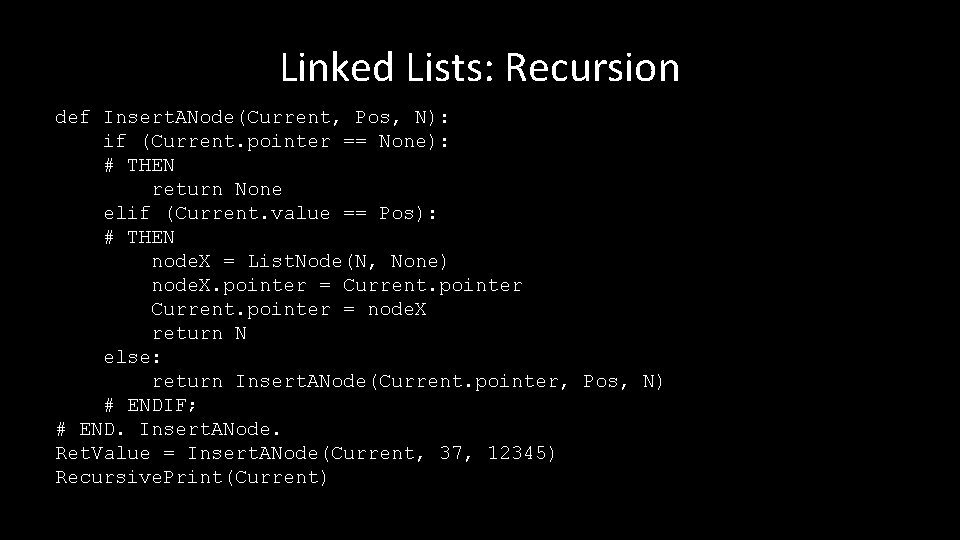
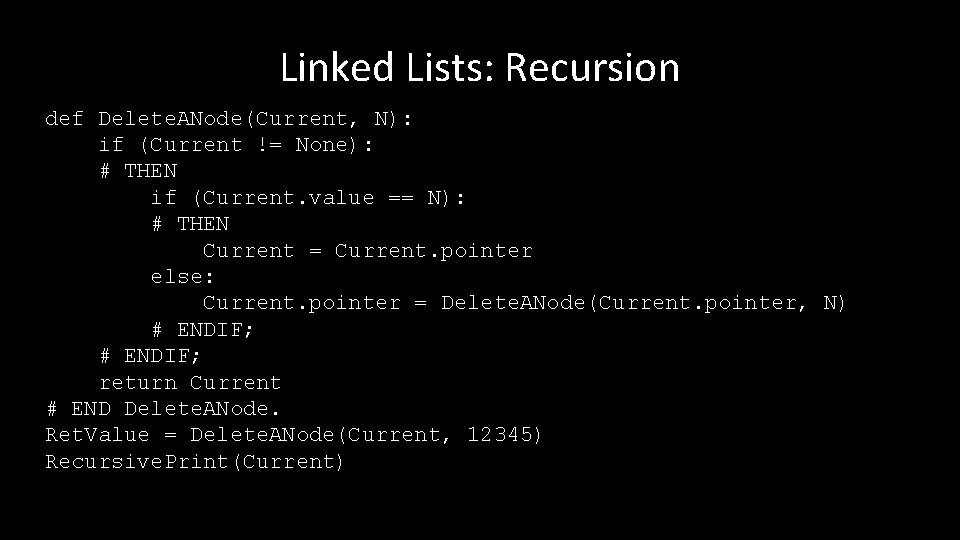
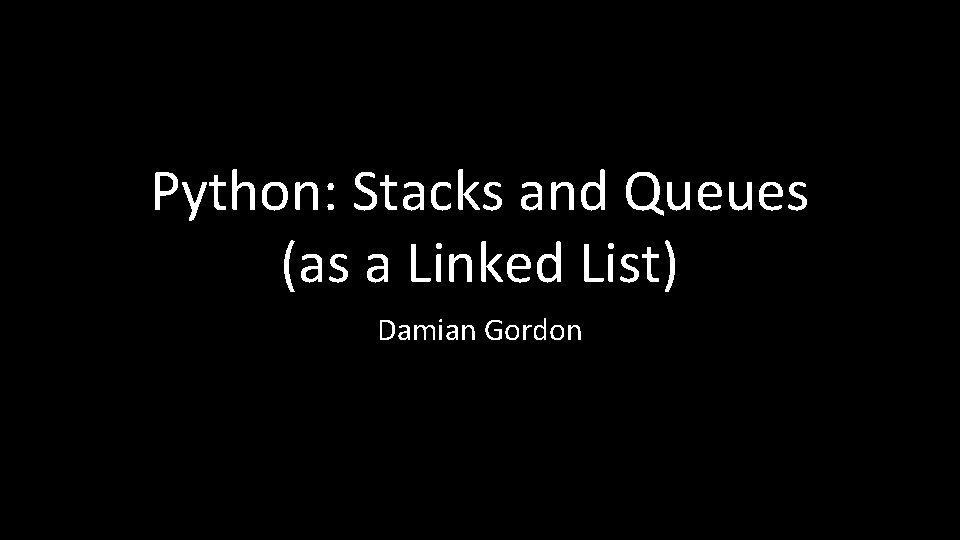
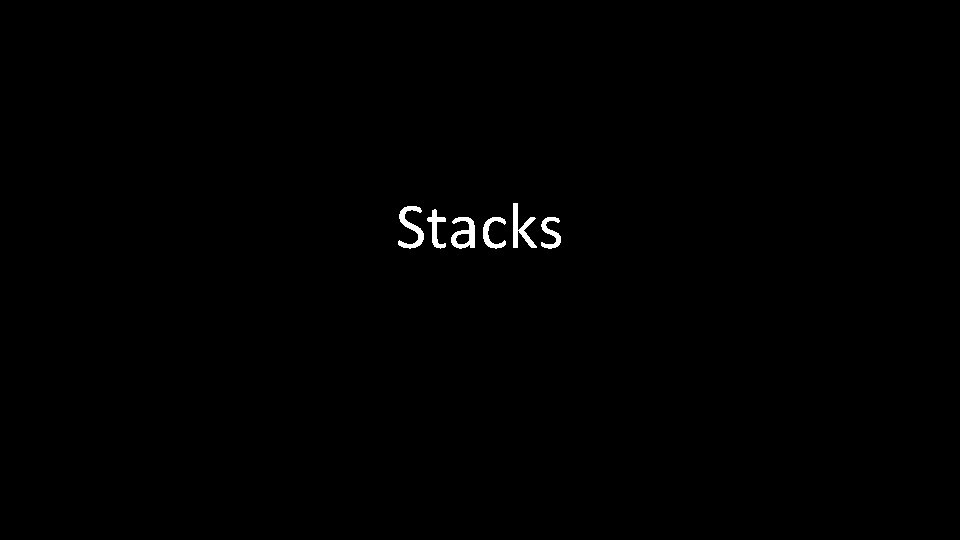
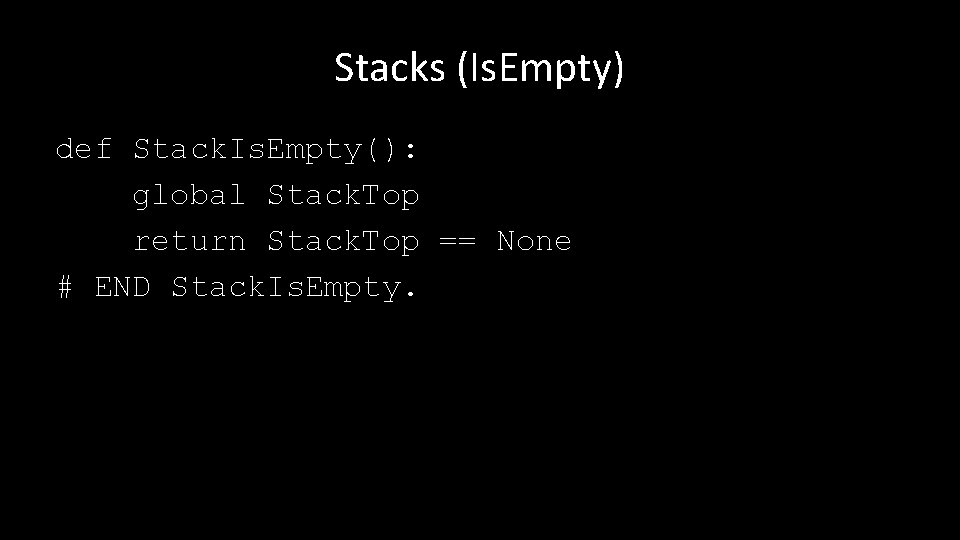
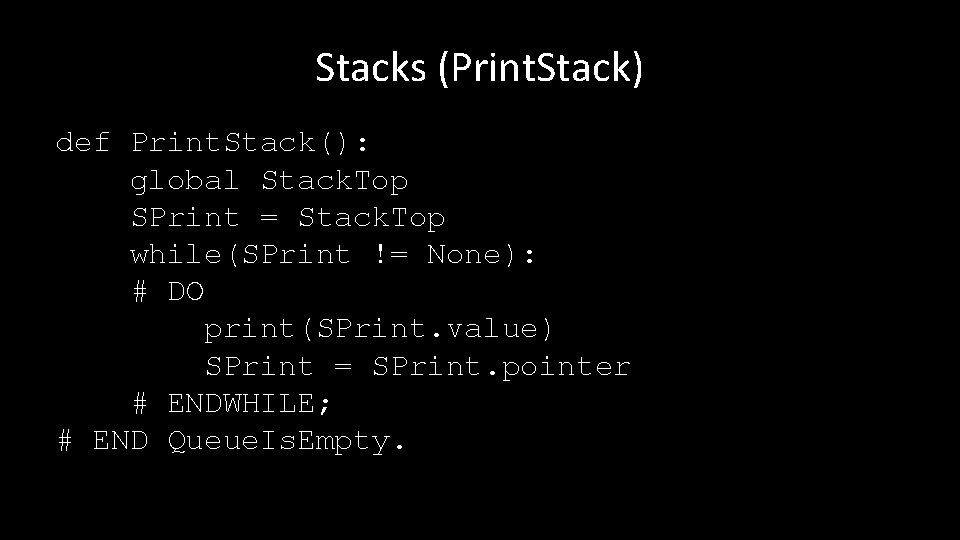
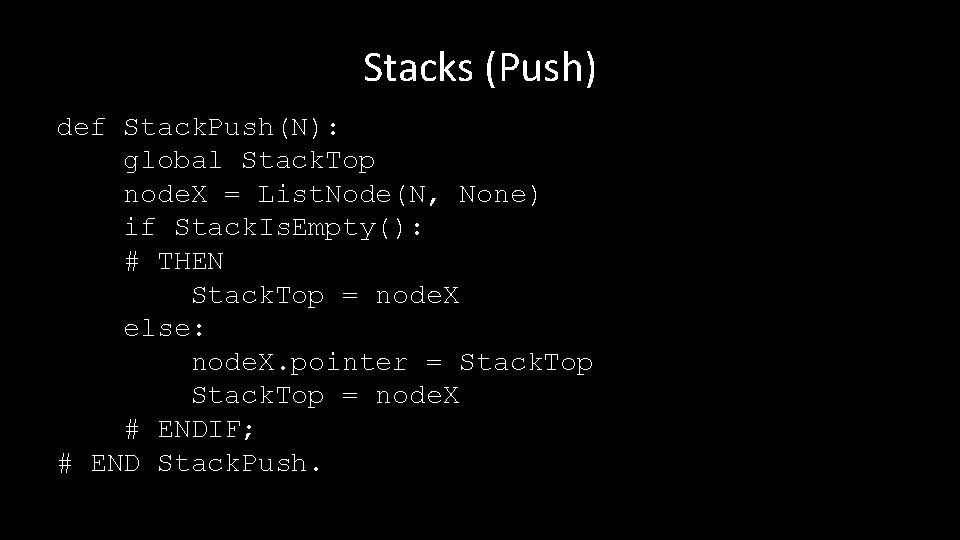
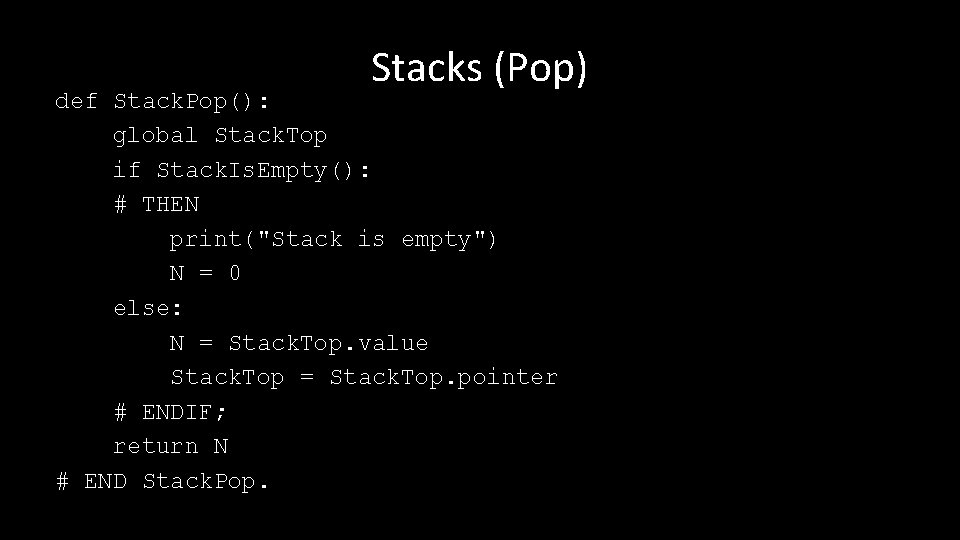
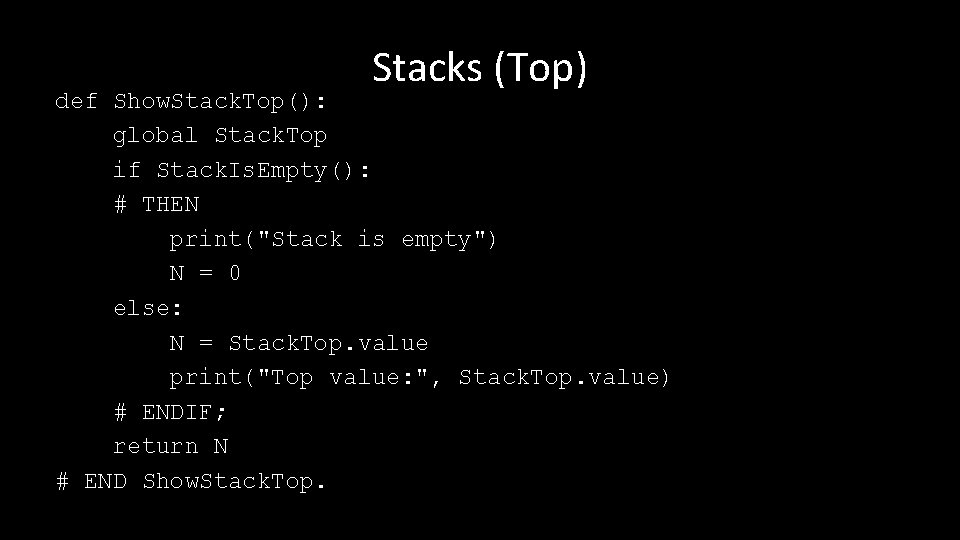
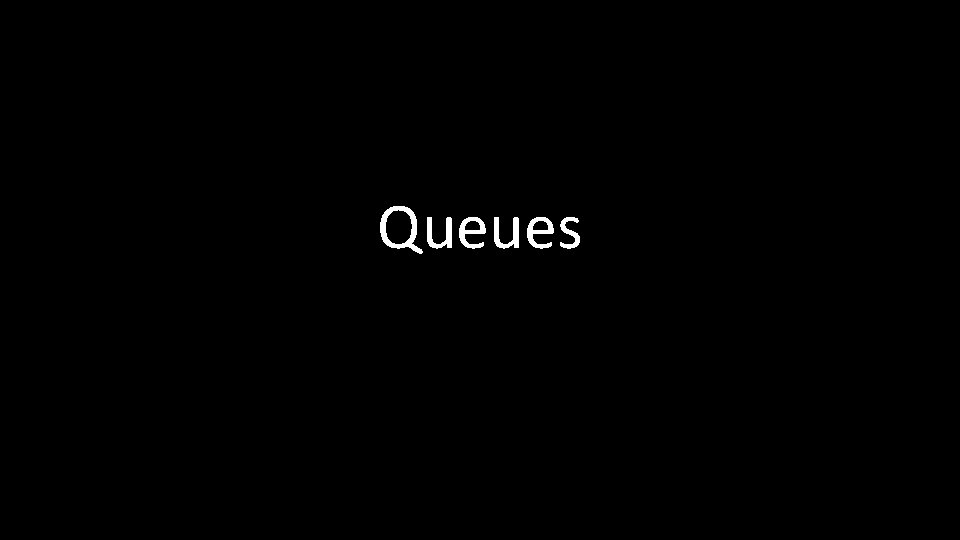
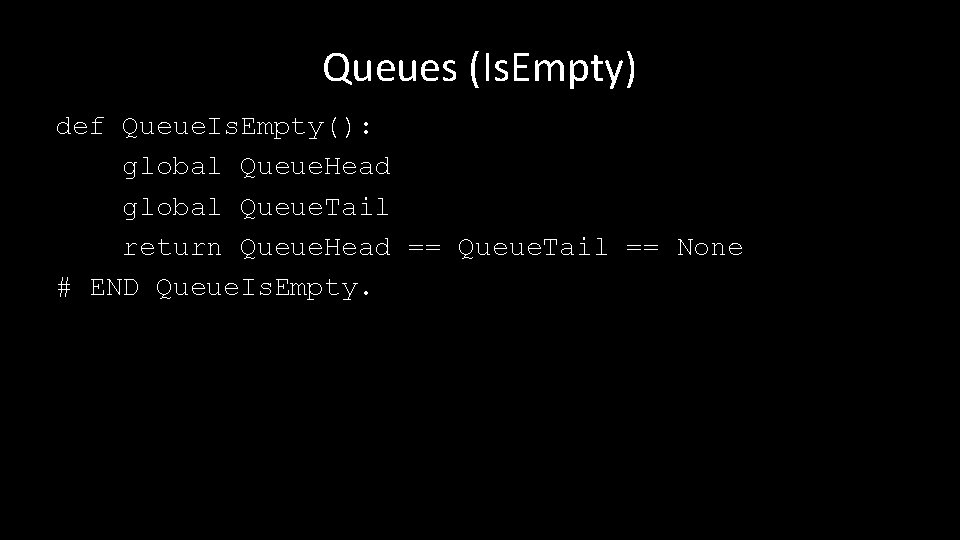
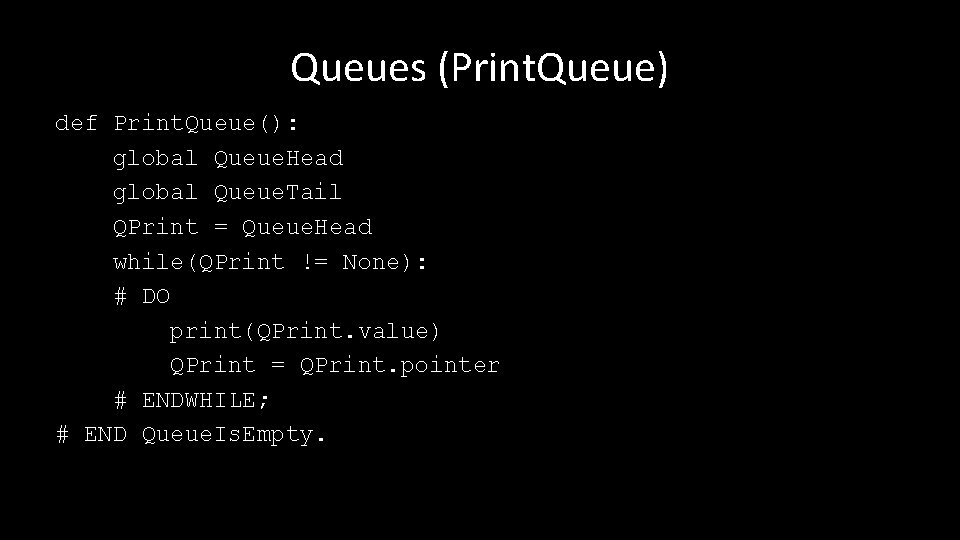
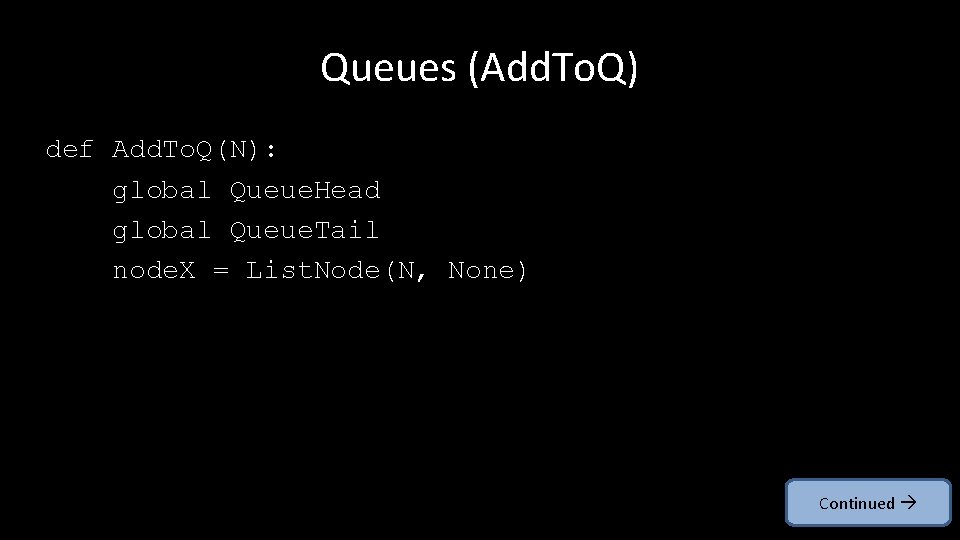
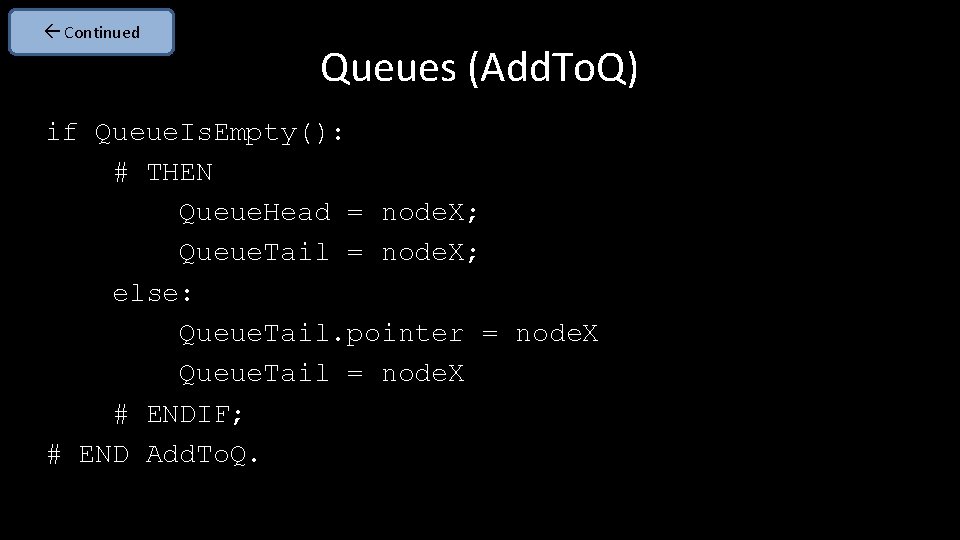
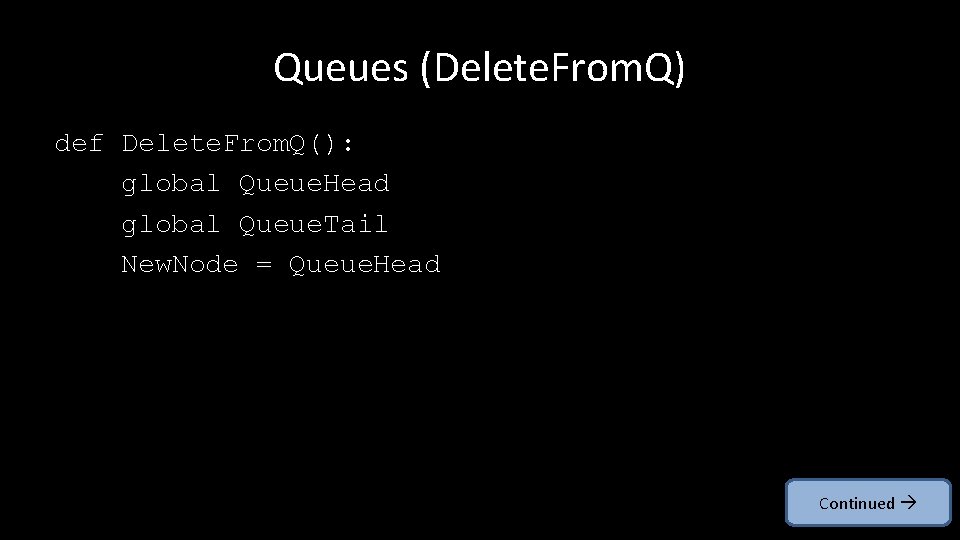
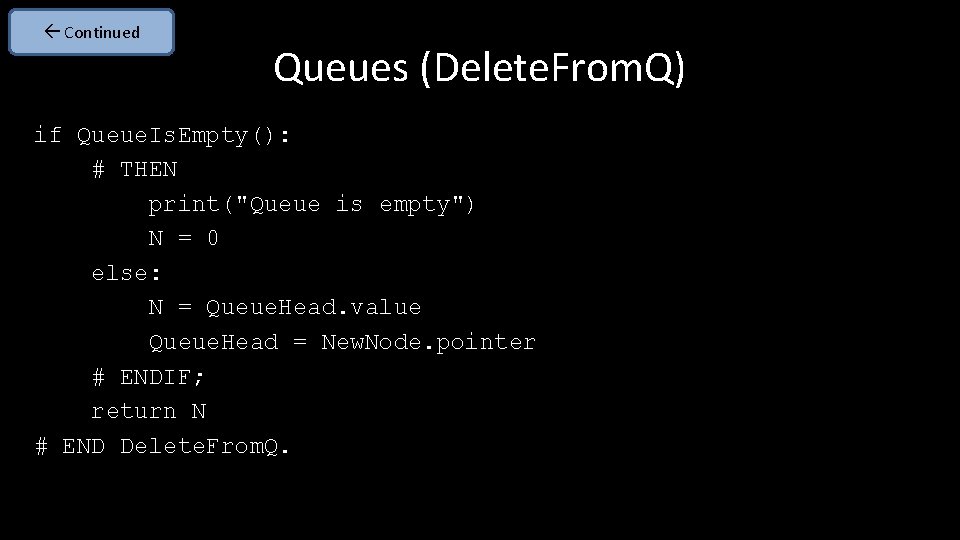
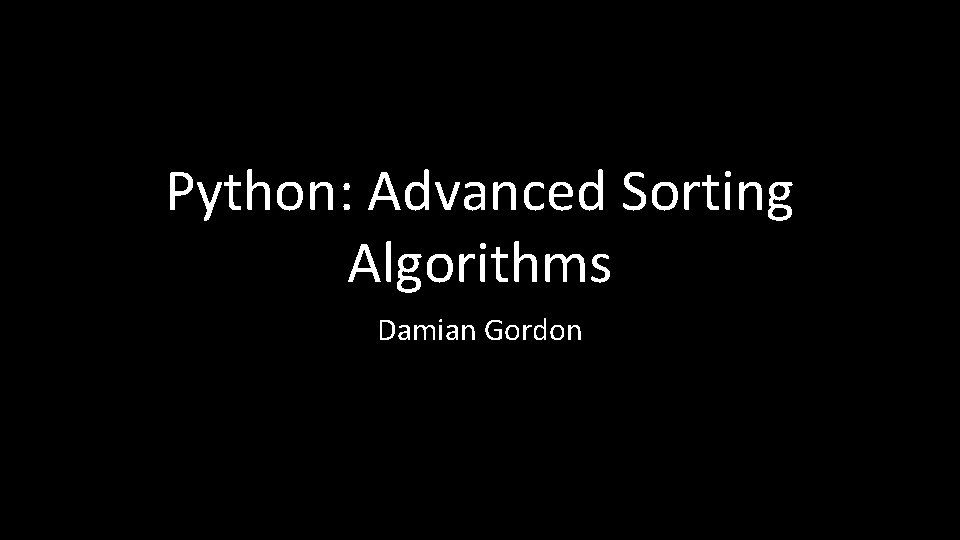
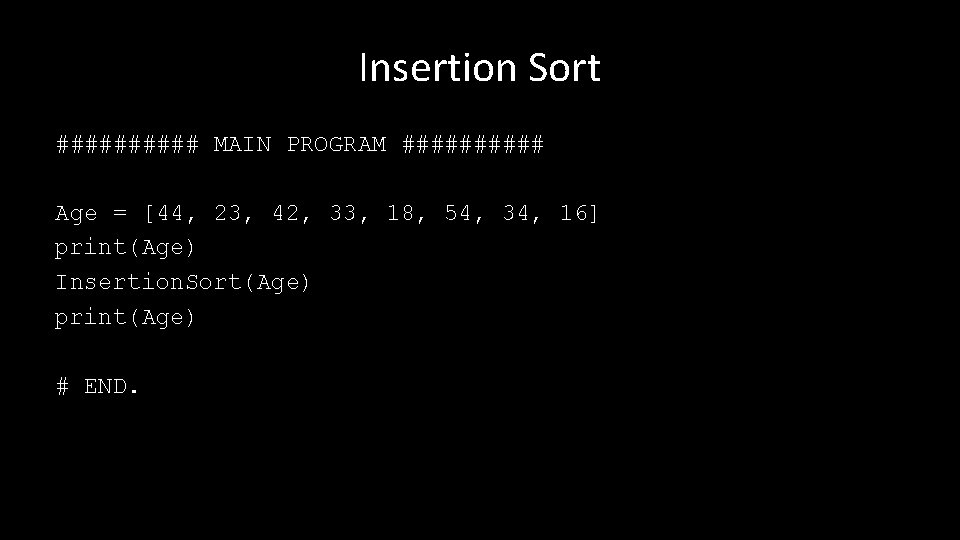
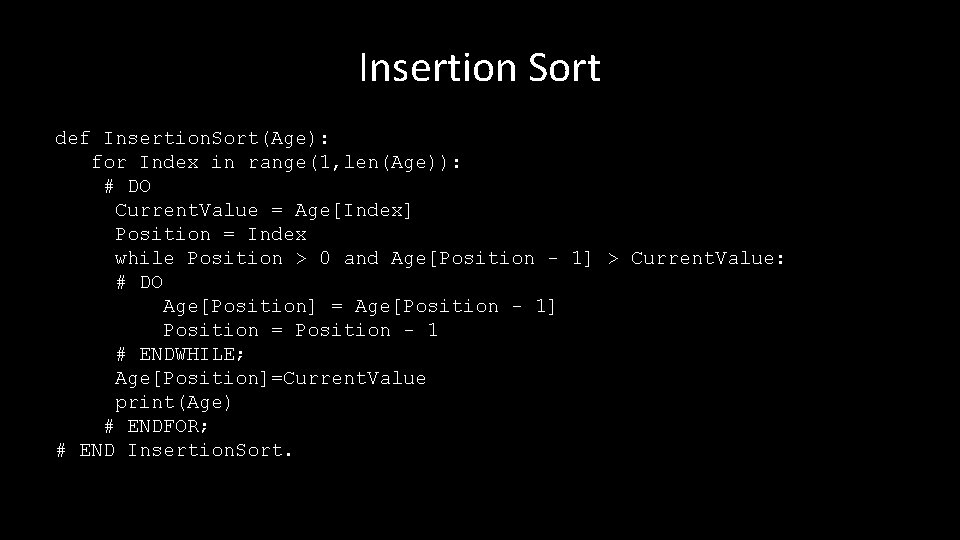
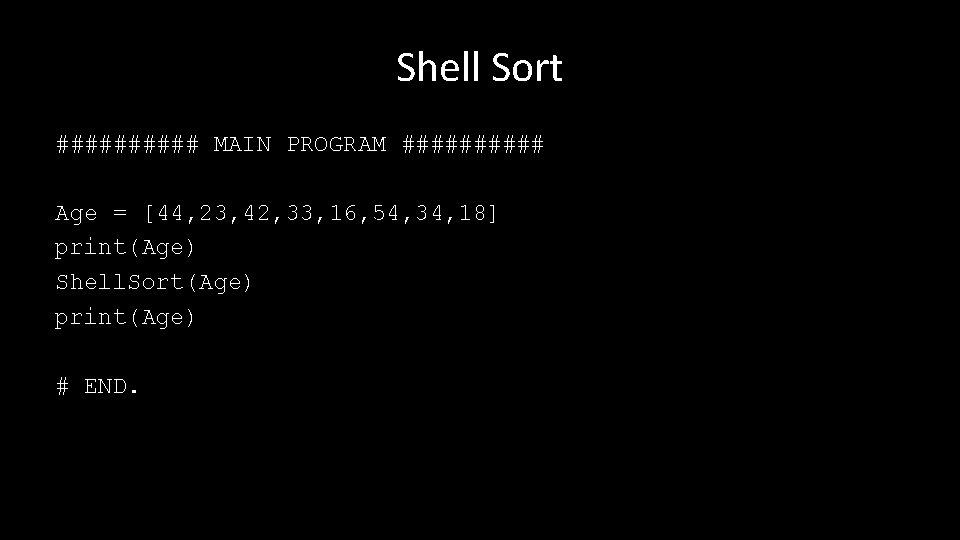
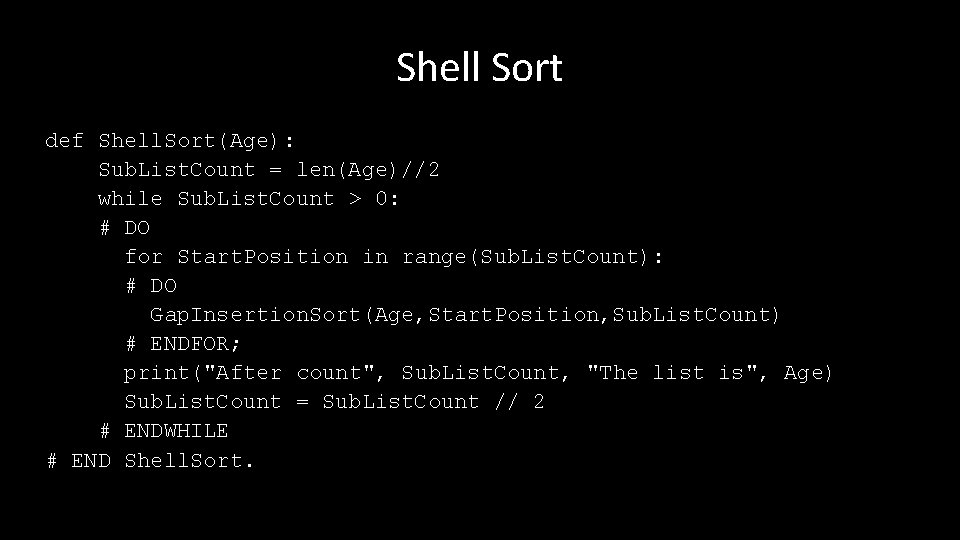
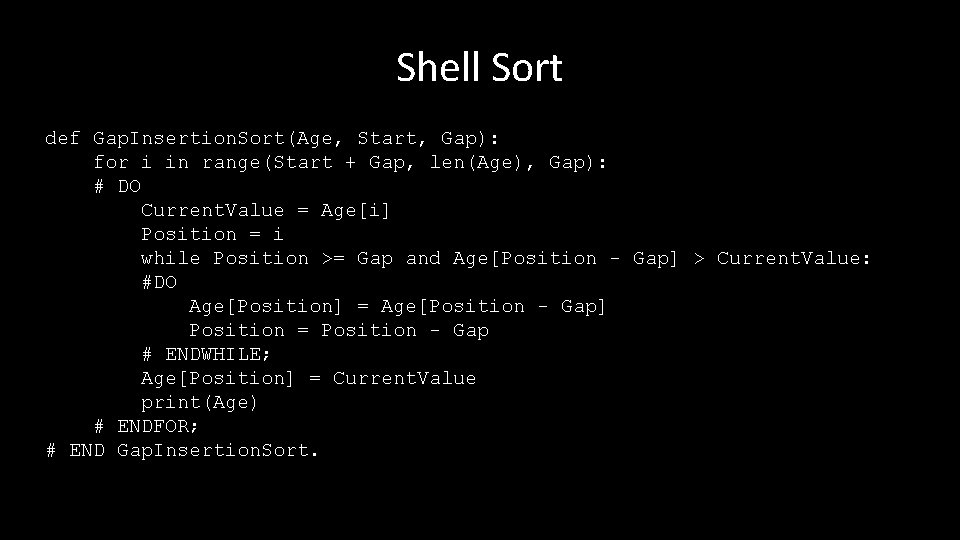
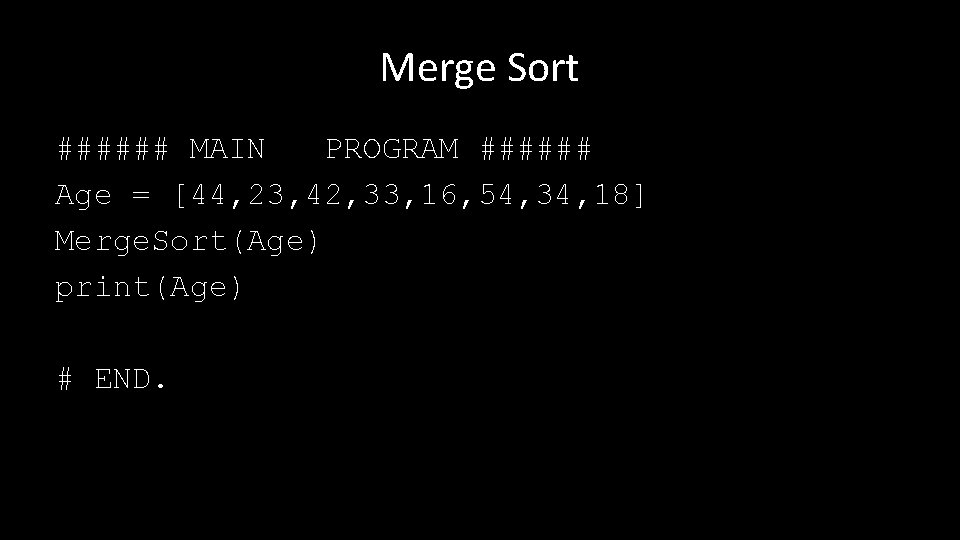
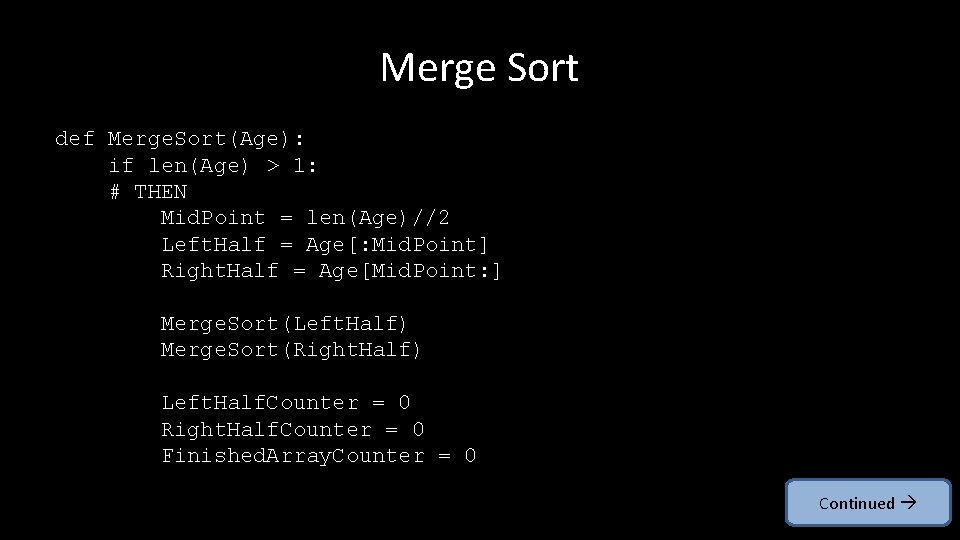
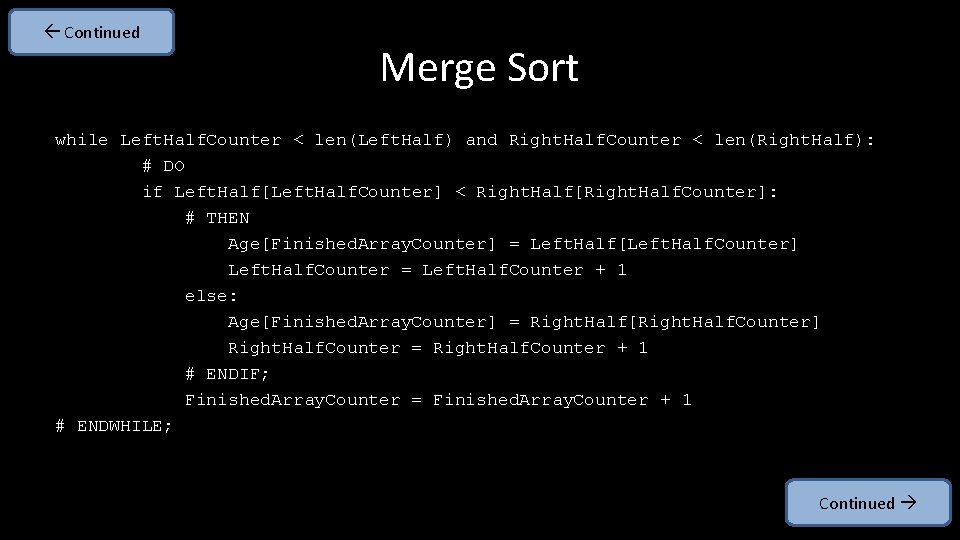
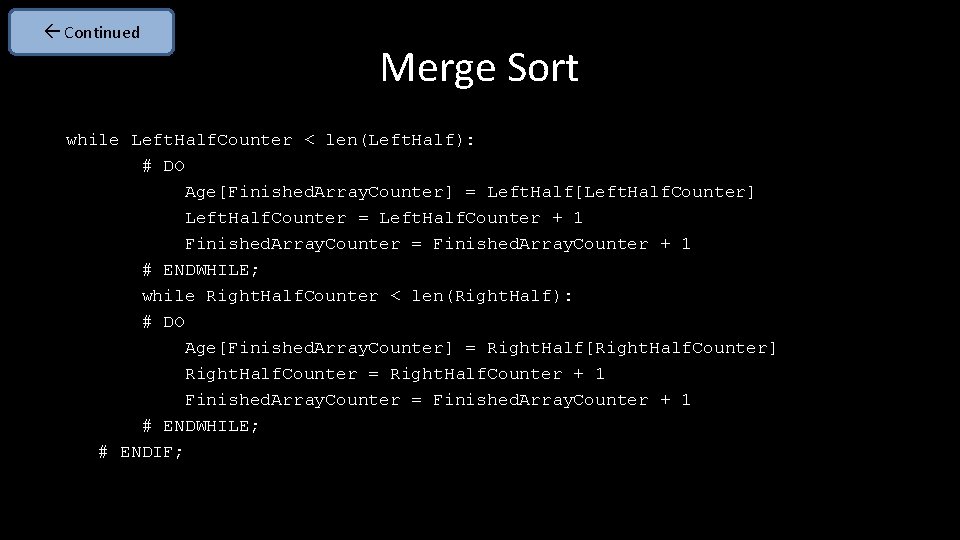
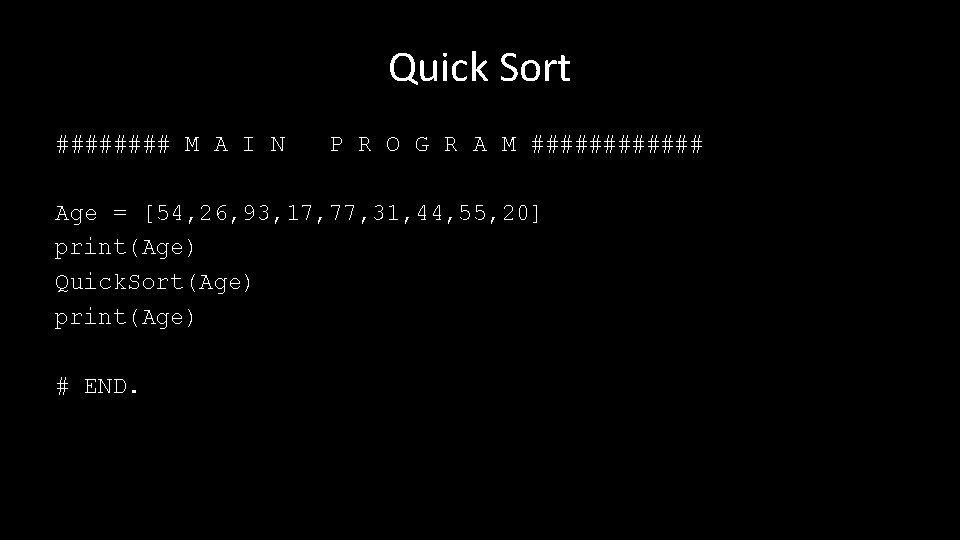
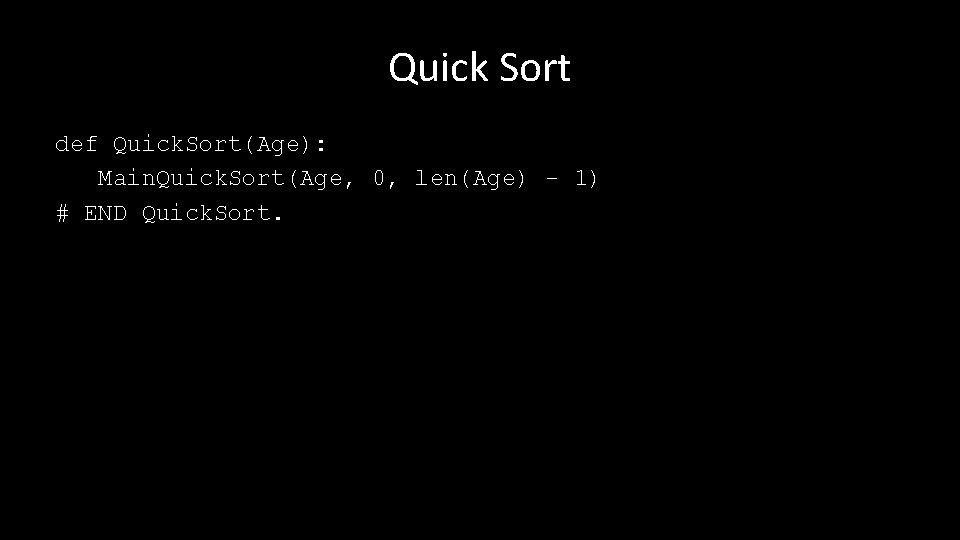
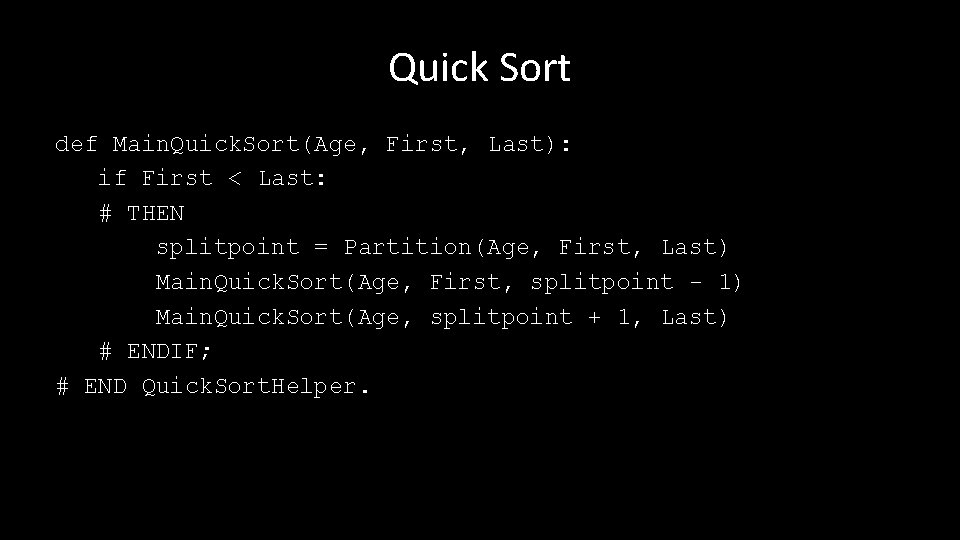
![Quick Sort def Partition(Age, First, Last): pivotvalue = Age[First] Finished = False Left. Pointer Quick Sort def Partition(Age, First, Last): pivotvalue = Age[First] Finished = False Left. Pointer](https://slidetodoc.com/presentation_image_h/aaf8f5358c1623ec5e9a26be12d8fb67/image-81.jpg)
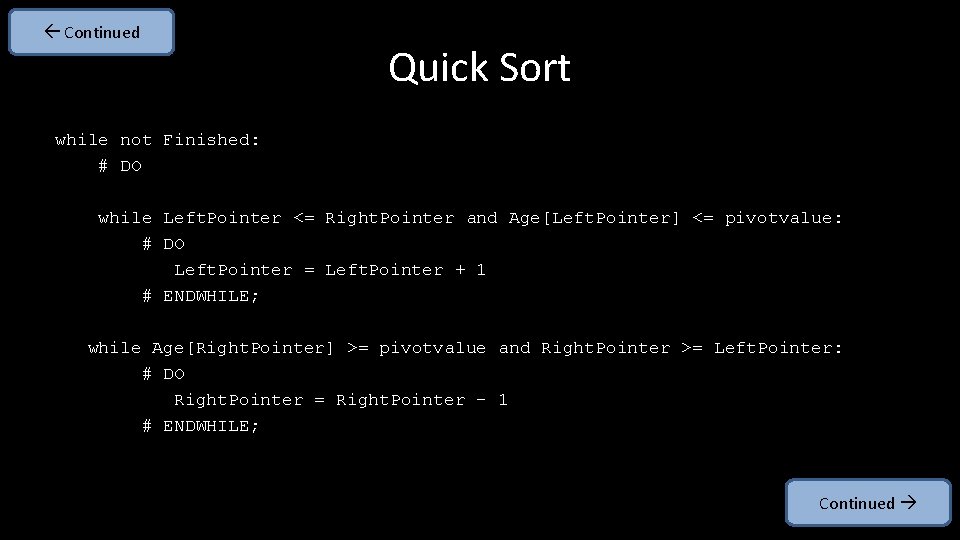
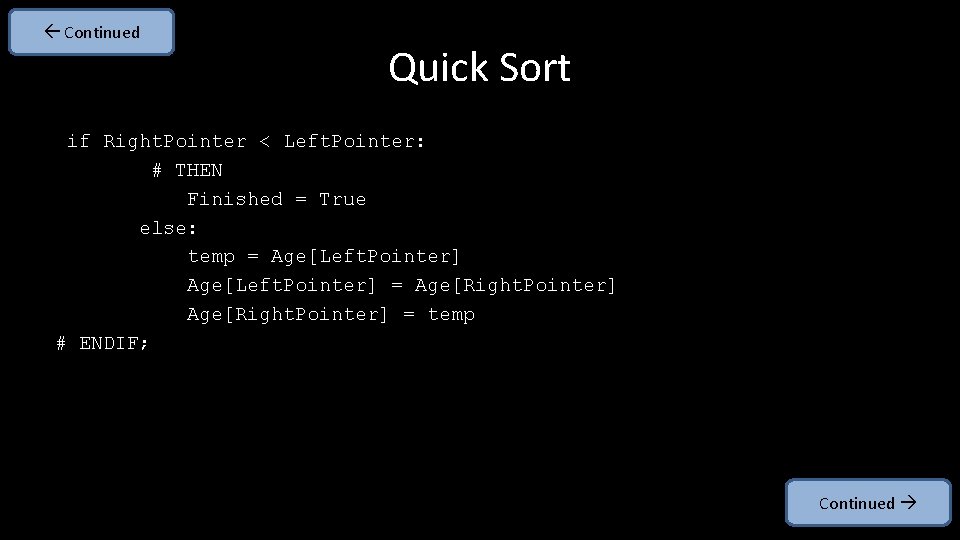
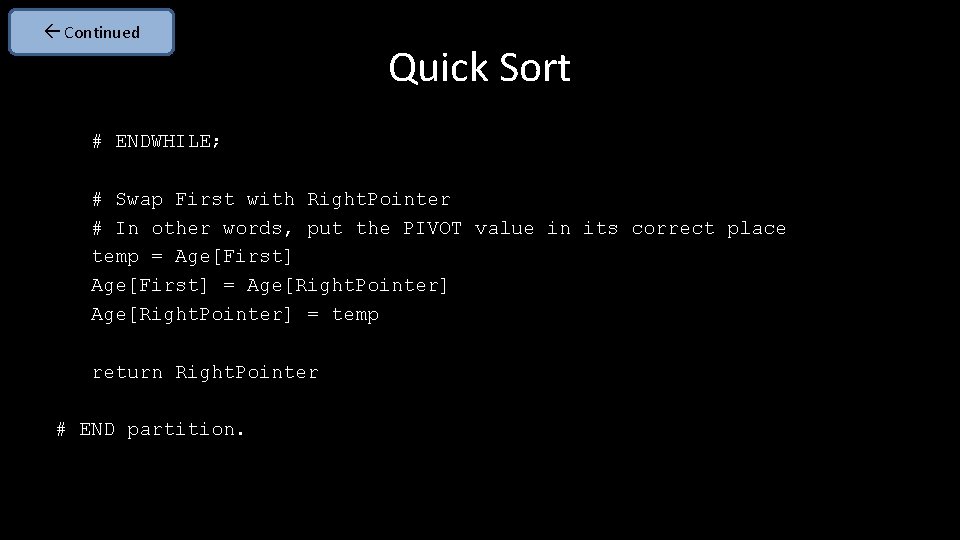
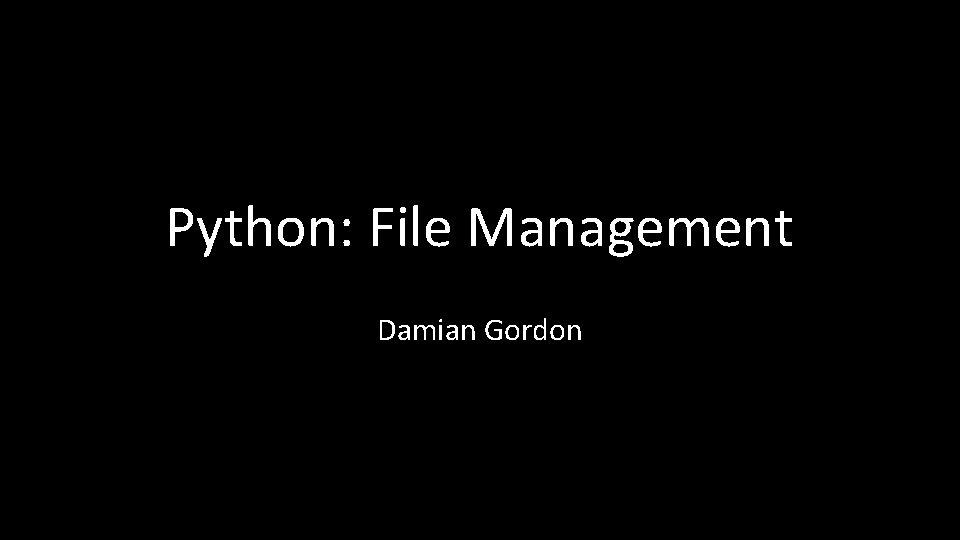
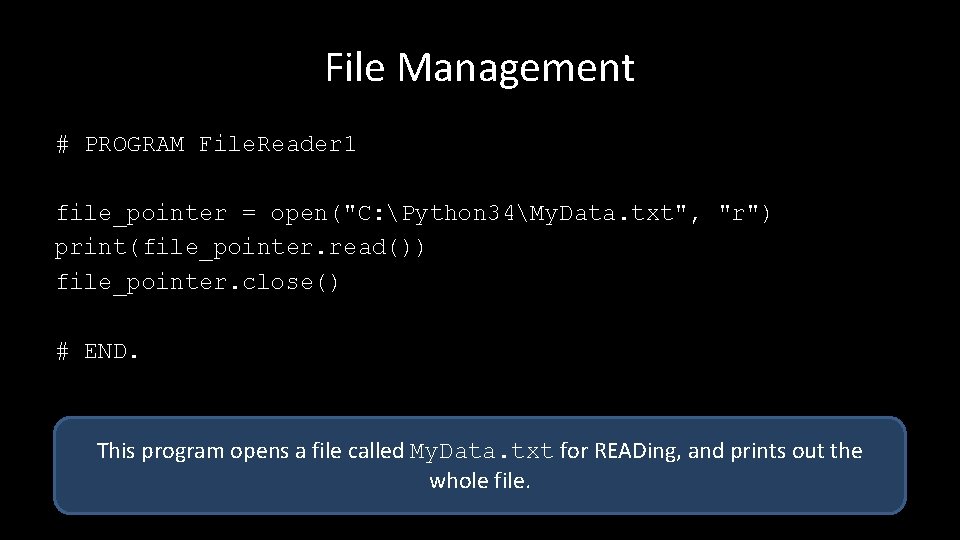
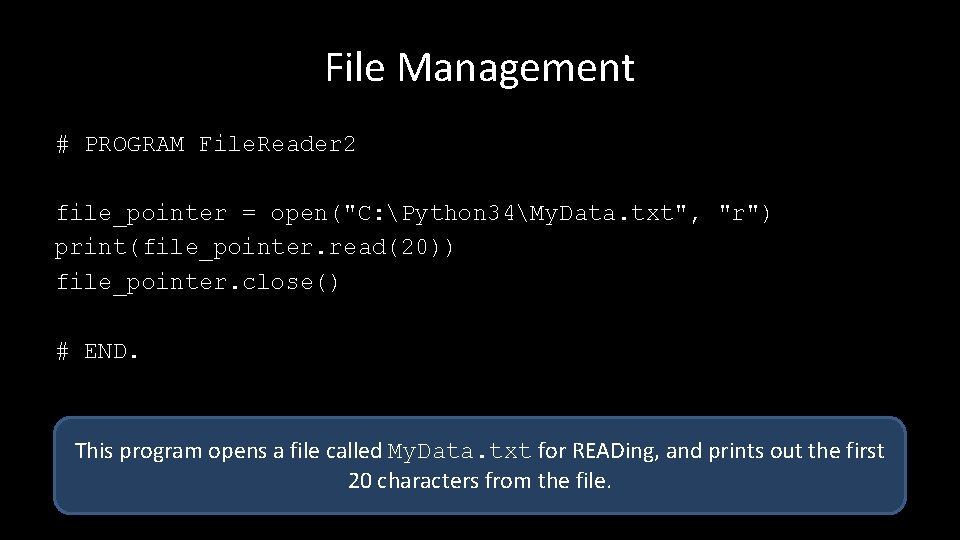
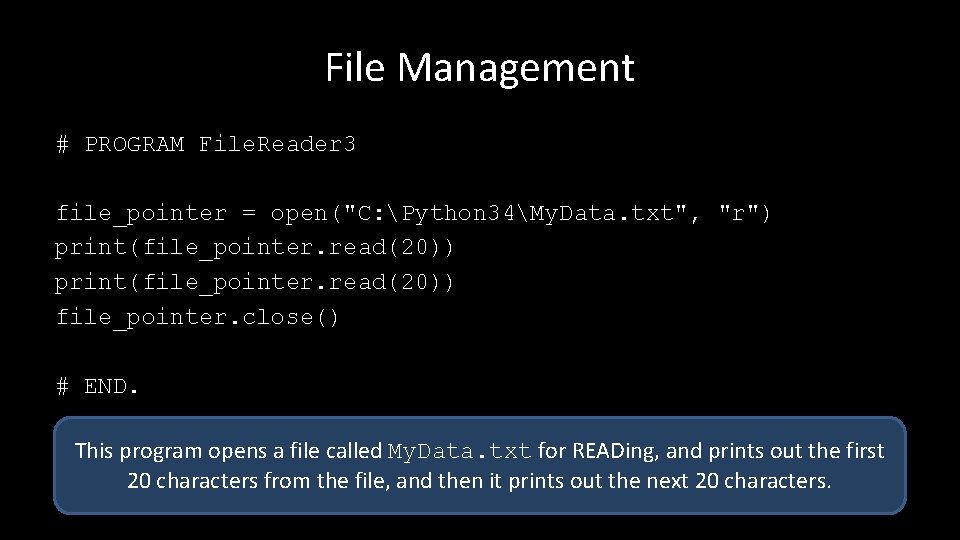
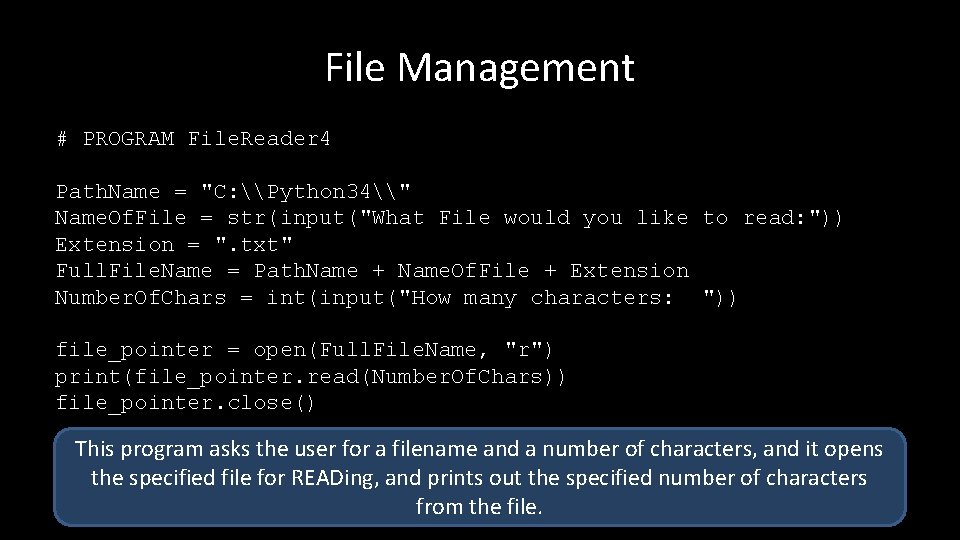
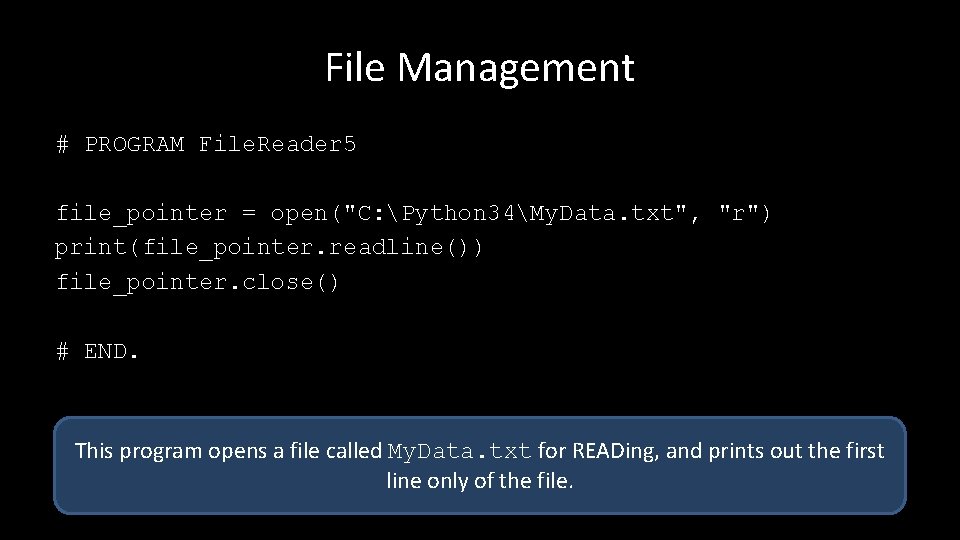
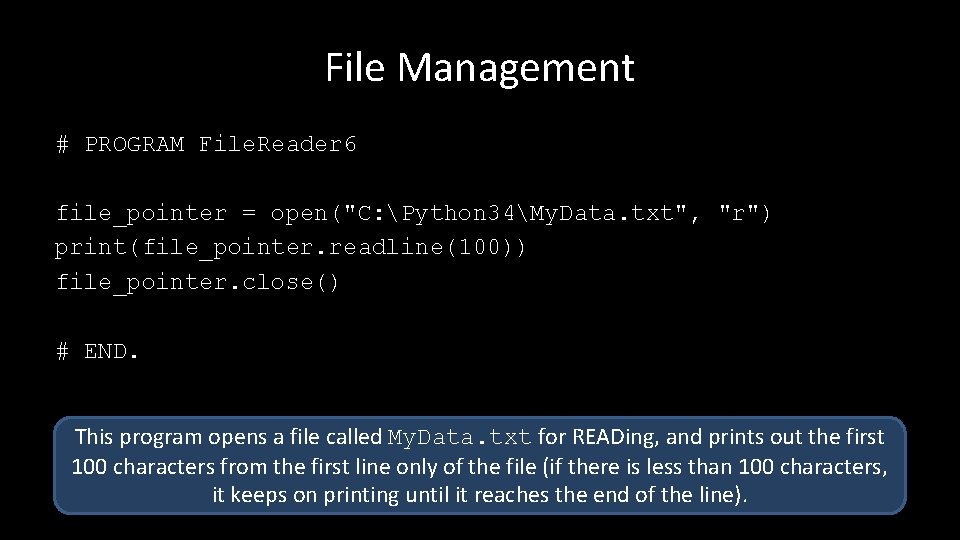
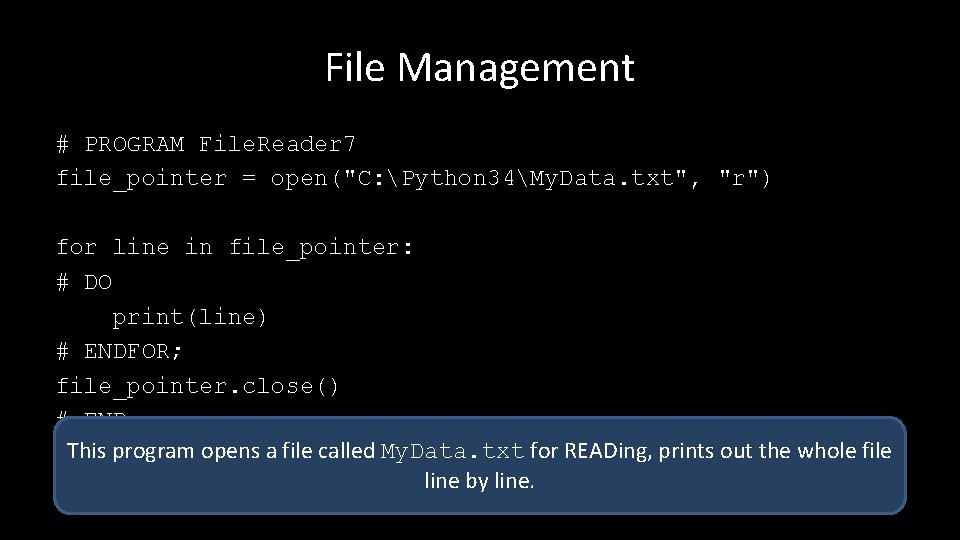
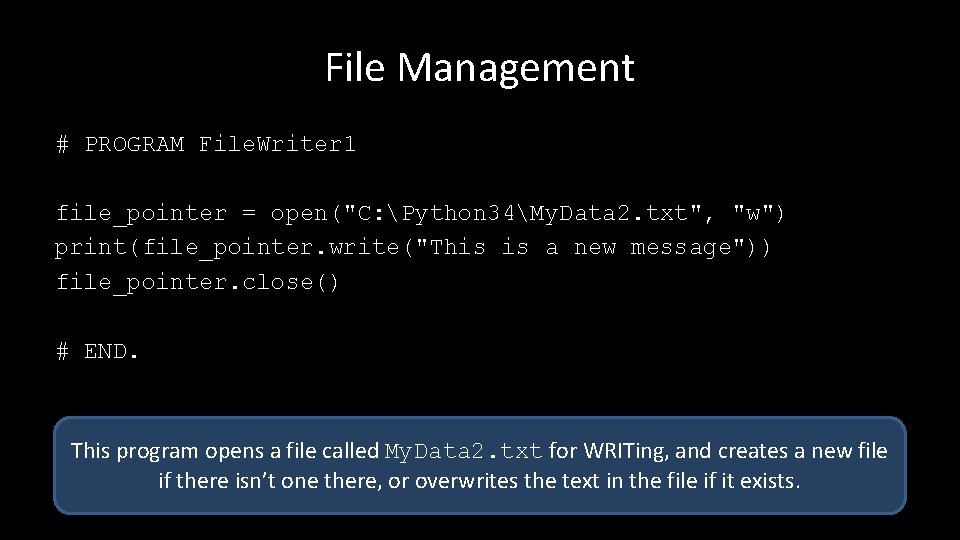
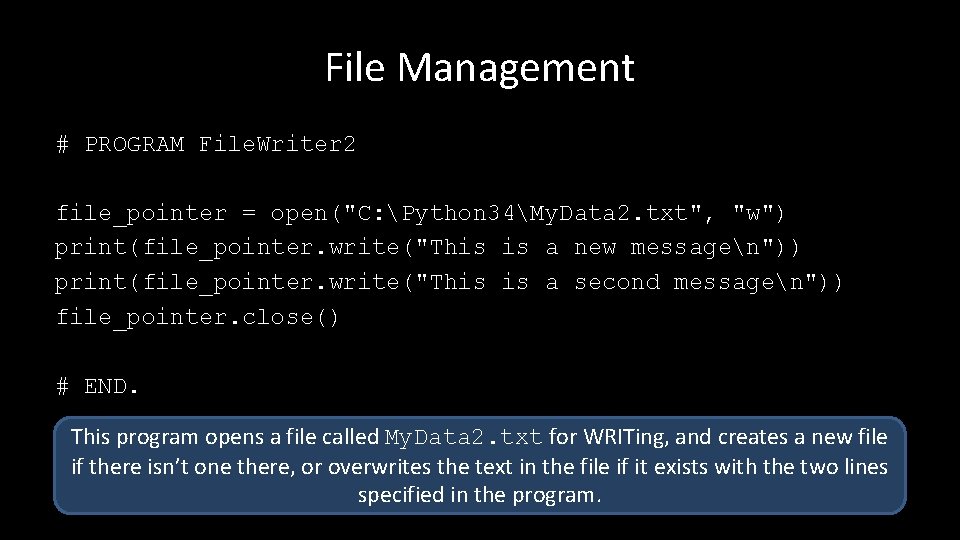
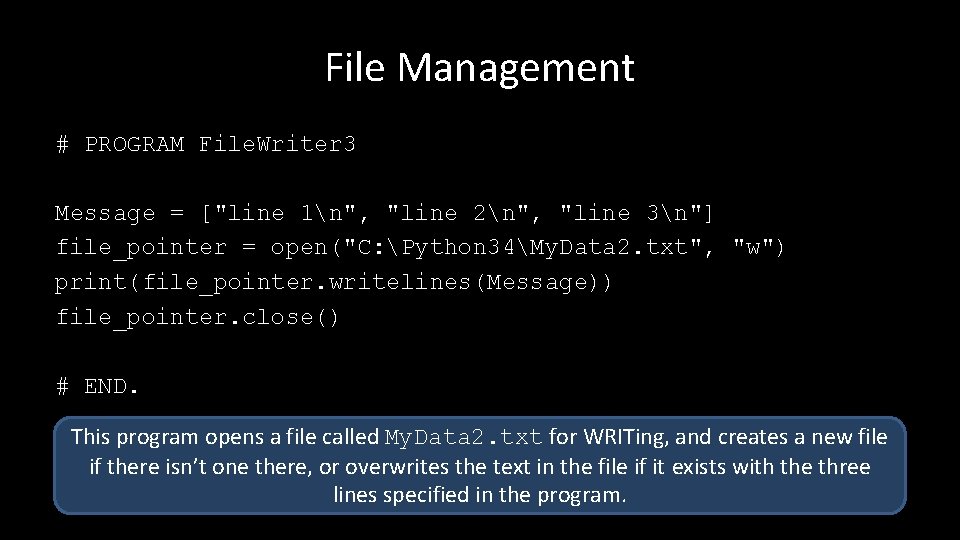
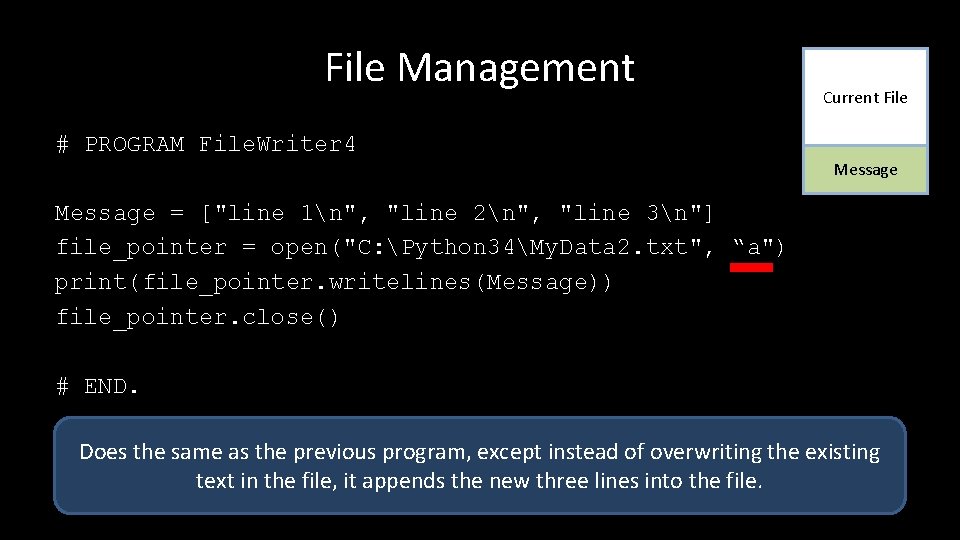
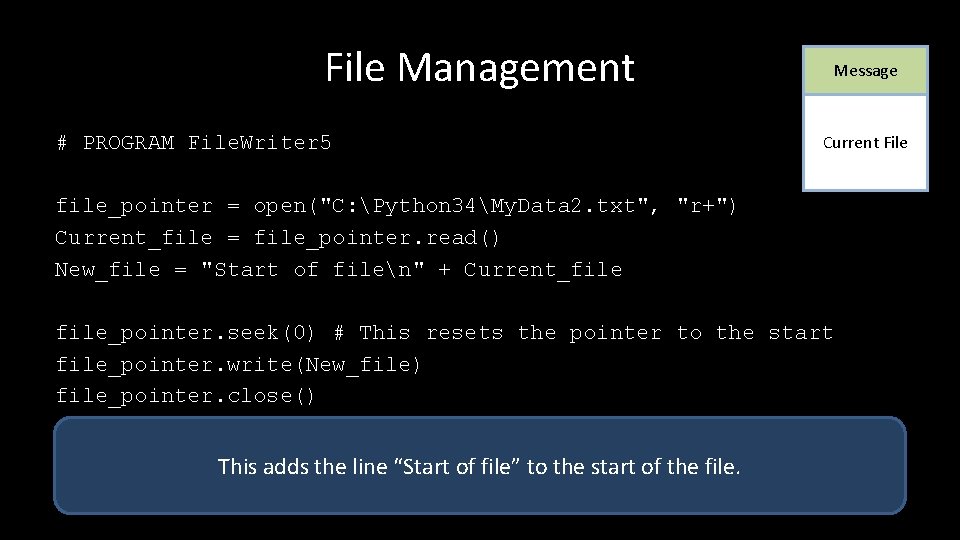
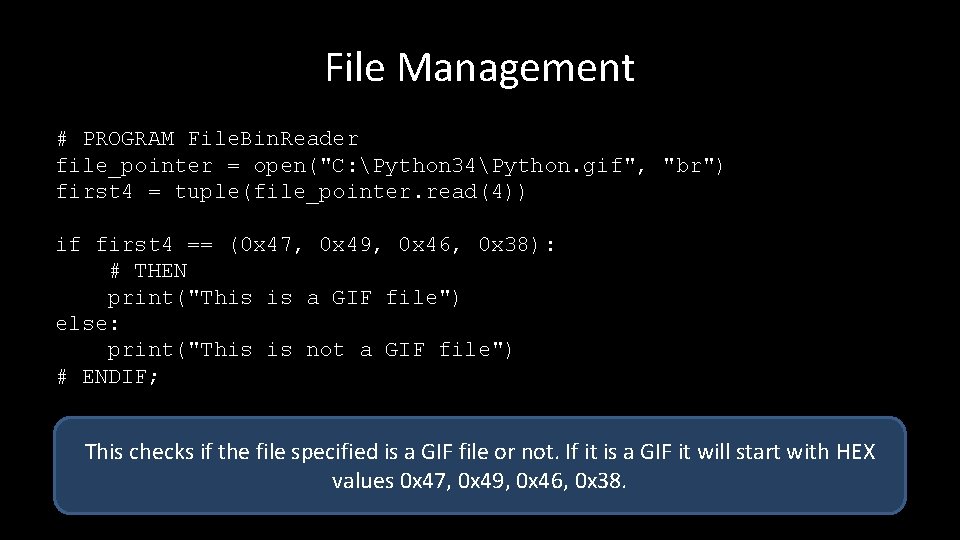
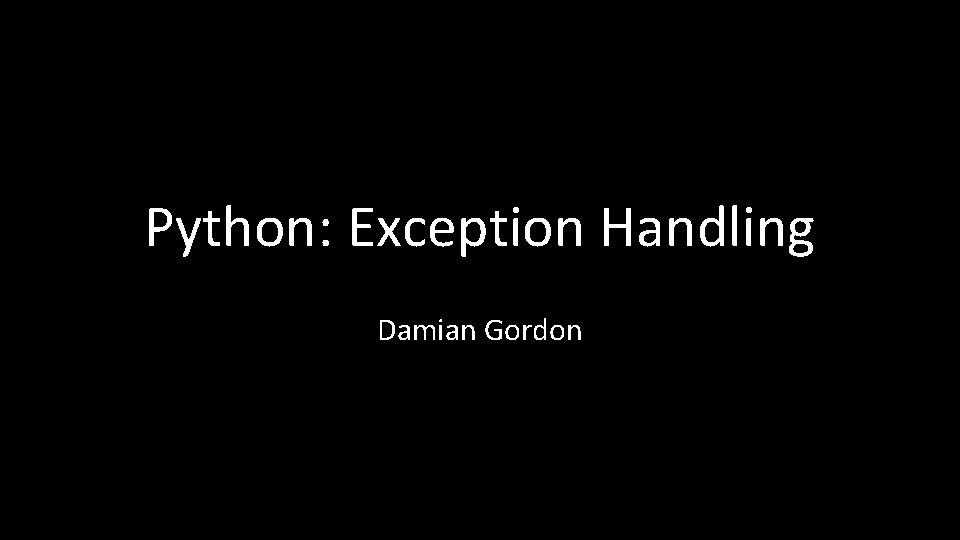
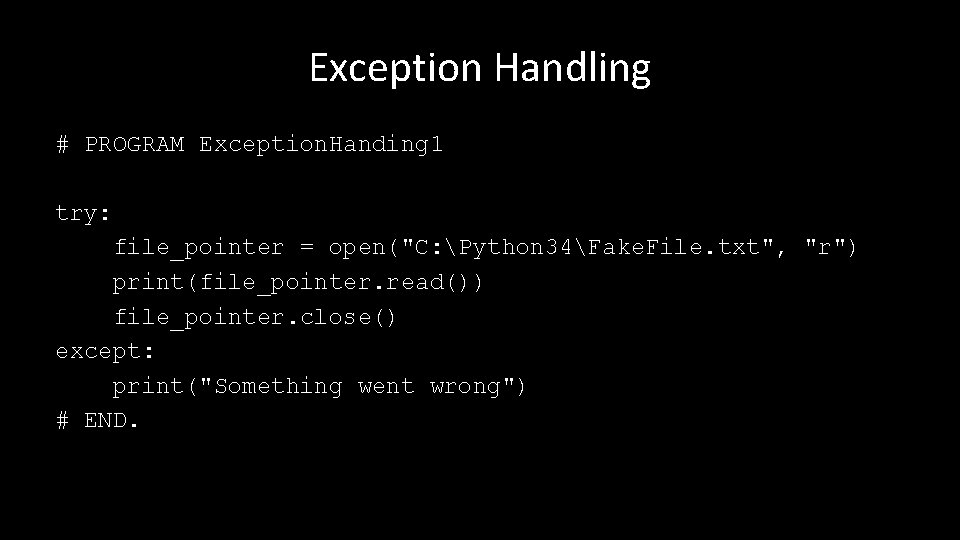
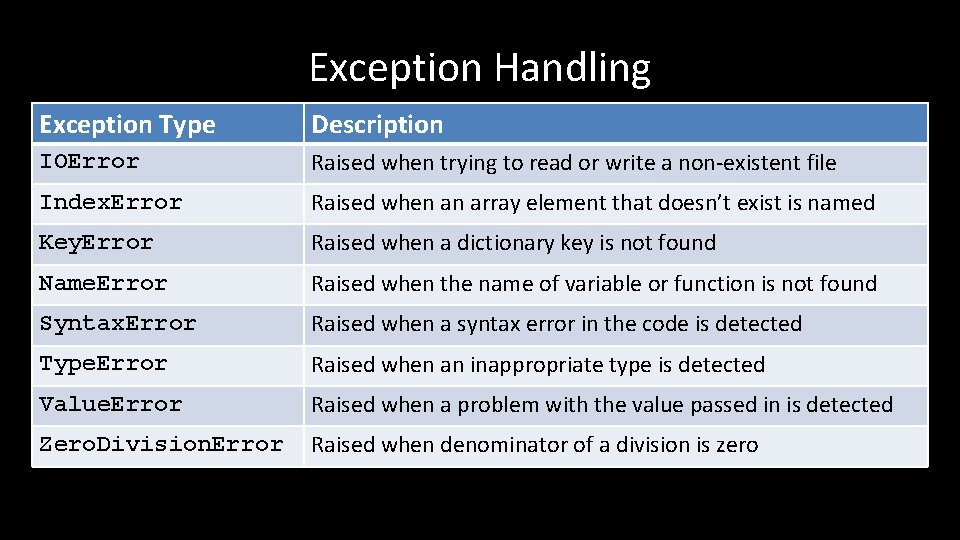
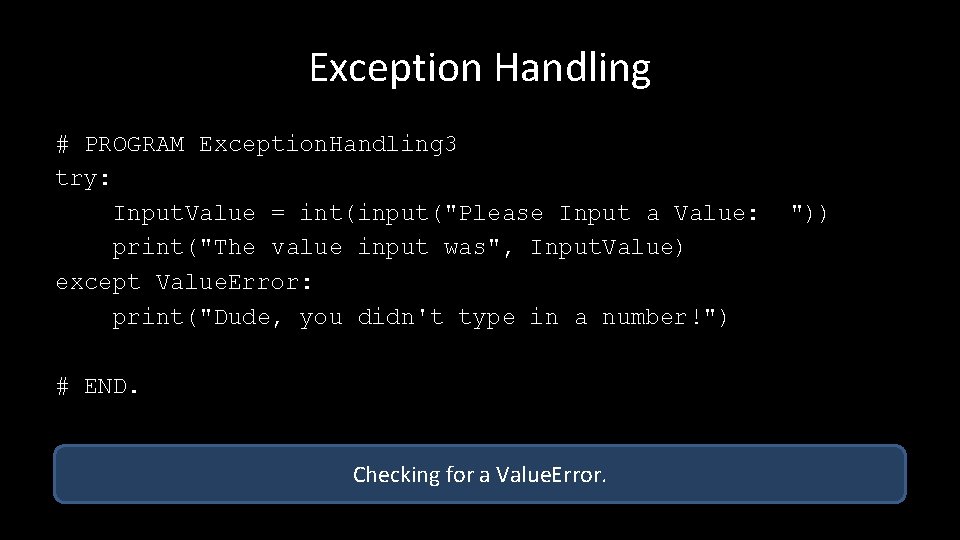
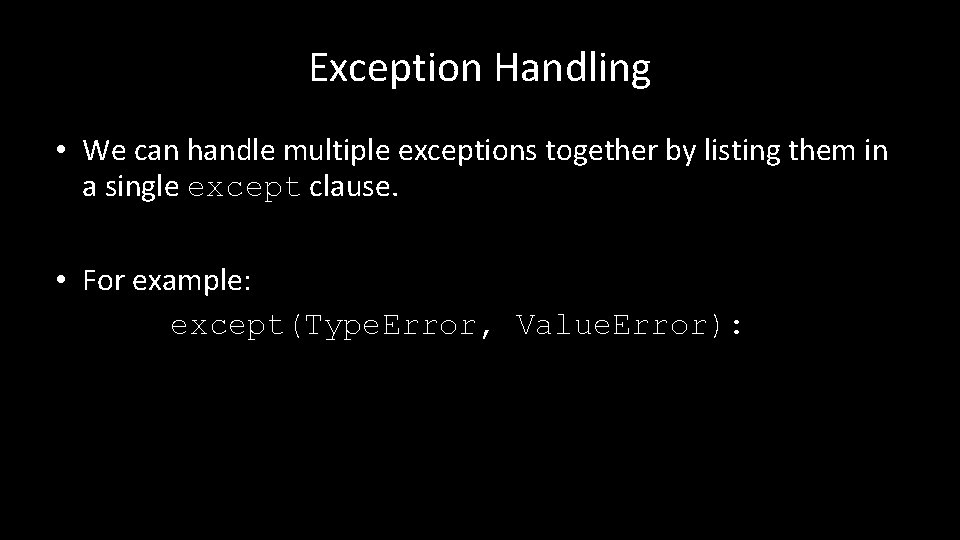
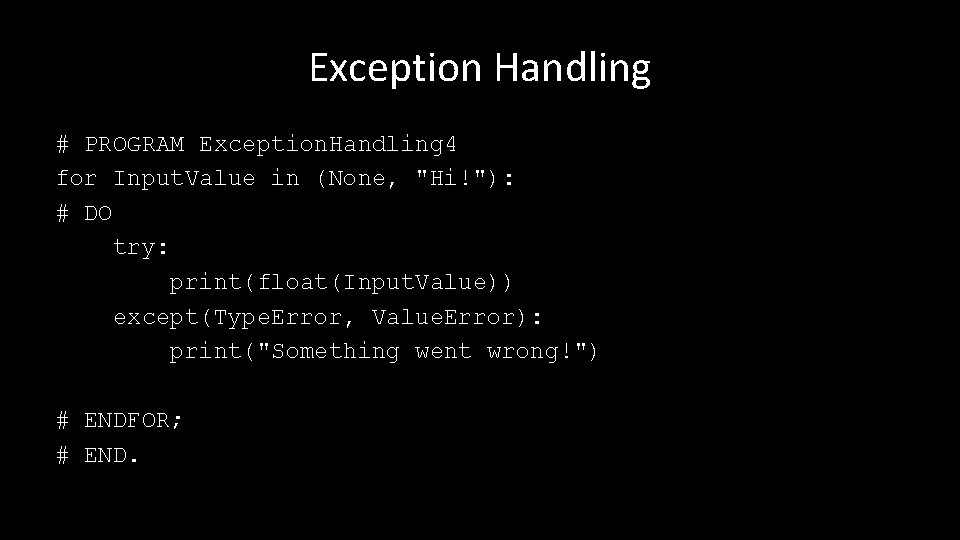
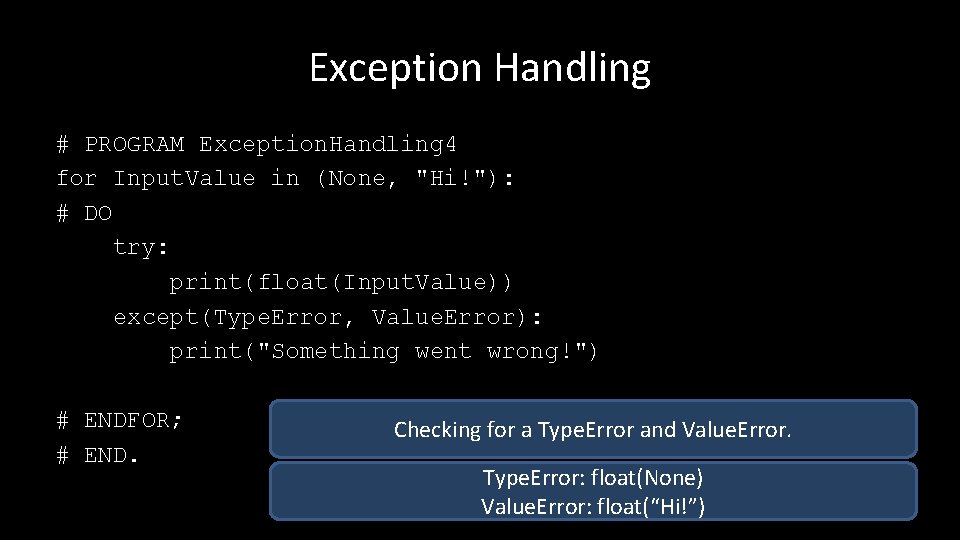
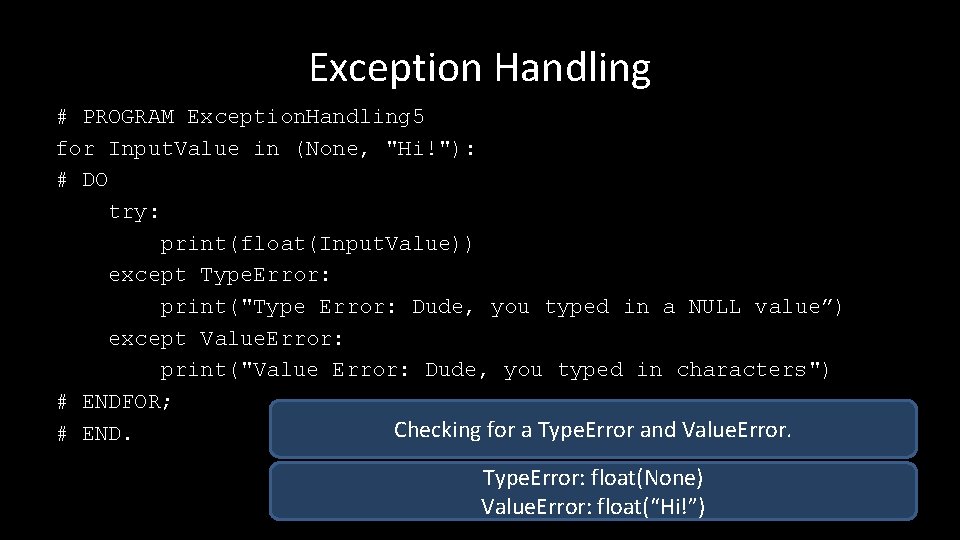
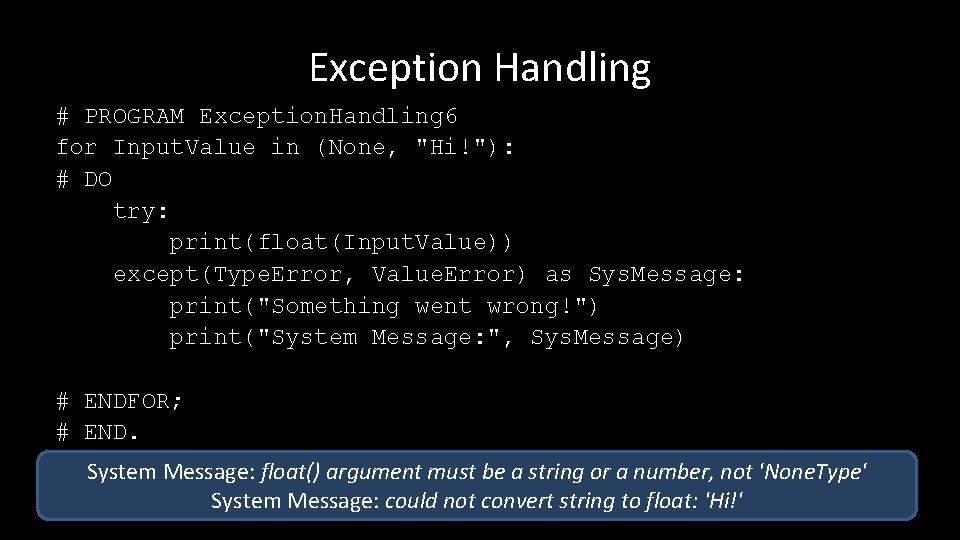
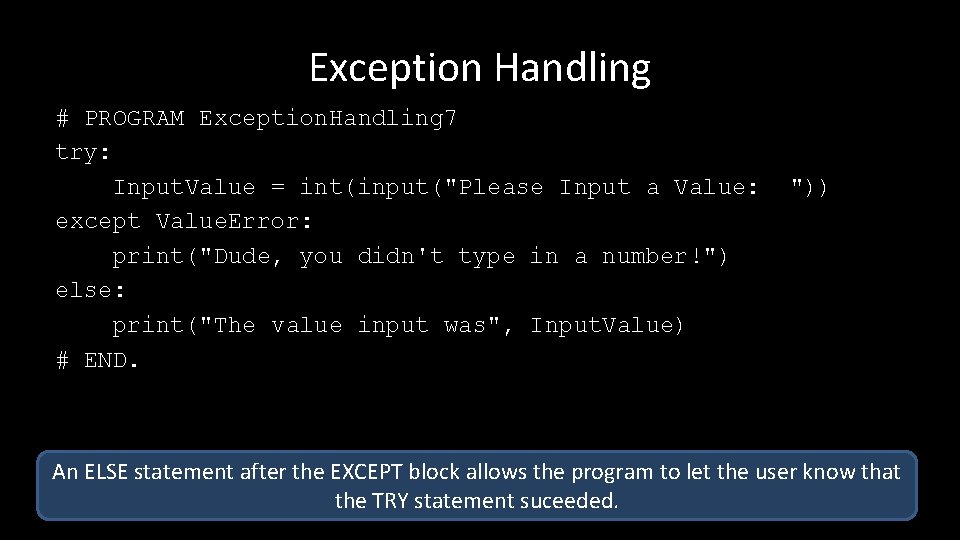
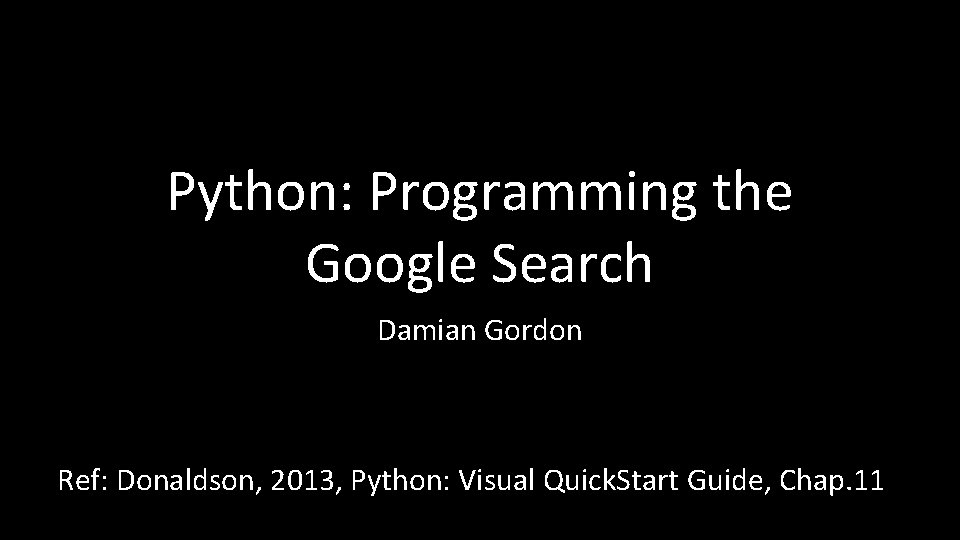
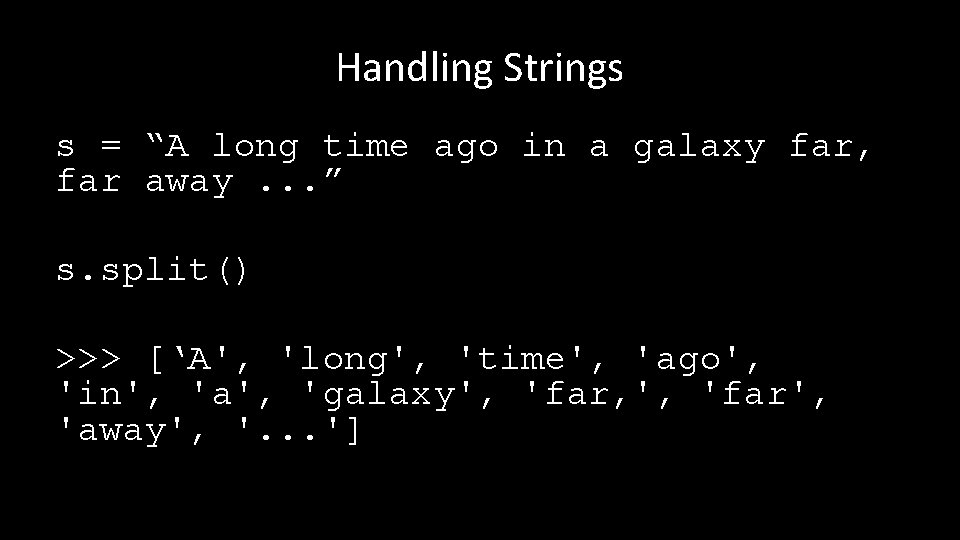
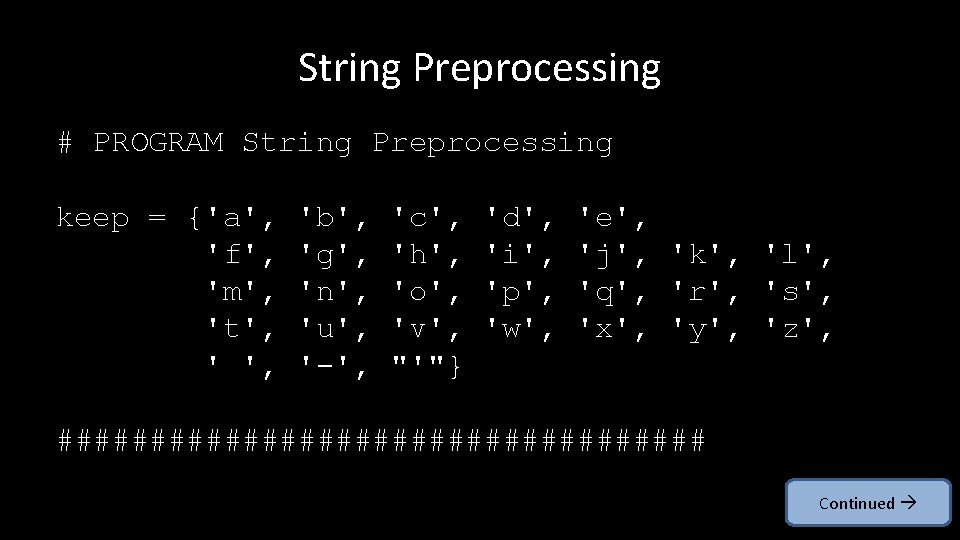
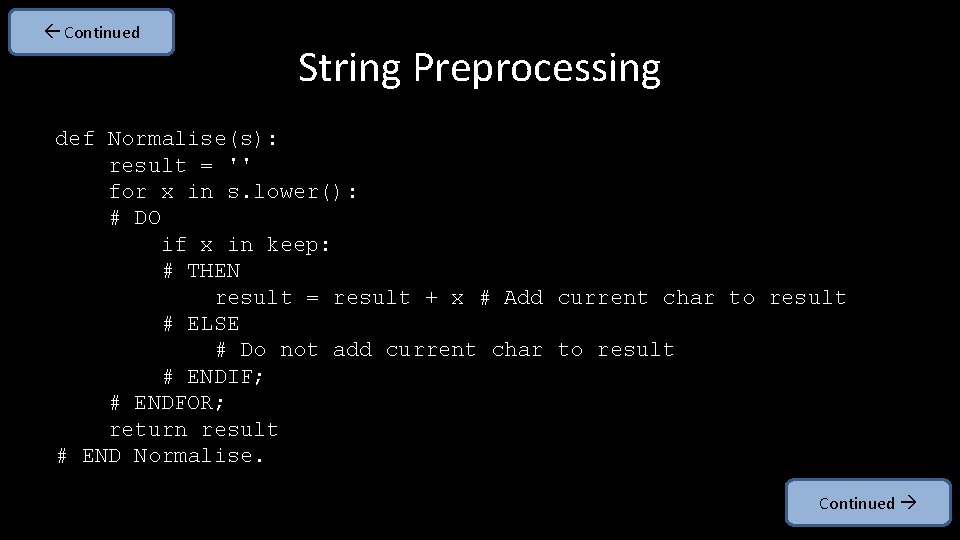
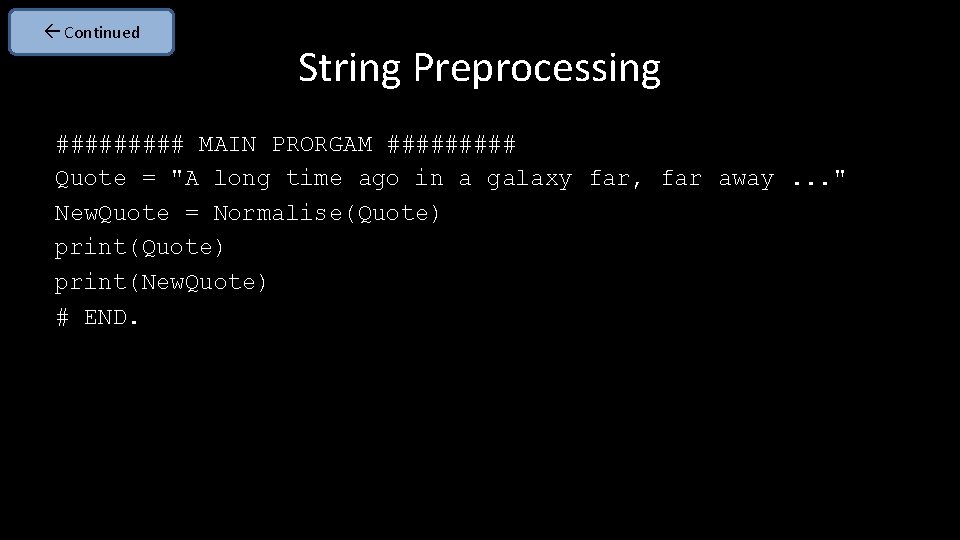
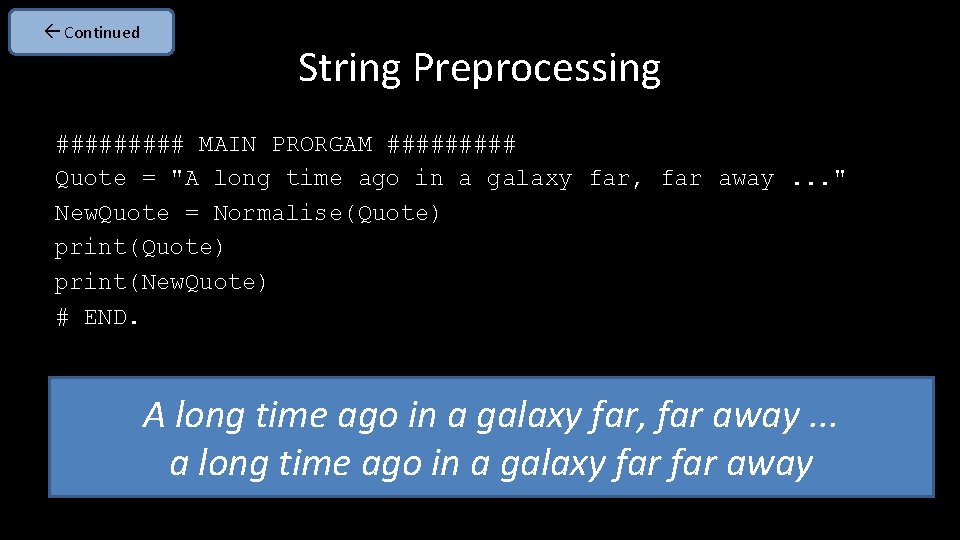
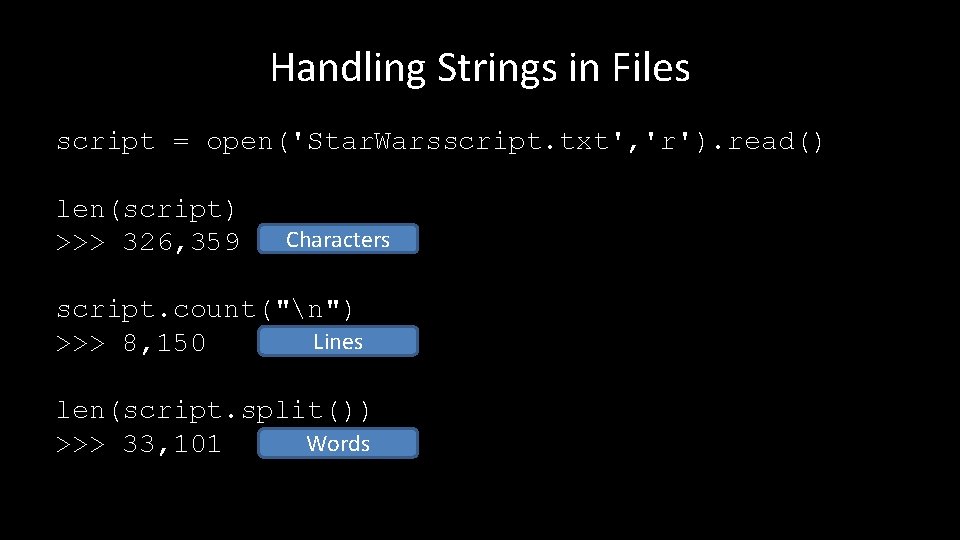
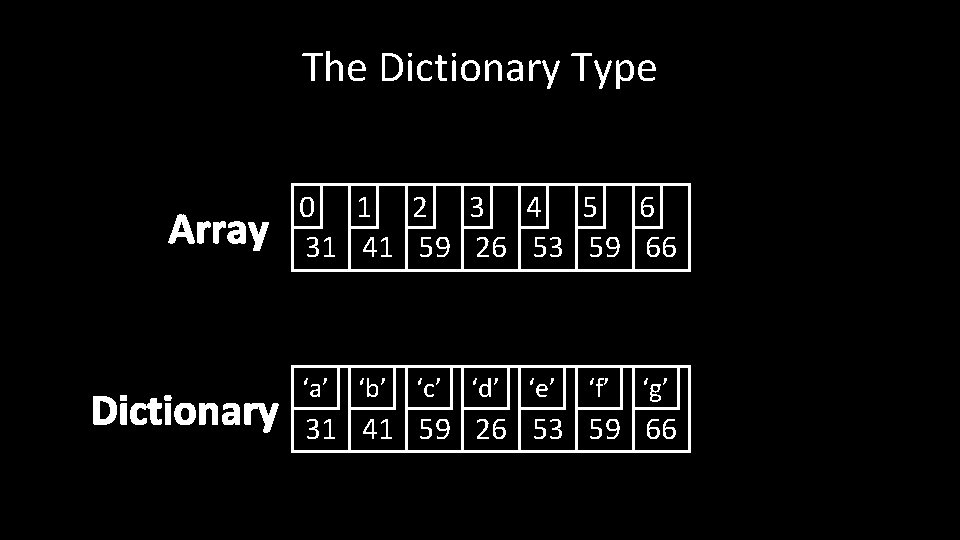
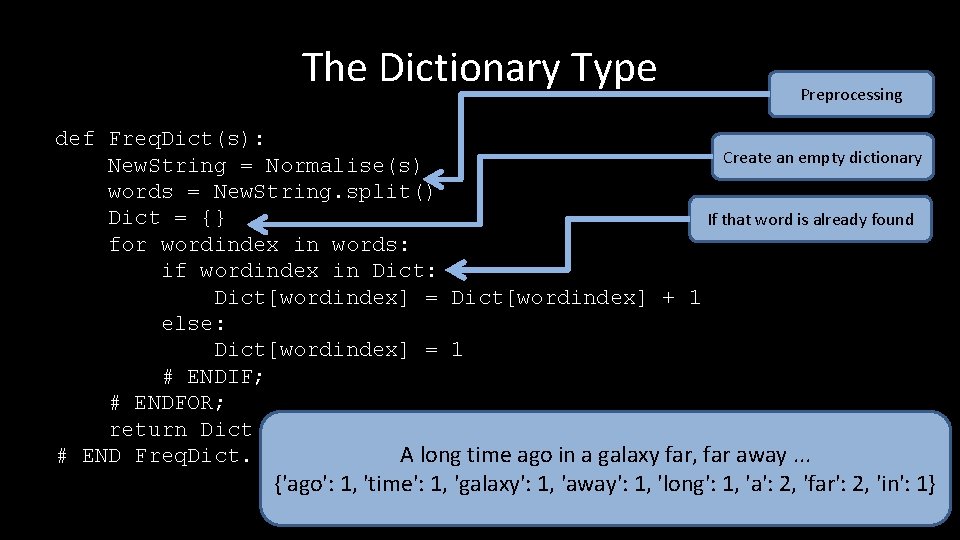
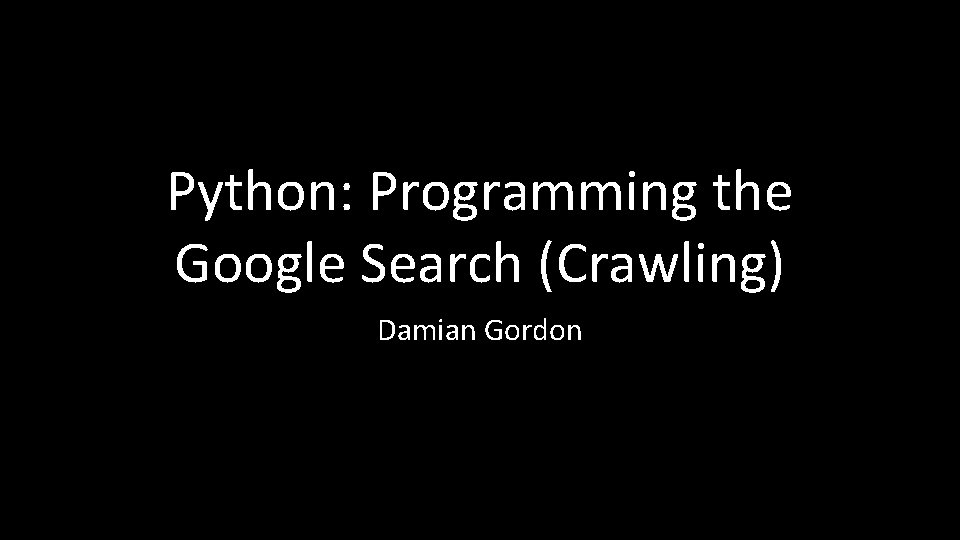
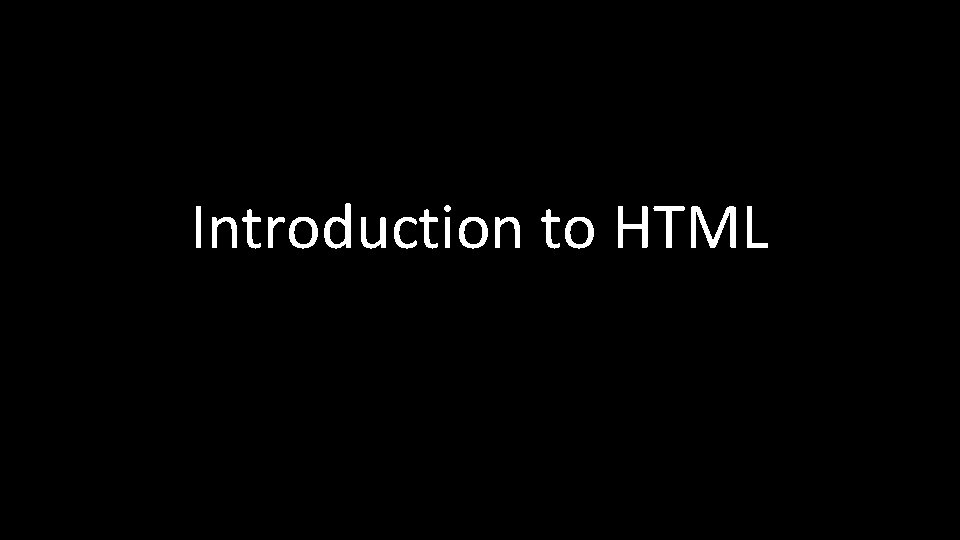
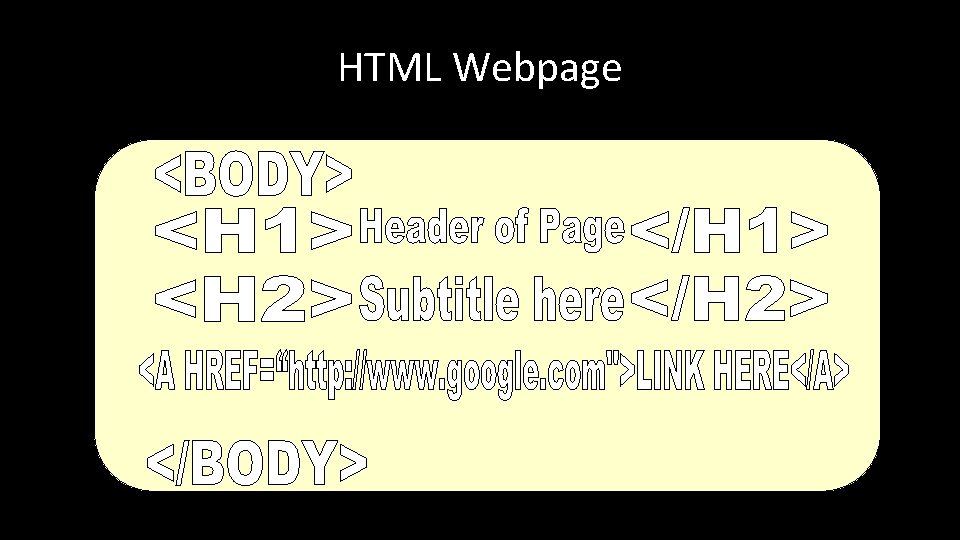
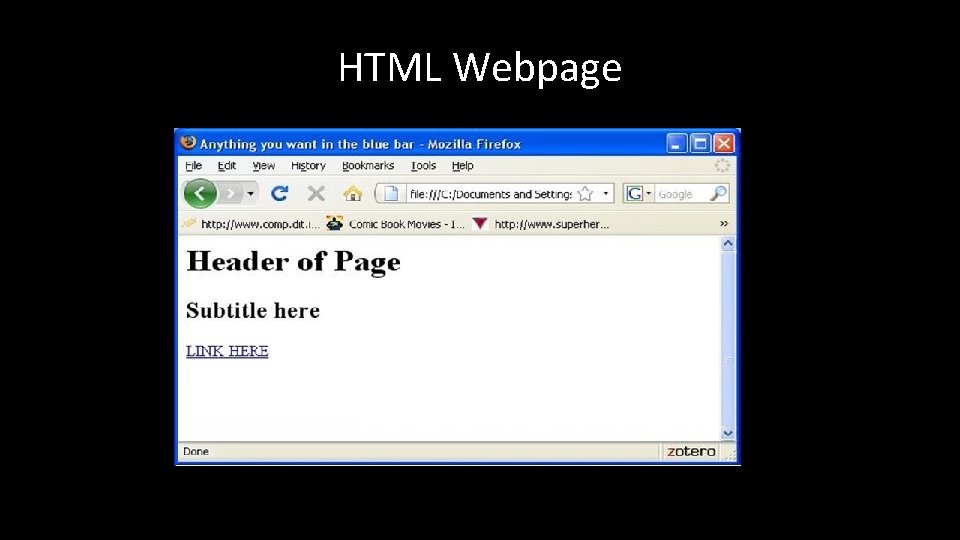
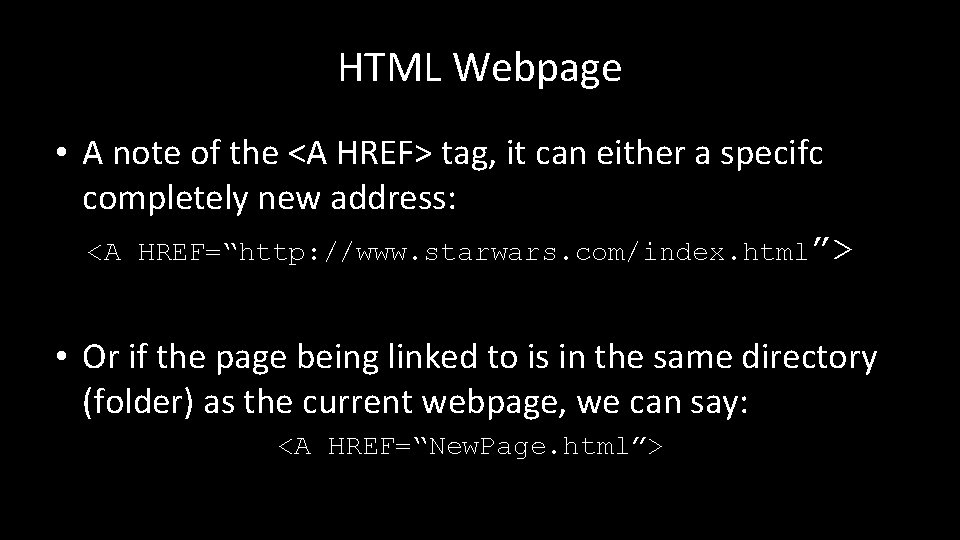
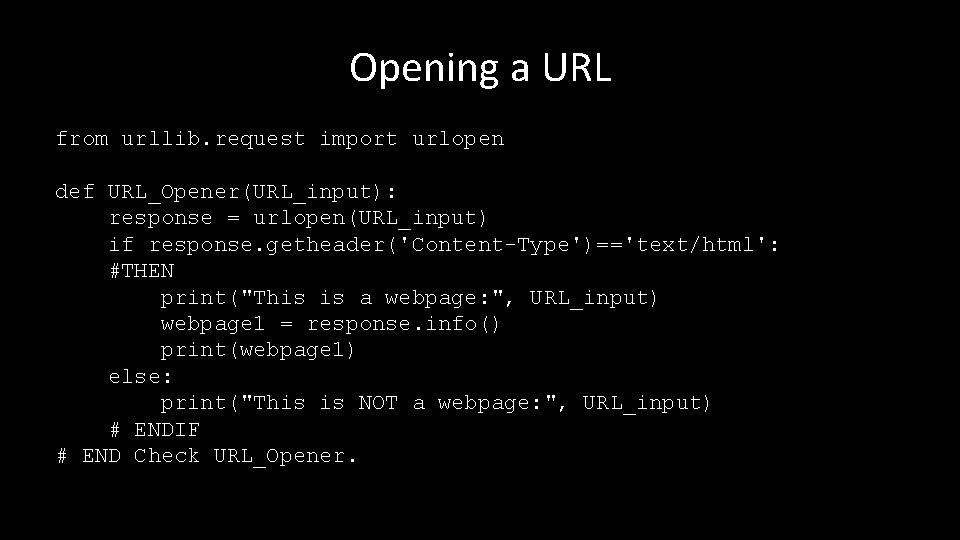
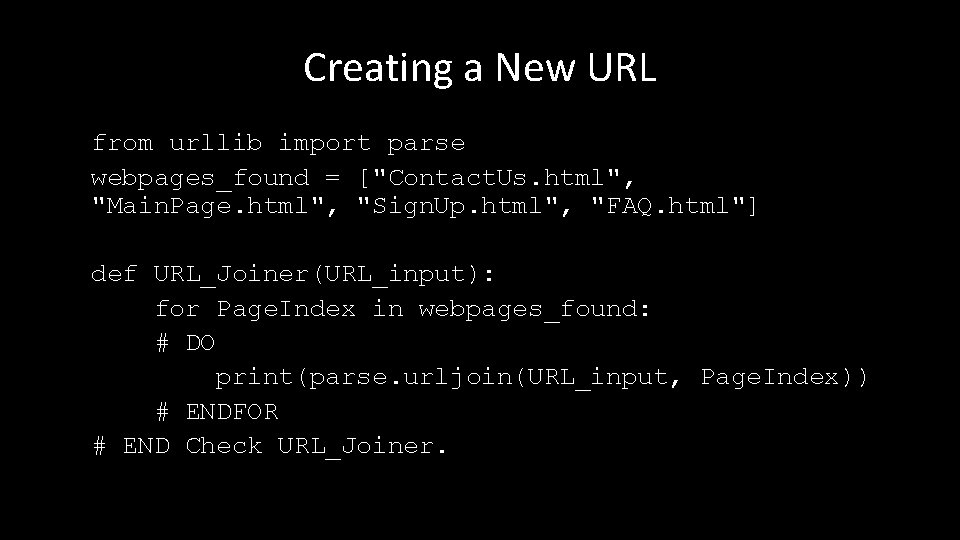
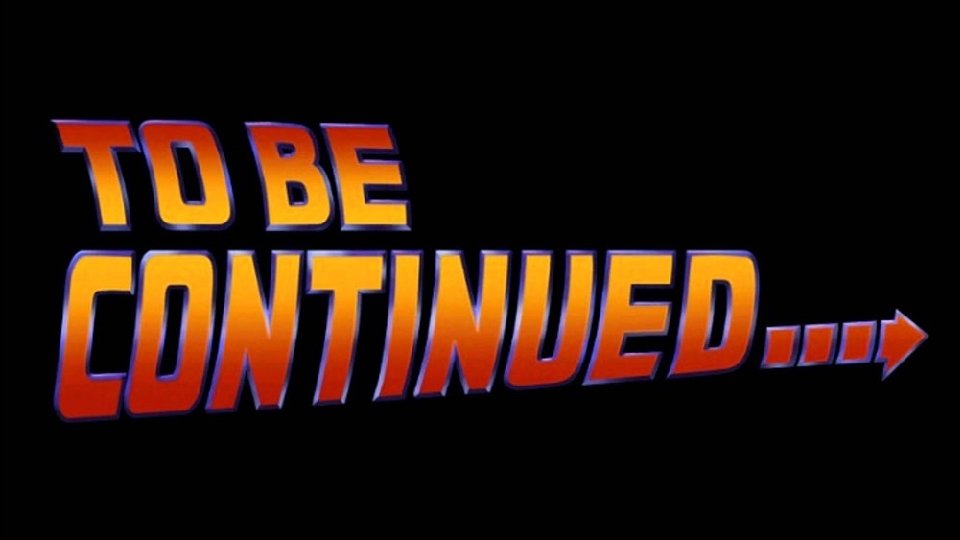
- Slides: 125
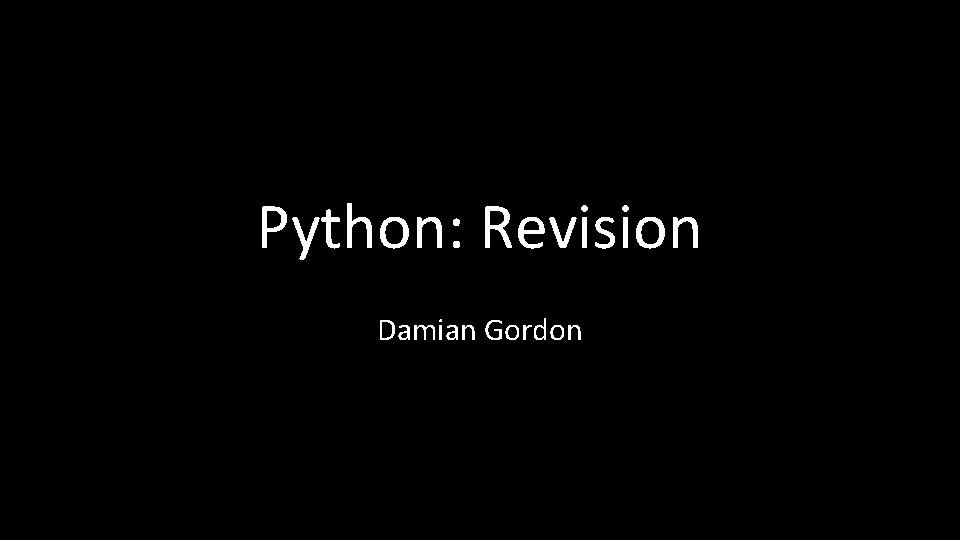
Python: Revision Damian Gordon
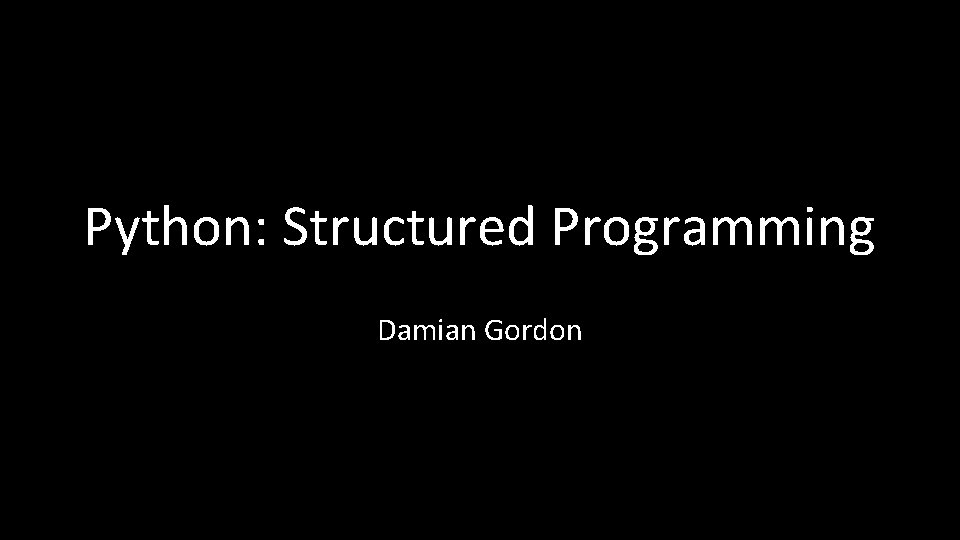
Python: Structured Programming Damian Gordon
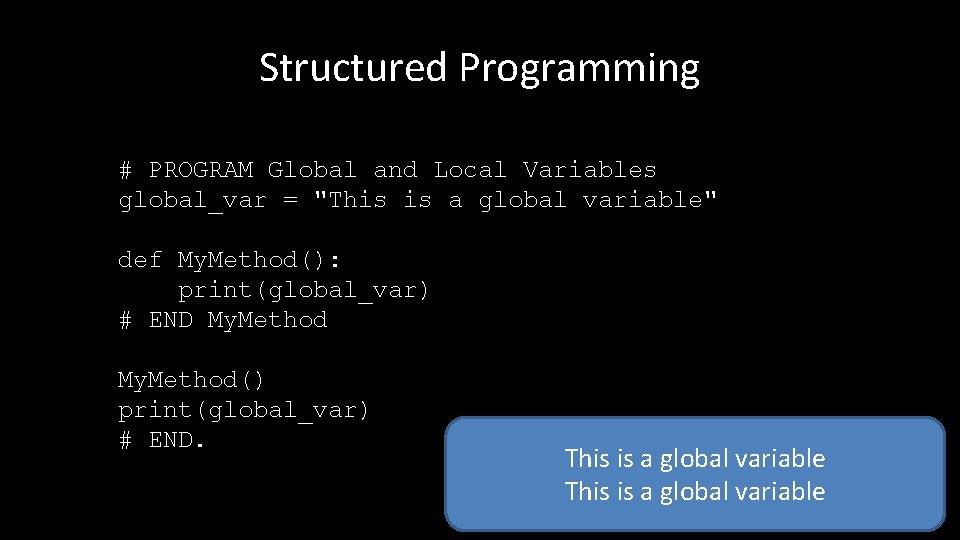
Structured Programming # PROGRAM Global and Local Variables global_var = "This is a global variable" def My. Method(): print(global_var) # END My. Method() print(global_var) # END. This is a global variable
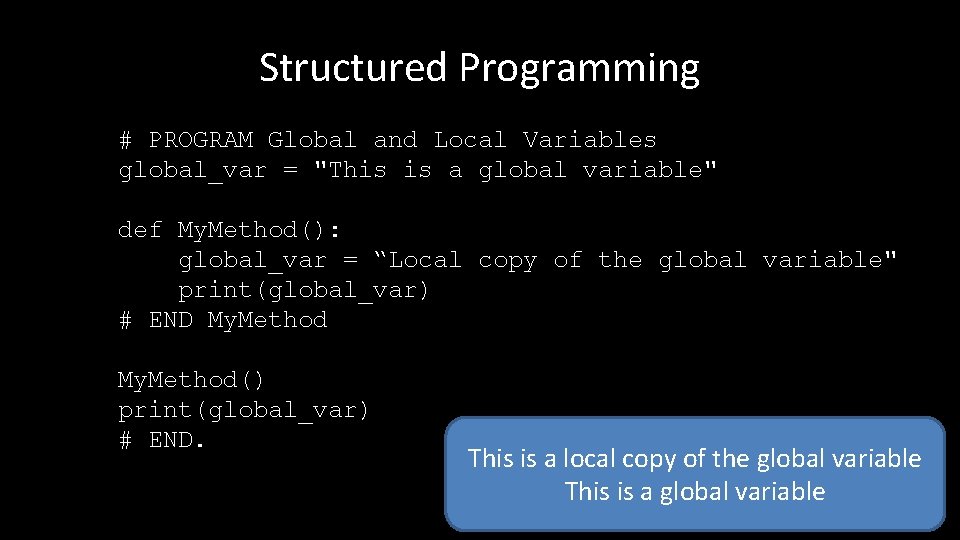
Structured Programming # PROGRAM Global and Local Variables global_var = "This is a global variable" def My. Method(): global_var = “Local copy of the global variable" print(global_var) # END My. Method() print(global_var) # END. This is a local copy of the global variable This is a global variable
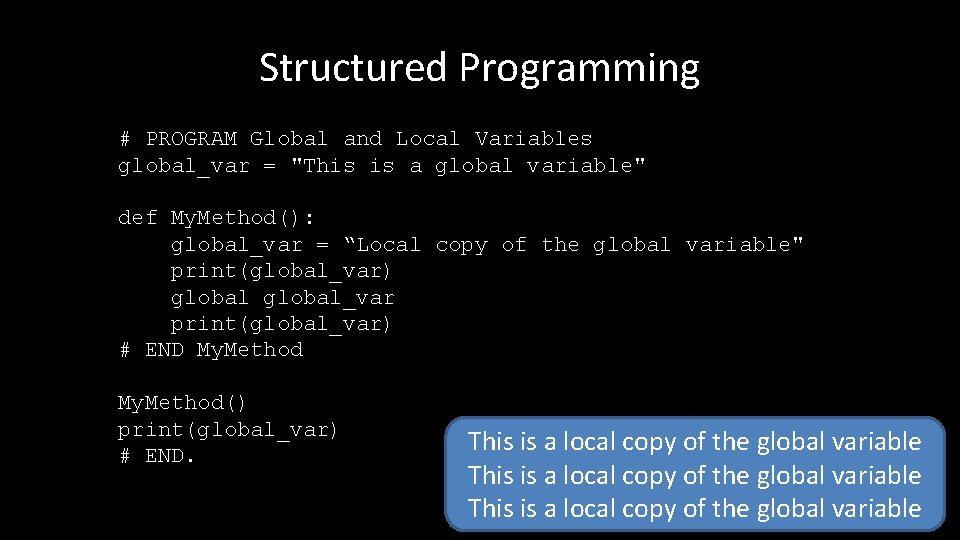
Structured Programming # PROGRAM Global and Local Variables global_var = "This is a global variable" def My. Method(): global_var = “Local copy of the global variable" print(global_var) global_var print(global_var) # END My. Method() print(global_var) # END. This is a local copy of the global variable
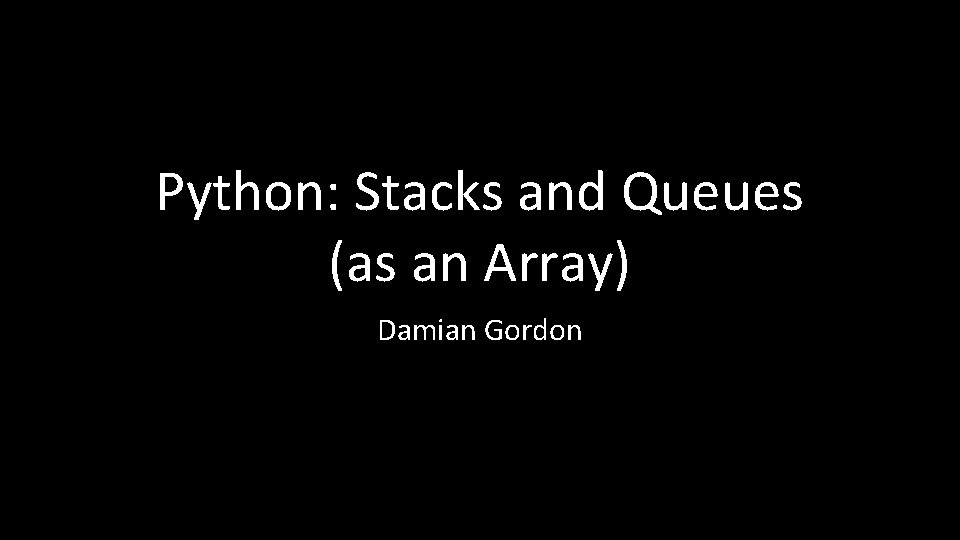
Python: Stacks and Queues (as an Array) Damian Gordon
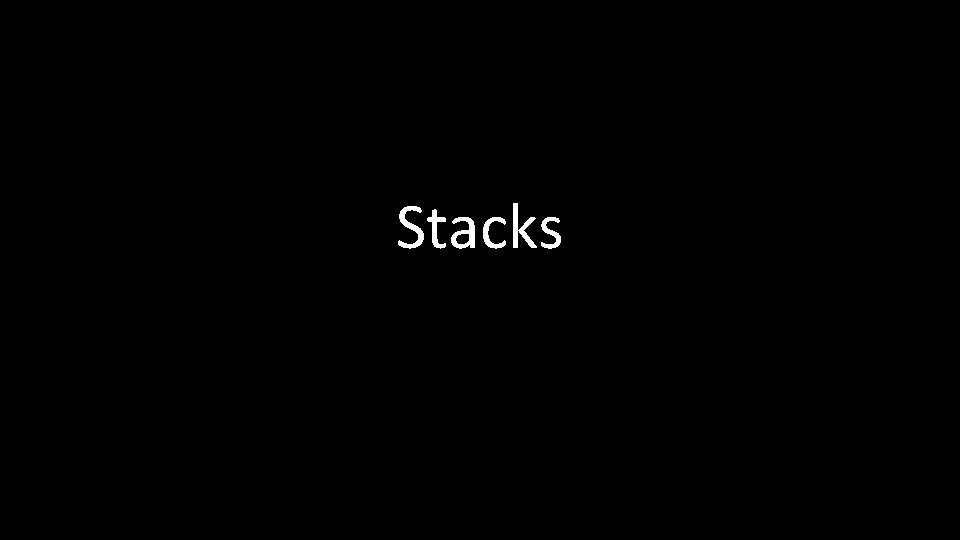
Stacks
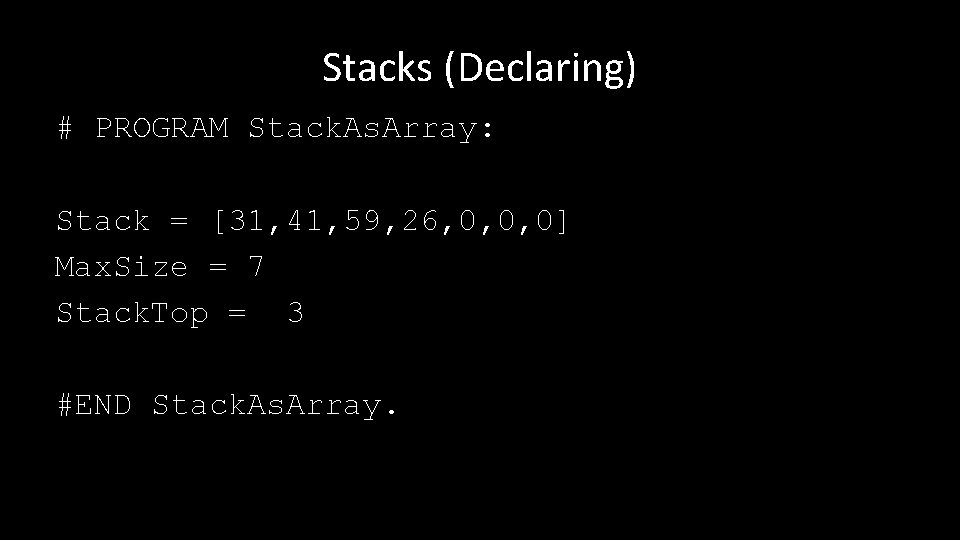
Stacks (Declaring) # PROGRAM Stack. As. Array: Stack = [31, 41, 59, 26, 0, 0, 0] Max. Size = 7 Stack. Top = 3 #END Stack. As. Array.
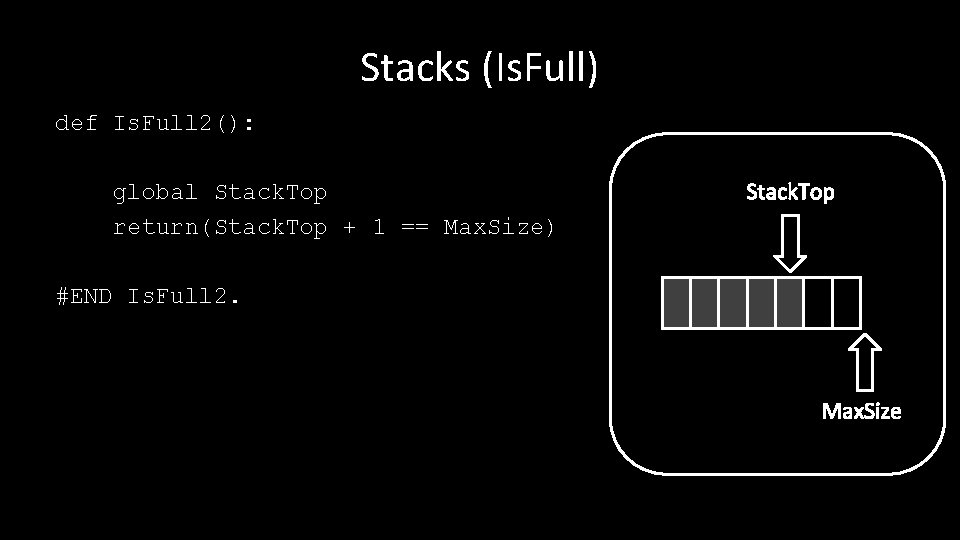
Stacks (Is. Full) def Is. Full 2(): global Stack. Top return(Stack. Top + 1 == Max. Size) Stack. Top #END Is. Full 2. Max. Size
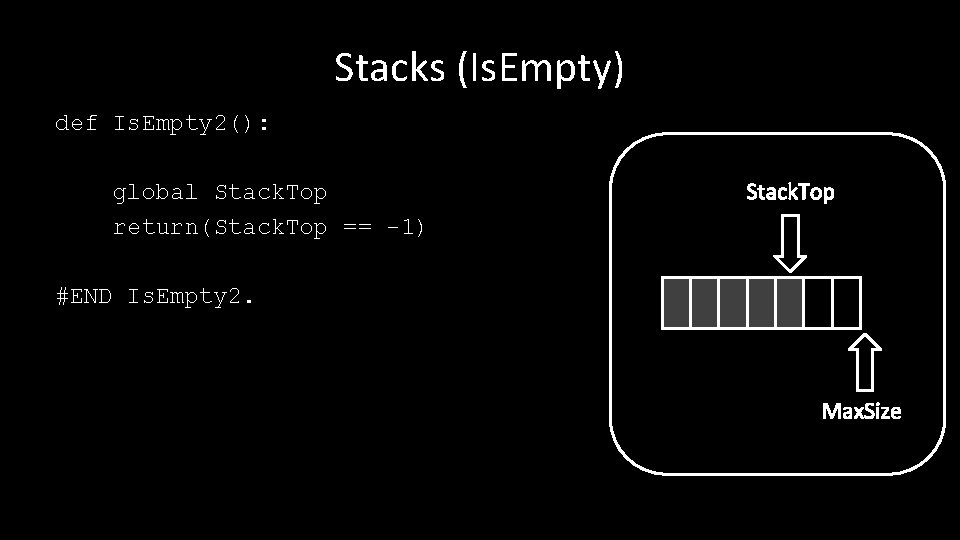
Stacks (Is. Empty) def Is. Empty 2(): global Stack. Top return(Stack. Top == -1) Stack. Top #END Is. Empty 2. Max. Size
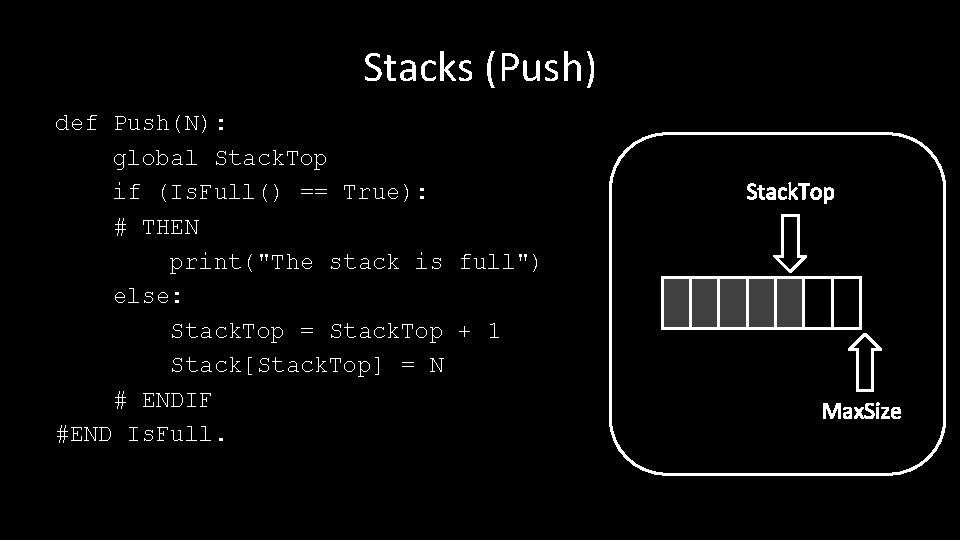
Stacks (Push) def Push(N): global Stack. Top if (Is. Full() == True): # THEN print("The stack is full") else: Stack. Top = Stack. Top + 1 Stack[Stack. Top] = N # ENDIF #END Is. Full. Stack. Top Max. Size
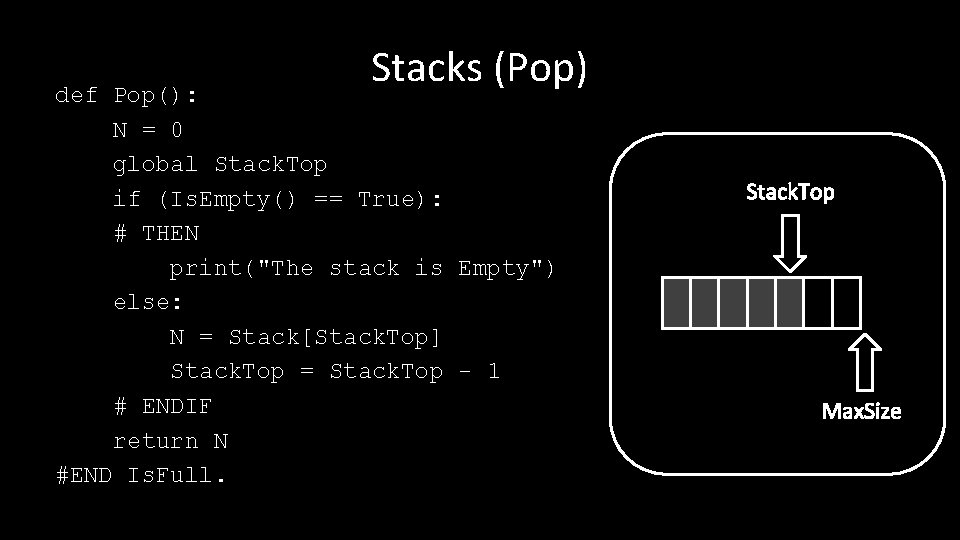
Stacks (Pop) def Pop(): N = 0 global Stack. Top if (Is. Empty() == True): # THEN print("The stack is Empty") else: N = Stack[Stack. Top] Stack. Top = Stack. Top - 1 # ENDIF return N #END Is. Full. Stack. Top Max. Size
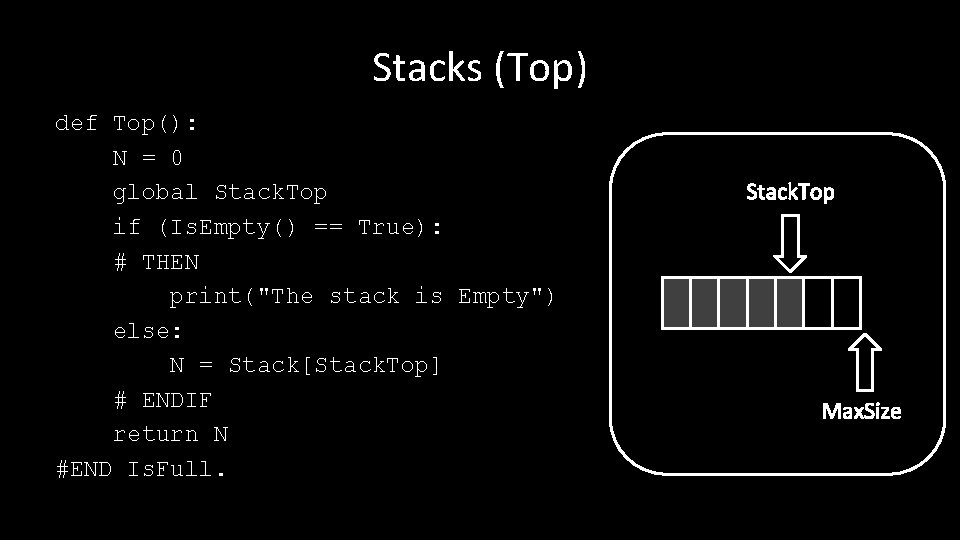
Stacks (Top) def Top(): N = 0 global Stack. Top if (Is. Empty() == True): # THEN print("The stack is Empty") else: N = Stack[Stack. Top] # ENDIF return N #END Is. Full. Stack. Top Max. Size
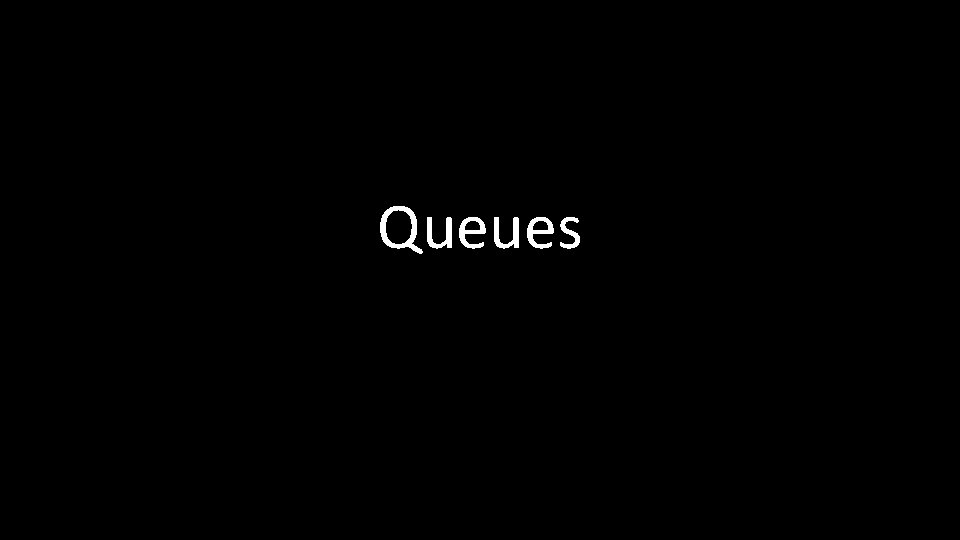
Queues
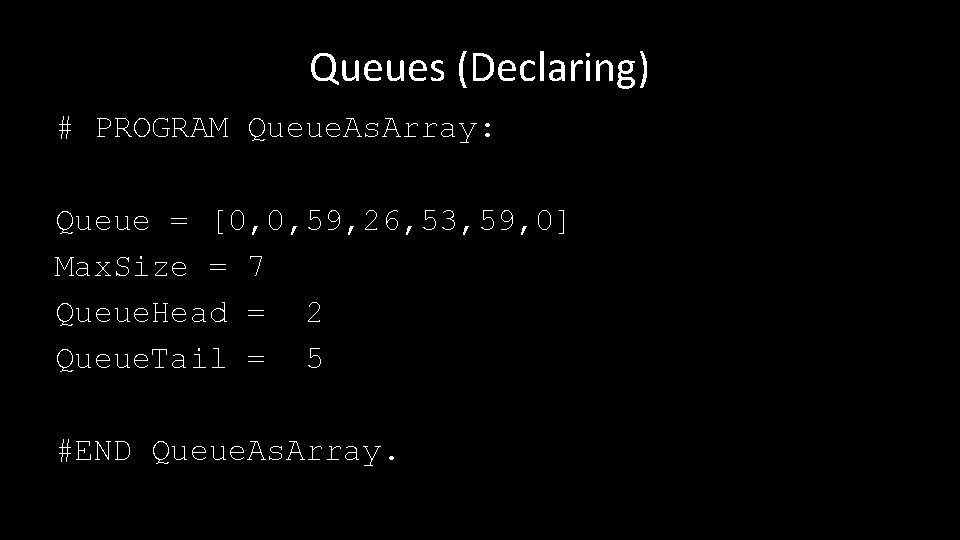
Queues (Declaring) # PROGRAM Queue. As. Array: Queue = [0, 0, 59, 26, 53, 59, 0] Max. Size = 7 Queue. Head = 2 Queue. Tail = 5 #END Queue. As. Array.
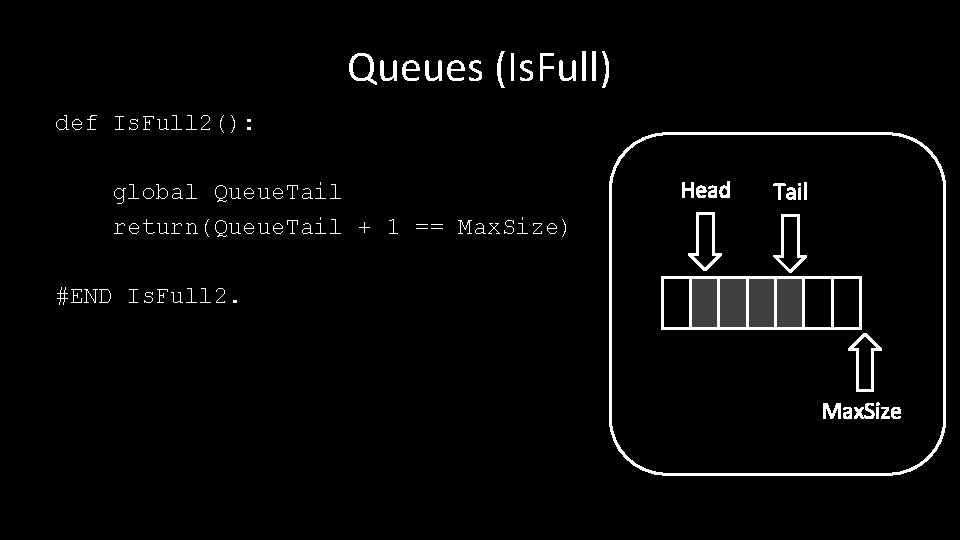
Queues (Is. Full) def Is. Full 2(): global Queue. Tail return(Queue. Tail + 1 == Max. Size) Head Tail #END Is. Full 2. Max. Size
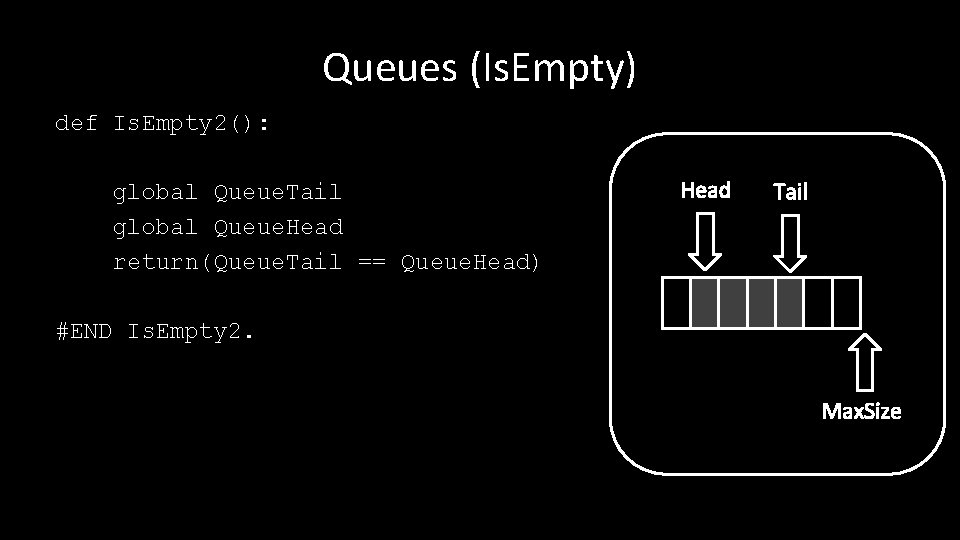
Queues (Is. Empty) def Is. Empty 2(): global Queue. Tail global Queue. Head return(Queue. Tail == Queue. Head) Head Tail #END Is. Empty 2. Max. Size
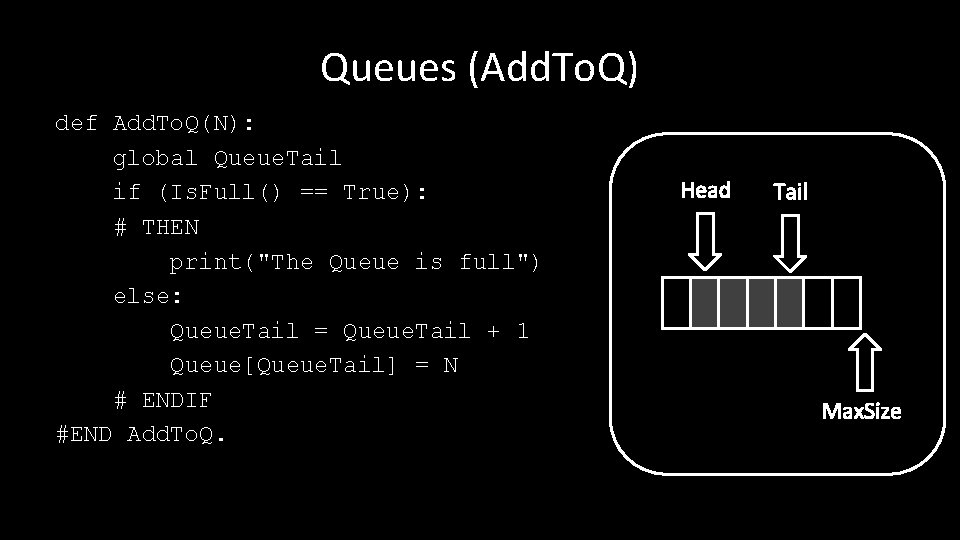
Queues (Add. To. Q) def Add. To. Q(N): global Queue. Tail if (Is. Full() == True): # THEN print("The Queue is full") else: Queue. Tail = Queue. Tail + 1 Queue[Queue. Tail] = N # ENDIF #END Add. To. Q. Head Tail Max. Size
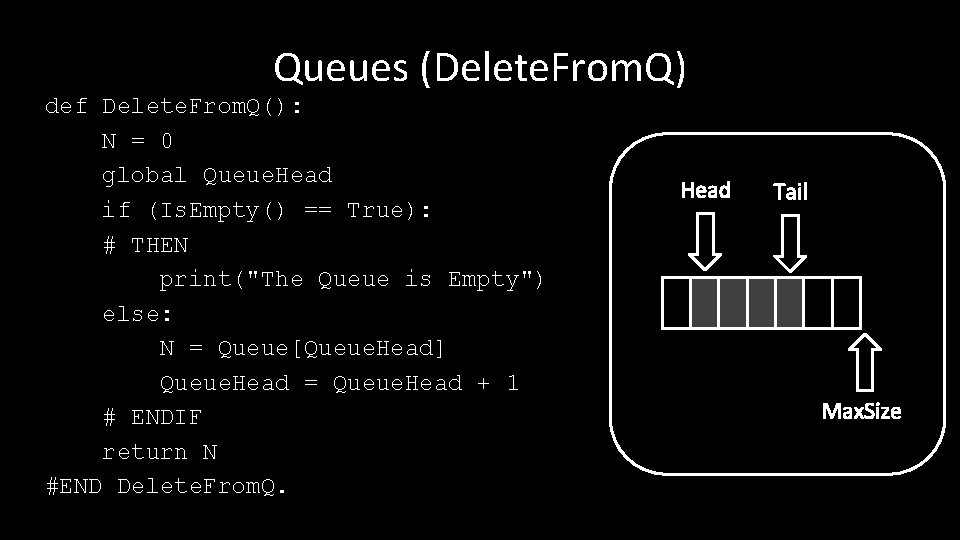
Queues (Delete. From. Q) def Delete. From. Q(): N = 0 global Queue. Head if (Is. Empty() == True): # THEN print("The Queue is Empty") else: N = Queue[Queue. Head] Queue. Head = Queue. Head + 1 # ENDIF return N #END Delete. From. Q. Head Tail Max. Size
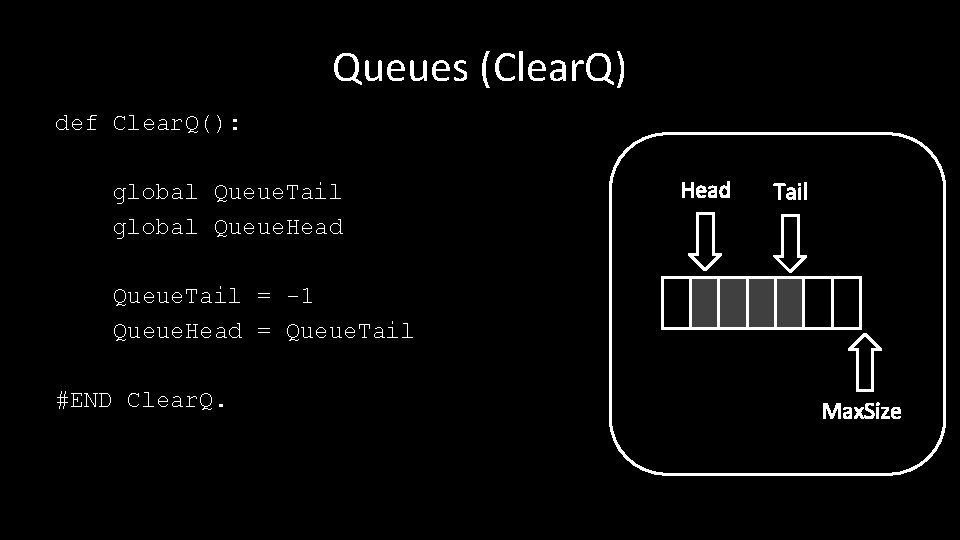
Queues (Clear. Q) def Clear. Q(): global Queue. Tail global Queue. Head Tail Queue. Tail = -1 Queue. Head = Queue. Tail #END Clear. Q. Max. Size
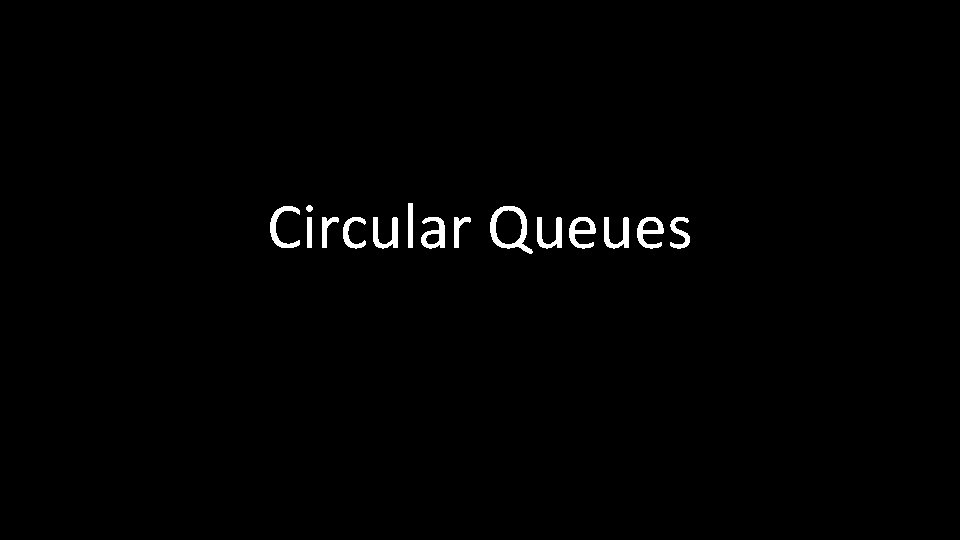
Circular Queues
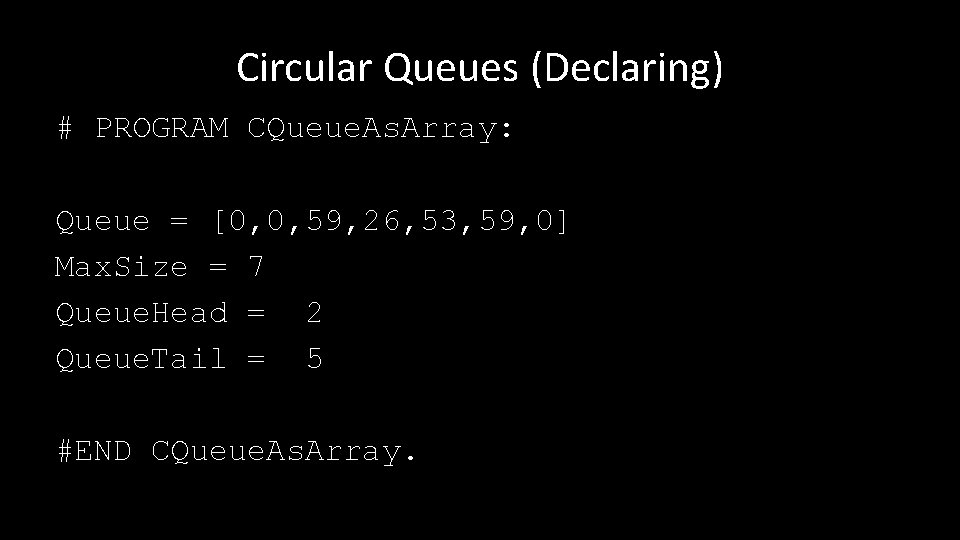
Circular Queues (Declaring) # PROGRAM CQueue. As. Array: Queue = [0, 0, 59, 26, 53, 59, 0] Max. Size = 7 Queue. Head = 2 Queue. Tail = 5 #END CQueue. As. Array.
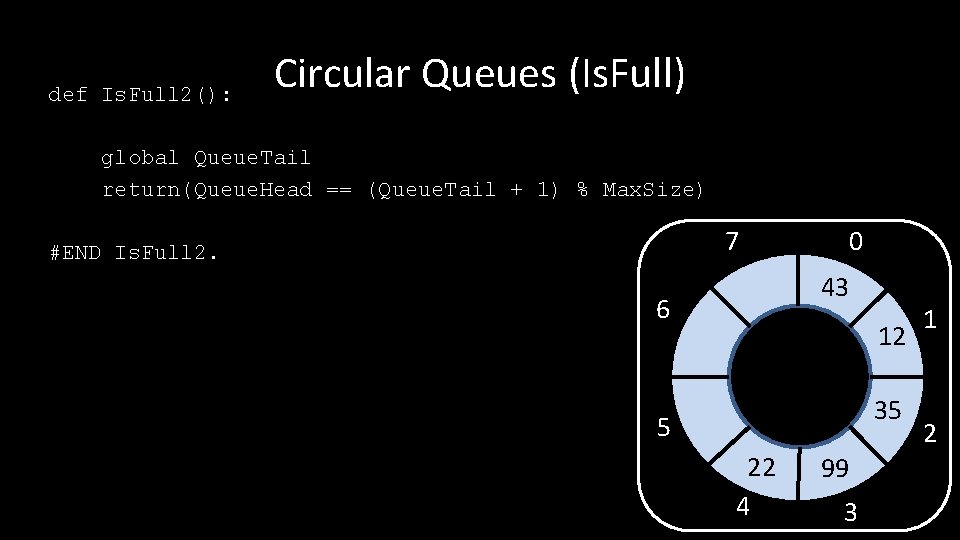
def Is. Full 2(): Circular Queues (Is. Full) global Queue. Tail return(Queue. Head == (Queue. Tail + 1) % Max. Size) 7 #END Is. Full 2. 0 43 6 12 35 5 22 4 99 3 1 2
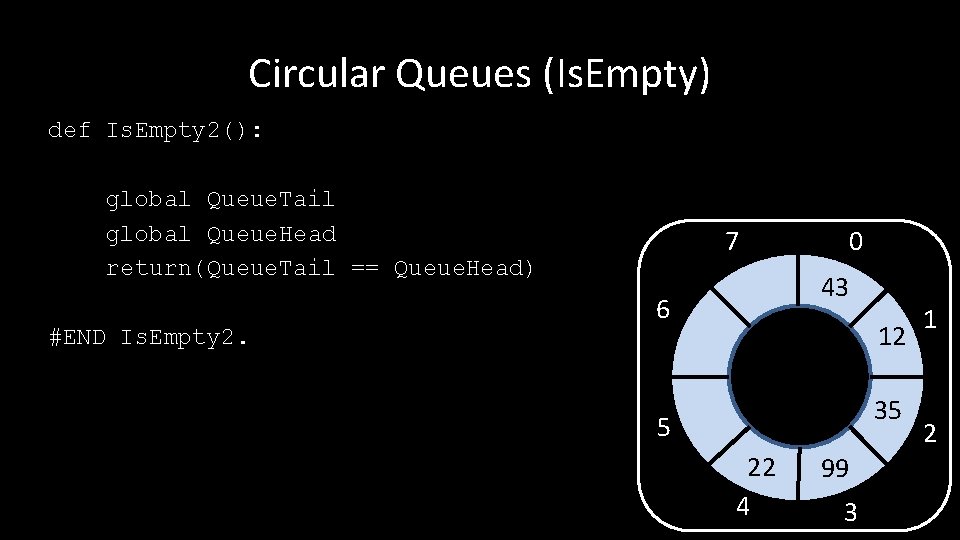
Circular Queues (Is. Empty) def Is. Empty 2(): global Queue. Tail global Queue. Head return(Queue. Tail == Queue. Head) #END Is. Empty 2. 7 0 43 6 12 35 5 22 4 99 3 1 2
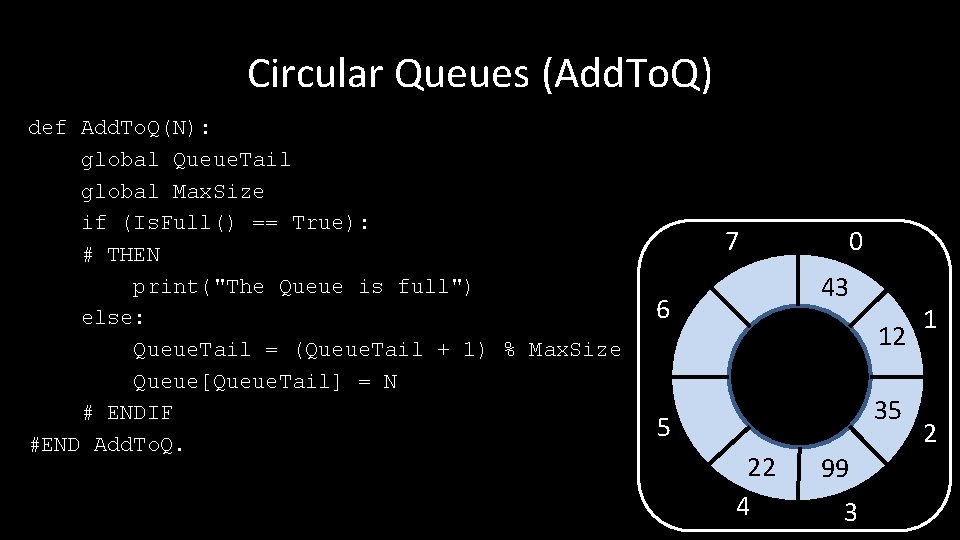
Circular Queues (Add. To. Q) def Add. To. Q(N): global Queue. Tail global Max. Size if (Is. Full() == True): # THEN print("The Queue is full") else: Queue. Tail = (Queue. Tail + 1) % Max. Size Queue[Queue. Tail] = N # ENDIF #END Add. To. Q. 7 0 43 6 12 35 5 22 4 99 3 1 2
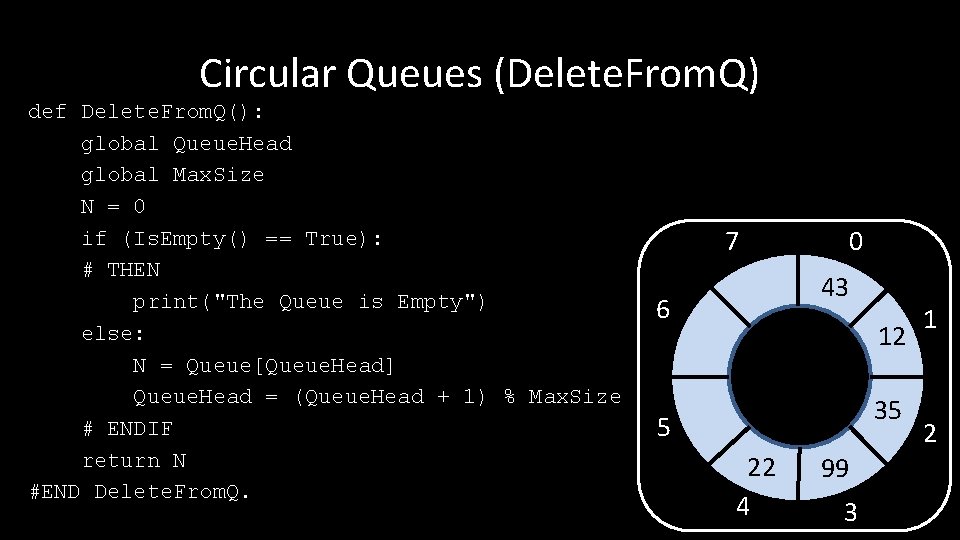
Circular Queues (Delete. From. Q) def Delete. From. Q(): global Queue. Head global Max. Size N = 0 if (Is. Empty() == True): # THEN print("The Queue is Empty") else: N = Queue[Queue. Head] Queue. Head = (Queue. Head + 1) % Max. Size # ENDIF return N #END Delete. From. Q. 7 0 43 6 12 35 5 22 4 99 3 1 2
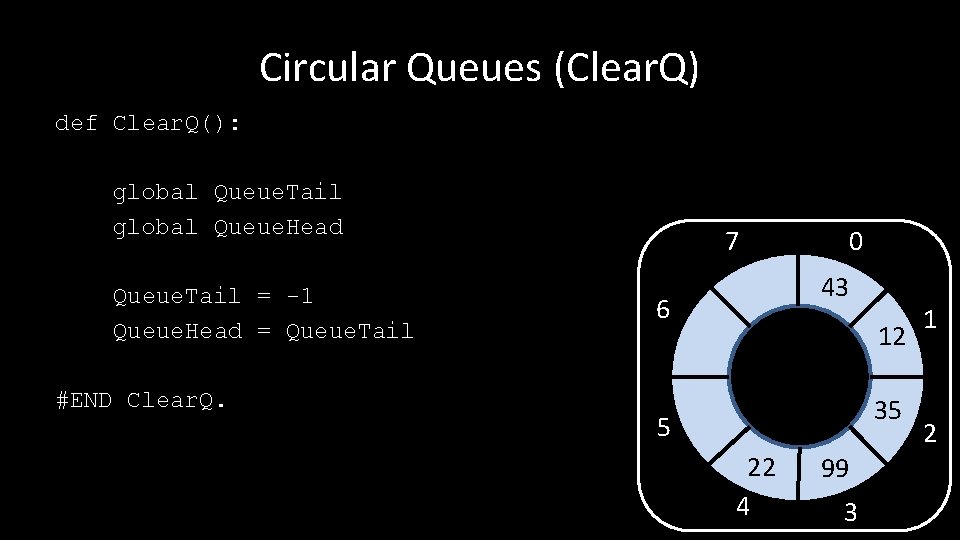
Circular Queues (Clear. Q) def Clear. Q(): global Queue. Tail global Queue. Head Queue. Tail = -1 Queue. Head = Queue. Tail #END Clear. Q. 7 0 43 6 12 35 5 22 4 99 3 1 2
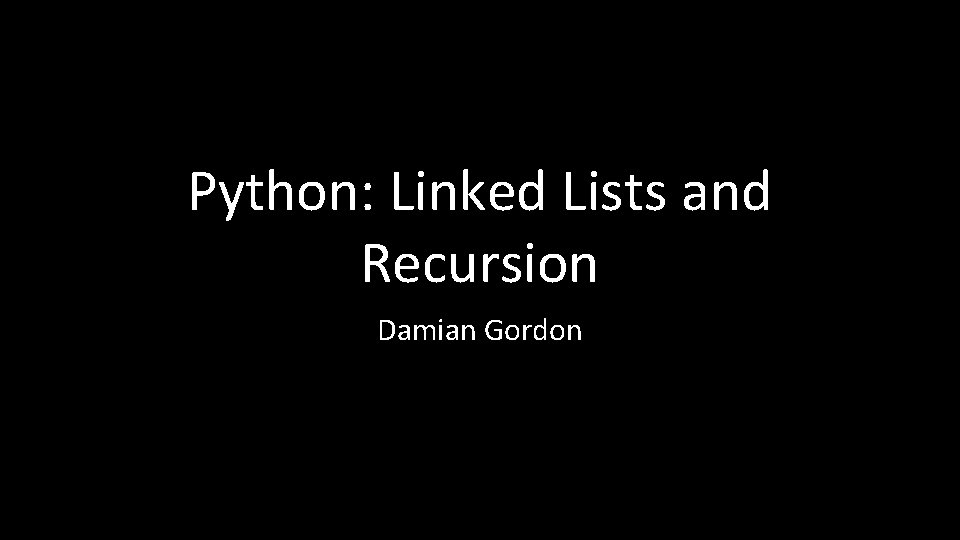
Python: Linked Lists and Recursion Damian Gordon
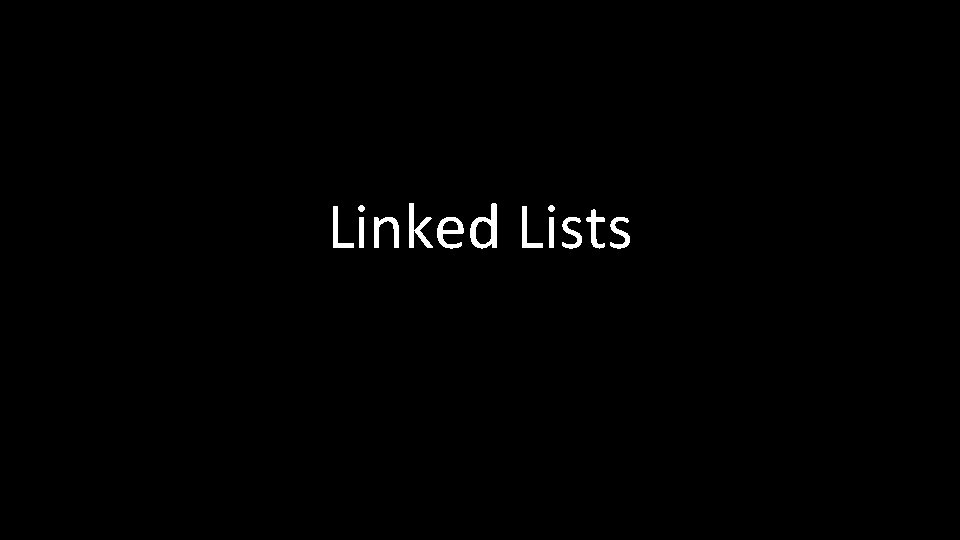
Linked Lists
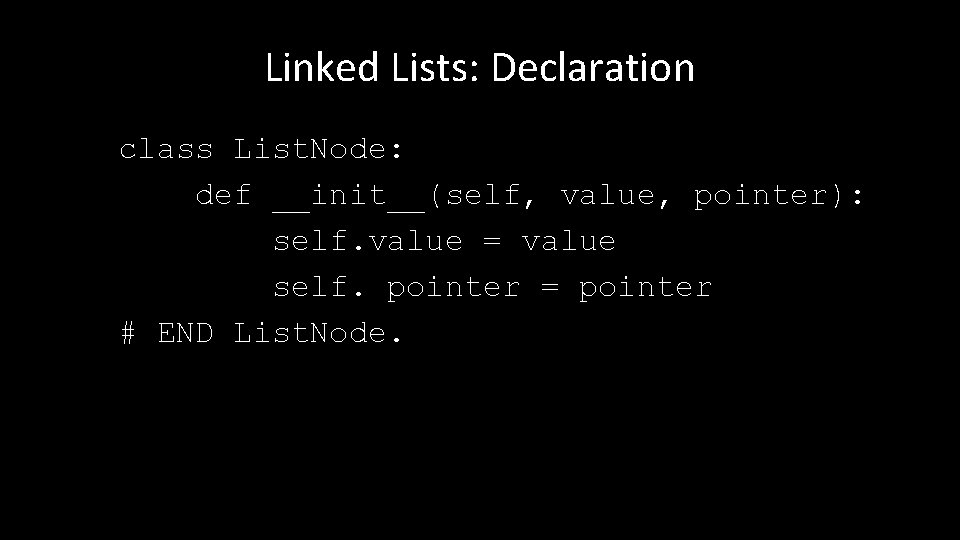
Linked Lists: Declaration class List. Node: def __init__(self, value, pointer): self. value = value self. pointer = pointer # END List. Node.
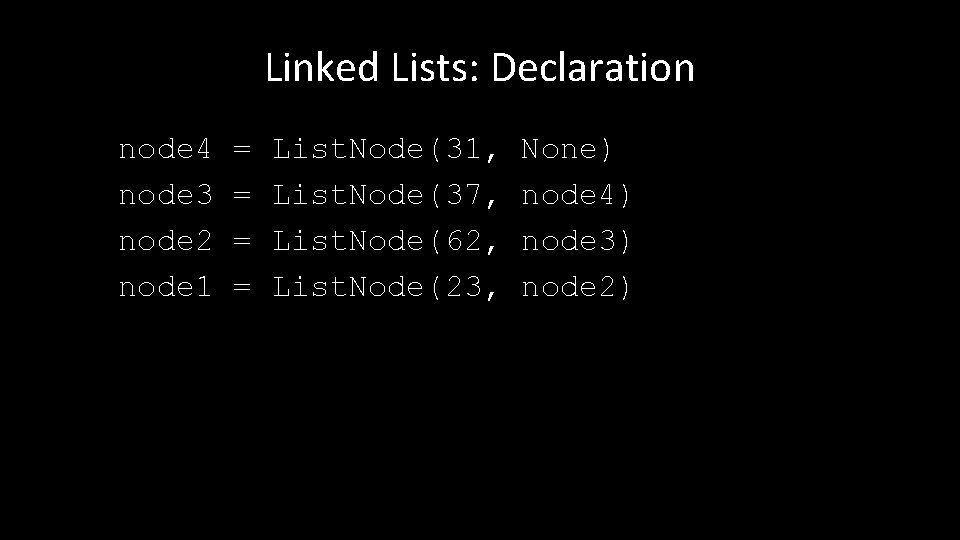
Linked Lists: Declaration node 4 node 3 node 2 node 1 = = List. Node(31, List. Node(37, List. Node(62, List. Node(23, None) node 4) node 3) node 2)
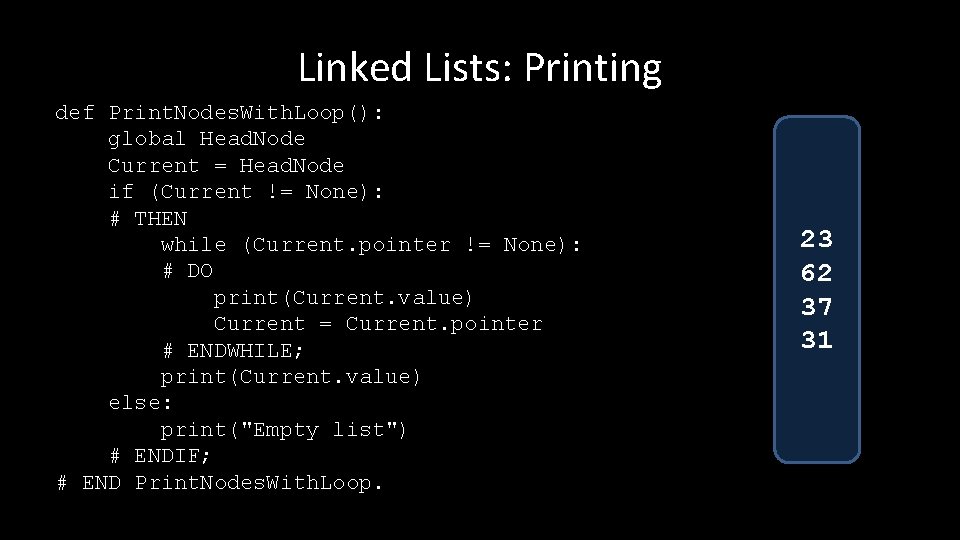
Linked Lists: Printing def Print. Nodes. With. Loop(): global Head. Node Current = Head. Node if (Current != None): # THEN while (Current. pointer != None): # DO print(Current. value) Current = Current. pointer # ENDWHILE; print(Current. value) else: print("Empty list") # ENDIF; # END Print. Nodes. With. Loop. 23 62 37 31
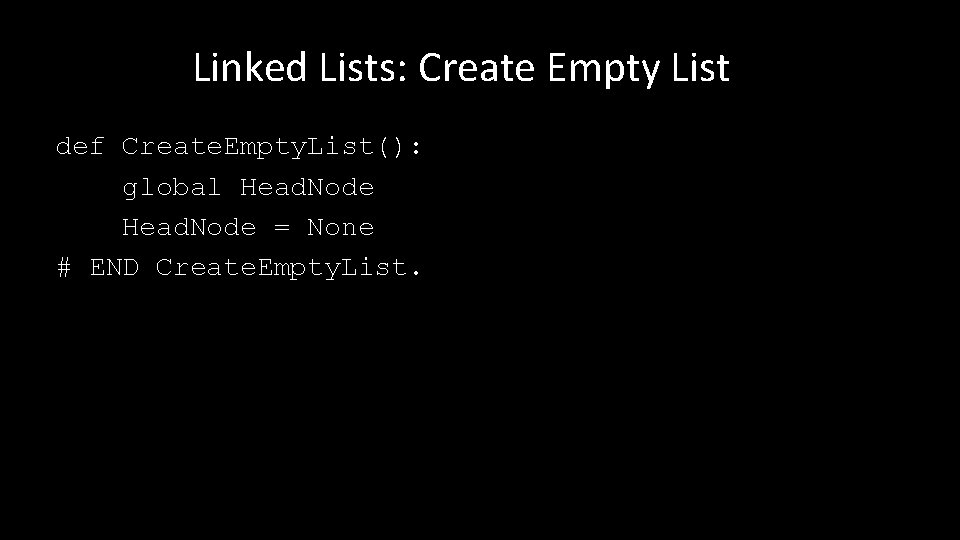
Linked Lists: Create Empty List def Create. Empty. List(): global Head. Node = None # END Create. Empty. List.
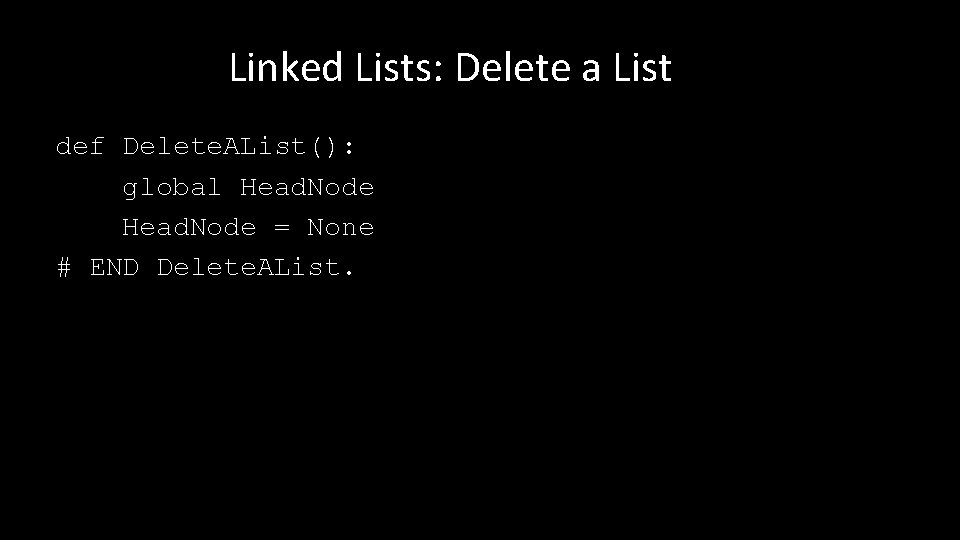
Linked Lists: Delete a List def Delete. AList(): global Head. Node = None # END Delete. AList.
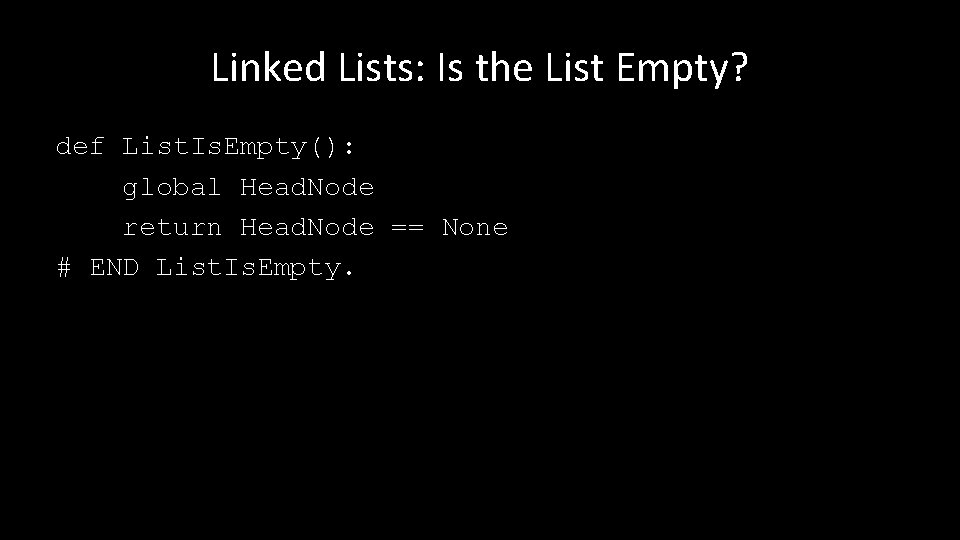
Linked Lists: Is the List Empty? def List. Is. Empty(): global Head. Node return Head. Node == None # END List. Is. Empty.
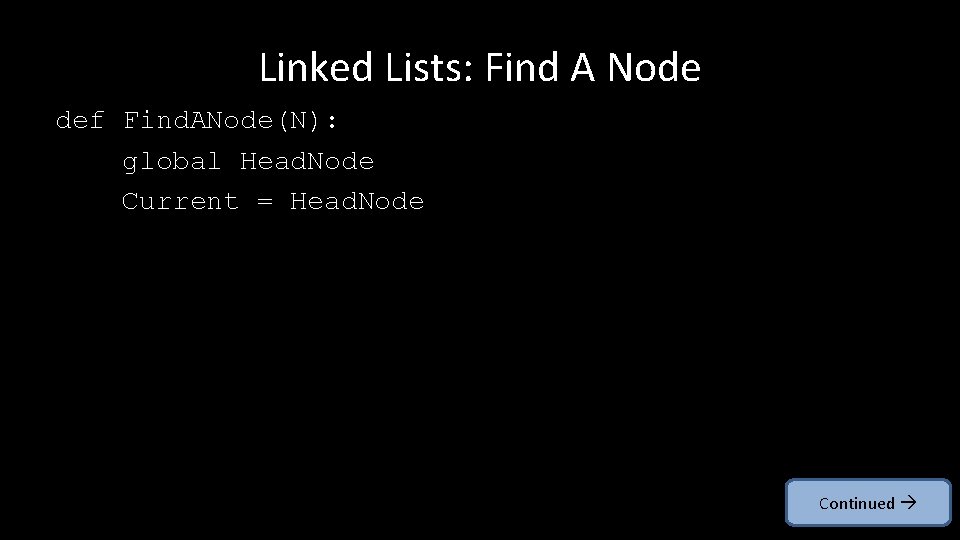
Linked Lists: Find A Node def Find. ANode(N): global Head. Node Current = Head. Node Continued
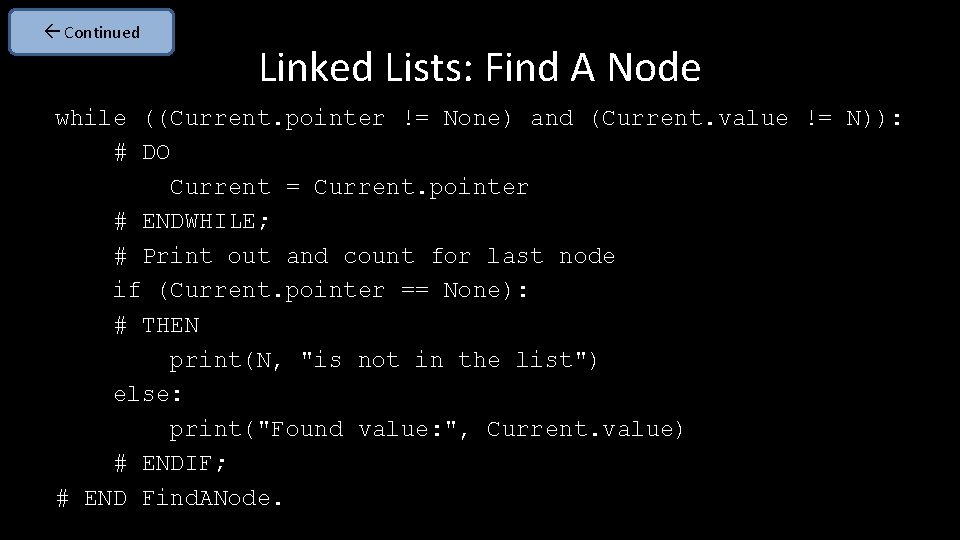
Continued Linked Lists: Find A Node while ((Current. pointer != None) and (Current. value != N)): # DO Current = Current. pointer # ENDWHILE; # Print out and count for last node if (Current. pointer == None): # THEN print(N, "is not in the list") else: print("Found value: ", Current. value) # ENDIF; # END Find. ANode.
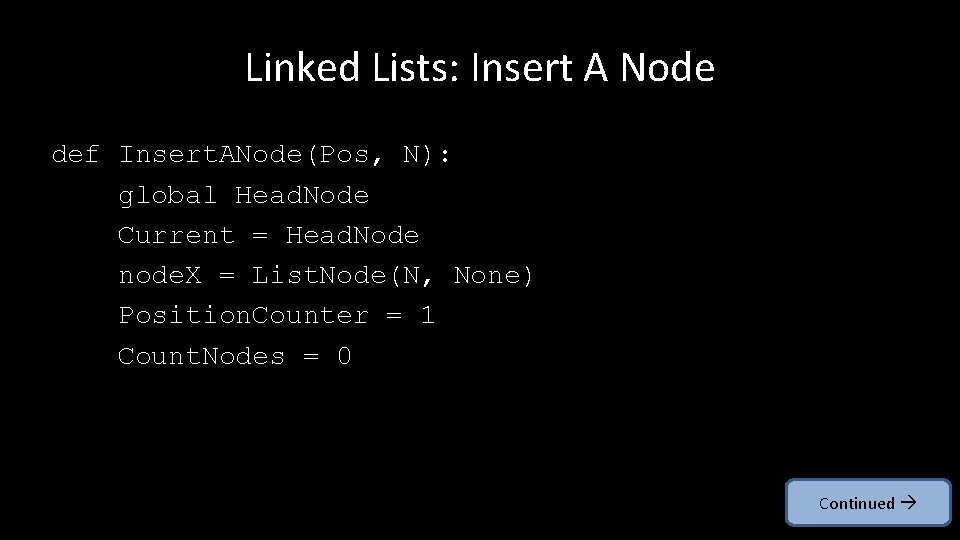
Linked Lists: Insert A Node def Insert. ANode(Pos, N): global Head. Node Current = Head. Node node. X = List. Node(N, None) Position. Counter = 1 Count. Nodes = 0 Continued
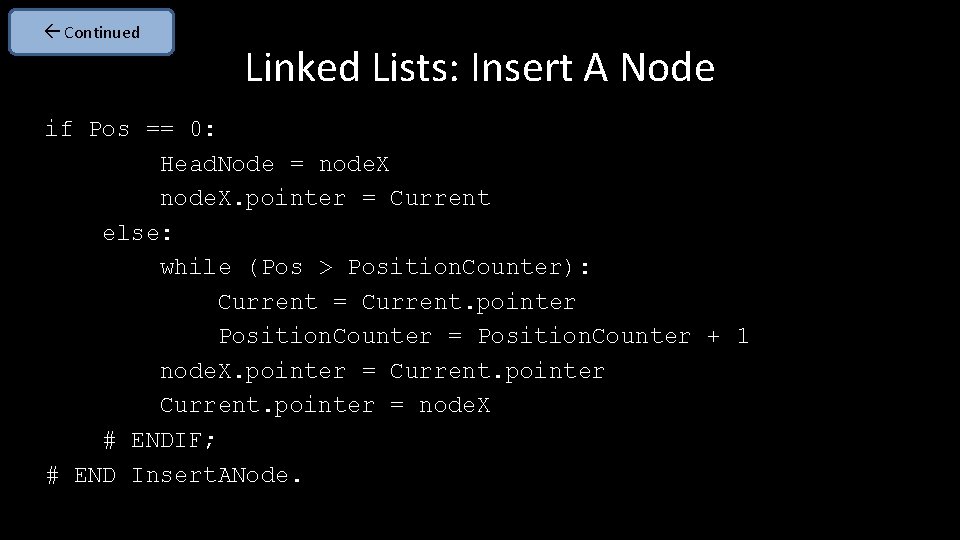
Continued Linked Lists: Insert A Node if Pos == 0: Head. Node = node. X. pointer = Current else: while (Pos > Position. Counter): Current = Current. pointer Position. Counter = Position. Counter + 1 node. X. pointer = Current. pointer = node. X # ENDIF; # END Insert. ANode.
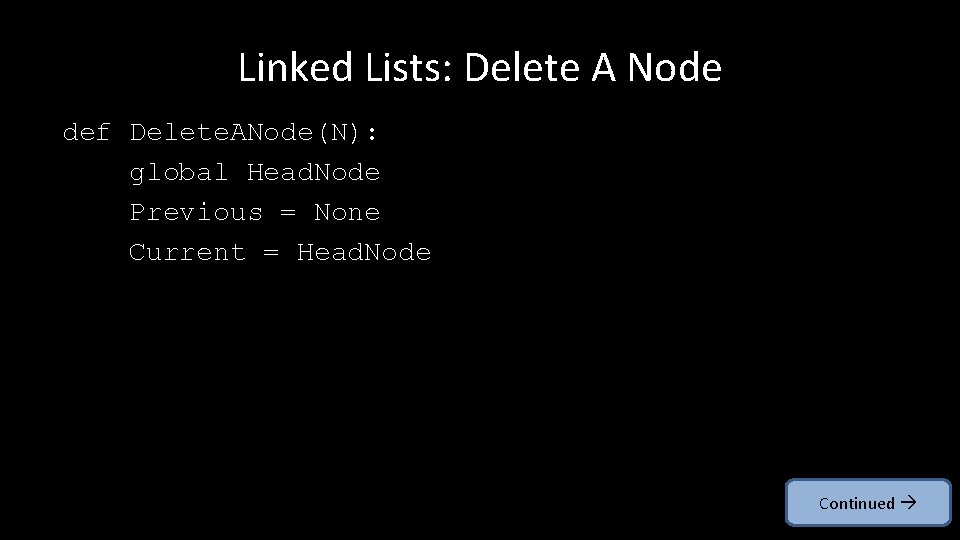
Linked Lists: Delete A Node def Delete. ANode(N): global Head. Node Previous = None Current = Head. Node Continued
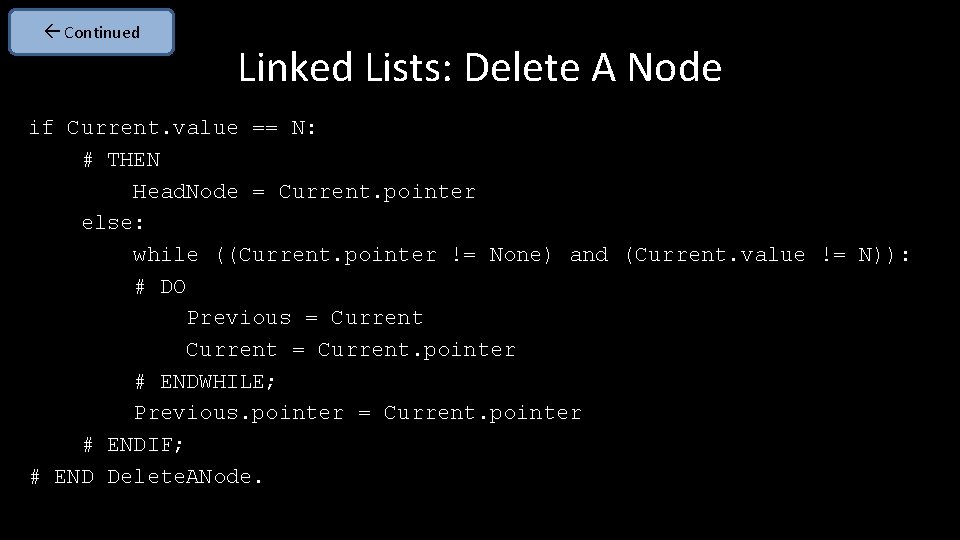
Continued Linked Lists: Delete A Node if Current. value == N: # THEN Head. Node = Current. pointer else: while ((Current. pointer != None) and (Current. value != N)): # DO Previous = Current. pointer # ENDWHILE; Previous. pointer = Current. pointer # ENDIF; # END Delete. ANode.
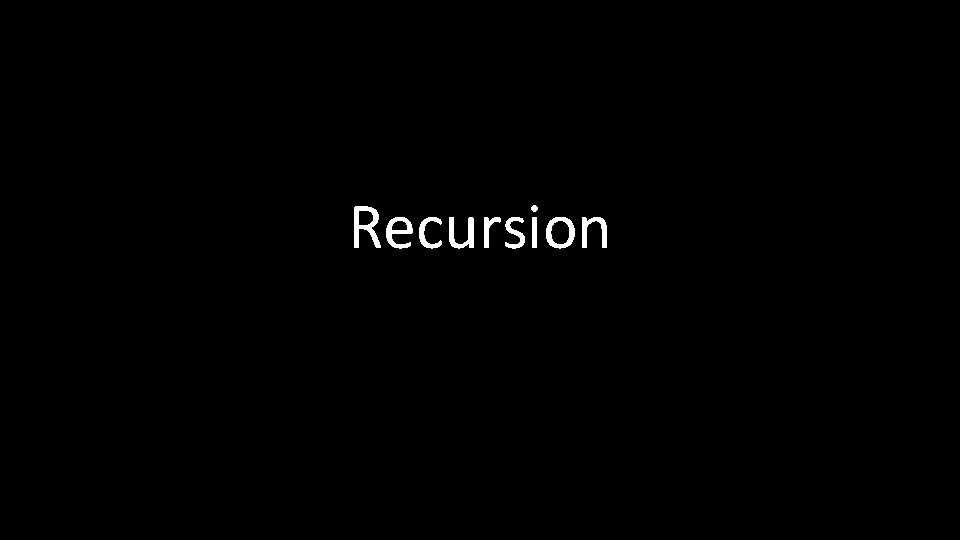
Recursion
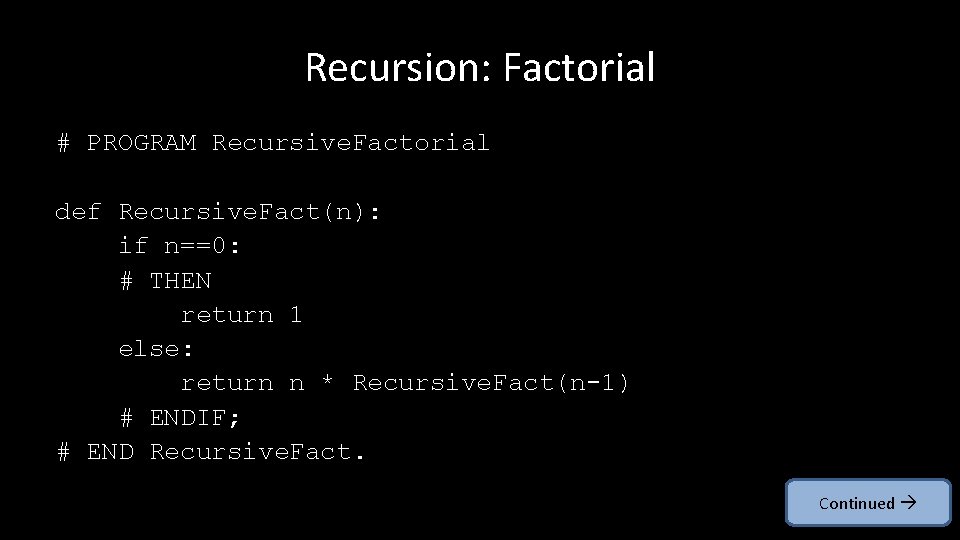
Recursion: Factorial # PROGRAM Recursive. Factorial def Recursive. Fact(n): if n==0: # THEN return 1 else: return n * Recursive. Fact(n-1) # ENDIF; # END Recursive. Fact. Continued
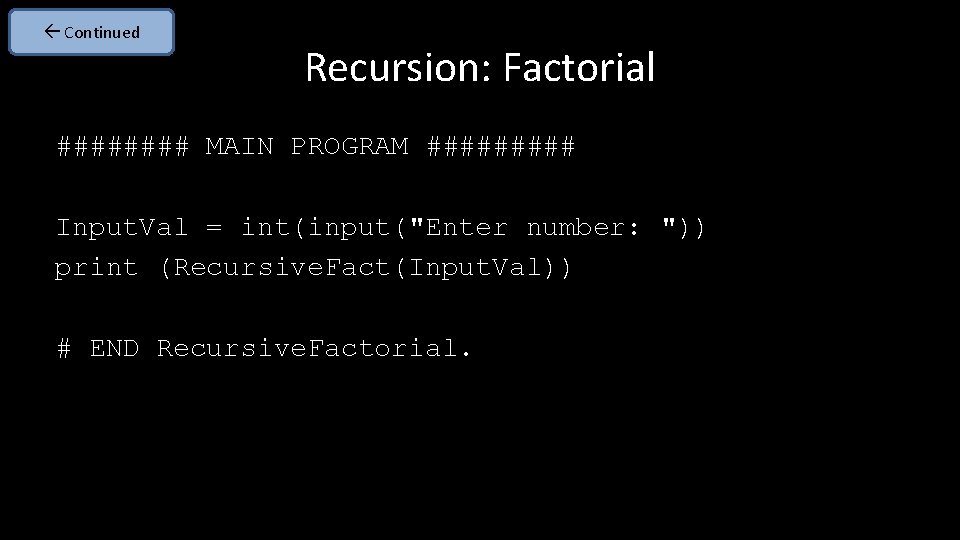
Continued Recursion: Factorial #### MAIN PROGRAM ##### Input. Val = int(input("Enter number: ")) print (Recursive. Fact(Input. Val)) # END Recursive. Factorial.
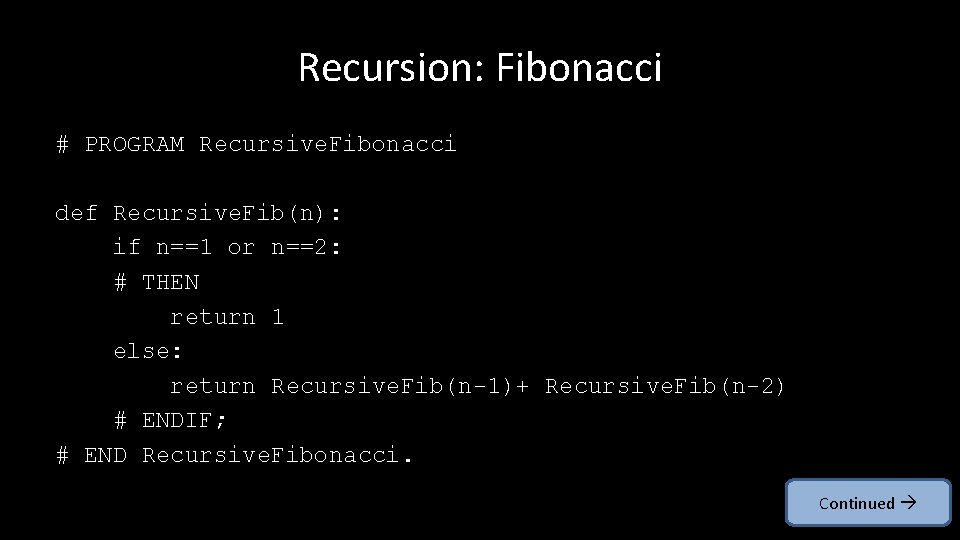
Recursion: Fibonacci # PROGRAM Recursive. Fibonacci def Recursive. Fib(n): if n==1 or n==2: # THEN return 1 else: return Recursive. Fib(n-1)+ Recursive. Fib(n-2) # ENDIF; # END Recursive. Fibonacci. Continued
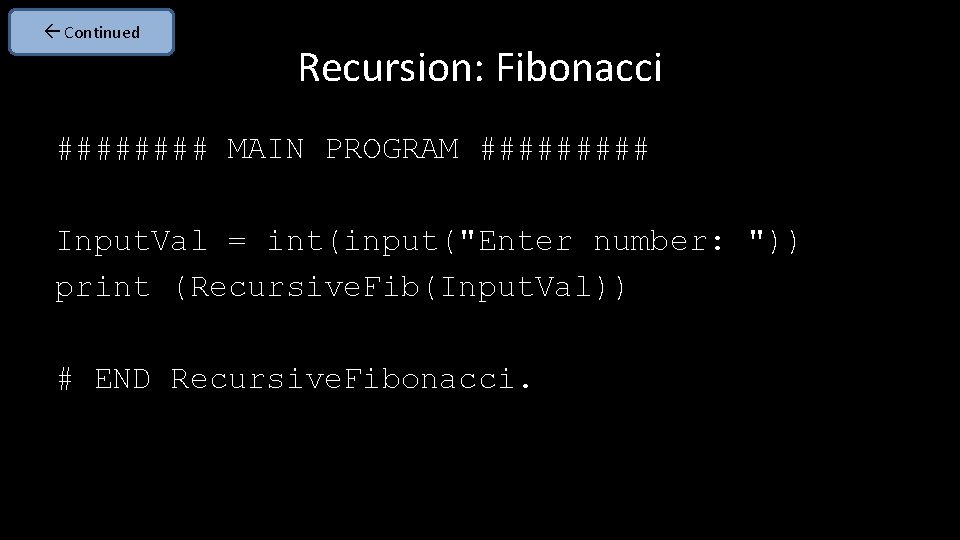
Continued Recursion: Fibonacci #### MAIN PROGRAM ##### Input. Val = int(input("Enter number: ")) print (Recursive. Fib(Input. Val)) # END Recursive. Fibonacci.
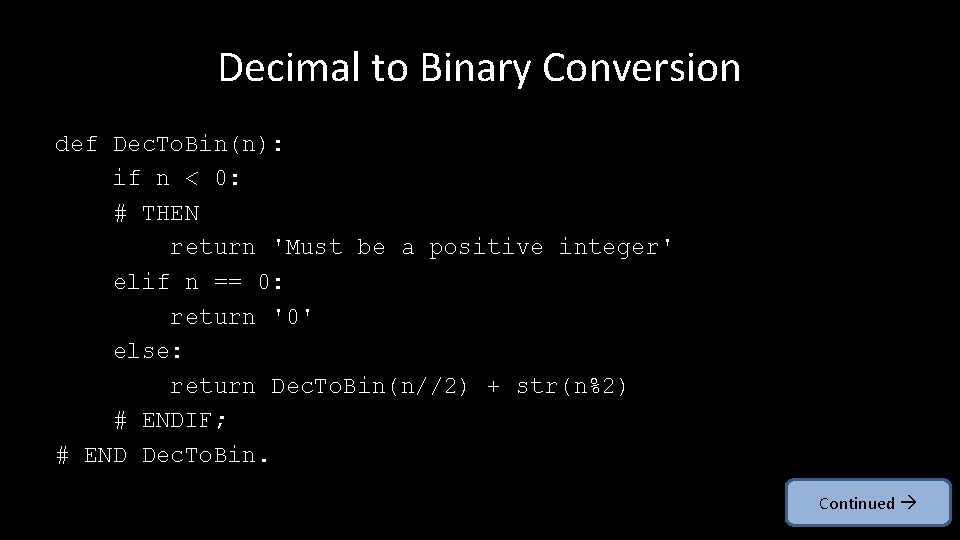
Decimal to Binary Conversion def Dec. To. Bin(n): if n < 0: # THEN return 'Must be a positive integer' elif n == 0: return '0' else: return Dec. To. Bin(n//2) + str(n%2) # ENDIF; # END Dec. To. Bin. Continued
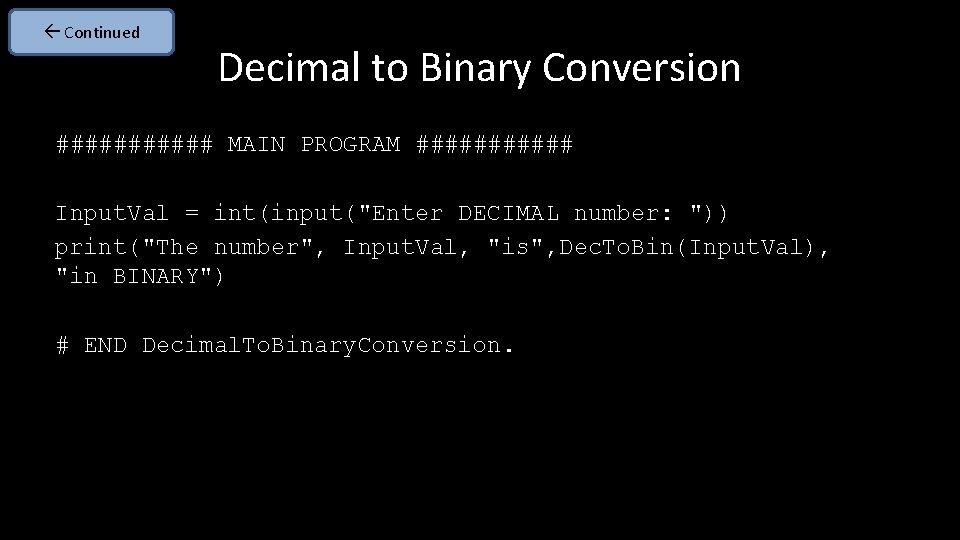
Continued Decimal to Binary Conversion ###### MAIN PROGRAM ###### Input. Val = int(input("Enter DECIMAL number: ")) print("The number", Input. Val, "is", Dec. To. Bin(Input. Val), "in BINARY") # END Decimal. To. Binary. Conversion.
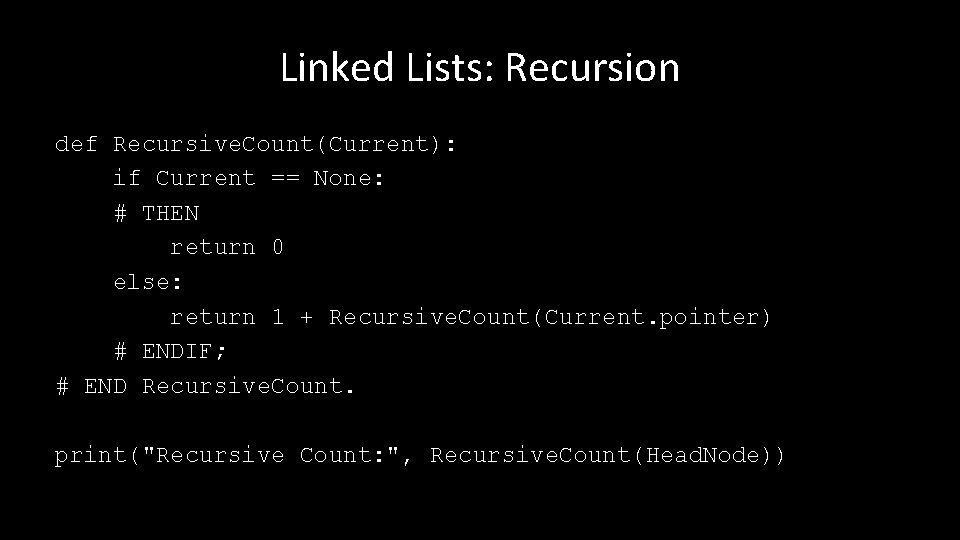
Linked Lists: Recursion def Recursive. Count(Current): if Current == None: # THEN return 0 else: return 1 + Recursive. Count(Current. pointer) # ENDIF; # END Recursive. Count. print("Recursive Count: ", Recursive. Count(Head. Node))
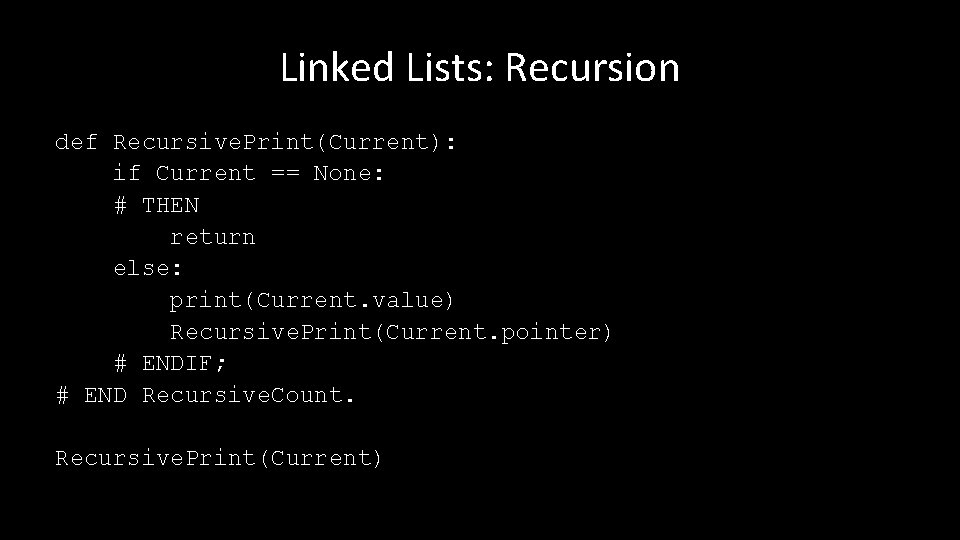
Linked Lists: Recursion def Recursive. Print(Current): if Current == None: # THEN return else: print(Current. value) Recursive. Print(Current. pointer) # ENDIF; # END Recursive. Count. Recursive. Print(Current)
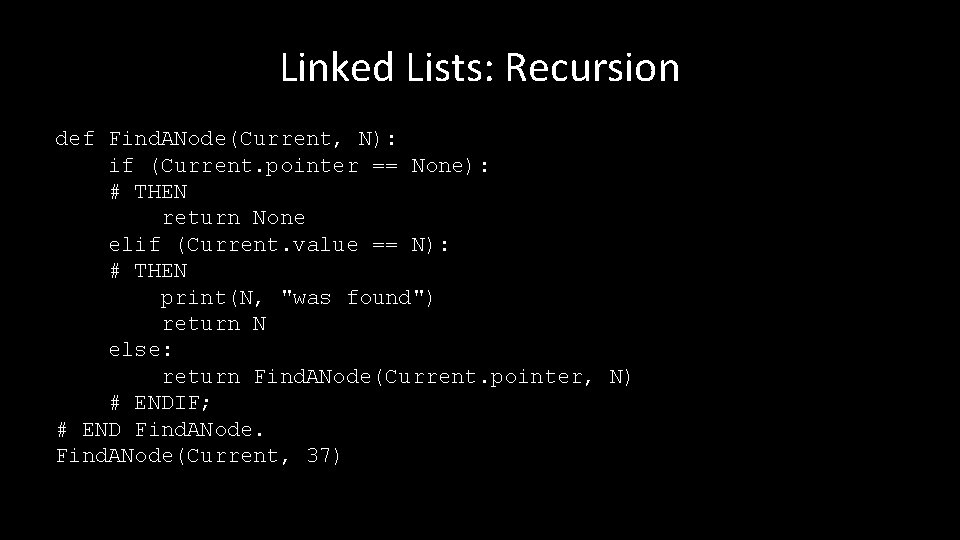
Linked Lists: Recursion def Find. ANode(Current, N): if (Current. pointer == None): # THEN return None elif (Current. value == N): # THEN print(N, "was found") return N else: return Find. ANode(Current. pointer, N) # ENDIF; # END Find. ANode(Current, 37)
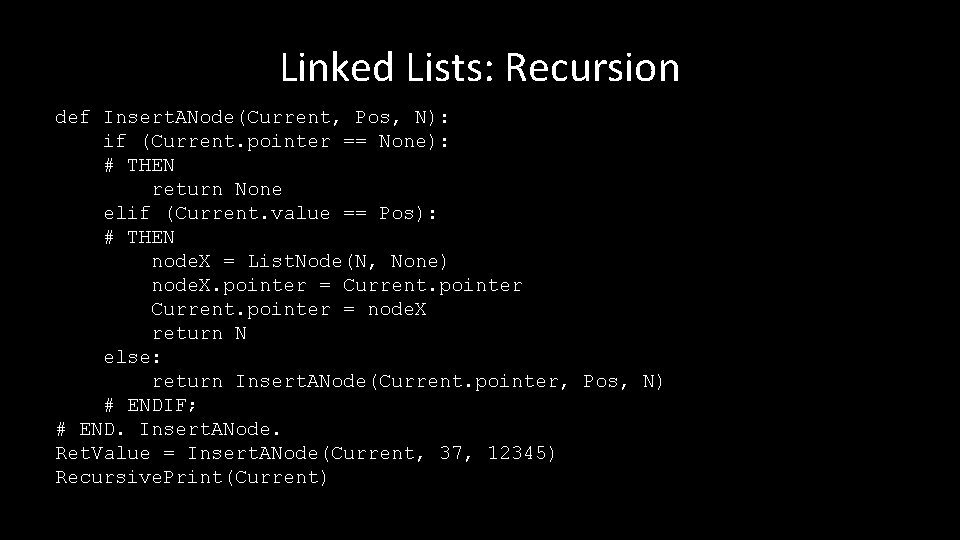
Linked Lists: Recursion def Insert. ANode(Current, Pos, N): if (Current. pointer == None): # THEN return None elif (Current. value == Pos): # THEN node. X = List. Node(N, None) node. X. pointer = Current. pointer = node. X return N else: return Insert. ANode(Current. pointer, Pos, N) # ENDIF; # END. Insert. ANode. Ret. Value = Insert. ANode(Current, 37, 12345) Recursive. Print(Current)
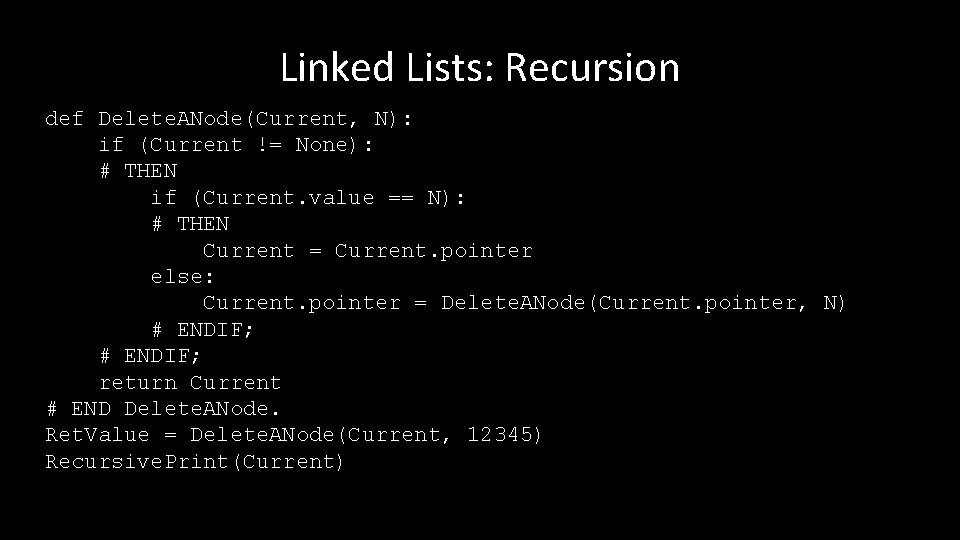
Linked Lists: Recursion def Delete. ANode(Current, N): if (Current != None): # THEN if (Current. value == N): # THEN Current = Current. pointer else: Current. pointer = Delete. ANode(Current. pointer, N) # ENDIF; return Current # END Delete. ANode. Ret. Value = Delete. ANode(Current, 12345) Recursive. Print(Current)
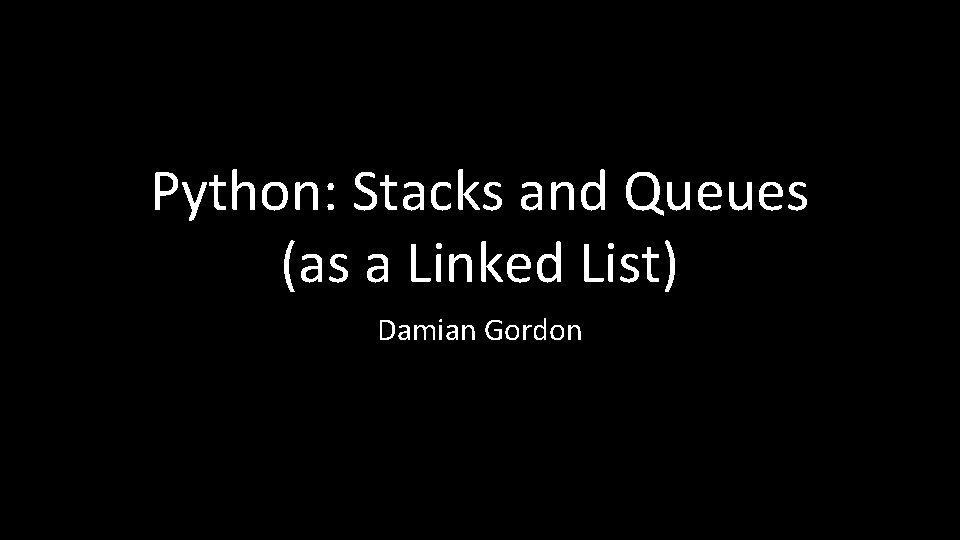
Python: Stacks and Queues (as a Linked List) Damian Gordon
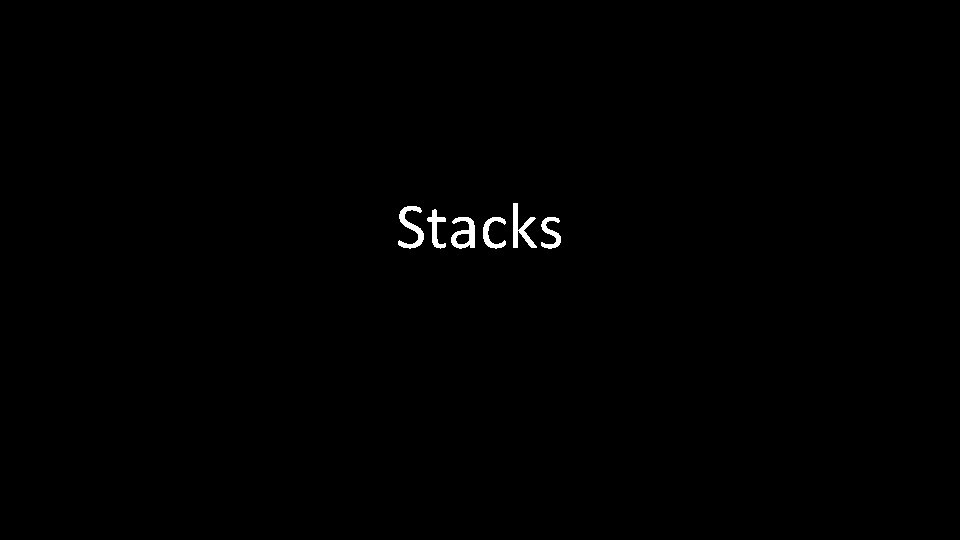
Stacks
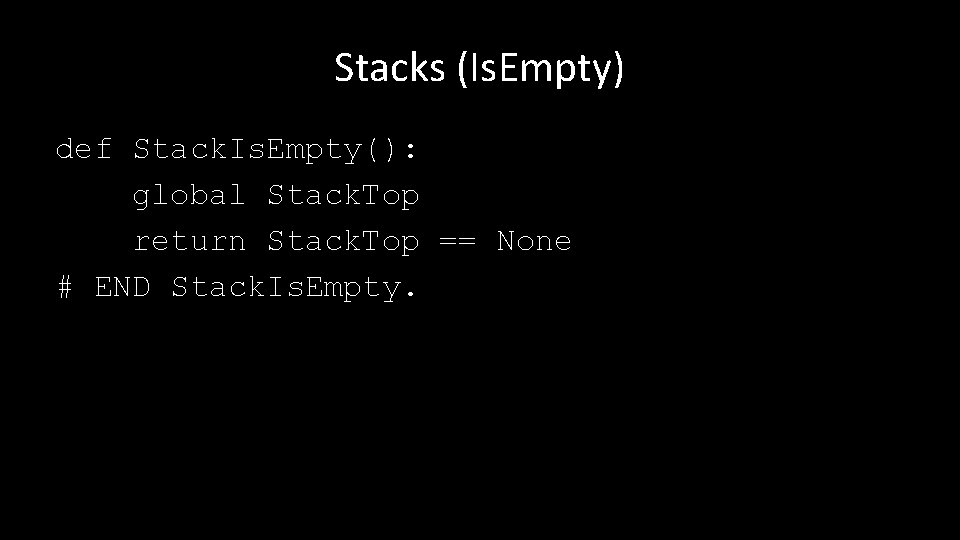
Stacks (Is. Empty) def Stack. Is. Empty(): global Stack. Top return Stack. Top == None # END Stack. Is. Empty.
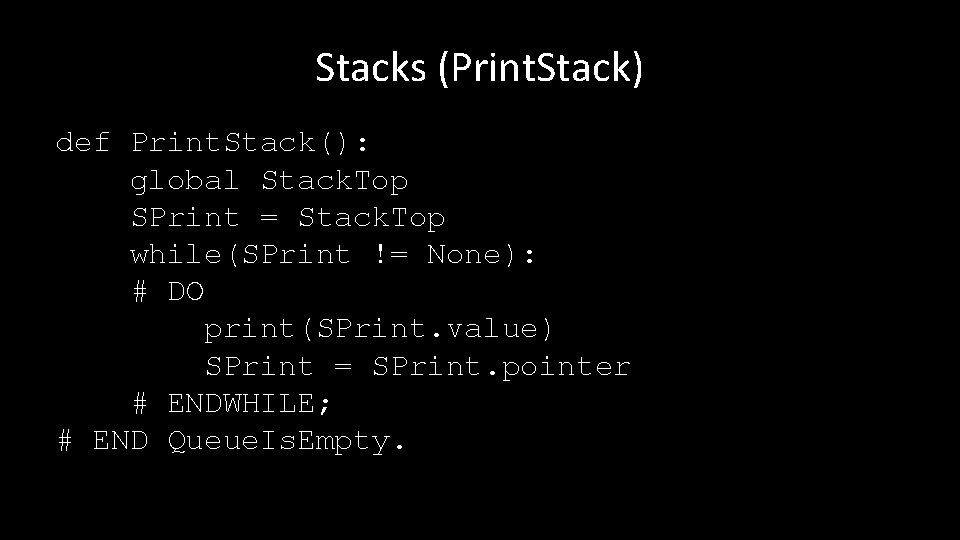
Stacks (Print. Stack) def Print. Stack(): global Stack. Top SPrint = Stack. Top while(SPrint != None): # DO print(SPrint. value) SPrint = SPrint. pointer # ENDWHILE; # END Queue. Is. Empty.
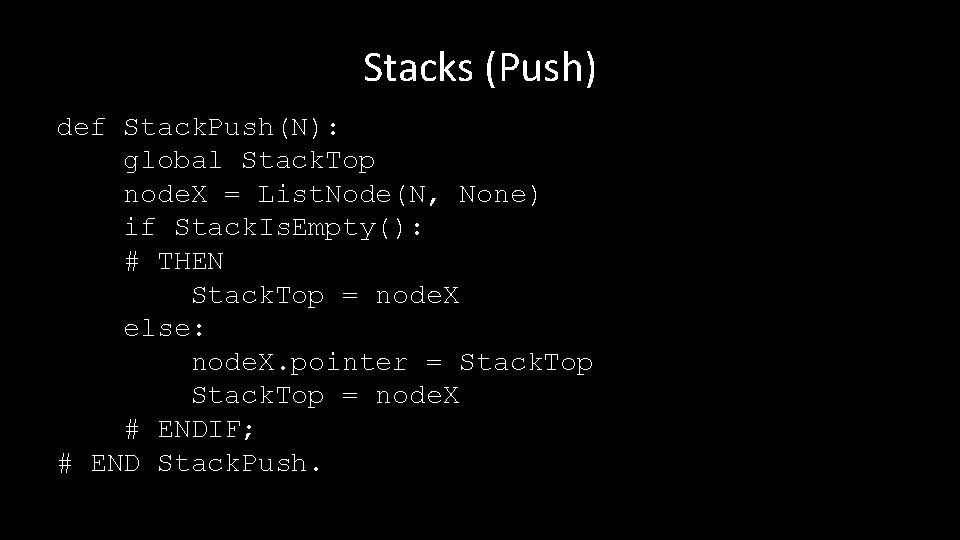
Stacks (Push) def Stack. Push(N): global Stack. Top node. X = List. Node(N, None) if Stack. Is. Empty(): # THEN Stack. Top = node. X else: node. X. pointer = Stack. Top = node. X # ENDIF; # END Stack. Push.
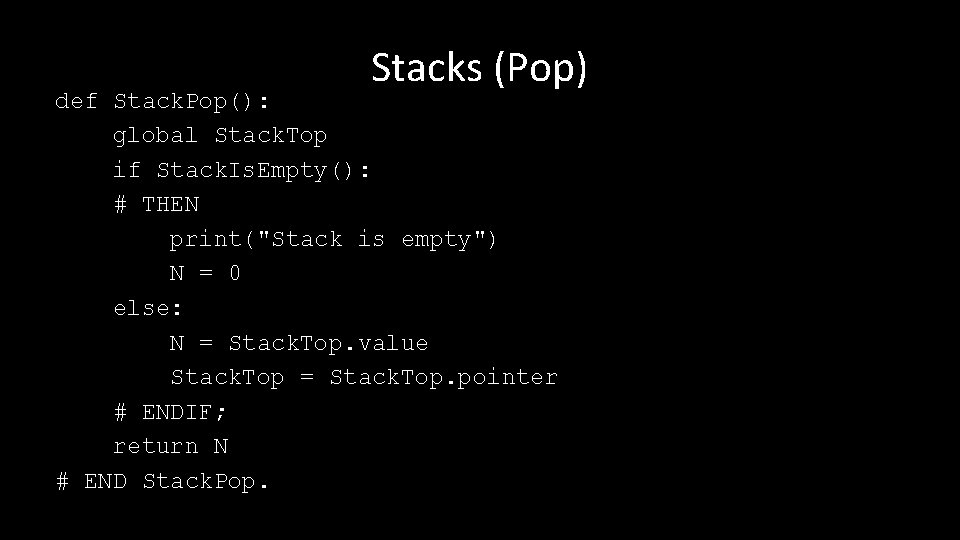
Stacks (Pop) def Stack. Pop(): global Stack. Top if Stack. Is. Empty(): # THEN print("Stack is empty") N = 0 else: N = Stack. Top. value Stack. Top = Stack. Top. pointer # ENDIF; return N # END Stack. Pop.
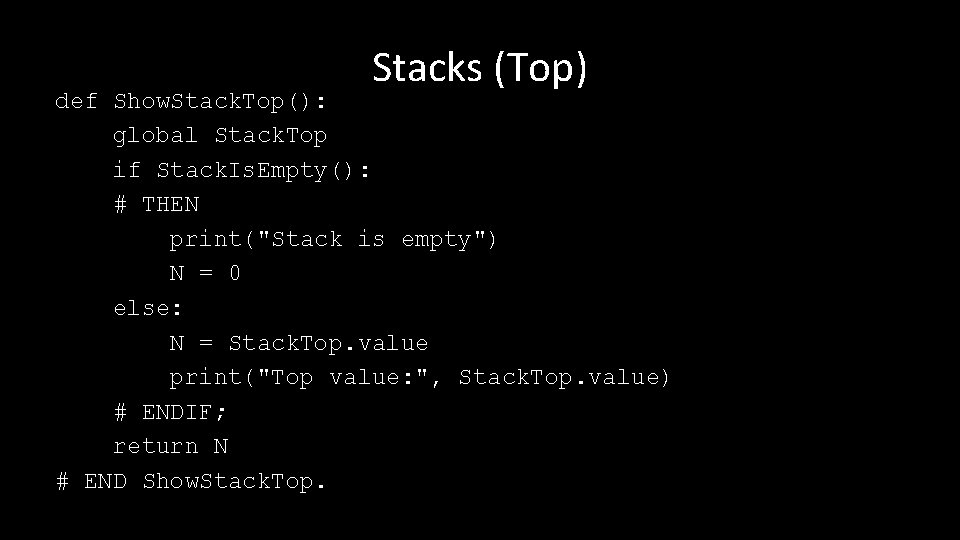
Stacks (Top) def Show. Stack. Top(): global Stack. Top if Stack. Is. Empty(): # THEN print("Stack is empty") N = 0 else: N = Stack. Top. value print("Top value: ", Stack. Top. value) # ENDIF; return N # END Show. Stack. Top.
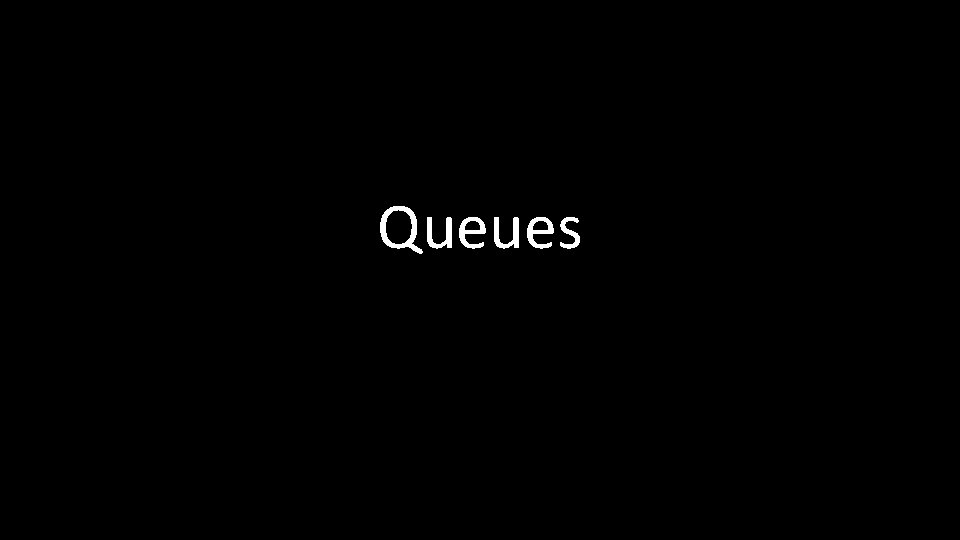
Queues
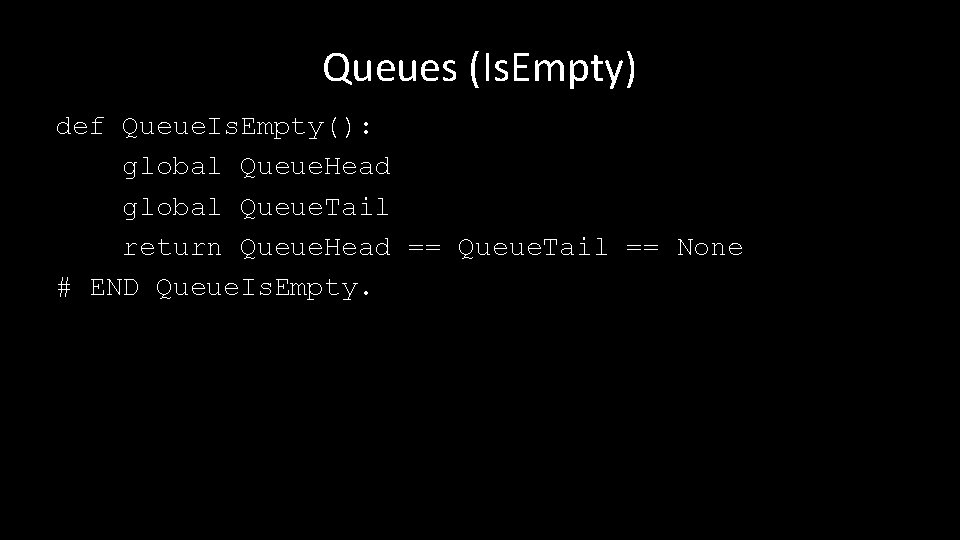
Queues (Is. Empty) def Queue. Is. Empty(): global Queue. Head global Queue. Tail return Queue. Head == Queue. Tail == None # END Queue. Is. Empty.
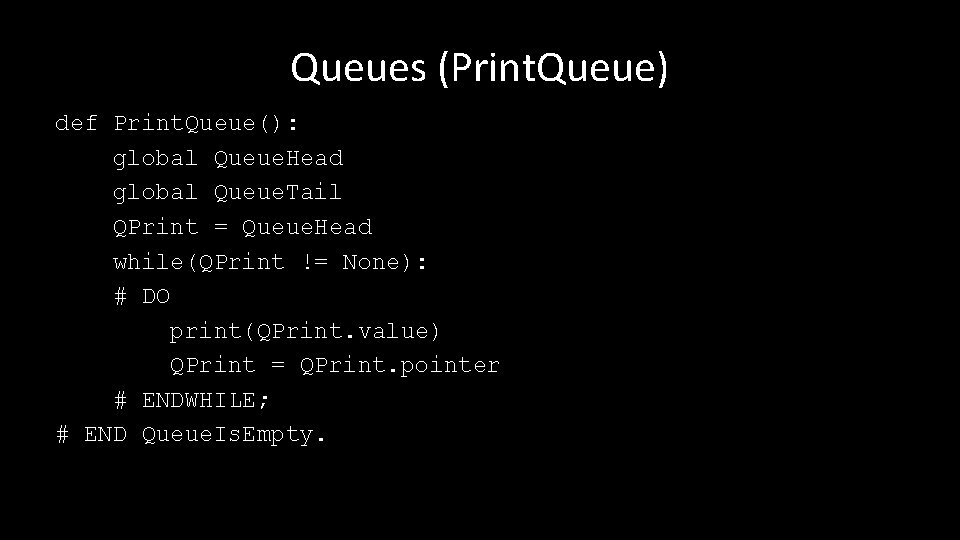
Queues (Print. Queue) def Print. Queue(): global Queue. Head global Queue. Tail QPrint = Queue. Head while(QPrint != None): # DO print(QPrint. value) QPrint = QPrint. pointer # ENDWHILE; # END Queue. Is. Empty.
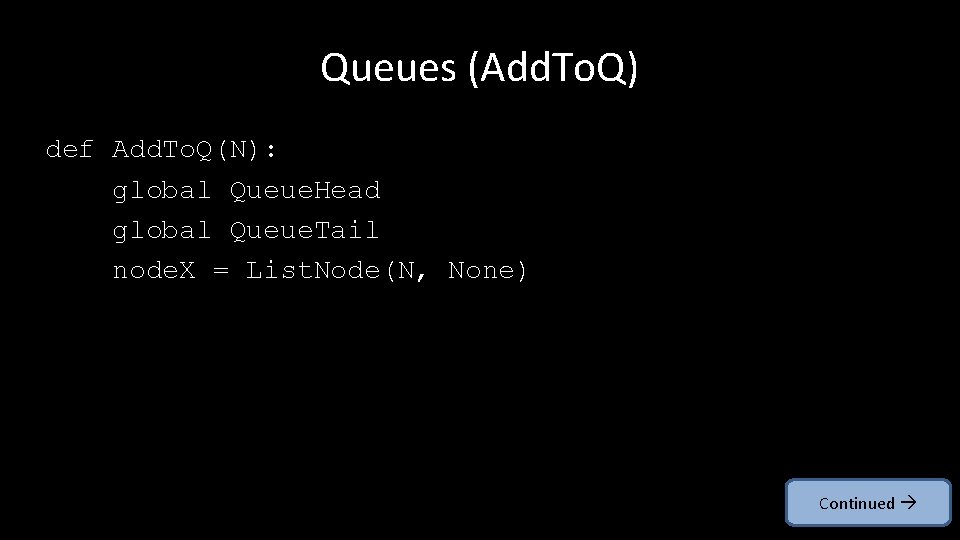
Queues (Add. To. Q) def Add. To. Q(N): global Queue. Head global Queue. Tail node. X = List. Node(N, None) Continued
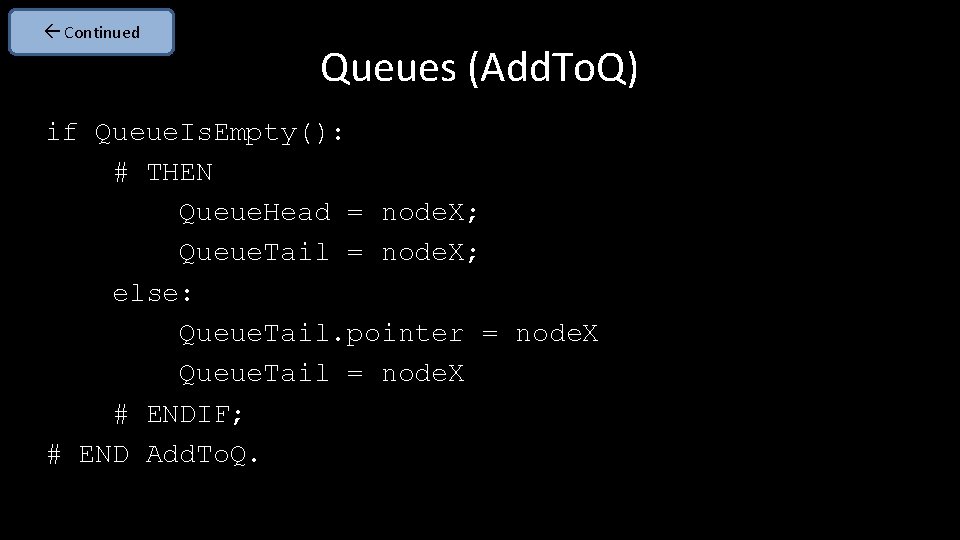
Continued Queues (Add. To. Q) if Queue. Is. Empty(): # THEN Queue. Head = node. X; Queue. Tail = node. X; else: Queue. Tail. pointer = node. X Queue. Tail = node. X # ENDIF; # END Add. To. Q.
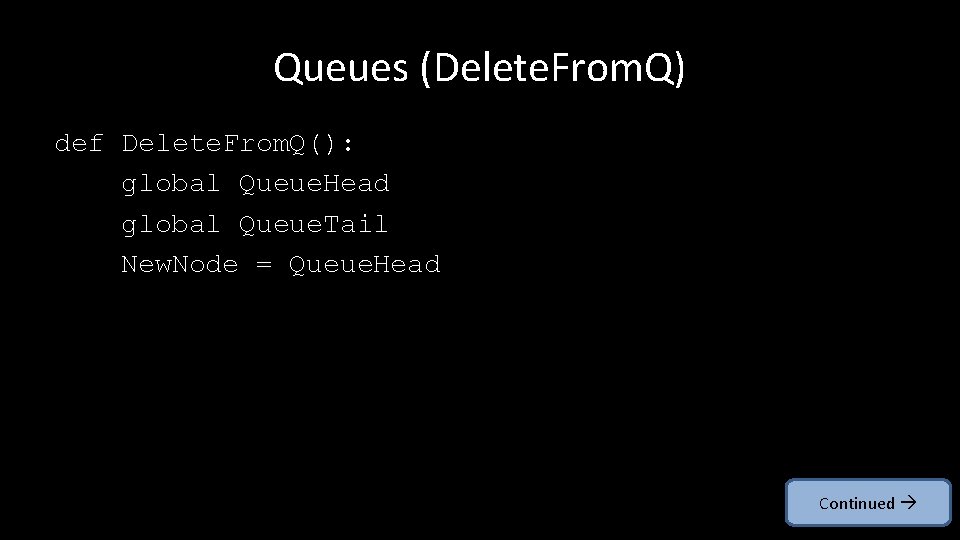
Queues (Delete. From. Q) def Delete. From. Q(): global Queue. Head global Queue. Tail New. Node = Queue. Head Continued
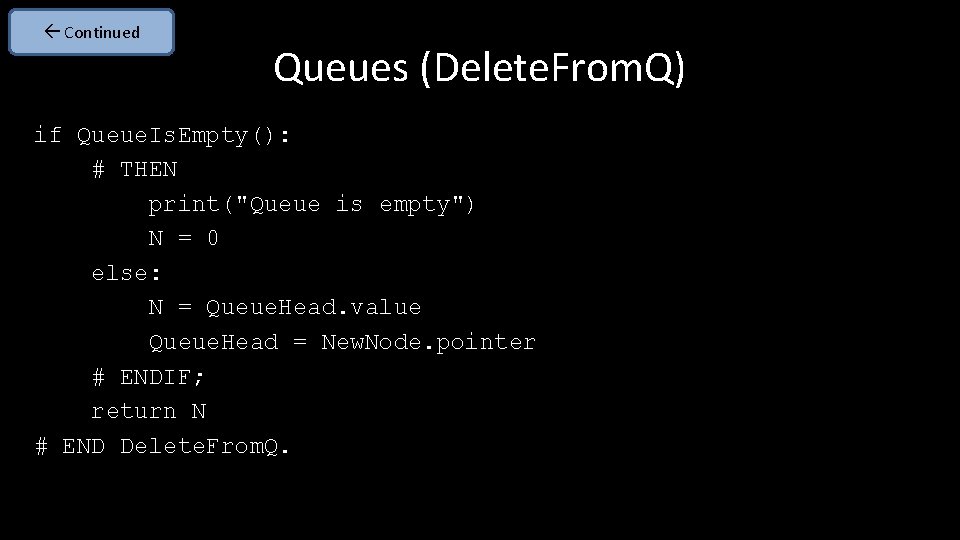
Continued Queues (Delete. From. Q) if Queue. Is. Empty(): # THEN print("Queue is empty") N = 0 else: N = Queue. Head. value Queue. Head = New. Node. pointer # ENDIF; return N # END Delete. From. Q.
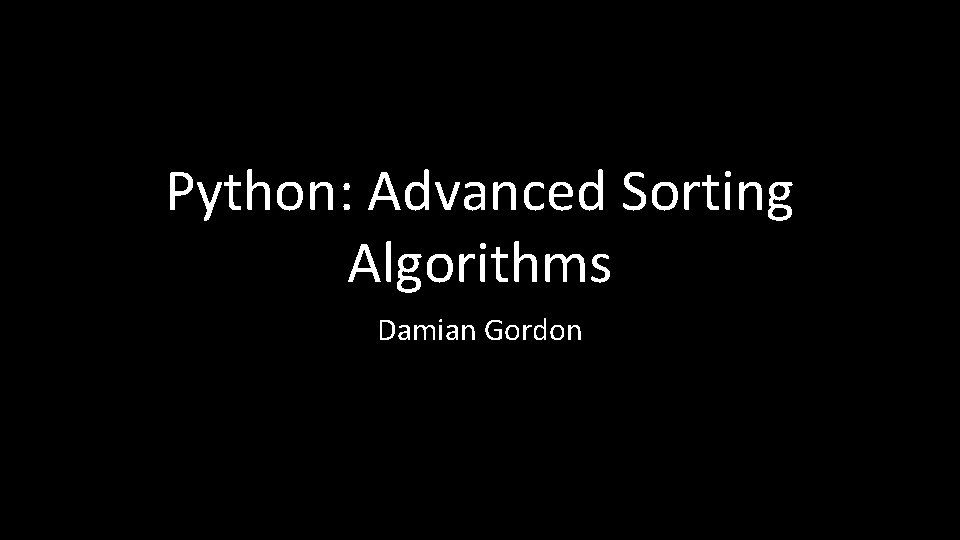
Python: Advanced Sorting Algorithms Damian Gordon
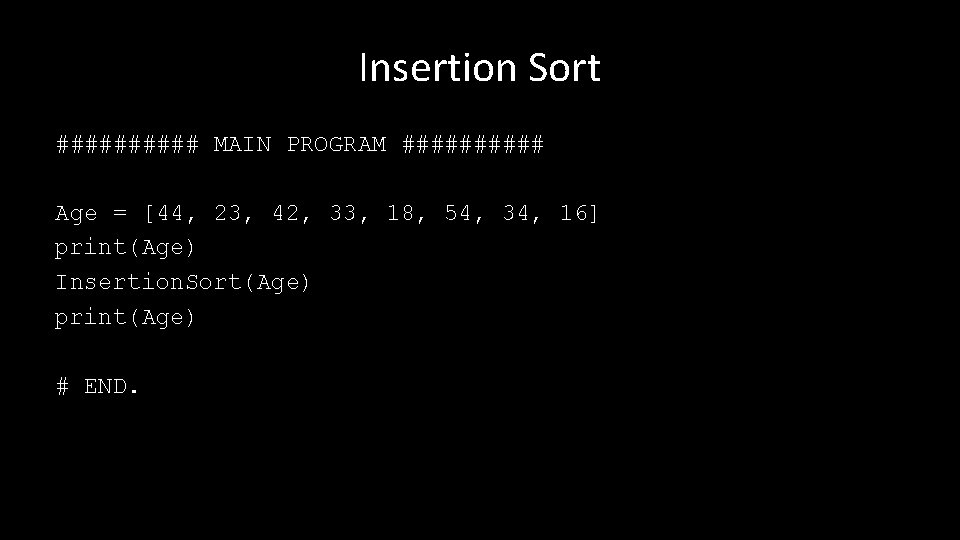
Insertion Sort ##### MAIN PROGRAM ##### Age = [44, 23, 42, 33, 18, 54, 34, 16] print(Age) Insertion. Sort(Age) print(Age) # END.
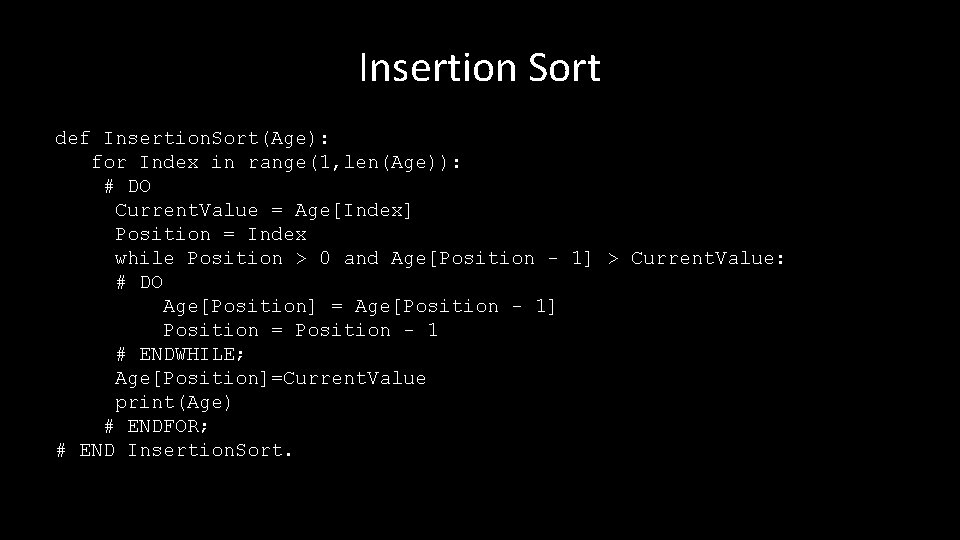
Insertion Sort def Insertion. Sort(Age): for Index in range(1, len(Age)): # DO Current. Value = Age[Index] Position = Index while Position > 0 and Age[Position - 1] > Current. Value: # DO Age[Position] = Age[Position - 1] Position = Position - 1 # ENDWHILE; Age[Position]=Current. Value print(Age) # ENDFOR; # END Insertion. Sort.
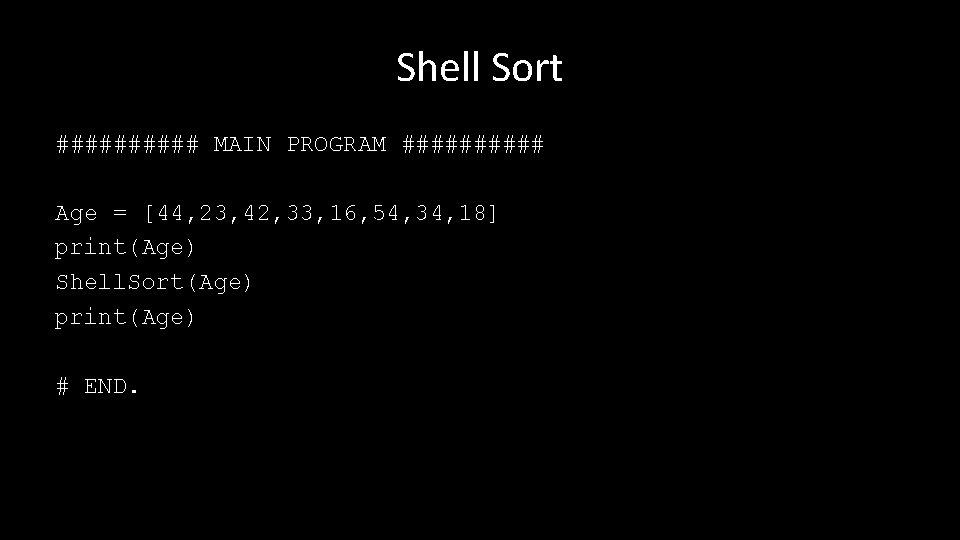
Shell Sort ##### MAIN PROGRAM ##### Age = [44, 23, 42, 33, 16, 54, 34, 18] print(Age) Shell. Sort(Age) print(Age) # END.
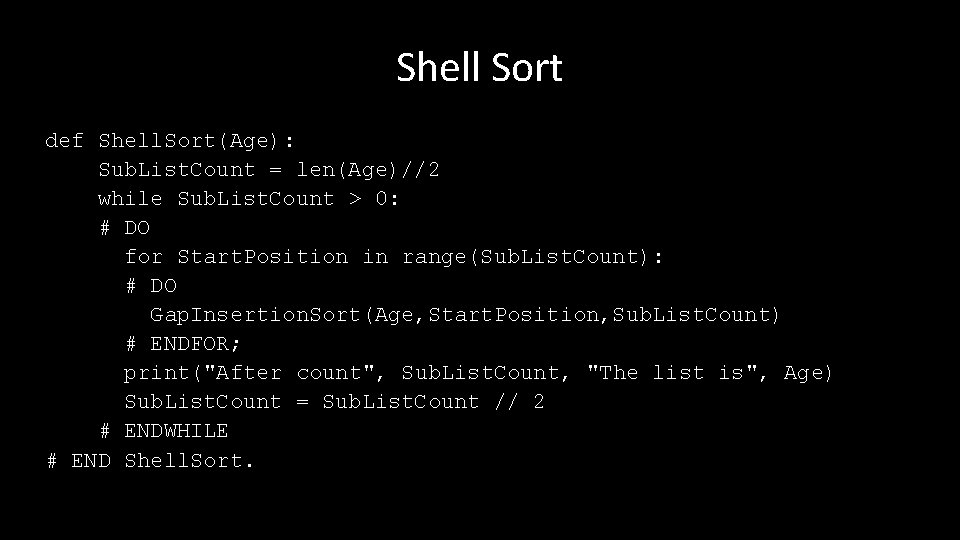
Shell Sort def Shell. Sort(Age): Sub. List. Count = len(Age)//2 while Sub. List. Count > 0: # DO for Start. Position in range(Sub. List. Count): # DO Gap. Insertion. Sort(Age, Start. Position, Sub. List. Count) # ENDFOR; print("After count", Sub. List. Count, "The list is", Age) Sub. List. Count = Sub. List. Count // 2 # ENDWHILE # END Shell. Sort.
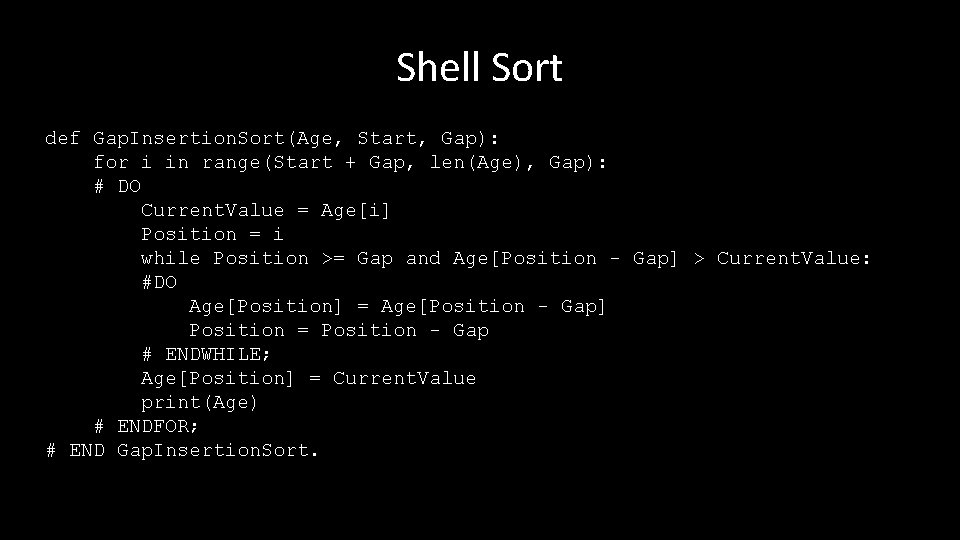
Shell Sort def Gap. Insertion. Sort(Age, Start, Gap): for i in range(Start + Gap, len(Age), Gap): # DO Current. Value = Age[i] Position = i while Position >= Gap and Age[Position - Gap] > Current. Value: #DO Age[Position] = Age[Position - Gap] Position = Position - Gap # ENDWHILE; Age[Position] = Current. Value print(Age) # ENDFOR; # END Gap. Insertion. Sort.
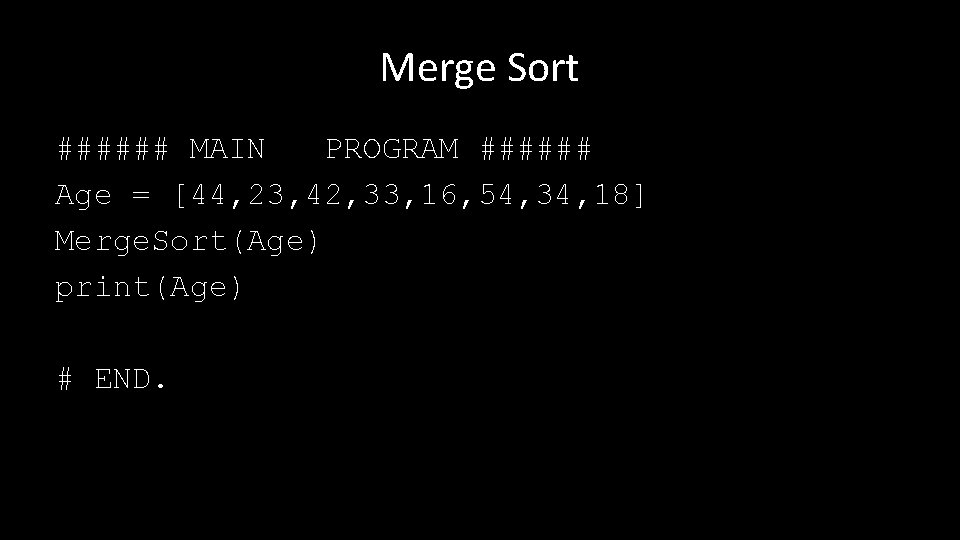
Merge Sort ###### MAIN PROGRAM ###### Age = [44, 23, 42, 33, 16, 54, 34, 18] Merge. Sort(Age) print(Age) # END.
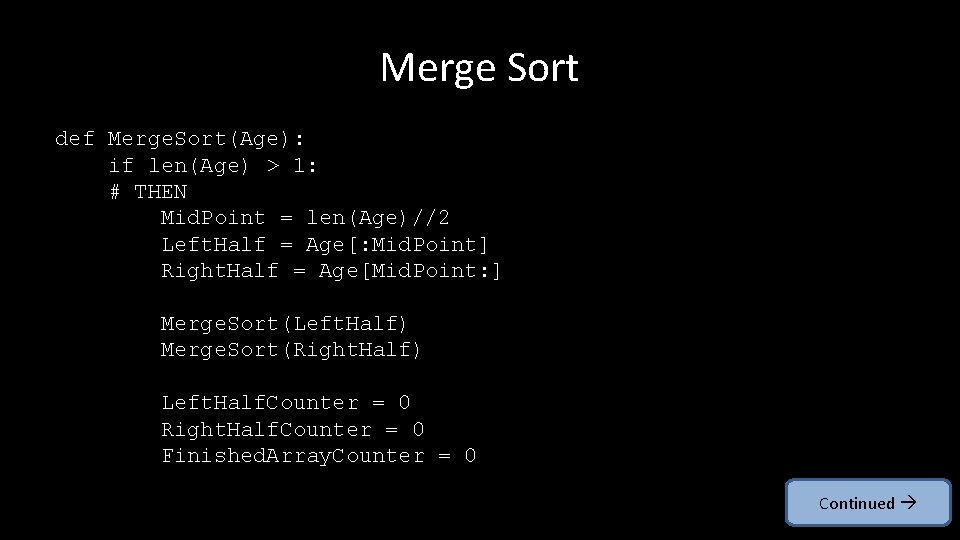
Merge Sort def Merge. Sort(Age): if len(Age) > 1: # THEN Mid. Point = len(Age)//2 Left. Half = Age[: Mid. Point] Right. Half = Age[Mid. Point: ] Merge. Sort(Left. Half) Merge. Sort(Right. Half) Left. Half. Counter = 0 Right. Half. Counter = 0 Finished. Array. Counter = 0 Continued
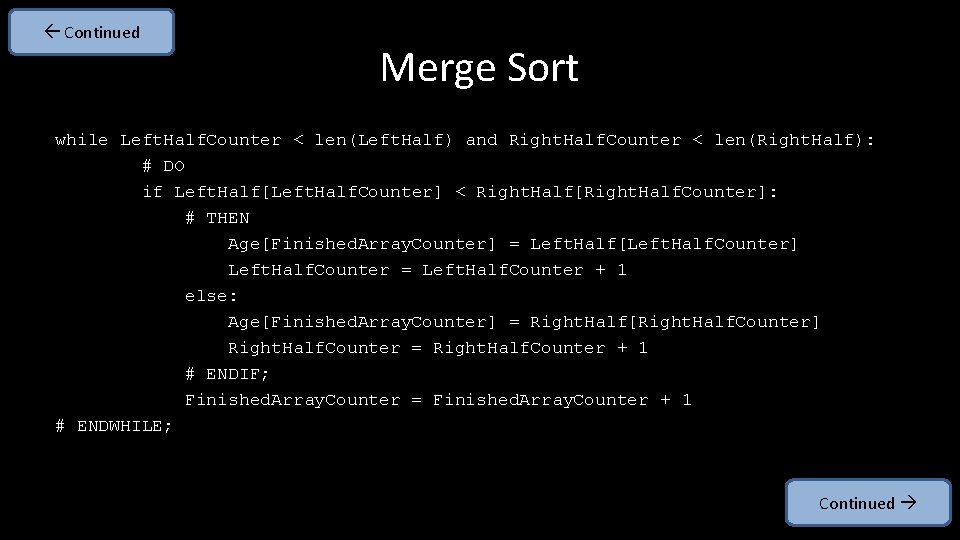
Continued Merge Sort while Left. Half. Counter < len(Left. Half) and Right. Half. Counter < len(Right. Half): # DO if Left. Half[Left. Half. Counter] < Right. Half[Right. Half. Counter]: # THEN Age[Finished. Array. Counter] = Left. Half[Left. Half. Counter] Left. Half. Counter = Left. Half. Counter + 1 else: Age[Finished. Array. Counter] = Right. Half[Right. Half. Counter] Right. Half. Counter = Right. Half. Counter + 1 # ENDIF; Finished. Array. Counter = Finished. Array. Counter + 1 # ENDWHILE; Continued
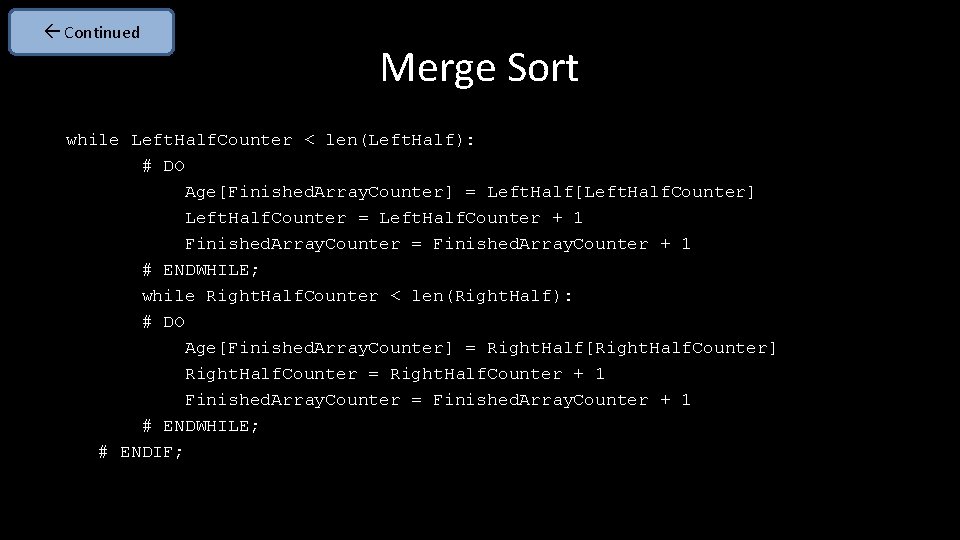
Continued Merge Sort while Left. Half. Counter < len(Left. Half): # DO Age[Finished. Array. Counter] = Left. Half[Left. Half. Counter] Left. Half. Counter = Left. Half. Counter + 1 Finished. Array. Counter = Finished. Array. Counter + 1 # ENDWHILE; while Right. Half. Counter < len(Right. Half): # DO Age[Finished. Array. Counter] = Right. Half[Right. Half. Counter] Right. Half. Counter = Right. Half. Counter + 1 Finished. Array. Counter = Finished. Array. Counter + 1 # ENDWHILE; # ENDIF;
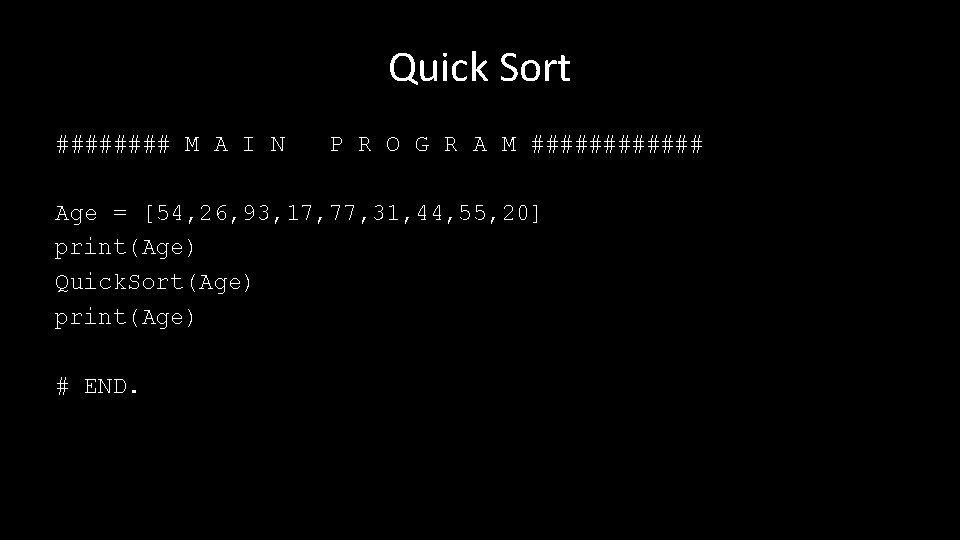
Quick Sort #### M A I N P R O G R A M ###### Age = [54, 26, 93, 17, 77, 31, 44, 55, 20] print(Age) Quick. Sort(Age) print(Age) # END.
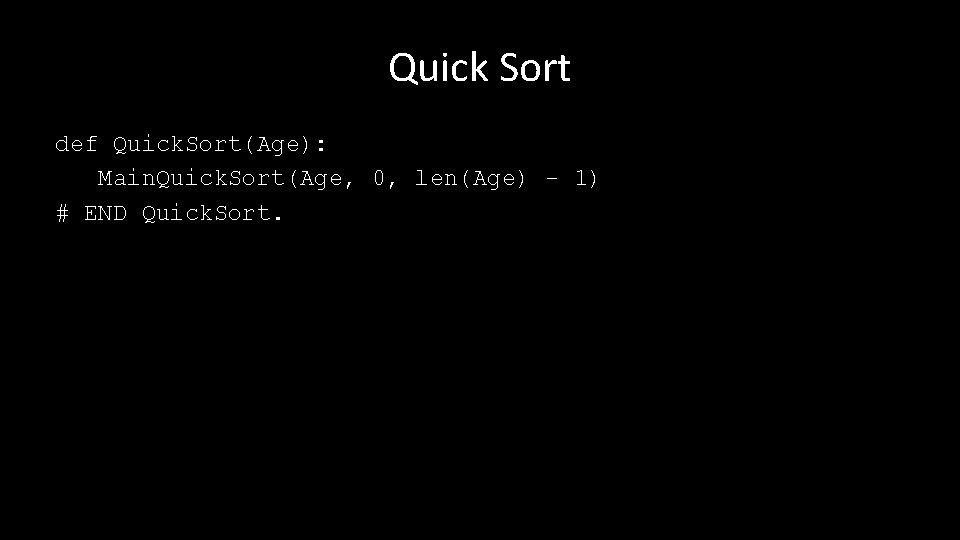
Quick Sort def Quick. Sort(Age): Main. Quick. Sort(Age, 0, len(Age) - 1) # END Quick. Sort.
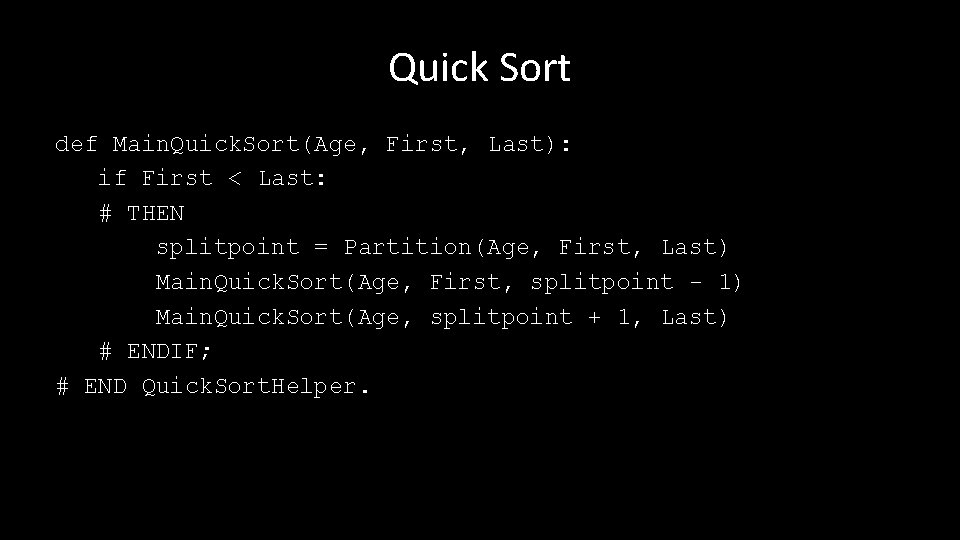
Quick Sort def Main. Quick. Sort(Age, First, Last): if First < Last: # THEN splitpoint = Partition(Age, First, Last) Main. Quick. Sort(Age, First, splitpoint - 1) Main. Quick. Sort(Age, splitpoint + 1, Last) # ENDIF; # END Quick. Sort. Helper.
![Quick Sort def PartitionAge First Last pivotvalue AgeFirst Finished False Left Pointer Quick Sort def Partition(Age, First, Last): pivotvalue = Age[First] Finished = False Left. Pointer](https://slidetodoc.com/presentation_image_h/aaf8f5358c1623ec5e9a26be12d8fb67/image-81.jpg)
Quick Sort def Partition(Age, First, Last): pivotvalue = Age[First] Finished = False Left. Pointer = First + 1 Right. Pointer = Last Continued
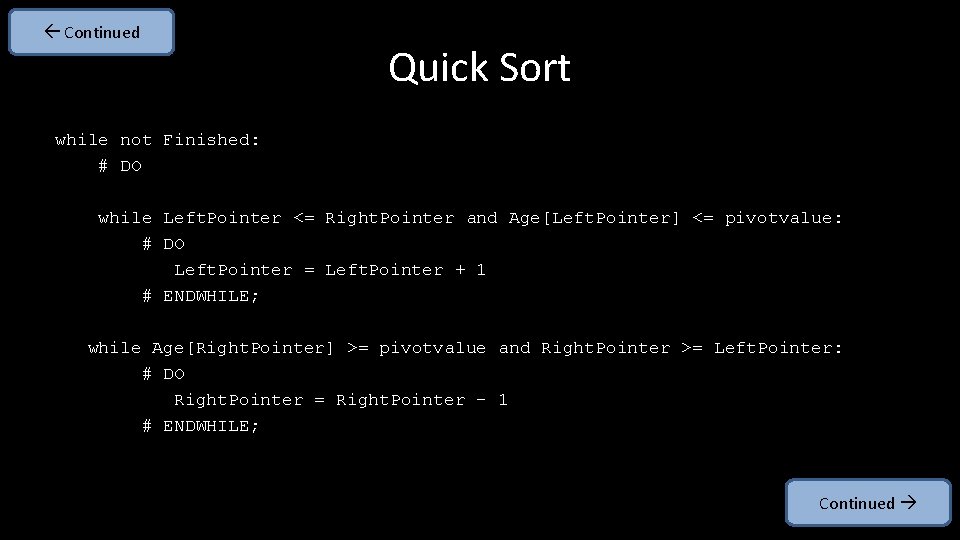
Continued Quick Sort while not Finished: # DO while Left. Pointer <= Right. Pointer and Age[Left. Pointer] <= pivotvalue: # DO Left. Pointer = Left. Pointer + 1 # ENDWHILE; while Age[Right. Pointer] >= pivotvalue and Right. Pointer >= Left. Pointer: # DO Right. Pointer = Right. Pointer - 1 # ENDWHILE; Continued
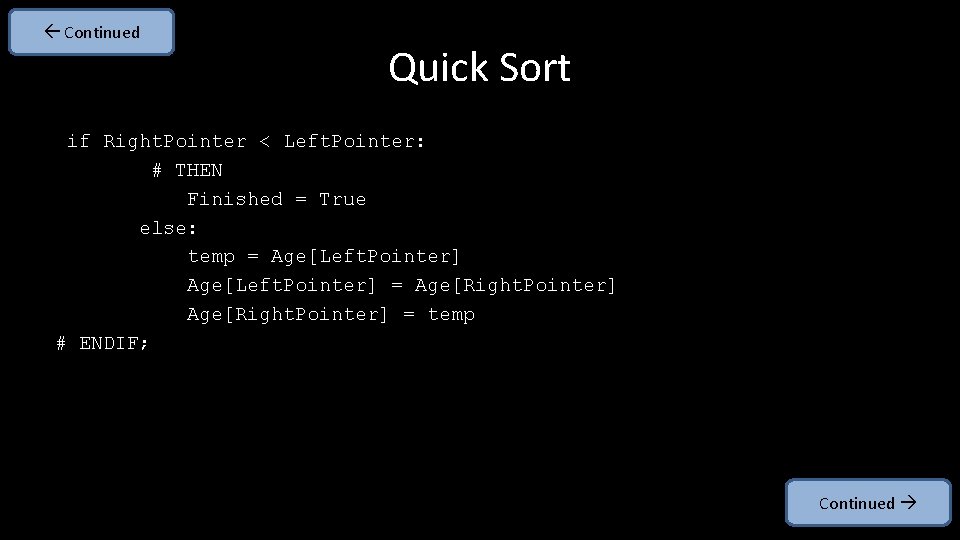
Continued Quick Sort if Right. Pointer < Left. Pointer: # THEN Finished = True else: temp = Age[Left. Pointer] = Age[Right. Pointer] = temp # ENDIF; Continued
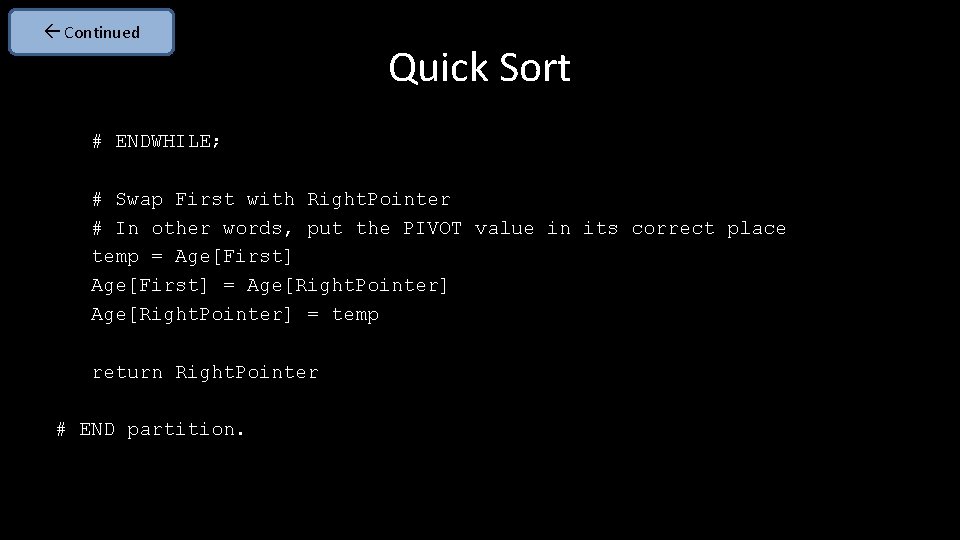
Continued Quick Sort # ENDWHILE; # Swap First with Right. Pointer # In other words, put the PIVOT value in its correct place temp = Age[First] = Age[Right. Pointer] = temp return Right. Pointer # END partition.
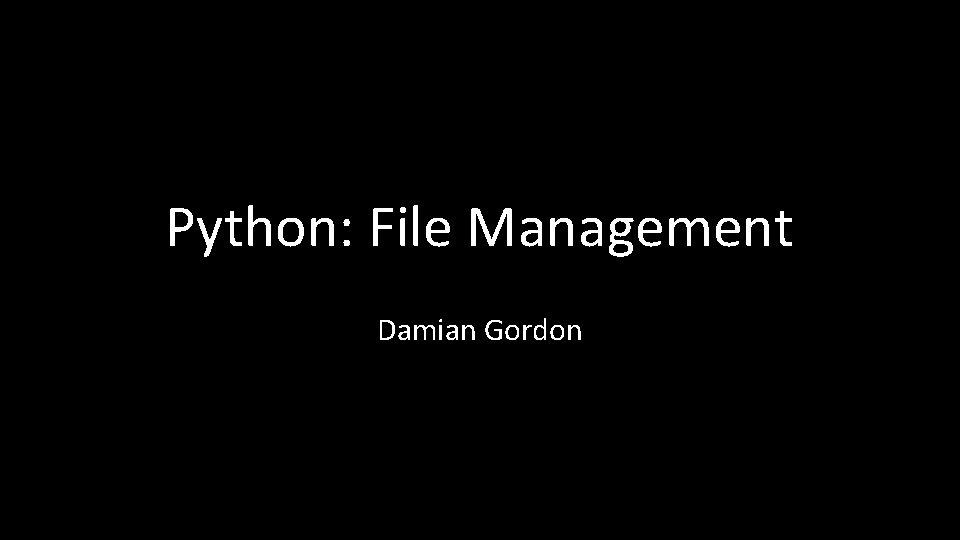
Python: File Management Damian Gordon
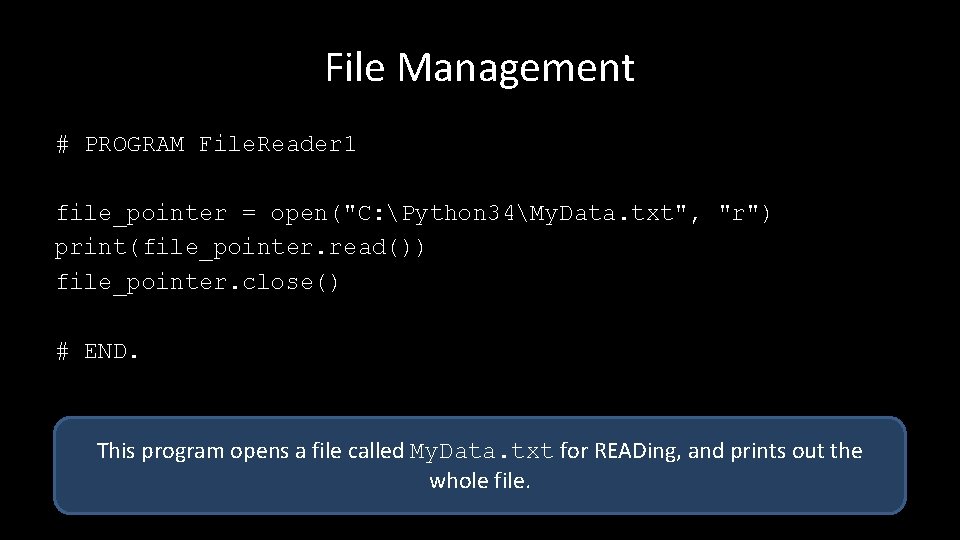
File Management # PROGRAM File. Reader 1 file_pointer = open("C: Python 34My. Data. txt", "r") print(file_pointer. read()) file_pointer. close() # END. This program opens a file called My. Data. txt for READing, and prints out the whole file.
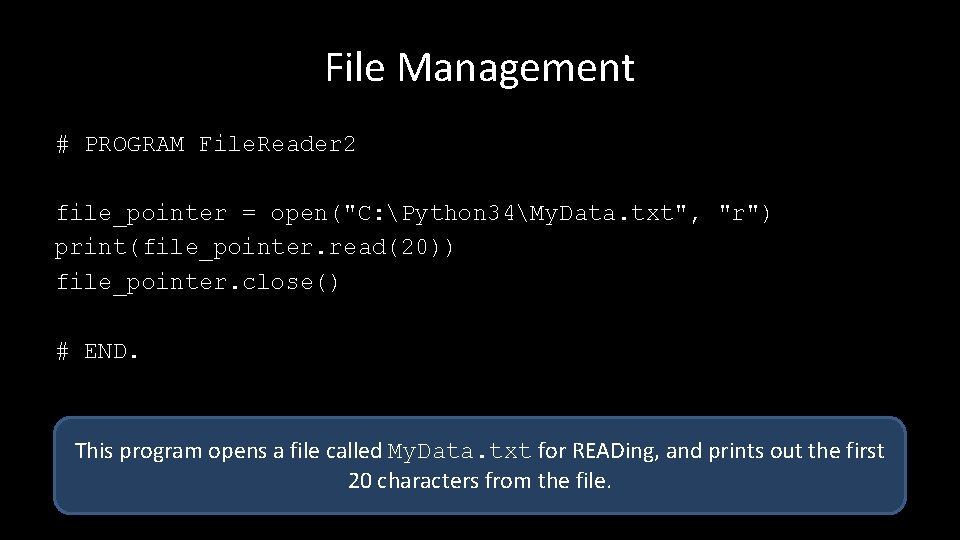
File Management # PROGRAM File. Reader 2 file_pointer = open("C: Python 34My. Data. txt", "r") print(file_pointer. read(20)) file_pointer. close() # END. This program opens a file called My. Data. txt for READing, and prints out the first 20 characters from the file.
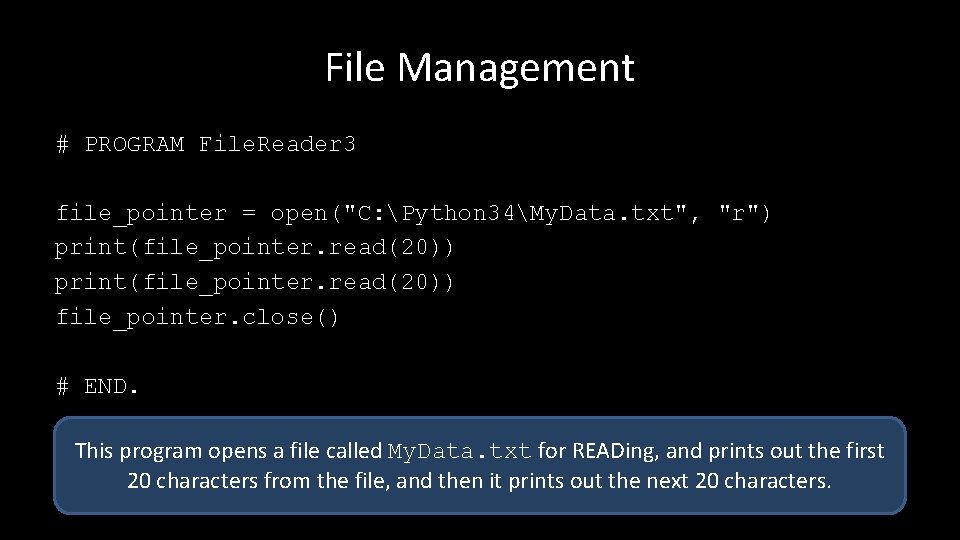
File Management # PROGRAM File. Reader 3 file_pointer = open("C: Python 34My. Data. txt", "r") print(file_pointer. read(20)) file_pointer. close() # END. This program opens a file called My. Data. txt for READing, and prints out the first 20 characters from the file, and then it prints out the next 20 characters.
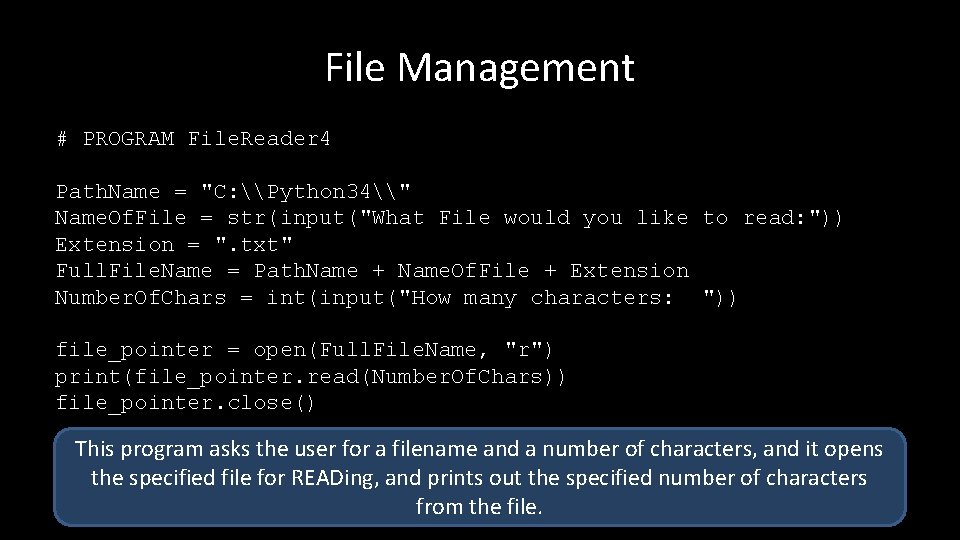
File Management # PROGRAM File. Reader 4 Path. Name = "C: \Python 34\" Name. Of. File = str(input("What File would you like to read: ")) Extension = ". txt" Full. File. Name = Path. Name + Name. Of. File + Extension Number. Of. Chars = int(input("How many characters: ")) file_pointer = open(Full. File. Name, "r") print(file_pointer. read(Number. Of. Chars)) file_pointer. close() program asks the user for a filename and a number of characters, and it opens # This END. the specified file for READing, and prints out the specified number of characters from the file.
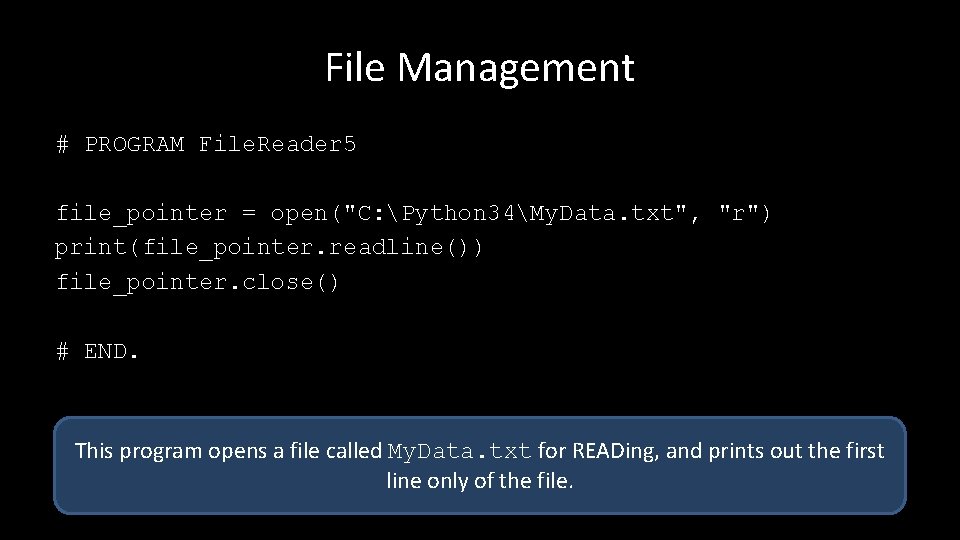
File Management # PROGRAM File. Reader 5 file_pointer = open("C: Python 34My. Data. txt", "r") print(file_pointer. readline()) file_pointer. close() # END. This program opens a file called My. Data. txt for READing, and prints out the first line only of the file.
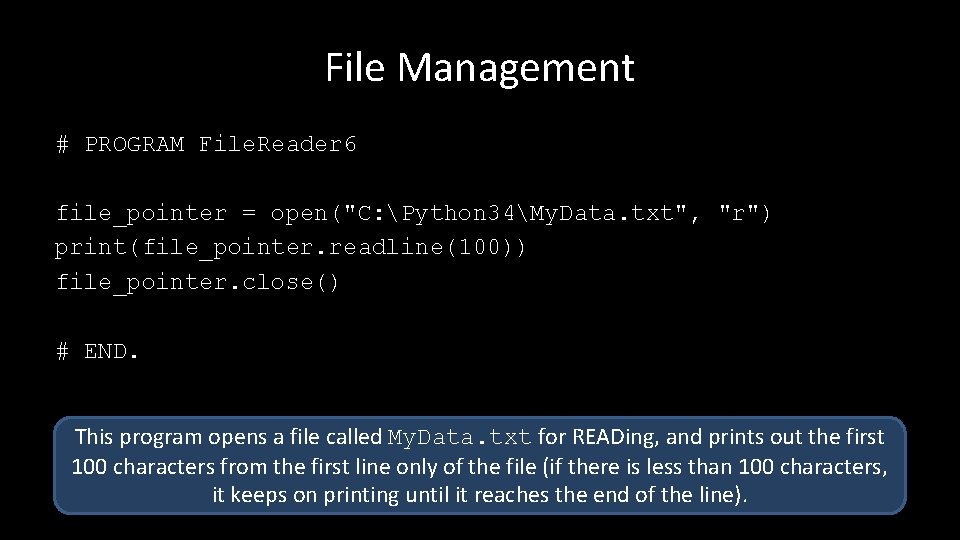
File Management # PROGRAM File. Reader 6 file_pointer = open("C: Python 34My. Data. txt", "r") print(file_pointer. readline(100)) file_pointer. close() # END. This program opens a file called My. Data. txt for READing, and prints out the first 100 characters from the first line only of the file (if there is less than 100 characters, it keeps on printing until it reaches the end of the line).
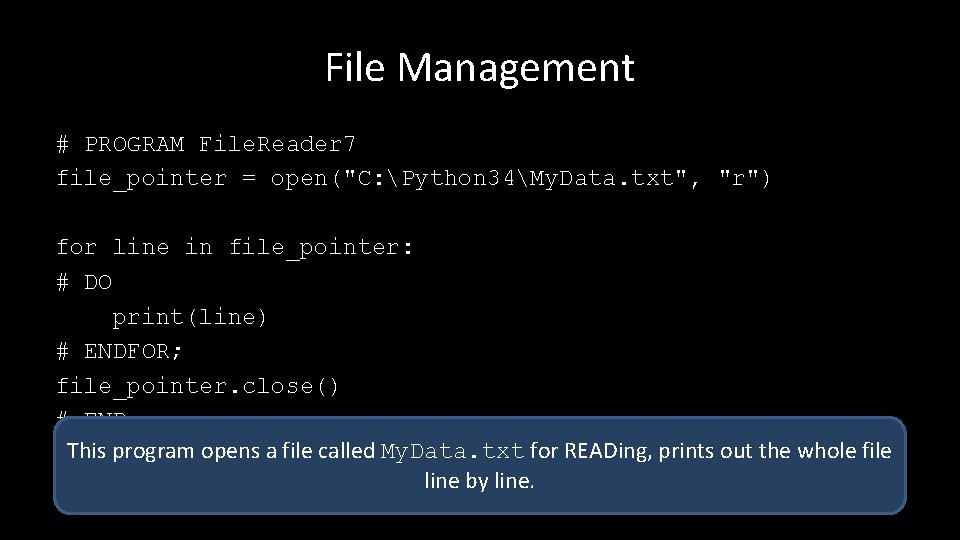
File Management # PROGRAM File. Reader 7 file_pointer = open("C: Python 34My. Data. txt", "r") for line in file_pointer: # DO print(line) # ENDFOR; file_pointer. close() # END. This program opens a file called My. Data. txt for READing, prints out the whole file line by line.
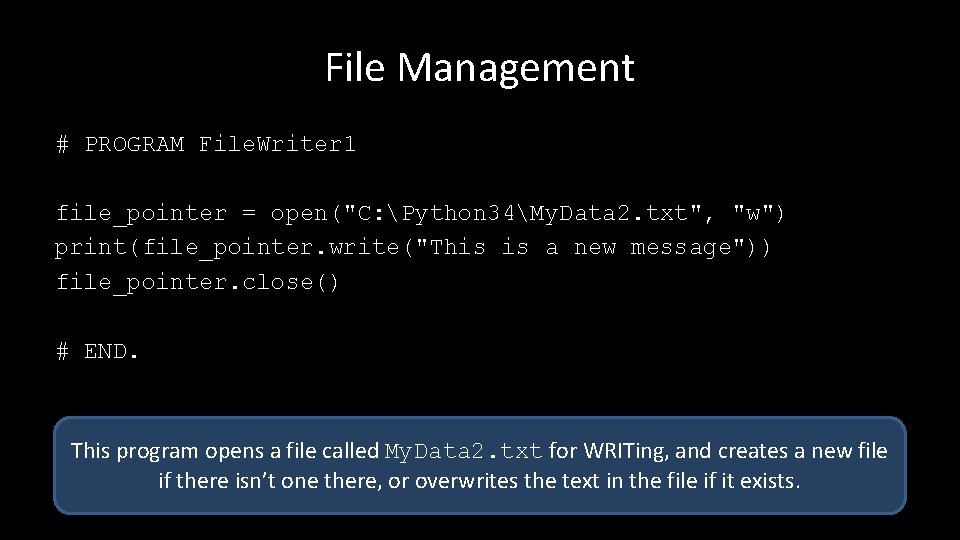
File Management # PROGRAM File. Writer 1 file_pointer = open("C: Python 34My. Data 2. txt", "w") print(file_pointer. write("This is a new message")) file_pointer. close() # END. This program opens a file called My. Data 2. txt for WRITing, and creates a new file if there isn’t one there, or overwrites the text in the file if it exists.
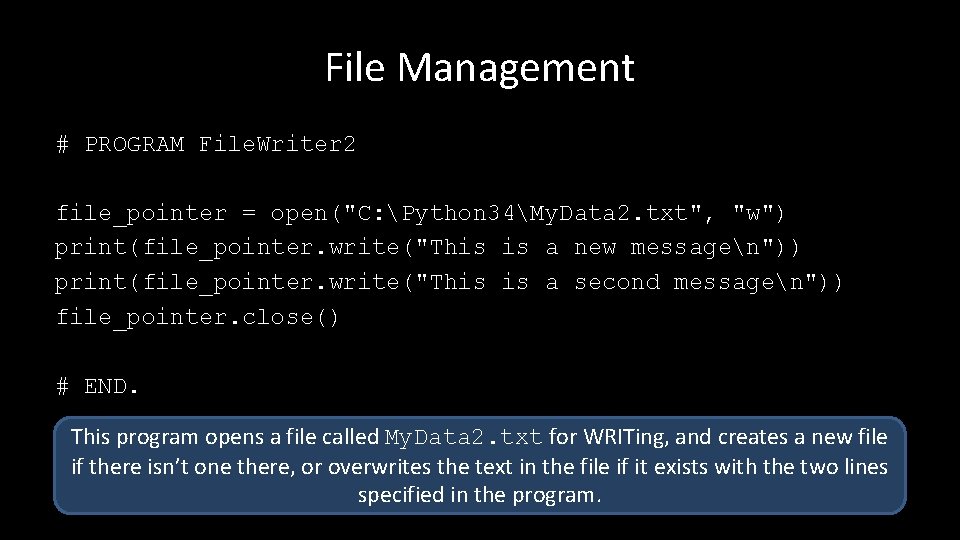
File Management # PROGRAM File. Writer 2 file_pointer = open("C: Python 34My. Data 2. txt", "w") print(file_pointer. write("This is a new messagen")) print(file_pointer. write("This is a second messagen")) file_pointer. close() # END. This program opens a file called My. Data 2. txt for WRITing, and creates a new file if there isn’t one there, or overwrites the text in the file if it exists with the two lines specified in the program.
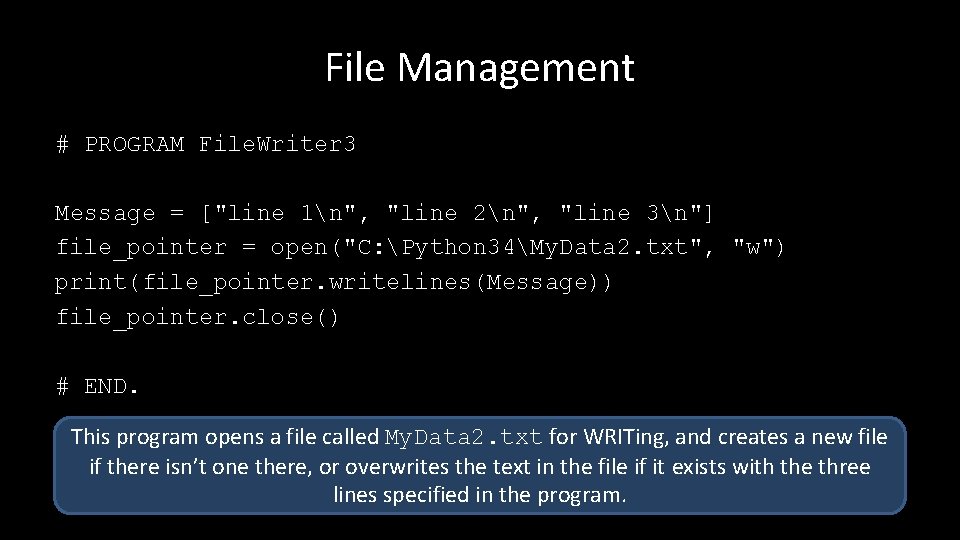
File Management # PROGRAM File. Writer 3 Message = ["line 1n", "line 2n", "line 3n"] file_pointer = open("C: Python 34My. Data 2. txt", "w") print(file_pointer. writelines(Message)) file_pointer. close() # END. This program opens a file called My. Data 2. txt for WRITing, and creates a new file if there isn’t one there, or overwrites the text in the file if it exists with the three lines specified in the program.
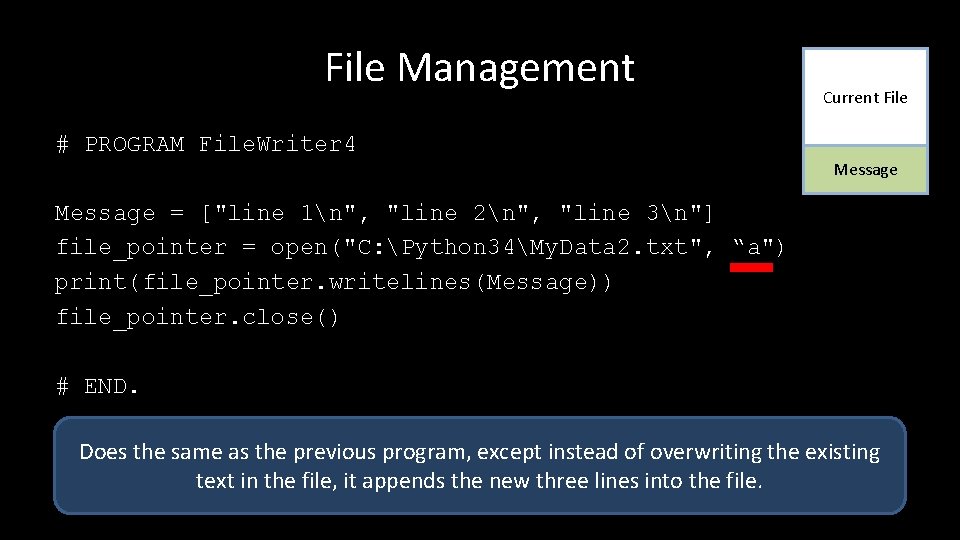
File Management Current File # PROGRAM File. Writer 4 Message = ["line 1n", "line 2n", "line 3n"] file_pointer = open("C: Python 34My. Data 2. txt", “a") print(file_pointer. writelines(Message)) file_pointer. close() # END. Does the same as the previous program, except instead of overwriting the existing text in the file, it appends the new three lines into the file.
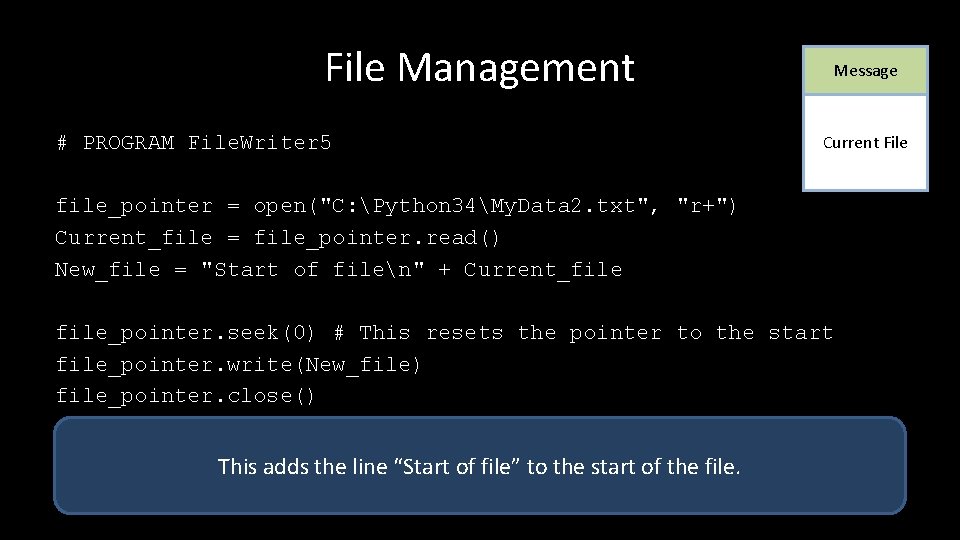
File Management # PROGRAM File. Writer 5 Message Current File file_pointer = open("C: Python 34My. Data 2. txt", "r+") Current_file = file_pointer. read() New_file = "Start of filen" + Current_file_pointer. seek(0) # This resets the pointer to the start file_pointer. write(New_file) file_pointer. close() # END. This adds the line “Start of file” to the start of the file.
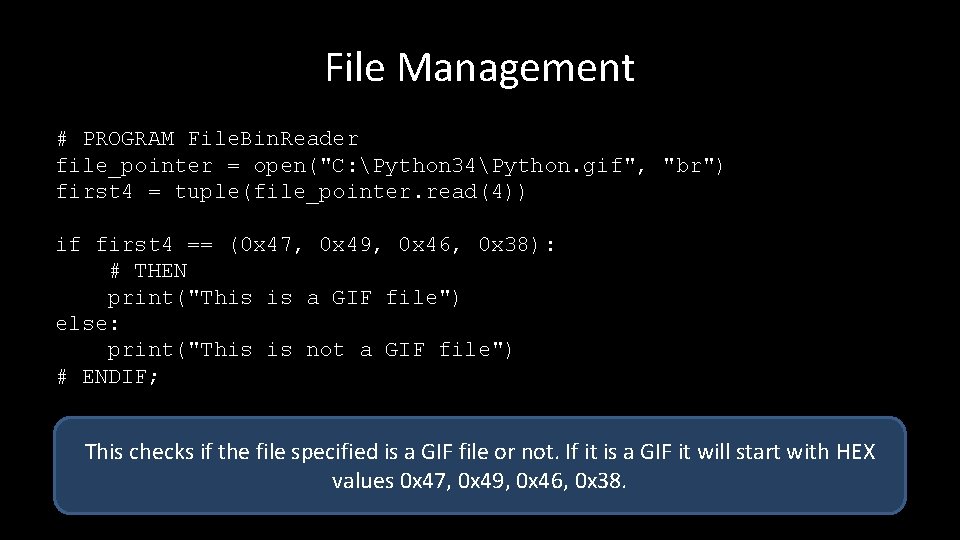
File Management # PROGRAM File. Bin. Reader file_pointer = open("C: Python 34Python. gif", "br") first 4 = tuple(file_pointer. read(4)) if first 4 == (0 x 47, 0 x 49, 0 x 46, 0 x 38): # THEN print("This is a GIF file") else: print("This is not a GIF file") # ENDIF; file_pointer. close() This checks if the file specified is a GIF file or not. If it is a GIF it will start with HEX # END. values 0 x 47, 0 x 49, 0 x 46, 0 x 38.
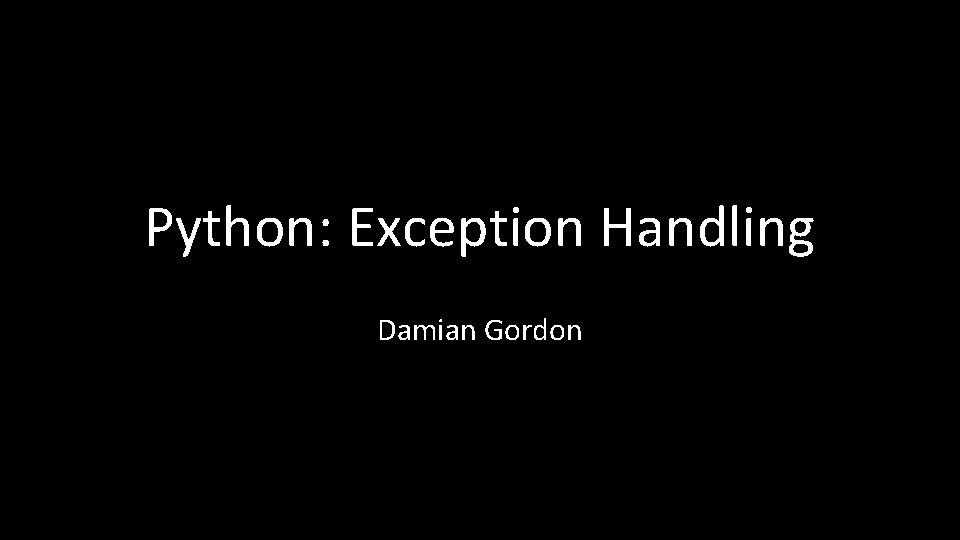
Python: Exception Handling Damian Gordon
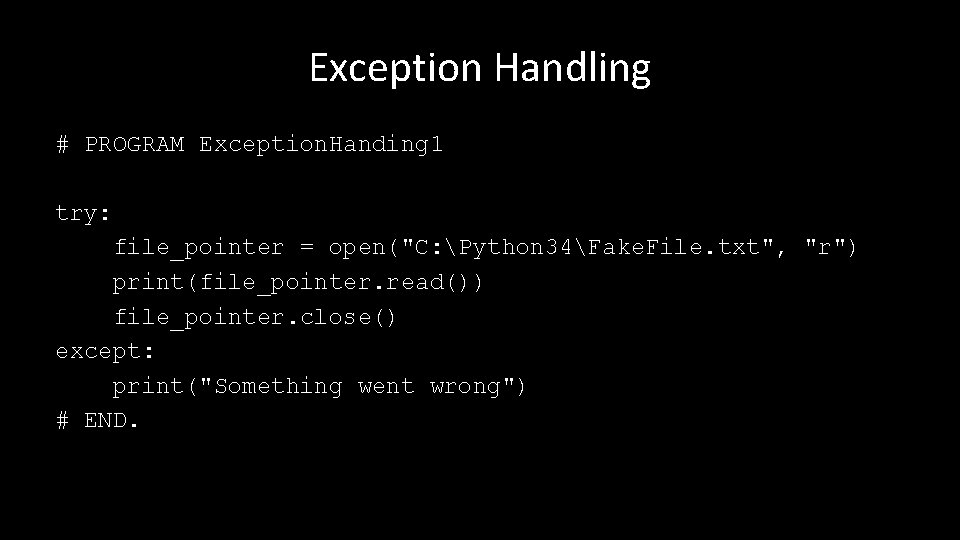
Exception Handling # PROGRAM Exception. Handing 1 try: file_pointer = open("C: Python 34Fake. File. txt", "r") print(file_pointer. read()) file_pointer. close() except: print("Something went wrong") # END.
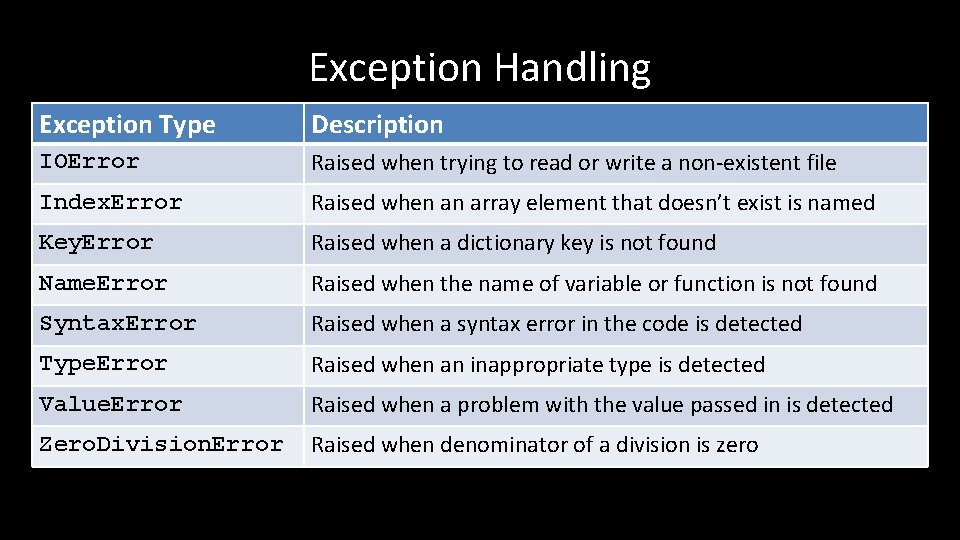
Exception Handling Exception Type Description IOError Raised when trying to read or write a non-existent file Index. Error Raised when an array element that doesn’t exist is named Key. Error Raised when a dictionary key is not found Name. Error Raised when the name of variable or function is not found Syntax. Error Raised when a syntax error in the code is detected Type. Error Raised when an inappropriate type is detected Value. Error Raised when a problem with the value passed in is detected Zero. Division. Error Raised when denominator of a division is zero
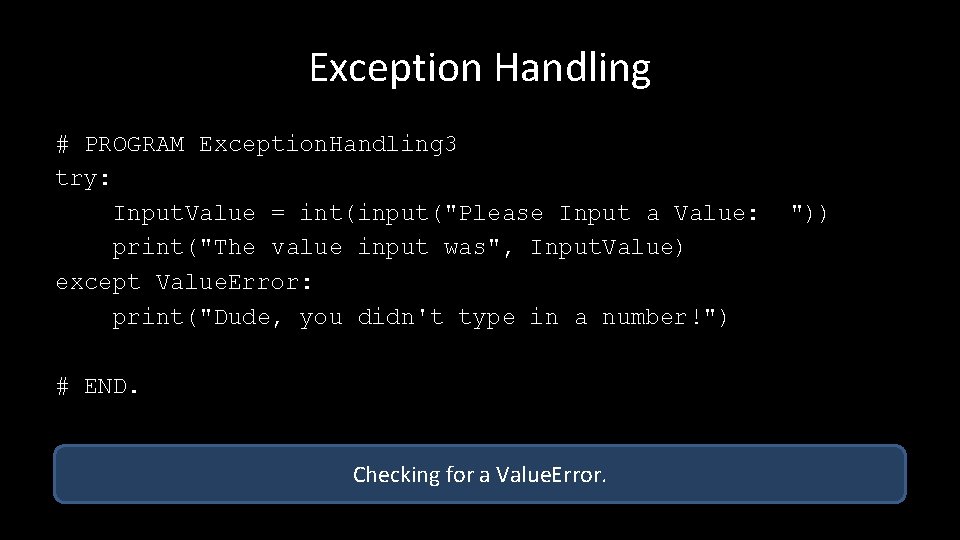
Exception Handling # PROGRAM Exception. Handling 3 try: Input. Value = int(input("Please Input a Value: print("The value input was", Input. Value) except Value. Error: print("Dude, you didn't type in a number!") # END. Checking for a Value. Error. "))
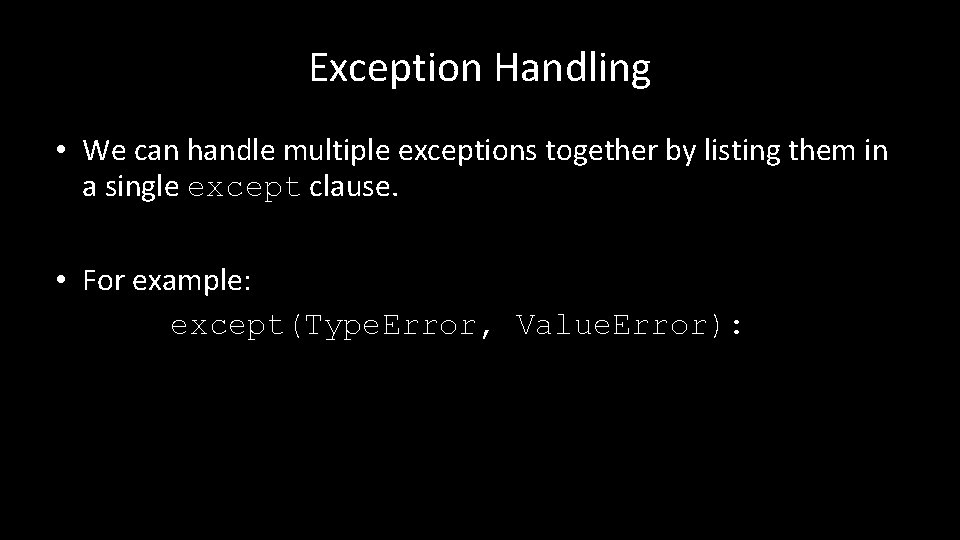
Exception Handling • We can handle multiple exceptions together by listing them in a single except clause. • For example: except(Type. Error, Value. Error):
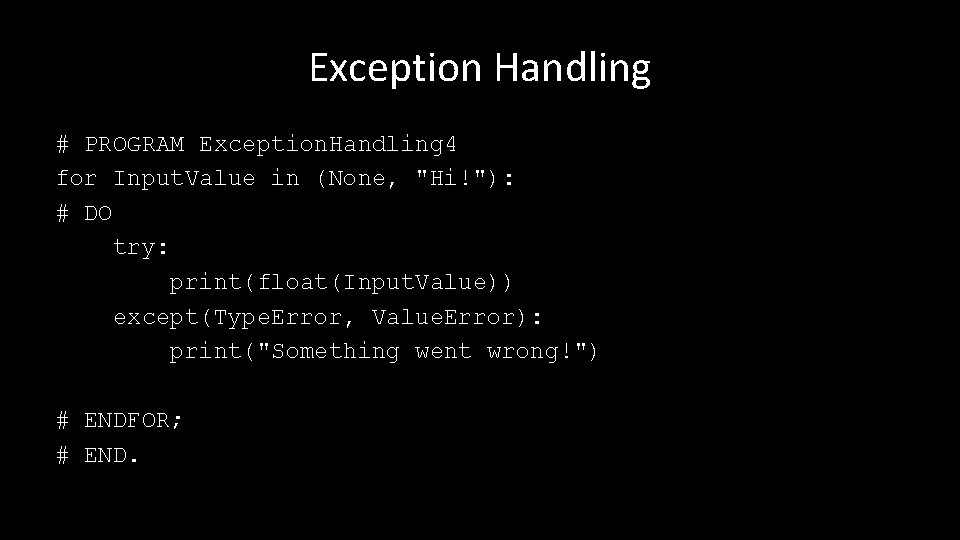
Exception Handling # PROGRAM Exception. Handling 4 for Input. Value in (None, "Hi!"): # DO try: print(float(Input. Value)) except(Type. Error, Value. Error): print("Something went wrong!") # ENDFOR; # END.
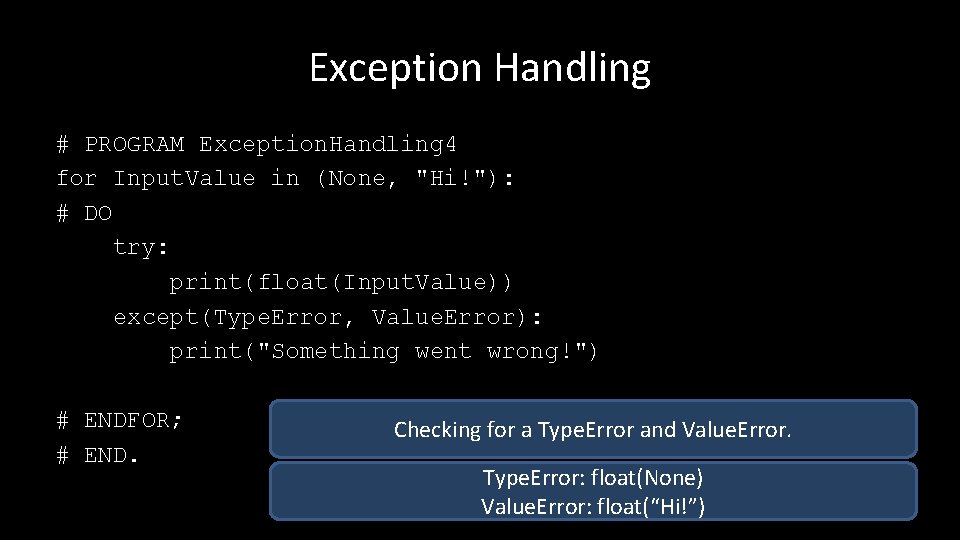
Exception Handling # PROGRAM Exception. Handling 4 for Input. Value in (None, "Hi!"): # DO try: print(float(Input. Value)) except(Type. Error, Value. Error): print("Something went wrong!") # ENDFOR; # END. Checking for a Type. Error and Value. Error. Type. Error: float(None) Value. Error: float(“Hi!”)
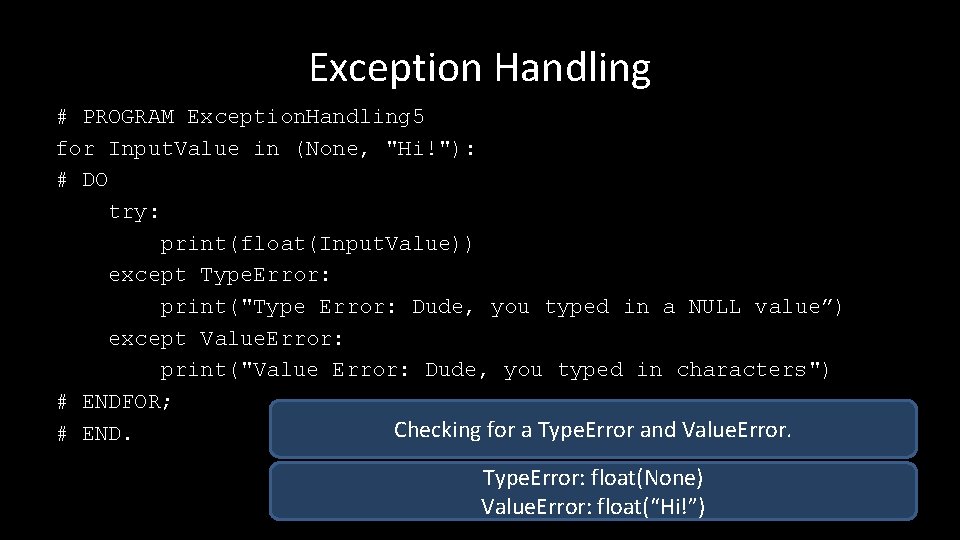
Exception Handling # PROGRAM Exception. Handling 5 for Input. Value in (None, "Hi!"): # DO try: print(float(Input. Value)) except Type. Error: print("Type Error: Dude, you typed in a NULL value”) except Value. Error: print("Value Error: Dude, you typed in characters") # ENDFOR; Checking for a Type. Error and Value. Error. # END. Type. Error: float(None) Value. Error: float(“Hi!”)
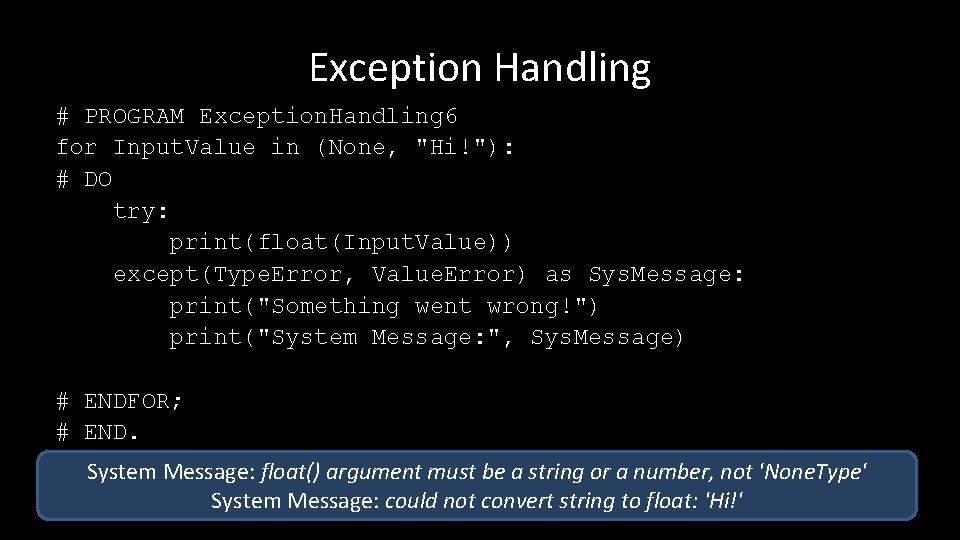
Exception Handling # PROGRAM Exception. Handling 6 for Input. Value in (None, "Hi!"): # DO try: print(float(Input. Value)) except(Type. Error, Value. Error) as Sys. Message: print("Something went wrong!") print("System Message: ", Sys. Message) # ENDFOR; # END. System Message: float() argument must be a string or a number, not 'None. Type' System Message: could not convert string to float: 'Hi!'
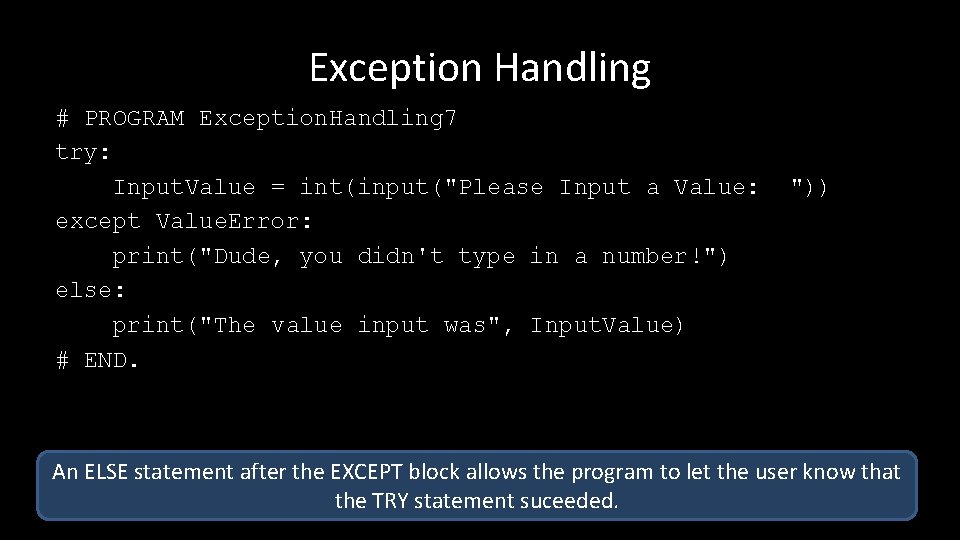
Exception Handling # PROGRAM Exception. Handling 7 try: Input. Value = int(input("Please Input a Value: except Value. Error: print("Dude, you didn't type in a number!") else: print("The value input was", Input. Value) # END. ")) An ELSE statement after the EXCEPT block allows the program to let the user know that the TRY statement suceeded.
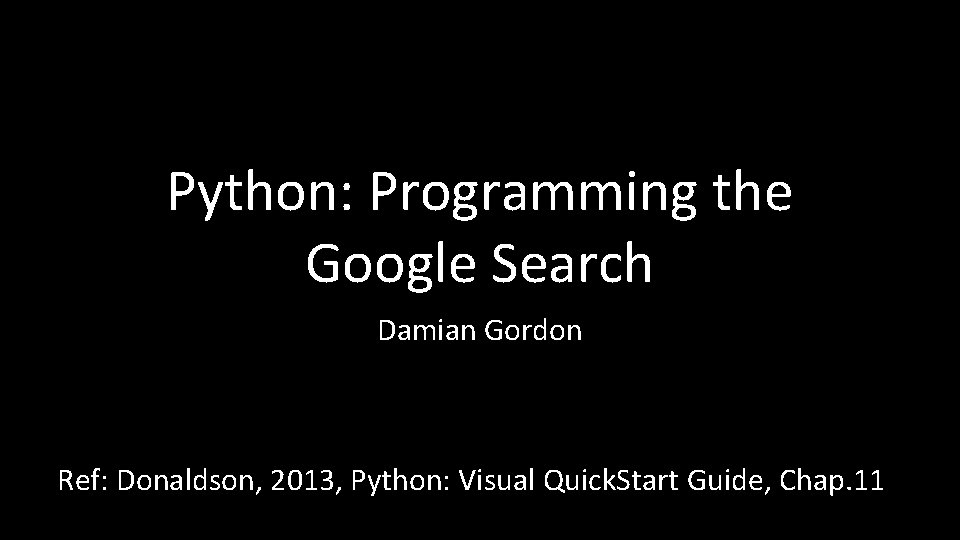
Python: Programming the Google Search Damian Gordon Ref: Donaldson, 2013, Python: Visual Quick. Start Guide, Chap. 11
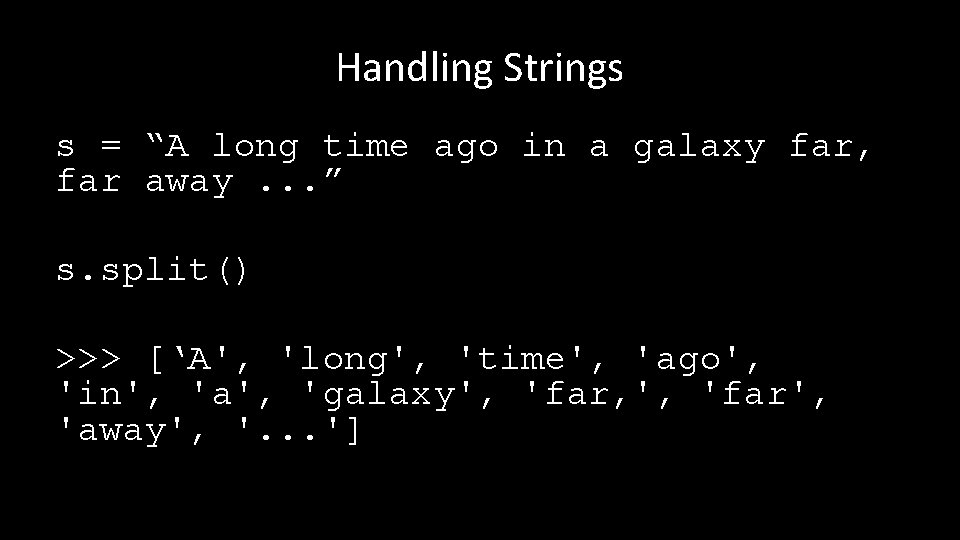
Handling Strings s = “A long time ago in a galaxy far, far away. . . ” s. split() >>> [‘A', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far, ', 'far', 'away', '. . . ']
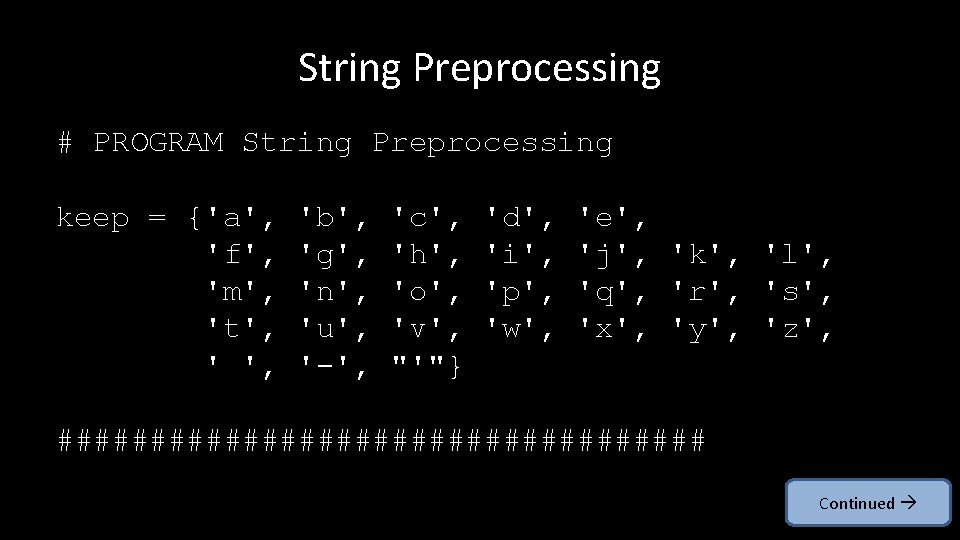
String Preprocessing # PROGRAM String Preprocessing keep = {'a', 'f', 'm', 't', ' ', 'b', 'g', 'n', 'u', '-', 'c', 'h', 'o', 'v', "'"} 'd', 'i', 'p', 'w', 'e', 'j', 'k', 'l', 'q', 'r', 's', 'x', 'y', 'z', ################## Continued
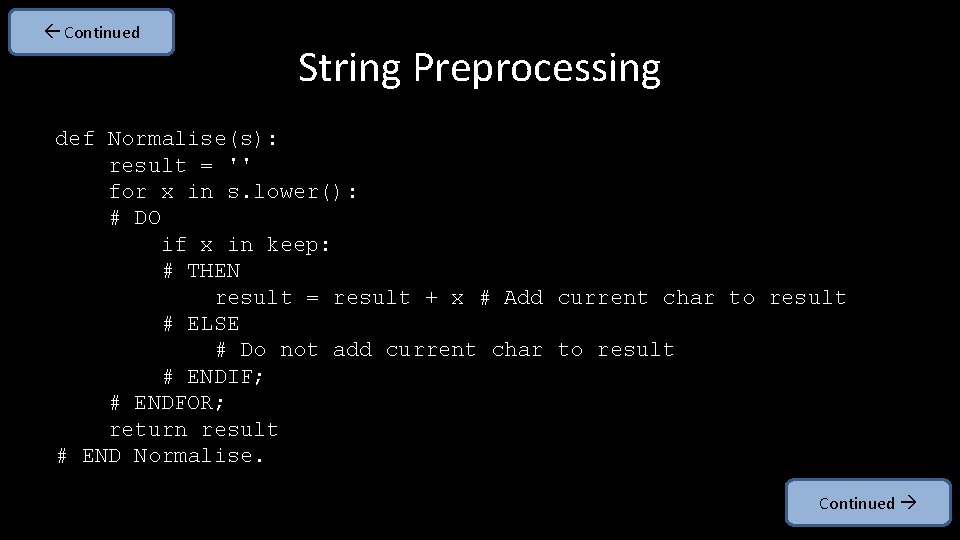
Continued String Preprocessing def Normalise(s): result = '' for x in s. lower(): # DO if x in keep: # THEN result = result + x # Add current char to result # ELSE # Do not add current char to result # ENDIF; # ENDFOR; return result # END Normalise. Continued
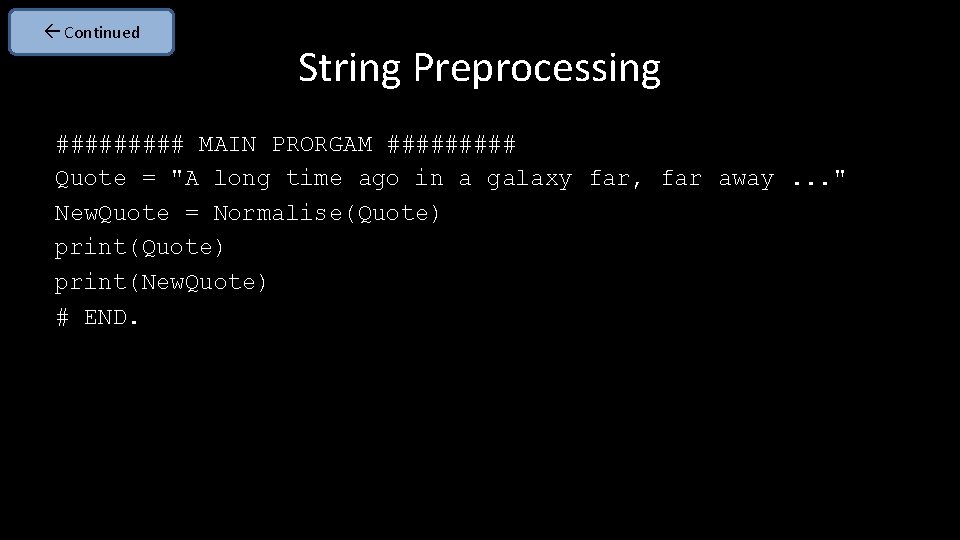
Continued String Preprocessing ##### MAIN PRORGAM ##### Quote = "A long time ago in a galaxy far, far away. . . " New. Quote = Normalise(Quote) print(New. Quote) # END.
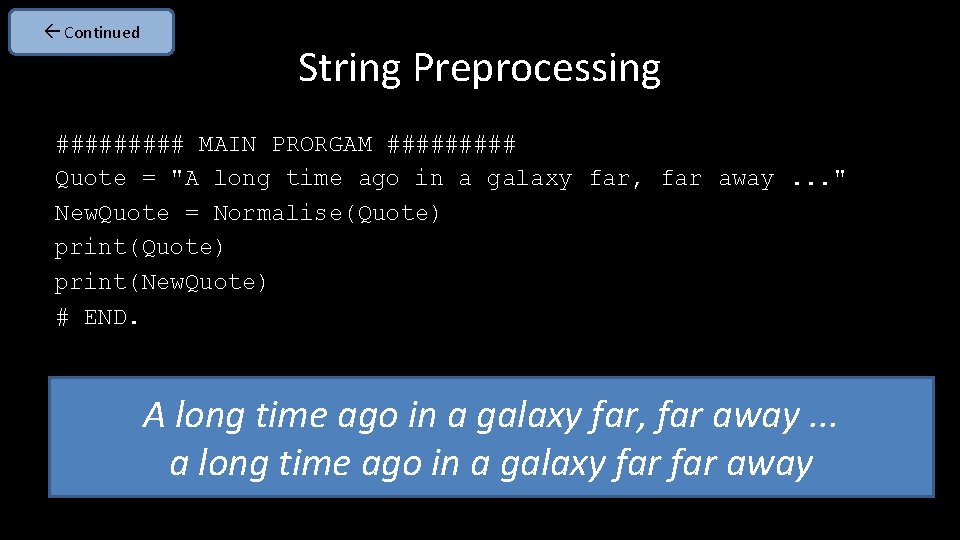
Continued String Preprocessing ##### MAIN PRORGAM ##### Quote = "A long time ago in a galaxy far, far away. . . " New. Quote = Normalise(Quote) print(New. Quote) # END. A long time ago in a galaxy far, far away. . . a long time ago in a galaxy far away
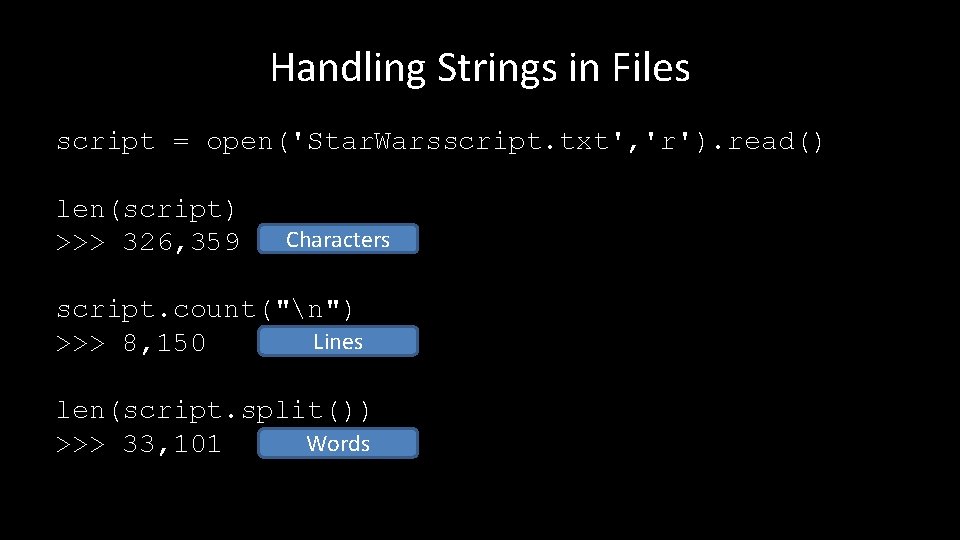
Handling Strings in Files script = open('Star. Warsscript. txt', 'r'). read() len(script) >>> 326, 359 Characters script. count("n") Lines >>> 8, 150 len(script. split()) Words >>> 33, 101
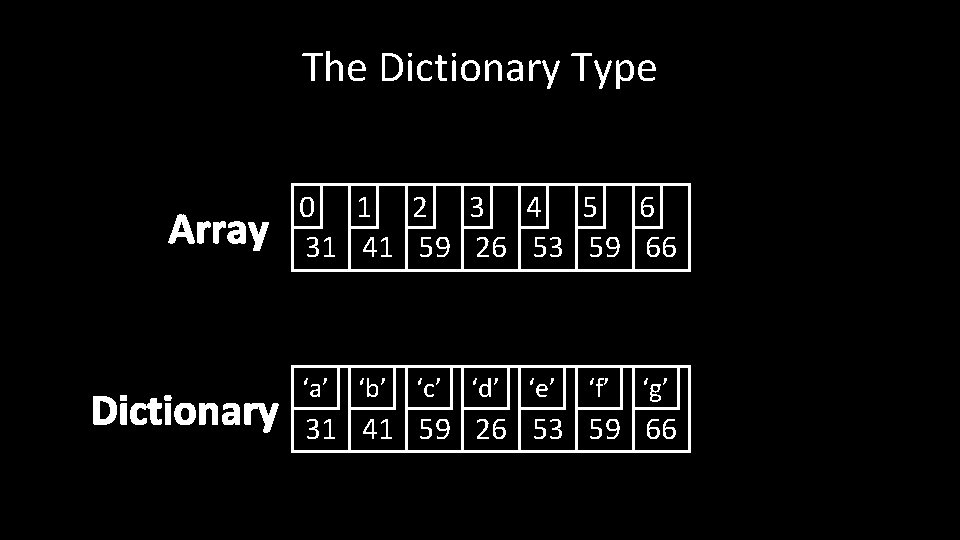
The Dictionary Type Array Dictionary 0 1 2 3 4 5 6 31 41 59 26 53 59 66 ‘a’ ‘b’ ‘c’ ‘d’ ‘e’ ‘f’ ‘g’ 31 41 59 26 53 59 66
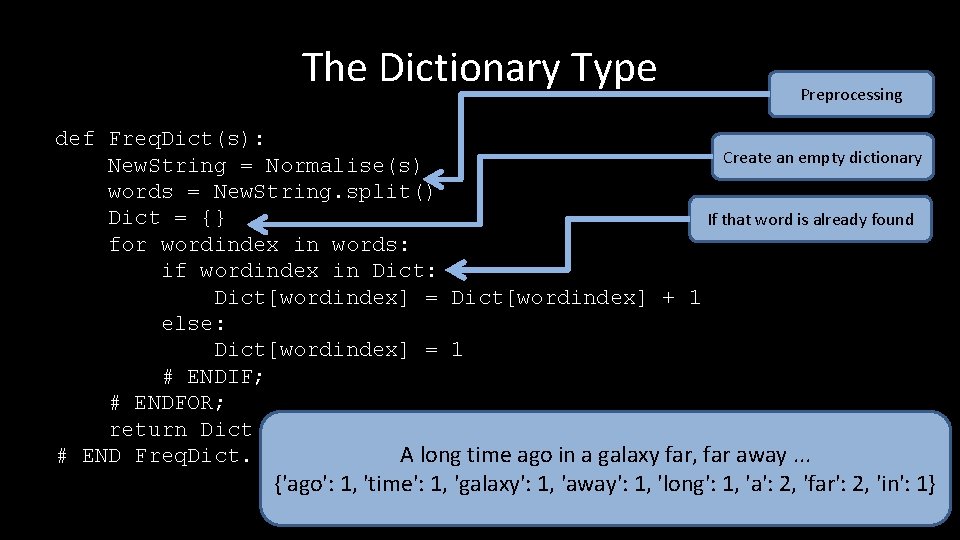
The Dictionary Type Preprocessing def Freq. Dict(s): Create an empty dictionary New. String = Normalise(s) words = New. String. split() Dict = {} If that word is already found for wordindex in words: if wordindex in Dict: Dict[wordindex] = Dict[wordindex] + 1 else: Dict[wordindex] = 1 # ENDIF; # ENDFOR; return Dict # END Freq. Dict. A long time ago in a galaxy far, far away. . . {'ago': 1, 'time': 1, 'galaxy': 1, 'away': 1, 'long': 1, 'a': 2, 'far': 2, 'in': 1}
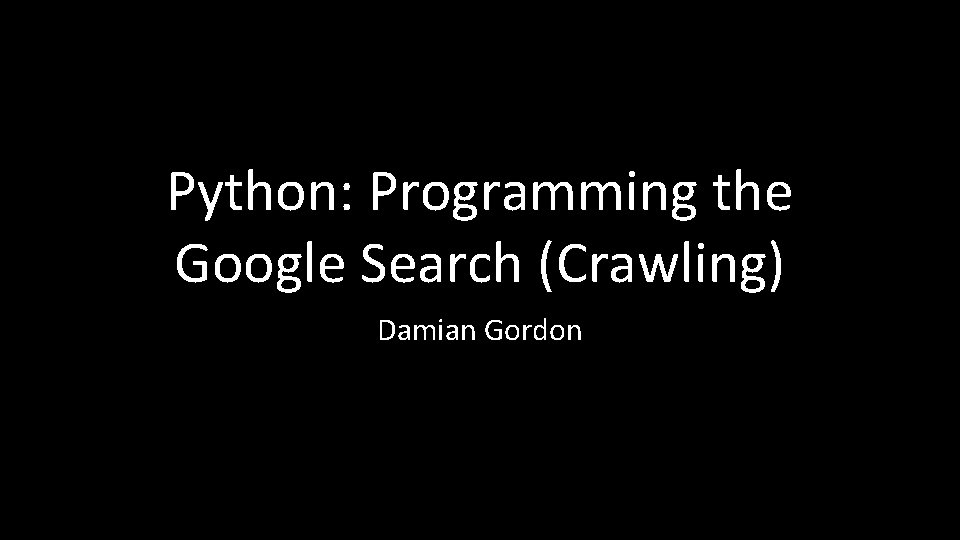
Python: Programming the Google Search (Crawling) Damian Gordon
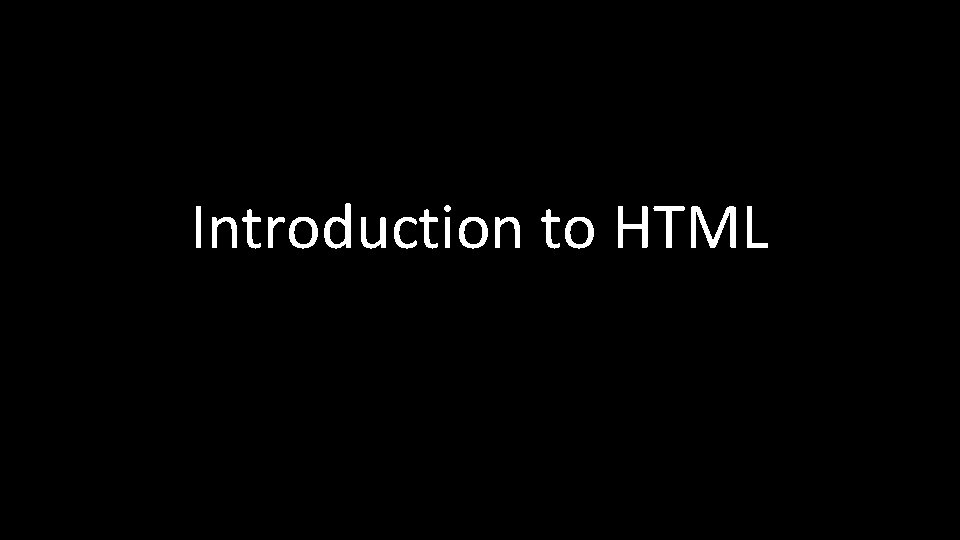
Introduction to HTML
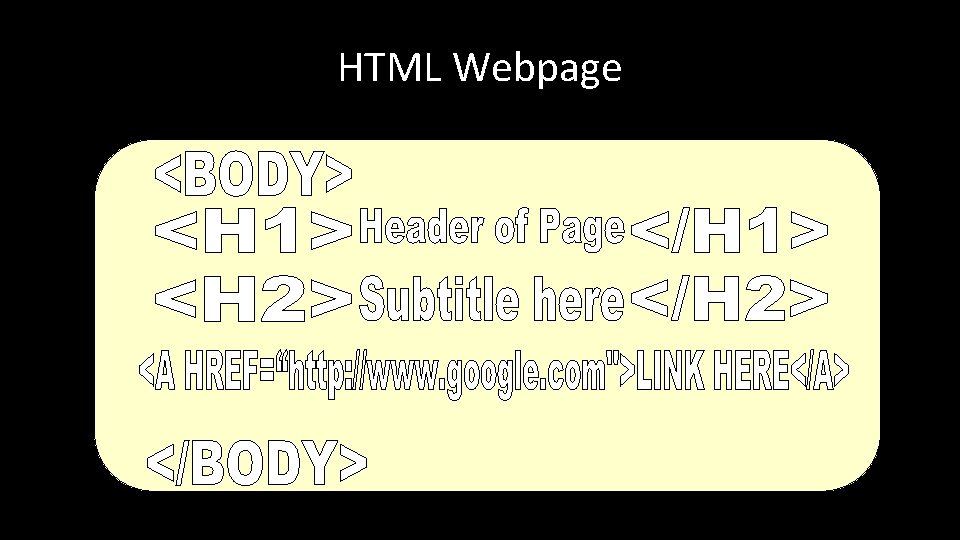
HTML Webpage
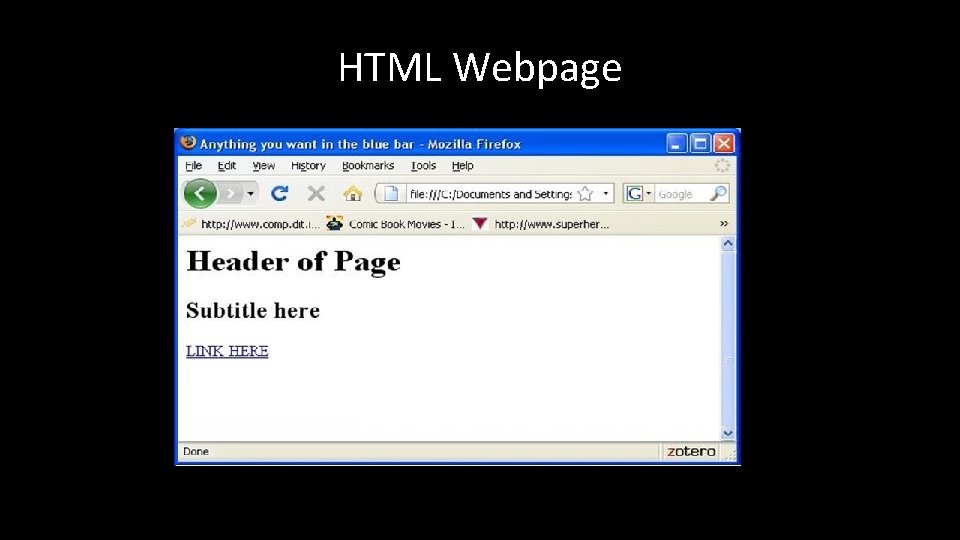
HTML Webpage
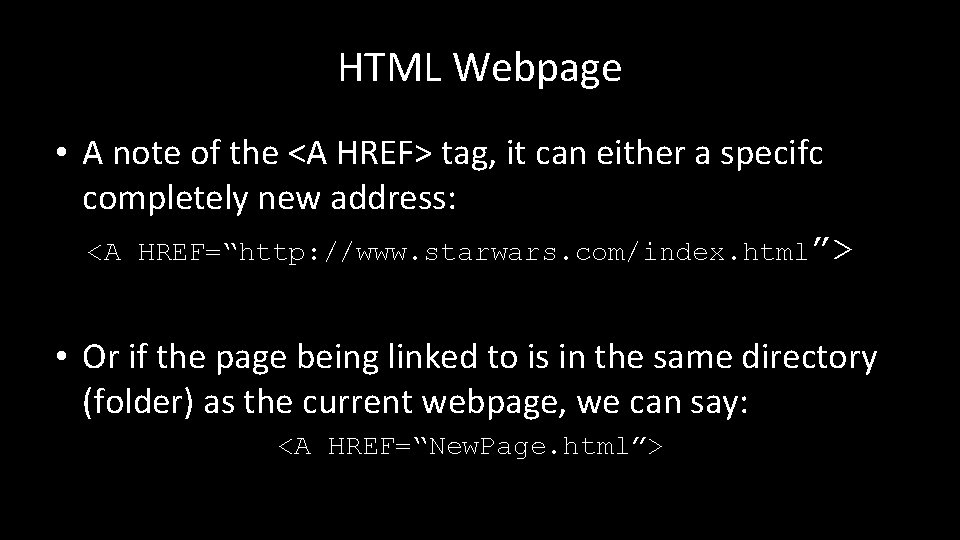
HTML Webpage • A note of the <A HREF> tag, it can either a specifc completely new address: <A HREF=“http: //www. starwars. com/index. html”> • Or if the page being linked to is in the same directory (folder) as the current webpage, we can say: <A HREF=“New. Page. html”>
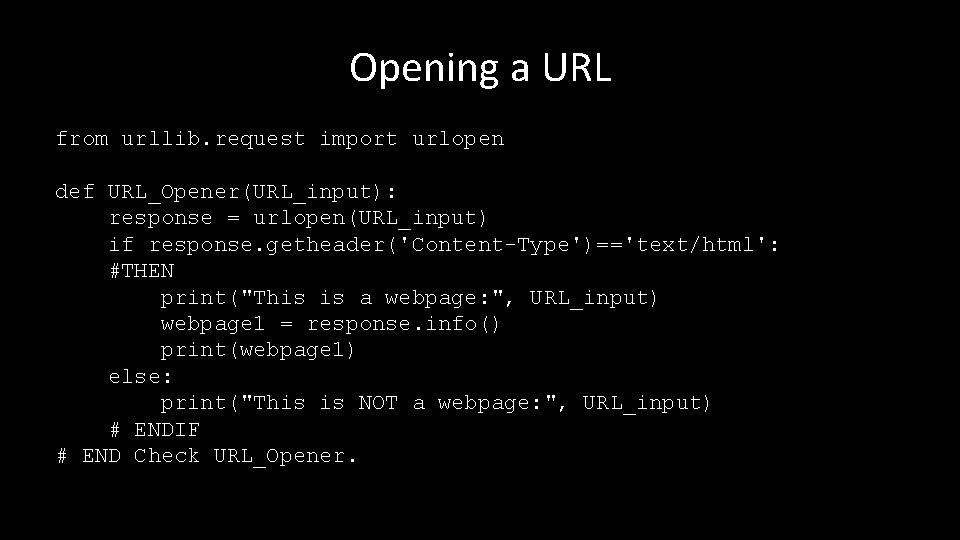
Opening a URL from urllib. request import urlopen def URL_Opener(URL_input): response = urlopen(URL_input) if response. getheader('Content-Type')=='text/html': #THEN print("This is a webpage: ", URL_input) webpage 1 = response. info() print(webpage 1) else: print("This is NOT a webpage: ", URL_input) # ENDIF # END Check URL_Opener.
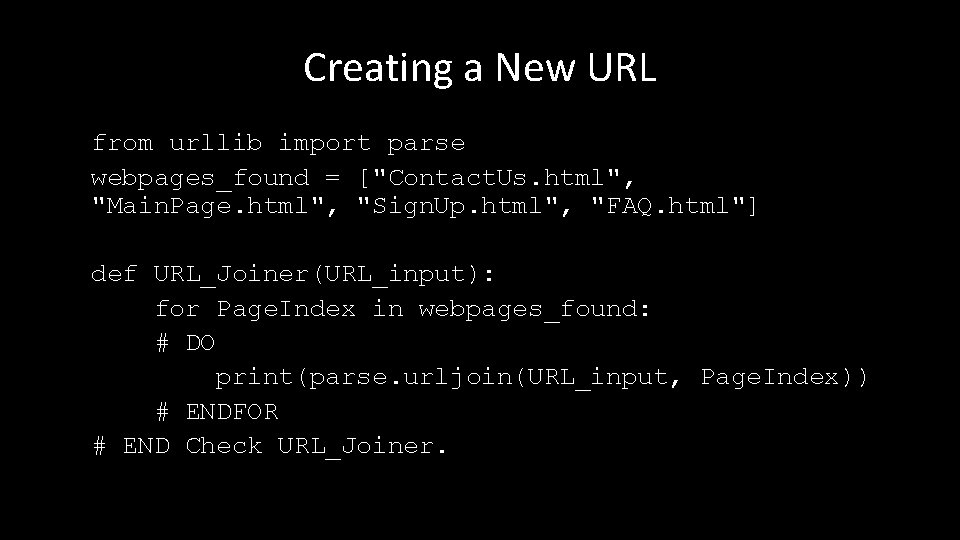
Creating a New URL from urllib import parse webpages_found = ["Contact. Us. html", "Main. Page. html", "Sign. Up. html", "FAQ. html"] def URL_Joiner(URL_input): for Page. Index in webpages_found: # DO print(parse. urljoin(URL_input, Page. Index)) # ENDFOR # END Check URL_Joiner.
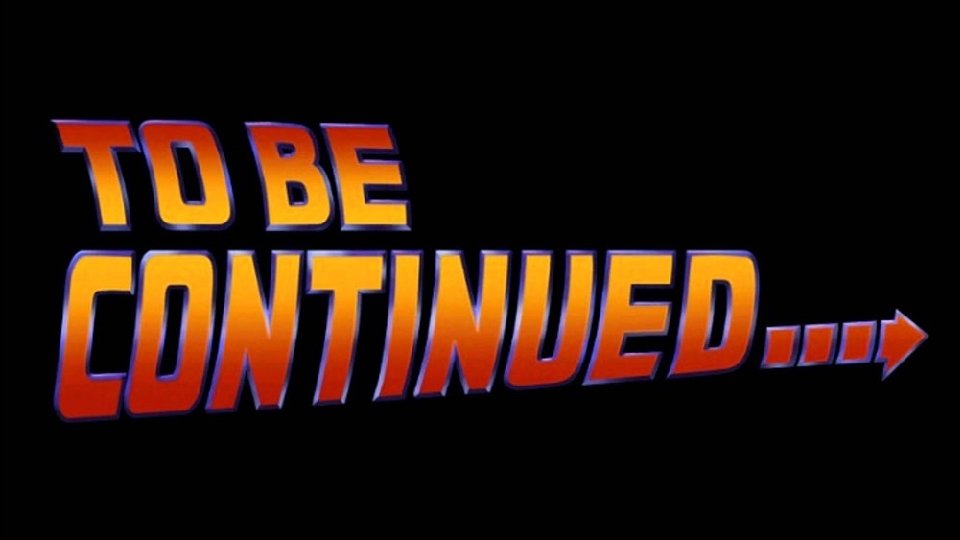
etc.
Damian gordon
Damian gordon
Index.of.secret
Unstructured interview
Sa/sd and jsd
Active revision vs passive revision
Percy jackson cda
Structured programming
Structured vs object oriented programming
Structured programming tutorial
Cobol search varying
Structured programming in c
Cobol area a and area b
Nature of cobol
Contoh pemrograman terstruktur
7-eleven
Literals in python
Perbedaan linear programming dan integer programming
Definisi linear
Greedy algorithm vs dynamic programming
System programming definition
Integer programming vs linear programming
Python programming in context
String python
Python programming in context
Python cgi form example
Programming essentials in python
Wiki
Constraint programming python
Blackjack python object oriented
Python chapter 5 programming exercises
Rapid gui programming with python and qt
Audiolab python
Python programming an introduction to computer science
Second order cone programming python
Damian czudek
Damian harding
Padre damian de veuster 2215
Damian sommerville
Damian heywood
Damian urbańczyk z żoną
Fray damian massanet picture
Damian sturzaker
Money money money team
Php podstawy
Damian clancy
Melissa damian
Christophe damian
Padre damian de veuster 2215 vitacura
Padre damian de veuster 2215 vitacura
Iru academy
Damian topolski
Damian krysztofik
дејмијан марли музичар
Damian mac
Padre damian de veuster 2215
Padre damian de veuster 2215
Logic gates and
Damian forbes
Dr damian folch
Gordon's skill development ladder
Gordon gillerman
Isportsman fort gordon
Gordon method in entrepreneurship
Gordon greenwood elementary
Gordon bell (qnx)
Gordon
Dr gordon jay strauss
Ryan c. gordon
Ian gordon lawyer
Differences between debt and equity
Nicholas gordon poet
Campo di dirac
Thomas gordon discipline as self control
Claire clairmont
Gordon alport
Putovanje cajlda harolda tema
Gordon stringer
Gordon richards drexel
Sine gordon
Gordon watson chemistry
Gordon cappelletty
Gordon college cts
Gordon rasp bars
Gordon lu
Gordon beattie nhs
Verpleegplan gordon
Fort gordon cabin rentals
Maxence mohr
Significato equazione di dirac
Fort gordon efmp office
Nara bridg
Mackenzie jean rowling murray
Gordon dunsire
Patrones de gordon
Difference between euler and rankine-gordon formula
George gordon lord byron
Atoms in space
Mô hình gordon
Sunusoidal
Rose gordon flowers for algernon
Gordon watson ap chemistry
Mick gordon gdc
Fazey and hardy (1988) activity worksheet
Hannahhlou
Gordon model of dividend policy
Anamnese gordon
*amdtoolsdev
Gordon schäfer modell
Thomas gordon model
Edmund t gordon
Gordon childe
Tecnica de grupo nominal
Gordon palmer hall
Personalidade segundo allport
Arusha gordon
Klein-gordon equation ppt
Gordon wetzstein
Gordon shakespear
Lippincott oliver wyman
Gordon handke
Rufus gordon
Gordon nichols
Gedragsraam
Dr craig gordon
Fort bliss levy packet