Python Advanced Sorting Algorithms Damian Gordon Insertion Sort
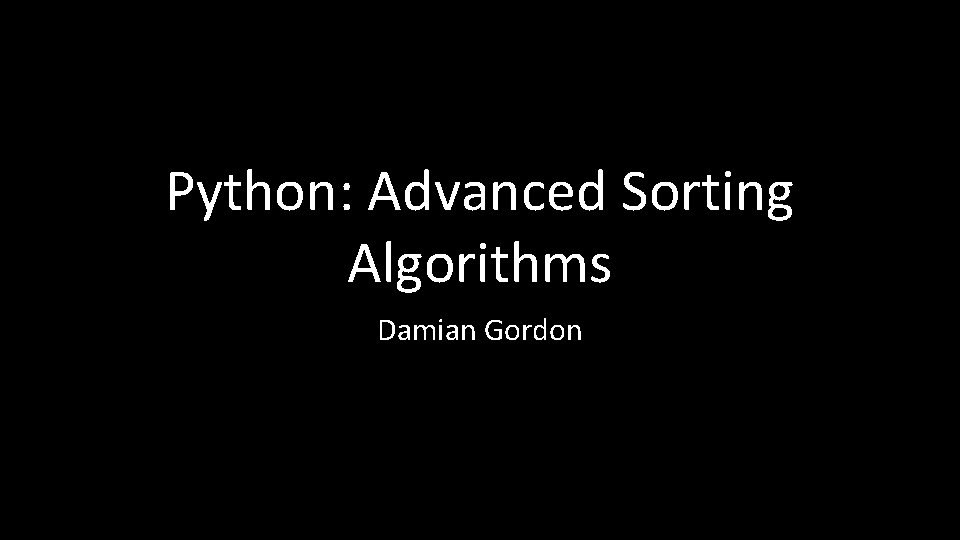
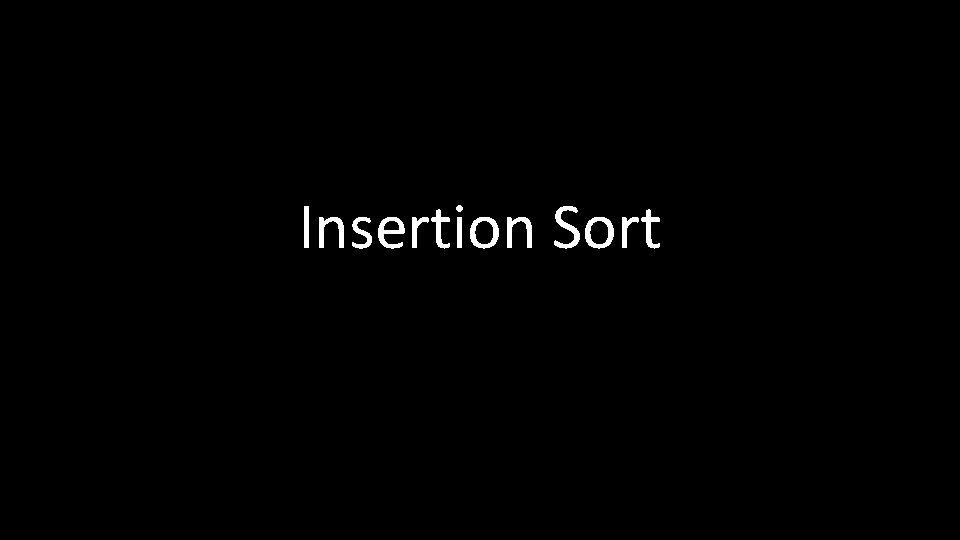
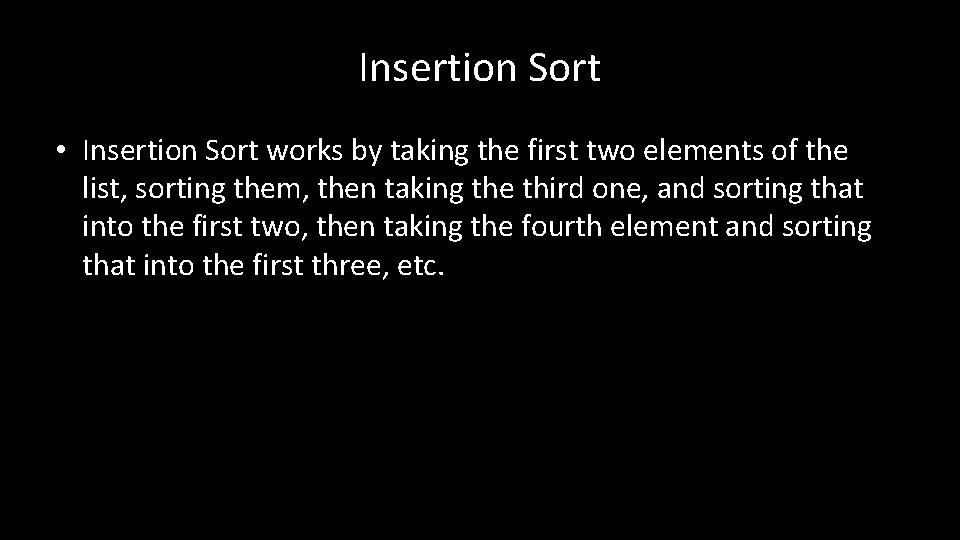
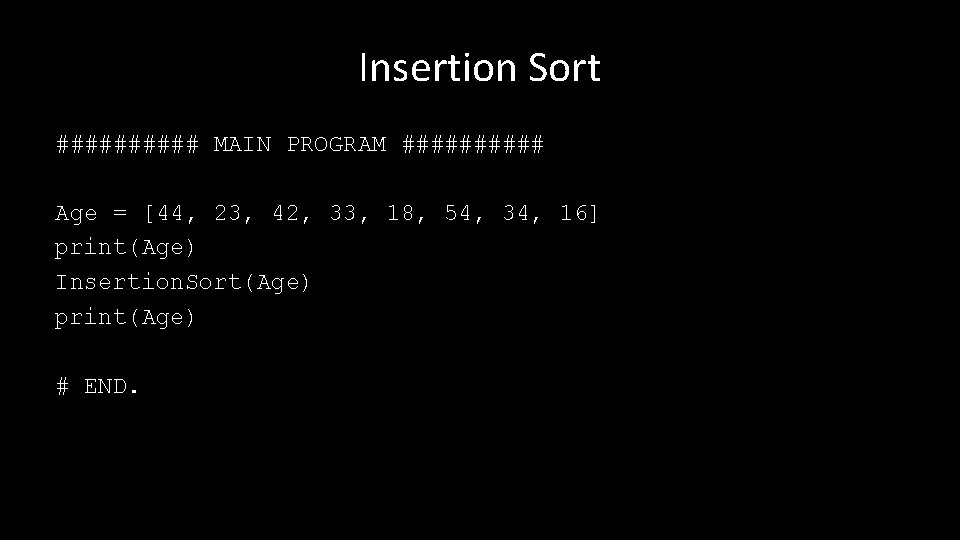
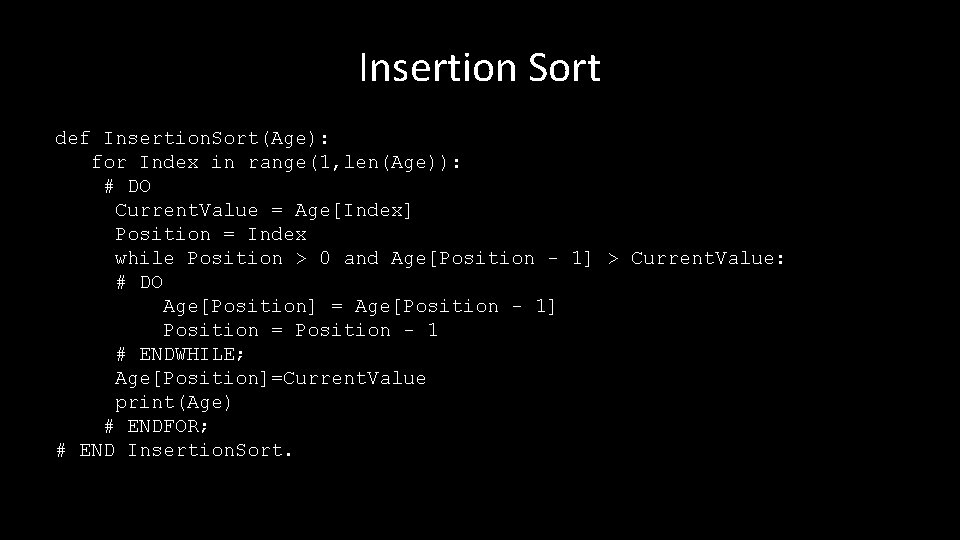
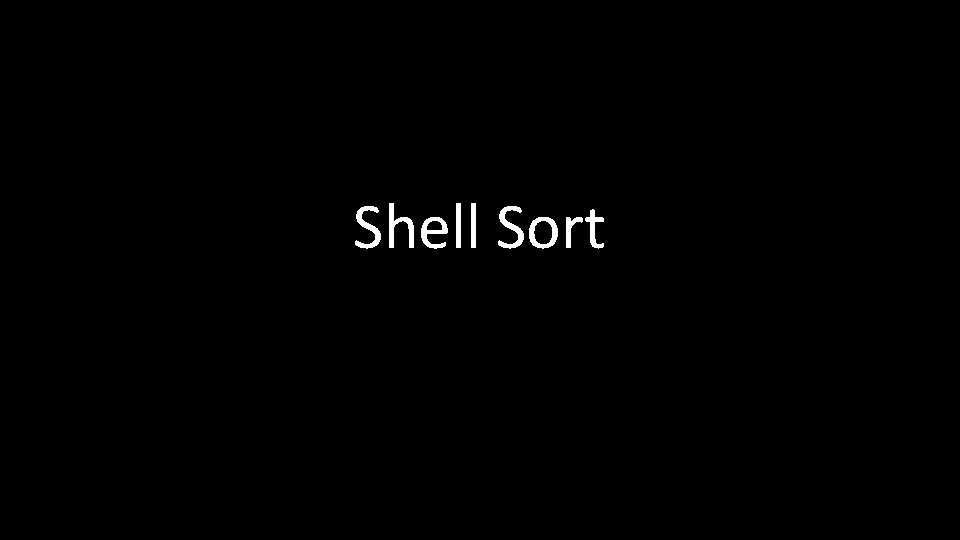
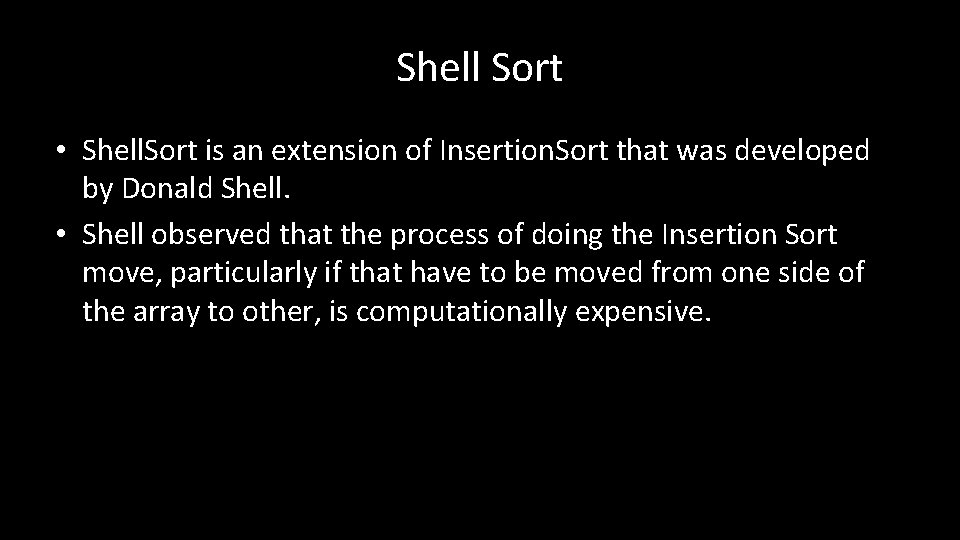
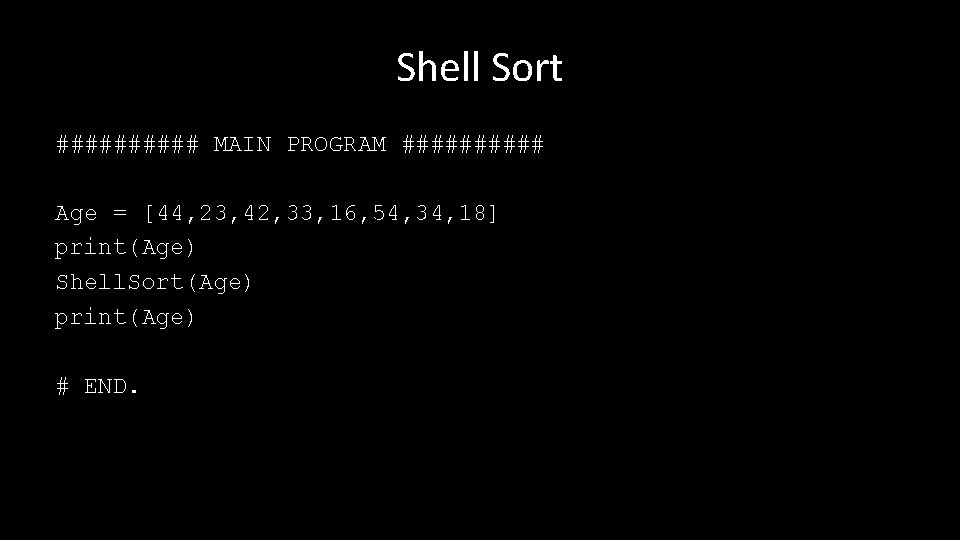
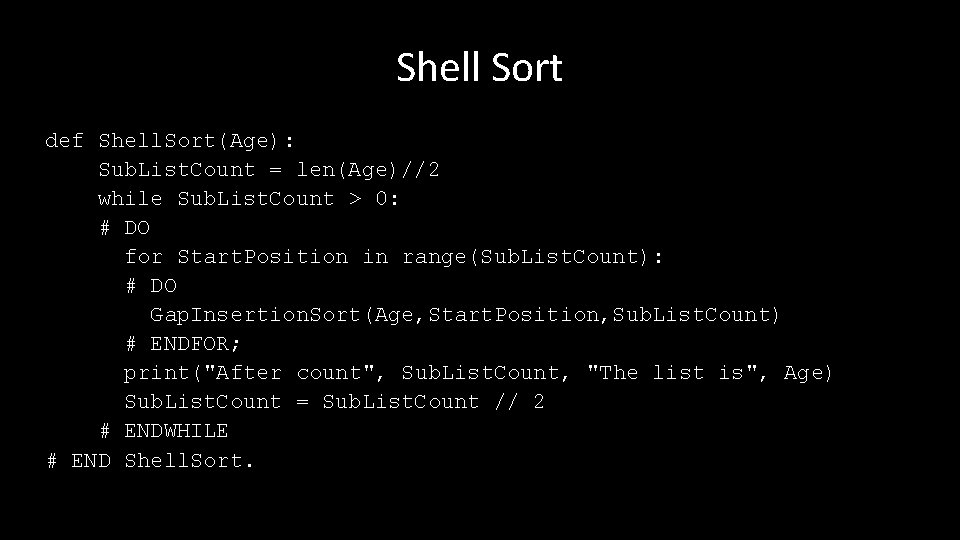
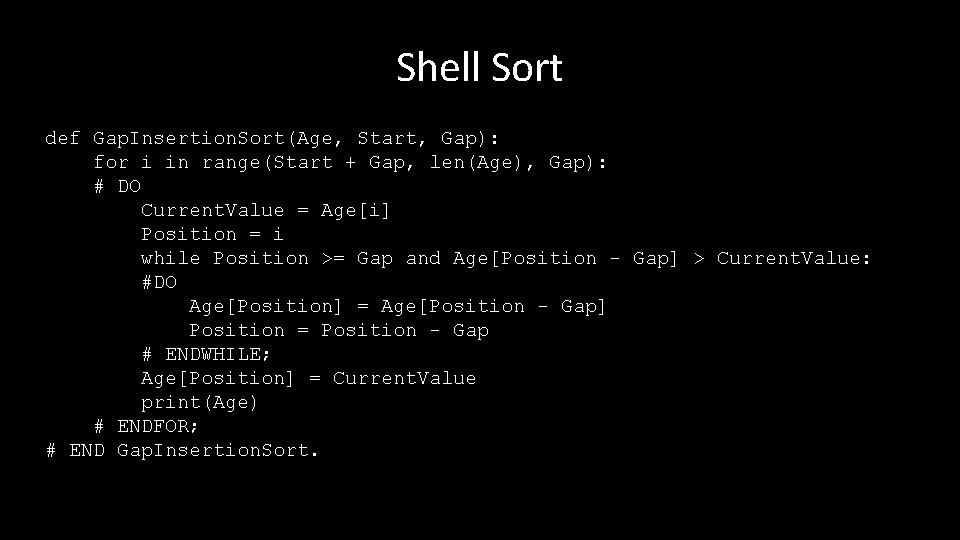
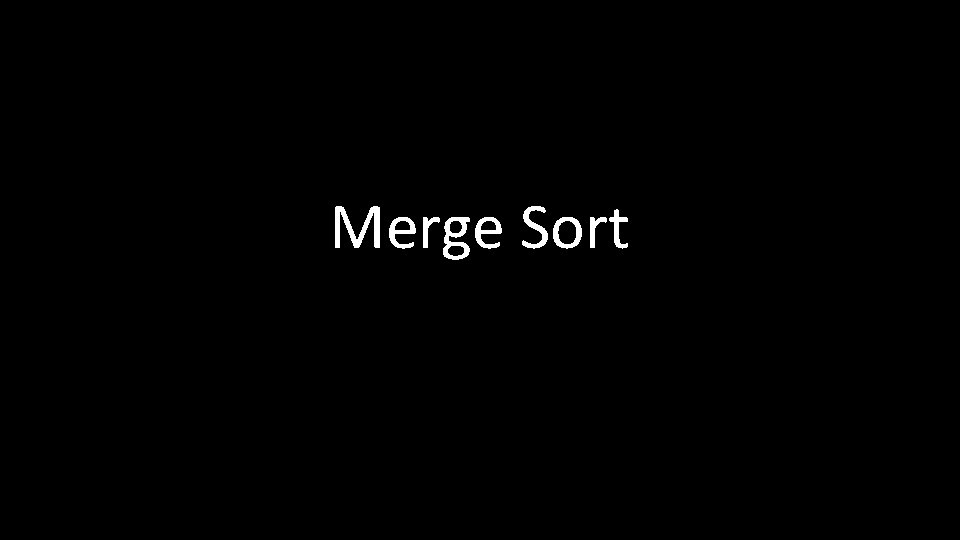
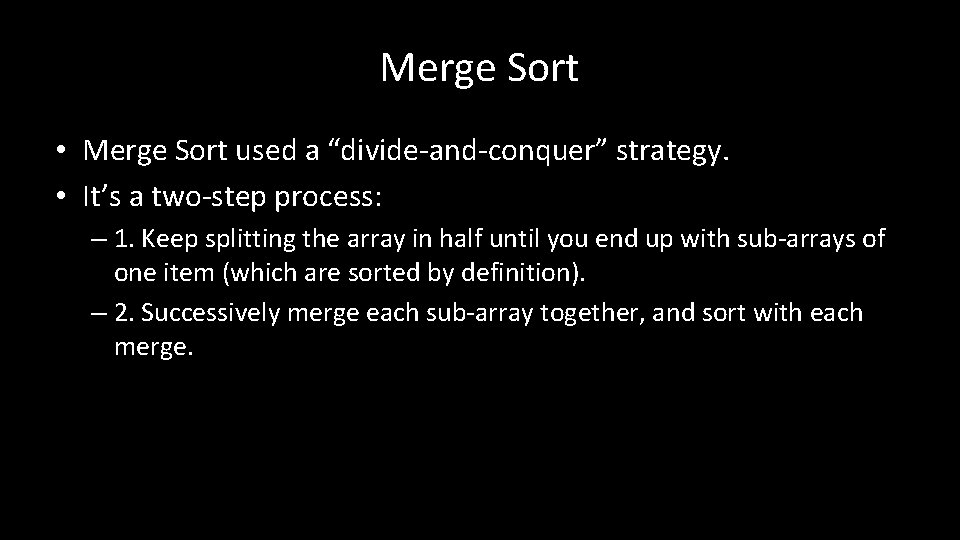
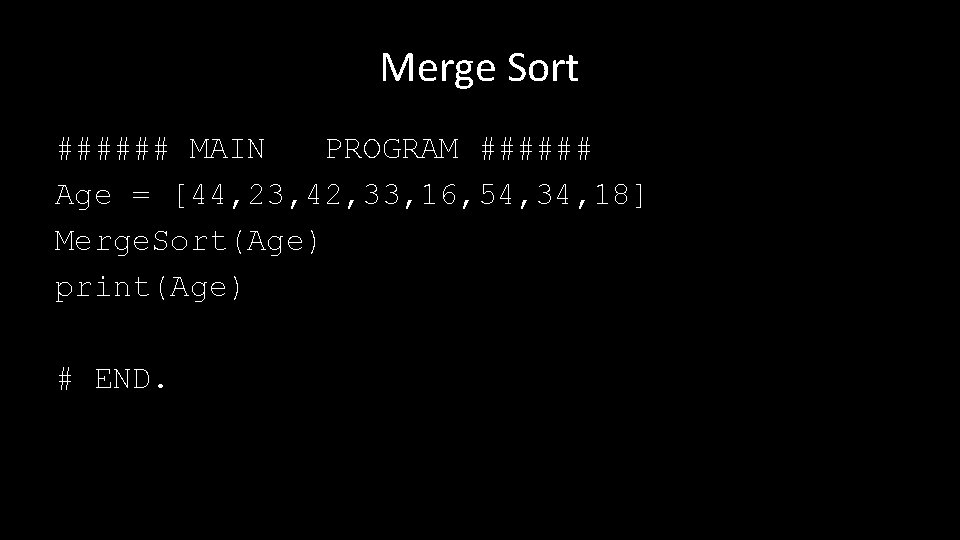
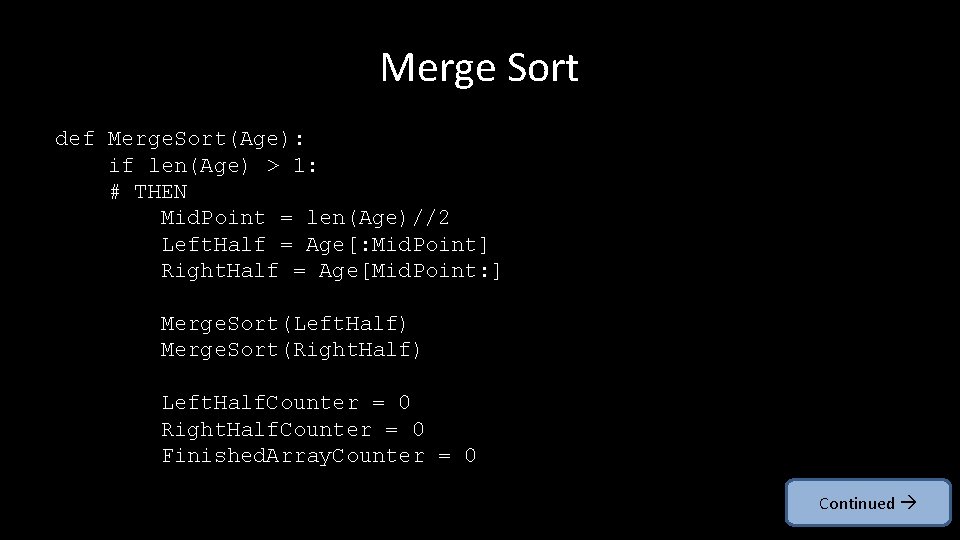
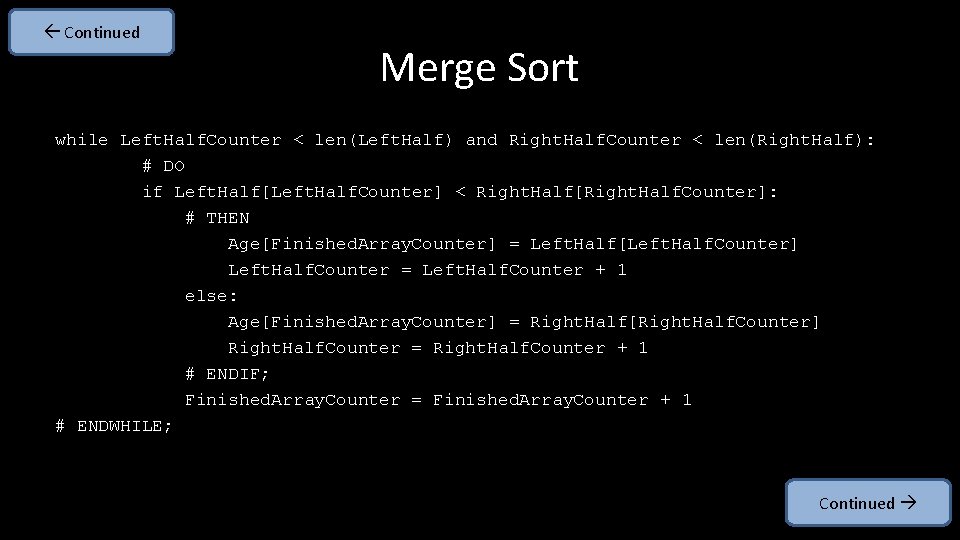
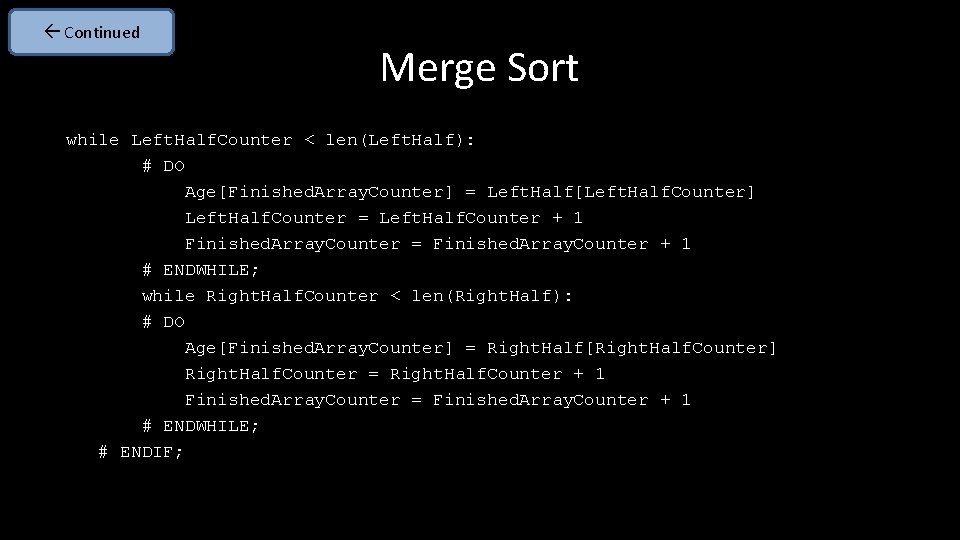
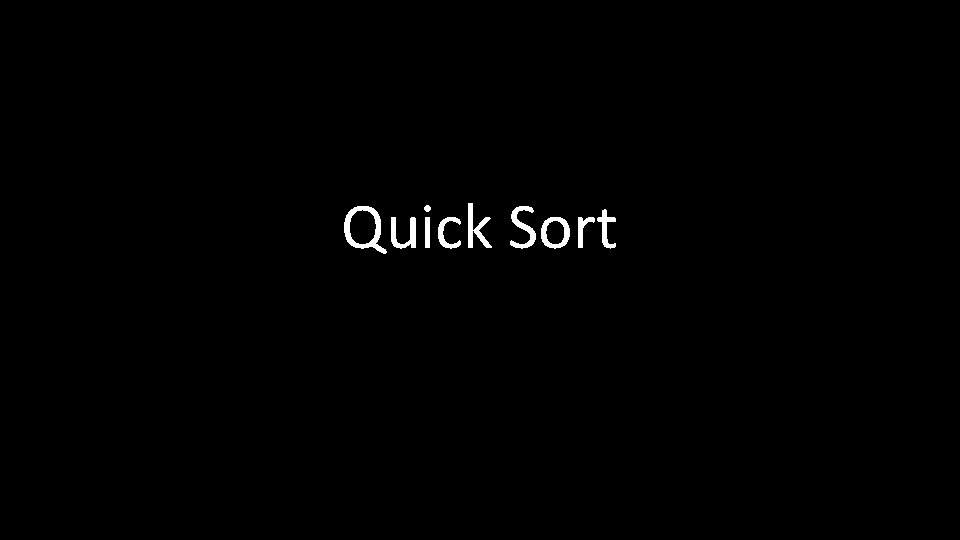
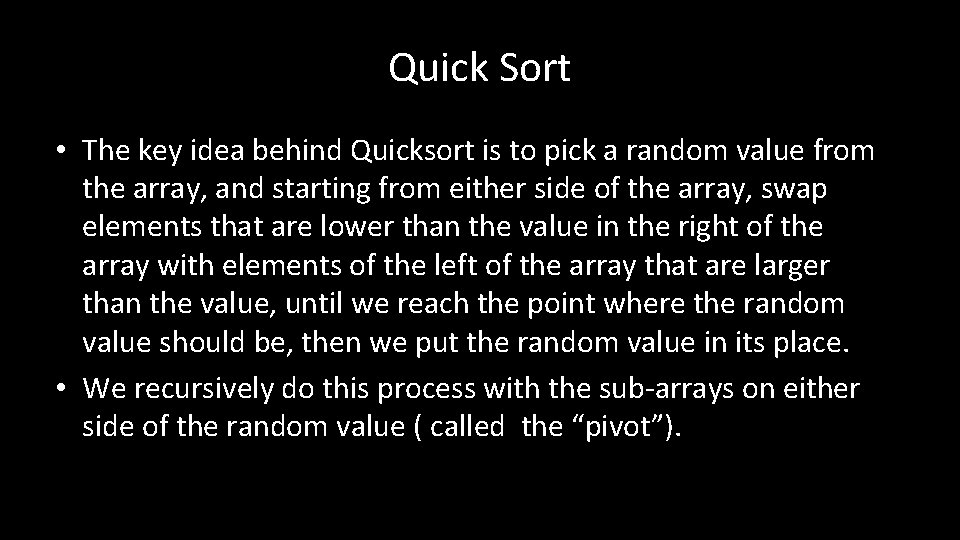
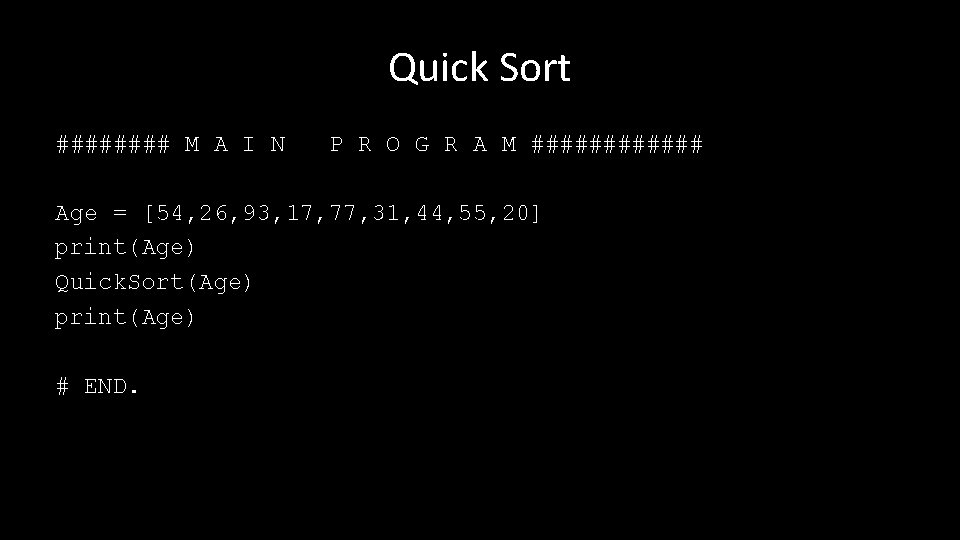
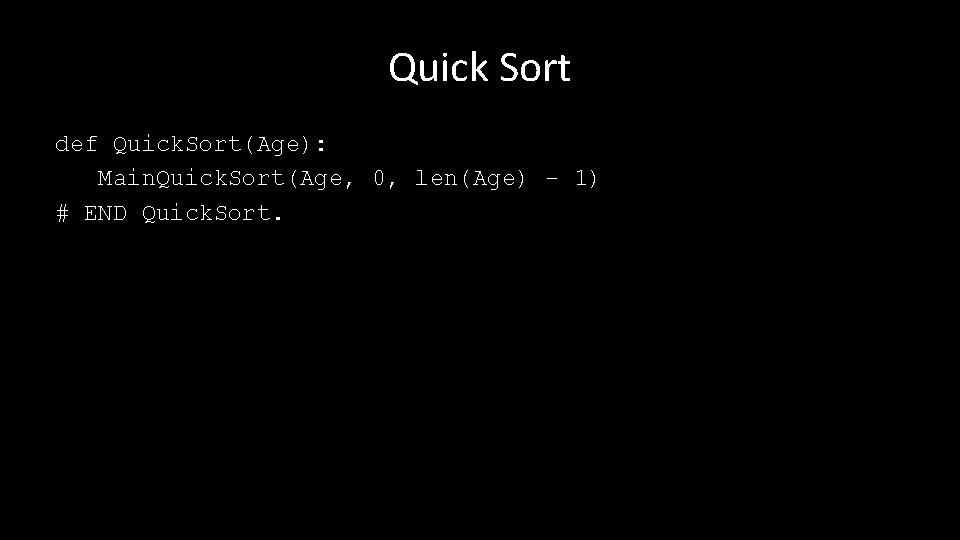
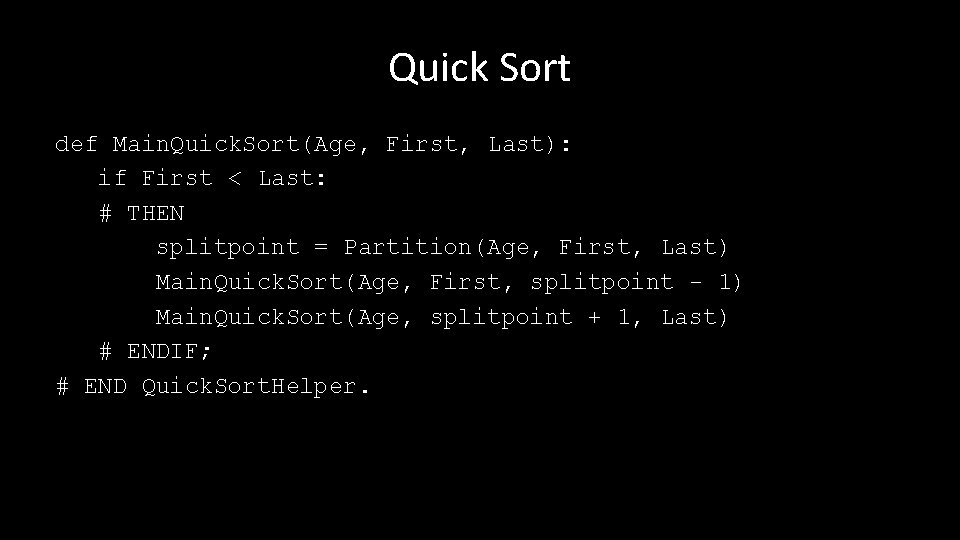
![Quick Sort def Partition(Age, First, Last): pivotvalue = Age[First] Finished = False Left. Pointer Quick Sort def Partition(Age, First, Last): pivotvalue = Age[First] Finished = False Left. Pointer](https://slidetodoc.com/presentation_image/bfa444933c3a68c315acf78192119096/image-22.jpg)
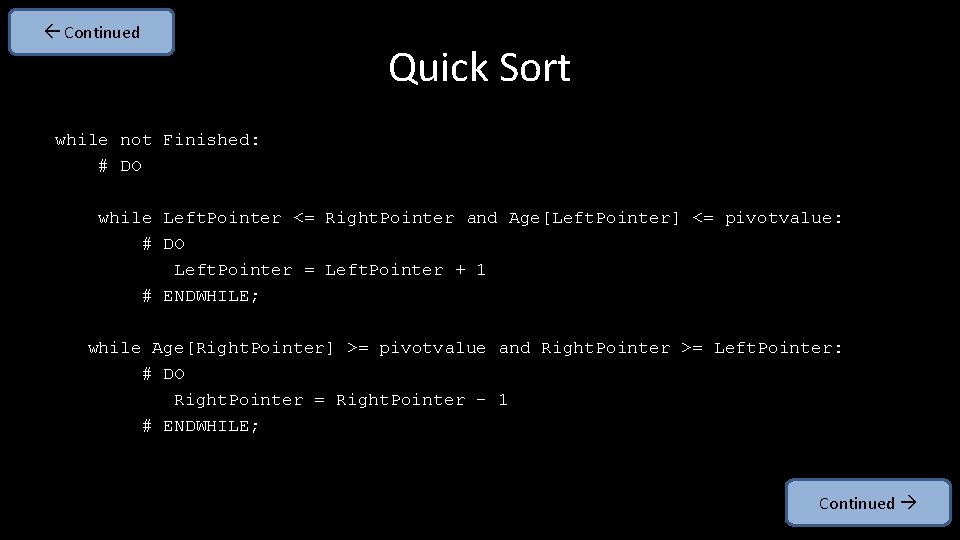
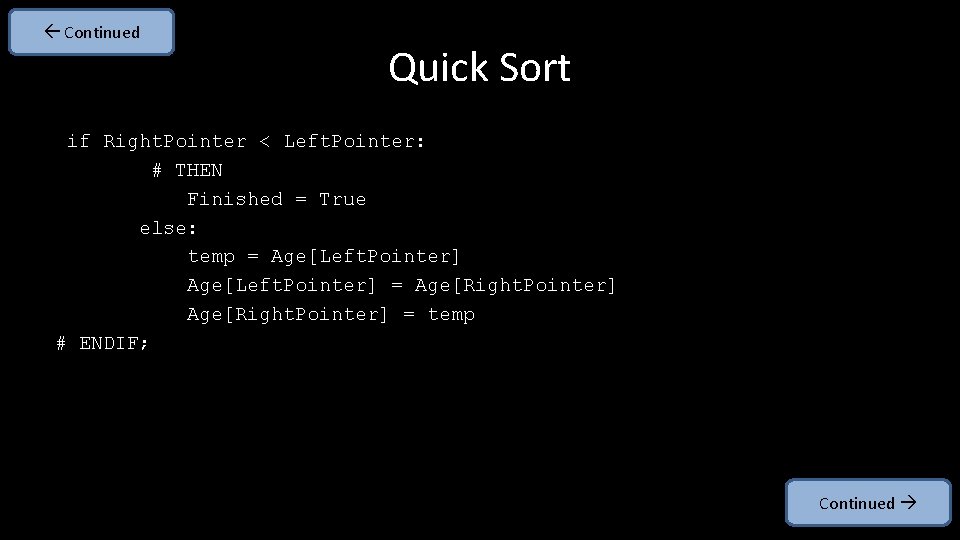
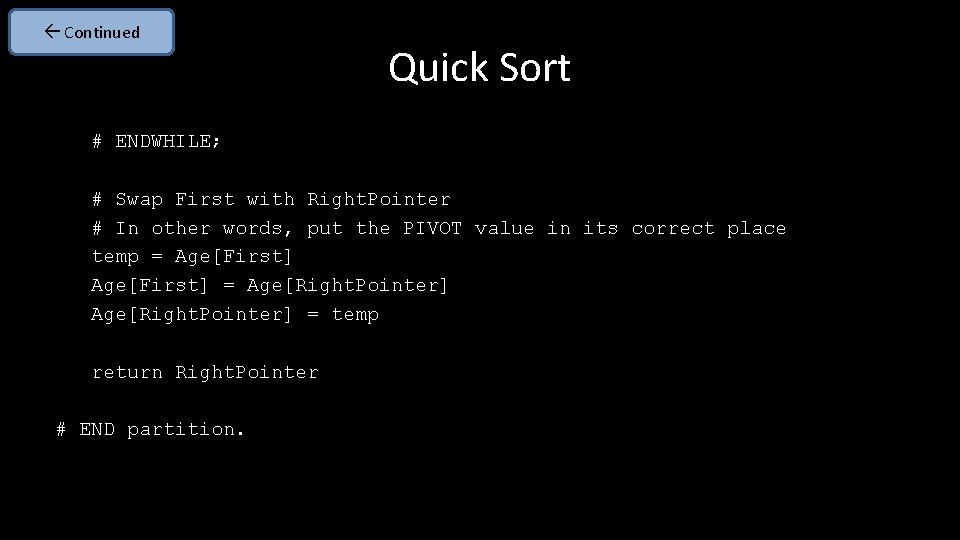
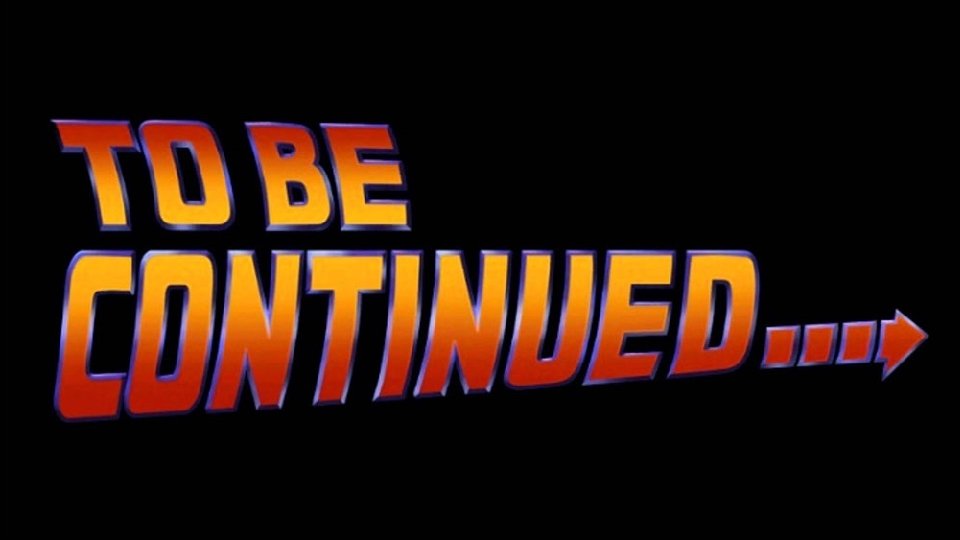
- Slides: 26
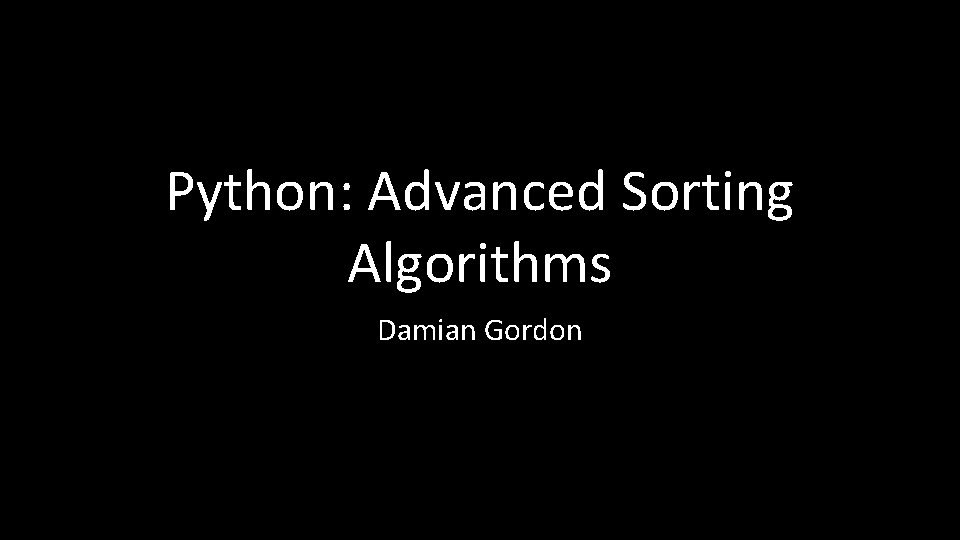
Python: Advanced Sorting Algorithms Damian Gordon
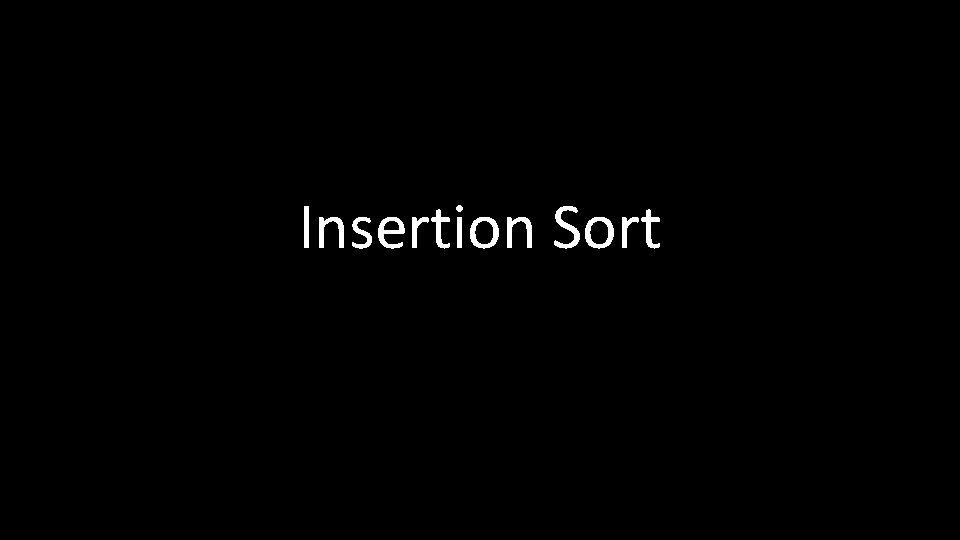
Insertion Sort
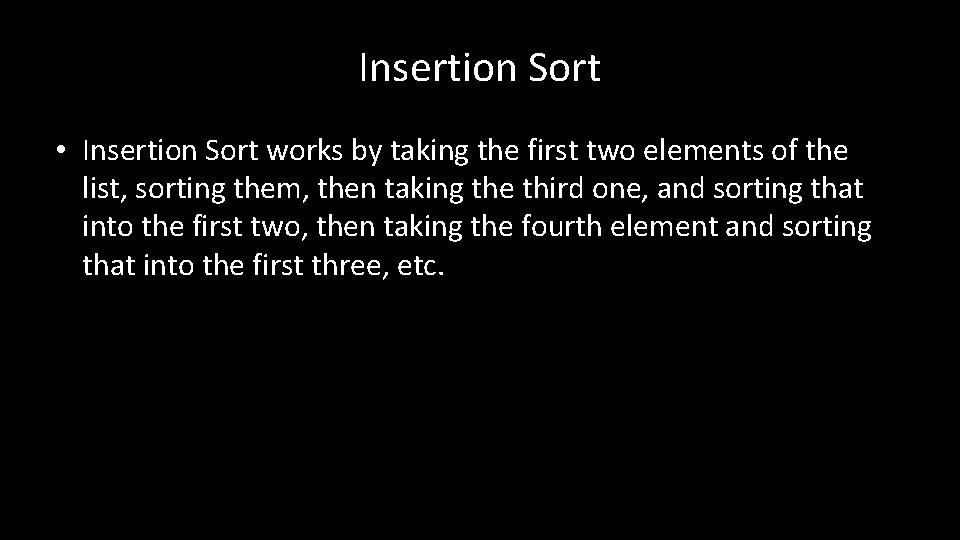
Insertion Sort • Insertion Sort works by taking the first two elements of the list, sorting them, then taking the third one, and sorting that into the first two, then taking the fourth element and sorting that into the first three, etc.
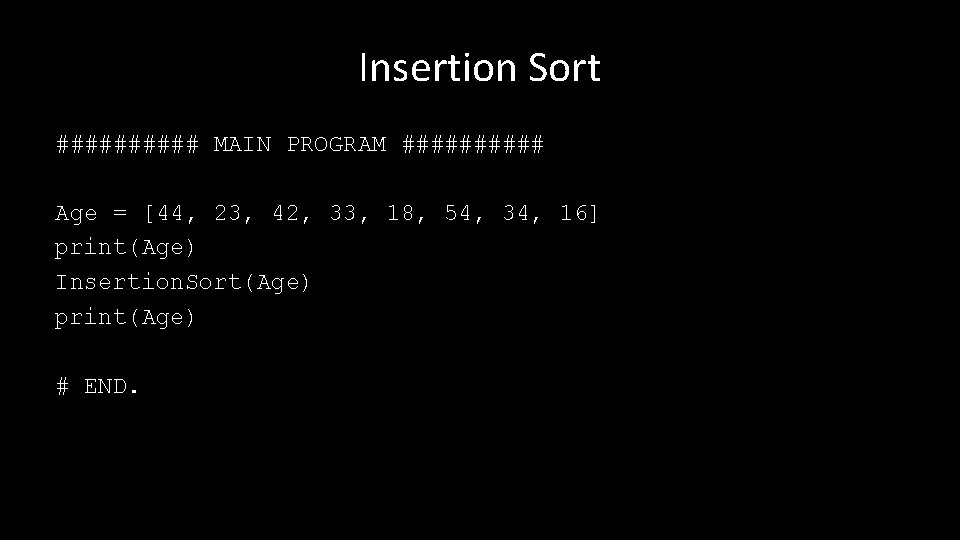
Insertion Sort ##### MAIN PROGRAM ##### Age = [44, 23, 42, 33, 18, 54, 34, 16] print(Age) Insertion. Sort(Age) print(Age) # END.
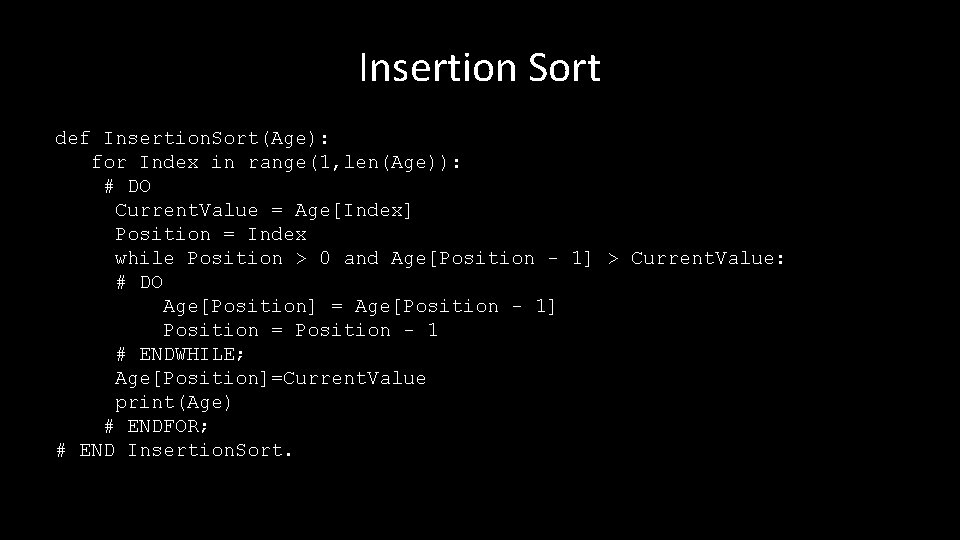
Insertion Sort def Insertion. Sort(Age): for Index in range(1, len(Age)): # DO Current. Value = Age[Index] Position = Index while Position > 0 and Age[Position - 1] > Current. Value: # DO Age[Position] = Age[Position - 1] Position = Position - 1 # ENDWHILE; Age[Position]=Current. Value print(Age) # ENDFOR; # END Insertion. Sort.
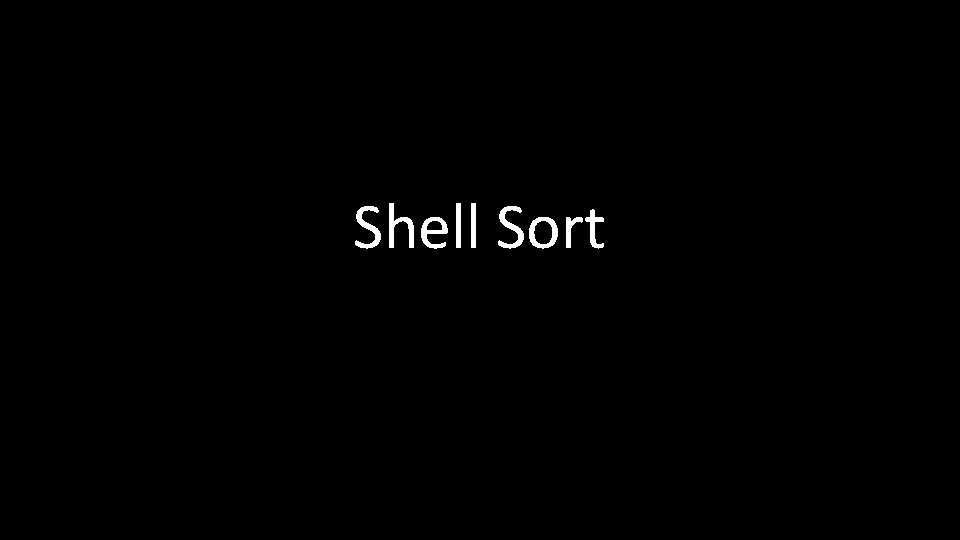
Shell Sort
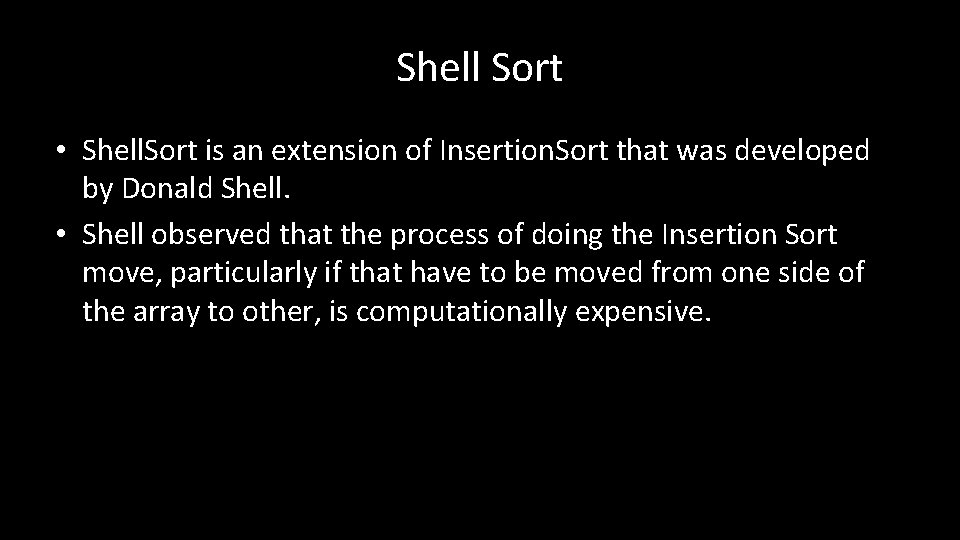
Shell Sort • Shell. Sort is an extension of Insertion. Sort that was developed by Donald Shell. • Shell observed that the process of doing the Insertion Sort move, particularly if that have to be moved from one side of the array to other, is computationally expensive.
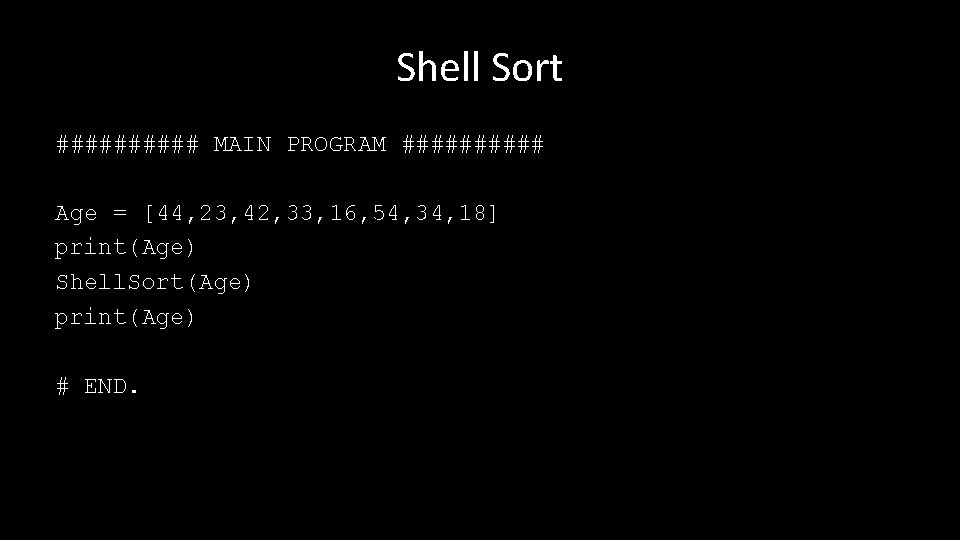
Shell Sort ##### MAIN PROGRAM ##### Age = [44, 23, 42, 33, 16, 54, 34, 18] print(Age) Shell. Sort(Age) print(Age) # END.
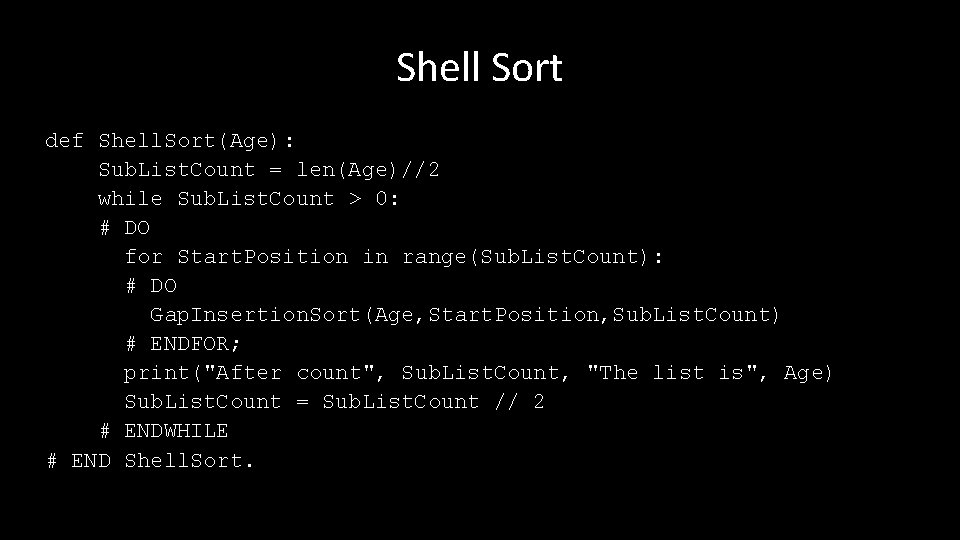
Shell Sort def Shell. Sort(Age): Sub. List. Count = len(Age)//2 while Sub. List. Count > 0: # DO for Start. Position in range(Sub. List. Count): # DO Gap. Insertion. Sort(Age, Start. Position, Sub. List. Count) # ENDFOR; print("After count", Sub. List. Count, "The list is", Age) Sub. List. Count = Sub. List. Count // 2 # ENDWHILE # END Shell. Sort.
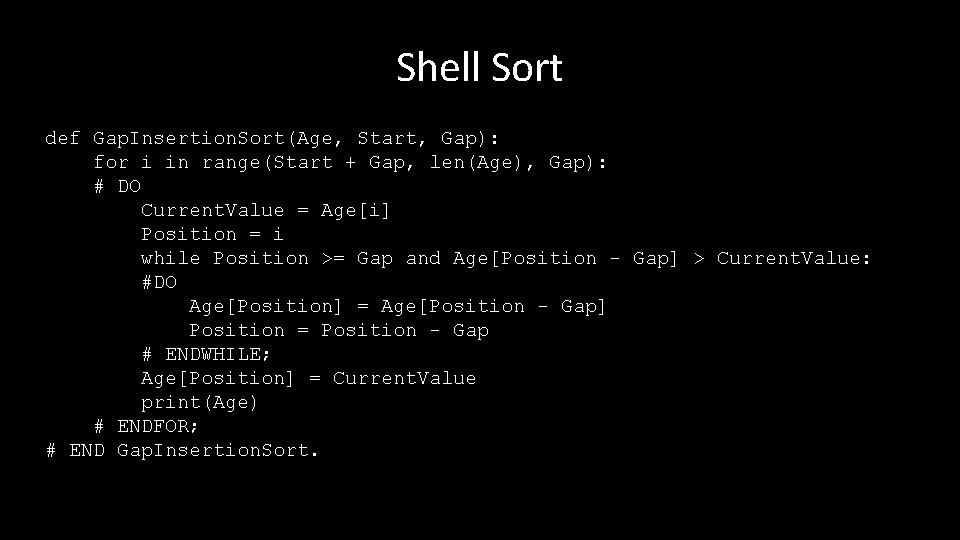
Shell Sort def Gap. Insertion. Sort(Age, Start, Gap): for i in range(Start + Gap, len(Age), Gap): # DO Current. Value = Age[i] Position = i while Position >= Gap and Age[Position - Gap] > Current. Value: #DO Age[Position] = Age[Position - Gap] Position = Position - Gap # ENDWHILE; Age[Position] = Current. Value print(Age) # ENDFOR; # END Gap. Insertion. Sort.
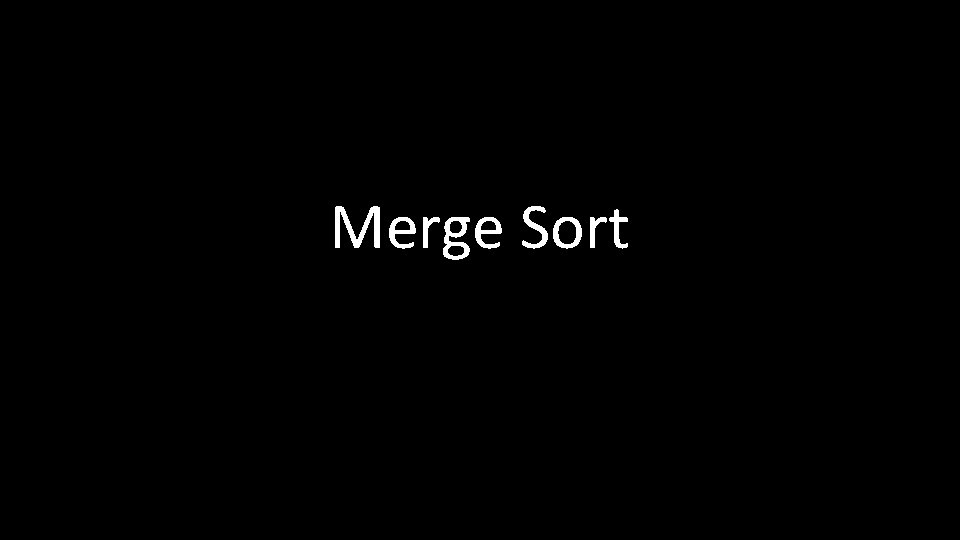
Merge Sort
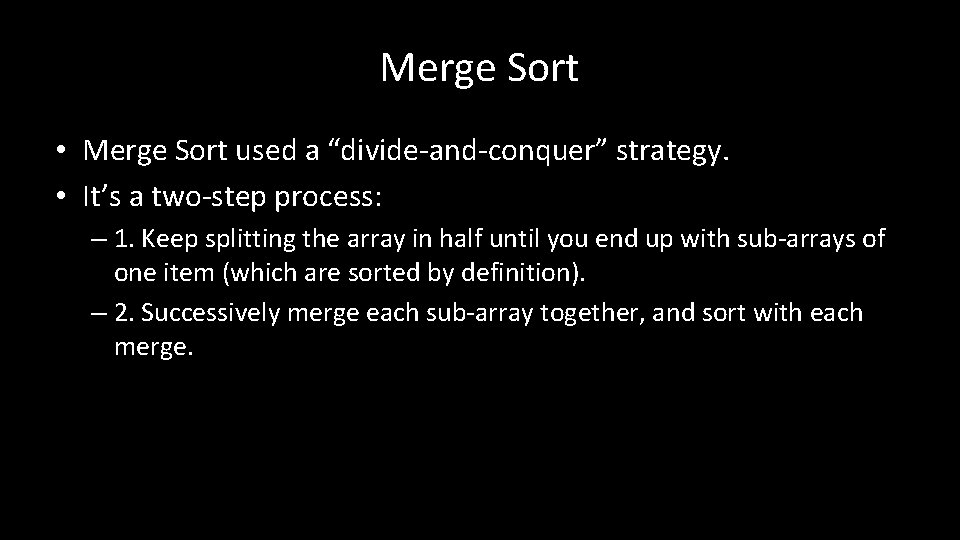
Merge Sort • Merge Sort used a “divide-and-conquer” strategy. • It’s a two-step process: – 1. Keep splitting the array in half until you end up with sub-arrays of one item (which are sorted by definition). – 2. Successively merge each sub-array together, and sort with each merge.
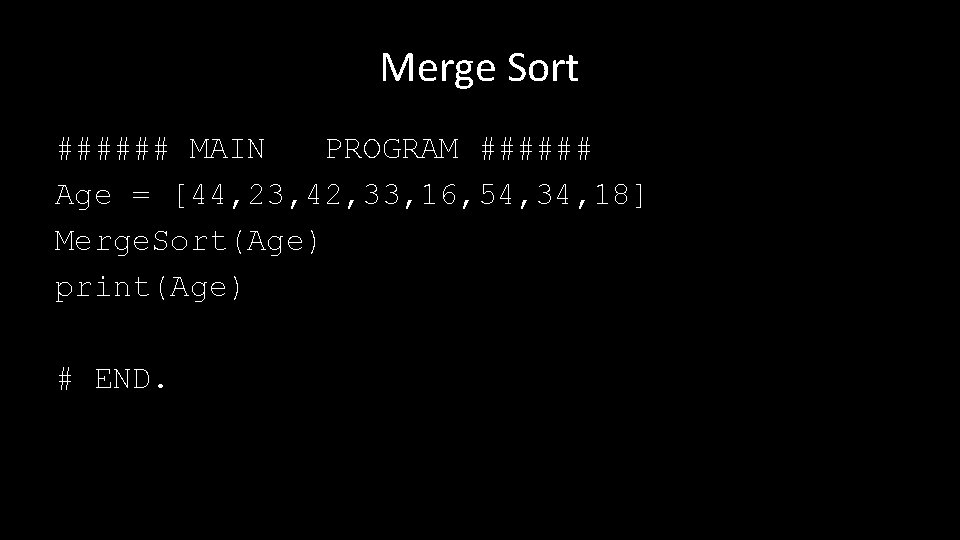
Merge Sort ###### MAIN PROGRAM ###### Age = [44, 23, 42, 33, 16, 54, 34, 18] Merge. Sort(Age) print(Age) # END.
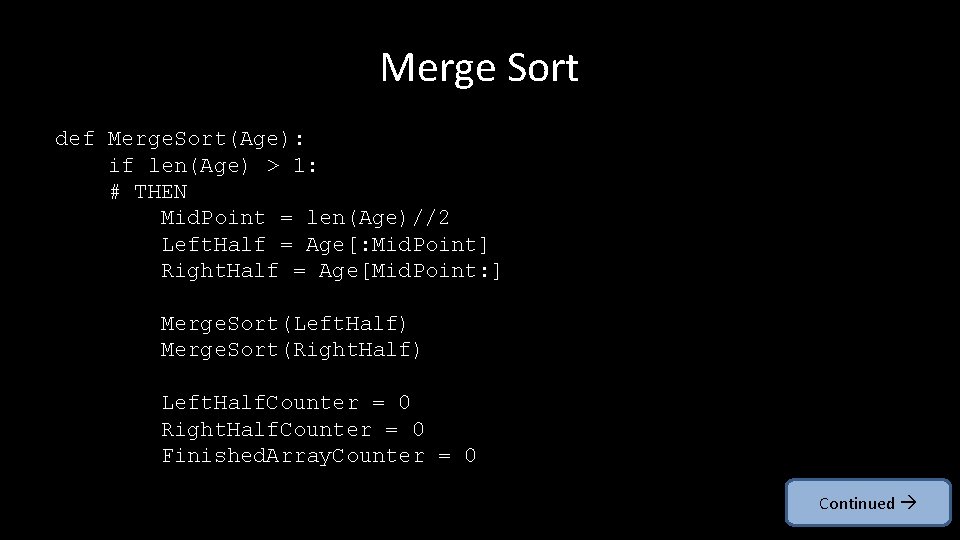
Merge Sort def Merge. Sort(Age): if len(Age) > 1: # THEN Mid. Point = len(Age)//2 Left. Half = Age[: Mid. Point] Right. Half = Age[Mid. Point: ] Merge. Sort(Left. Half) Merge. Sort(Right. Half) Left. Half. Counter = 0 Right. Half. Counter = 0 Finished. Array. Counter = 0 Continued
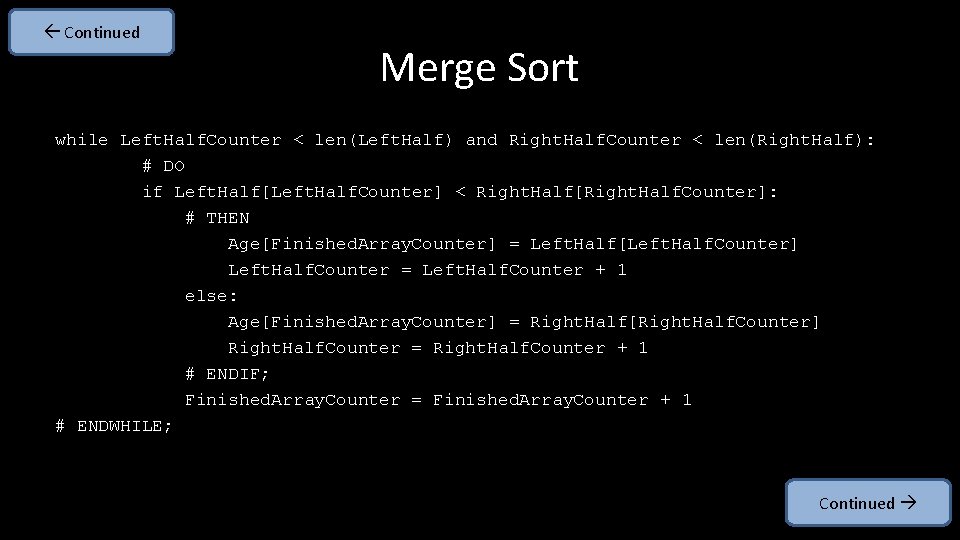
Continued Merge Sort while Left. Half. Counter < len(Left. Half) and Right. Half. Counter < len(Right. Half): # DO if Left. Half[Left. Half. Counter] < Right. Half[Right. Half. Counter]: # THEN Age[Finished. Array. Counter] = Left. Half[Left. Half. Counter] Left. Half. Counter = Left. Half. Counter + 1 else: Age[Finished. Array. Counter] = Right. Half[Right. Half. Counter] Right. Half. Counter = Right. Half. Counter + 1 # ENDIF; Finished. Array. Counter = Finished. Array. Counter + 1 # ENDWHILE; Continued
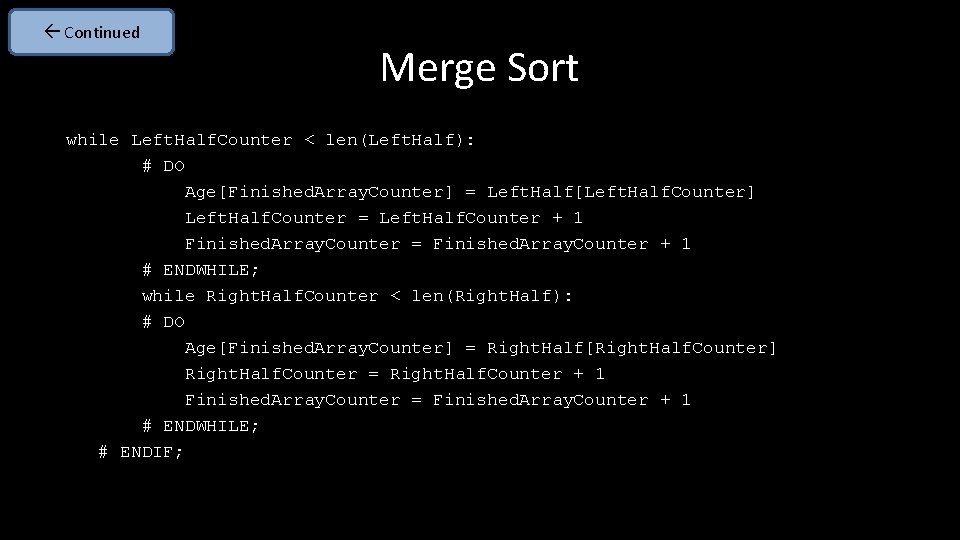
Continued Merge Sort while Left. Half. Counter < len(Left. Half): # DO Age[Finished. Array. Counter] = Left. Half[Left. Half. Counter] Left. Half. Counter = Left. Half. Counter + 1 Finished. Array. Counter = Finished. Array. Counter + 1 # ENDWHILE; while Right. Half. Counter < len(Right. Half): # DO Age[Finished. Array. Counter] = Right. Half[Right. Half. Counter] Right. Half. Counter = Right. Half. Counter + 1 Finished. Array. Counter = Finished. Array. Counter + 1 # ENDWHILE; # ENDIF;
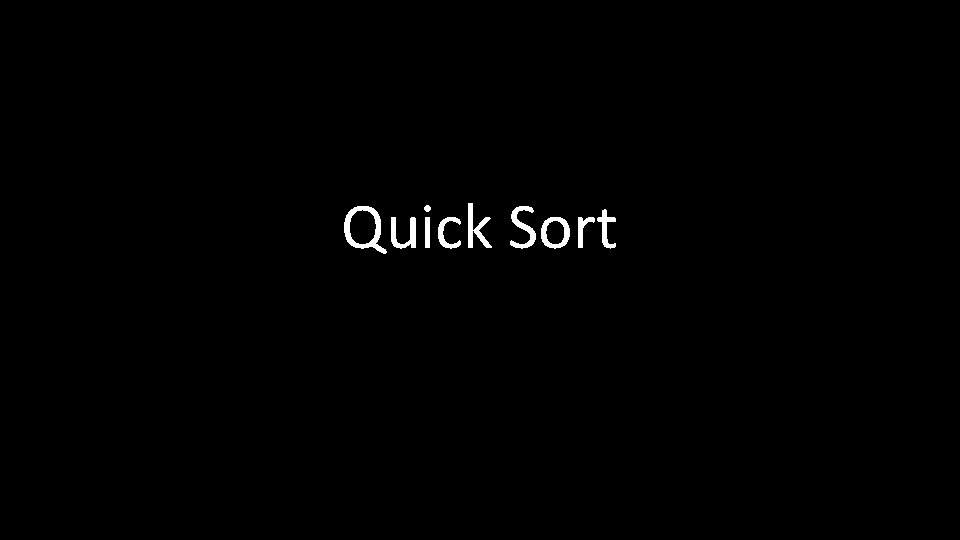
Quick Sort
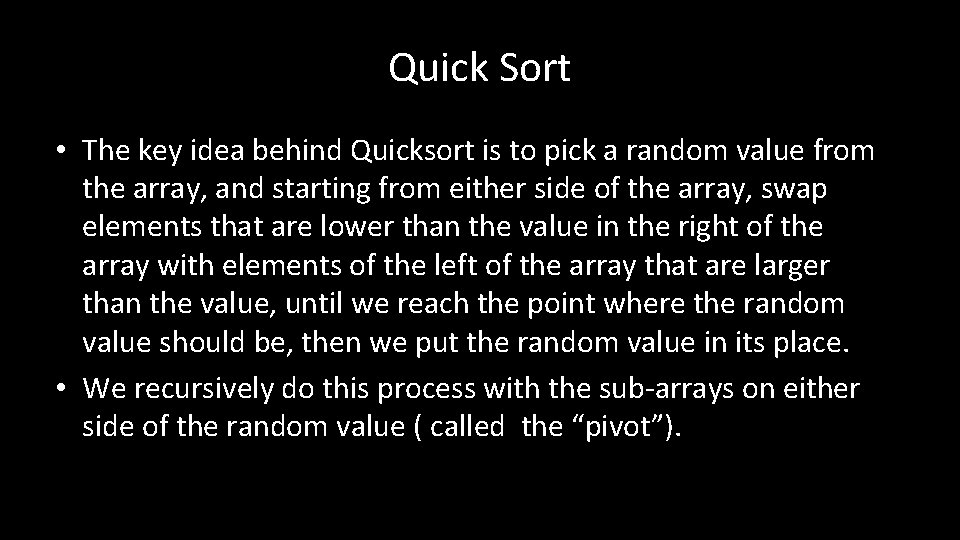
Quick Sort • The key idea behind Quicksort is to pick a random value from the array, and starting from either side of the array, swap elements that are lower than the value in the right of the array with elements of the left of the array that are larger than the value, until we reach the point where the random value should be, then we put the random value in its place. • We recursively do this process with the sub-arrays on either side of the random value ( called the “pivot”).
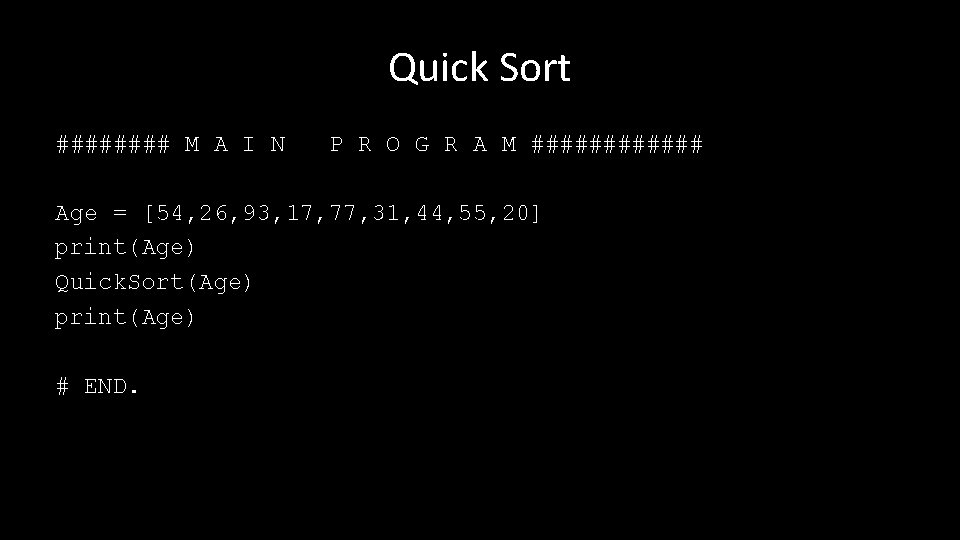
Quick Sort #### M A I N P R O G R A M ###### Age = [54, 26, 93, 17, 77, 31, 44, 55, 20] print(Age) Quick. Sort(Age) print(Age) # END.
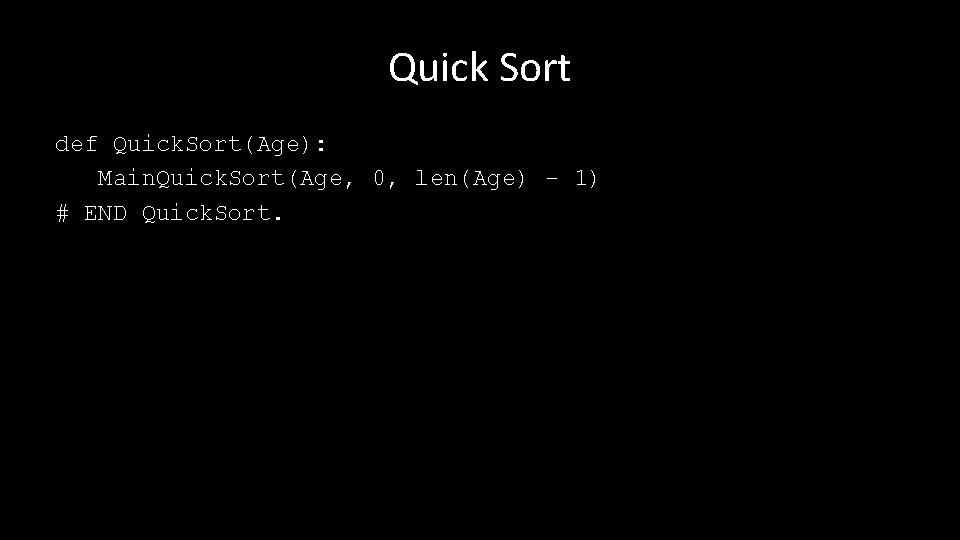
Quick Sort def Quick. Sort(Age): Main. Quick. Sort(Age, 0, len(Age) - 1) # END Quick. Sort.
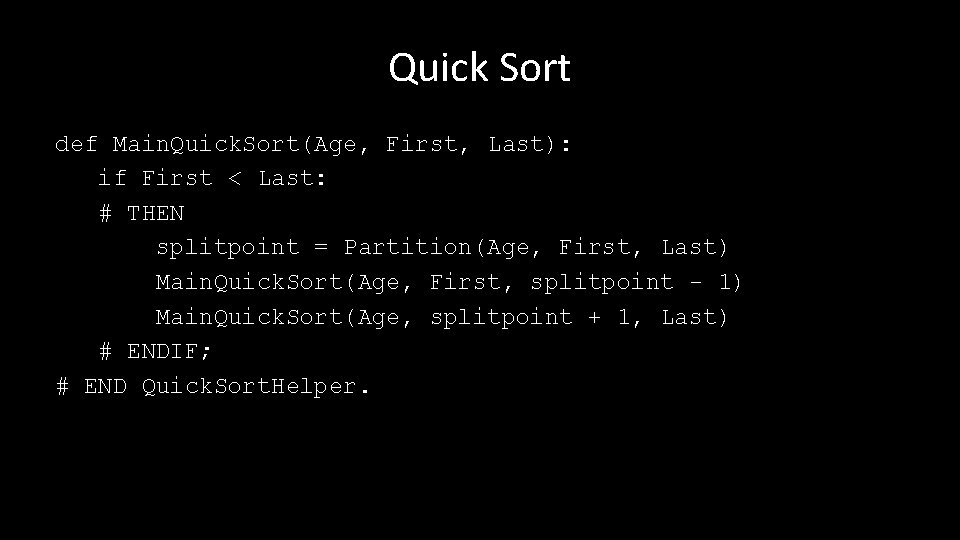
Quick Sort def Main. Quick. Sort(Age, First, Last): if First < Last: # THEN splitpoint = Partition(Age, First, Last) Main. Quick. Sort(Age, First, splitpoint - 1) Main. Quick. Sort(Age, splitpoint + 1, Last) # ENDIF; # END Quick. Sort. Helper.
![Quick Sort def PartitionAge First Last pivotvalue AgeFirst Finished False Left Pointer Quick Sort def Partition(Age, First, Last): pivotvalue = Age[First] Finished = False Left. Pointer](https://slidetodoc.com/presentation_image/bfa444933c3a68c315acf78192119096/image-22.jpg)
Quick Sort def Partition(Age, First, Last): pivotvalue = Age[First] Finished = False Left. Pointer = First + 1 Right. Pointer = Last Continued
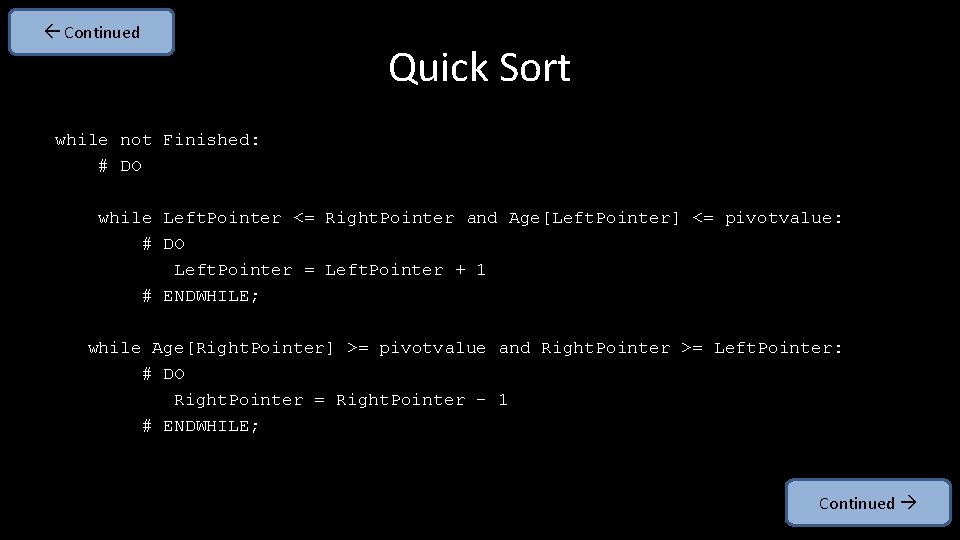
Continued Quick Sort while not Finished: # DO while Left. Pointer <= Right. Pointer and Age[Left. Pointer] <= pivotvalue: # DO Left. Pointer = Left. Pointer + 1 # ENDWHILE; while Age[Right. Pointer] >= pivotvalue and Right. Pointer >= Left. Pointer: # DO Right. Pointer = Right. Pointer - 1 # ENDWHILE; Continued
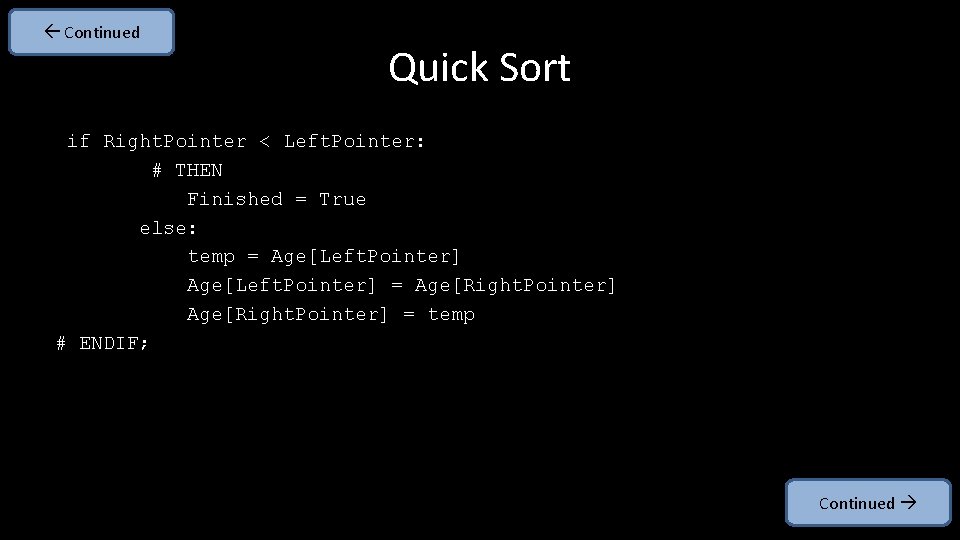
Continued Quick Sort if Right. Pointer < Left. Pointer: # THEN Finished = True else: temp = Age[Left. Pointer] = Age[Right. Pointer] = temp # ENDIF; Continued
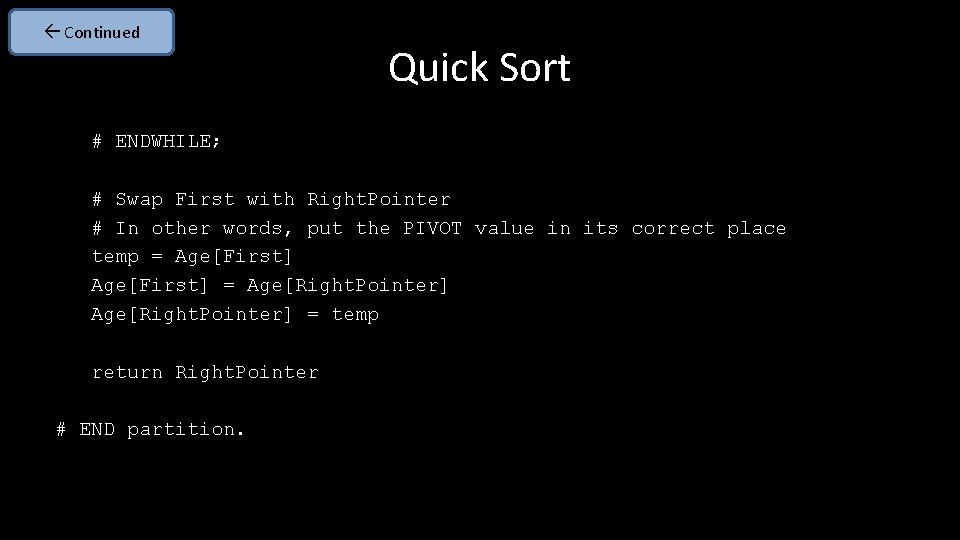
Continued Quick Sort # ENDWHILE; # Swap First with Right. Pointer # In other words, put the PIVOT value in its correct place temp = Age[First] = Age[Right. Pointer] = temp return Right. Pointer # END partition.
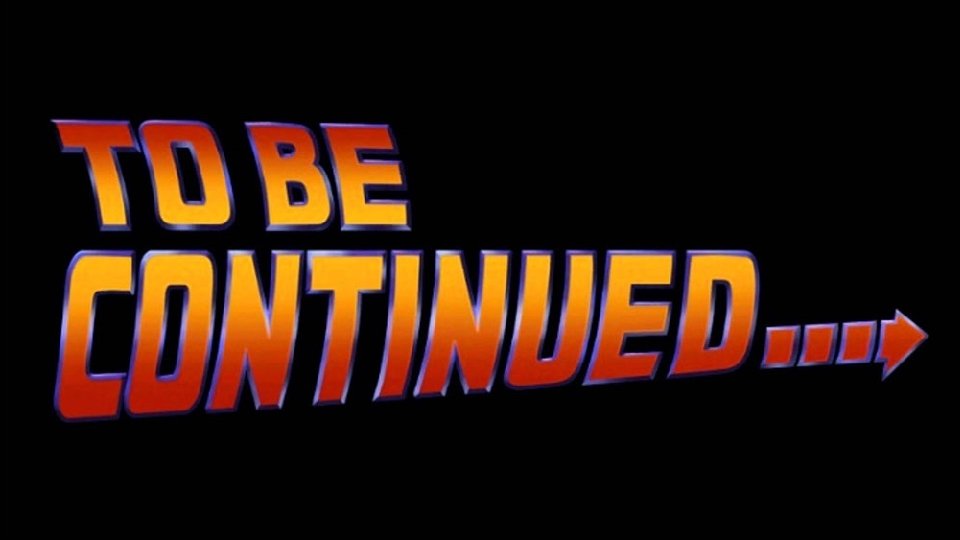
etc.
Difference between insertion sort and bubble sort
How selection sort works
排序
Insertion sort advanced analysis
Insertion sort
Damian gordon
Damian gordon
Damian hacks
Internal and external sorting
10 sorting algorithms
Insertion sort decision tree 4 elements
Efficiency of sorting algorithms
External sorting algorithms
Sorting algorithms in c
Most common sorting algorithms
Clhelse
Big o functions
Introduction to sorting algorithms
Quadratic sorting algorithms
Bsort
Sort meaning
Clickpivot
Space complexity of insertion sort
Dfs algorithm
Selection sort mips
Selection sort proof by induction
Mips selection sort