Introduction to Sorting Algorithms Sort arrange values into
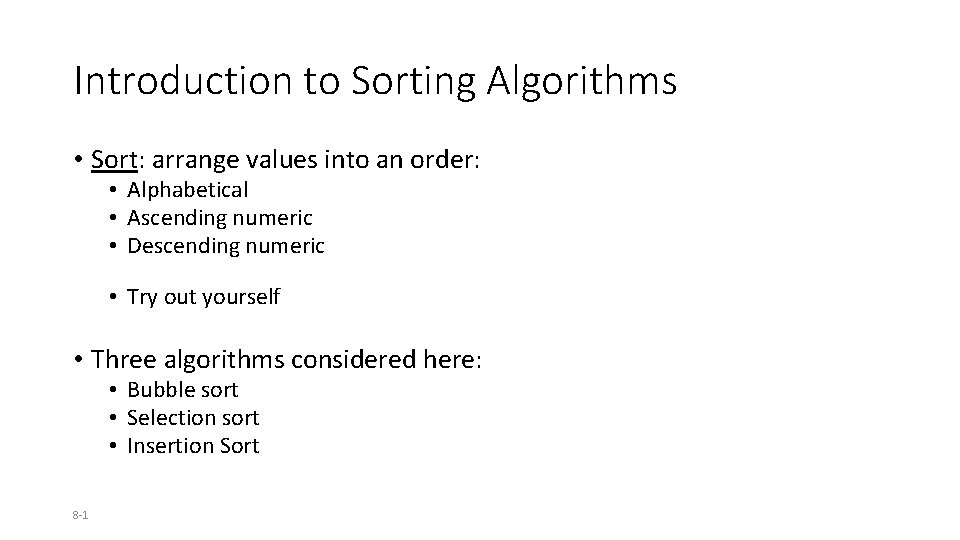
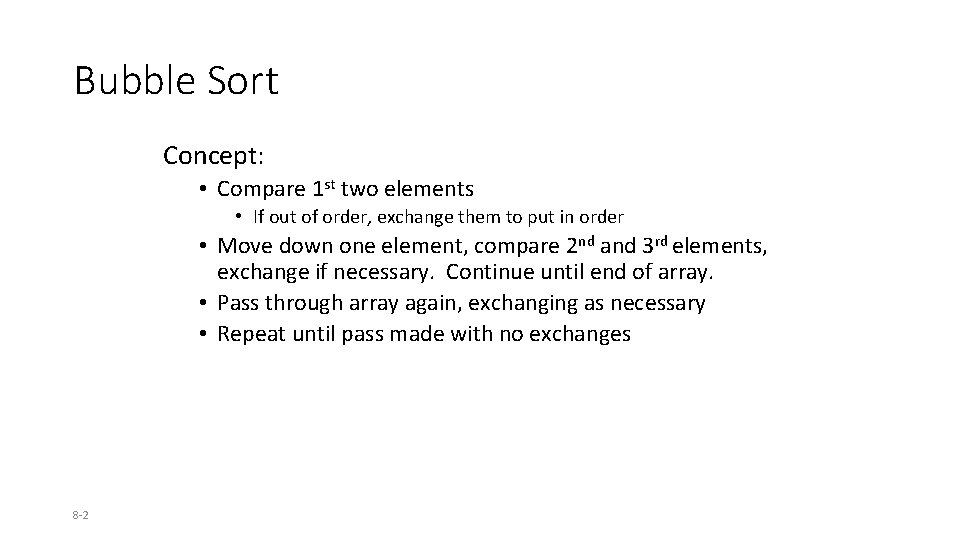
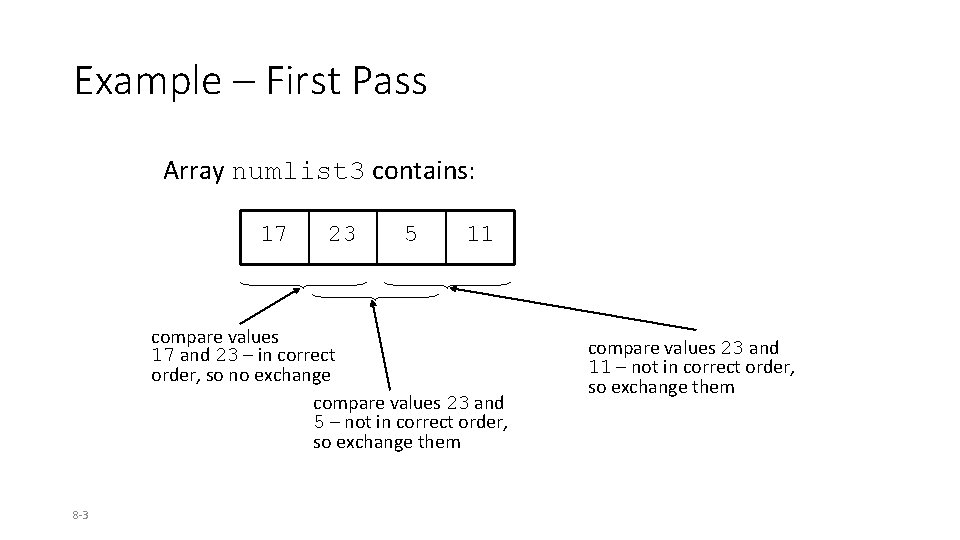
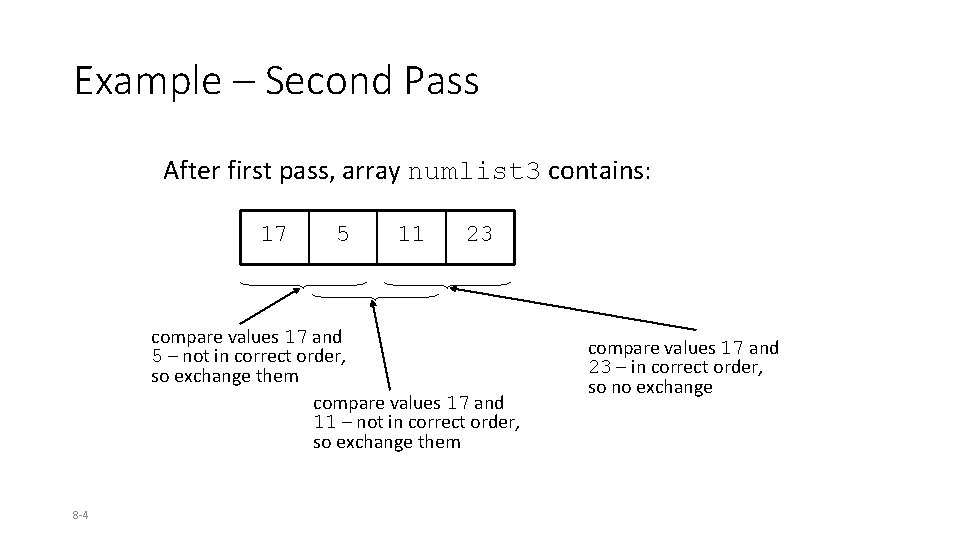
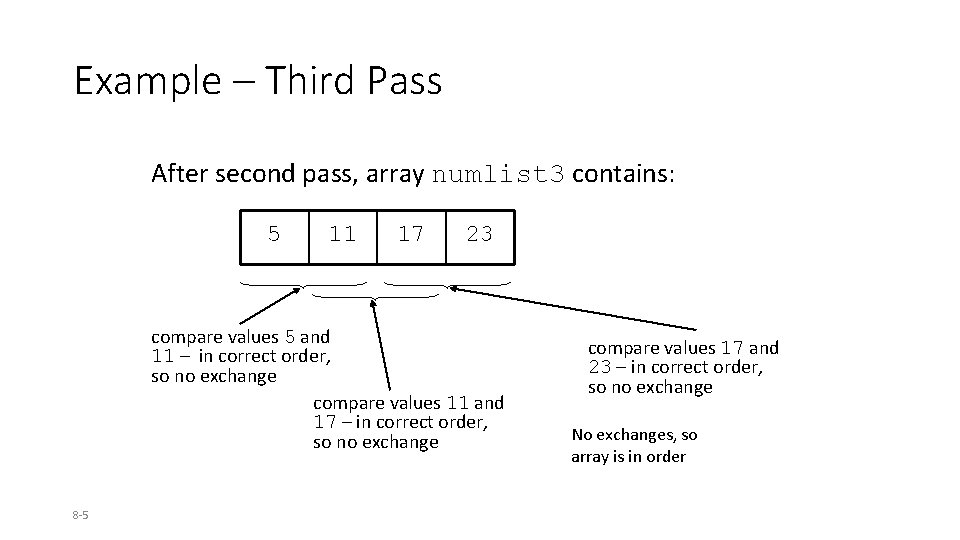
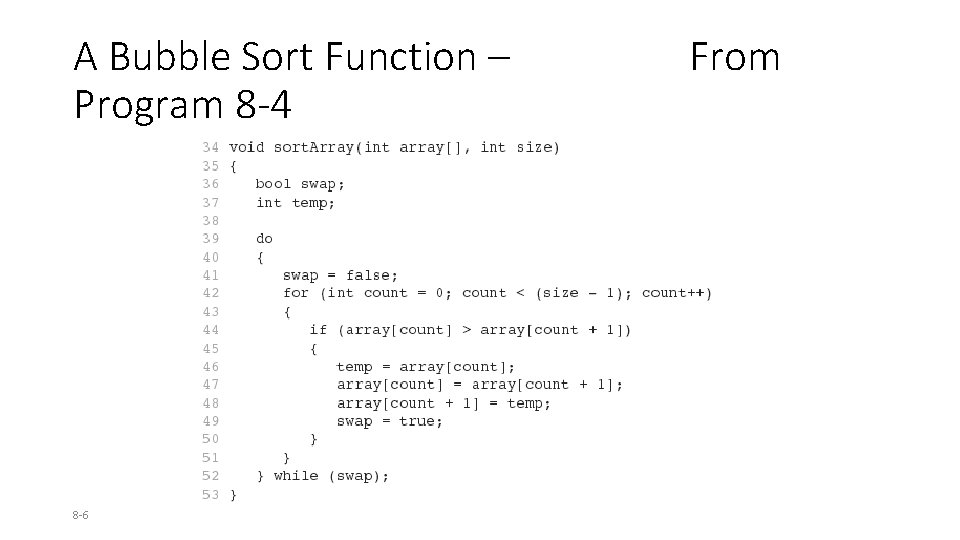
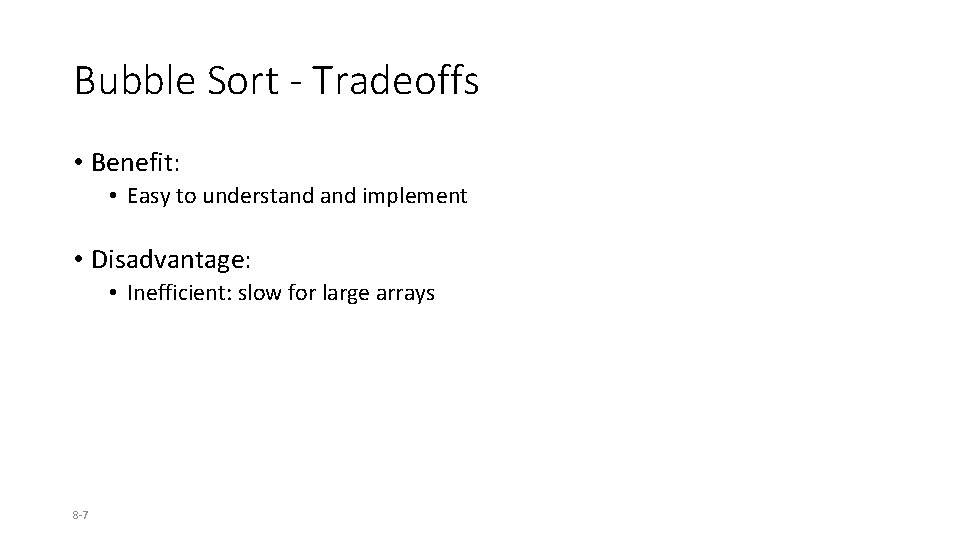
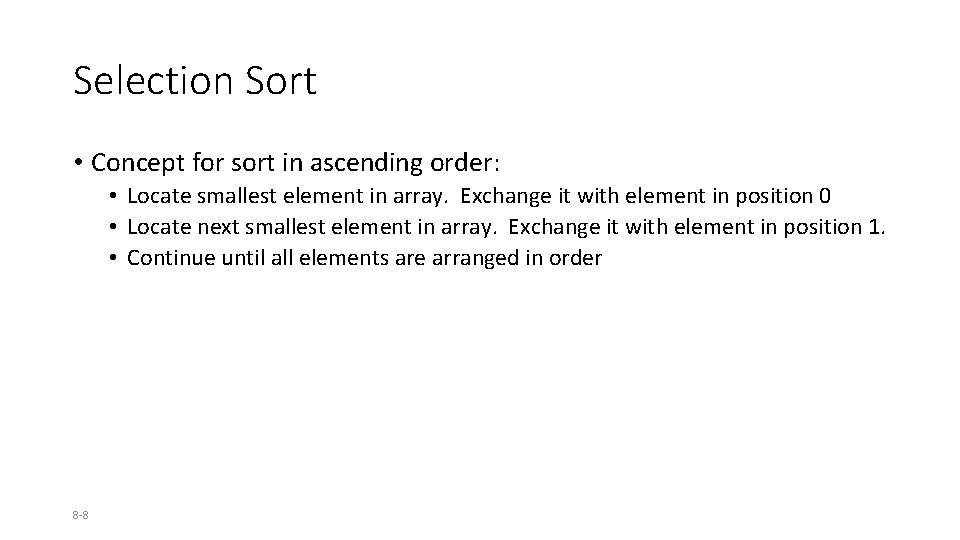
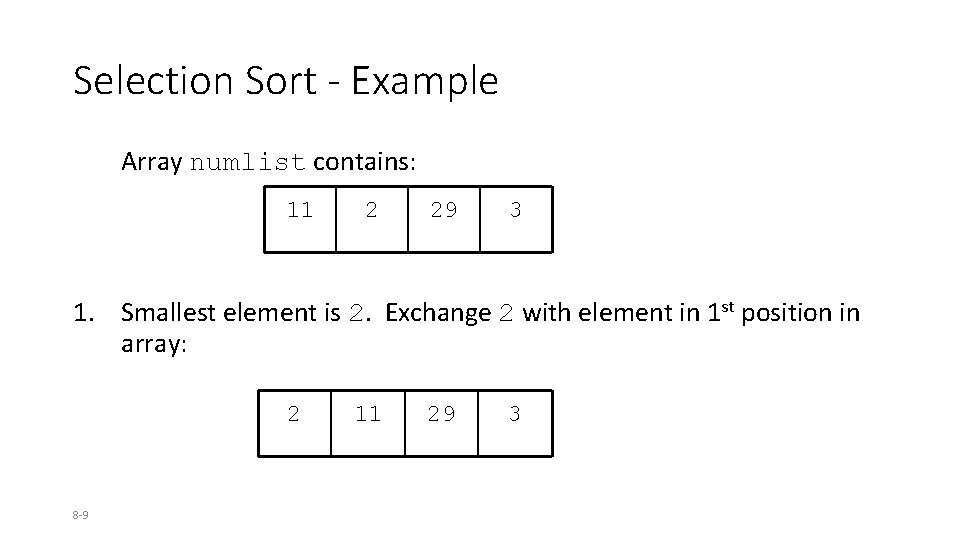
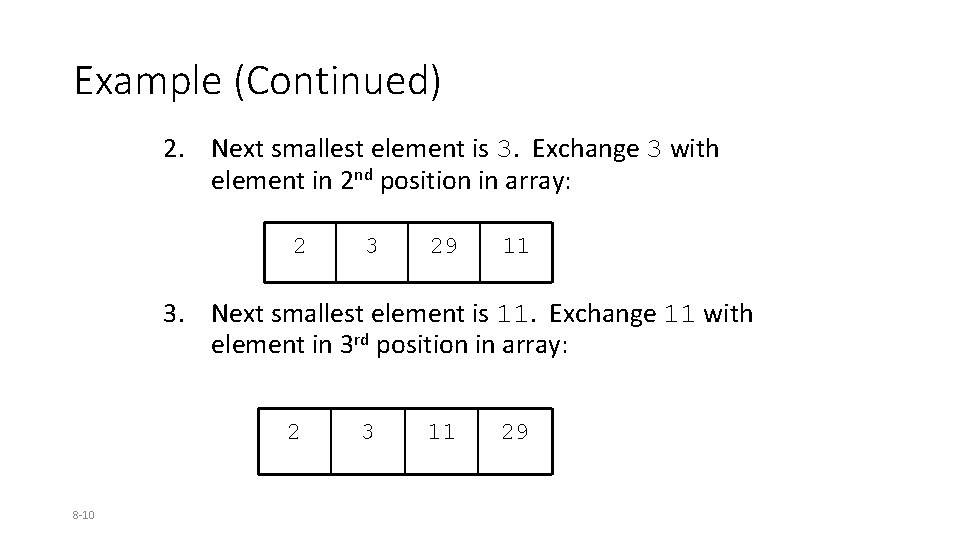
![A Selection Sort Function – 8 -5 From Program 35 void selection. Sort(int array[], A Selection Sort Function – 8 -5 From Program 35 void selection. Sort(int array[],](https://slidetodoc.com/presentation_image/6bdeefd0e1651c14a5d0d4e1b8c11ec7/image-11.jpg)
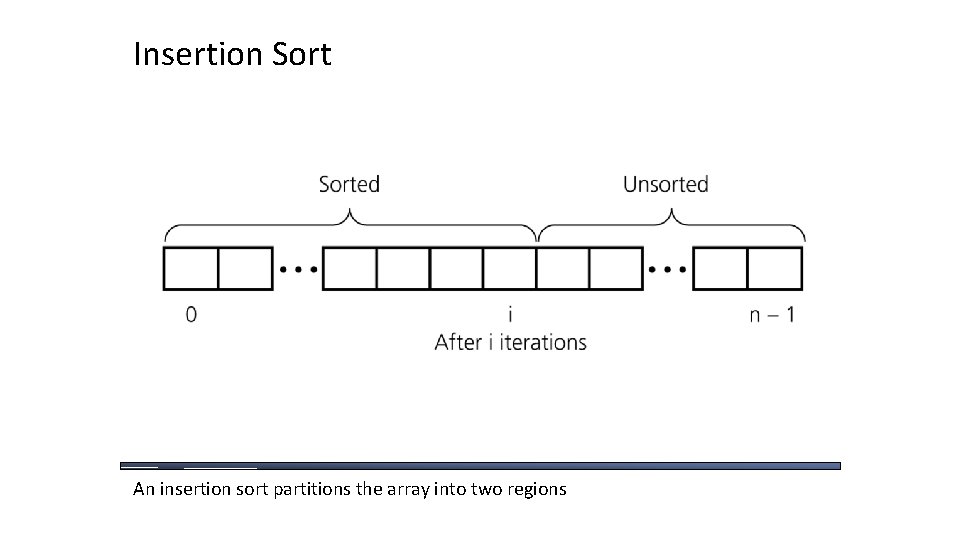
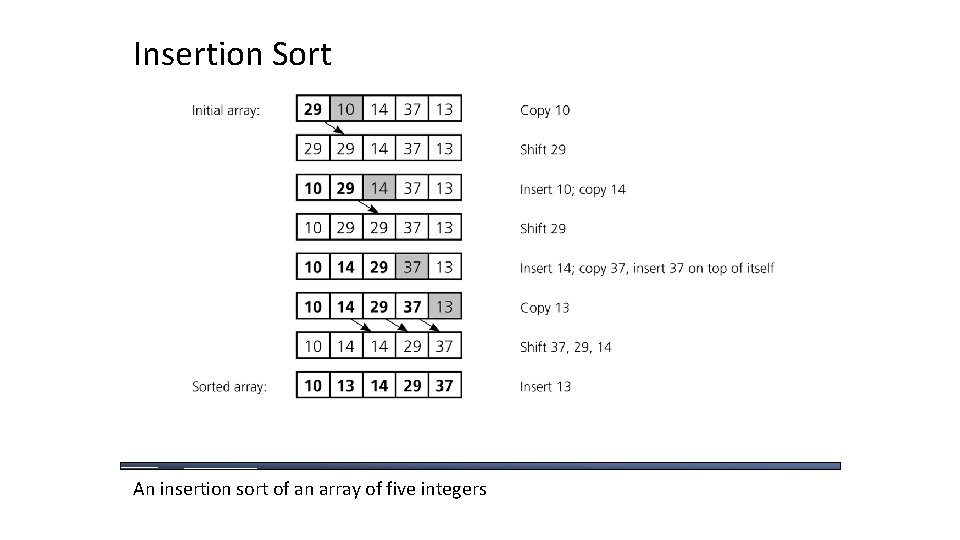
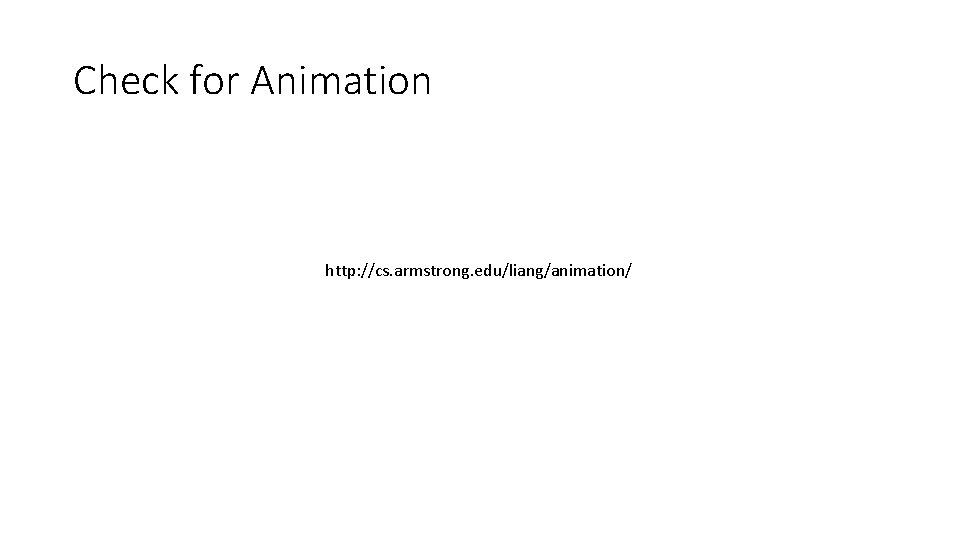
- Slides: 14
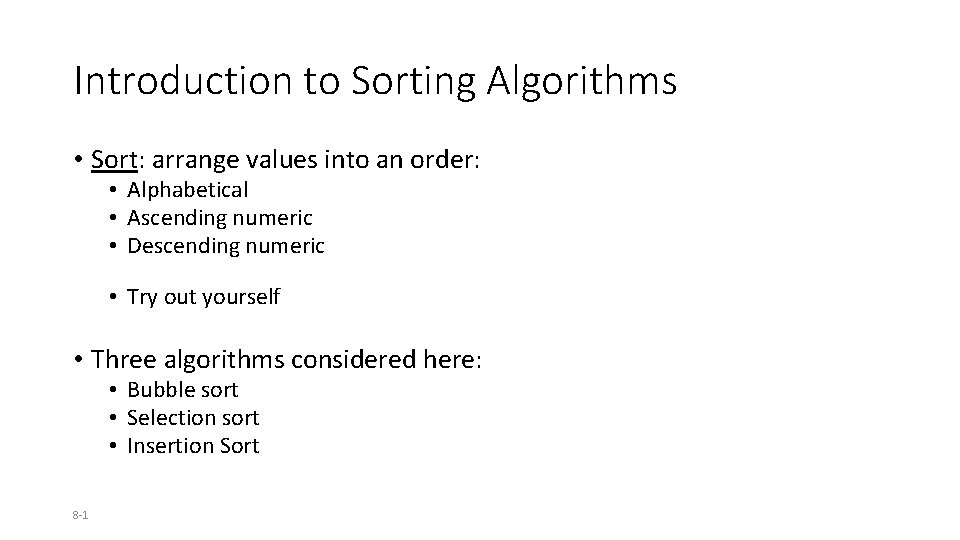
Introduction to Sorting Algorithms • Sort: arrange values into an order: • Alphabetical • Ascending numeric • Descending numeric • Try out yourself • Three algorithms considered here: • Bubble sort • Selection sort • Insertion Sort 8 -1
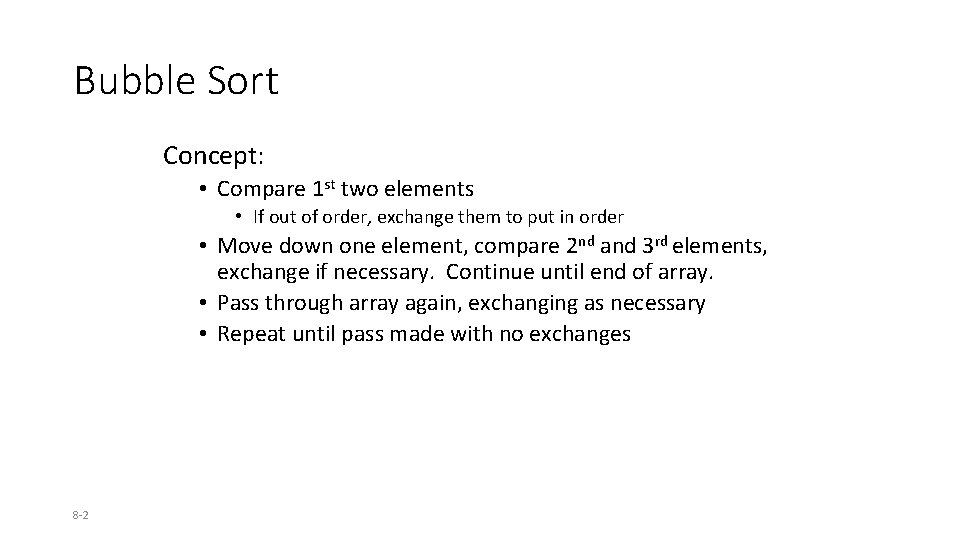
Bubble Sort Concept: • Compare 1 st two elements • If out of order, exchange them to put in order • Move down one element, compare 2 nd and 3 rd elements, exchange if necessary. Continue until end of array. • Pass through array again, exchanging as necessary • Repeat until pass made with no exchanges 8 -2
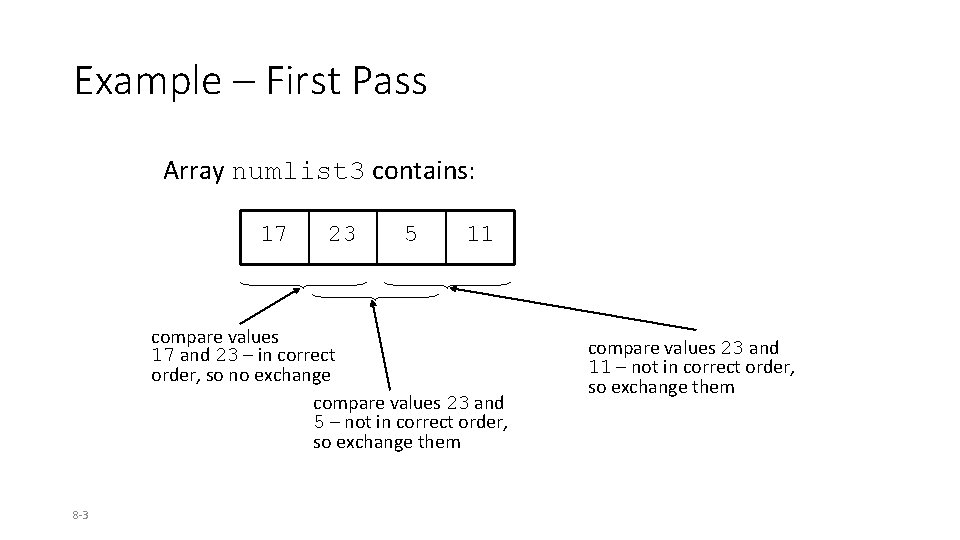
Example – First Pass Array numlist 3 contains: 17 23 5 11 compare values 17 and 23 – in correct order, so no exchange compare values 23 and 5 – not in correct order, so exchange them 8 -3 compare values 23 and 11 – not in correct order, so exchange them
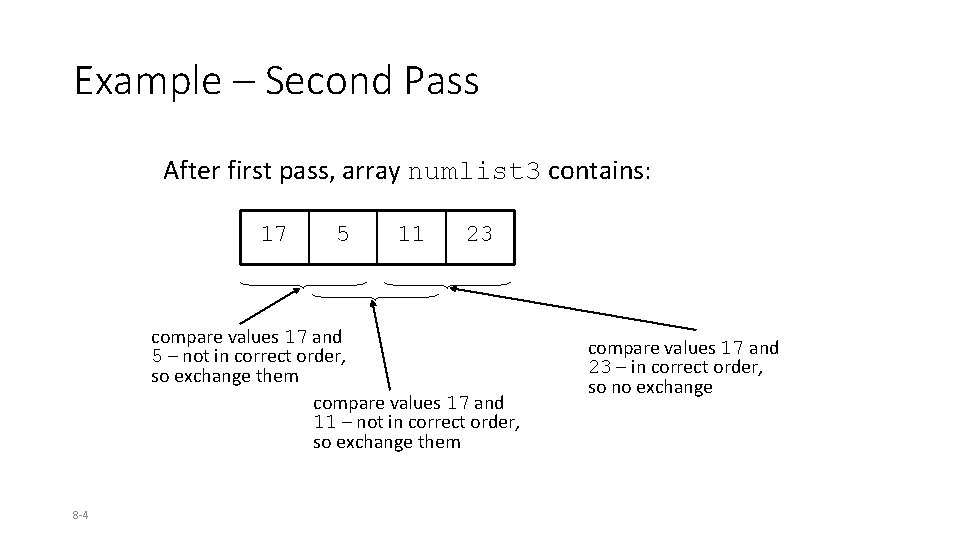
Example – Second Pass After first pass, array numlist 3 contains: 17 5 11 23 compare values 17 and 5 – not in correct order, so exchange them compare values 17 and 11 – not in correct order, so exchange them 8 -4 compare values 17 and 23 – in correct order, so no exchange
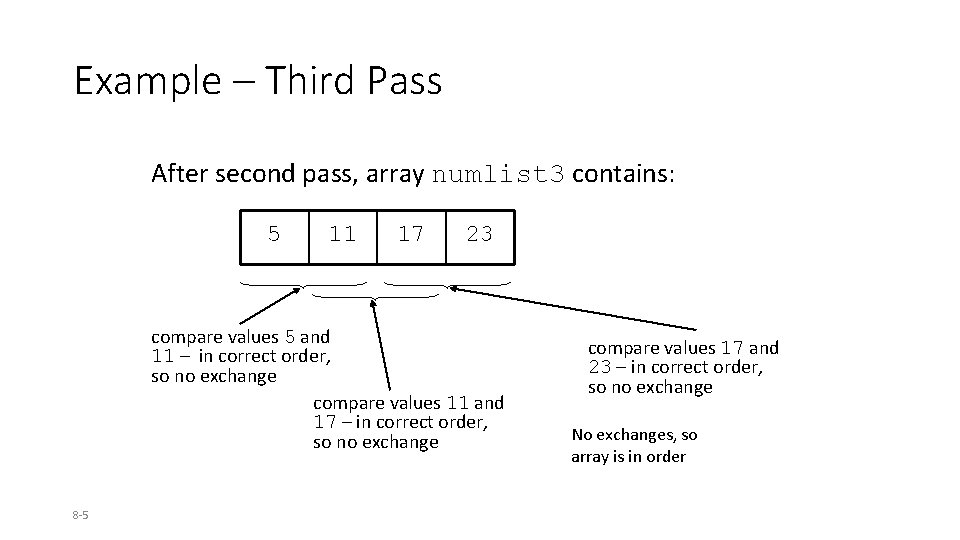
Example – Third Pass After second pass, array numlist 3 contains: 5 11 17 23 compare values 5 and 11 – in correct order, so no exchange compare values 11 and 17 – in correct order, so no exchange 8 -5 compare values 17 and 23 – in correct order, so no exchange No exchanges, so array is in order
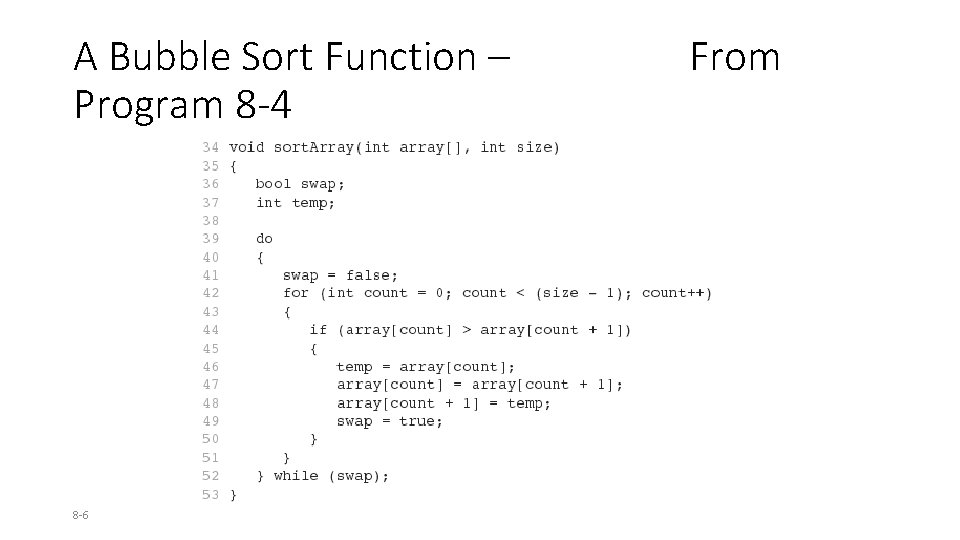
A Bubble Sort Function – Program 8 -4 8 -6 From
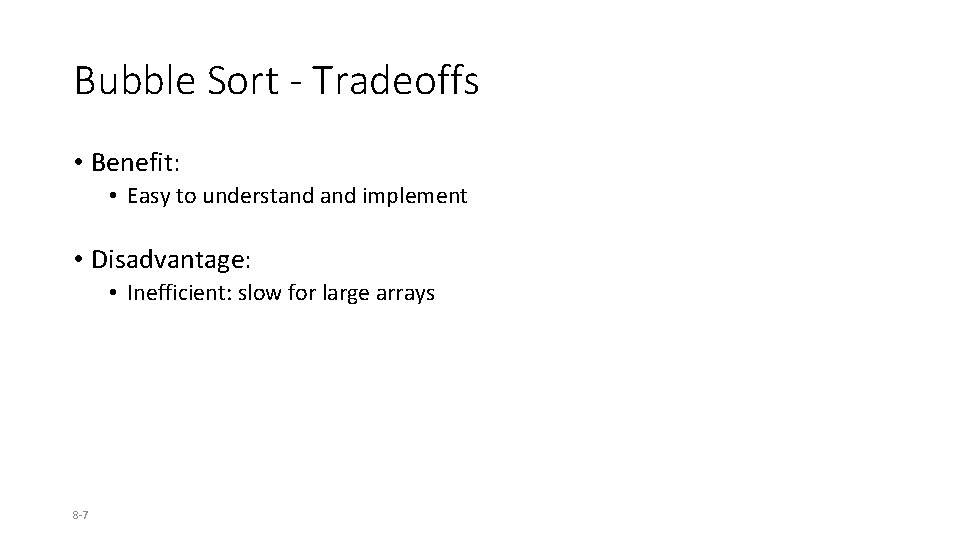
Bubble Sort - Tradeoffs • Benefit: • Easy to understand implement • Disadvantage: • Inefficient: slow for large arrays 8 -7
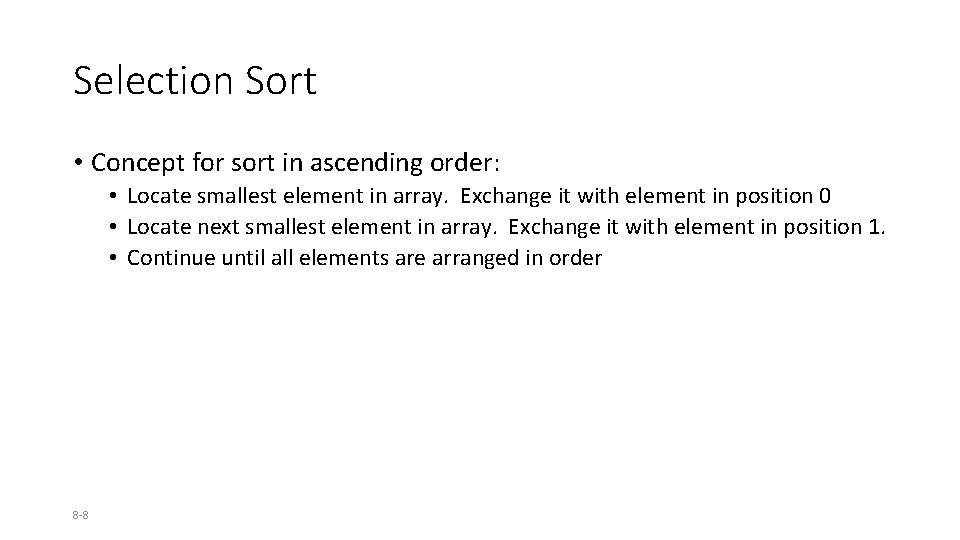
Selection Sort • Concept for sort in ascending order: • Locate smallest element in array. Exchange it with element in position 0 • Locate next smallest element in array. Exchange it with element in position 1. • Continue until all elements are arranged in order 8 -8
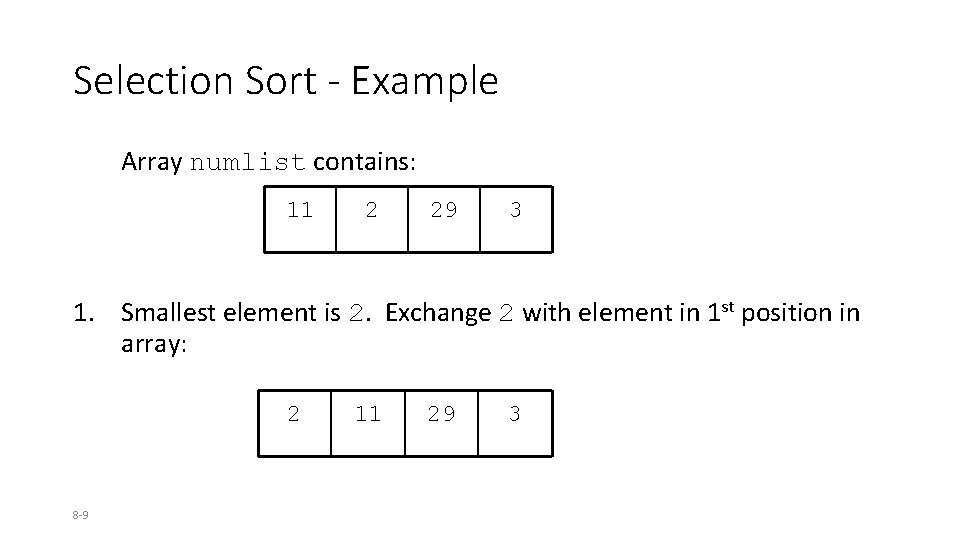
Selection Sort - Example Array numlist contains: 11 2 29 3 1. Smallest element is 2. Exchange 2 with element in 1 st position in array: 2 8 -9 11 29 3
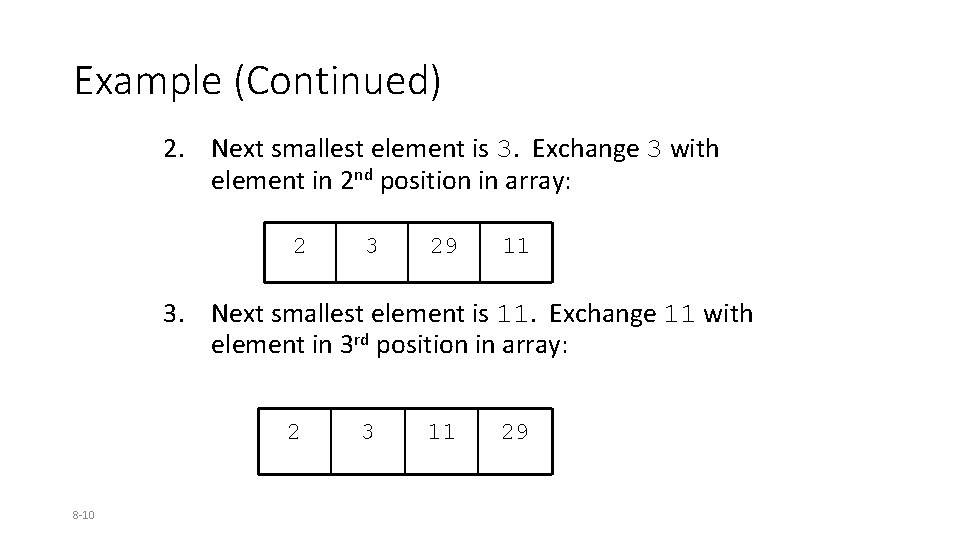
Example (Continued) 2. Next smallest element is 3. Exchange 3 with element in 2 nd position in array: 2 3 29 11 3. Next smallest element is 11. Exchange 11 with element in 3 rd position in array: 2 8 -10 3 11 29
![A Selection Sort Function 8 5 From Program 35 void selection Sortint array A Selection Sort Function – 8 -5 From Program 35 void selection. Sort(int array[],](https://slidetodoc.com/presentation_image/6bdeefd0e1651c14a5d0d4e1b8c11ec7/image-11.jpg)
A Selection Sort Function – 8 -5 From Program 35 void selection. Sort(int array[], int size) 36 { 37 int start. Scan, min. Index, min. Value; 38 39 for (start. Scan = 0; start. Scan < (size - 1); start. Scan++) 40 { 41 min. Index = start. Scan; 42 min. Value = array[start. Scan]; 43 for(int index = start. Scan + 1; index < size; index++) 44 { 45 if (array[index] < min. Value) 46 { 47 min. Value = array[index]; 48 min. Index = index; 49 } 50 } 51 array[min. Index] = array[start. Scan]; 52 array[start. Scan] = min. Value; 53 } 54 } 8 -11
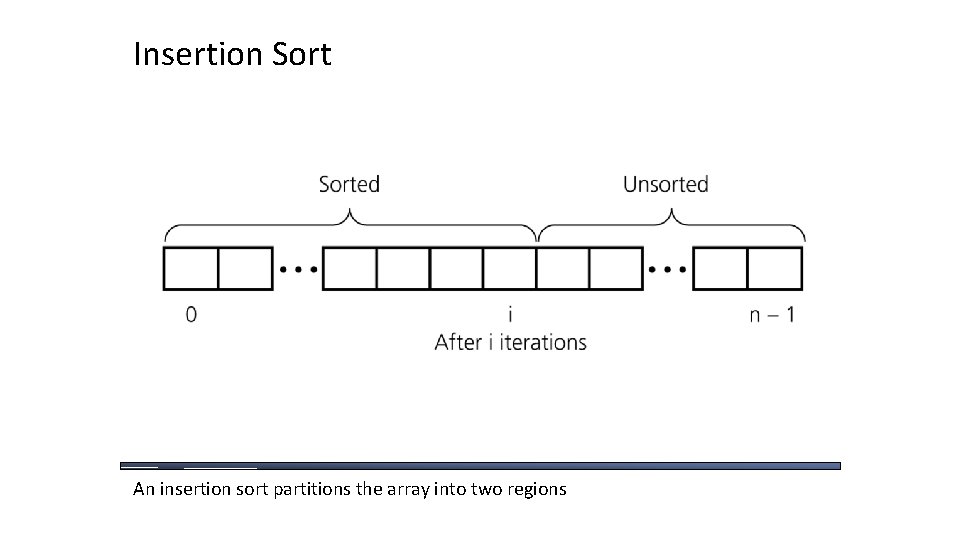
Insertion Sort An insertion sort partitions the array into two regions
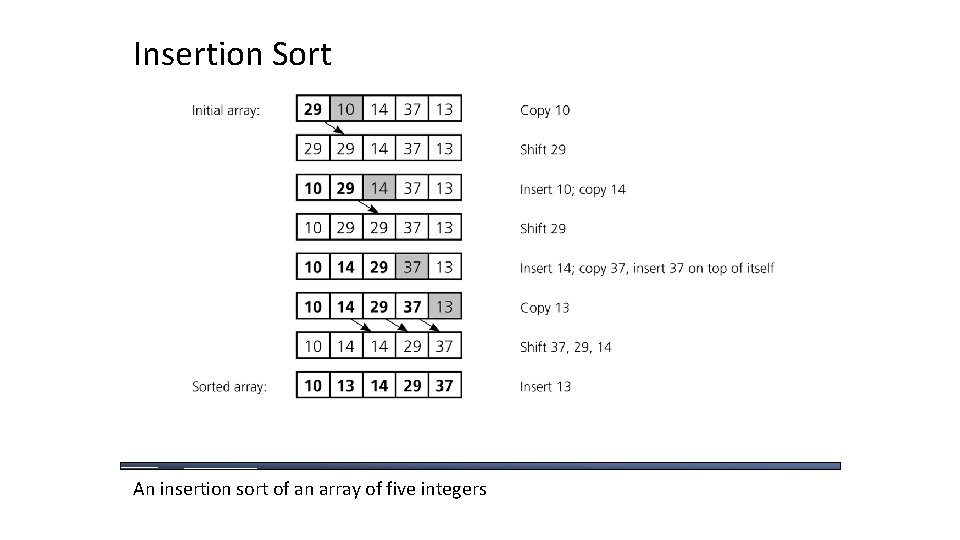
Insertion Sort An insertion sort of an array of five integers
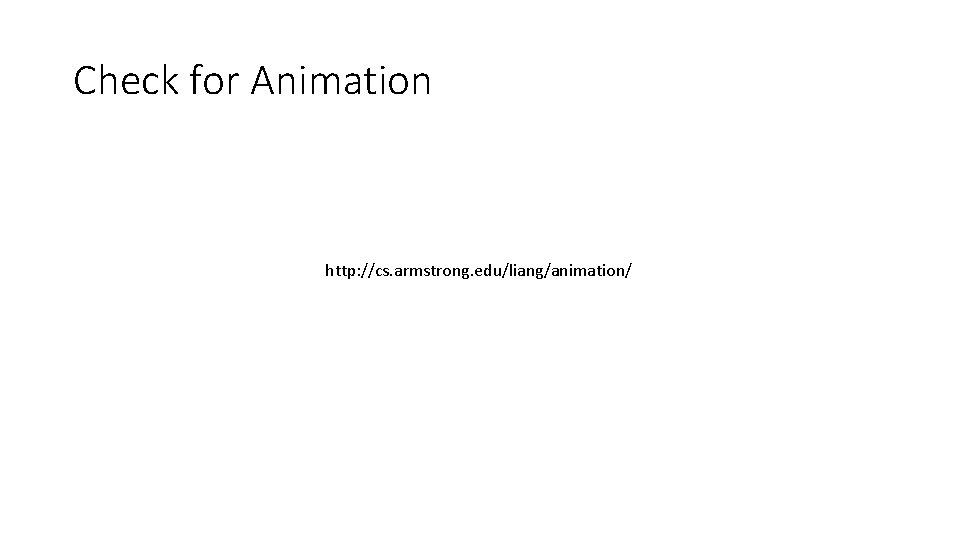
Check for Animation http: //cs. armstrong. edu/liang/animation/