Python Programming the Google Search Damian Gordon Ref
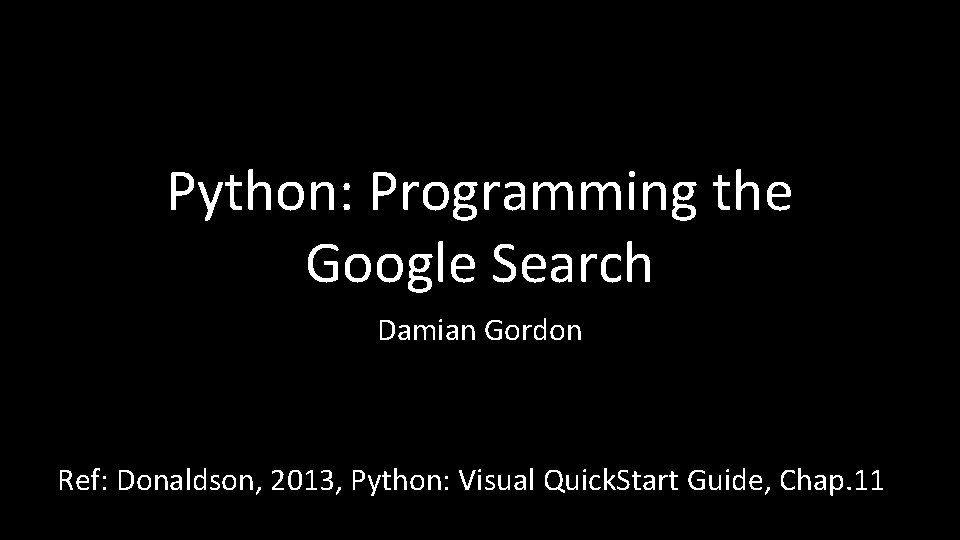
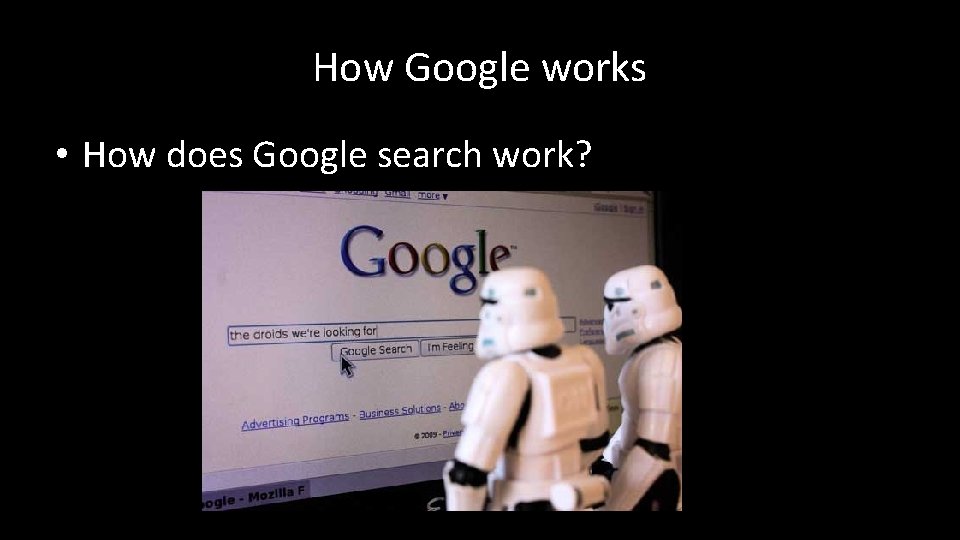
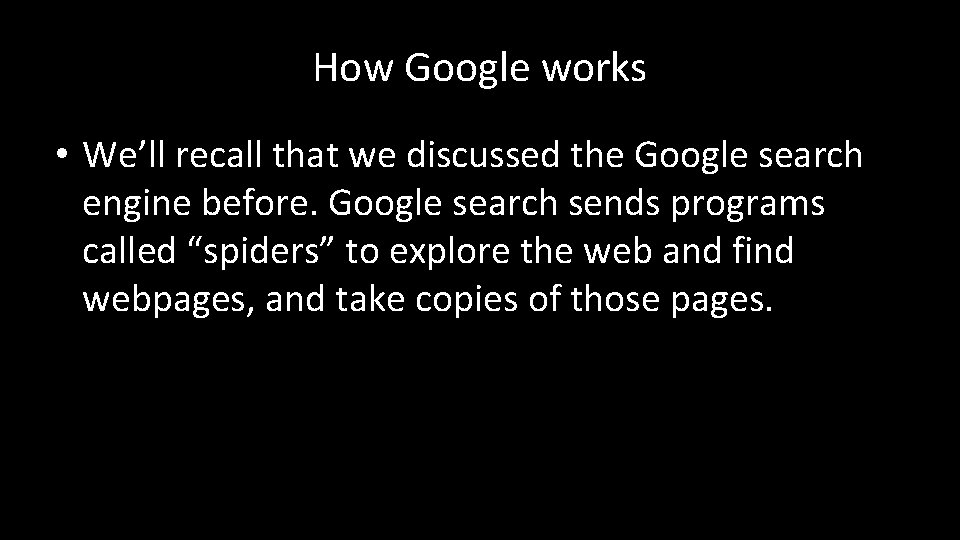
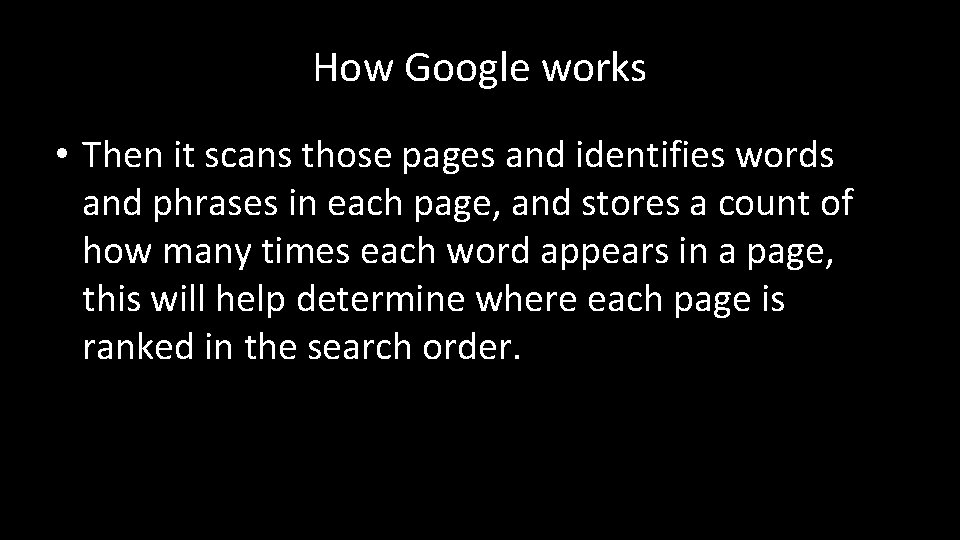
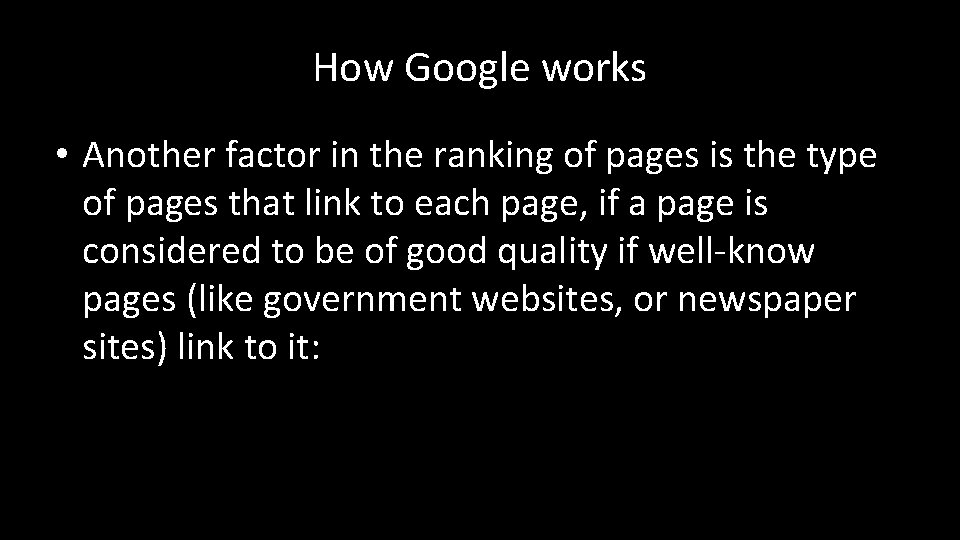
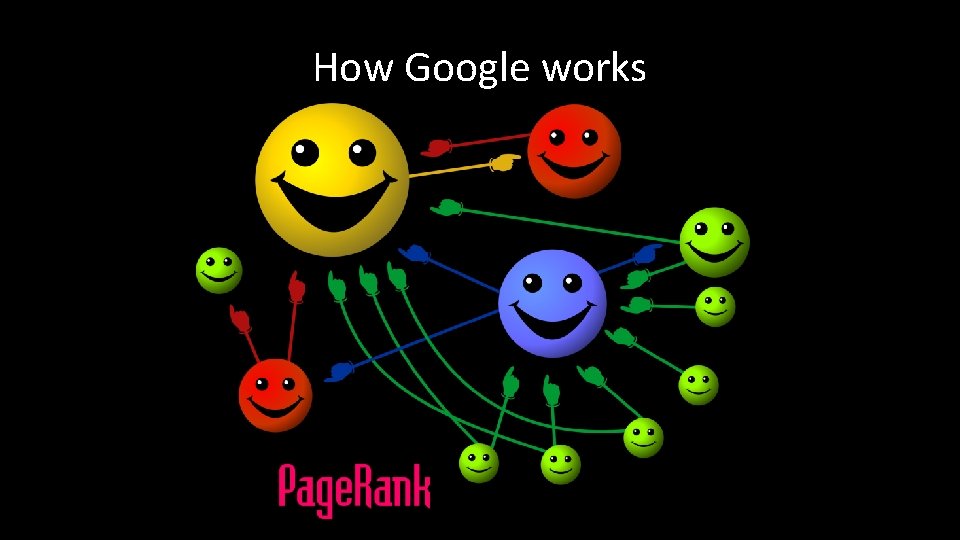
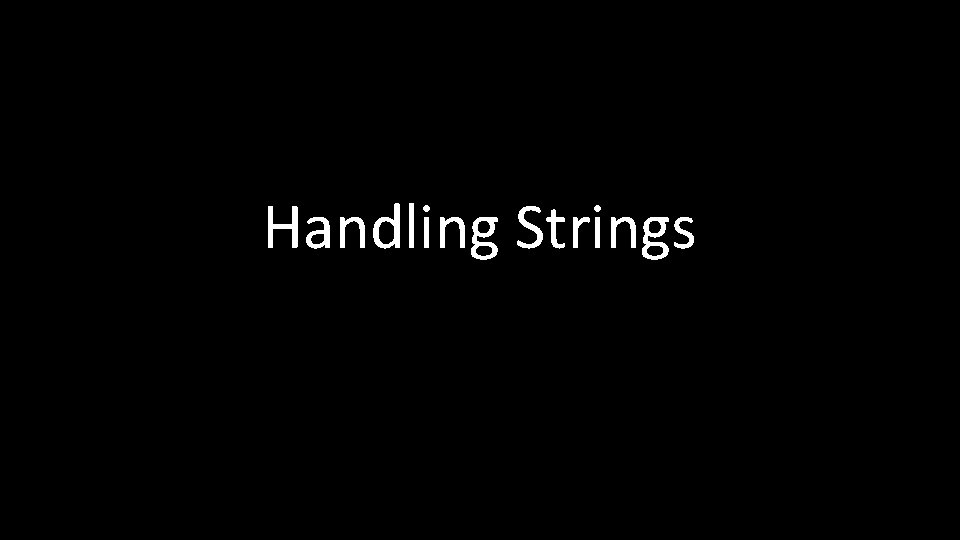
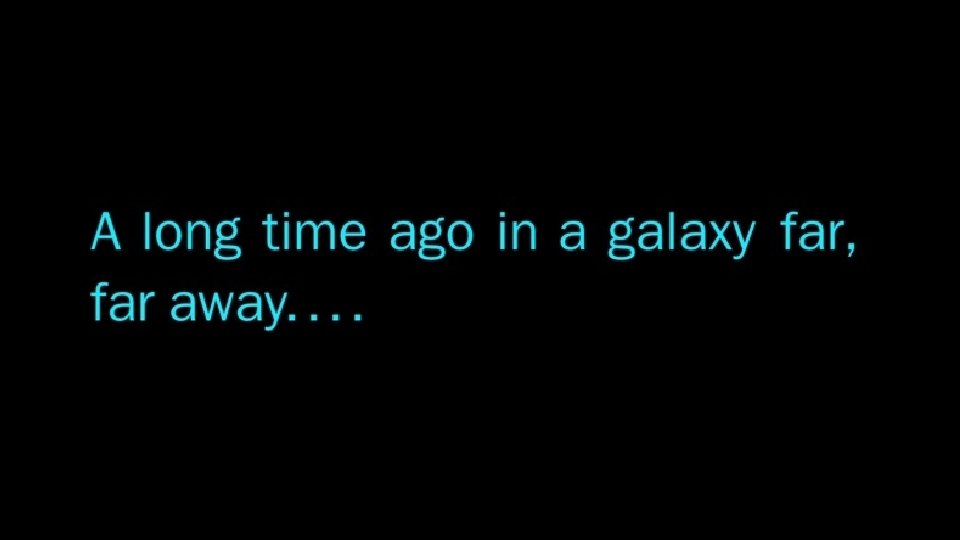
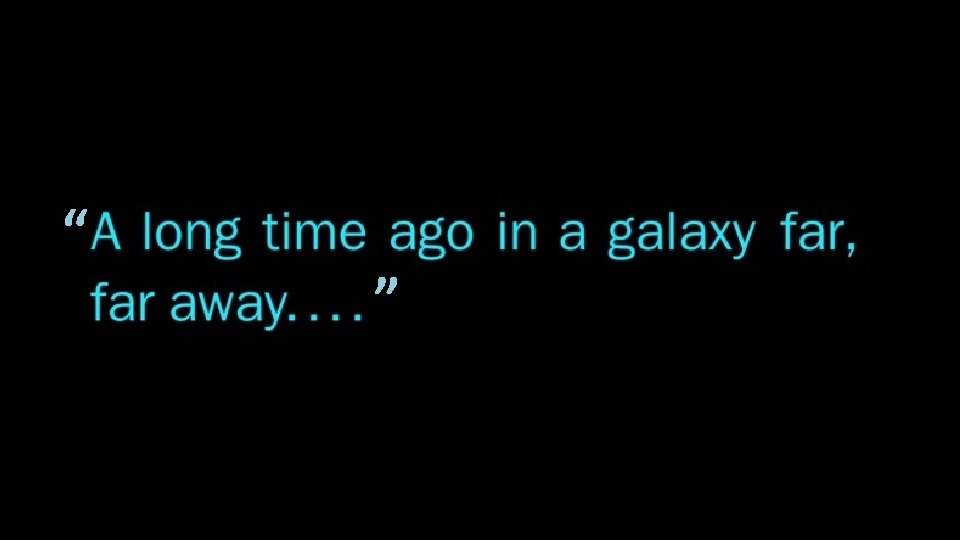
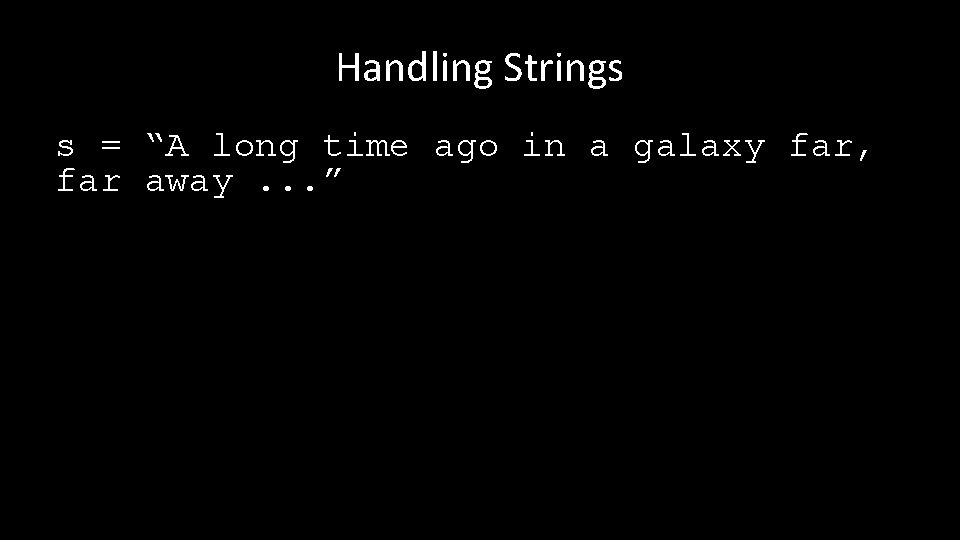
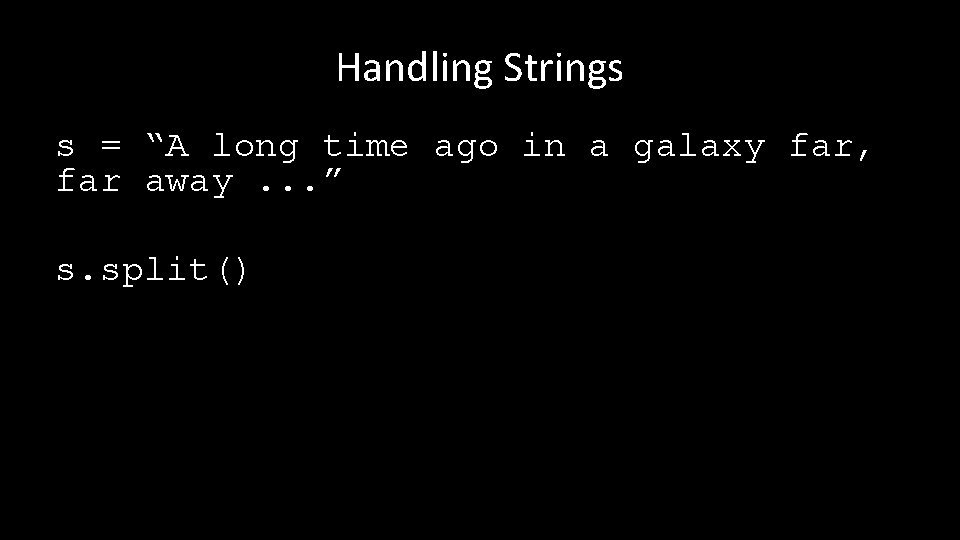
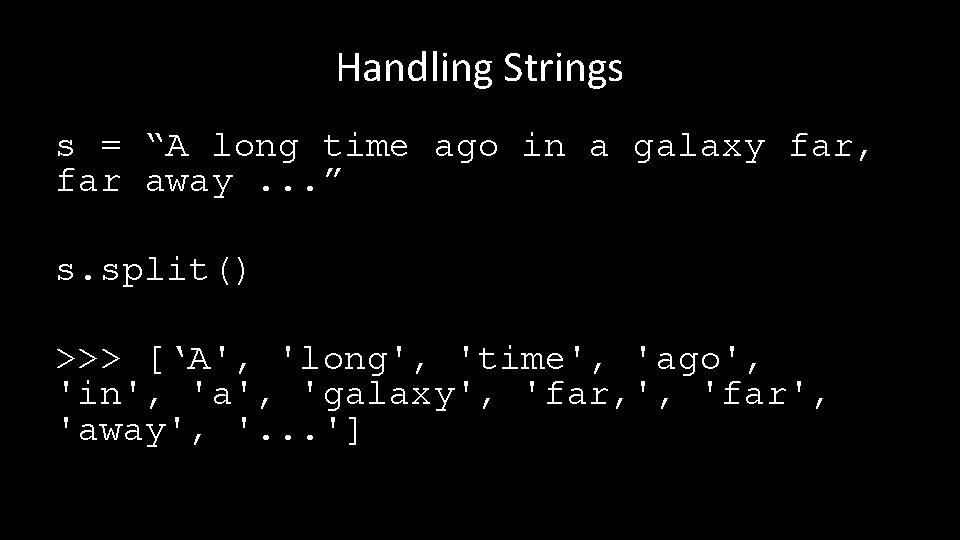
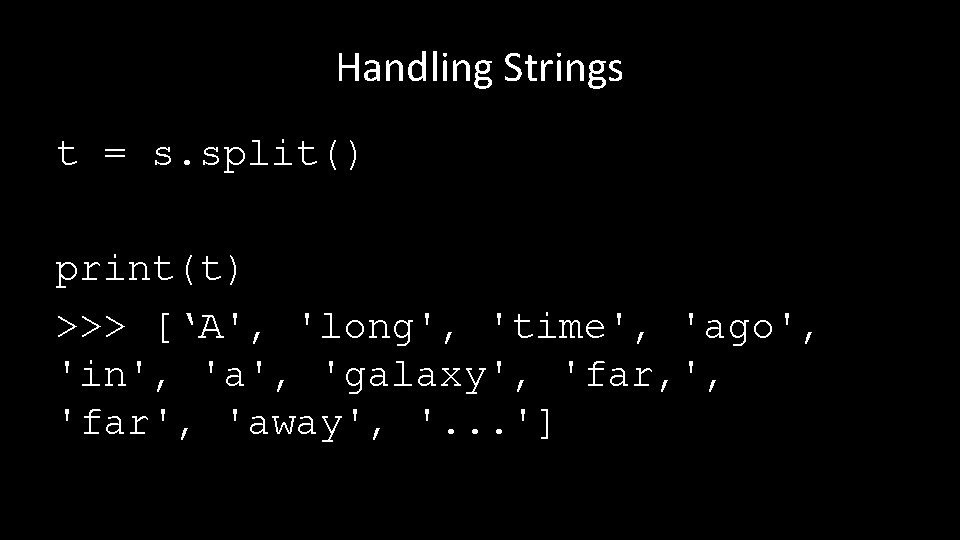
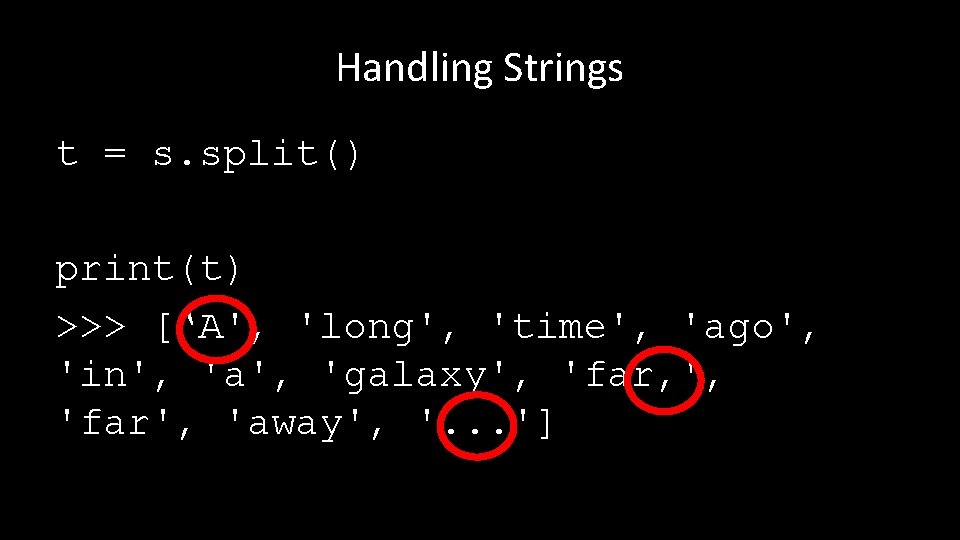
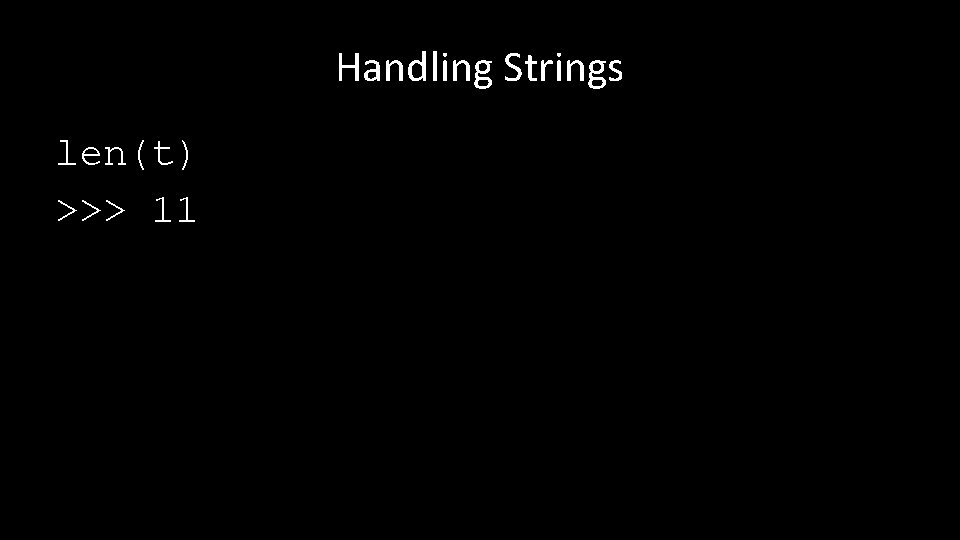
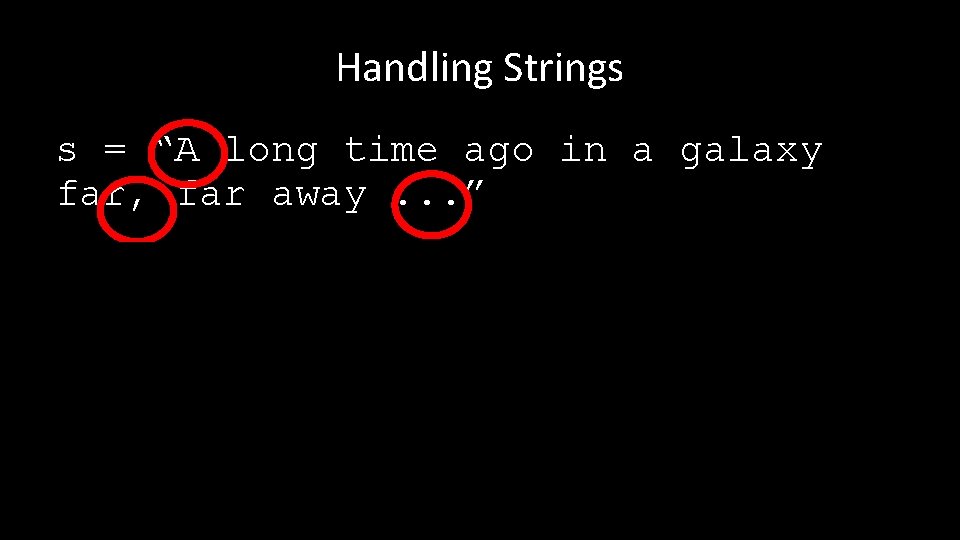
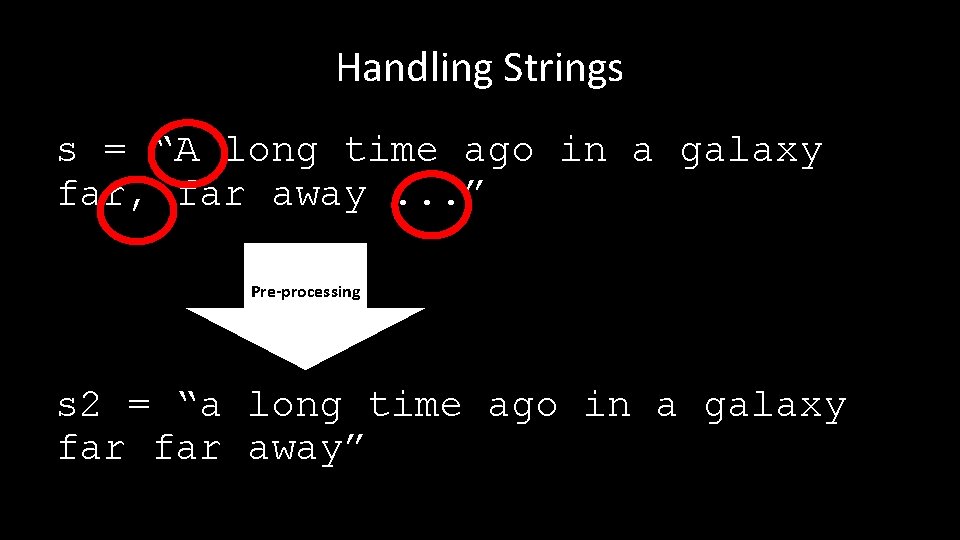
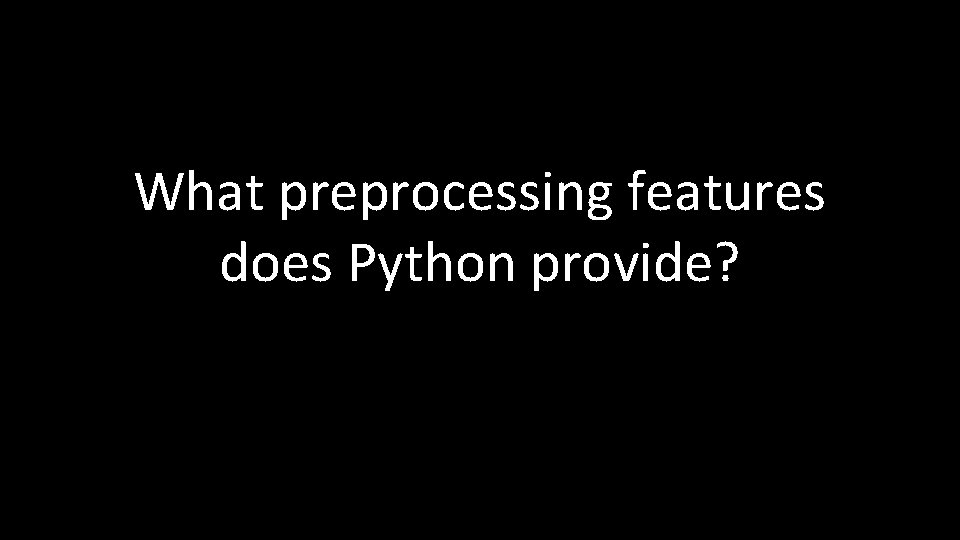
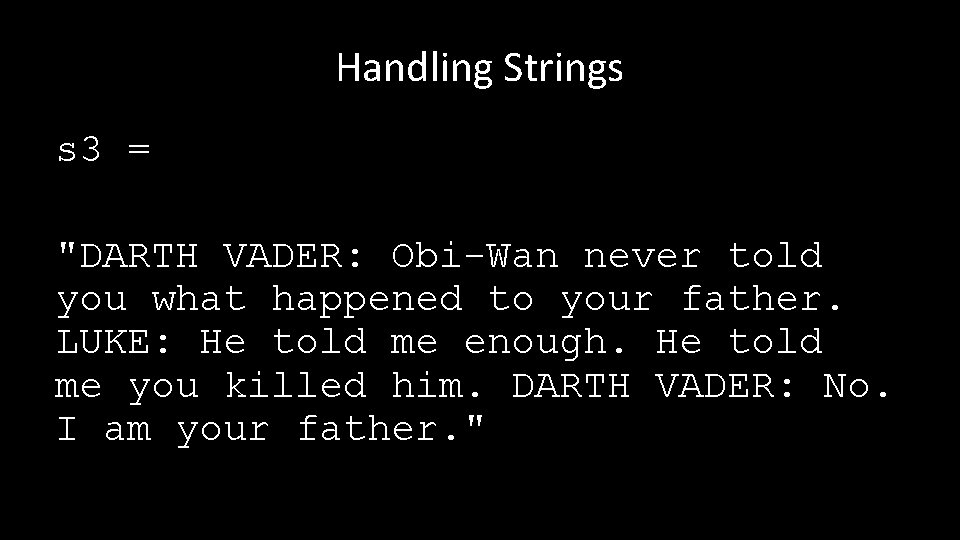
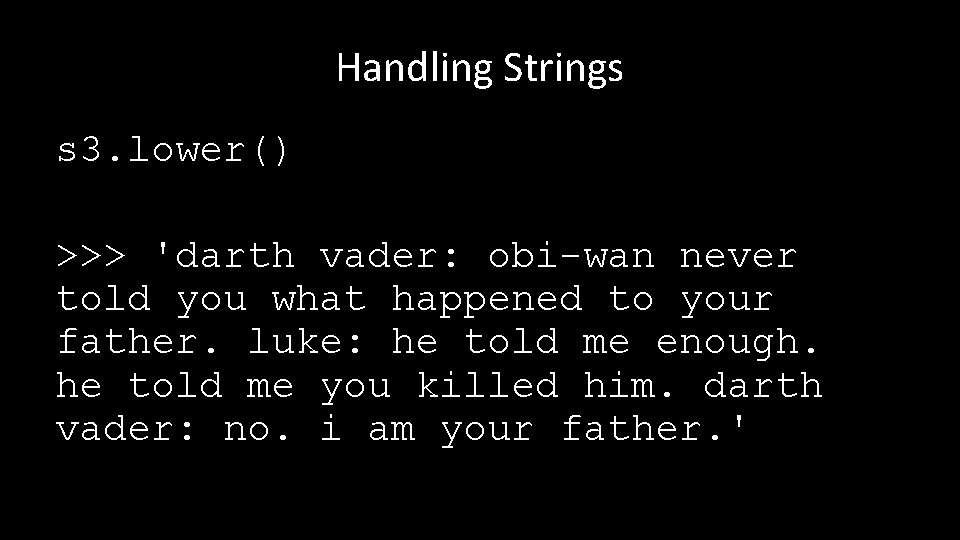
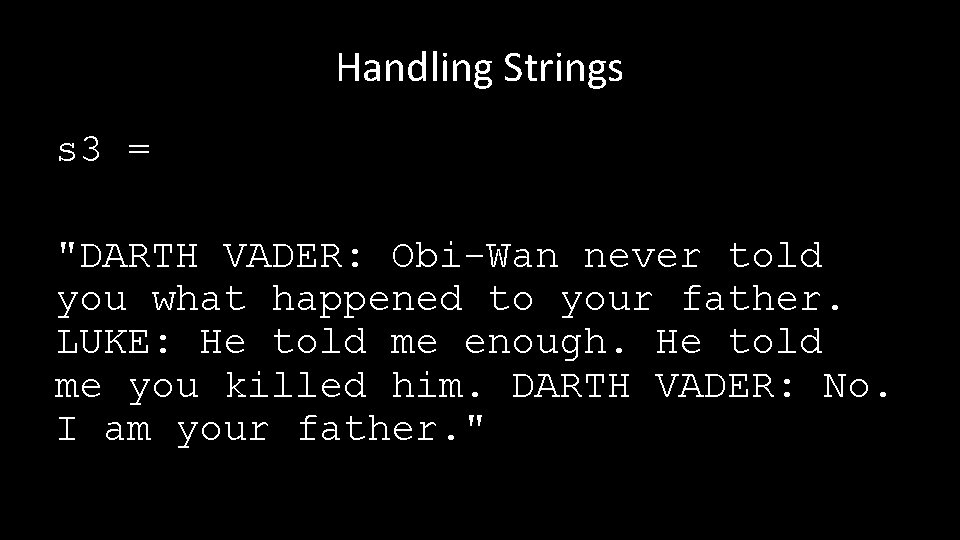
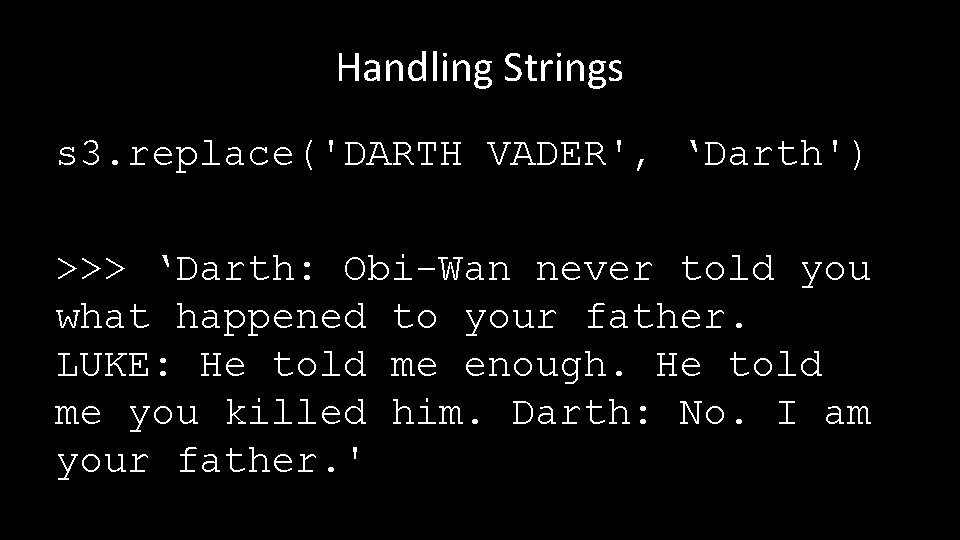
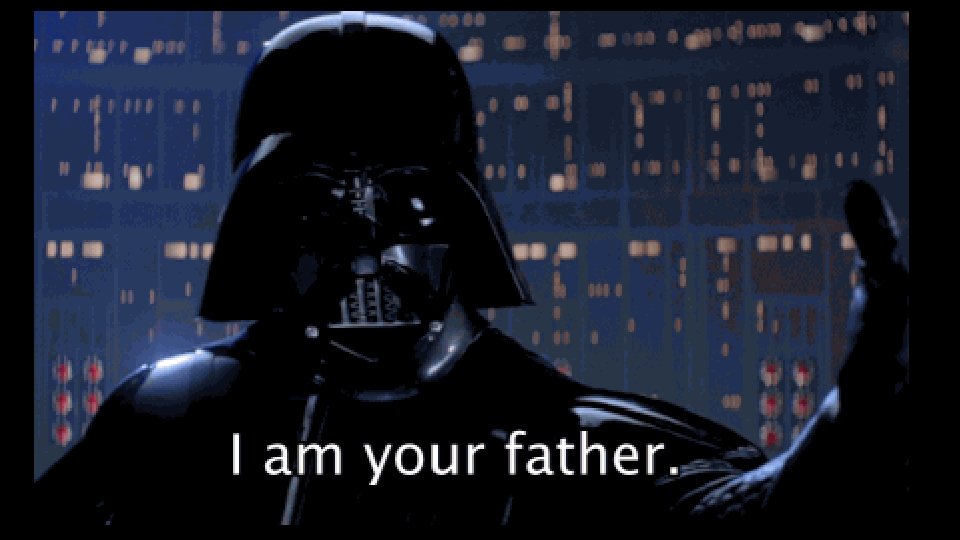
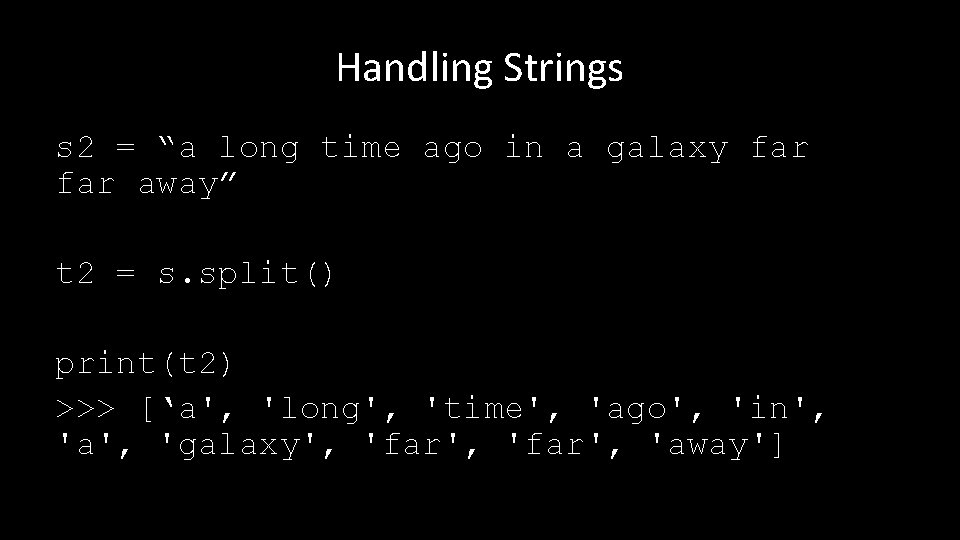
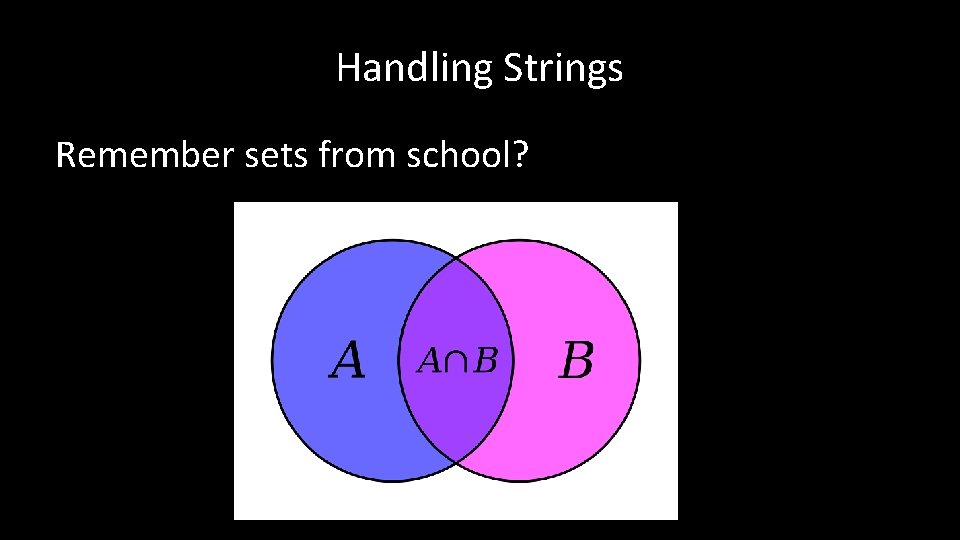
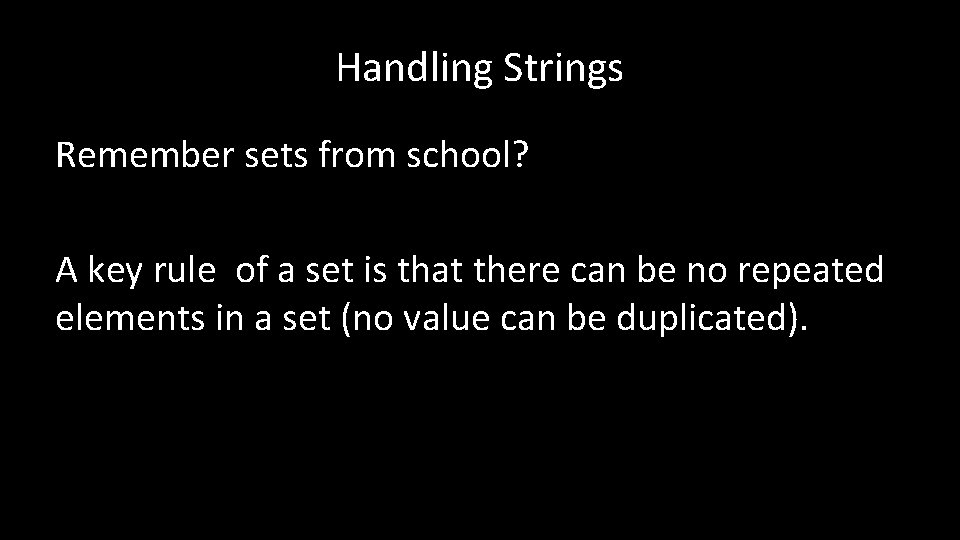
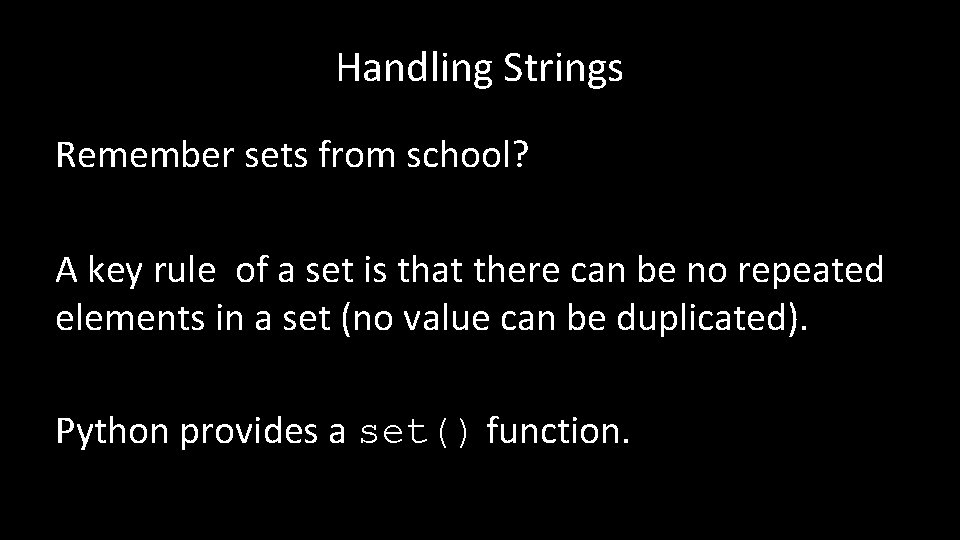
![Handling Strings print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away'] Handling Strings print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away']](https://slidetodoc.com/presentation_image_h2/64478e146d3074f94ebdedaded710bc8/image-28.jpg)
![Handling Strings print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away'] Handling Strings print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away']](https://slidetodoc.com/presentation_image_h2/64478e146d3074f94ebdedaded710bc8/image-29.jpg)
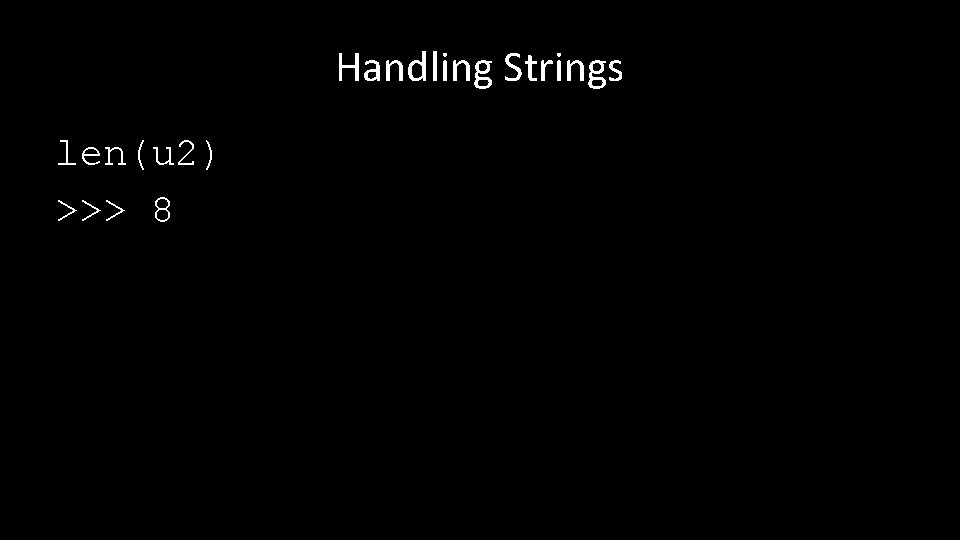
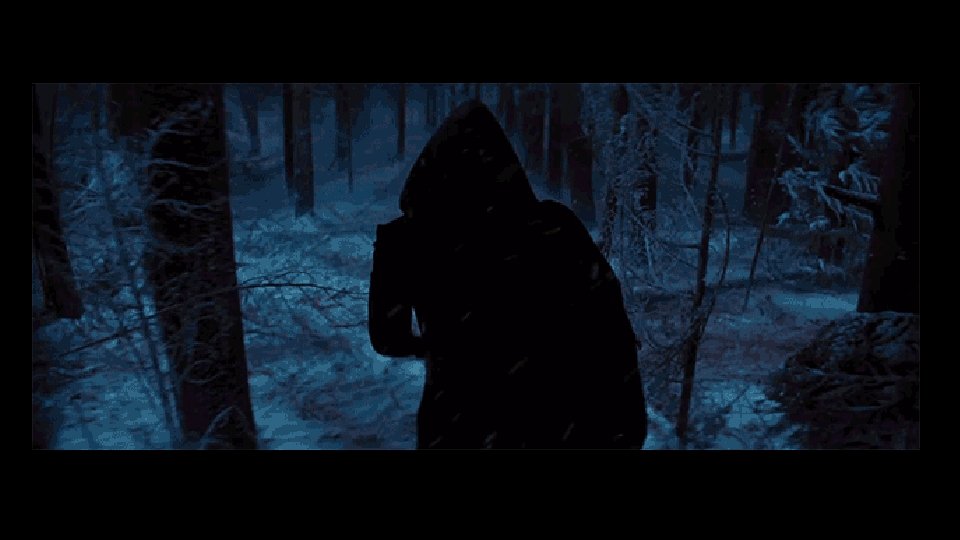
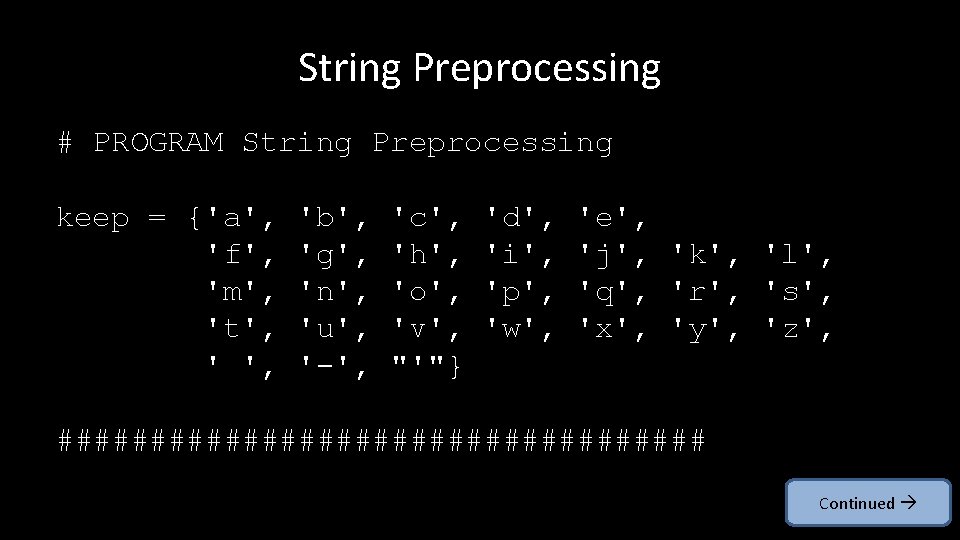
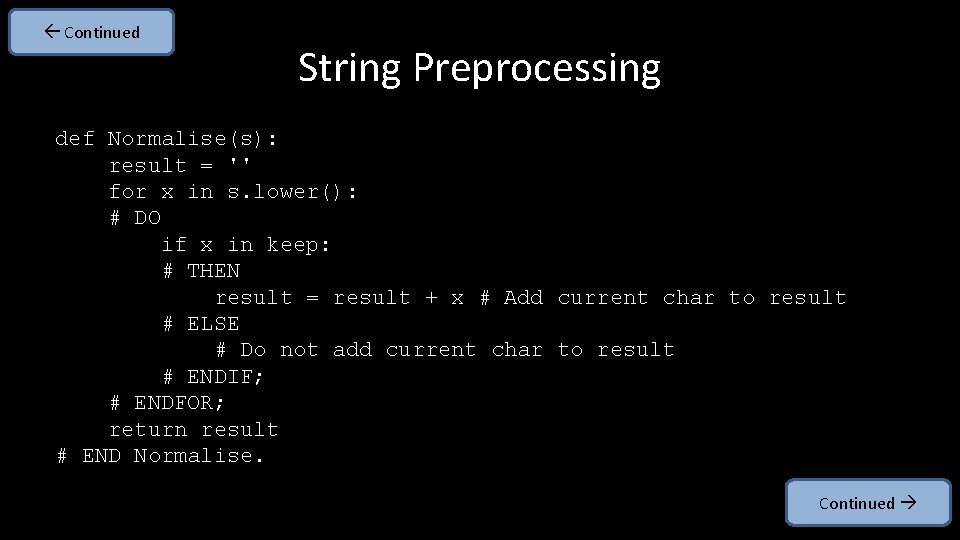
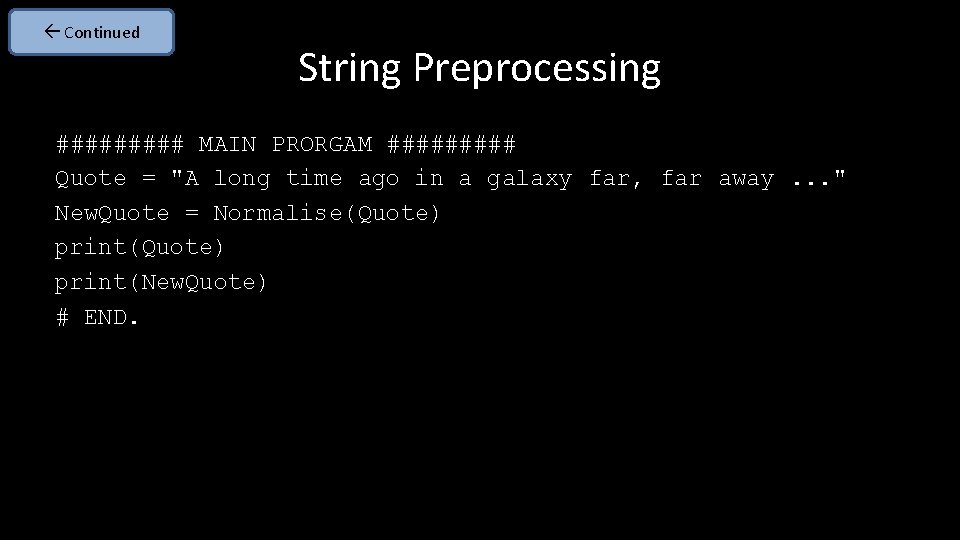
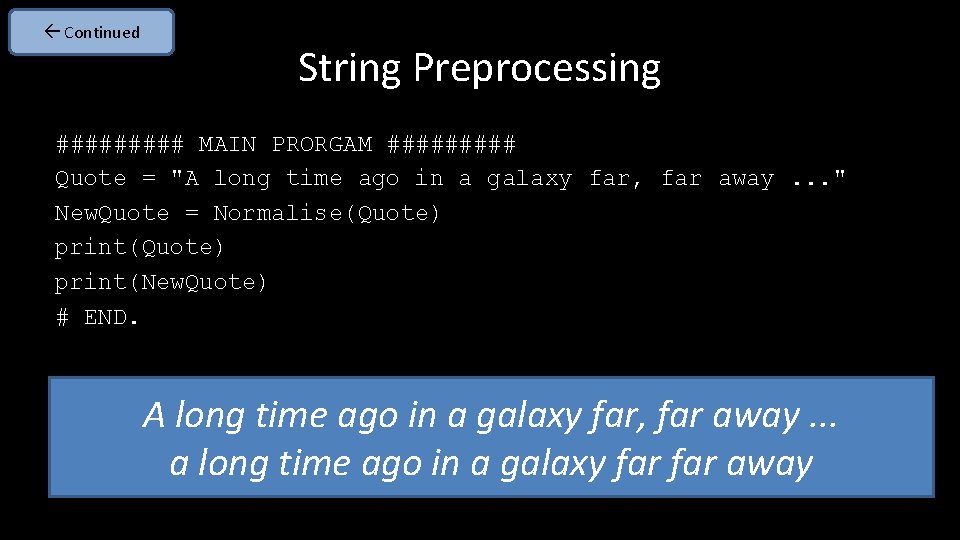
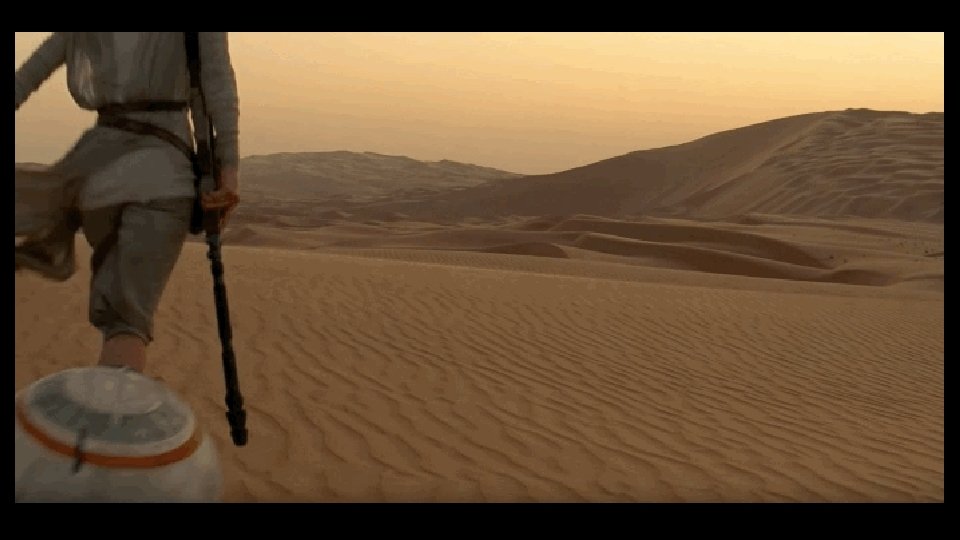
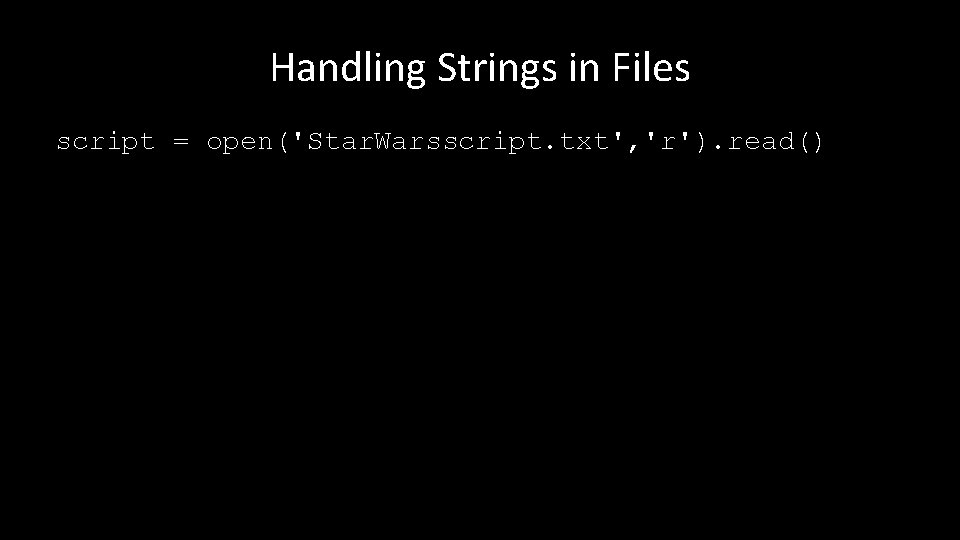
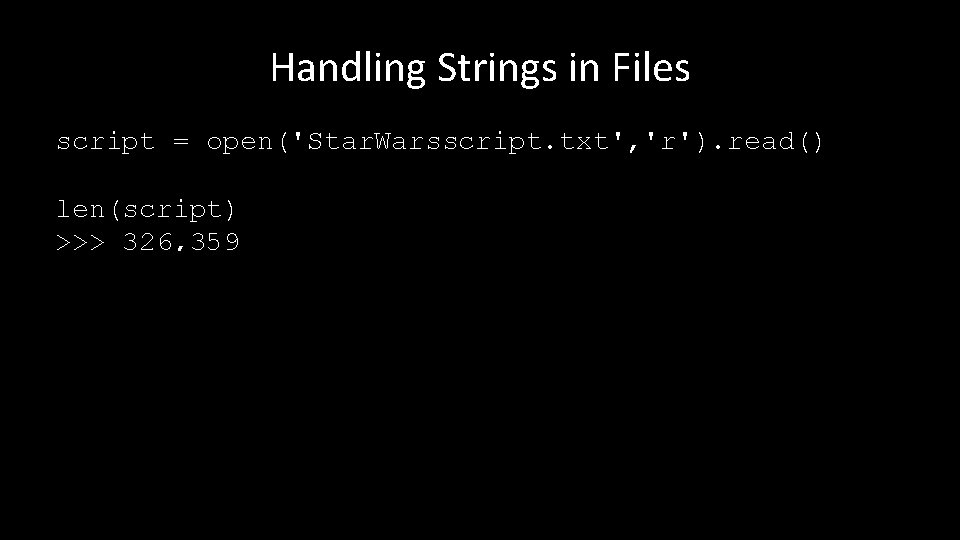
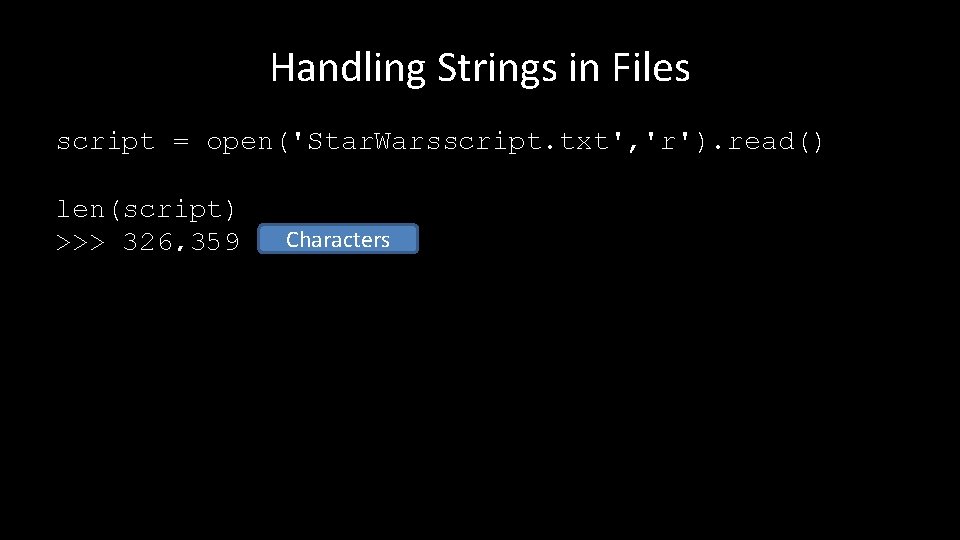
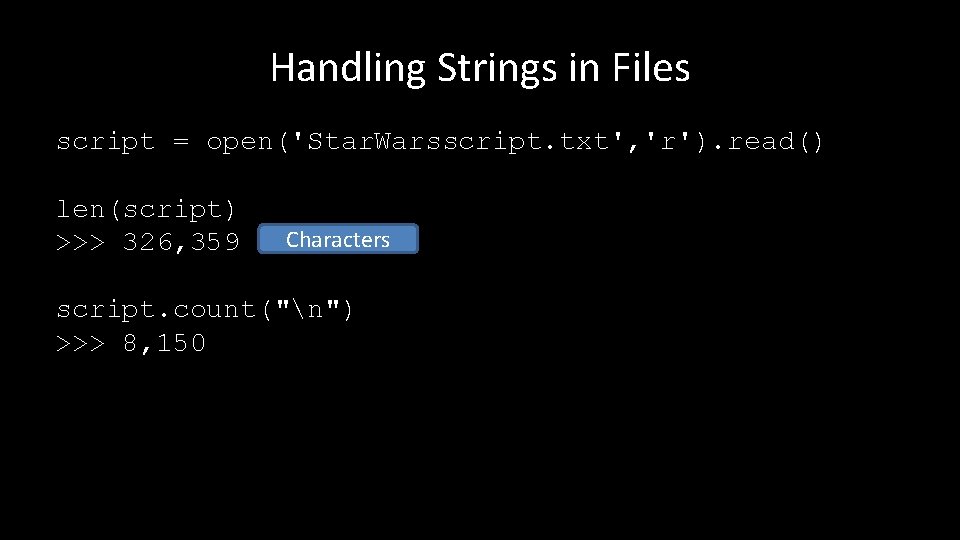
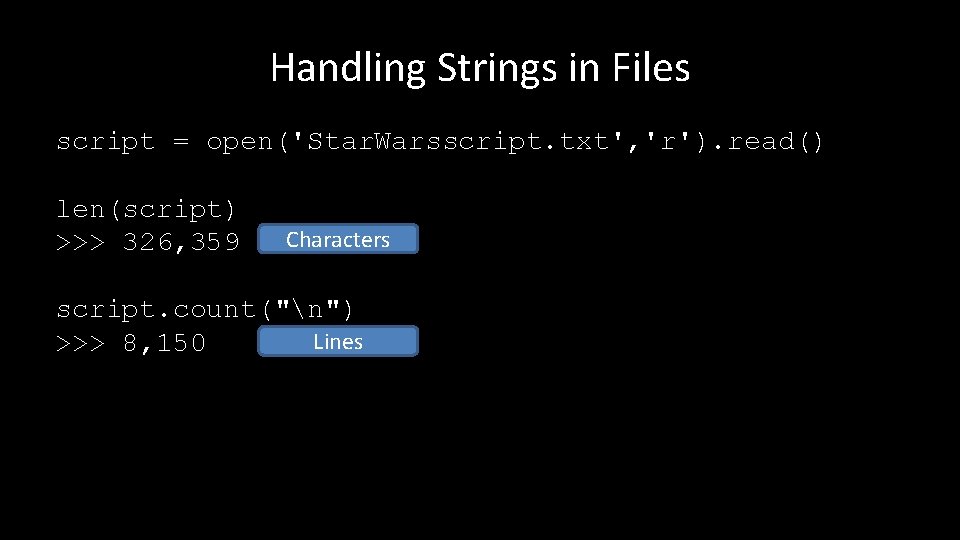
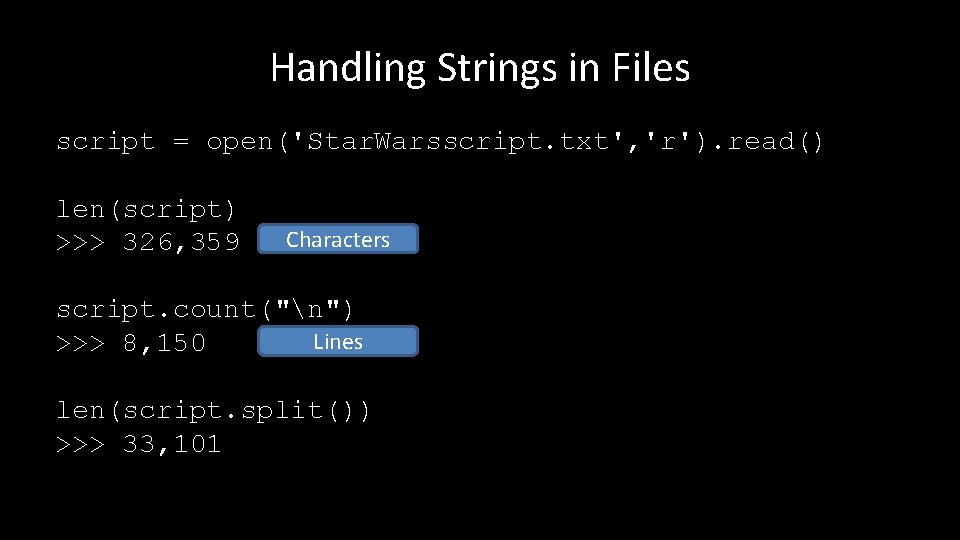
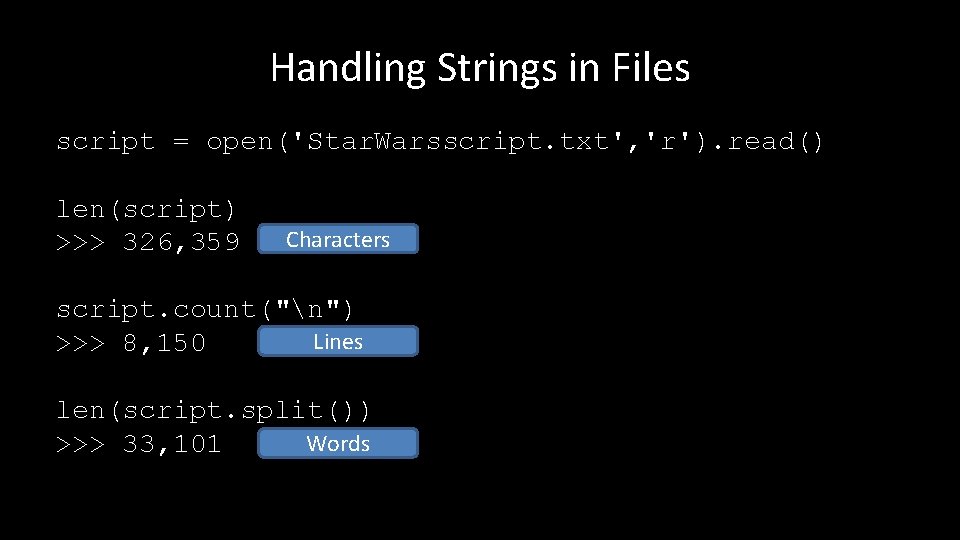
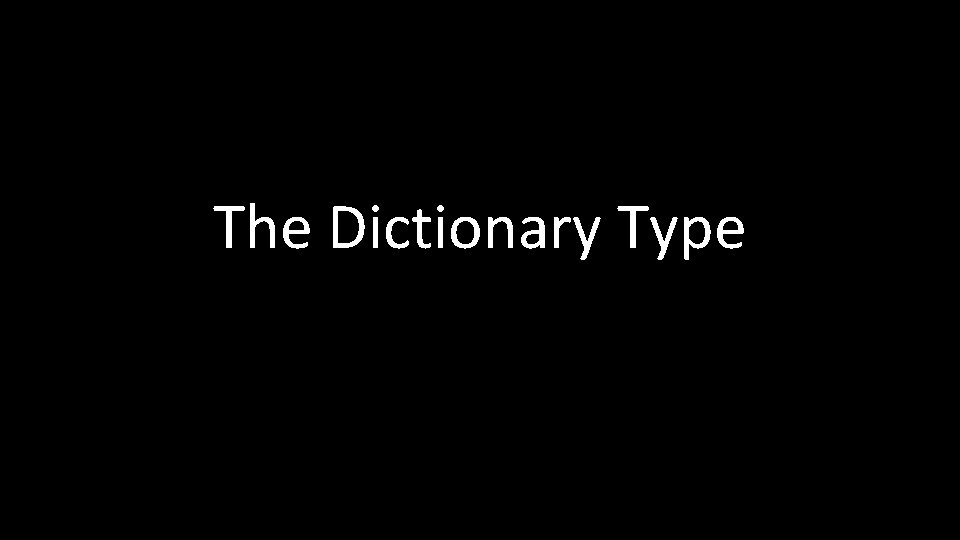
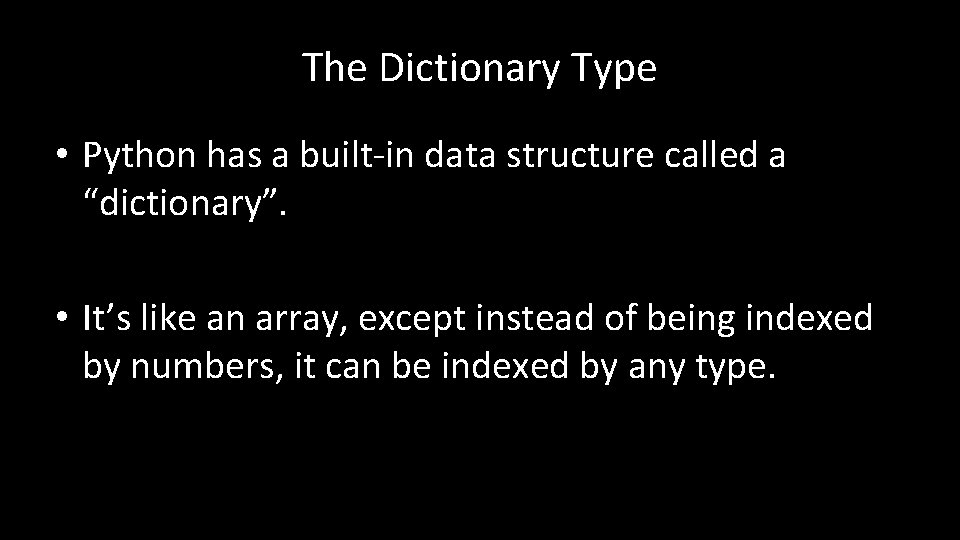
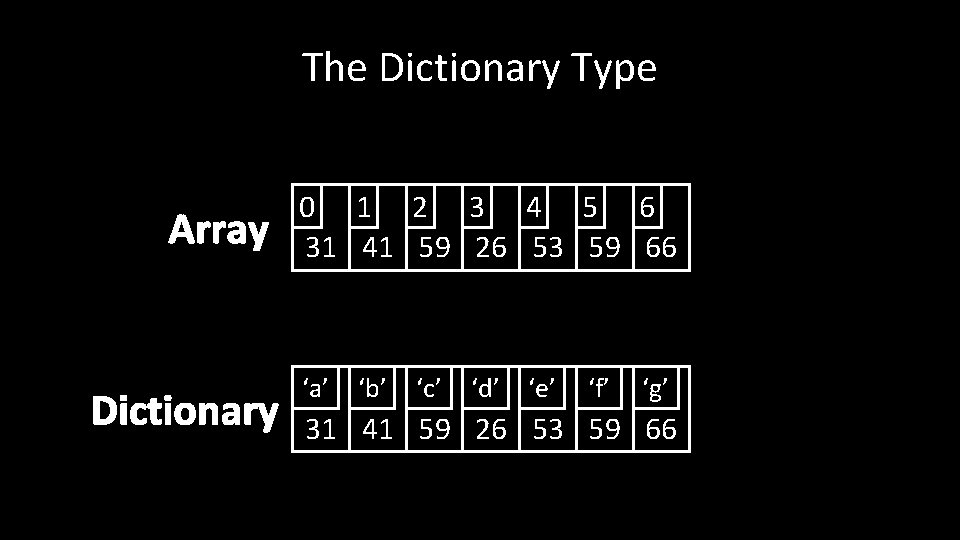
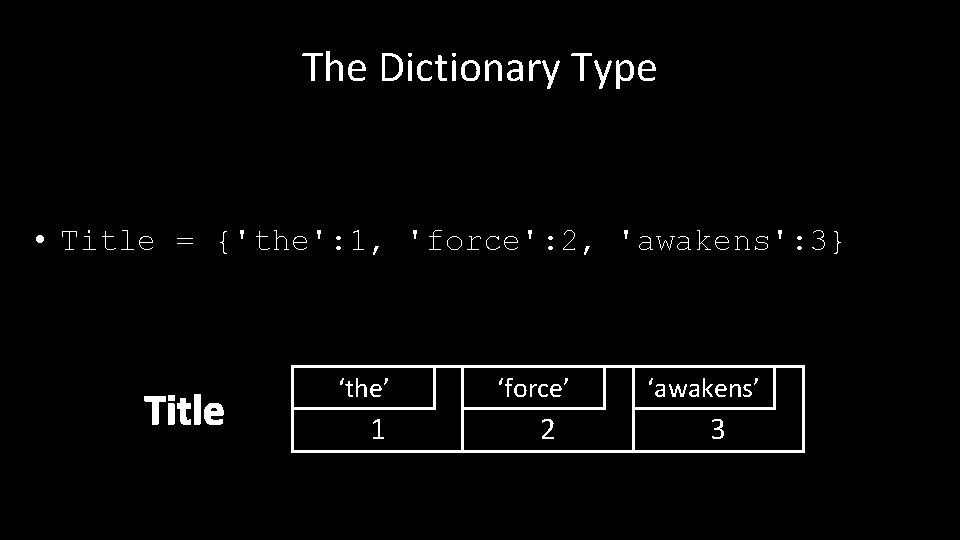
![The Dictionary Type • Title. keys() >>> dict_keys(['the', 'force', 'awakens']) Title ‘the’ 1 ‘force’ The Dictionary Type • Title. keys() >>> dict_keys(['the', 'force', 'awakens']) Title ‘the’ 1 ‘force’](https://slidetodoc.com/presentation_image_h2/64478e146d3074f94ebdedaded710bc8/image-48.jpg)
![The Dictionary Type Title['Star Wars'] = 0 print(Title) >>> {'Star Wars': 0, 'the': 1, The Dictionary Type Title['Star Wars'] = 0 print(Title) >>> {'Star Wars': 0, 'the': 1,](https://slidetodoc.com/presentation_image_h2/64478e146d3074f94ebdedaded710bc8/image-49.jpg)
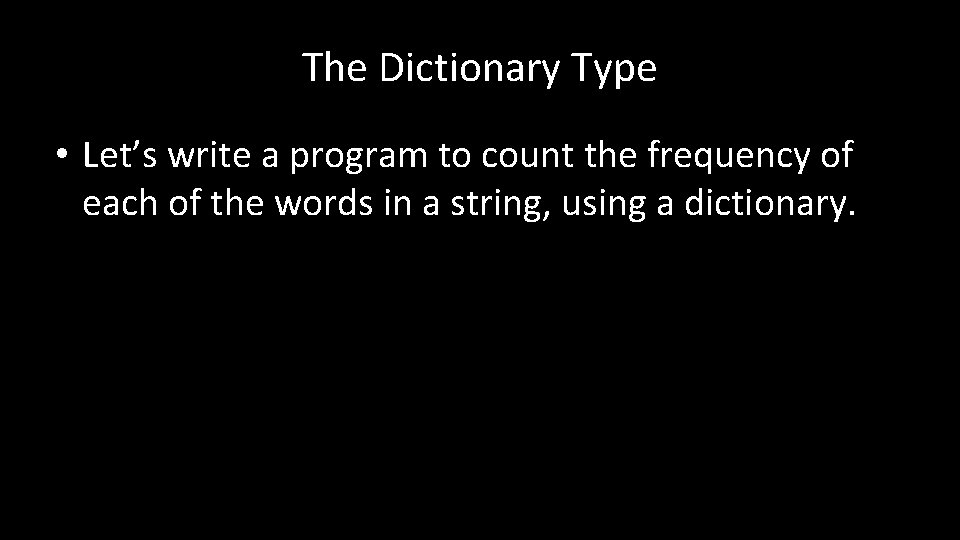
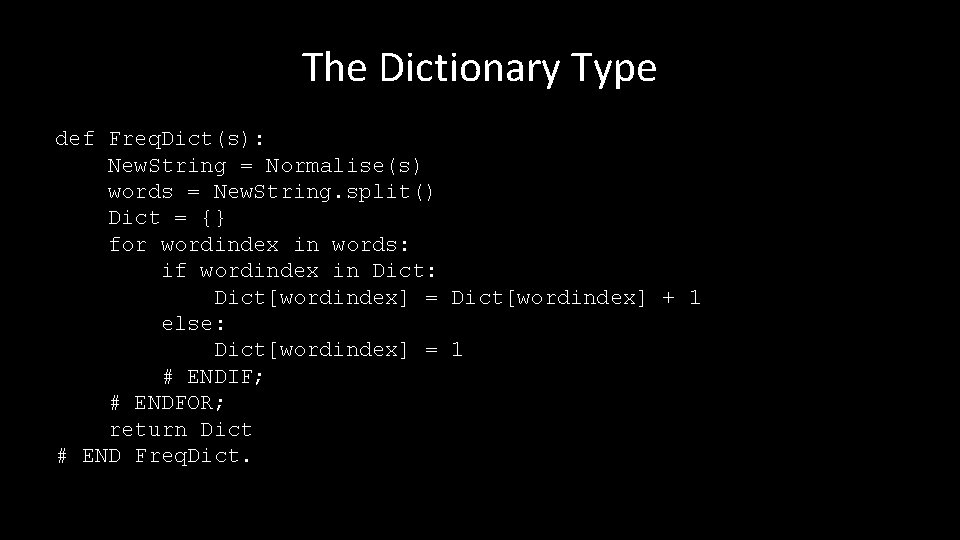
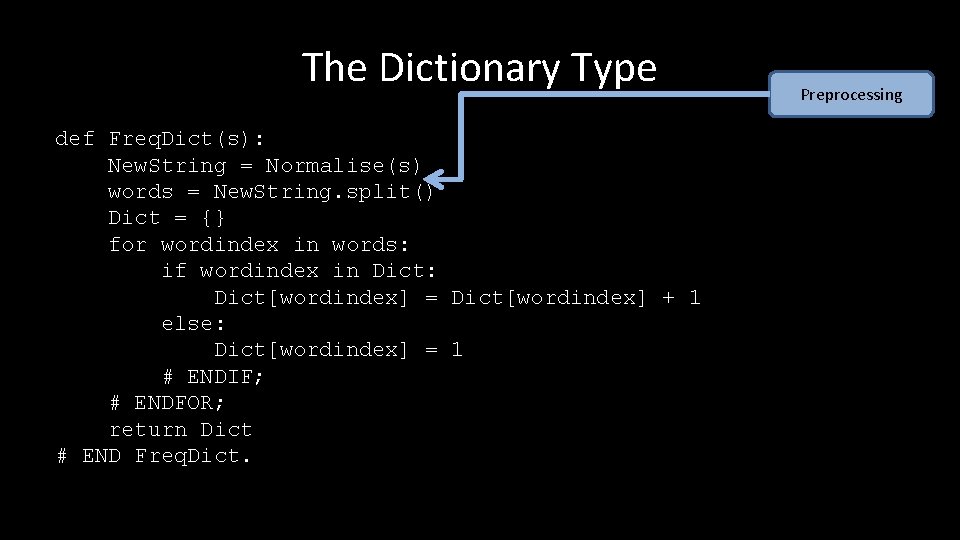
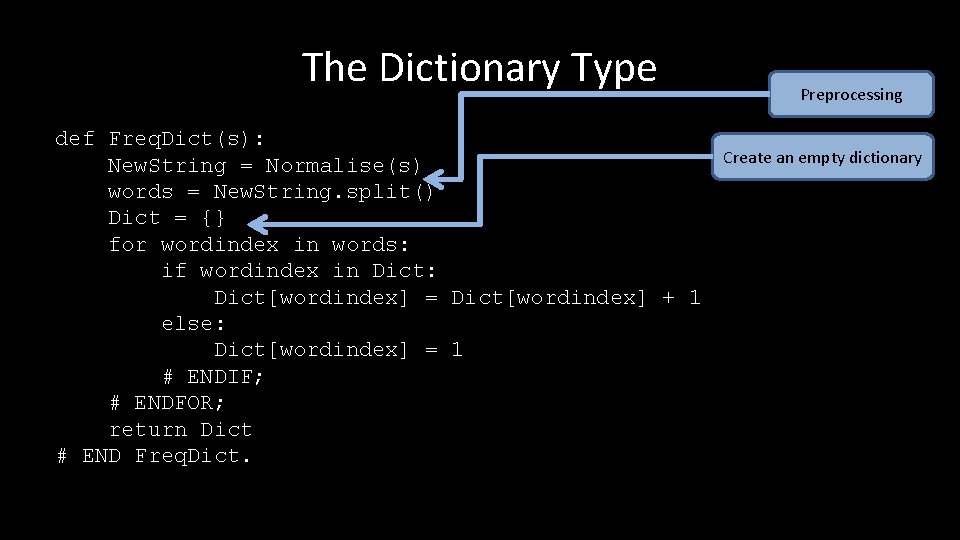
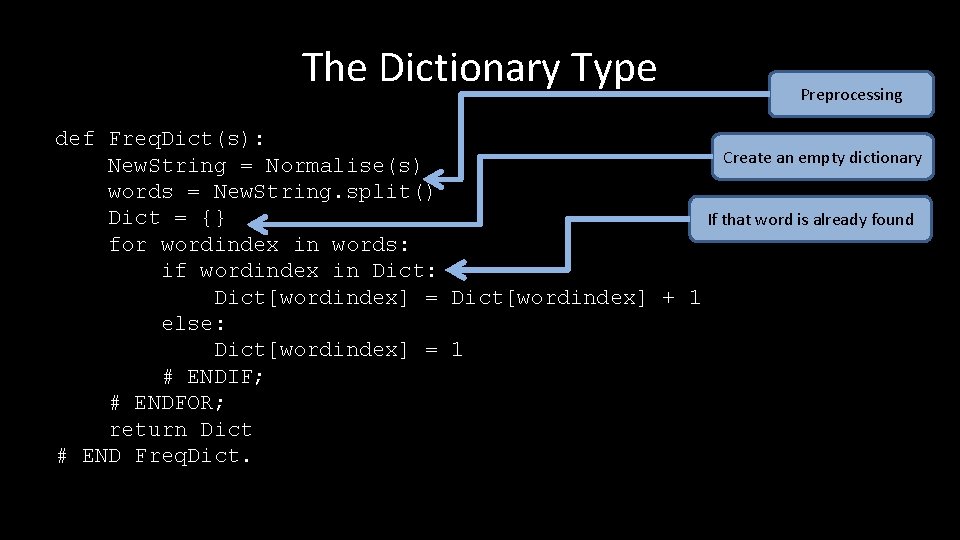
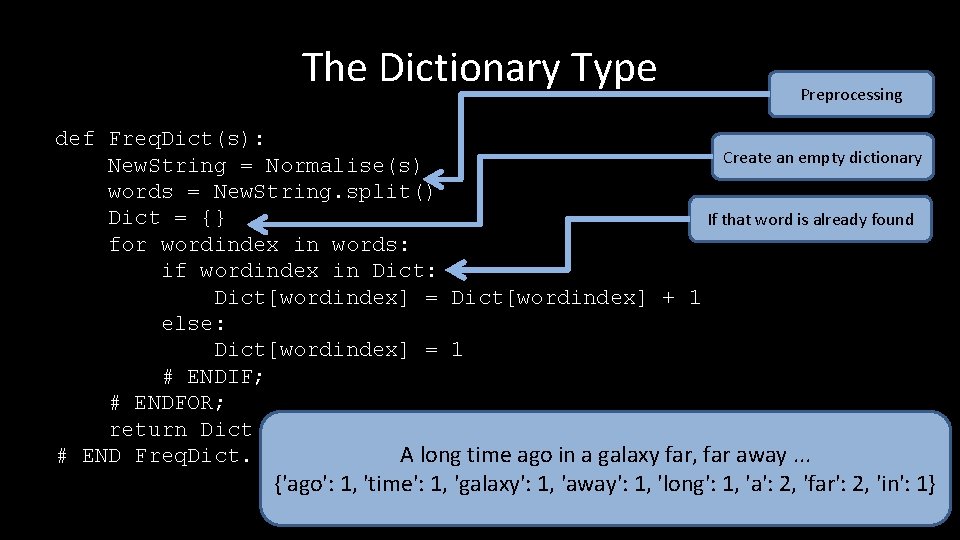
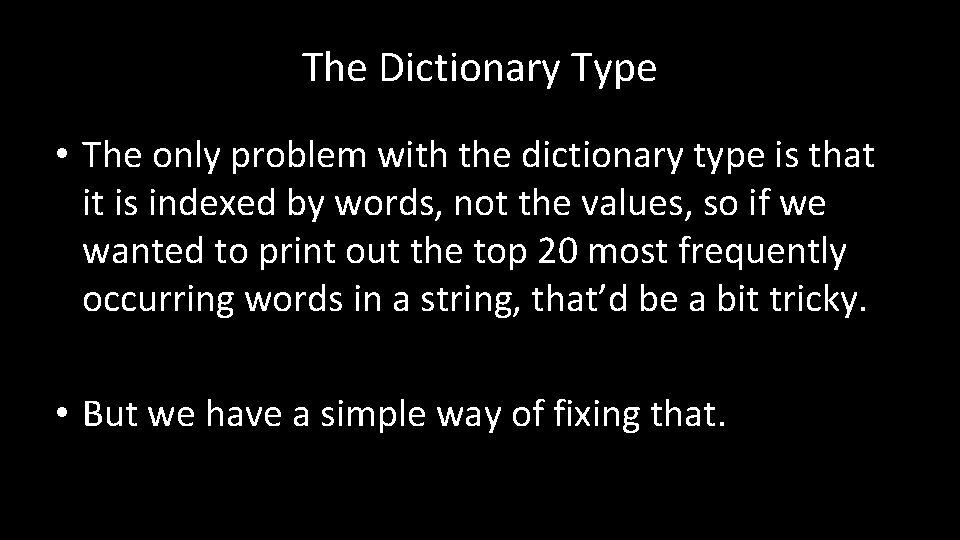
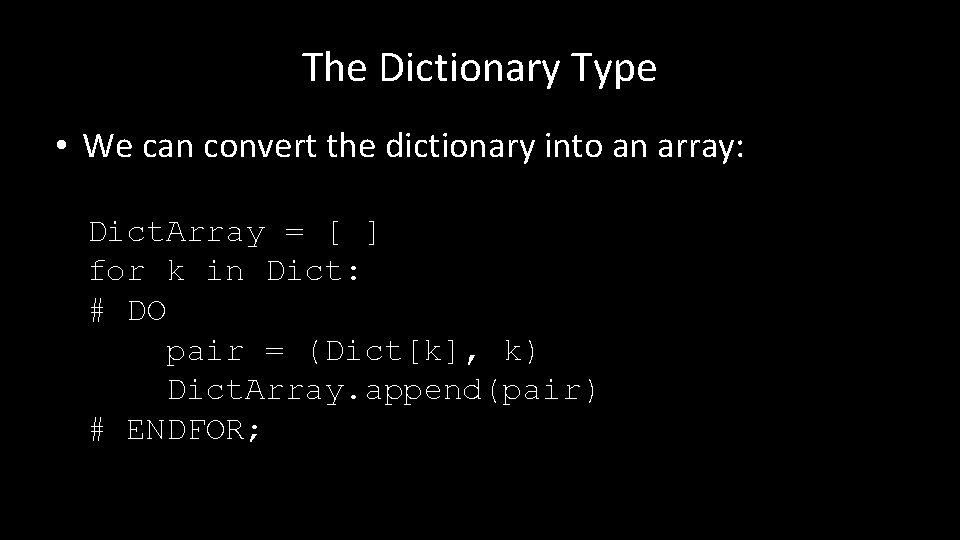
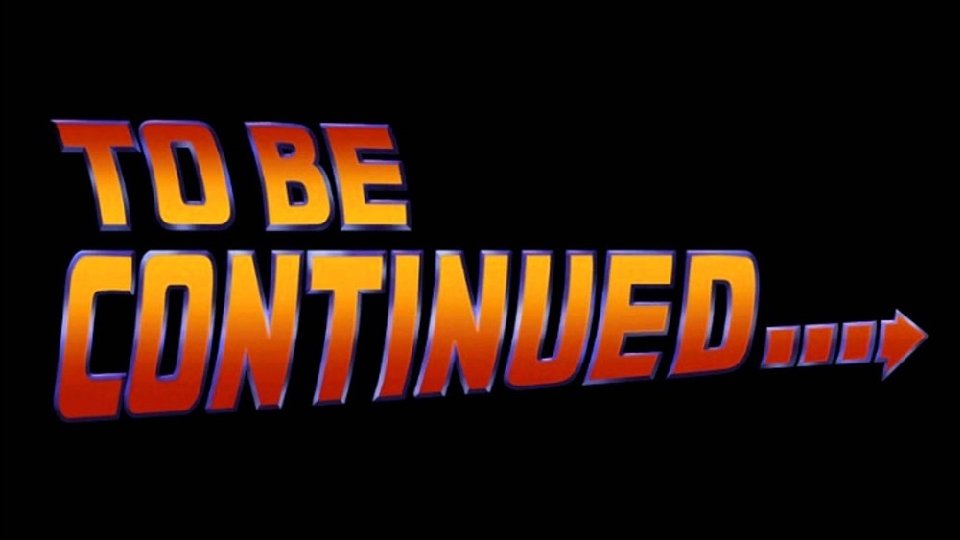
- Slides: 58
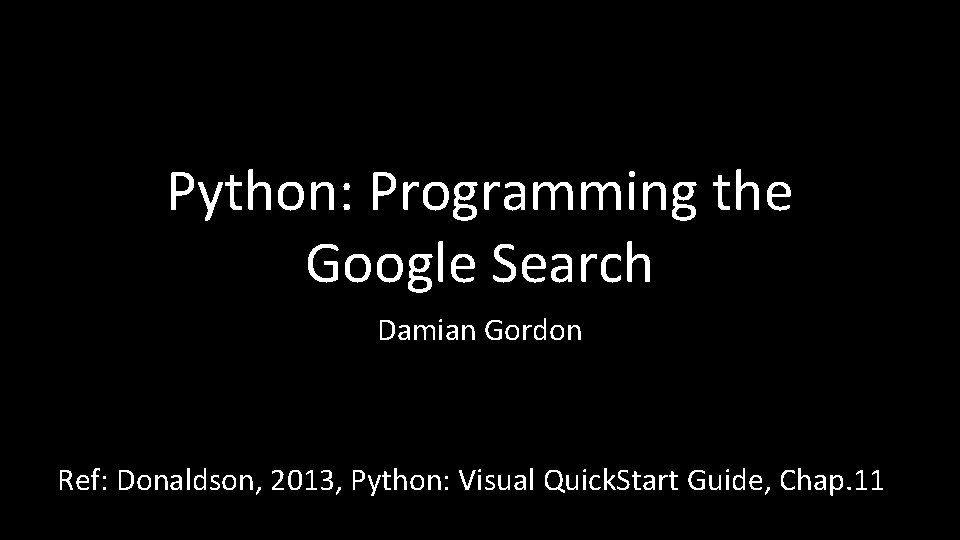
Python: Programming the Google Search Damian Gordon Ref: Donaldson, 2013, Python: Visual Quick. Start Guide, Chap. 11
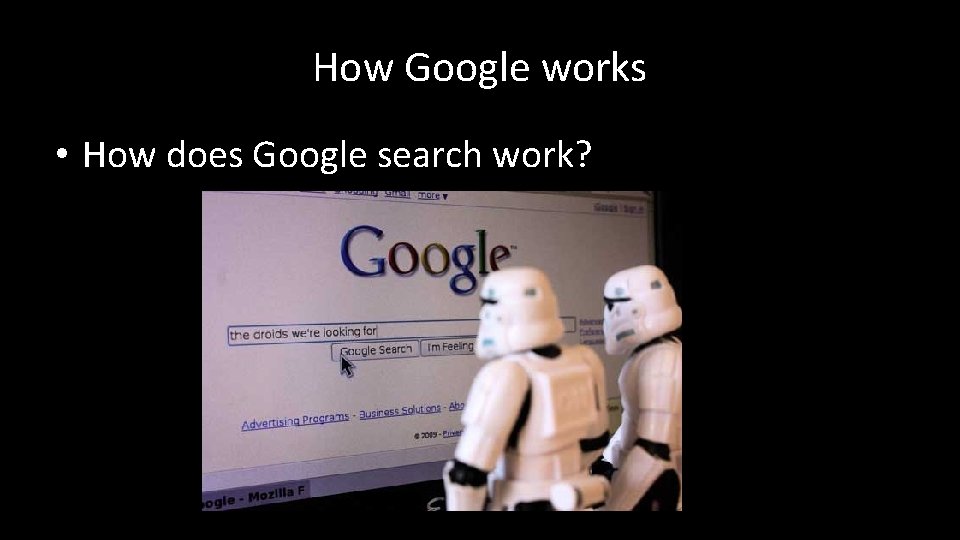
How Google works • How does Google search work?
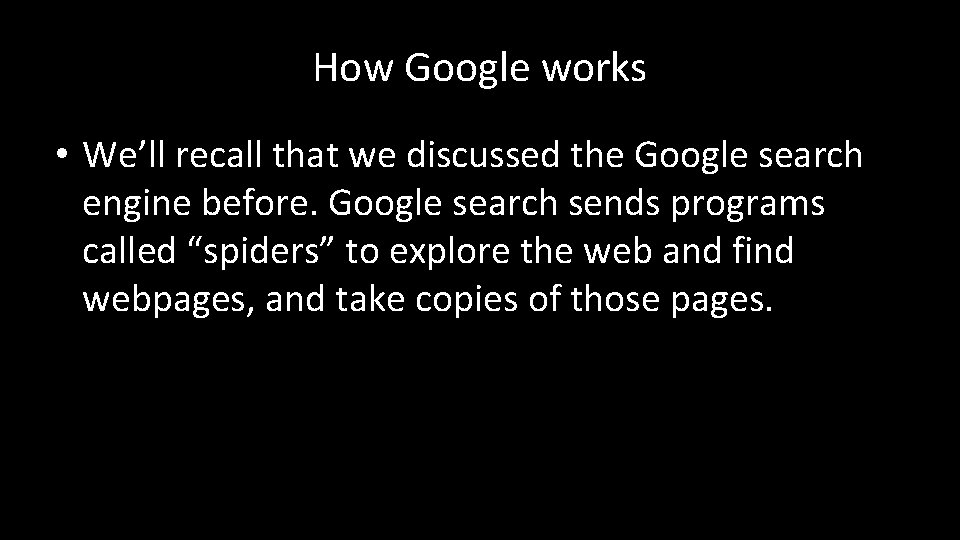
How Google works • We’ll recall that we discussed the Google search engine before. Google search sends programs called “spiders” to explore the web and find webpages, and take copies of those pages.
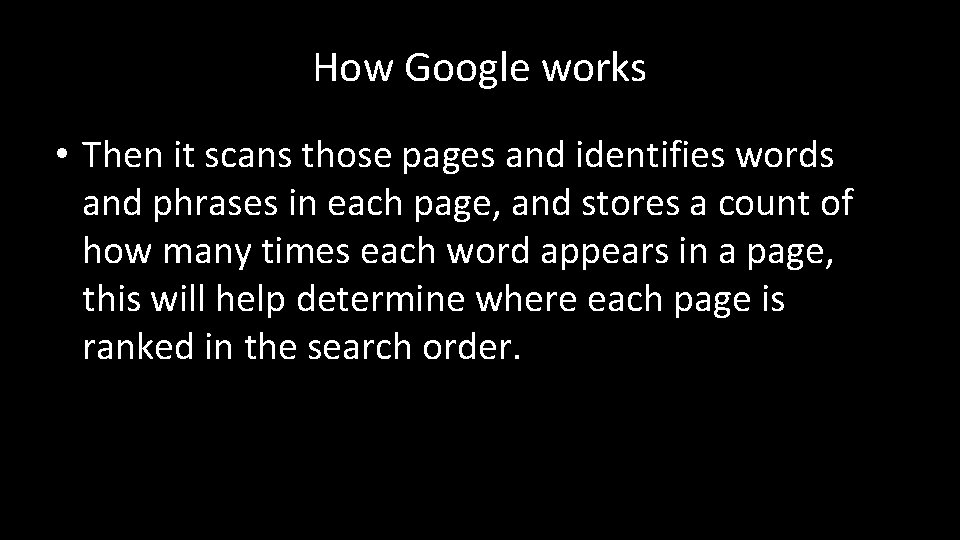
How Google works • Then it scans those pages and identifies words and phrases in each page, and stores a count of how many times each word appears in a page, this will help determine where each page is ranked in the search order.
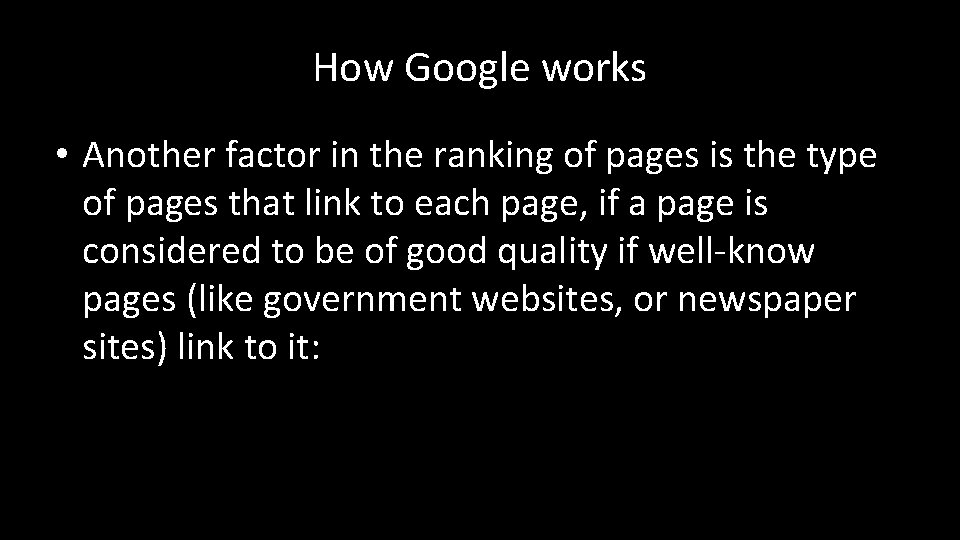
How Google works • Another factor in the ranking of pages is the type of pages that link to each page, if a page is considered to be of good quality if well-know pages (like government websites, or newspaper sites) link to it:
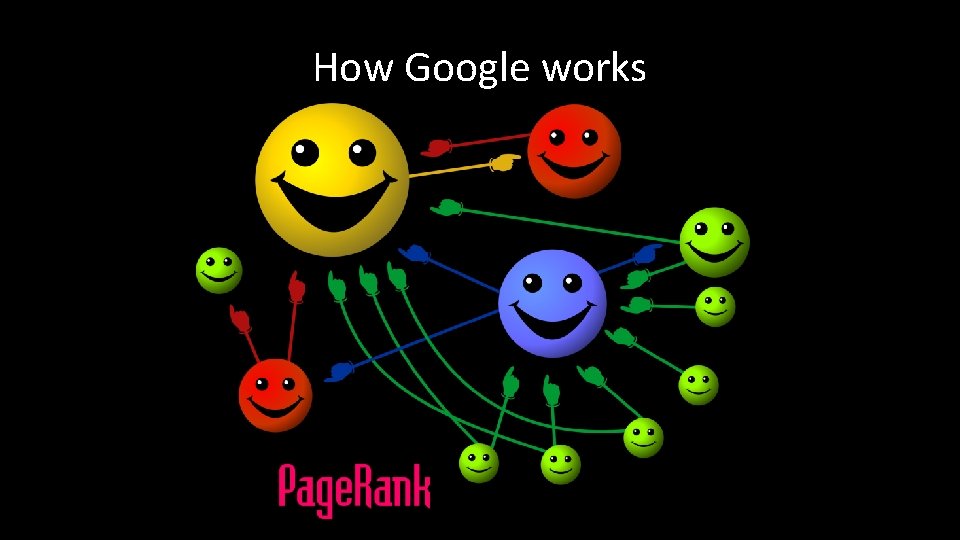
How Google works
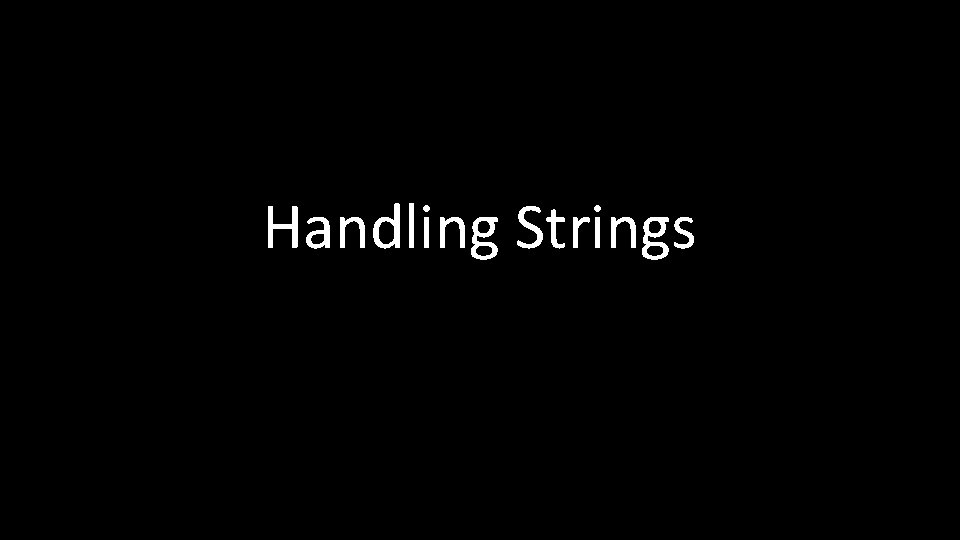
Handling Strings
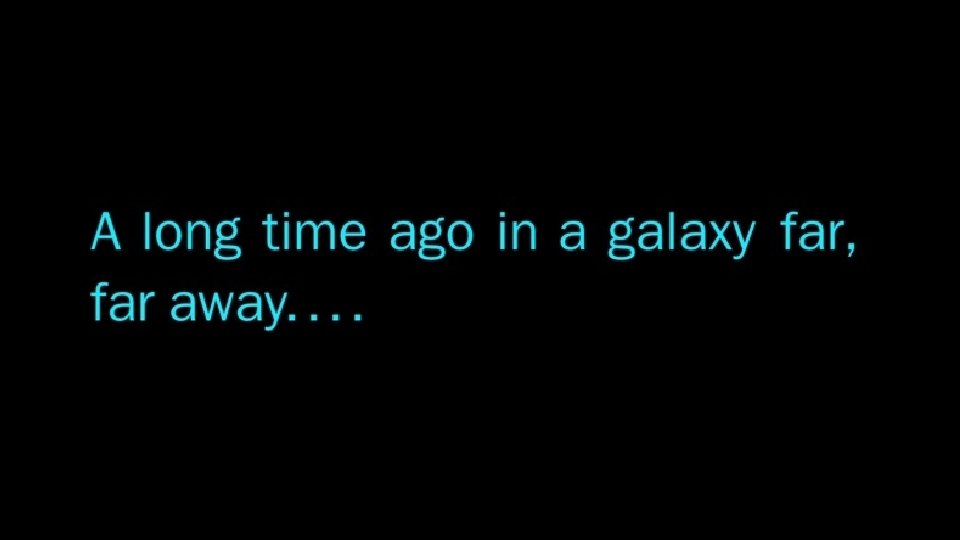
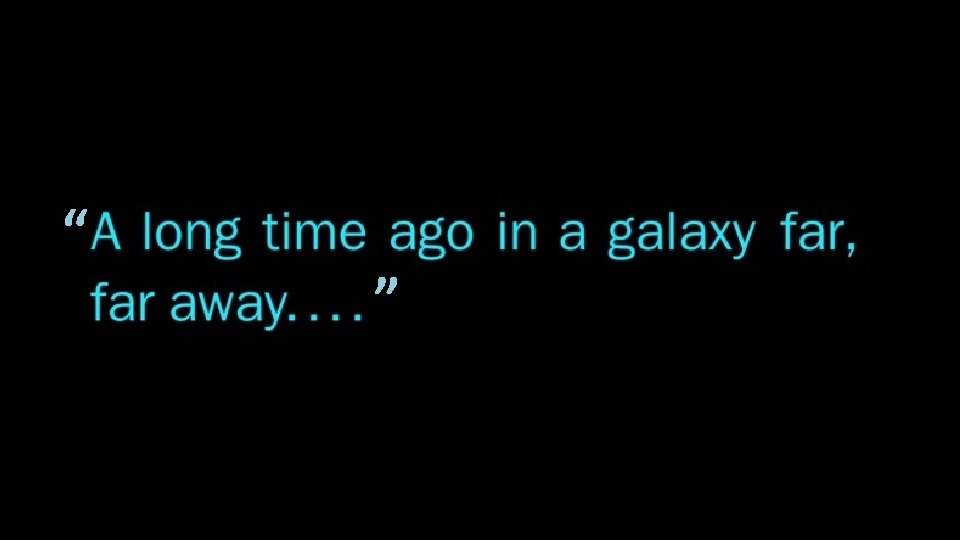
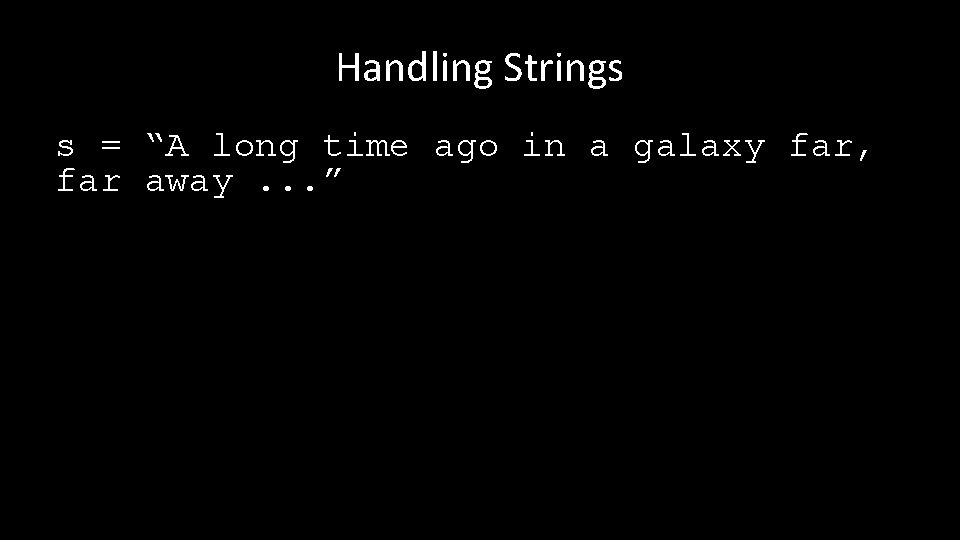
Handling Strings s = “A long time ago in a galaxy far, far away. . . ” s. split() ['a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far, ', 'far', 'away', '. . . ']
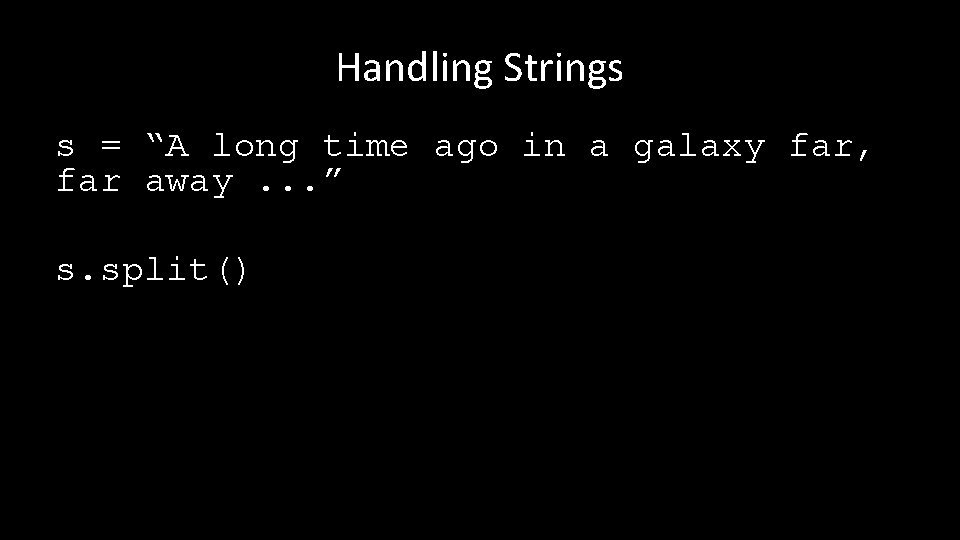
Handling Strings s = “A long time ago in a galaxy far, far away. . . ” s. split() ['a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far, ', 'far', 'away', '. . . ']
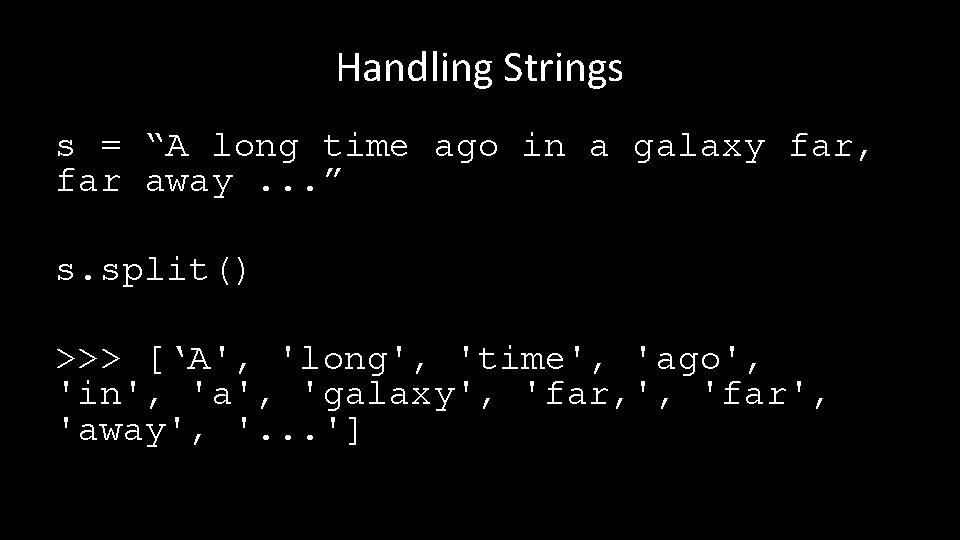
Handling Strings s = “A long time ago in a galaxy far, far away. . . ” s. split() >>> [‘A', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far, ', 'far', 'away', '. . . ']
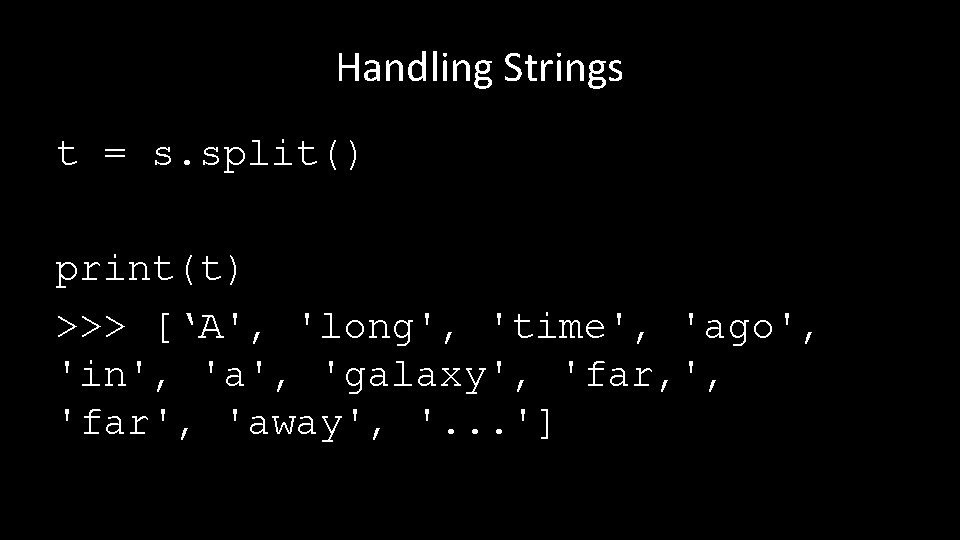
Handling Strings t = s. split() print(t) >>> [‘A', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far, ', 'far', 'away', '. . . ']
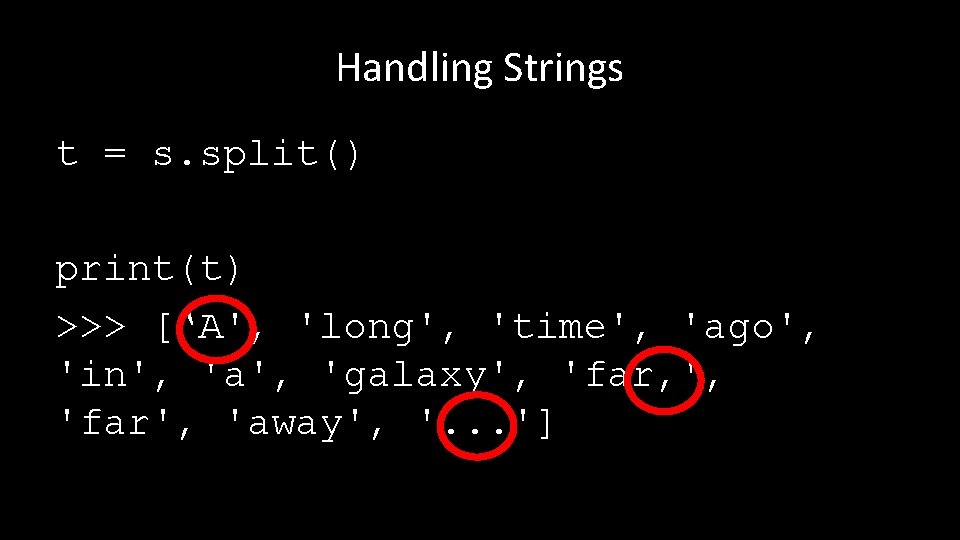
Handling Strings t = s. split() print(t) >>> [‘A', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far, ', 'far', 'away', '. . . ']
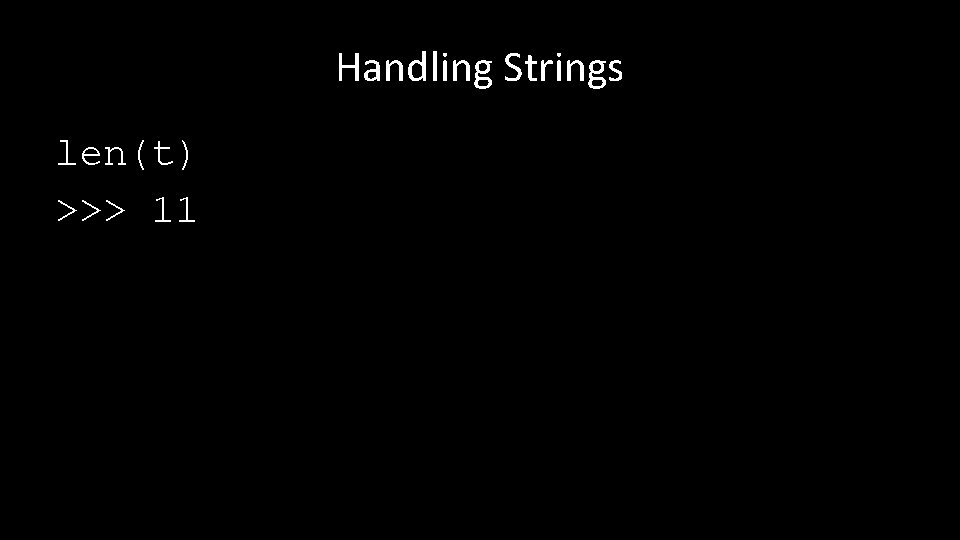
Handling Strings len(t) >>> 11
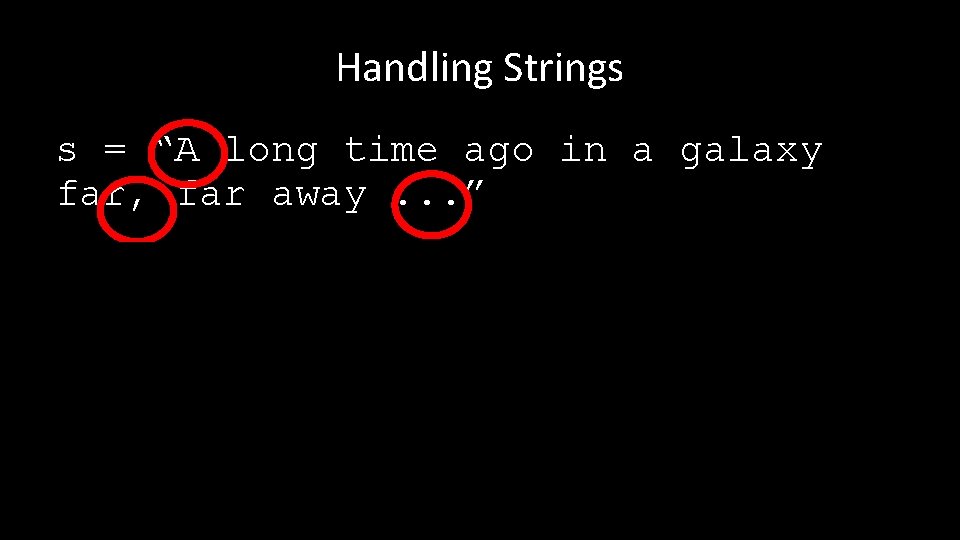
Handling Strings s = “A long time ago in a galaxy far, far away. . . ” Pre-processing s 2 = “a long time ago in a galaxy far away”
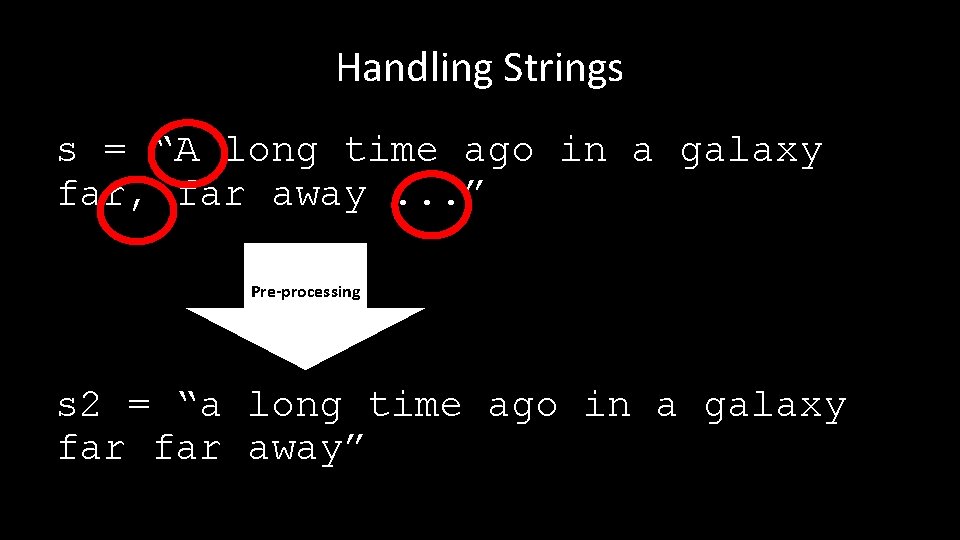
Handling Strings s = “A long time ago in a galaxy far, far away. . . ” Pre-processing s 2 = “a long time ago in a galaxy far away”
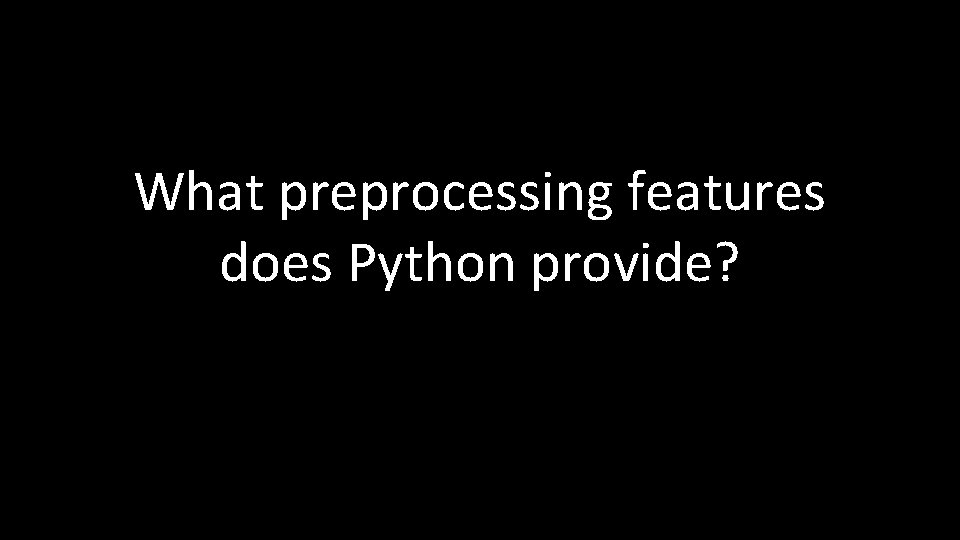
What preprocessing features does Python provide?
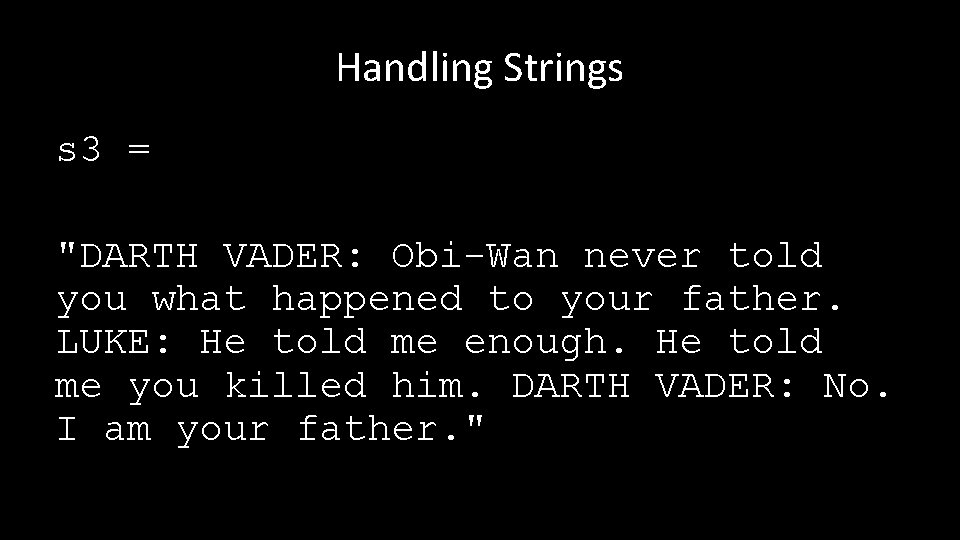
Handling Strings s 3 = "DARTH VADER: Obi-Wan never told you what happened to your father. LUKE: He told me enough. He told me you killed him. DARTH VADER: No. I am your father. "
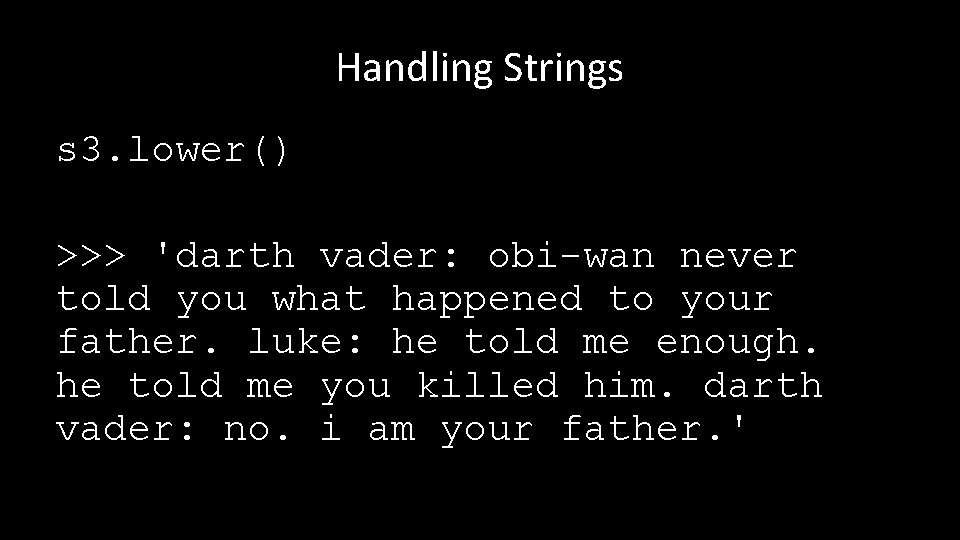
Handling Strings s 3. lower() >>> 'darth vader: obi-wan never told you what happened to your father. luke: he told me enough. he told me you killed him. darth vader: no. i am your father. '
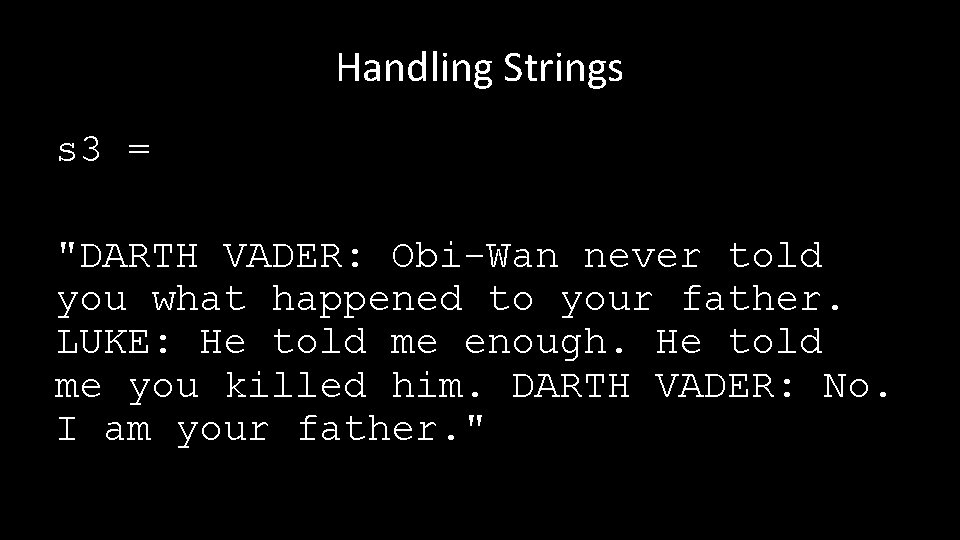
Handling Strings s 3 = "DARTH VADER: Obi-Wan never told you what happened to your father. LUKE: He told me enough. He told me you killed him. DARTH VADER: No. I am your father. "
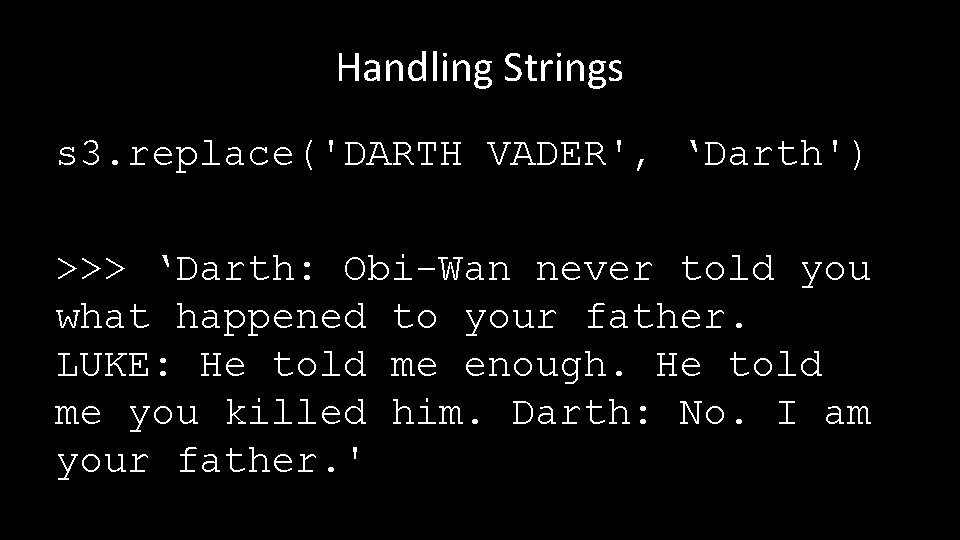
Handling Strings s 3. replace('DARTH VADER', ‘Darth') >>> ‘Darth: Obi-Wan never told you what happened to your father. LUKE: He told me enough. He told me you killed him. Darth: No. I am your father. '
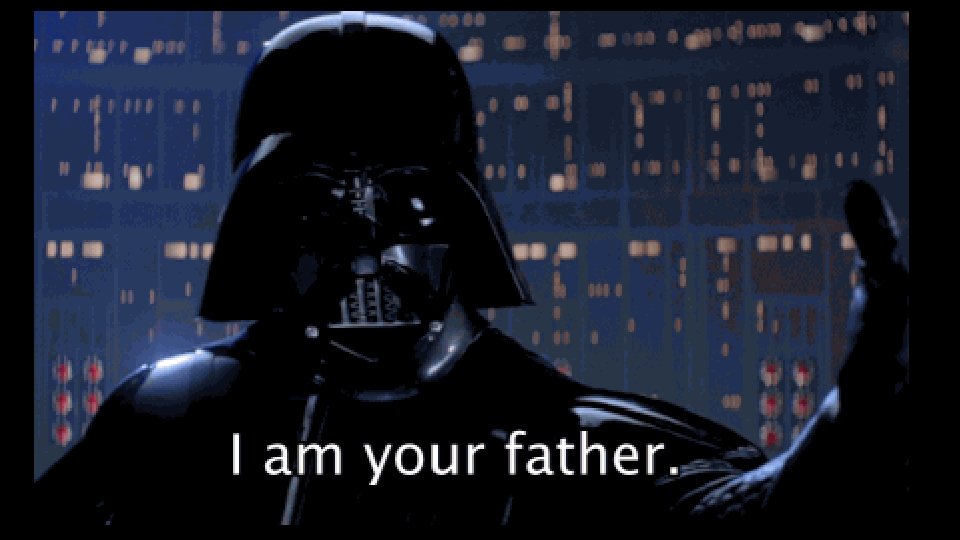
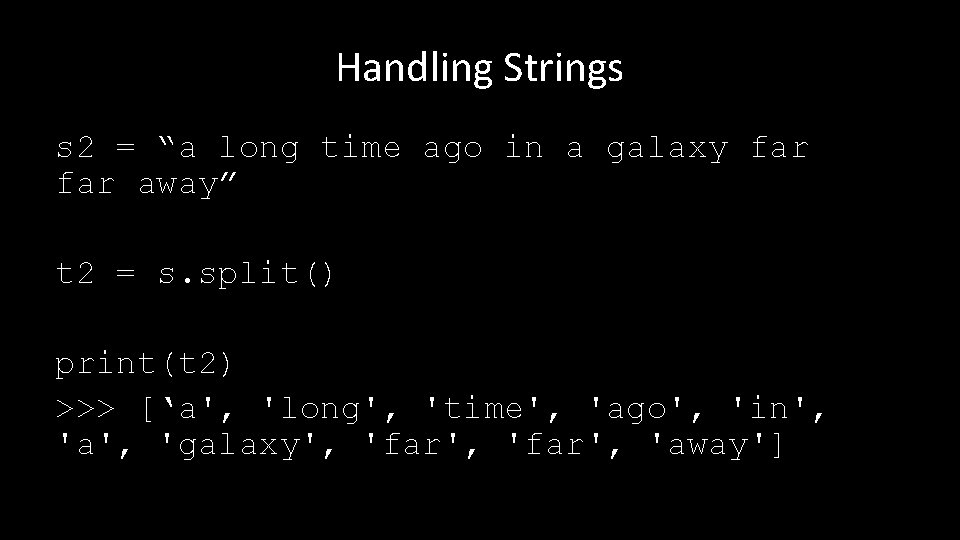
Handling Strings s 2 = “a long time ago in a galaxy far away” t 2 = s. split() print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away']
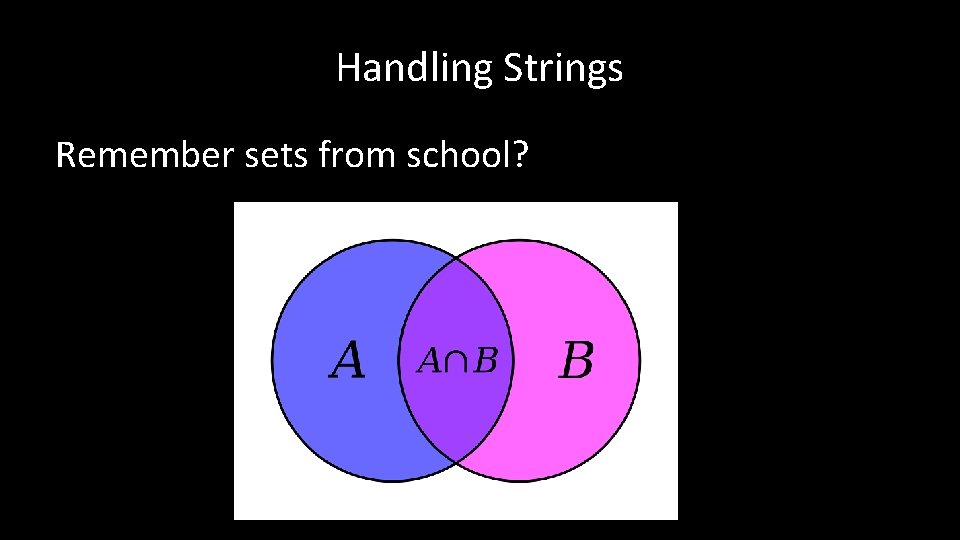
Handling Strings Remember sets from school?
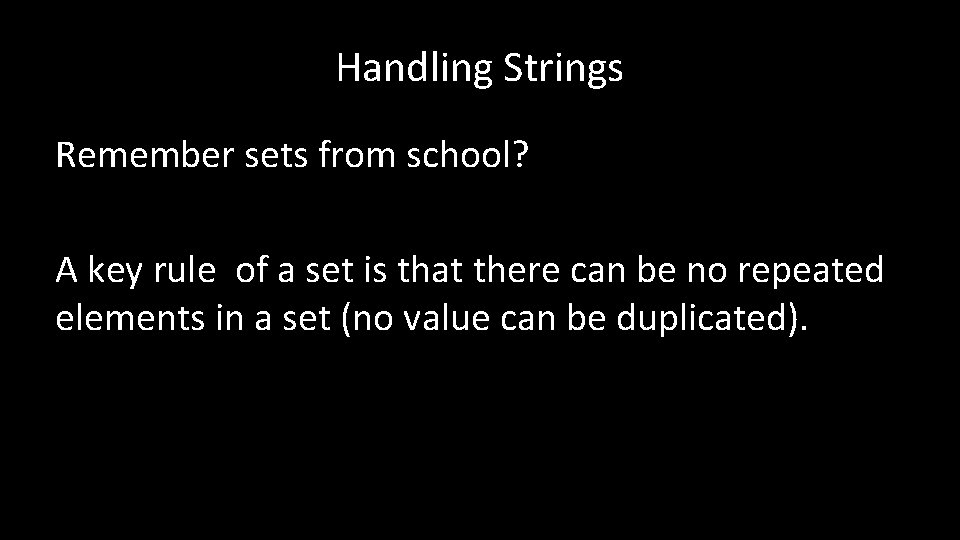
Handling Strings Remember sets from school? A key rule of a set is that there can be no repeated elements in a set (no value can be duplicated).
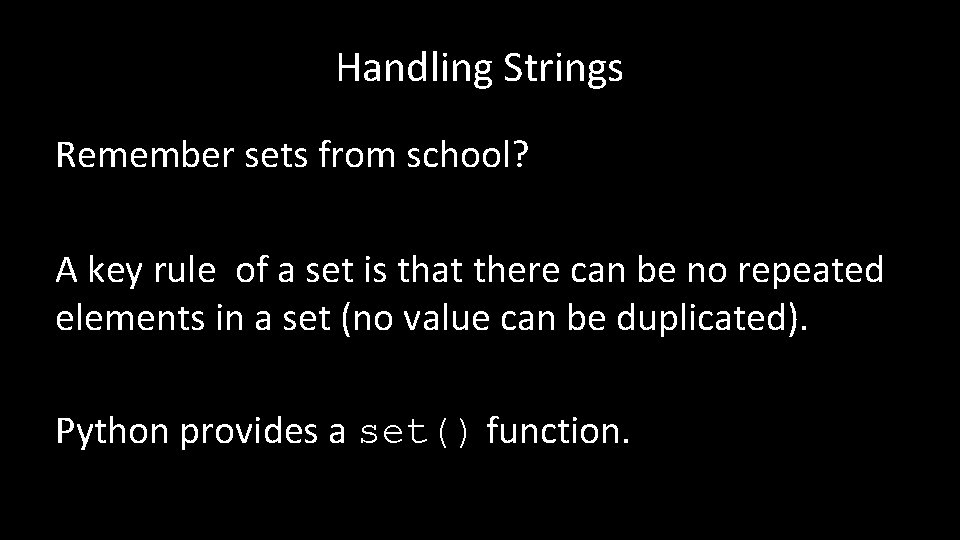
Handling Strings Remember sets from school? A key rule of a set is that there can be no repeated elements in a set (no value can be duplicated). Python provides a set() function.
![Handling Strings printt 2 a long time ago in a galaxy far away Handling Strings print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away']](https://slidetodoc.com/presentation_image_h2/64478e146d3074f94ebdedaded710bc8/image-28.jpg)
Handling Strings print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away'] u 2 =set(t 2) Print(u 2) {'away', 'long', 'far', 'ago', 'galaxy', 'in', 'a', 'time'}
![Handling Strings printt 2 a long time ago in a galaxy far away Handling Strings print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away']](https://slidetodoc.com/presentation_image_h2/64478e146d3074f94ebdedaded710bc8/image-29.jpg)
Handling Strings print(t 2) >>> [‘a', 'long', 'time', 'ago', 'in', 'a', 'galaxy', 'far', 'away'] u 2 =set(t 2) Print(u 2) >>> {'away', 'long', 'far', 'ago', 'galaxy', 'in', 'a', 'time'}
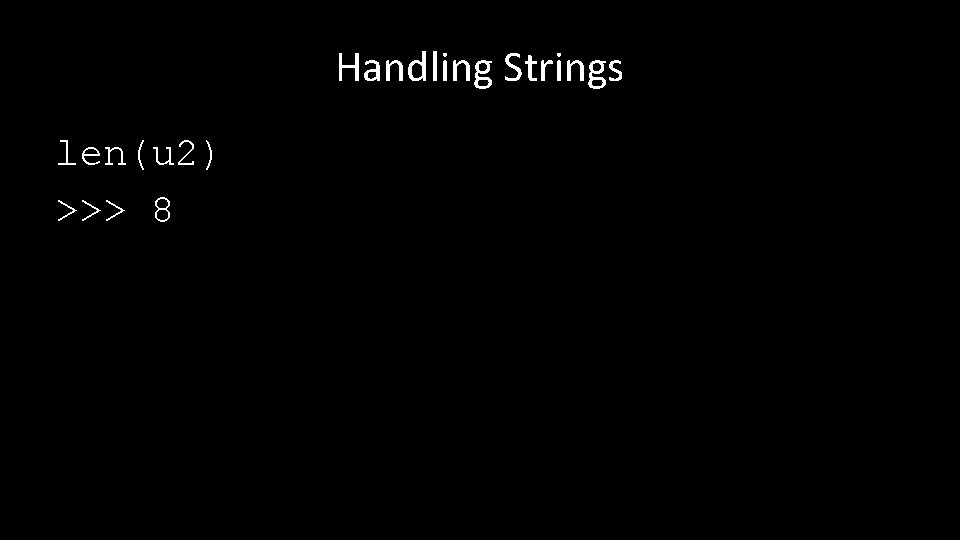
Handling Strings len(u 2) >>> 8
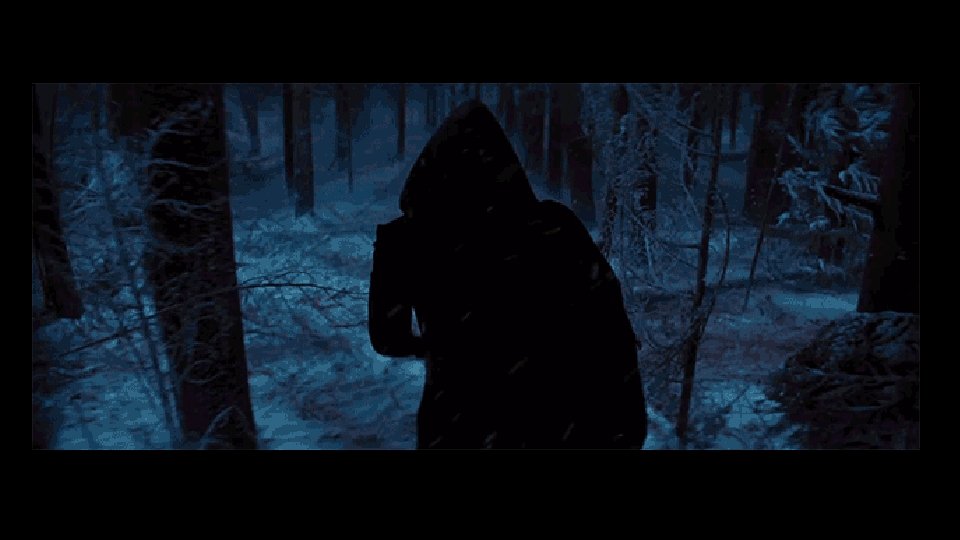
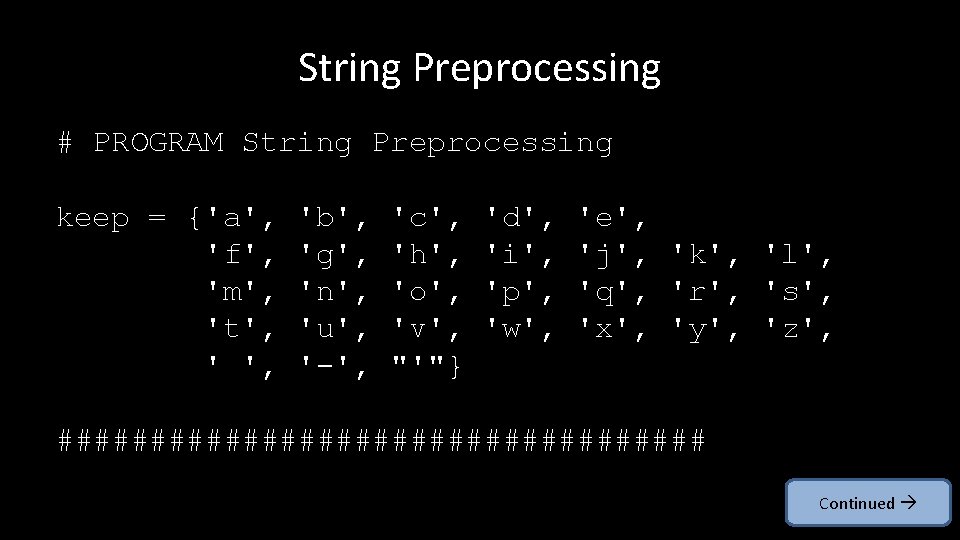
String Preprocessing # PROGRAM String Preprocessing keep = {'a', 'f', 'm', 't', ' ', 'b', 'g', 'n', 'u', '-', 'c', 'h', 'o', 'v', "'"} 'd', 'i', 'p', 'w', 'e', 'j', 'k', 'l', 'q', 'r', 's', 'x', 'y', 'z', ################## Continued
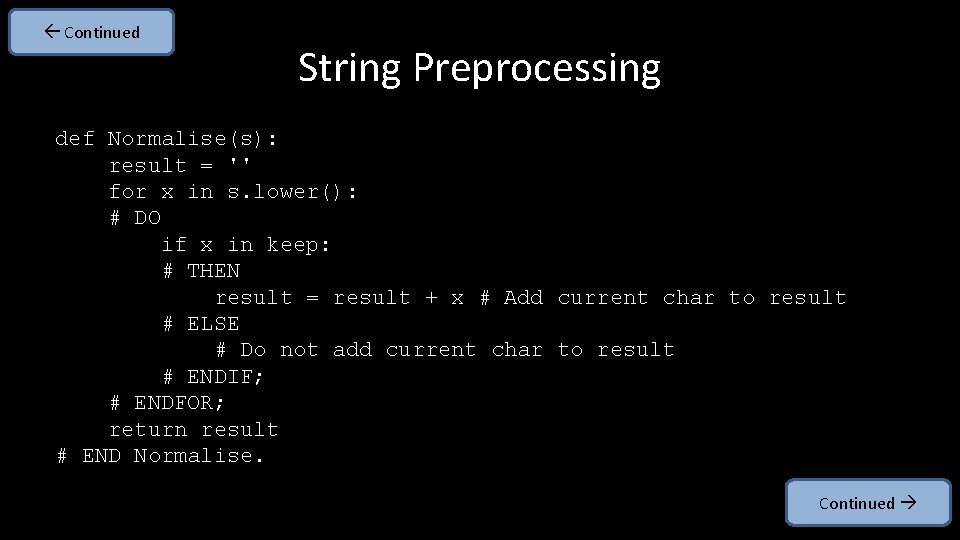
Continued String Preprocessing def Normalise(s): result = '' for x in s. lower(): # DO if x in keep: # THEN result = result + x # Add current char to result # ELSE # Do not add current char to result # ENDIF; # ENDFOR; return result # END Normalise. Continued
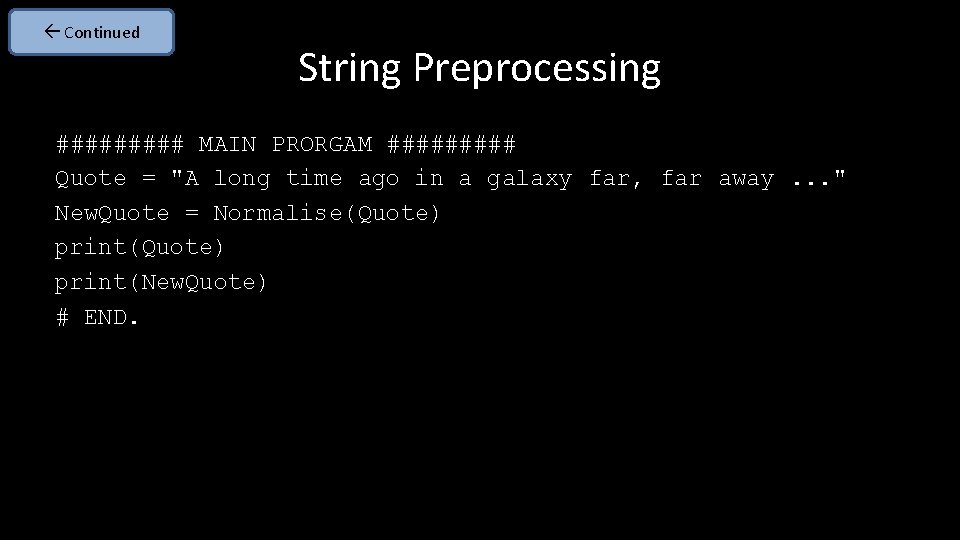
Continued String Preprocessing ##### MAIN PRORGAM ##### Quote = "A long time ago in a galaxy far, far away. . . " New. Quote = Normalise(Quote) print(New. Quote) # END.
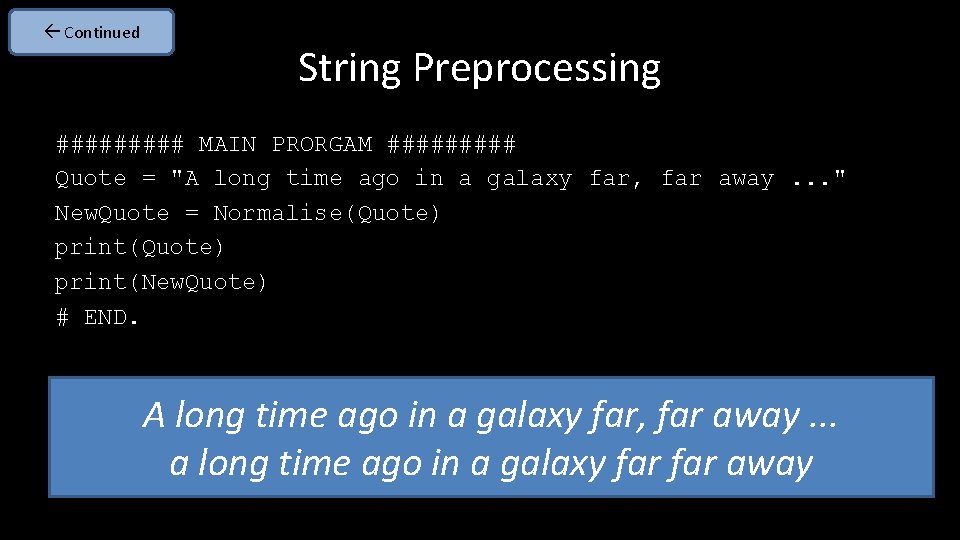
Continued String Preprocessing ##### MAIN PRORGAM ##### Quote = "A long time ago in a galaxy far, far away. . . " New. Quote = Normalise(Quote) print(New. Quote) # END. A long time ago in a galaxy far, far away. . . a long time ago in a galaxy far away
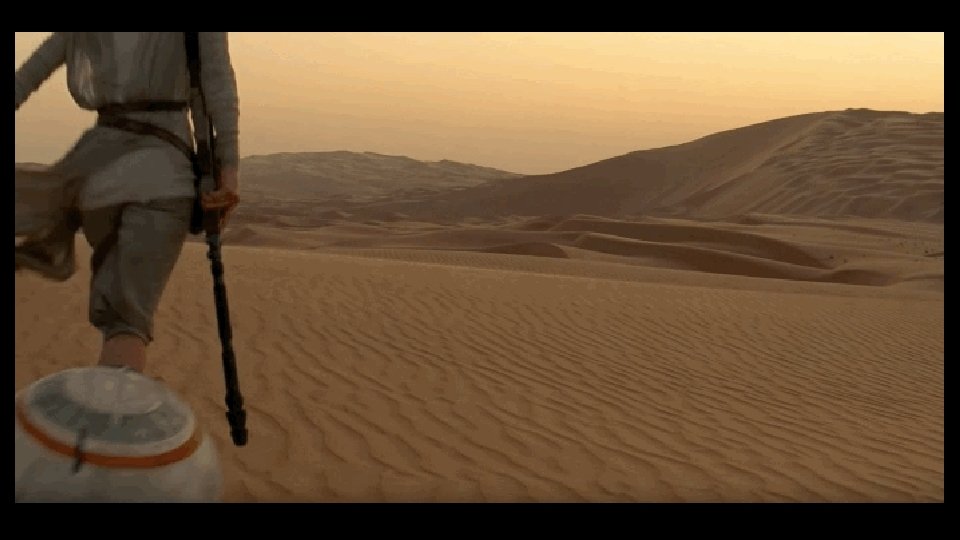
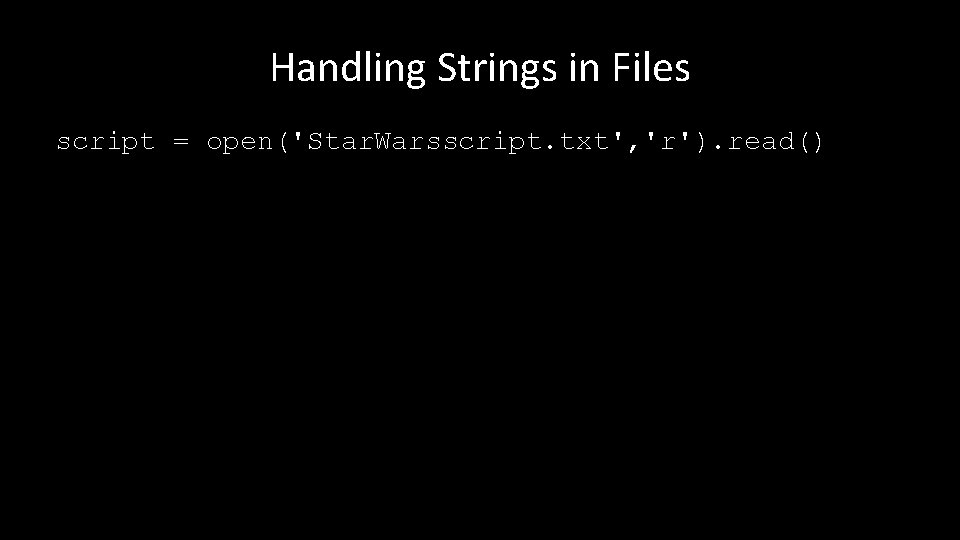
Handling Strings in Files script = open('Star. Warsscript. txt', 'r'). read() len(script) 326, 359 script. count("n") 8, 150 len(script. split()) 33, 101
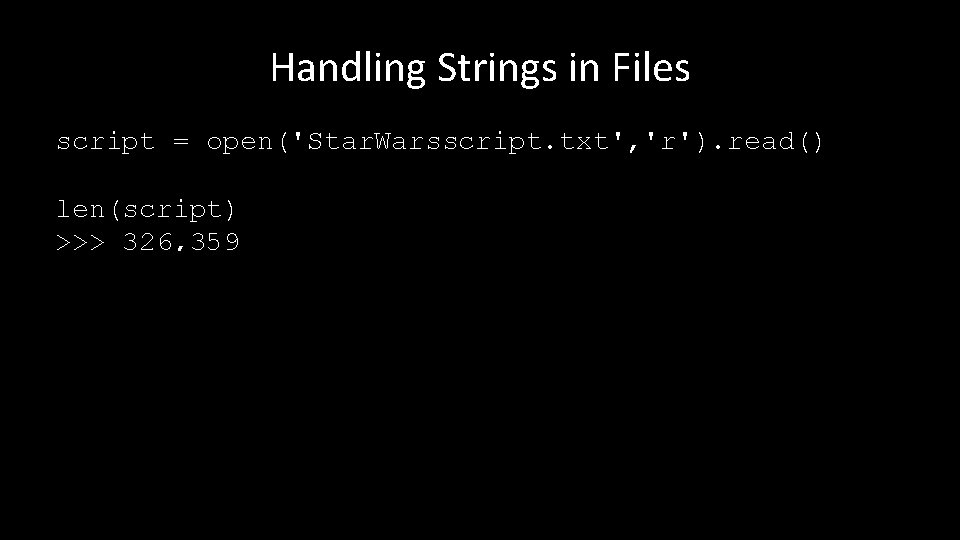
Handling Strings in Files script = open('Star. Warsscript. txt', 'r'). read() len(script) >>> 326, 359 script. count("n") 8, 150 len(script. split()) 33, 101
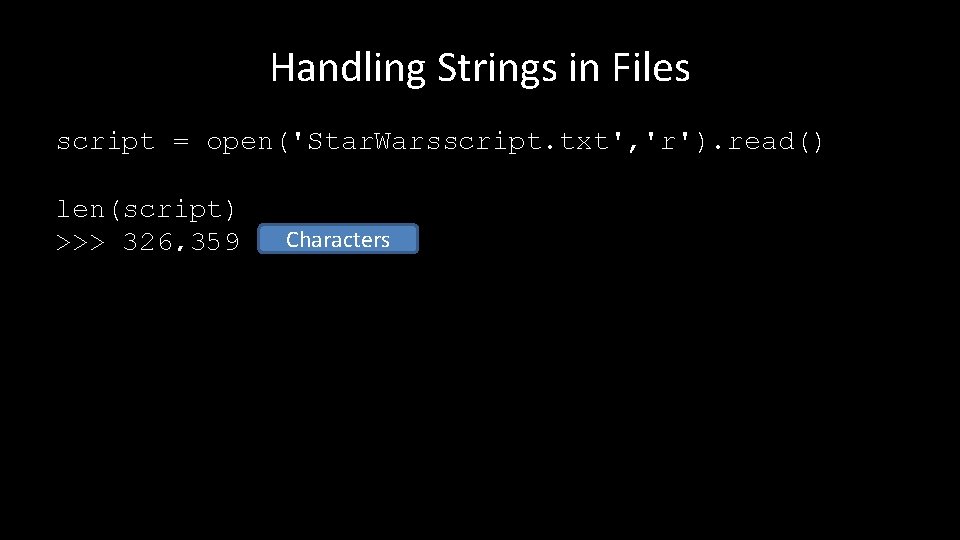
Handling Strings in Files script = open('Star. Warsscript. txt', 'r'). read() len(script) >>> 326, 359 Characters script. count("n") 8, 150 len(script. split()) 33, 101
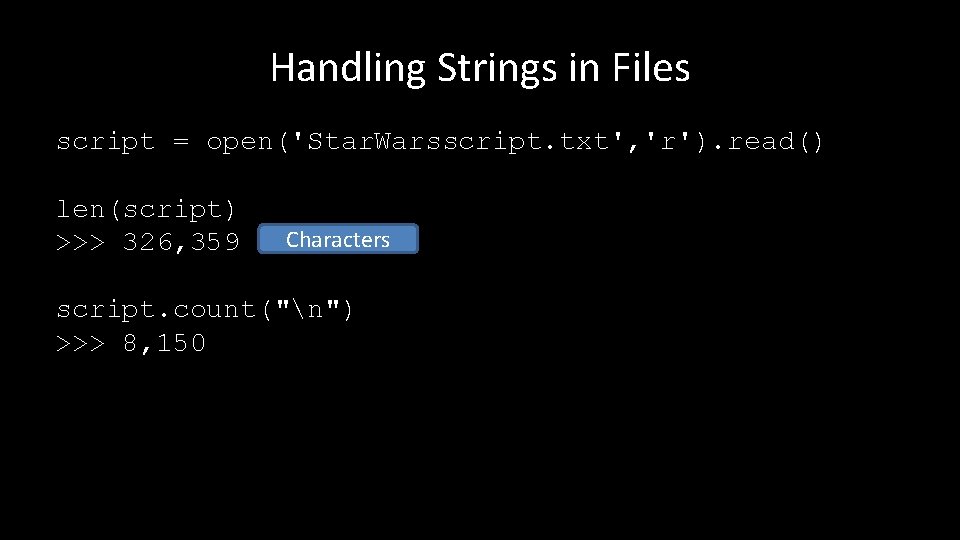
Handling Strings in Files script = open('Star. Warsscript. txt', 'r'). read() len(script) >>> 326, 359 Characters script. count("n") >>> 8, 150 len(script. split()) 33, 101
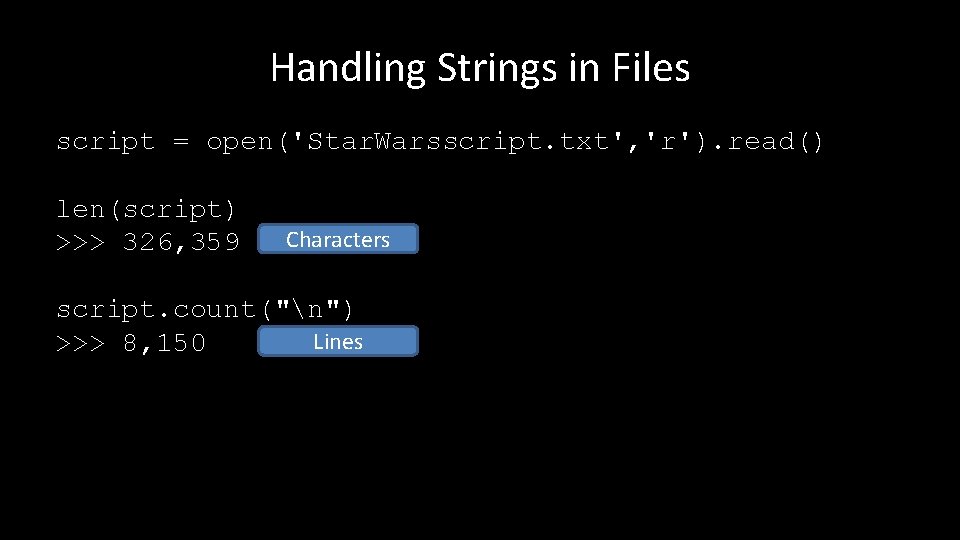
Handling Strings in Files script = open('Star. Warsscript. txt', 'r'). read() len(script) >>> 326, 359 Characters script. count("n") Lines >>> 8, 150 len(script. split()) 33, 101
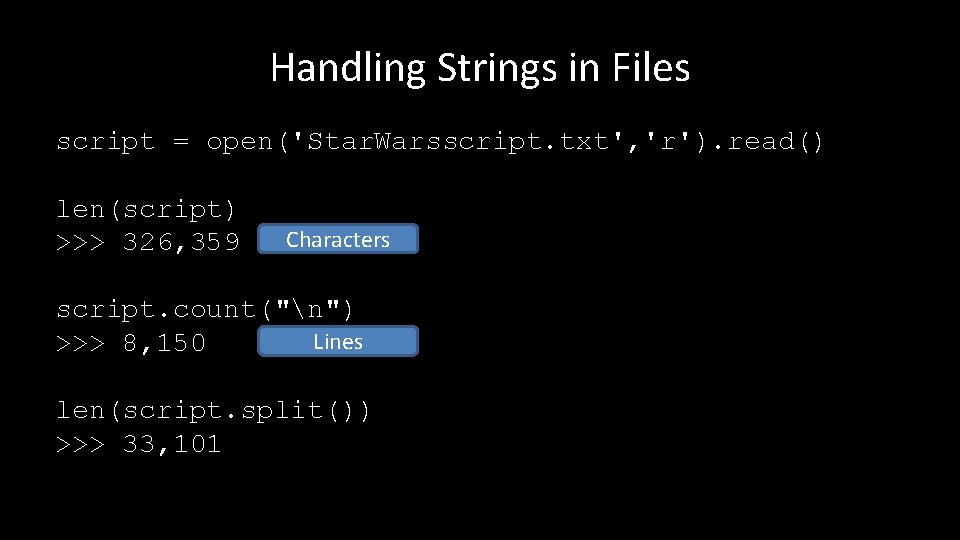
Handling Strings in Files script = open('Star. Warsscript. txt', 'r'). read() len(script) >>> 326, 359 Characters script. count("n") Lines >>> 8, 150 len(script. split()) >>> 33, 101
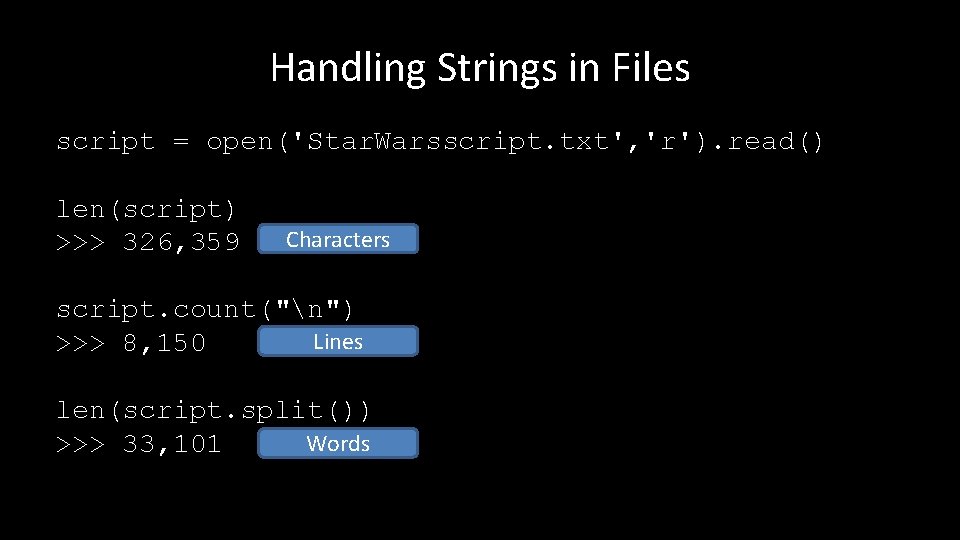
Handling Strings in Files script = open('Star. Warsscript. txt', 'r'). read() len(script) >>> 326, 359 Characters script. count("n") Lines >>> 8, 150 len(script. split()) Words >>> 33, 101
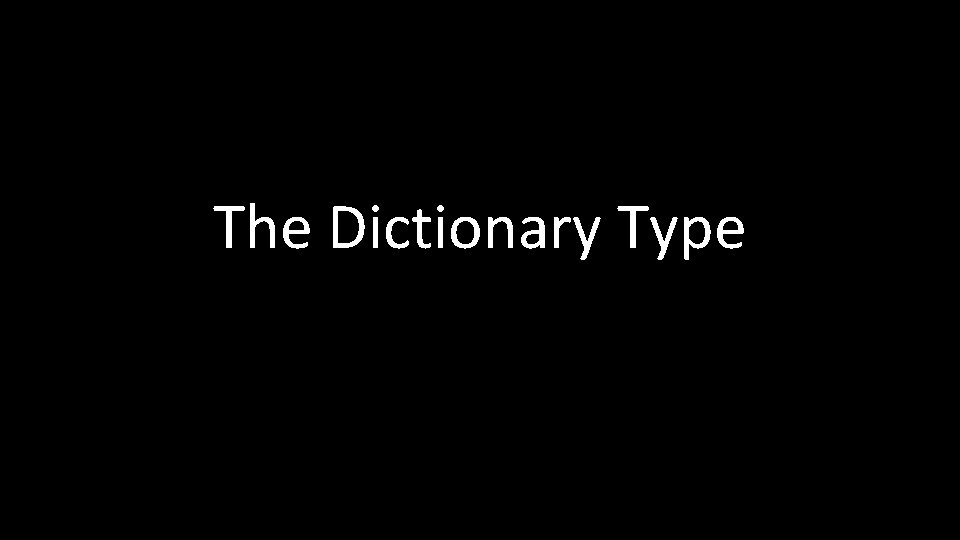
The Dictionary Type
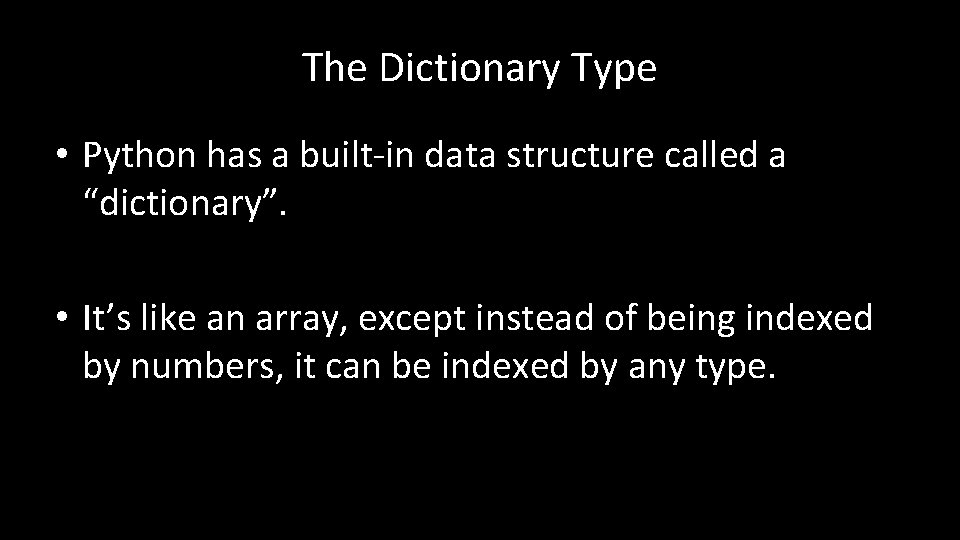
The Dictionary Type • Python has a built-in data structure called a “dictionary”. • It’s like an array, except instead of being indexed by numbers, it can be indexed by any type.
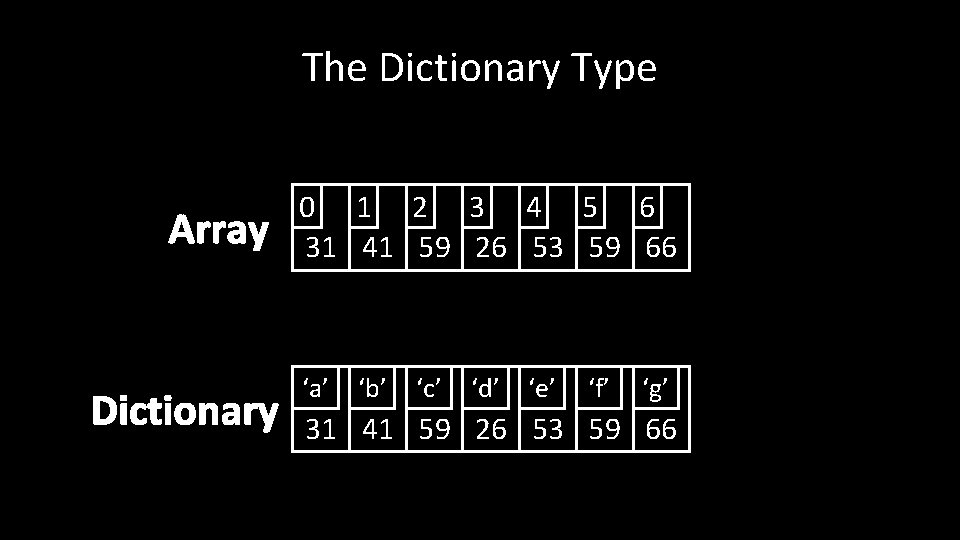
The Dictionary Type Array Dictionary 0 1 2 3 4 5 6 31 41 59 26 53 59 66 ‘a’ ‘b’ ‘c’ ‘d’ ‘e’ ‘f’ ‘g’ 31 41 59 26 53 59 66
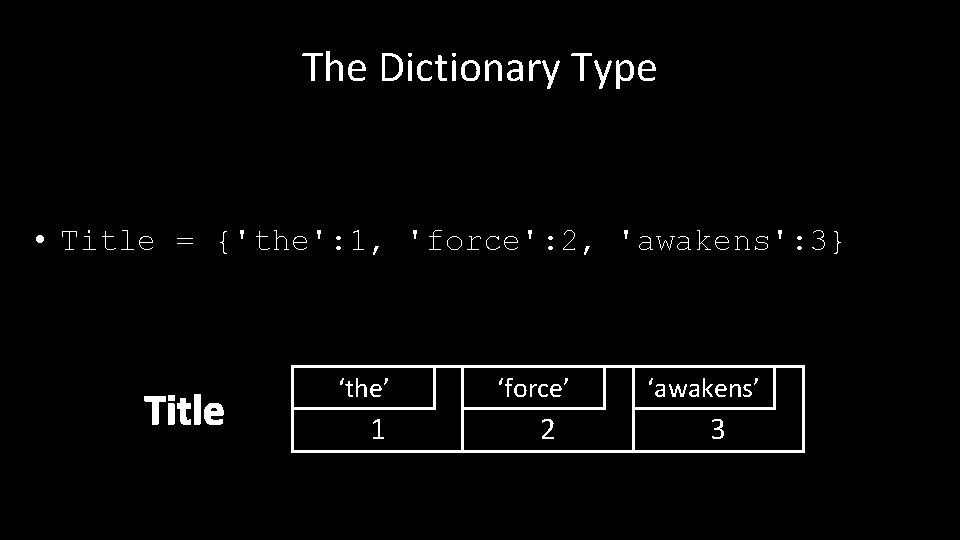
The Dictionary Type • Title = {'the': 1, 'force': 2, 'awakens': 3} Title ‘the’ 1 ‘force’ 2 ‘awakens’ 3
![The Dictionary Type Title keys dictkeysthe force awakens Title the 1 force The Dictionary Type • Title. keys() >>> dict_keys(['the', 'force', 'awakens']) Title ‘the’ 1 ‘force’](https://slidetodoc.com/presentation_image_h2/64478e146d3074f94ebdedaded710bc8/image-48.jpg)
The Dictionary Type • Title. keys() >>> dict_keys(['the', 'force', 'awakens']) Title ‘the’ 1 ‘force’ 2 ‘awakens’ 3
![The Dictionary Type TitleStar Wars 0 printTitle Star Wars 0 the 1 The Dictionary Type Title['Star Wars'] = 0 print(Title) >>> {'Star Wars': 0, 'the': 1,](https://slidetodoc.com/presentation_image_h2/64478e146d3074f94ebdedaded710bc8/image-49.jpg)
The Dictionary Type Title['Star Wars'] = 0 print(Title) >>> {'Star Wars': 0, 'the': 1, 'force': 2, 'awakens': 3} Title ‘Star Wars’ 0 ‘the’ 1 ‘force’ 2 ‘awakens’ 3
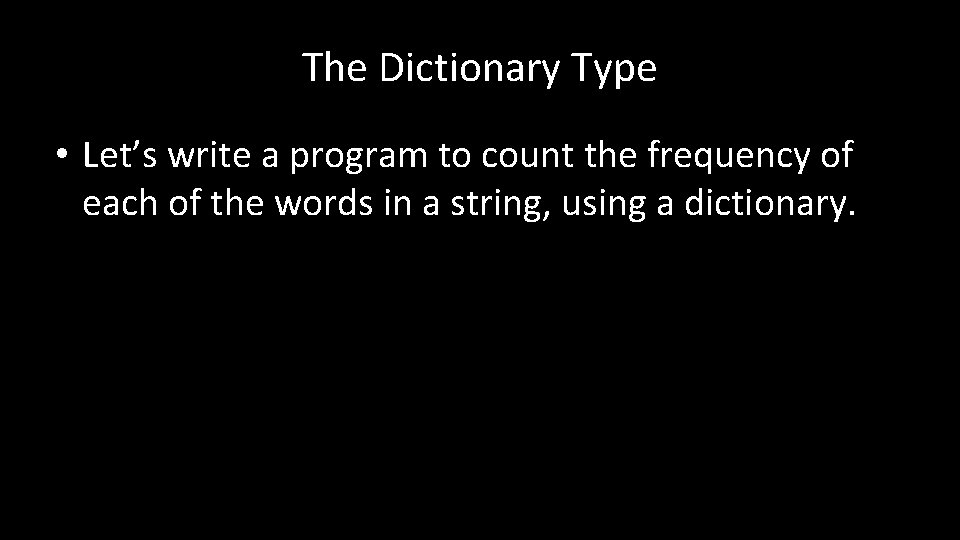
The Dictionary Type • Let’s write a program to count the frequency of each of the words in a string, using a dictionary.
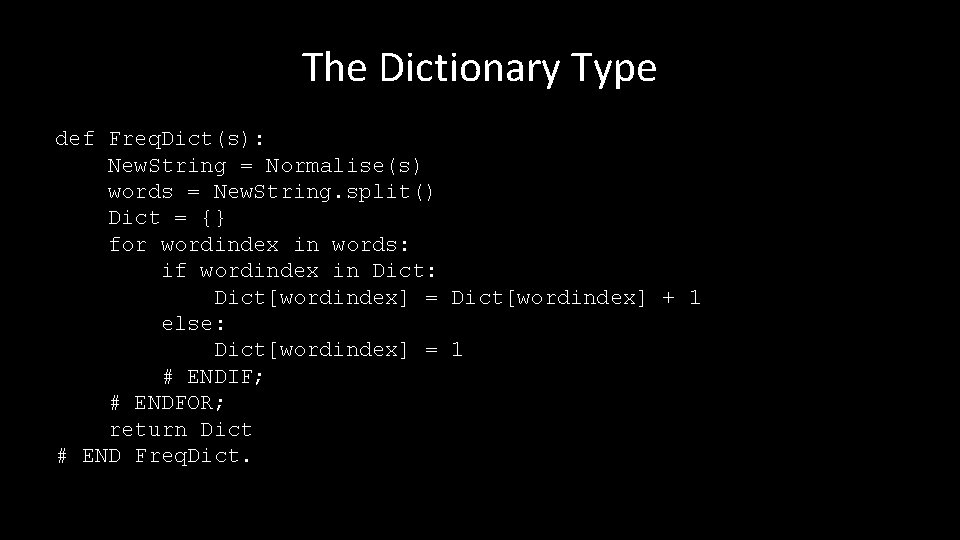
The Dictionary Type def Freq. Dict(s): New. String = Normalise(s) words = New. String. split() Dict = {} for wordindex in words: if wordindex in Dict: Dict[wordindex] = Dict[wordindex] + 1 else: Dict[wordindex] = 1 # ENDIF; # ENDFOR; return Dict # END Freq. Dict.
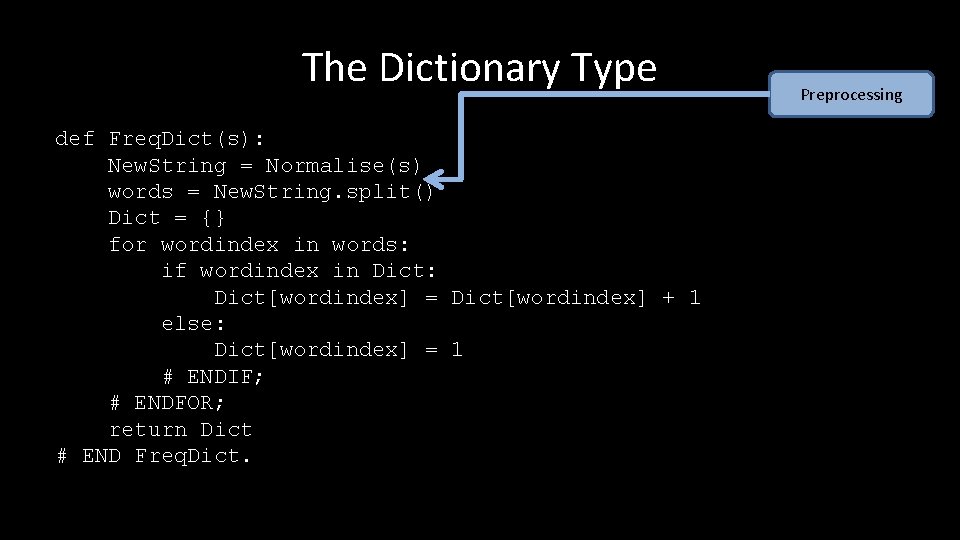
The Dictionary Type def Freq. Dict(s): New. String = Normalise(s) words = New. String. split() Dict = {} for wordindex in words: if wordindex in Dict: Dict[wordindex] = Dict[wordindex] + 1 else: Dict[wordindex] = 1 # ENDIF; # ENDFOR; return Dict # END Freq. Dict. Preprocessing
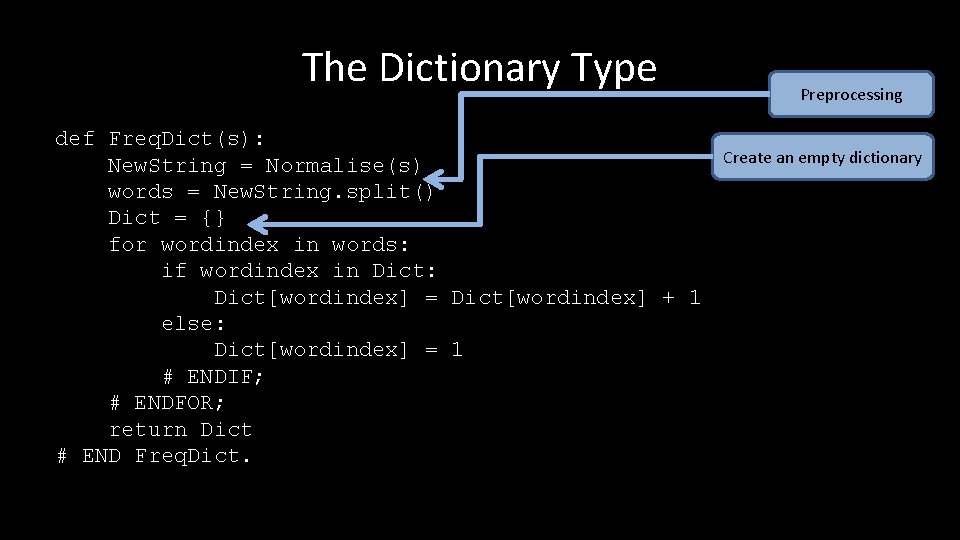
The Dictionary Type Preprocessing def Freq. Dict(s): Create an empty dictionary New. String = Normalise(s) words = New. String. split() Dict = {} for wordindex in words: if wordindex in Dict: Dict[wordindex] = Dict[wordindex] + 1 else: Dict[wordindex] = 1 # ENDIF; # ENDFOR; return Dict # END Freq. Dict.
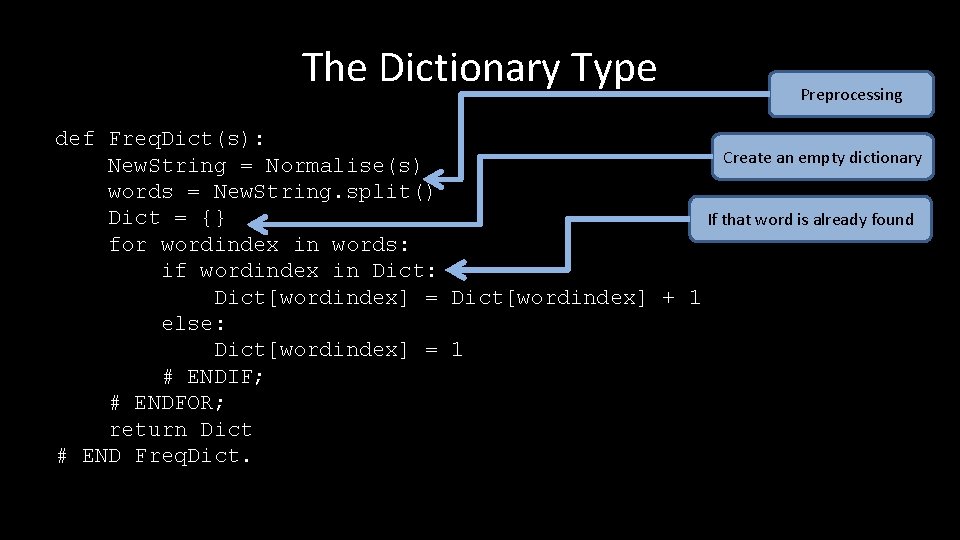
The Dictionary Type Preprocessing def Freq. Dict(s): Create an empty dictionary New. String = Normalise(s) words = New. String. split() Dict = {} If that word is already found for wordindex in words: if wordindex in Dict: Dict[wordindex] = Dict[wordindex] + 1 else: Dict[wordindex] = 1 # ENDIF; # ENDFOR; return Dict # END Freq. Dict.
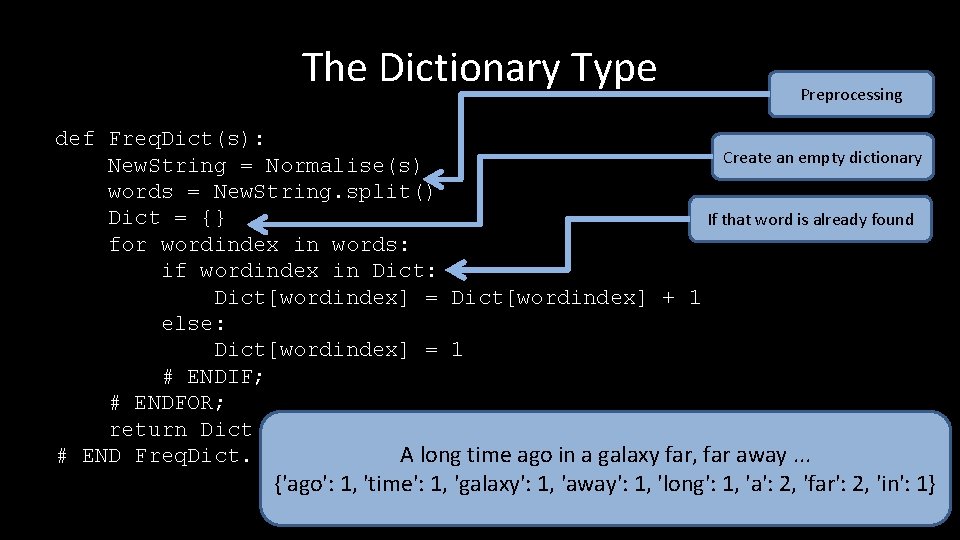
The Dictionary Type Preprocessing def Freq. Dict(s): Create an empty dictionary New. String = Normalise(s) words = New. String. split() Dict = {} If that word is already found for wordindex in words: if wordindex in Dict: Dict[wordindex] = Dict[wordindex] + 1 else: Dict[wordindex] = 1 # ENDIF; # ENDFOR; return Dict # END Freq. Dict. A long time ago in a galaxy far, far away. . . {'ago': 1, 'time': 1, 'galaxy': 1, 'away': 1, 'long': 1, 'a': 2, 'far': 2, 'in': 1}
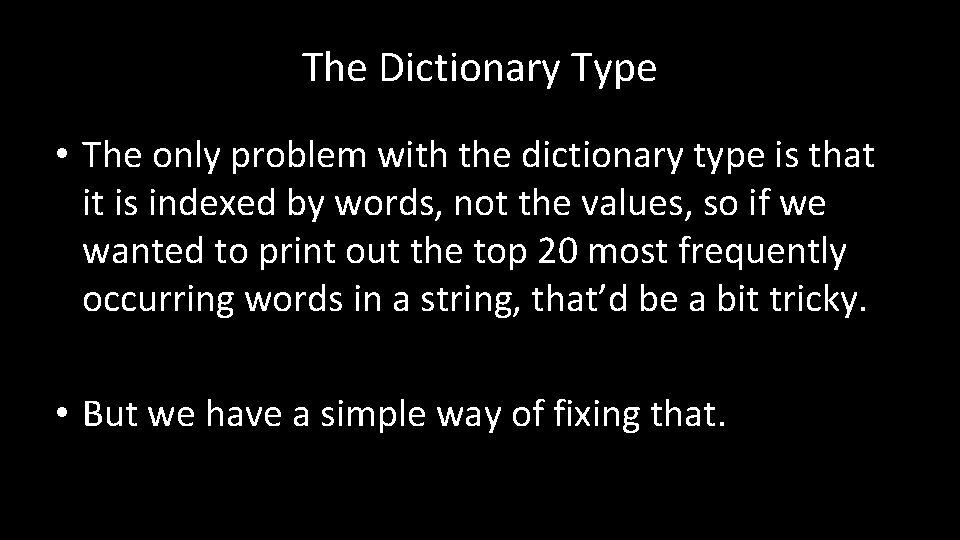
The Dictionary Type • The only problem with the dictionary type is that it is indexed by words, not the values, so if we wanted to print out the top 20 most frequently occurring words in a string, that’d be a bit tricky. • But we have a simple way of fixing that.
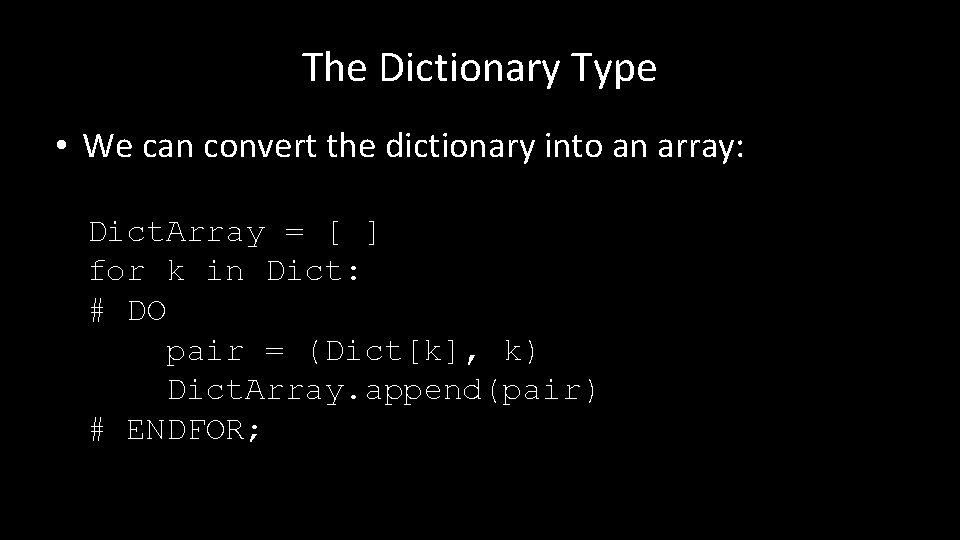
The Dictionary Type • We can convert the dictionary into an array: Dict. Array = [ ] for k in Dict: # DO pair = (Dict[k], k) Dict. Array. append(pair) # ENDFOR;
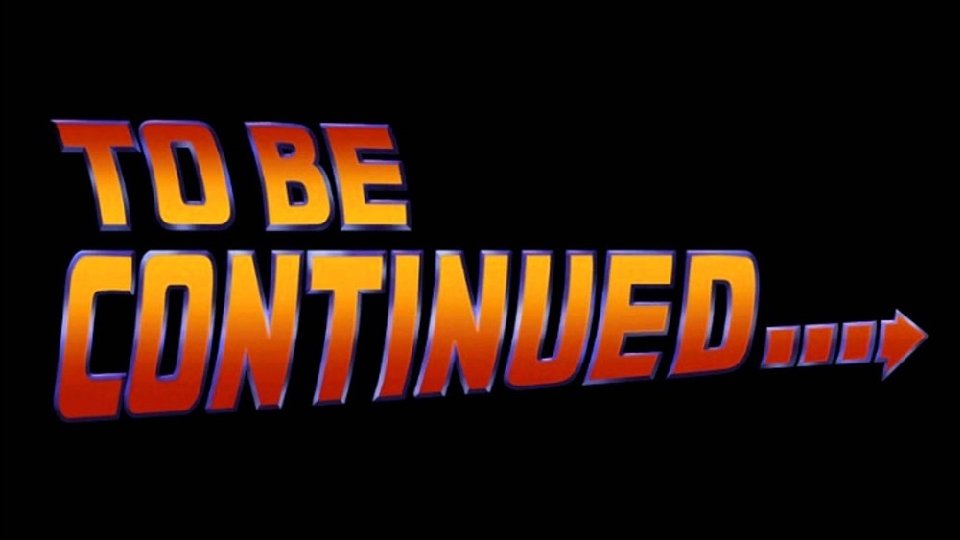
etc.
Damian gordon
Damian gordon
Damian gordon
Http://प
Neraca saldo pt sejahtera
Ref 2021 twitter
General ledger post ref
Ref dalam jurnal umum
Twitter ref 2021
Hockey ref hand signals
Ref criteria
Linesman positioning hockey
Usa hockey ref signals
Ref et idaho
Ref kirche rothrist
Packages_display.php?ref=
Volleyball ref stand
Divider slide
X ref
Ref 2026
X ref
Ref impact case studies
Ref
Erguns equation
Ref jur
Ref-qualified member functions
Irinyiref
Post. ref. accounting
Damian sturzaker
Padre damian de veuster 2215
Estructura de una biografia
Padre damian de veuster 2215 vitacura
Damian clancy
Damian krysztofik
Damian folch
Damian urbańczyk żona
Christophe damian
Damian mac
Fray damian massanet picture
Padre damian de veuster 2215 vitacura
Padre damian de veuster 2215
Damian harding
Damian urbańczyk wikipedia
Toczenie bez poślizgu
Oscar sandoval md
A 1017
Melissa damian
Damian marli
Damian czudek
Money money money team
Iru academy
Xor gate python
666 rule presentation
Procedural python
Python chapter 5
Second order cone programming python
Python programming in context
Constraint programming python
Rapid gui programming with python and qt