Programming for Art Algorithms ART 315 Dr J
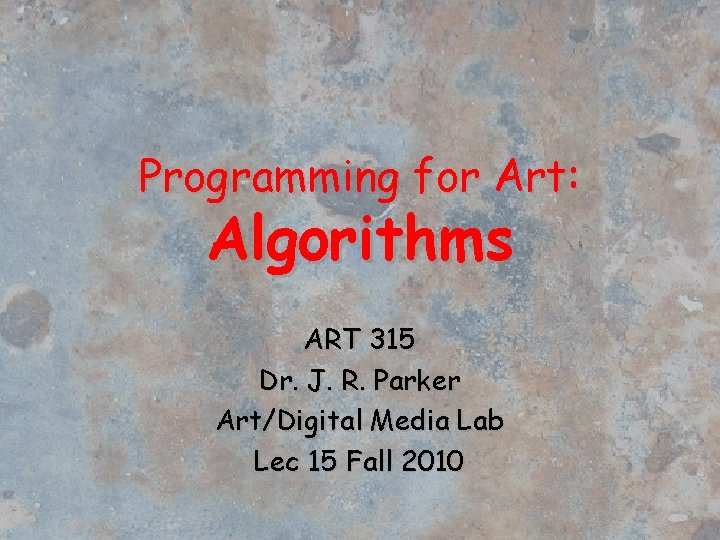
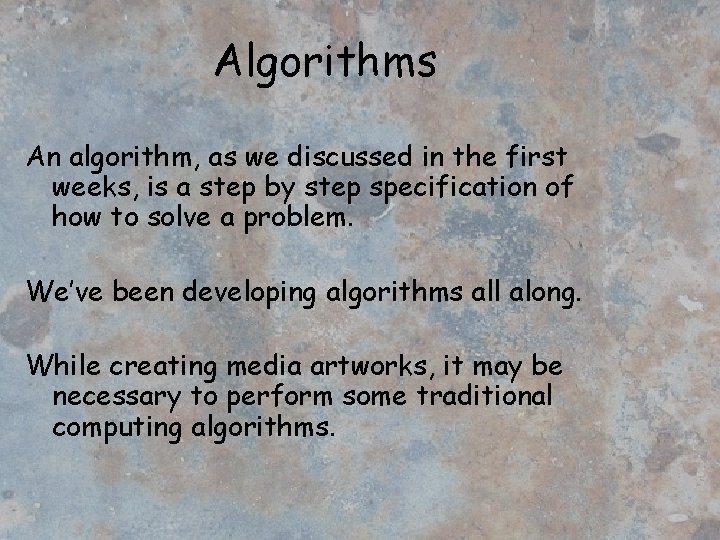
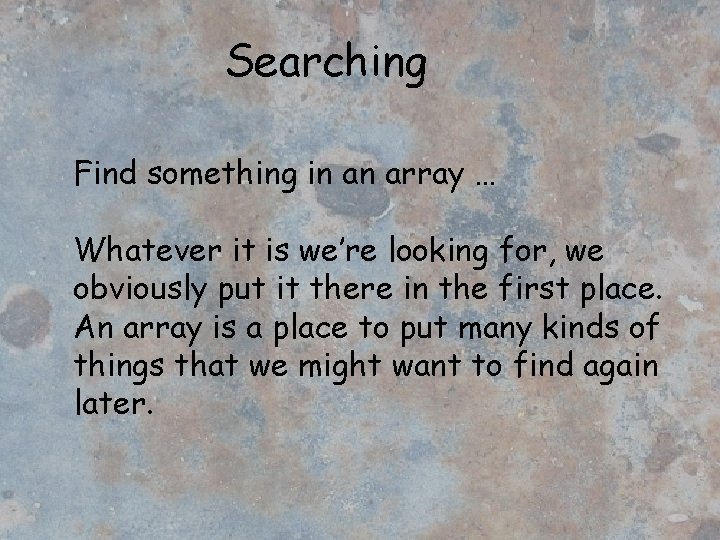
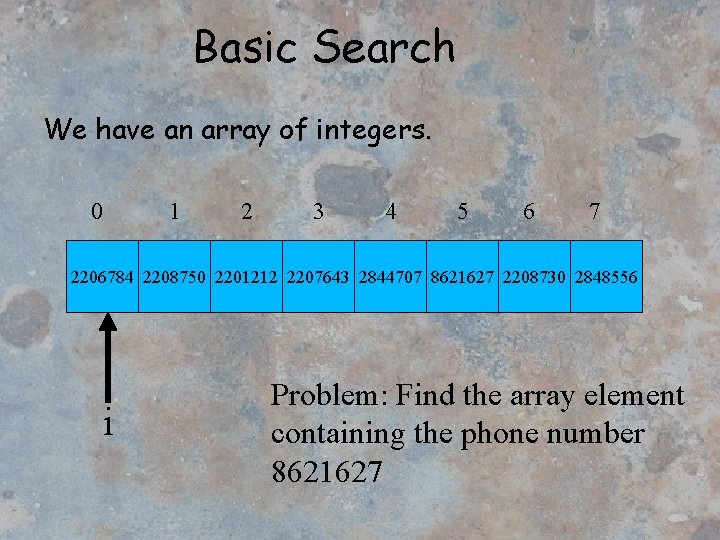
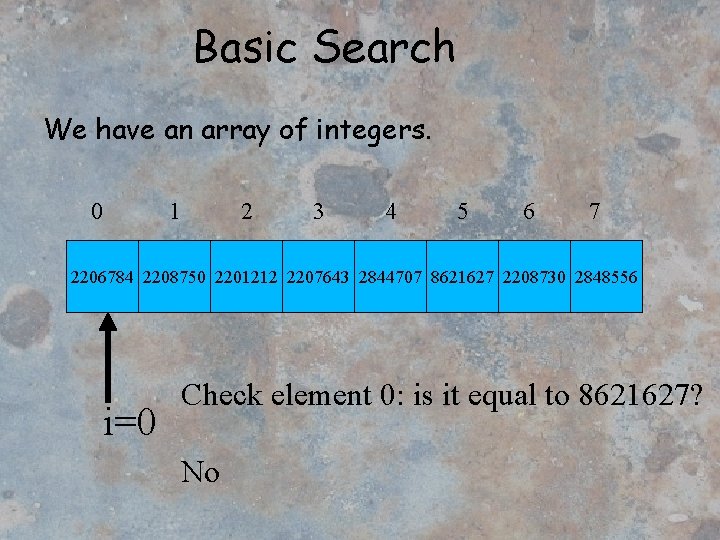
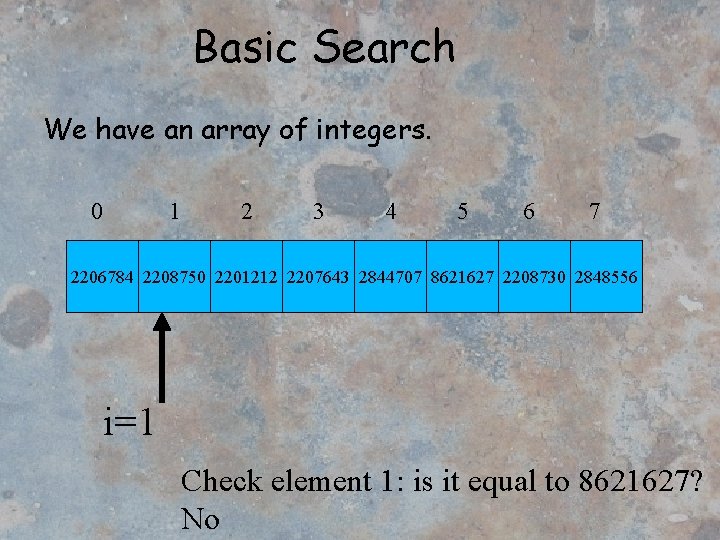
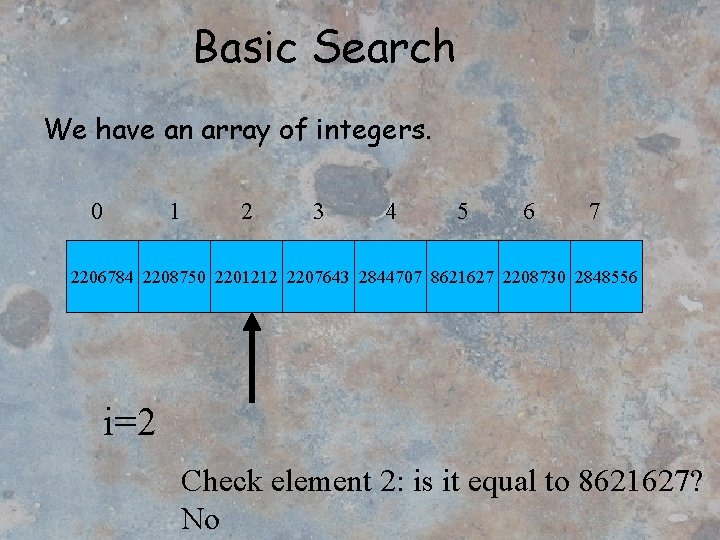
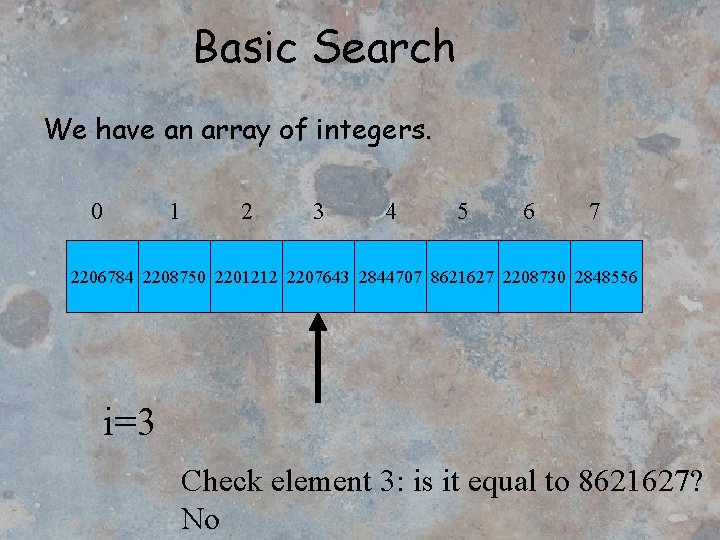
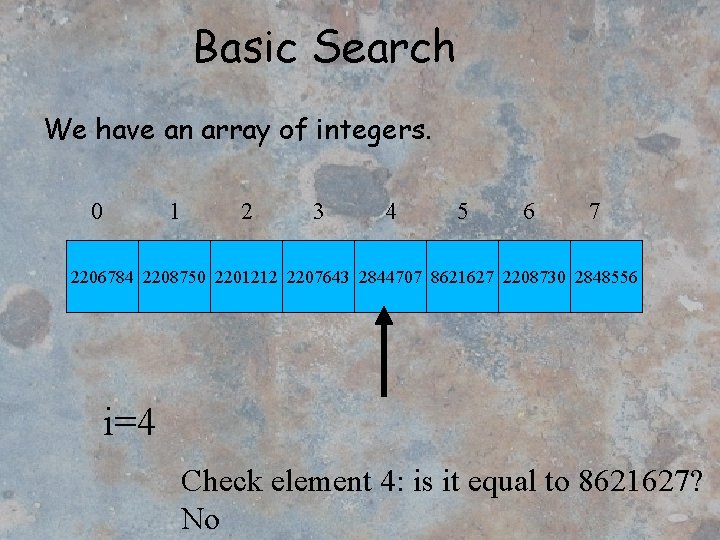
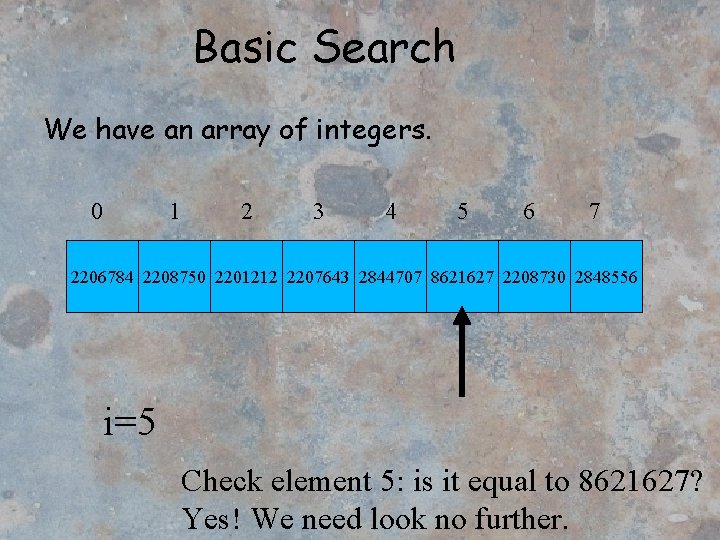
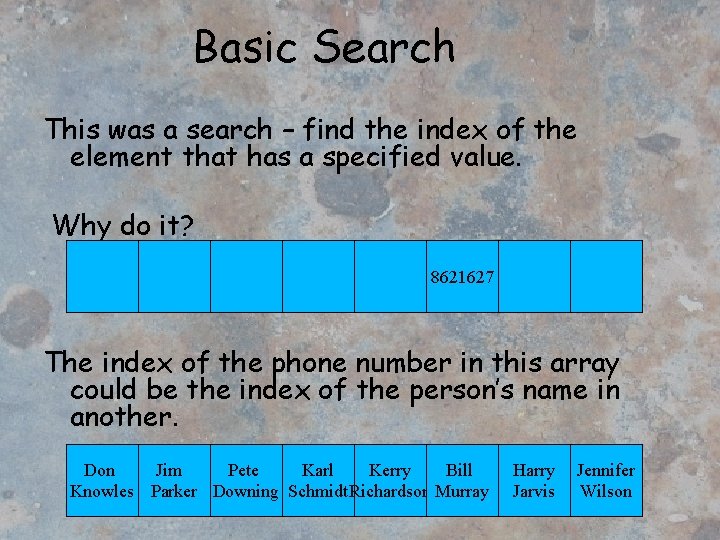
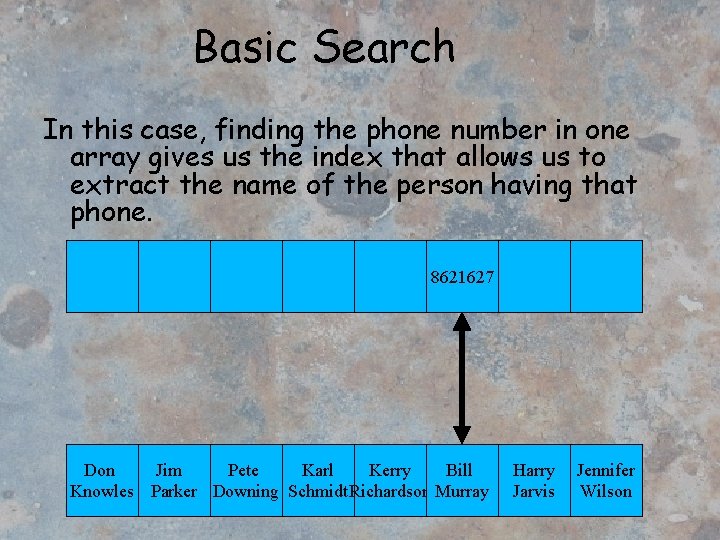
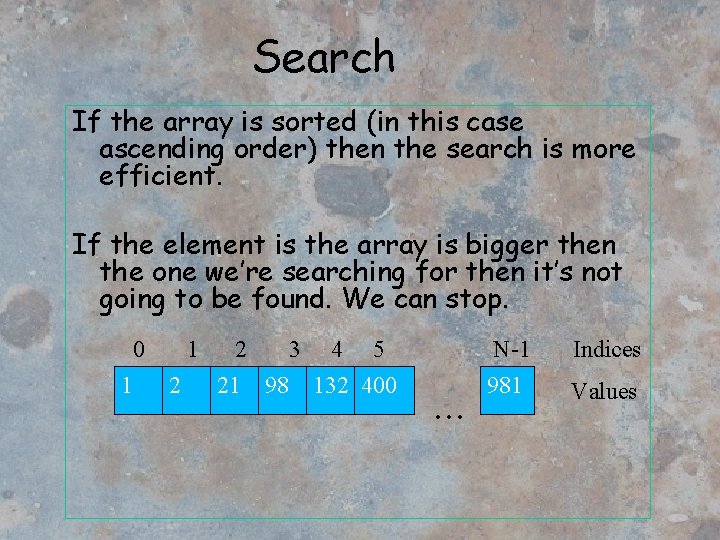
![Search for (i=0; i<N; i++) { if (array[i] == target) // The value has Search for (i=0; i<N; i++) { if (array[i] == target) // The value has](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-14.jpg)
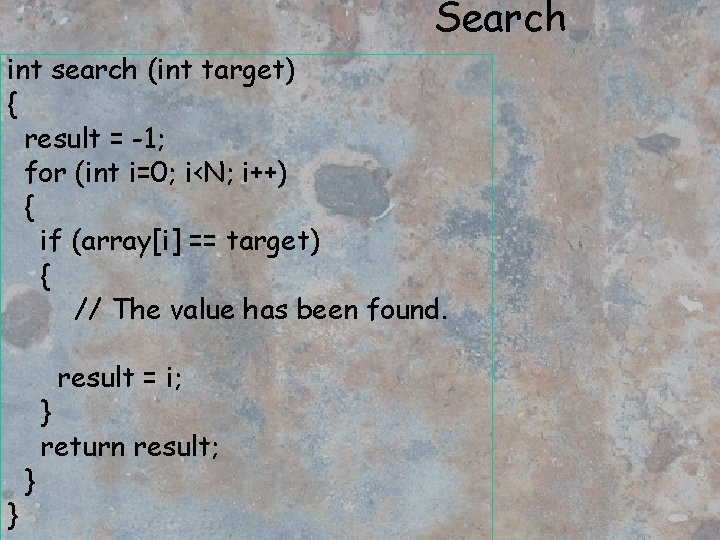
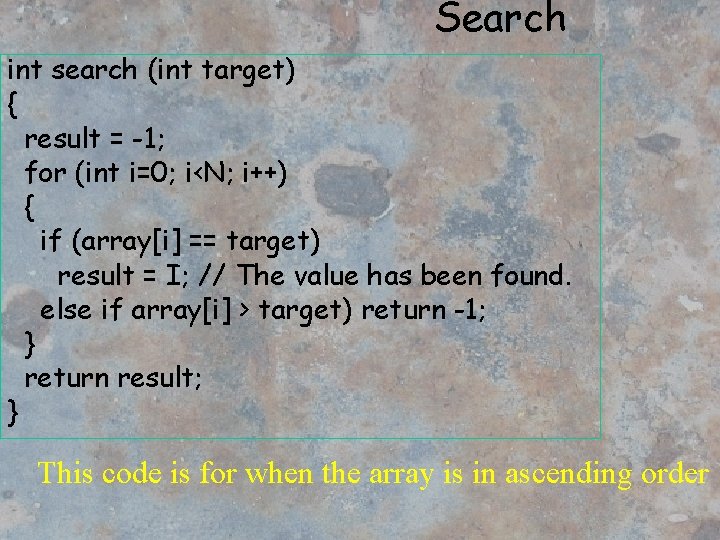
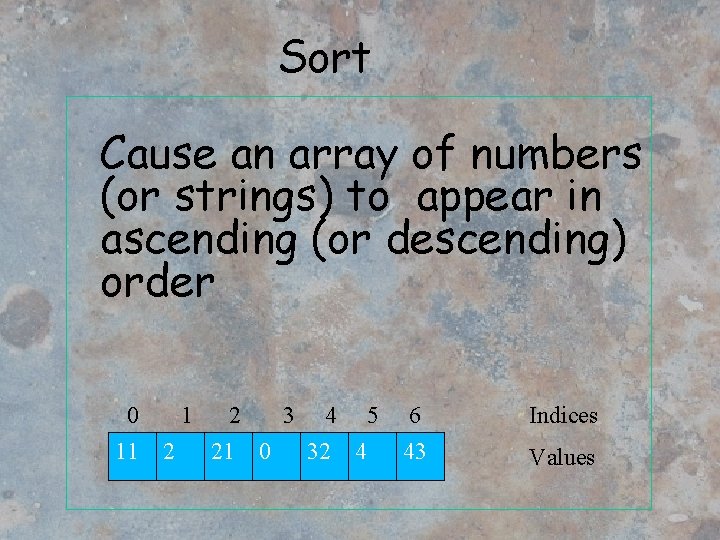
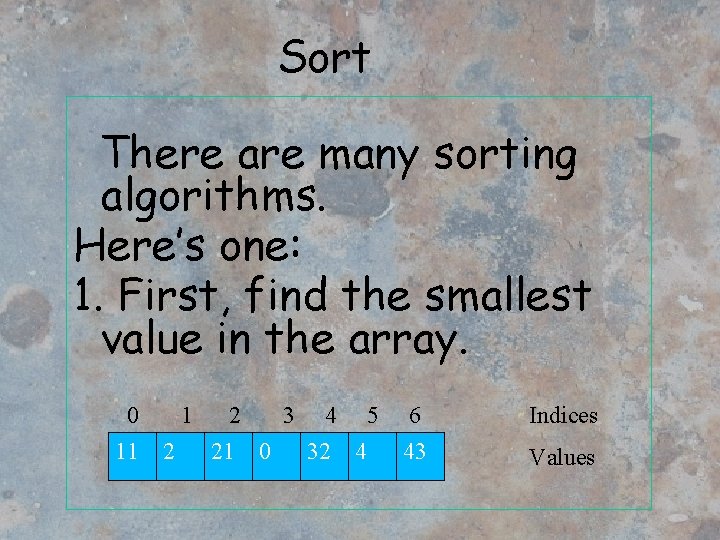
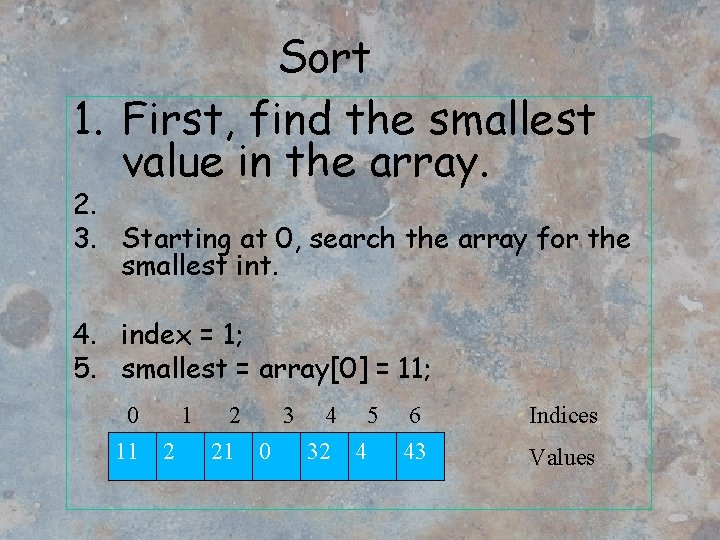
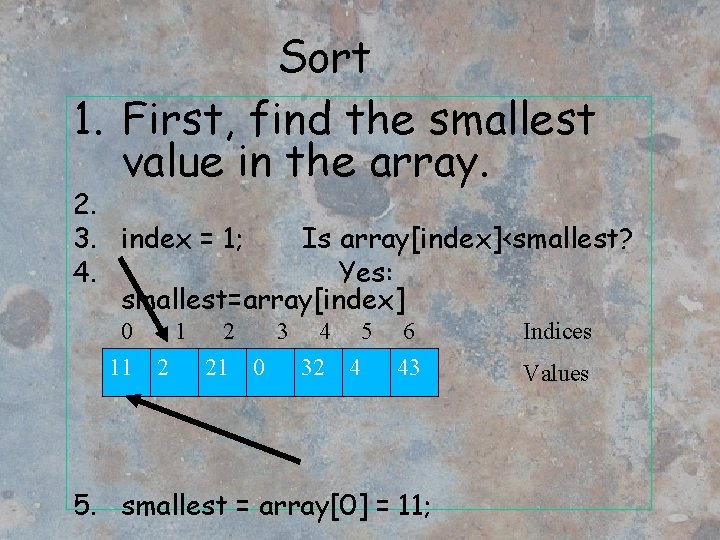
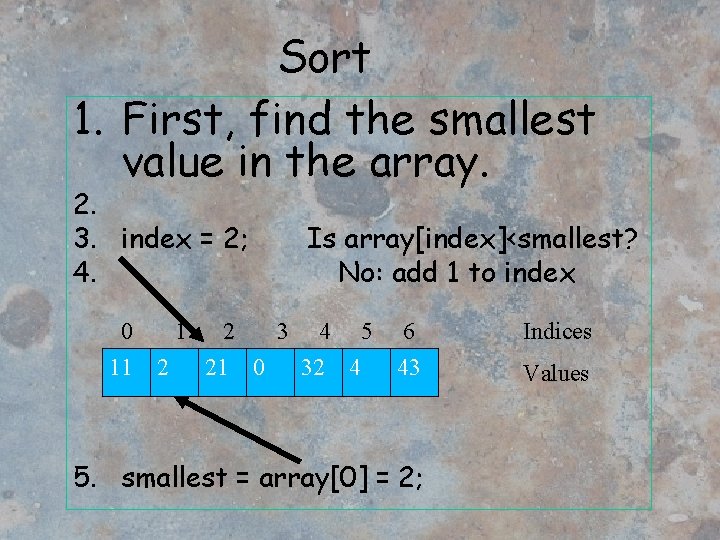
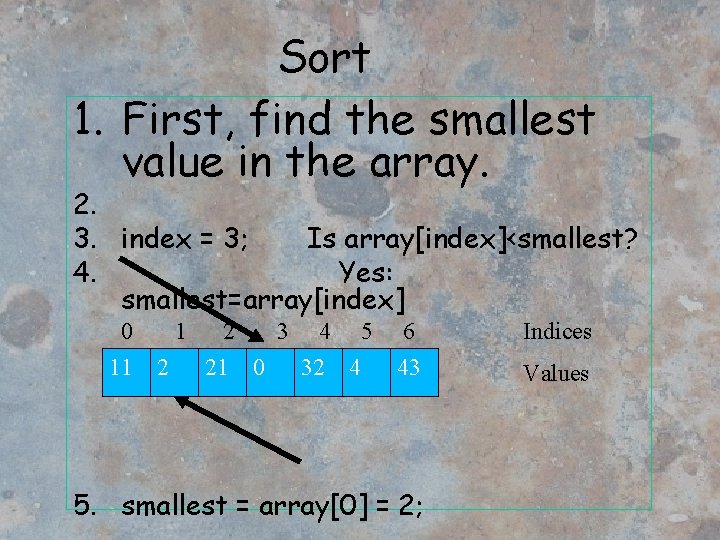
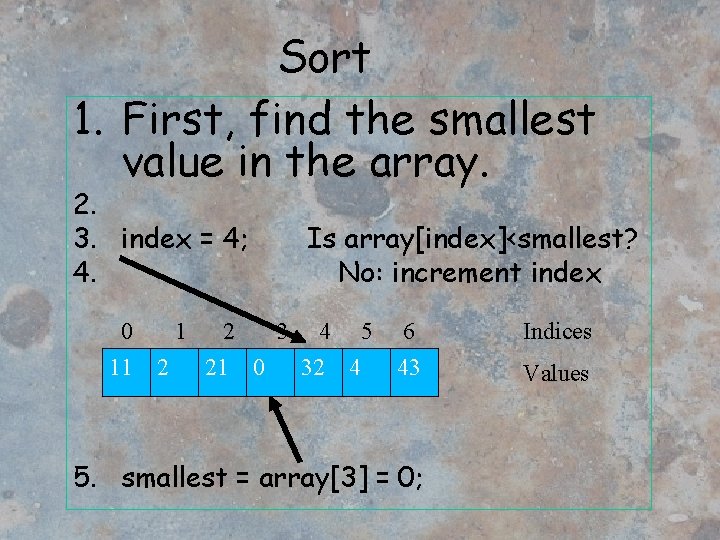
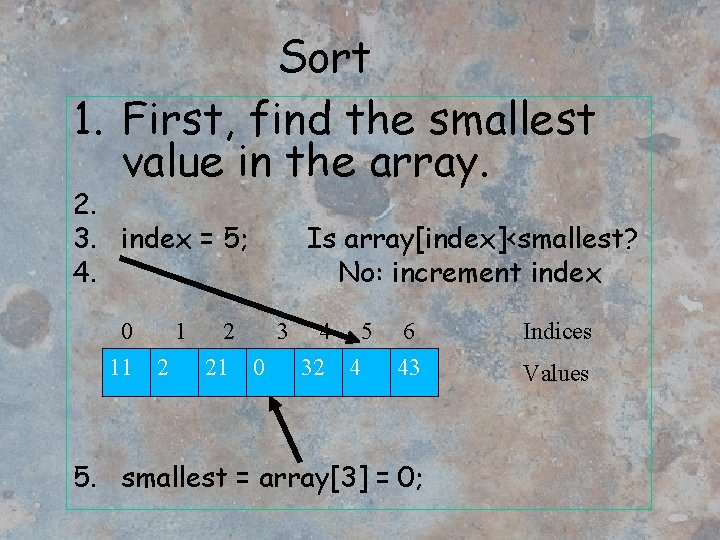
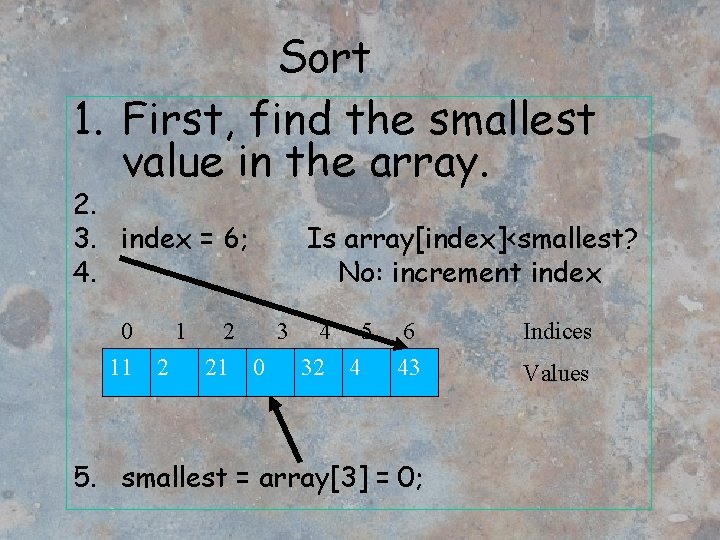
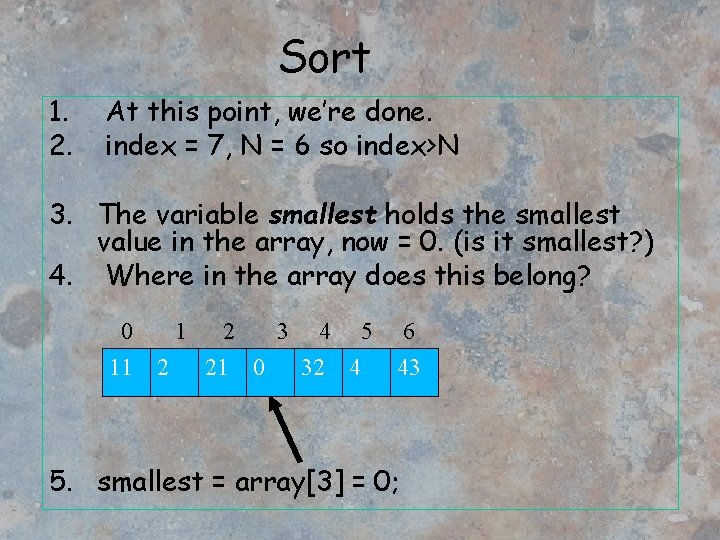
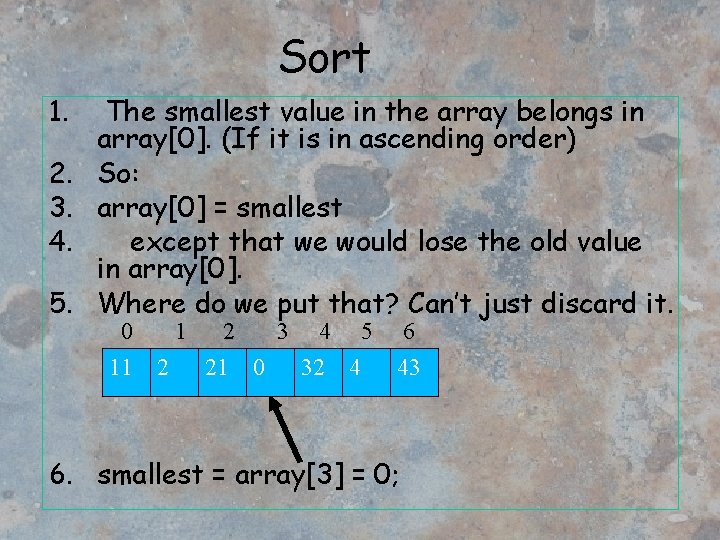
![Sort 1. The smallest value in the array belongs in array[0]. (If it is Sort 1. The smallest value in the array belongs in array[0]. (If it is](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-28.jpg)
![Sort 1. We could put the smallest value in array[0] and the old value Sort 1. We could put the smallest value in array[0] and the old value](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-29.jpg)
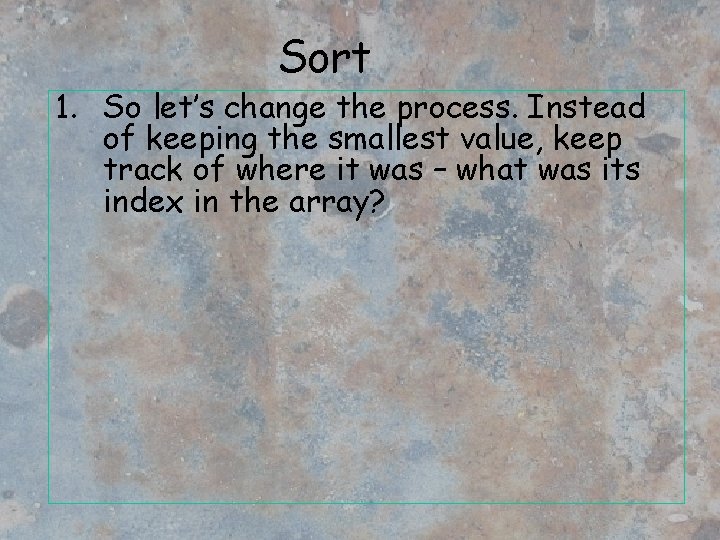
![Sort 1. smallest initially 0 2. index = 1; Is array[index]<array[smallest]? 3. Yes: smallest=index Sort 1. smallest initially 0 2. index = 1; Is array[index]<array[smallest]? 3. Yes: smallest=index](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-31.jpg)
![Sort 1. 2. index = 2; Is array[index]<array[smallest]? 3. No: add 1 to index Sort 1. 2. index = 2; Is array[index]<array[smallest]? 3. No: add 1 to index](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-32.jpg)
![Sort 1. 2. index = 3; Is array[index]<array[smallest]? 3. Yes: smallest=index 0 11 4. Sort 1. 2. index = 3; Is array[index]<array[smallest]? 3. Yes: smallest=index 0 11 4.](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-33.jpg)
![Sort 1. 2. index = 4; Is array[index]<array[smallest]? 3. No: increment index 0 11 Sort 1. 2. index = 4; Is array[index]<array[smallest]? 3. No: increment index 0 11](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-34.jpg)
![Sort 1. 2. index = 5; Is array[index]<array[smallest]? 3. No: increment index 0 11 Sort 1. 2. index = 5; Is array[index]<array[smallest]? 3. No: increment index 0 11](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-35.jpg)
![Sort 1. 2. index = 6; Is array[index]<array[smallest]? 3. No: increment index 0 11 Sort 1. 2. index = 6; Is array[index]<array[smallest]? 3. No: increment index 0 11](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-36.jpg)
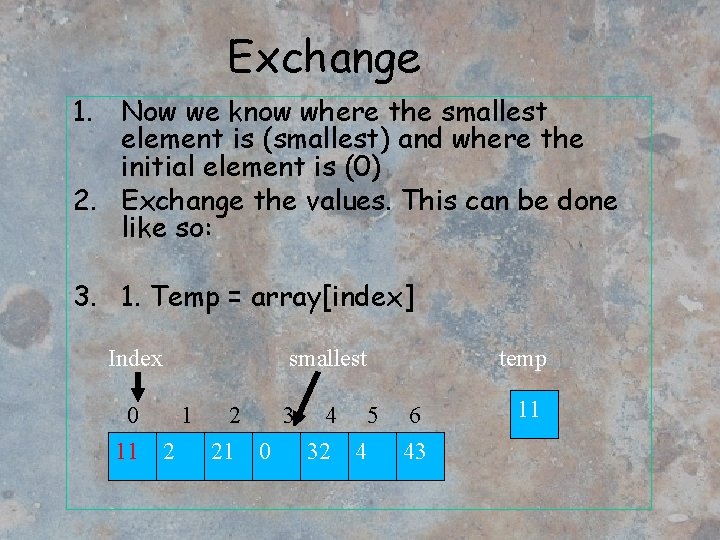
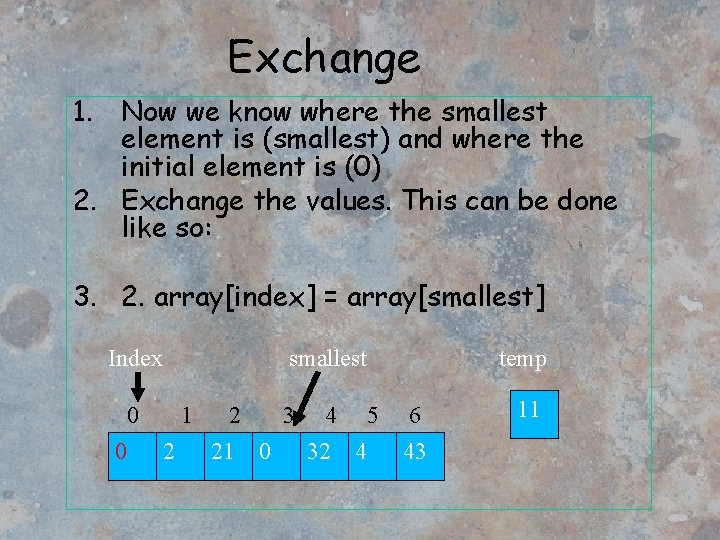
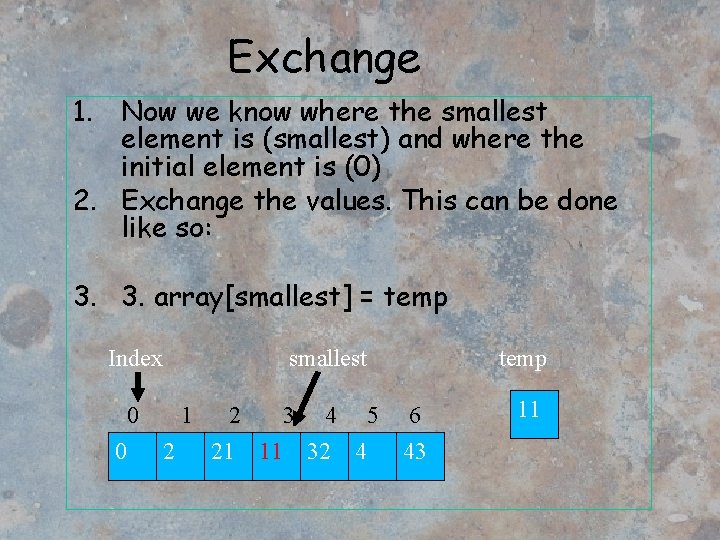
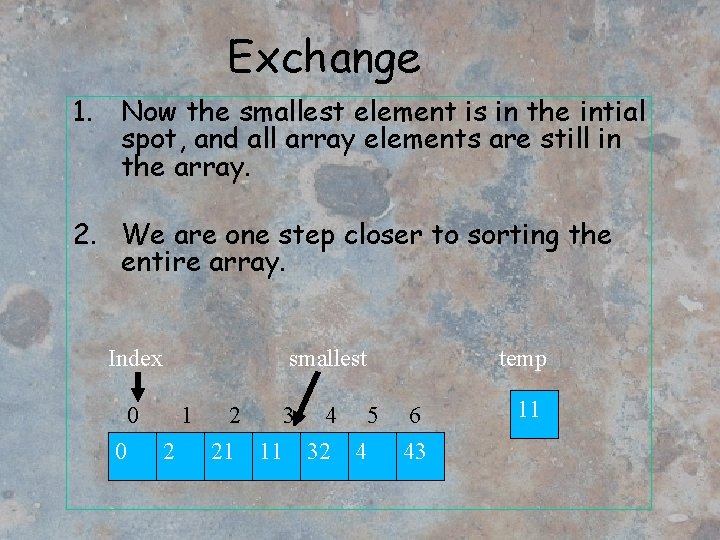
![Code smallest=0; for (i=1; i<N; i++) // Find smallest if (array[i] < array[smallest]) smallest Code smallest=0; for (i=1; i<N; i++) // Find smallest if (array[i] < array[smallest]) smallest](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-41.jpg)
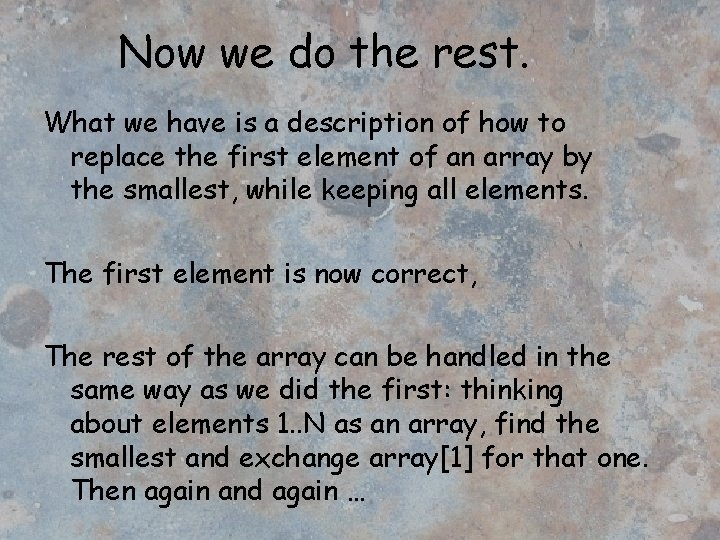
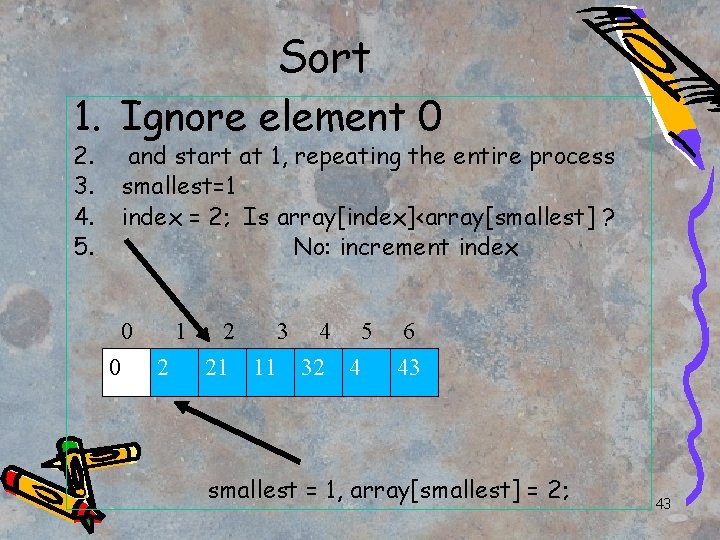
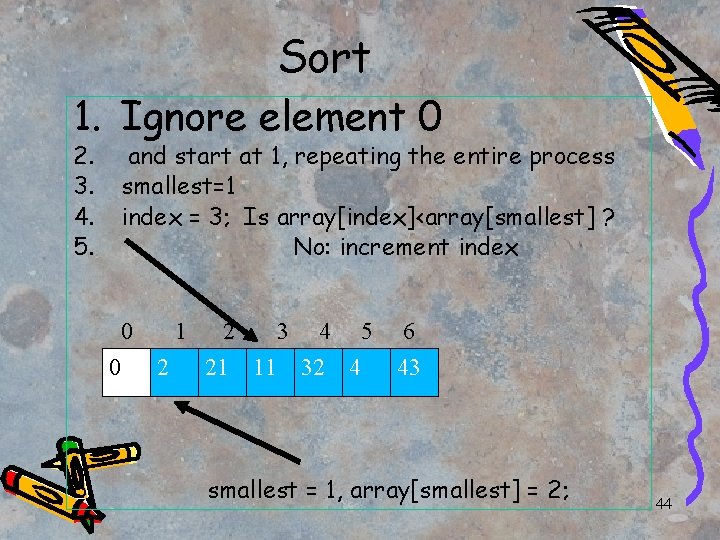
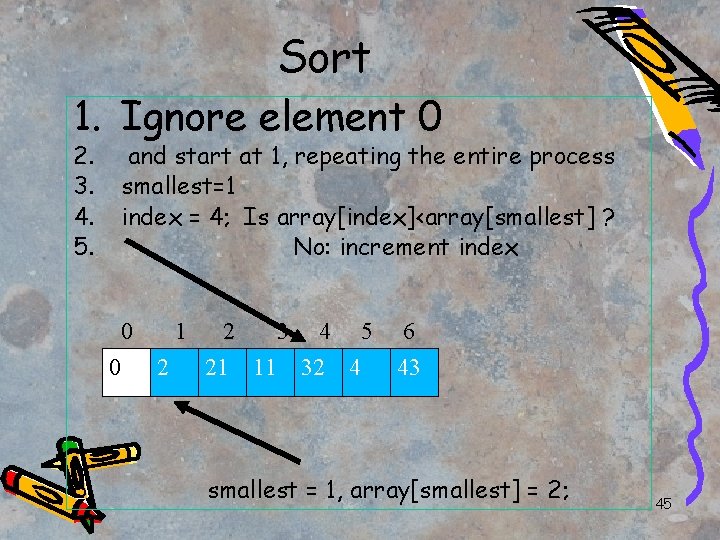
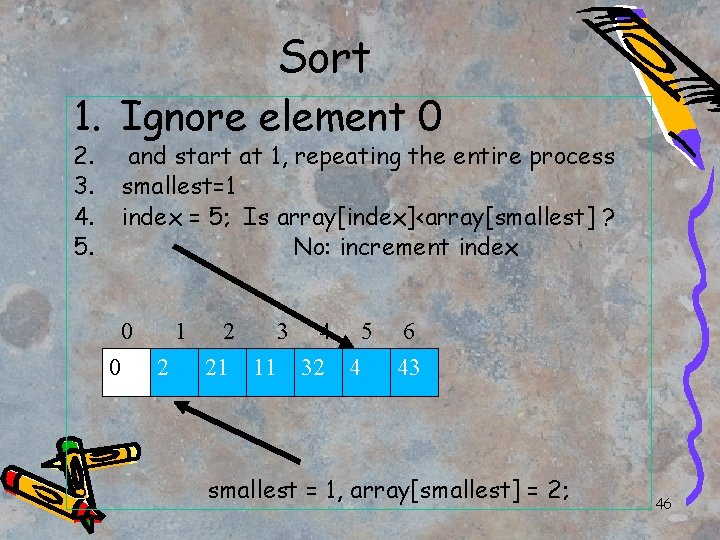
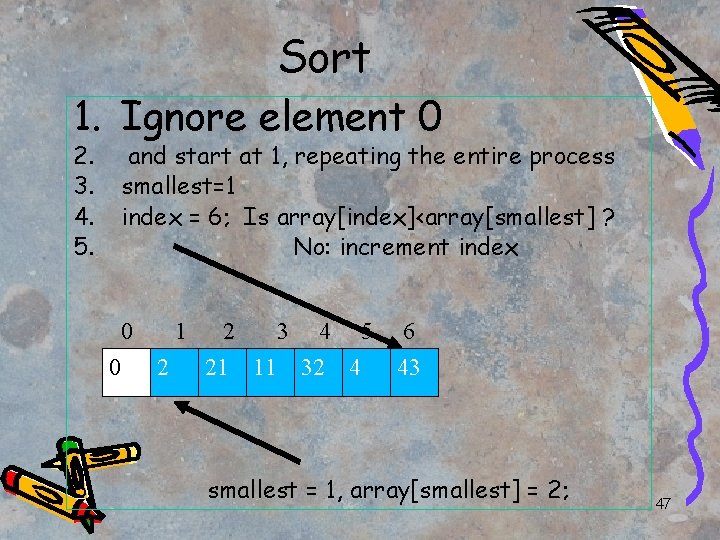
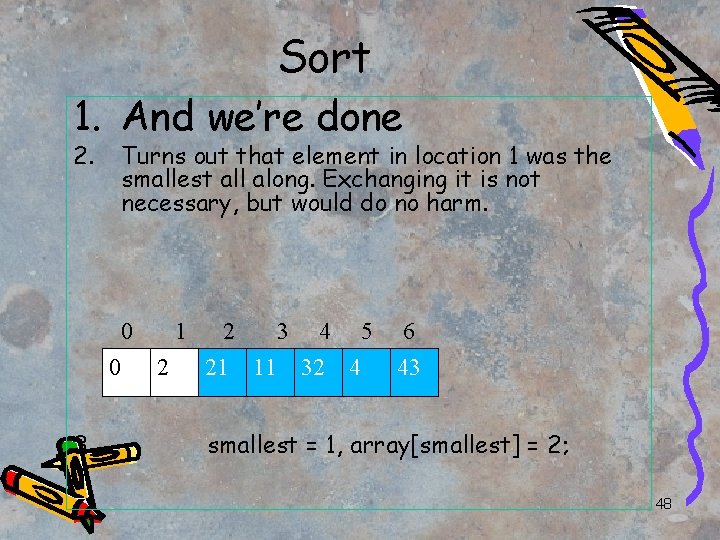
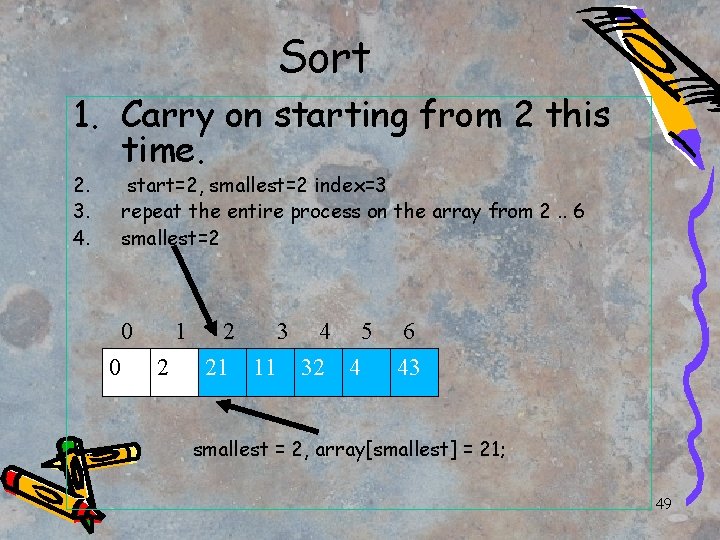
![Sort 1. Ignore elements 0 and 1 2. 3. index = 3; Is array[index]<array[smallest] Sort 1. Ignore elements 0 and 1 2. 3. index = 3; Is array[index]<array[smallest]](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-50.jpg)
![Sort 1. Ignore elements 0 and 1 2. 3. index = 4; Is array[index]<array[smallest] Sort 1. Ignore elements 0 and 1 2. 3. index = 4; Is array[index]<array[smallest]](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-51.jpg)
![Sort 1. Ignore elements 0 and 1 2. 3. index = 5; Is array[index]<array[smallest] Sort 1. Ignore elements 0 and 1 2. 3. index = 5; Is array[index]<array[smallest]](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-52.jpg)
![Sort 1. Ignore elements 0 and 1 2. 3. index = 5; Is array[index]<array[smallest] Sort 1. Ignore elements 0 and 1 2. 3. index = 5; Is array[index]<array[smallest]](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-53.jpg)
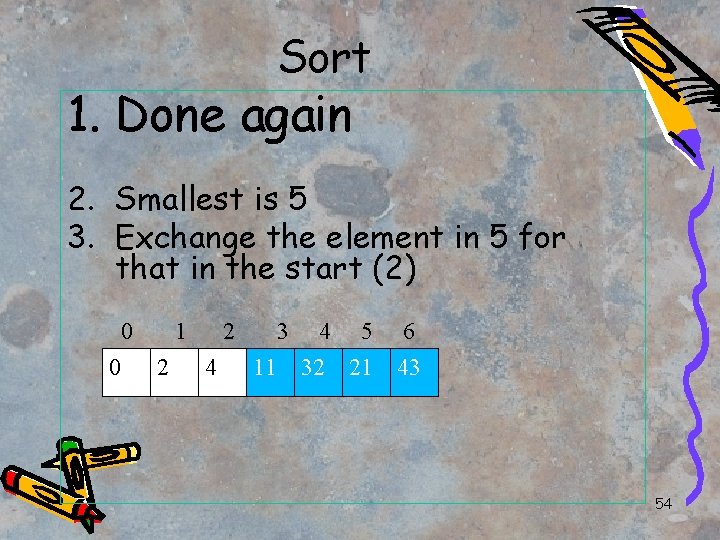
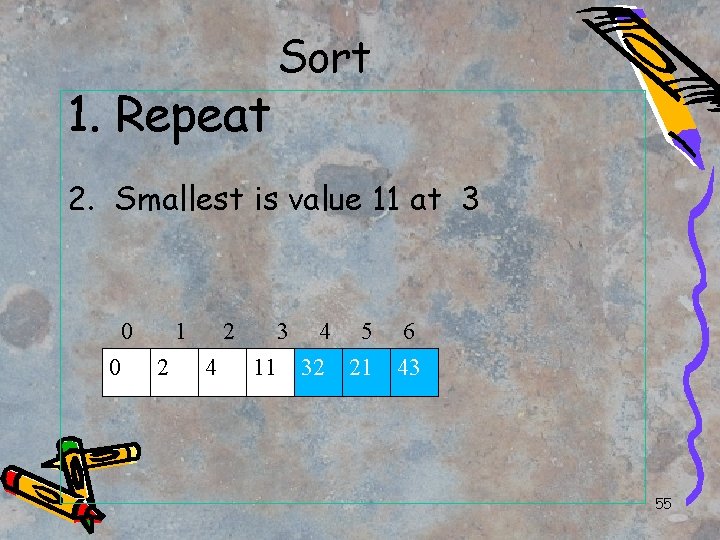
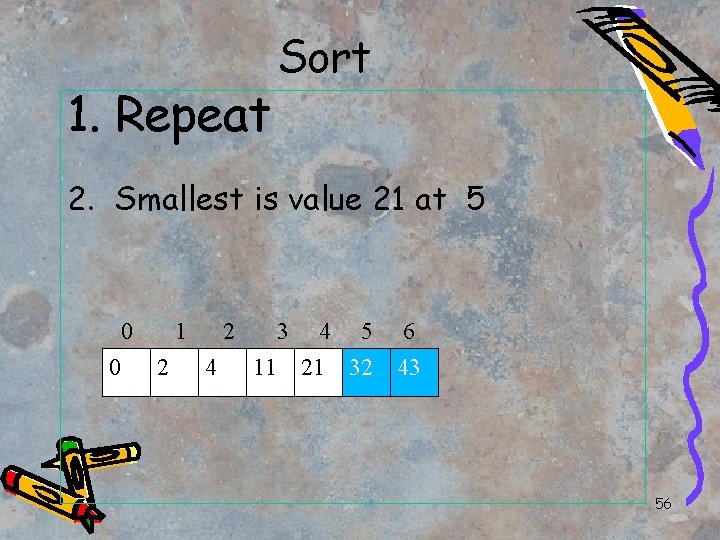
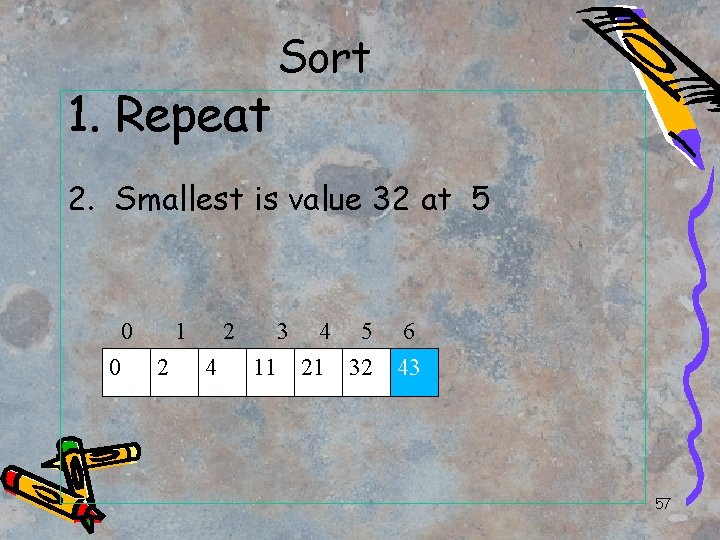
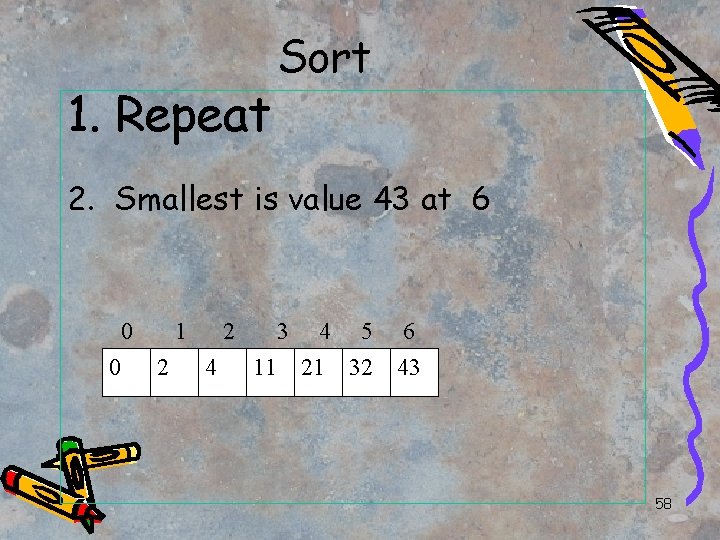
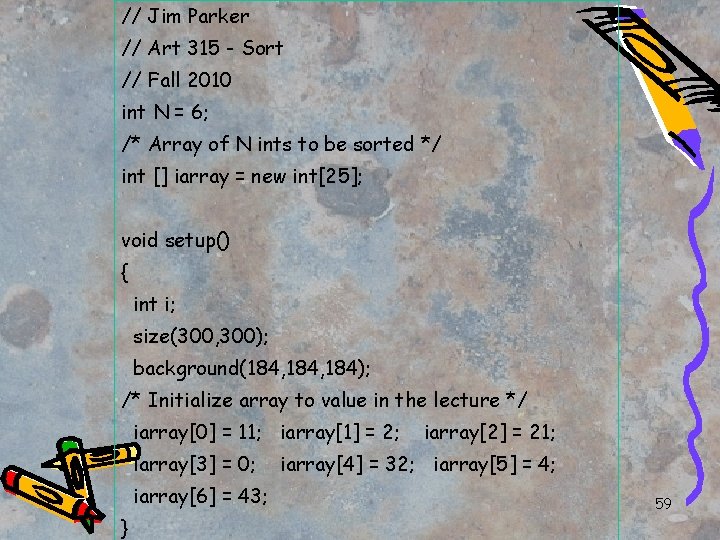
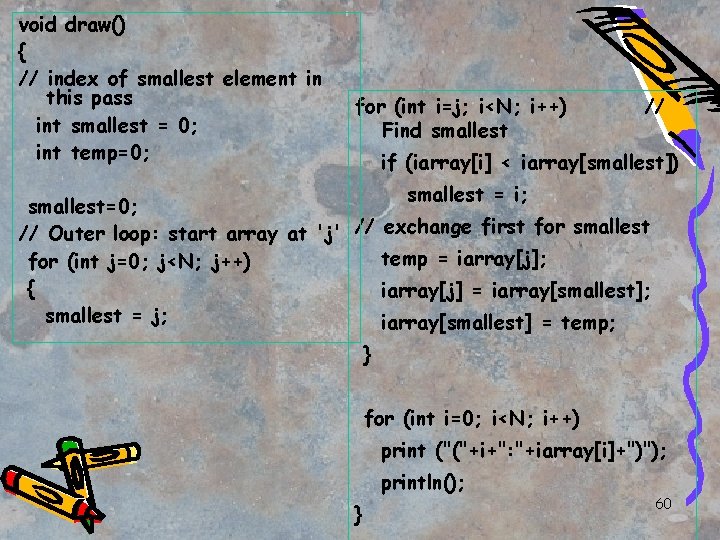
- Slides: 60
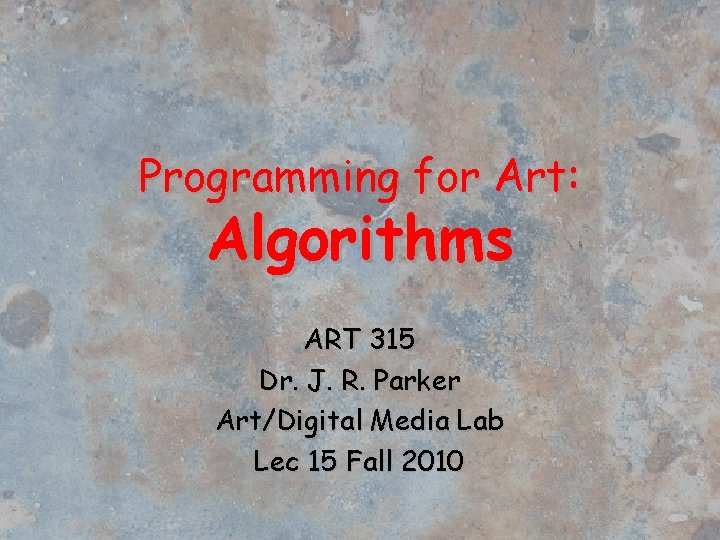
Programming for Art: Algorithms ART 315 Dr. J. R. Parker Art/Digital Media Lab Lec 15 Fall 2010
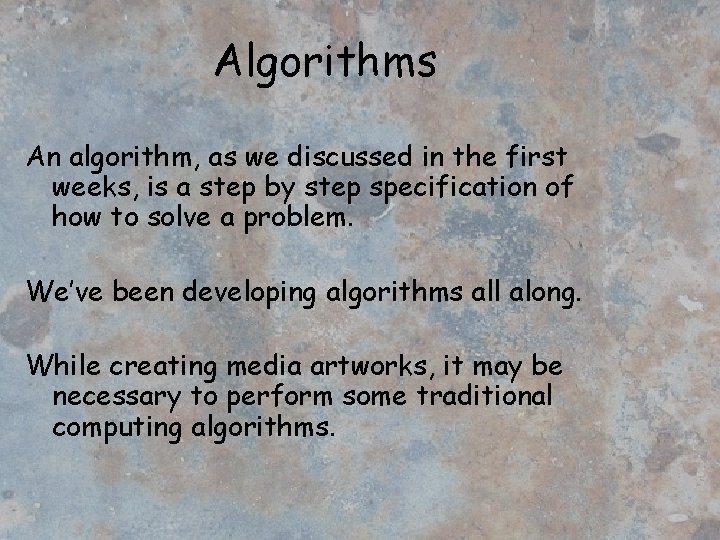
Algorithms An algorithm, as we discussed in the first weeks, is a step by step specification of how to solve a problem. We’ve been developing algorithms all along. While creating media artworks, it may be necessary to perform some traditional computing algorithms.
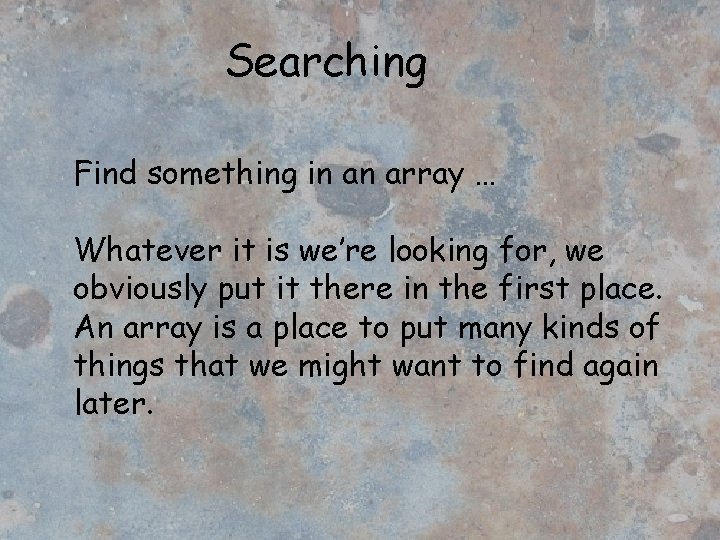
Searching Find something in an array … Whatever it is we’re looking for, we obviously put it there in the first place. An array is a place to put many kinds of things that we might want to find again later.
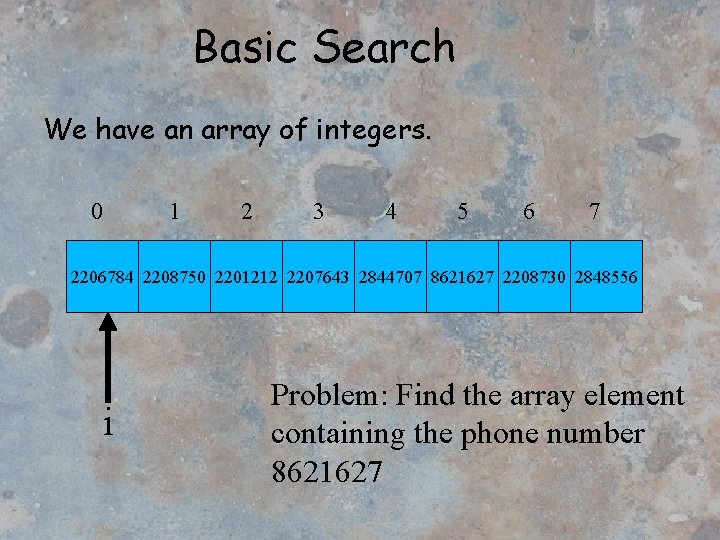
Basic Search We have an array of integers. 0 1 2 3 4 5 6 7 2206784 2208750 2201212 2207643 2844707 8621627 2208730 2848556 i Problem: Find the array element containing the phone number 8621627
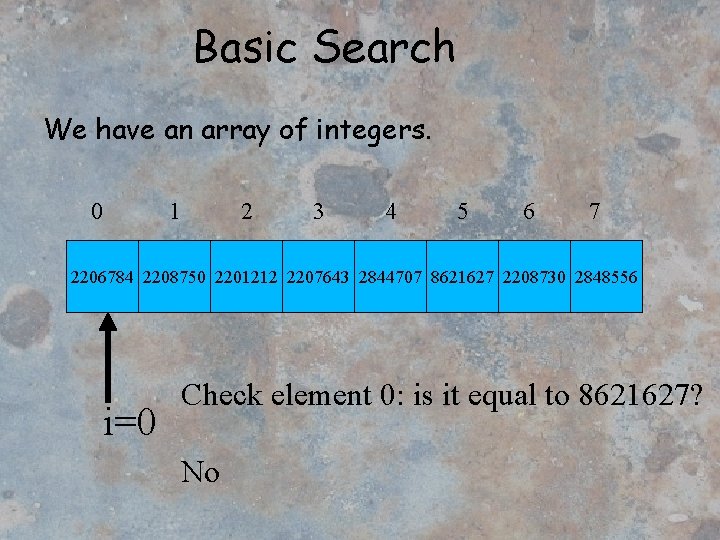
Basic Search We have an array of integers. 0 1 2 3 4 5 6 7 2206784 2208750 2201212 2207643 2844707 8621627 2208730 2848556 i=0 Check element 0: is it equal to 8621627? No
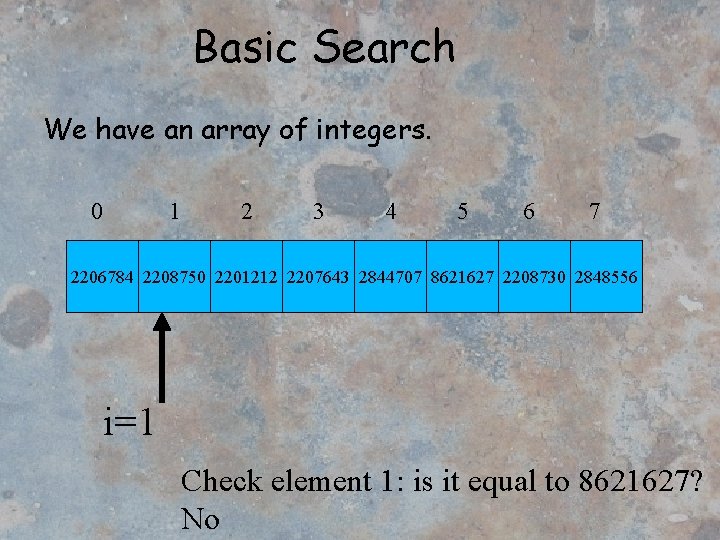
Basic Search We have an array of integers. 0 1 2 3 4 5 6 7 2206784 2208750 2201212 2207643 2844707 8621627 2208730 2848556 i=1 Check element 1: is it equal to 8621627? No
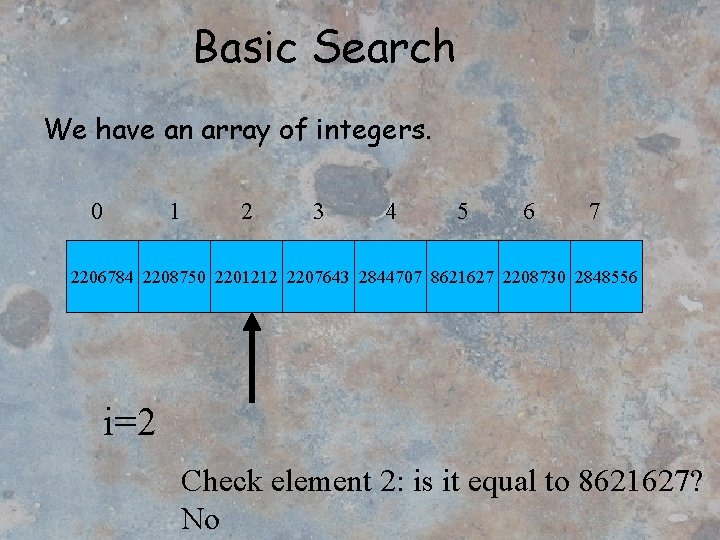
Basic Search We have an array of integers. 0 1 2 3 4 5 6 7 2206784 2208750 2201212 2207643 2844707 8621627 2208730 2848556 i=2 Check element 2: is it equal to 8621627? No
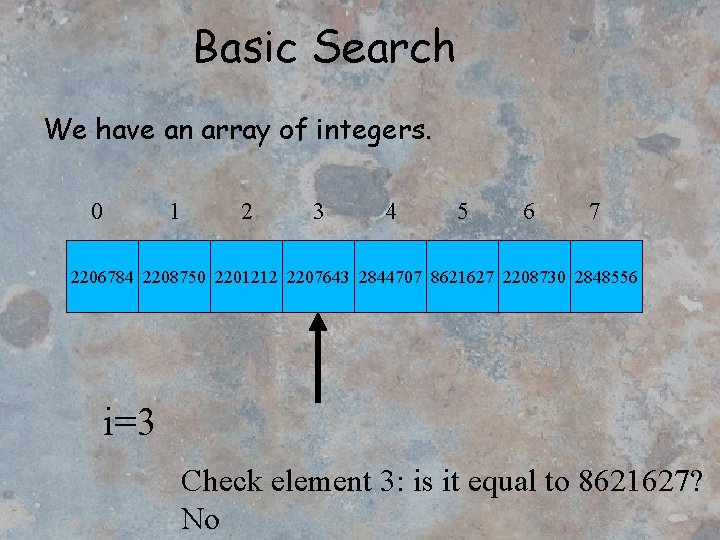
Basic Search We have an array of integers. 0 1 2 3 4 5 6 7 2206784 2208750 2201212 2207643 2844707 8621627 2208730 2848556 i=3 Check element 3: is it equal to 8621627? No
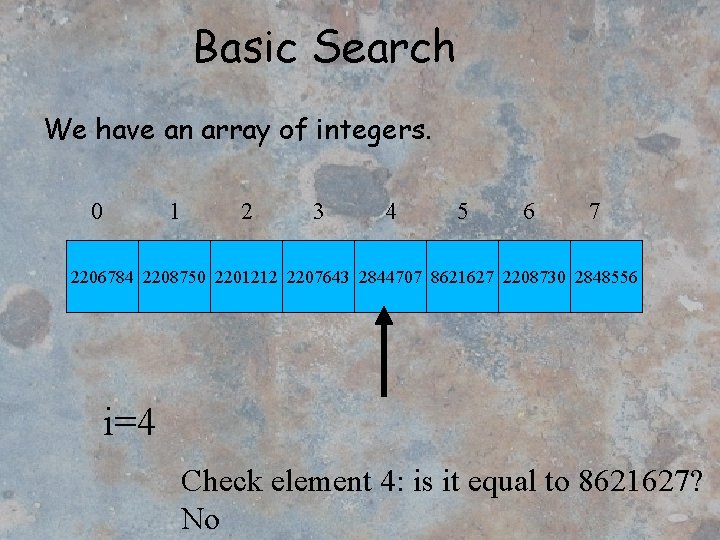
Basic Search We have an array of integers. 0 1 2 3 4 5 6 7 2206784 2208750 2201212 2207643 2844707 8621627 2208730 2848556 i=4 Check element 4: is it equal to 8621627? No
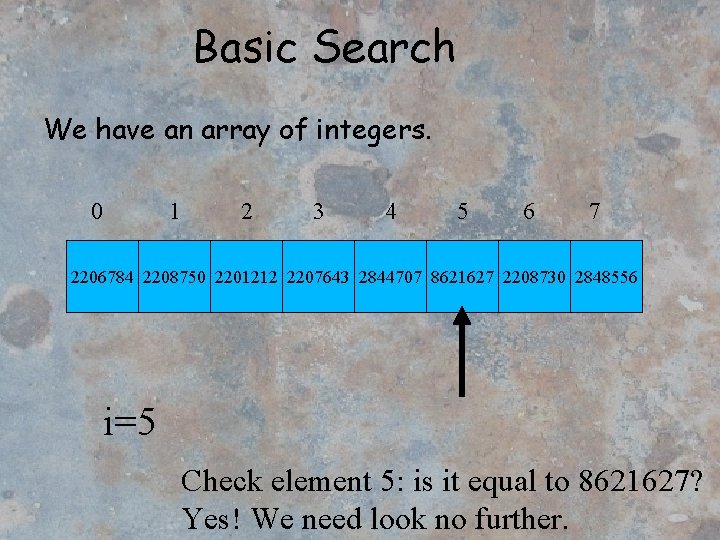
Basic Search We have an array of integers. 0 1 2 3 4 5 6 7 2206784 2208750 2201212 2207643 2844707 8621627 2208730 2848556 i=5 Check element 5: is it equal to 8621627? Yes! We need look no further.
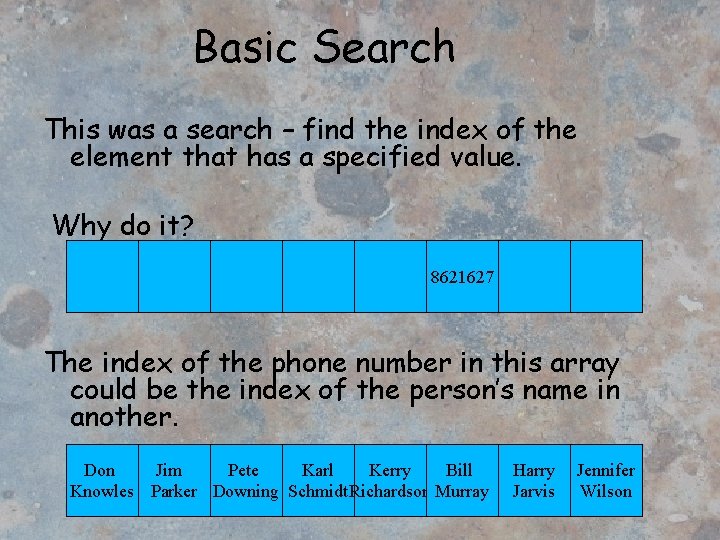
Basic Search This was a search – find the index of the element that has a specified value. Why do it? 8621627 The index of the phone number in this array could be the index of the person’s name in another. Don Jim Pete Karl Kerry Bill Knowles Parker Downing Schmidt Richardson Murray Harry Jarvis Jennifer Wilson
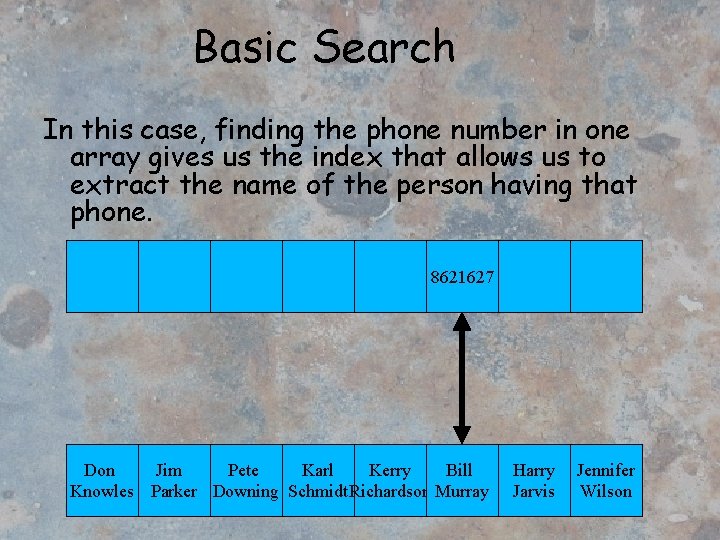
Basic Search In this case, finding the phone number in one array gives us the index that allows us to extract the name of the person having that phone. 8621627 Don Jim Pete Karl Kerry Bill Knowles Parker Downing Schmidt Richardson Murray Harry Jarvis Jennifer Wilson
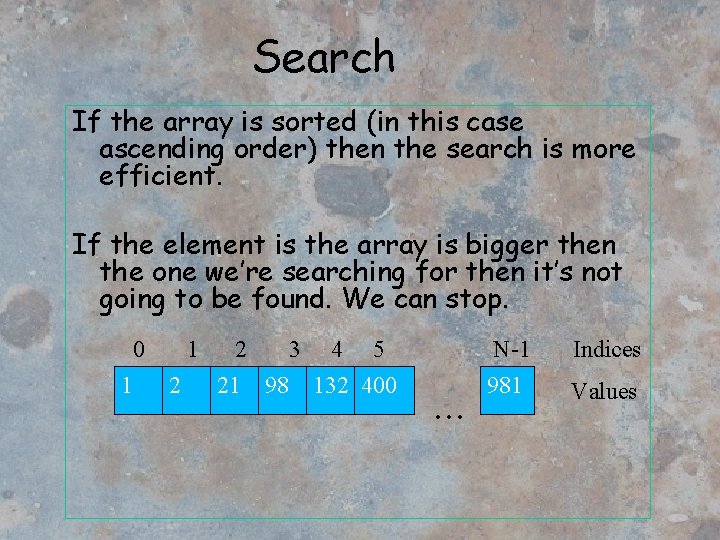
Search If the array is sorted (in this case ascending order) then the search is more efficient. If the element is the array is bigger then the one we’re searching for then it’s not going to be found. We can stop. 0 1 1 2 2 3 4 5 21 98 132 400 . . . N-1 981 Indices Values
![Search for i0 iN i if arrayi target The value has Search for (i=0; i<N; i++) { if (array[i] == target) // The value has](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-14.jpg)
Search for (i=0; i<N; i++) { if (array[i] == target) // The value has been found. } result = i;
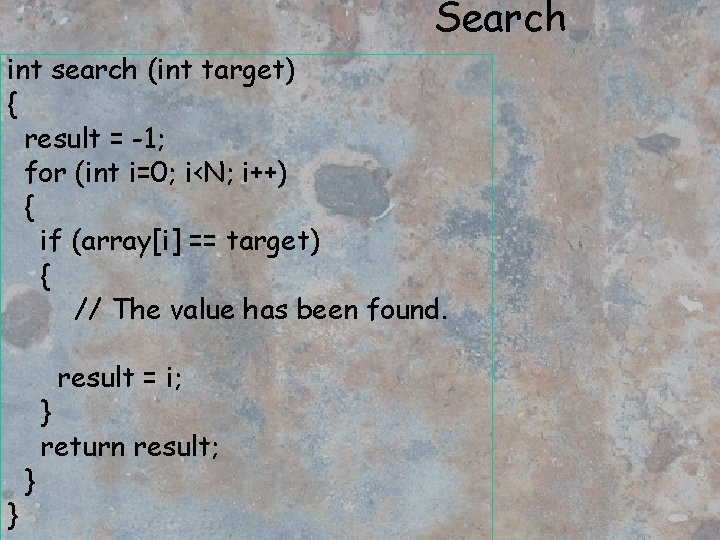
Search int search (int target) { result = -1; for (int i=0; i<N; i++) { if (array[i] == target) { // The value has been found. result = i; } } } return result;
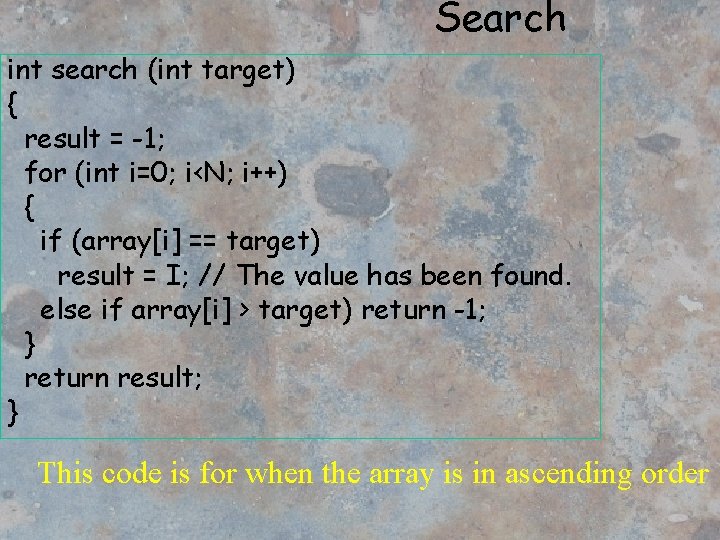
Search int search (int target) { result = -1; for (int i=0; i<N; i++) { if (array[i] == target) result = I; // The value has been found. else if array[i] > target) return -1; } return result; } This code is for when the array is in ascending order
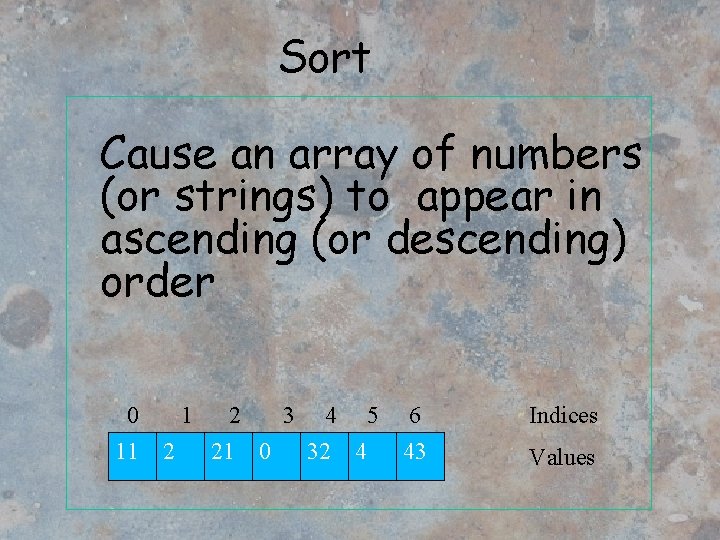
Sort Cause an array of numbers (or strings) to appear in ascending (or descending) order 0 11 1 2 2 3 4 5 21 0 32 4 6 43 Indices Values
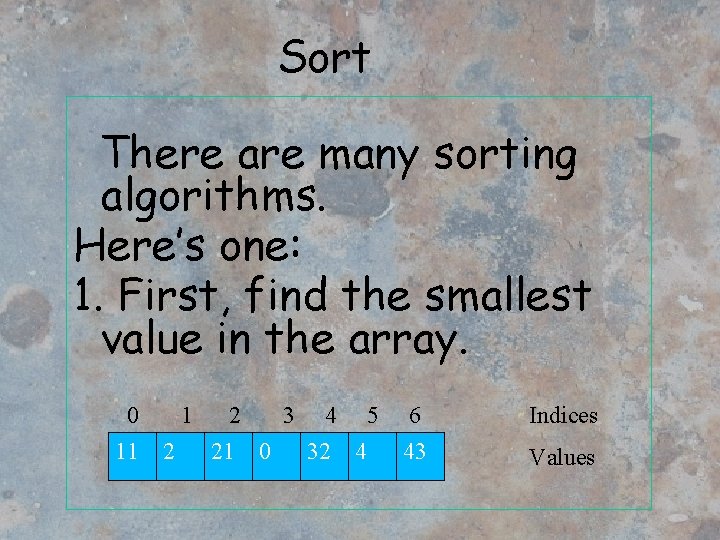
Sort There are many sorting algorithms. Here’s one: 1. First, find the smallest value in the array. 0 11 1 2 2 3 4 5 21 0 32 4 6 43 Indices Values
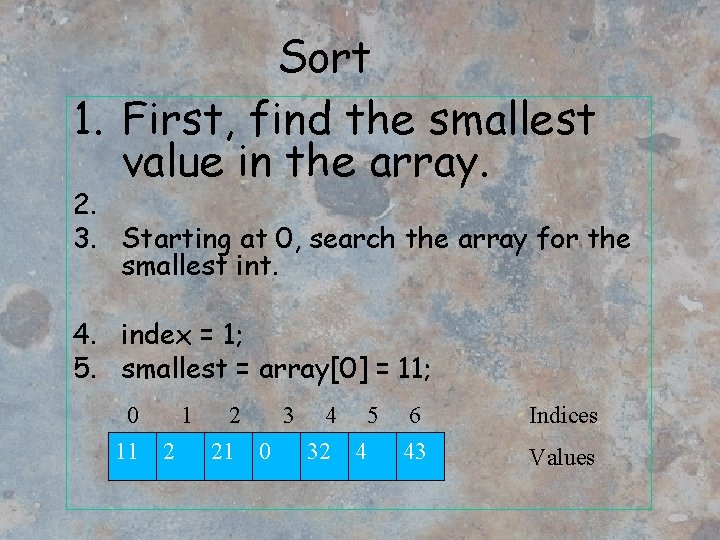
Sort 1. First, find the smallest value in the array. 2. 3. Starting at 0, search the array for the smallest int. 4. index = 1; 5. smallest = array[0] = 11; 0 11 1 2 2 3 4 5 21 0 32 4 6 43 Indices Values
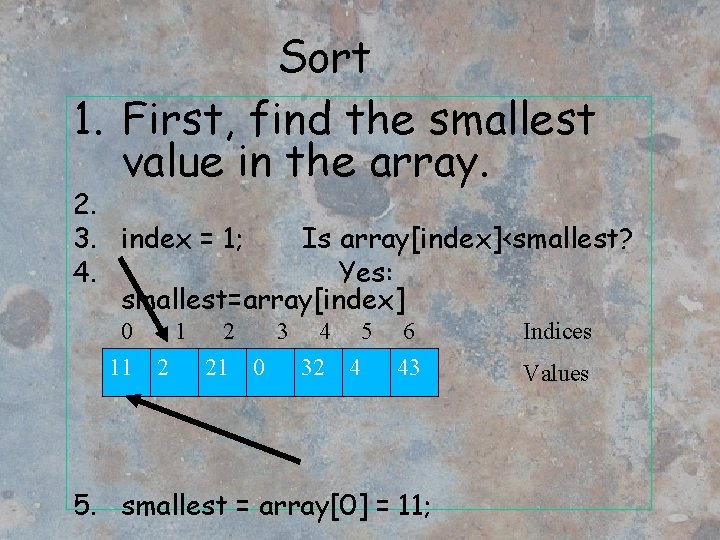
Sort 1. First, find the smallest value in the array. 2. 3. index = 1; Is array[index]<smallest? 4. Yes: smallest=array[index] 0 11 1 2 2 3 4 5 21 0 32 4 6 43 5. smallest = array[0] = 11; Indices Values
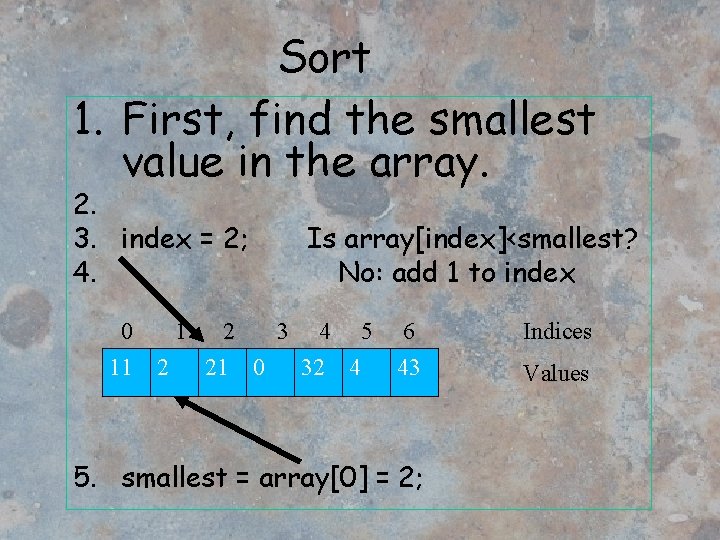
Sort 1. First, find the smallest value in the array. 2. 3. index = 2; 4. 0 11 1 2 Is array[index]<smallest? No: add 1 to index 2 3 4 5 21 0 32 4 6 43 5. smallest = array[0] = 2; Indices Values
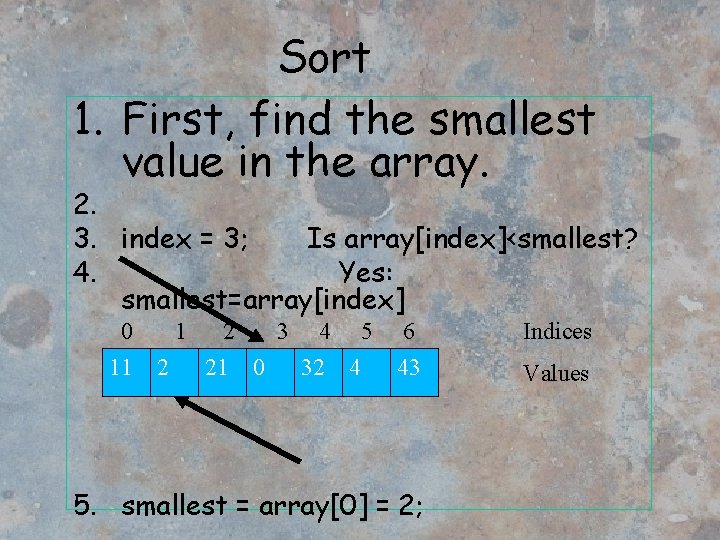
Sort 1. First, find the smallest value in the array. 2. 3. index = 3; Is array[index]<smallest? 4. Yes: smallest=array[index] 0 11 1 2 2 3 4 5 21 0 32 4 6 43 5. smallest = array[0] = 2; Indices Values
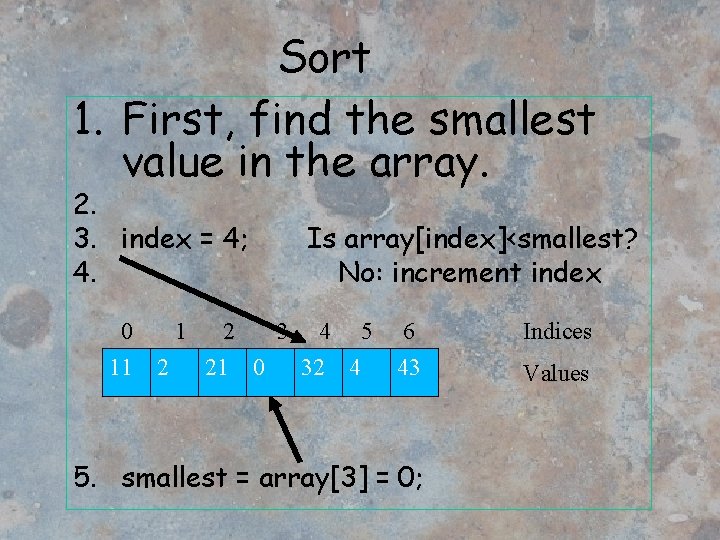
Sort 1. First, find the smallest value in the array. 2. 3. index = 4; 4. 0 11 1 2 Is array[index]<smallest? No: increment index 2 3 4 5 21 0 32 4 6 43 5. smallest = array[3] = 0; Indices Values
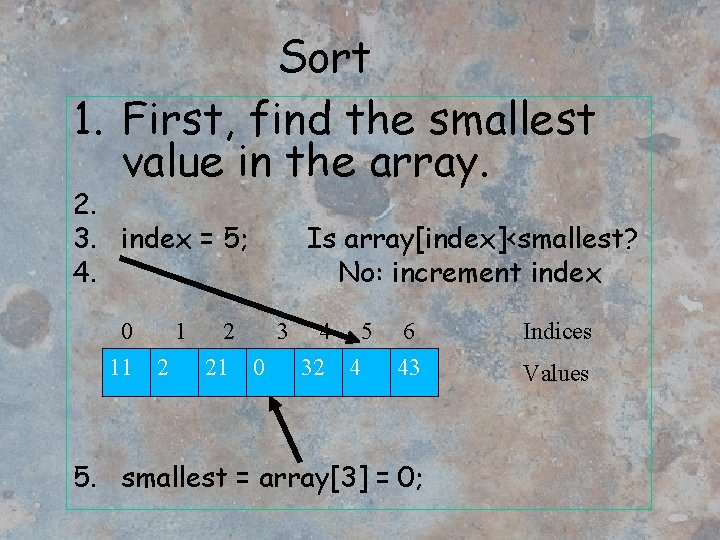
Sort 1. First, find the smallest value in the array. 2. 3. index = 5; 4. 0 11 1 2 Is array[index]<smallest? No: increment index 2 3 4 5 21 0 32 4 6 43 5. smallest = array[3] = 0; Indices Values
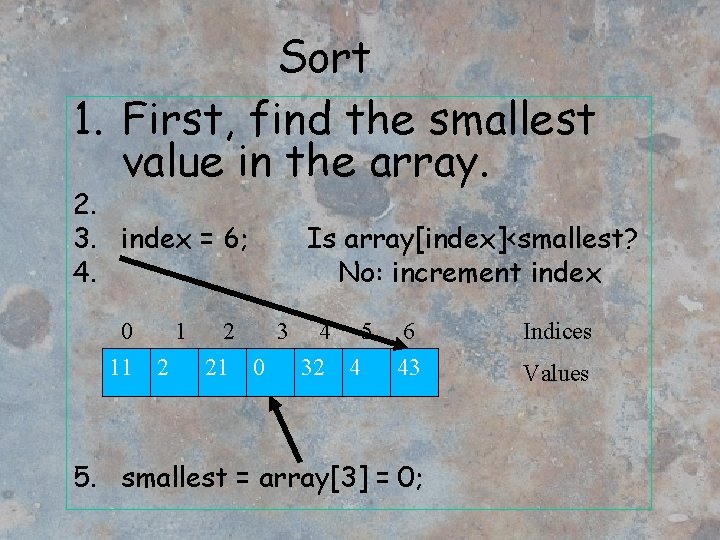
Sort 1. First, find the smallest value in the array. 2. 3. index = 6; 4. 0 11 1 2 Is array[index]<smallest? No: increment index 2 3 4 5 21 0 32 4 6 43 5. smallest = array[3] = 0; Indices Values
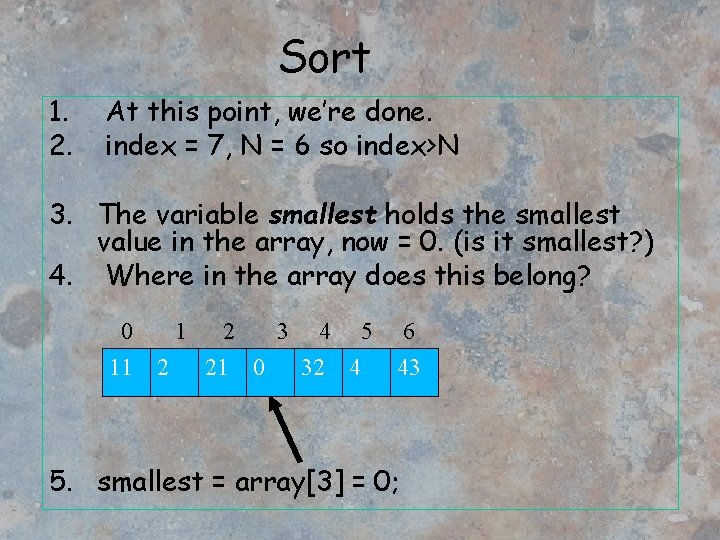
Sort 1. 2. At this point, we’re done. index = 7, N = 6 so index>N 3. The variable smallest holds the smallest value in the array, now = 0. (is it smallest? ) 4. Where in the array does this belong? 0 11 1 2 2 3 4 5 21 0 32 4 6 43 5. smallest = array[3] = 0;
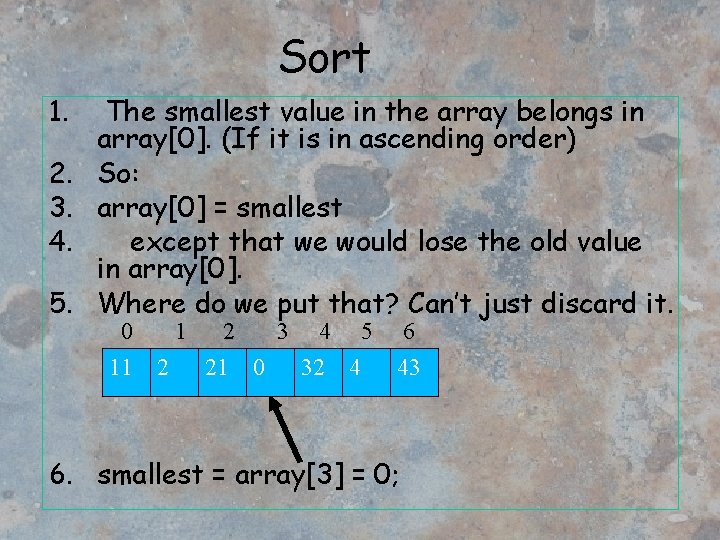
Sort 1. 2. 3. 4. 5. The smallest value in the array belongs in array[0]. (If it is in ascending order) So: array[0] = smallest except that we would lose the old value in array[0]. Where do we put that? Can’t just discard it. 0 11 1 2 2 3 4 5 21 0 32 4 6 43 6. smallest = array[3] = 0;
![Sort 1 The smallest value in the array belongs in array0 If it is Sort 1. The smallest value in the array belongs in array[0]. (If it is](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-28.jpg)
Sort 1. The smallest value in the array belongs in array[0]. (If it is in ascending order) 2. So: 3. array[0] = smallest 4. except that we would lose the old value in array[0]. 0 11 1 2 2 21 3 4 5 32 4 6 43 0 5. Where do we put that? Can’t just discard it.
![Sort 1 We could put the smallest value in array0 and the old value Sort 1. We could put the smallest value in array[0] and the old value](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-29.jpg)
Sort 1. We could put the smallest value in array[0] and the old value that was in array[0] into the place where the smallest came from. 2. … but we goofed. We know what the smallest value is, but not where we got it. We did not save the index of where we 11 found it. 0 0 1 2 2 21 3 4 5 32 4 6 43 3. Where do we put that? Can’t just discard it.
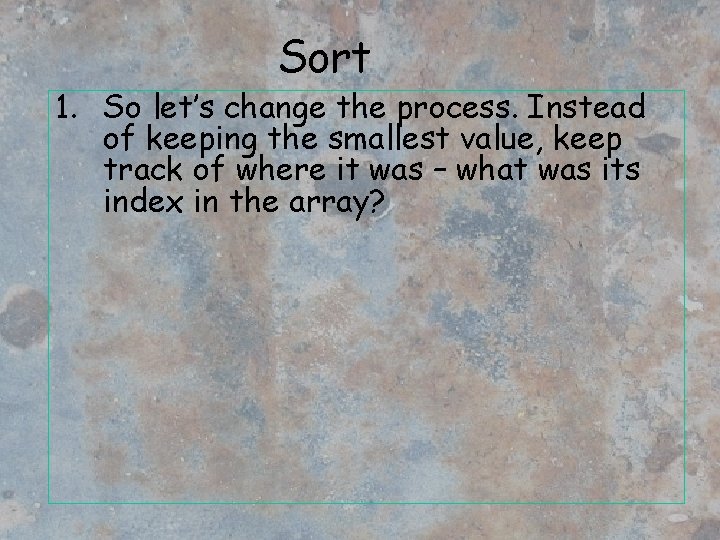
Sort 1. So let’s change the process. Instead of keeping the smallest value, keep track of where it was – what was its index in the array?
![Sort 1 smallest initially 0 2 index 1 Is arrayindexarraysmallest 3 Yes smallestindex Sort 1. smallest initially 0 2. index = 1; Is array[index]<array[smallest]? 3. Yes: smallest=index](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-31.jpg)
Sort 1. smallest initially 0 2. index = 1; Is array[index]<array[smallest]? 3. Yes: smallest=index 0 11 4. 11; 1 2 2 3 4 5 21 0 32 4 6 43 smallest = 0, array[smallest] = 31
![Sort 1 2 index 2 Is arrayindexarraysmallest 3 No add 1 to index Sort 1. 2. index = 2; Is array[index]<array[smallest]? 3. No: add 1 to index](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-32.jpg)
Sort 1. 2. index = 2; Is array[index]<array[smallest]? 3. No: add 1 to index 0 11 4. 1 2 2 3 4 5 21 0 32 4 6 43 smallest = 1 array[1] = 2; 32
![Sort 1 2 index 3 Is arrayindexarraysmallest 3 Yes smallestindex 0 11 4 Sort 1. 2. index = 3; Is array[index]<array[smallest]? 3. Yes: smallest=index 0 11 4.](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-33.jpg)
Sort 1. 2. index = 3; Is array[index]<array[smallest]? 3. Yes: smallest=index 0 11 4. 1 2 2 3 4 5 21 0 32 4 6 43 smallest = 1 array[1] = 2; 33
![Sort 1 2 index 4 Is arrayindexarraysmallest 3 No increment index 0 11 Sort 1. 2. index = 4; Is array[index]<array[smallest]? 3. No: increment index 0 11](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-34.jpg)
Sort 1. 2. index = 4; Is array[index]<array[smallest]? 3. No: increment index 0 11 4. 1 2 2 3 4 5 21 0 32 4 smallest =3 6 43 array[3]= 0; 34
![Sort 1 2 index 5 Is arrayindexarraysmallest 3 No increment index 0 11 Sort 1. 2. index = 5; Is array[index]<array[smallest]? 3. No: increment index 0 11](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-35.jpg)
Sort 1. 2. index = 5; Is array[index]<array[smallest]? 3. No: increment index 0 11 4. 5. 1 2 2 3 4 5 21 0 32 4 smallest =3 6 43 Indices Values array[3] = 0; 35
![Sort 1 2 index 6 Is arrayindexarraysmallest 3 No increment index 0 11 Sort 1. 2. index = 6; Is array[index]<array[smallest]? 3. No: increment index 0 11](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-36.jpg)
Sort 1. 2. index = 6; Is array[index]<array[smallest]? 3. No: increment index 0 11 4. 1 2 2 3 4 5 21 0 32 4 6 43 smallest =3 array[3] = 0; 36
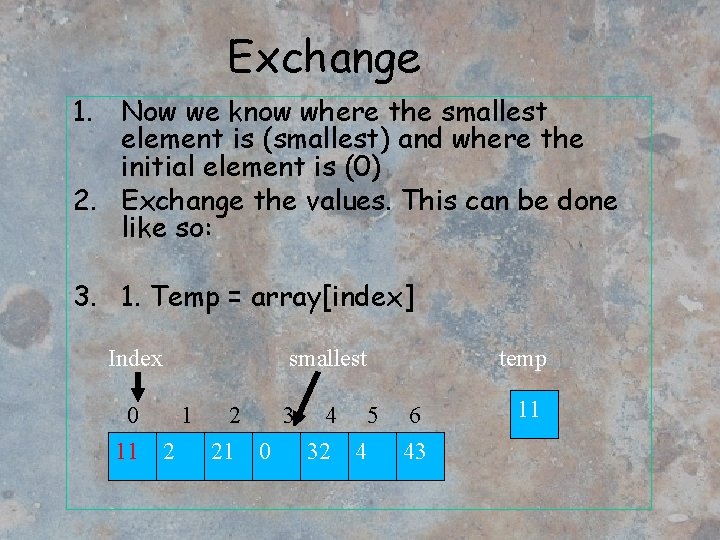
Exchange 1. Now we know where the smallest element is (smallest) and where the initial element is (0) 2. Exchange the values. This can be done like so: 3. 1. Temp = array[index] Index 0 11 smallest 1 2 2 3 4 5 21 0 32 4 temp 6 43 11
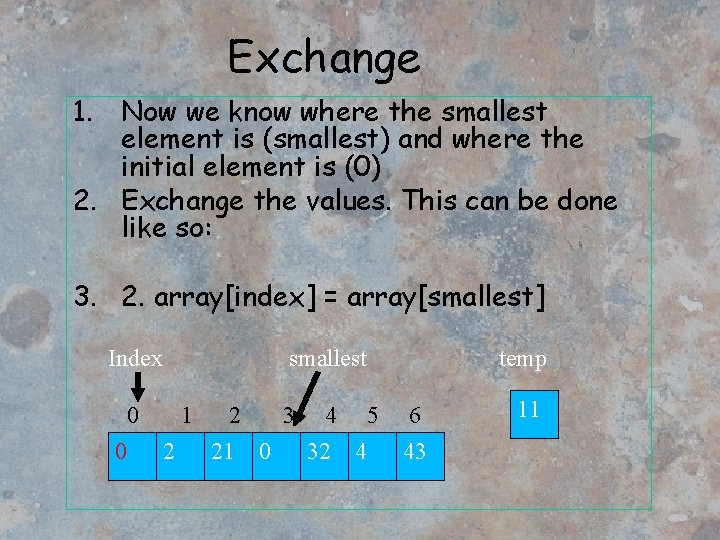
Exchange 1. Now we know where the smallest element is (smallest) and where the initial element is (0) 2. Exchange the values. This can be done like so: 3. 2. array[index] = array[smallest] Index smallest 0 0 1 2 2 3 4 5 21 0 32 4 temp 6 43 11
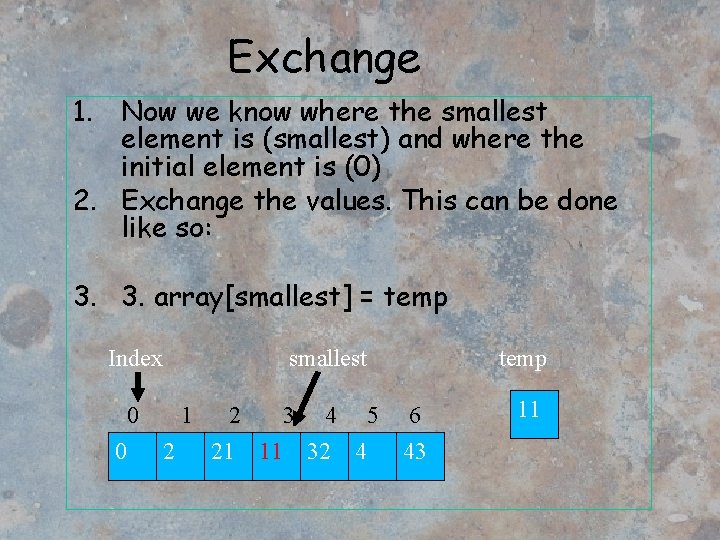
Exchange 1. Now we know where the smallest element is (smallest) and where the initial element is (0) 2. Exchange the values. This can be done like so: 3. 3. array[smallest] = temp Index smallest 0 0 1 2 2 3 4 5 21 11 32 4 temp 6 43 11
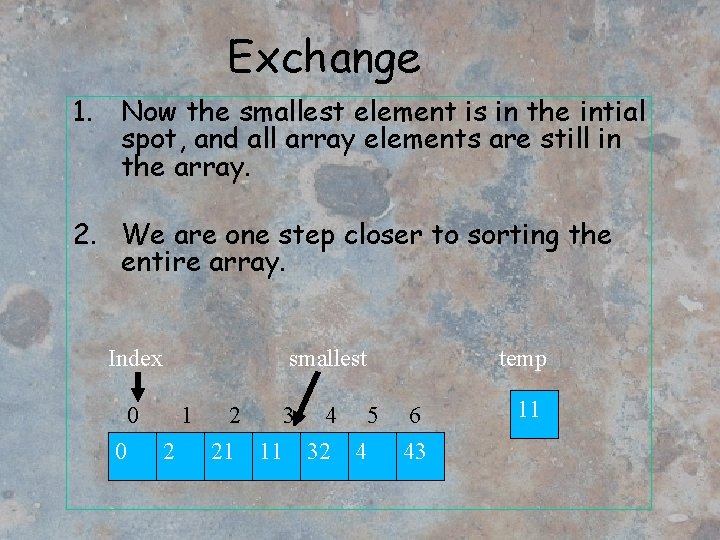
Exchange 1. Now the smallest element is in the intial spot, and all array elements are still in the array. 2. We are one step closer to sorting the entire array. Index smallest 0 0 1 2 2 3 4 5 21 11 32 4 temp 6 43 11
![Code smallest0 for i1 iN i Find smallest if arrayi arraysmallest smallest Code smallest=0; for (i=1; i<N; i++) // Find smallest if (array[i] < array[smallest]) smallest](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-41.jpg)
Code smallest=0; for (i=1; i<N; i++) // Find smallest if (array[i] < array[smallest]) smallest = i; // exchange first for smallest temp = array[i]; array[i] = array[smallest]; array[smallest] = temp; This only puts one element in order (the first). Now what?
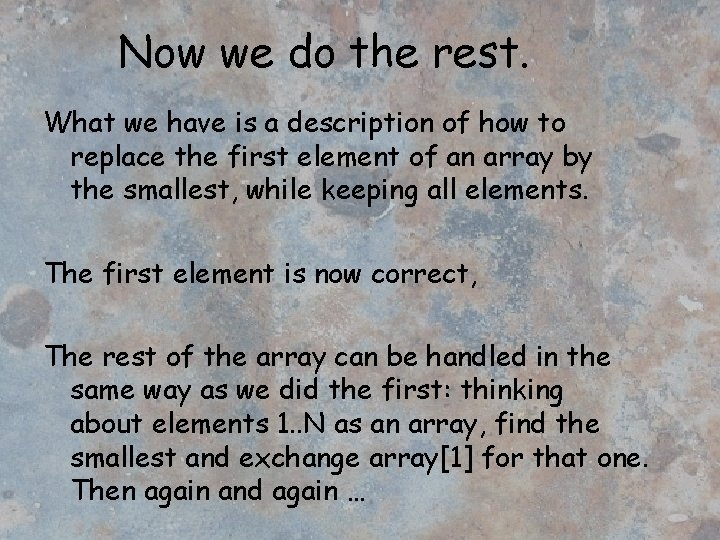
Now we do the rest. What we have is a description of how to replace the first element of an array by the smallest, while keeping all elements. The first element is now correct, The rest of the array can be handled in the same way as we did the first: thinking about elements 1. . N as an array, find the smallest and exchange array[1] for that one. Then again and again …
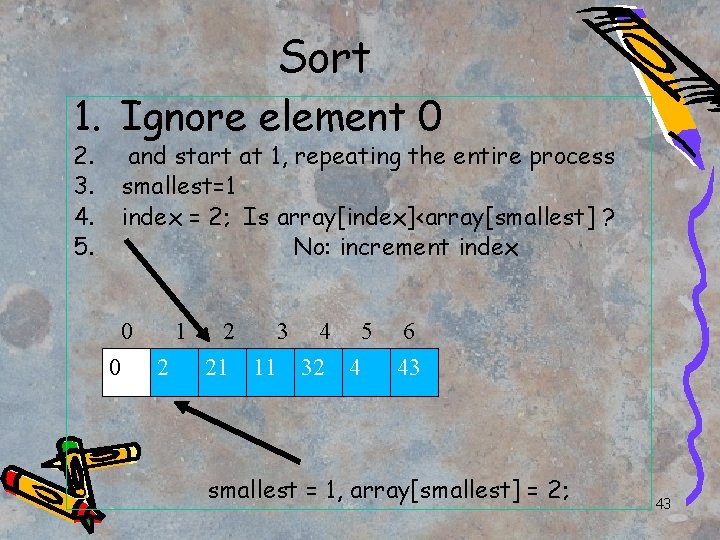
Sort 1. Ignore element 0 2. 3. 4. 5. and start at 1, repeating the entire process smallest=1 index = 2; Is array[index]<array[smallest] ? No: increment index 0 0 6. 1 2 2 3 4 5 21 11 32 4 6 43 smallest = 1, array[smallest] = 2; 43
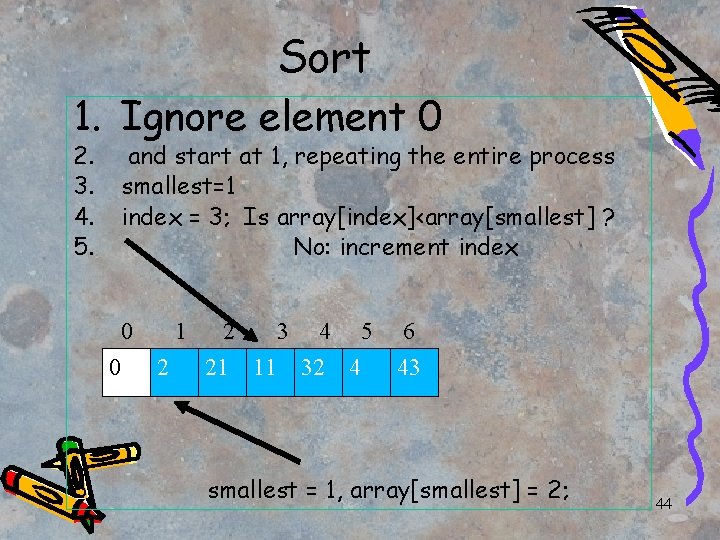
Sort 1. Ignore element 0 2. 3. 4. 5. and start at 1, repeating the entire process smallest=1 index = 3; Is array[index]<array[smallest] ? No: increment index 0 0 6. 1 2 2 3 4 5 21 11 32 4 6 43 smallest = 1, array[smallest] = 2; 44
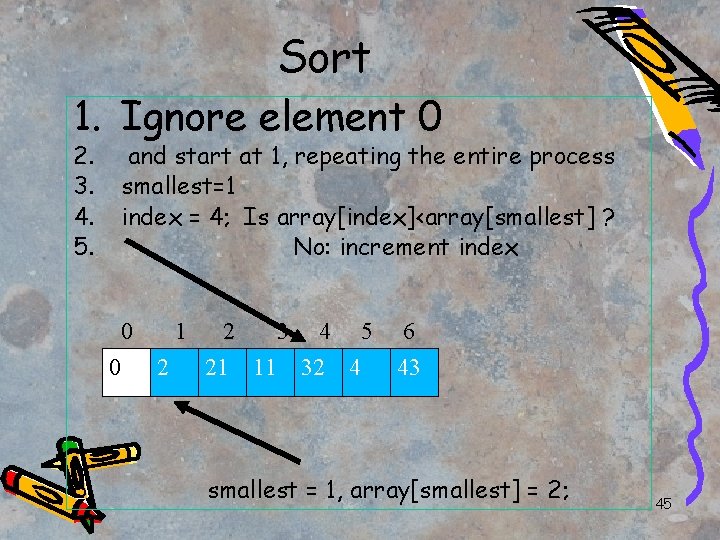
Sort 1. Ignore element 0 2. 3. 4. 5. and start at 1, repeating the entire process smallest=1 index = 4; Is array[index]<array[smallest] ? No: increment index 0 0 6. 1 2 2 3 4 5 21 11 32 4 6 43 smallest = 1, array[smallest] = 2; 45
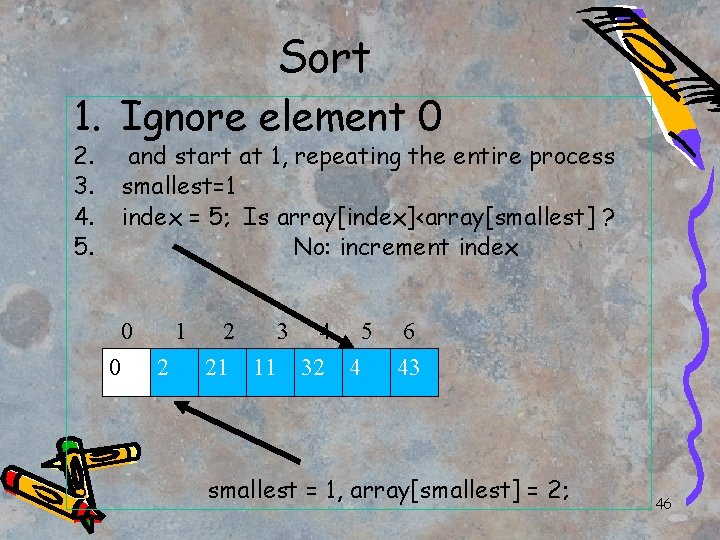
Sort 1. Ignore element 0 2. 3. 4. 5. and start at 1, repeating the entire process smallest=1 index = 5; Is array[index]<array[smallest] ? No: increment index 0 0 6. 1 2 2 3 4 5 21 11 32 4 6 43 smallest = 1, array[smallest] = 2; 46
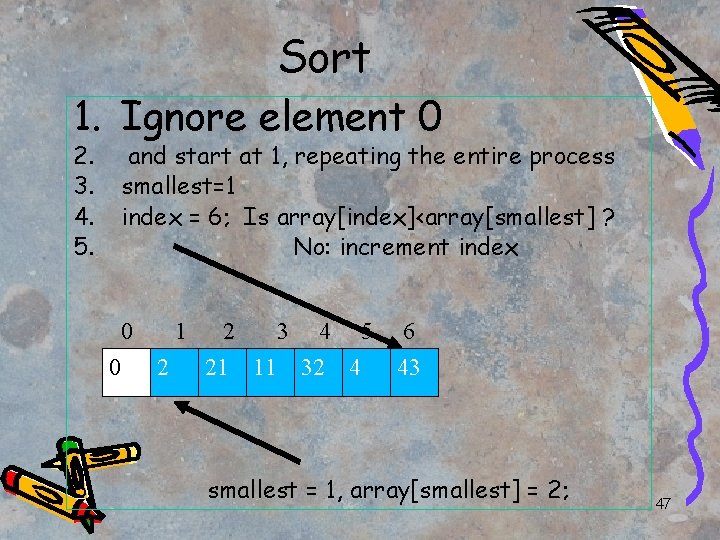
Sort 1. Ignore element 0 2. 3. 4. 5. and start at 1, repeating the entire process smallest=1 index = 6; Is array[index]<array[smallest] ? No: increment index 0 0 6. 1 2 2 3 4 5 21 11 32 4 6 43 smallest = 1, array[smallest] = 2; 47
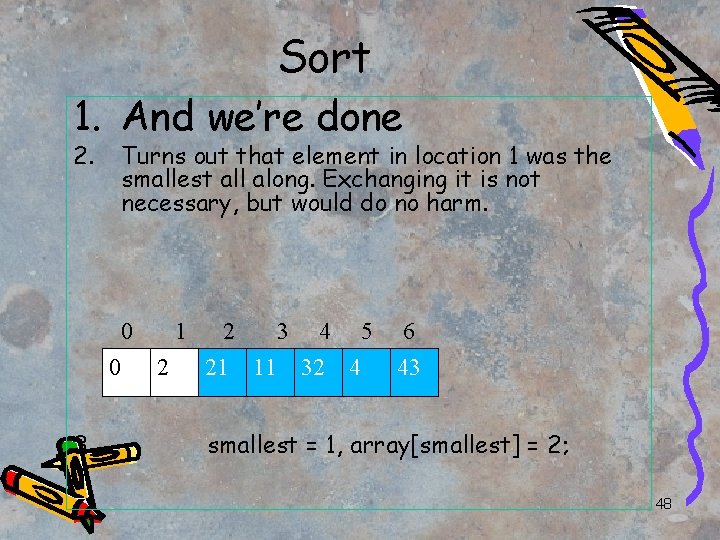
Sort 1. And we’re done 2. Turns out that element in location 1 was the smallest all along. Exchanging it is not necessary, but would do no harm. 0 0 3. 1 2 2 3 4 5 21 11 32 4 6 43 smallest = 1, array[smallest] = 2; 48
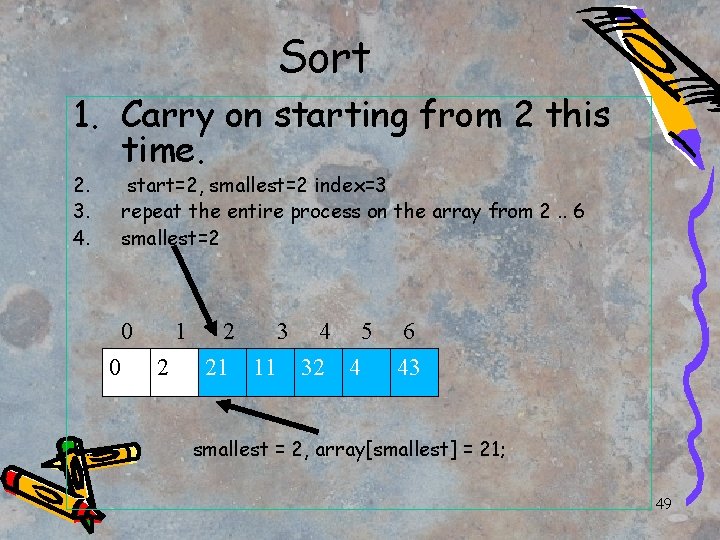
Sort 1. Carry on starting from 2 this time. 2. 3. 4. start=2, smallest=2 index=3 repeat the entire process on the array from 2. . 6 smallest=2 0 0 5. 1 2 2 3 4 5 21 11 32 4 6 43 smallest = 2, array[smallest] = 21; 49
![Sort 1 Ignore elements 0 and 1 2 3 index 3 Is arrayindexarraysmallest Sort 1. Ignore elements 0 and 1 2. 3. index = 3; Is array[index]<array[smallest]](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-50.jpg)
Sort 1. Ignore elements 0 and 1 2. 3. index = 3; Is array[index]<array[smallest] ? Yes: smallest = index 0 4. 1 2 3 4 5 21 11 32 4 6 43 smallest = 2, array[smallest] = 21; 50
![Sort 1 Ignore elements 0 and 1 2 3 index 4 Is arrayindexarraysmallest Sort 1. Ignore elements 0 and 1 2. 3. index = 4; Is array[index]<array[smallest]](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-51.jpg)
Sort 1. Ignore elements 0 and 1 2. 3. index = 4; Is array[index]<array[smallest] ? No: increment index 0 4. 1 2 3 4 5 21 11 32 4 6 43 smallest =3, array[smallest] = 11; 51
![Sort 1 Ignore elements 0 and 1 2 3 index 5 Is arrayindexarraysmallest Sort 1. Ignore elements 0 and 1 2. 3. index = 5; Is array[index]<array[smallest]](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-52.jpg)
Sort 1. Ignore elements 0 and 1 2. 3. index = 5; Is array[index]<array[smallest] ? yes: smallest = index 0 4. 1 2 3 4 5 21 11 32 4 6 43 smallest =3, array[smallest] = 11; 52
![Sort 1 Ignore elements 0 and 1 2 3 index 5 Is arrayindexarraysmallest Sort 1. Ignore elements 0 and 1 2. 3. index = 5; Is array[index]<array[smallest]](https://slidetodoc.com/presentation_image_h2/bc577f7fa7ffa1d661178232ac607989/image-53.jpg)
Sort 1. Ignore elements 0 and 1 2. 3. index = 5; Is array[index]<array[smallest] ? no: increment index 0 4. 1 2 3 4 5 21 11 32 4 6 43 smallest =5, array[smallest] = 4; 53
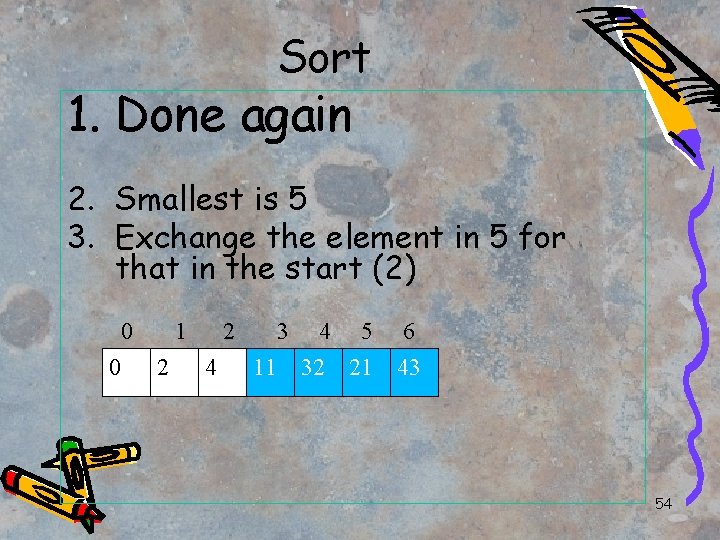
Sort 1. Done again 2. Smallest is 5 3. Exchange the element in 5 for that in the start (2) 0 0 1 2 2 4 3 11 4 5 32 21 6 43 54
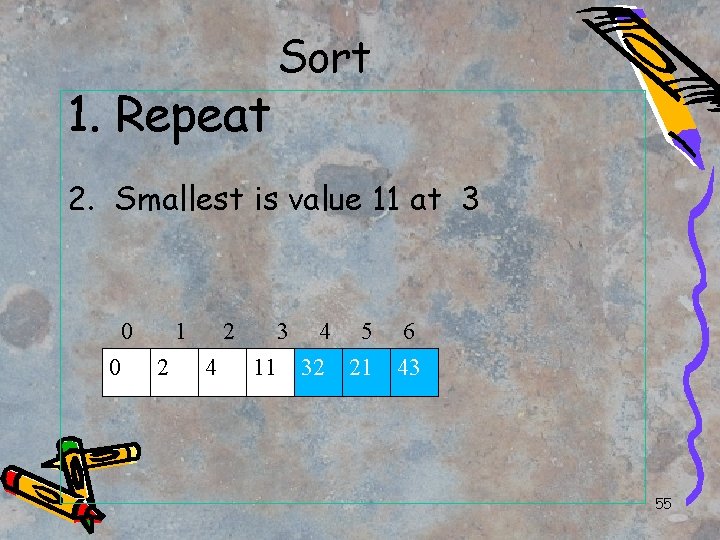
1. Repeat Sort 2. Smallest is value 11 at 3 0 0 1 2 2 4 3 11 4 5 32 21 6 43 55
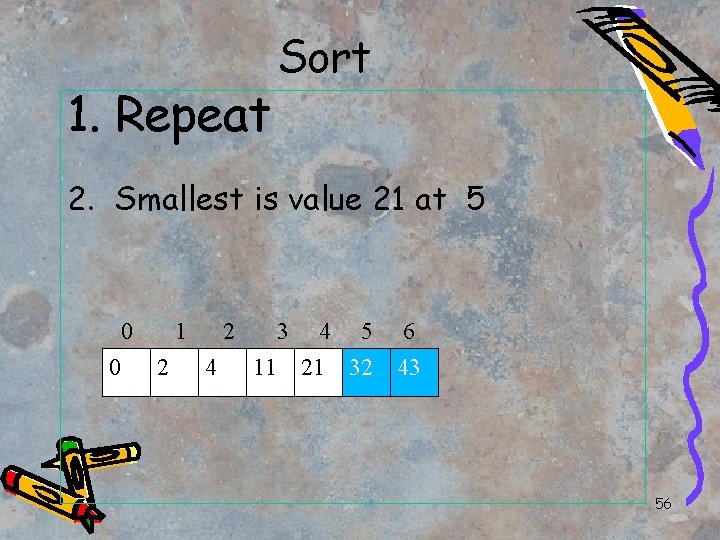
1. Repeat Sort 2. Smallest is value 21 at 5 0 0 1 2 2 4 3 11 4 5 21 32 6 43 56
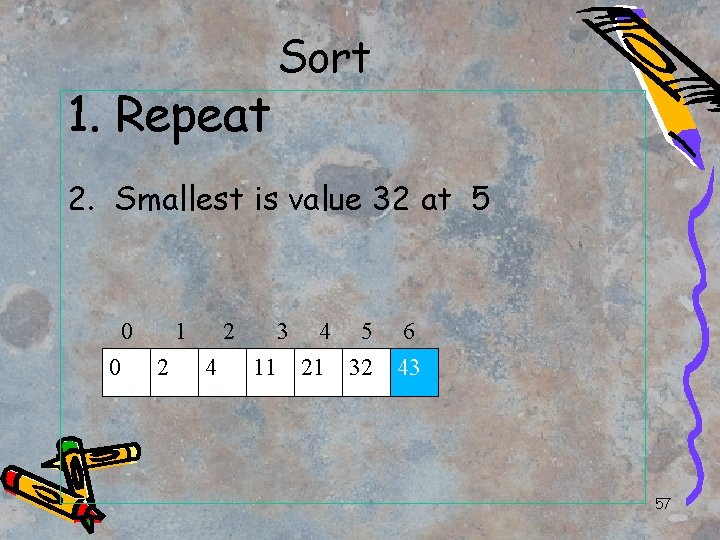
1. Repeat Sort 2. Smallest is value 32 at 5 0 0 1 2 2 4 3 11 4 5 21 32 6 43 57
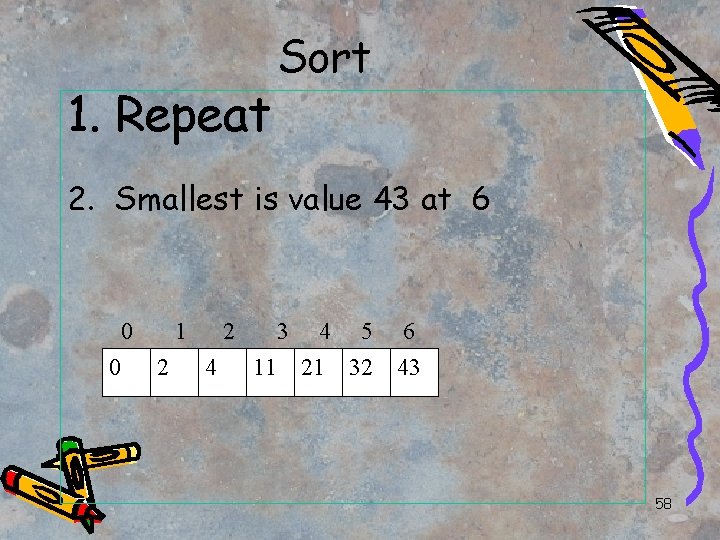
1. Repeat Sort 2. Smallest is value 43 at 6 0 0 1 2 2 4 3 11 4 5 21 32 6 43 58
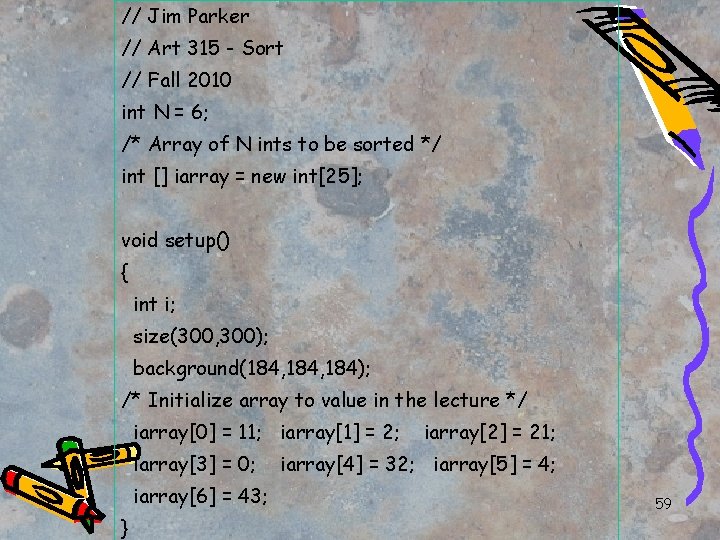
// Jim Parker // Art 315 - Sort // Fall 2010 int N = 6; /* Array of N ints to be sorted */ int [] iarray = new int[25]; void setup() { int i; size(300, 300); background(184, 184); /* Initialize array to value in the lecture */ iarray[0] = 11; iarray[1] = 2; iarray[3] = 0; iarray[6] = 43; } iarray[2] = 21; iarray[4] = 32; iarray[5] = 4; 59
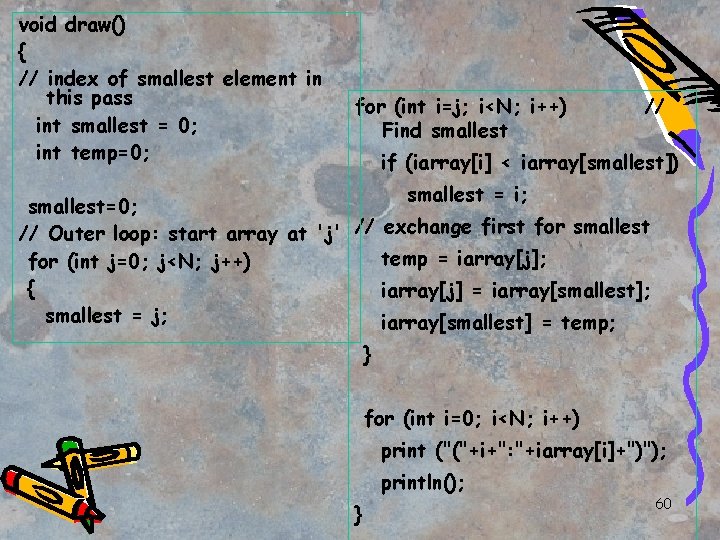
void draw() { // index of smallest element in this pass int smallest = 0; int temp=0; for (int i=j; i<N; i++) Find smallest // if (iarray[i] < iarray[smallest]) smallest = i; smallest=0; // Outer loop: start array at 'j' // exchange first for smallest temp = iarray[j]; for (int j=0; j<N; j++) { iarray[j] = iarray[smallest]; smallest = j; iarray[smallest] = temp; } for (int i=0; i<N; i++) print ("("+i+": "+iarray[i]+")"); println(); } 60
Computational thinking algorithms and programming
Synchronization algorithms and concurrent programming
Lerp
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Runtime programming
Linear vs integer programming
Definisi linear
Isa 315 revised
Isa 315 revised
Cvp graph
Find the exact value tan(315)
Pbb matematika
Nep 315
All students take calculus rule
Nilai sin 315 adalah
315
Economic 315
Operating system slides
Ece 451
Economic 315
Ba 315
Tentukan volume bola berikut a b c d e f
315 production
Kontinuitetshantering i praktiken
Typiska drag för en novell
Tack för att ni lyssnade bild
Returpilarna
Varför kallas perioden 1918-1939 för mellankrigstiden?
En lathund för arbete med kontinuitetshantering
Särskild löneskatt för pensionskostnader
Personlig tidbok för yrkesförare
A gastrica
Densitet vatten
Datorkunskap för nybörjare
Stig kerman
Debattartikel mall
Magnetsjukhus
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Vätsketryck formel
Publik sektor
Lyckans minut erik lindorm analys
Presentera för publik crossboss
Teckenspråk minoritetsspråk argument
Plats för toran ark
Treserva lathund
Luftstrupen för medicinare
Claes martinsson
Cks
Programskede byggprocessen
Mat för unga idrottare
Verktyg för automatisering av utbetalningar
Rutin för avvikelsehantering
Smärtskolan kunskap för livet
Ministerstyre för och nackdelar
Tack för att ni har lyssnat
Referatmarkeringar
Redogör för vad psykologi är
Borstål, egenskaper
Atmosfr