INTRODUCTION TO C PROGRAMMING Curso Propedeutico 2 CINVESTAVGuadalajara
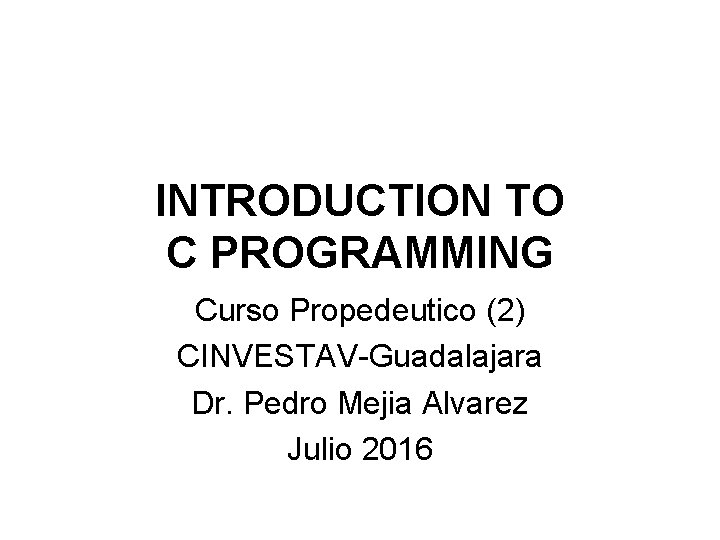
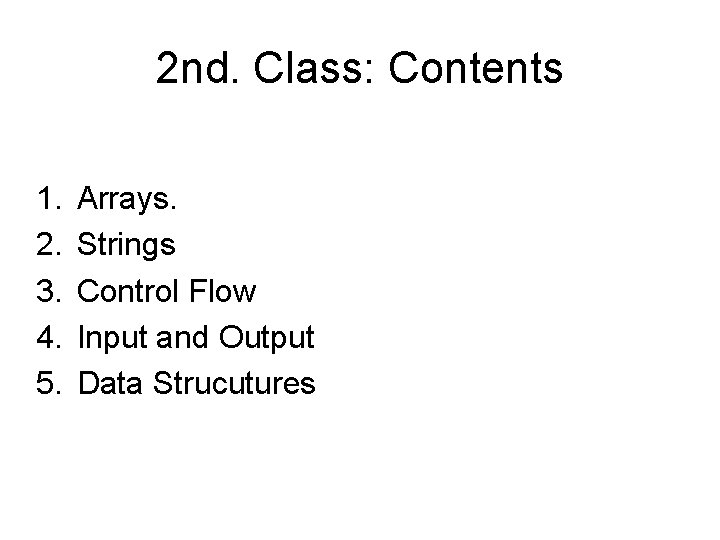
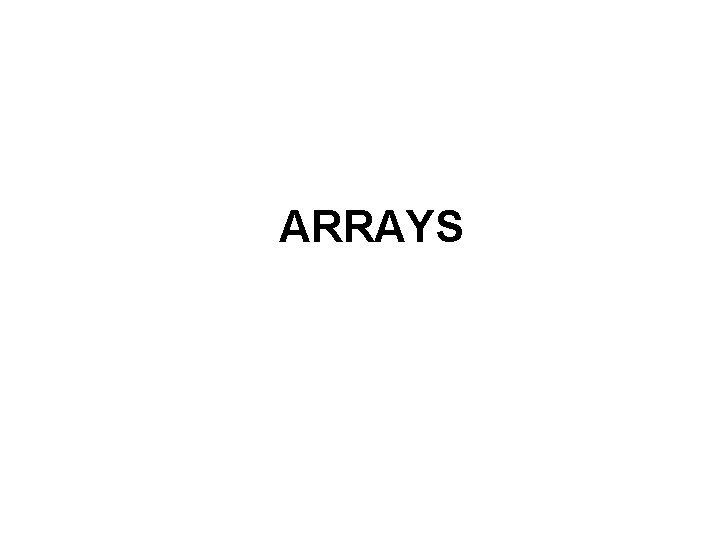
![One-Dimensional Arrays • A one-dimensional array is declared in the following way: int a[10]; One-Dimensional Arrays • A one-dimensional array is declared in the following way: int a[10];](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-4.jpg)
![Array Subscripting • To select an element of an array, use the [] operator: Array Subscripting • To select an element of an array, use the [] operator:](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-5.jpg)
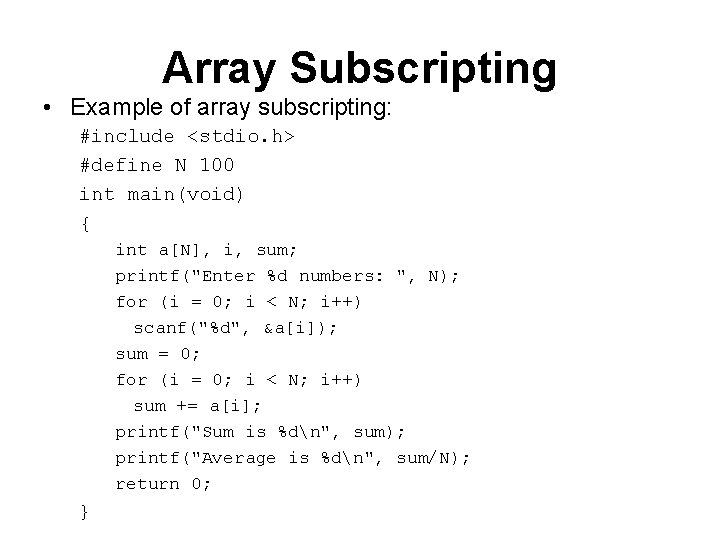
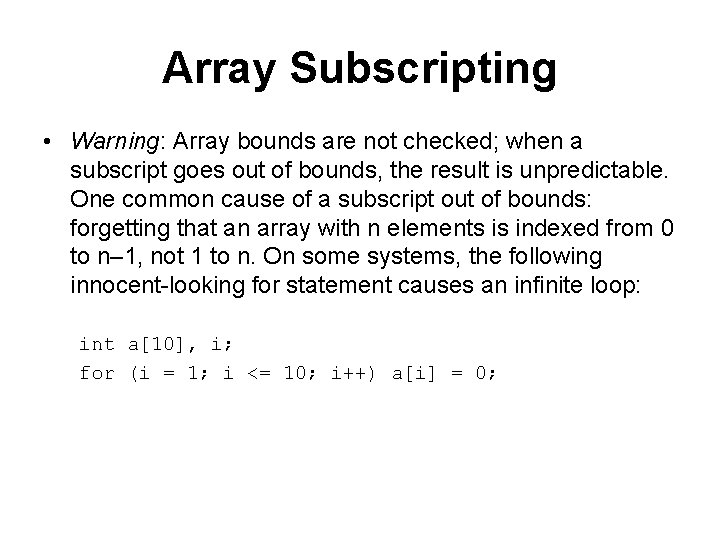
![Multidimensional Arrays • Arrays may have more than one dimension: int m[5][9]; m has Multidimensional Arrays • Arrays may have more than one dimension: int m[5][9]; m has](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-8.jpg)
![Multidimensional Arrays • An element of m is accessed by writing m[i][j]. • Example Multidimensional Arrays • An element of m is accessed by writing m[i][j]. • Example](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-9.jpg)
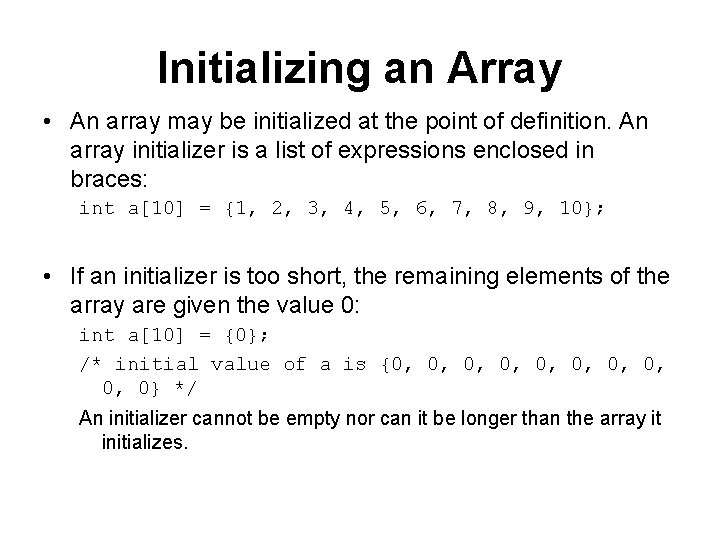
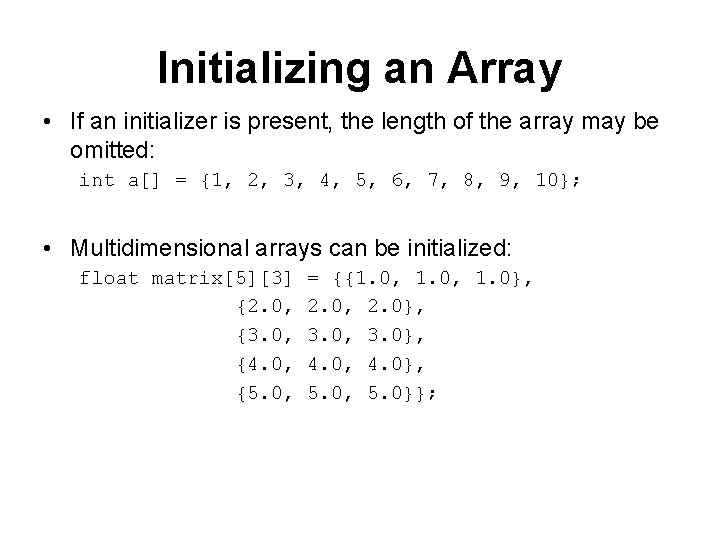
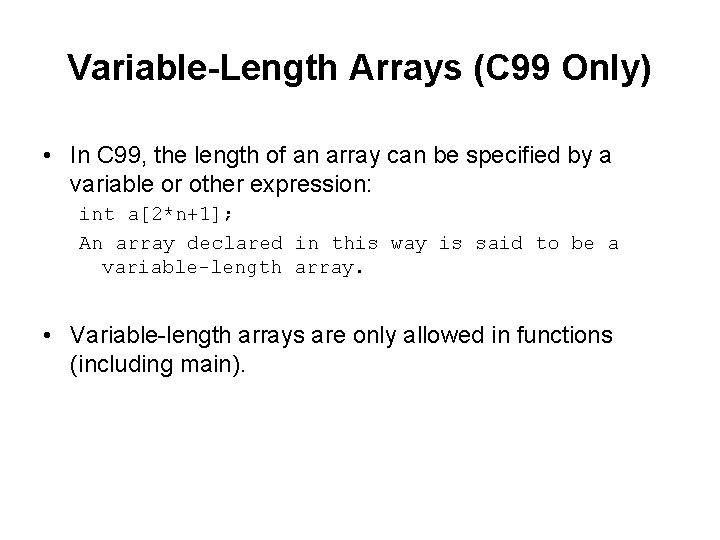
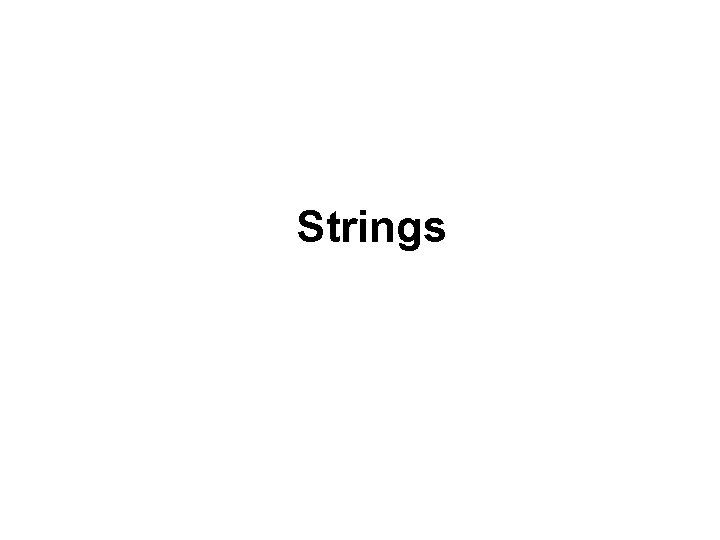
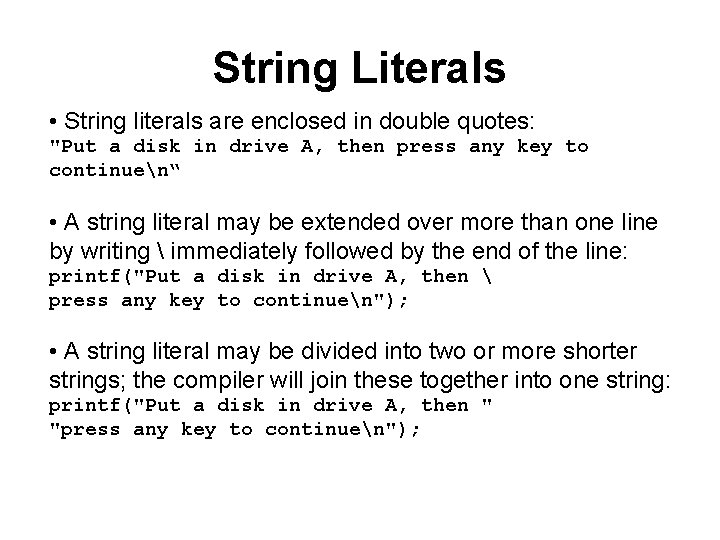
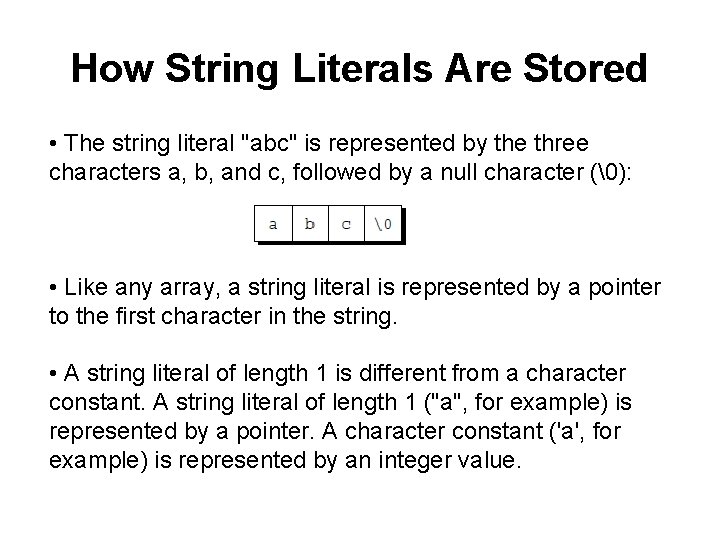
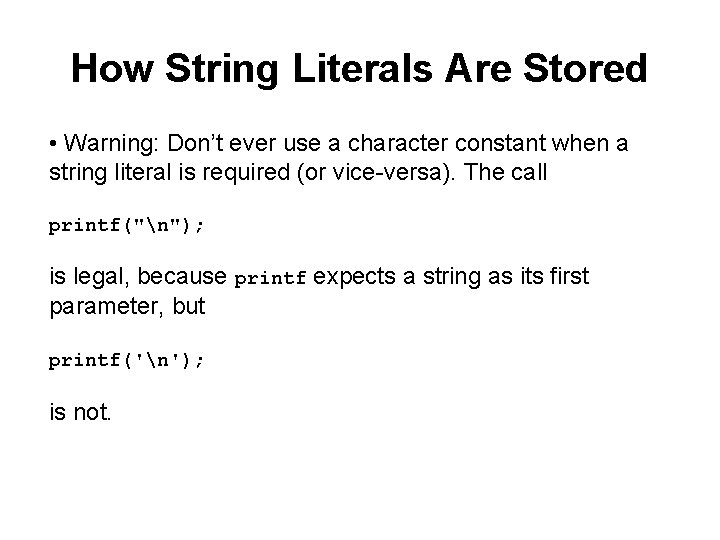
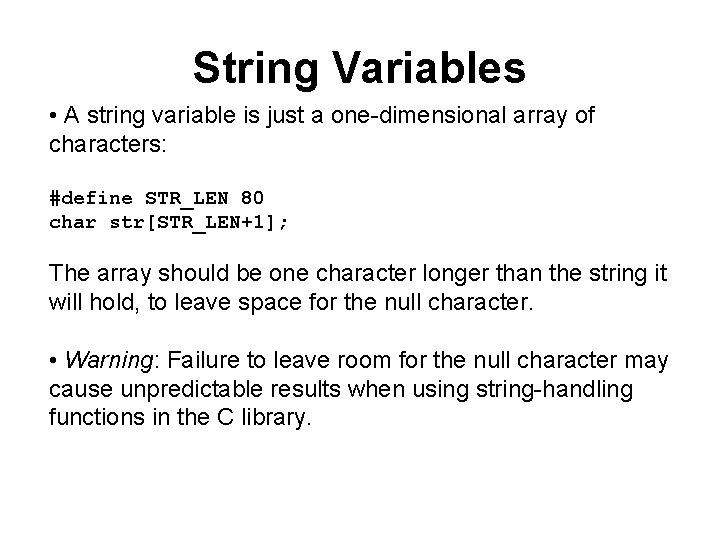
![String Variables • A string variable can be initialized: char date 1[8] = "June String Variables • A string variable can be initialized: char date 1[8] = "June](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-18.jpg)
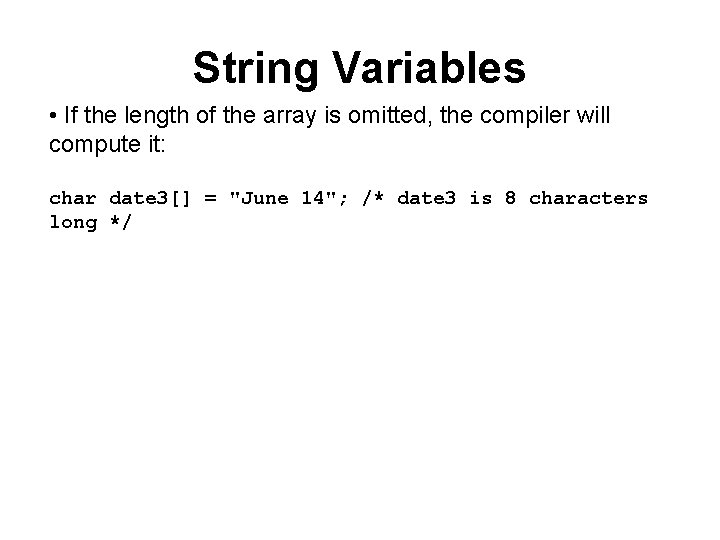
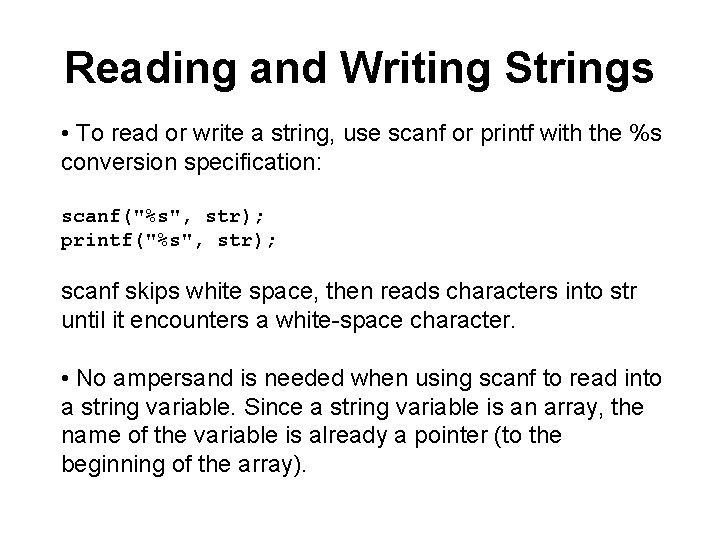
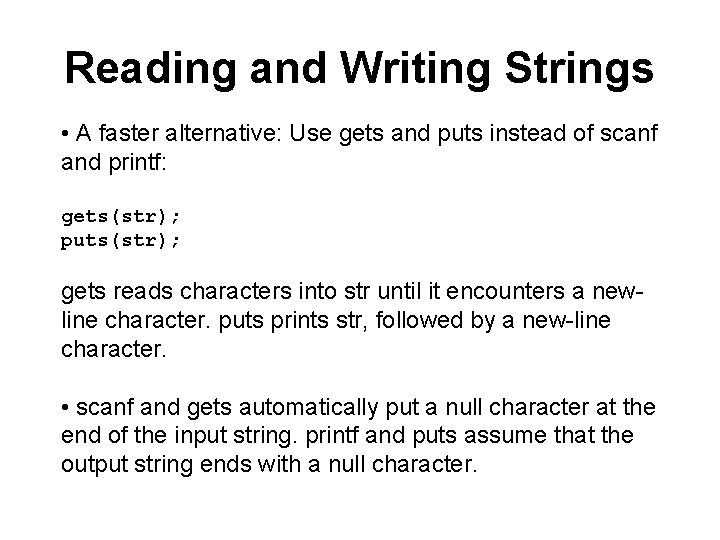
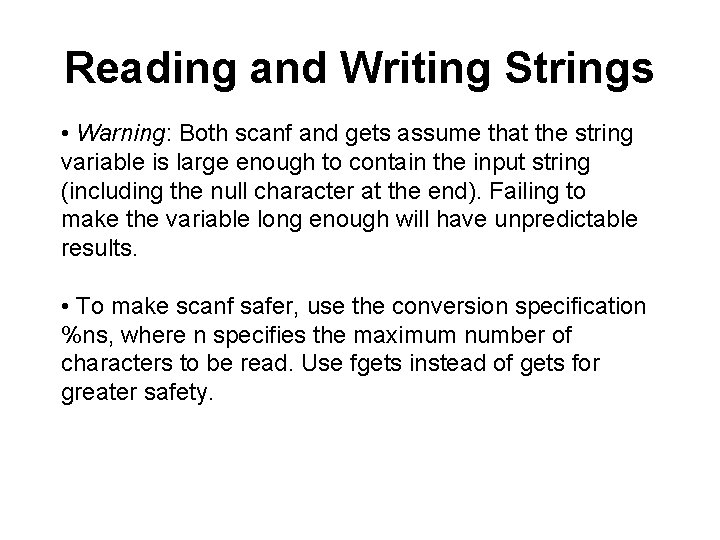
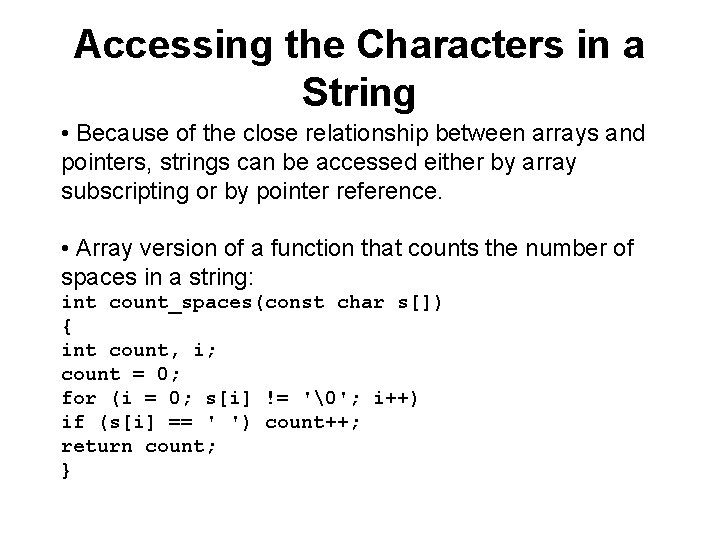
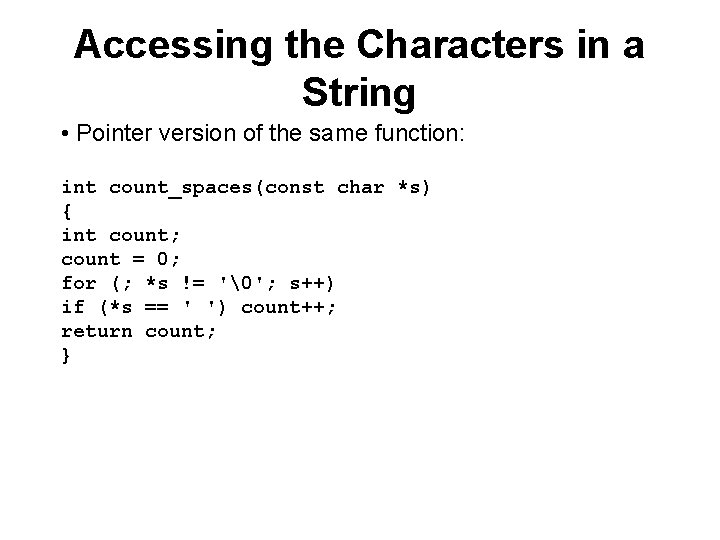
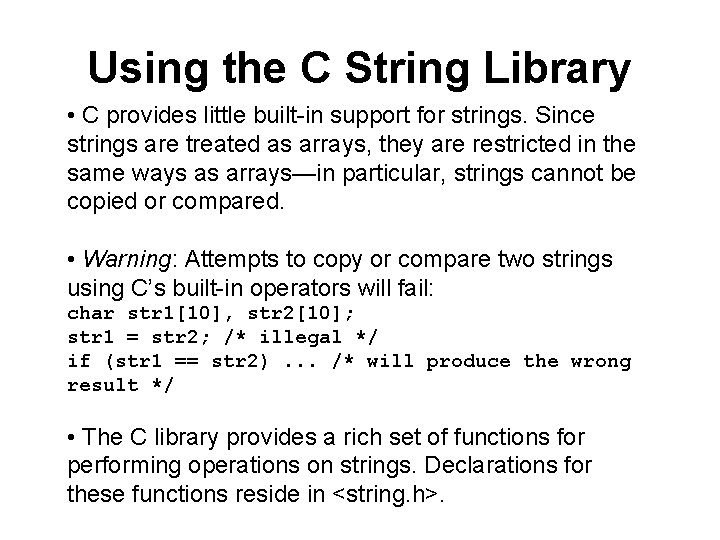
![The strcpy Function • strcpy copies one string into another: char str 1[10], str The strcpy Function • strcpy copies one string into another: char str 1[10], str](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-26.jpg)
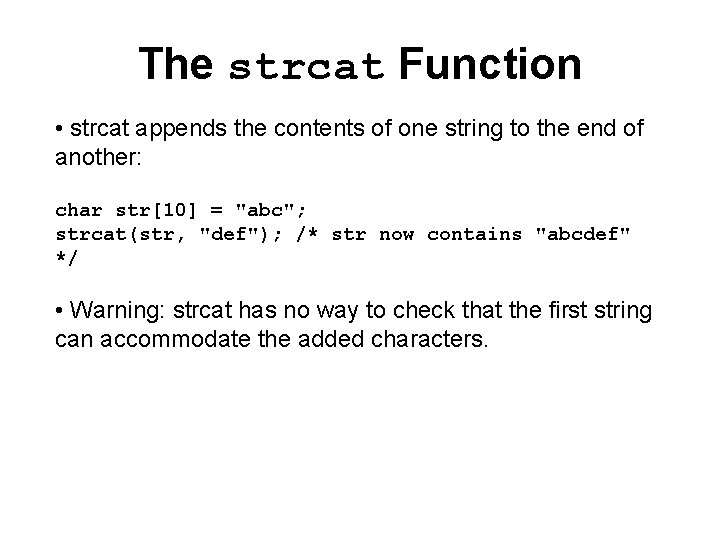
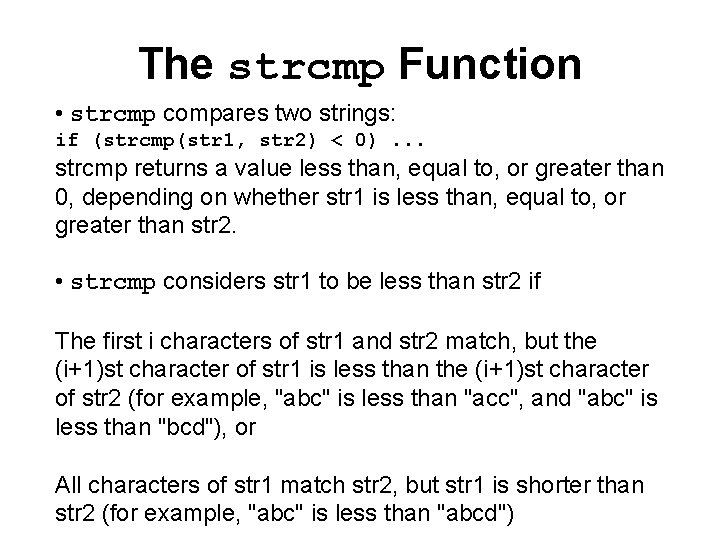
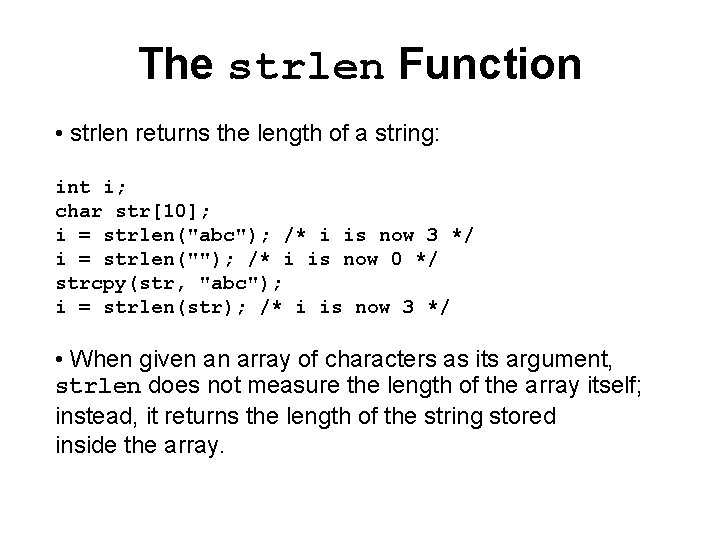
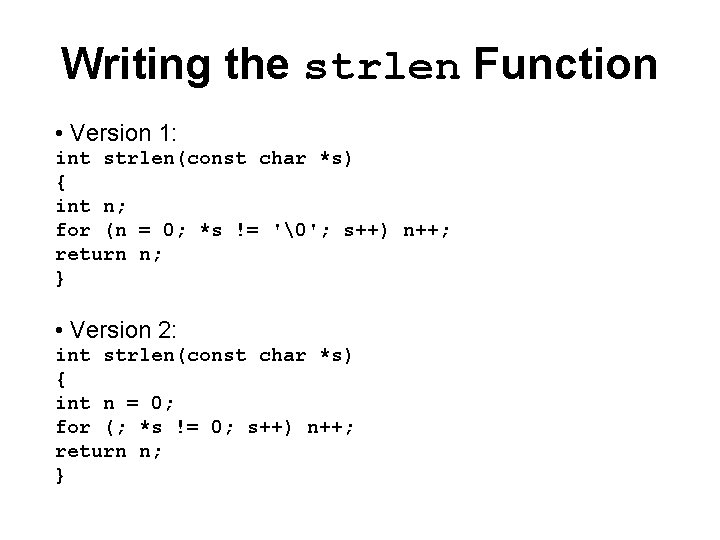
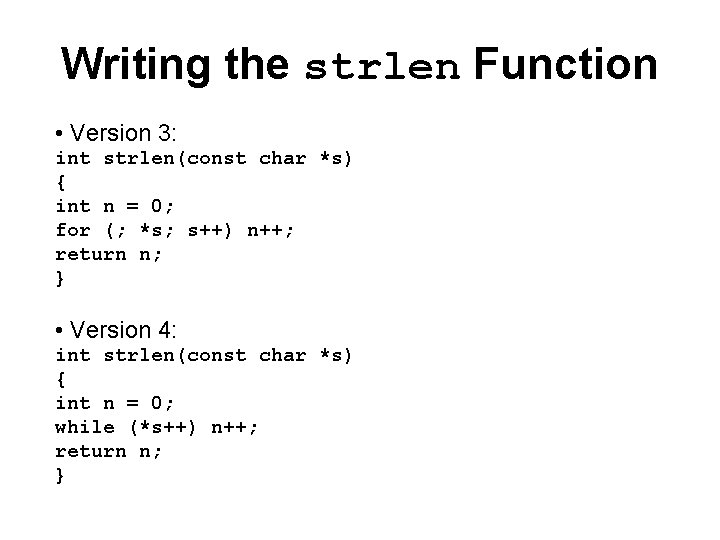
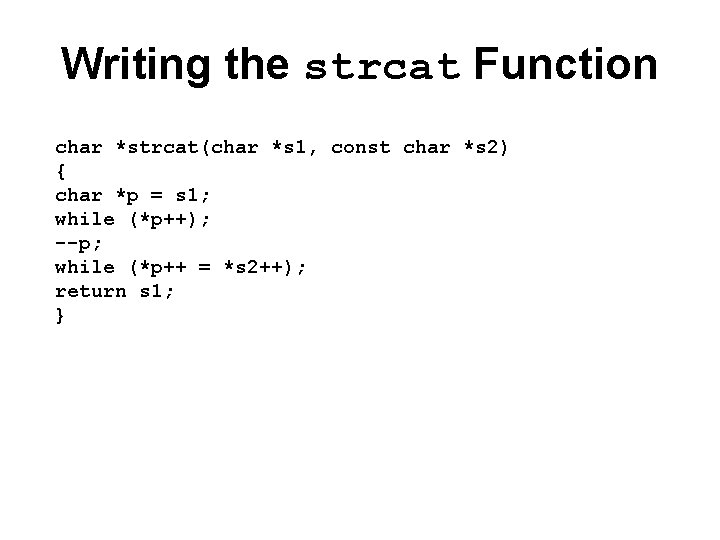
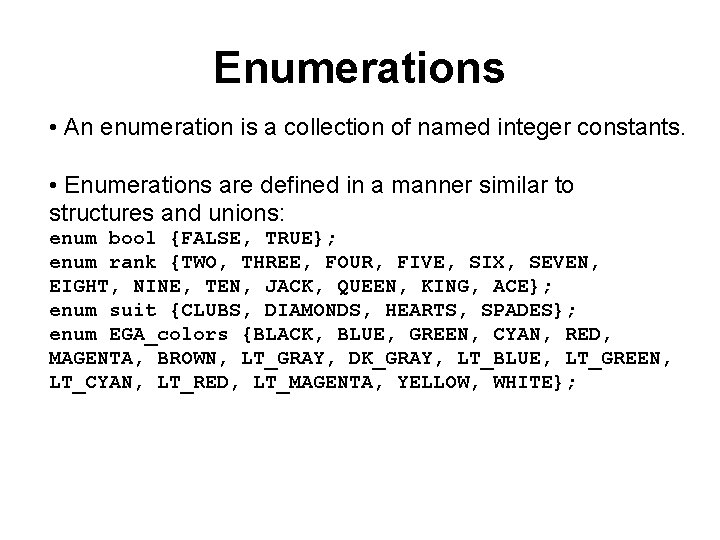
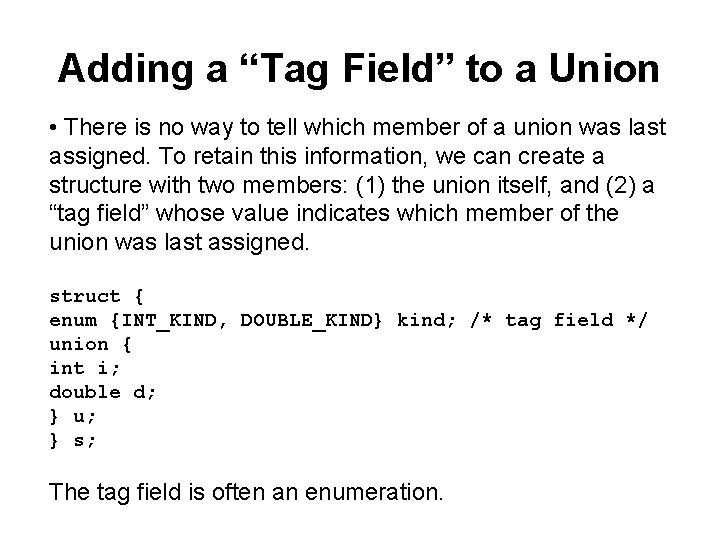
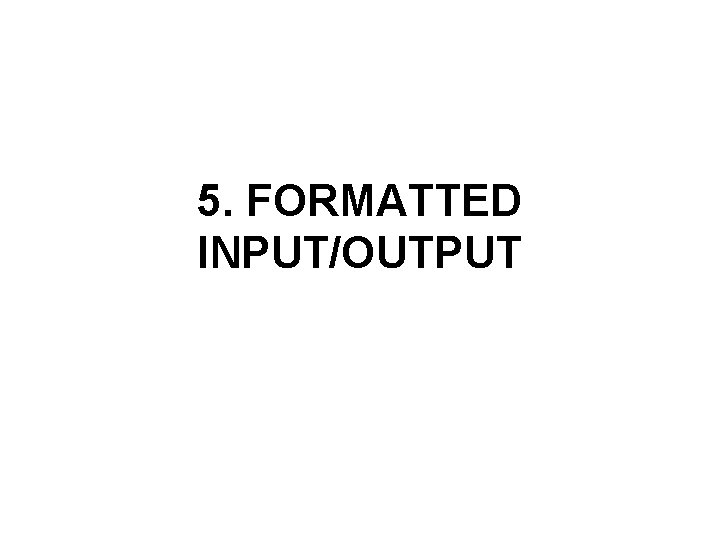
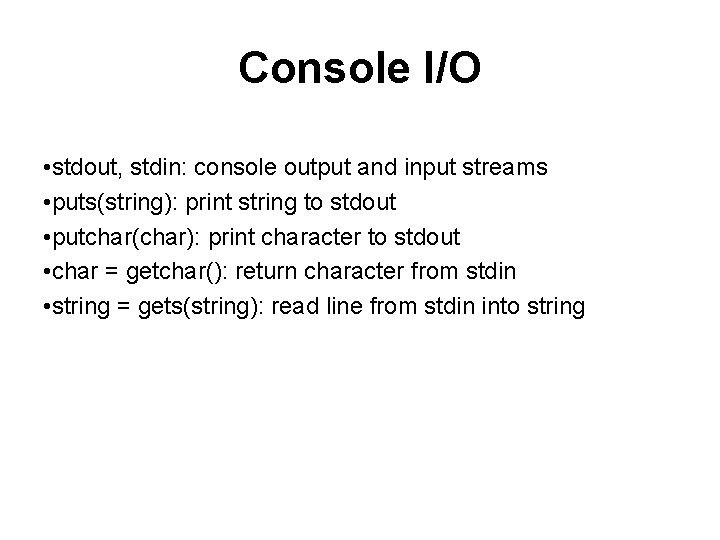
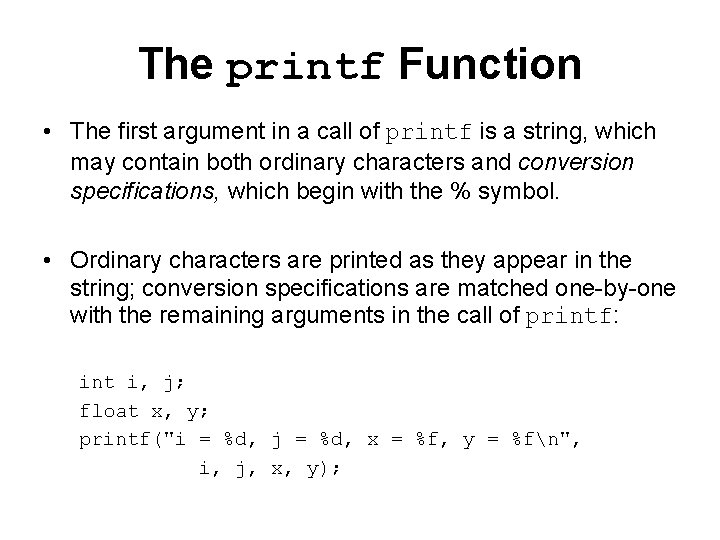
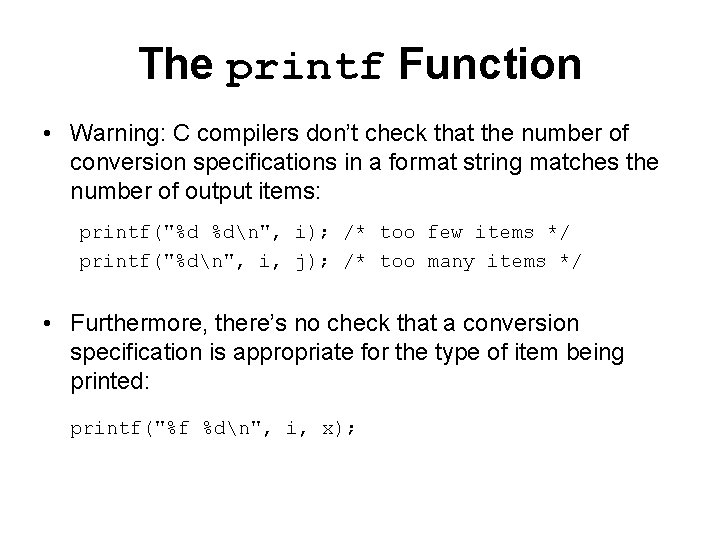
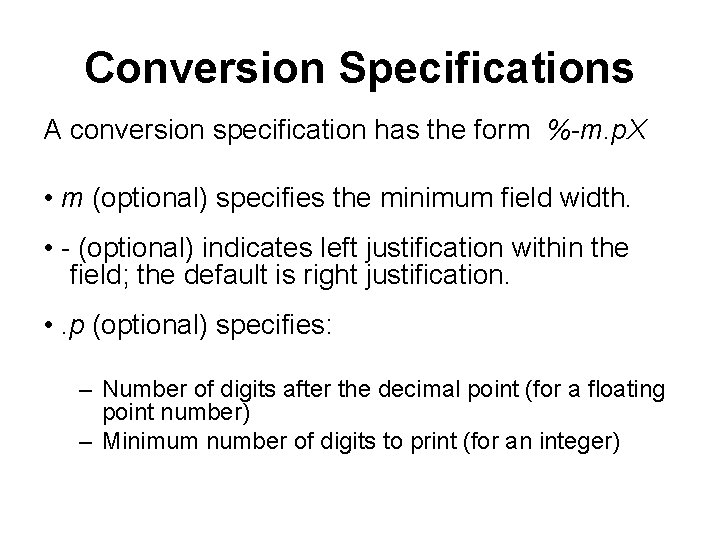
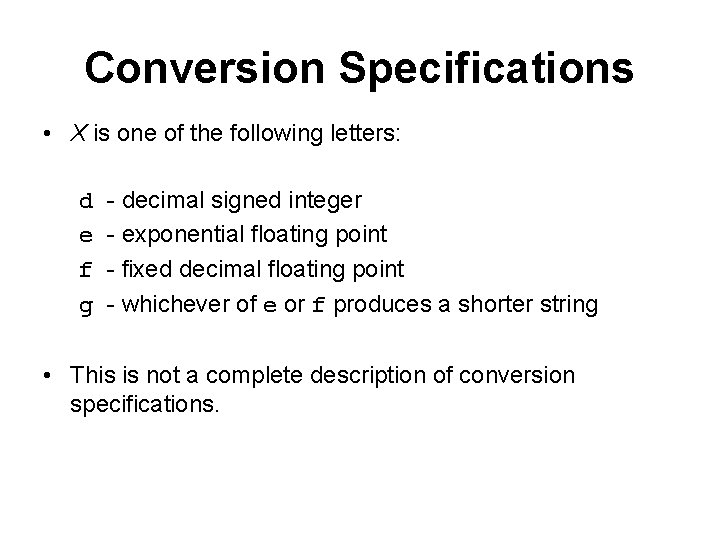
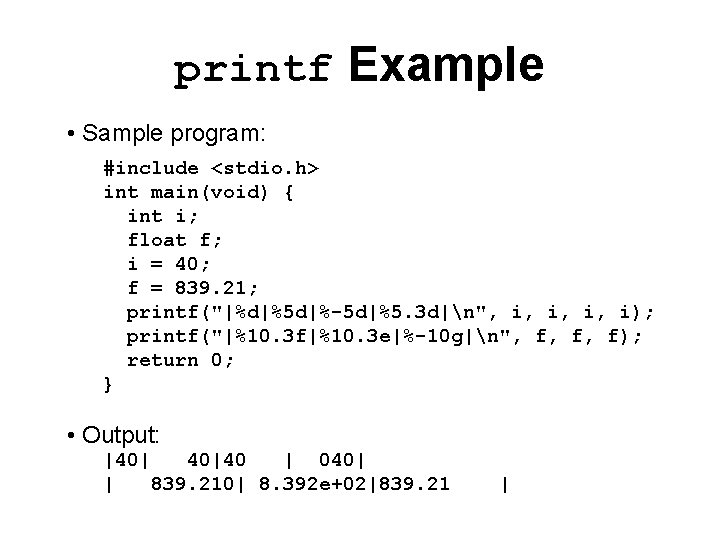
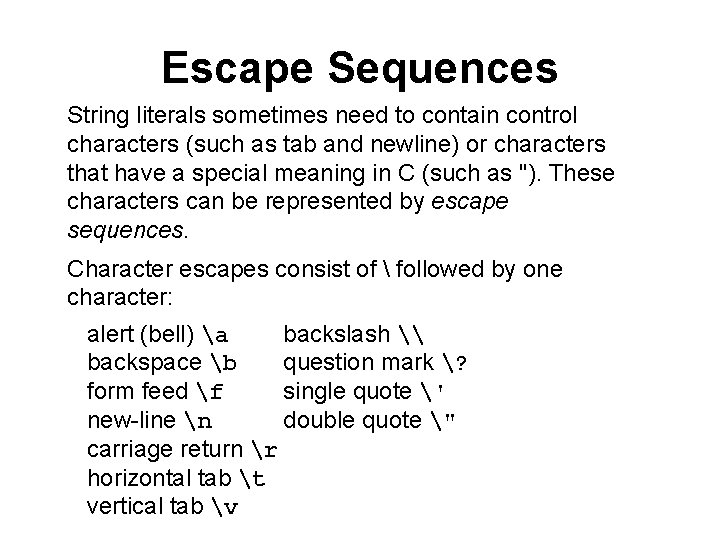
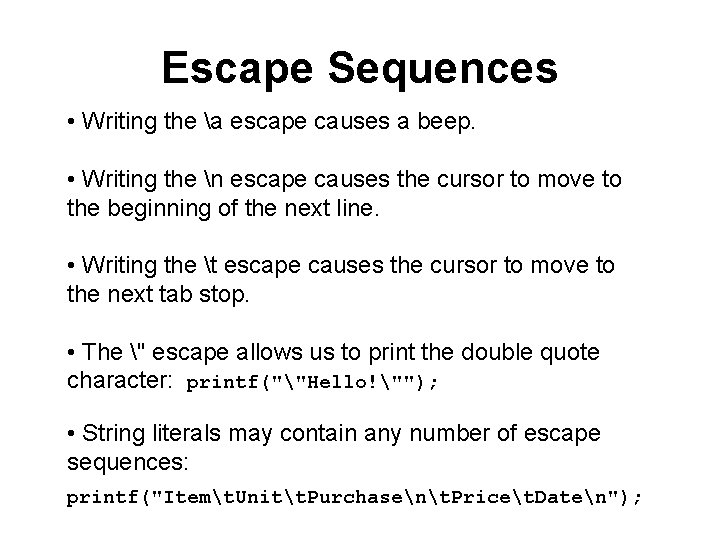
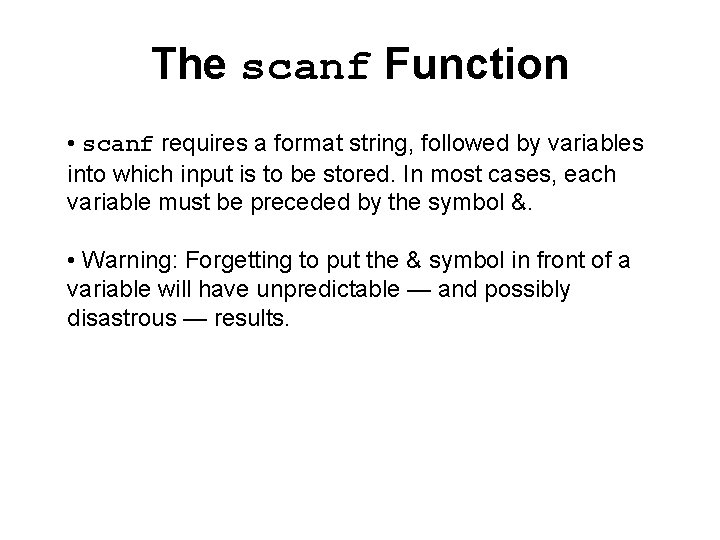
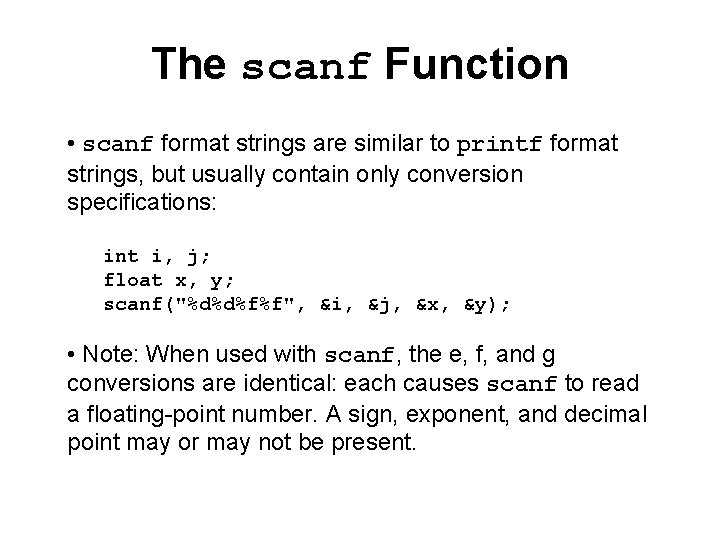
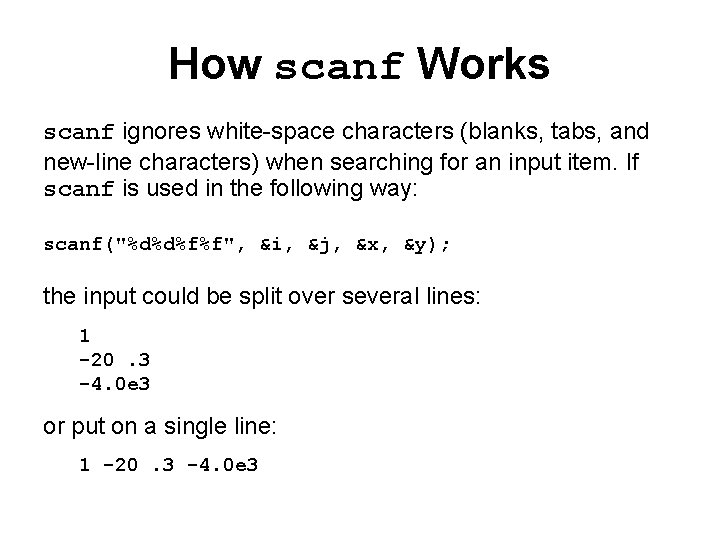
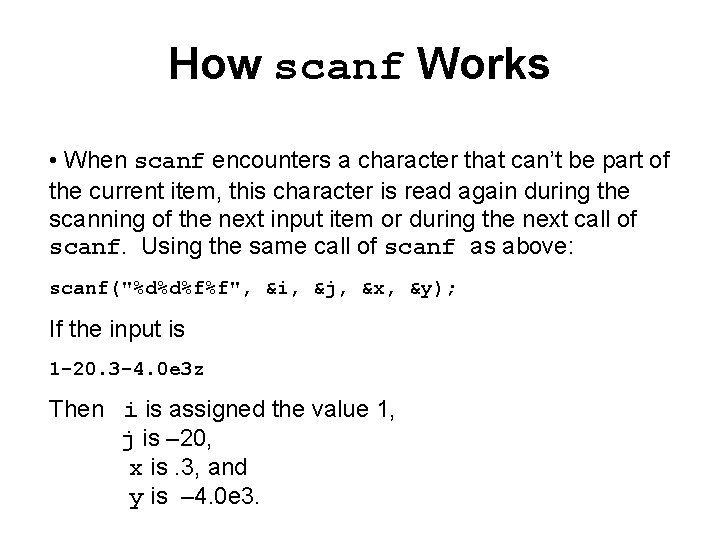
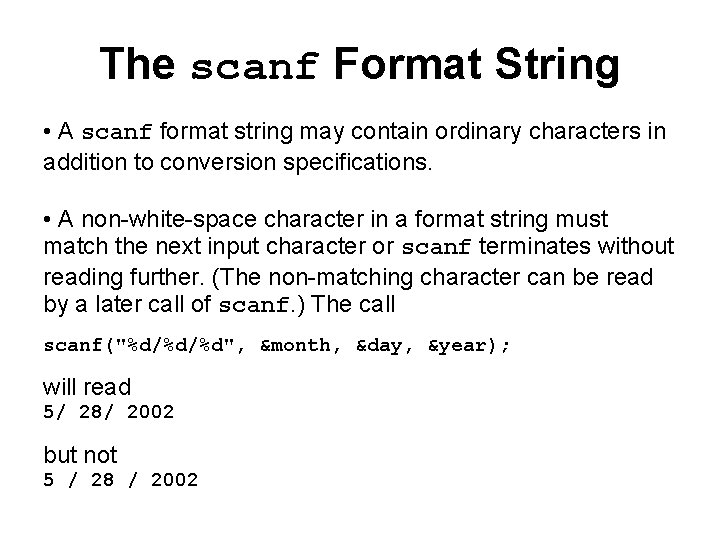
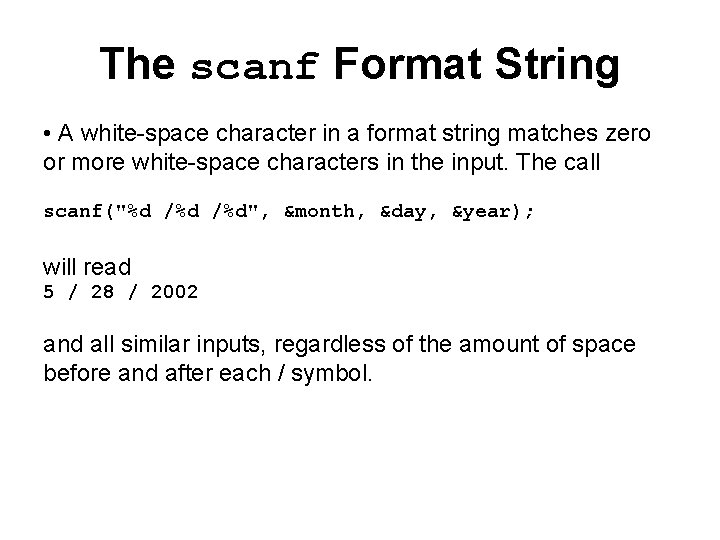
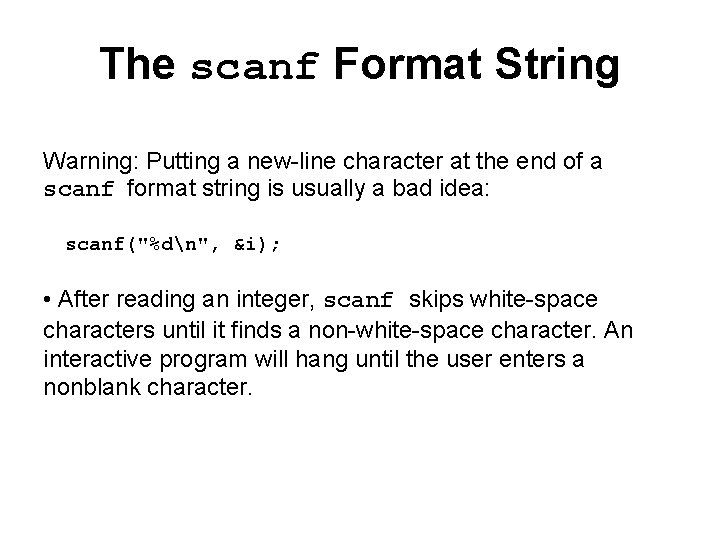
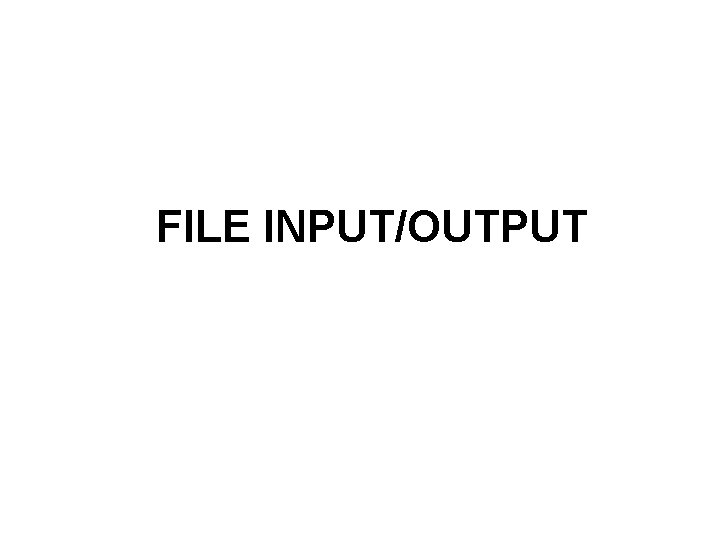
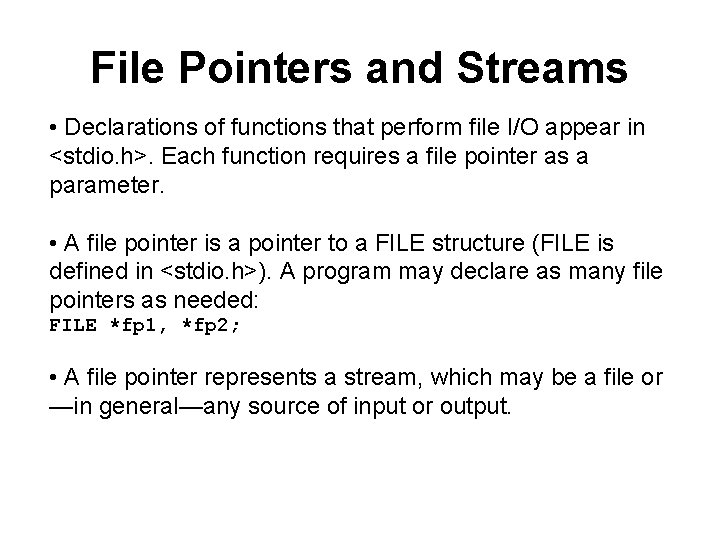
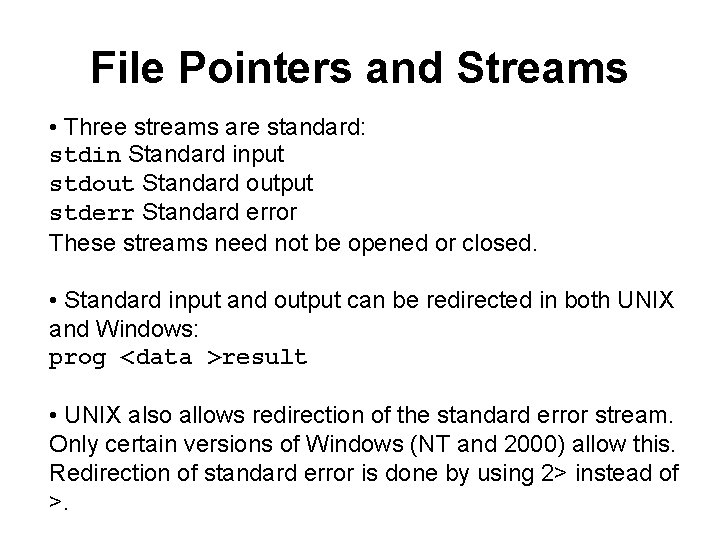
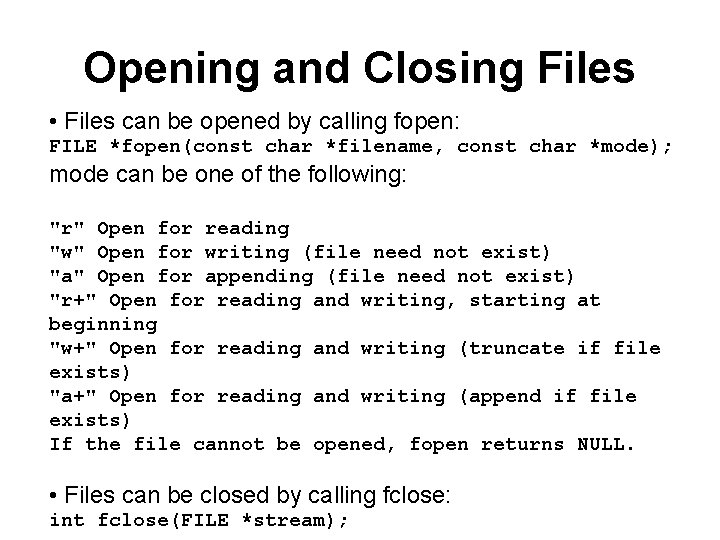
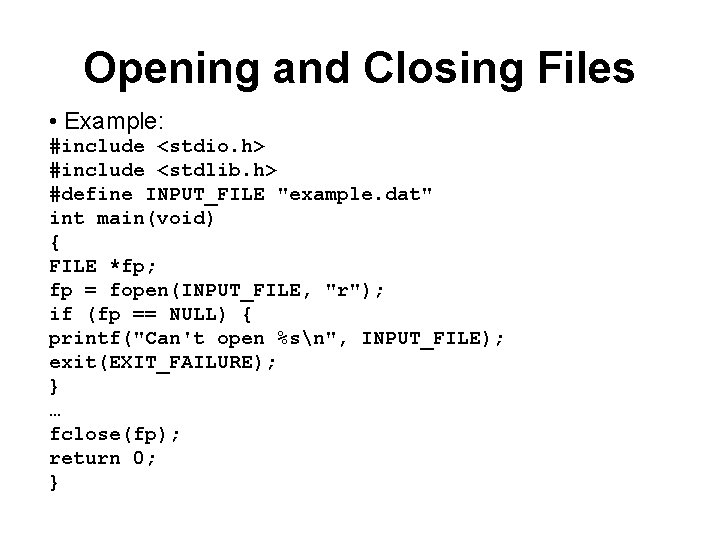
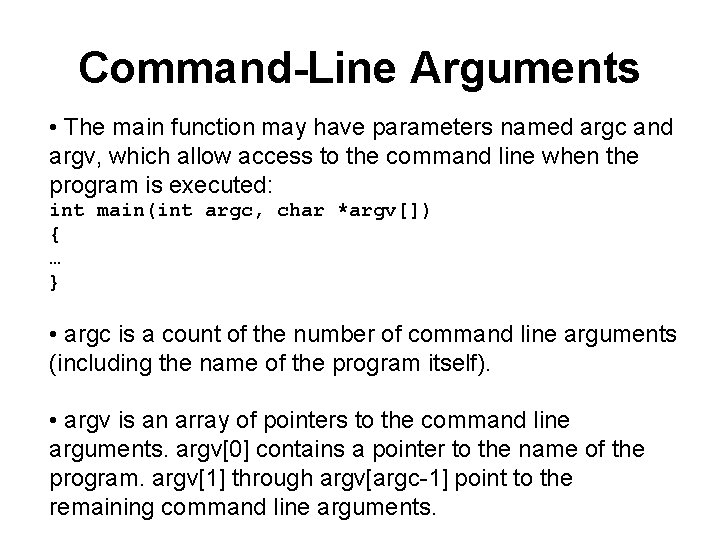
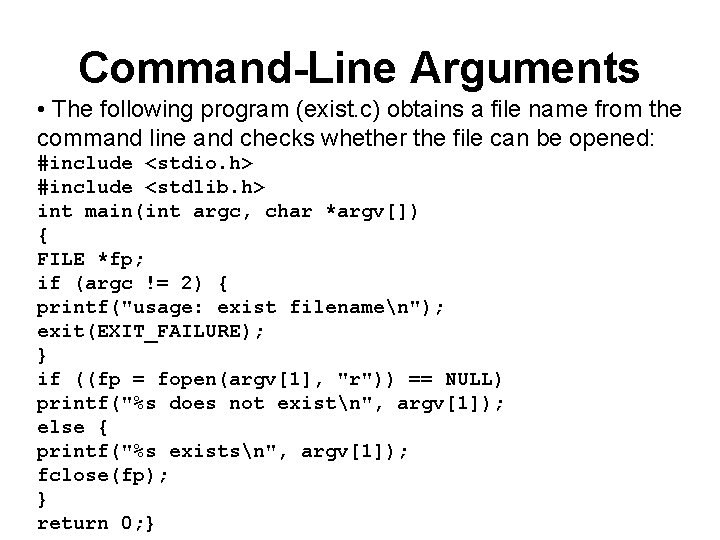
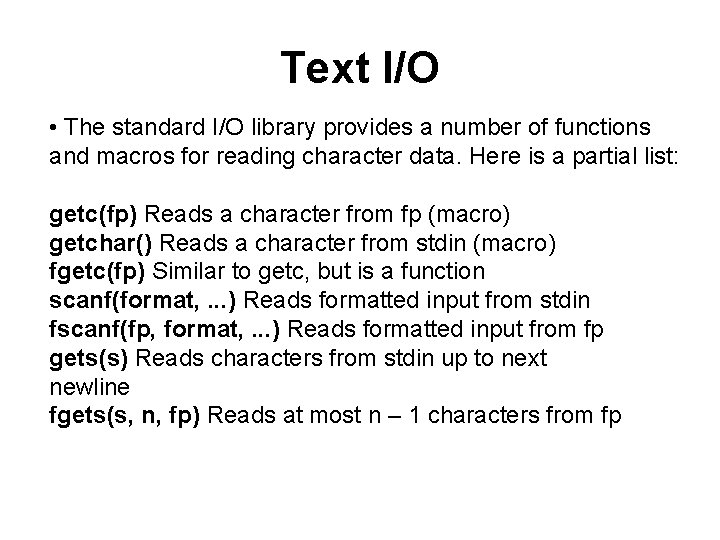
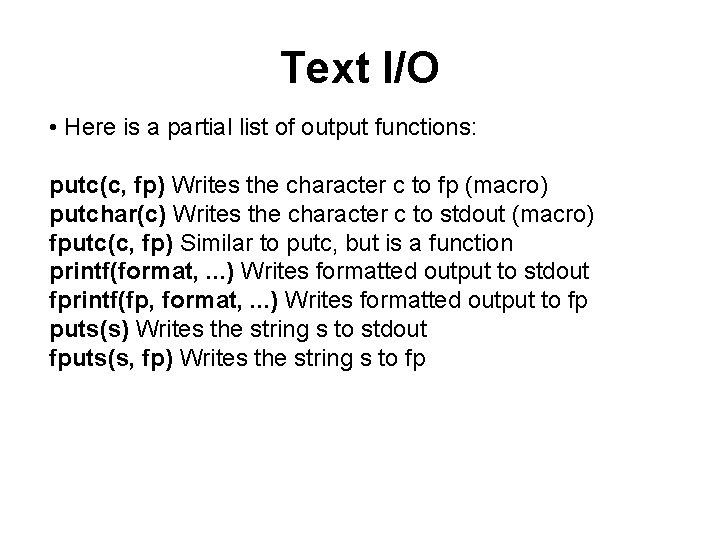
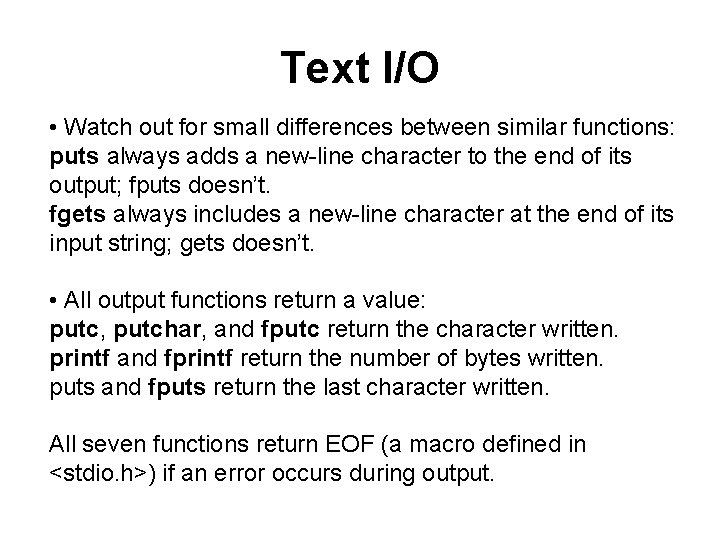
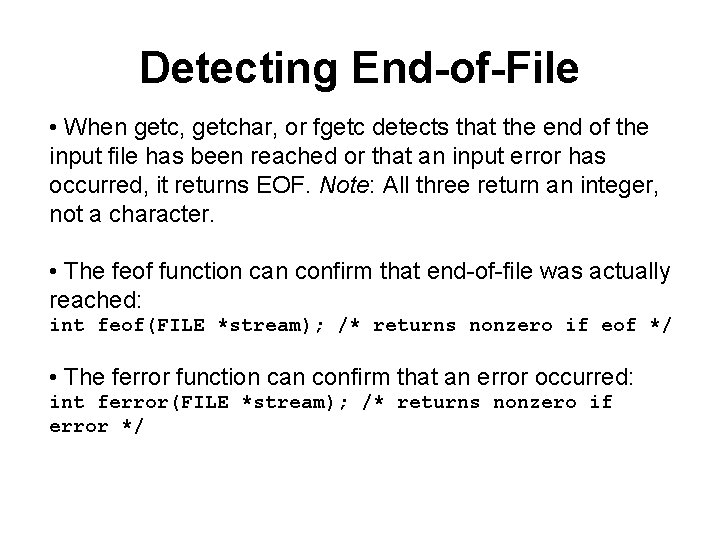
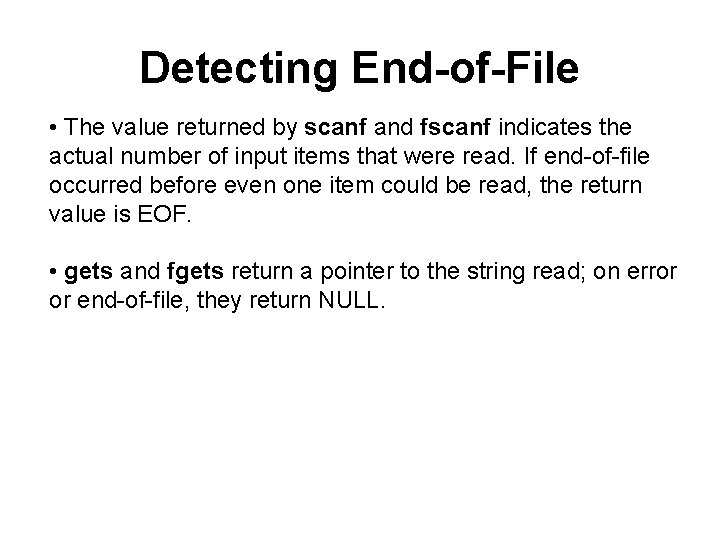
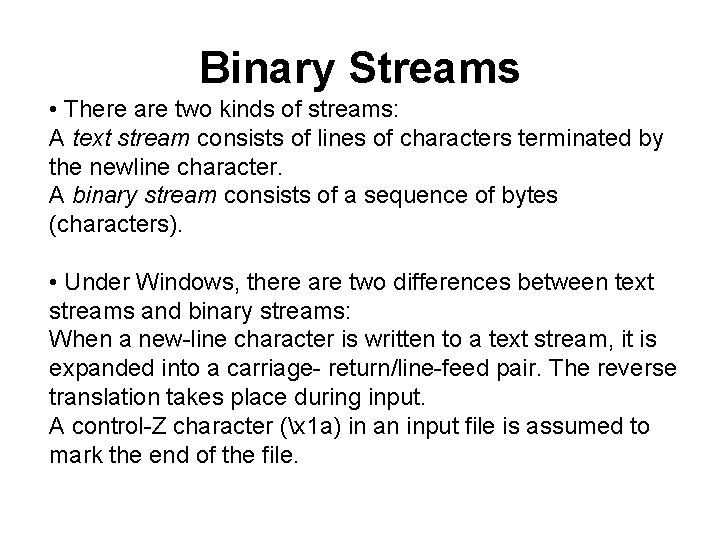
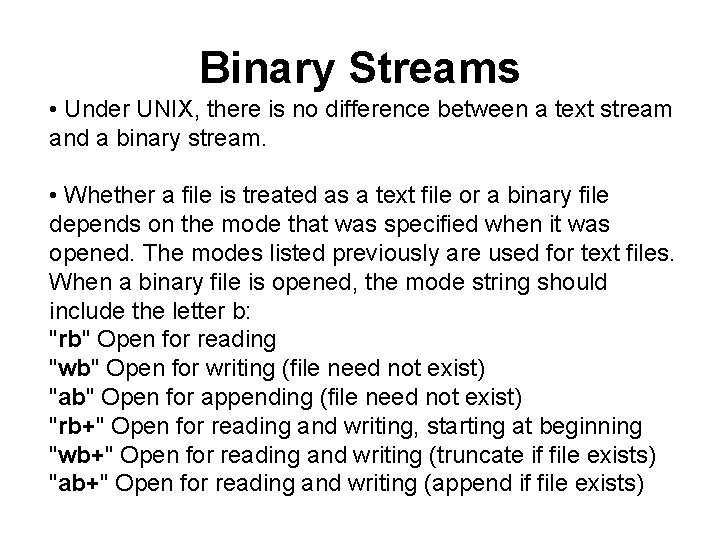
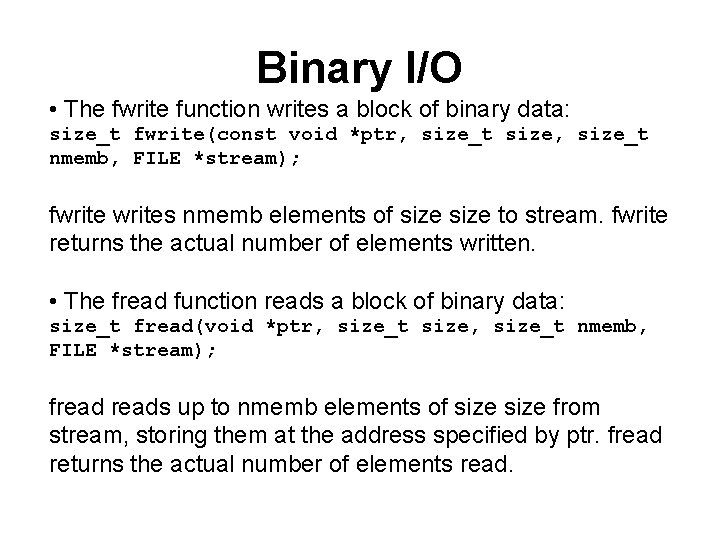
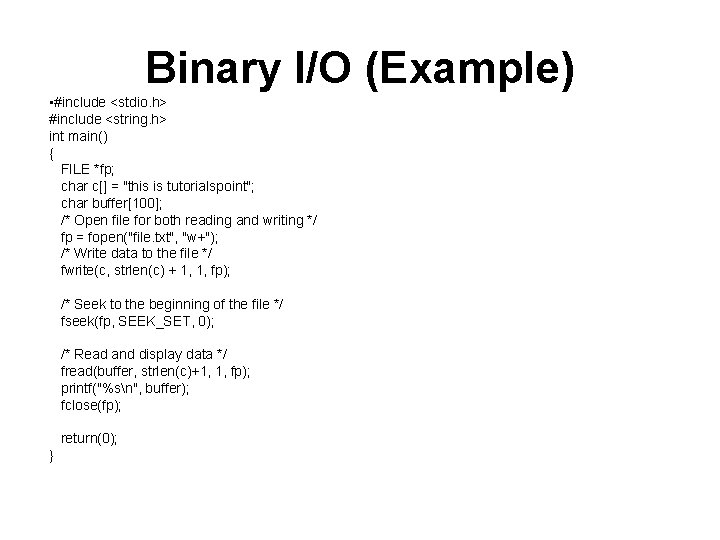
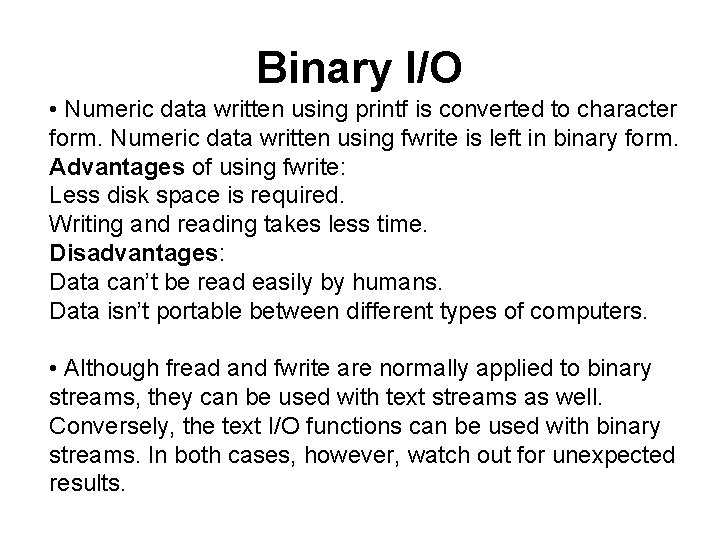
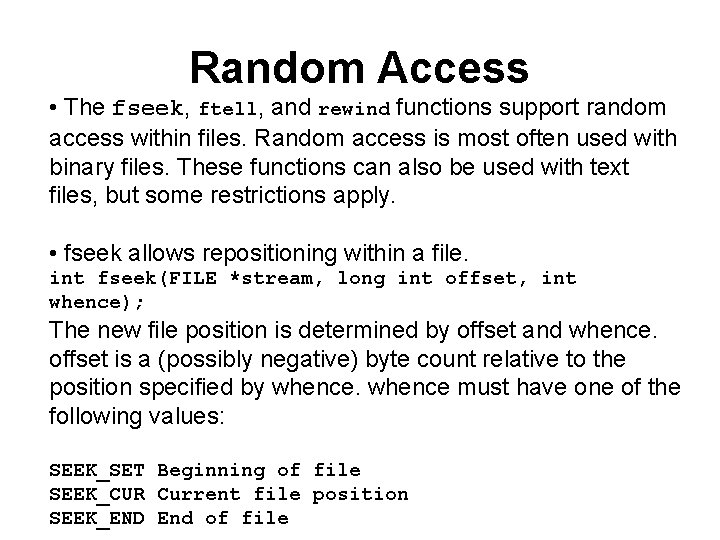
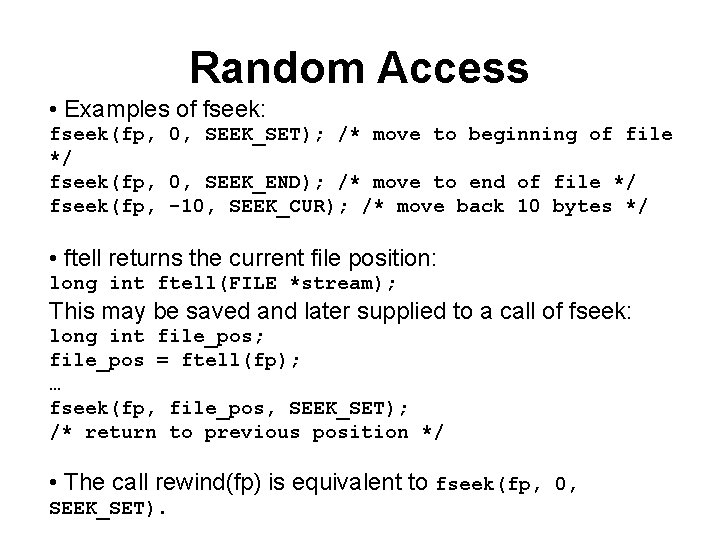
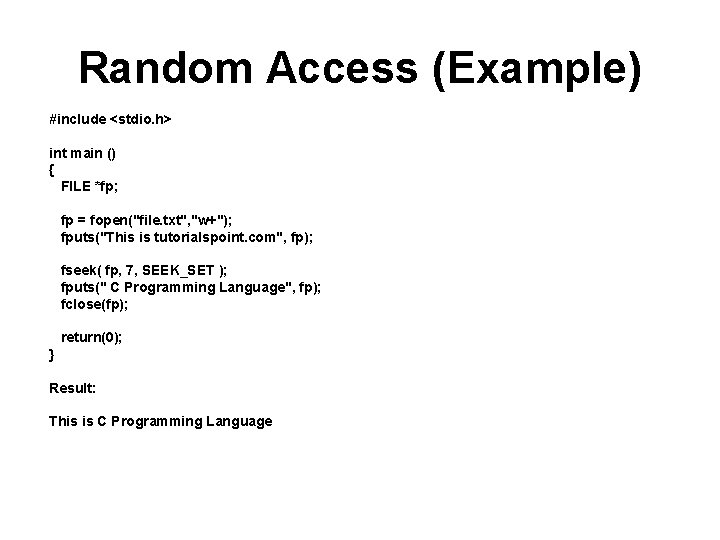
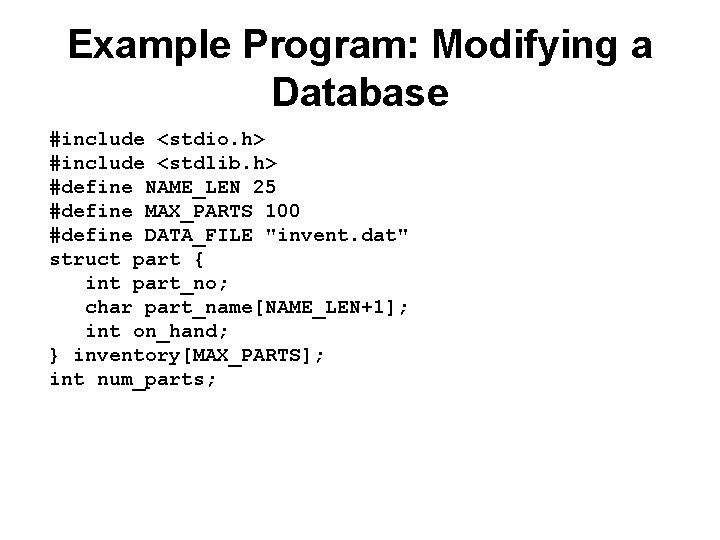
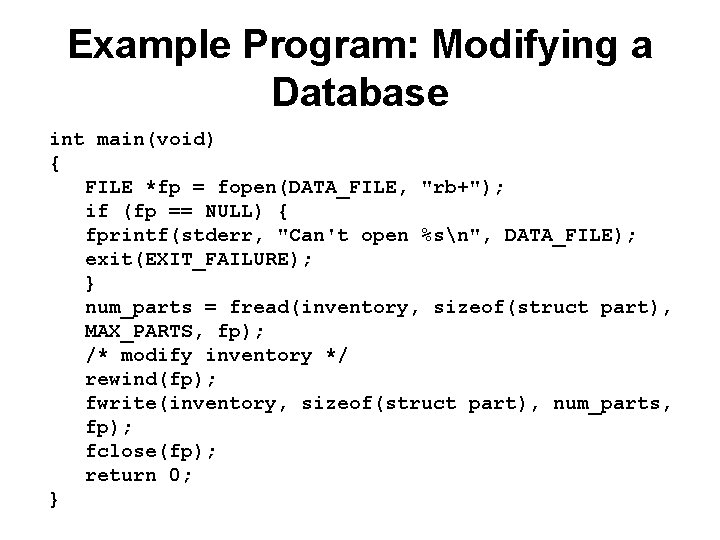
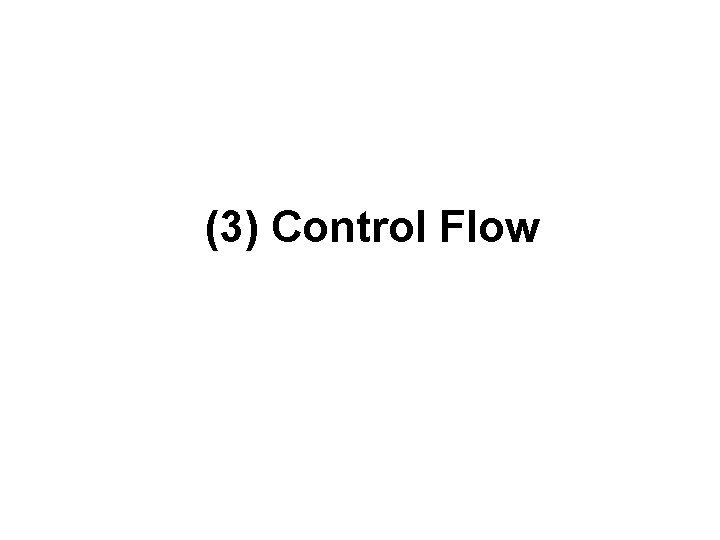
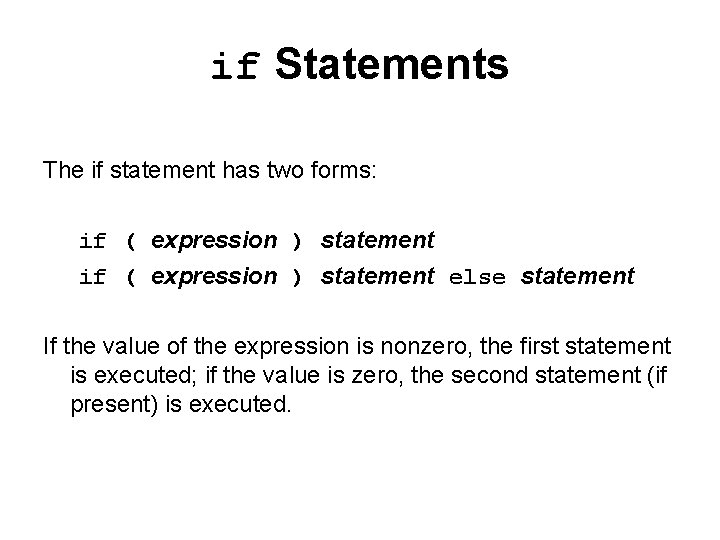
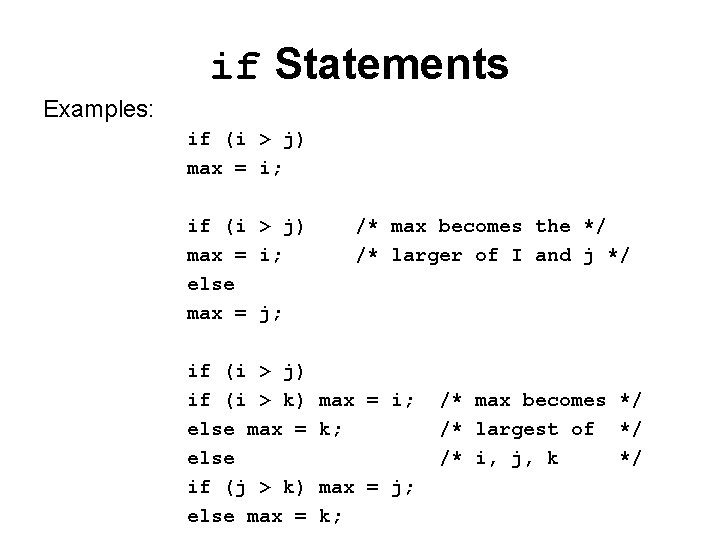
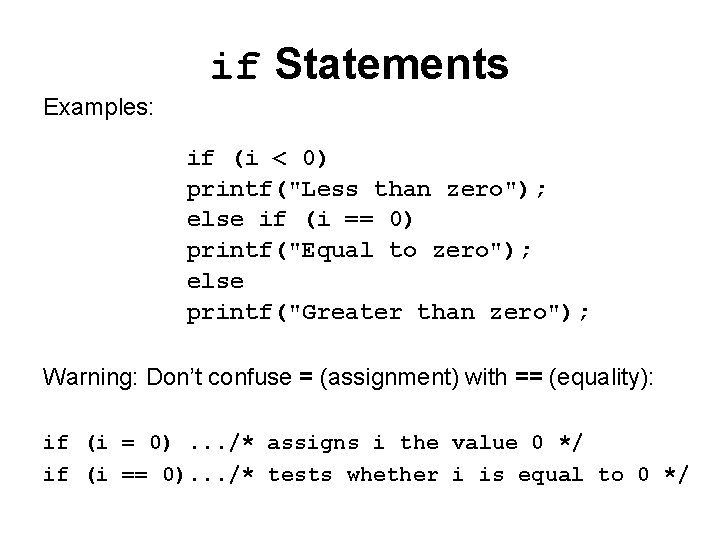
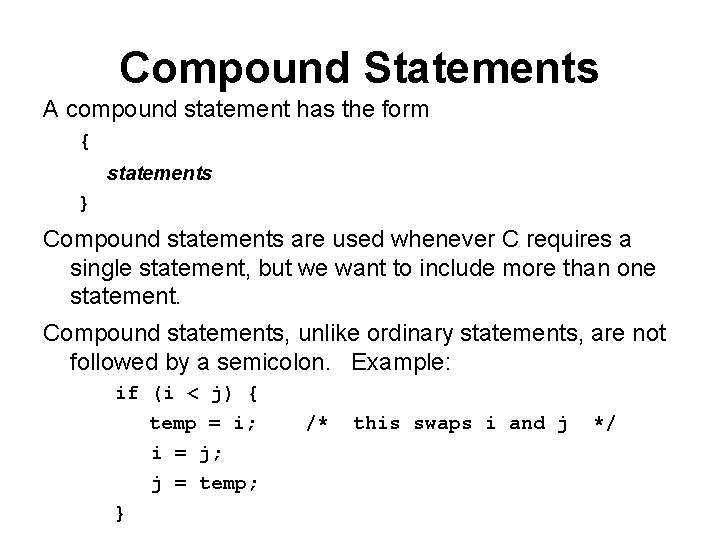
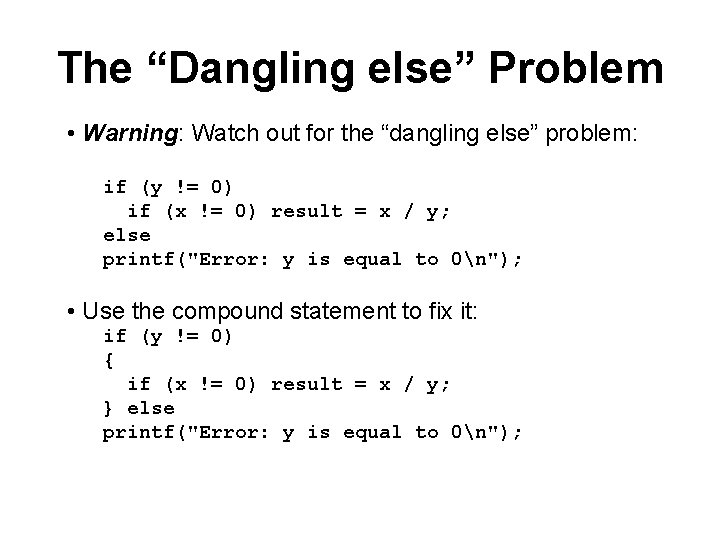
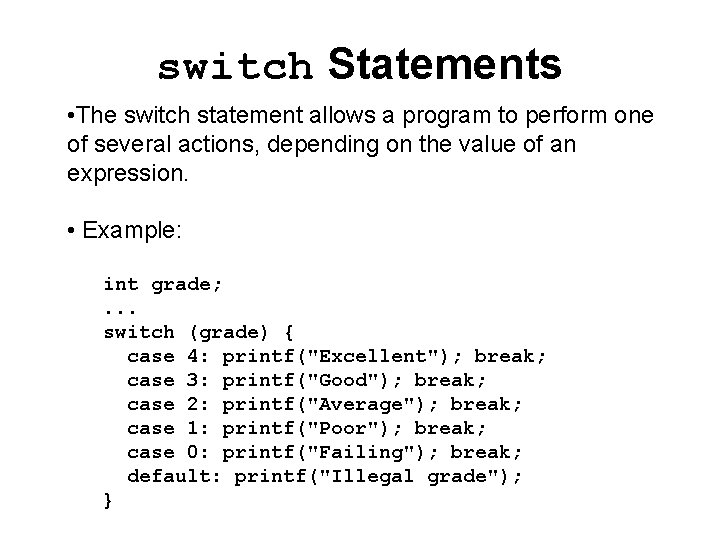
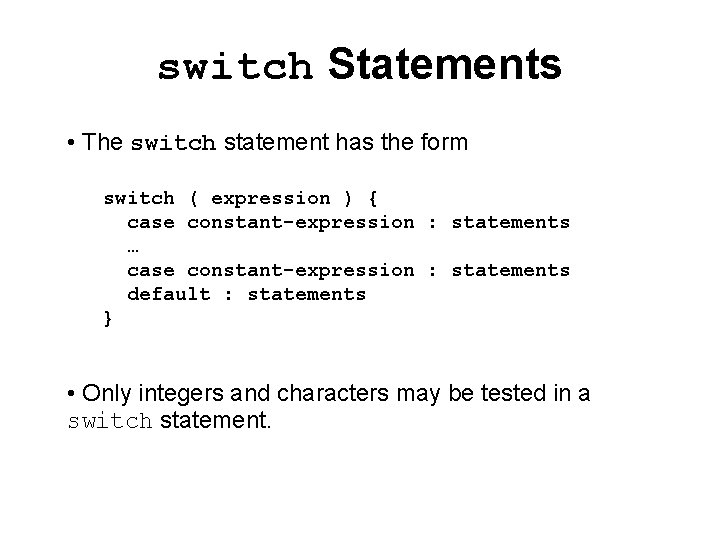
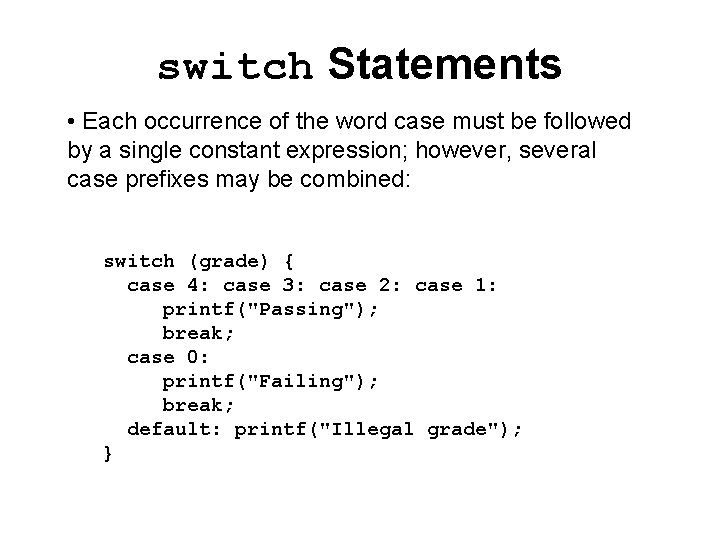
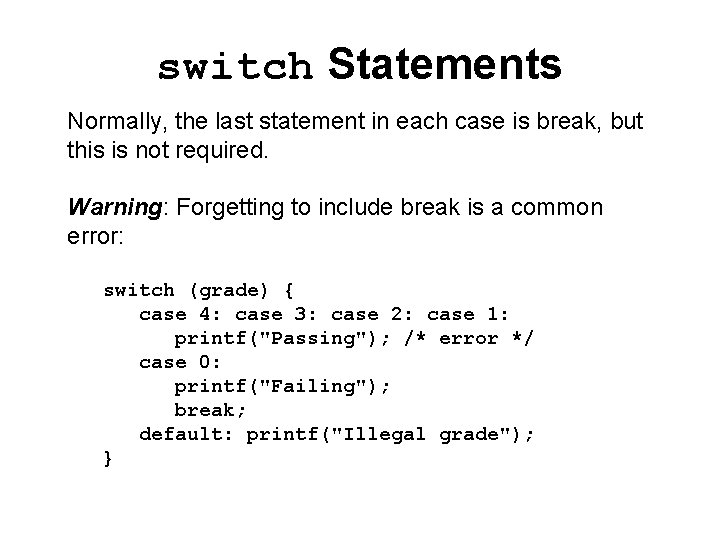
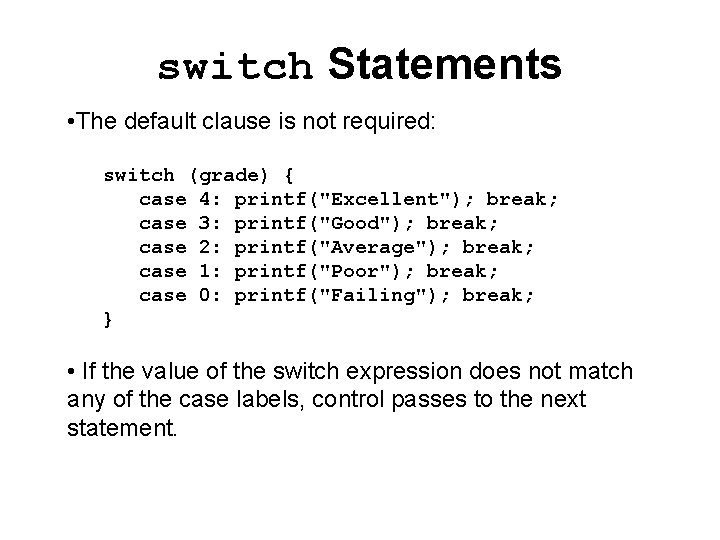
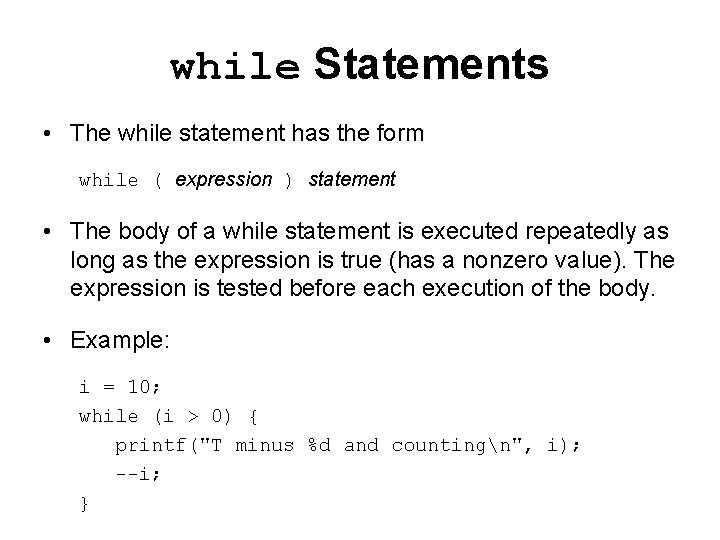
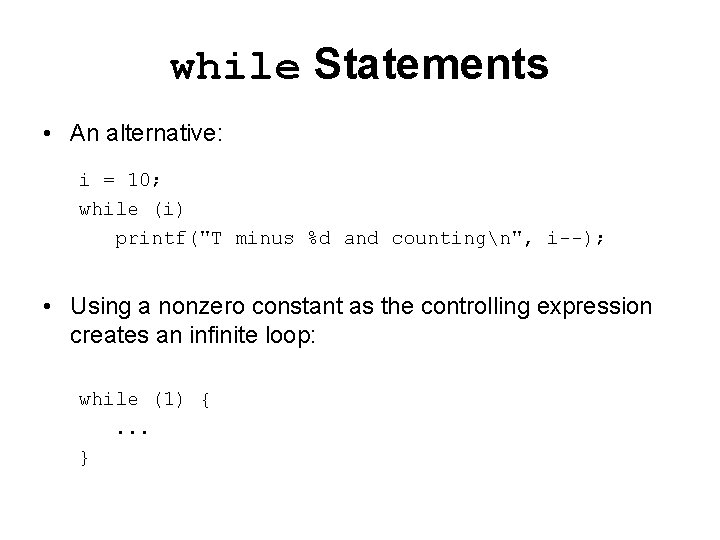
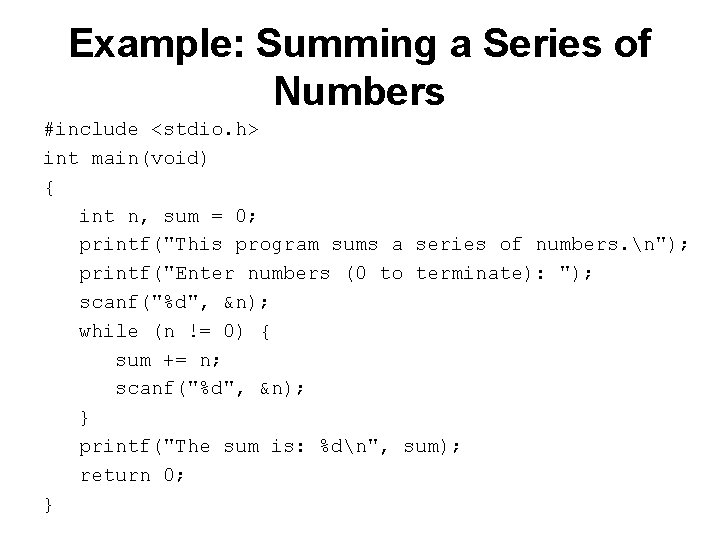
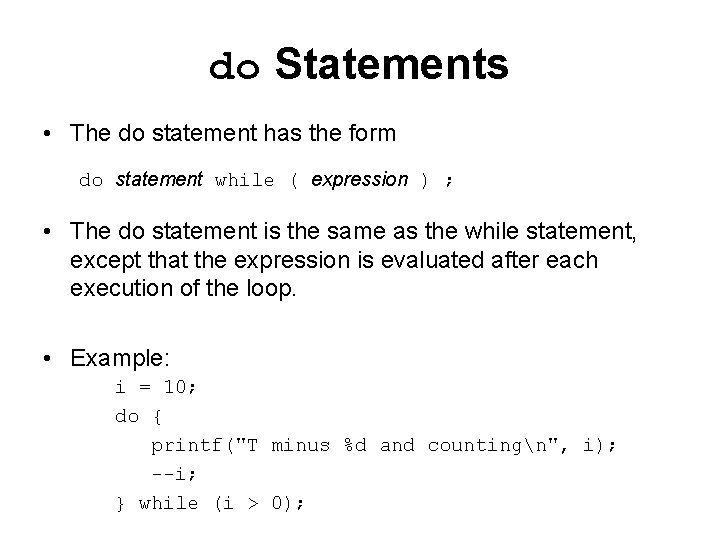
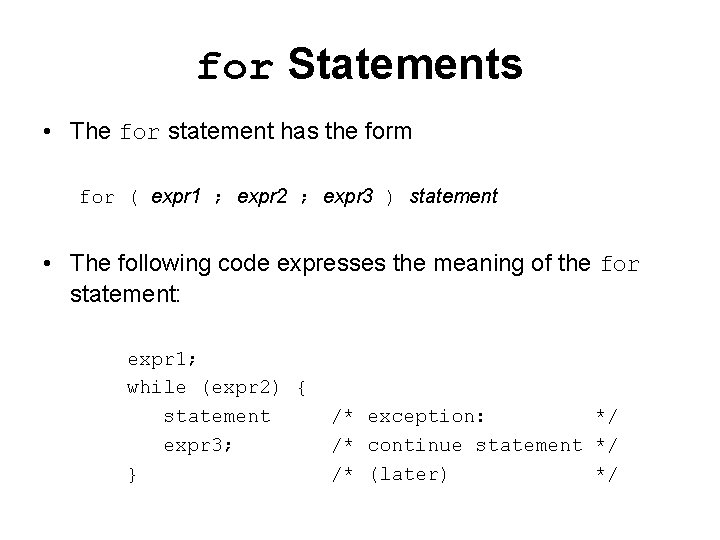
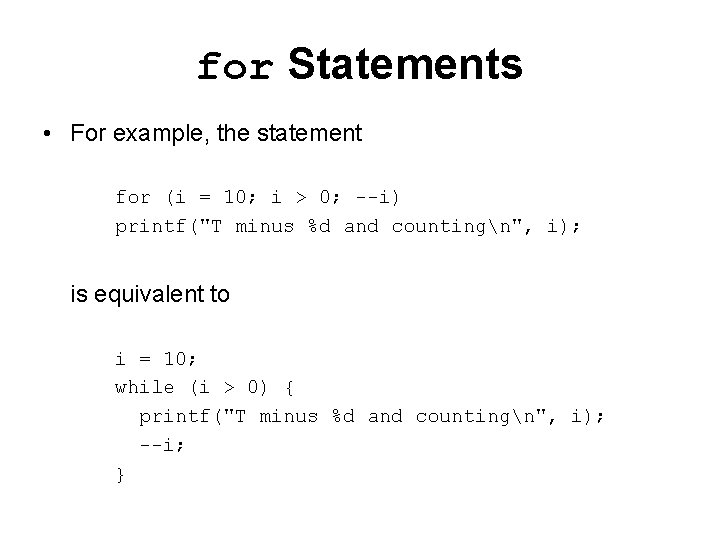
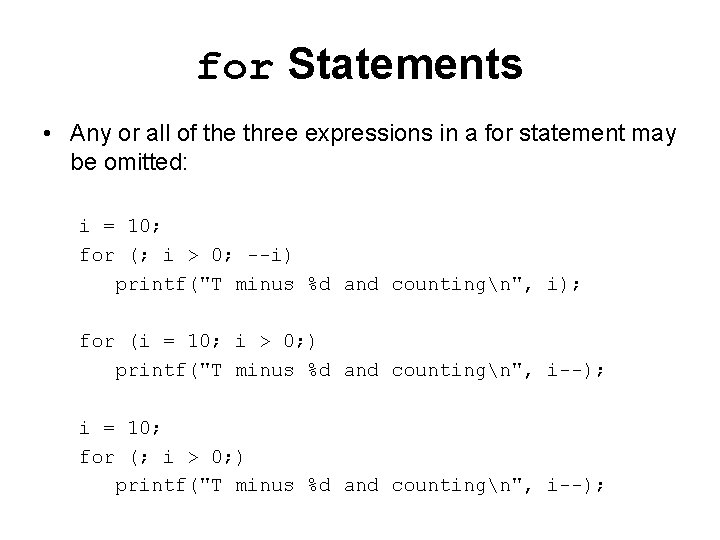
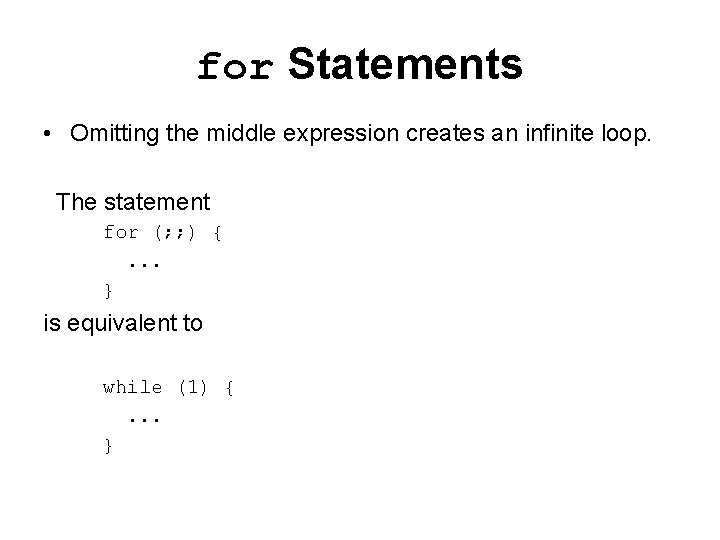
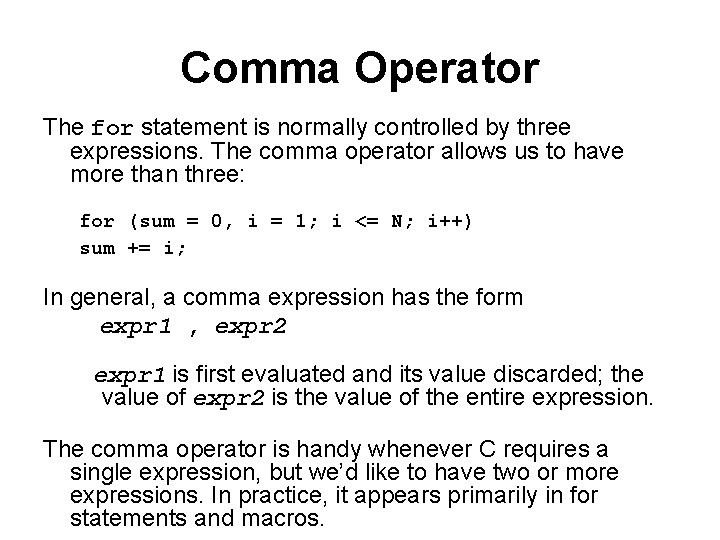
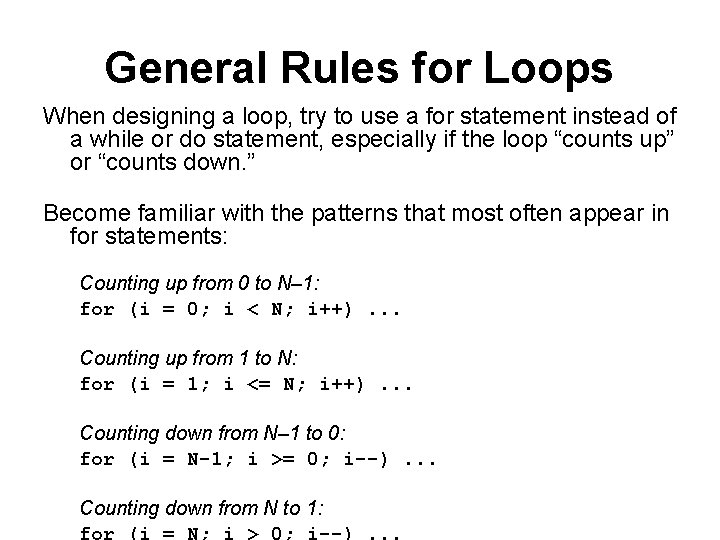
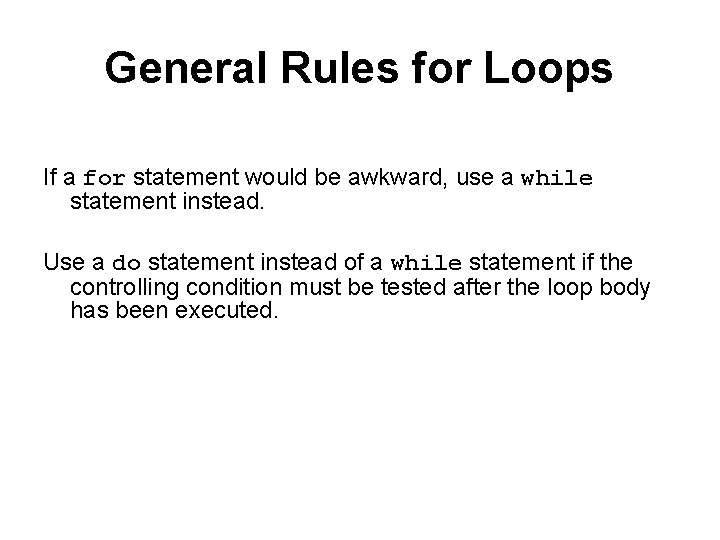
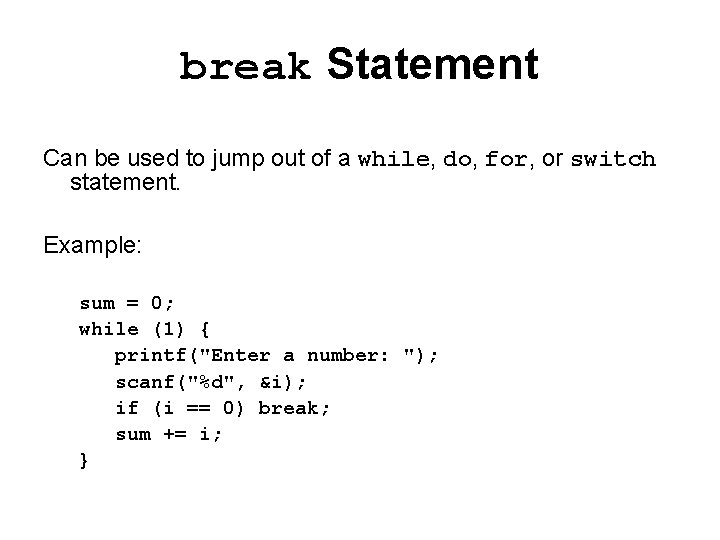
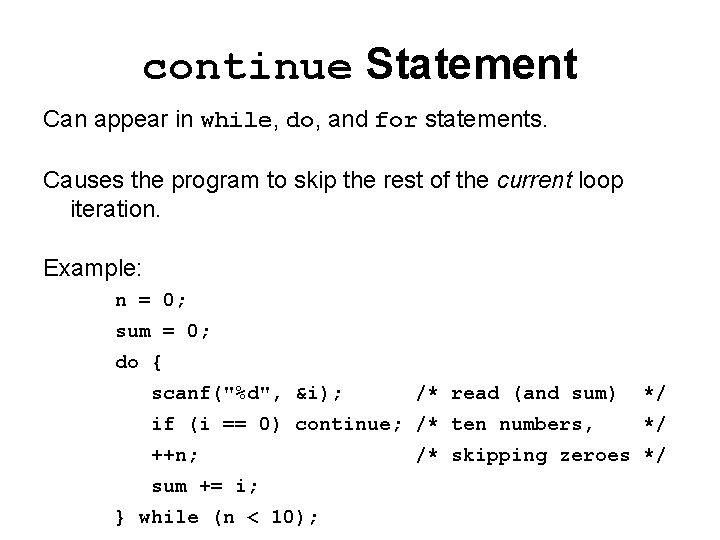
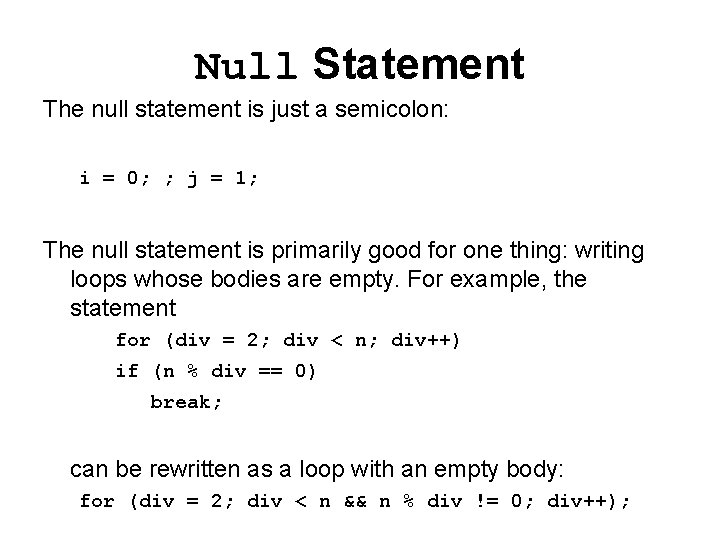
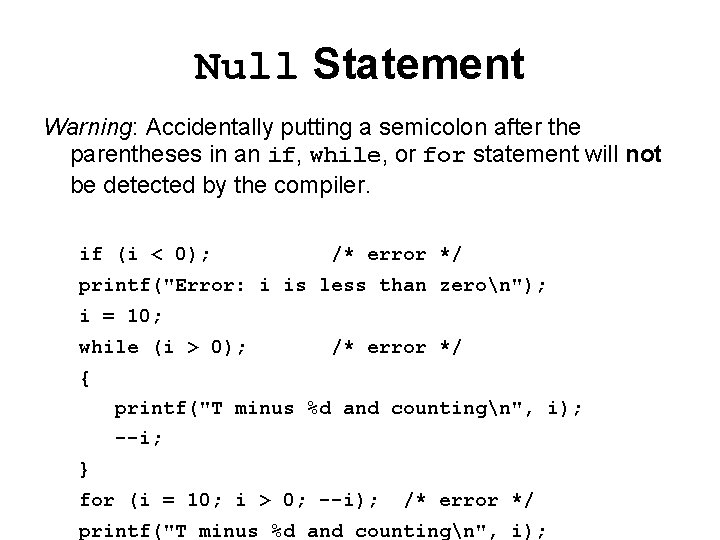
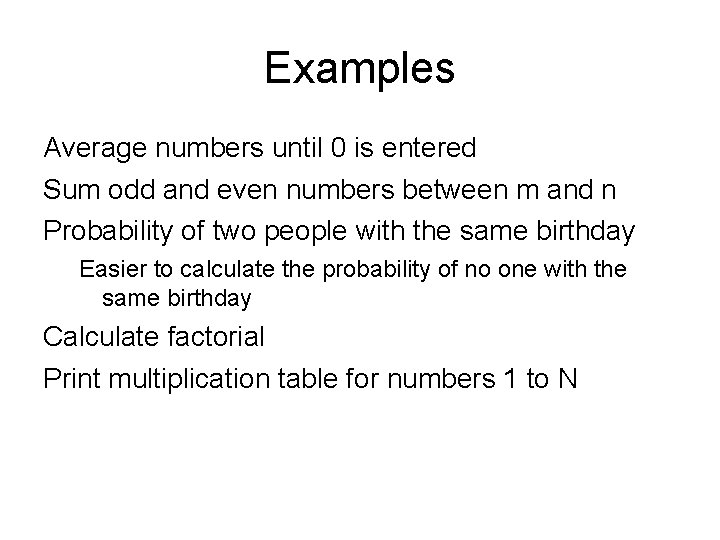
- Slides: 99
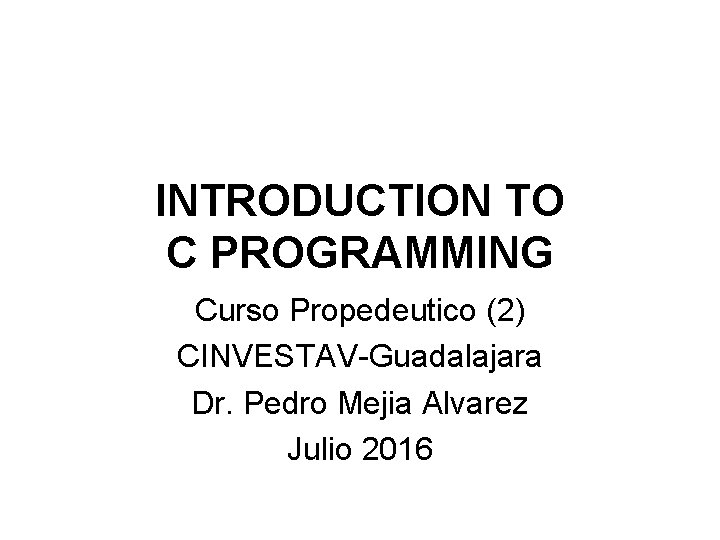
INTRODUCTION TO C PROGRAMMING Curso Propedeutico (2) CINVESTAV-Guadalajara Dr. Pedro Mejia Alvarez Julio 2016
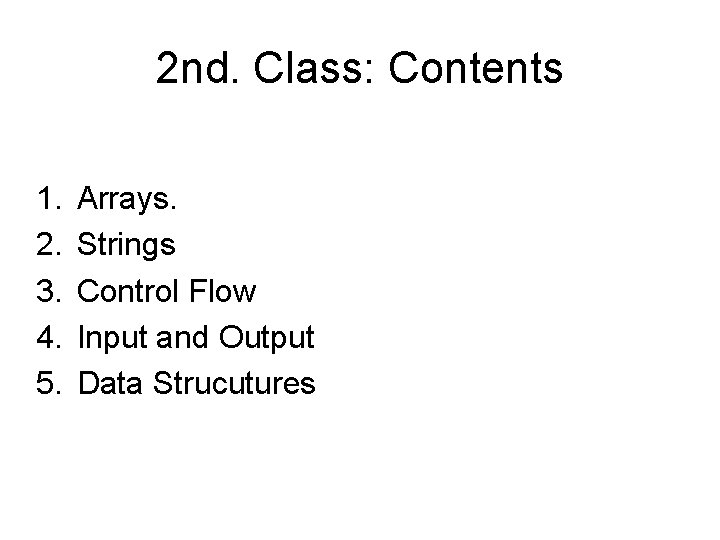
2 nd. Class: Contents 1. 2. 3. 4. 5. Arrays. Strings Control Flow Input and Output Data Strucutures
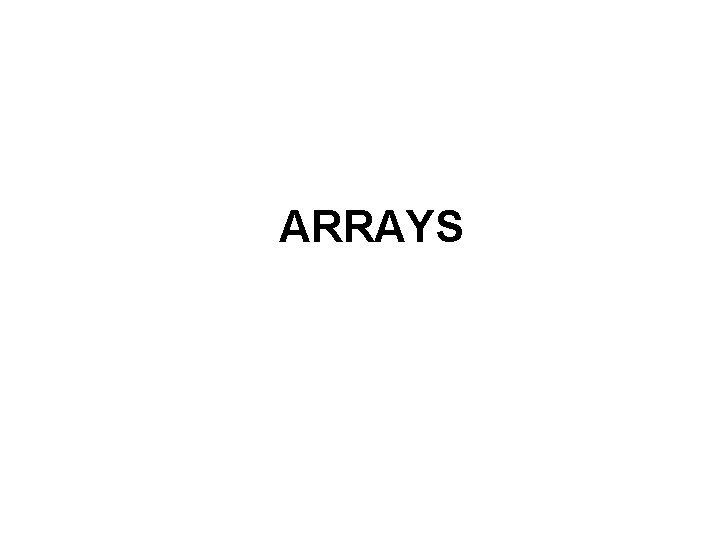
ARRAYS
![OneDimensional Arrays A onedimensional array is declared in the following way int a10 One-Dimensional Arrays • A one-dimensional array is declared in the following way: int a[10];](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-4.jpg)
One-Dimensional Arrays • A one-dimensional array is declared in the following way: int a[10]; The number 10 declares that a will have ten elements. • Array bounds always start at 0, so a has the following appearance: Notice that 10 is the length of the array, not the array’s upper bound.
![Array Subscripting To select an element of an array use the operator Array Subscripting • To select an element of an array, use the [] operator:](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-5.jpg)
Array Subscripting • To select an element of an array, use the [] operator: a[0] a[i*2+1] This operation is called array subscripting.
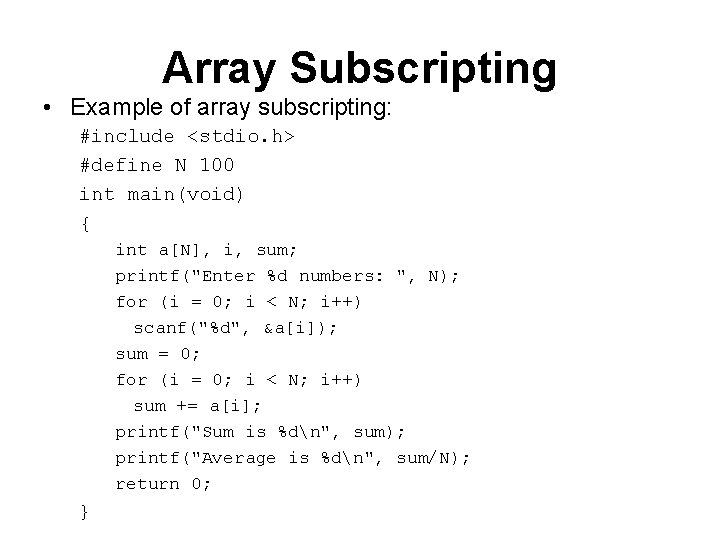
Array Subscripting • Example of array subscripting: #include <stdio. h> #define N 100 int main(void) { int a[N], i, sum; printf("Enter %d numbers: ", N); for (i = 0; i < N; i++) scanf("%d", &a[i]); sum = 0; for (i = 0; i < N; i++) sum += a[i]; printf("Sum is %dn", sum); printf("Average is %dn", sum/N); return 0; }
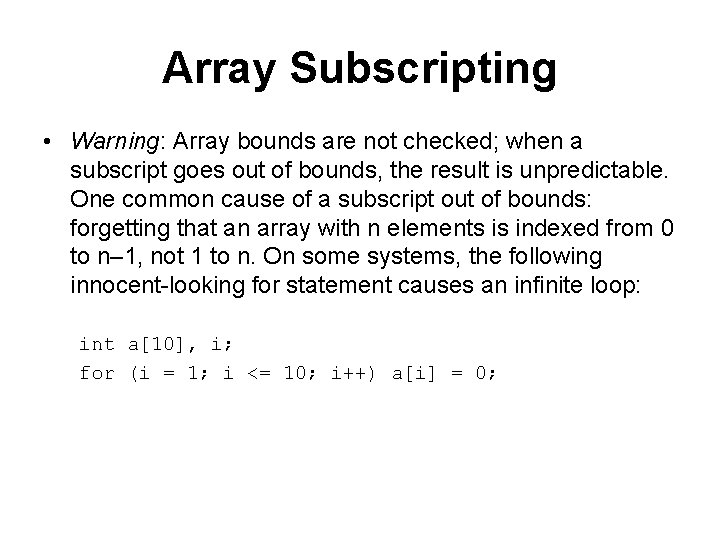
Array Subscripting • Warning: Array bounds are not checked; when a subscript goes out of bounds, the result is unpredictable. One common cause of a subscript out of bounds: forgetting that an array with n elements is indexed from 0 to n– 1, not 1 to n. On some systems, the following innocent-looking for statement causes an infinite loop: int a[10], i; for (i = 1; i <= 10; i++) a[i] = 0;
![Multidimensional Arrays Arrays may have more than one dimension int m59 m has Multidimensional Arrays • Arrays may have more than one dimension: int m[5][9]; m has](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-8.jpg)
Multidimensional Arrays • Arrays may have more than one dimension: int m[5][9]; m has the following appearance:
![Multidimensional Arrays An element of m is accessed by writing mij Example Multidimensional Arrays • An element of m is accessed by writing m[i][j]. • Example](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-9.jpg)
Multidimensional Arrays • An element of m is accessed by writing m[i][j]. • Example of multidimensional array use: #define NUM_ROWS 10 #define NUM_COLS 10 int m[NUM_ROWS][NUM_COLS], row, col; for (row = 0; row < NUM_ROWS; row++) for (col = 0; col < NUM_COLS; col++) if (row == col) m[row][col] = 1; else m[row][col] = 0;
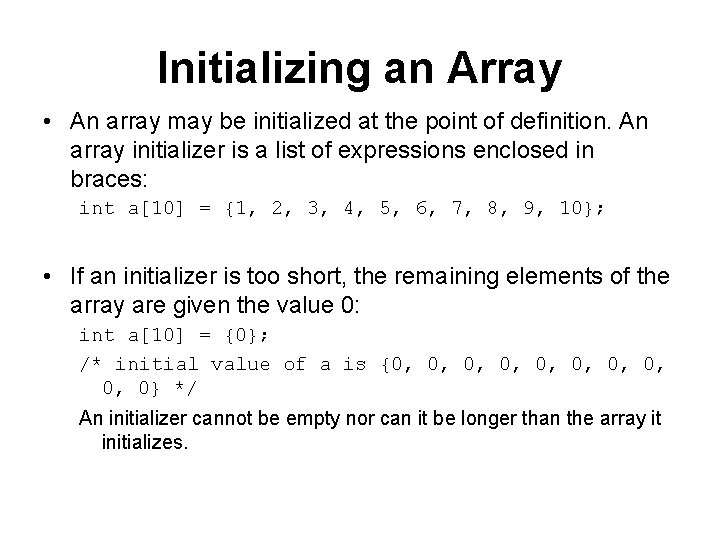
Initializing an Array • An array may be initialized at the point of definition. An array initializer is a list of expressions enclosed in braces: int a[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; • If an initializer is too short, the remaining elements of the array are given the value 0: int a[10] = {0}; /* initial value of a is {0, 0, 0, 0} */ An initializer cannot be empty nor can it be longer than the array it initializes.
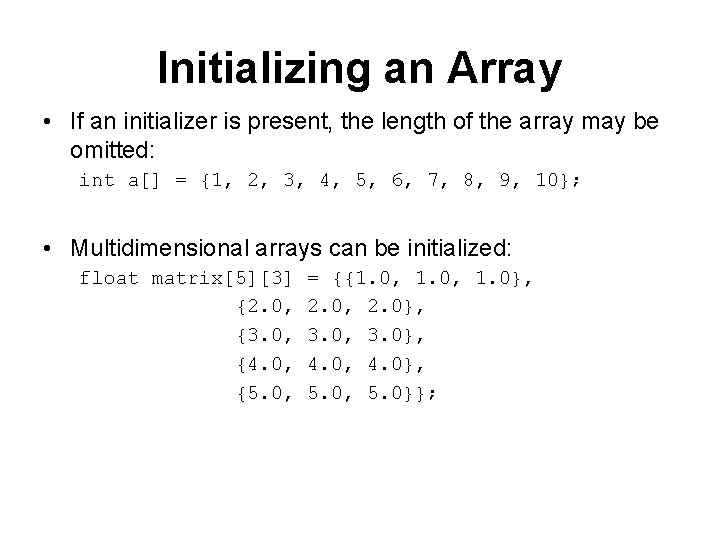
Initializing an Array • If an initializer is present, the length of the array may be omitted: int a[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; • Multidimensional arrays can be initialized: float matrix[5][3] {2. 0, {3. 0, {4. 0, {5. 0, = {{1. 0, 1. 0}, 2. 0}, 3. 0}, 4. 0}, 5. 0}};
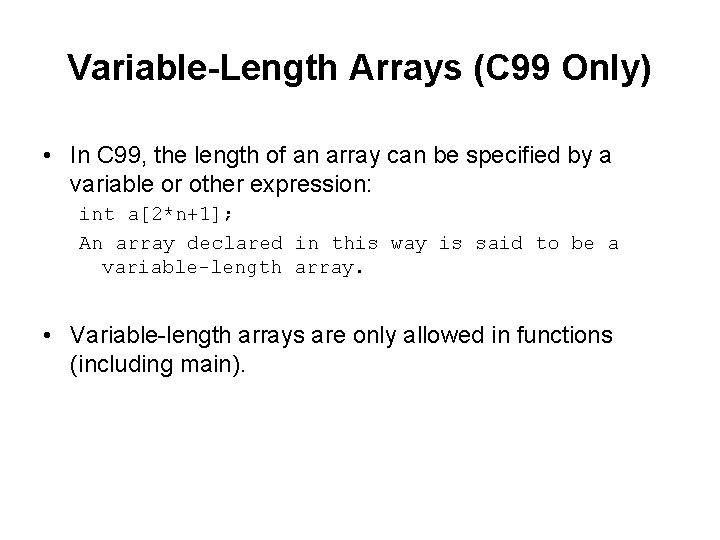
Variable-Length Arrays (C 99 Only) • In C 99, the length of an array can be specified by a variable or other expression: int a[2*n+1]; An array declared in this way is said to be a variable-length array. • Variable-length arrays are only allowed in functions (including main).
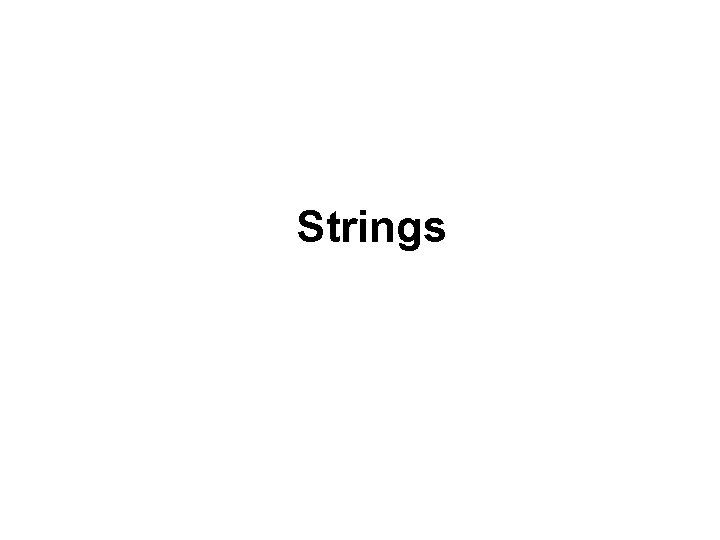
Strings
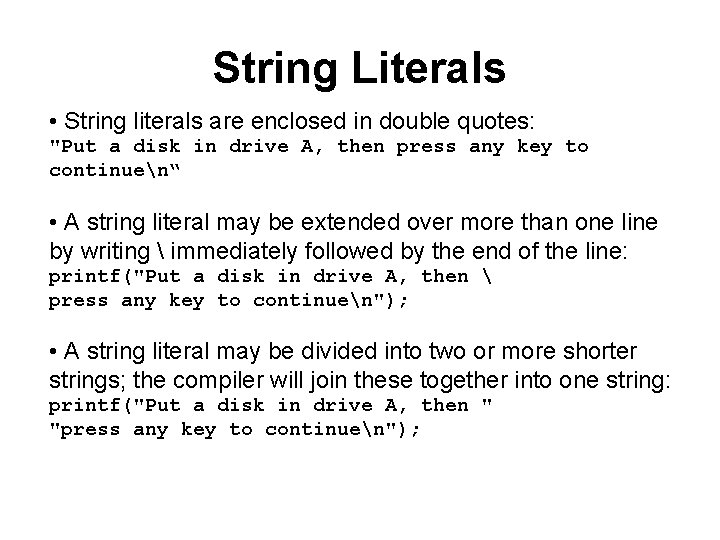
String Literals • String literals are enclosed in double quotes: "Put a disk in drive A, then press any key to continuen“ • A string literal may be extended over more than one line by writing immediately followed by the end of the line: printf("Put a disk in drive A, then press any key to continuen"); • A string literal may be divided into two or more shorter strings; the compiler will join these together into one string: printf("Put a disk in drive A, then " "press any key to continuen");
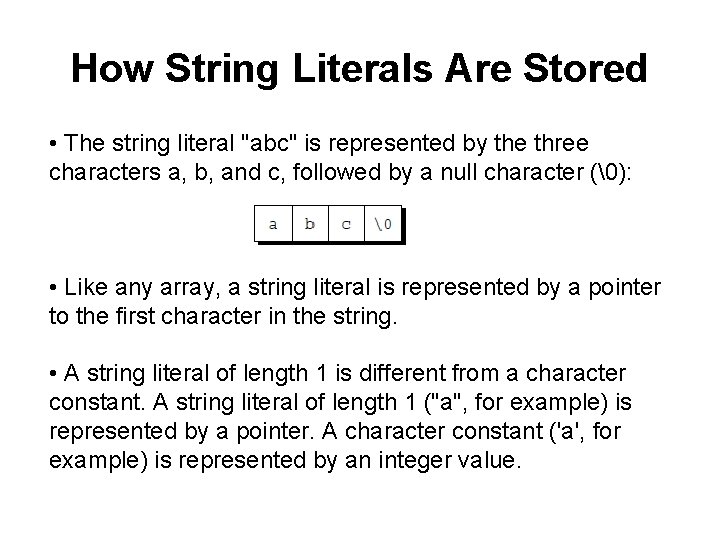
How String Literals Are Stored • The string literal "abc" is represented by the three characters a, b, and c, followed by a null character ( ): • Like any array, a string literal is represented by a pointer to the first character in the string. • A string literal of length 1 is different from a character constant. A string literal of length 1 ("a", for example) is represented by a pointer. A character constant ('a', for example) is represented by an integer value.
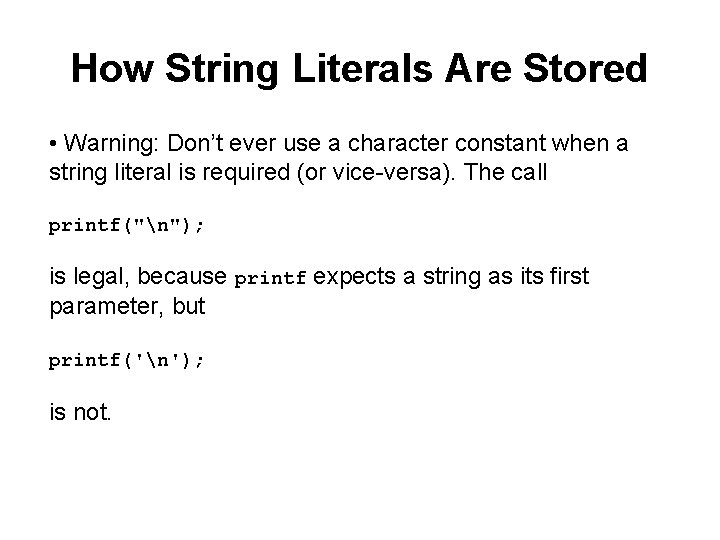
How String Literals Are Stored • Warning: Don’t ever use a character constant when a string literal is required (or vice-versa). The call printf("n"); is legal, because printf expects a string as its first parameter, but printf('n'); is not.
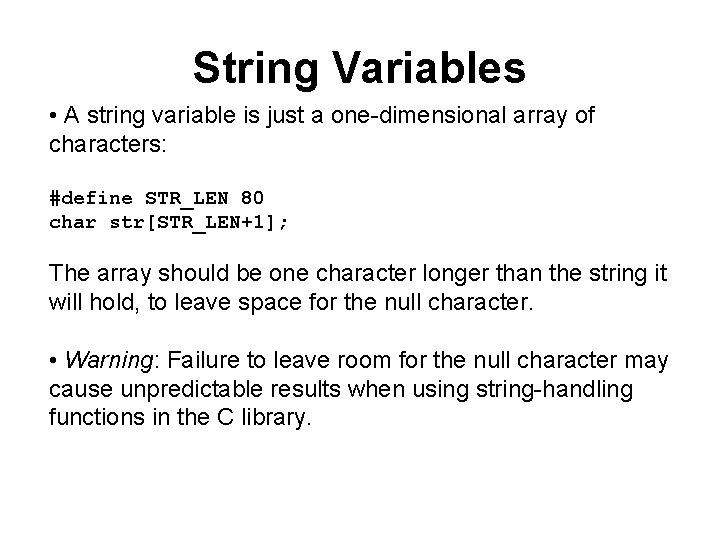
String Variables • A string variable is just a one-dimensional array of characters: #define STR_LEN 80 char str[STR_LEN+1]; The array should be one character longer than the string it will hold, to leave space for the null character. • Warning: Failure to leave room for the null character may cause unpredictable results when using string-handling functions in the C library.
![String Variables A string variable can be initialized char date 18 June String Variables • A string variable can be initialized: char date 1[8] = "June](https://slidetodoc.com/presentation_image_h2/6267d05df94eb03e7ca8f30975cc3c7d/image-18.jpg)
String Variables • A string variable can be initialized: char date 1[8] = "June 14"; The date array will have the following appearance: • A string initializer need not completely fill the array: char date 2[9] = "June 14"; The leftover array elements are filled with null characters:
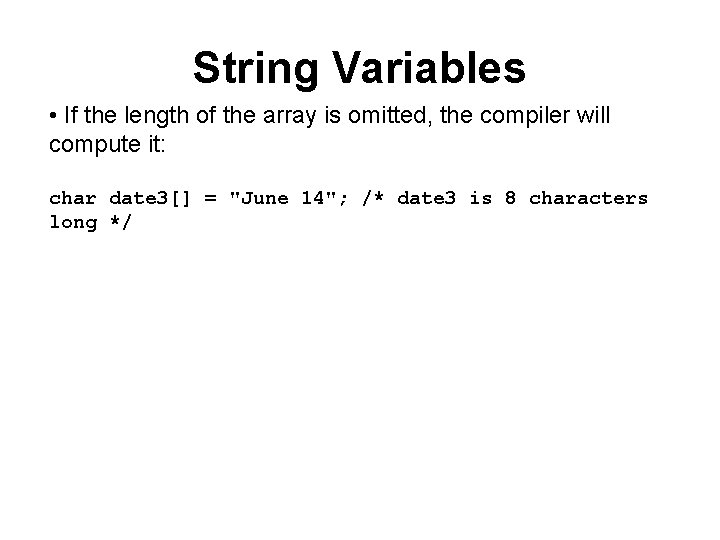
String Variables • If the length of the array is omitted, the compiler will compute it: char date 3[] = "June 14"; /* date 3 is 8 characters long */
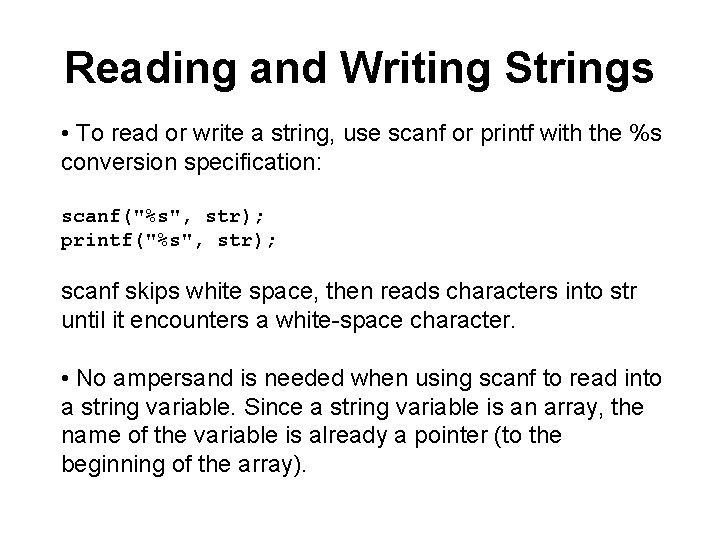
Reading and Writing Strings • To read or write a string, use scanf or printf with the %s conversion specification: scanf("%s", str); printf("%s", str); scanf skips white space, then reads characters into str until it encounters a white-space character. • No ampersand is needed when using scanf to read into a string variable. Since a string variable is an array, the name of the variable is already a pointer (to the beginning of the array).
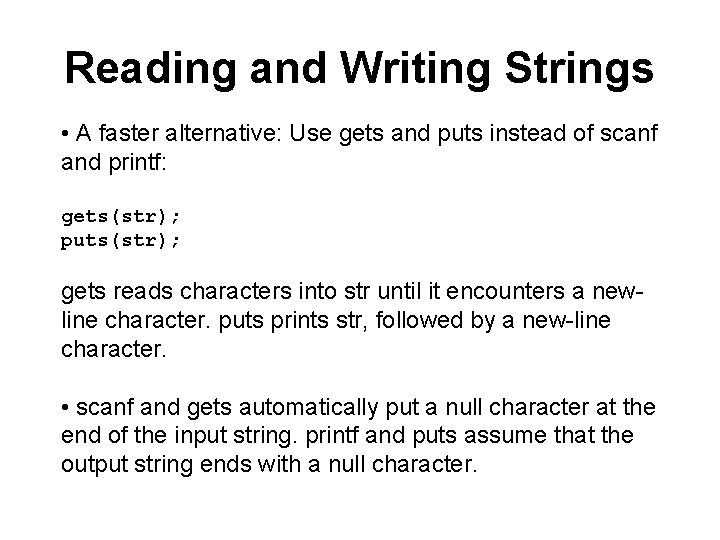
Reading and Writing Strings • A faster alternative: Use gets and puts instead of scanf and printf: gets(str); puts(str); gets reads characters into str until it encounters a newline character. puts prints str, followed by a new-line character. • scanf and gets automatically put a null character at the end of the input string. printf and puts assume that the output string ends with a null character.
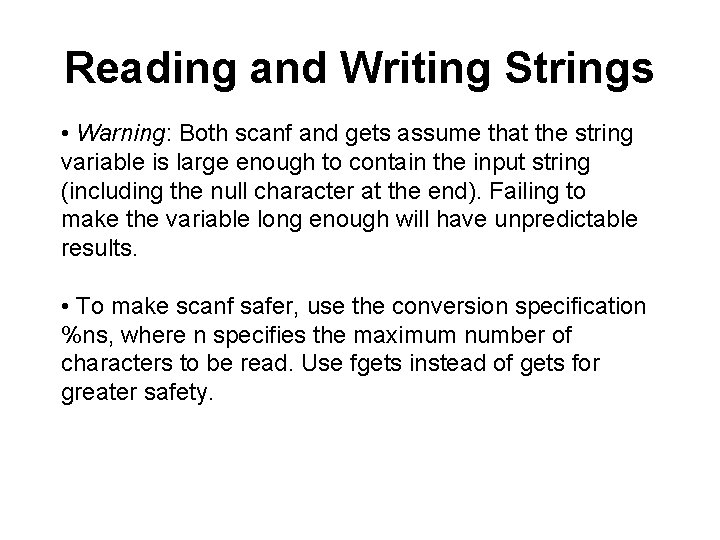
Reading and Writing Strings • Warning: Both scanf and gets assume that the string variable is large enough to contain the input string (including the null character at the end). Failing to make the variable long enough will have unpredictable results. • To make scanf safer, use the conversion specification %ns, where n specifies the maximum number of characters to be read. Use fgets instead of gets for greater safety.
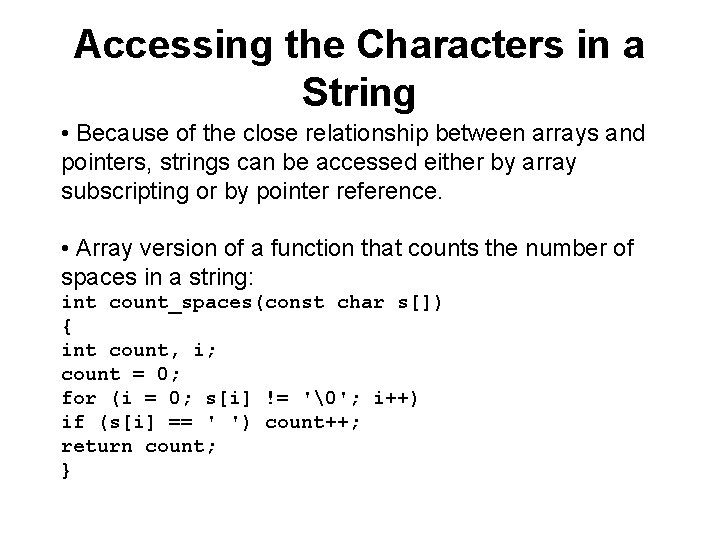
Accessing the Characters in a String • Because of the close relationship between arrays and pointers, strings can be accessed either by array subscripting or by pointer reference. • Array version of a function that counts the number of spaces in a string: int count_spaces(const char s[]) { int count, i; count = 0; for (i = 0; s[i] != '