AspectOriented Programming Programming paradigms n Procedural programming n
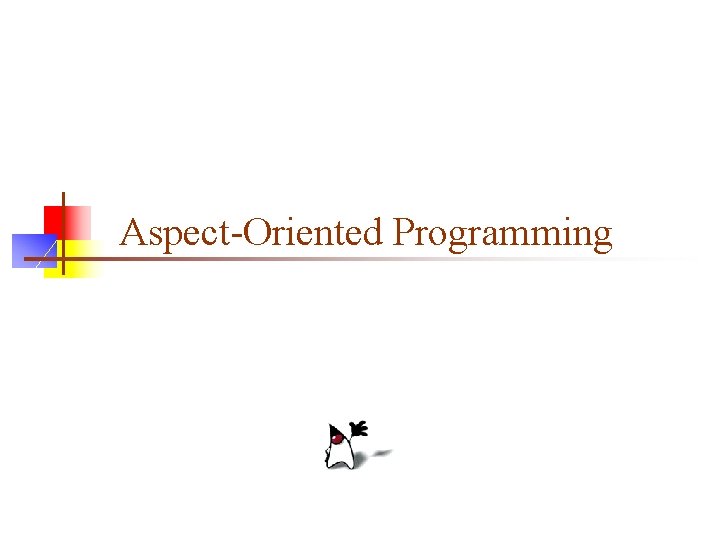
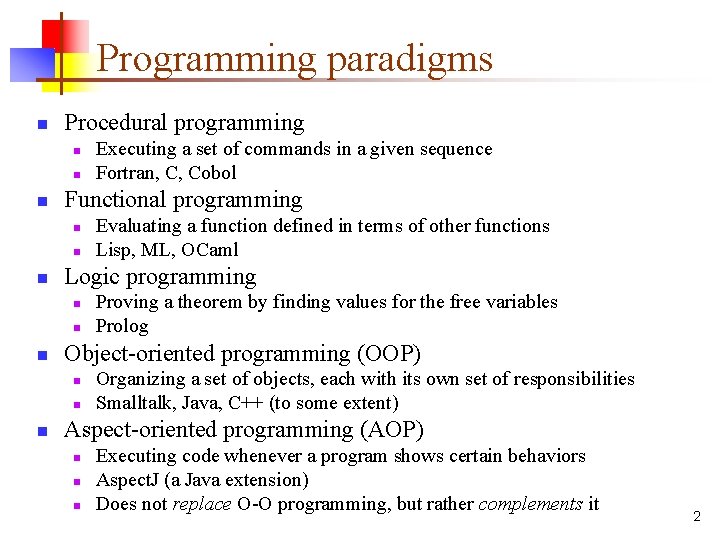
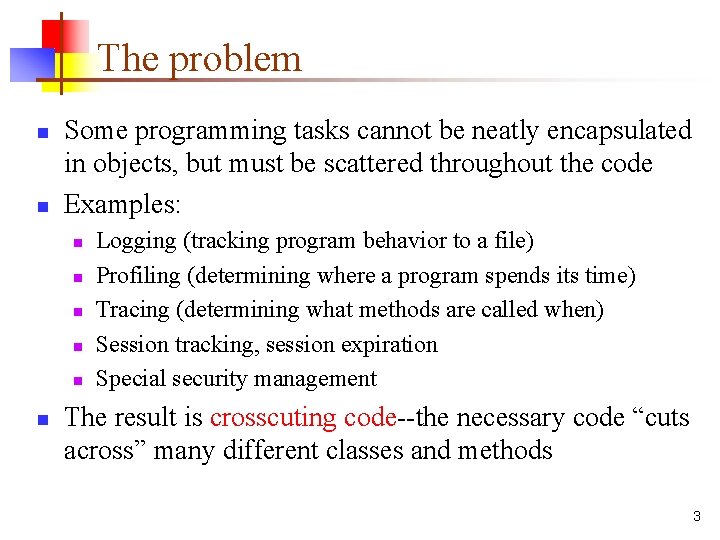
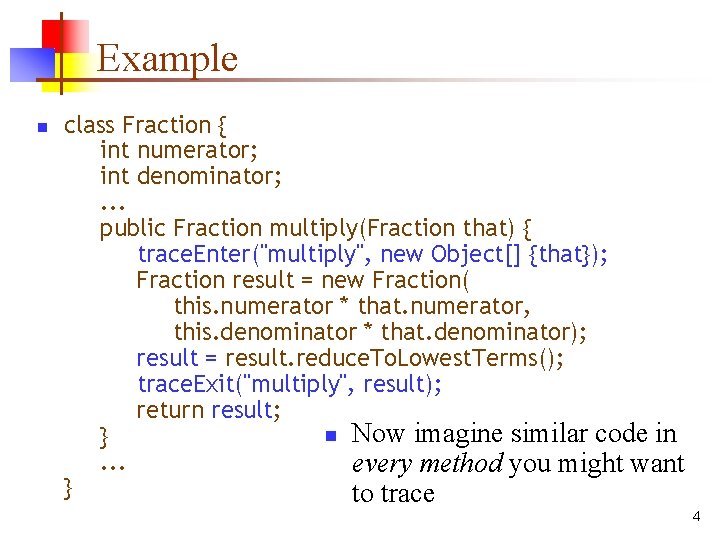
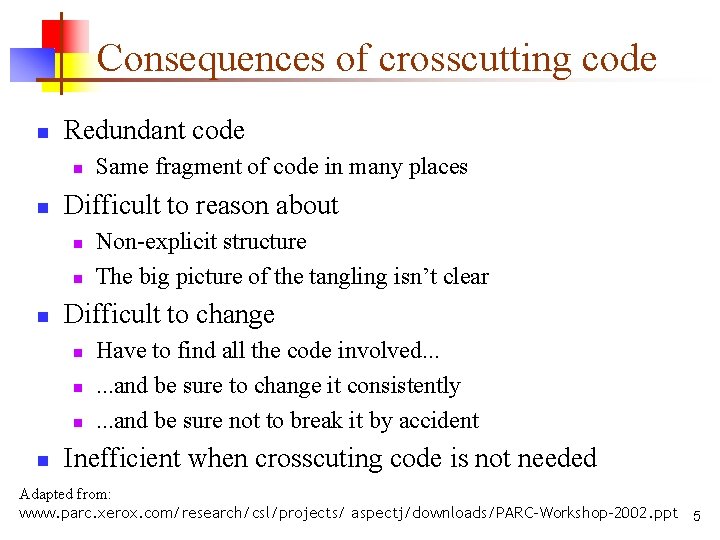
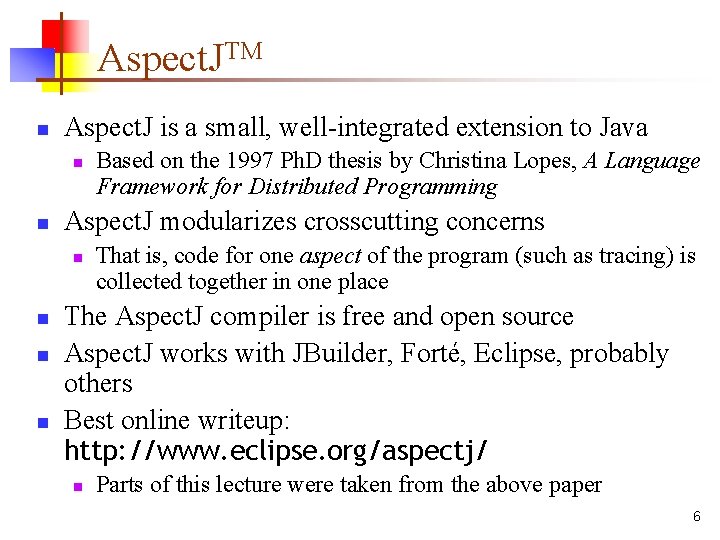
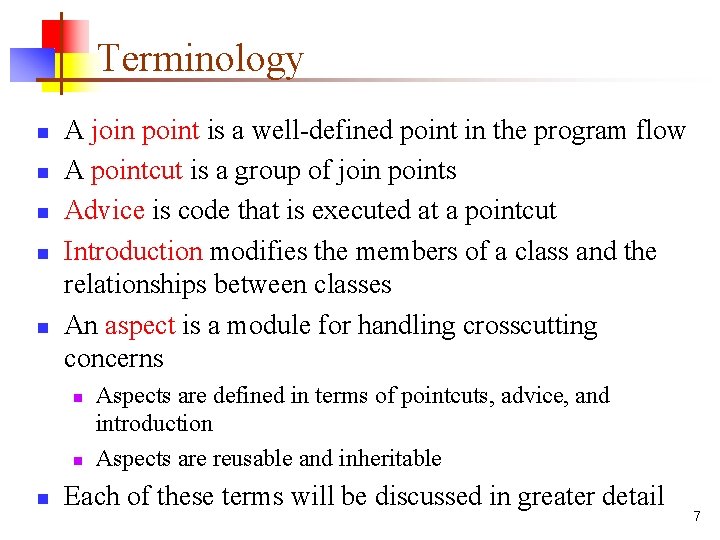
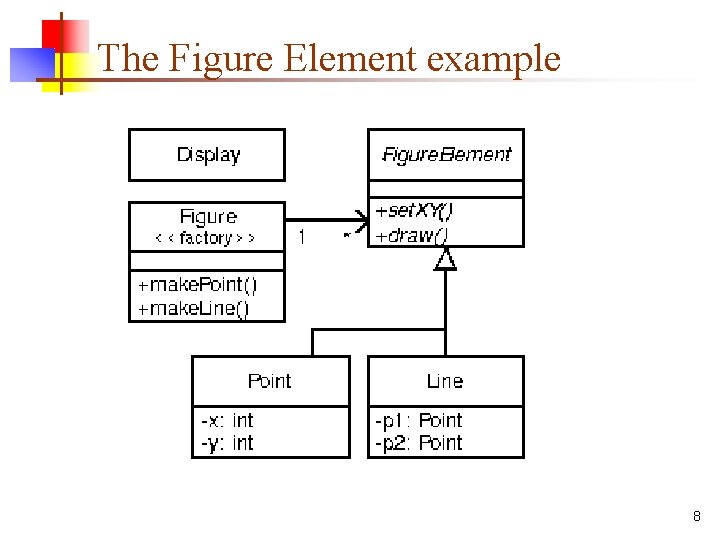
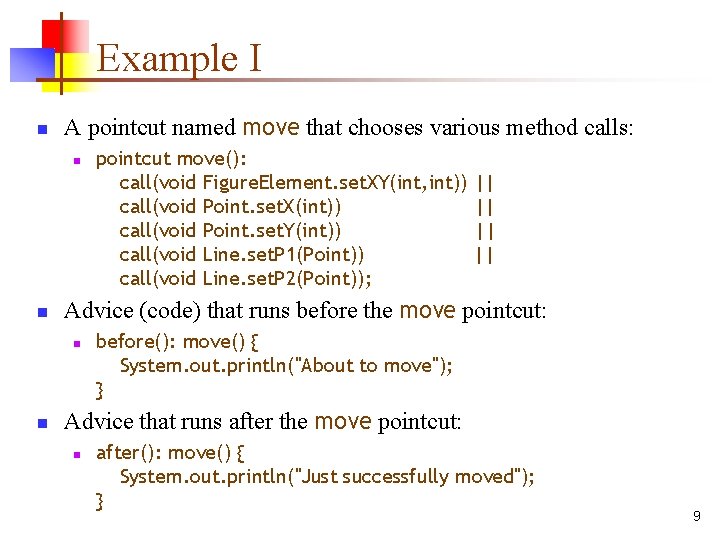
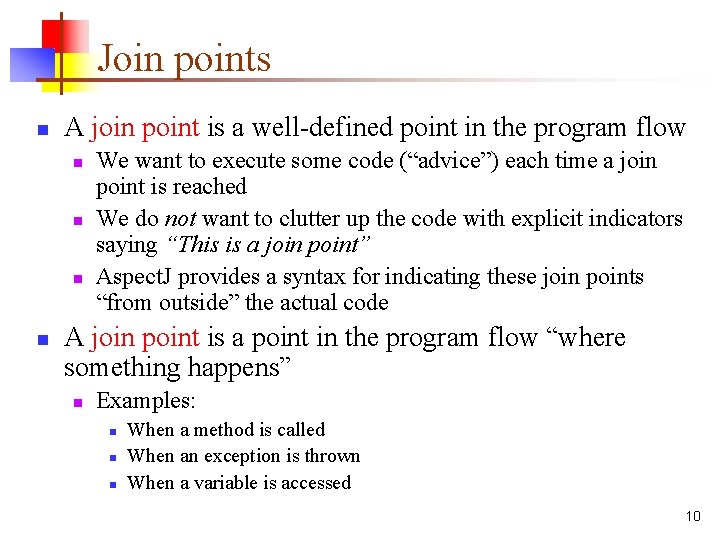
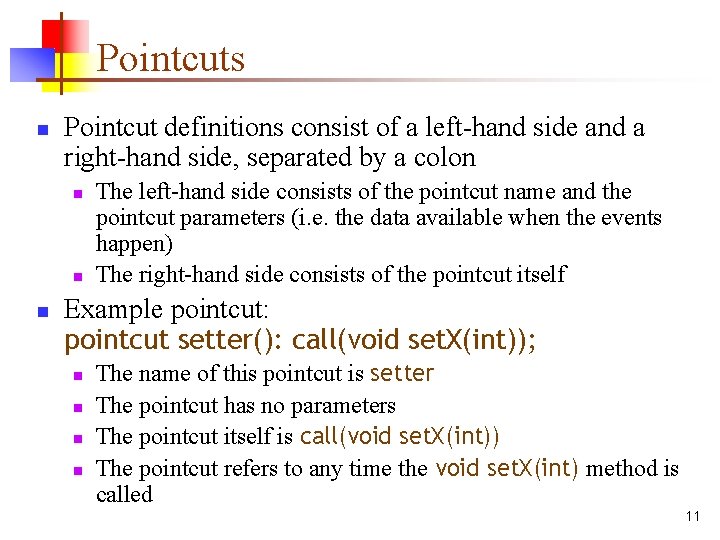
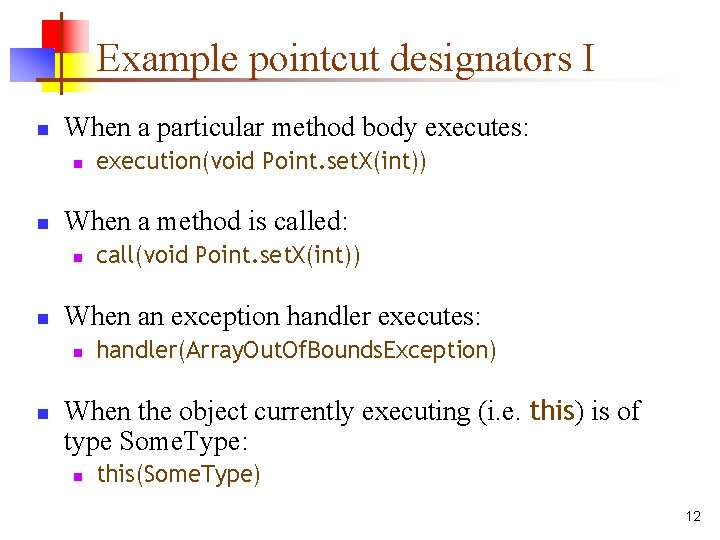
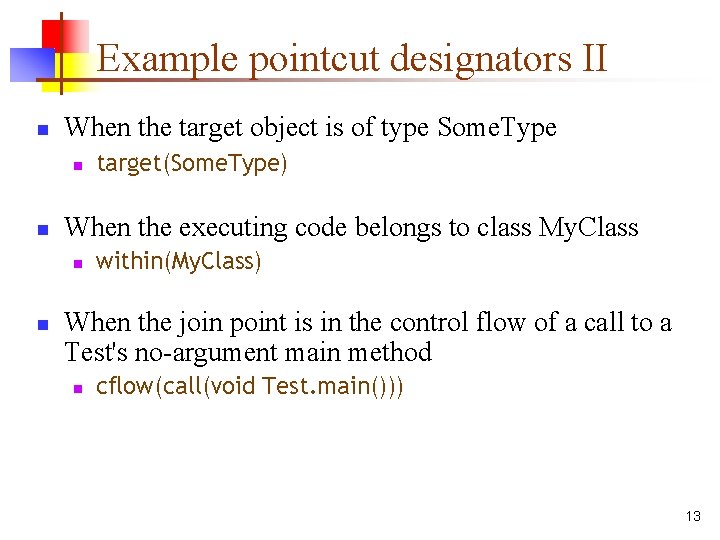
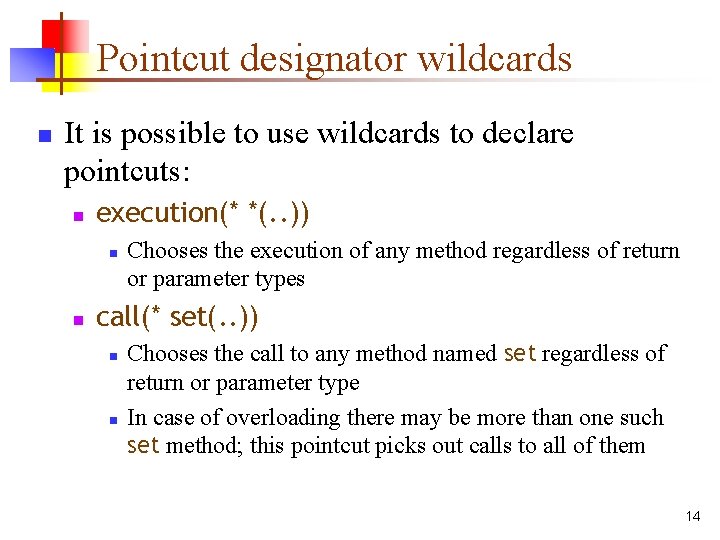
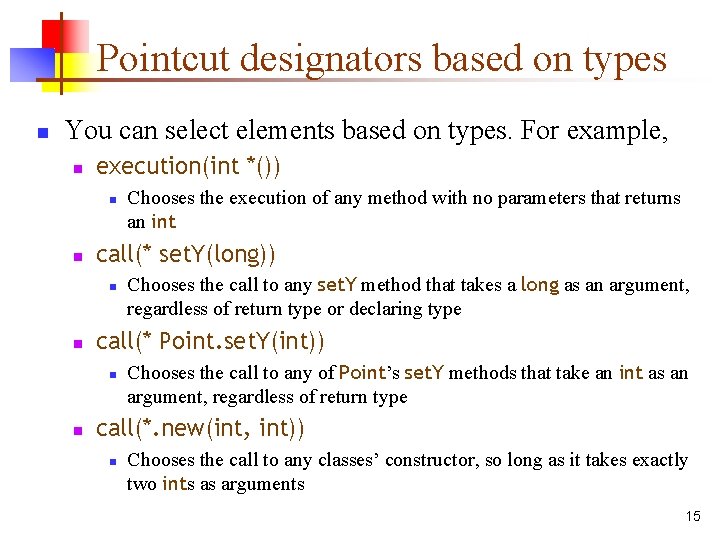
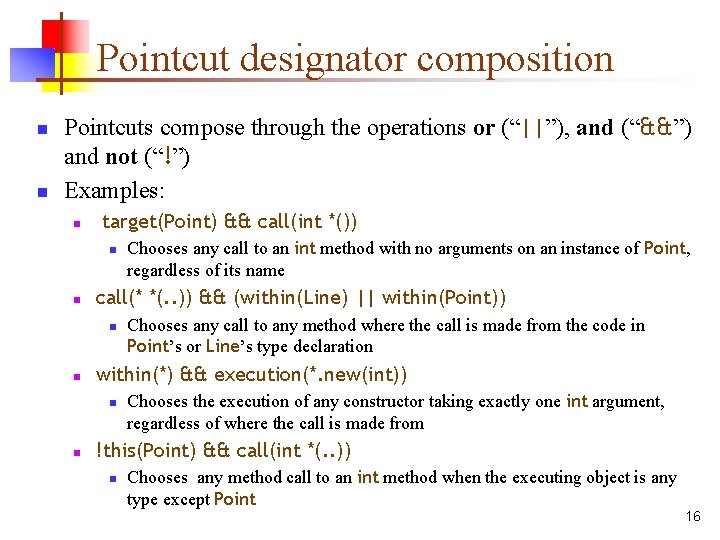
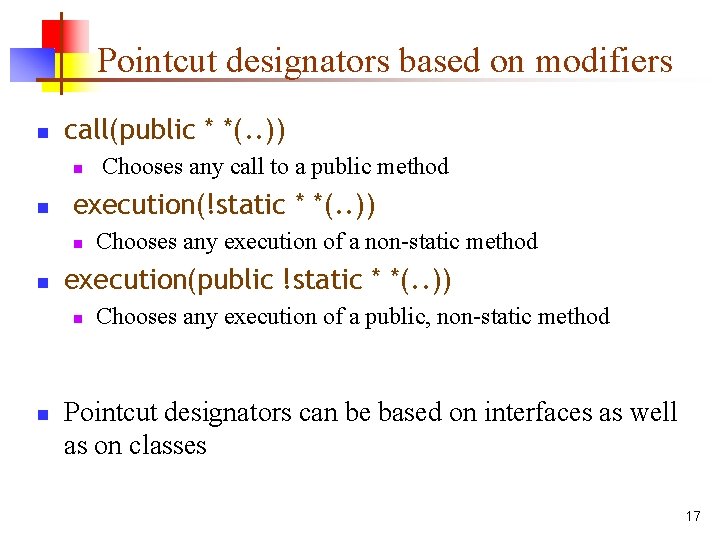
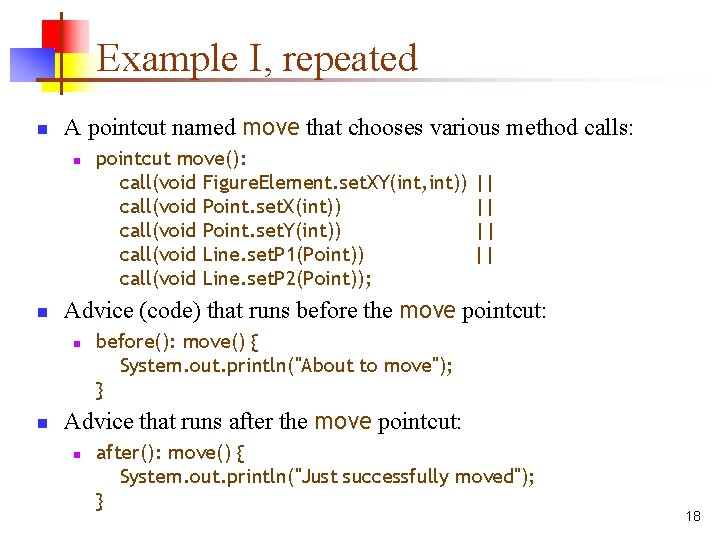
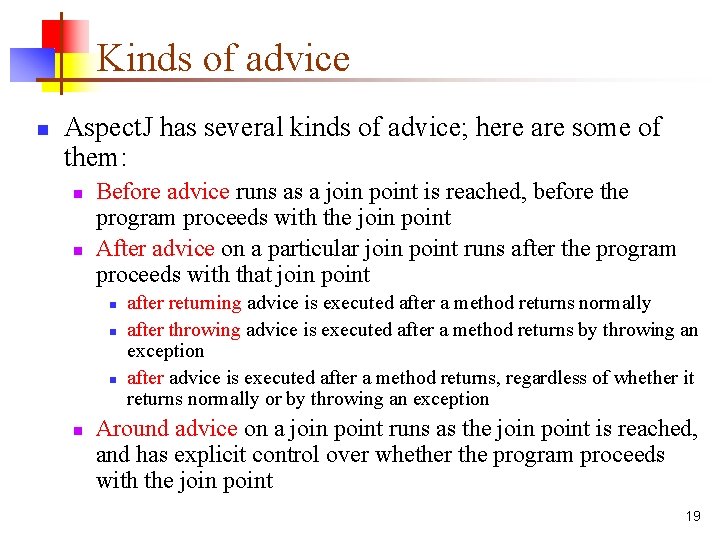
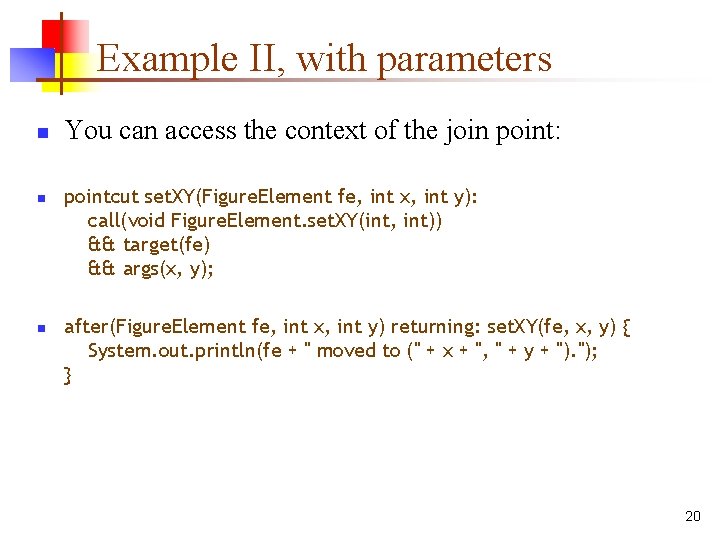
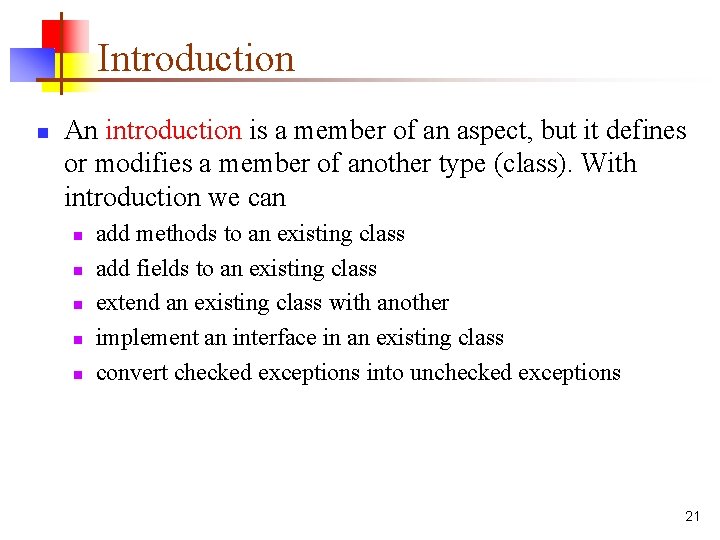
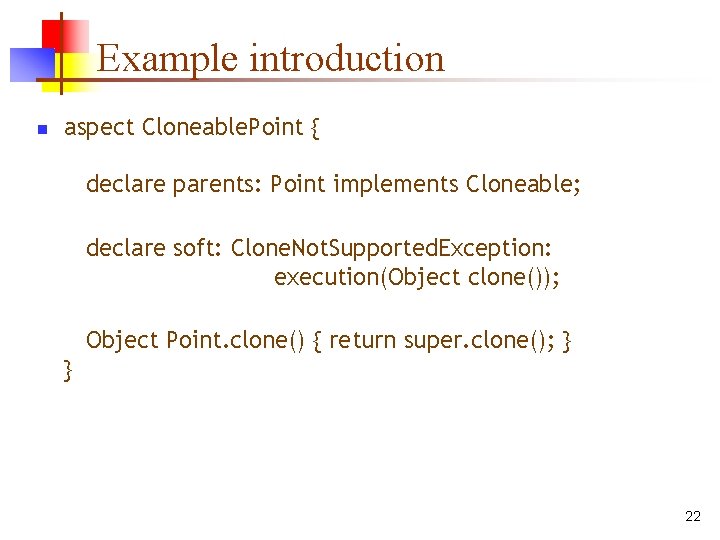
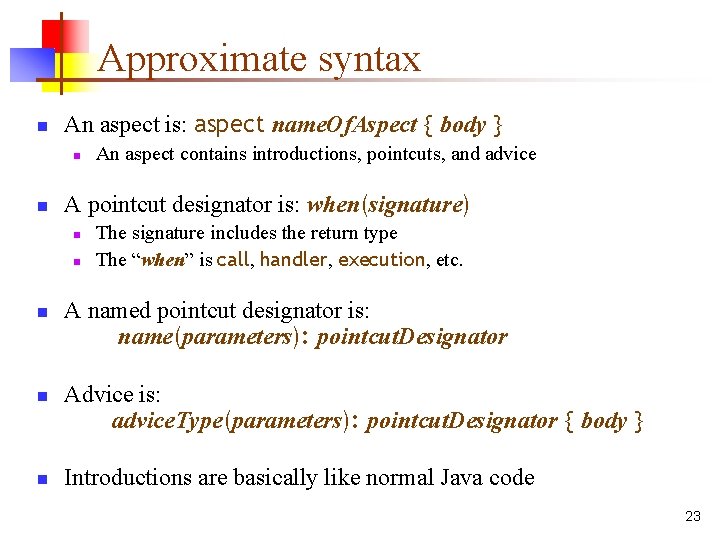
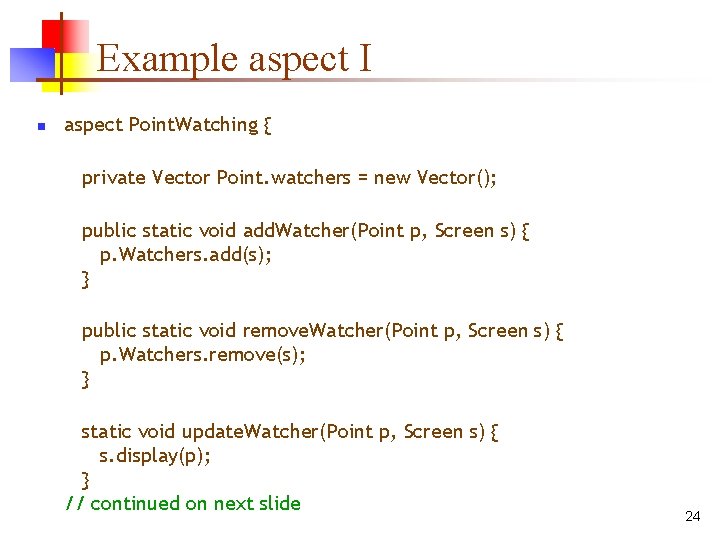
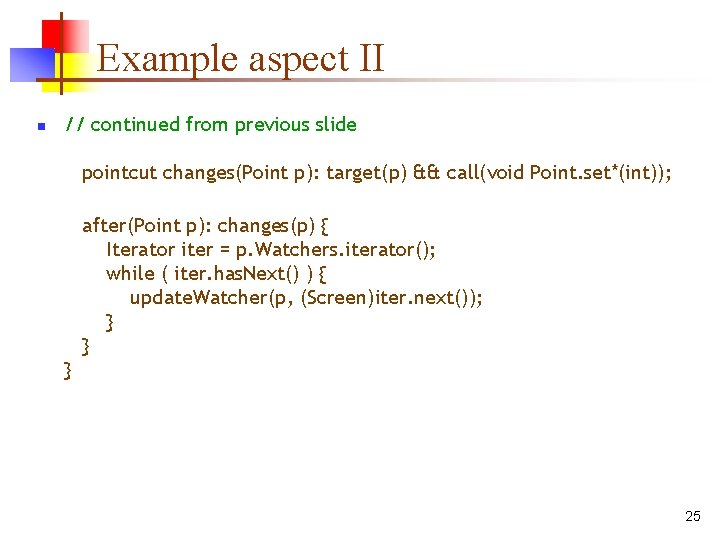
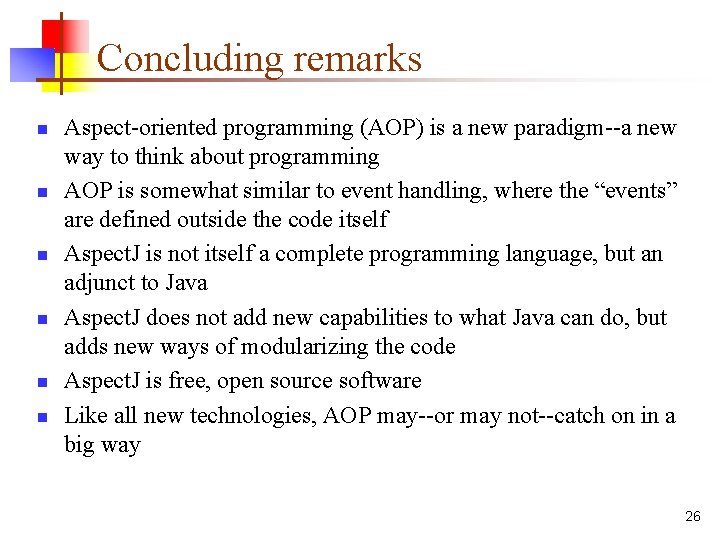
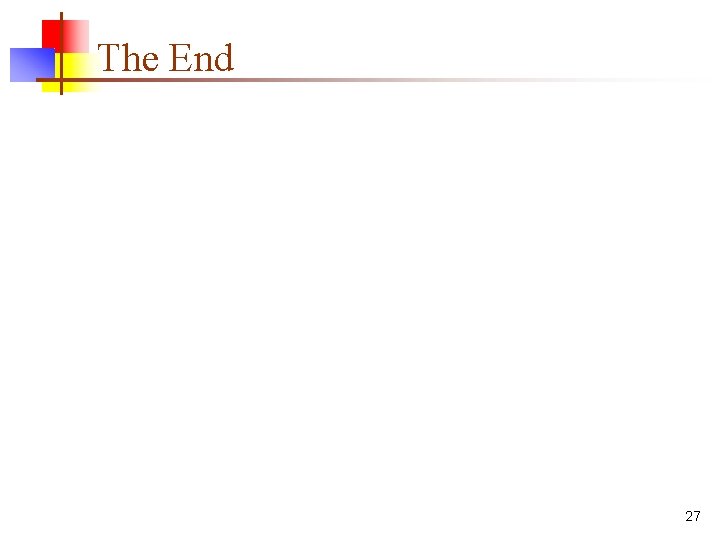
- Slides: 27
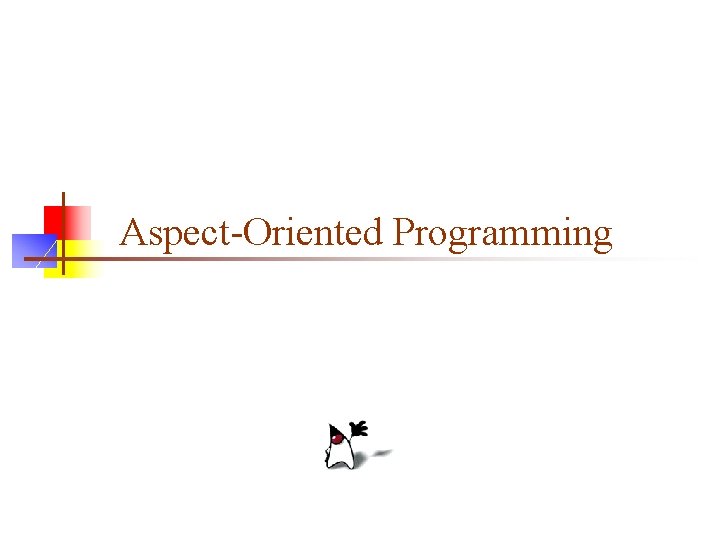
Aspect-Oriented Programming
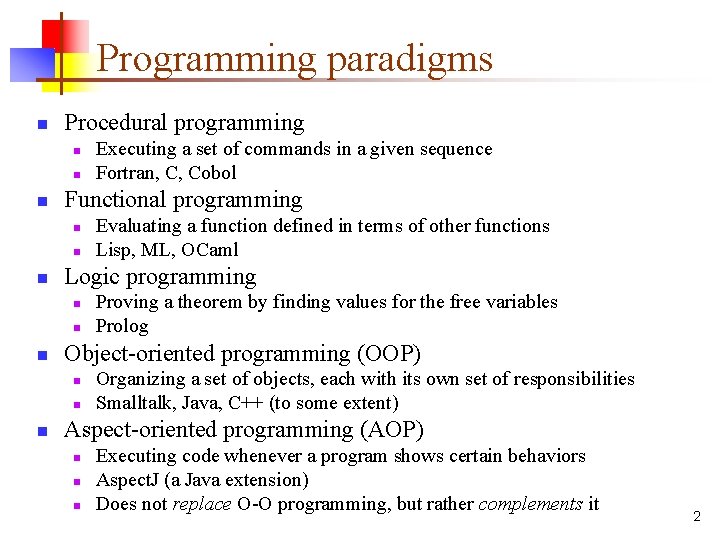
Programming paradigms n Procedural programming n n n Functional programming n n Proving a theorem by finding values for the free variables Prolog Object-oriented programming (OOP) n n n Evaluating a function defined in terms of other functions Lisp, ML, OCaml Logic programming n n Executing a set of commands in a given sequence Fortran, C, Cobol Organizing a set of objects, each with its own set of responsibilities Smalltalk, Java, C++ (to some extent) Aspect-oriented programming (AOP) n n n Executing code whenever a program shows certain behaviors Aspect. J (a Java extension) Does not replace O-O programming, but rather complements it 2
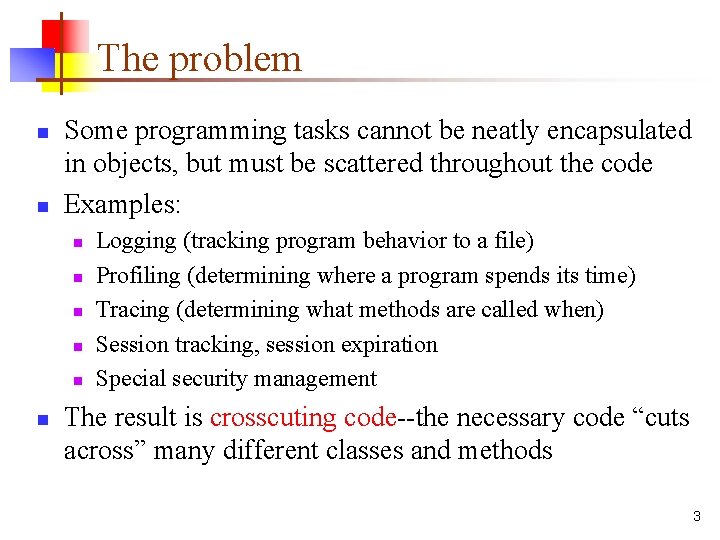
The problem n n Some programming tasks cannot be neatly encapsulated in objects, but must be scattered throughout the code Examples: n n n Logging (tracking program behavior to a file) Profiling (determining where a program spends its time) Tracing (determining what methods are called when) Session tracking, session expiration Special security management The result is crosscuting code--the necessary code “cuts across” many different classes and methods 3
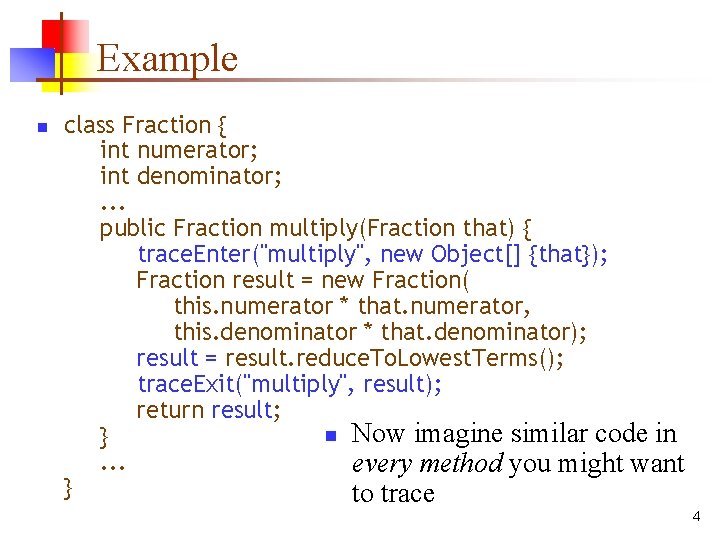
Example n class Fraction { int numerator; int denominator; . . . public Fraction multiply(Fraction that) { trace. Enter("multiply", new Object[] {that}); Fraction result = new Fraction( this. numerator * that. numerator, this. denominator * that. denominator); result = result. reduce. To. Lowest. Terms(); trace. Exit("multiply", result); return result; n Now imagine similar code in }. . . every method you might want } to trace 4
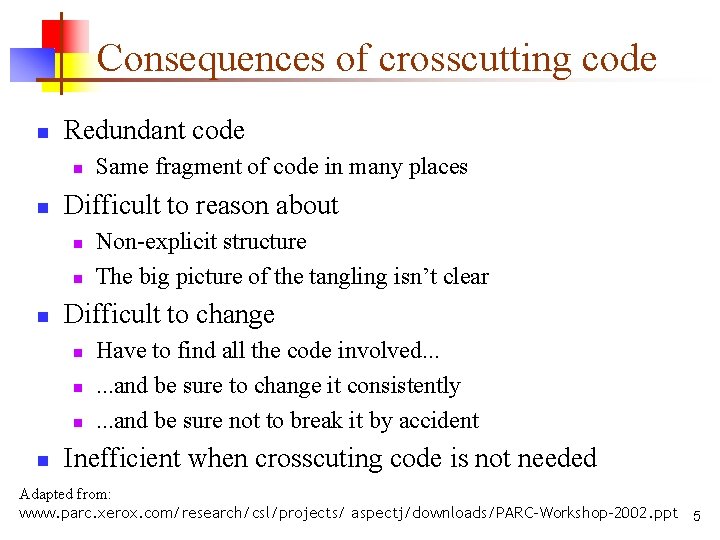
Consequences of crosscutting code n Redundant code n n Difficult to reason about n n n Non-explicit structure The big picture of the tangling isn’t clear Difficult to change n n Same fragment of code in many places Have to find all the code involved. . . and be sure to change it consistently. . . and be sure not to break it by accident Inefficient when crosscuting code is not needed Adapted from: www. parc. xerox. com/research/csl/projects/ aspectj/downloads/PARC-Workshop-2002. ppt 5
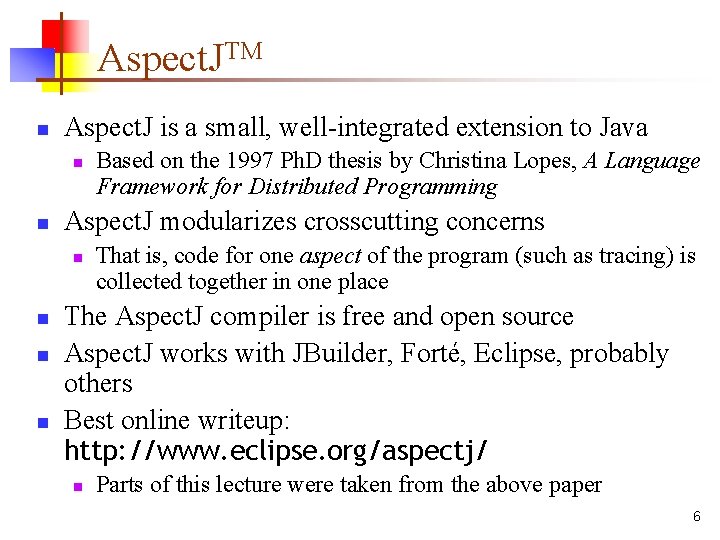
Aspect. JTM n Aspect. J is a small, well-integrated extension to Java n n Aspect. J modularizes crosscutting concerns n n Based on the 1997 Ph. D thesis by Christina Lopes, A Language Framework for Distributed Programming That is, code for one aspect of the program (such as tracing) is collected together in one place The Aspect. J compiler is free and open source Aspect. J works with JBuilder, Forté, Eclipse, probably others Best online writeup: http: //www. eclipse. org/aspectj/ n Parts of this lecture were taken from the above paper 6
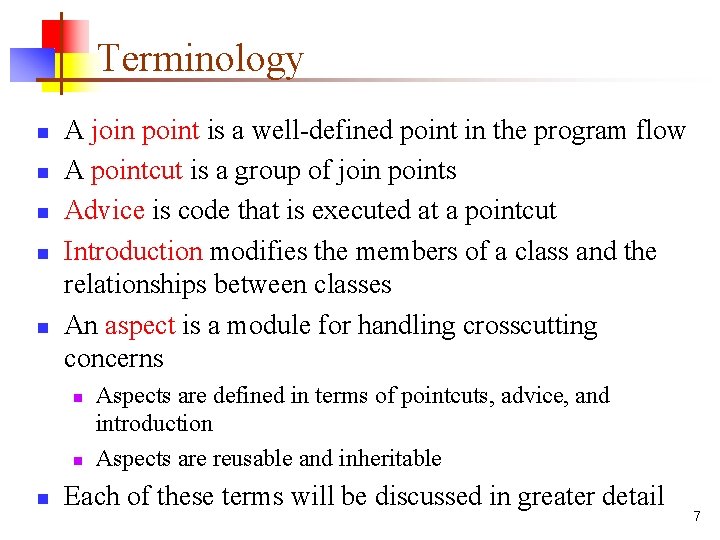
Terminology n n n A join point is a well-defined point in the program flow A pointcut is a group of join points Advice is code that is executed at a pointcut Introduction modifies the members of a class and the relationships between classes An aspect is a module for handling crosscutting concerns n n n Aspects are defined in terms of pointcuts, advice, and introduction Aspects are reusable and inheritable Each of these terms will be discussed in greater detail 7
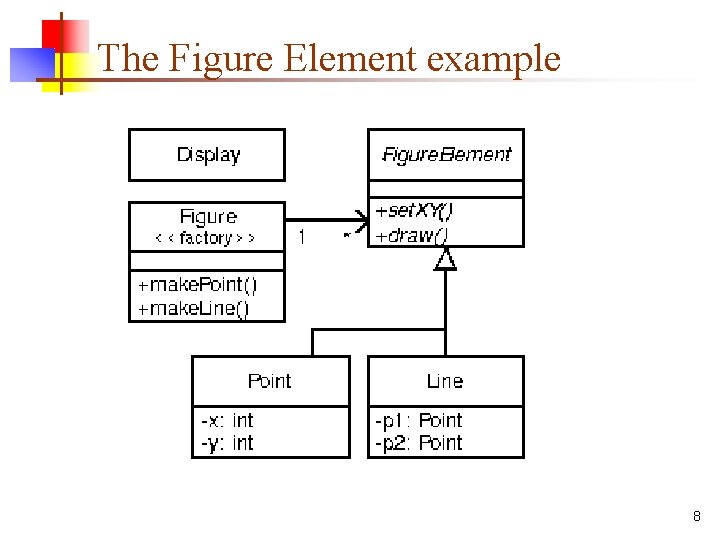
The Figure Element example 8
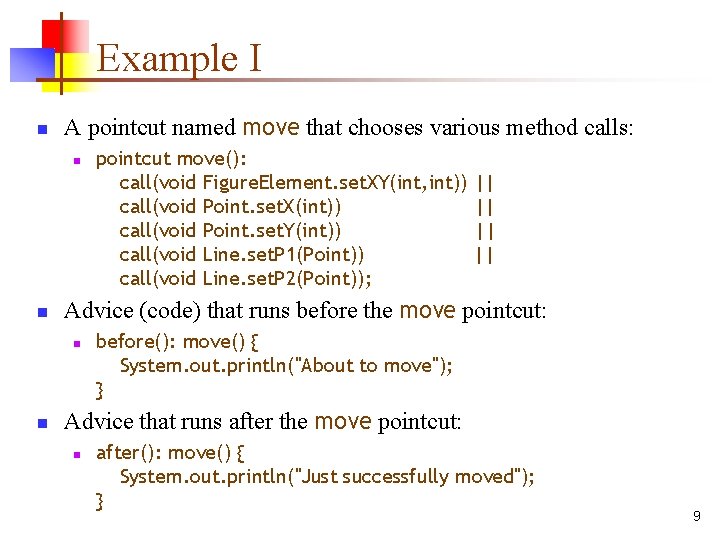
Example I n A pointcut named move that chooses various method calls: n n Advice (code) that runs before the move pointcut: n n pointcut move(): call(void Figure. Element. set. XY(int, int)) || call(void Point. set. X(int)) || call(void Point. set. Y(int)) || call(void Line. set. P 1(Point)) || call(void Line. set. P 2(Point)); before(): move() { System. out. println("About to move"); } Advice that runs after the move pointcut: n after(): move() { System. out. println("Just successfully moved"); } 9
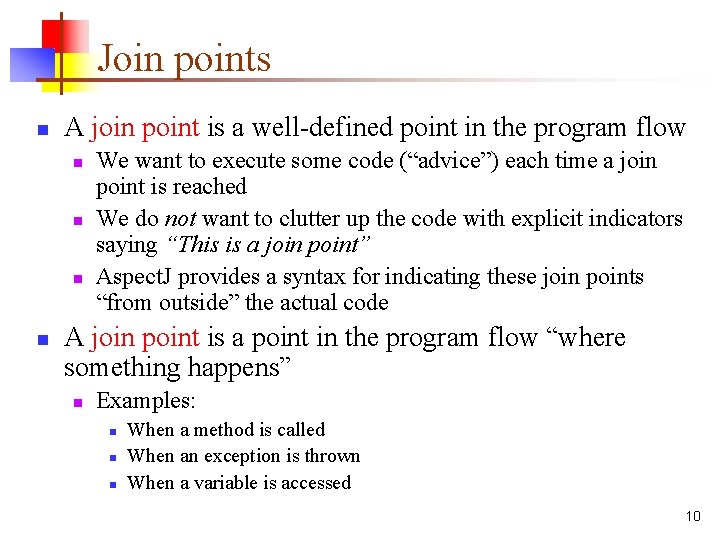
Join points n A join point is a well-defined point in the program flow n n We want to execute some code (“advice”) each time a join point is reached We do not want to clutter up the code with explicit indicators saying “This is a join point” Aspect. J provides a syntax for indicating these join points “from outside” the actual code A join point is a point in the program flow “where something happens” n Examples: n n n When a method is called When an exception is thrown When a variable is accessed 10
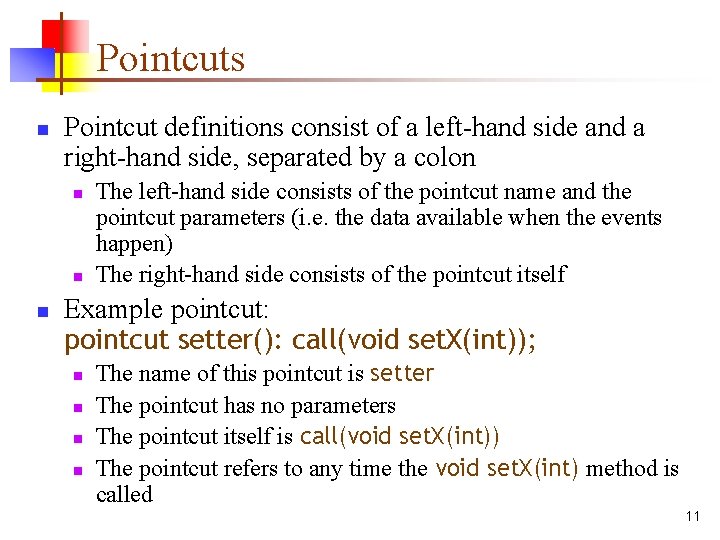
Pointcuts n Pointcut definitions consist of a left-hand side and a right-hand side, separated by a colon n The left-hand side consists of the pointcut name and the pointcut parameters (i. e. the data available when the events happen) The right-hand side consists of the pointcut itself Example pointcut: pointcut setter(): call(void set. X(int)); n n The name of this pointcut is setter The pointcut has no parameters The pointcut itself is call(void set. X(int)) The pointcut refers to any time the void set. X(int) method is called 11
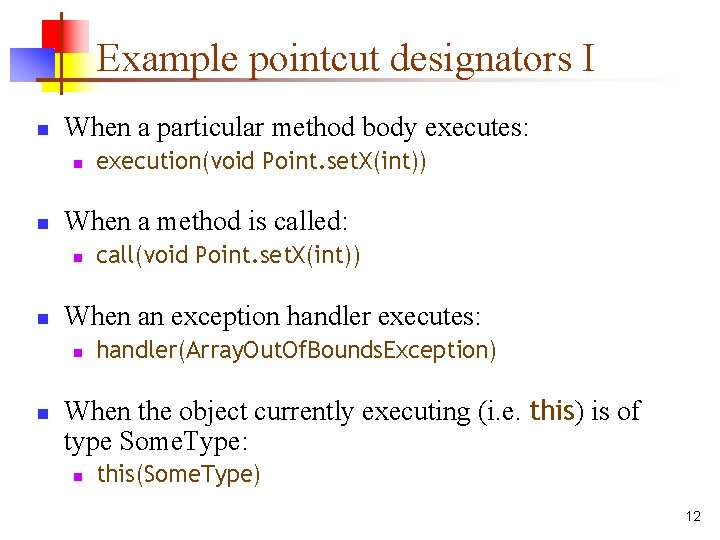
Example pointcut designators I n When a particular method body executes: n n When a method is called: n n call(void Point. set. X(int)) When an exception handler executes: n n execution(void Point. set. X(int)) handler(Array. Out. Of. Bounds. Exception) When the object currently executing (i. e. this) is of type Some. Type: n this(Some. Type) 12
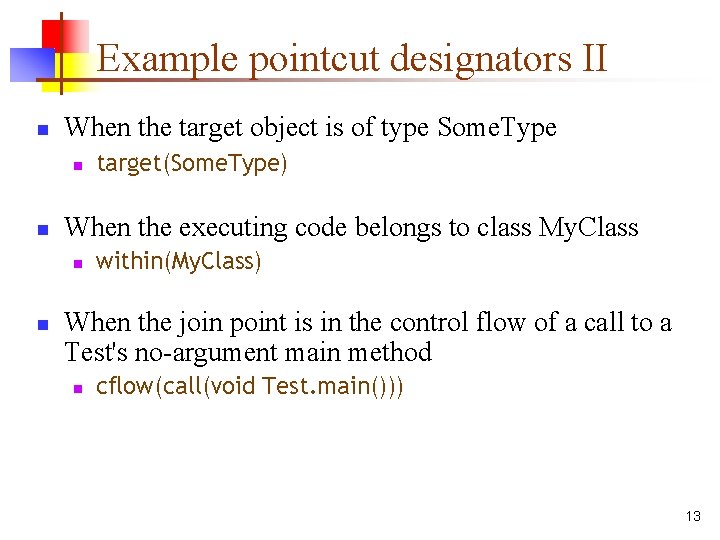
Example pointcut designators II n When the target object is of type Some. Type n n When the executing code belongs to class My. Class n n target(Some. Type) within(My. Class) When the join point is in the control flow of a call to a Test's no-argument main method n cflow(call(void Test. main())) 13
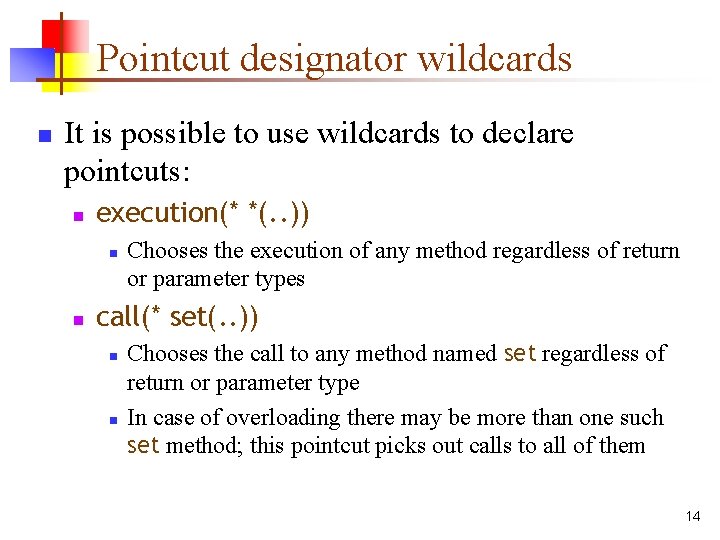
Pointcut designator wildcards n It is possible to use wildcards to declare pointcuts: n execution(* *(. . )) n n Chooses the execution of any method regardless of return or parameter types call(* set(. . )) n n Chooses the call to any method named set regardless of return or parameter type In case of overloading there may be more than one such set method; this pointcut picks out calls to all of them 14
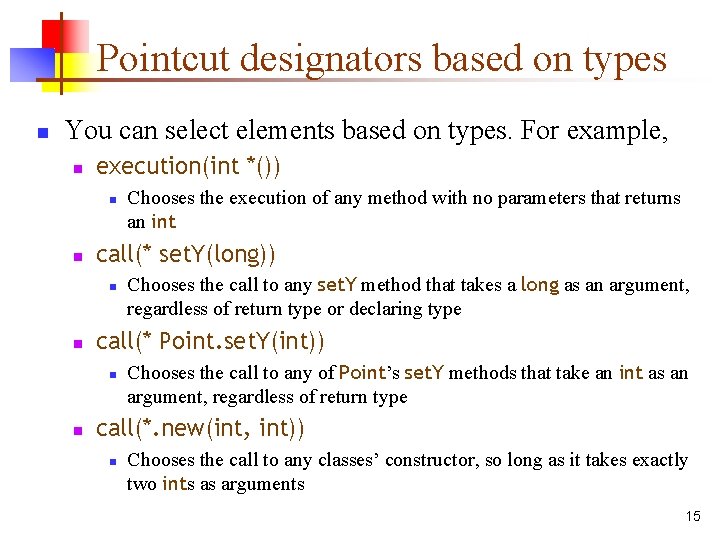
Pointcut designators based on types n You can select elements based on types. For example, n execution(int *()) n n call(* set. Y(long)) n n Chooses the call to any set. Y method that takes a long as an argument, regardless of return type or declaring type call(* Point. set. Y(int)) n n Chooses the execution of any method with no parameters that returns an int Chooses the call to any of Point’s set. Y methods that take an int as an argument, regardless of return type call(*. new(int, int)) n Chooses the call to any classes’ constructor, so long as it takes exactly two ints as arguments 15
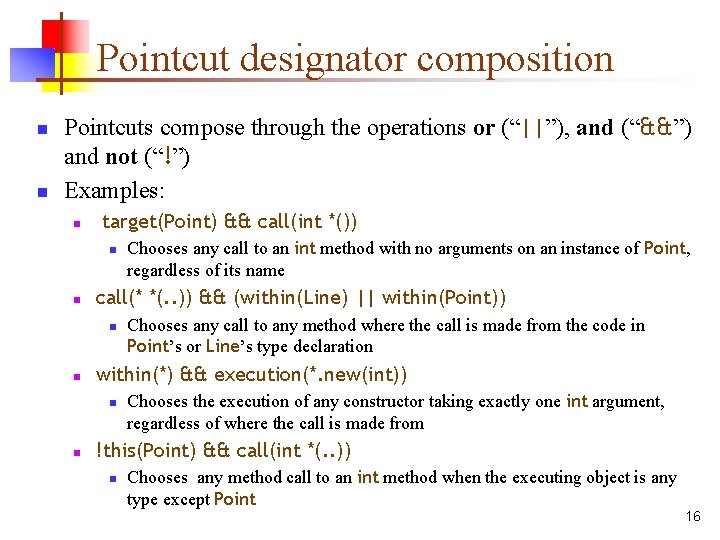
Pointcut designator composition n n Pointcuts compose through the operations or (“||”), and (“&&”) and not (“!”) Examples: n target(Point) && call(int *()) n n call(* *(. . )) && (within(Line) || within(Point)) n n Chooses any call to any method where the call is made from the code in Point’s or Line’s type declaration within(*) && execution(*. new(int)) n n Chooses any call to an int method with no arguments on an instance of Point, regardless of its name Chooses the execution of any constructor taking exactly one int argument, regardless of where the call is made from !this(Point) && call(int *(. . )) n Chooses any method call to an int method when the executing object is any type except Point 16
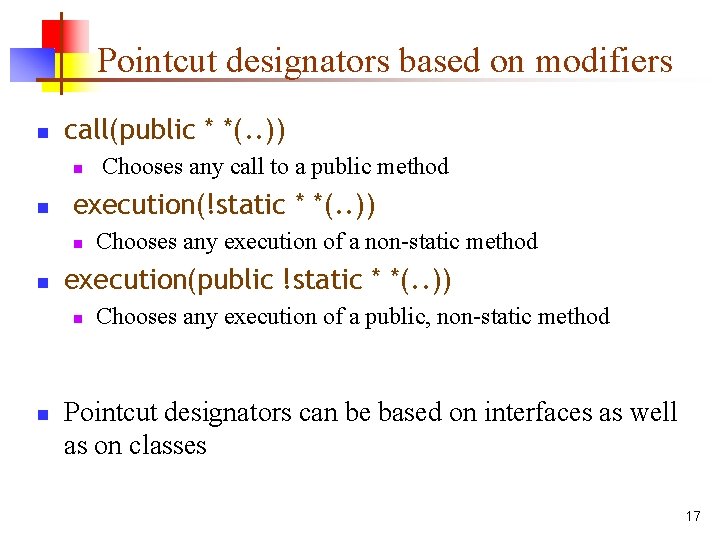
Pointcut designators based on modifiers n call(public * *(. . )) n n execution(!static * *(. . )) n n Chooses any execution of a non-static method execution(public !static * *(. . )) n n Chooses any call to a public method Chooses any execution of a public, non-static method Pointcut designators can be based on interfaces as well as on classes 17
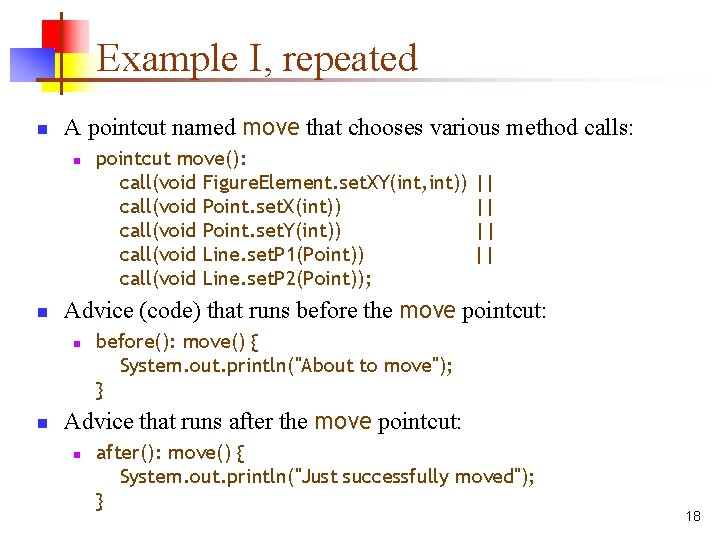
Example I, repeated n A pointcut named move that chooses various method calls: n n Advice (code) that runs before the move pointcut: n n pointcut move(): call(void Figure. Element. set. XY(int, int)) || call(void Point. set. X(int)) || call(void Point. set. Y(int)) || call(void Line. set. P 1(Point)) || call(void Line. set. P 2(Point)); before(): move() { System. out. println("About to move"); } Advice that runs after the move pointcut: n after(): move() { System. out. println("Just successfully moved"); } 18
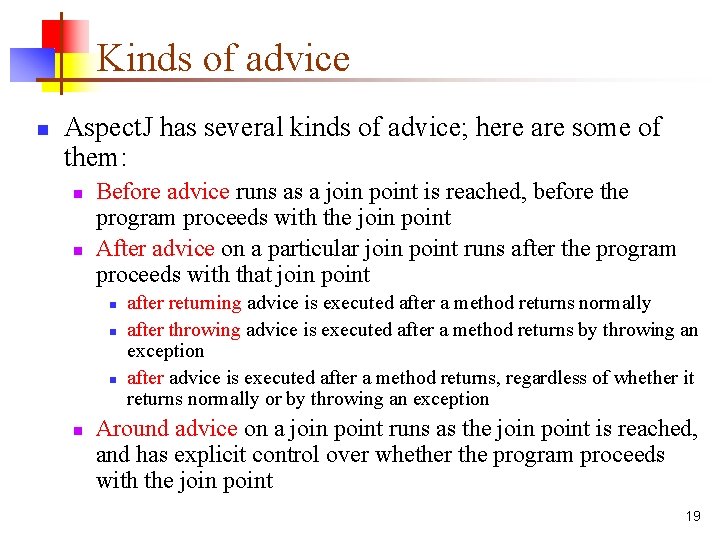
Kinds of advice n Aspect. J has several kinds of advice; here are some of them: n n Before advice runs as a join point is reached, before the program proceeds with the join point After advice on a particular join point runs after the program proceeds with that join point n n after returning advice is executed after a method returns normally after throwing advice is executed after a method returns by throwing an exception after advice is executed after a method returns, regardless of whether it returns normally or by throwing an exception Around advice on a join point runs as the join point is reached, and has explicit control over whether the program proceeds with the join point 19
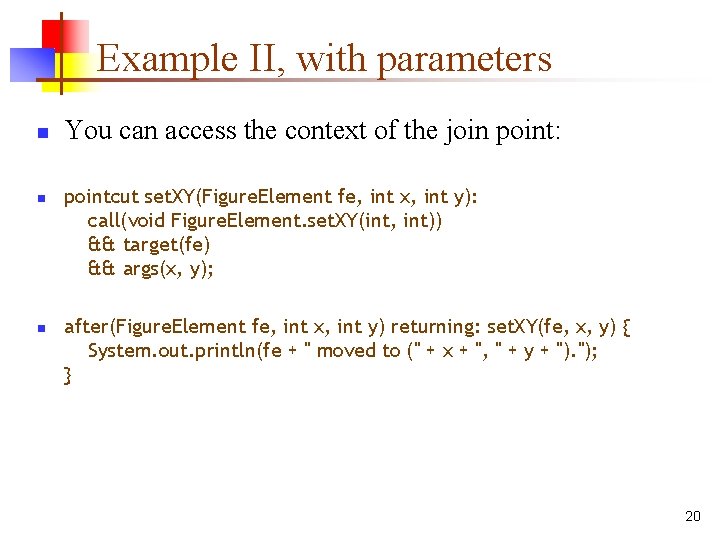
Example II, with parameters n n n You can access the context of the join point: pointcut set. XY(Figure. Element fe, int x, int y): call(void Figure. Element. set. XY(int, int)) && target(fe) && args(x, y); after(Figure. Element fe, int x, int y) returning: set. XY(fe, x, y) { System. out. println(fe + " moved to (" + x + ", " + y + "). "); } 20
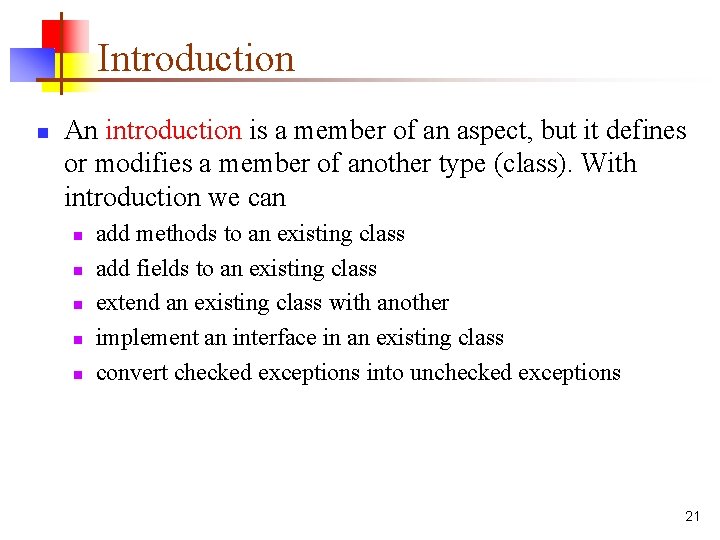
Introduction n An introduction is a member of an aspect, but it defines or modifies a member of another type (class). With introduction we can n n add methods to an existing class add fields to an existing class extend an existing class with another implement an interface in an existing class convert checked exceptions into unchecked exceptions 21
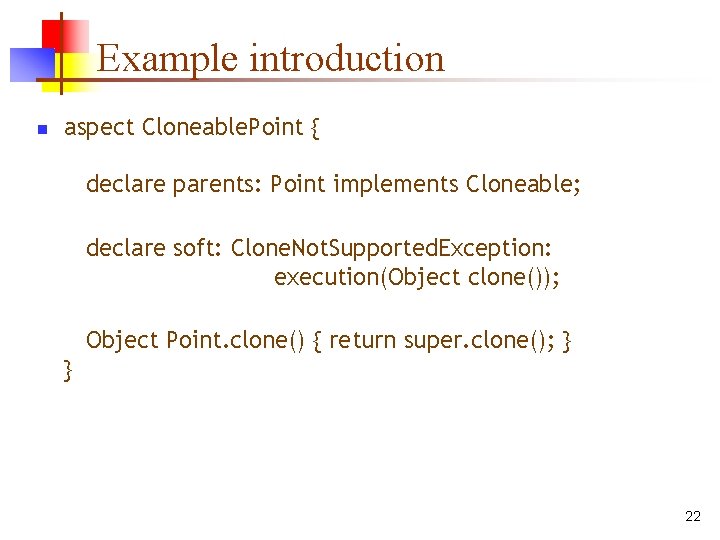
Example introduction n aspect Cloneable. Point { declare parents: Point implements Cloneable; declare soft: Clone. Not. Supported. Exception: execution(Object clone()); Object Point. clone() { return super. clone(); } } 22
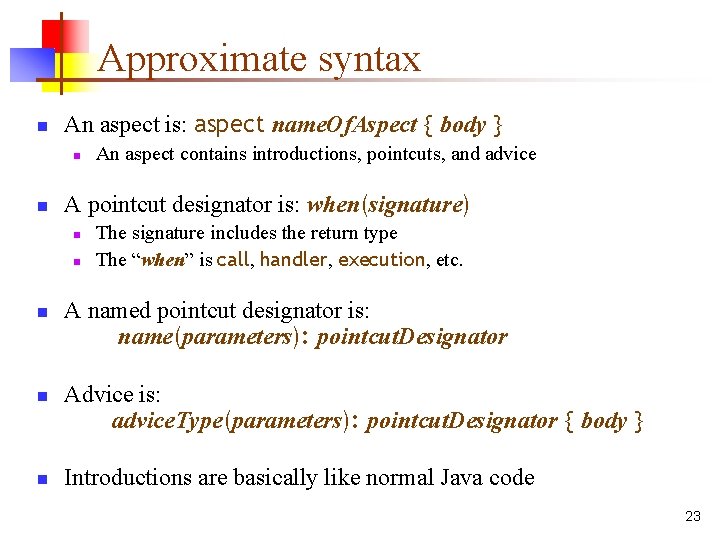
Approximate syntax n An aspect is: aspect name. Of. Aspect { body } n n A pointcut designator is: when(signature) n n n An aspect contains introductions, pointcuts, and advice The signature includes the return type The “when” is call, handler, execution, etc. A named pointcut designator is: name(parameters): pointcut. Designator Advice is: advice. Type(parameters): pointcut. Designator { body } Introductions are basically like normal Java code 23
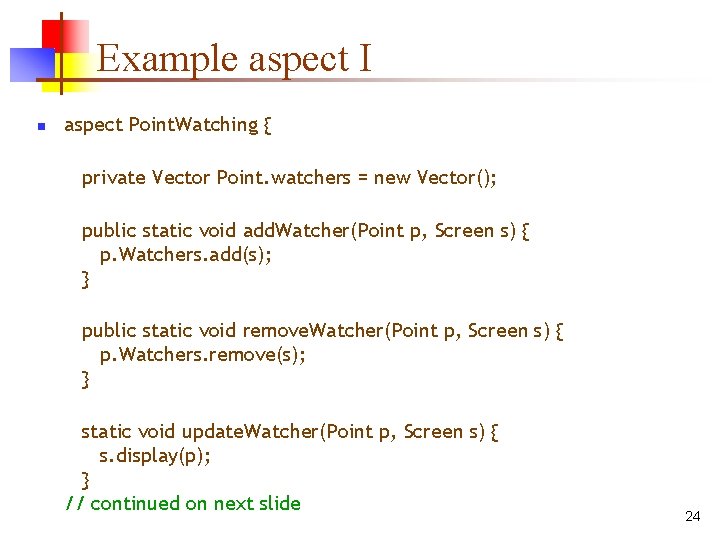
Example aspect I n aspect Point. Watching { private Vector Point. watchers = new Vector(); public static void add. Watcher(Point p, Screen s) { p. Watchers. add(s); } public static void remove. Watcher(Point p, Screen s) { p. Watchers. remove(s); } static void update. Watcher(Point p, Screen s) { s. display(p); } // continued on next slide 24
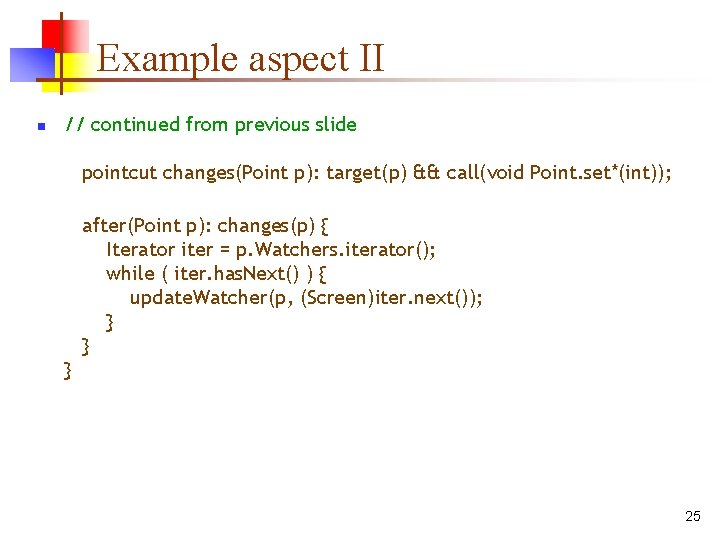
Example aspect II n // continued from previous slide pointcut changes(Point p): target(p) && call(void Point. set*(int)); after(Point p): changes(p) { Iterator iter = p. Watchers. iterator(); while ( iter. has. Next() ) { update. Watcher(p, (Screen)iter. next()); } } } 25
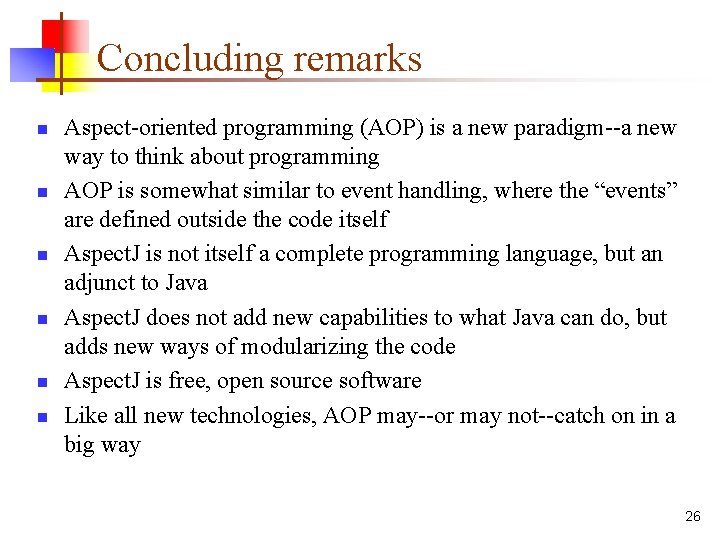
Concluding remarks n n n Aspect-oriented programming (AOP) is a new paradigm--a new way to think about programming AOP is somewhat similar to event handling, where the “events” are defined outside the code itself Aspect. J is not itself a complete programming language, but an adjunct to Java Aspect. J does not add new capabilities to what Java can do, but adds new ways of modularizing the code Aspect. J is free, open source software Like all new technologies, AOP may--or may not--catch on in a big way 26
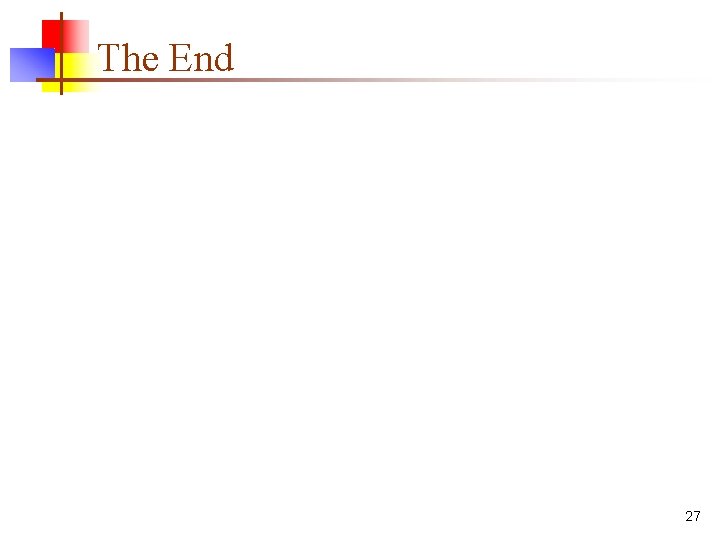
The End 27