INTRODUCTION TO C PROGRAMMING Curso Propedeutico 1 CINVESTAVGuadalajara
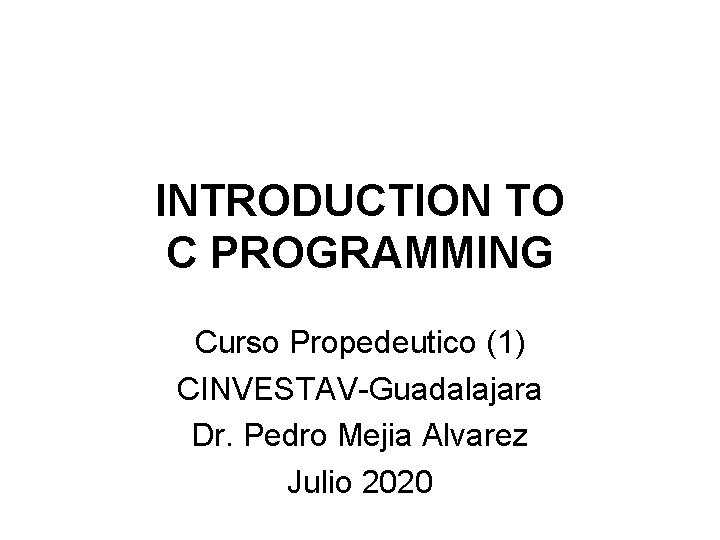
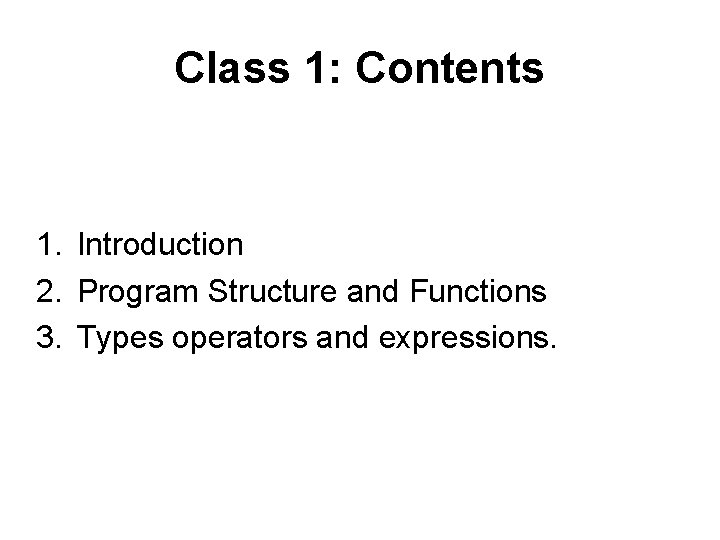
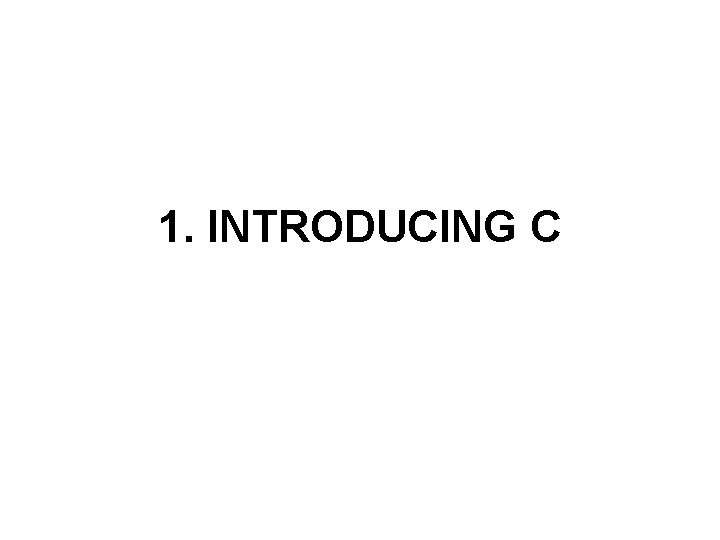
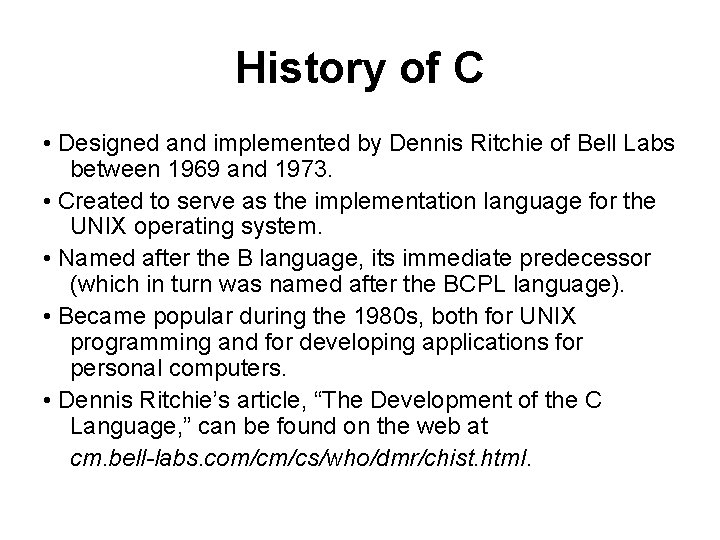
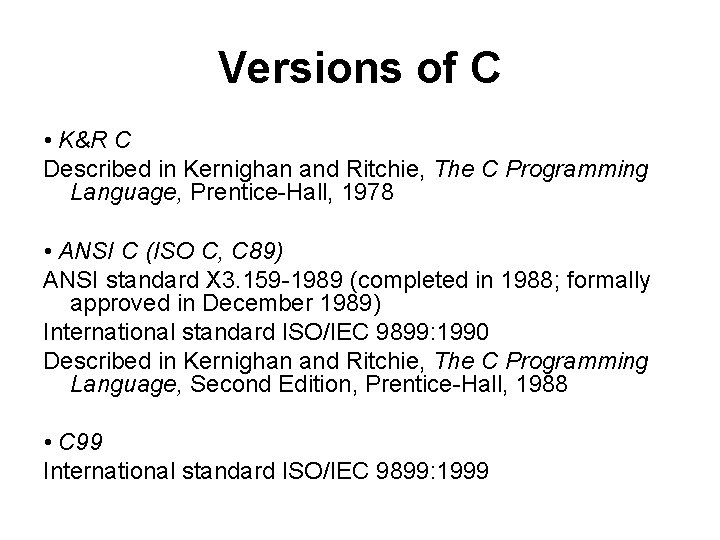
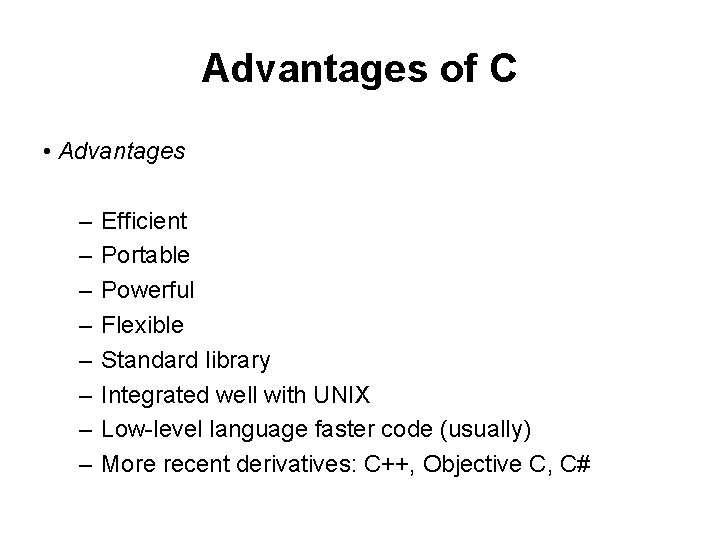
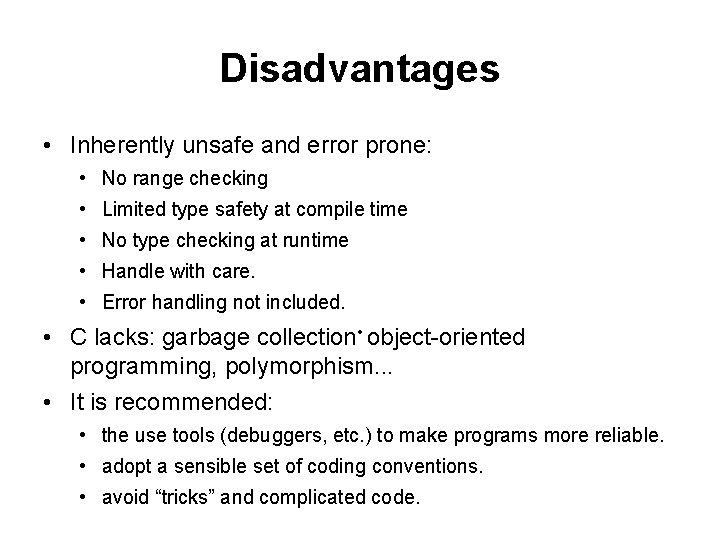
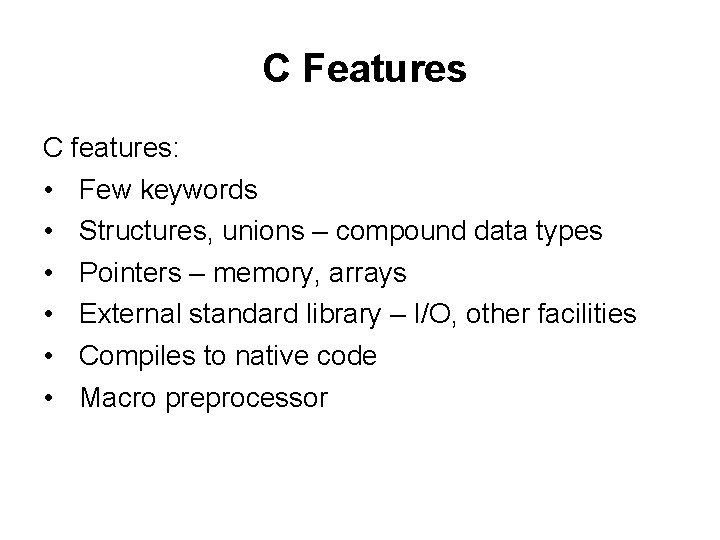
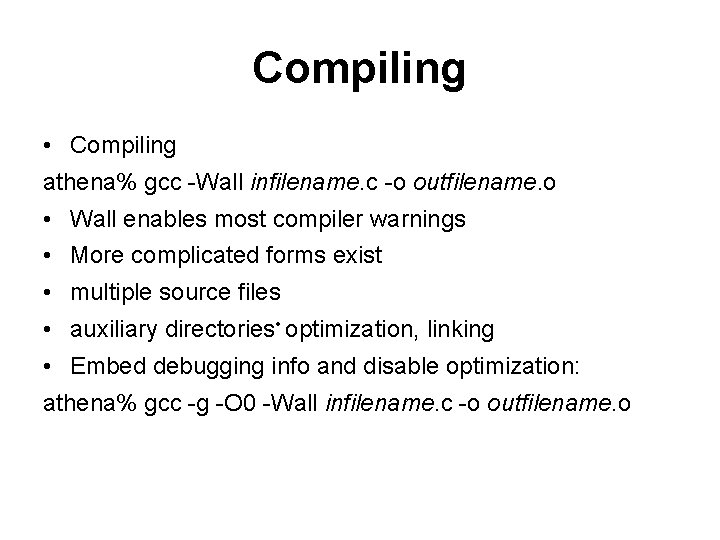
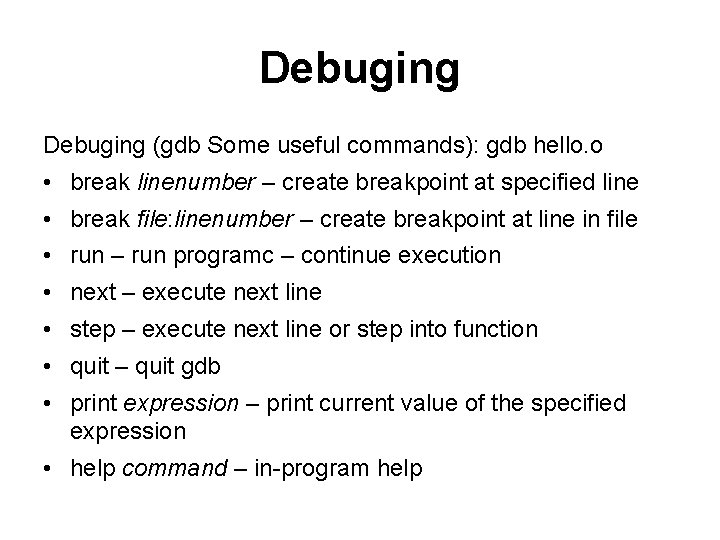
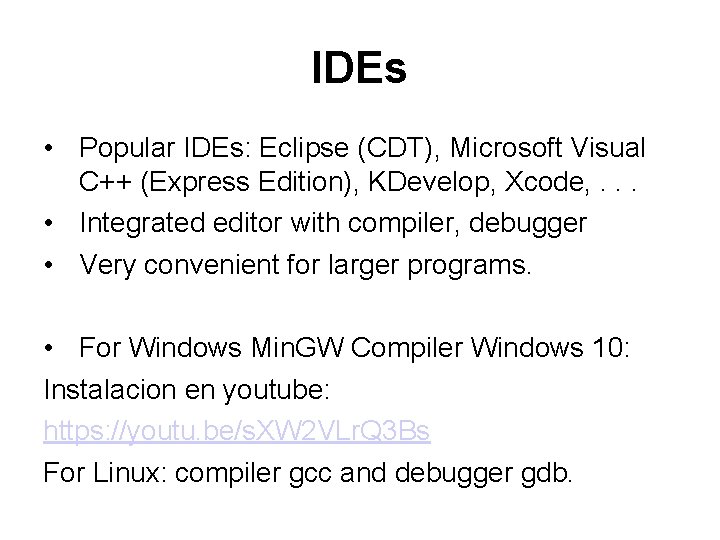
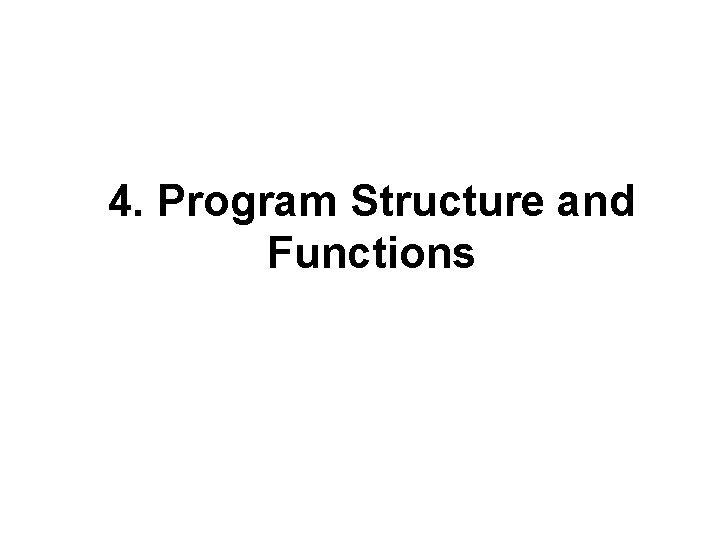
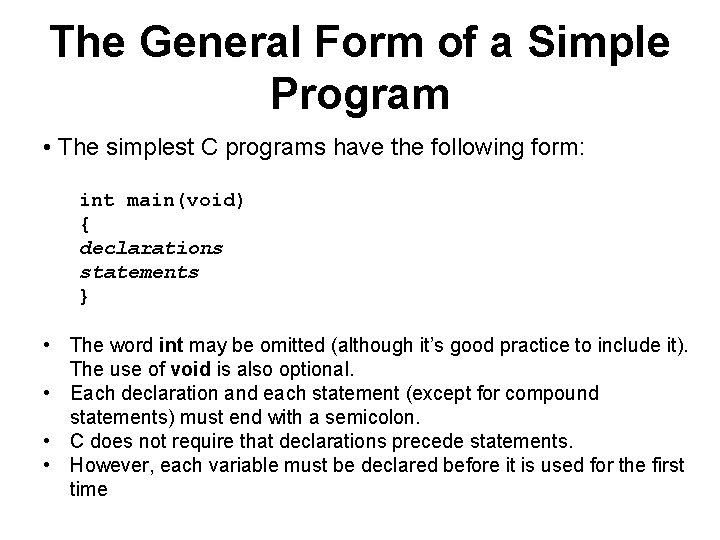
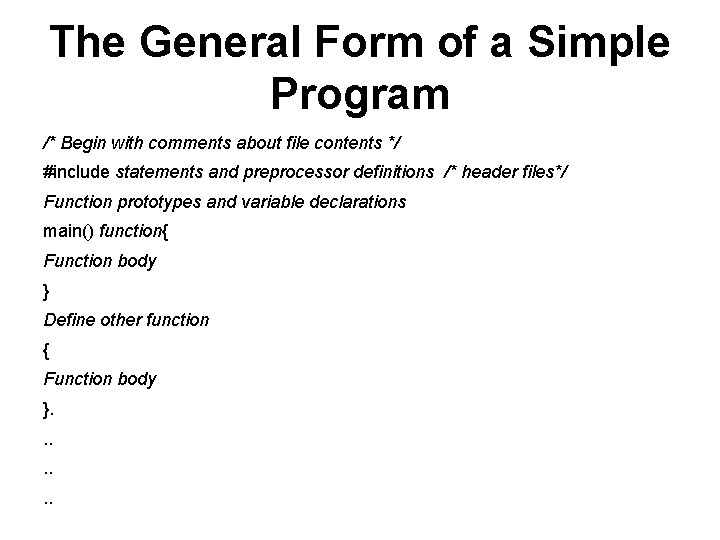
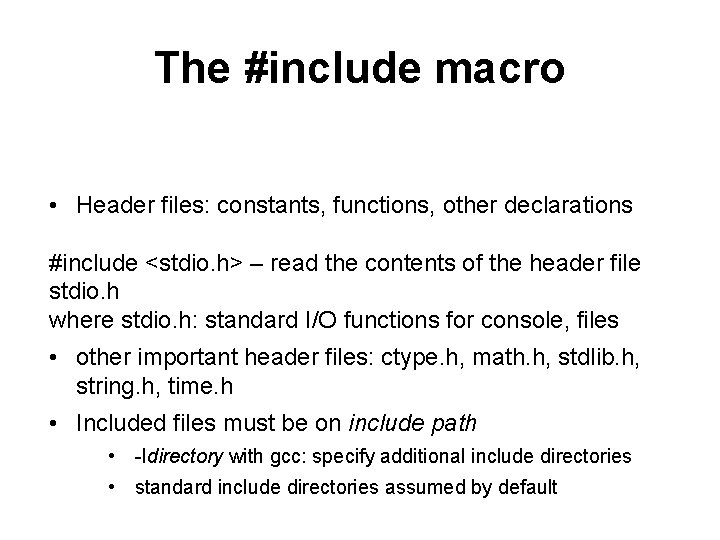
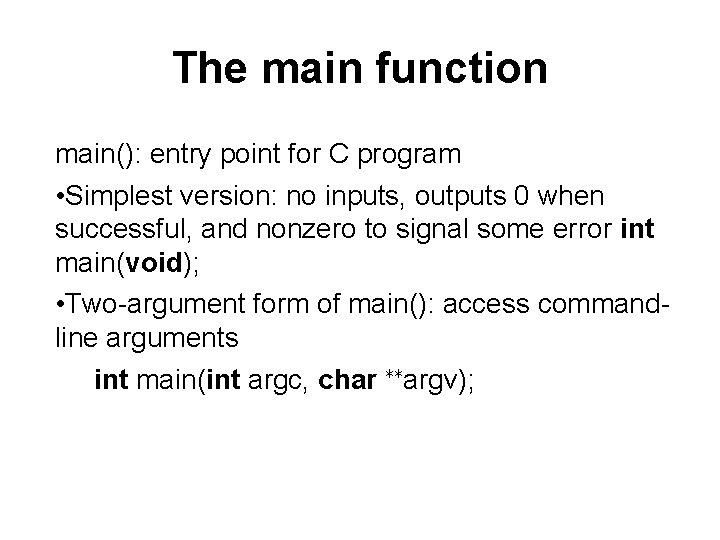
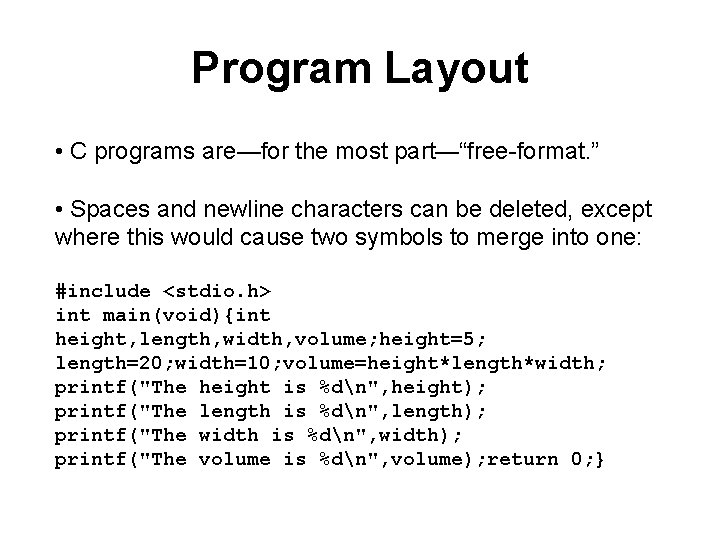
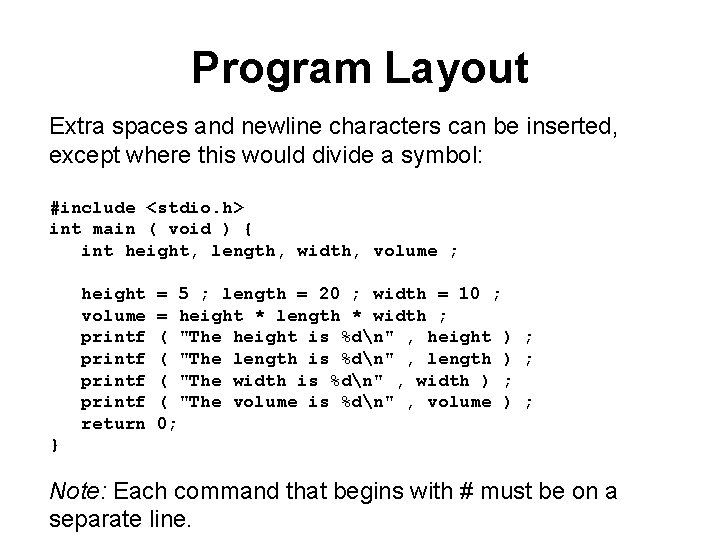
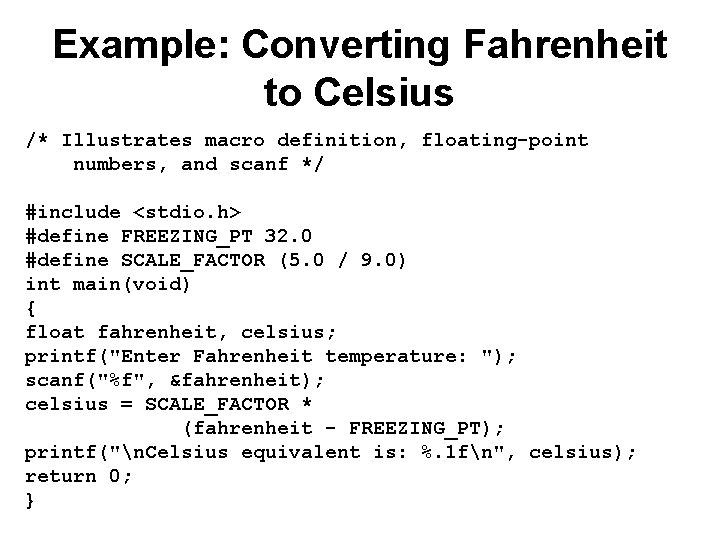
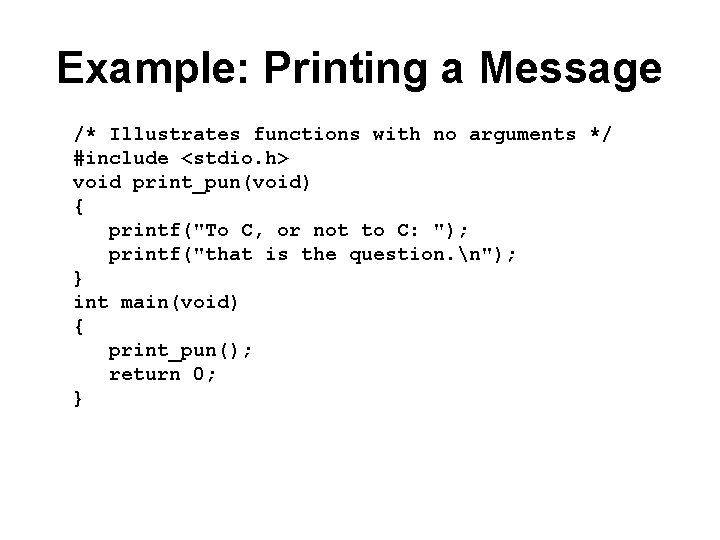
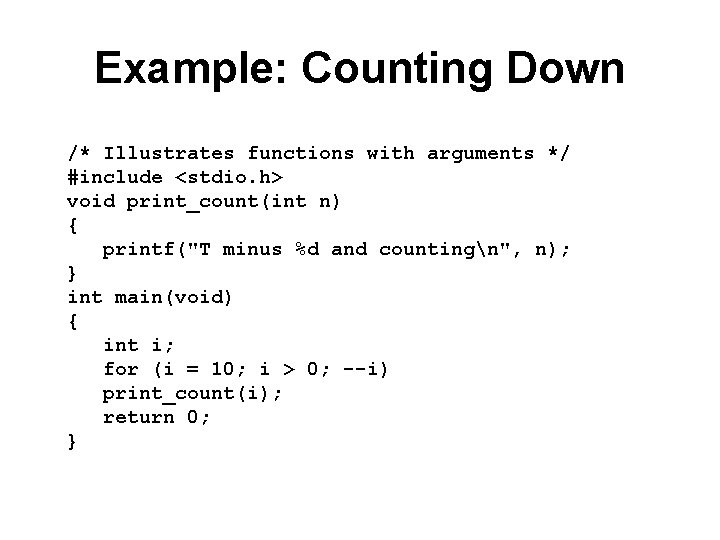
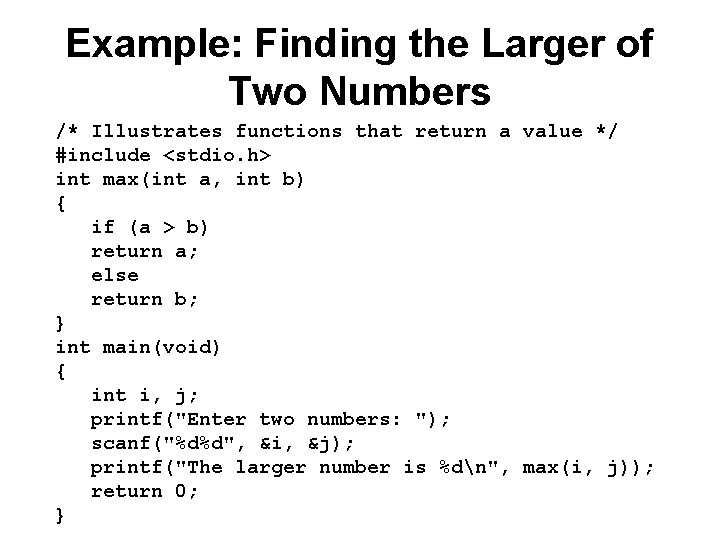
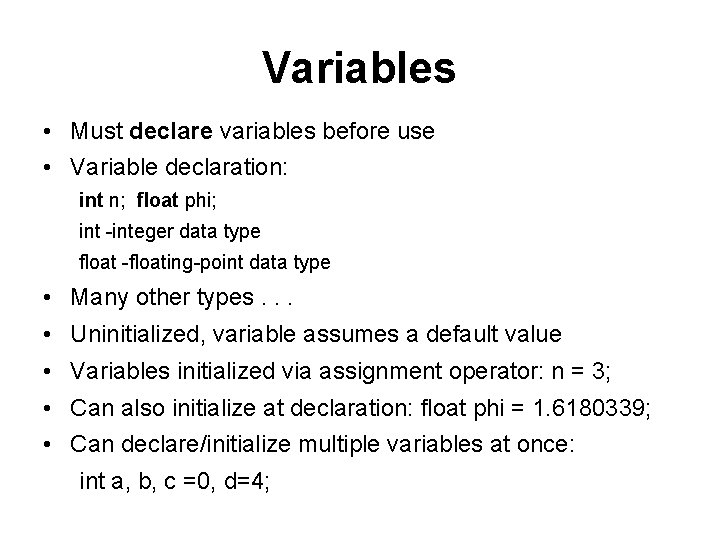
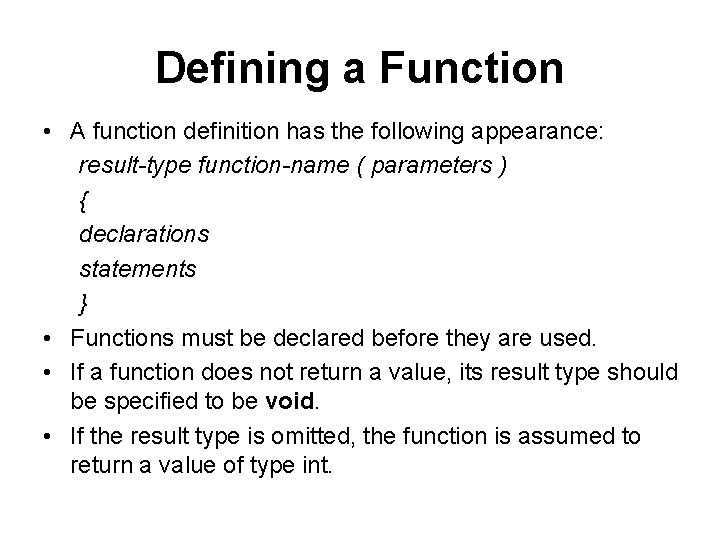
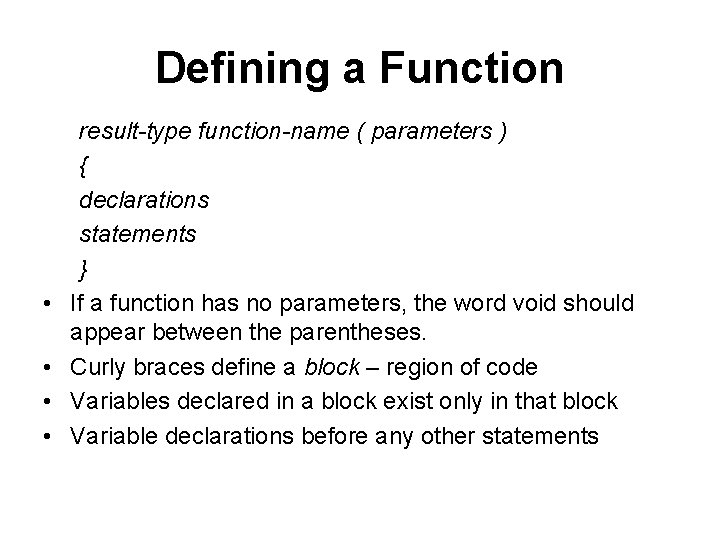
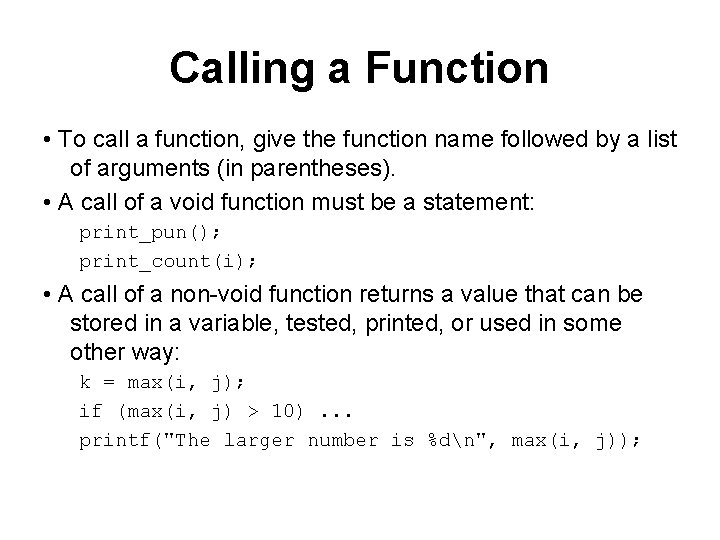
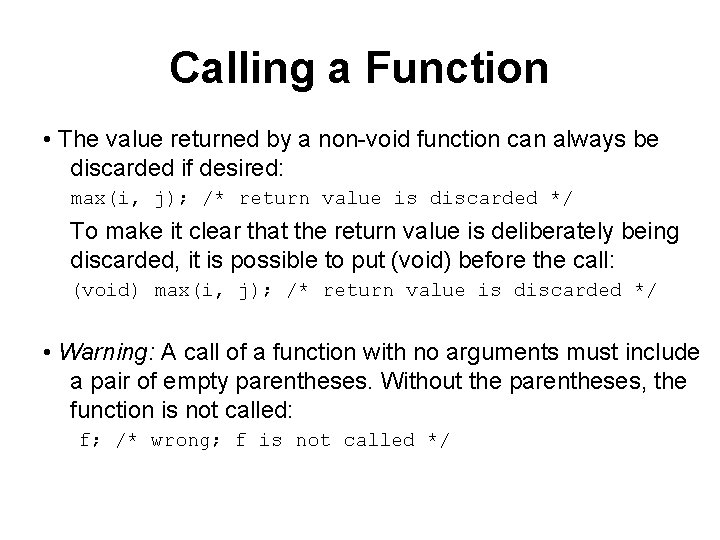
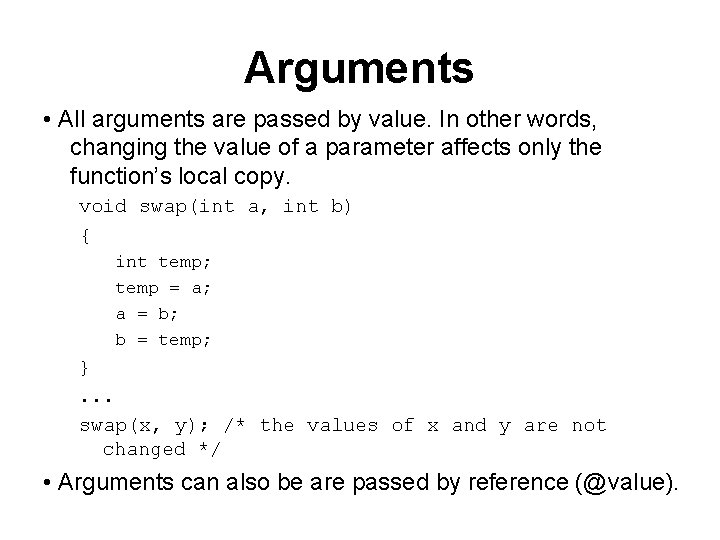
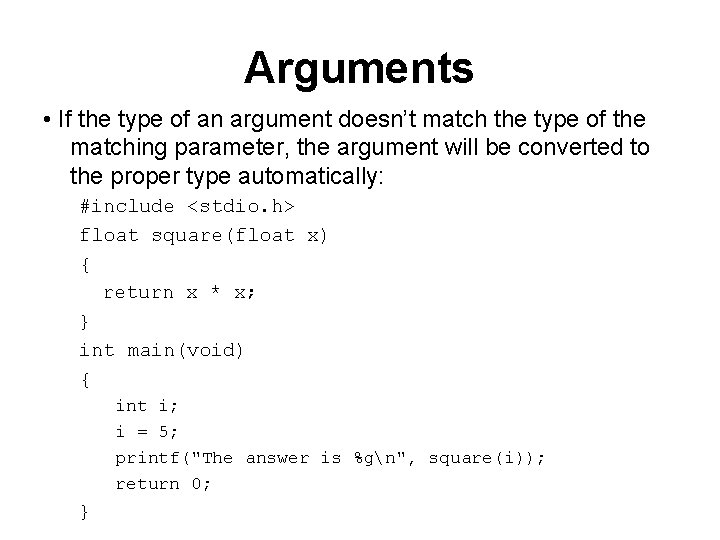
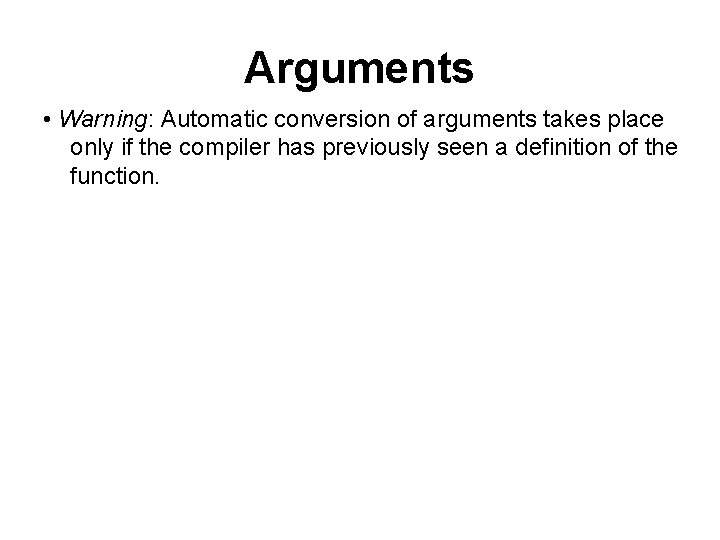
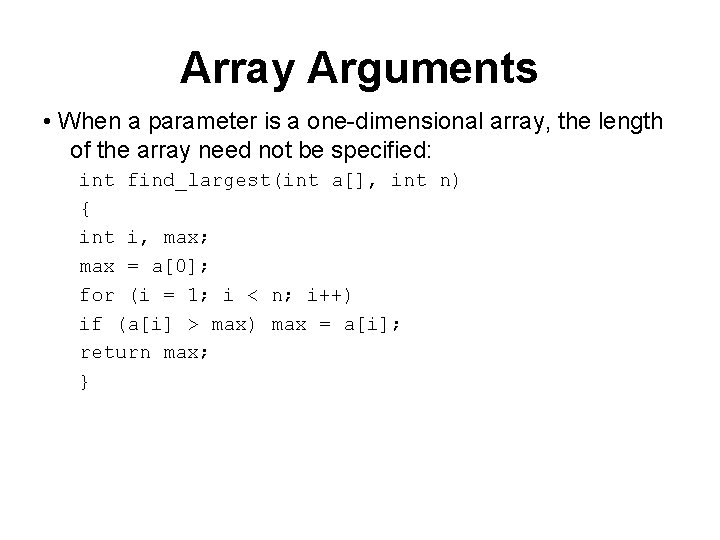
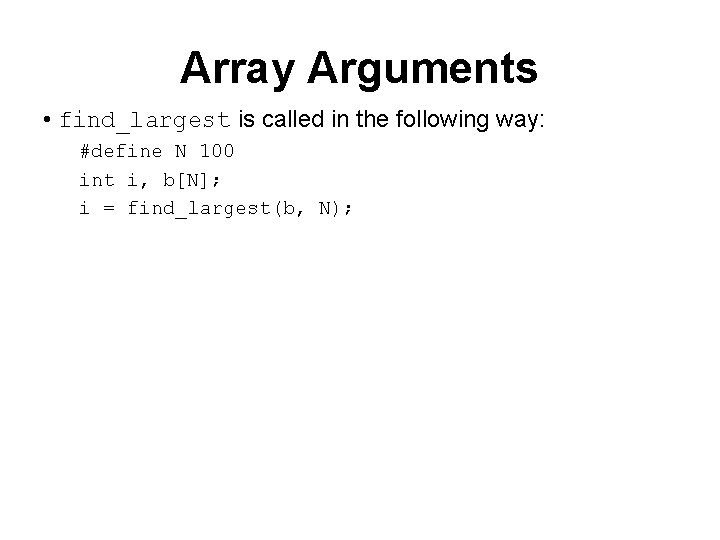
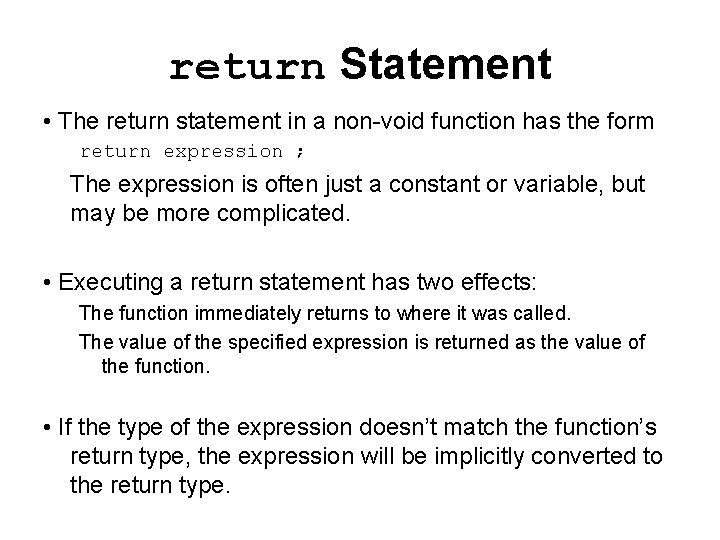
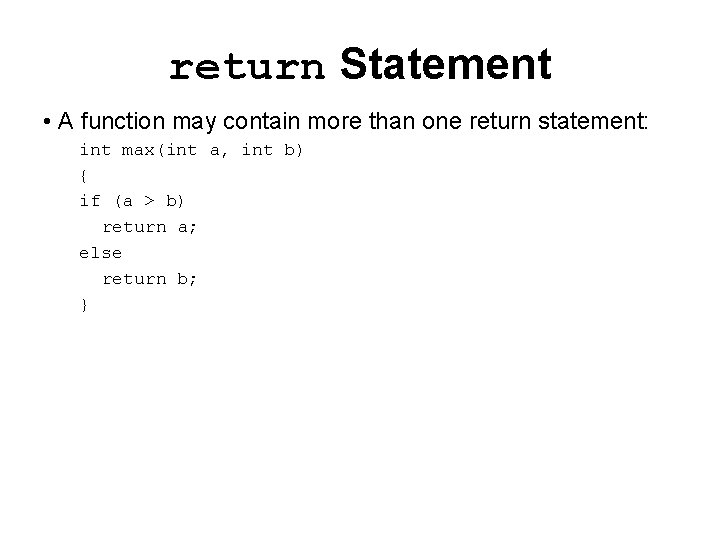
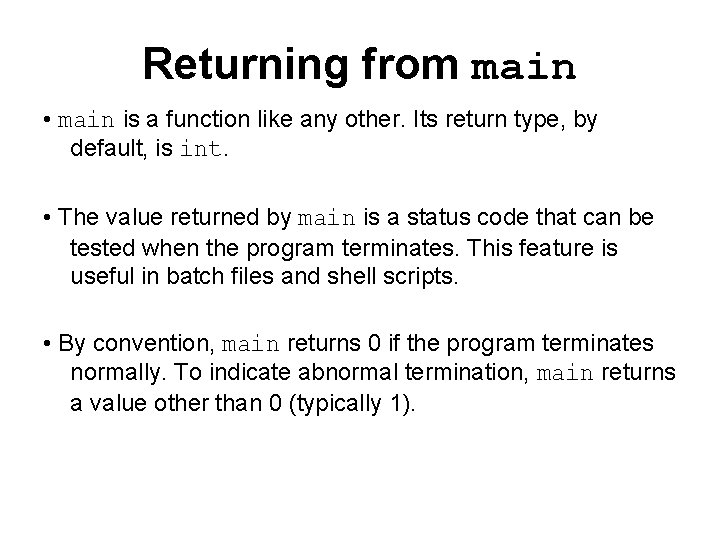
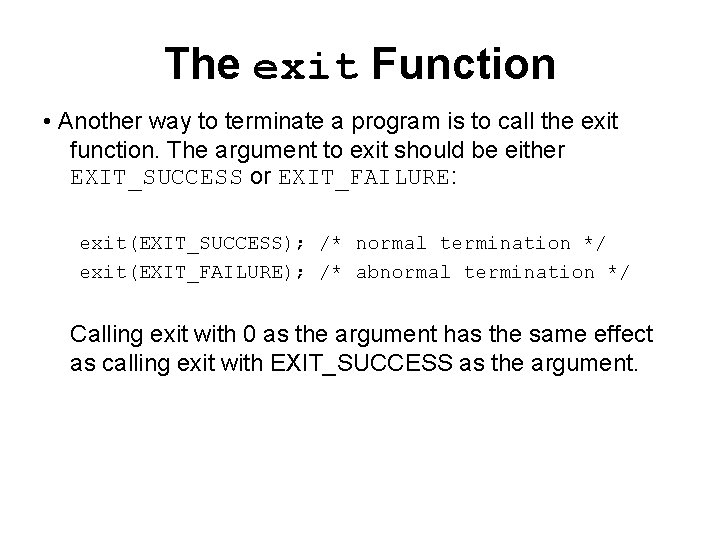
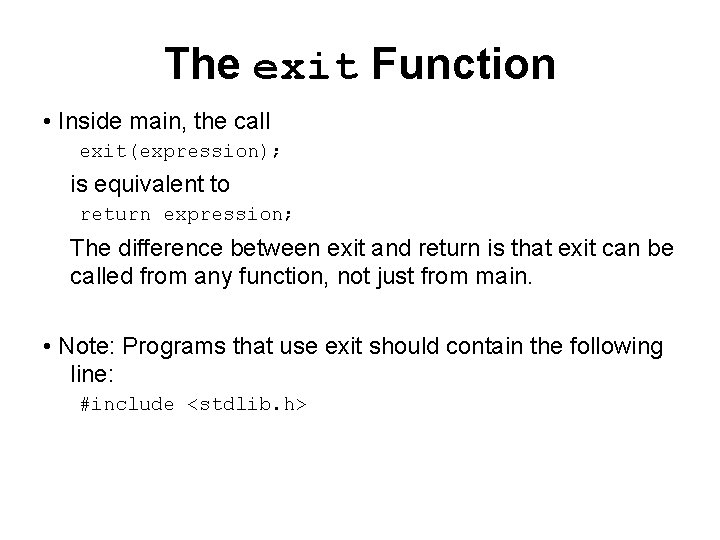
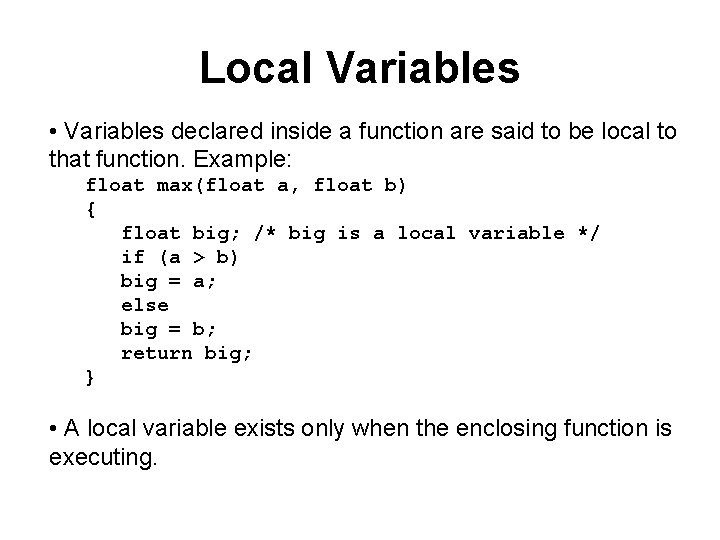
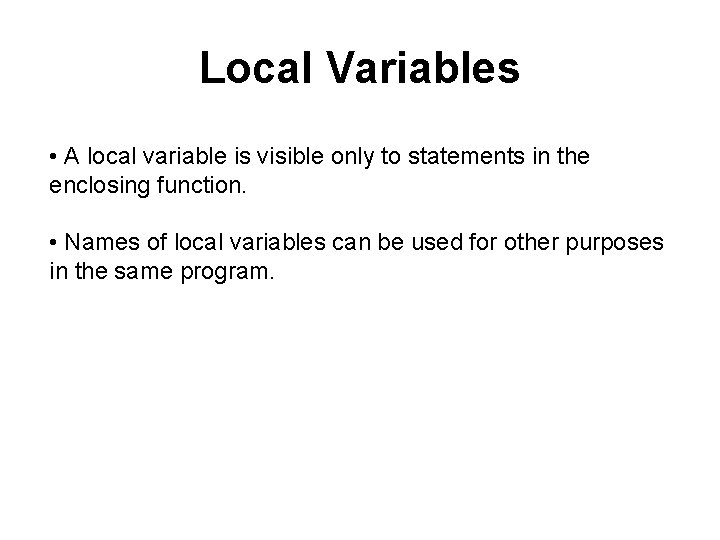
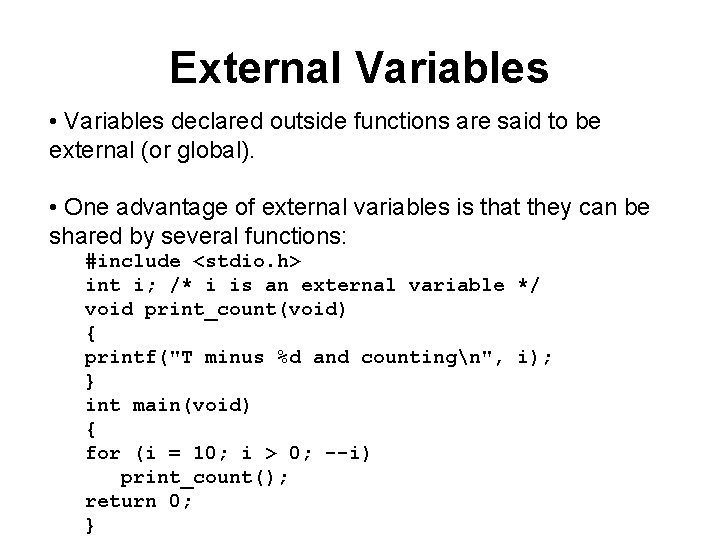
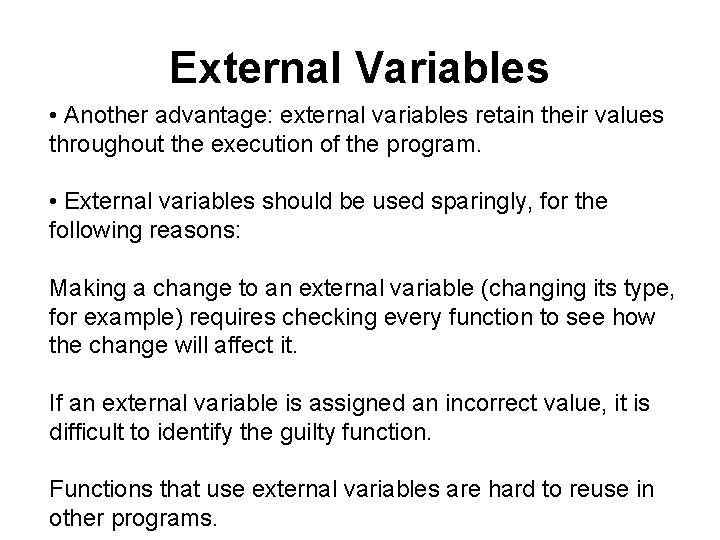
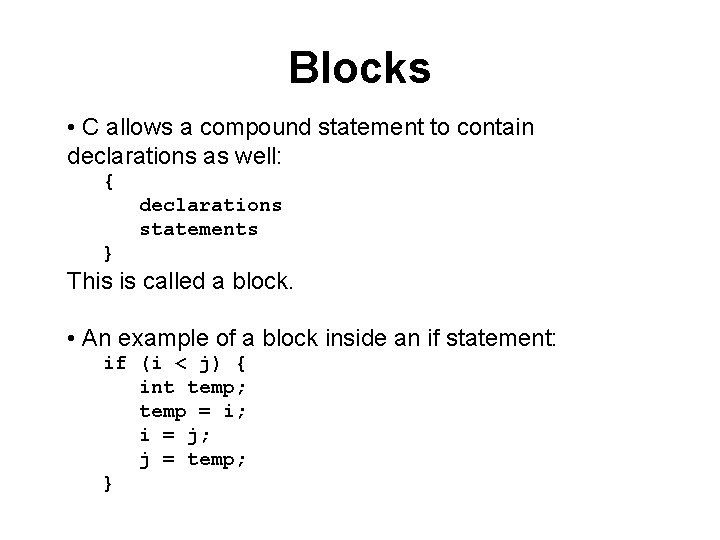
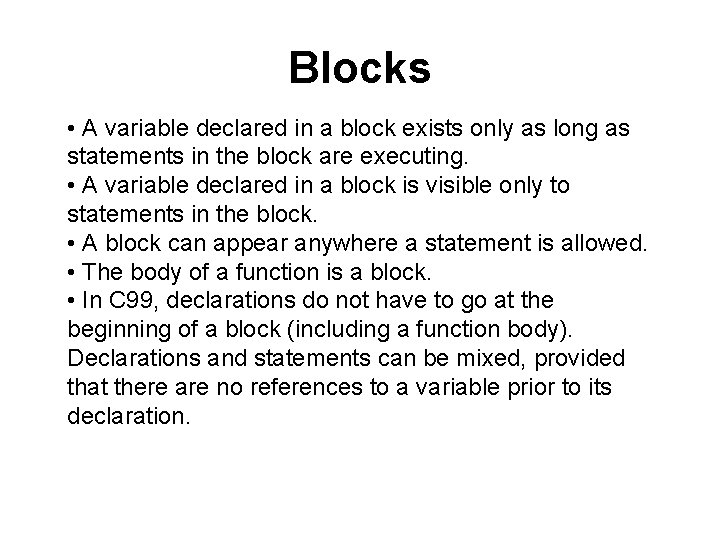
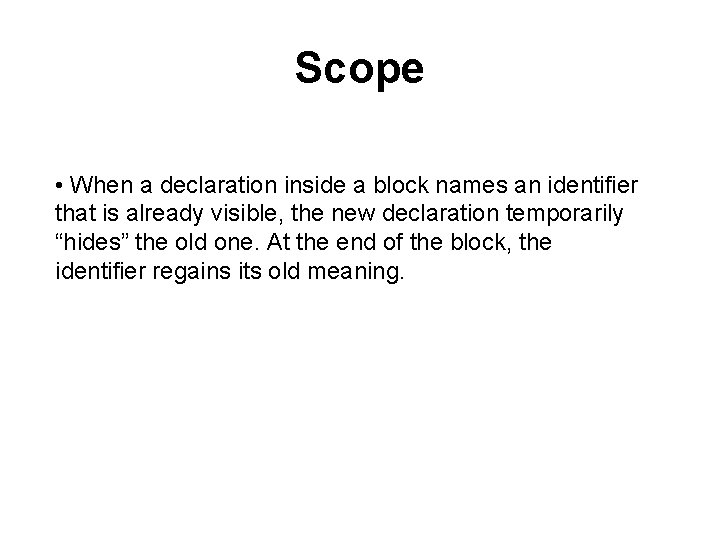
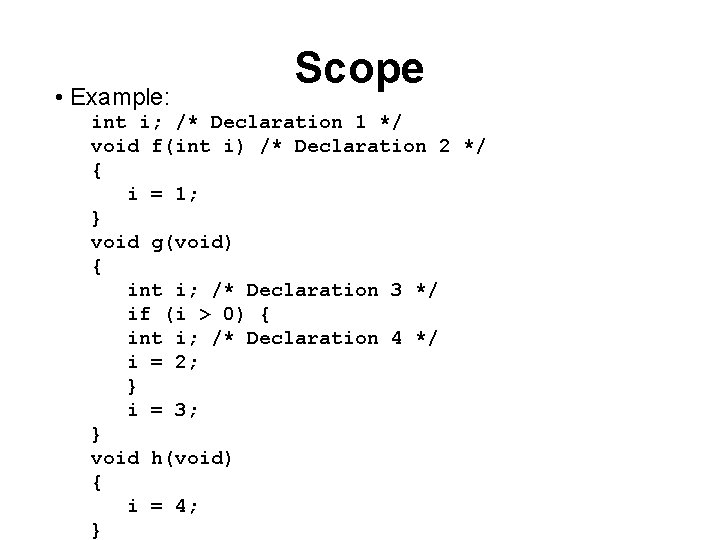
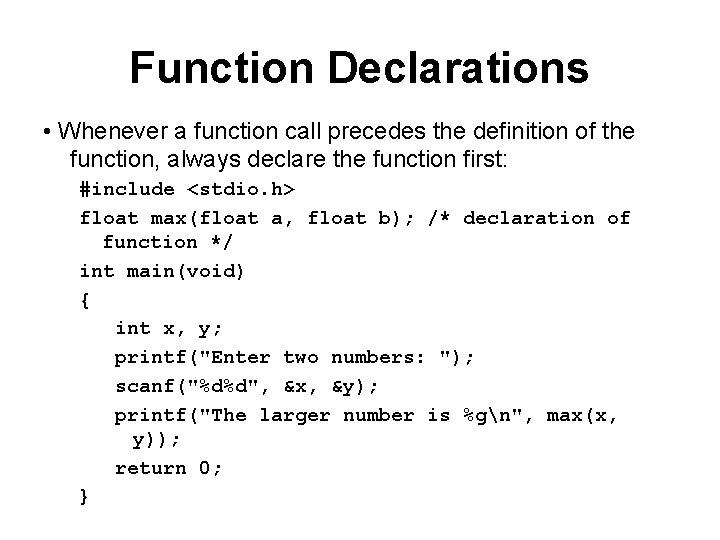
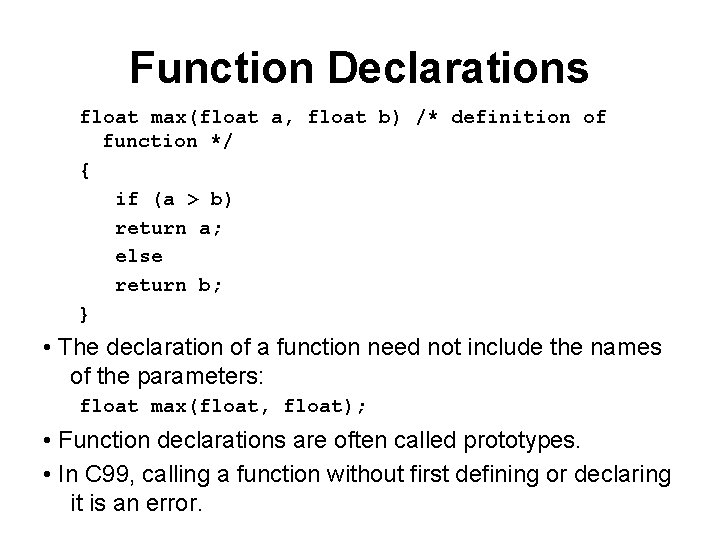
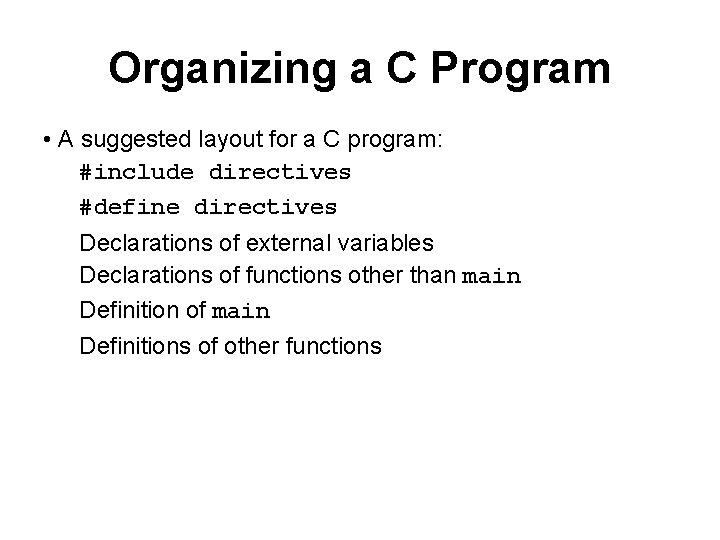
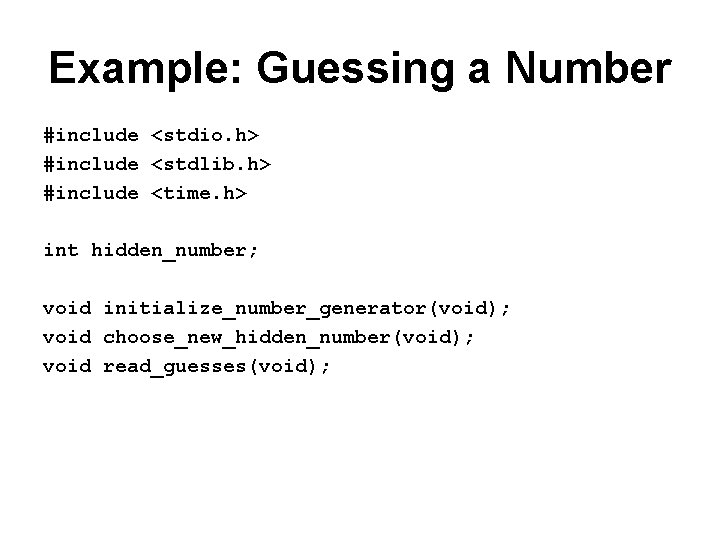
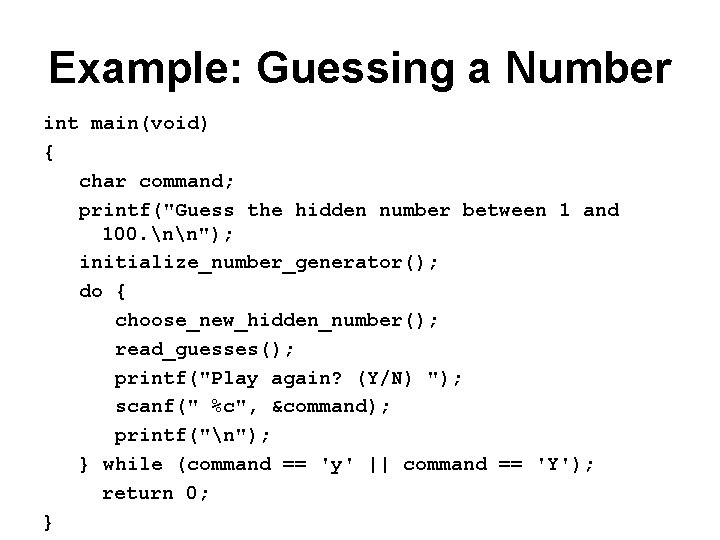
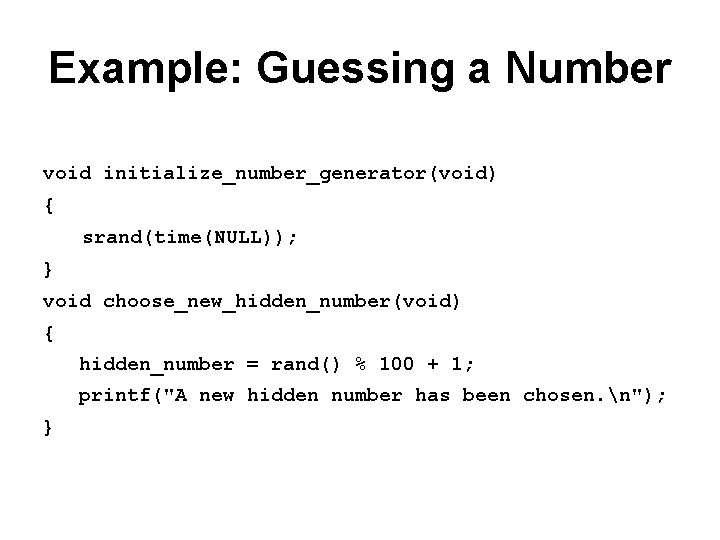
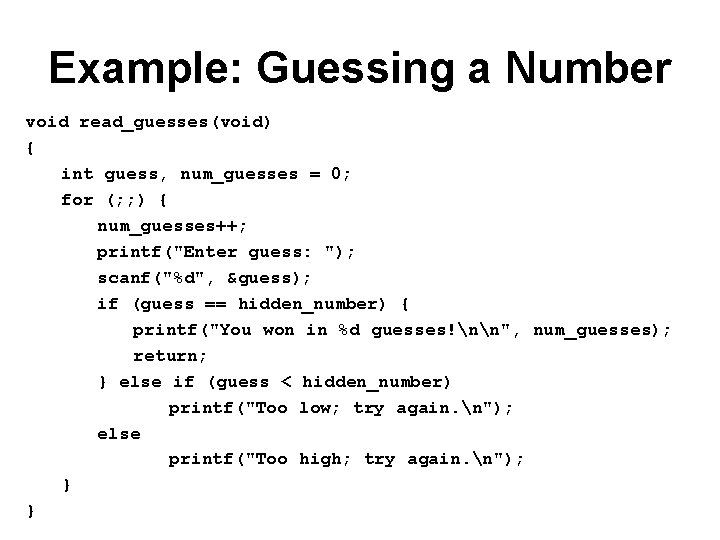
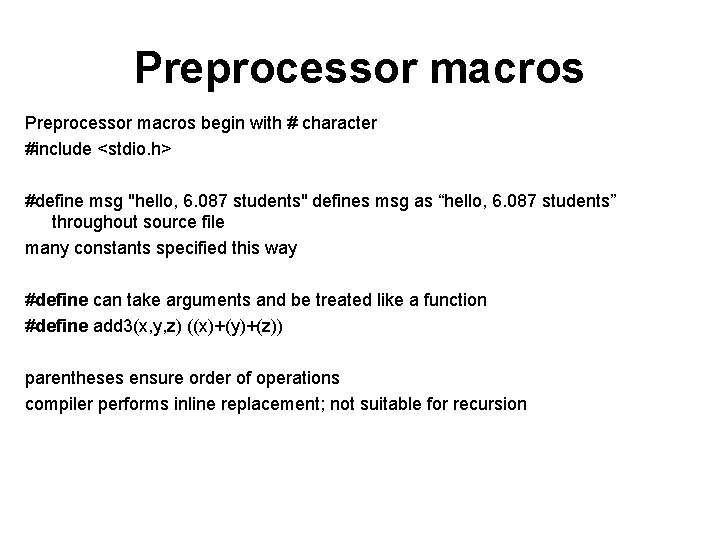
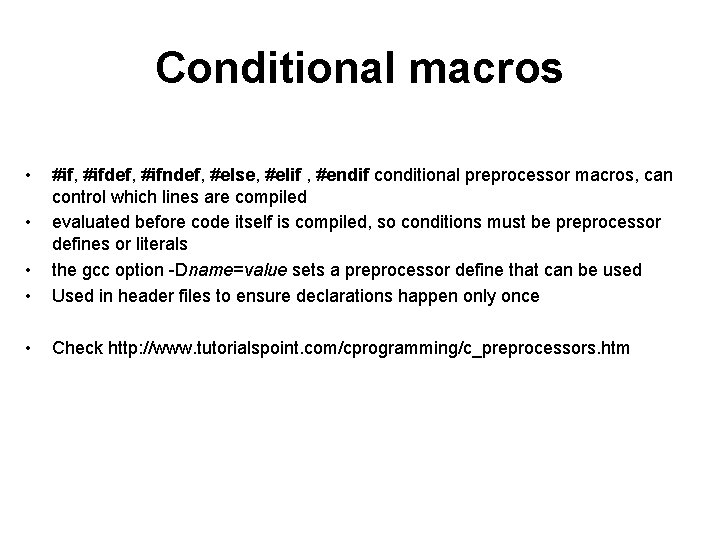
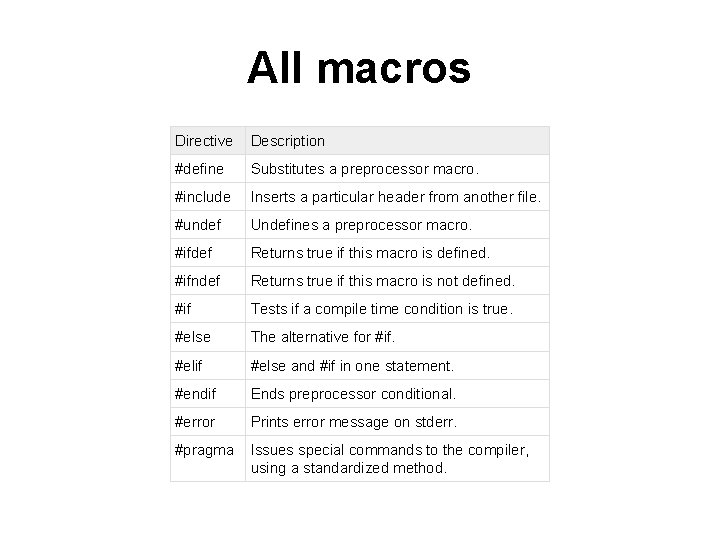
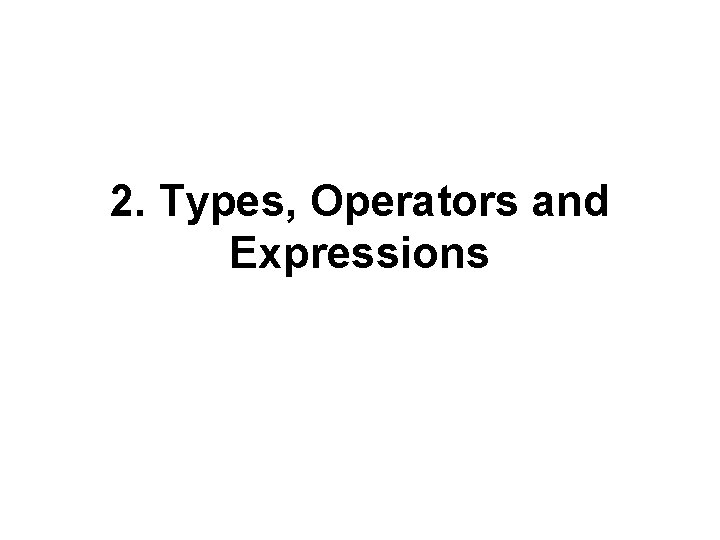
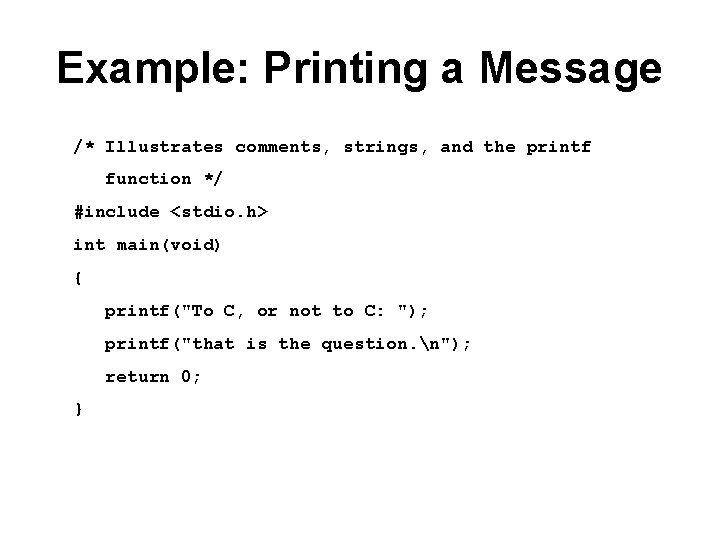
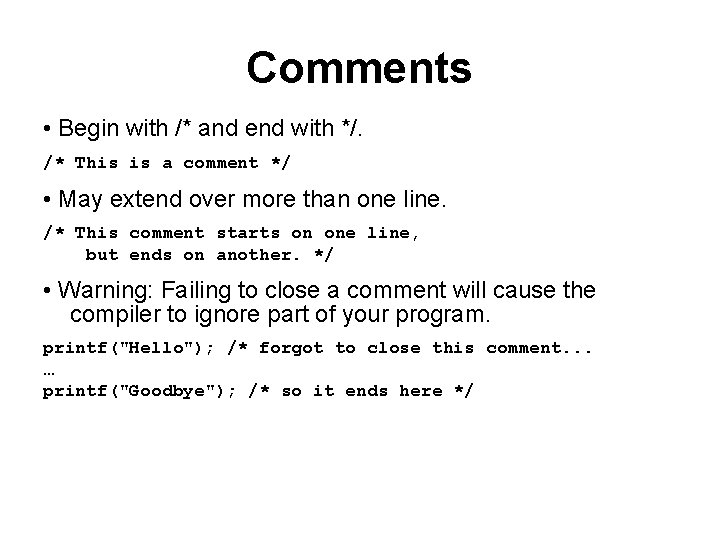
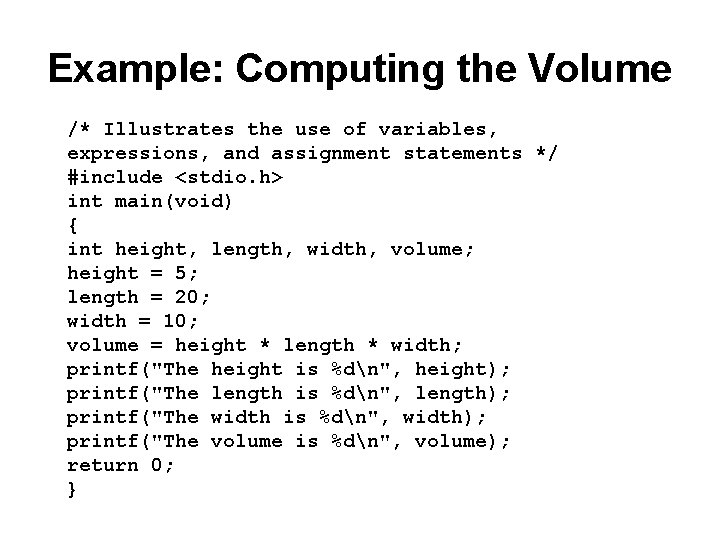
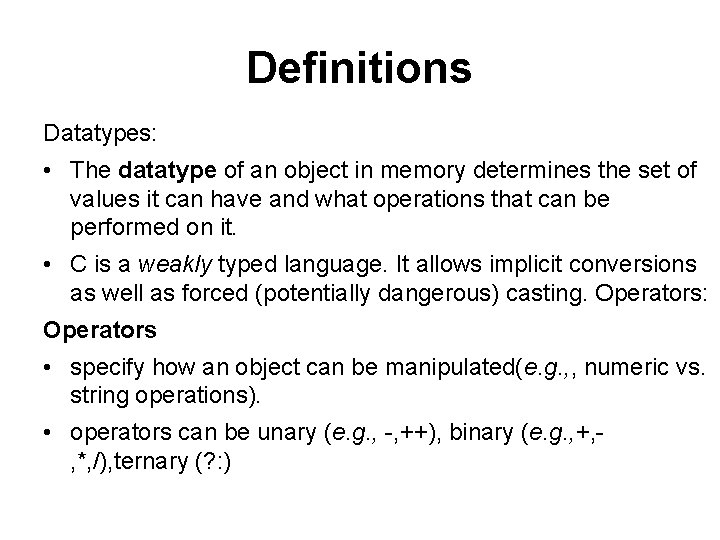
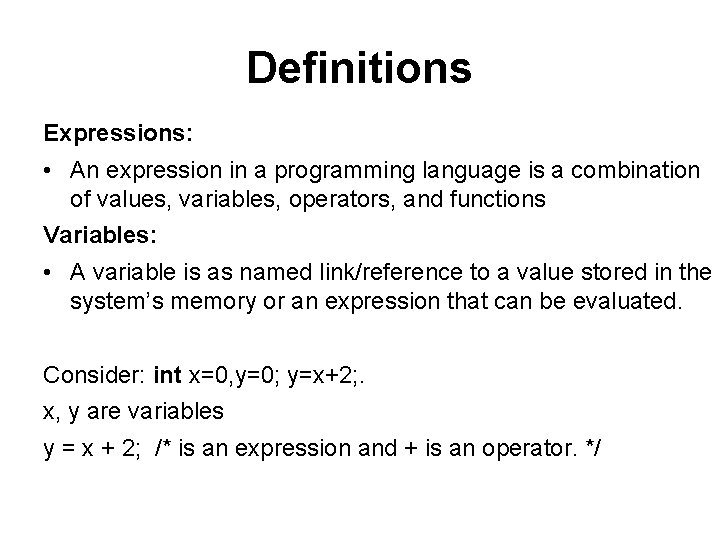
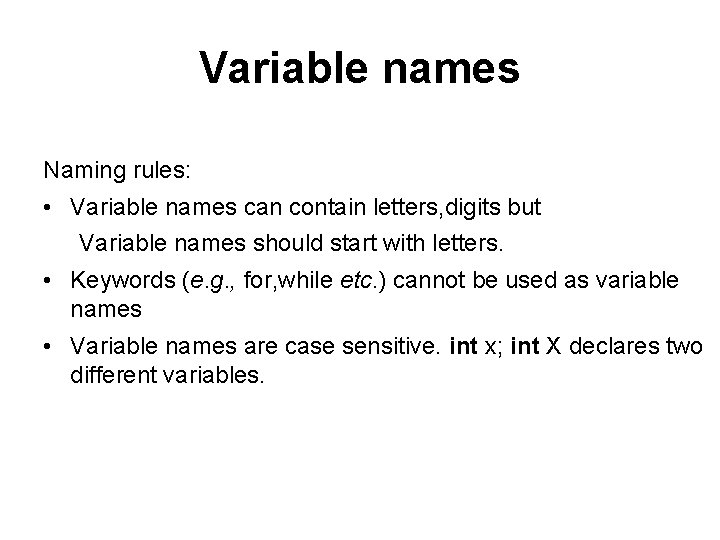
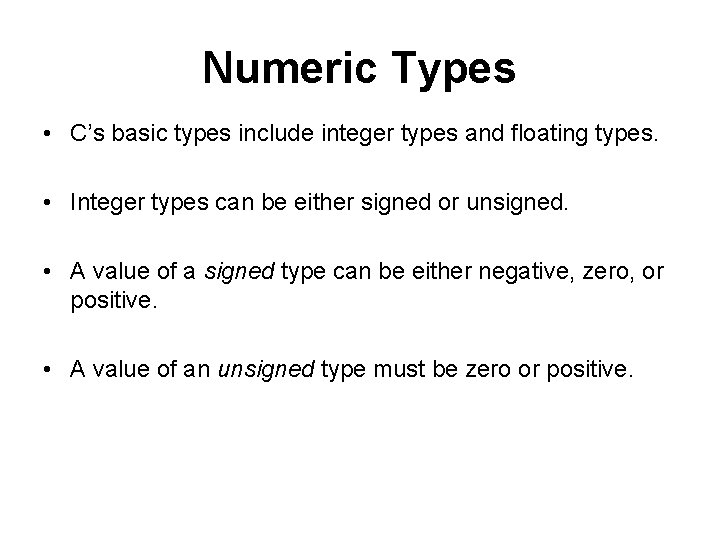
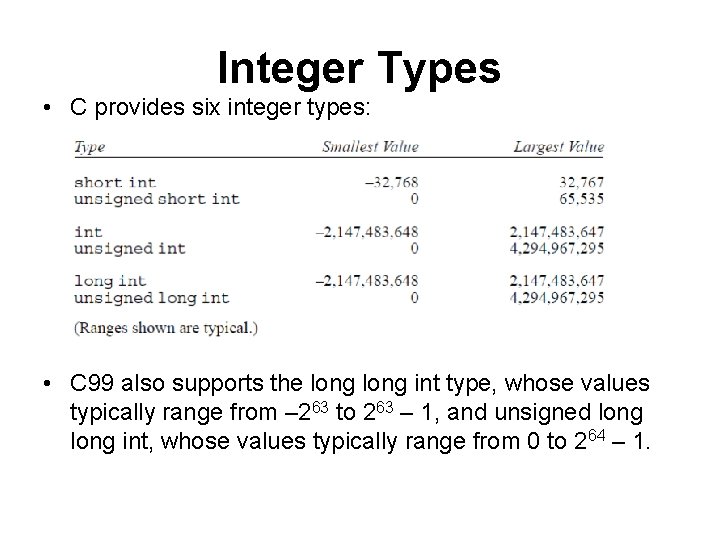
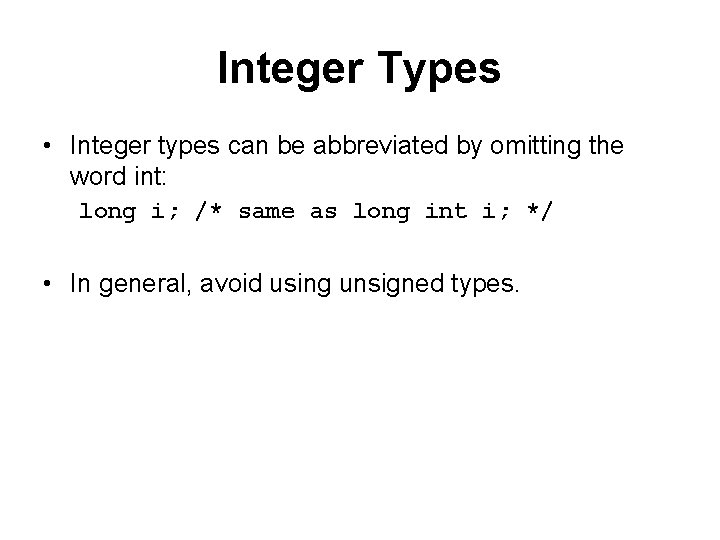
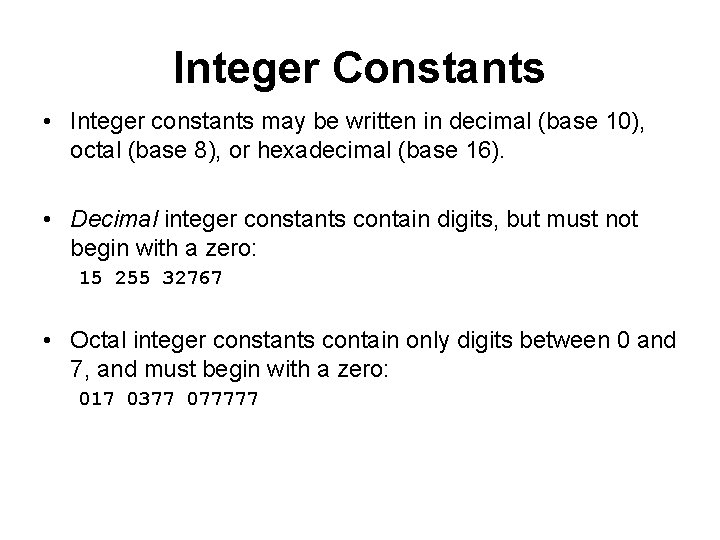
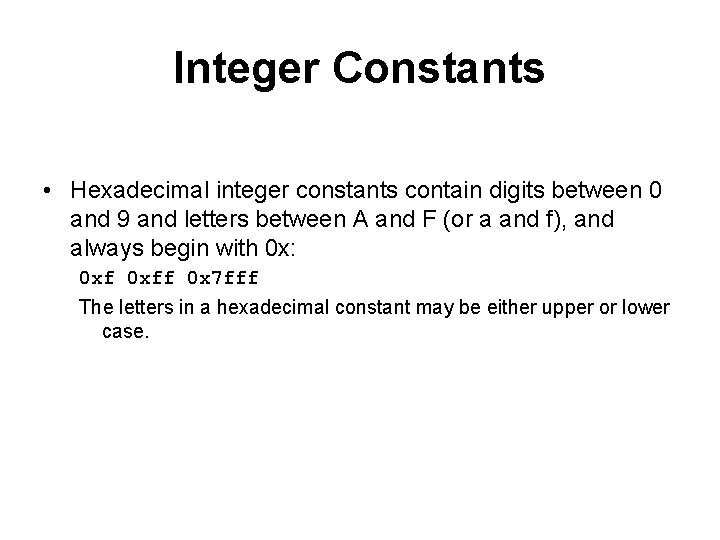
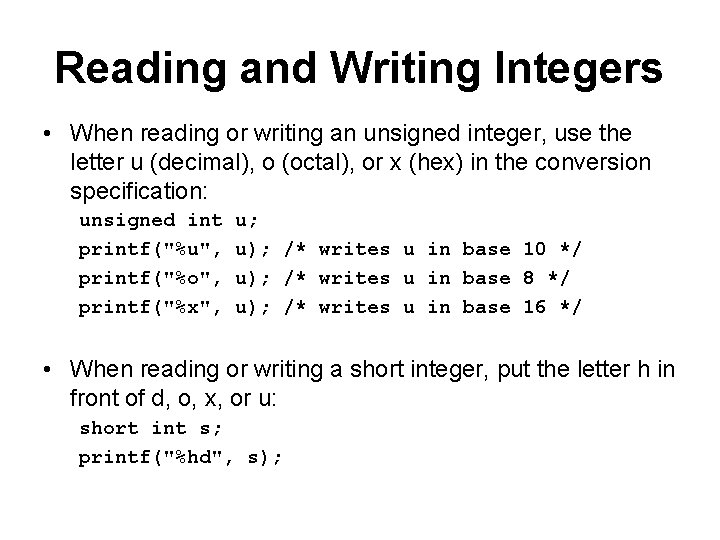
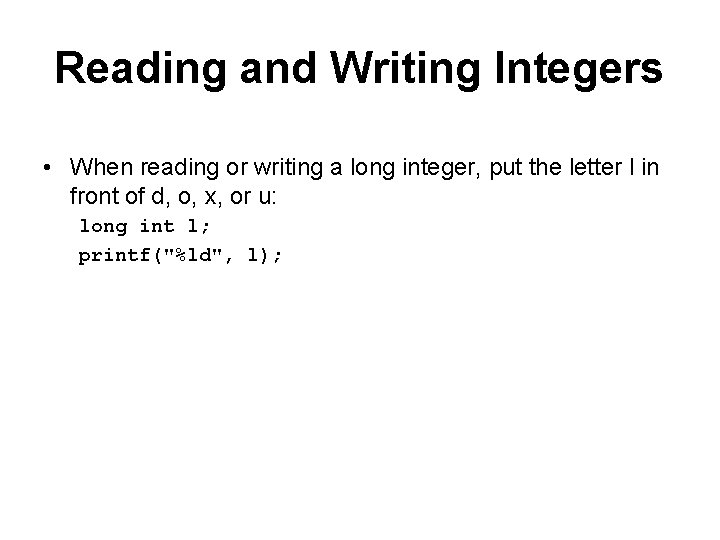
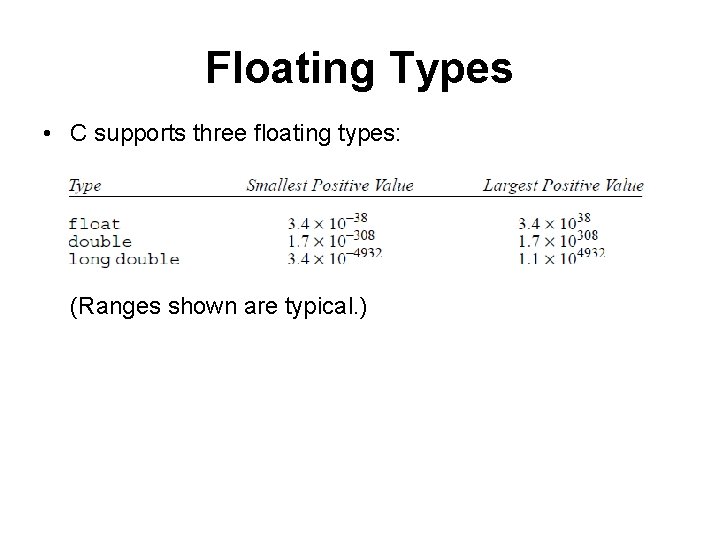
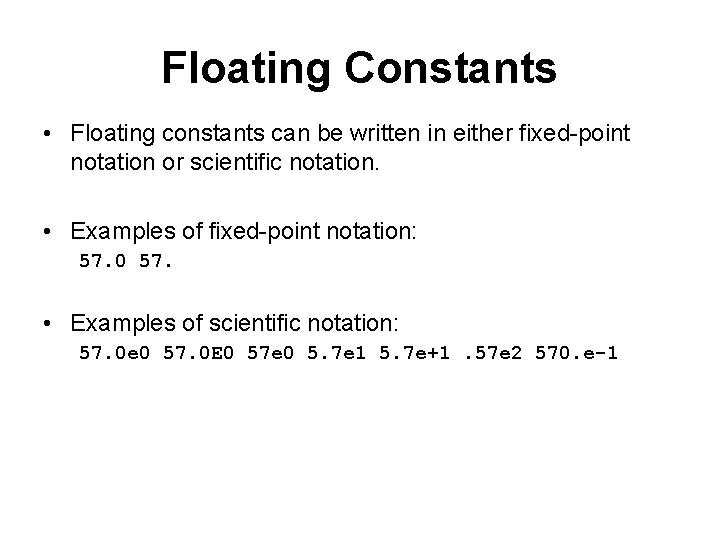
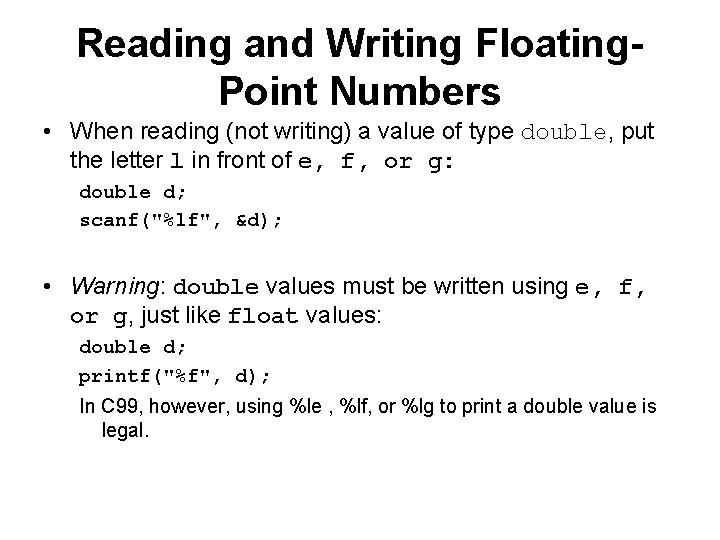
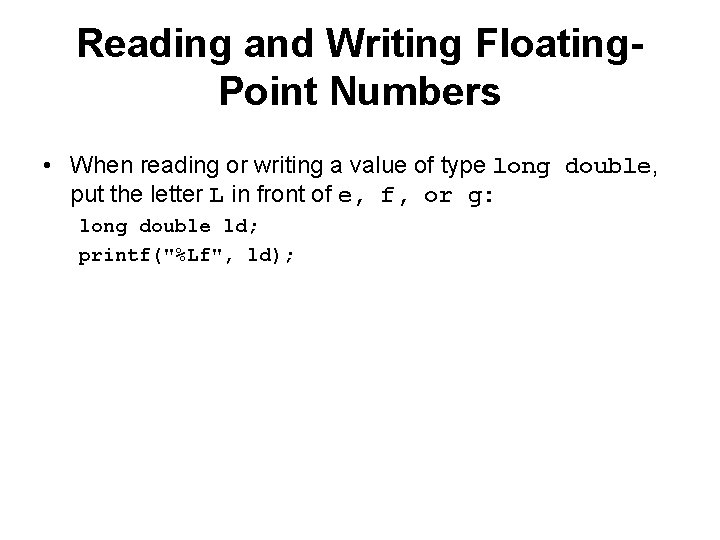
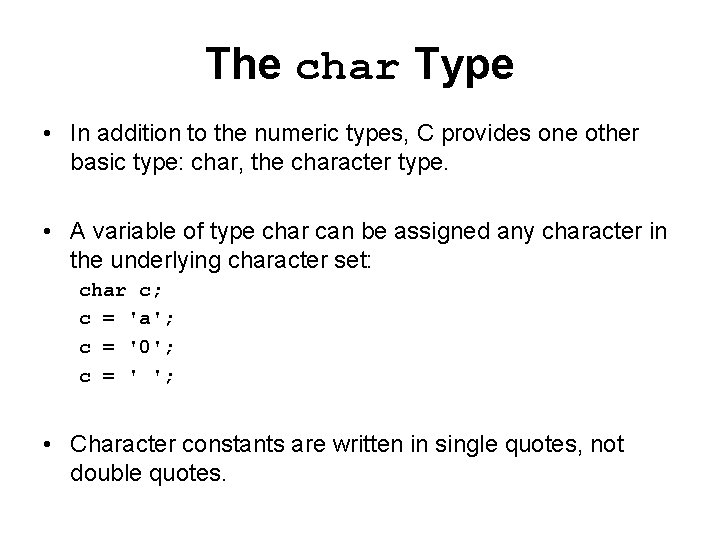
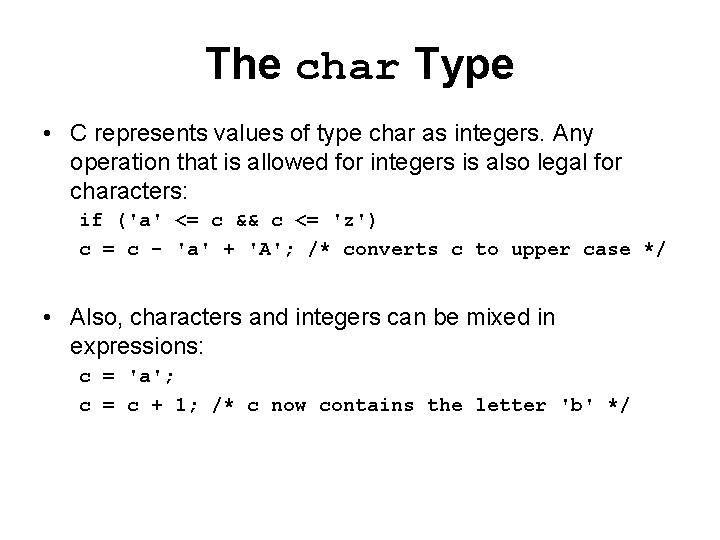
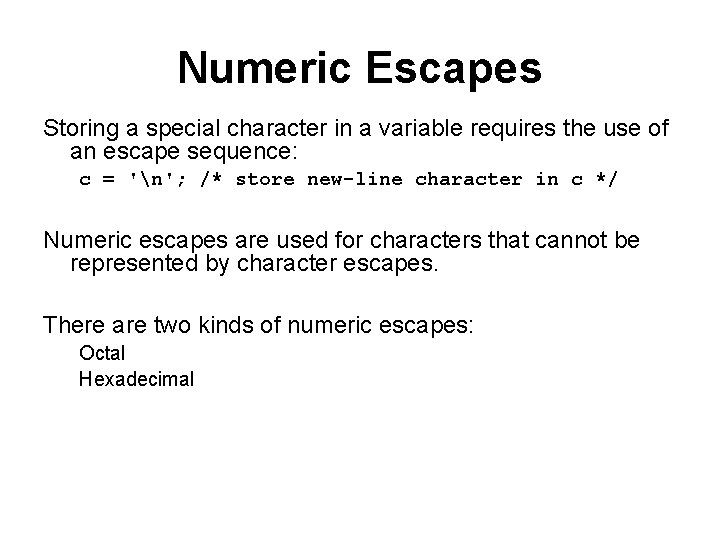
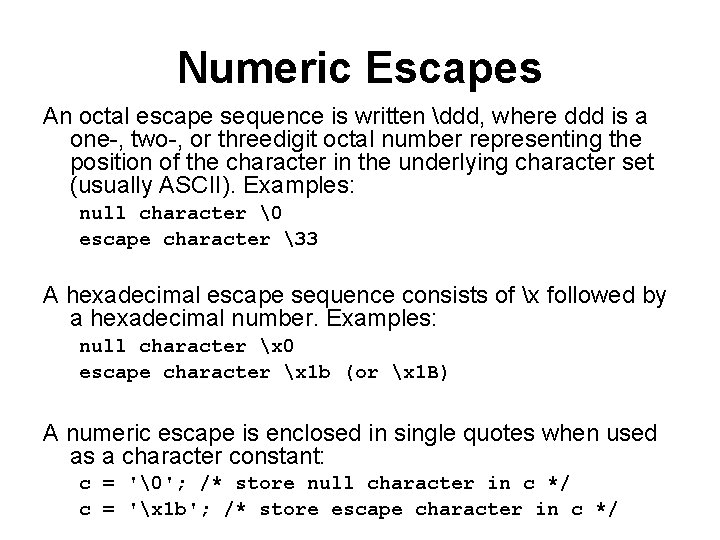
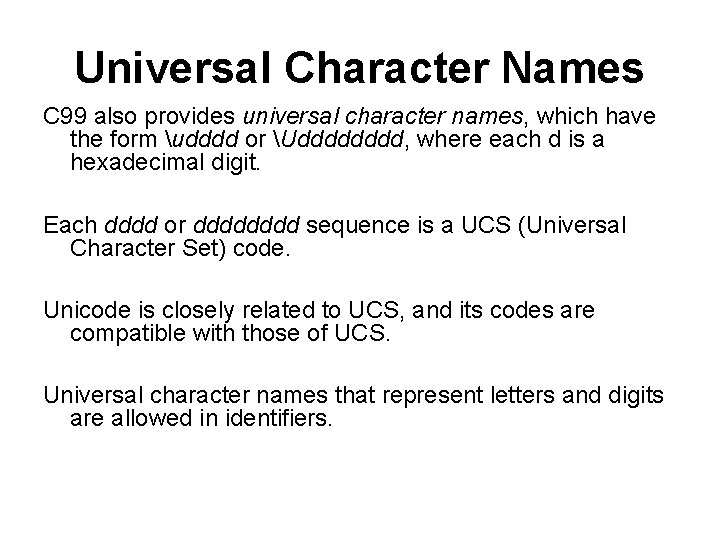
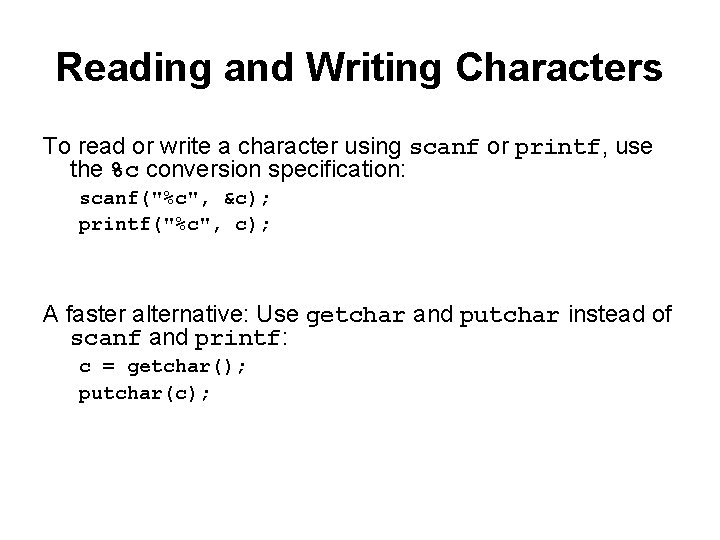
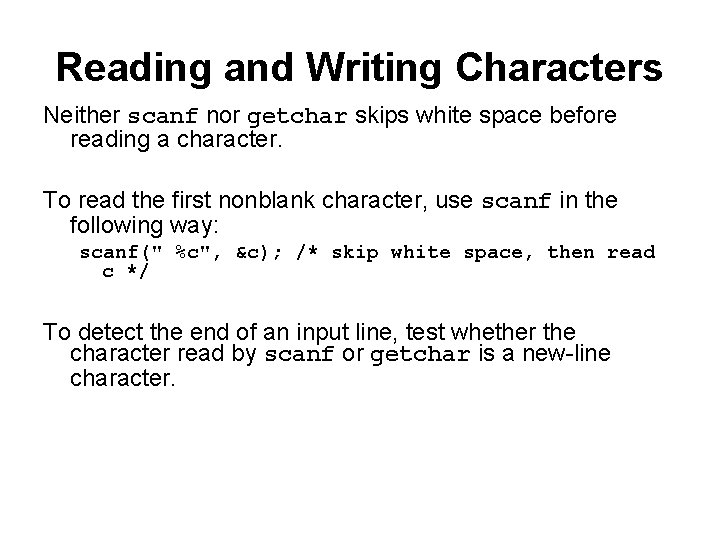
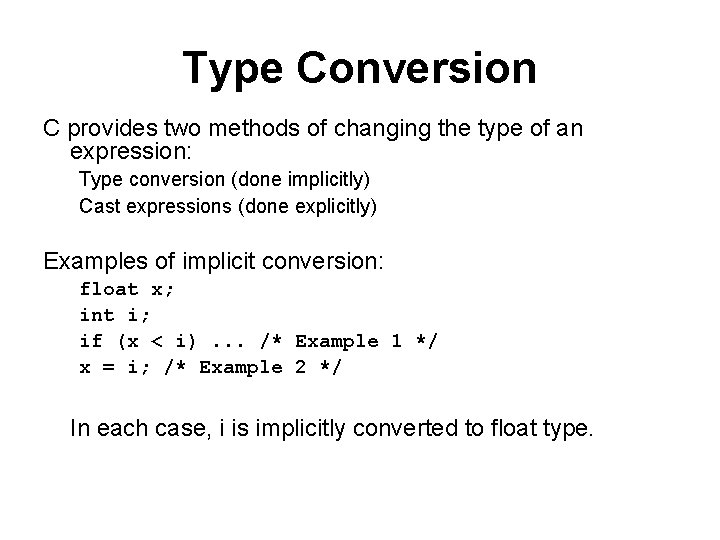
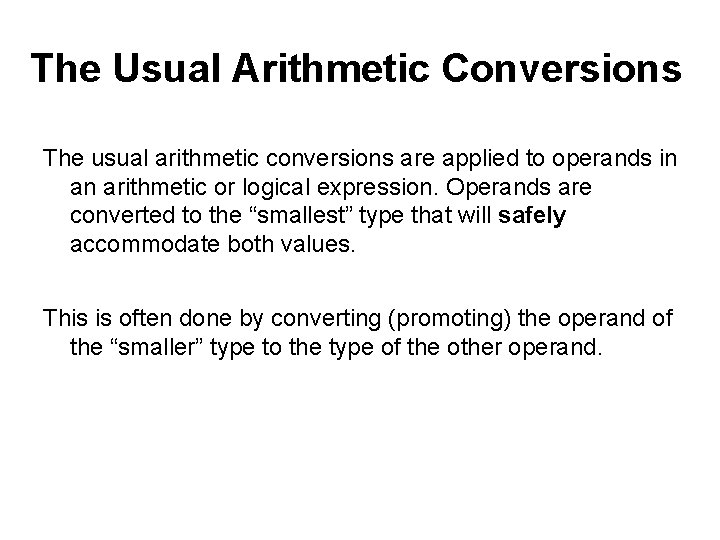
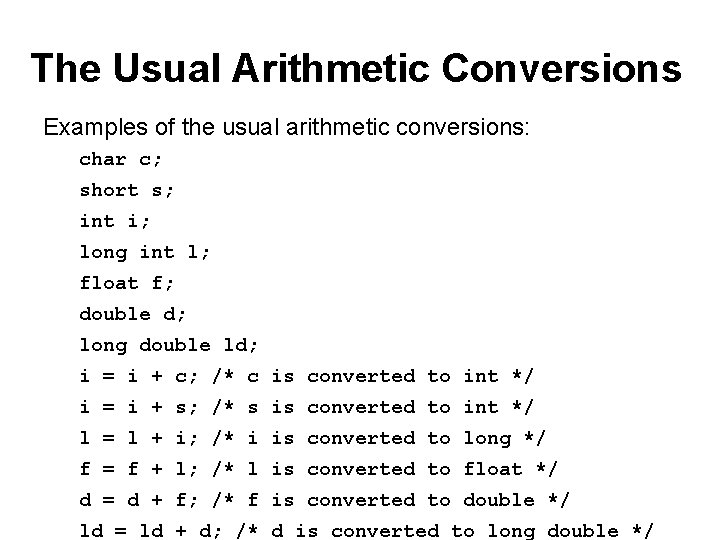
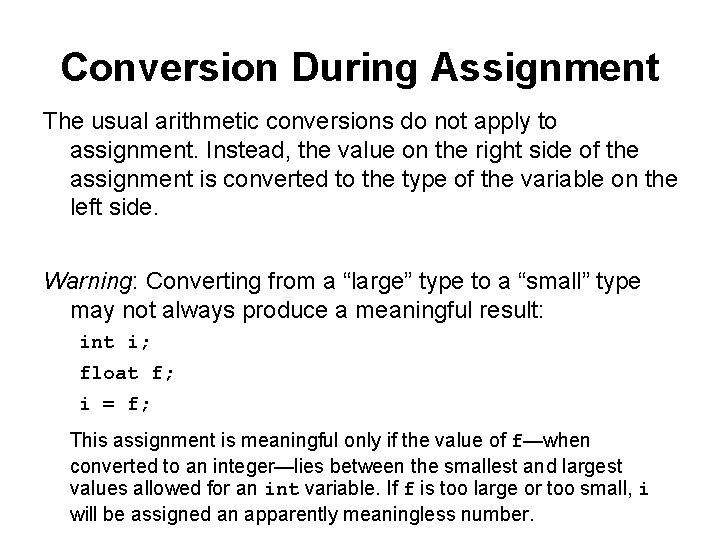
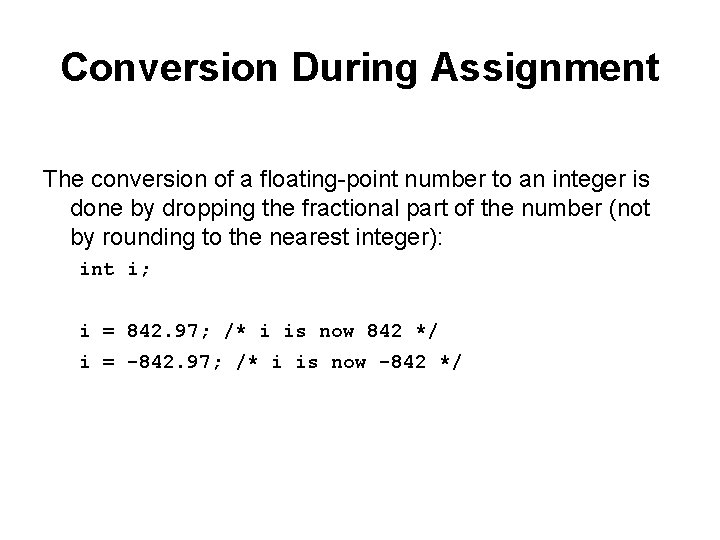
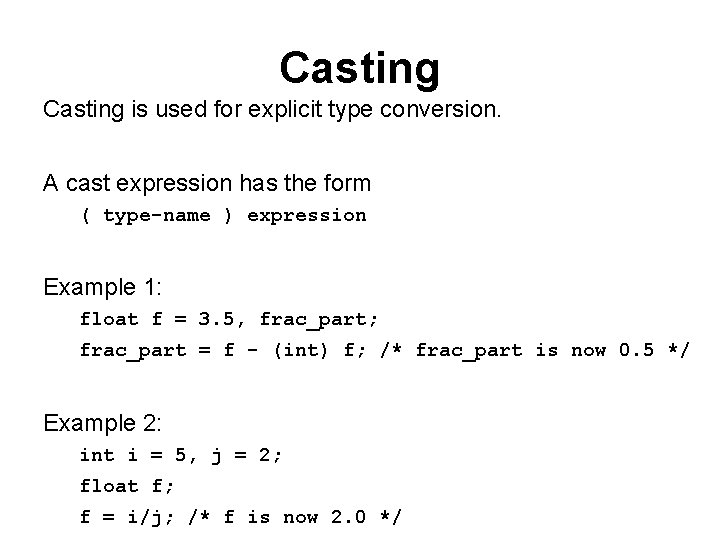
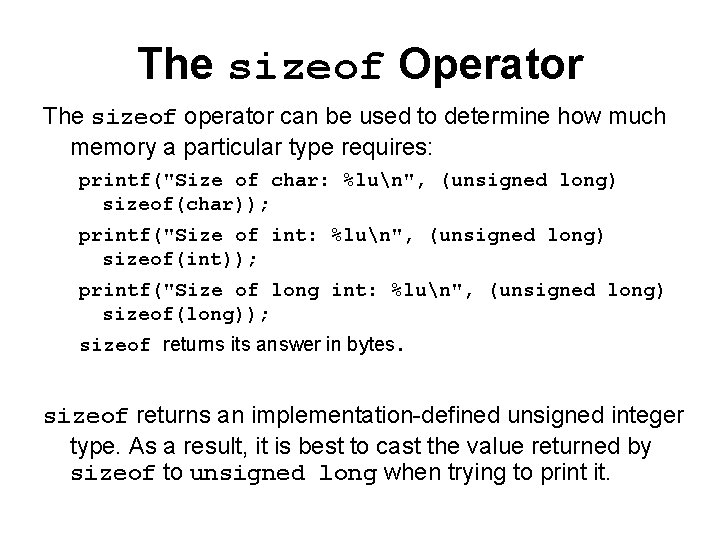
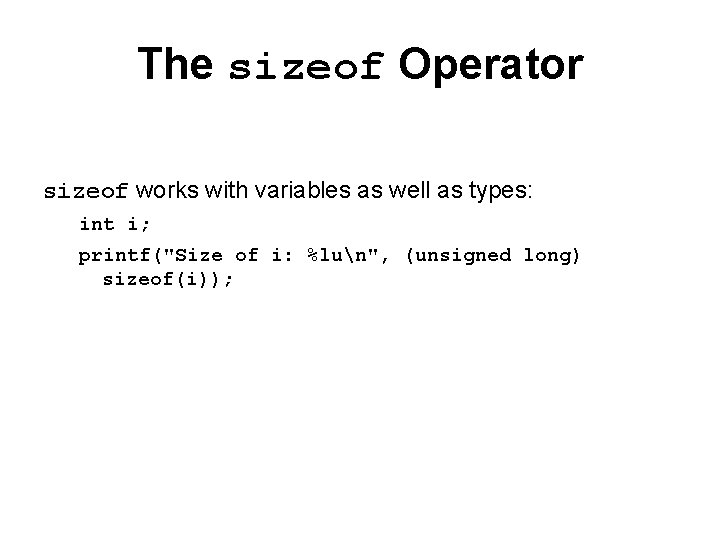
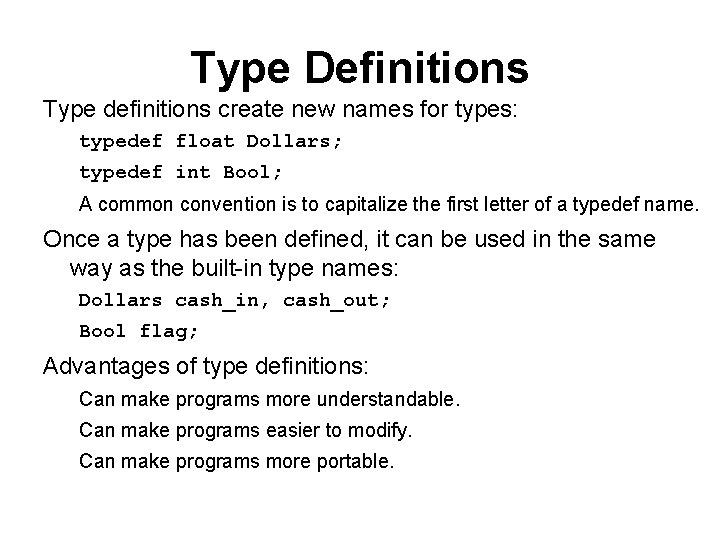
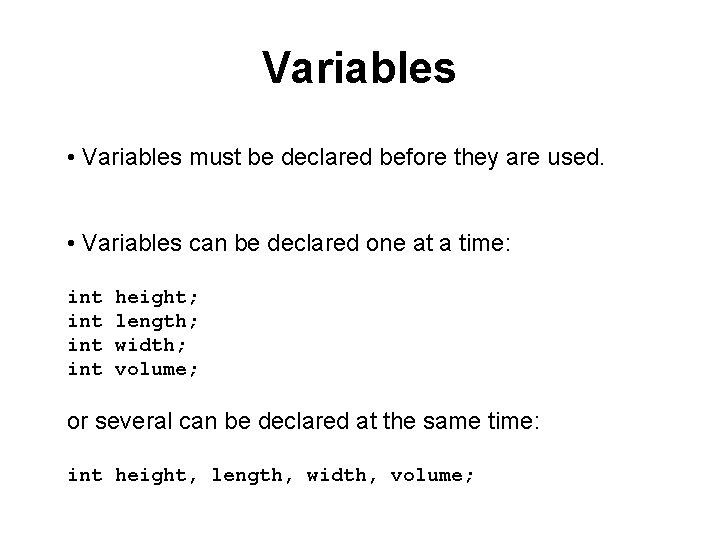
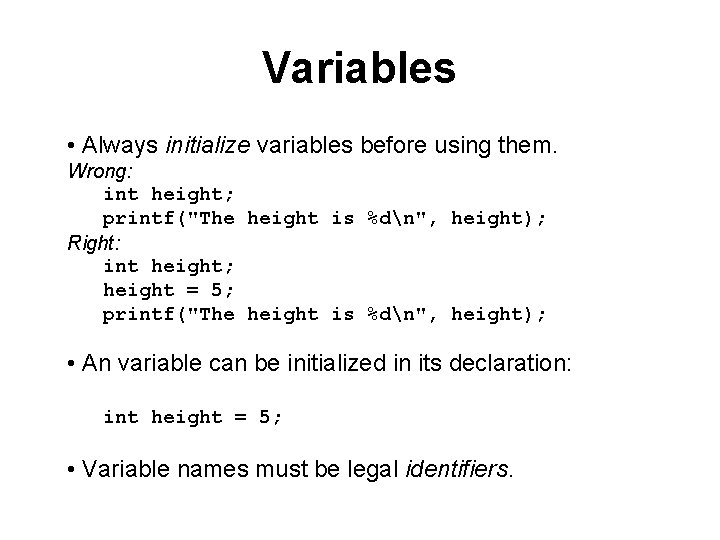
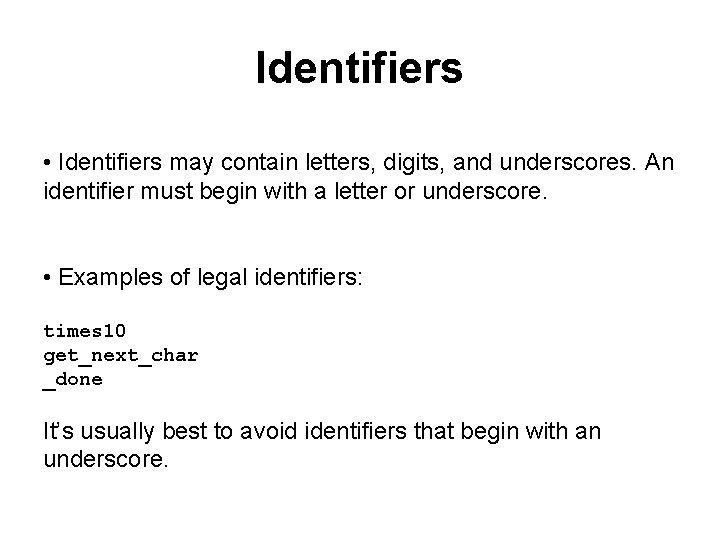
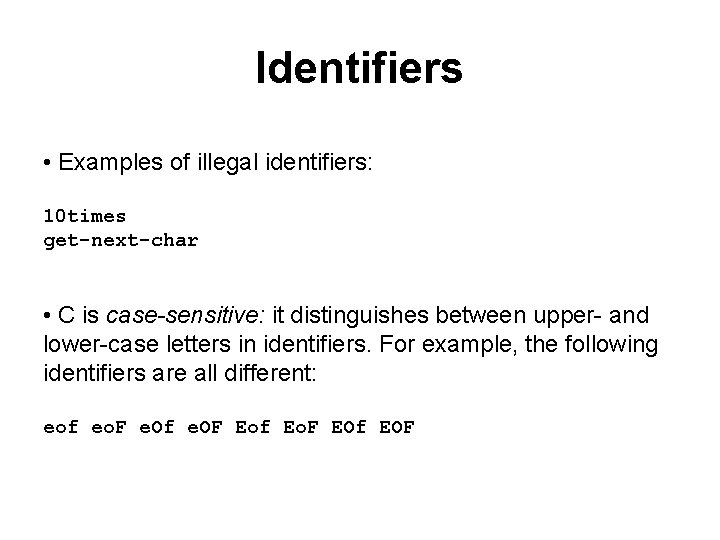
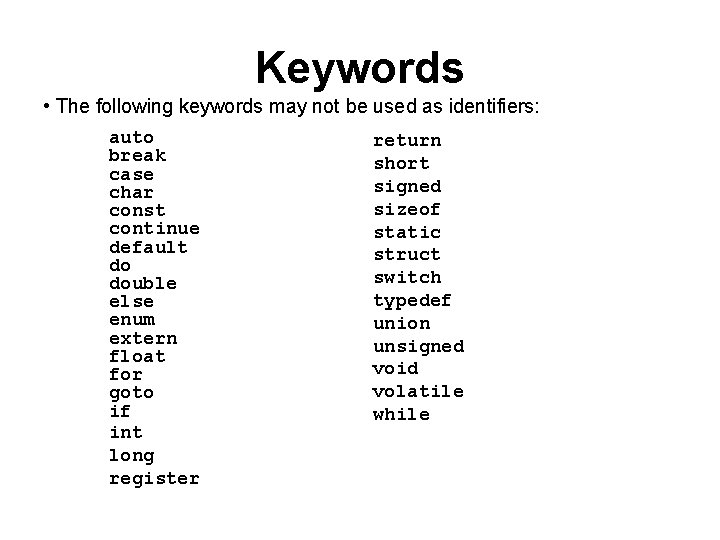
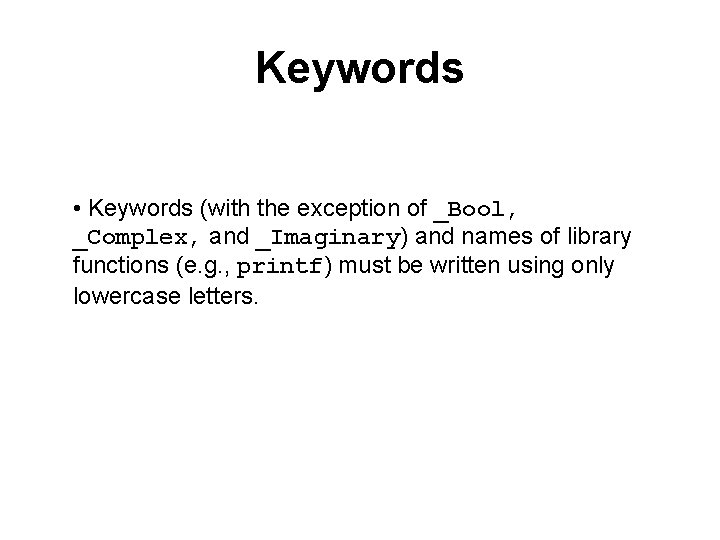
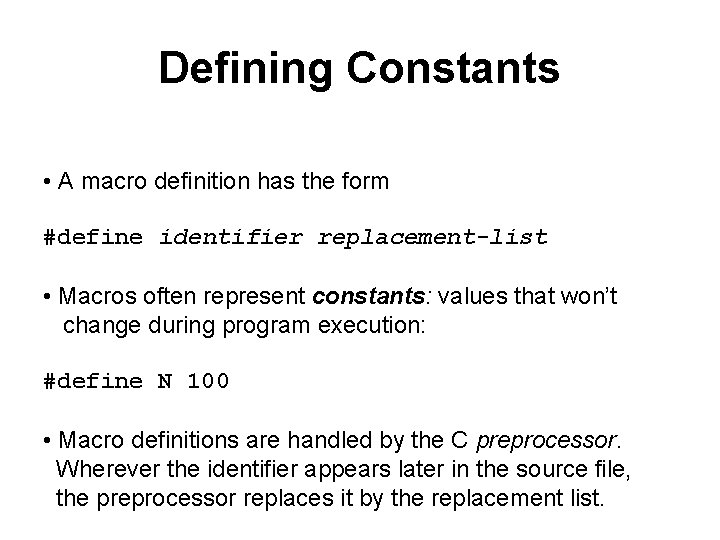
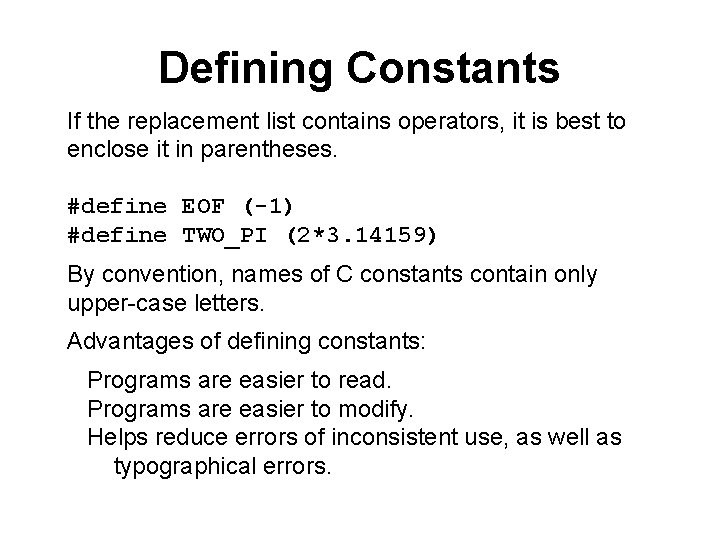
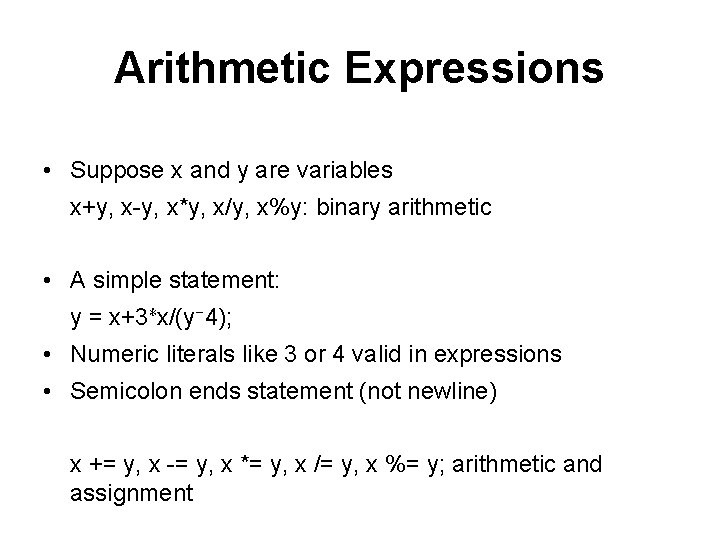
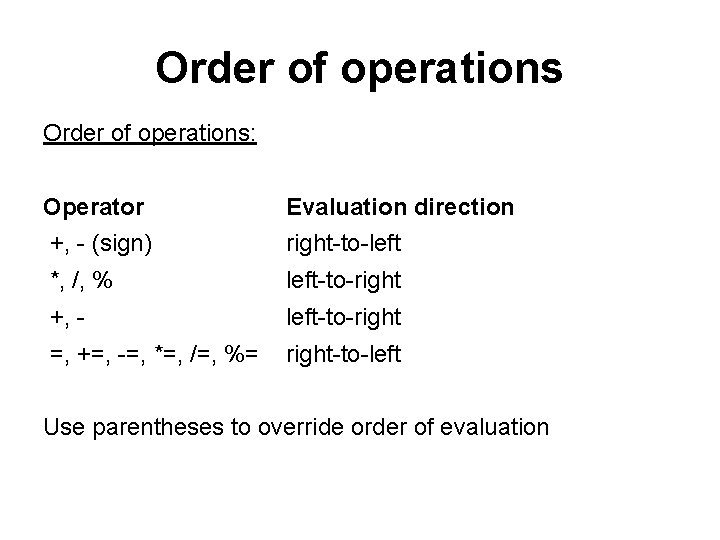
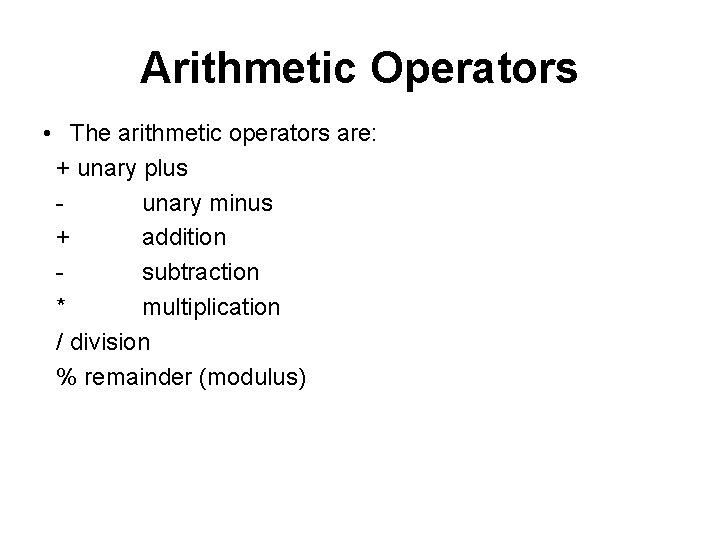
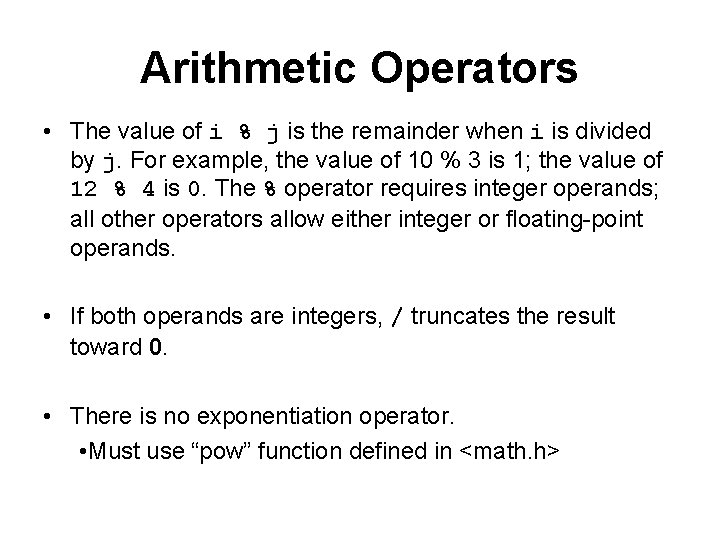
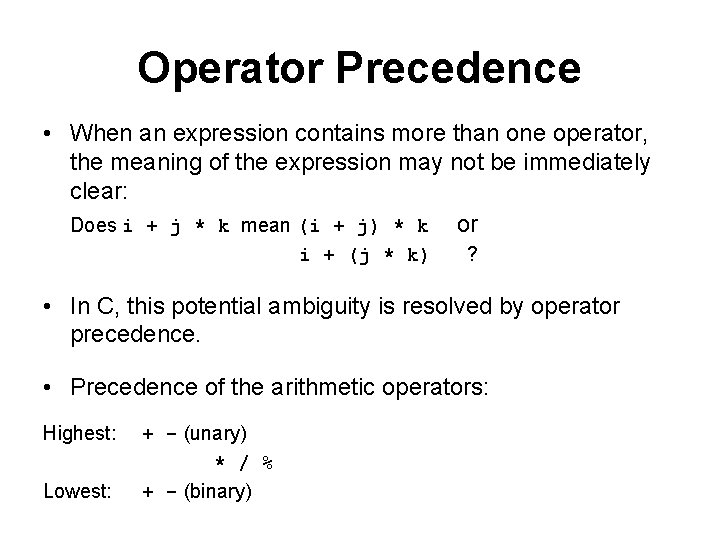
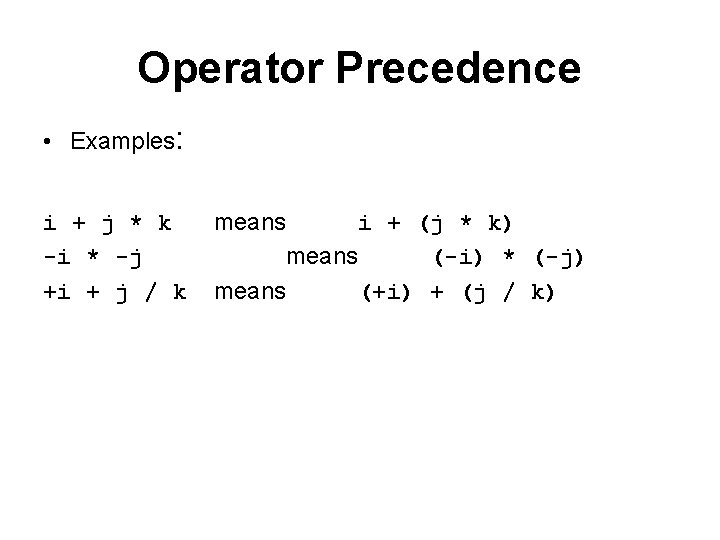
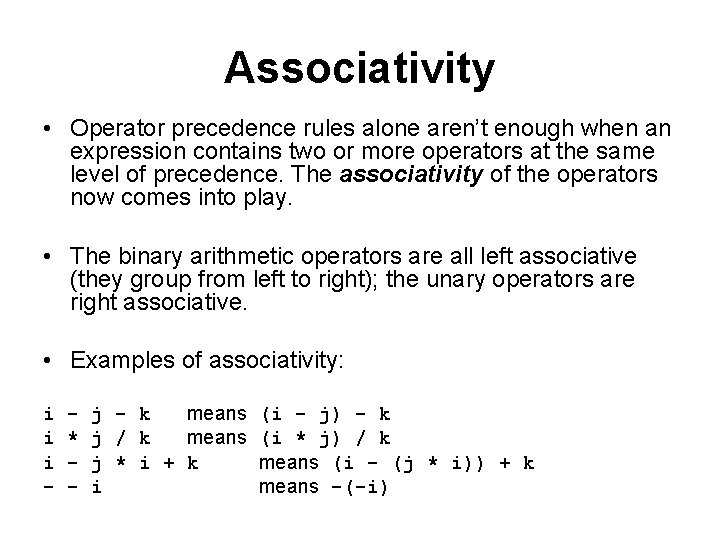
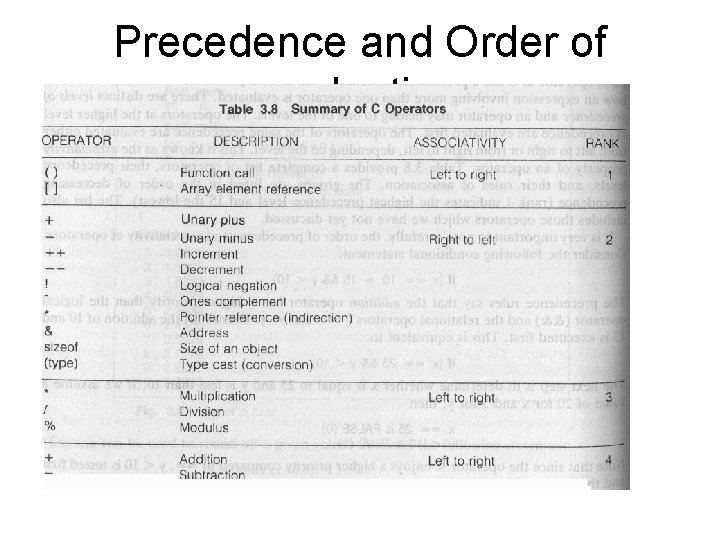
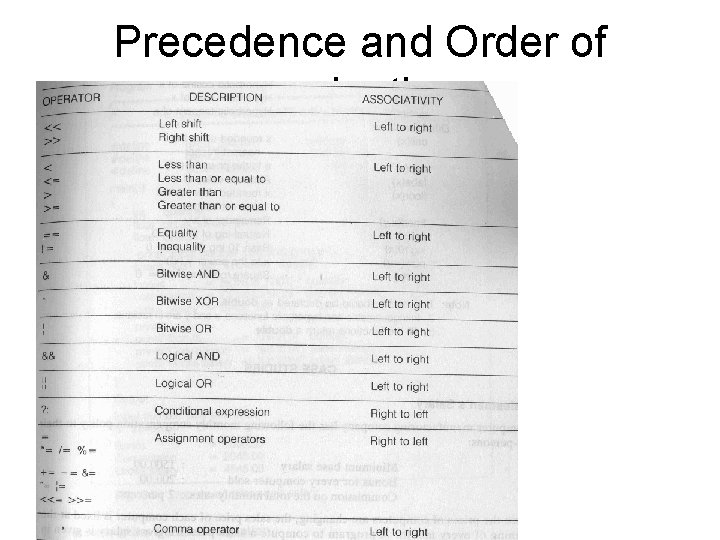
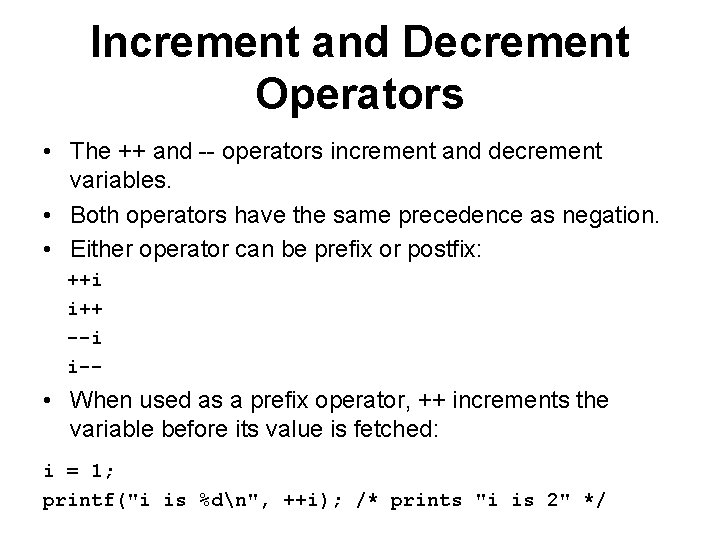
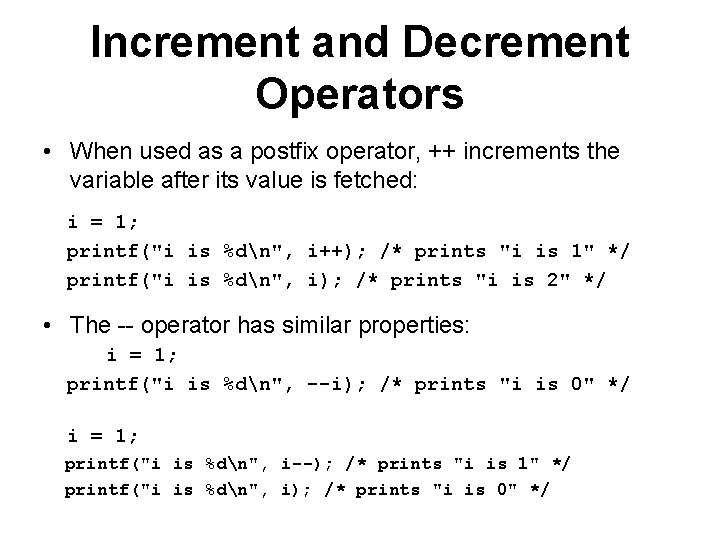
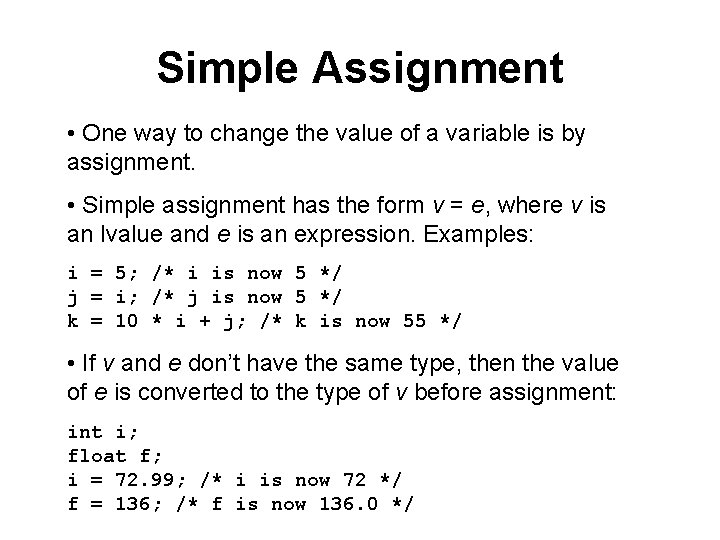
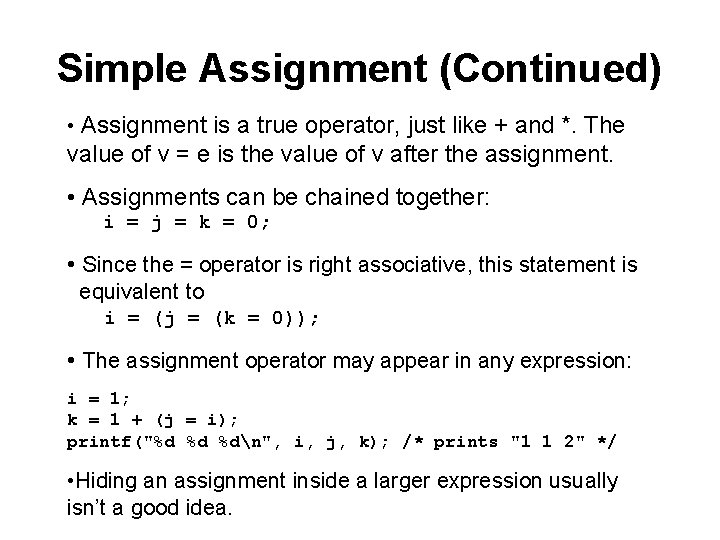
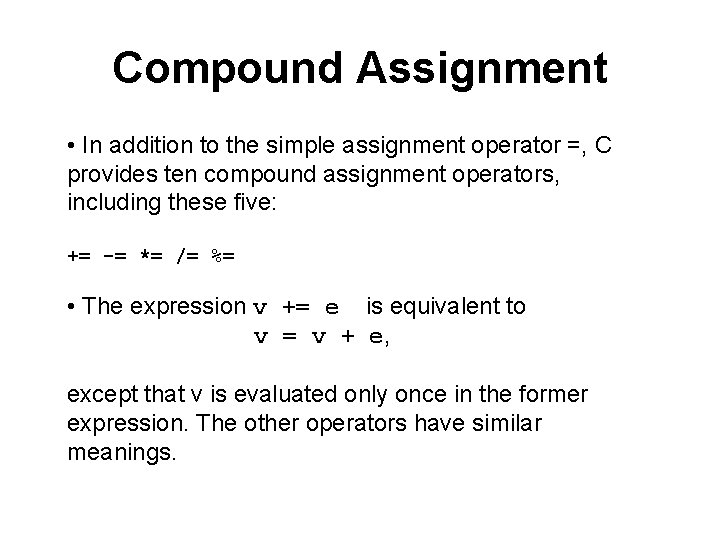
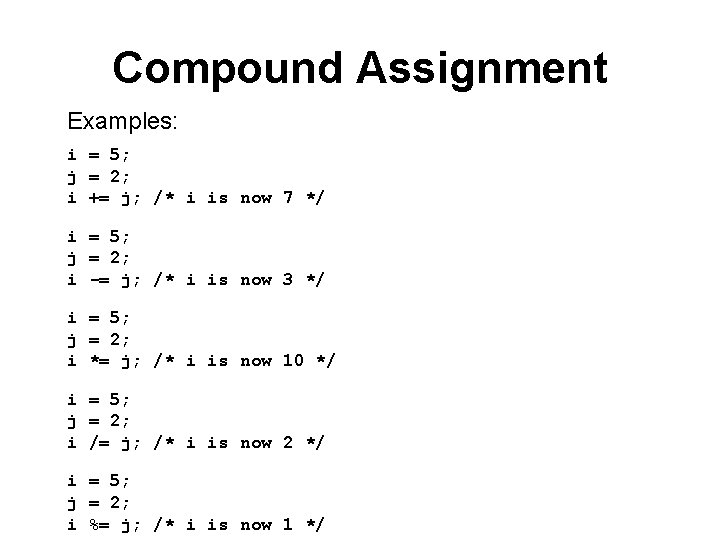
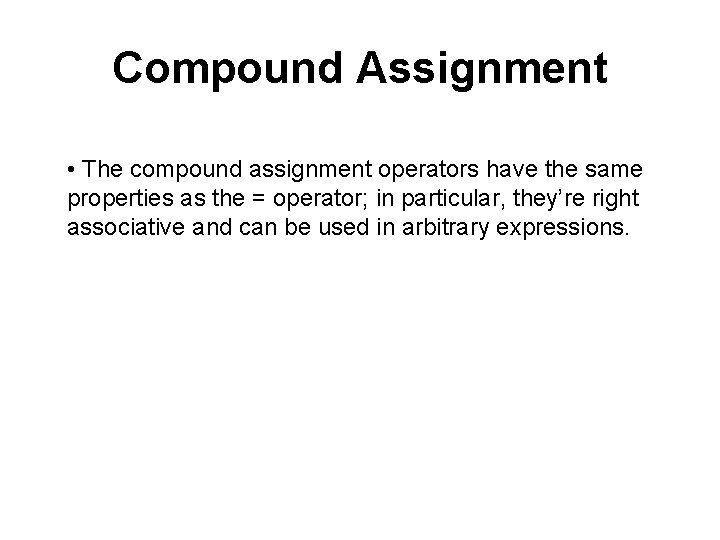
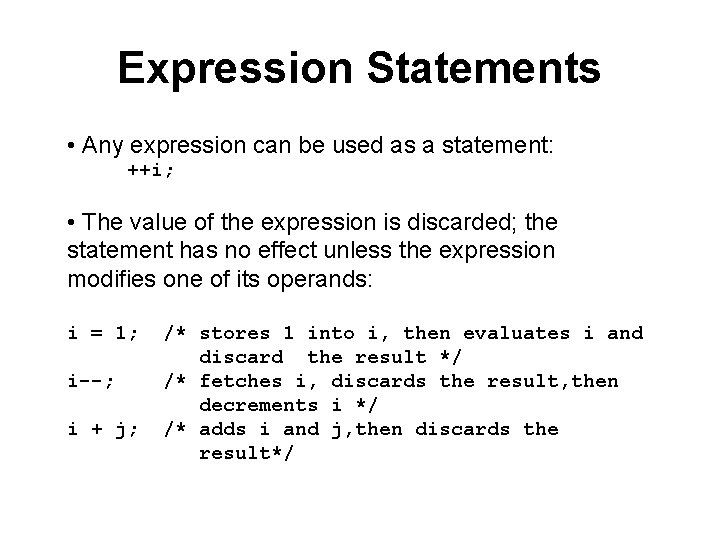
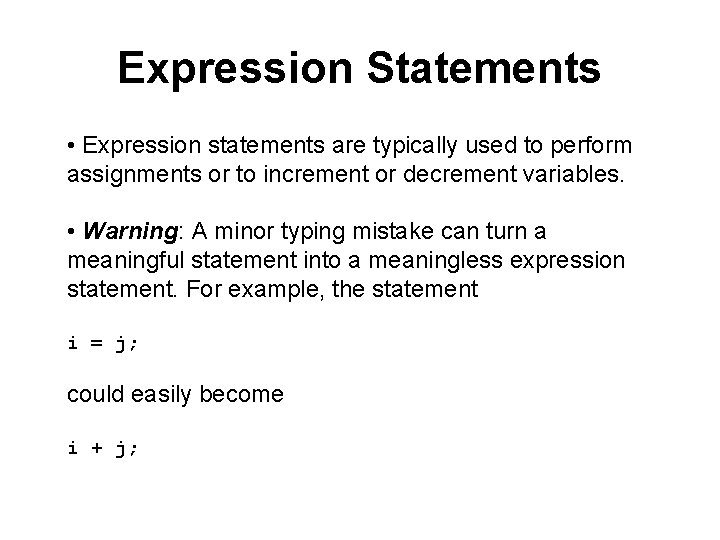
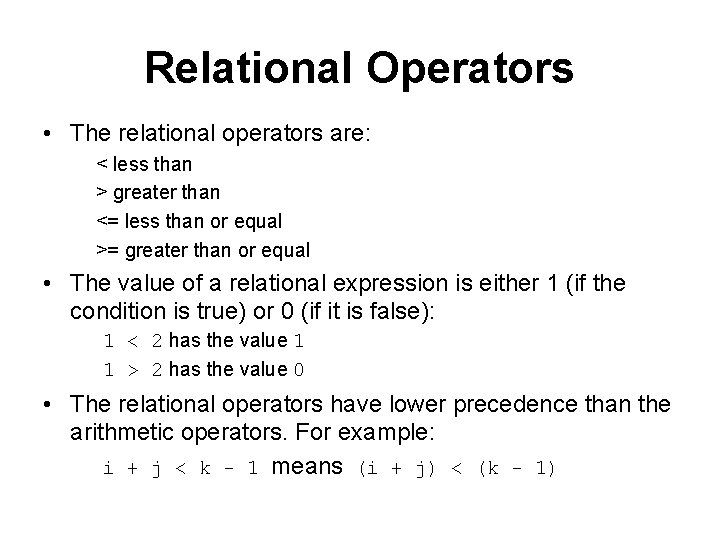
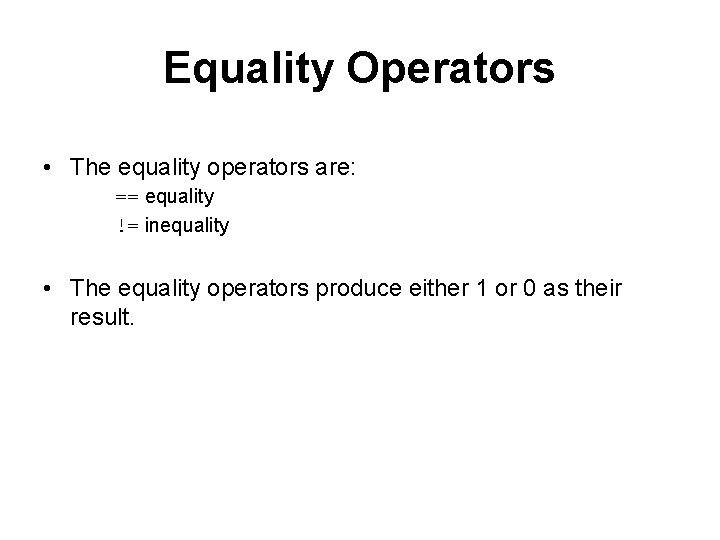
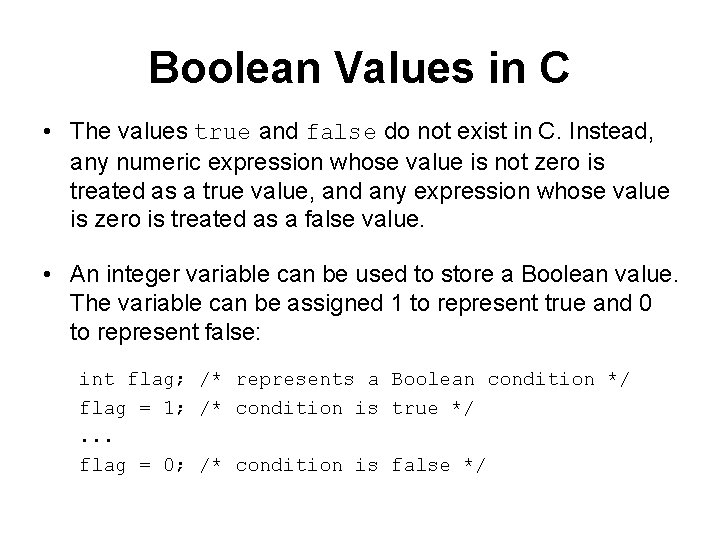
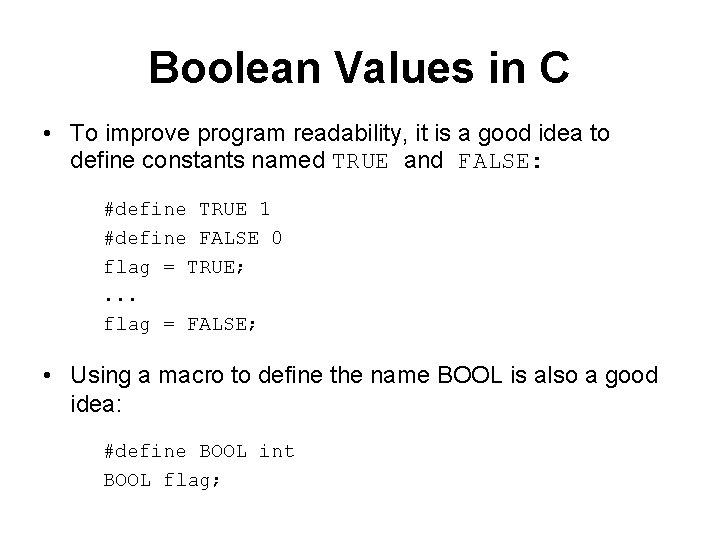
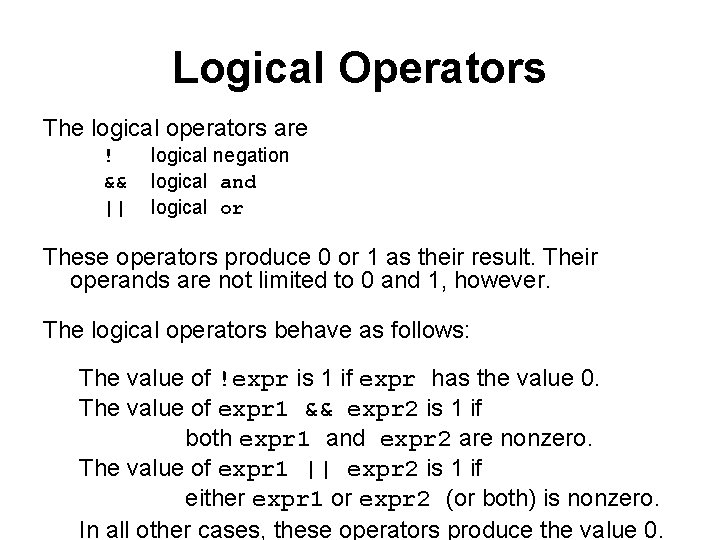
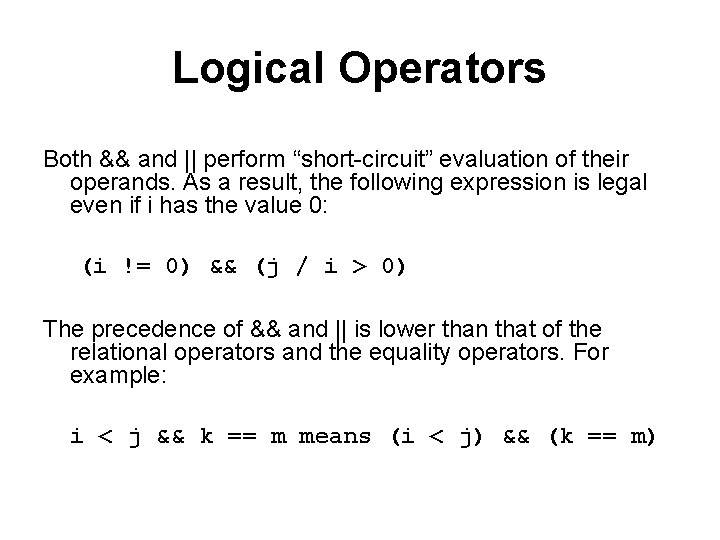
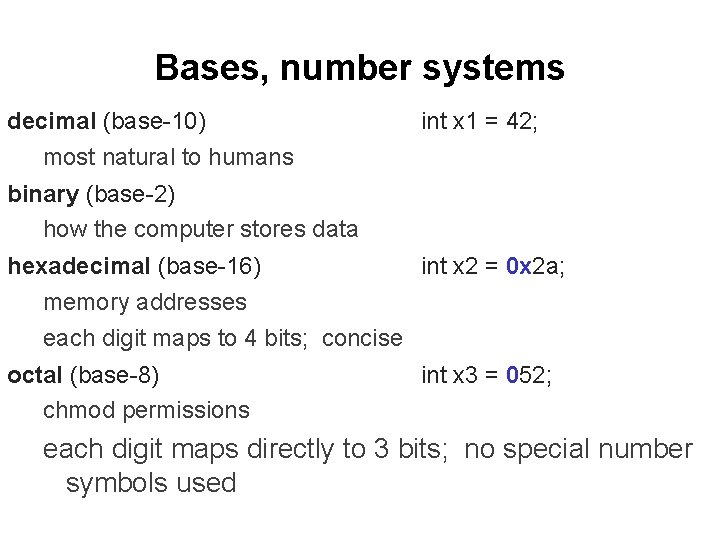
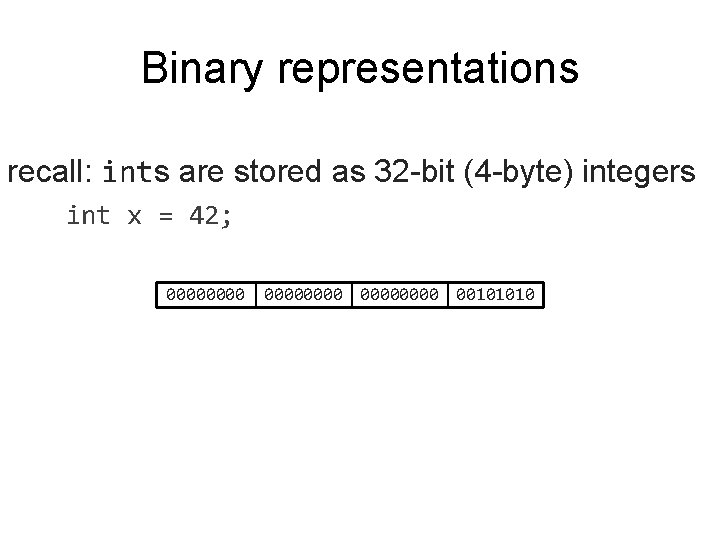
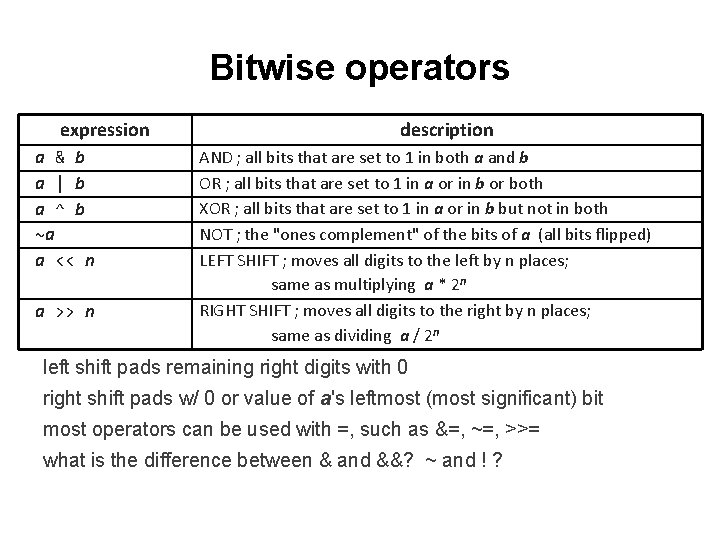
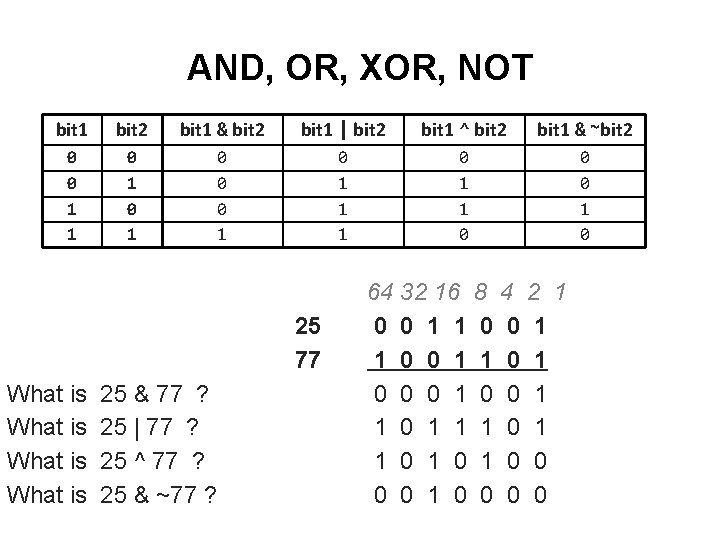
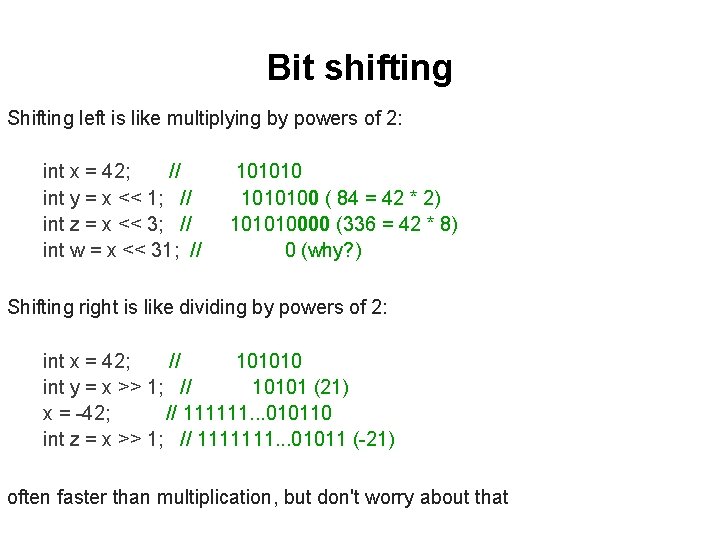
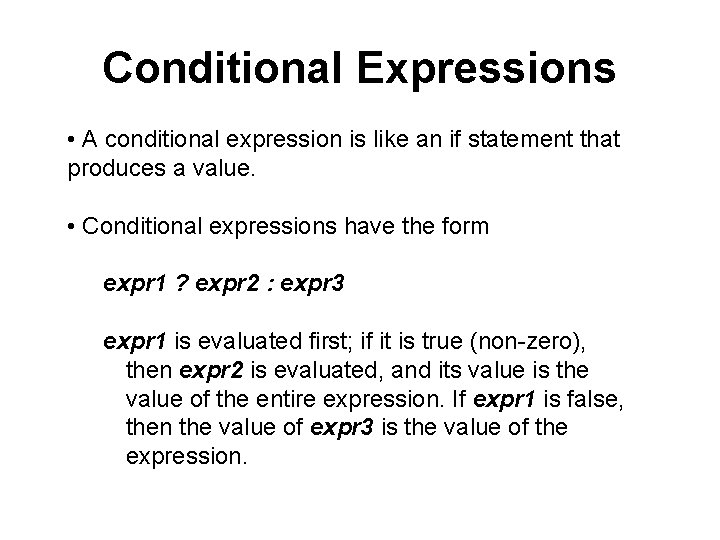
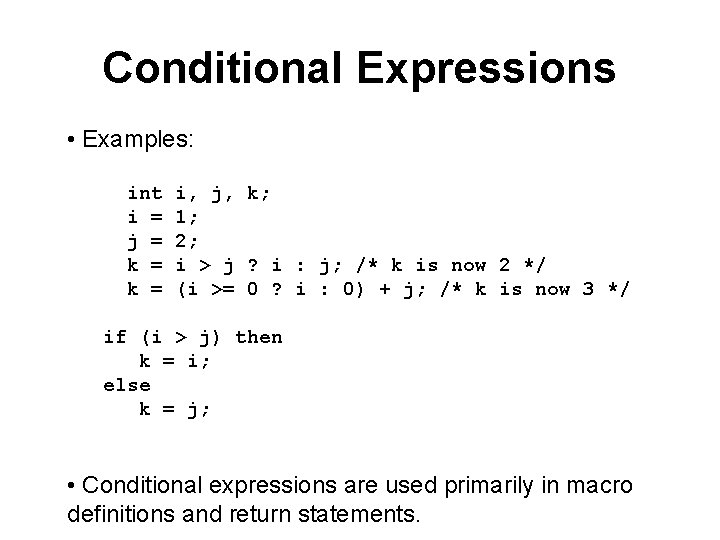
- Slides: 128
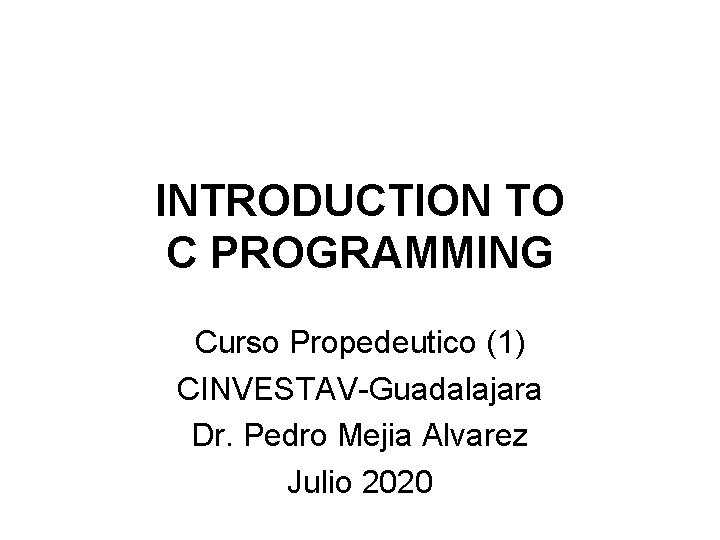
INTRODUCTION TO C PROGRAMMING Curso Propedeutico (1) CINVESTAV-Guadalajara Dr. Pedro Mejia Alvarez Julio 2020
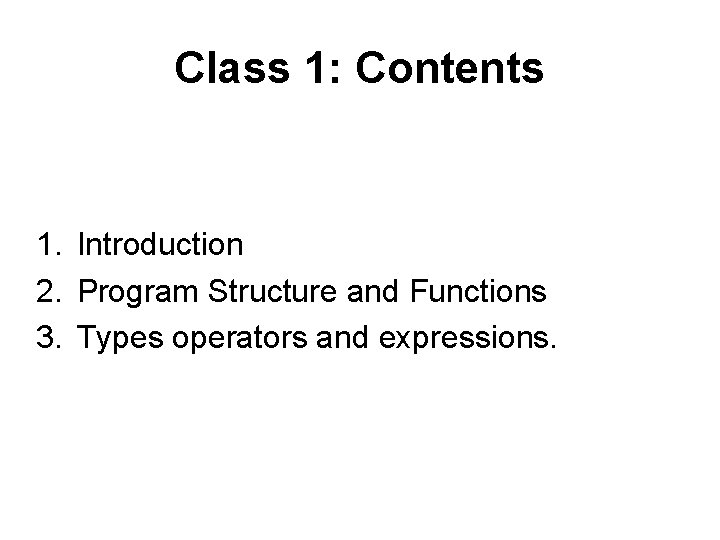
Class 1: Contents 1. Introduction 2. Program Structure and Functions 3. Types operators and expressions.
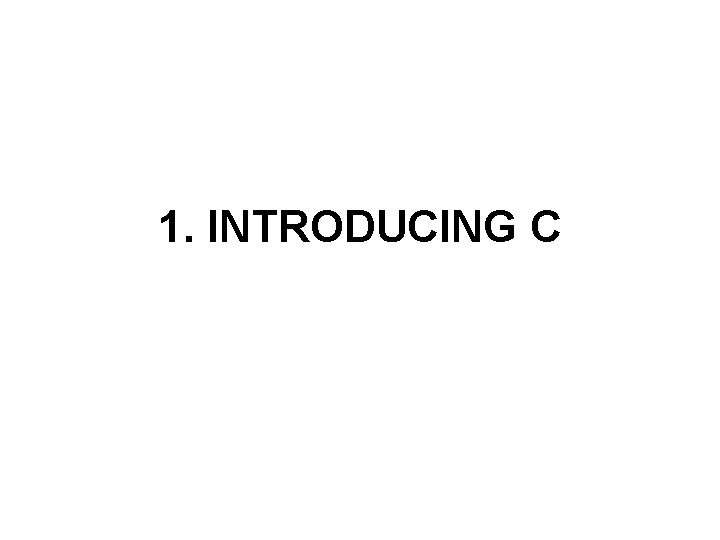
1. INTRODUCING C
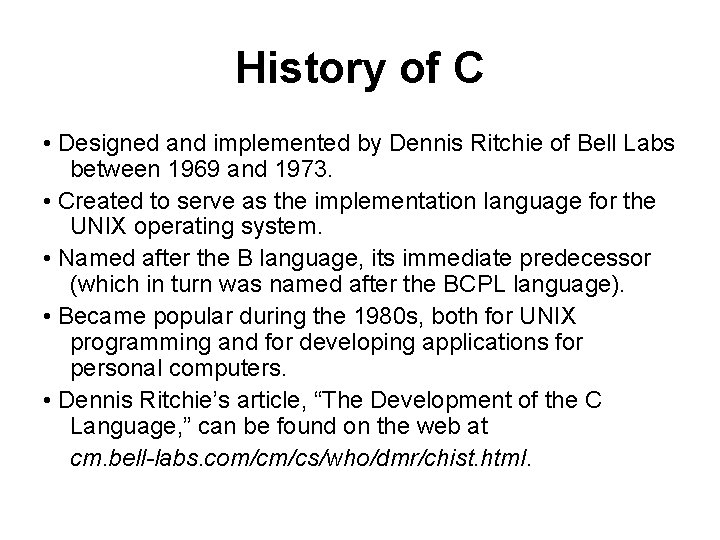
History of C • Designed and implemented by Dennis Ritchie of Bell Labs between 1969 and 1973. • Created to serve as the implementation language for the UNIX operating system. • Named after the B language, its immediate predecessor (which in turn was named after the BCPL language). • Became popular during the 1980 s, both for UNIX programming and for developing applications for personal computers. • Dennis Ritchie’s article, “The Development of the C Language, ” can be found on the web at cm. bell-labs. com/cm/cs/who/dmr/chist. html.
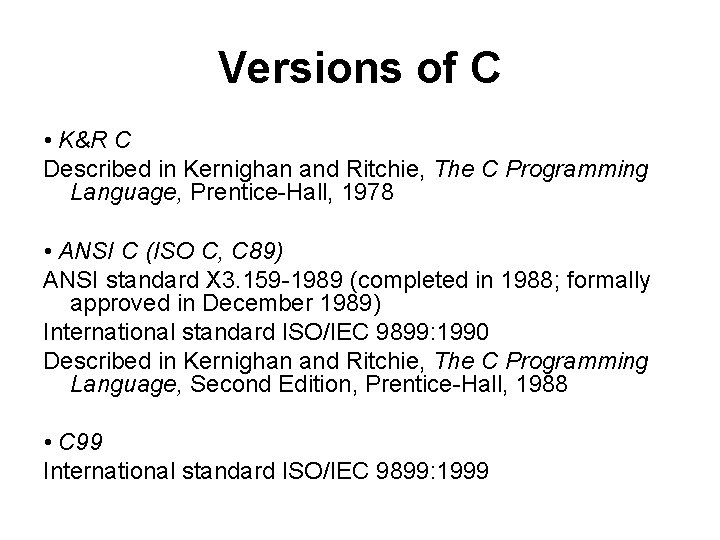
Versions of C • K&R C Described in Kernighan and Ritchie, The C Programming Language, Prentice-Hall, 1978 • ANSI C (ISO C, C 89) ANSI standard X 3. 159 -1989 (completed in 1988; formally approved in December 1989) International standard ISO/IEC 9899: 1990 Described in Kernighan and Ritchie, The C Programming Language, Second Edition, Prentice-Hall, 1988 • C 99 International standard ISO/IEC 9899: 1999
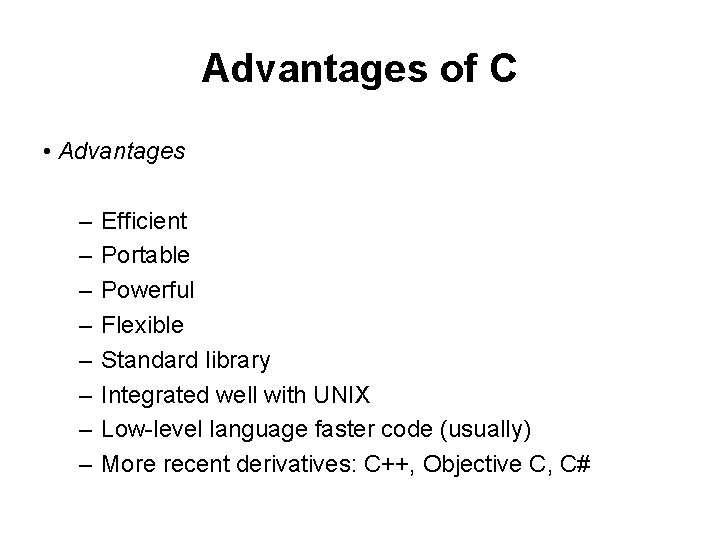
Advantages of C • Advantages – – – – Efficient Portable Powerful Flexible Standard library Integrated well with UNIX Low-level language faster code (usually) More recent derivatives: C++, Objective C, C#
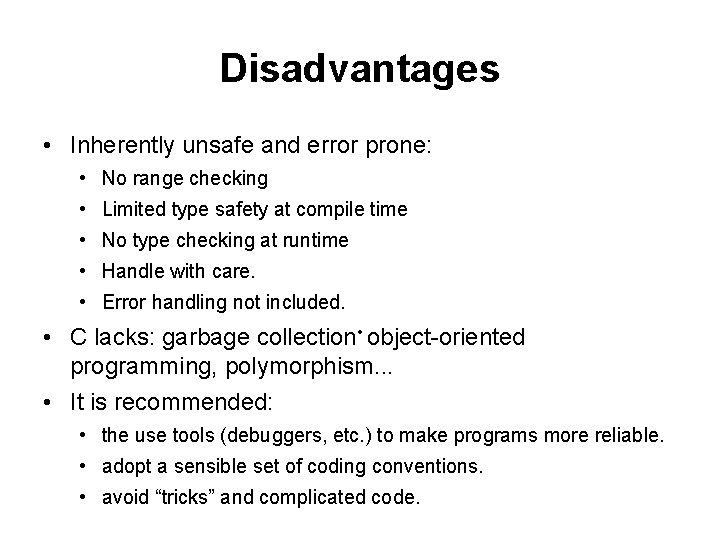
Disadvantages • Inherently unsafe and error prone: • No range checking • Limited type safety at compile time • No type checking at runtime • Handle with care. • Error handling not included. • C lacks: garbage collection • object-oriented programming, polymorphism. . . • It is recommended: • the use tools (debuggers, etc. ) to make programs more reliable. • adopt a sensible set of coding conventions. • avoid “tricks” and complicated code.
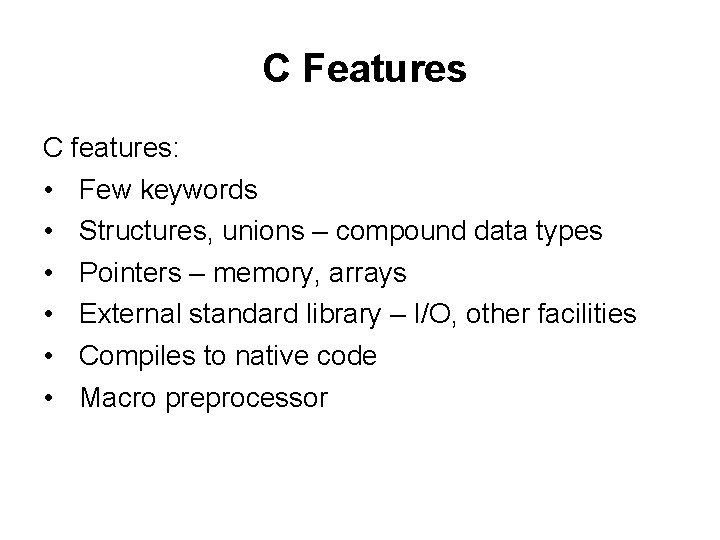
C Features C features: • Few keywords • Structures, unions – compound data types • Pointers – memory, arrays • External standard library – I/O, other facilities • Compiles to native code • Macro preprocessor
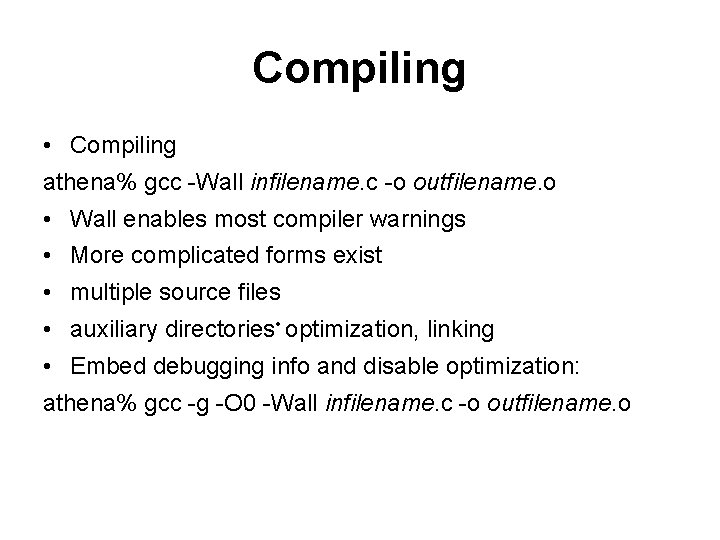
Compiling • Compiling athena% gcc -Wall infilename. c -o outfilename. o • Wall enables most compiler warnings • More complicated forms exist • multiple source files • auxiliary directories • optimization, linking • Embed debugging info and disable optimization: athena% gcc -g -O 0 -Wall infilename. c -o outfilename. o
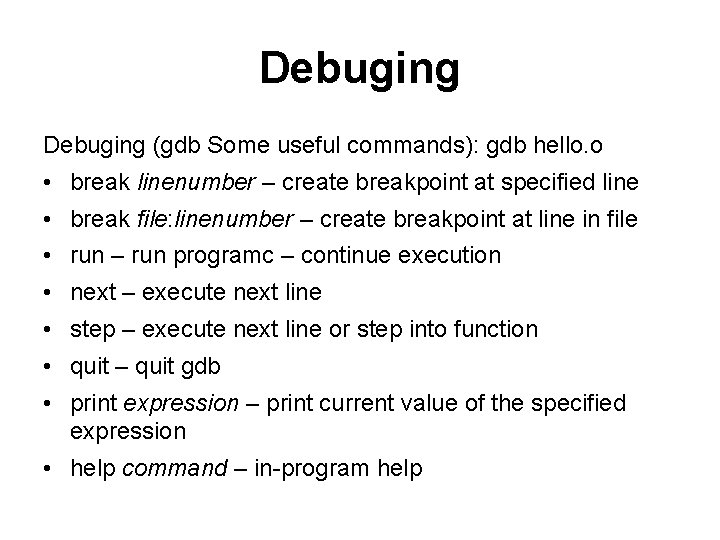
Debuging (gdb Some useful commands): gdb hello. o • break linenumber – create breakpoint at specified line • break file: linenumber – create breakpoint at line in file • run – run programc – continue execution • next – execute next line • step – execute next line or step into function • quit – quit gdb • print expression – print current value of the specified expression • help command – in-program help
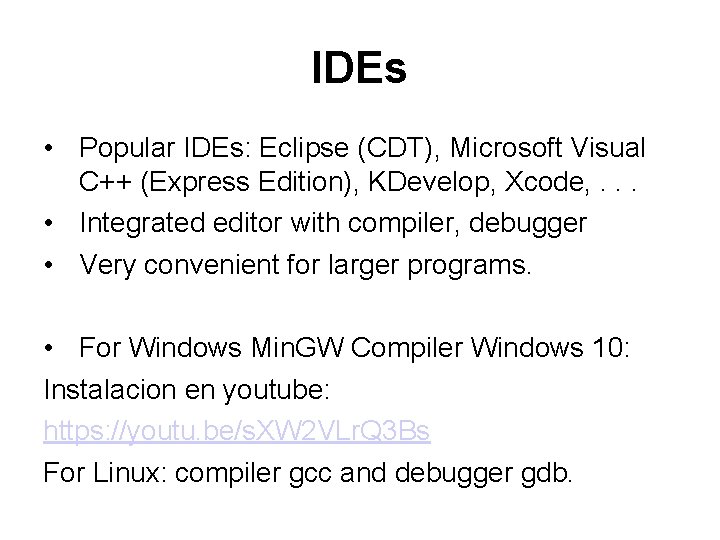
IDEs • Popular IDEs: Eclipse (CDT), Microsoft Visual C++ (Express Edition), KDevelop, Xcode, . . . • Integrated editor with compiler, debugger • Very convenient for larger programs. • For Windows Min. GW Compiler Windows 10: Instalacion en youtube: https: //youtu. be/s. XW 2 VLr. Q 3 Bs For Linux: compiler gcc and debugger gdb.
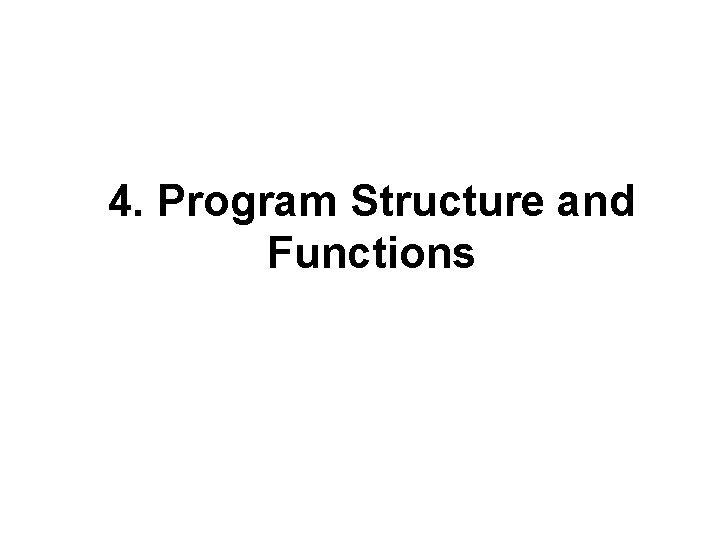
4. Program Structure and Functions
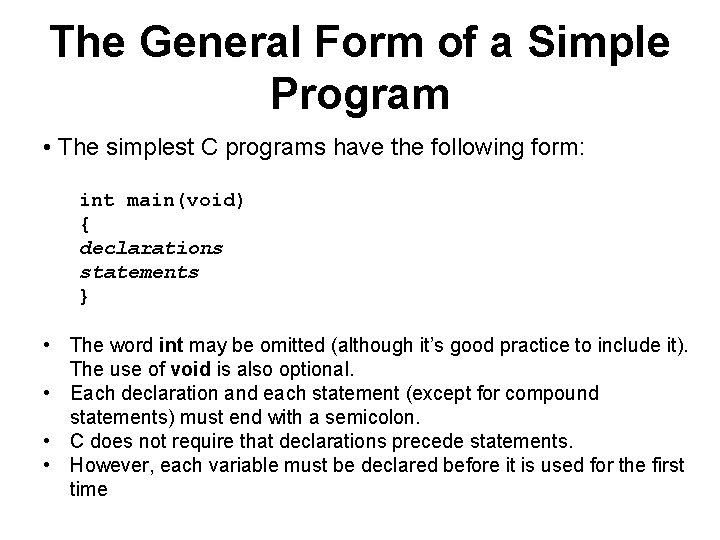
The General Form of a Simple Program • The simplest C programs have the following form: int main(void) { declarations statements } • The word int may be omitted (although it’s good practice to include it). The use of void is also optional. • Each declaration and each statement (except for compound statements) must end with a semicolon. • C does not require that declarations precede statements. • However, each variable must be declared before it is used for the first time
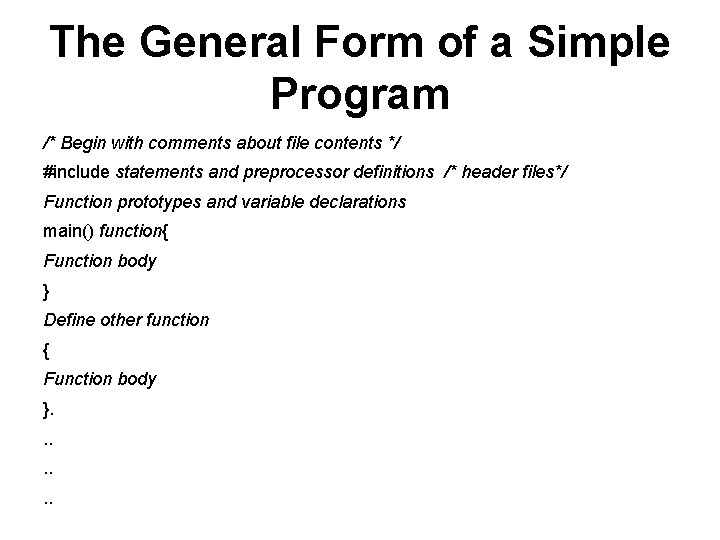
The General Form of a Simple Program /* Begin with comments about file contents */ #include statements and preprocessor definitions /* header files*/ Function prototypes and variable declarations main() function{ Function body } Define other function { Function body }. . . .
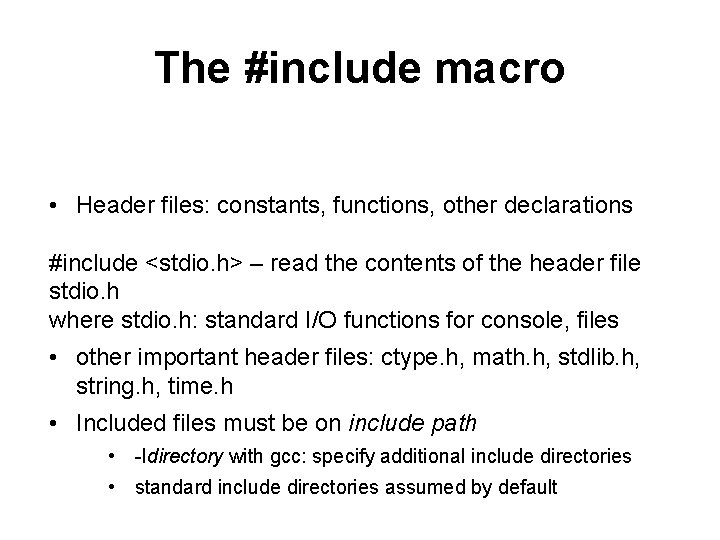
The #include macro • Header files: constants, functions, other declarations #include <stdio. h> – read the contents of the header file stdio. h where stdio. h: standard I/O functions for console, files • other important header files: ctype. h, math. h, stdlib. h, string. h, time. h • Included files must be on include path • -Idirectory with gcc: specify additional include directories • standard include directories assumed by default
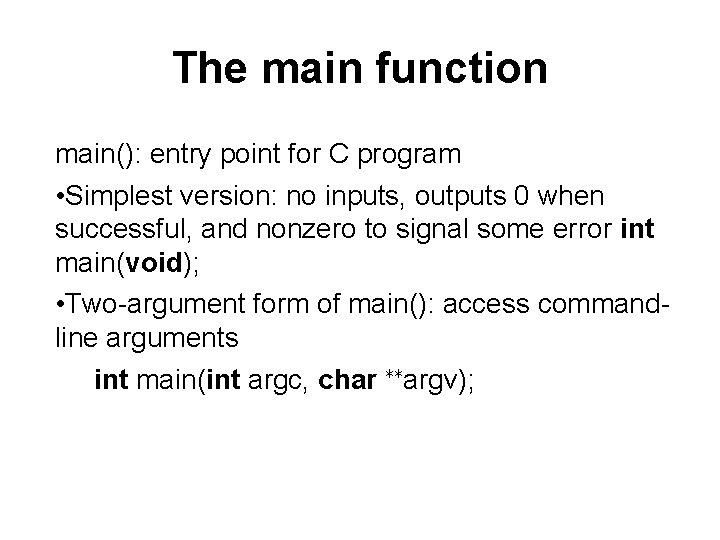
The main function main(): entry point for C program • Simplest version: no inputs, outputs 0 when successful, and nonzero to signal some error int main(void); • Two-argument form of main(): access commandline arguments int main(int argc, char ∗∗argv);
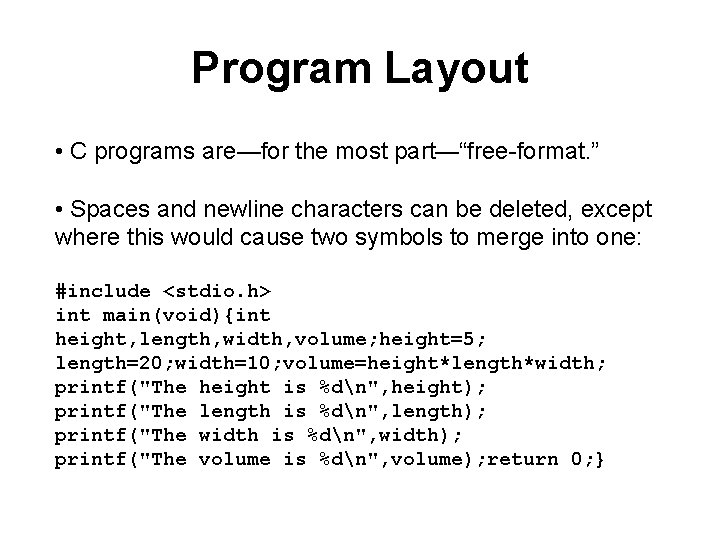
Program Layout • C programs are—for the most part—“free-format. ” • Spaces and newline characters can be deleted, except where this would cause two symbols to merge into one: #include <stdio. h> int main(void){int height, length, width, volume; height=5; length=20; width=10; volume=height*length*width; printf("The height is %dn", height); printf("The length is %dn", length); printf("The width is %dn", width); printf("The volume is %dn", volume); return 0; }
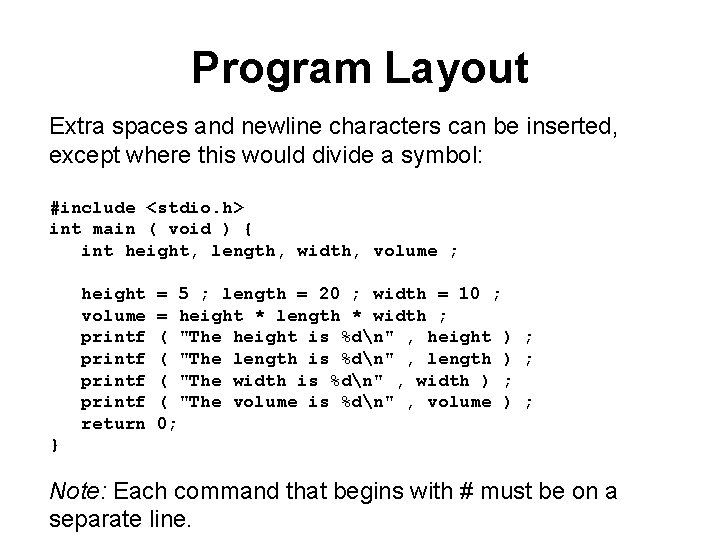
Program Layout Extra spaces and newline characters can be inserted, except where this would divide a symbol: #include <stdio. h> int main ( void ) { int height, length, width, volume ; height volume printf return = 5 ; length = 20 ; width = 10 ; = height * length * width ; ( "The height is %dn" , height ) ; ( "The length is %dn" , length ) ; ( "The width is %dn" , width ) ; ( "The volume is %dn" , volume ) ; 0; } Note: Each command that begins with # must be on a separate line.
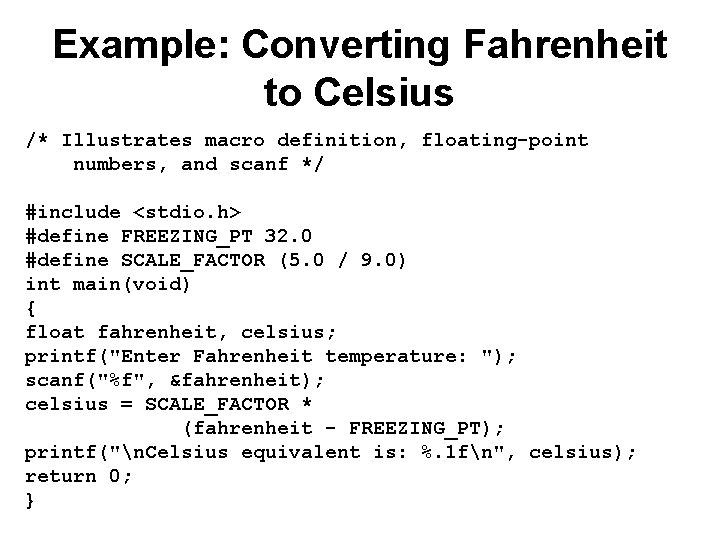
Example: Converting Fahrenheit to Celsius /* Illustrates macro definition, floating-point numbers, and scanf */ #include <stdio. h> #define FREEZING_PT 32. 0 #define SCALE_FACTOR (5. 0 / 9. 0) int main(void) { float fahrenheit, celsius; printf("Enter Fahrenheit temperature: "); scanf("%f", &fahrenheit); celsius = SCALE_FACTOR * (fahrenheit - FREEZING_PT); printf("n. Celsius equivalent is: %. 1 fn", celsius); return 0; }
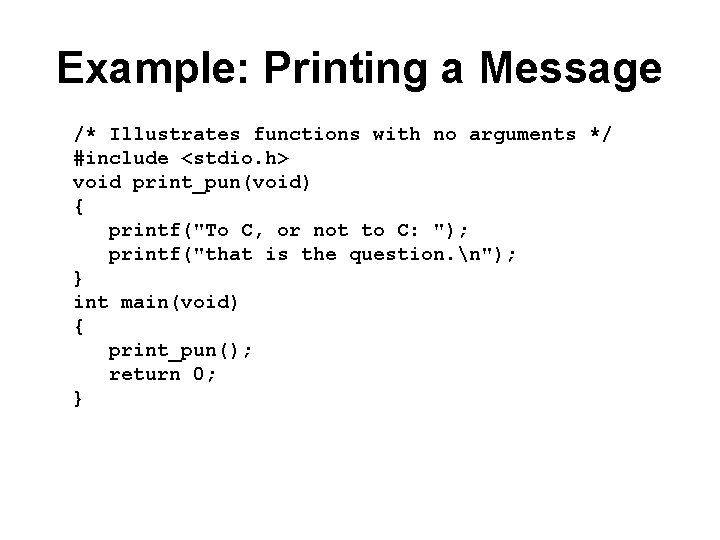
Example: Printing a Message /* Illustrates functions with no arguments */ #include <stdio. h> void print_pun(void) { printf("To C, or not to C: "); printf("that is the question. n"); } int main(void) { print_pun(); return 0; }
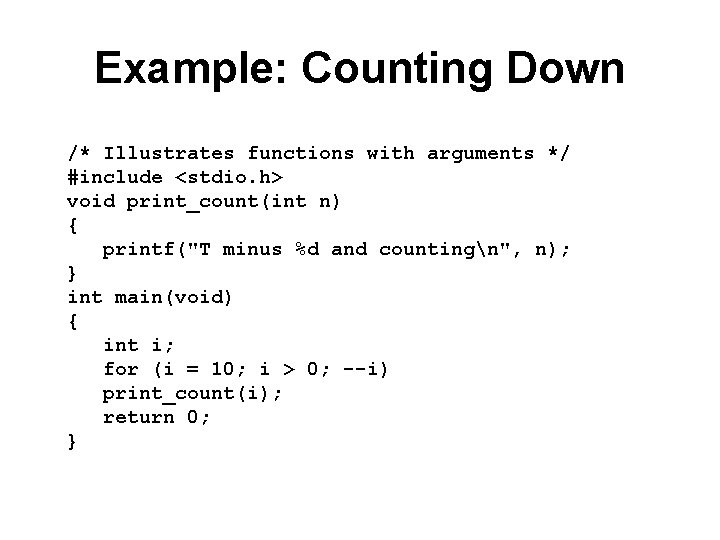
Example: Counting Down /* Illustrates functions with arguments */ #include <stdio. h> void print_count(int n) { printf("T minus %d and countingn", n); } int main(void) { int i; for (i = 10; i > 0; --i) print_count(i); return 0; }
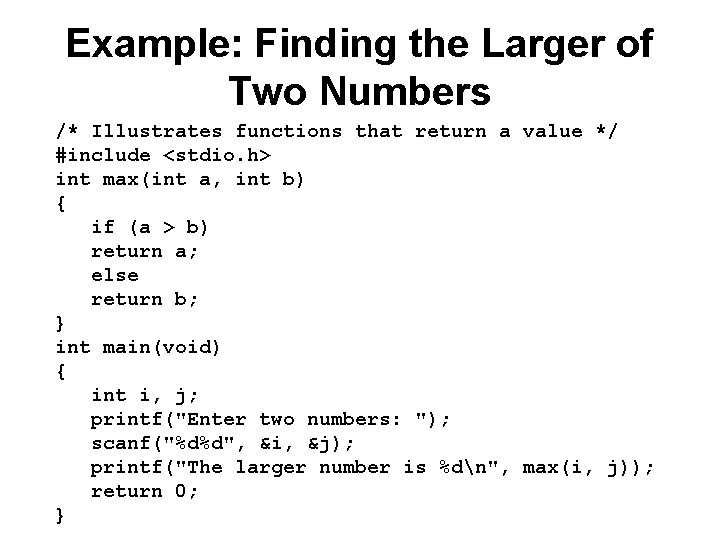
Example: Finding the Larger of Two Numbers /* Illustrates functions that return a value */ #include <stdio. h> int max(int a, int b) { if (a > b) return a; else return b; } int main(void) { int i, j; printf("Enter two numbers: "); scanf("%d%d", &i, &j); printf("The larger number is %dn", max(i, j)); return 0; }
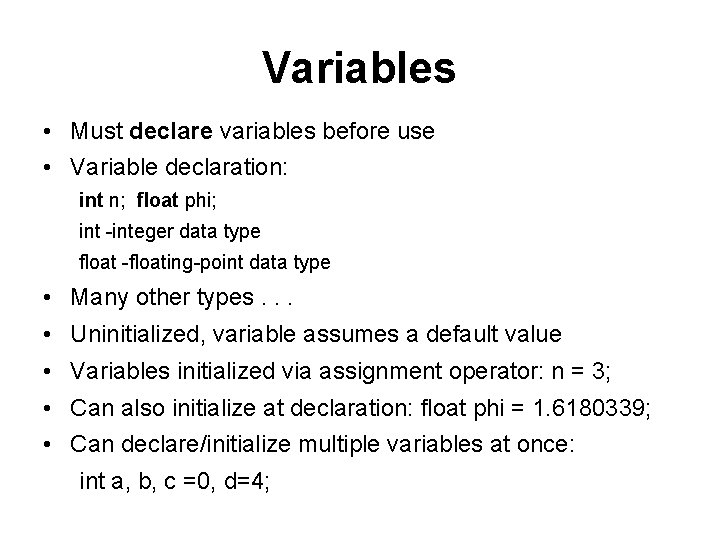
Variables • Must declare variables before use • Variable declaration: int n; float phi; int -integer data type float -floating-point data type • Many other types. . . • Uninitialized, variable assumes a default value • Variables initialized via assignment operator: n = 3; • Can also initialize at declaration: float phi = 1. 6180339; • Can declare/initialize multiple variables at once: int a, b, c =0, d=4;
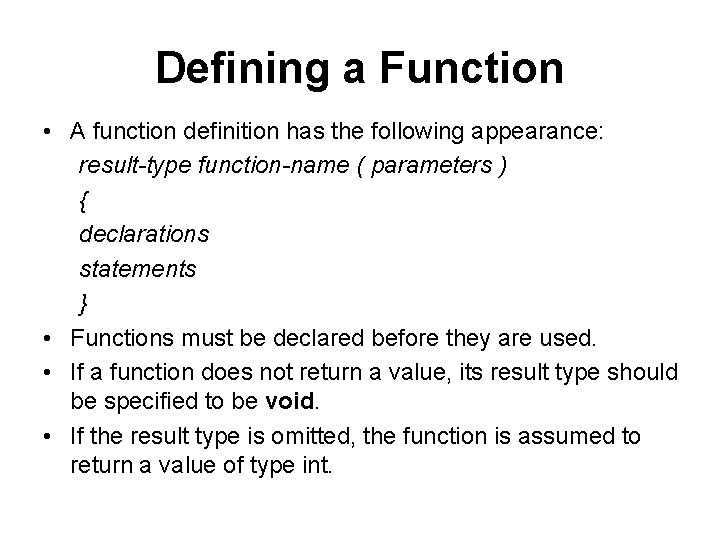
Defining a Function • A function definition has the following appearance: result-type function-name ( parameters ) { declarations statements } • Functions must be declared before they are used. • If a function does not return a value, its result type should be specified to be void. • If the result type is omitted, the function is assumed to return a value of type int.
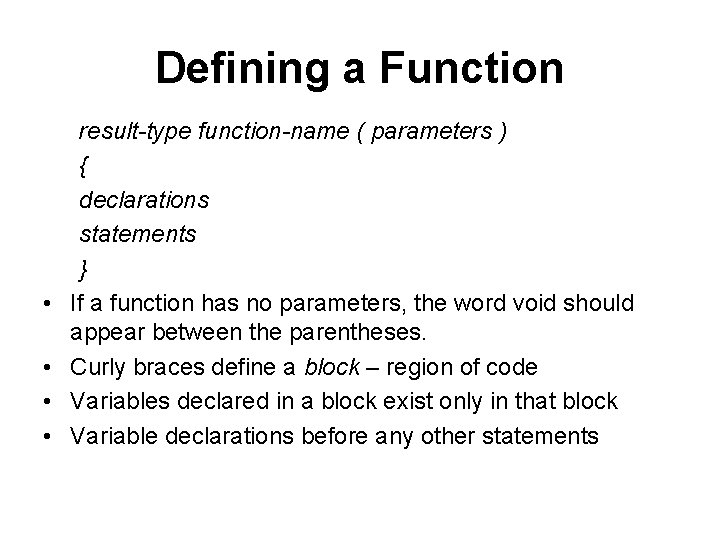
Defining a Function • • result-type function-name ( parameters ) { declarations statements } If a function has no parameters, the word void should appear between the parentheses. Curly braces define a block – region of code Variables declared in a block exist only in that block Variable declarations before any other statements
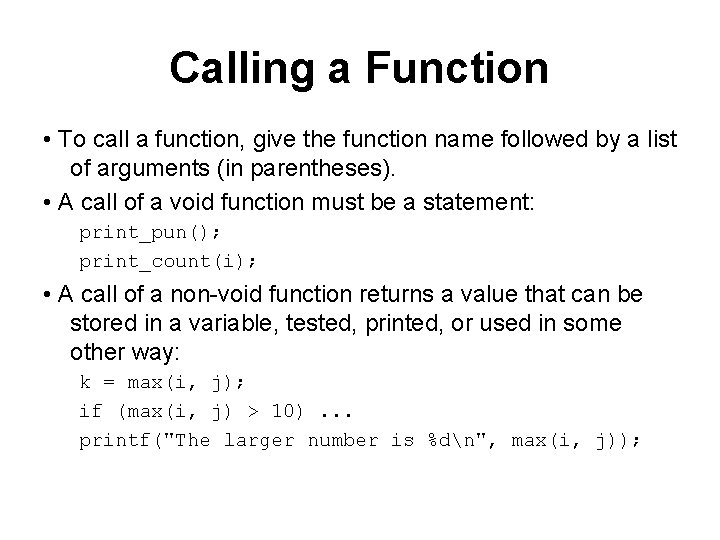
Calling a Function • To call a function, give the function name followed by a list of arguments (in parentheses). • A call of a void function must be a statement: print_pun(); print_count(i); • A call of a non-void function returns a value that can be stored in a variable, tested, printed, or used in some other way: k = max(i, j); if (max(i, j) > 10). . . printf("The larger number is %dn", max(i, j));
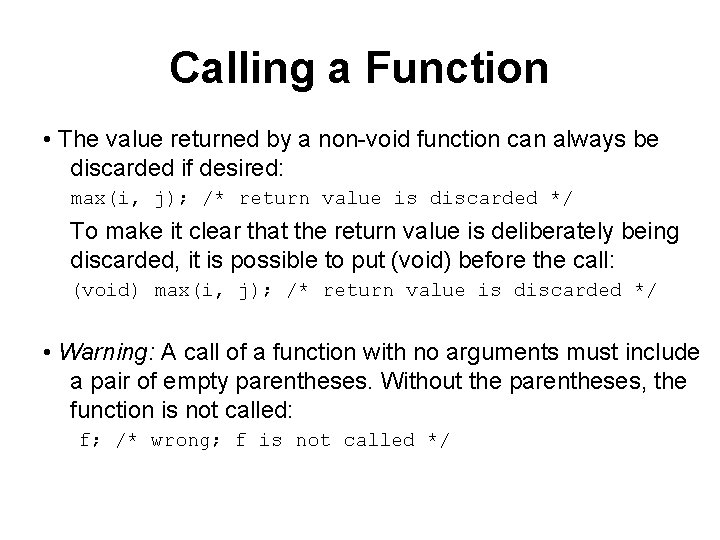
Calling a Function • The value returned by a non-void function can always be discarded if desired: max(i, j); /* return value is discarded */ To make it clear that the return value is deliberately being discarded, it is possible to put (void) before the call: (void) max(i, j); /* return value is discarded */ • Warning: A call of a function with no arguments must include a pair of empty parentheses. Without the parentheses, the function is not called: f; /* wrong; f is not called */
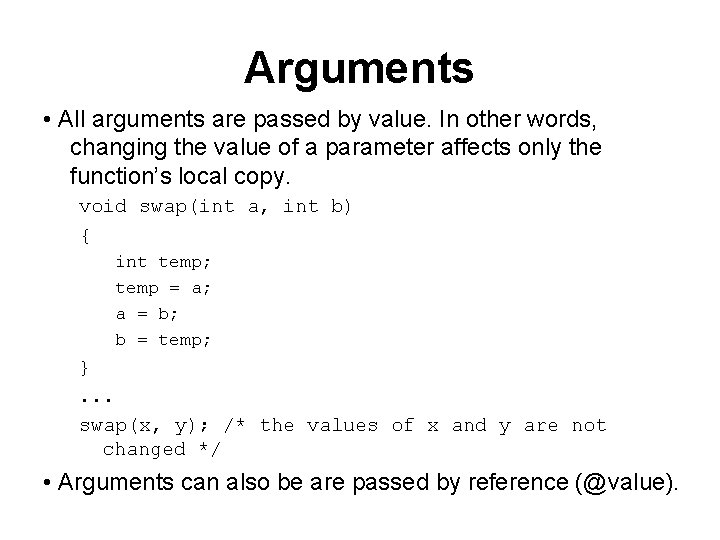
Arguments • All arguments are passed by value. In other words, changing the value of a parameter affects only the function’s local copy. void swap(int a, int b) { int temp; temp = a; a = b; b = temp; }. . . swap(x, y); /* the values of x and y are not changed */ • Arguments can also be are passed by reference (@value).
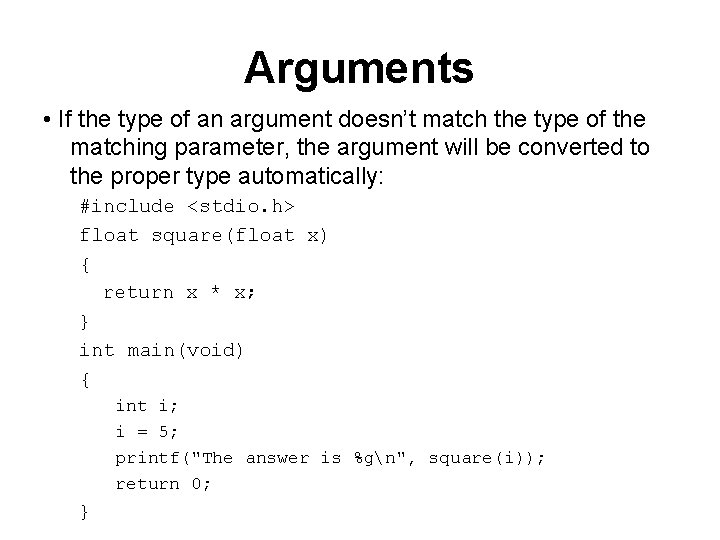
Arguments • If the type of an argument doesn’t match the type of the matching parameter, the argument will be converted to the proper type automatically: #include <stdio. h> float square(float x) { return x * x; } int main(void) { int i; i = 5; printf("The answer is %gn", square(i)); return 0; }
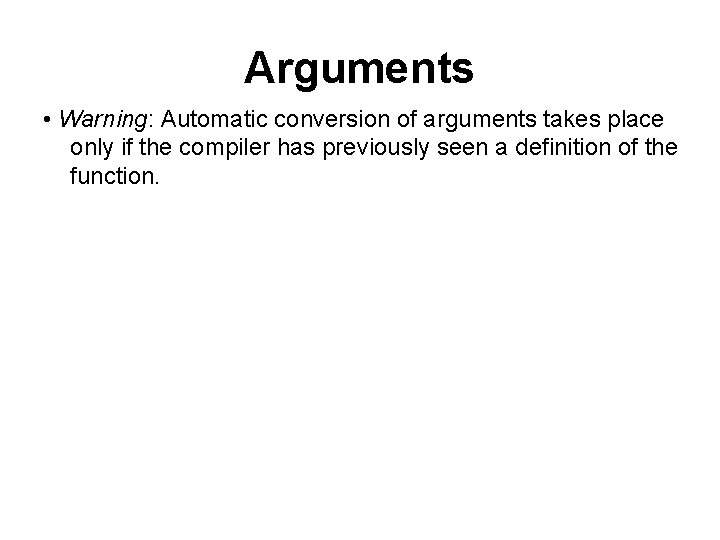
Arguments • Warning: Automatic conversion of arguments takes place only if the compiler has previously seen a definition of the function.
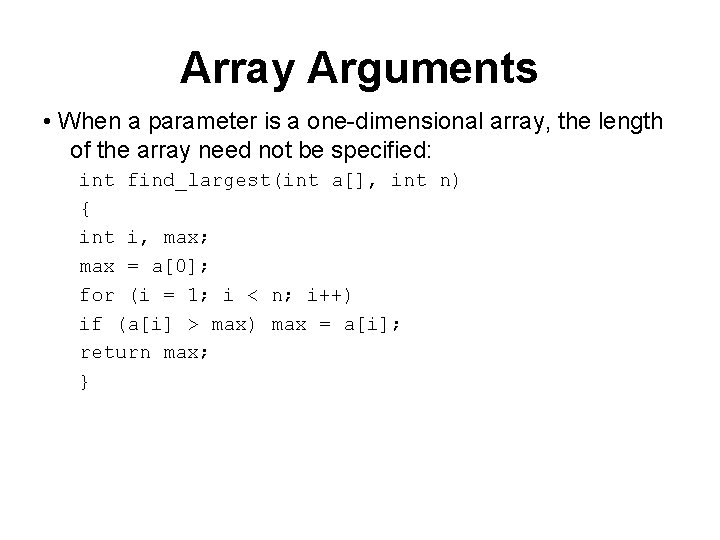
Array Arguments • When a parameter is a one-dimensional array, the length of the array need not be specified: int find_largest(int a[], int n) { int i, max; max = a[0]; for (i = 1; i < n; i++) if (a[i] > max) max = a[i]; return max; }
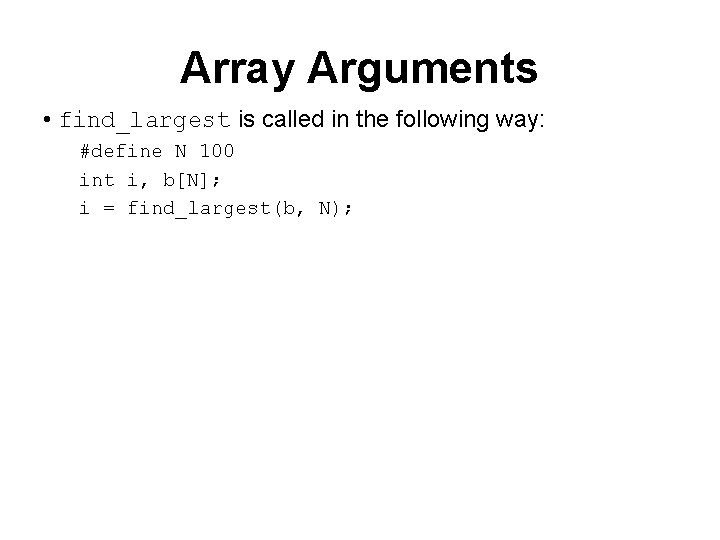
Array Arguments • find_largest is called in the following way: #define N 100 int i, b[N]; i = find_largest(b, N);
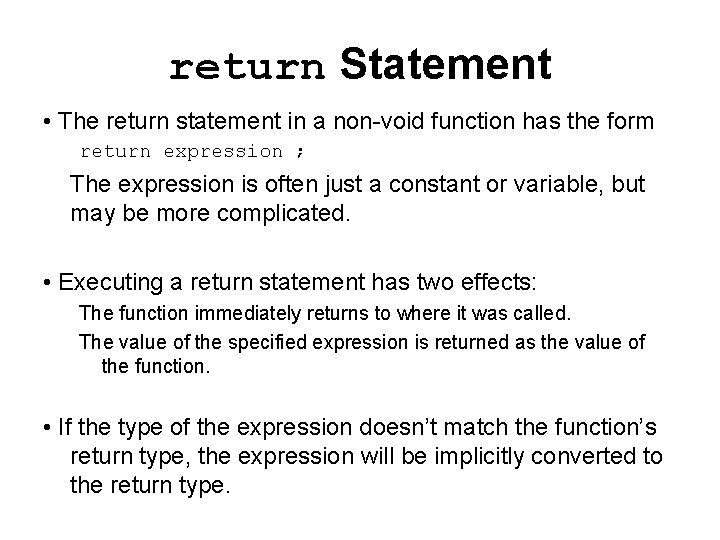
return Statement • The return statement in a non-void function has the form return expression ; The expression is often just a constant or variable, but may be more complicated. • Executing a return statement has two effects: The function immediately returns to where it was called. The value of the specified expression is returned as the value of the function. • If the type of the expression doesn’t match the function’s return type, the expression will be implicitly converted to the return type.
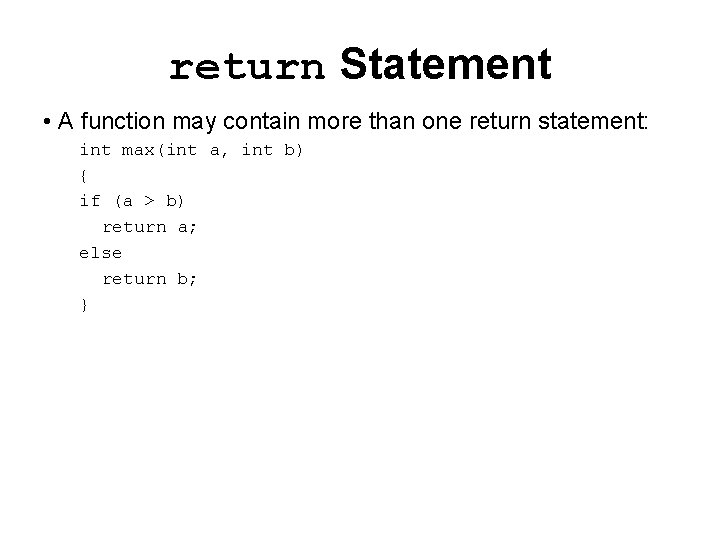
return Statement • A function may contain more than one return statement: int max(int a, int b) { if (a > b) return a; else return b; }
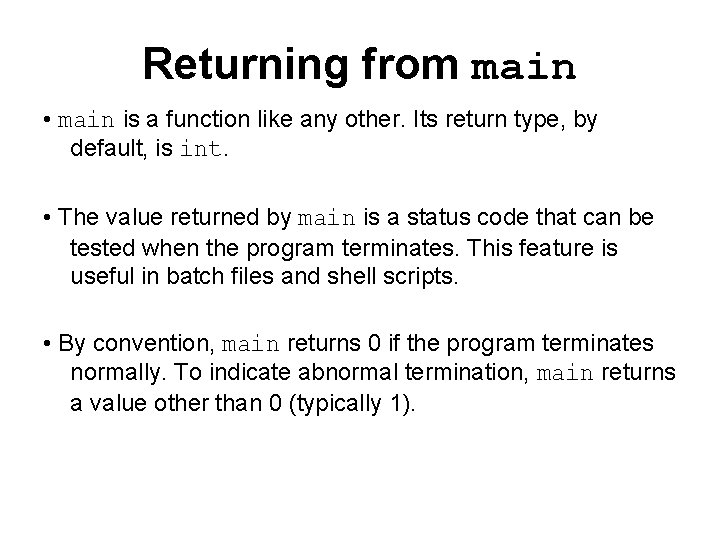
Returning from main • main is a function like any other. Its return type, by default, is int. • The value returned by main is a status code that can be tested when the program terminates. This feature is useful in batch files and shell scripts. • By convention, main returns 0 if the program terminates normally. To indicate abnormal termination, main returns a value other than 0 (typically 1).
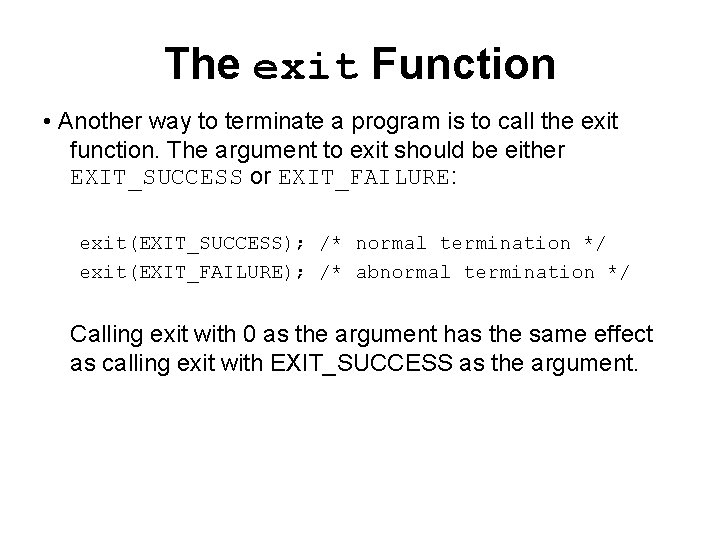
The exit Function • Another way to terminate a program is to call the exit function. The argument to exit should be either EXIT_SUCCESS or EXIT_FAILURE: exit(EXIT_SUCCESS); /* normal termination */ exit(EXIT_FAILURE); /* abnormal termination */ Calling exit with 0 as the argument has the same effect as calling exit with EXIT_SUCCESS as the argument.
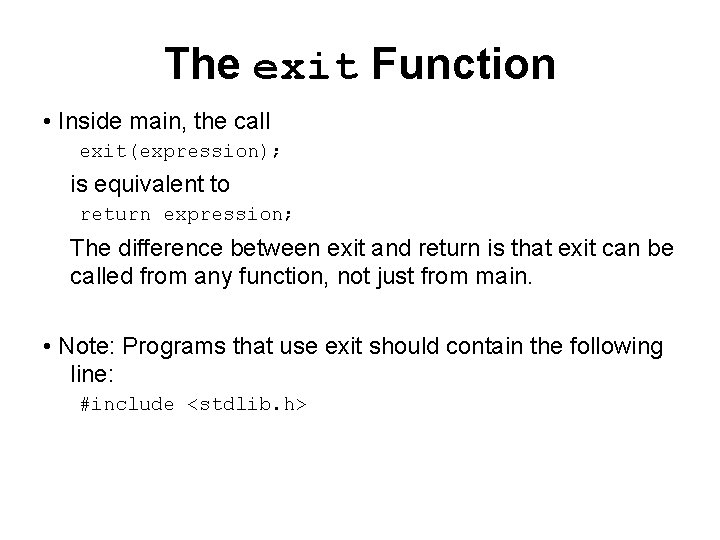
The exit Function • Inside main, the call exit(expression); is equivalent to return expression; The difference between exit and return is that exit can be called from any function, not just from main. • Note: Programs that use exit should contain the following line: #include <stdlib. h>
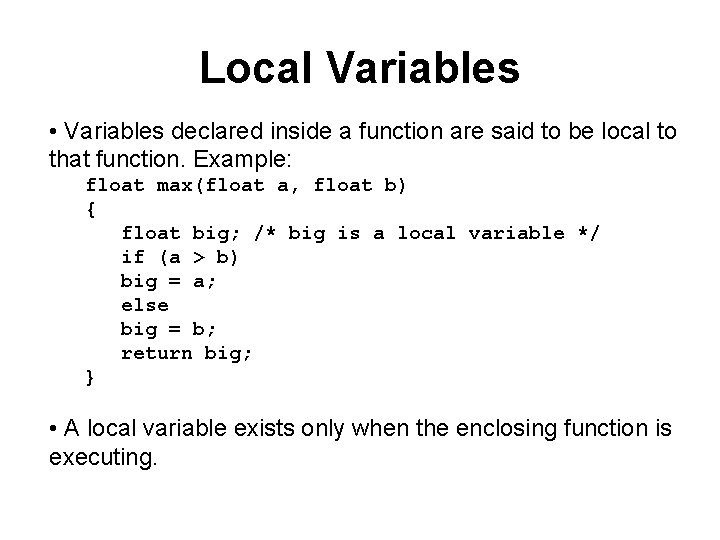
Local Variables • Variables declared inside a function are said to be local to that function. Example: float max(float a, float b) { float big; /* big is a local variable */ if (a > b) big = a; else big = b; return big; } • A local variable exists only when the enclosing function is executing.
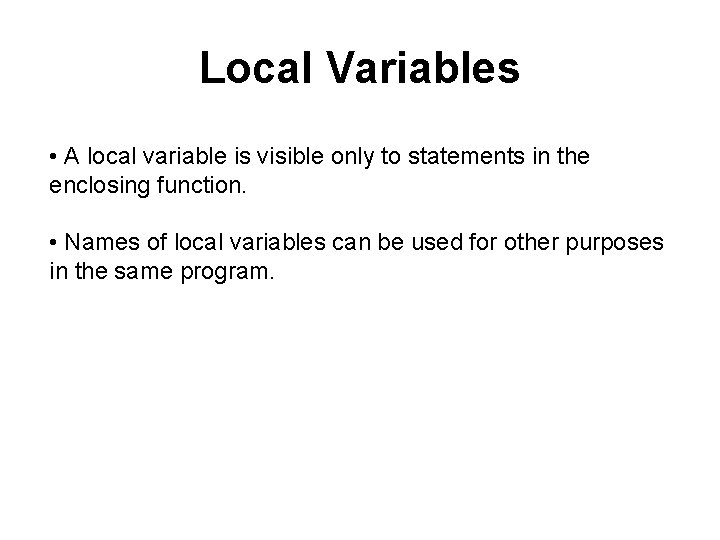
Local Variables • A local variable is visible only to statements in the enclosing function. • Names of local variables can be used for other purposes in the same program.
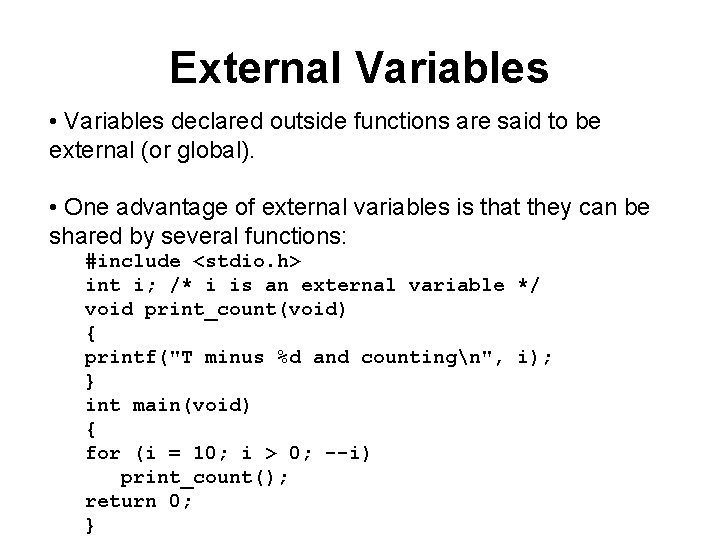
External Variables • Variables declared outside functions are said to be external (or global). • One advantage of external variables is that they can be shared by several functions: #include <stdio. h> int i; /* i is an external variable */ void print_count(void) { printf("T minus %d and countingn", i); } int main(void) { for (i = 10; i > 0; --i) print_count(); return 0; }
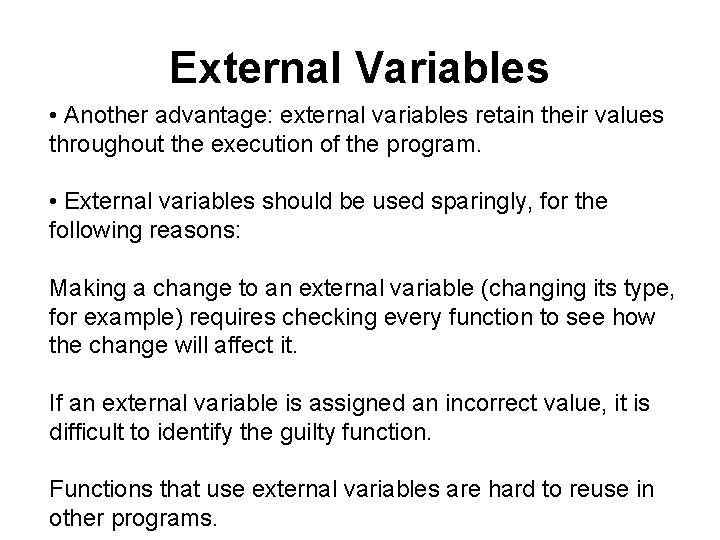
External Variables • Another advantage: external variables retain their values throughout the execution of the program. • External variables should be used sparingly, for the following reasons: Making a change to an external variable (changing its type, for example) requires checking every function to see how the change will affect it. If an external variable is assigned an incorrect value, it is difficult to identify the guilty function. Functions that use external variables are hard to reuse in other programs.
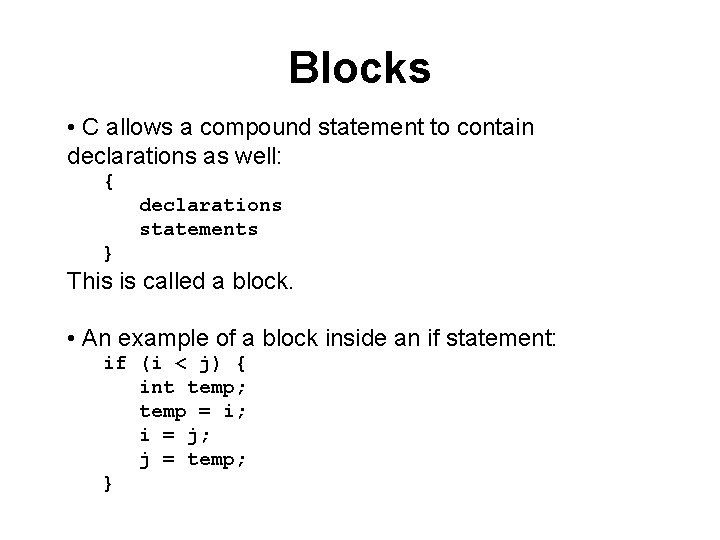
Blocks • C allows a compound statement to contain declarations as well: { declarations statements } This is called a block. • An example of a block inside an if statement: if (i < j) { int temp; temp = i; i = j; j = temp; }
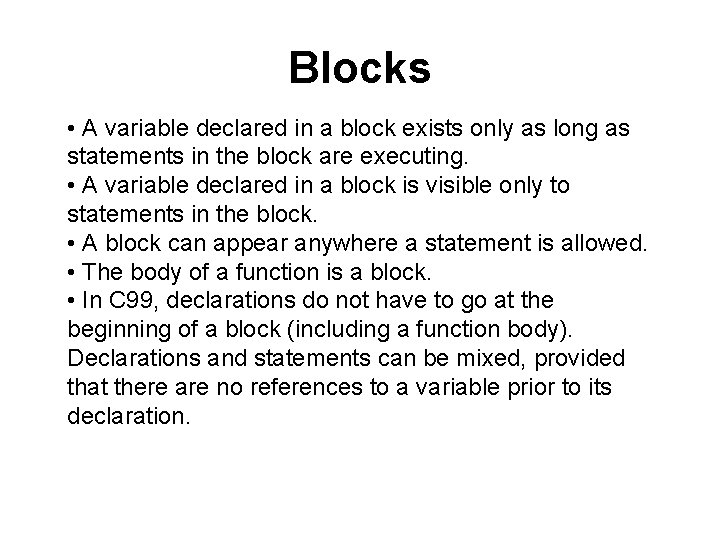
Blocks • A variable declared in a block exists only as long as statements in the block are executing. • A variable declared in a block is visible only to statements in the block. • A block can appear anywhere a statement is allowed. • The body of a function is a block. • In C 99, declarations do not have to go at the beginning of a block (including a function body). Declarations and statements can be mixed, provided that there are no references to a variable prior to its declaration.
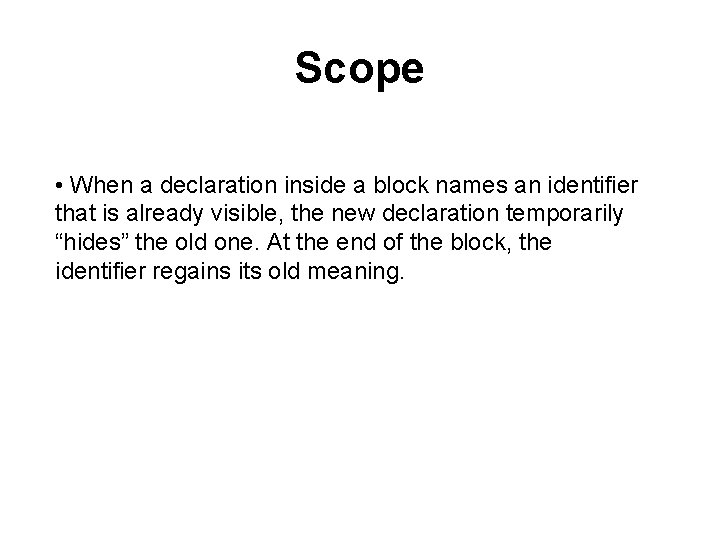
Scope • When a declaration inside a block names an identifier that is already visible, the new declaration temporarily “hides” the old one. At the end of the block, the identifier regains its old meaning.
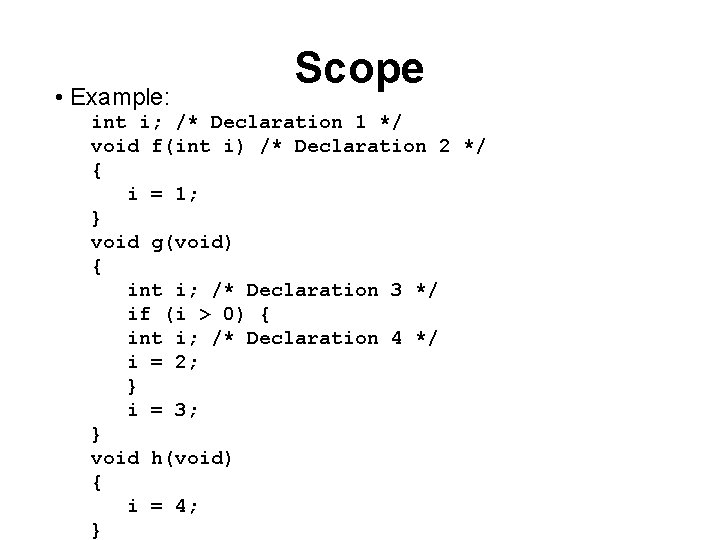
• Example: Scope int i; /* Declaration 1 */ void f(int i) /* Declaration 2 */ { i = 1; } void g(void) { int i; /* Declaration 3 */ if (i > 0) { int i; /* Declaration 4 */ i = 2; } i = 3; } void h(void) { i = 4; }
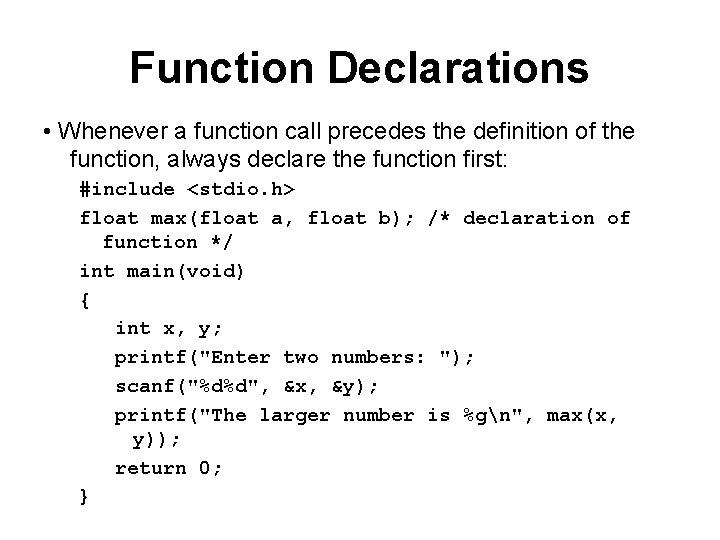
Function Declarations • Whenever a function call precedes the definition of the function, always declare the function first: #include <stdio. h> float max(float a, float b); /* declaration of function */ int main(void) { int x, y; printf("Enter two numbers: "); scanf("%d%d", &x, &y); printf("The larger number is %gn", max(x, y)); return 0; }
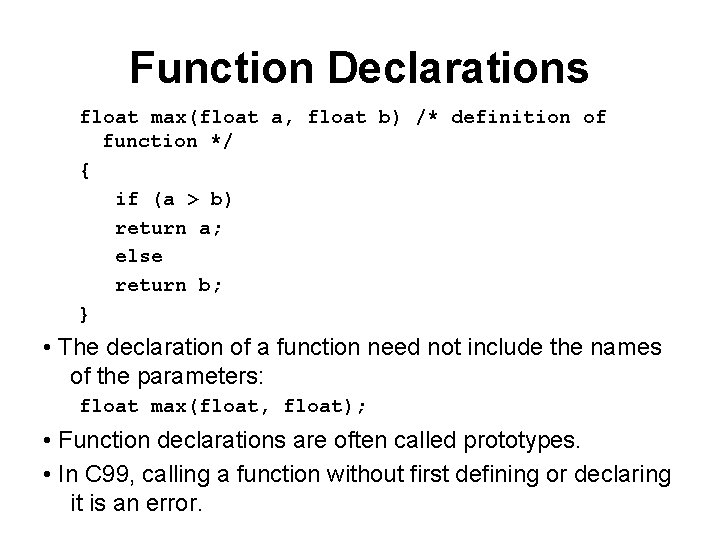
Function Declarations float max(float a, float b) /* definition of function */ { if (a > b) return a; else return b; } • The declaration of a function need not include the names of the parameters: float max(float, float); • Function declarations are often called prototypes. • In C 99, calling a function without first defining or declaring it is an error.
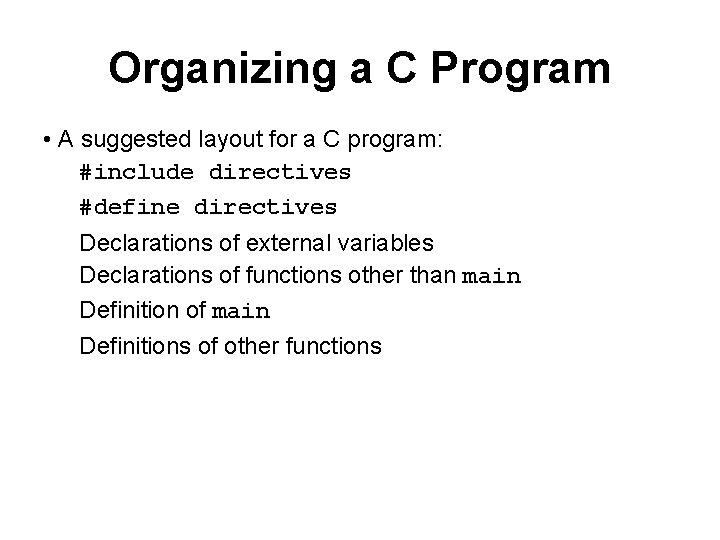
Organizing a C Program • A suggested layout for a C program: #include directives #define directives Declarations of external variables Declarations of functions other than main Definition of main Definitions of other functions
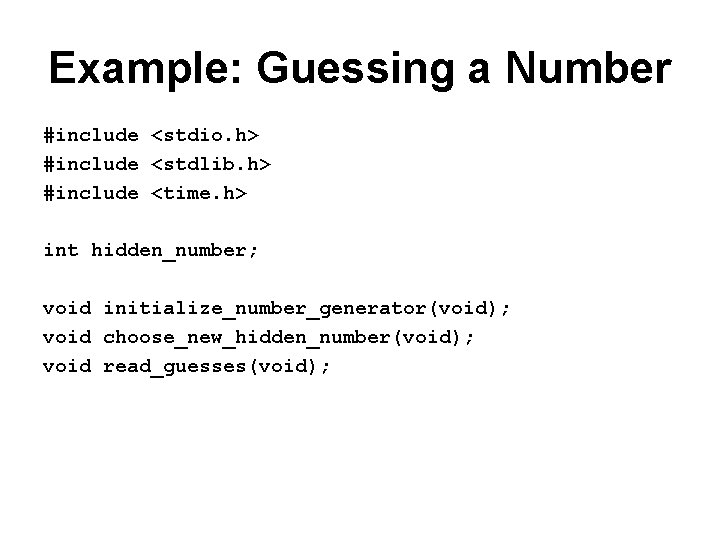
Example: Guessing a Number #include <stdio. h> #include <stdlib. h> #include <time. h> int hidden_number; void initialize_number_generator(void); void choose_new_hidden_number(void); void read_guesses(void);
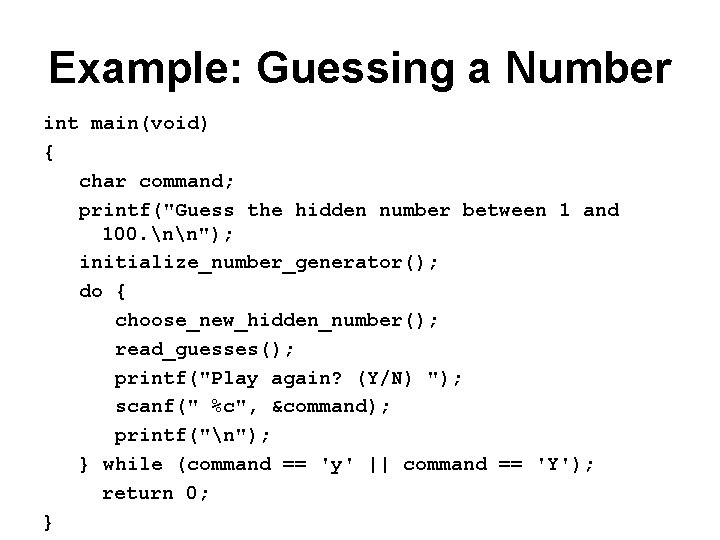
Example: Guessing a Number int main(void) { char command; printf("Guess the hidden number between 1 and 100. nn"); initialize_number_generator(); do { choose_new_hidden_number(); read_guesses(); printf("Play again? (Y/N) "); scanf(" %c", &command); printf("n"); } while (command == 'y' || command == 'Y'); return 0; }
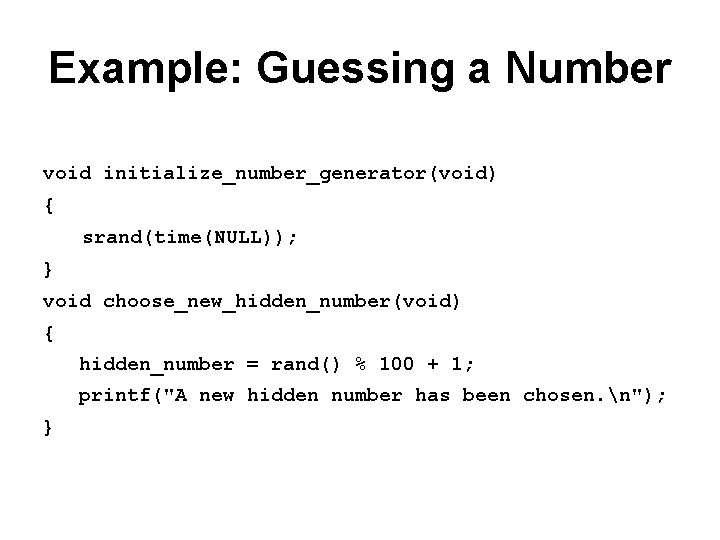
Example: Guessing a Number void initialize_number_generator(void) { srand(time(NULL)); } void choose_new_hidden_number(void) { hidden_number = rand() % 100 + 1; printf("A new hidden number has been chosen. n"); }
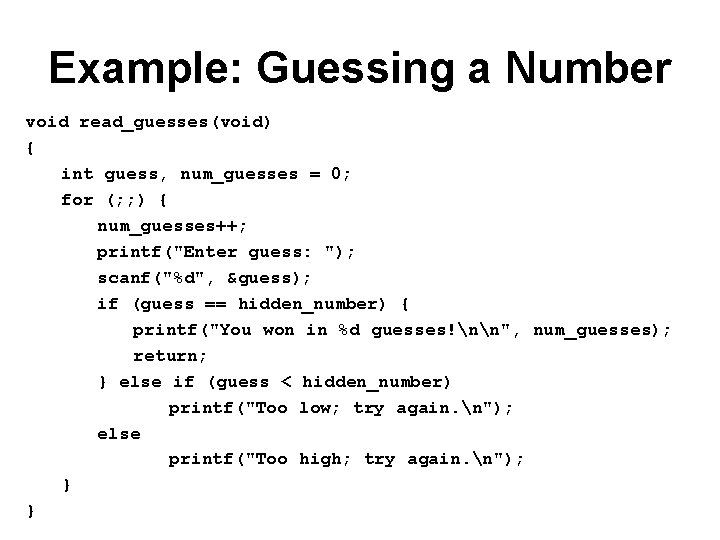
Example: Guessing a Number void read_guesses(void) { int guess, num_guesses = 0; for (; ; ) { num_guesses++; printf("Enter guess: "); scanf("%d", &guess); if (guess == hidden_number) { printf("You won in %d guesses!nn", num_guesses); return; } else if (guess < hidden_number) printf("Too low; try again. n"); else printf("Too high; try again. n"); } }
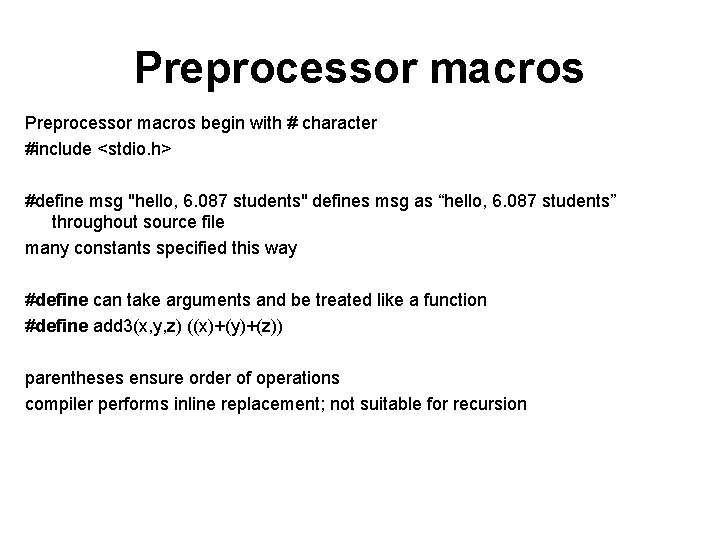
Preprocessor macros begin with # character #include <stdio. h> #define msg "hello, 6. 087 students" defines msg as “hello, 6. 087 students” throughout source file many constants specified this way #define can take arguments and be treated like a function #define add 3(x, y, z) ((x)+(y)+(z)) parentheses ensure order of operations compiler performs inline replacement; not suitable for recursion
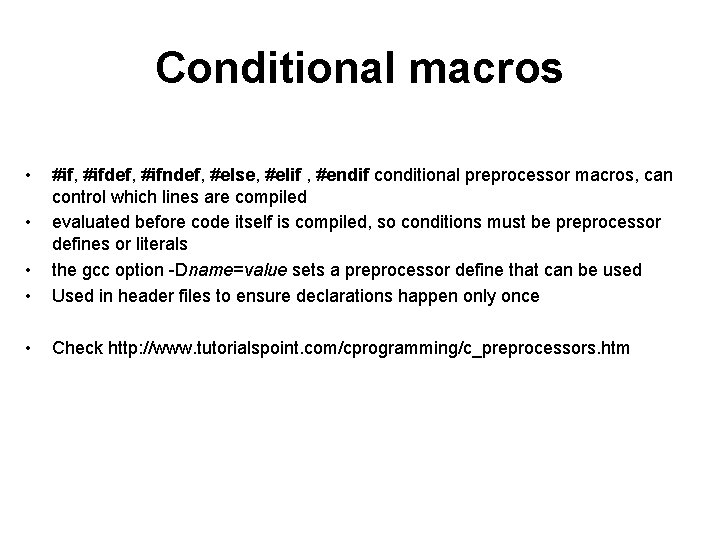
Conditional macros • • • #if, #ifdef, #ifndef, #else, #elif , #endif conditional preprocessor macros, can control which lines are compiled evaluated before code itself is compiled, so conditions must be preprocessor defines or literals the gcc option -Dname=value sets a preprocessor define that can be used Used in header files to ensure declarations happen only once • Check http: //www. tutorialspoint. com/cprogramming/c_preprocessors. htm •
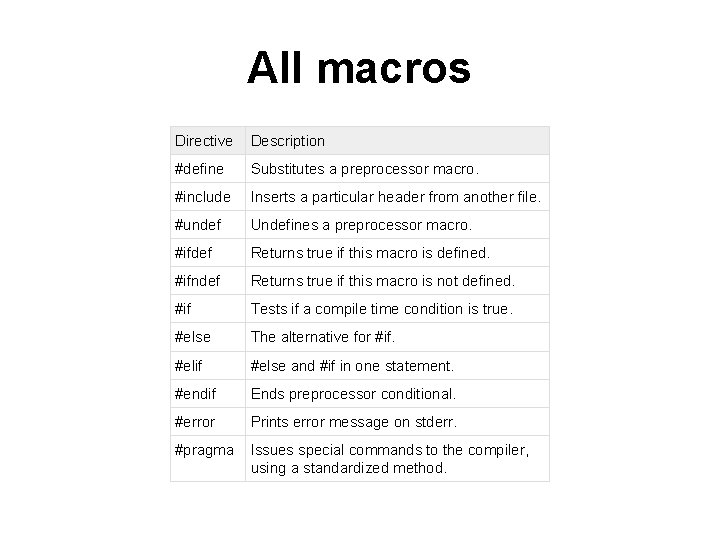
All macros Directive Description #define Substitutes a preprocessor macro. #include Inserts a particular header from another file. #undef Undefines a preprocessor macro. #ifdef Returns true if this macro is defined. #ifndef Returns true if this macro is not defined. #if Tests if a compile time condition is true. #else The alternative for #if. #elif #else and #if in one statement. #endif Ends preprocessor conditional. #error Prints error message on stderr. #pragma Issues special commands to the compiler, using a standardized method.
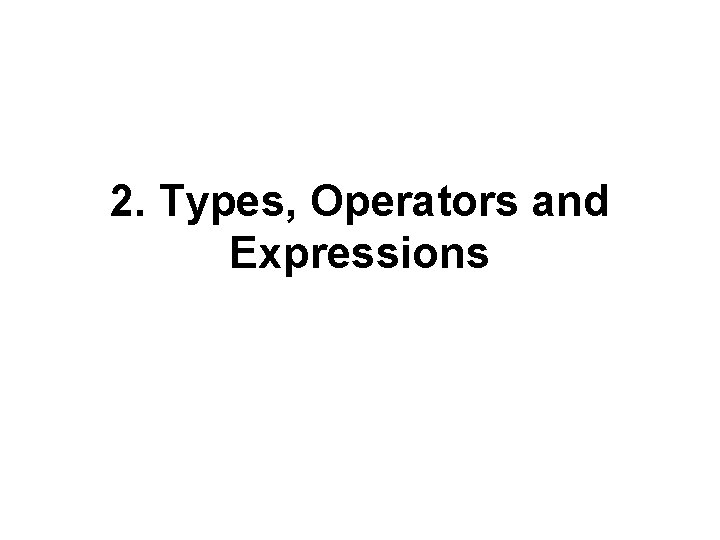
2. Types, Operators and Expressions
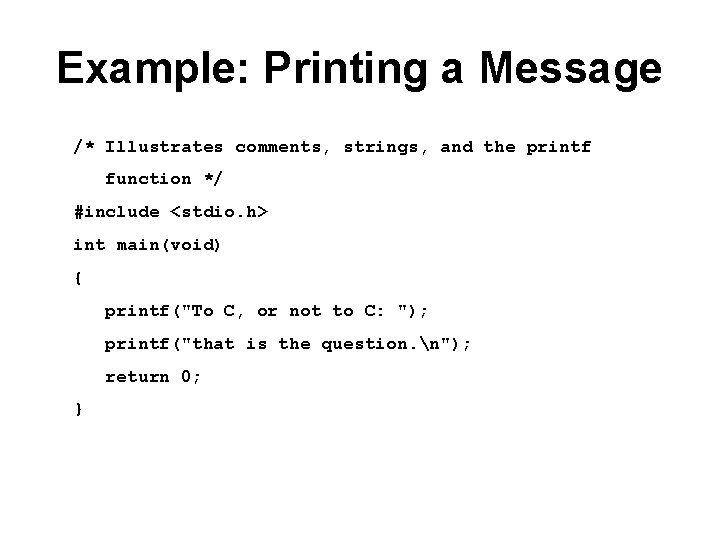
Example: Printing a Message /* Illustrates comments, strings, and the printf function */ #include <stdio. h> int main(void) { printf("To C, or not to C: "); printf("that is the question. n"); return 0; }
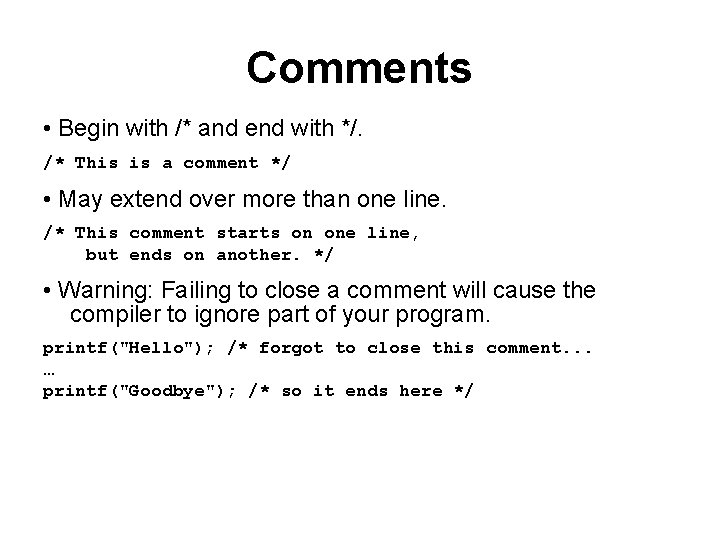
Comments • Begin with /* and end with */. /* This is a comment */ • May extend over more than one line. /* This comment starts on one line, but ends on another. */ • Warning: Failing to close a comment will cause the compiler to ignore part of your program. printf("Hello"); /* forgot to close this comment. . . … printf("Goodbye"); /* so it ends here */
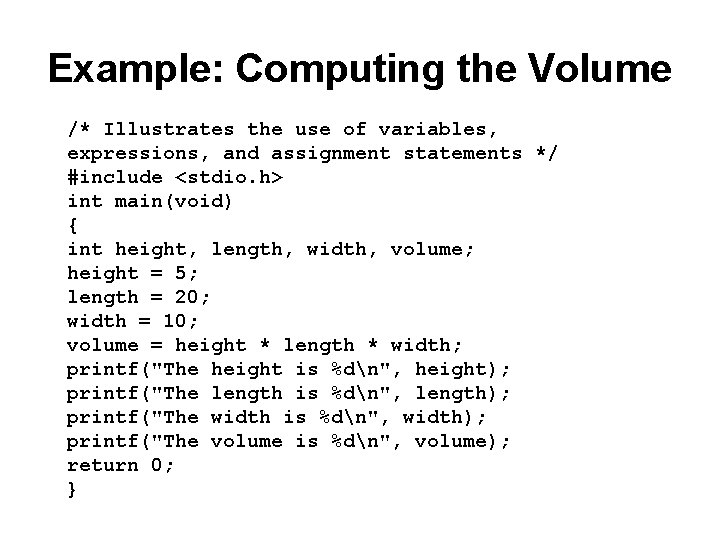
Example: Computing the Volume /* Illustrates the use of variables, expressions, and assignment statements */ #include <stdio. h> int main(void) { int height, length, width, volume; height = 5; length = 20; width = 10; volume = height * length * width; printf("The height is %dn", height); printf("The length is %dn", length); printf("The width is %dn", width); printf("The volume is %dn", volume); return 0; }
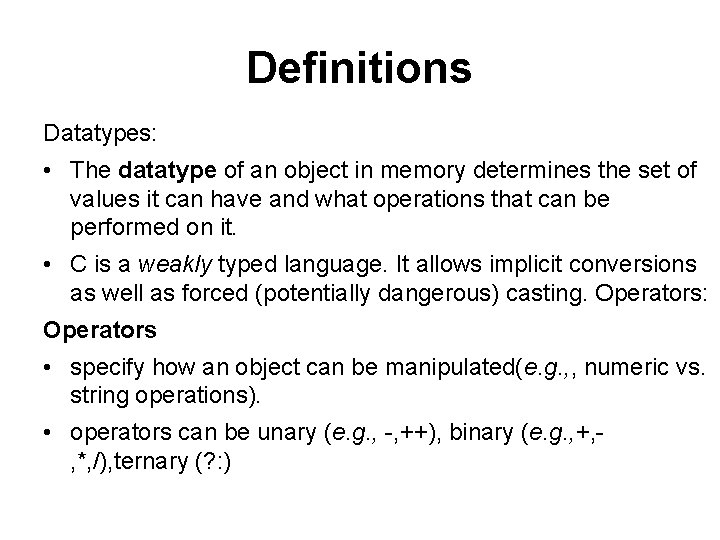
Definitions Datatypes: • The datatype of an object in memory determines the set of values it can have and what operations that can be performed on it. • C is a weakly typed language. It allows implicit conversions as well as forced (potentially dangerous) casting. Operators: Operators • specify how an object can be manipulated(e. g. , , numeric vs. string operations). • operators can be unary (e. g. , -, ++), binary (e. g. , +, , *, /), ternary (? : )
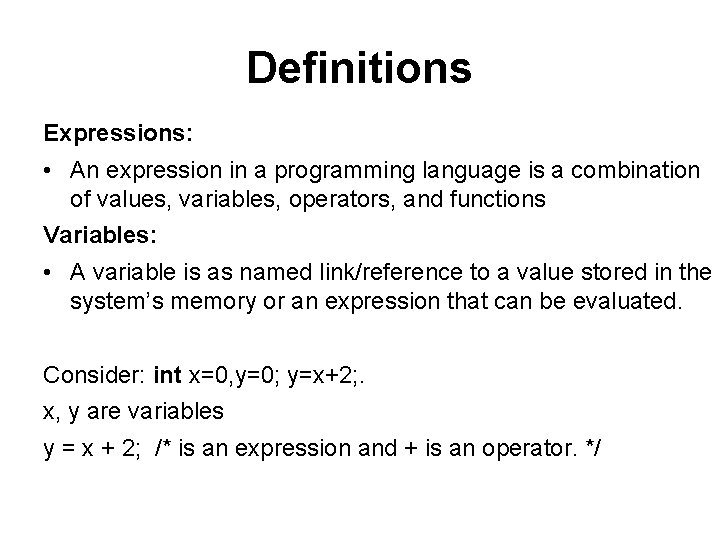
Definitions Expressions: • An expression in a programming language is a combination of values, variables, operators, and functions Variables: • A variable is as named link/reference to a value stored in the system’s memory or an expression that can be evaluated. Consider: int x=0, y=0; y=x+2; . x, y are variables y = x + 2; /* is an expression and + is an operator. */
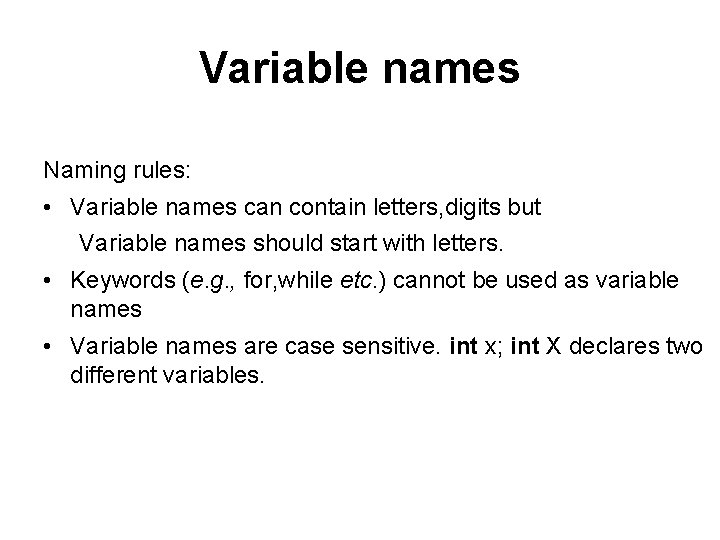
Variable names Naming rules: • Variable names can contain letters, digits but Variable names should start with letters. • Keywords (e. g. , for, while etc. ) cannot be used as variable names • Variable names are case sensitive. int x; int X declares two different variables.
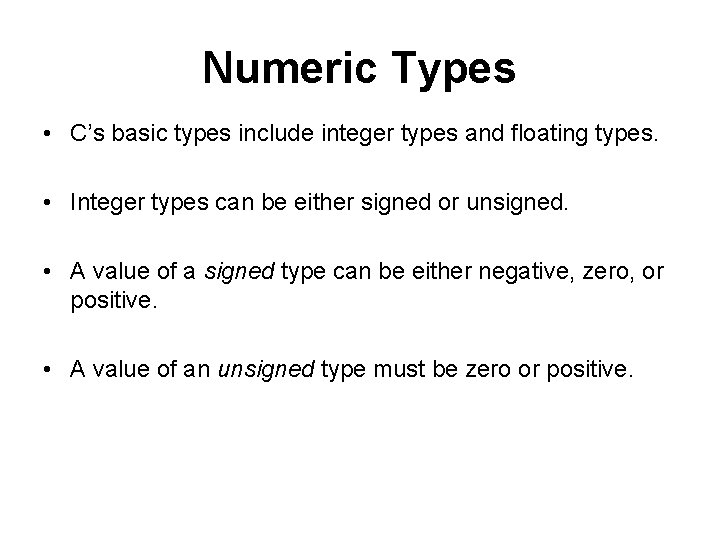
Numeric Types • C’s basic types include integer types and floating types. • Integer types can be either signed or unsigned. • A value of a signed type can be either negative, zero, or positive. • A value of an unsigned type must be zero or positive.
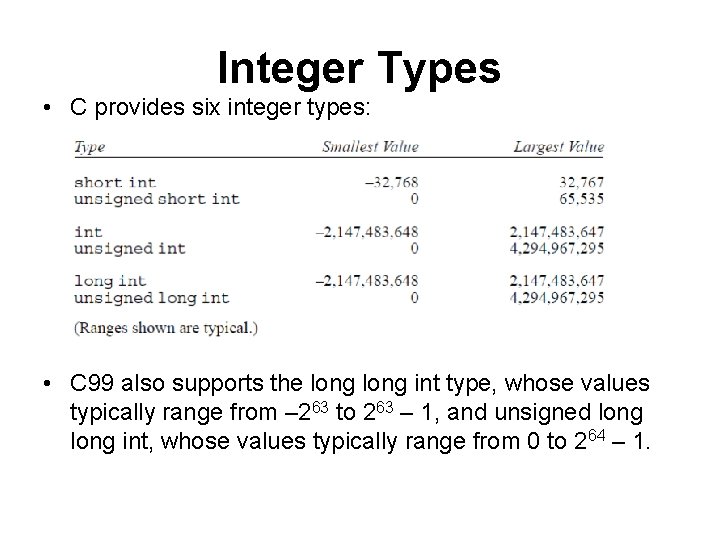
Integer Types • C provides six integer types: • C 99 also supports the long int type, whose values typically range from – 263 to 263 – 1, and unsigned long int, whose values typically range from 0 to 264 – 1.
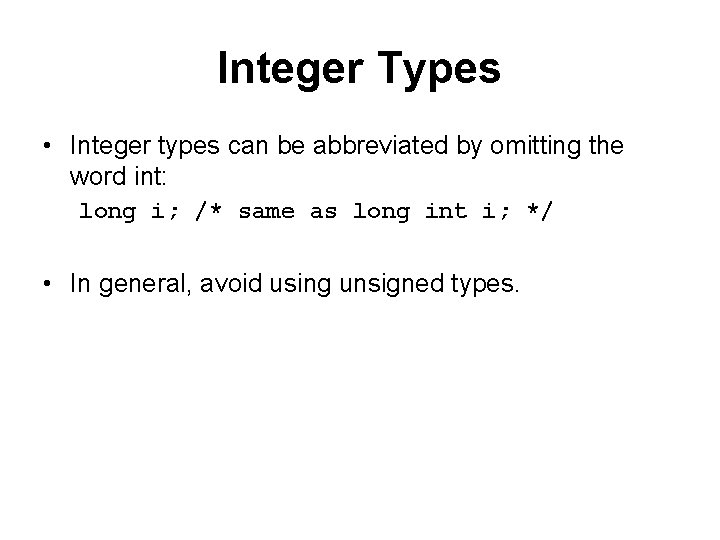
Integer Types • Integer types can be abbreviated by omitting the word int: long i; /* same as long int i; */ • In general, avoid using unsigned types.
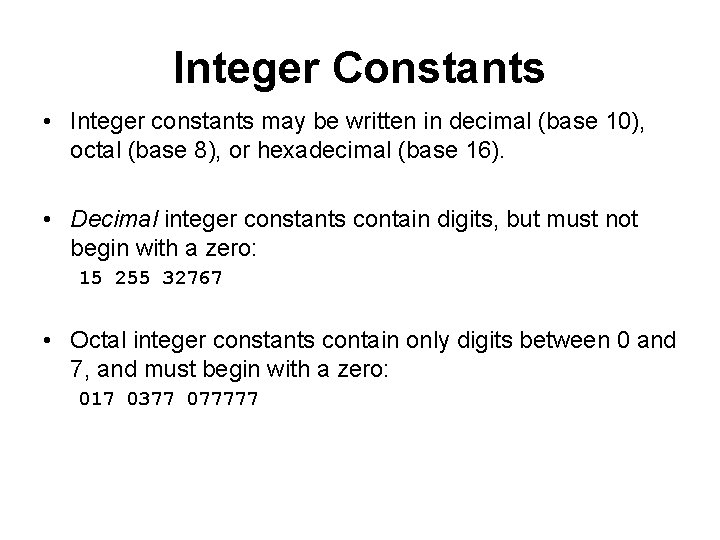
Integer Constants • Integer constants may be written in decimal (base 10), octal (base 8), or hexadecimal (base 16). • Decimal integer constants contain digits, but must not begin with a zero: 15 255 32767 • Octal integer constants contain only digits between 0 and 7, and must begin with a zero: 017 0377 077777
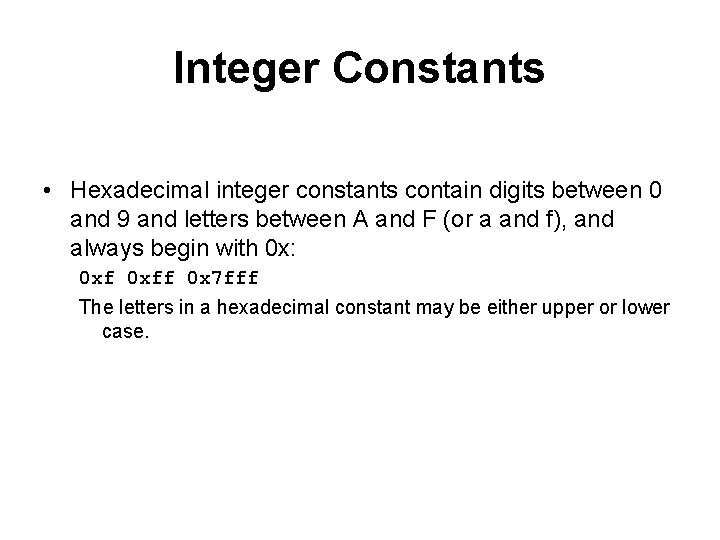
Integer Constants • Hexadecimal integer constants contain digits between 0 and 9 and letters between A and F (or a and f), and always begin with 0 x: 0 xff 0 x 7 fff The letters in a hexadecimal constant may be either upper or lower case.
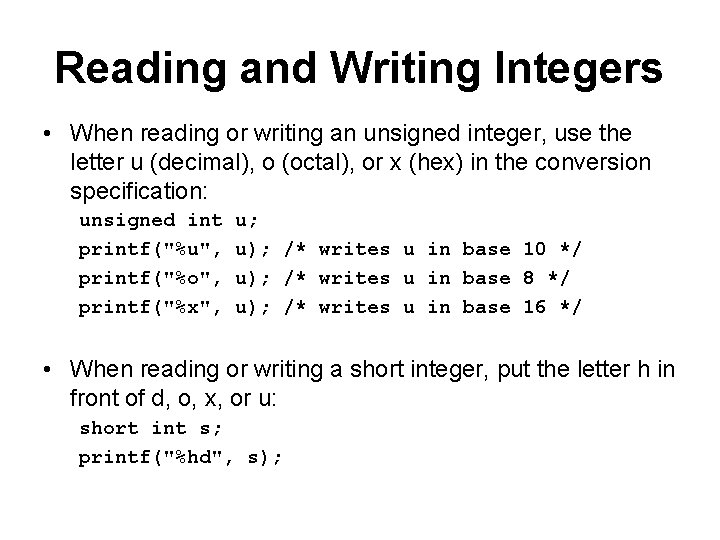
Reading and Writing Integers • When reading or writing an unsigned integer, use the letter u (decimal), o (octal), or x (hex) in the conversion specification: unsigned int printf("%u", printf("%o", printf("%x", u; u); /* writes u in base 10 */ u); /* writes u in base 8 */ u); /* writes u in base 16 */ • When reading or writing a short integer, put the letter h in front of d, o, x, or u: short int s; printf("%hd", s);
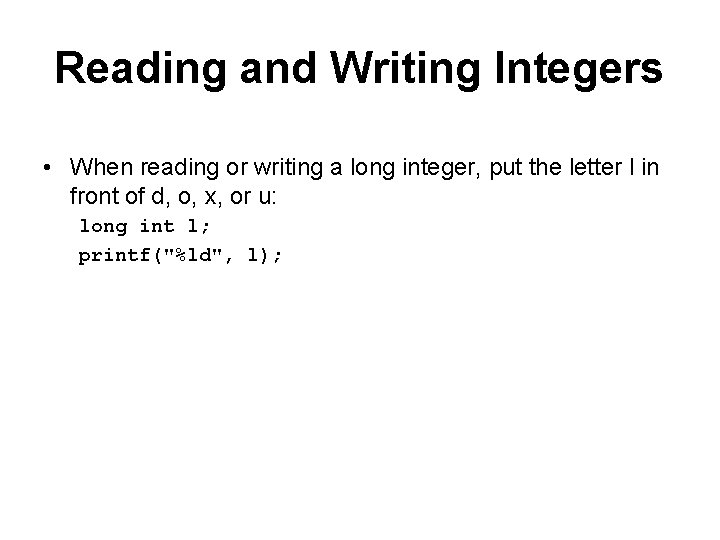
Reading and Writing Integers • When reading or writing a long integer, put the letter l in front of d, o, x, or u: long int l; printf("%ld", l);
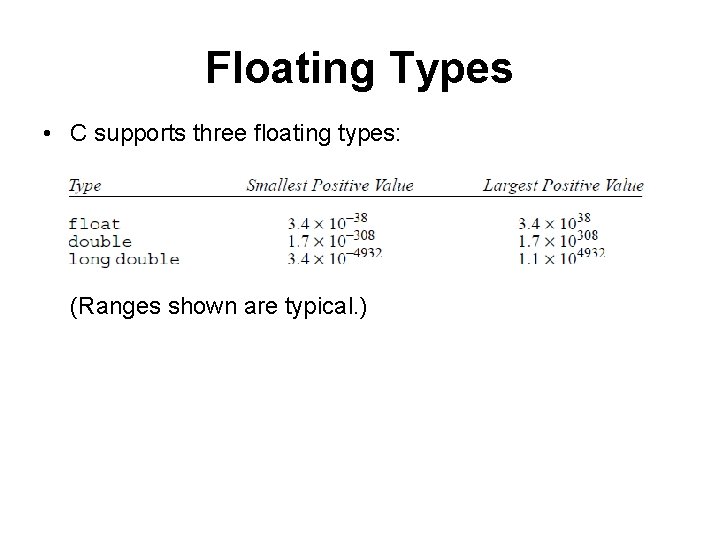
Floating Types • C supports three floating types: (Ranges shown are typical. )
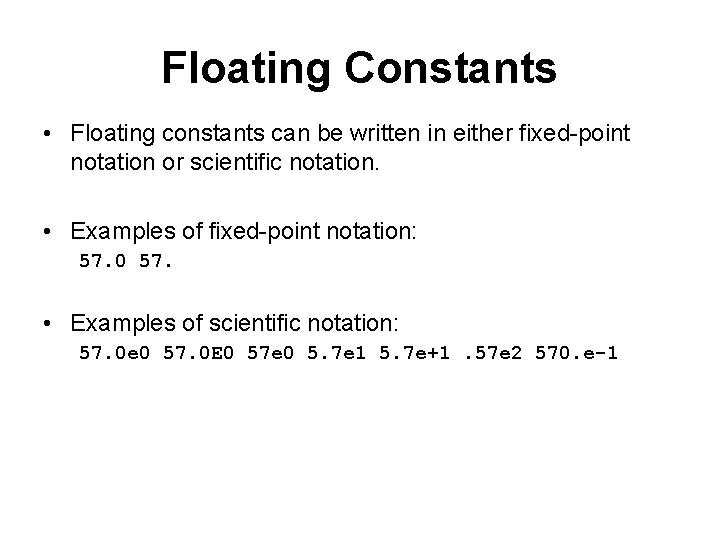
Floating Constants • Floating constants can be written in either fixed-point notation or scientific notation. • Examples of fixed-point notation: 57. 0 57. • Examples of scientific notation: 57. 0 e 0 57. 0 E 0 57 e 0 5. 7 e 1 5. 7 e+1. 57 e 2 570. e-1
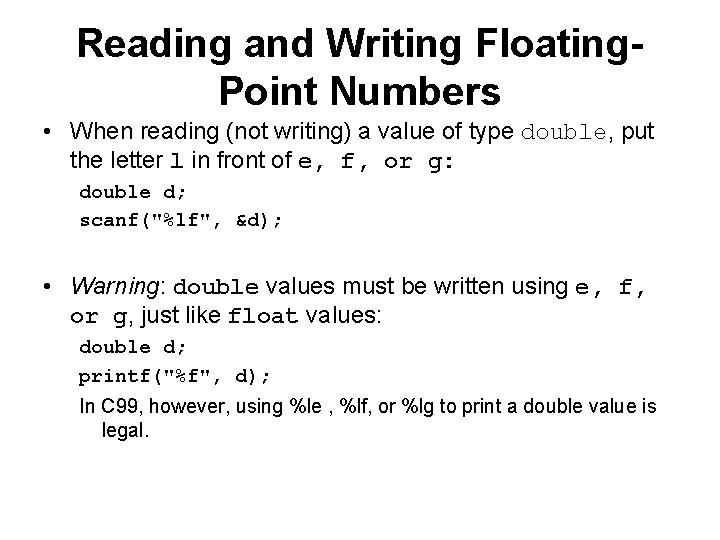
Reading and Writing Floating. Point Numbers • When reading (not writing) a value of type double, put the letter l in front of e, f, or g: double d; scanf("%lf", &d); • Warning: double values must be written using e, f, or g, just like float values: double d; printf("%f", d); In C 99, however, using %le , %lf, or %lg to print a double value is legal.
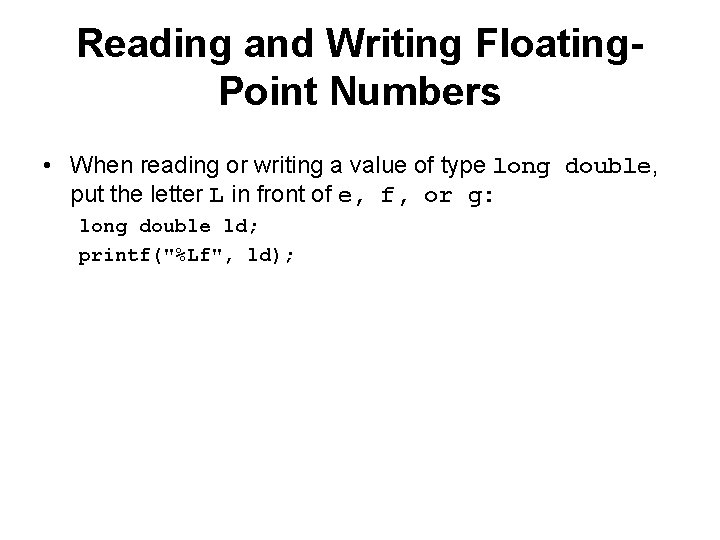
Reading and Writing Floating. Point Numbers • When reading or writing a value of type long double, put the letter L in front of e, f, or g: long double ld; printf("%Lf", ld);
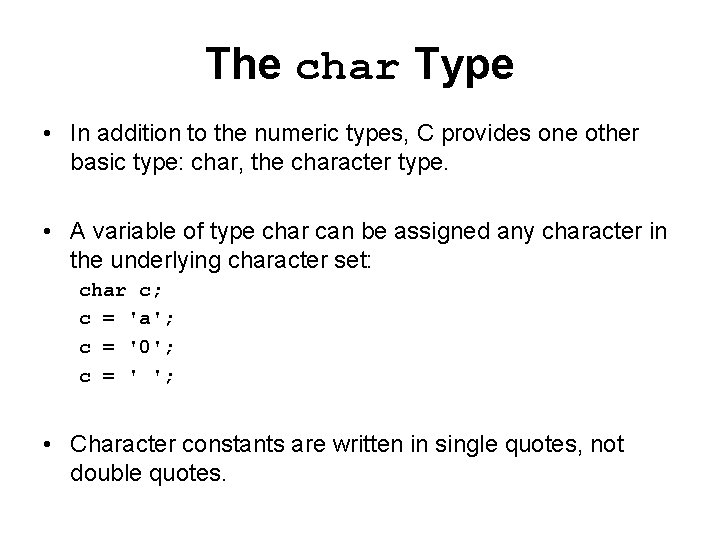
The char Type • In addition to the numeric types, C provides one other basic type: char, the character type. • A variable of type char can be assigned any character in the underlying character set: char c; c = 'a'; c = '0'; c = ' '; • Character constants are written in single quotes, not double quotes.
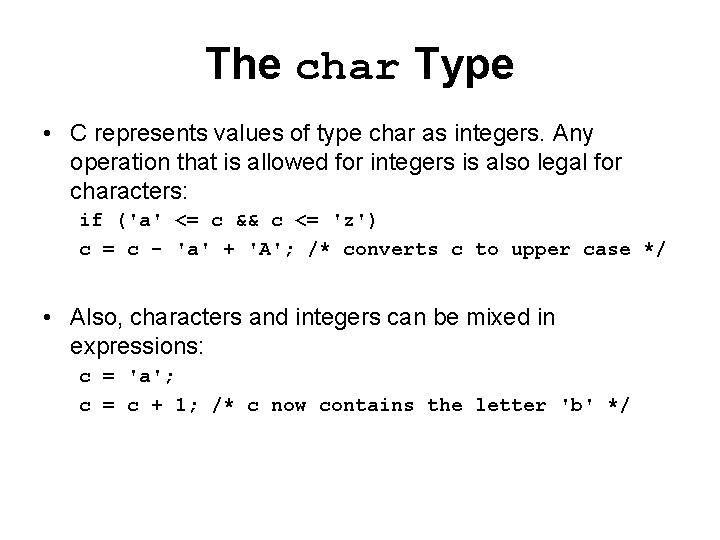
The char Type • C represents values of type char as integers. Any operation that is allowed for integers is also legal for characters: if ('a' <= c && c <= 'z') c = c - 'a' + 'A'; /* converts c to upper case */ • Also, characters and integers can be mixed in expressions: c = 'a'; c = c + 1; /* c now contains the letter 'b' */
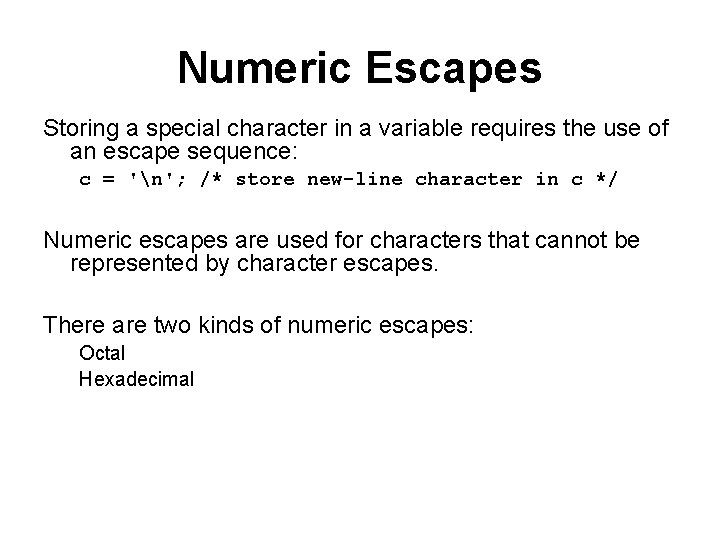
Numeric Escapes Storing a special character in a variable requires the use of an escape sequence: c = 'n'; /* store new-line character in c */ Numeric escapes are used for characters that cannot be represented by character escapes. There are two kinds of numeric escapes: Octal Hexadecimal
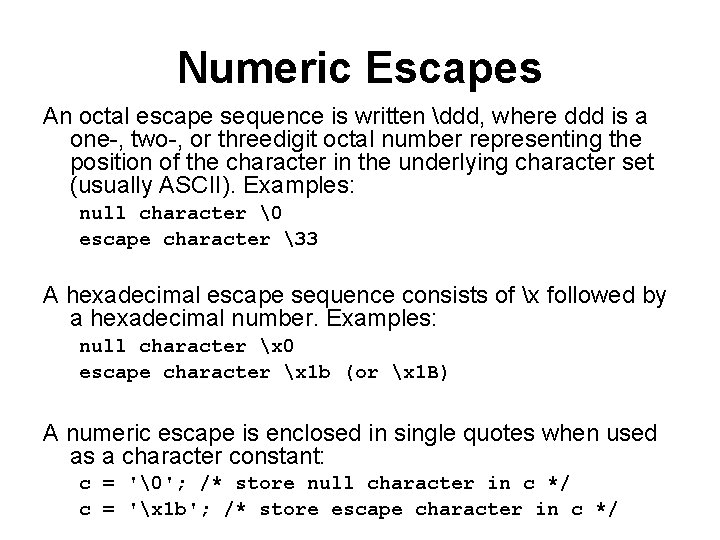
Numeric Escapes An octal escape sequence is written ddd, where ddd is a one-, two-, or threedigit octal number representing the position of the character in the underlying character set (usually ASCII). Examples: null character escape character 33 A hexadecimal escape sequence consists of x followed by a hexadecimal number. Examples: null character x 0 escape character x 1 b (or x 1 B) A numeric escape is enclosed in single quotes when used as a character constant: c = '