Data structures D 1 Data structures The features
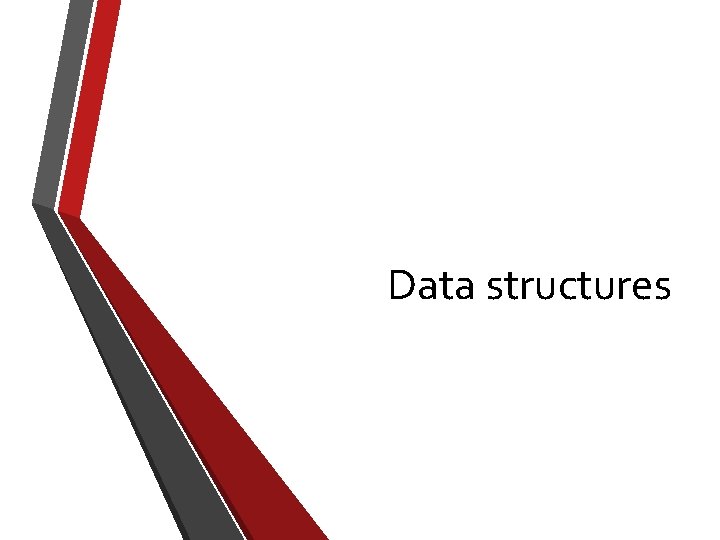
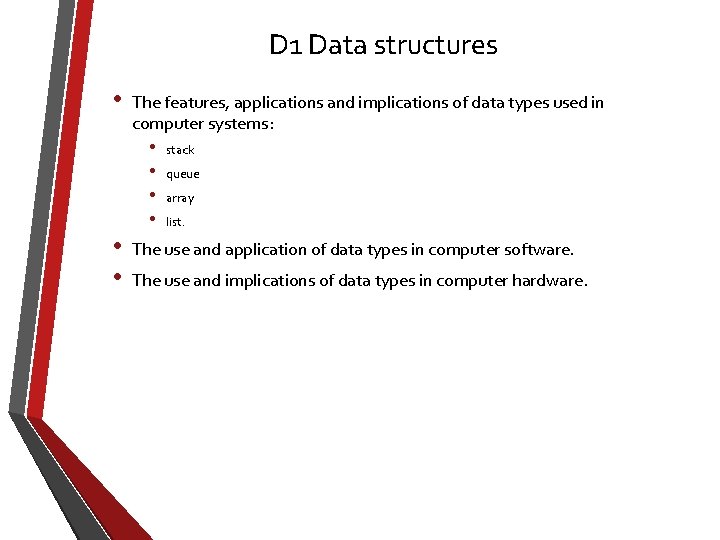
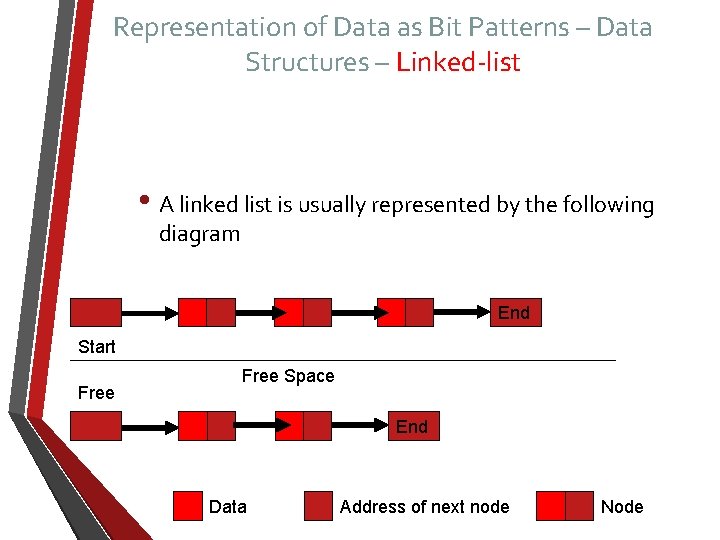
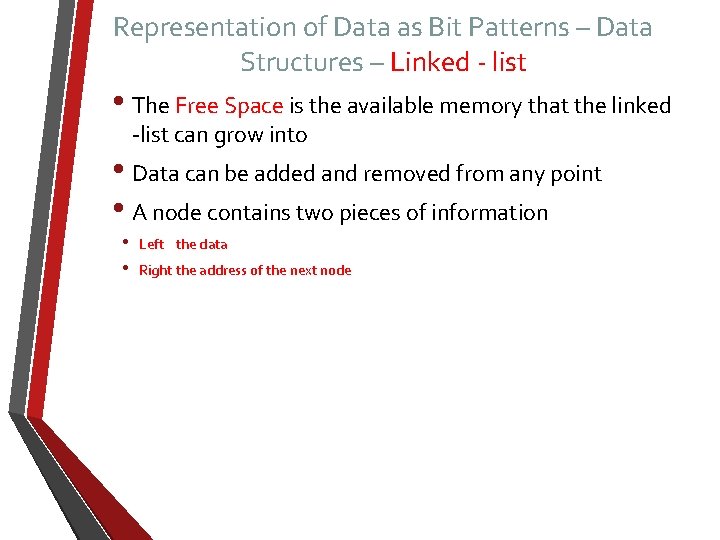
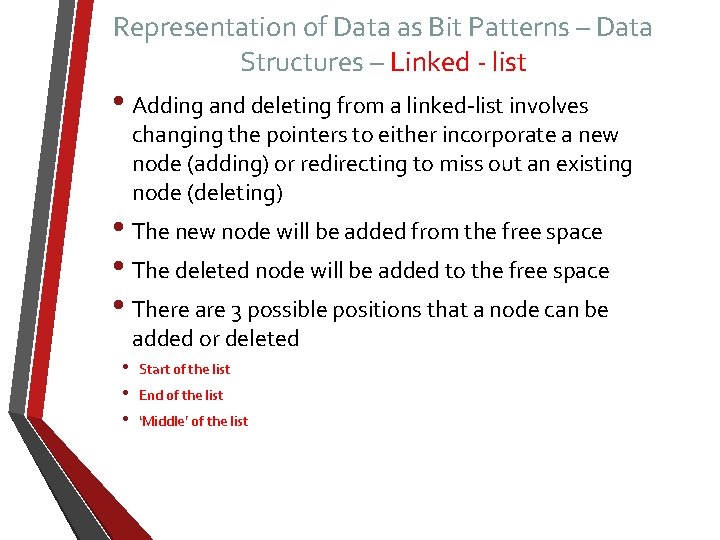
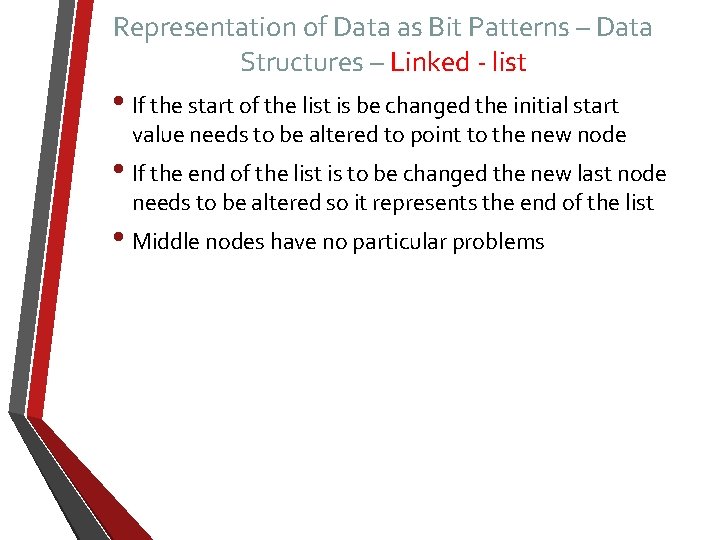
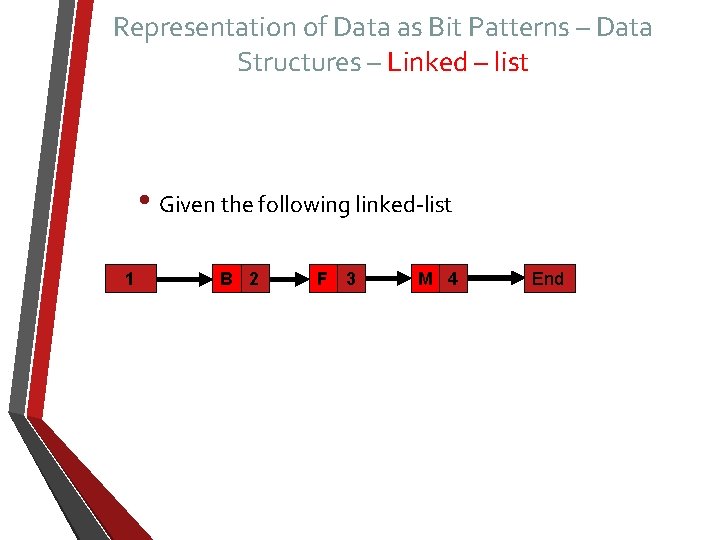
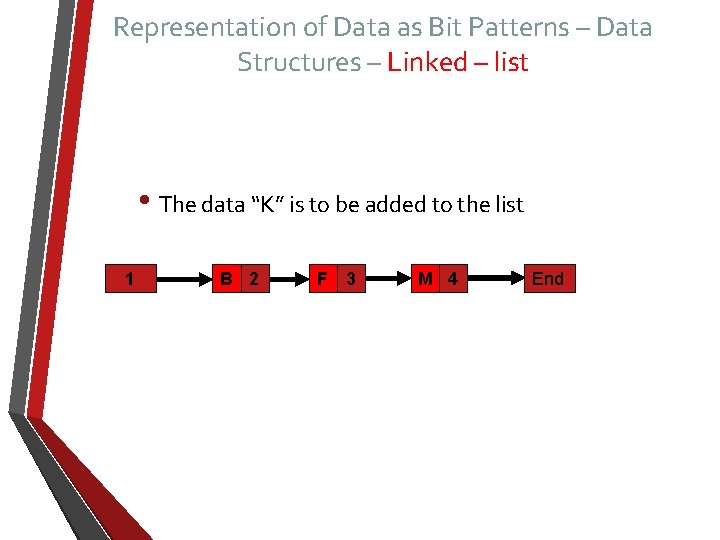
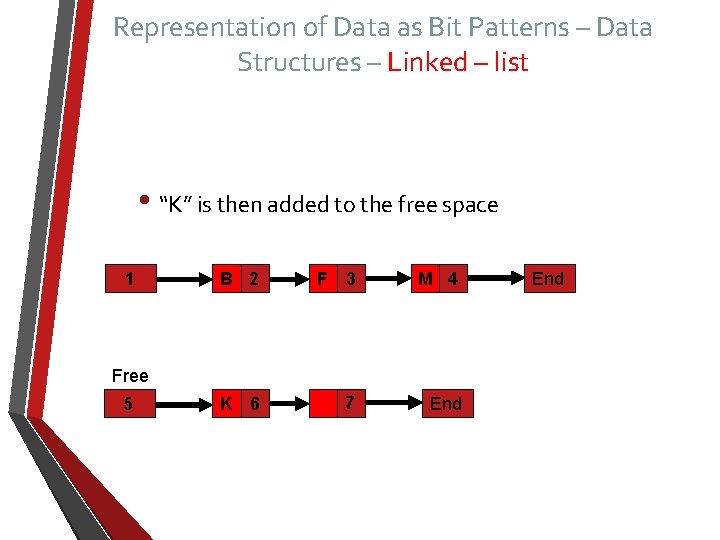
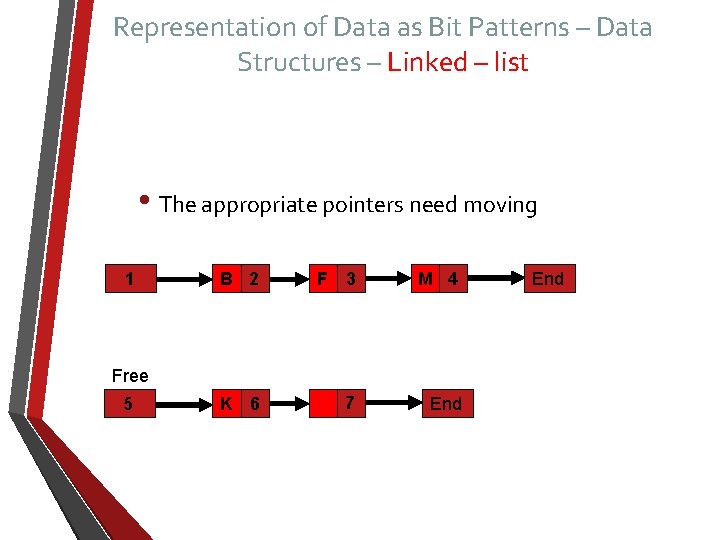
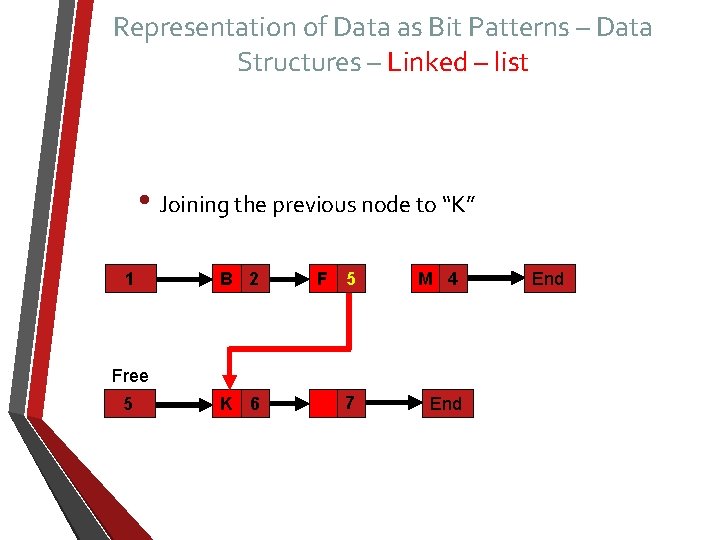
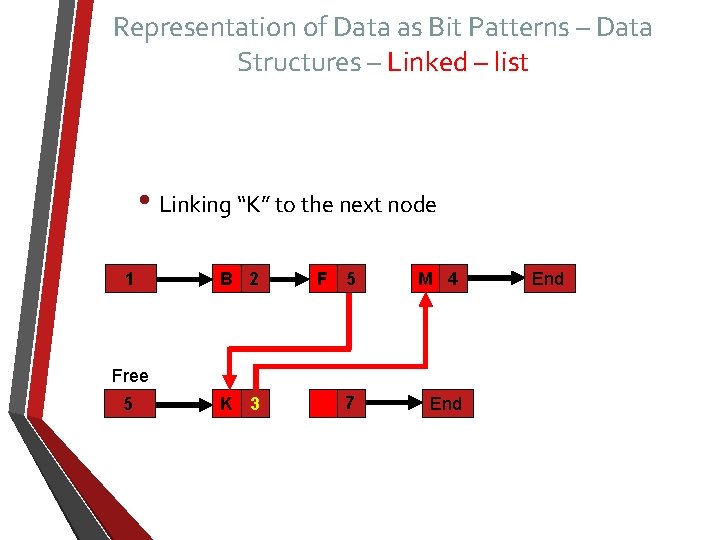
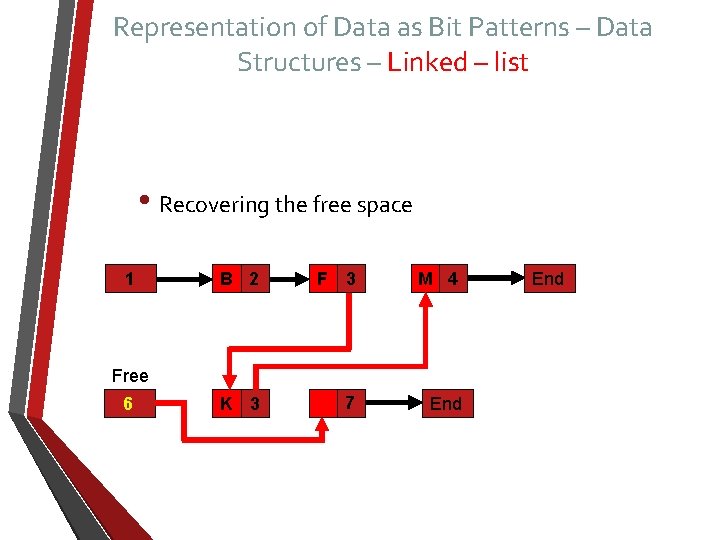
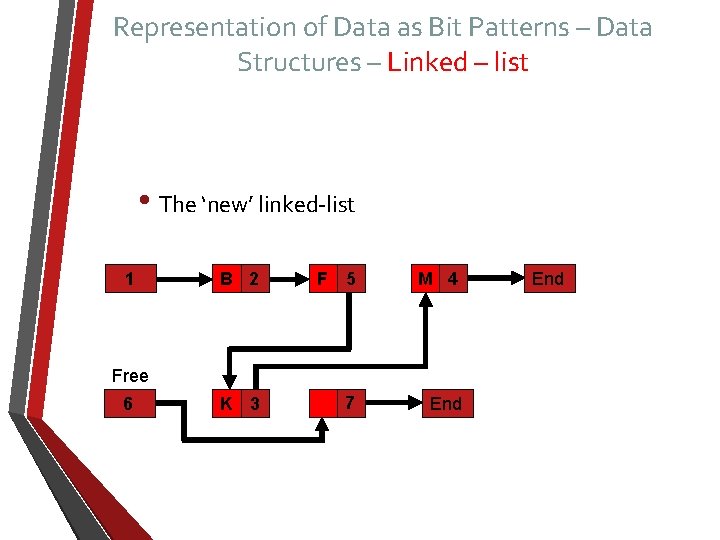
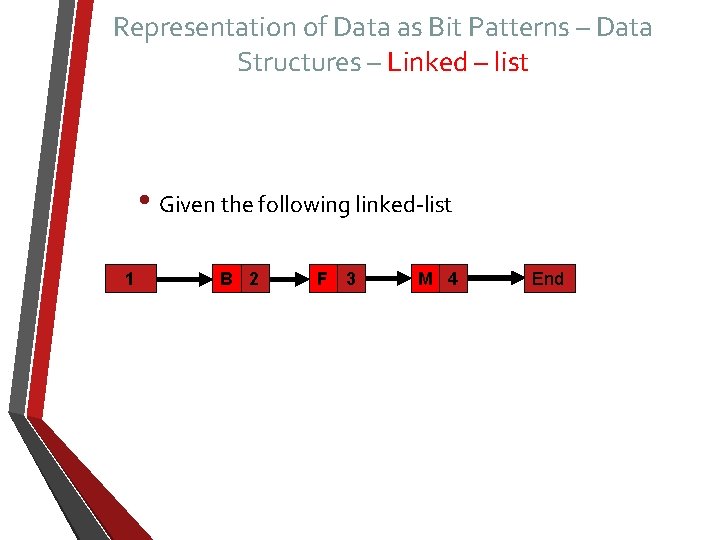
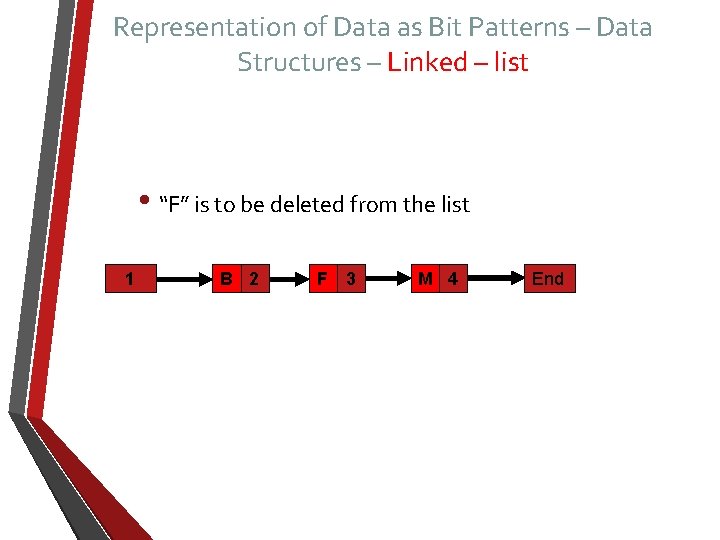
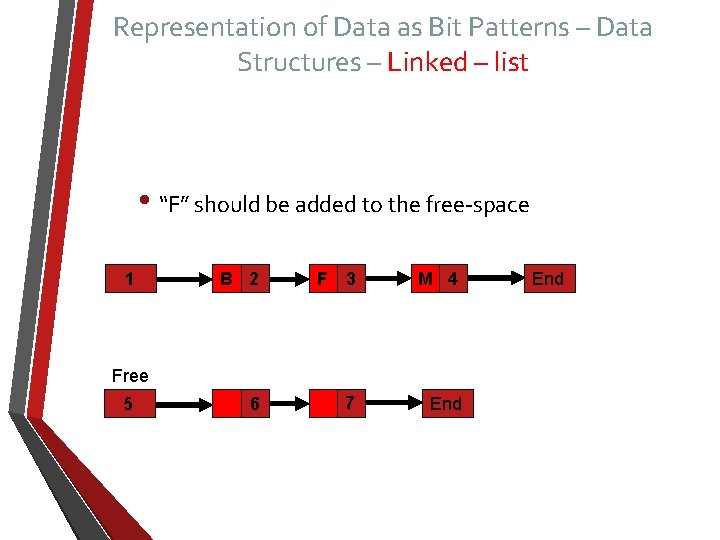
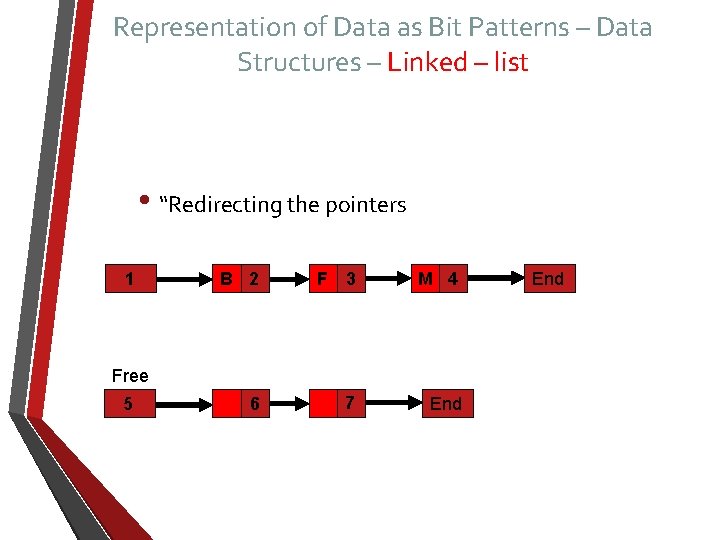
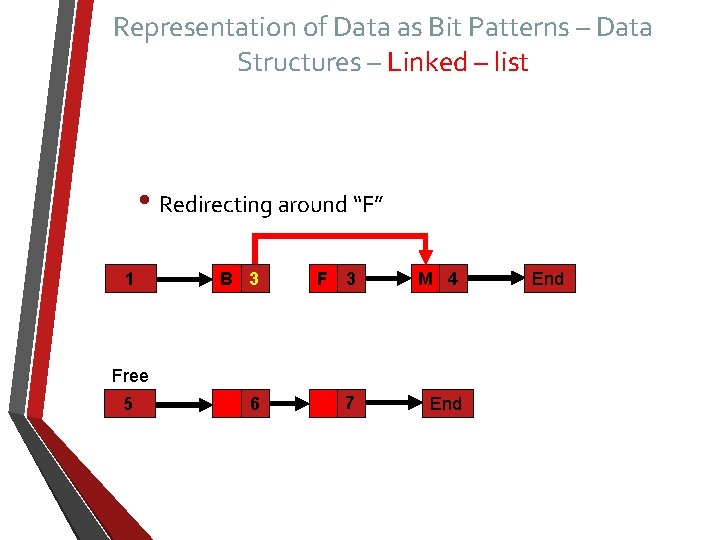
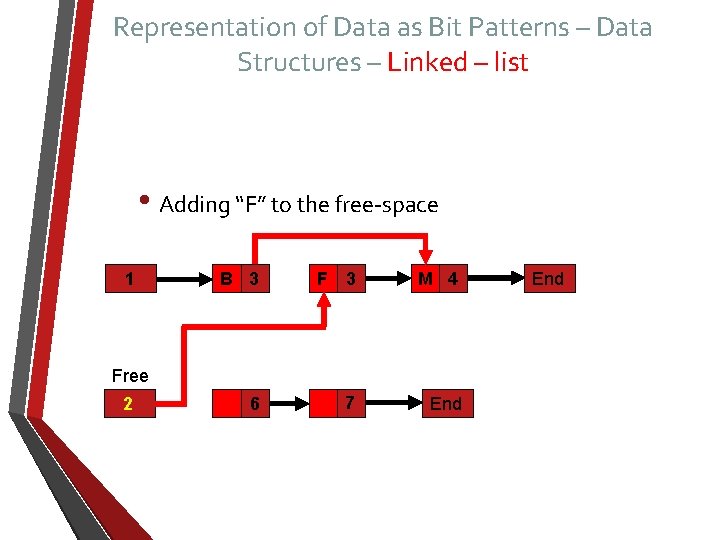
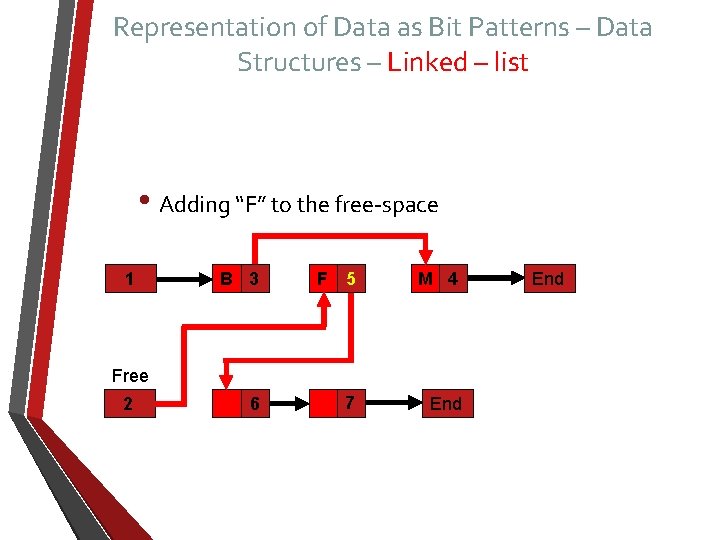
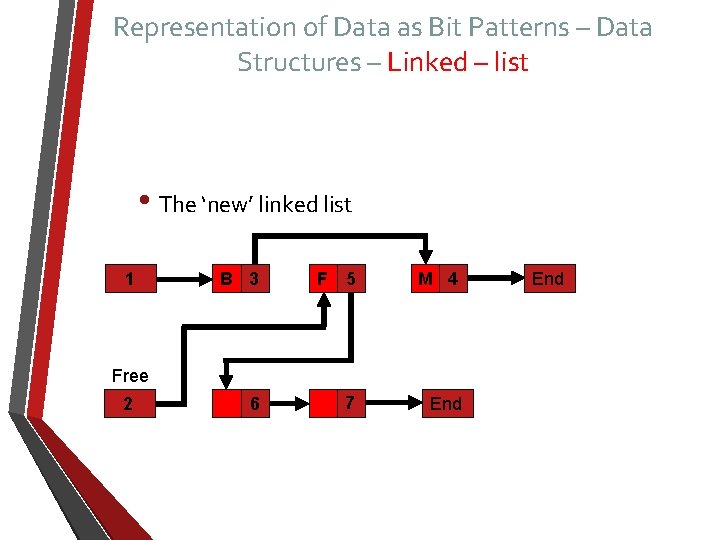
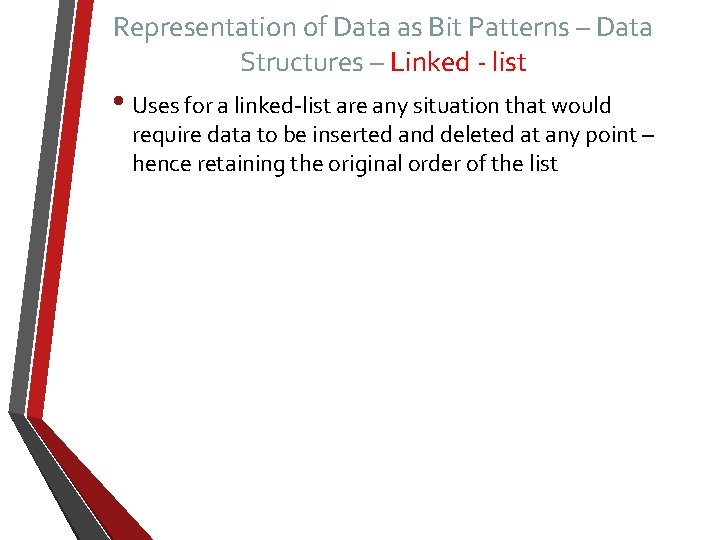
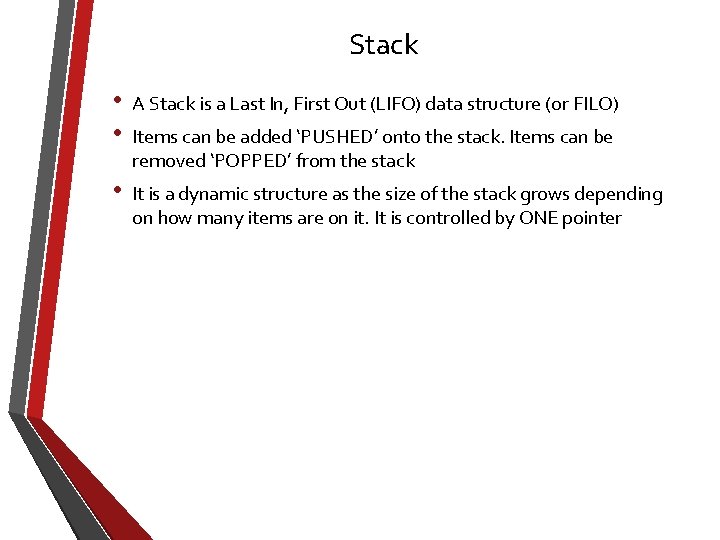
![Stacks [6] [5] [4] [3] [2] [1] • Items can be pushed (add), • Stacks [6] [5] [4] [3] [2] [1] • Items can be pushed (add), •](https://slidetodoc.com/presentation_image_h/1f219c66a8e1a7e9201f3c19e3c2e4d4/image-25.jpg)
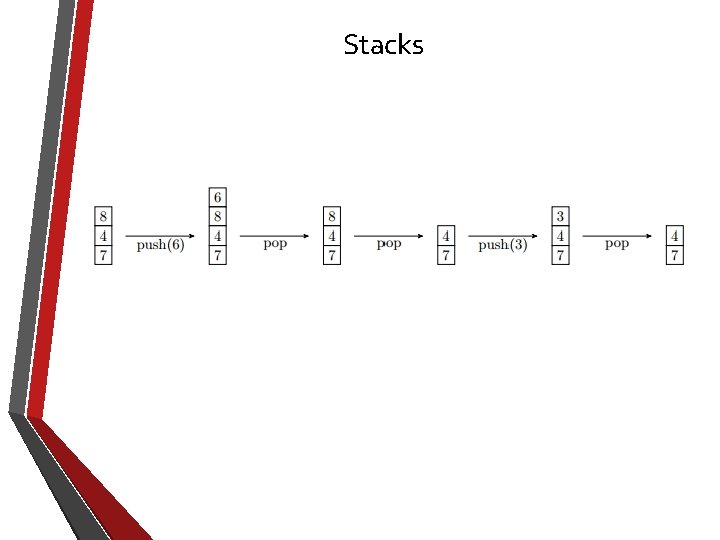
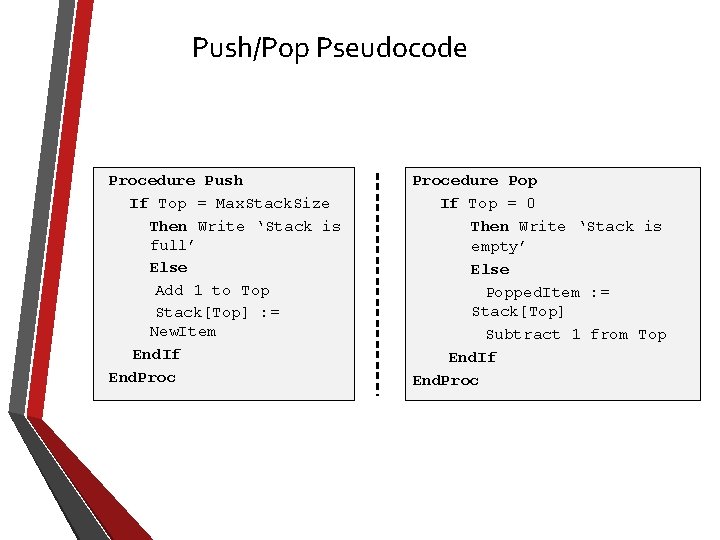
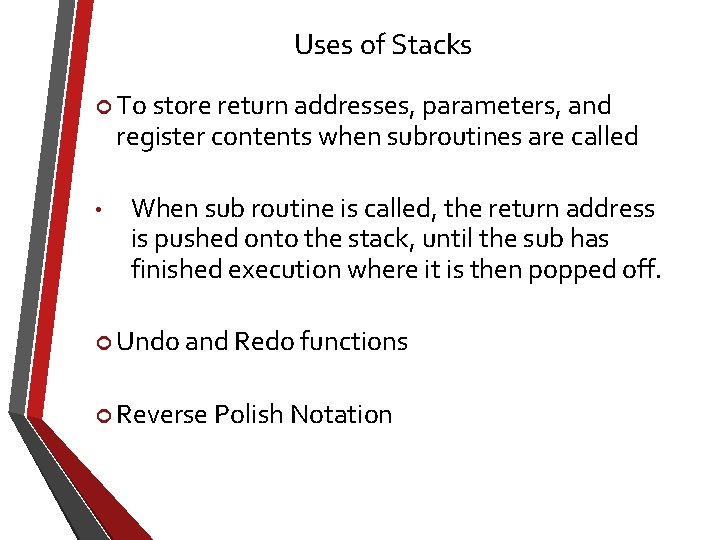
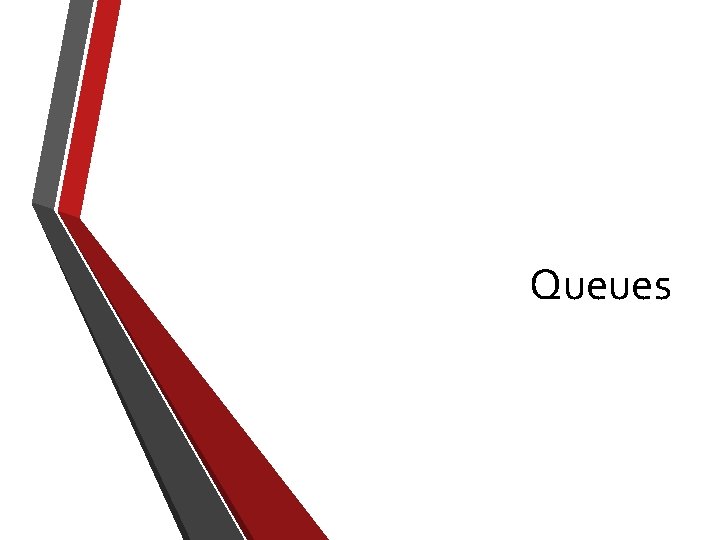
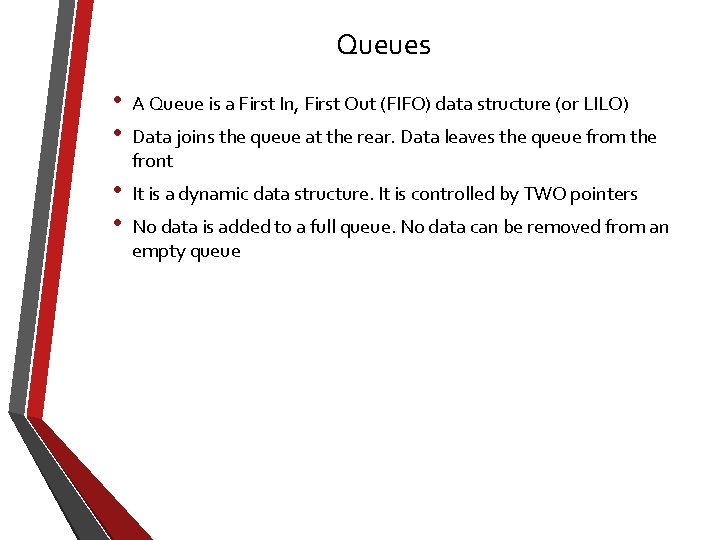
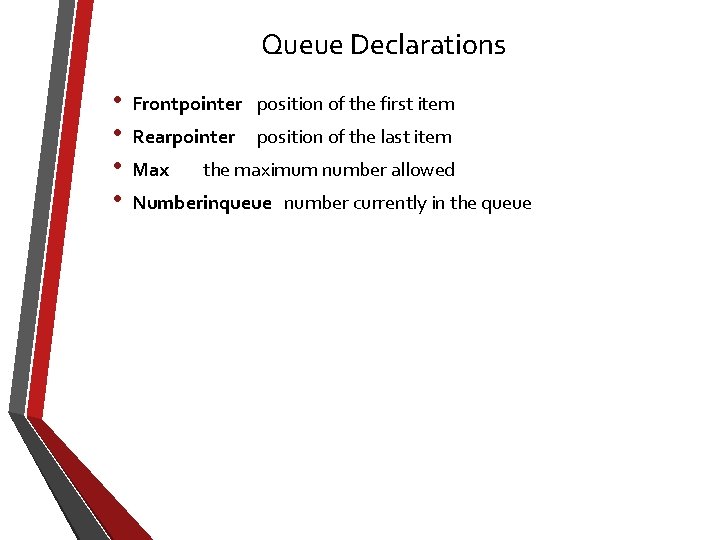
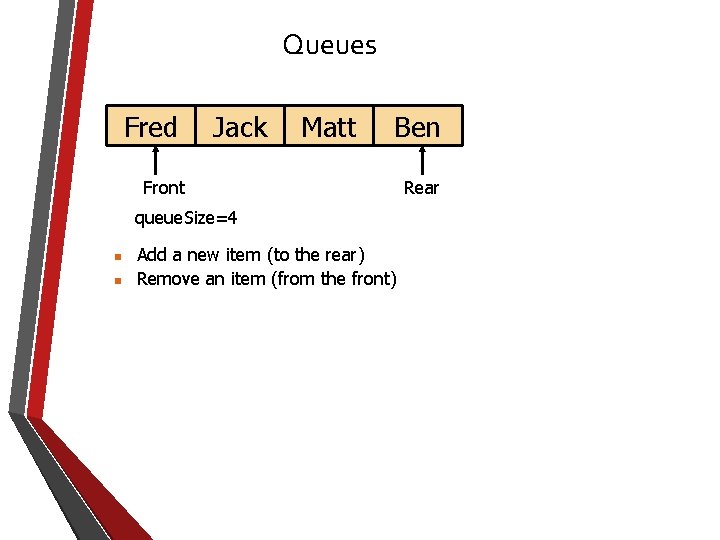
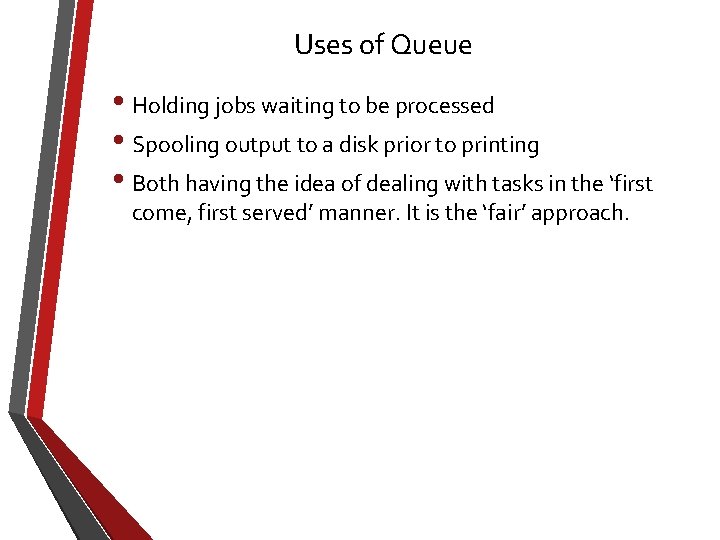
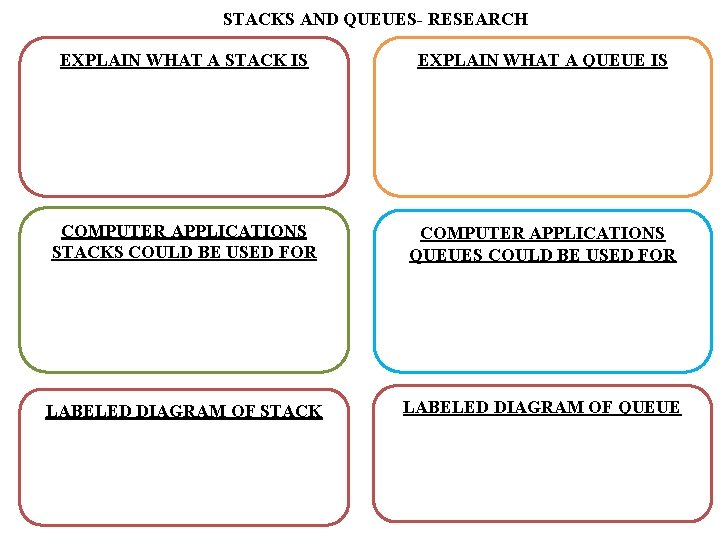
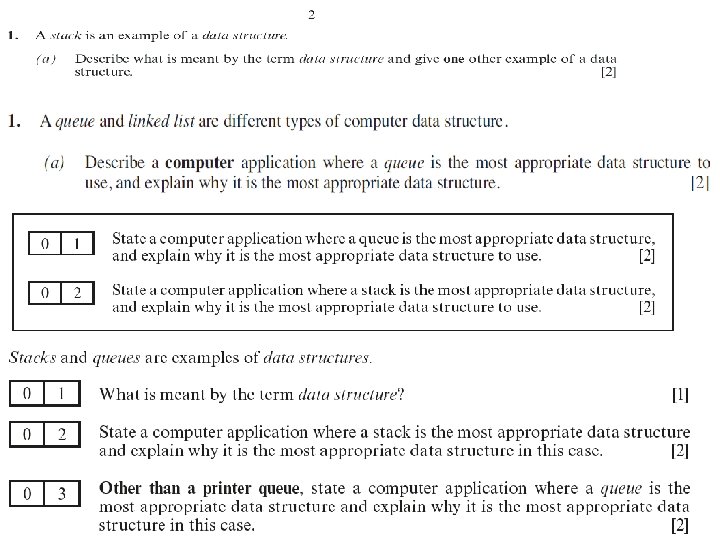
- Slides: 35
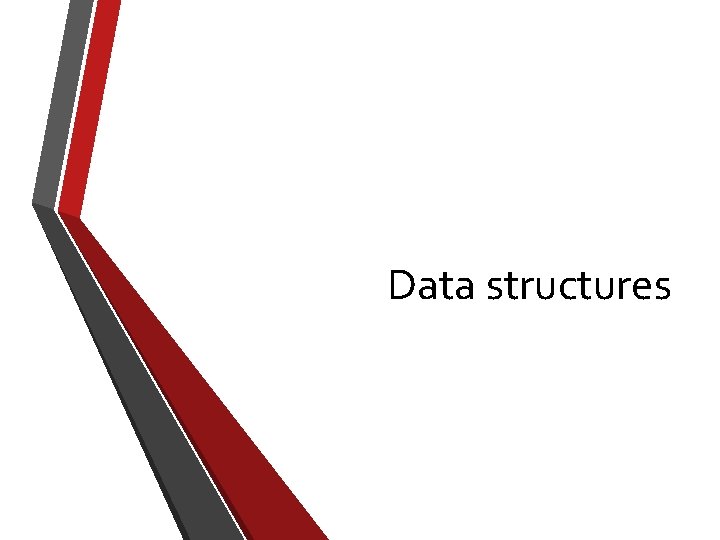
Data structures
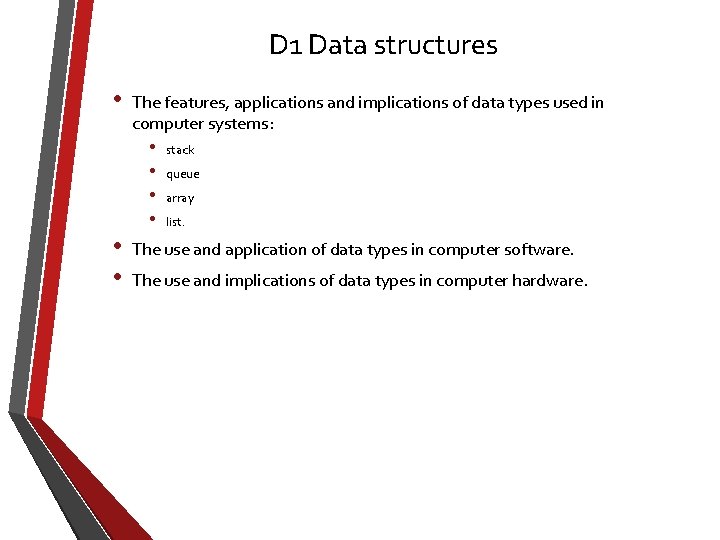
D 1 Data structures • The features, applications and implications of data types used in computer systems: • • • stack queue array list. The use and application of data types in computer software. The use and implications of data types in computer hardware.
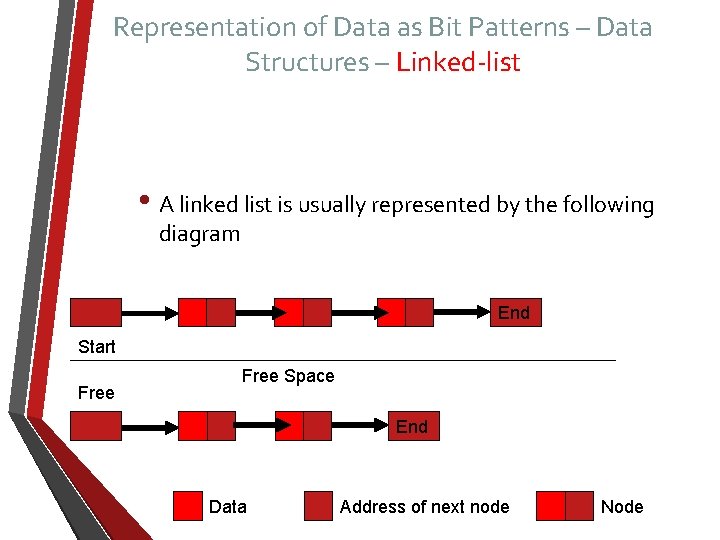
Representation of Data as Bit Patterns – Data Structures – Linked-list • A linked list is usually represented by the following diagram End Start Free Space End Data Address of next node Node
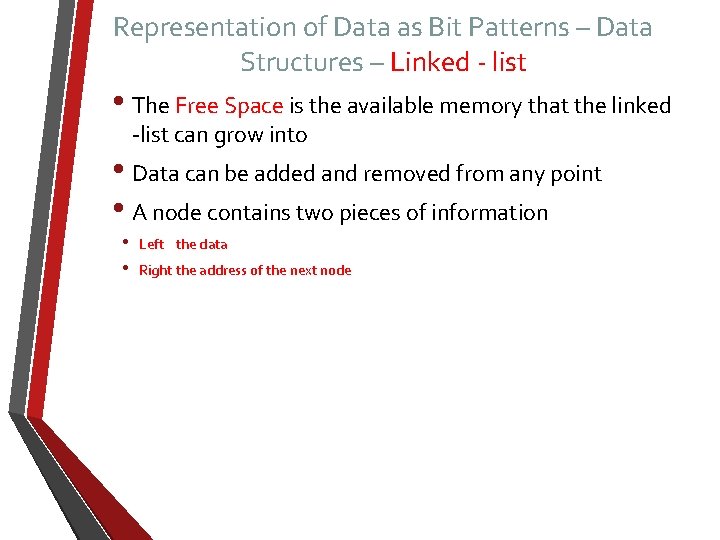
Representation of Data as Bit Patterns – Data Structures – Linked - list • The Free Space is the available memory that the linked -list can grow into • Data can be added and removed from any point • A node contains two pieces of information • • Left the data Right the address of the next node
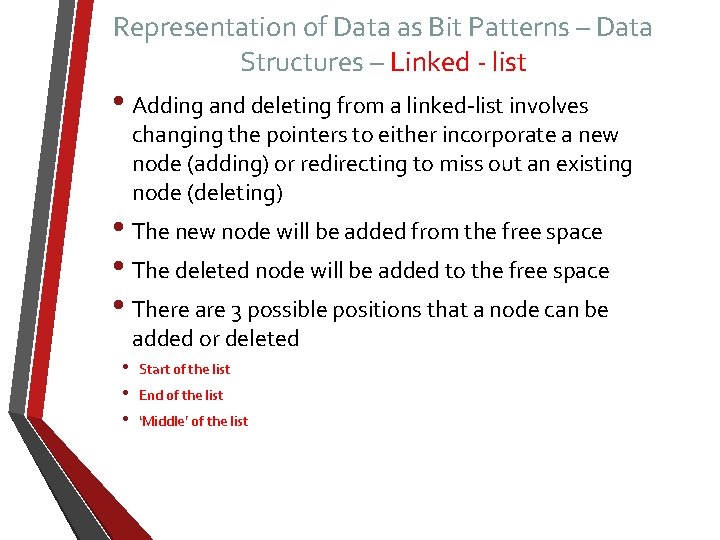
Representation of Data as Bit Patterns – Data Structures – Linked - list • Adding and deleting from a linked-list involves changing the pointers to either incorporate a new node (adding) or redirecting to miss out an existing node (deleting) • The new node will be added from the free space • The deleted node will be added to the free space • There are 3 possible positions that a node can be added or deleted • • • Start of the list End of the list ‘Middle’ of the list
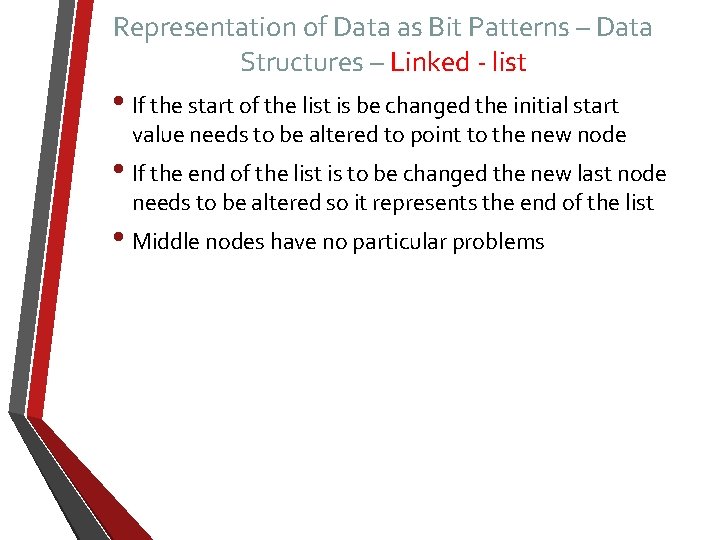
Representation of Data as Bit Patterns – Data Structures – Linked - list • If the start of the list is be changed the initial start value needs to be altered to point to the new node • If the end of the list is to be changed the new last node needs to be altered so it represents the end of the list • Middle nodes have no particular problems
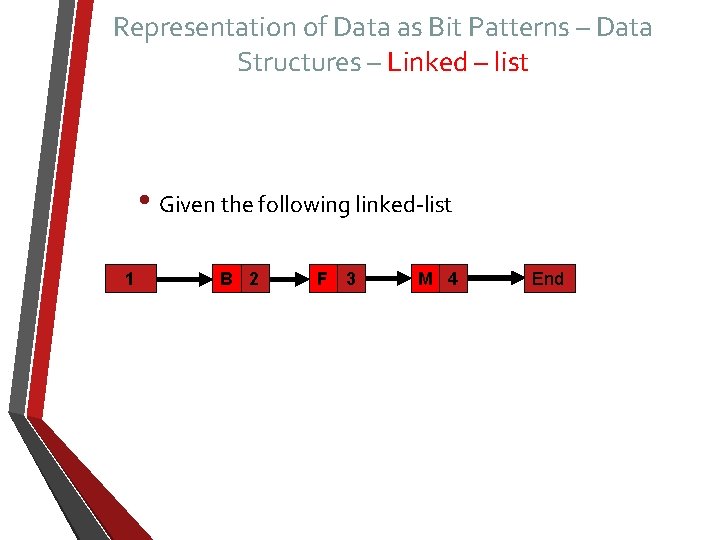
Representation of Data as Bit Patterns – Data Structures – Linked – list • Given the following linked-list 1 B 2 F 3 M 4 End
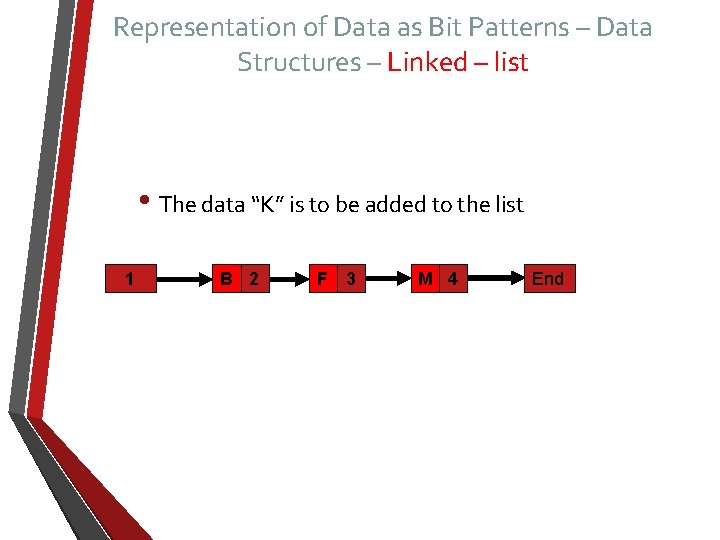
Representation of Data as Bit Patterns – Data Structures – Linked – list • The data “K” is to be added to the list 1 B 2 F 3 M 4 End
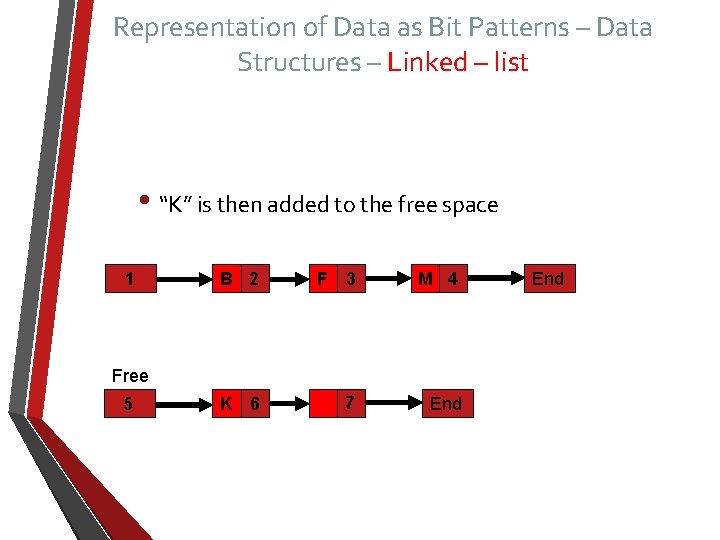
Representation of Data as Bit Patterns – Data Structures – Linked – list • “K” is then added to the free space 1 B 2 F 3 M 4 K 6 7 End Free 5 End
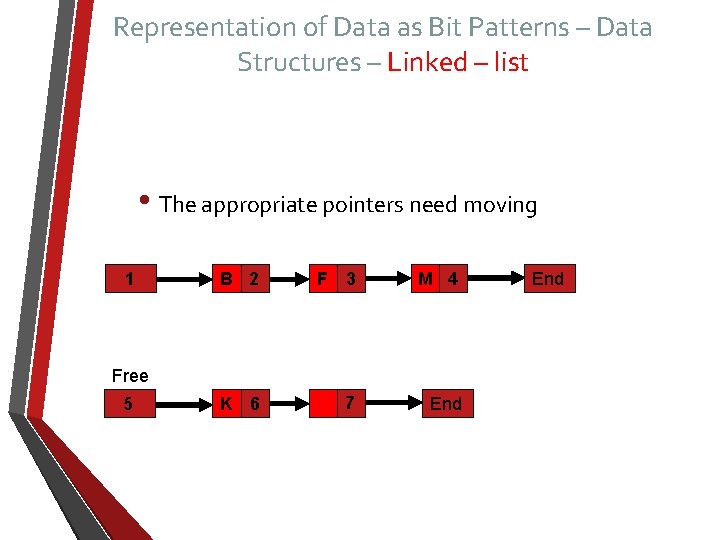
Representation of Data as Bit Patterns – Data Structures – Linked – list • The appropriate pointers need moving 1 B 2 F 3 M 4 K 6 7 End Free 5 End
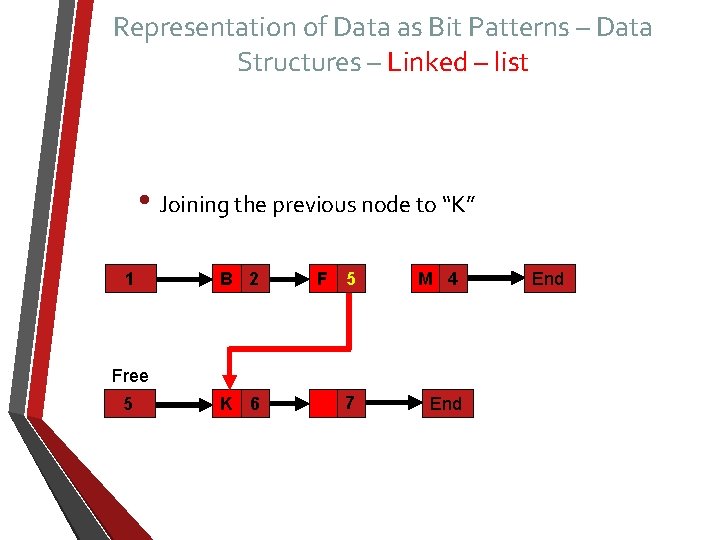
Representation of Data as Bit Patterns – Data Structures – Linked – list • Joining the previous node to “K” 1 B 2 F 5 M 4 K 6 7 End Free 5 End
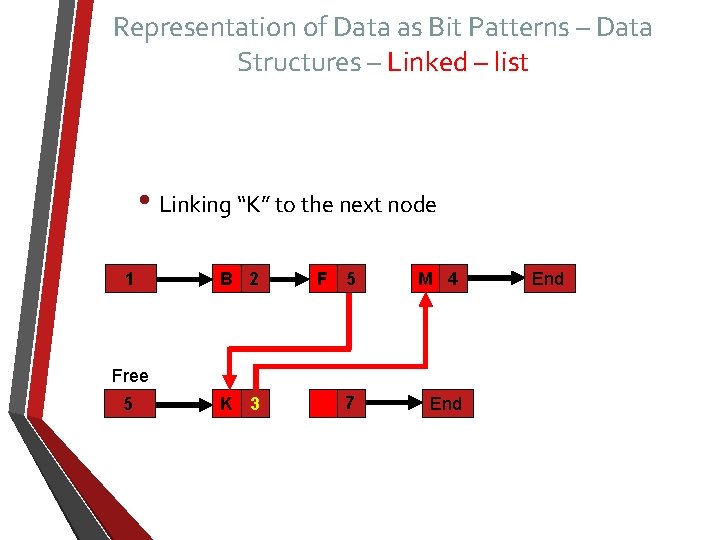
Representation of Data as Bit Patterns – Data Structures – Linked – list • Linking “K” to the next node 1 B 2 F 5 M 4 K 3 7 End Free 5 End
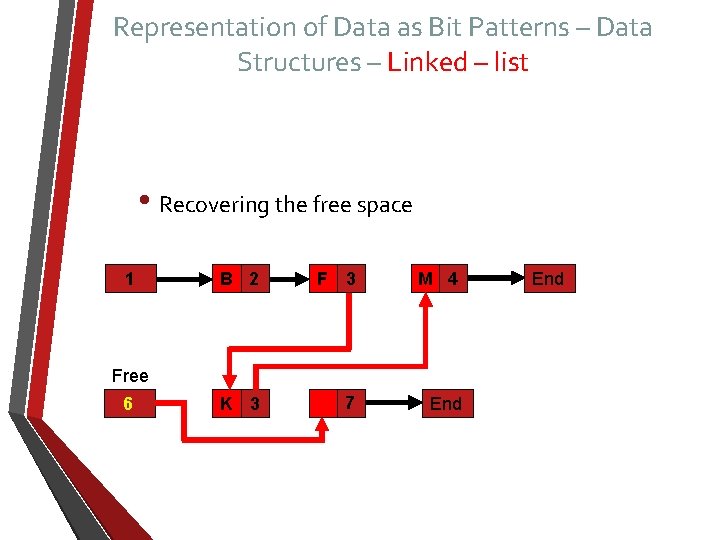
Representation of Data as Bit Patterns – Data Structures – Linked – list • Recovering the free space 1 B 2 F 3 M 4 K 3 7 End Free 6 End
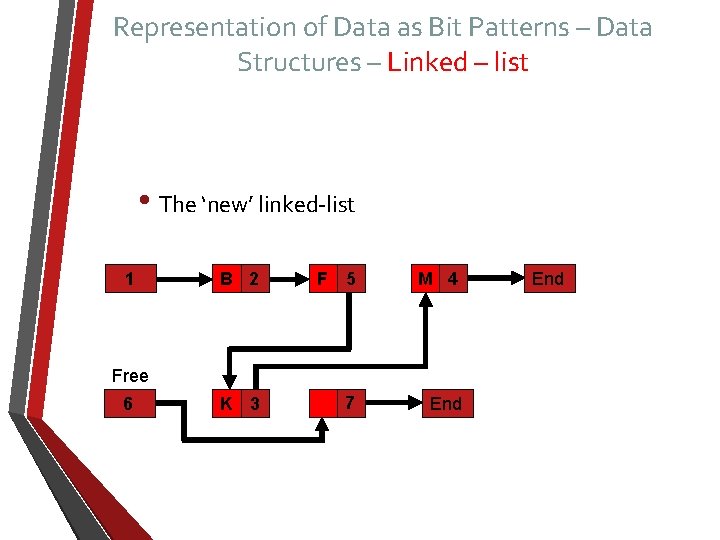
Representation of Data as Bit Patterns – Data Structures – Linked – list • The ‘new’ linked-list 1 B 2 F 5 M 4 K 3 7 End Free 6 End
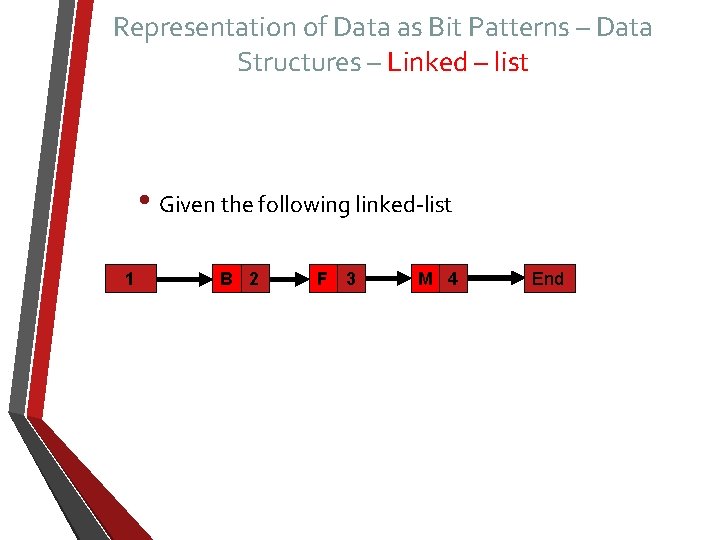
Representation of Data as Bit Patterns – Data Structures – Linked – list • Given the following linked-list 1 B 2 F 3 M 4 End
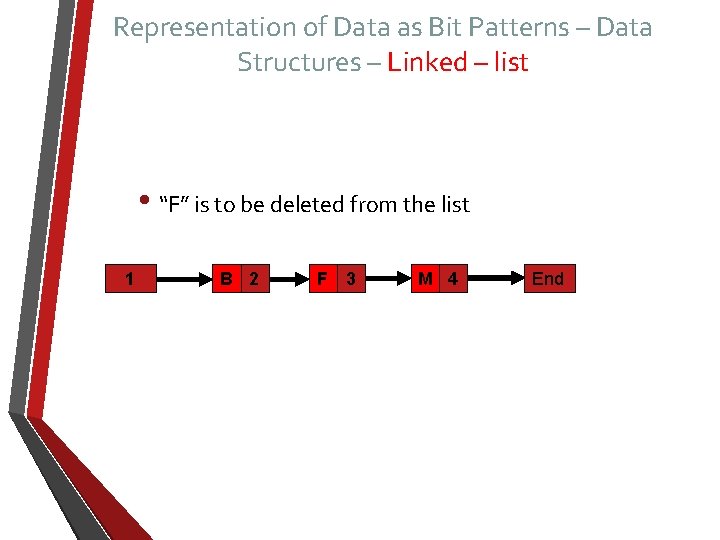
Representation of Data as Bit Patterns – Data Structures – Linked – list • “F” is to be deleted from the list 1 B 2 F 3 M 4 End
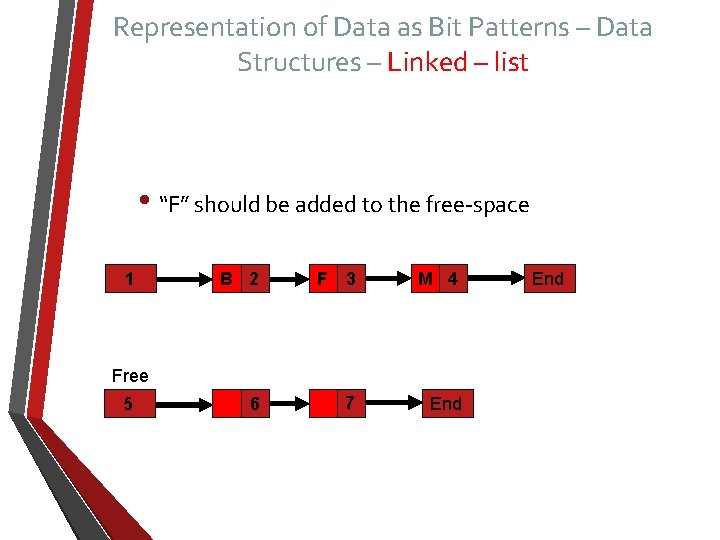
Representation of Data as Bit Patterns – Data Structures – Linked – list • “F” should be added to the free-space 1 B 2 F 3 M 4 6 7 End Free 5 End
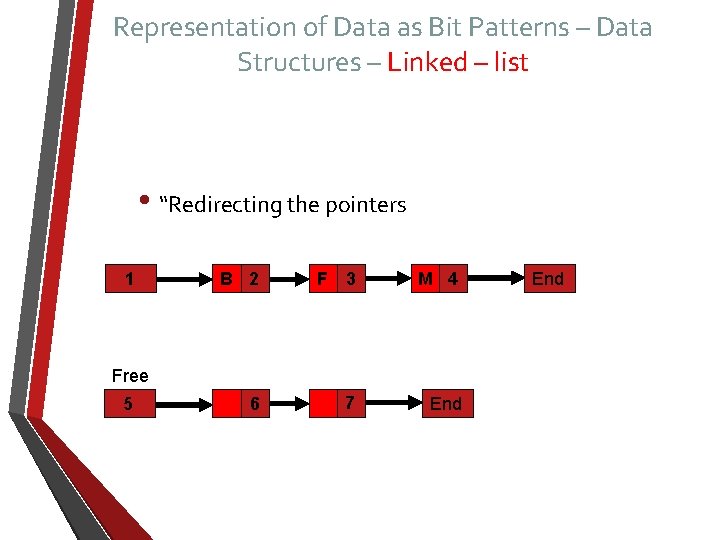
Representation of Data as Bit Patterns – Data Structures – Linked – list • “Redirecting the pointers 1 B 2 F 3 M 4 6 7 End Free 5 End
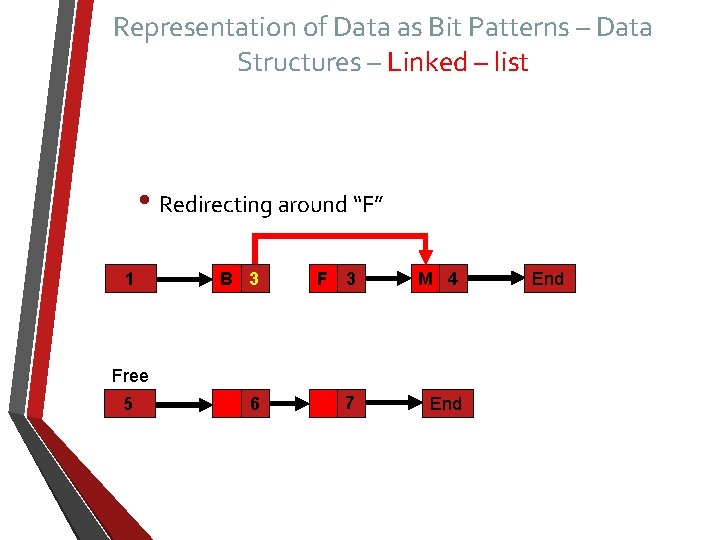
Representation of Data as Bit Patterns – Data Structures – Linked – list • Redirecting around “F” 1 B 3 F 3 M 4 6 7 End Free 5 End
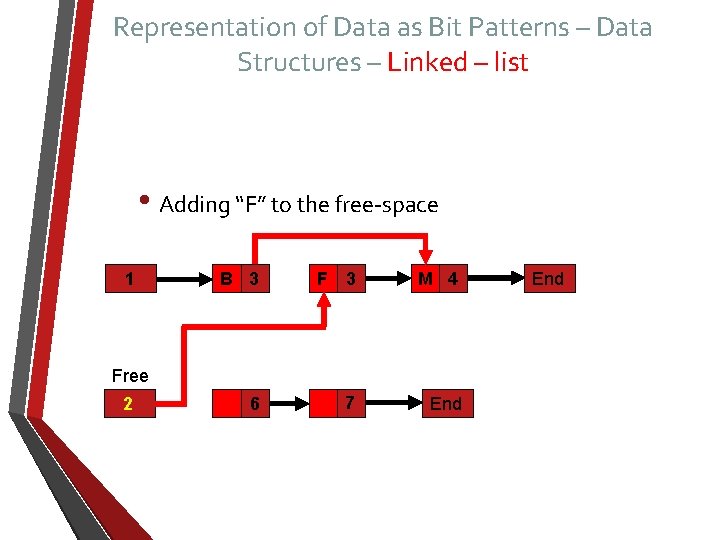
Representation of Data as Bit Patterns – Data Structures – Linked – list • Adding “F” to the free-space 1 B 3 F 3 M 4 6 7 End Free 2 End
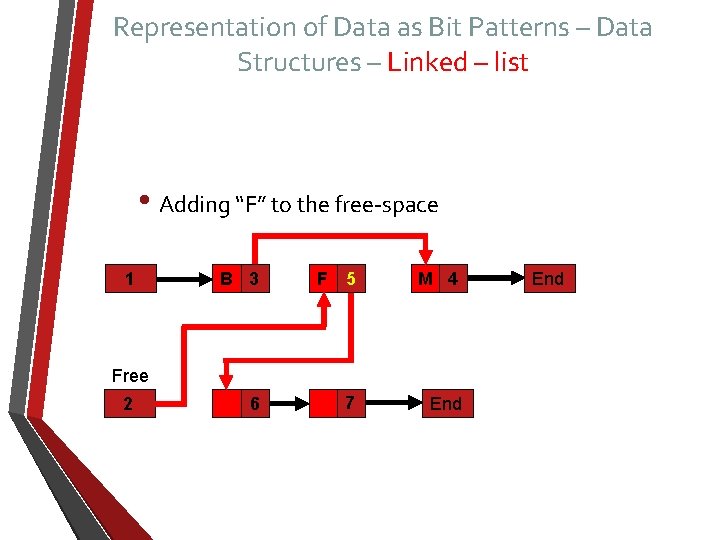
Representation of Data as Bit Patterns – Data Structures – Linked – list • Adding “F” to the free-space 1 B 3 F 5 M 4 6 7 End Free 2 End
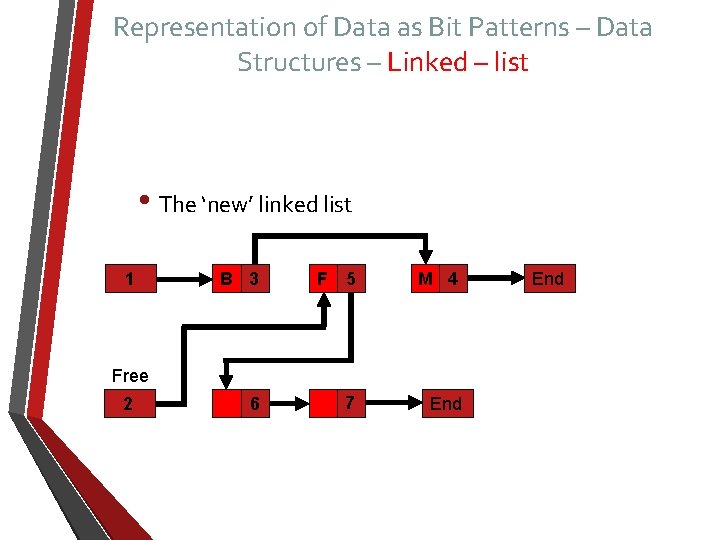
Representation of Data as Bit Patterns – Data Structures – Linked – list • The ‘new’ linked list 1 B 3 F 5 M 4 6 7 End Free 2 End
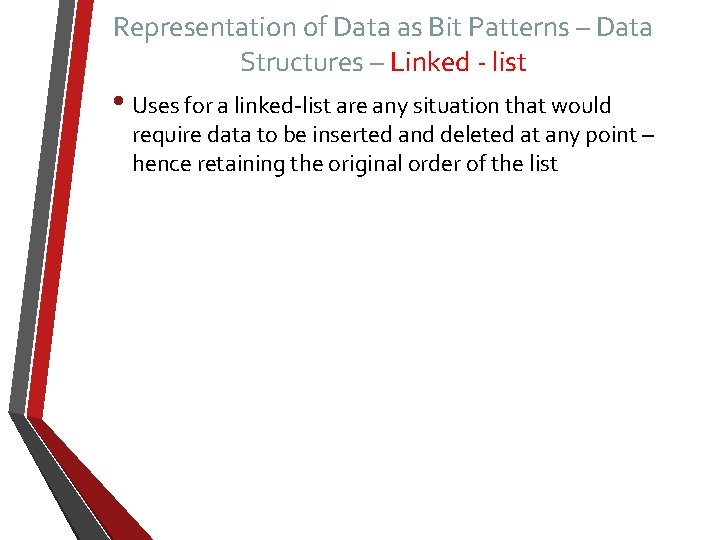
Representation of Data as Bit Patterns – Data Structures – Linked - list • Uses for a linked-list are any situation that would require data to be inserted and deleted at any point – hence retaining the original order of the list
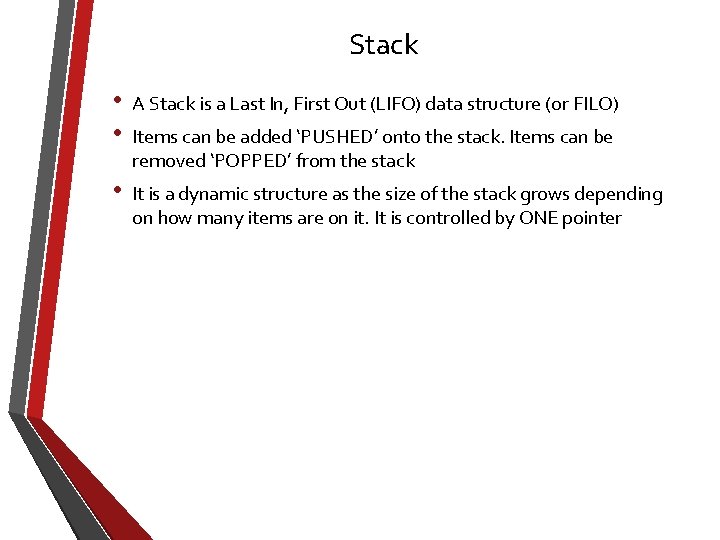
Stack • • A Stack is a Last In, First Out (LIFO) data structure (or FILO) • It is a dynamic structure as the size of the stack grows depending on how many items are on it. It is controlled by ONE pointer Items can be added ‘PUSHED’ onto the stack. Items can be removed ‘POPPED’ from the stack
![Stacks 6 5 4 3 2 1 Items can be pushed add Stacks [6] [5] [4] [3] [2] [1] • Items can be pushed (add), •](https://slidetodoc.com/presentation_image_h/1f219c66a8e1a7e9201f3c19e3c2e4d4/image-25.jpg)
Stacks [6] [5] [4] [3] [2] [1] • Items can be pushed (add), • Its can be popped (remove) Katie Millie Joe max. Stack. Size = 6 top =3 • Can only be added to the top of the Stack
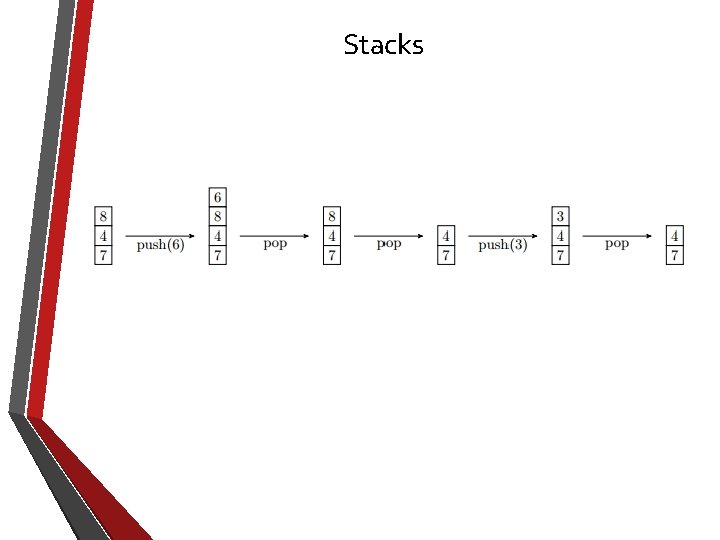
Stacks
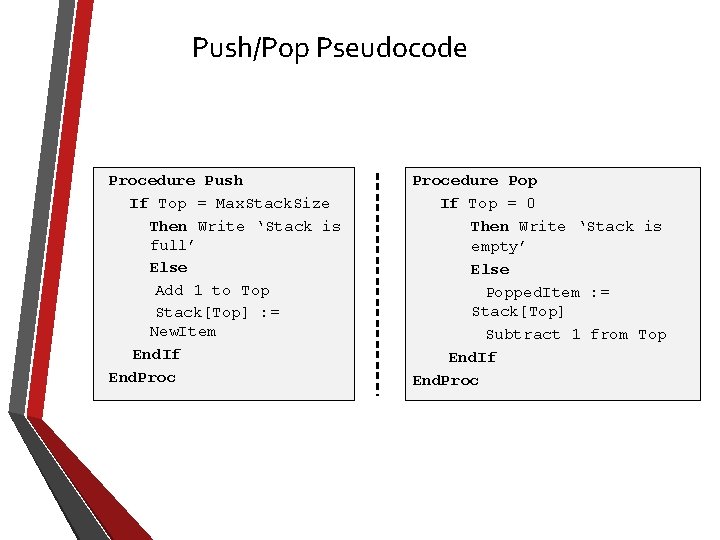
Push/Pop Pseudocode Procedure Push If Top = Max. Stack. Size Then Write ‘Stack is full’ Else Add 1 to Top Stack[Top] : = New. Item End. If End. Procedure Pop If Top = 0 Then Write ‘Stack is empty’ Else Popped. Item : = Stack[Top] Subtract 1 from Top End. If End. Proc
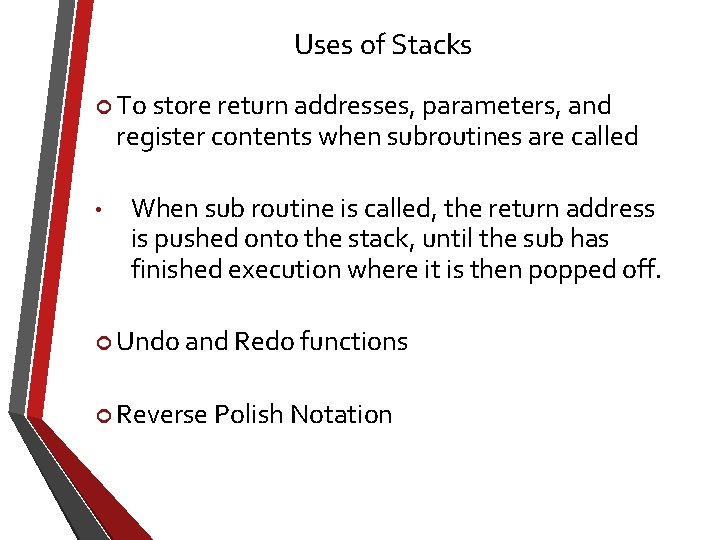
Uses of Stacks To store return addresses, parameters, and register contents when subroutines are called • When sub routine is called, the return address is pushed onto the stack, until the sub has finished execution where it is then popped off. Undo and Redo functions Reverse Polish Notation
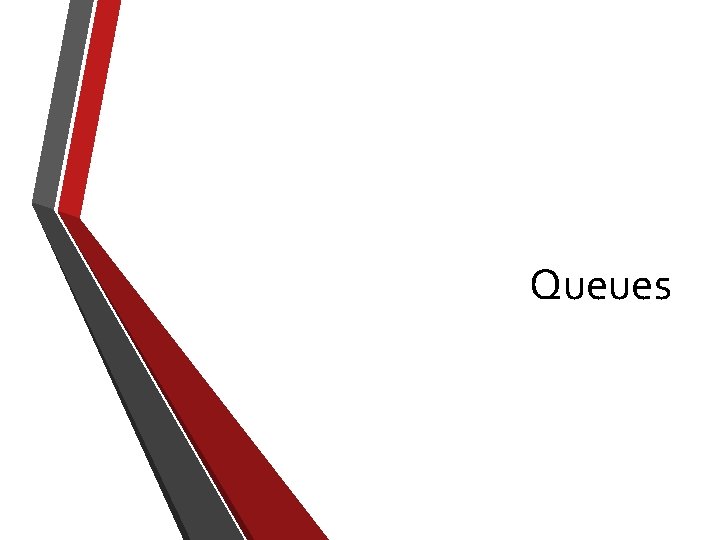
Queues
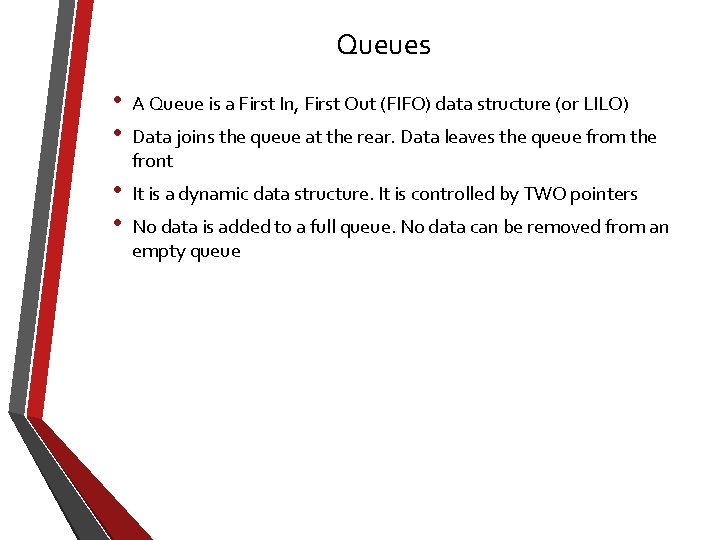
Queues • • A Queue is a First In, First Out (FIFO) data structure (or LILO) • • It is a dynamic data structure. It is controlled by TWO pointers Data joins the queue at the rear. Data leaves the queue from the front No data is added to a full queue. No data can be removed from an empty queue
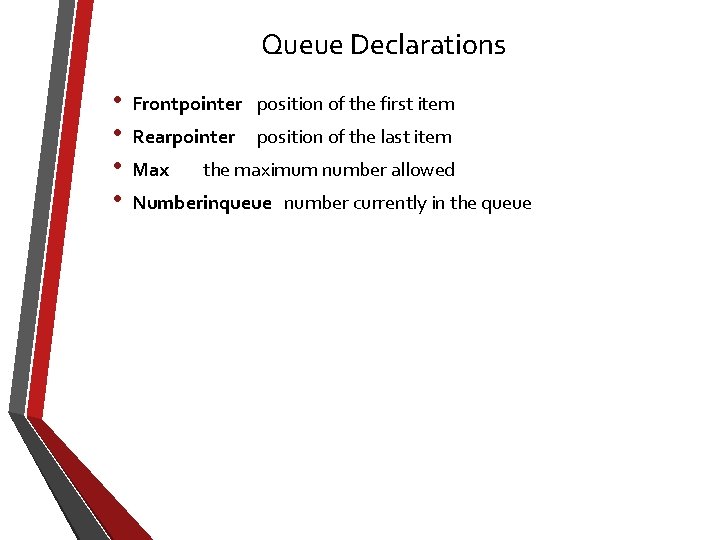
Queue Declarations • • Frontpointer position of the first item Rearpointer Max position of the last item the maximum number allowed Numberinqueue number currently in the queue
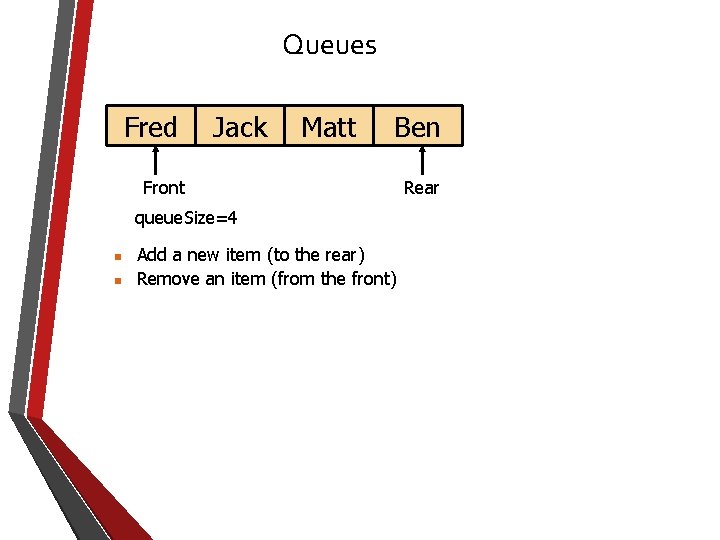
Queues Fred Jack Matt Ben Front queue. Size=4 n n Add a new item (to the rear) Remove an item (from the front) Rear
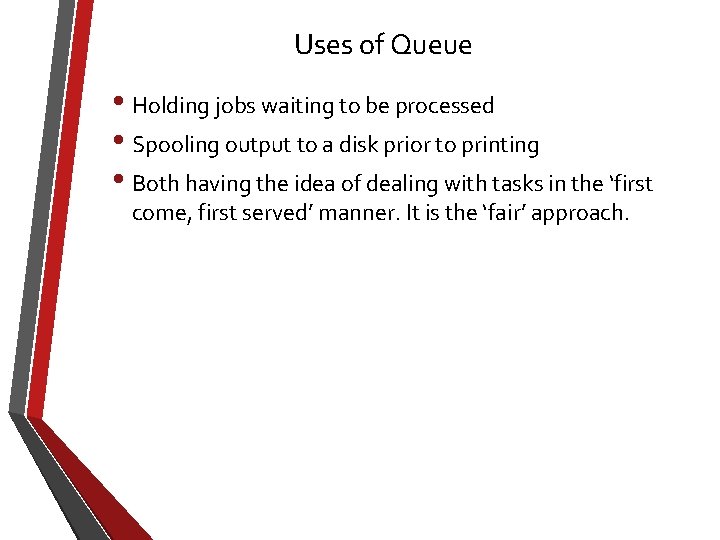
Uses of Queue • Holding jobs waiting to be processed • Spooling output to a disk prior to printing • Both having the idea of dealing with tasks in the ‘first come, first served’ manner. It is the ‘fair’ approach.
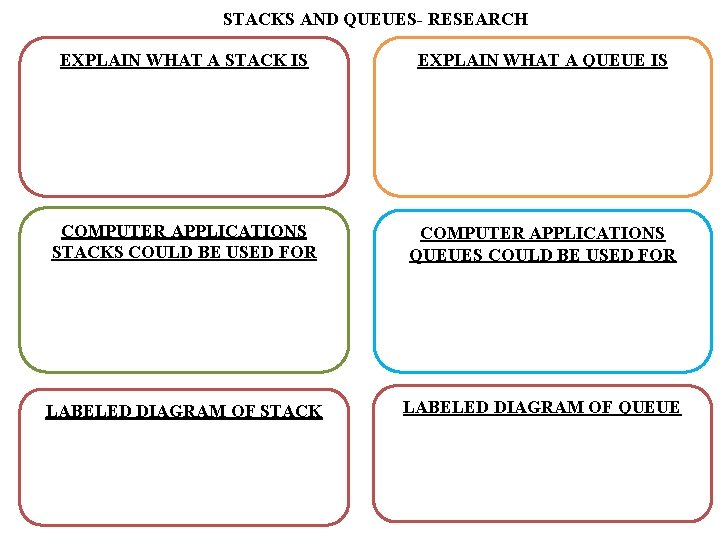
STACKS AND QUEUES- RESEARCH EXPLAIN WHAT A STACK IS EXPLAIN WHAT A QUEUE IS COMPUTER APPLICATIONS STACKS COULD BE USED FOR COMPUTER APPLICATIONS QUEUES COULD BE USED FOR LABELED DIAGRAM OF STACK LABELED DIAGRAM OF QUEUE
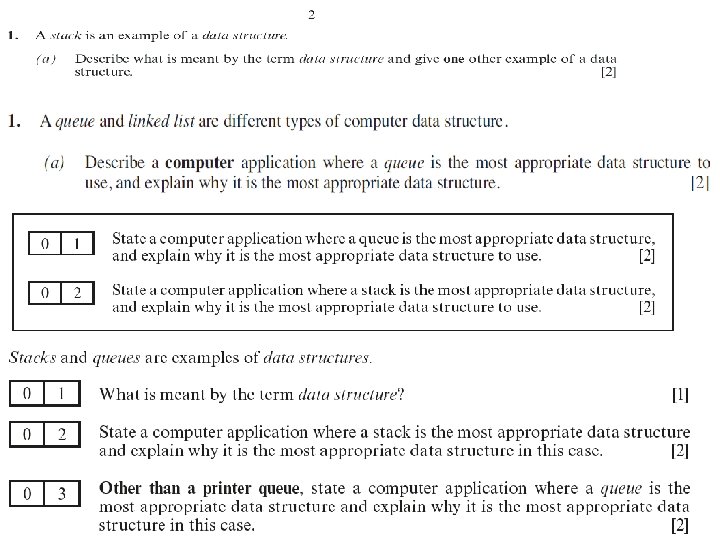
Text structures vs text features
Structure of magazine article
Example of homologous structure
Hát kết hợp bộ gõ cơ thể
Ng-html
Bổ thể
Tỉ lệ cơ thể trẻ em
Voi kéo gỗ như thế nào
Thang điểm glasgow
Hát lên người ơi
Các môn thể thao bắt đầu bằng tiếng đua
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công của trọng lực
Trời xanh đây là của chúng ta thể thơ
Cách giải mật thư tọa độ
Phép trừ bù
độ dài liên kết
Các châu lục và đại dương trên thế giới
Thơ thất ngôn tứ tuyệt đường luật
Quá trình desamine hóa có thể tạo ra
Một số thể thơ truyền thống
Cái miệng bé xinh thế chỉ nói điều hay thôi
Vẽ hình chiếu vuông góc của vật thể sau
Nguyên nhân của sự mỏi cơ sinh 8
đặc điểm cơ thể của người tối cổ
Thế nào là giọng cùng tên?
Vẽ hình chiếu đứng bằng cạnh của vật thể
Phối cảnh
Thẻ vin
đại từ thay thế
điện thế nghỉ
Tư thế ngồi viết
Diễn thế sinh thái là
Dạng đột biến một nhiễm là
Số nguyên tố là gì