Complex Structures Nested Structures Self referential structures A
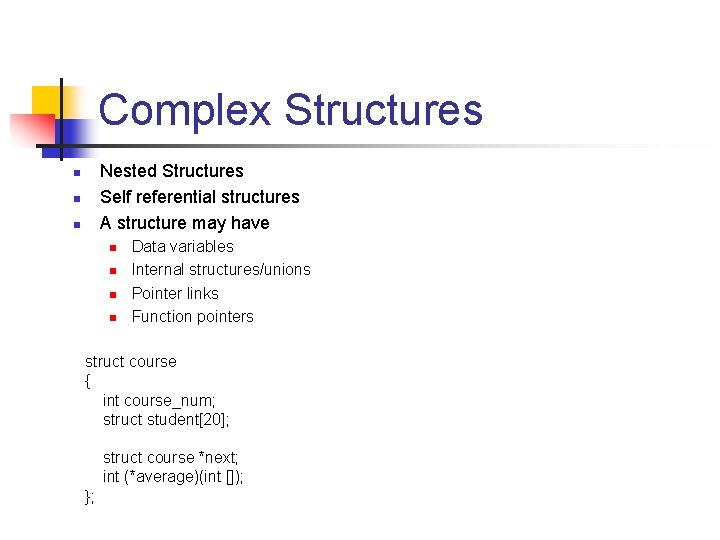
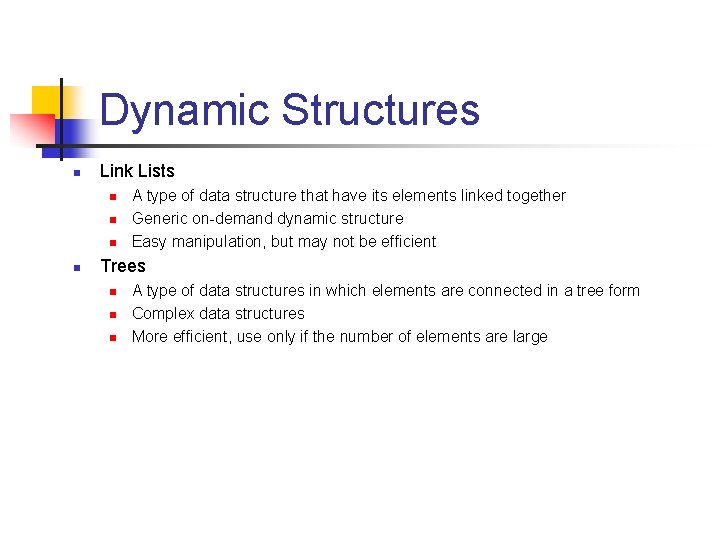
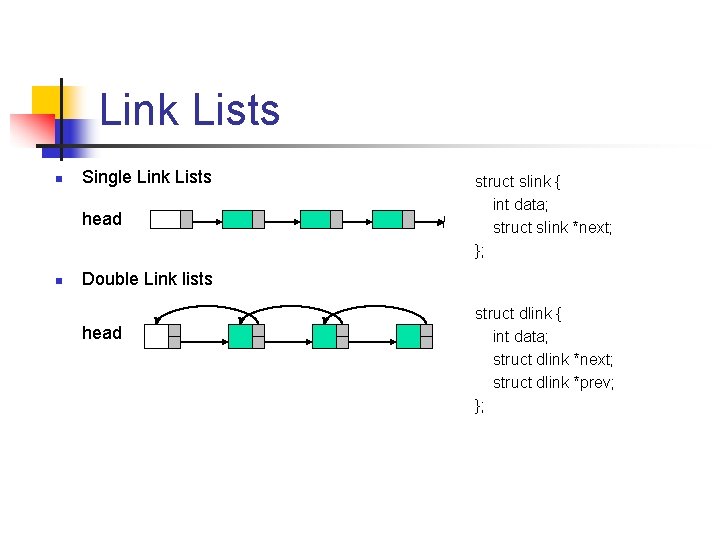
![Other Link Lists n Hash link lists bin[size] n Other variants: queues and stacks Other Link Lists n Hash link lists bin[size] n Other variants: queues and stacks](https://slidetodoc.com/presentation_image_h/033dbe7e48ea59d650ec3793e38f3431/image-4.jpg)
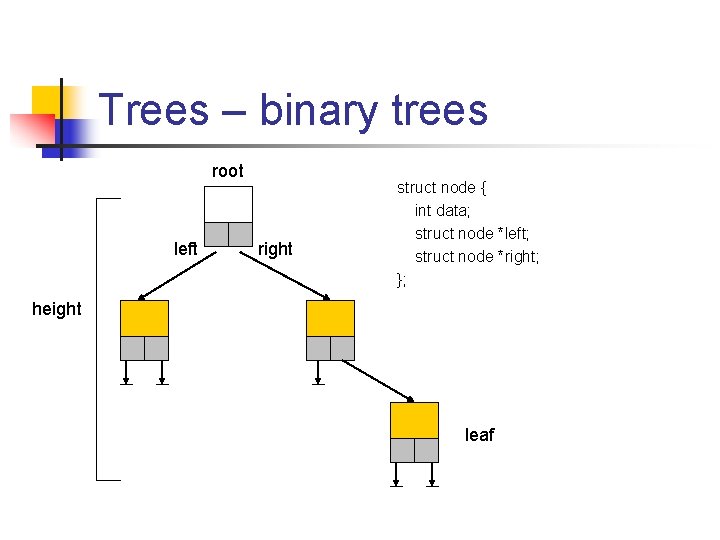
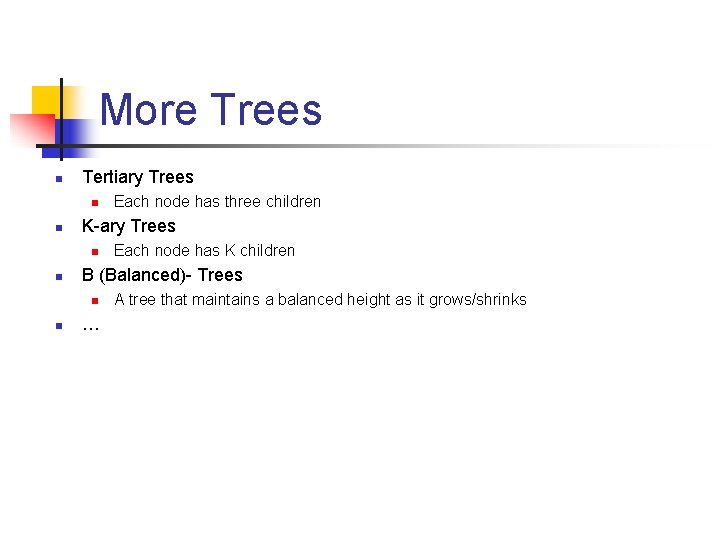
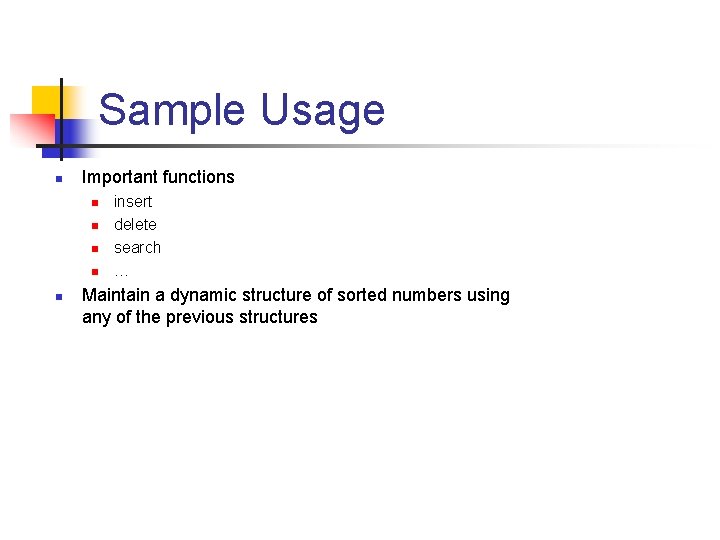
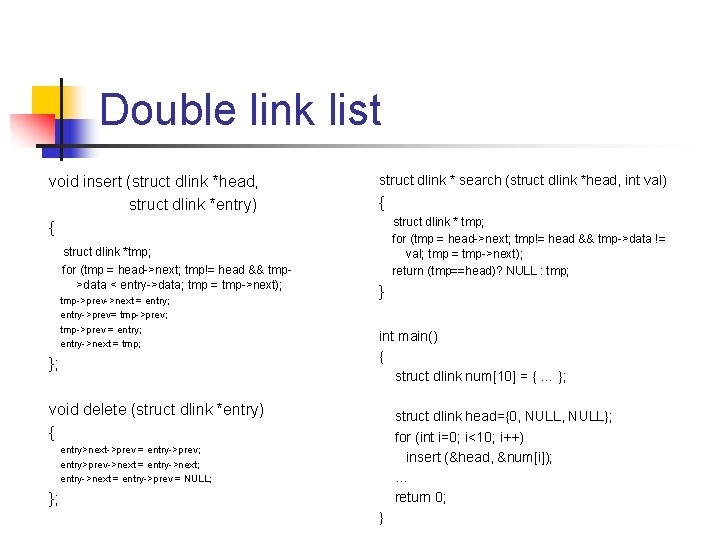
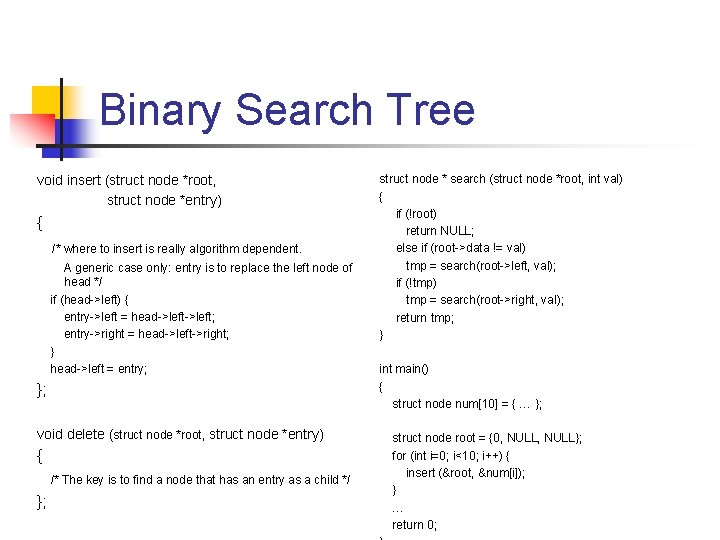
- Slides: 9
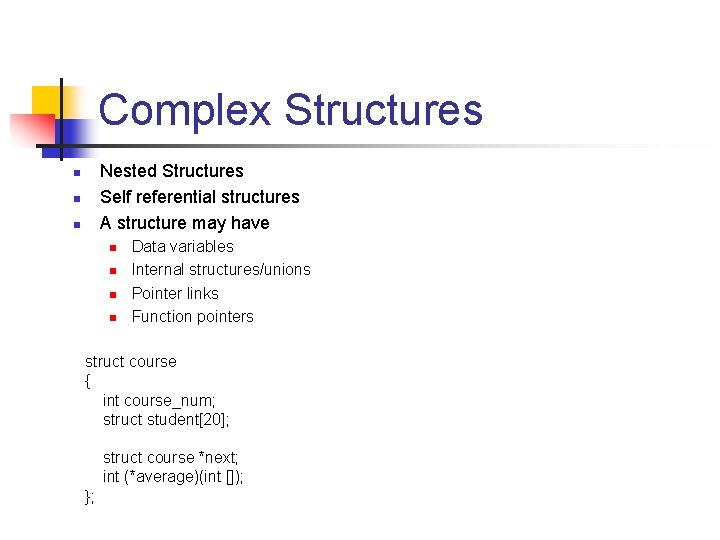
Complex Structures Nested Structures Self referential structures A structure may have n n n n Data variables Internal structures/unions Pointer links Function pointers struct course { int course_num; struct student[20]; struct course *next; int (*average)(int []); };
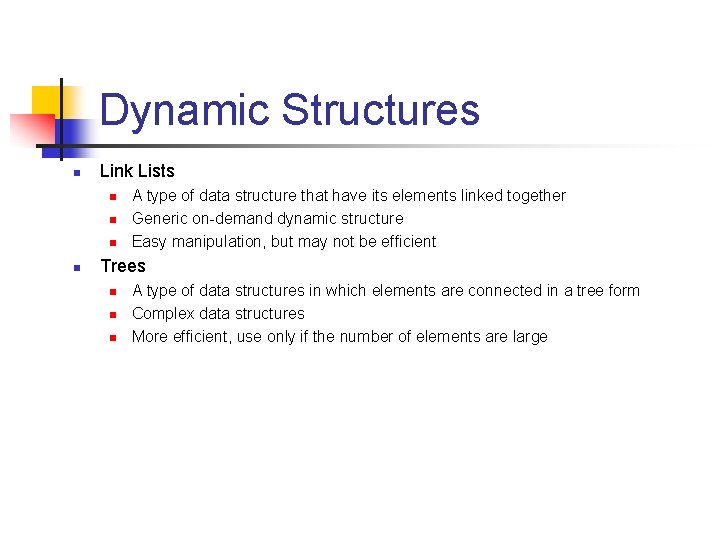
Dynamic Structures n Link Lists n n A type of data structure that have its elements linked together Generic on-demand dynamic structure Easy manipulation, but may not be efficient Trees n n n A type of data structures in which elements are connected in a tree form Complex data structures More efficient, use only if the number of elements are large
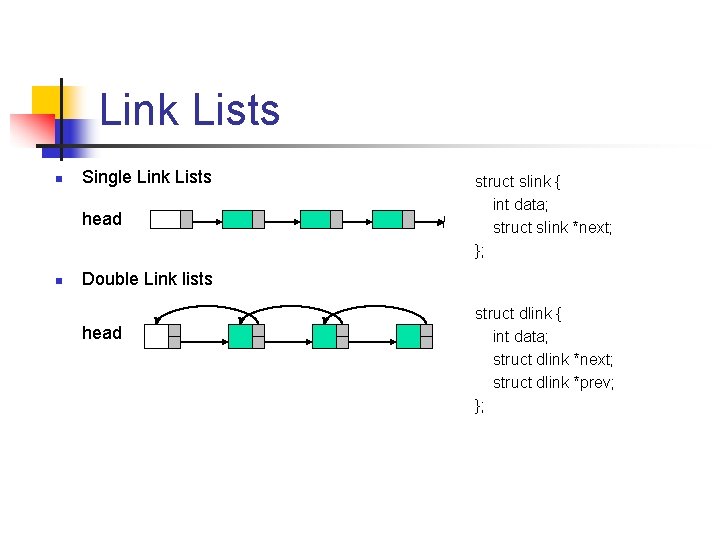
Link Lists n Single Link Lists head n struct slink { int data; struct slink *next; }; Double Link lists head struct dlink { int data; struct dlink *next; struct dlink *prev; };
![Other Link Lists n Hash link lists binsize n Other variants queues and stacks Other Link Lists n Hash link lists bin[size] n Other variants: queues and stacks](https://slidetodoc.com/presentation_image_h/033dbe7e48ea59d650ec3793e38f3431/image-4.jpg)
Other Link Lists n Hash link lists bin[size] n Other variants: queues and stacks n n Queue: a link list grown at one end, shrink at another, i. e. FIFO Stack: a LIFO link list
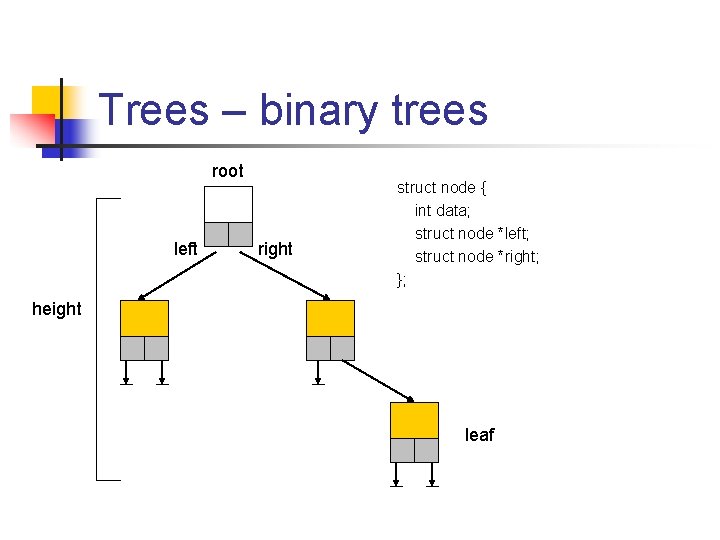
Trees – binary trees root left right struct node { int data; struct node *left; struct node *right; }; height leaf
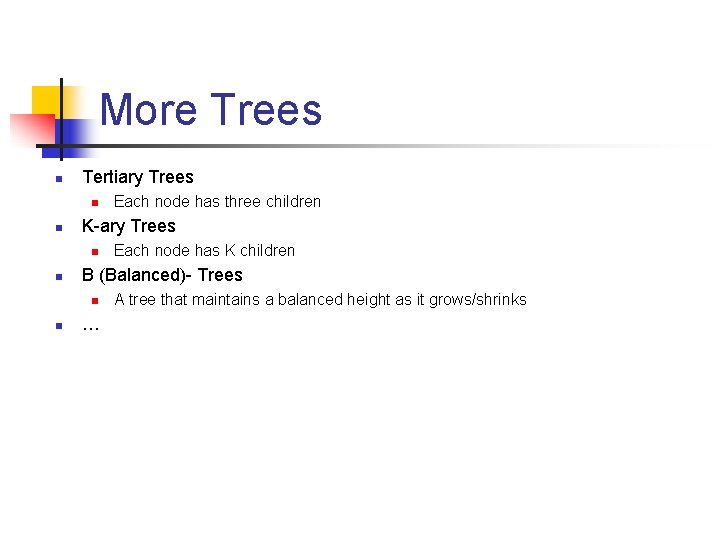
More Trees n Tertiary Trees n n K-ary Trees n n Each node has K children B (Balanced)- Trees n n Each node has three children … A tree that maintains a balanced height as it grows/shrinks
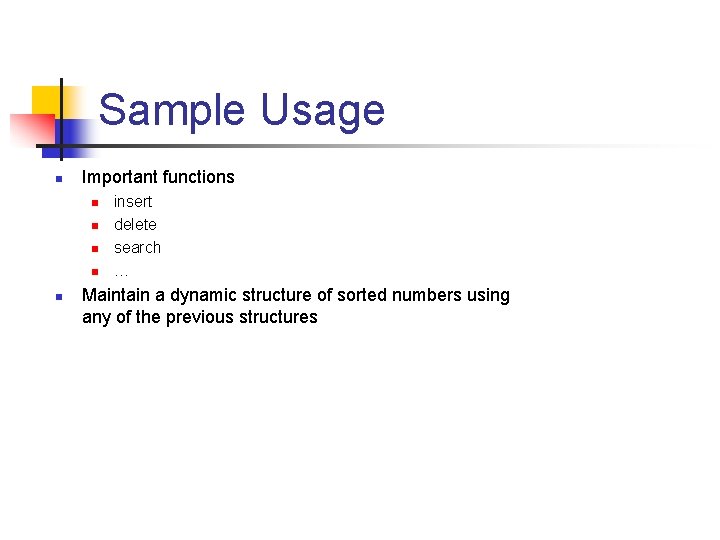
Sample Usage n Important functions n n n insert delete search … Maintain a dynamic structure of sorted numbers using any of the previous structures
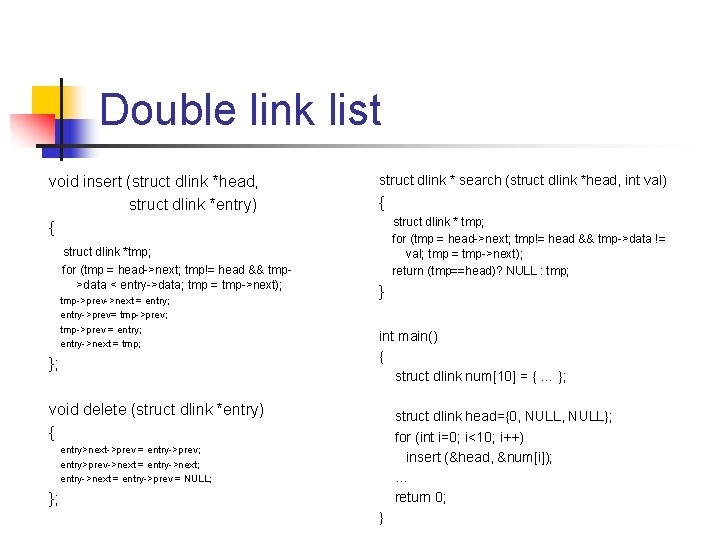
Double link list void insert (struct dlink *head, struct dlink *entry) { struct dlink * search (struct dlink *head, int val) { struct dlink * tmp; for (tmp = head->next; tmp!= head && tmp->data != val; tmp = tmp->next); return (tmp==head)? NULL : tmp; struct dlink *tmp; for (tmp = head->next; tmp!= head && tmp>data < entry->data; tmp = tmp->next); tmp->prev->next = entry; entry->prev= tmp->prev; tmp->prev = entry; entry->next = tmp; } int main() { struct dlink num[10] = { … }; void delete (struct dlink *entry) { struct dlink head={0, NULL}; for (int i=0; i<10; i++) insert (&head, &num[i]); … return 0; entry>next->prev = entry->prev; entry>prev->next = entry->next; entry->next = entry->prev = NULL; }; }
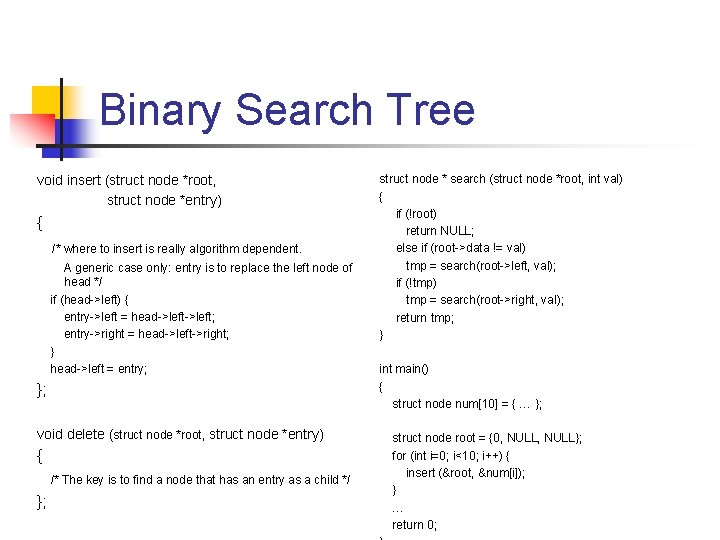
Binary Search Tree void insert (struct node *root, struct node *entry) { /* where to insert is really algorithm dependent. A generic case only: entry is to replace the left node of head */ if (head->left) { entry->left = head->left; entry->right = head->left->right; } head->left = entry; }; void delete (struct node *root, struct node *entry) { /* The key is to find a node that has an entry as a child */ }; struct node * search (struct node *root, int val) { if (!root) return NULL; else if (root->data != val) tmp = search(root->left, val); if (!tmp) tmp = search(root->right, val); return tmp; } int main() { struct node num[10] = { … }; struct node root = {0, NULL}; for (int i=0; i<10; i++) { insert (&root, &num[i]); } … return 0;