Contents Introduction n Computer Architecture n ARM Architecture
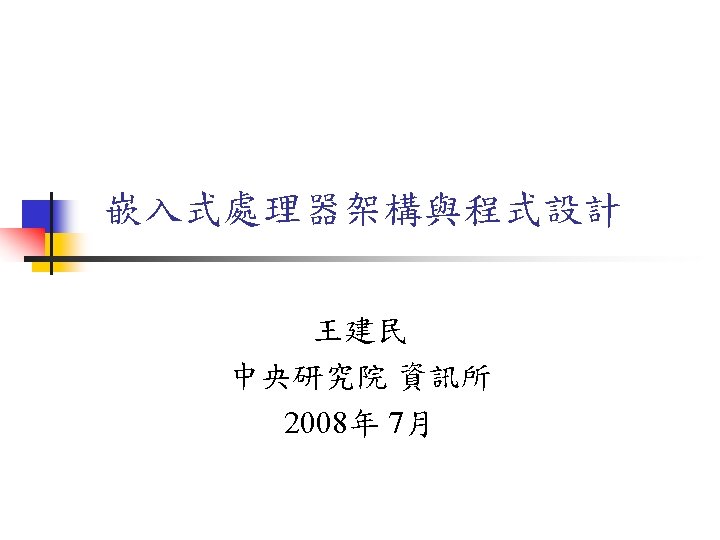
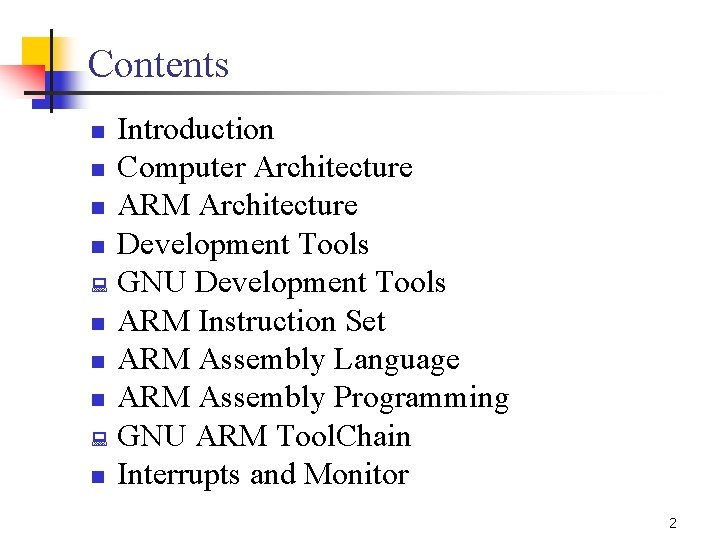
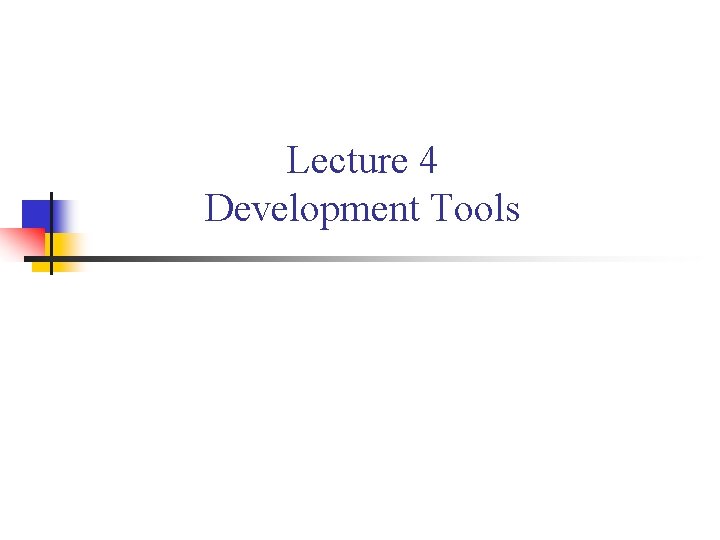
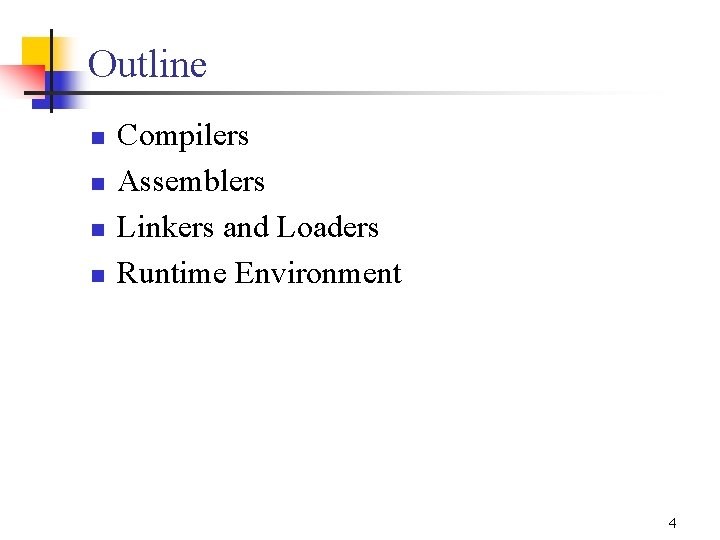
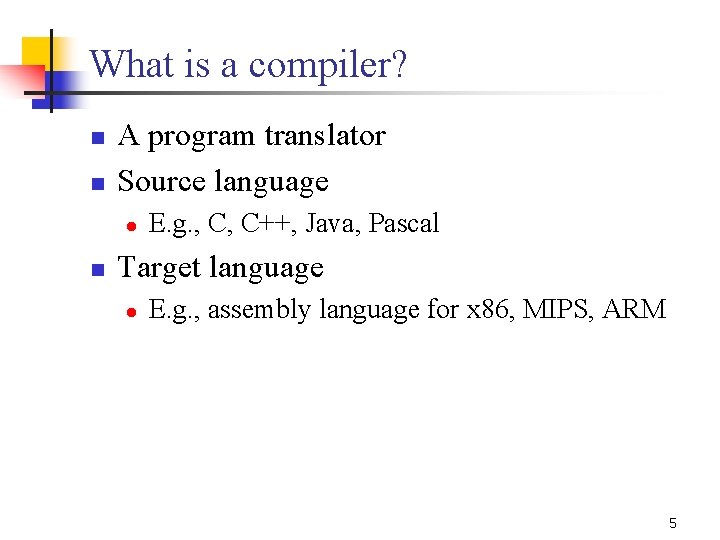
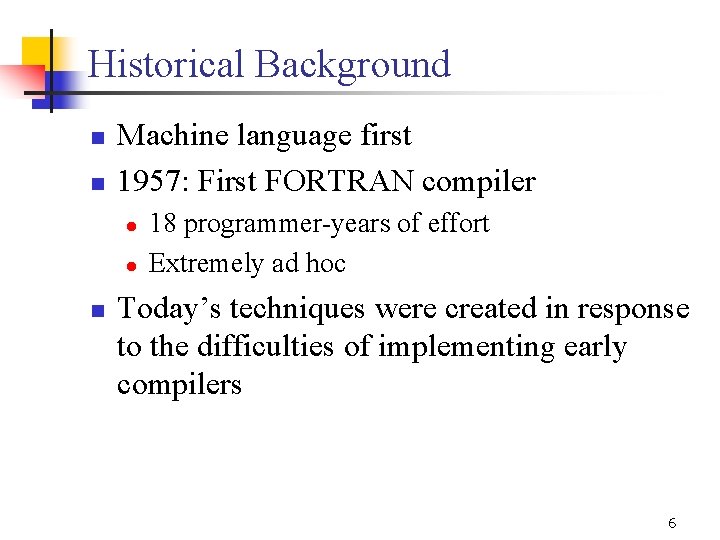
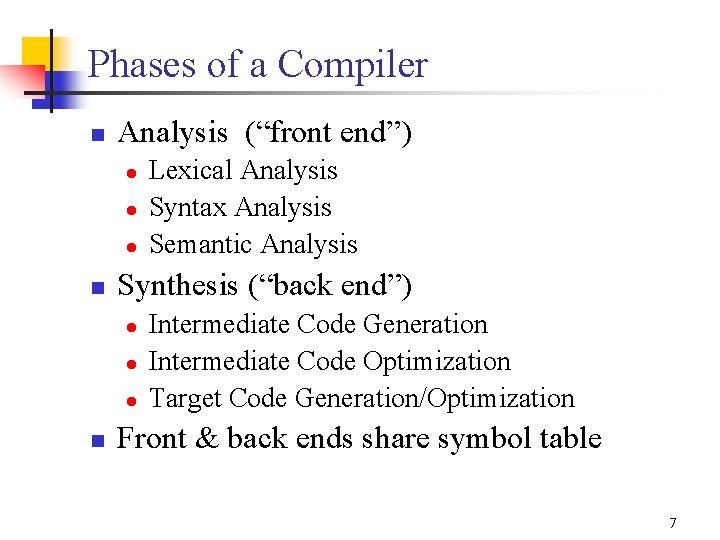
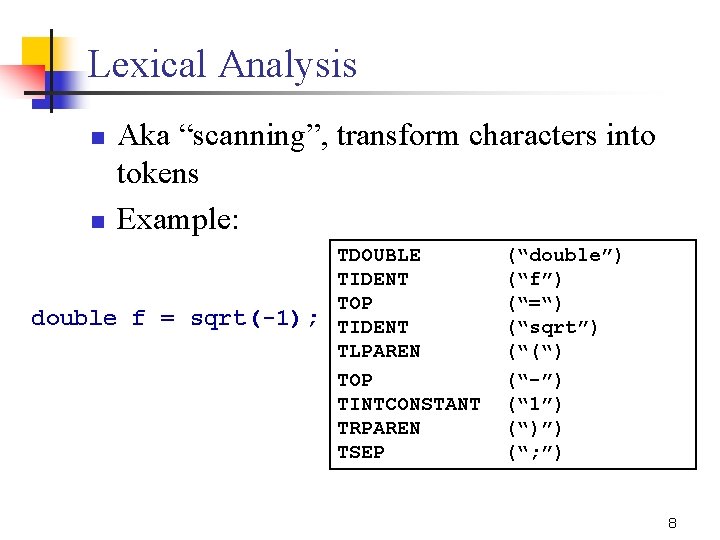
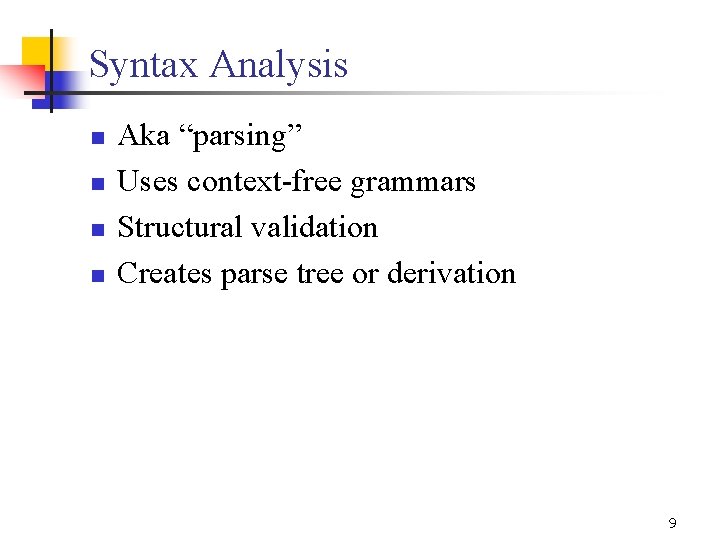
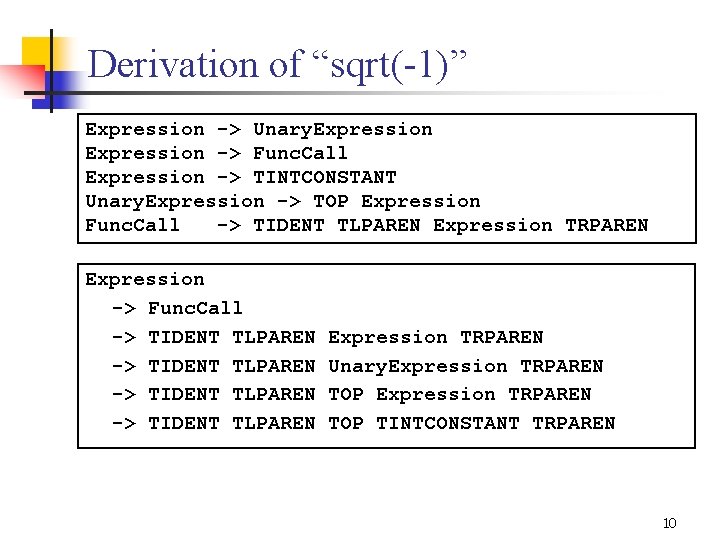
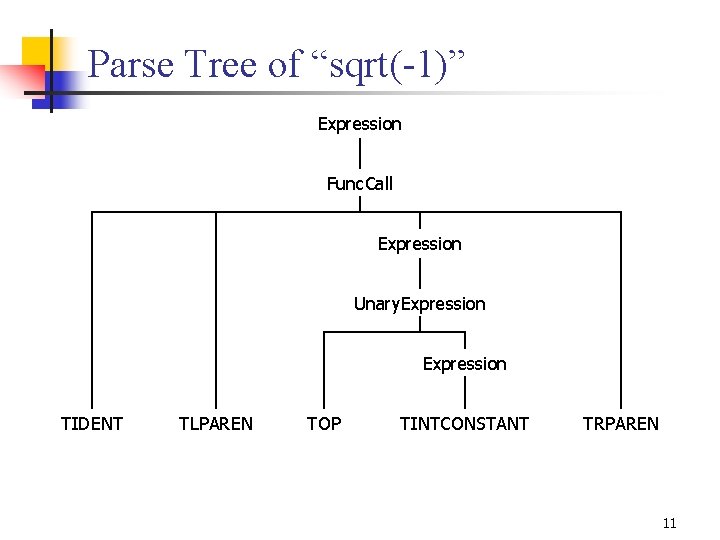
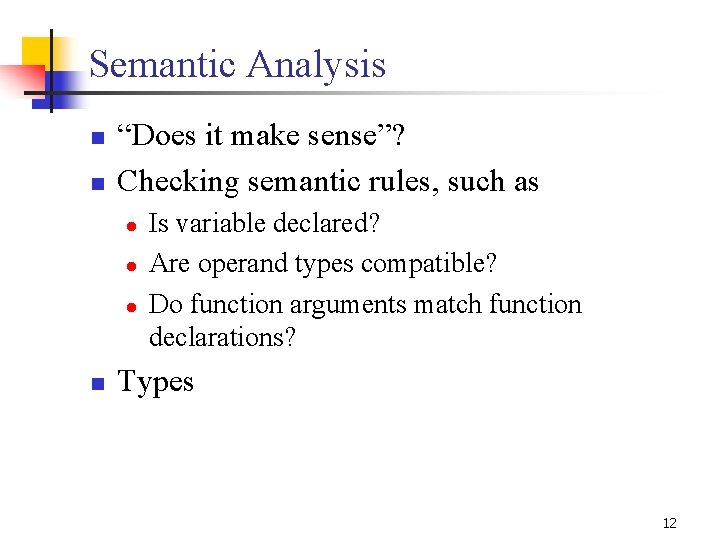
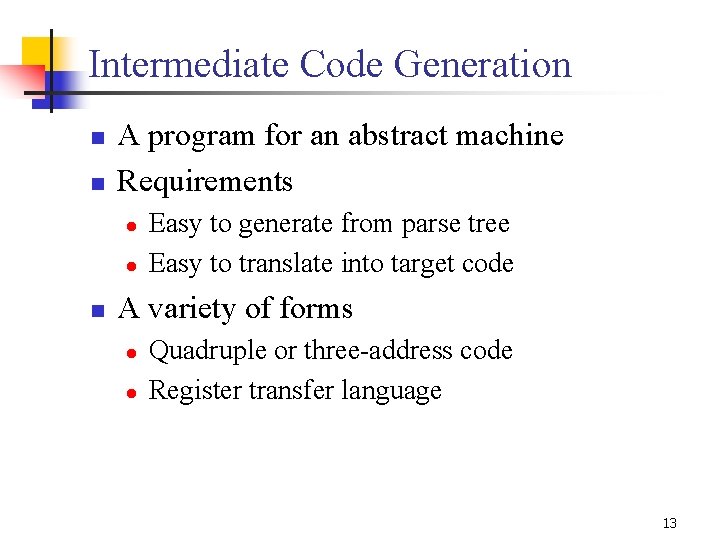
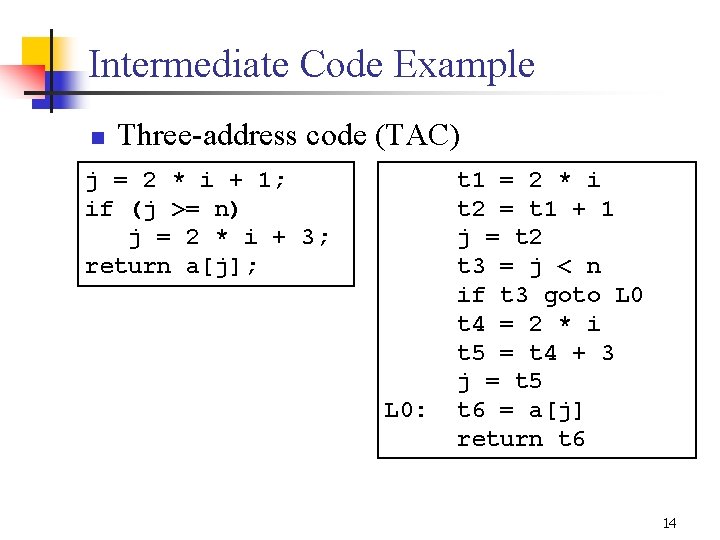
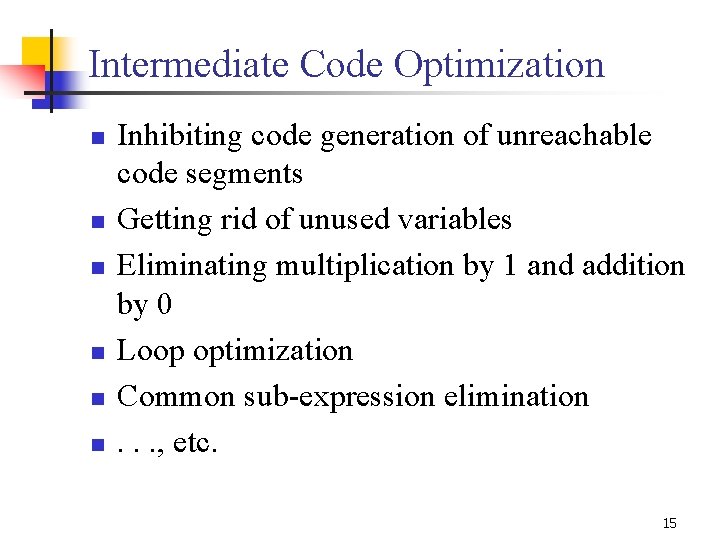
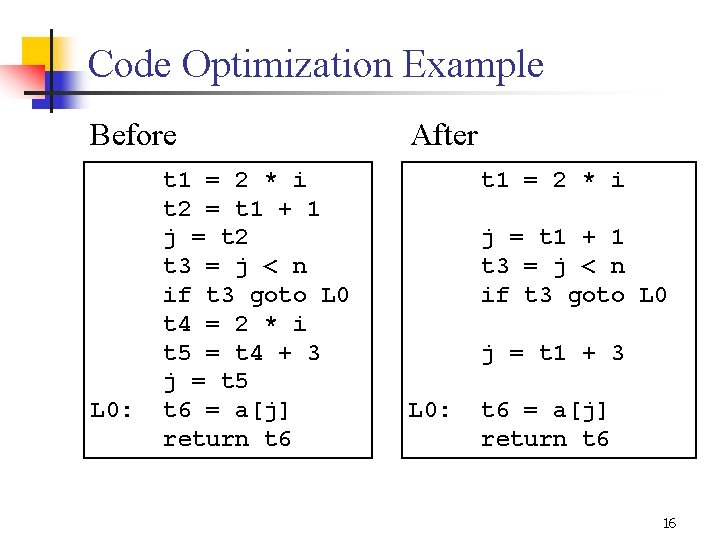
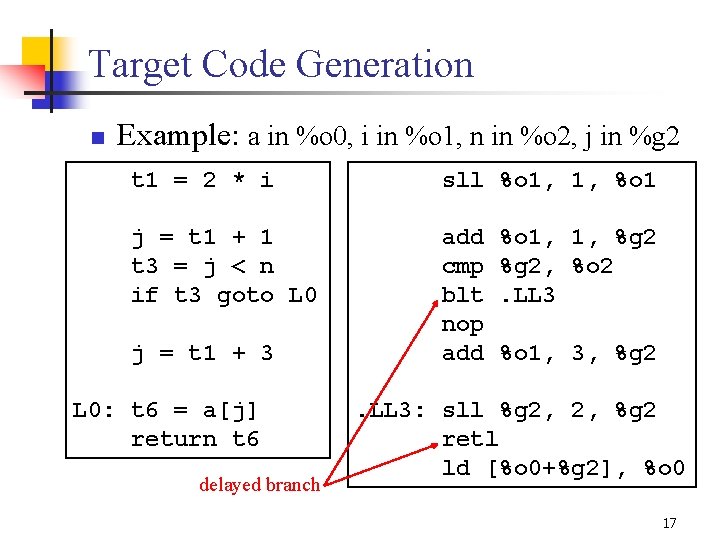
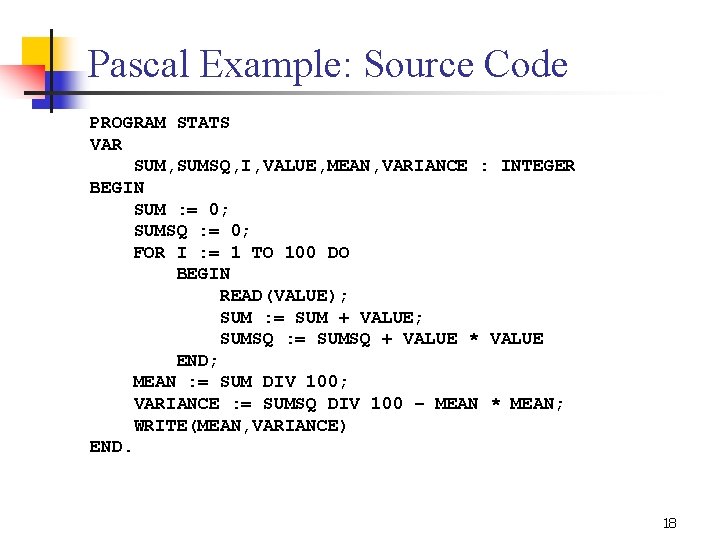
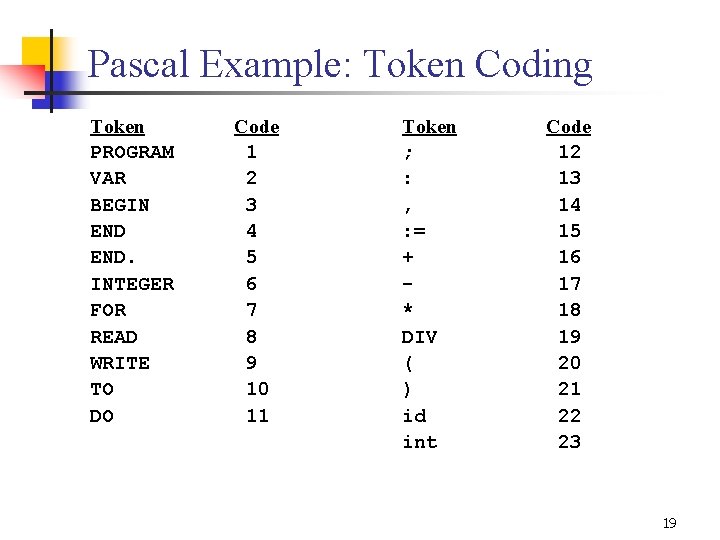
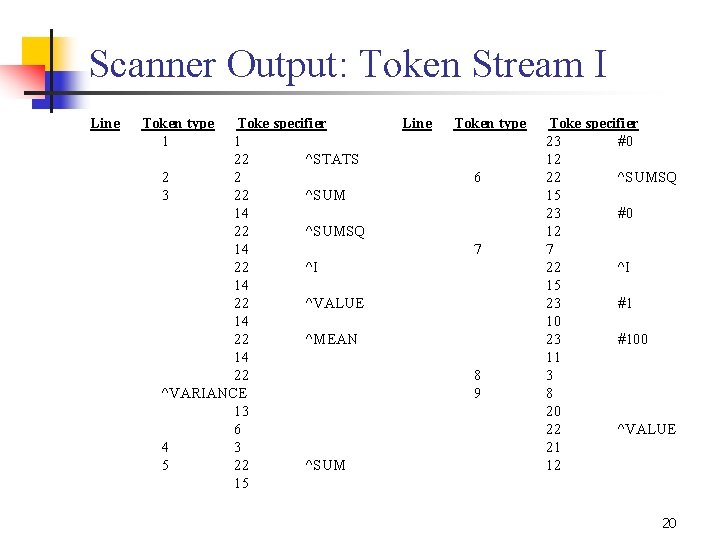
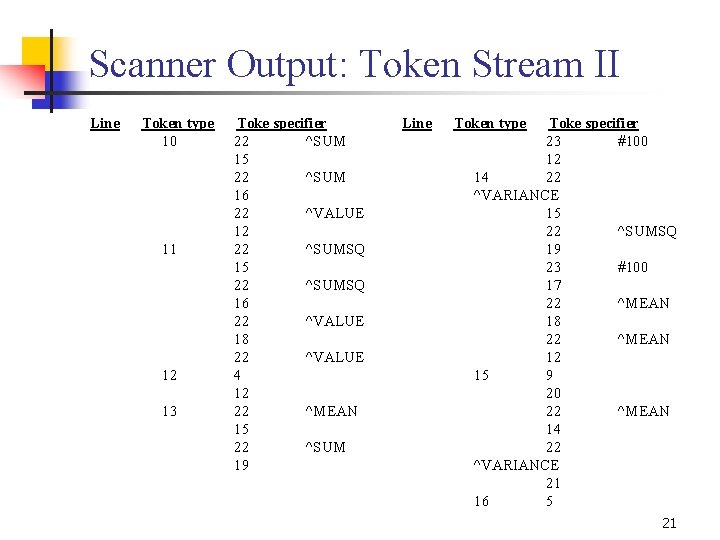
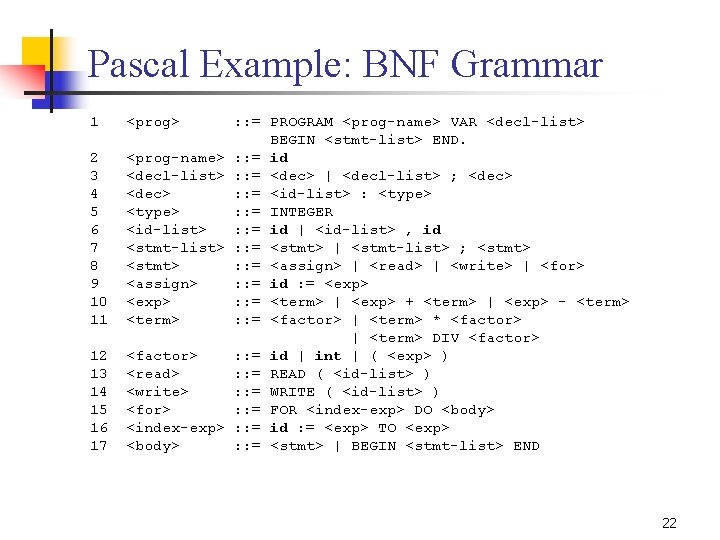
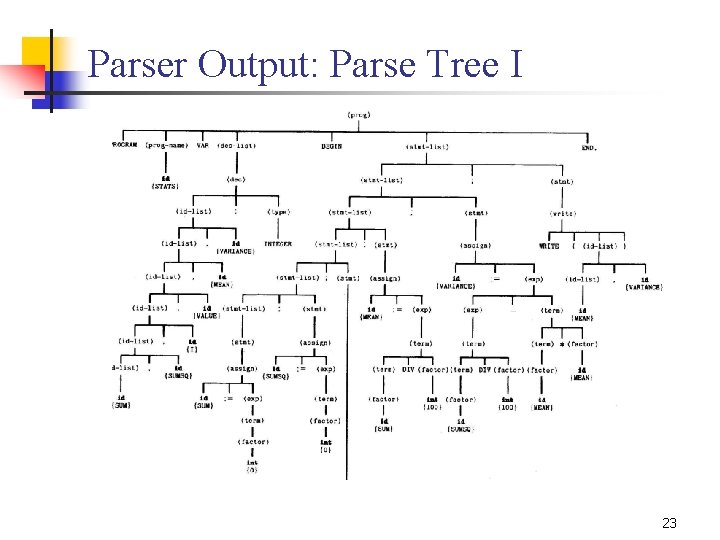
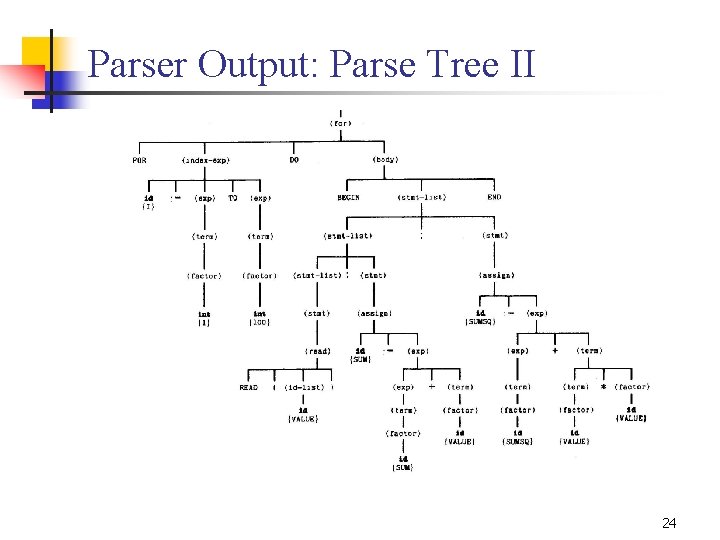
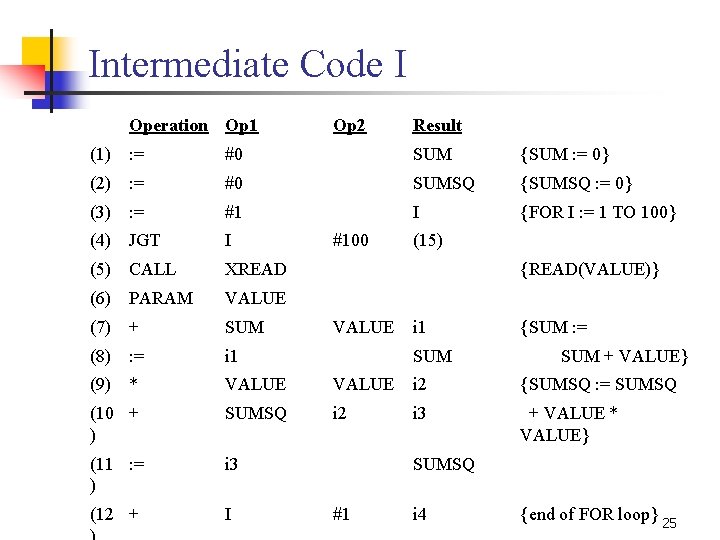
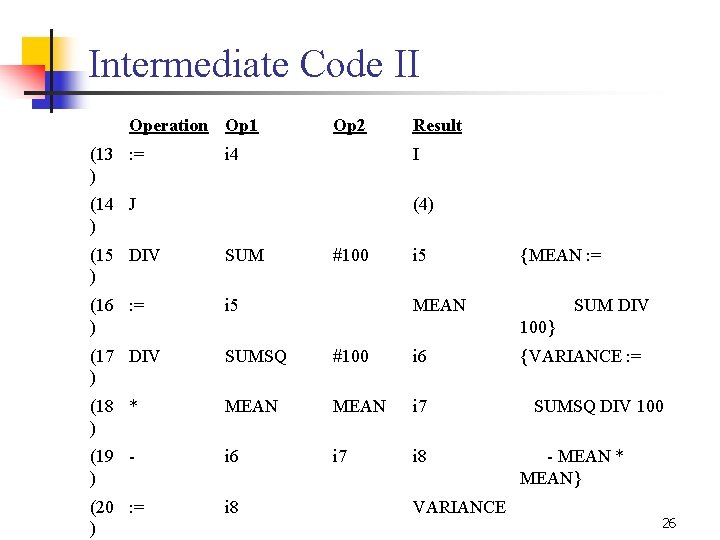
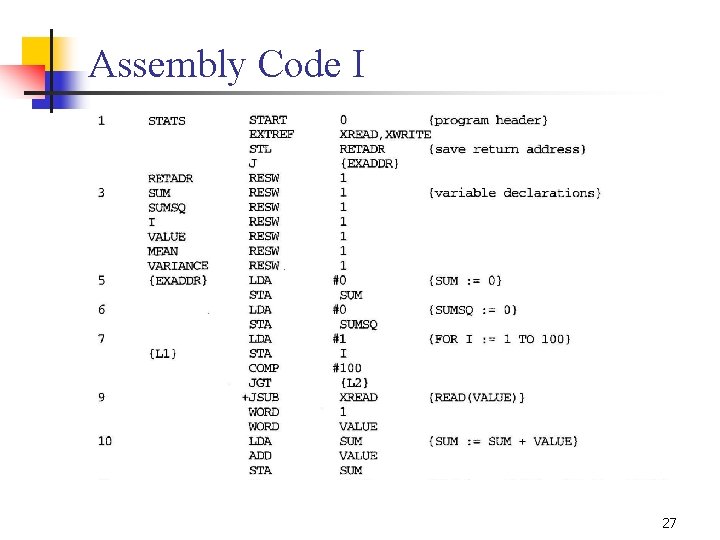
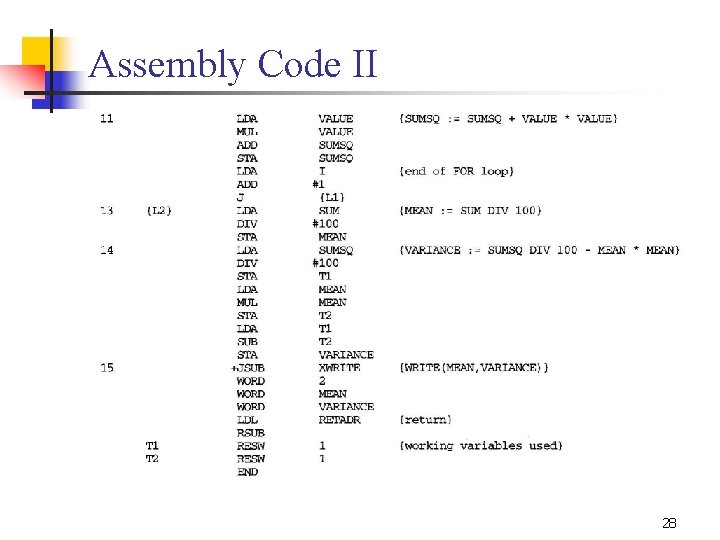
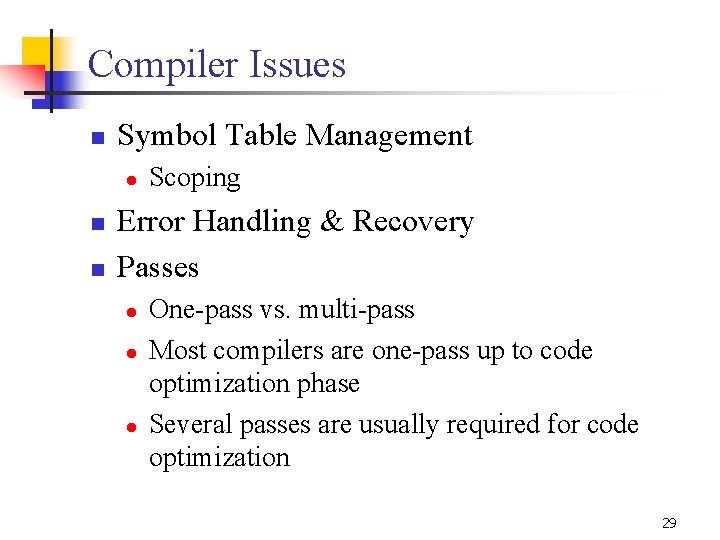
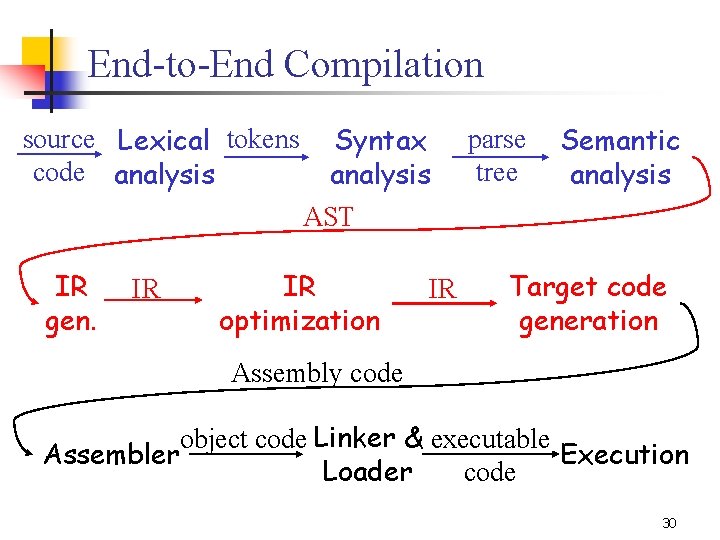
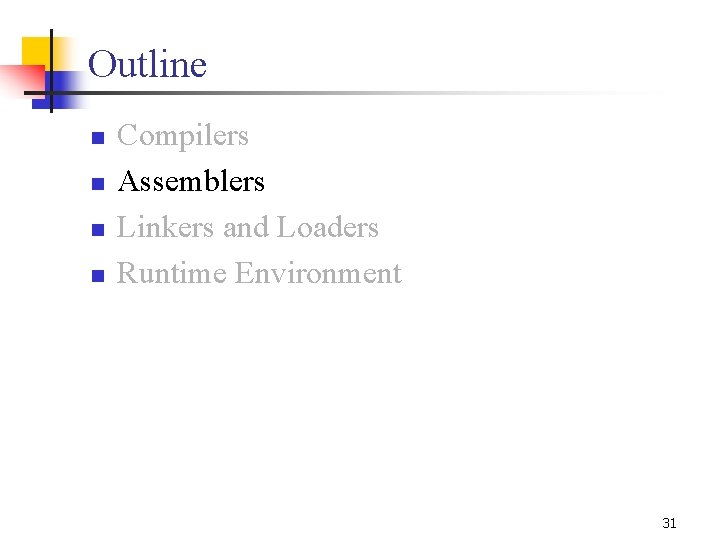
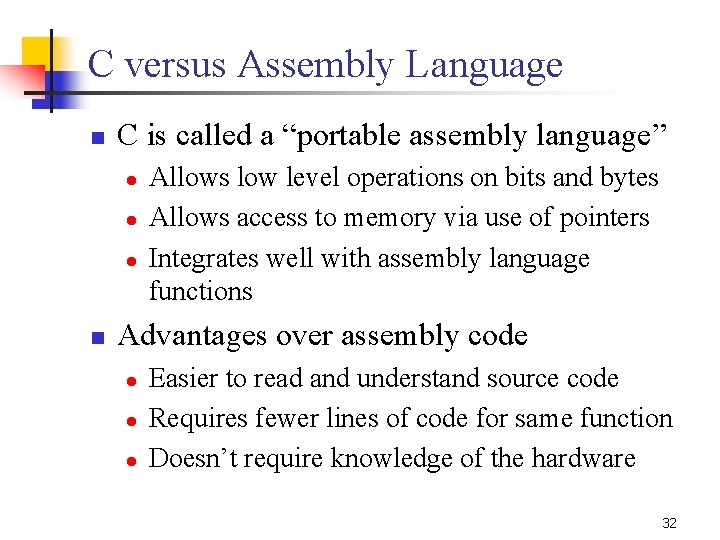
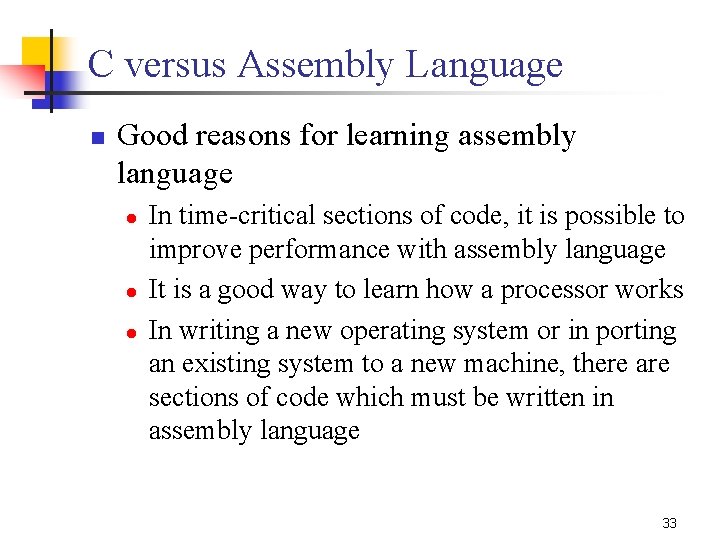
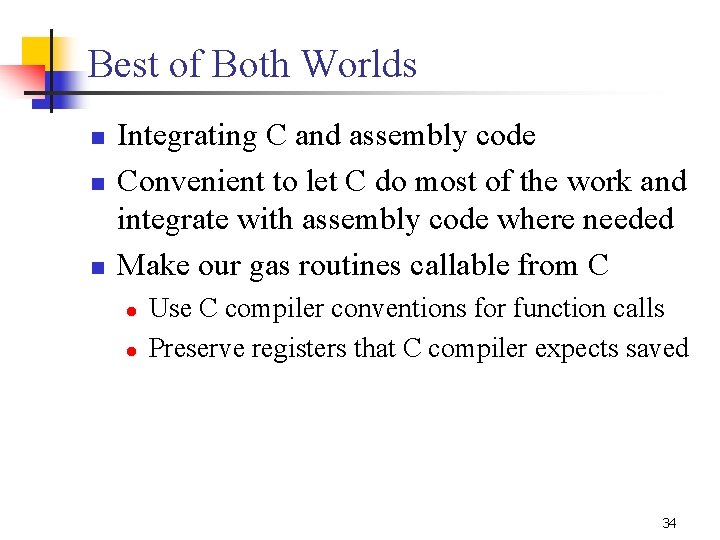
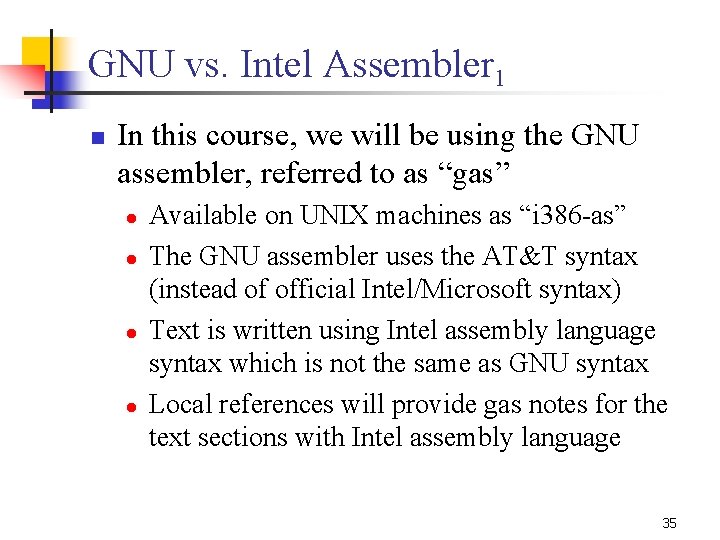
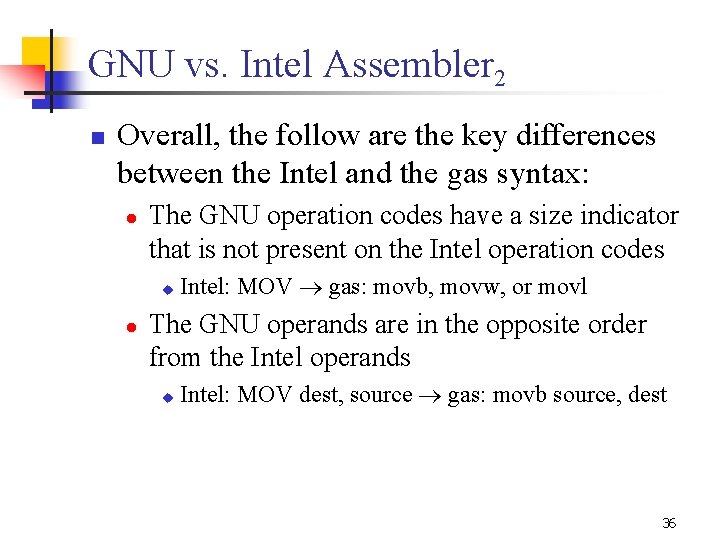
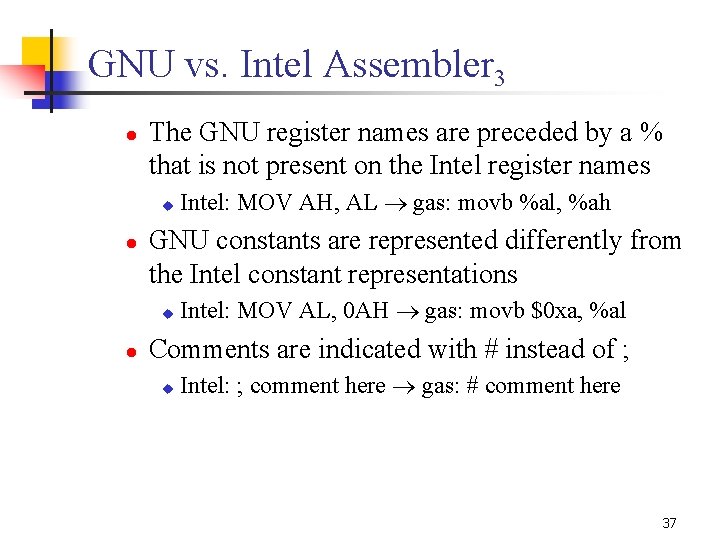
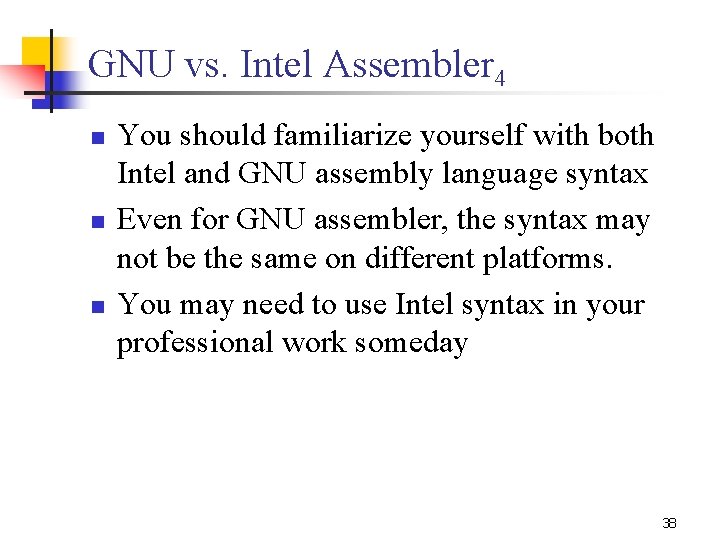
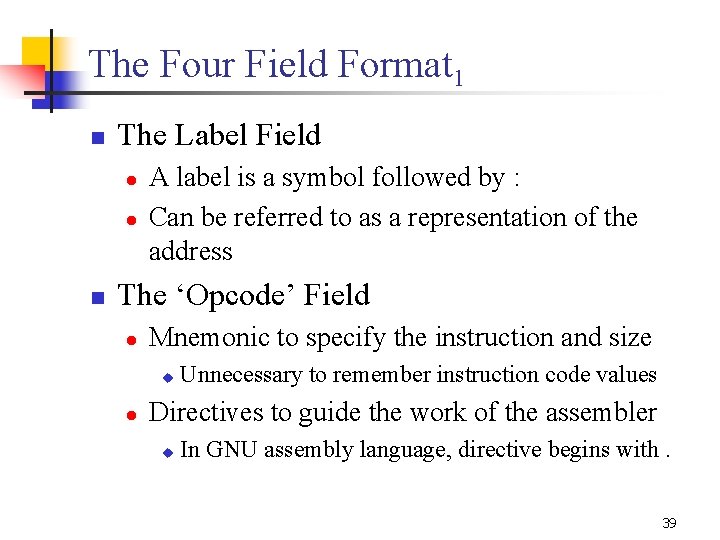
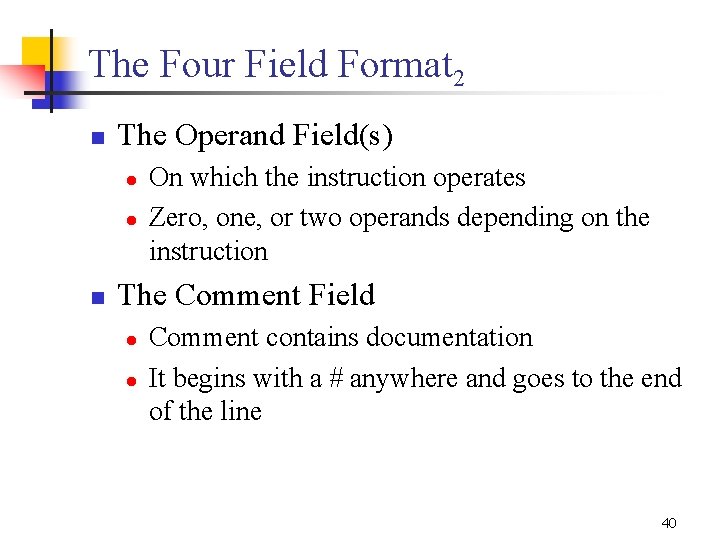
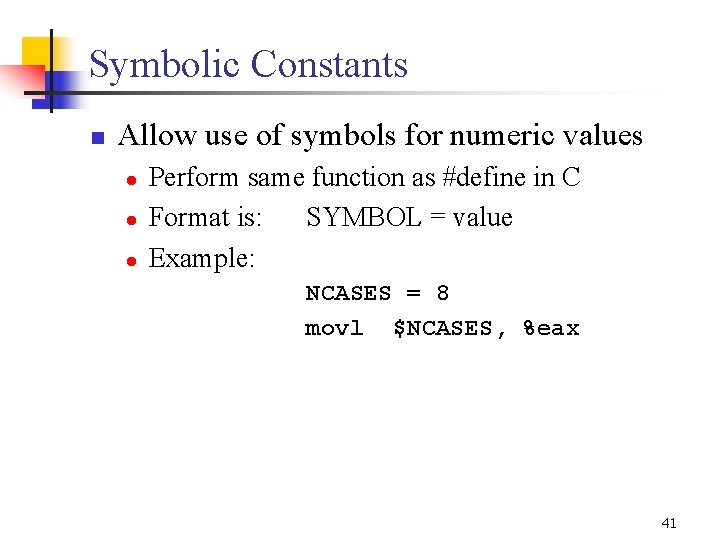
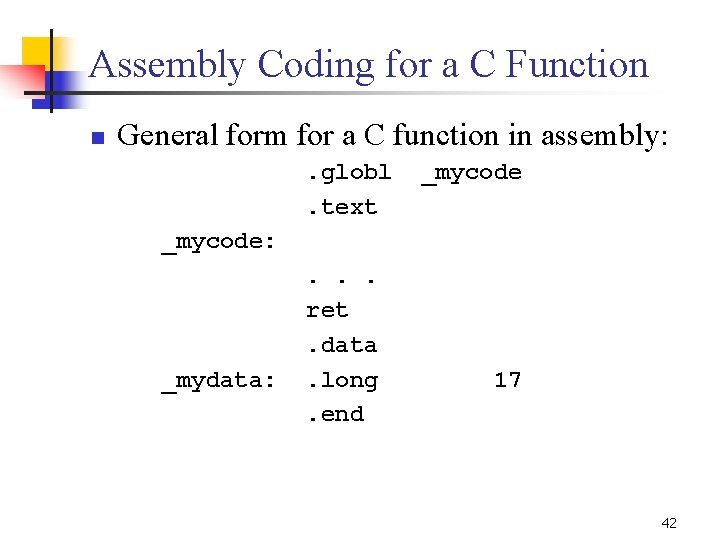
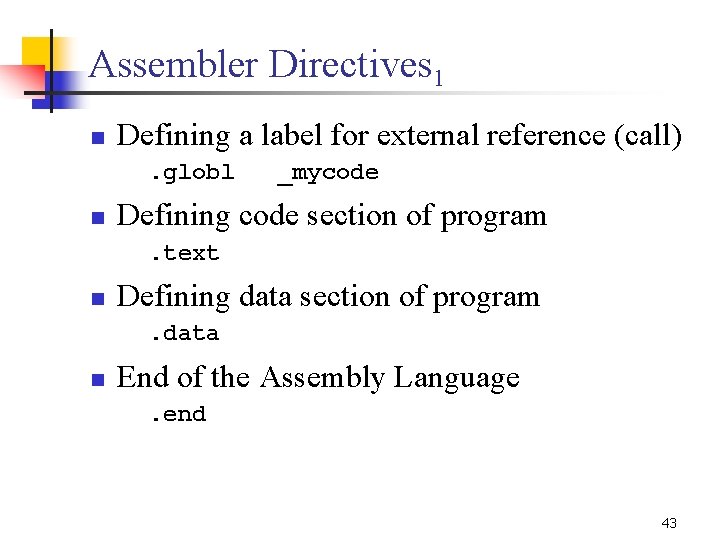
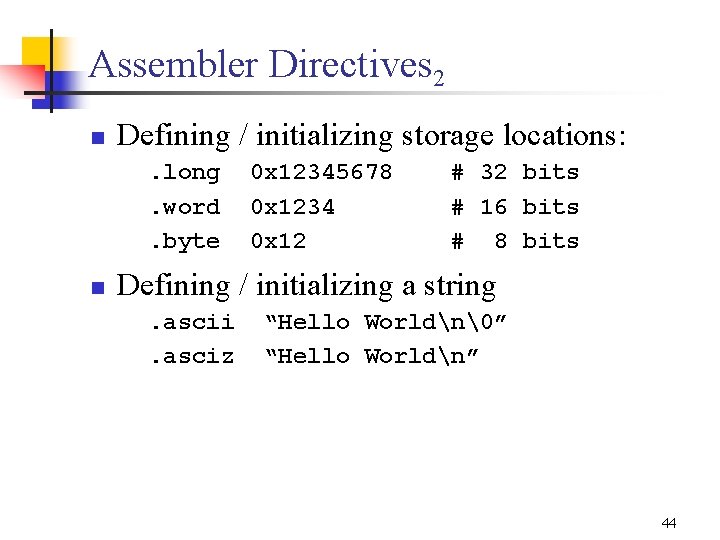
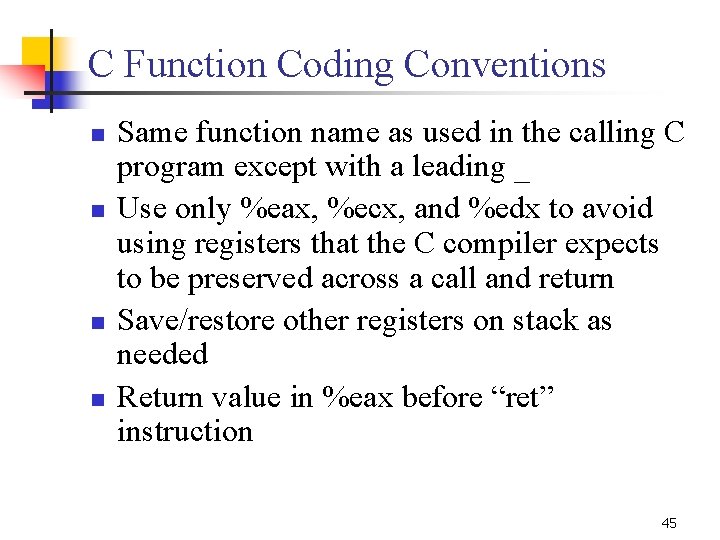
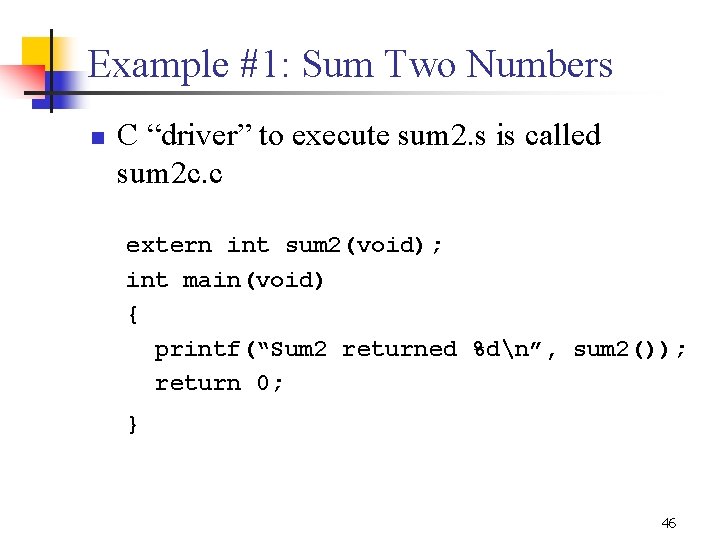
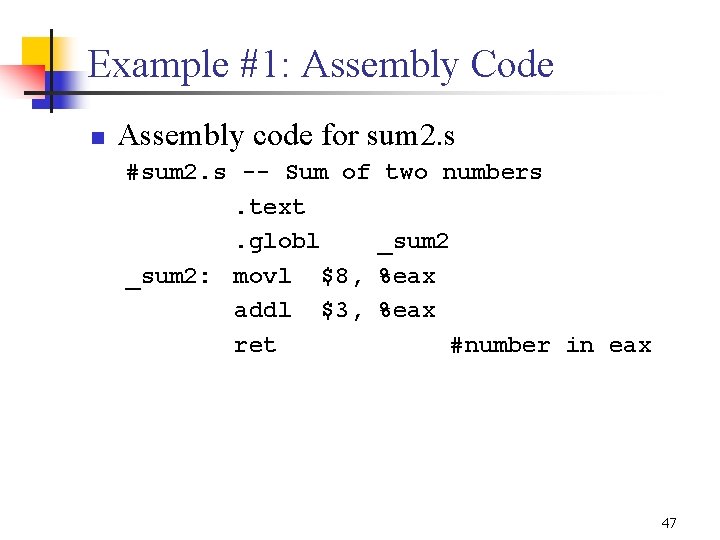
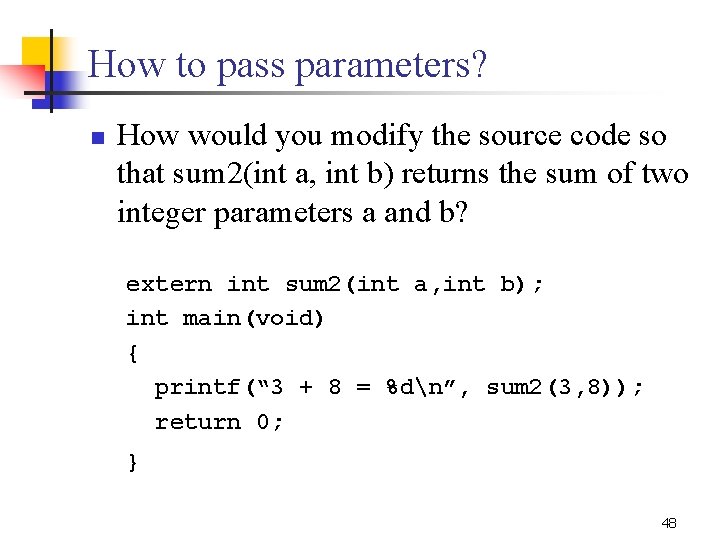
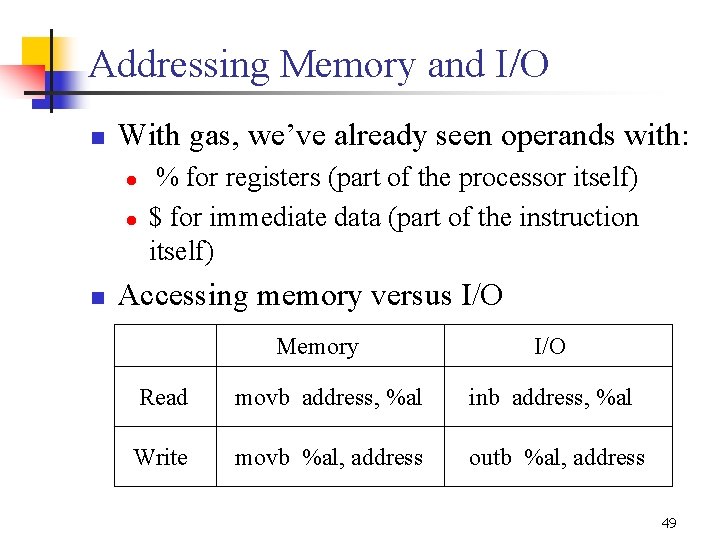
![Addressing Memory 1 n Direct addressing for memory l l Intel uses [ ] Addressing Memory 1 n Direct addressing for memory l l Intel uses [ ]](https://slidetodoc.com/presentation_image_h/d6e6f5f51917c4973116f9f00071c180/image-50.jpg)
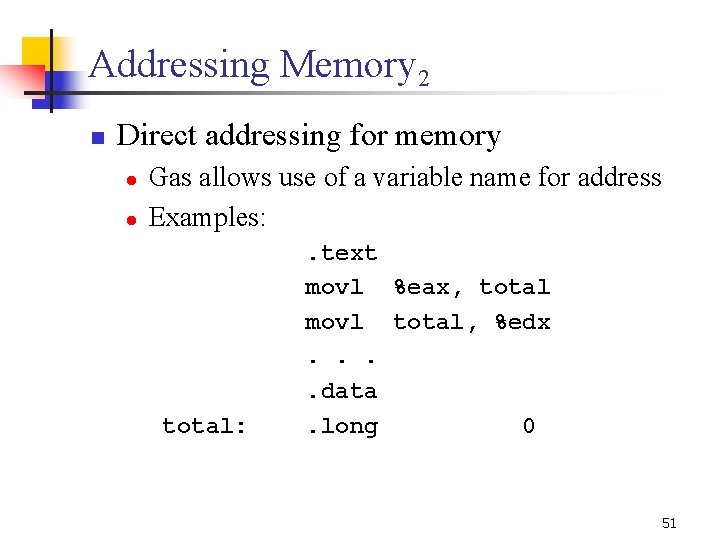
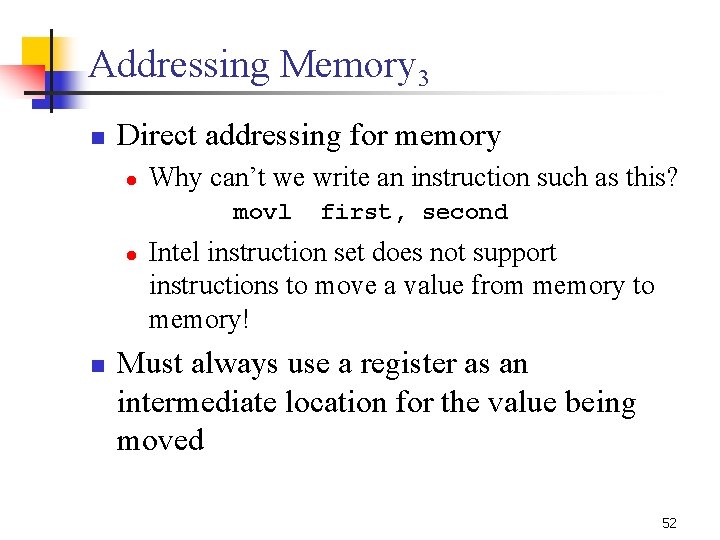
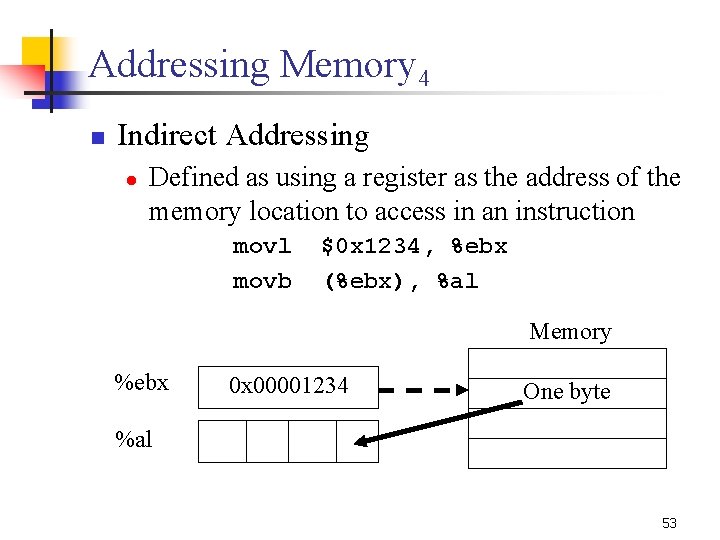
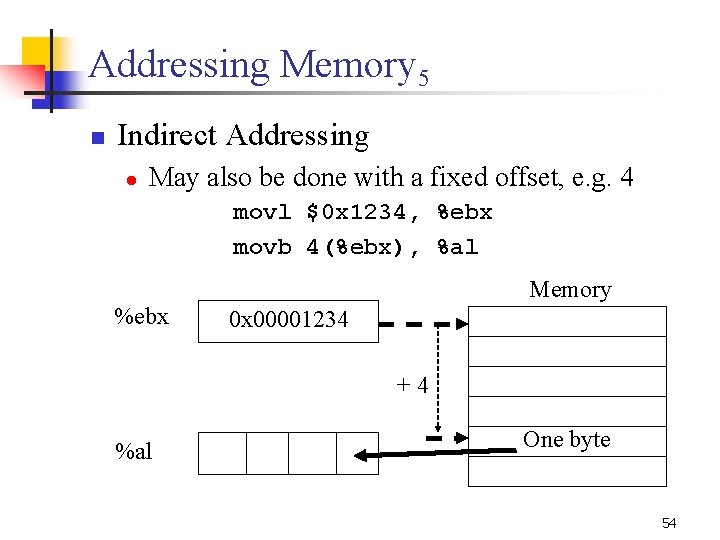
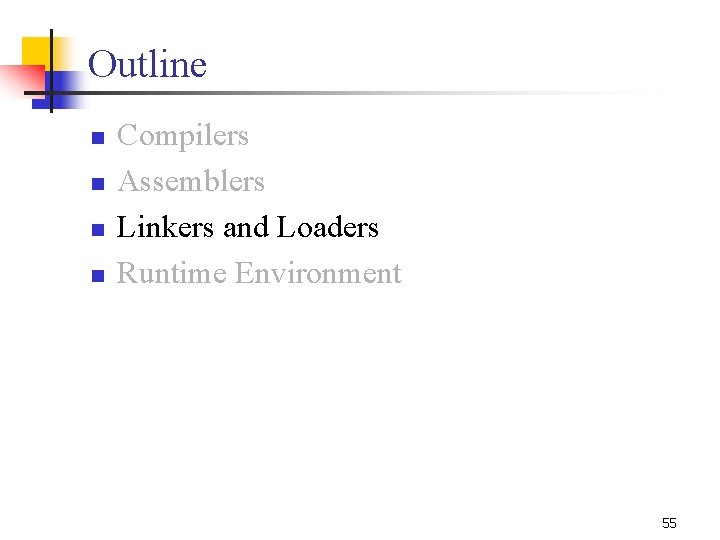
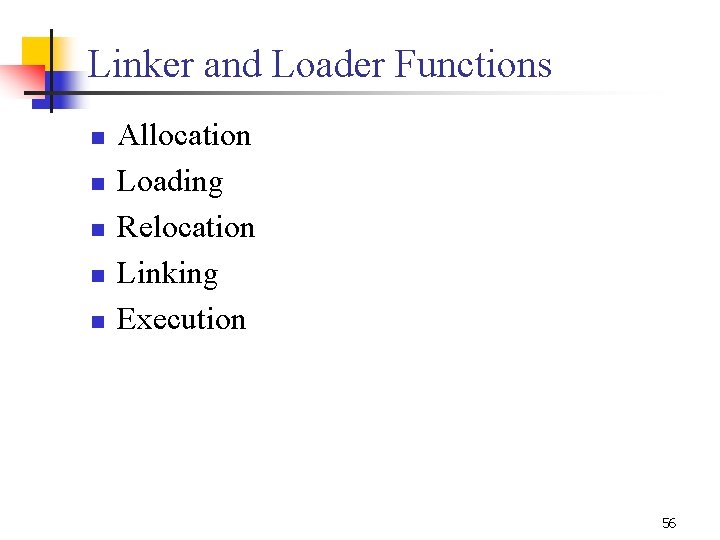
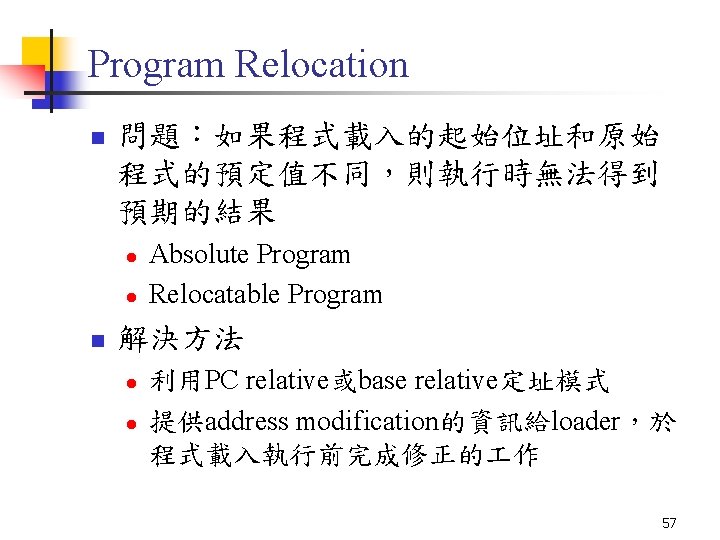
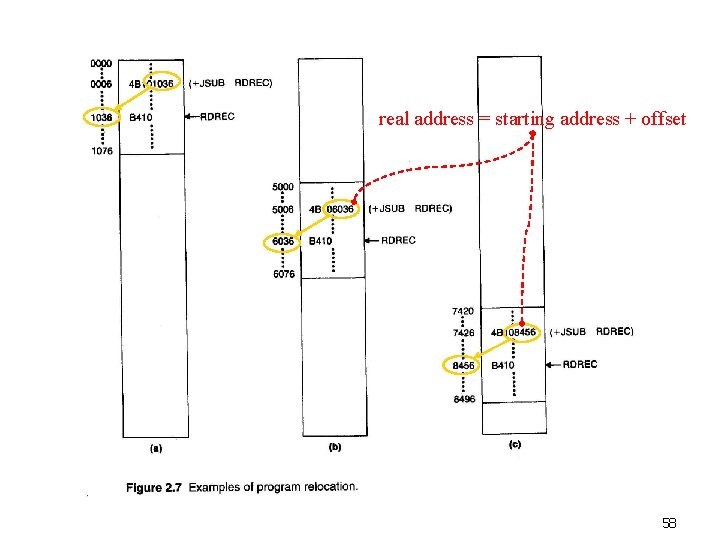
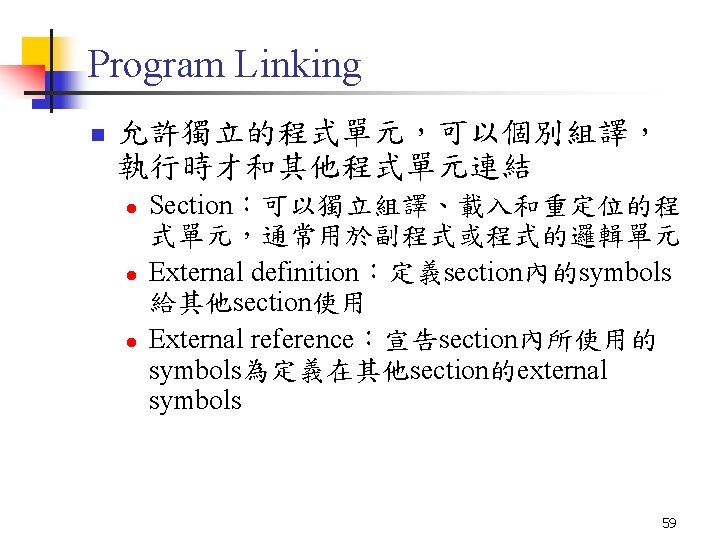
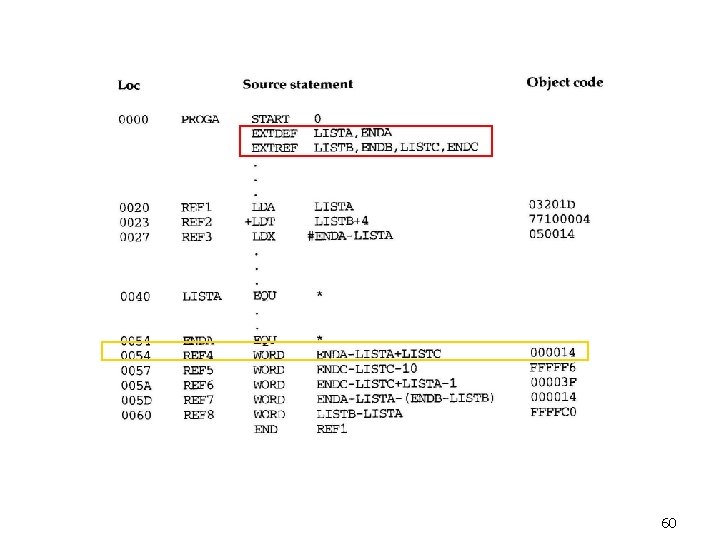
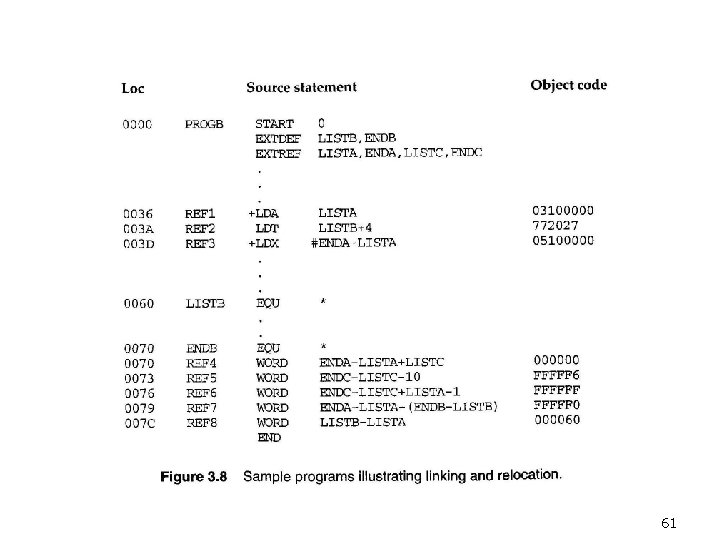
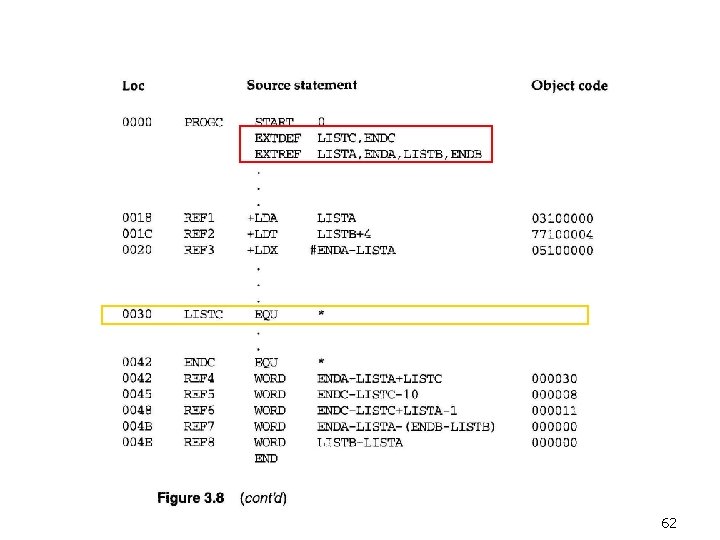
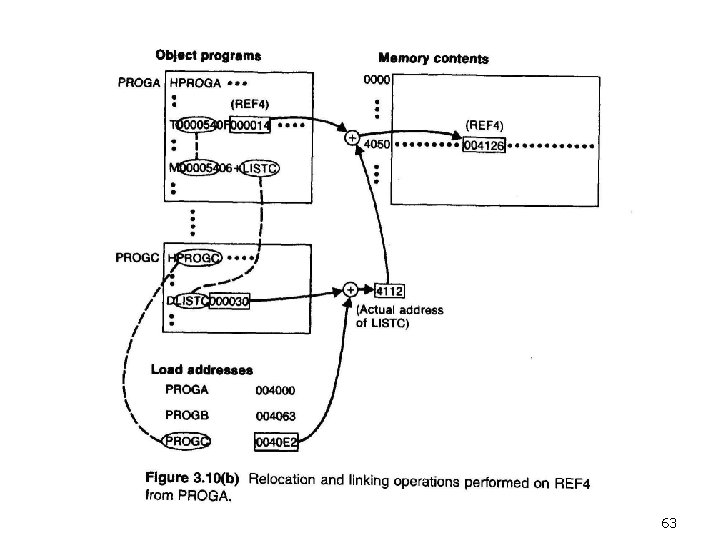
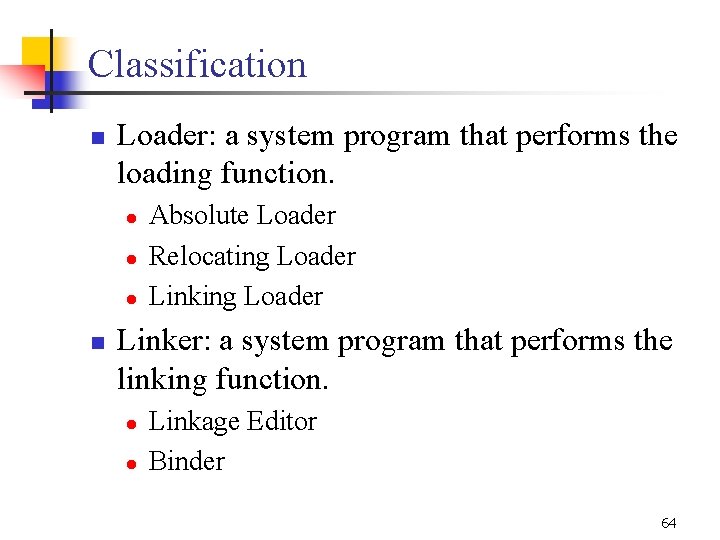
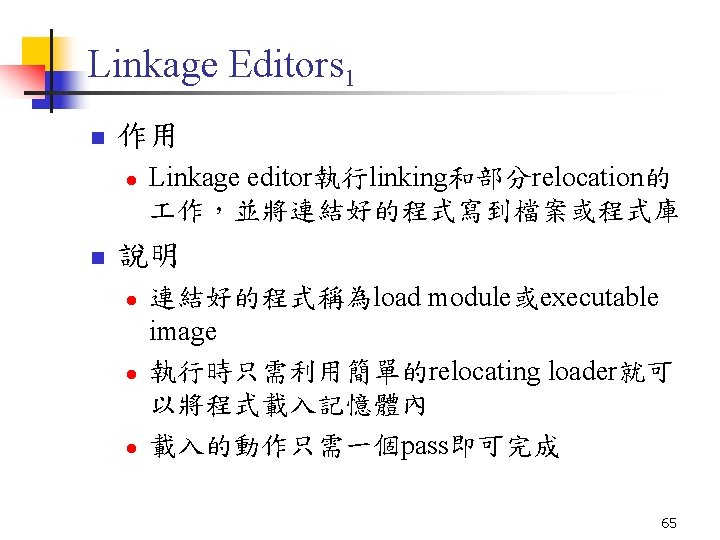
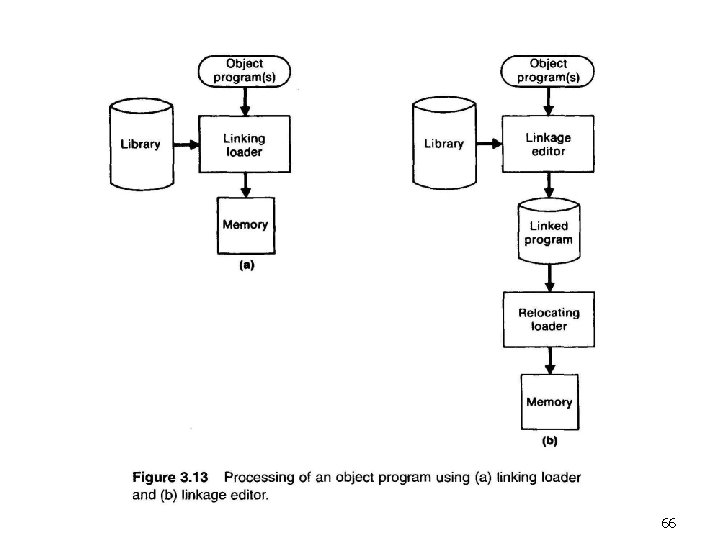
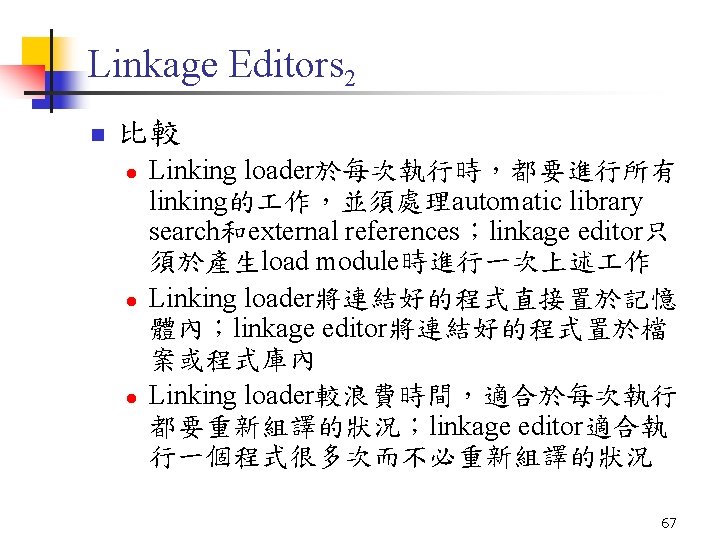
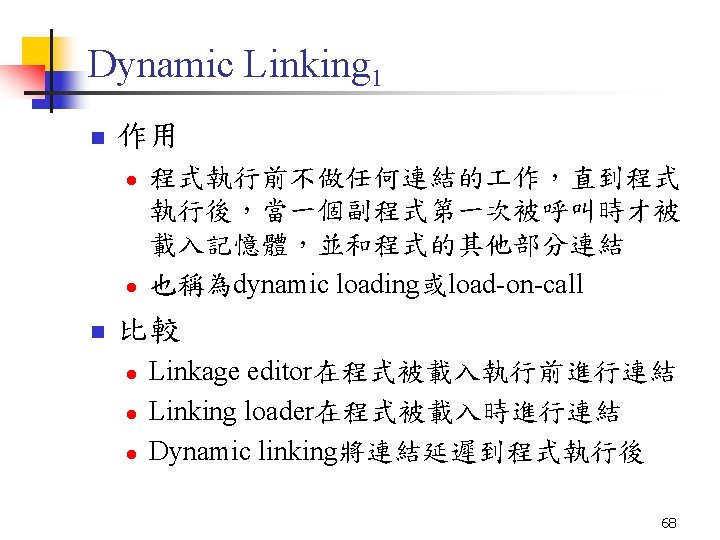
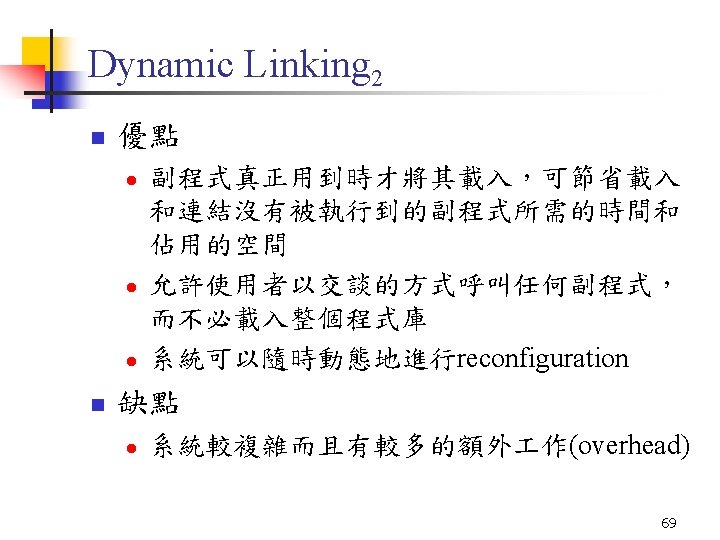
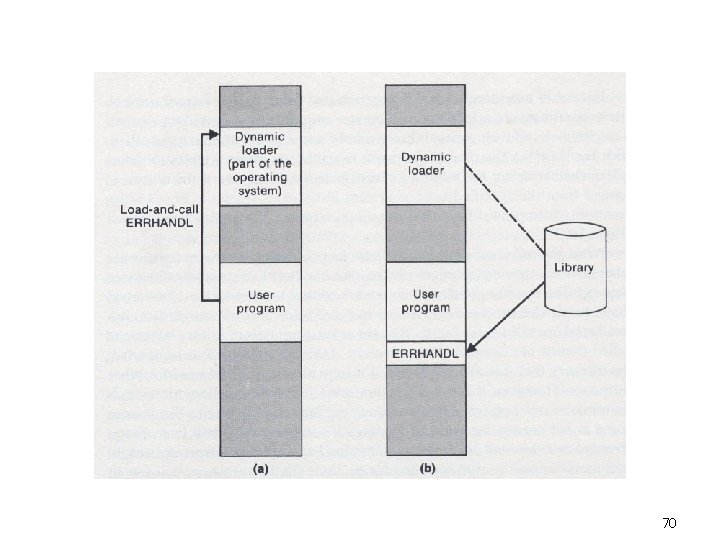
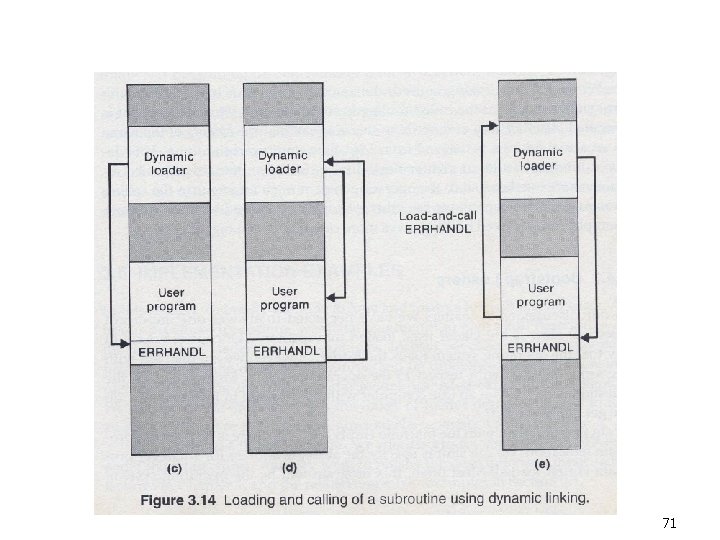
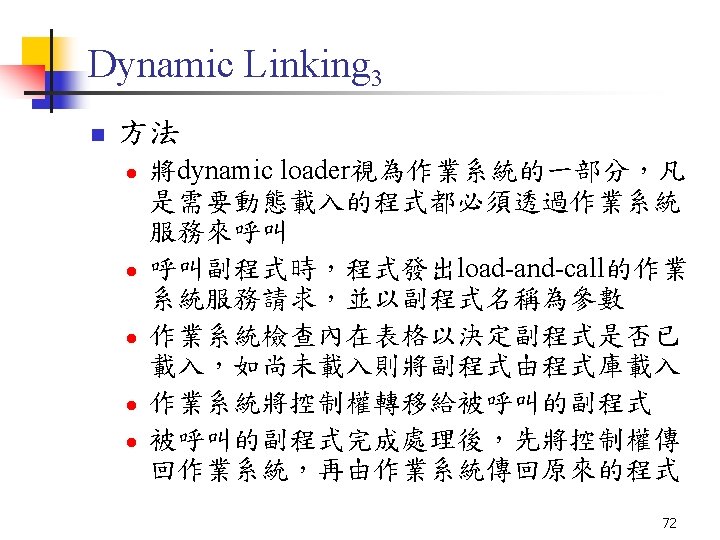
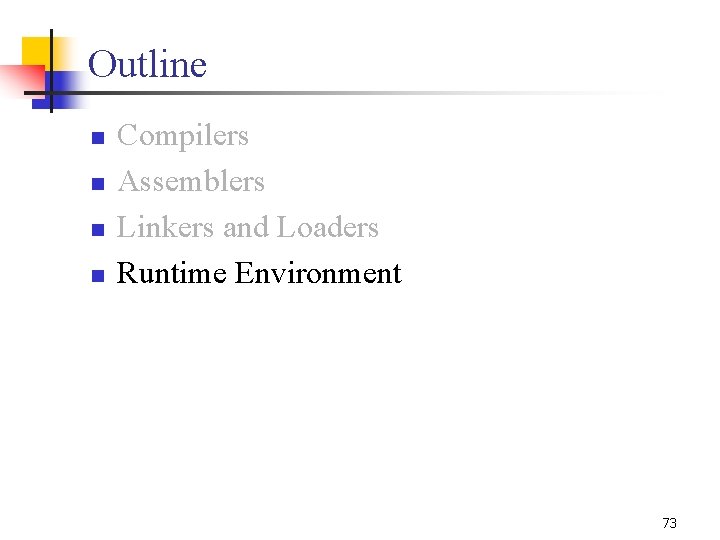
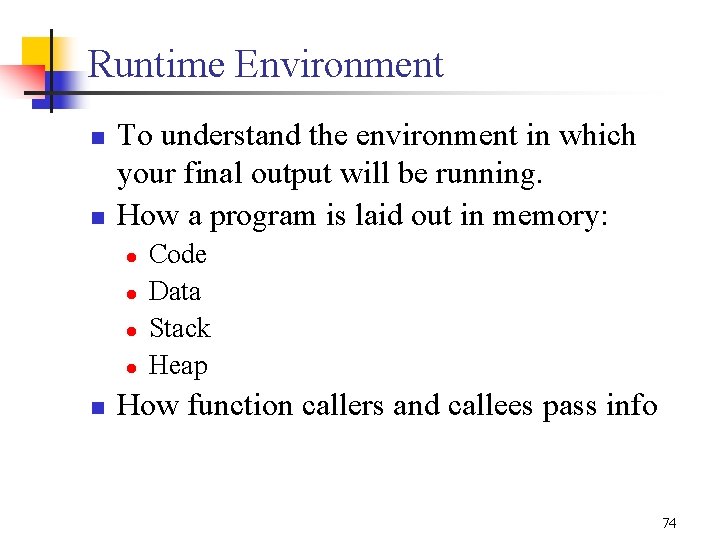
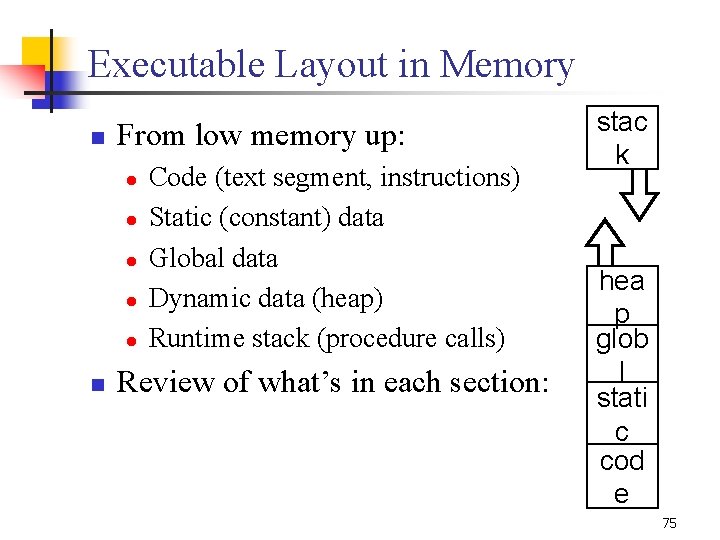
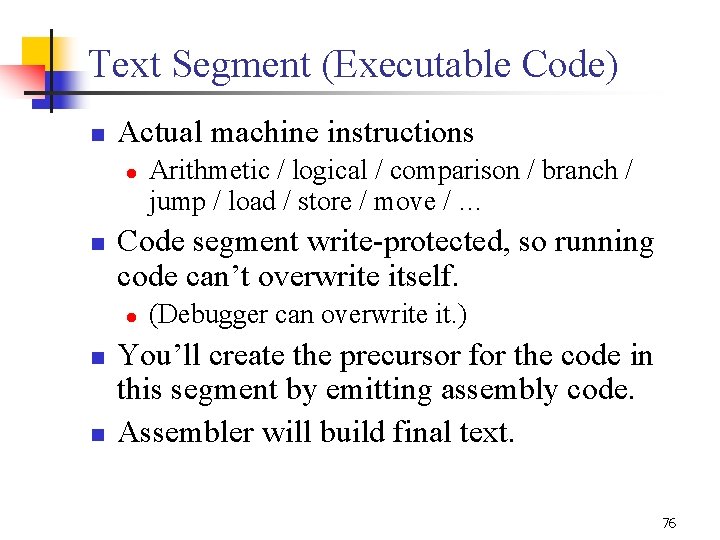
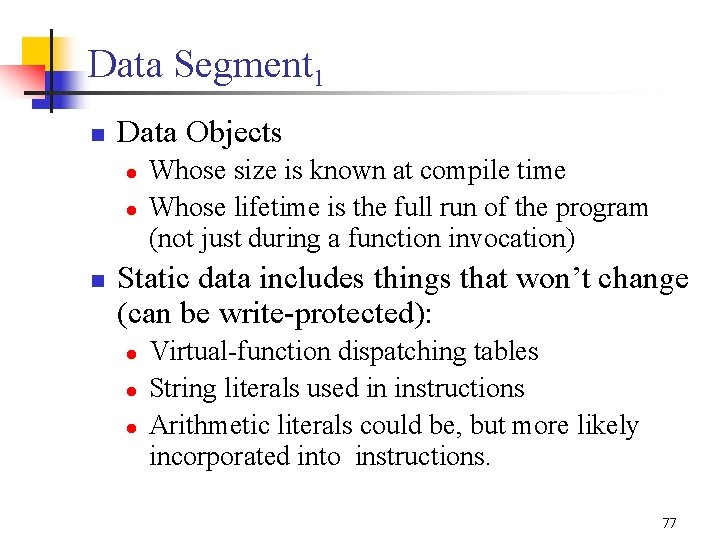
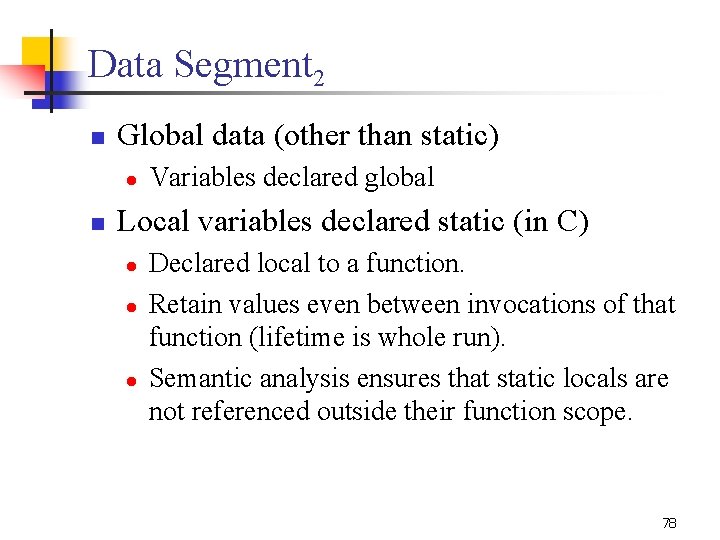
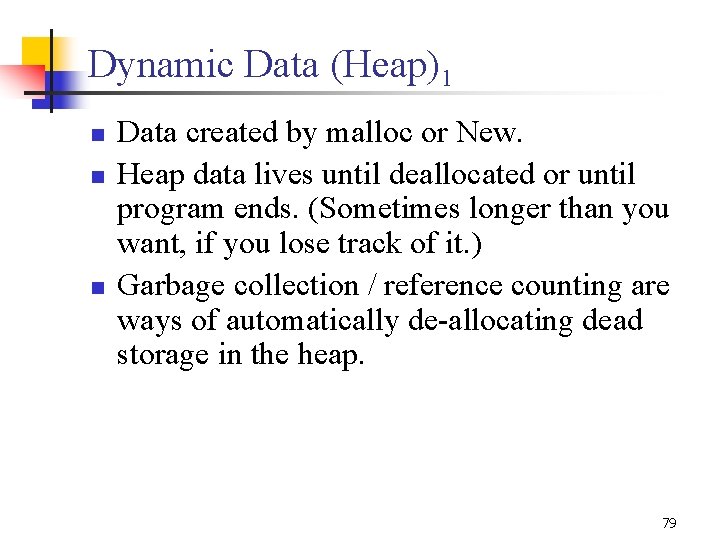
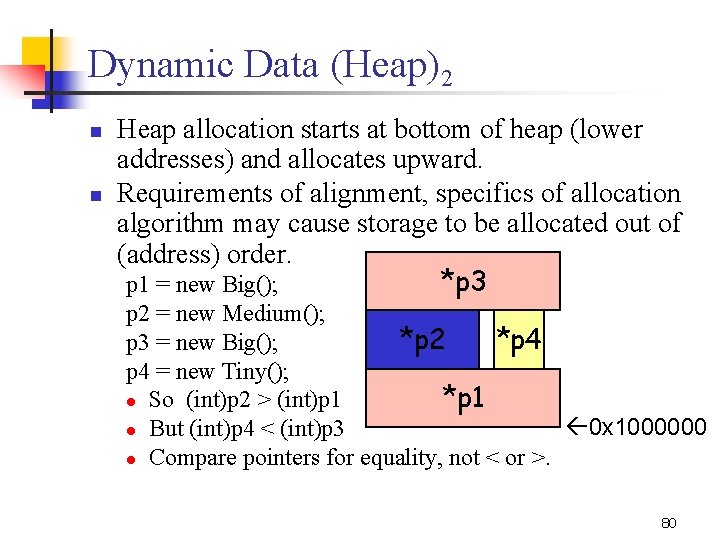
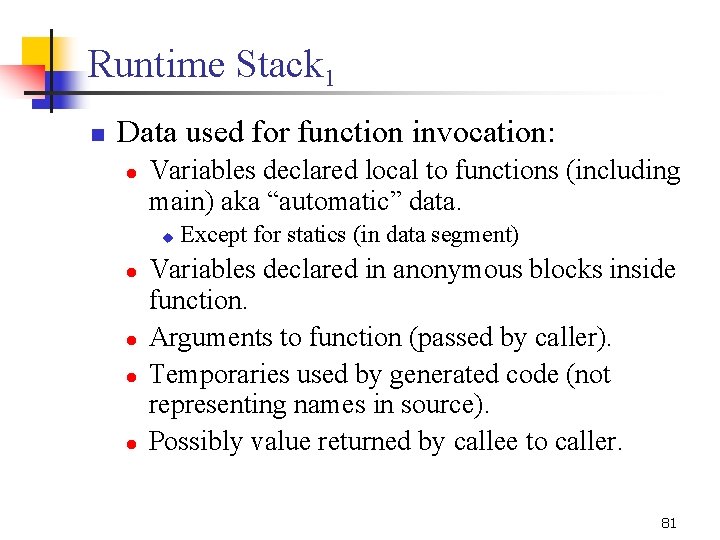
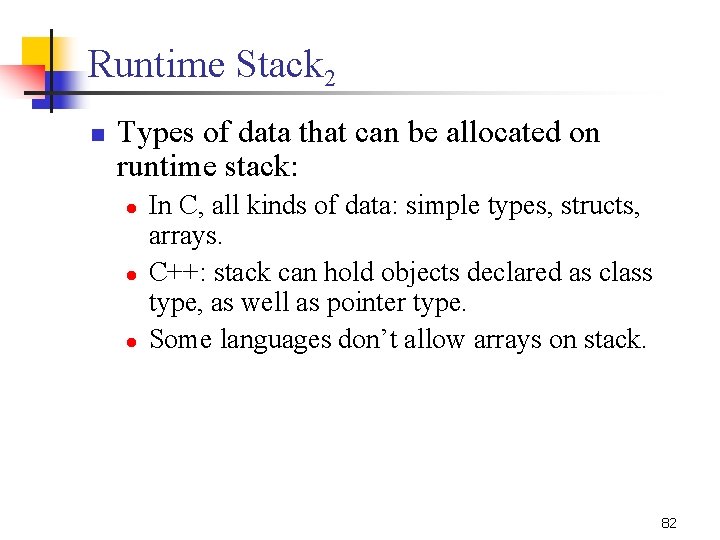
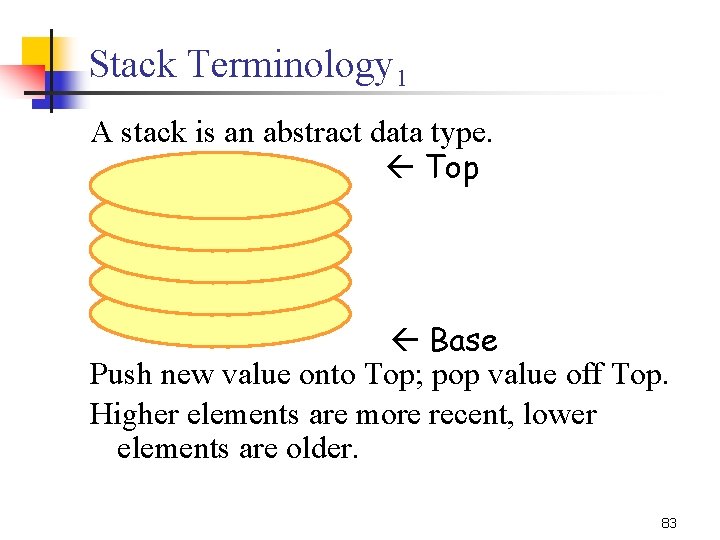
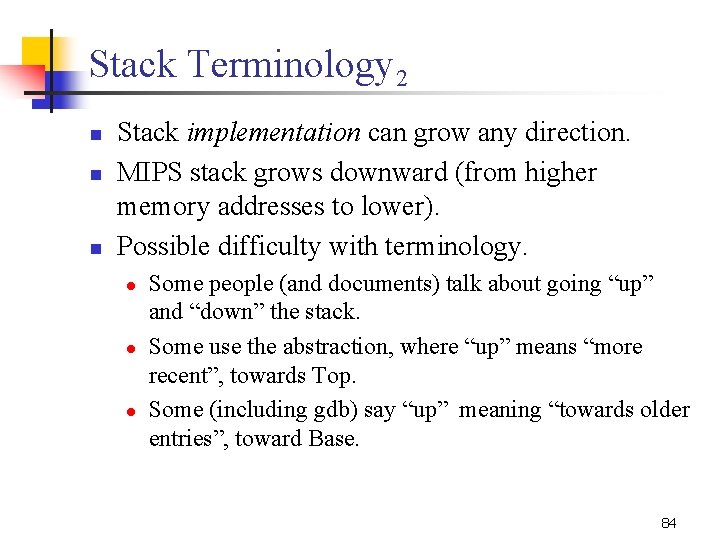
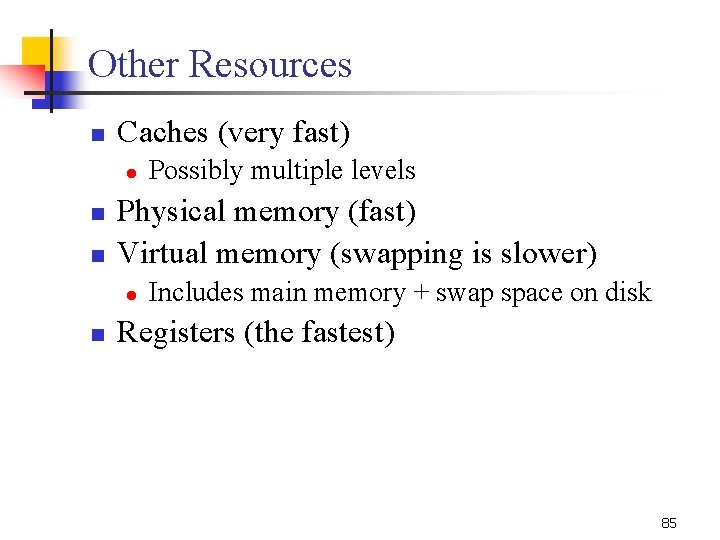
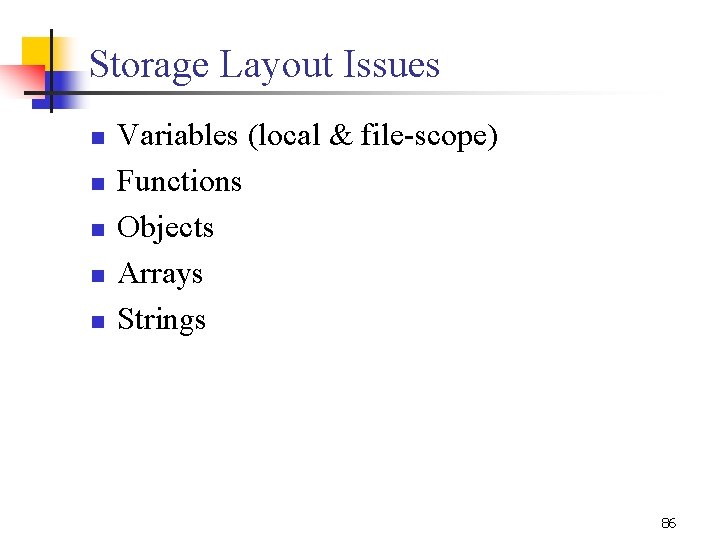
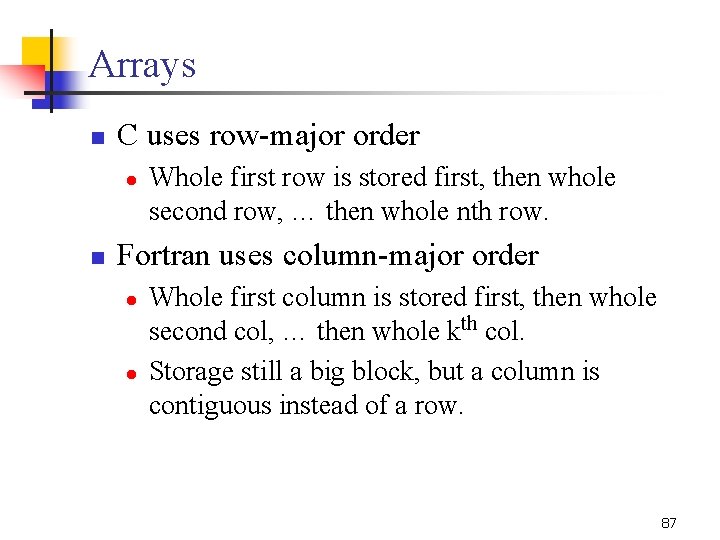
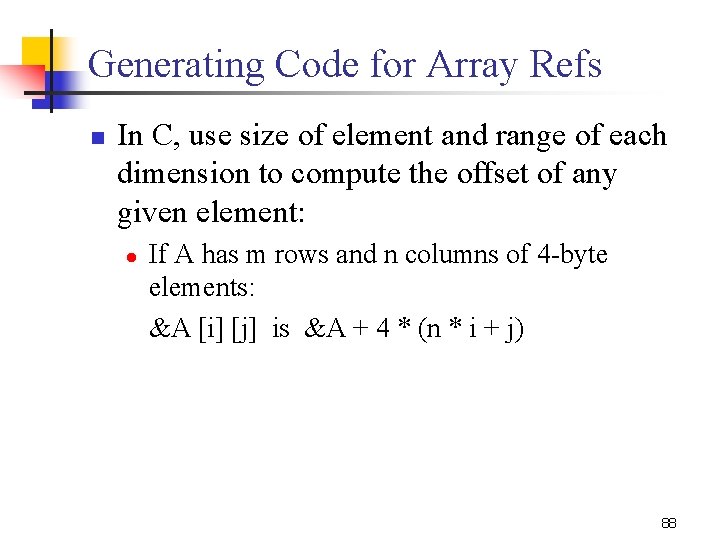
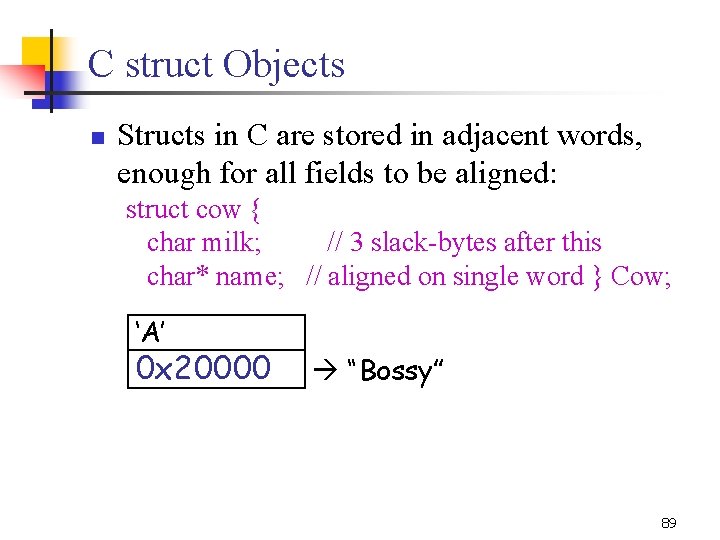
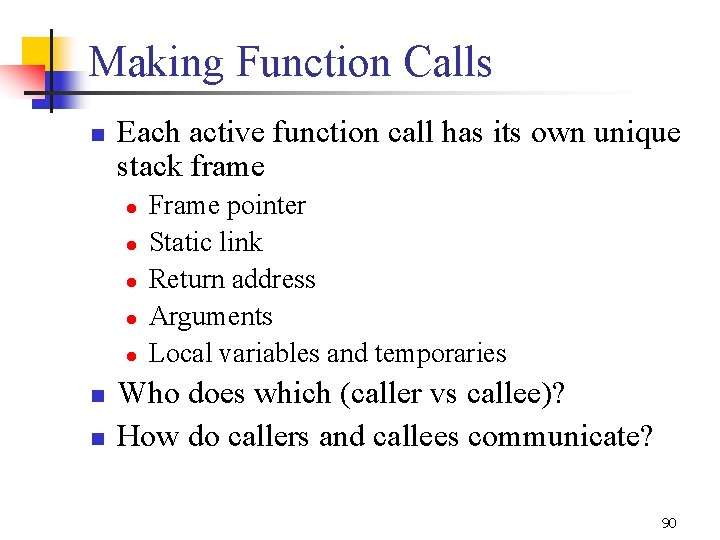
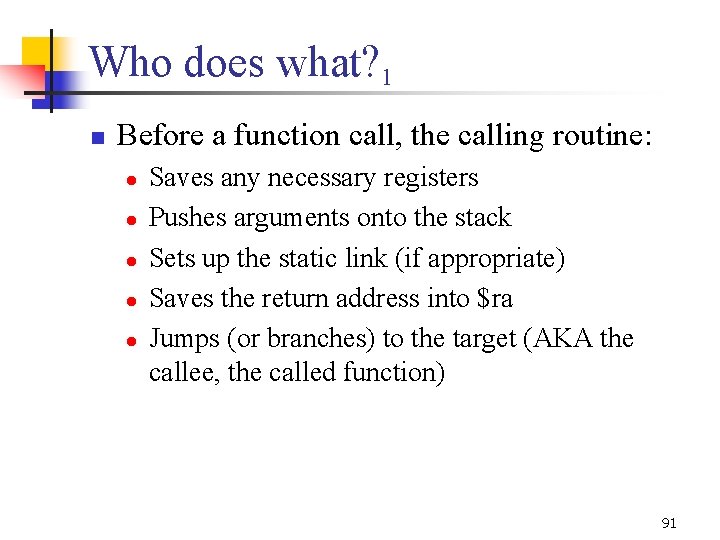
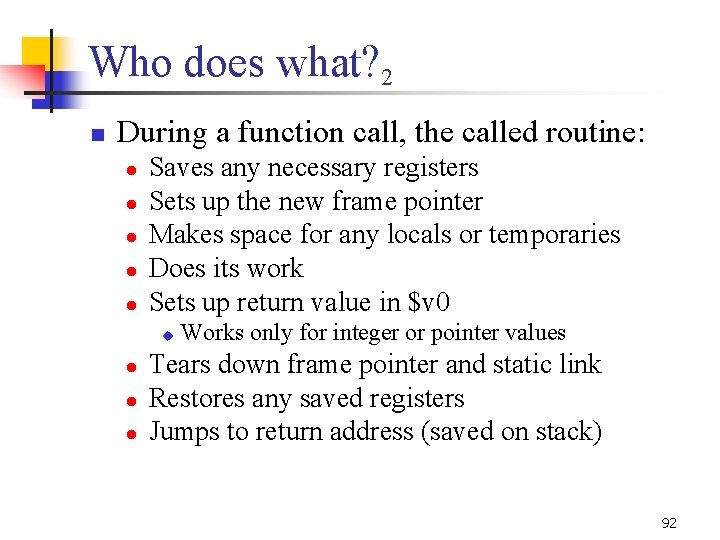
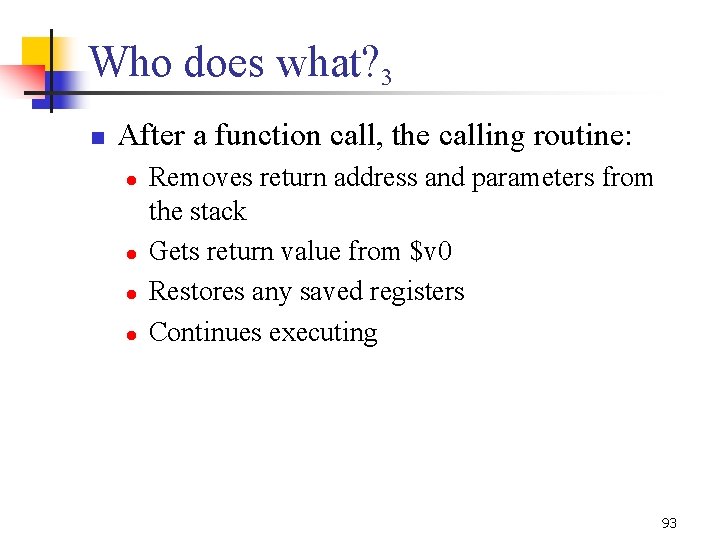
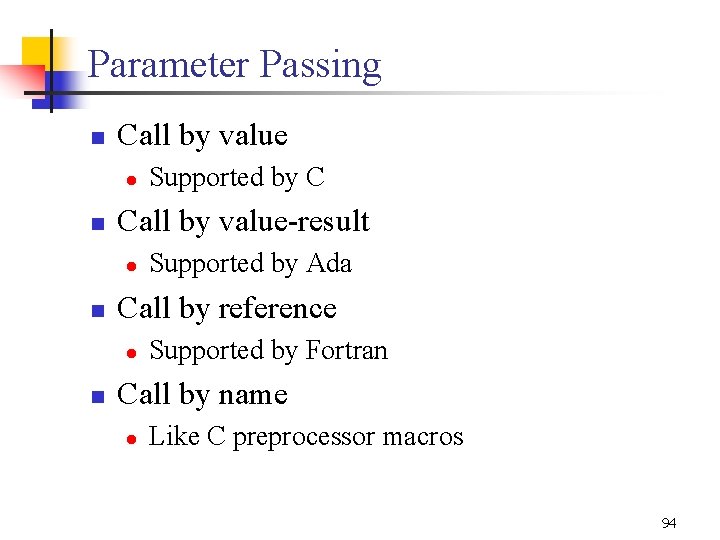
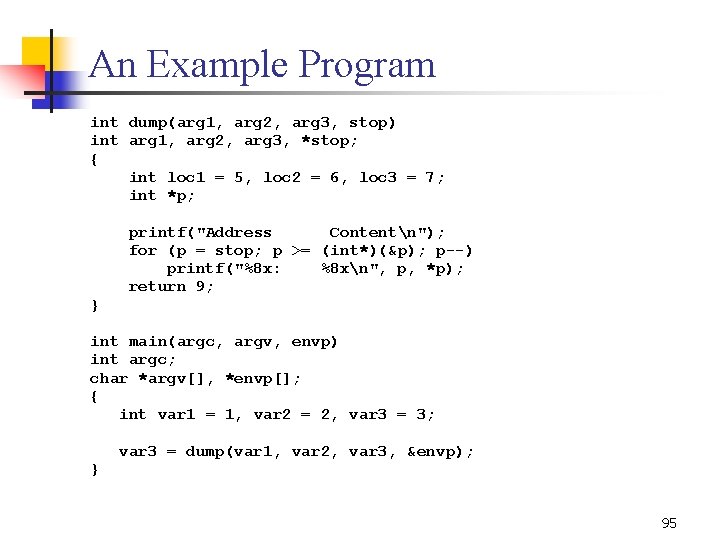
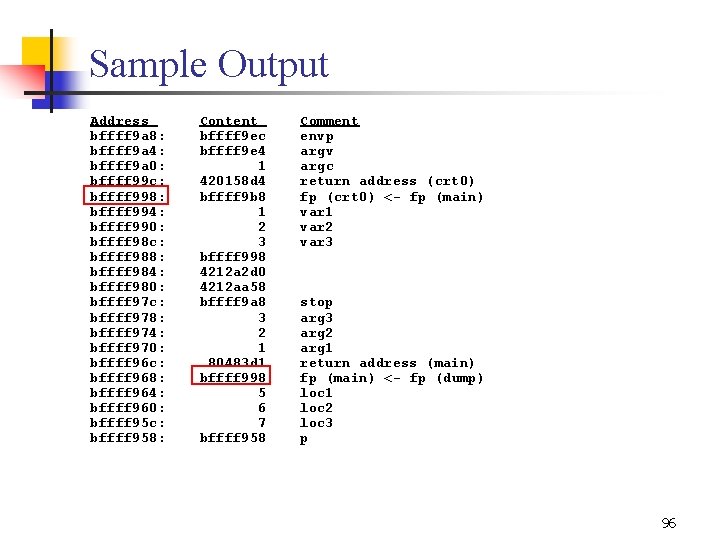
- Slides: 96
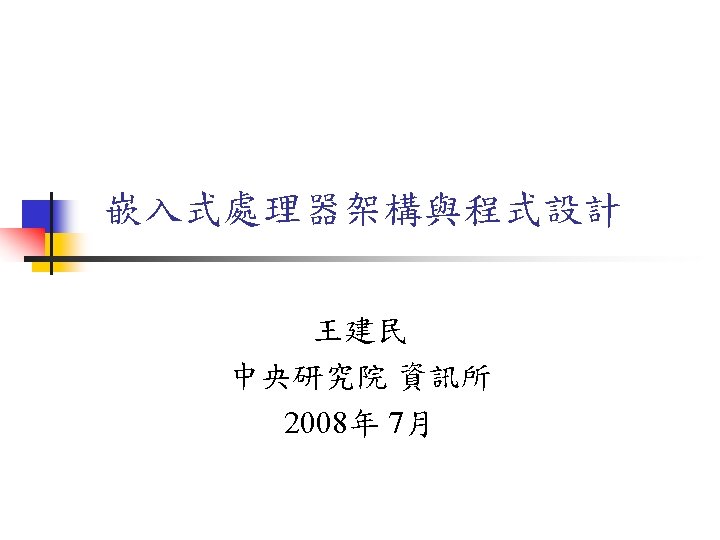
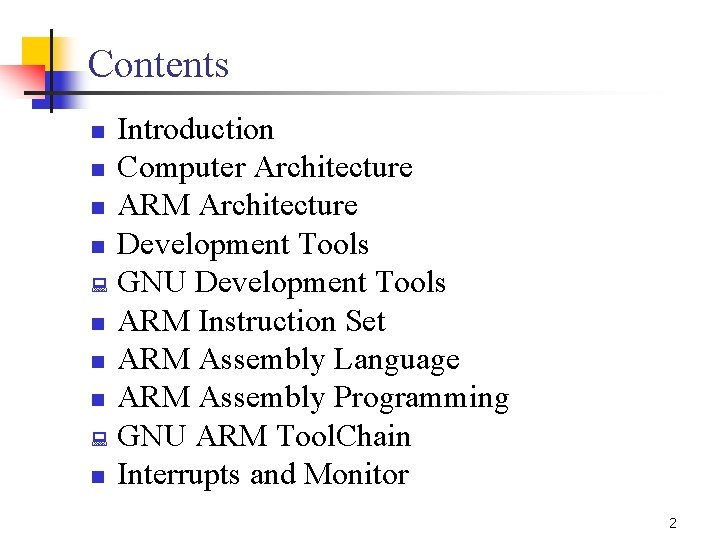
Contents Introduction n Computer Architecture n ARM Architecture n Development Tools : GNU Development Tools n ARM Instruction Set n ARM Assembly Language n ARM Assembly Programming : GNU ARM Tool. Chain n Interrupts and Monitor n 2
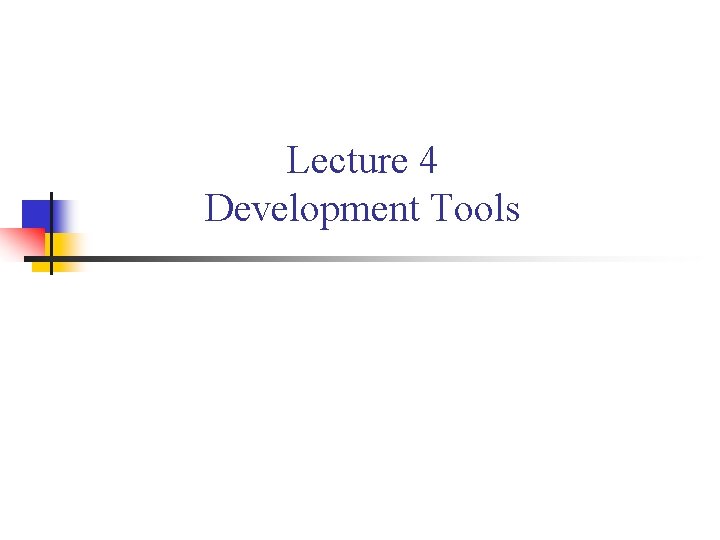
Lecture 4 Development Tools
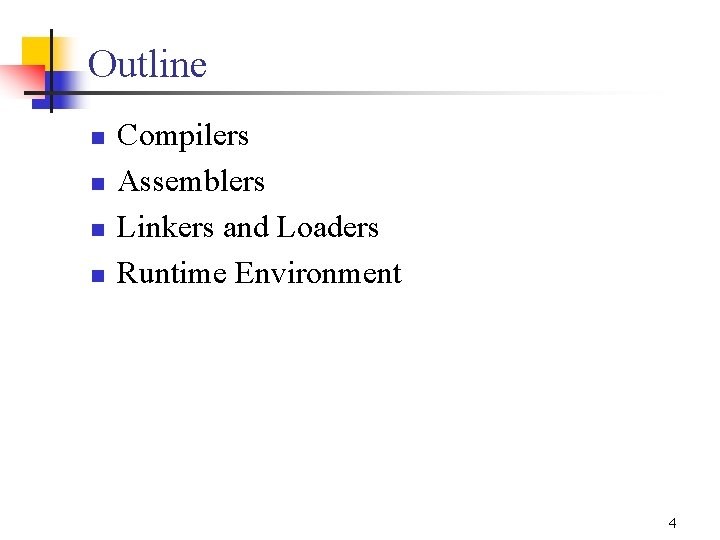
Outline n n Compilers Assemblers Linkers and Loaders Runtime Environment 4
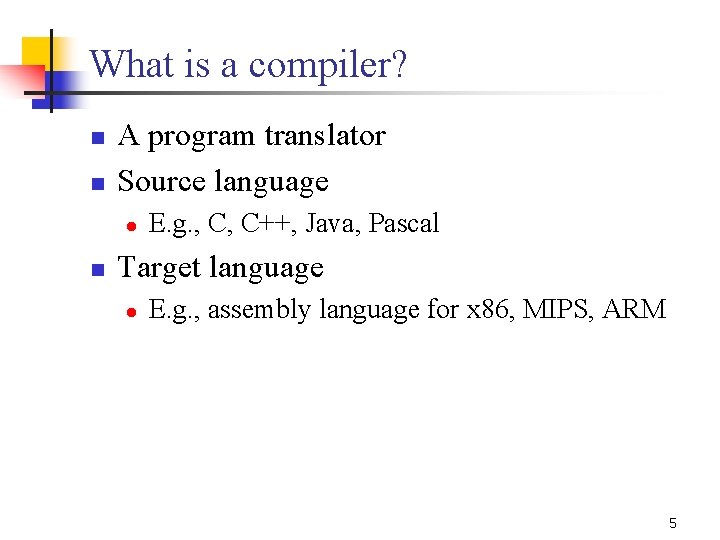
What is a compiler? n n A program translator Source language l n E. g. , C, C++, Java, Pascal Target language l E. g. , assembly language for x 86, MIPS, ARM 5
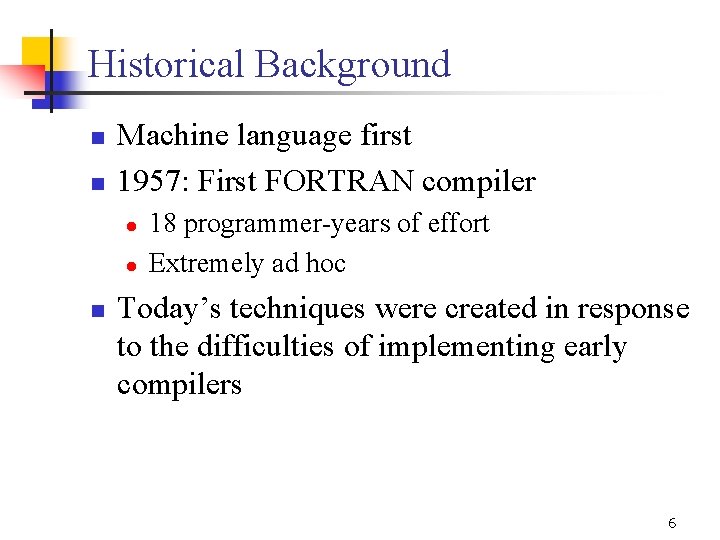
Historical Background n n Machine language first 1957: First FORTRAN compiler l l n 18 programmer-years of effort Extremely ad hoc Today’s techniques were created in response to the difficulties of implementing early compilers 6
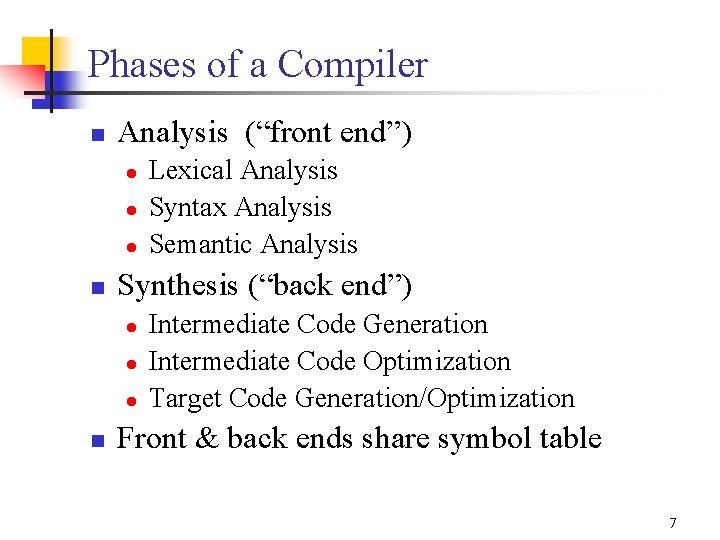
Phases of a Compiler n Analysis (“front end”) l l l n Synthesis (“back end”) l l l n Lexical Analysis Syntax Analysis Semantic Analysis Intermediate Code Generation Intermediate Code Optimization Target Code Generation/Optimization Front & back ends share symbol table 7
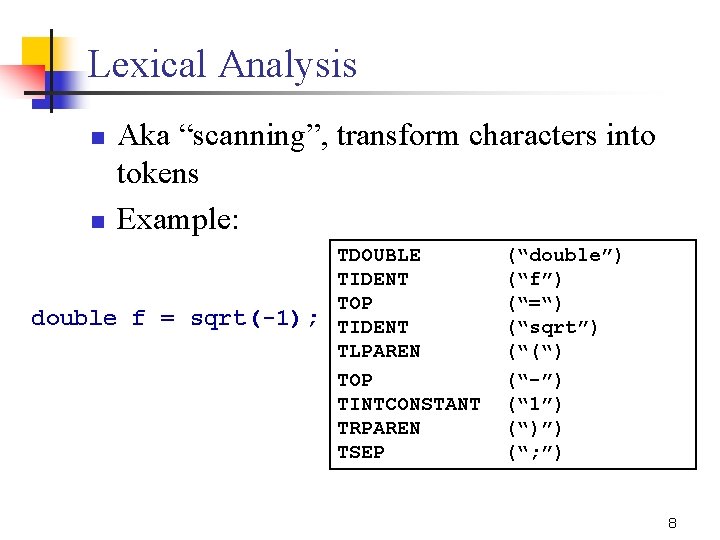
Lexical Analysis n n Aka “scanning”, transform characters into tokens Example: double f = sqrt(-1); TDOUBLE TIDENT TOP TIDENT TLPAREN TOP TINTCONSTANT TRPAREN TSEP (“double”) (“f”) (“=“) (“sqrt”) (“(“) (“-”) (“ 1”) (“)”) (“; ”) 8
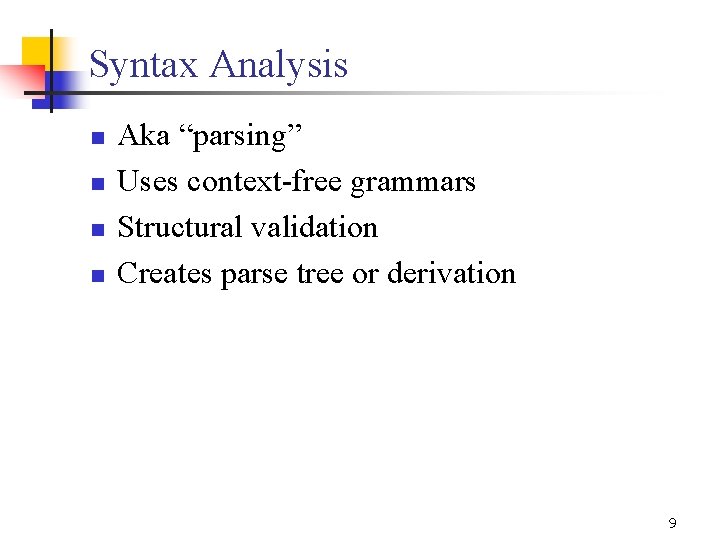
Syntax Analysis n n Aka “parsing” Uses context-free grammars Structural validation Creates parse tree or derivation 9
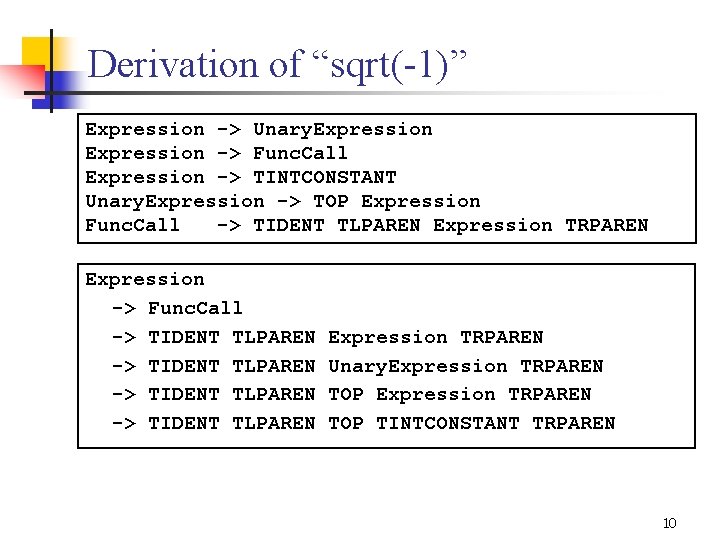
Derivation of “sqrt(-1)” Expression -> Unary. Expression -> Func. Call Expression -> TINTCONSTANT Unary. Expression -> TOP Expression Func. Call -> TIDENT TLPAREN Expression TRPAREN Expression -> Func. Call -> TIDENT TLPAREN Expression TRPAREN Unary. Expression TRPAREN TOP TINTCONSTANT TRPAREN 10
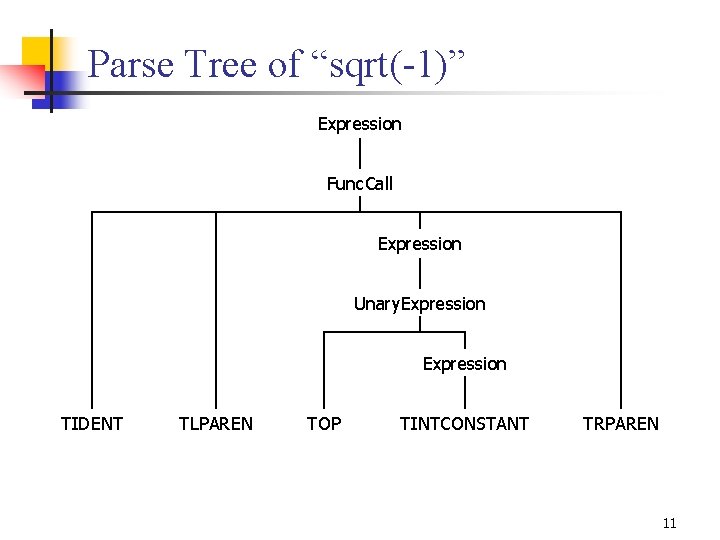
Parse Tree of “sqrt(-1)” Expression Func. Call Expression Unary. Expression TIDENT TLPAREN TOP TINTCONSTANT TRPAREN 11
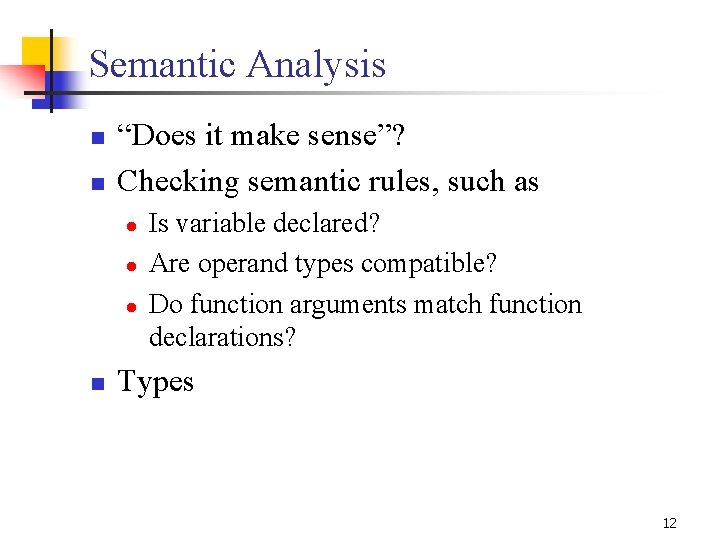
Semantic Analysis n n “Does it make sense”? Checking semantic rules, such as l l l n Is variable declared? Are operand types compatible? Do function arguments match function declarations? Types 12
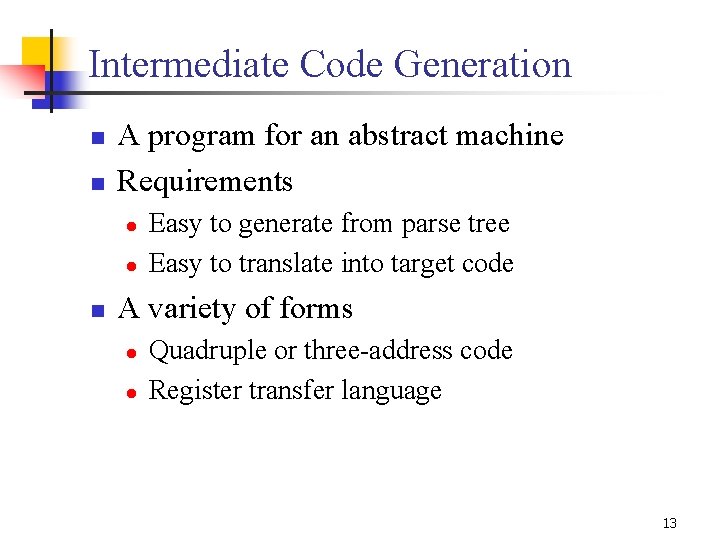
Intermediate Code Generation n n A program for an abstract machine Requirements l l n Easy to generate from parse tree Easy to translate into target code A variety of forms l l Quadruple or three-address code Register transfer language 13
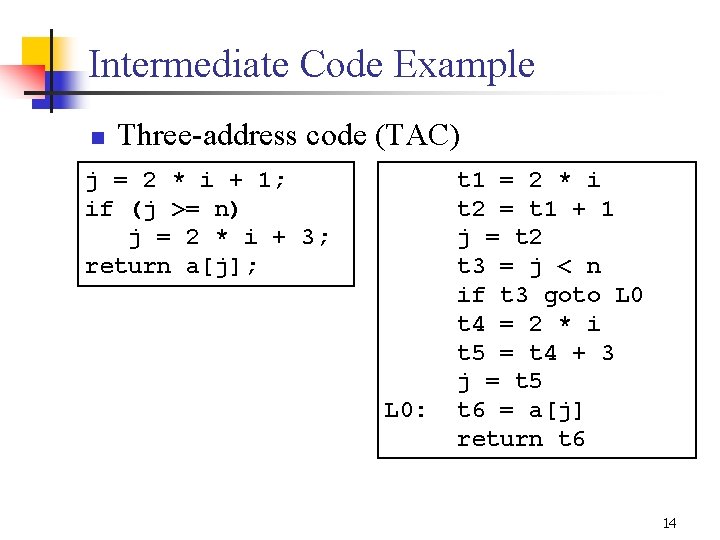
Intermediate Code Example n Three-address code (TAC) j = 2 * i + 1; if (j >= n) j = 2 * i + 3; return a[j]; L 0: t 1 = 2 * i t 2 = t 1 + 1 j = t 2 t 3 = j < n if t 3 goto L 0 t 4 = 2 * i t 5 = t 4 + 3 j = t 5 t 6 = a[j] return t 6 14
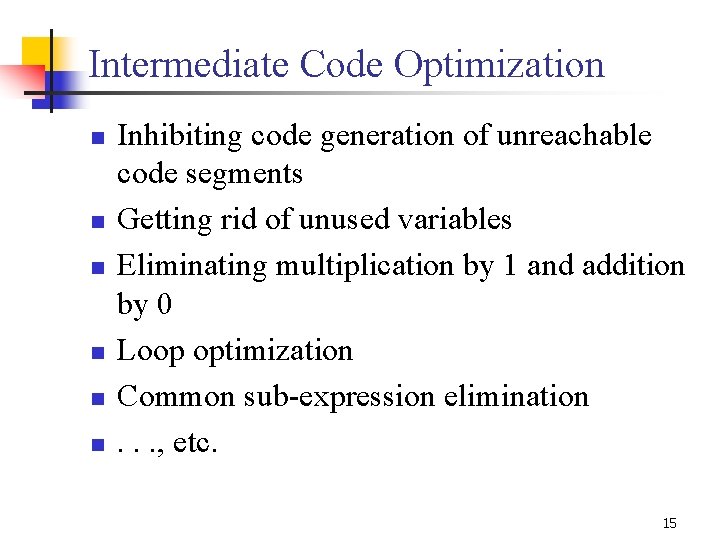
Intermediate Code Optimization n n n Inhibiting code generation of unreachable code segments Getting rid of unused variables Eliminating multiplication by 1 and addition by 0 Loop optimization Common sub-expression elimination. . . , etc. 15
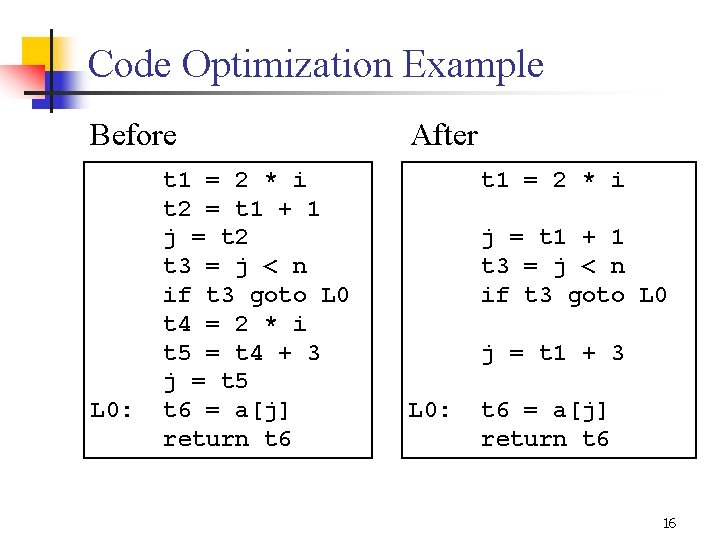
Code Optimization Example Before L 0: t 1 = 2 * i t 2 = t 1 + 1 j = t 2 t 3 = j < n if t 3 goto L 0 t 4 = 2 * i t 5 = t 4 + 3 j = t 5 t 6 = a[j] return t 6 After t 1 = 2 * i j = t 1 + 1 t 3 = j < n if t 3 goto L 0 j = t 1 + 3 L 0: t 6 = a[j] return t 6 16
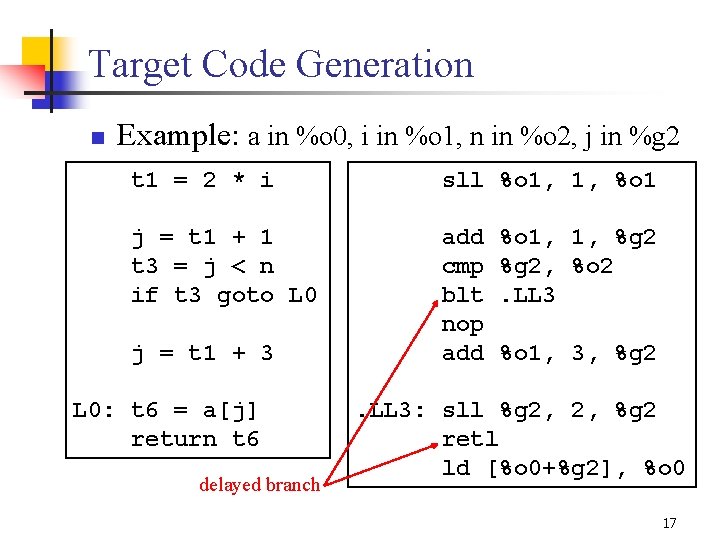
Target Code Generation n Example: a in %o 0, i in %o 1, n in %o 2, j in %g 2 t 1 = 2 * i sll %o 1, 1, %o 1 j = t 1 + 1 t 3 = j < n if t 3 goto L 0 add cmp blt nop add j = t 1 + 3 L 0: t 6 = a[j] return t 6 delayed branch %o 1, 1, %g 2, %o 2. LL 3 %o 1, 3, %g 2 . LL 3: sll %g 2, 2, %g 2 retl ld [%o 0+%g 2], %o 0 17
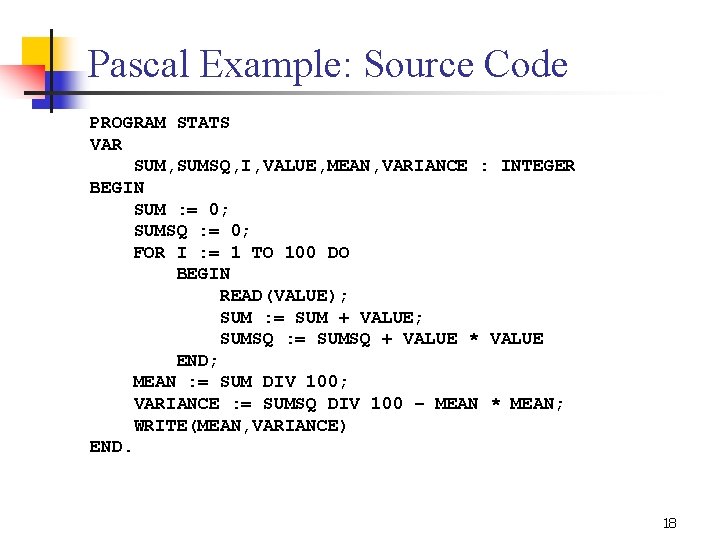
Pascal Example: Source Code PROGRAM STATS VAR SUM, SUMSQ, I, VALUE, MEAN, VARIANCE : INTEGER BEGIN SUM : = 0; SUMSQ : = 0; FOR I : = 1 TO 100 DO BEGIN READ(VALUE); SUM : = SUM + VALUE; SUMSQ : = SUMSQ + VALUE * VALUE END; MEAN : = SUM DIV 100; VARIANCE : = SUMSQ DIV 100 – MEAN * MEAN; WRITE(MEAN, VARIANCE) END. 18
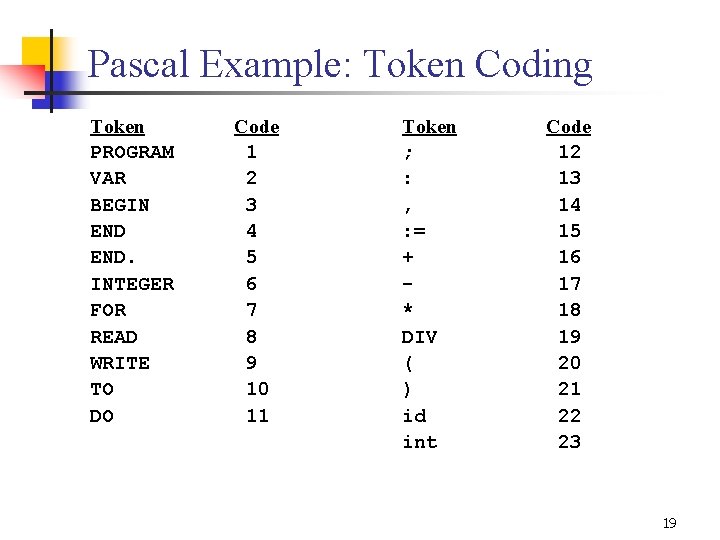
Pascal Example: Token Coding Token PROGRAM VAR BEGIN END. INTEGER FOR READ WRITE TO DO Code 1 2 3 4 5 6 7 8 9 10 11 Token ; : , : = + * DIV ( ) id int Code 12 13 14 15 16 17 18 19 20 21 22 23 19
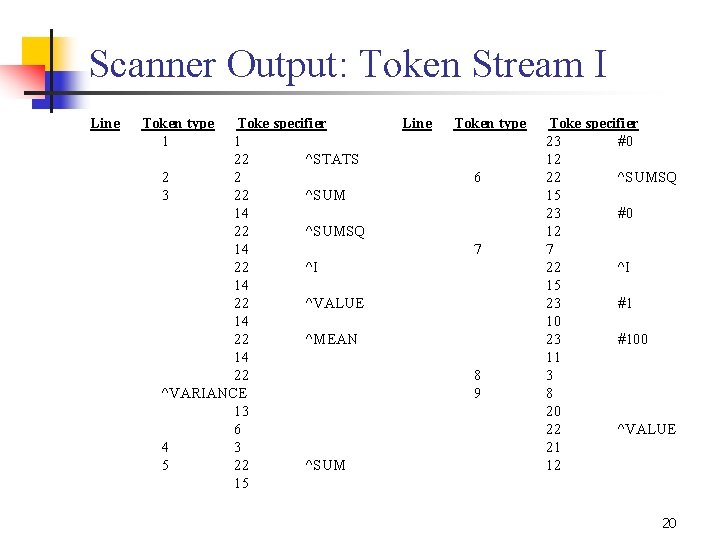
Scanner Output: Token Stream I Line Token type 1 Toke specifier 1 22 ^STATS 2 2 3 22 ^SUM 14 22 ^SUMSQ 14 22 ^I 14 22 ^VALUE 14 22 ^MEAN 14 22 ^VARIANCE 13 6 4 3 5 22 ^SUM 15 Line Token type 6 7 8 9 Toke specifier 23 #0 12 22 ^SUMSQ 15 23 #0 12 7 22 ^I 15 23 #1 10 23 #100 11 3 8 20 22 ^VALUE 21 12 20
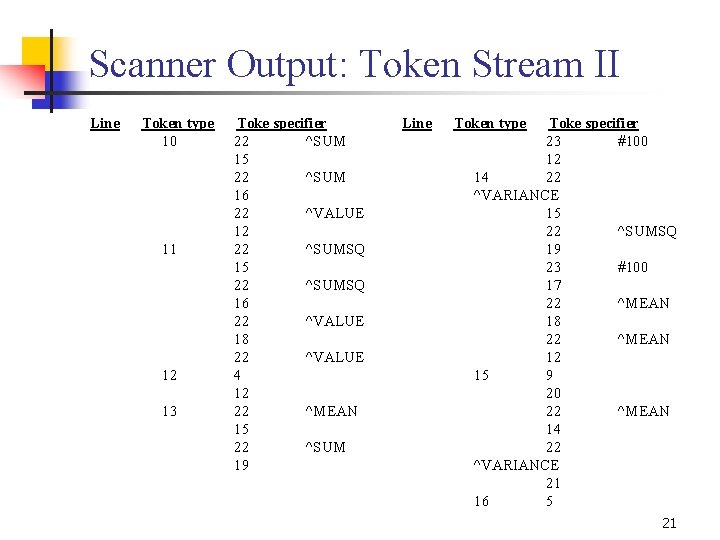
Scanner Output: Token Stream II Line Token type 10 11 12 13 Toke specifier 22 ^SUM 15 22 ^SUM 16 22 ^VALUE 12 22 ^SUMSQ 15 22 ^SUMSQ 16 22 ^VALUE 18 22 ^VALUE 4 12 22 ^MEAN 15 22 ^SUM 19 Line Token type Toke specifier 23 #100 12 14 22 ^VARIANCE 15 22 ^SUMSQ 19 23 #100 17 22 ^MEAN 18 22 ^MEAN 12 15 9 20 22 ^MEAN 14 22 ^VARIANCE 21 16 5 21
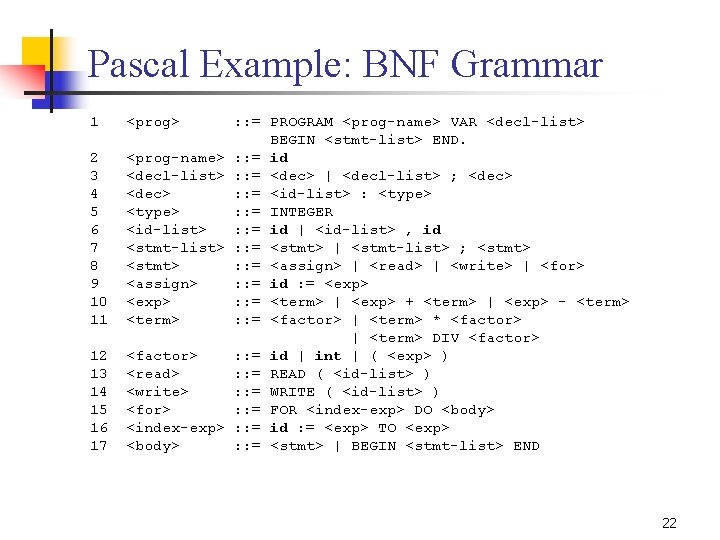
Pascal Example: BNF Grammar 1 <prog> 2 3 4 5 6 7 8 9 10 11 <prog-name> <decl-list> <dec> <type> <id-list> <stmt> <assign> <exp> <term> 12 13 14 15 16 17 <factor> <read> <write> <for> <index-exp> <body> : : = PROGRAM <prog-name> VAR <decl-list> BEGIN <stmt-list> END. : : = id : : = <dec> | <decl-list> ; <dec> : : = <id-list> : <type> : : = INTEGER : : = id | <id-list> , id : : = <stmt> | <stmt-list> ; <stmt> : : = <assign> | <read> | <write> | <for> : : = id : = <exp> : : = <term> | <exp> + <term> | <exp> - <term> : : = <factor> | <term> * <factor> | <term> DIV <factor> : : = id | int | ( <exp> ) : : = READ ( <id-list> ) : : = WRITE ( <id-list> ) : : = FOR <index-exp> DO <body> : : = id : = <exp> TO <exp> : : = <stmt> | BEGIN <stmt-list> END 22
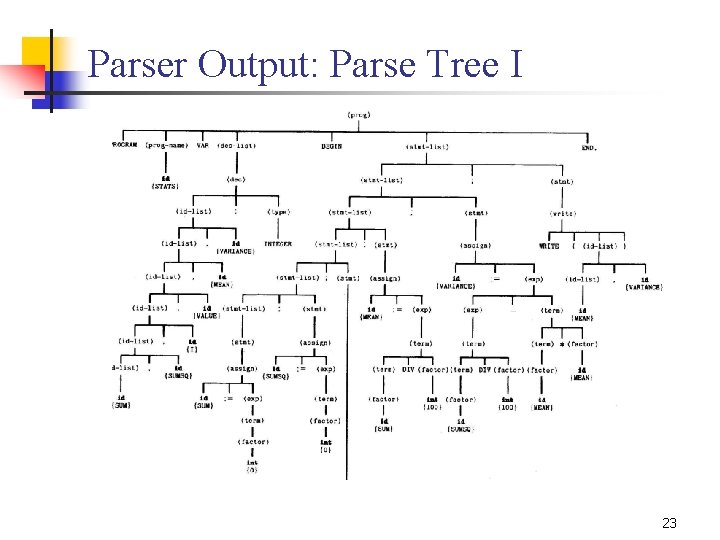
Parser Output: Parse Tree I 23
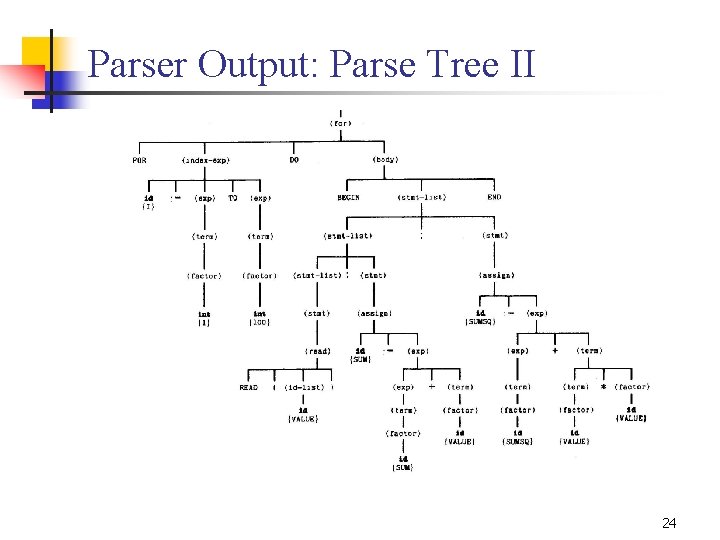
Parser Output: Parse Tree II 24
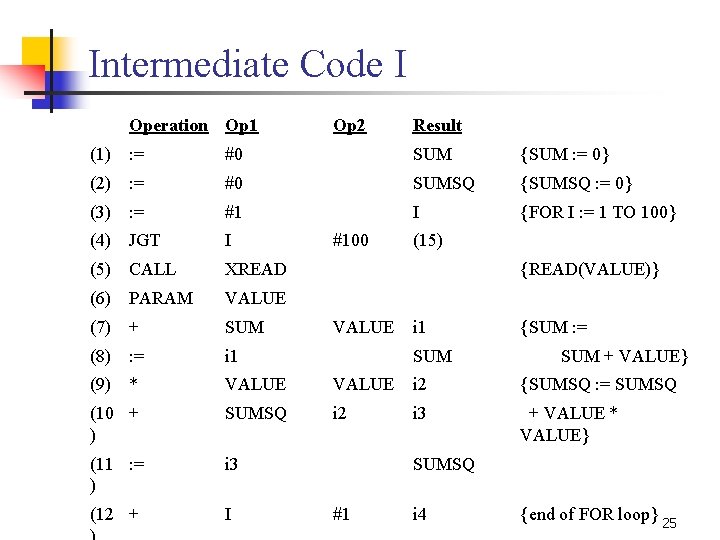
Intermediate Code I Operation Op 1 Op 2 Result (1) : = #0 SUM {SUM : = 0} (2) : = #0 SUMSQ {SUMSQ : = 0} (3) : = #1 I {FOR I : = 1 TO 100} (4) JGT I (5) CALL XREAD (6) PARAM VALUE (7) + SUM (8) : = i 1 (9) * VALUE i 2 {SUMSQ : = SUMSQ (10 + ) SUMSQ i 2 i 3 + VALUE * VALUE} (11 : = ) i 3 (12 + I #100 (15) {READ(VALUE)} VALUE i 1 SUM {SUM : = SUM + VALUE} SUMSQ #1 i 4 {end of FOR loop} 25
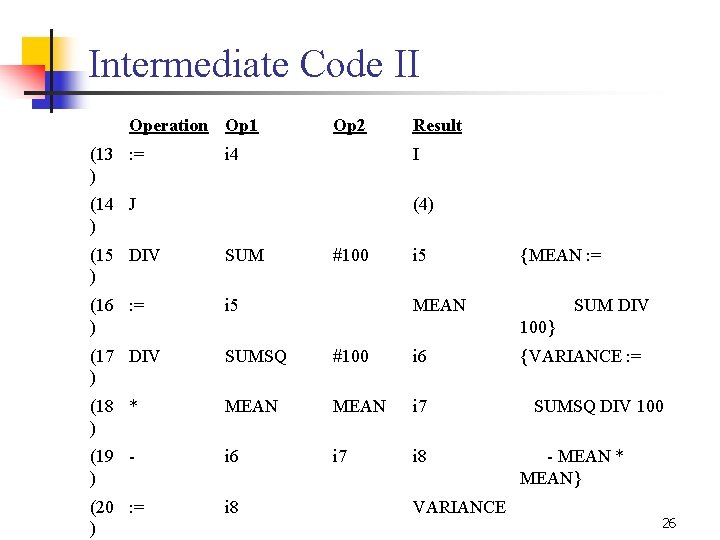
Intermediate Code II Operation Op 1 (13 : = ) Op 2 i 4 Result I (14 J ) (4) (15 DIV ) SUM #100 i 5 (16 : = ) i 5 (17 DIV ) SUMSQ #100 i 6 (18 * ) MEAN i 7 (19 ) i 6 i 7 i 8 (20 : = ) i 8 {MEAN : = MEAN SUM DIV 100} VARIANCE {VARIANCE : = SUMSQ DIV 100 - MEAN * MEAN} 26
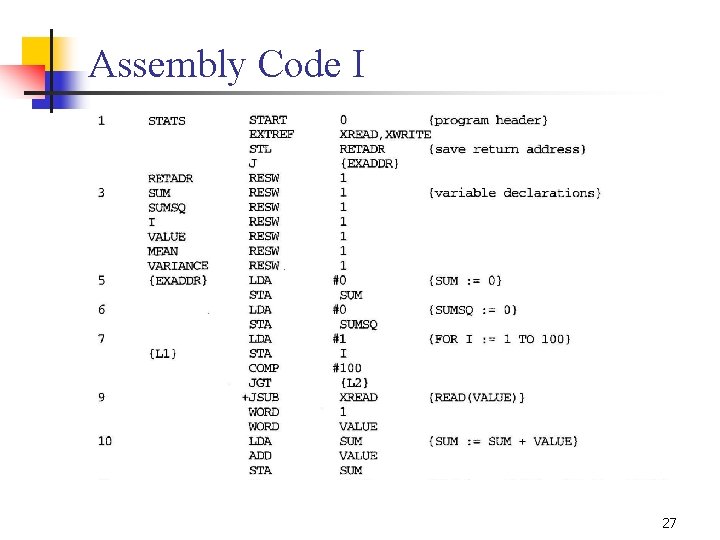
Assembly Code I 27
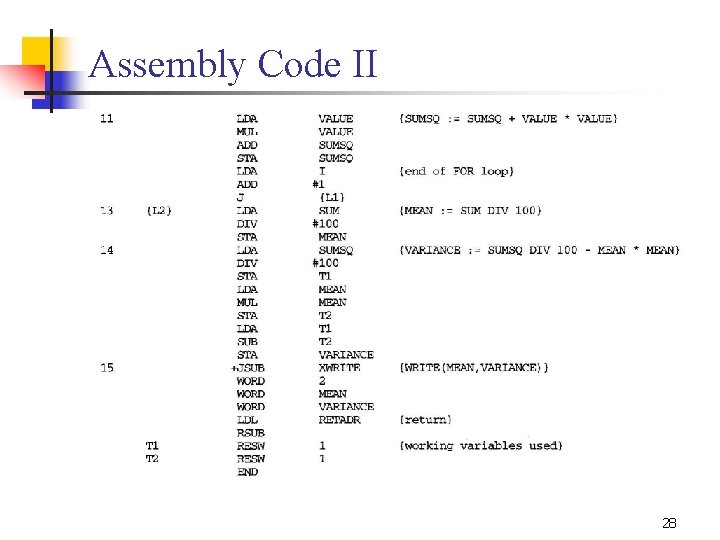
Assembly Code II 28
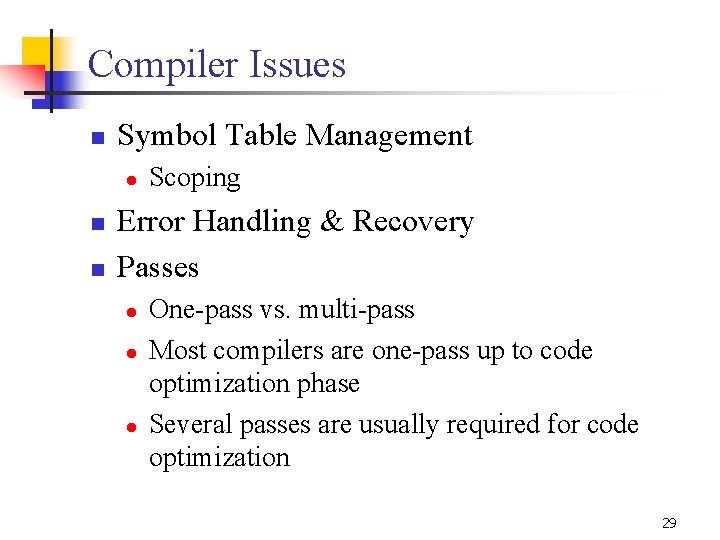
Compiler Issues n Symbol Table Management l n n Scoping Error Handling & Recovery Passes l l l One-pass vs. multi-pass Most compilers are one-pass up to code optimization phase Several passes are usually required for code optimization 29
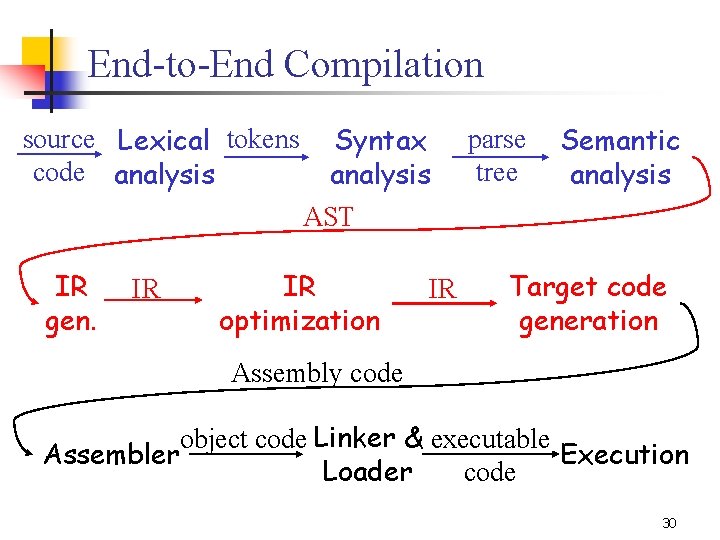
End-to-End Compilation source Lexical tokens code analysis IR gen. IR Syntax analysis AST IR optimization IR parse tree Semantic analysis Target code generation Assembly code object code Linker & executable Assembler Execution Loader code 30
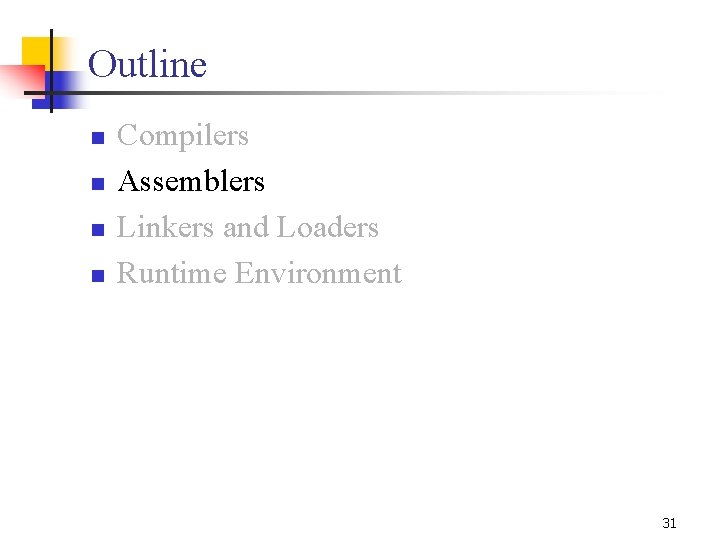
Outline n n Compilers Assemblers Linkers and Loaders Runtime Environment 31
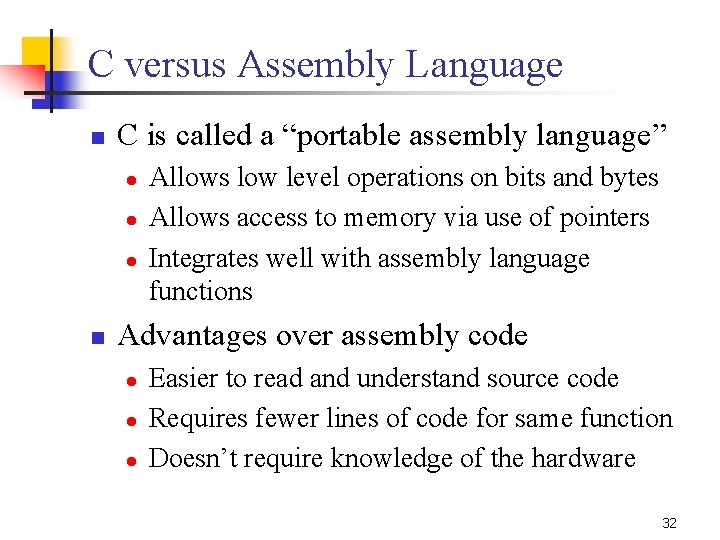
C versus Assembly Language n C is called a “portable assembly language” l l l n Allows low level operations on bits and bytes Allows access to memory via use of pointers Integrates well with assembly language functions Advantages over assembly code l l l Easier to read and understand source code Requires fewer lines of code for same function Doesn’t require knowledge of the hardware 32
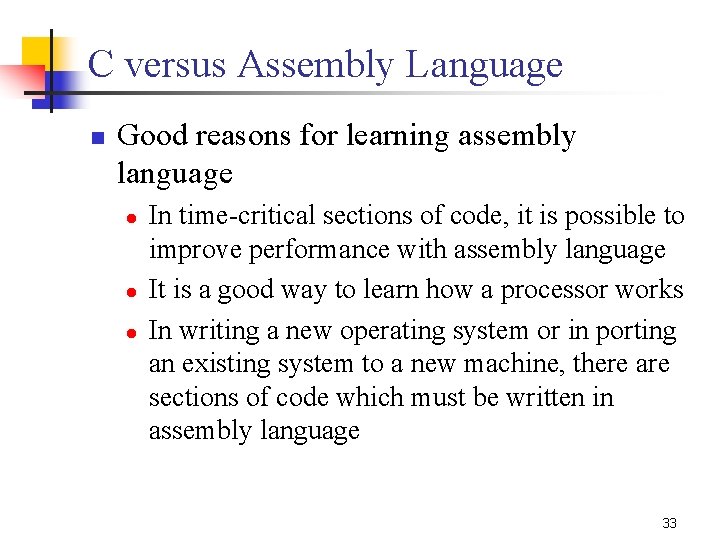
C versus Assembly Language n Good reasons for learning assembly language l l l In time-critical sections of code, it is possible to improve performance with assembly language It is a good way to learn how a processor works In writing a new operating system or in porting an existing system to a new machine, there are sections of code which must be written in assembly language 33
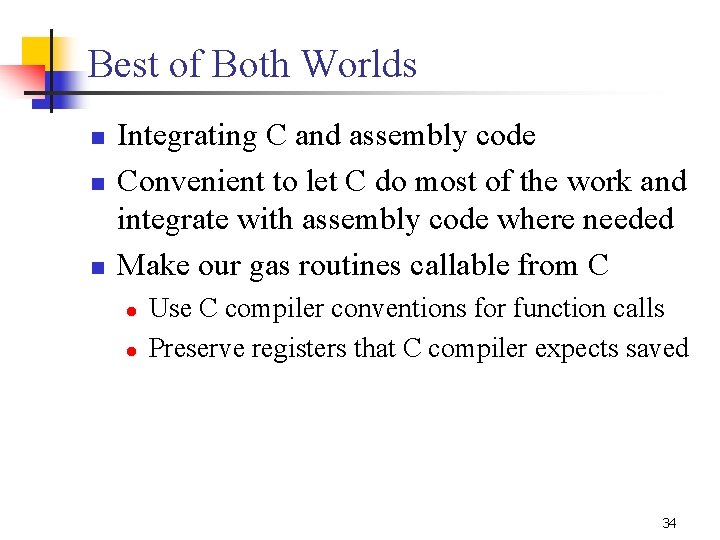
Best of Both Worlds n n n Integrating C and assembly code Convenient to let C do most of the work and integrate with assembly code where needed Make our gas routines callable from C l l Use C compiler conventions for function calls Preserve registers that C compiler expects saved 34
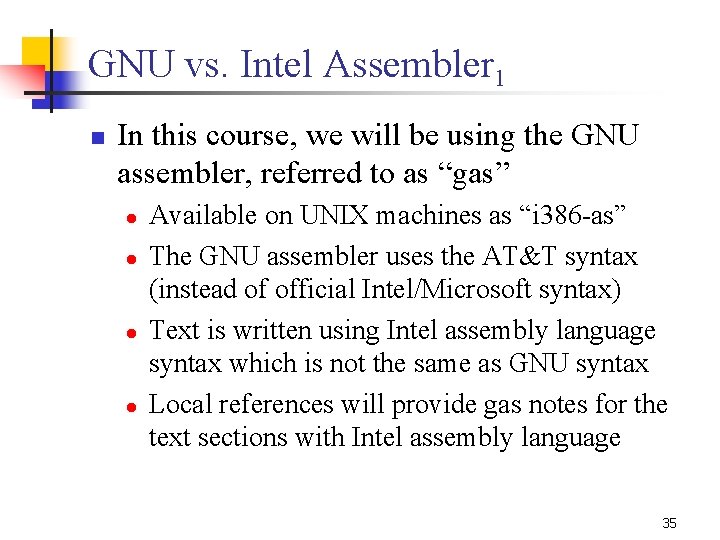
GNU vs. Intel Assembler 1 n In this course, we will be using the GNU assembler, referred to as “gas” l l Available on UNIX machines as “i 386 -as” The GNU assembler uses the AT&T syntax (instead of official Intel/Microsoft syntax) Text is written using Intel assembly language syntax which is not the same as GNU syntax Local references will provide gas notes for the text sections with Intel assembly language 35
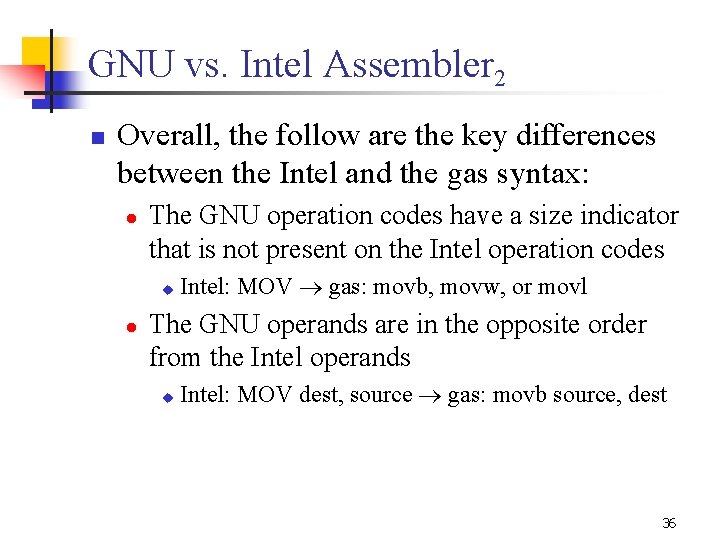
GNU vs. Intel Assembler 2 n Overall, the follow are the key differences between the Intel and the gas syntax: l The GNU operation codes have a size indicator that is not present on the Intel operation codes u l Intel: MOV gas: movb, movw, or movl The GNU operands are in the opposite order from the Intel operands u Intel: MOV dest, source gas: movb source, dest 36
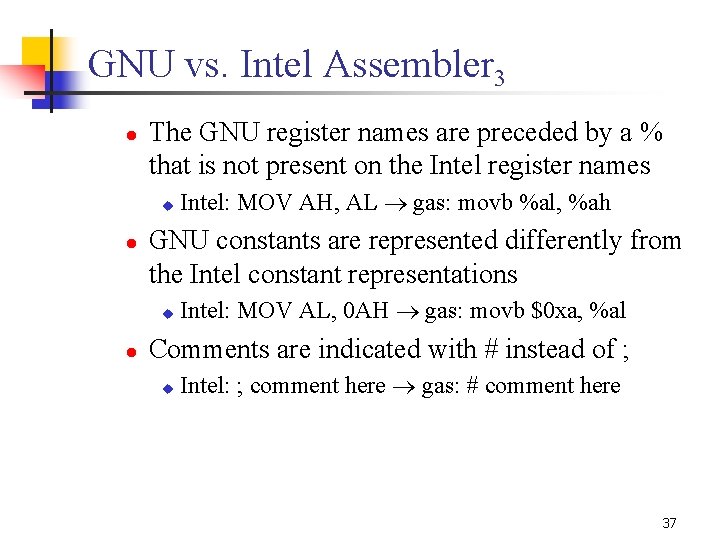
GNU vs. Intel Assembler 3 l The GNU register names are preceded by a % that is not present on the Intel register names u l GNU constants are represented differently from the Intel constant representations u l Intel: MOV AH, AL gas: movb %al, %ah Intel: MOV AL, 0 AH gas: movb $0 xa, %al Comments are indicated with # instead of ; u Intel: ; comment here gas: # comment here 37
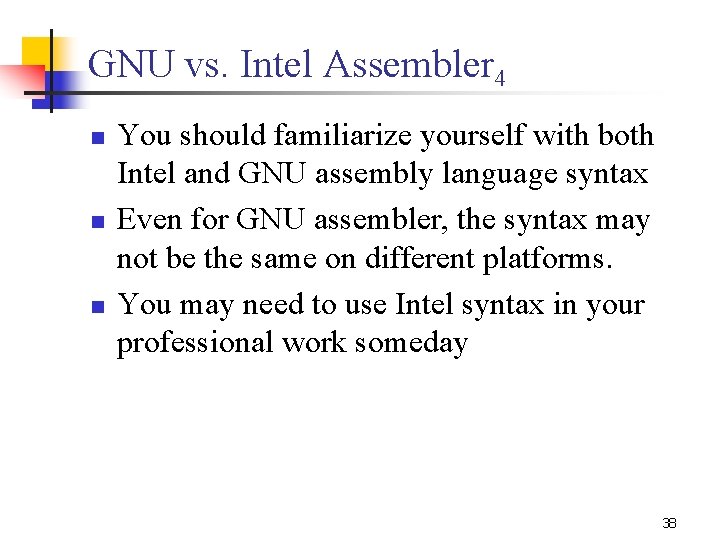
GNU vs. Intel Assembler 4 n n n You should familiarize yourself with both Intel and GNU assembly language syntax Even for GNU assembler, the syntax may not be the same on different platforms. You may need to use Intel syntax in your professional work someday 38
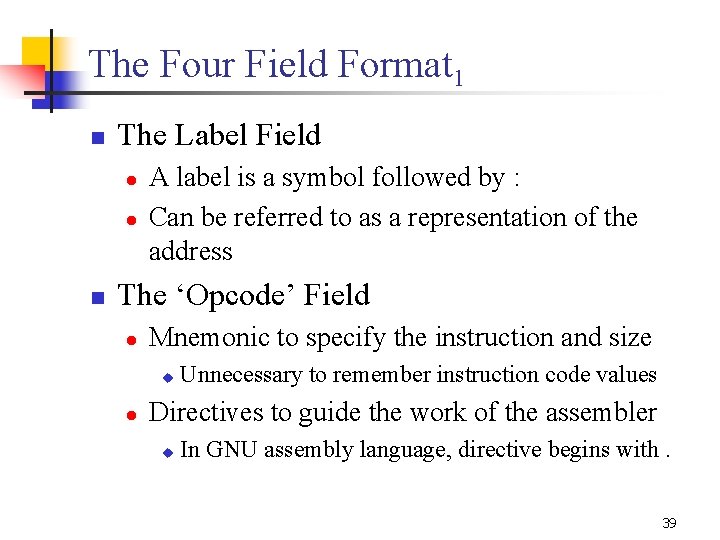
The Four Field Format 1 n The Label Field l l n A label is a symbol followed by : Can be referred to as a representation of the address The ‘Opcode’ Field l Mnemonic to specify the instruction and size u l Unnecessary to remember instruction code values Directives to guide the work of the assembler u In GNU assembly language, directive begins with. 39
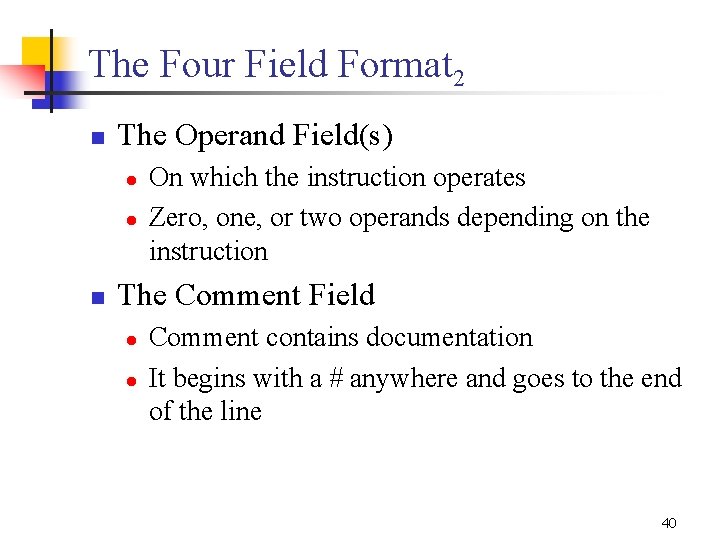
The Four Field Format 2 n The Operand Field(s) l l n On which the instruction operates Zero, one, or two operands depending on the instruction The Comment Field l l Comment contains documentation It begins with a # anywhere and goes to the end of the line 40
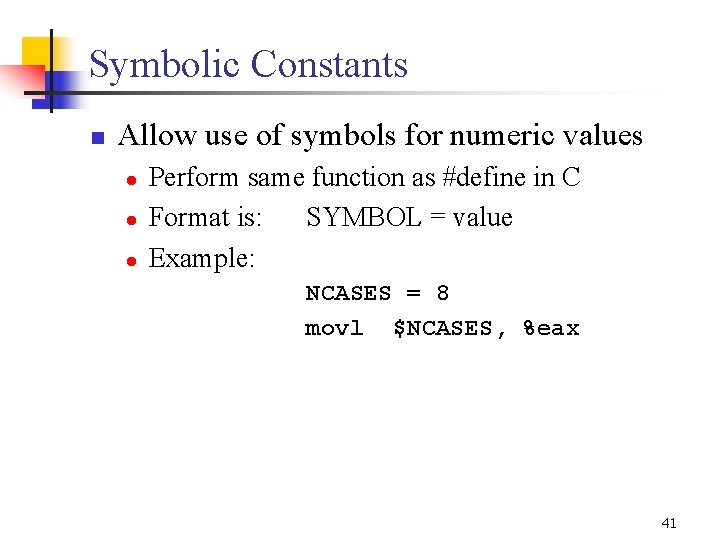
Symbolic Constants n Allow use of symbols for numeric values l l l Perform same function as #define in C Format is: SYMBOL = value Example: NCASES = 8 movl $NCASES, %eax 41
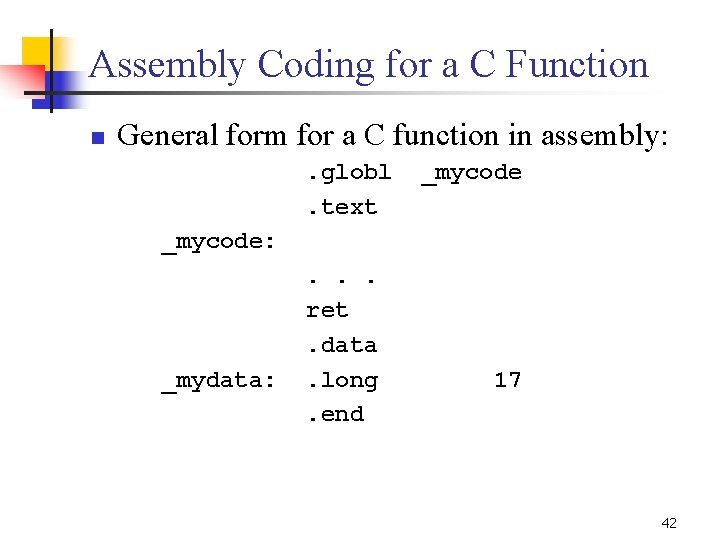
Assembly Coding for a C Function n General form for a C function in assembly: . globl. text _mycode: _mydata: . . . ret. data. long. end 17 42
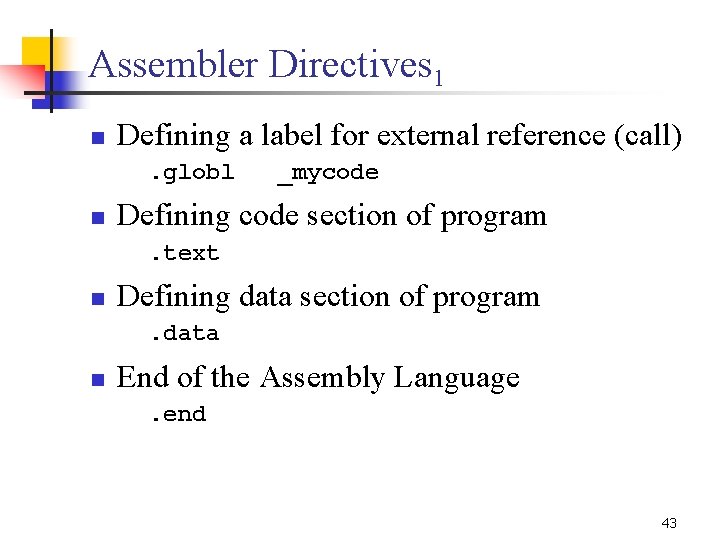
Assembler Directives 1 n Defining a label for external reference (call). globl n _mycode Defining code section of program. text n Defining data section of program. data n End of the Assembly Language. end 43
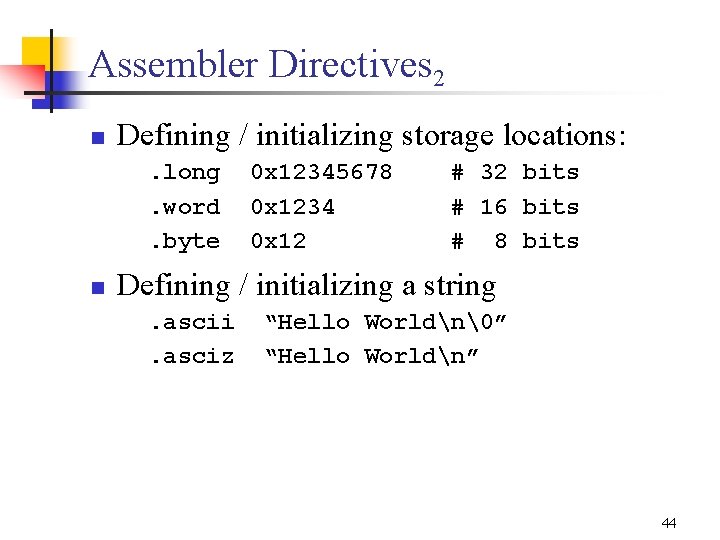
Assembler Directives 2 n Defining / initializing storage locations: . long. word. byte n 0 x 12345678 0 x 1234 0 x 12 # 32 bits # 16 bits # 8 bits Defining / initializing a string. ascii. asciz “Hello Worldn ” “Hello Worldn” 44
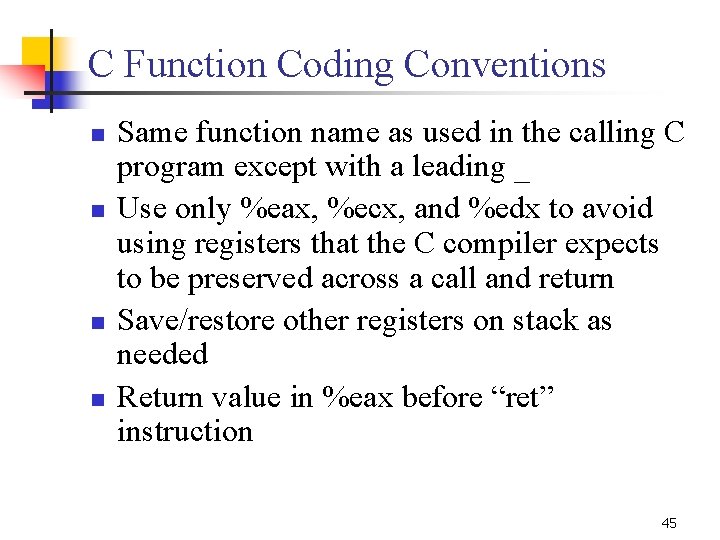
C Function Coding Conventions n n Same function name as used in the calling C program except with a leading _ Use only %eax, %ecx, and %edx to avoid using registers that the C compiler expects to be preserved across a call and return Save/restore other registers on stack as needed Return value in %eax before “ret” instruction 45
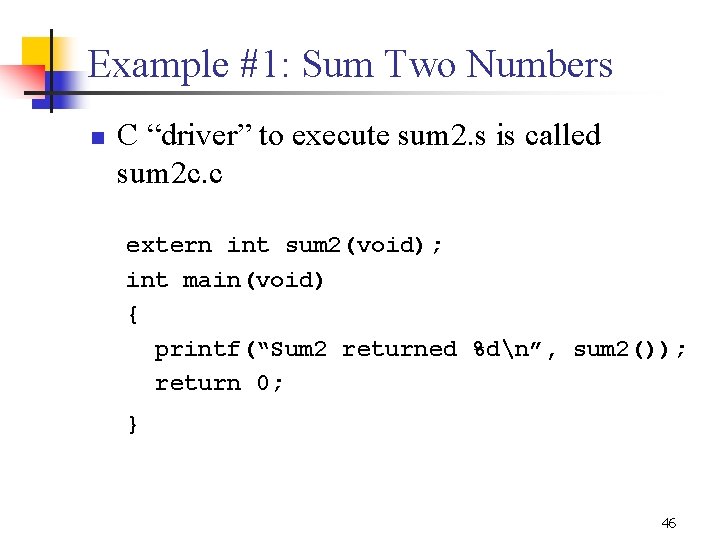
Example #1: Sum Two Numbers n C “driver” to execute sum 2. s is called sum 2 c. c extern int sum 2(void); int main(void) { printf(“Sum 2 returned %dn”, sum 2()); return 0; } 46
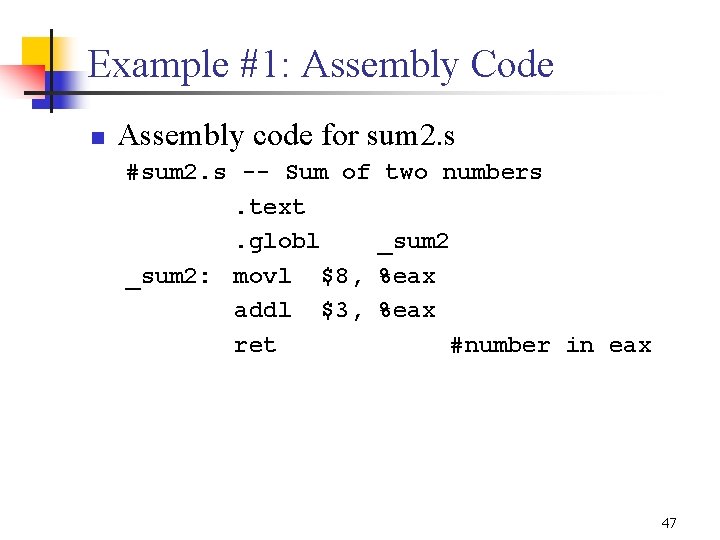
Example #1: Assembly Code n Assembly code for sum 2. s #sum 2. s -- Sum of two numbers. text. globl _sum 2: movl $8, %eax addl $3, %eax ret #number in eax 47
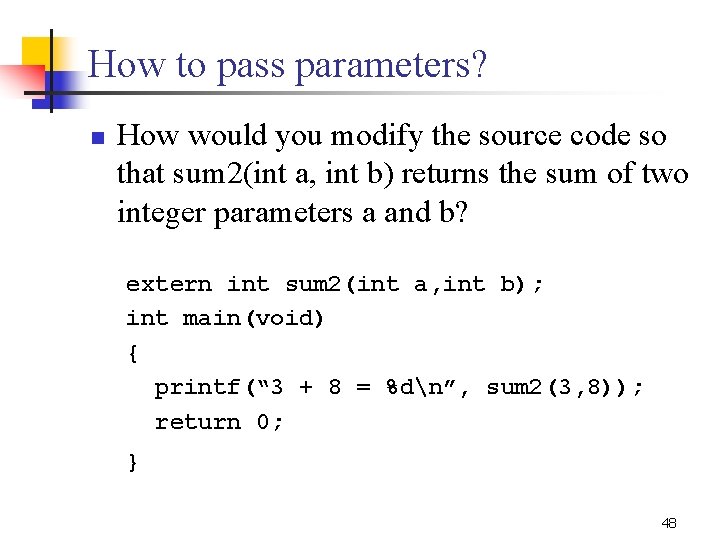
How to pass parameters? n How would you modify the source code so that sum 2(int a, int b) returns the sum of two integer parameters a and b? extern int sum 2(int a, int b); int main(void) { printf(“ 3 + 8 = %dn”, sum 2(3, 8)); return 0; } 48
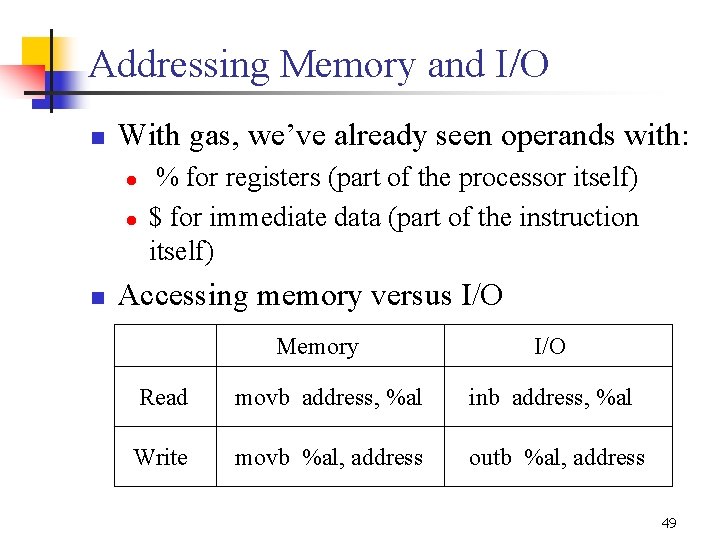
Addressing Memory and I/O n With gas, we’ve already seen operands with: l l n % for registers (part of the processor itself) $ for immediate data (part of the instruction itself) Accessing memory versus I/O Memory I/O Read movb address, %al inb address, %al Write movb %al, address outb %al, address 49
![Addressing Memory 1 n Direct addressing for memory l l Intel uses Addressing Memory 1 n Direct addressing for memory l l Intel uses [ ]](https://slidetodoc.com/presentation_image_h/d6e6f5f51917c4973116f9f00071c180/image-50.jpg)
Addressing Memory 1 n Direct addressing for memory l l Intel uses [ ] gas does not use any “operator” Example: . text movl %eax, 0 x 1234 movl 0 x 1234, %edx. . . 50
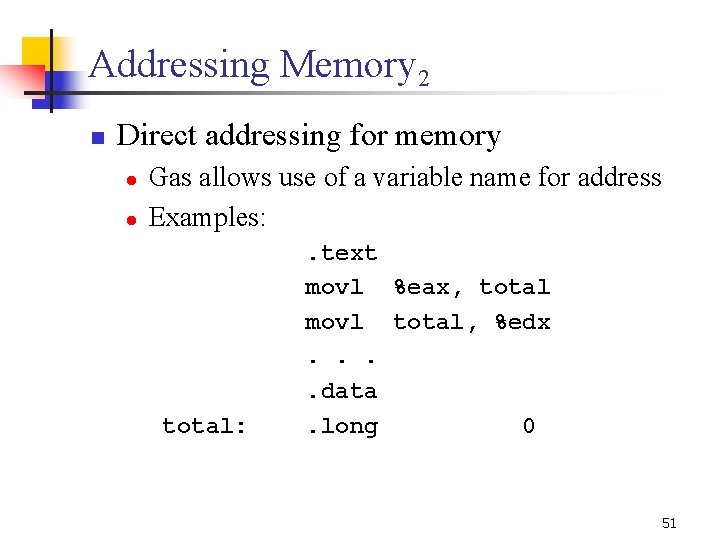
Addressing Memory 2 n Direct addressing for memory l l Gas allows use of a variable name for address Examples: total: . text movl %eax, total movl total, %edx. . data. long 0 51
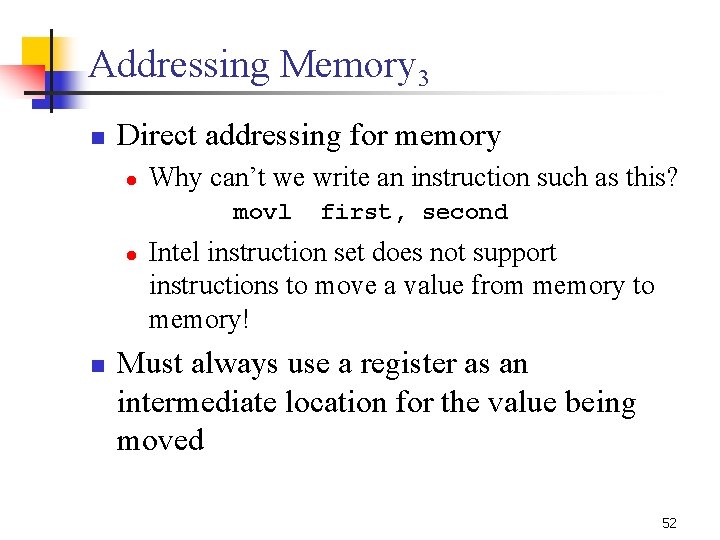
Addressing Memory 3 n Direct addressing for memory l Why can’t we write an instruction such as this? movl l n first, second Intel instruction set does not support instructions to move a value from memory to memory! Must always use a register as an intermediate location for the value being moved 52
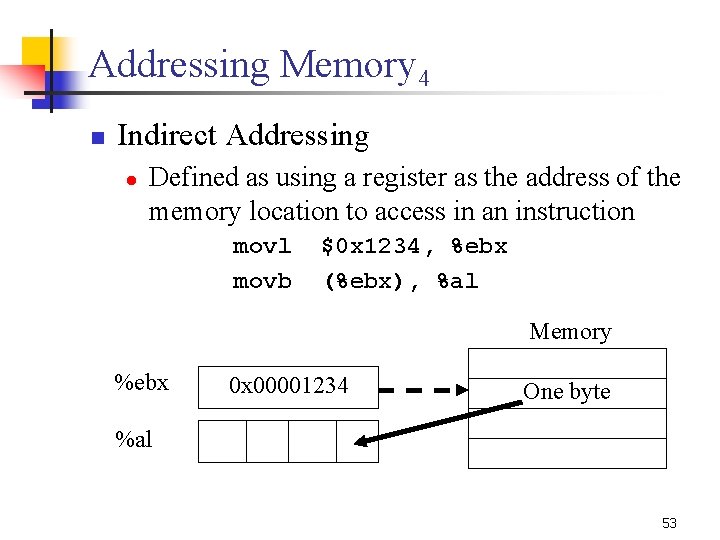
Addressing Memory 4 n Indirect Addressing l Defined as using a register as the address of the memory location to access in an instruction movl movb $0 x 1234, %ebx (%ebx), %al Memory %ebx 0 x 00001234 One byte %al 53
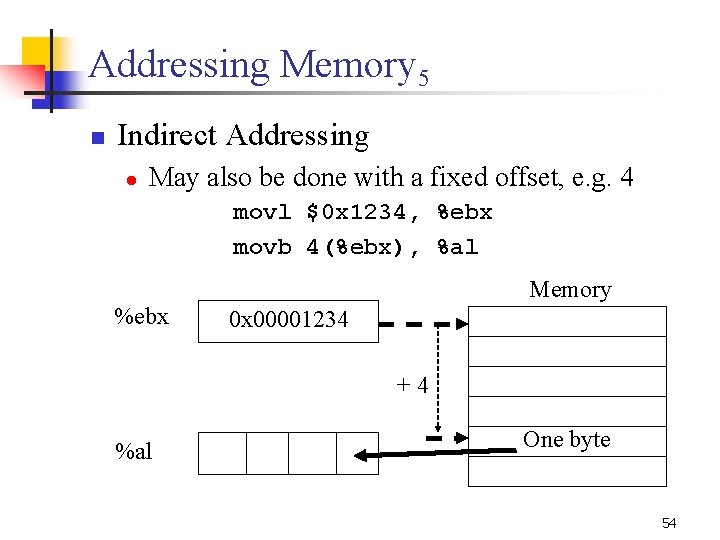
Addressing Memory 5 n Indirect Addressing l May also be done with a fixed offset, e. g. 4 movl $0 x 1234, %ebx movb 4(%ebx), %al Memory %ebx 0 x 00001234 +4 %al One byte 54
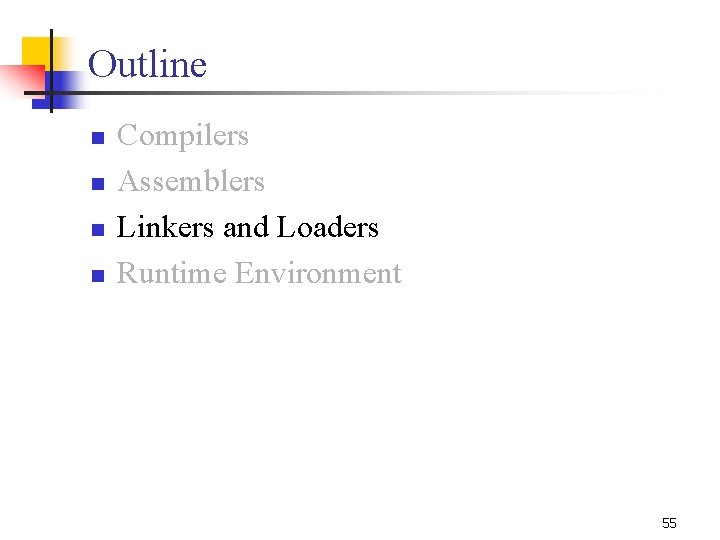
Outline n n Compilers Assemblers Linkers and Loaders Runtime Environment 55
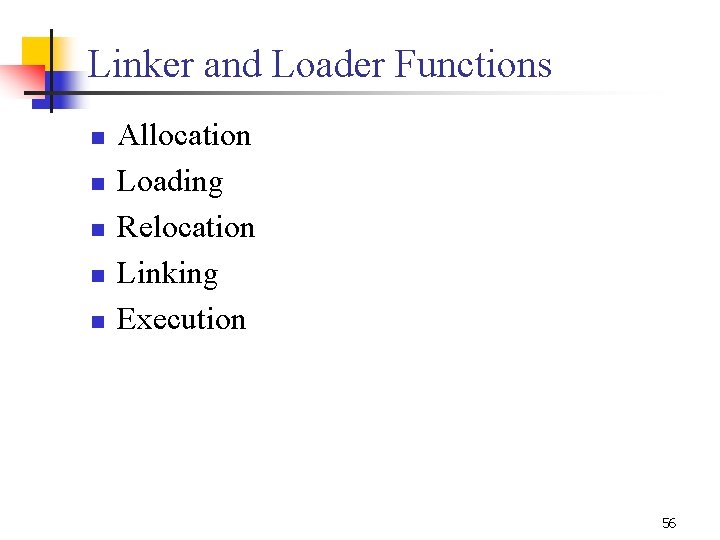
Linker and Loader Functions n n n Allocation Loading Relocation Linking Execution 56
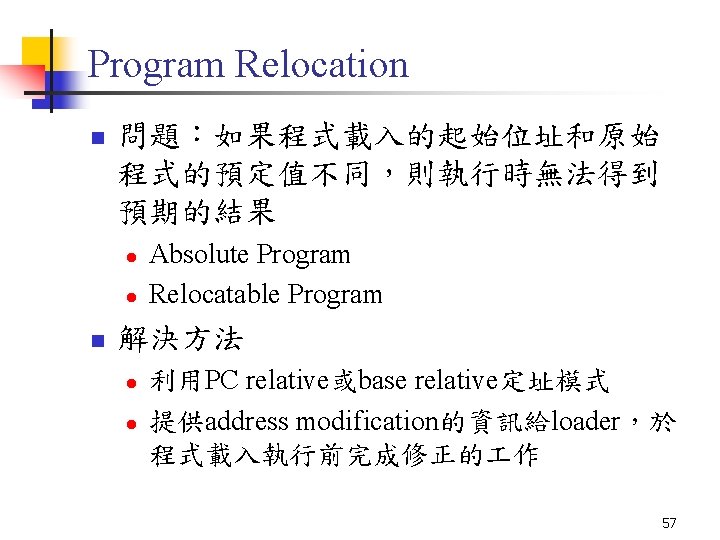
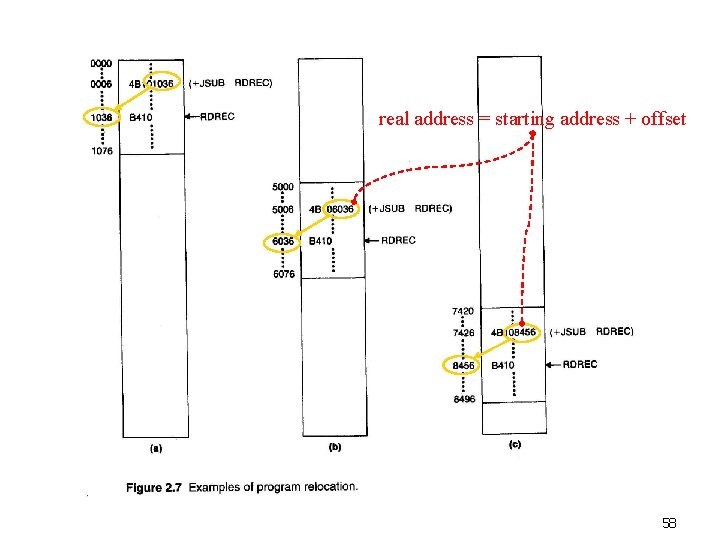
real address = starting address + offset 58
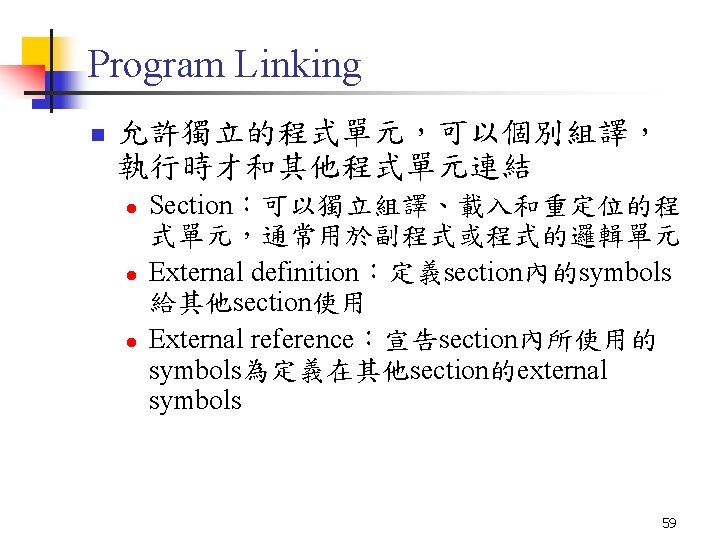
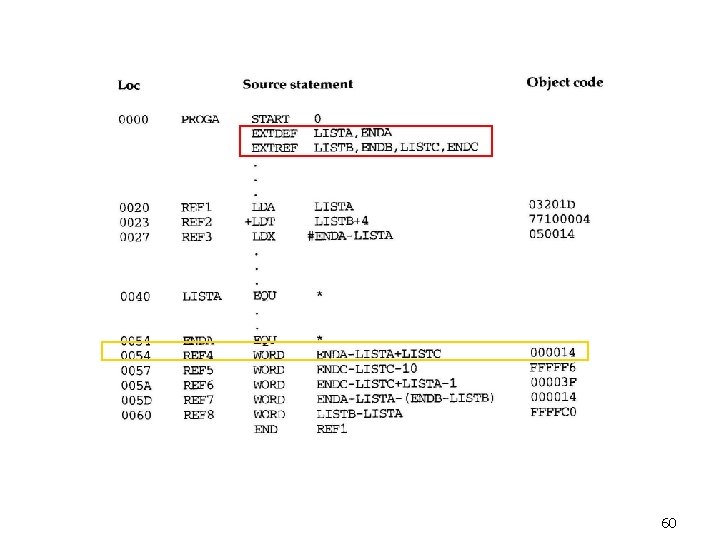
60
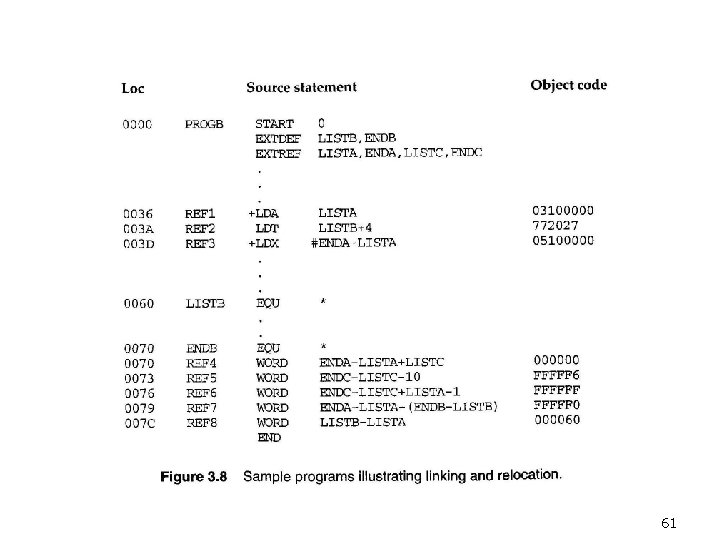
61
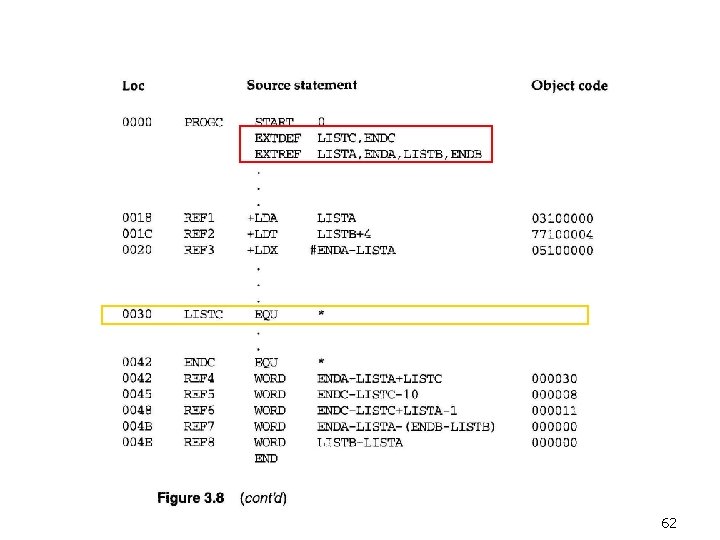
62
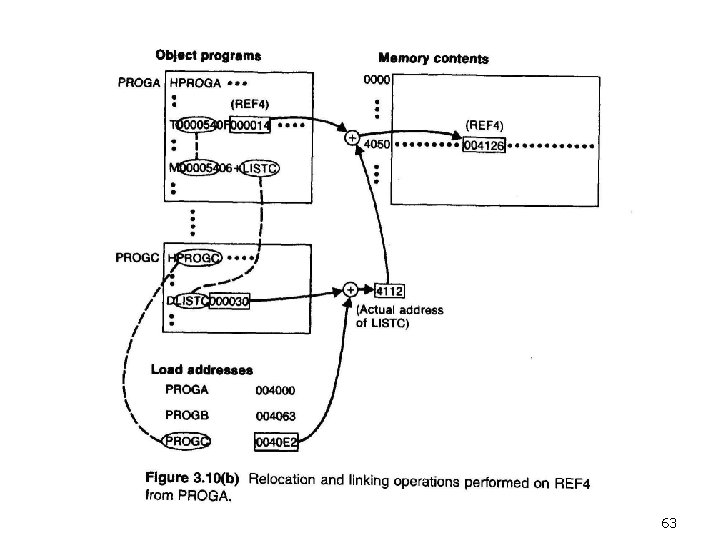
63
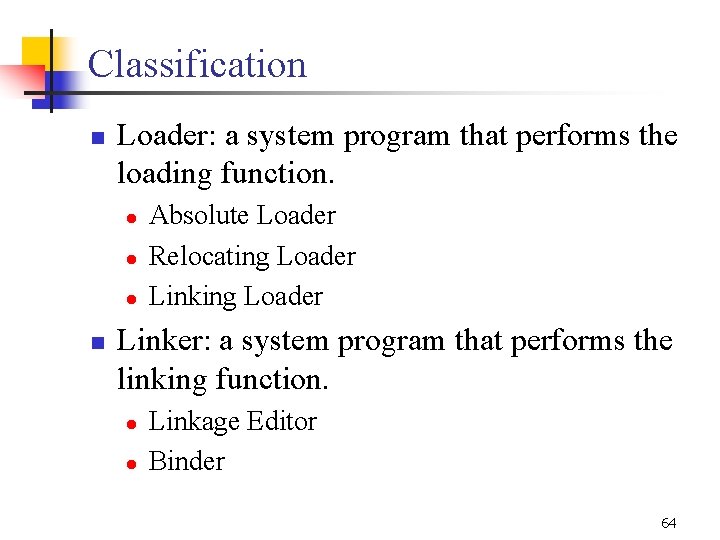
Classification n Loader: a system program that performs the loading function. l l l n Absolute Loader Relocating Loader Linker: a system program that performs the linking function. l l Linkage Editor Binder 64
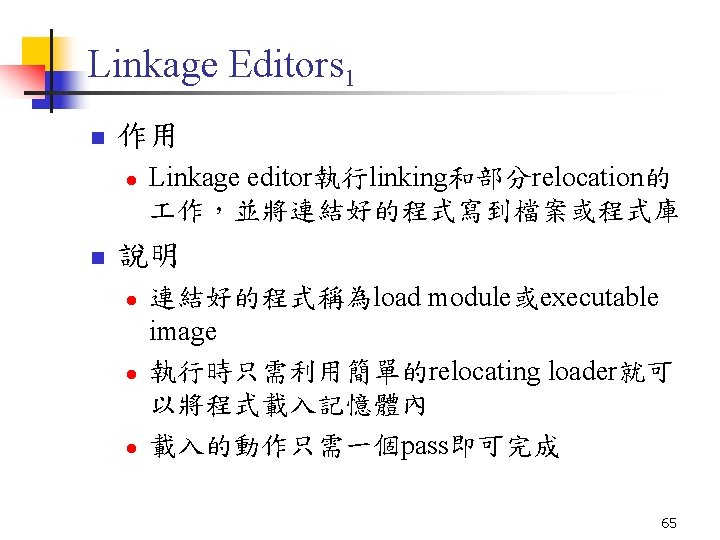
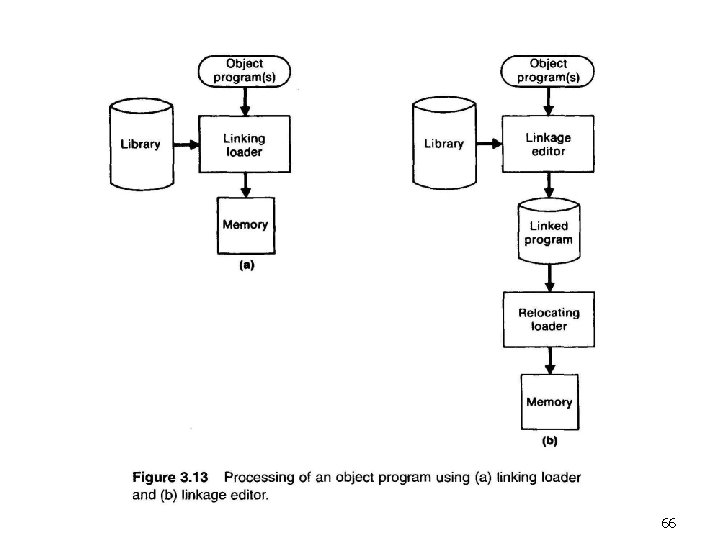
66
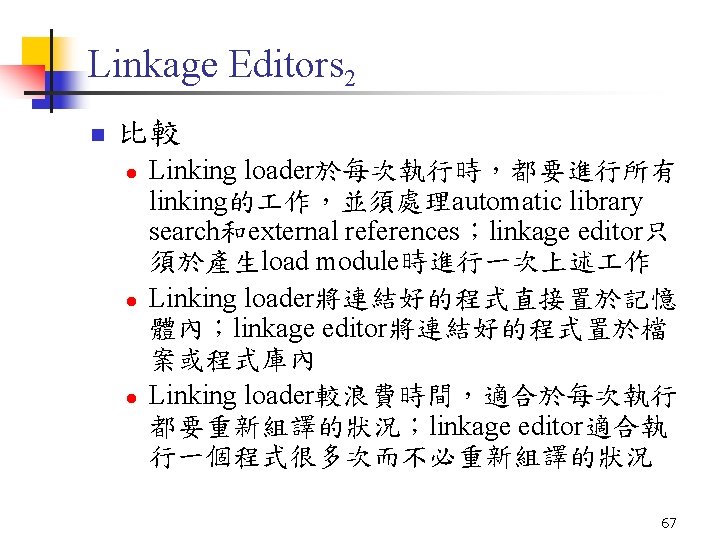
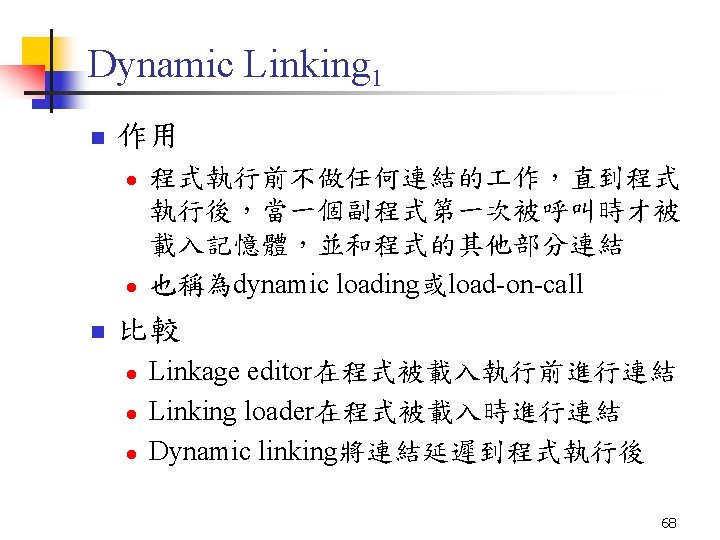
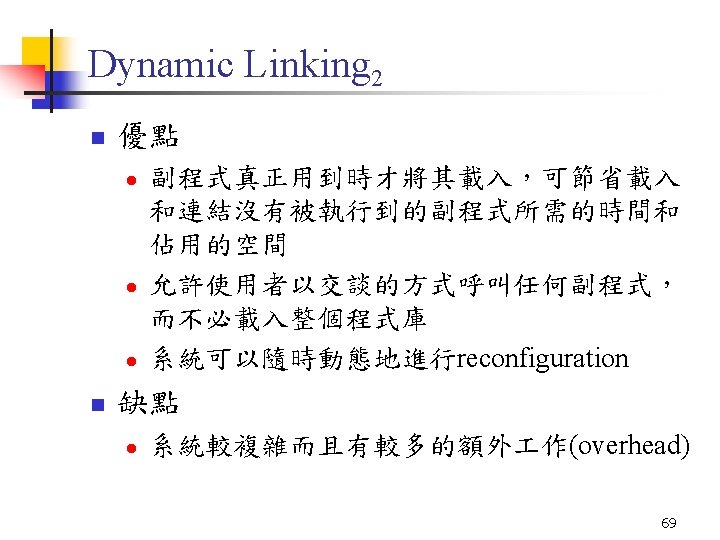
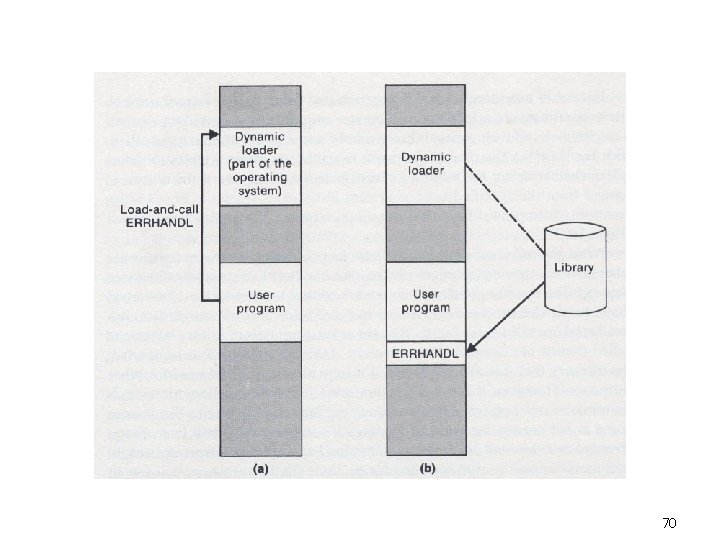
70
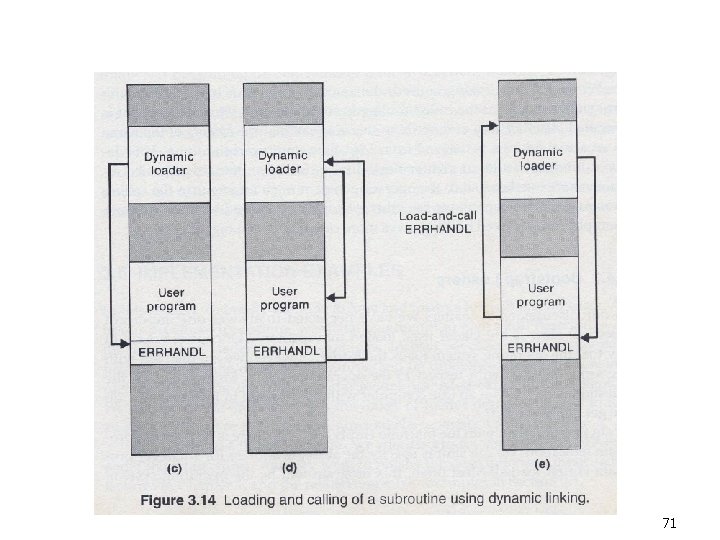
71
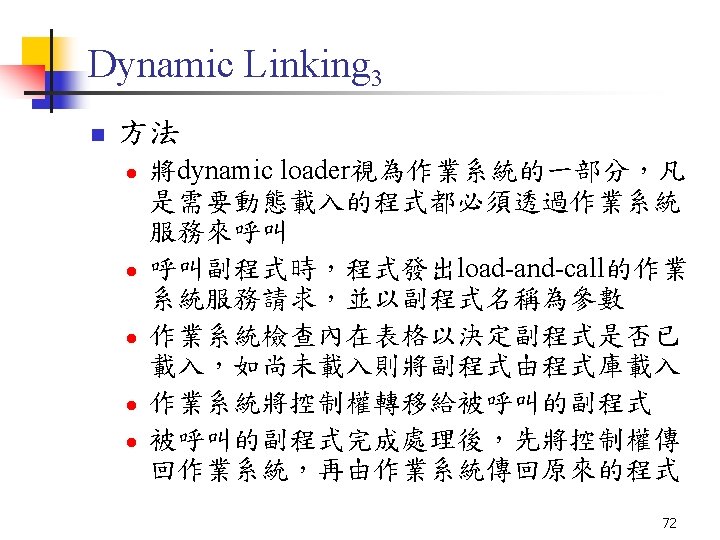
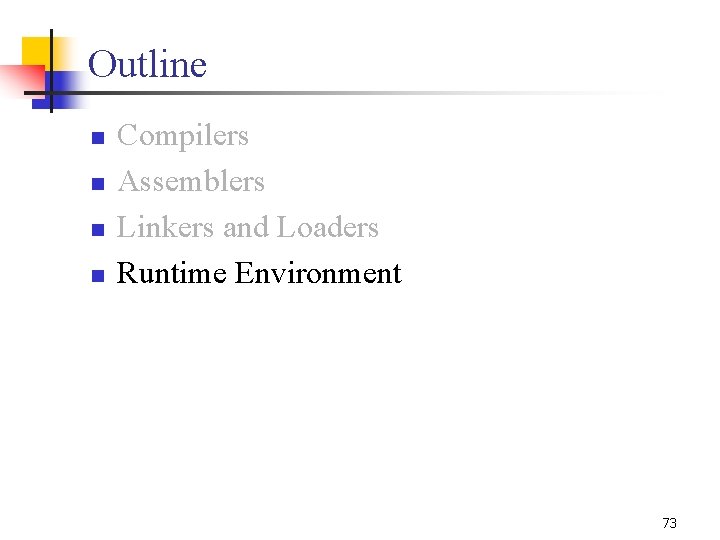
Outline n n Compilers Assemblers Linkers and Loaders Runtime Environment 73
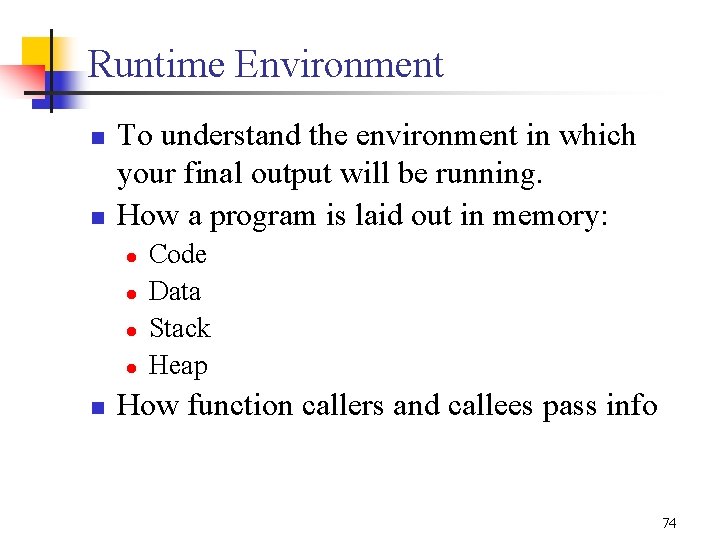
Runtime Environment n n To understand the environment in which your final output will be running. How a program is laid out in memory: l l n Code Data Stack Heap How function callers and callees pass info 74
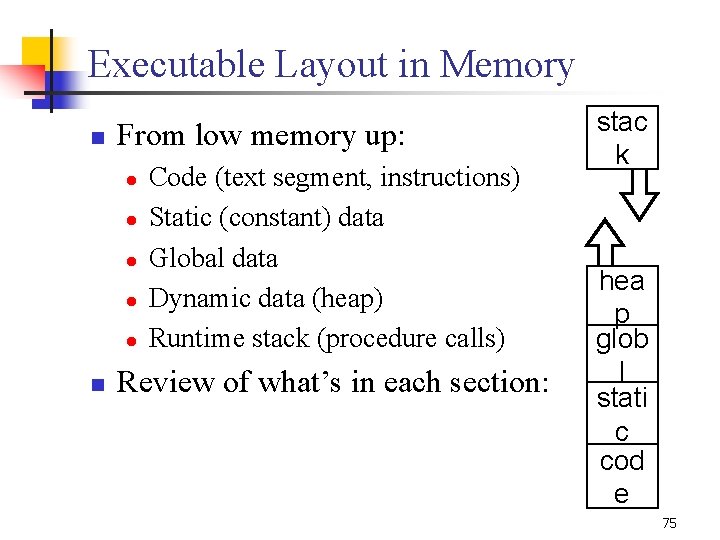
Executable Layout in Memory n From low memory up: l l l n Code (text segment, instructions) Static (constant) data Global data Dynamic data (heap) Runtime stack (procedure calls) Review of what’s in each section: stac k hea p glob l stati c cod e 75
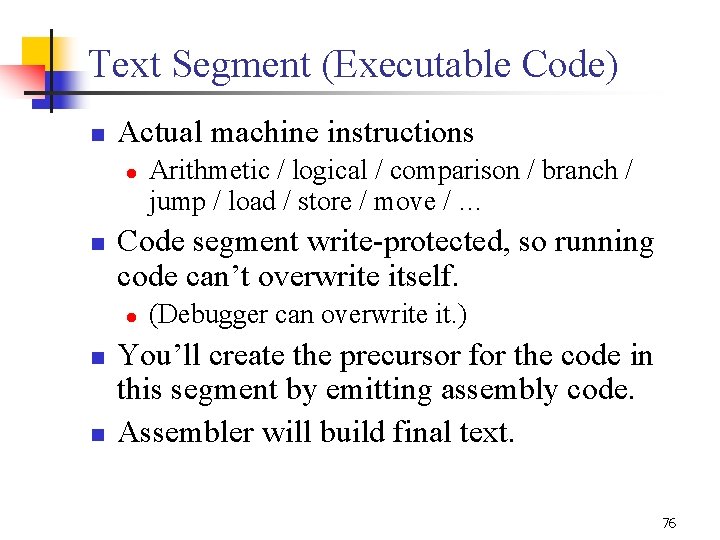
Text Segment (Executable Code) n Actual machine instructions l n Code segment write-protected, so running code can’t overwrite itself. l n n Arithmetic / logical / comparison / branch / jump / load / store / move / … (Debugger can overwrite it. ) You’ll create the precursor for the code in this segment by emitting assembly code. Assembler will build final text. 76
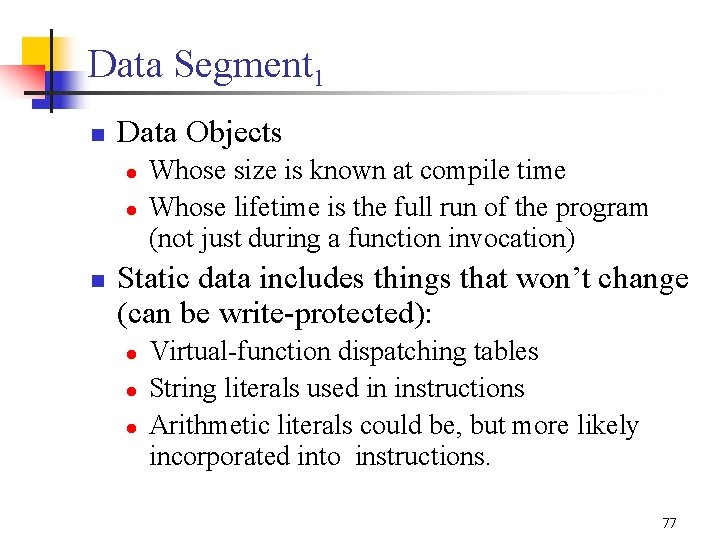
Data Segment 1 n Data Objects l l n Whose size is known at compile time Whose lifetime is the full run of the program (not just during a function invocation) Static data includes things that won’t change (can be write-protected): l l l Virtual-function dispatching tables String literals used in instructions Arithmetic literals could be, but more likely incorporated into instructions. 77
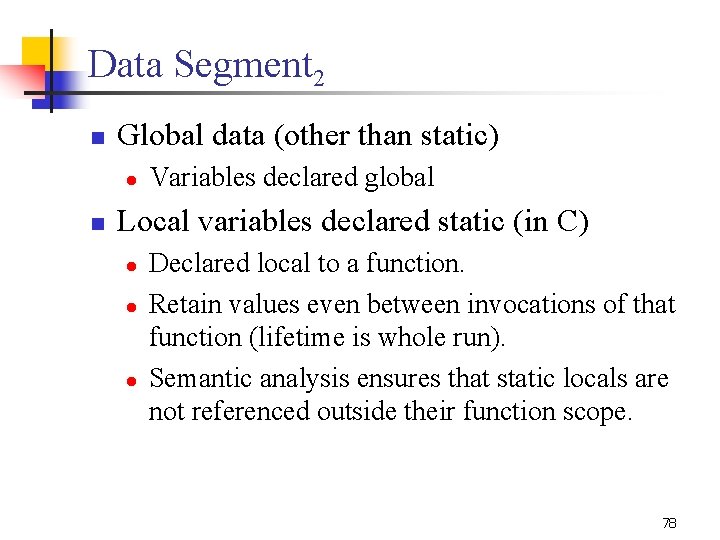
Data Segment 2 n Global data (other than static) l n Variables declared global Local variables declared static (in C) l l l Declared local to a function. Retain values even between invocations of that function (lifetime is whole run). Semantic analysis ensures that static locals are not referenced outside their function scope. 78
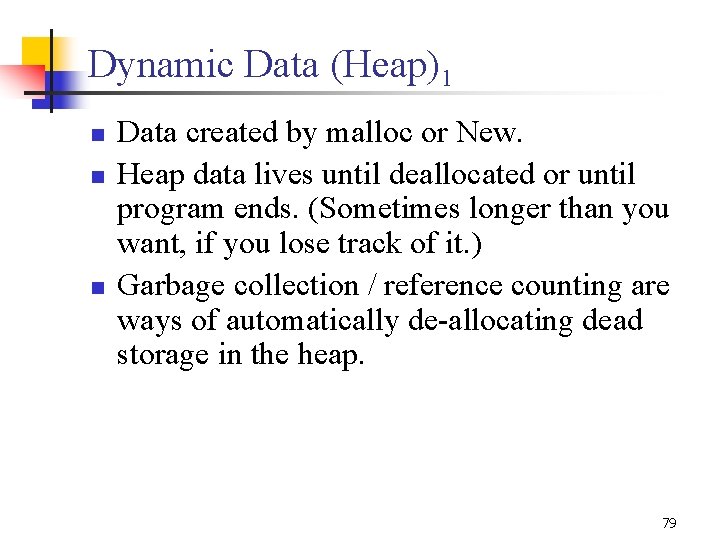
Dynamic Data (Heap)1 n n n Data created by malloc or New. Heap data lives until deallocated or until program ends. (Sometimes longer than you want, if you lose track of it. ) Garbage collection / reference counting are ways of automatically de-allocating dead storage in the heap. 79
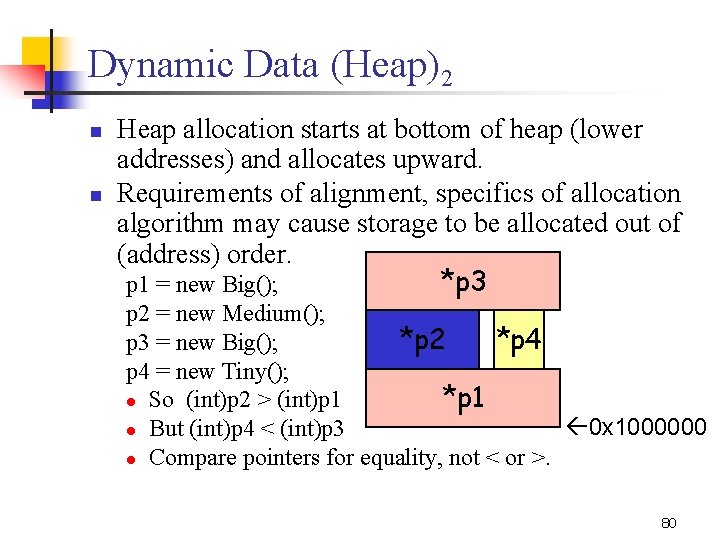
Dynamic Data (Heap)2 n n Heap allocation starts at bottom of heap (lower addresses) and allocates upward. Requirements of alignment, specifics of allocation algorithm may cause storage to be allocated out of (address) order. *p 3 p 1 = new Big(); p 2 = new Medium(); *p 2 *p 4 p 3 = new Big(); p 4 = new Tiny(); l So (int)p 2 > (int)p 1 *p 1 0 x 1000000 l But (int)p 4 < (int)p 3 l Compare pointers for equality, not < or >. 80
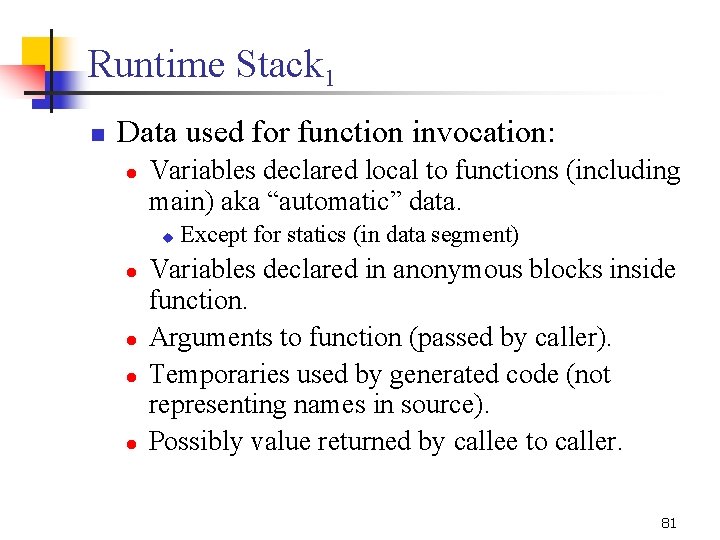
Runtime Stack 1 n Data used for function invocation: l Variables declared local to functions (including main) aka “automatic” data. u l l Except for statics (in data segment) Variables declared in anonymous blocks inside function. Arguments to function (passed by caller). Temporaries used by generated code (not representing names in source). Possibly value returned by callee to caller. 81
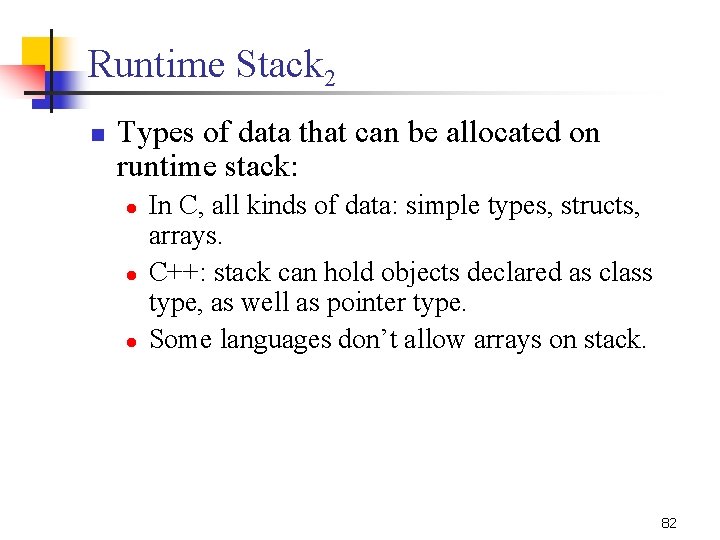
Runtime Stack 2 n Types of data that can be allocated on runtime stack: l l l In C, all kinds of data: simple types, structs, arrays. C++: stack can hold objects declared as class type, as well as pointer type. Some languages don’t allow arrays on stack. 82
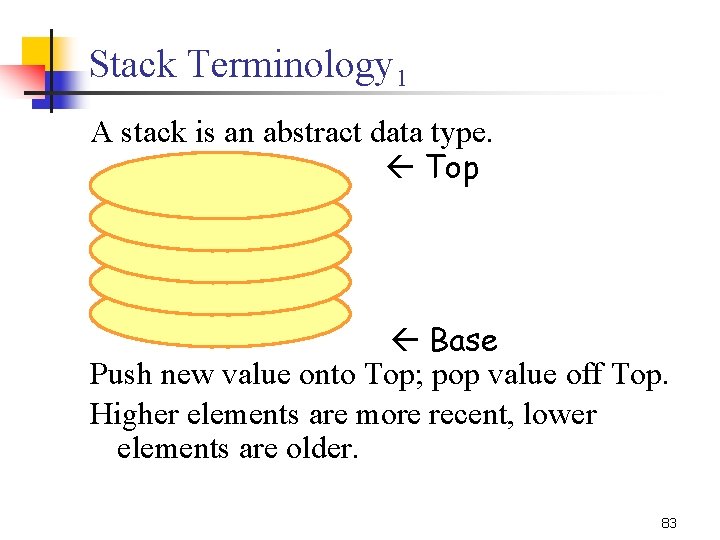
Stack Terminology 1 A stack is an abstract data type. Top Base Push new value onto Top; pop value off Top. Higher elements are more recent, lower elements are older. 83
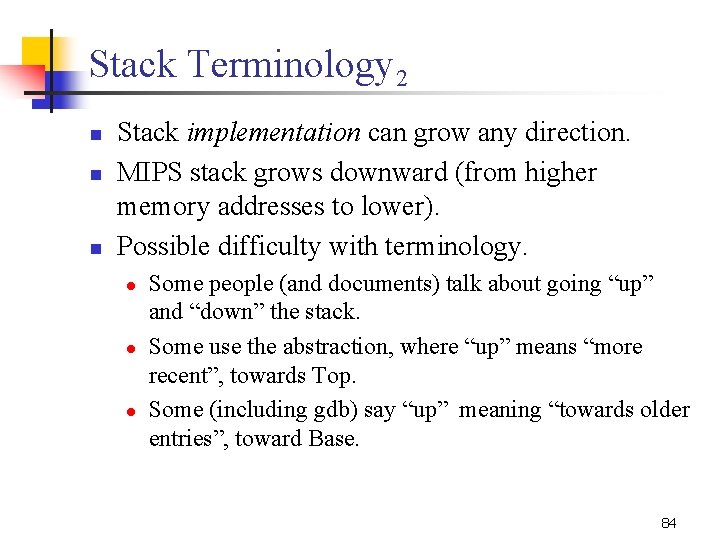
Stack Terminology 2 n n n Stack implementation can grow any direction. MIPS stack grows downward (from higher memory addresses to lower). Possible difficulty with terminology. l l l Some people (and documents) talk about going “up” and “down” the stack. Some use the abstraction, where “up” means “more recent”, towards Top. Some (including gdb) say “up” meaning “towards older entries”, toward Base. 84
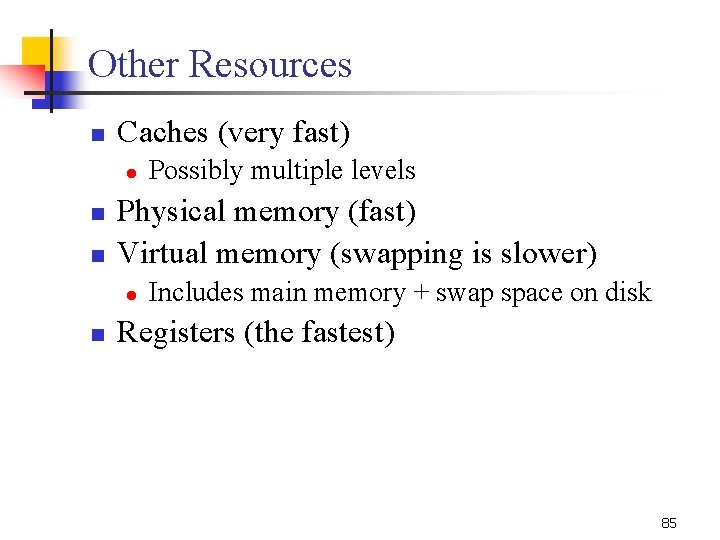
Other Resources n Caches (very fast) l n n Physical memory (fast) Virtual memory (swapping is slower) l n Possibly multiple levels Includes main memory + swap space on disk Registers (the fastest) 85
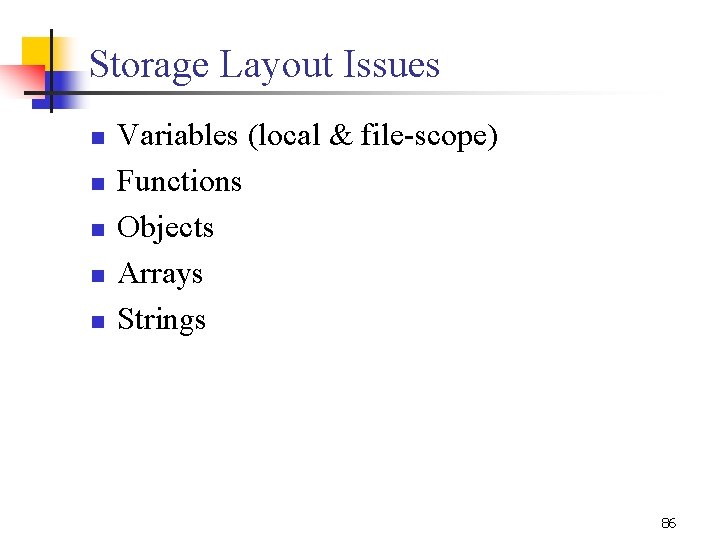
Storage Layout Issues n n n Variables (local & file-scope) Functions Objects Arrays Strings 86
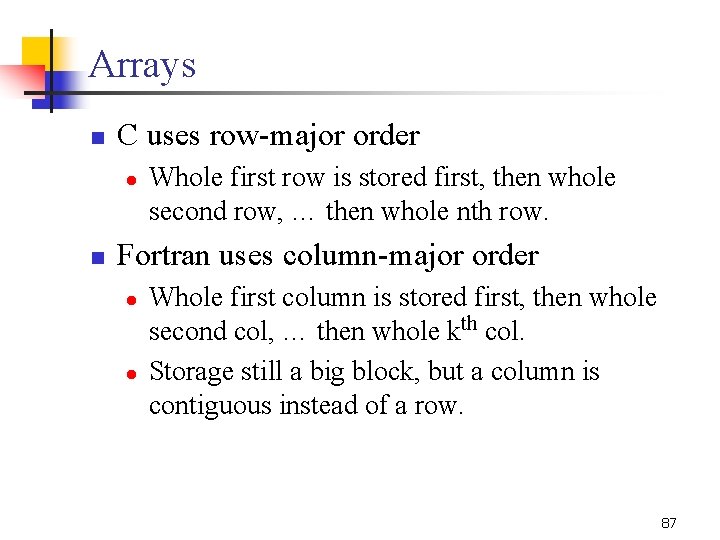
Arrays n C uses row-major order l n Whole first row is stored first, then whole second row, … then whole nth row. Fortran uses column-major order l l Whole first column is stored first, then whole second col, … then whole kth col. Storage still a big block, but a column is contiguous instead of a row. 87
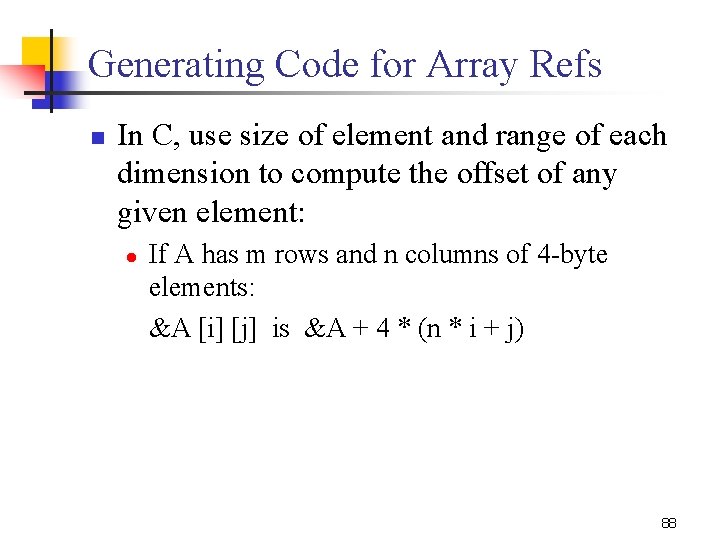
Generating Code for Array Refs n In C, use size of element and range of each dimension to compute the offset of any given element: l If A has m rows and n columns of 4 -byte elements: &A [i] [j] is &A + 4 * (n * i + j) 88
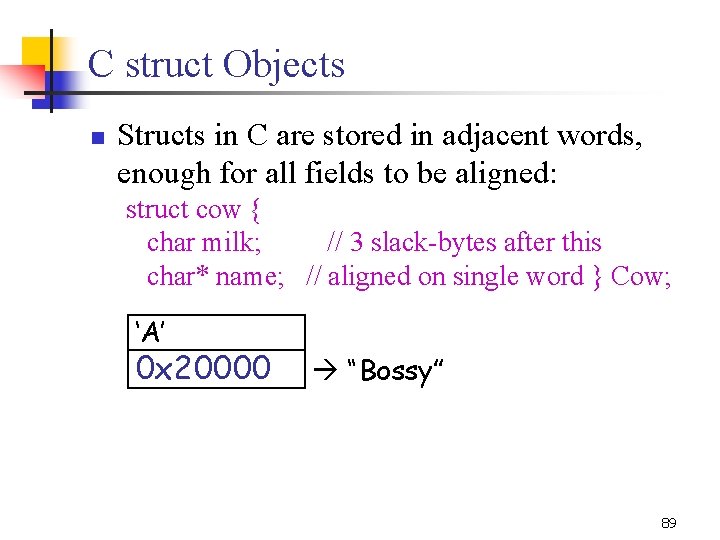
C struct Objects n Structs in C are stored in adjacent words, enough for all fields to be aligned: struct cow { char milk; // 3 slack-bytes after this char* name; // aligned on single word } Cow; ‘A’ 0 x 20000 “Bossy” 89
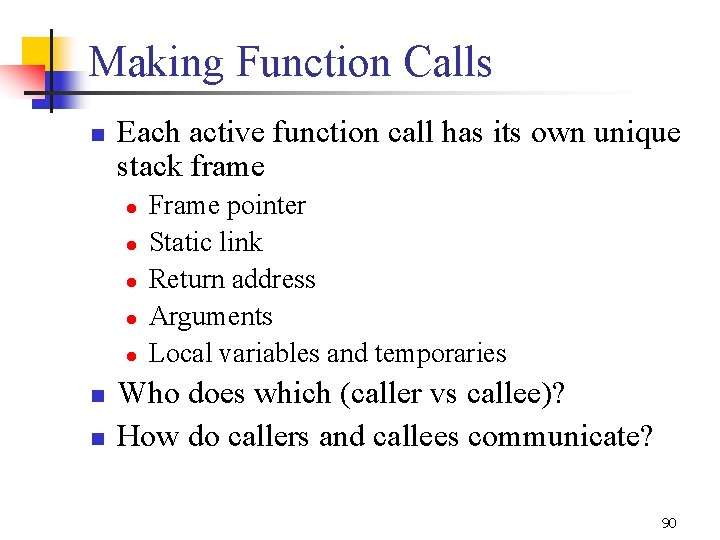
Making Function Calls n Each active function call has its own unique stack frame l l l n n Frame pointer Static link Return address Arguments Local variables and temporaries Who does which (caller vs callee)? How do callers and callees communicate? 90
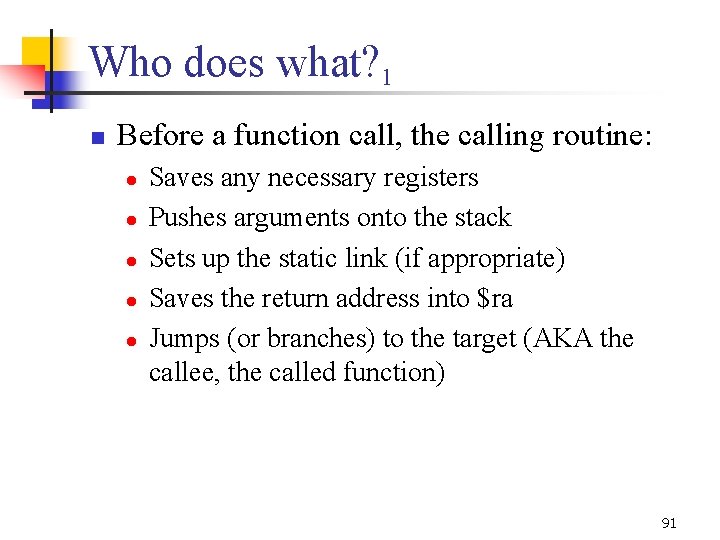
Who does what? 1 n Before a function call, the calling routine: l l l Saves any necessary registers Pushes arguments onto the stack Sets up the static link (if appropriate) Saves the return address into $ra Jumps (or branches) to the target (AKA the callee, the called function) 91
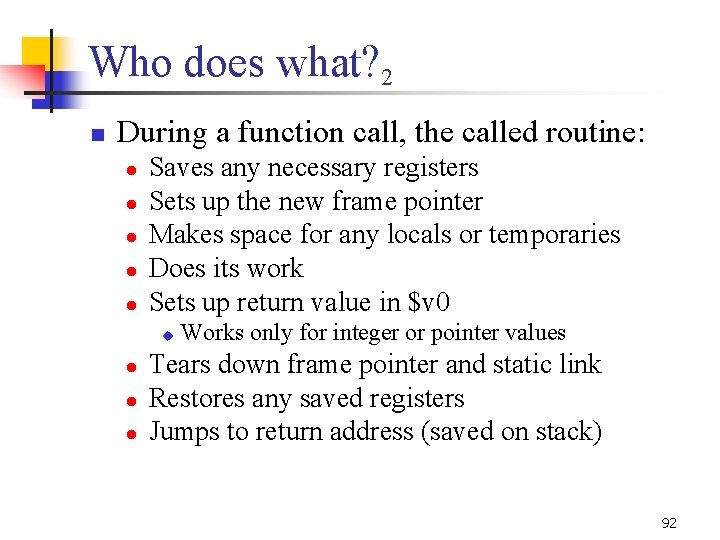
Who does what? 2 n During a function call, the called routine: l l l Saves any necessary registers Sets up the new frame pointer Makes space for any locals or temporaries Does its work Sets up return value in $v 0 u l l l Works only for integer or pointer values Tears down frame pointer and static link Restores any saved registers Jumps to return address (saved on stack) 92
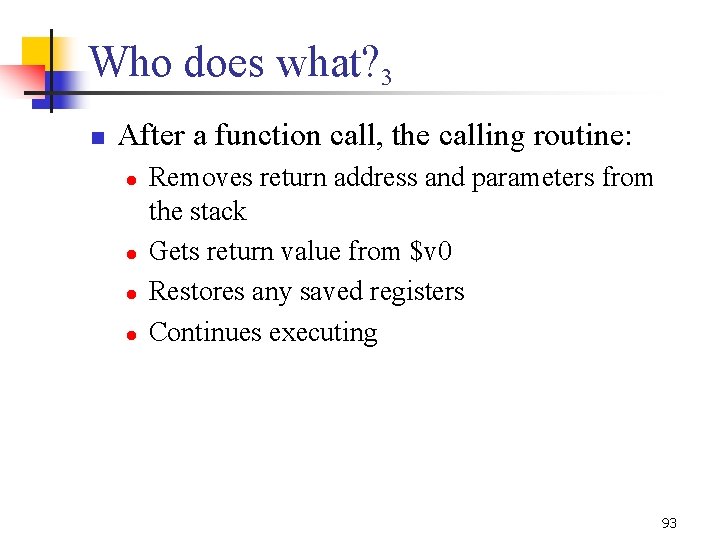
Who does what? 3 n After a function call, the calling routine: l l Removes return address and parameters from the stack Gets return value from $v 0 Restores any saved registers Continues executing 93
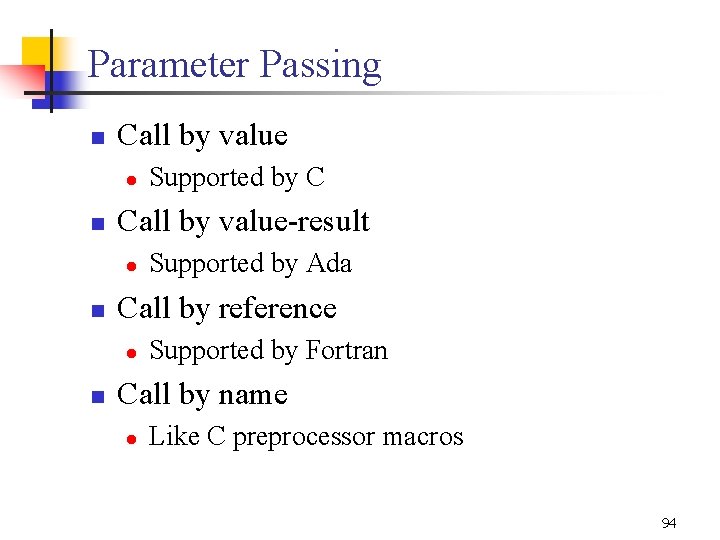
Parameter Passing n Call by value l n Call by value-result l n Supported by Ada Call by reference l n Supported by C Supported by Fortran Call by name l Like C preprocessor macros 94
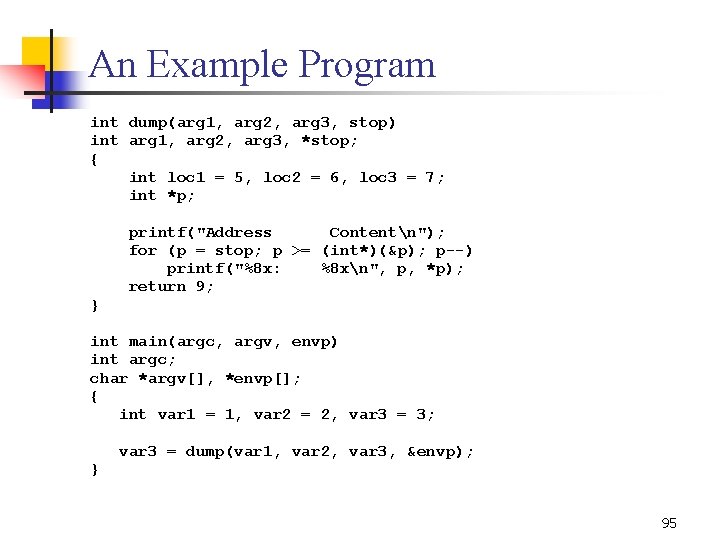
An Example Program int dump(arg 1, arg 2, arg 3, stop) int arg 1, arg 2, arg 3, *stop; { int loc 1 = 5, loc 2 = 6, loc 3 = 7; int *p; printf("Address Contentn"); for (p = stop; p >= (int*)(&p); p--) printf("%8 x: %8 xn", p, *p); return 9; } int main(argc, argv, envp) int argc; char *argv[], *envp[]; { int var 1 = 1, var 2 = 2, var 3 = 3; var 3 = dump(var 1, var 2, var 3, &envp); } 95
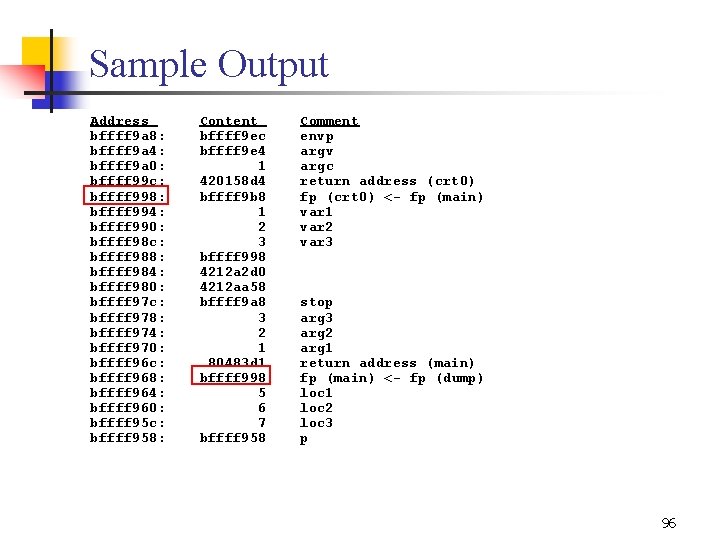
Sample Output Address bffff 9 a 8: bffff 9 a 4: bffff 9 a 0: bffff 99 c: bffff 998: bffff 994: bffff 990: bffff 98 c: bffff 988: bffff 984: bffff 980: bffff 97 c: bffff 978: bffff 974: bffff 970: bffff 96 c: bffff 968: bffff 964: bffff 960: bffff 95 c: bffff 958: Content bffff 9 ec bffff 9 e 4 1 420158 d 4 bffff 9 b 8 1 2 3 bffff 998 4212 a 2 d 0 4212 aa 58 bffff 9 a 8 3 2 1 80483 d 1 bffff 998 5 6 7 bffff 958 Comment envp argv argc return address (crt 0) fp (crt 0) <- fp (main) var 1 var 2 var 3 stop arg 3 arg 2 arg 1 return address (main) fp (main) <- fp (dump) loc 1 loc 2 loc 3 p 96
Disadvantages of second class levers
Rpd clasp types
How do machines make work easier
Classes of levers
Linker arm left arm
Intracoronal retainer
Digital design and computer architecture arm edition
Computer architecture arm edition
Digital design and computer architecture: arm edition
Contents introduction
Arm in computer organization
Architecture and organization difference
Design of basic computer with flowchart
Introduction to computer organization and architecture
Arm architecture
Arm architecture logo
Arm system on chip architecture
Arm architecture history
Implement carry arbitration encoding in arm9tdmi
3 bus architecture
Portfolio of evidence example
Deep perineal pouch contents
Fresh frozen plasma contents
Ffp vs platelets
Posterior mediastinum contents
Femoral triangle anatomy
Identify
The immortal life of henrietta lacks table of contents
Contents of internal capsule
Subscapularis insertion and origin
Ark of the covenant lampstand
So we meet again my dear doctor
Ligament of treitz
Mla table of contents
Stylistic synonyms lexicology
Front
Continuous variable example
Writing appendix in a report
Ffp vs platelets
Superior mediastinum contents
Mediastinum vs pericardium
Abstract and introduction
Persepolis table
Persepolis table
Interactive notebook table of contents
Cryoprecipitate dose
Interpeduncular fossa
Spermatic cord covering
Greater sciatic foramen contents
Events management team structure
Contents of the middle mediastinum
Posterior mediastinum
Carotid sheath contents
Triangular space contents
Curriculum vitaem
Ctd modules
Conclusion of cost audit
Civics interactive notebook
Jasdeep dhaliwal
Fresh frozen plasma contents
Air pollution contents
Magazine contents page analysis
Nerve in cubital fossa
Relations of elbow joint
Boundaries of anterior triangle
Quadriceps femoral
Medial aspect of antecubital fossa
Quad origin
Rotator cuff interval
Hamlet history background
Optab in assembler
Ctd module 5 table of contents
Urogenital triangle
Entrepreneurship training and development
Scrapbook table of contents
Infrahyoid region
Table of contents science
Styloid apparatus
Science project setup
Basic principles of pharmacokinetics
Clipart table of contents
Transverse perineal muscle
Interpalatine suture
Trial master file contents
Define term health education
Ctd triangle
Bibliography for chemistry project
Cruropopliteal canal
Contents of a dead man's pocket conflict
Blood components
Table of contents interactive notebook
Table of contents science
All of the following accurately describe hadoop
Literature review table of contents
Sds software development
Epq exemplars
Science fair logbook table of contents