Sorting and Insertion Sort Sorting techniques Ideally when
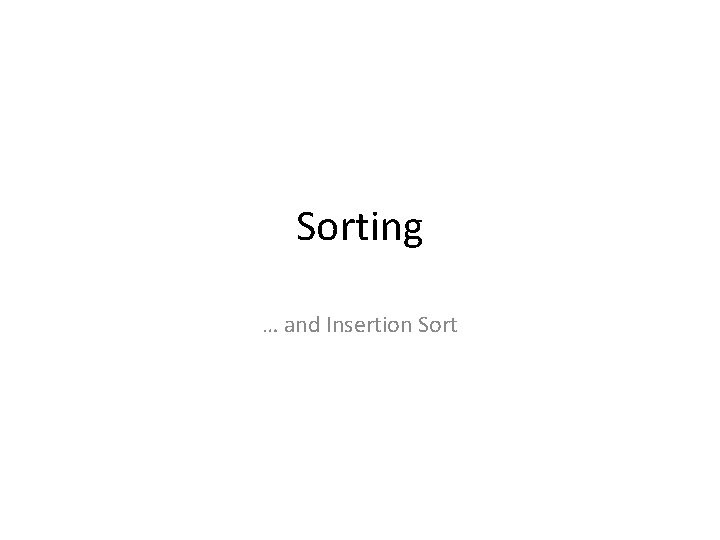
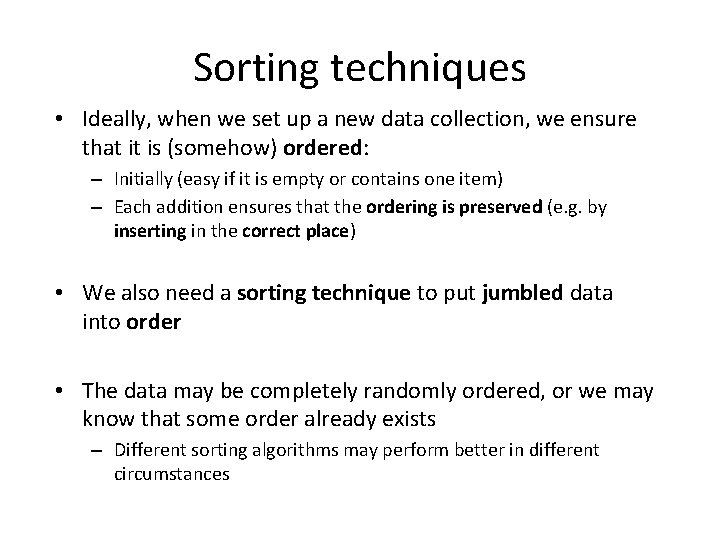
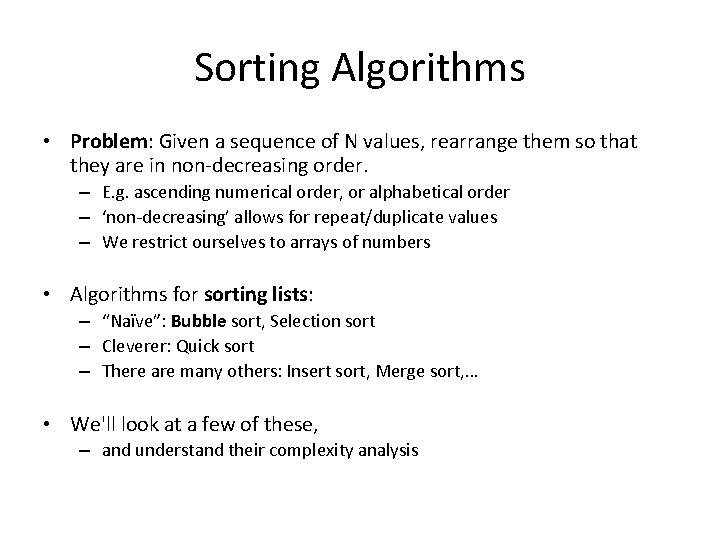
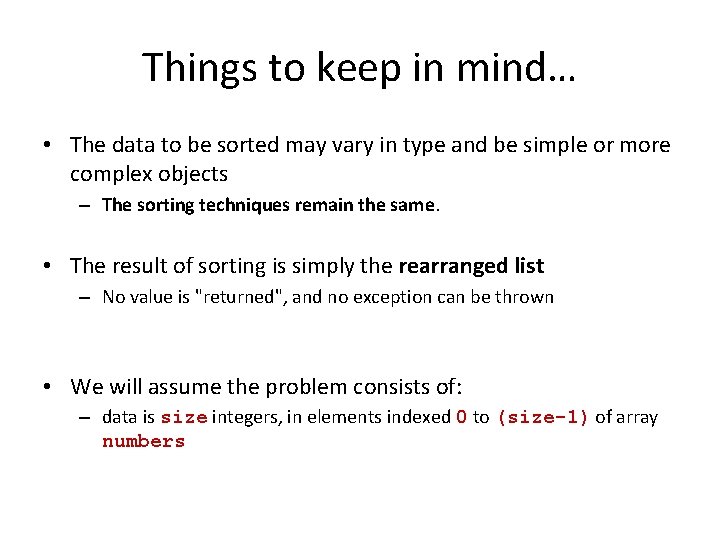
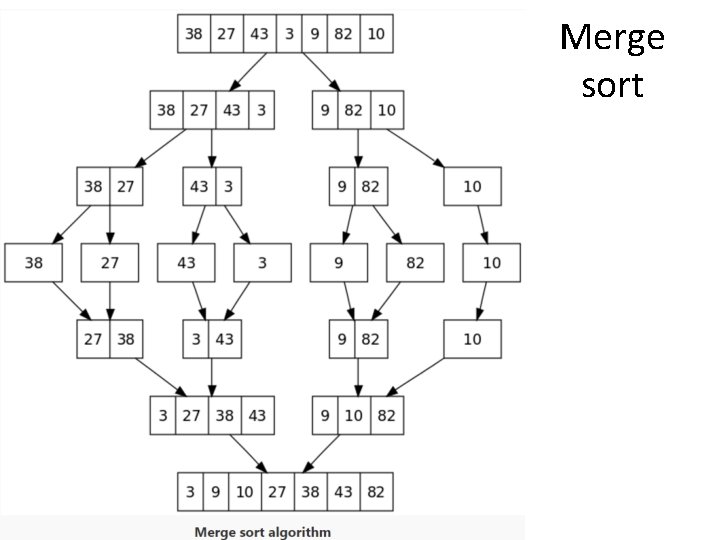
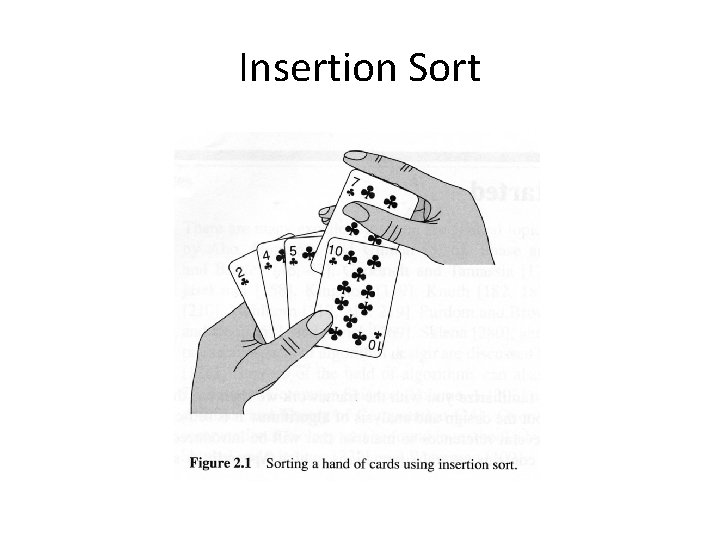
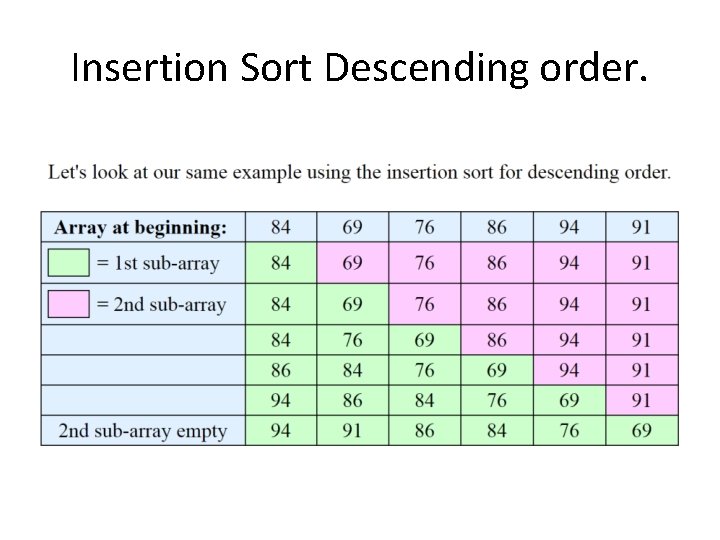
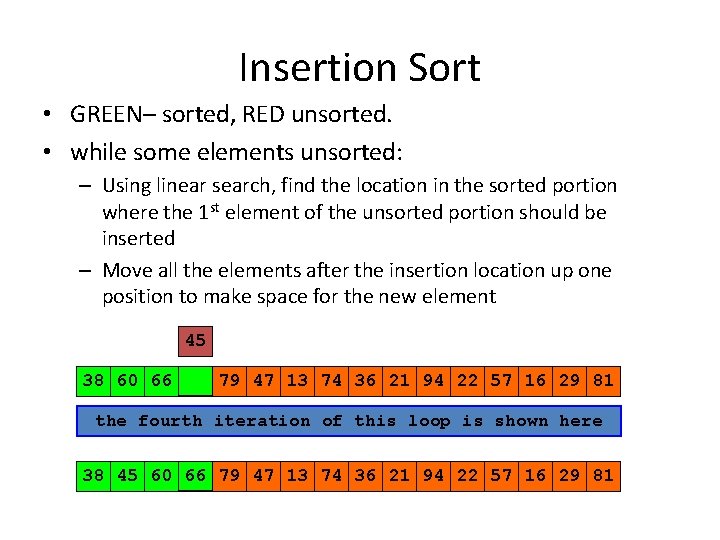
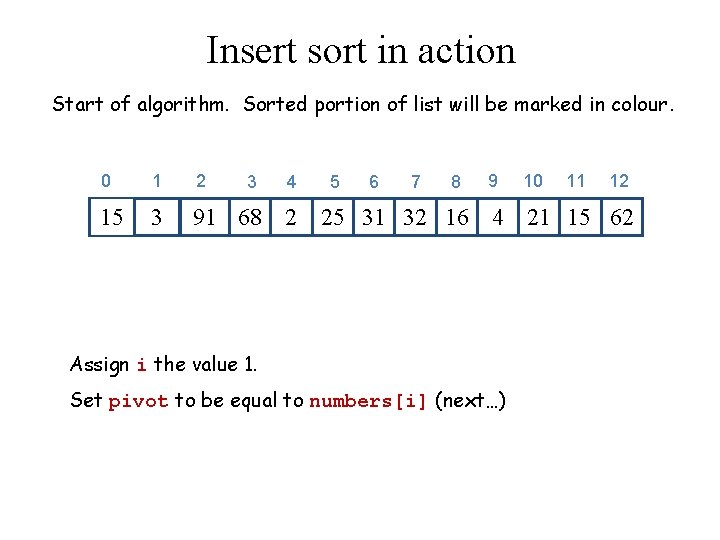
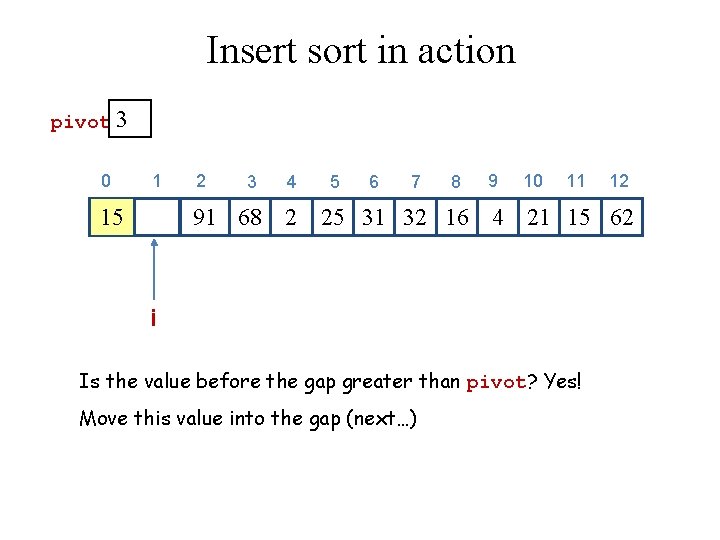
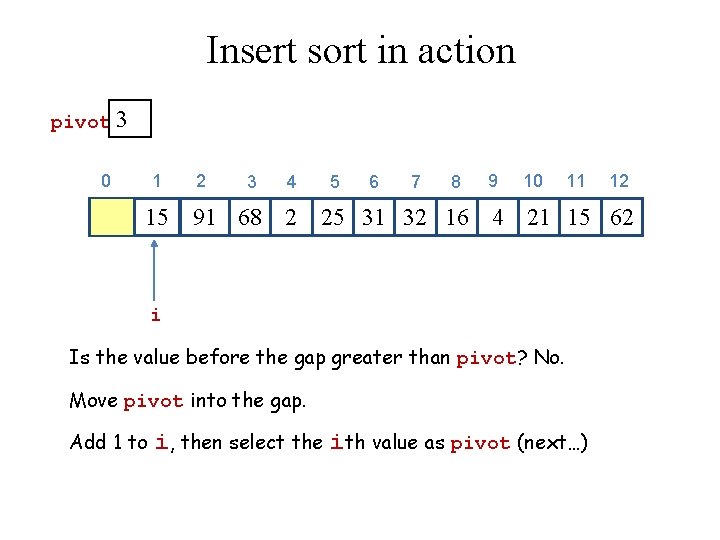
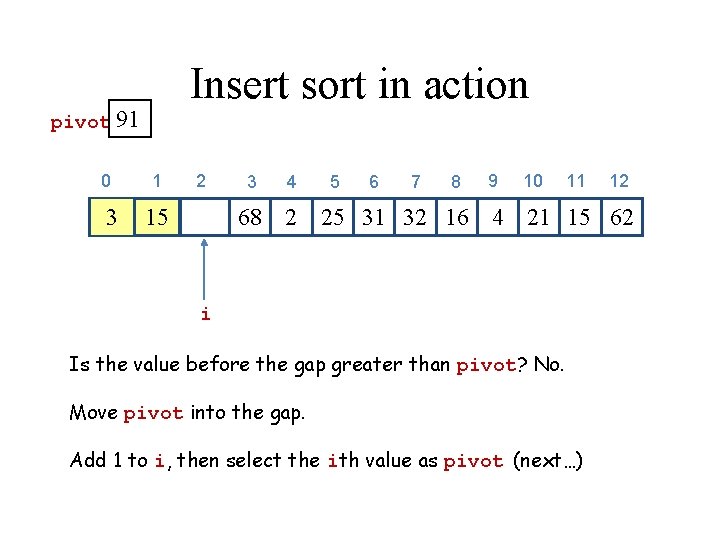
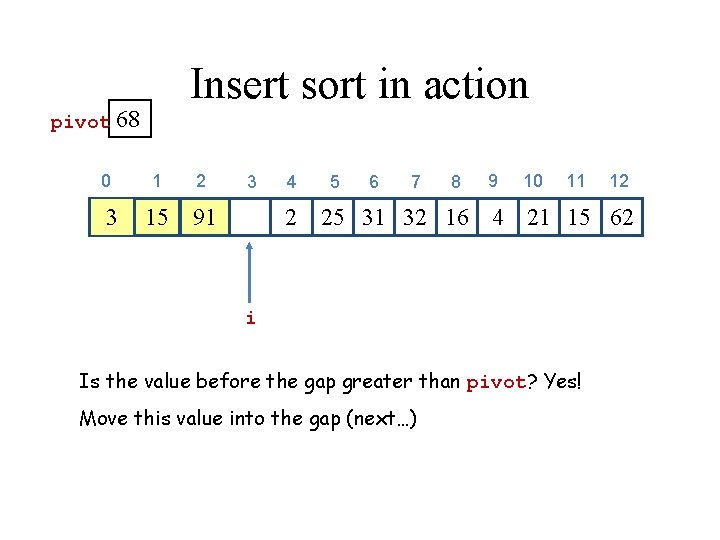
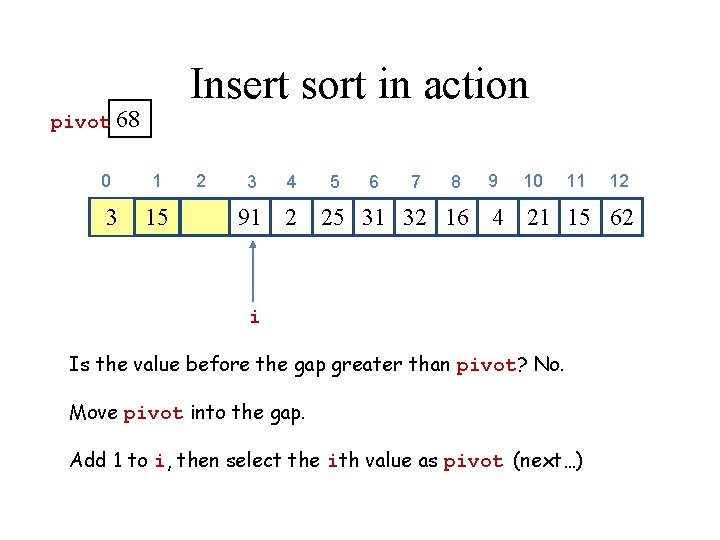
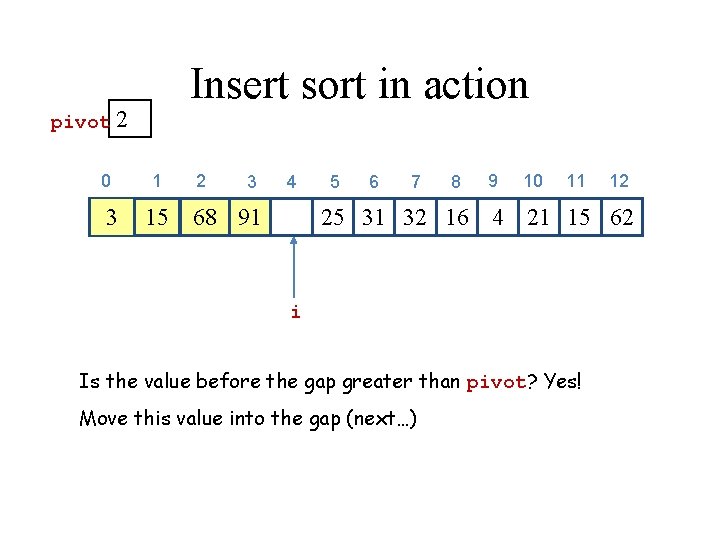
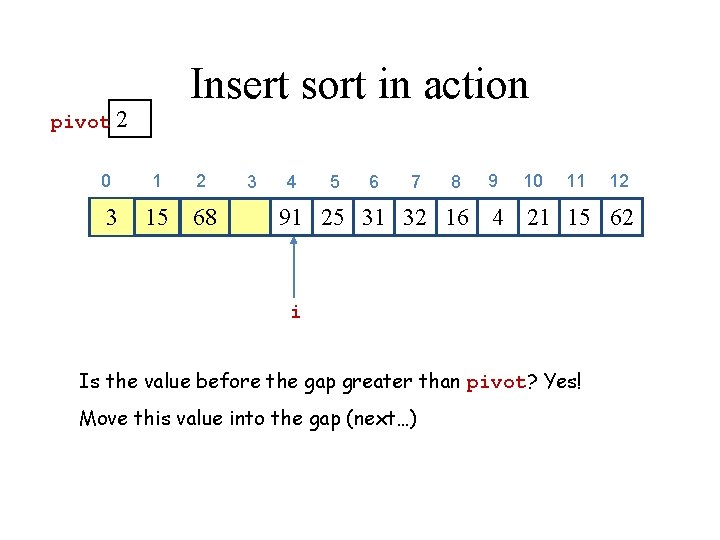
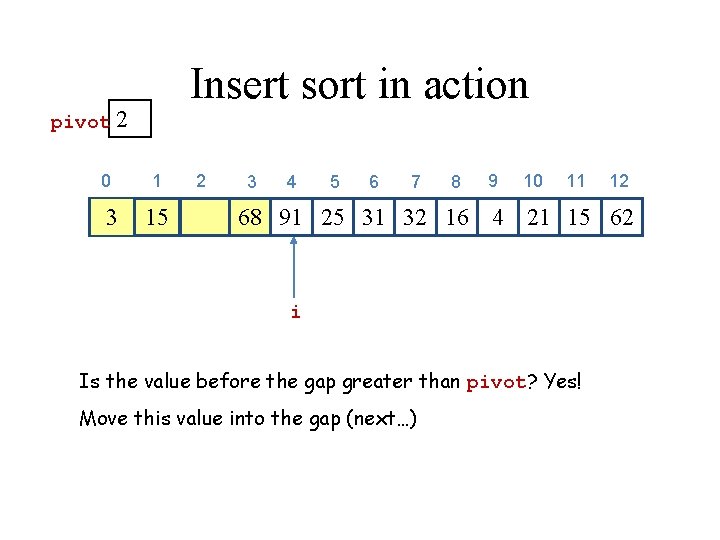
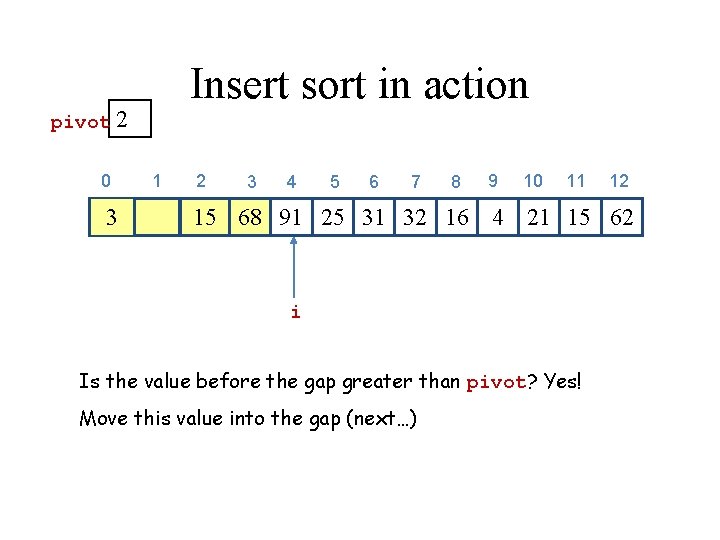
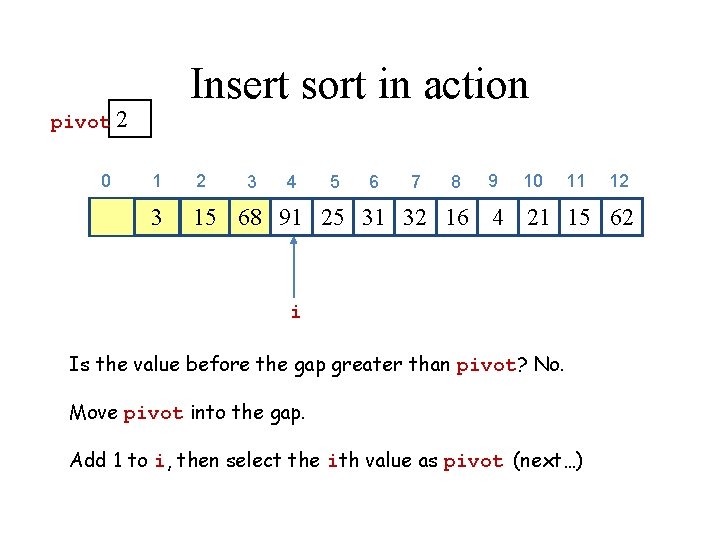
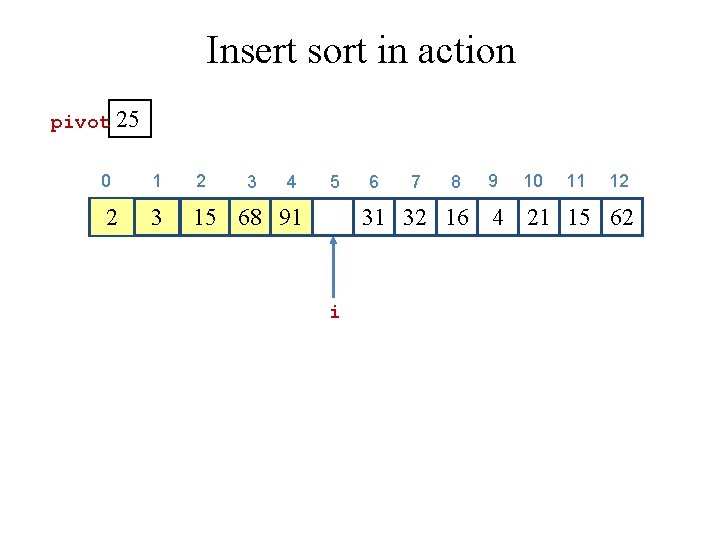
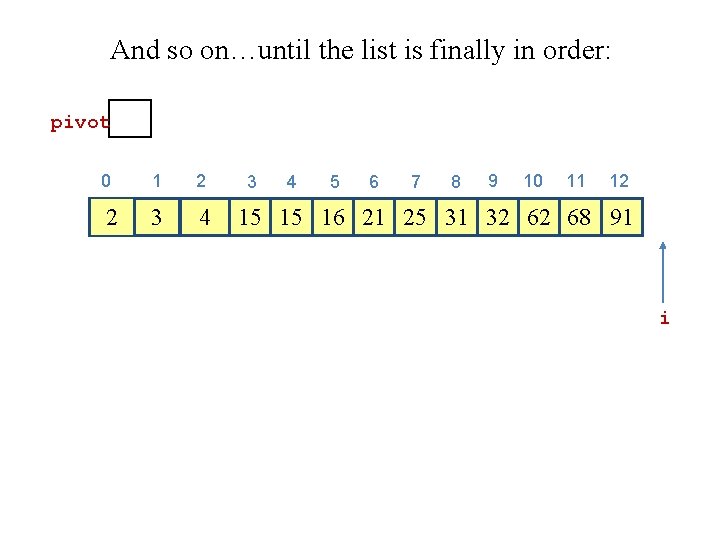
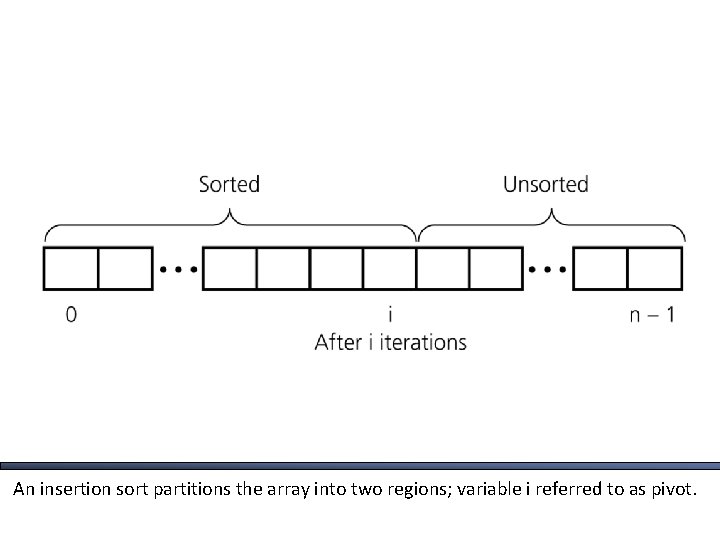
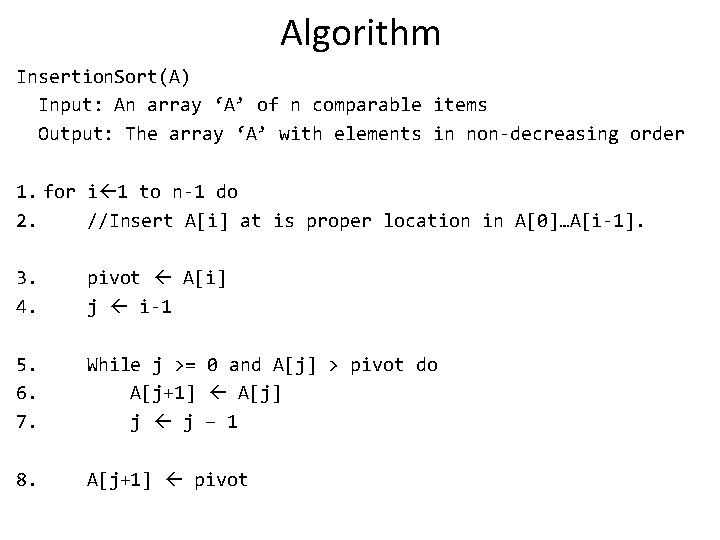
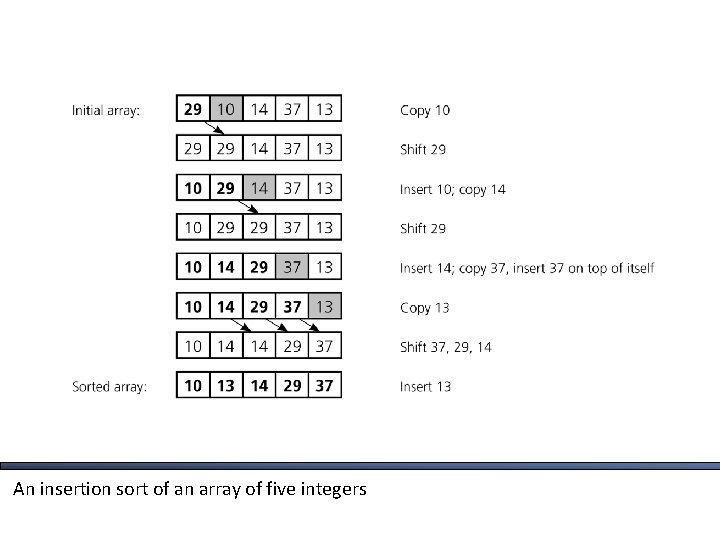
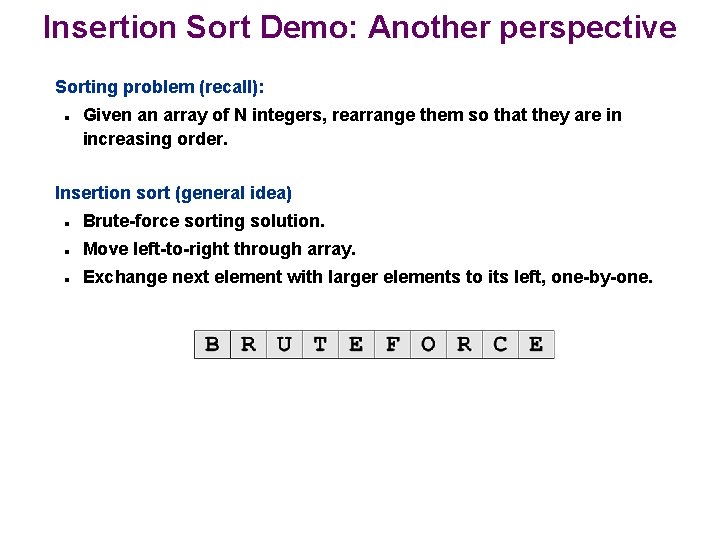
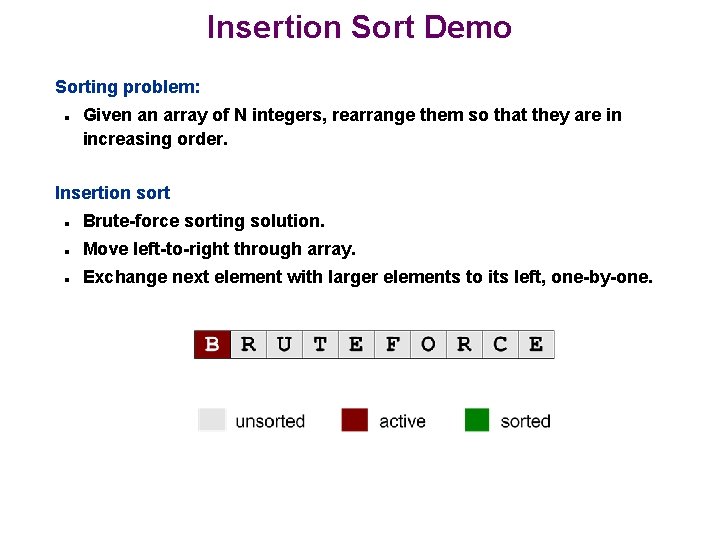
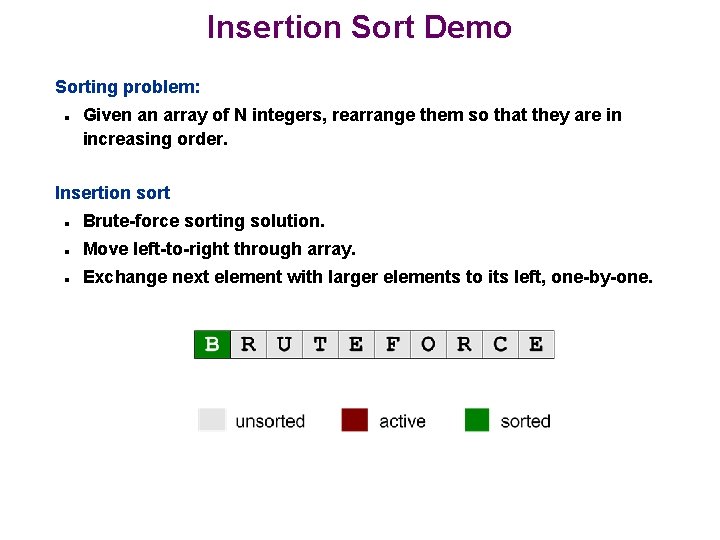
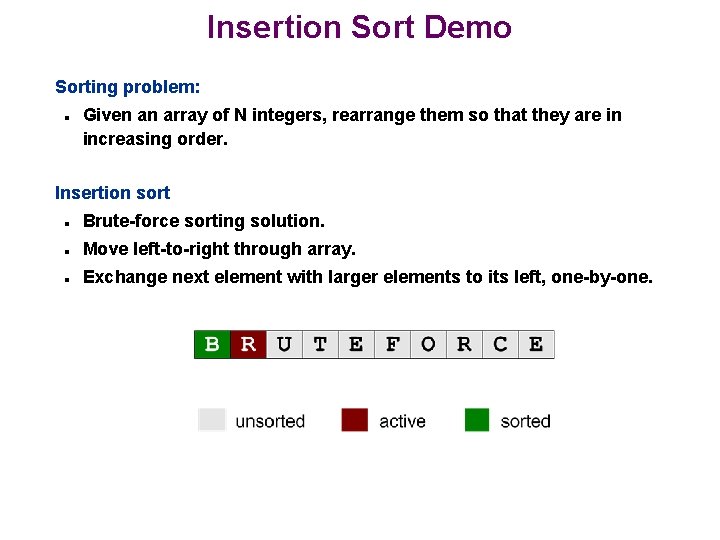
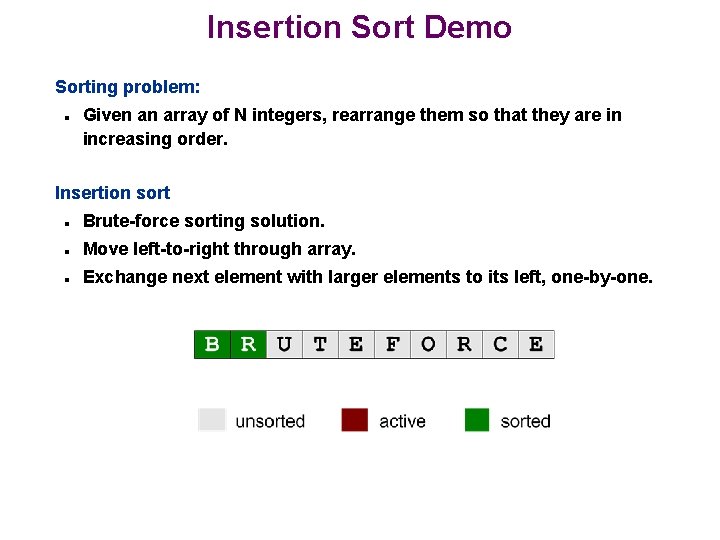
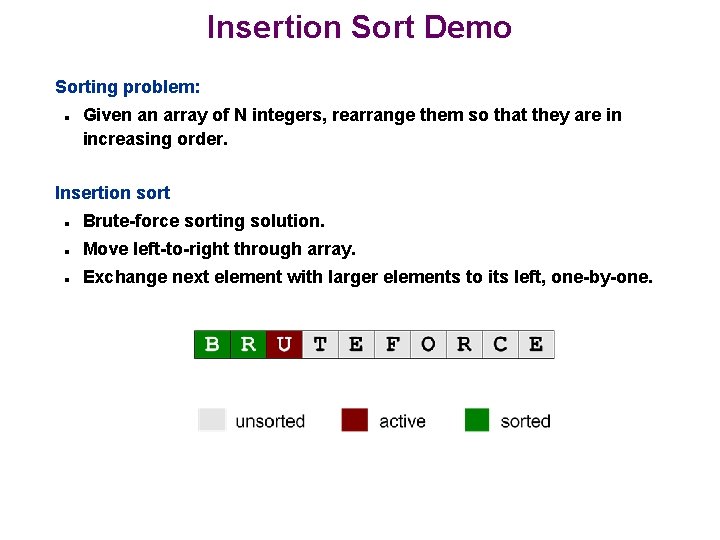
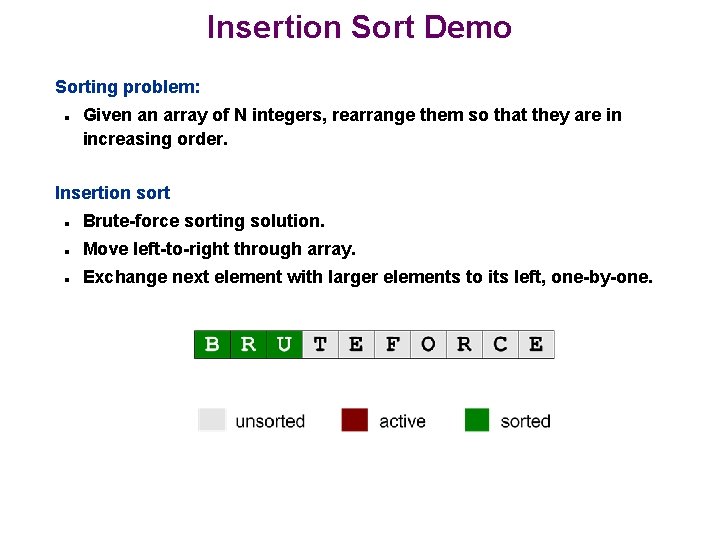
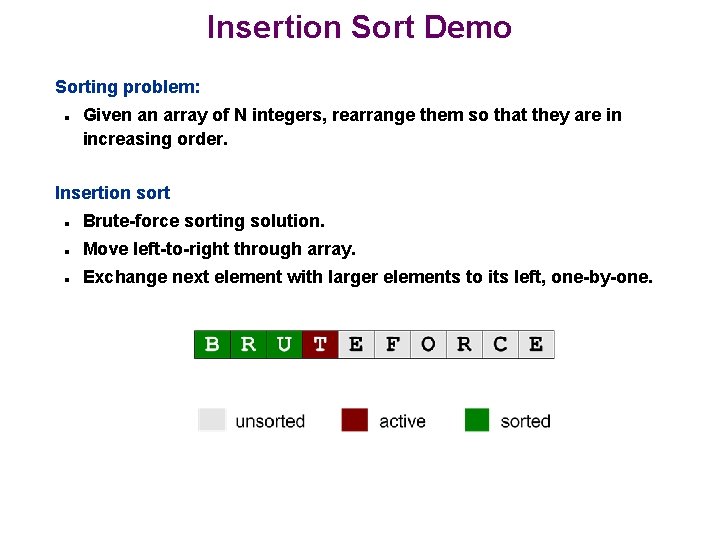
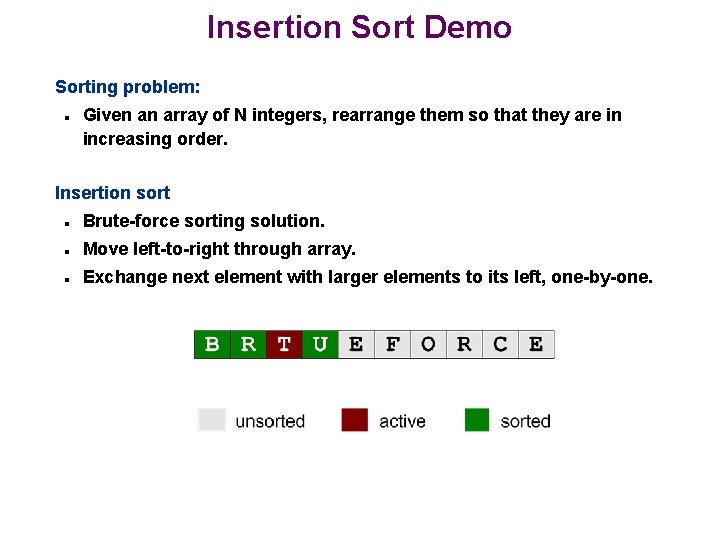
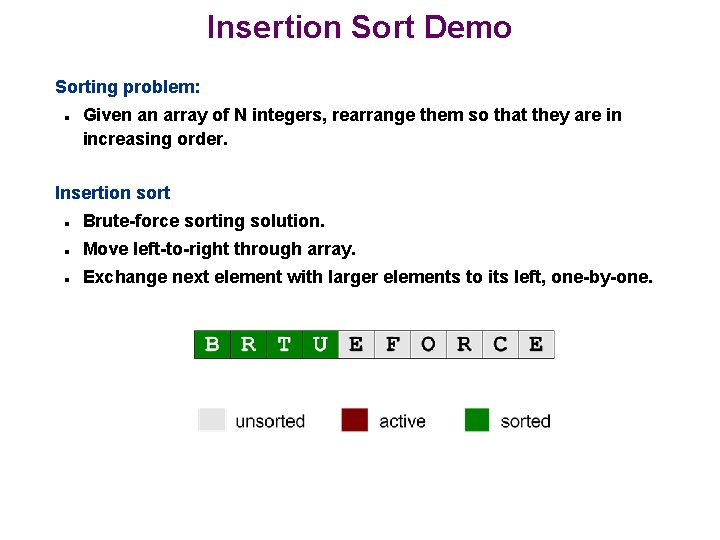
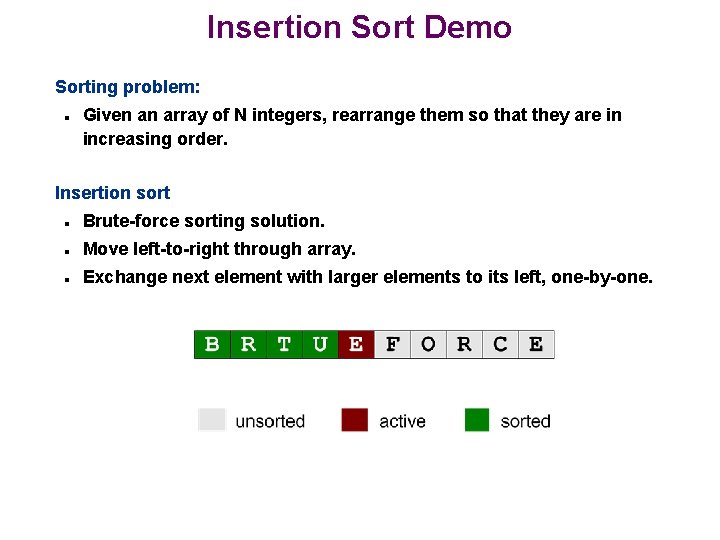
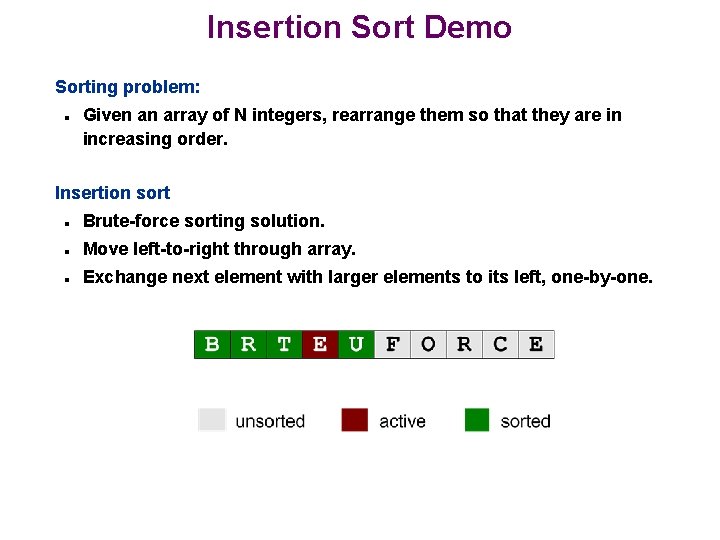
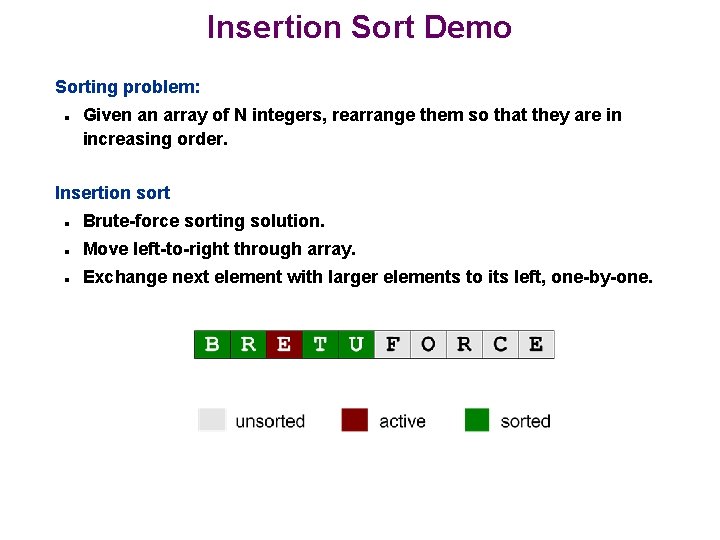
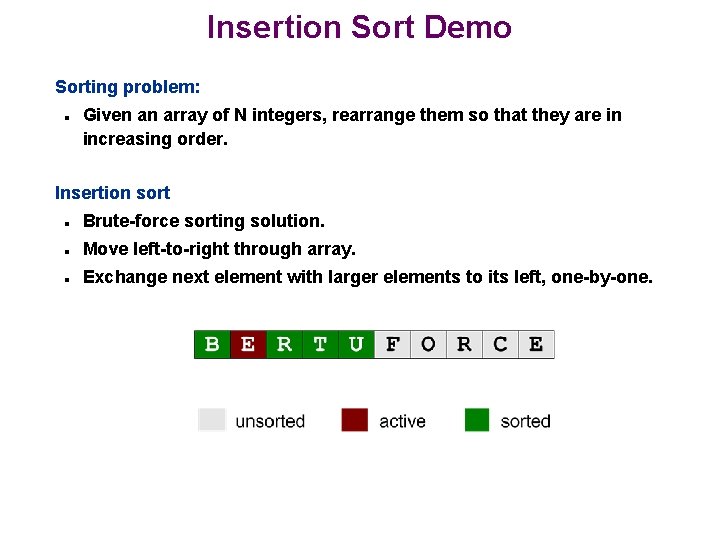
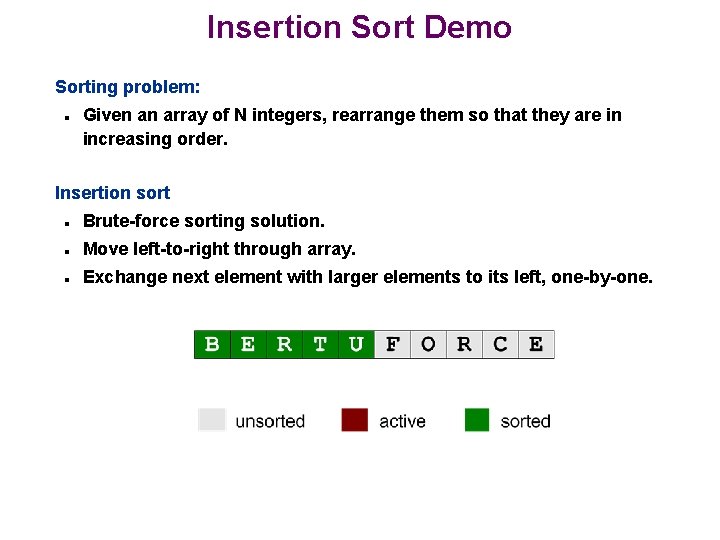
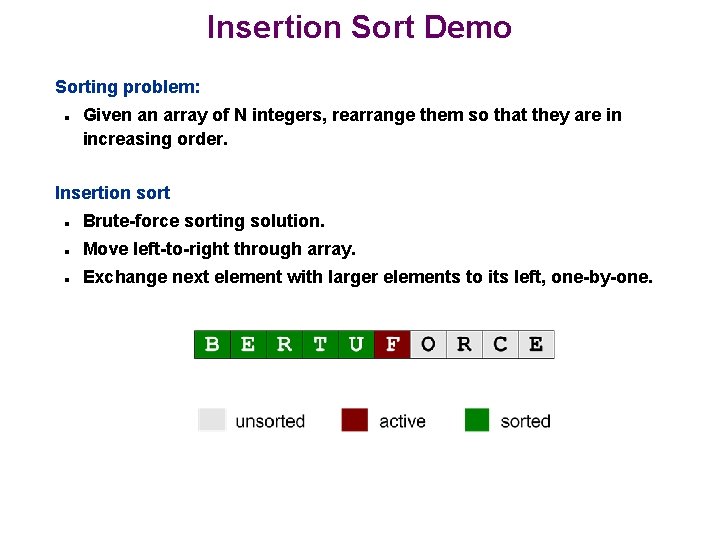
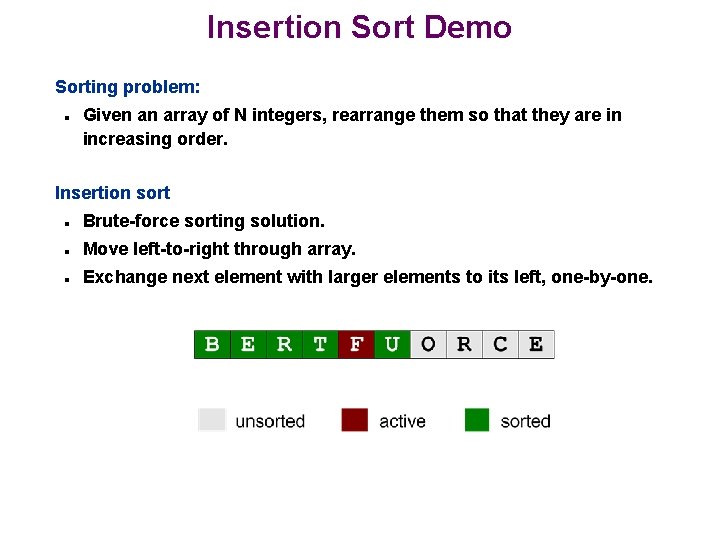
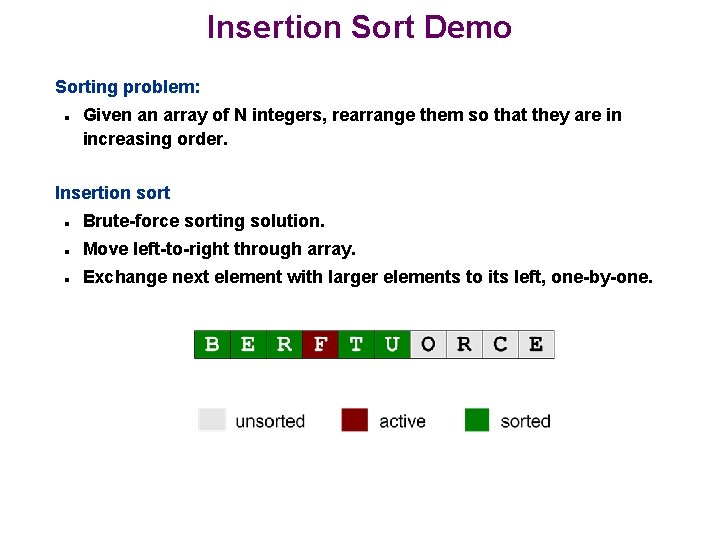
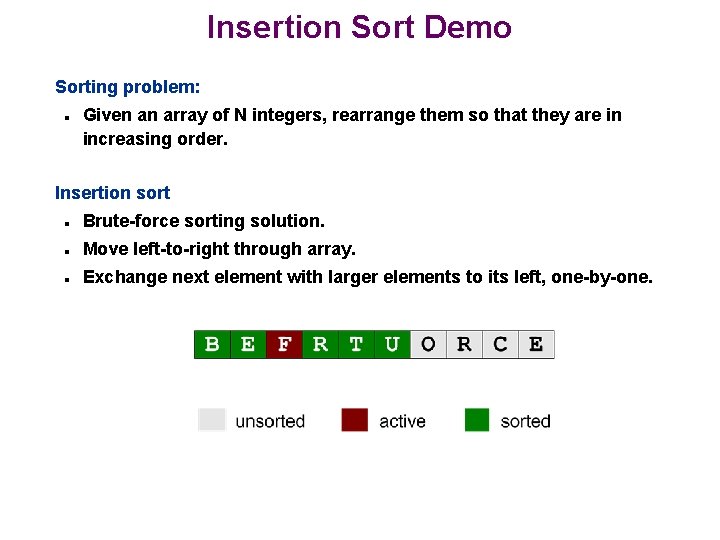
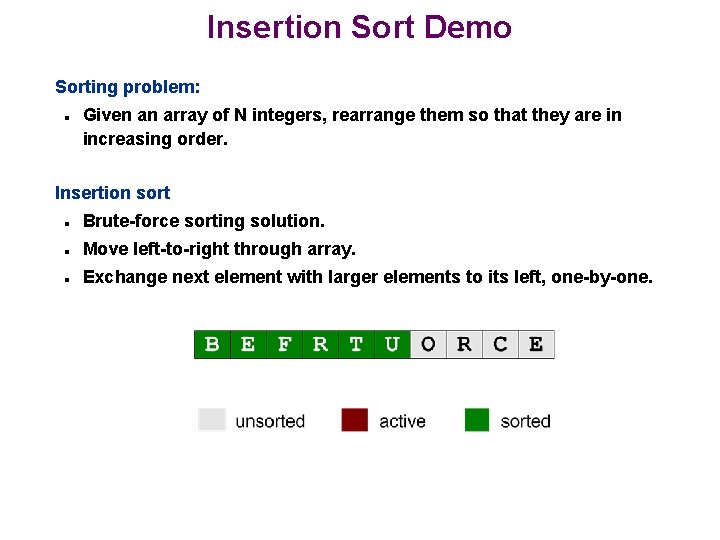
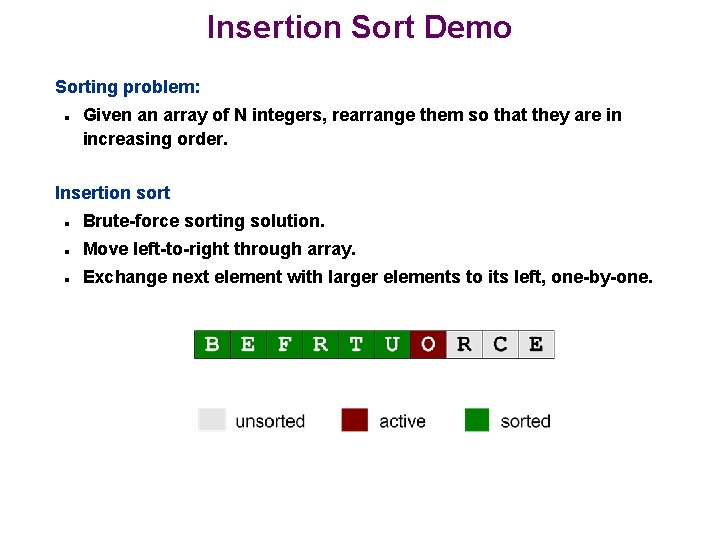
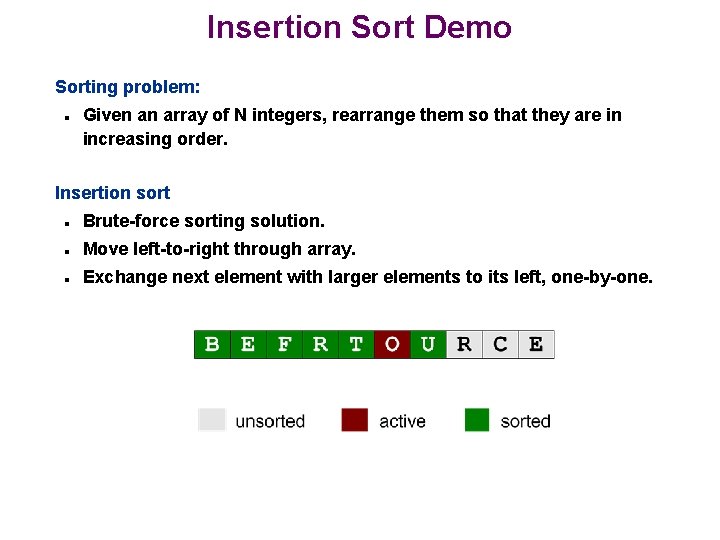
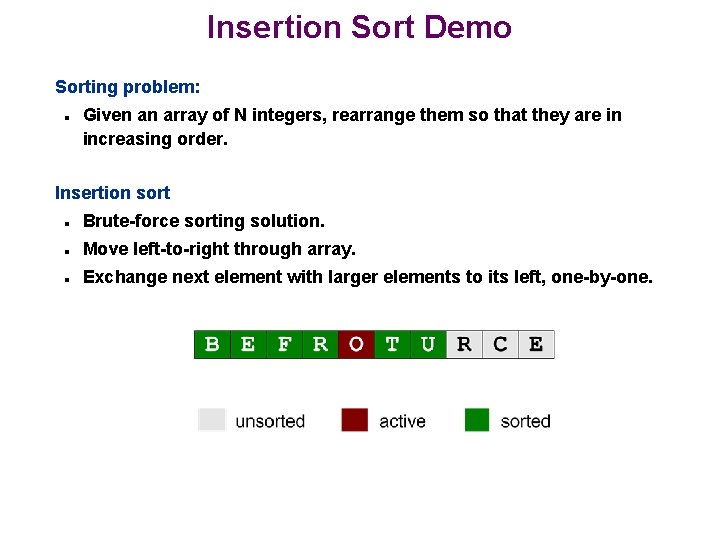
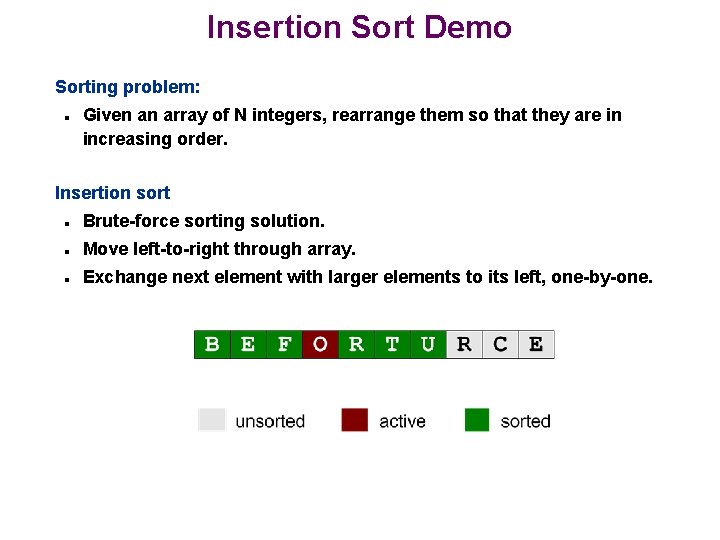
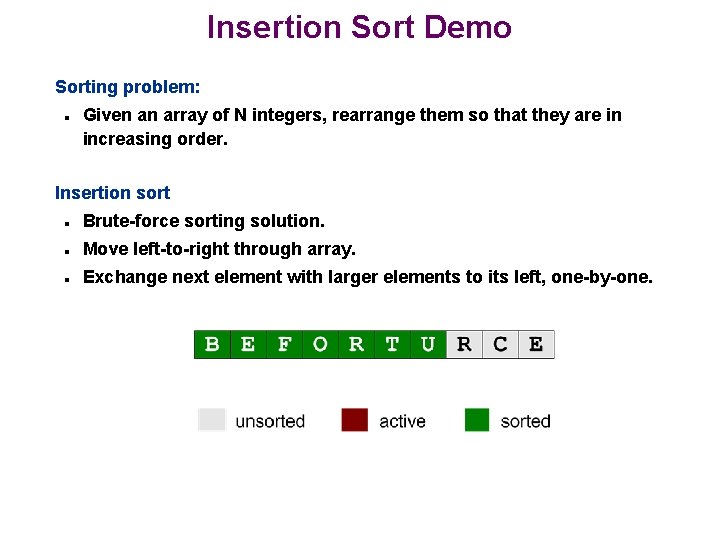
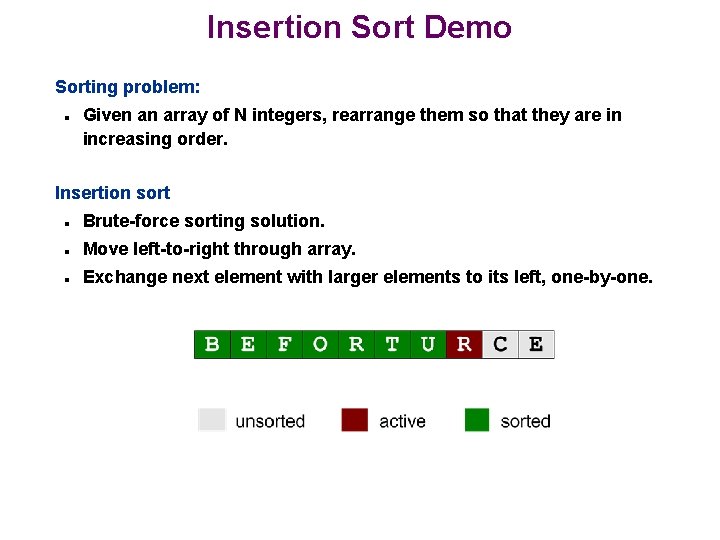
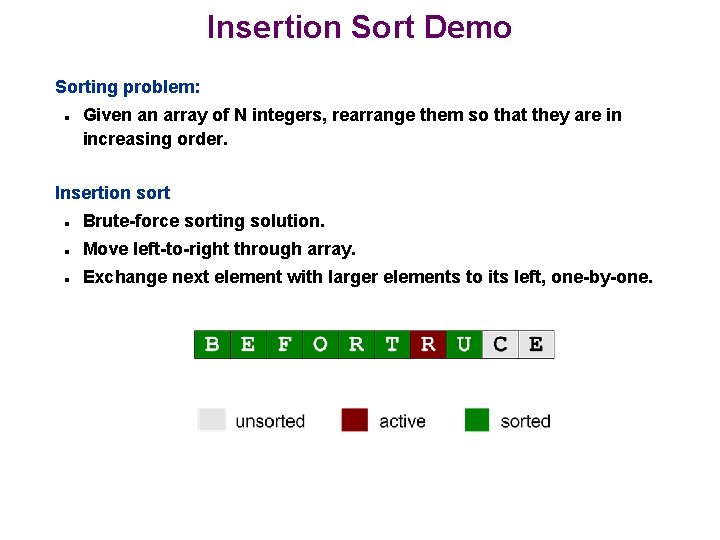
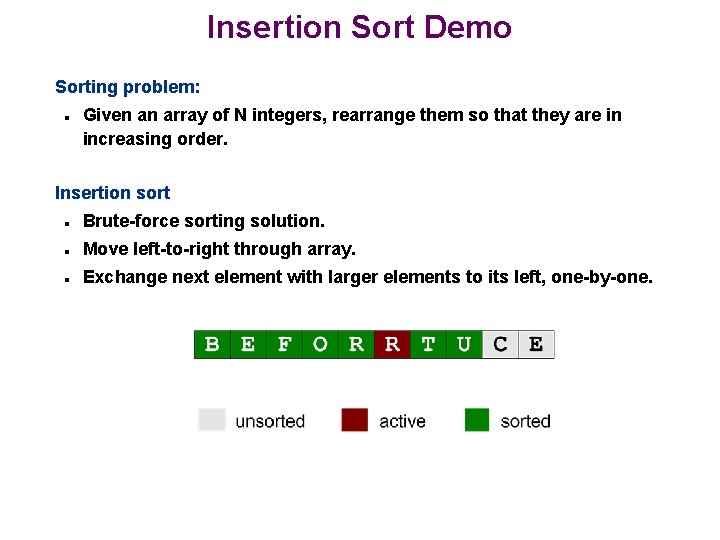
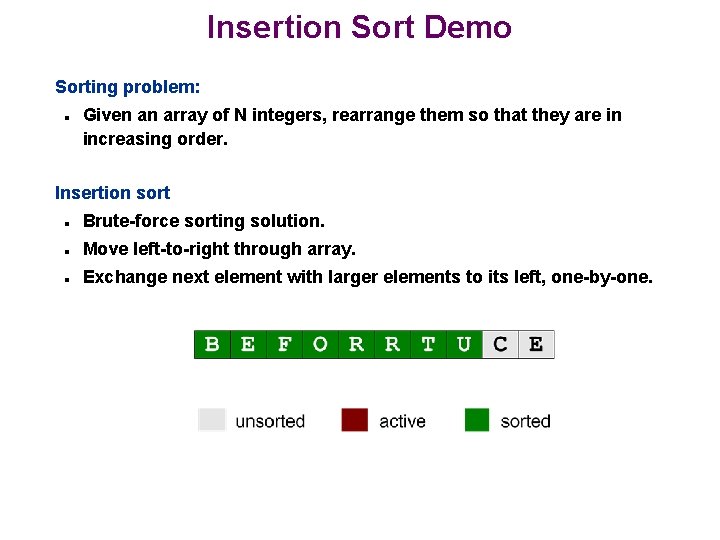
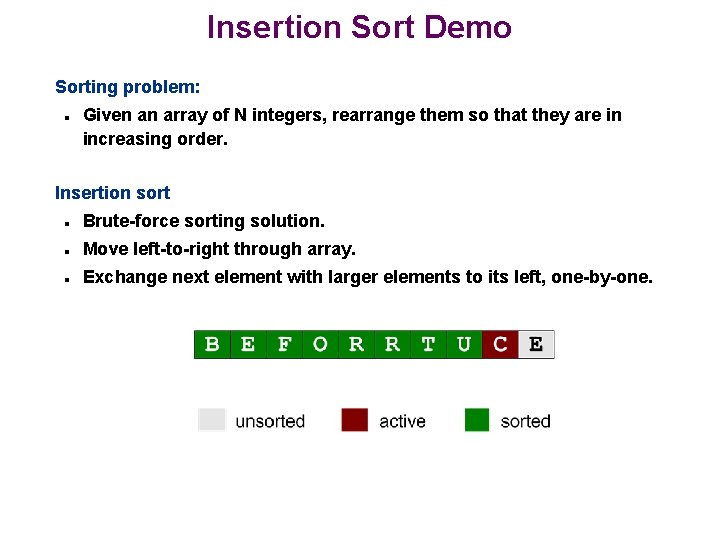
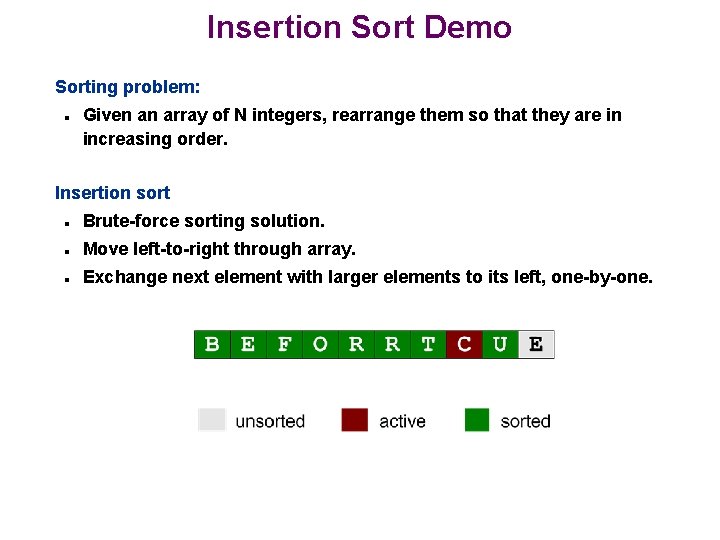
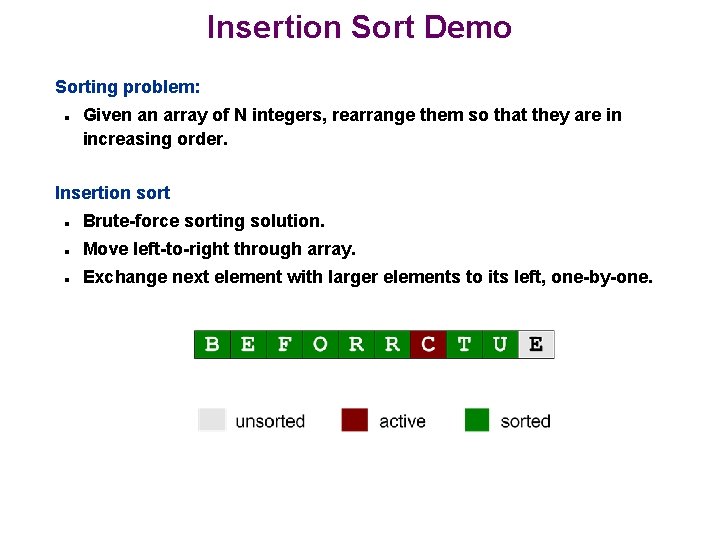
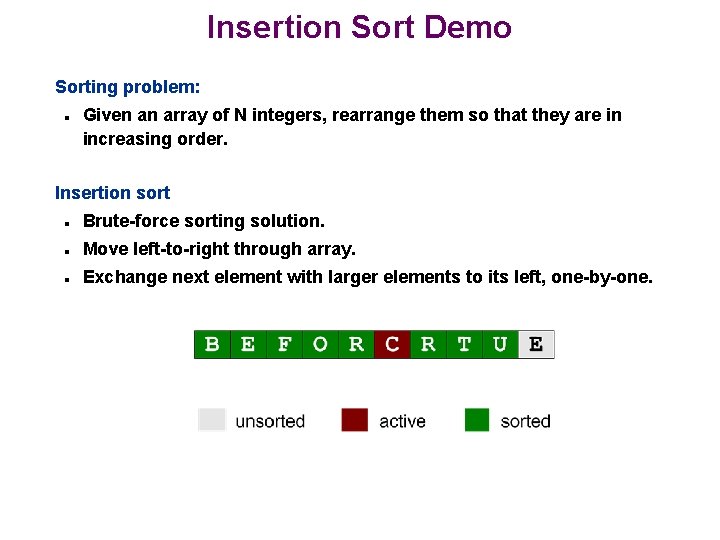
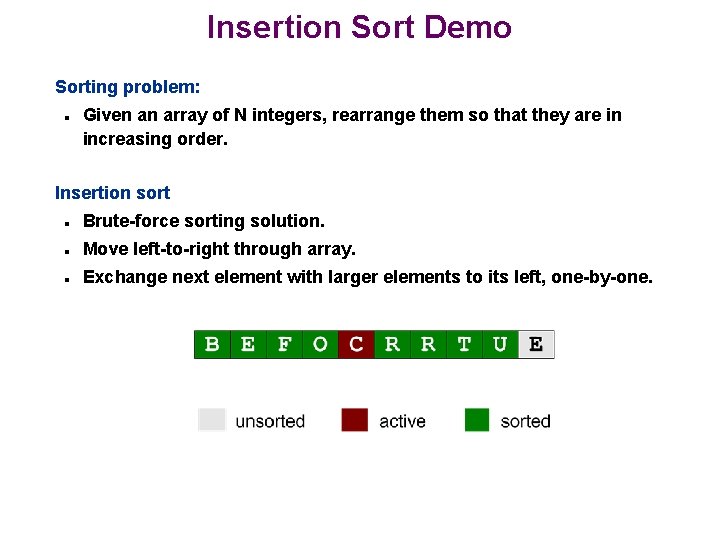
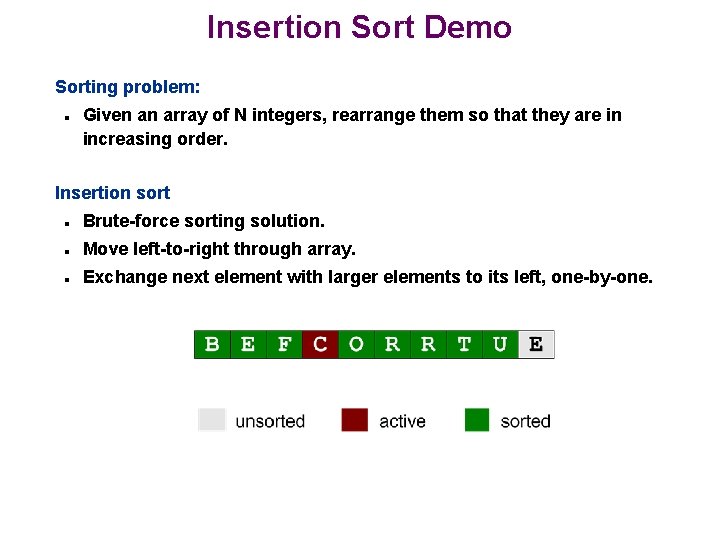
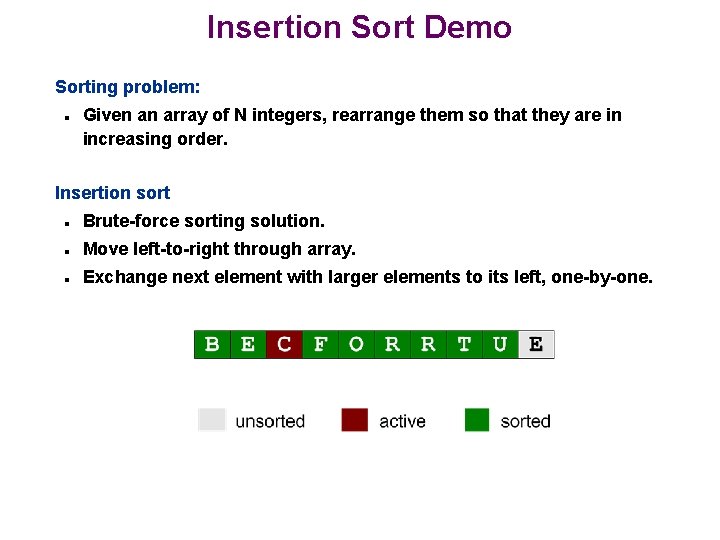
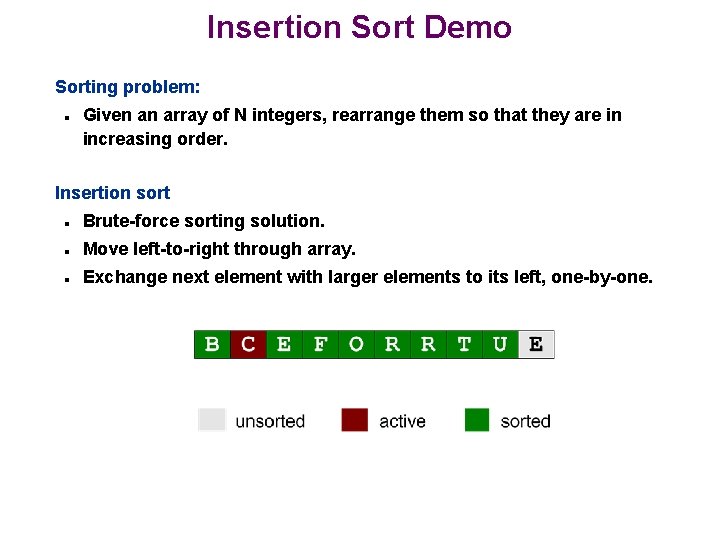
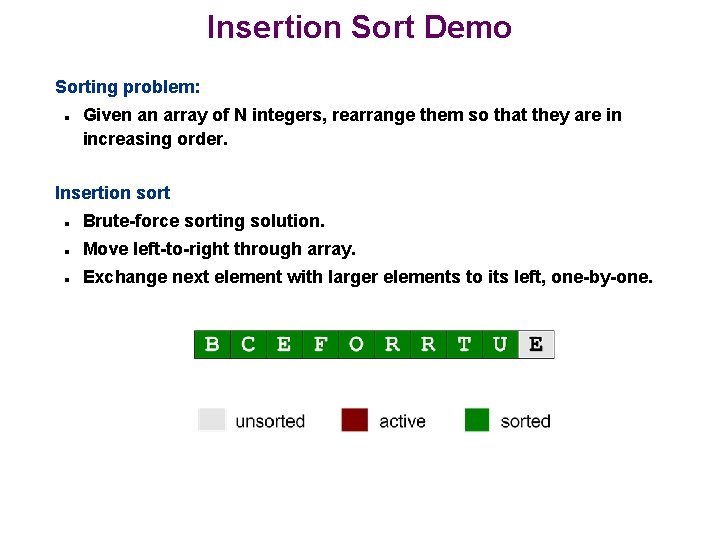
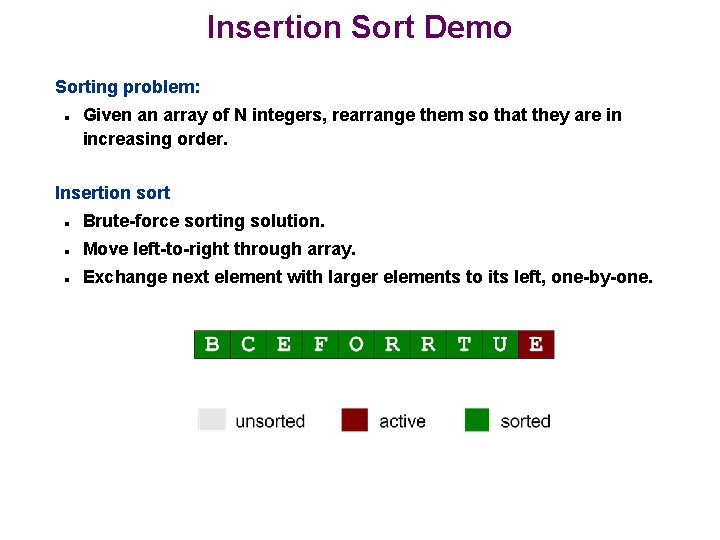
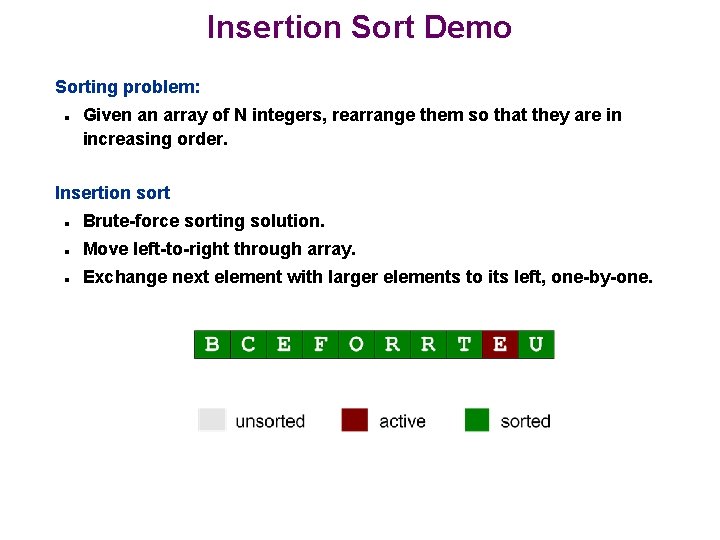
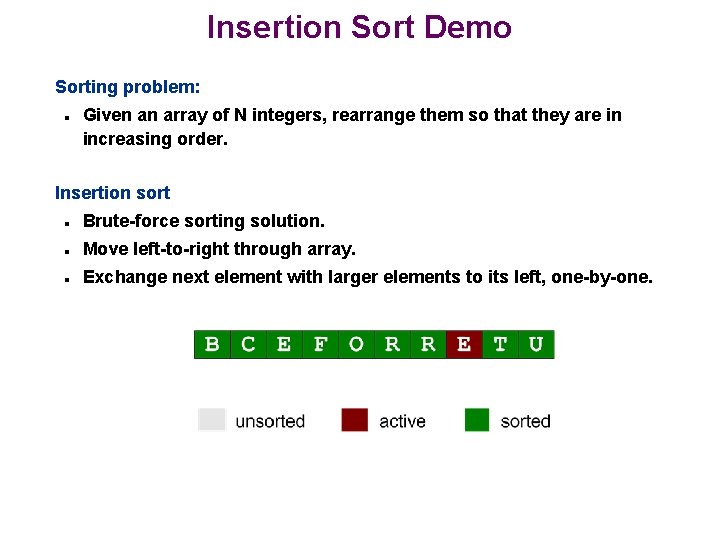
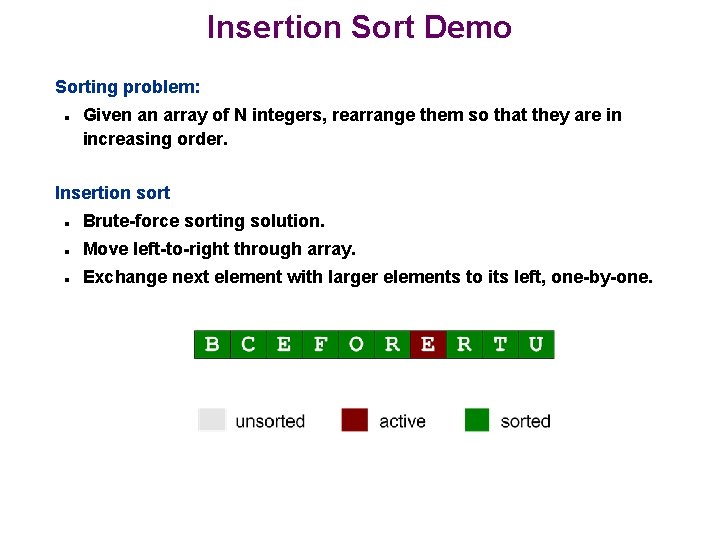
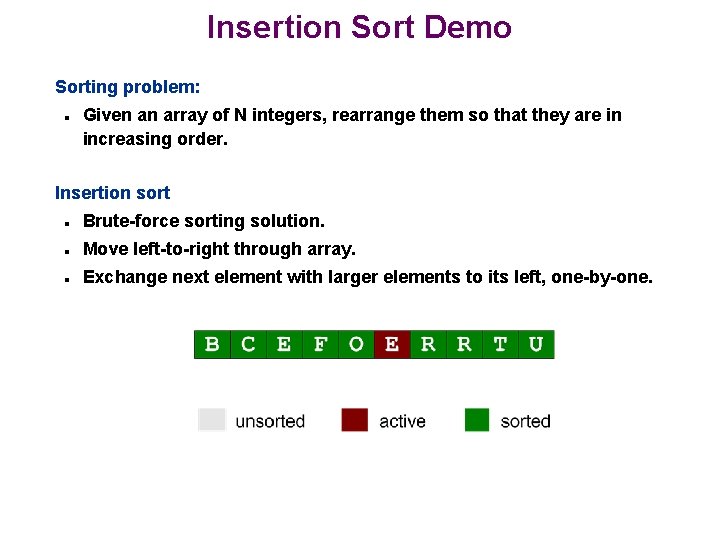
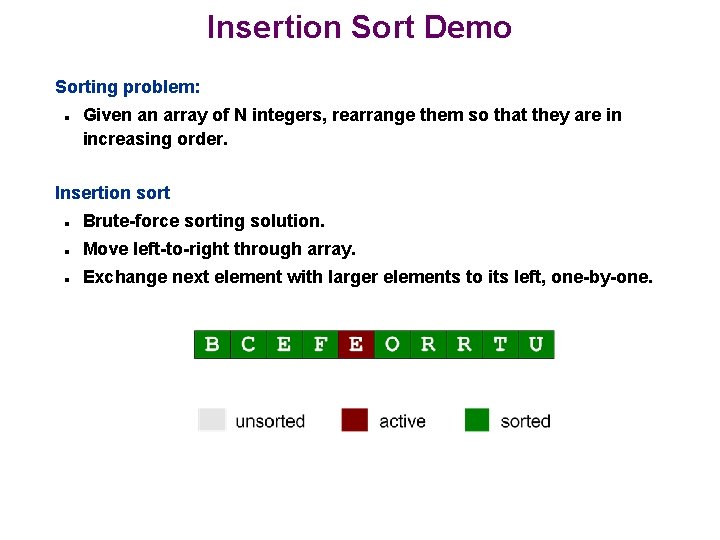
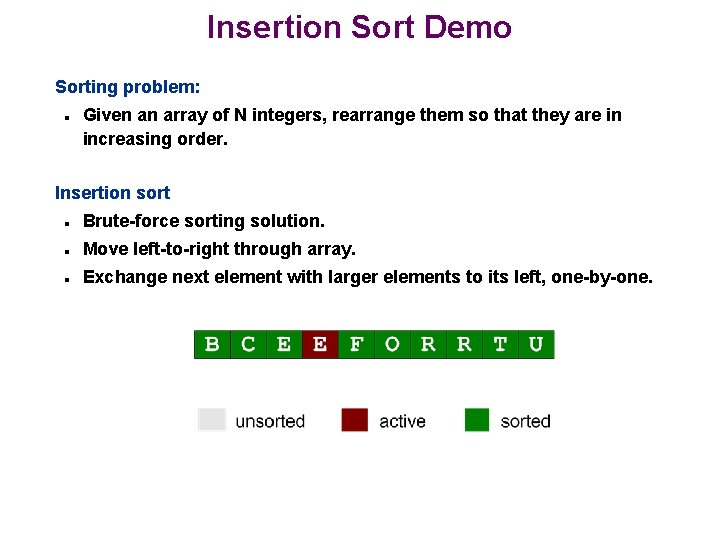
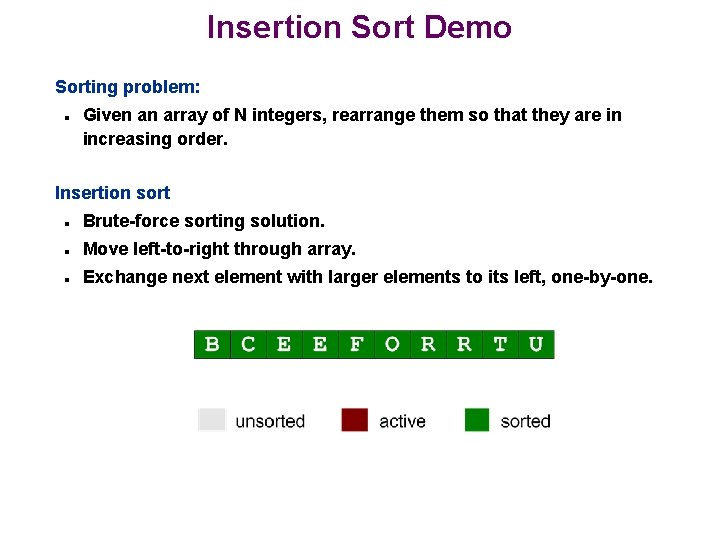
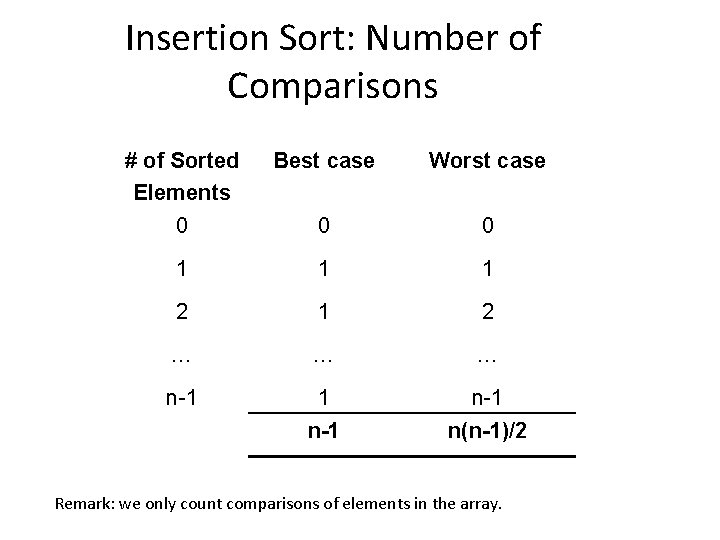
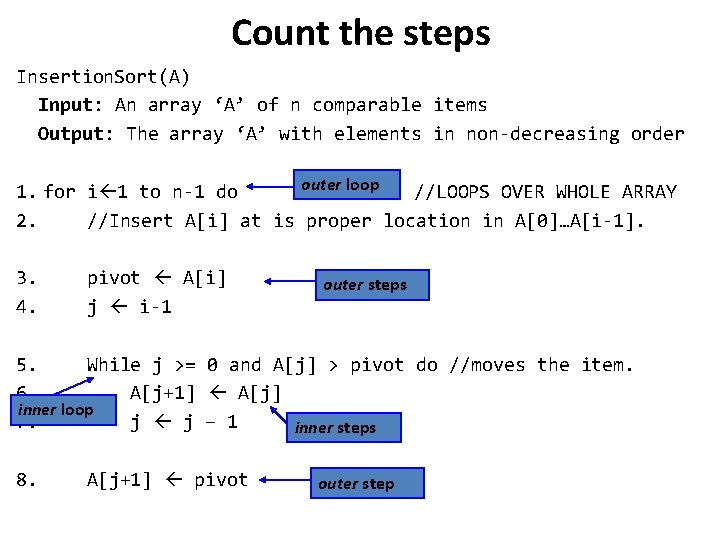
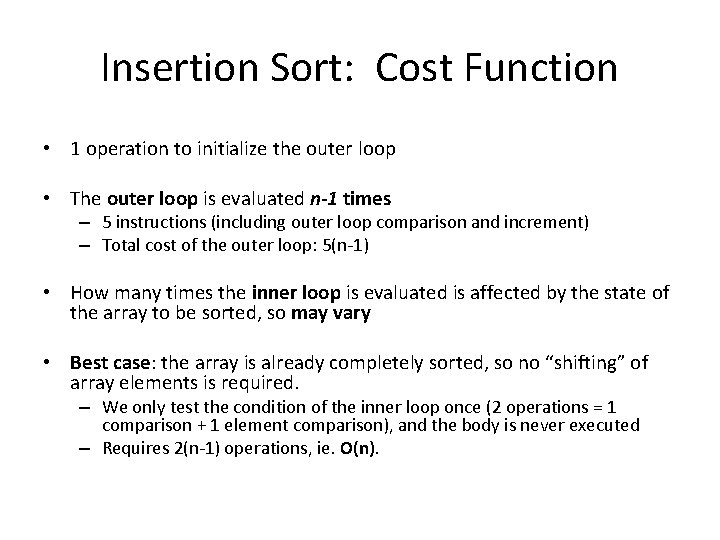
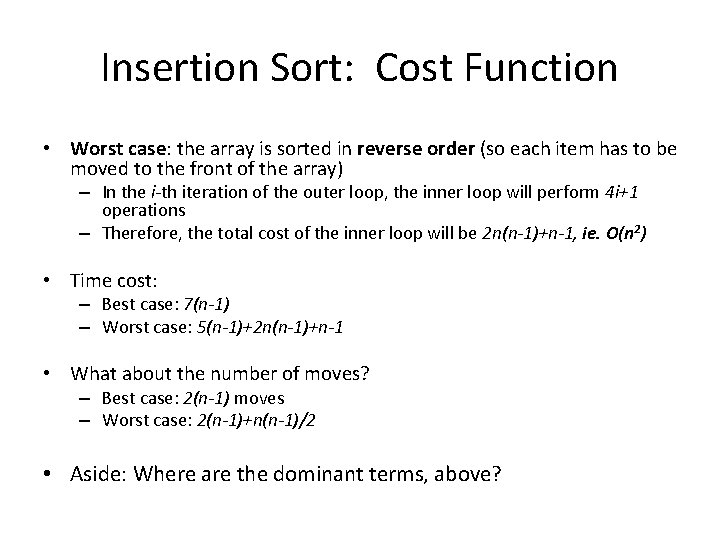
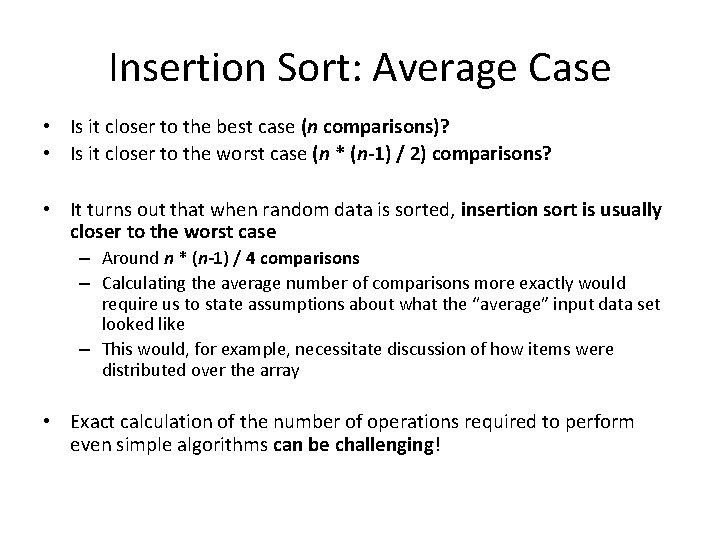
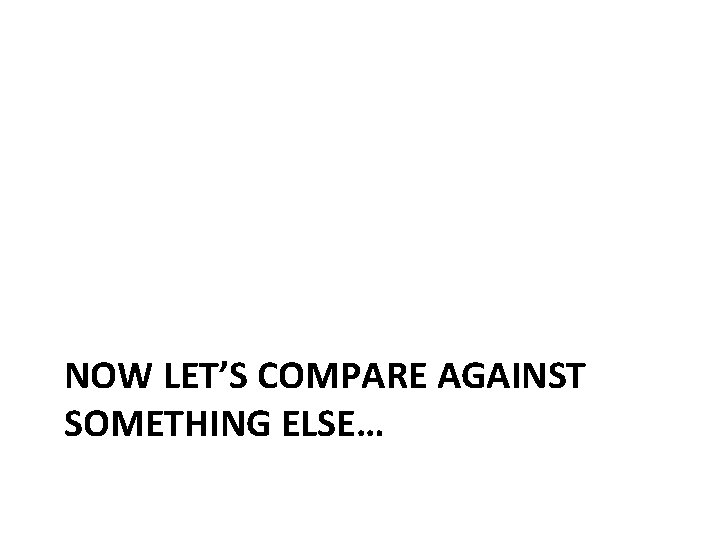
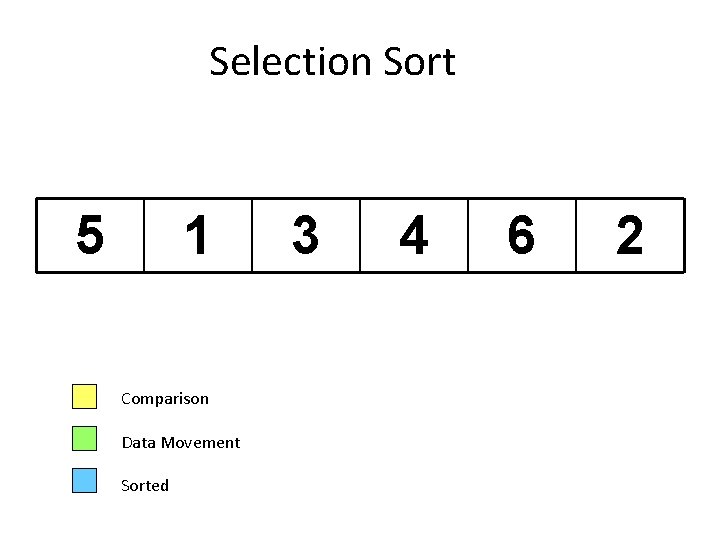
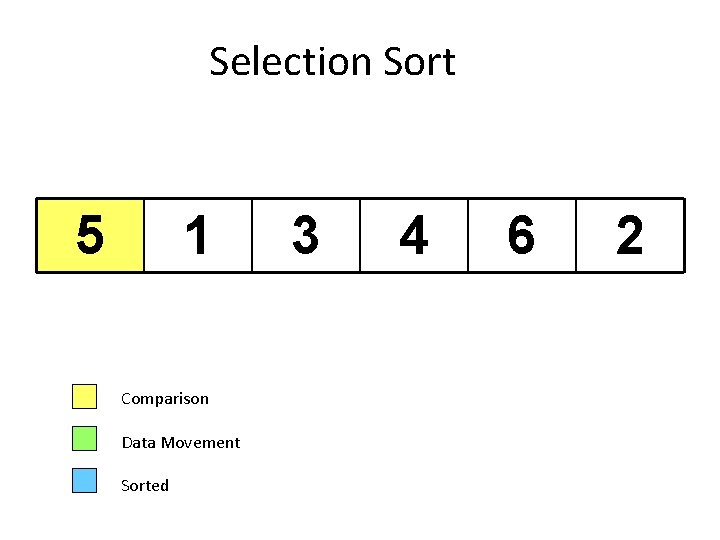
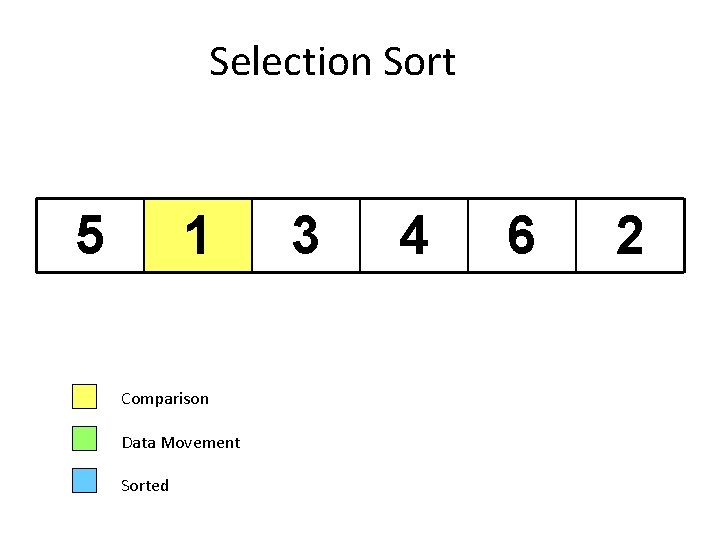
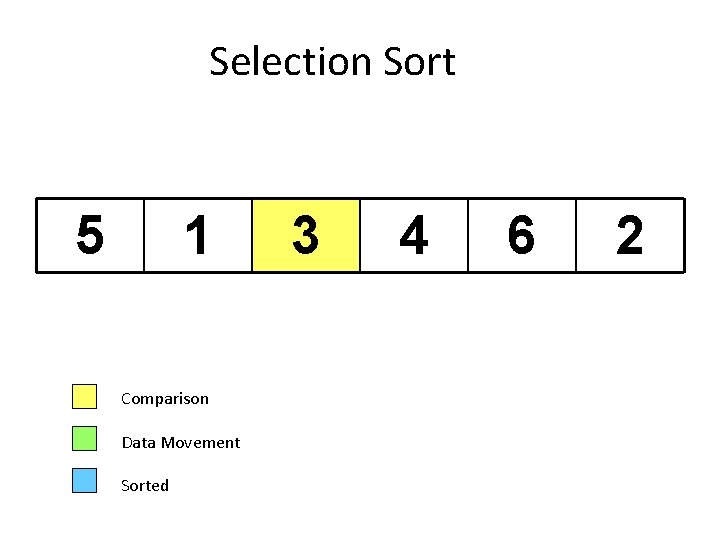
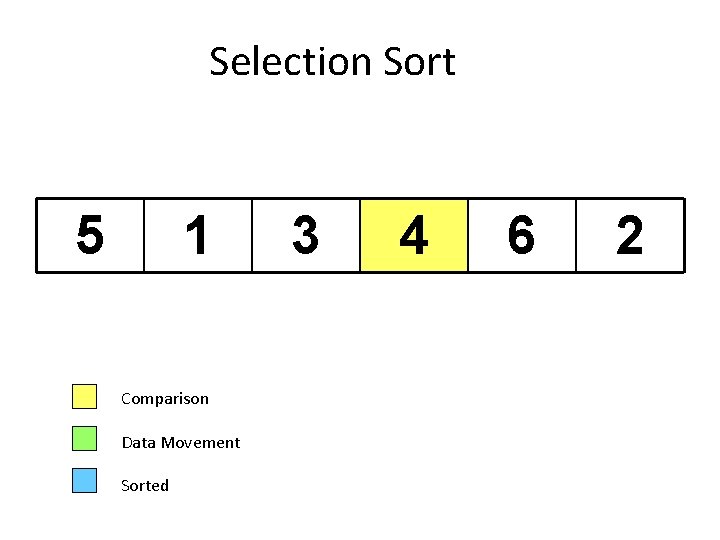
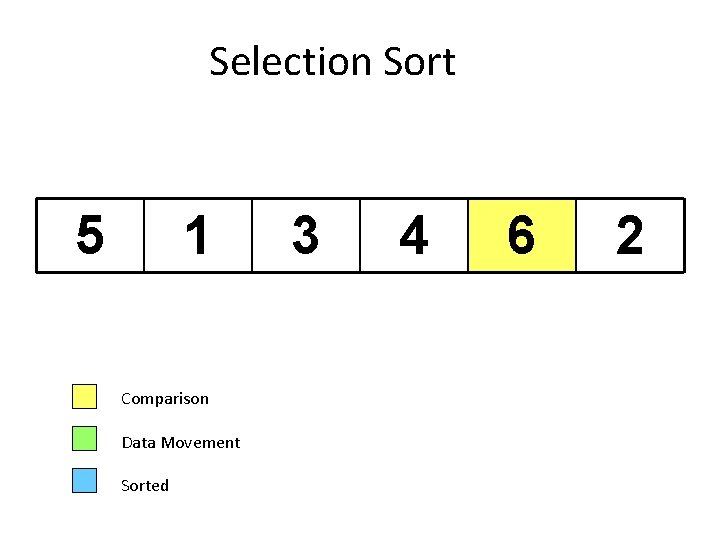
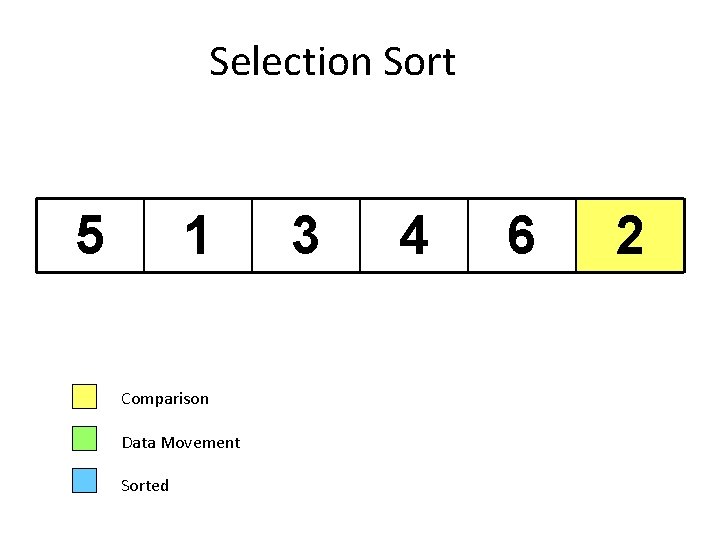
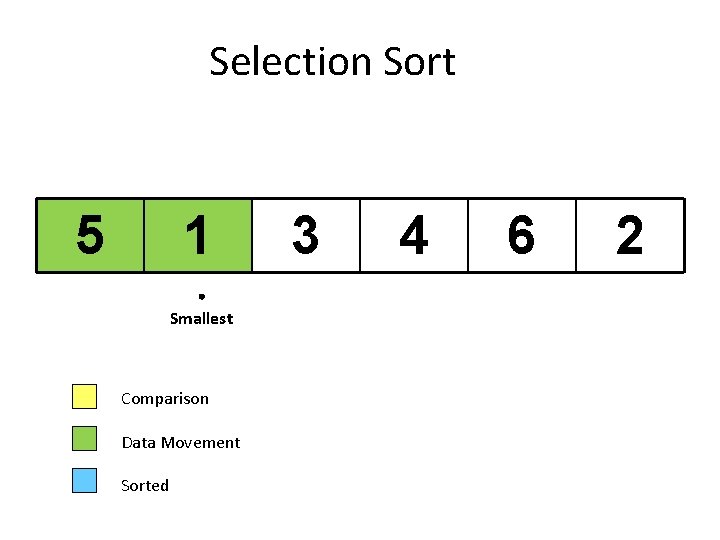
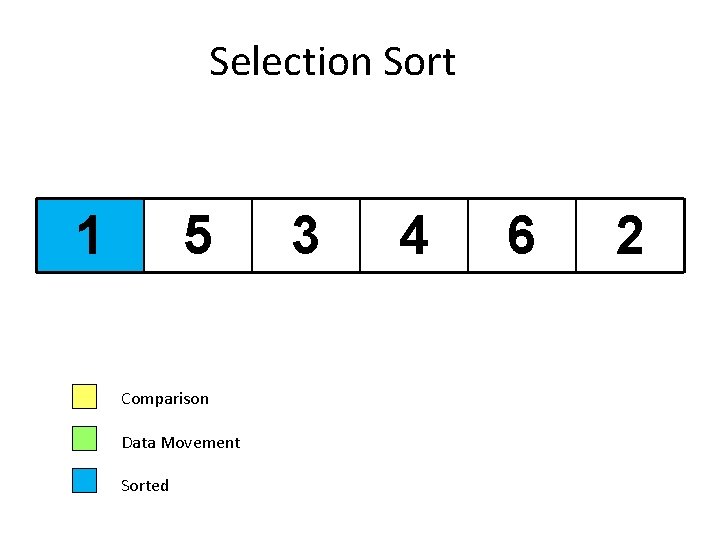
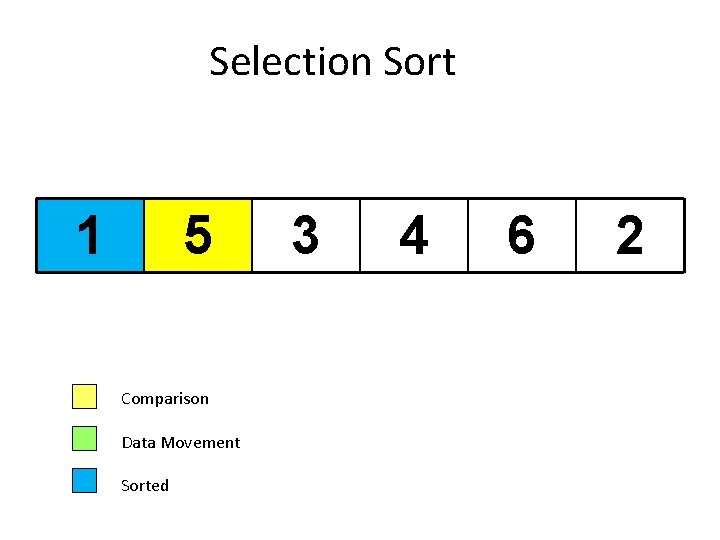
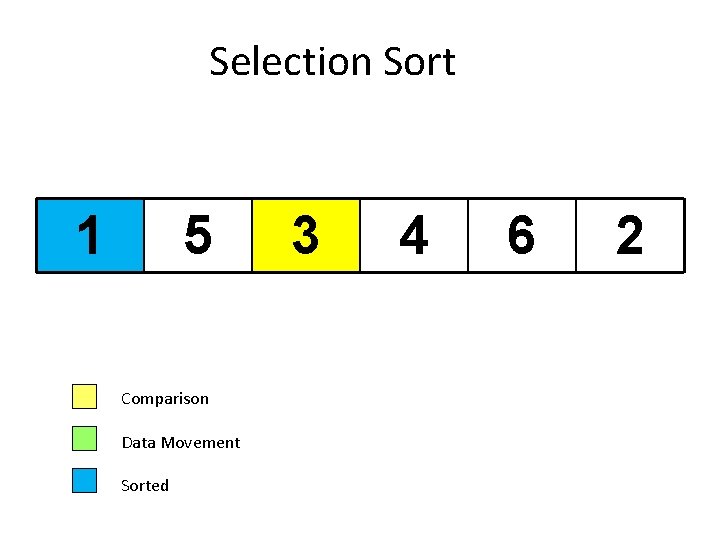
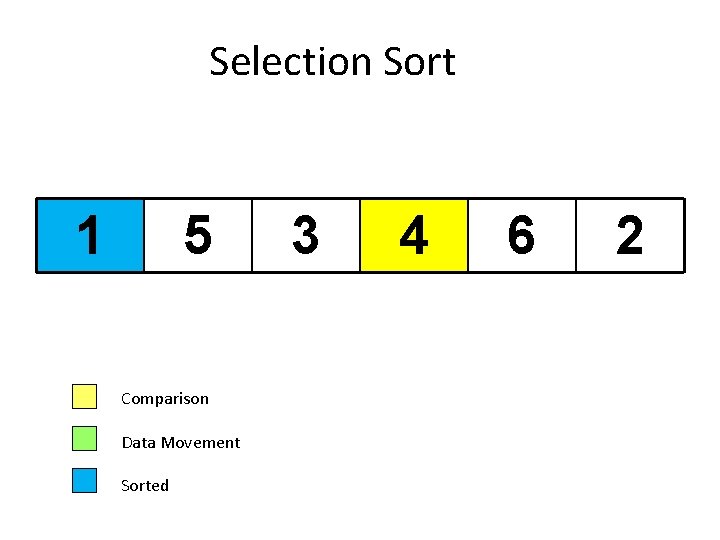
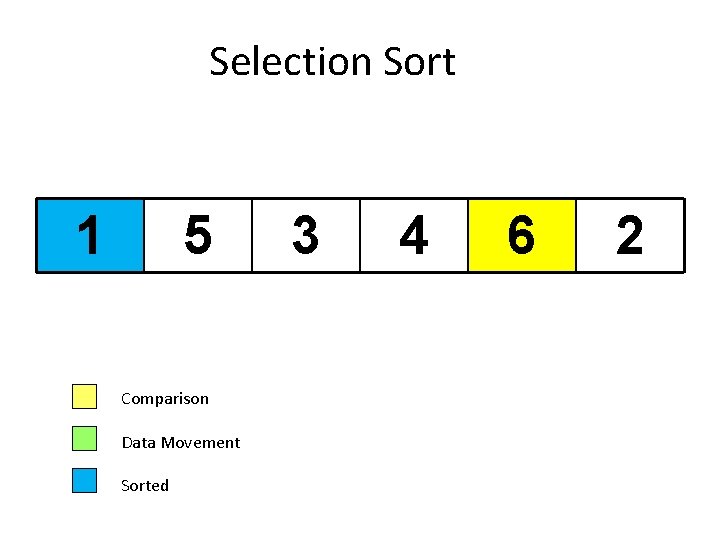
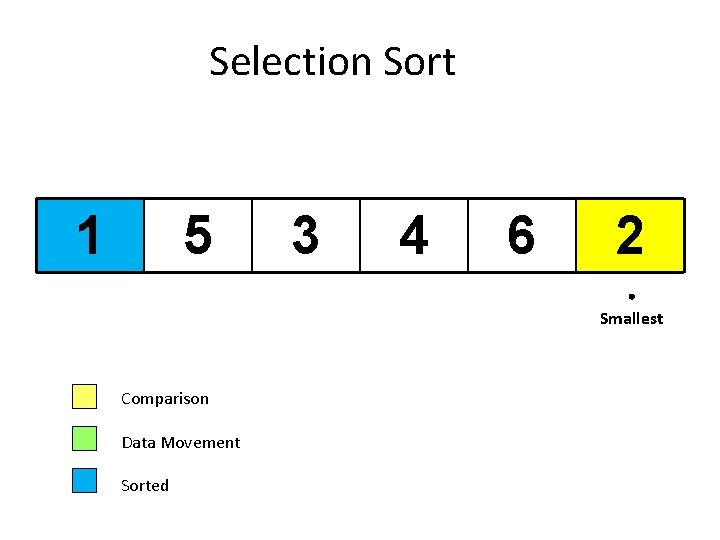
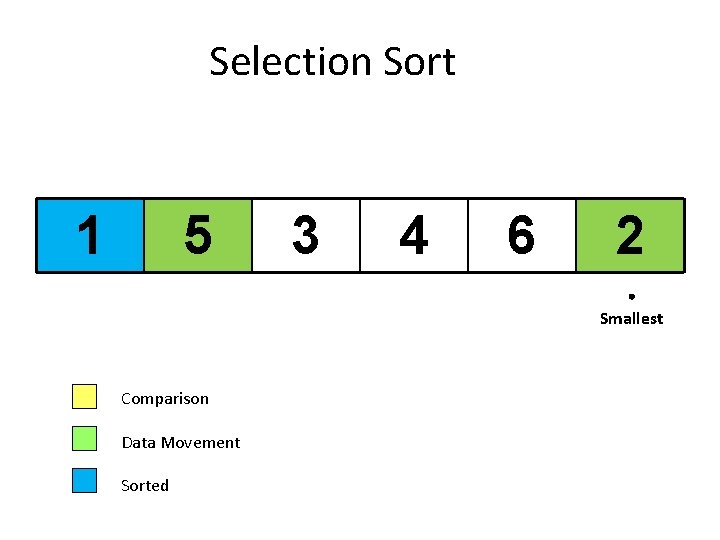
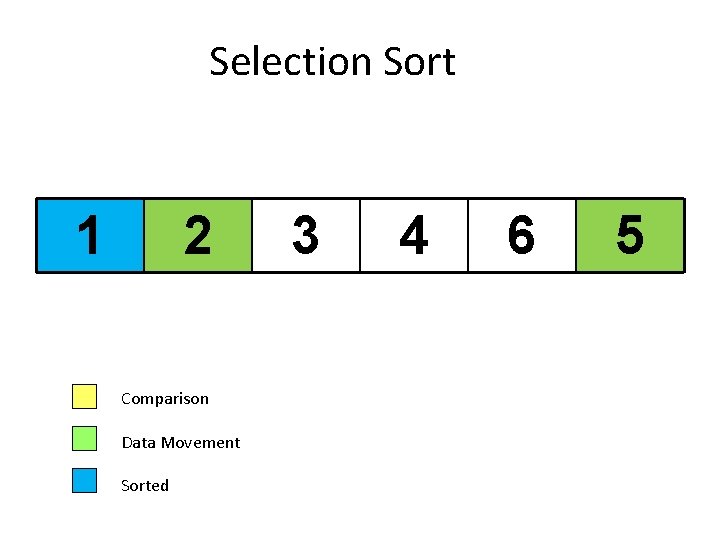
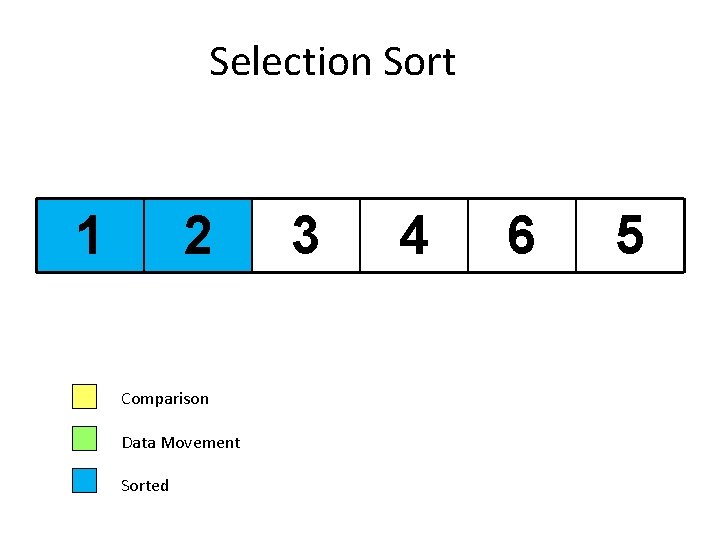
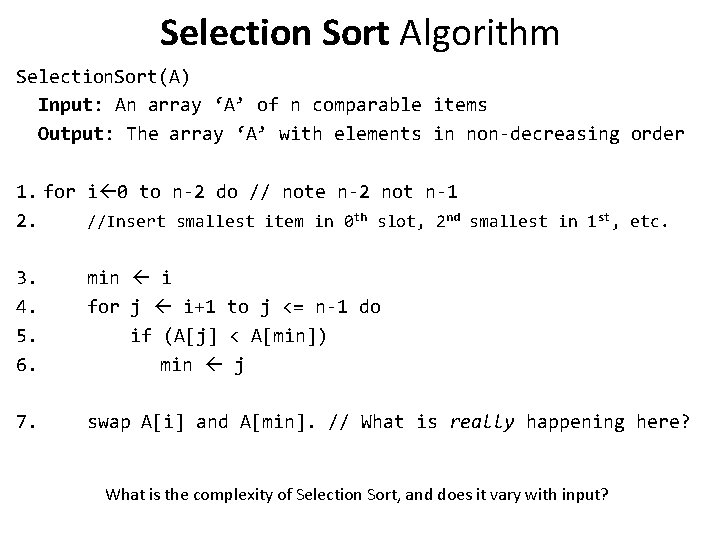
- Slides: 94
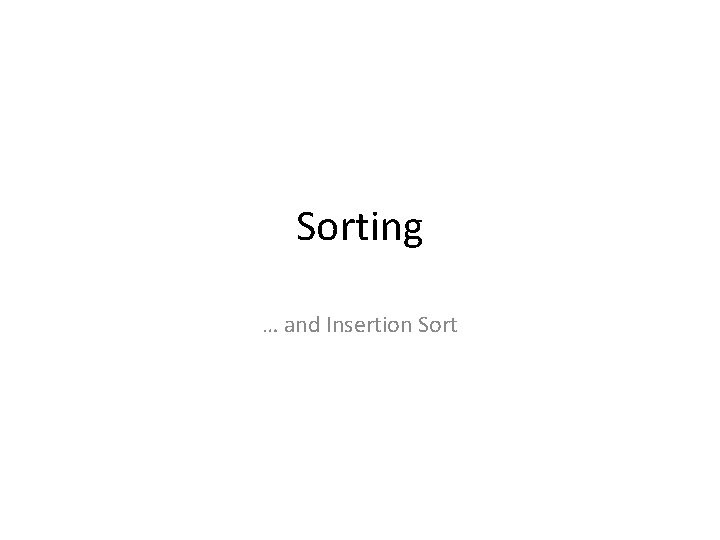
Sorting … and Insertion Sort
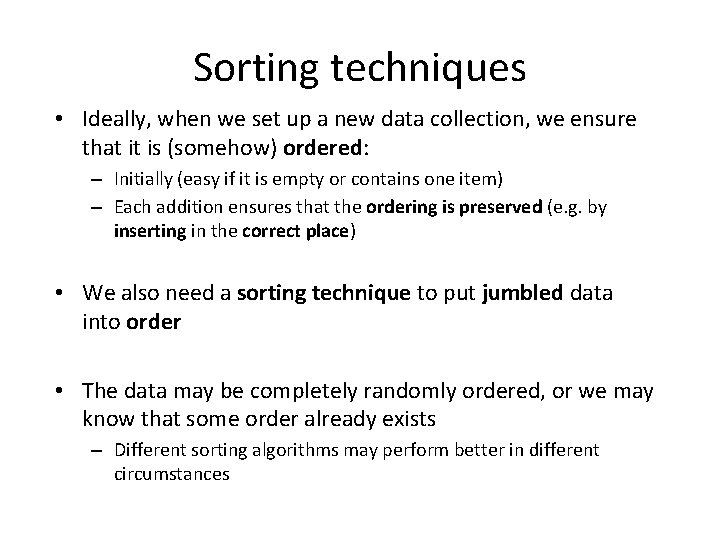
Sorting techniques • Ideally, when we set up a new data collection, we ensure that it is (somehow) ordered: – Initially (easy if it is empty or contains one item) – Each addition ensures that the ordering is preserved (e. g. by inserting in the correct place) • We also need a sorting technique to put jumbled data into order • The data may be completely randomly ordered, or we may know that some order already exists – Different sorting algorithms may perform better in different circumstances
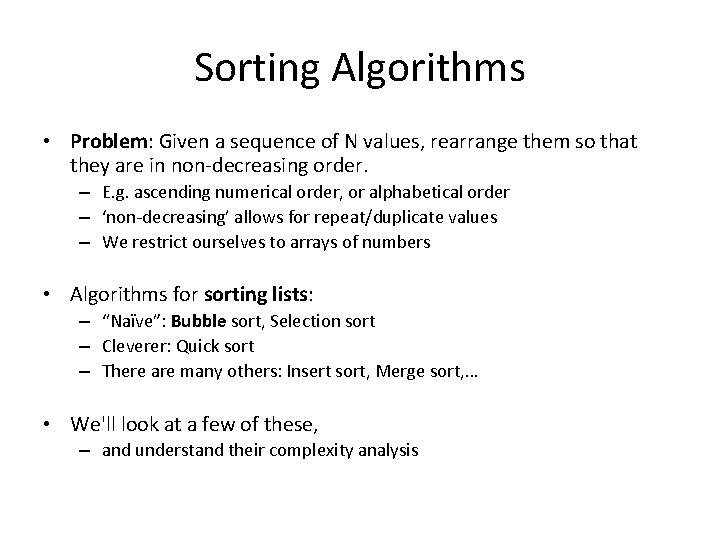
Sorting Algorithms • Problem: Given a sequence of N values, rearrange them so that they are in non-decreasing order. – E. g. ascending numerical order, or alphabetical order – ‘non-decreasing’ allows for repeat/duplicate values – We restrict ourselves to arrays of numbers • Algorithms for sorting lists: – “Naïve”: Bubble sort, Selection sort – Cleverer: Quick sort – There are many others: Insert sort, Merge sort, . . . • We'll look at a few of these, – and understand their complexity analysis
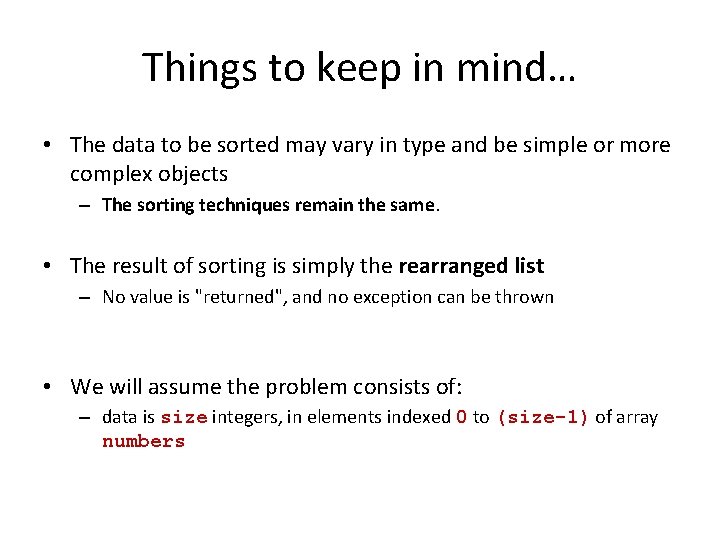
Things to keep in mind… • The data to be sorted may vary in type and be simple or more complex objects – The sorting techniques remain the same. • The result of sorting is simply the rearranged list – No value is "returned", and no exception can be thrown • We will assume the problem consists of: – data is size integers, in elements indexed 0 to (size-1) of array numbers
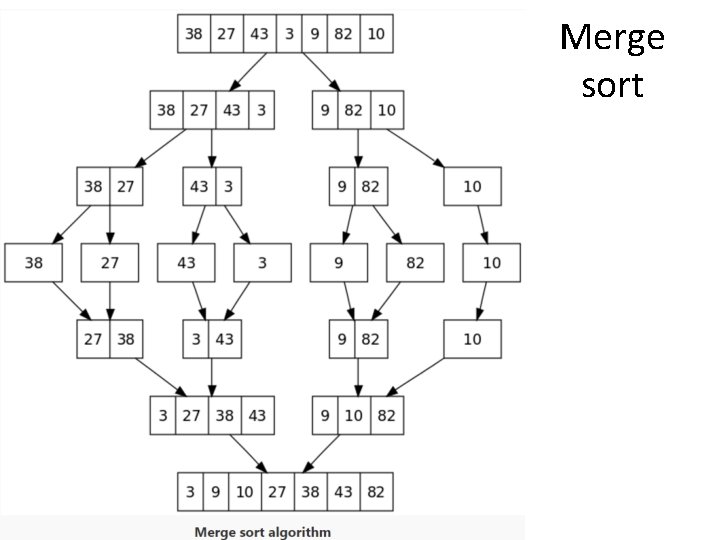
Merge sort
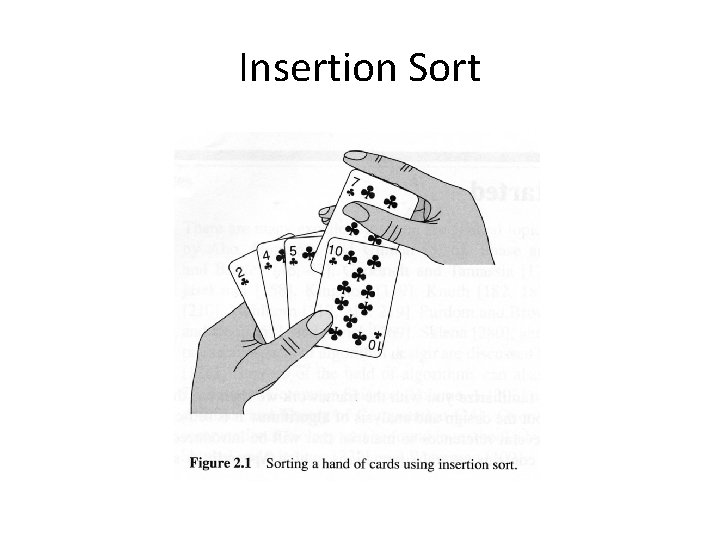
Insertion Sort
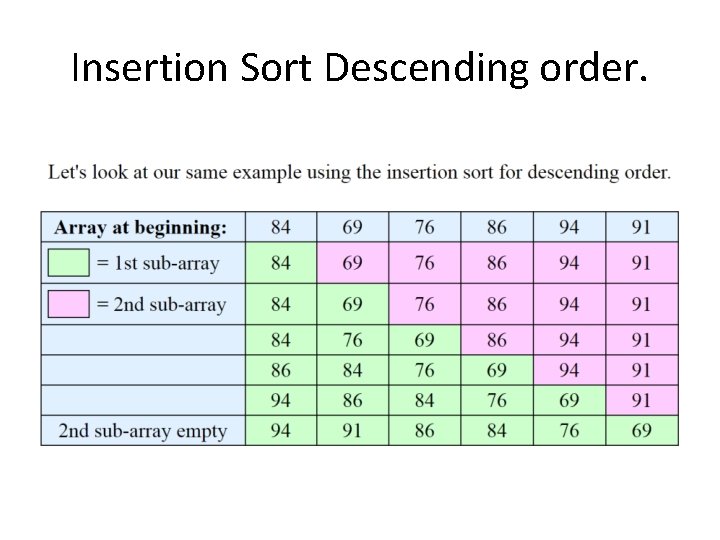
Insertion Sort Descending order.
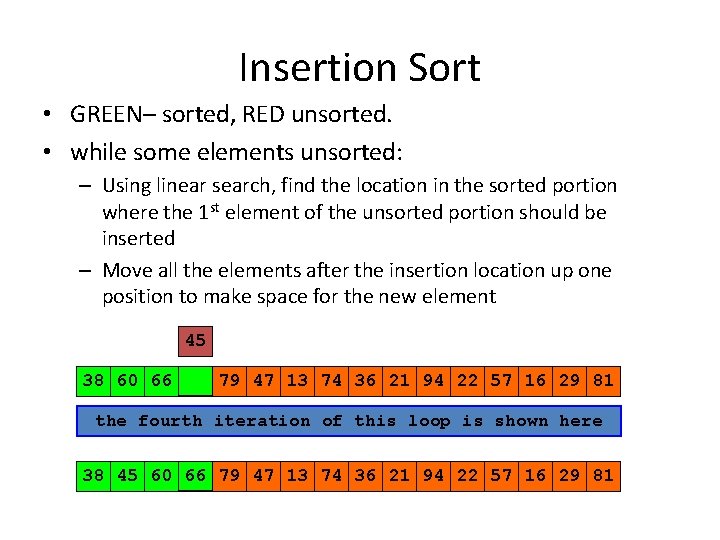
Insertion Sort • GREEN– sorted, RED unsorted. • while some elements unsorted: – Using linear search, find the location in the sorted portion where the 1 st element of the unsorted portion should be inserted – Move all the elements after the insertion location up one position to make space for the new element 45 38 60 66 45 79 47 13 74 36 21 94 22 57 16 29 81 the fourth iteration of this loop is shown here 38 45 60 60 66 45 66 79 47 13 74 36 21 94 22 57 16 29 81
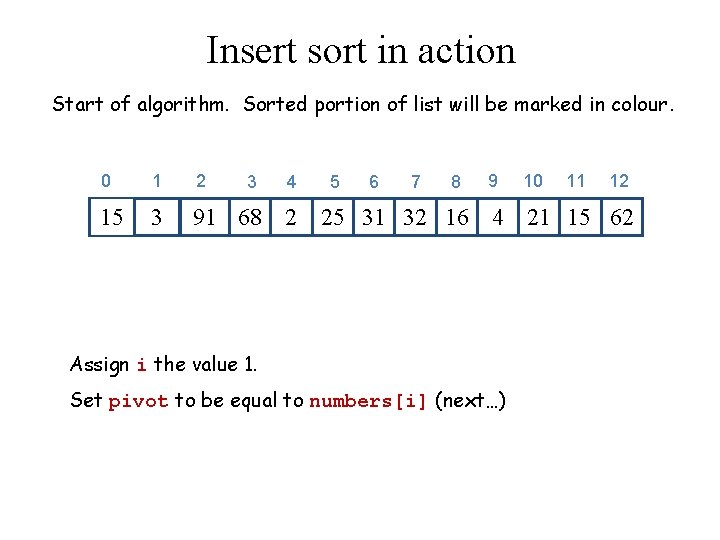
Insert sort in action Start of algorithm. Sorted portion of list will be marked in colour. 0 1 2 15 3 91 68 2 25 31 32 16 4 21 15 62 3 4 5 6 7 8 9 Assign i the value 1. Set pivot to be equal to numbers[i] (next…) 10 11 12
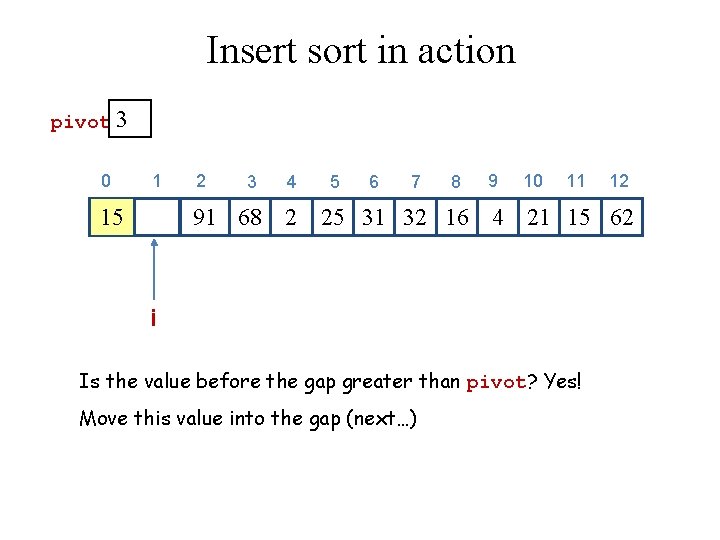
Insert sort in action pivot 3 0 1 15 2 3 4 5 6 7 8 9 10 11 12 91 68 2 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? Yes! Move this value into the gap (next…)
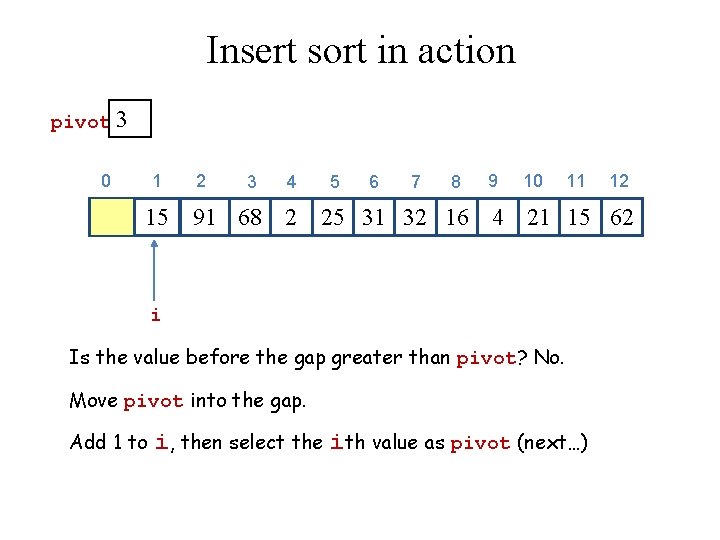
Insert sort in action pivot 3 0 1 2 3 4 5 6 7 8 9 10 11 12 15 91 68 2 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? No. Move pivot into the gap. Add 1 to i, then select the ith value as pivot (next…)
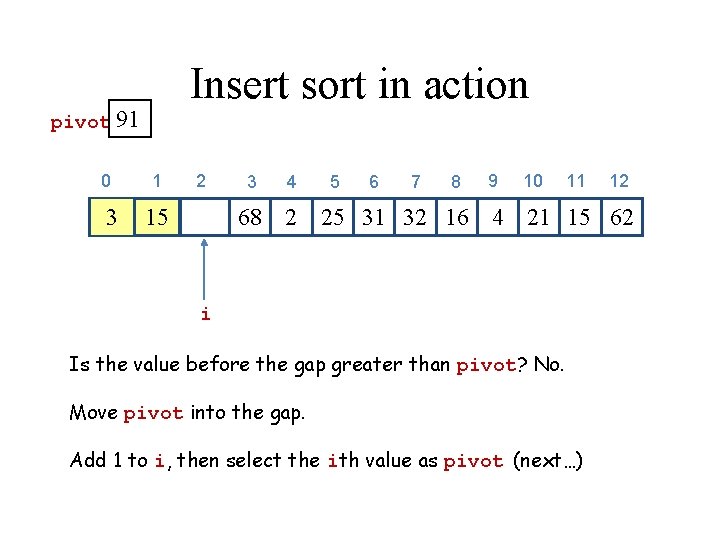
Insert sort in action pivot 91 0 3 1 2 15 3 4 5 6 7 8 9 10 11 12 68 2 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? No. Move pivot into the gap. Add 1 to i, then select the ith value as pivot (next…)
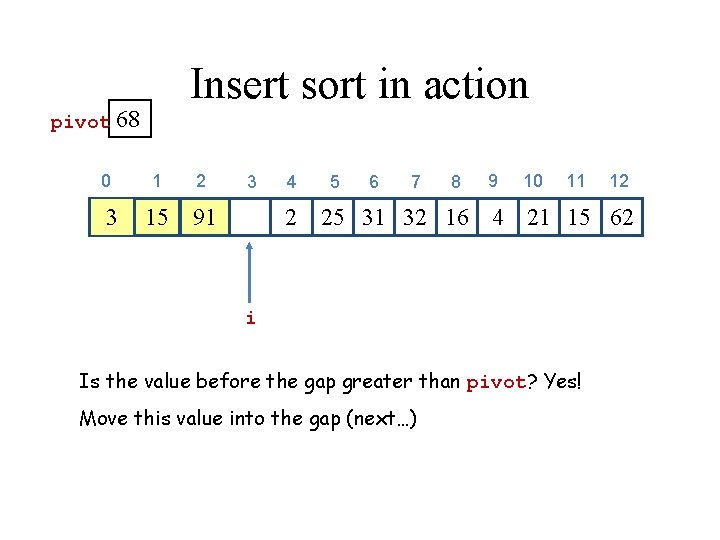
Insert sort in action pivot 68 0 3 1 2 3 15 91 4 5 6 7 8 9 10 11 12 2 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? Yes! Move this value into the gap (next…)
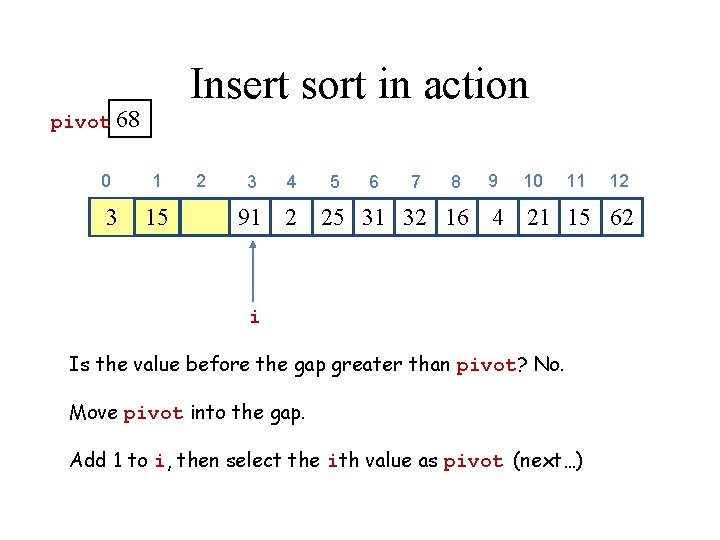
Insert sort in action pivot 68 0 3 1 15 2 3 4 5 6 7 8 9 10 11 12 91 2 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? No. Move pivot into the gap. Add 1 to i, then select the ith value as pivot (next…)
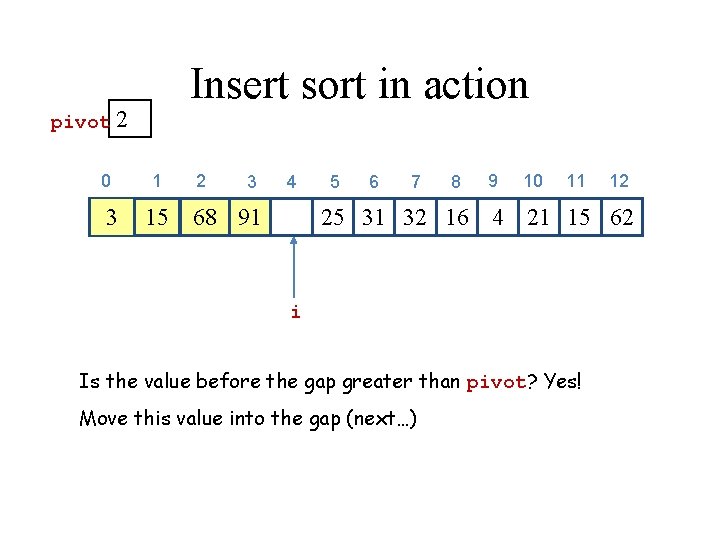
Insert sort in action pivot 2 0 3 1 2 3 4 15 68 91 5 6 7 8 9 10 11 12 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? Yes! Move this value into the gap (next…)
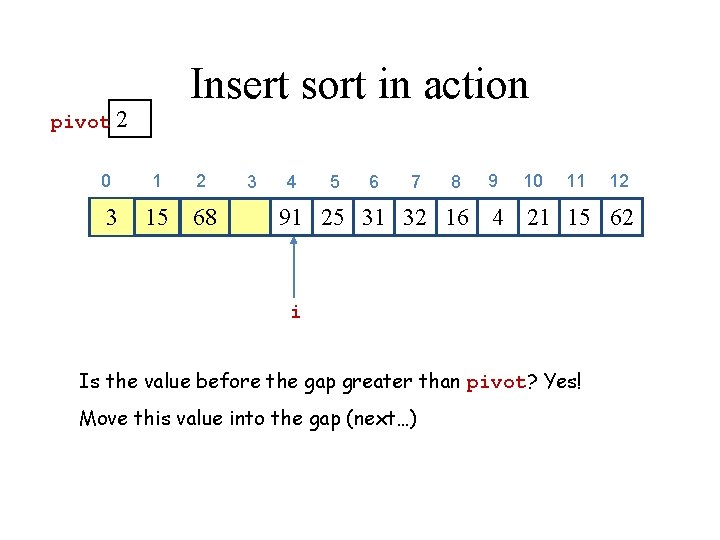
Insert sort in action pivot 2 0 3 1 2 15 68 3 4 5 6 7 8 9 10 11 12 91 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? Yes! Move this value into the gap (next…)
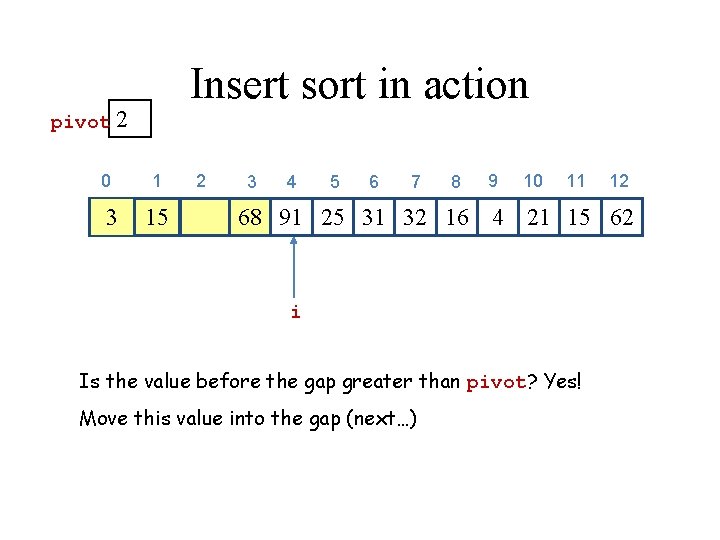
Insert sort in action pivot 2 0 3 1 15 2 3 4 5 6 7 8 9 10 11 12 68 91 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? Yes! Move this value into the gap (next…)
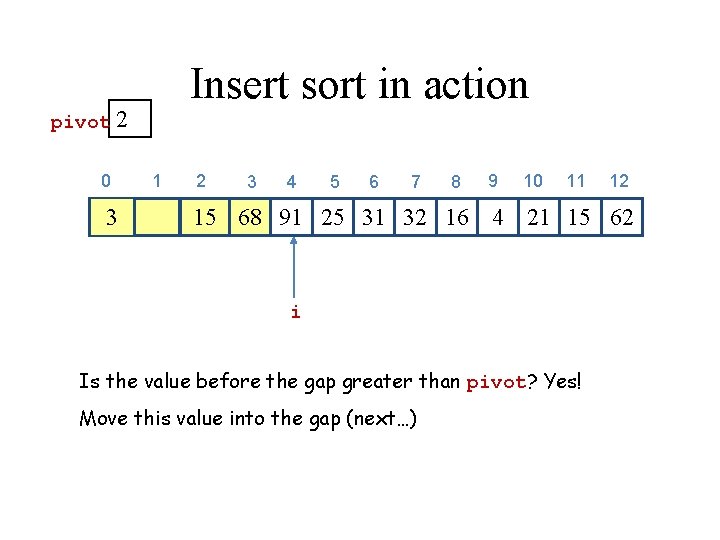
Insert sort in action pivot 2 0 3 1 2 3 4 5 6 7 8 9 10 11 12 15 68 91 25 31 32 16 4 21 15 62 i Is the value before the gap greater than pivot? Yes! Move this value into the gap (next…)
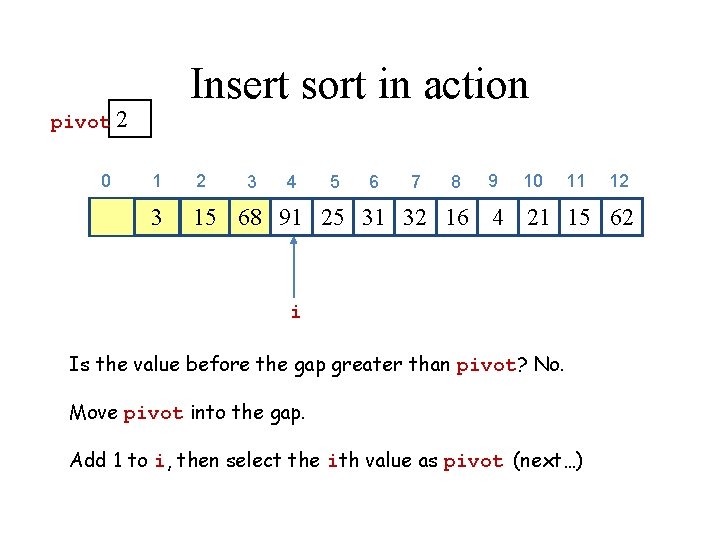
Insert sort in action pivot 2 0 1 2 3 15 68 91 25 31 32 16 4 21 15 62 3 4 5 6 7 8 9 10 11 i Is the value before the gap greater than pivot? No. Move pivot into the gap. Add 1 to i, then select the ith value as pivot (next…) 12
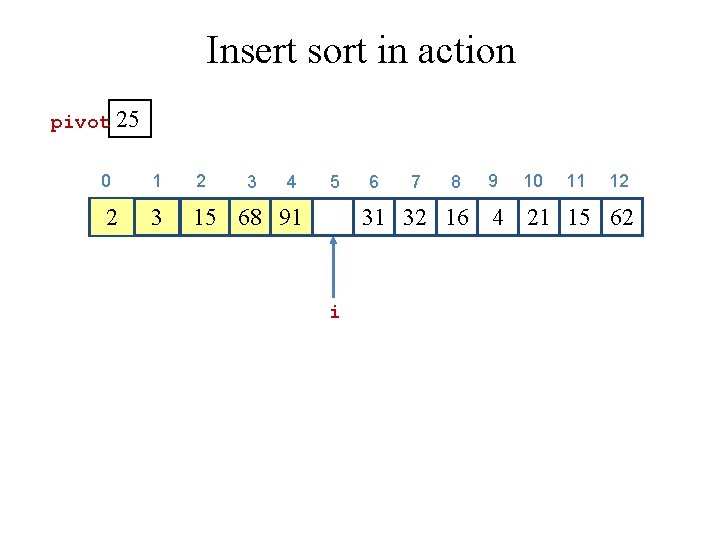
Insert sort in action pivot 25 0 1 2 2 3 15 68 91 3 4 5 6 7 8 9 10 11 12 31 32 16 4 21 15 62 i
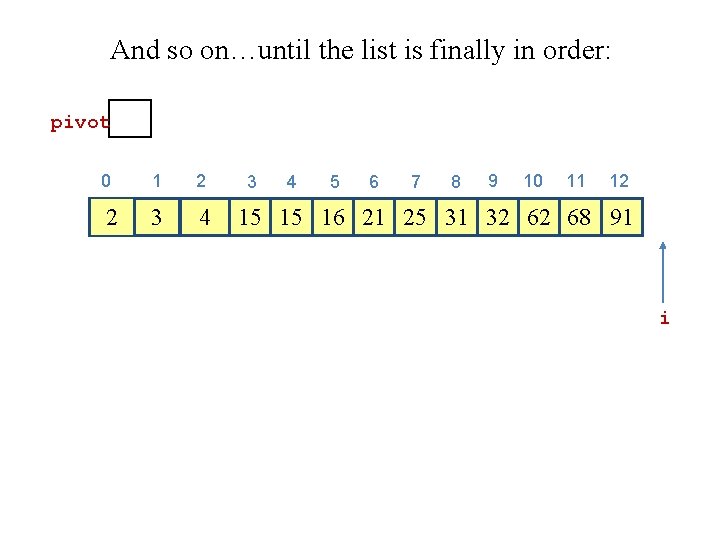
And so on…until the list is finally in order: pivot 0 1 2 2 3 4 5 6 7 8 9 10 11 12 15 15 16 21 25 31 32 62 68 91 i
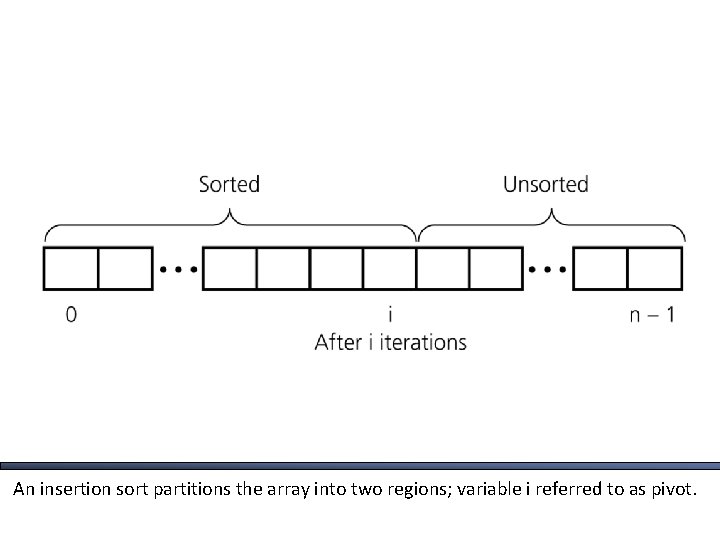
An insertion sort partitions the array into two regions; variable i referred to as pivot.
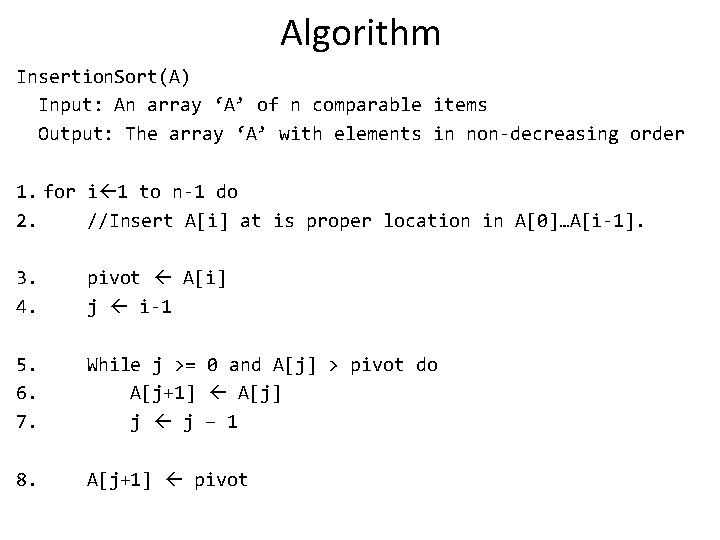
Algorithm Insertion. Sort(A) Input: An array ‘A’ of n comparable items Output: The array ‘A’ with elements in non-decreasing order 1. for i 1 to n-1 do 2. //Insert A[i] at is proper location in A[0]…A[i-1]. 3. 4. pivot A[i] j i-1 5. 6. 7. While j >= 0 and A[j] > pivot do A[j+1] A[j] j j – 1 8. A[j+1] pivot
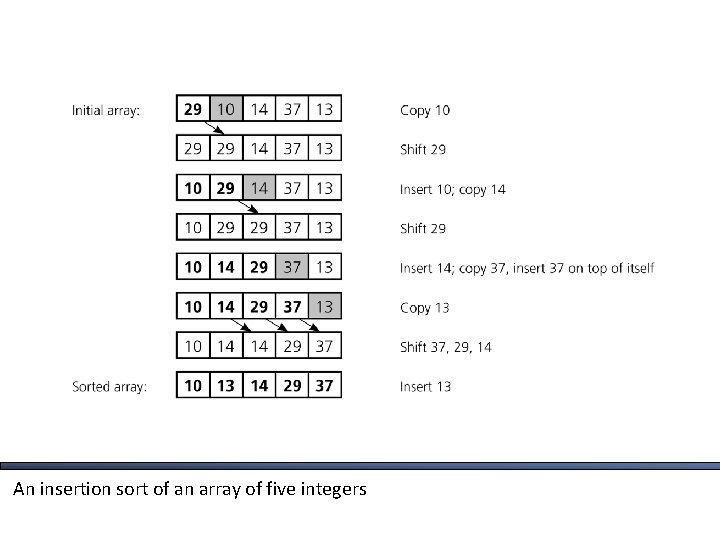
An insertion sort of an array of five integers
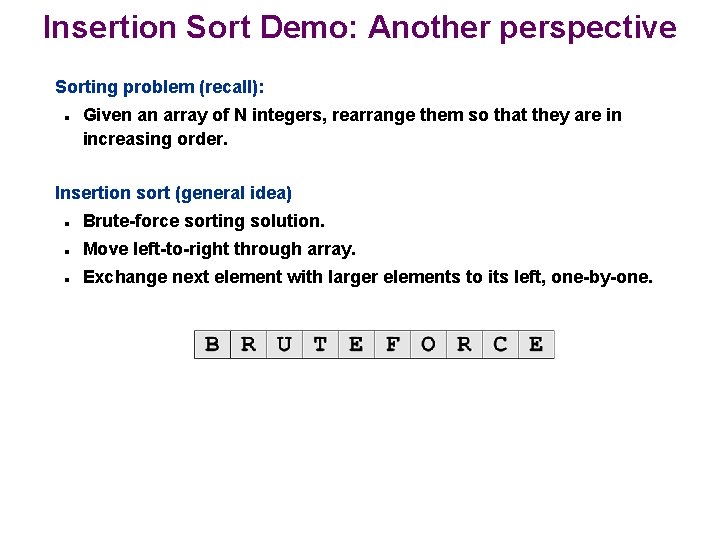
Insertion Sort Demo: Another perspective Sorting problem (recall): n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort (general idea) n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
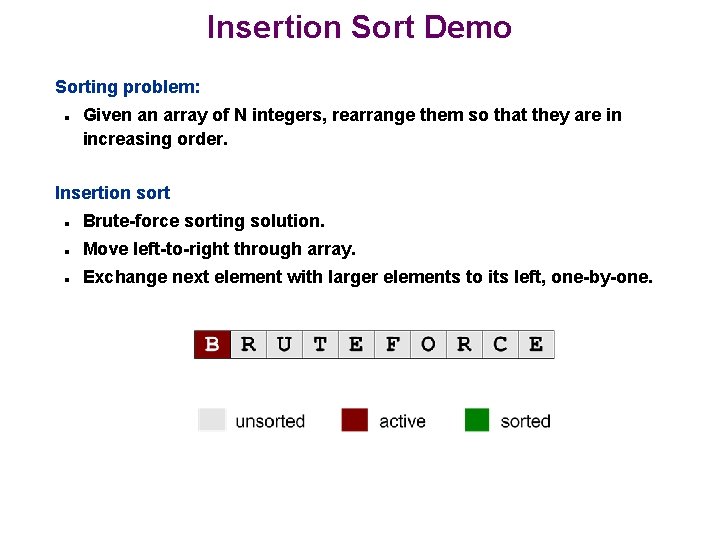
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
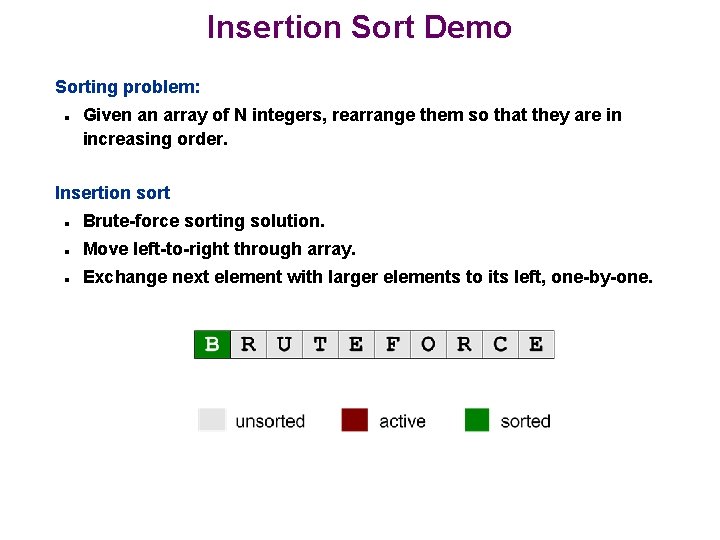
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
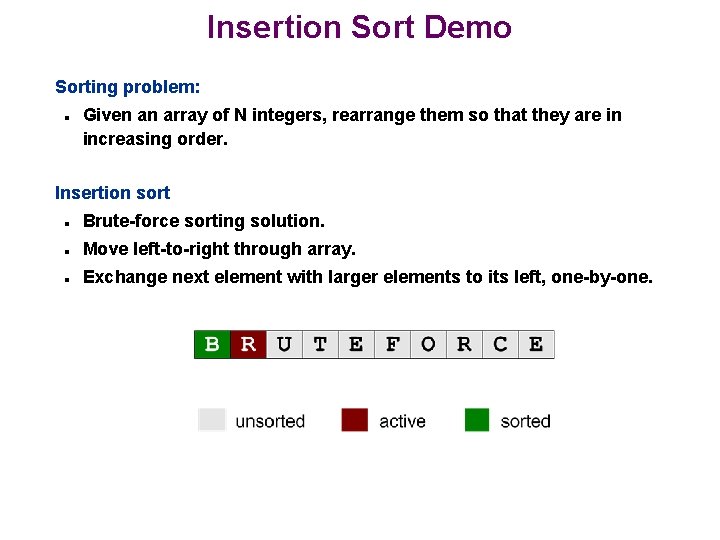
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
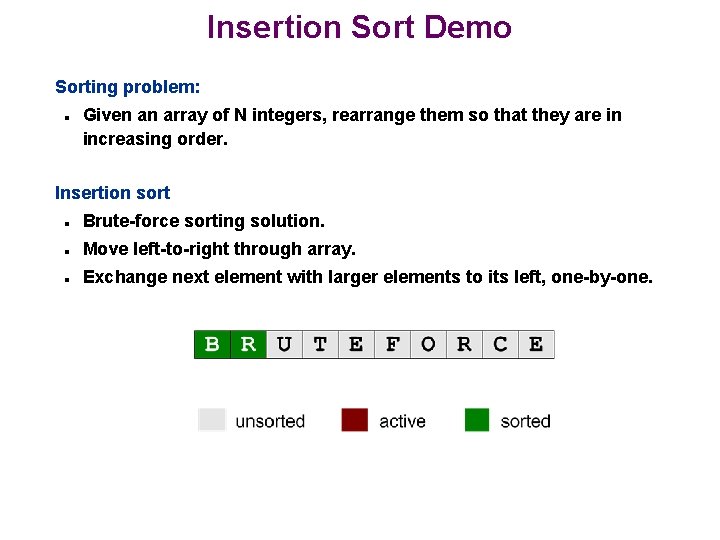
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
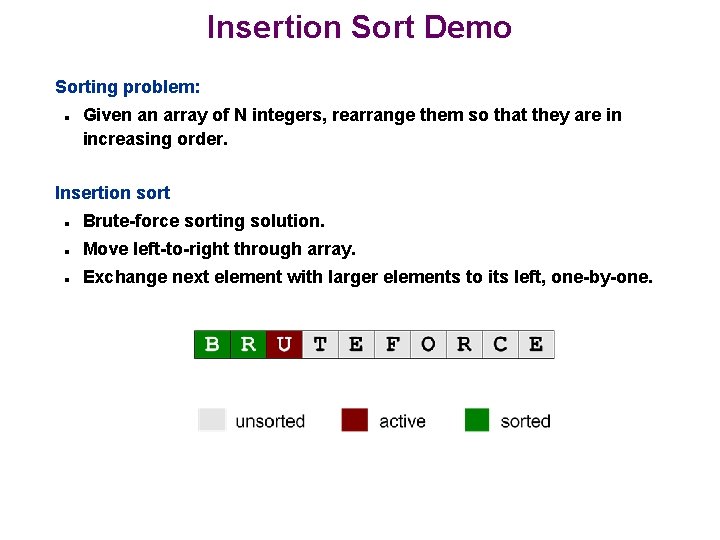
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
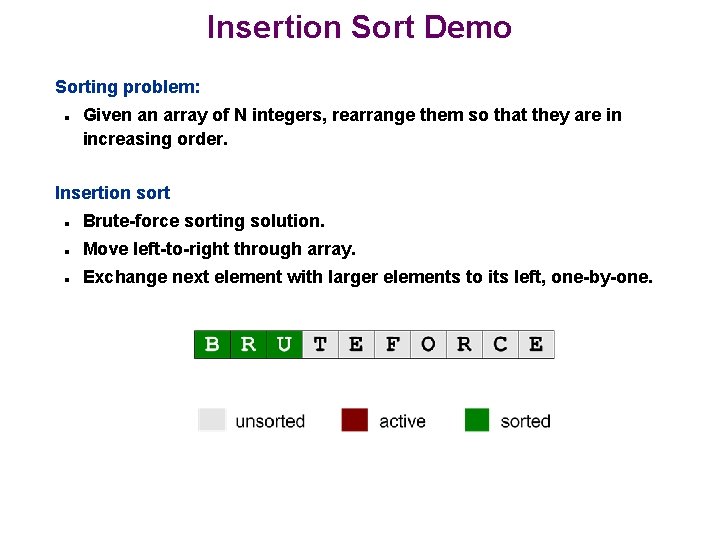
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
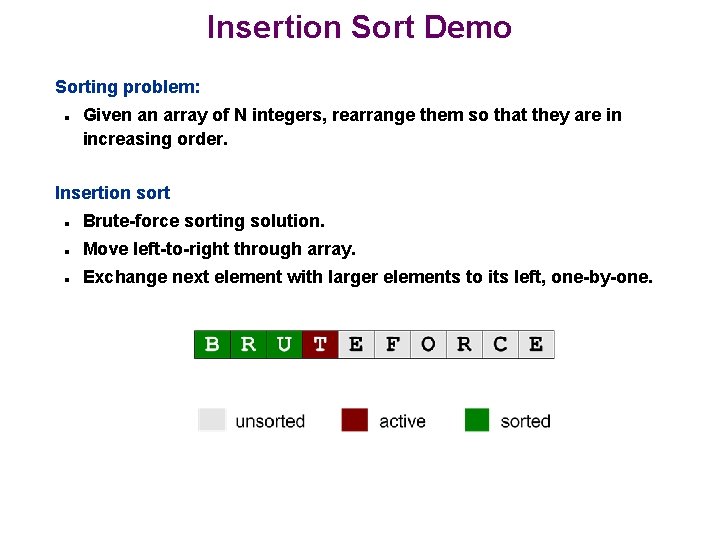
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
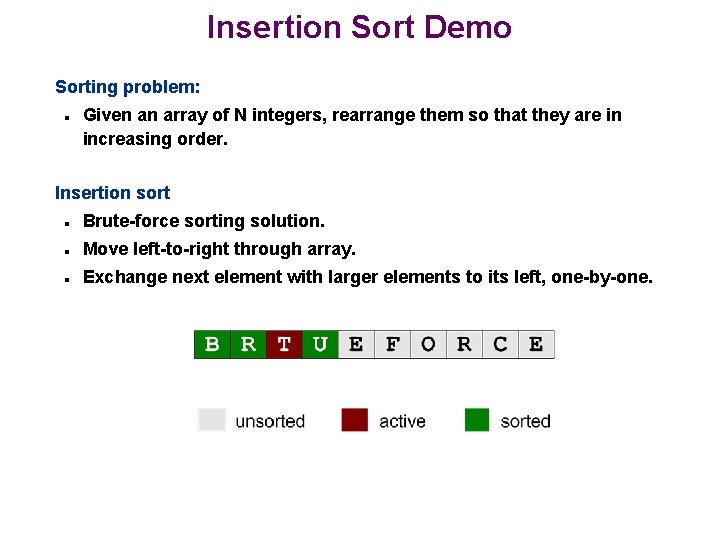
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
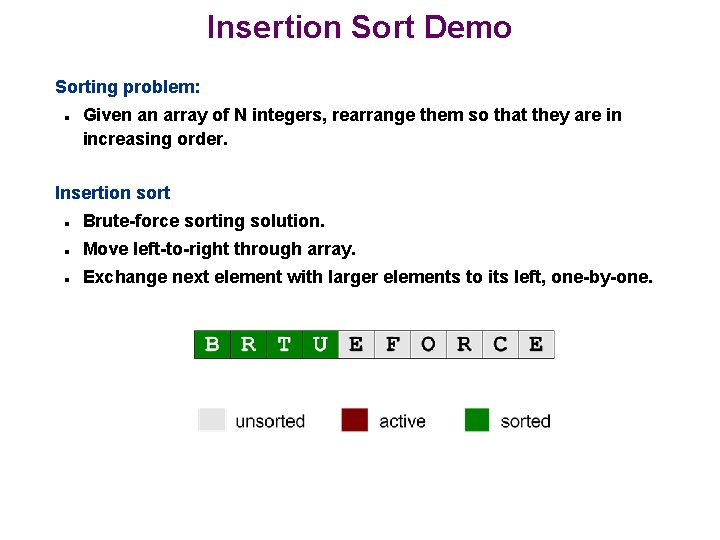
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
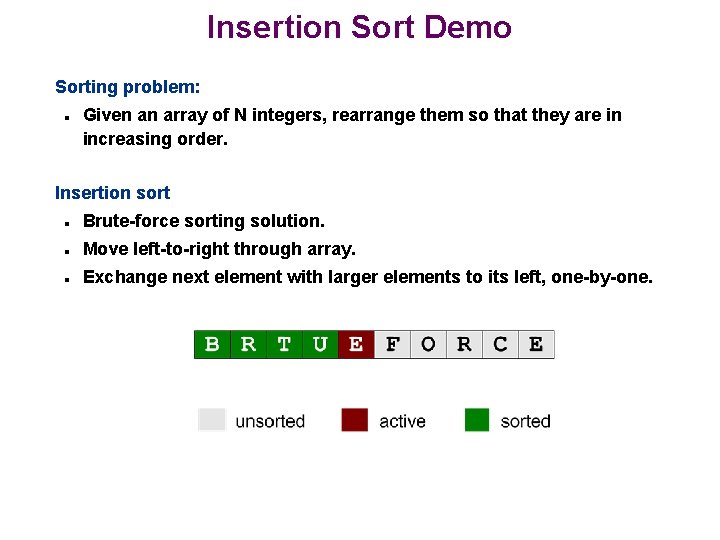
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
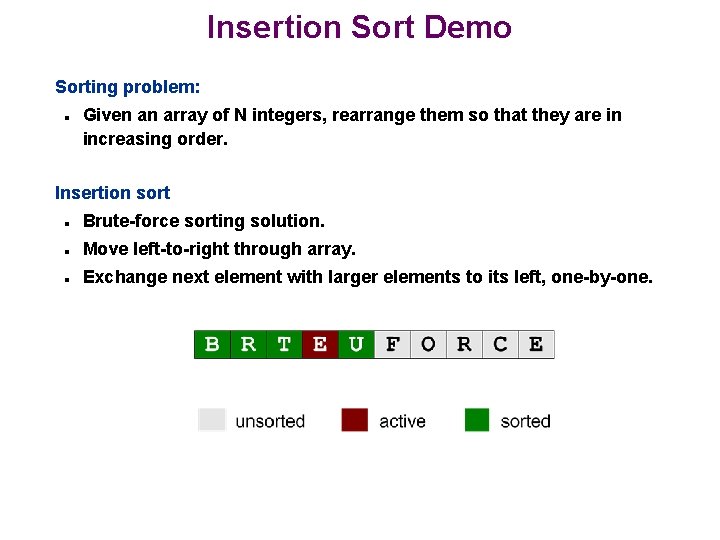
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
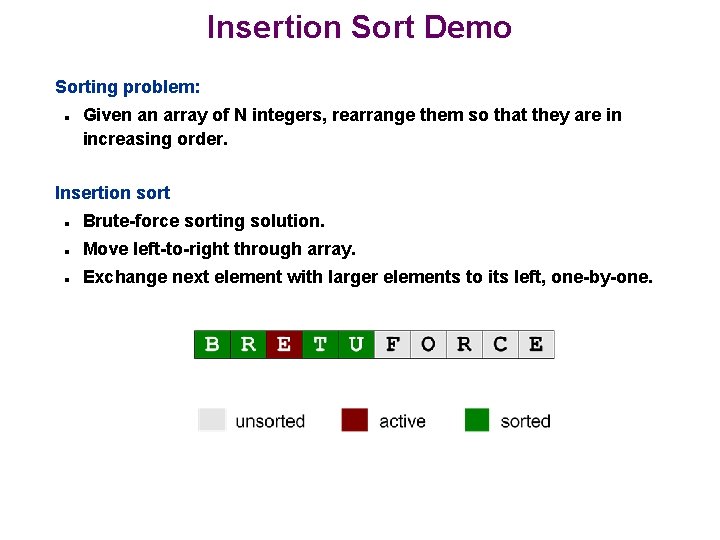
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
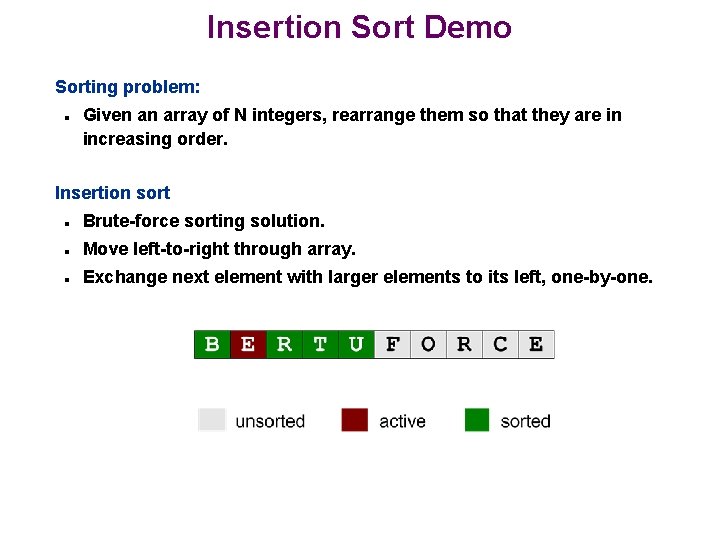
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
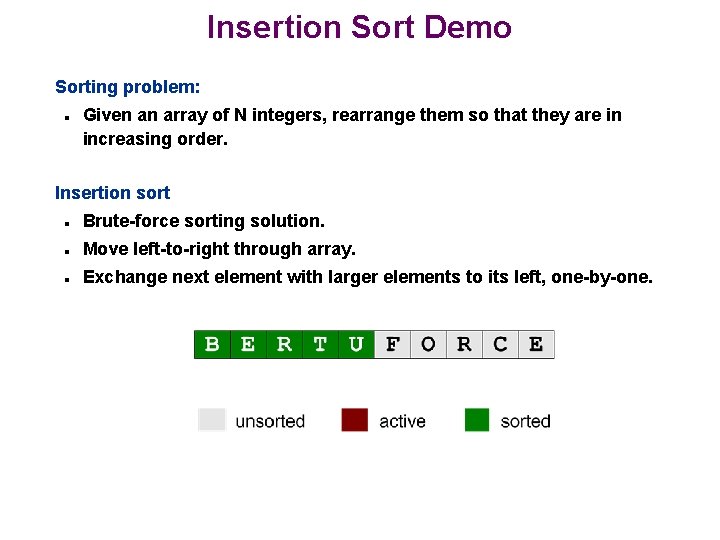
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
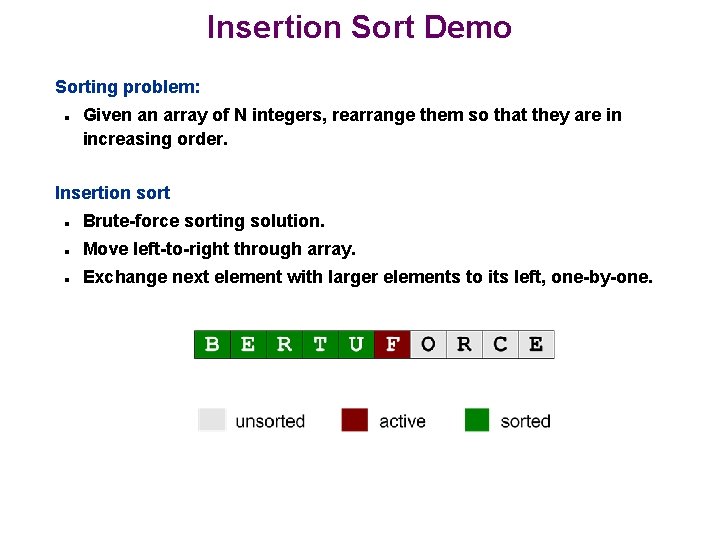
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
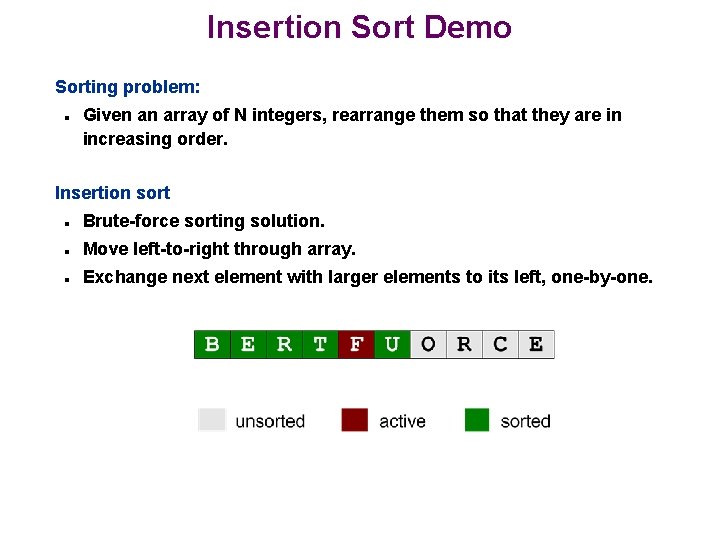
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
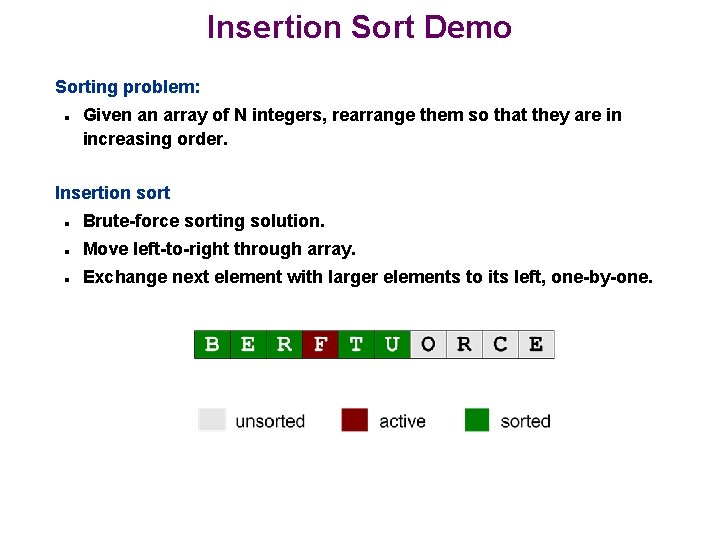
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
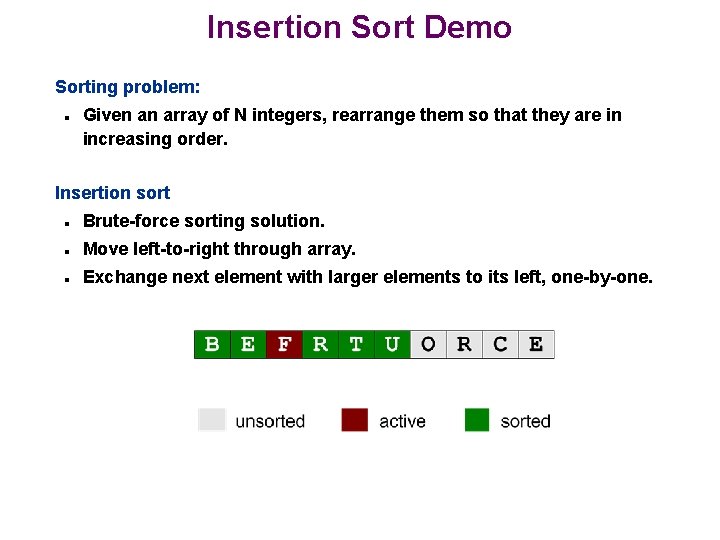
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
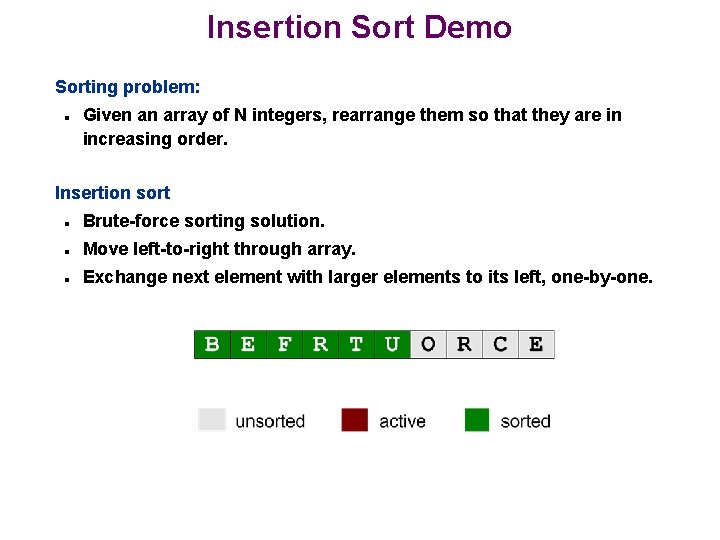
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
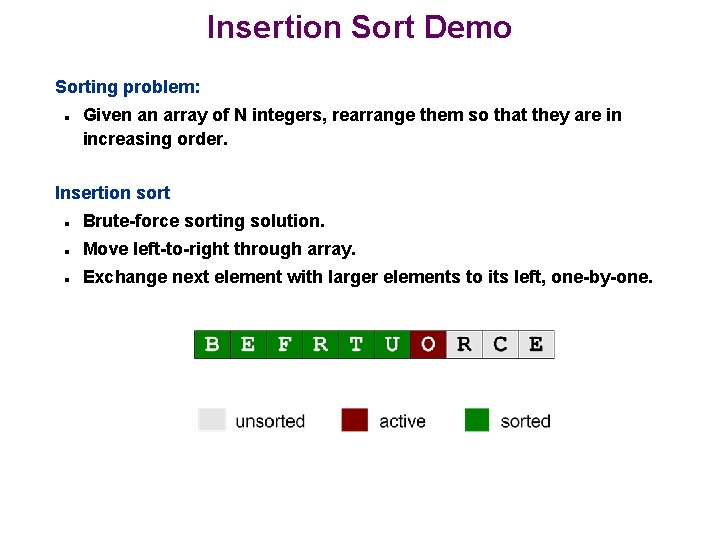
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
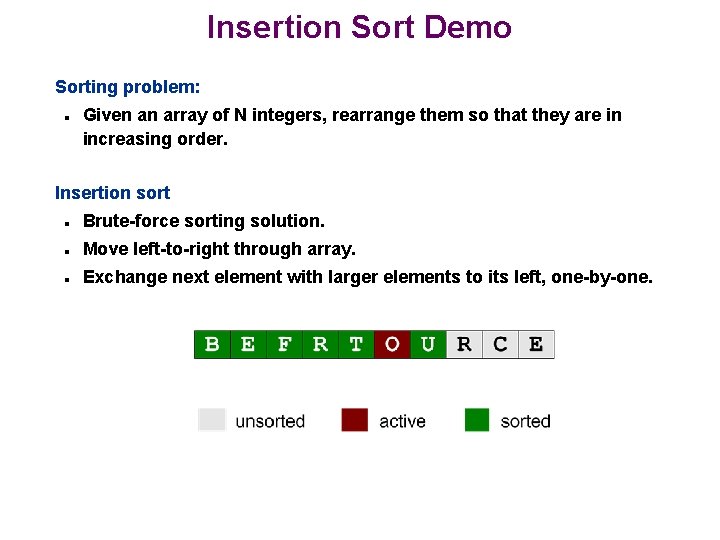
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
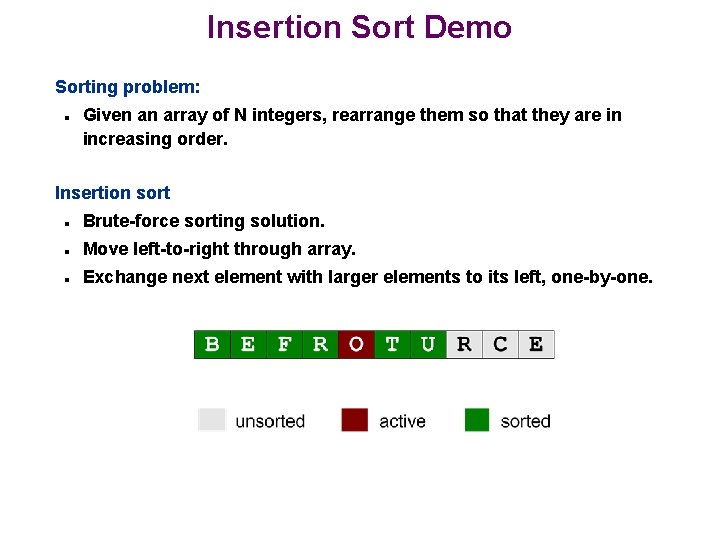
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
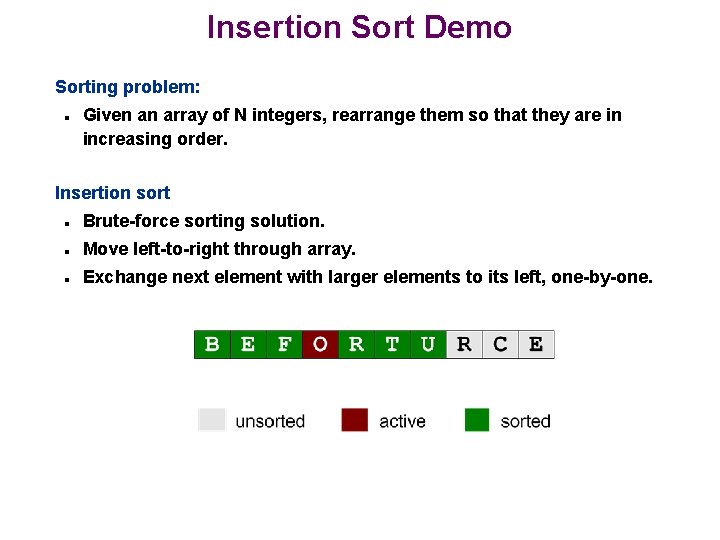
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
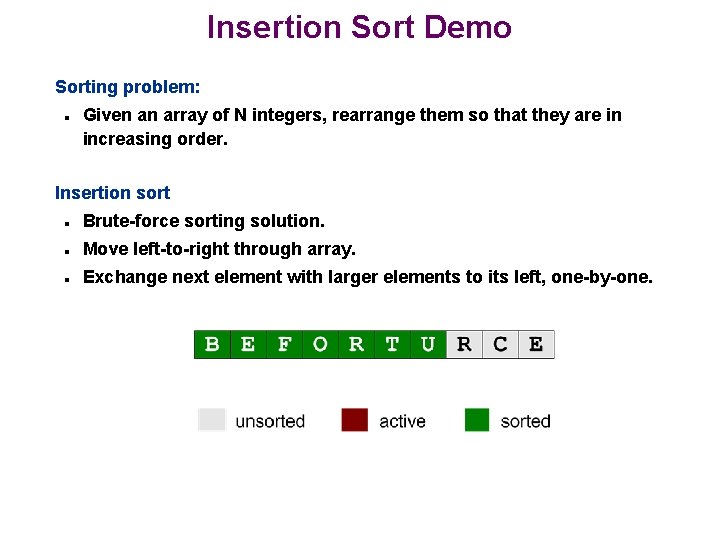
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
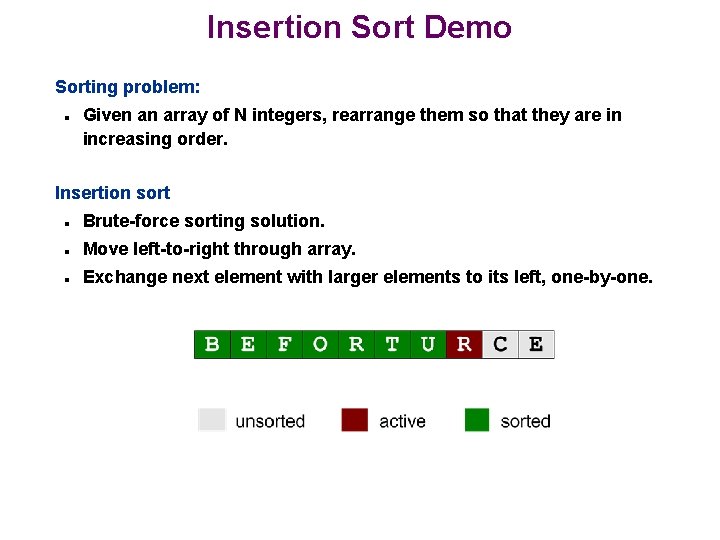
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
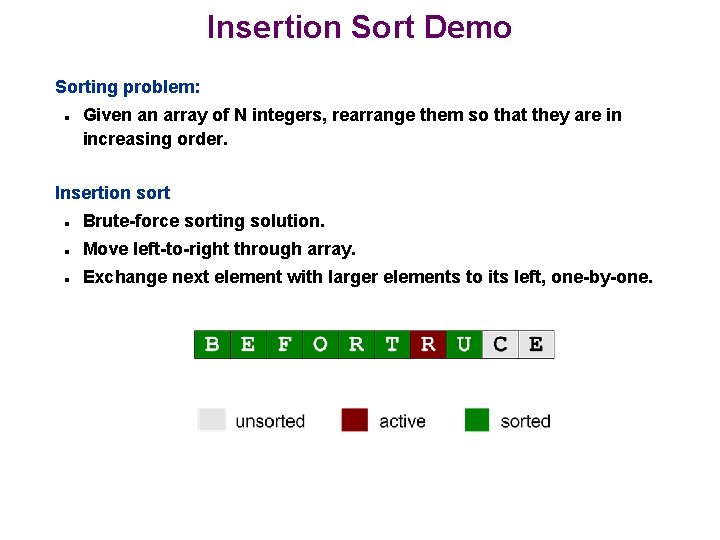
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
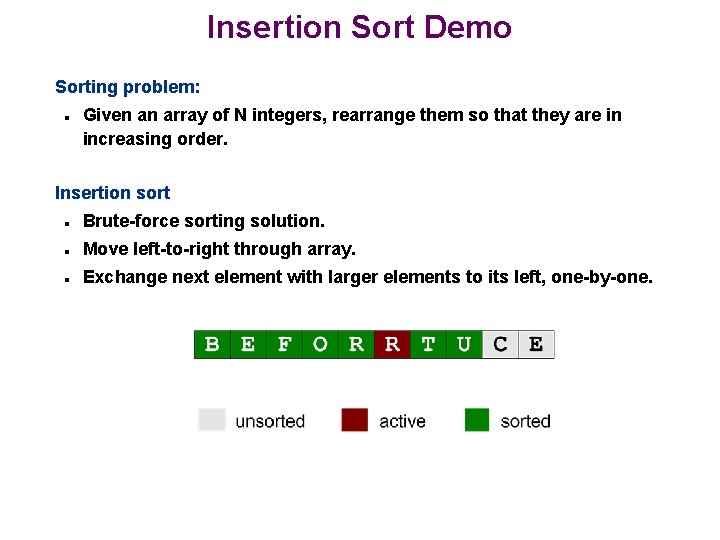
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
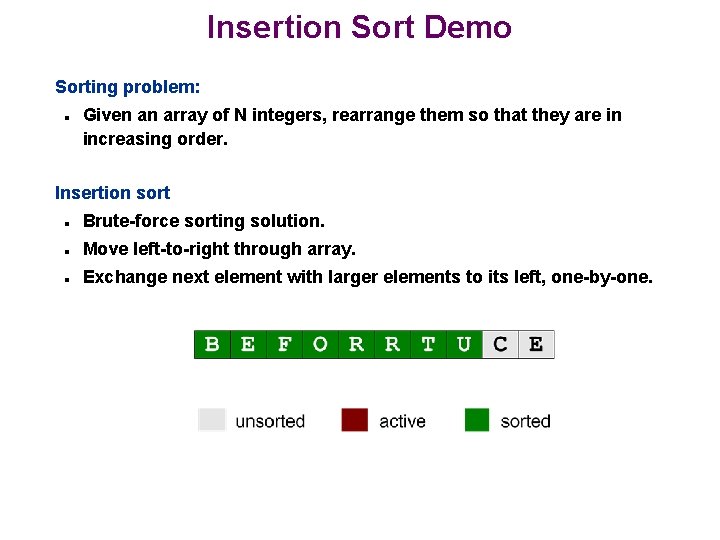
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
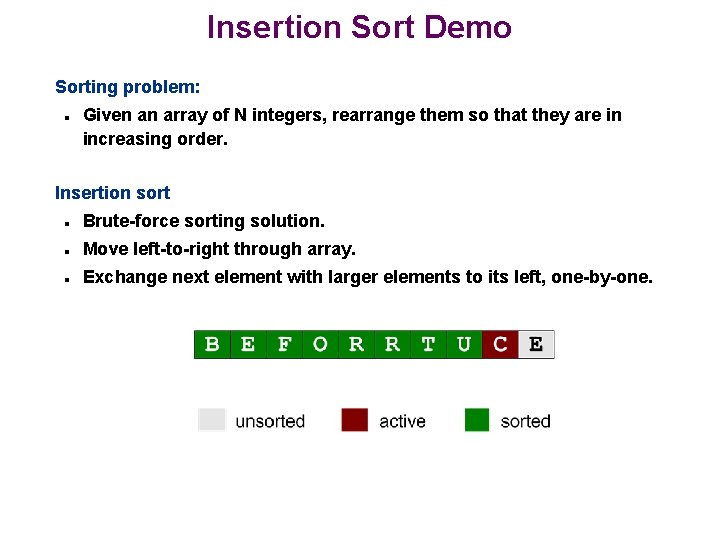
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
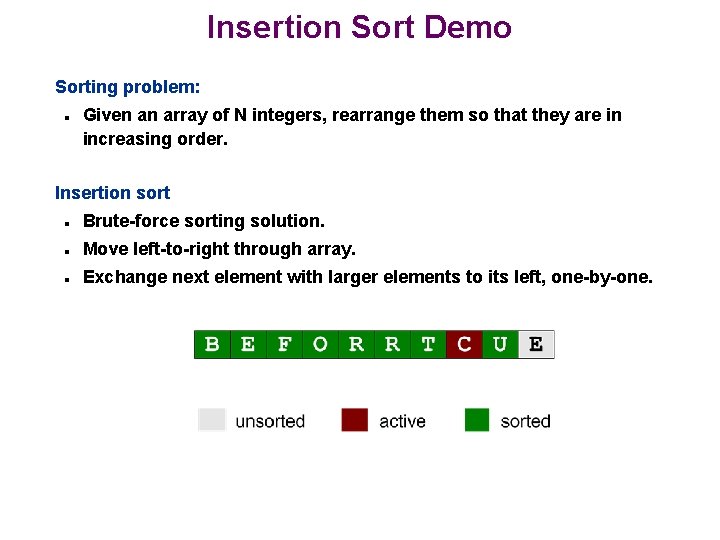
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
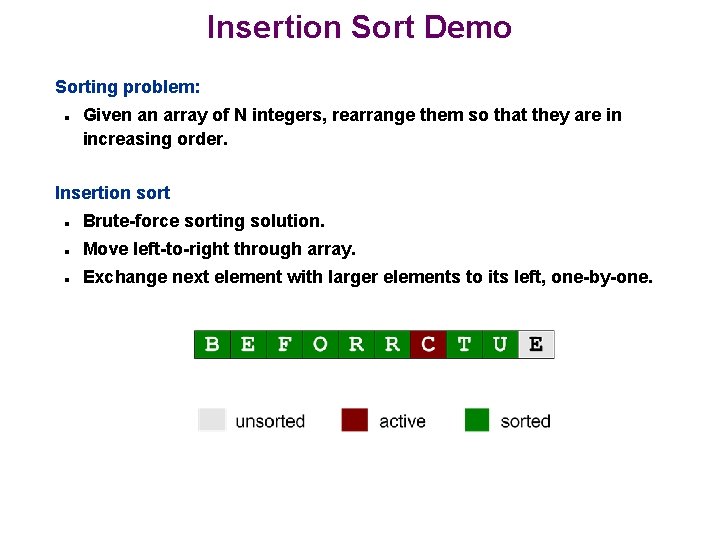
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
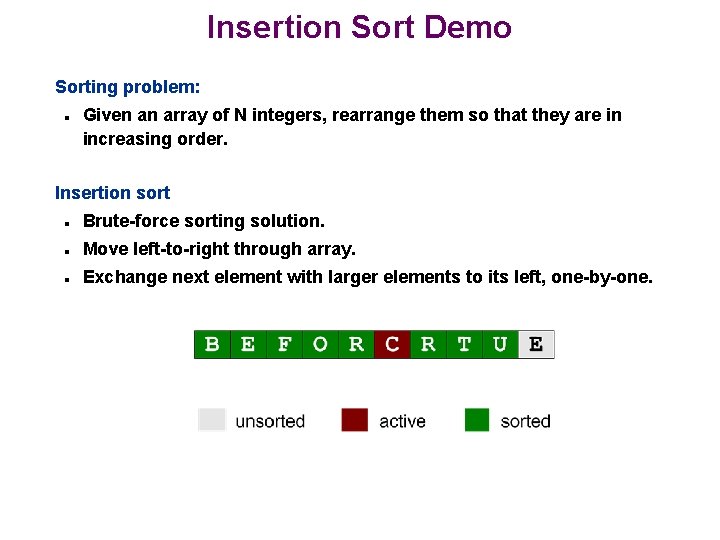
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
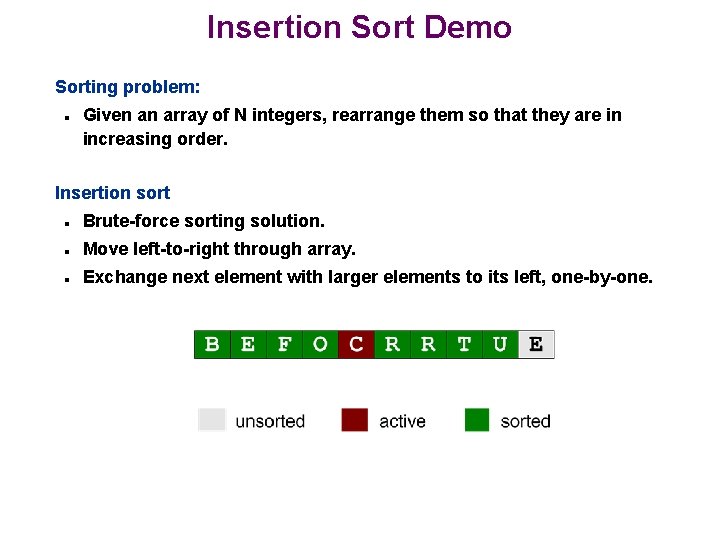
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
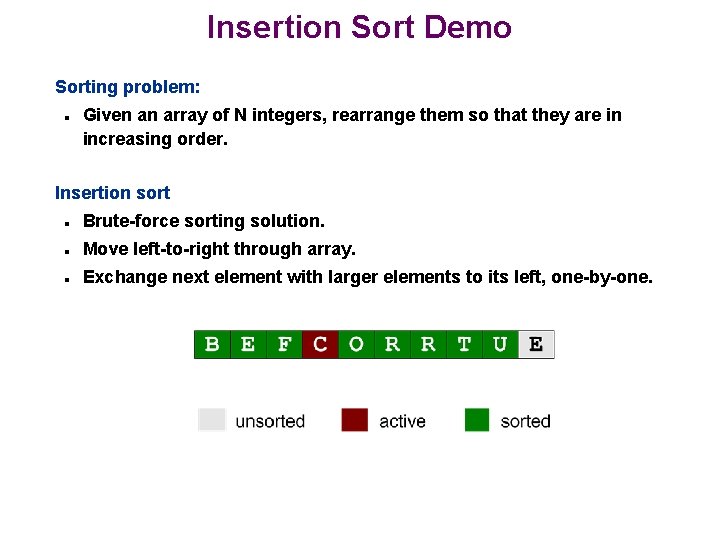
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
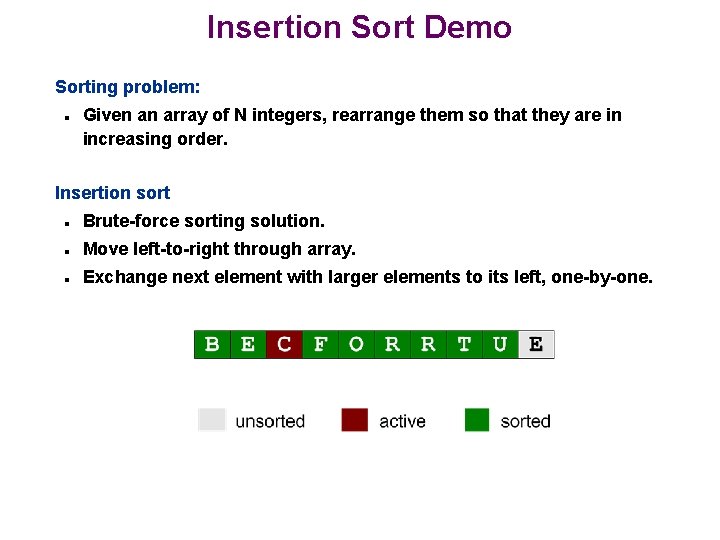
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
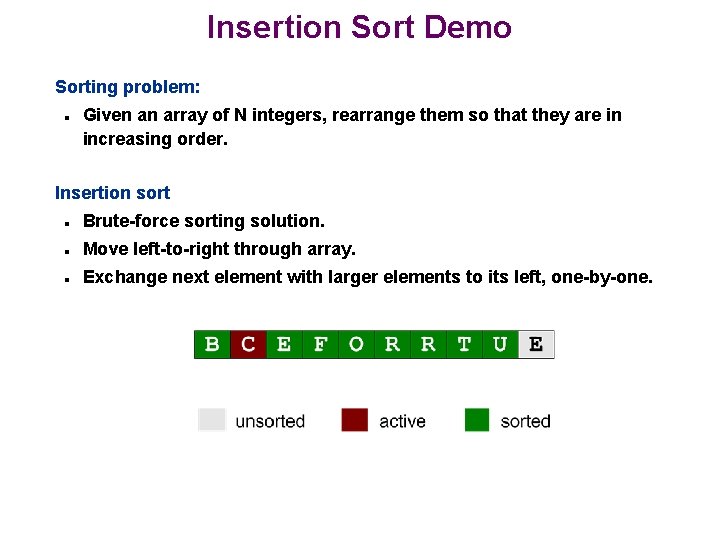
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
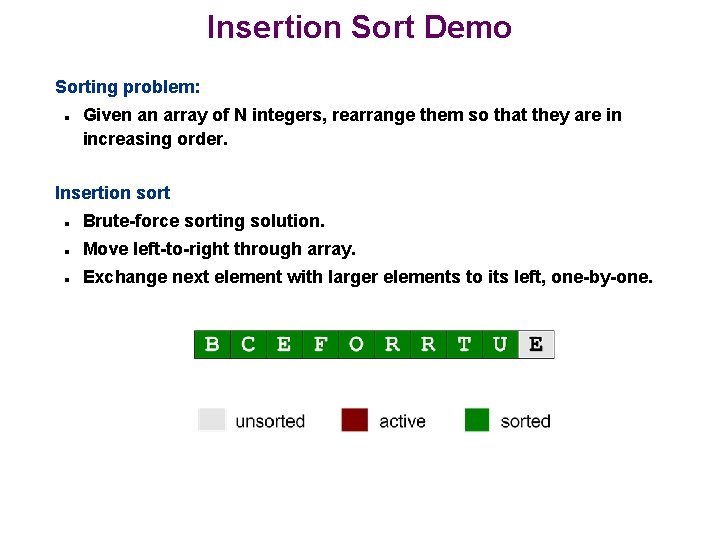
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
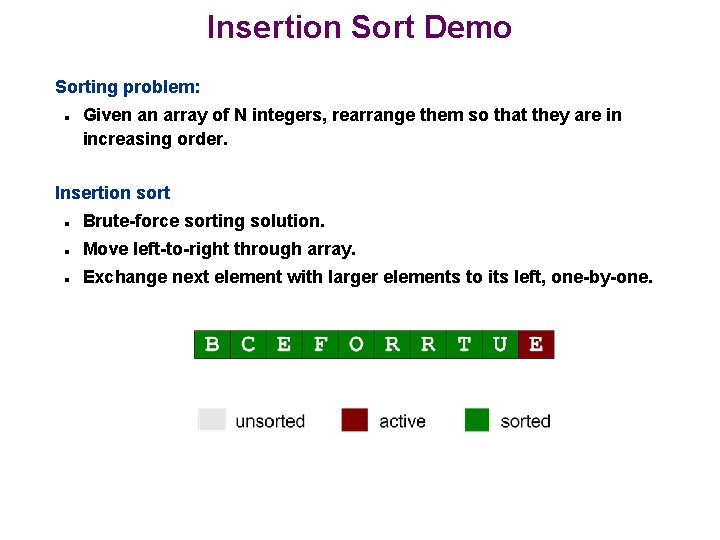
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
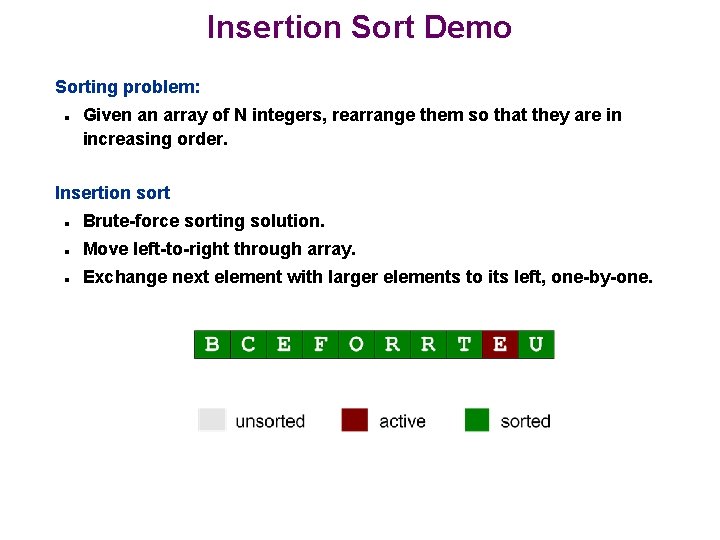
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
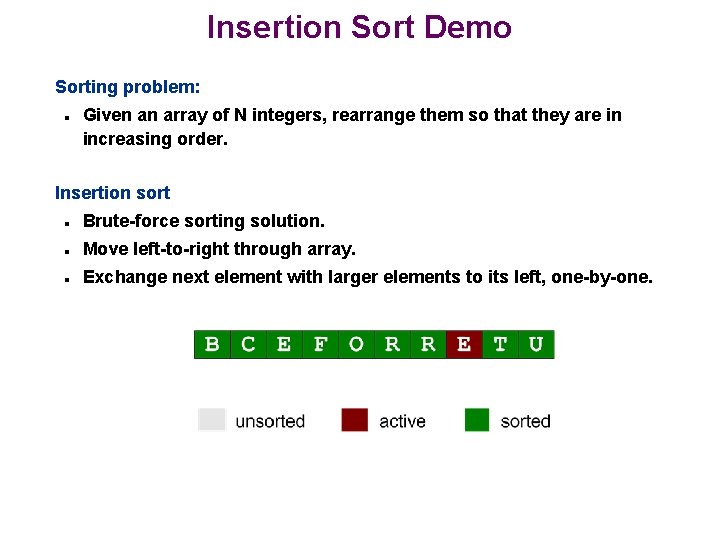
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
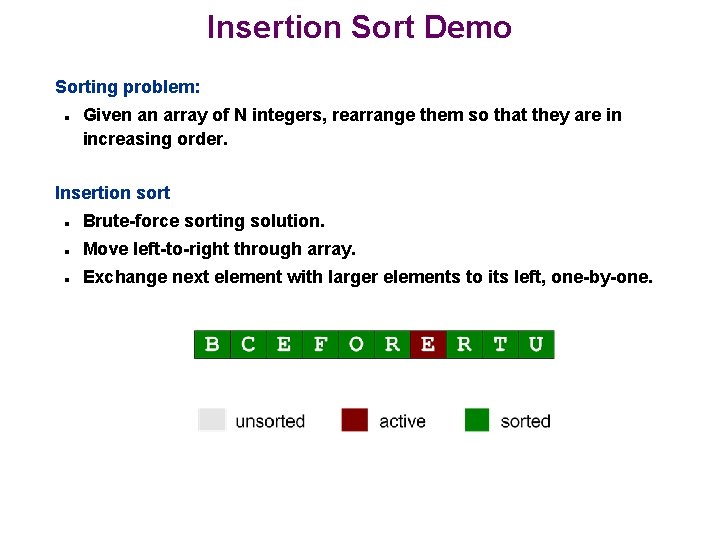
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
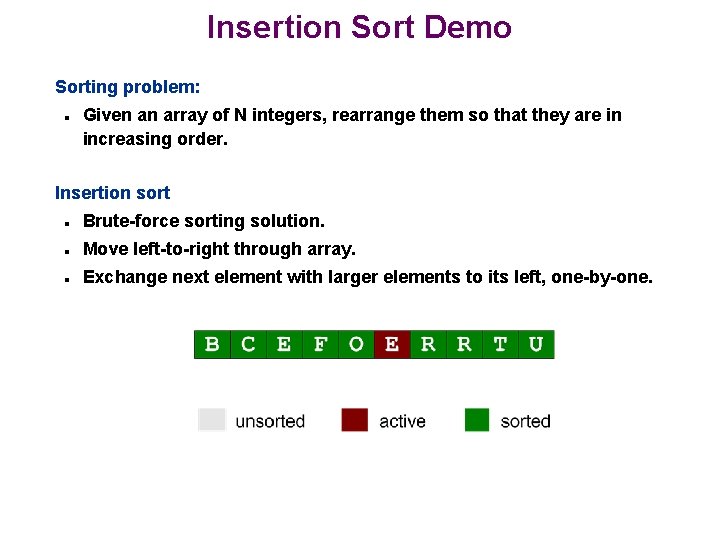
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
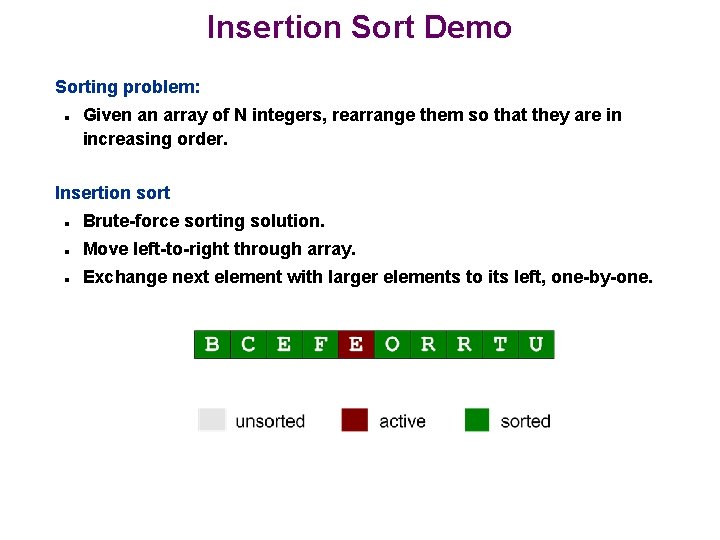
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
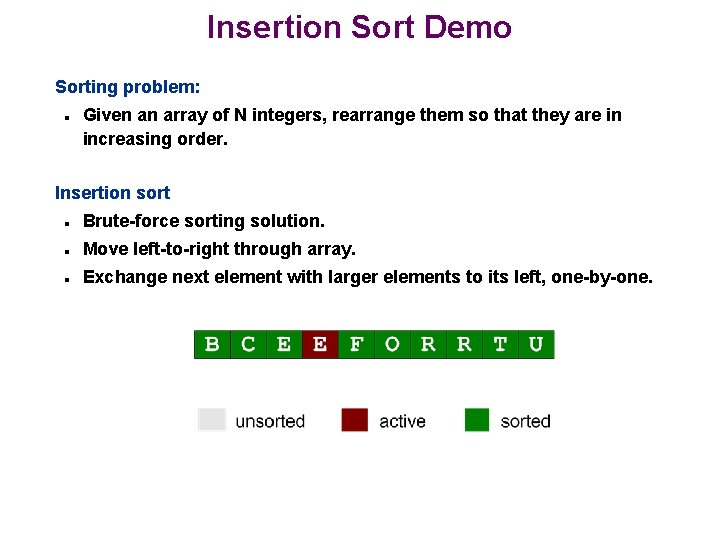
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
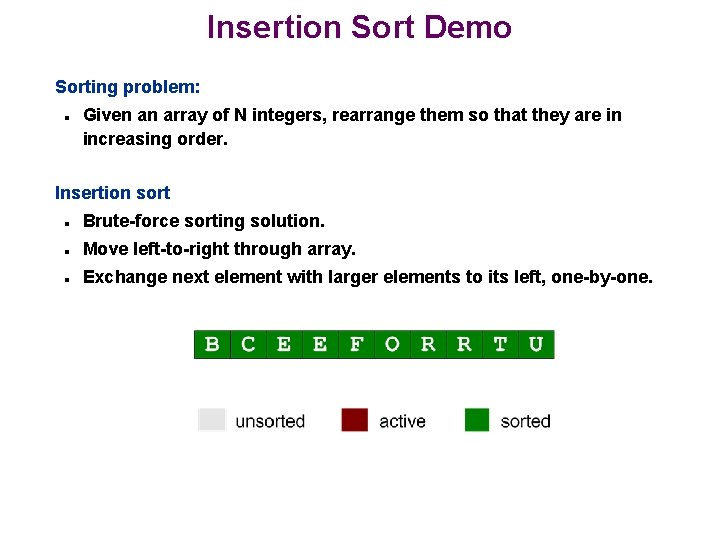
Insertion Sort Demo Sorting problem: n Given an array of N integers, rearrange them so that they are in increasing order. Insertion sort n Brute-force sorting solution. n Move left-to-right through array. n Exchange next element with larger elements to its left, one-by-one.
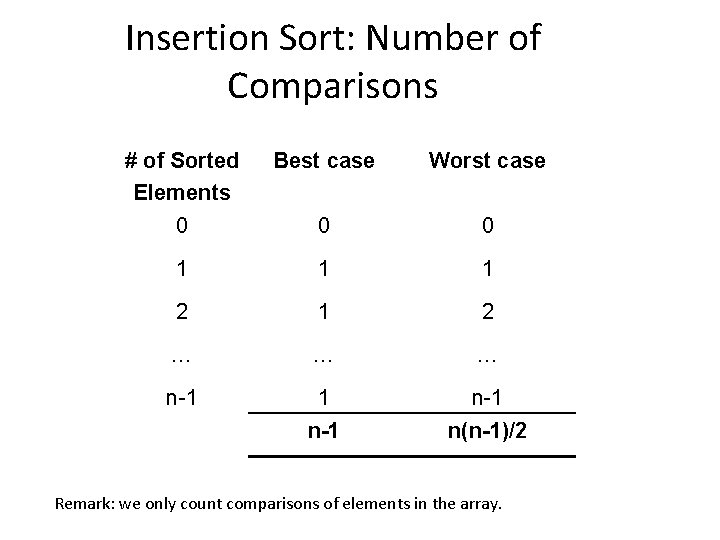
Insertion Sort: Number of Comparisons # of Sorted Elements Best case Worst case 0 0 0 1 1 1 2 … … … n-1 1 n-1 n(n-1)/2 Remark: we only count comparisons of elements in the array.
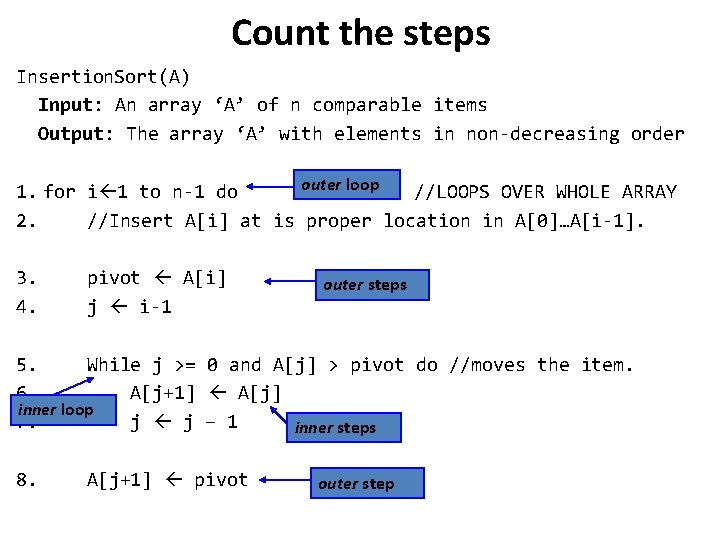
Count the steps Insertion. Sort(A) Input: An array ‘A’ of n comparable items Output: The array ‘A’ with elements in non-decreasing order outer loop 1. for i 1 to n-1 do //LOOPS OVER WHOLE ARRAY 2. //Insert A[i] at is proper location in A[0]…A[i-1]. 3. 4. pivot A[i] j i-1 outer steps 5. While j >= 0 and A[j] > pivot do //moves the item. 6. A[j+1] A[j] inner loop 7. j j – 1 inner steps 8. A[j+1] pivot outer step
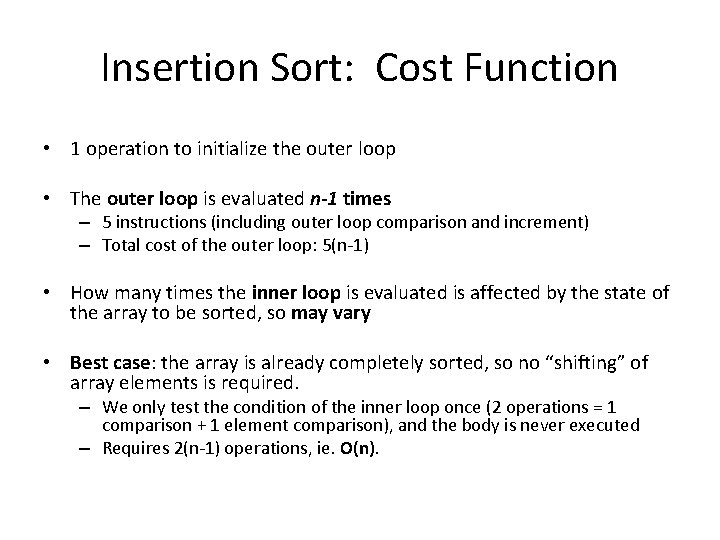
Insertion Sort: Cost Function • 1 operation to initialize the outer loop • The outer loop is evaluated n-1 times – 5 instructions (including outer loop comparison and increment) – Total cost of the outer loop: 5(n-1) • How many times the inner loop is evaluated is affected by the state of the array to be sorted, so may vary • Best case: the array is already completely sorted, so no “shifting” of array elements is required. – We only test the condition of the inner loop once (2 operations = 1 comparison + 1 element comparison), and the body is never executed – Requires 2(n-1) operations, ie. O(n).
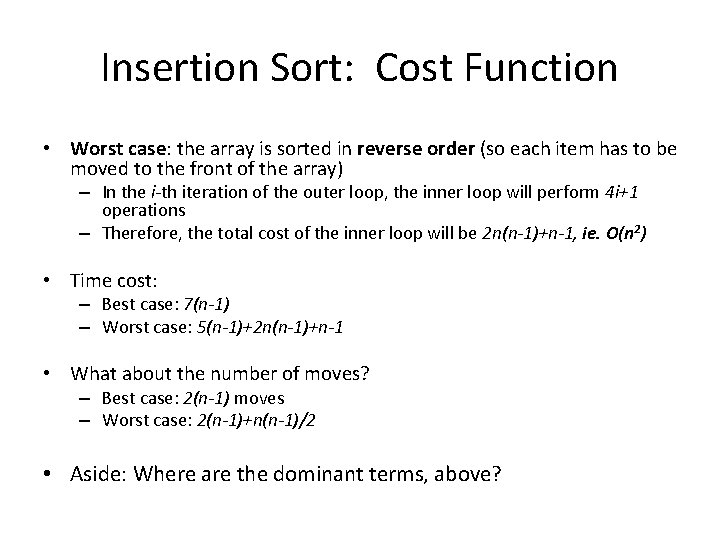
Insertion Sort: Cost Function • Worst case: the array is sorted in reverse order (so each item has to be moved to the front of the array) – In the i-th iteration of the outer loop, the inner loop will perform 4 i+1 operations – Therefore, the total cost of the inner loop will be 2 n(n-1)+n-1, ie. O(n 2) • Time cost: – Best case: 7(n-1) – Worst case: 5(n-1)+2 n(n-1)+n-1 • What about the number of moves? – Best case: 2(n-1) moves – Worst case: 2(n-1)+n(n-1)/2 • Aside: Where are the dominant terms, above?
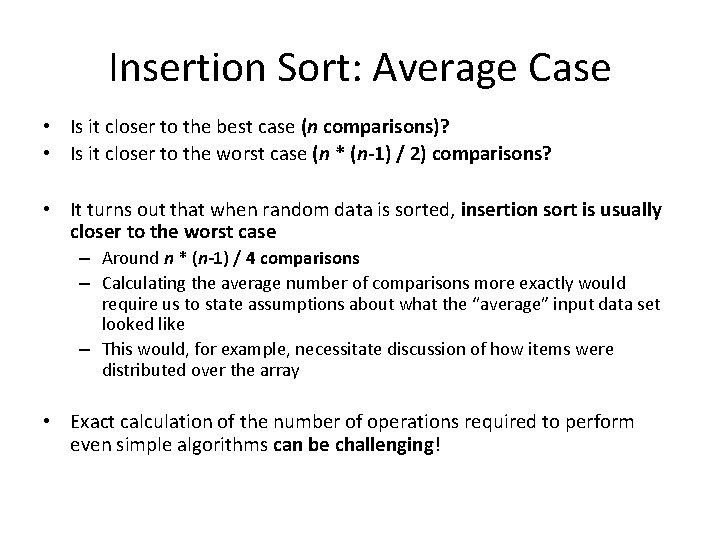
Insertion Sort: Average Case • Is it closer to the best case (n comparisons)? • Is it closer to the worst case (n * (n-1) / 2) comparisons? • It turns out that when random data is sorted, insertion sort is usually closer to the worst case – Around n * (n-1) / 4 comparisons – Calculating the average number of comparisons more exactly would require us to state assumptions about what the “average” input data set looked like – This would, for example, necessitate discussion of how items were distributed over the array • Exact calculation of the number of operations required to perform even simple algorithms can be challenging!
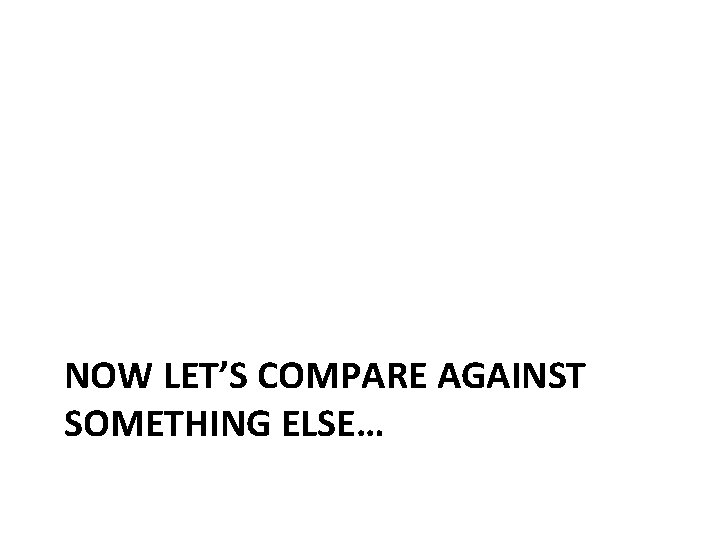
NOW LET’S COMPARE AGAINST SOMETHING ELSE…
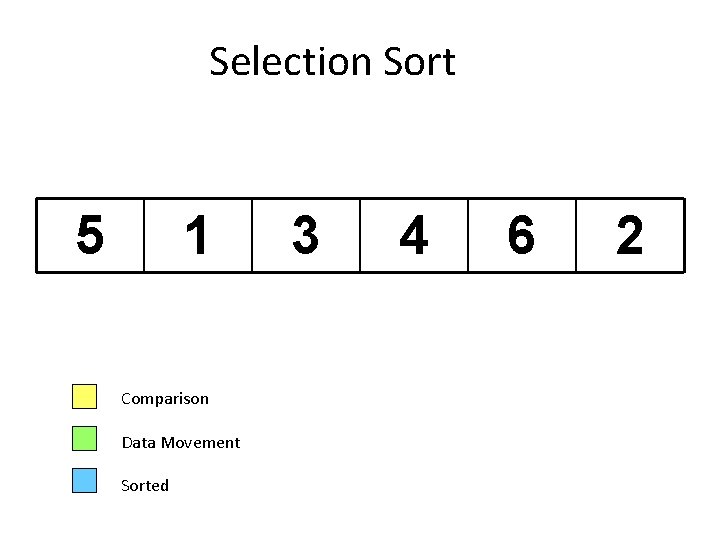
Selection Sort 5 1 Comparison Data Movement Sorted 3 4 6 2
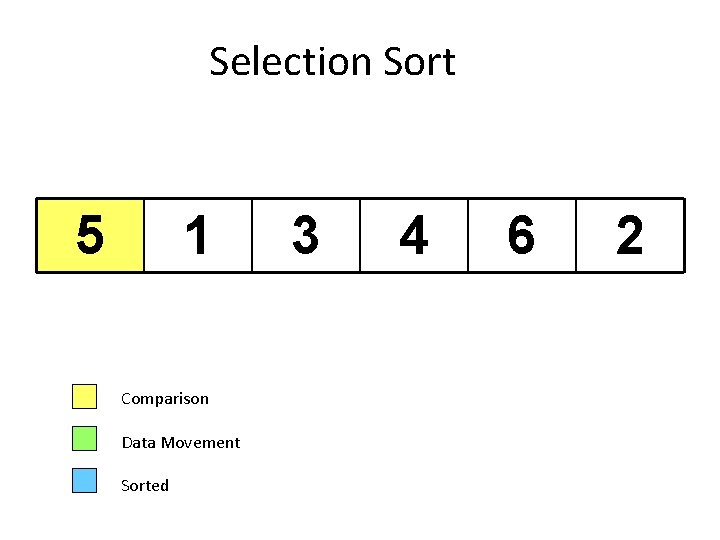
Selection Sort 5 1 Comparison Data Movement Sorted 3 4 6 2
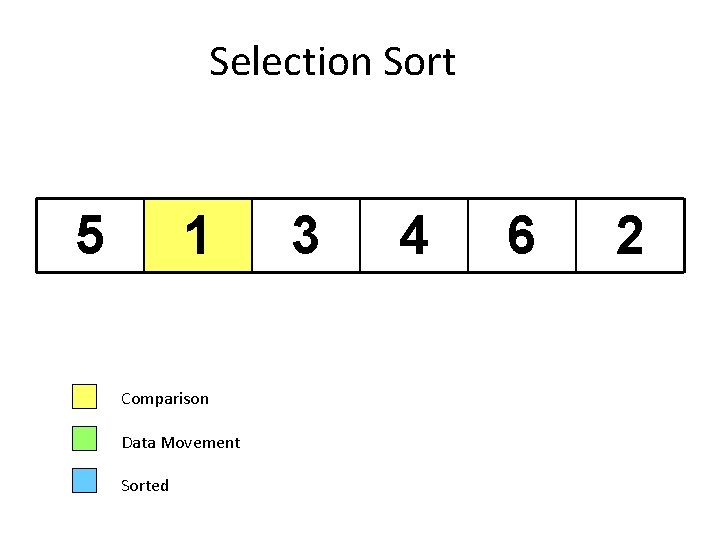
Selection Sort 5 1 Comparison Data Movement Sorted 3 4 6 2
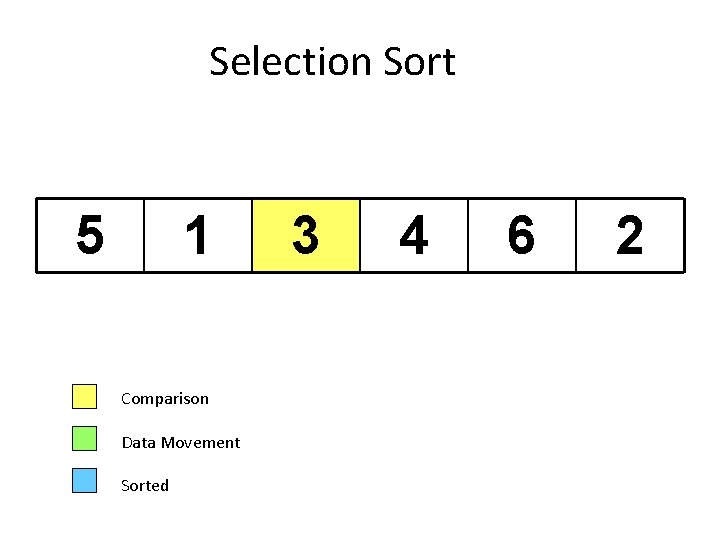
Selection Sort 5 1 Comparison Data Movement Sorted 3 4 6 2
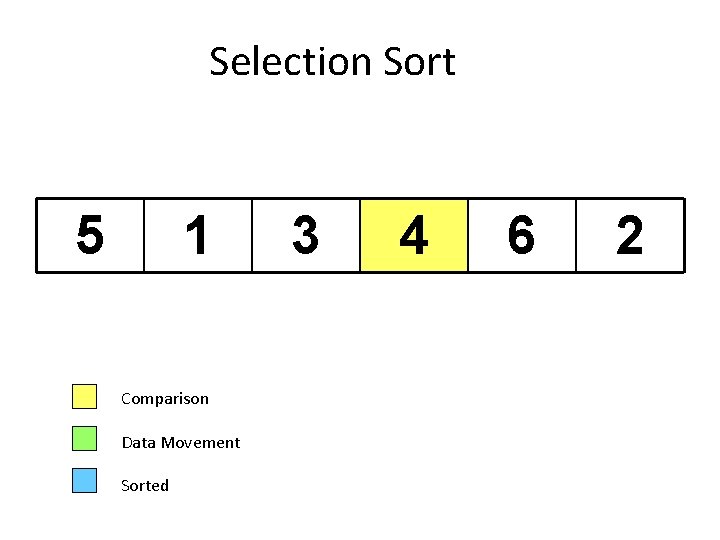
Selection Sort 5 1 Comparison Data Movement Sorted 3 4 6 2
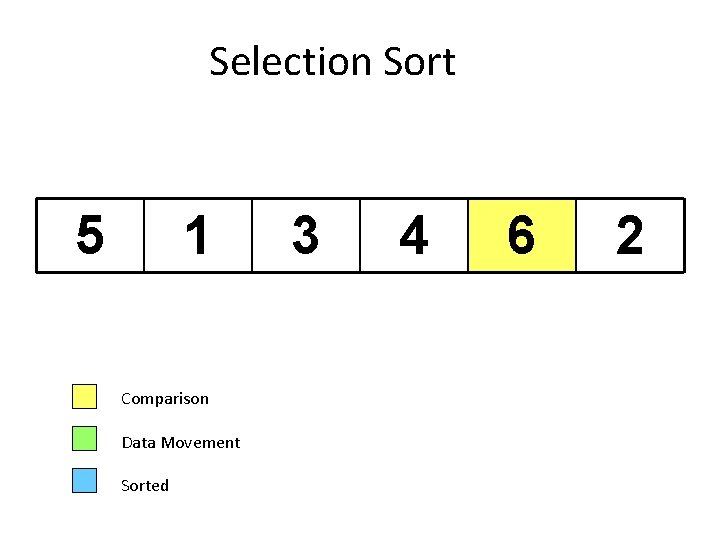
Selection Sort 5 1 Comparison Data Movement Sorted 3 4 6 2
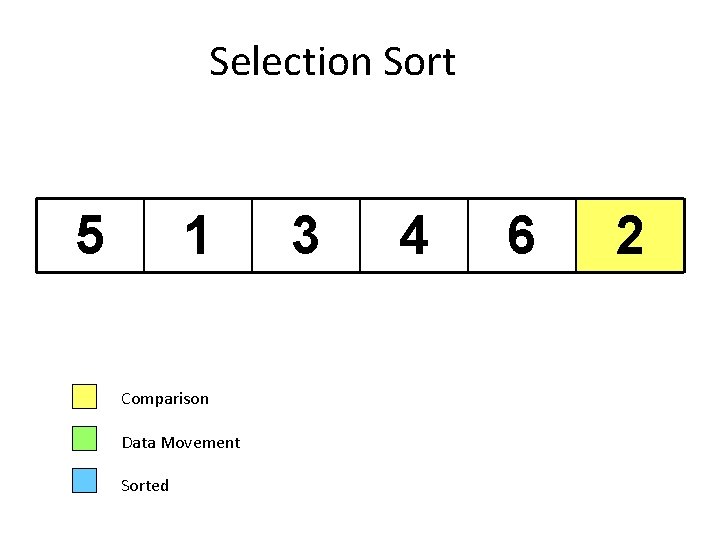
Selection Sort 5 1 Comparison Data Movement Sorted 3 4 6 2
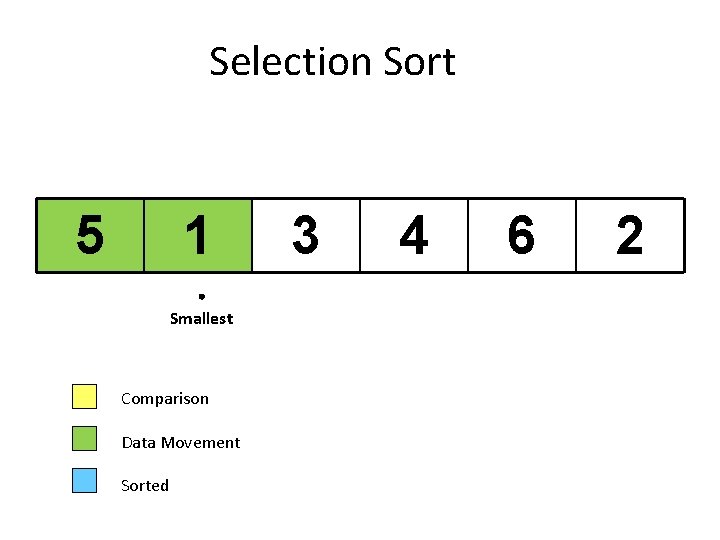
Selection Sort 5 1 Smallest Comparison Data Movement Sorted 3 4 6 2
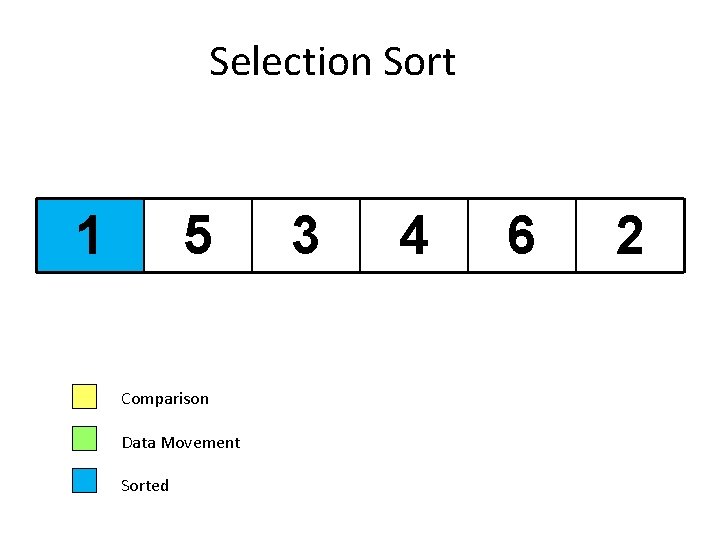
Selection Sort 1 5 Comparison Data Movement Sorted 3 4 6 2
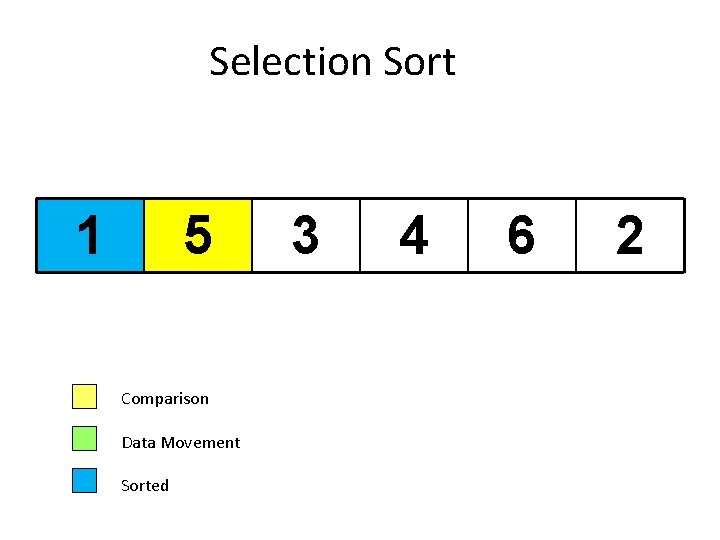
Selection Sort 1 5 Comparison Data Movement Sorted 3 4 6 2
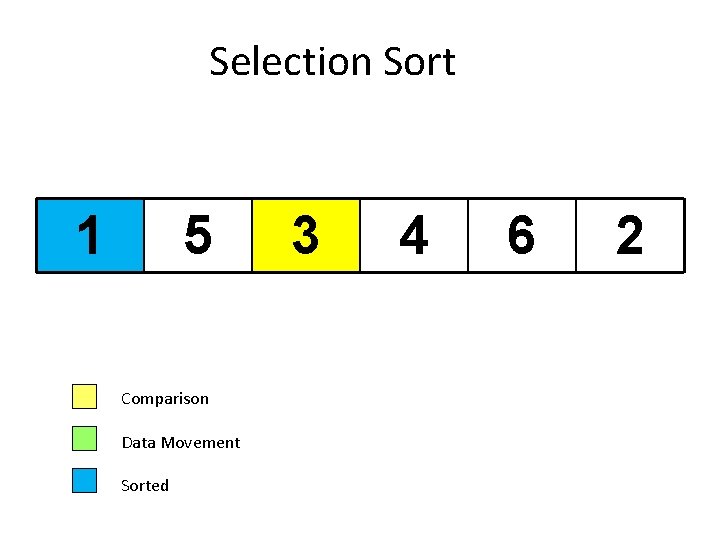
Selection Sort 1 5 Comparison Data Movement Sorted 3 4 6 2
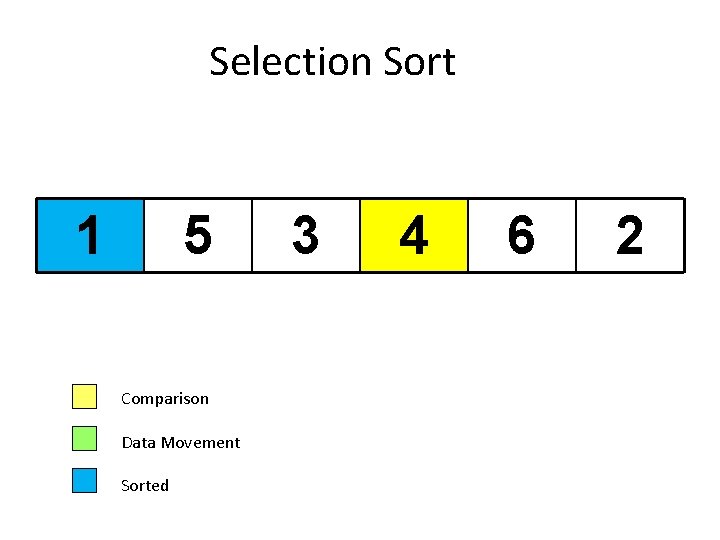
Selection Sort 1 5 Comparison Data Movement Sorted 3 4 6 2
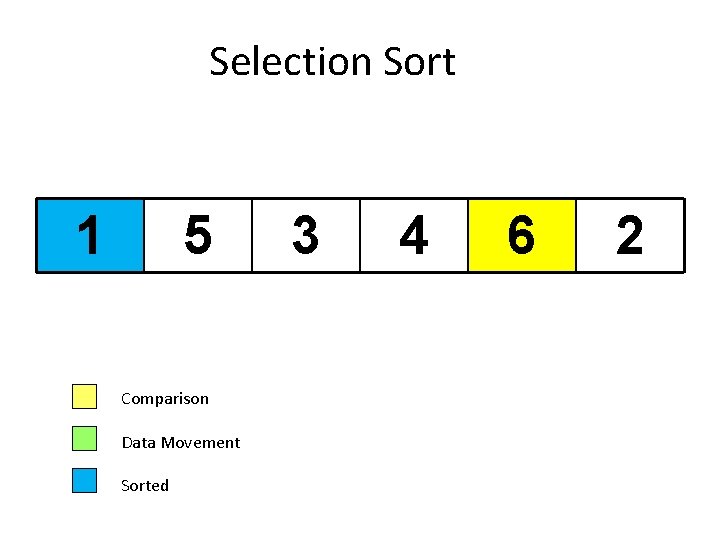
Selection Sort 1 5 Comparison Data Movement Sorted 3 4 6 2
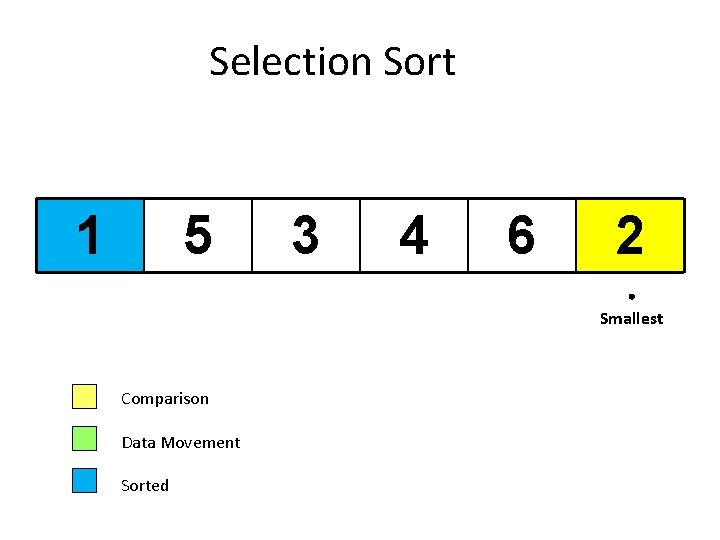
Selection Sort 1 5 3 4 6 2 Smallest Comparison Data Movement Sorted
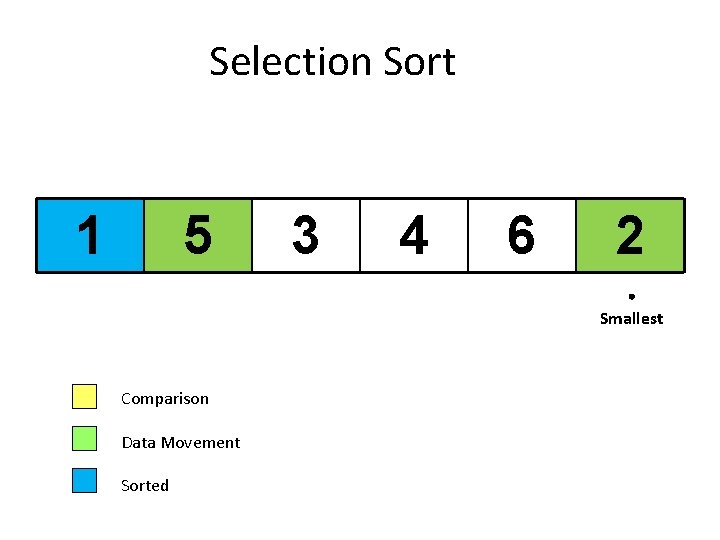
Selection Sort 1 5 3 4 6 2 Smallest Comparison Data Movement Sorted
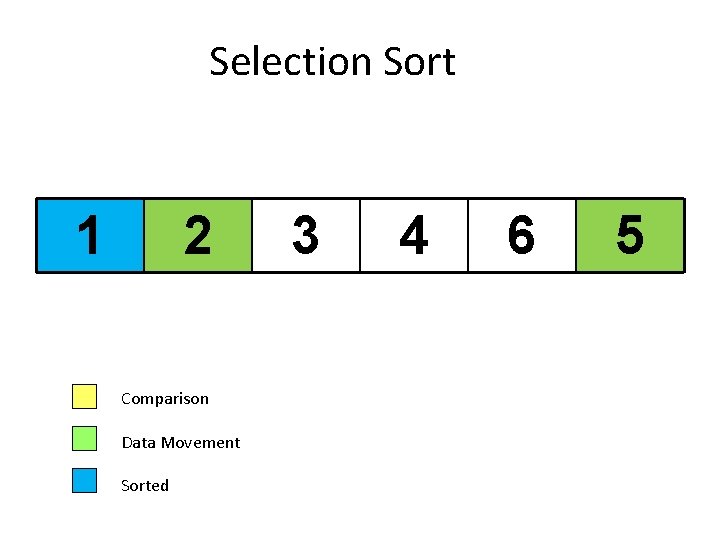
Selection Sort 1 2 Comparison Data Movement Sorted 3 4 6 5
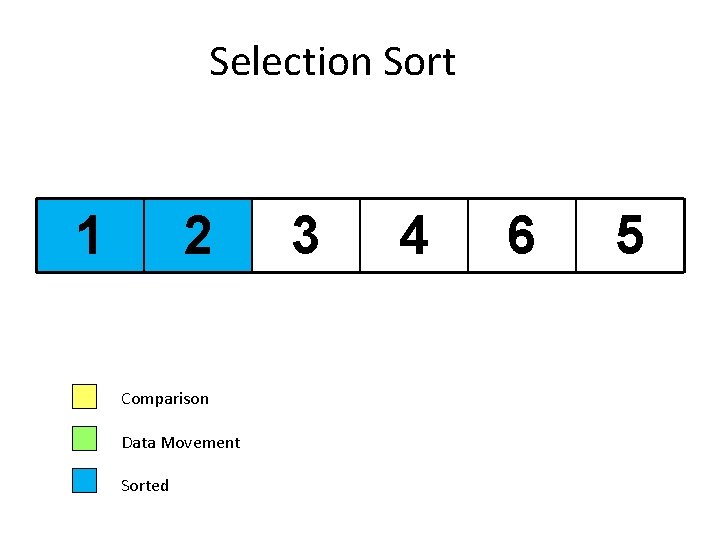
Selection Sort 1 2 Comparison Data Movement Sorted 3 4 6 5
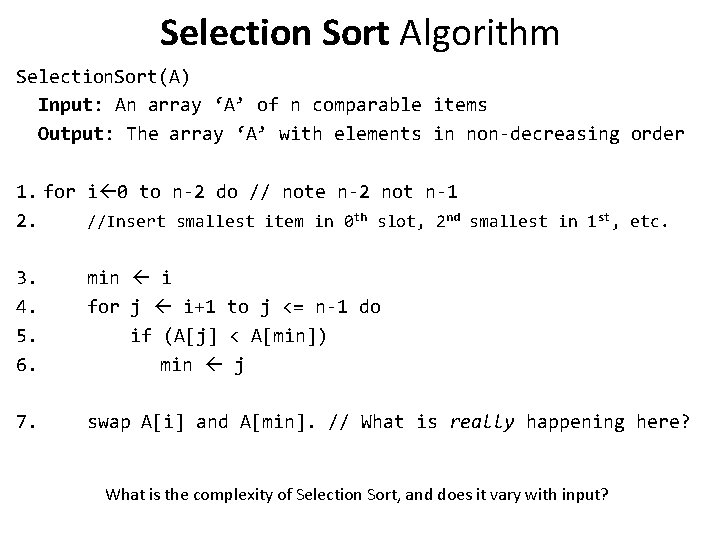
Selection Sort Algorithm Selection. Sort(A) Input: An array ‘A’ of n comparable items Output: The array ‘A’ with elements in non-decreasing order 1. for i 0 to n-2 do // note n-2 not n-1 2. //Insert smallest item in 0 th slot, 2 nd smallest in 1 st, etc. 3. 4. 5. 6. min i for j i+1 to j <= n-1 do if (A[j] < A[min]) min j 7. swap A[i] and A[min]. // What is really happening here? What is the complexity of Selection Sort, and does it vary with input?
Difference between insertion sort and bubble sort
Disadvantage of insertion sort
Bubble sort insertion sort
What is internal and external sorting
Decrease and conquer
Difference between selection and insertion sort
Is shell sort divide and conquer
Sort meaning
Bubble sort vs selection sort
Selection sort vs heap sort
Recurrence relation for selection sort
Merge sort mips
Insertion sort pseudocode
Insertion sort flow chart
Insertion sort flowchart in c
Wikipedia insertion sort
Pengertian insertion sort
Shellsort
Insertion sort pseudocode
Proof by induction calculator
Insertion sort cost
Specifies the way to arrange data in a particular order.
Merge insertion sort python
Space complexity of insertion sort
Insertion sort demo
Insertion of idea
Lower bound for comparison based sorting algorithms
Insertion sort
Insertion sort mips
Insertion sort advanced analysis
Insertion sort comparison counter
Insertion sort bbc bitesize
Bubble sort vs selection sort
Selection sort best case
Quick sort merge sort
Quick sort merge sort
Radix sort animation
Radix bucket sort
Erd vs erm
Ideally insurable risk
Polarizable and non polarizable electrode
Ideally the input bias current should be__________ . *
Attribute repeatability and reproducibility
Examples of external sorting
Fonction technique scooter
Vastus lateralis origin insertion action
Serratus attachments
Oral cavity
Biceps brachii action
Protract retract
Anconeus origin and insertion
Brachioradialos
Gastrocnemius muscle origin and insertion
Boundaries of axilla
Muscles of pectoral region
Origin and insertion
Diaphragm origin insertion
Middle scalene origin and insertion
Gracilis origin and insertion
Gastrocnemius muscle origin and insertion
Temporalis muscle
Medial epicondyle
Abdominal origin and insertion
Omohyoid muscle origin and insertion
Peroneus tertius origin and insertion
Adductor pollicis origin and insertion
Sternocostalis
Muscles of forearm posterior compartment
Normal shoulder extension
Diaphragm openings
Adductor pollicis origin and insertion
Phonology analysis
Outlet pelvis
Compartments of forearm
Psoas insertion and origin
Muscle origin and insertion flashcards with pictures
Medial rotation of hip muscles
Triangular space contents
External occipital protuberance
Type of sternoclavicular joint
Abductor hallucis
Kt tape tensor fascia lata
Intrinsic muscles of tongue
Serratus anterior origin and insertion
L
Tibialis muscle function
Nuchal line muscle attachment
Cims&h
Nerve supply to pectineus
Flexor pollicis longus origin and insertion
Superior oblique action
Differentiate between sorting and grading
Intracellular compartments and protein sorting
Searching and sorting arrays in c++
Searching and sorting in java