Programming Fundamentals I COSC 1336 Lecture 7 prepared
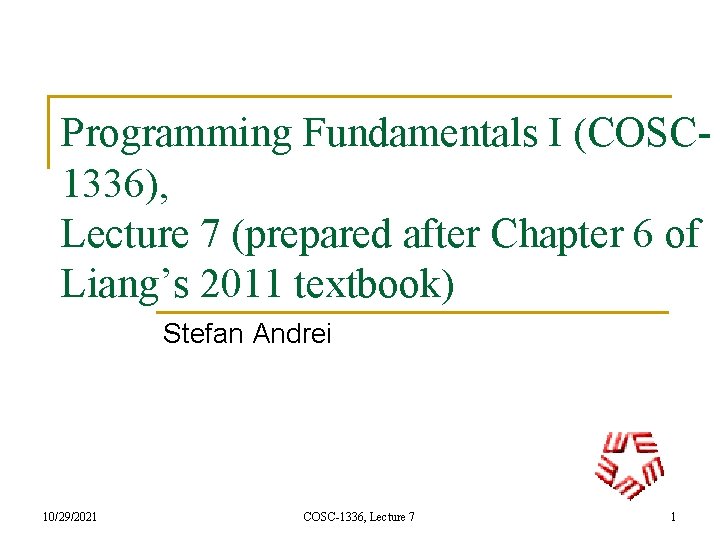
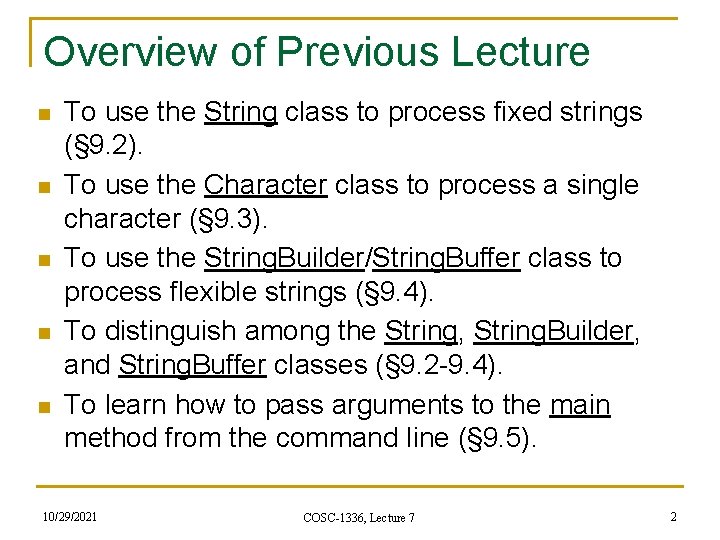
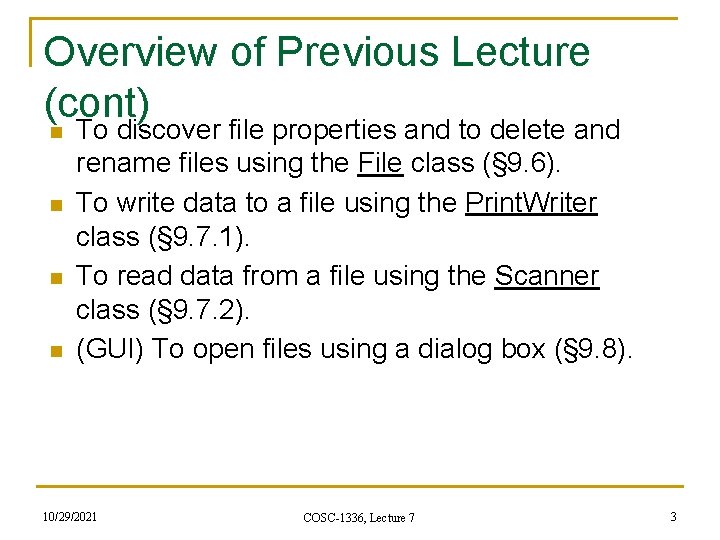
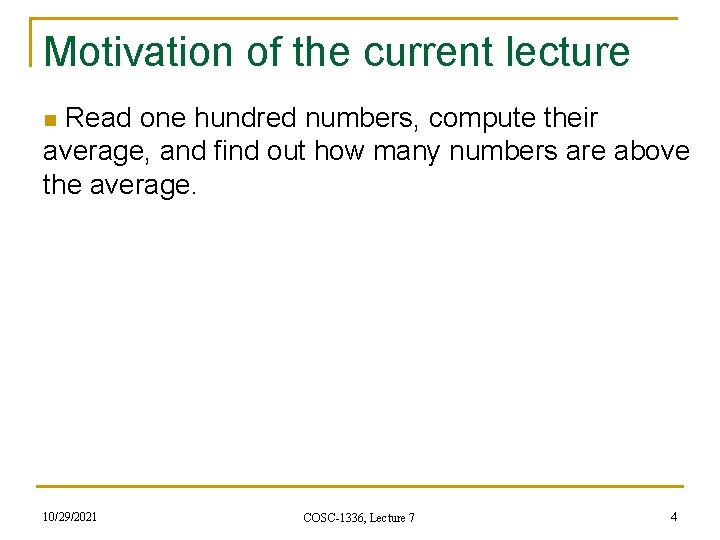
![Solution public class Analyze. Numbers { public static void main(String[] args) { final int Solution public class Analyze. Numbers { public static void main(String[] args) { final int](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-5.jpg)
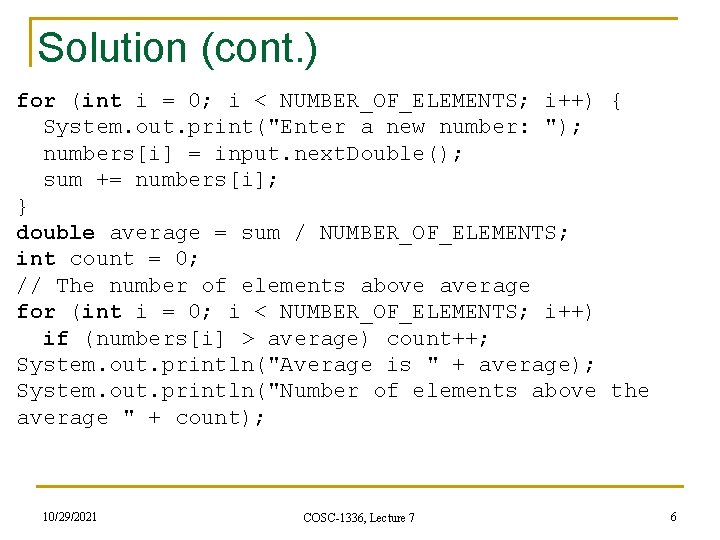
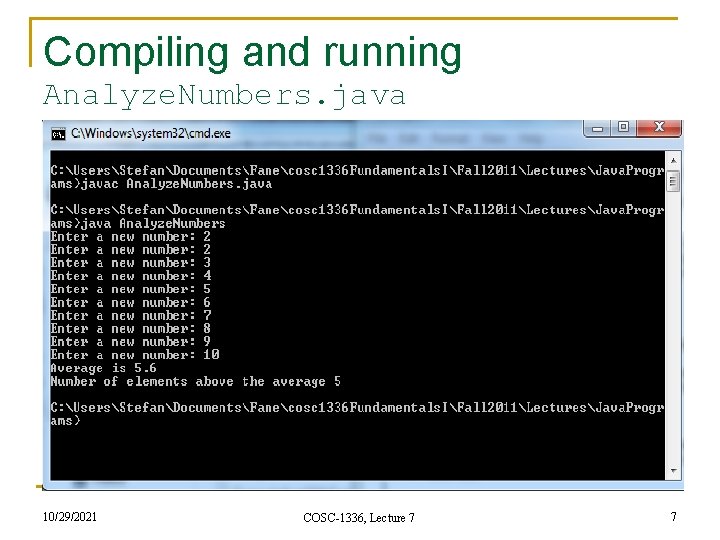
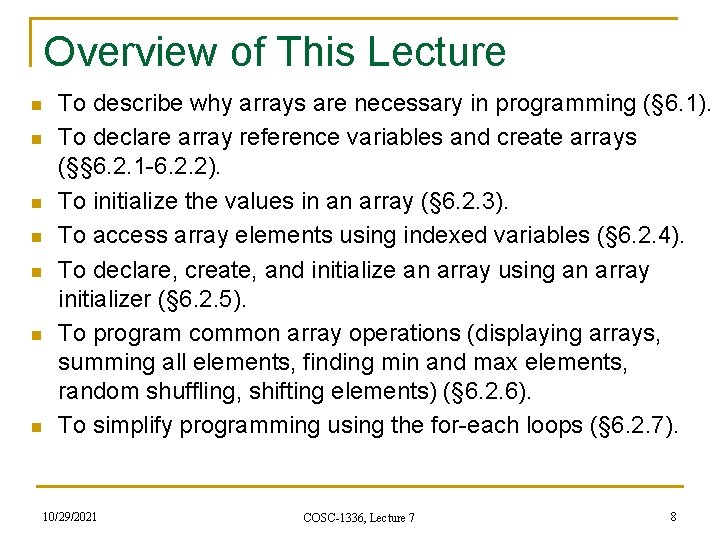
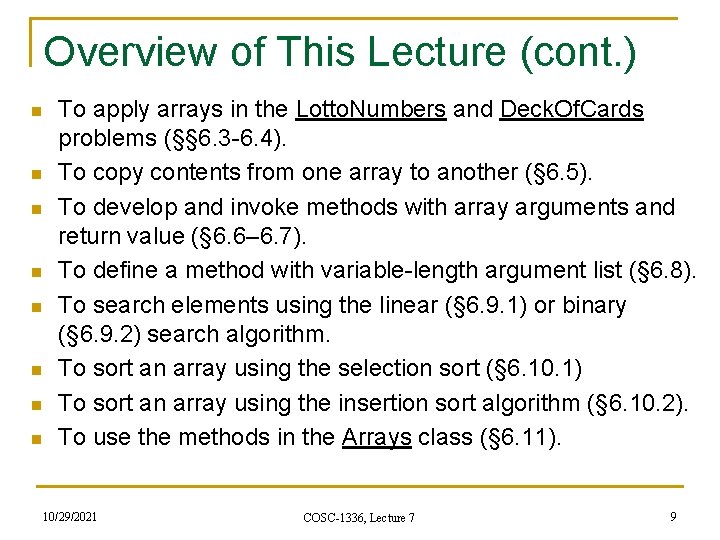
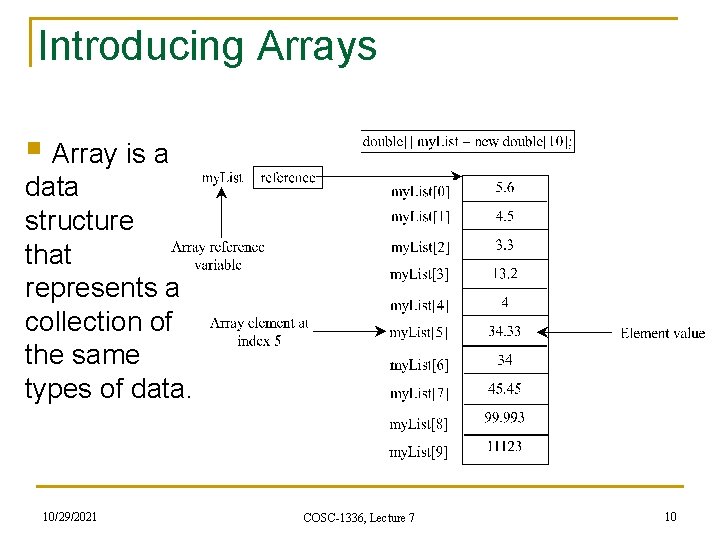
![Declaring Array Variables n General syntax: datatype[] array. Ref. Var; Example: double[] my. List; Declaring Array Variables n General syntax: datatype[] array. Ref. Var; Example: double[] my. List;](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-11.jpg)
![Creating Arrays n General syntax: array. Ref. Var = new datatype[array. Size]; n Example: Creating Arrays n General syntax: array. Ref. Var = new datatype[array. Size]; n Example:](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-12.jpg)
![Declaring and Creating in One Step n n General syntax: datatype[] array. Ref. Var Declaring and Creating in One Step n n General syntax: datatype[] array. Ref. Var](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-13.jpg)
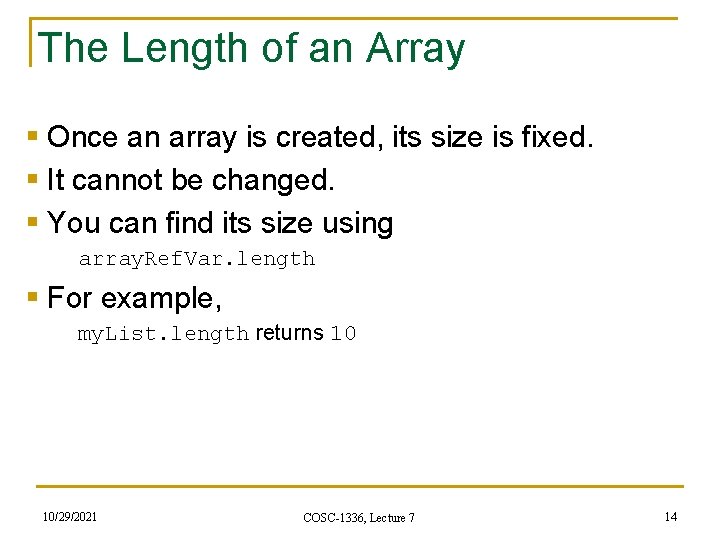
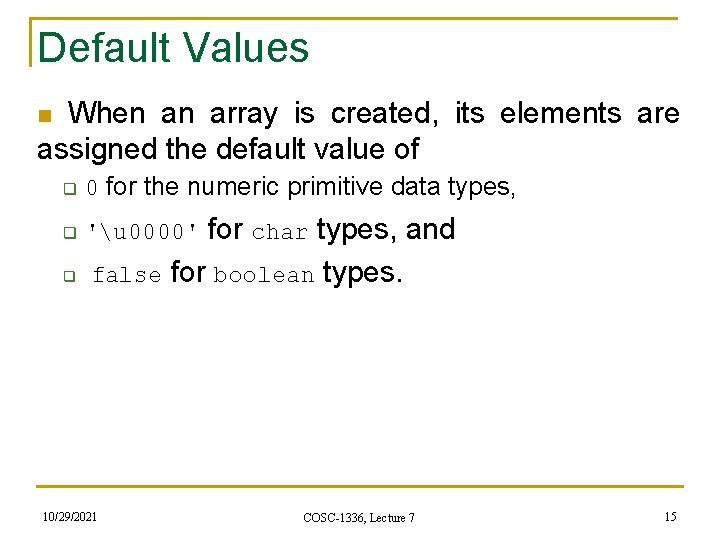
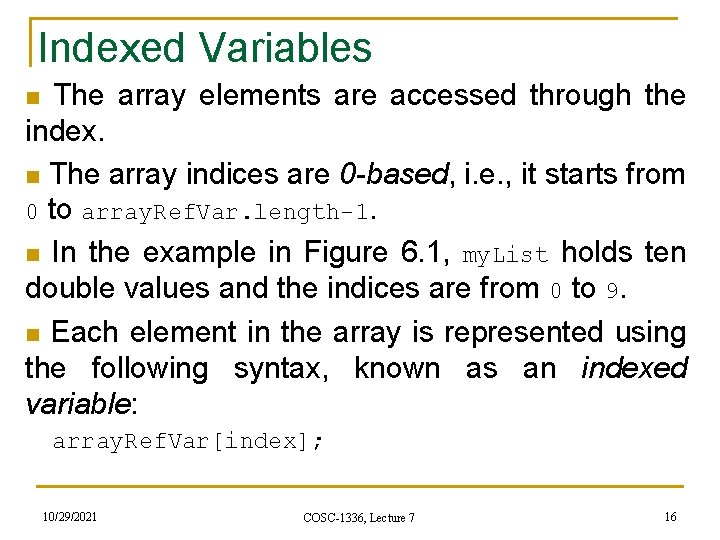
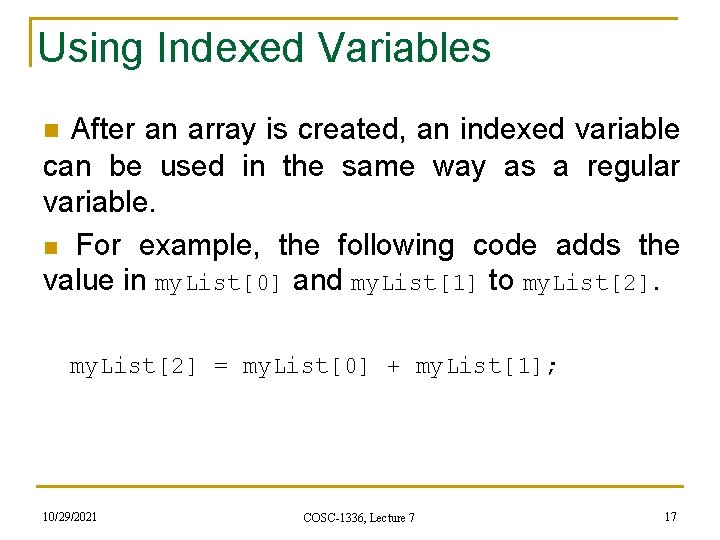
![Array Initializers n Declaring, creating, initializing in one step: double[] my. List = {1. Array Initializers n Declaring, creating, initializing in one step: double[] my. List = {1.](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-18.jpg)
![Declaring, Creating, Initializing using the Shorthand Notation double[] my. List = {1. 9, 2. Declaring, Creating, Initializing using the Shorthand Notation double[] my. List = {1. 9, 2.](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-19.jpg)
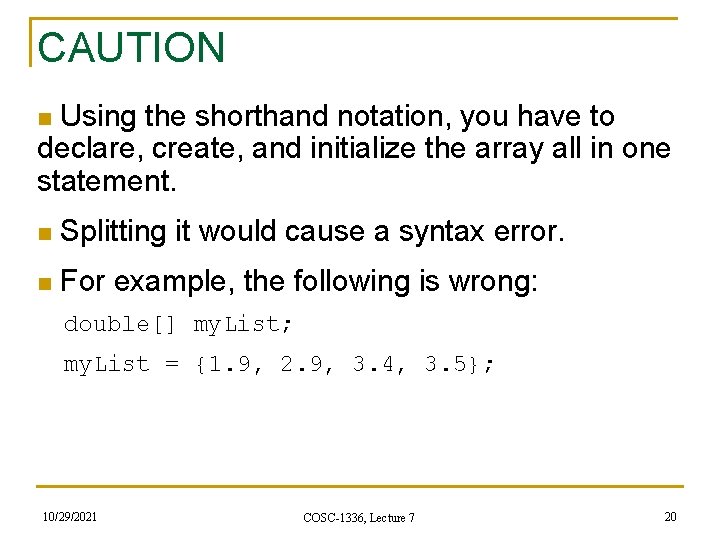
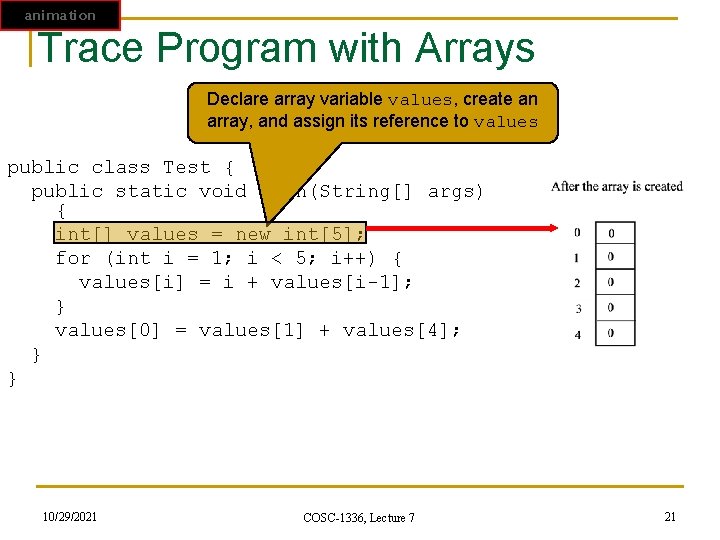
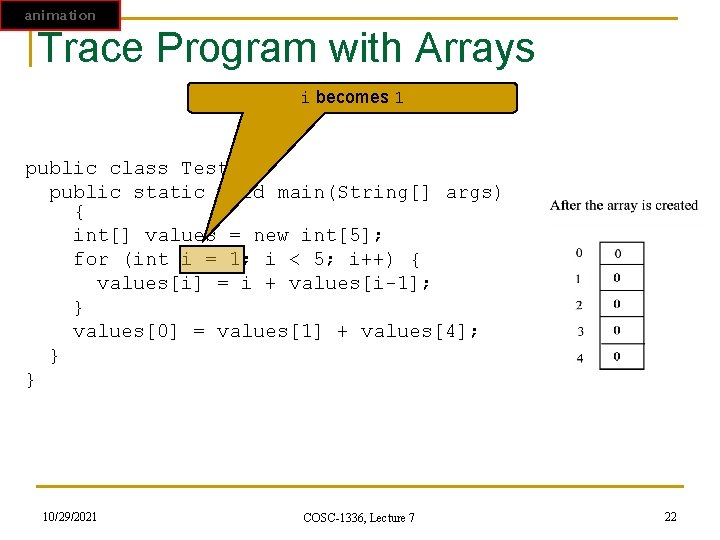
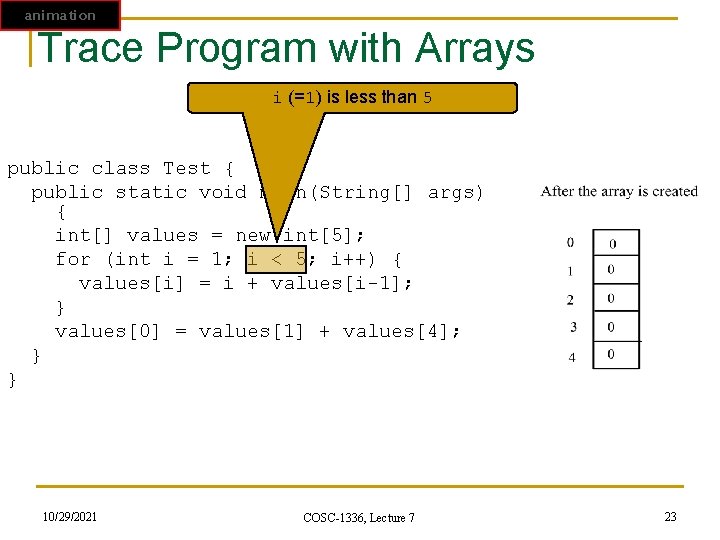
![animation Trace Program with Arrays After this line is executed, value[1] is 1 public animation Trace Program with Arrays After this line is executed, value[1] is 1 public](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-24.jpg)
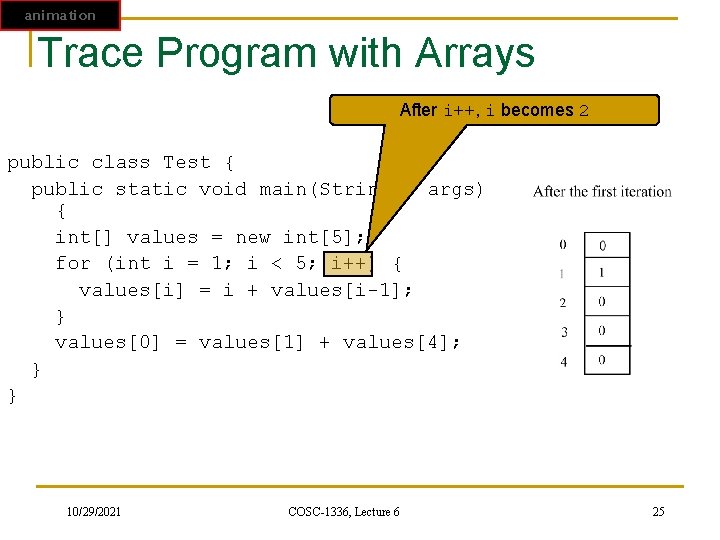
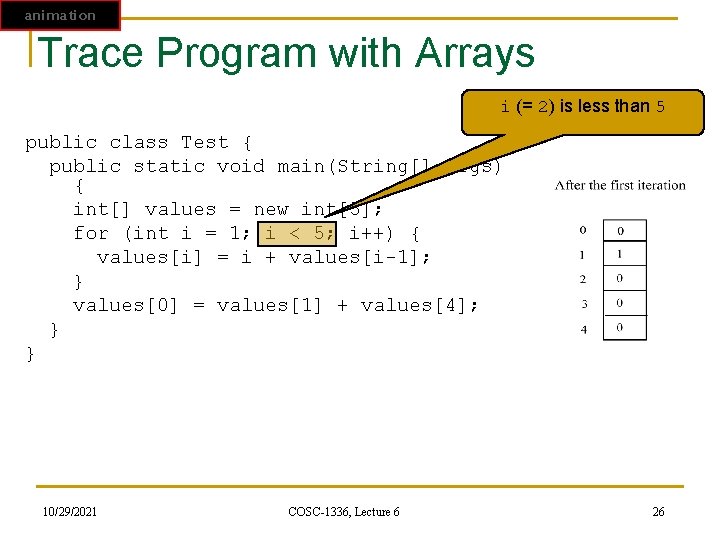
![animation Trace Program with Arrays After this line is executed, values[2] is 3 (2 animation Trace Program with Arrays After this line is executed, values[2] is 3 (2](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-27.jpg)
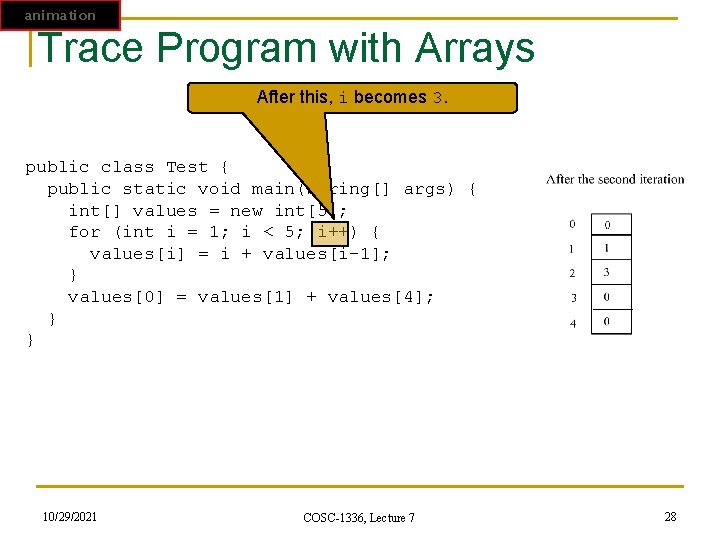
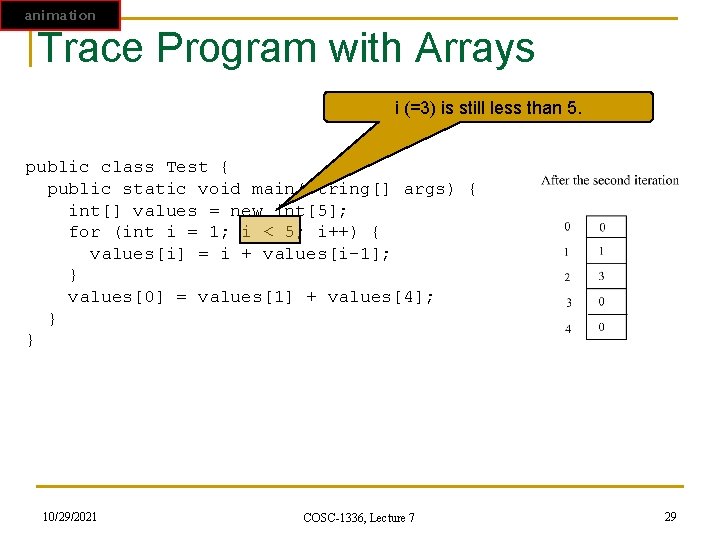
![animation Trace Program with Arrays After this line, values[3] becomes 6 (3 + 3) animation Trace Program with Arrays After this line, values[3] becomes 6 (3 + 3)](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-30.jpg)
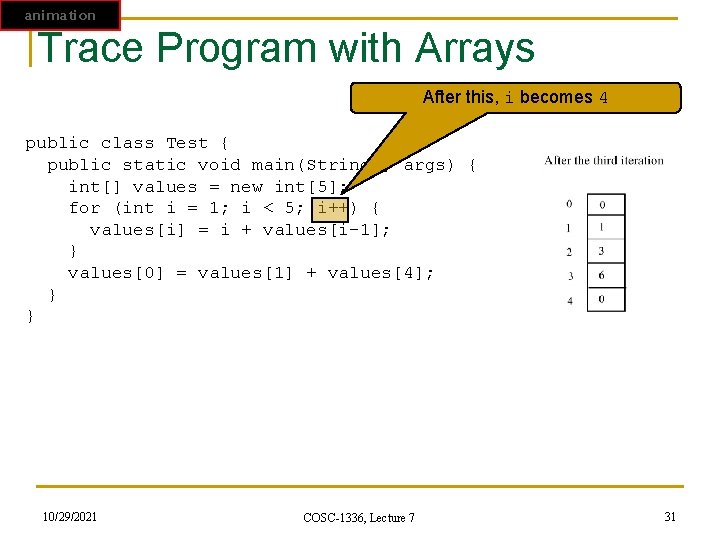
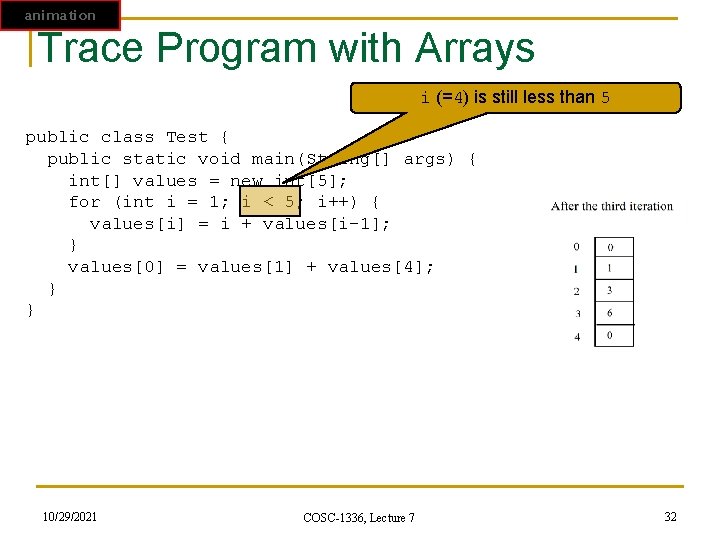
![animation Trace Program with Arrays After this, values[4] becomes 10 (4 + 6) public animation Trace Program with Arrays After this, values[4] becomes 10 (4 + 6) public](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-33.jpg)
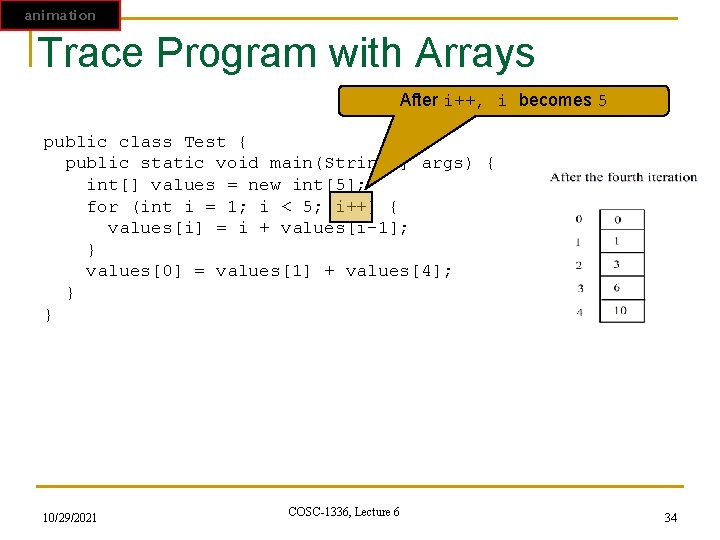
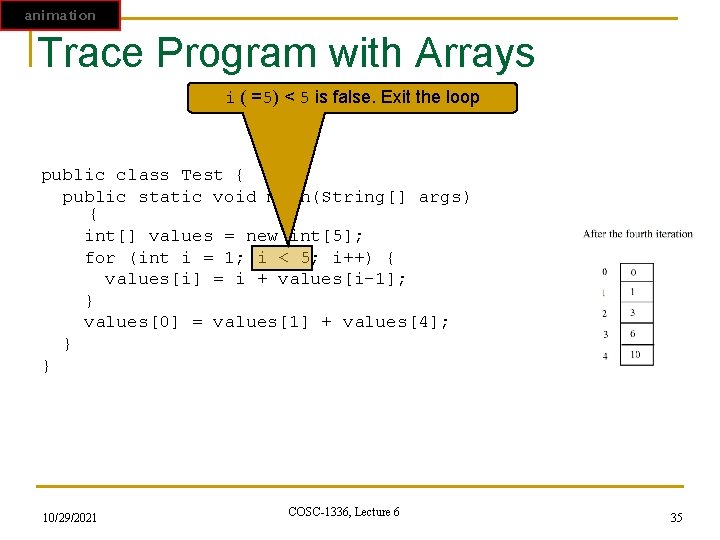
![animation Trace Program with Arrays After this line, values[0] is 11 (1 + 10) animation Trace Program with Arrays After this line, values[0] is 11 (1 + 10)](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-36.jpg)
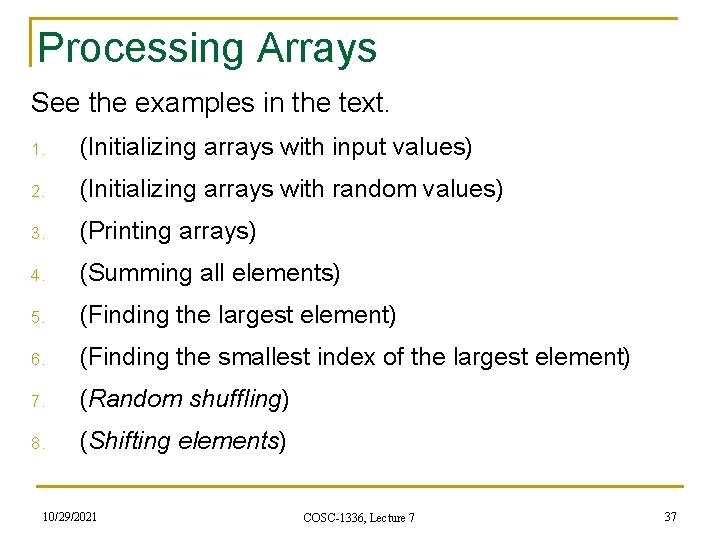
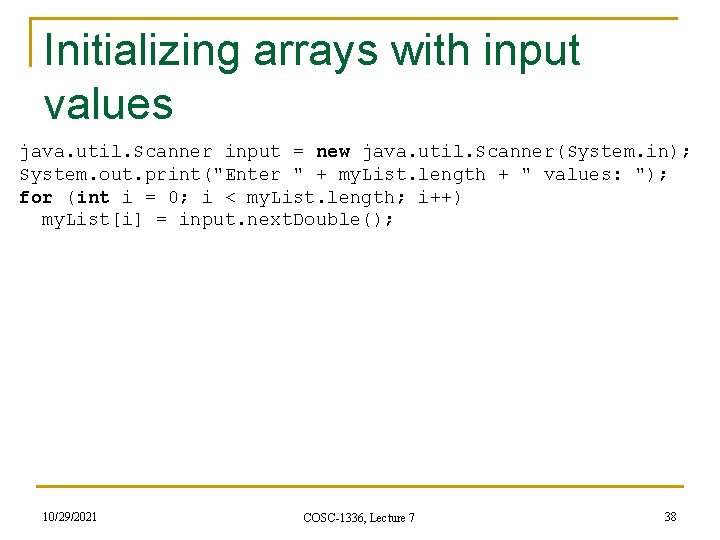
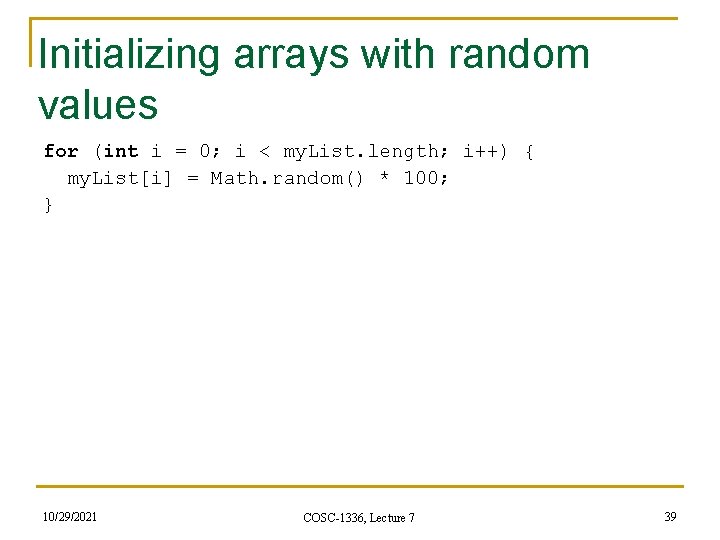
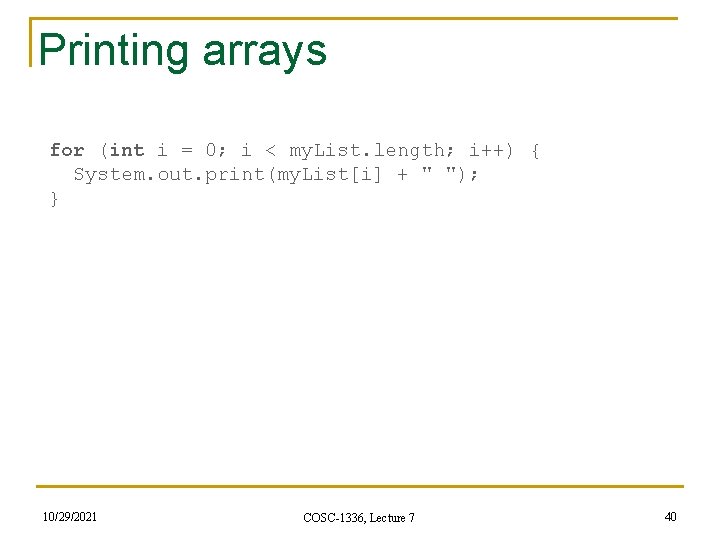
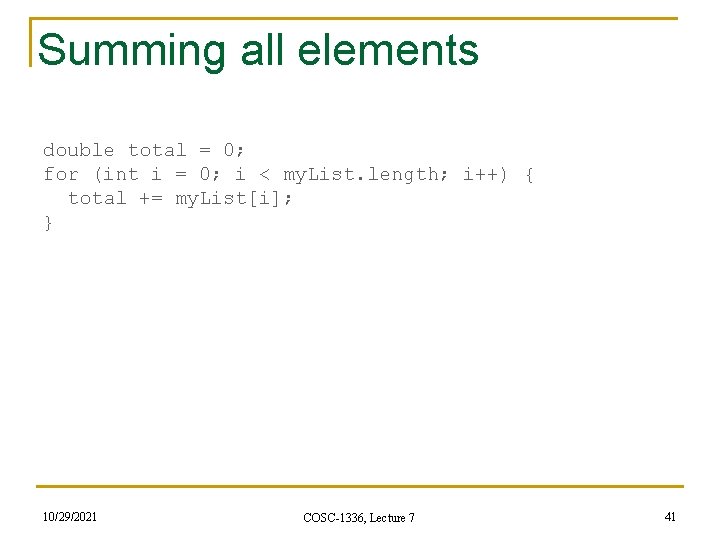
![Finding the largest element double max = my. List[0]; for (int i = 1; Finding the largest element double max = my. List[0]; for (int i = 1;](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-42.jpg)
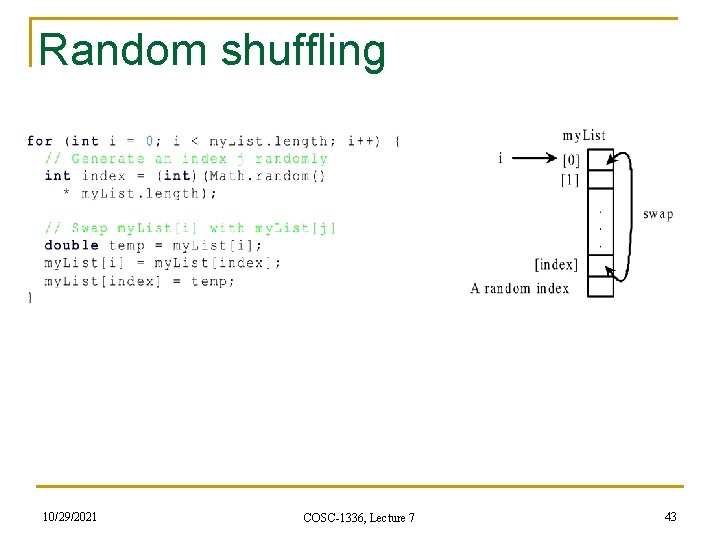
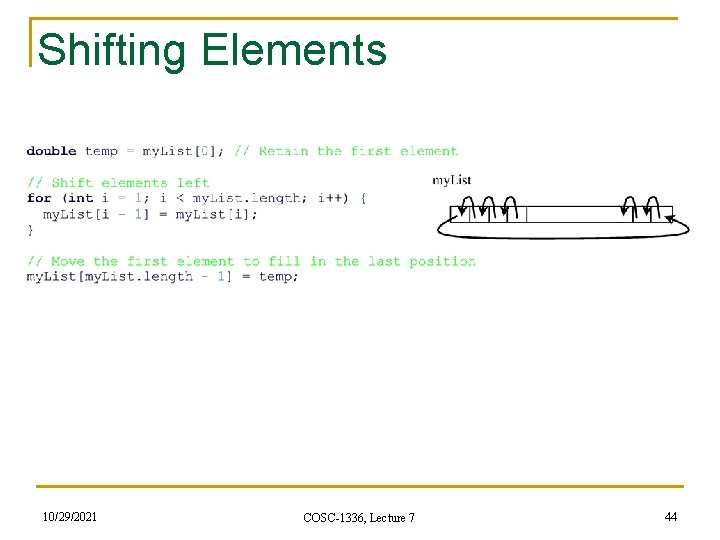
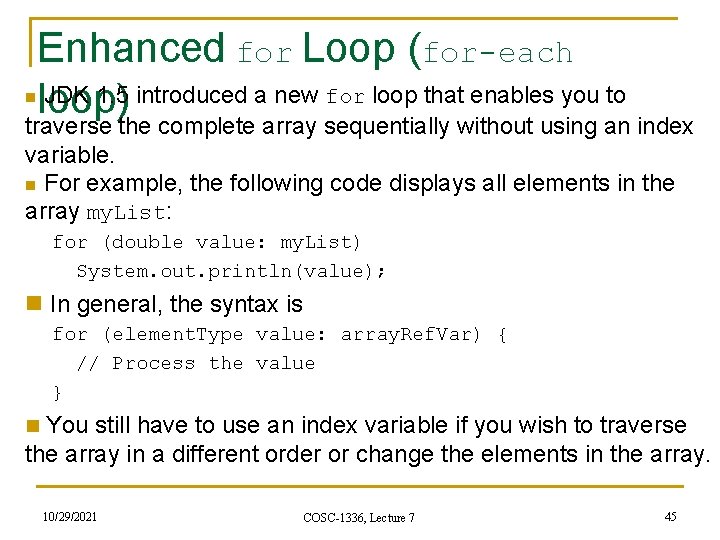
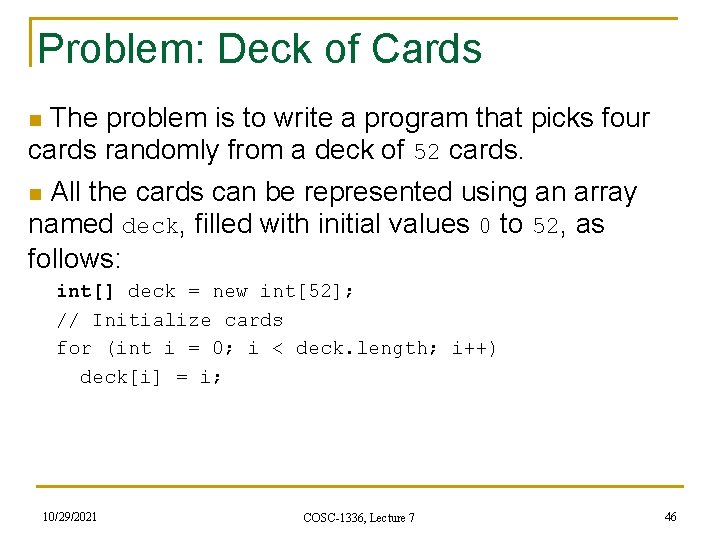
![Deck. Of. Cards. java public class Deck. Of. Cards { public static void main(String[] Deck. Of. Cards. java public class Deck. Of. Cards { public static void main(String[]](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-47.jpg)
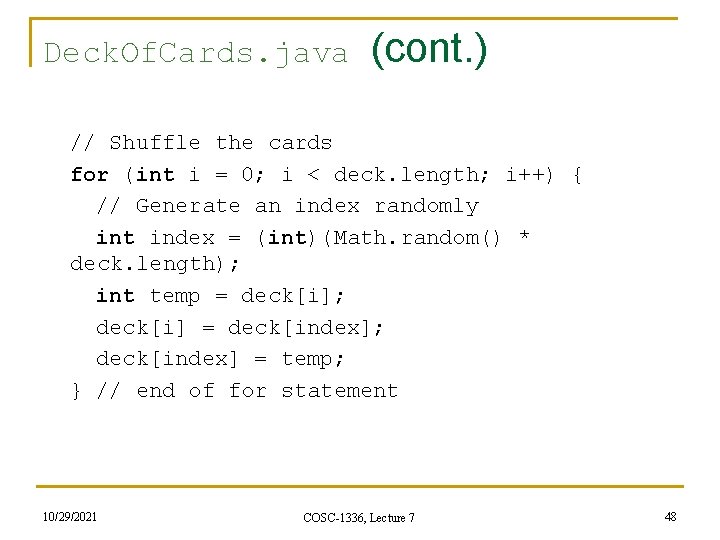
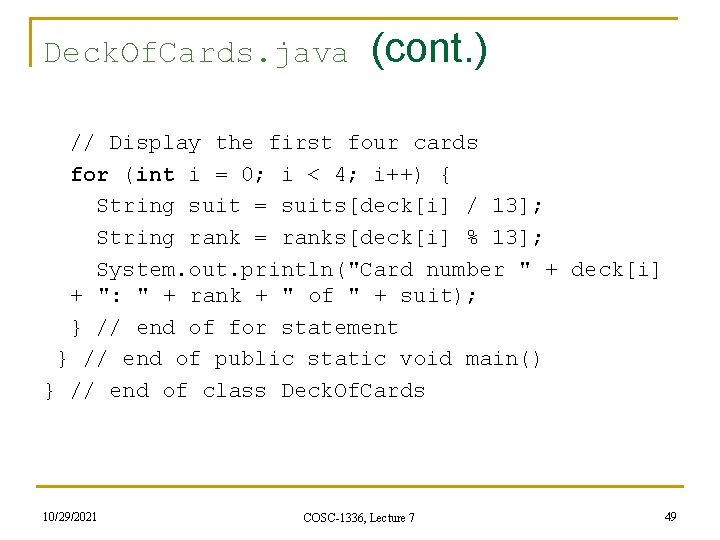
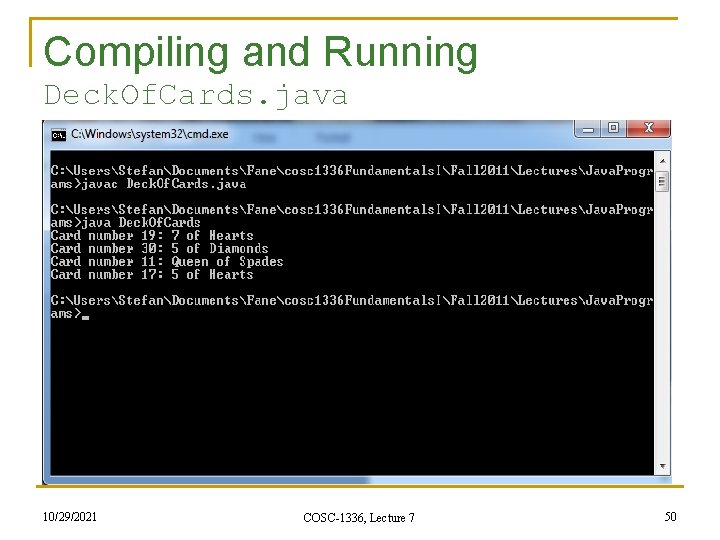
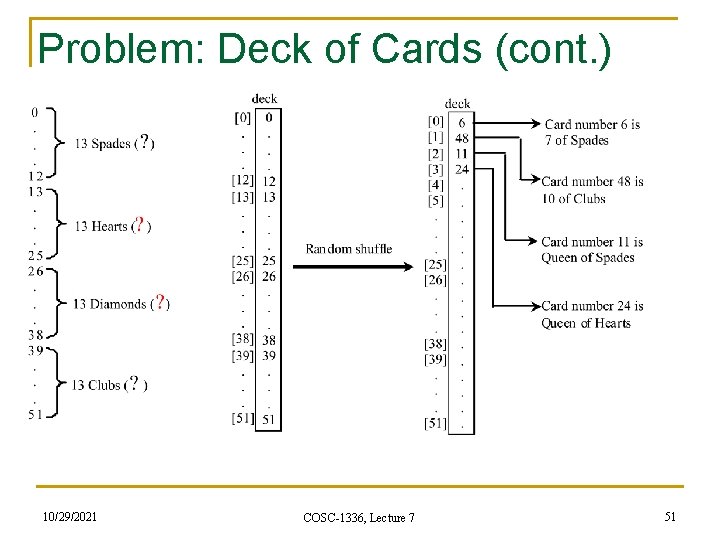
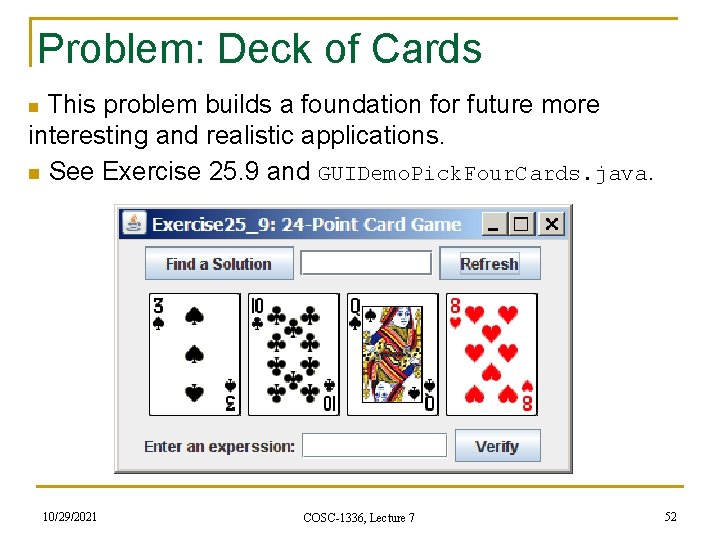
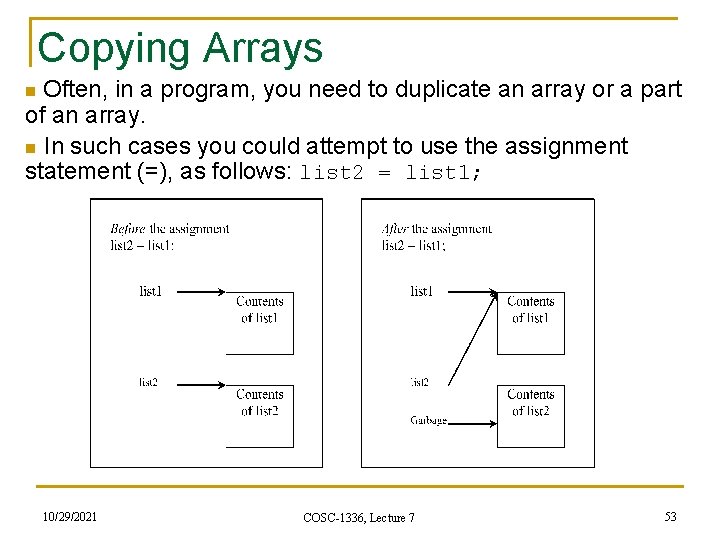
![Copying Arrays n Using a loop: int[] source. Array = {2, 3, 1, 5, Copying Arrays n Using a loop: int[] source. Array = {2, 3, 1, 5,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-54.jpg)
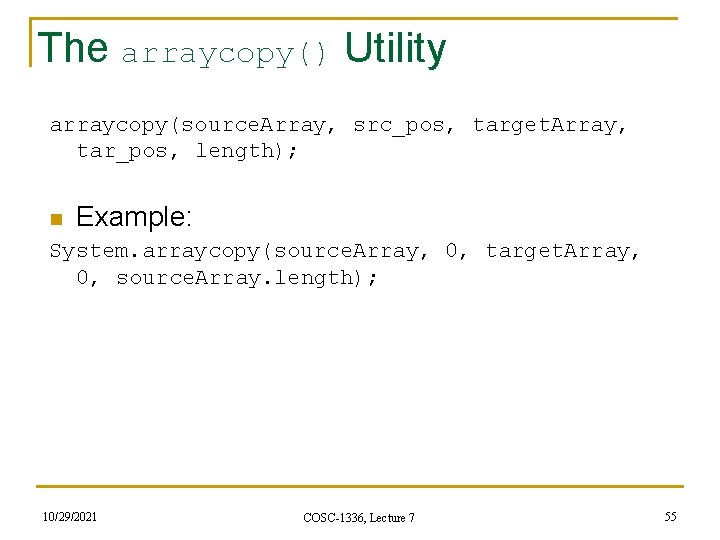
![Passing Arrays to Methods public static void print. Array(int[] array) { for (int i Passing Arrays to Methods public static void print. Array(int[] array) { for (int i](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-56.jpg)
![Anonymous Array § The statement print. Array(new int[]{3, 1, 2, 6, 4, 2}); § Anonymous Array § The statement print. Array(new int[]{3, 1, 2, 6, 4, 2}); §](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-57.jpg)
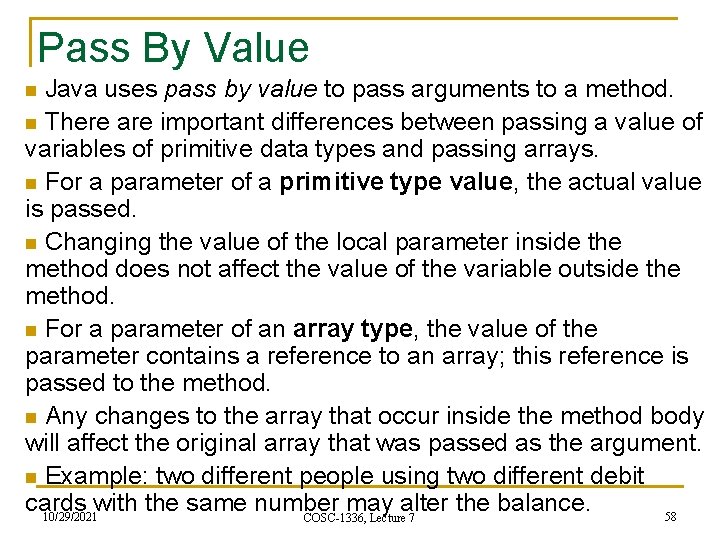
![Simple Example public class Test { public static void main(String[] args) { int x Simple Example public class Test { public static void main(String[] args) { int x](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-59.jpg)
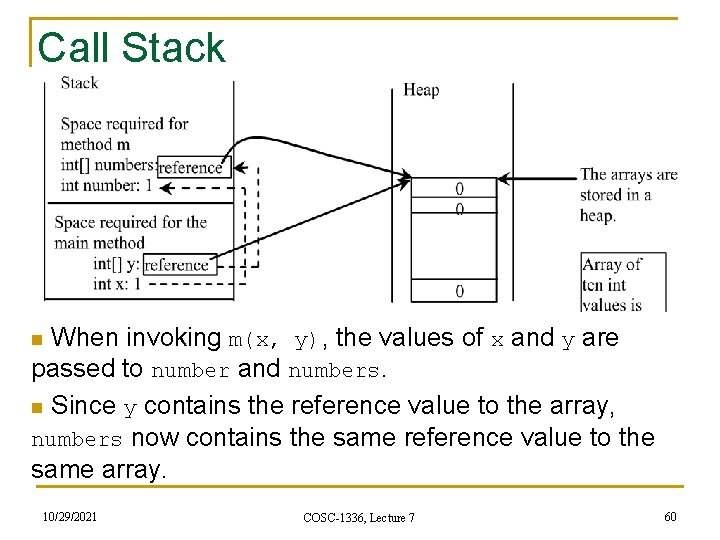
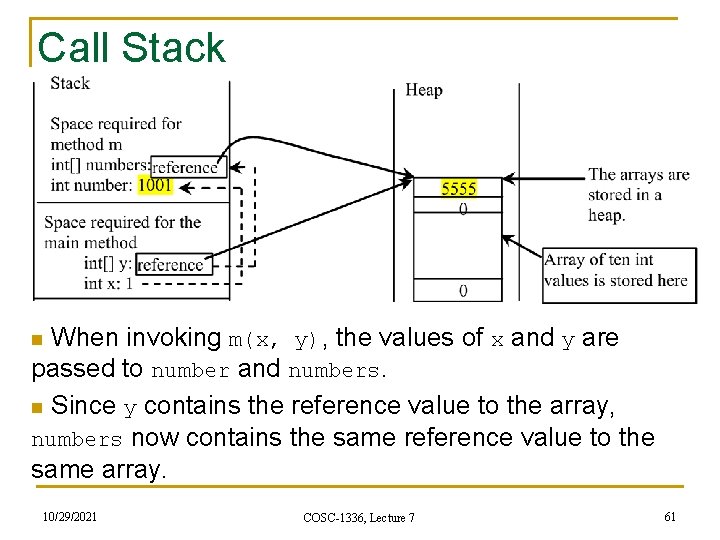
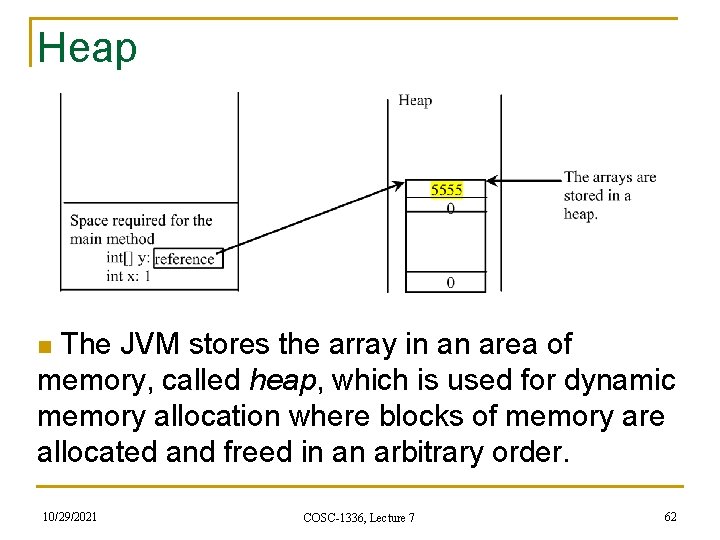
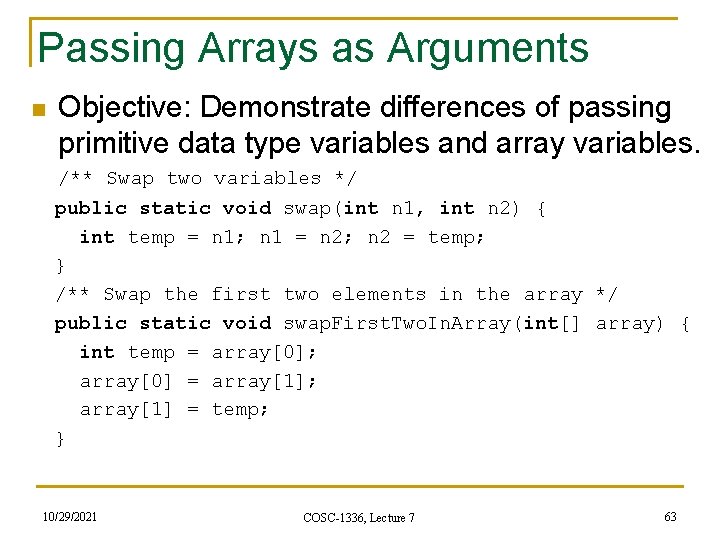
![public class Test. Pass. Array { public static void main(String[] args) { int[] a public class Test. Pass. Array { public static void main(String[] args) { int[] a](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-64.jpg)
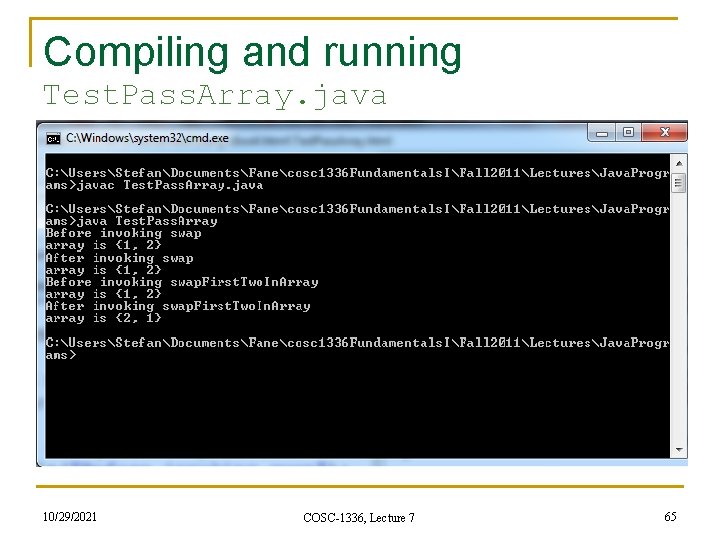
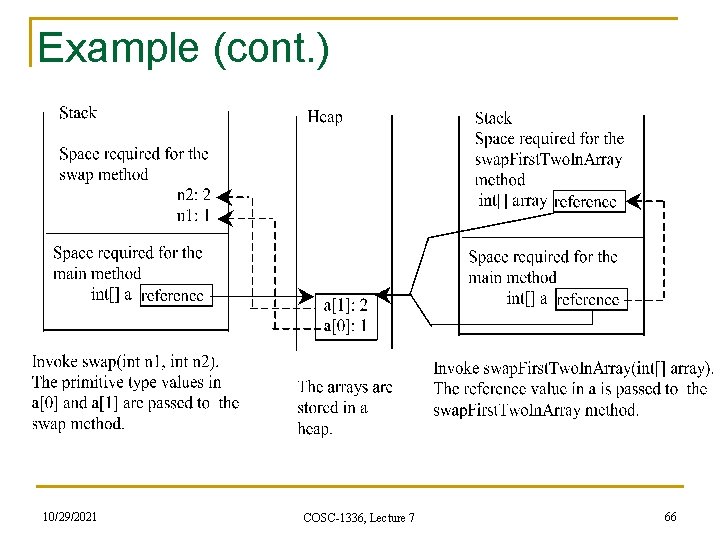
![Returning an Array from a public static int[] reverse(int[] list) Method int[] result = Returning an Array from a public static int[] reverse(int[] list) Method int[] result =](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-67.jpg)
![animation Trace the reverse() Method int[] list 1 = {1, 2, 3, 4, 5, animation Trace the reverse() Method int[] list 1 = {1, 2, 3, 4, 5,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-68.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-69.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-70.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-71.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-72.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-73.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-74.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-75.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-76.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-77.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-78.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-79.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-80.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-81.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-82.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-83.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-84.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-85.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-86.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-87.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-88.jpg)
![animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-89.jpg)
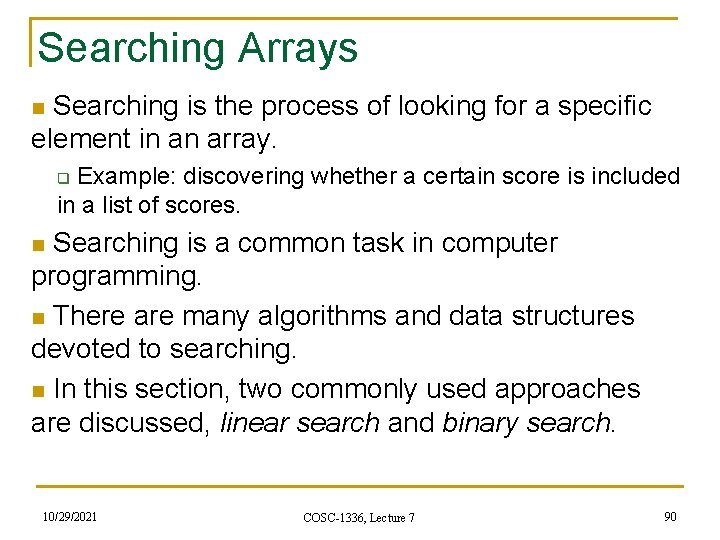
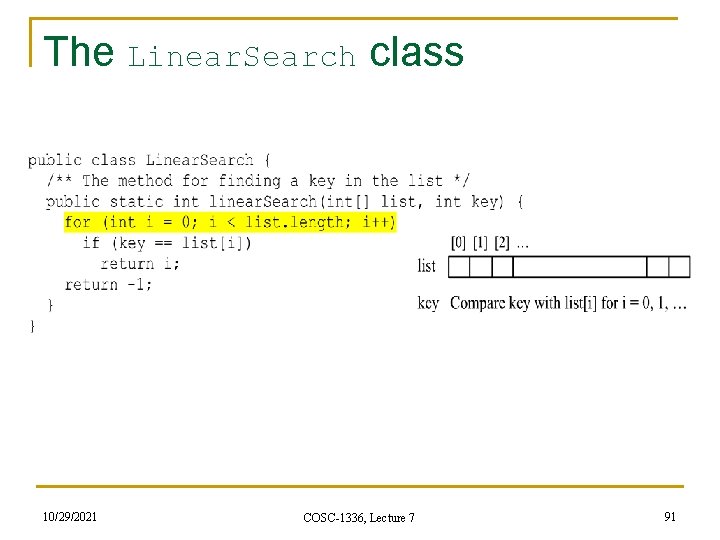
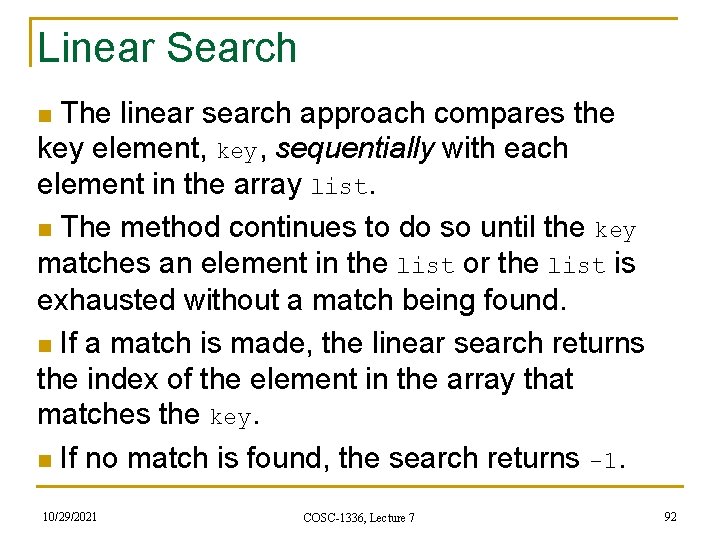
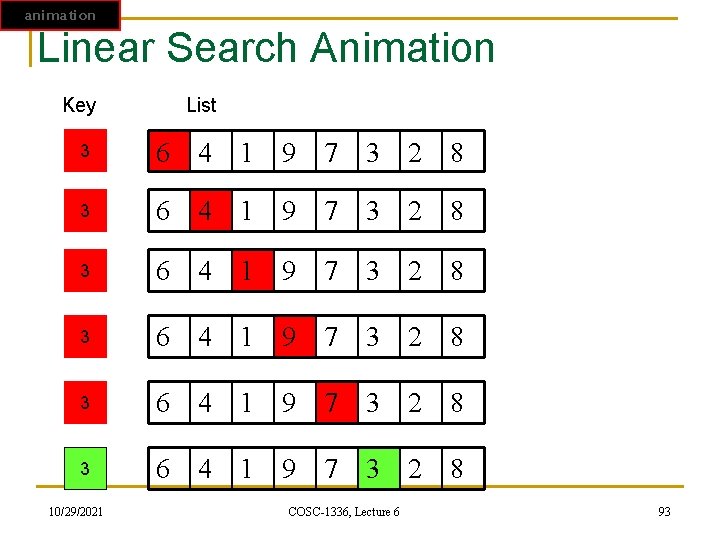
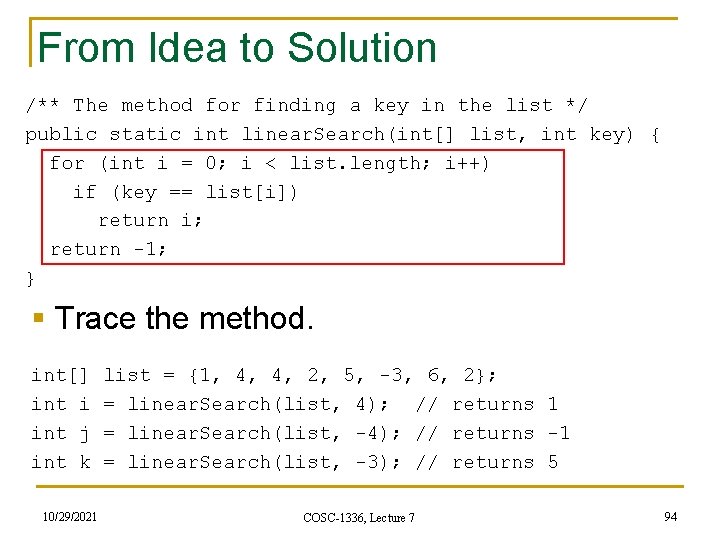
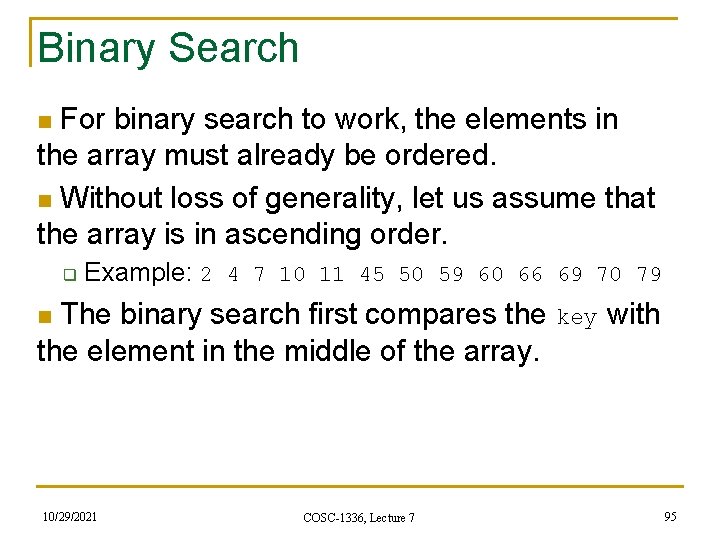
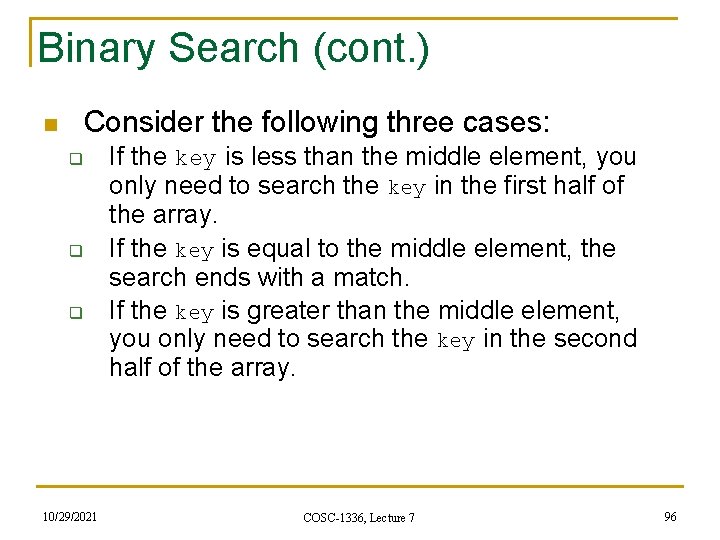
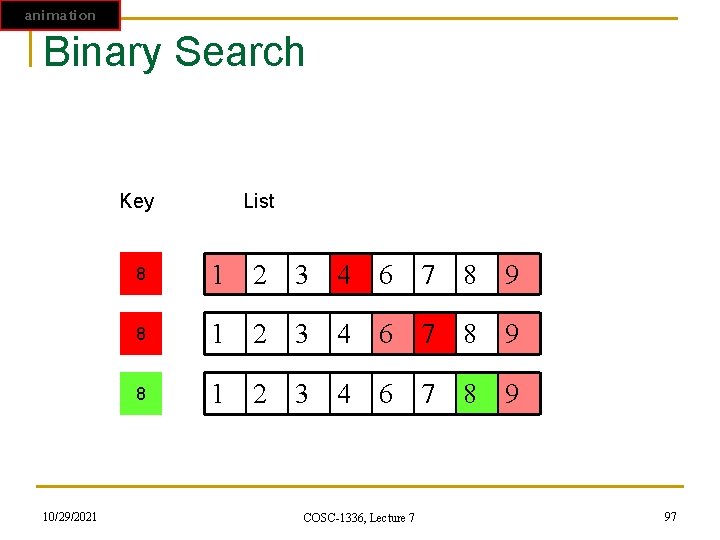
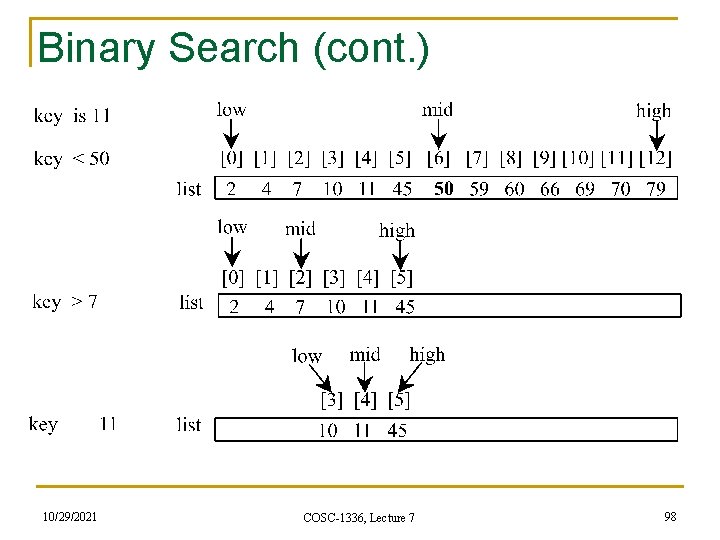
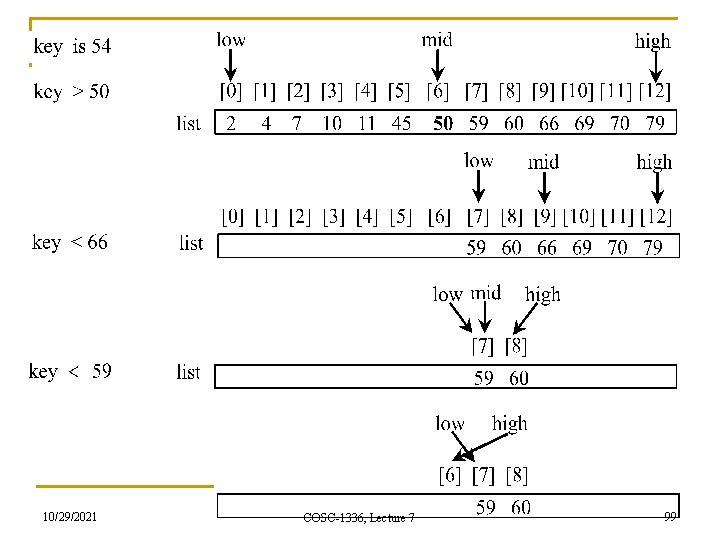
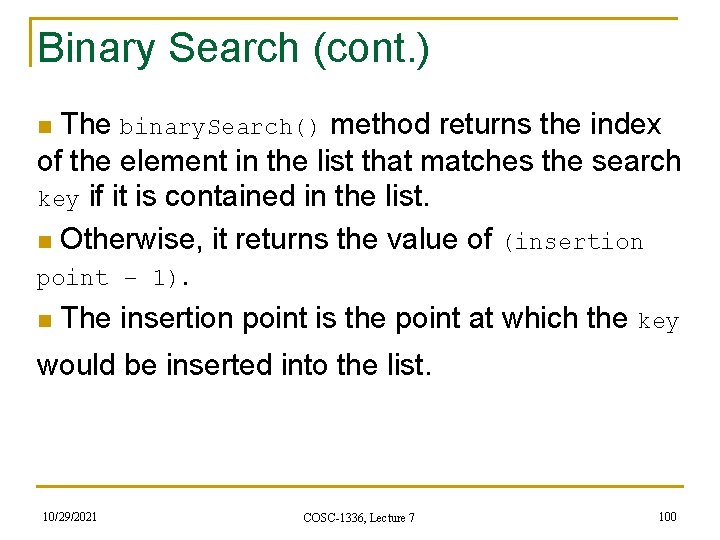
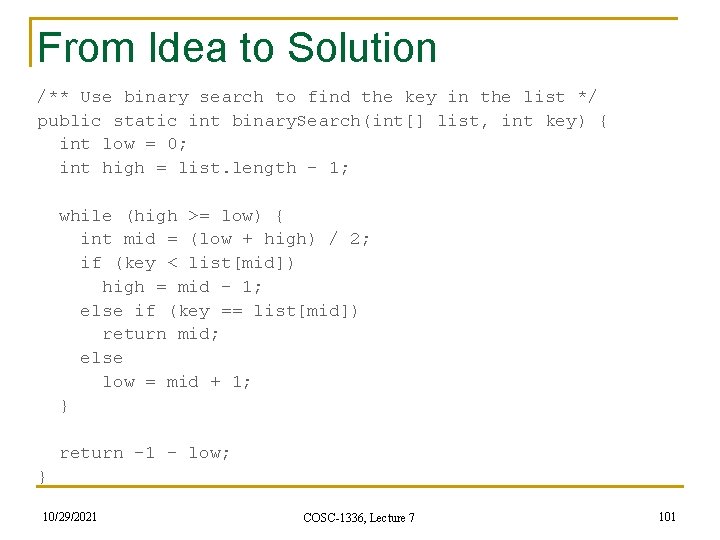
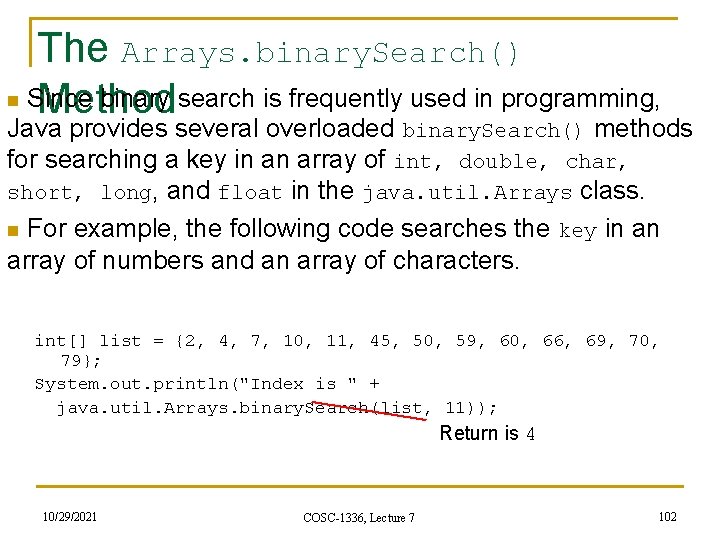
![The Arrays. binary. Search() Method char[] chars = {'a', 'c', 'g', 'x', 'y', 'z'}; The Arrays. binary. Search() Method char[] chars = {'a', 'c', 'g', 'x', 'y', 'z'};](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-103.jpg)
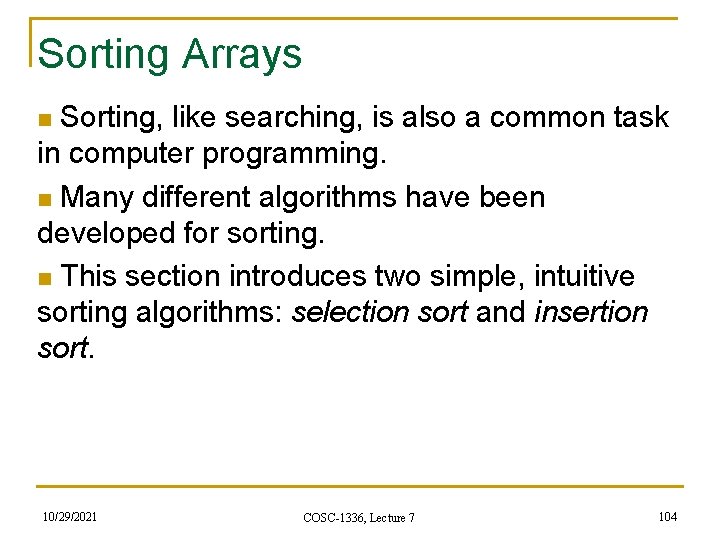
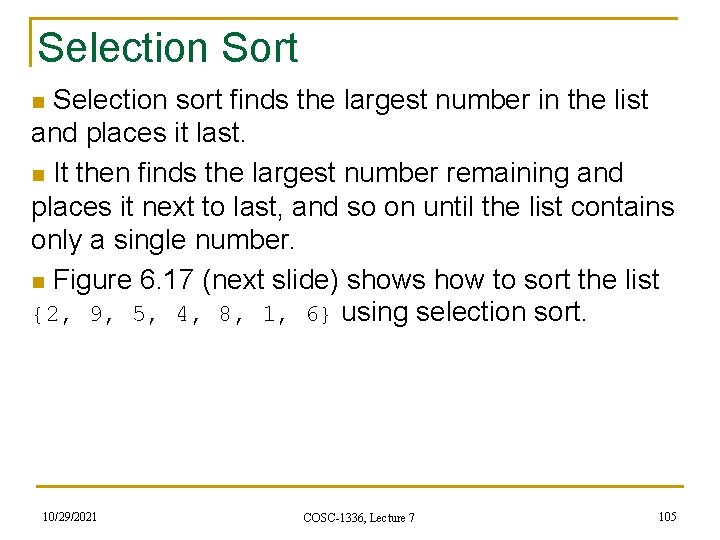
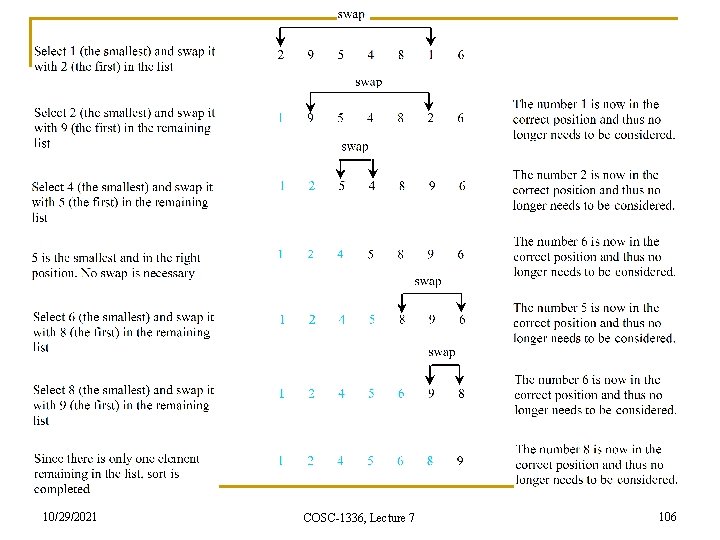
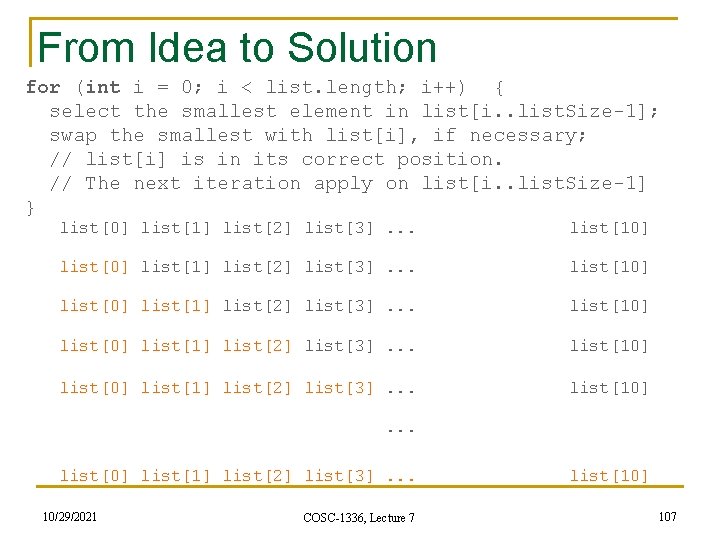
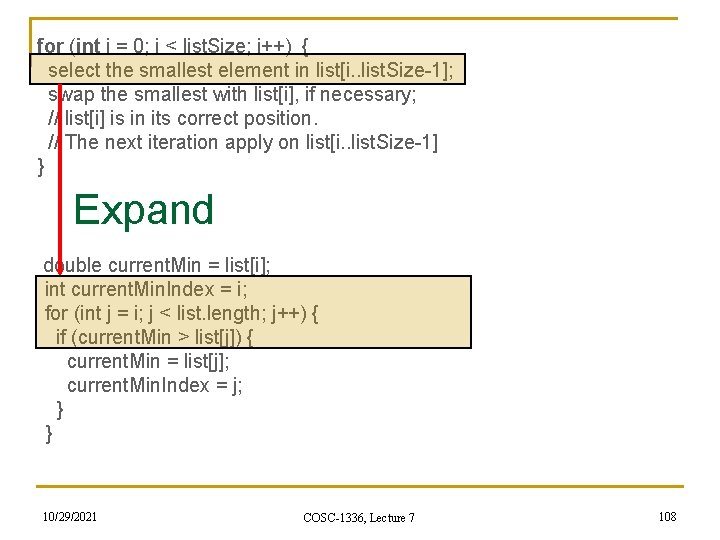
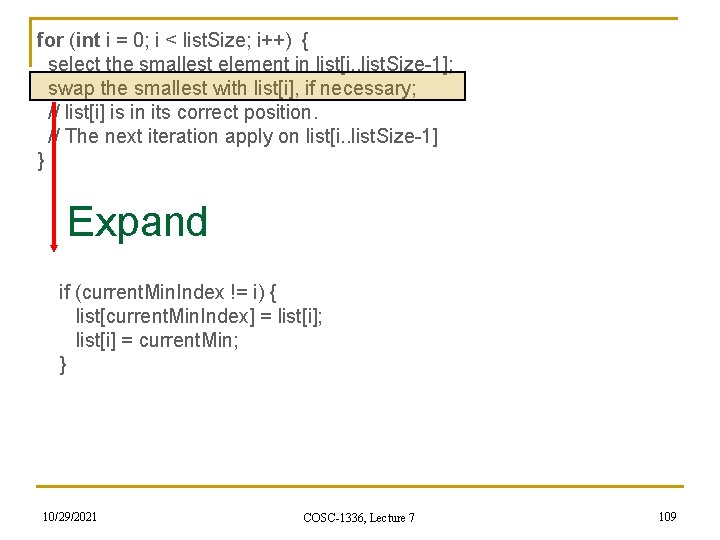
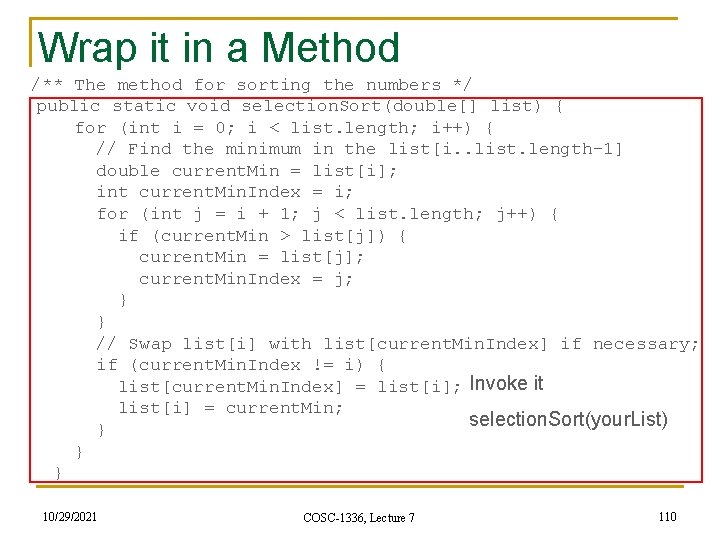
![Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; // Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; //](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-111.jpg)
![animation Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; animation Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6};](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-112.jpg)
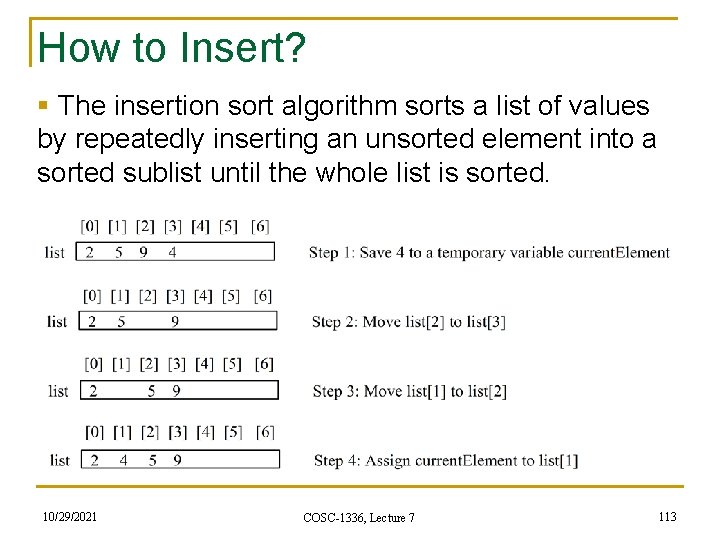
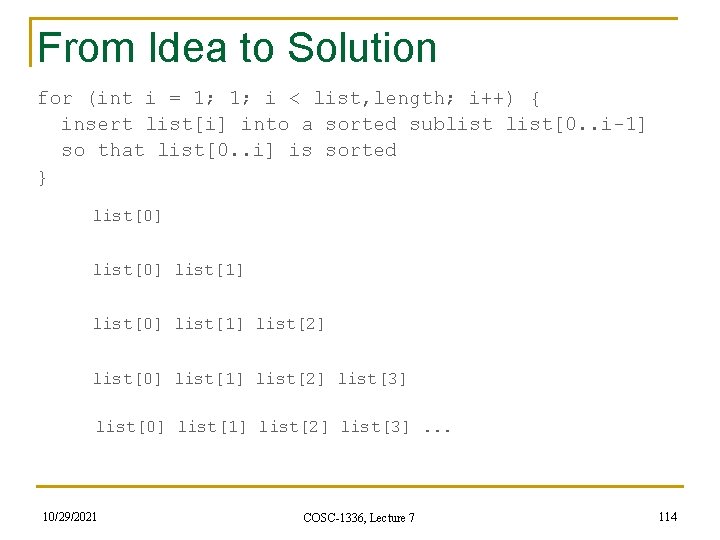
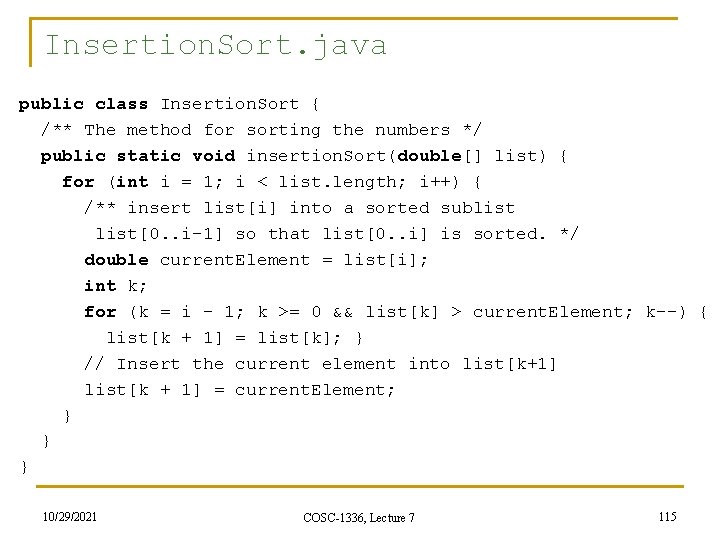
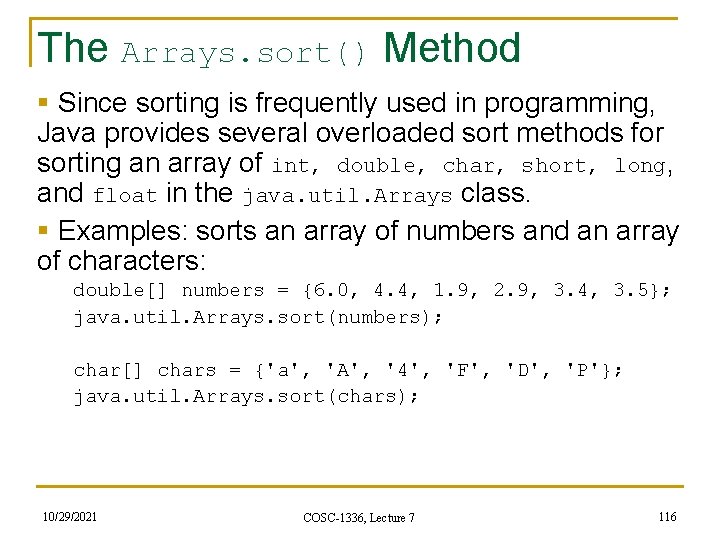
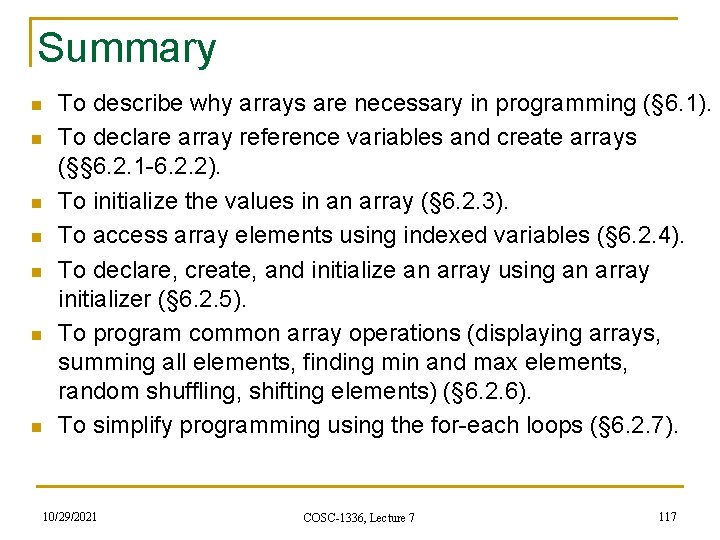
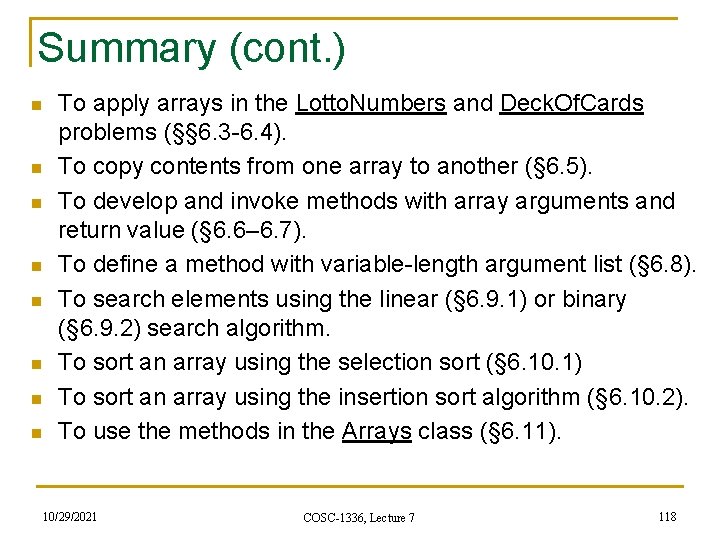
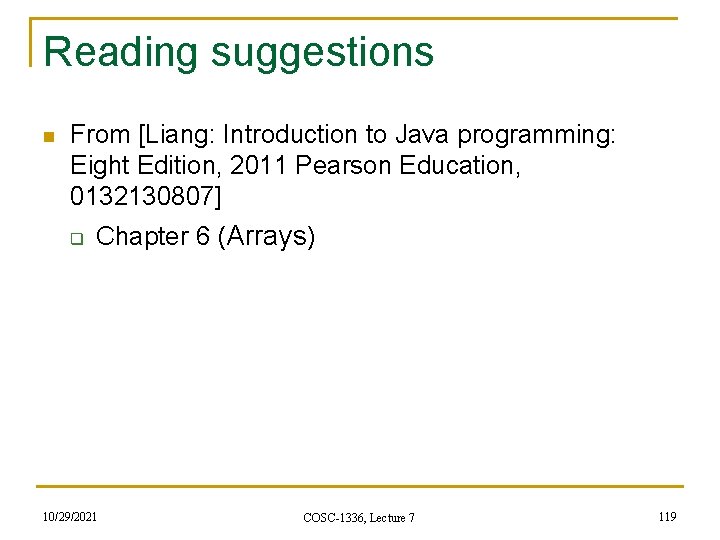
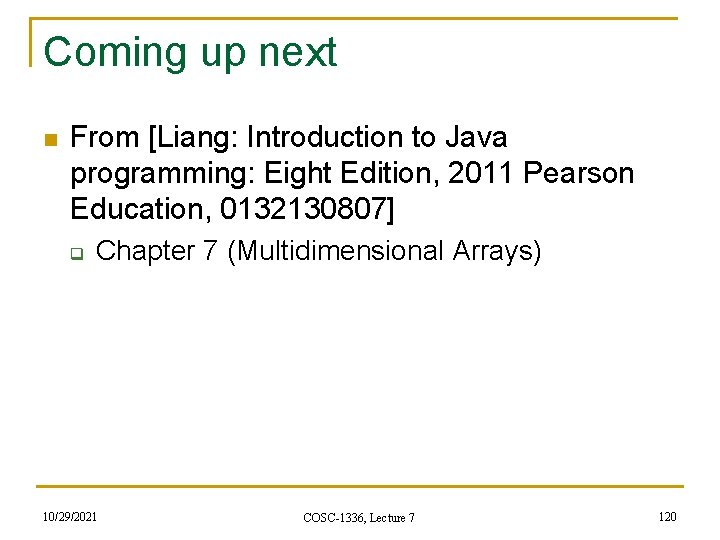
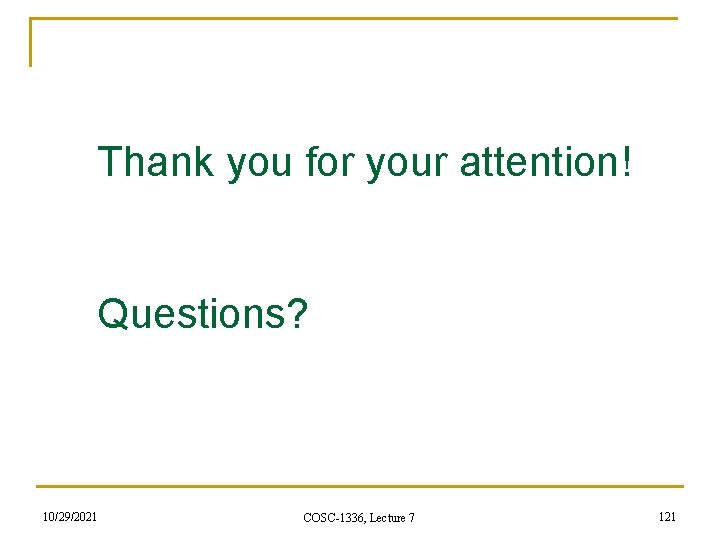
- Slides: 121
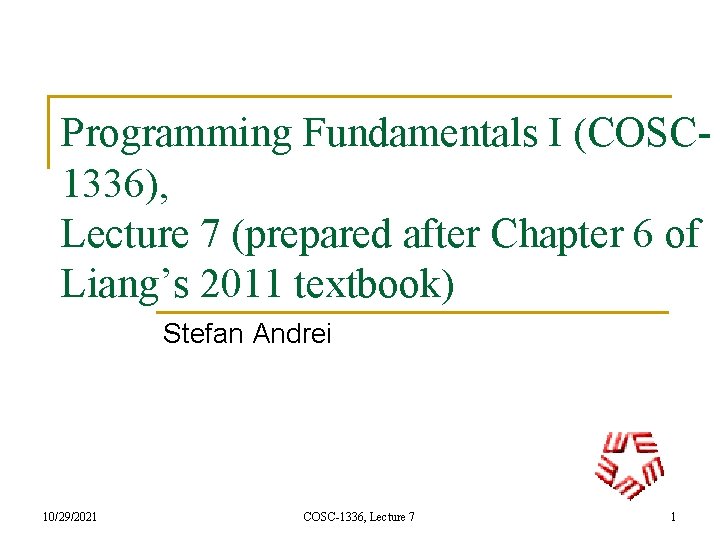
Programming Fundamentals I (COSC 1336), Lecture 7 (prepared after Chapter 6 of Liang’s 2011 textbook) Stefan Andrei 10/29/2021 COSC-1336, Lecture 7 1
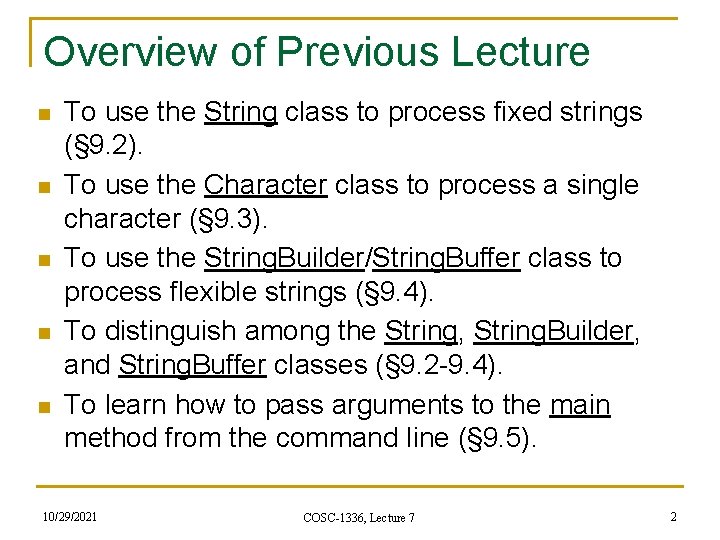
Overview of Previous Lecture n n n To use the String class to process fixed strings (§ 9. 2). To use the Character class to process a single character (§ 9. 3). To use the String. Builder/String. Buffer class to process flexible strings (§ 9. 4). To distinguish among the String, String. Builder, and String. Buffer classes (§ 9. 2 -9. 4). To learn how to pass arguments to the main method from the command line (§ 9. 5). 10/29/2021 COSC-1336, Lecture 7 2
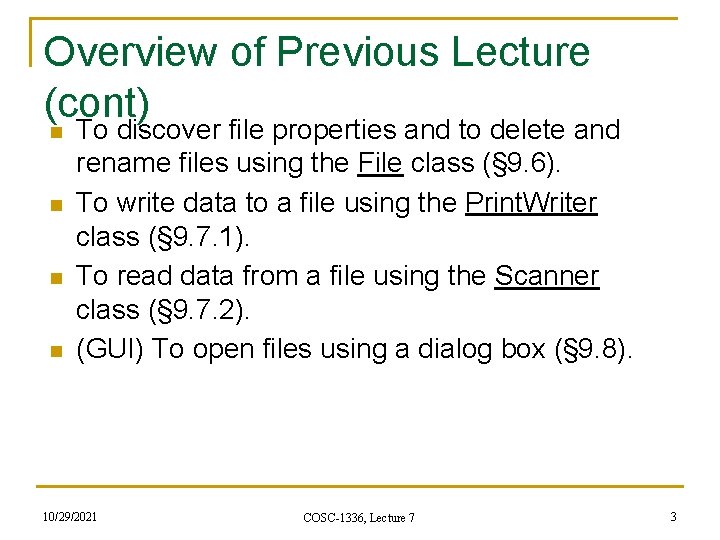
Overview of Previous Lecture (cont) n n To discover file properties and to delete and rename files using the File class (§ 9. 6). To write data to a file using the Print. Writer class (§ 9. 7. 1). To read data from a file using the Scanner class (§ 9. 7. 2). (GUI) To open files using a dialog box (§ 9. 8). 10/29/2021 COSC-1336, Lecture 7 3
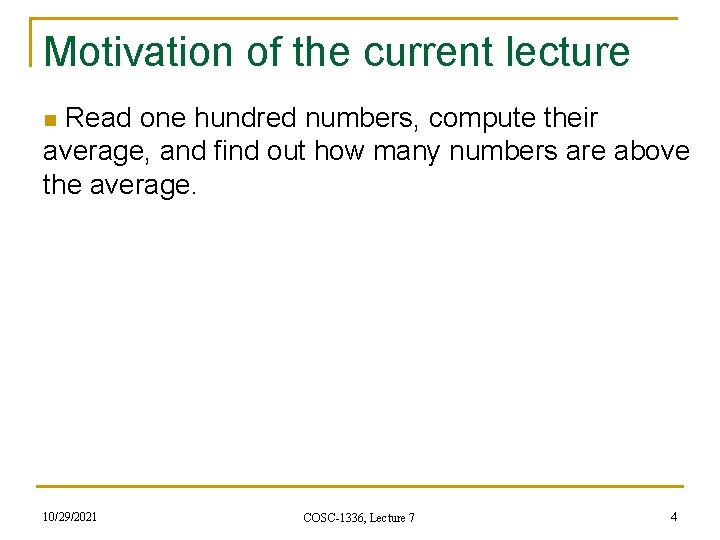
Motivation of the current lecture Read one hundred numbers, compute their average, and find out how many numbers are above the average. n 10/29/2021 COSC-1336, Lecture 7 4
![Solution public class Analyze Numbers public static void mainString args final int Solution public class Analyze. Numbers { public static void main(String[] args) { final int](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-5.jpg)
Solution public class Analyze. Numbers { public static void main(String[] args) { final int NUMBER_OF_ELEMENTS = 10; double[] numbers = new double[NUMBER_OF_ELEMENTS]; double sum = 0; java. util. Scanner input = new java. util. Scanner(System. in); //. . . The for statement is next slide } } 10/29/2021 COSC-1336, Lecture 7 5
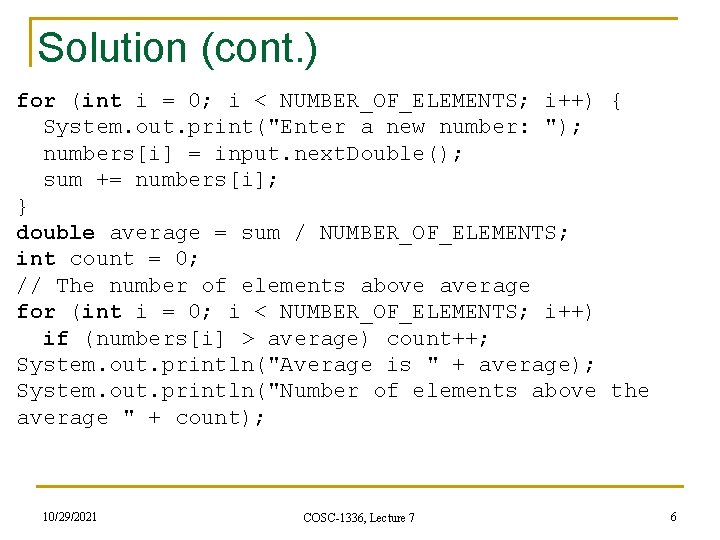
Solution (cont. ) for (int i = 0; i < NUMBER_OF_ELEMENTS; i++) { System. out. print("Enter a new number: "); numbers[i] = input. next. Double(); sum += numbers[i]; } double average = sum / NUMBER_OF_ELEMENTS; int count = 0; // The number of elements above average for (int i = 0; i < NUMBER_OF_ELEMENTS; i++) if (numbers[i] > average) count++; System. out. println("Average is " + average); System. out. println("Number of elements above the average " + count); 10/29/2021 COSC-1336, Lecture 7 6
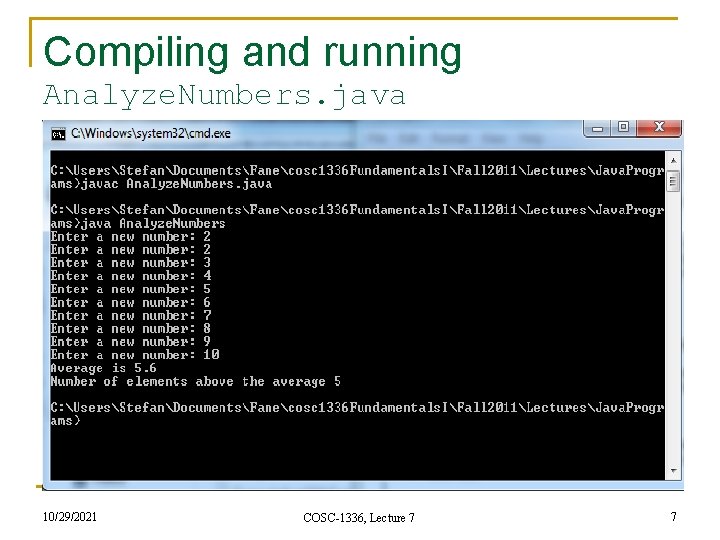
Compiling and running Analyze. Numbers. java 10/29/2021 COSC-1336, Lecture 7 7
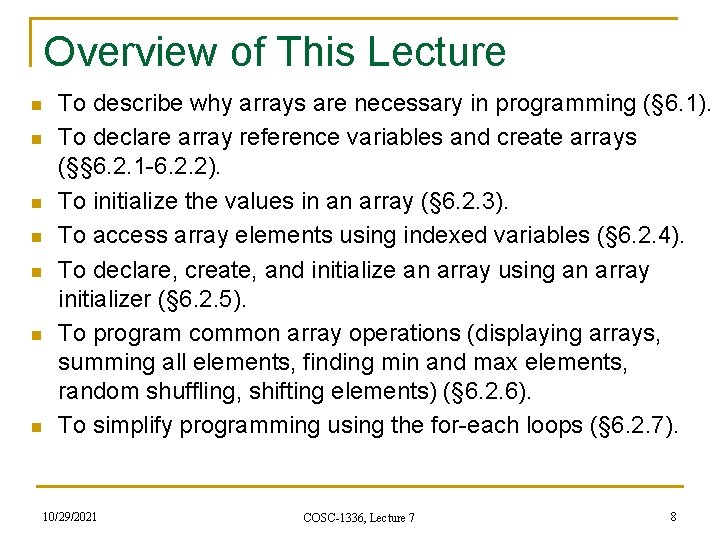
Overview of This Lecture n n n n To describe why arrays are necessary in programming (§ 6. 1). To declare array reference variables and create arrays (§§ 6. 2. 1 -6. 2. 2). To initialize the values in an array (§ 6. 2. 3). To access array elements using indexed variables (§ 6. 2. 4). To declare, create, and initialize an array using an array initializer (§ 6. 2. 5). To program common array operations (displaying arrays, summing all elements, finding min and max elements, random shuffling, shifting elements) (§ 6. 2. 6). To simplify programming using the for-each loops (§ 6. 2. 7). 10/29/2021 COSC-1336, Lecture 7 8
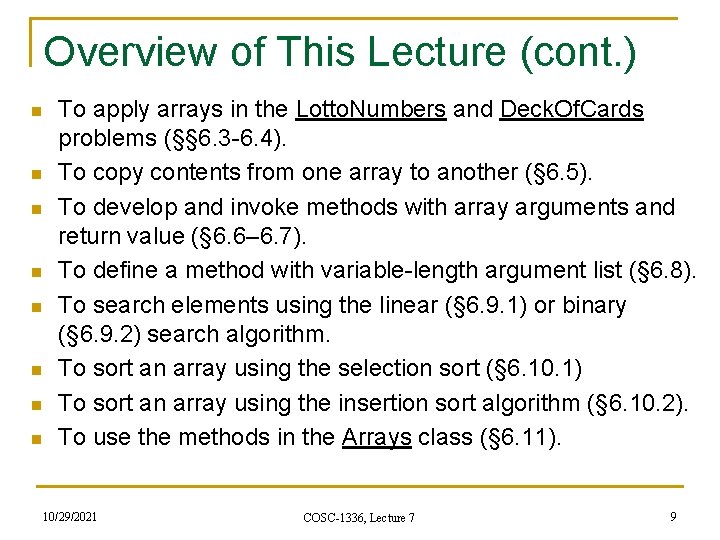
Overview of This Lecture (cont. ) n n n n To apply arrays in the Lotto. Numbers and Deck. Of. Cards problems (§§ 6. 3 -6. 4). To copy contents from one array to another (§ 6. 5). To develop and invoke methods with array arguments and return value (§ 6. 6– 6. 7). To define a method with variable-length argument list (§ 6. 8). To search elements using the linear (§ 6. 9. 1) or binary (§ 6. 9. 2) search algorithm. To sort an array using the selection sort (§ 6. 10. 1) To sort an array using the insertion sort algorithm (§ 6. 10. 2). To use the methods in the Arrays class (§ 6. 11). 10/29/2021 COSC-1336, Lecture 7 9
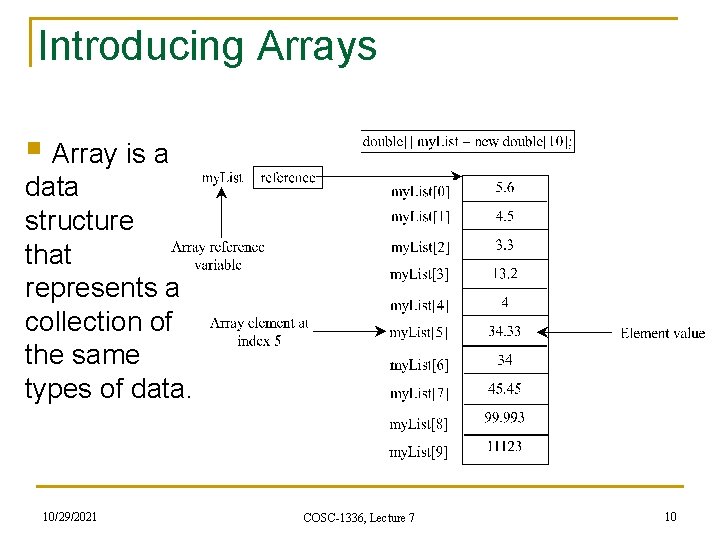
Introducing Arrays § Array is a data structure that represents a collection of the same types of data. 10/29/2021 COSC-1336, Lecture 7 10
![Declaring Array Variables n General syntax datatype array Ref Var Example double my List Declaring Array Variables n General syntax: datatype[] array. Ref. Var; Example: double[] my. List;](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-11.jpg)
Declaring Array Variables n General syntax: datatype[] array. Ref. Var; Example: double[] my. List; n General syntax: datatype array. Ref. Var[]; n Note: This style is allowed, but not preferred. Example: double my. List[]; n n 10/29/2021 COSC-1336, Lecture 7 11
![Creating Arrays n General syntax array Ref Var new datatypearray Size n Example Creating Arrays n General syntax: array. Ref. Var = new datatype[array. Size]; n Example:](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-12.jpg)
Creating Arrays n General syntax: array. Ref. Var = new datatype[array. Size]; n Example: my. List = new double[10]; my. List[0] references the first element in the array. my. List[9] references the last element in the array. n n 10/29/2021 COSC-1336, Lecture 7 12
![Declaring and Creating in One Step n n General syntax datatype array Ref Var Declaring and Creating in One Step n n General syntax: datatype[] array. Ref. Var](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-13.jpg)
Declaring and Creating in One Step n n General syntax: datatype[] array. Ref. Var = new datatype[array. Size]; Example: double[] my. List = new double[10]; General syntax: datatype array. Ref. Var[] = new datatype[array. Size]; Example: double my. List[] = new double[10]; 10/29/2021 COSC-1336, Lecture 7 13
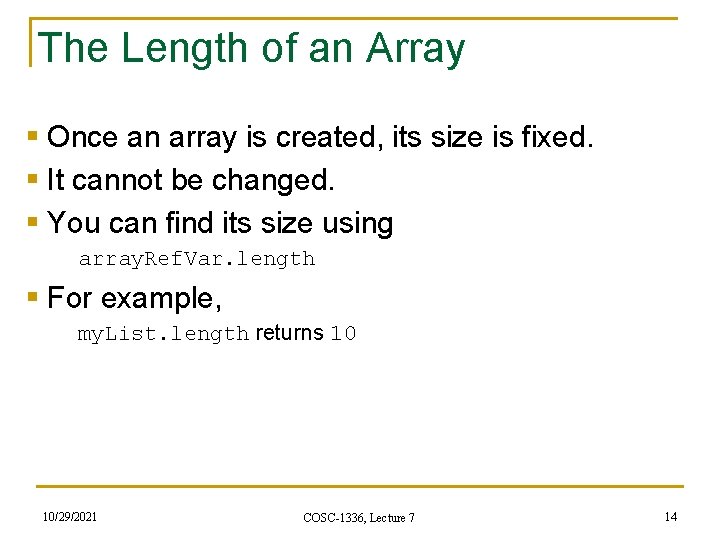
The Length of an Array § Once an array is created, its size is fixed. § It cannot be changed. § You can find its size using array. Ref. Var. length § For example, my. List. length returns 10 10/29/2021 COSC-1336, Lecture 7 14
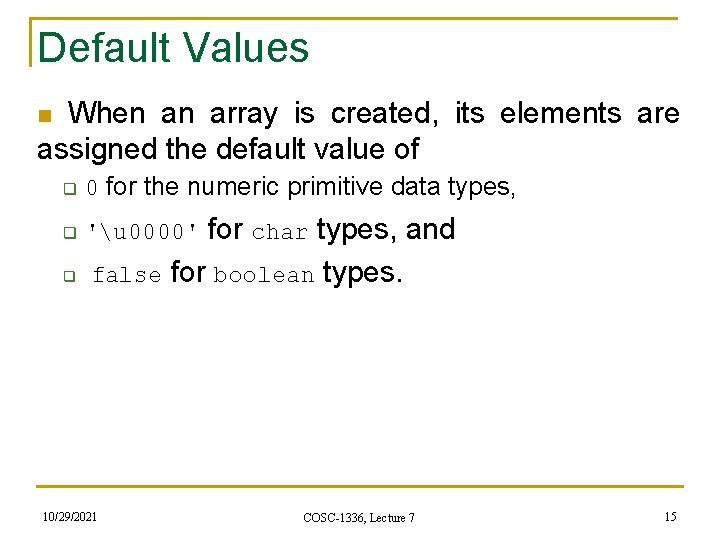
Default Values When an array is created, its elements are assigned the default value of n q 0 for the numeric primitive data types, q 'u 0000' q for char types, and false for boolean types. 10/29/2021 COSC-1336, Lecture 7 15
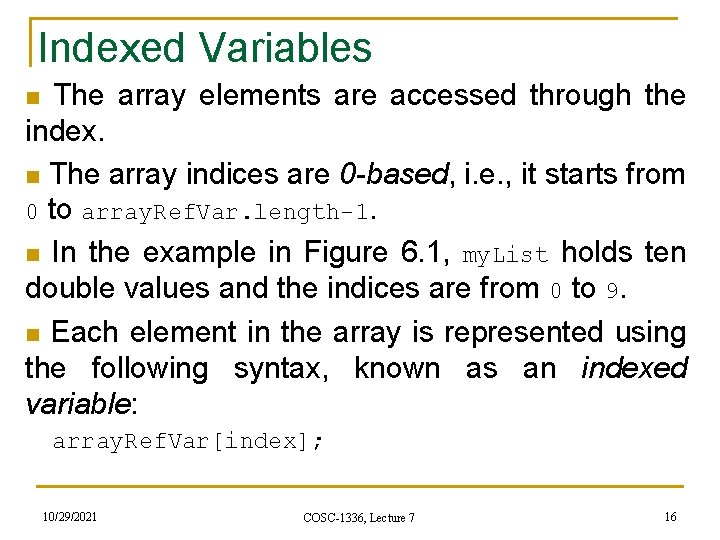
Indexed Variables The array elements are accessed through the index. n The array indices are 0 -based, i. e. , it starts from 0 to array. Ref. Var. length-1. n In the example in Figure 6. 1, my. List holds ten double values and the indices are from 0 to 9. n Each element in the array is represented using the following syntax, known as an indexed variable: n array. Ref. Var[index]; 10/29/2021 COSC-1336, Lecture 7 16
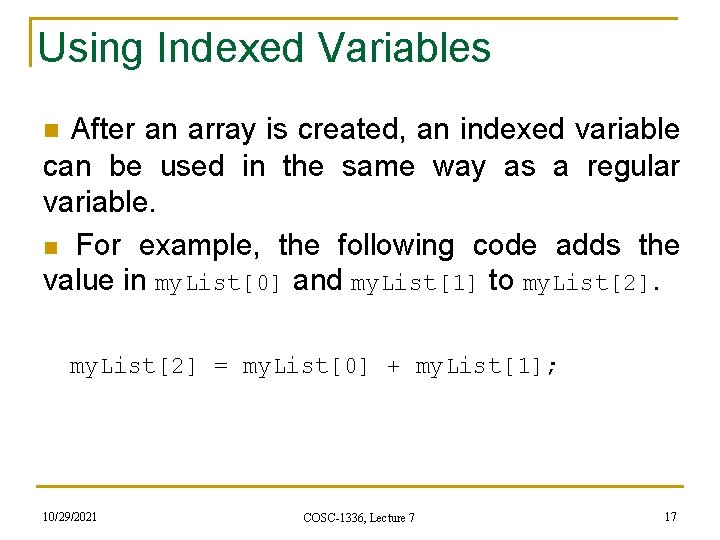
Using Indexed Variables After an array is created, an indexed variable can be used in the same way as a regular variable. n For example, the following code adds the value in my. List[0] and my. List[1] to my. List[2]. n my. List[2] = my. List[0] + my. List[1]; 10/29/2021 COSC-1336, Lecture 7 17
![Array Initializers n Declaring creating initializing in one step double my List 1 Array Initializers n Declaring, creating, initializing in one step: double[] my. List = {1.](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-18.jpg)
Array Initializers n Declaring, creating, initializing in one step: double[] my. List = {1. 9, 2. 9, 3. 4, 3. 5}; n This shorthand syntax must be in one statement. 10/29/2021 COSC-1336, Lecture 7 18
![Declaring Creating Initializing using the Shorthand Notation double my List 1 9 2 Declaring, Creating, Initializing using the Shorthand Notation double[] my. List = {1. 9, 2.](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-19.jpg)
Declaring, Creating, Initializing using the Shorthand Notation double[] my. List = {1. 9, 2. 9, 3. 4, 3. 5}; This shorthand notation is equivalent to the following statements: n double[] my. List = new double[4]; my. List[0] = 1. 9; my. List[1] = 2. 9; my. List[2] = 3. 4; my. List[3] = 3. 5; 10/29/2021 COSC-1336, Lecture 7 19
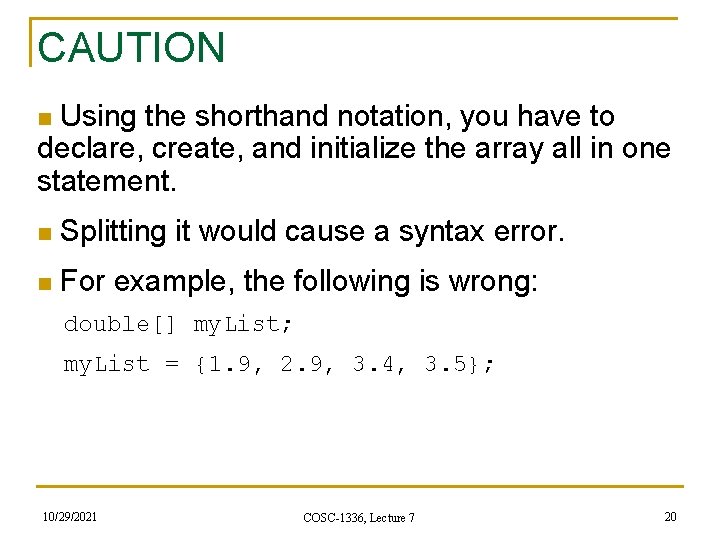
CAUTION Using the shorthand notation, you have to declare, create, and initialize the array all in one statement. n n Splitting it would cause a syntax error. n For example, the following is wrong: double[] my. List; my. List = {1. 9, 2. 9, 3. 4, 3. 5}; 10/29/2021 COSC-1336, Lecture 7 20
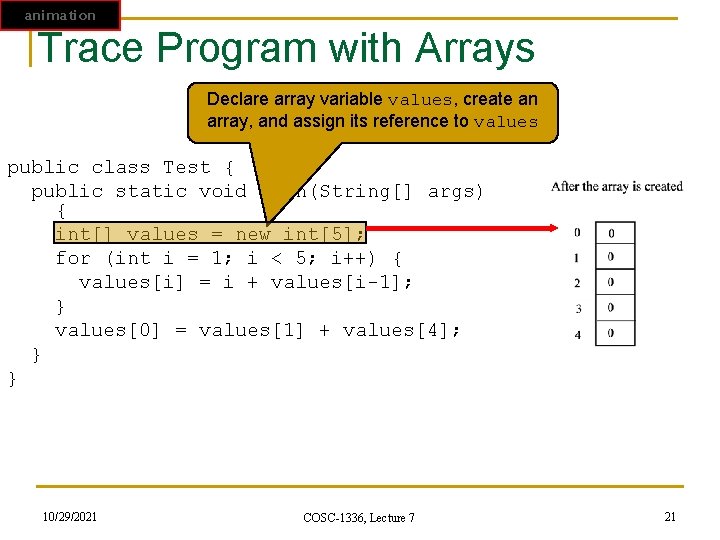
animation Trace Program with Arrays Declare array variable values, create an array, and assign its reference to values public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 21
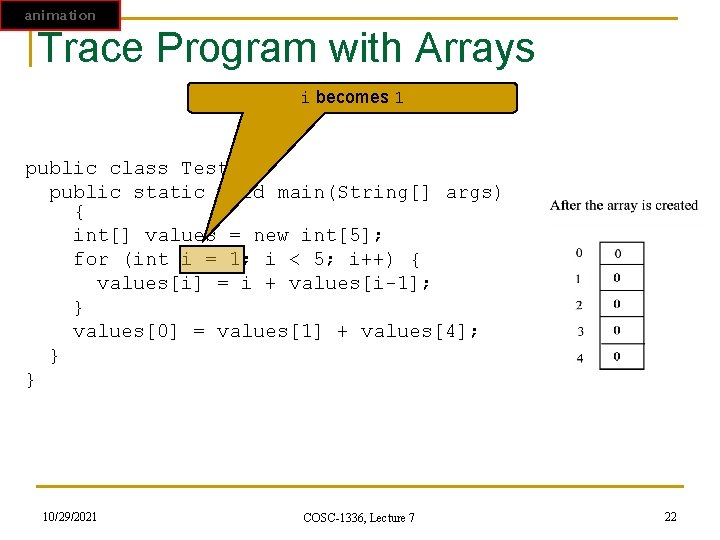
animation Trace Program with Arrays i becomes 1 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 22
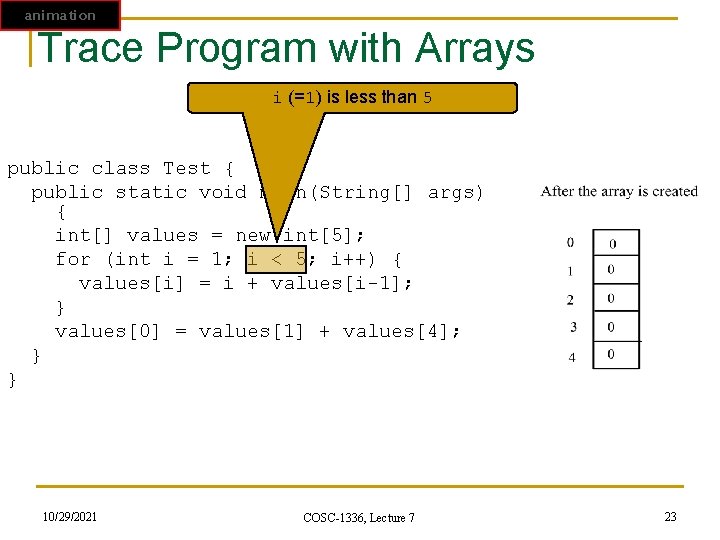
animation Trace Program with Arrays i (=1) is less than 5 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 23
![animation Trace Program with Arrays After this line is executed value1 is 1 public animation Trace Program with Arrays After this line is executed, value[1] is 1 public](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-24.jpg)
animation Trace Program with Arrays After this line is executed, value[1] is 1 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 24
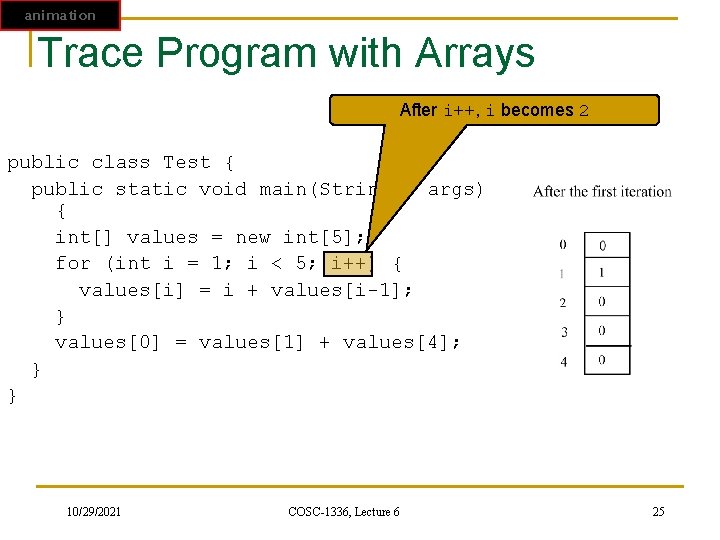
animation Trace Program with Arrays After i++, i becomes 2 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 6 25
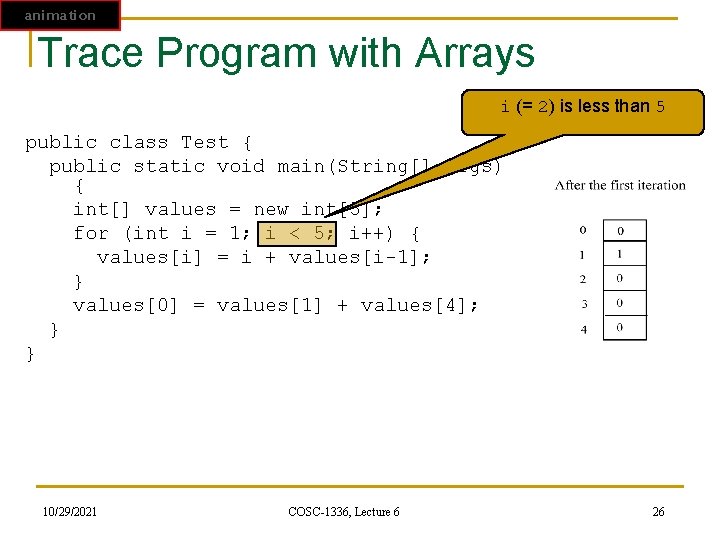
animation Trace Program with Arrays i (= 2) is less than 5 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 6 26
![animation Trace Program with Arrays After this line is executed values2 is 3 2 animation Trace Program with Arrays After this line is executed, values[2] is 3 (2](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-27.jpg)
animation Trace Program with Arrays After this line is executed, values[2] is 3 (2 + 1) public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 27
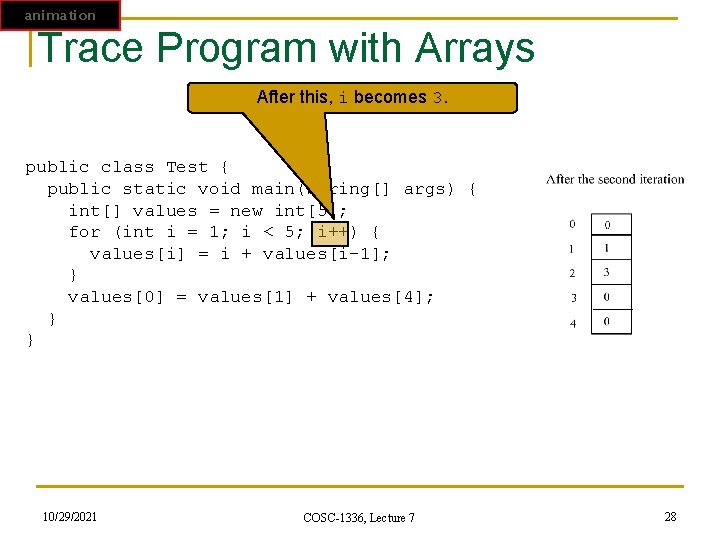
animation Trace Program with Arrays After this, i becomes 3. public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 28
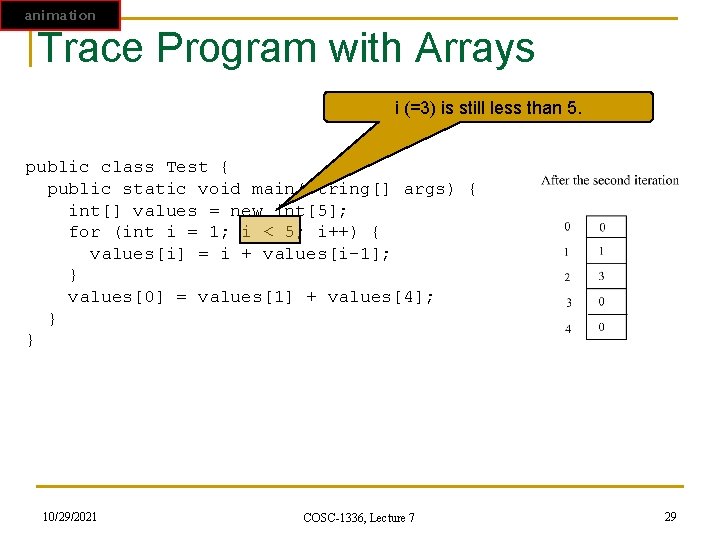
animation Trace Program with Arrays i (=3) is still less than 5. public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 29
![animation Trace Program with Arrays After this line values3 becomes 6 3 3 animation Trace Program with Arrays After this line, values[3] becomes 6 (3 + 3)](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-30.jpg)
animation Trace Program with Arrays After this line, values[3] becomes 6 (3 + 3) public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 30
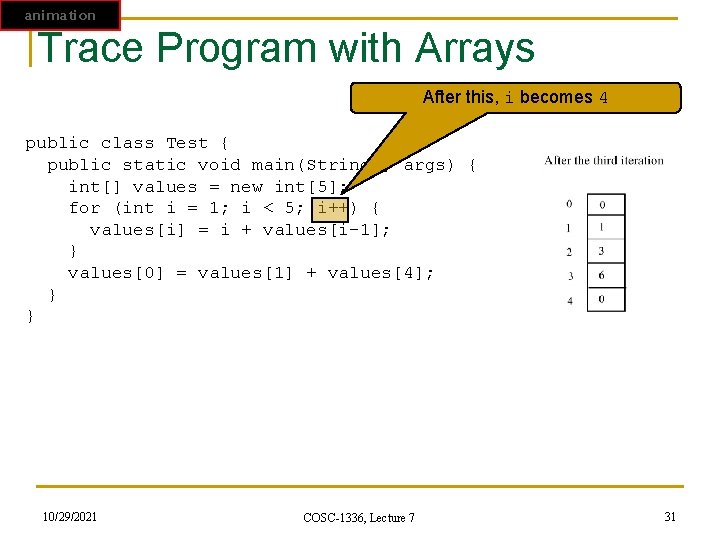
animation Trace Program with Arrays After this, i becomes 4 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 31
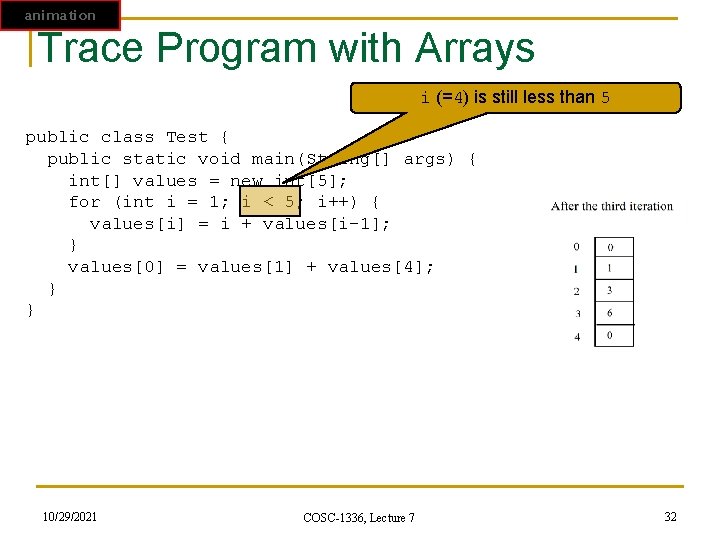
animation Trace Program with Arrays i (=4) is still less than 5 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 32
![animation Trace Program with Arrays After this values4 becomes 10 4 6 public animation Trace Program with Arrays After this, values[4] becomes 10 (4 + 6) public](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-33.jpg)
animation Trace Program with Arrays After this, values[4] becomes 10 (4 + 6) public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 33
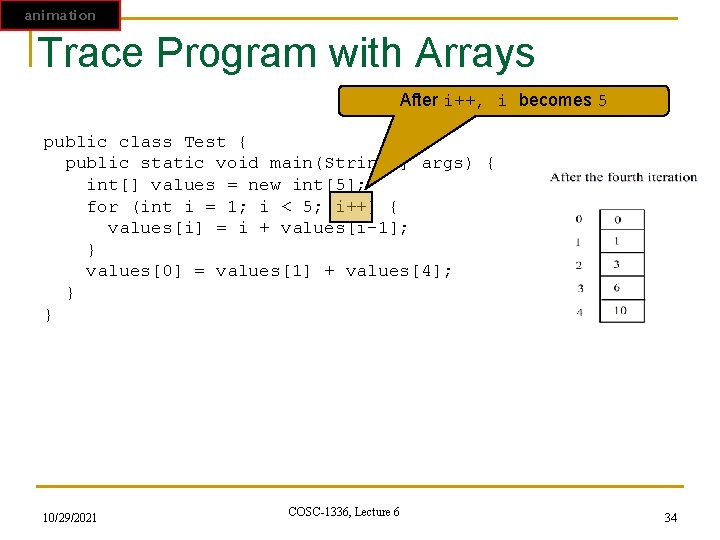
animation Trace Program with Arrays After i++, i becomes 5 public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 6 34
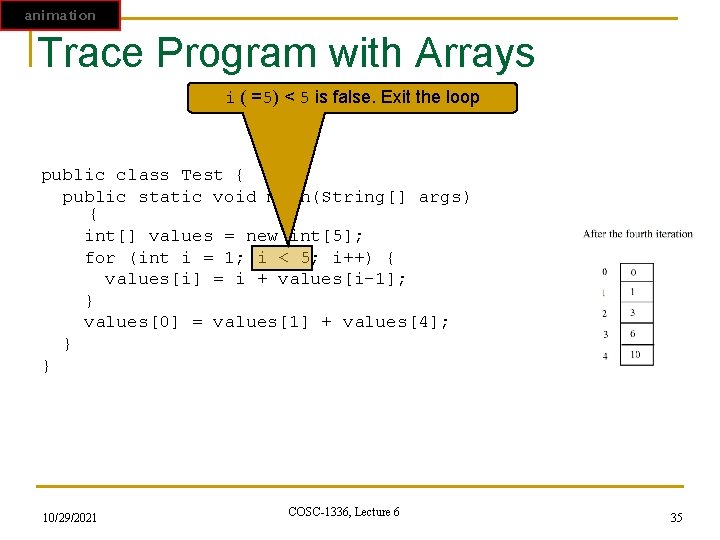
animation Trace Program with Arrays i ( =5) < 5 is false. Exit the loop public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 6 35
![animation Trace Program with Arrays After this line values0 is 11 1 10 animation Trace Program with Arrays After this line, values[0] is 11 (1 + 10)](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-36.jpg)
animation Trace Program with Arrays After this line, values[0] is 11 (1 + 10) public class Test { public static void main(String[] args) { int[] values = new int[5]; for (int i = 1; i < 5; i++) { values[i] = i + values[i-1]; } values[0] = values[1] + values[4]; } } 10/29/2021 COSC-1336, Lecture 7 36
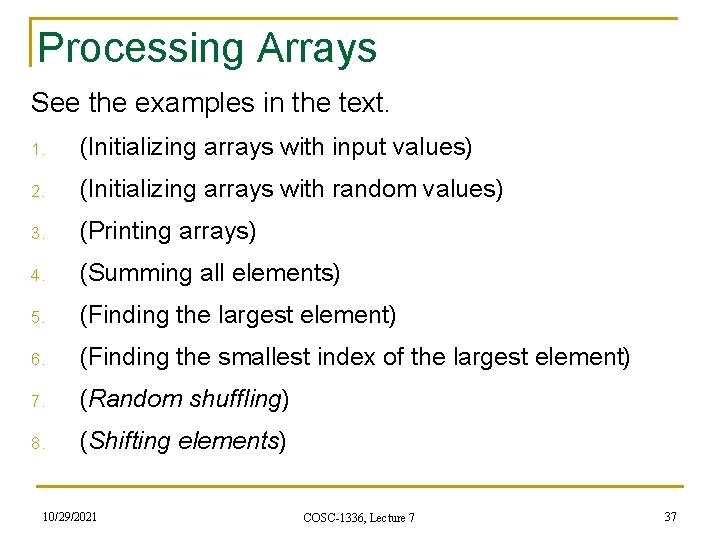
Processing Arrays See the examples in the text. 1. (Initializing arrays with input values) 2. (Initializing arrays with random values) 3. (Printing arrays) 4. (Summing all elements) 5. (Finding the largest element) 6. (Finding the smallest index of the largest element) 7. (Random shuffling) 8. (Shifting elements) 10/29/2021 COSC-1336, Lecture 7 37
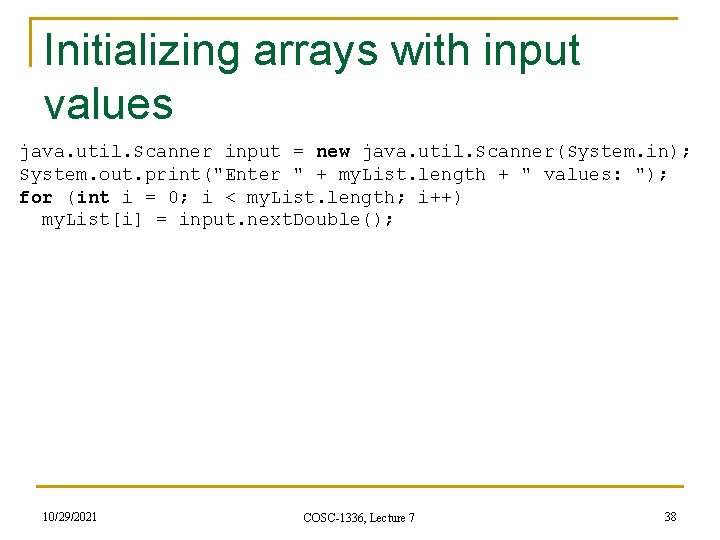
Initializing arrays with input values java. util. Scanner input = new java. util. Scanner(System. in); System. out. print("Enter " + my. List. length + " values: "); for (int i = 0; i < my. List. length; i++) my. List[i] = input. next. Double(); 10/29/2021 COSC-1336, Lecture 7 38
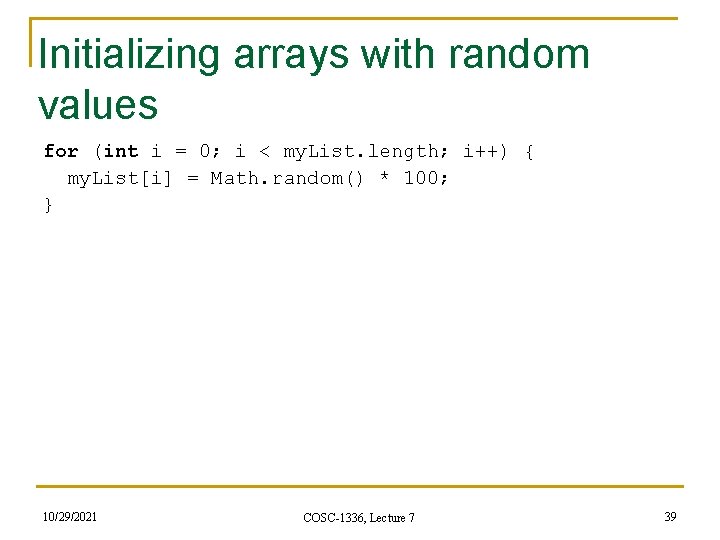
Initializing arrays with random values for (int i = 0; i < my. List. length; i++) { my. List[i] = Math. random() * 100; } 10/29/2021 COSC-1336, Lecture 7 39
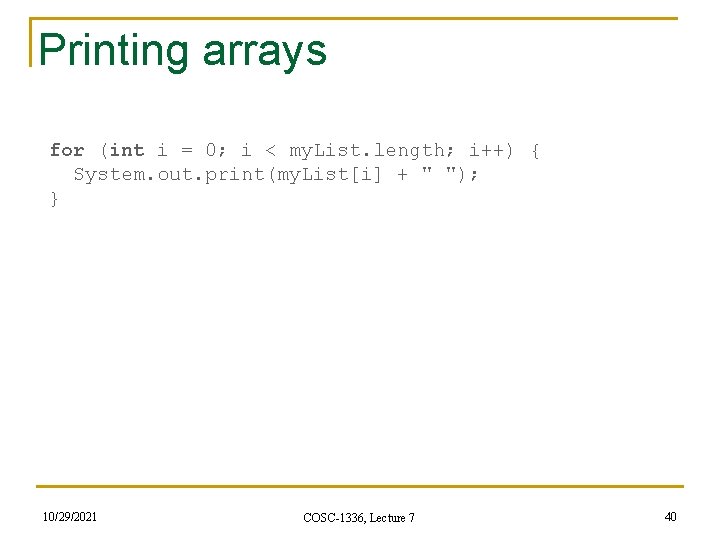
Printing arrays for (int i = 0; i < my. List. length; i++) { System. out. print(my. List[i] + " "); } 10/29/2021 COSC-1336, Lecture 7 40
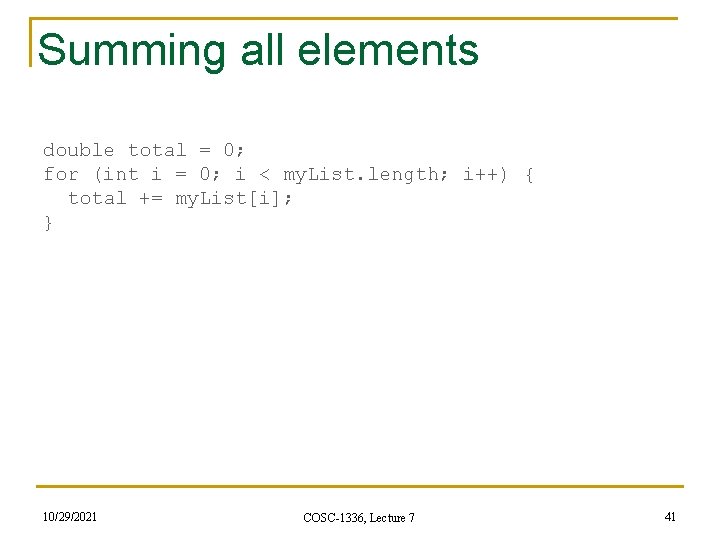
Summing all elements double total = 0; for (int i = 0; i < my. List. length; i++) { total += my. List[i]; } 10/29/2021 COSC-1336, Lecture 7 41
![Finding the largest element double max my List0 for int i 1 Finding the largest element double max = my. List[0]; for (int i = 1;](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-42.jpg)
Finding the largest element double max = my. List[0]; for (int i = 1; i < my. List. length; i++) { if (my. List[i] > max) max = my. List[i]; } 10/29/2021 COSC-1336, Lecture 7 42
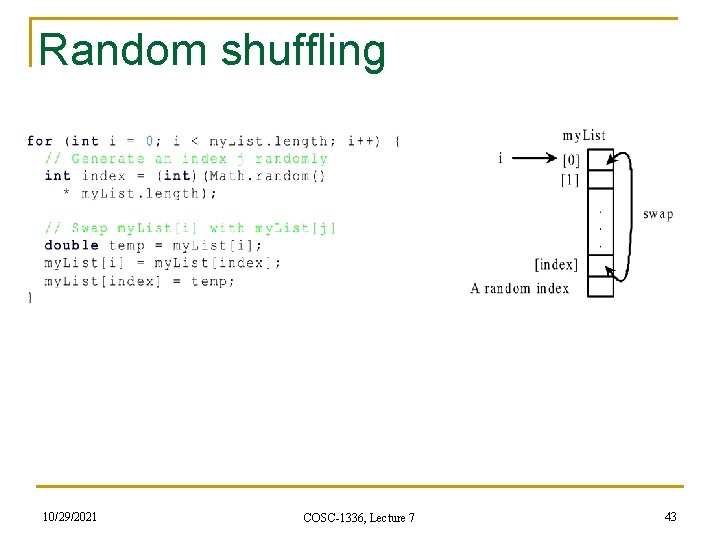
Random shuffling 10/29/2021 COSC-1336, Lecture 7 43
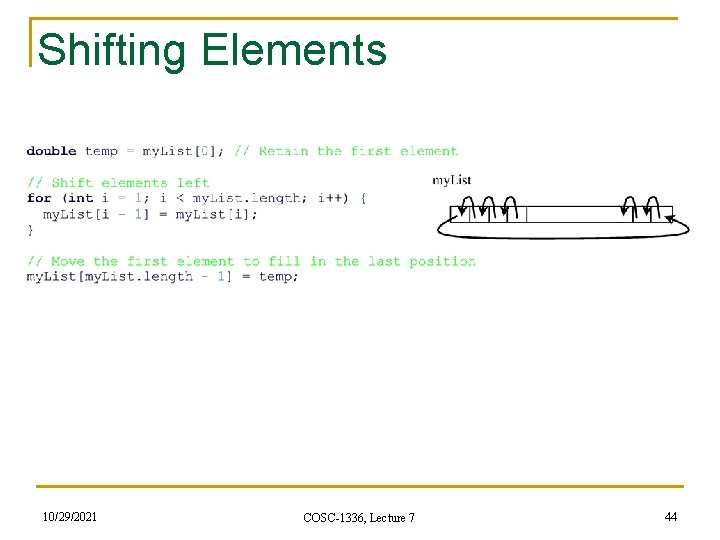
Shifting Elements 10/29/2021 COSC-1336, Lecture 7 44
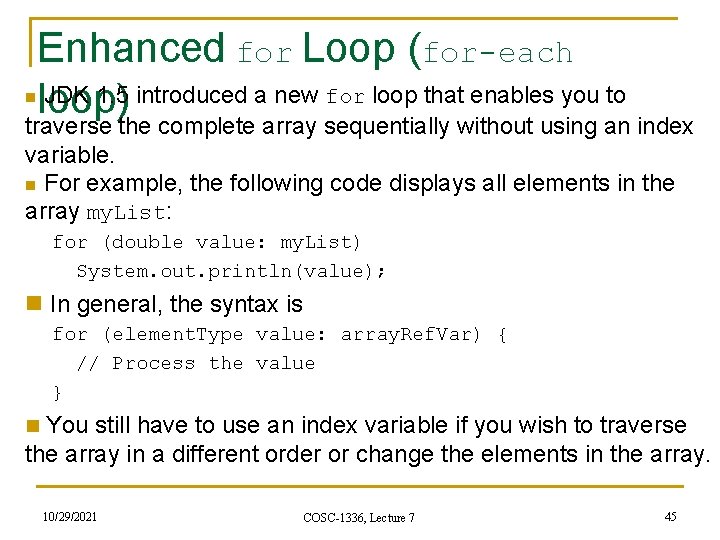
Enhanced for Loop (for-each JDK 1. 5 introduced a new for loop that enables you to loop) traverse the complete array sequentially without using an index n variable. n For example, the following code displays all elements in the array my. List: for (double value: my. List) System. out. println(value); n In general, the syntax is for (element. Type value: array. Ref. Var) { // Process the value } n You still have to use an index variable if you wish to traverse the array in a different order or change the elements in the array. 10/29/2021 COSC-1336, Lecture 7 45
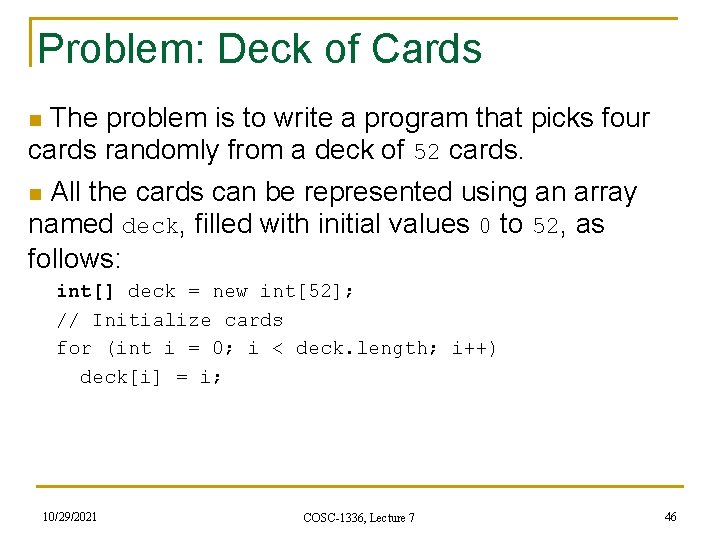
Problem: Deck of Cards The problem is to write a program that picks four cards randomly from a deck of 52 cards. n All the cards can be represented using an array named deck, filled with initial values 0 to 52, as follows: n int[] deck = new int[52]; // Initialize cards for (int i = 0; i < deck. length; i++) deck[i] = i; 10/29/2021 COSC-1336, Lecture 7 46
![Deck Of Cards java public class Deck Of Cards public static void mainString Deck. Of. Cards. java public class Deck. Of. Cards { public static void main(String[]](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-47.jpg)
Deck. Of. Cards. java public class Deck. Of. Cards { public static void main(String[] args) { int[] deck = new int[52]; String[] suits = {"Spades", "Hearts", "Diamonds", "Clubs"}; String[] ranks = {"Ace", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King"}; // Initialize cards for (int i = 0; i < deck. length; i++) deck[i] = i; 10/29/2021 COSC-1336, Lecture 7 47
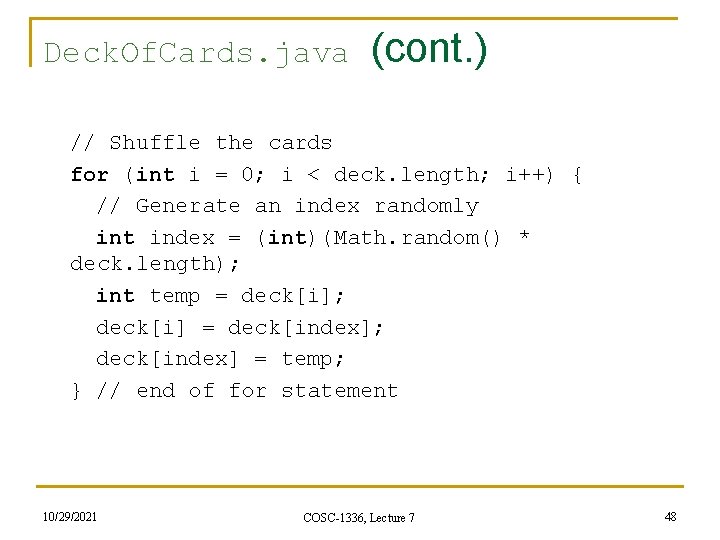
Deck. Of. Cards. java (cont. ) // Shuffle the cards for (int i = 0; i < deck. length; i++) { // Generate an index randomly int index = (int)(Math. random() * deck. length); int temp = deck[i]; deck[i] = deck[index]; deck[index] = temp; } // end of for statement 10/29/2021 COSC-1336, Lecture 7 48
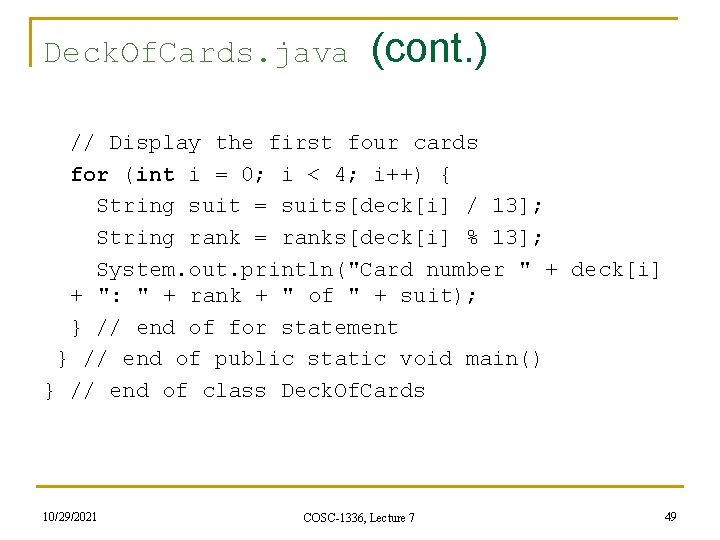
Deck. Of. Cards. java (cont. ) // Display the first four cards for (int i = 0; i < 4; i++) { String suit = suits[deck[i] / 13]; String rank = ranks[deck[i] % 13]; System. out. println("Card number " + deck[i] + ": " + rank + " of " + suit); } // end of for statement } // end of public static void main() } // end of class Deck. Of. Cards 10/29/2021 COSC-1336, Lecture 7 49
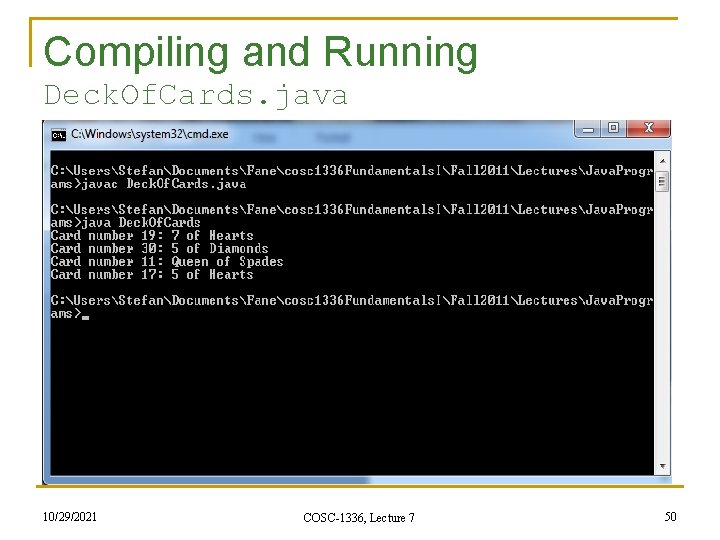
Compiling and Running Deck. Of. Cards. java 10/29/2021 COSC-1336, Lecture 7 50
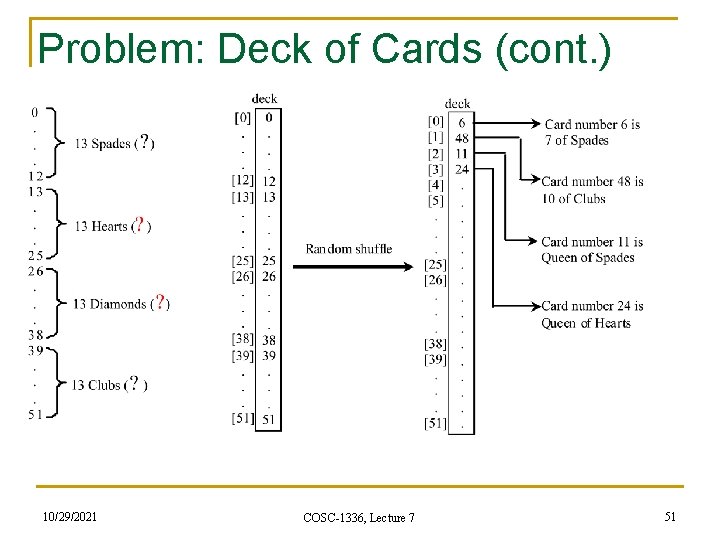
Problem: Deck of Cards (cont. ) 10/29/2021 COSC-1336, Lecture 7 51
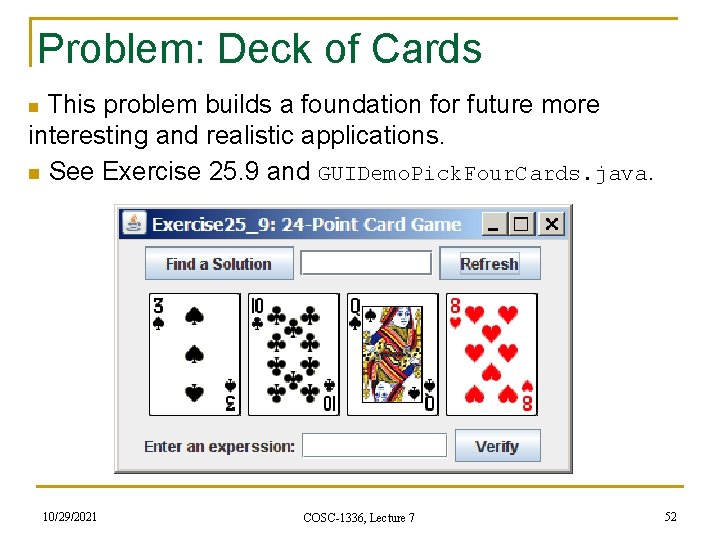
Problem: Deck of Cards This problem builds a foundation for future more interesting and realistic applications. n See Exercise 25. 9 and GUIDemo. Pick. Four. Cards. java. n 10/29/2021 COSC-1336, Lecture 7 52
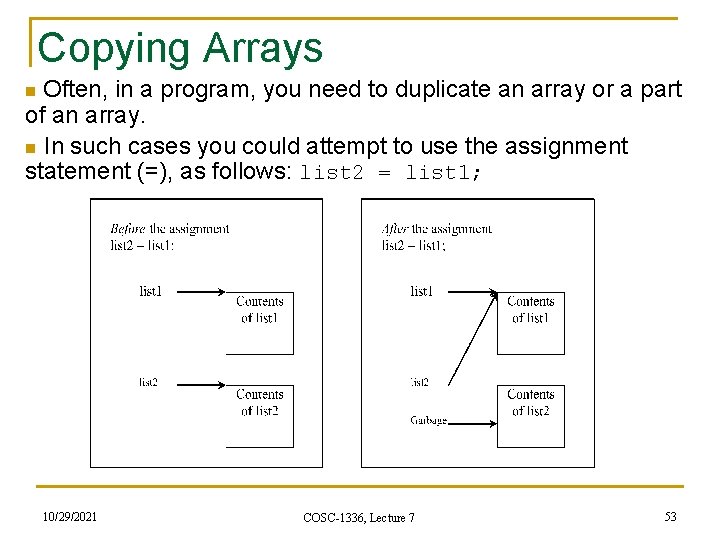
Copying Arrays Often, in a program, you need to duplicate an array or a part of an array. n In such cases you could attempt to use the assignment statement (=), as follows: list 2 = list 1; n 10/29/2021 COSC-1336, Lecture 7 53
![Copying Arrays n Using a loop int source Array 2 3 1 5 Copying Arrays n Using a loop: int[] source. Array = {2, 3, 1, 5,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-54.jpg)
Copying Arrays n Using a loop: int[] source. Array = {2, 3, 1, 5, 10}; int[] target. Array = new int[source. Array. length]; for (int i = 0; i < source. Arrays. length; i++) target. Array[i] = source. Array[i]; 10/29/2021 COSC-1336, Lecture 7 54
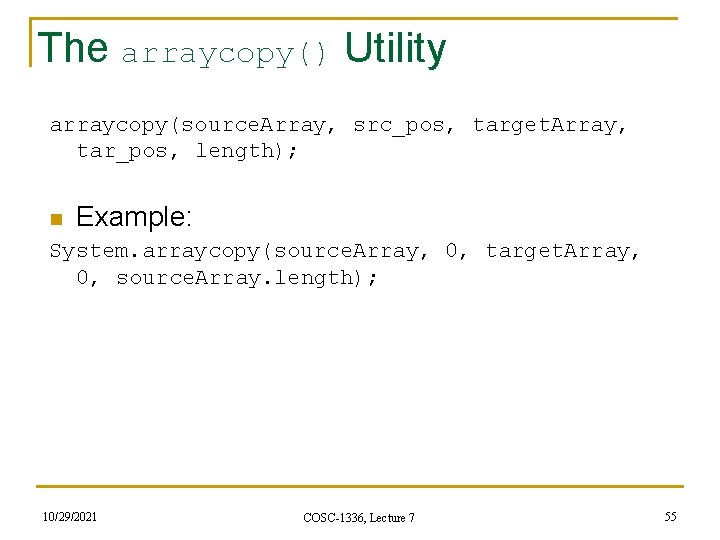
The arraycopy() Utility arraycopy(source. Array, src_pos, target. Array, tar_pos, length); n Example: System. arraycopy(source. Array, 0, target. Array, 0, source. Array. length); 10/29/2021 COSC-1336, Lecture 7 55
![Passing Arrays to Methods public static void print Arrayint array for int i Passing Arrays to Methods public static void print. Array(int[] array) { for (int i](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-56.jpg)
Passing Arrays to Methods public static void print. Array(int[] array) { for (int i = 0; i < array. length; i++) { System. out. print(array[i] + " "); } } Invoke the method int[] list = {3, 1, 2, 6, 4, 2}; print. Array(list); Invoke the method print. Array(new int[]{3, 1, 2, 6, 4, 2}); Anonymous array 10/29/2021 COSC-1336, Lecture 7 56
![Anonymous Array The statement print Arraynew int3 1 2 6 4 2 Anonymous Array § The statement print. Array(new int[]{3, 1, 2, 6, 4, 2}); §](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-57.jpg)
Anonymous Array § The statement print. Array(new int[]{3, 1, 2, 6, 4, 2}); § creates an array using the following syntax: new data. Type[]{literal 0, literal 1, . . . , literalk}; §There is no explicit reference variable for the array. § Such array is called an anonymous array. 10/29/2021 COSC-1336, Lecture 7 57
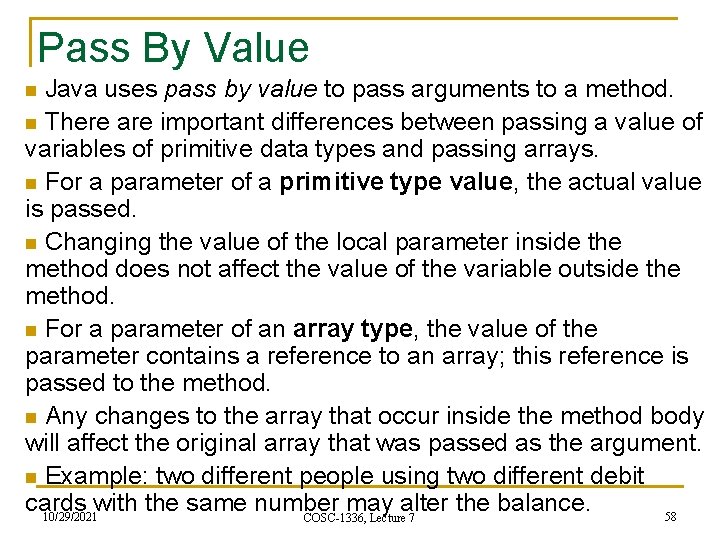
Pass By Value Java uses pass by value to pass arguments to a method. n There are important differences between passing a value of variables of primitive data types and passing arrays. n For a parameter of a primitive type value, the actual value is passed. n Changing the value of the local parameter inside the method does not affect the value of the variable outside the method. n For a parameter of an array type, the value of the parameter contains a reference to an array; this reference is passed to the method. n Any changes to the array that occur inside the method body will affect the original array that was passed as the argument. n Example: two different people using two different debit cards with the same number may alter the balance. 10/29/2021 58 COSC-1336, Lecture 7 n
![Simple Example public class Test public static void mainString args int x Simple Example public class Test { public static void main(String[] args) { int x](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-59.jpg)
Simple Example public class Test { public static void main(String[] args) { int x = 1; // x represents an int value int[] y = new int[10]; // y represents an array of int values m(x, y); // Invoke m with arguments x and y System. out. println("x is " + x); System. out. println("y[0] is " + y[0]); } public static void m(int number, int[] numbers) { number = 1001; // Assign a new value to numbers[0] = 5555; // Assign a new value to numbers[0] } } 10/29/2021 COSC-1336, Lecture 7 59
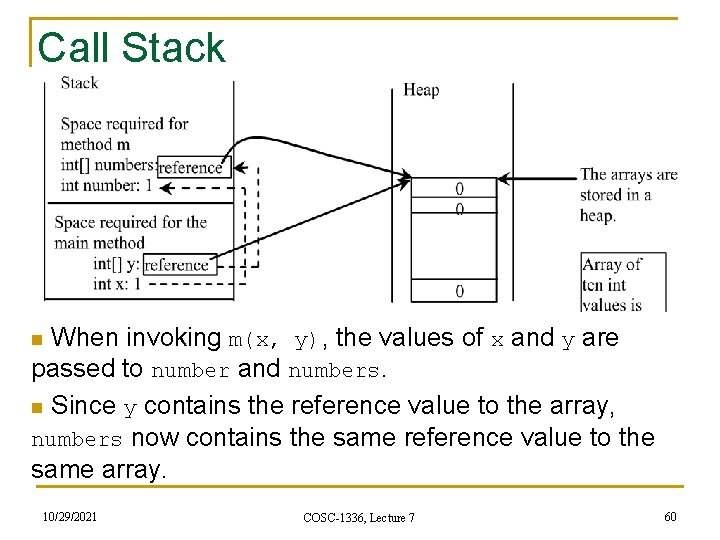
Call Stack When invoking m(x, y), the values of x and y are passed to number and numbers. n Since y contains the reference value to the array, numbers now contains the same reference value to the same array. n 10/29/2021 COSC-1336, Lecture 7 60
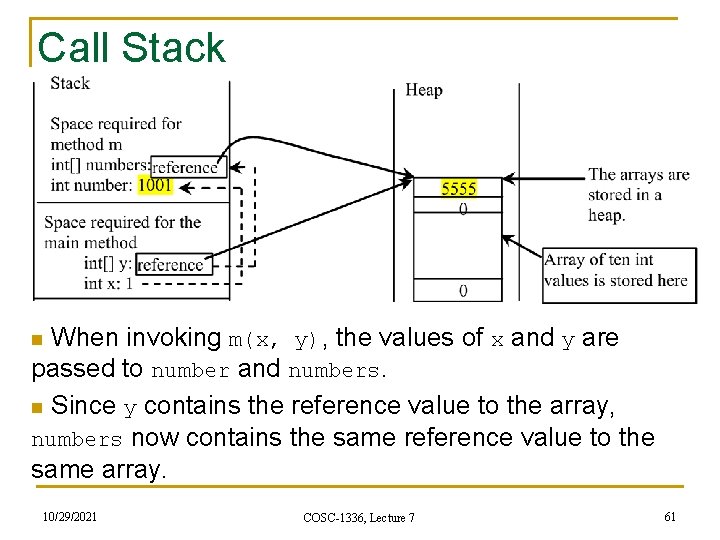
Call Stack When invoking m(x, y), the values of x and y are passed to number and numbers. n Since y contains the reference value to the array, numbers now contains the same reference value to the same array. n 10/29/2021 COSC-1336, Lecture 7 61
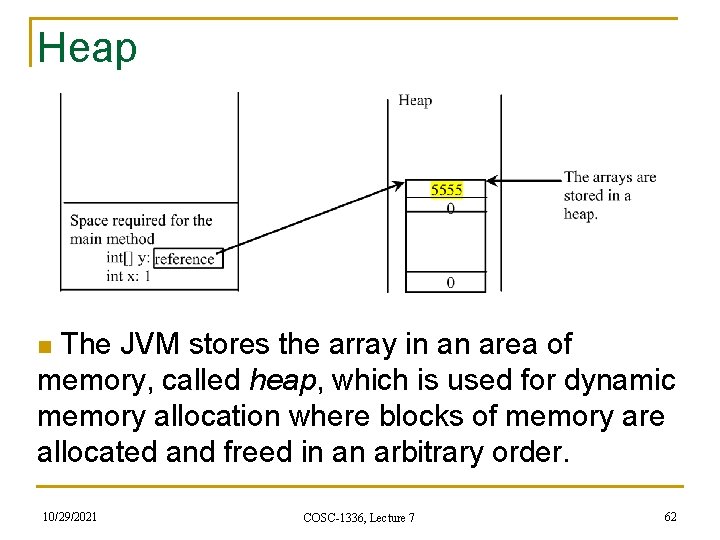
Heap The JVM stores the array in an area of memory, called heap, which is used for dynamic memory allocation where blocks of memory are allocated and freed in an arbitrary order. n 10/29/2021 COSC-1336, Lecture 7 62
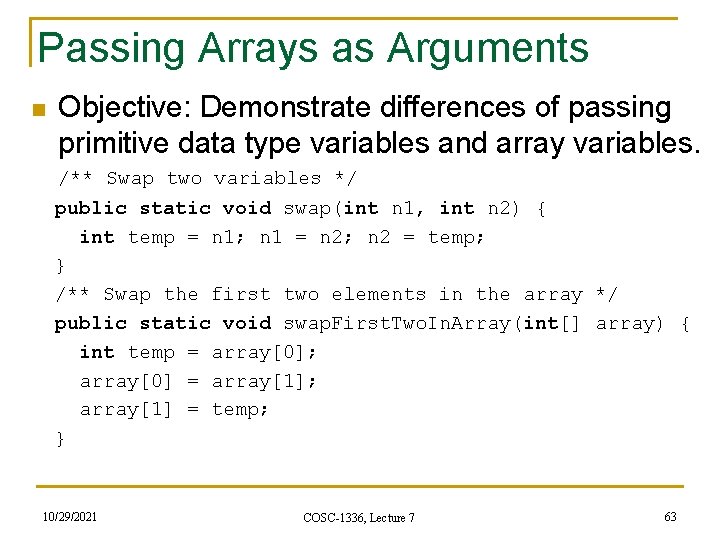
Passing Arrays as Arguments n Objective: Demonstrate differences of passing primitive data type variables and array variables. /** Swap two variables */ public static void swap(int n 1, int n 2) { int temp = n 1; n 1 = n 2; n 2 = temp; } /** Swap the first two elements in the array */ public static void swap. First. Two. In. Array(int[] array) { int temp = array[0]; array[0] = array[1]; array[1] = temp; } 10/29/2021 COSC-1336, Lecture 7 63
![public class Test Pass Array public static void mainString args int a public class Test. Pass. Array { public static void main(String[] args) { int[] a](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-64.jpg)
public class Test. Pass. Array { public static void main(String[] args) { int[] a = {1, 2}; // Swap elements using the swap() method System. out. println("Before invoking swap"); System. out. println("array is {" + a[0] + ", " + a[1] + "}"); swap(a[0], a[1]); System. out. println("After invoking swap"); System. out. println("array is {" + a[0] + ", " + a[1] + "}"); // Swap elements using the swap. First. Two. In. Array() method System. out. println("Before invoking swap. First. Two. In. Array"); System. out. println("array is {" + a[0] + ", " + a[1] + "}"); swap. First. Two. In. Array(a); System. out. println("After invoking swap. First. Two. In. Array"); System. out. println("array is {" + a[0] + ", " + a[1] + "}"); } } 10/29/2021 COSC-1336, Lecture 7 64
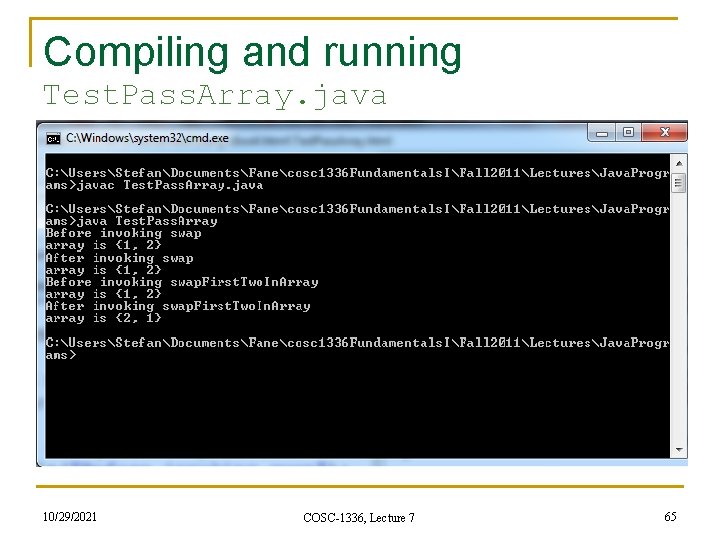
Compiling and running Test. Pass. Array. java 10/29/2021 COSC-1336, Lecture 7 65
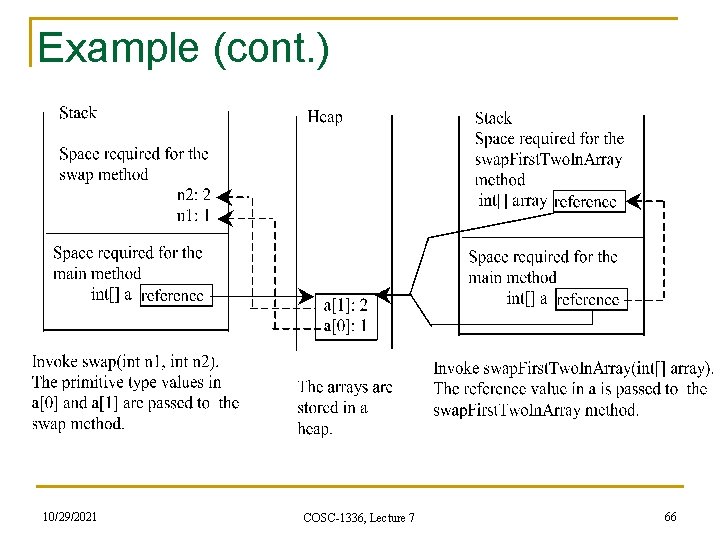
Example (cont. ) 10/29/2021 COSC-1336, Lecture 7 66
![Returning an Array from a public static int reverseint list Method int result Returning an Array from a public static int[] reverse(int[] list) Method int[] result =](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-67.jpg)
Returning an Array from a public static int[] reverse(int[] list) Method int[] result = new int[list. length]; { for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } list return result; } result int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; int[] list 2 = reverse(list 1); 10/29/2021 COSC-1336, Lecture 7 67
![animation Trace the reverse Method int list 1 1 2 3 4 5 animation Trace the reverse() Method int[] list 1 = {1, 2, 3, 4, 5,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-68.jpg)
animation Trace the reverse() Method int[] list 1 = {1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); Declare result and create array public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 COSC-1336, Lecture 7 68
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-69.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i = 0 and j = 5 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 COSC-1336, Lecture 7 69
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-70.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i (= 0) is less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 COSC-1336, Lecture 7 70
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-71.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i = 0 and j = 5 Assign list[0] to result[5] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 1 COSC-1336, Lecture 7 71
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-72.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); After this, i becomes 1 and j becomes 4 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 1 COSC-1336, Lecture 7 72
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-73.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i (=1) is less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 1 COSC-1336, Lecture 7 73
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-74.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i = 1 and j = 4 Assign list[1] to result[4] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 2 1 COSC-1336, Lecture 7 74
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-75.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); After this, i becomes 2 and j becomes 3 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 2 1 COSC-1336, Lecture 7 75
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-76.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i (=2) is still less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 2 1 COSC-1336, Lecture 7 76
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-77.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i = 2 and j = 3 Assign list[i] to result[j] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 3 2 1 COSC-1336, Lecture 7 77
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-78.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); After this, i becomes 3 and j becomes 2 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 3 2 1 COSC-1336, Lecture 7 78
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-79.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i (=3) is still less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 0 3 2 1 COSC-1336, Lecture 7 79
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-80.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i = 3 and j = 2 Assign list[i] to result[j] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 4 3 2 1 COSC-1336, Lecture 7 80
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-81.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); After this, i becomes 4 and j becomes 1 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 4 3 2 1 COSC-1336, Lecture 7 81
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-82.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i (=4) is still less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 0 4 3 2 1 COSC-1336, Lecture 7 82
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-83.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i = 4 and j = 1 Assign list[i] to result[j] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 5 4 3 2 1 COSC-1336, Lecture 7 83
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-84.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); After this, i becomes 5 and j becomes 0 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 5 4 3 2 1 COSC-1336, Lecture 7 84
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-85.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i (=5) is still less than 6 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 0 5 4 3 2 1 COSC-1336, Lecture 7 85
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-86.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i = 5 and j = 0 Assign list[i] to result[j] public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 6 5 4 3 2 1 COSC-1336, Lecture 7 86
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-87.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); After this, i becomes 6 and j becomes -1 public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 6 5 4 3 2 1 COSC-1336, Lecture 7 87
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-88.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); i (=6) < 6 is false. So exit the loop. public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list result 10/29/2021 1 2 3 4 5 6 6 5 4 3 2 1 COSC-1336, Lecture 7 88
![animation Trace the reverse Method int list 1 new int1 2 3 4 animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4,](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-89.jpg)
animation Trace the reverse() Method int[] list 1 = new int[]{1, 2, 3, 4, 5, 6}; (cont. ) int[] list 2 = reverse(list 1); Return result public static int[] reverse(int[] list) { int[] result = new int[list. length]; for (int i = 0, j = result. length - 1; i < list. length; i++, j--) { result[j] = list[i]; } return result; } list 1 2 3 4 5 6 6 5 4 3 2 1 list 2 10/29/2021 resul t COSC-1336, Lecture 7 89
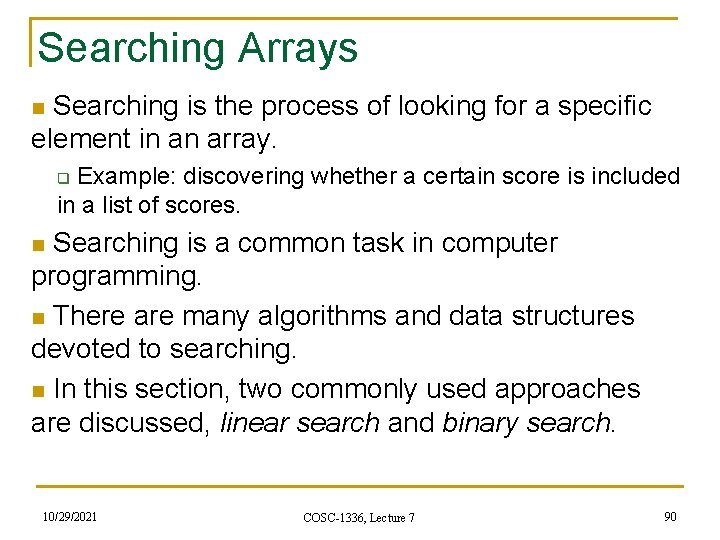
Searching Arrays Searching is the process of looking for a specific element in an array. n Example: discovering whether a certain score is included in a list of scores. q Searching is a common task in computer programming. n There are many algorithms and data structures devoted to searching. n In this section, two commonly used approaches are discussed, linear search and binary search. n 10/29/2021 COSC-1336, Lecture 7 90
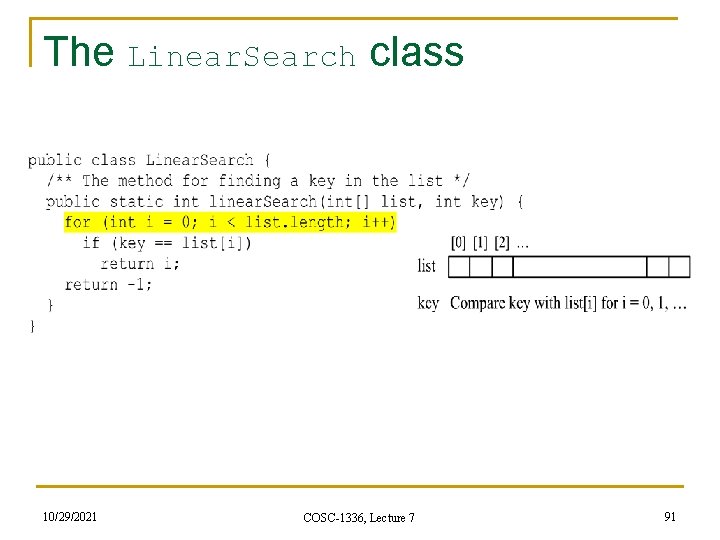
The Linear. Search class 10/29/2021 COSC-1336, Lecture 7 91
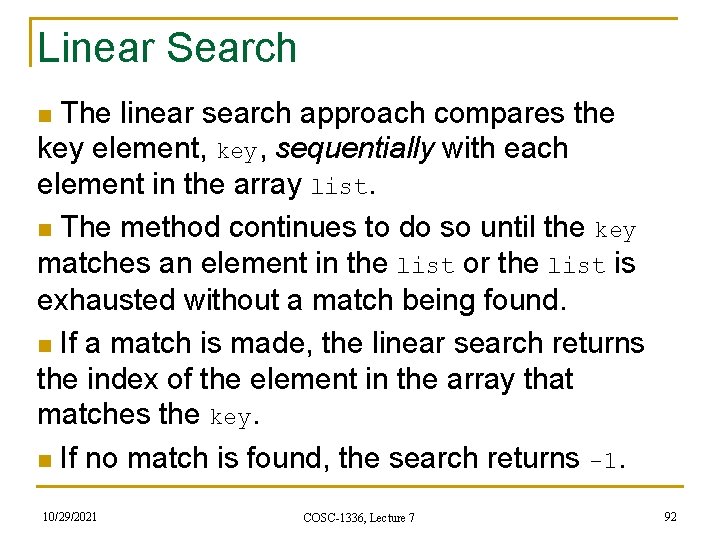
Linear Search The linear search approach compares the key element, key, sequentially with each element in the array list. n The method continues to do so until the key matches an element in the list or the list is exhausted without a match being found. n If a match is made, the linear search returns the index of the element in the array that matches the key. n If no match is found, the search returns -1. n 10/29/2021 COSC-1336, Lecture 7 92
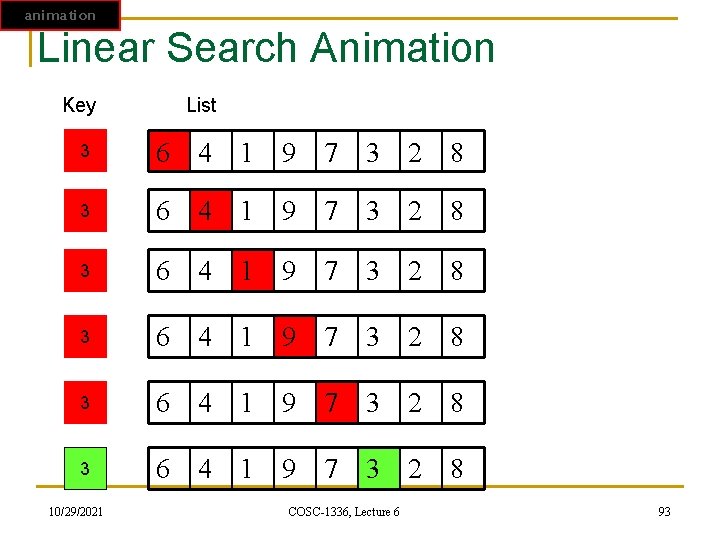
animation Linear Search Animation Key List 3 6 4 1 9 7 3 2 8 3 6 4 1 9 7 3 2 8 10/29/2021 COSC-1336, Lecture 6 93
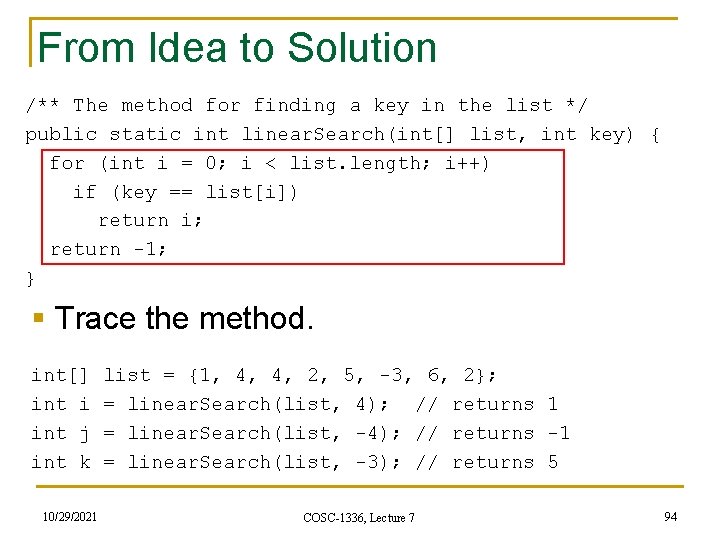
From Idea to Solution /** The method for finding a key in the list */ public static int linear. Search(int[] list, int key) { for (int i = 0; i < list. length; i++) if (key == list[i]) return i; return -1; } § Trace the method. int[] int i int j int k 10/29/2021 list = {1, 4, 4, 2, 5, -3, 6, 2}; = linear. Search(list, 4); // returns 1 = linear. Search(list, -4); // returns -1 = linear. Search(list, -3); // returns 5 COSC-1336, Lecture 7 94
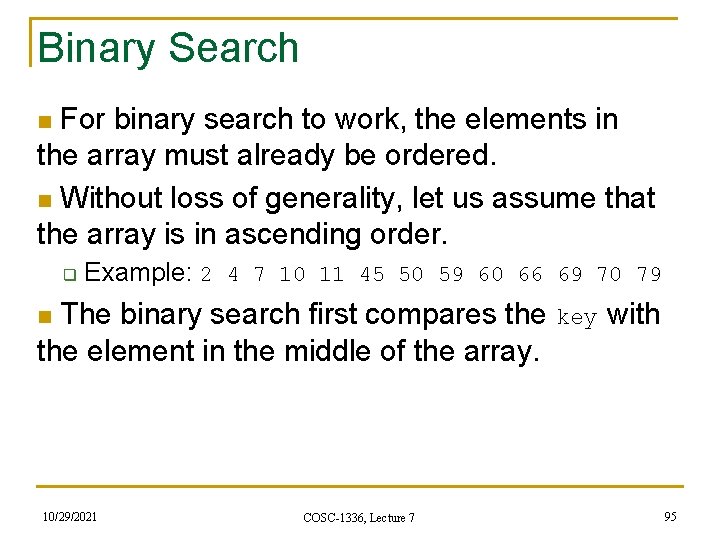
Binary Search For binary search to work, the elements in the array must already be ordered. n Without loss of generality, let us assume that the array is in ascending order. n q Example: 2 4 7 10 11 45 50 59 60 66 69 70 79 The binary search first compares the key with the element in the middle of the array. n 10/29/2021 COSC-1336, Lecture 7 95
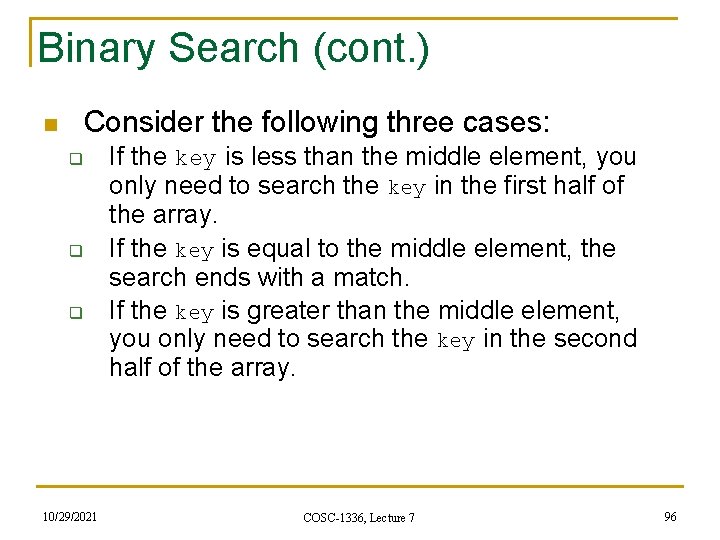
Binary Search (cont. ) Consider the following three cases: n q q q 10/29/2021 If the key is less than the middle element, you only need to search the key in the first half of the array. If the key is equal to the middle element, the search ends with a match. If the key is greater than the middle element, you only need to search the key in the second half of the array. COSC-1336, Lecture 7 96
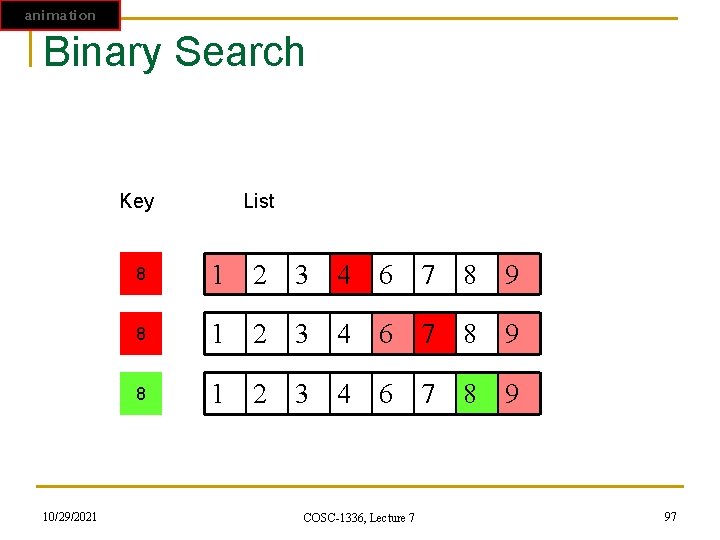
animation Binary Search Key 10/29/2021 List 8 1 2 3 4 6 7 8 9 COSC-1336, Lecture 7 97
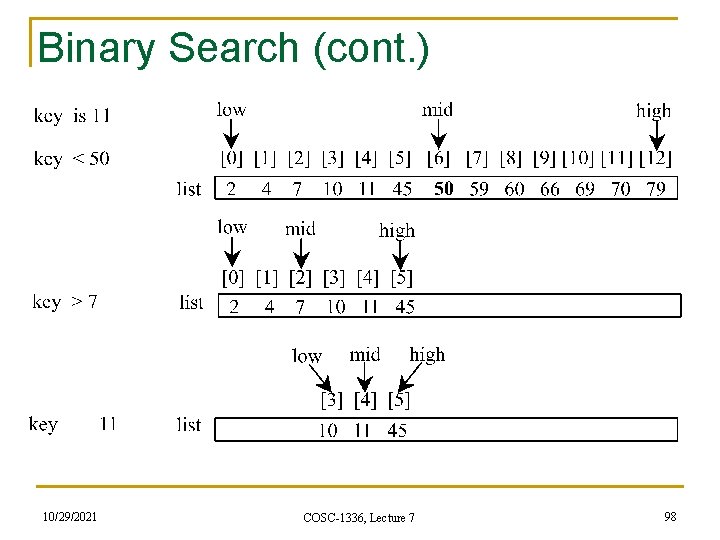
Binary Search (cont. ) 10/29/2021 COSC-1336, Lecture 7 98
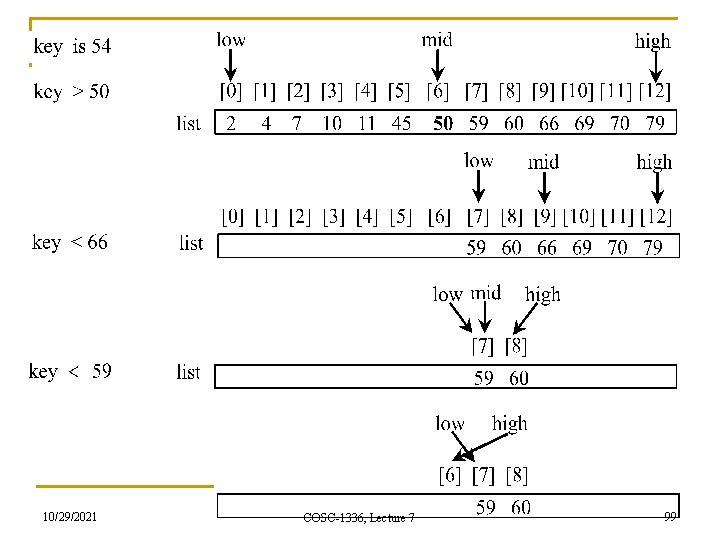
10/29/2021 COSC-1336, Lecture 7 99
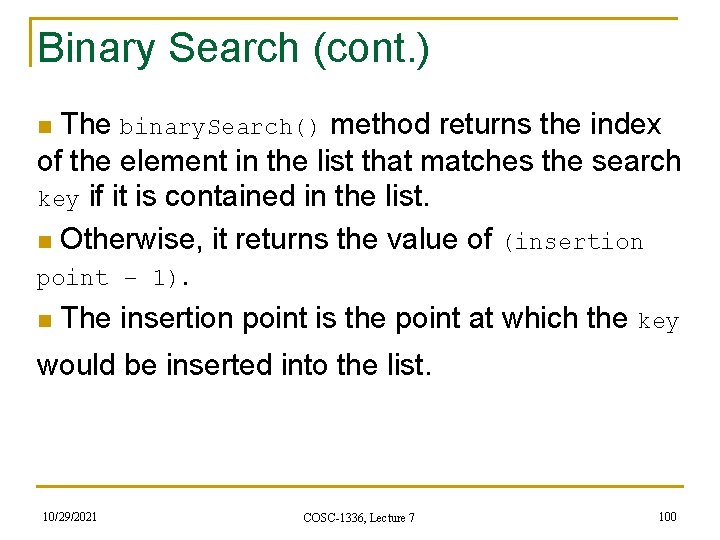
Binary Search (cont. ) The binary. Search() method returns the index of the element in the list that matches the search key if it is contained in the list. n Otherwise, it returns the value of (insertion point – 1). n The insertion point is the point at which the key n would be inserted into the list. 10/29/2021 COSC-1336, Lecture 7 100
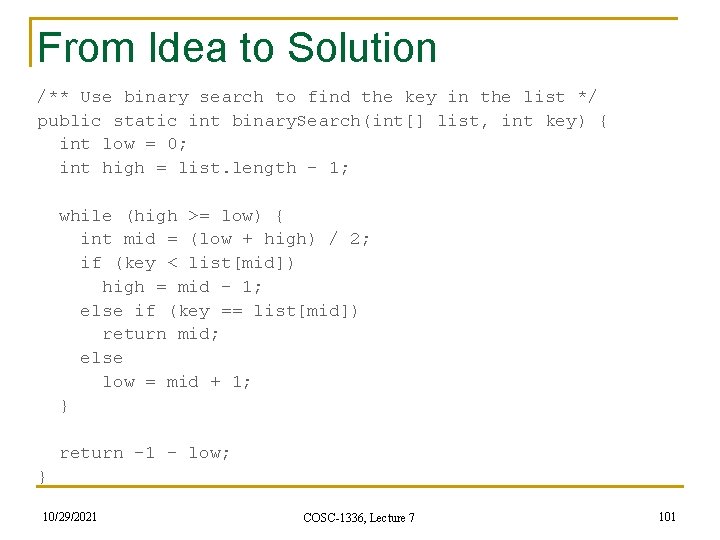
From Idea to Solution /** Use binary search to find the key in the list */ public static int binary. Search(int[] list, int key) { int low = 0; int high = list. length - 1; while (high >= low) { int mid = (low + high) / 2; if (key < list[mid]) high = mid - 1; else if (key == list[mid]) return mid; else low = mid + 1; } return -1 - low; } 10/29/2021 COSC-1336, Lecture 7 101
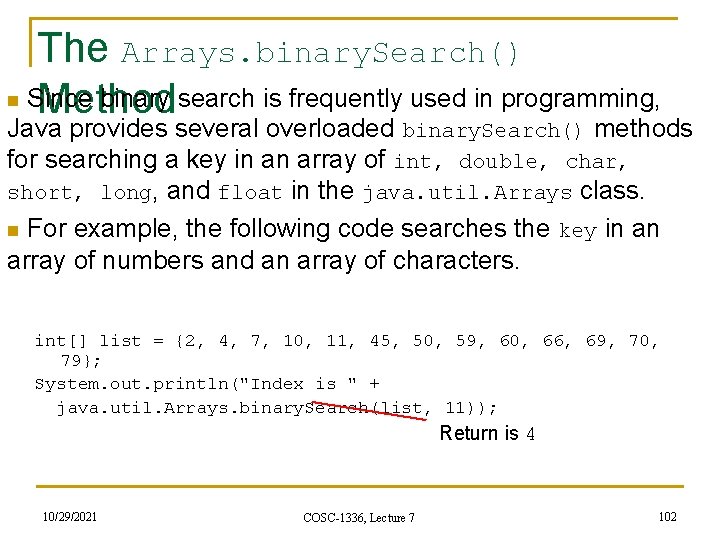
The Arrays. binary. Search() n Since binary search is frequently used in programming, Method Java provides several overloaded binary. Search() methods for searching a key in an array of int, double, char, short, long, and float in the java. util. Arrays class. n For example, the following code searches the key in an array of numbers and an array of characters. int[] list = {2, 4, 7, 10, 11, 45, 50, 59, 60, 66, 69, 70, 79}; System. out. println("Index is " + java. util. Arrays. binary. Search(list, 11)); Return is 4 10/29/2021 COSC-1336, Lecture 7 102
![The Arrays binary Search Method char chars a c g x y z The Arrays. binary. Search() Method char[] chars = {'a', 'c', 'g', 'x', 'y', 'z'};](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-103.jpg)
The Arrays. binary. Search() Method char[] chars = {'a', 'c', 'g', 'x', 'y', 'z'}; System. out. println("Index is " + java. util. Arrays. binary. Search(chars, 't')); Return is – 4 (insertion point is 3, so return is -3 -1). For the binary. Search() method to work, the array must be pre-sorted in increasing order. n 10/29/2021 COSC-1336, Lecture 7 103
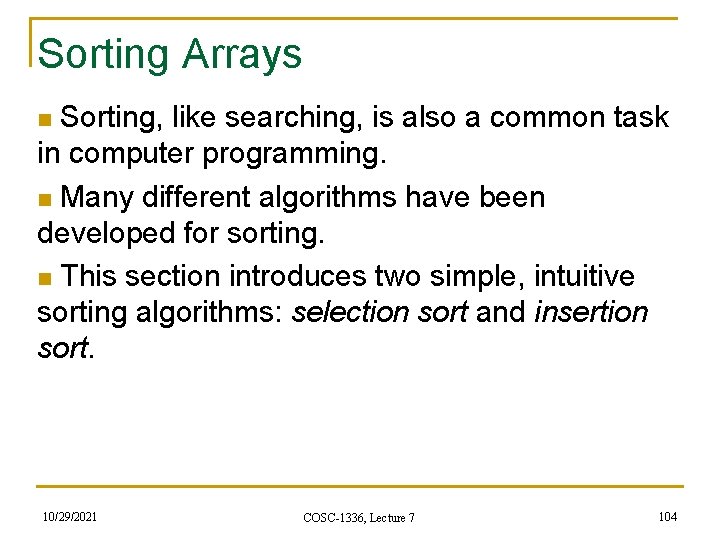
Sorting Arrays Sorting, like searching, is also a common task in computer programming. n Many different algorithms have been developed for sorting. n This section introduces two simple, intuitive sorting algorithms: selection sort and insertion sort. n 10/29/2021 COSC-1336, Lecture 7 104
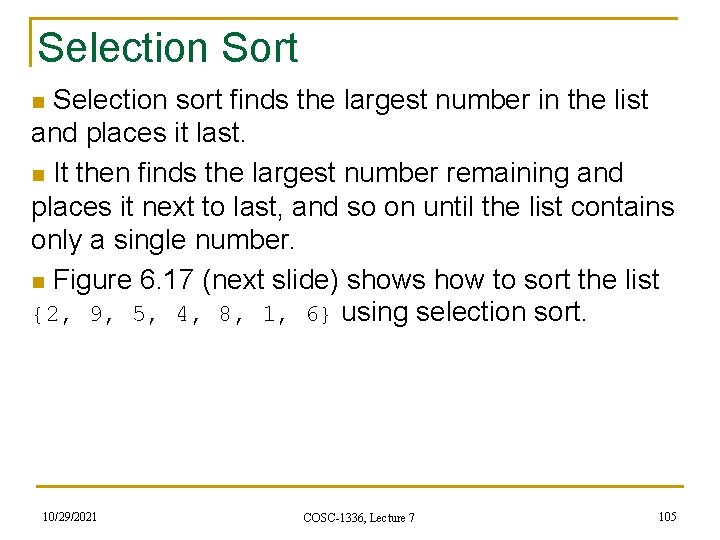
Selection Sort Selection sort finds the largest number in the list and places it last. n It then finds the largest number remaining and places it next to last, and so on until the list contains only a single number. n Figure 6. 17 (next slide) shows how to sort the list {2, 9, 5, 4, 8, 1, 6} using selection sort. n 10/29/2021 COSC-1336, Lecture 7 105
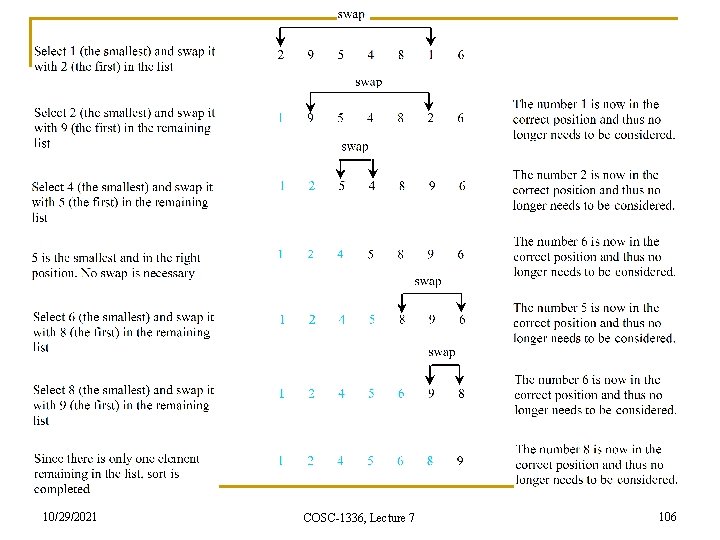
10/29/2021 COSC-1336, Lecture 7 106
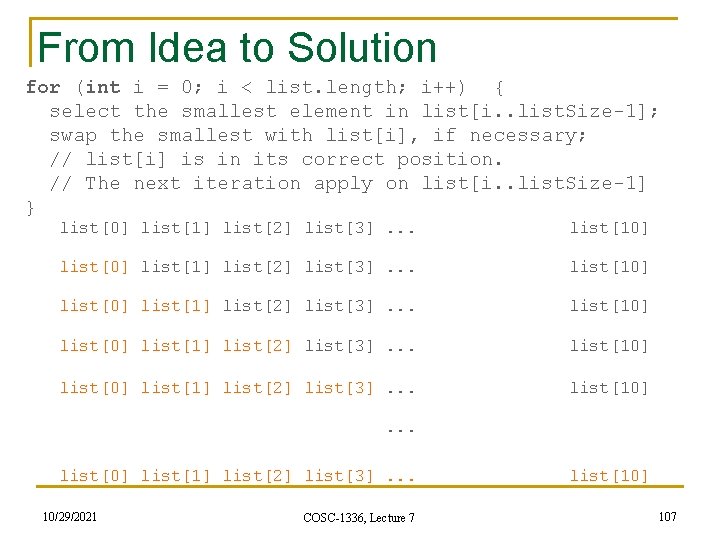
From Idea to Solution for (int i = 0; i < list. length; i++) { select the smallest element in list[i. . list. Size-1]; swap the smallest with list[i], if necessary; // list[i] is in its correct position. // The next iteration apply on list[i. . list. Size-1] } list[0] list[1] list[2] list[3]. . . list[10] . . . list[0] list[1] list[2] list[3]. . . 10/29/2021 COSC-1336, Lecture 7 list[10] 107
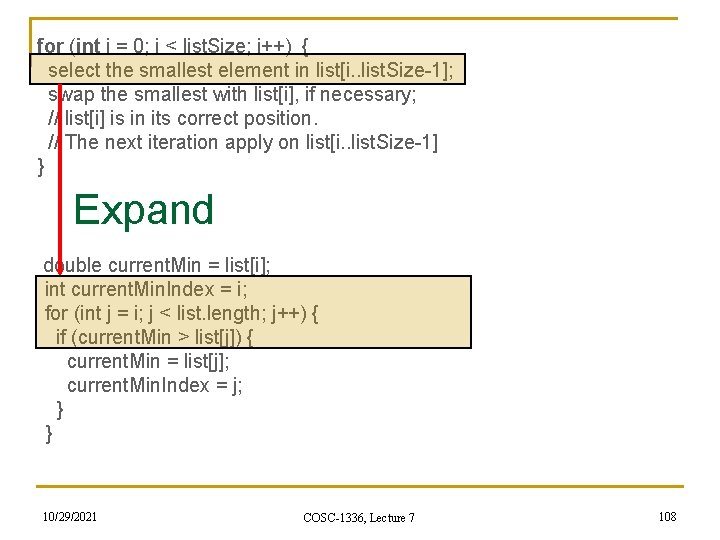
for (int i = 0; i < list. Size; i++) { select the smallest element in list[i. . list. Size-1]; swap the smallest with list[i], if necessary; // list[i] is in its correct position. // The next iteration apply on list[i. . list. Size-1] } Expand double current. Min = list[i]; int current. Min. Index = i; for (int j = i; j < list. length; j++) { if (current. Min > list[j]) { current. Min = list[j]; current. Min. Index = j; } } 10/29/2021 COSC-1336, Lecture 7 108
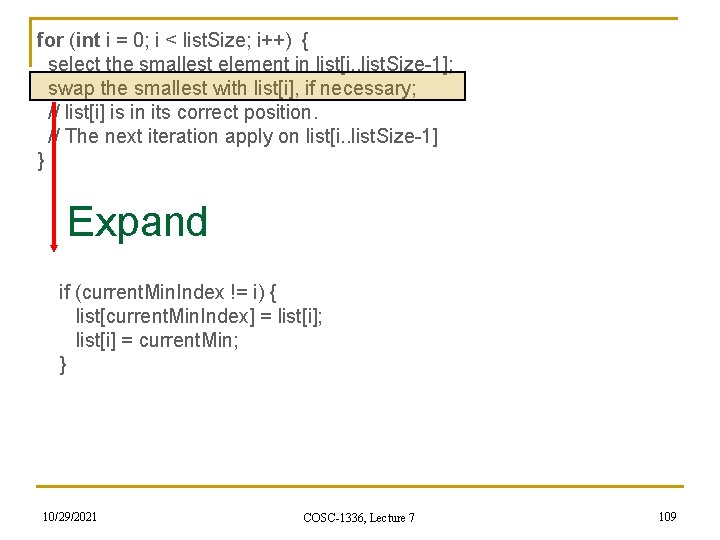
for (int i = 0; i < list. Size; i++) { select the smallest element in list[i. . list. Size-1]; swap the smallest with list[i], if necessary; // list[i] is in its correct position. // The next iteration apply on list[i. . list. Size-1] } Expand if (current. Min. Index != i) { list[current. Min. Index] = list[i]; list[i] = current. Min; } 10/29/2021 COSC-1336, Lecture 7 109
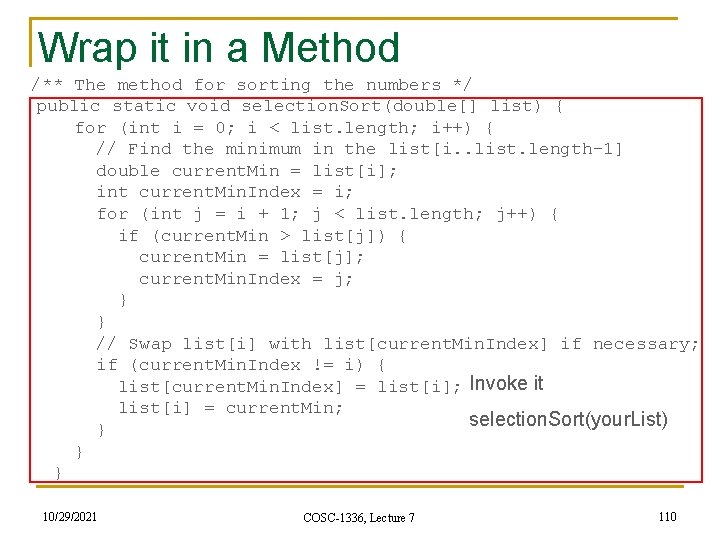
Wrap it in a Method /** The method for sorting the numbers */ public static void selection. Sort(double[] list) { for (int i = 0; i < list. length; i++) { // Find the minimum in the list[i. . list. length-1] double current. Min = list[i]; int current. Min. Index = i; for (int j = i + 1; j < list. length; j++) { if (current. Min > list[j]) { current. Min = list[j]; current. Min. Index = j; } } // Swap list[i] with list[current. Min. Index] if necessary; if (current. Min. Index != i) { list[current. Min. Index] = list[i]; Invoke it list[i] = current. Min; selection. Sort(your. List) } } } 10/29/2021 COSC-1336, Lecture 7 110
![Insertion Sort int my List 2 9 5 4 8 1 6 Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; //](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-111.jpg)
Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; // Unsorted §The insertion sort algorithm sorts a list of values by repeatedly inserting an unsorted element into a sorted sublist until the whole list is sorted. 10/29/2021 COSC-1336, Lecture 7 111
![animation Insertion Sort int my List 2 9 5 4 8 1 6 animation Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6};](https://slidetodoc.com/presentation_image_h2/f7c23bb2fc99aa7ec6b81982a0f9d9bd/image-112.jpg)
animation Insertion Sort int[] my. List = {2, 9, 5, 4, 8, 1, 6}; // Unsorted 2 9 5 4 8 1 6 2 5 9 4 8 1 6 2 4 1 2 10/29/2021 5 4 8 5 9 1 6 8 2 9 5 4 8 1 6 2 4 5 9 8 1 6 1 2 4 5 8 9 6 6 9 COSC-1336, Lecture 7 112
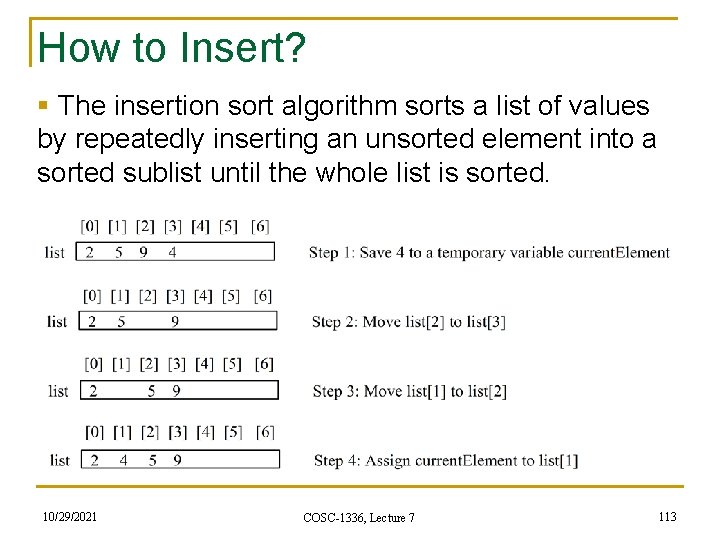
How to Insert? § The insertion sort algorithm sorts a list of values by repeatedly inserting an unsorted element into a sorted sublist until the whole list is sorted. 10/29/2021 COSC-1336, Lecture 7 113
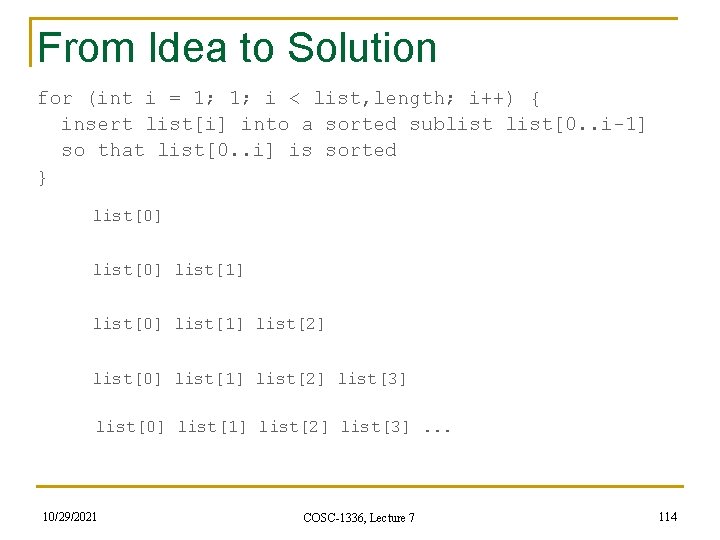
From Idea to Solution for (int i = 1; 1; i < list, length; i++) { insert list[i] into a sorted sublist[0. . i-1] so that list[0. . i] is sorted } list[0] list[1] list[2] list[3] list[0] list[1] list[2] list[3]. . . 10/29/2021 COSC-1336, Lecture 7 114
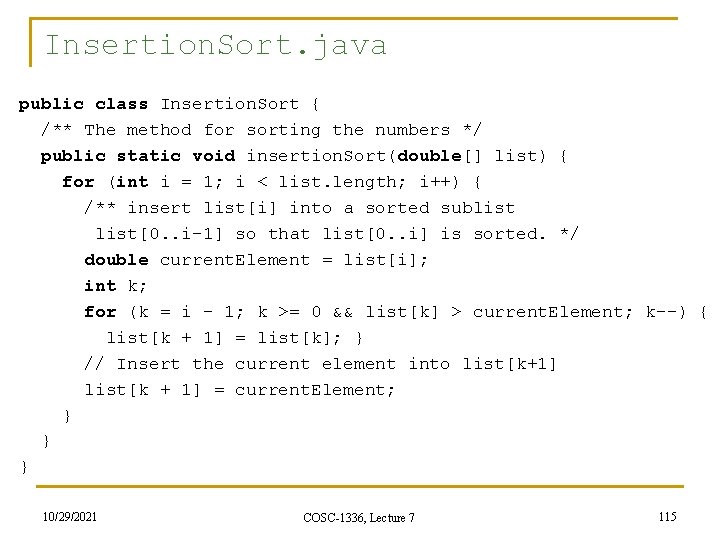
Insertion. Sort. java public class Insertion. Sort { /** The method for sorting the numbers */ public static void insertion. Sort(double[] list) { for (int i = 1; i < list. length; i++) { /** insert list[i] into a sorted sublist[0. . i-1] so that list[0. . i] is sorted. */ double current. Element = list[i]; int k; for (k = i - 1; k >= 0 && list[k] > current. Element; k--) { list[k + 1] = list[k]; } // Insert the current element into list[k+1] list[k + 1] = current. Element; } } } 10/29/2021 COSC-1336, Lecture 7 115
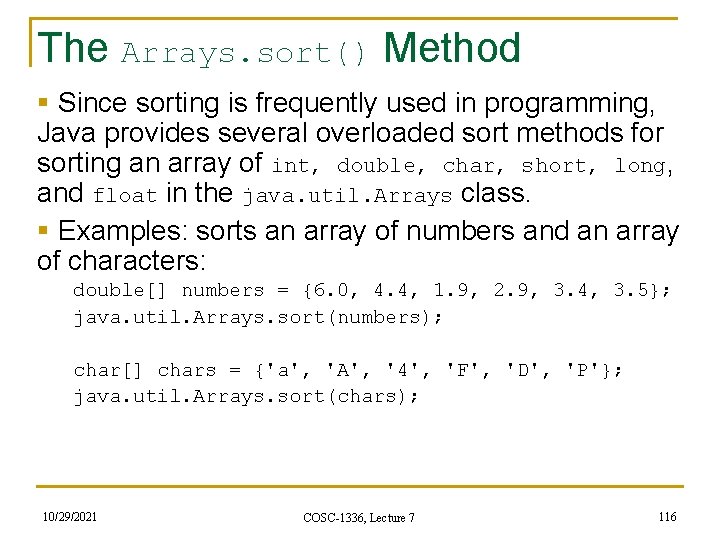
The Arrays. sort() Method § Since sorting is frequently used in programming, Java provides several overloaded sort methods for sorting an array of int, double, char, short, long, and float in the java. util. Arrays class. § Examples: sorts an array of numbers and an array of characters: double[] numbers = {6. 0, 4. 4, 1. 9, 2. 9, 3. 4, 3. 5}; java. util. Arrays. sort(numbers); char[] chars = {'a', 'A', '4', 'F', 'D', 'P'}; java. util. Arrays. sort(chars); 10/29/2021 COSC-1336, Lecture 7 116
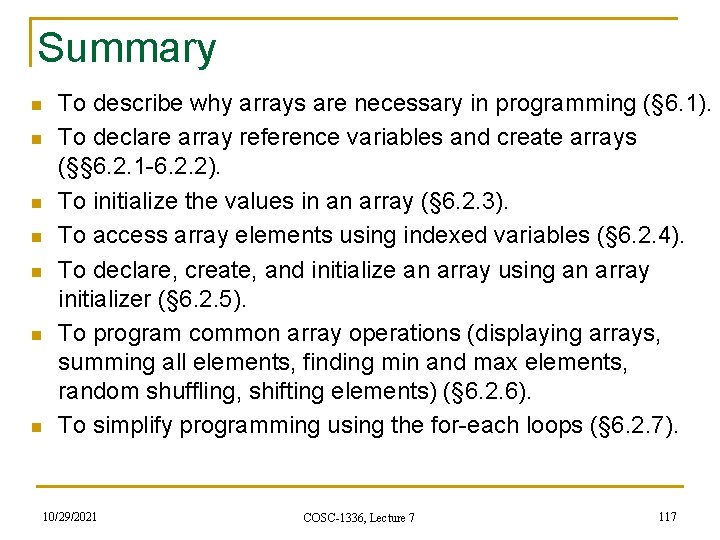
Summary n n n n To describe why arrays are necessary in programming (§ 6. 1). To declare array reference variables and create arrays (§§ 6. 2. 1 -6. 2. 2). To initialize the values in an array (§ 6. 2. 3). To access array elements using indexed variables (§ 6. 2. 4). To declare, create, and initialize an array using an array initializer (§ 6. 2. 5). To program common array operations (displaying arrays, summing all elements, finding min and max elements, random shuffling, shifting elements) (§ 6. 2. 6). To simplify programming using the for-each loops (§ 6. 2. 7). 10/29/2021 COSC-1336, Lecture 7 117
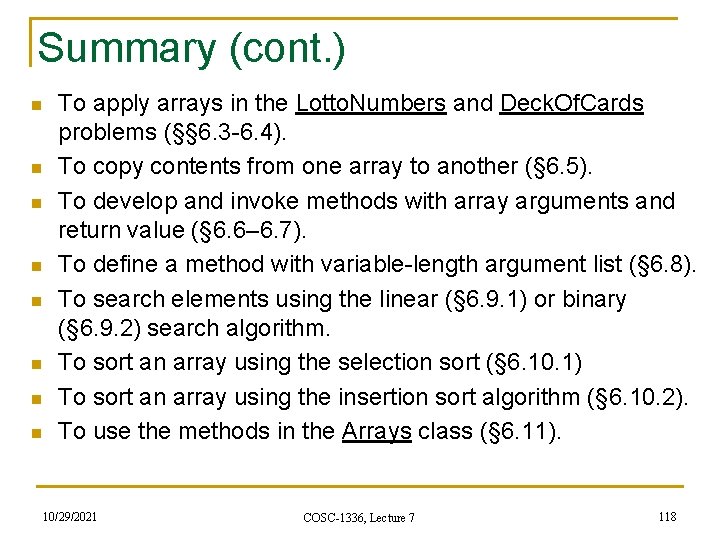
Summary (cont. ) n n n n To apply arrays in the Lotto. Numbers and Deck. Of. Cards problems (§§ 6. 3 -6. 4). To copy contents from one array to another (§ 6. 5). To develop and invoke methods with array arguments and return value (§ 6. 6– 6. 7). To define a method with variable-length argument list (§ 6. 8). To search elements using the linear (§ 6. 9. 1) or binary (§ 6. 9. 2) search algorithm. To sort an array using the selection sort (§ 6. 10. 1) To sort an array using the insertion sort algorithm (§ 6. 10. 2). To use the methods in the Arrays class (§ 6. 11). 10/29/2021 COSC-1336, Lecture 7 118
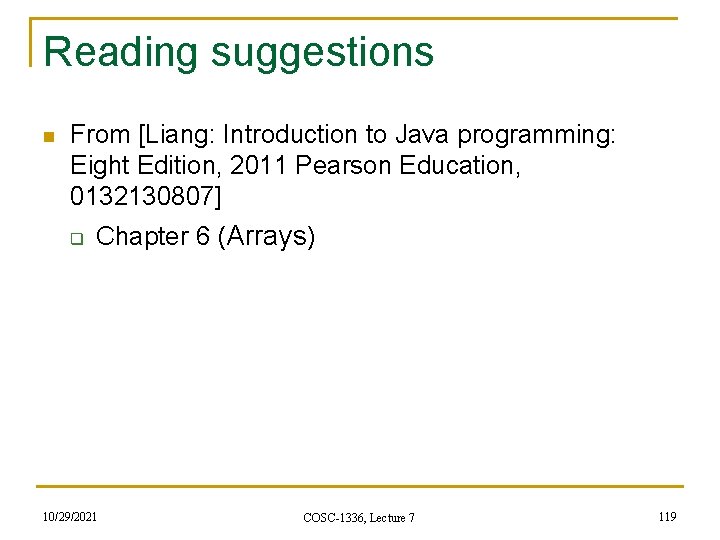
Reading suggestions n From [Liang: Introduction to Java programming: Eight Edition, 2011 Pearson Education, 0132130807] q Chapter 6 (Arrays) 10/29/2021 COSC-1336, Lecture 7 119
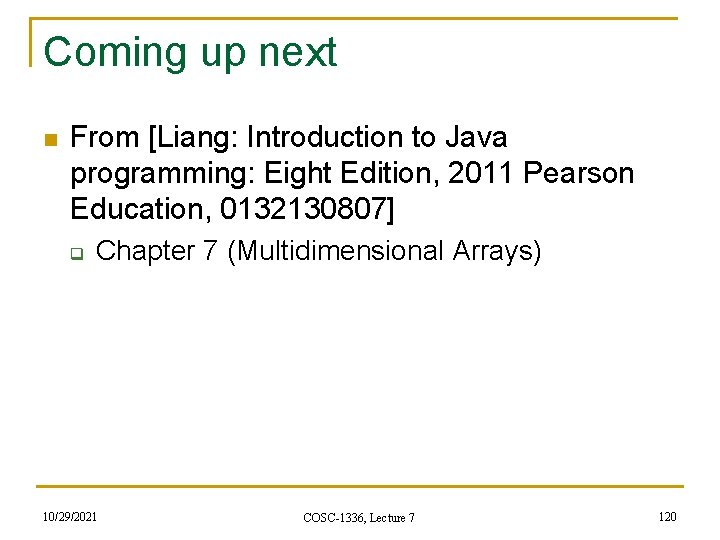
Coming up next n From [Liang: Introduction to Java programming: Eight Edition, 2011 Pearson Education, 0132130807] q Chapter 7 (Multidimensional Arrays) 10/29/2021 COSC-1336, Lecture 7 120
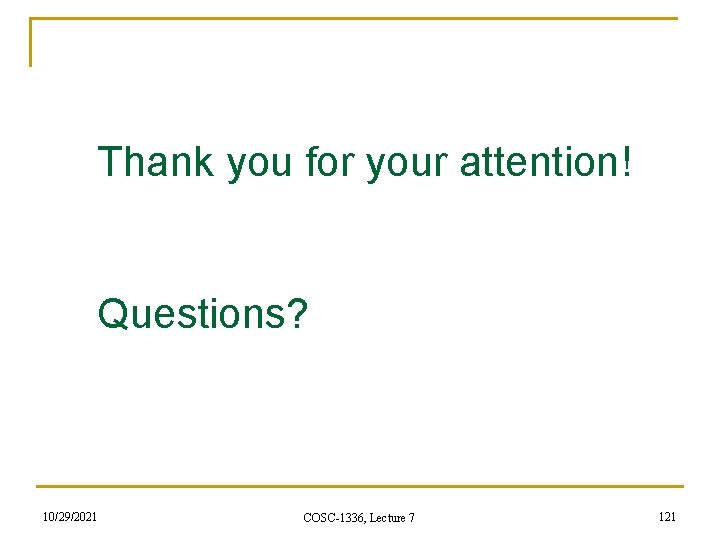
Thank you for your attention! Questions? 10/29/2021 COSC-1336, Lecture 7 121
Sohibidan
Prepared programming
Prepared programming
01:640:244 lecture notes - lecture 15: plat, idah, farad
Cs 1101 programming fundamentals
Fundamentals of functional programming
Salute report example
Aaron tan tuck choy
Cs 1101 final
Fundamentals of functional programming language
Cs 1101 programming fundamentals
Fundamentals of programming 1
C data types with examples
Cosc 4p41
Cosc 3p94
Cosc 3p92
Cosc 320
Cosc 1306
Cosc 121
Cosc 3340
Cosc 1p02
Cosc 4p41
Cosc 101
Cosc 121
Cosc parameters
Cosc 1306
Cosc -cos d
68030 pinout
Cosc 2p12
Cosc
Cosc 3340
Cosc 4p61
Cosc 121
Cosc 2p12
Cosc 4368
Cosc 1306
Cosc 121
Cosc 3p92
Cosc 3340
Cosc 3p91
Cosc 3340
Cosc 4p42
Trap vector table lc3
Adt functional programming
Perbedaan linear programming dan integer programming
Programing adalah
Greedy programming vs dynamic programming
Definition of system programming
Linear vs integer programming
Fusion method pharmacy
Wilson company prepared the following preliminary budget
Monophasic liquid dosage example
The accepted standard of sound and rhythm is
Summary of act 4 and 5 romeo and juliet
Knock 2.hali
Control accounts in accounting
An advance cash distribution plan is prepared
Let's get prepared
Guide planes in rpd
Department account
Salvage title cars
Institutional food service
Example of expository sermon outline
Pictures of
Preparatory reviewing
Prepared exclusively for
How is periscope prepared
Wake county prepared food tax
Be prepared to share the hope
Client name example
Benefits of bsn-prepared nurses
Business of making and serving prepared food and drink
The business plan should be prepared by
Why is carcase meat prepared into cuts joints and mince
Iodometric titration
Galenical prepared by extraction
Periscope bbc bitesize
Be prepared to give an answer
Food and fun evs class 4
Which type of melanin lends black and brown colors to hair?
Prepared exclusively for
Award prepared
Standard cost operating statement
Past tense chart
Backhand writing
The flung spray hits the very windows
Two node loop instability
Descion tree
δwc
Ezra the scribe
In williamson’s synthesis, ethoxyethane is prepared by-
Always be ready to give an answer
Sharon milgram
Wet land preparation
Method of preparation of ointment
Trading account purpose
Fundamentals of subprograms
Fundamentals of thermal-fluidsciences chapter 1 problem 16p
5 principle of patrolling
Tire wheel and wheel bearing fundamentals
Forensic science fundamentals and investigations chapter 6
[author] java message service - jms fundamentals course
Mta security fundamentals exam questions and answers
Cardinality and modality in database
Activity and exercise fundamentals of nursing
Fluid mechanics fundamentals and applications
Fundamentals of nursing chapter 15 critical thinking
Sli.do softuni
Image
"language fundamentals"
Data warehouse fundamentals
The fundamentals of care framework
Fundamentals of artificial intelligence
Difference between bulk deformation and sheet metal forming
14 fundamentals of following the prophet
Fundamentals of data structures in c
Spatial and temporal redundancy in digital image processing
Thermal conduction resistance
Fundamentals of metal casting
Unit 1 fundamentals of it
Database processing fundamentals design and implementation
Fundamentals of information systems 9th edition