Programming Fundamentals I COSC 1336 Lecture 2 prepared
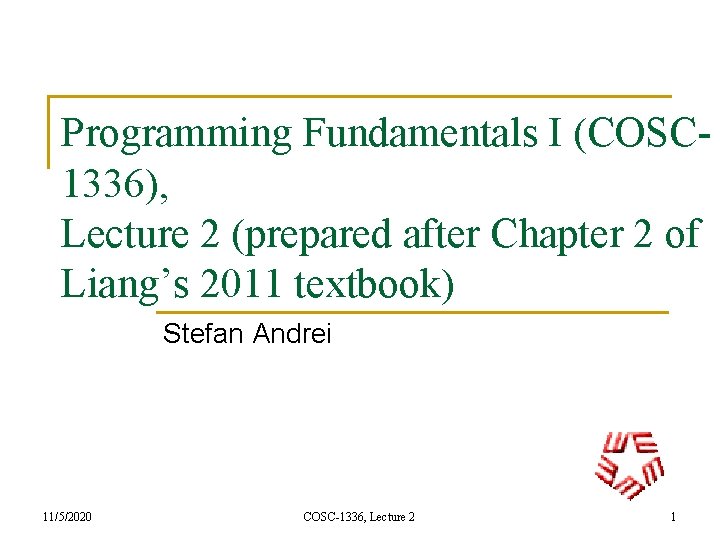
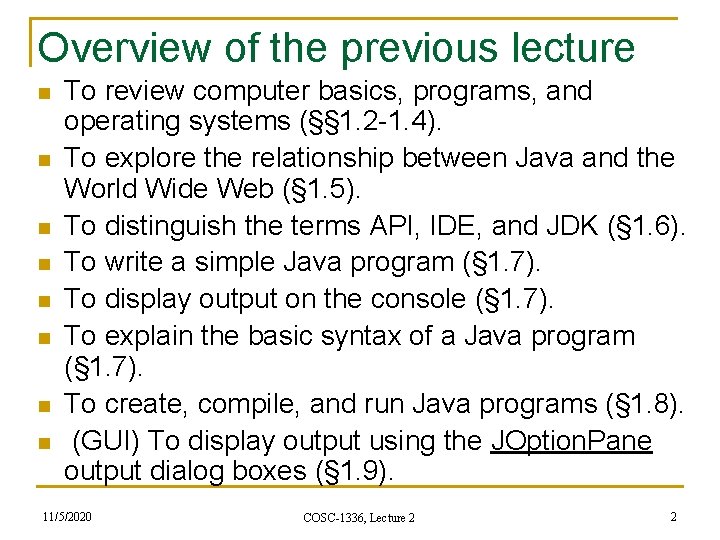
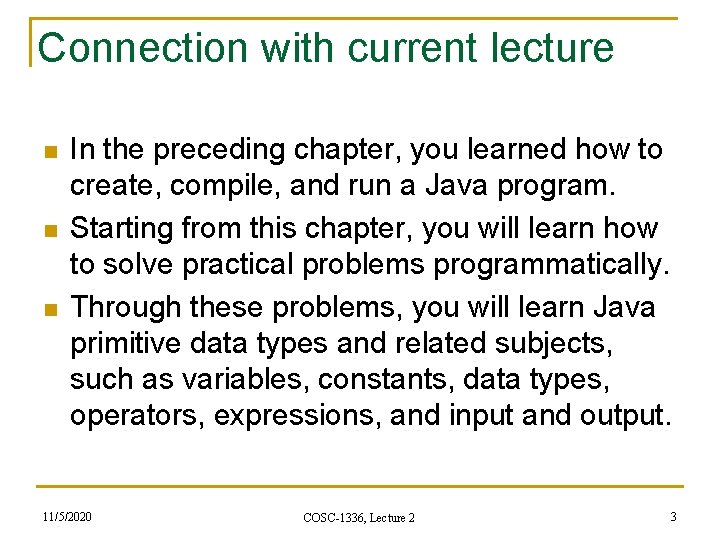
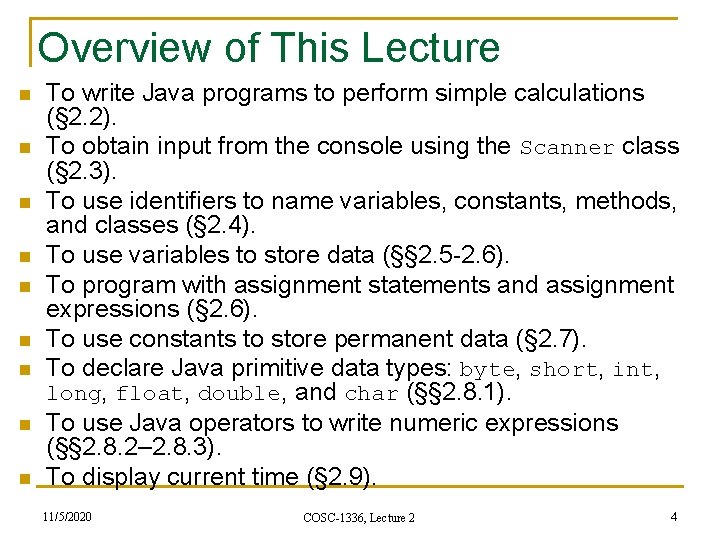
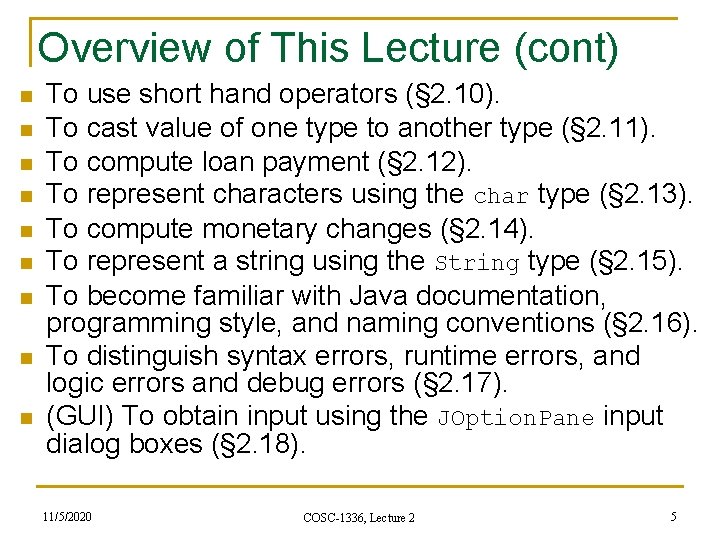
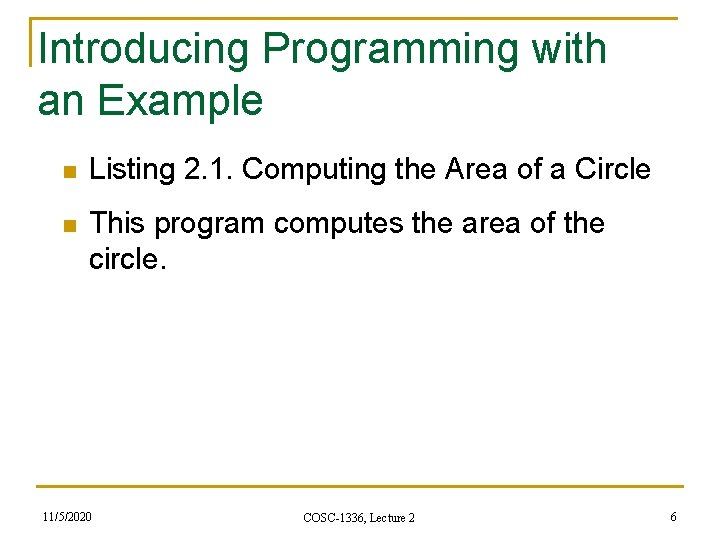
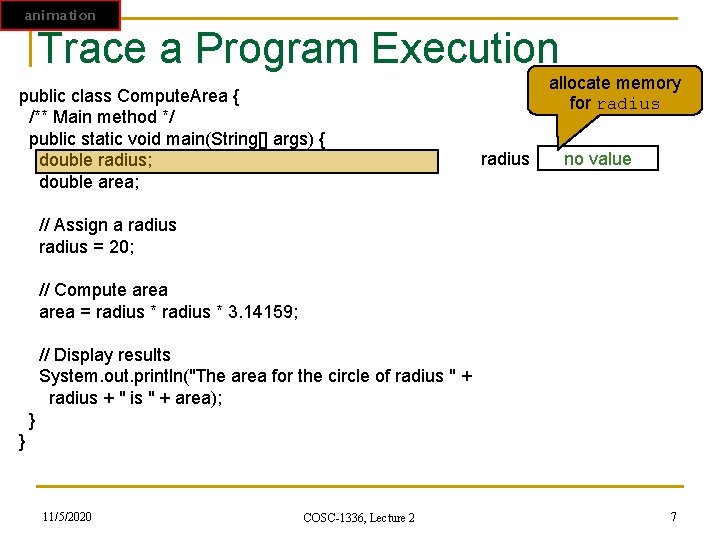
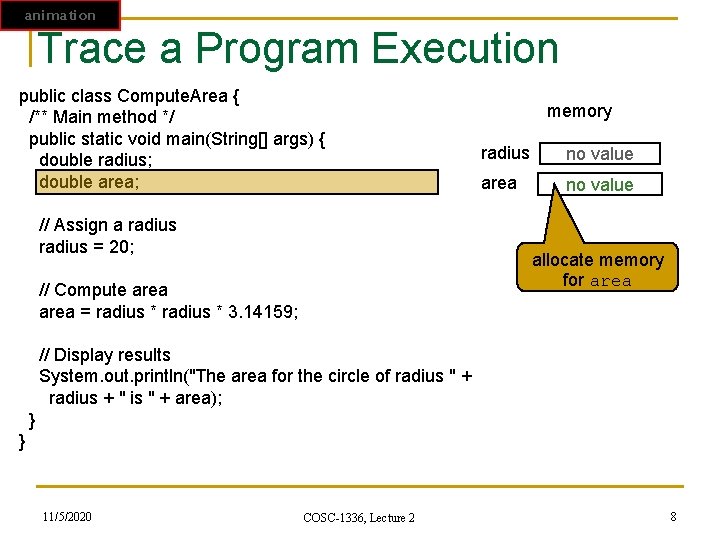
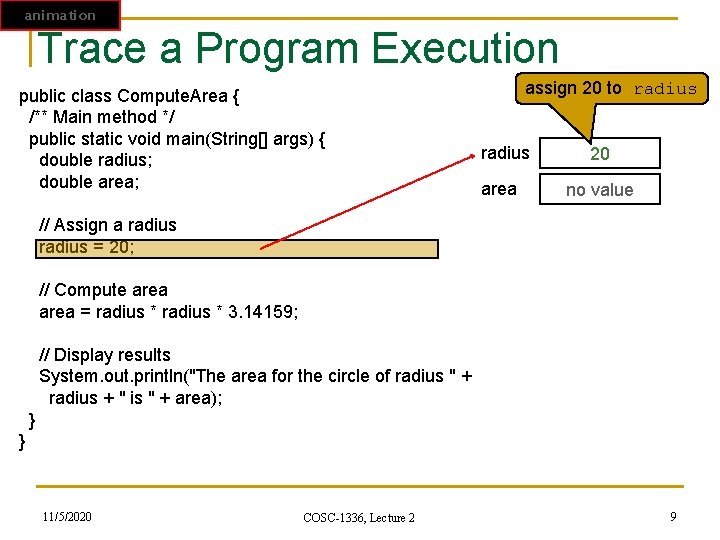
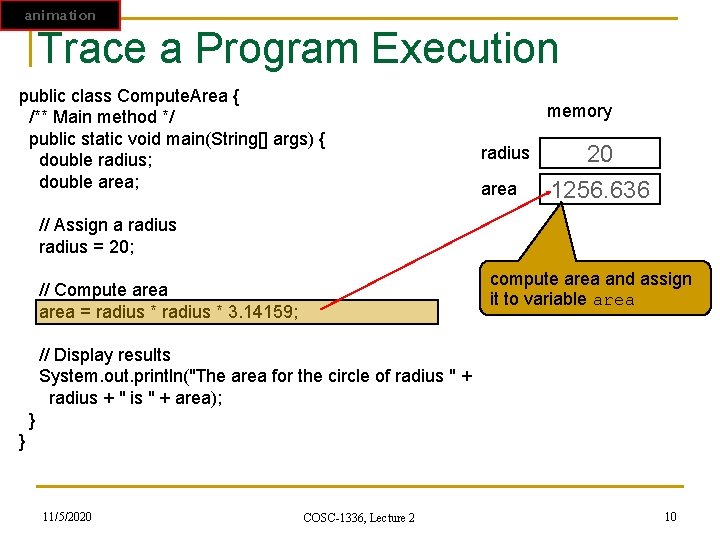
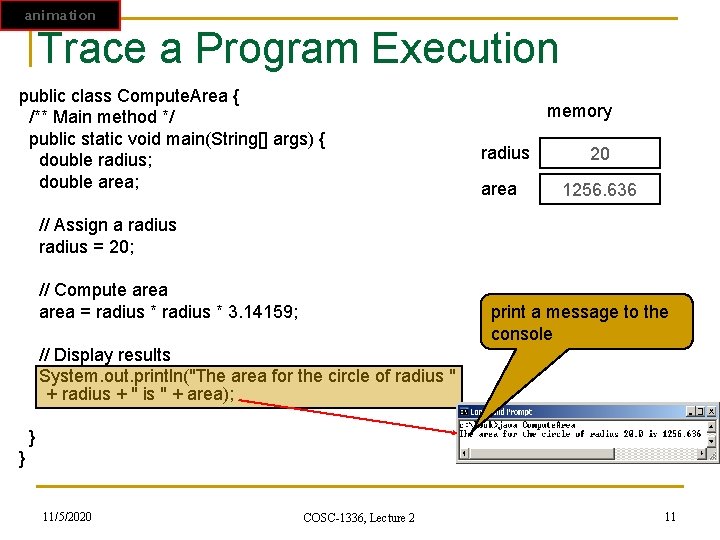
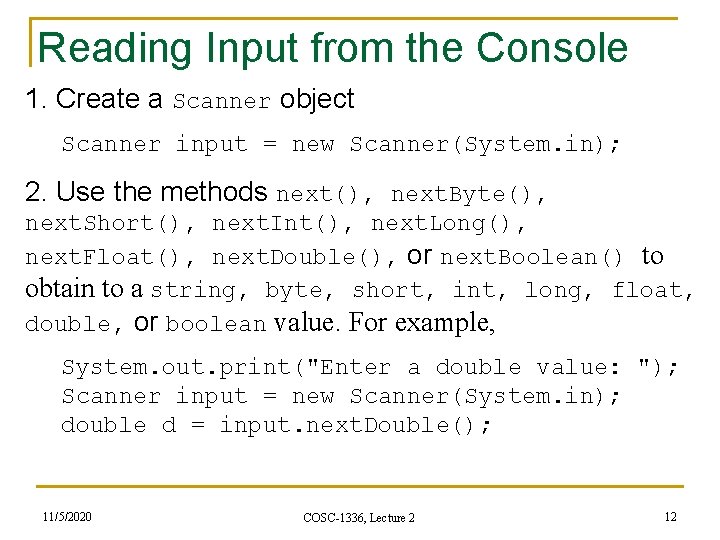
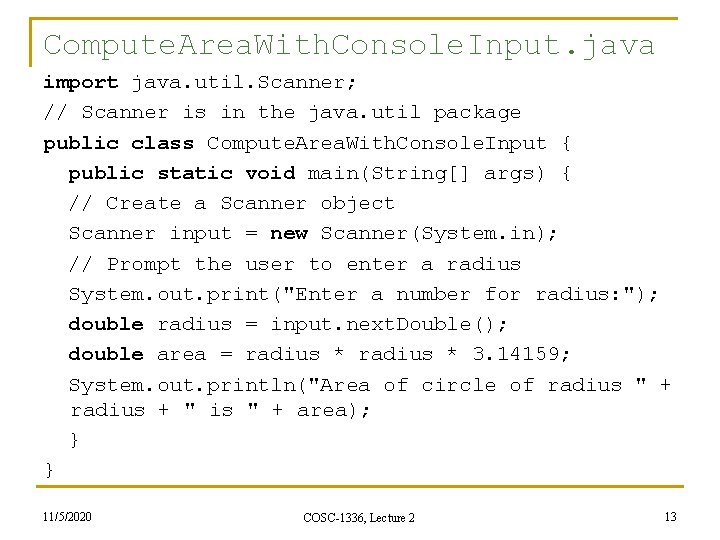
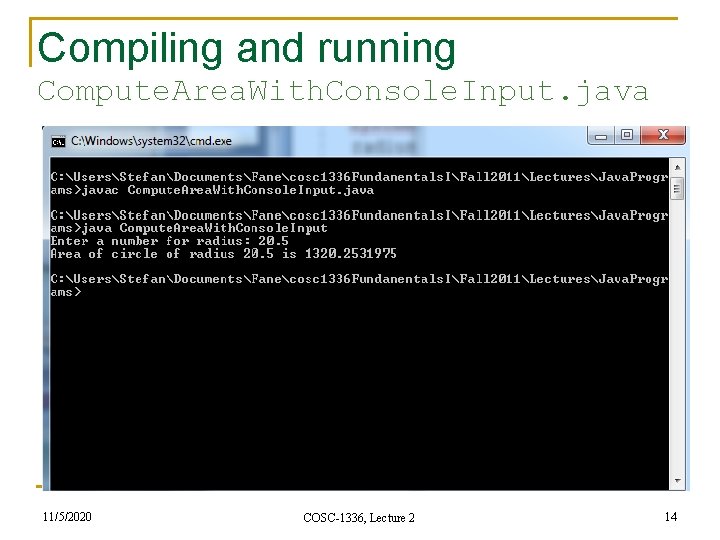
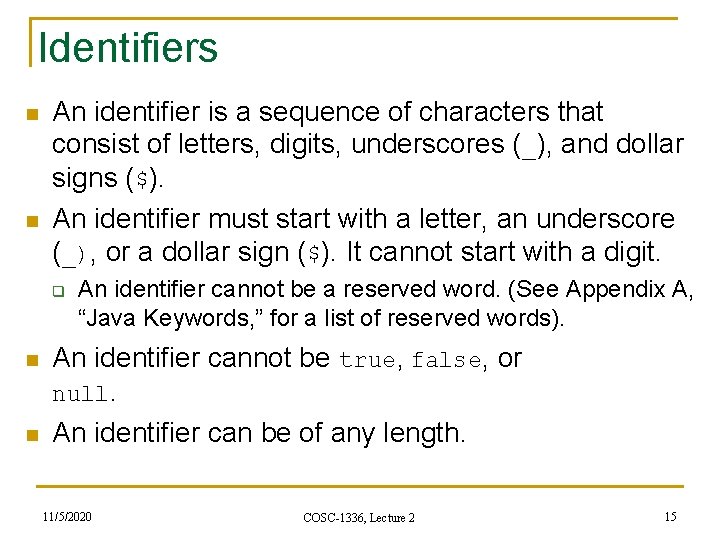
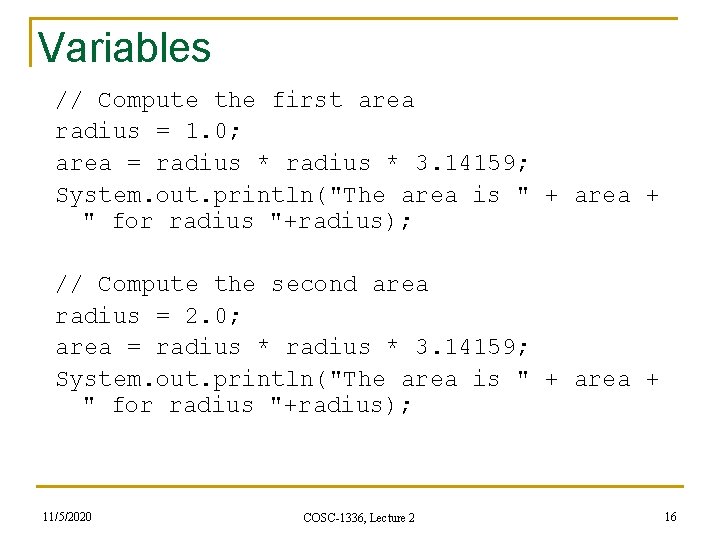
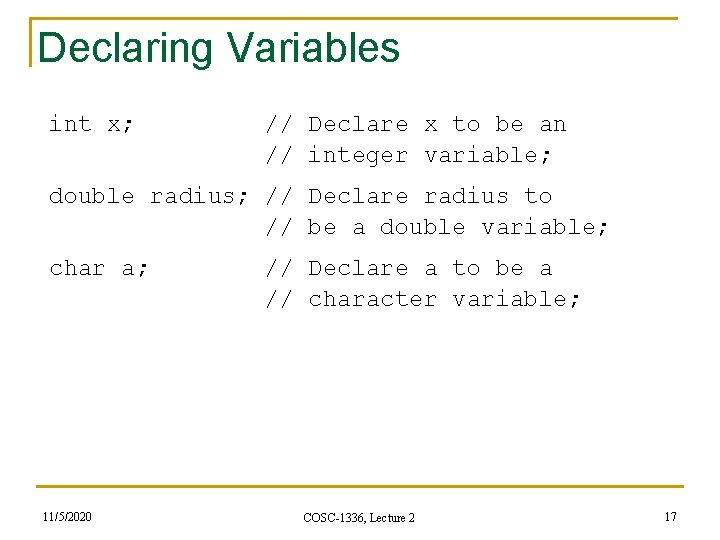
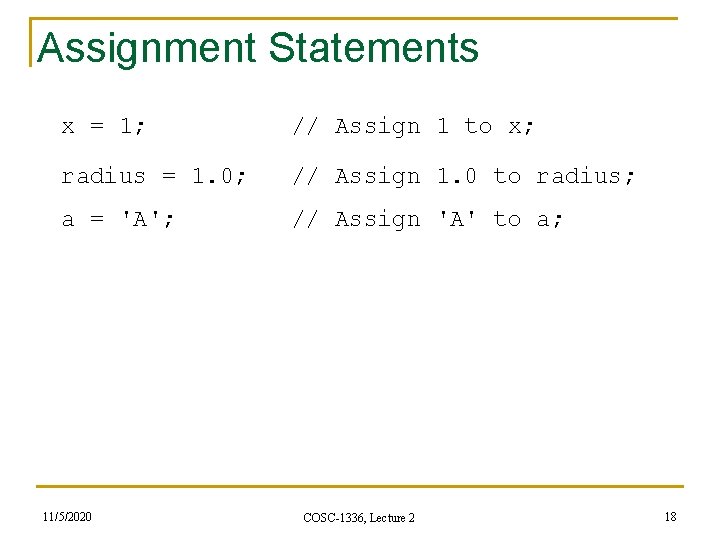
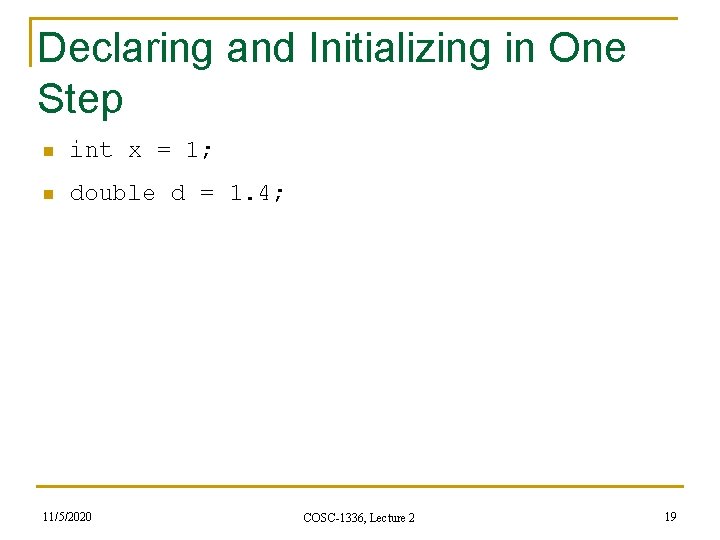
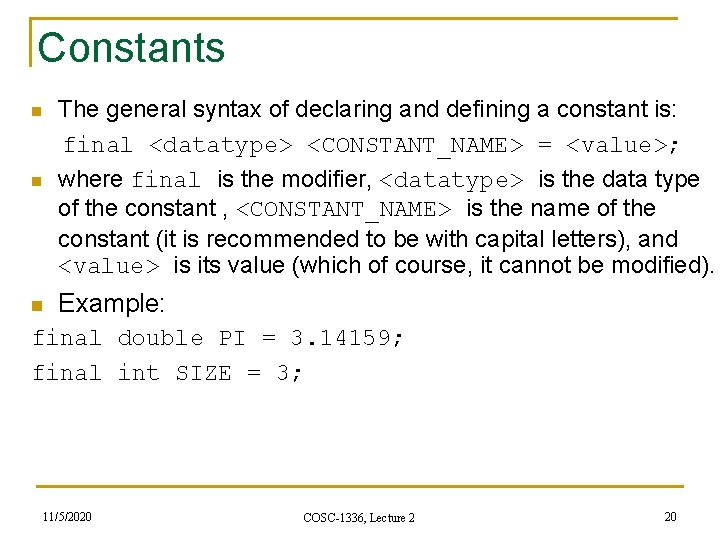
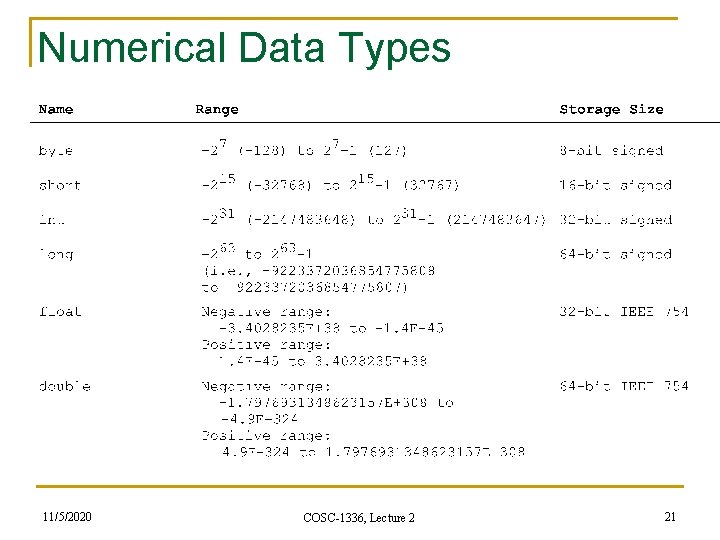
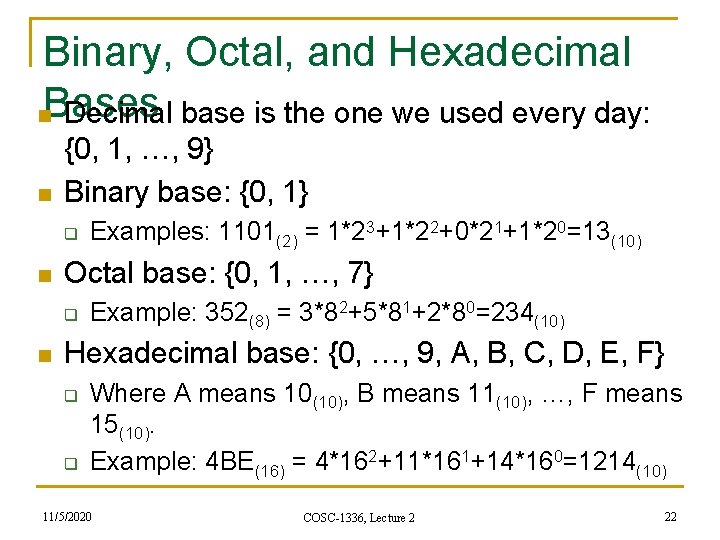
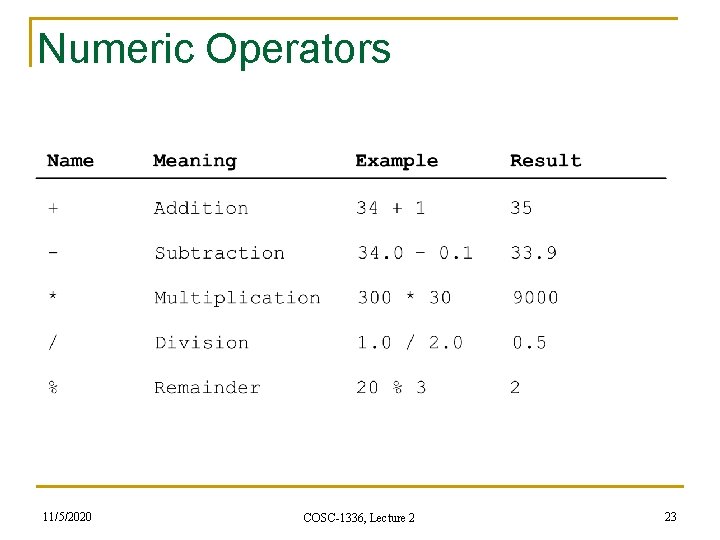
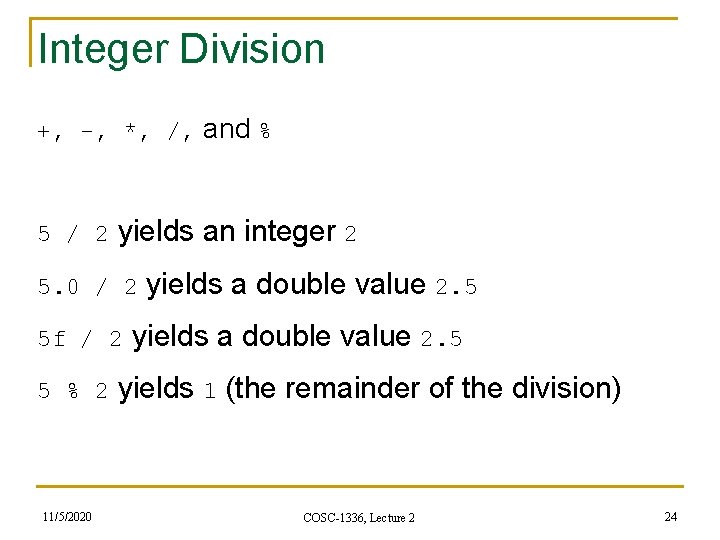
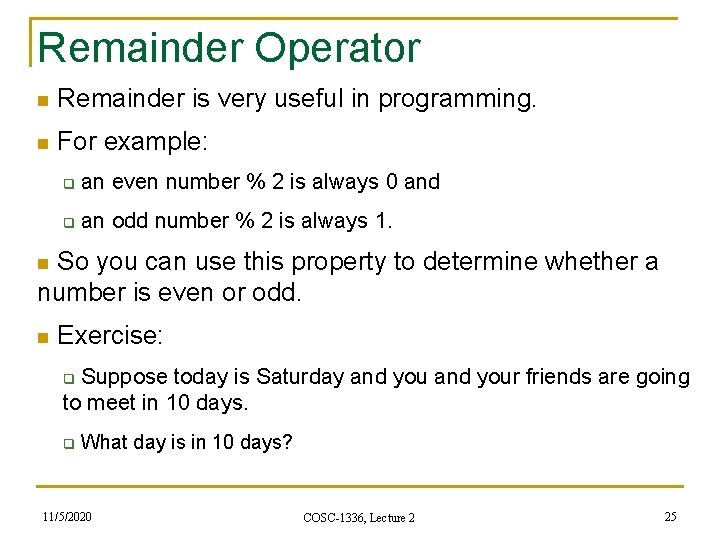
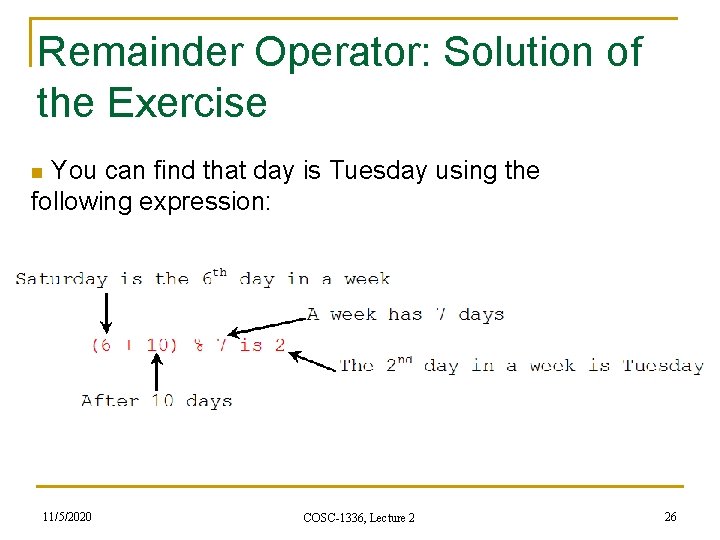
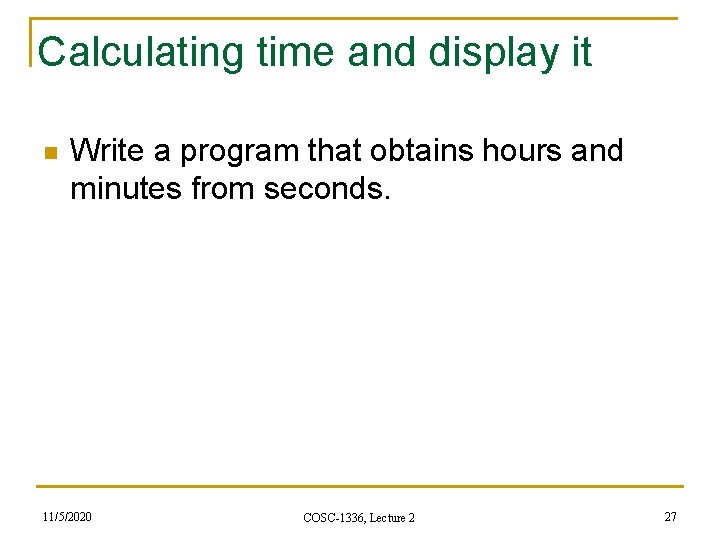
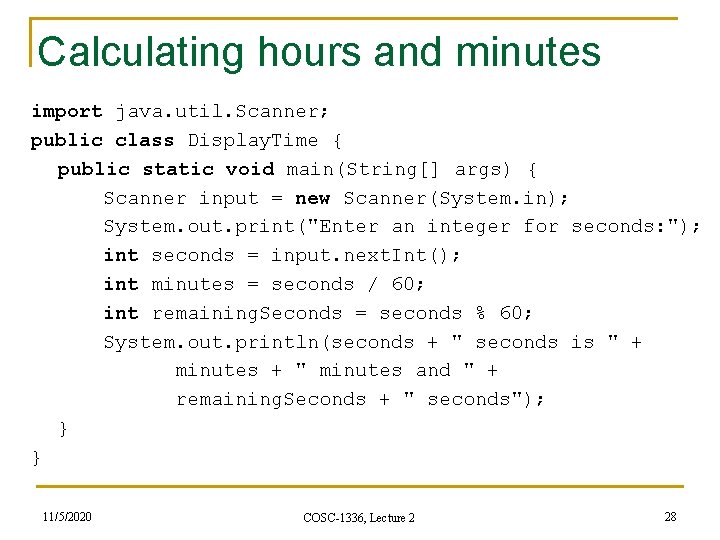
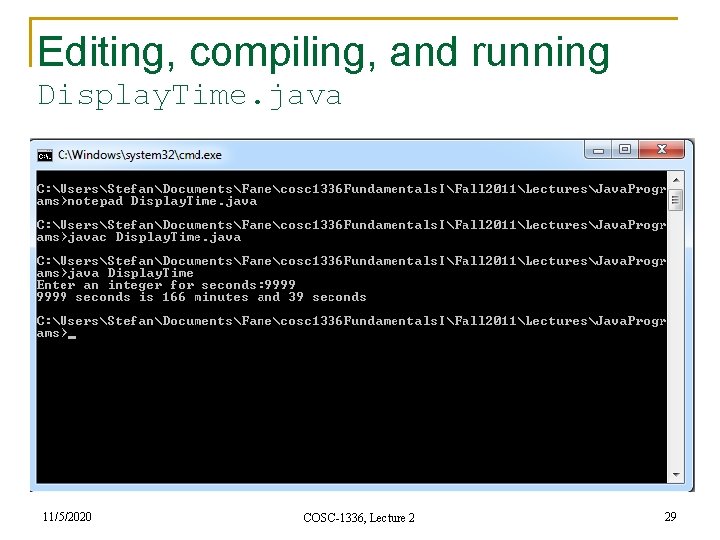
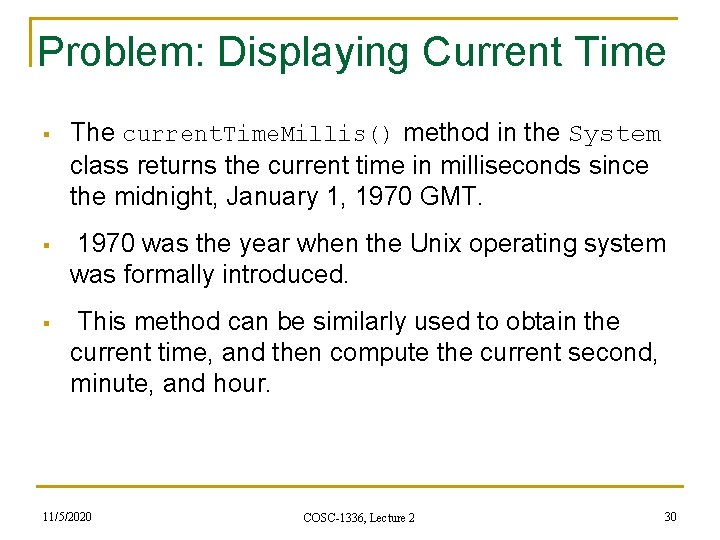
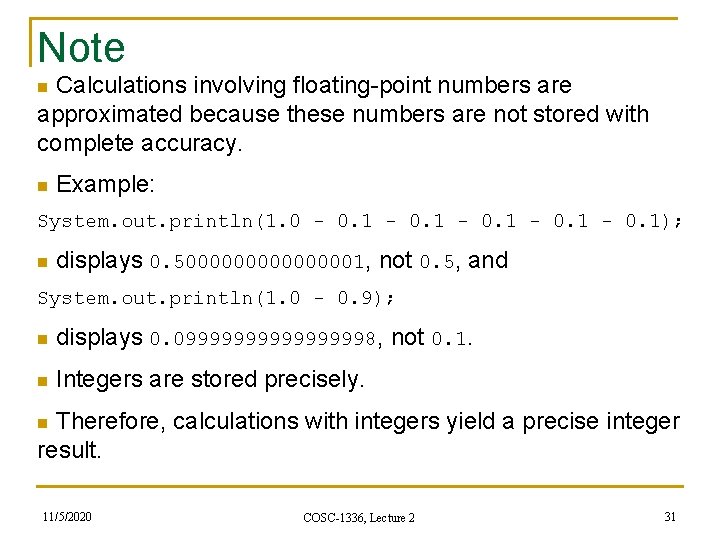
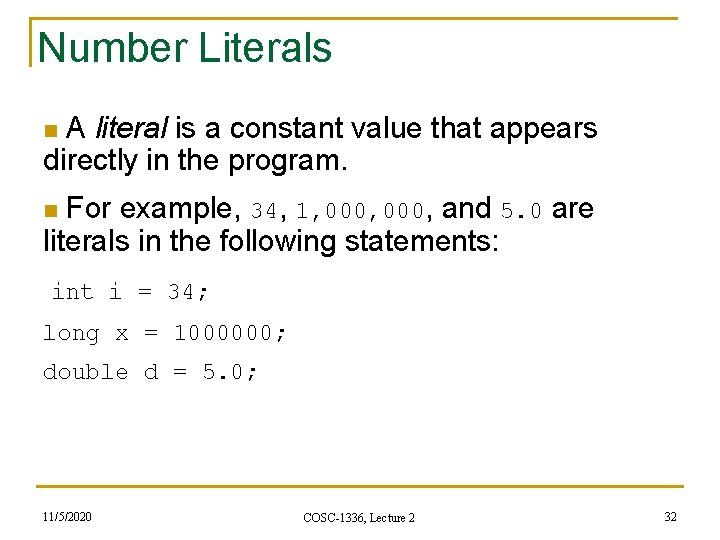
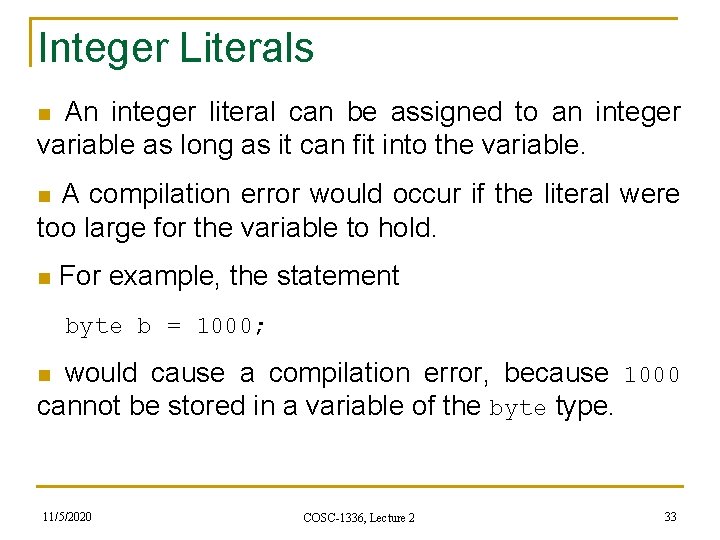
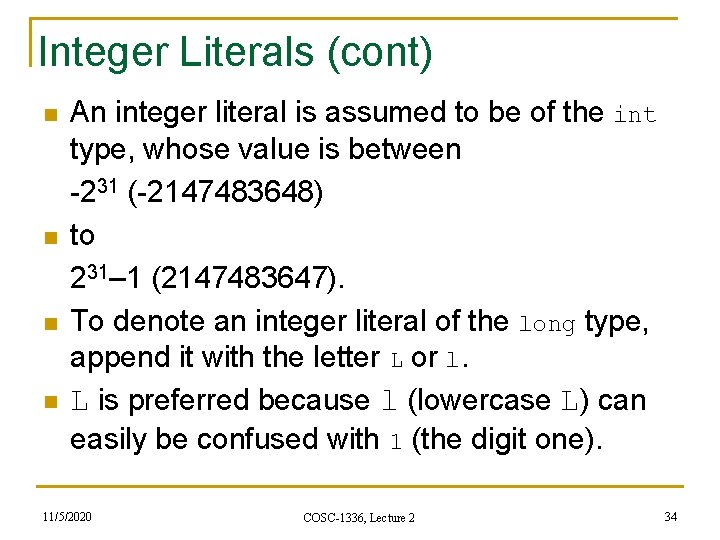
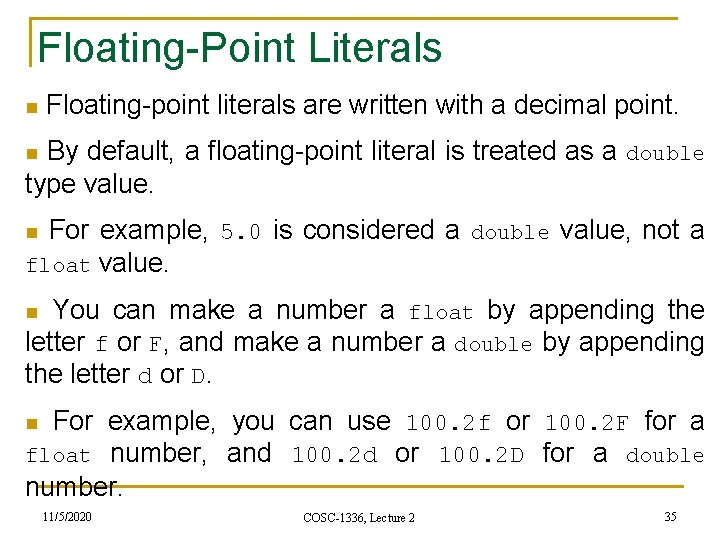
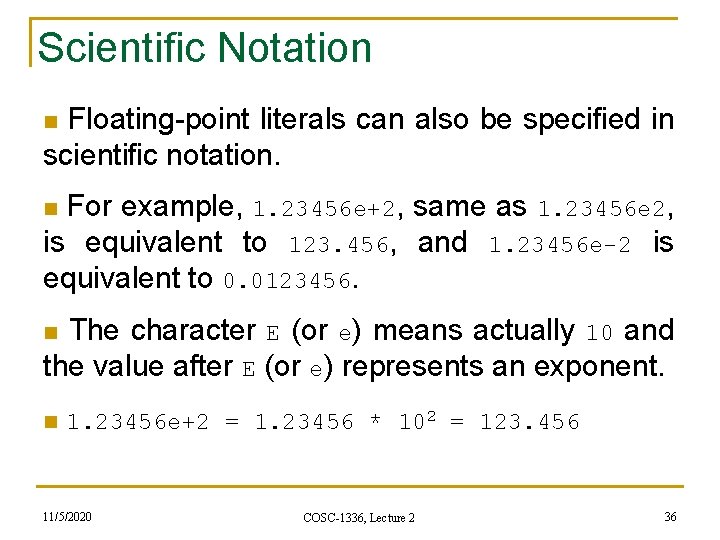
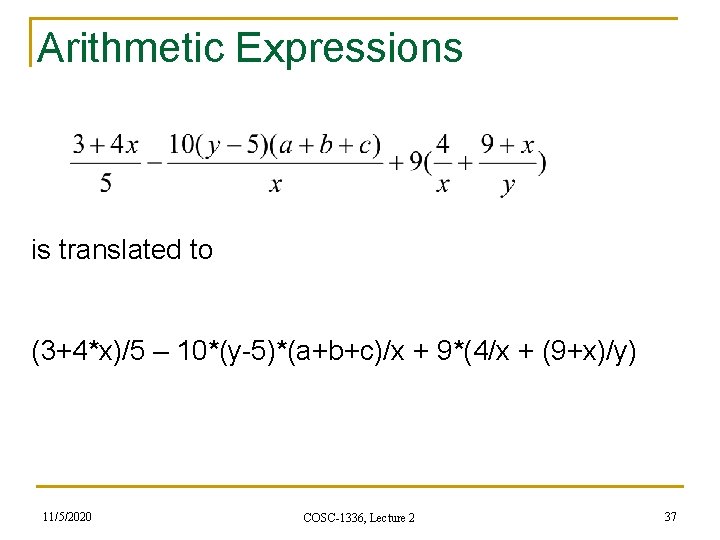
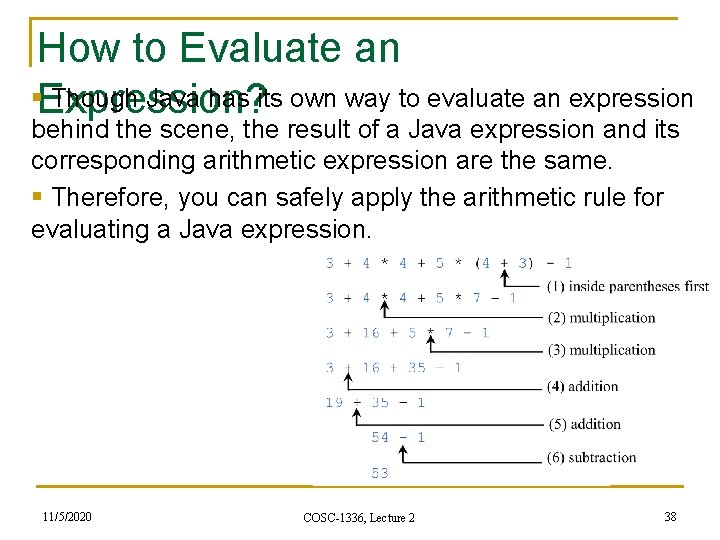
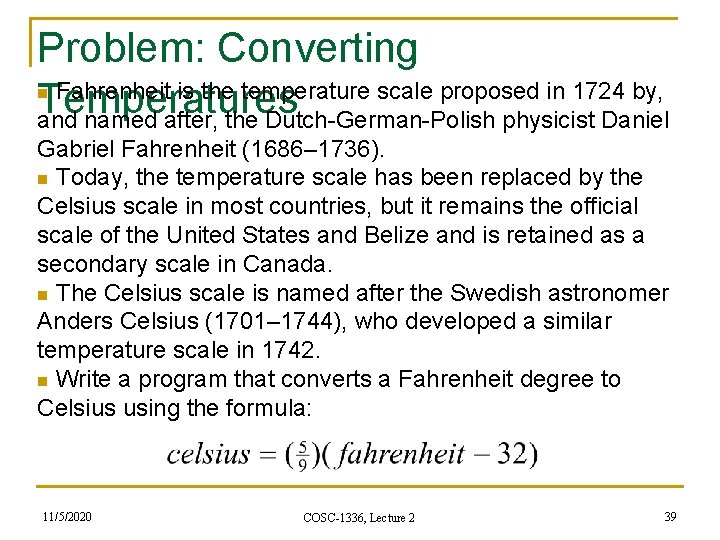
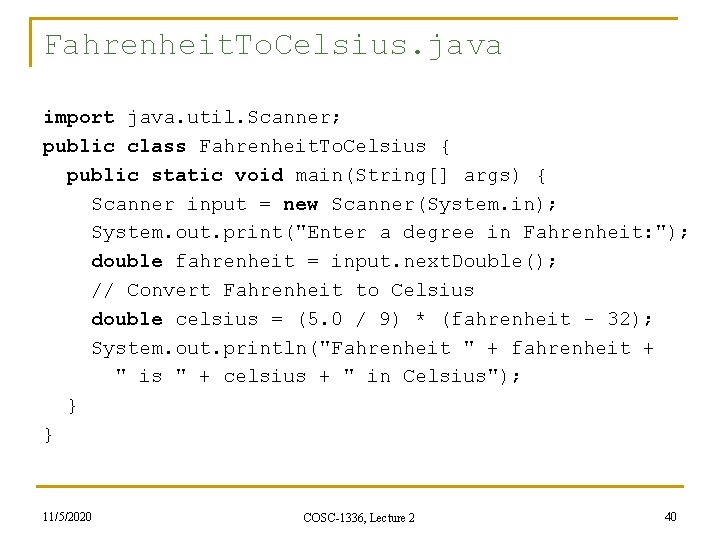
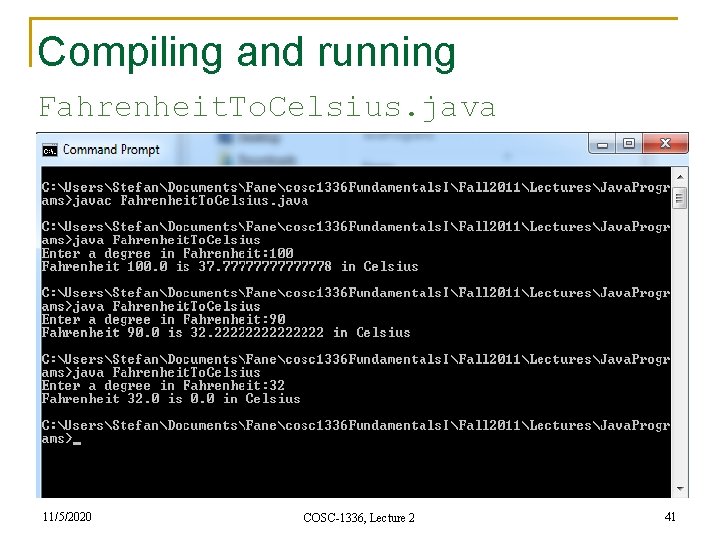
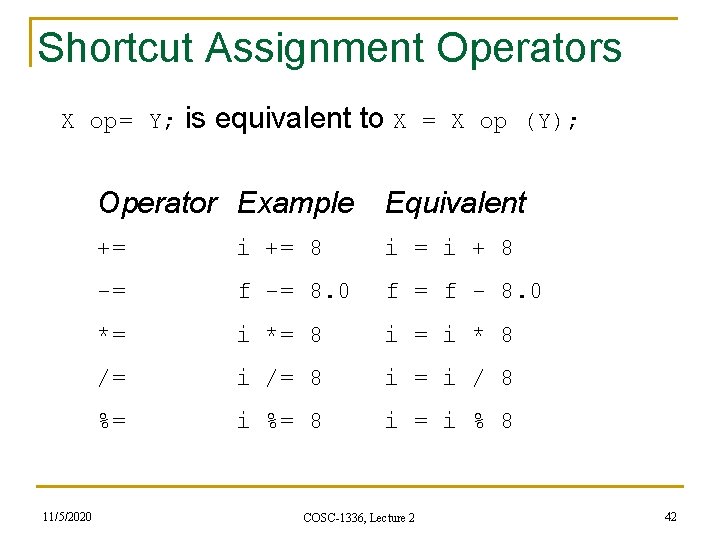
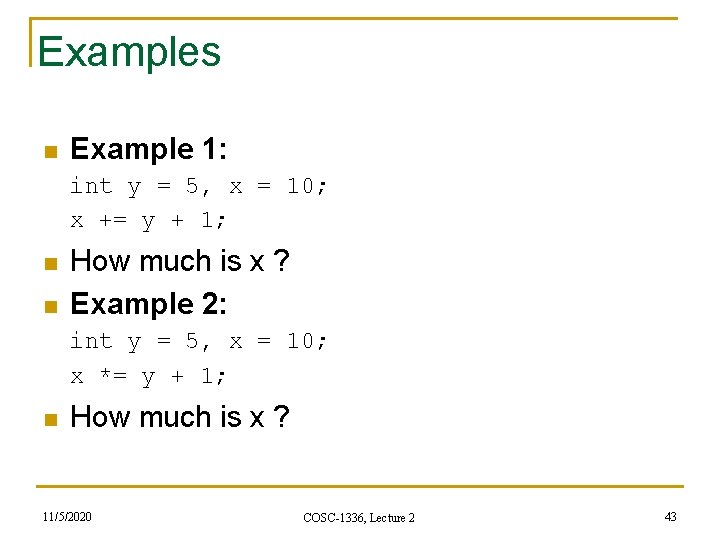
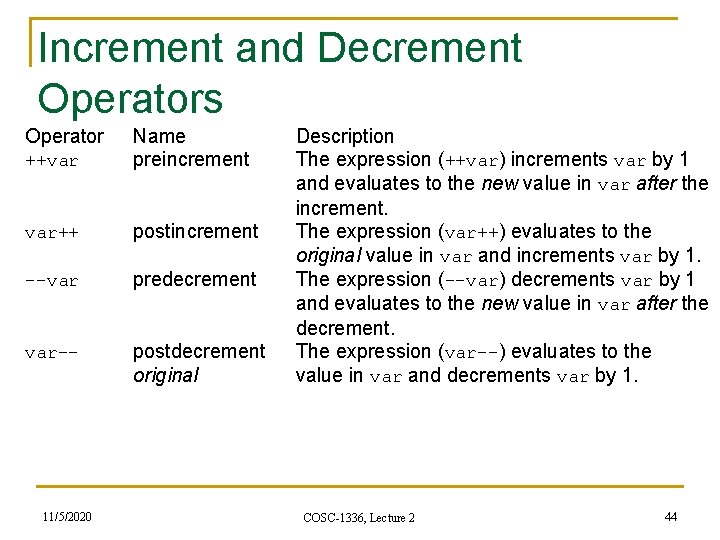
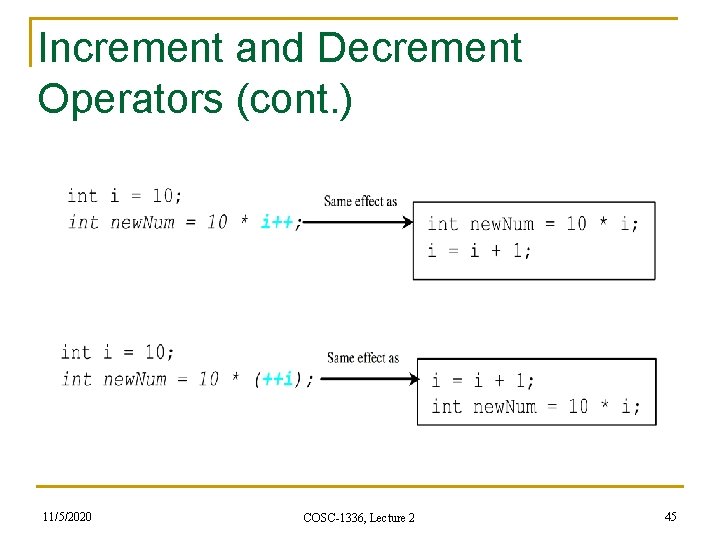
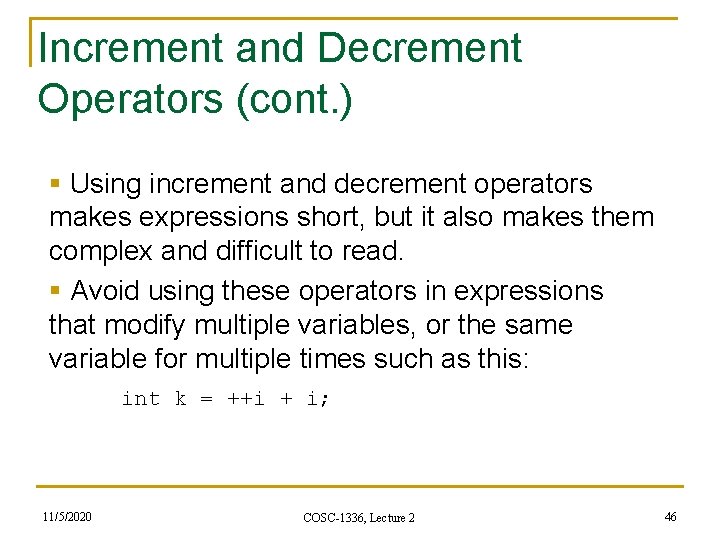
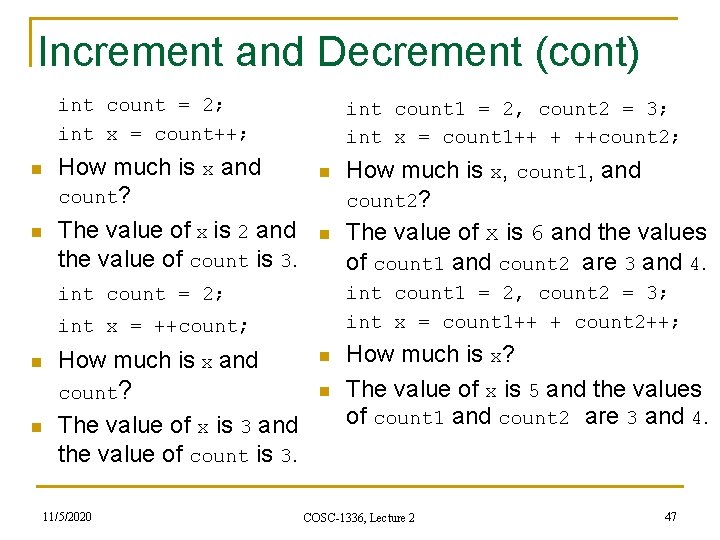
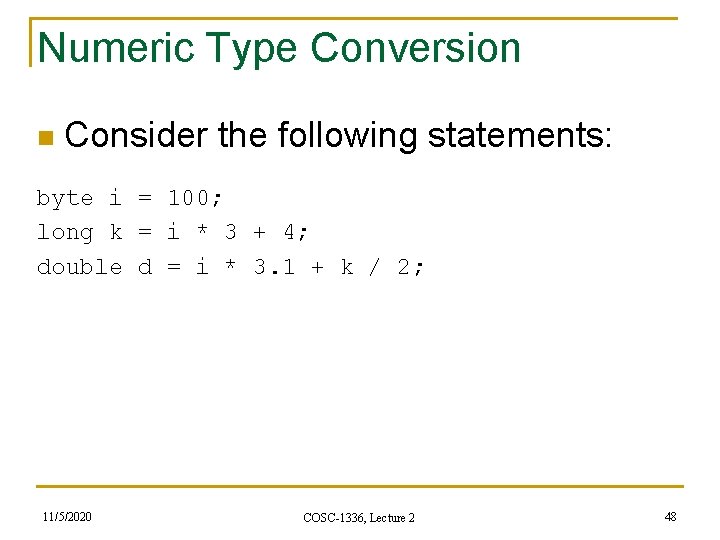
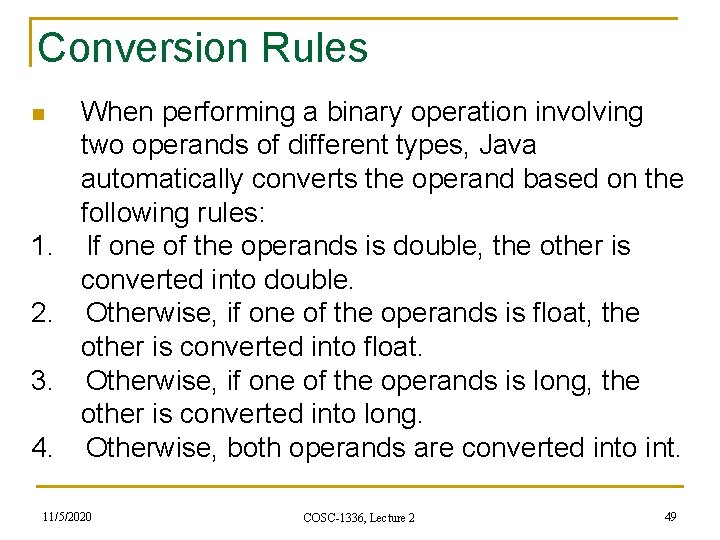
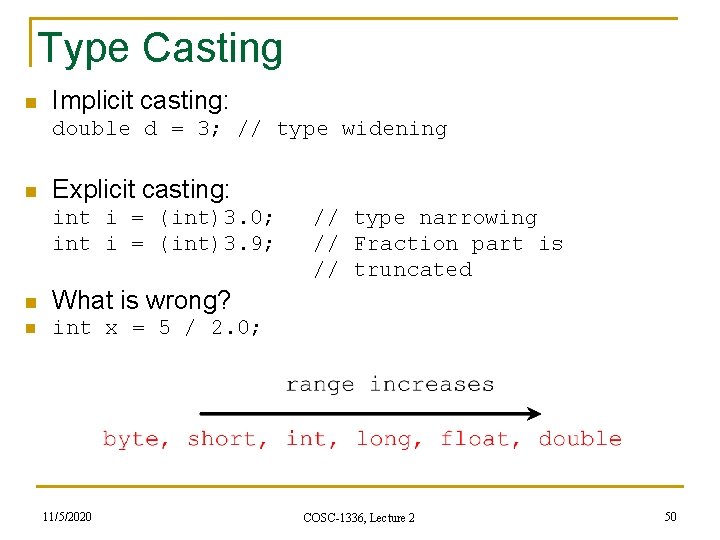
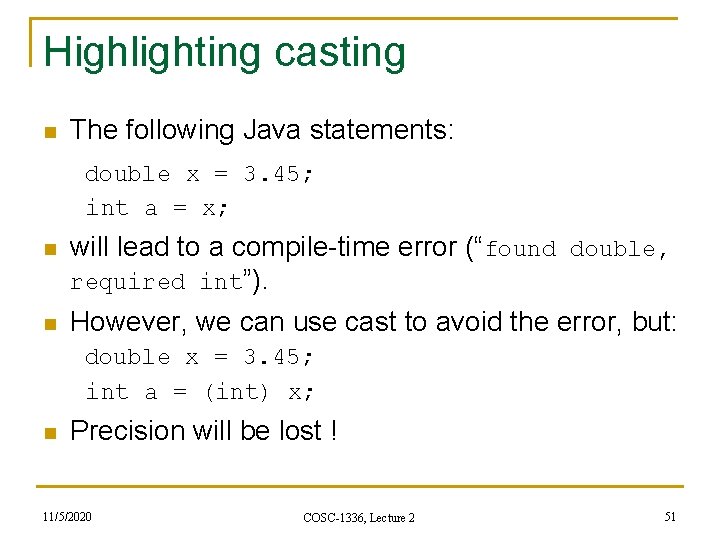
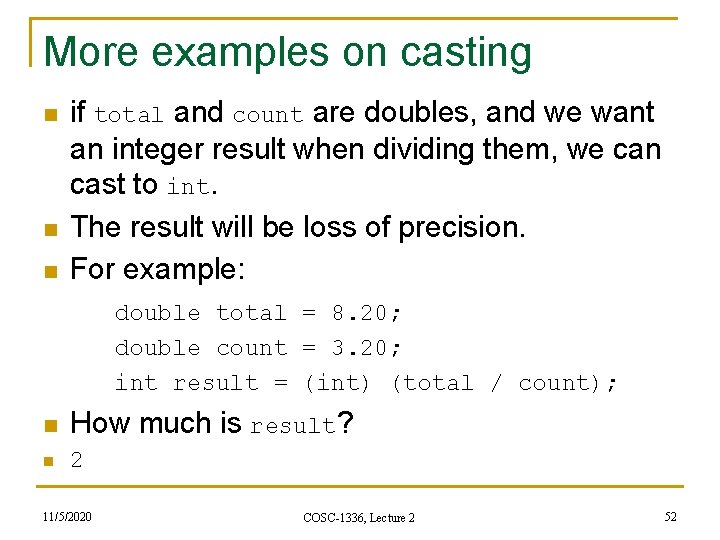
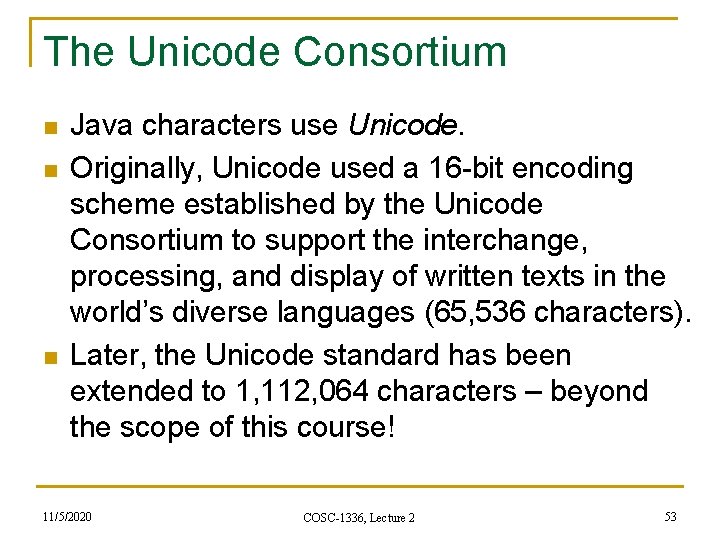
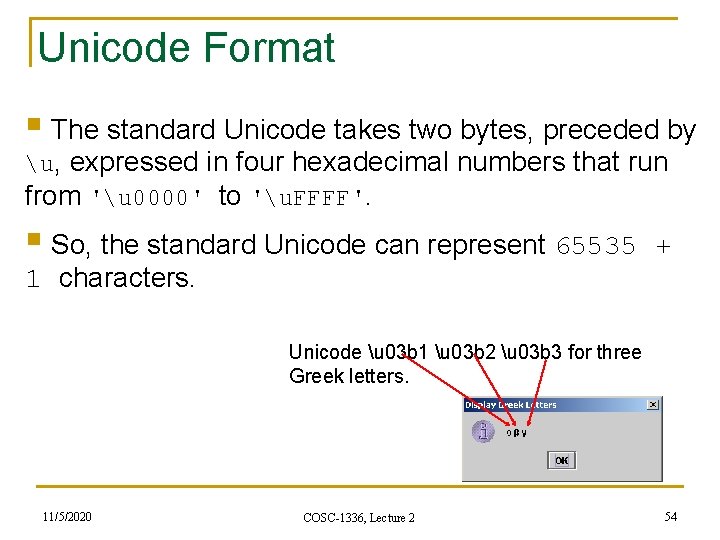
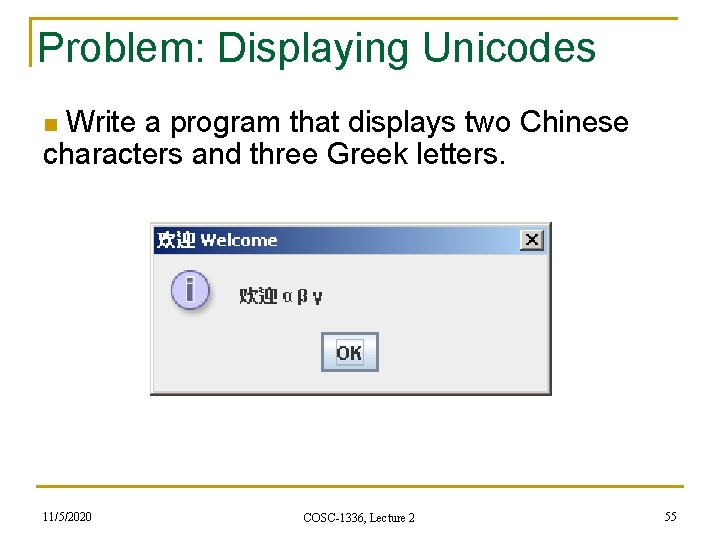
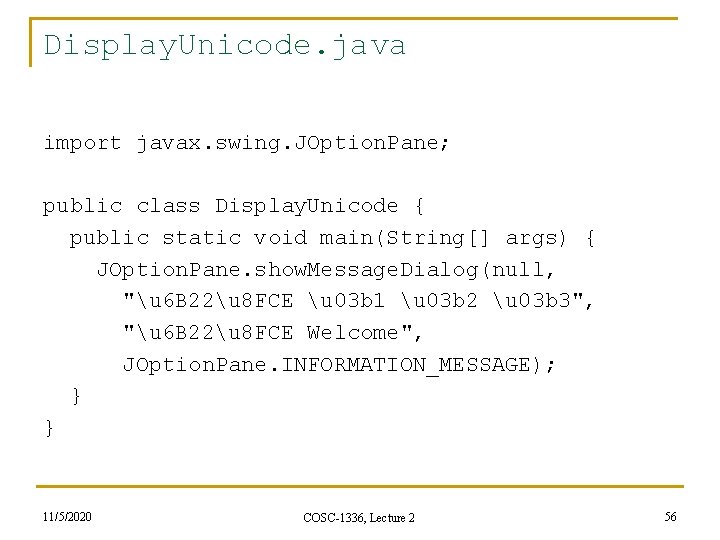
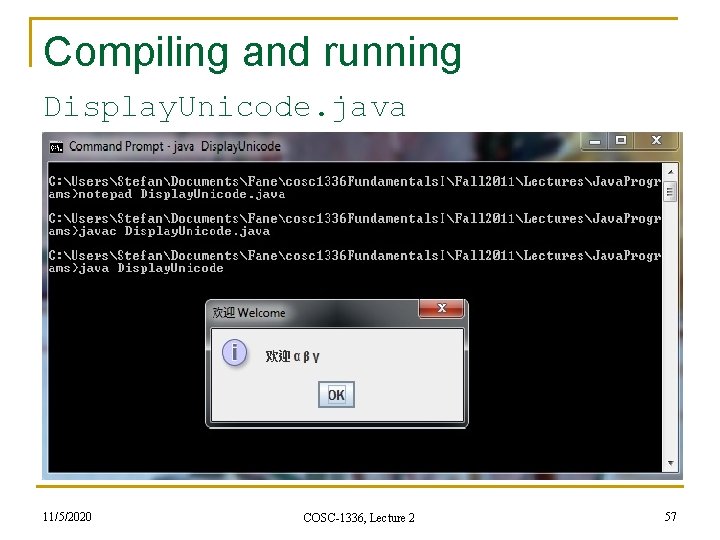
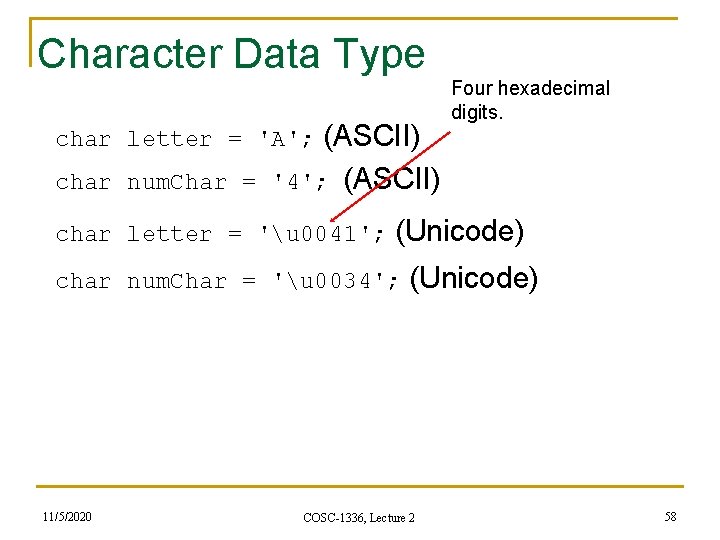
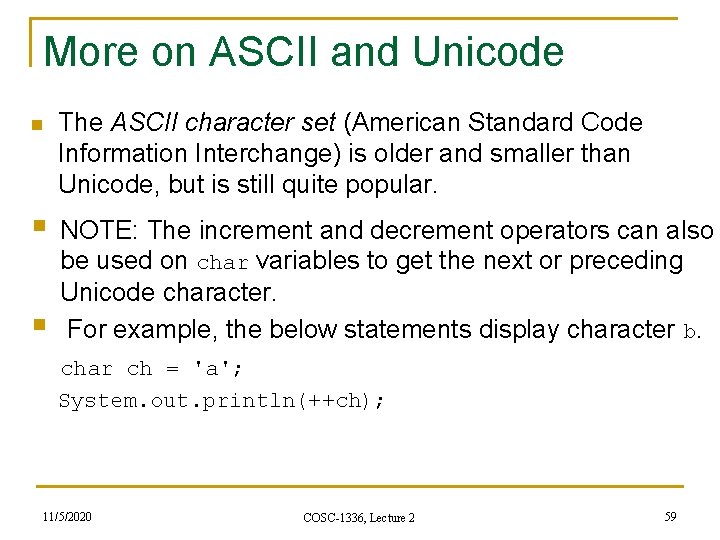
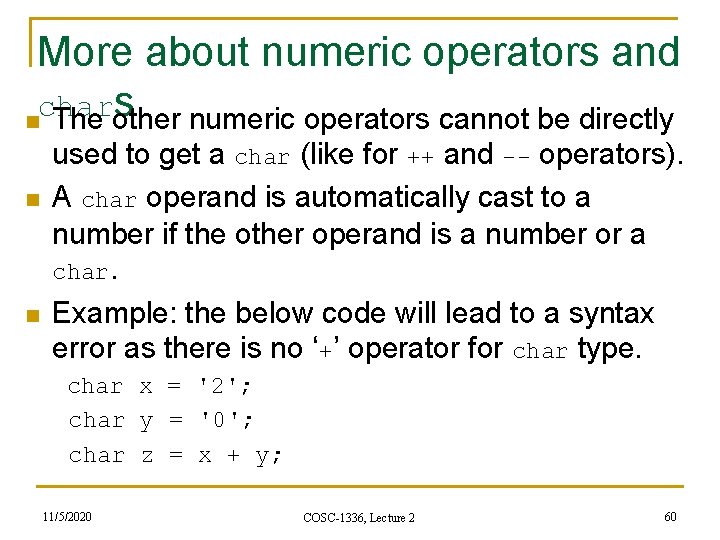
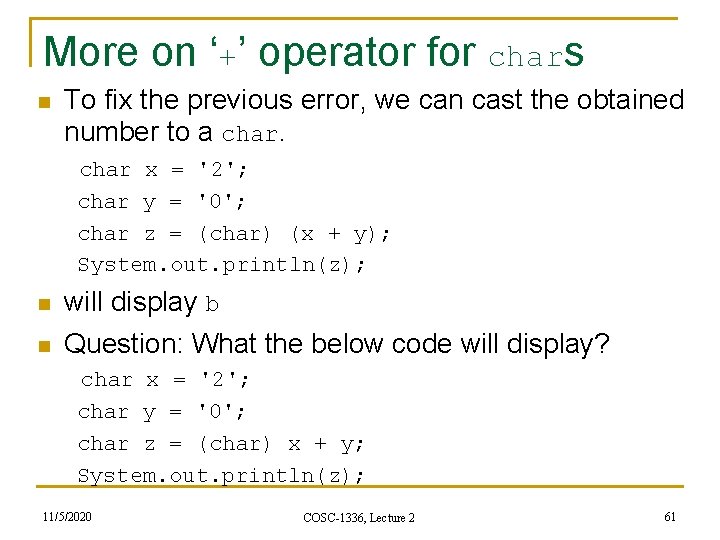
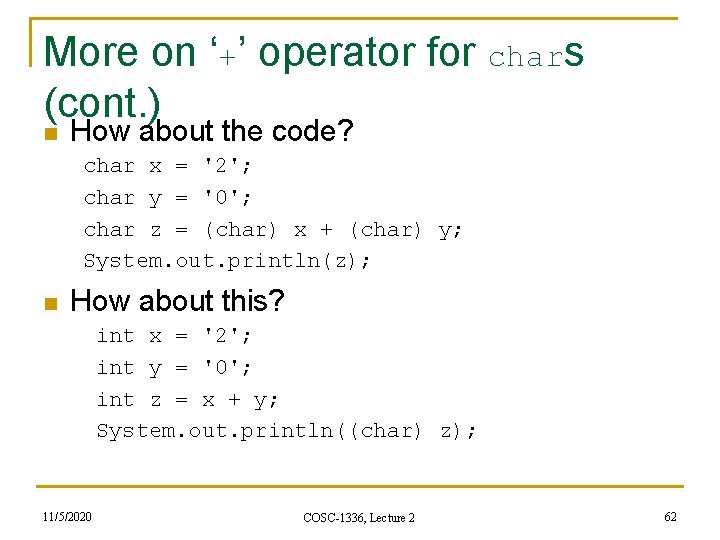
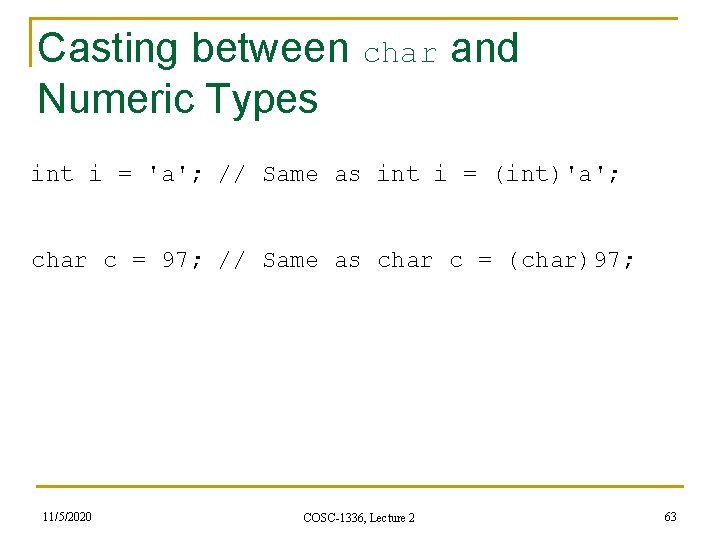
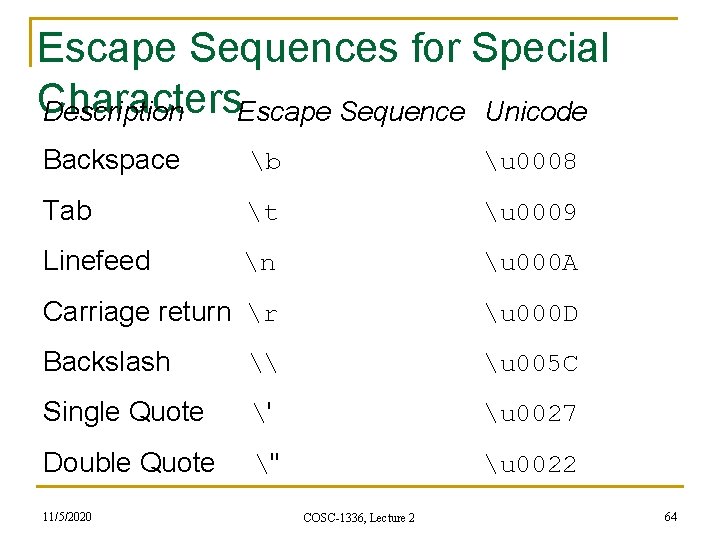
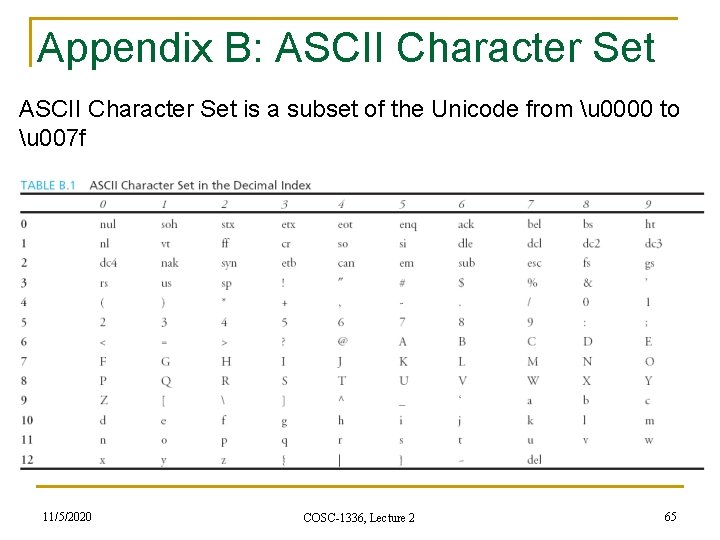
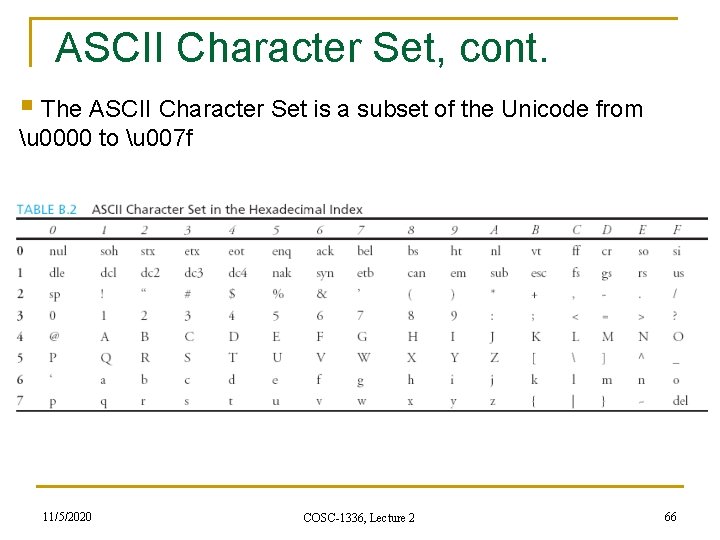
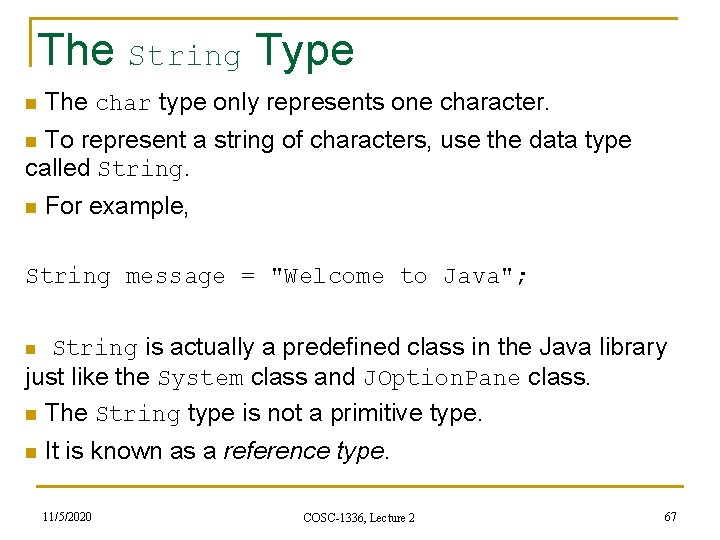
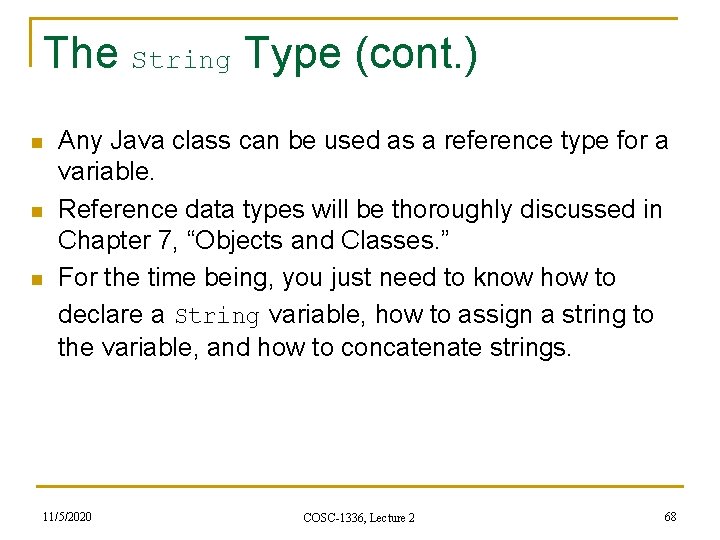
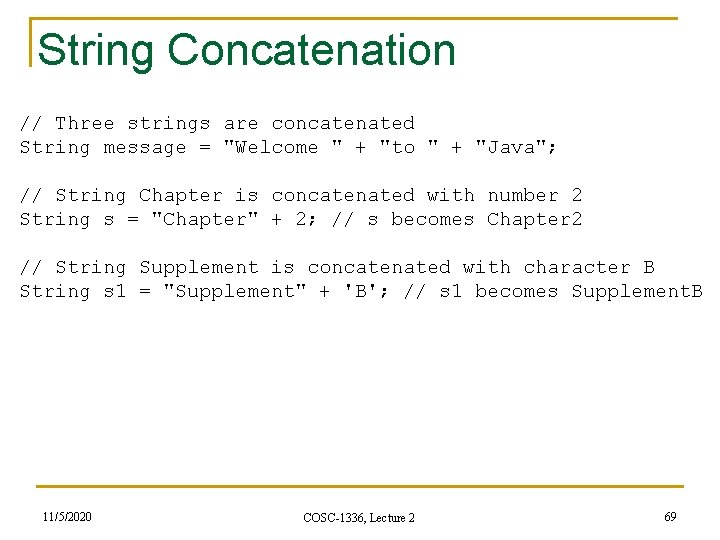
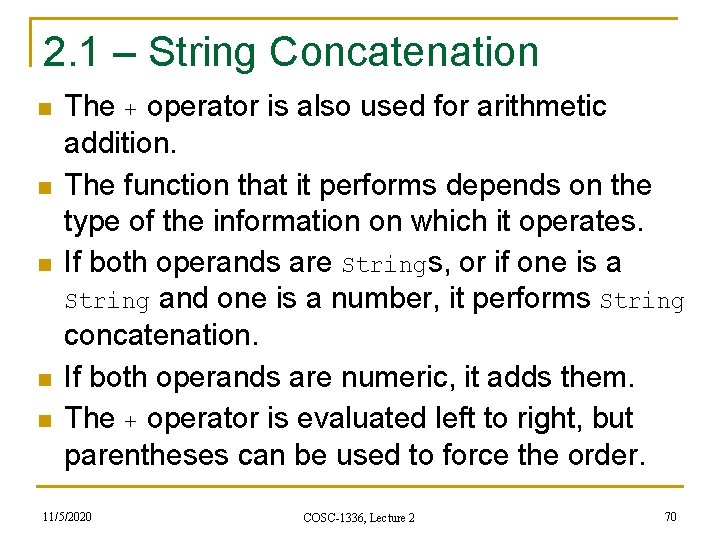
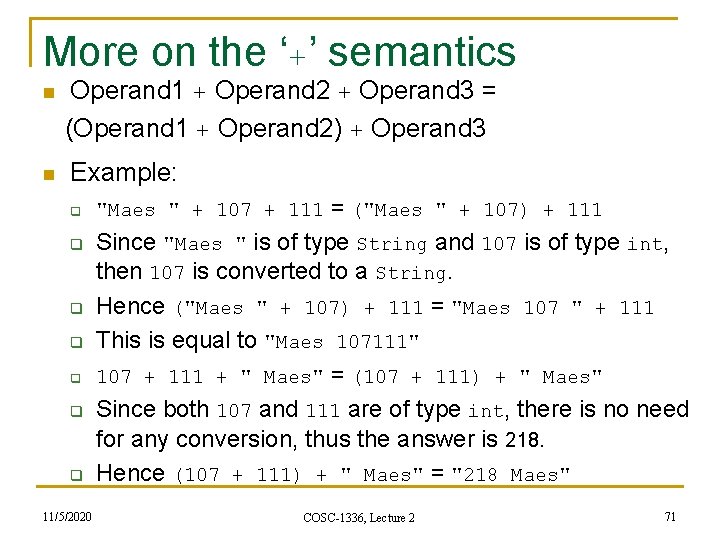
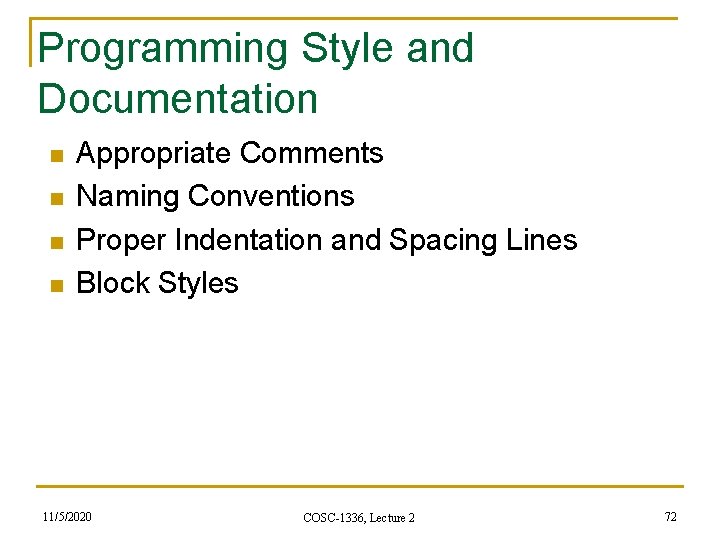
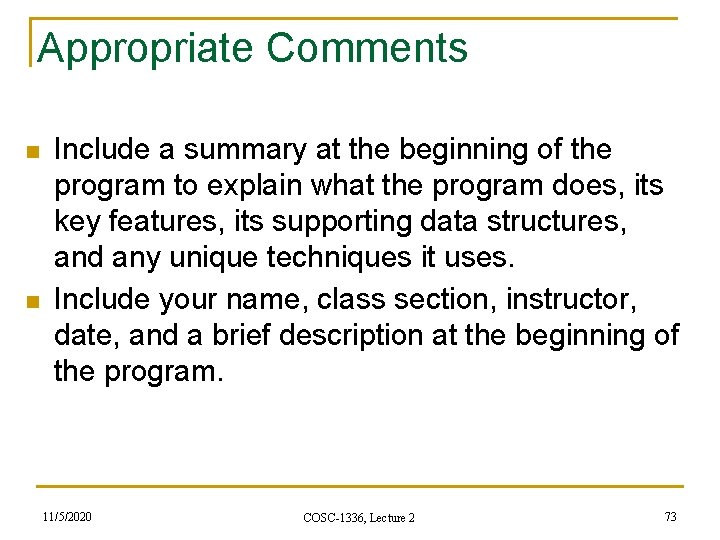
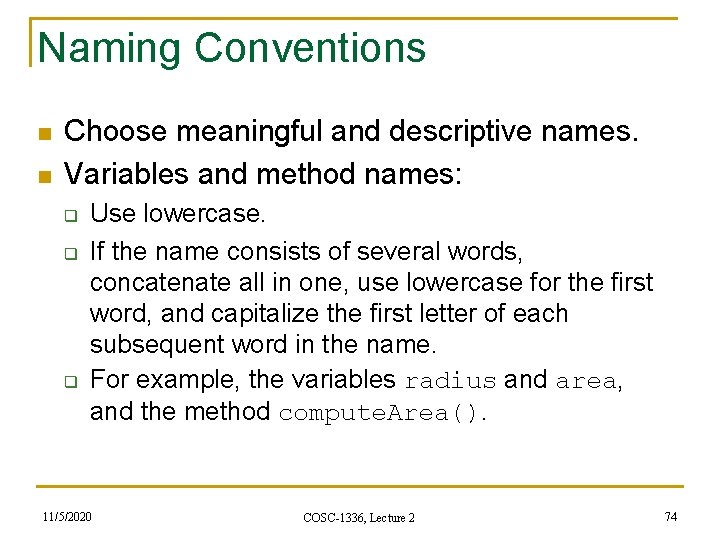
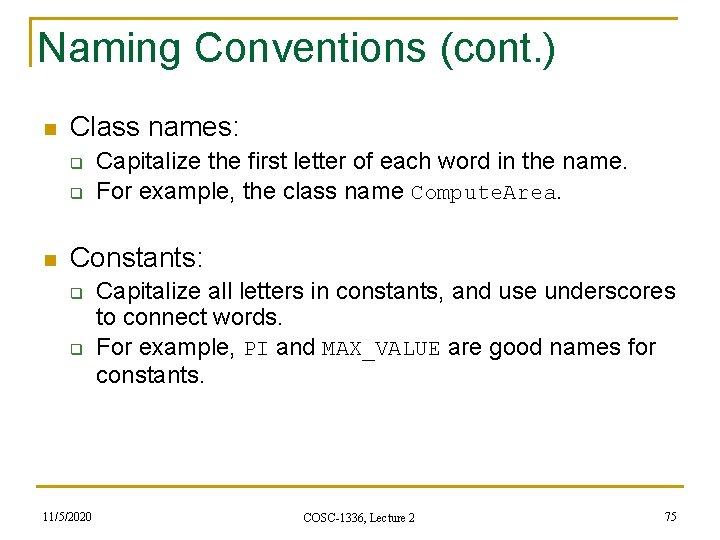
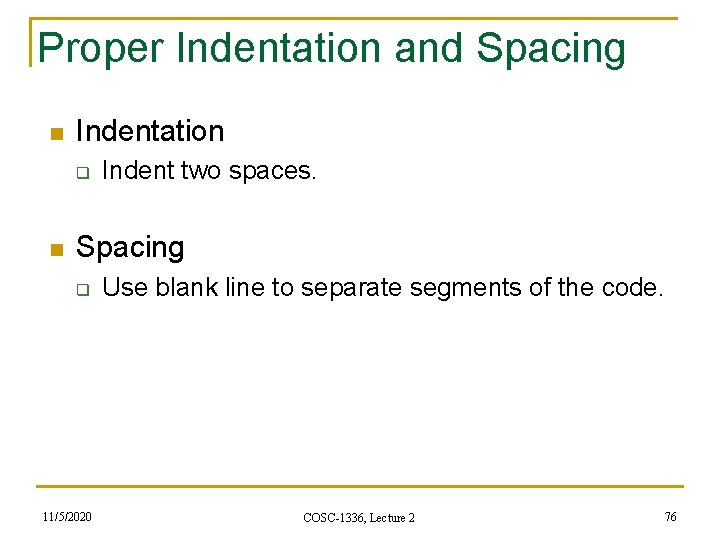
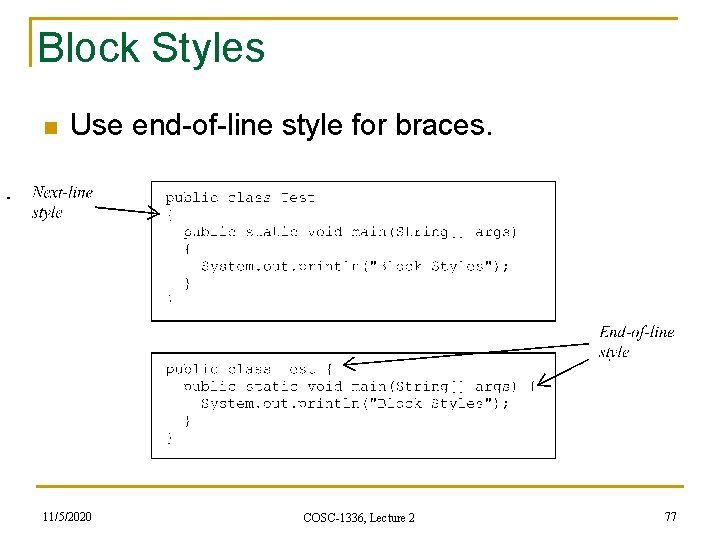
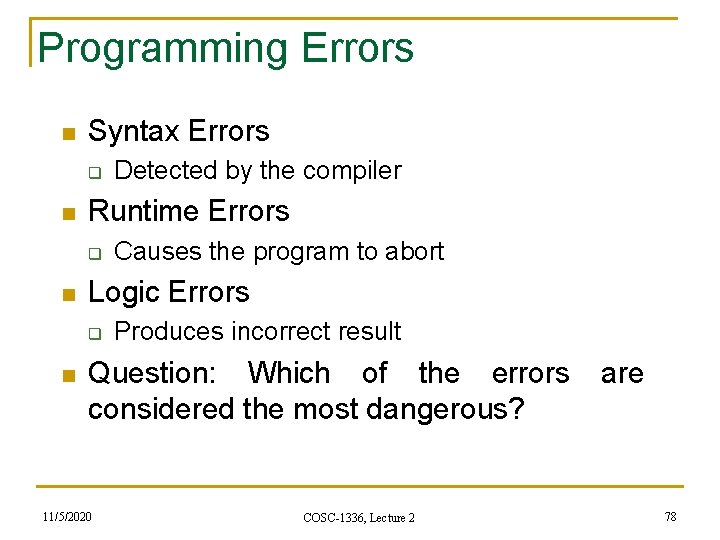
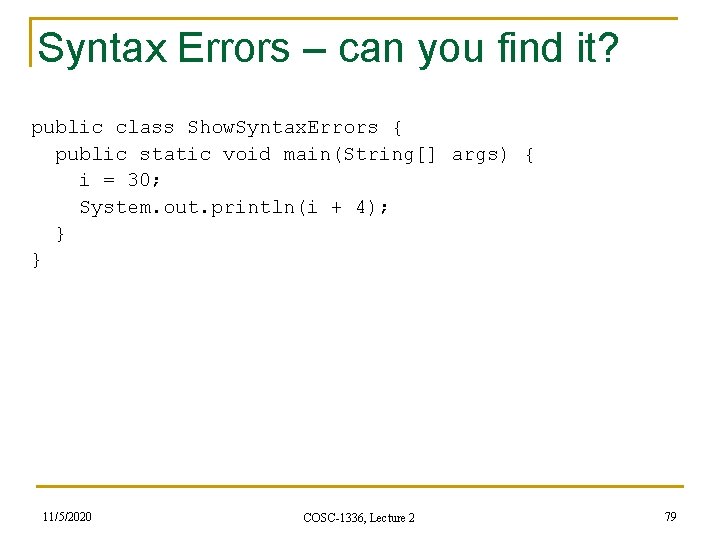
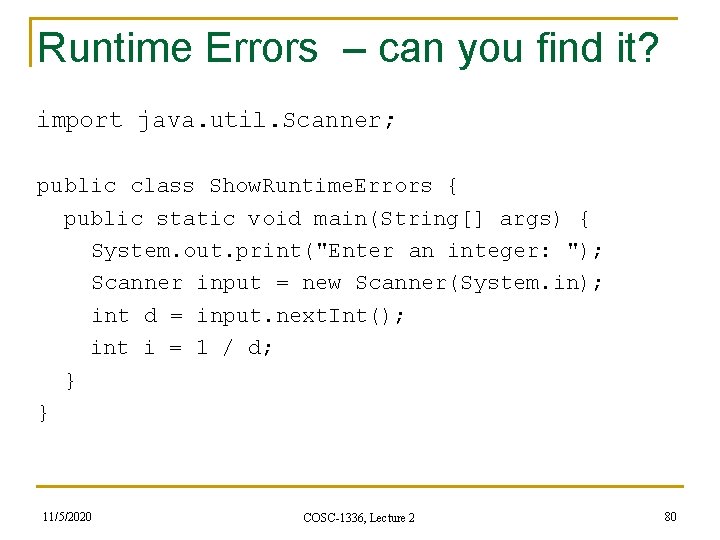
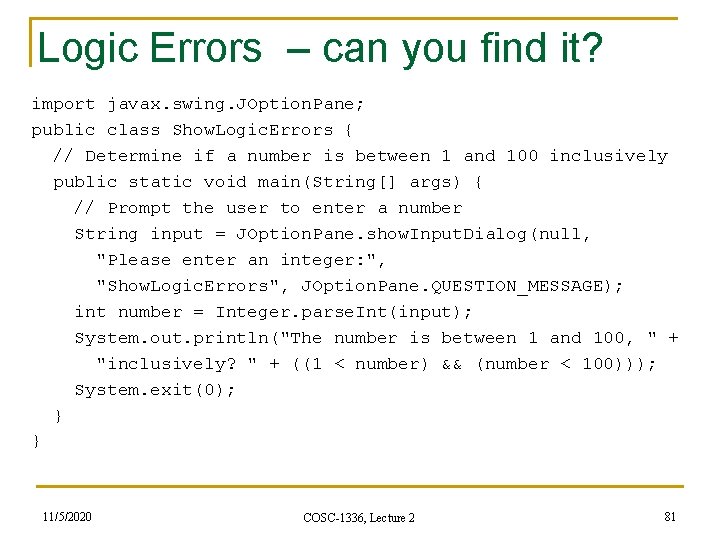
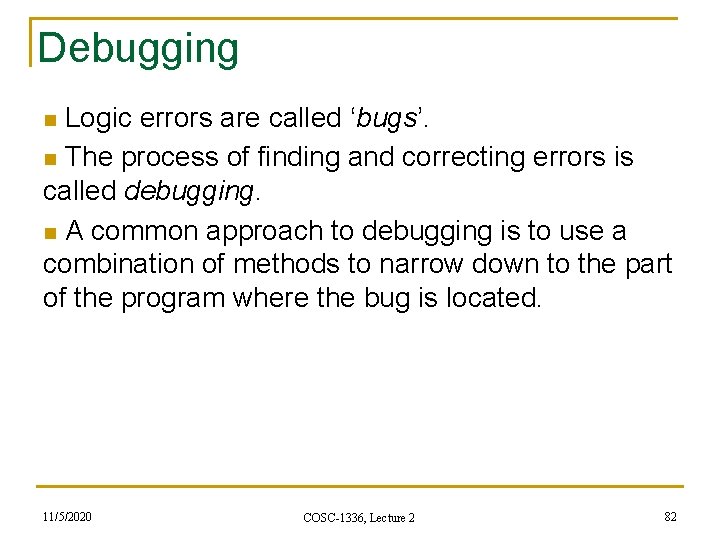
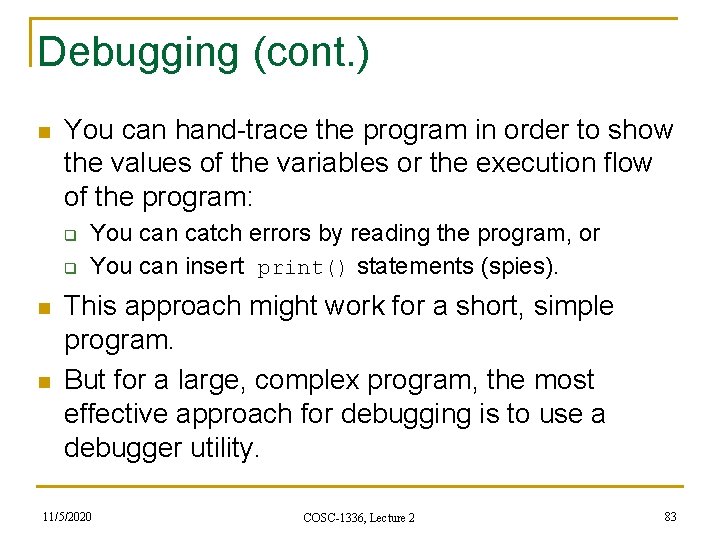
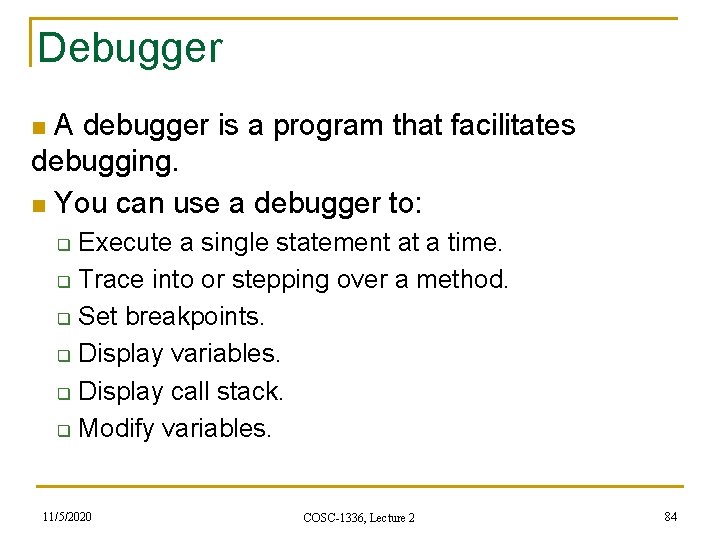
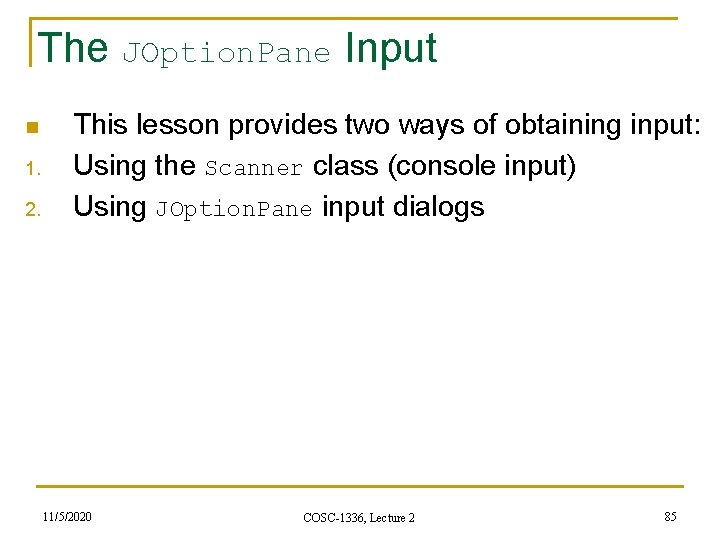
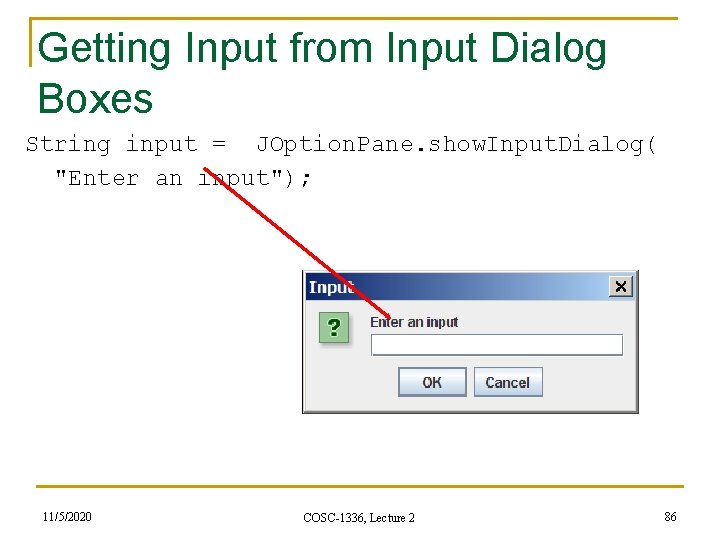
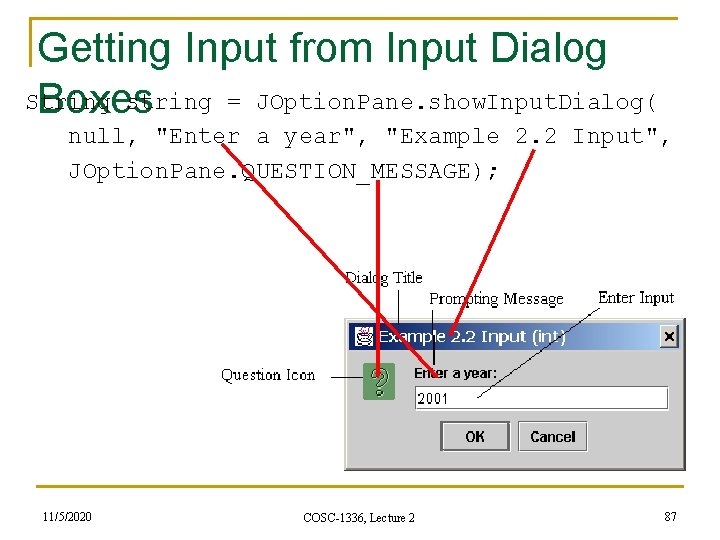
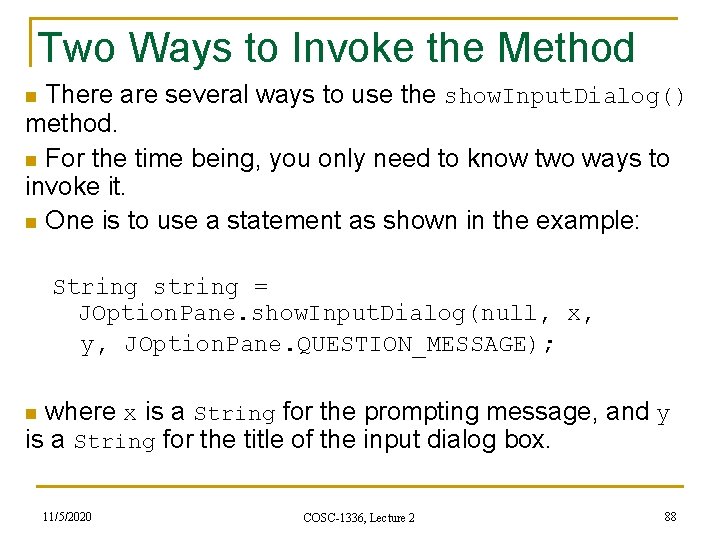
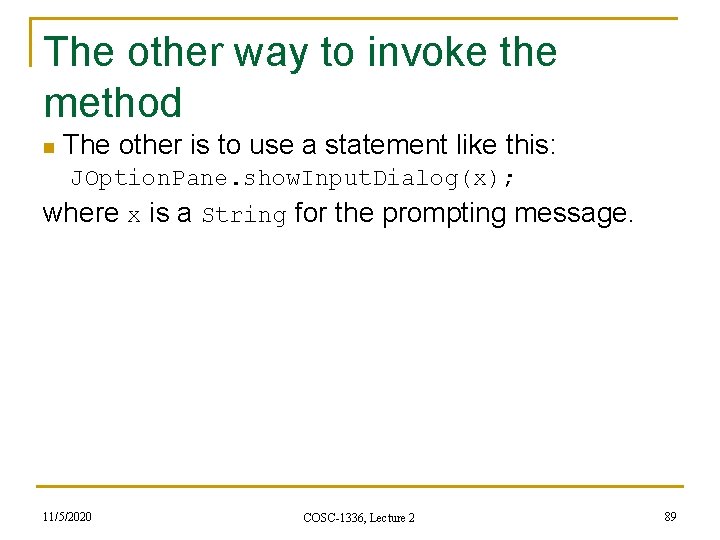
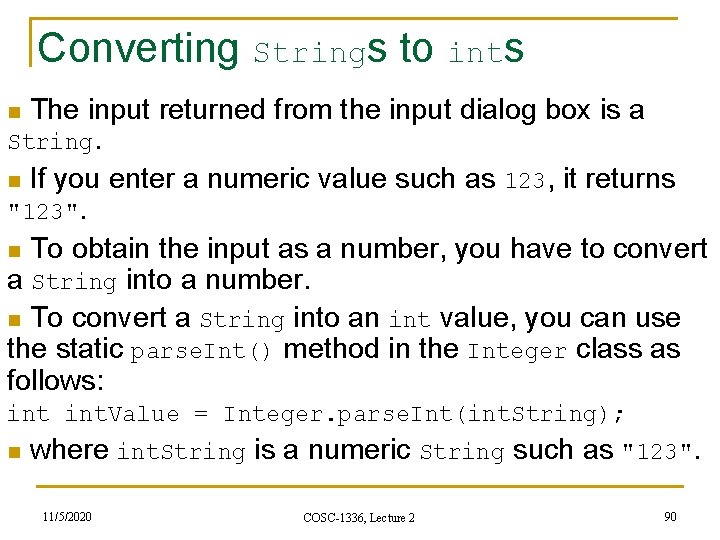
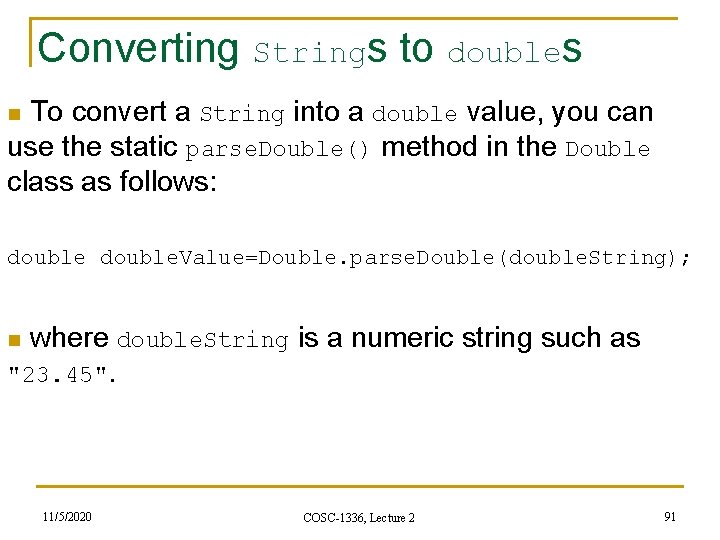
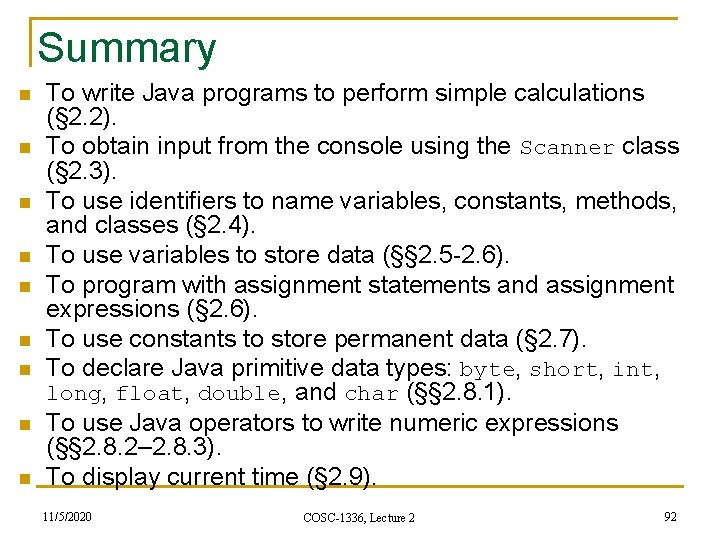
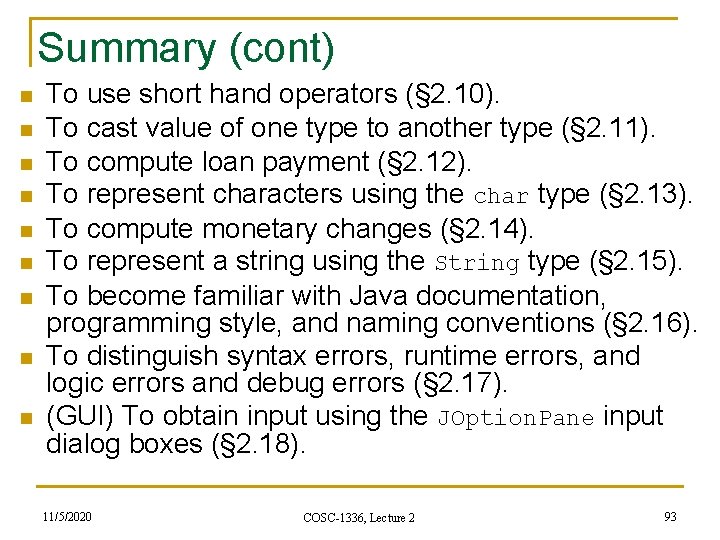
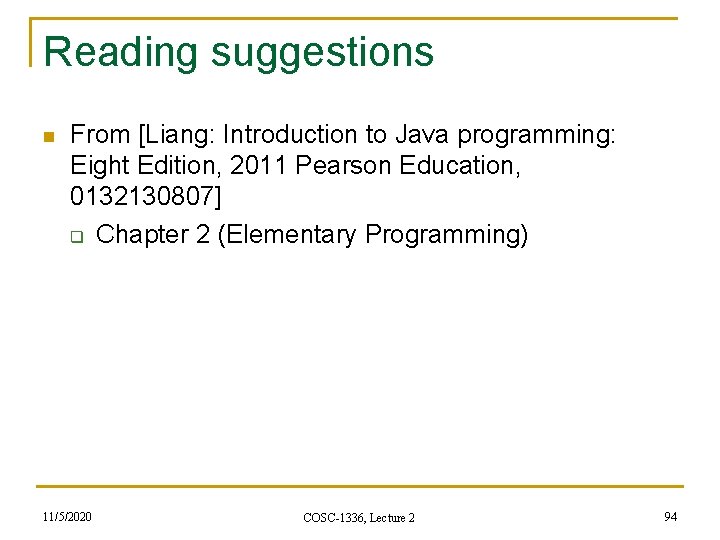
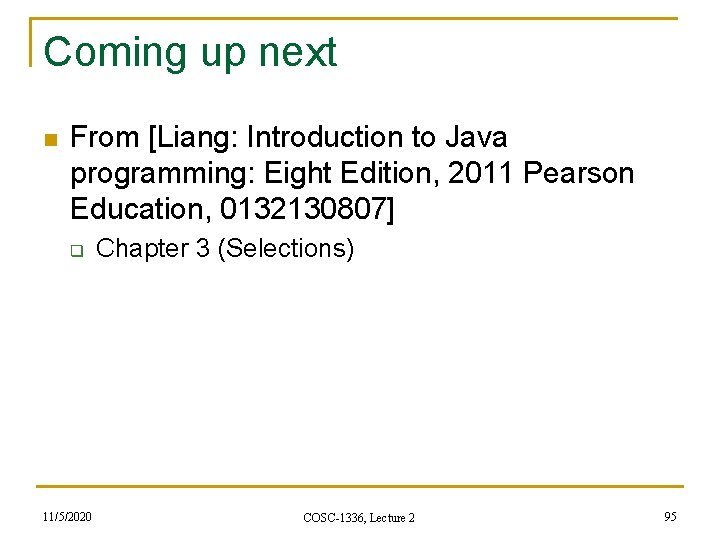
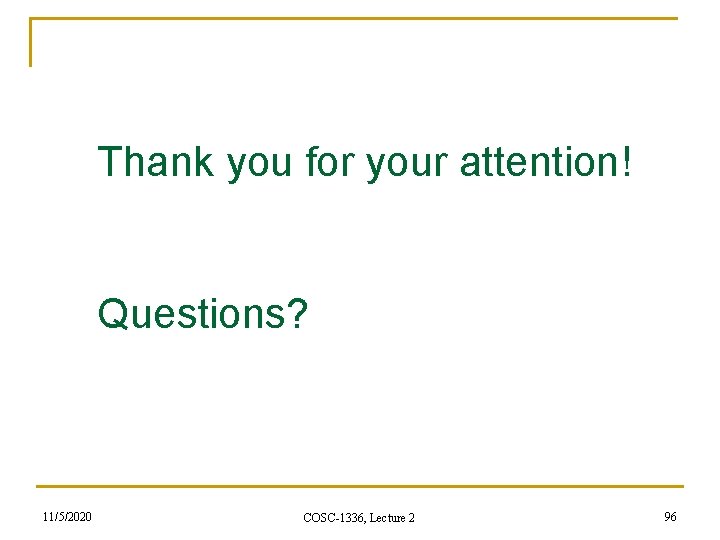
- Slides: 96
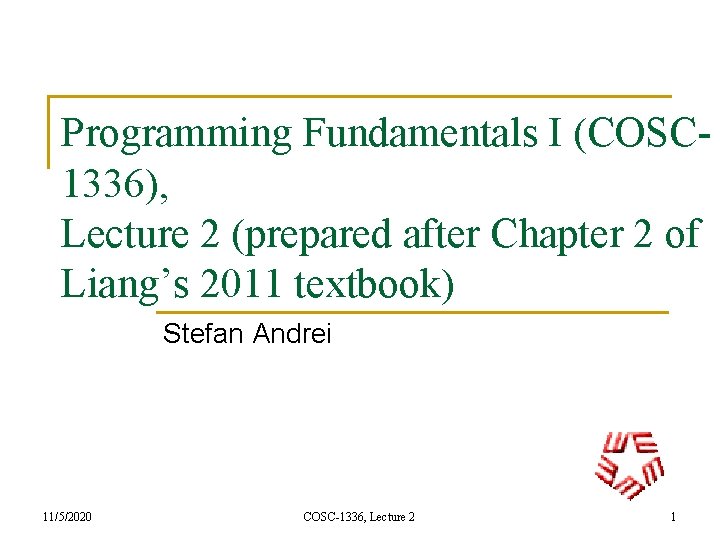
Programming Fundamentals I (COSC 1336), Lecture 2 (prepared after Chapter 2 of Liang’s 2011 textbook) Stefan Andrei 11/5/2020 COSC-1336, Lecture 2 1
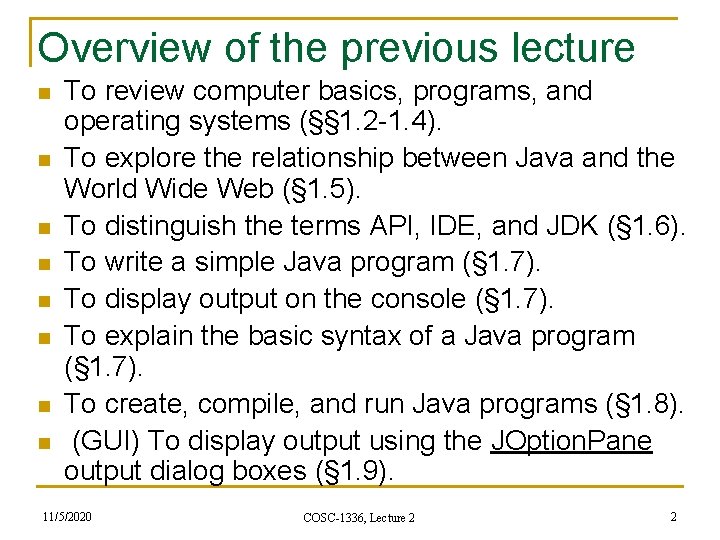
Overview of the previous lecture n n n n To review computer basics, programs, and operating systems (§§ 1. 2 -1. 4). To explore the relationship between Java and the World Wide Web (§ 1. 5). To distinguish the terms API, IDE, and JDK (§ 1. 6). To write a simple Java program (§ 1. 7). To display output on the console (§ 1. 7). To explain the basic syntax of a Java program (§ 1. 7). To create, compile, and run Java programs (§ 1. 8). (GUI) To display output using the JOption. Pane output dialog boxes (§ 1. 9). 11/5/2020 COSC-1336, Lecture 2 2
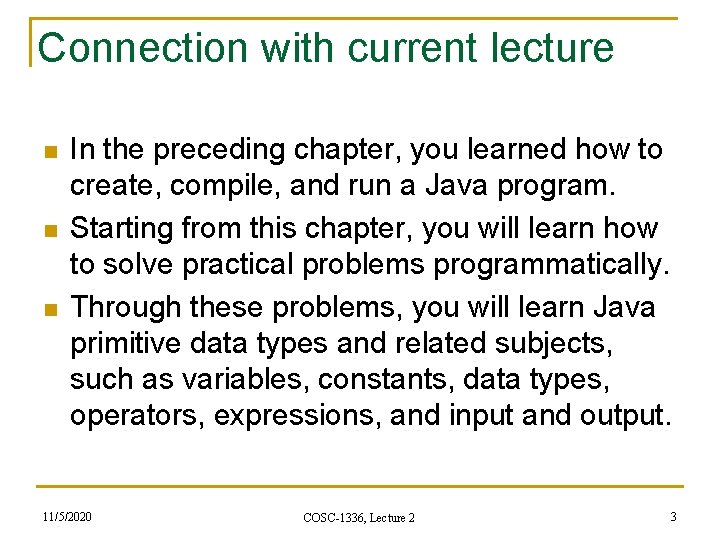
Connection with current lecture n n n In the preceding chapter, you learned how to create, compile, and run a Java program. Starting from this chapter, you will learn how to solve practical problems programmatically. Through these problems, you will learn Java primitive data types and related subjects, such as variables, constants, data types, operators, expressions, and input and output. 11/5/2020 COSC-1336, Lecture 2 3
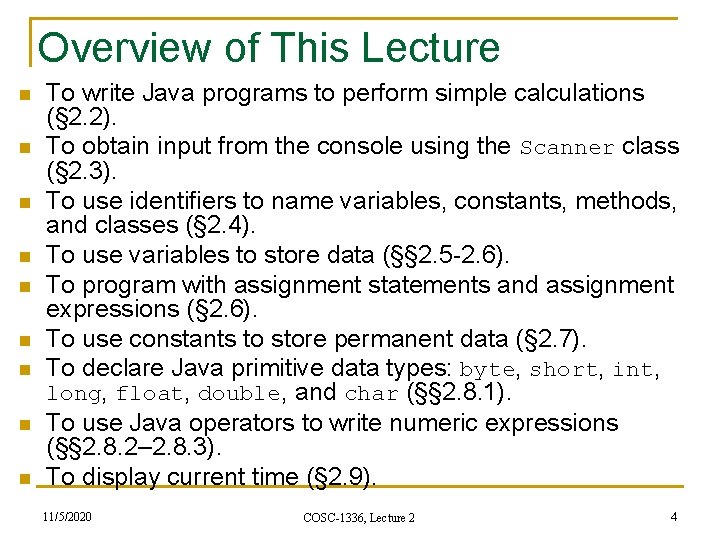
Overview of This Lecture n n n n n To write Java programs to perform simple calculations (§ 2. 2). To obtain input from the console using the Scanner class (§ 2. 3). To use identifiers to name variables, constants, methods, and classes (§ 2. 4). To use variables to store data (§§ 2. 5 -2. 6). To program with assignment statements and assignment expressions (§ 2. 6). To use constants to store permanent data (§ 2. 7). To declare Java primitive data types: byte, short, int, long, float, double, and char (§§ 2. 8. 1). To use Java operators to write numeric expressions (§§ 2. 8. 2– 2. 8. 3). To display current time (§ 2. 9). 11/5/2020 COSC-1336, Lecture 2 4
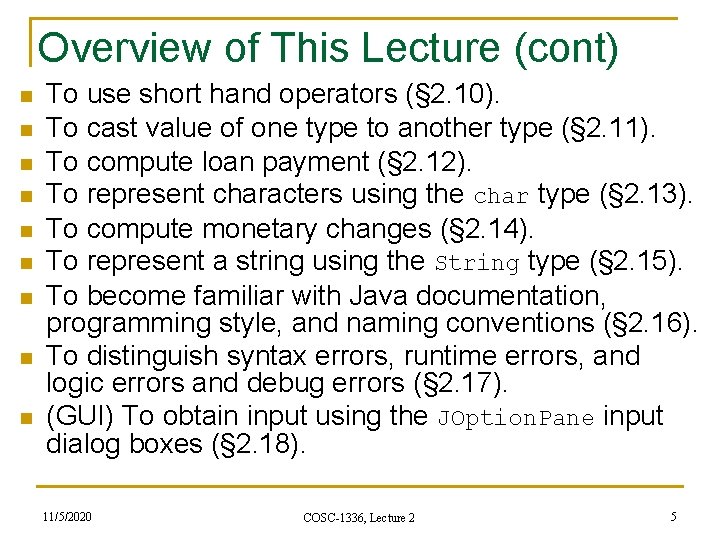
Overview of This Lecture (cont) n n n n n To use short hand operators (§ 2. 10). To cast value of one type to another type (§ 2. 11). To compute loan payment (§ 2. 12). To represent characters using the char type (§ 2. 13). To compute monetary changes (§ 2. 14). To represent a string using the String type (§ 2. 15). To become familiar with Java documentation, programming style, and naming conventions (§ 2. 16). To distinguish syntax errors, runtime errors, and logic errors and debug errors (§ 2. 17). (GUI) To obtain input using the JOption. Pane input dialog boxes (§ 2. 18). 11/5/2020 COSC-1336, Lecture 2 5
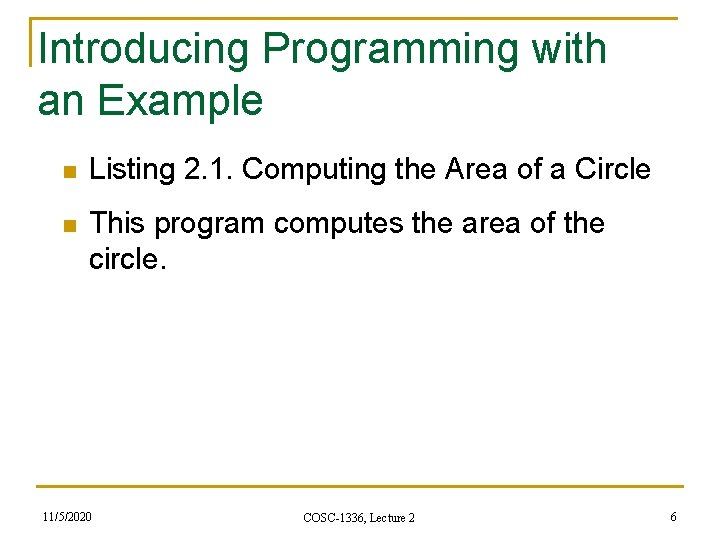
Introducing Programming with an Example n Listing 2. 1. Computing the Area of a Circle n This program computes the area of the circle. 11/5/2020 COSC-1336, Lecture 2 6
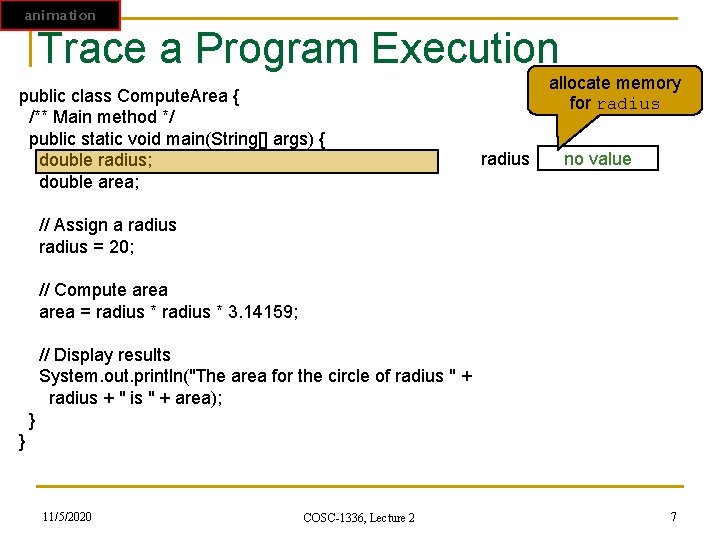
animation Trace a Program Execution public class Compute. Area { /** Main method */ public static void main(String[] args) { radius double radius; double area; // Assign a radius = 20; // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } 11/5/2020 COSC-1336, Lecture 2 allocate memory for radius no value 7
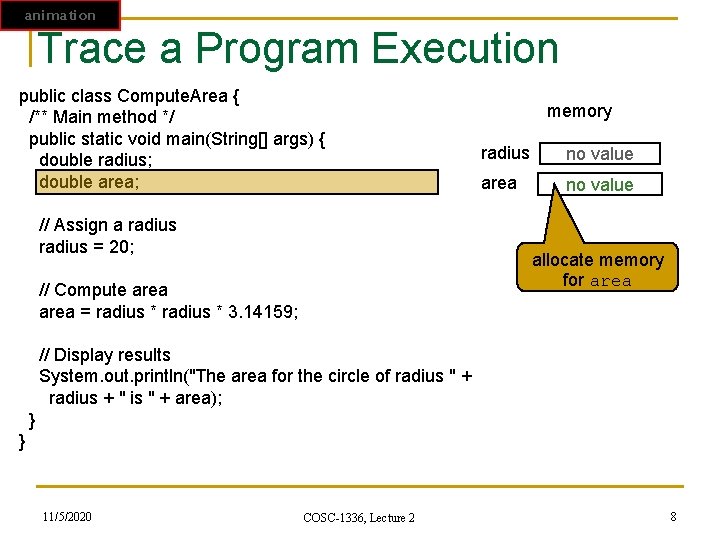
animation Trace a Program Execution public class Compute. Area { memory /** Main method */ public static void main(String[] args) { radius no value double radius; double area; area no value // Assign a radius = 20; allocate memory for area // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } 11/5/2020 COSC-1336, Lecture 2 8
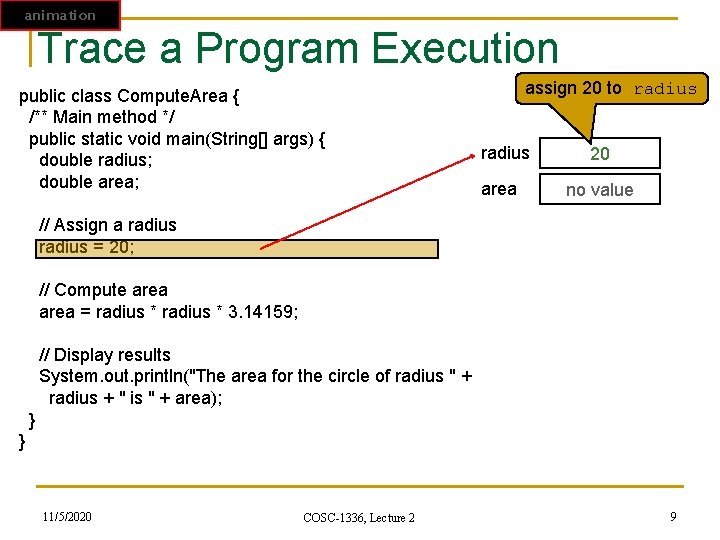
animation Trace a Program Execution assign 20 to radius public class Compute. Area { /** Main method */ public static void main(String[] args) { radius 20 double radius; double area; area no value // Assign a radius = 20; // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } 11/5/2020 COSC-1336, Lecture 2 9
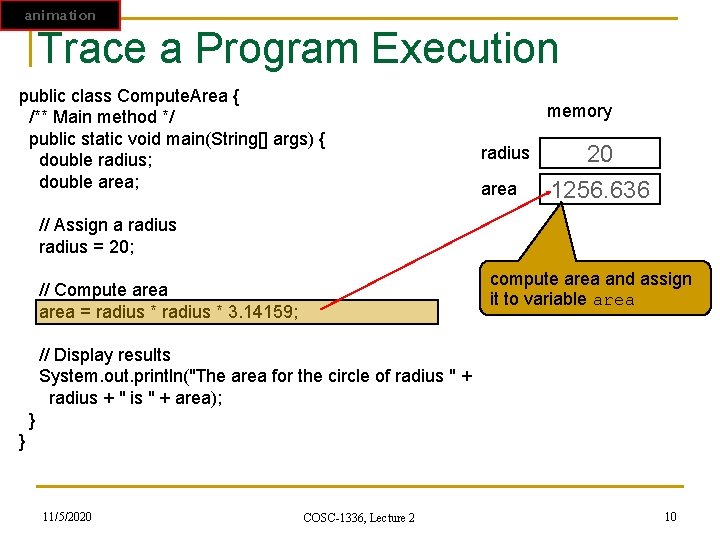
animation Trace a Program Execution public class Compute. Area { memory /** Main method */ public static void main(String[] args) { radius 20 double radius; double area; area 1256. 636 // Assign a radius = 20; compute area and assign // Compute area it to variable area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } 11/5/2020 COSC-1336, Lecture 2 10
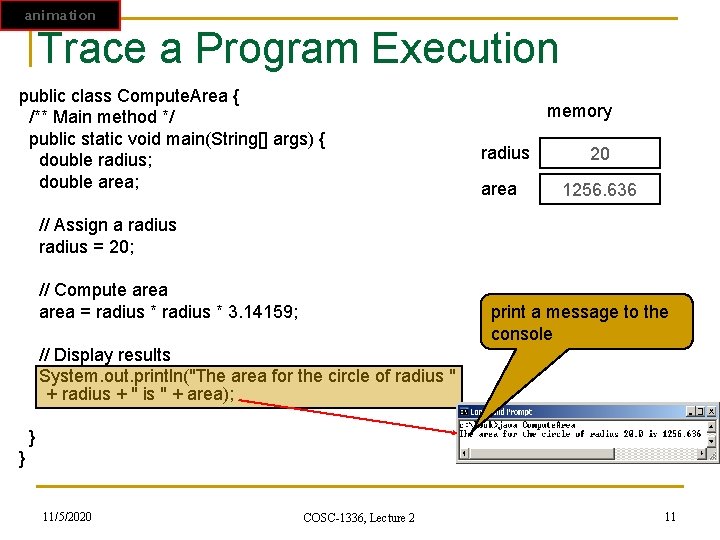
animation Trace a Program Execution public class Compute. Area { /** Main method */ public static void main(String[] args) { double radius; double area; // Assign a radius = 20; // Compute area = radius * 3. 14159; // Display results System. out. println("The area for the circle of radius " + radius + " is " + area); } } 11/5/2020 COSC-1336, Lecture 2 memory radius area 20 1256. 636 print a message to the console 11
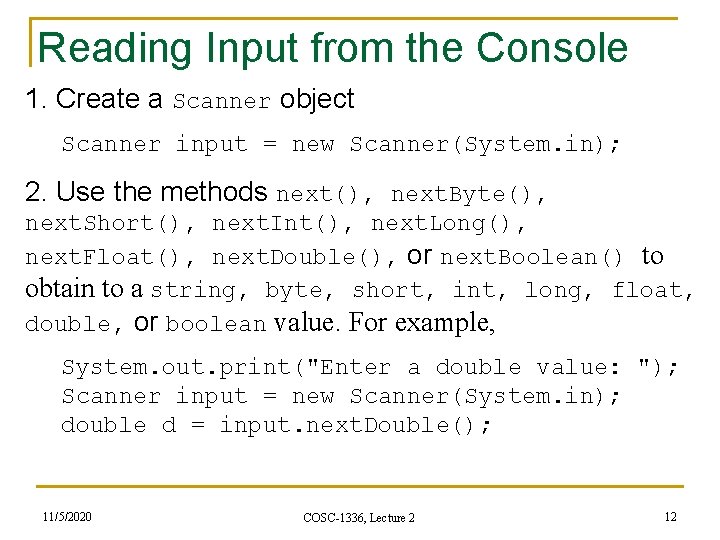
Reading Input from the Console 1. Create a Scanner object Scanner input = new Scanner(System. in); 2. Use the methods next(), next. Byte(), next. Short(), next. Int(), next. Long(), next. Float(), next. Double(), or next. Boolean() to obtain to a string, byte, short, int, long, float, double, or boolean value. For example, System. out. print("Enter a double value: "); Scanner input = new Scanner(System. in); double d = input. next. Double(); 11/5/2020 COSC-1336, Lecture 2 12
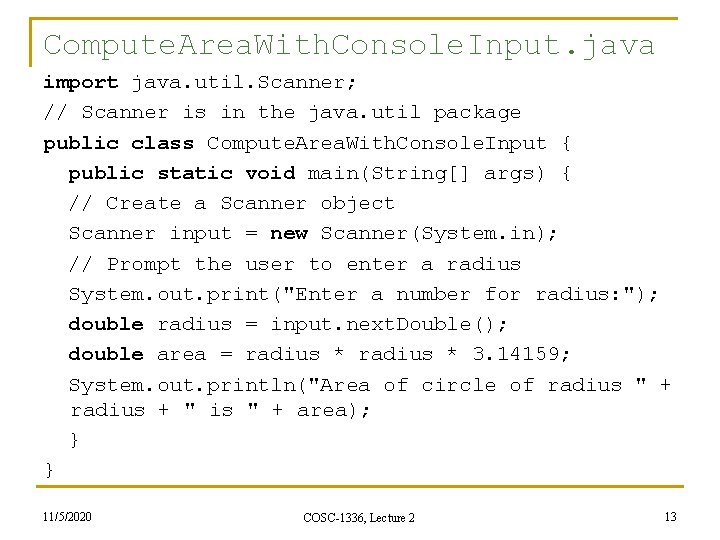
Compute. Area. With. Console. Input. java import java. util. Scanner; // Scanner is in the java. util package public class Compute. Area. With. Console. Input { public static void main(String[] args) { // Create a Scanner object Scanner input = new Scanner(System. in); // Prompt the user to enter a radius System. out. print("Enter a number for radius: "); double radius = input. next. Double(); double area = radius * 3. 14159; System. out. println("Area of circle of radius " + radius + " is " + area); } } 11/5/2020 COSC-1336, Lecture 2 13
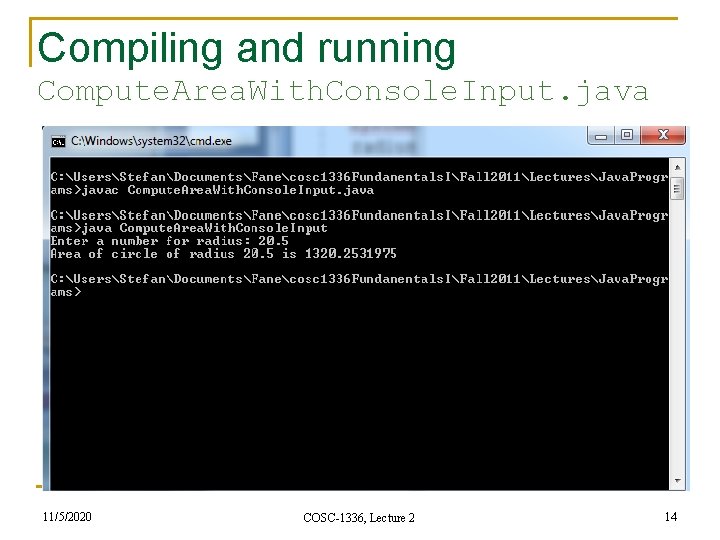
Compiling and running Compute. Area. With. Console. Input. java 11/5/2020 COSC-1336, Lecture 2 14
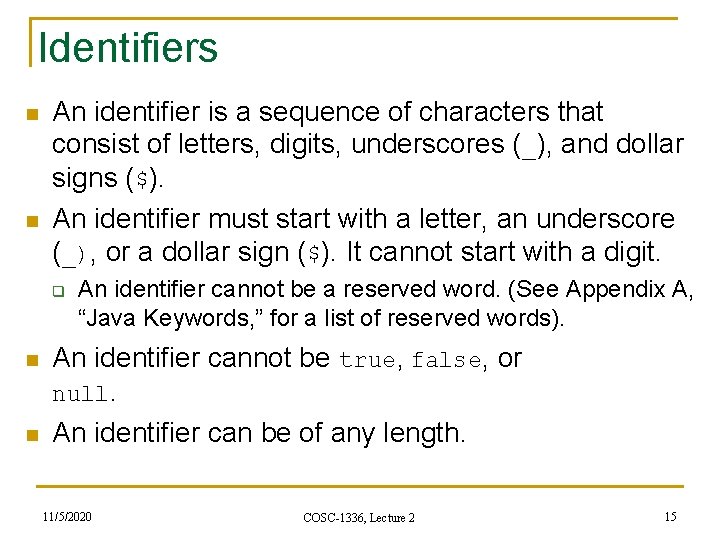
Identifiers n n An identifier is a sequence of characters that consist of letters, digits, underscores (_), and dollar signs ($). An identifier must start with a letter, an underscore (_), or a dollar sign ($). It cannot start with a digit. q n n An identifier cannot be a reserved word. (See Appendix A, “Java Keywords, ” for a list of reserved words). An identifier cannot be true, false, or null. An identifier can be of any length. 11/5/2020 COSC-1336, Lecture 2 15
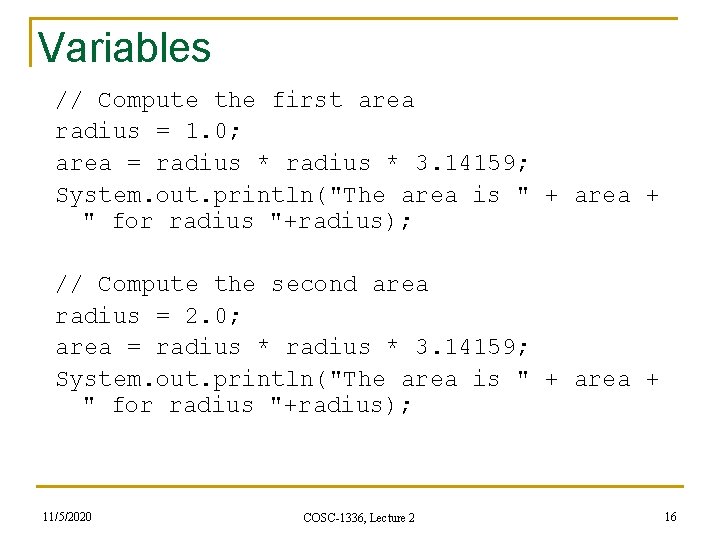
Variables // Compute the first area radius = 1. 0; area = radius * 3. 14159; System. out. println("The area is " + area + " for radius "+radius); // Compute the second area radius = 2. 0; area = radius * 3. 14159; System. out. println("The area is " + area + " for radius "+radius); 11/5/2020 COSC-1336, Lecture 2 16
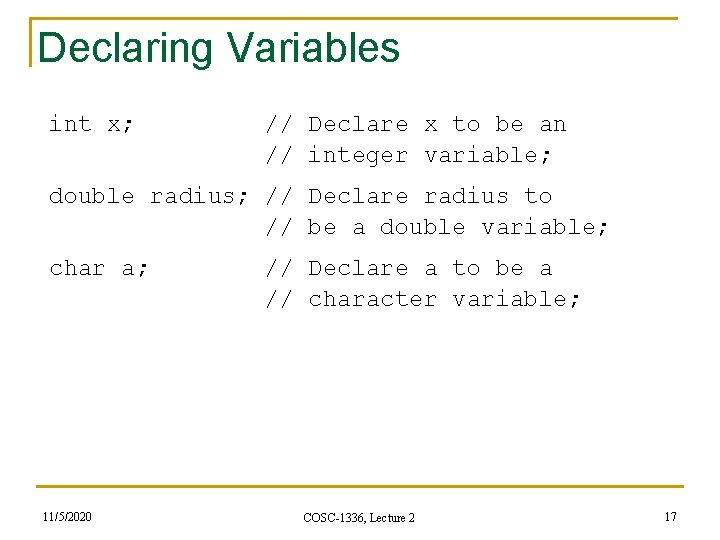
Declaring Variables int x; // Declare x to be an // integer variable; double radius; // Declare radius to // be a double variable; char a; // Declare a to be a // character variable; 11/5/2020 COSC-1336, Lecture 2 17
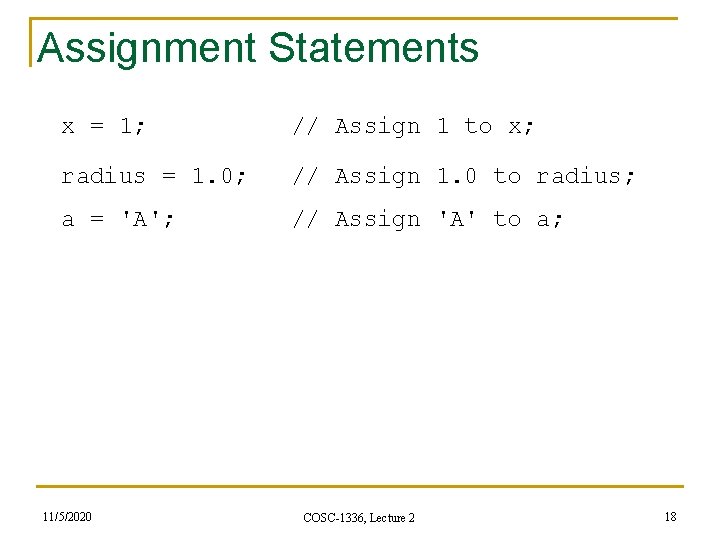
Assignment Statements x = 1; // Assign 1 to x; radius = 1. 0; // Assign 1. 0 to radius; a = 'A'; // Assign 'A' to a; 11/5/2020 COSC-1336, Lecture 2 18
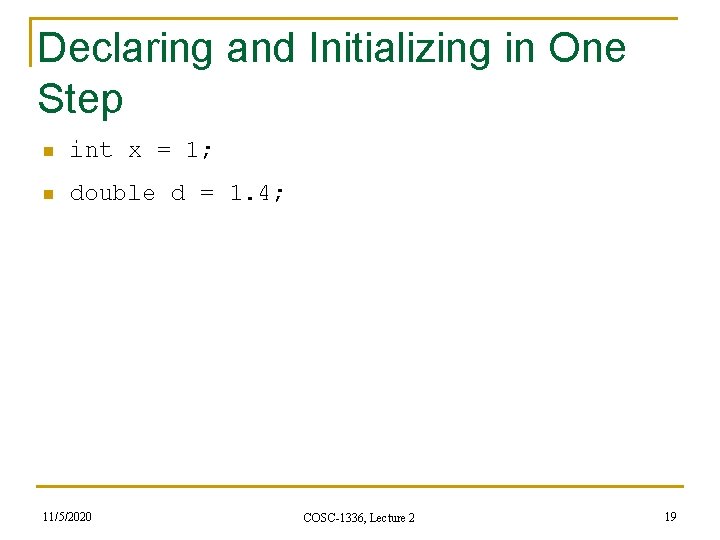
Declaring and Initializing in One Step n int x = 1; n double d = 1. 4; 11/5/2020 COSC-1336, Lecture 2 19
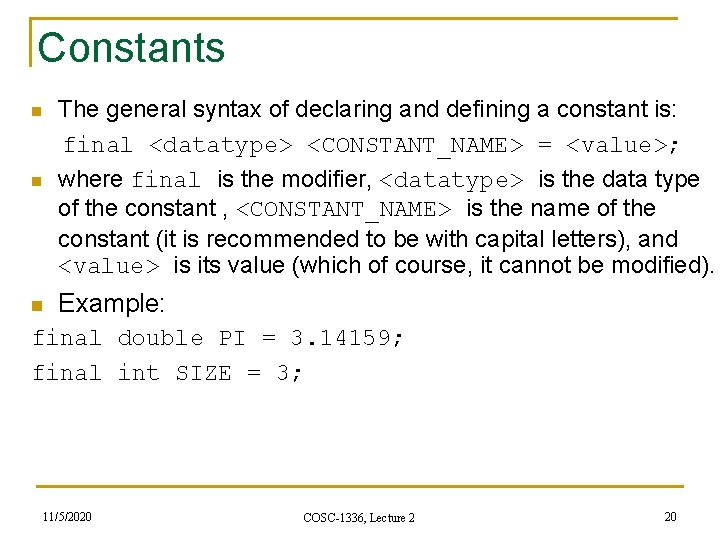
Constants The general syntax of declaring and defining a constant is: final <datatype> <CONSTANT_NAME> = <value>; n where final is the modifier, <datatype> is the data type of the constant , <CONSTANT_NAME> is the name of the constant (it is recommended to be with capital letters), and <value> is its value (which of course, it cannot be modified). n n Example: final double PI = 3. 14159; final int SIZE = 3; 11/5/2020 COSC-1336, Lecture 2 20
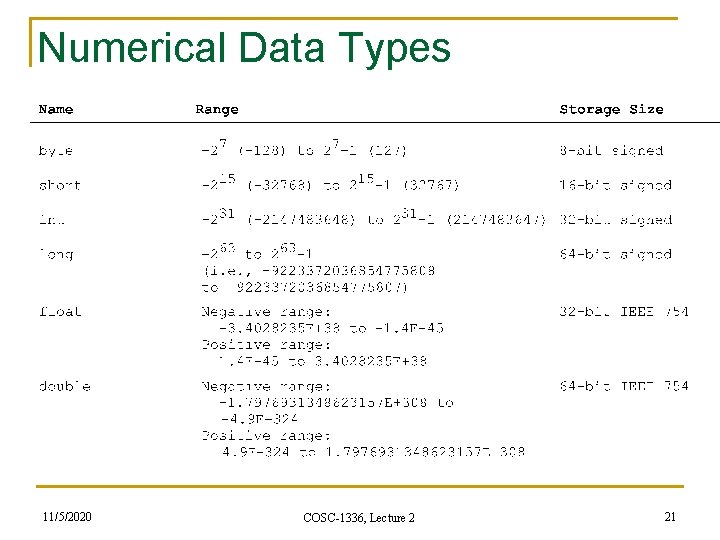
Numerical Data Types 11/5/2020 COSC-1336, Lecture 2 21
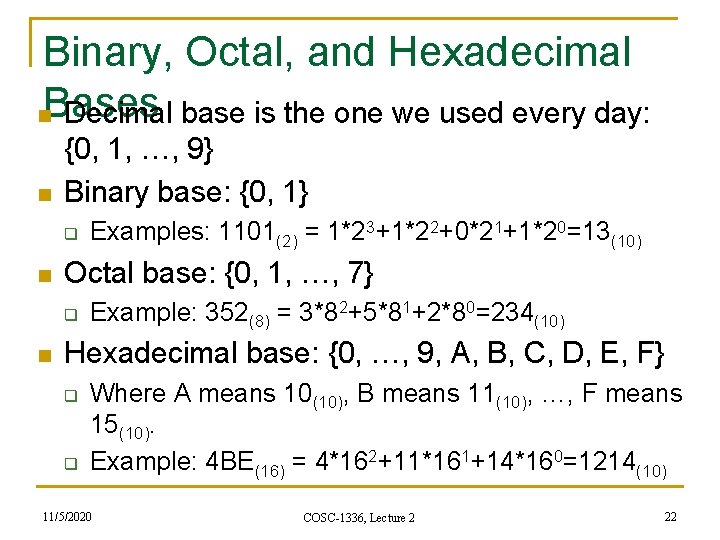
Binary, Octal, and Hexadecimal n. Bases Decimal base is the one we used every day: n {0, 1, …, 9} Binary base: {0, 1} q n Octal base: {0, 1, …, 7} q n Examples: 1101(2) = 1*23+1*22+0*21+1*20=13(10) Example: 352(8) = 3*82+5*81+2*80=234(10) Hexadecimal base: {0, …, 9, A, B, C, D, E, F} q q Where A means 10(10), B means 11(10), …, F means 15(10). Example: 4 BE(16) = 4*162+11*161+14*160=1214(10) 11/5/2020 COSC-1336, Lecture 2 22
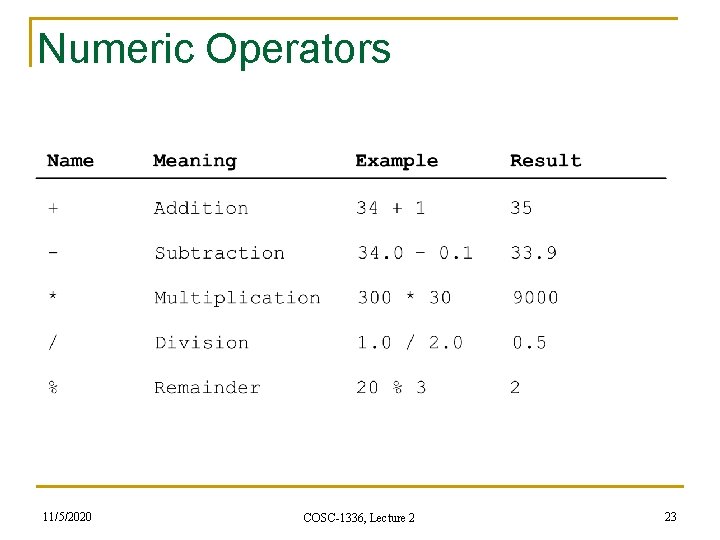
Numeric Operators 11/5/2020 COSC-1336, Lecture 2 23
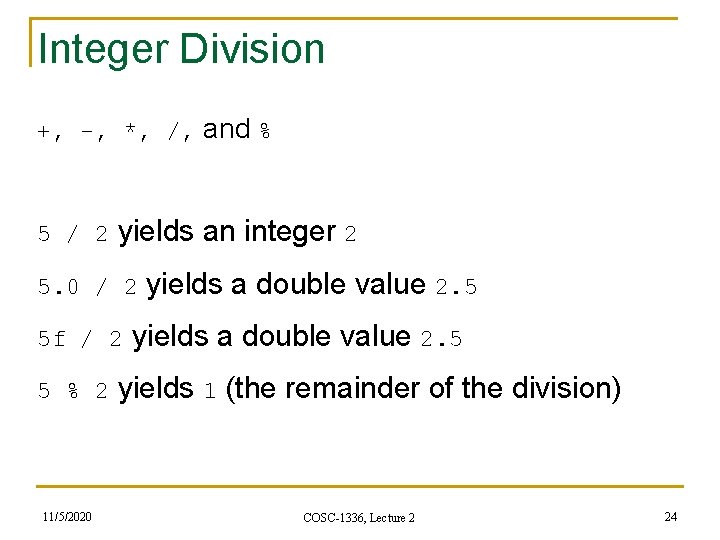
Integer Division +, -, *, /, and % 5 / 2 yields an integer 2 5. 0 / 2 yields a double value 2. 5 5 f / 2 yields a double value 2. 5 5 % 2 yields 1 (the remainder of the division) 11/5/2020 COSC-1336, Lecture 2 24
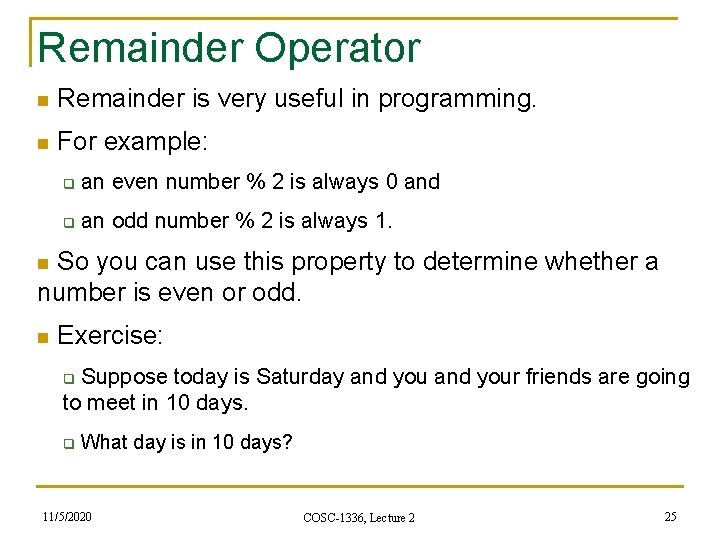
Remainder Operator n Remainder is very useful in programming. n For example: q an even number % 2 is always 0 and q an odd number % 2 is always 1. n So you can use this property to determine whether a number is even or odd. n Exercise: Suppose today is Saturday and your friends are going to meet in 10 days. q q What day is in 10 days? 11/5/2020 COSC-1336, Lecture 2 25
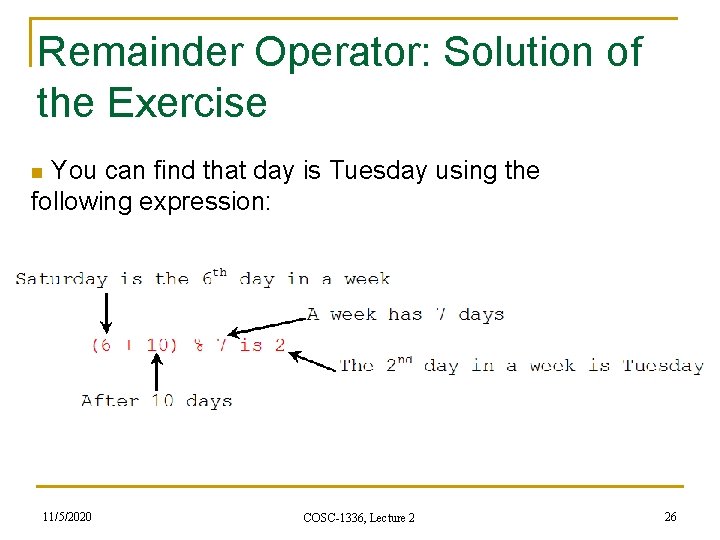
Remainder Operator: Solution of the Exercise n You can find that day is Tuesday using the following expression: 11/5/2020 COSC-1336, Lecture 2 26
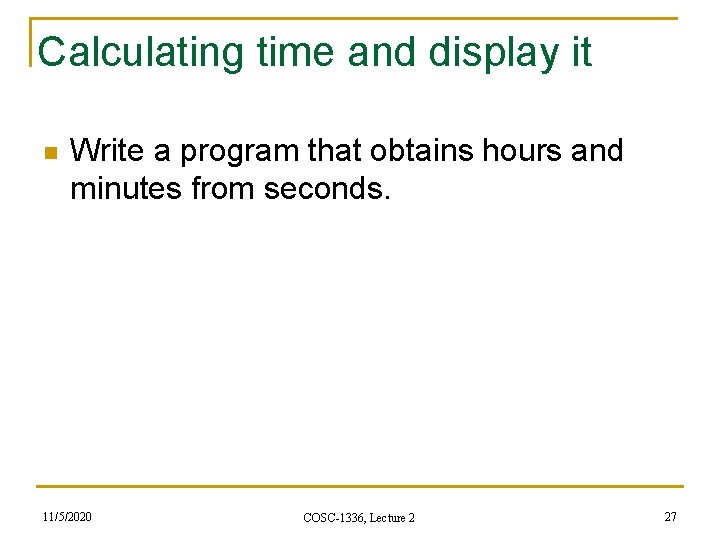
Calculating time and display it n Write a program that obtains hours and minutes from seconds. 11/5/2020 COSC-1336, Lecture 2 27
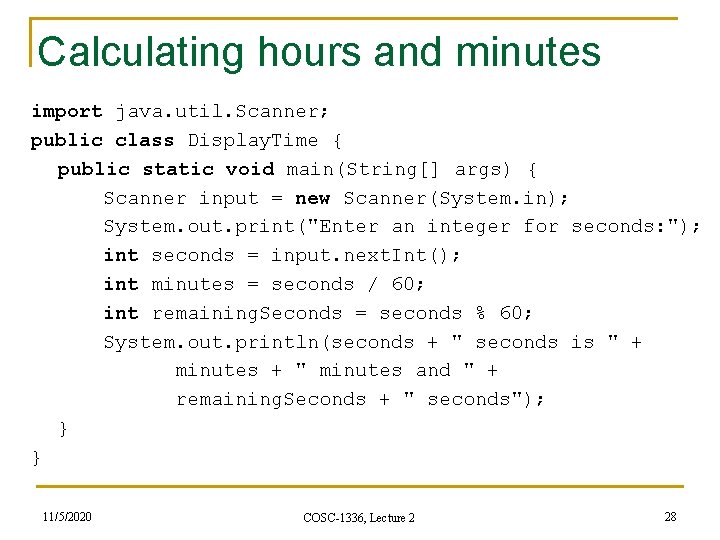
Calculating hours and minutes import java. util. Scanner; public class Display. Time { public static void main(String[] args) { Scanner input = new Scanner(System. in); System. out. print("Enter an integer for seconds: "); int seconds = input. next. Int(); int minutes = seconds / 60; int remaining. Seconds = seconds % 60; System. out. println(seconds + " seconds is " + minutes + " minutes and " + remaining. Seconds + " seconds"); } } 11/5/2020 COSC-1336, Lecture 2 28
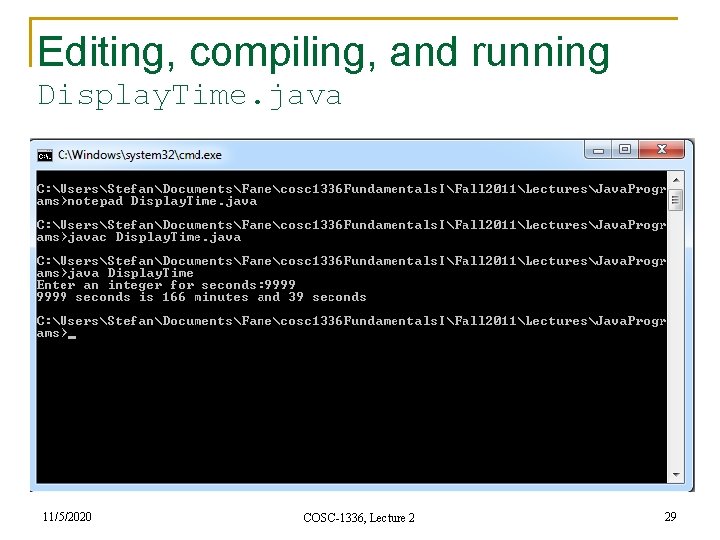
Editing, compiling, and running Display. Time. java 11/5/2020 COSC-1336, Lecture 2 29
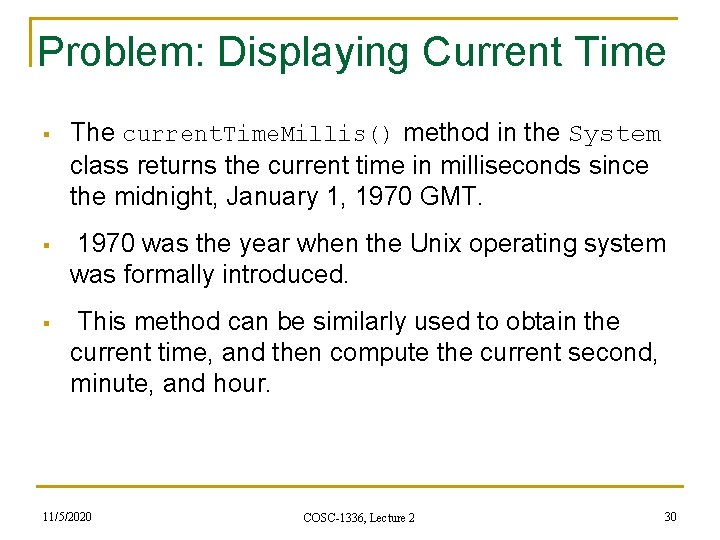
Problem: Displaying Current Time § The current. Time. Millis() method in the System class returns the current time in milliseconds since the midnight, January 1, 1970 GMT. § 1970 was the year when the Unix operating system was formally introduced. § This method can be similarly used to obtain the current time, and then compute the current second, minute, and hour. 11/5/2020 COSC-1336, Lecture 2 30
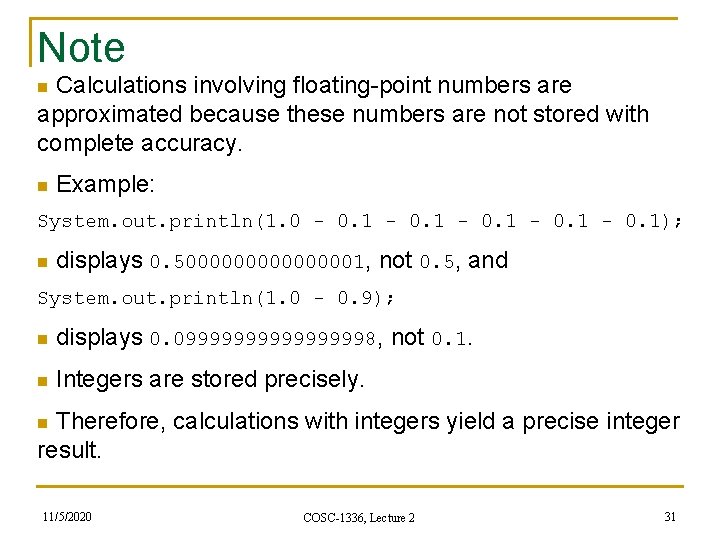
Note n Calculations involving floating-point numbers are approximated because these numbers are not stored with complete accuracy. n Example: System. out. println(1. 0 - 0. 1); n displays 0. 500000001, not 0. 5, and System. out. println(1. 0 - 0. 9); n displays 0. 0999999998, not 0. 1. n Integers are stored precisely. n Therefore, calculations with integers yield a precise integer result. 11/5/2020 COSC-1336, Lecture 2 31
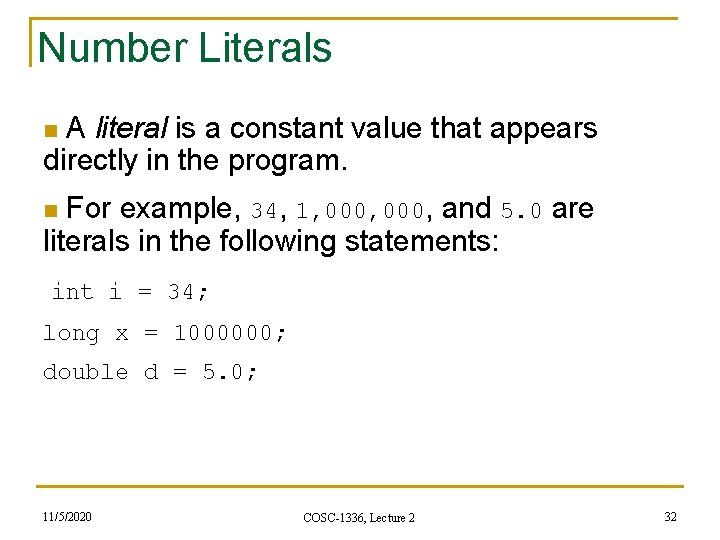
Number Literals n A literal is a constant value that appears directly in the program. n For example, 34, 1, 000, and 5. 0 are literals in the following statements: int i = 34; long x = 1000000; double d = 5. 0; 11/5/2020 COSC-1336, Lecture 2 32
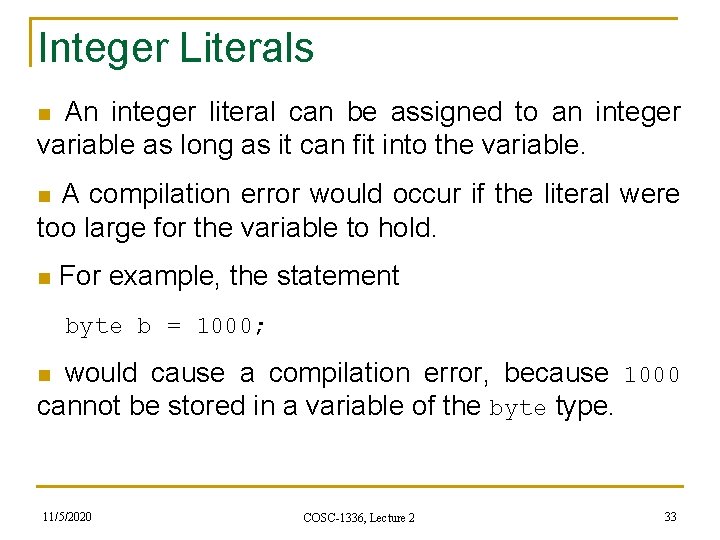
Integer Literals n An integer literal can be assigned to an integer variable as long as it can fit into the variable. n A compilation error would occur if the literal were too large for the variable to hold. n For example, the statement byte b = 1000; n would cause a compilation error, because 1000 cannot be stored in a variable of the byte type. 11/5/2020 COSC-1336, Lecture 2 33
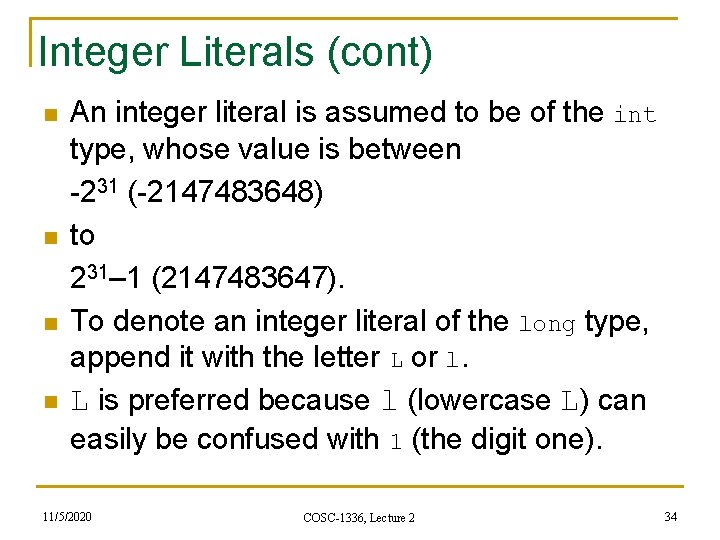
Integer Literals (cont) n n An integer literal is assumed to be of the int type, whose value is between -231 (-2147483648) to 231– 1 (2147483647). To denote an integer literal of the long type, append it with the letter L or l. L is preferred because l (lowercase L) can easily be confused with 1 (the digit one). 11/5/2020 COSC-1336, Lecture 2 34
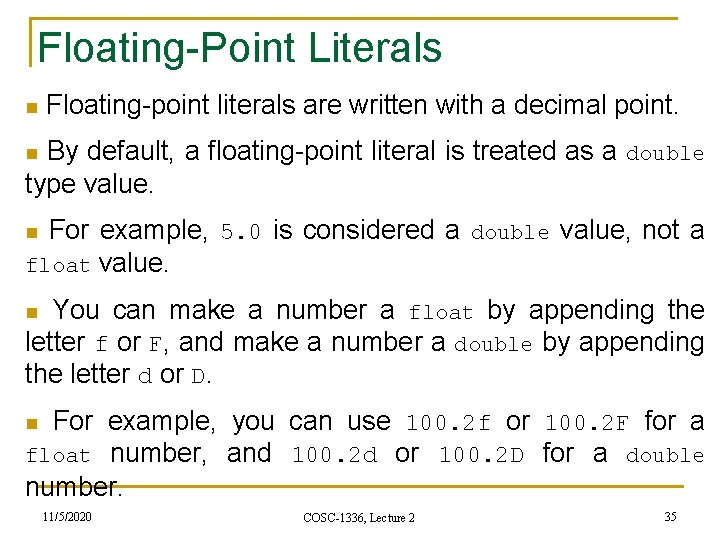
Floating-Point Literals n Floating-point literals are written with a decimal point. n By default, a floating-point literal is treated as a double type value. n For example, 5. 0 is considered a double value, not a float value. n You can make a number a float by appending the letter f or F, and make a number a double by appending the letter d or D. n For example, you can use 100. 2 f or 100. 2 F for a float number, and 100. 2 d or 100. 2 D for a double number. 11/5/2020 COSC-1336, Lecture 2 35
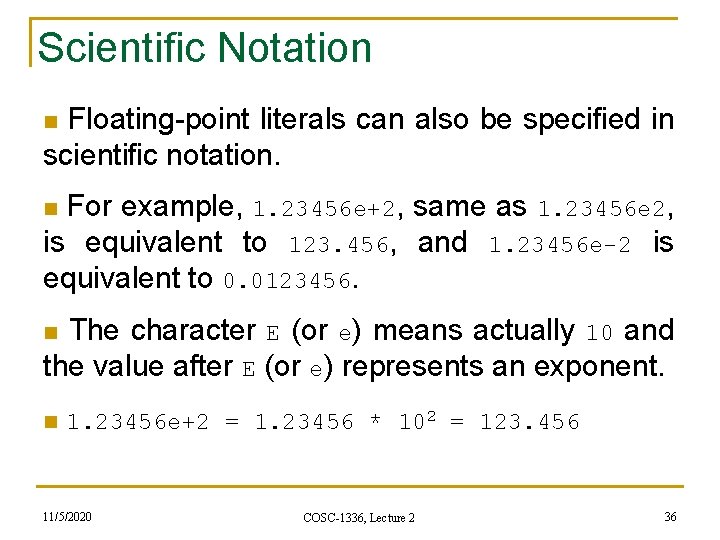
Scientific Notation n Floating-point literals can also be specified in scientific notation. n For example, 1. 23456 e+2, same as 1. 23456 e 2, is equivalent to 123. 456, and equivalent to 0. 0123456. 1. 23456 e-2 is n The character E (or e) means actually 10 and the value after E (or e) represents an exponent. n 1. 23456 e+2 = 1. 23456 * 102 = 123. 456 11/5/2020 COSC-1336, Lecture 2 36
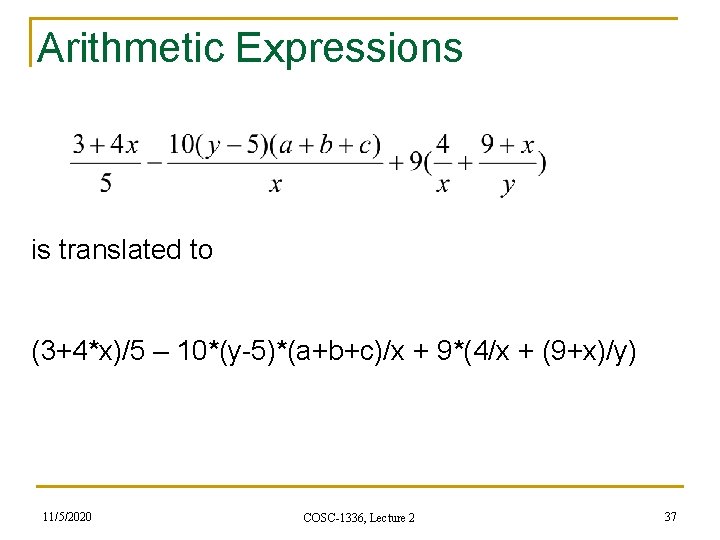
Arithmetic Expressions is translated to (3+4*x)/5 – 10*(y-5)*(a+b+c)/x + 9*(4/x + (9+x)/y) 11/5/2020 COSC-1336, Lecture 2 37
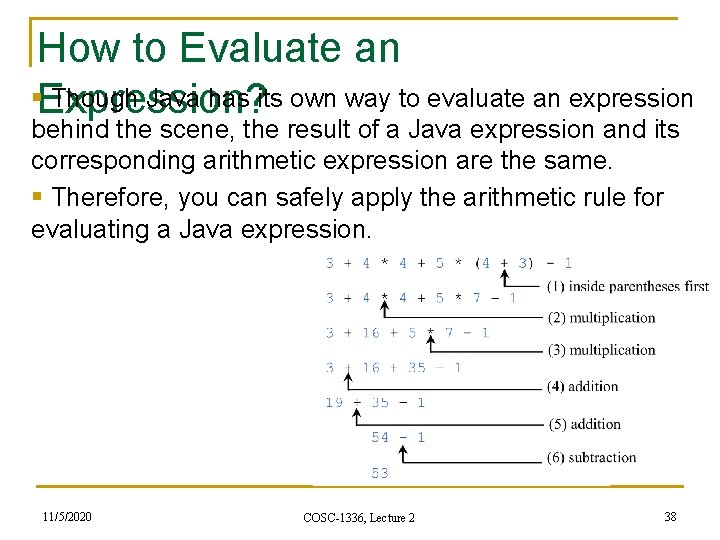
How to Evaluate an §Expression? Though Java has its own way to evaluate an expression behind the scene, the result of a Java expression and its corresponding arithmetic expression are the same. § Therefore, you can safely apply the arithmetic rule for evaluating a Java expression. 11/5/2020 COSC-1336, Lecture 2 38
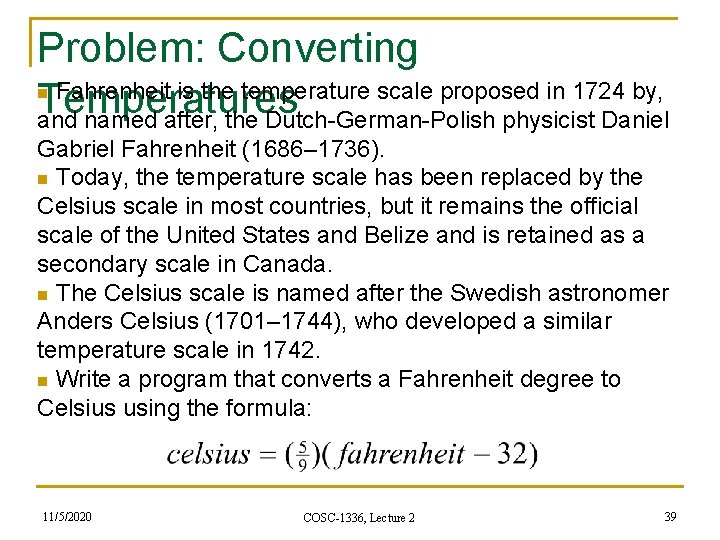
Problem: Converting Fahrenheit is the temperature scale proposed in 1724 by, Temperatures and named after, the Dutch-German-Polish physicist Daniel n Gabriel Fahrenheit (1686– 1736). n Today, the temperature scale has been replaced by the Celsius scale in most countries, but it remains the official scale of the United States and Belize and is retained as a secondary scale in Canada. n The Celsius scale is named after the Swedish astronomer Anders Celsius (1701– 1744), who developed a similar temperature scale in 1742. n Write a program that converts a Fahrenheit degree to Celsius using the formula: 11/5/2020 COSC-1336, Lecture 2 39
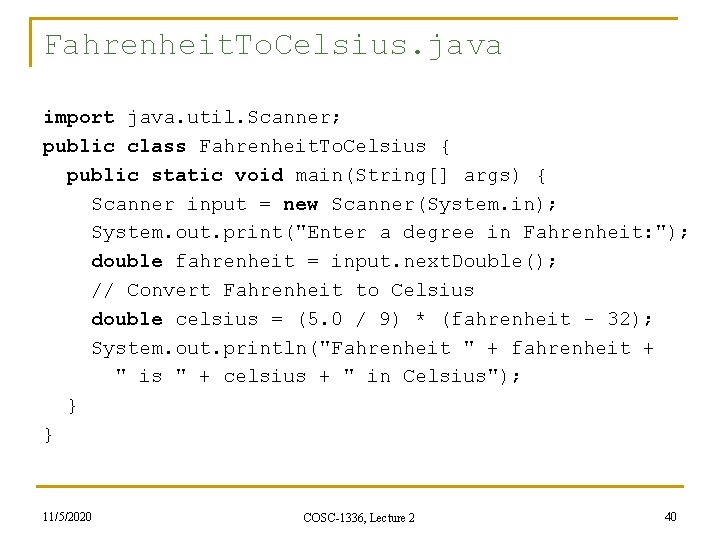
Fahrenheit. To. Celsius. java import java. util. Scanner; public class Fahrenheit. To. Celsius { public static void main(String[] args) { Scanner input = new Scanner(System. in); System. out. print("Enter a degree in Fahrenheit: "); double fahrenheit = input. next. Double(); // Convert Fahrenheit to Celsius double celsius = (5. 0 / 9) * (fahrenheit - 32); System. out. println("Fahrenheit " + fahrenheit + " is " + celsius + " in Celsius"); } } 11/5/2020 COSC-1336, Lecture 2 40
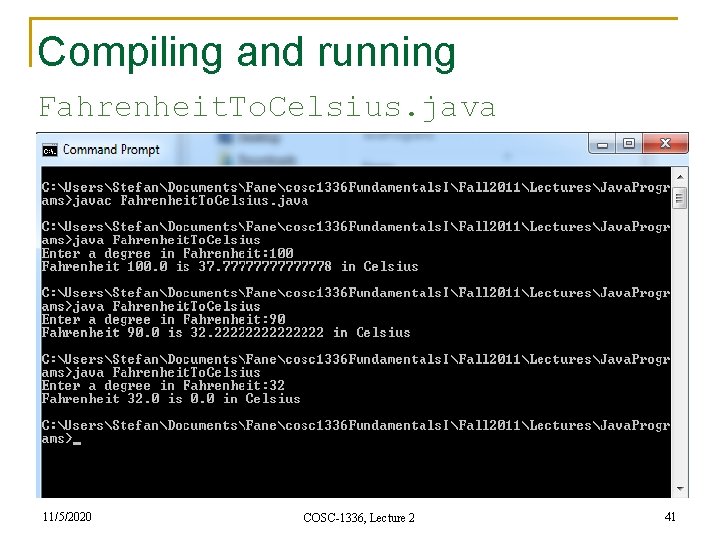
Compiling and running Fahrenheit. To. Celsius. java 11/5/2020 COSC-1336, Lecture 2 41
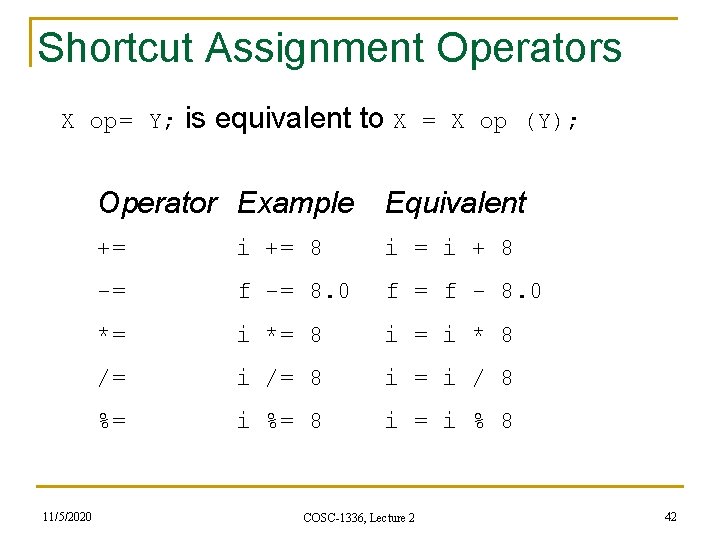
Shortcut Assignment Operators X op= Y; is equivalent to X = X op (Y); 11/5/2020 Operator Example Equivalent += i += 8 i = i + 8 -= f -= 8. 0 f = f - 8. 0 *= i *= 8 i = i * 8 /= i /= 8 i = i / 8 %= i %= 8 i = i % 8 COSC-1336, Lecture 2 42
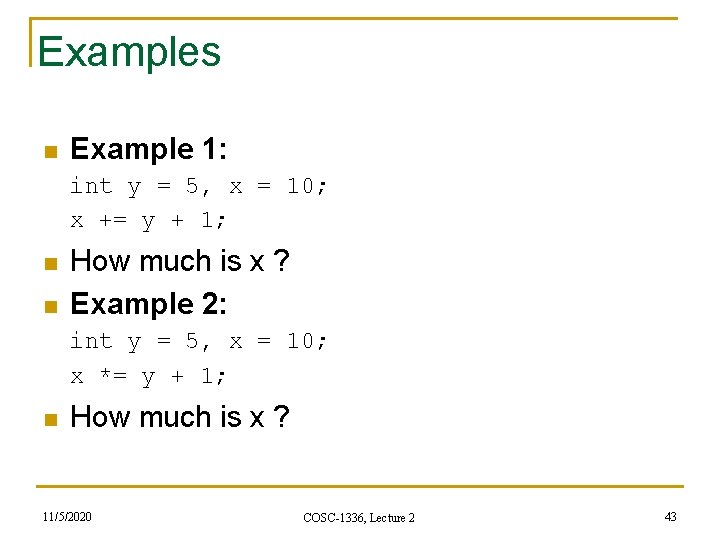
Examples n Example 1: int y = 5, x = 10; x += y + 1; n n How much is x ? Example 2: int y = 5, x = 10; x *= y + 1; n How much is x ? 11/5/2020 COSC-1336, Lecture 2 43
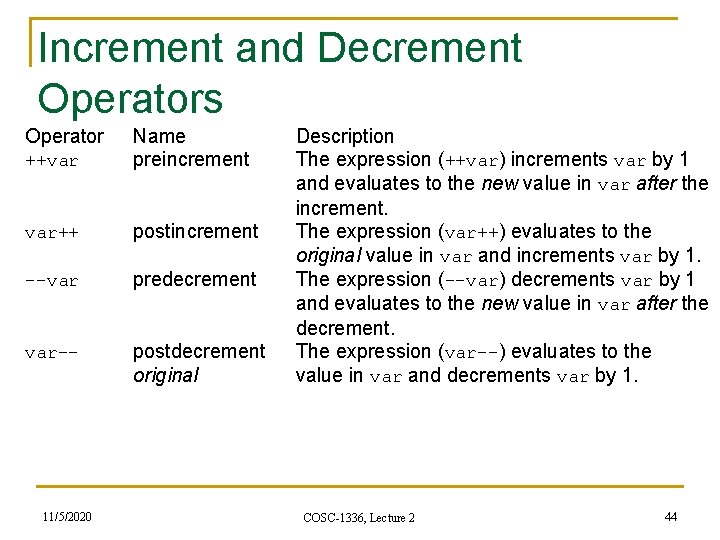
Increment and Decrement Operators Operator ++var var++ --var var-- 11/5/2020 Name preincrement Description The expression (++var) increments var by 1 and evaluates to the new value in var after the increment. postincrement The expression (var++) evaluates to the original value in var and increments var by 1. predecrement The expression (--var) decrements var by 1 and evaluates to the new value in var after the decrement. postdecrement The expression (var--) evaluates to the original value in var and decrements var by 1. COSC-1336, Lecture 2 44
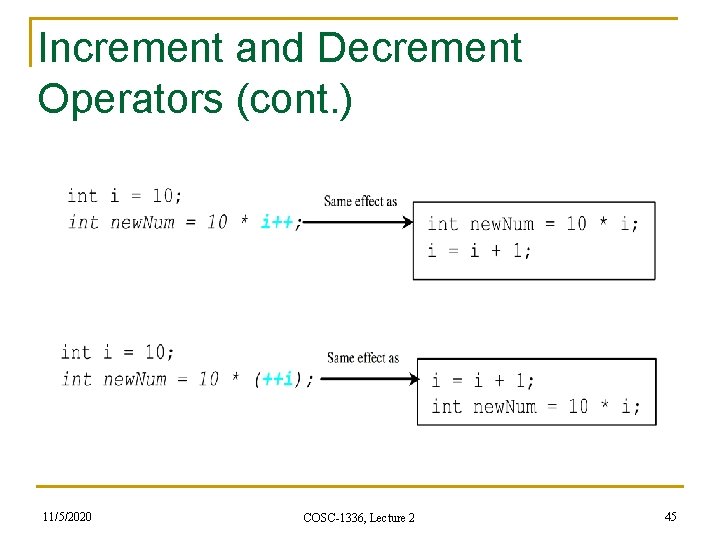
Increment and Decrement Operators (cont. ) 11/5/2020 COSC-1336, Lecture 2 45
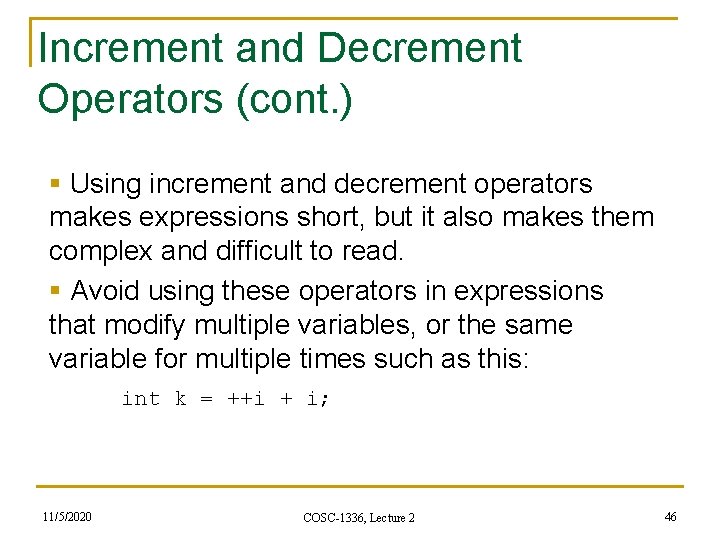
Increment and Decrement Operators (cont. ) § Using increment and decrement operators makes expressions short, but it also makes them complex and difficult to read. § Avoid using these operators in expressions that modify multiple variables, or the same variable for multiple times such as this: int k = ++i + i; 11/5/2020 COSC-1336, Lecture 2 46
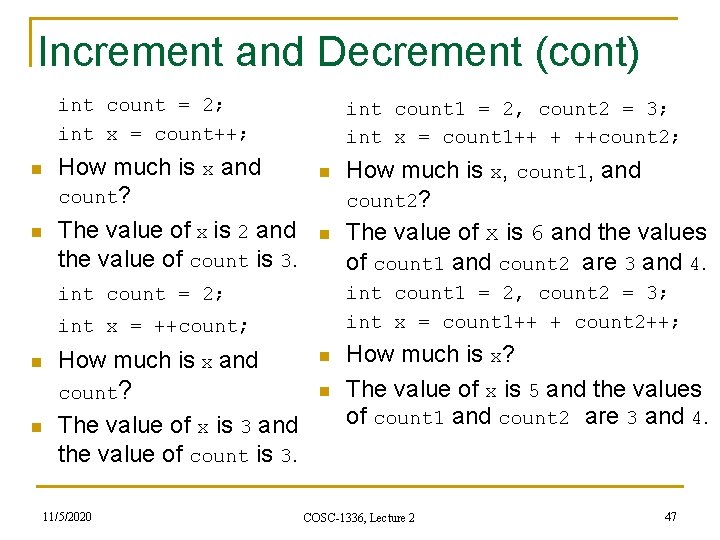
Increment and Decrement (cont) int count = 2; int x = count++; n n int count 1 = 2, count 2 = 3; int x = count 1++ + ++count 2; How much is x and count? The value of x is 2 and the value of count is 3. n n int count 1 = 2, count 2 = 3; int x = count 1++ + count 2++; int count = 2; int x = ++count; n n How much is x and n count? n The value of x is 3 and the value of count is 3. 11/5/2020 How much is x, count 1, and count 2? The value of x is 6 and the values of count 1 and count 2 are 3 and 4. How much is x? The value of x is 5 and the values of count 1 and count 2 are 3 and 4. COSC-1336, Lecture 2 47
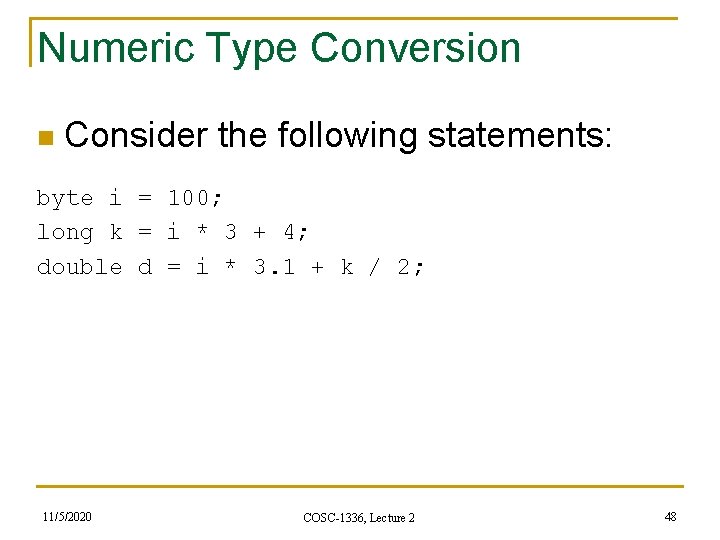
Numeric Type Conversion n Consider the following statements: byte i = 100; long k = i * 3 + 4; double d = i * 3. 1 + k / 2; 11/5/2020 COSC-1336, Lecture 2 48
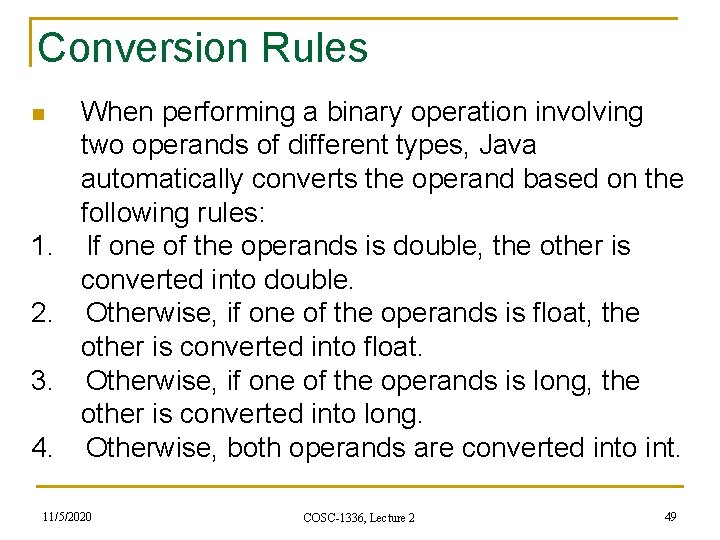
Conversion Rules When performing a binary operation involving two operands of different types, Java automatically converts the operand based on the following rules: 1. If one of the operands is double, the other is converted into double. 2. Otherwise, if one of the operands is float, the other is converted into float. 3. Otherwise, if one of the operands is long, the other is converted into long. 4. Otherwise, both operands are converted into int. n 11/5/2020 COSC-1336, Lecture 2 49
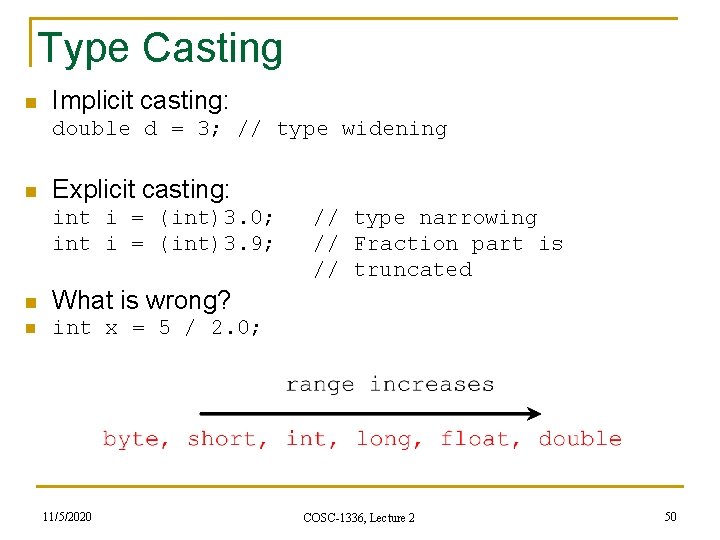
Type Casting n Implicit casting: double d = 3; // type widening n Explicit casting: int i = (int)3. 0; // type narrowing int i = (int)3. 9; // Fraction part is // truncated n What is wrong? n int x = 5 / 2. 0; 11/5/2020 COSC-1336, Lecture 2 50
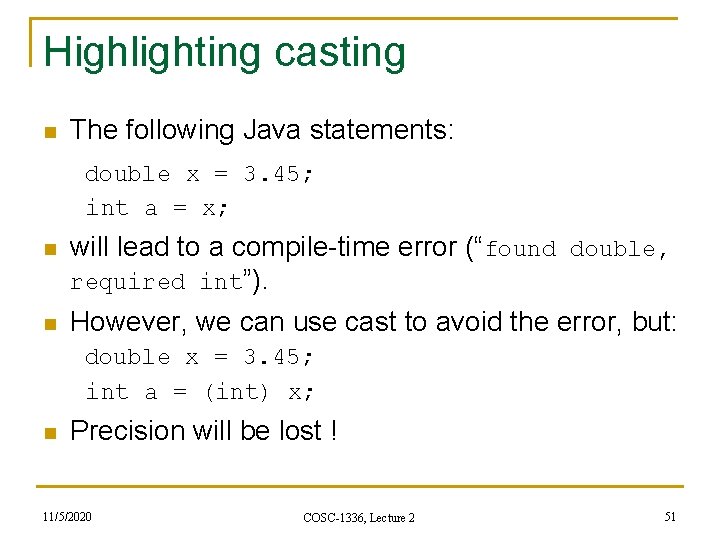
Highlighting casting n The following Java statements: double x = 3. 45; int a = x; n n will lead to a compile-time error (“found double, required int”). However, we can use cast to avoid the error, but: double x = 3. 45; int a = (int) x; n Precision will be lost ! 11/5/2020 COSC-1336, Lecture 2 51
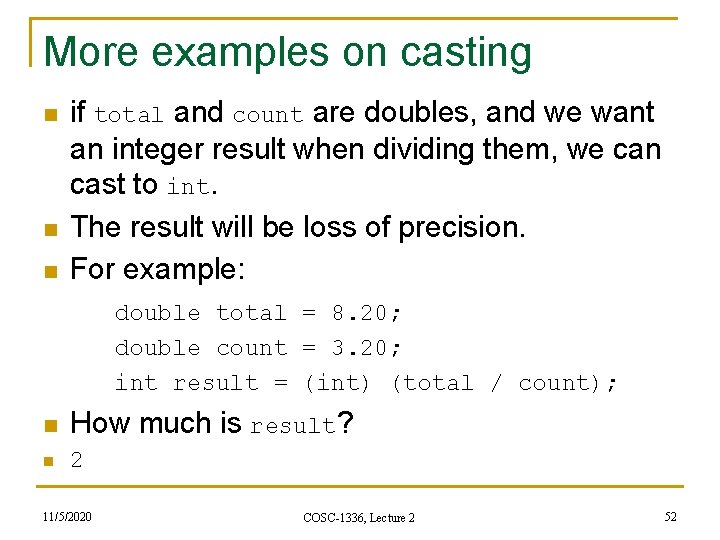
More examples on casting n n n if total and count are doubles, and we want an integer result when dividing them, we can cast to int. The result will be loss of precision. For example: double total = 8. 20; double count = 3. 20; int result = (int) (total / count); n How much is result? n 2 11/5/2020 COSC-1336, Lecture 2 52
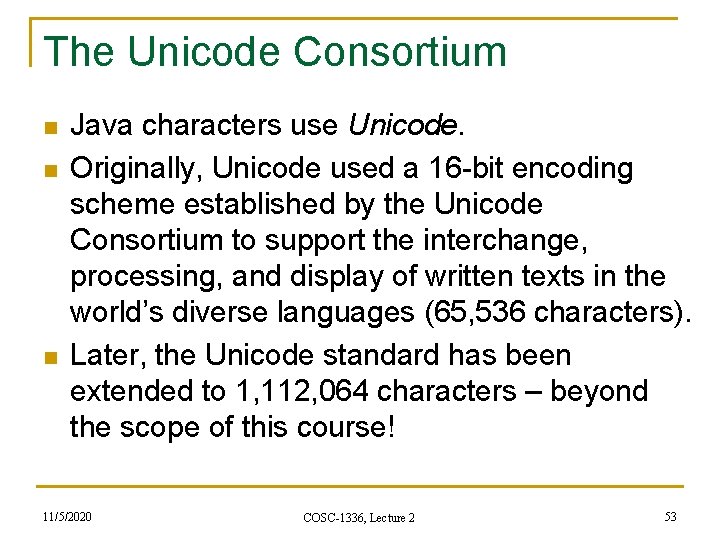
The Unicode Consortium n n n Java characters use Unicode. Originally, Unicode used a 16 -bit encoding scheme established by the Unicode Consortium to support the interchange, processing, and display of written texts in the world’s diverse languages (65, 536 characters). Later, the Unicode standard has been extended to 1, 112, 064 characters – beyond the scope of this course! 11/5/2020 COSC-1336, Lecture 2 53
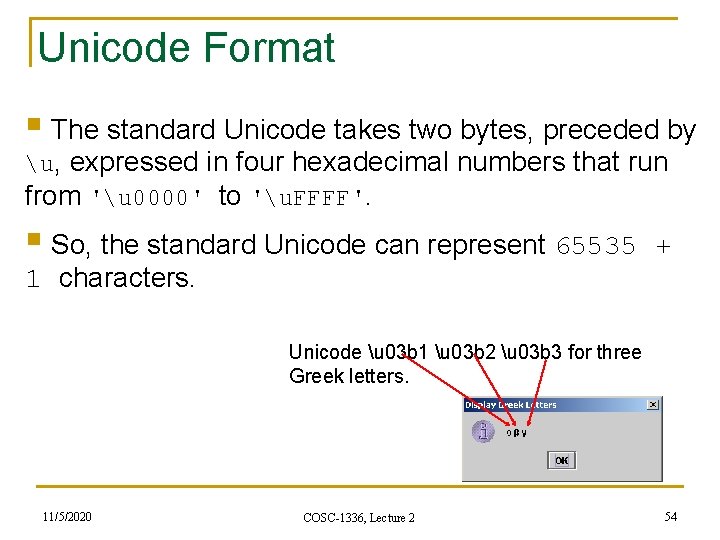
Unicode Format § The standard Unicode takes two bytes, preceded by u, expressed in four hexadecimal numbers that run from 'u 0000' to 'u. FFFF'. § So, the standard Unicode can represent 65535 + 1 characters. Unicode u 03 b 1 u 03 b 2 u 03 b 3 for three Greek letters. 11/5/2020 COSC-1336, Lecture 2 54
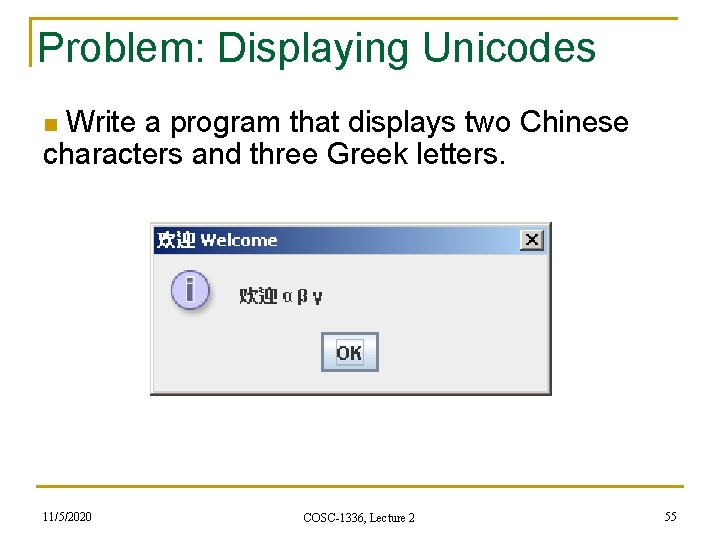
Problem: Displaying Unicodes n Write a program that displays two Chinese characters and three Greek letters. 11/5/2020 COSC-1336, Lecture 2 55
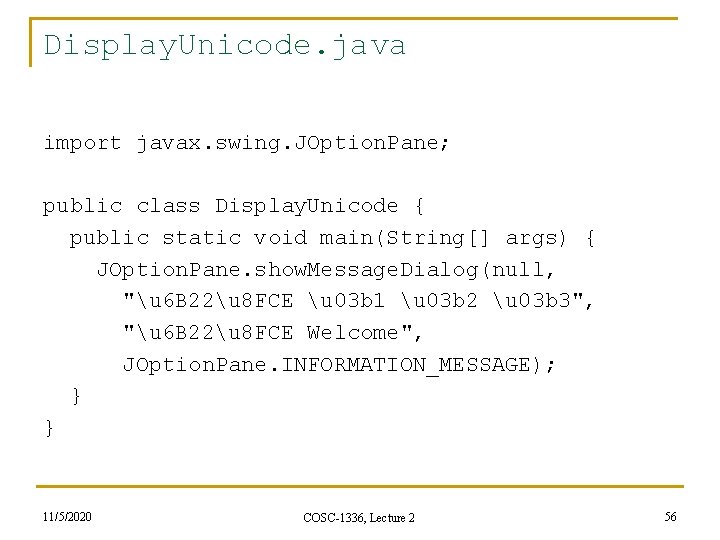
Display. Unicode. java import javax. swing. JOption. Pane; public class Display. Unicode { public static void main(String[] args) { JOption. Pane. show. Message. Dialog(null, "u 6 B 22u 8 FCE u 03 b 1 u 03 b 2 u 03 b 3", "u 6 B 22u 8 FCE Welcome", JOption. Pane. INFORMATION_MESSAGE); } } 11/5/2020 COSC-1336, Lecture 2 56
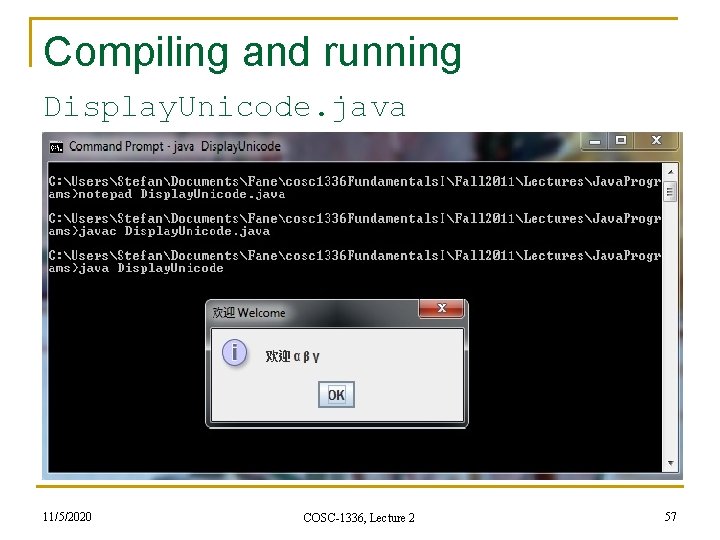
Compiling and running Display. Unicode. java 11/5/2020 COSC-1336, Lecture 2 57
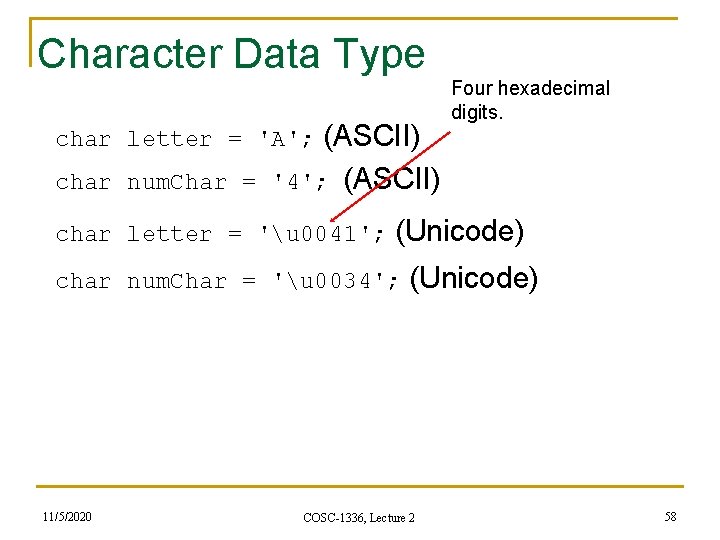
Character Data Type Four hexadecimal digits. char letter = 'A'; (ASCII) char num. Char = '4'; (ASCII) char letter = 'u 0041'; (Unicode) char num. Char = 'u 0034'; (Unicode) 11/5/2020 COSC-1336, Lecture 2 58
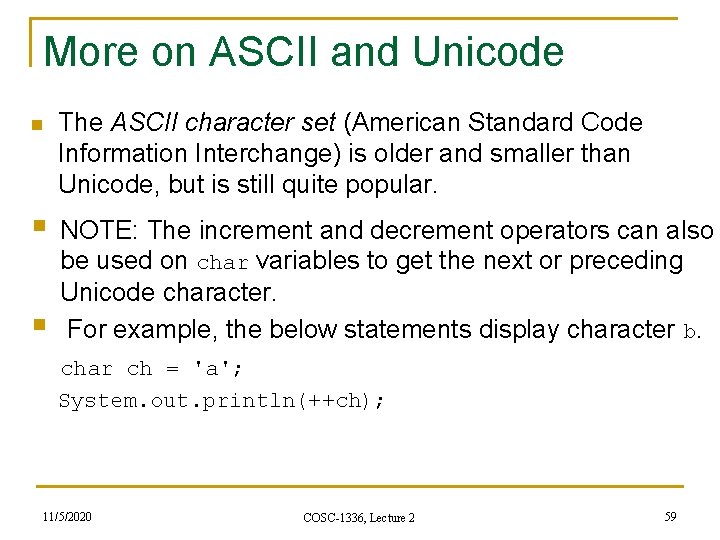
More on ASCII and Unicode n The ASCII character set (American Standard Code Information Interchange) is older and smaller than Unicode, but is still quite popular. § NOTE: The increment and decrement operators can also be used on char variables to get the next or preceding Unicode character. § For example, the below statements display character b. char ch = 'a'; System. out. println(++ch); 11/5/2020 COSC-1336, Lecture 2 59
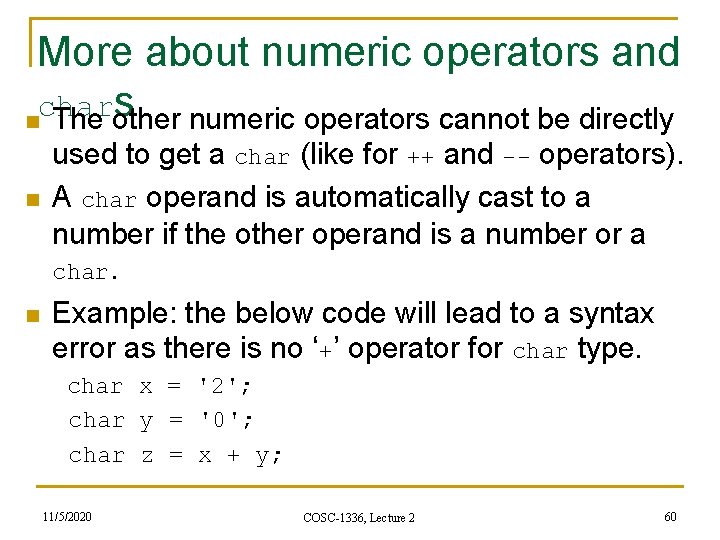
More about numeric operators and chars n The other numeric operators cannot be directly n n used to get a char (like for ++ and -- operators). A char operand is automatically cast to a number if the other operand is a number or a char. Example: the below code will lead to a syntax error as there is no ‘+’ operator for char type. char x = '2'; char y = '0'; char z = x + y; 11/5/2020 COSC-1336, Lecture 2 60
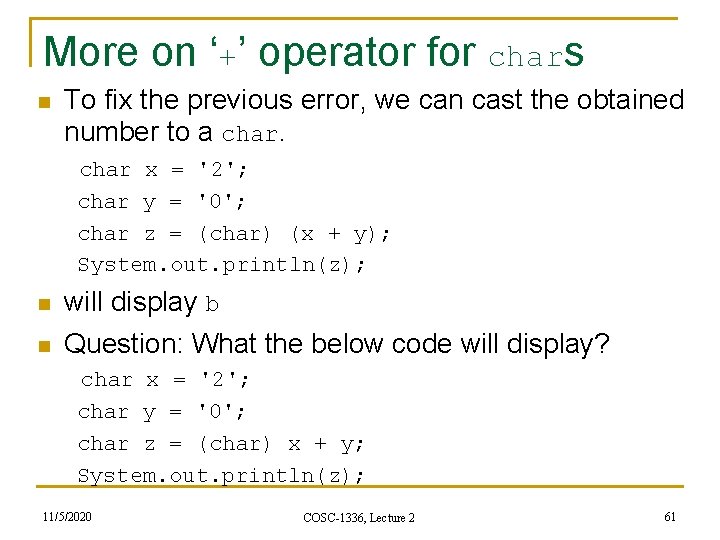
More on ‘+’ operator for chars n To fix the previous error, we can cast the obtained number to a char x = '2'; char y = '0'; char z = (char) (x + y); System. out. println(z); n n will display b Question: What the below code will display? char x = '2'; char y = '0'; char z = (char) x + y; System. out. println(z); 11/5/2020 COSC-1336, Lecture 2 61
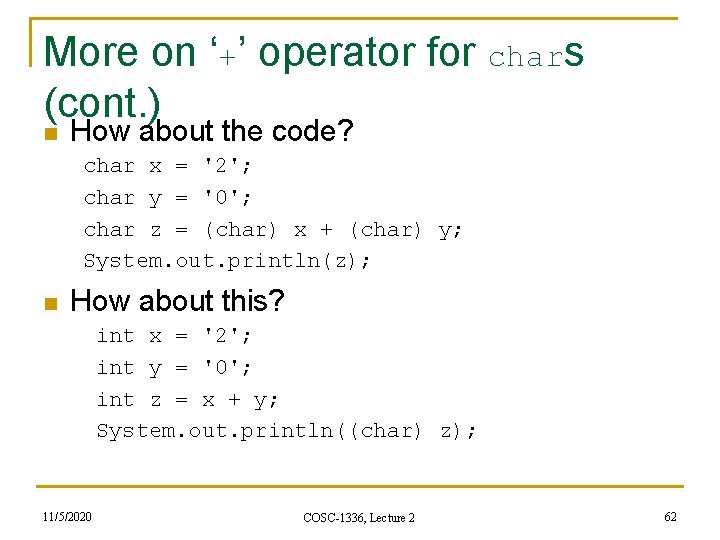
More on ‘+’ operator for chars (cont. ) n How about the code? char x = '2'; char y = '0'; char z = (char) x + (char) y; System. out. println(z); n How about this? int x = '2'; int y = '0'; int z = x + y; System. out. println((char) z); 11/5/2020 COSC-1336, Lecture 2 62
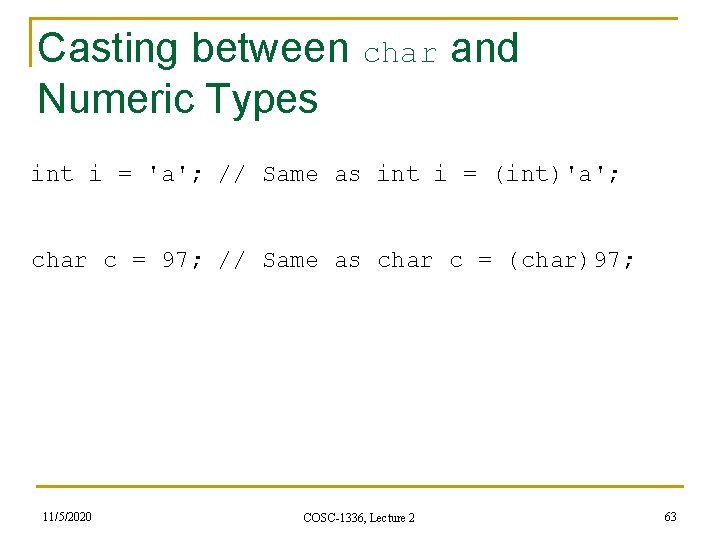
Casting between char and Numeric Types int i = 'a'; // Same as int i = (int)'a'; char c = 97; // Same as char c = (char)97; 11/5/2020 COSC-1336, Lecture 2 63
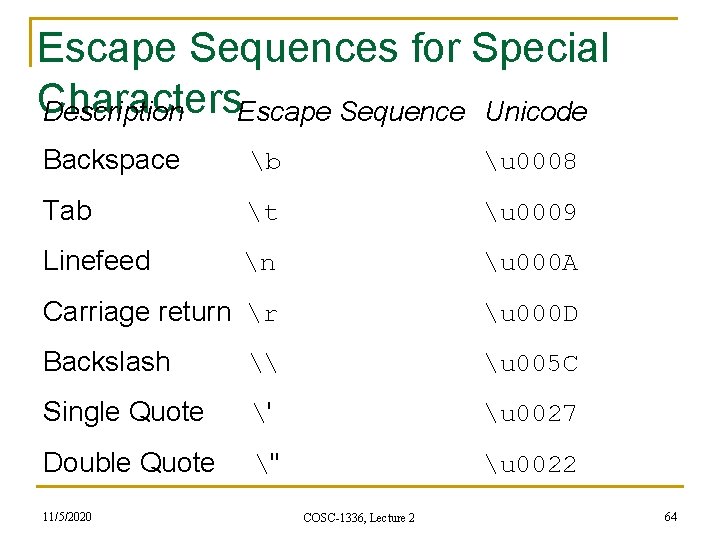
Escape Sequences for Special Characters Description Escape Sequence Unicode Backspace b u 0008 Tab t u 0009 Linefeed n u 000 A Carriage return r u 000 D Backslash \ u 005 C Single Quote ' u 0027 Double Quote " u 0022 11/5/2020 COSC-1336, Lecture 2 64
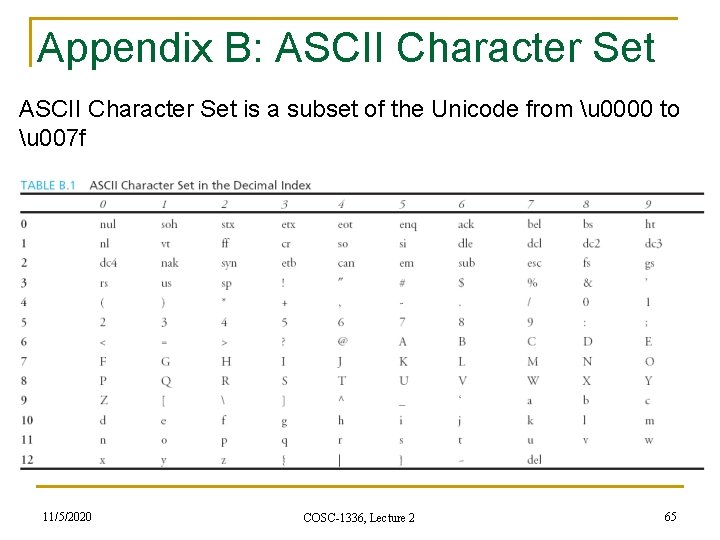
Appendix B: ASCII Character Set is a subset of the Unicode from u 0000 to u 007 f 11/5/2020 COSC-1336, Lecture 2 65
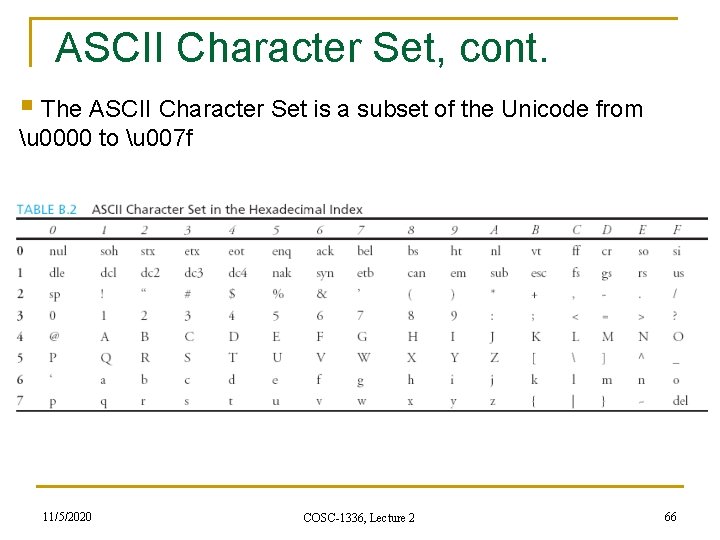
ASCII Character Set, cont. § The ASCII Character Set is a subset of the Unicode from u 0000 to u 007 f 11/5/2020 COSC-1336, Lecture 2 66
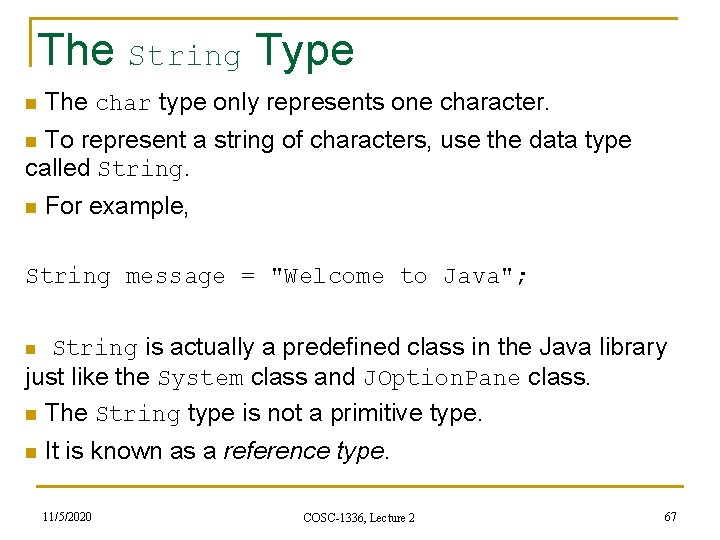
The String Type n The char type only represents one character. n To represent a string of characters, use the data type called String. n For example, String message = "Welcome to Java"; n String is actually a predefined class in the Java library just like the System class and JOption. Pane class. n The String type is not a primitive type. n It is known as a reference 11/5/2020 type. COSC-1336, Lecture 2 67
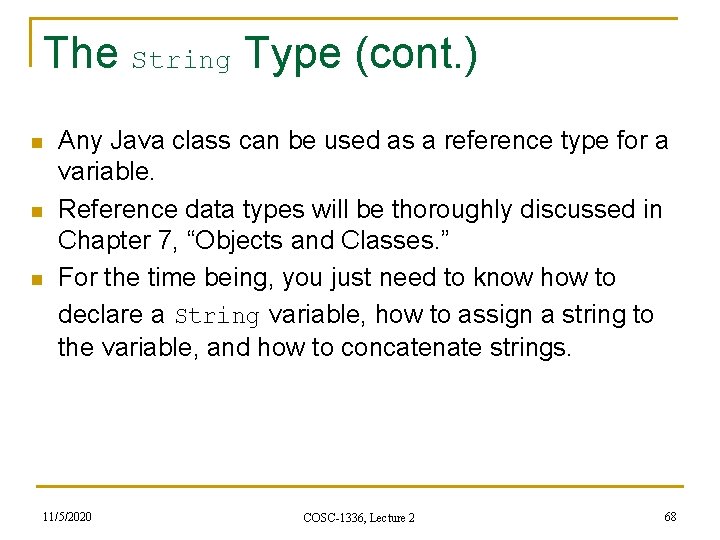
The String Type (cont. ) n n n Any Java class can be used as a reference type for a variable. Reference data types will be thoroughly discussed in Chapter 7, “Objects and Classes. ” For the time being, you just need to know how to declare a String variable, how to assign a string to the variable, and how to concatenate strings. 11/5/2020 COSC-1336, Lecture 2 68
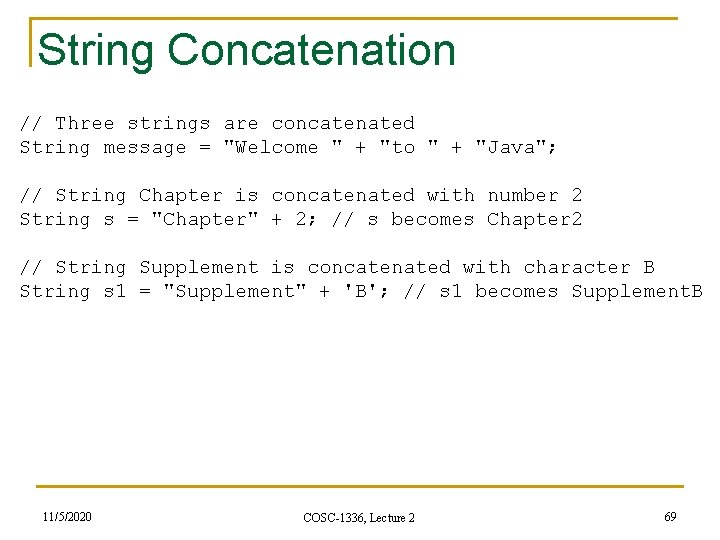
String Concatenation // Three strings are concatenated String message = "Welcome " + "to " + "Java"; // String Chapter is concatenated with number 2 String s = "Chapter" + 2; // s becomes Chapter 2 // String Supplement is concatenated with character B String s 1 = "Supplement" + 'B'; // s 1 becomes Supplement. B 11/5/2020 COSC-1336, Lecture 2 69
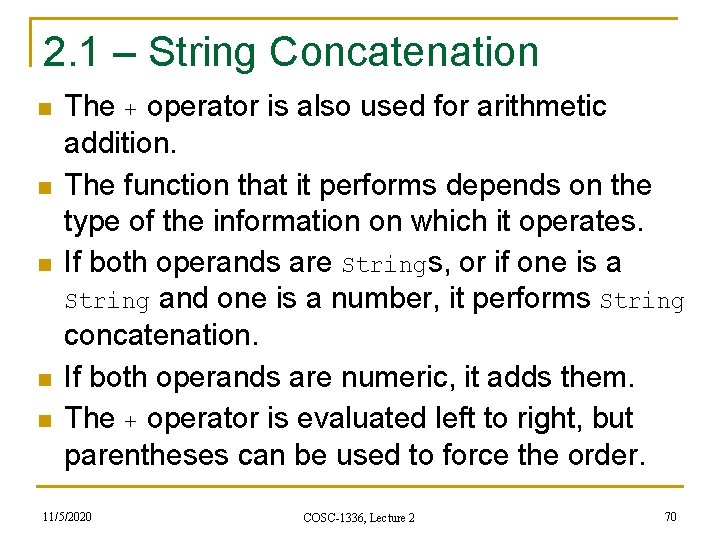
2. 1 – String Concatenation n n The + operator is also used for arithmetic addition. The function that it performs depends on the type of the information on which it operates. If both operands are Strings, or if one is a String and one is a number, it performs String concatenation. If both operands are numeric, it adds them. The + operator is evaluated left to right, but parentheses can be used to force the order. 11/5/2020 COSC-1336, Lecture 2 70
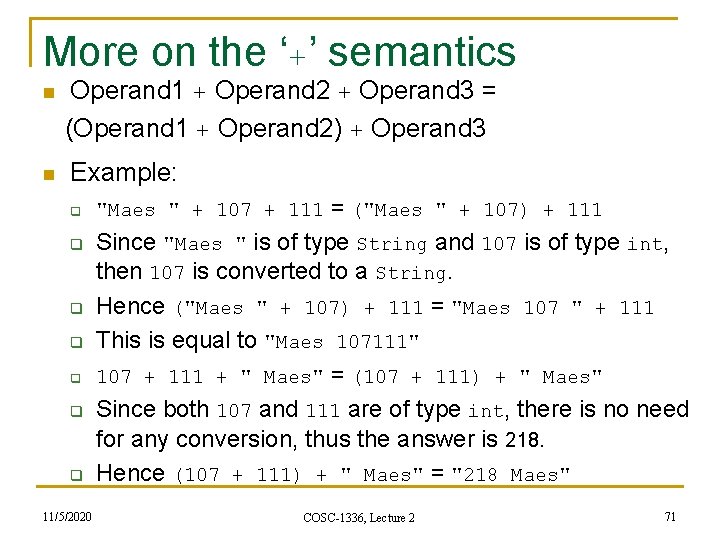
More on the ‘+’ semantics Operand 1 + Operand 2 + Operand 3 = (Operand 1 + Operand 2) + Operand 3 n n Example: q q q q 11/5/2020 "Maes " + 107 + 111 = ("Maes " + 107) + 111 Since "Maes " is of type String and 107 is of type int, then 107 is converted to a String. Hence ("Maes " + 107) + 111 = "Maes 107 " + 111 This is equal to "Maes 107111" 107 + 111 + " Maes" = (107 + 111) + " Maes" Since both 107 and 111 are of type int, there is no need for any conversion, thus the answer is 218. Hence (107 + 111) + " Maes" = "218 Maes" COSC-1336, Lecture 2 71
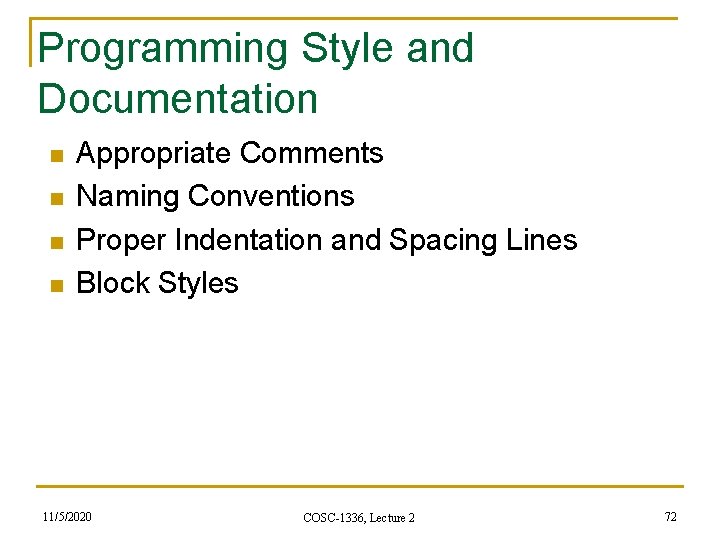
Programming Style and Documentation n n Appropriate Comments Naming Conventions Proper Indentation and Spacing Lines Block Styles 11/5/2020 COSC-1336, Lecture 2 72
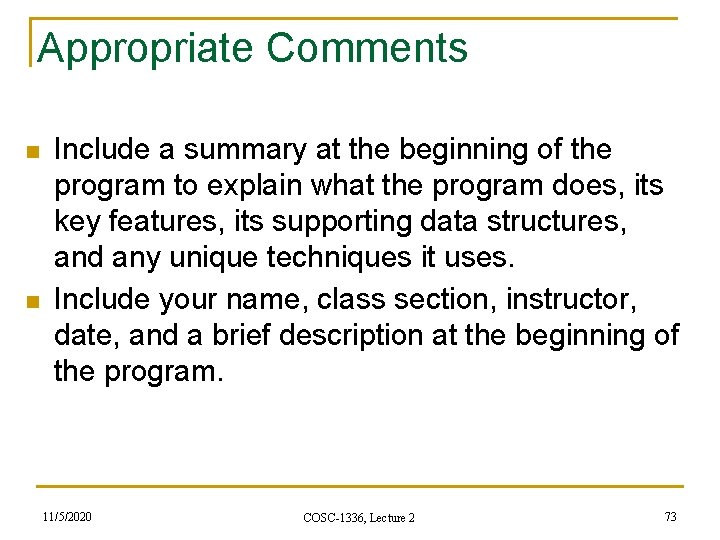
Appropriate Comments n n Include a summary at the beginning of the program to explain what the program does, its key features, its supporting data structures, and any unique techniques it uses. Include your name, class section, instructor, date, and a brief description at the beginning of the program. 11/5/2020 COSC-1336, Lecture 2 73
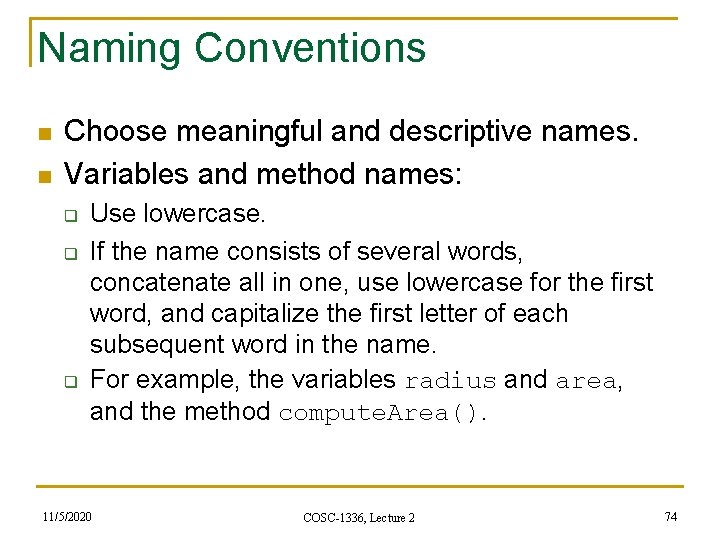
Naming Conventions n n Choose meaningful and descriptive names. Variables and method names: q q q Use lowercase. If the name consists of several words, concatenate all in one, use lowercase for the first word, and capitalize the first letter of each subsequent word in the name. For example, the variables radius and area, and the method compute. Area(). 11/5/2020 COSC-1336, Lecture 2 74
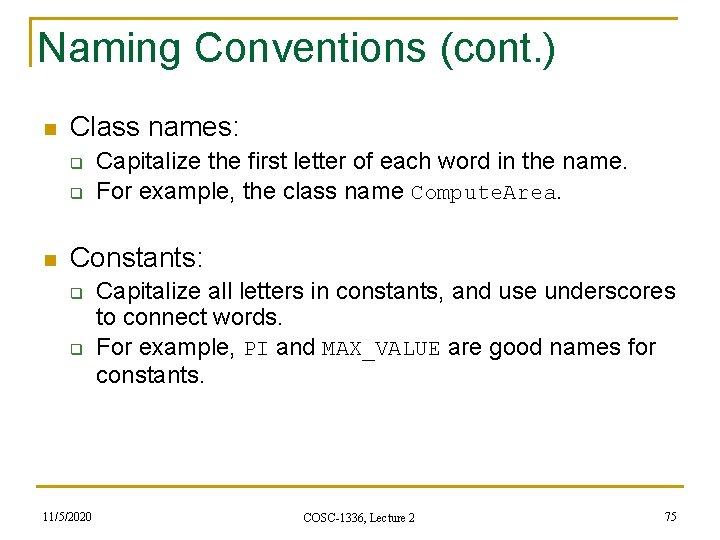
Naming Conventions (cont. ) n Class names: q q n Capitalize the first letter of each word in the name. For example, the class name Compute. Area. Constants: q q 11/5/2020 Capitalize all letters in constants, and use underscores to connect words. For example, PI and MAX_VALUE are good names for constants. COSC-1336, Lecture 2 75
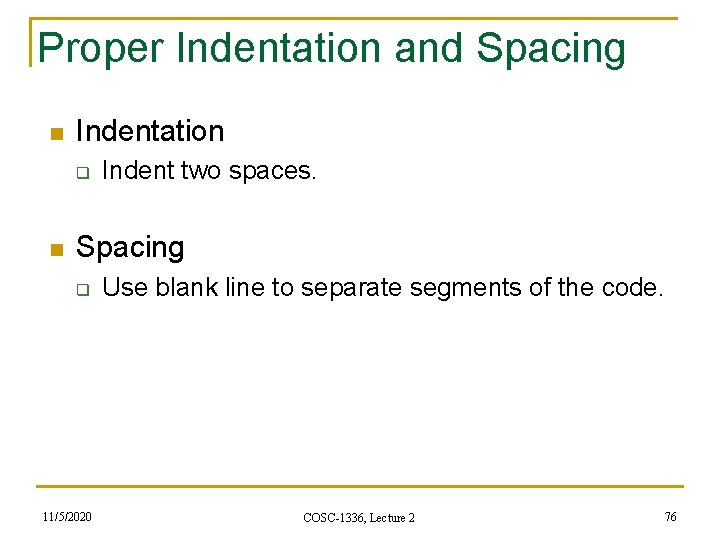
Proper Indentation and Spacing n Indentation q n Indent two spaces. Spacing q 11/5/2020 Use blank line to separate segments of the code. COSC-1336, Lecture 2 76
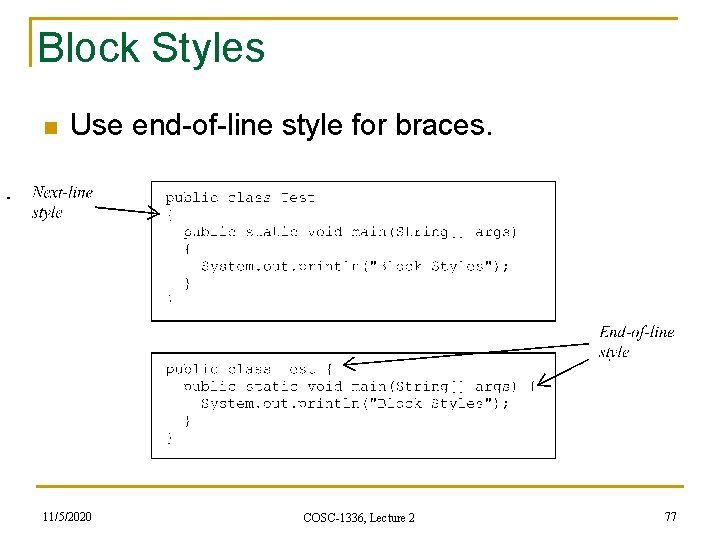
Block Styles n Use end-of-line style for braces. 11/5/2020 COSC-1336, Lecture 2 77
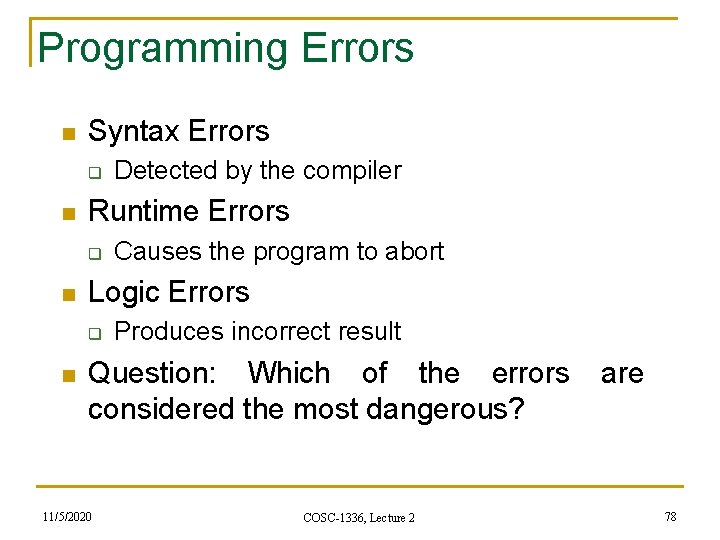
Programming Errors n Syntax Errors q n Runtime Errors q n Causes the program to abort Logic Errors q n Detected by the compiler Produces incorrect result Question: Which of the errors are considered the most dangerous? 11/5/2020 COSC-1336, Lecture 2 78
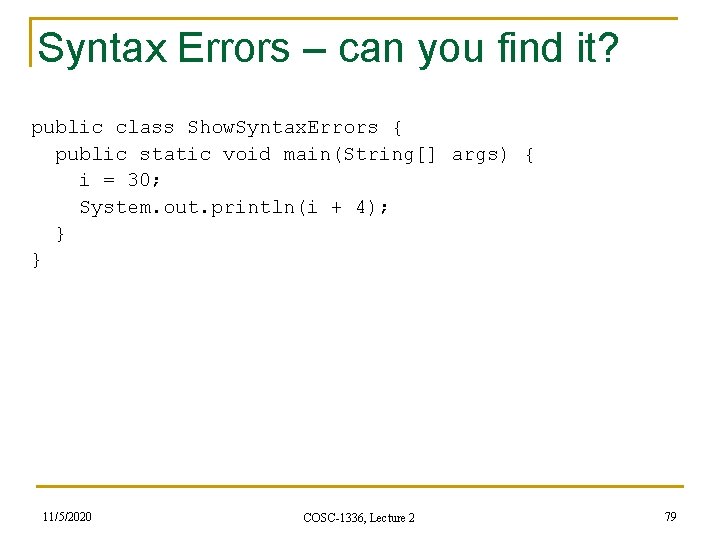
Syntax Errors – can you find it? public class Show. Syntax. Errors { public static void main(String[] args) { i = 30; System. out. println(i + 4); } } 11/5/2020 COSC-1336, Lecture 2 79
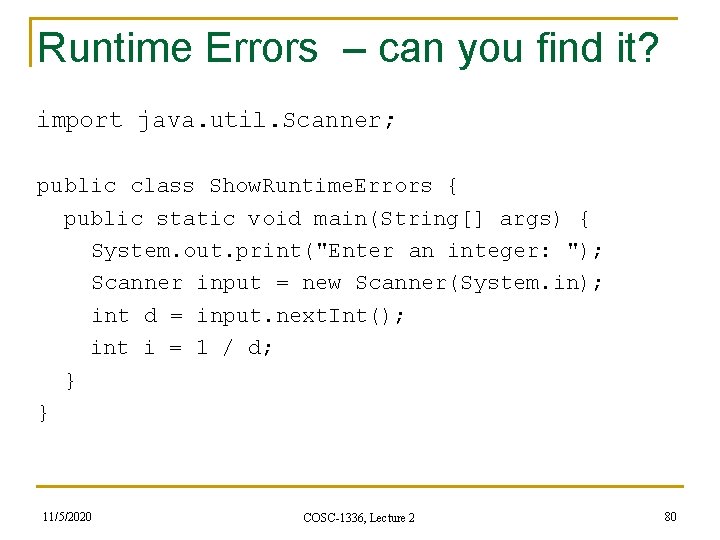
Runtime Errors – can you find it? import java. util. Scanner; public class Show. Runtime. Errors { public static void main(String[] args) { System. out. print("Enter an integer: "); Scanner input = new Scanner(System. in); int d = input. next. Int(); int i = 1 / d; } } 11/5/2020 COSC-1336, Lecture 2 80
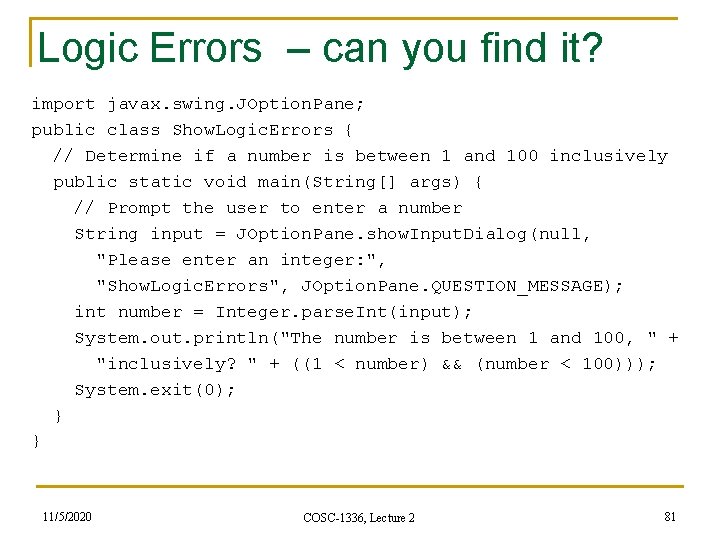
Logic Errors – can you find it? import javax. swing. JOption. Pane; public class Show. Logic. Errors { // Determine if a number is between 1 and 100 inclusively public static void main(String[] args) { // Prompt the user to enter a number String input = JOption. Pane. show. Input. Dialog(null, "Please enter an integer: ", "Show. Logic. Errors", JOption. Pane. QUESTION_MESSAGE); int number = Integer. parse. Int(input); System. out. println("The number is between 1 and 100, " + "inclusively? " + ((1 < number) && (number < 100))); System. exit(0); } } 11/5/2020 COSC-1336, Lecture 2 81
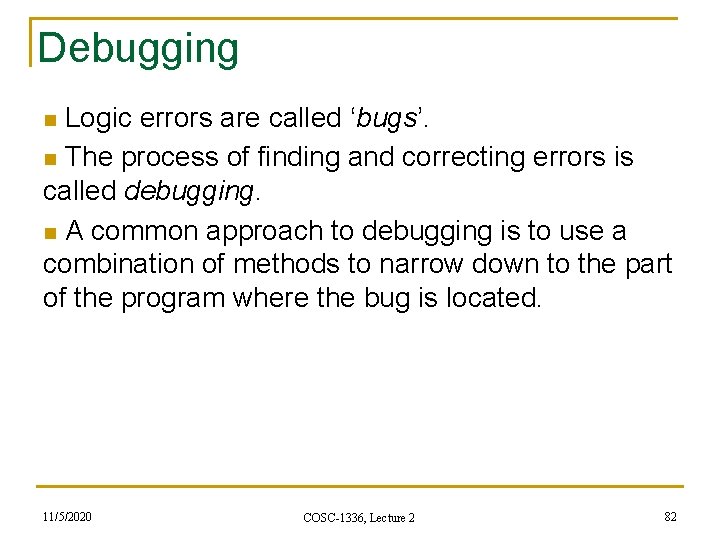
Debugging n Logic errors are called ‘bugs’. n The process of finding and correcting errors is called debugging. n A common approach to debugging is to use a combination of methods to narrow down to the part of the program where the bug is located. 11/5/2020 COSC-1336, Lecture 2 82
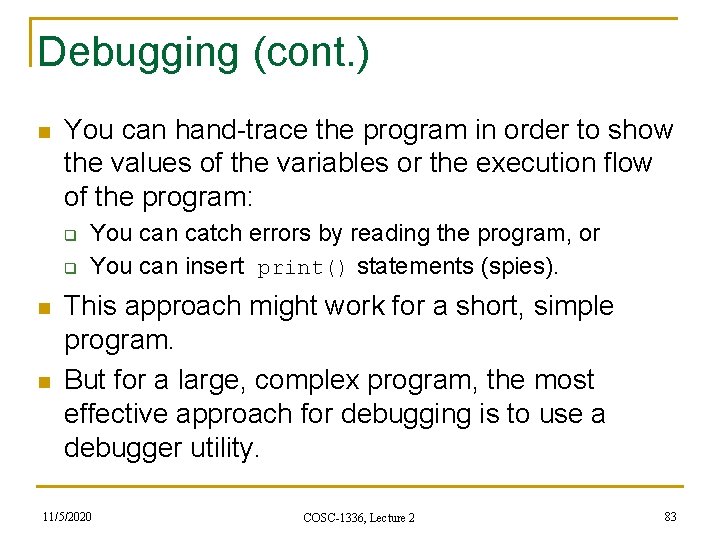
Debugging (cont. ) n You can hand-trace the program in order to show the values of the variables or the execution flow of the program: q q n n You can catch errors by reading the program, or You can insert print() statements (spies). This approach might work for a short, simple program. But for a large, complex program, the most effective approach for debugging is to use a debugger utility. 11/5/2020 COSC-1336, Lecture 2 83
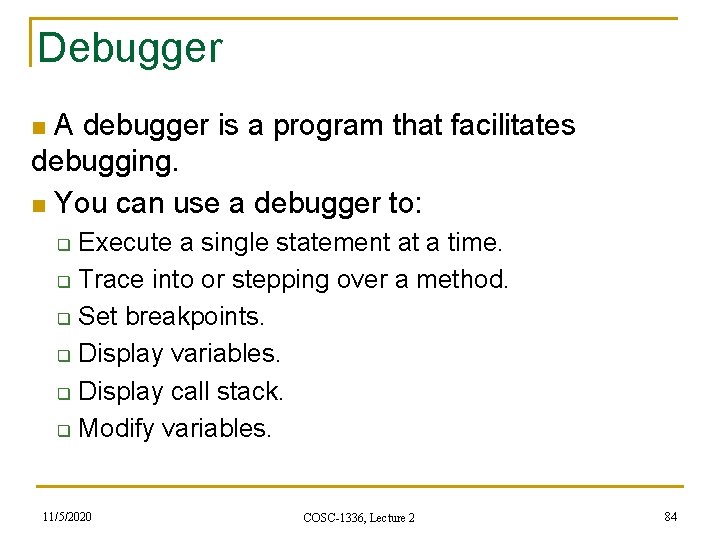
Debugger n A debugger is a program that facilitates debugging. n You can use a debugger to: Execute a single statement at a time. q Trace into or stepping over a method. q Set breakpoints. q Display variables. q Display call stack. q Modify variables. q 11/5/2020 COSC-1336, Lecture 2 84
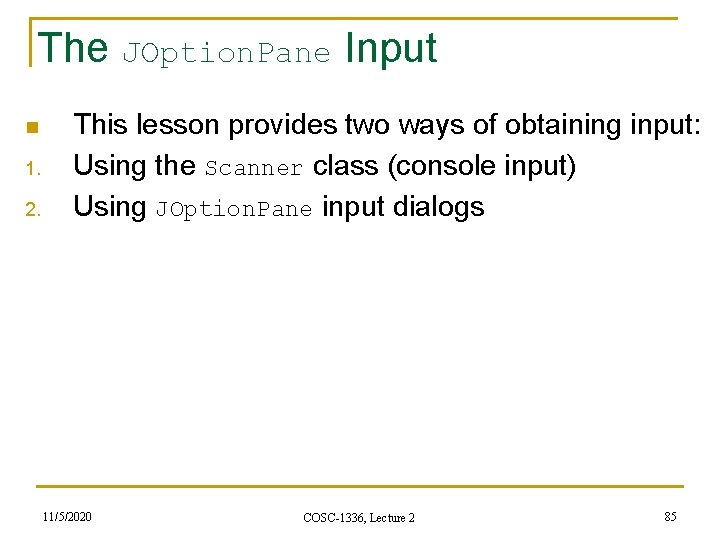
The JOption. Pane Input n 1. 2. This lesson provides two ways of obtaining input: Using the Scanner class (console input) Using JOption. Pane input dialogs 11/5/2020 COSC-1336, Lecture 2 85
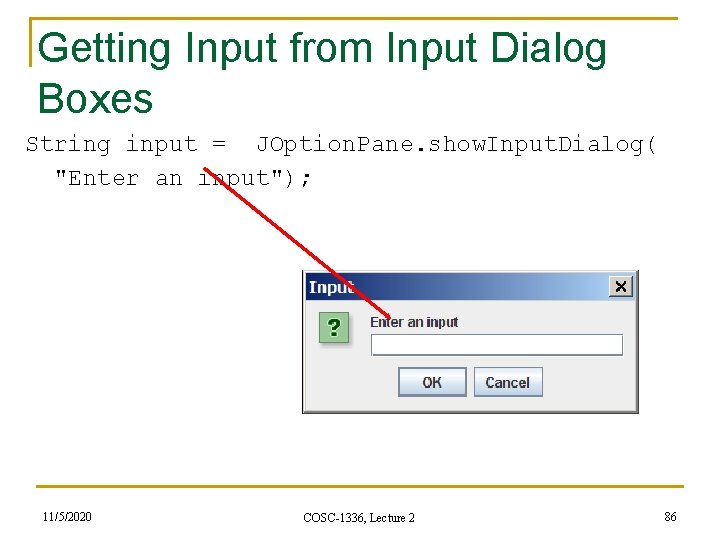
Getting Input from Input Dialog Boxes String input = JOption. Pane. show. Input. Dialog( "Enter an input"); 11/5/2020 COSC-1336, Lecture 2 86
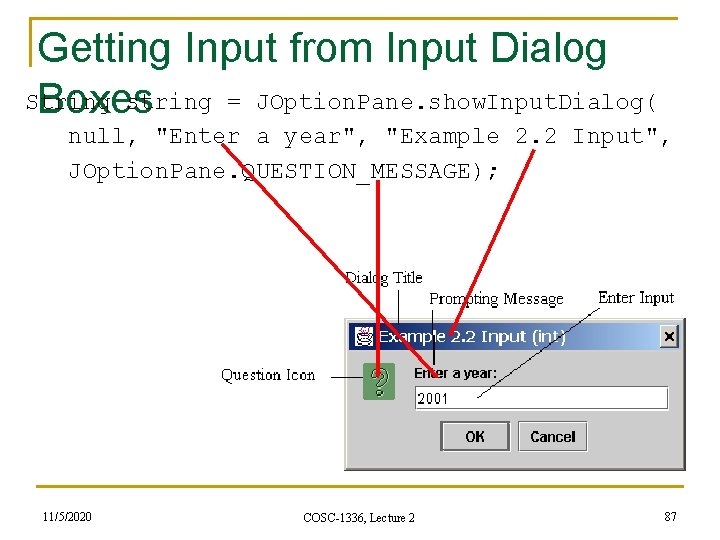
Getting Input from Input Dialog String string = JOption. Pane. show. Input. Dialog( Boxes null, "Enter a year", "Example 2. 2 Input", JOption. Pane. QUESTION_MESSAGE); 11/5/2020 COSC-1336, Lecture 2 87
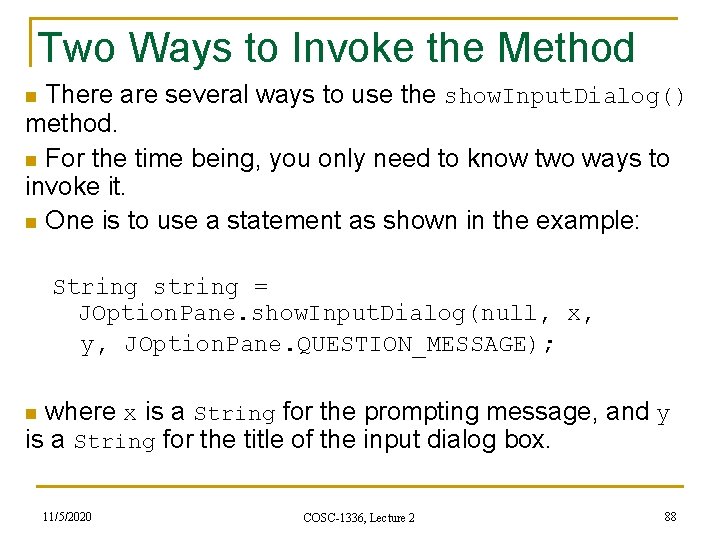
Two Ways to Invoke the Method n There are several ways to use the show. Input. Dialog() method. n For the time being, you only need to know two ways to invoke it. n One is to use a statement as shown in the example: String string = JOption. Pane. show. Input. Dialog(null, x, y, JOption. Pane. QUESTION_MESSAGE); n where x is a String for the prompting message, and y is a String for the title of the input dialog box. 11/5/2020 COSC-1336, Lecture 2 88
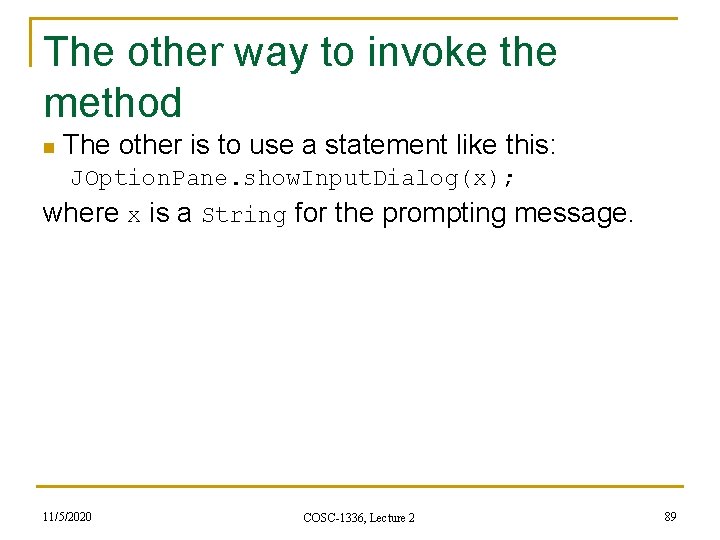
The other way to invoke the method n The other is to use a statement like this: JOption. Pane. show. Input. Dialog(x); where x is a String for the prompting message. 11/5/2020 COSC-1336, Lecture 2 89
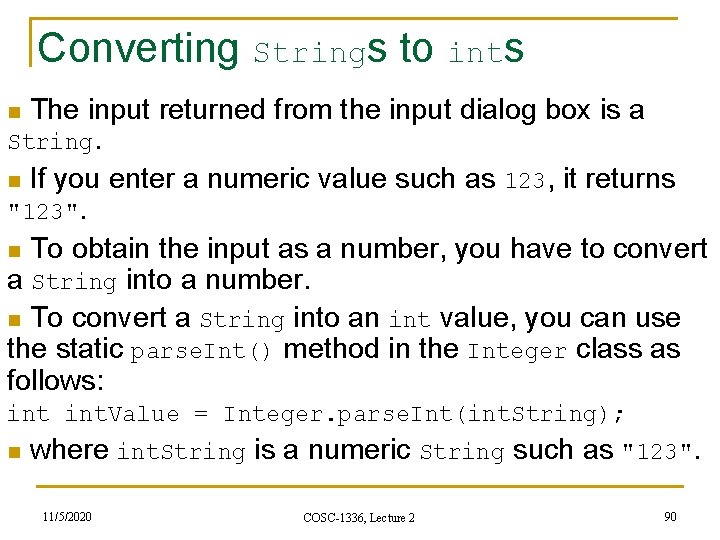
Converting Strings to ints n The input returned from the input dialog box is a String. n If you enter a numeric value such as 123, it returns "123". n To obtain the input as a number, you have to convert a String into a number. n To convert a String into an int value, you can use the static parse. Int() method in the Integer class as follows: int. Value = Integer. parse. Int(int. String); n where int. String is a numeric String such as "123". 11/5/2020 COSC-1336, Lecture 2 90
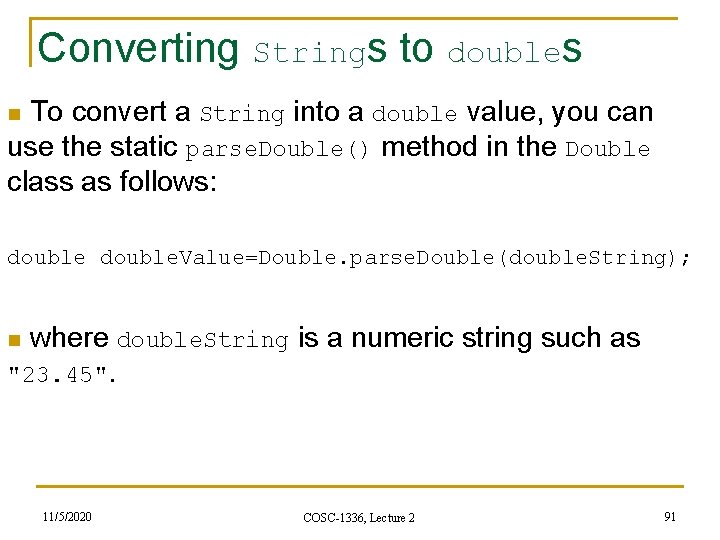
Converting Strings to doubles n To convert a String into a double value, you can use the static parse. Double() method in the Double class as follows: double. Value=Double. parse. Double(double. String); n where double. String is a numeric string such as "23. 45". 11/5/2020 COSC-1336, Lecture 2 91
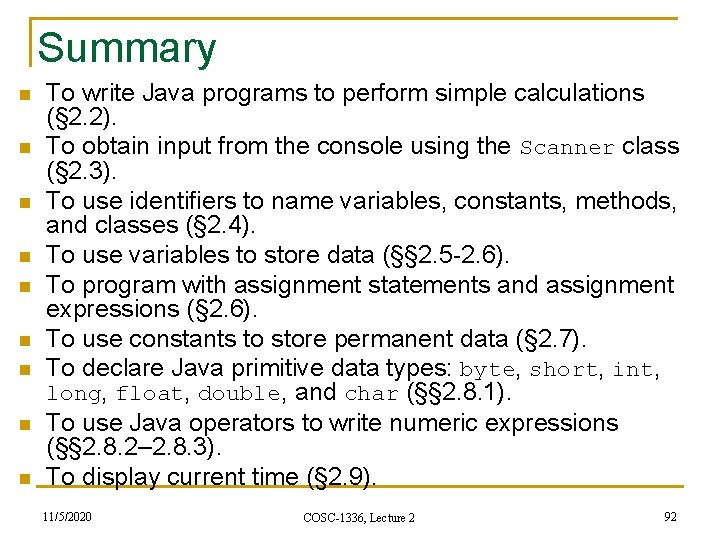
Summary n n n n n To write Java programs to perform simple calculations (§ 2. 2). To obtain input from the console using the Scanner class (§ 2. 3). To use identifiers to name variables, constants, methods, and classes (§ 2. 4). To use variables to store data (§§ 2. 5 -2. 6). To program with assignment statements and assignment expressions (§ 2. 6). To use constants to store permanent data (§ 2. 7). To declare Java primitive data types: byte, short, int, long, float, double, and char (§§ 2. 8. 1). To use Java operators to write numeric expressions (§§ 2. 8. 2– 2. 8. 3). To display current time (§ 2. 9). 11/5/2020 COSC-1336, Lecture 2 92
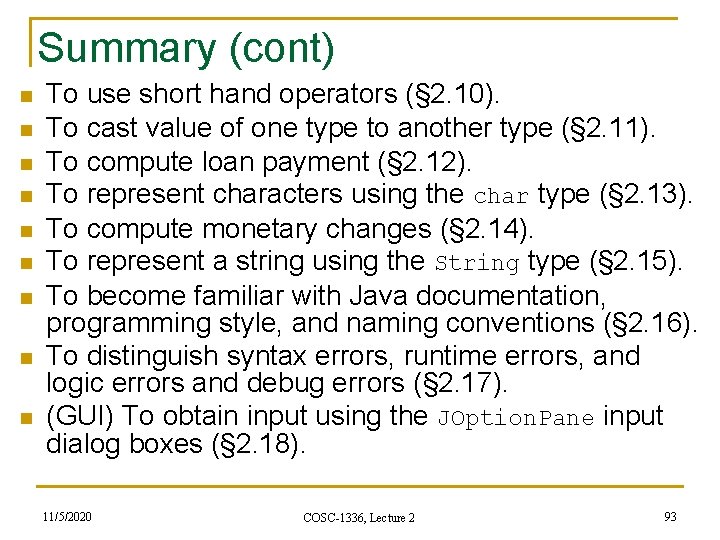
Summary (cont) n n n n n To use short hand operators (§ 2. 10). To cast value of one type to another type (§ 2. 11). To compute loan payment (§ 2. 12). To represent characters using the char type (§ 2. 13). To compute monetary changes (§ 2. 14). To represent a string using the String type (§ 2. 15). To become familiar with Java documentation, programming style, and naming conventions (§ 2. 16). To distinguish syntax errors, runtime errors, and logic errors and debug errors (§ 2. 17). (GUI) To obtain input using the JOption. Pane input dialog boxes (§ 2. 18). 11/5/2020 COSC-1336, Lecture 2 93
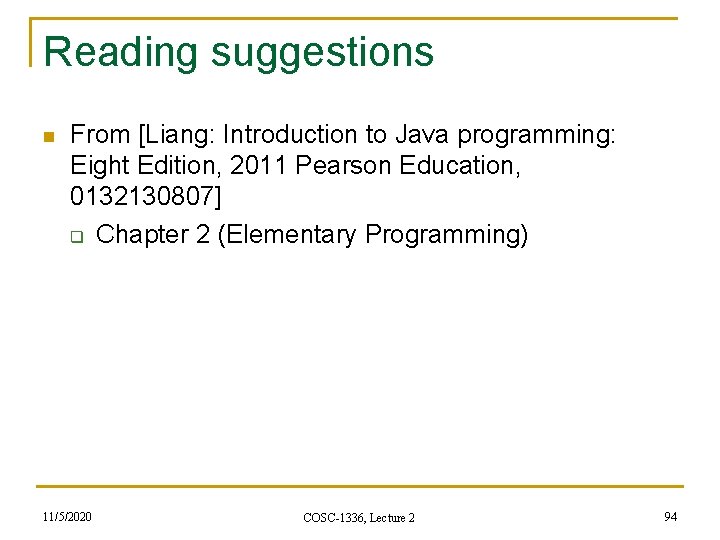
Reading suggestions n From [Liang: Introduction to Java programming: Eight Edition, 2011 Pearson Education, 0132130807] q Chapter 2 (Elementary Programming) 11/5/2020 COSC-1336, Lecture 2 94
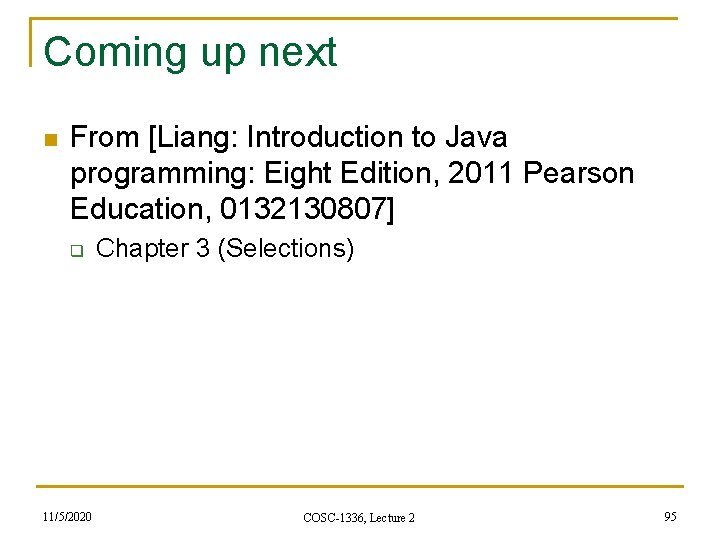
Coming up next n From [Liang: Introduction to Java programming: Eight Edition, 2011 Pearson Education, 0132130807] q 11/5/2020 Chapter 3 (Selections) COSC-1336, Lecture 2 95
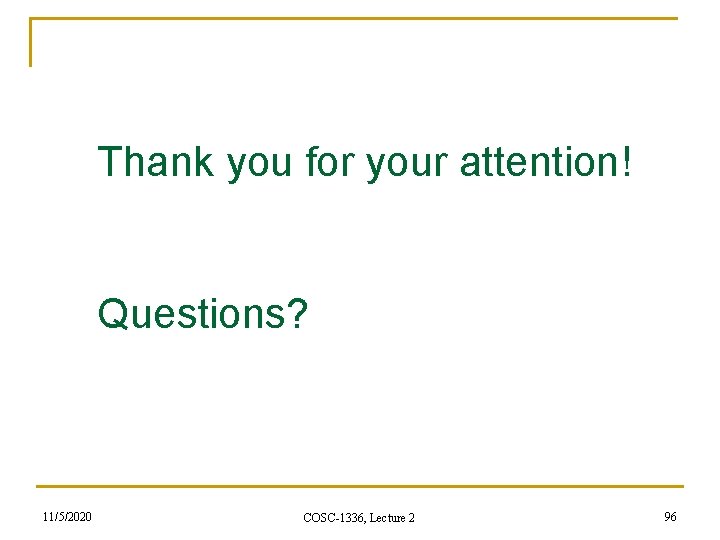
Thank you for your attention! Questions? 11/5/2020 COSC-1336, Lecture 2 96