Fundamental of Programming C Lecturer Omid Jafarinezhad Lecture
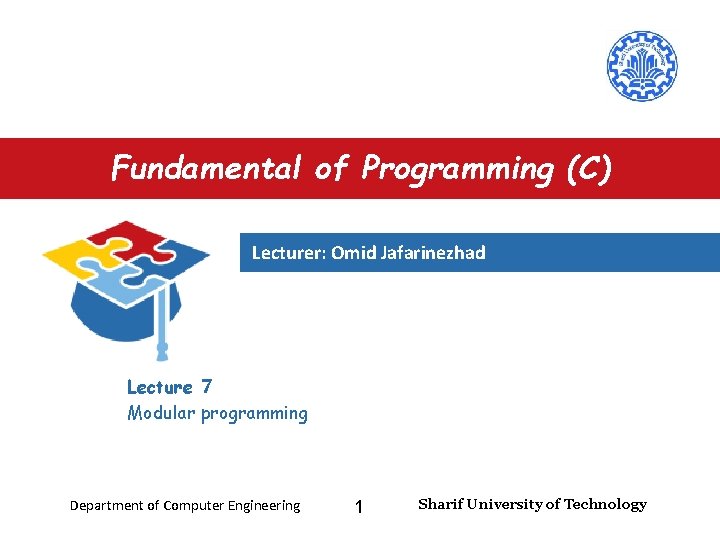
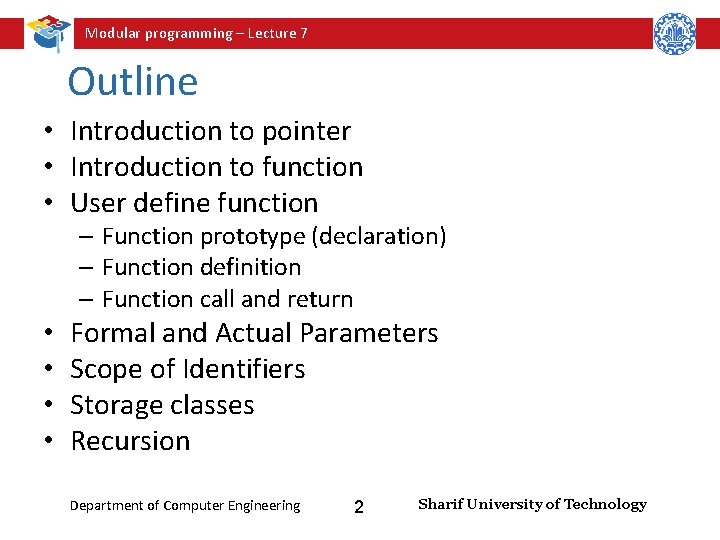
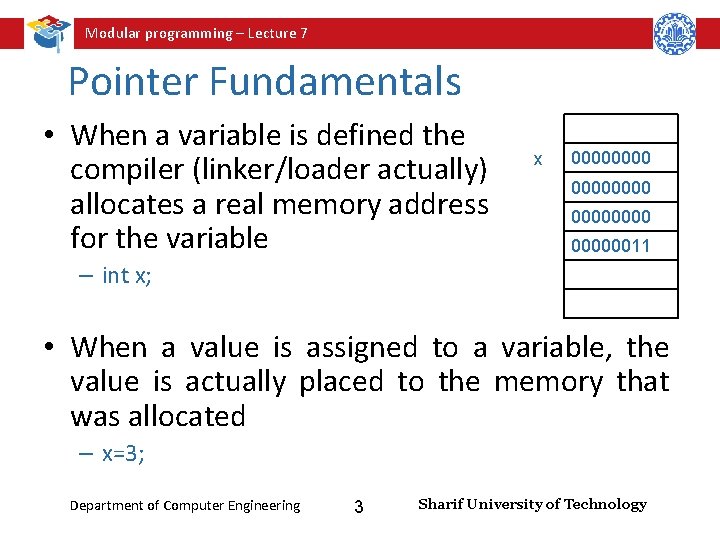
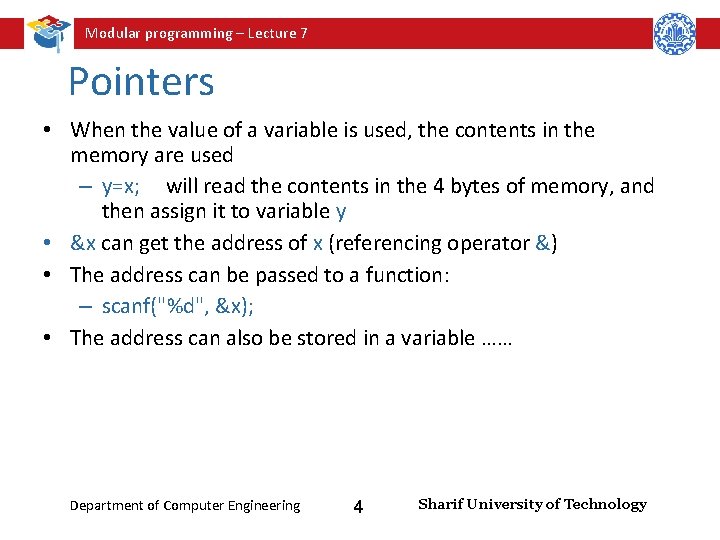
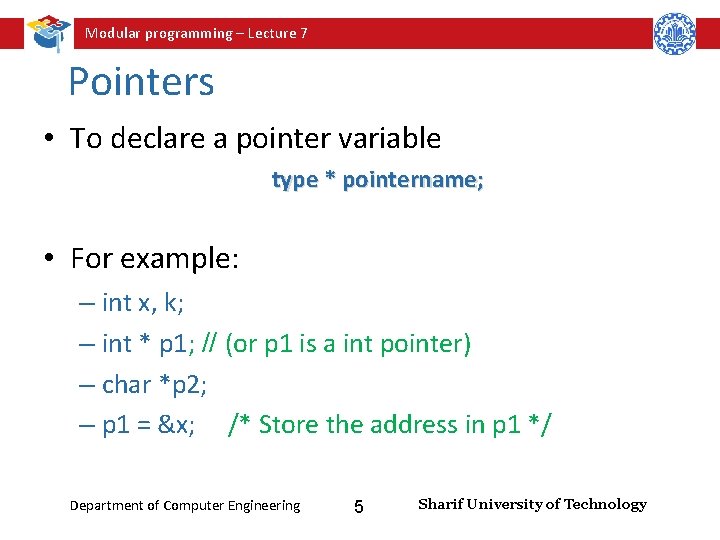
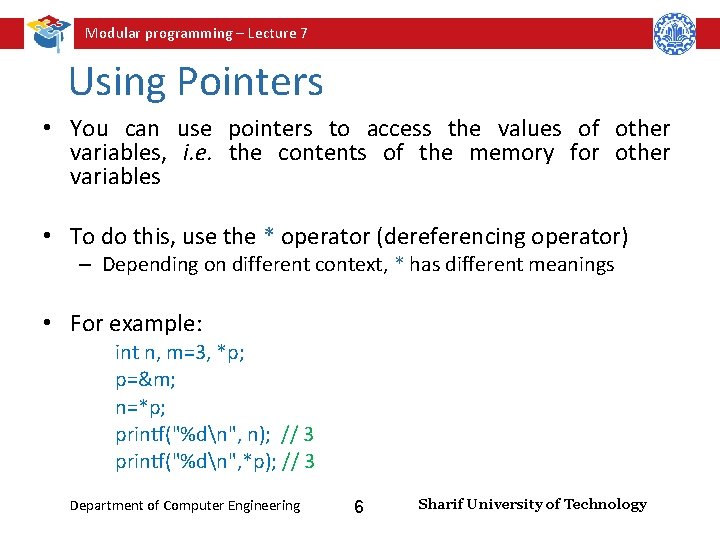
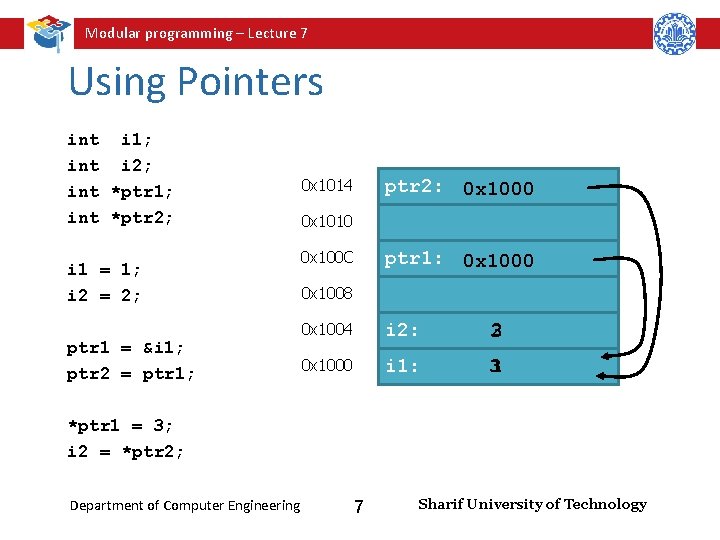
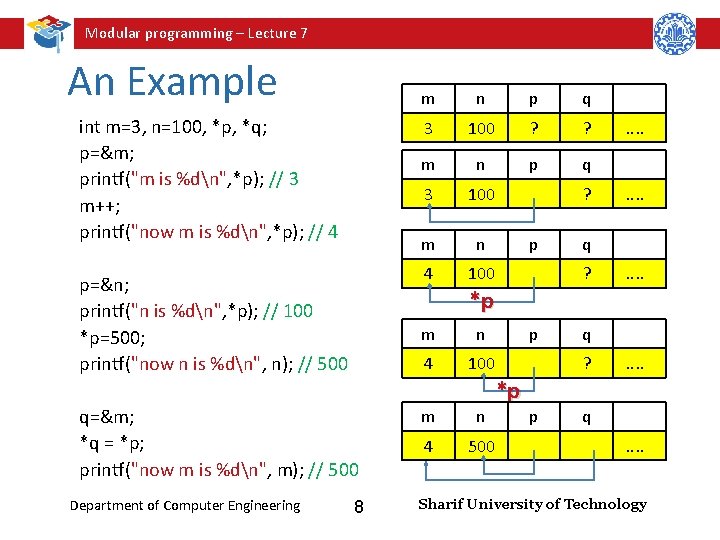
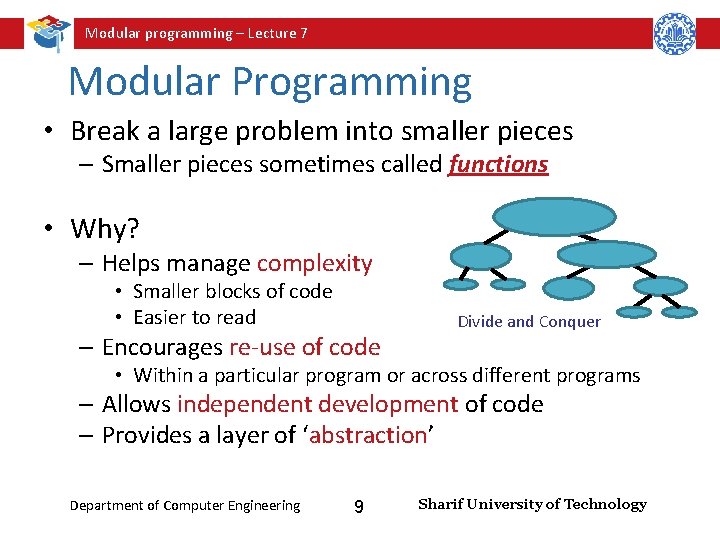
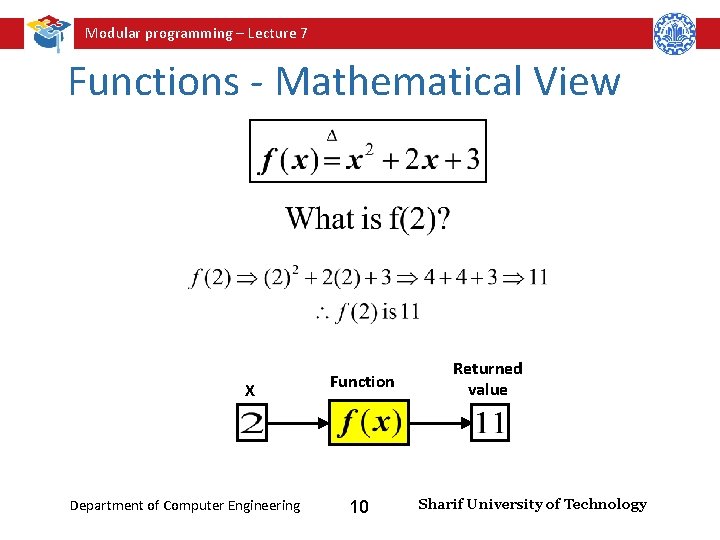
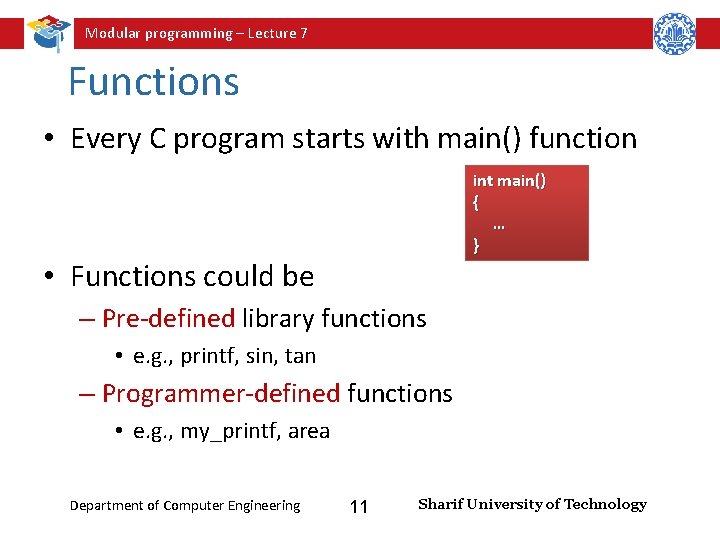
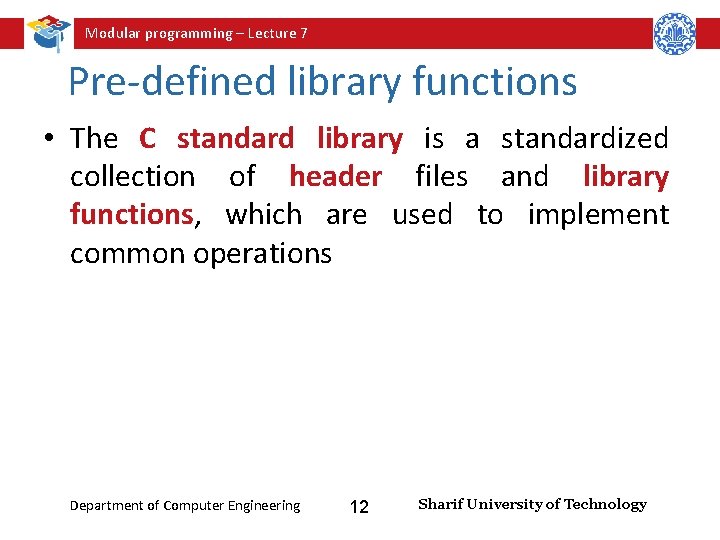
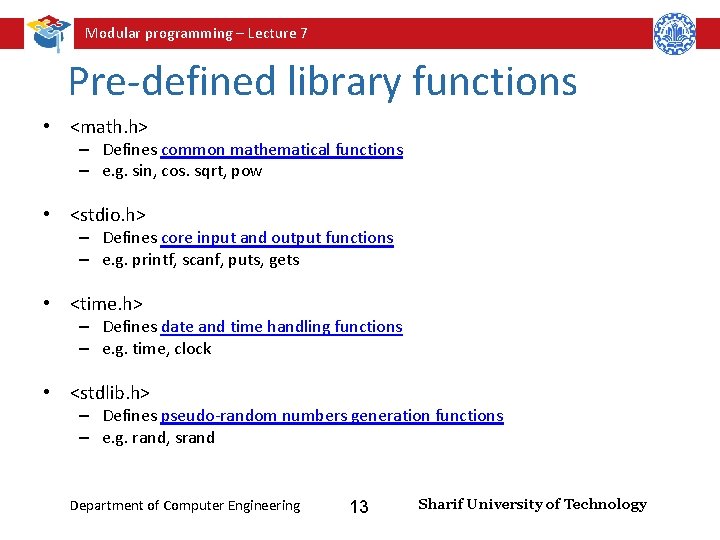
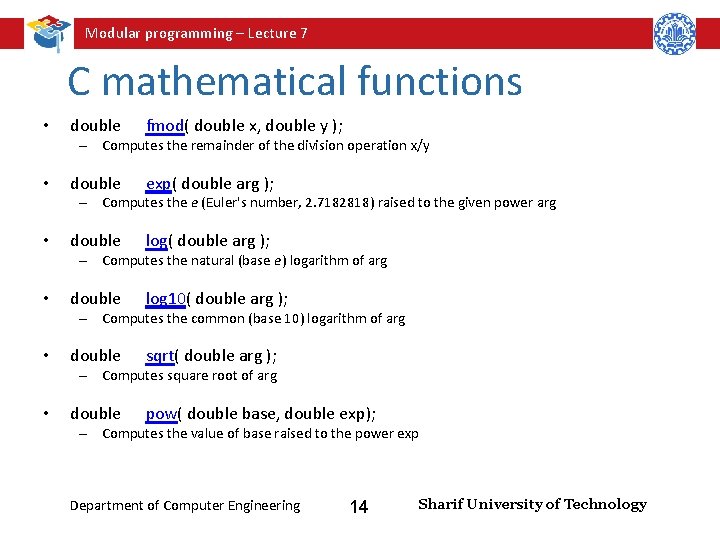
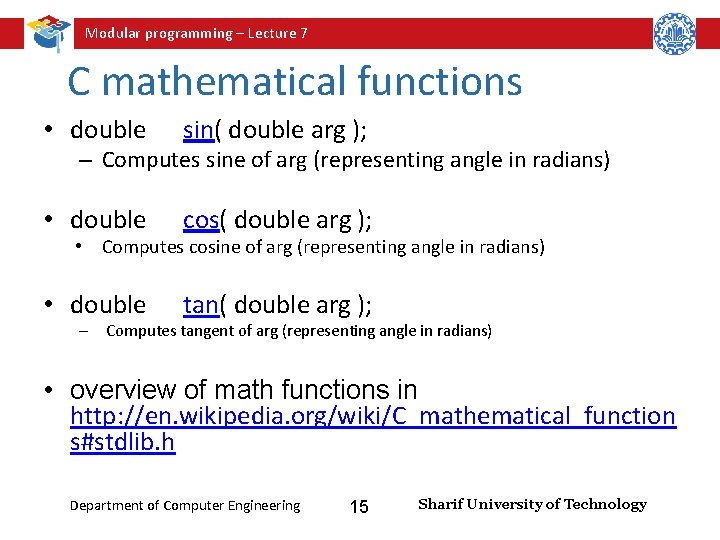
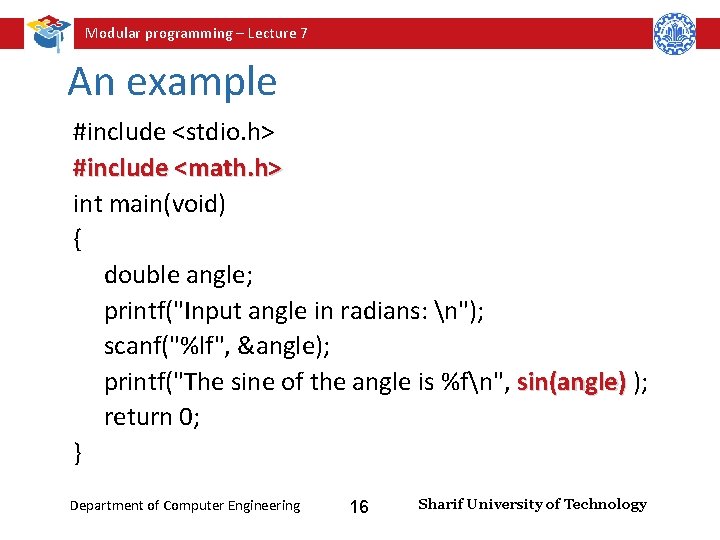
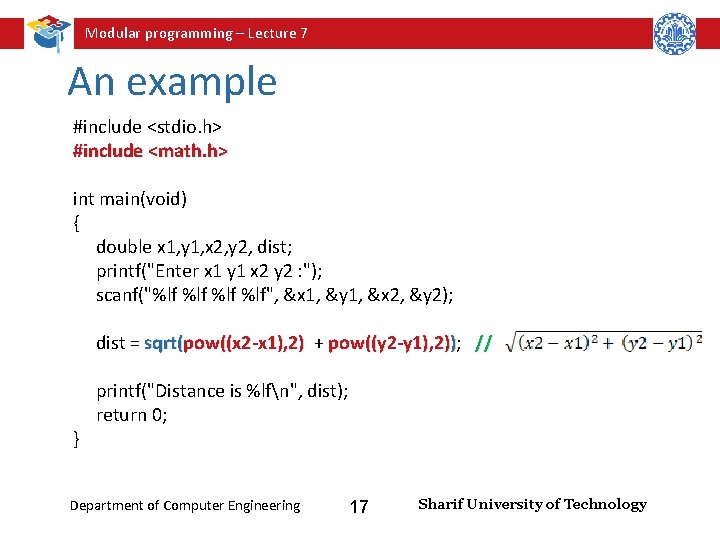
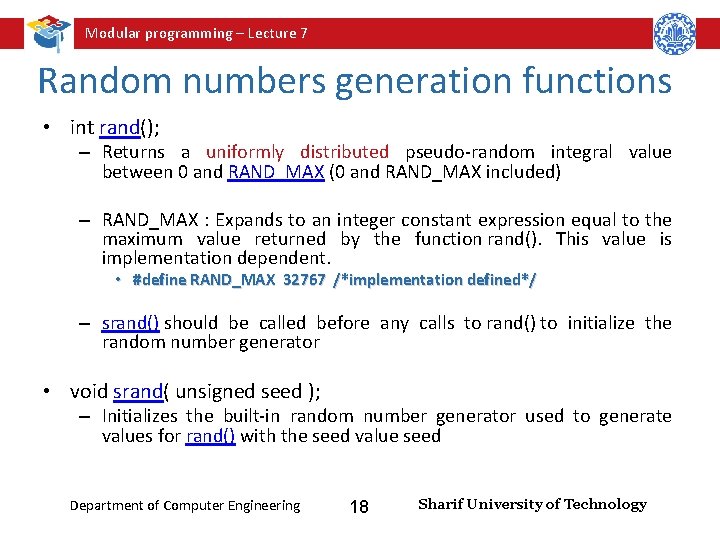
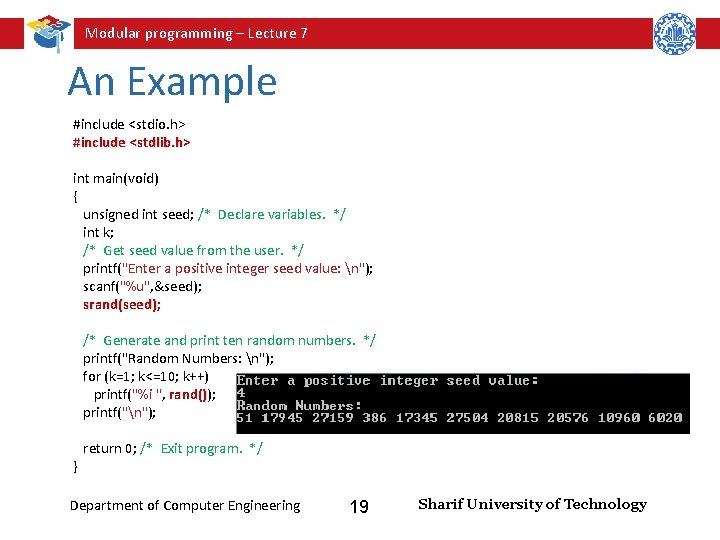
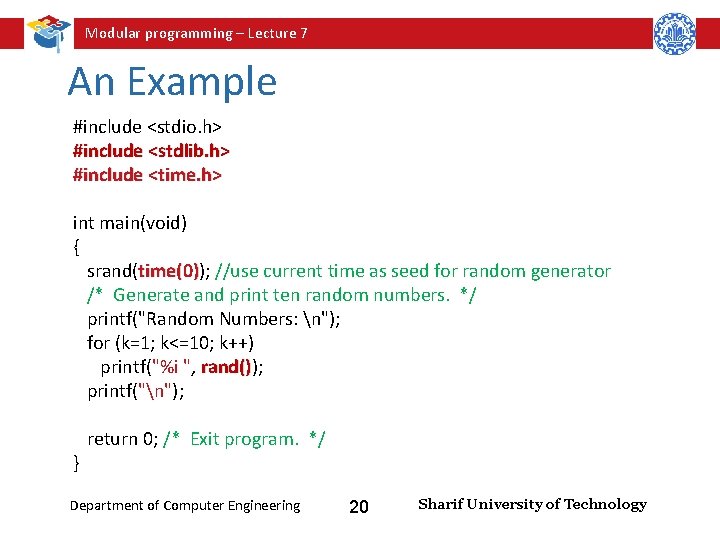
![Modular programming – Lecture 7 Random Numbers in [a b] • Generate a random Modular programming – Lecture 7 Random Numbers in [a b] • Generate a random](https://slidetodoc.com/presentation_image_h2/8b33ae5fc9fb43e9977c7f9025e6a4cf/image-21.jpg)
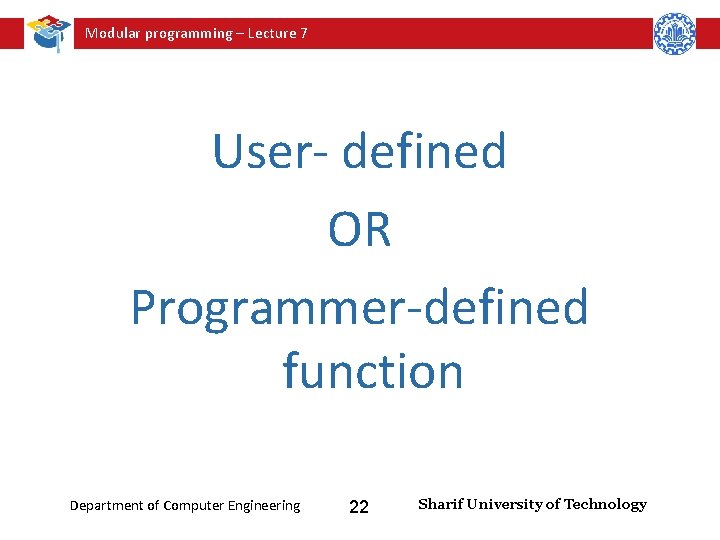
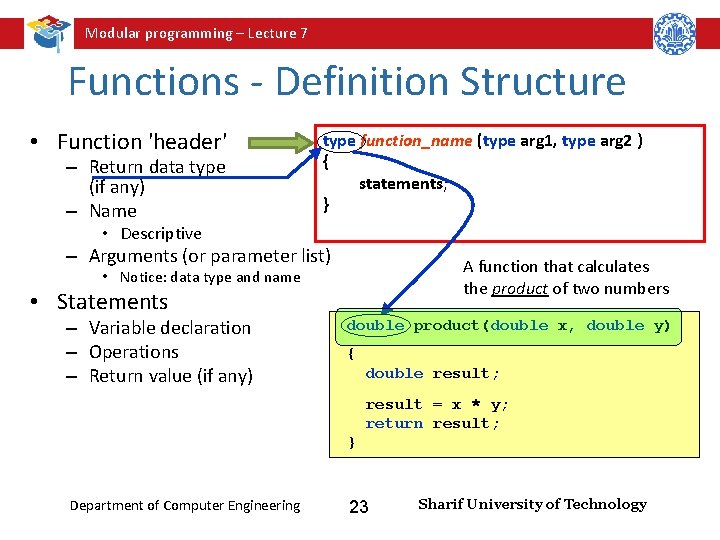
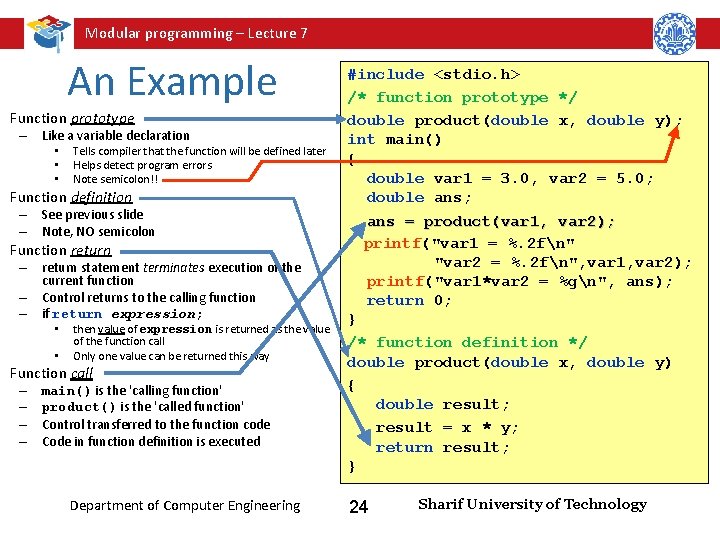
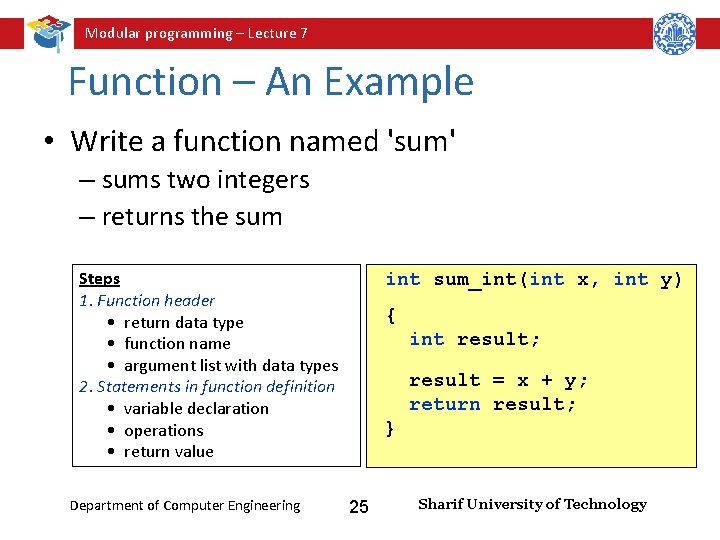
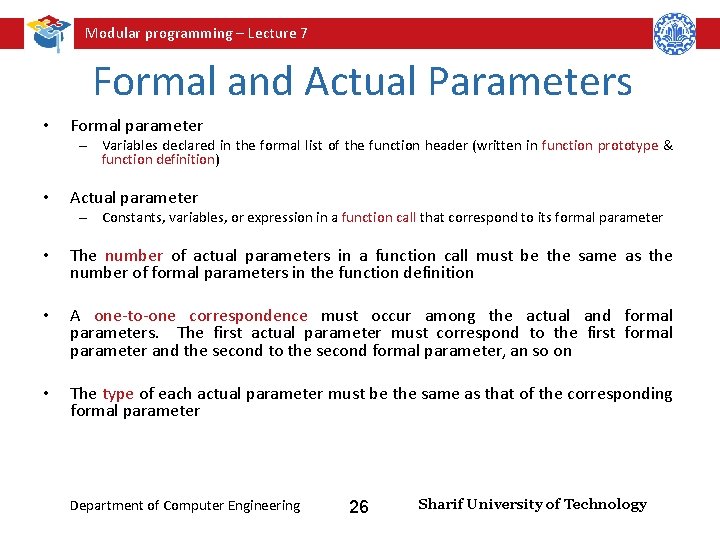
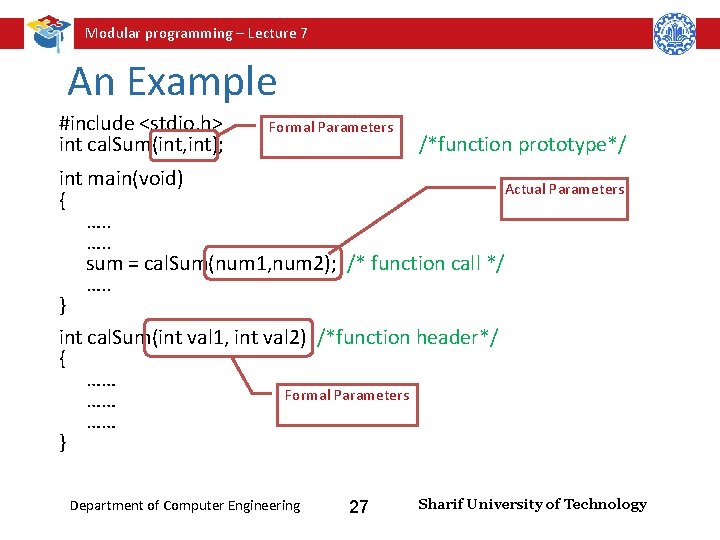
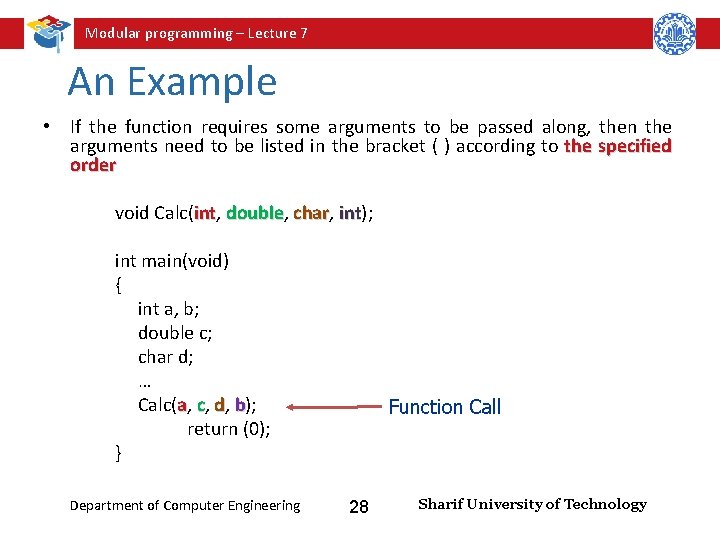
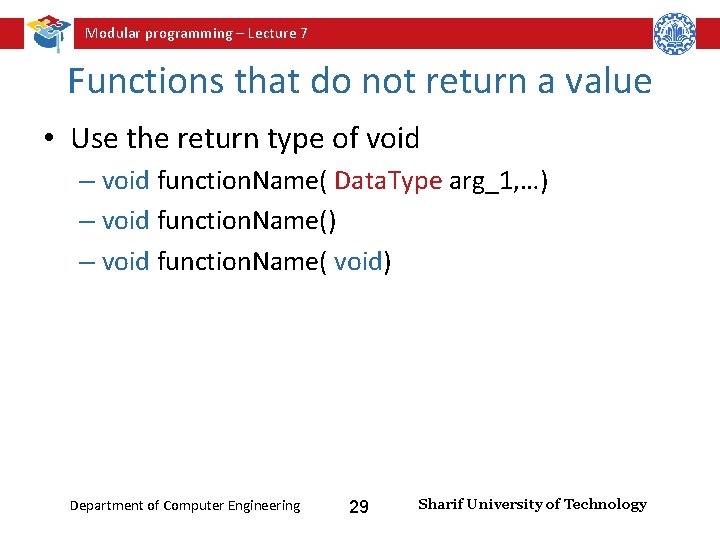
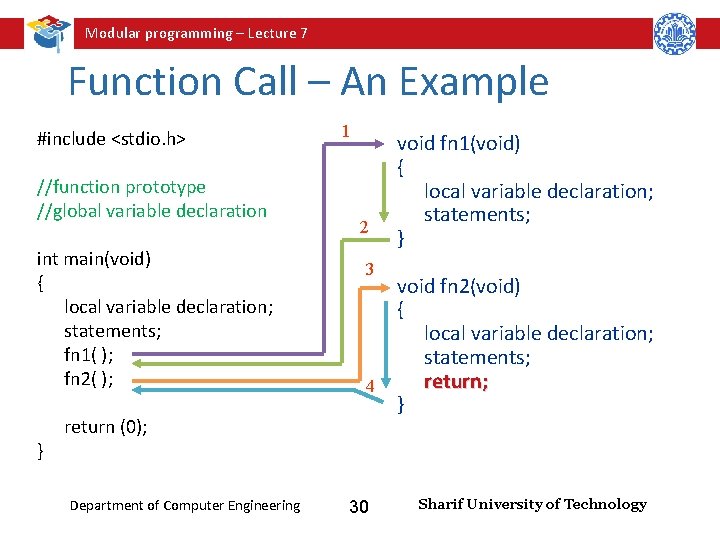
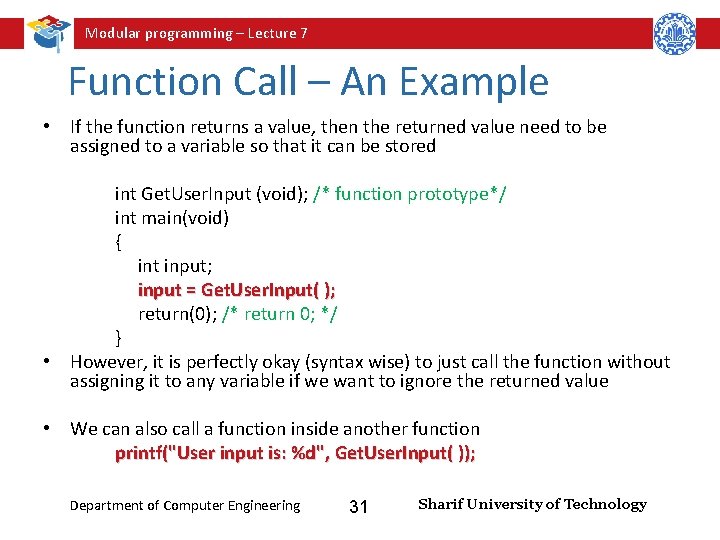
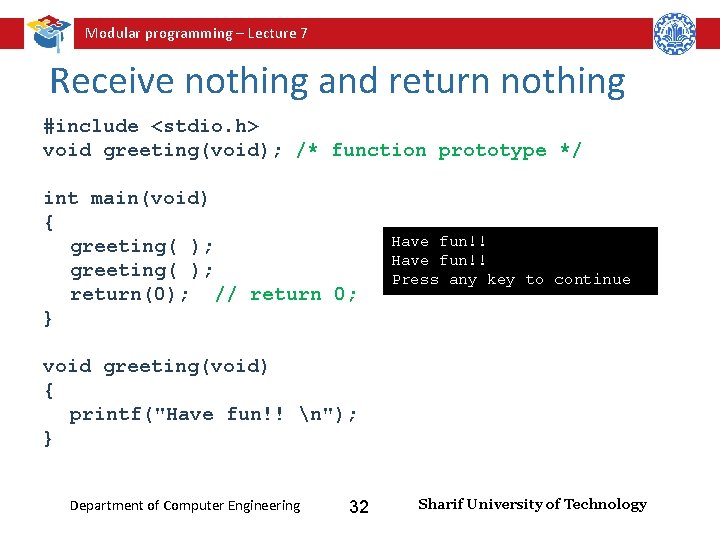
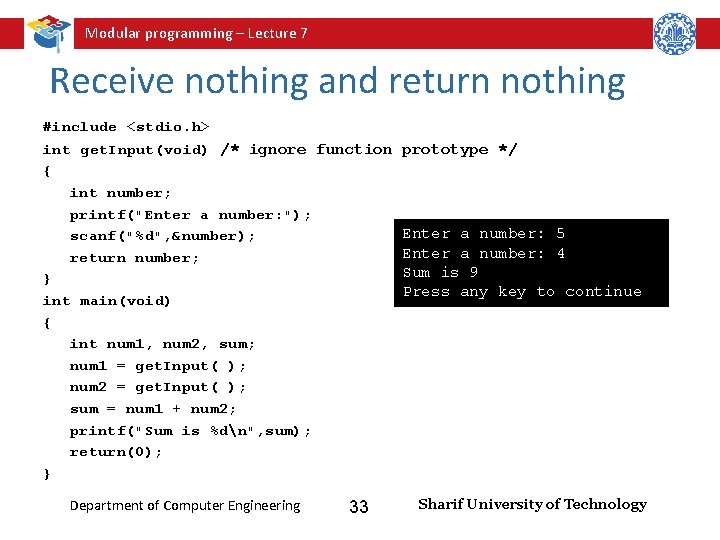
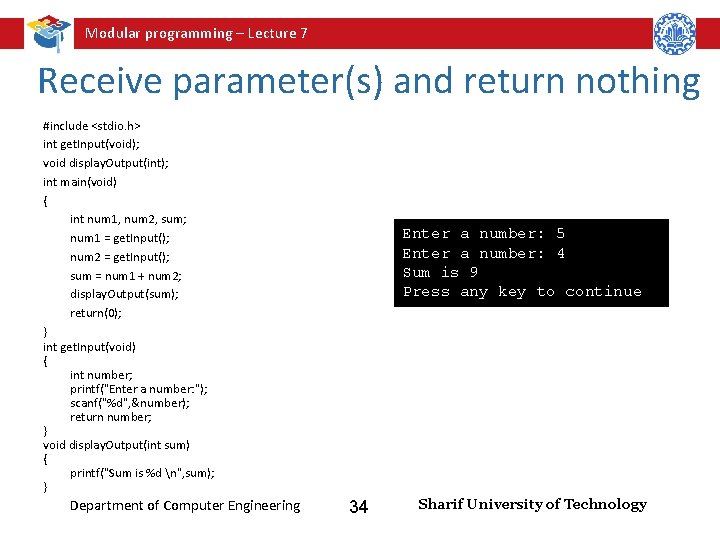
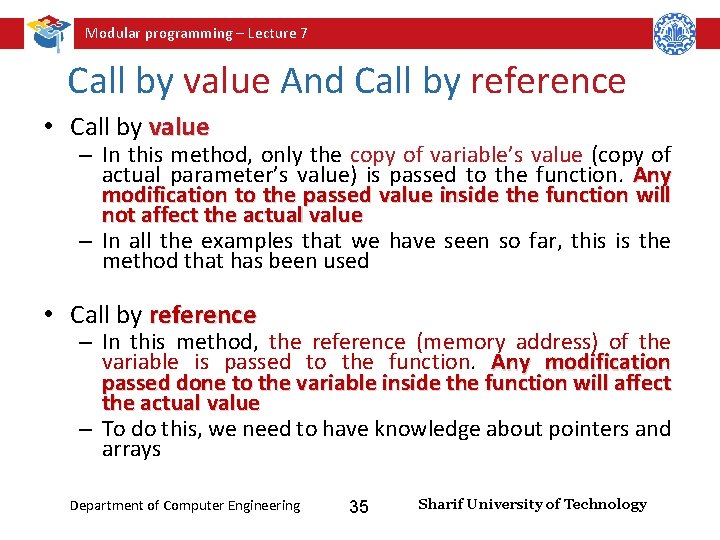
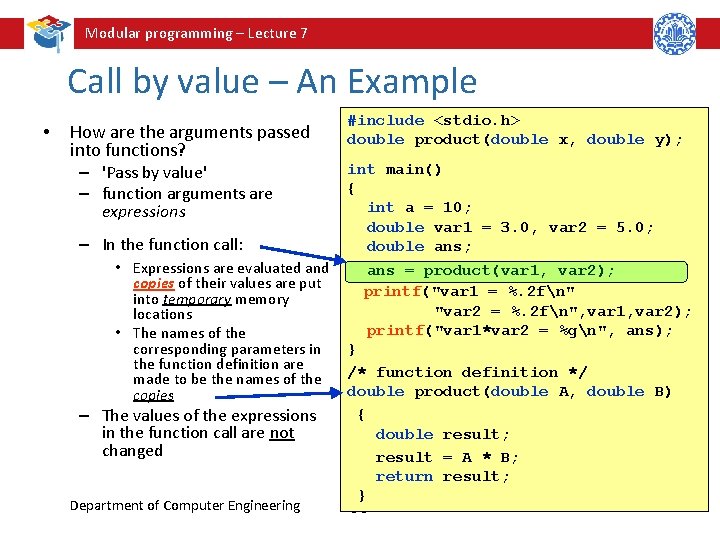
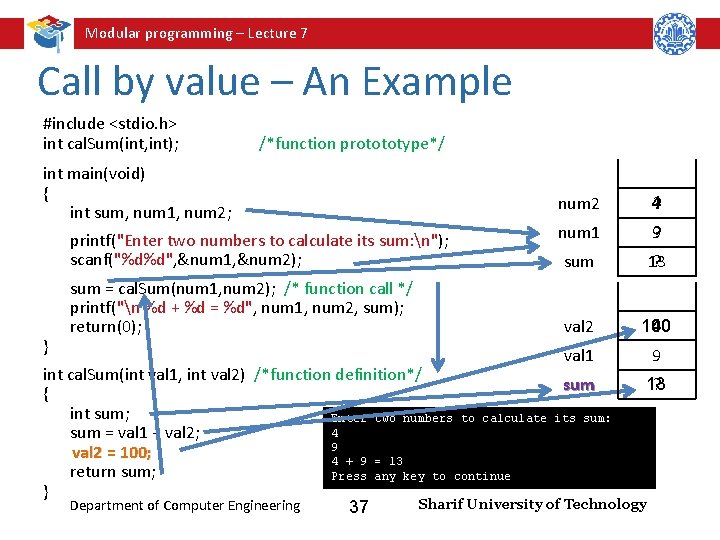
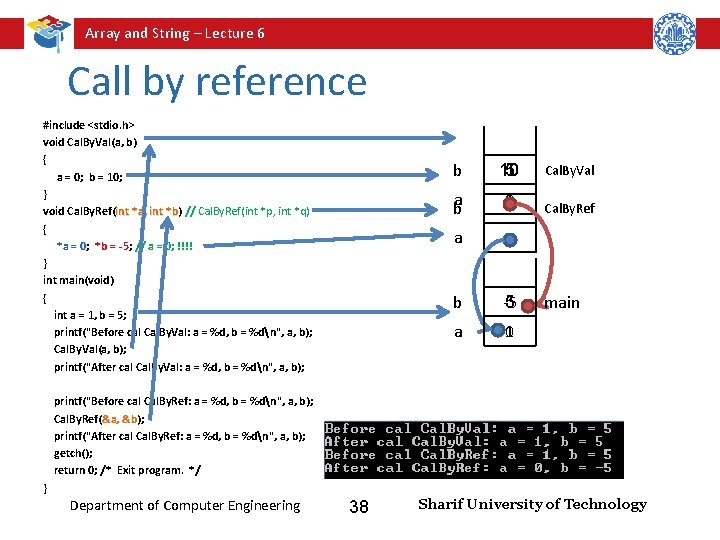
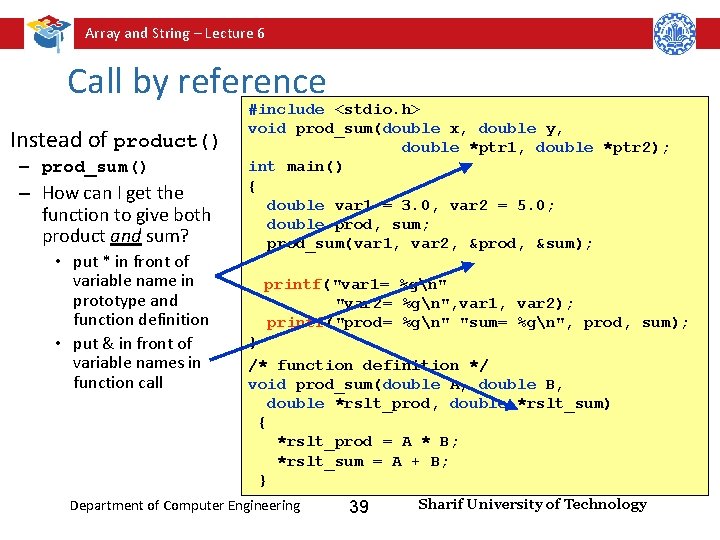
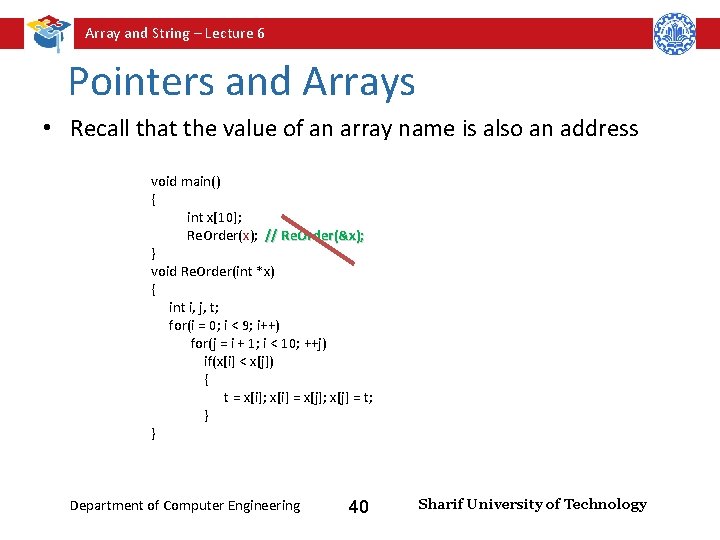
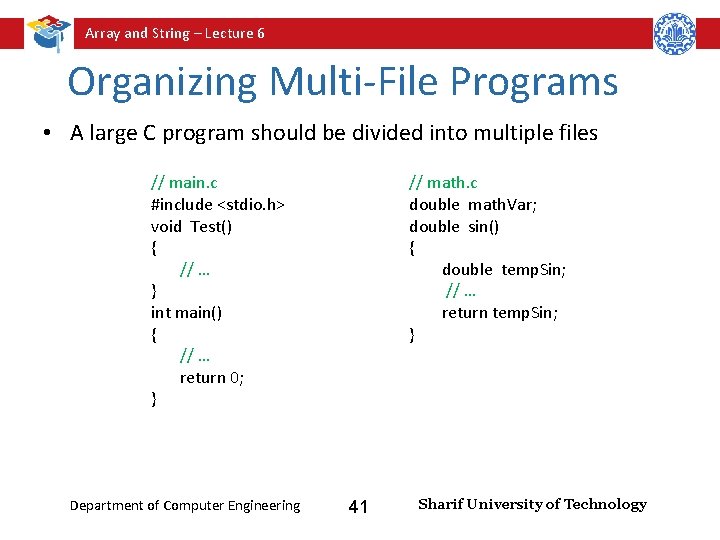
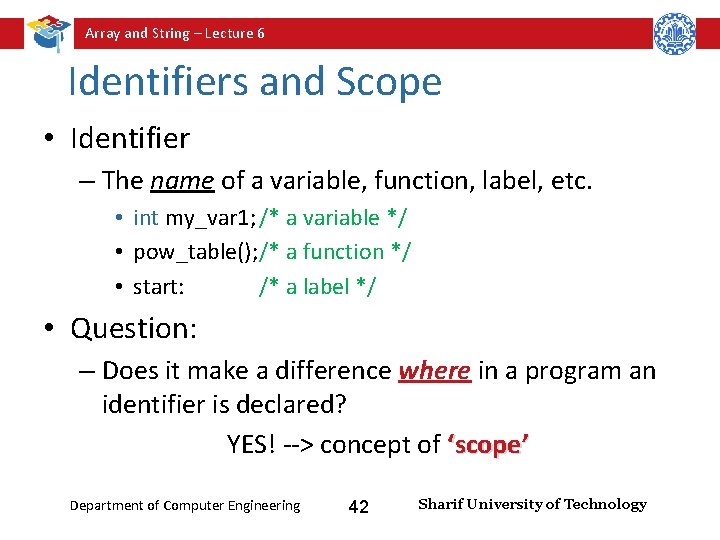
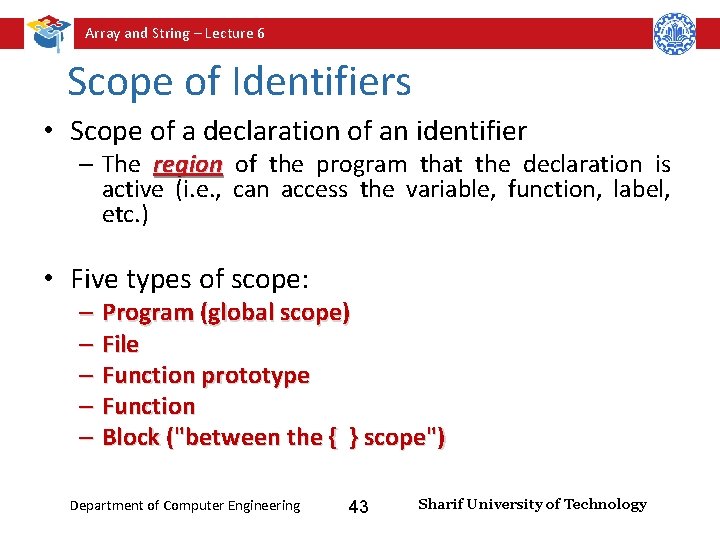
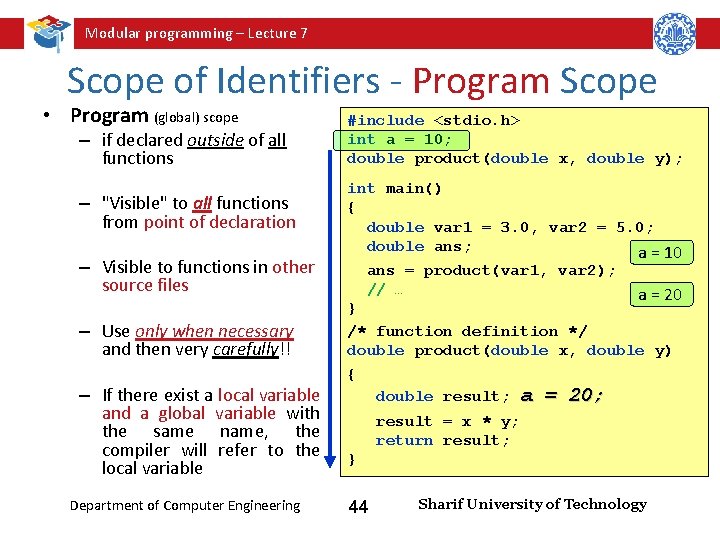
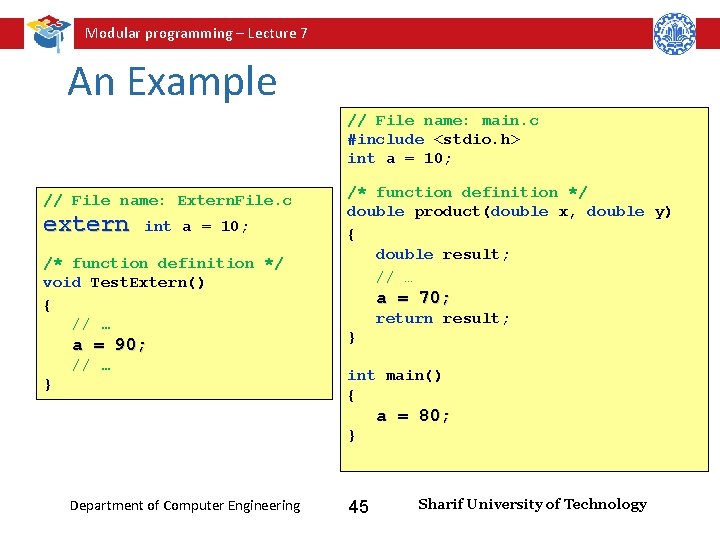
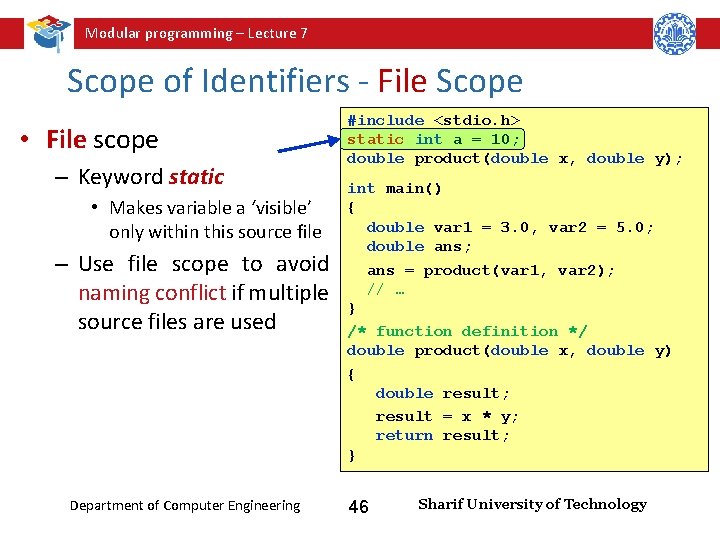
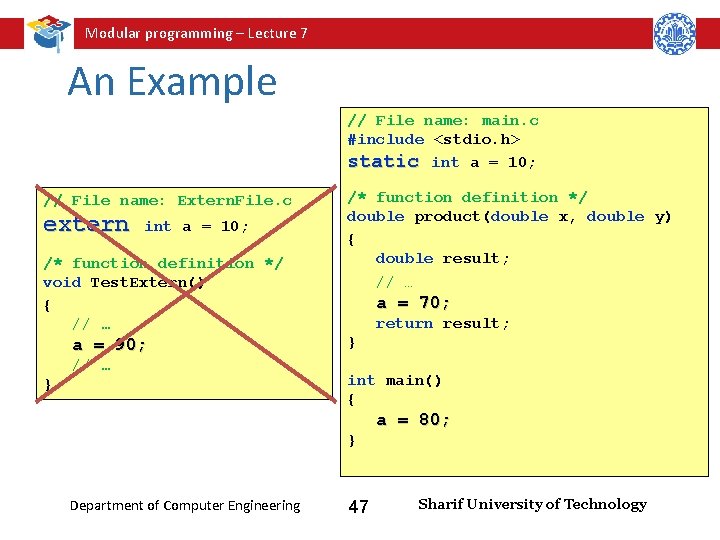
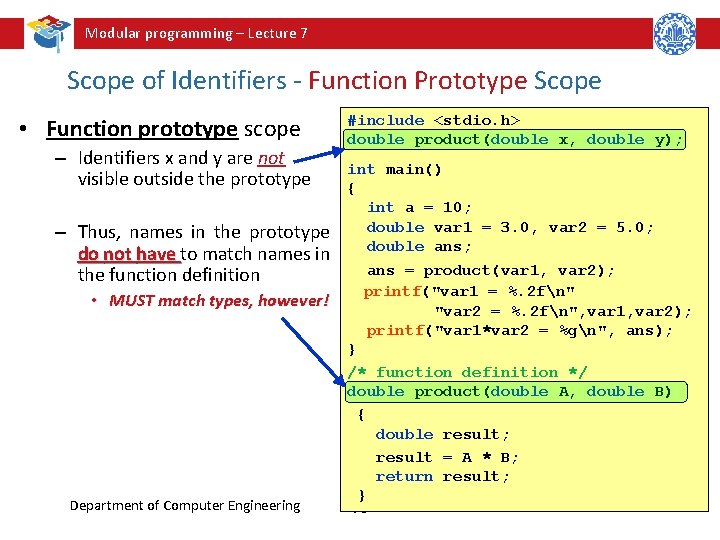
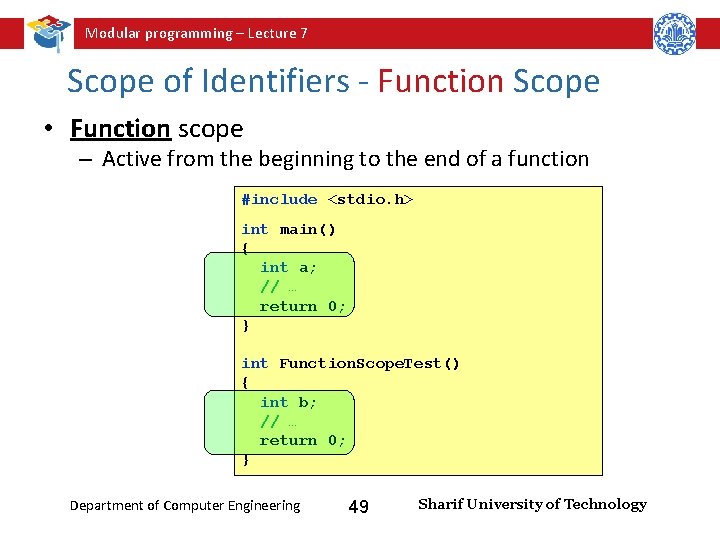
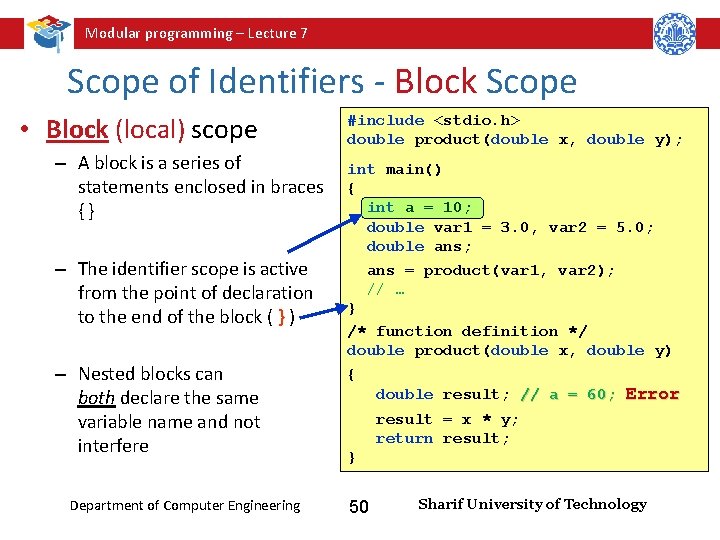
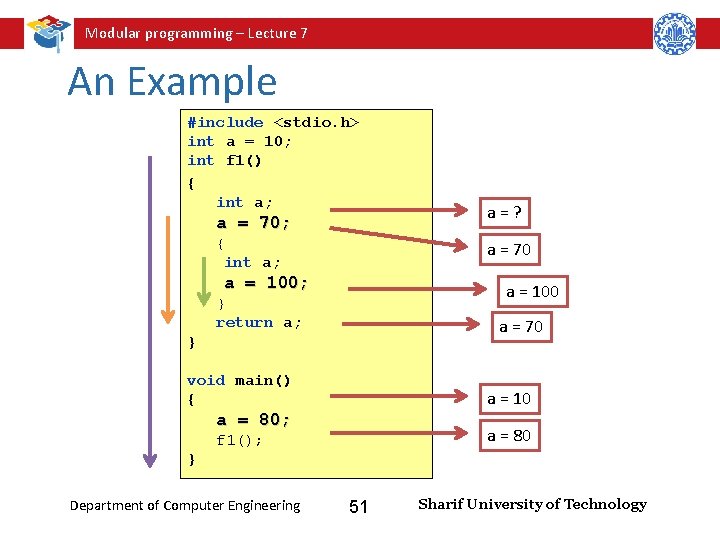
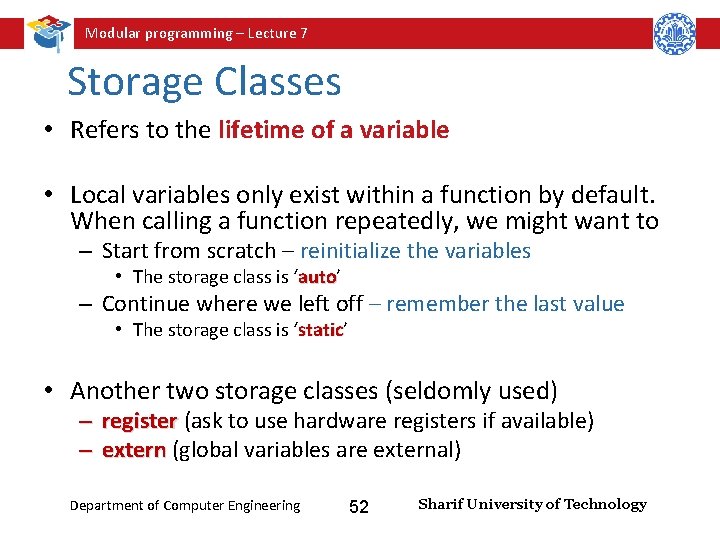
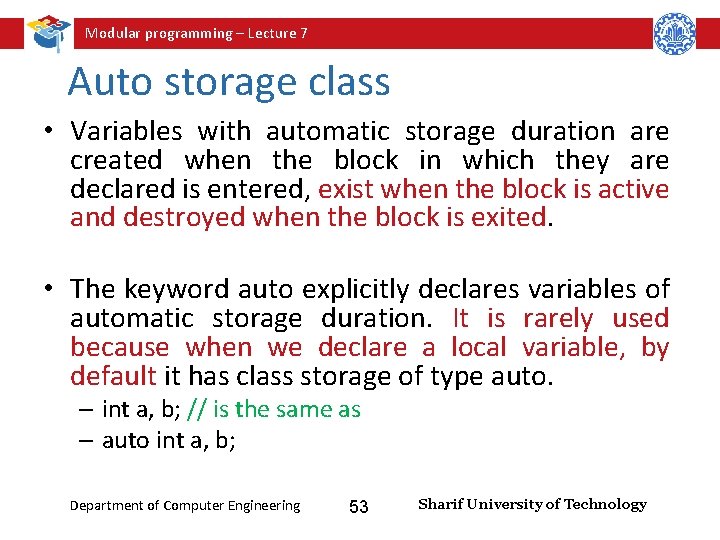
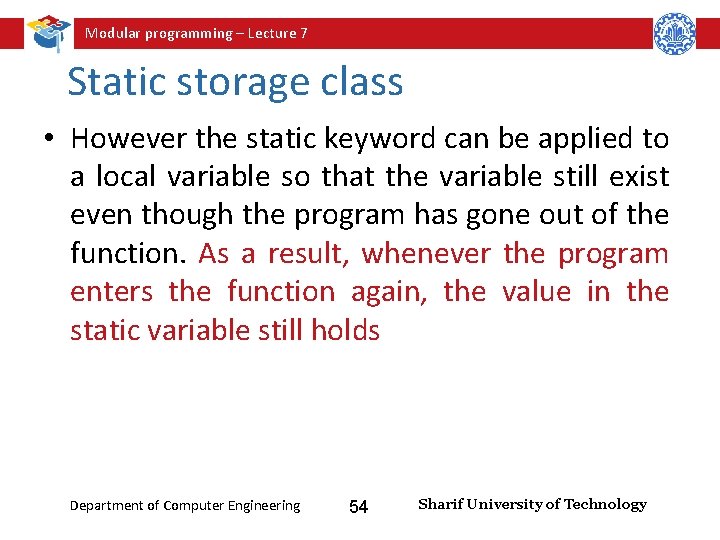
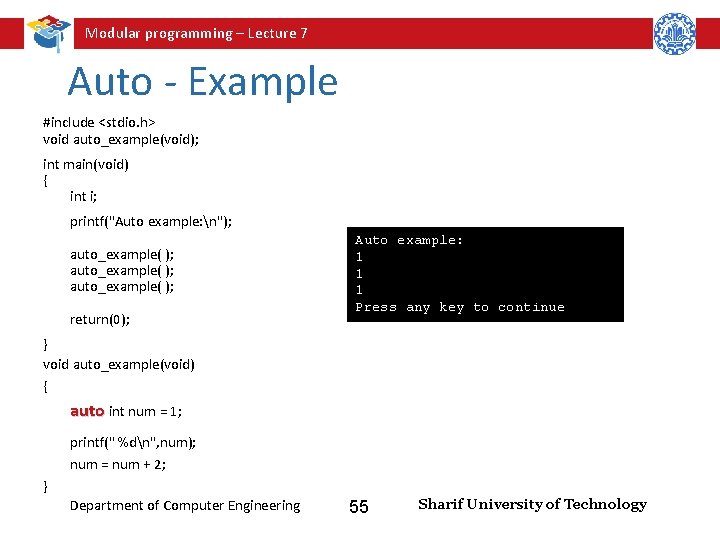
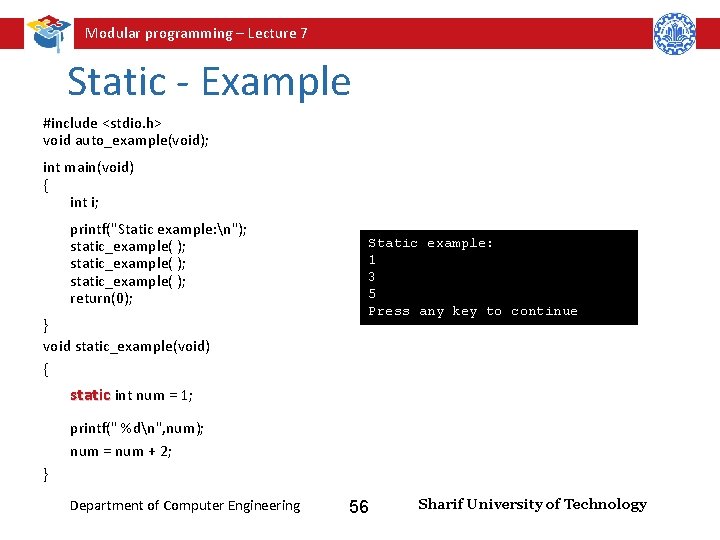
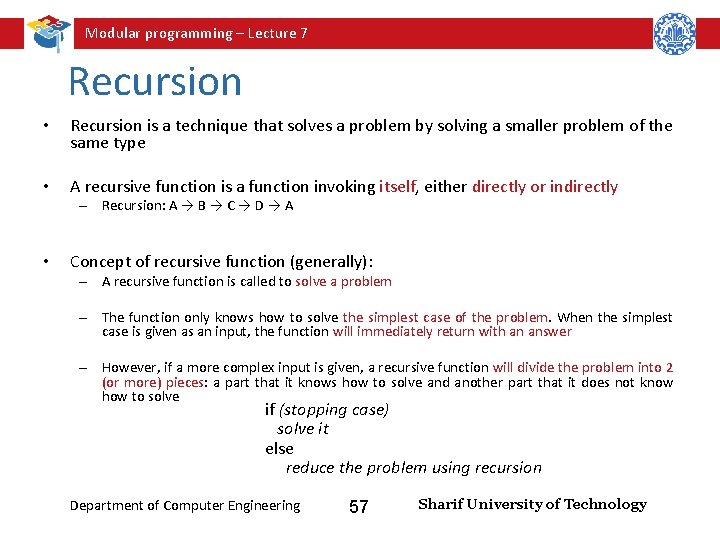
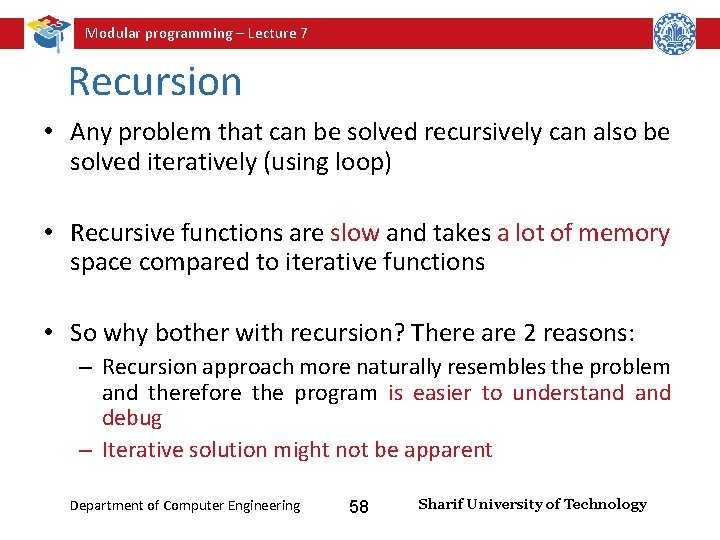
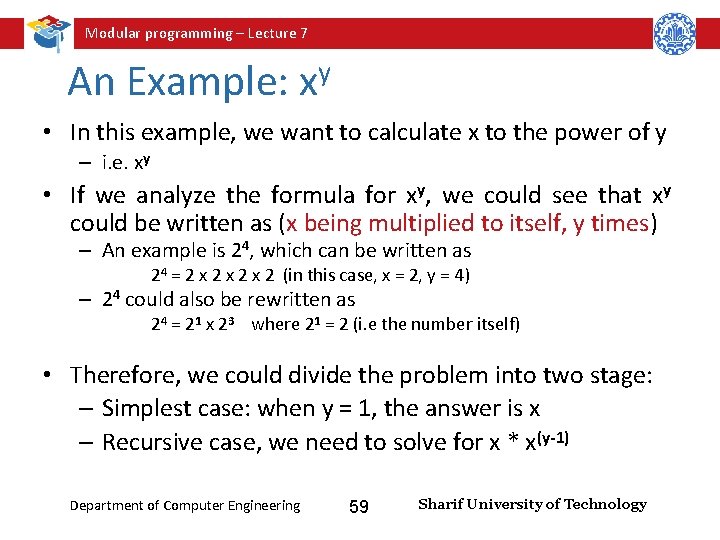
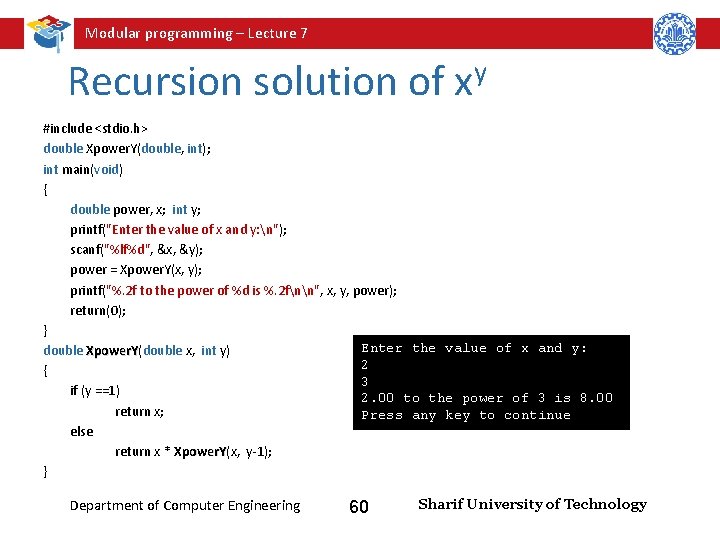
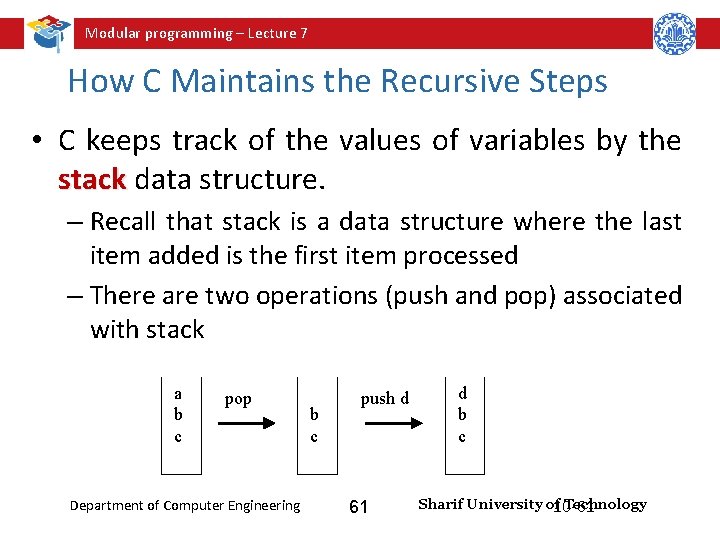
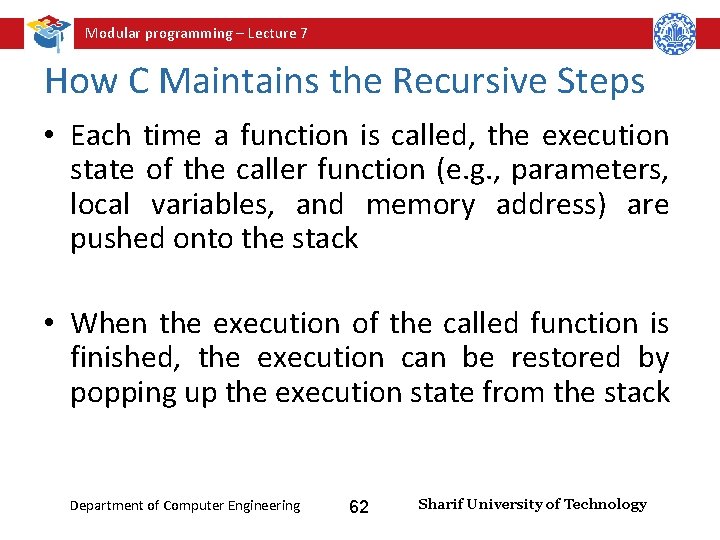
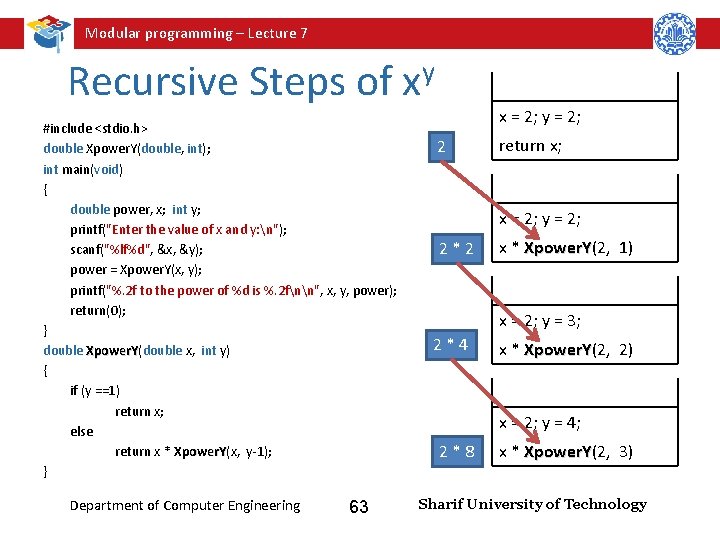
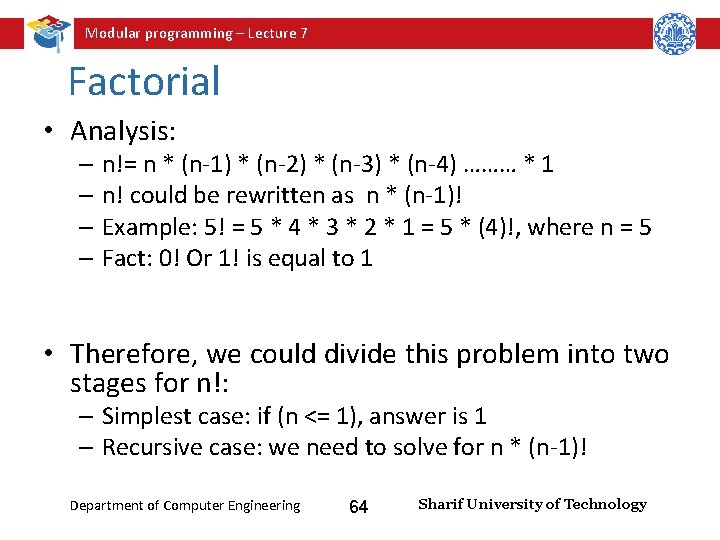
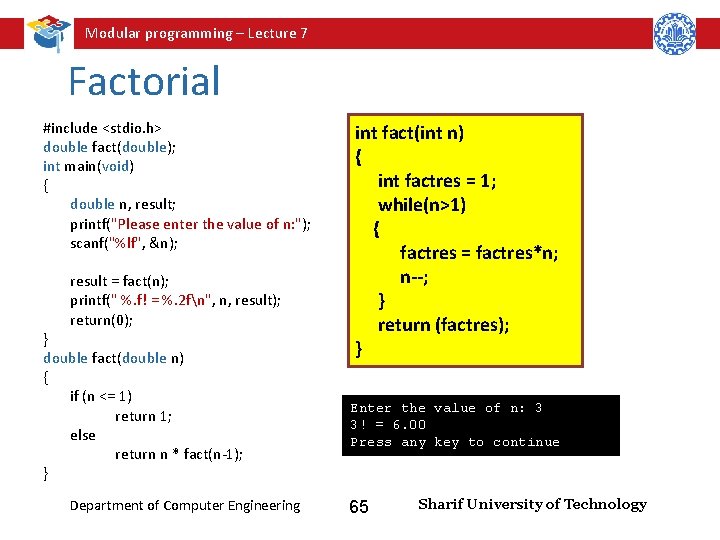
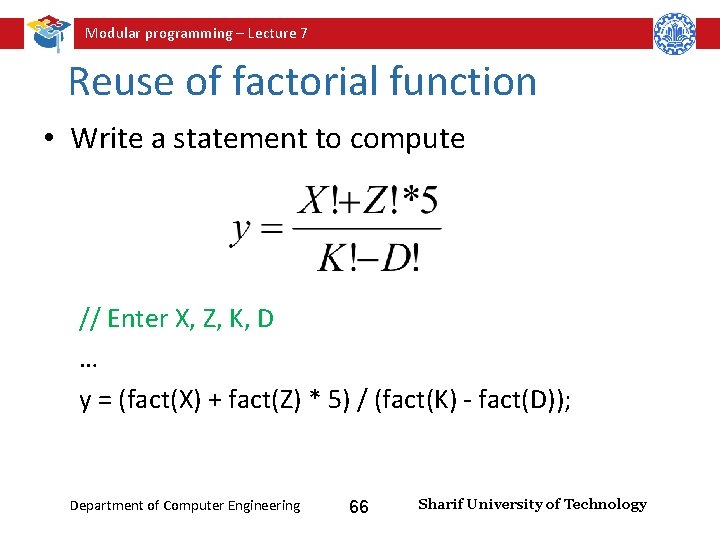
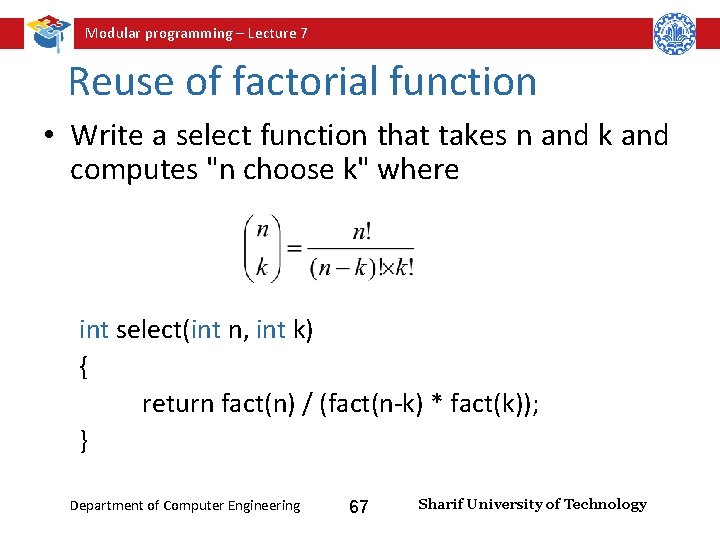
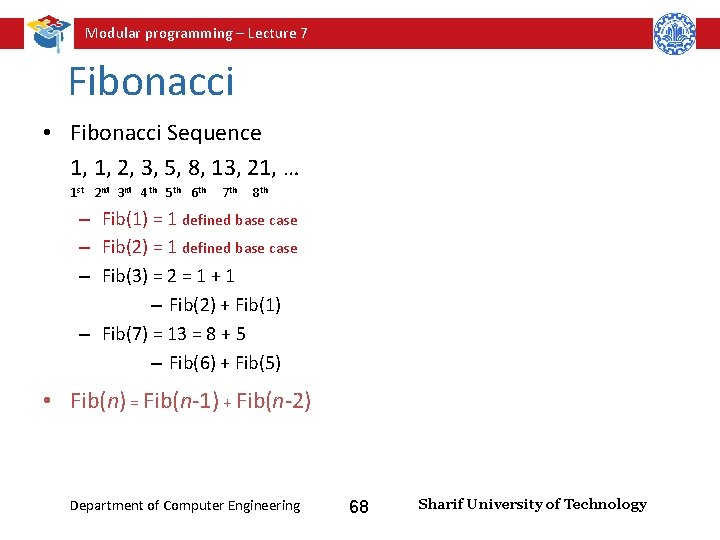
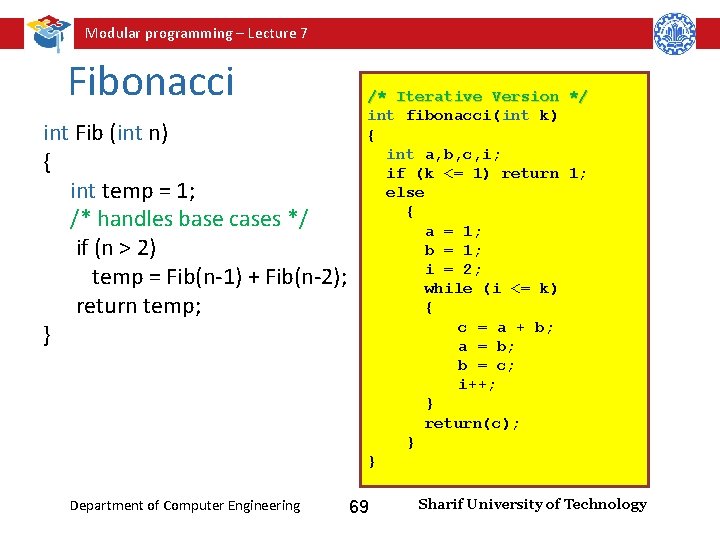
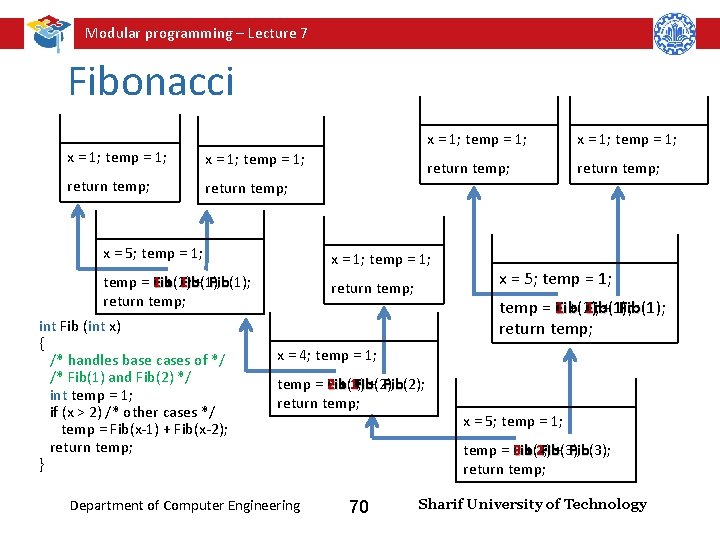
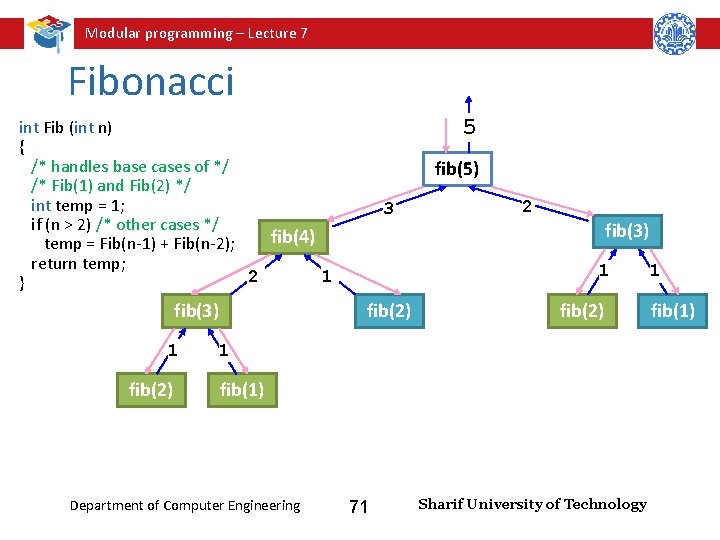
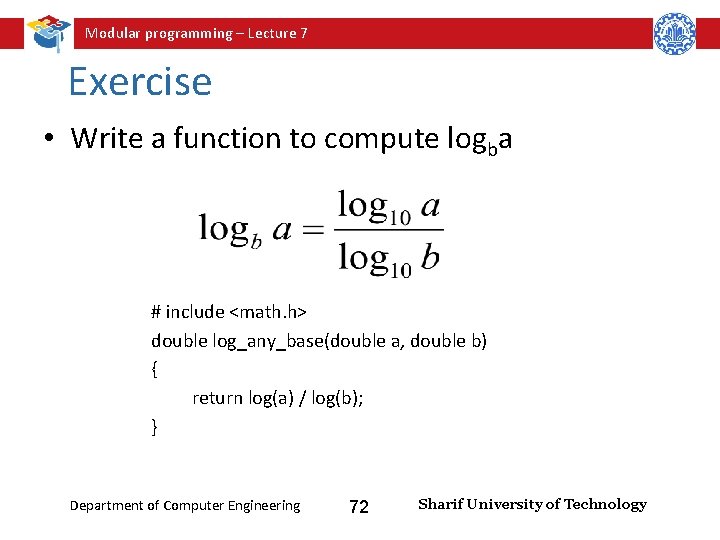
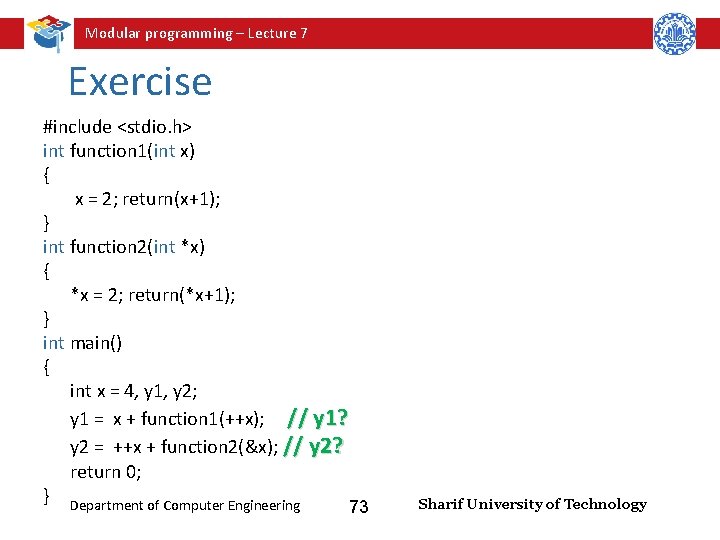
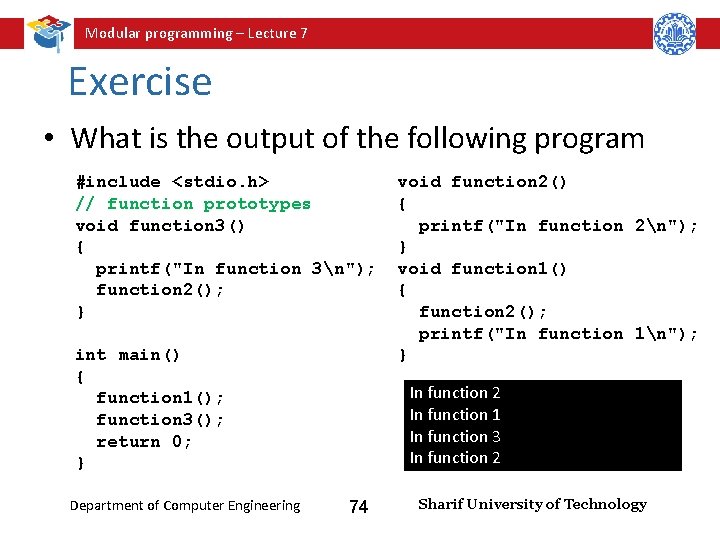
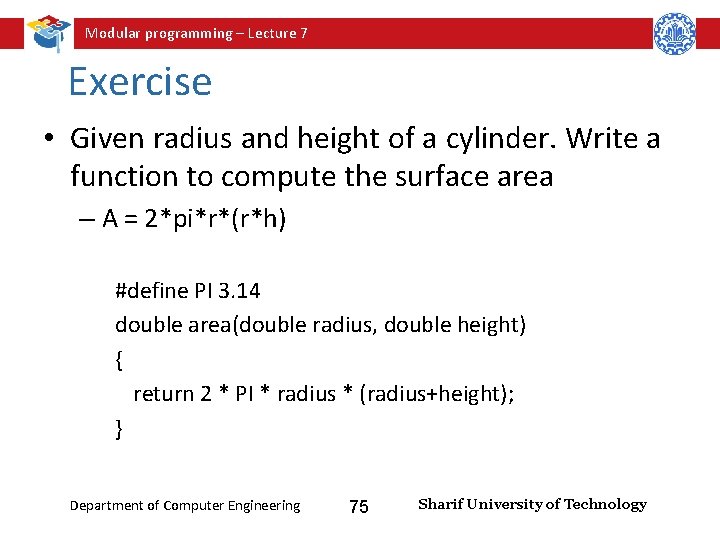
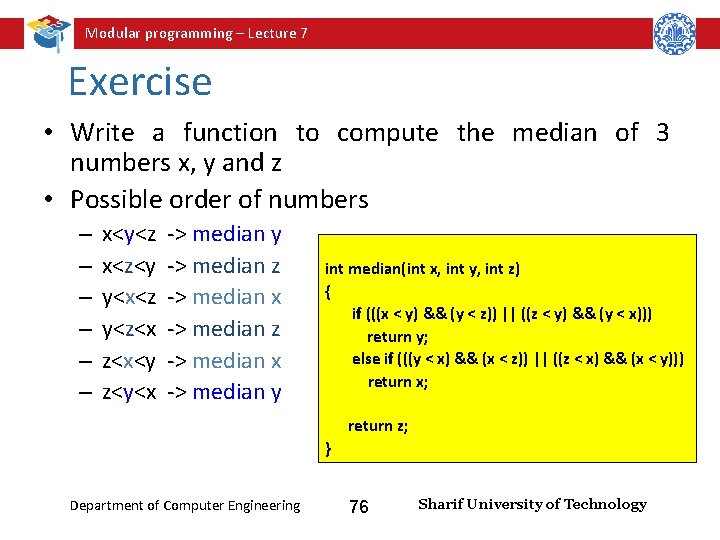
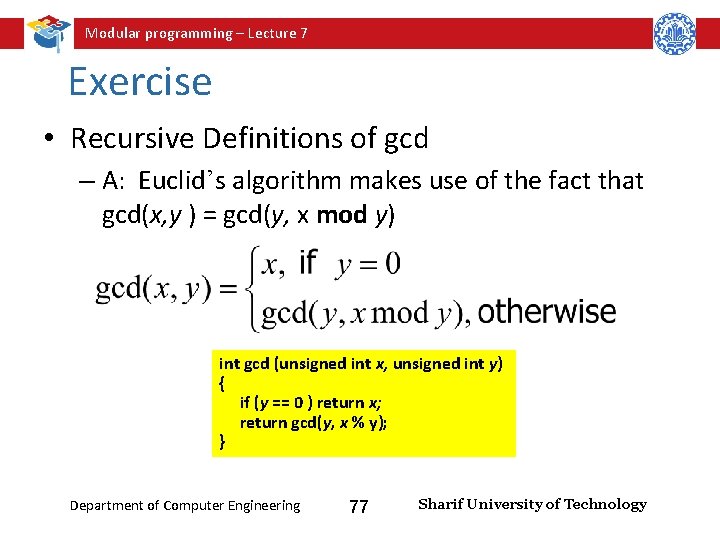
- Slides: 77
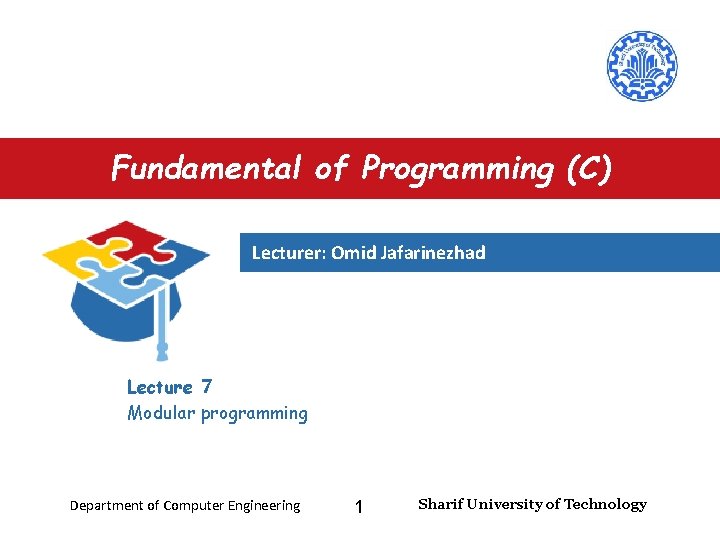
Fundamental of Programming (C) Lecturer: Omid Jafarinezhad Lecture 7 Modular programming Department of Computer Engineering 1 Sharif University of Technology
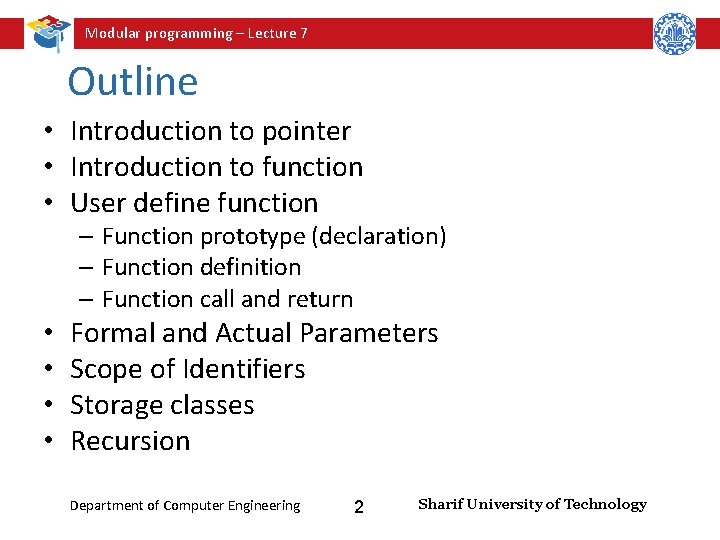
Modular programming – Lecture 7 Outline • Introduction to pointer • Introduction to function • User define function – Function prototype (declaration) – Function definition – Function call and return • • Formal and Actual Parameters Scope of Identifiers Storage classes Recursion Department of Computer Engineering 2 Sharif University of Technology
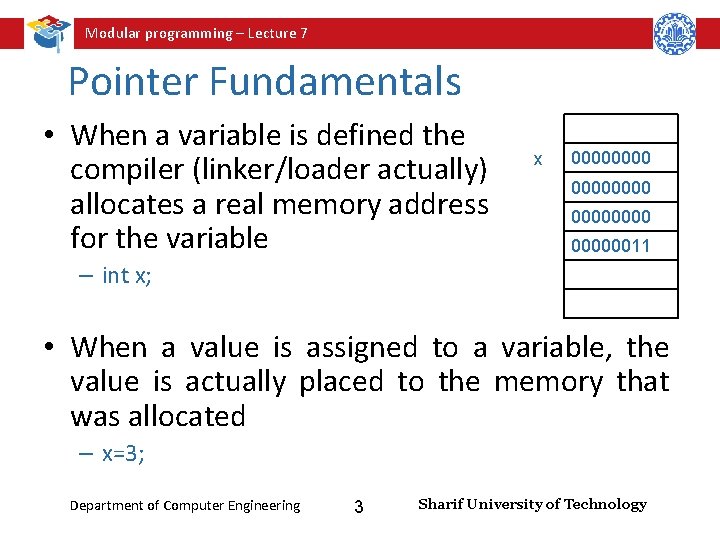
Modular programming – Lecture 7 Pointer Fundamentals • When a variable is defined the compiler (linker/loader actually) allocates a real memory address for the variable x 00000000 00000011 – int x; • When a value is assigned to a variable, the value is actually placed to the memory that was allocated – x=3; Department of Computer Engineering 3 Sharif University of Technology
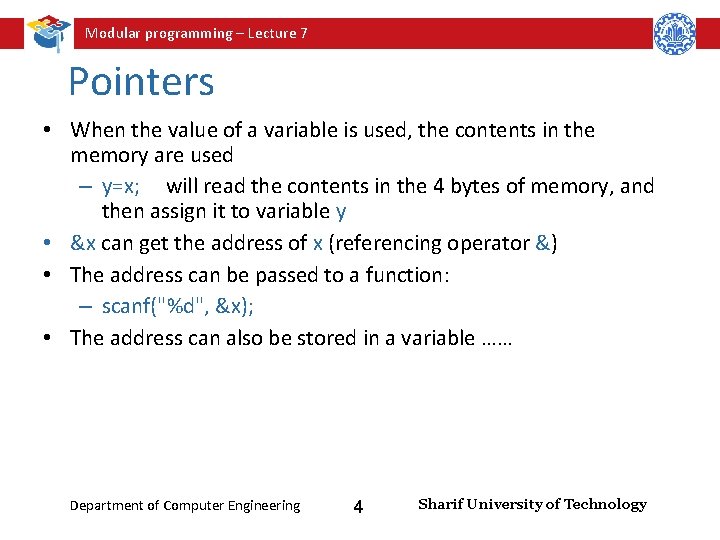
Modular programming – Lecture 7 Pointers • When the value of a variable is used, the contents in the memory are used – y=x; will read the contents in the 4 bytes of memory, and then assign it to variable y • &x can get the address of x (referencing operator &) • The address can be passed to a function: – scanf("%d", &x); • The address can also be stored in a variable …… Department of Computer Engineering 4 Sharif University of Technology
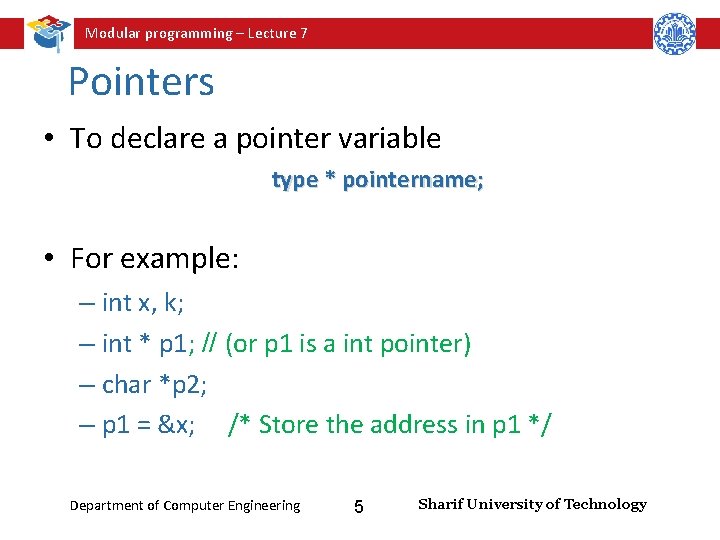
Modular programming – Lecture 7 Pointers • To declare a pointer variable type * pointername; • For example: – int x, k; – int * p 1; // (or p 1 is a int pointer) – char *p 2; – p 1 = &x; /* Store the address in p 1 */ Department of Computer Engineering 5 Sharif University of Technology
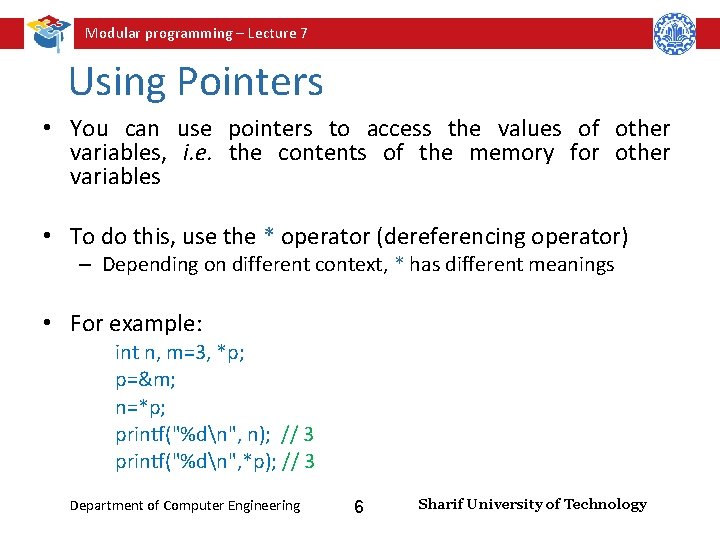
Modular programming – Lecture 7 Using Pointers • You can use pointers to access the values of other variables, i. e. the contents of the memory for other variables • To do this, use the * operator (dereferencing operator) – Depending on different context, * has different meanings • For example: int n, m=3, *p; p=&m; n=*p; printf("%dn", n); // 3 printf("%dn", *p); // 3 Department of Computer Engineering 6 Sharif University of Technology
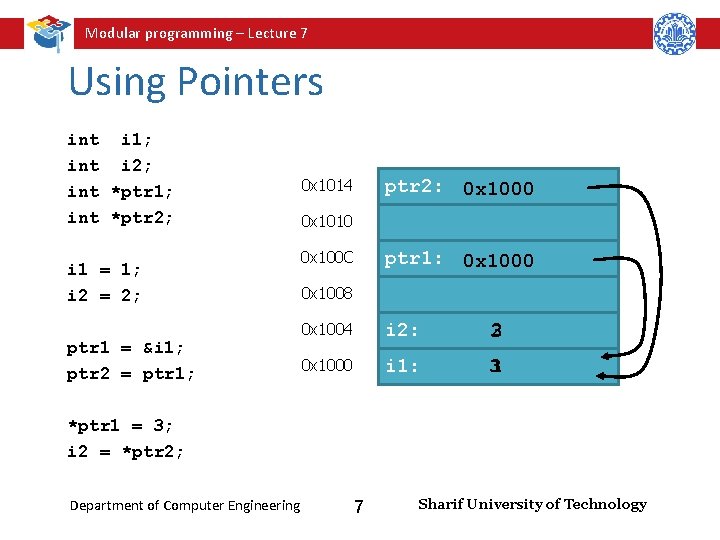
Modular programming – Lecture 7 Using Pointers int i 1; int i 2; int *ptr 1; int *ptr 2; i 1 = 1; i 2 = 2; ptr 1 = &i 1; ptr 2 = ptr 1; ptr 2: 0 x 1000 0 x 1014 0 x 1010 ptr 1: 0 x 1000 0 x 100 C 0 x 1008 0 x 1004 i 2: 2 3 0 x 1000 i 1: 3 1 *ptr 1 = 3; i 2 = *ptr 2; Department of Computer Engineering 7 Sharif University of Technology
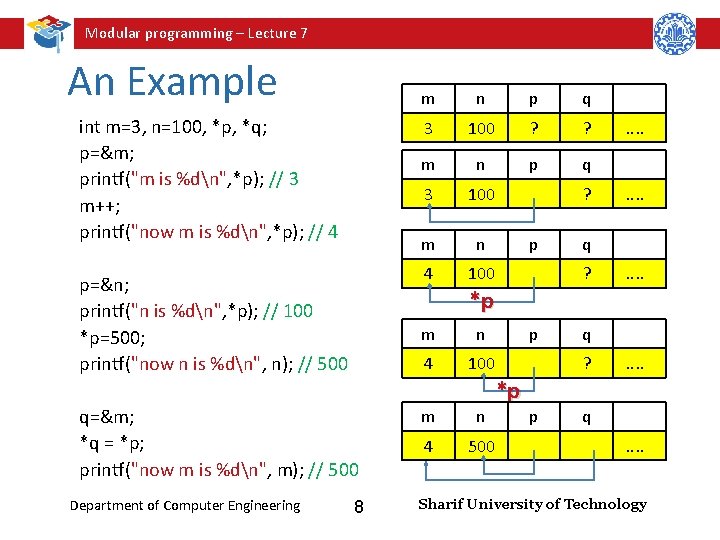
Modular programming – Lecture 7 An Example int m=3, n=100, *p, *q; p=&m; printf("m is %dn", *p); // 3 m++; printf("now m is %dn", *p); // 4 p=&n; printf("n is %dn", *p); // 100 *p=500; printf("now n is %dn", n); // 500 n p q 3 100 ? ? m n p q 3 100 m n 4 100 ? p . . . . q ? . . *p q=&m; *q = *p; printf("now m is %dn", m); // 500 Department of Computer Engineering m 8 m n 4 100 p q ? . . *p m n 4 500 p q. . Sharif University of Technology
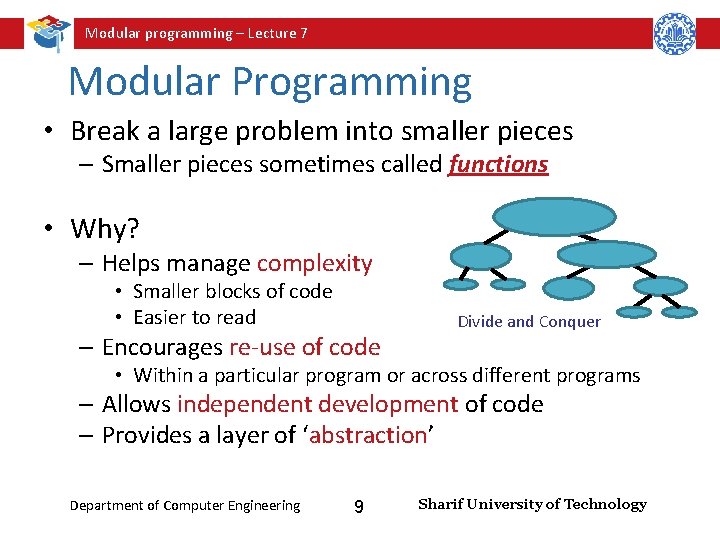
Modular programming – Lecture 7 Modular Programming • Break a large problem into smaller pieces – Smaller pieces sometimes called functions • Why? – Helps manage complexity • Smaller blocks of code • Easier to read – Encourages re-use of code Divide and Conquer • Within a particular program or across different programs – Allows independent development of code – Provides a layer of ‘abstraction’ Department of Computer Engineering 9 Sharif University of Technology
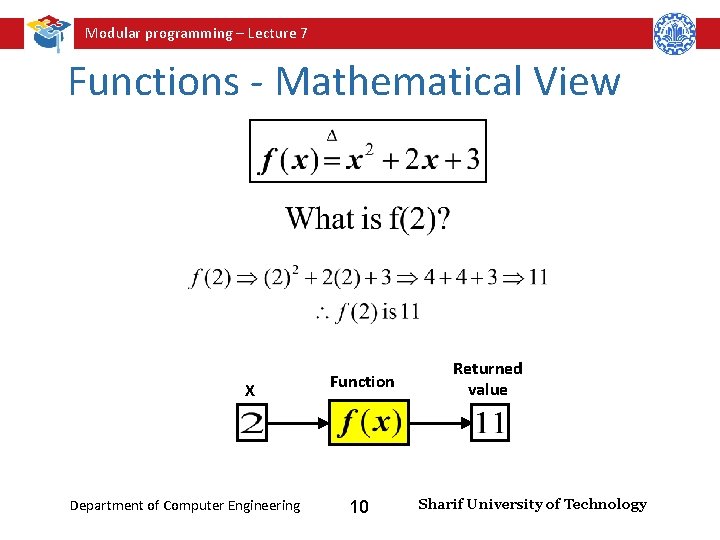
Modular programming – Lecture 7 Functions - Mathematical View X Department of Computer Engineering Function 10 Returned value Sharif University of Technology
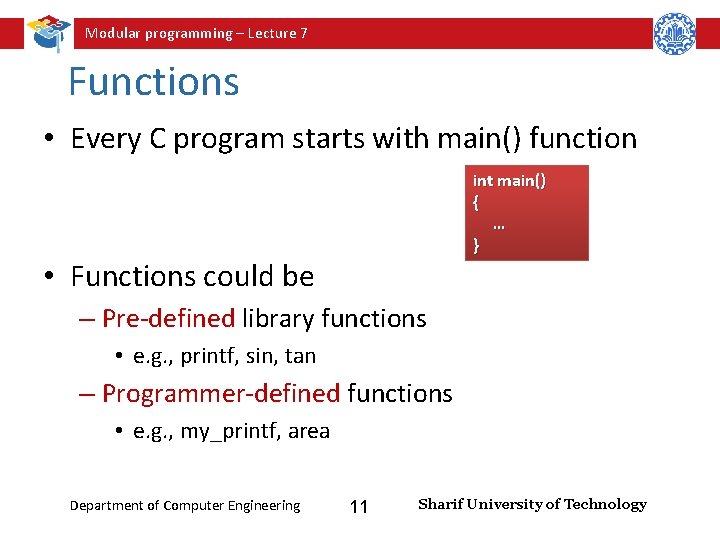
Modular programming – Lecture 7 Functions • Every C program starts with main() function int main() { … } • Functions could be – Pre-defined library functions • e. g. , printf, sin, tan – Programmer-defined functions • e. g. , my_printf, area Department of Computer Engineering 11 Sharif University of Technology
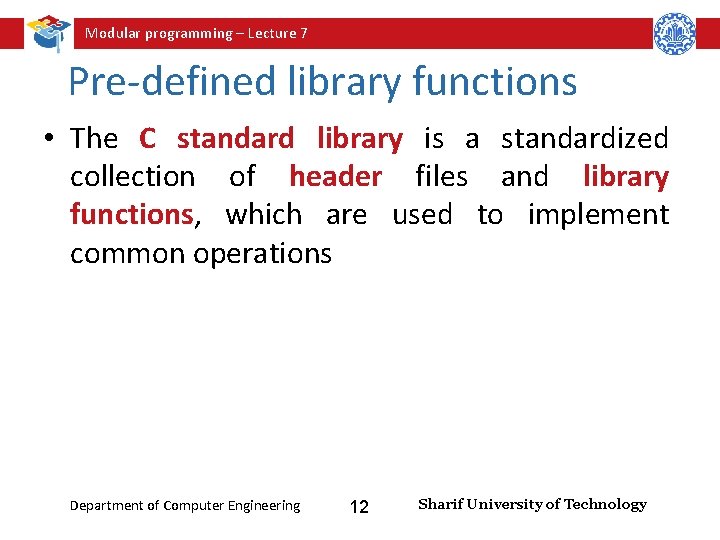
Modular programming – Lecture 7 Pre-defined library functions • The C standard library is a standardized collection of header files and library functions, which are used to implement common operations Department of Computer Engineering 12 Sharif University of Technology
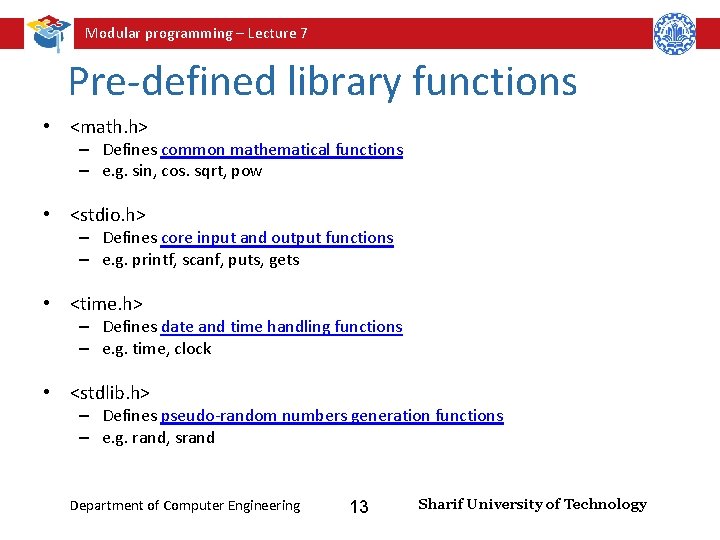
Modular programming – Lecture 7 Pre-defined library functions • <math. h> – Defines common mathematical functions – e. g. sin, cos. sqrt, pow • <stdio. h> – Defines core input and output functions – e. g. printf, scanf, puts, gets • <time. h> – Defines date and time handling functions – e. g. time, clock • <stdlib. h> – Defines pseudo-random numbers generation functions – e. g. rand, srand Department of Computer Engineering 13 Sharif University of Technology
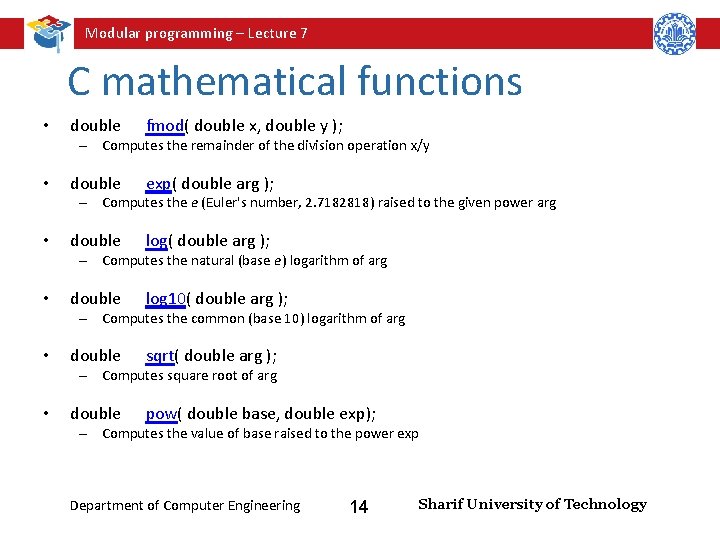
Modular programming – Lecture 7 C mathematical functions • double fmod( double x, double y ); double exp( double arg ); double log 10( double arg ); double sqrt( double arg ); double pow( double base, double exp); – Computes the remainder of the division operation x/y • – Computes the e (Euler's number, 2. 7182818) raised to the given power arg • – Computes the natural (base e) logarithm of arg • – Computes the common (base 10) logarithm of arg • – Computes square root of arg • – Computes the value of base raised to the power exp Department of Computer Engineering 14 Sharif University of Technology
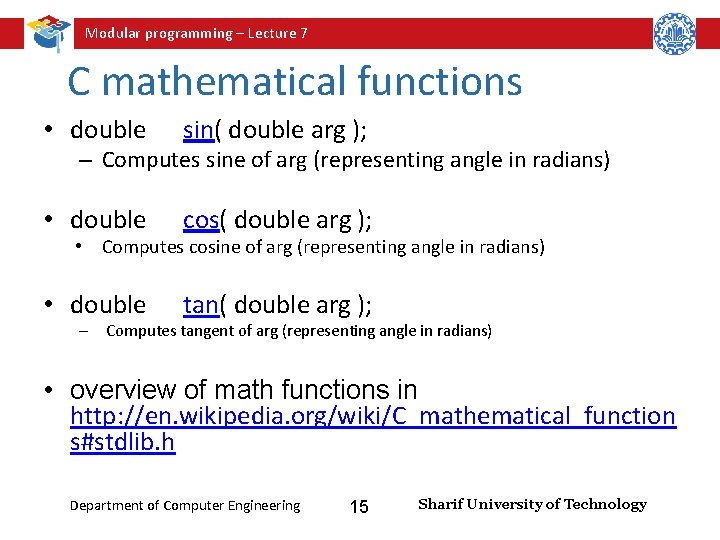
Modular programming – Lecture 7 C mathematical functions • double sin( double arg ); • double cos( double arg ); • double tan( double arg ); – Computes sine of arg (representing angle in radians) • Computes cosine of arg (representing angle in radians) – Computes tangent of arg (representing angle in radians) • overview of math functions in http: //en. wikipedia. org/wiki/C_mathematical_function s#stdlib. h Department of Computer Engineering 15 Sharif University of Technology
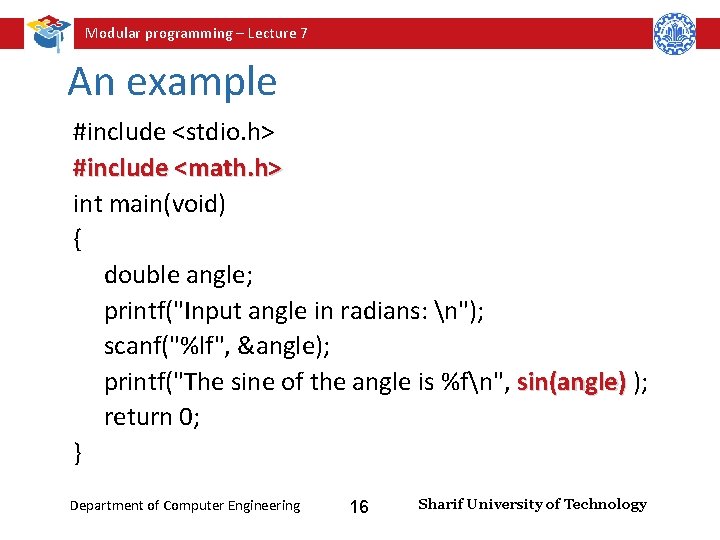
Modular programming – Lecture 7 An example #include <stdio. h> #include <math. h> int main(void) { double angle; printf("Input angle in radians: n"); scanf("%lf", &angle); printf("The sine of the angle is %fn", sin(angle) ); return 0; } Department of Computer Engineering 16 Sharif University of Technology
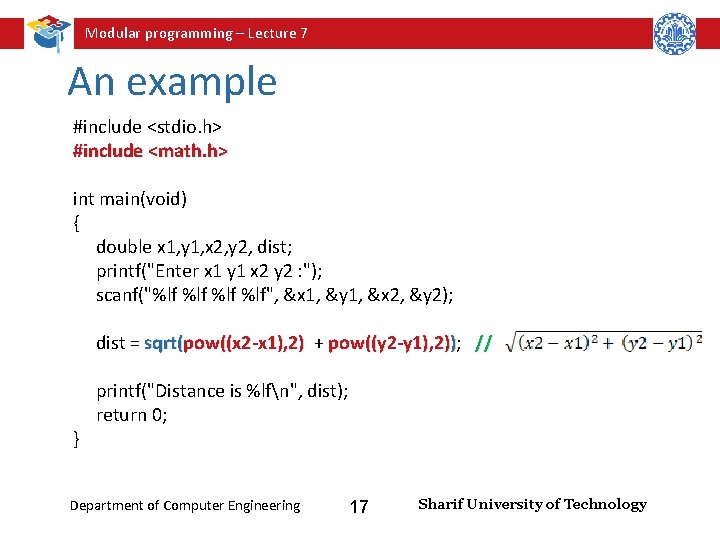
Modular programming – Lecture 7 An example #include <stdio. h> #include <math. h> int main(void) { double x 1, y 1, x 2, y 2, dist; printf("Enter x 1 y 1 x 2 y 2 : "); scanf("%lf %lf %lf", &x 1, &y 1, &x 2, &y 2); dist = sqrt(pow((x 2 -x 1), 2) + pow((y 2 -y 1), 2)); // } printf("Distance is %lfn", dist); return 0; Department of Computer Engineering 17 Sharif University of Technology
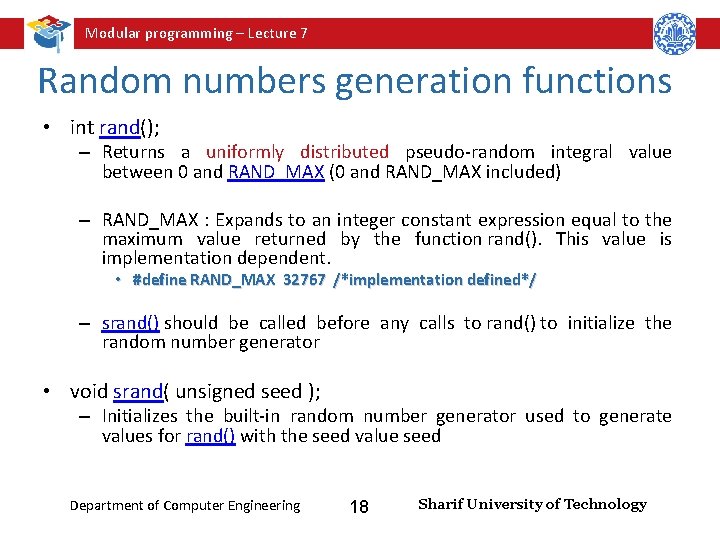
Modular programming – Lecture 7 Random numbers generation functions • int rand(); – Returns a uniformly distributed pseudo-random integral value between 0 and RAND_MAX (0 and RAND_MAX included) – RAND_MAX : Expands to an integer constant expression equal to the maximum value returned by the function rand(). This value is implementation dependent. • #define RAND_MAX 32767 /*implementation defined*/ – srand() should be called before any calls to rand() to initialize the random number generator • void srand( unsigned seed ); – Initializes the built-in random number generator used to generate values for rand() with the seed value seed Department of Computer Engineering 18 Sharif University of Technology
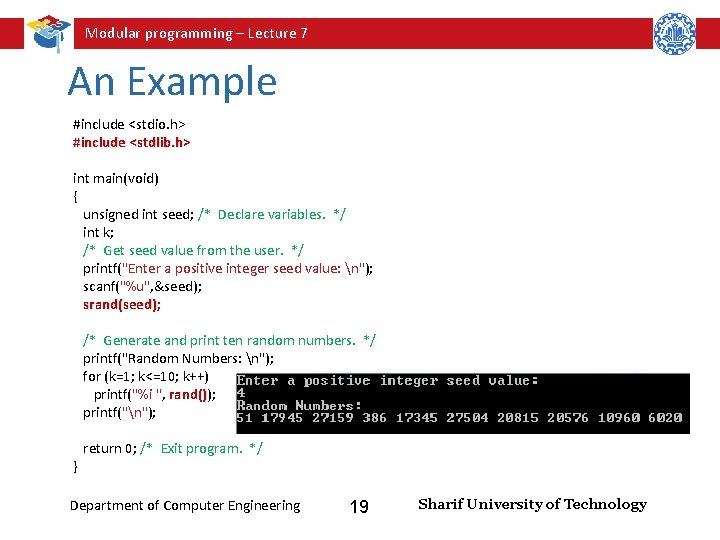
Modular programming – Lecture 7 An Example #include <stdio. h> #include <stdlib. h> int main(void) { unsigned int seed; /* Declare variables. */ int k; /* Get seed value from the user. */ printf("Enter a positive integer seed value: n"); scanf("%u", &seed); srand(seed); /* Generate and print ten random numbers. */ printf("Random Numbers: n"); for (k=1; k<=10; k++) printf("%i ", rand()); rand() printf("n"); } return 0; /* Exit program. */ Department of Computer Engineering 19 Sharif University of Technology
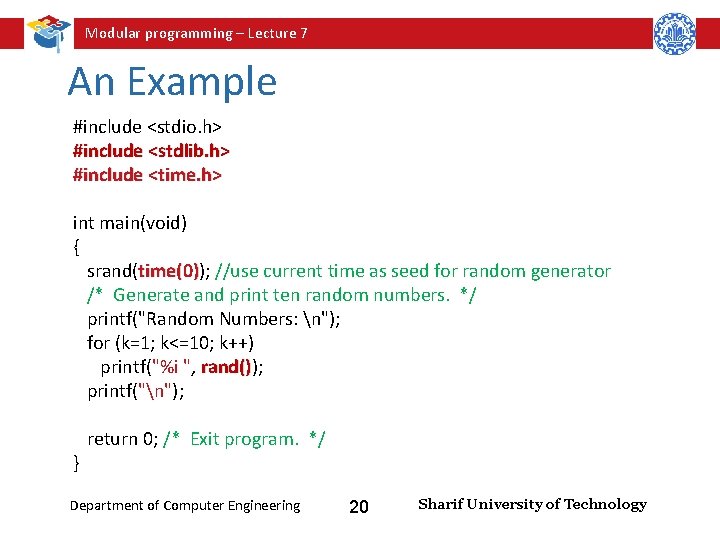
Modular programming – Lecture 7 An Example #include <stdio. h> #include <stdlib. h> #include <time. h> int main(void) { srand(time(0)); time(0) //use current time as seed for random generator /* Generate and print ten random numbers. */ printf("Random Numbers: n"); for (k=1; k<=10; k++) printf("%i ", rand()); rand() printf("n"); } return 0; /* Exit program. */ Department of Computer Engineering 20 Sharif University of Technology
![Modular programming Lecture 7 Random Numbers in a b Generate a random Modular programming – Lecture 7 Random Numbers in [a b] • Generate a random](https://slidetodoc.com/presentation_image_h2/8b33ae5fc9fb43e9977c7f9025e6a4cf/image-21.jpg)
Modular programming – Lecture 7 Random Numbers in [a b] • Generate a random number [0. . 7] – x = rand() % 8; • Generate a random number [10. . 17] – x = 10 + rand() % 8; • rand() % (b-a+1) + a; Department of Computer Engineering 21 Sharif University of 21 Technology
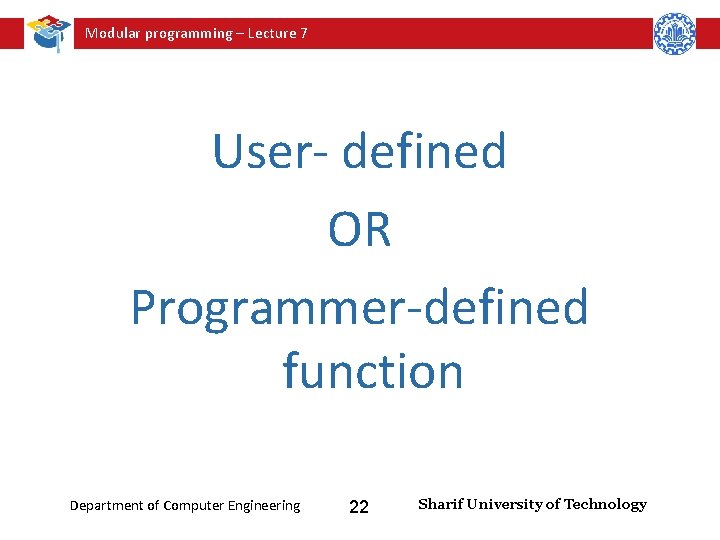
Modular programming – Lecture 7 User- defined OR Programmer-defined function Department of Computer Engineering 22 Sharif University of Technology
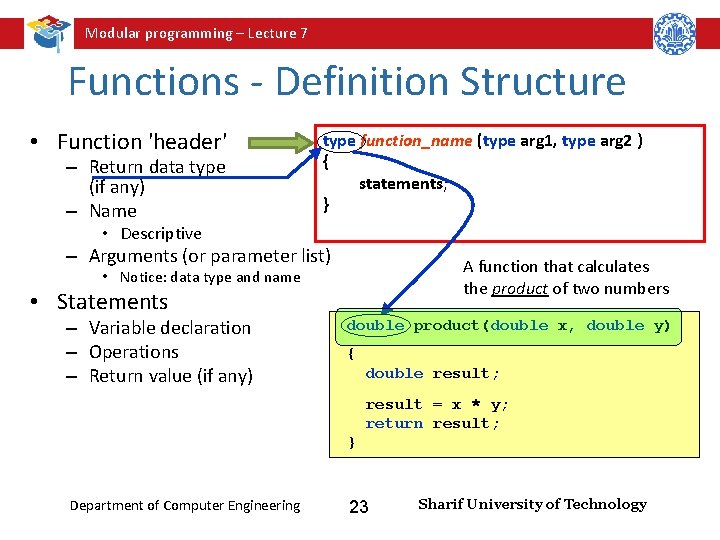
Modular programming – Lecture 7 Functions - Definition Structure • Function 'header' – Return data type (if any) – Name type function_name (type arg 1, type arg 2 ) { statements; } • Descriptive – Arguments (or parameter list) A function that calculates the product of two numbers • Notice: data type and name • Statements – Variable declaration – Operations – Return value (if any) double product(double x, double y) { double result; result = x * y; return result; } Department of Computer Engineering 23 Sharif University of Technology
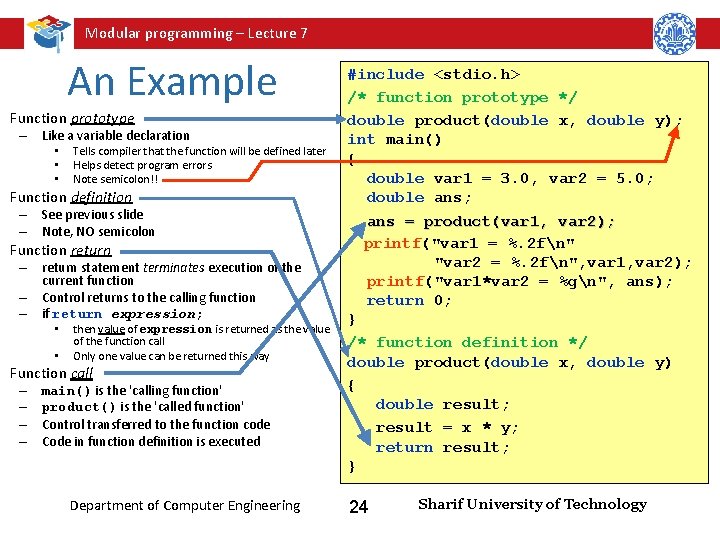
Modular programming – Lecture 7 An Example Function prototype – Like a variable declaration • • • Tells compiler that the function will be defined later Helps detect program errors Note semicolon!! Function definition – See previous slide – Note, NO semicolon Function return – return statement terminates execution of the current function – Control returns to the calling function – if return expression; • • then value of expression is returned as the value of the function call Only one value can be returned this way Function call – – main() is the 'calling function' product() is the 'called function' Control transferred to the function code Code in function definition is executed Department of Computer Engineering #include <stdio. h> /* function prototype */ double product(double x, double y); int main() { double var 1 = 3. 0, var 2 = 5. 0; double ans; ans = product(var 1, var 2); printf("var 1 = %. 2 fn" "var 2 = %. 2 fn", var 1, var 2); printf("var 1*var 2 = %gn", ans); return 0; } /* function definition */ double product(double x, double y) { double result; result = x * y; return result; } 24 Sharif University of Technology
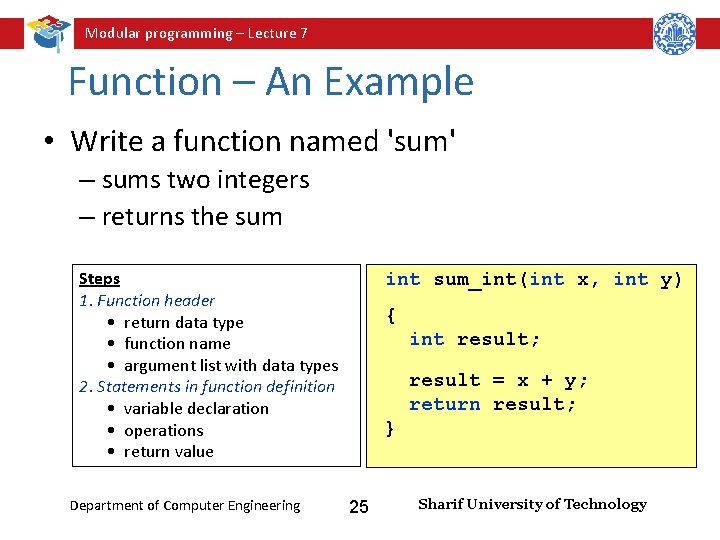
Modular programming – Lecture 7 Function – An Example • Write a function named 'sum' – sums two integers – returns the sum Steps 1. Function header • return data type • function name • argument list with data types 2. Statements in function definition • variable declaration • operations • return value Department of Computer Engineering int sum_int(int x, int y) { int result; result = x + y; return result; } 25 Sharif University of Technology
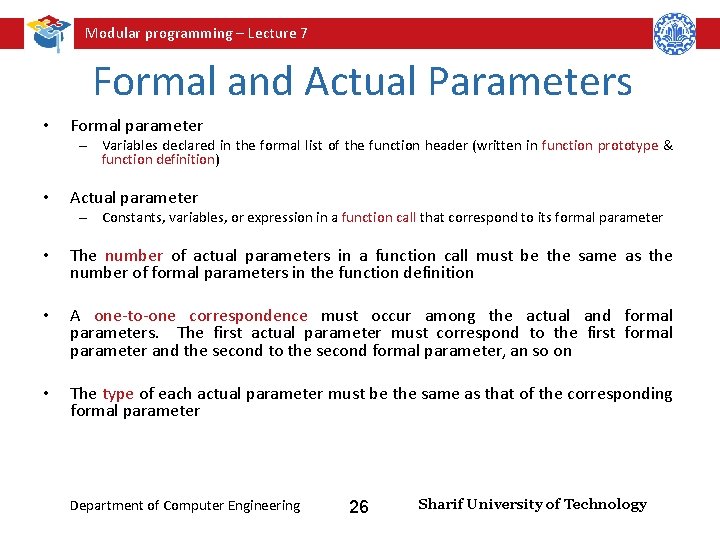
Modular programming – Lecture 7 Formal and Actual Parameters • Formal parameter – Variables declared in the formal list of the function header (written in function prototype & function definition) • Actual parameter – Constants, variables, or expression in a function call that correspond to its formal parameter • The number of actual parameters in a function call must be the same as the number of formal parameters in the function definition • A one-to-one correspondence must occur among the actual and formal parameters. The first actual parameter must correspond to the first formal parameter and the second to the second formal parameter, an so on • The type of each actual parameter must be the same as that of the corresponding formal parameter Department of Computer Engineering 26 Sharif University of Technology
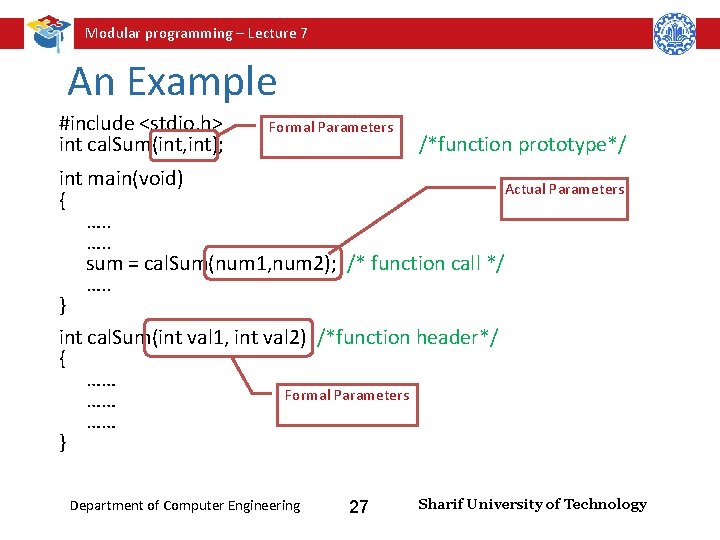
Modular programming – Lecture 7 An Example #include <stdio. h> int cal. Sum(int, int); Formal Parameters /*function prototype*/ int main(void) Actual Parameters { …. . sum = cal. Sum(num 1, num 2); /* function call */ …. . } int cal. Sum(int val 1, int val 2) /*function header*/ { …… Formal Parameters …… …… } Department of Computer Engineering 27 Sharif University of Technology
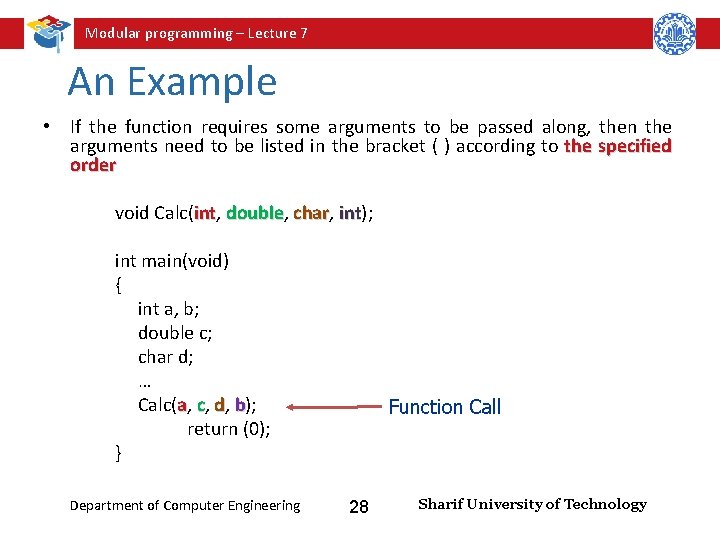
Modular programming – Lecture 7 An Example • If the function requires some arguments to be passed along, then the arguments need to be listed in the bracket ( ) according to the specified order void Calc(int, int double, double char, char int); int main(void) { int a, b; double c; char d; … Calc(a, c, d, b); return (0); } Department of Computer Engineering Function Call 28 Sharif University of Technology
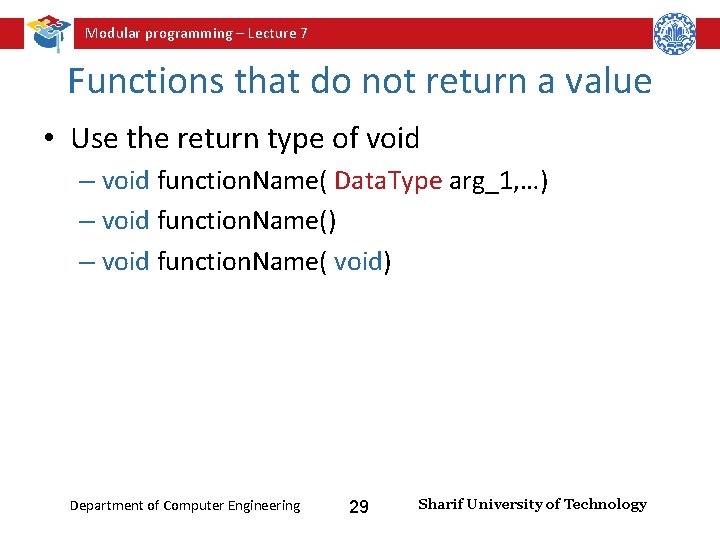
Modular programming – Lecture 7 Functions that do not return a value • Use the return type of void – void function. Name( Data. Type arg_1, …) – void function. Name( void) Department of Computer Engineering 29 Sharif University of Technology
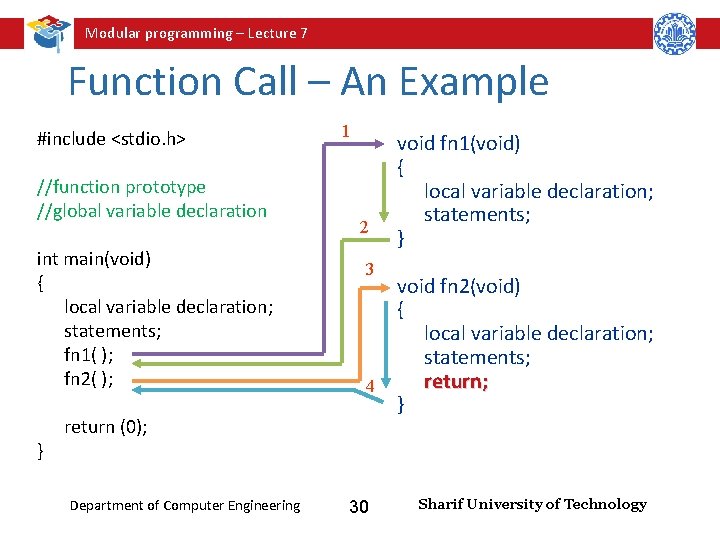
Modular programming – Lecture 7 Function Call – An Example #include <stdio. h> //function prototype //global variable declaration int main(void) { local variable declaration; statements; fn 1( ); fn 2( ); } 1 2 3 4 return (0); Department of Computer Engineering 30 void fn 1(void) { local variable declaration; statements; } void fn 2(void) { local variable declaration; statements; return; } Sharif University of Technology
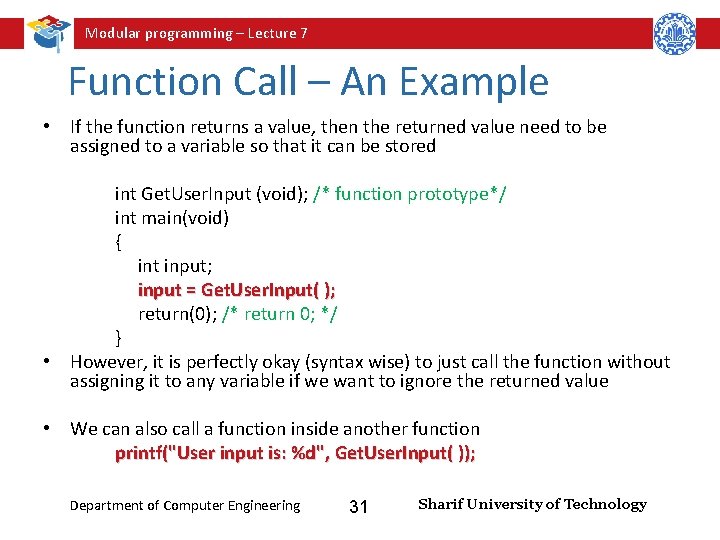
Modular programming – Lecture 7 Function Call – An Example • If the function returns a value, then the returned value need to be assigned to a variable so that it can be stored int Get. User. Input (void); /* function prototype*/ int main(void) { int input; input = Get. User. Input( ); return(0); /* return 0; */ } • However, it is perfectly okay (syntax wise) to just call the function without assigning it to any variable if we want to ignore the returned value • We can also call a function inside another function printf("User input is: %d", Get. User. Input( )); Department of Computer Engineering 31 Sharif University of Technology
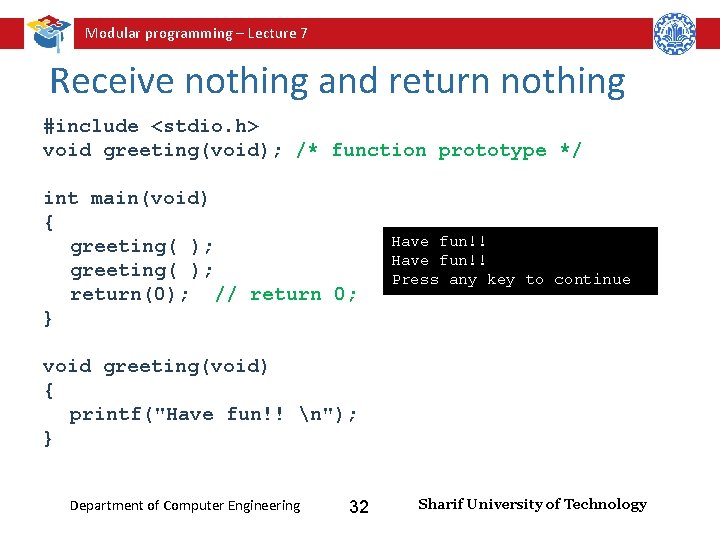
Modular programming – Lecture 7 Receive nothing and return nothing #include <stdio. h> void greeting(void); /* function prototype */ int main(void) { greeting( ); return(0); // return 0; } Have fun!! Press any key to continue void greeting(void) { printf("Have fun!! n"); } Department of Computer Engineering 32 Sharif University of Technology
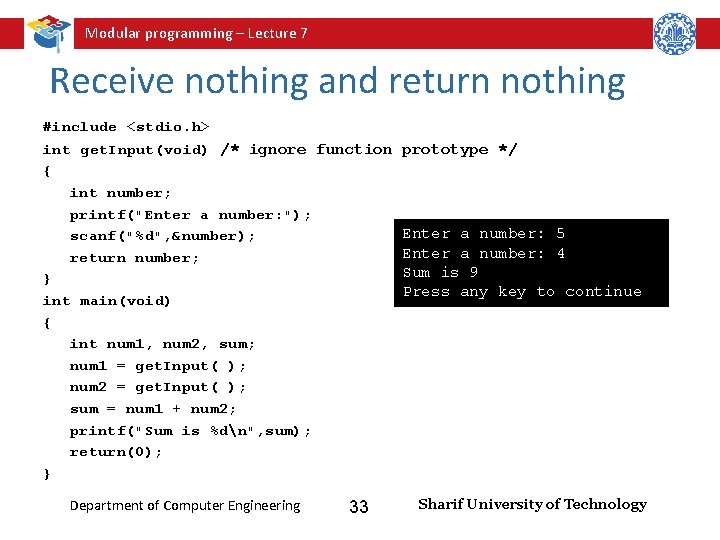
Modular programming – Lecture 7 Receive nothing and return nothing #include <stdio. h> int get. Input(void) /* ignore function { int number; printf("Enter a number: "); scanf("%d", &number); return number; } int main(void) { int num 1, num 2, sum; num 1 = get. Input( ); num 2 = get. Input( ); sum = num 1 + num 2; printf("Sum is %dn", sum); return(0); } Department of Computer Engineering 33 prototype */ Enter a number: 5 Enter a number: 4 Sum is 9 Press any key to continue Sharif University of Technology
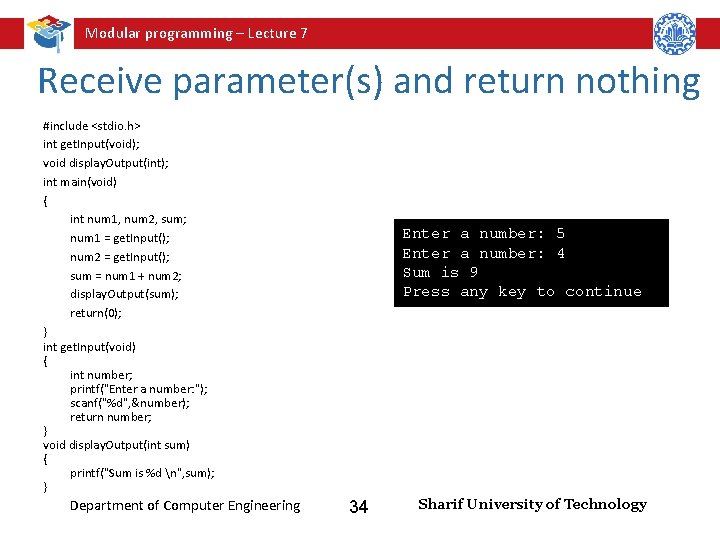
Modular programming – Lecture 7 Receive parameter(s) and return nothing #include <stdio. h> int get. Input(void); void display. Output(int); int main(void) { int num 1, num 2, sum; num 1 = get. Input(); num 2 = get. Input(); sum = num 1 + num 2; display. Output(sum); return(0); } int get. Input(void) { int number; printf("Enter a number: "); scanf("%d", &number); return number; } void display. Output(int sum) { printf("Sum is %d n", sum); } Department of Computer Engineering Enter a number: 5 Enter a number: 4 Sum is 9 Press any key to continue 34 Sharif University of Technology
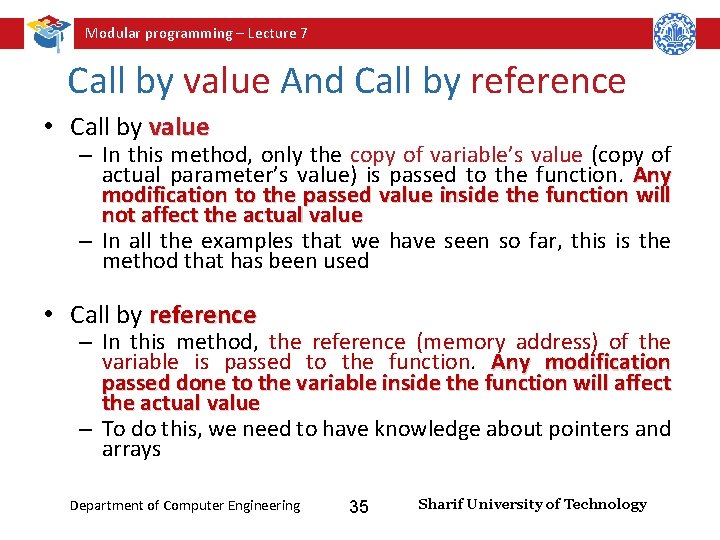
Modular programming – Lecture 7 Call by value And Call by reference • Call by value – In this method, only the copy of variable’s value (copy of actual parameter’s value) is passed to the function. Any modification to the passed value inside the function will not affect the actual value – In all the examples that we have seen so far, this is the method that has been used • Call by reference – In this method, the reference (memory address) of the variable is passed to the function. Any modification passed done to the variable inside the function will affect the actual value – To do this, we need to have knowledge about pointers and arrays Department of Computer Engineering 35 Sharif University of Technology
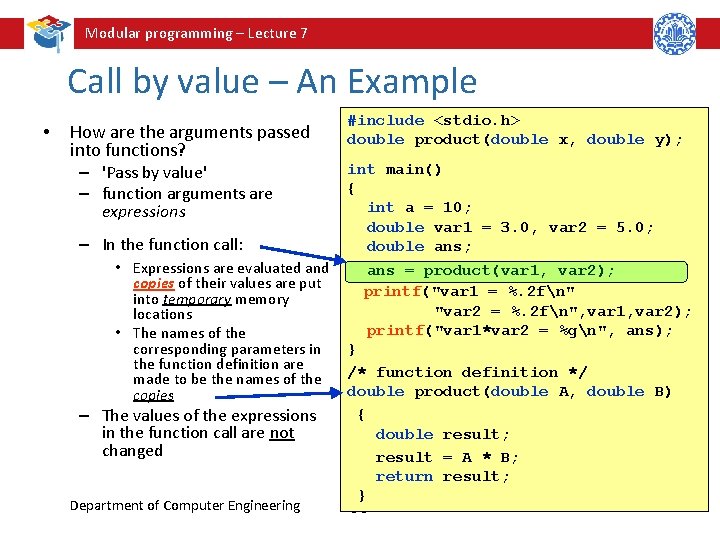
Modular programming – Lecture 7 Call by value – An Example • How are the arguments passed into functions? – 'Pass by value' – function arguments are expressions – In the function call: • Expressions are evaluated and copies of their values are put into temporary memory locations • The names of the corresponding parameters in the function definition are made to be the names of the copies – The values of the expressions in the function call are not changed Department of Computer Engineering #include <stdio. h> double product(double x, double y); int main() { int a = 10; double var 1 = 3. 0, var 2 = 5. 0; double ans; ans = product(var 1, var 2); printf("var 1 = %. 2 fn" "var 2 = %. 2 fn", var 1, var 2); printf("var 1*var 2 = %gn", ans); } /* function definition */ double product(double A, double B) { double result; result = A * B; return result; } 36 Sharif University of Technology
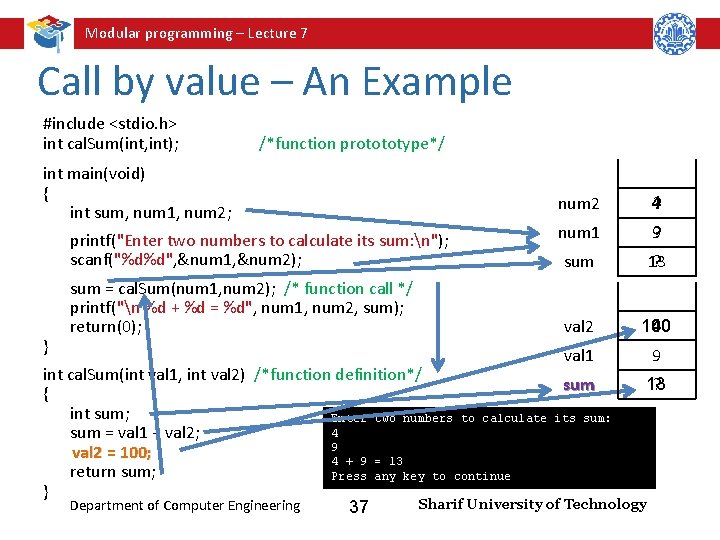
Modular programming – Lecture 7 Call by value – An Example #include <stdio. h> int cal. Sum(int, int); /*function protototype*/ int main(void) { int sum, num 1, num 2; printf("Enter two numbers to calculate its sum: n"); scanf("%d%d", &num 1, &num 2); } sum = cal. Sum(num 1, num 2); /* function call */ printf("n %d + %d = %d", num 1, num 2, sum); return(0); num 2 4? num 1 9? sum 13 ? val 2 100 4 val 1 9 int cal. Sum(int val 1, int val 2) /*function definition*/ sum 13 ? { int sum; Enter two numbers to calculate its sum: 4 sum = val 1 + val 2; 9 val 2 = 100; 4 + 9 = 13 return sum; Press any key to continue } Sharif University of Technology Department of Computer Engineering 37
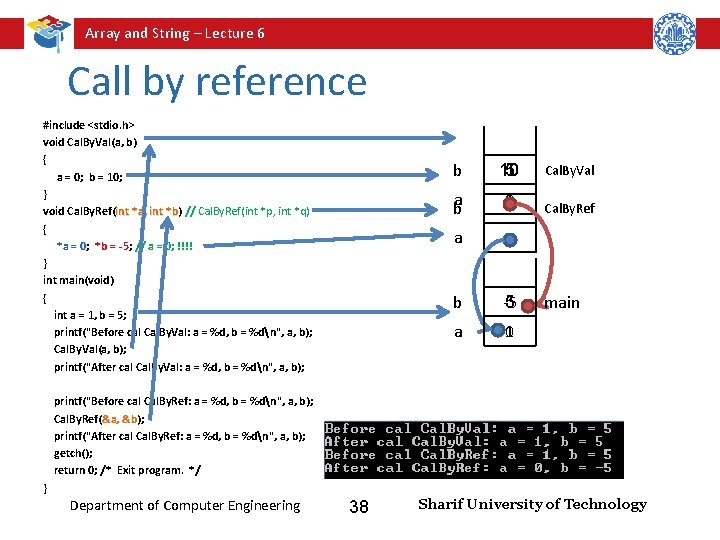
Array and String – Lecture 6 Call by reference #include <stdio. h> void Cal. By. Val(a, b) { a = 0; b = 10; } void Cal. By. Ref(int *a, int *b) *b // Cal. By. Ref(int *p, int *q) { *a = 0; 0 *b = -5; -5 // a = 0; !!!! } int main(void) { int a = 1, b = 5; printf("Before cal Cal. By. Val: a = %d, b = %dn", a, b); Cal. By. Val(a, b); printf("After cal Cal. By. Val: a = %d, b = %dn", a, b); b 10 5 Cal. By. Val a b 1 0 Cal. By. Ref b -5 5 main a 1 0 a printf("Before cal Cal. By. Ref: a = %d, b = %dn", a, b); Cal. By. Ref(&a, &b); &b printf("After cal Cal. By. Ref: a = %d, b = %dn", a, b); getch(); return 0; /* Exit program. */ } Department of Computer Engineering 38 Sharif University of Technology
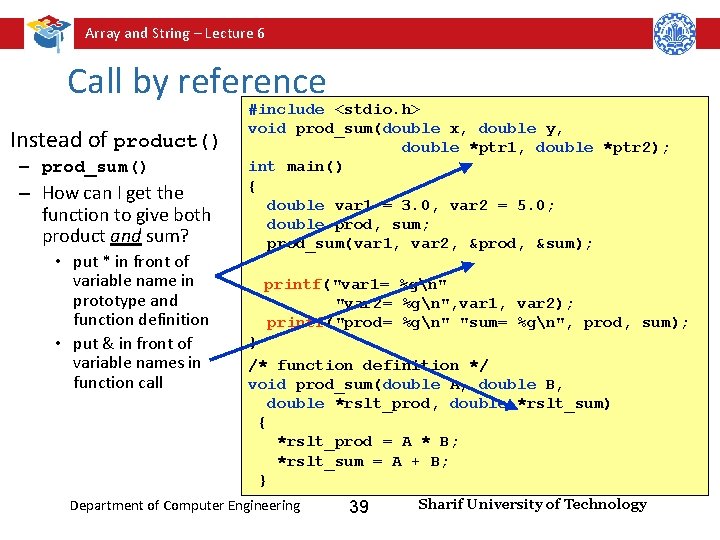
Array and String – Lecture 6 Call by reference Instead of product() – prod_sum() – How can I get the function to give both product and sum? • put * in front of variable name in prototype and function definition • put & in front of variable names in function call #include <stdio. h> void prod_sum(double x, double y, double *ptr 1, double *ptr 2); int main() { double var 1 = 3. 0, var 2 = 5. 0; double prod, sum; prod_sum(var 1, var 2, &prod, &sum); printf("var 1= %gn" "var 2= %gn", var 1, var 2); printf("prod= %gn" "sum= %gn", prod, sum); } /* function definition */ void prod_sum(double A, double B, double *rslt_prod, double *rslt_sum) { *rslt_prod = A * B; *rslt_sum = A + B; } Department of Computer Engineering 39 Sharif University of Technology
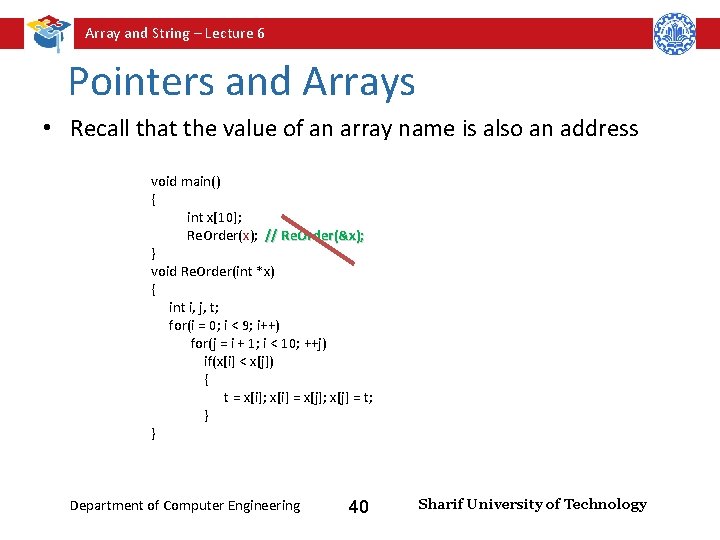
Array and String – Lecture 6 Pointers and Arrays • Recall that the value of an array name is also an address void main() { int x[10]; Re. Order(x); // Re. Order(&x); } void Re. Order(int *x) { int i, j, t; for(i = 0; i < 9; i++) for(j = i + 1; i < 10; ++j) if(x[i] < x[j]) { t = x[i]; x[i] = x[j]; x[j] = t; } } Department of Computer Engineering 40 Sharif University of Technology
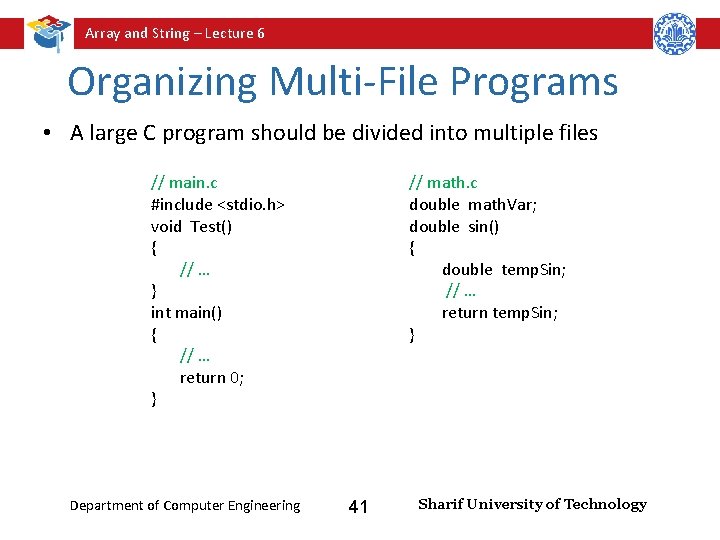
Array and String – Lecture 6 Organizing Multi-File Programs • A large C program should be divided into multiple files // main. c #include <stdio. h> void Test() { // … } int main() { // … return 0; } Department of Computer Engineering // math. c double math. Var; double sin() { double temp. Sin; // … return temp. Sin; } 41 Sharif University of Technology
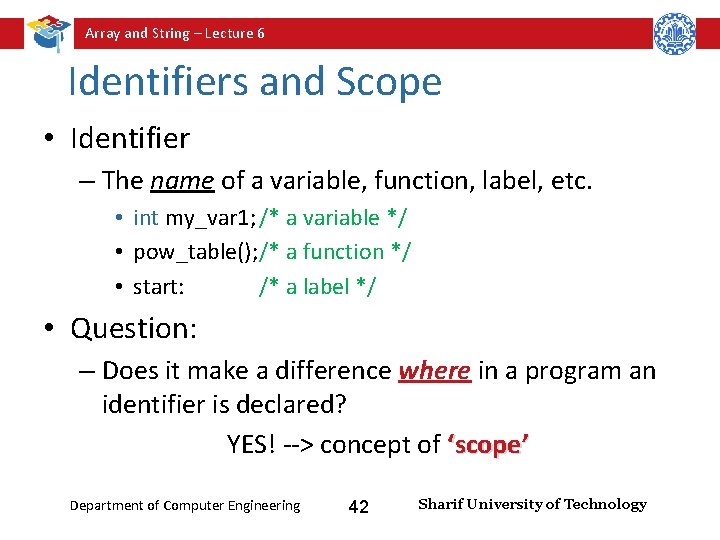
Array and String – Lecture 6 Identifiers and Scope • Identifier – The name of a variable, function, label, etc. • int my_var 1; /* a variable */ • pow_table(); /* a function */ • start: /* a label */ • Question: – Does it make a difference where in a program an identifier is declared? YES! --> concept of ‘scope’ Department of Computer Engineering 42 Sharif University of Technology
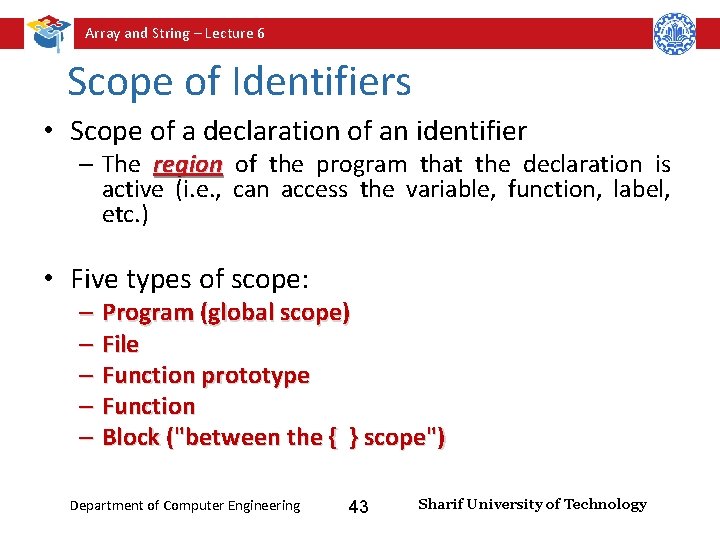
Array and String – Lecture 6 Scope of Identifiers • Scope of a declaration of an identifier – The region of the program that the declaration is active (i. e. , can access the variable, function, label, etc. ) • Five types of scope: – Program (global scope) – File – Function prototype – Function – Block ("between the { } scope") Department of Computer Engineering 43 Sharif University of Technology
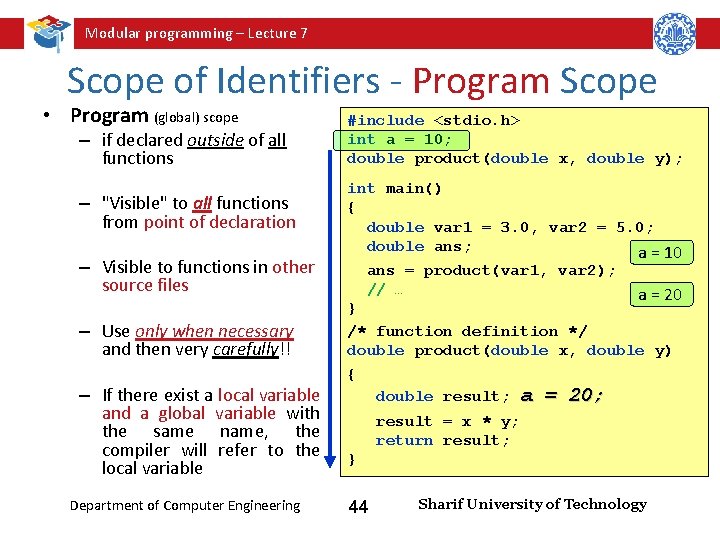
Modular programming – Lecture 7 Scope of Identifiers - Program Scope • Program (global) scope – if declared outside of all functions – "Visible" to all functions from point of declaration – Visible to functions in other source files – Use only when necessary and then very carefully!! – If there exist a local variable and a global variable with the same name, the compiler will refer to the local variable Department of Computer Engineering #include <stdio. h> int a = 10; double product(double x, double y); int main() { double var 1 = 3. 0, var 2 = 5. 0; double ans; a = 10 ans = product(var 1, var 2); // … a = 20 } /* function definition */ double product(double x, double y) { double result; a = 20; result = x * y; return result; } 44 Sharif University of Technology
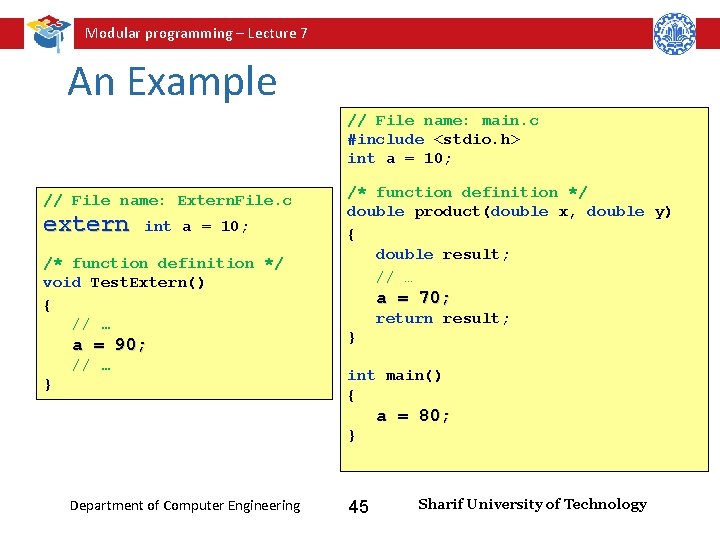
Modular programming – Lecture 7 An Example // File name: main. c #include <stdio. h> int a = 10; // File name: Extern. File. c extern int a = 10; /* function definition */ void Test. Extern() { // … a = 90; // … } /* function definition */ double product(double x, double y) { double result; // … a = 70; return result; } int main() { a = 80; } Department of Computer Engineering 45 Sharif University of Technology
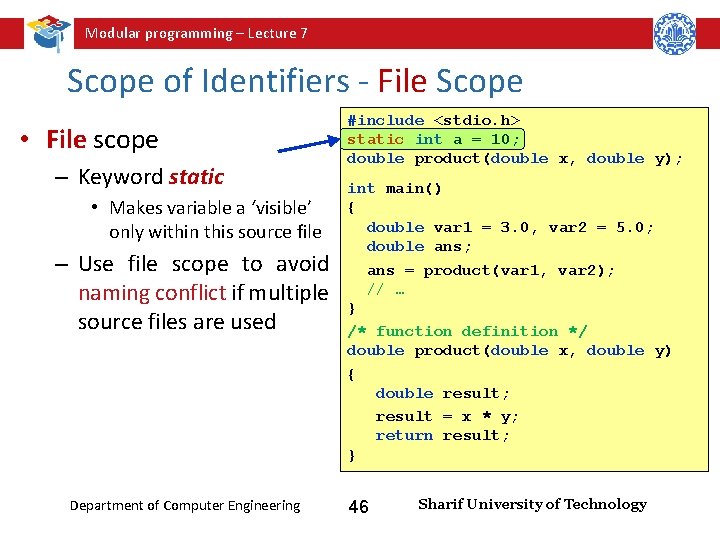
Modular programming – Lecture 7 Scope of Identifiers - File Scope • File scope – Keyword static • Makes variable a ‘visible’ only within this source file – Use file scope to avoid naming conflict if multiple source files are used Department of Computer Engineering #include <stdio. h> static int a = 10; double product(double x, double y); int main() { double var 1 = 3. 0, var 2 = 5. 0; double ans; ans = product(var 1, var 2); // … } /* function definition */ double product(double x, double y) { double result; result = x * y; return result; } 46 Sharif University of Technology
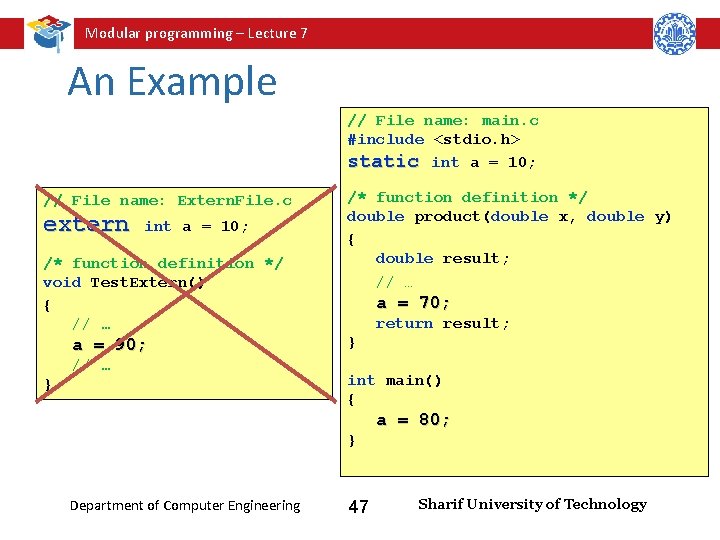
Modular programming – Lecture 7 An Example // File name: main. c #include <stdio. h> static int a = 10; // File name: Extern. File. c extern int a = 10; /* function definition */ void Test. Extern() { // … a = 90; // … } /* function definition */ double product(double x, double y) { double result; // … a = 70; return result; } int main() { a = 80; } Department of Computer Engineering 47 Sharif University of Technology
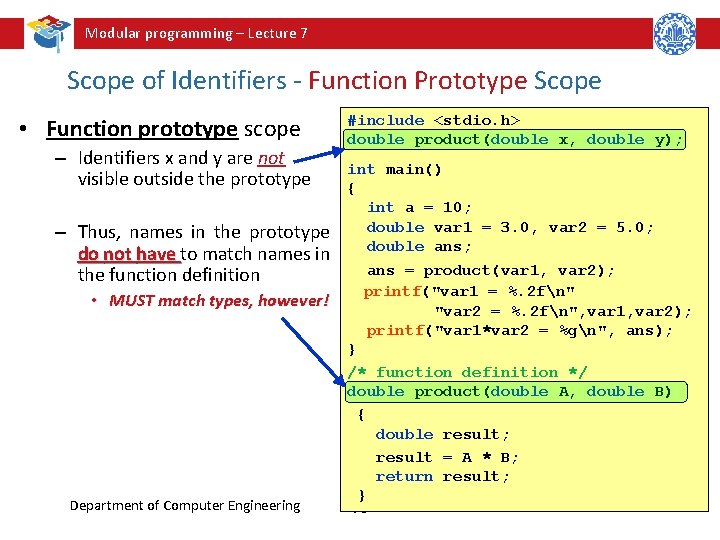
Modular programming – Lecture 7 Scope of Identifiers - Function Prototype Scope • Function prototype scope – Identifiers x and y are not visible outside the prototype – Thus, names in the prototype do not have to match names in the function definition • MUST match types, however! Department of Computer Engineering #include <stdio. h> double product(double x, double y); int main() { int a = 10; double var 1 = 3. 0, var 2 = 5. 0; double ans; ans = product(var 1, var 2); printf("var 1 = %. 2 fn" "var 2 = %. 2 fn", var 1, var 2); printf("var 1*var 2 = %gn", ans); } /* function definition */ double product(double A, double B) { double result; result = A * B; return result; } 48 Sharif University of Technology
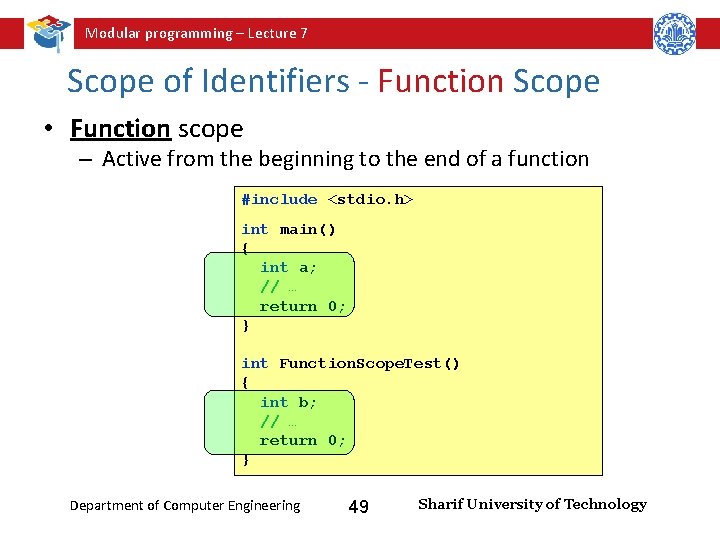
Modular programming – Lecture 7 Scope of Identifiers - Function Scope • Function scope – Active from the beginning to the end of a function #include <stdio. h> int main() { int a; // … return 0; } int Function. Scope. Test() { int b; // … return 0; } Department of Computer Engineering 49 Sharif University of Technology
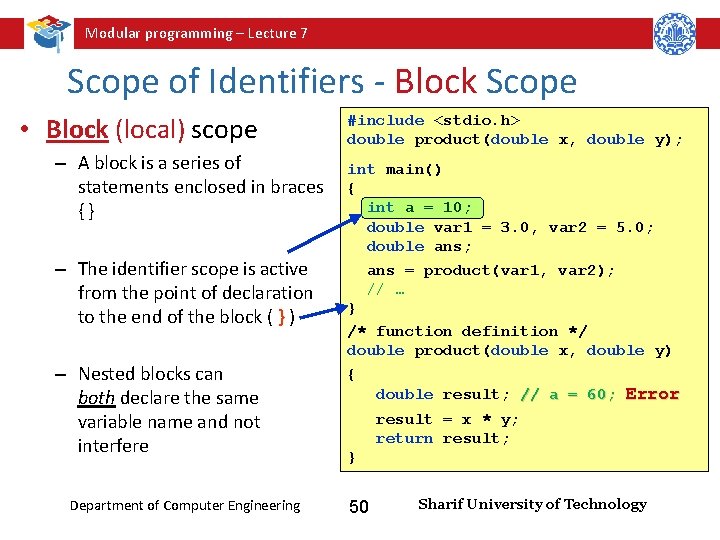
Modular programming – Lecture 7 Scope of Identifiers - Block Scope • Block (local) scope – A block is a series of statements enclosed in braces {} – The identifier scope is active from the point of declaration to the end of the block ( } ) – Nested blocks can both declare the same variable name and not interfere Department of Computer Engineering #include <stdio. h> double product(double x, double y); int main() { int a = 10; double var 1 = 3. 0, var 2 = 5. 0; double ans; ans = product(var 1, var 2); // … } /* function definition */ double product(double x, double y) { double result; // a = 60; Error result = x * y; return result; } 50 Sharif University of Technology
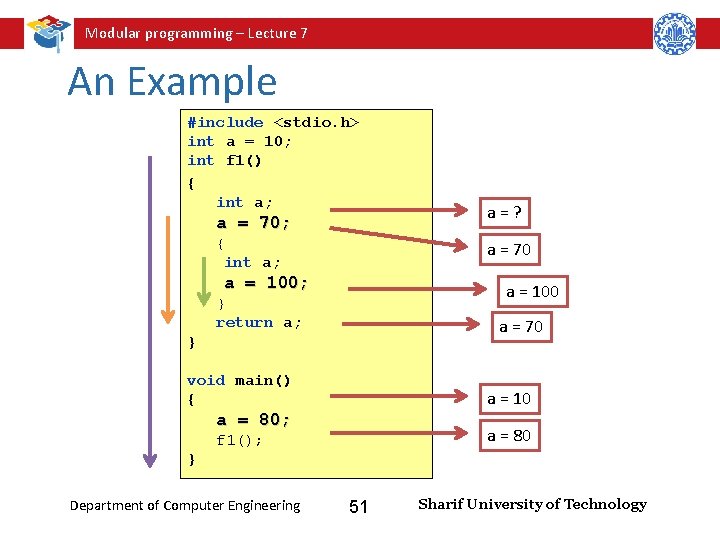
Modular programming – Lecture 7 An Example #include <stdio. h> int a = 10; int f 1() { int a; a = 70; { int a; a=? a = 70 a = 100; a = 100 } return a; a = 70 } void main() { a = 10 a = 80; a = 80 f 1(); } Department of Computer Engineering 51 Sharif University of Technology
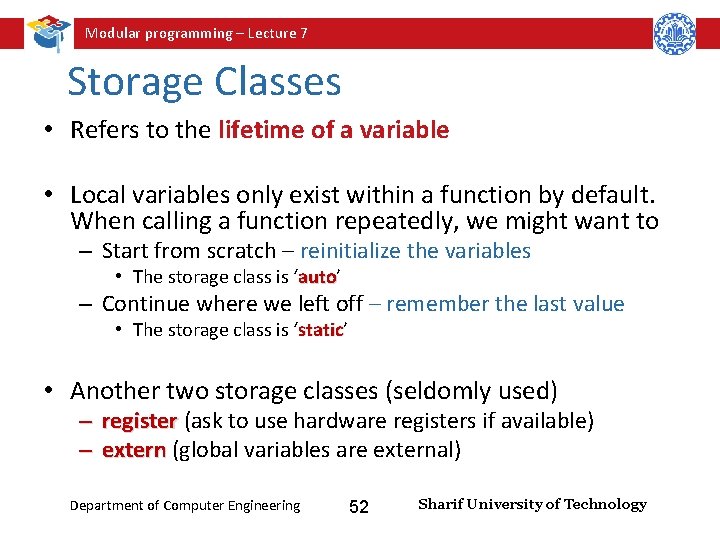
Modular programming – Lecture 7 Storage Classes • Refers to the lifetime of a variable • Local variables only exist within a function by default. When calling a function repeatedly, we might want to – Start from scratch – reinitialize the variables • The storage class is ‘auto’ auto – Continue where we left off – remember the last value • The storage class is ‘static’ static • Another two storage classes (seldomly used) – register (ask to use hardware registers if available) – extern (global variables are external) Department of Computer Engineering 52 Sharif University of Technology
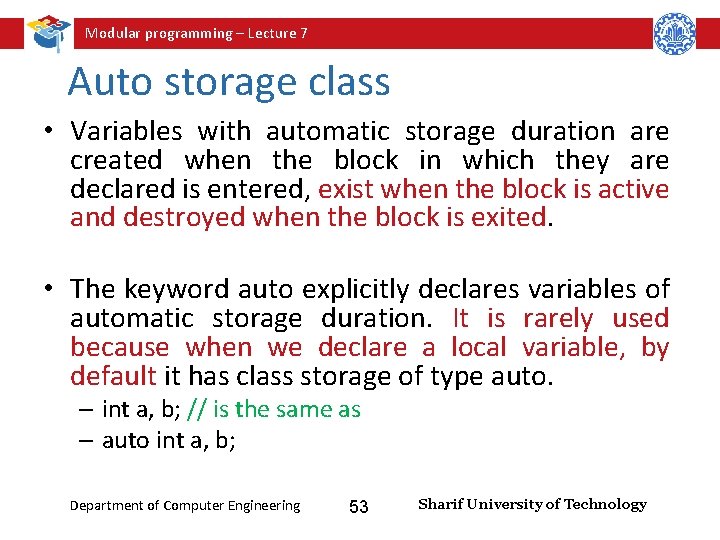
Modular programming – Lecture 7 Auto storage class • Variables with automatic storage duration are created when the block in which they are declared is entered, exist when the block is active and destroyed when the block is exited. • The keyword auto explicitly declares variables of automatic storage duration. It is rarely used because when we declare a local variable, by default it has class storage of type auto. – int a, b; // is the same as – auto int a, b; Department of Computer Engineering 53 Sharif University of Technology
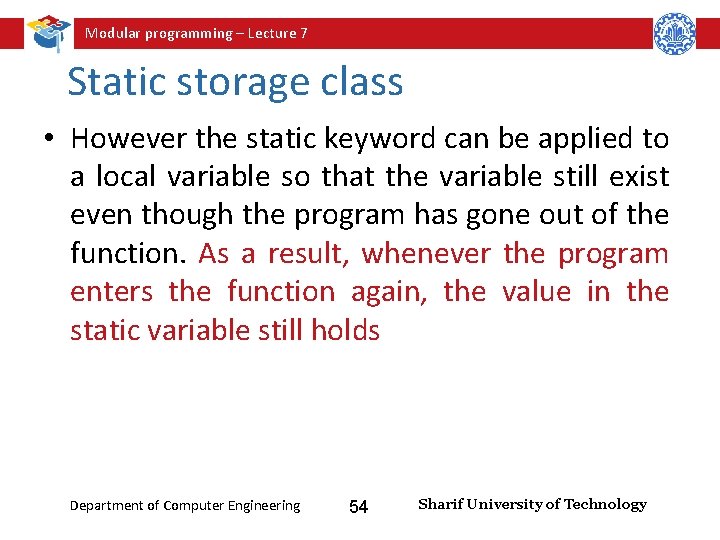
Modular programming – Lecture 7 Static storage class • However the static keyword can be applied to a local variable so that the variable still exist even though the program has gone out of the function. As a result, whenever the program enters the function again, the value in the static variable still holds Department of Computer Engineering 54 Sharif University of Technology
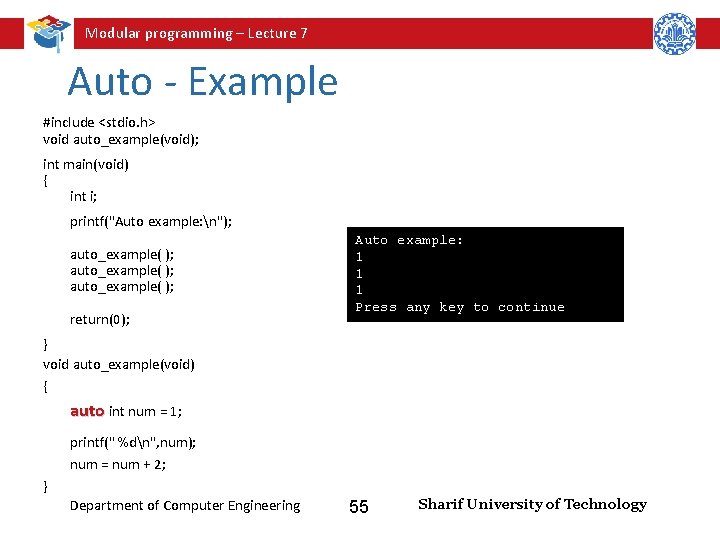
Modular programming – Lecture 7 Auto - Example #include <stdio. h> void auto_example(void); int main(void) { int i; printf("Auto example: n"); auto_example( ); return(0); Auto example: 1 1 1 Press any key to continue } void auto_example(void) { auto int num = 1; printf(" %dn", num); num = num + 2; } Department of Computer Engineering 55 Sharif University of Technology
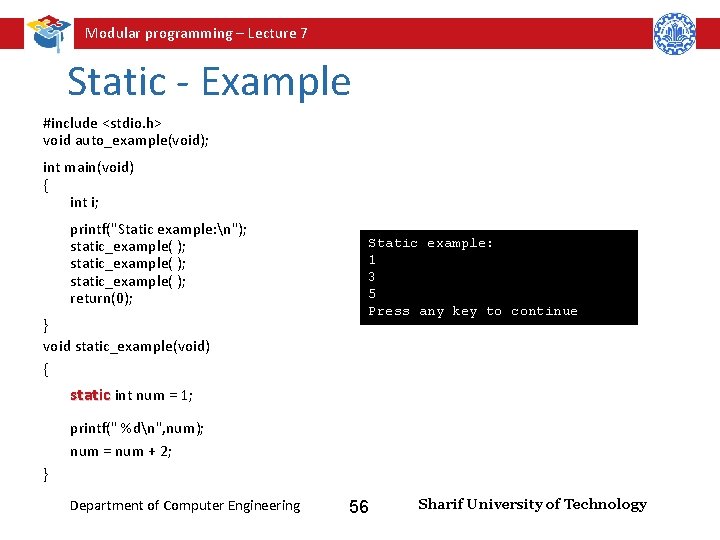
Modular programming – Lecture 7 Static - Example #include <stdio. h> void auto_example(void); int main(void) { int i; printf("Static example: n"); static_example( ); return(0); } void static_example(void) { Static example: 1 3 5 Press any key to continue static int num = 1; printf(" %dn", num); num = num + 2; } Department of Computer Engineering 56 Sharif University of Technology
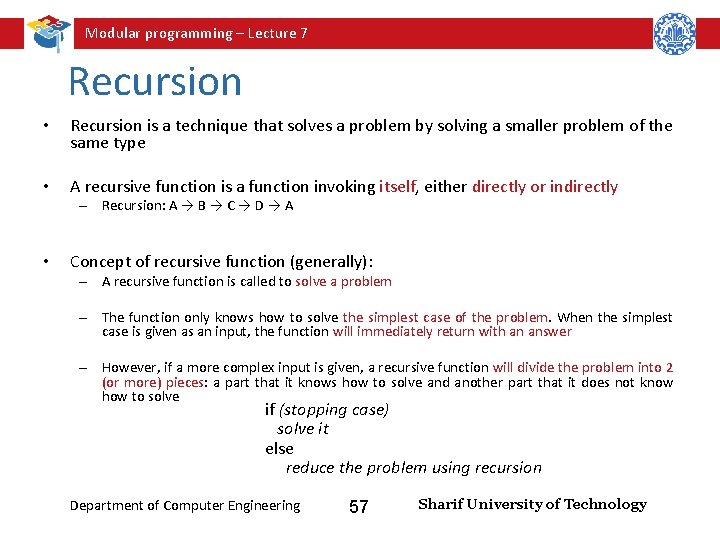
Modular programming – Lecture 7 Recursion • Recursion is a technique that solves a problem by solving a smaller problem of the same type • A recursive function is a function invoking itself, either directly or indirectly – Recursion: A → B → C → D → A • Concept of recursive function (generally): – A recursive function is called to solve a problem – The function only knows how to solve the simplest case of the problem. When the simplest case is given as an input, the function will immediately return with an answer – However, if a more complex input is given, a recursive function will divide the problem into 2 (or more) pieces: a part that it knows how to solve and another part that it does not know how to solve if (stopping case) solve it else reduce the problem using recursion Department of Computer Engineering 57 Sharif University of Technology
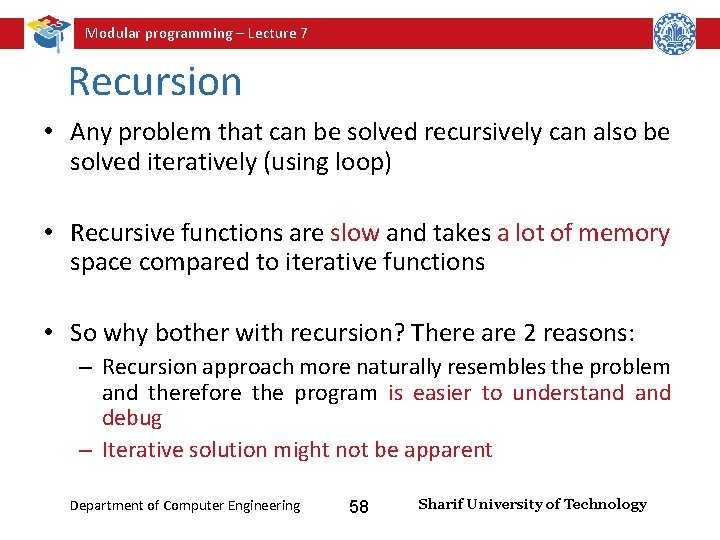
Modular programming – Lecture 7 Recursion • Any problem that can be solved recursively can also be solved iteratively (using loop) • Recursive functions are slow and takes a lot of memory space compared to iterative functions • So why bother with recursion? There are 2 reasons: – Recursion approach more naturally resembles the problem and therefore the program is easier to understand debug – Iterative solution might not be apparent Department of Computer Engineering 58 Sharif University of Technology
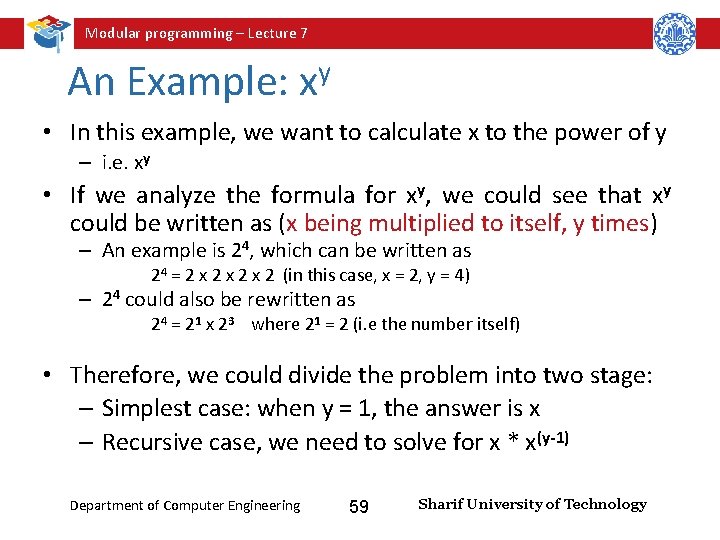
Modular programming – Lecture 7 An Example: xy • In this example, we want to calculate x to the power of y – i. e. xy • If we analyze the formula for xy, we could see that xy could be written as (x being multiplied to itself, y times) – An example is 24, which can be written as 24 = 2 x 2 x 2 (in this case, x = 2, y = 4) – 24 could also be rewritten as 24 = 21 x 23 where 21 = 2 (i. e the number itself) • Therefore, we could divide the problem into two stage: – Simplest case: when y = 1, the answer is x – Recursive case, we need to solve for x * x(y-1) Department of Computer Engineering 59 Sharif University of Technology
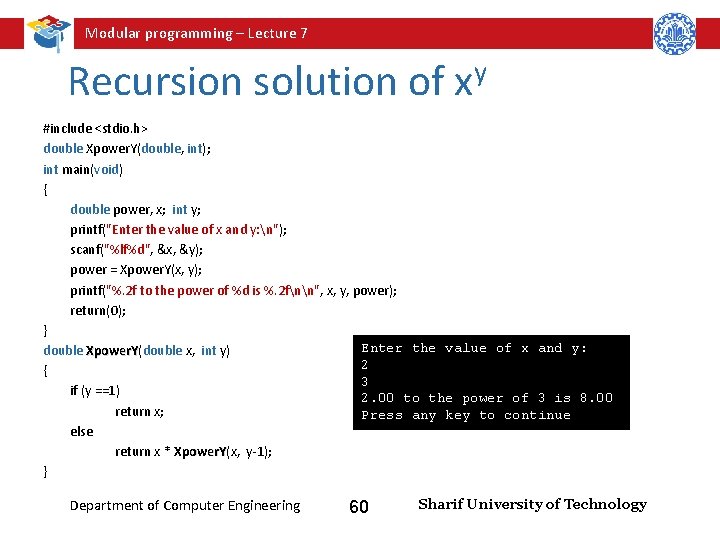
Modular programming – Lecture 7 Recursion solution of xy #include <stdio. h> double Xpower. Y(double, int); int main(void) { double power, x; int y; printf("Enter the value of x and y: n"); scanf("%lf%d", &x, &y); power = Xpower. Y(x, y); printf("%. 2 f to the power of %d is %. 2 fnn", x, y, power); return(0); } Enter the value of x and y: double Xpower. Y(double x, int y) Xpower. Y 2 { 3 if (y ==1) 2. 00 to the power of 3 is 8. 00 return x; Press any key to continue else return x * Xpower. Y(x, Xpower. Y y-1); } Department of Computer Engineering 60 Sharif University of Technology
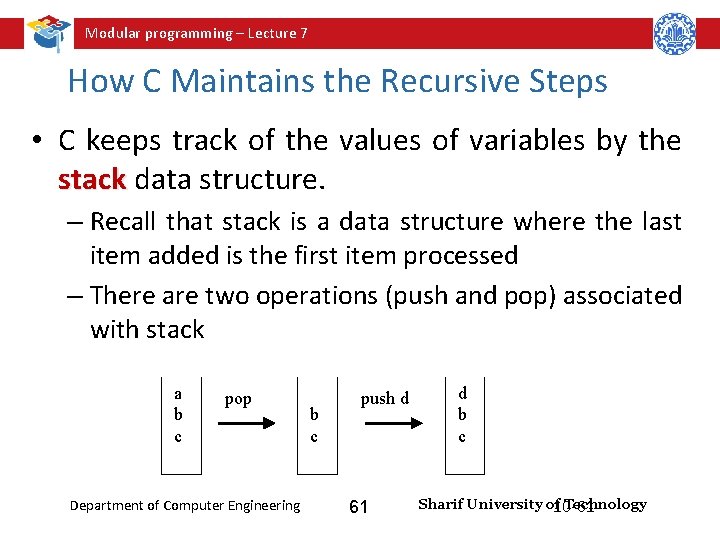
Modular programming – Lecture 7 How C Maintains the Recursive Steps • C keeps track of the values of variables by the stack data structure. – Recall that stack is a data structure where the last item added is the first item processed – There are two operations (push and pop) associated with stack a b c pop Department of Computer Engineering b c push d 61 d b c Sharif University of Technology 10 -61
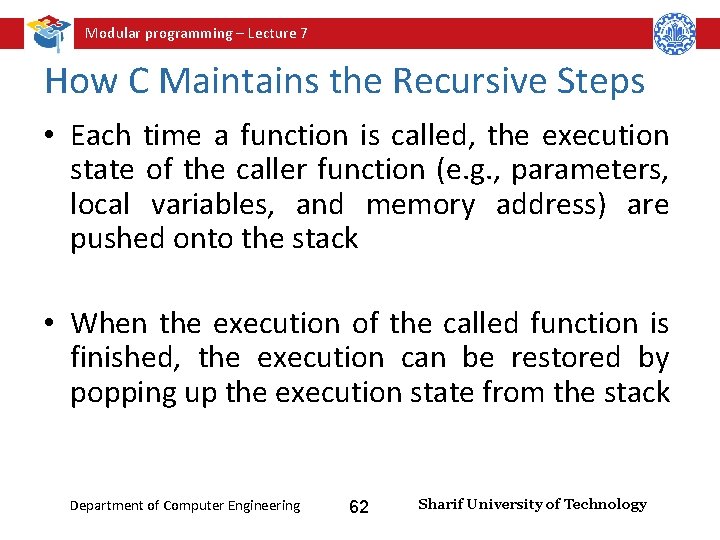
Modular programming – Lecture 7 How C Maintains the Recursive Steps • Each time a function is called, the execution state of the caller function (e. g. , parameters, local variables, and memory address) are pushed onto the stack • When the execution of the called function is finished, the execution can be restored by popping up the execution state from the stack Department of Computer Engineering 62 Sharif University of Technology
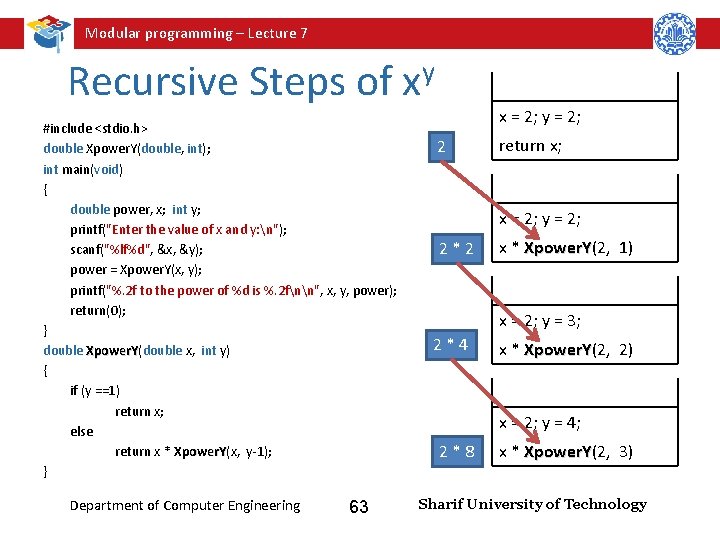
Modular programming – Lecture 7 Recursive Steps of xy #include <stdio. h> double Xpower. Y(double, int); int main(void) { double power, x; int y; printf("Enter the value of x and y: n"); scanf("%lf%d", &x, &y); power = Xpower. Y(x, y); printf("%. 2 f to the power of %d is %. 2 fnn", x, y, power); return(0); } double Xpower. Y(double x, int y) Xpower. Y { if (y ==1) return x; else return x * Xpower. Y(x, Xpower. Y y-1); } Department of Computer Engineering 63 x = 2; y = 2; 2 return x; x = 2; y = 2; 2*2 x * Xpower. Y(2, 1) x = 2; y = 3; 2*4 x * Xpower. Y(2, 2) x = 2; y = 4; 2*8 x * Xpower. Y(2, 3) Sharif University of Technology
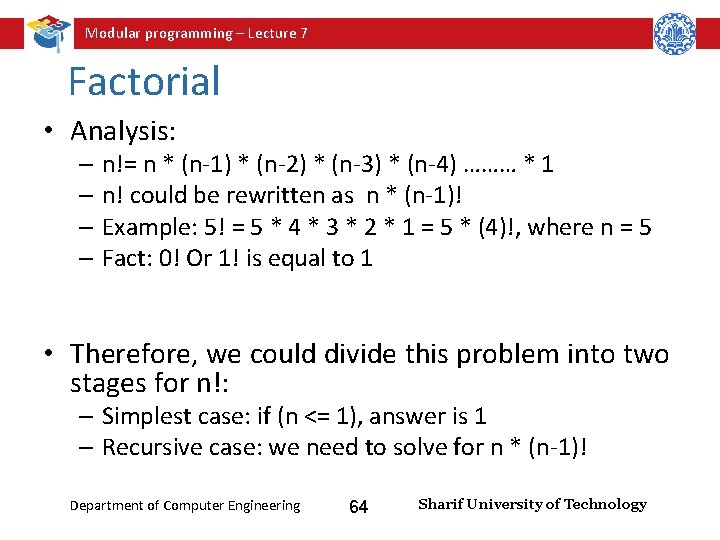
Modular programming – Lecture 7 Factorial • Analysis: – n!= n * (n-1) * (n-2) * (n-3) * (n-4) ……… * 1 – n! could be rewritten as n * (n-1)! – Example: 5! = 5 * 4 * 3 * 2 * 1 = 5 * (4)!, where n = 5 – Fact: 0! Or 1! is equal to 1 • Therefore, we could divide this problem into two stages for n!: – Simplest case: if (n <= 1), answer is 1 – Recursive case: we need to solve for n * (n-1)! Department of Computer Engineering 64 Sharif University of Technology
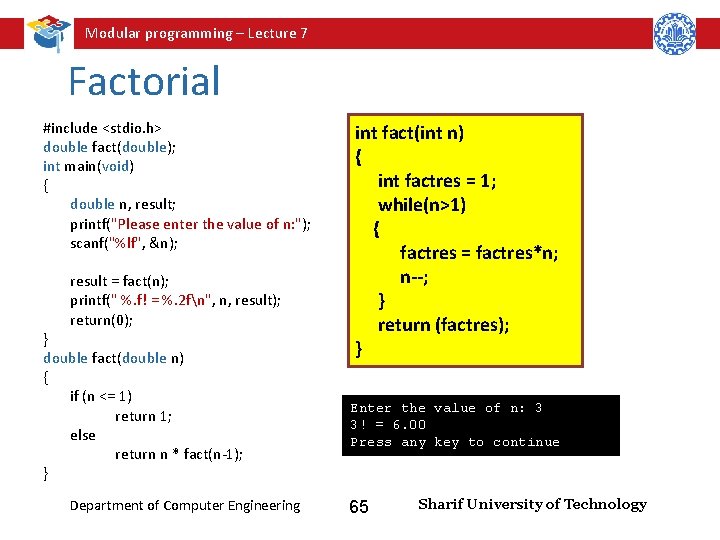
Modular programming – Lecture 7 Factorial #include <stdio. h> double fact(double); int main(void) { double n, result; printf("Please enter the value of n: "); scanf("%lf", &n); result = fact(n); printf(" %. f! = %. 2 fn", n, result); return(0); } double fact(double n) { if (n <= 1) return 1; else return n * fact(n-1); } Department of Computer Engineering int fact(int n) { int factres = 1; while(n>1) { factres = factres*n; n--; } return (factres); } Enter the value of n: 3 3! = 6. 00 Press any key to continue 65 Sharif University of Technology
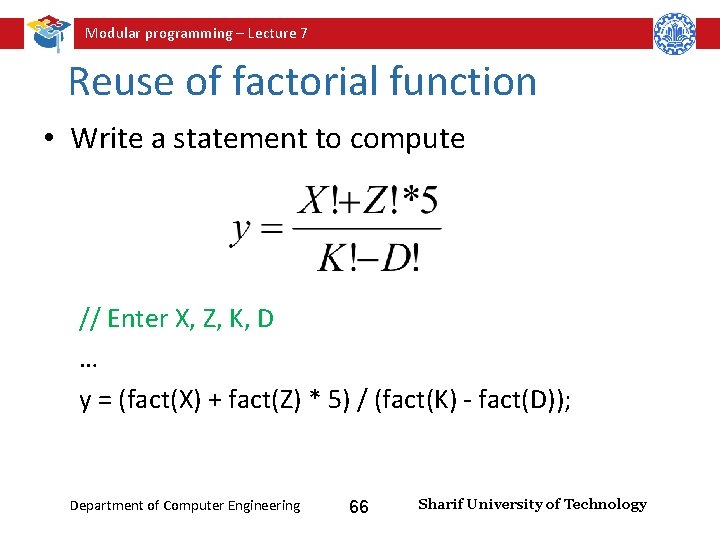
Modular programming – Lecture 7 Reuse of factorial function • Write a statement to compute // Enter X, Z, K, D … y = (fact(X) + fact(Z) * 5) / (fact(K) - fact(D)); Department of Computer Engineering 66 Sharif University of Technology
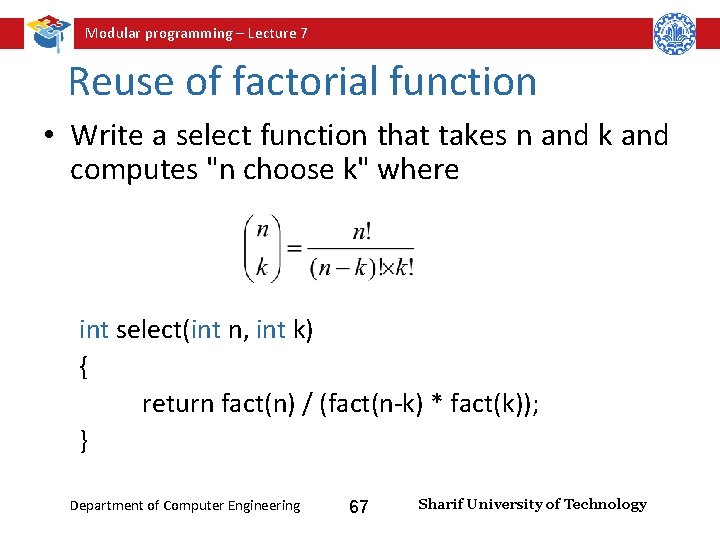
Modular programming – Lecture 7 Reuse of factorial function • Write a select function that takes n and k and computes "n choose k" where int select(int n, int k) { return fact(n) / (fact(n-k) * fact(k)); } Department of Computer Engineering 67 Sharif University of Technology
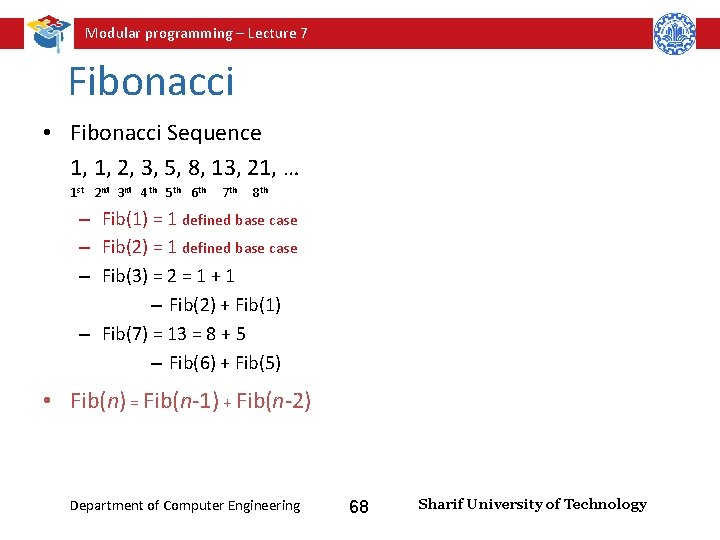
Modular programming – Lecture 7 Fibonacci • Fibonacci Sequence 1, 1, 2, 3, 5, 8, 13, 21, … 1 st 2 nd 3 rd 4 th 5 th 6 th 7 th 8 th – Fib(1) = 1 defined base case – Fib(2) = 1 defined base case – Fib(3) = 2 = 1 + 1 – Fib(2) + Fib(1) – Fib(7) = 13 = 8 + 5 – Fib(6) + Fib(5) • Fib(n) = Fib(n-1) + Fib(n-2) Department of Computer Engineering 68 Sharif University of Technology
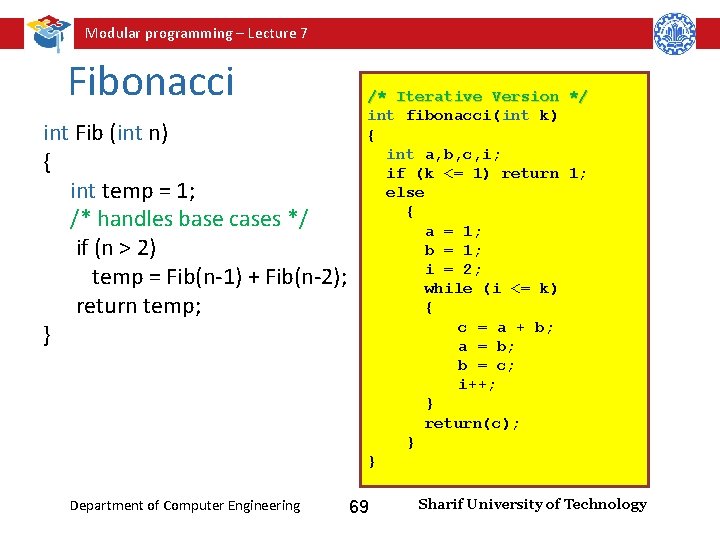
Modular programming – Lecture 7 Fibonacci int Fib (int n) { int temp = 1; /* handles base cases */ if (n > 2) temp = Fib(n-1) + Fib(n-2); return temp; } Department of Computer Engineering /* Iterative Version */ int fibonacci(int k) { int a, b, c, i; if (k <= 1) return 1; else { a = 1; b = 1; i = 2; while (i <= k) { c = a + b; a = b; b = c; i++; } return(c); } } 69 Sharif University of Technology
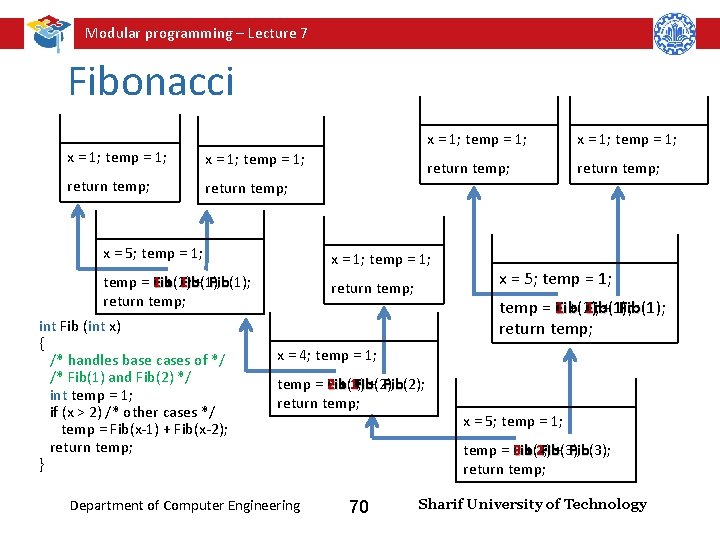
Modular programming – Lecture 7 Fibonacci x = 1; temp = 1; return temp; x = 5; temp = 1; x = 1; temp = 1; temp = 1 Fib(2) + 1 Fib(1); ; + Fib(1); Fib Fib return temp; int Fib (int x) { /* handles base cases of */ /* Fib(1) and Fib(2) */ int temp = 1; if (x > 2) /* other cases */ temp = Fib(x-1) + Fib(x-2); return temp; } x = 5; temp = 1 Fib(2) + 1 Fib(1); ; + Fib(1); Fib Fib return temp; x = 4; temp = 1; temp = 2 Fib(3) + 1 Fib(2); ; + Fib(2); Fib Fib return temp; Department of Computer Engineering x = 5; temp = 1; temp = 3 Fib(4) + 2 Fib(3); ; + Fib(3); Fib Fib return temp; 70 Sharif University of Technology
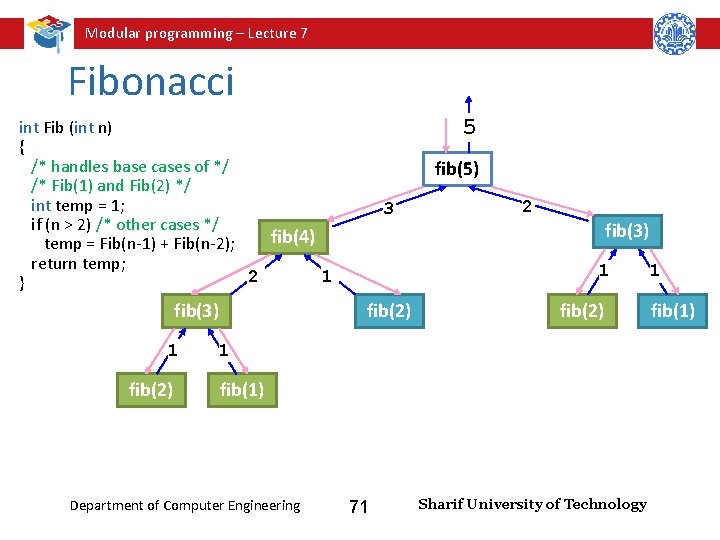
Modular programming – Lecture 7 Fibonacci 5 int Fib (int n) { /* handles base cases of */ /* Fib(1) and Fib(2) */ int temp = 1; if (n > 2) /* other cases */ fib(4) temp = Fib(n-1) + Fib(n-2); return temp; 2 1 } fib(3) 1 fib(2) fib(5) 3 2 fib(3) 1 fib(2) 1 fib(1) Department of Computer Engineering 71 Sharif University of Technology 1 fib(1)
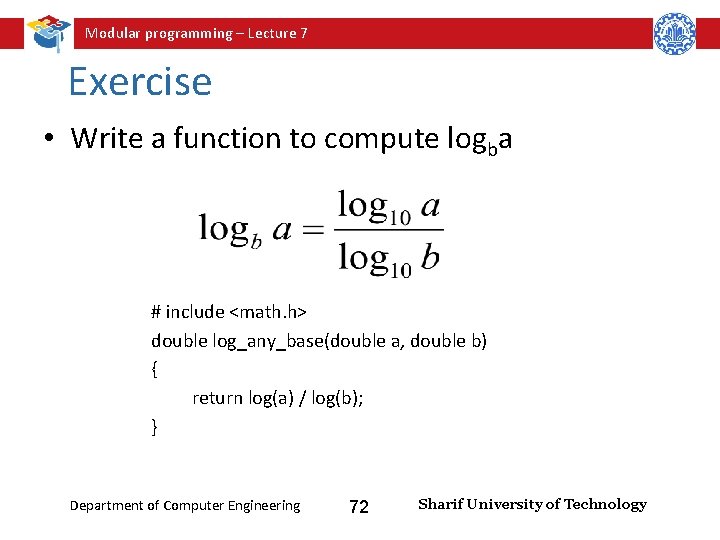
Modular programming – Lecture 7 Exercise • Write a function to compute logba # include <math. h> double log_any_base(double a, double b) { return log(a) / log(b); } Department of Computer Engineering 72 Sharif University of Technology
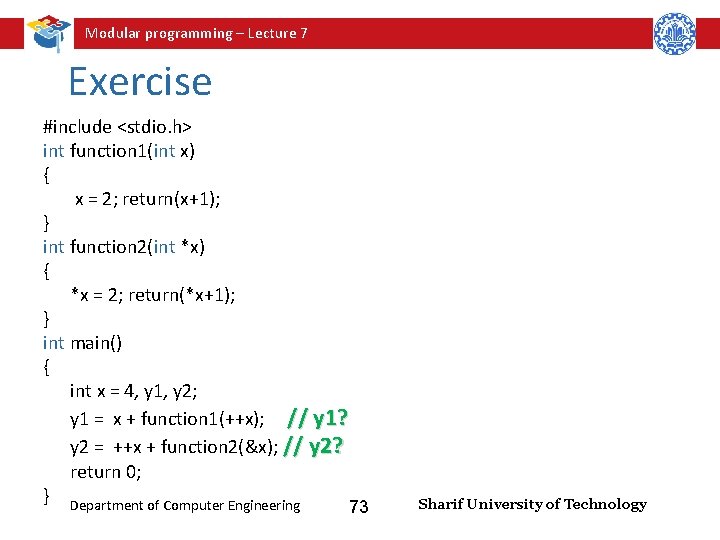
Modular programming – Lecture 7 Exercise #include <stdio. h> int function 1(int x) { x = 2; return(x+1); } int function 2(int *x) { *x = 2; return(*x+1); } int main() { int x = 4, y 1, y 2; y 1 = x + function 1(++x); // y 1? y 2 = ++x + function 2(&x); // y 2? return 0; } Department of Computer Engineering 73 Sharif University of Technology
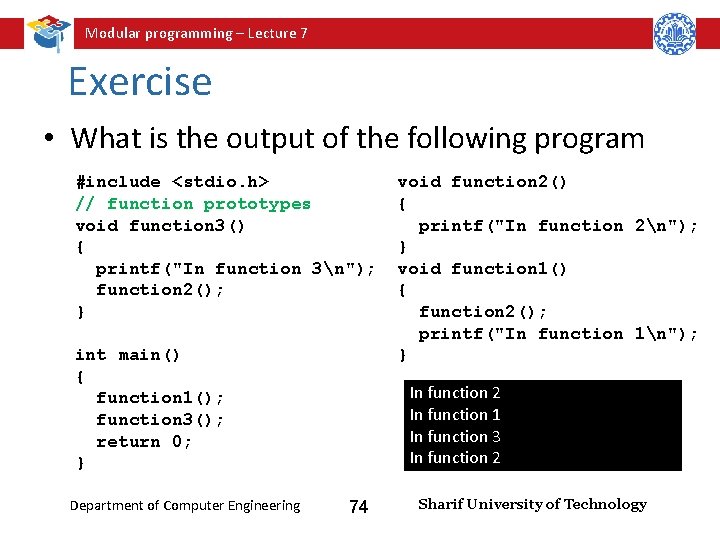
Modular programming – Lecture 7 Exercise • What is the output of the following program #include <stdio. h> // function prototypes void function 3() { printf("In function 3n"); function 2(); } int main() { function 1(); function 3(); return 0; } Department of Computer Engineering void function 2() { printf("In function 2n"); } void function 1() { function 2(); printf("In function 1n"); } In function 2 In function 1 In function 3 In function 2 74 Sharif University of Technology
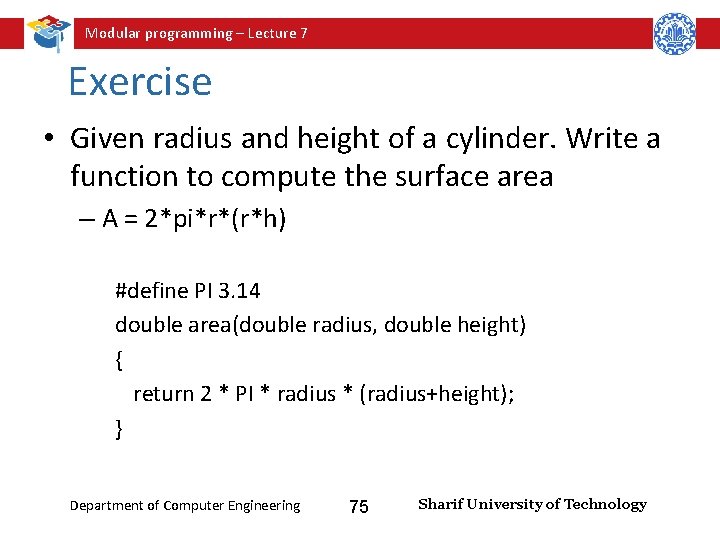
Modular programming – Lecture 7 Exercise • Given radius and height of a cylinder. Write a function to compute the surface area – A = 2*pi*r*(r*h) #define PI 3. 14 double area(double radius, double height) { return 2 * PI * radius * (radius+height); } Department of Computer Engineering 75 Sharif University of Technology
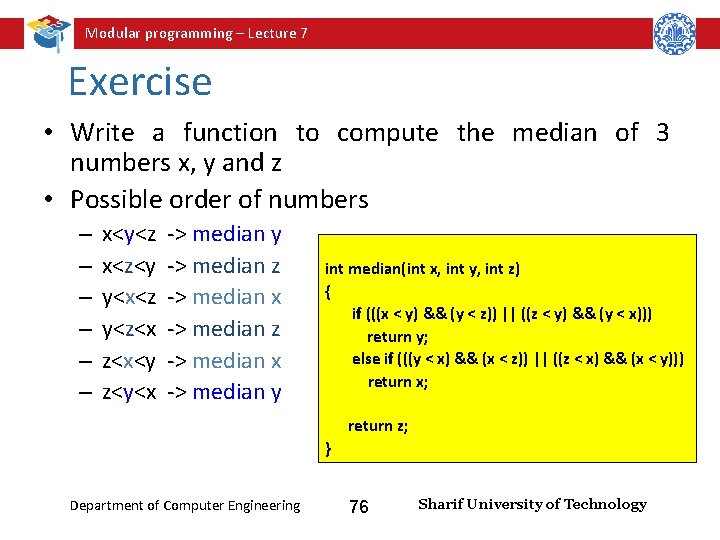
Modular programming – Lecture 7 Exercise • Write a function to compute the median of 3 numbers x, y and z • Possible order of numbers – – – x<y<z x<z<y y<x<z y<z<x z<x<y z<y<x -> median y -> median z -> median x -> median y int median(int x, int y, int z) { if (((x < y) && (y < z)) || ((z < y) && (y < x))) return y; else if (((y < x) && (x < z)) || ((z < x) && (x < y))) return x; return z; } Department of Computer Engineering 76 Sharif University of Technology
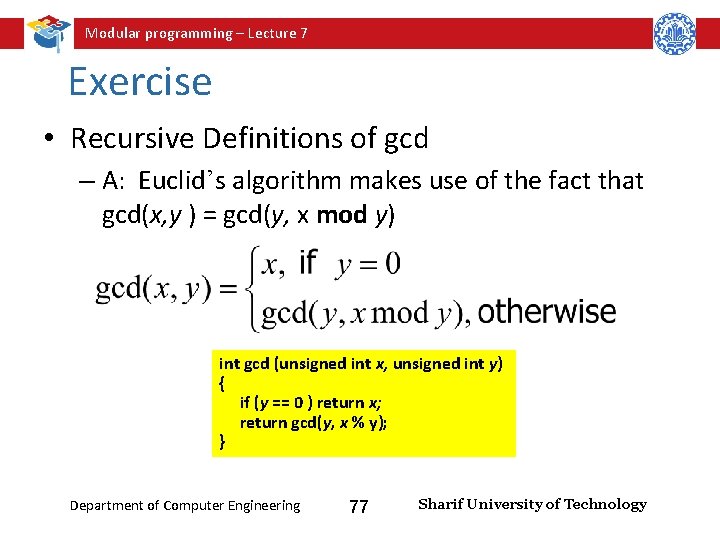
Modular programming – Lecture 7 Exercise • Recursive Definitions of gcd – A: Euclid’s algorithm makes use of the fact that gcd(x, y ) = gcd(y, x mod y) int gcd (unsigned int x, unsigned int y) { if (y == 0 ) return x; return gcd(y, x % y); } Department of Computer Engineering 77 Sharif University of Technology
Omid jafarinezhad
Omid jafarinezhad
Lecturer's name
01:640:244 lecture notes - lecture 15: plat, idah, farad
Lecturer in charge
Lecturer name
Spe distinguished lecturer
Cfa lecturer handbook
Designation lecturer
Pearson lecturer resources
Teacher good morning students
Lecturer asad ali
Designation of lecturer
Lector vs lecturer
Spe distinguished lecturer
Photography lecturer
Why himalayan rivers are pernnial in nature
Physician associate lecturer
Lecturer in charge
Omid aghazadeh
Omid askari
Omid ahangar
Omid asadi artist
Martin ordonez
Omid fatahi valilai
Omid fatahi valilai
Omid mirmotahari
Omid parhizkar
Omid kashefi
Omid kashefi
C data types with examples
Linear vs integer programming
Perbedaan linear programming dan integer programming
Programing adalah
Greedy programming vs dynamic programming
System programming vs application programming
Carbohydrates lecture notes
Lecture about social media
Fiche de lecture corrigé
Les dictions
Ic fabrication lecture notes
Matlab lecture
What is aerodynamics
Strategic management lecture
Persuasive book review example
Punctuated lecture
Research methods notes kenyatta university
In text citation for a lecture
Tarsals lecture
End of lecture
Comprhension
Hugh et leifson
Https developer android com studio index html
Simpler syllables
Magnetically coupled circuits lecture notes
Gsm network overview
Lecture about sport
Lecture outline meaning
A friendly introduction to machine learning
Introduction to machine learning ethem alpaydın
Corporate governance lecture
Franck-condon principle lecture notes
Pride and prejudice lecture
Kay haugaard
According to walter pauk, 10 weeks after lecture
Patella lecture
Bayesian decision theory lecture notes
Ros lecture
Compiler lecture
Stern-gerlach experiment lecture notes
Katus lighting
Data mining lecture notes
Siege lecture
English language lecture
Drawing lecture
Divine principle 3 hour lecture
Practical transformer equivalent circuit
Lecture is derived from