Fundamental Programming Introduction to Functions Fundamental Programming 310201
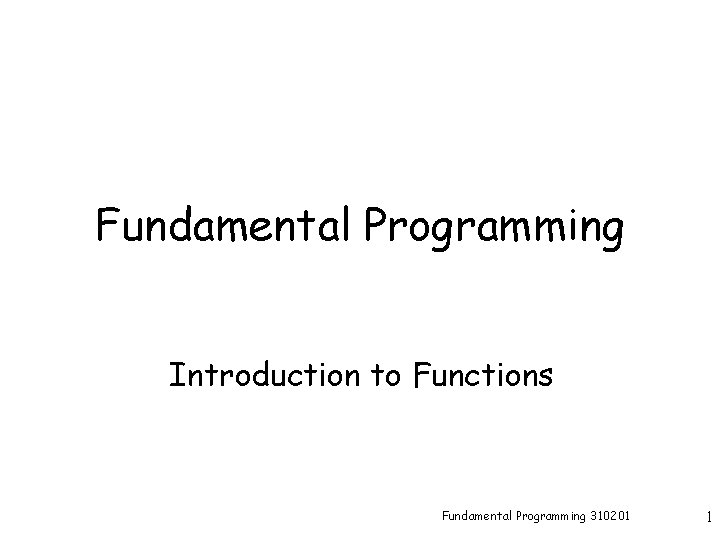
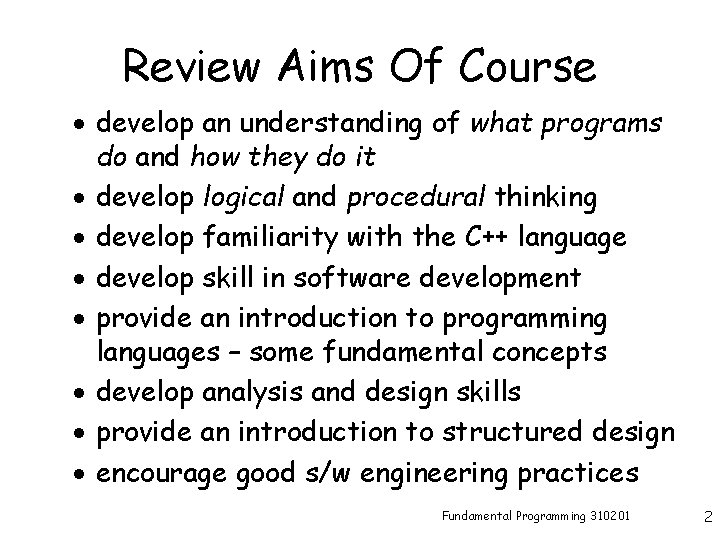
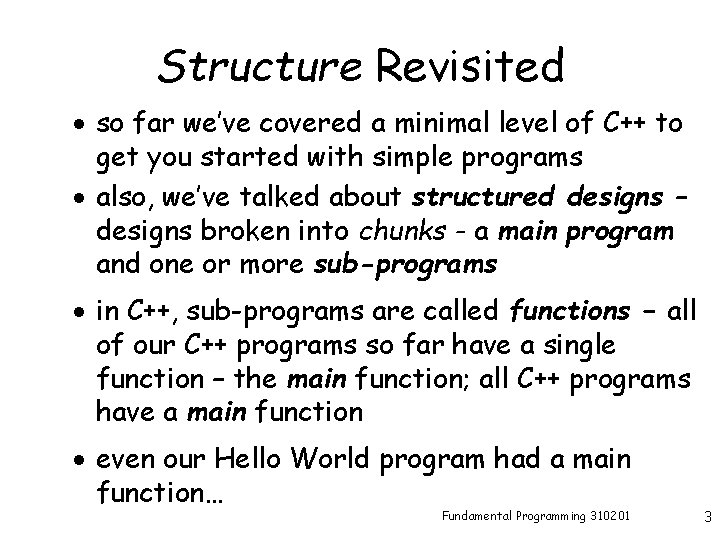
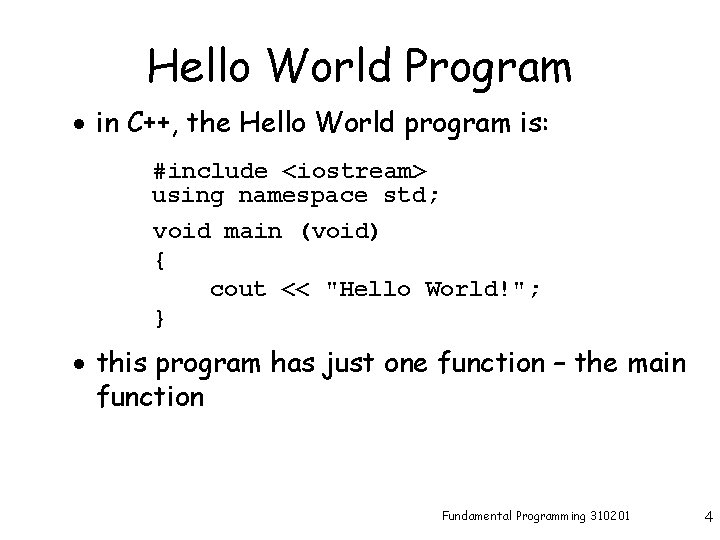
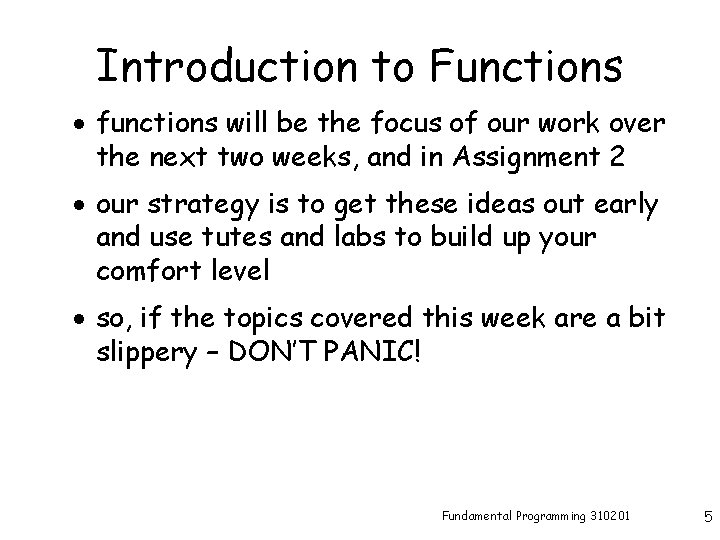
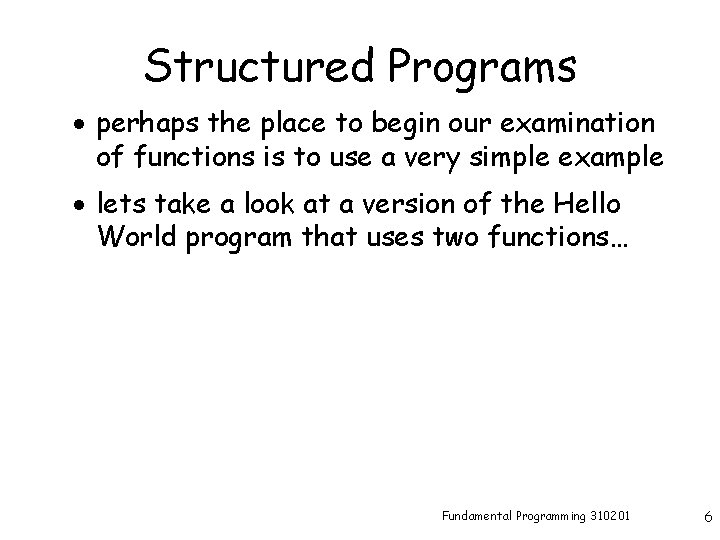
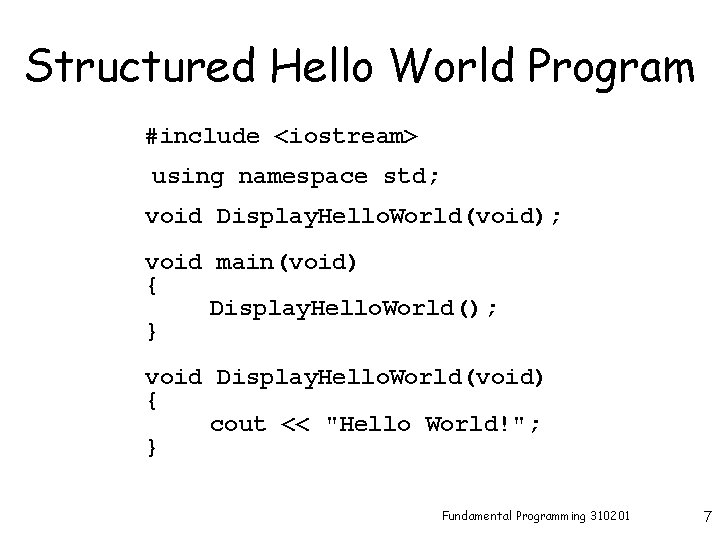
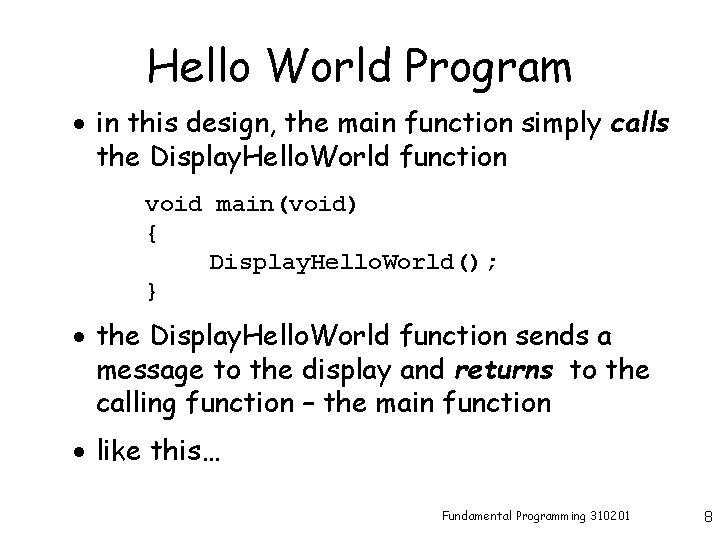
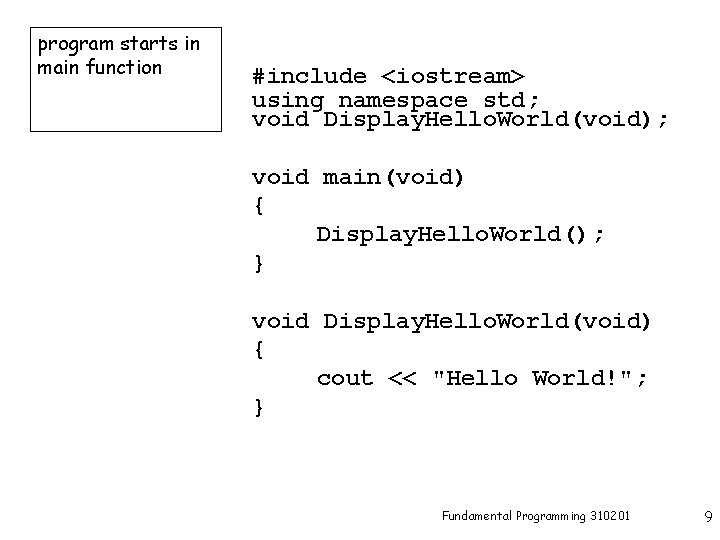
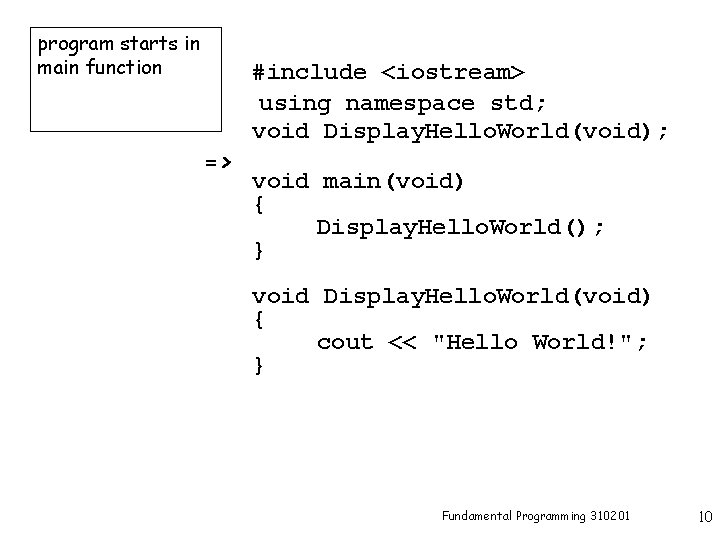
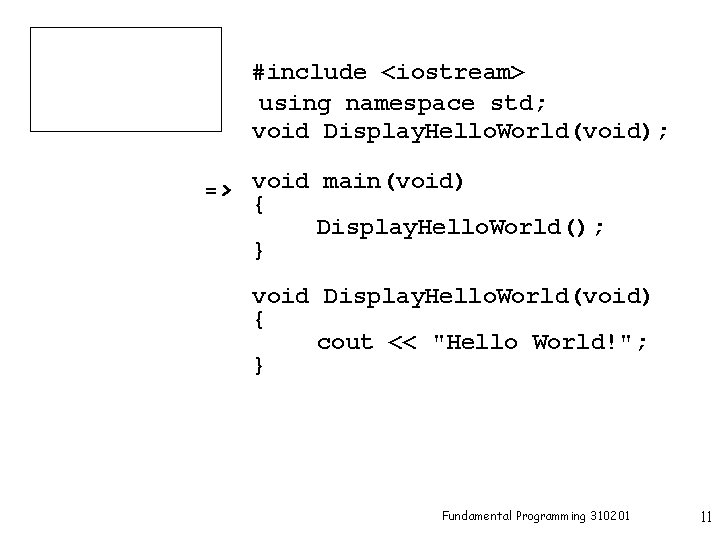
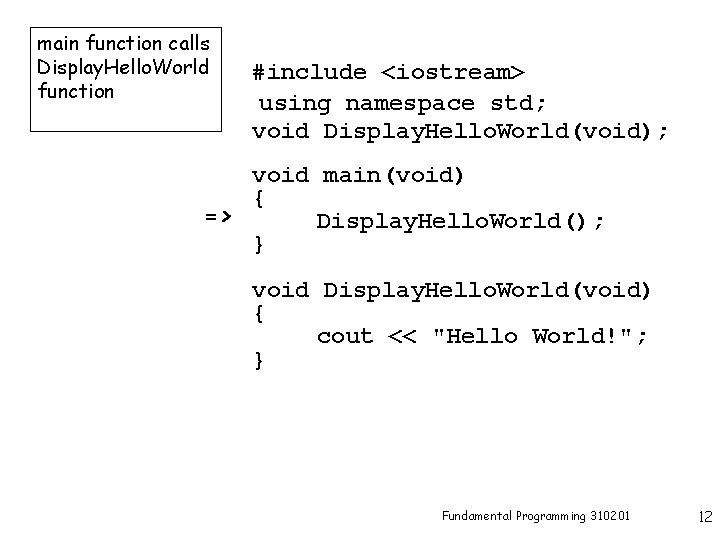
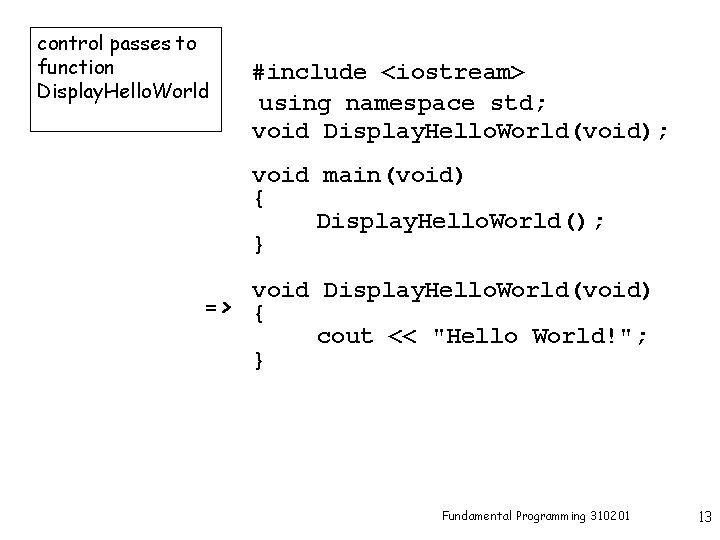
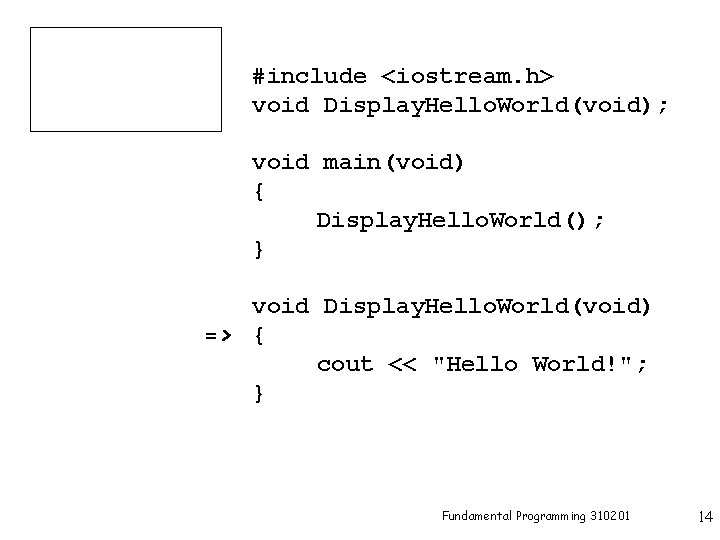
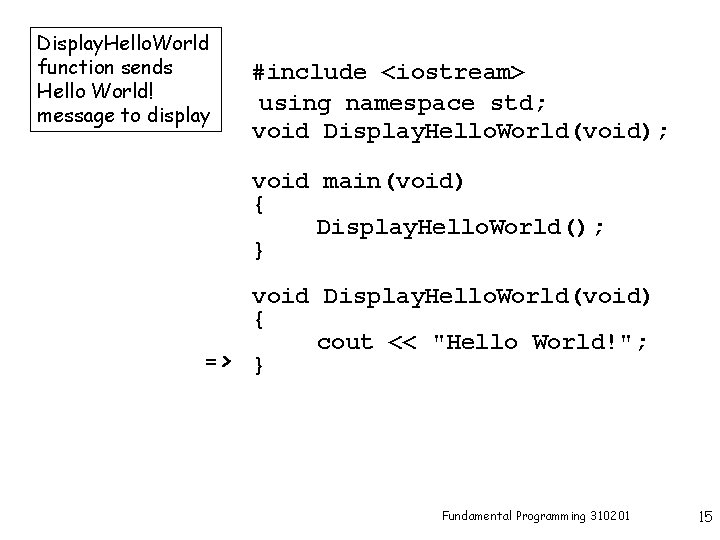
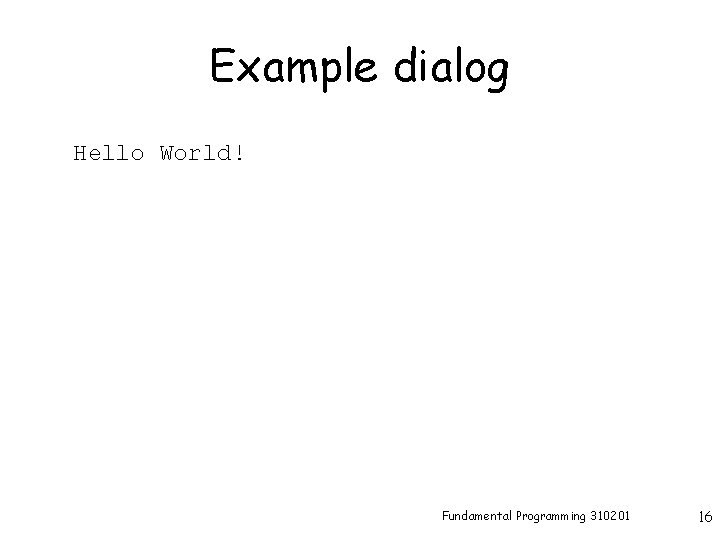
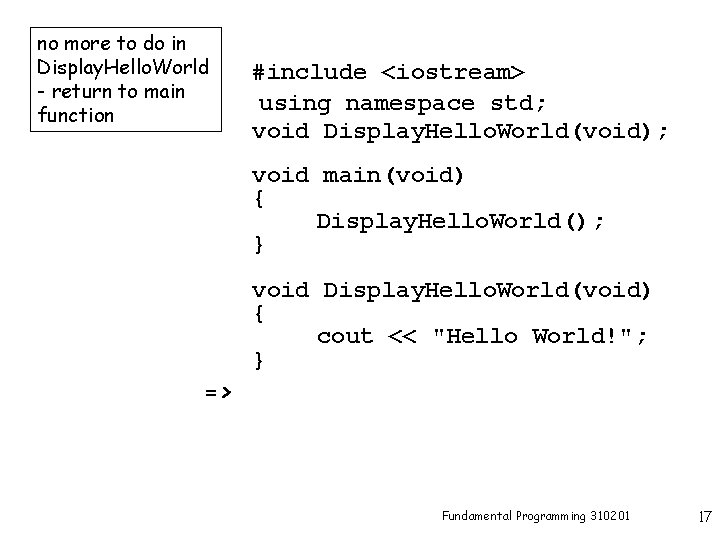
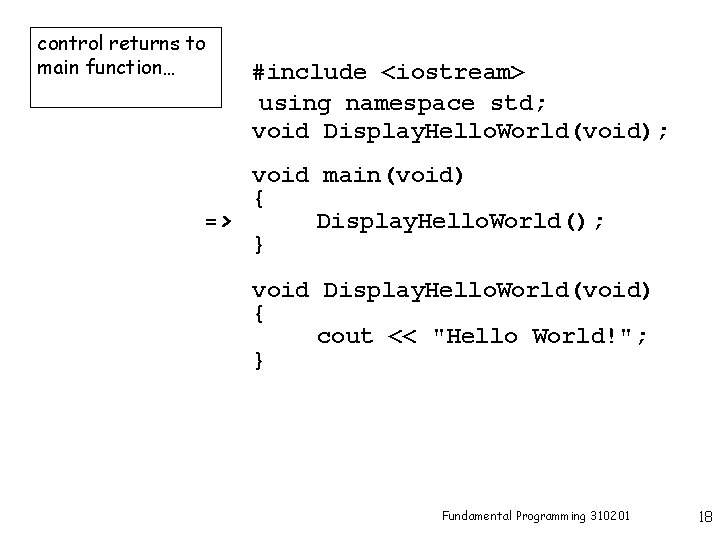
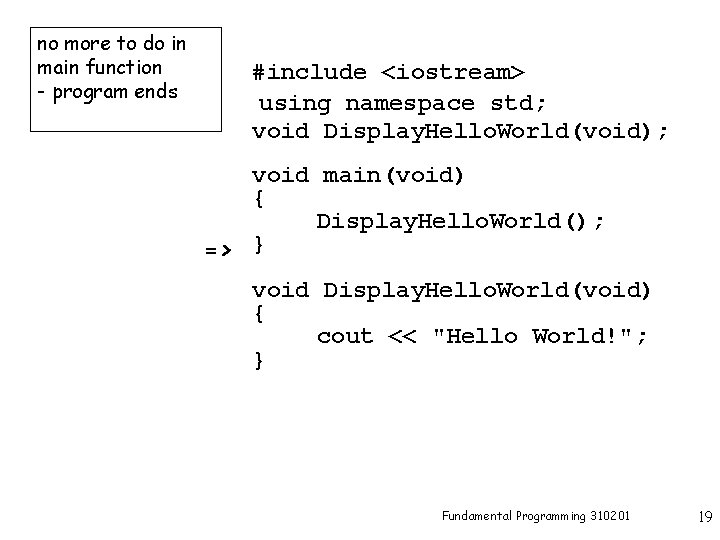
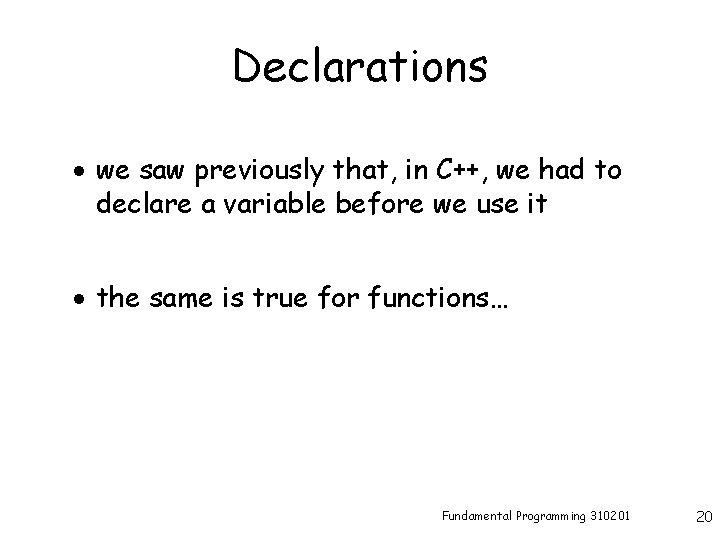
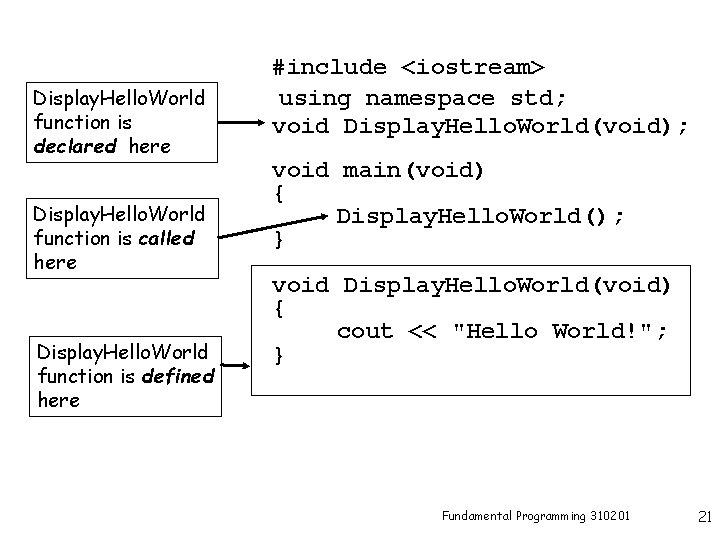
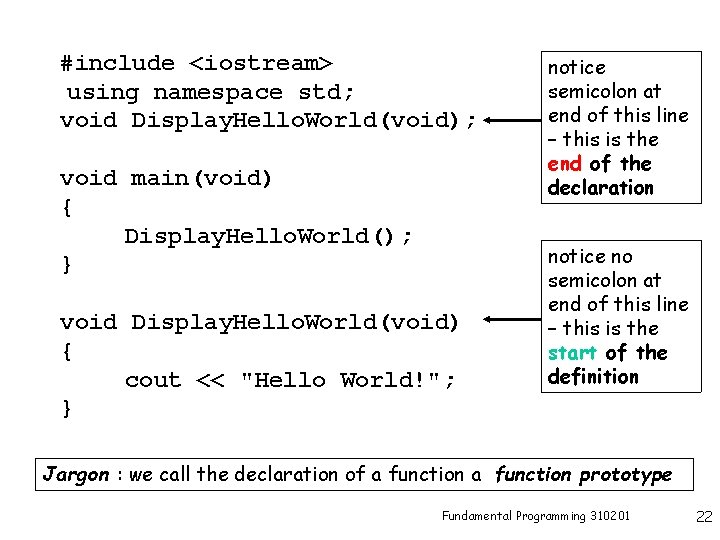
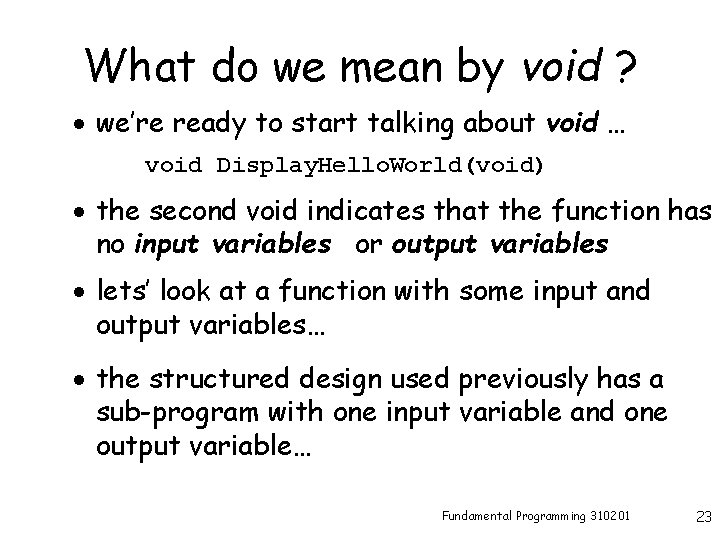
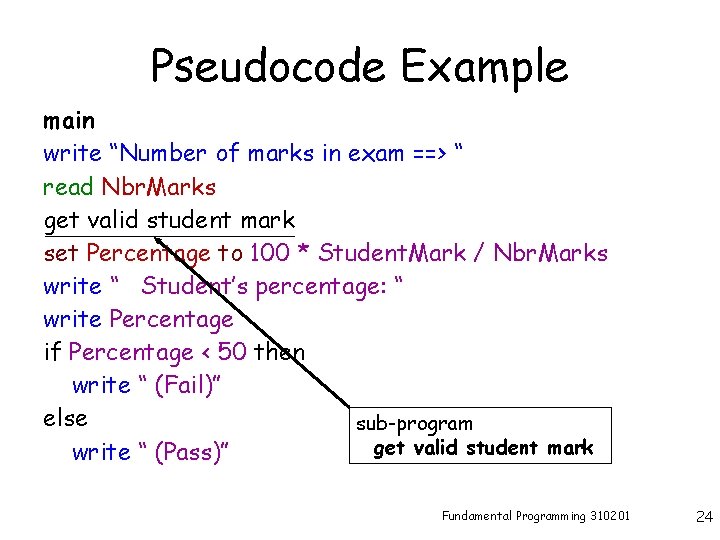
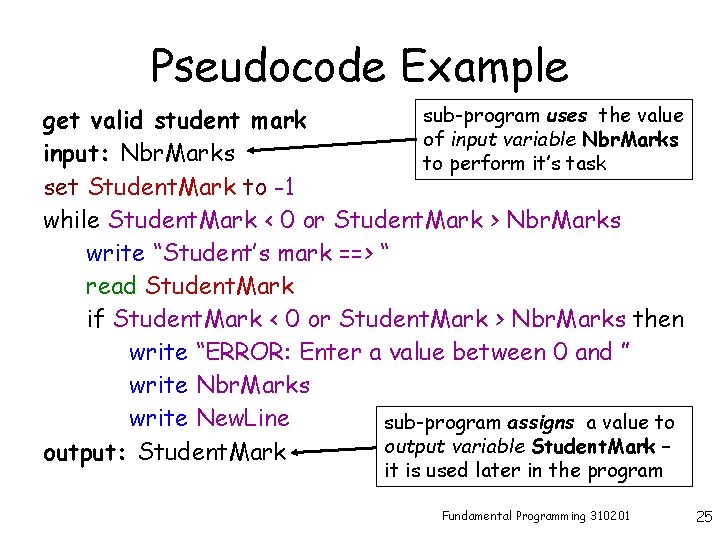
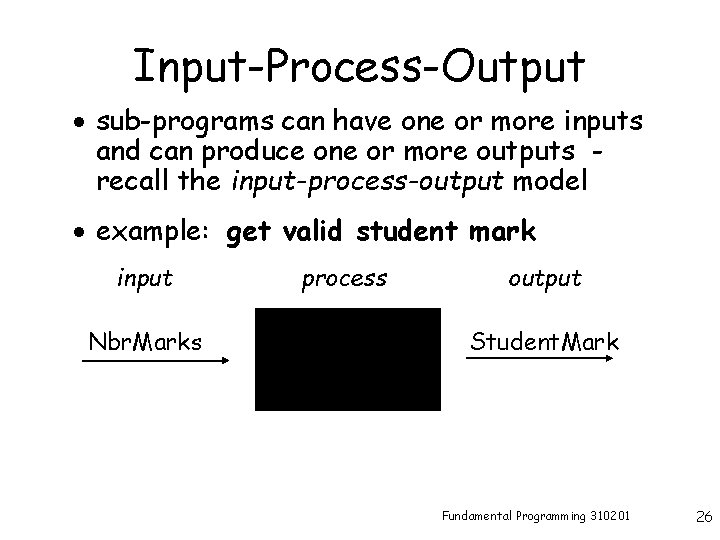
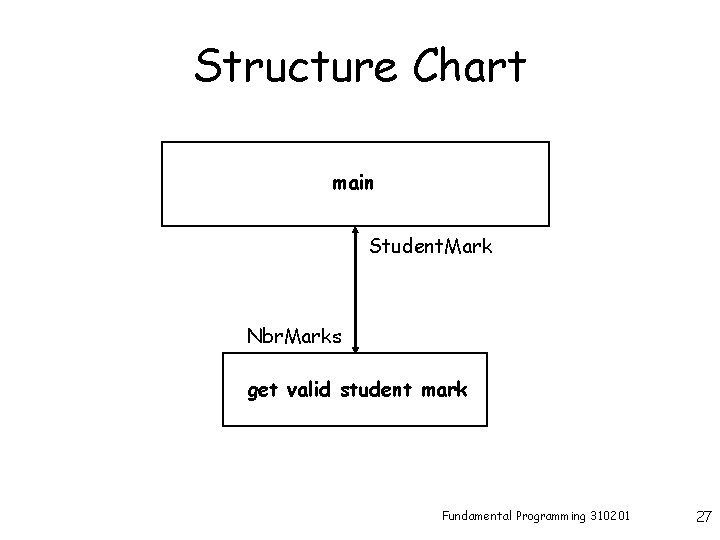
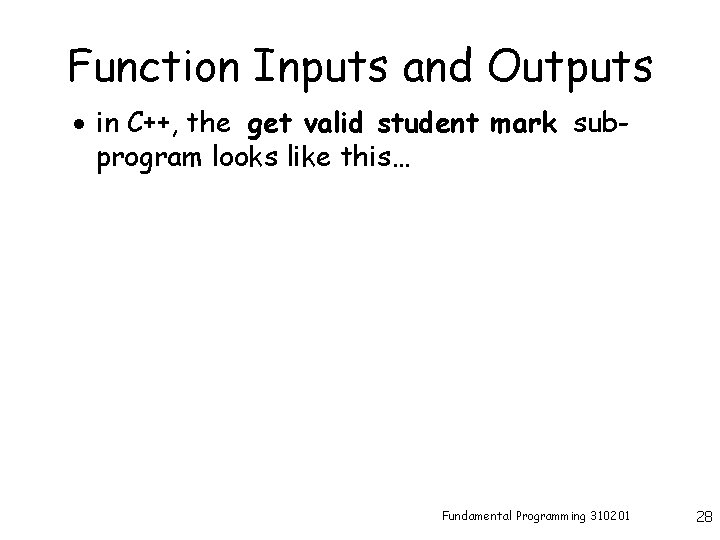
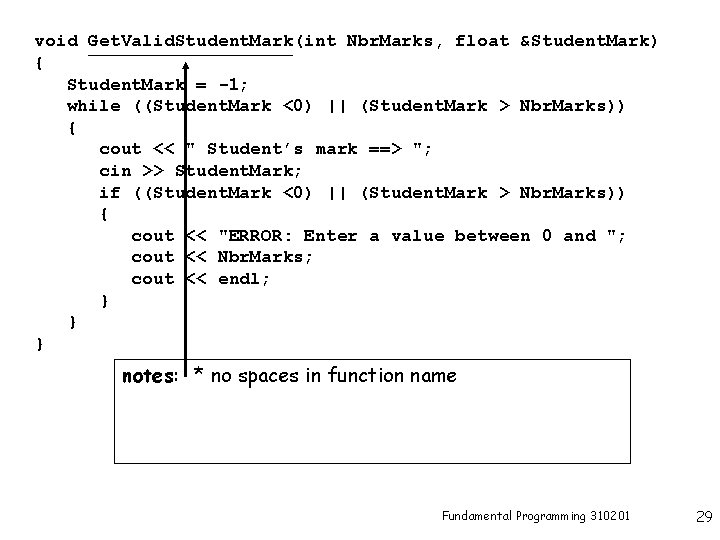
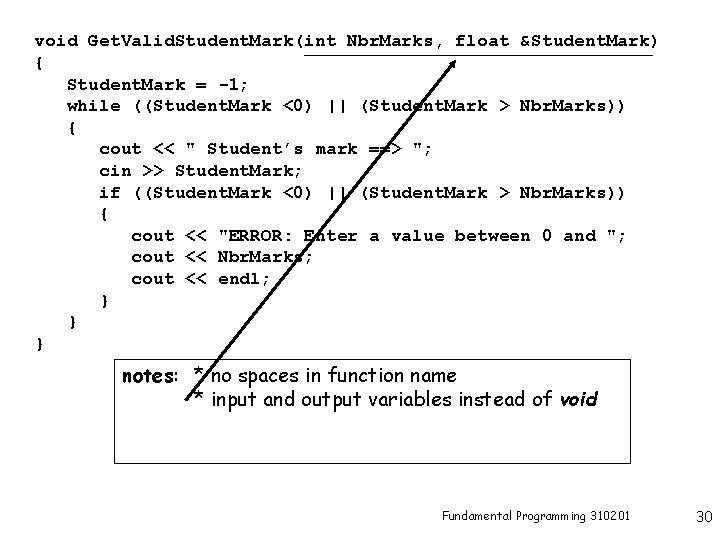
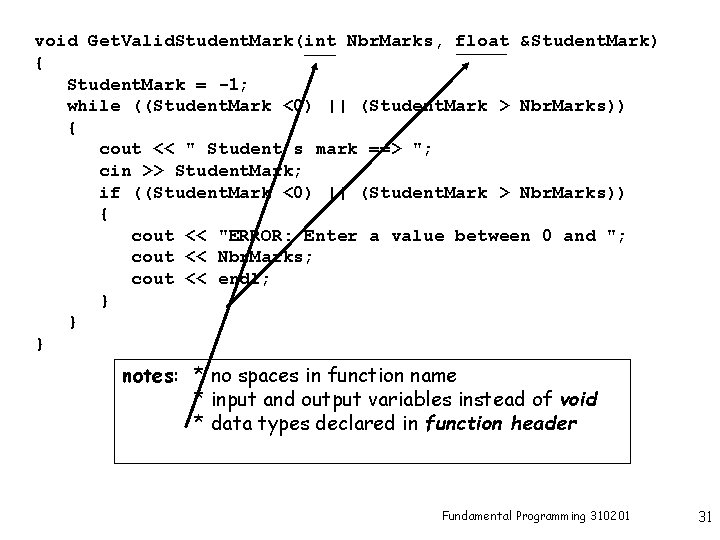
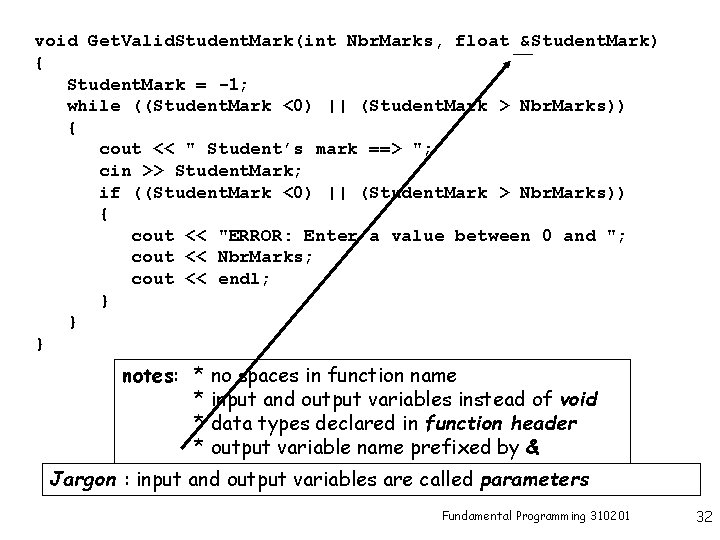
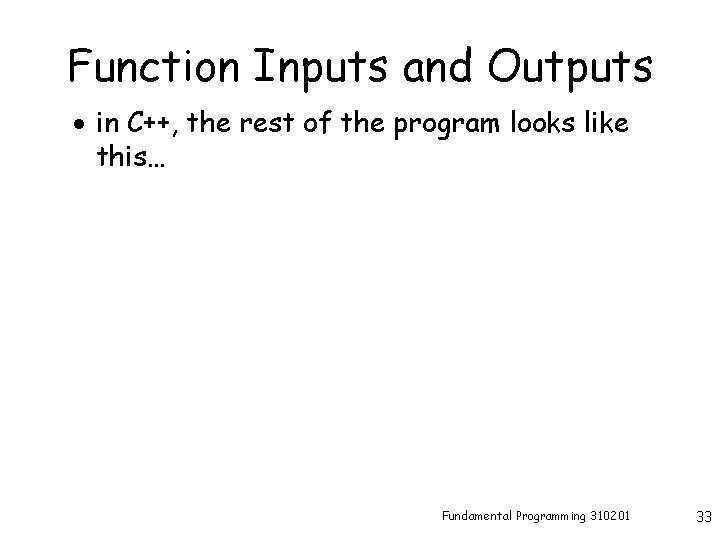
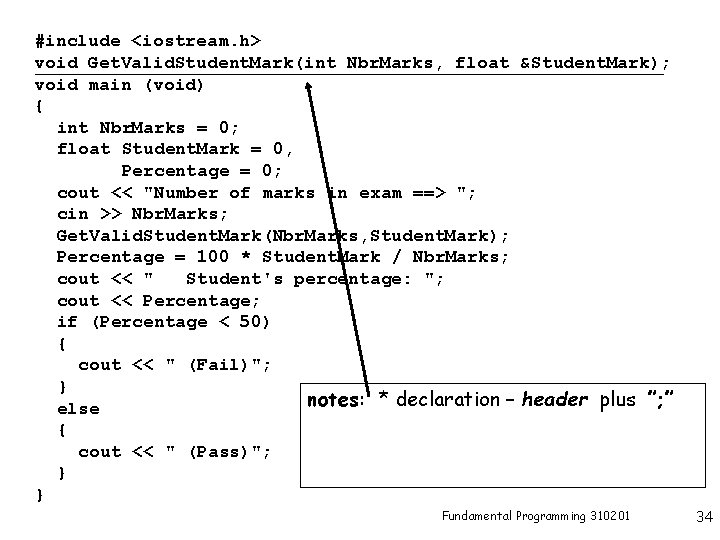
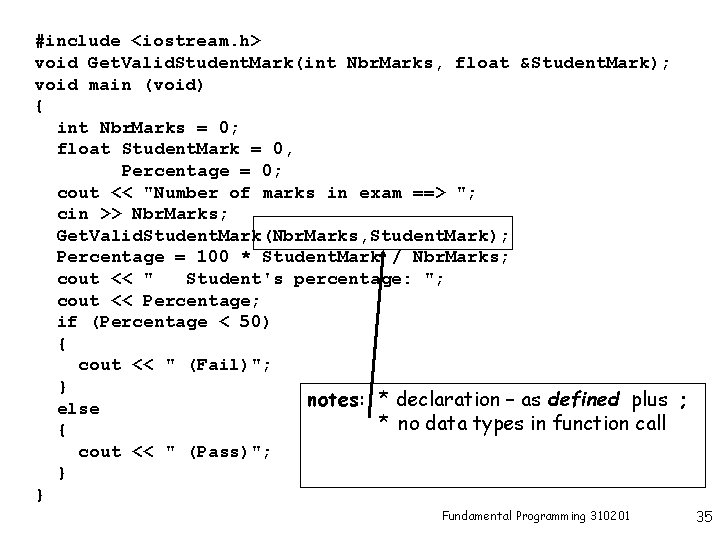
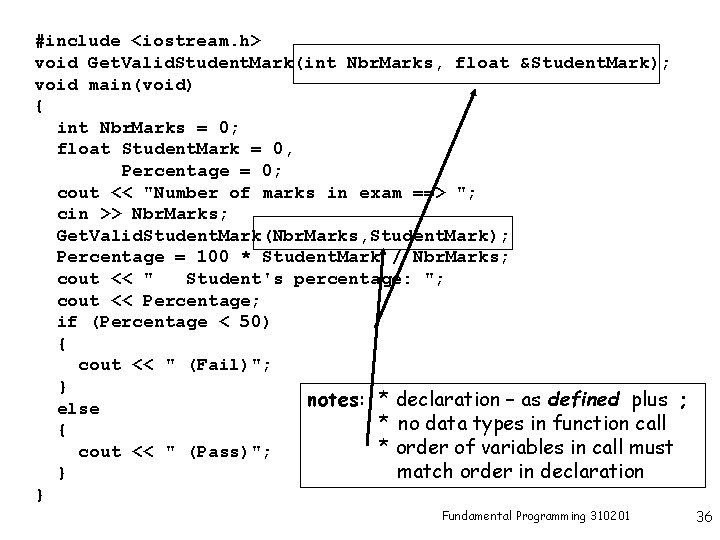
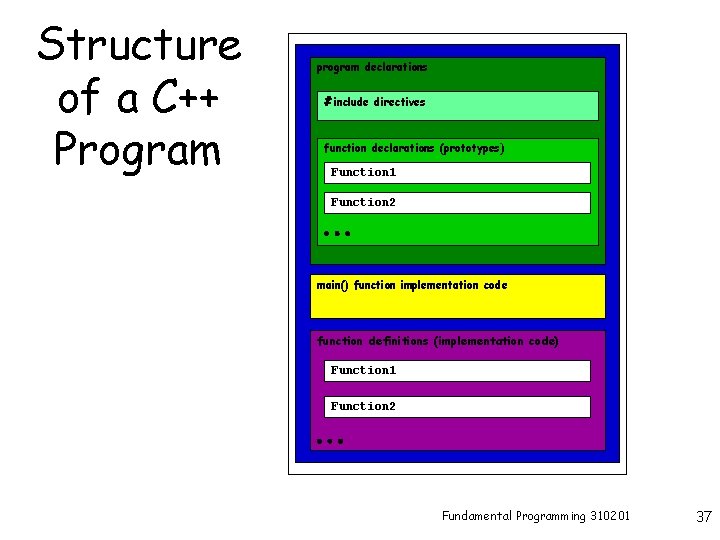
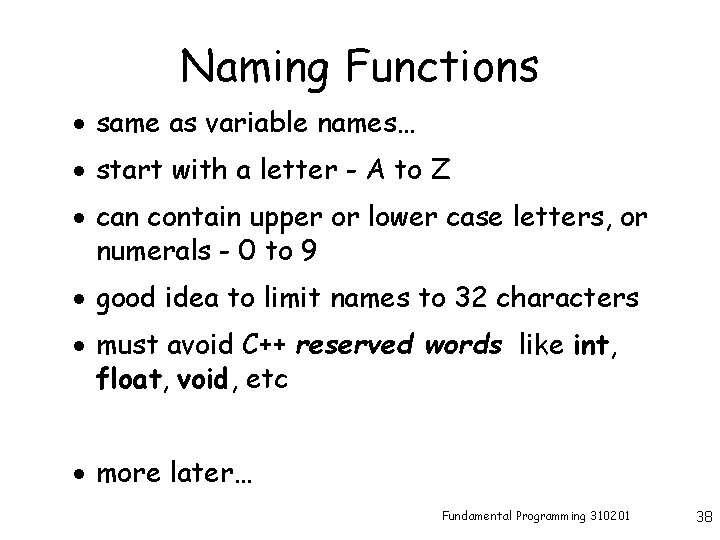
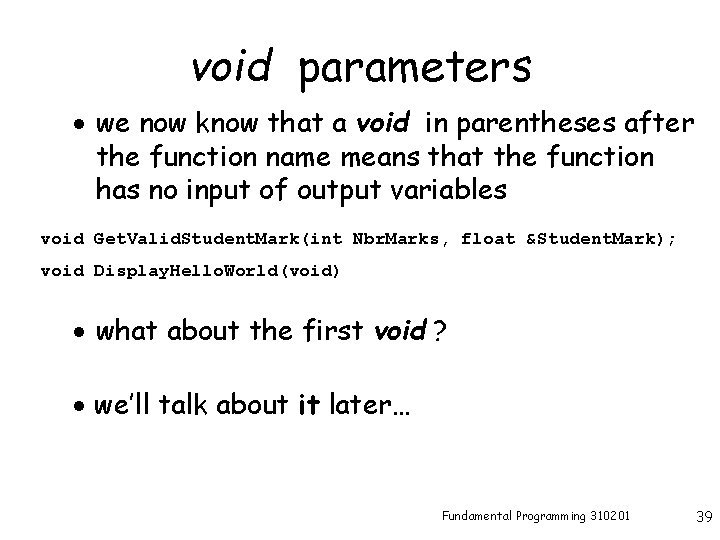
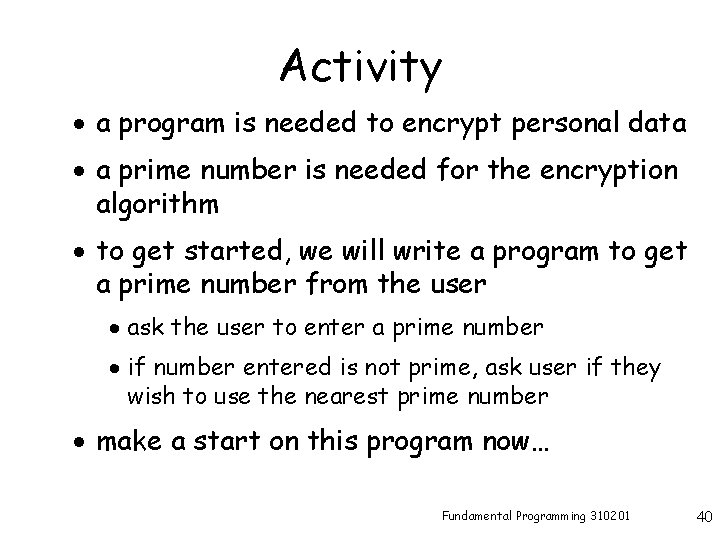
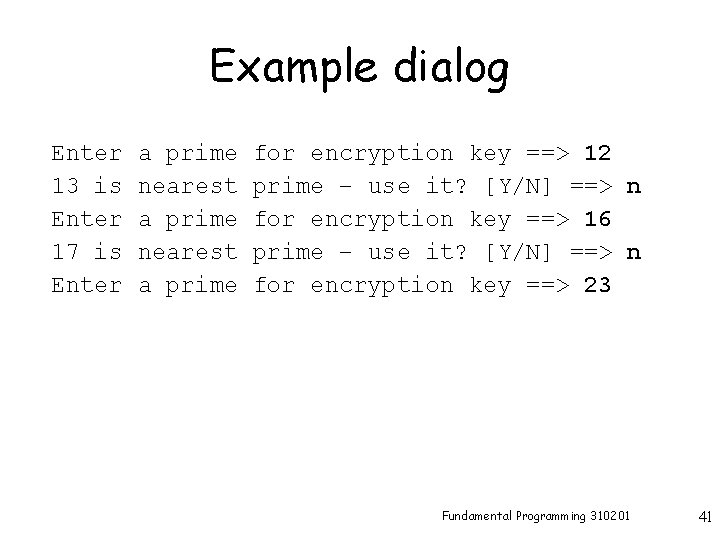
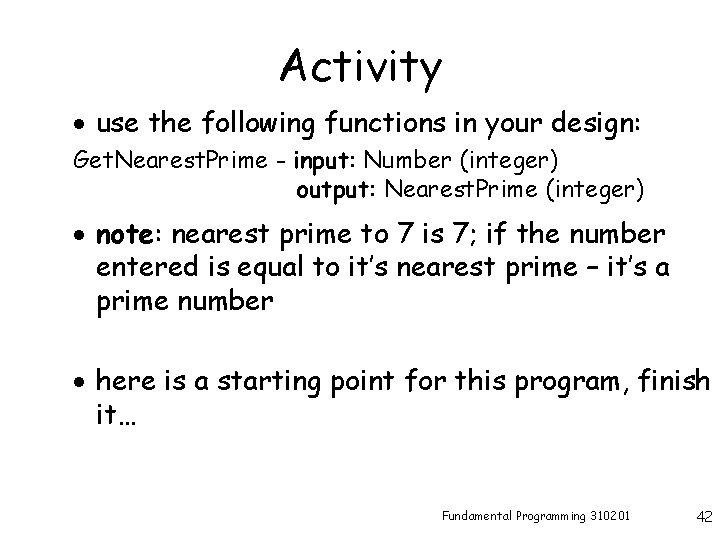
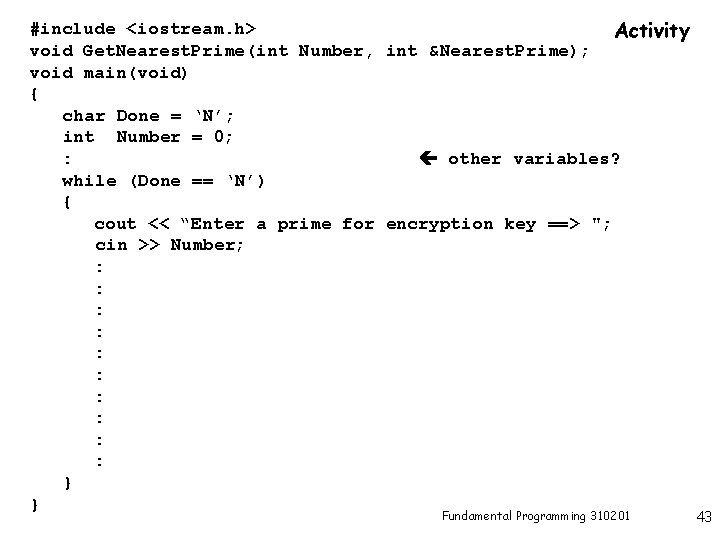
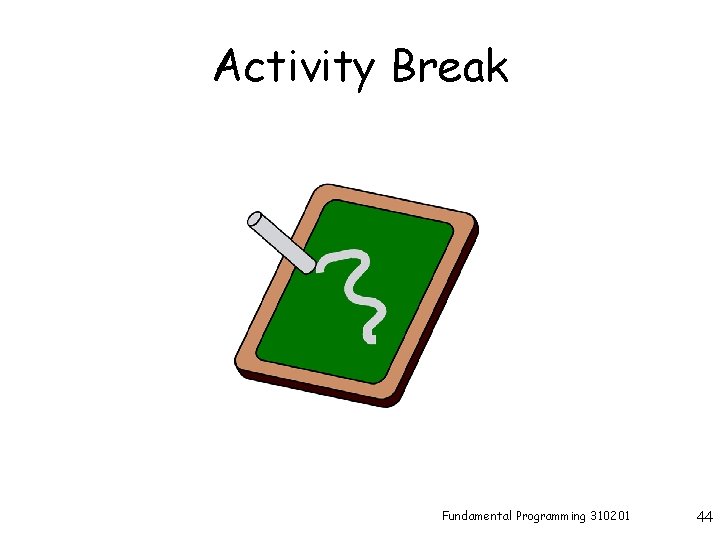
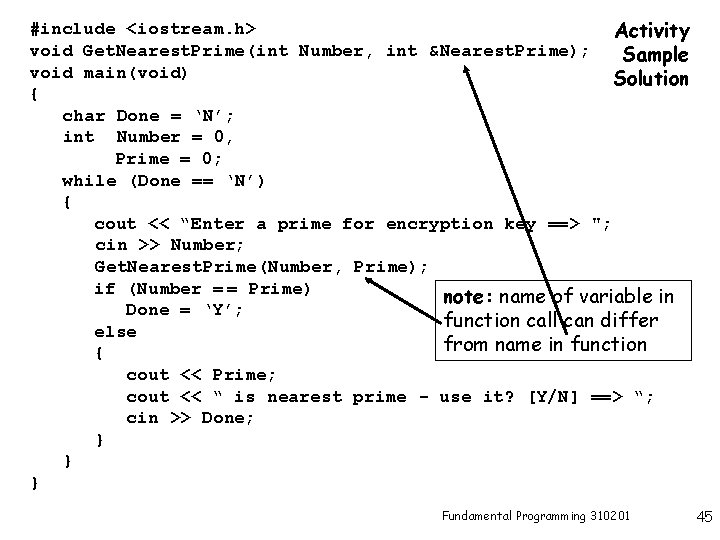
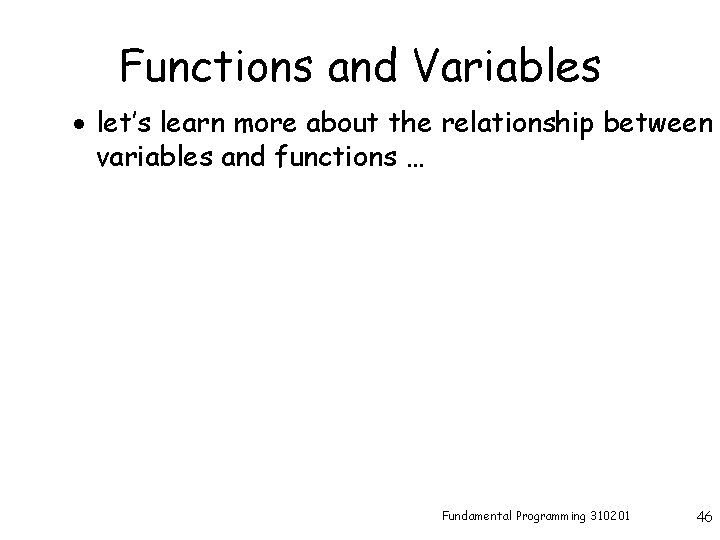
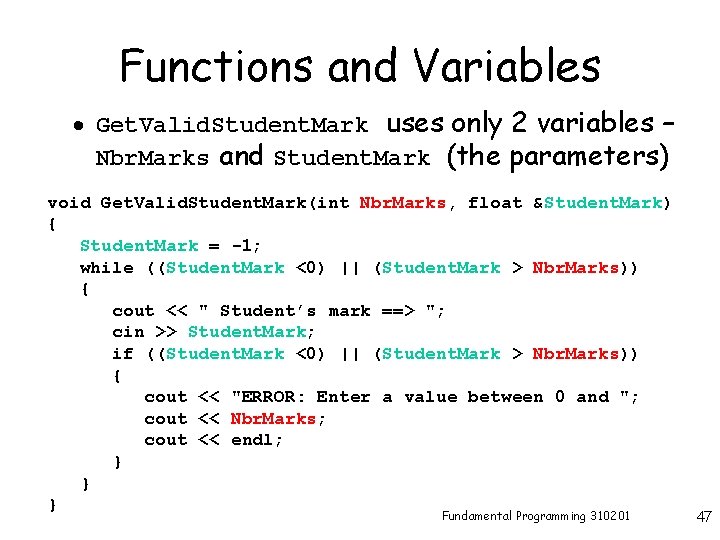
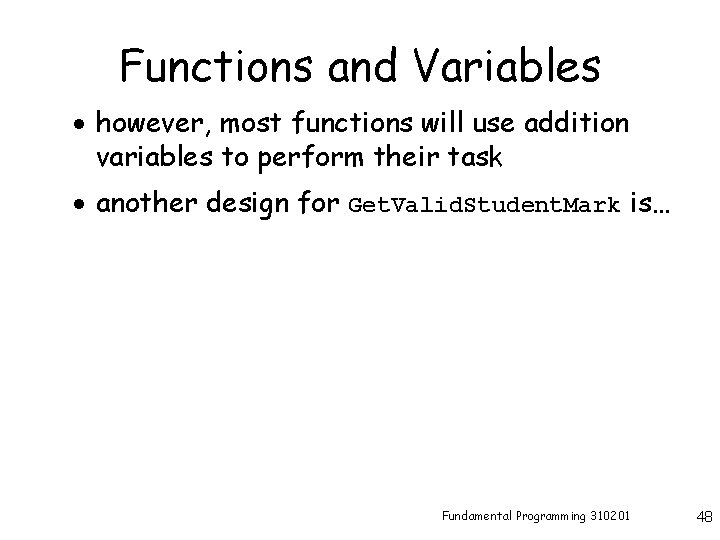
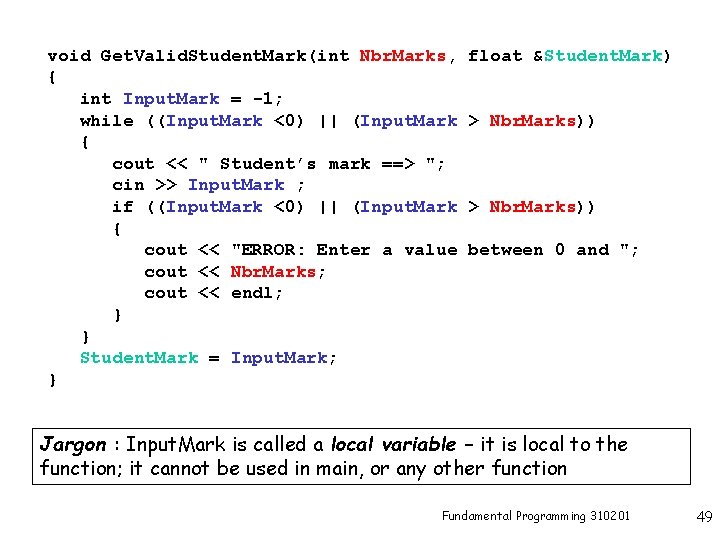
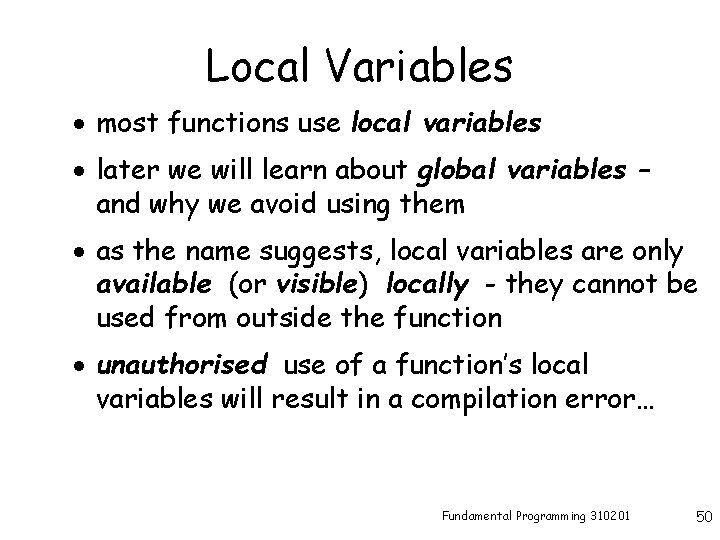
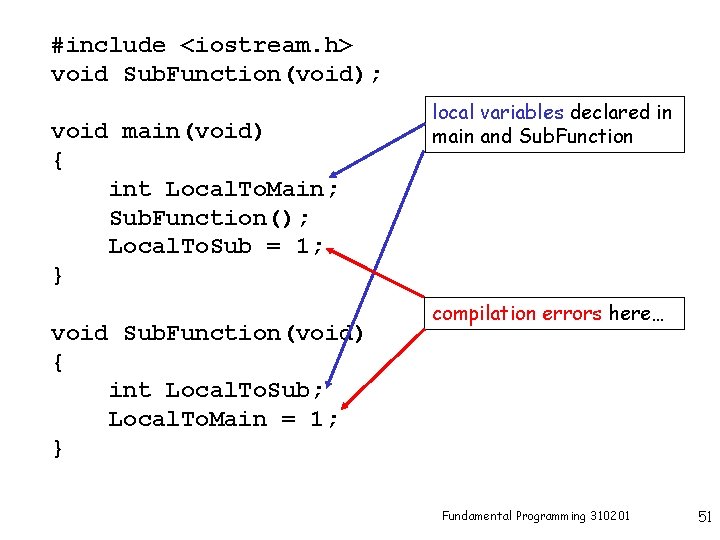
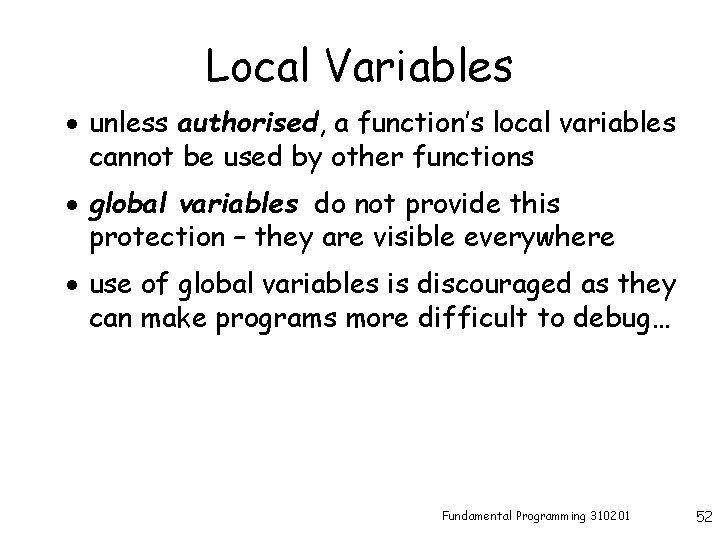
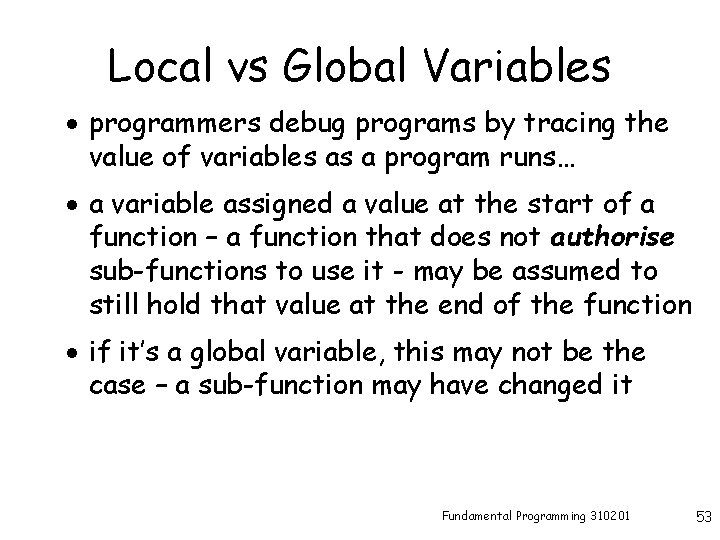
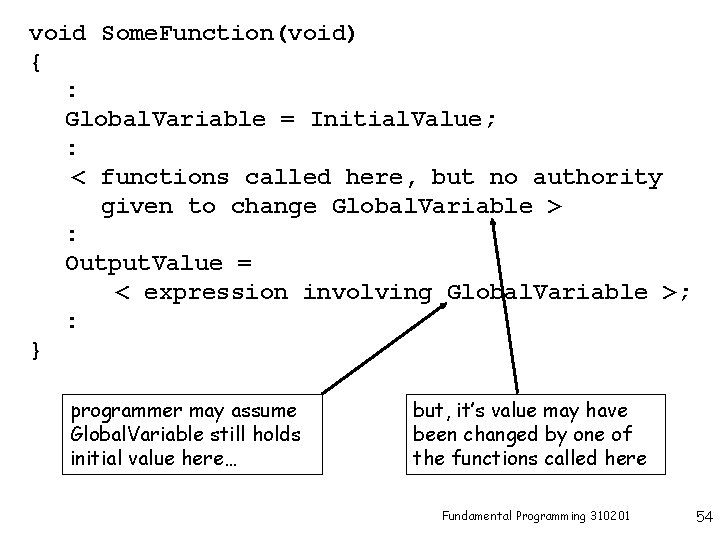
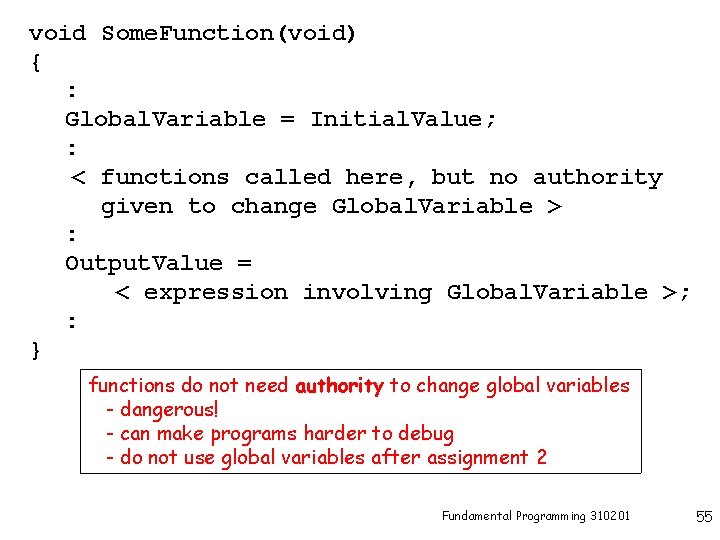
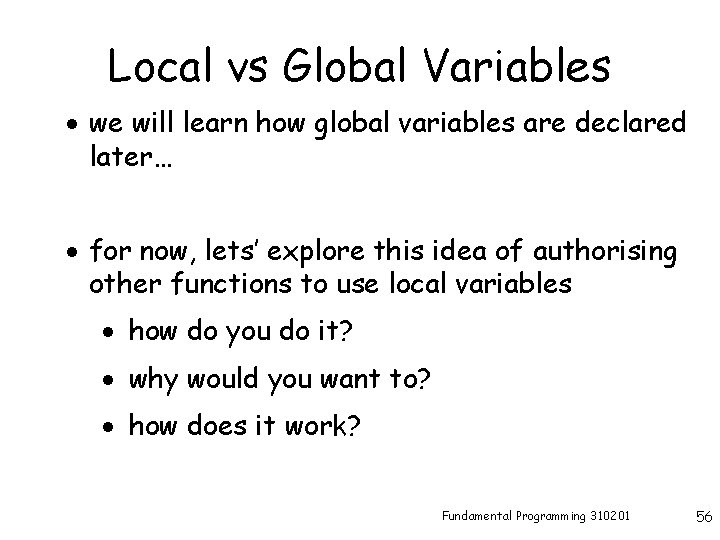
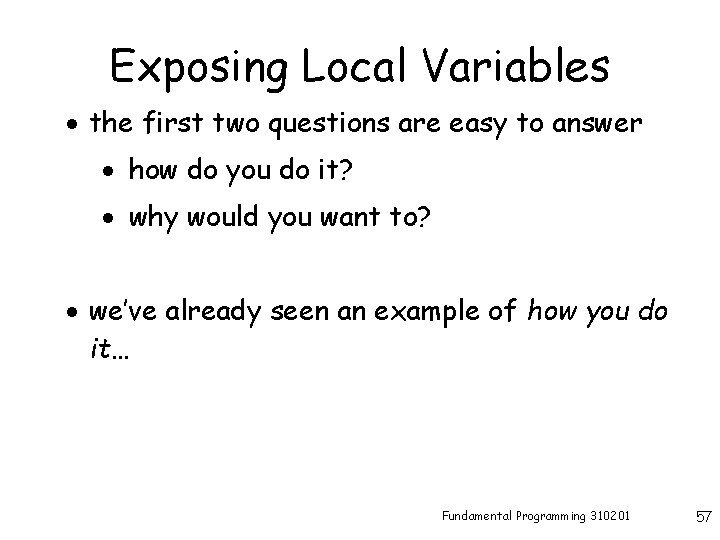
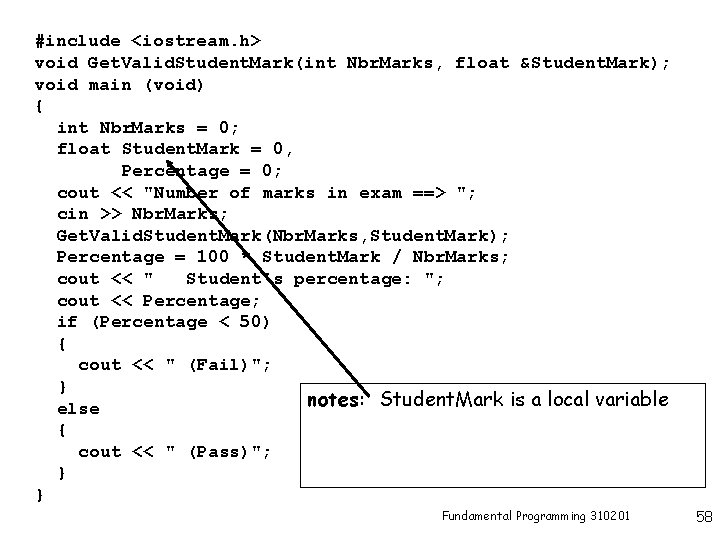
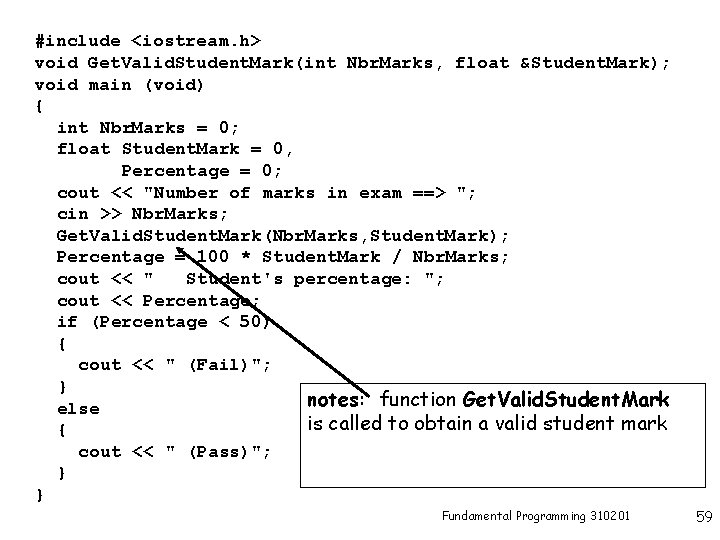
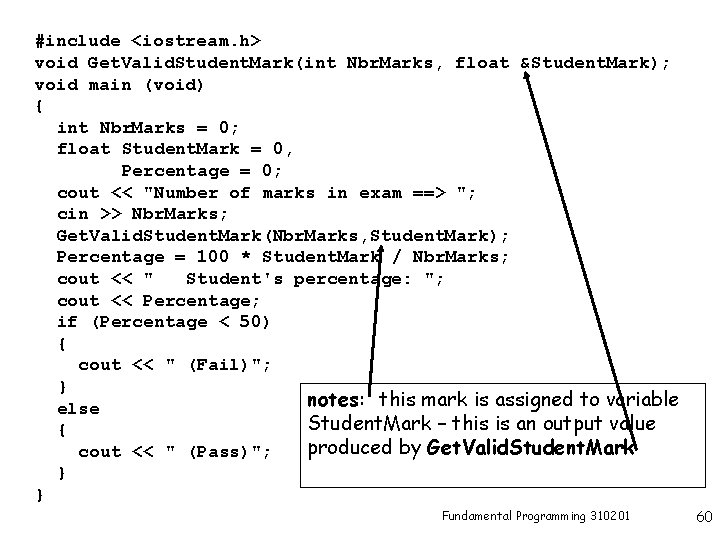
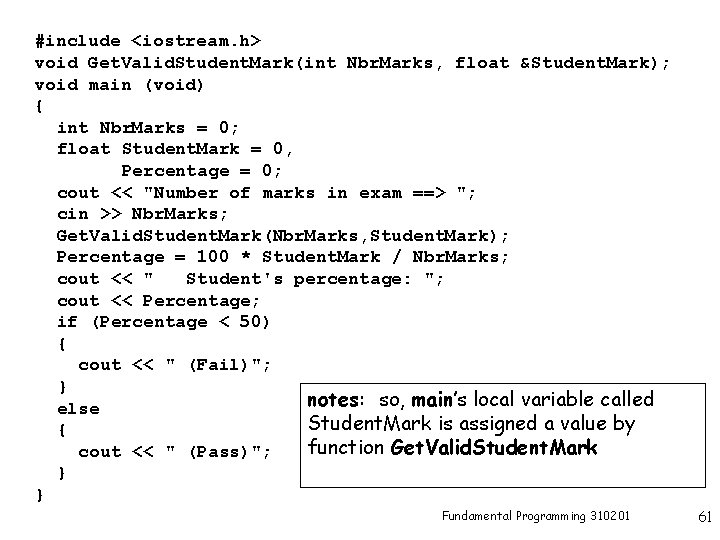
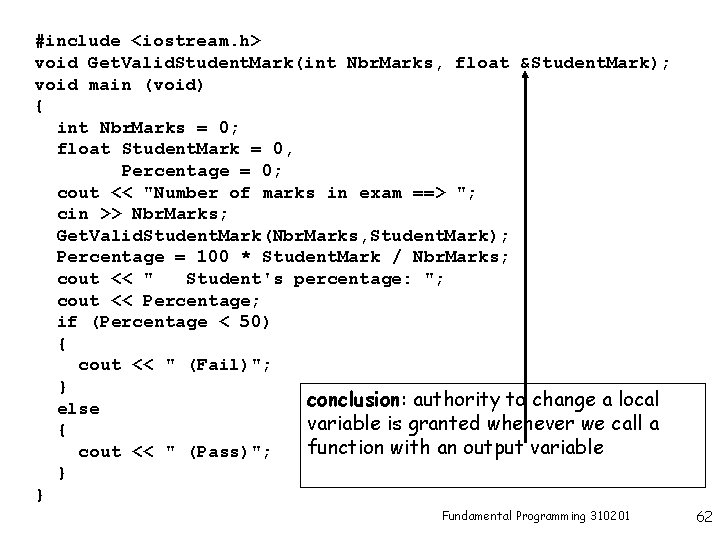
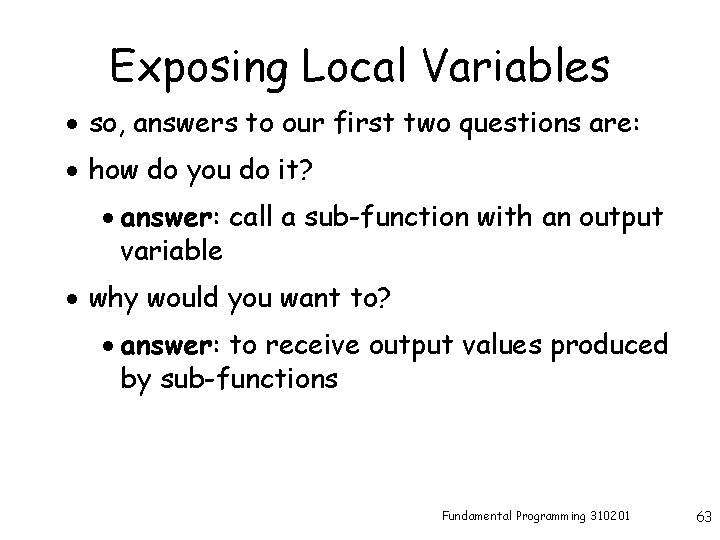
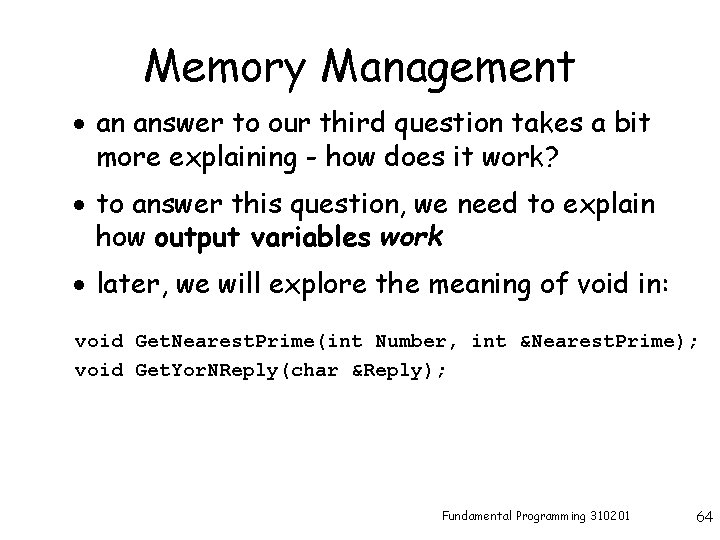
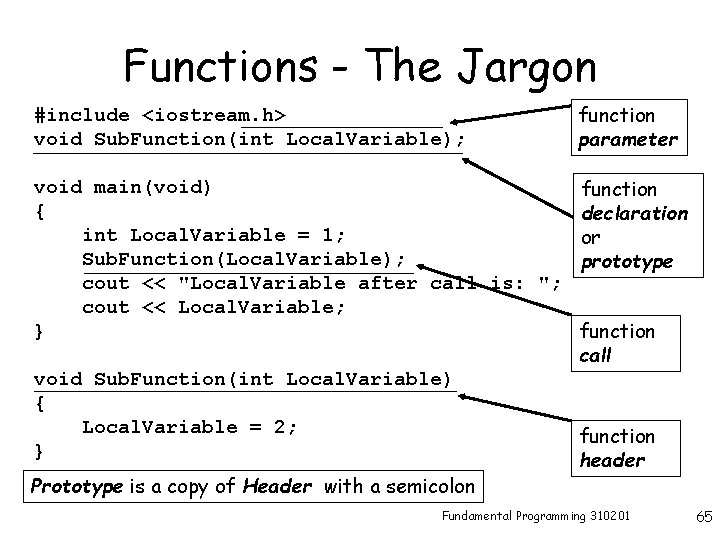
- Slides: 65
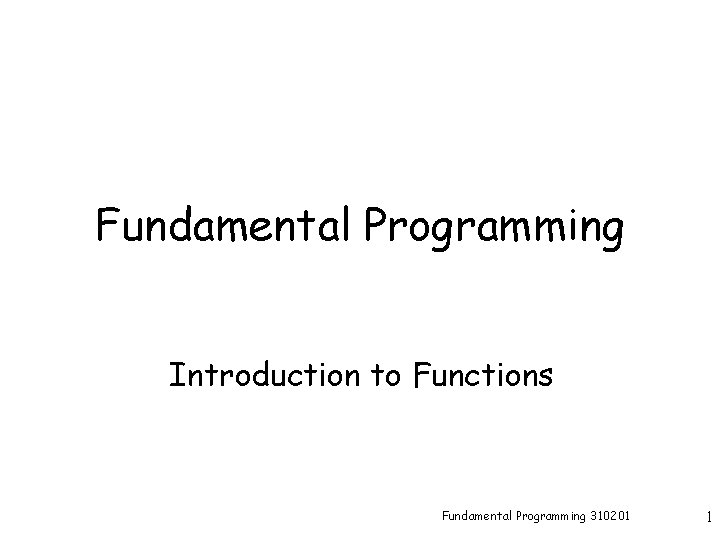
Fundamental Programming Introduction to Functions Fundamental Programming 310201 1
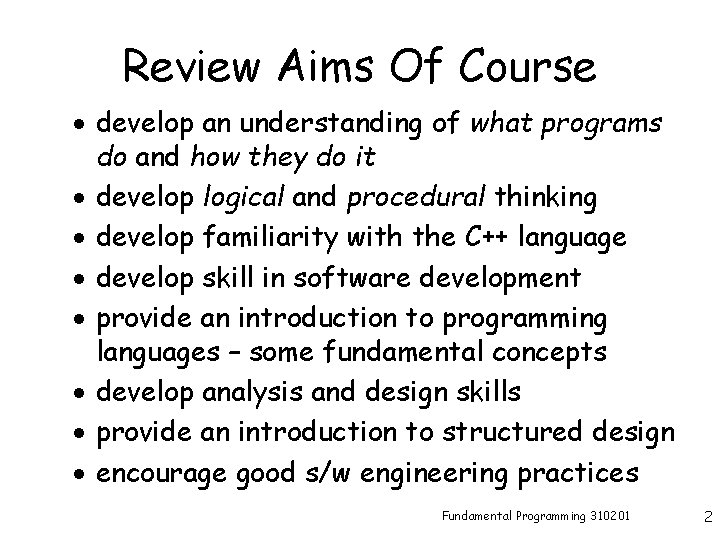
Review Aims Of Course · develop an understanding of what programs do and how they do it · develop logical and procedural thinking · develop familiarity with the C++ language · develop skill in software development · provide an introduction to programming languages – some fundamental concepts · develop analysis and design skills · provide an introduction to structured design · encourage good s/w engineering practices Fundamental Programming 310201 2
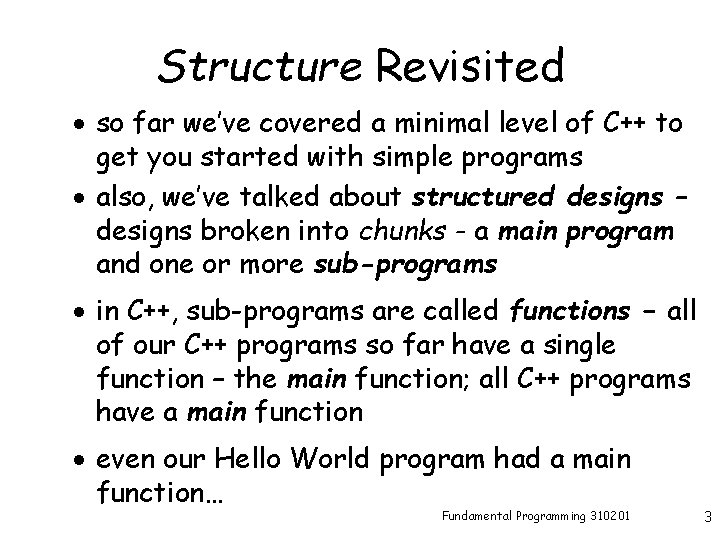
Structure Revisited · so far we’ve covered a minimal level of C++ to get you started with simple programs · also, we’ve talked about structured designs – designs broken into chunks - a main program and one or more sub-programs · in C++, sub-programs are called functions – all of our C++ programs so far have a single function – the main function; all C++ programs have a main function · even our Hello World program had a main function… Fundamental Programming 310201 3
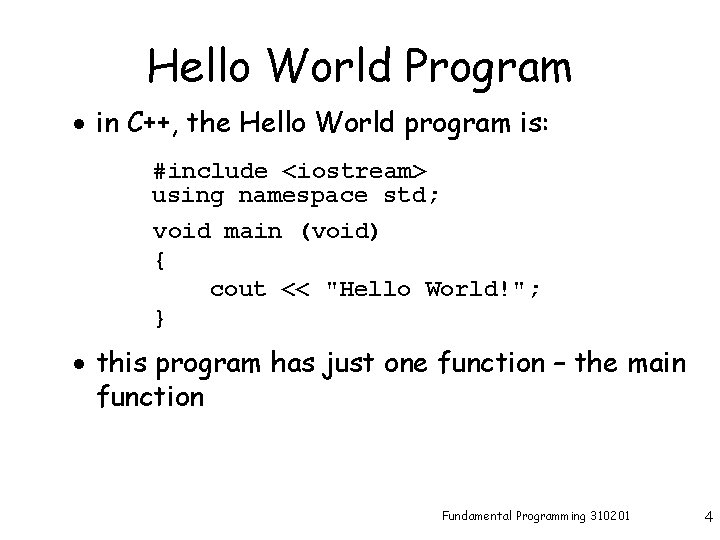
Hello World Program · in C++, the Hello World program is: #include <iostream> using namespace std; void main (void) { cout << "Hello World!"; } · this program has just one function – the main function Fundamental Programming 310201 4
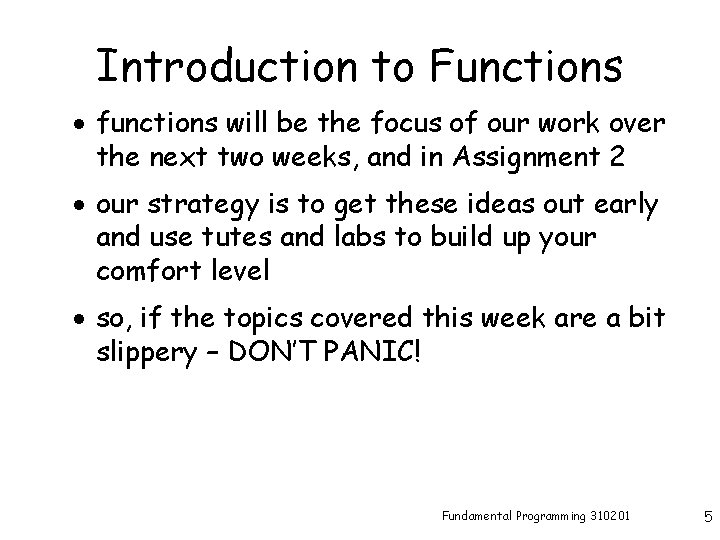
Introduction to Functions · functions will be the focus of our work over the next two weeks, and in Assignment 2 · our strategy is to get these ideas out early and use tutes and labs to build up your comfort level · so, if the topics covered this week are a bit slippery – DON’T PANIC! Fundamental Programming 310201 5
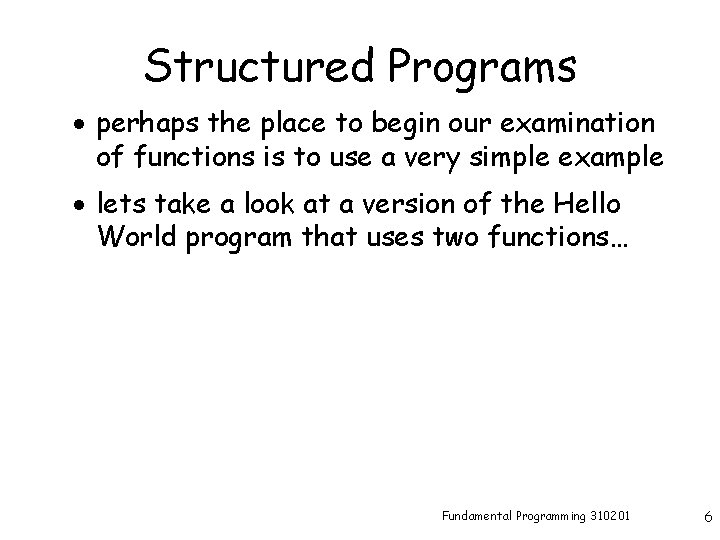
Structured Programs · perhaps the place to begin our examination of functions is to use a very simple example · lets take a look at a version of the Hello World program that uses two functions… Fundamental Programming 310201 6
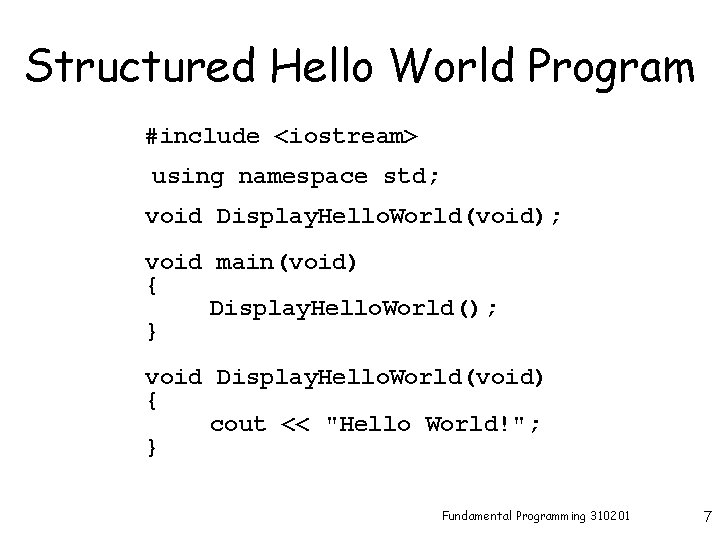
Structured Hello World Program #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); } void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 7
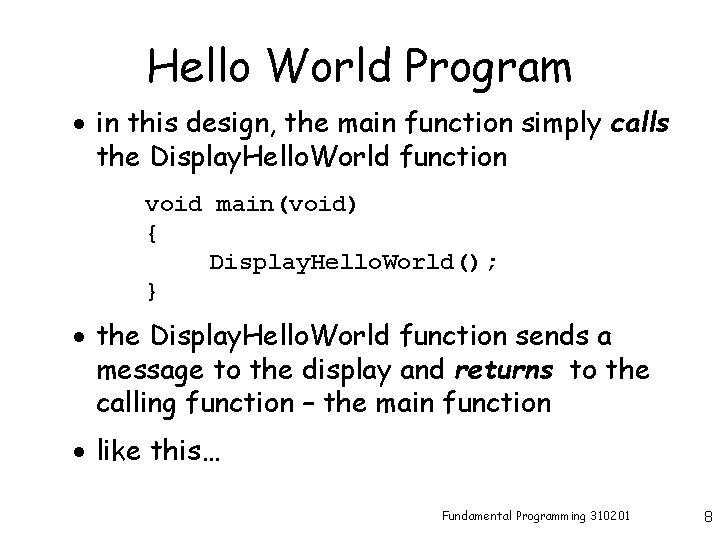
Hello World Program · in this design, the main function simply calls the Display. Hello. World function void main(void) { Display. Hello. World(); } · the Display. Hello. World function sends a message to the display and returns to the calling function – the main function · like this… Fundamental Programming 310201 8
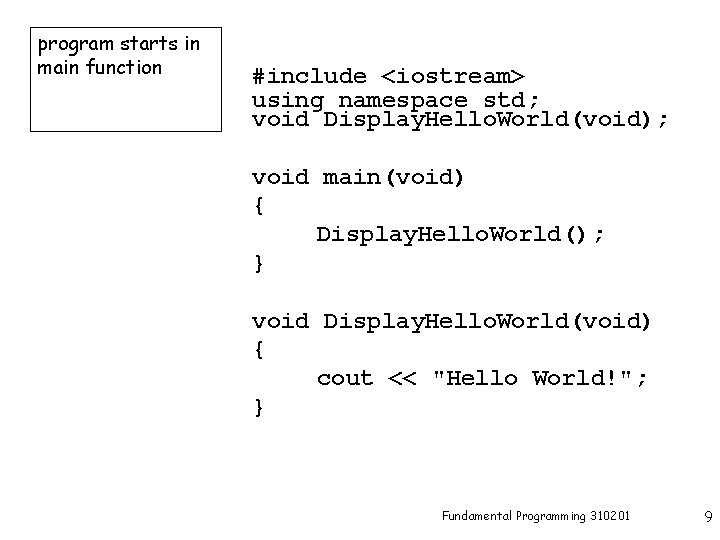
program starts in main function #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); } void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 9
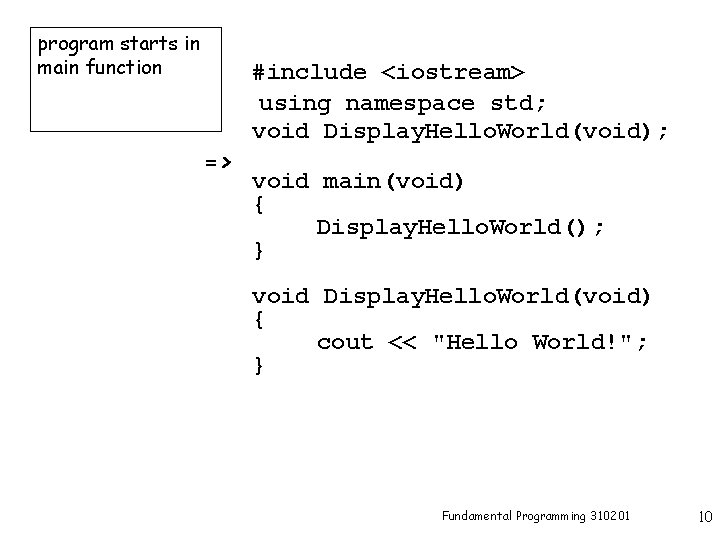
program starts in main function => #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); } void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 10
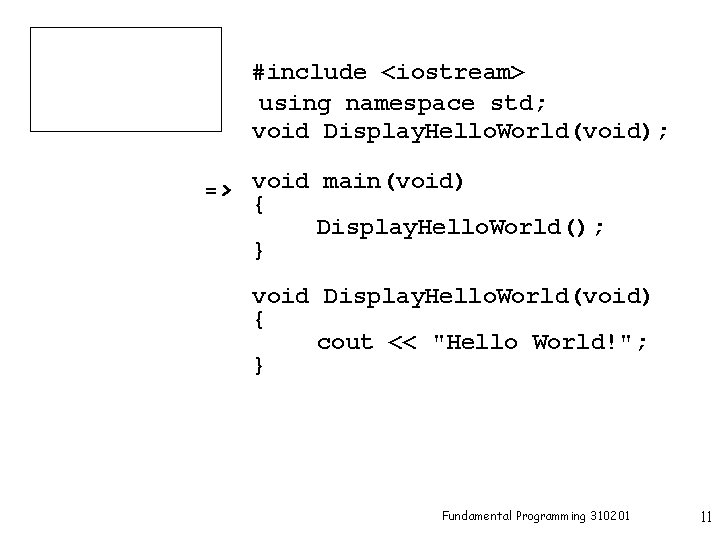
#include <iostream> using namespace std; void Display. Hello. World(void); => void main(void) { Display. Hello. World(); } void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 11
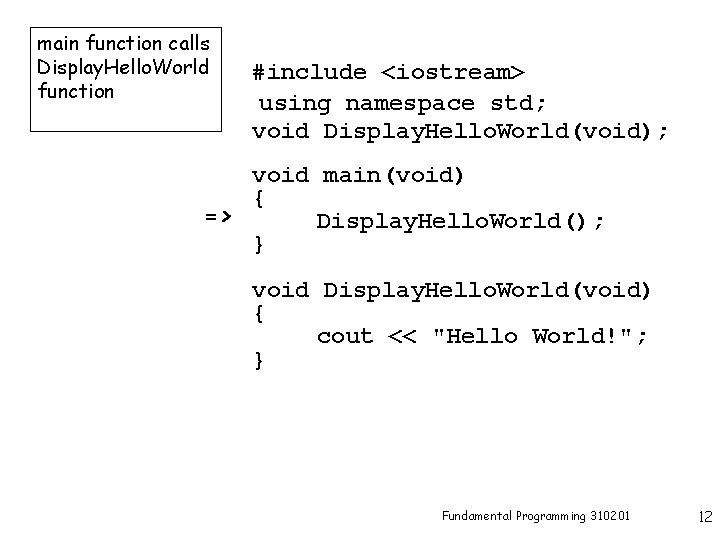
main function calls Display. Hello. World function #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { => Display. Hello. World(); } void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 12
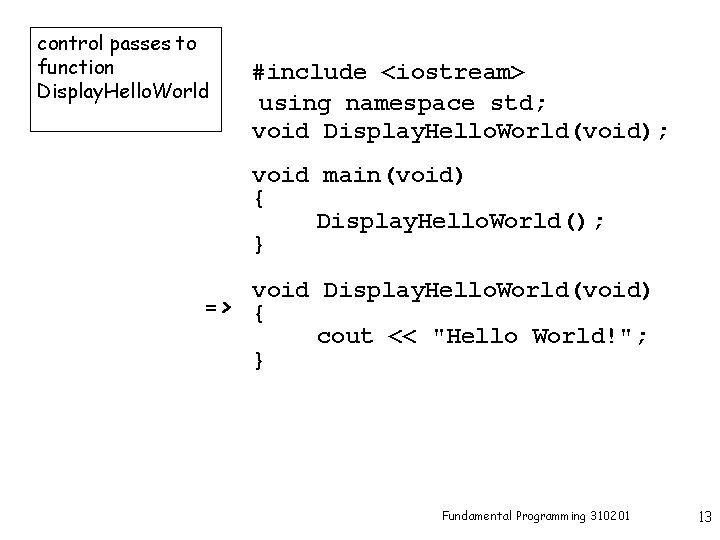
control passes to function Display. Hello. World #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); } void Display. Hello. World(void) => { cout << "Hello World!"; } Fundamental Programming 310201 13
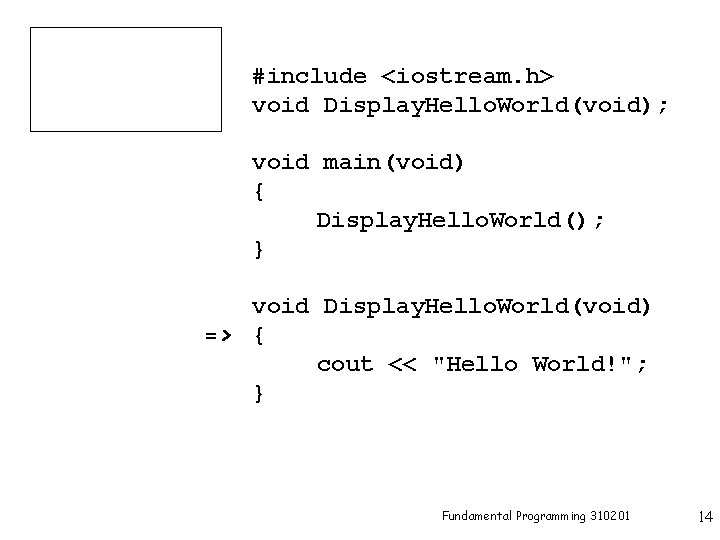
#include <iostream. h> void Display. Hello. World(void); void main(void) { Display. Hello. World(); } void Display. Hello. World(void) => { cout << "Hello World!"; } Fundamental Programming 310201 14
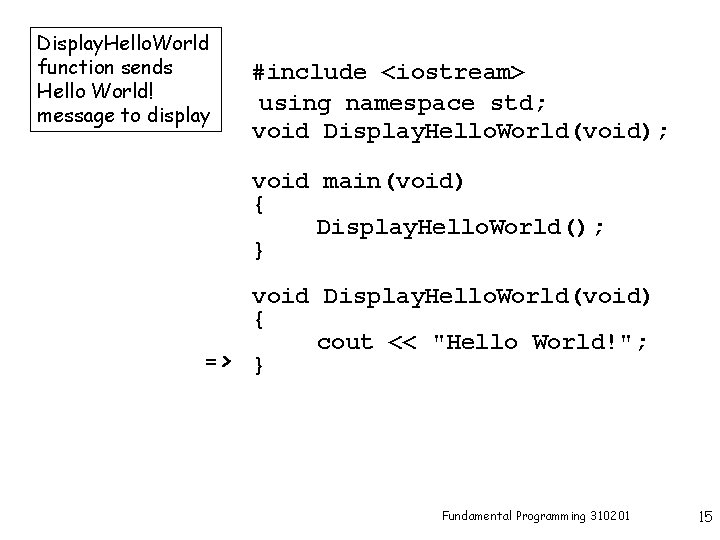
Display. Hello. World function sends Hello World! message to display #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); } void Display. Hello. World(void) { cout << "Hello World!"; => } Fundamental Programming 310201 15
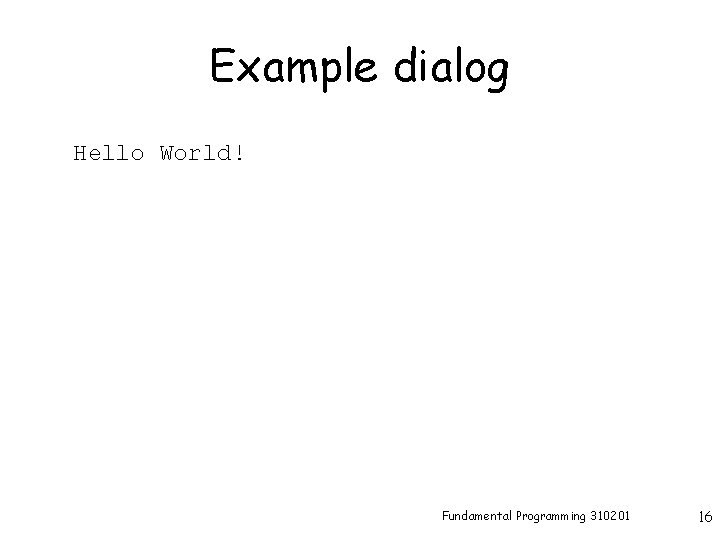
Example dialog Hello World! Fundamental Programming 310201 16
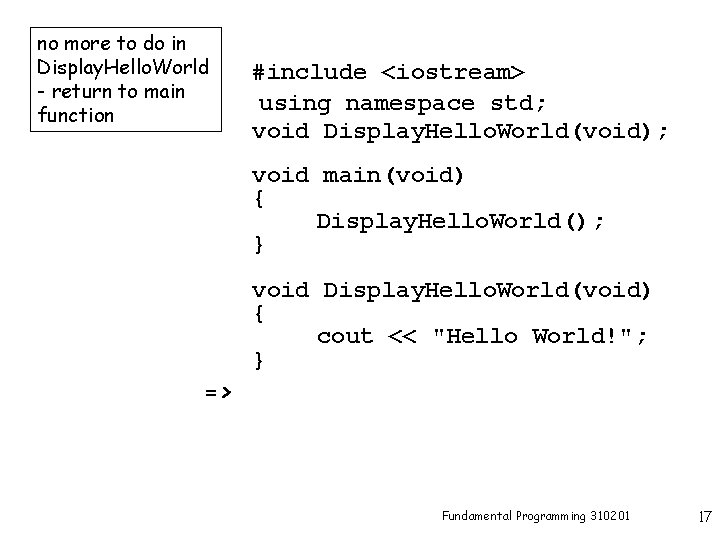
no more to do in Display. Hello. World - return to main function #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); } => void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 17
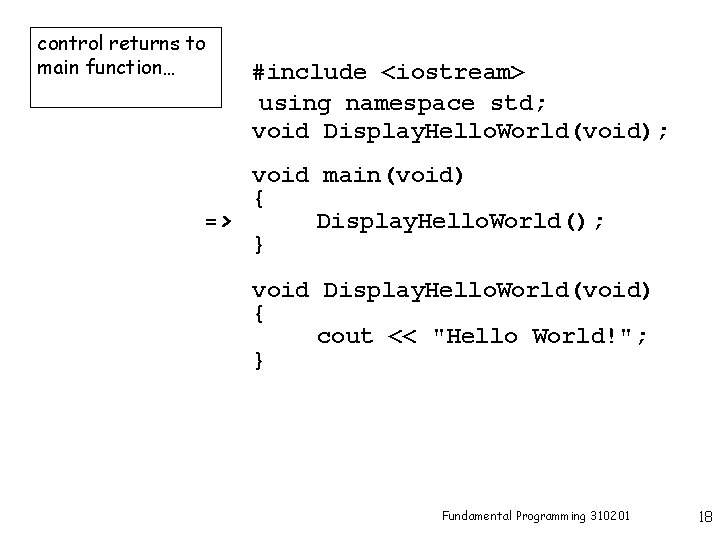
control returns to main function… #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); => } void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 18
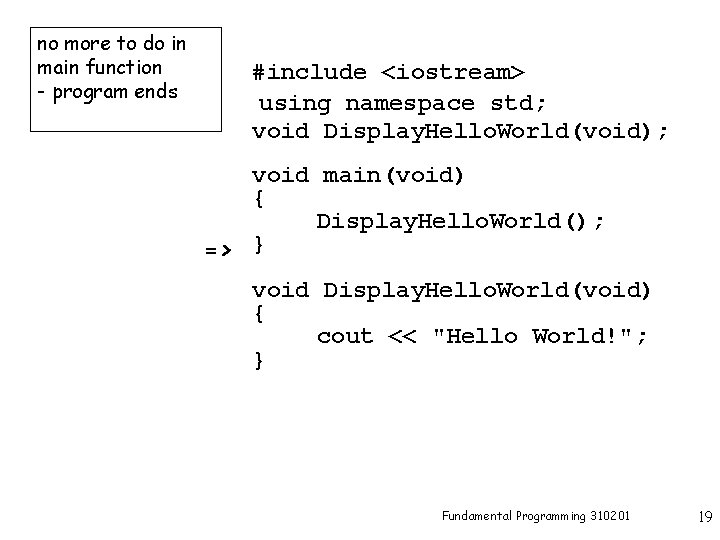
no more to do in main function - program ends #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); => } void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 19
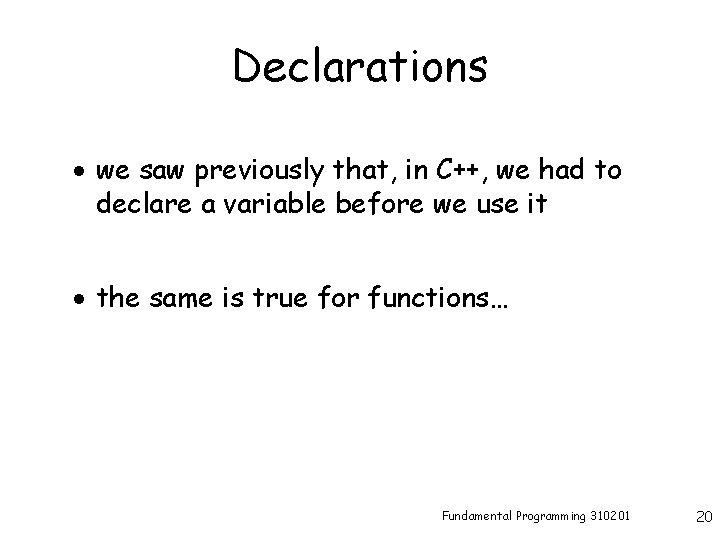
Declarations · we saw previously that, in C++, we had to declare a variable before we use it · the same is true for functions… Fundamental Programming 310201 20
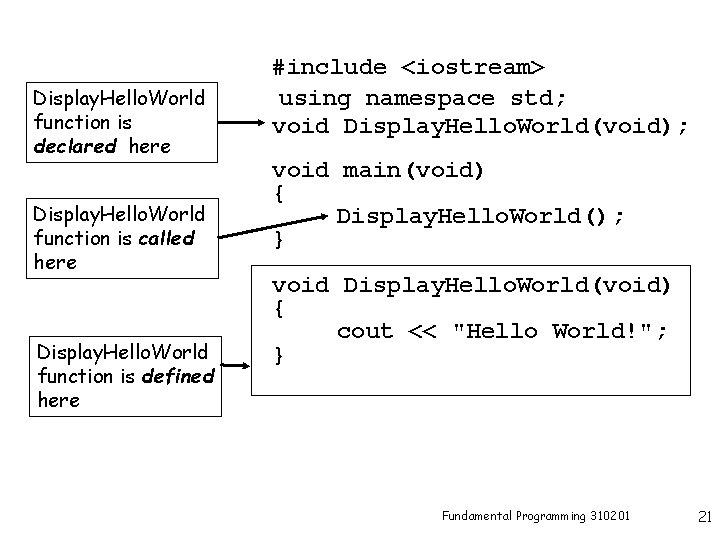
Display. Hello. World function is declared here Display. Hello. World function is called here Display. Hello. World function is defined here #include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); } void Display. Hello. World(void) { cout << "Hello World!"; } Fundamental Programming 310201 21
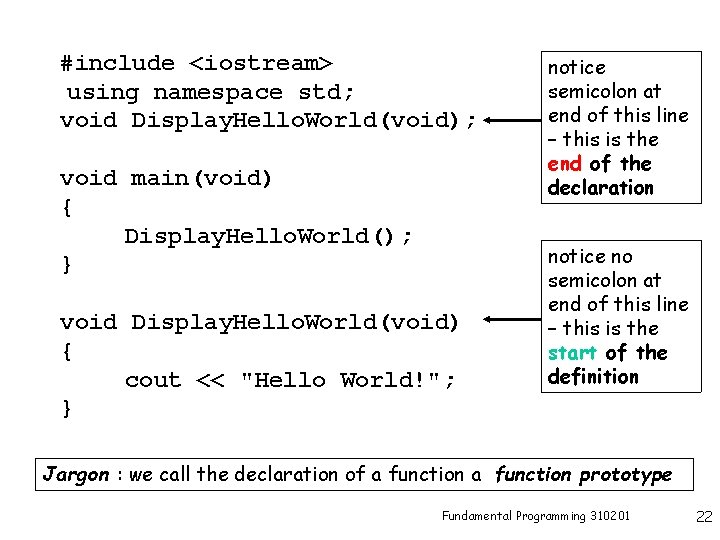
#include <iostream> using namespace std; void Display. Hello. World(void); void main(void) { Display. Hello. World(); } void Display. Hello. World(void) { cout << "Hello World!"; } notice semicolon at end of this line – this is the end of the declaration notice no semicolon at end of this line – this is the start of the definition Jargon : we call the declaration of a function prototype Fundamental Programming 310201 22
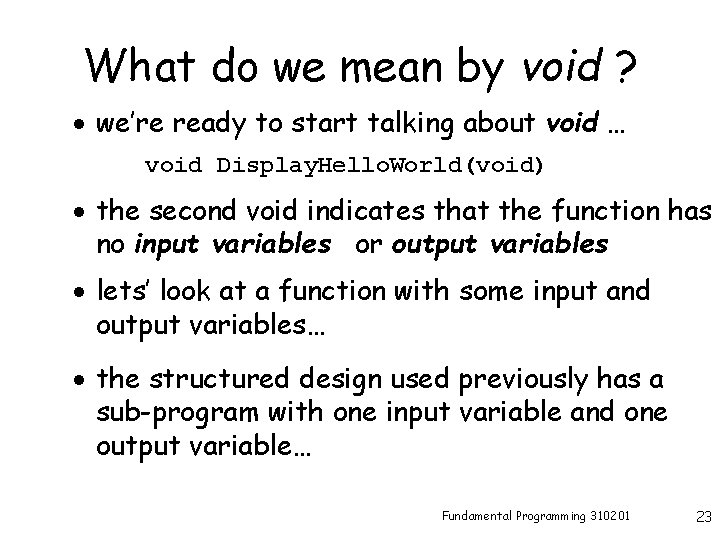
What do we mean by void ? · we’re ready to start talking about void … void Display. Hello. World(void) · the second void indicates that the function has no input variables or output variables · lets’ look at a function with some input and output variables… · the structured design used previously has a sub-program with one input variable and one output variable… Fundamental Programming 310201 23
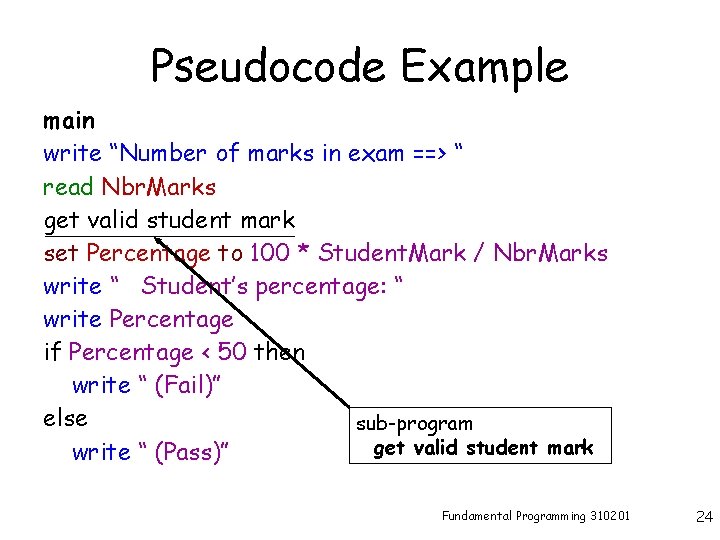
Pseudocode Example main write “Number of marks in exam ==> “ read Nbr. Marks get valid student mark set Percentage to 100 * Student. Mark / Nbr. Marks write “ Student’s percentage: “ write Percentage if Percentage < 50 then write “ (Fail)” else sub-program get valid student mark write “ (Pass)” Fundamental Programming 310201 24
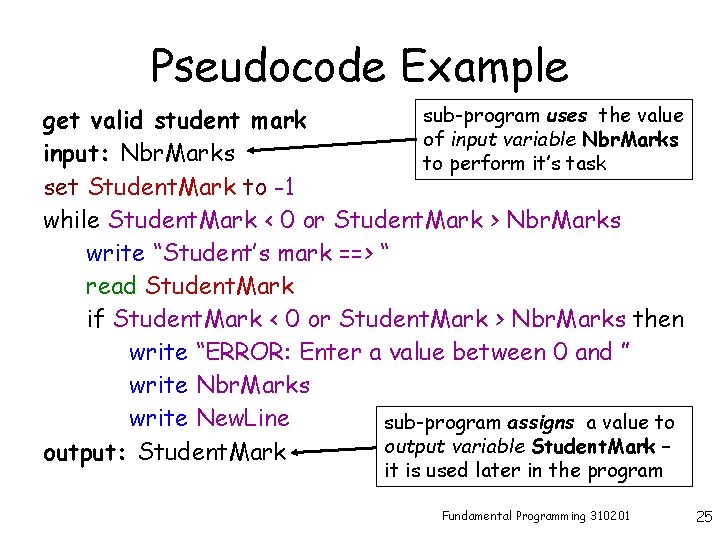
Pseudocode Example sub-program uses the value get valid student mark of input variable Nbr. Marks input: Nbr. Marks to perform it’s task set Student. Mark to -1 while Student. Mark < 0 or Student. Mark > Nbr. Marks write “Student’s mark ==> “ read Student. Mark if Student. Mark < 0 or Student. Mark > Nbr. Marks then write “ERROR: Enter a value between 0 and ” write Nbr. Marks write New. Line sub-program assigns a value to output variable Student. Mark – output: Student. Mark it is used later in the program Fundamental Programming 310201 25
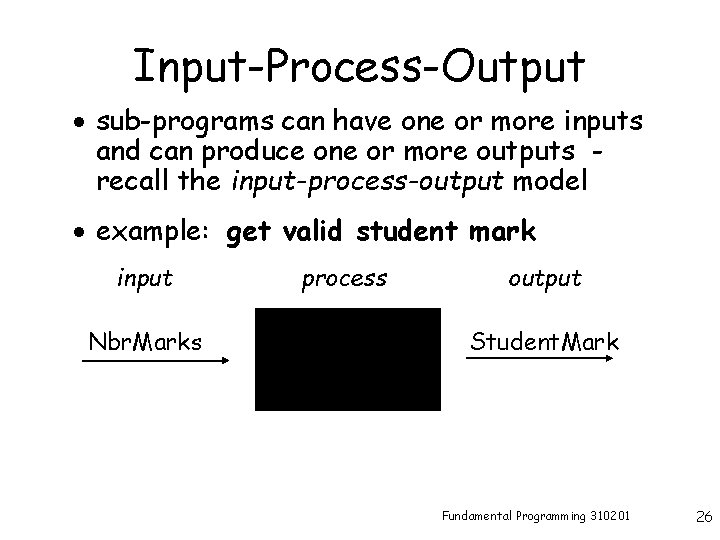
Input-Process-Output · sub-programs can have one or more inputs and can produce one or more outputs recall the input-process-output model · example: get valid student mark input Nbr. Marks process output Student. Mark Fundamental Programming 310201 26
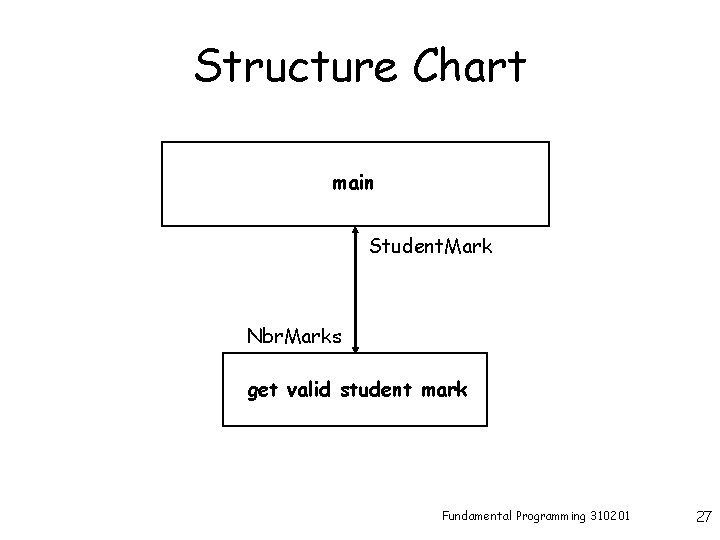
Structure Chart main Student. Mark Nbr. Marks get valid student mark Fundamental Programming 310201 27
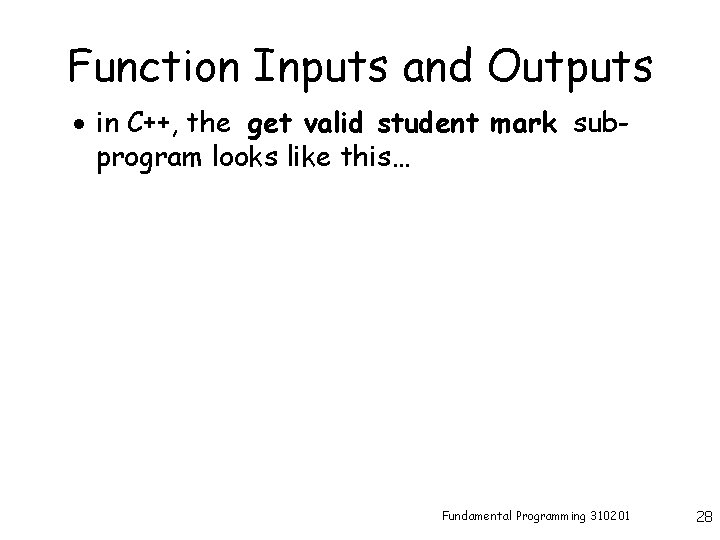
Function Inputs and Outputs · in C++, the get valid student mark subprogram looks like this… Fundamental Programming 310201 28
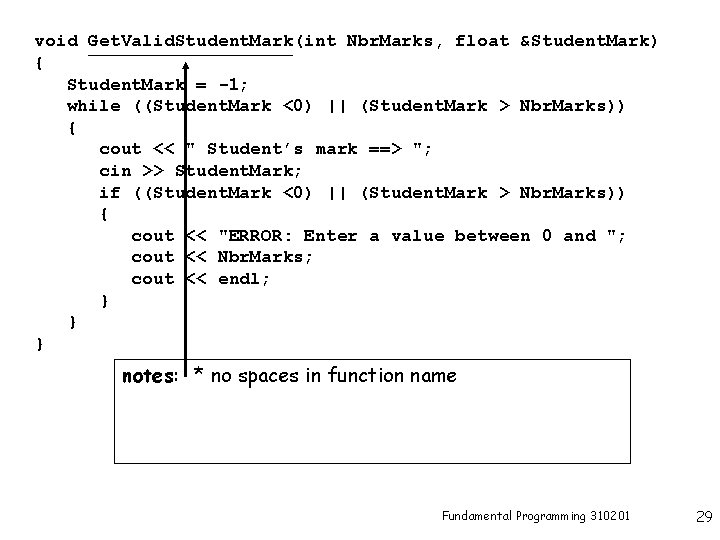
void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark) { Student. Mark = -1; while ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << " Student’s mark ==> "; cin >> Student. Mark; if ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << "ERROR: Enter a value between 0 and "; cout << Nbr. Marks; cout << endl; } } } notes: * no spaces in function name Fundamental Programming 310201 29
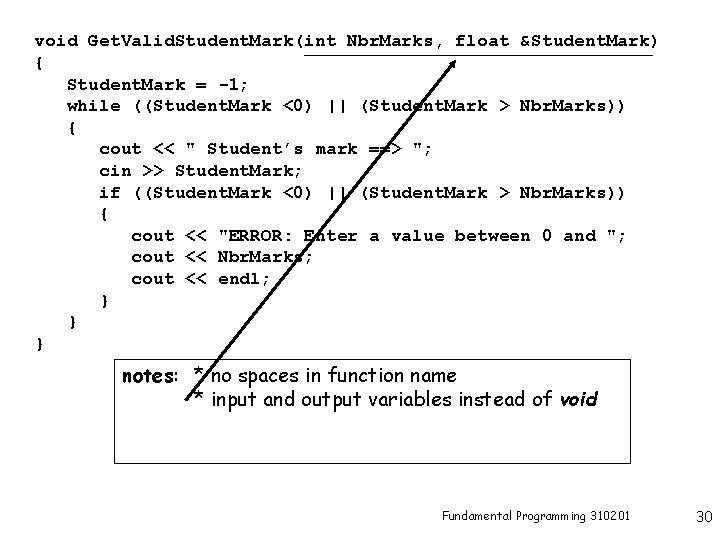
void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark) { Student. Mark = -1; while ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << " Student’s mark ==> "; cin >> Student. Mark; if ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << "ERROR: Enter a value between 0 and "; cout << Nbr. Marks; cout << endl; } } } notes: * no spaces in function name * input and output variables instead of void Fundamental Programming 310201 30
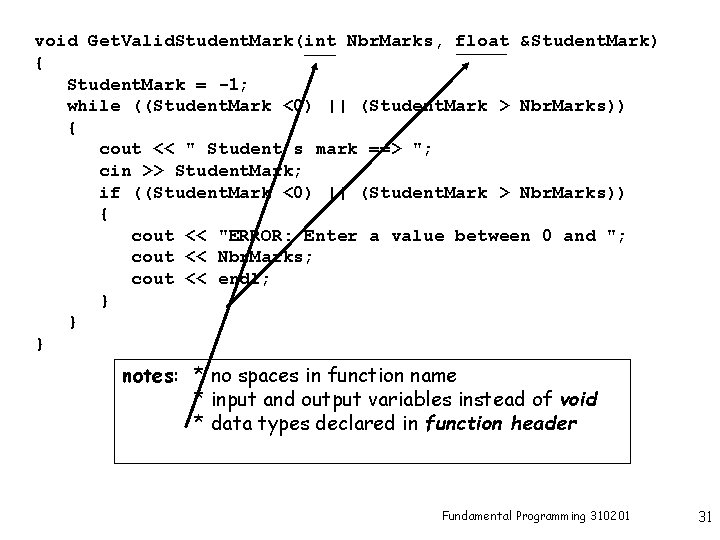
void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark) { Student. Mark = -1; while ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << " Student’s mark ==> "; cin >> Student. Mark; if ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << "ERROR: Enter a value between 0 and "; cout << Nbr. Marks; cout << endl; } } } notes: * no spaces in function name * input and output variables instead of void * data types declared in function header Fundamental Programming 310201 31
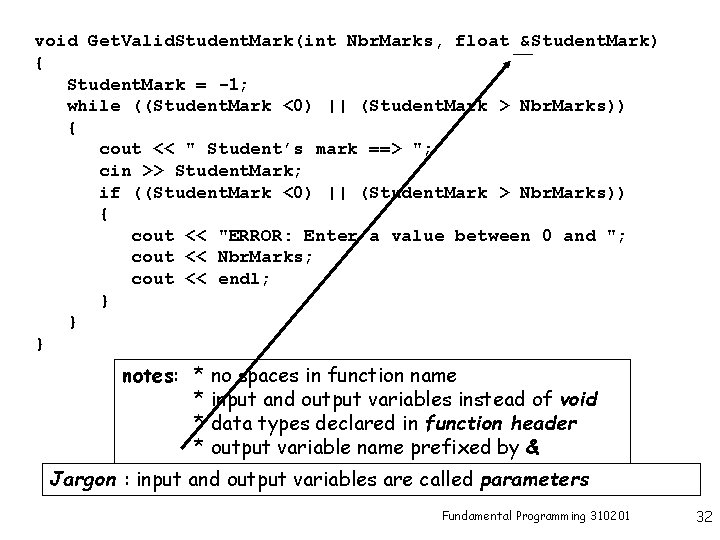
void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark) { Student. Mark = -1; while ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << " Student’s mark ==> "; cin >> Student. Mark; if ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << "ERROR: Enter a value between 0 and "; cout << Nbr. Marks; cout << endl; } } } notes: * no spaces in function name * input and output variables instead of void * data types declared in function header * output variable name prefixed by & Jargon : input and output variables are called parameters Fundamental Programming 310201 32
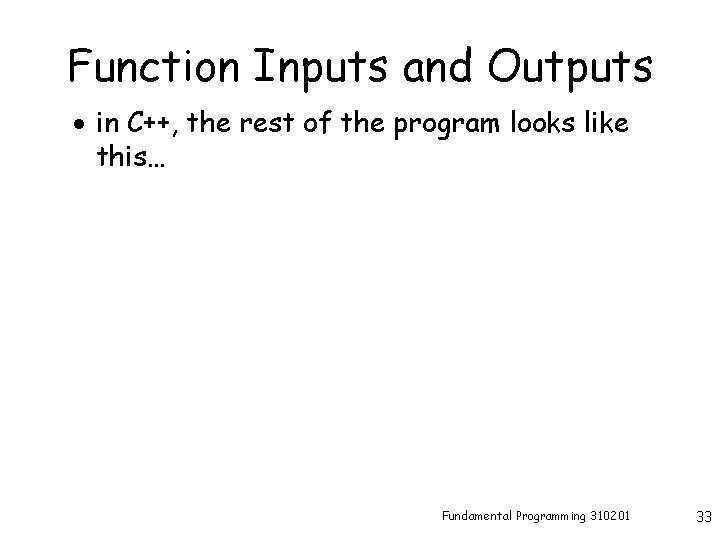
Function Inputs and Outputs · in C++, the rest of the program looks like this… Fundamental Programming 310201 33
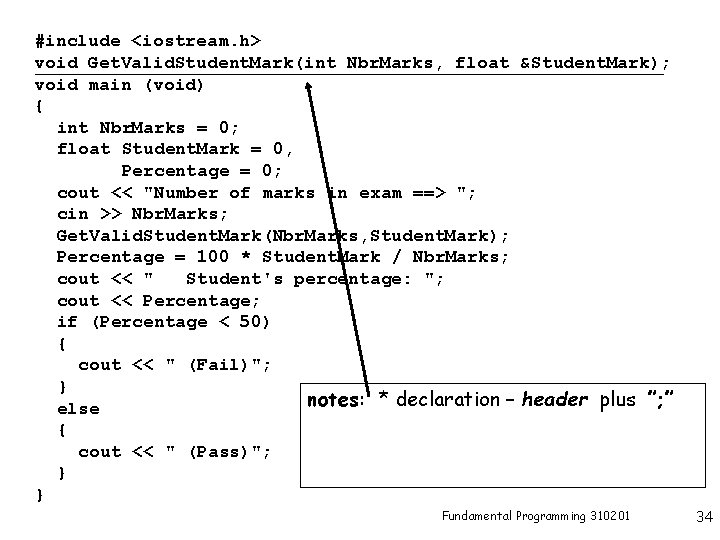
#include <iostream. h> void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void main (void) { int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; Get. Valid. Student. Mark(Nbr. Marks, Student. Mark); Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student's percentage: "; cout << Percentage; if (Percentage < 50) { cout << " (Fail)"; } notes: * declaration – header plus ”; ” else { cout << " (Pass)"; } } Fundamental Programming 310201 34
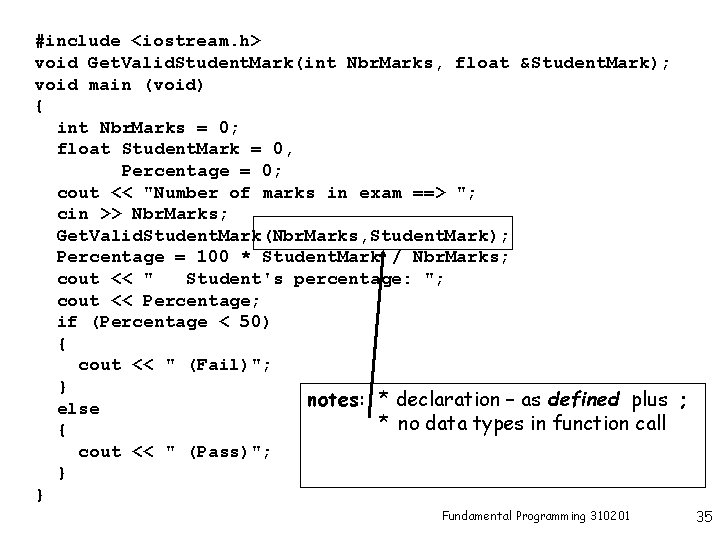
#include <iostream. h> void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void main (void) { int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; Get. Valid. Student. Mark(Nbr. Marks, Student. Mark); Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student's percentage: "; cout << Percentage; if (Percentage < 50) { cout << " (Fail)"; } notes: * declaration – as defined plus ; else * no data types in function call { cout << " (Pass)"; } } Fundamental Programming 310201 35
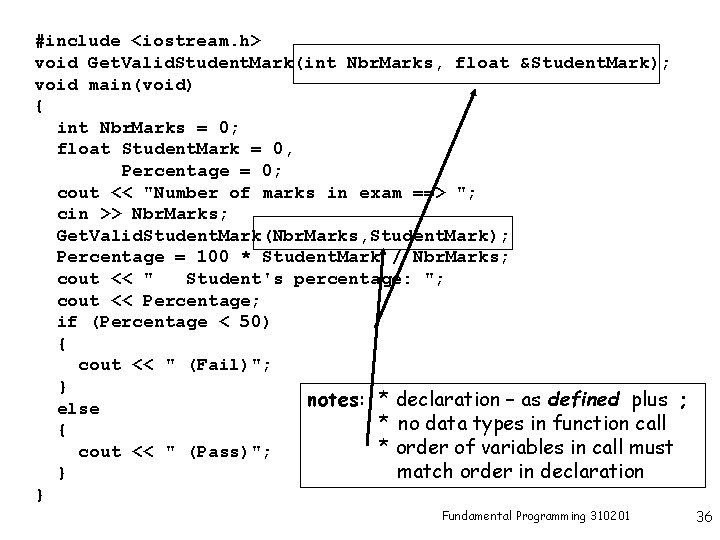
#include <iostream. h> void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void main(void) { int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; Get. Valid. Student. Mark(Nbr. Marks, Student. Mark); Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student's percentage: "; cout << Percentage; if (Percentage < 50) { cout << " (Fail)"; } notes: * declaration – as defined plus ; else * no data types in function call { * order of variables in call must cout << " (Pass)"; match order in declaration } } Fundamental Programming 310201 36
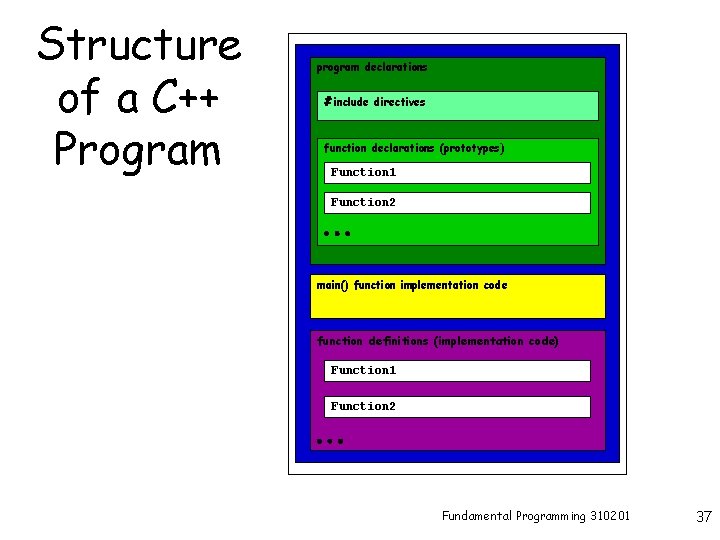
Structure of a C++ Program program declarations #include directives function declarations (prototypes) Function 1 Function 2 main() function implementation code function definitions (implementation code) Function 1 Function 2 Fundamental Programming 310201 37
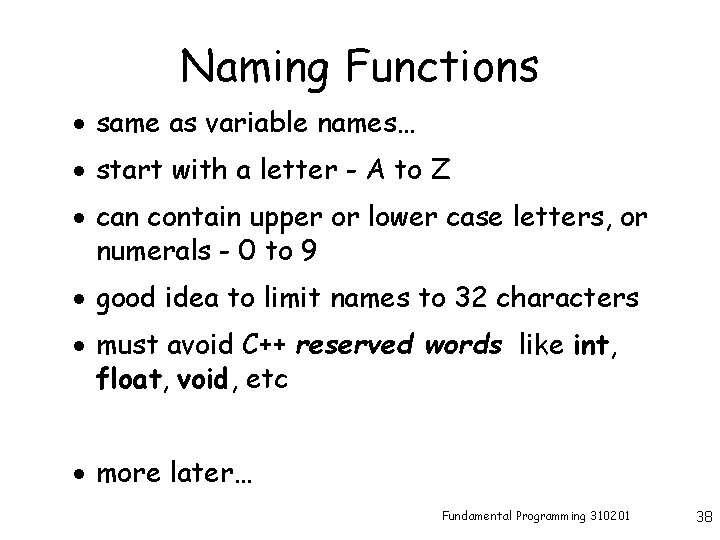
Naming Functions · same as variable names… · start with a letter - A to Z · can contain upper or lower case letters, or numerals - 0 to 9 · good idea to limit names to 32 characters · must avoid C++ reserved words like int, float, void, etc · more later… Fundamental Programming 310201 38
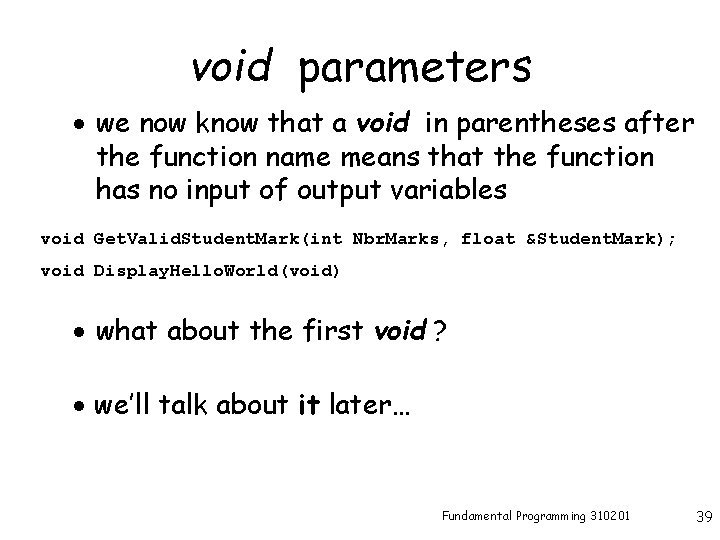
void parameters · we now know that a void in parentheses after the function name means that the function has no input of output variables void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void Display. Hello. World(void) · what about the first void ? · we’ll talk about it later… Fundamental Programming 310201 39
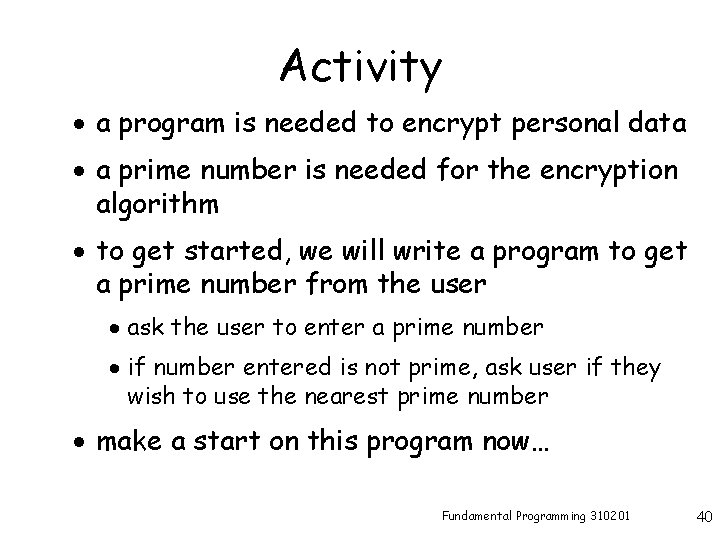
Activity · a program is needed to encrypt personal data · a prime number is needed for the encryption algorithm · to get started, we will write a program to get a prime number from the user · ask the user to enter a prime number · if number entered is not prime, ask user if they wish to use the nearest prime number · make a start on this program now… Fundamental Programming 310201 40
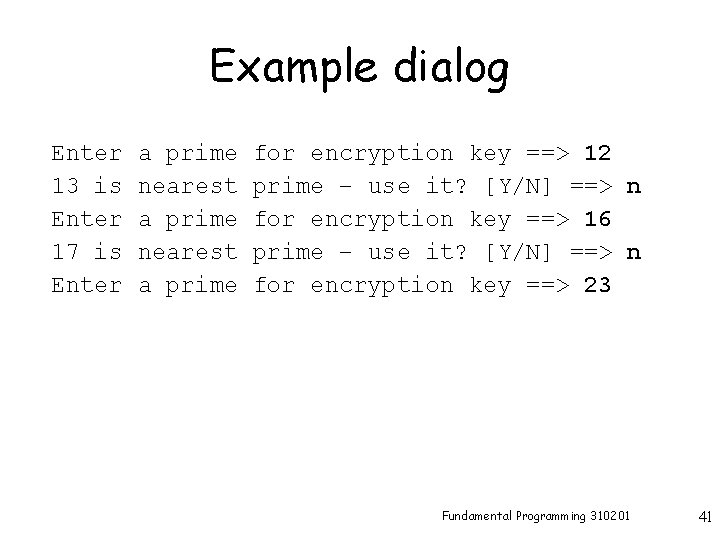
Example dialog Enter 13 is Enter 17 is Enter a prime nearest a prime for encryption key ==> 12 prime – use it? [Y/N] ==> n for encryption key ==> 16 prime – use it? [Y/N] ==> n for encryption key ==> 23 Fundamental Programming 310201 41
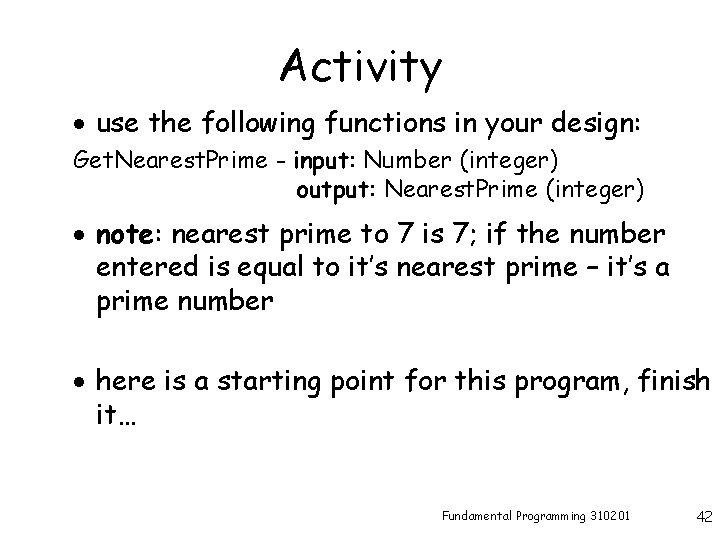
Activity · use the following functions in your design: Get. Nearest. Prime - input: Number (integer) output: Nearest. Prime (integer) · note: nearest prime to 7 is 7; if the number entered is equal to it’s nearest prime – it’s a prime number · here is a starting point for this program, finish it… Fundamental Programming 310201 42
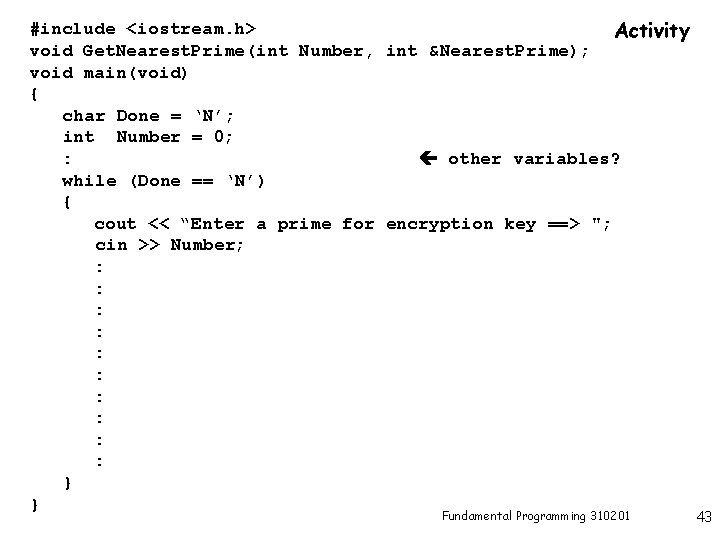
#include <iostream. h> Activity void Get. Nearest. Prime(int Number, int &Nearest. Prime); void main(void) { char Done = ‘N’; int Number = 0; : other variables? while (Done == ‘N’) { cout << “Enter a prime for encryption key ==> "; cin >> Number; : : : : : } } Fundamental Programming 310201 43
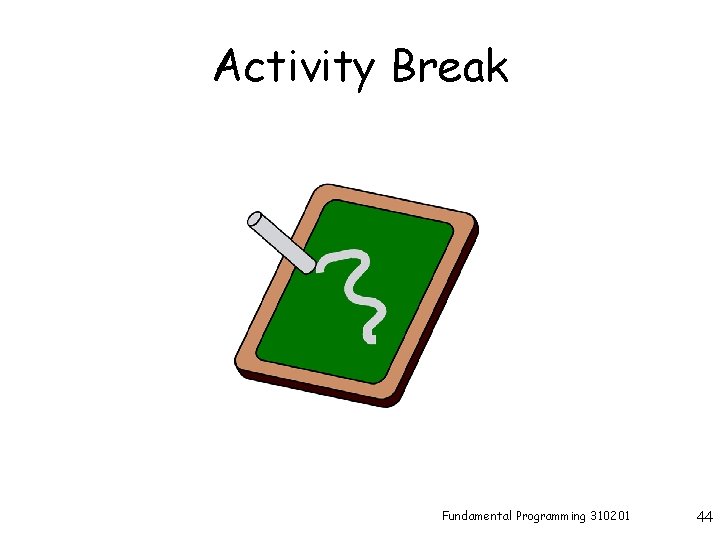
Activity Break Fundamental Programming 310201 44
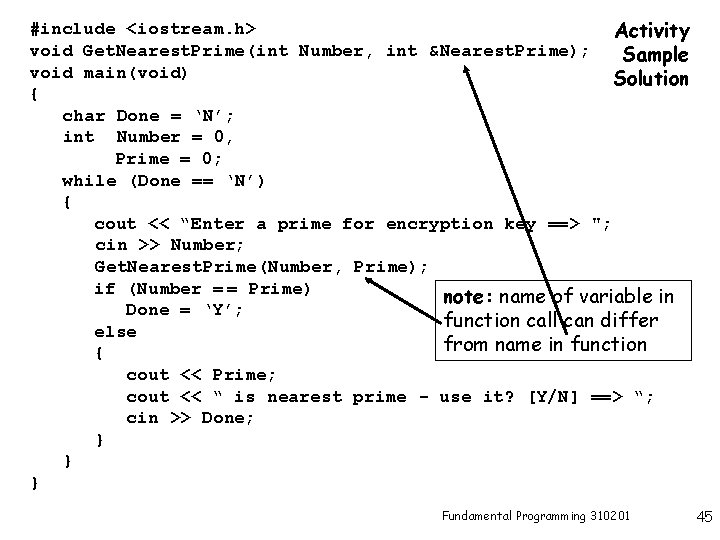
#include <iostream. h> Activity void Get. Nearest. Prime(int Number, int &Nearest. Prime); Sample void main(void) Solution { char Done = ‘N’; int Number = 0, Prime = 0; while (Done == ‘N’) { cout << “Enter a prime for encryption key ==> "; cin >> Number; Get. Nearest. Prime(Number, Prime); if (Number = = Prime) note: name of variable in Done = ‘Y’; function call can differ else from name in function { cout << Prime; cout << “ is nearest prime - use it? [Y/N] ==> “; cin >> Done; } } } Fundamental Programming 310201 45
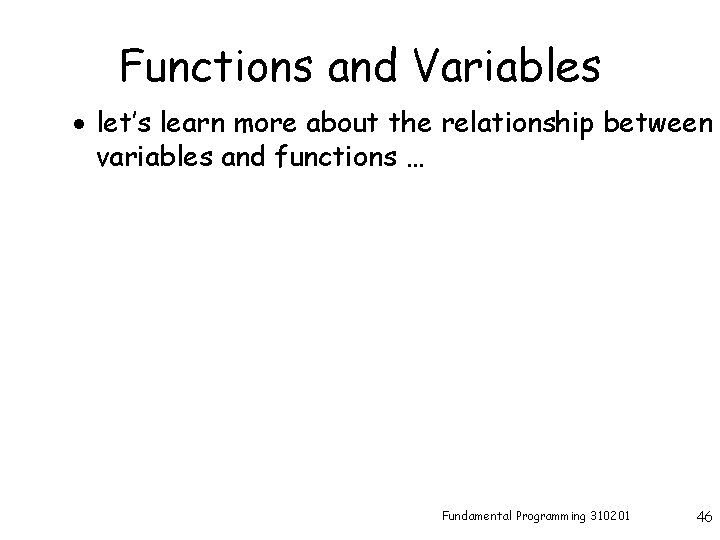
Functions and Variables · let’s learn more about the relationship between variables and functions … Fundamental Programming 310201 46
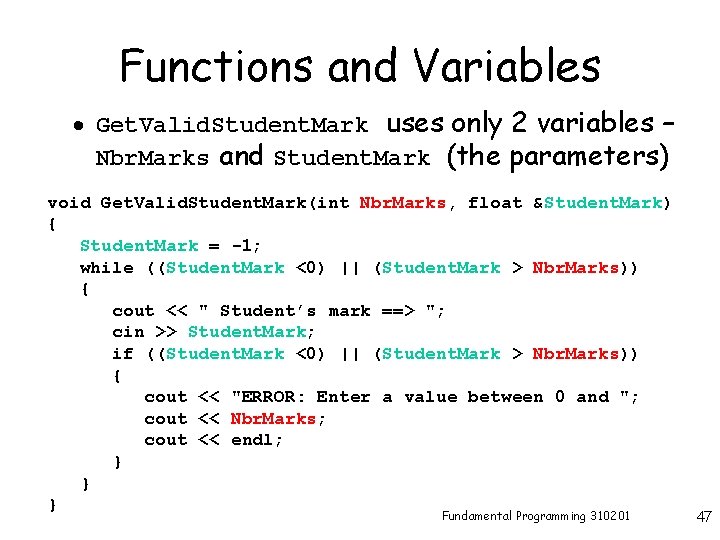
Functions and Variables · Get. Valid. Student. Mark uses only 2 variables – Nbr. Marks and Student. Mark (the parameters) void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark) { Student. Mark = -1; while ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << " Student’s mark ==> "; cin >> Student. Mark; if ((Student. Mark <0) || (Student. Mark > Nbr. Marks)) { cout << "ERROR: Enter a value between 0 and "; cout << Nbr. Marks; cout << endl; } } } Fundamental Programming 310201 47
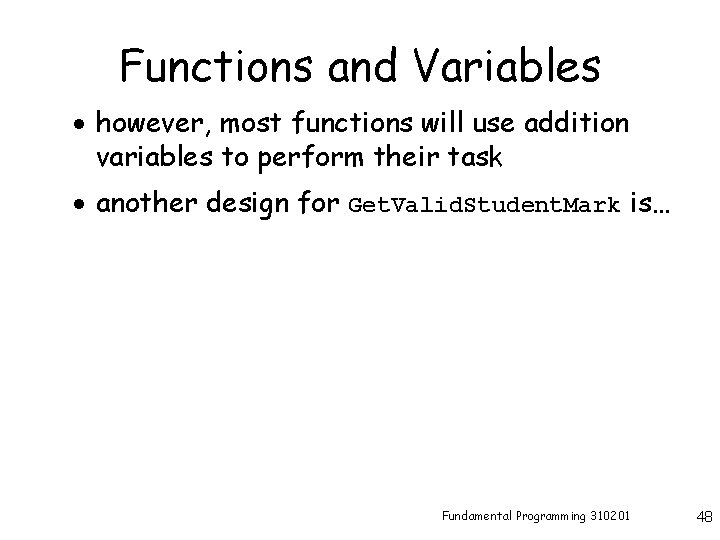
Functions and Variables · however, most functions will use addition variables to perform their task · another design for Get. Valid. Student. Mark is… Fundamental Programming 310201 48
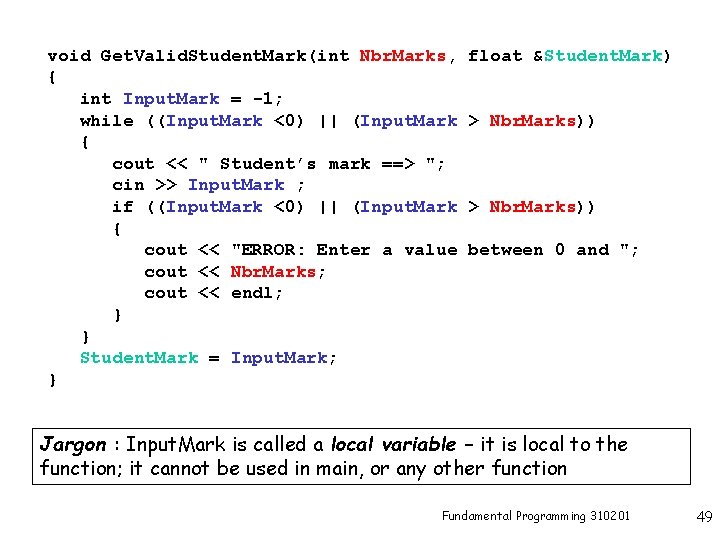
void Get. Valid. Student. Mark(int Nbr. Marks, { int Input. Mark = -1; while ((Input. Mark <0) || (Input. Mark { cout << " Student’s mark ==> "; cin >> Input. Mark ; if ((Input. Mark <0) || (Input. Mark { cout << "ERROR: Enter a value cout << Nbr. Marks; cout << endl; } } Student. Mark = Input. Mark; } float &Student. Mark) > Nbr. Marks)) between 0 and "; Jargon : Input. Mark is called a local variable – it is local to the function; it cannot be used in main, or any other function Fundamental Programming 310201 49
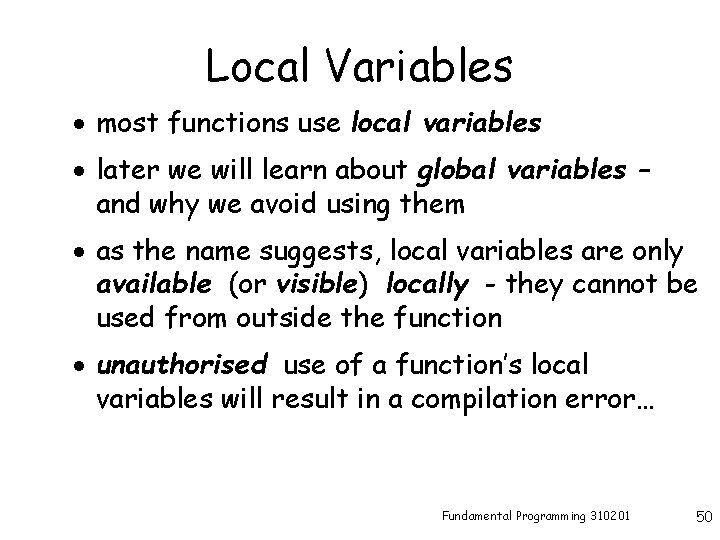
Local Variables · most functions use local variables · later we will learn about global variables – and why we avoid using them · as the name suggests, local variables are only available (or visible) locally - they cannot be used from outside the function · unauthorised use of a function’s local variables will result in a compilation error… Fundamental Programming 310201 50
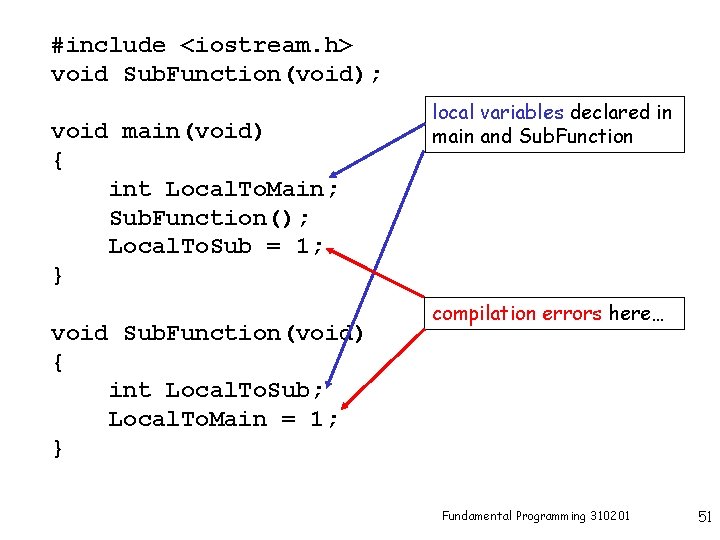
#include <iostream. h> void Sub. Function(void); void main(void) { int Local. To. Main; Sub. Function(); Local. To. Sub = 1; } void Sub. Function(void) { int Local. To. Sub; Local. To. Main = 1; } local variables declared in main and Sub. Function compilation errors here… Fundamental Programming 310201 51
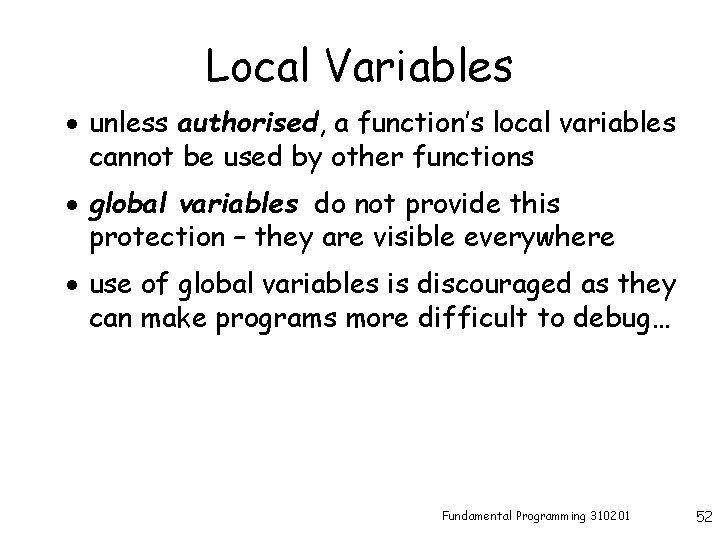
Local Variables · unless authorised, a function’s local variables cannot be used by other functions · global variables do not provide this protection – they are visible everywhere · use of global variables is discouraged as they can make programs more difficult to debug… Fundamental Programming 310201 52
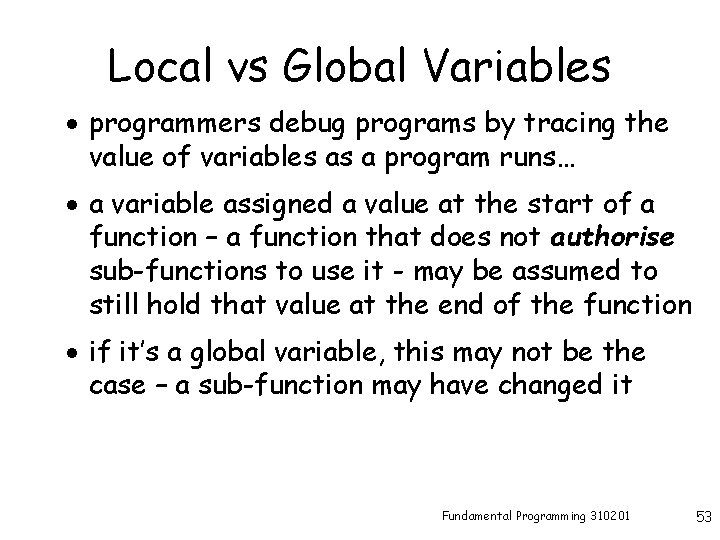
Local vs Global Variables · programmers debug programs by tracing the value of variables as a program runs… · a variable assigned a value at the start of a function – a function that does not authorise sub-functions to use it - may be assumed to still hold that value at the end of the function · if it’s a global variable, this may not be the case – a sub-function may have changed it Fundamental Programming 310201 53
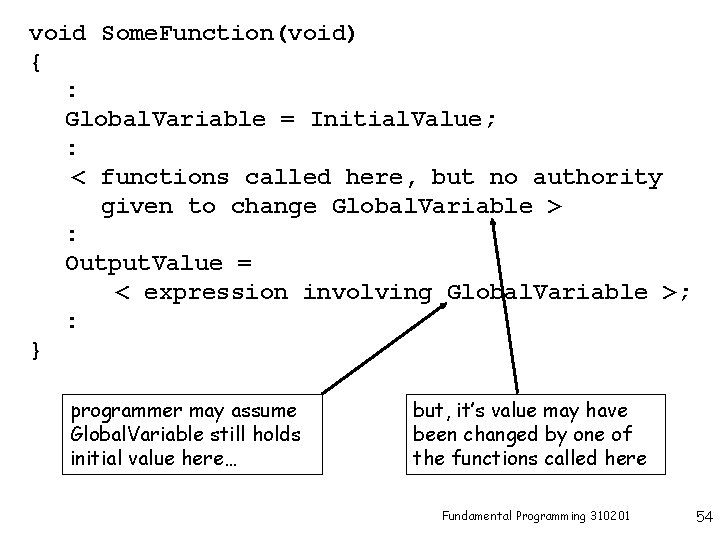
void Some. Function(void) { : Global. Variable = Initial. Value; : < functions called here, but no authority given to change Global. Variable > : Output. Value = < expression involving Global. Variable >; : } programmer may assume Global. Variable still holds initial value here… but, it’s value may have been changed by one of the functions called here Fundamental Programming 310201 54
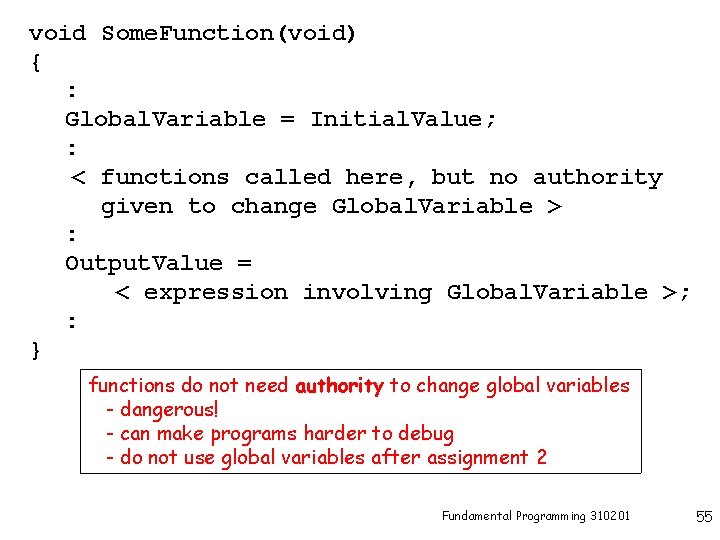
void Some. Function(void) { : Global. Variable = Initial. Value; : < functions called here, but no authority given to change Global. Variable > : Output. Value = < expression involving Global. Variable >; : } functions do not need authority to change global variables - dangerous! - can make programs harder to debug - do not use global variables after assignment 2 Fundamental Programming 310201 55
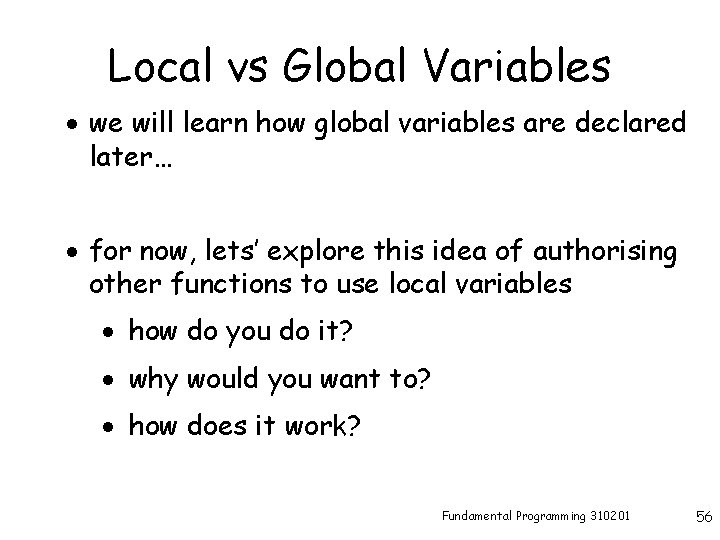
Local vs Global Variables · we will learn how global variables are declared later… · for now, lets’ explore this idea of authorising other functions to use local variables · how do you do it? · why would you want to? · how does it work? Fundamental Programming 310201 56
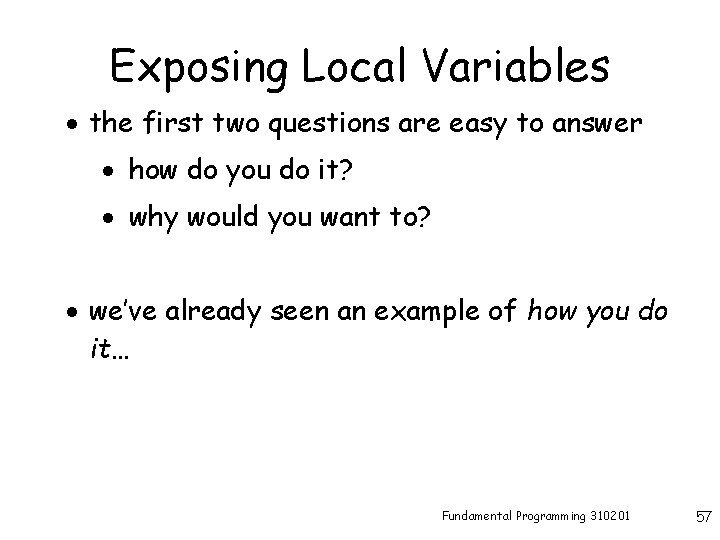
Exposing Local Variables · the first two questions are easy to answer · how do you do it? · why would you want to? · we’ve already seen an example of how you do it… Fundamental Programming 310201 57
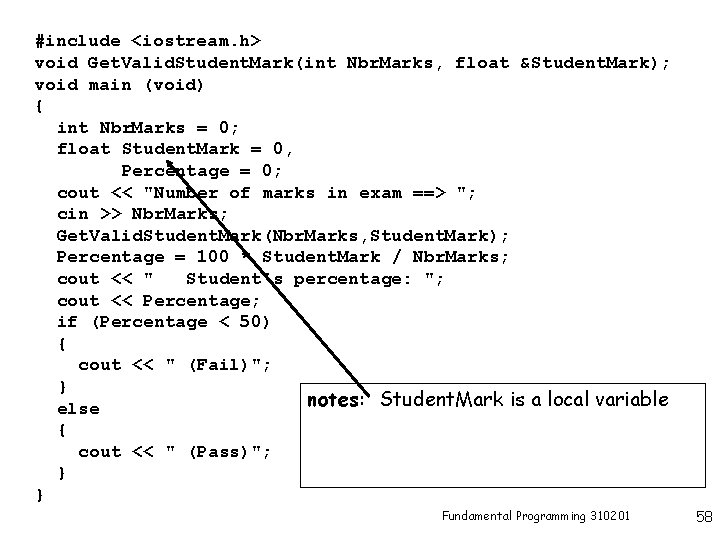
#include <iostream. h> void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void main (void) { int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; Get. Valid. Student. Mark(Nbr. Marks, Student. Mark); Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student's percentage: "; cout << Percentage; if (Percentage < 50) { cout << " (Fail)"; } notes: Student. Mark is a local variable else { cout << " (Pass)"; } } Fundamental Programming 310201 58
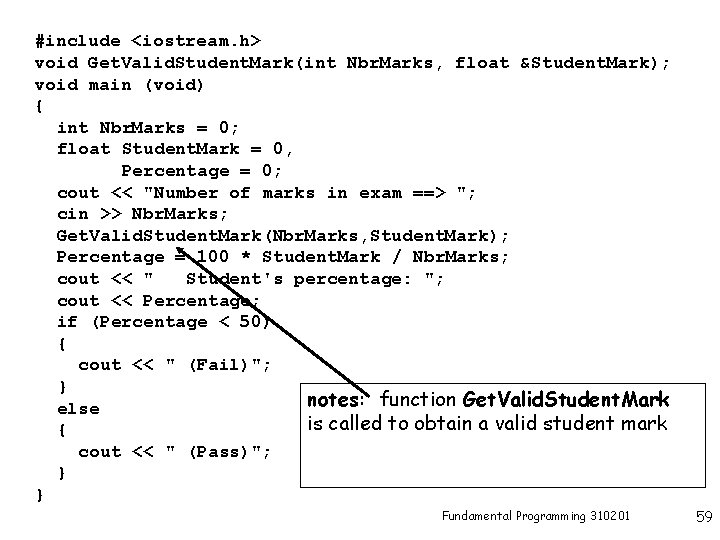
#include <iostream. h> void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void main (void) { int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; Get. Valid. Student. Mark(Nbr. Marks, Student. Mark); Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student's percentage: "; cout << Percentage; if (Percentage < 50) { cout << " (Fail)"; } notes: function Get. Valid. Student. Mark else is called to obtain a valid student mark { cout << " (Pass)"; } } Fundamental Programming 310201 59
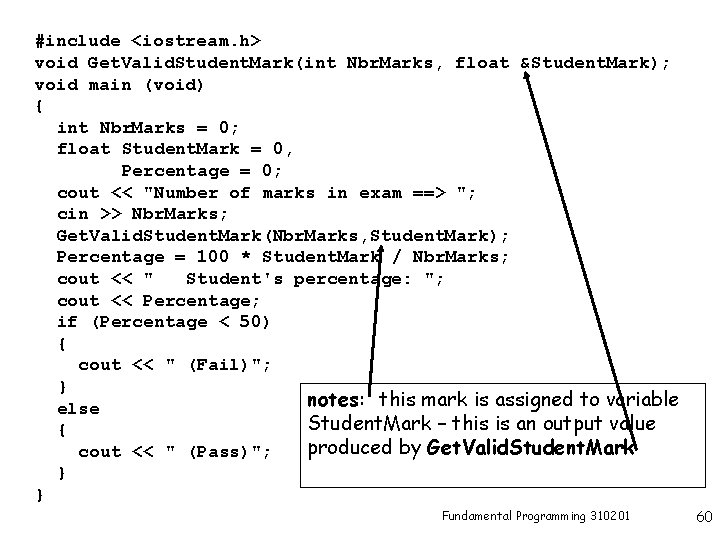
#include <iostream. h> void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void main (void) { int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; Get. Valid. Student. Mark(Nbr. Marks, Student. Mark); Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student's percentage: "; cout << Percentage; if (Percentage < 50) { cout << " (Fail)"; } notes: this mark is assigned to variable else Student. Mark – this is an output value { produced by Get. Valid. Student. Mark cout << " (Pass)"; } } Fundamental Programming 310201 60
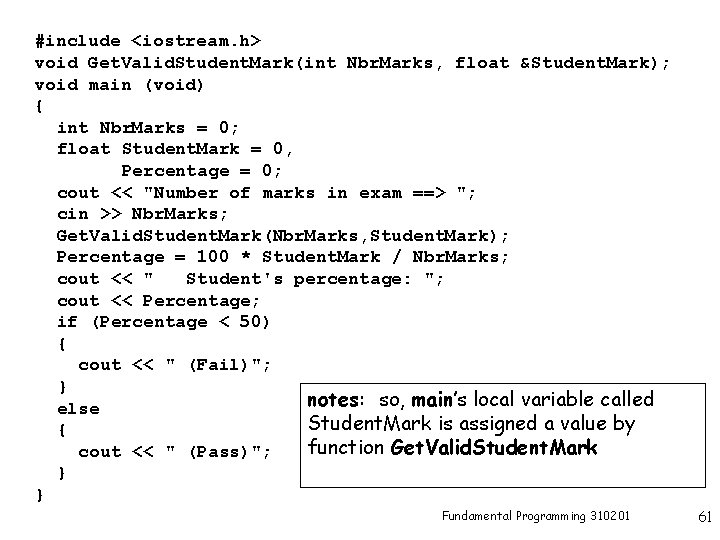
#include <iostream. h> void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void main (void) { int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; Get. Valid. Student. Mark(Nbr. Marks, Student. Mark); Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student's percentage: "; cout << Percentage; if (Percentage < 50) { cout << " (Fail)"; } notes: so, main’s local variable called else Student. Mark is assigned a value by { function Get. Valid. Student. Mark cout << " (Pass)"; } } Fundamental Programming 310201 61
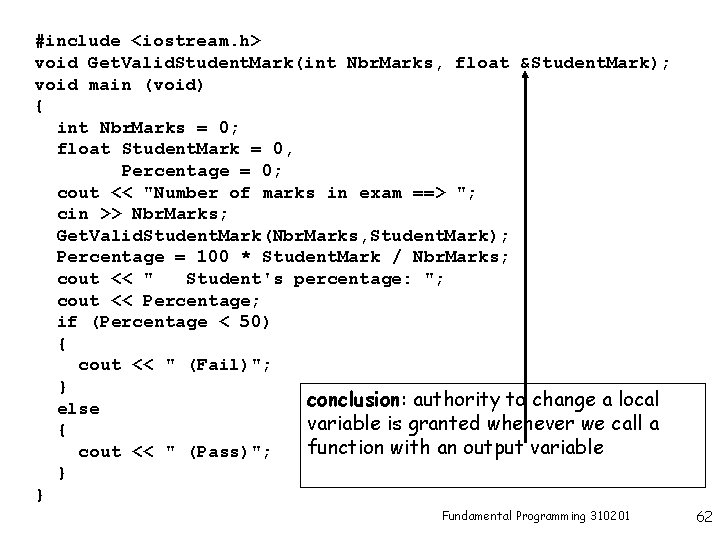
#include <iostream. h> void Get. Valid. Student. Mark(int Nbr. Marks, float &Student. Mark); void main (void) { int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; Get. Valid. Student. Mark(Nbr. Marks, Student. Mark); Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student's percentage: "; cout << Percentage; if (Percentage < 50) { cout << " (Fail)"; } conclusion: authority to change a local else variable is granted whenever we call a { function with an output variable cout << " (Pass)"; } } Fundamental Programming 310201 62
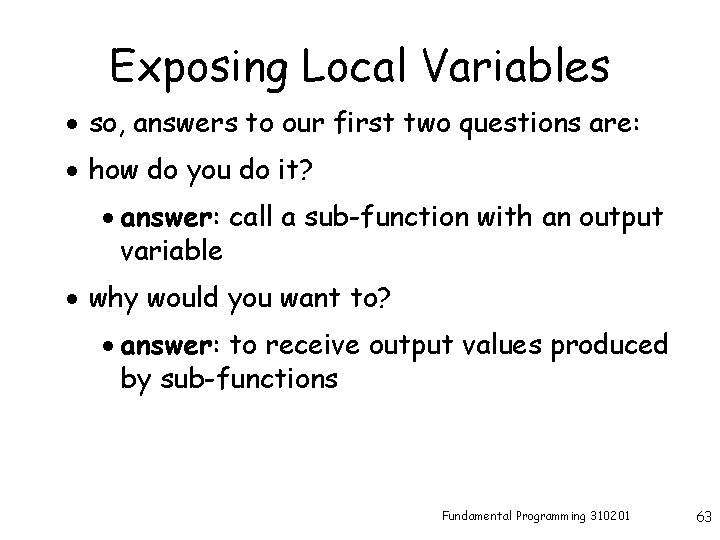
Exposing Local Variables · so, answers to our first two questions are: · how do you do it? · answer: call a sub-function with an output variable · why would you want to? · answer: to receive output values produced by sub-functions Fundamental Programming 310201 63
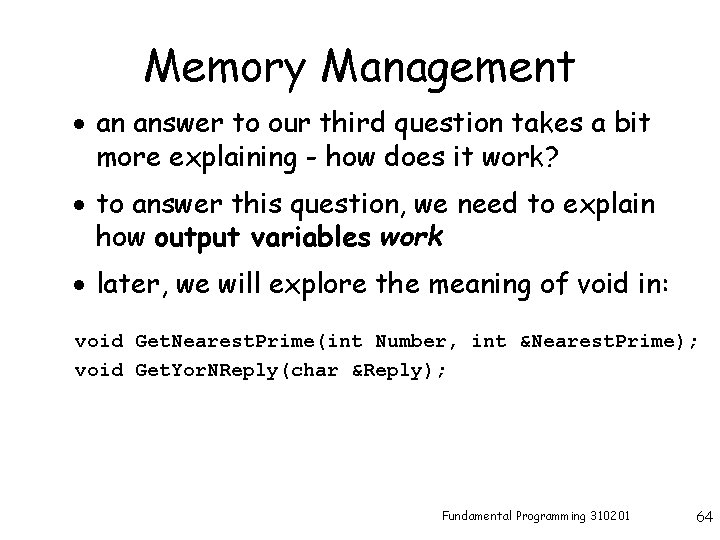
Memory Management · an answer to our third question takes a bit more explaining - how does it work? · to answer this question, we need to explain how output variables work · later, we will explore the meaning of void in: void Get. Nearest. Prime(int Number, int &Nearest. Prime); void Get. Yor. NReply(char &Reply); Fundamental Programming 310201 64
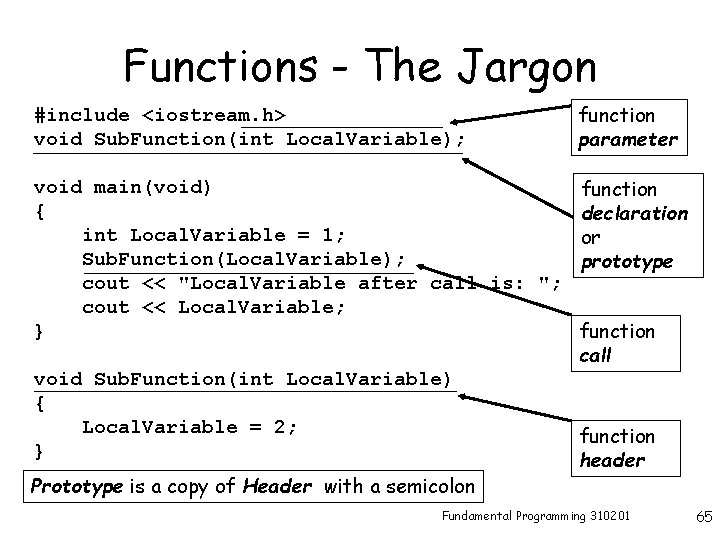
Functions - The Jargon #include <iostream. h> void Sub. Function(int Local. Variable); function parameter void main(void) function { declaration int Local. Variable = 1; or Sub. Function(Local. Variable); prototype cout << "Local. Variable after call is: "; cout << Local. Variable; } function call void Sub. Function(int Local. Variable) { Local. Variable = 2; function } header Prototype is a copy of Header with a semicolon Fundamental Programming 310201 65
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
What is system program
Linear vs integer programming
Definisi linear
Types of functions in programming
Absolute value piecewise function
Evaluating functions
Evaluating functions and operations on functions
Introduction to server side programming
Java introduction to problem solving and programming
Introduction to programming languages
Elementary programming in java
An introduction to parallel programming peter pacheco
Intro to visual basic
What does plc stands for
Java introduction to problem solving and programming
Console programming
Programming language
Csc102
A web based introduction to programming
Sic/xe programming examples
Computer programming chapter 1
C programming and numerical analysis an introduction
Introduction to visual basic programming
Scratch programming concepts
Python programming an introduction to computer science
Java introduction to problem solving and programming
Computer programming chapter 1
Introduction to java programming 10th edition quizzes
Introduction to sql programming techniques
Introduction to sql programming techniques
Chapter 1 introduction to computers and programming
Chapter 1 introduction to computers and programming
Introduction to quadratic function
Piecewise function generator
Horizontal domain
Quiz 2-3 parent functions transformations graphing
Introduction to functions (review game)
Section 3 introduction to functions
Identify the parent function.
Introduction to logarithmic functions
Conclusion paragraph format
Image formation model in digital image processing ppt
7 fundamental units
Antecedents of modern quality management
40h6 tolerance
Fundamental theorem of algebra
Calculus theorems
Fundamental theorem
Four fundamental forces
Four fundamental forces
Teoremas de la circunferencia
Teorema fundamental kalkulus 2
Teorema fundamental del algebra
Cos 90
Tabela de entes trigonométricos
Projeto de vida - tecendo relações
Principio fundamental del conteo
Fundamental theorem of statistics
Si standard units
Fundamental deviation
Fundamental theorem of calculus
Basic understanging of the problem is established in
Aktivitas fundamental dari proses perangkat lunak
Revisión fundamental