310201 Fundamental Programming Introduction to Multidimensional Arrays Slide
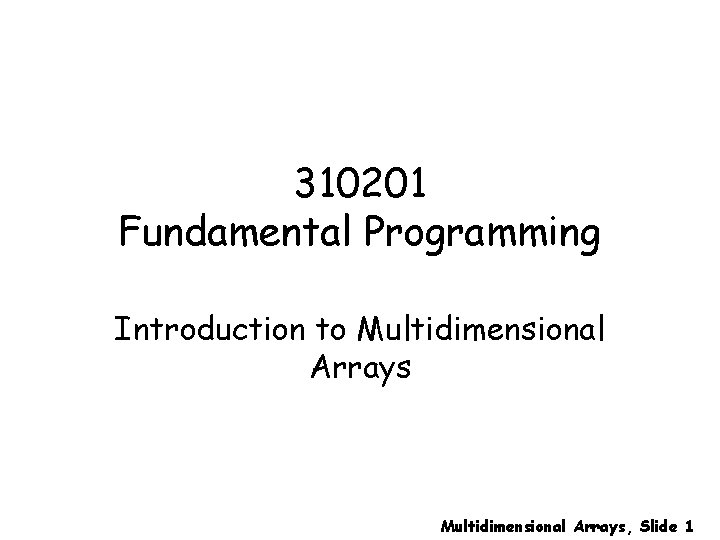
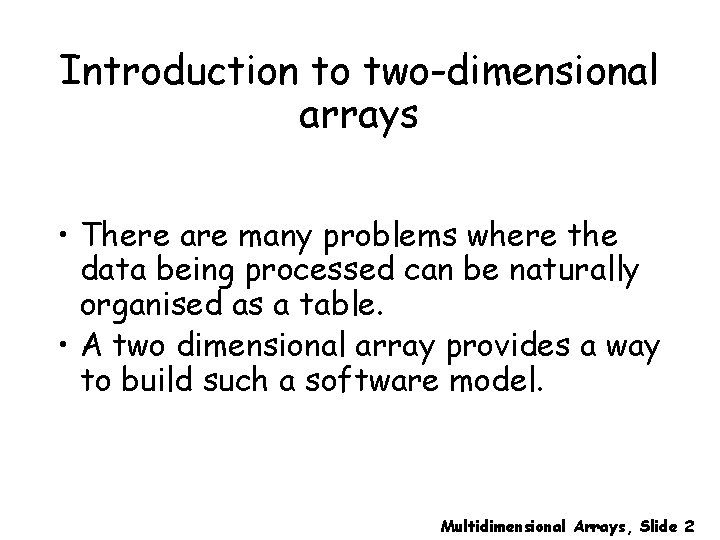
![A 2 D Array <Type of array> <Array Name> [<num rows>] [<num columns>] • A 2 D Array <Type of array> <Array Name> [<num rows>] [<num columns>] •](https://slidetodoc.com/presentation_image_h2/42bc1676f3178279065fd4389c926b83/image-3.jpg)
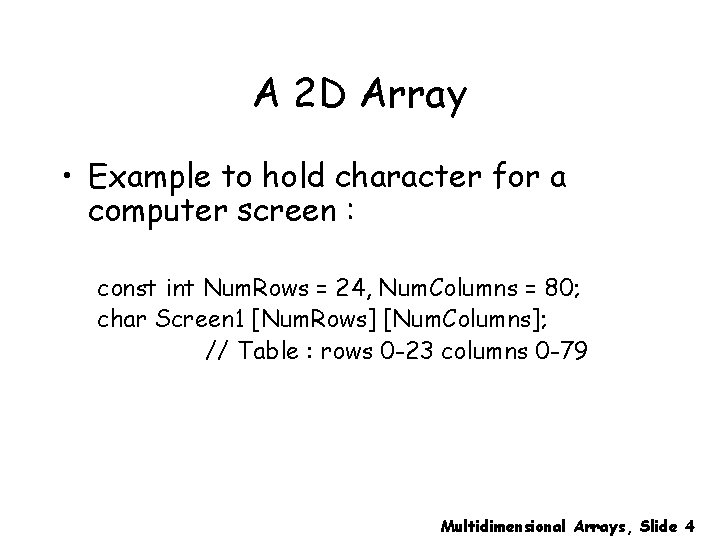
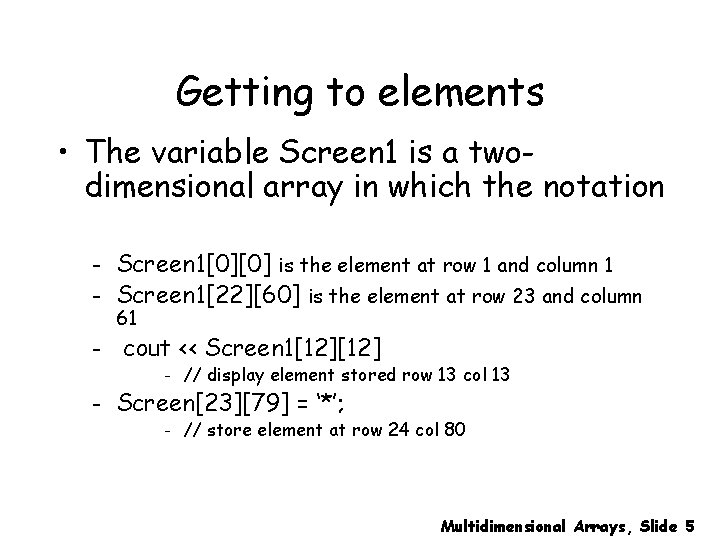
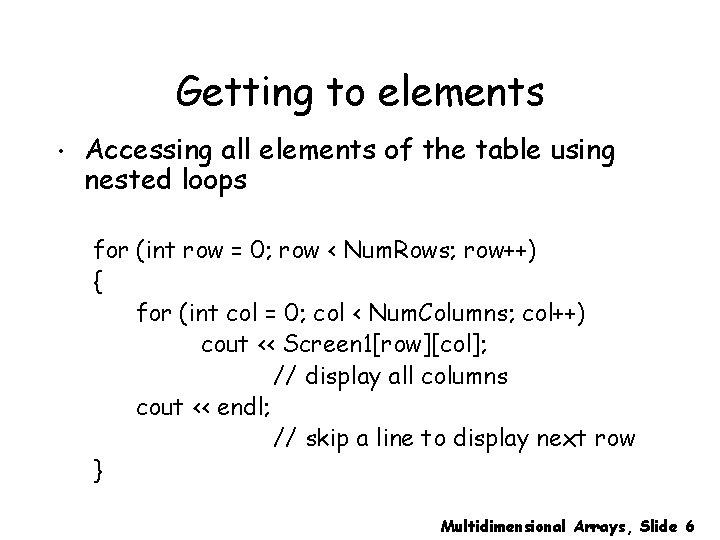
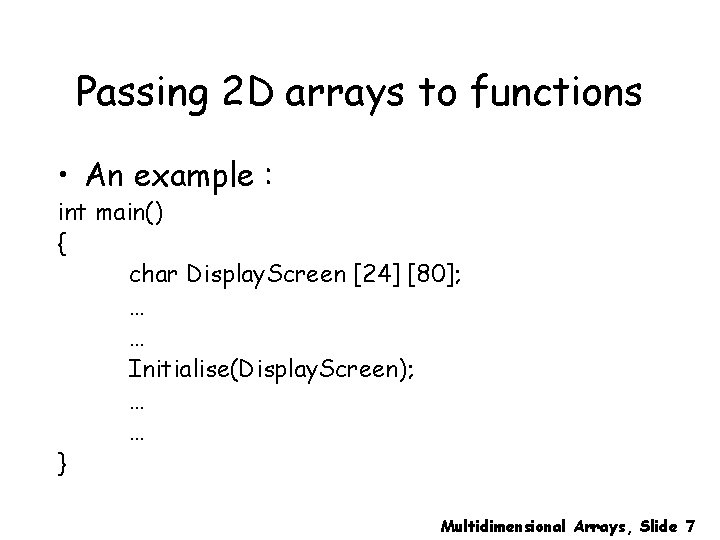
![Passing 2 D arrays to functions void Initialise(Screen [ ]) // Initialise the Screen Passing 2 D arrays to functions void Initialise(Screen [ ]) // Initialise the Screen](https://slidetodoc.com/presentation_image_h2/42bc1676f3178279065fd4389c926b83/image-8.jpg)
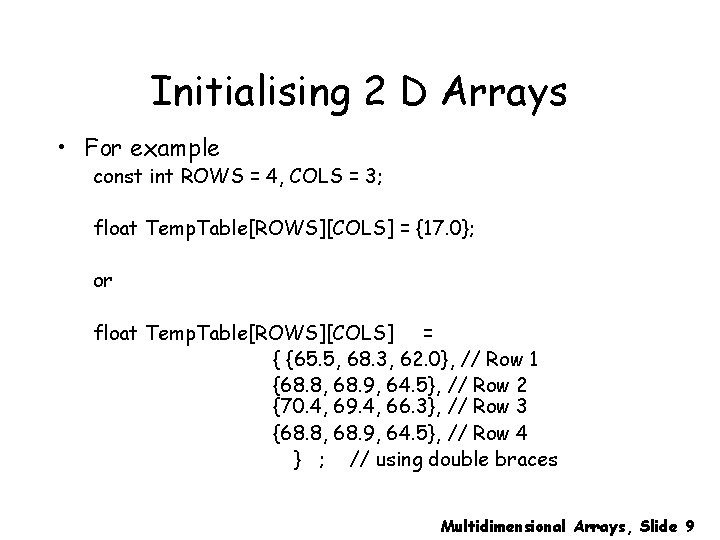
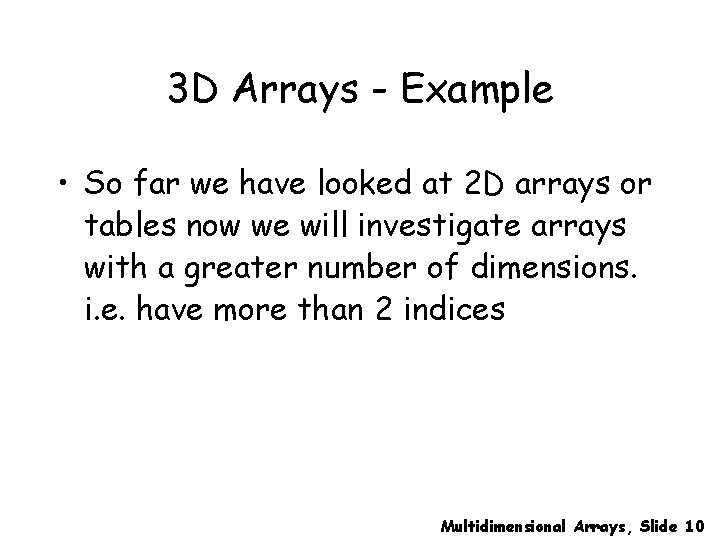
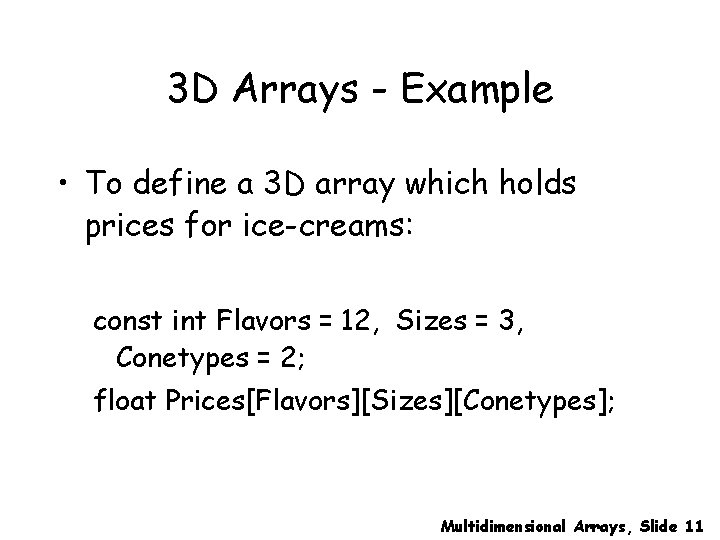
![3 D Arrays - Example • To address individual elements cout << Prices[8][2][1]; // 3 D Arrays - Example • To address individual elements cout << Prices[8][2][1]; //](https://slidetodoc.com/presentation_image_h2/42bc1676f3178279065fd4389c926b83/image-12.jpg)
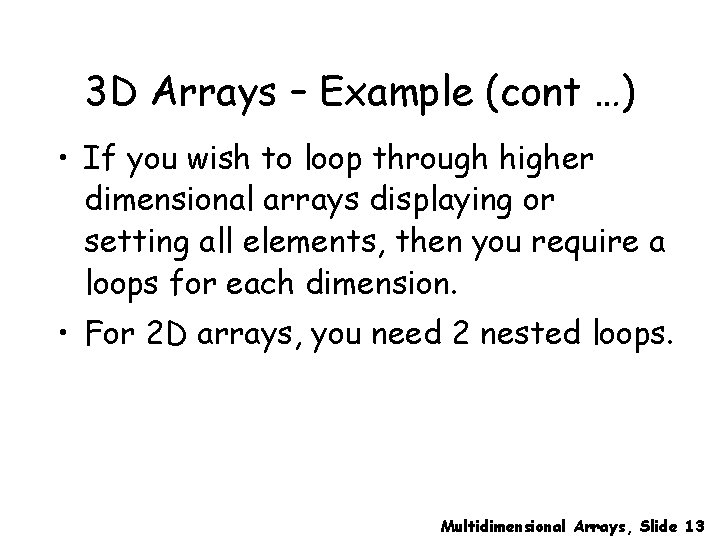
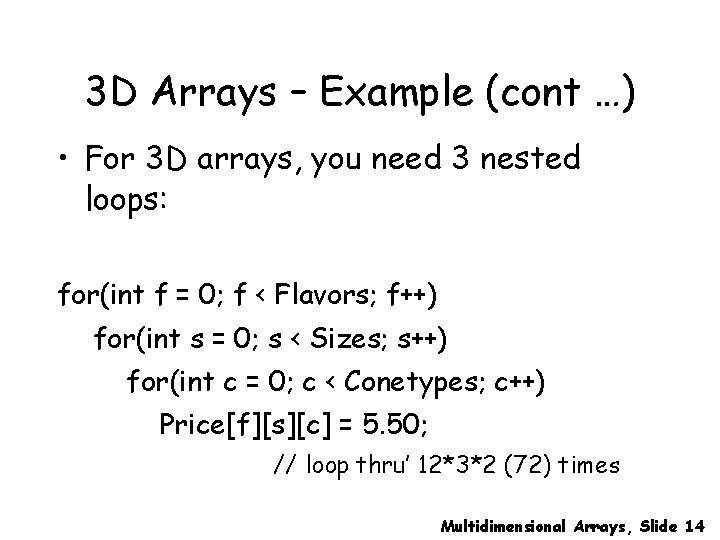
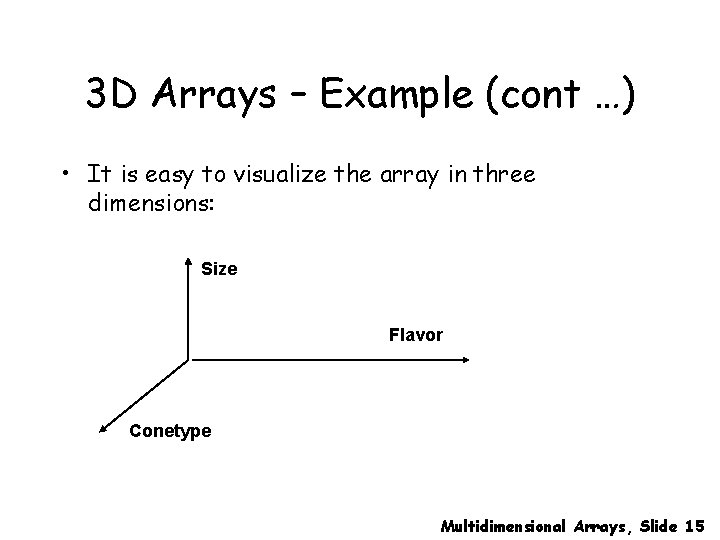
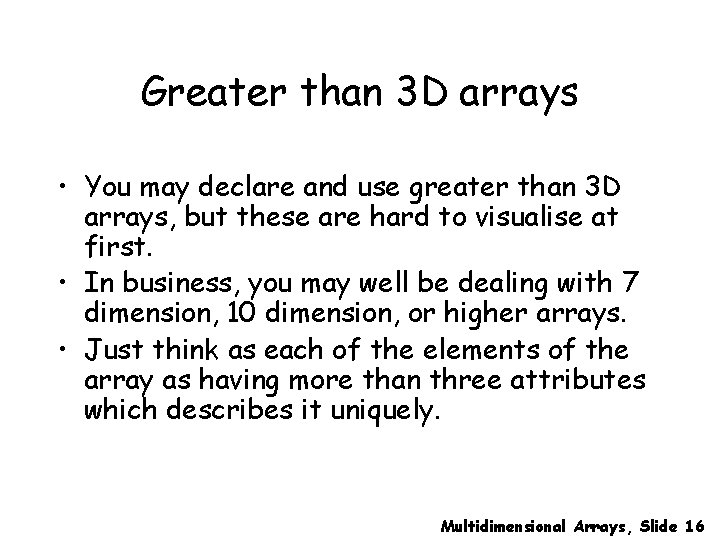
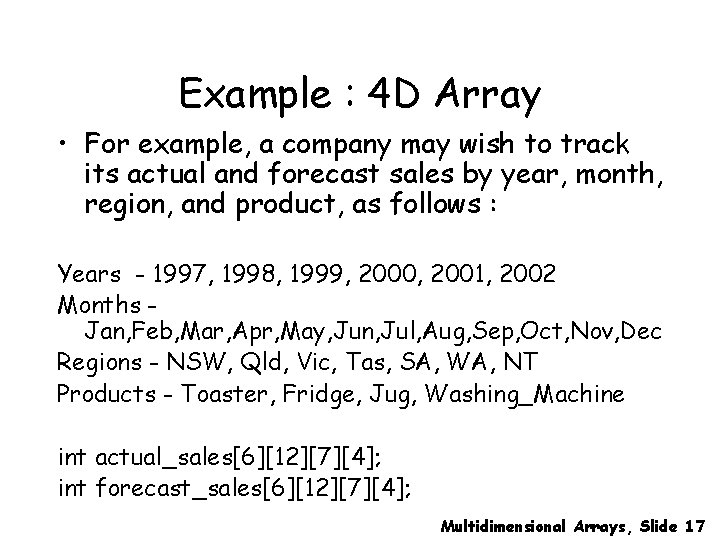
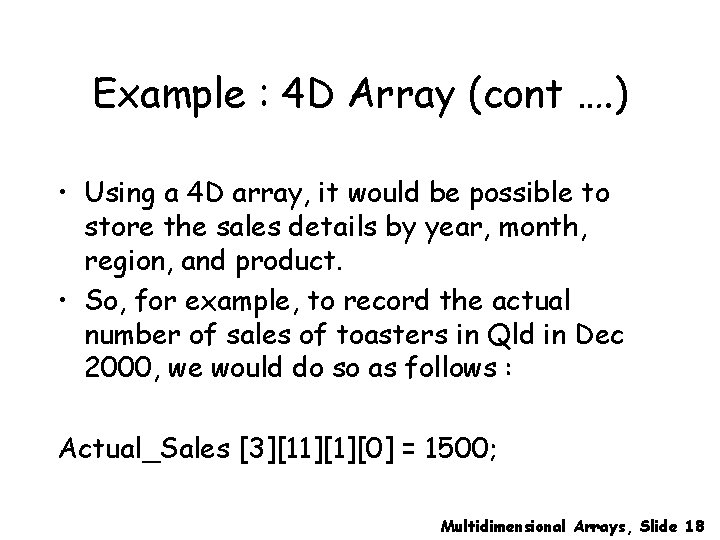
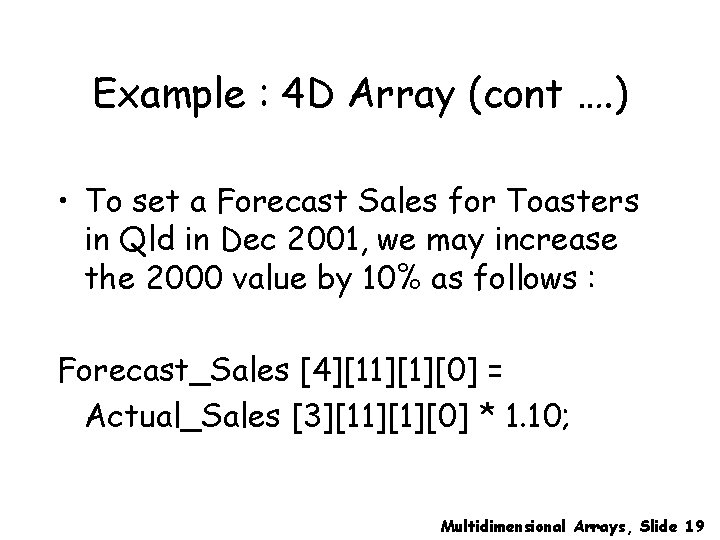
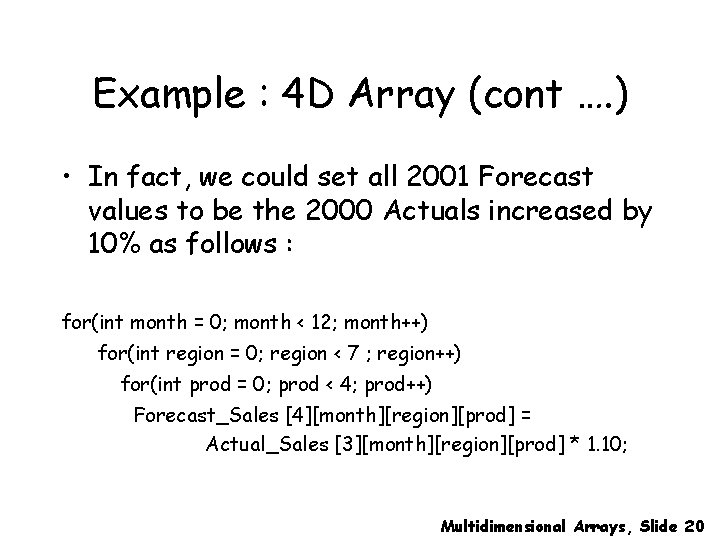
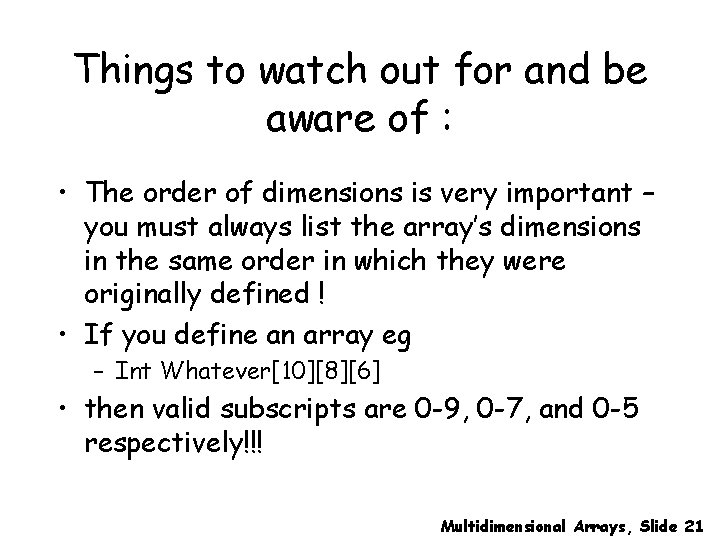
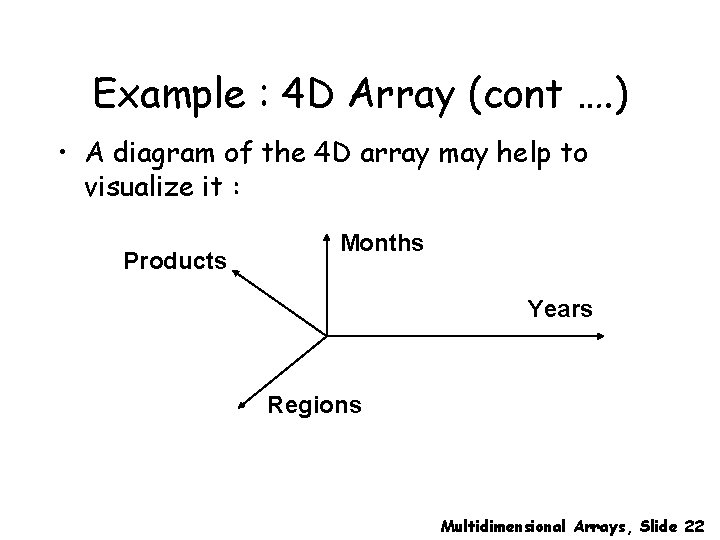
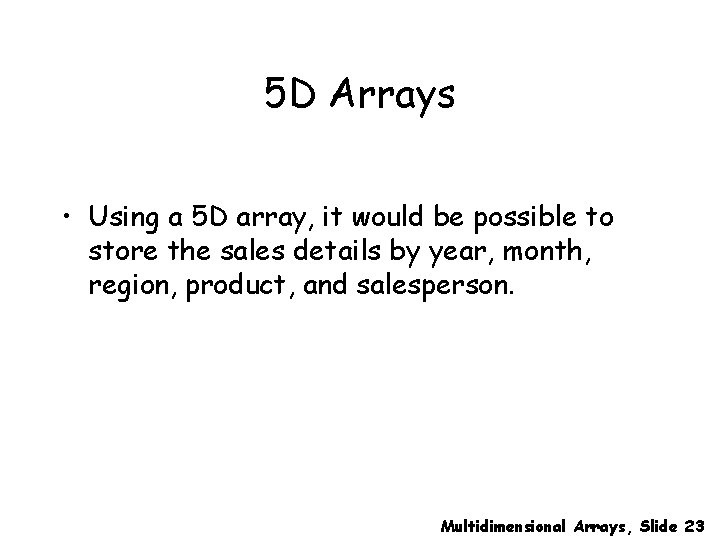
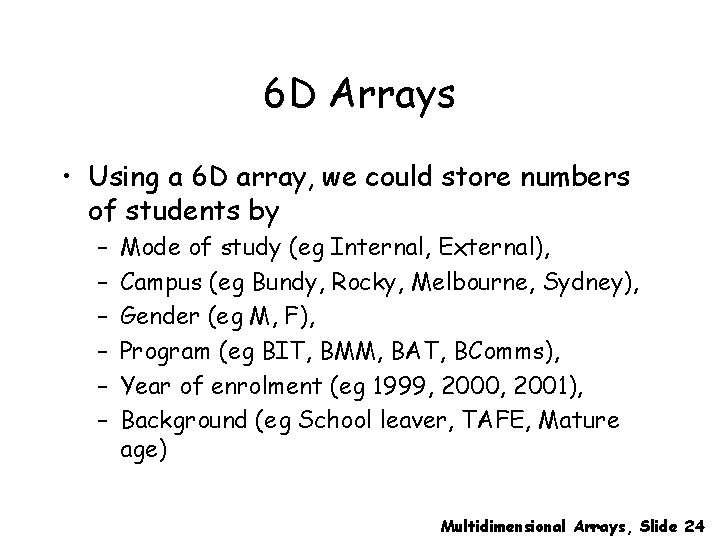
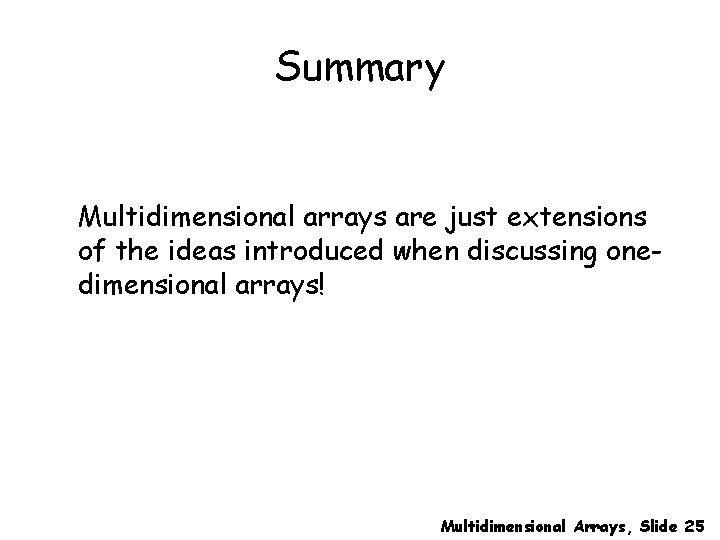
- Slides: 25
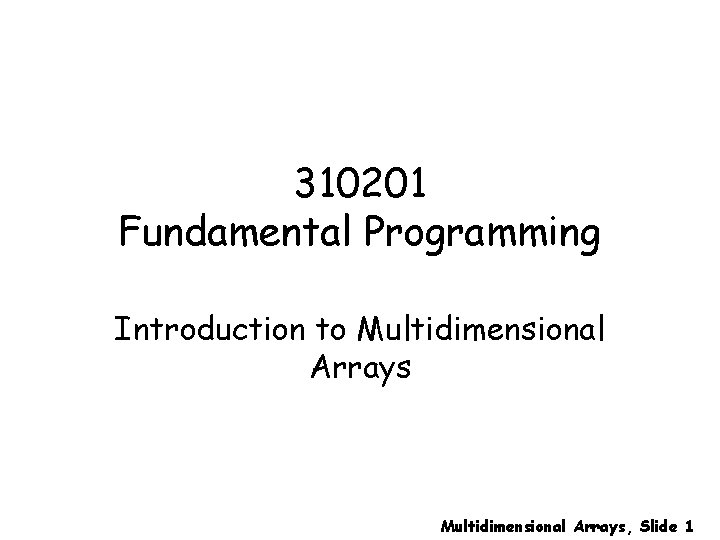
310201 Fundamental Programming Introduction to Multidimensional Arrays, Slide 1
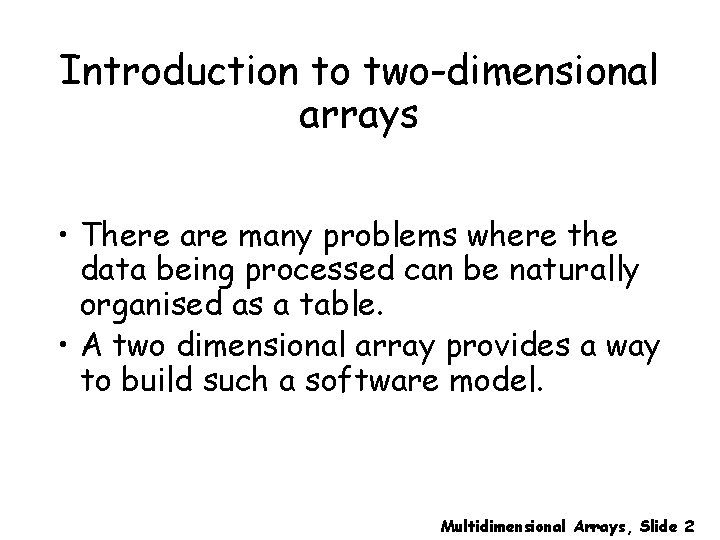
Introduction to two-dimensional arrays • There are many problems where the data being processed can be naturally organised as a table. • A two dimensional array provides a way to build such a software model. Multidimensional Arrays, Slide 2
![A 2 D Array Type of array Array Name num rows num columns A 2 D Array <Type of array> <Array Name> [<num rows>] [<num columns>] •](https://slidetodoc.com/presentation_image_h2/42bc1676f3178279065fd4389c926b83/image-3.jpg)
A 2 D Array <Type of array> <Array Name> [<num rows>] [<num columns>] • Example : float Sales_Array [3] [12] // Hold 3 years of monthly sales data. Multidimensional Arrays, Slide 3
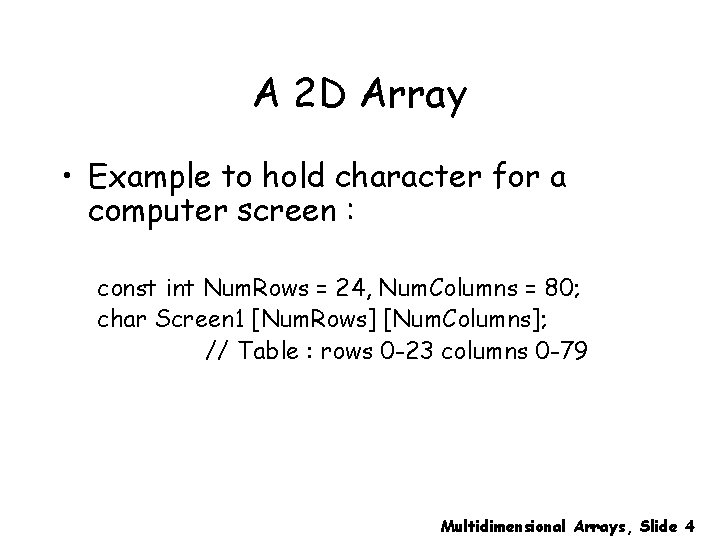
A 2 D Array • Example to hold character for a computer screen : const int Num. Rows = 24, Num. Columns = 80; char Screen 1 [Num. Rows] [Num. Columns]; // Table : rows 0 -23 columns 0 -79 Multidimensional Arrays, Slide 4
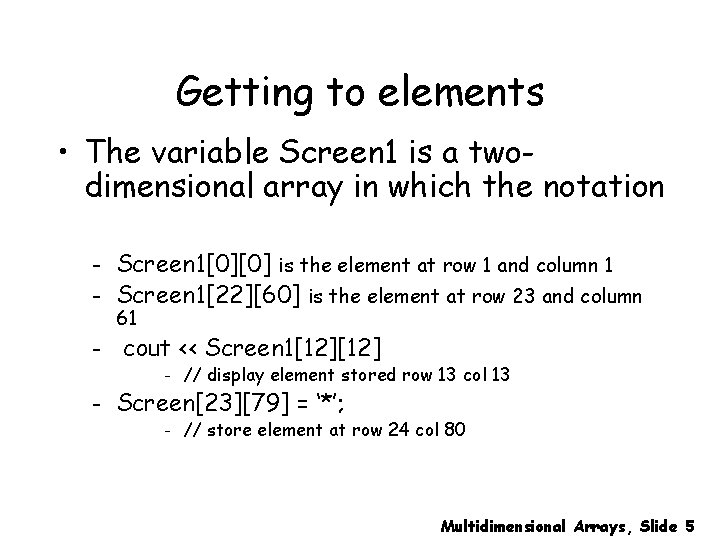
Getting to elements • The variable Screen 1 is a twodimensional array in which the notation – – Screen 1[0][0] is the element at row 1 and column 1 Screen 1[22][60] is the element at row 23 and column 61 cout << Screen 1[12] – // display element stored row 13 col 13 – // store element at row 24 col 80 Screen[23][79] = ‘*’; Multidimensional Arrays, Slide 5
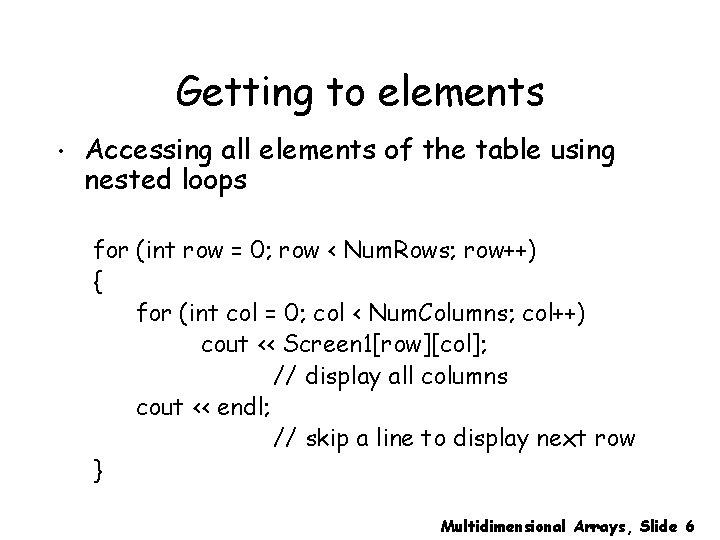
Getting to elements • Accessing all elements of the table using nested loops for (int row = 0; row < Num. Rows; row++) { for (int col = 0; col < Num. Columns; col++) cout << Screen 1[row][col]; // display all columns cout << endl; // skip a line to display next row } Multidimensional Arrays, Slide 6
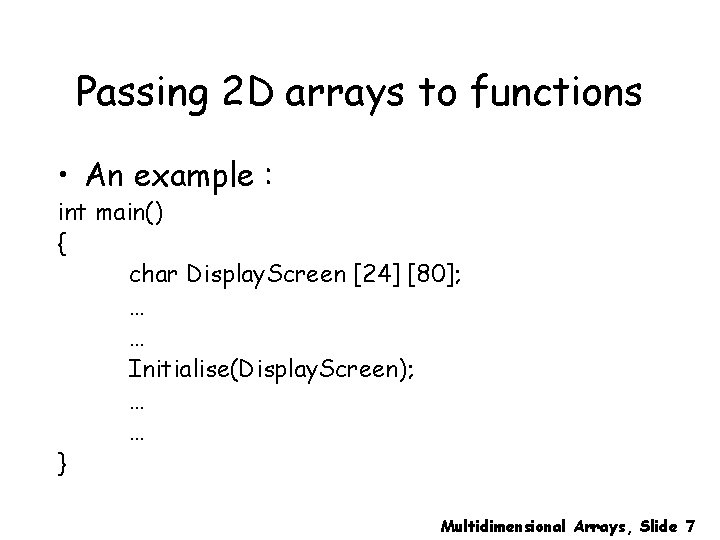
Passing 2 D arrays to functions • An example : int main() { char Display. Screen [24] [80]; … … Initialise(Display. Screen); … … } Multidimensional Arrays, Slide 7
![Passing 2 D arrays to functions void InitialiseScreen Initialise the Screen Passing 2 D arrays to functions void Initialise(Screen [ ]) // Initialise the Screen](https://slidetodoc.com/presentation_image_h2/42bc1676f3178279065fd4389c926b83/image-8.jpg)
Passing 2 D arrays to functions void Initialise(Screen [ ]) // Initialise the Screen Array – set all values to spaces. { } for (int row = 0; row < Num. Rows; row++) for (int col = 0; col < Num. Columns; col++) Screen[row][col] = ‘ ‘; Multidimensional Arrays, Slide 8
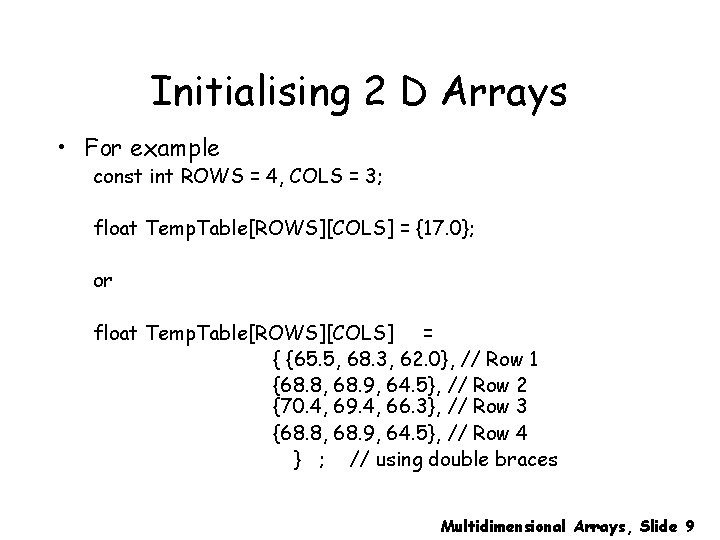
Initialising 2 D Arrays • For example const int ROWS = 4, COLS = 3; float Temp. Table[ROWS][COLS] = {17. 0}; or float Temp. Table[ROWS][COLS] = { {65. 5, 68. 3, 62. 0}, // Row 1 {68. 8, 68. 9, 64. 5}, // Row 2 {70. 4, 69. 4, 66. 3}, // Row 3 {68. 8, 68. 9, 64. 5}, // Row 4 } ; // using double braces Multidimensional Arrays, Slide 9
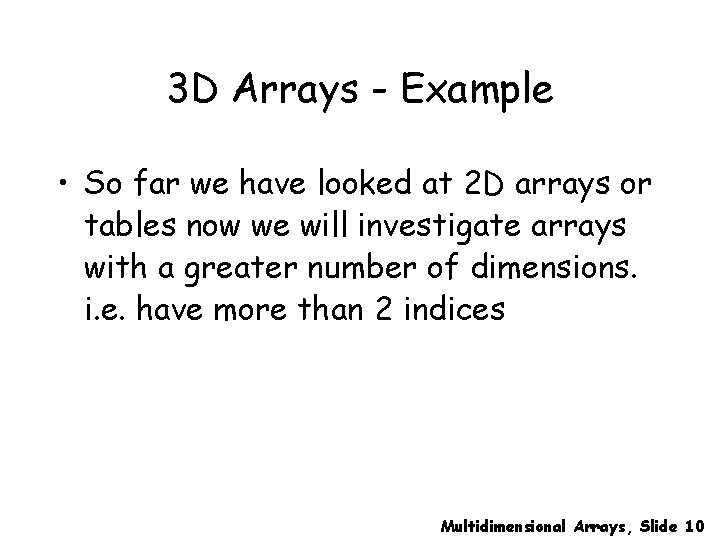
3 D Arrays - Example • So far we have looked at 2 D arrays or tables now we will investigate arrays with a greater number of dimensions. i. e. have more than 2 indices Multidimensional Arrays, Slide 10
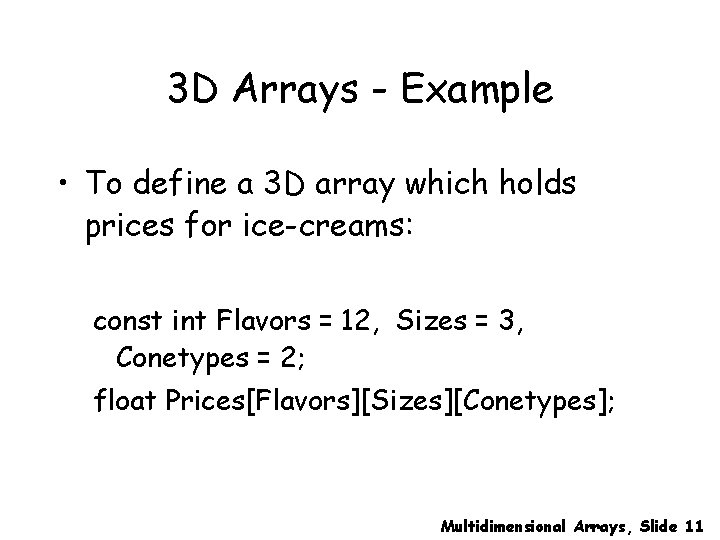
3 D Arrays - Example • To define a 3 D array which holds prices for ice-creams: const int Flavors = 12, Sizes = 3, Conetypes = 2; float Prices[Flavors][Sizes][Conetypes]; Multidimensional Arrays, Slide 11
![3 D Arrays Example To address individual elements cout Prices821 3 D Arrays - Example • To address individual elements cout << Prices[8][2][1]; //](https://slidetodoc.com/presentation_image_h2/42bc1676f3178279065fd4389c926b83/image-12.jpg)
3 D Arrays - Example • To address individual elements cout << Prices[8][2][1]; // price of flavor 9, size 3, conetype 2. Price[10][0][1] = 4. 75; // price of flavor 11, size 1, conetype 2. Multidimensional Arrays, Slide 12
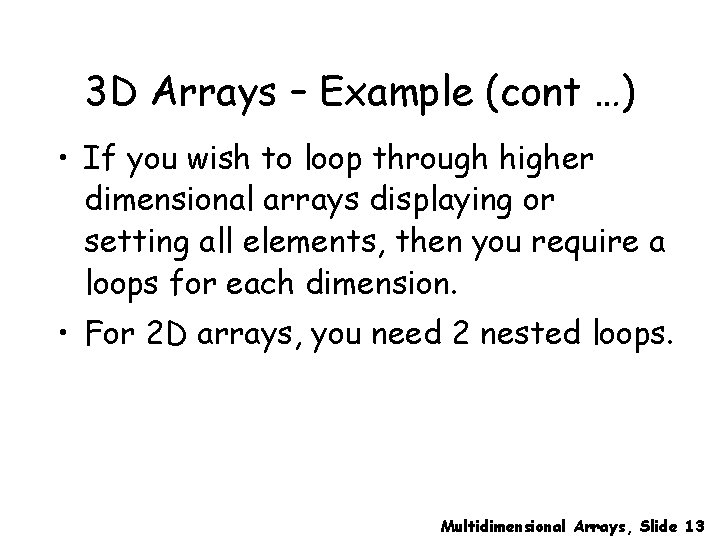
3 D Arrays – Example (cont …) • If you wish to loop through higher dimensional arrays displaying or setting all elements, then you require a loops for each dimension. • For 2 D arrays, you need 2 nested loops. Multidimensional Arrays, Slide 13
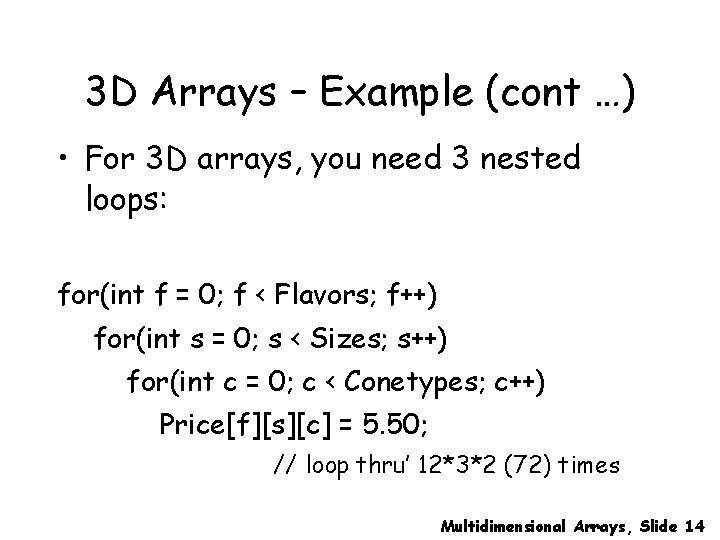
3 D Arrays – Example (cont …) • For 3 D arrays, you need 3 nested loops: for(int f = 0; f < Flavors; f++) for(int s = 0; s < Sizes; s++) for(int c = 0; c < Conetypes; c++) Price[f][s][c] = 5. 50; // loop thru’ 12*3*2 (72) times Multidimensional Arrays, Slide 14
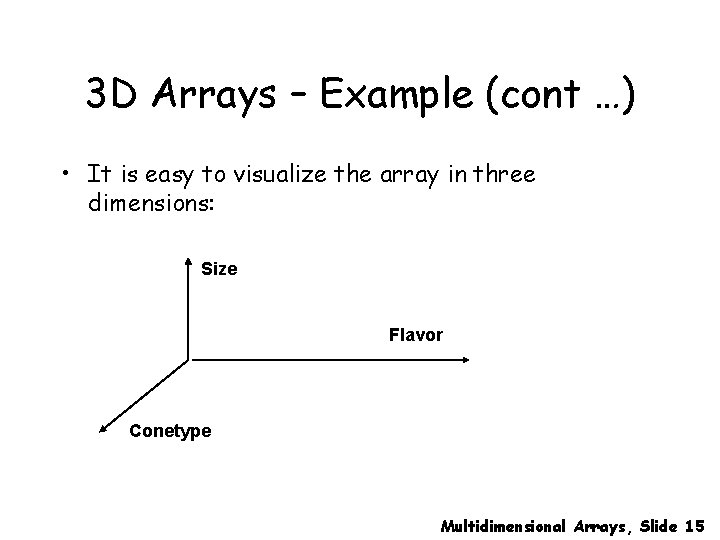
3 D Arrays – Example (cont …) • It is easy to visualize the array in three dimensions: Size Flavor Conetype Multidimensional Arrays, Slide 15
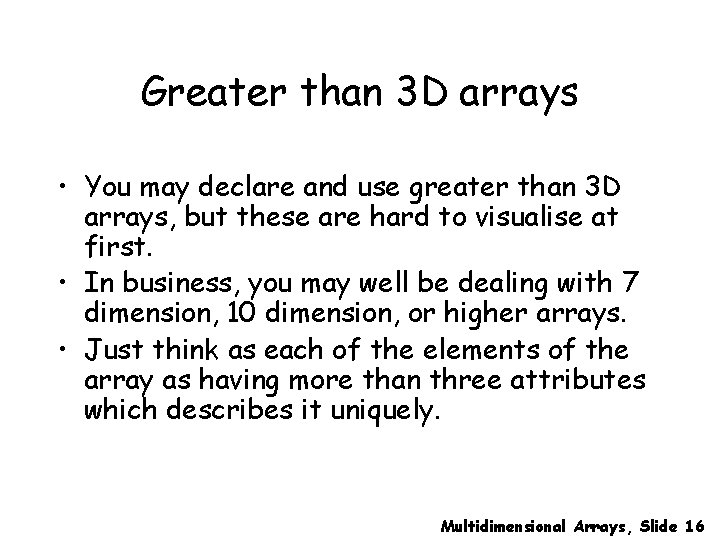
Greater than 3 D arrays • You may declare and use greater than 3 D arrays, but these are hard to visualise at first. • In business, you may well be dealing with 7 dimension, 10 dimension, or higher arrays. • Just think as each of the elements of the array as having more than three attributes which describes it uniquely. Multidimensional Arrays, Slide 16
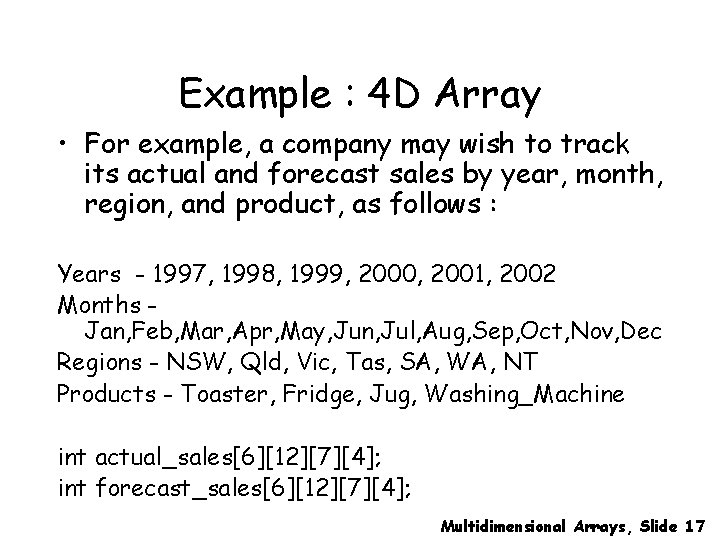
Example : 4 D Array • For example, a company may wish to track its actual and forecast sales by year, month, region, and product, as follows : Years - 1997, 1998, 1999, 2000, 2001, 2002 Months Jan, Feb, Mar, Apr, May, Jun, Jul, Aug, Sep, Oct, Nov, Dec Regions - NSW, Qld, Vic, Tas, SA, WA, NT Products - Toaster, Fridge, Jug, Washing_Machine int actual_sales[6][12][7][4]; int forecast_sales[6][12][7][4]; Multidimensional Arrays, Slide 17
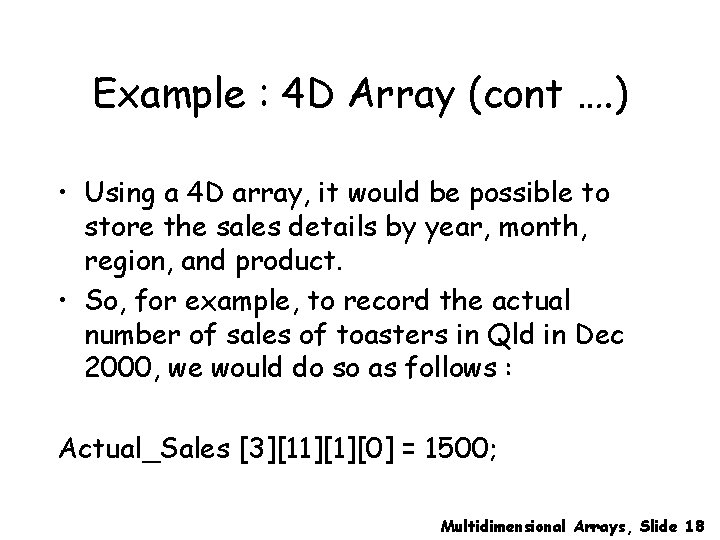
Example : 4 D Array (cont …. ) • Using a 4 D array, it would be possible to store the sales details by year, month, region, and product. • So, for example, to record the actual number of sales of toasters in Qld in Dec 2000, we would do so as follows : Actual_Sales [3][11][1][0] = 1500; Multidimensional Arrays, Slide 18
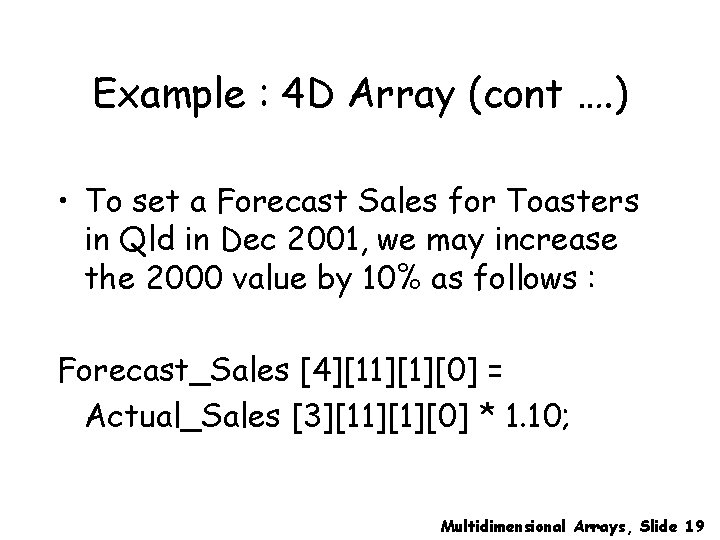
Example : 4 D Array (cont …. ) • To set a Forecast Sales for Toasters in Qld in Dec 2001, we may increase the 2000 value by 10% as follows : Forecast_Sales [4][11][1][0] = Actual_Sales [3][11][1][0] * 1. 10; Multidimensional Arrays, Slide 19
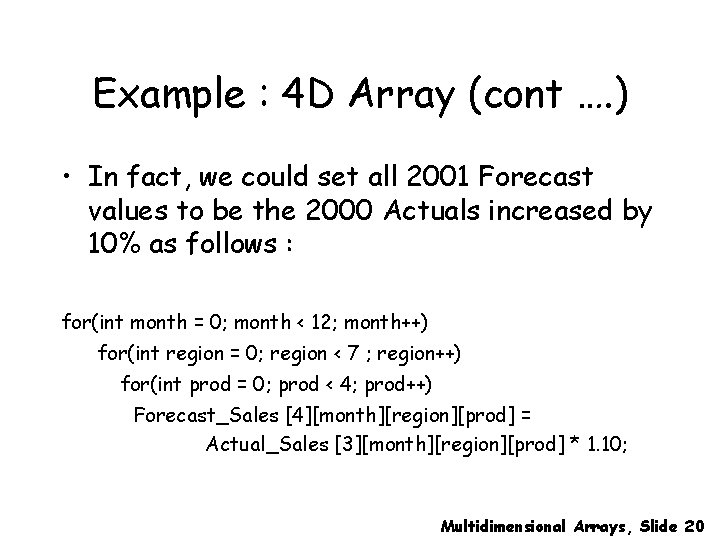
Example : 4 D Array (cont …. ) • In fact, we could set all 2001 Forecast values to be the 2000 Actuals increased by 10% as follows : for(int month = 0; month < 12; month++) for(int region = 0; region < 7 ; region++) for(int prod = 0; prod < 4; prod++) Forecast_Sales [4][month][region][prod] = Actual_Sales [3][month][region][prod] * 1. 10; Multidimensional Arrays, Slide 20
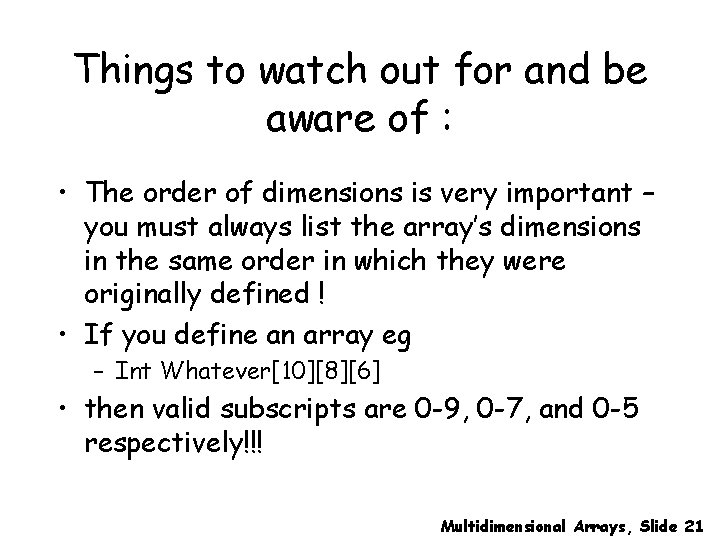
Things to watch out for and be aware of : • The order of dimensions is very important – you must always list the array’s dimensions in the same order in which they were originally defined ! • If you define an array eg – Int Whatever[10][8][6] • then valid subscripts are 0 -9, 0 -7, and 0 -5 respectively!!! Multidimensional Arrays, Slide 21
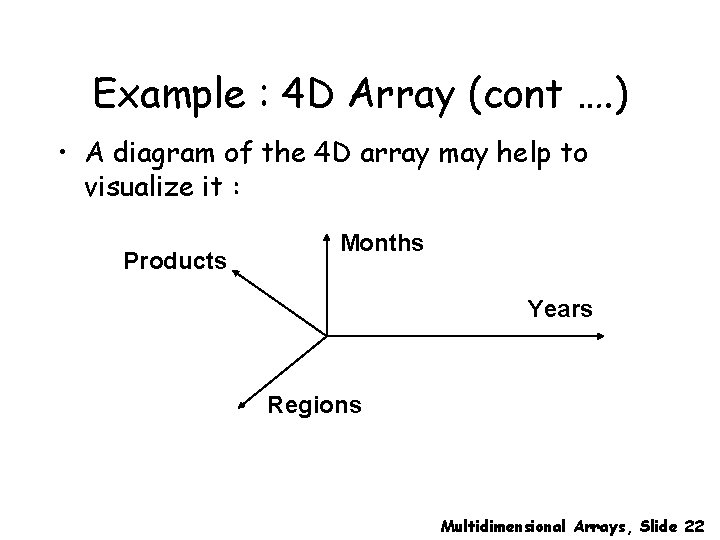
Example : 4 D Array (cont …. ) • A diagram of the 4 D array may help to visualize it : Products Months Years Regions Multidimensional Arrays, Slide 22
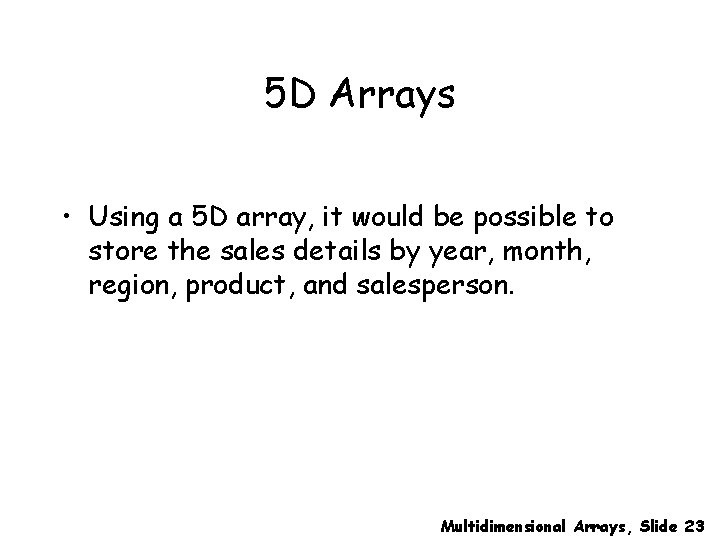
5 D Arrays • Using a 5 D array, it would be possible to store the sales details by year, month, region, product, and salesperson. Multidimensional Arrays, Slide 23
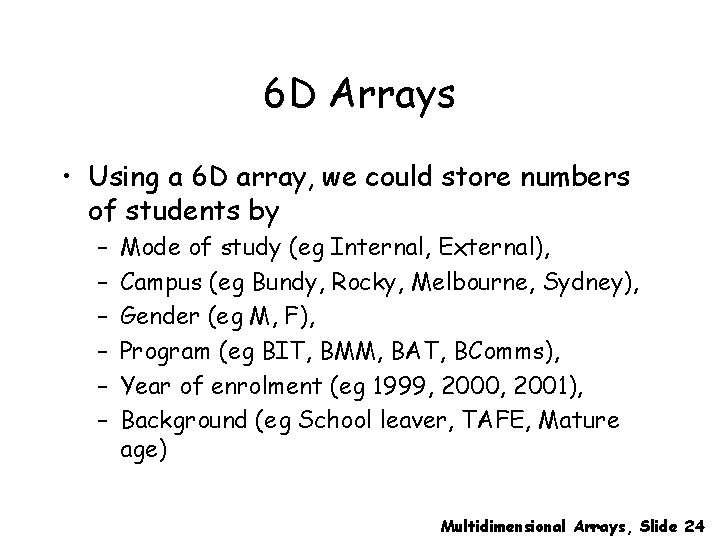
6 D Arrays • Using a 6 D array, we could store numbers of students by – – – Mode of study (eg Internal, External), Campus (eg Bundy, Rocky, Melbourne, Sydney), Gender (eg M, F), Program (eg BIT, BMM, BAT, BComms), Year of enrolment (eg 1999, 2000, 2001), Background (eg School leaver, TAFE, Mature age) Multidimensional Arrays, Slide 24
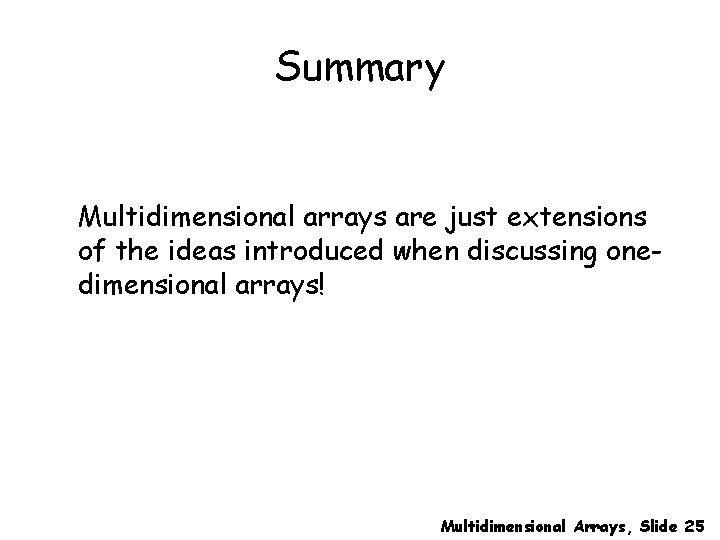
Summary Multidimensional arrays are just extensions of the ideas introduced when discussing onedimensional arrays! Multidimensional Arrays, Slide 25