310201 Fundamental Programming More on Arrays Slide 1
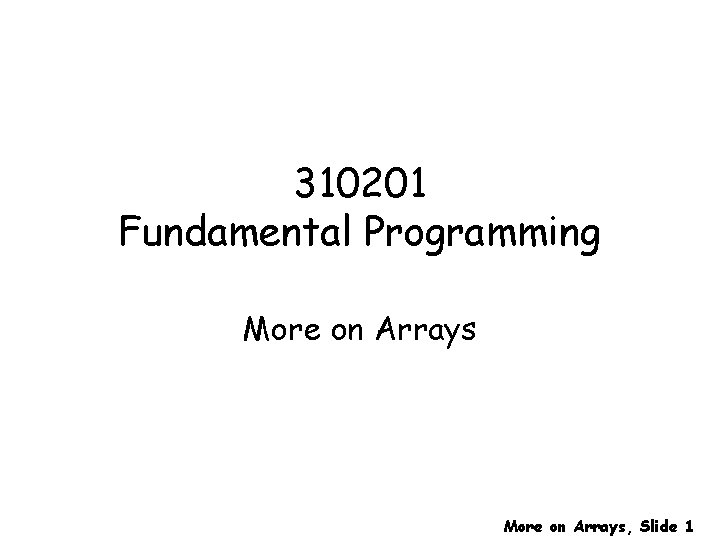
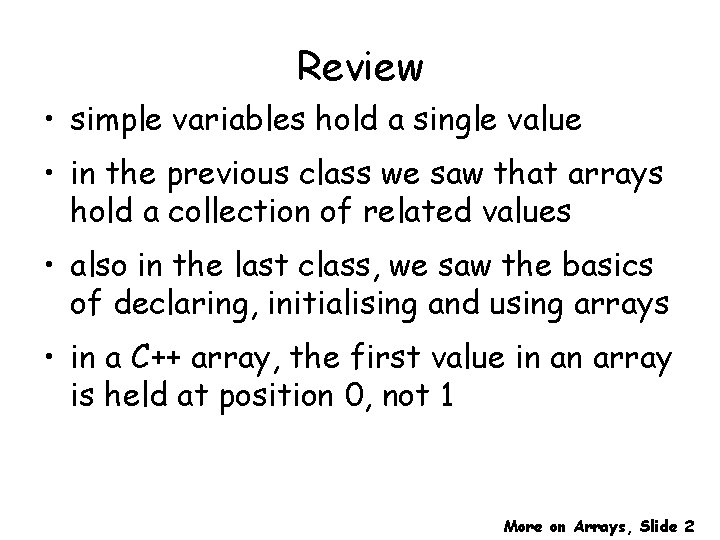
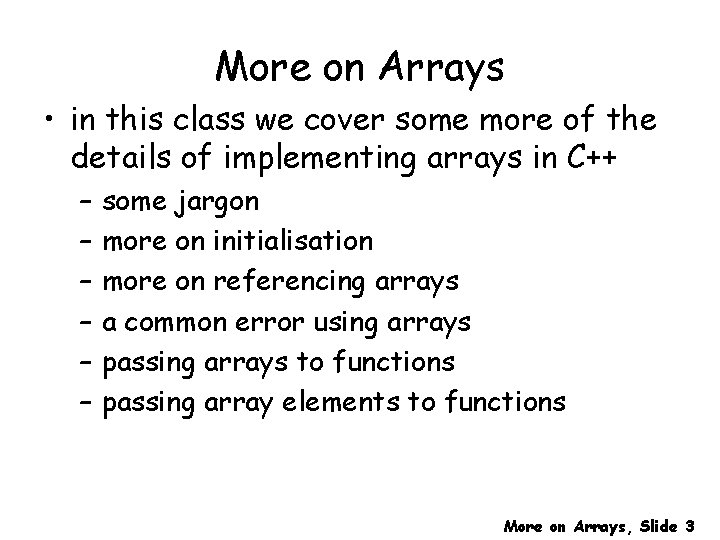
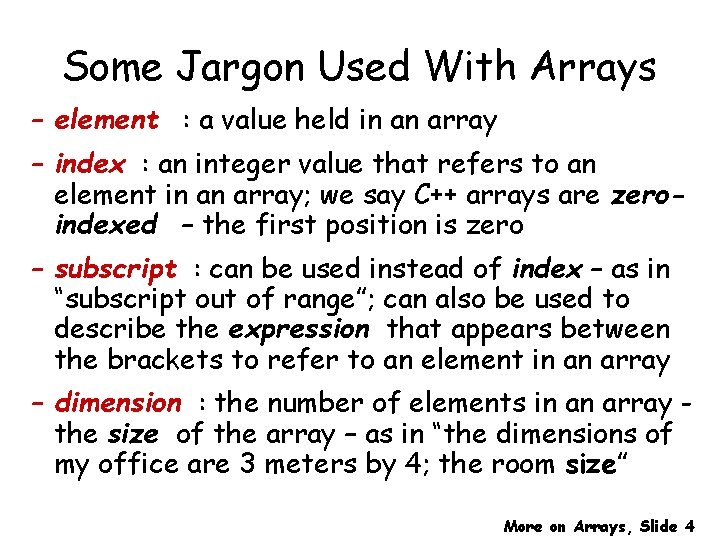
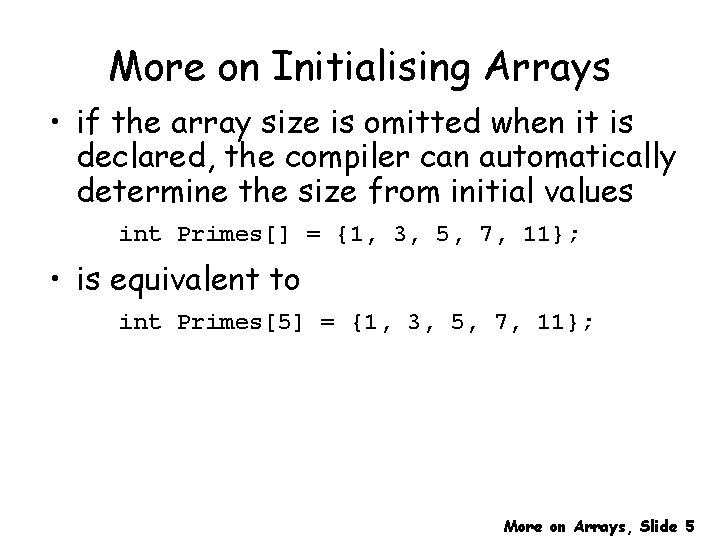
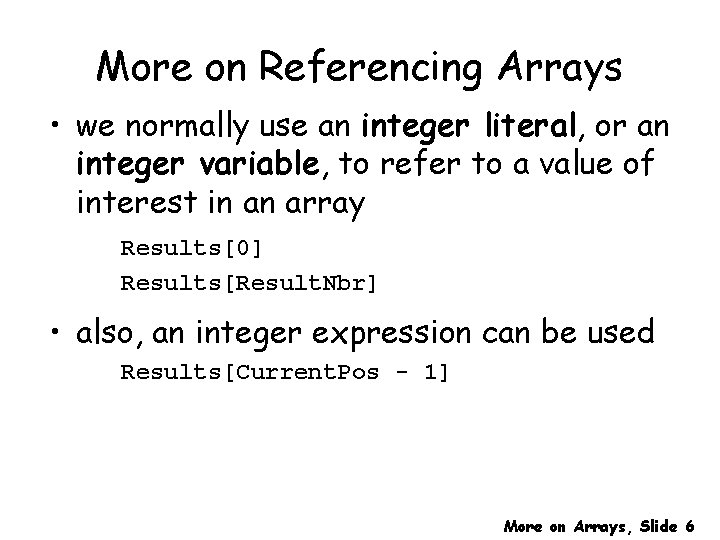
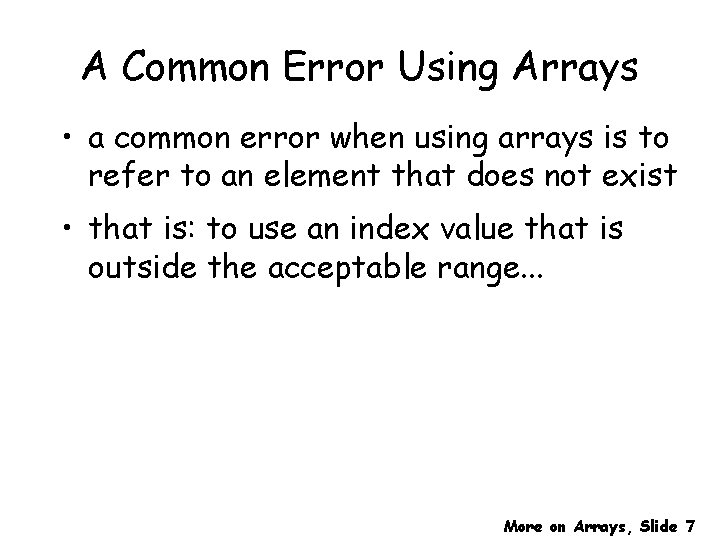
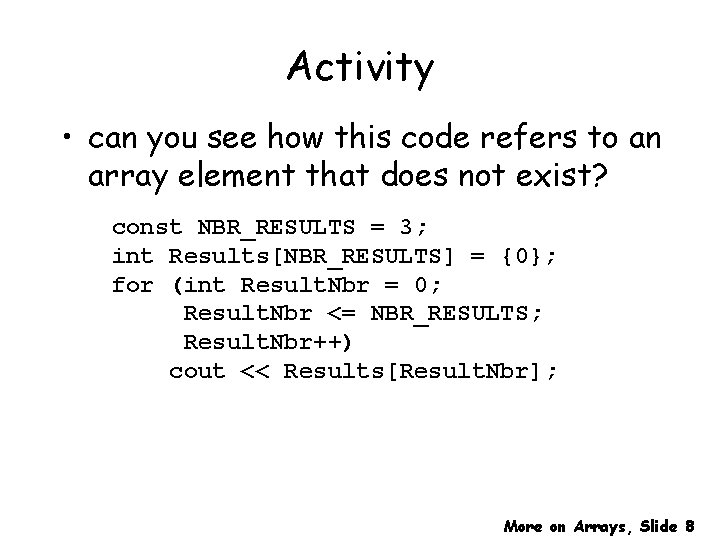
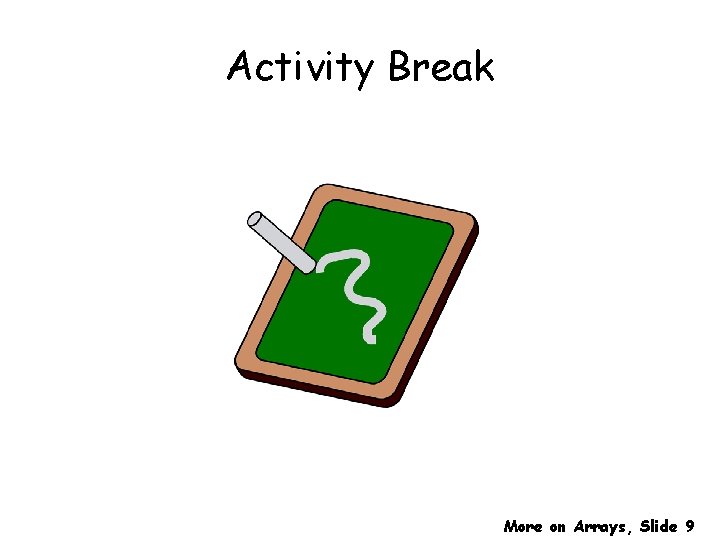
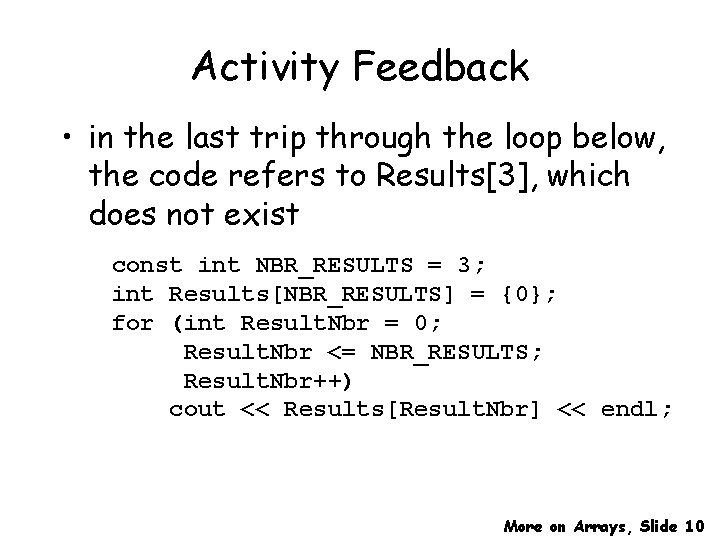
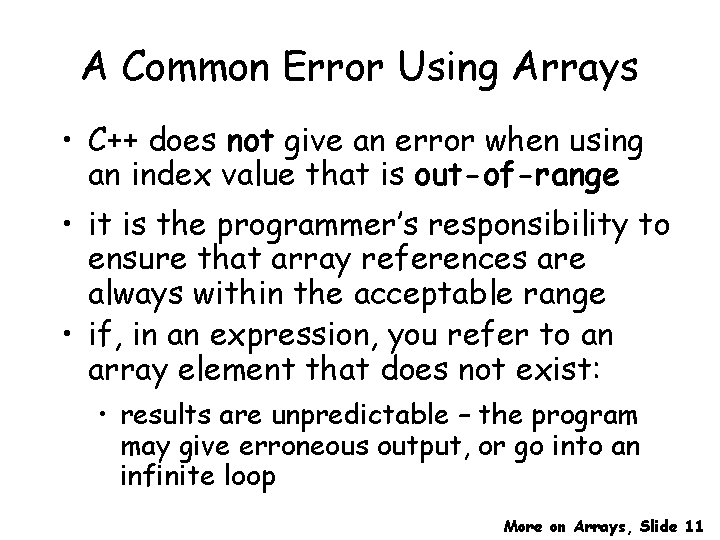
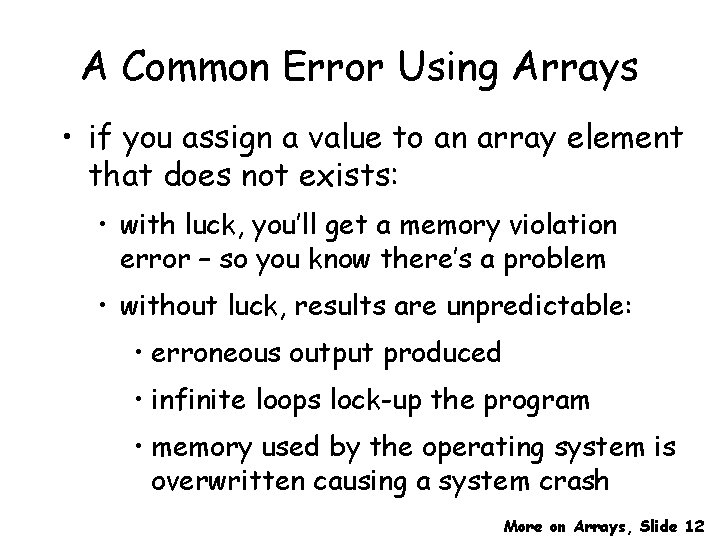
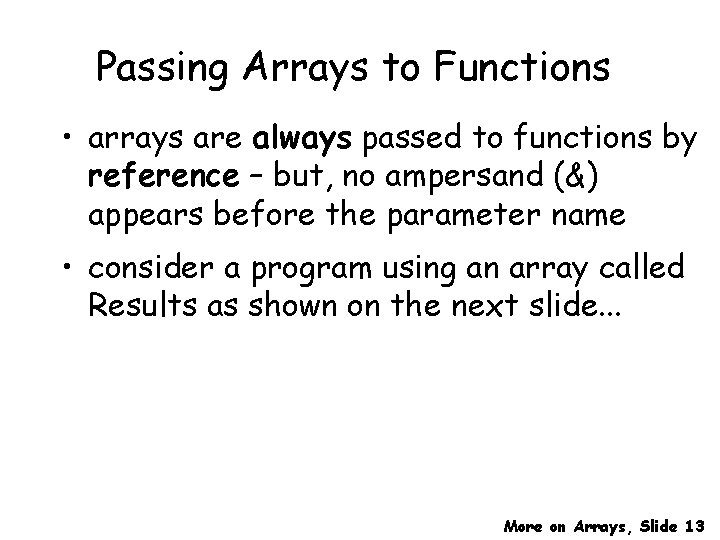
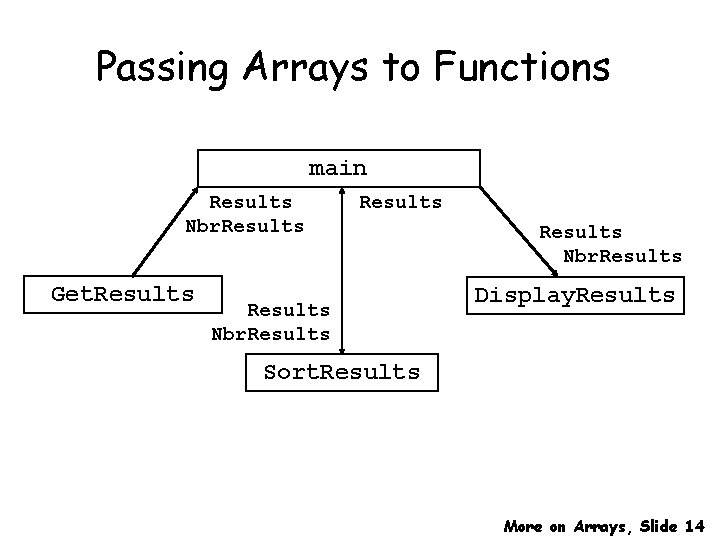
![void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-15.jpg)
![void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-16.jpg)
![void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-17.jpg)
![void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-18.jpg)
![void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-19.jpg)
![void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-20.jpg)
![void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-21.jpg)
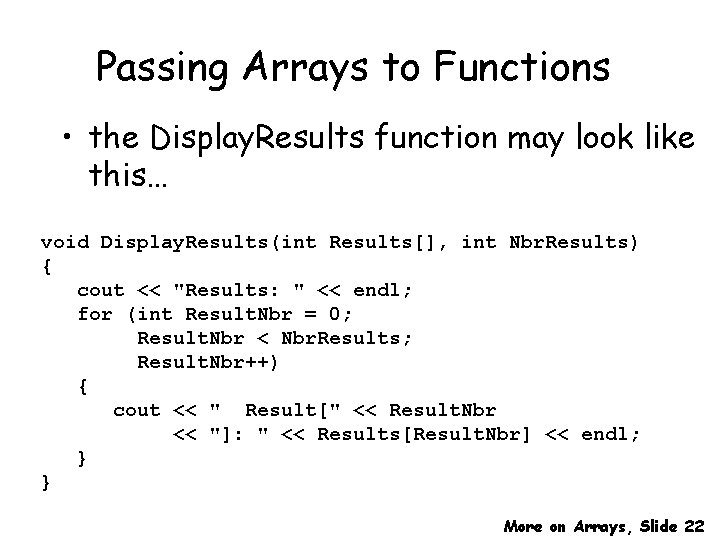
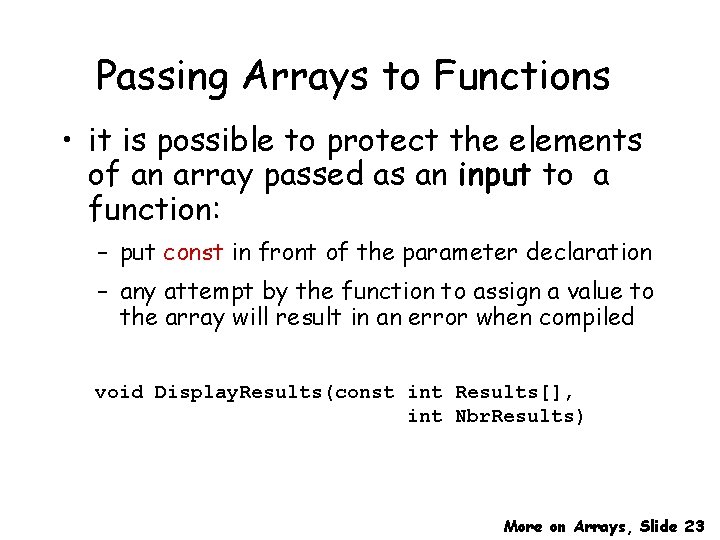
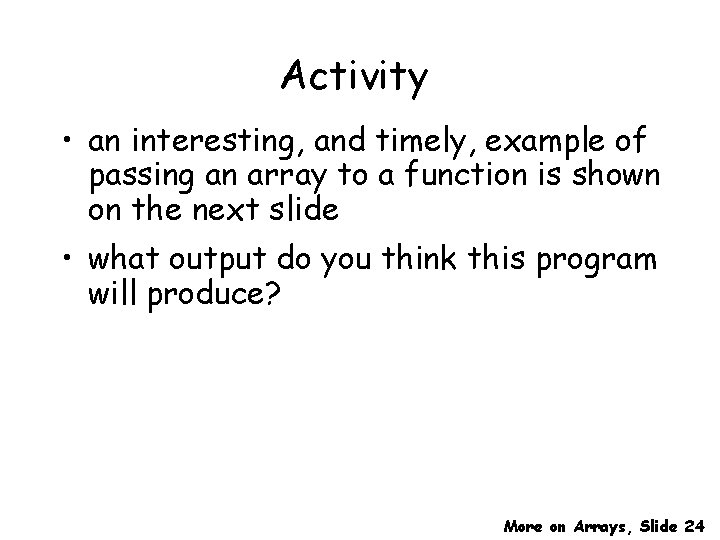
![#include <iostream. h> void Transform(char Array[], int Nbr. Elements); void main (void) { const #include <iostream. h> void Transform(char Array[], int Nbr. Elements); void main (void) { const](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-25.jpg)
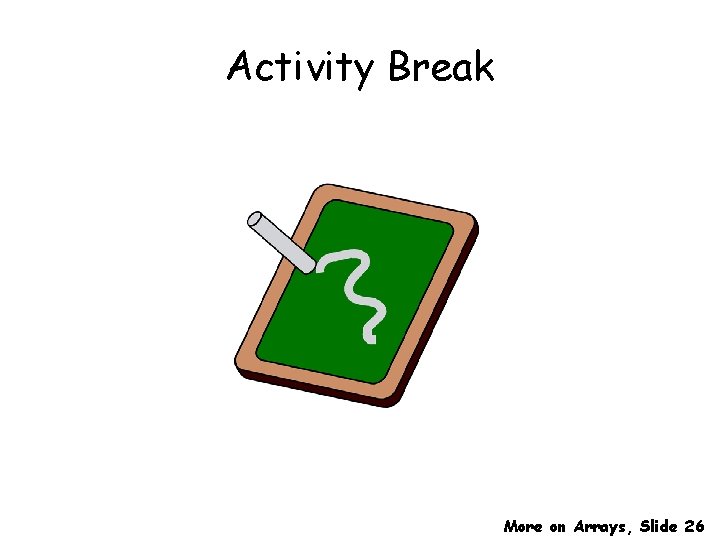
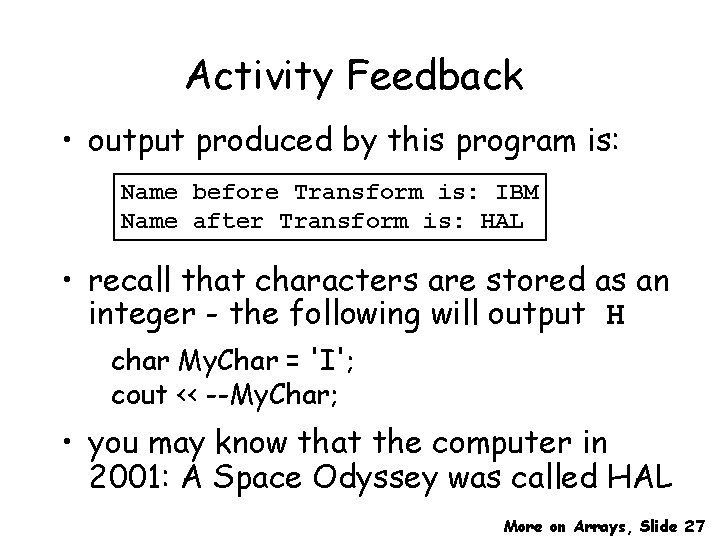
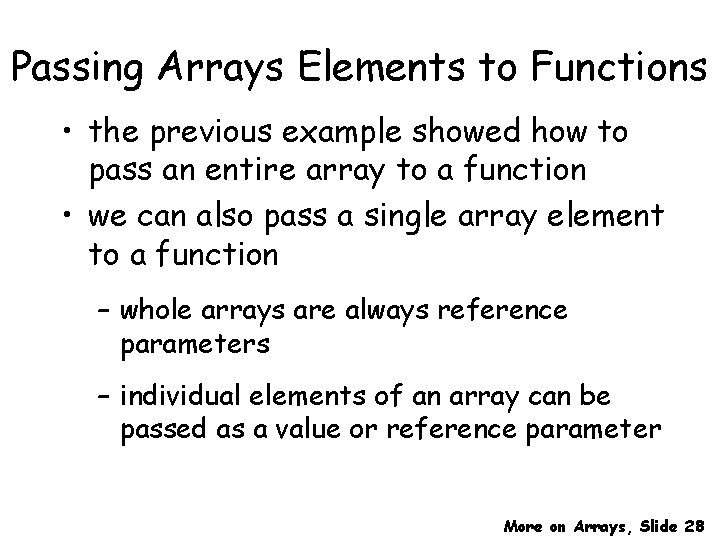
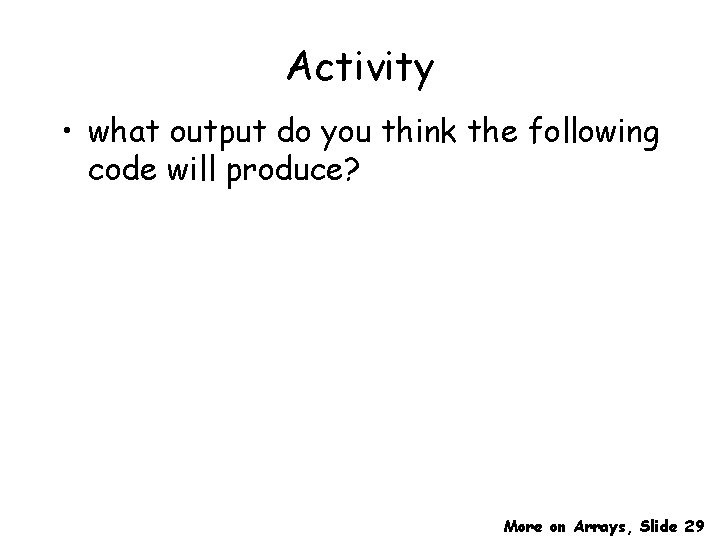
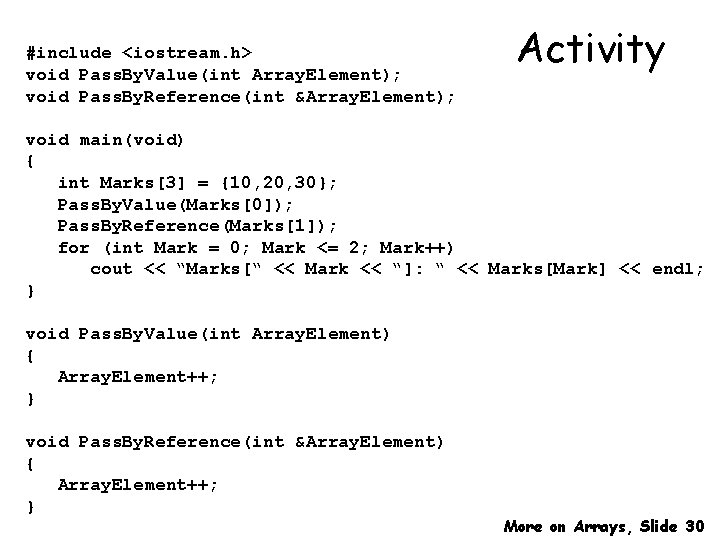
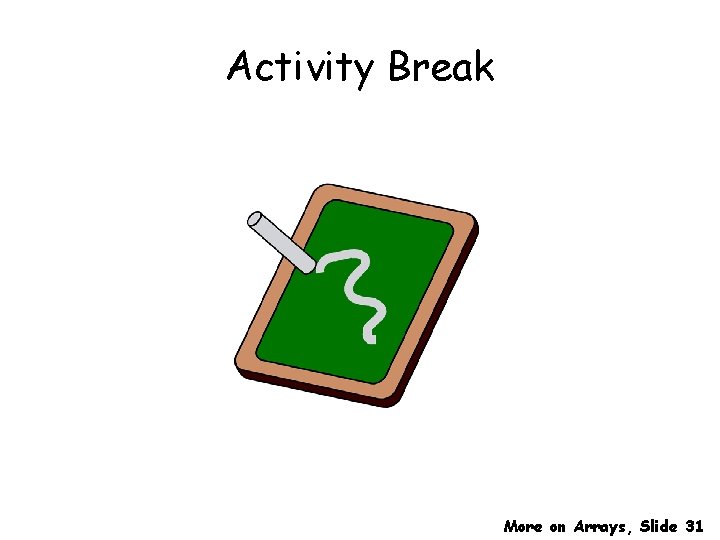
![Activity Feedback • output produced by this program is: Marks[0]: 10 Marks[1]: 21 Marks[2]: Activity Feedback • output produced by this program is: Marks[0]: 10 Marks[1]: 21 Marks[2]:](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-32.jpg)
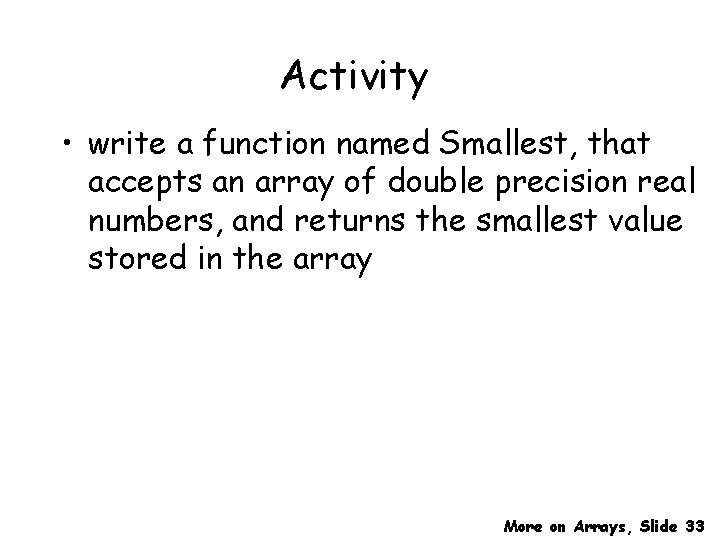
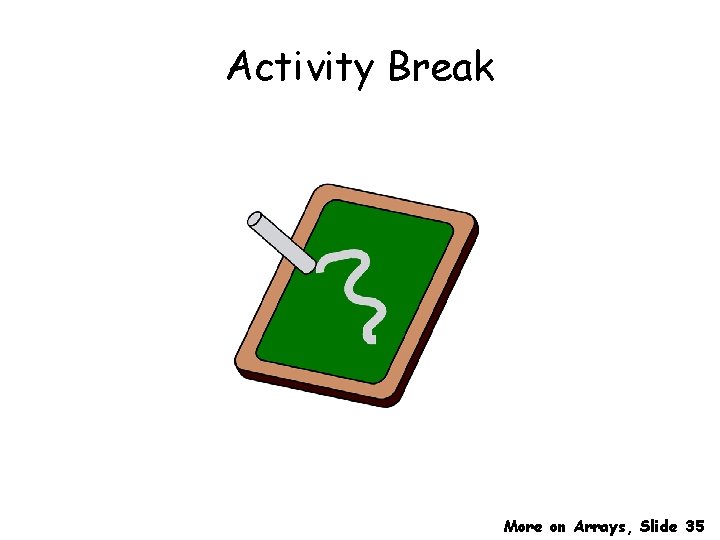
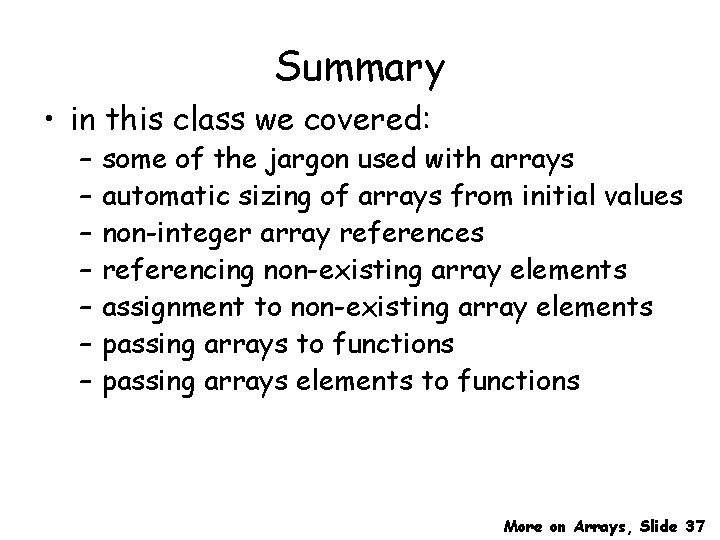
- Slides: 35
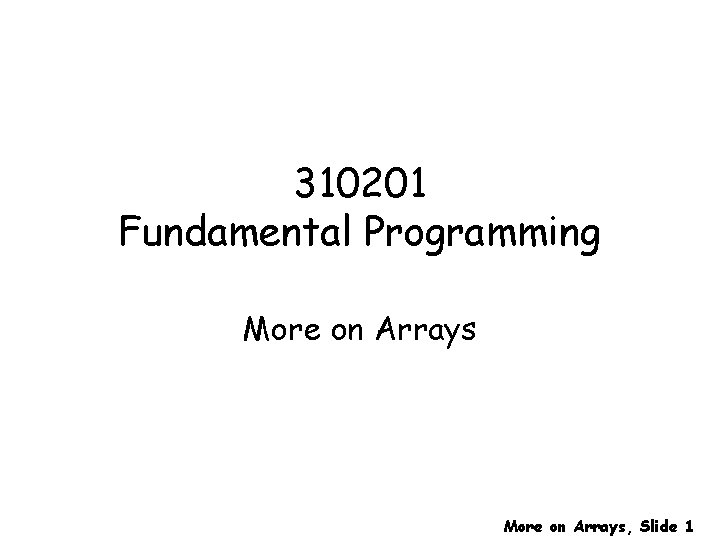
310201 Fundamental Programming More on Arrays, Slide 1
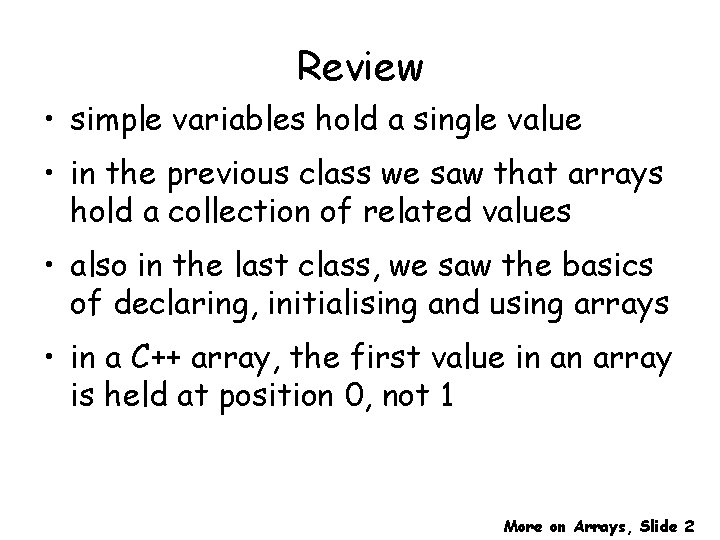
Review • simple variables hold a single value • in the previous class we saw that arrays hold a collection of related values • also in the last class, we saw the basics of declaring, initialising and using arrays • in a C++ array, the first value in an array is held at position 0, not 1 More on Arrays, Slide 2
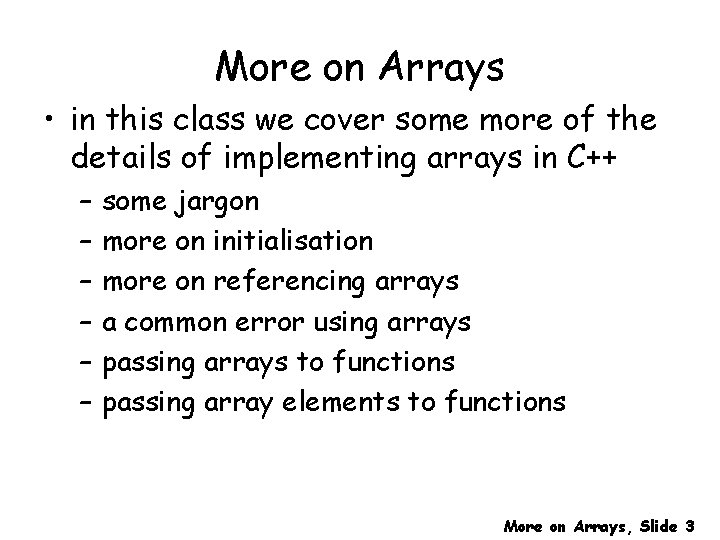
More on Arrays • in this class we cover some more of the details of implementing arrays in C++ – – – some jargon more on initialisation more on referencing arrays a common error using arrays passing arrays to functions passing array elements to functions More on Arrays, Slide 3
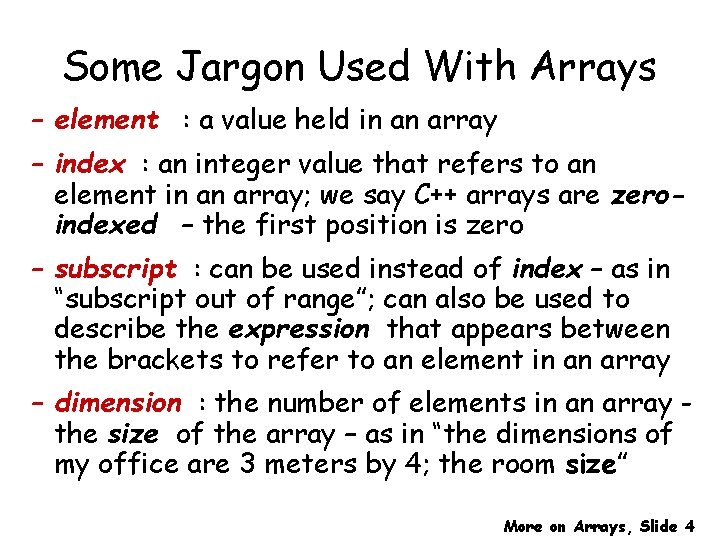
Some Jargon Used With Arrays – element : a value held in an array – index : an integer value that refers to an element in an array; we say C++ arrays are zeroindexed – the first position is zero – subscript : can be used instead of index – as in “subscript out of range”; can also be used to describe the expression that appears between the brackets to refer to an element in an array – dimension : the number of elements in an array the size of the array – as in “the dimensions of my office are 3 meters by 4; the room size” More on Arrays, Slide 4
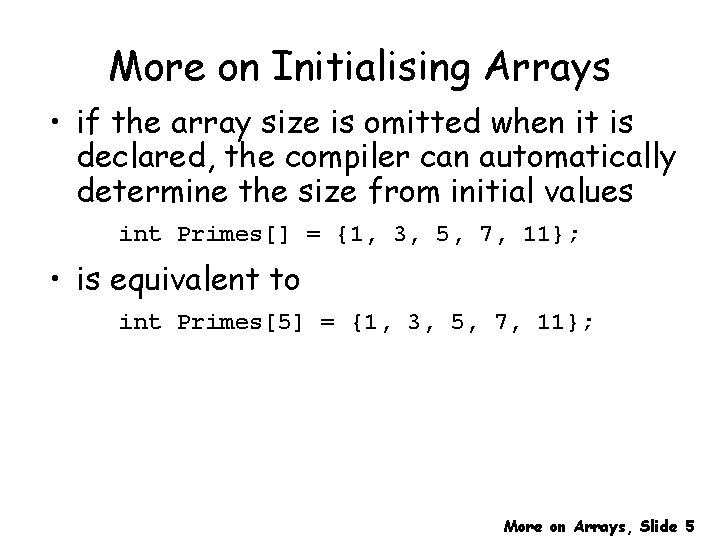
More on Initialising Arrays • if the array size is omitted when it is declared, the compiler can automatically determine the size from initial values int Primes[] = {1, 3, 5, 7, 11}; • is equivalent to int Primes[5] = {1, 3, 5, 7, 11}; More on Arrays, Slide 5
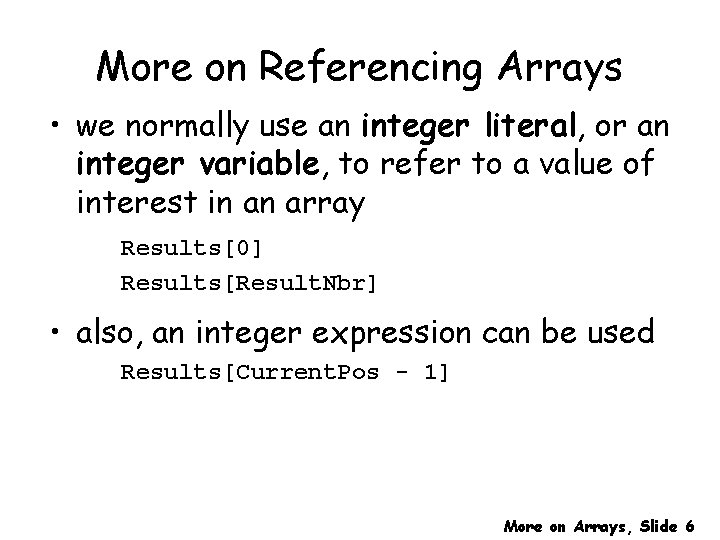
More on Referencing Arrays • we normally use an integer literal, or an integer variable, to refer to a value of interest in an array Results[0] Results[Result. Nbr] • also, an integer expression can be used Results[Current. Pos - 1] More on Arrays, Slide 6
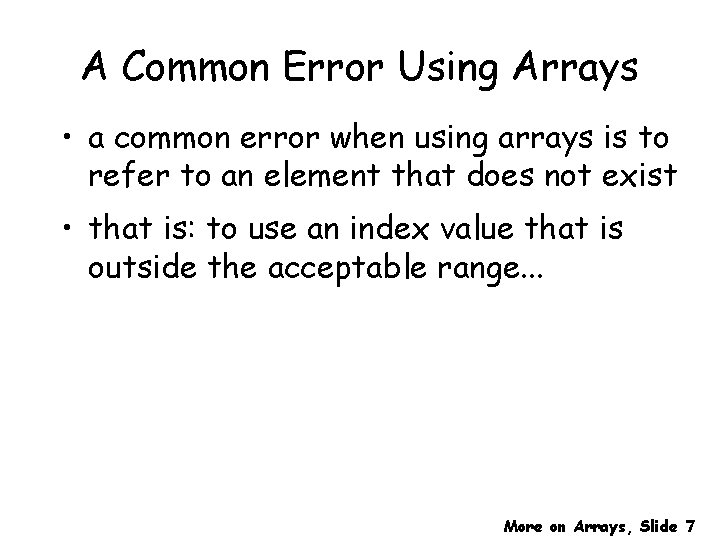
A Common Error Using Arrays • a common error when using arrays is to refer to an element that does not exist • that is: to use an index value that is outside the acceptable range. . . More on Arrays, Slide 7
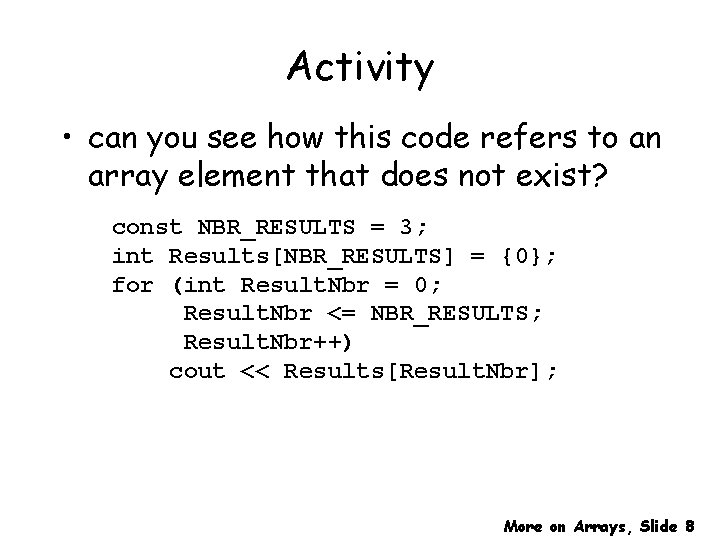
Activity • can you see how this code refers to an array element that does not exist? const NBR_RESULTS = 3; int Results[NBR_RESULTS] = {0}; for (int Result. Nbr = 0; Result. Nbr <= NBR_RESULTS; Result. Nbr++) cout << Results[Result. Nbr]; More on Arrays, Slide 8
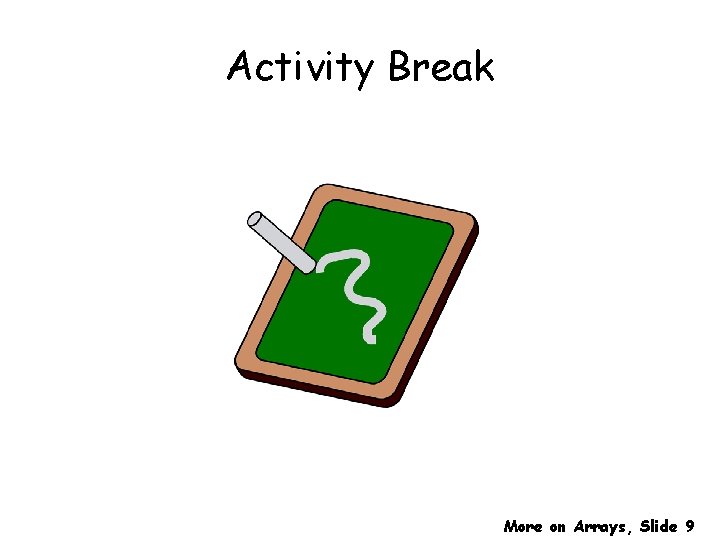
Activity Break More on Arrays, Slide 9
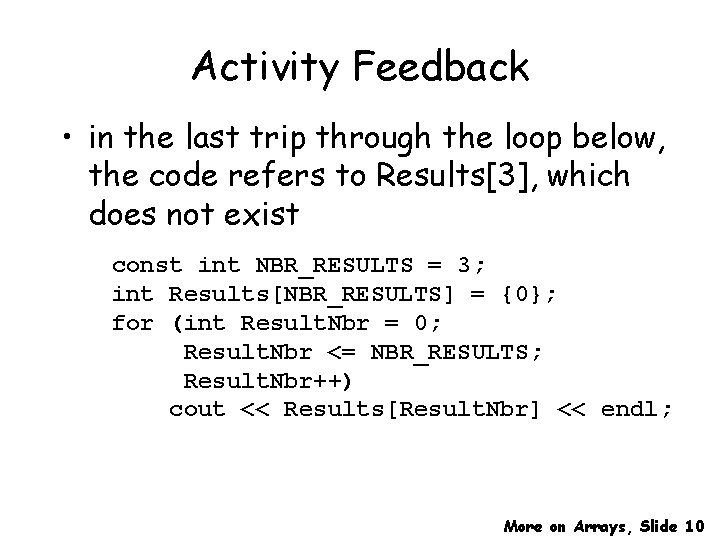
Activity Feedback • in the last trip through the loop below, the code refers to Results[3], which does not exist const int NBR_RESULTS = 3; int Results[NBR_RESULTS] = {0}; for (int Result. Nbr = 0; Result. Nbr <= NBR_RESULTS; Result. Nbr++) cout << Results[Result. Nbr] << endl; More on Arrays, Slide 10
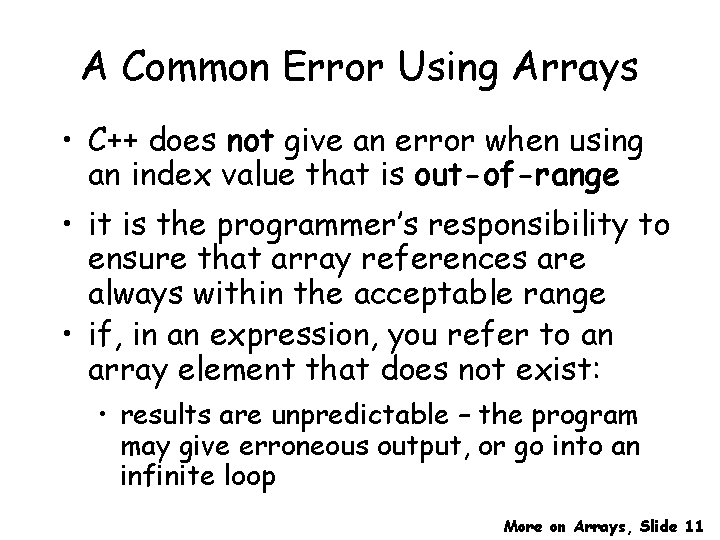
A Common Error Using Arrays • C++ does not give an error when using an index value that is out-of-range • it is the programmer’s responsibility to ensure that array references are always within the acceptable range • if, in an expression, you refer to an array element that does not exist: • results are unpredictable – the program may give erroneous output, or go into an infinite loop More on Arrays, Slide 11
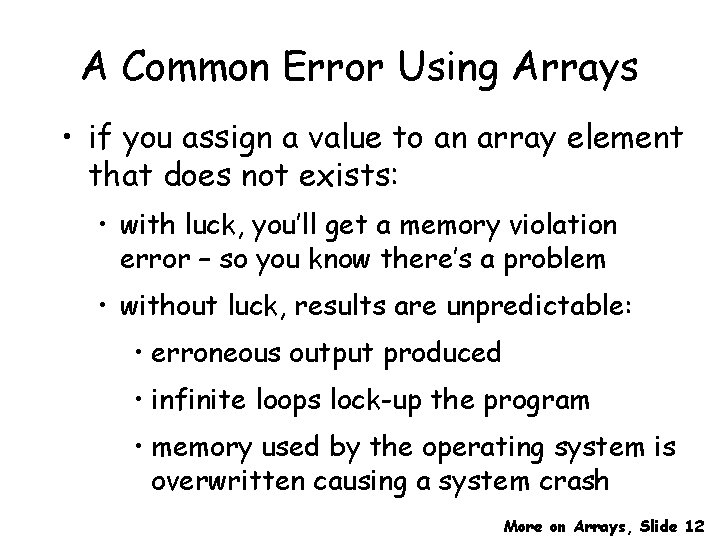
A Common Error Using Arrays • if you assign a value to an array element that does not exists: • with luck, you’ll get a memory violation error – so you know there’s a problem • without luck, results are unpredictable: • erroneous output produced • infinite loops lock-up the program • memory used by the operating system is overwritten causing a system crash More on Arrays, Slide 12
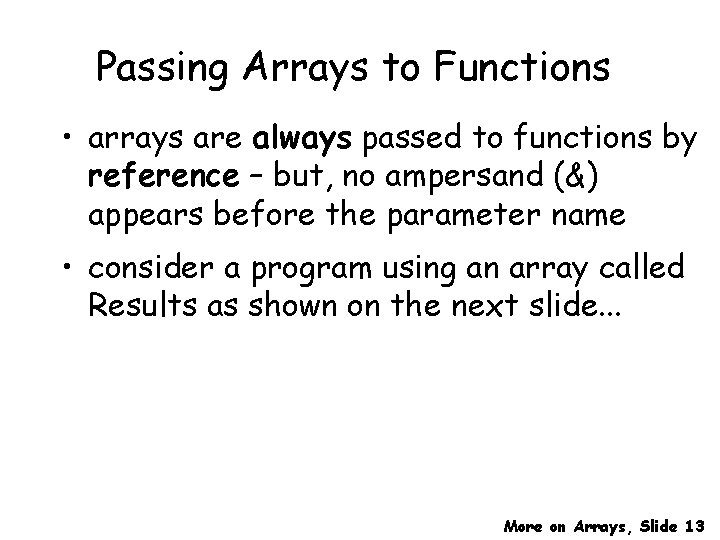
Passing Arrays to Functions • arrays are always passed to functions by reference – but, no ampersand (&) appears before the parameter name • consider a program using an array called Results as shown on the next slide. . . More on Arrays, Slide 13
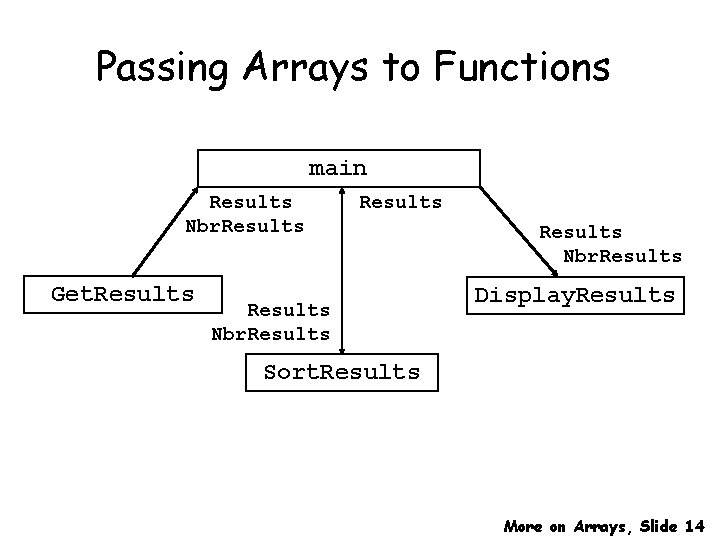
Passing Arrays to Functions main Results Nbr. Results Get. Results Nbr. Results Display. Results Sort. Results More on Arrays, Slide 14
![void Get Resultsint Results int Nbr Results void Sort Resultsint Results int Nbr Results void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-15.jpg)
void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Display. Results(int Results[], int Nbr. Results); const int MAX_NBR_RESULTS = 10; void main (void) { int Results[MAX_NBR_RESULTS] = {0}, Nbr. Results = 0; Get. Results(Results, Nbr. Results); Sort. Results(Results, Nbr. Results); Display. Results(Results, Nbr. Results); } notes: here, the maximum number of results is defined as a global constant – it is used to define the size of the Results array and will also be used to avoid an out-of-range error in the Get. Results function More on Arrays, Slide 15
![void Get Resultsint Results int Nbr Results void Sort Resultsint Results int Nbr Results void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-16.jpg)
void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Display. Results(int Results[], int Nbr. Results); const int MAX_NBR_RESULTS = 10; void main (void) { int Results[MAX_NBR_RESULTS] = {0}, Nbr. Results = 0; Get. Results(Results, Nbr. Results); Sort. Results(Results, Nbr. Results); Display. Results(Results, Nbr. Results); } notes: the Nbr. Results parameter is an output of the Get. Results function, so an ampersand appears before its name in the Get. Results header and declaration More on Arrays, Slide 16
![void Get Resultsint Results int Nbr Results void Sort Resultsint Results int Nbr Results void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-17.jpg)
void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Display. Results(int Results[], int Nbr. Results); const int MAX_NBR_RESULTS = 10; void main (void) { int Results[MAX_NBR_RESULTS] = {0}, Nbr. Results = 0; Get. Results(Results, Nbr. Results); Sort. Results(Results, Nbr. Results); Display. Results(Results, Nbr. Results); } notes: the Results array is also an output of the Get. Results function, but an ampersand does not appear before its name in Get. Results header and declaration More on Arrays, Slide 17
![void Get Resultsint Results int Nbr Results void Sort Resultsint Results int Nbr Results void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-18.jpg)
void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Display. Results(int Results[], int Nbr. Results); const int MAX_NBR_RESULTS = 10; void main (void) { int Results[MAX_NBR_RESULTS] = {0}, Nbr. Results = 0; Get. Results(Results, Nbr. Results); Sort. Results(Results, Nbr. Results); Display. Results(Results, Nbr. Results); } notes: square brackets appear in header and declaration to show that a parameter is an array More on Arrays, Slide 18
![void Get Resultsint Results int Nbr Results void Sort Resultsint Results int Nbr Results void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-19.jpg)
void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Display. Results(int Results[], int Nbr. Results); const int MAX_NBR_RESULTS = 10; void main (void) { int Results[MAX_NBR_RESULTS] = {0}, Nbr. Results = 0; Get. Results(Results, Nbr. Results); Sort. Results(Results, Nbr. Results); Display. Results(Results, Nbr. Results); } notes: square brackets do not appear in the function call More on Arrays, Slide 19
![void Get Resultsint Results int Nbr Results void Sort Resultsint Results int Nbr Results void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-20.jpg)
void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Display. Results(int Results[], int Nbr. Results); const int MAX_NBR_RESULTS = 10; void main (void) { int Results[MAX_NBR_RESULTS] = {0}, Nbr. Results = 0; Get. Results(Results, Nbr. Results); Sort. Results(Results, Nbr. Results); Display. Results(Results, Nbr. Results); } notes: one does not need to specify the size of an array that appears as a parameter in a function header or declaration – it is normal practice to not specify the size of a parameter that is an array More on Arrays, Slide 20
![void Get Resultsint Results int Nbr Results void Sort Resultsint Results int Nbr Results void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results);](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-21.jpg)
void Get. Results(int Results[], int &Nbr. Results); void Sort. Results(int Results[], int Nbr. Results); void Display. Results(int Results[], int Nbr. Results); const int MAX_NBR_RESULTS = 10; void main (void) { int Results[MAX_NBR_RESULTS] = {0}, Nbr. Results = 0; Get. Results(Results, Nbr. Results); Sort. Results(Results, Nbr. Results); Display. Results(Results, Nbr. Results); } notes: however, called functions need to know how many values have been stored in the array More on Arrays, Slide 21
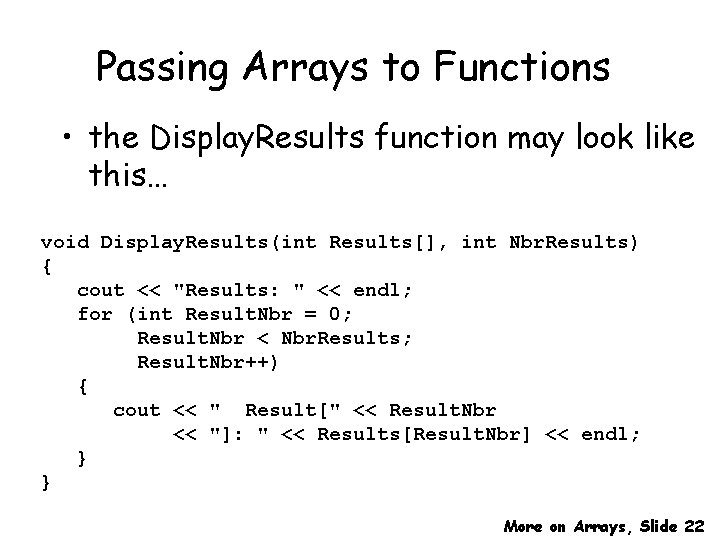
Passing Arrays to Functions • the Display. Results function may look like this… void Display. Results(int Results[], int Nbr. Results) { cout << "Results: " << endl; for (int Result. Nbr = 0; Result. Nbr < Nbr. Results; Result. Nbr++) { cout << " Result[" << Result. Nbr << "]: " << Results[Result. Nbr] << endl; } } More on Arrays, Slide 22
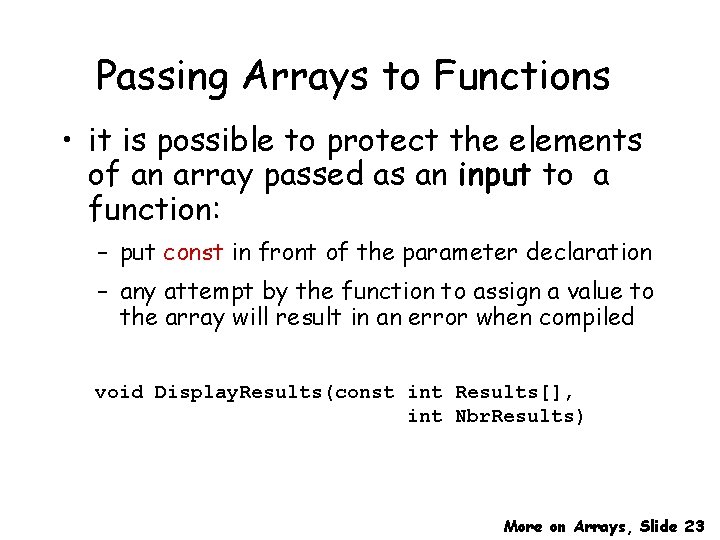
Passing Arrays to Functions • it is possible to protect the elements of an array passed as an input to a function: – put const in front of the parameter declaration – any attempt by the function to assign a value to the array will result in an error when compiled void Display. Results(const int Results[], int Nbr. Results) More on Arrays, Slide 23
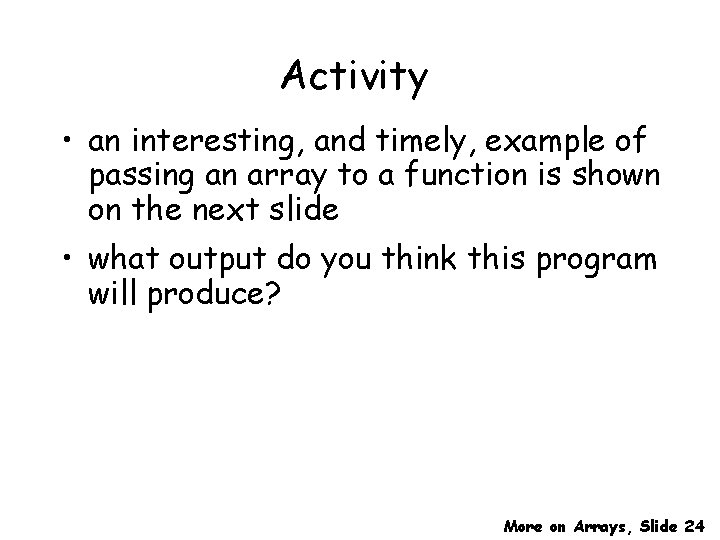
Activity • an interesting, and timely, example of passing an array to a function is shown on the next slide • what output do you think this program will produce? More on Arrays, Slide 24
![include iostream h void Transformchar Array int Nbr Elements void main void const #include <iostream. h> void Transform(char Array[], int Nbr. Elements); void main (void) { const](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-25.jpg)
#include <iostream. h> void Transform(char Array[], int Nbr. Elements); void main (void) { const int NBR_CHARS = 3; char Name[NBR_CHARS] = {'I', 'B', 'M'}; int Index; cout << "Name before Transform is: " ; for (Index = 0; Index < NBR_CHARS; Index++) cout << Name[Index]; Transform(Name, NBR_CHARS); cout << endl << "Name after Transform is: " ; for (Index = 0; Index < NBR_CHARS; Index++) cout<<Name[Index]; } void Transform(char Array[], int Nbr. Elements) { for (int Index = 0; Index < Nbr. Elements; Index++) --Array[Index]; } More on Arrays, Slide 25
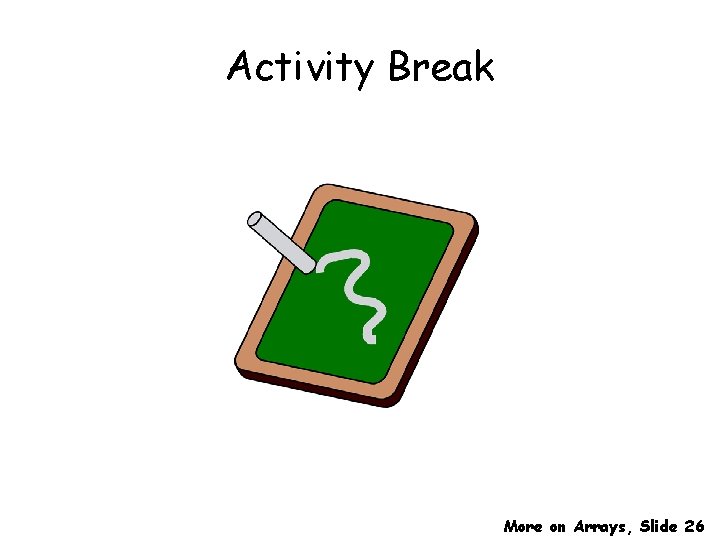
Activity Break More on Arrays, Slide 26
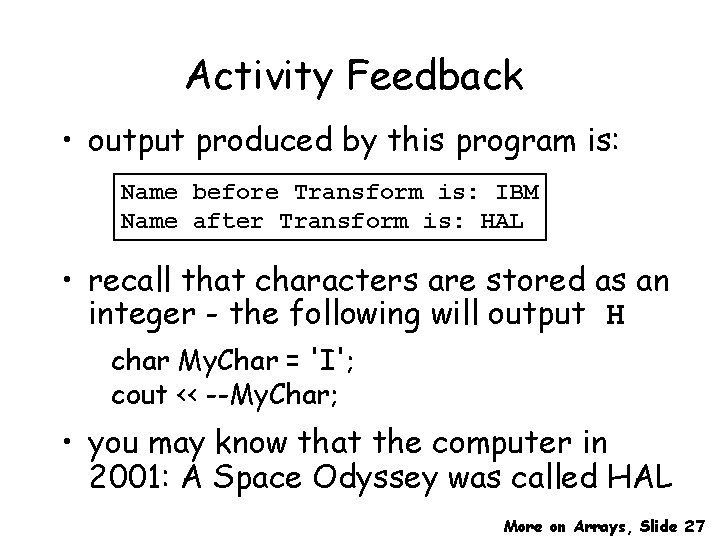
Activity Feedback • output produced by this program is: Name before Transform is: IBM Name after Transform is: HAL • recall that characters are stored as an integer - the following will output H char My. Char = 'I'; cout << --My. Char; • you may know that the computer in 2001: A Space Odyssey was called HAL More on Arrays, Slide 27
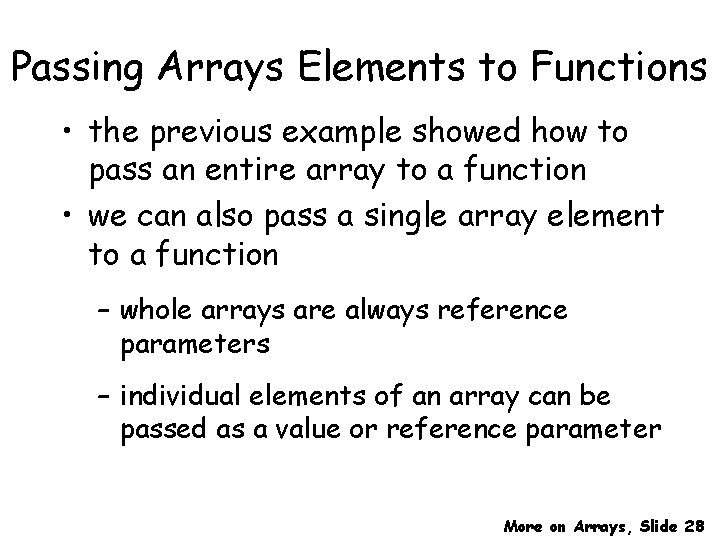
Passing Arrays Elements to Functions • the previous example showed how to pass an entire array to a function • we can also pass a single array element to a function – whole arrays are always reference parameters – individual elements of an array can be passed as a value or reference parameter More on Arrays, Slide 28
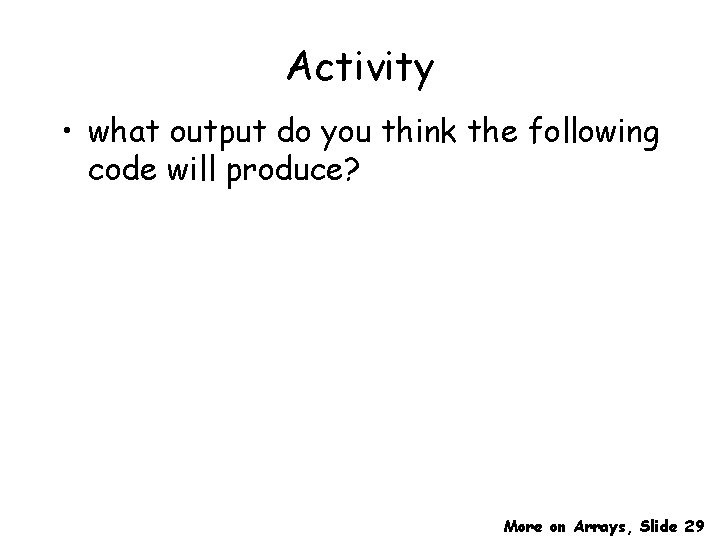
Activity • what output do you think the following code will produce? More on Arrays, Slide 29
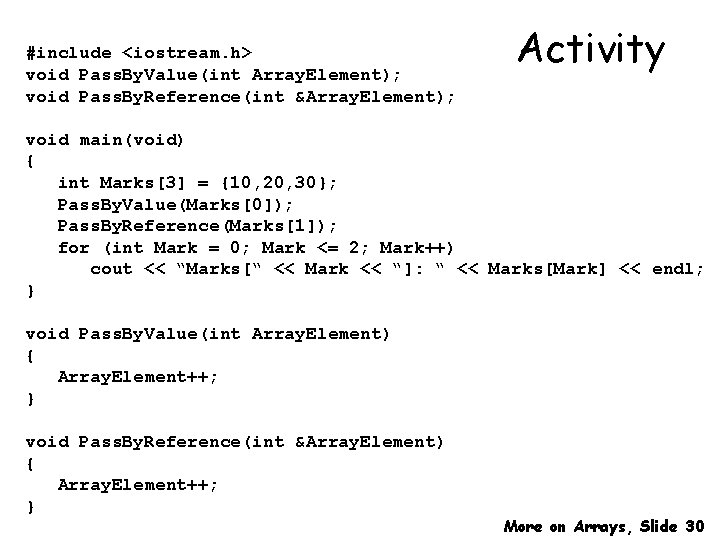
#include <iostream. h> void Pass. By. Value(int Array. Element); void Pass. By. Reference(int &Array. Element); Activity void main(void) { int Marks[3] = {10, 20, 30}; Pass. By. Value(Marks[0]); Pass. By. Reference(Marks[1]); for (int Mark = 0; Mark <= 2; Mark++) cout << “Marks[“ << Mark << “]: “ << Marks[Mark] << endl; } void Pass. By. Value(int Array. Element) { Array. Element++; } void Pass. By. Reference(int &Array. Element) { Array. Element++; } More on Arrays, Slide 30
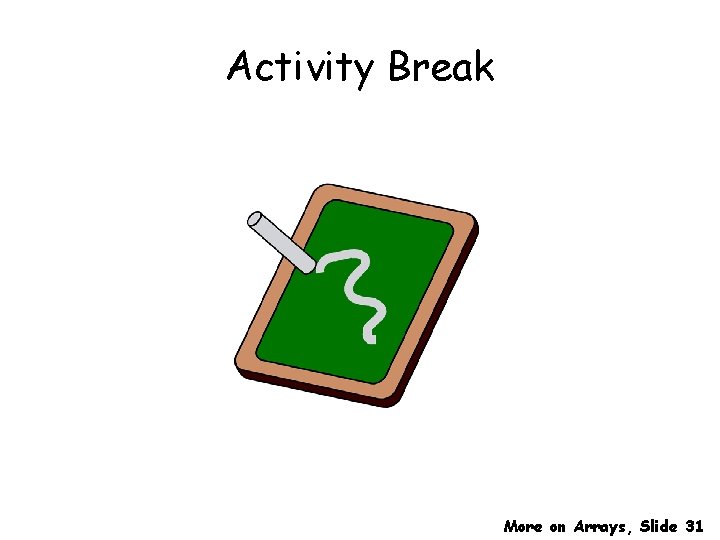
Activity Break More on Arrays, Slide 31
![Activity Feedback output produced by this program is Marks0 10 Marks1 21 Marks2 Activity Feedback • output produced by this program is: Marks[0]: 10 Marks[1]: 21 Marks[2]:](https://slidetodoc.com/presentation_image_h2/4b7ff7516a33b3b242708c4e8c5bee60/image-32.jpg)
Activity Feedback • output produced by this program is: Marks[0]: 10 Marks[1]: 21 Marks[2]: 30 More on Arrays, Slide 32
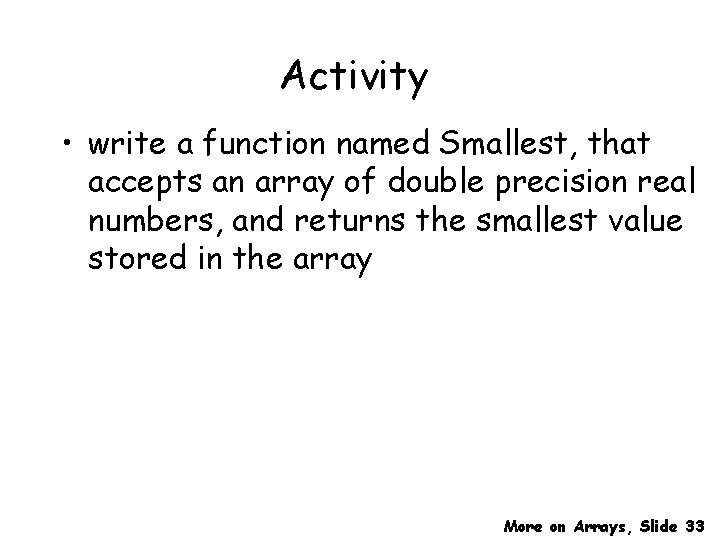
Activity • write a function named Smallest, that accepts an array of double precision real numbers, and returns the smallest value stored in the array More on Arrays, Slide 33
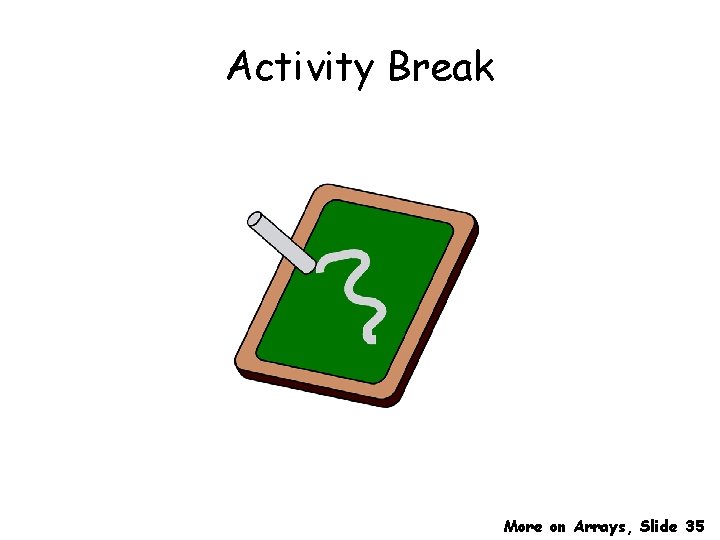
Activity Break More on Arrays, Slide 35
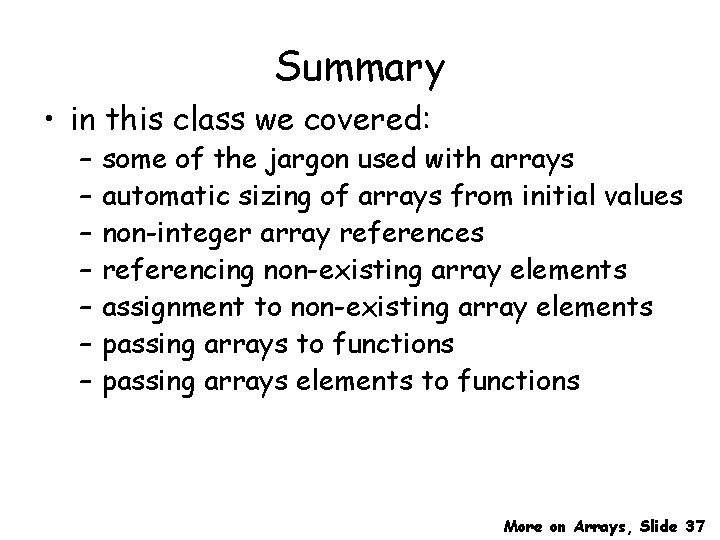
Summary • in this class we covered: – – – – some of the jargon used with arrays automatic sizing of arrays from initial values non-integer array references referencing non-existing array elements assignment to non-existing array elements passing arrays to functions passing arrays elements to functions More on Arrays, Slide 37
More more more i want more more more more we praise you
More more more i want more more more more we praise you
Heel and toe heel and toe slide slide slide lyrics
Parallel arrays in c
Array of arrays c++
Parallel arrays
Veteork
C++ parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Arreglos unidimensionales en java ejemplos
Arreglo java
Mips array example
Polynomial representation using array in c
Arrays in arm assembly
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++
Arrays visual basic
Python parallel arrays
Advantages and disadvantages of arrays in data structure
I wonder is it possible
Pascal 2d array
Mips dynamic array
Creating arrays matlab
Arrays as adt
Java partially filled array
Redundant arrays of independent disks
Python list of arrays
Arrays
Day 3: arrays
A case for redundant arrays of inexpensive disks
Small basic array
Disadvantages of arrays
Microled arrays
Are vectors dynamic arrays