310201 Fundamental Programming Introduction to Arrays 310201 Fundamental
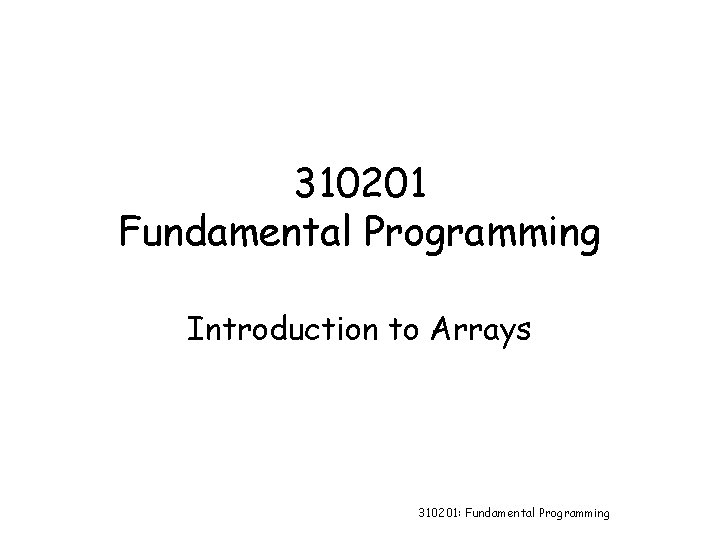
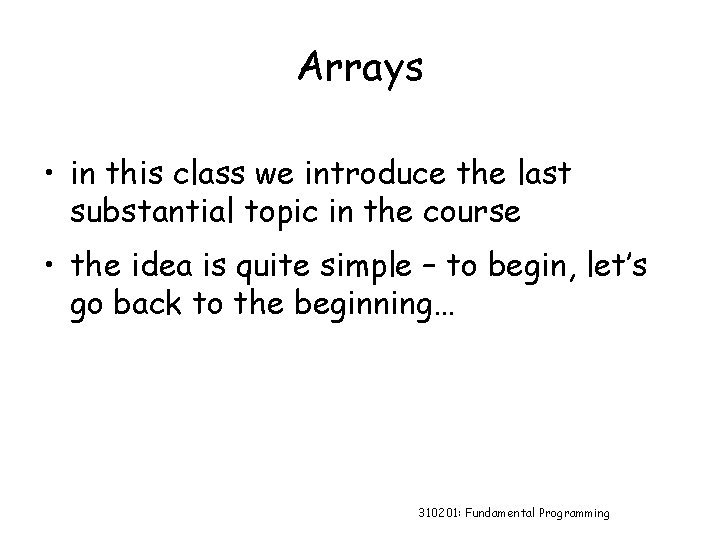
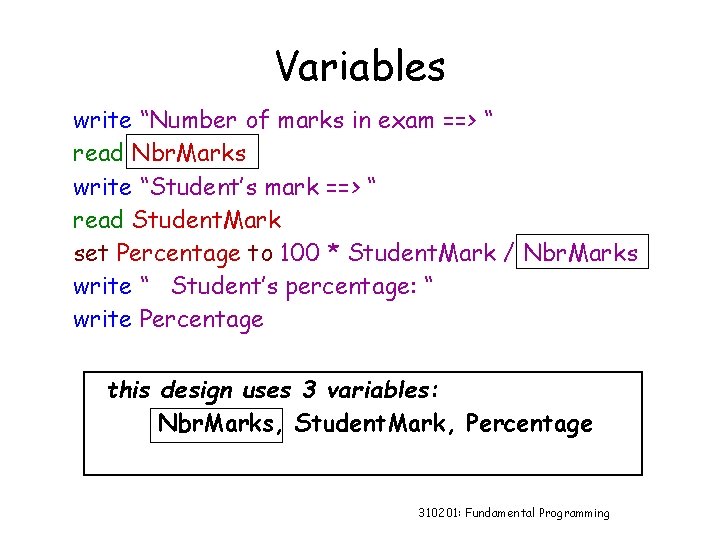
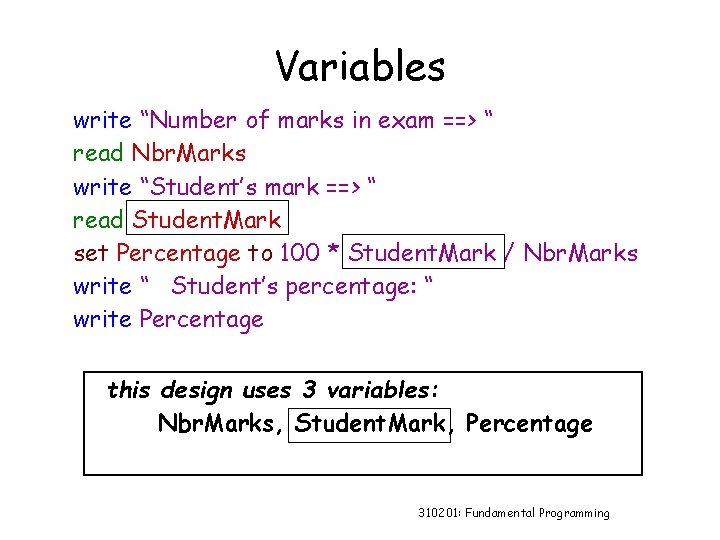
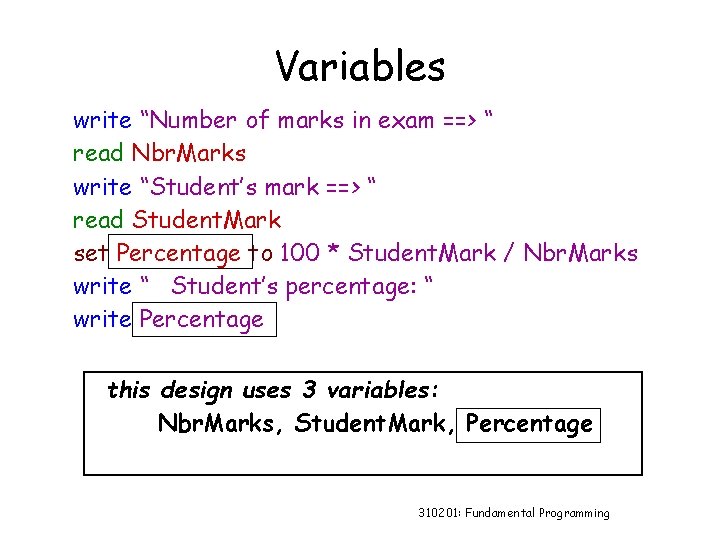
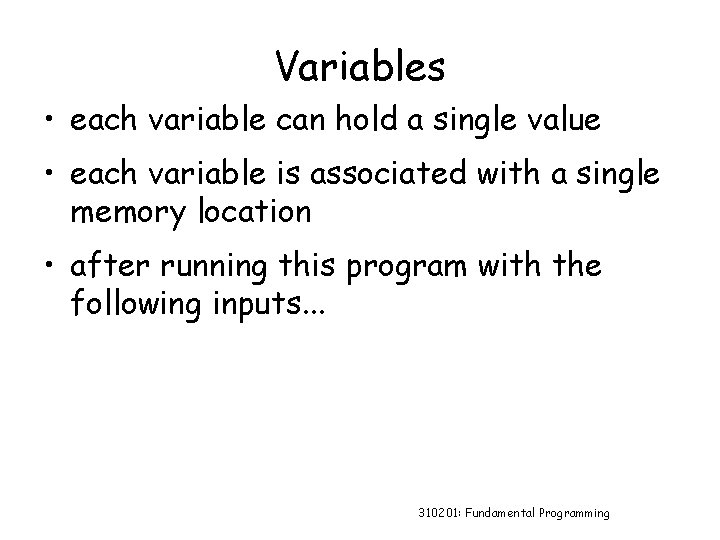
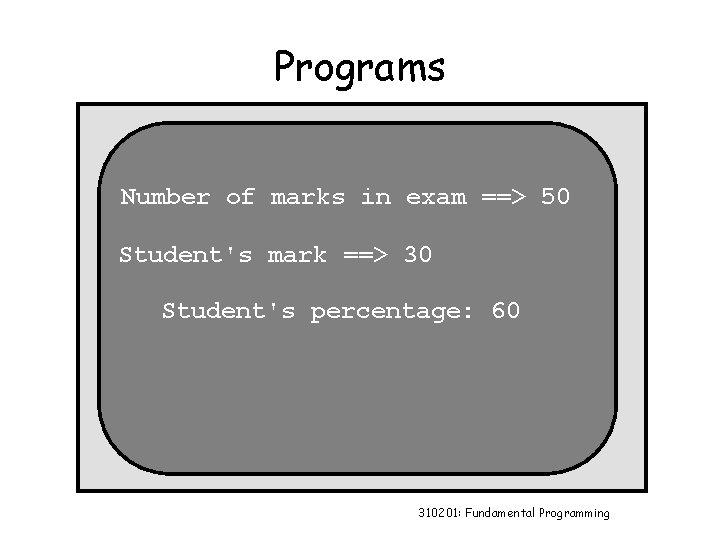
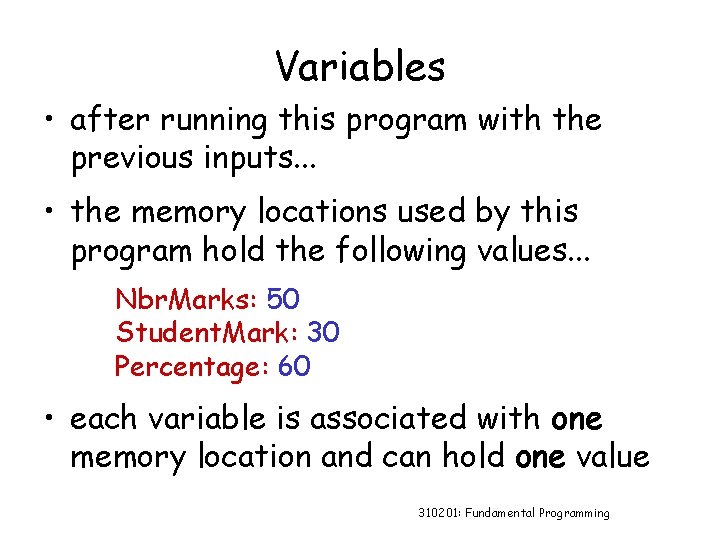
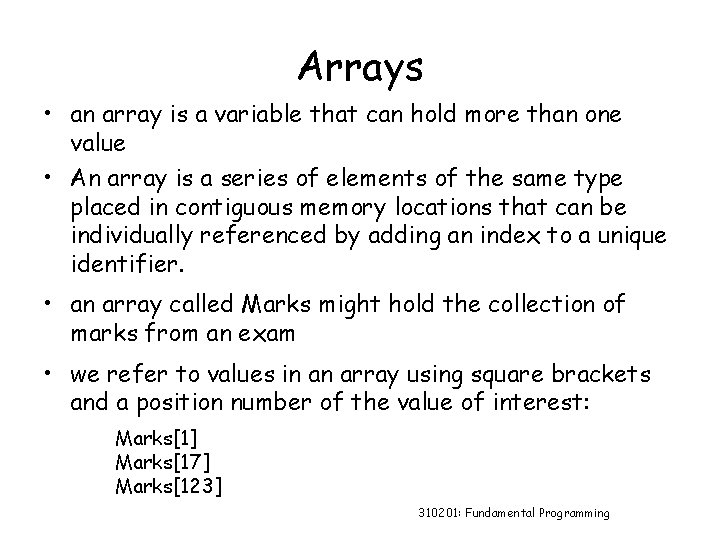
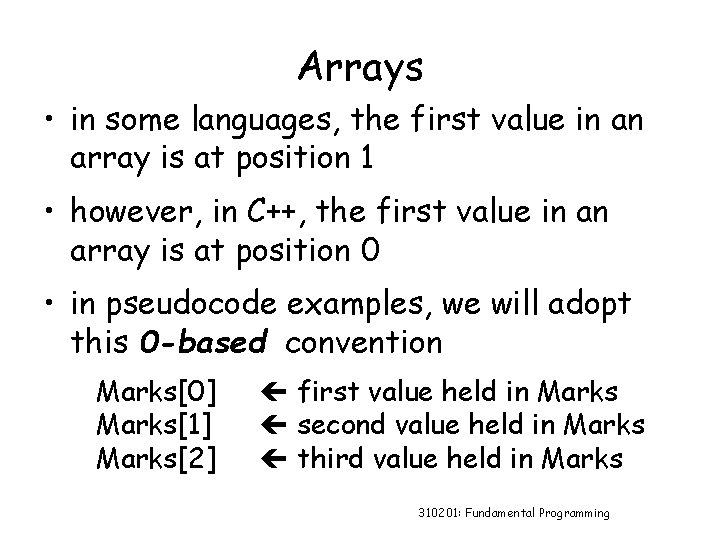
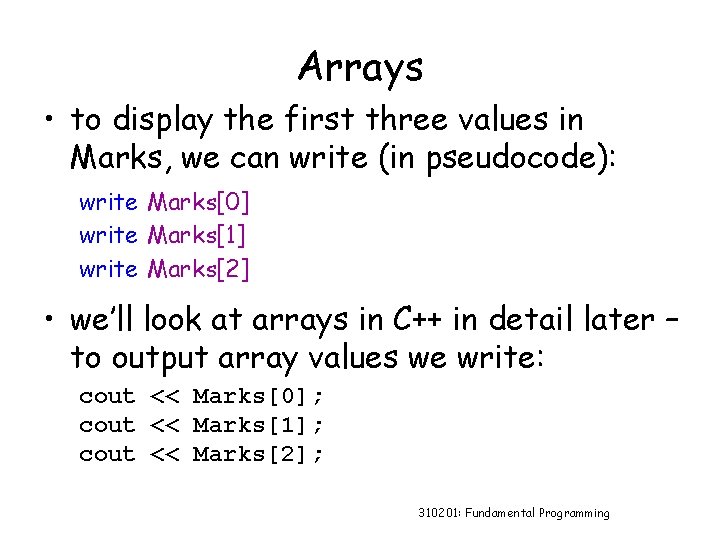
![Arrays • we assign values to an array using the same notation: read Marks[0] Arrays • we assign values to an array using the same notation: read Marks[0]](https://slidetodoc.com/presentation_image_h2/57d040b26985fd6581efb580eeca89ce/image-12.jpg)
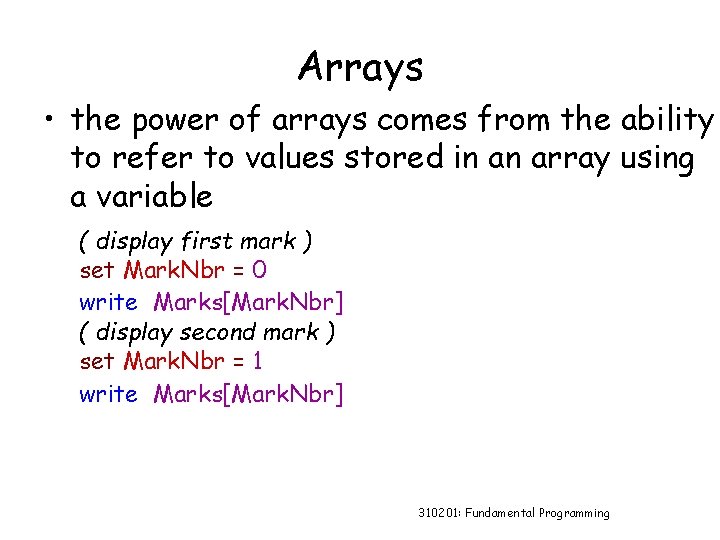
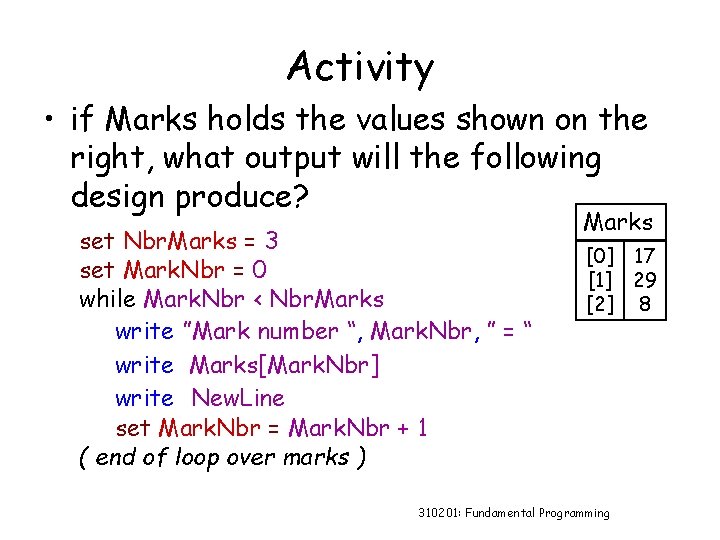
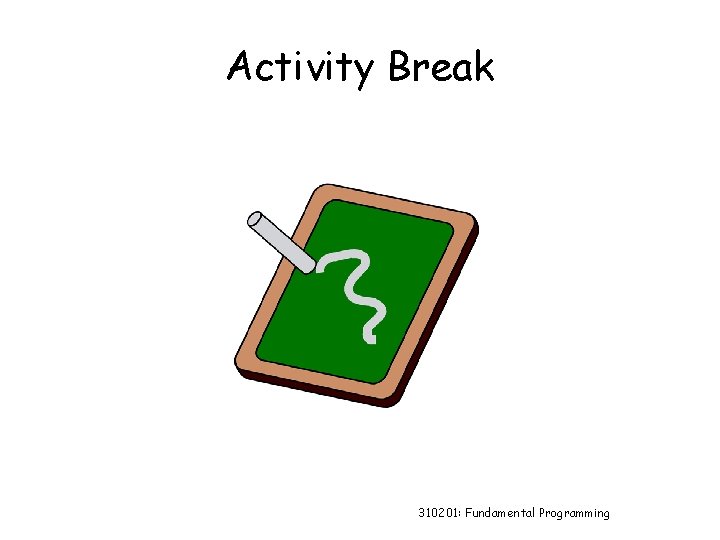
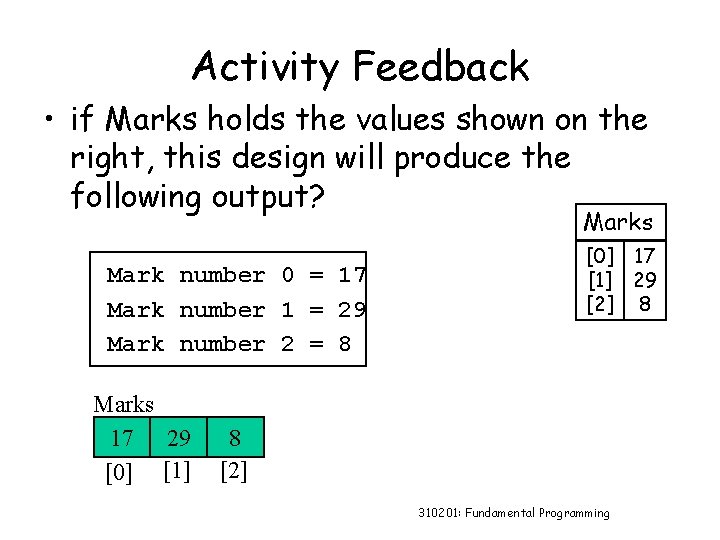
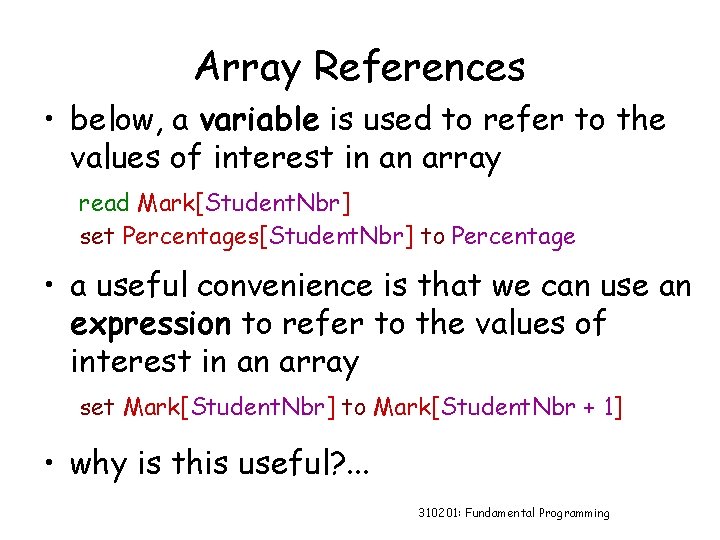
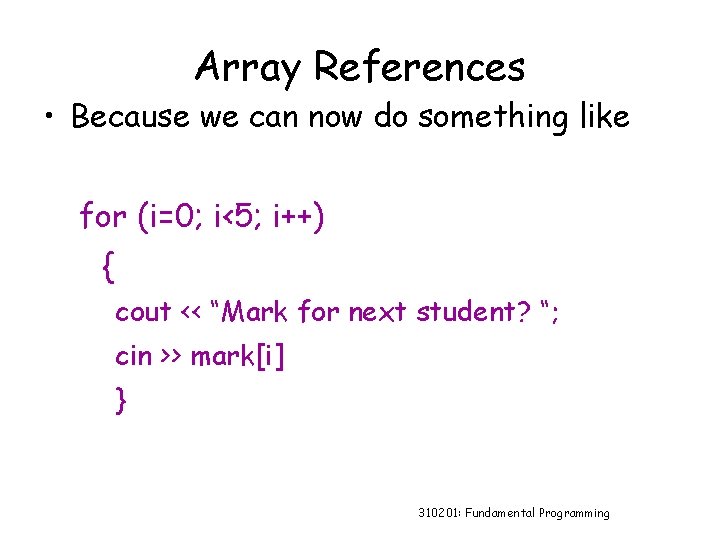
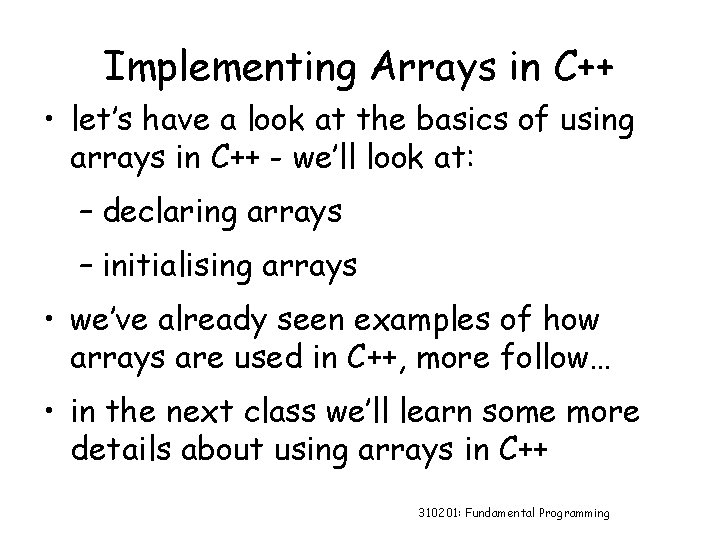
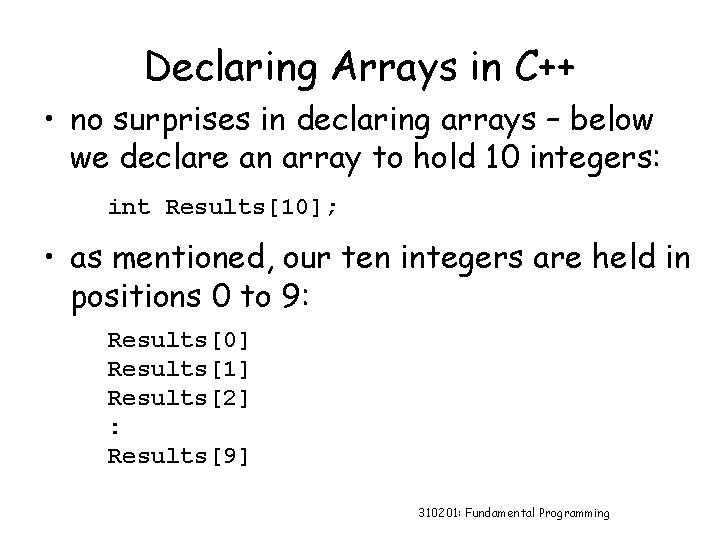
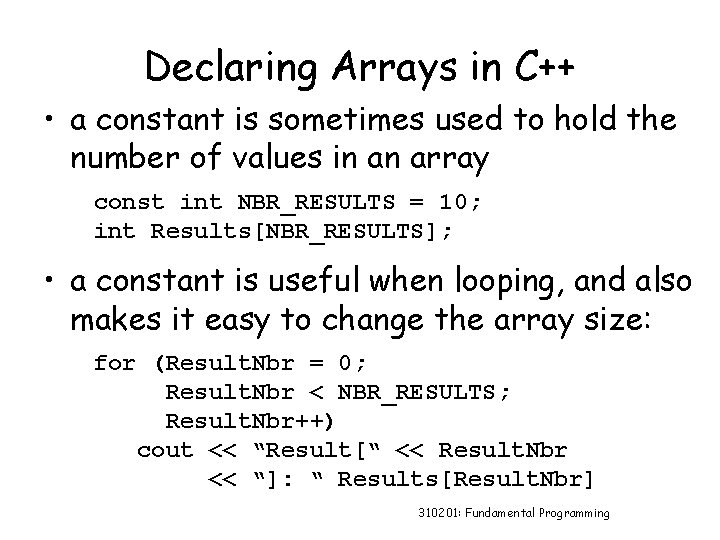
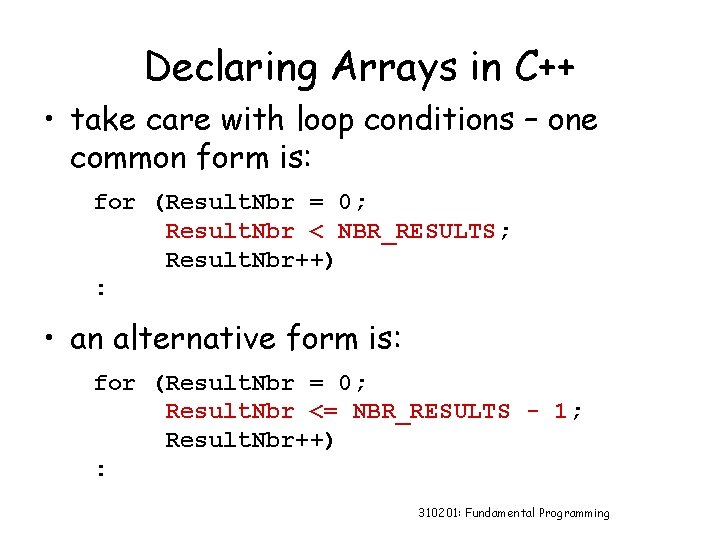
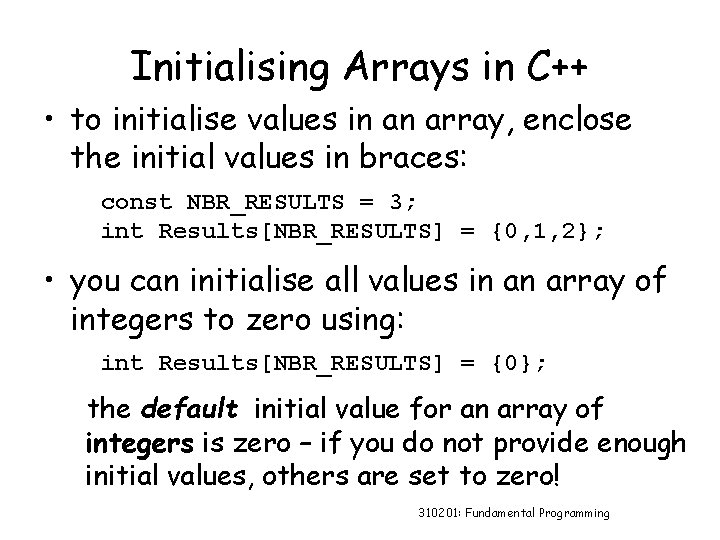
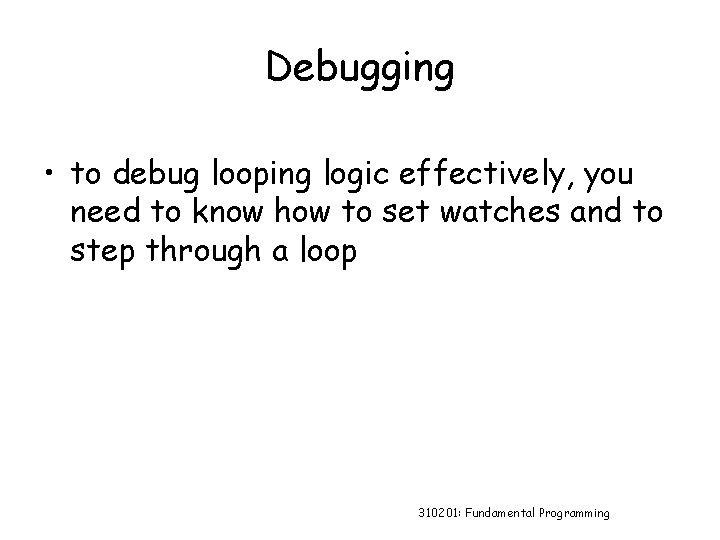
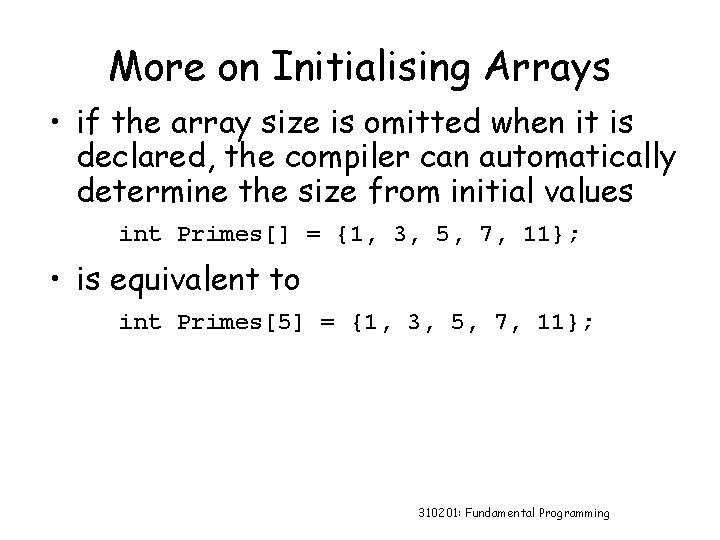
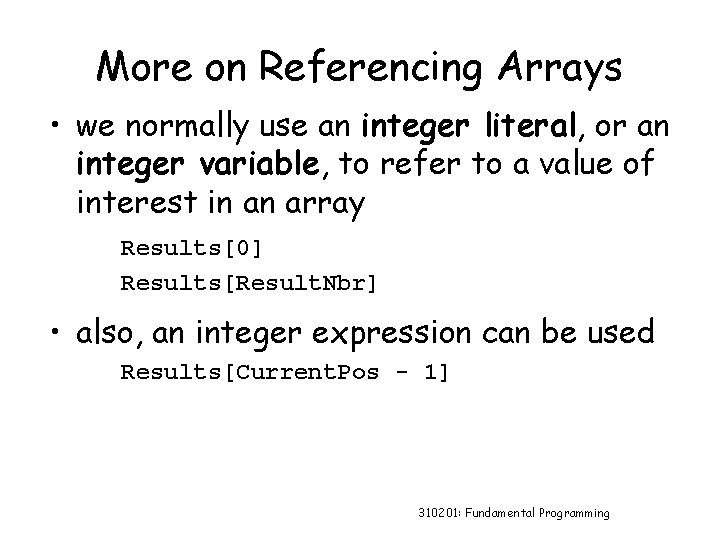
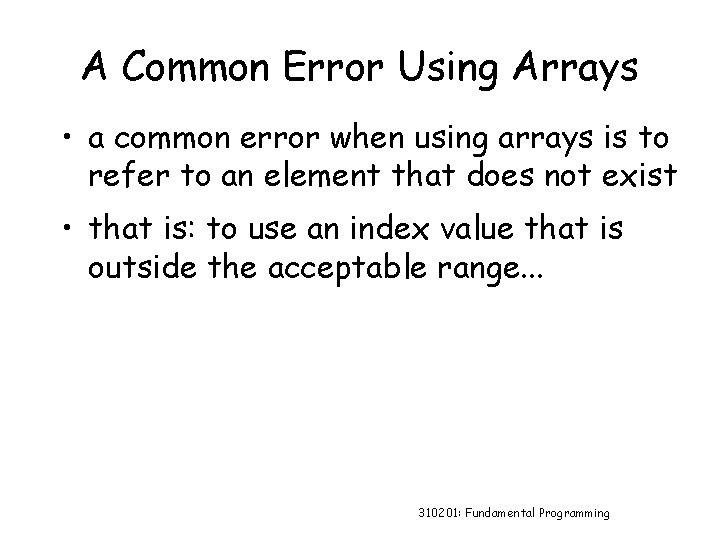
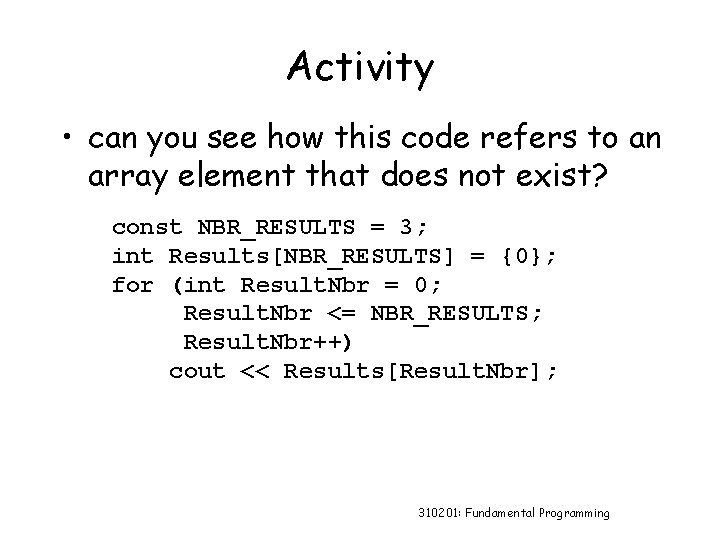
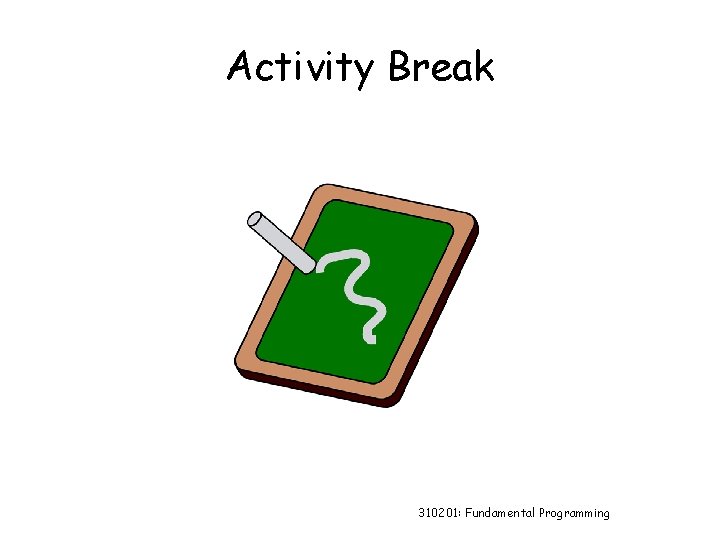
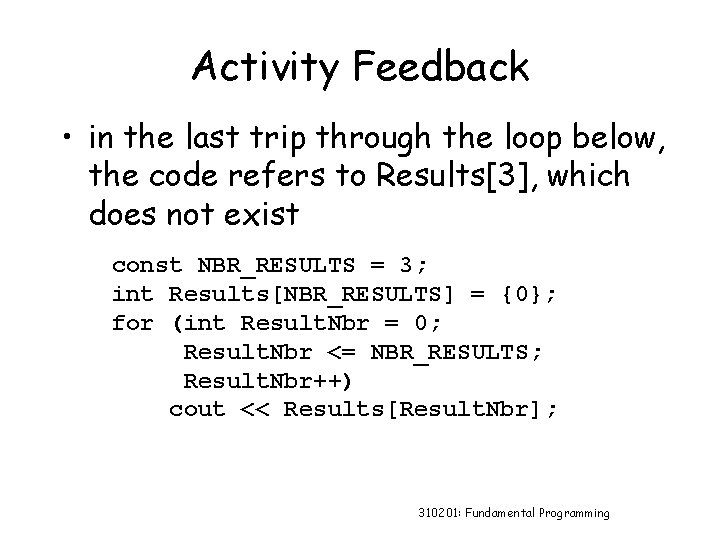
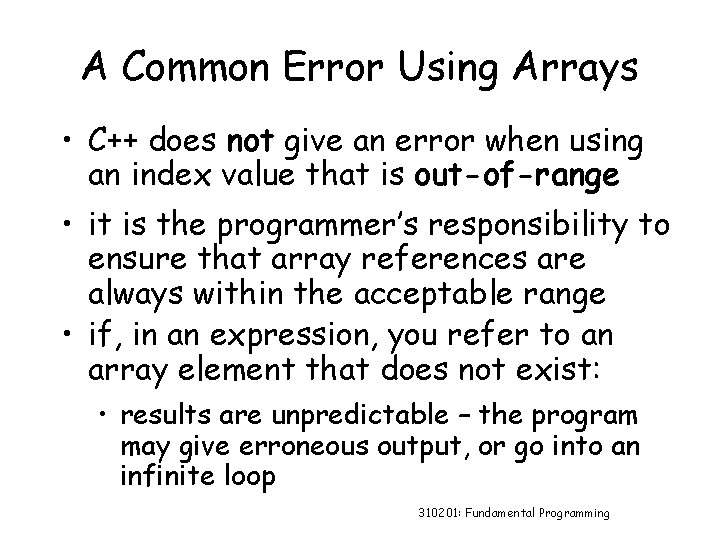
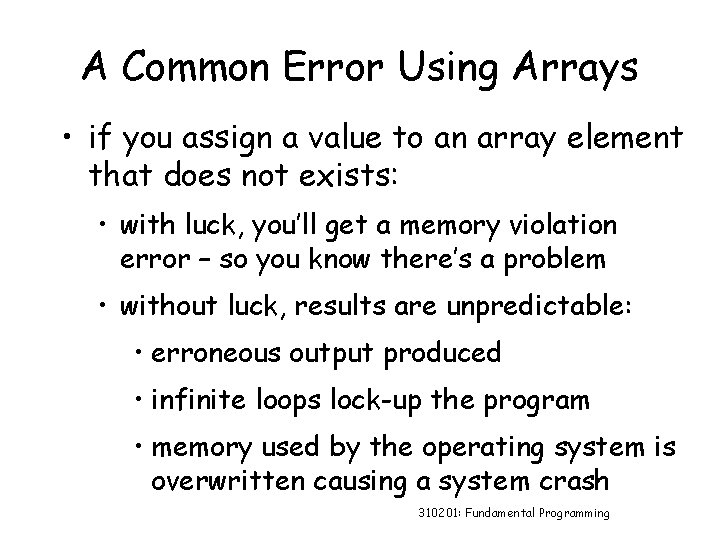
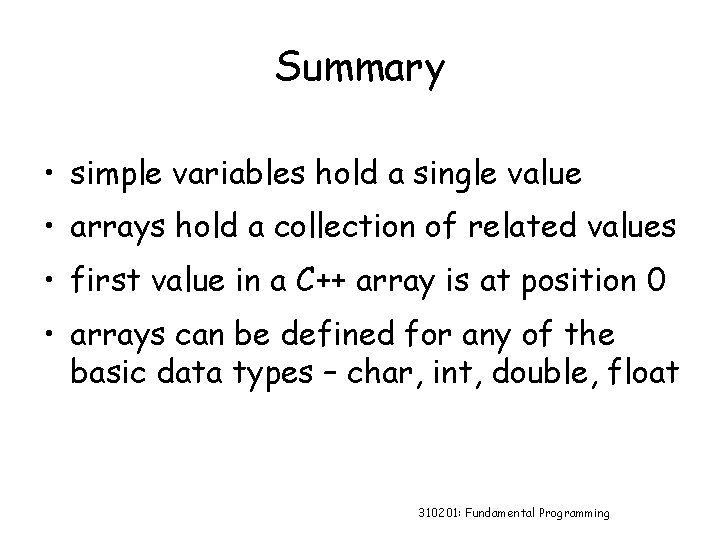
- Slides: 33
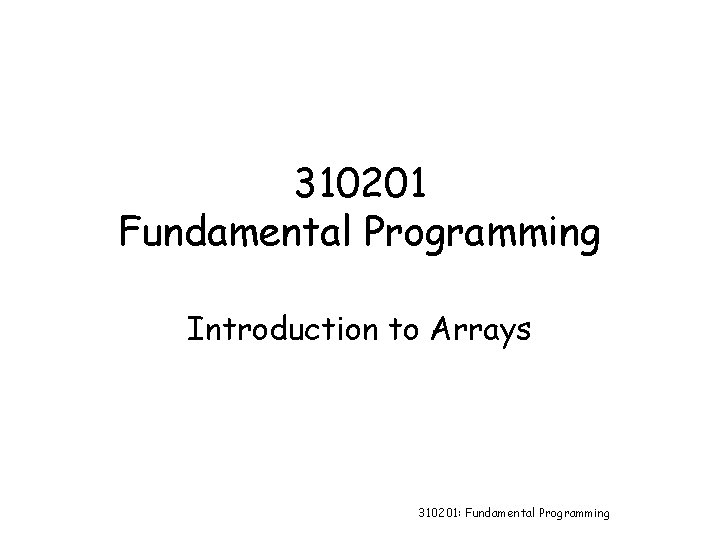
310201 Fundamental Programming Introduction to Arrays 310201: Fundamental Programming
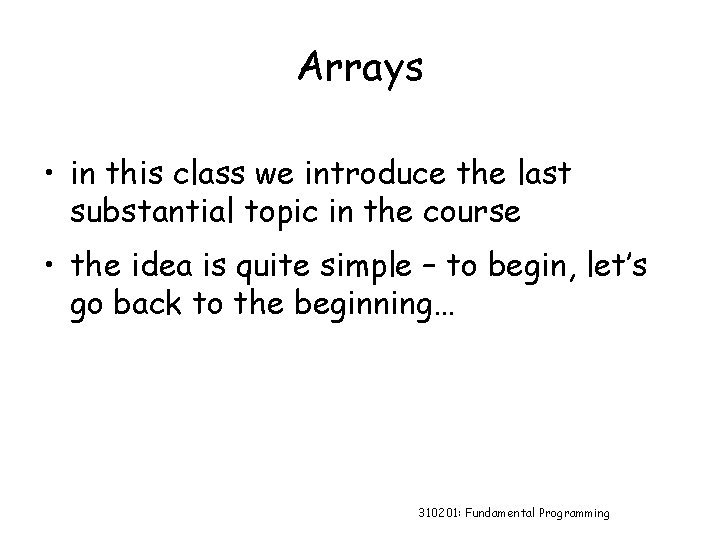
Arrays • in this class we introduce the last substantial topic in the course • the idea is quite simple – to begin, let’s go back to the beginning… 310201: Fundamental Programming
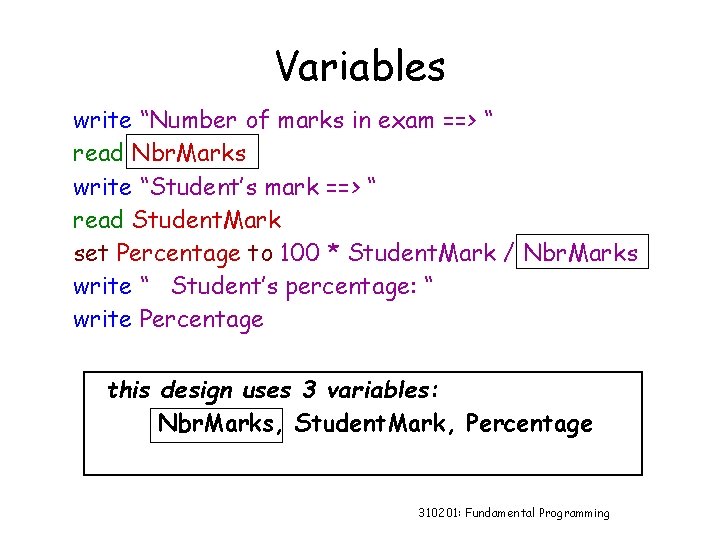
Variables write “Number of marks in exam ==> “ read Nbr. Marks write “Student’s mark ==> “ read Student. Mark set Percentage to 100 * Student. Mark / Nbr. Marks write “ Student’s percentage: “ write Percentage this design uses 3 variables: Nbr. Marks, Student. Mark, Percentage 310201: Fundamental Programming
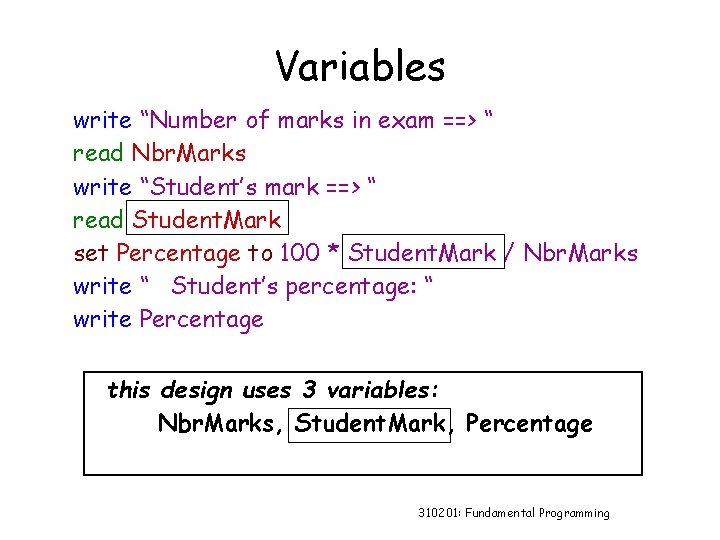
Variables write “Number of marks in exam ==> “ read Nbr. Marks write “Student’s mark ==> “ read Student. Mark set Percentage to 100 * Student. Mark / Nbr. Marks write “ Student’s percentage: “ write Percentage this design uses 3 variables: Nbr. Marks, Student. Mark, Percentage 310201: Fundamental Programming
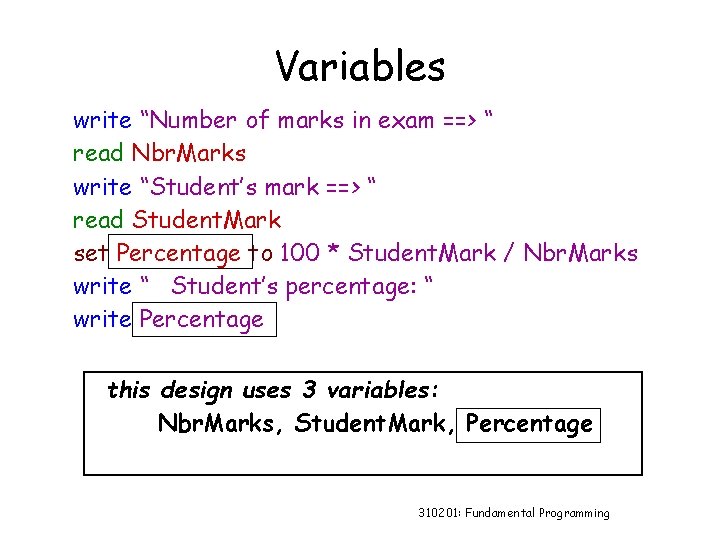
Variables write “Number of marks in exam ==> “ read Nbr. Marks write “Student’s mark ==> “ read Student. Mark set Percentage to 100 * Student. Mark / Nbr. Marks write “ Student’s percentage: “ write Percentage this design uses 3 variables: Nbr. Marks, Student. Mark, Percentage 310201: Fundamental Programming
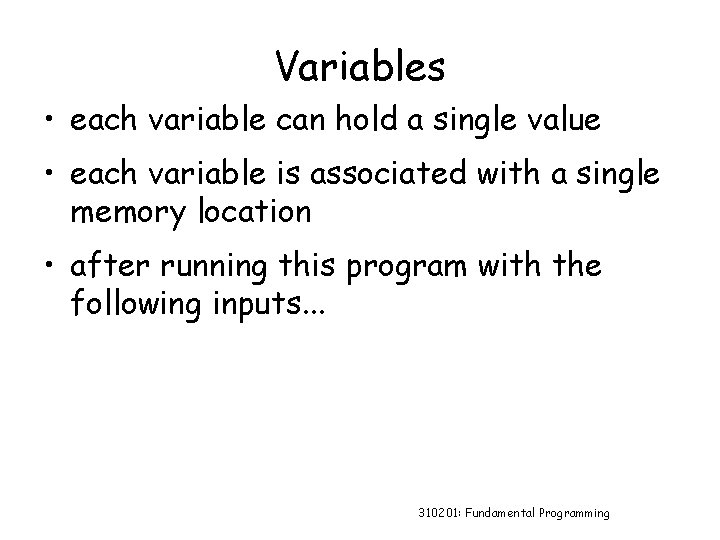
Variables • each variable can hold a single value • each variable is associated with a single memory location • after running this program with the following inputs. . . 310201: Fundamental Programming
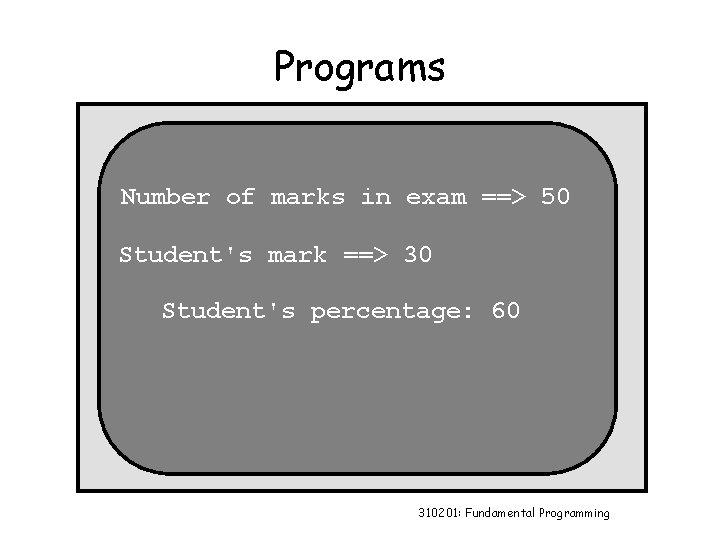
Programs Number of marks in exam ==> 50 Student's mark ==> 30 Student's percentage: 60 310201: Fundamental Programming
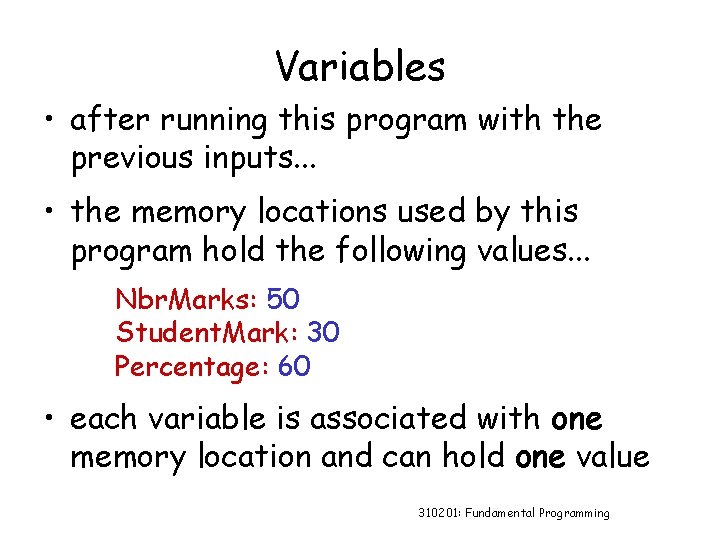
Variables • after running this program with the previous inputs. . . • the memory locations used by this program hold the following values. . . Nbr. Marks: 50 Student. Mark: 30 Percentage: 60 • each variable is associated with one memory location and can hold one value 310201: Fundamental Programming
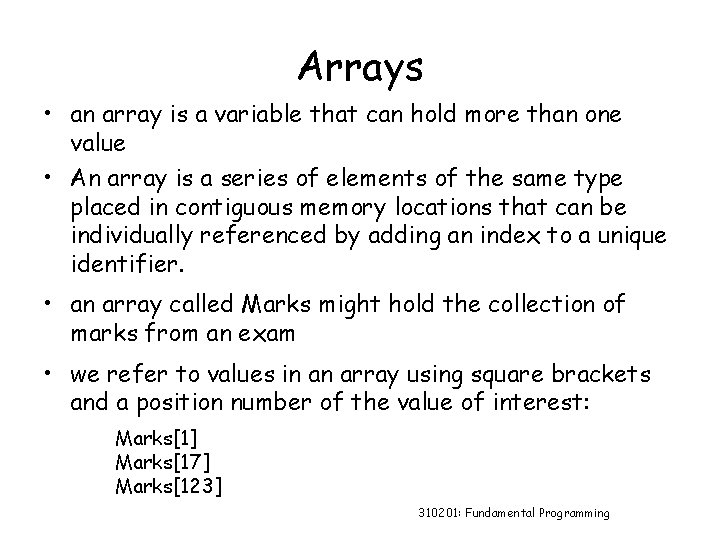
Arrays • an array is a variable that can hold more than one value • An array is a series of elements of the same type placed in contiguous memory locations that can be individually referenced by adding an index to a unique identifier. • an array called Marks might hold the collection of marks from an exam • we refer to values in an array using square brackets and a position number of the value of interest: Marks[1] Marks[17] Marks[123] 310201: Fundamental Programming
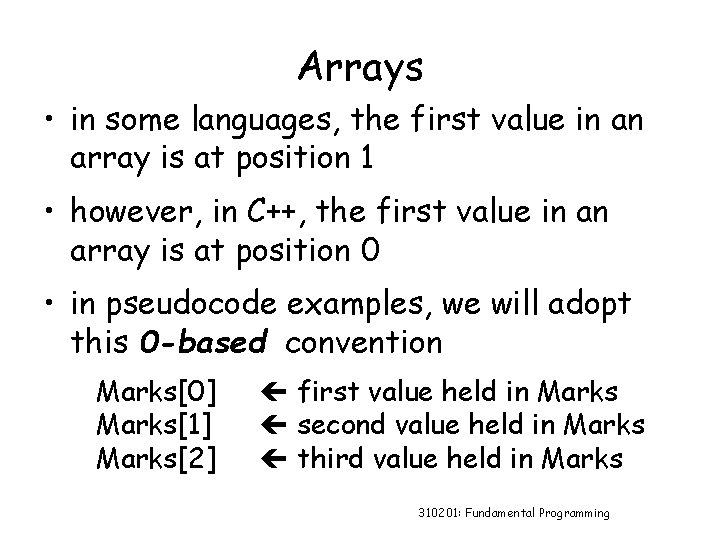
Arrays • in some languages, the first value in an array is at position 1 • however, in C++, the first value in an array is at position 0 • in pseudocode examples, we will adopt this 0 -based convention Marks[0] Marks[1] Marks[2] first value held in Marks second value held in Marks third value held in Marks 310201: Fundamental Programming
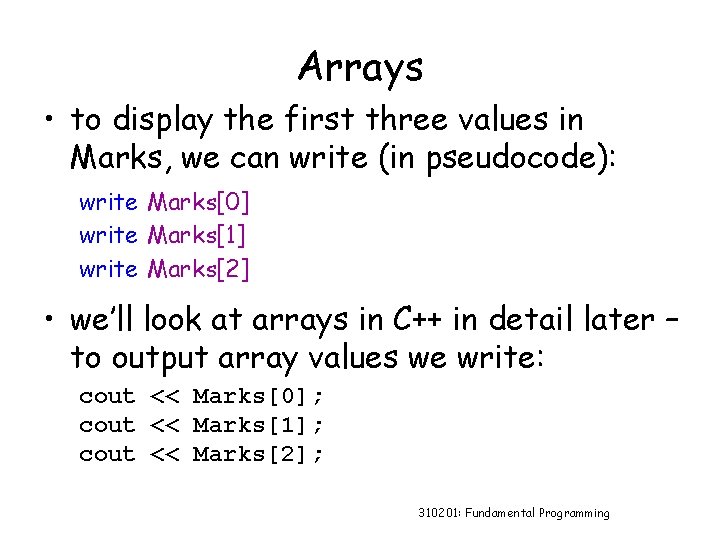
Arrays • to display the first three values in Marks, we can write (in pseudocode): write Marks[0] write Marks[1] write Marks[2] • we’ll look at arrays in C++ in detail later – to output array values we write: cout << Marks[0]; cout << Marks[1]; cout << Marks[2]; 310201: Fundamental Programming
![Arrays we assign values to an array using the same notation read Marks0 Arrays • we assign values to an array using the same notation: read Marks[0]](https://slidetodoc.com/presentation_image_h2/57d040b26985fd6581efb580eeca89ce/image-12.jpg)
Arrays • we assign values to an array using the same notation: read Marks[0] (read value and assign as first in Marks) set Marks[5] = 0 (assign sixth value in Marks to 0) • no surprises here in C++: cin >> Marks[0]; Marks[5] = 0; 310201: Fundamental Programming
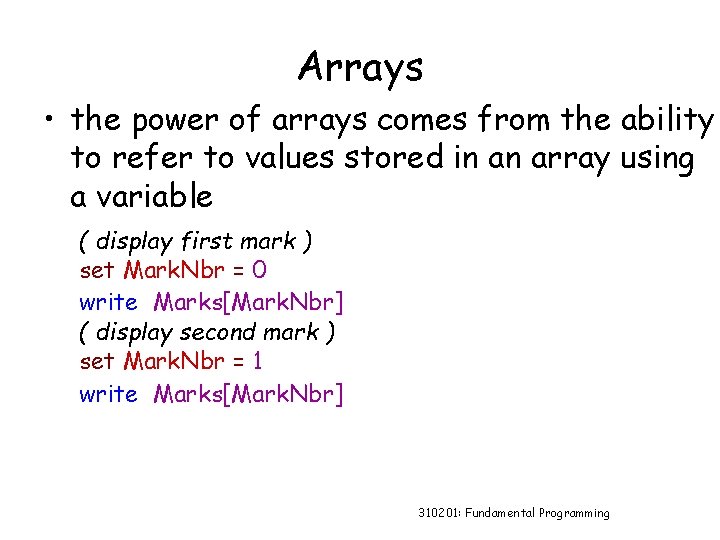
Arrays • the power of arrays comes from the ability to refer to values stored in an array using a variable ( display first mark ) set Mark. Nbr = 0 write Marks[Mark. Nbr] ( display second mark ) set Mark. Nbr = 1 write Marks[Mark. Nbr] 310201: Fundamental Programming
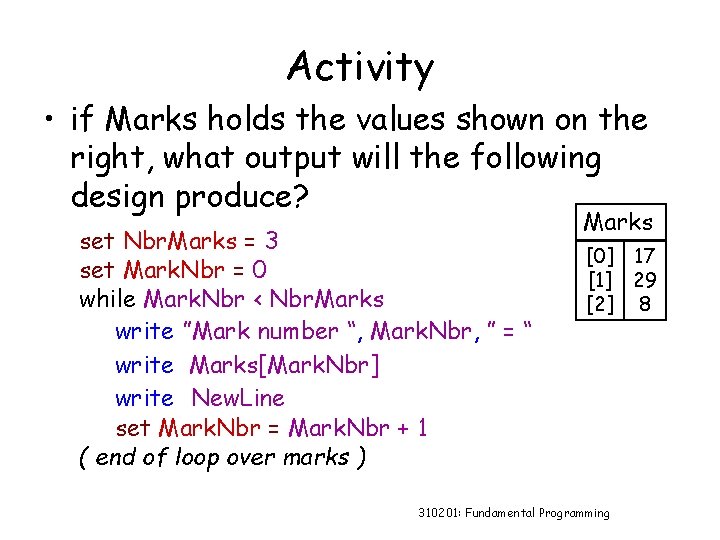
Activity • if Marks holds the values shown on the right, what output will the following design produce? set Nbr. Marks = 3 set Mark. Nbr = 0 while Mark. Nbr < Nbr. Marks write ”Mark number “, Mark. Nbr, ” = “ write Marks[Mark. Nbr] write New. Line set Mark. Nbr = Mark. Nbr + 1 ( end of loop over marks ) Marks [0] 17 [1] 29 [2] 8 310201: Fundamental Programming
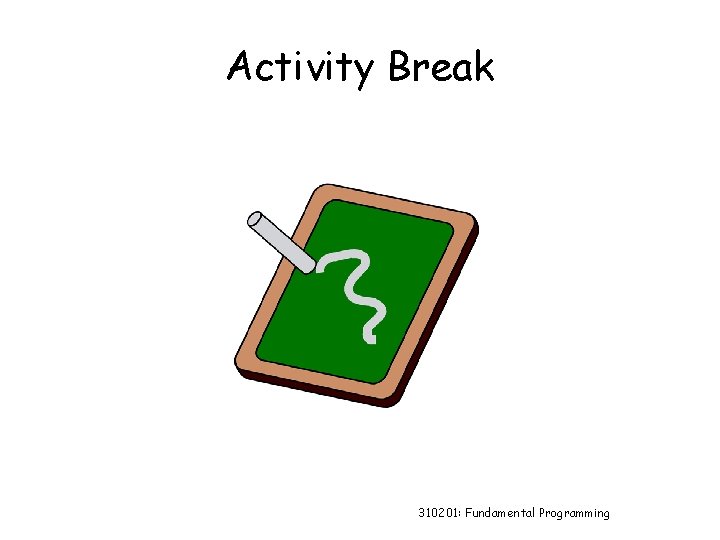
Activity Break 310201: Fundamental Programming
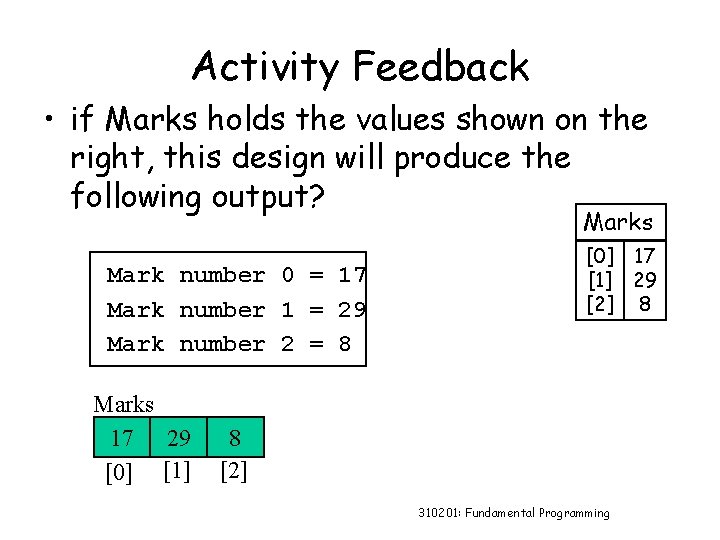
Activity Feedback • if Marks holds the values shown on the right, this design will produce the following output? Marks Mark number 0 = 17 Mark number 1 = 29 Mark number 2 = 8 Marks 17 29 [0] [1] [0] 17 [1] 29 [2] 8 8 [2] 310201: Fundamental Programming
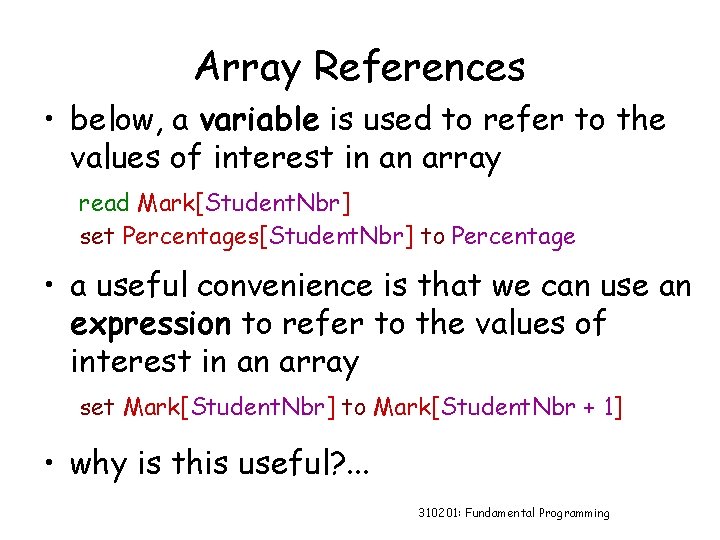
Array References • below, a variable is used to refer to the values of interest in an array read Mark[Student. Nbr] set Percentages[Student. Nbr] to Percentage • a useful convenience is that we can use an expression to refer to the values of interest in an array set Mark[Student. Nbr] to Mark[Student. Nbr + 1] • why is this useful? . . . 310201: Fundamental Programming
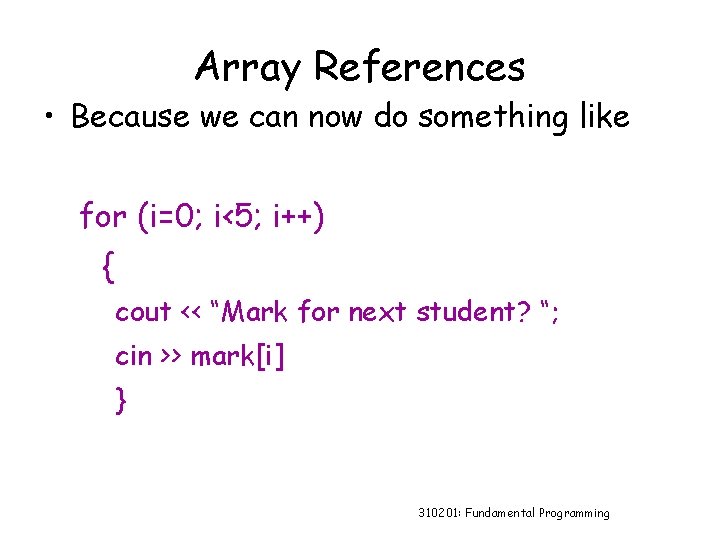
Array References • Because we can now do something like for (i=0; i<5; i++) { cout << “Mark for next student? “; cin >> mark[i] } 310201: Fundamental Programming
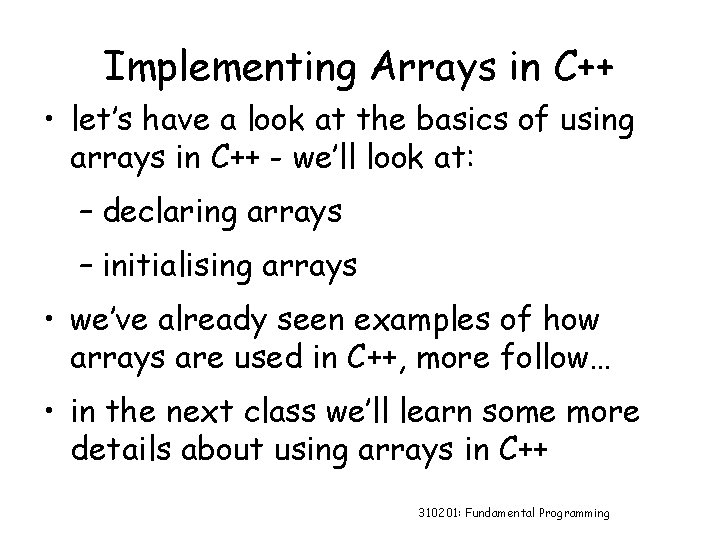
Implementing Arrays in C++ • let’s have a look at the basics of using arrays in C++ - we’ll look at: – declaring arrays – initialising arrays • we’ve already seen examples of how arrays are used in C++, more follow… • in the next class we’ll learn some more details about using arrays in C++ 310201: Fundamental Programming
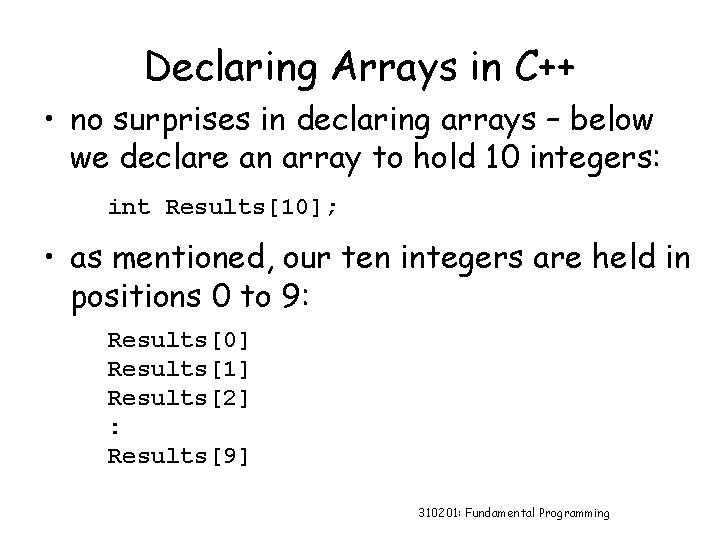
Declaring Arrays in C++ • no surprises in declaring arrays – below we declare an array to hold 10 integers: int Results[10]; • as mentioned, our ten integers are held in positions 0 to 9: Results[0] Results[1] Results[2] : Results[9] 310201: Fundamental Programming
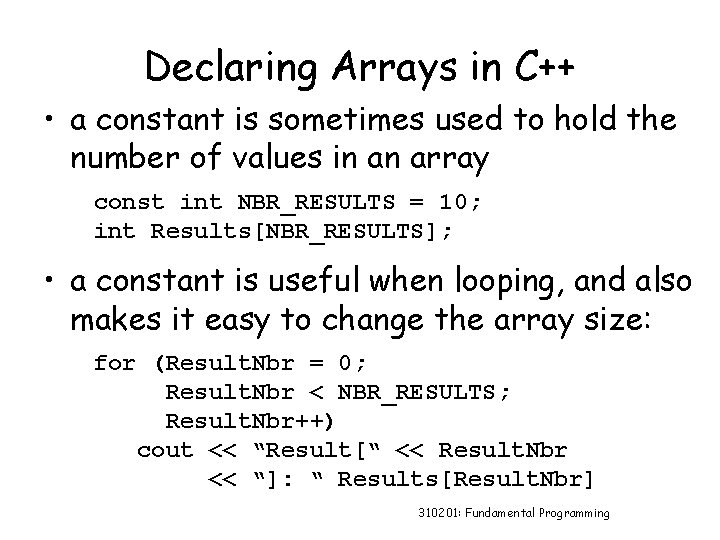
Declaring Arrays in C++ • a constant is sometimes used to hold the number of values in an array const int NBR_RESULTS = 10; int Results[NBR_RESULTS]; • a constant is useful when looping, and also makes it easy to change the array size: for (Result. Nbr = 0; Result. Nbr < NBR_RESULTS; Result. Nbr++) cout << “Result[“ << Result. Nbr << “]: “ Results[Result. Nbr] 310201: Fundamental Programming
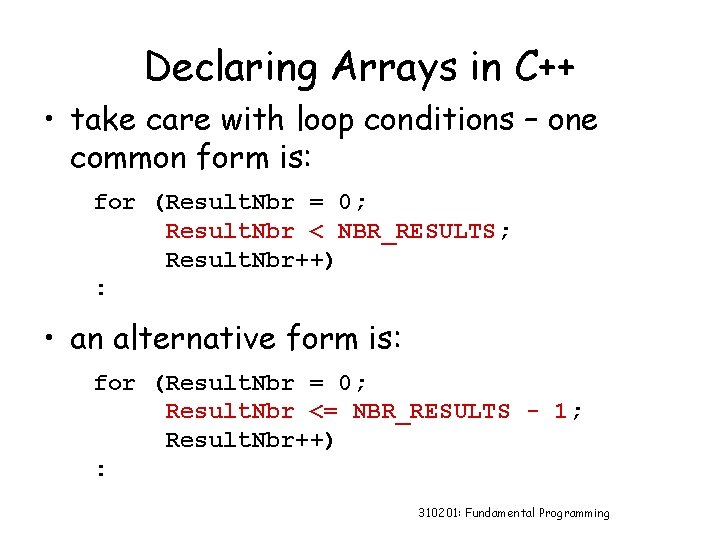
Declaring Arrays in C++ • take care with loop conditions – one common form is: for (Result. Nbr = 0; Result. Nbr < NBR_RESULTS; Result. Nbr++) : • an alternative form is: for (Result. Nbr = 0; Result. Nbr <= NBR_RESULTS - 1; Result. Nbr++) : 310201: Fundamental Programming
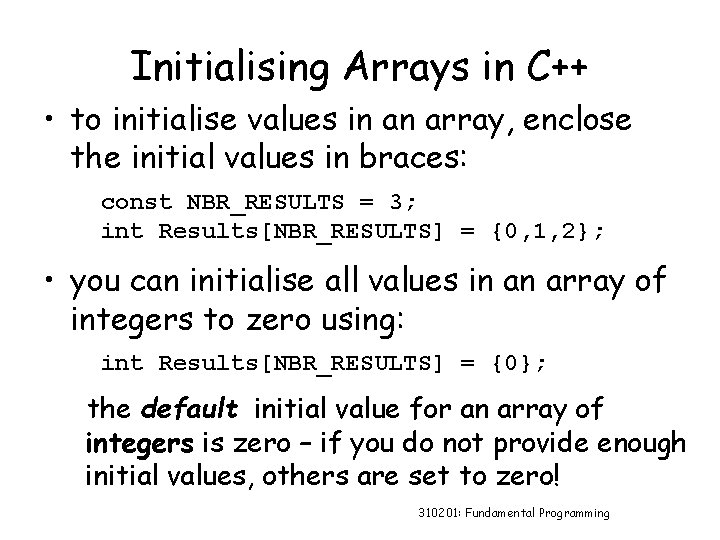
Initialising Arrays in C++ • to initialise values in an array, enclose the initial values in braces: const NBR_RESULTS = 3; int Results[NBR_RESULTS] = {0, 1, 2}; • you can initialise all values in an array of integers to zero using: int Results[NBR_RESULTS] = {0}; the default initial value for an array of integers is zero – if you do not provide enough initial values, others are set to zero! 310201: Fundamental Programming
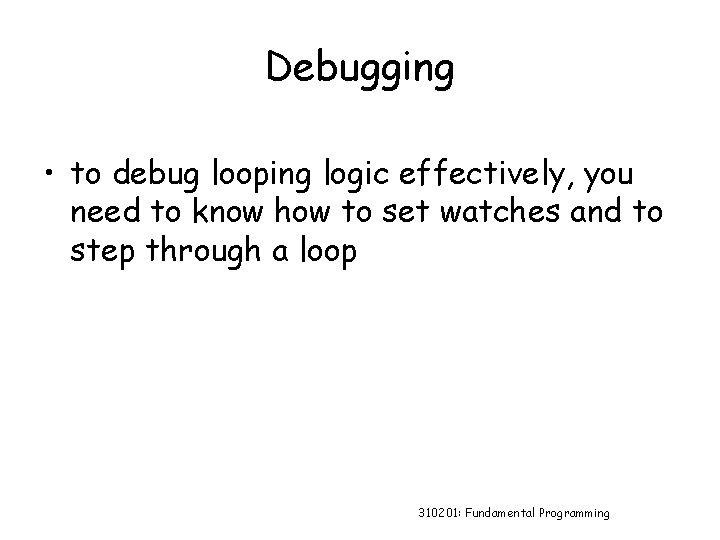
Debugging • to debug looping logic effectively, you need to know how to set watches and to step through a loop 310201: Fundamental Programming
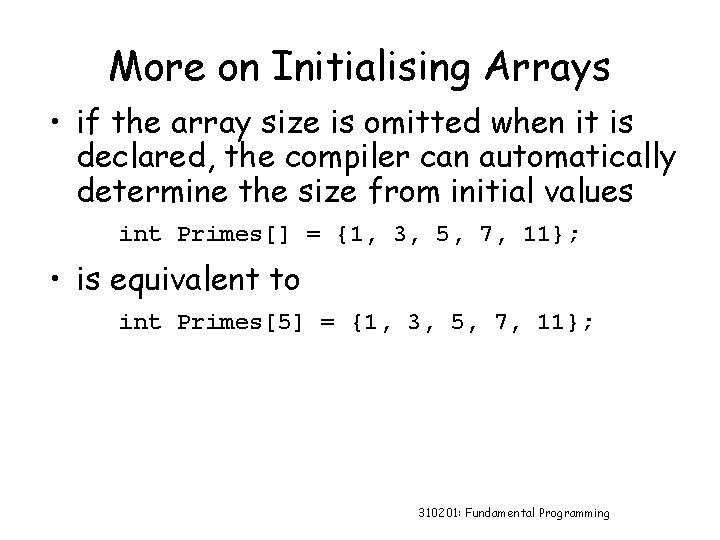
More on Initialising Arrays • if the array size is omitted when it is declared, the compiler can automatically determine the size from initial values int Primes[] = {1, 3, 5, 7, 11}; • is equivalent to int Primes[5] = {1, 3, 5, 7, 11}; 310201: Fundamental Programming
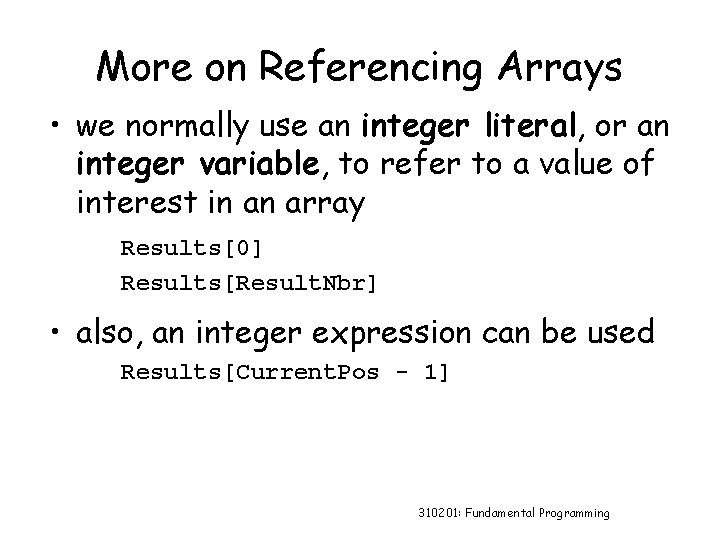
More on Referencing Arrays • we normally use an integer literal, or an integer variable, to refer to a value of interest in an array Results[0] Results[Result. Nbr] • also, an integer expression can be used Results[Current. Pos - 1] 310201: Fundamental Programming
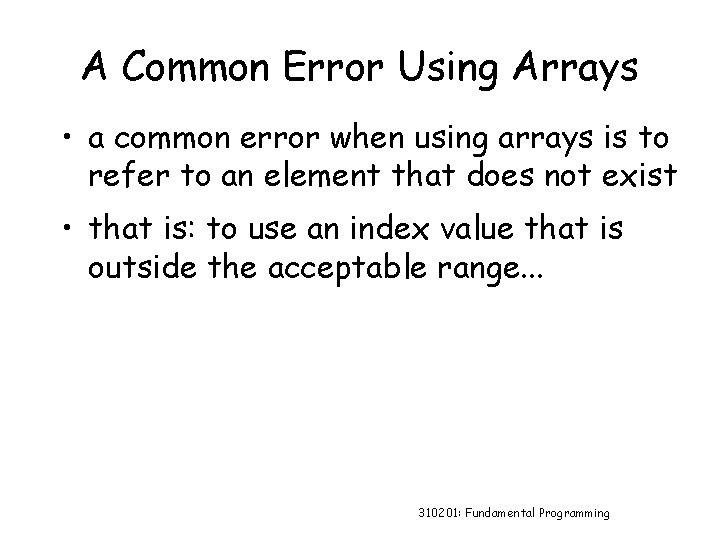
A Common Error Using Arrays • a common error when using arrays is to refer to an element that does not exist • that is: to use an index value that is outside the acceptable range. . . 310201: Fundamental Programming
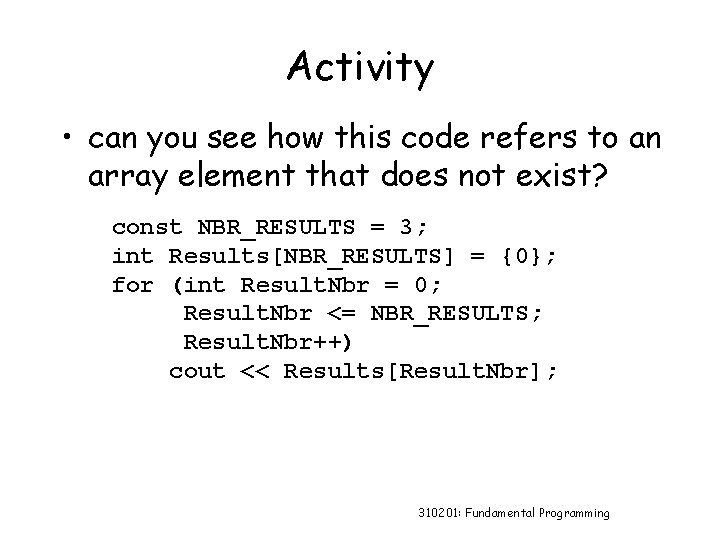
Activity • can you see how this code refers to an array element that does not exist? const NBR_RESULTS = 3; int Results[NBR_RESULTS] = {0}; for (int Result. Nbr = 0; Result. Nbr <= NBR_RESULTS; Result. Nbr++) cout << Results[Result. Nbr]; 310201: Fundamental Programming
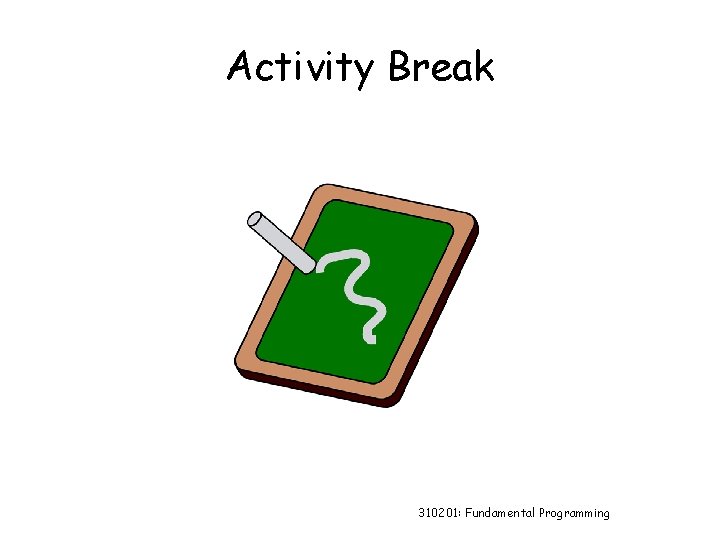
Activity Break 310201: Fundamental Programming
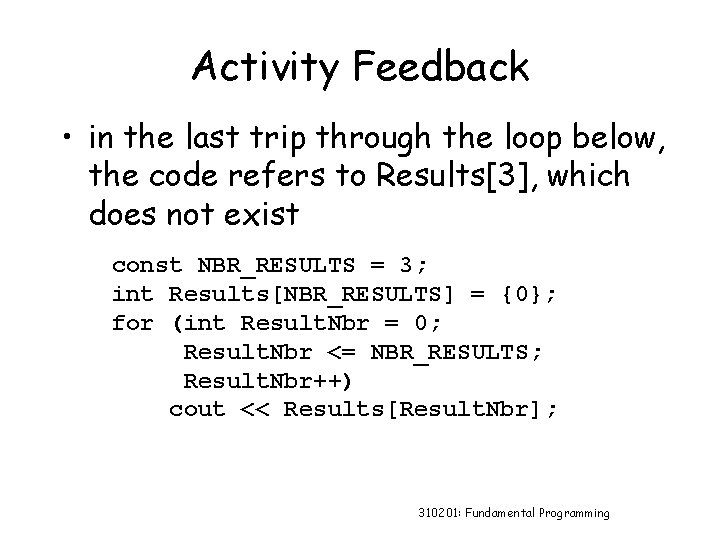
Activity Feedback • in the last trip through the loop below, the code refers to Results[3], which does not exist const NBR_RESULTS = 3; int Results[NBR_RESULTS] = {0}; for (int Result. Nbr = 0; Result. Nbr <= NBR_RESULTS; Result. Nbr++) cout << Results[Result. Nbr]; 310201: Fundamental Programming
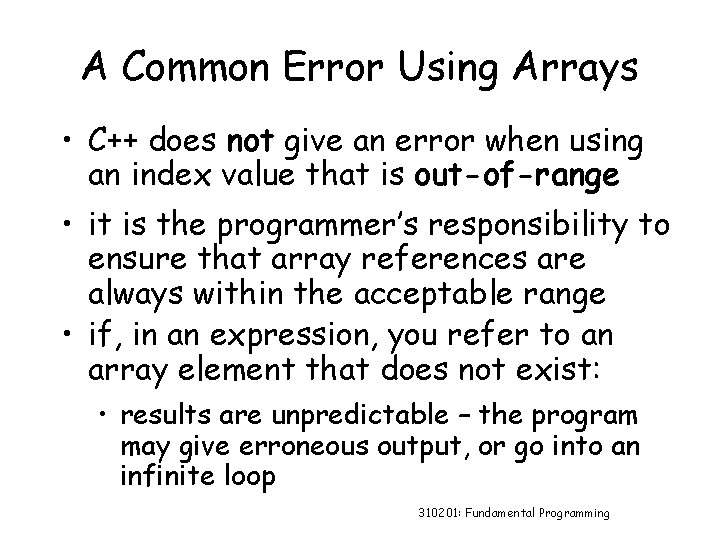
A Common Error Using Arrays • C++ does not give an error when using an index value that is out-of-range • it is the programmer’s responsibility to ensure that array references are always within the acceptable range • if, in an expression, you refer to an array element that does not exist: • results are unpredictable – the program may give erroneous output, or go into an infinite loop 310201: Fundamental Programming
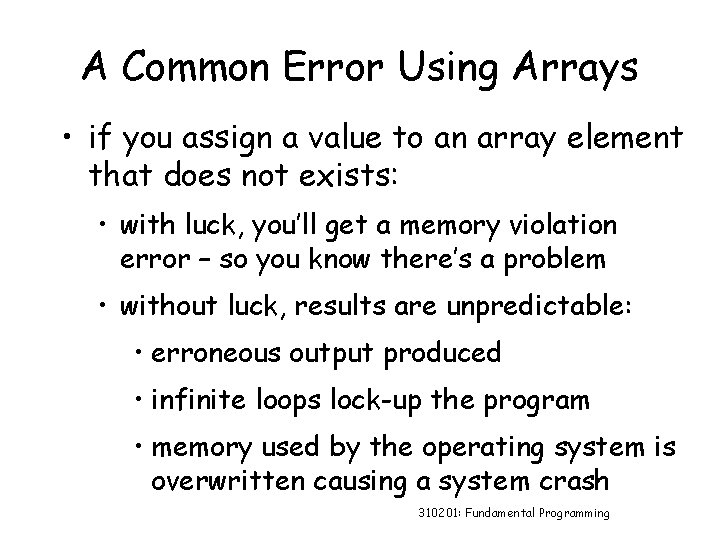
A Common Error Using Arrays • if you assign a value to an array element that does not exists: • with luck, you’ll get a memory violation error – so you know there’s a problem • without luck, results are unpredictable: • erroneous output produced • infinite loops lock-up the program • memory used by the operating system is overwritten causing a system crash 310201: Fundamental Programming
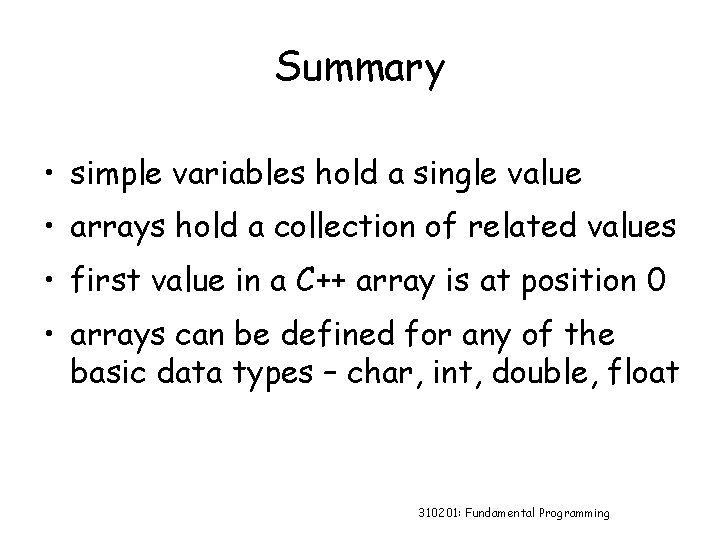
Summary • simple variables hold a single value • arrays hold a collection of related values • first value in a C++ array is at position 0 • arrays can be defined for any of the basic data types – char, int, double, float 310201: Fundamental Programming
Parallel arrays python
Array of arrays c++
Parallel arrays
Partially filled arrays
C++ parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Arreglos unidimensionales en java
Java arreglos bidimensionales
Arrays mips
Polynomial representation using array in c
Arrays in arm assembly
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++
Arrays visual basic
Python find index of max
Array advantage and disadvantage
I wonder is it possible
2d array pascal
Dynamic array mips
Creating arrays matlab
Array adt
Partially filled array
Redundancy array of independent disk
Python list of arrays
Arrays
Day 3: arrays
Redundant arrays of inexpensive disks
Small basic array examples
Dynamic p table
Microled arrays
Are vectors dynamic arrays
Facts about arrays