Arrays and Structures Arrays Polynomial representation Polynomial add
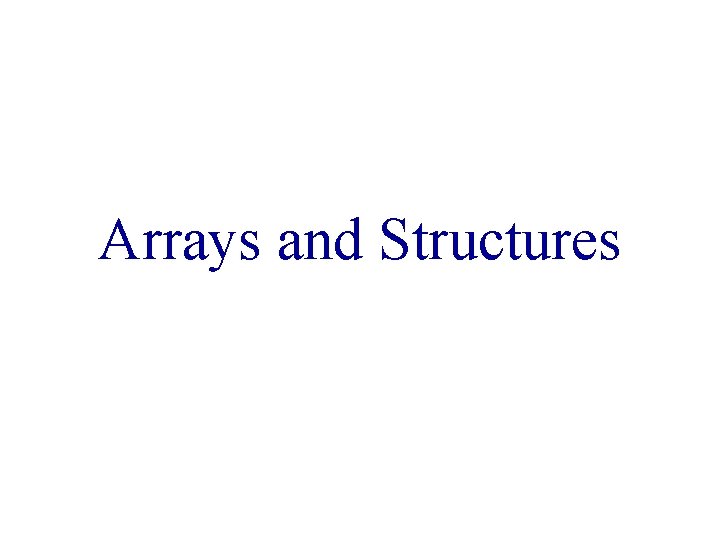
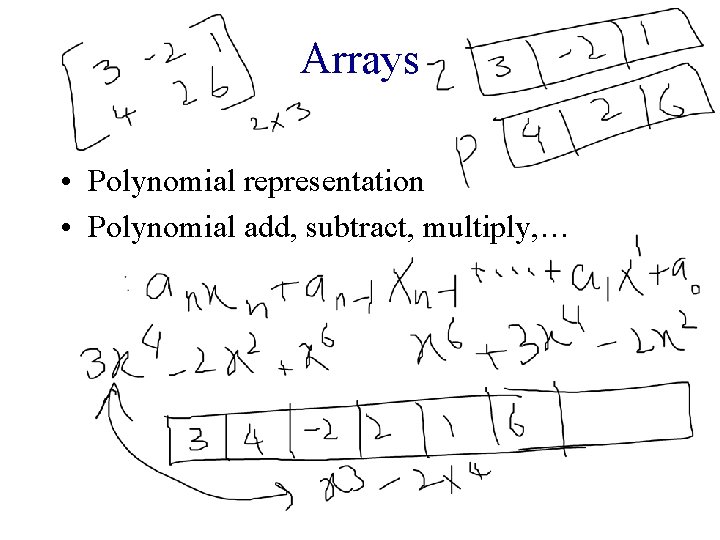
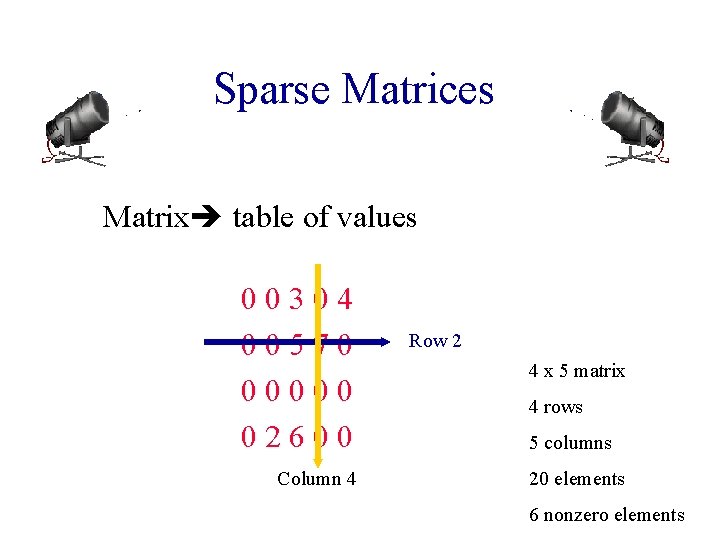
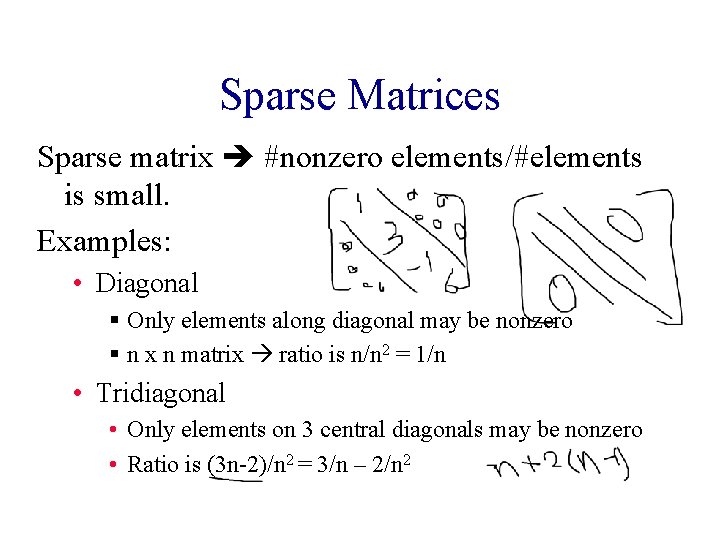
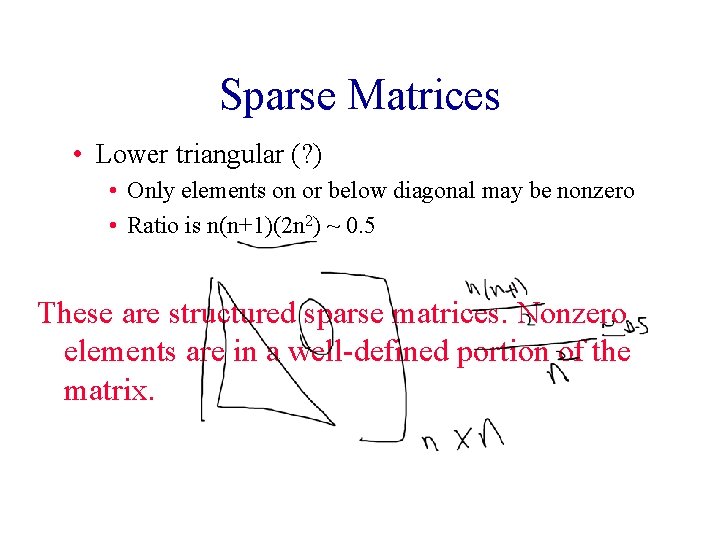
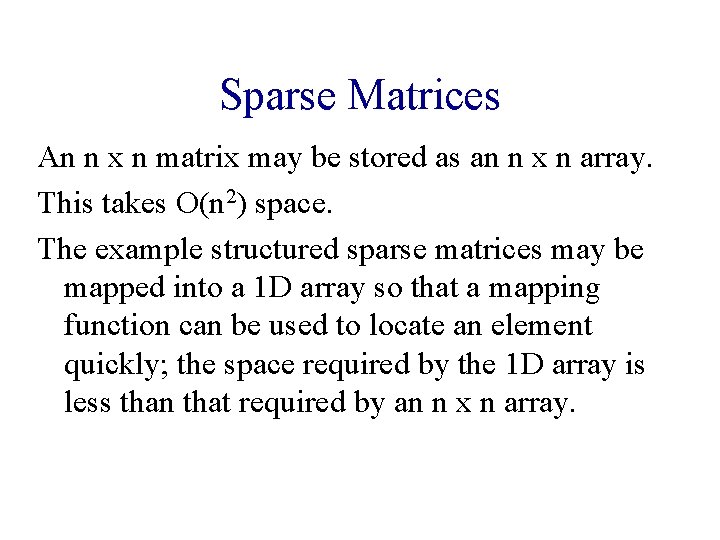
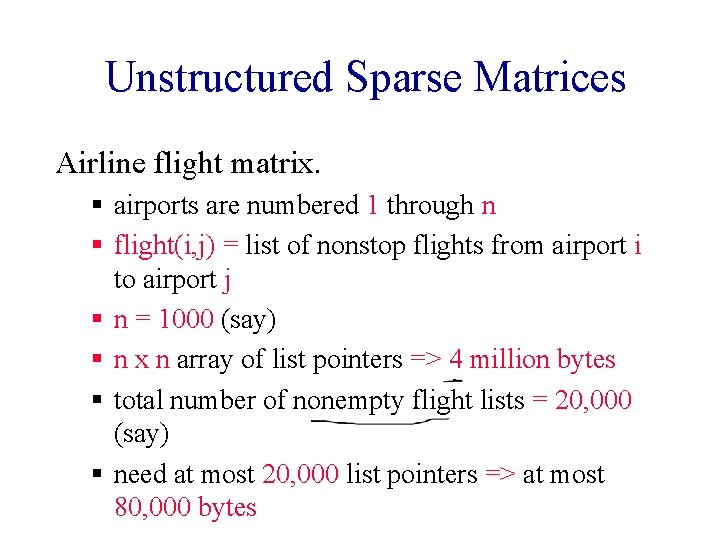
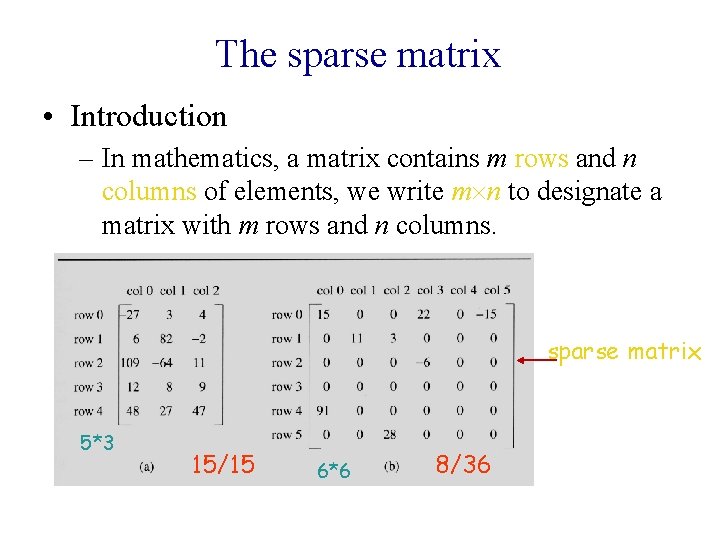
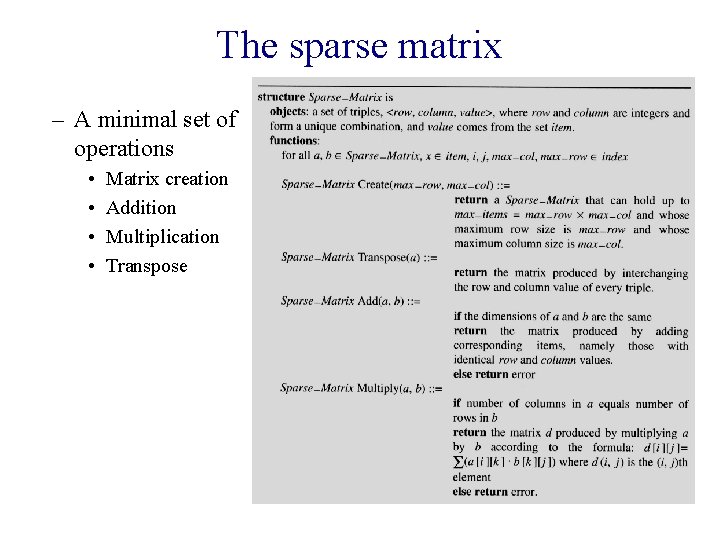
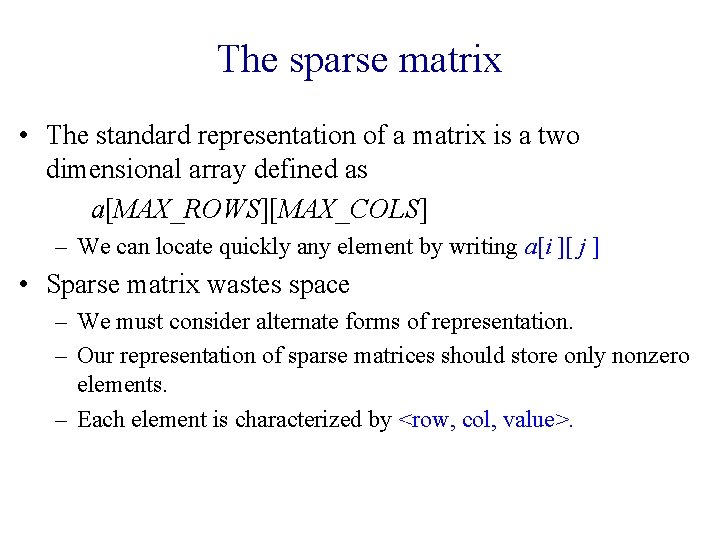
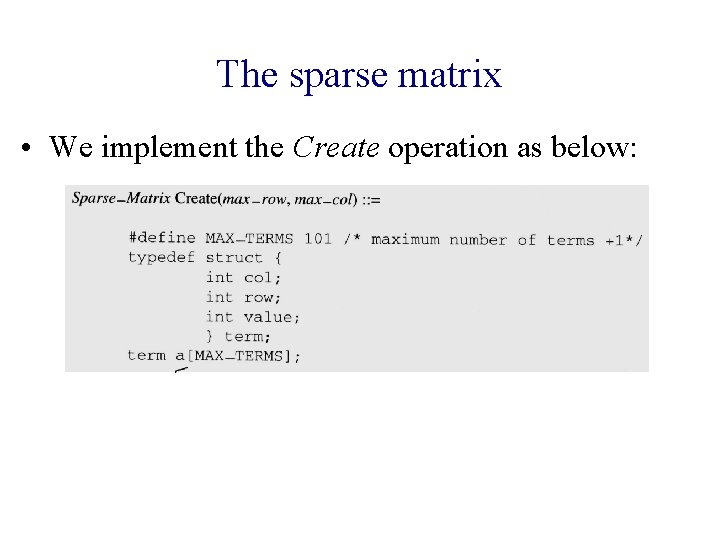
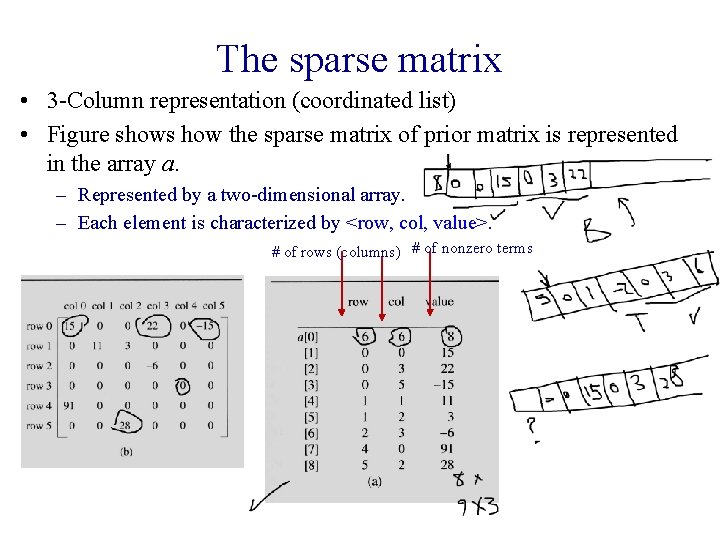
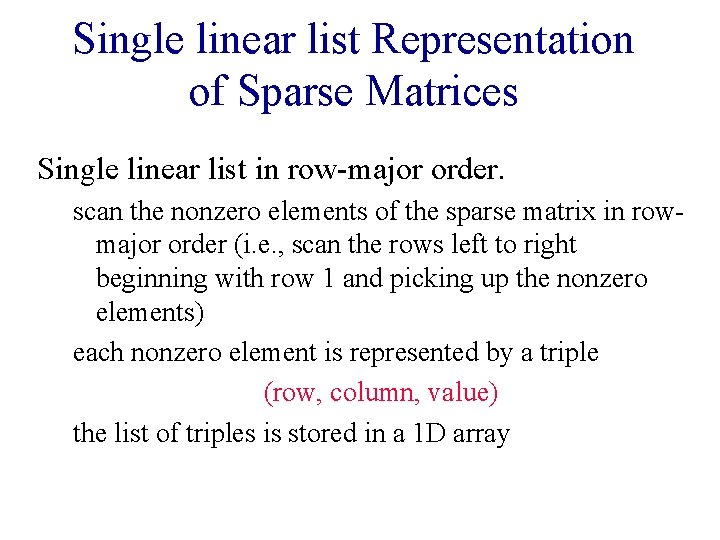
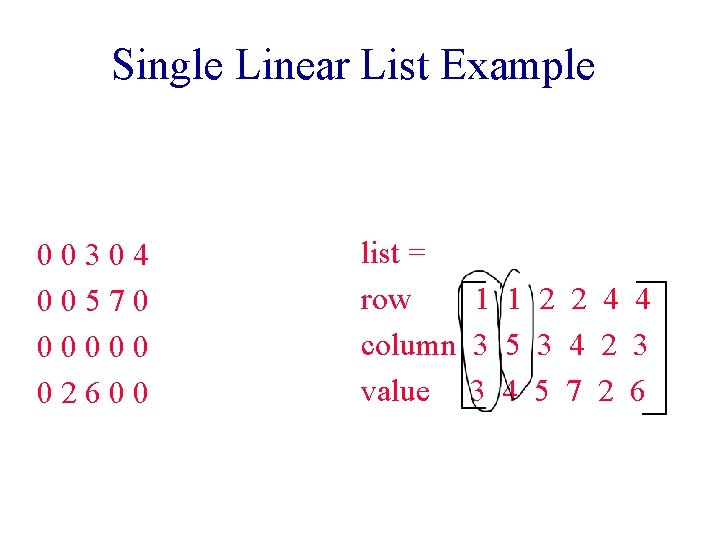
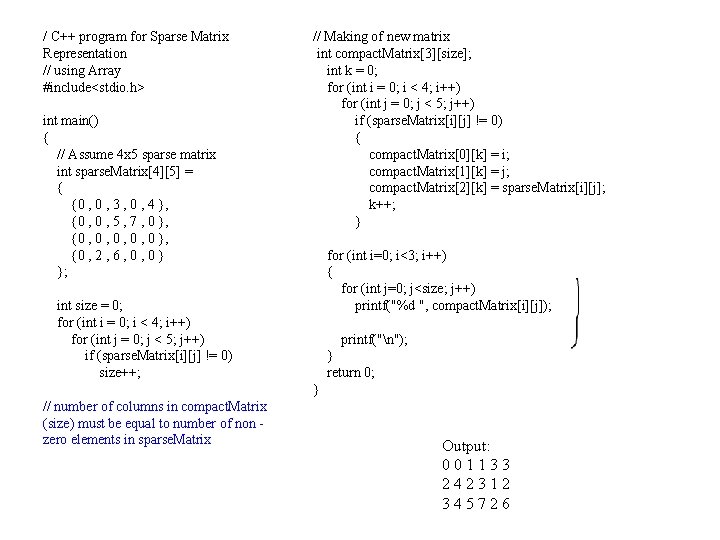
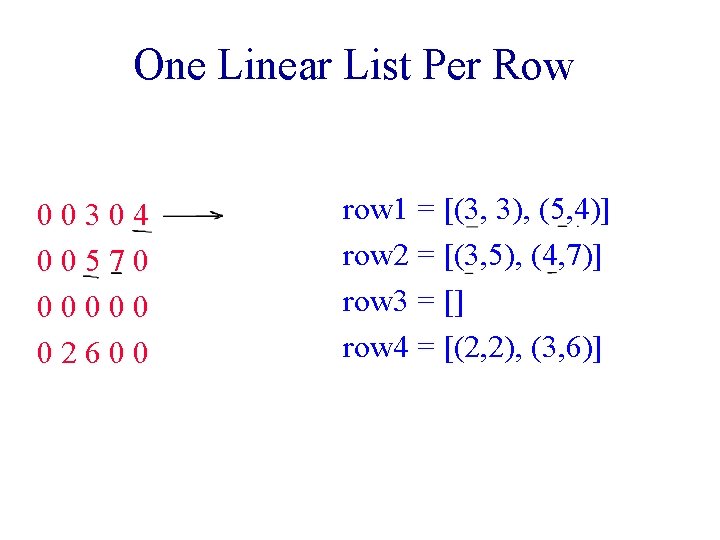
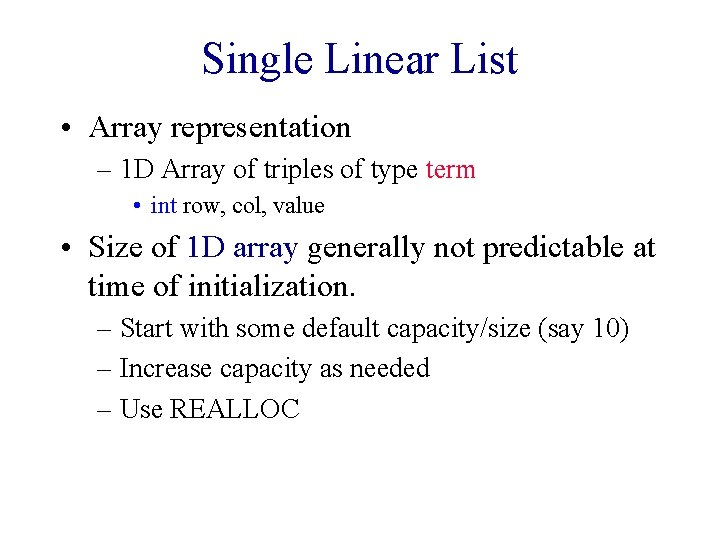
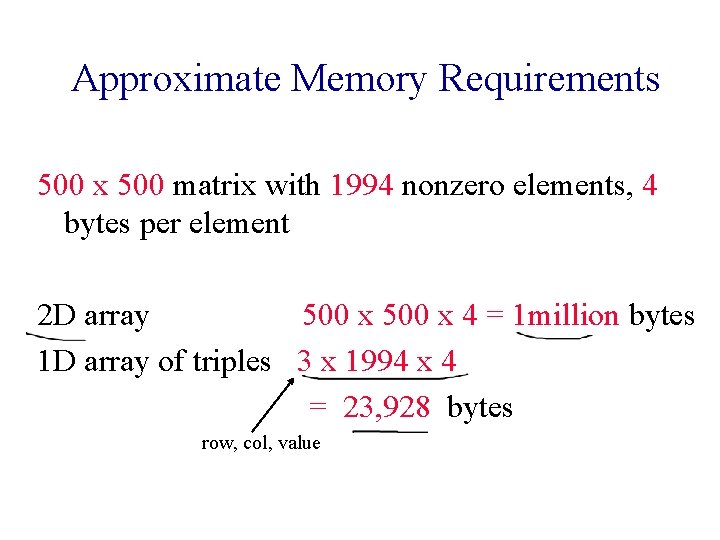
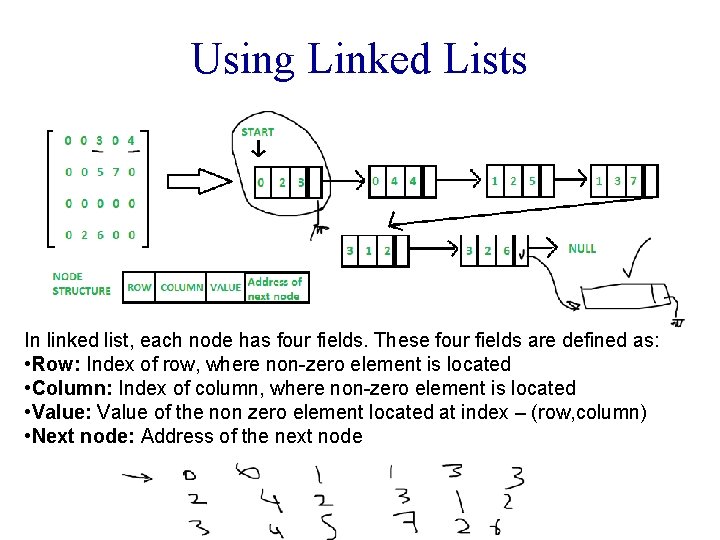
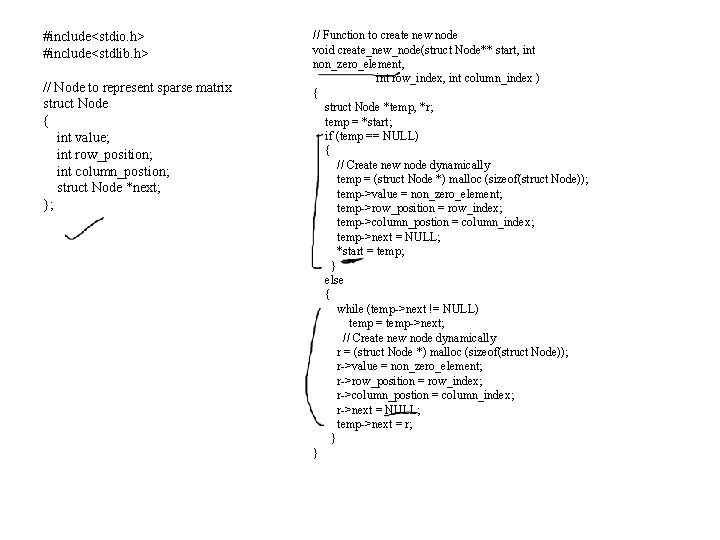
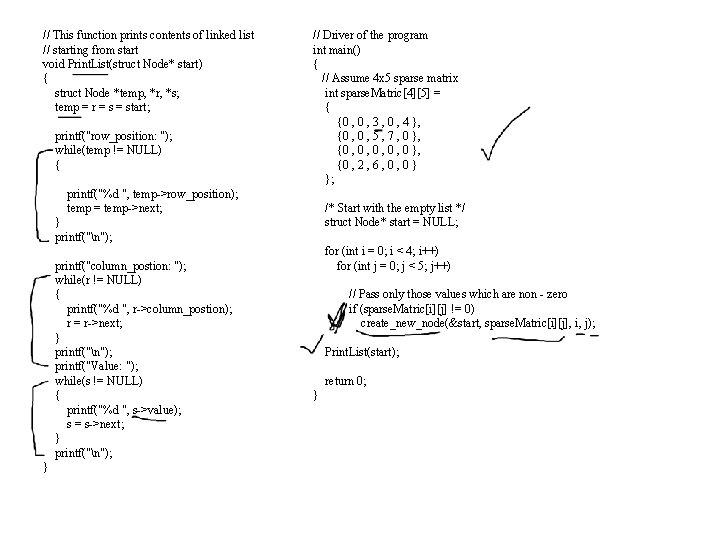
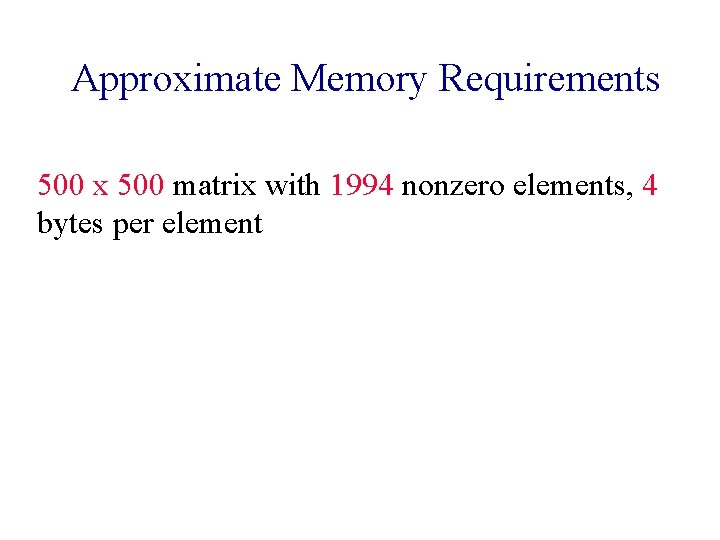
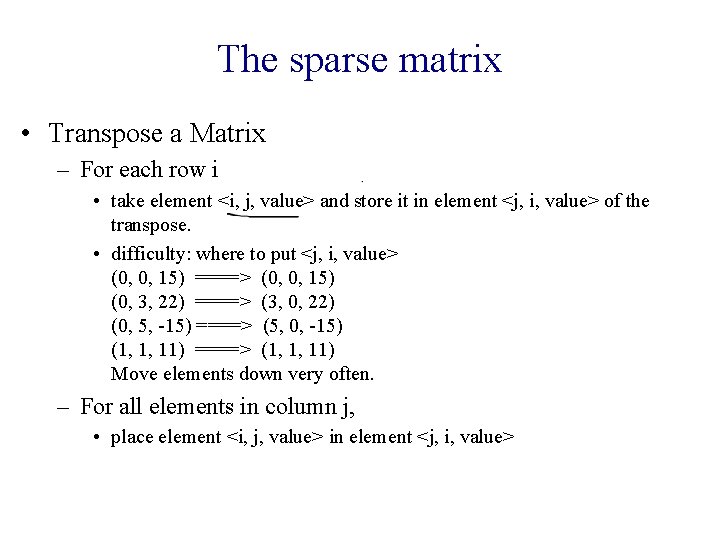
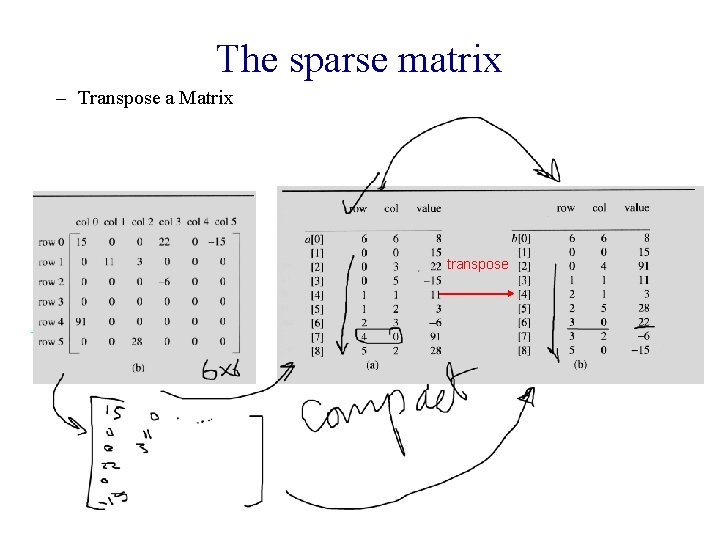
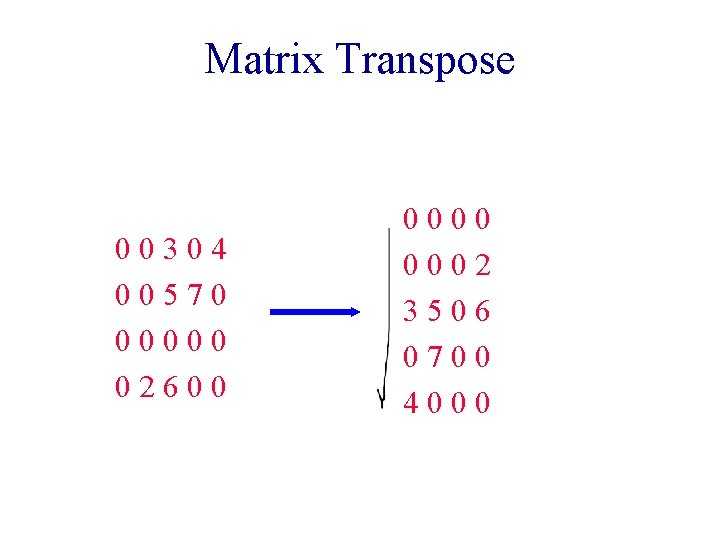
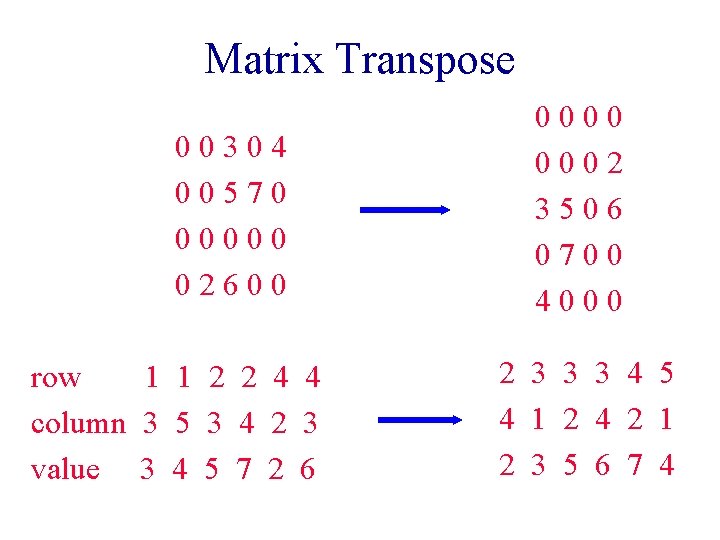
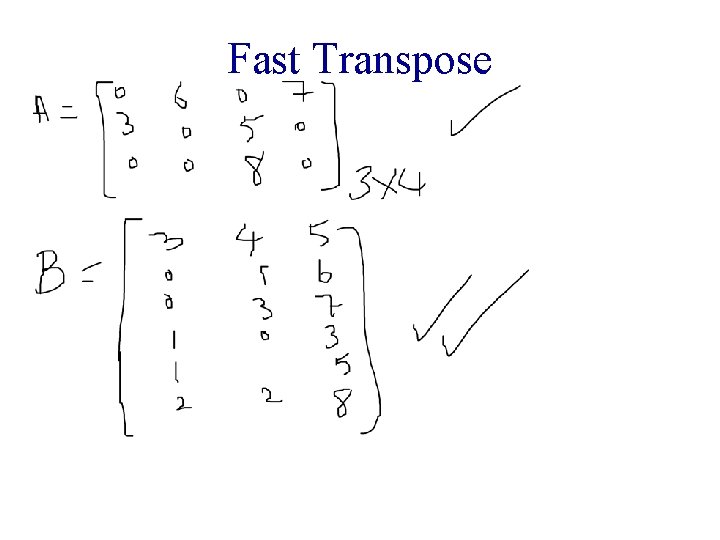
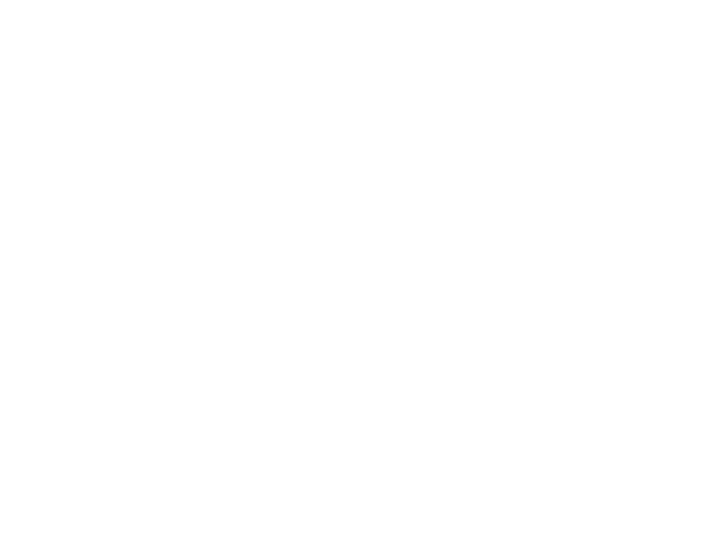
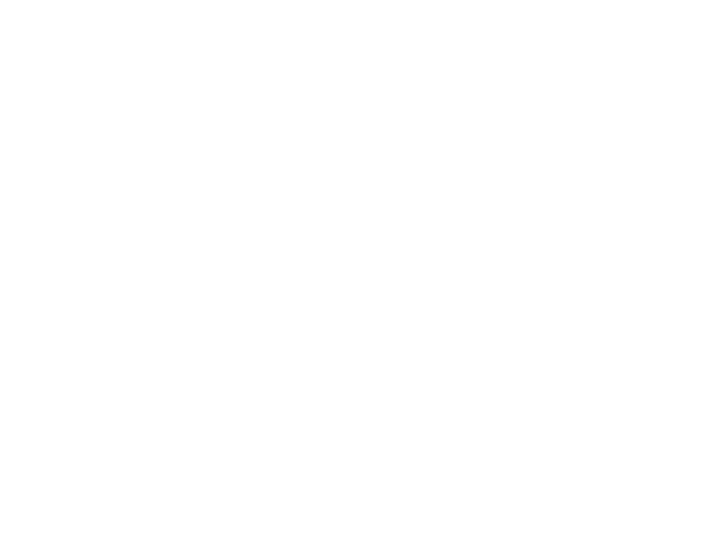
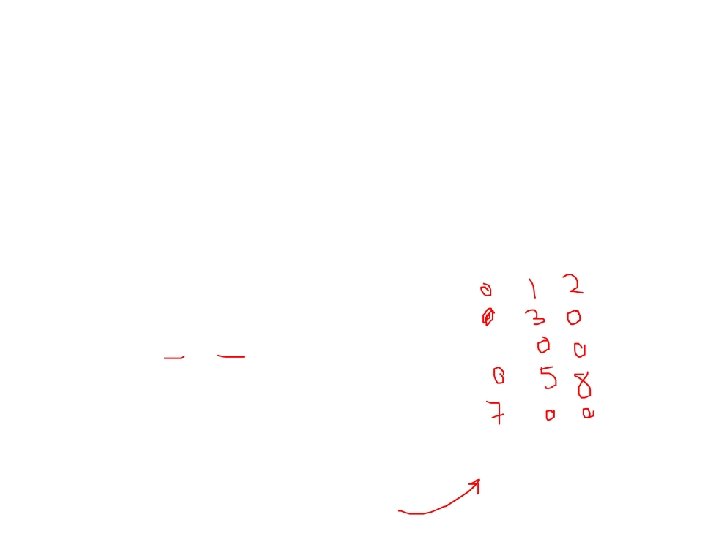
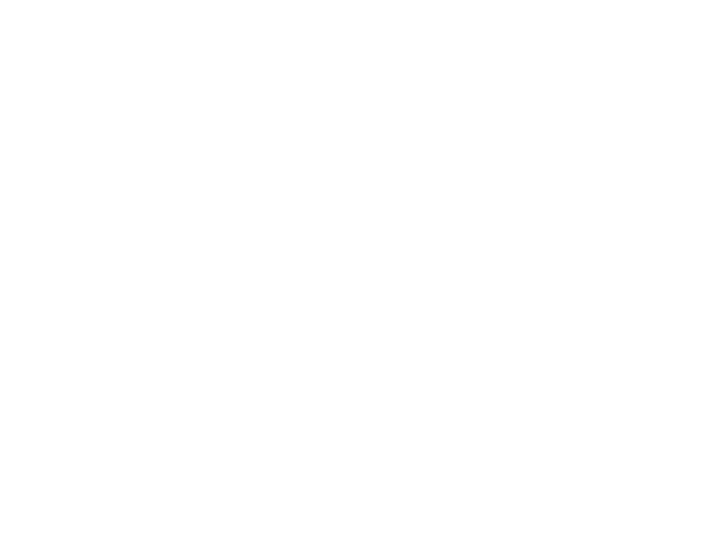
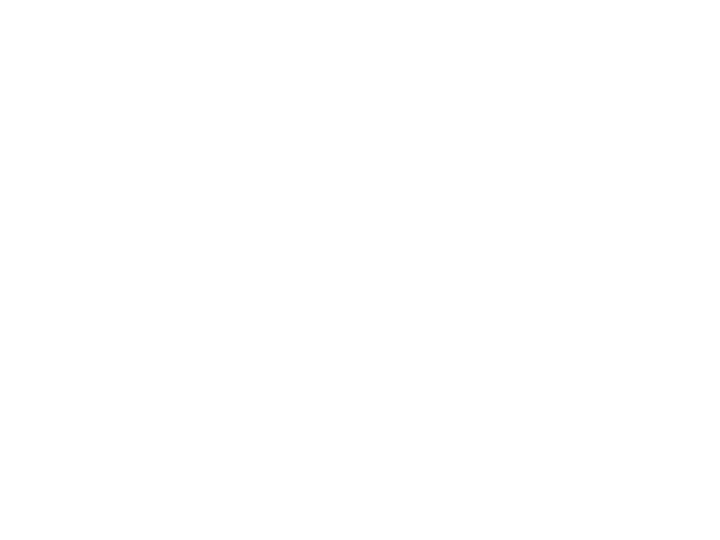
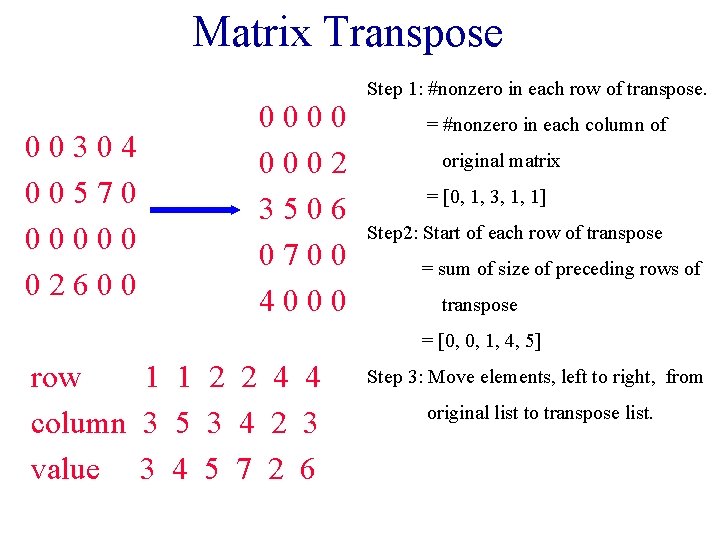
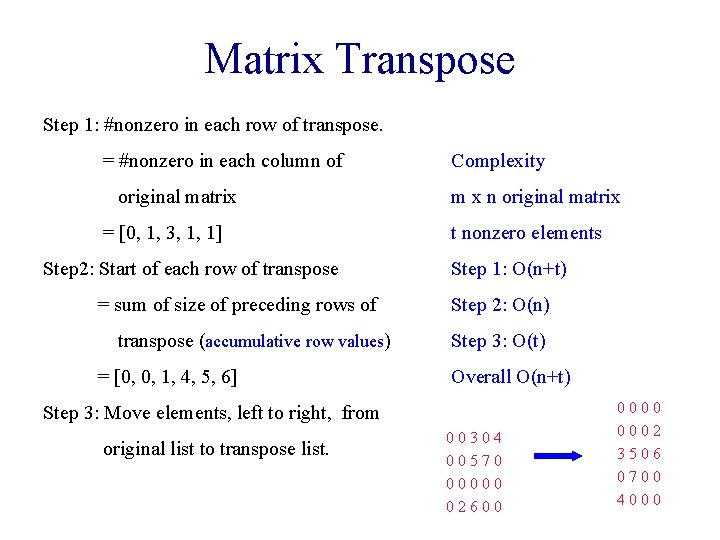
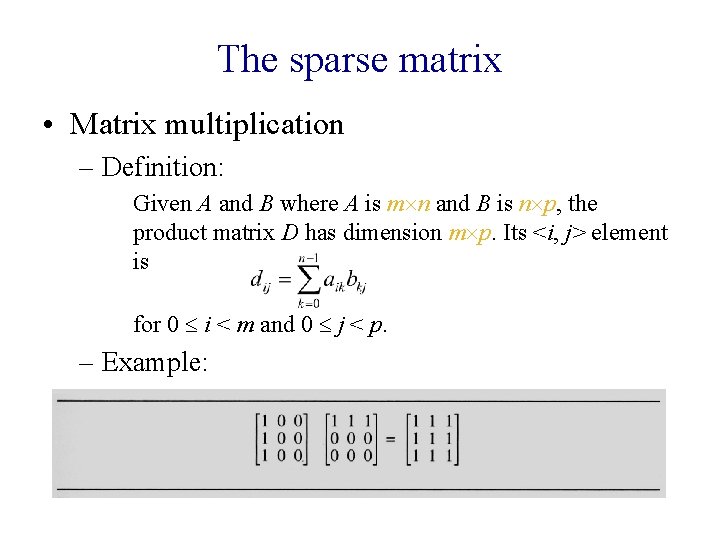
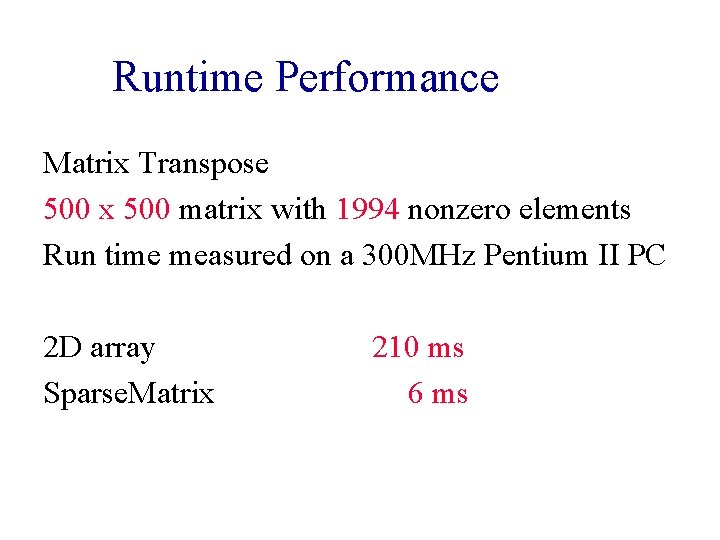
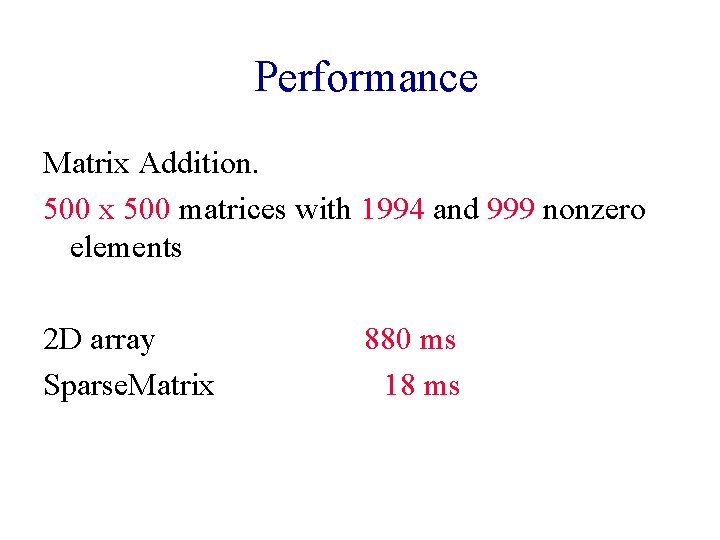
- Slides: 37
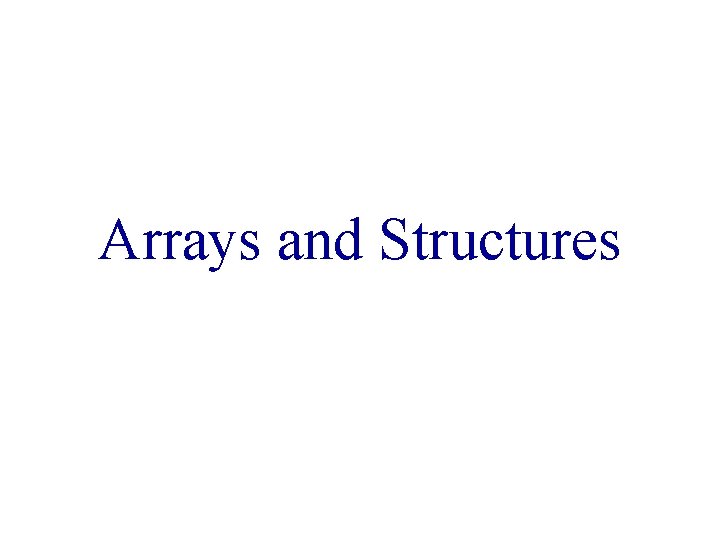
Arrays and Structures
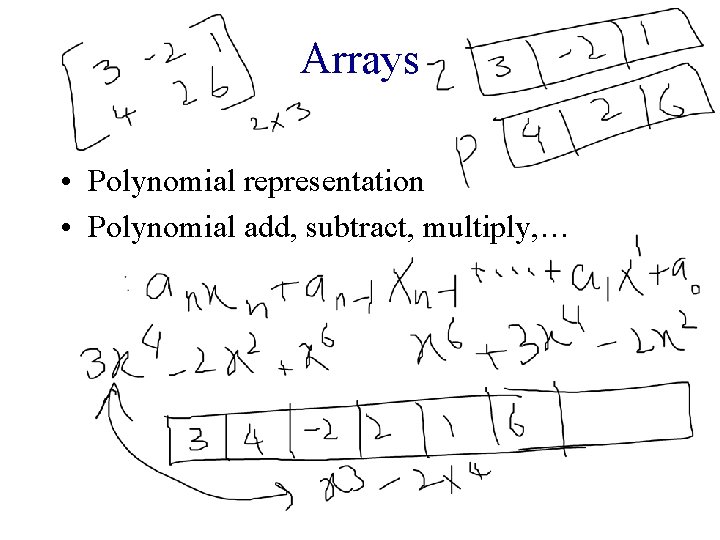
Arrays • Polynomial representation • Polynomial add, subtract, multiply, …
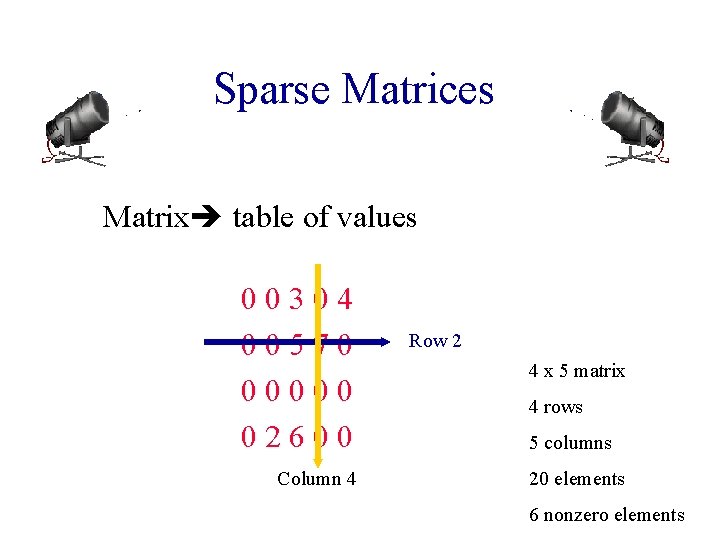
Sparse Matrices Matrix table of values 00304 00570 00000 02600 Column 4 Row 2 4 x 5 matrix 4 rows 5 columns 20 elements 6 nonzero elements
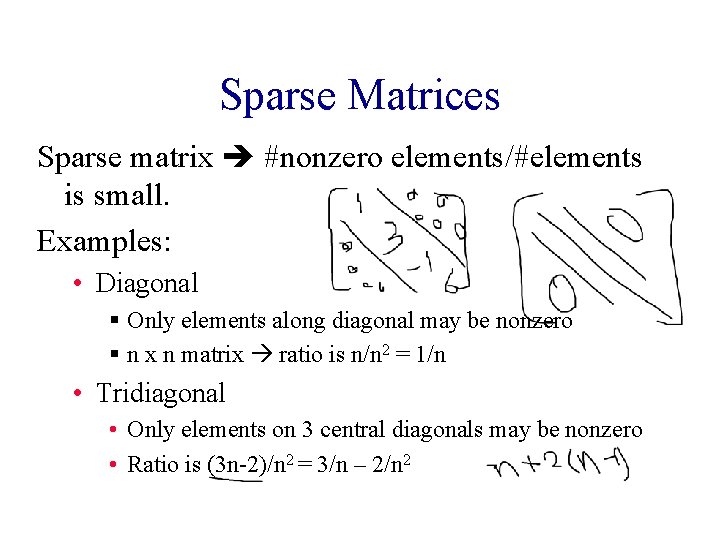
Sparse Matrices Sparse matrix #nonzero elements/#elements is small. Examples: • Diagonal § Only elements along diagonal may be nonzero § n x n matrix ratio is n/n 2 = 1/n • Tridiagonal • Only elements on 3 central diagonals may be nonzero • Ratio is (3 n-2)/n 2 = 3/n – 2/n 2
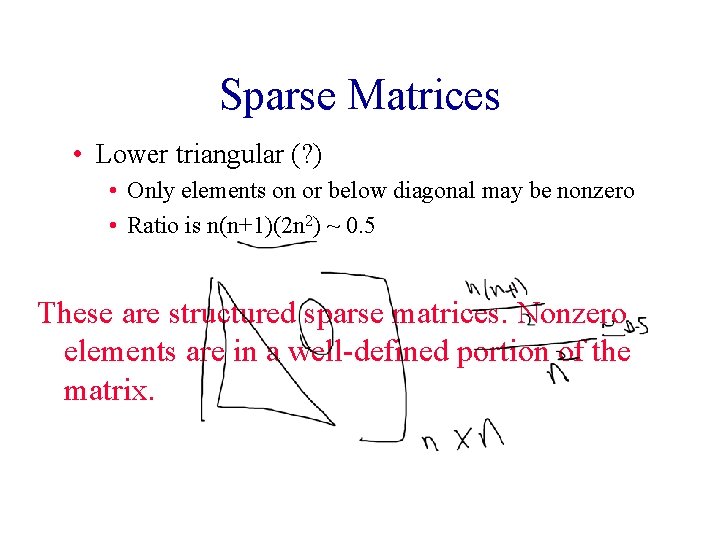
Sparse Matrices • Lower triangular (? ) • Only elements on or below diagonal may be nonzero • Ratio is n(n+1)(2 n 2) ~ 0. 5 These are structured sparse matrices. Nonzero elements are in a well-defined portion of the matrix.
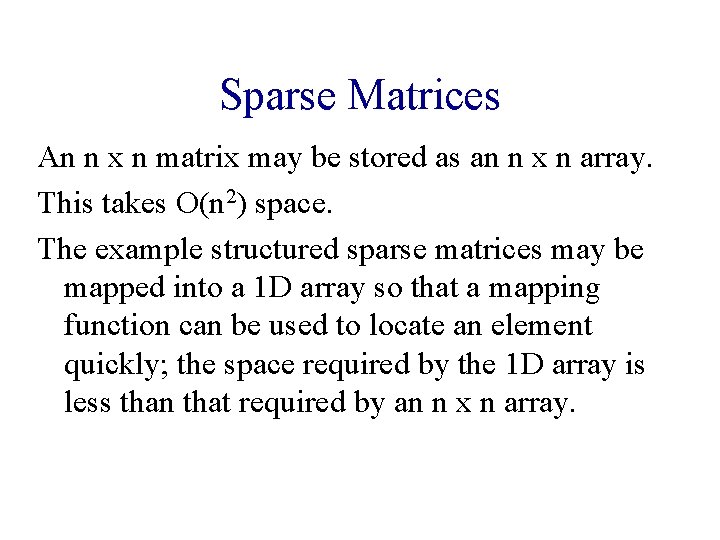
Sparse Matrices An n x n matrix may be stored as an n x n array. This takes O(n 2) space. The example structured sparse matrices may be mapped into a 1 D array so that a mapping function can be used to locate an element quickly; the space required by the 1 D array is less than that required by an n x n array.
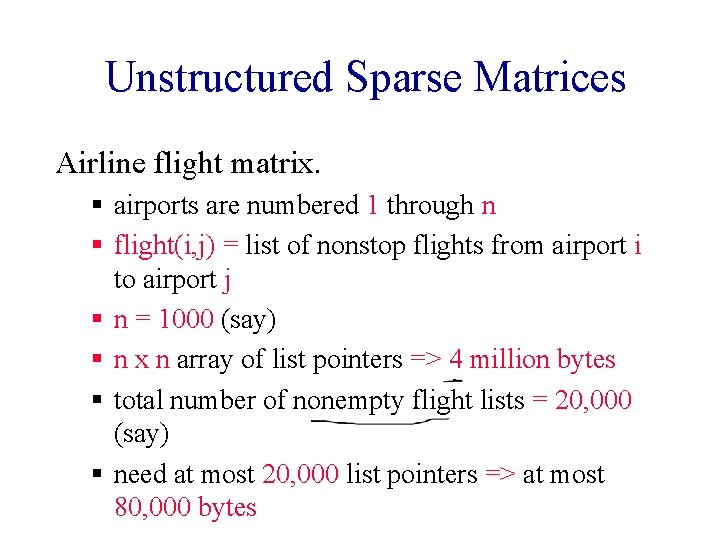
Unstructured Sparse Matrices Airline flight matrix. § airports are numbered 1 through n § flight(i, j) = list of nonstop flights from airport i to airport j § n = 1000 (say) § n x n array of list pointers => 4 million bytes § total number of nonempty flight lists = 20, 000 (say) § need at most 20, 000 list pointers => at most 80, 000 bytes
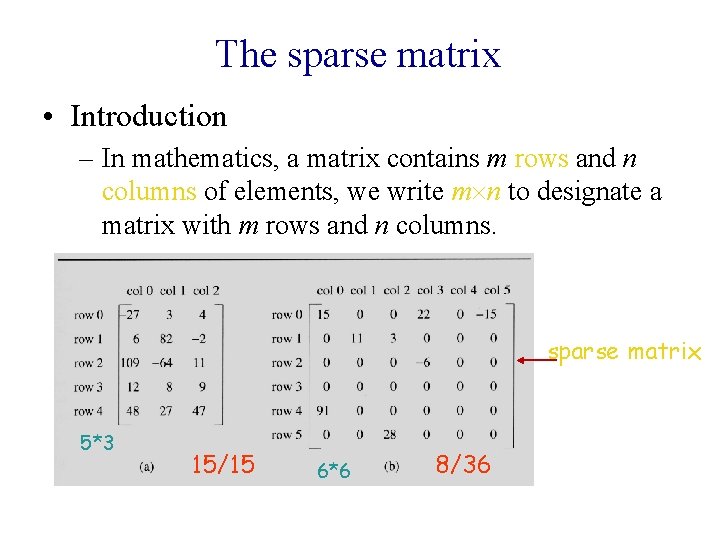
The sparse matrix • Introduction – In mathematics, a matrix contains m rows and n columns of elements, we write m n to designate a matrix with m rows and n columns. sparse matrix 5*3 15/15 6*6 8/36
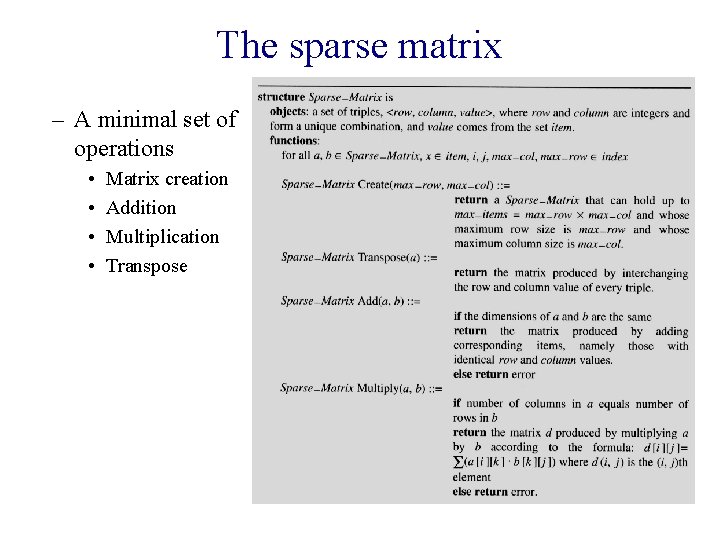
The sparse matrix – A minimal set of operations • • Matrix creation Addition Multiplication Transpose
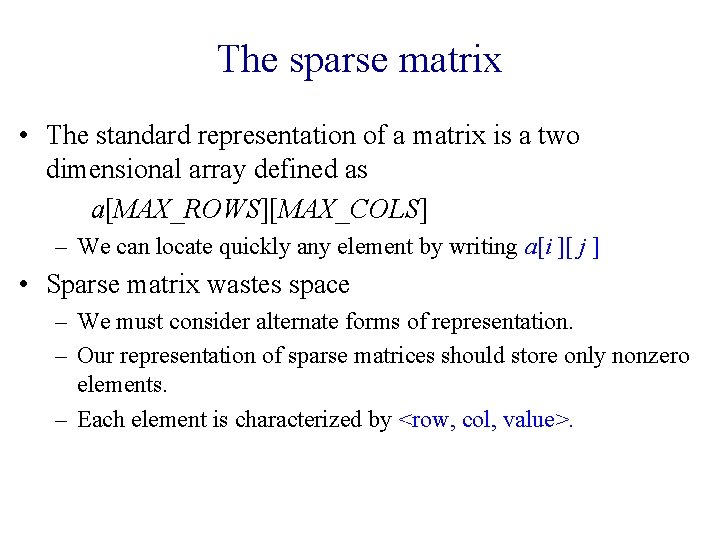
The sparse matrix • The standard representation of a matrix is a two dimensional array defined as a[MAX_ROWS][MAX_COLS] – We can locate quickly any element by writing a[i ][ j ] • Sparse matrix wastes space – We must consider alternate forms of representation. – Our representation of sparse matrices should store only nonzero elements. – Each element is characterized by <row, col, value>.
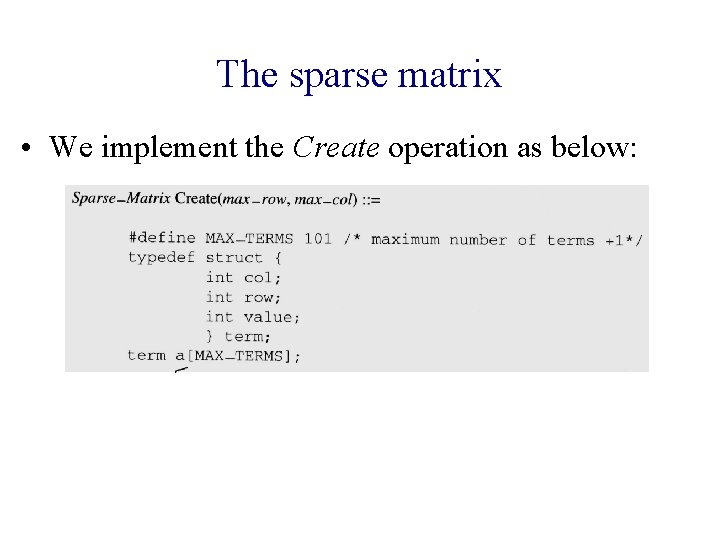
The sparse matrix • We implement the Create operation as below:
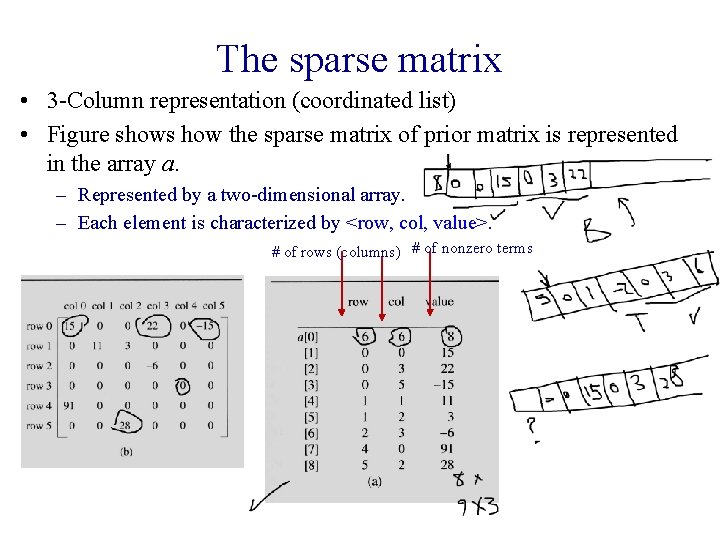
The sparse matrix • 3 -Column representation (coordinated list) • Figure shows how the sparse matrix of prior matrix is represented in the array a. – Represented by a two-dimensional array. – Each element is characterized by <row, col, value>. # of rows (columns) # of nonzero terms
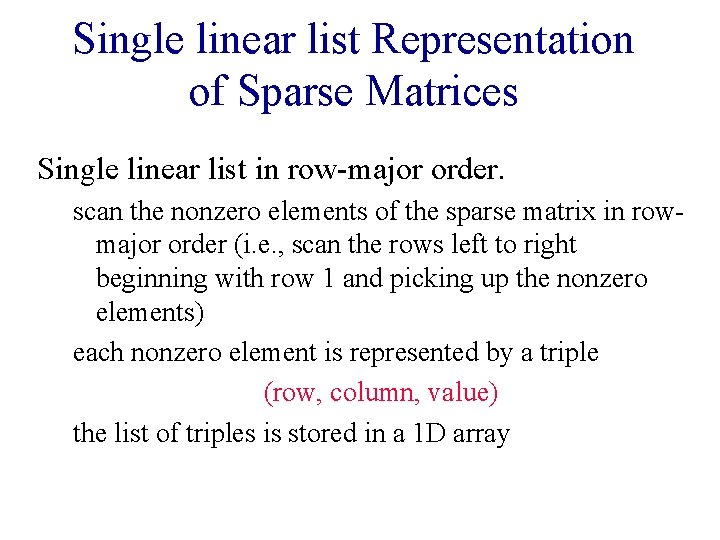
Single linear list Representation of Sparse Matrices Single linear list in row-major order. scan the nonzero elements of the sparse matrix in rowmajor order (i. e. , scan the rows left to right beginning with row 1 and picking up the nonzero elements) each nonzero element is represented by a triple (row, column, value) the list of triples is stored in a 1 D array
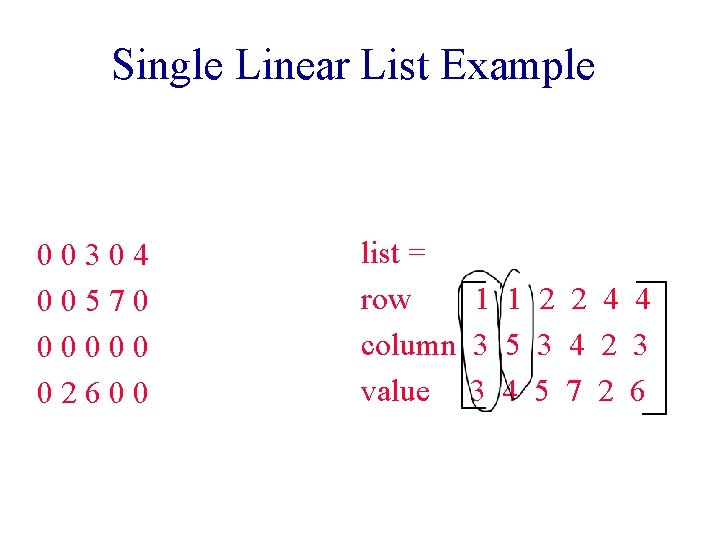
Single Linear List Example 00304 00570 00000 02600 list = row 1 1 2 2 4 4 column 3 5 3 4 2 3 value 3 4 5 7 2 6
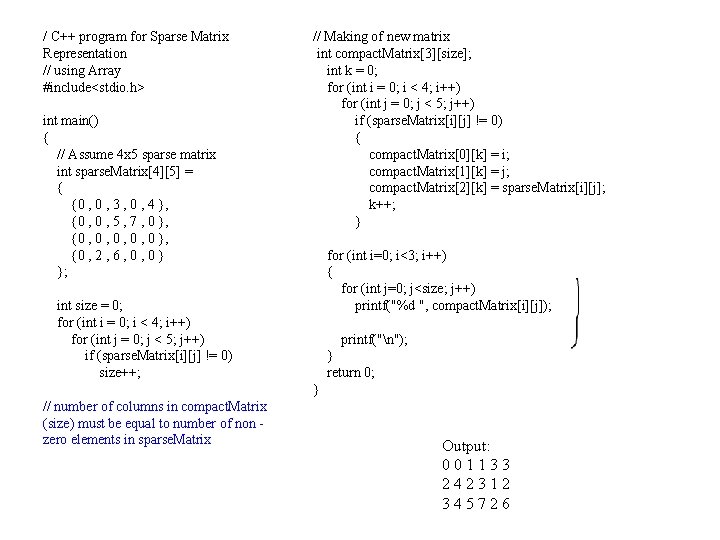
/ C++ program for Sparse Matrix Representation // using Array #include<stdio. h> int main() { // Assume 4 x 5 sparse matrix int sparse. Matrix[4][5] = { {0 , 3 , 0 , 4 }, {0 , 5 , 7 , 0 }, {0 , 0 , 0 }, {0 , 2 , 6 , 0 } }; // Making of new matrix int compact. Matrix[3][size]; int k = 0; for (int i = 0; i < 4; i++) for (int j = 0; j < 5; j++) if (sparse. Matrix[i][j] != 0) { compact. Matrix[0][k] = i; compact. Matrix[1][k] = j; compact. Matrix[2][k] = sparse. Matrix[i][j]; k++; } for (int i=0; i<3; i++) { for (int j=0; j<size; j++) printf("%d ", compact. Matrix[i][j]); int size = 0; for (int i = 0; i < 4; i++) for (int j = 0; j < 5; j++) if (sparse. Matrix[i][j] != 0) size++; printf("n"); } return 0; } // number of columns in compact. Matrix (size) must be equal to number of non zero elements in sparse. Matrix Output: 001133 242312 345726
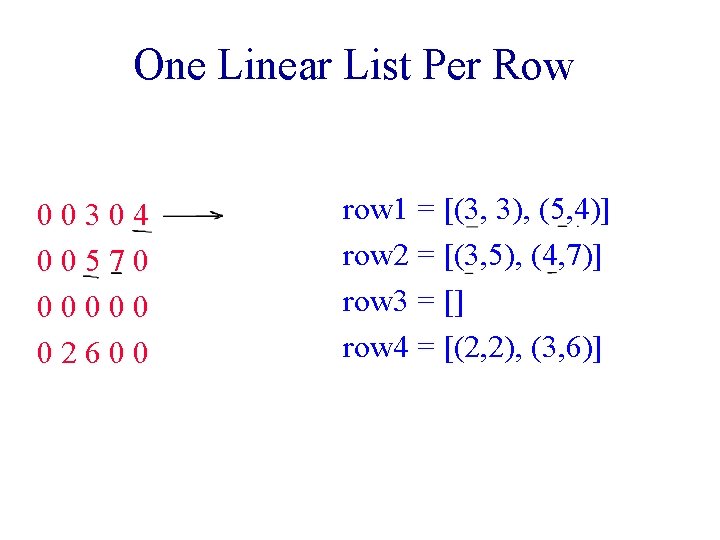
One Linear List Per Row 00304 00570 00000 02600 row 1 = [(3, 3), (5, 4)] row 2 = [(3, 5), (4, 7)] row 3 = [] row 4 = [(2, 2), (3, 6)]
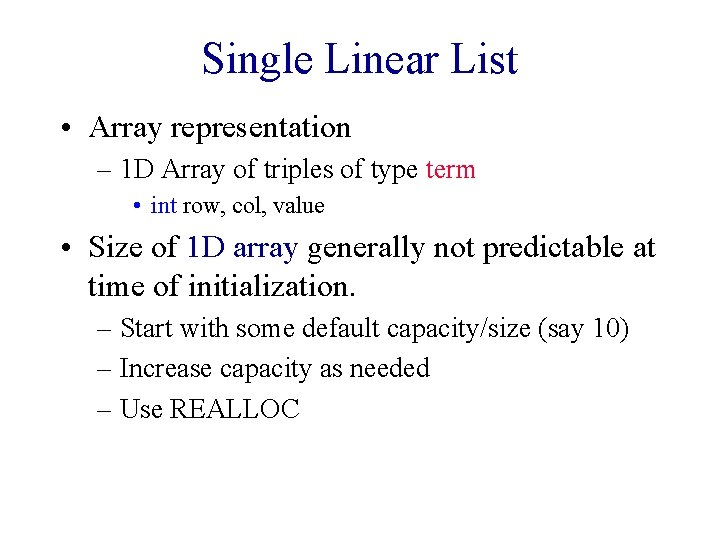
Single Linear List • Array representation – 1 D Array of triples of type term • int row, col, value • Size of 1 D array generally not predictable at time of initialization. – Start with some default capacity/size (say 10) – Increase capacity as needed – Use REALLOC
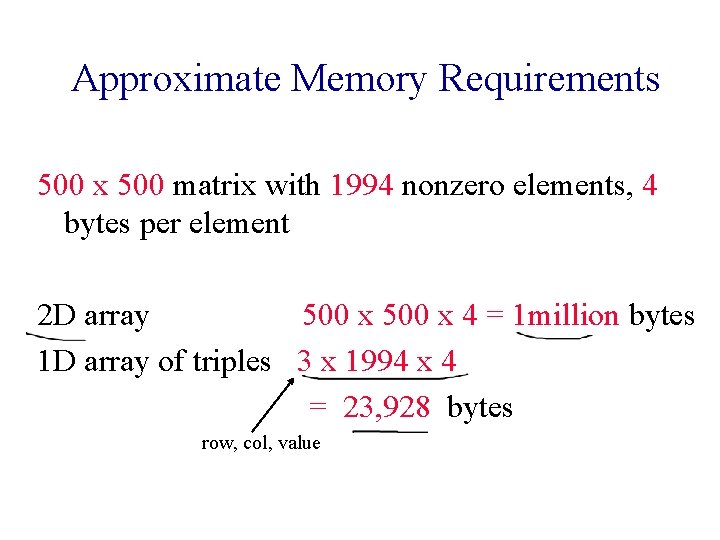
Approximate Memory Requirements 500 x 500 matrix with 1994 nonzero elements, 4 bytes per element 2 D array 500 x 4 = 1 million bytes 1 D array of triples 3 x 1994 x 4 = 23, 928 bytes row, col, value
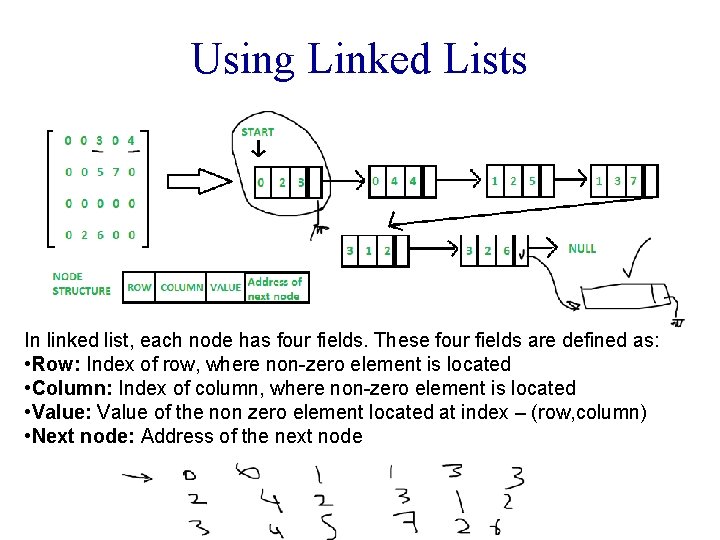
Using Linked Lists In linked list, each node has four fields. These four fields are defined as: • Row: Index of row, where non-zero element is located • Column: Index of column, where non-zero element is located • Value: Value of the non zero element located at index – (row, column) • Next node: Address of the next node
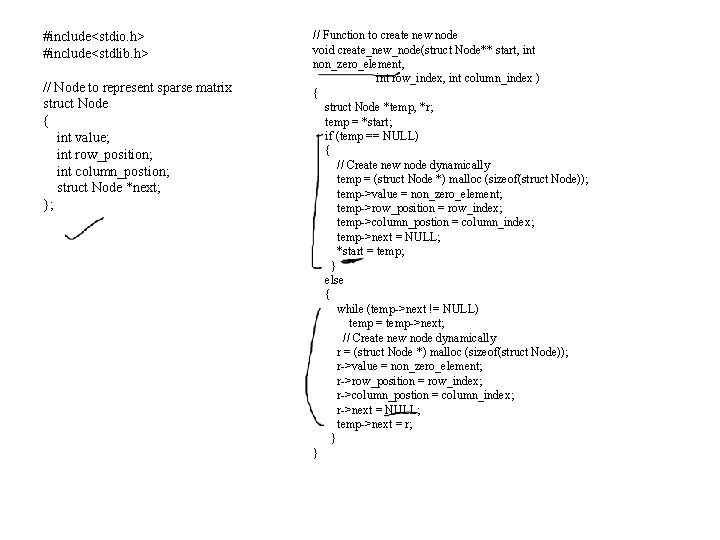
#include<stdio. h> #include<stdlib. h> // Node to represent sparse matrix struct Node { int value; int row_position; int column_postion; struct Node *next; }; // Function to create new node void create_new_node(struct Node** start, int non_zero_element, int row_index, int column_index ) { struct Node *temp, *r; temp = *start; if (temp == NULL) { // Create new node dynamically temp = (struct Node *) malloc (sizeof(struct Node)); temp->value = non_zero_element; temp->row_position = row_index; temp->column_postion = column_index; temp->next = NULL; *start = temp; } else { while (temp->next != NULL) temp = temp->next; // Create new node dynamically r = (struct Node *) malloc (sizeof(struct Node)); r->value = non_zero_element; r->row_position = row_index; r->column_postion = column_index; r->next = NULL; temp->next = r; } }
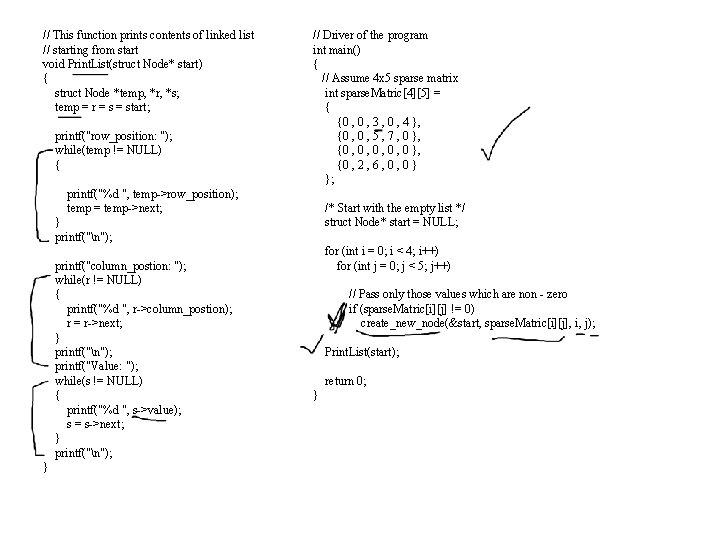
// This function prints contents of linked list // starting from start void Print. List(struct Node* start) { struct Node *temp, *r, *s; temp = r = start; printf("row_position: "); while(temp != NULL) { // Driver of the program int main() { // Assume 4 x 5 sparse matrix int sparse. Matric[4][5] = { {0 , 3 , 0 , 4 }, {0 , 5 , 7 , 0 }, {0 , 0 , 0 }, {0 , 2 , 6 , 0 } }; printf("%d ", temp->row_position); temp = temp->next; /* Start with the empty list */ struct Node* start = NULL; } printf("n"); printf("column_postion: "); while(r != NULL) { printf("%d ", r->column_postion); r = r->next; } printf("n"); printf("Value: "); while(s != NULL) { printf("%d ", s->value); s = s->next; } printf("n"); } for (int i = 0; i < 4; i++) for (int j = 0; j < 5; j++) // Pass only those values which are non - zero if (sparse. Matric[i][j] != 0) create_new_node(&start, sparse. Matric[i][j], i, j); Print. List(start); return 0; }
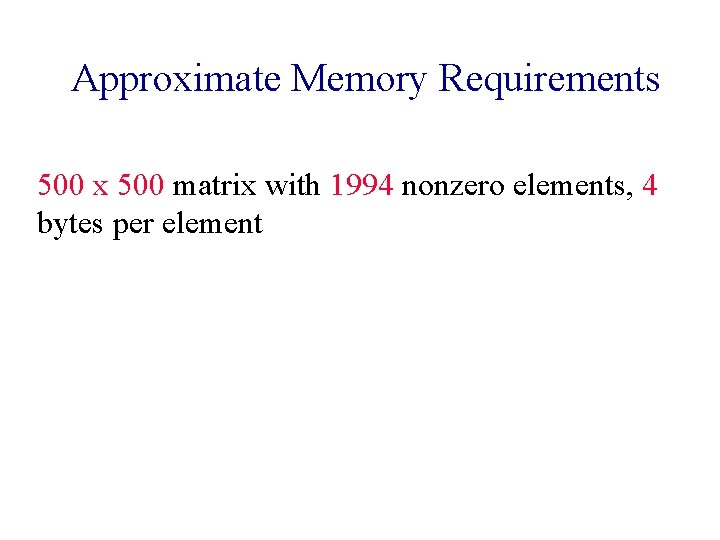
Approximate Memory Requirements 500 x 500 matrix with 1994 nonzero elements, 4 bytes per element
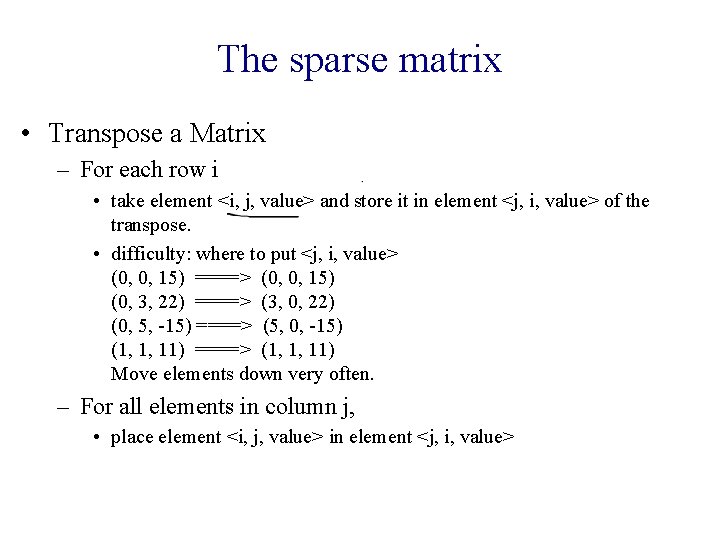
The sparse matrix • Transpose a Matrix – For each row i • take element <i, j, value> and store it in element <j, i, value> of the transpose. • difficulty: where to put <j, i, value> (0, 0, 15) ====> (0, 0, 15) (0, 3, 22) ====> (3, 0, 22) (0, 5, -15) ====> (5, 0, -15) (1, 1, 11) ====> (1, 1, 11) Move elements down very often. – For all elements in column j, • place element <i, j, value> in element <j, i, value>
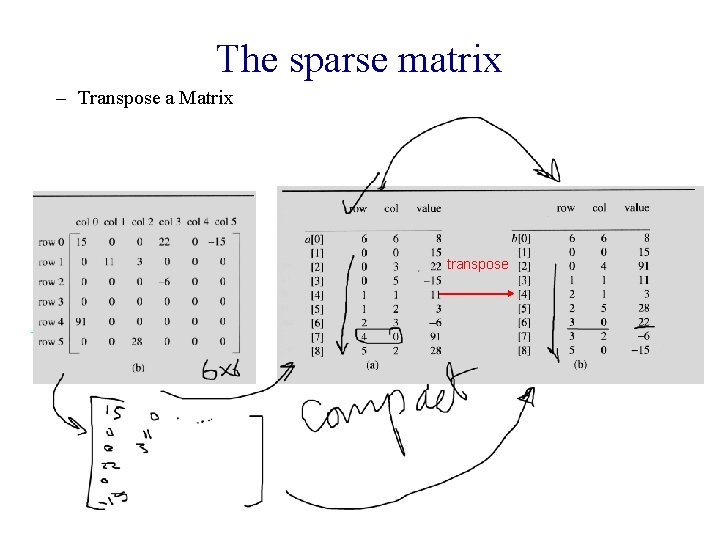
The sparse matrix – Transpose a Matrix transpose
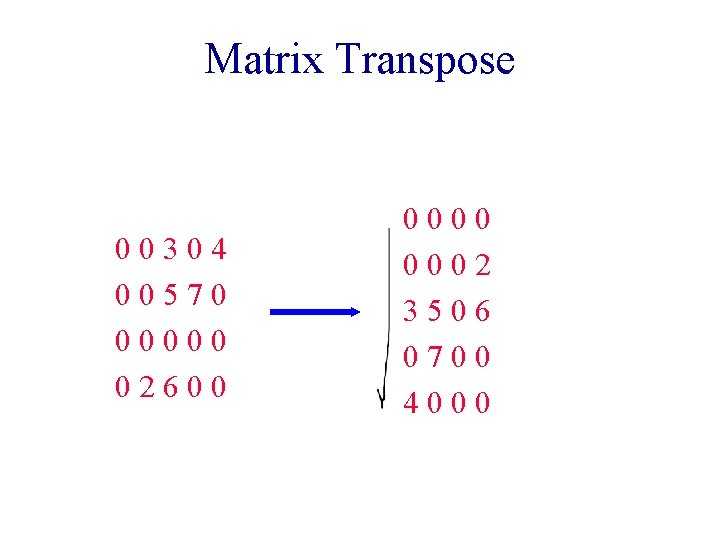
Matrix Transpose 00304 00570 00000 02600 0002 3506 0700 4000
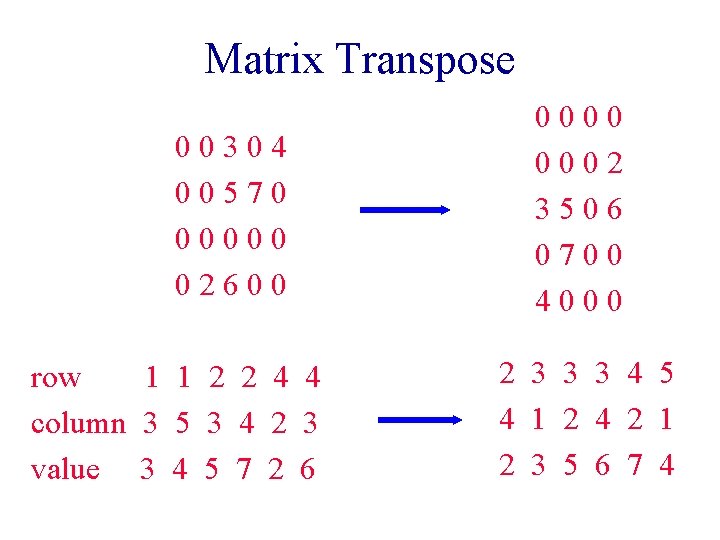
Matrix Transpose 00304 00570 00000 02600 row 1 1 2 2 4 4 column 3 5 3 4 2 3 value 3 4 5 7 2 6 0000 0002 3506 0700 4000 2 3 3 3 4 5 4 1 2 4 2 1 2 3 5 6 7 4
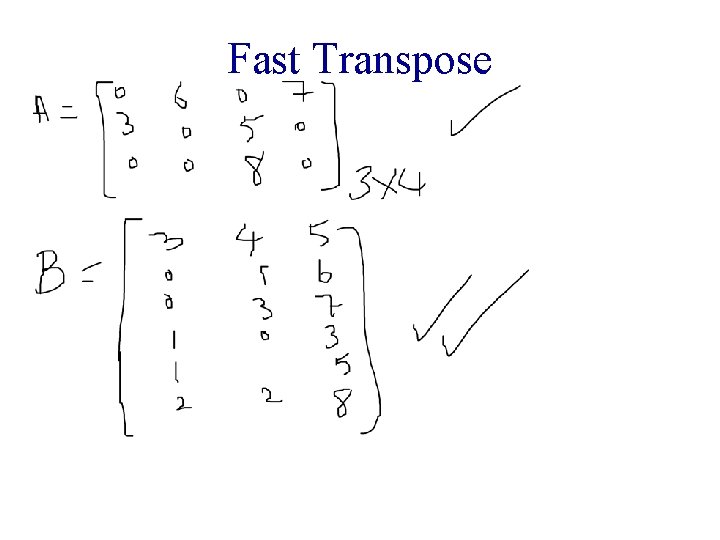
Fast Transpose
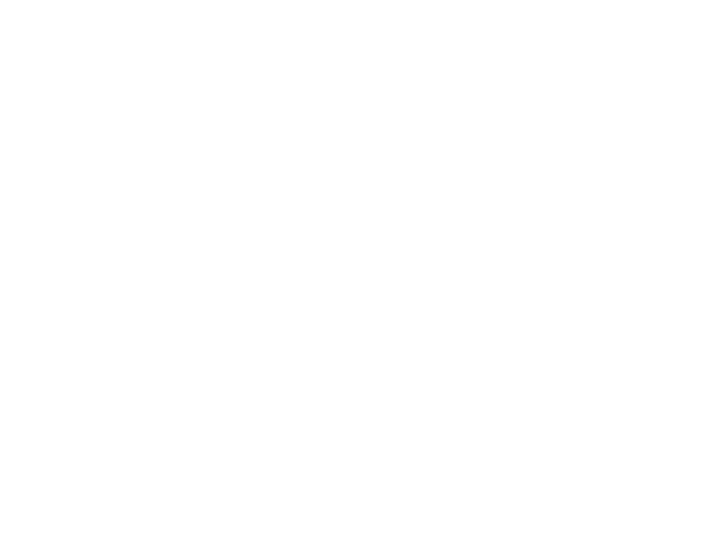
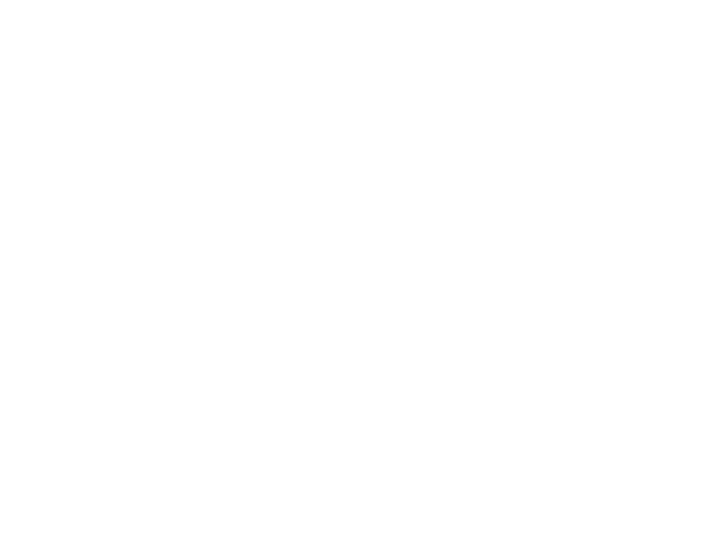
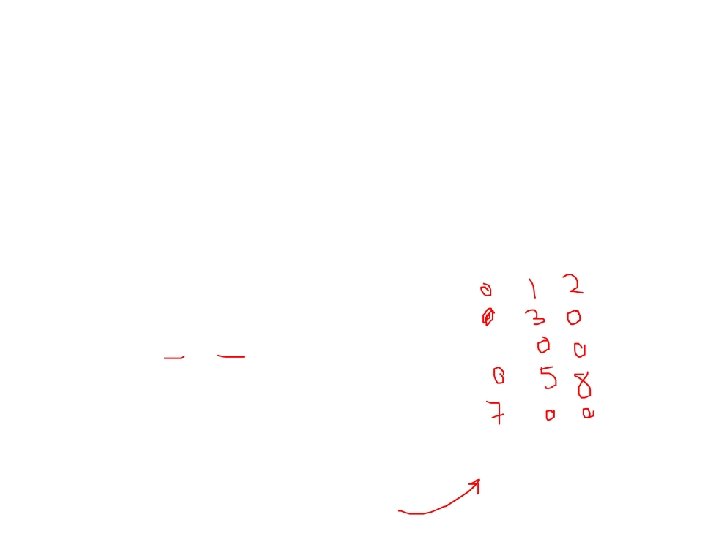
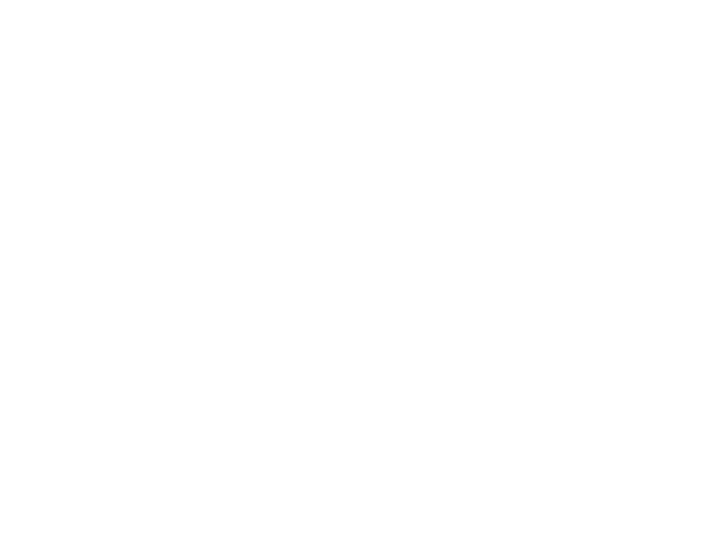
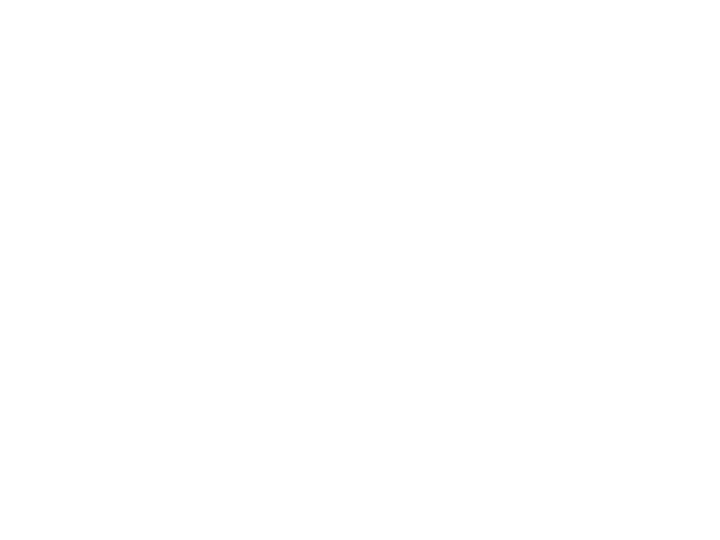
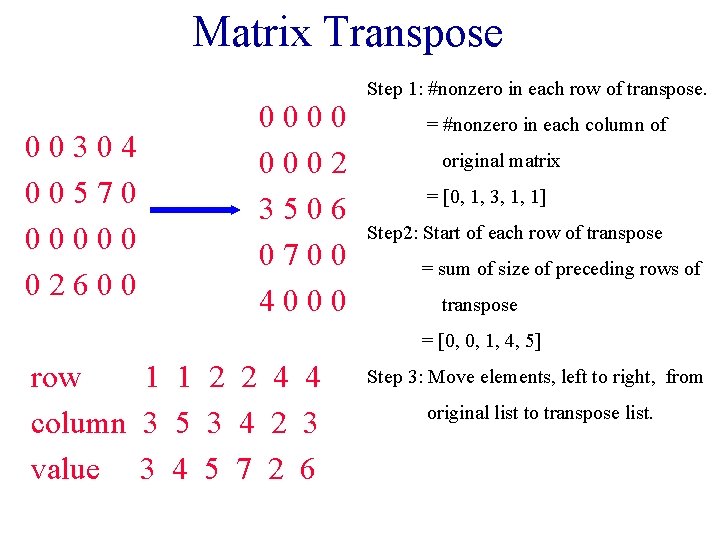
Matrix Transpose 00304 00570 00000 02600 0002 3506 0700 4000 Step 1: #nonzero in each row of transpose. = #nonzero in each column of original matrix = [0, 1, 3, 1, 1] Step 2: Start of each row of transpose = sum of size of preceding rows of transpose = [0, 0, 1, 4, 5] row 1 1 2 2 4 4 column 3 5 3 4 2 3 value 3 4 5 7 2 6 Step 3: Move elements, left to right, from original list to transpose list.
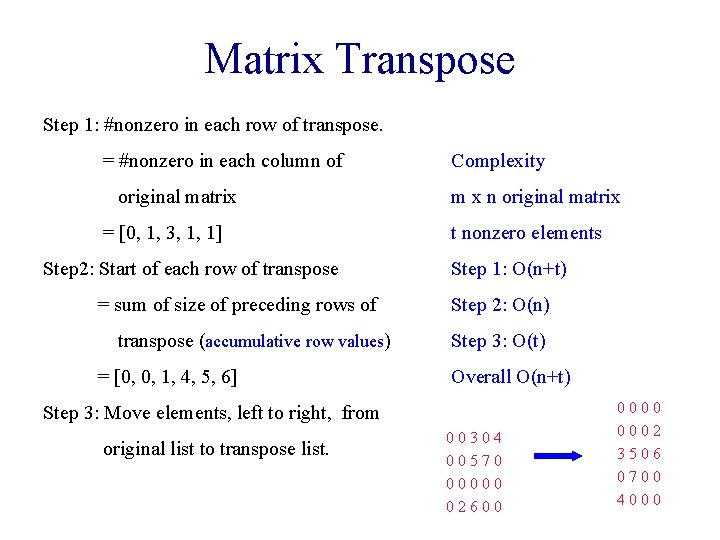
Matrix Transpose Step 1: #nonzero in each row of transpose. = #nonzero in each column of original matrix = [0, 1, 3, 1, 1] Step 2: Start of each row of transpose = sum of size of preceding rows of transpose (accumulative row values) = [0, 0, 1, 4, 5, 6] Complexity m x n original matrix t nonzero elements Step 1: O(n+t) Step 2: O(n) Step 3: O(t) Overall O(n+t) Step 3: Move elements, left to right, from original list to transpose list. 00304 00570 00000 02600 0002 3506 0700 4000
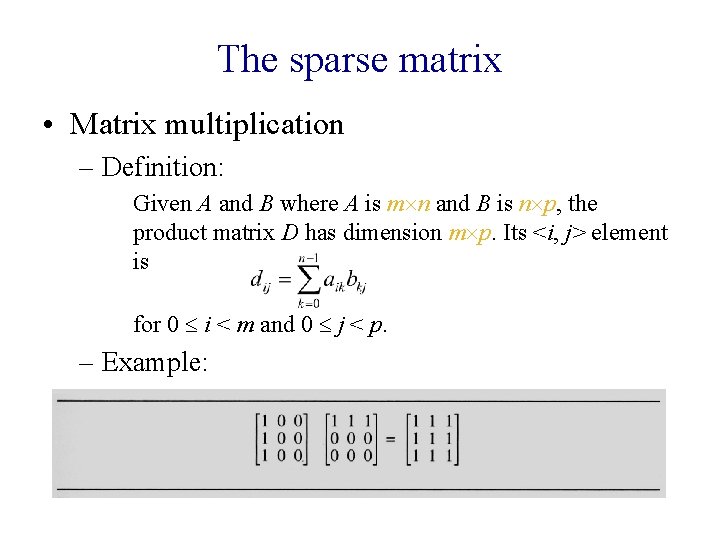
The sparse matrix • Matrix multiplication – Definition: Given A and B where A is m n and B is n p, the product matrix D has dimension m p. Its <i, j> element is for 0 i < m and 0 j < p. – Example:
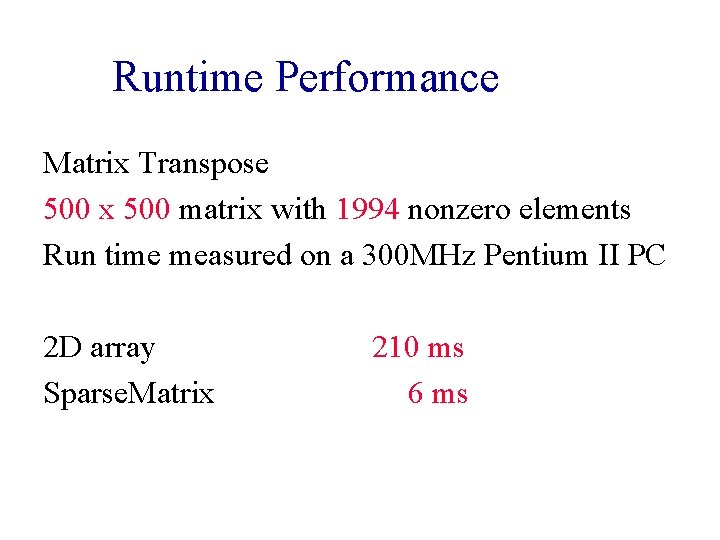
Runtime Performance Matrix Transpose 500 x 500 matrix with 1994 nonzero elements Run time measured on a 300 MHz Pentium II PC 2 D array Sparse. Matrix 210 ms 6 ms
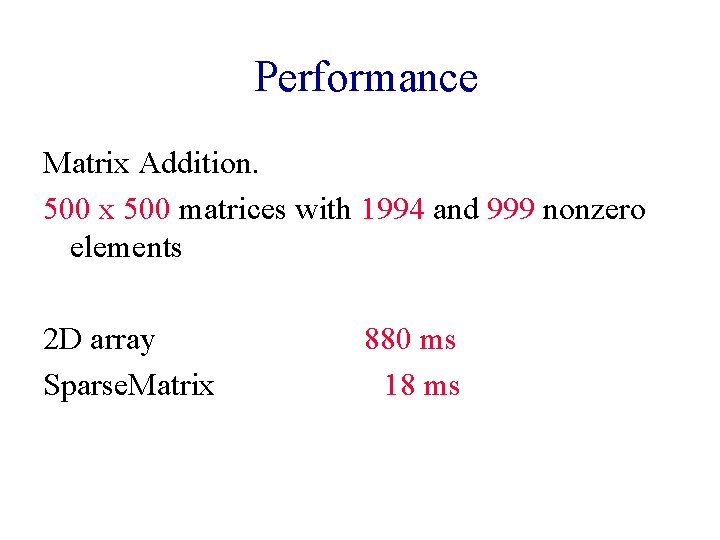
Performance Matrix Addition. 500 x 500 matrices with 1994 and 999 nonzero elements 2 D array Sparse. Matrix 880 ms 18 ms
Polynomial representation using arrays
Click to add titleclick to add subtitle
Click to add titleclick to add subtitle
Click to add titleclick to add subtitle
How to subtract fractions with polynomials
Human arm and whale flipper function
Polynomial addition in linked list
Binary to polynomial
Dynamic arrays and amortized analysis
Searching and sorting arrays in c++
Advantages of array
Matplotlib inline
How to divide a polynomial by another polynomial
Parallel arrays in data structure
Array of arrays c++
Ragged array
Partially filled arrays
C++ parallel arrays
Why do we need arrays?
Suma de arreglos unidimensionales en c
Java arreglos bidimensionales
Arrays in mips
Array of strings assembly
Global arrays in c
Computer science arrays
Arrays visual basic
Python find index of max
How many arrays in 24
Arrays in pascal
Mips arrays
Creating arrays matlab
Array adt
Partially filled array
Redundancy array of independent disk
Python list of arrays
Arrays
Day 3: arrays
Redundant array of inexpensive disk