Fundamental Programming More Expressions and Data Types Fundamental
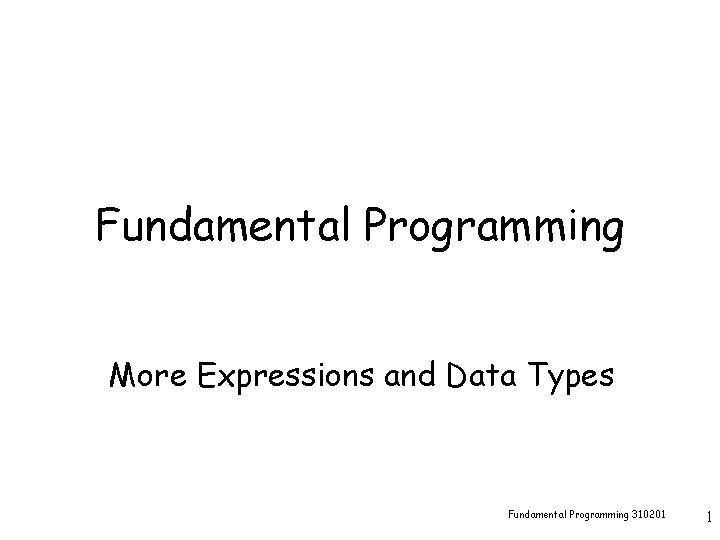
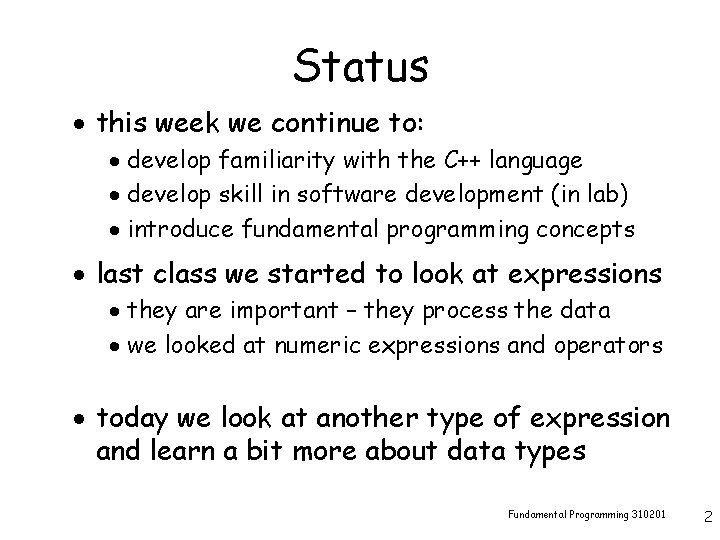
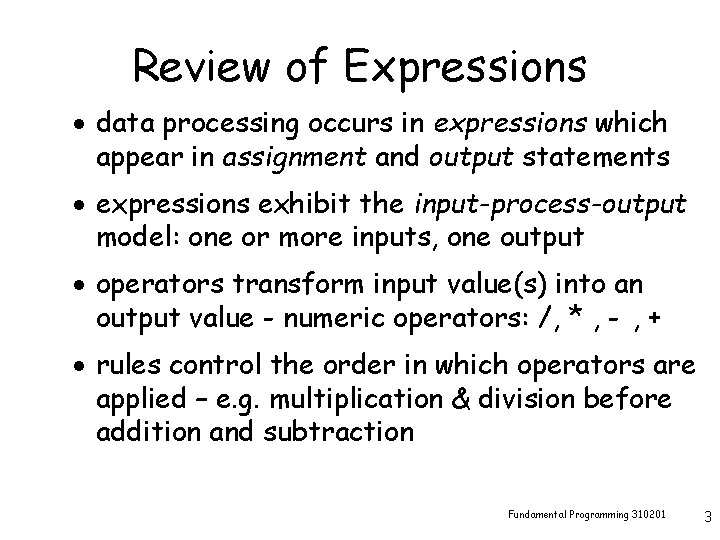
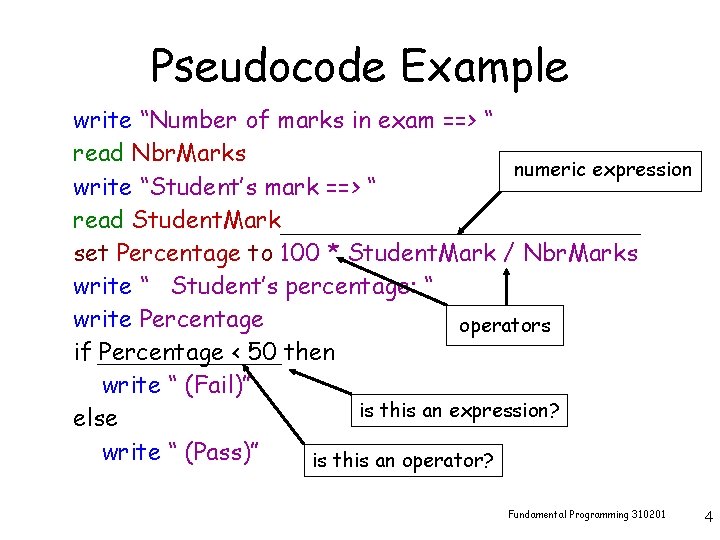
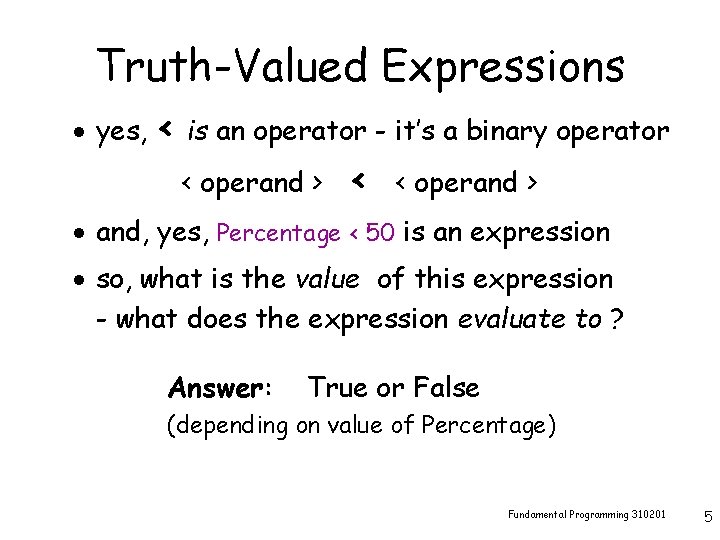
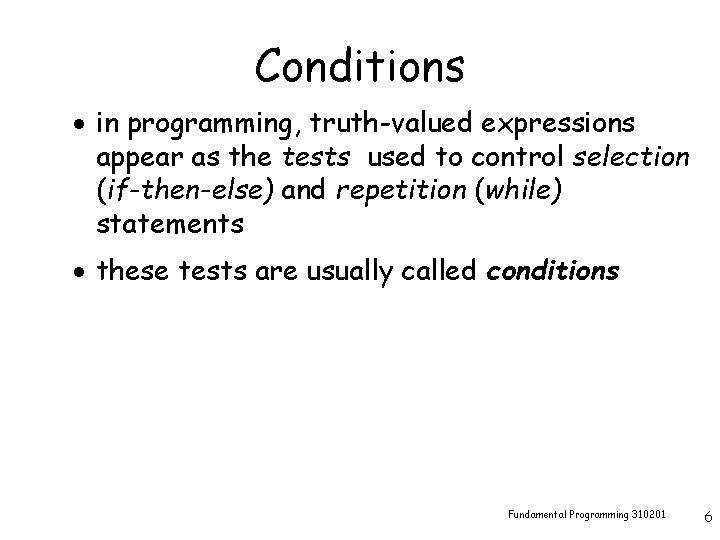
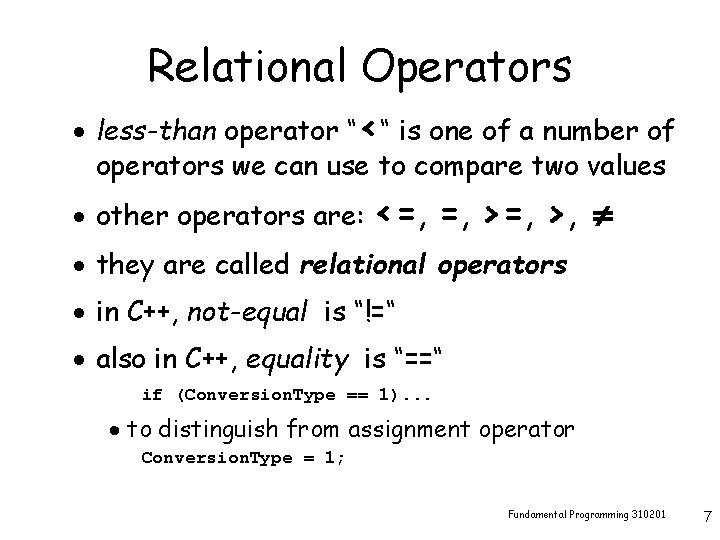
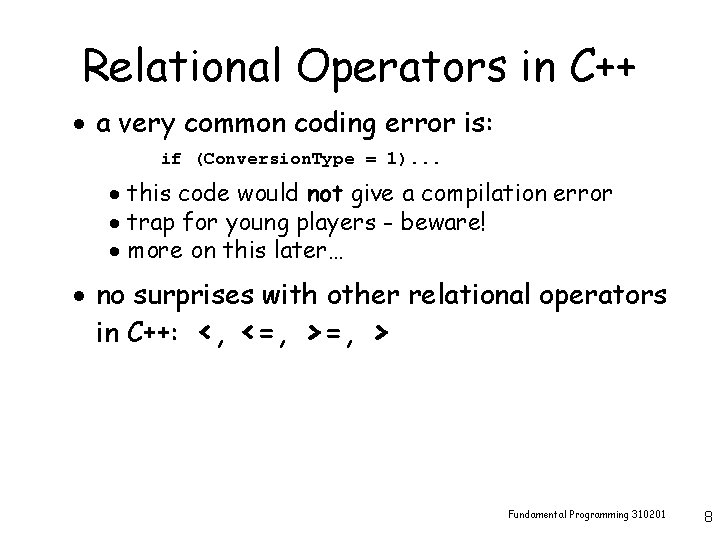
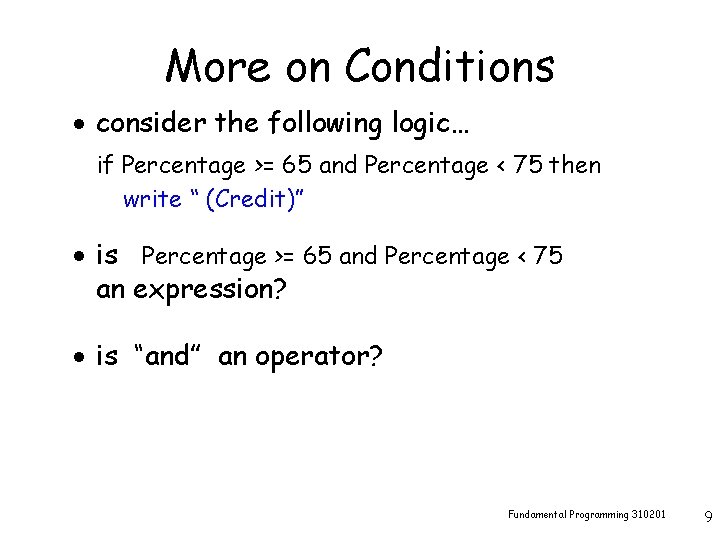
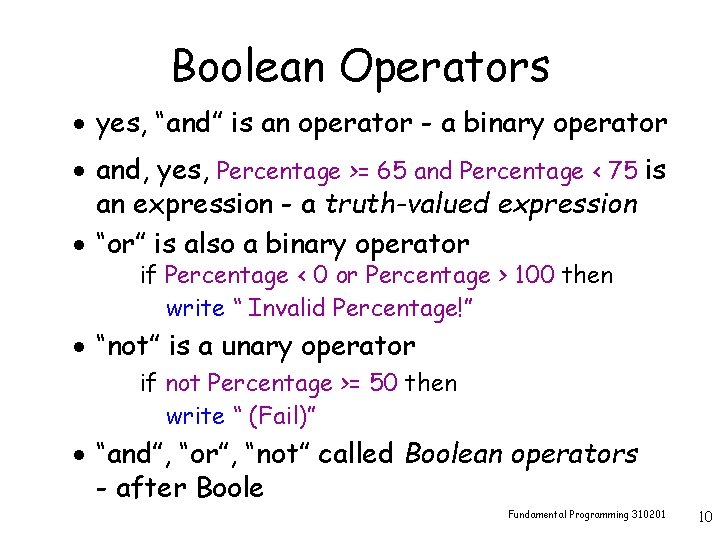
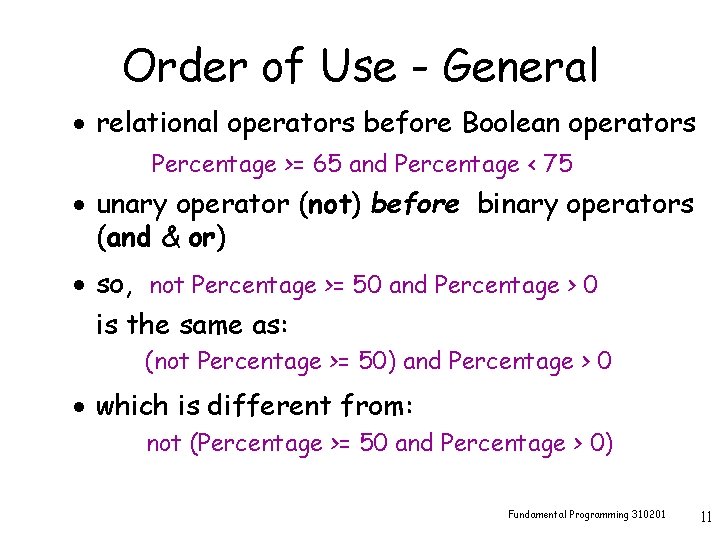
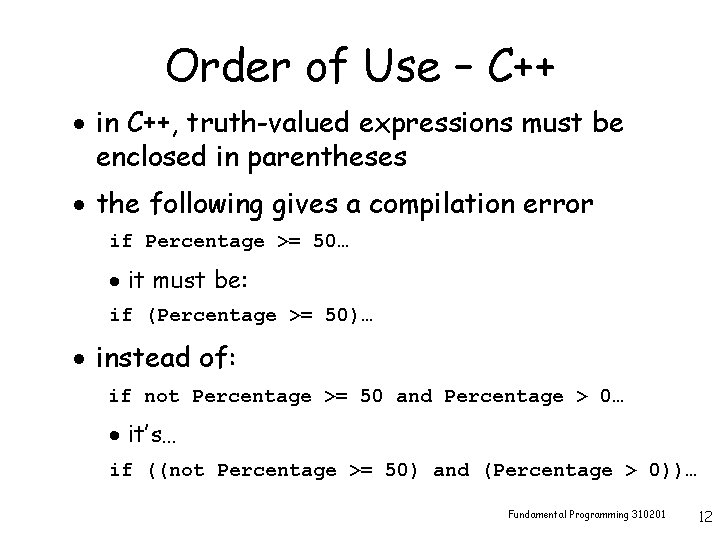
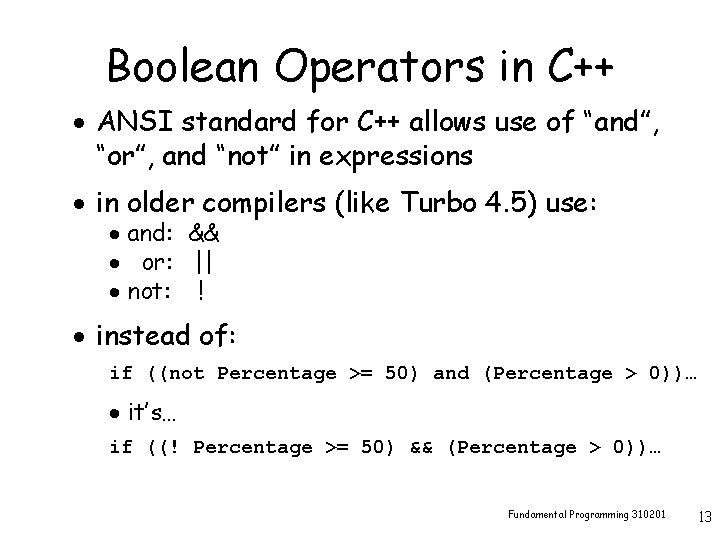
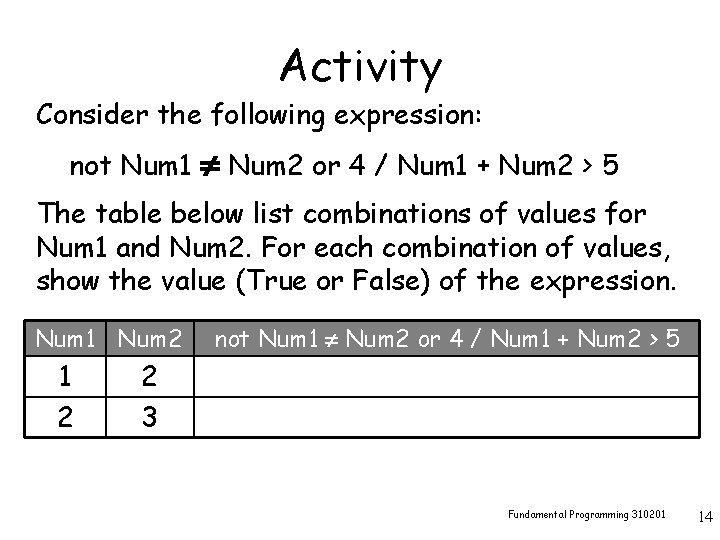
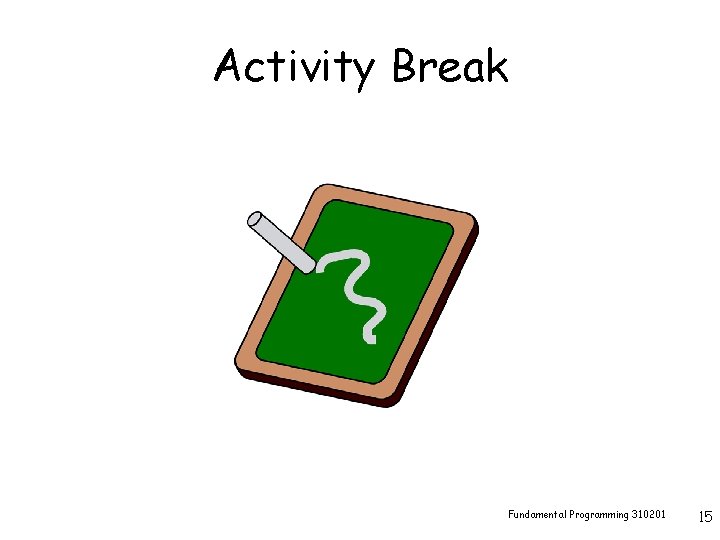
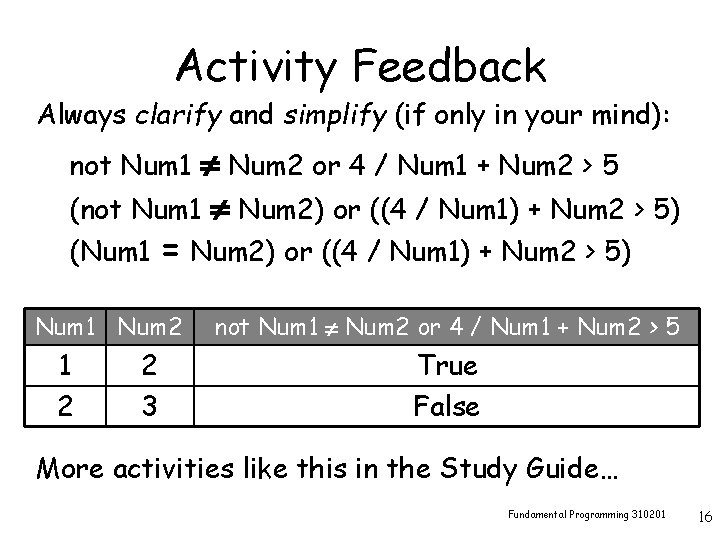
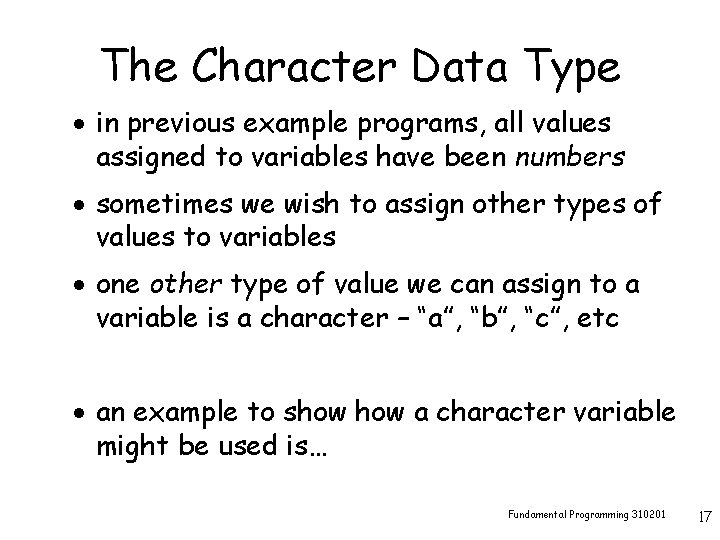
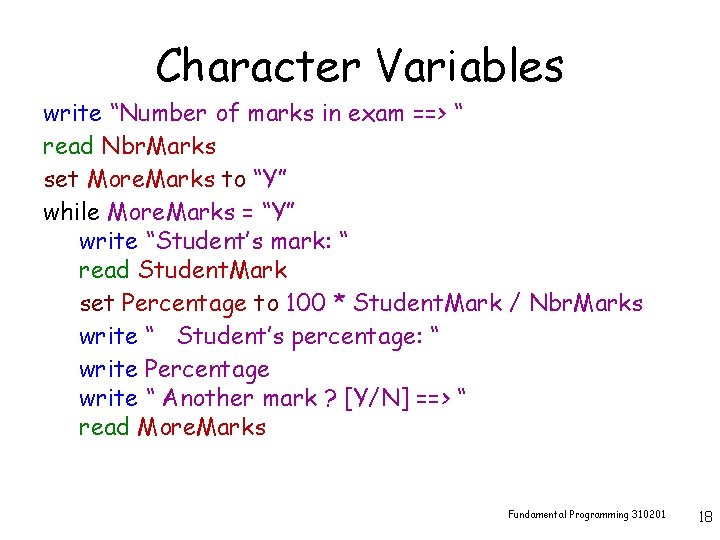
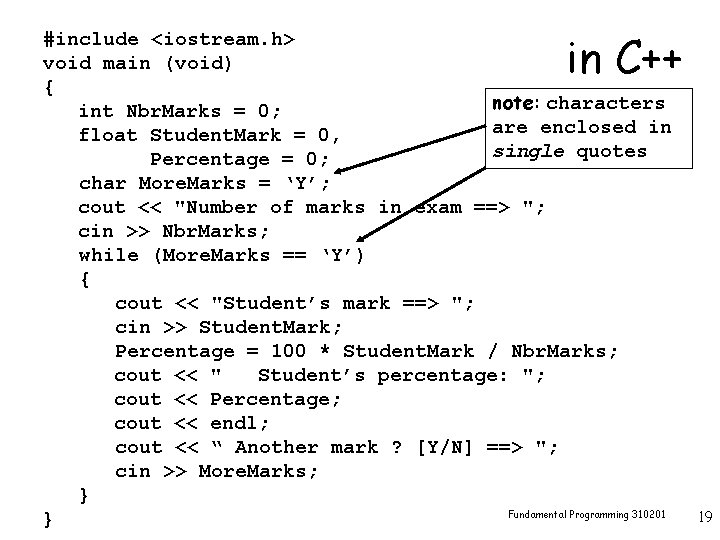
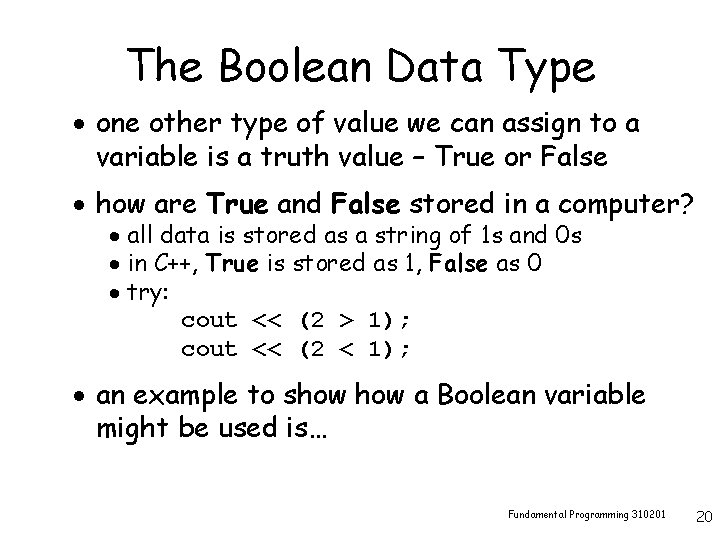
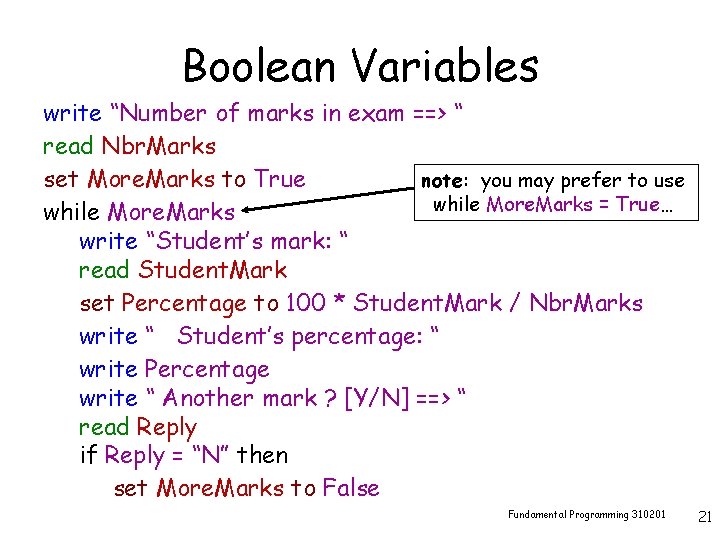
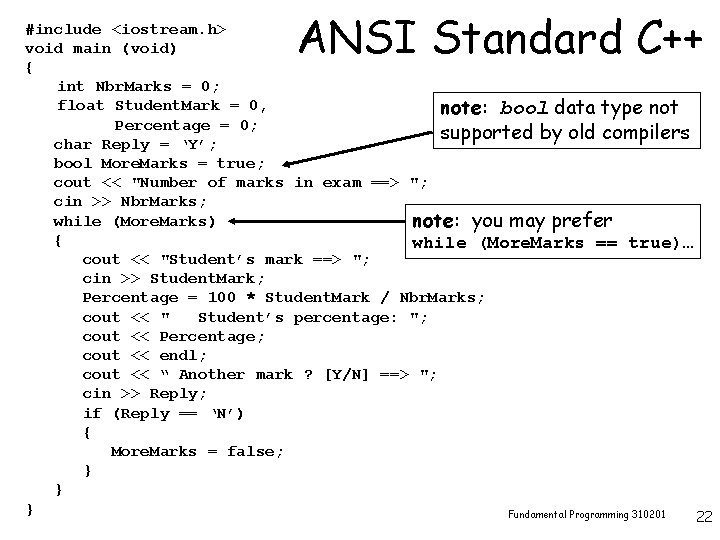
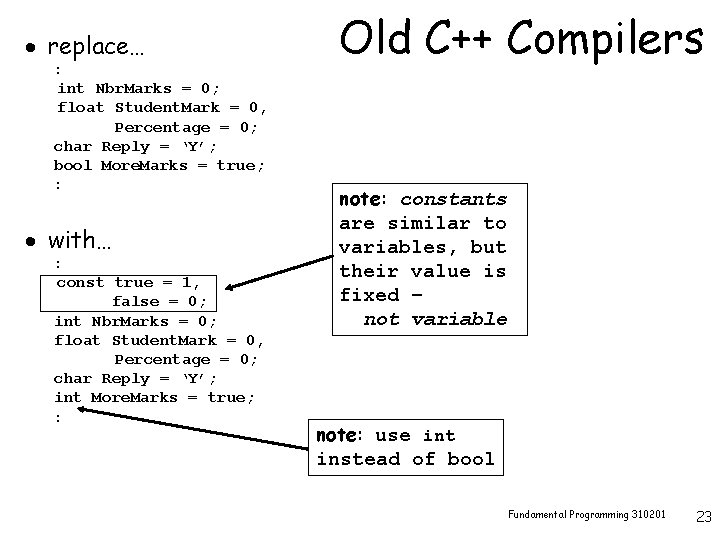
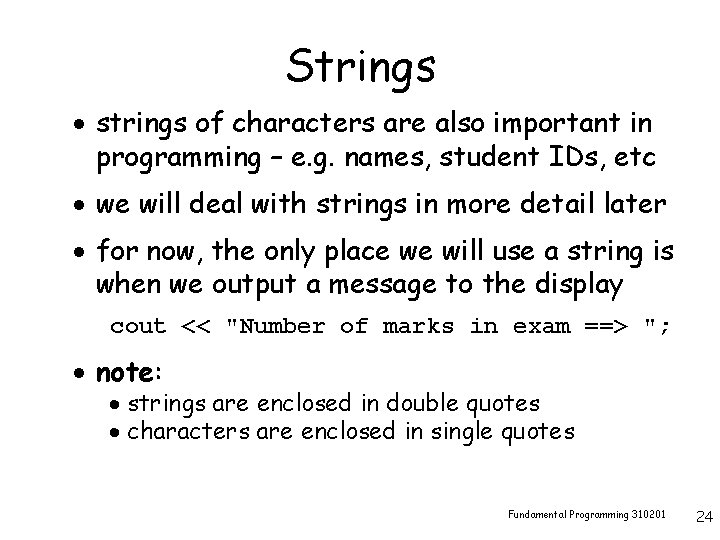
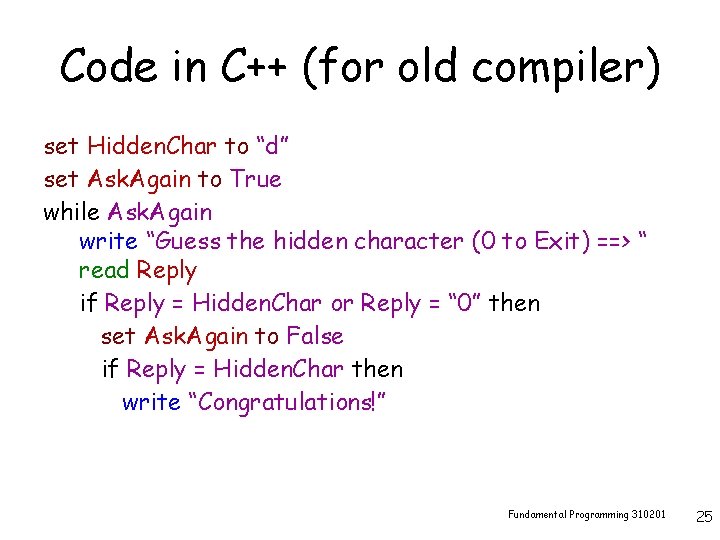
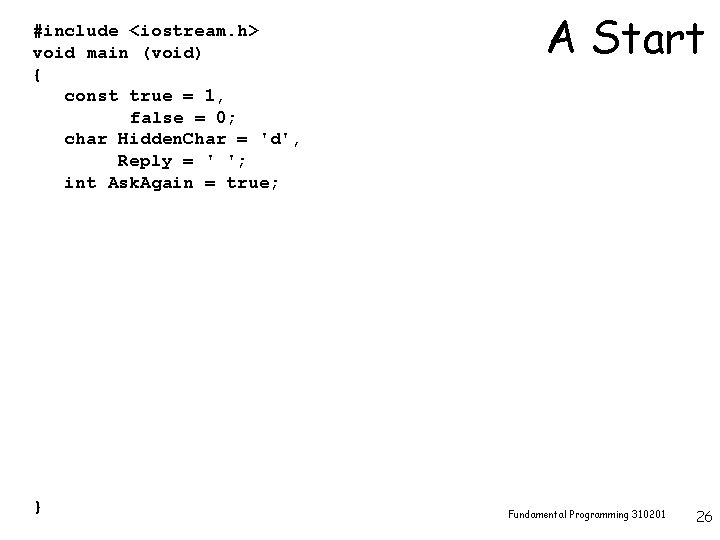
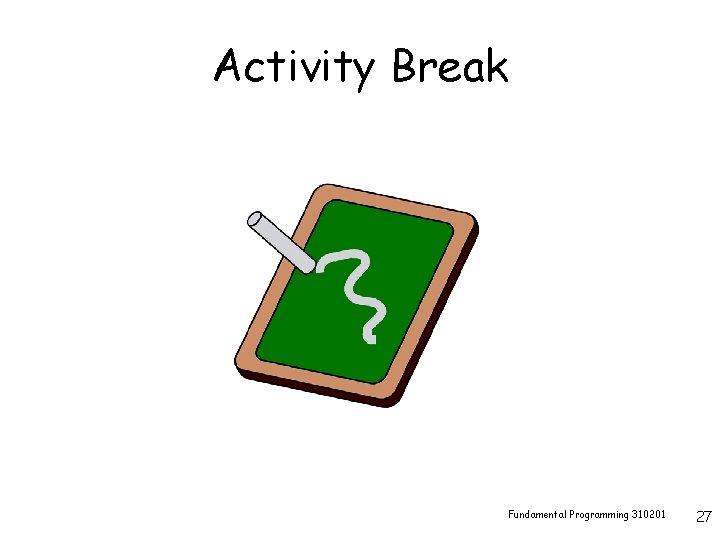
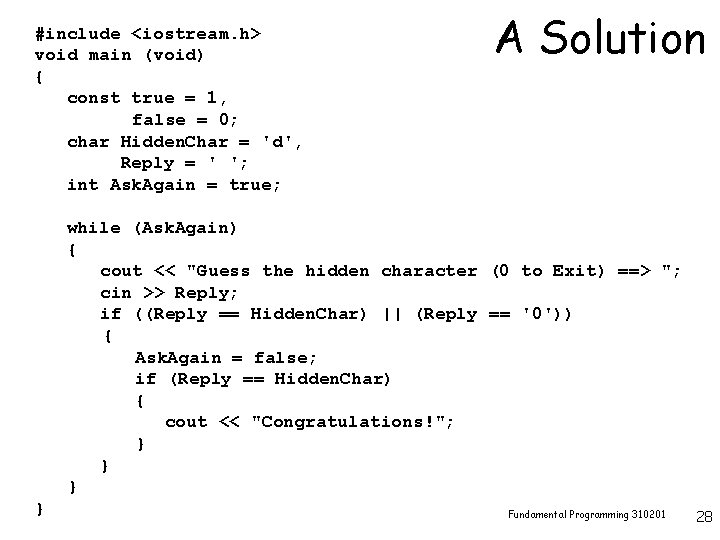
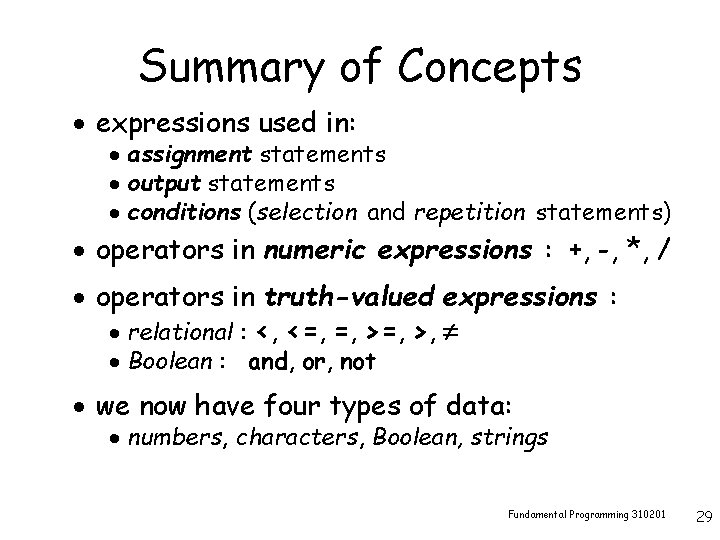
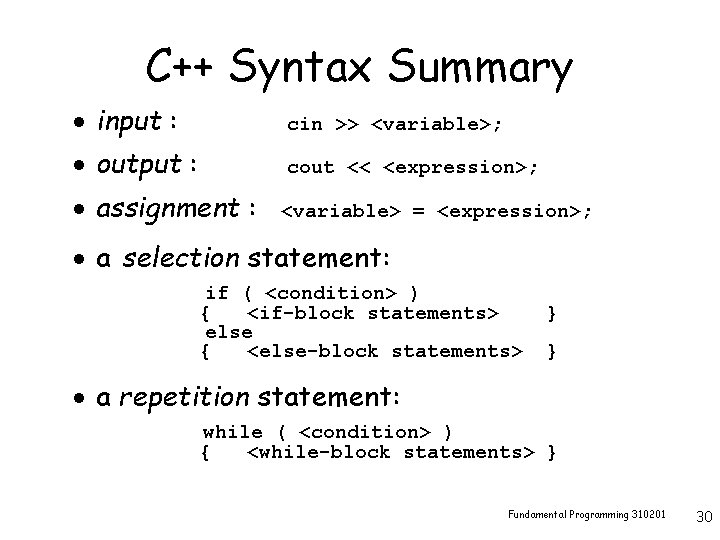
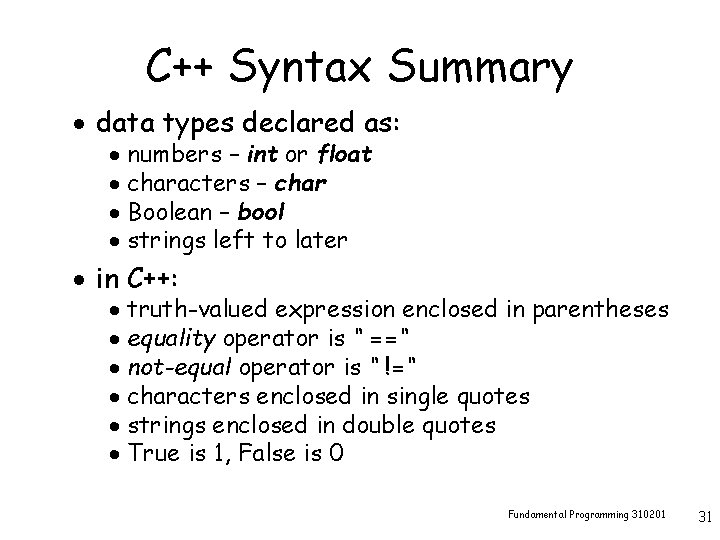
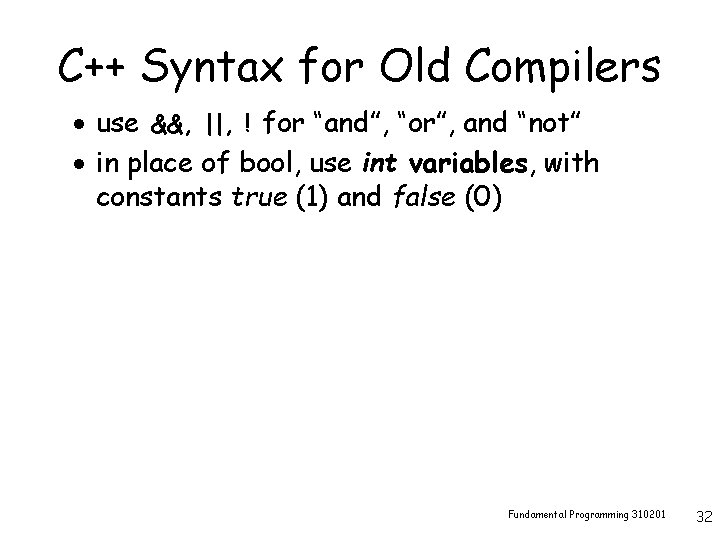
- Slides: 32
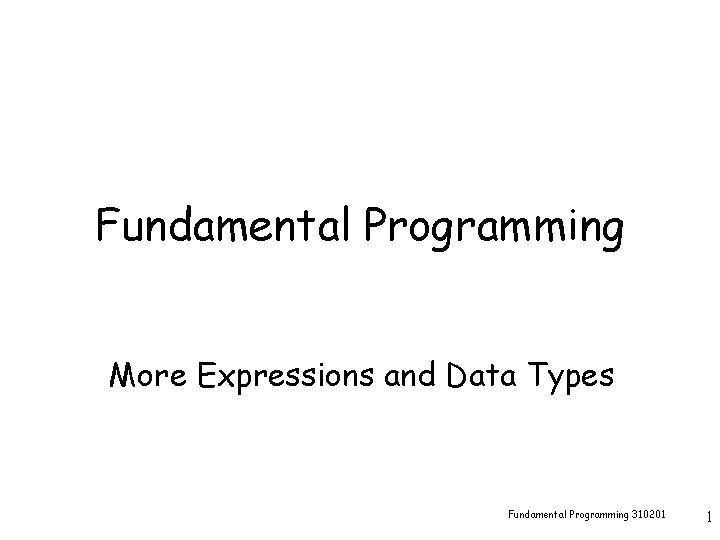
Fundamental Programming More Expressions and Data Types Fundamental Programming 310201 1
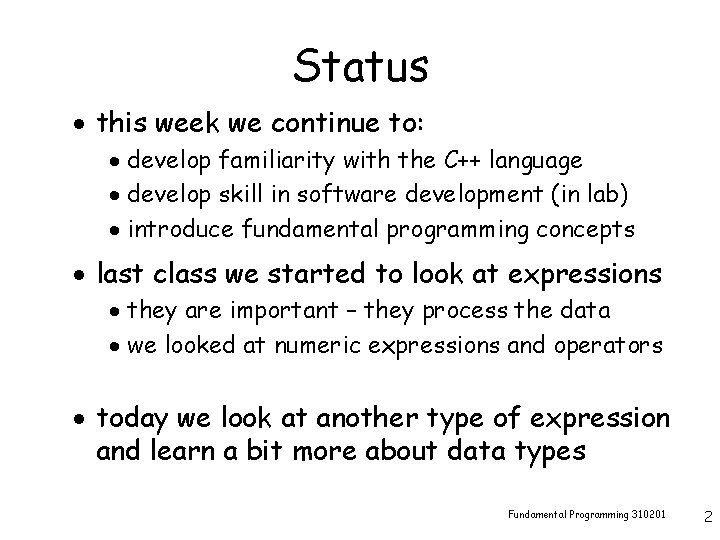
Status · this week we continue to: · develop familiarity with the C++ language · develop skill in software development (in lab) · introduce fundamental programming concepts · last class we started to look at expressions · they are important – they process the data · we looked at numeric expressions and operators · today we look at another type of expression and learn a bit more about data types Fundamental Programming 310201 2
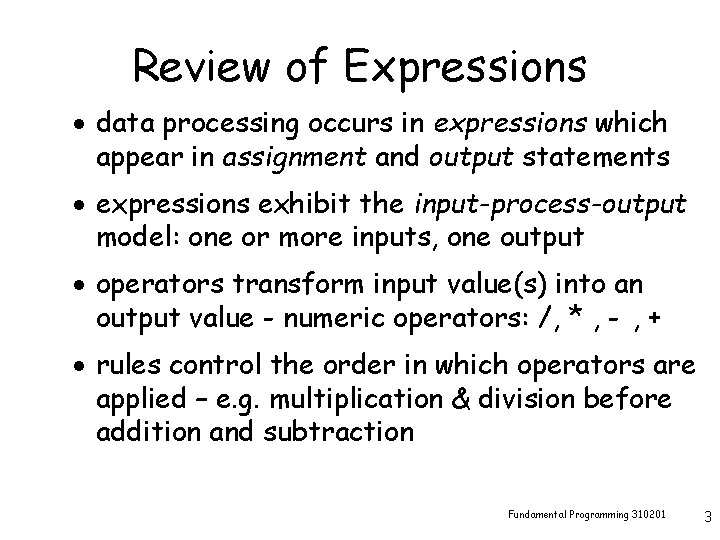
Review of Expressions · data processing occurs in expressions which appear in assignment and output statements · expressions exhibit the input-process-output model: one or more inputs, one output · operators transform input value(s) into an output value - numeric operators: /, * , - , + · rules control the order in which operators are applied – e. g. multiplication & division before addition and subtraction Fundamental Programming 310201 3
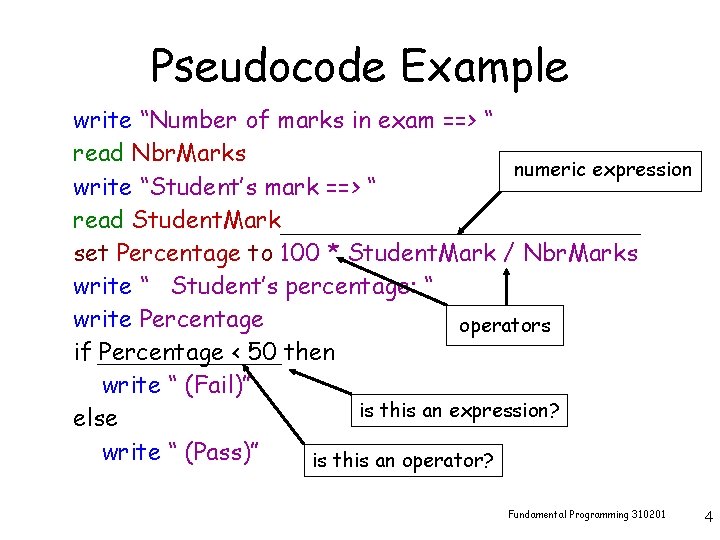
Pseudocode Example write “Number of marks in exam ==> “ read Nbr. Marks numeric expression write “Student’s mark ==> “ read Student. Mark set Percentage to 100 * Student. Mark / Nbr. Marks write “ Student’s percentage: “ write Percentage operators if Percentage < 50 then write “ (Fail)” is this an expression? else write “ (Pass)” is this an operator? Fundamental Programming 310201 4
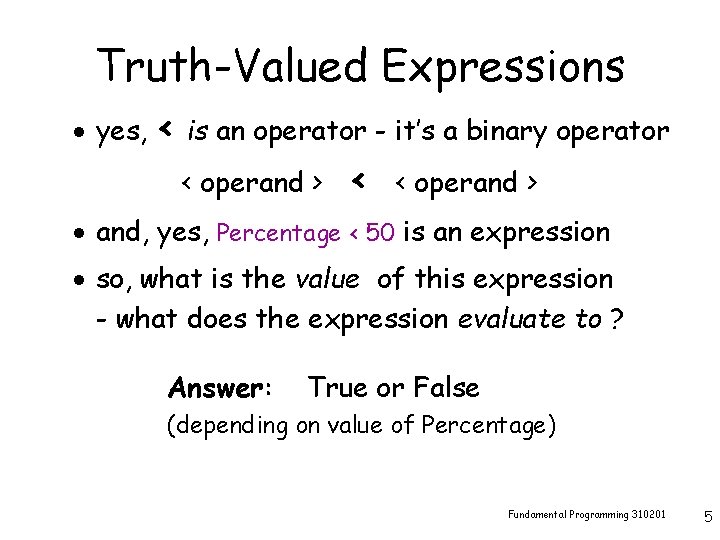
Truth-Valued Expressions · yes, < is an operator - it’s a binary operator < operand > < < operand > · and, yes, Percentage < 50 is an expression · so, what is the value of this expression - what does the expression evaluate to ? Answer: True or False (depending on value of Percentage) Fundamental Programming 310201 5
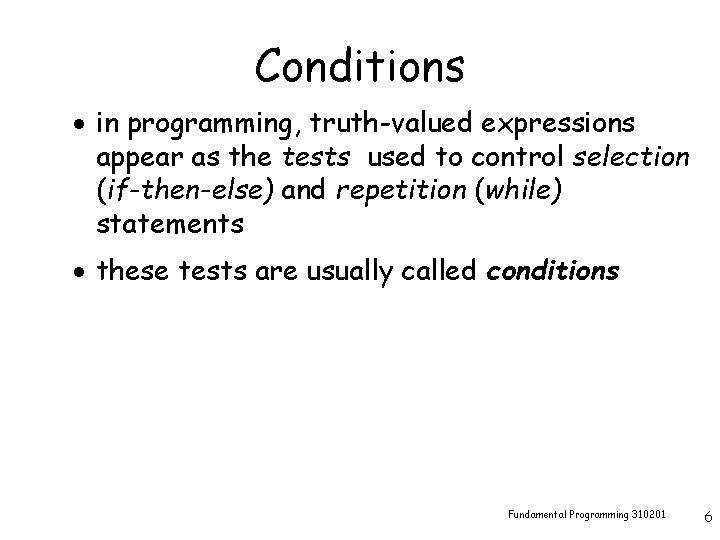
Conditions · in programming, truth-valued expressions appear as the tests used to control selection (if-then-else) and repetition (while) statements · these tests are usually called conditions Fundamental Programming 310201 6
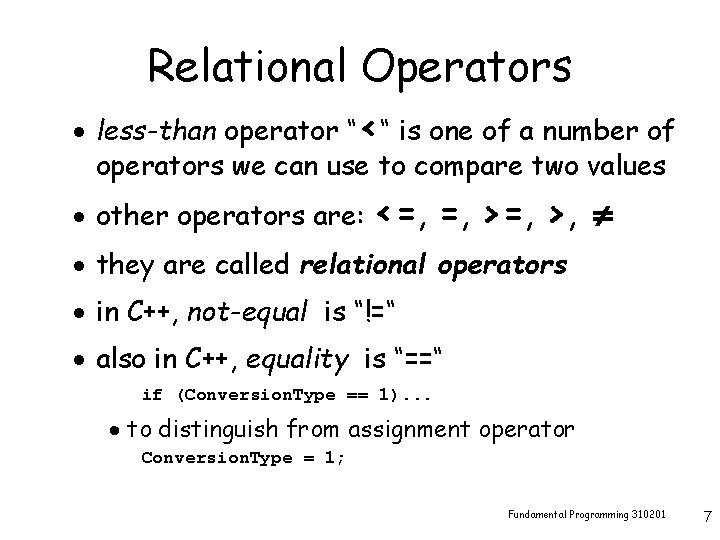
Relational Operators · less-than operator “<“ is one of a number of operators we can use to compare two values · other operators are: <=, =, >, · they are called relational operators · in C++, not-equal is “!=“ · also in C++, equality is “==“ if (Conversion. Type == 1). . . · to distinguish from assignment operator Conversion. Type = 1; Fundamental Programming 310201 7
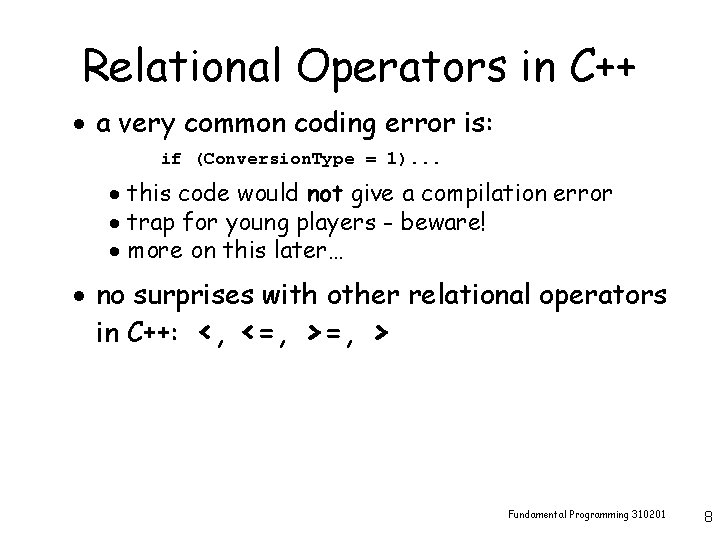
Relational Operators in C++ · a very common coding error is: if (Conversion. Type = 1). . . · this code would not give a compilation error · trap for young players - beware! · more on this later… · no surprises with other relational operators in C++: <, <=, > Fundamental Programming 310201 8
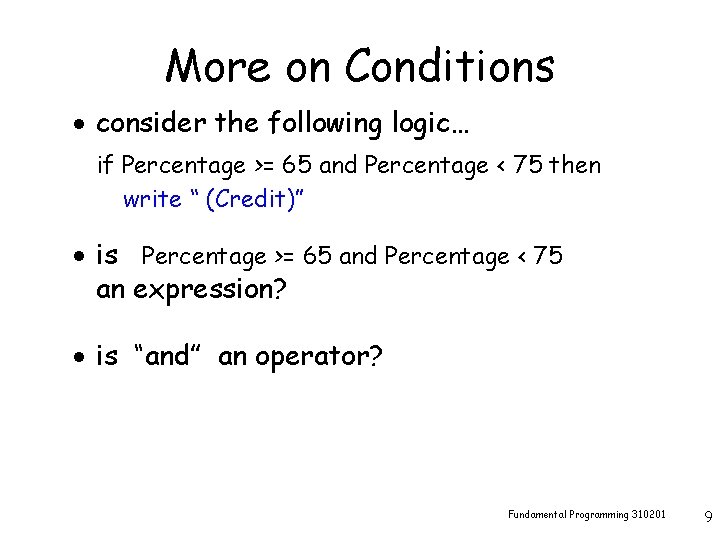
More on Conditions · consider the following logic… if Percentage >= 65 and Percentage < 75 then write “ (Credit)” · is Percentage >= 65 and Percentage < 75 an expression? · is “and” an operator? Fundamental Programming 310201 9
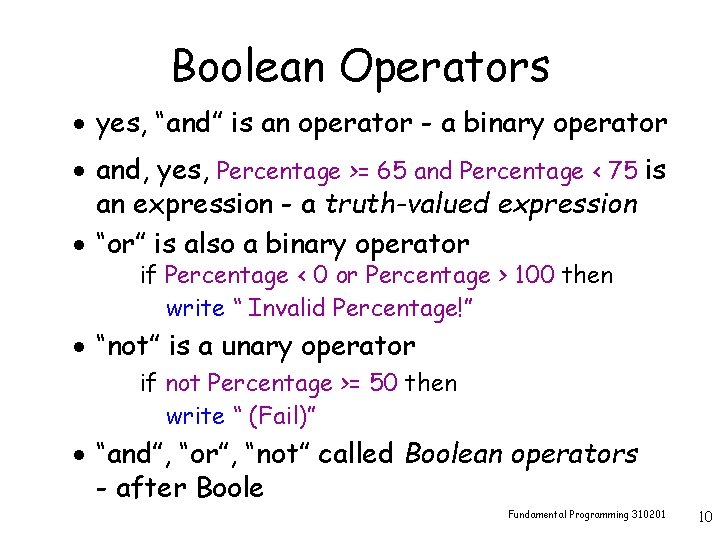
Boolean Operators · yes, “and” is an operator - a binary operator · and, yes, Percentage >= 65 and Percentage < 75 is an expression - a truth-valued expression · “or” is also a binary operator if Percentage < 0 or Percentage > 100 then write “ Invalid Percentage!” · “not” is a unary operator if not Percentage >= 50 then write “ (Fail)” · “and”, “or”, “not” called Boolean operators - after Boole Fundamental Programming 310201 10
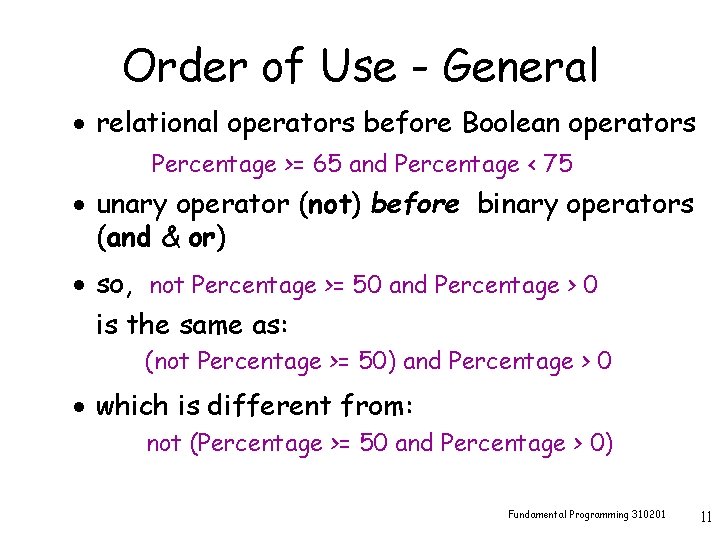
Order of Use - General · relational operators before Boolean operators Percentage >= 65 and Percentage < 75 · unary operator (not) before binary operators (and & or) · so, not Percentage >= 50 and Percentage > 0 is the same as: (not Percentage >= 50) and Percentage > 0 · which is different from: not (Percentage >= 50 and Percentage > 0) Fundamental Programming 310201 11
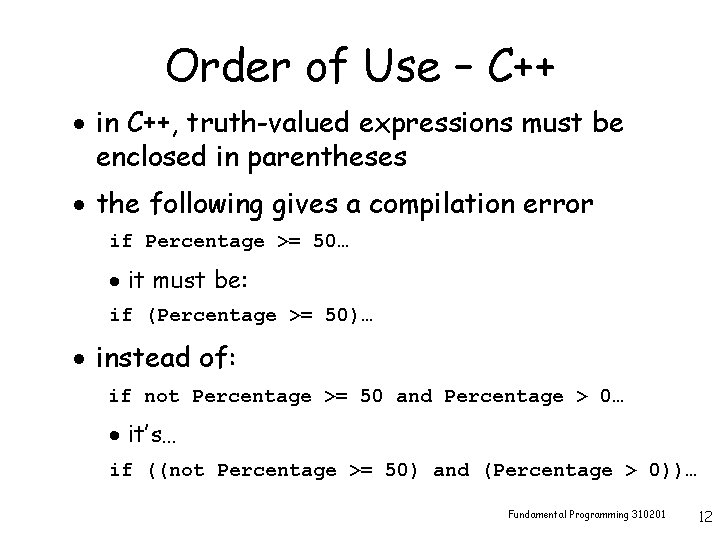
Order of Use – C++ · in C++, truth-valued expressions must be enclosed in parentheses · the following gives a compilation error if Percentage >= 50… · it must be: if (Percentage >= 50)… · instead of: if not Percentage >= 50 and Percentage > 0… · it’s… if ((not Percentage >= 50) and (Percentage > 0))… Fundamental Programming 310201 12
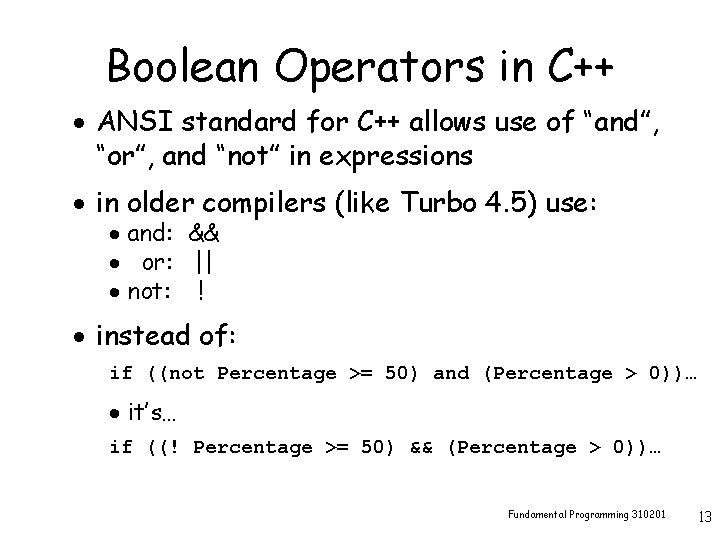
Boolean Operators in C++ · ANSI standard for C++ allows use of “and”, “or”, and “not” in expressions · in older compilers (like Turbo 4. 5) use: · and: && · or: || · not: ! · instead of: if ((not Percentage >= 50) and (Percentage > 0))… · it’s… if ((! Percentage >= 50) && (Percentage > 0))… Fundamental Programming 310201 13
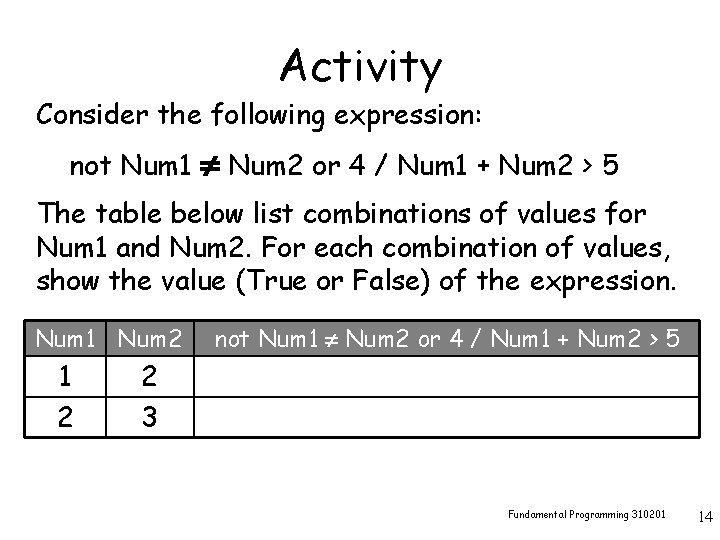
Activity Consider the following expression: not Num 1 Num 2 or 4 / Num 1 + Num 2 > 5 The table below list combinations of values for Num 1 and Num 2. For each combination of values, show the value (True or False) of the expression. Num 1 Num 2 1 2 not Num 1 Num 2 or 4 / Num 1 + Num 2 > 5 2 3 Fundamental Programming 310201 14
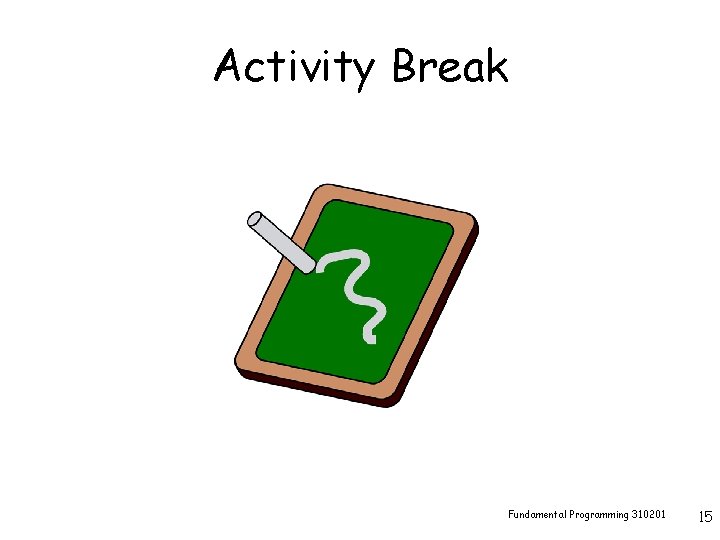
Activity Break Fundamental Programming 310201 15
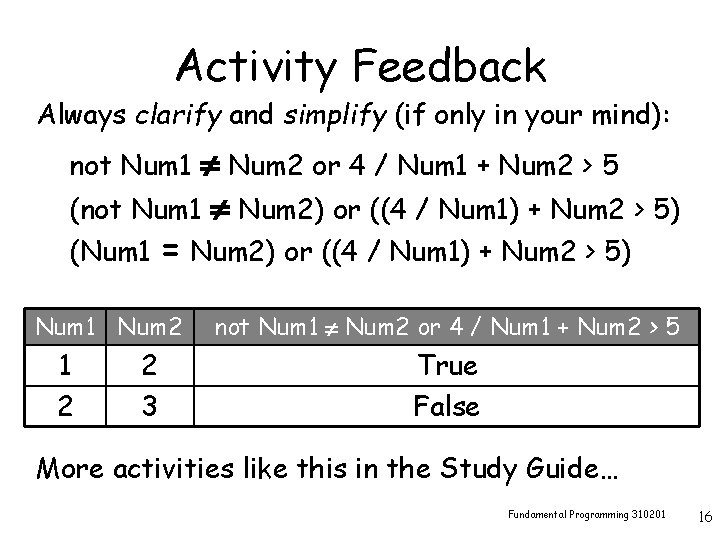
Activity Feedback Always clarify and simplify (if only in your mind): Num 2 or 4 / Num 1 + Num 2 > 5 (not Num 1 Num 2) or ((4 / Num 1) + Num 2 > 5) (Num 1 = Num 2) or ((4 / Num 1) + Num 2 > 5) not Num 1 Num 2 1 2 2 3 not Num 1 Num 2 or 4 / Num 1 + Num 2 > 5 True False More activities like this in the Study Guide… Fundamental Programming 310201 16
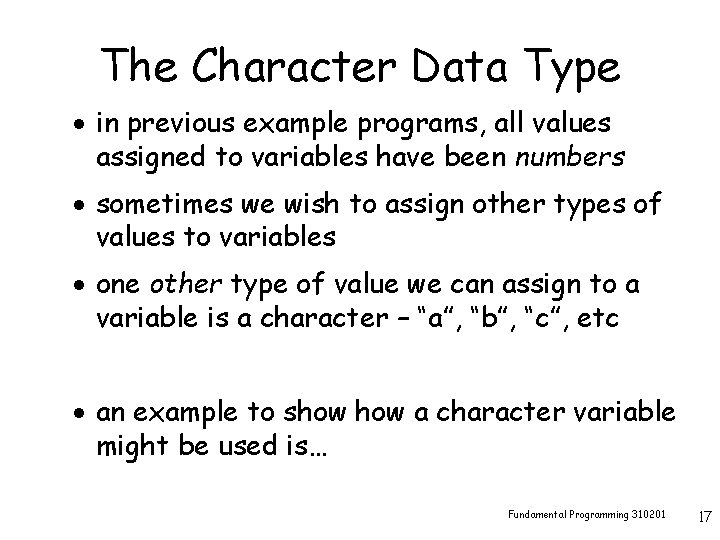
The Character Data Type · in previous example programs, all values assigned to variables have been numbers · sometimes we wish to assign other types of values to variables · one other type of value we can assign to a variable is a character – “a”, “b”, “c”, etc · an example to show a character variable might be used is… Fundamental Programming 310201 17
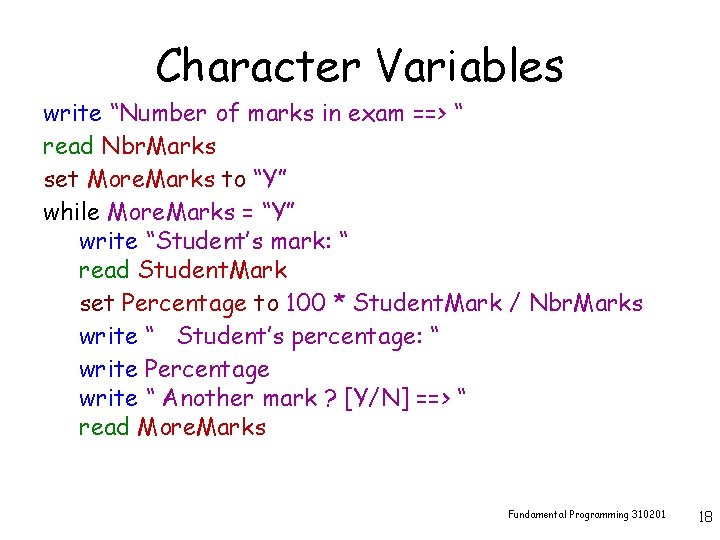
Character Variables write “Number of marks in exam ==> “ read Nbr. Marks set More. Marks to “Y” while More. Marks = “Y” write “Student’s mark: “ read Student. Mark set Percentage to 100 * Student. Mark / Nbr. Marks write “ Student’s percentage: “ write Percentage write “ Another mark ? [Y/N] ==> “ read More. Marks Fundamental Programming 310201 18
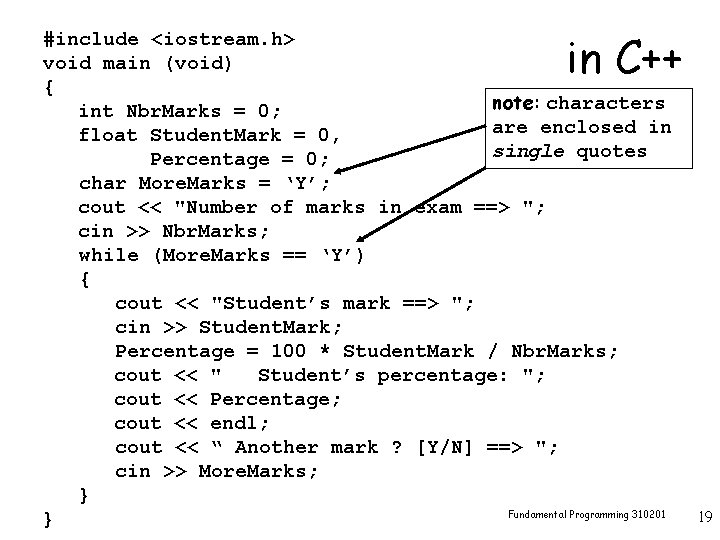
in C++ #include <iostream. h> void main (void) { note: characters int Nbr. Marks = 0; are enclosed in float Student. Mark = 0, single quotes Percentage = 0; char More. Marks = ‘Y’; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; while (More. Marks == ‘Y’) { cout << "Student’s mark ==> "; cin >> Student. Mark; Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student’s percentage: "; cout << Percentage; cout << endl; cout << “ Another mark ? [Y/N] ==> "; cin >> More. Marks; } Fundamental Programming 310201 } 19
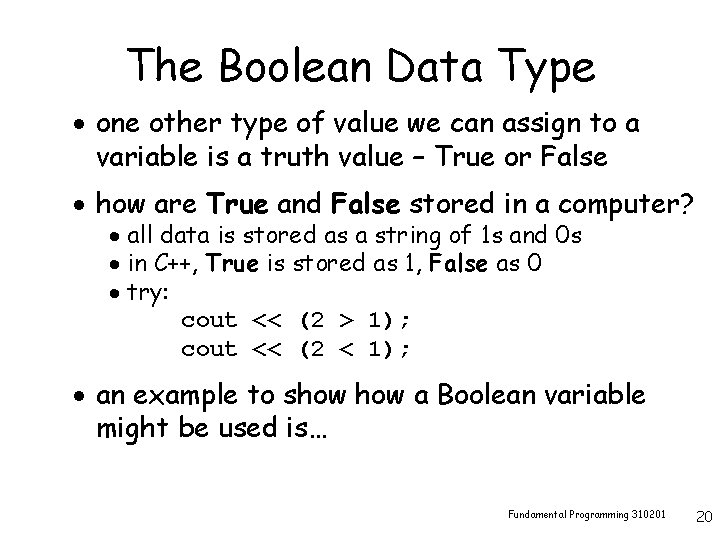
The Boolean Data Type · one other type of value we can assign to a variable is a truth value – True or False · how are True and False stored in a computer? · all data is stored as a string of 1 s and 0 s · in C++, True is stored as 1, False as 0 · try: cout << (2 > 1); cout << (2 < 1); · an example to show a Boolean variable might be used is… Fundamental Programming 310201 20
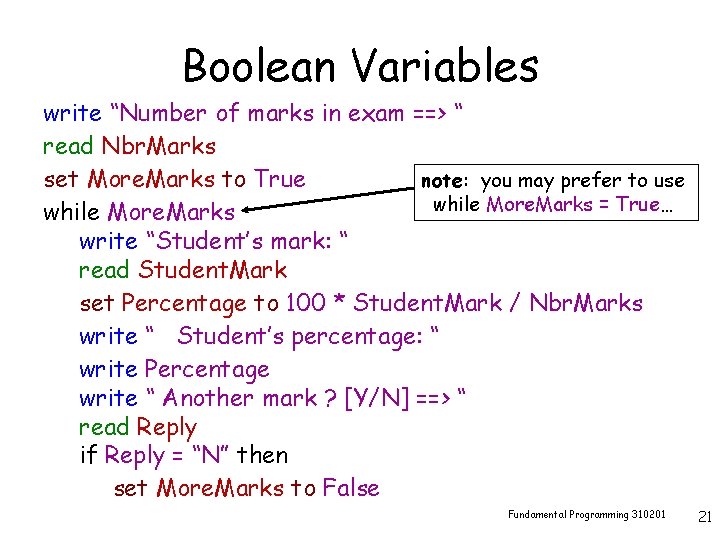
Boolean Variables write “Number of marks in exam ==> “ read Nbr. Marks note: you may prefer to use set More. Marks to True while More. Marks = True… while More. Marks write “Student’s mark: “ read Student. Mark set Percentage to 100 * Student. Mark / Nbr. Marks write “ Student’s percentage: “ write Percentage write “ Another mark ? [Y/N] ==> “ read Reply if Reply = “N” then set More. Marks to False Fundamental Programming 310201 21
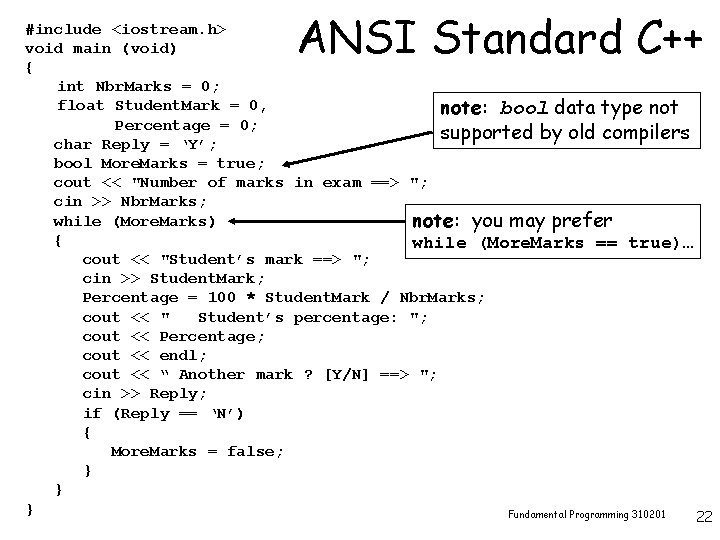
ANSI Standard C++ #include <iostream. h> void main (void) { int Nbr. Marks = 0; float Student. Mark = 0, note: bool data type not Percentage = 0; supported by old compilers char Reply = ‘Y’; bool More. Marks = true; cout << "Number of marks in exam ==> "; cin >> Nbr. Marks; while (More. Marks) note: you may prefer { while (More. Marks == true)… cout << "Student’s mark ==> "; cin >> Student. Mark; Percentage = 100 * Student. Mark / Nbr. Marks; cout << " Student’s percentage: "; cout << Percentage; cout << endl; cout << “ Another mark ? [Y/N] ==> "; cin >> Reply; if (Reply == ‘N’) { More. Marks = false; } } } Fundamental Programming 310201 22
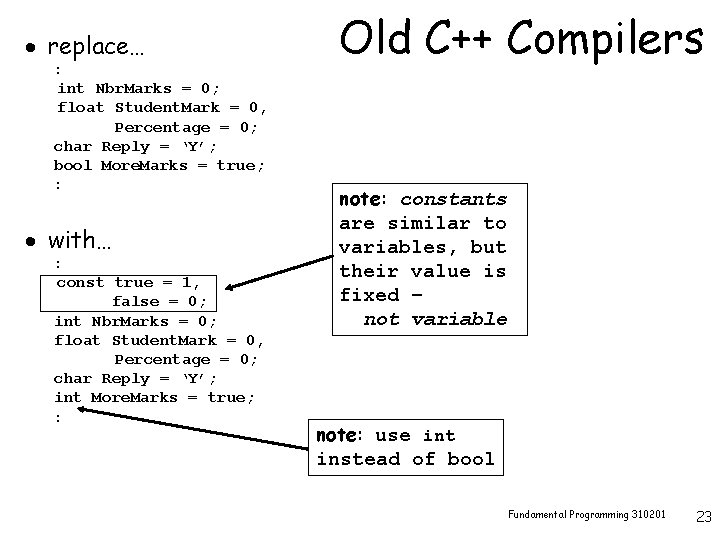
· replace… : int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; char Reply = ‘Y’; bool More. Marks = true; : · with… : const true = 1, false = 0; int Nbr. Marks = 0; float Student. Mark = 0, Percentage = 0; char Reply = ‘Y’; int More. Marks = true; : Old C++ Compilers note: constants are similar to variables, but their value is fixed – not variable note: use int instead of bool Fundamental Programming 310201 23
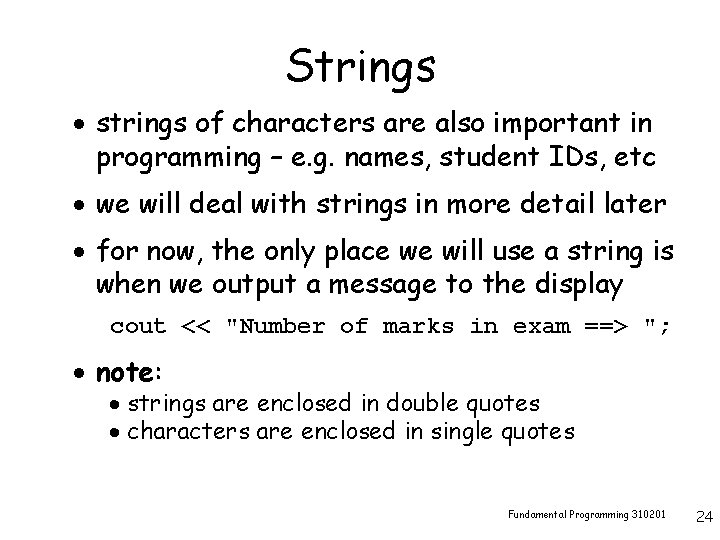
Strings · strings of characters are also important in programming – e. g. names, student IDs, etc · we will deal with strings in more detail later · for now, the only place we will use a string is when we output a message to the display cout << "Number of marks in exam ==> "; · note: · strings are enclosed in double quotes · characters are enclosed in single quotes Fundamental Programming 310201 24
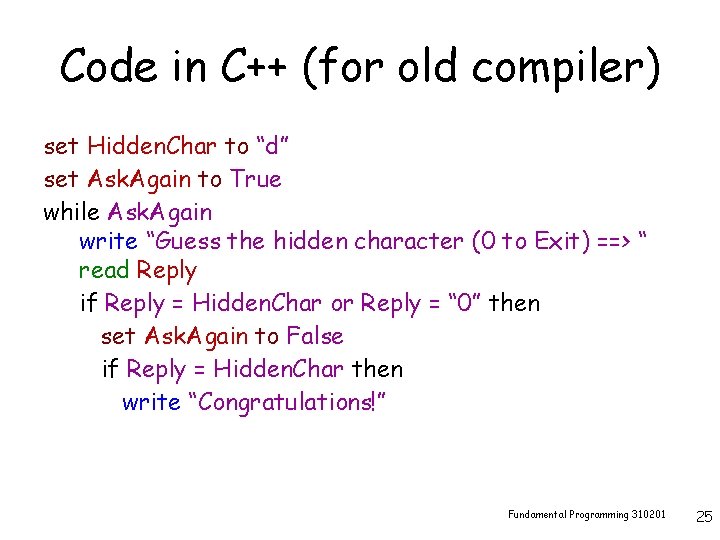
Code in C++ (for old compiler) set Hidden. Char to “d” set Ask. Again to True while Ask. Again write “Guess the hidden character (0 to Exit) ==> “ read Reply if Reply = Hidden. Char or Reply = “ 0” then set Ask. Again to False if Reply = Hidden. Char then write “Congratulations!” Fundamental Programming 310201 25
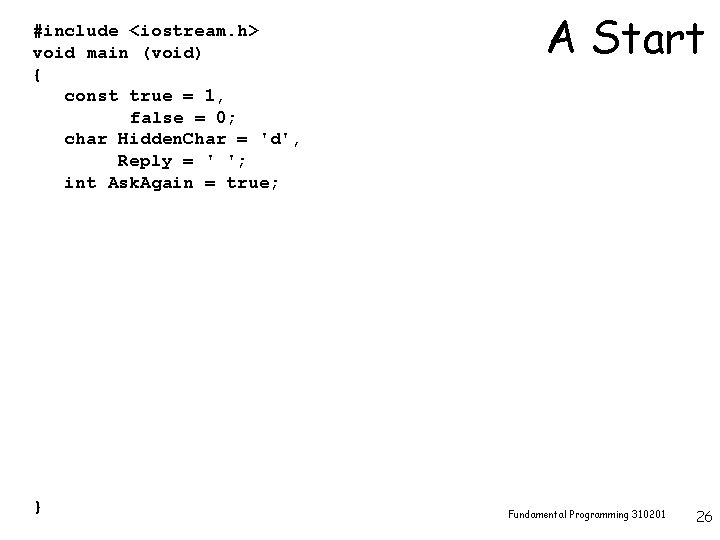
#include <iostream. h> void main (void) { const true = 1, false = 0; char Hidden. Char = 'd', Reply = ' '; int Ask. Again = true; } A Start Fundamental Programming 310201 26
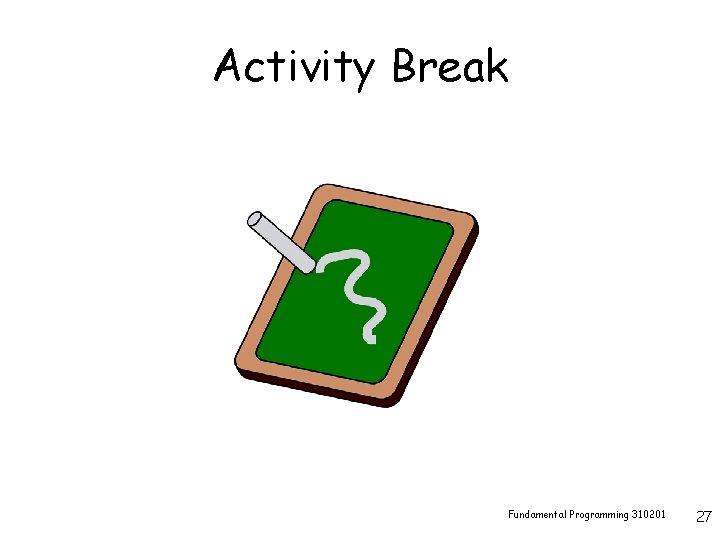
Activity Break Fundamental Programming 310201 27
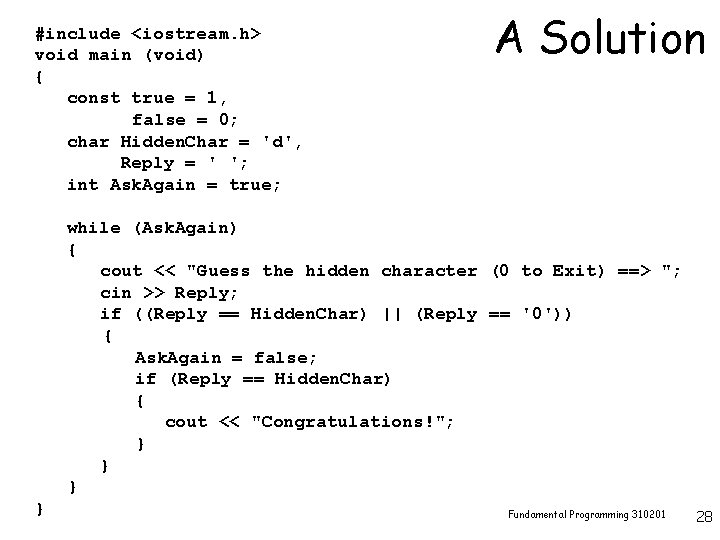
#include <iostream. h> void main (void) { const true = 1, false = 0; char Hidden. Char = 'd', Reply = ' '; int Ask. Again = true; A Solution while (Ask. Again) { cout << "Guess the hidden character (0 to Exit) ==> "; cin >> Reply; if ((Reply == Hidden. Char) || (Reply == '0')) { Ask. Again = false; if (Reply == Hidden. Char) { cout << "Congratulations!"; } } Fundamental Programming 310201 28
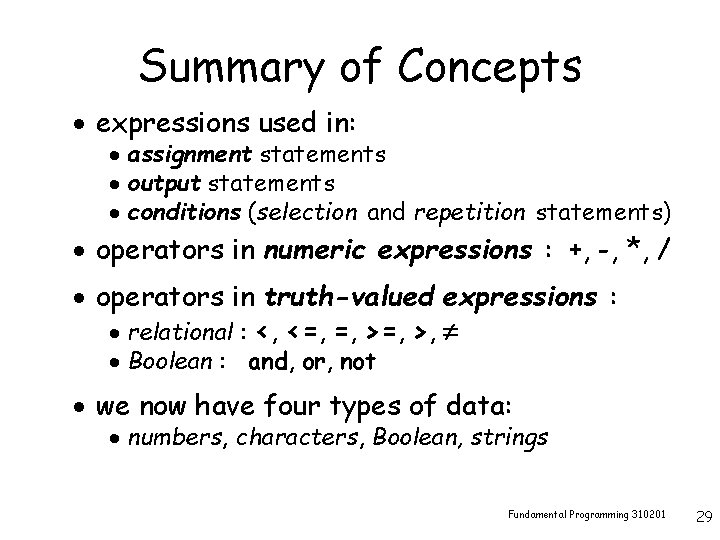
Summary of Concepts · expressions used in: · assignment statements · output statements · conditions (selection and repetition statements) · operators in numeric expressions : +, -, *, / · operators in truth-valued expressions : · relational : <, <=, =, >, · Boolean : and, or, not · we now have four types of data: · numbers, characters, Boolean, strings Fundamental Programming 310201 29
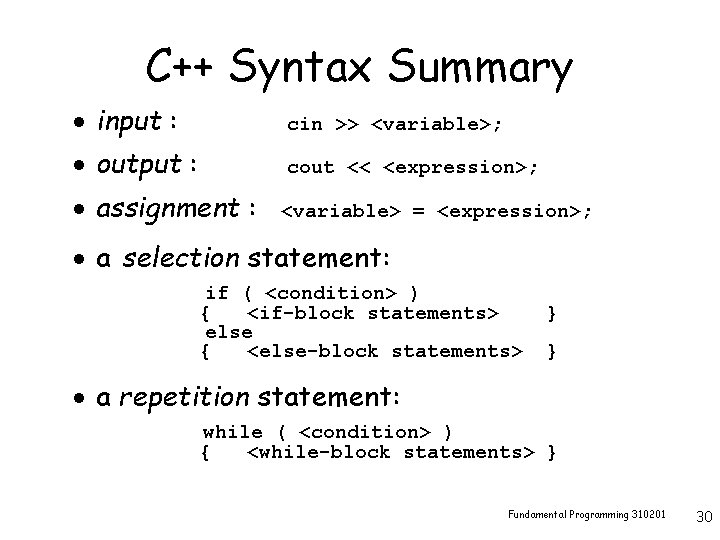
C++ Syntax Summary · input : cin >> <variable>; · output : cout << <expression>; · assignment : <variable> = <expression>; · a selection statement: if ( <condition> ) { <if-block statements> else { <else-block statements> } } · a repetition statement: while ( <condition> ) { <while-block statements> } Fundamental Programming 310201 30
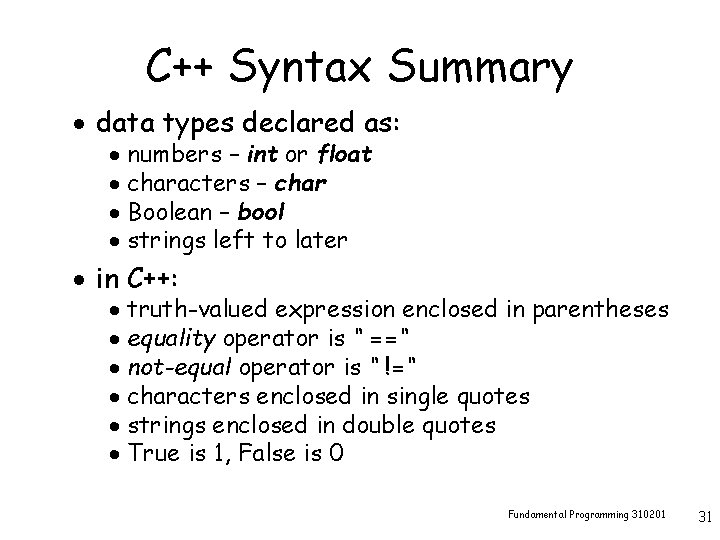
C++ Syntax Summary · data types declared as: · numbers – int or float · characters – char · Boolean – bool · strings left to later · in C++: · truth-valued expression enclosed in parentheses · equality operator is “ ==“ · not-equal operator is “ !=“ · characters enclosed in single quotes · strings enclosed in double quotes · True is 1, False is 0 Fundamental Programming 310201 31
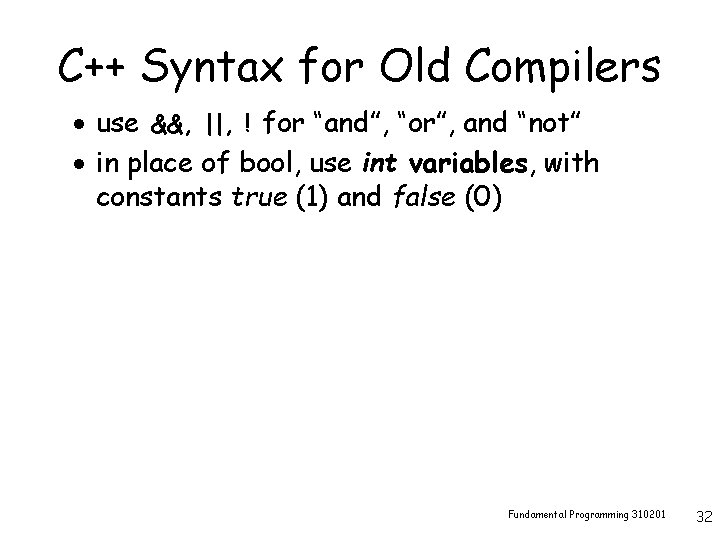
C++ Syntax for Old Compilers · use &&, ||, ! for “and”, “or”, and “not” · in place of bool, use int variables, with constants true (1) and false (0) Fundamental Programming 310201 32