Expressions and Control Flow in PHP Expressions Expressions
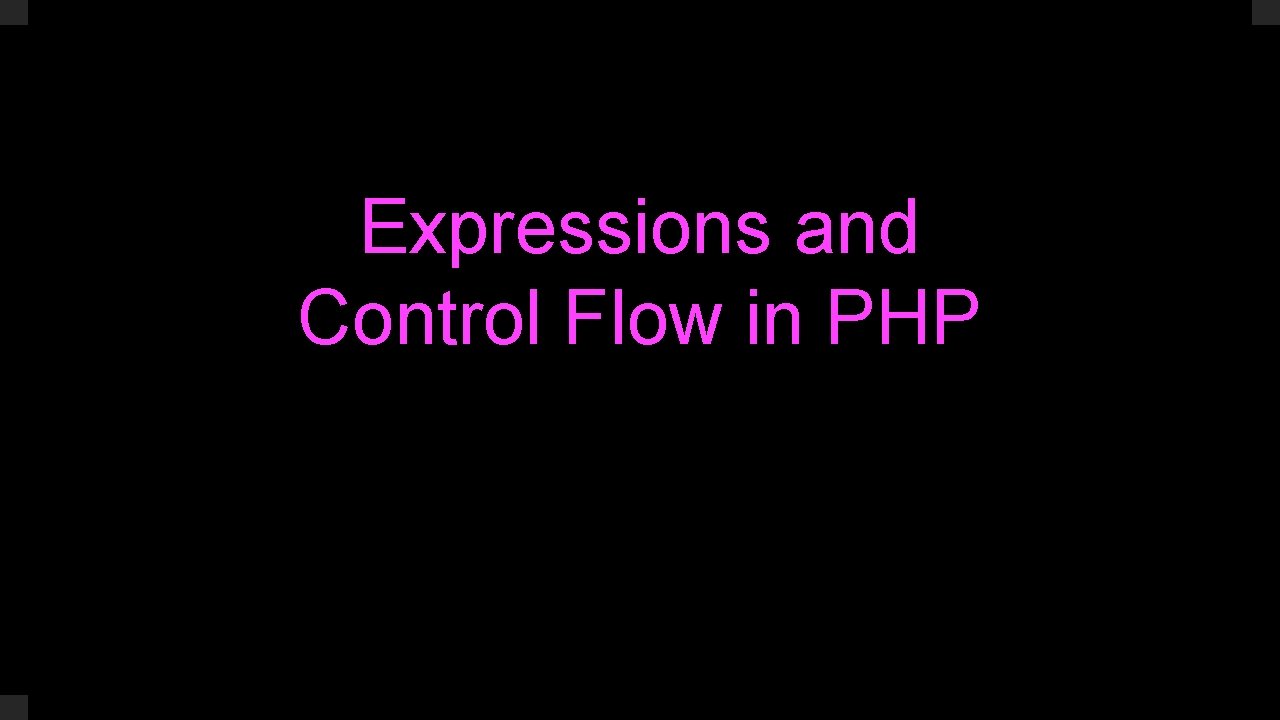
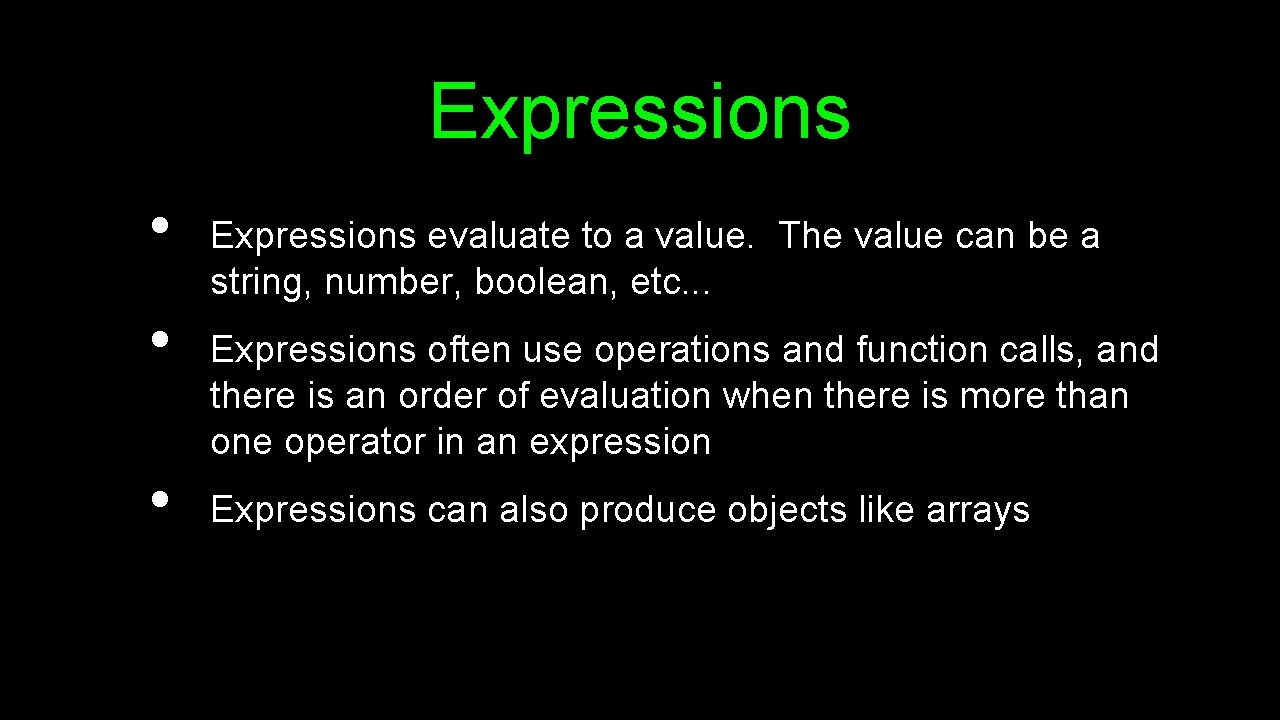
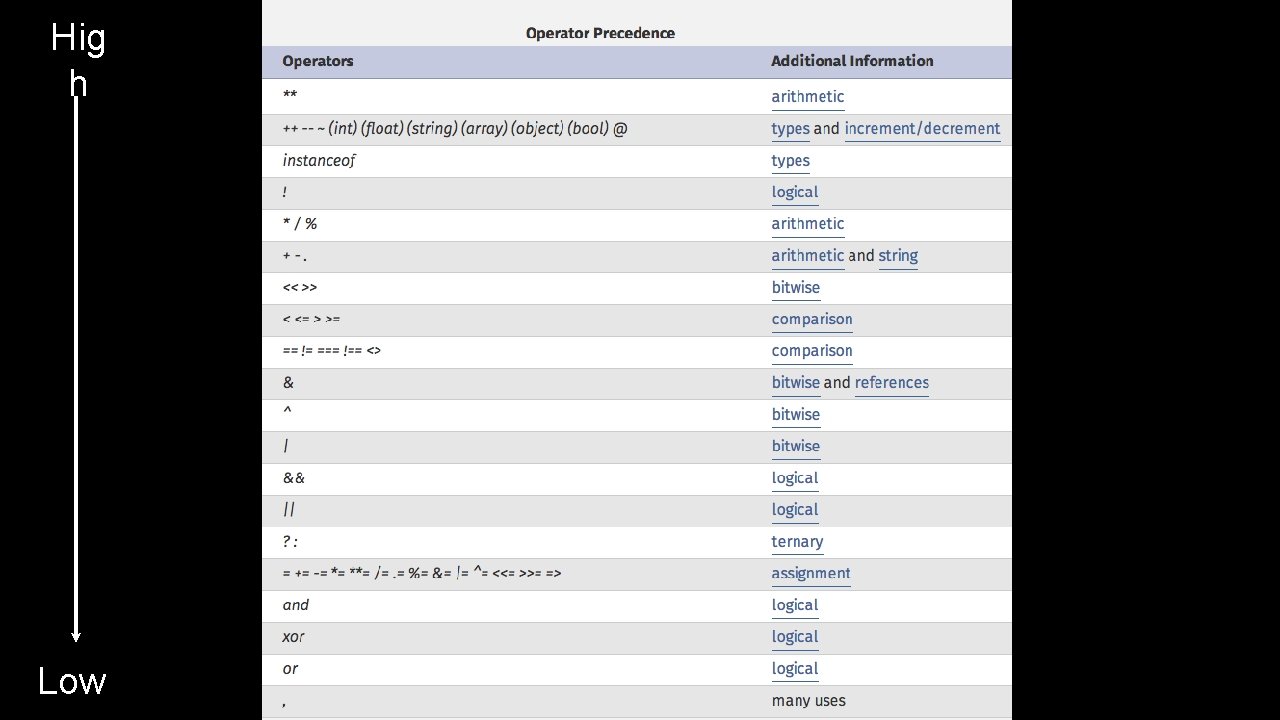
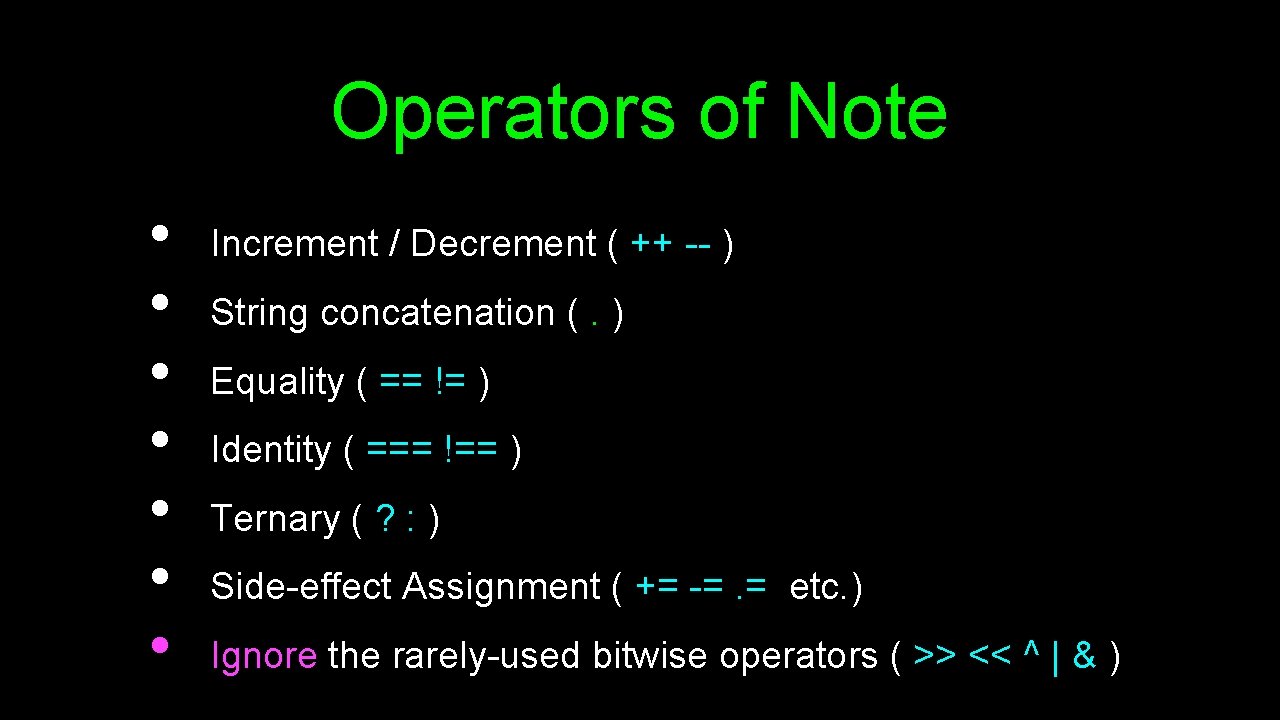
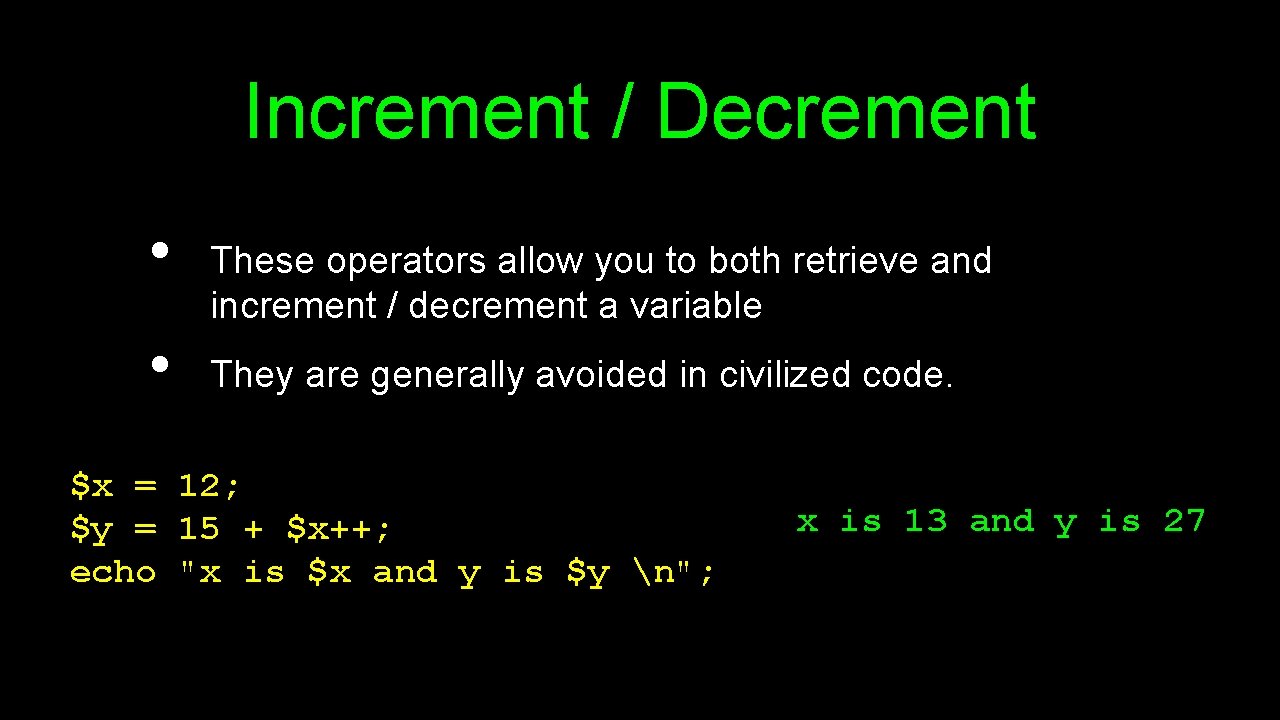
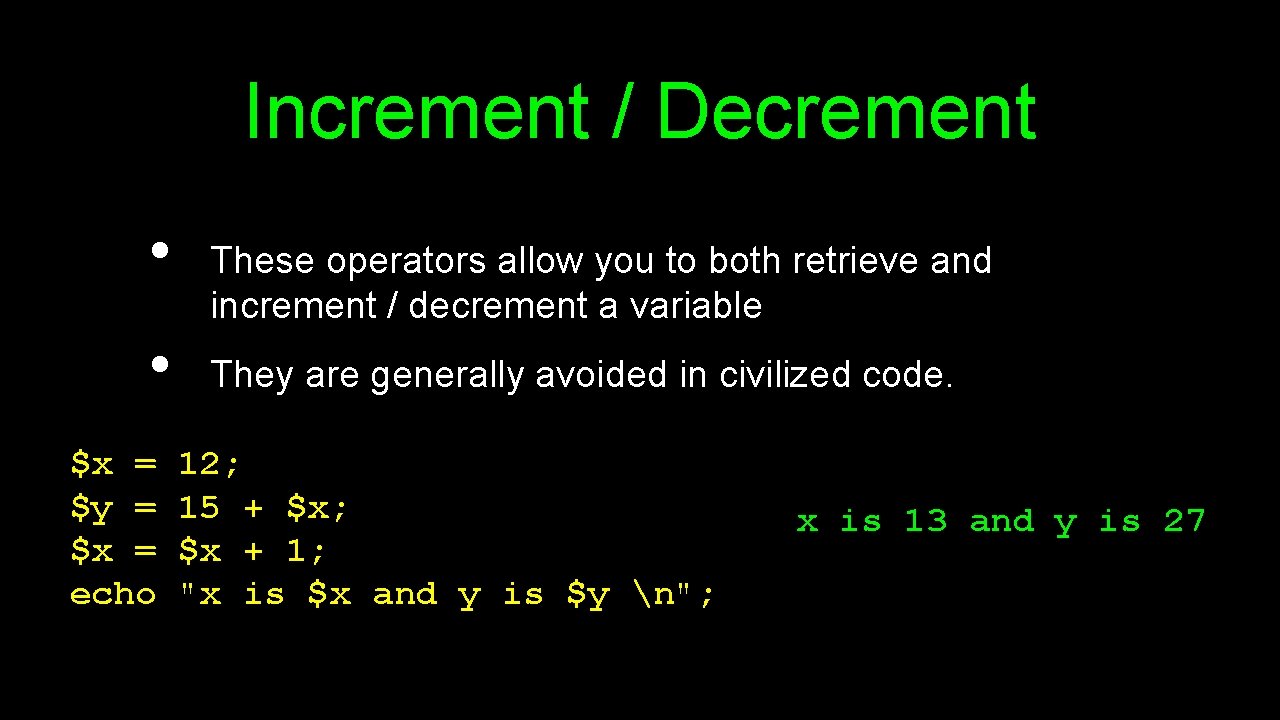
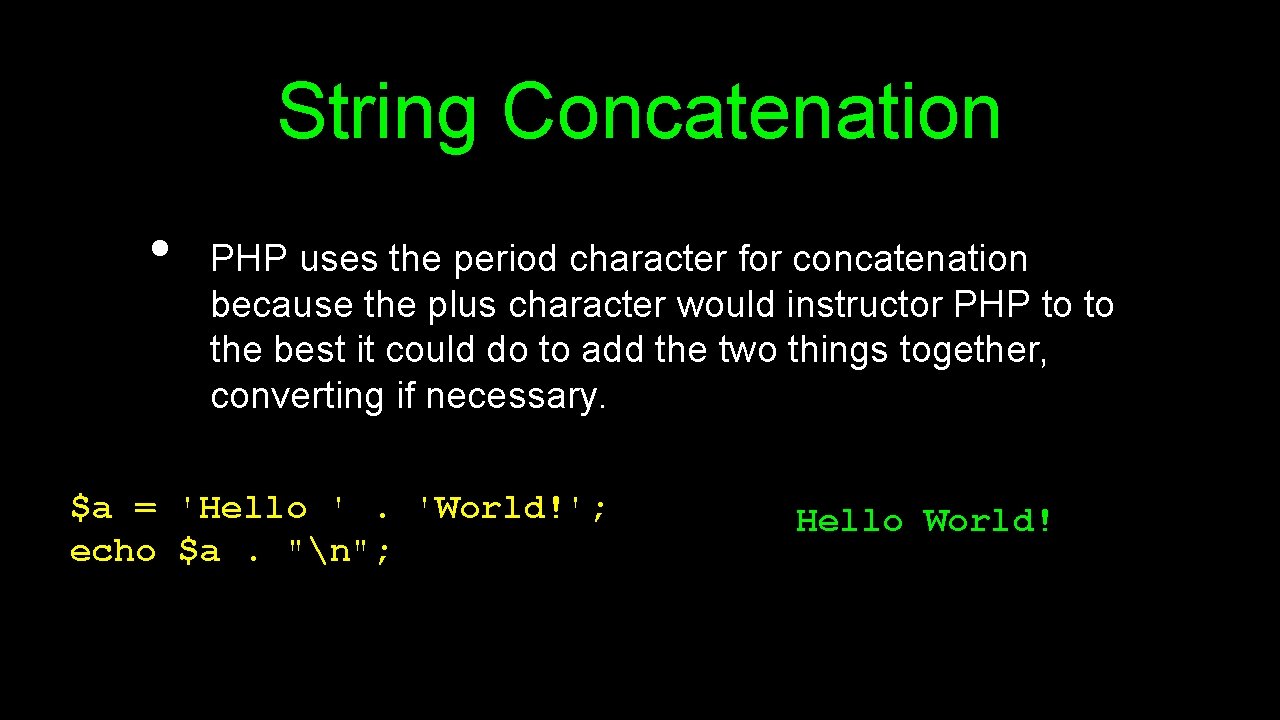
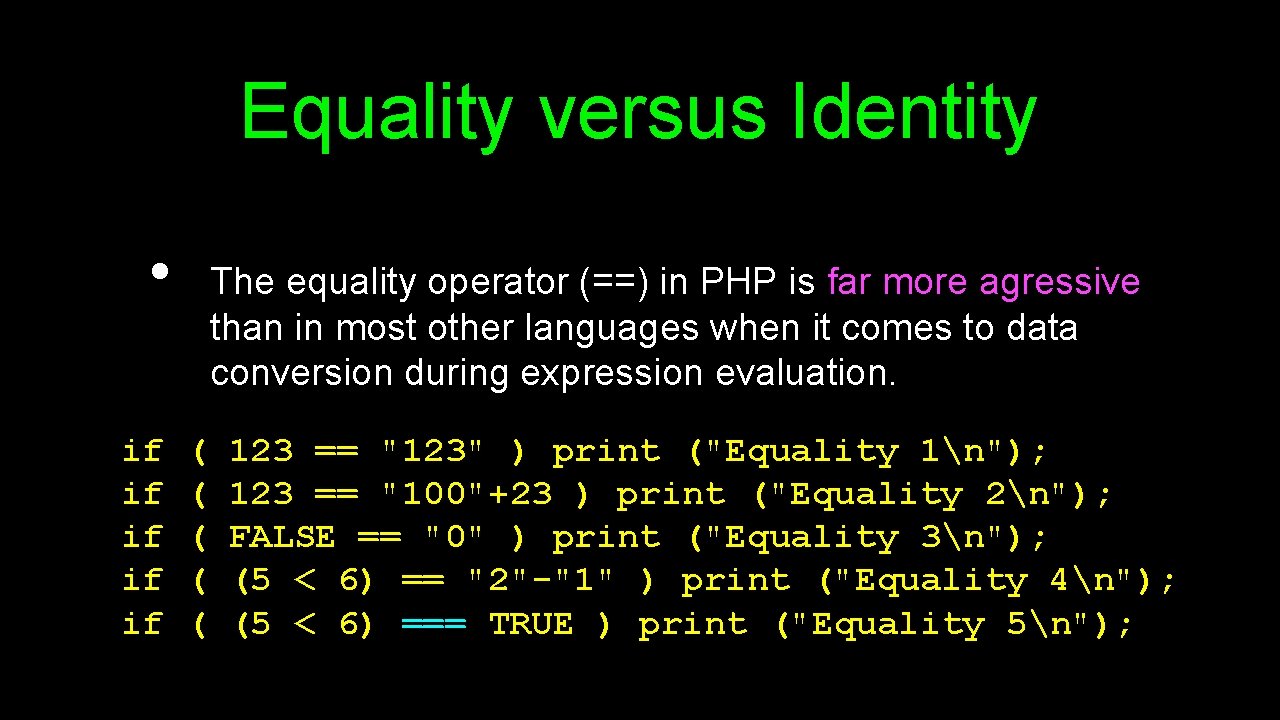
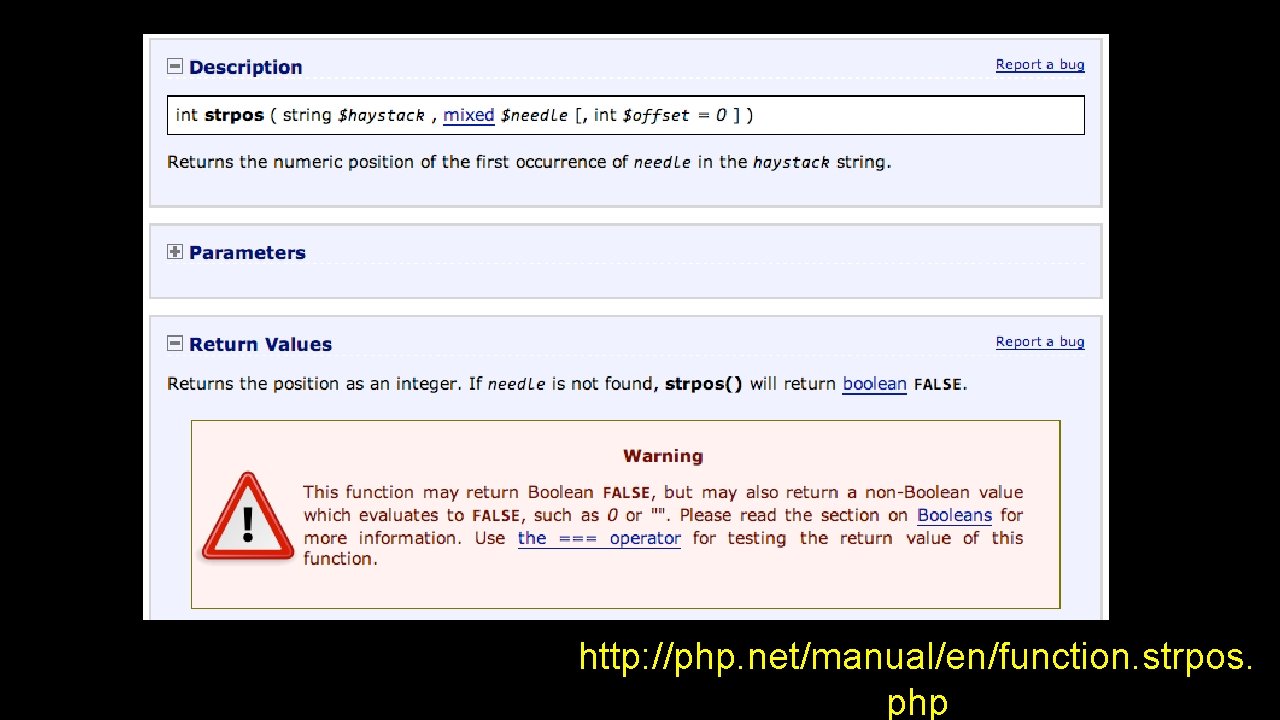
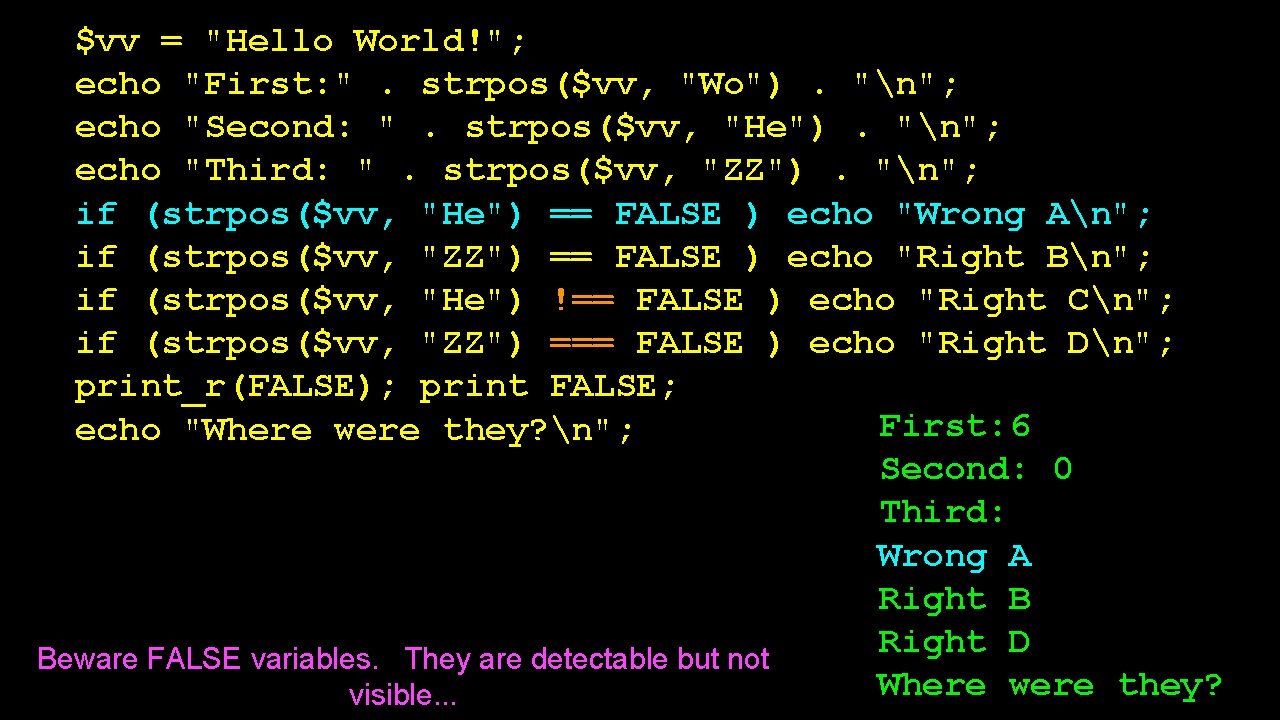
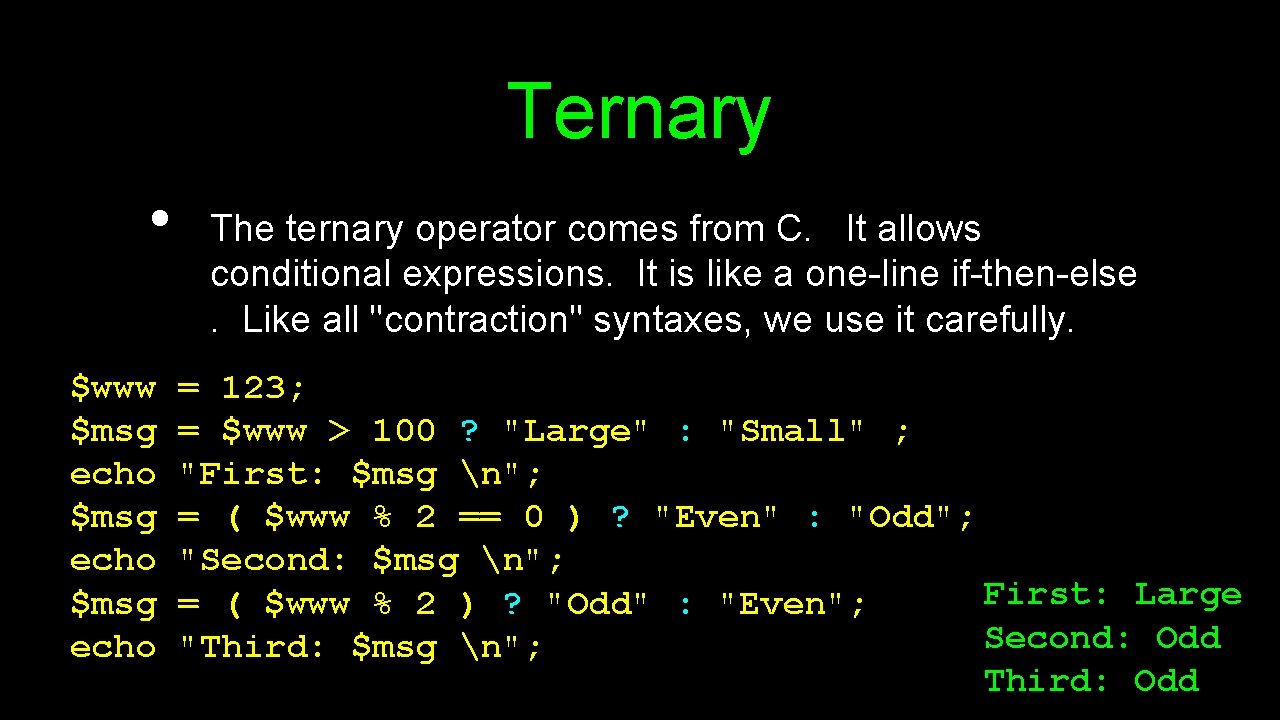
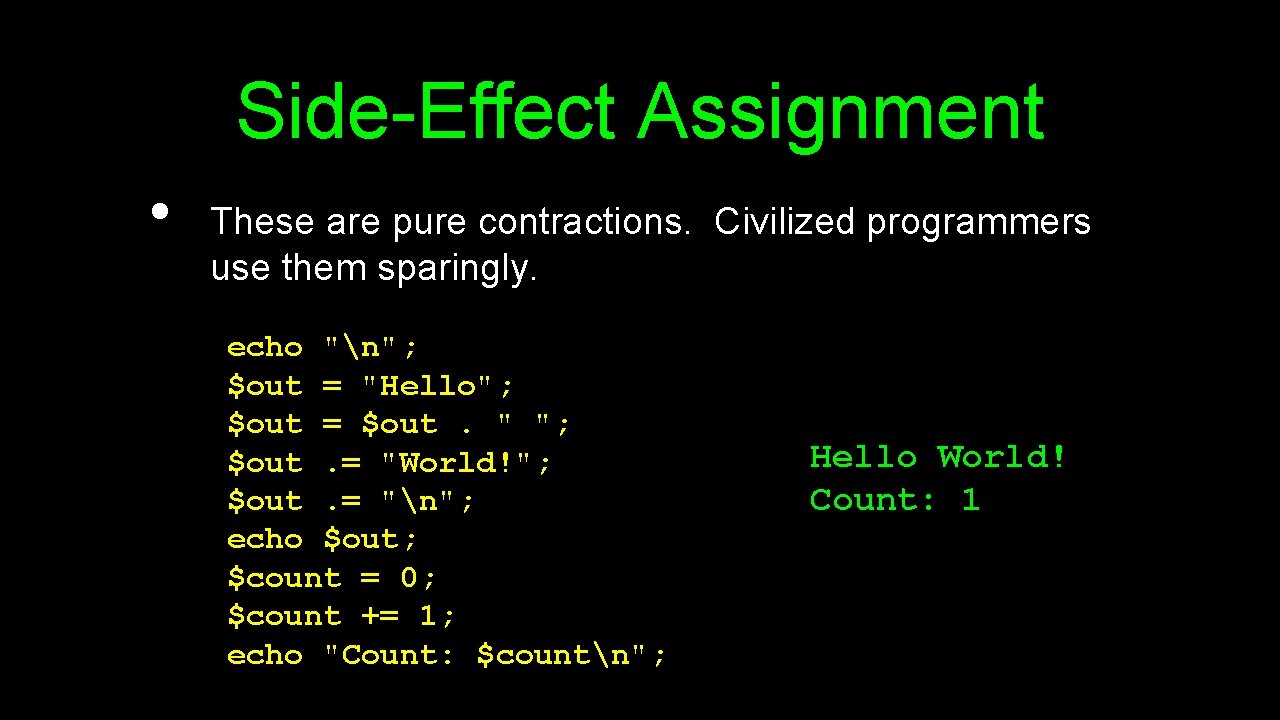
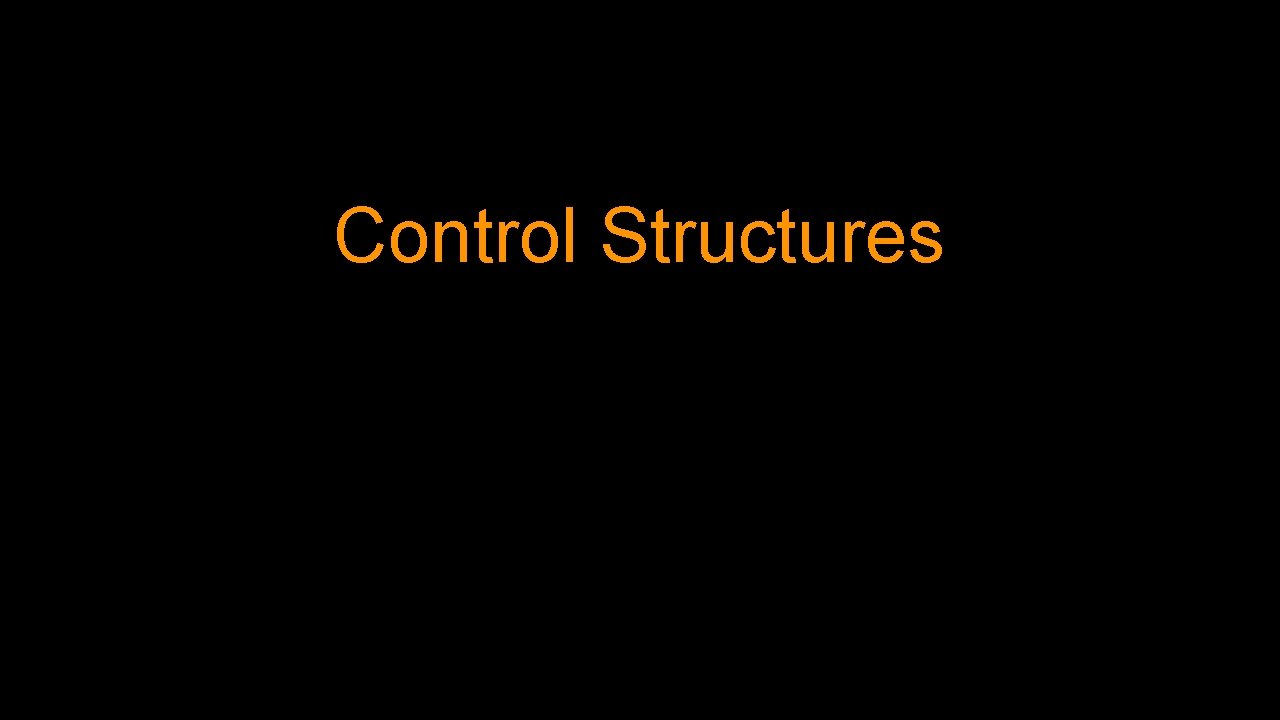
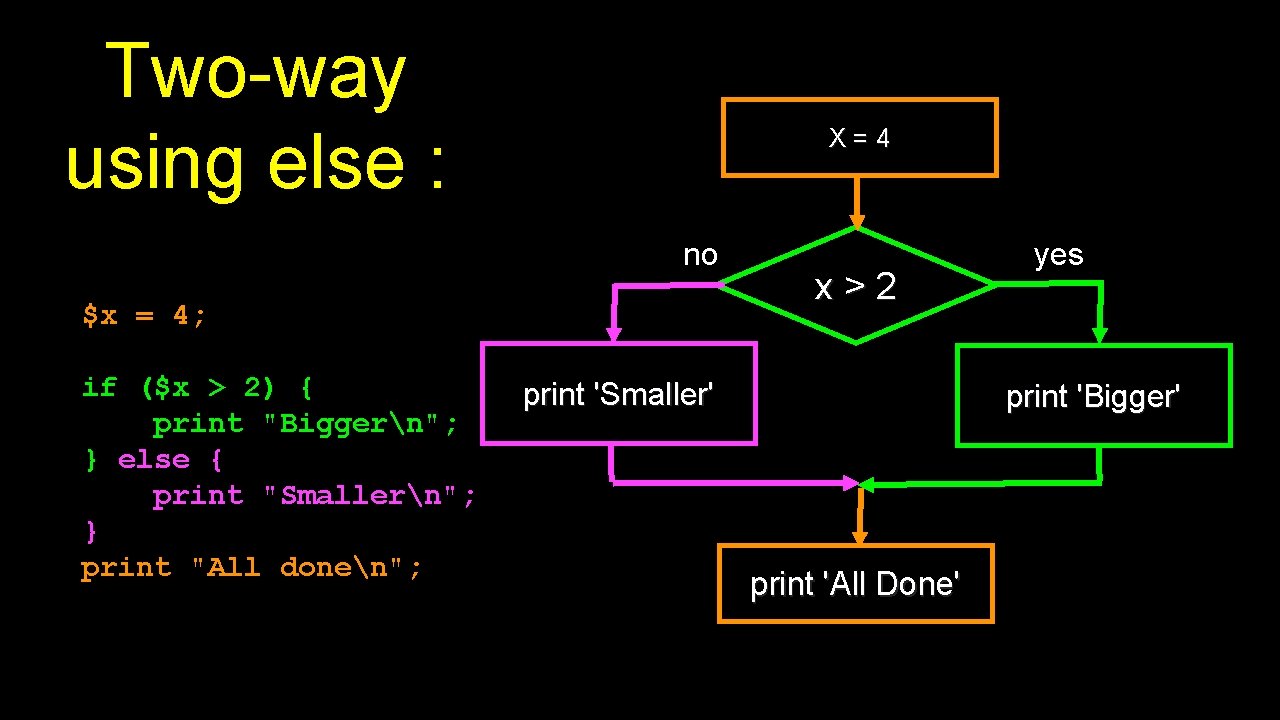
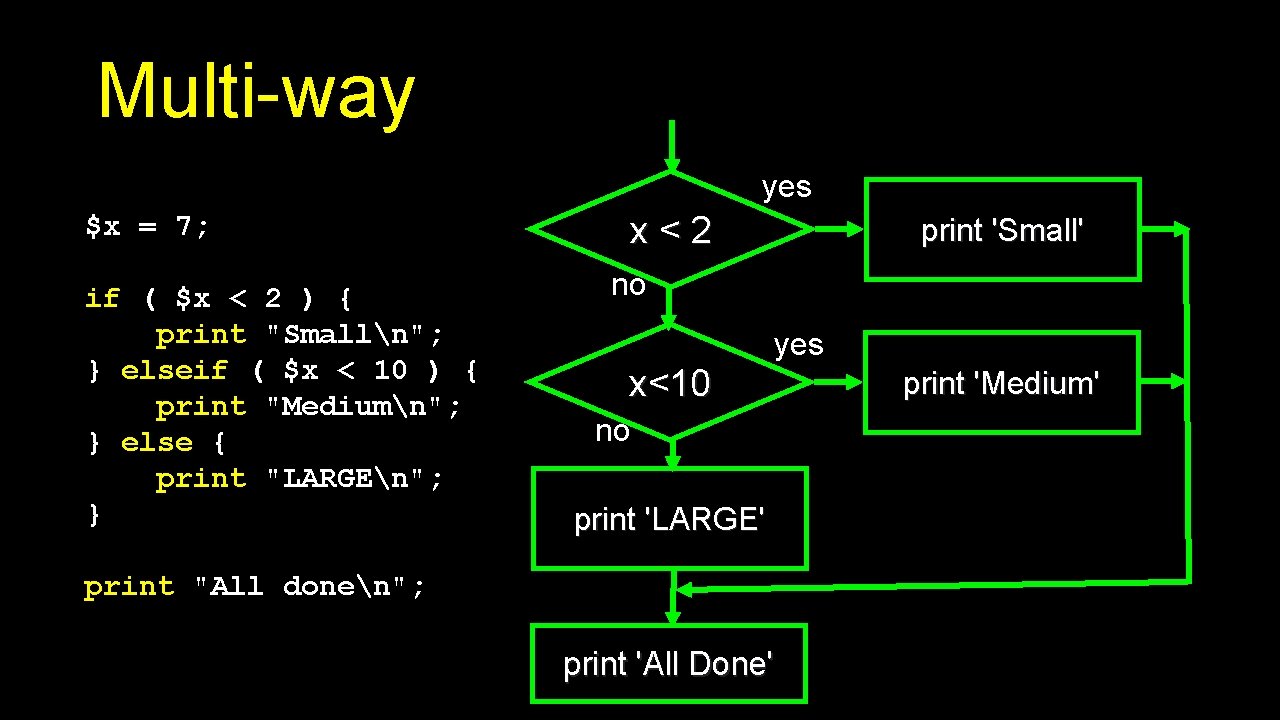
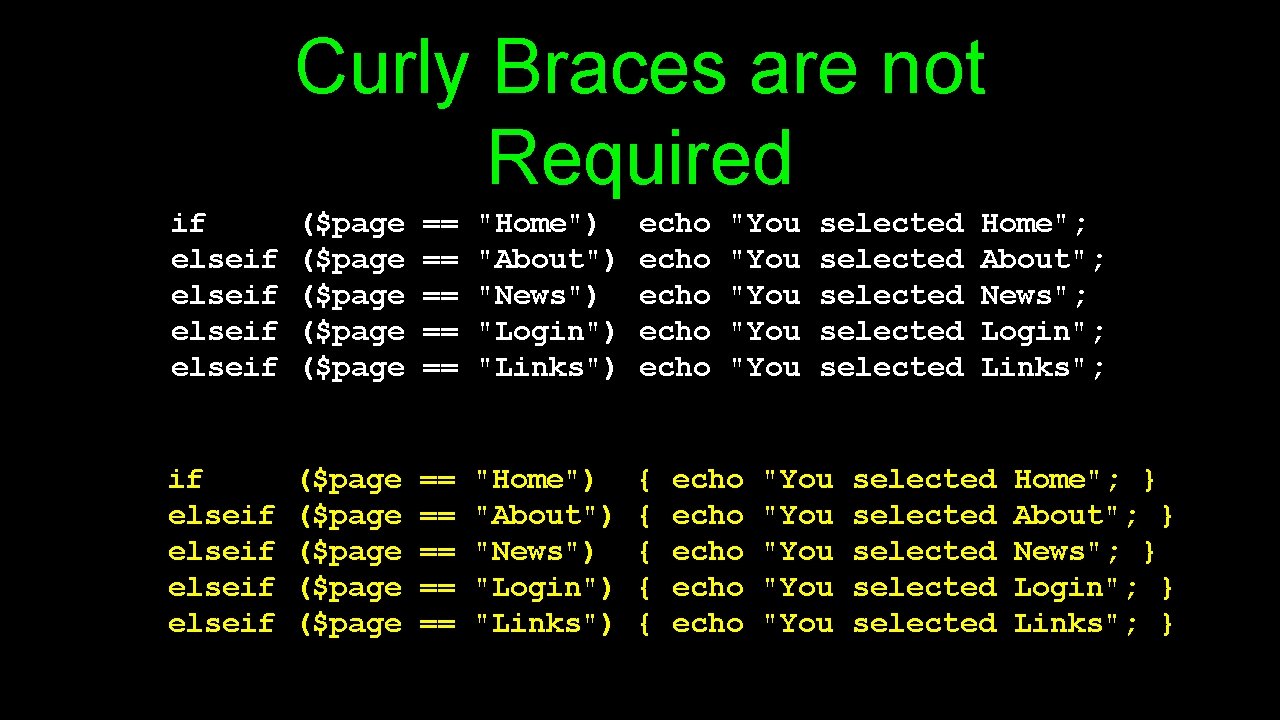
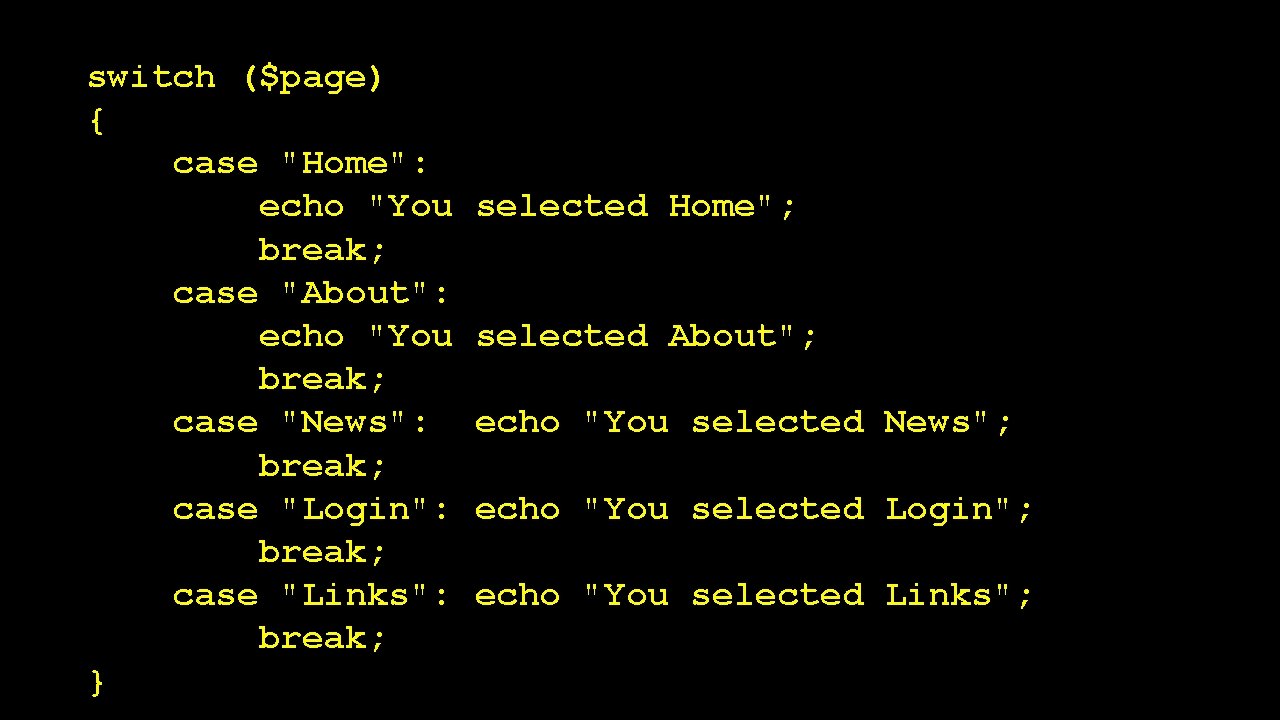
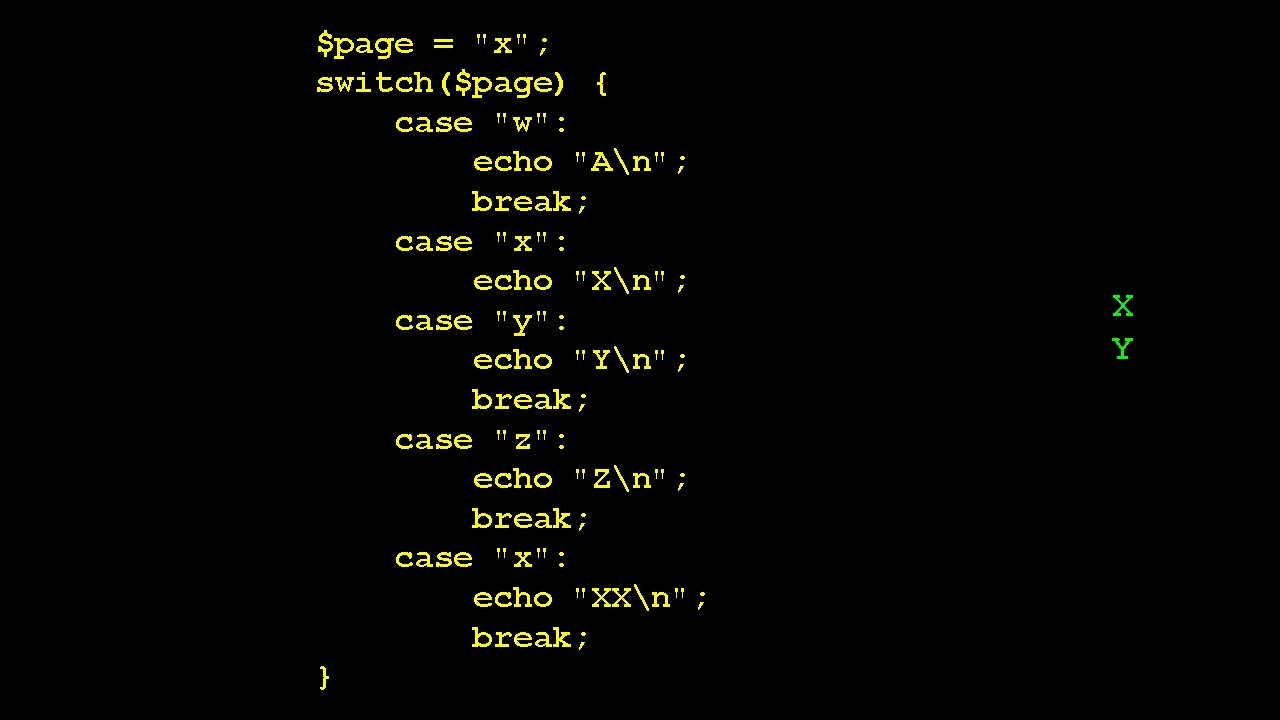
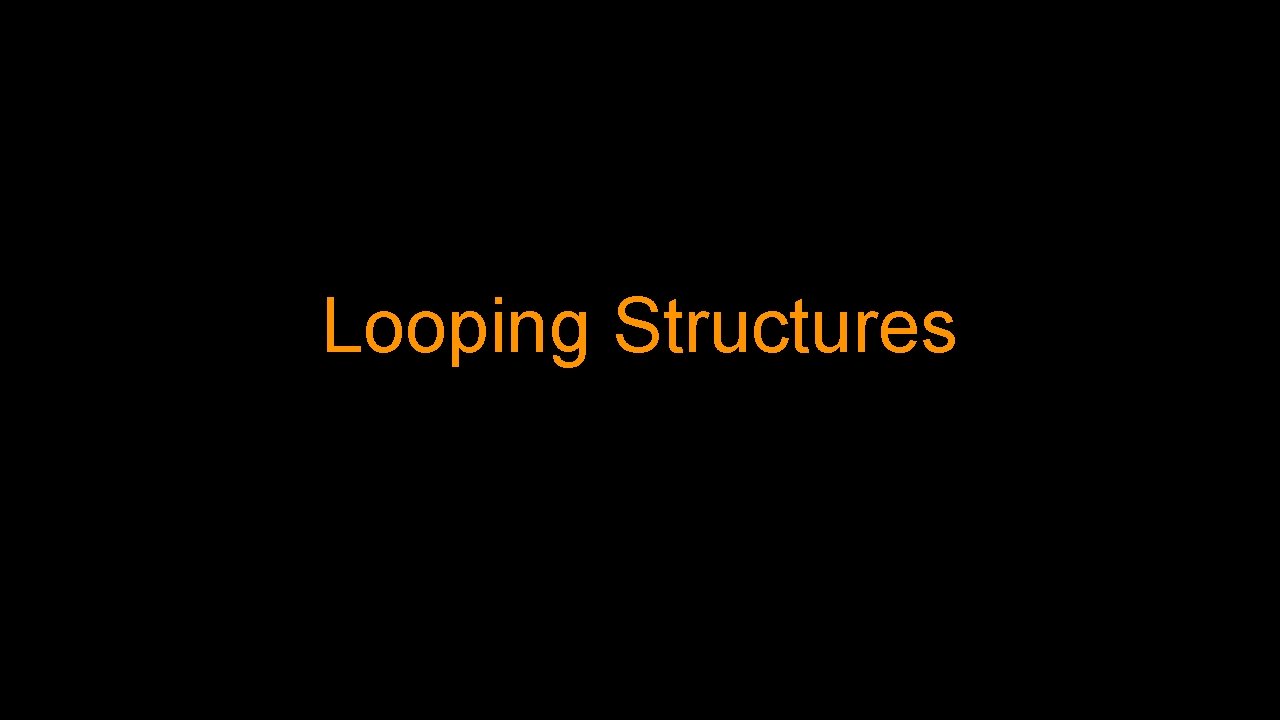
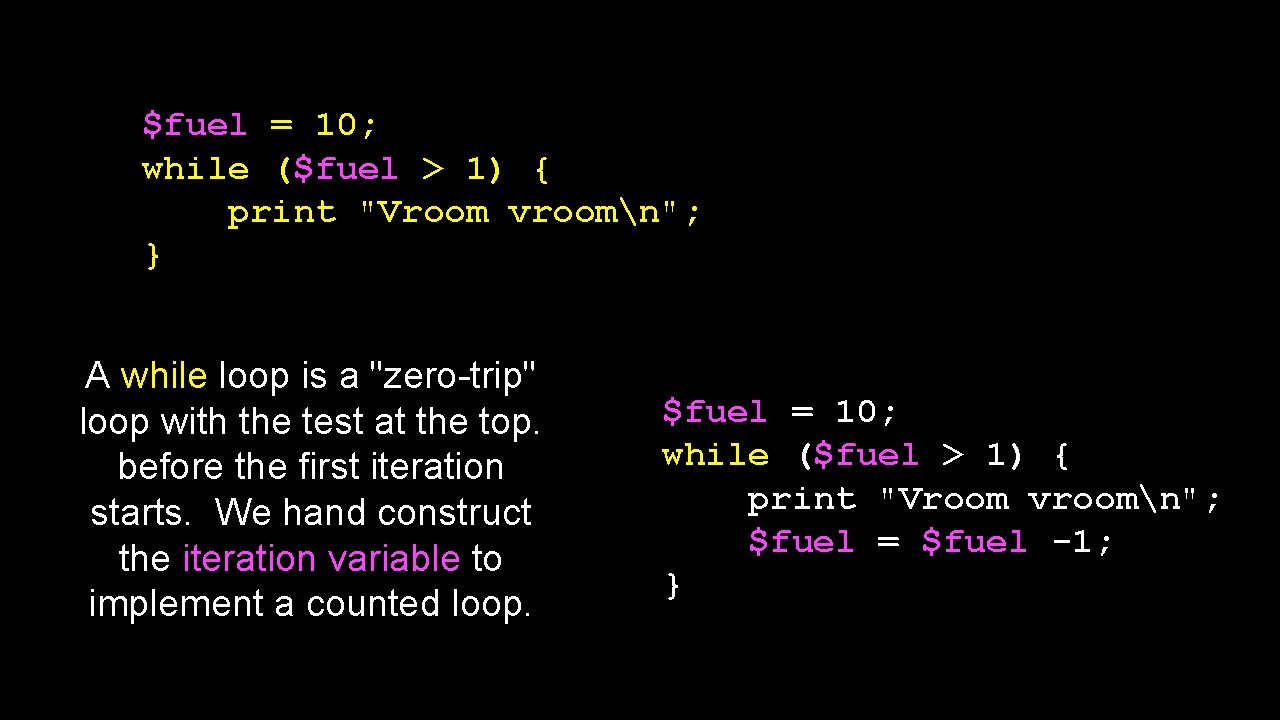
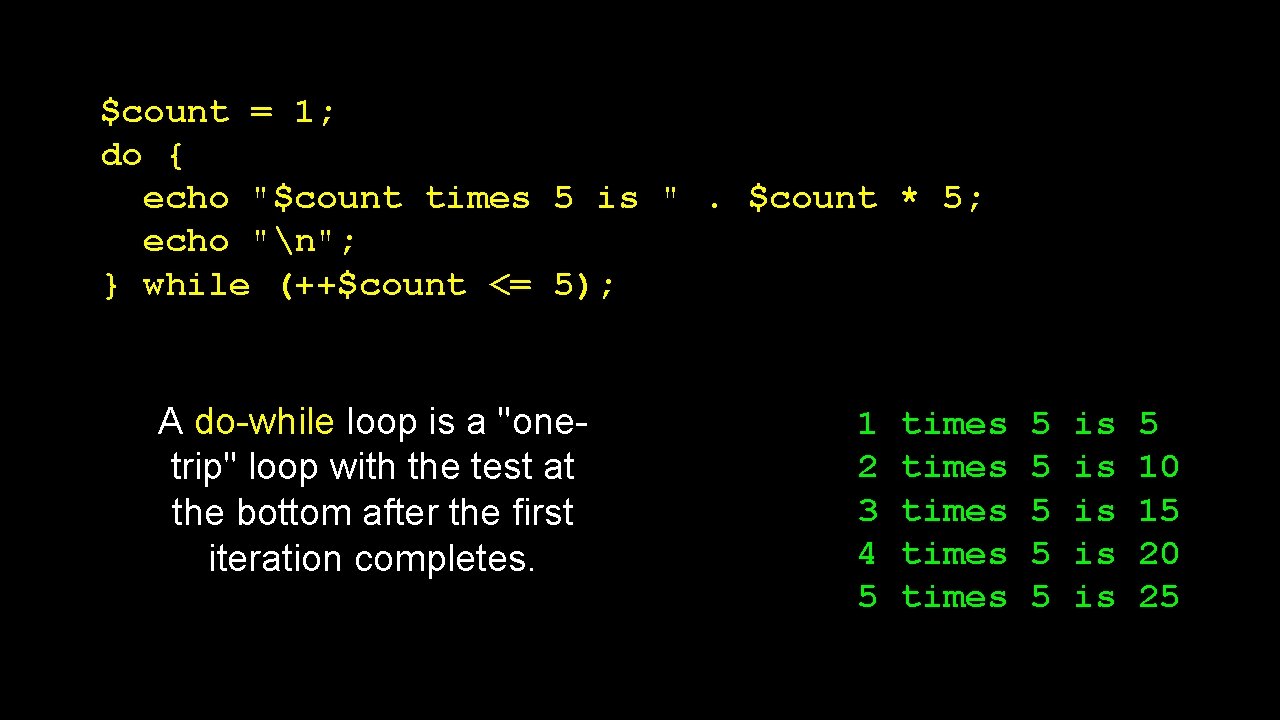
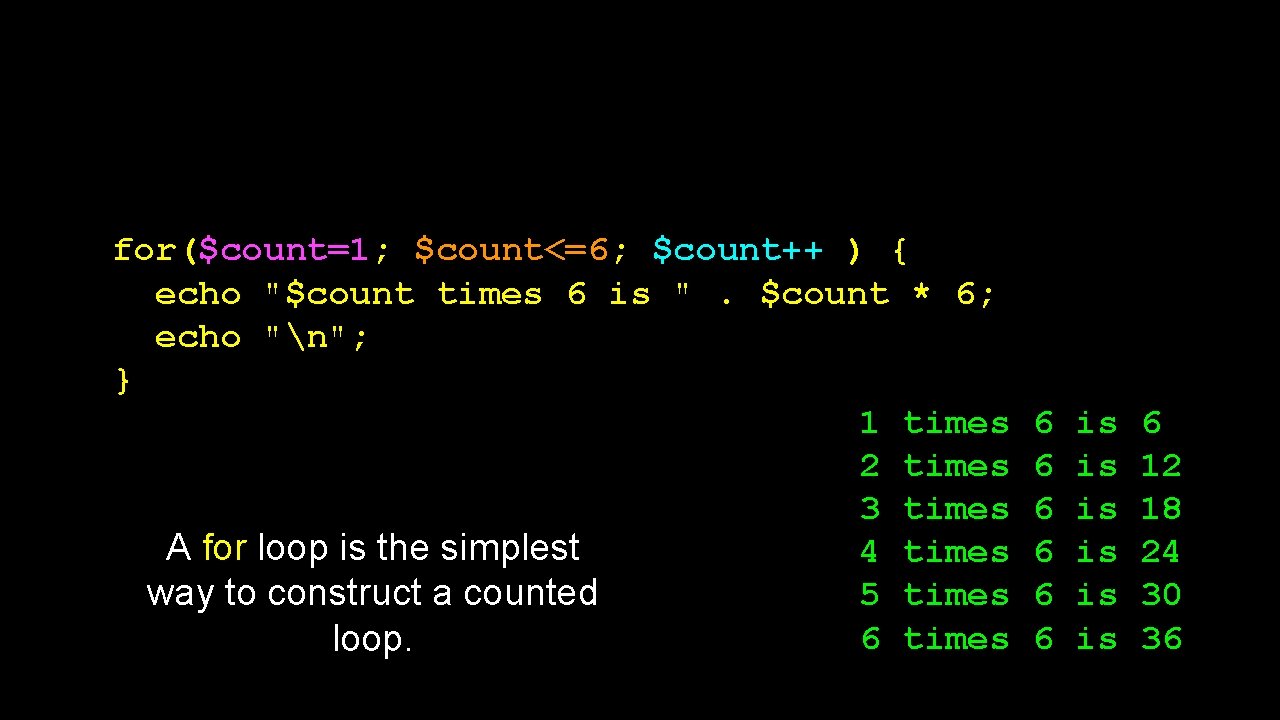
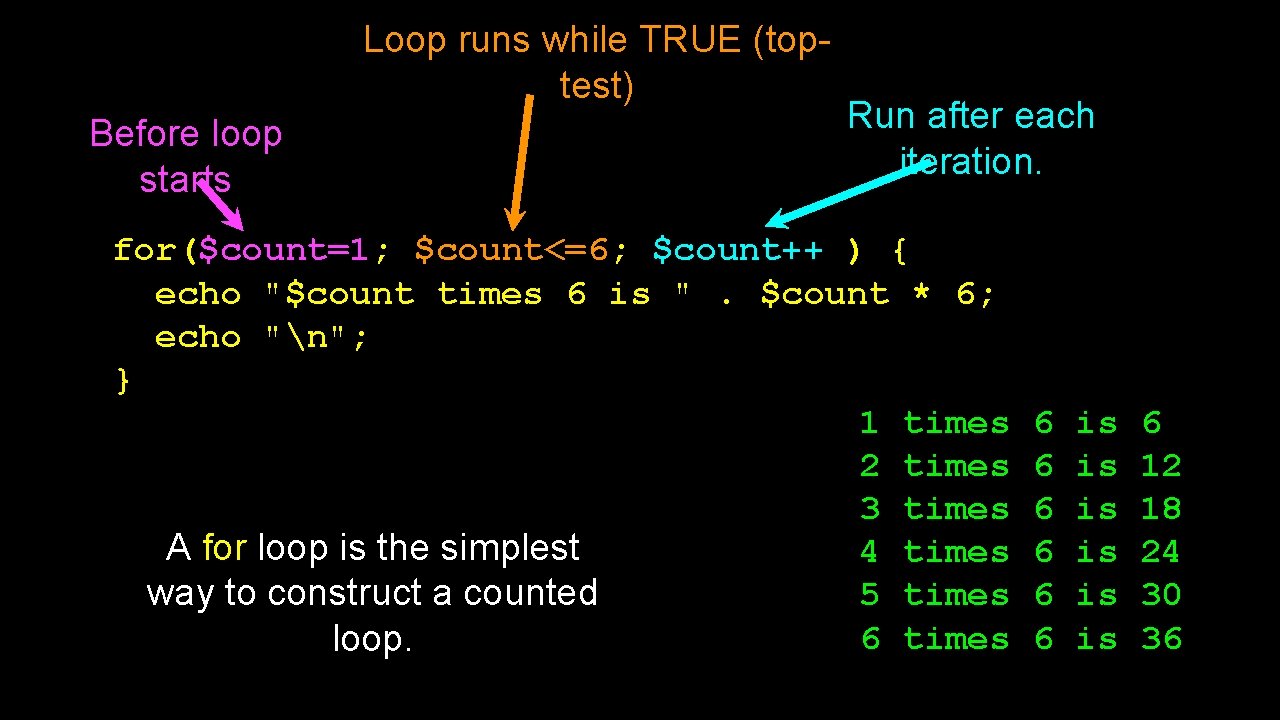
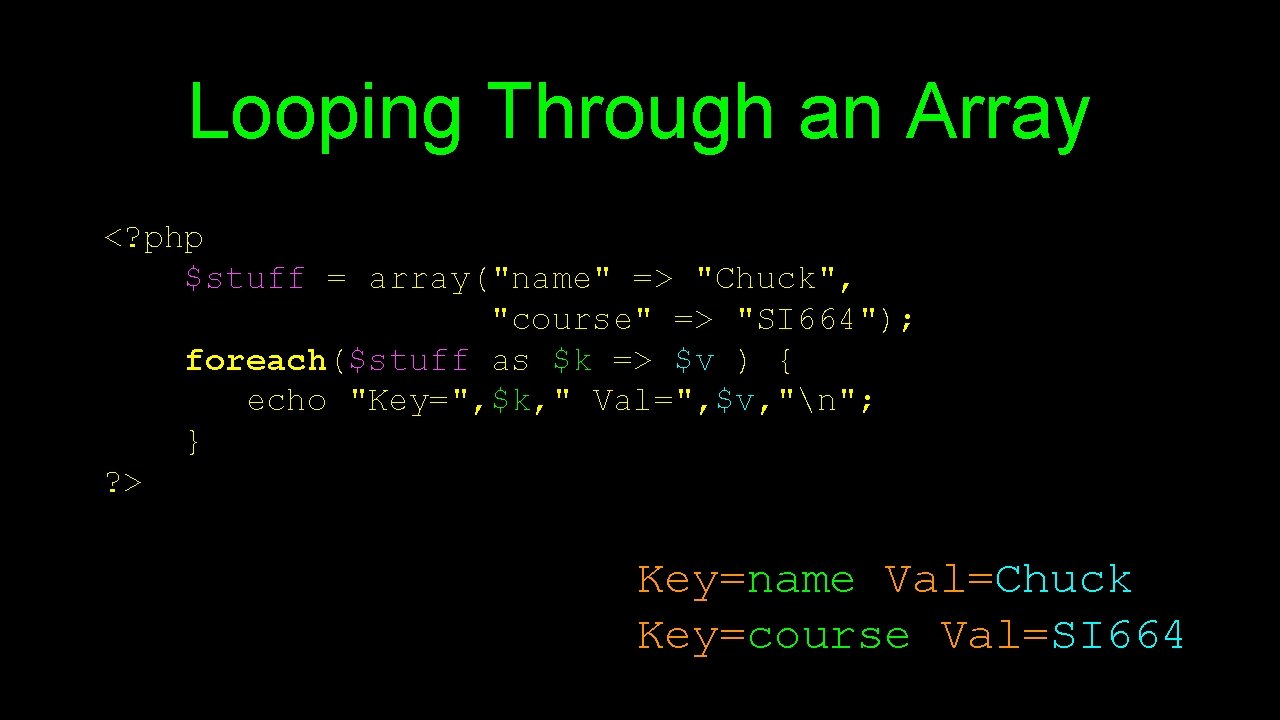
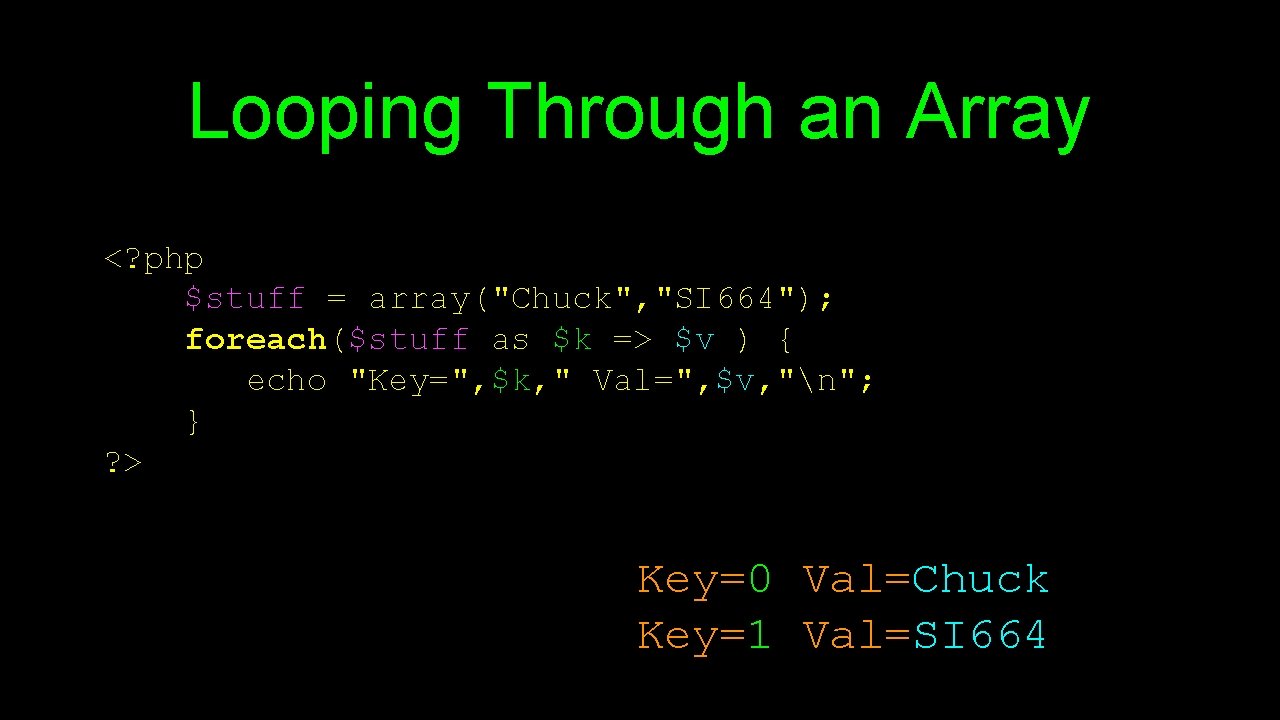
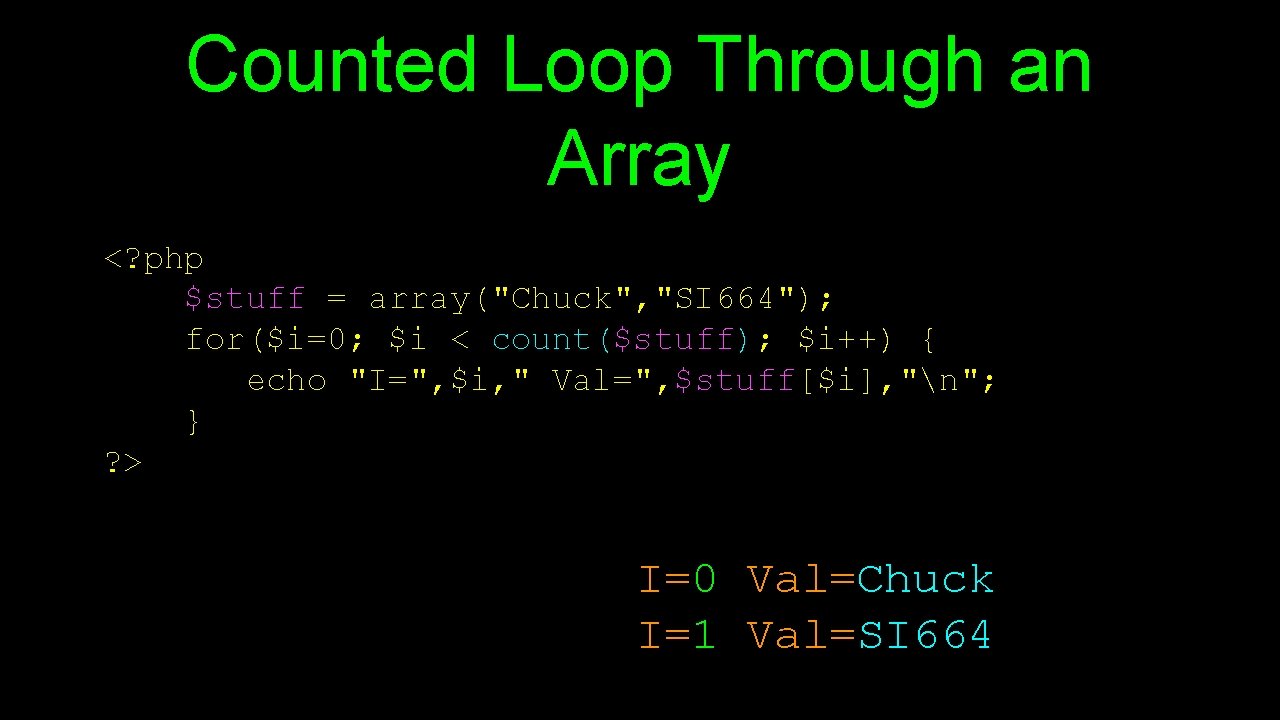
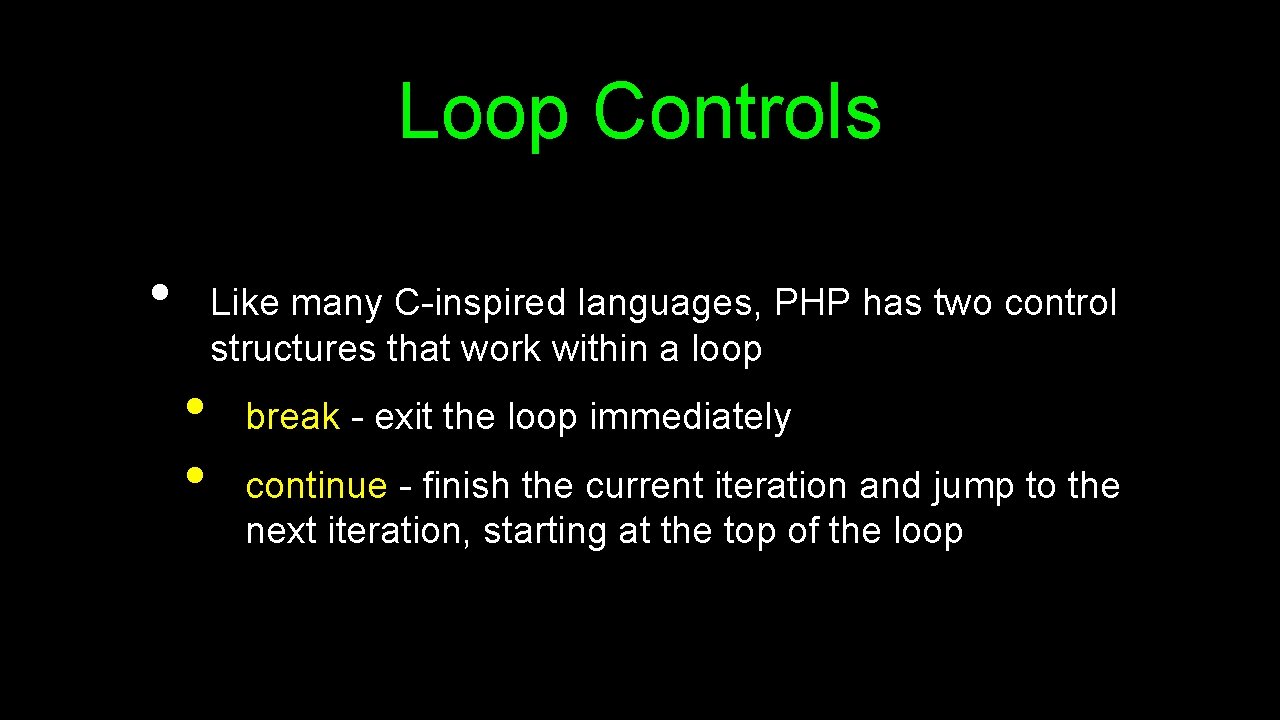
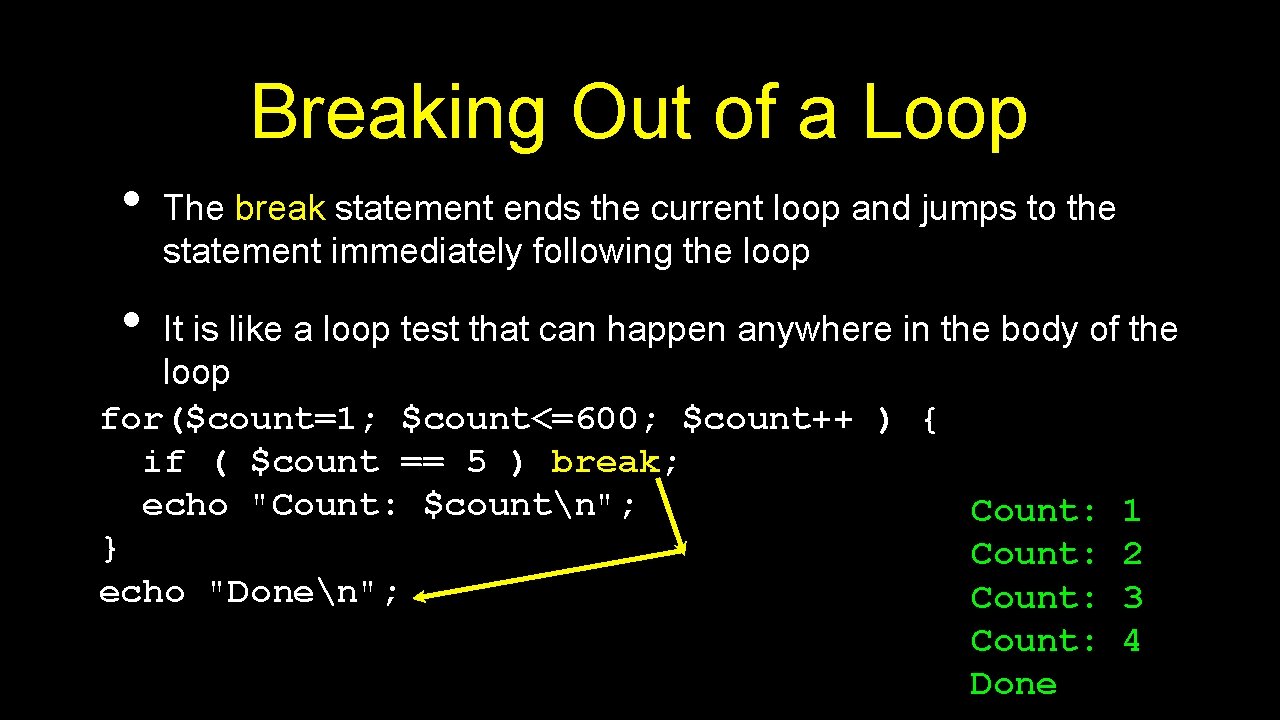
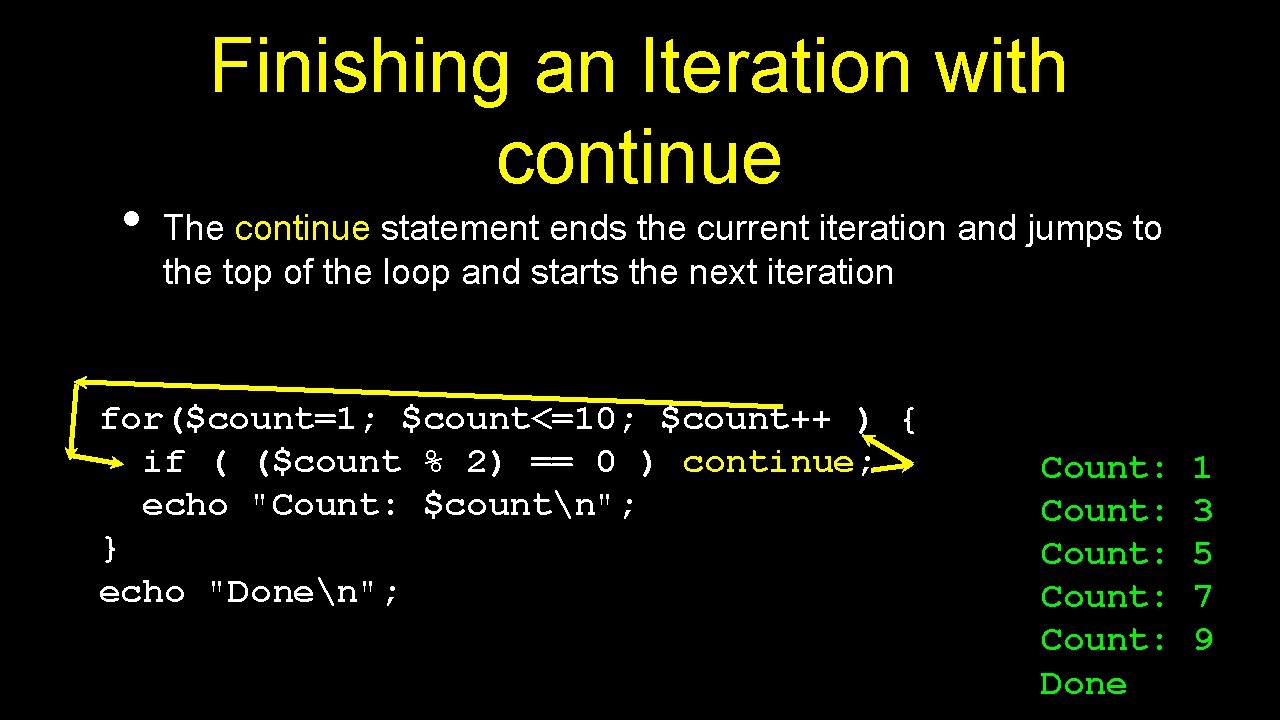
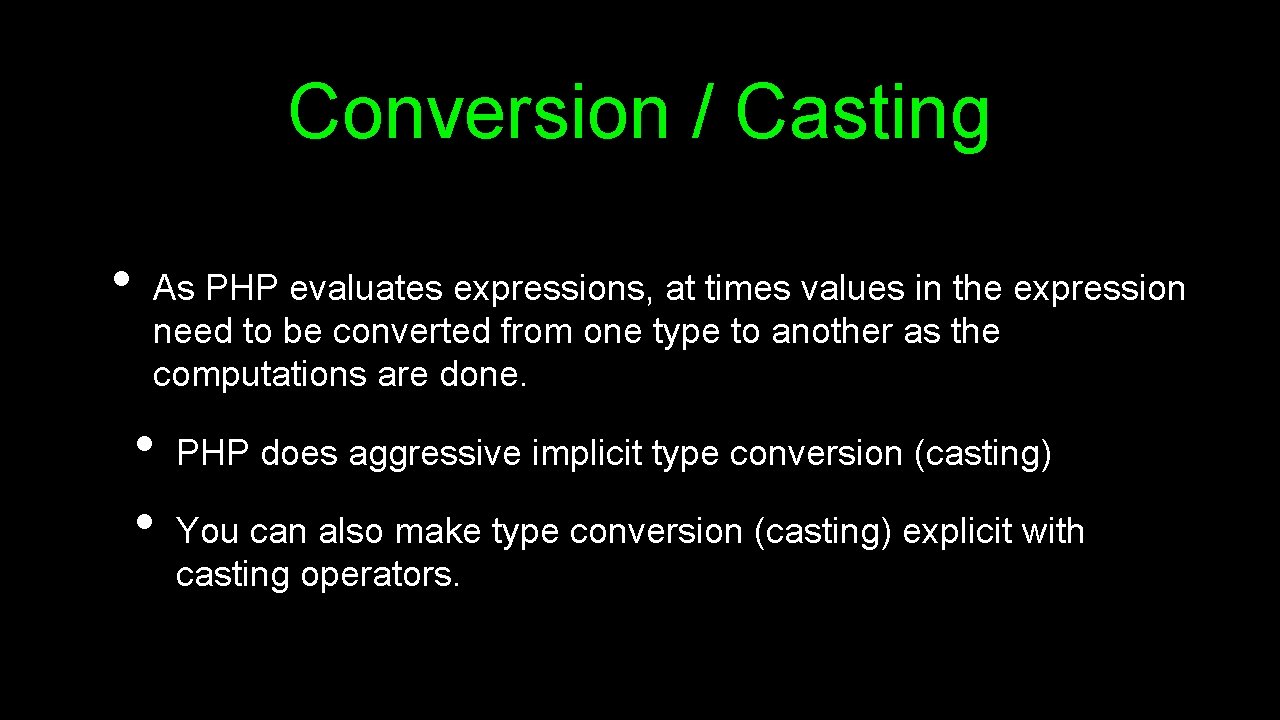
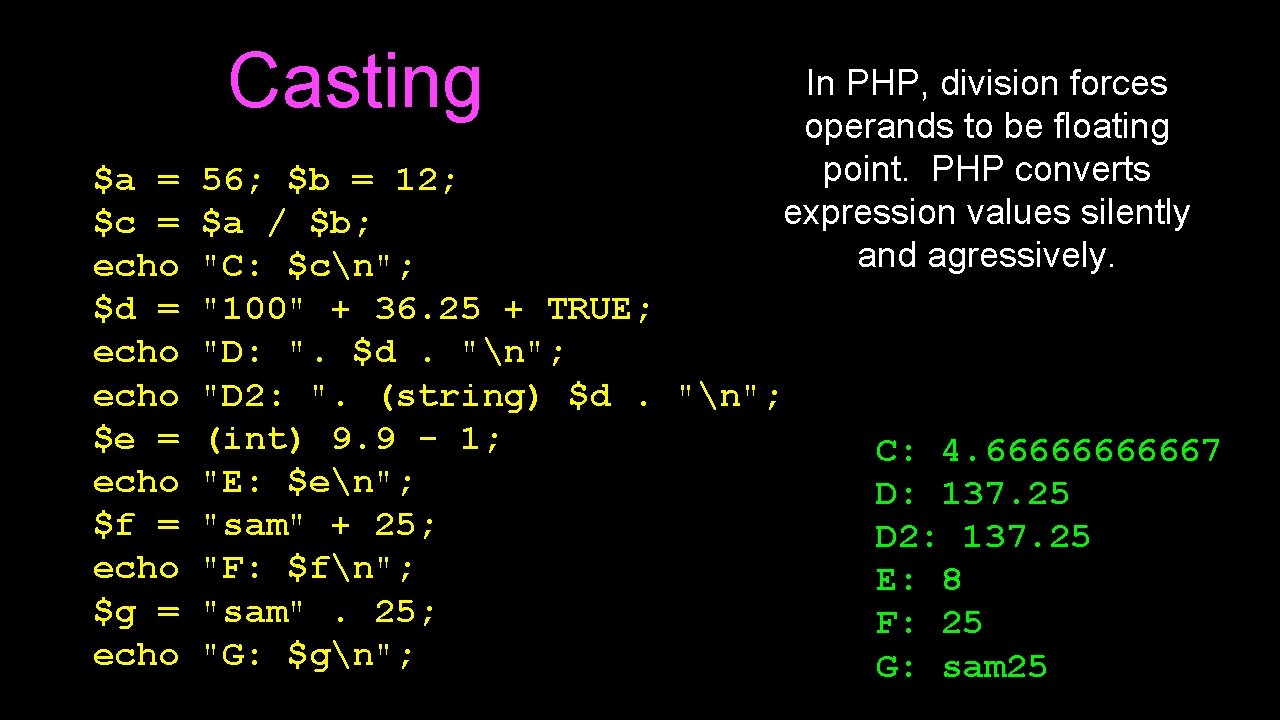
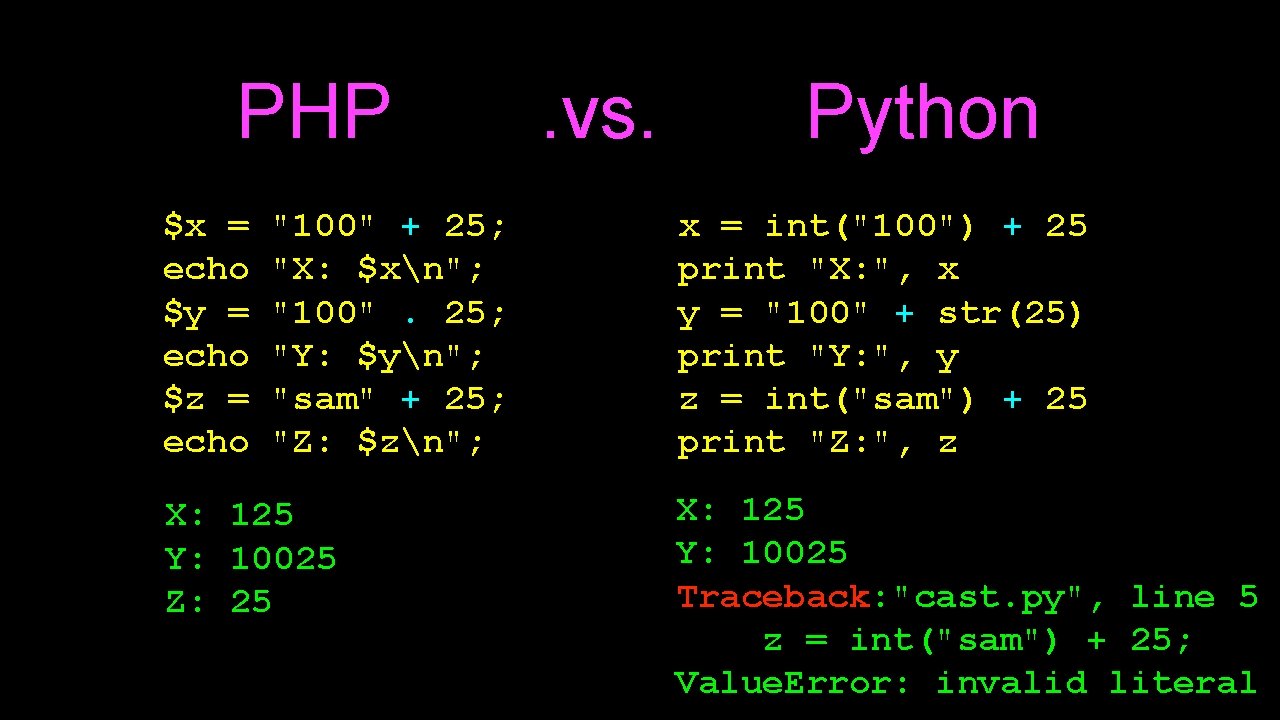
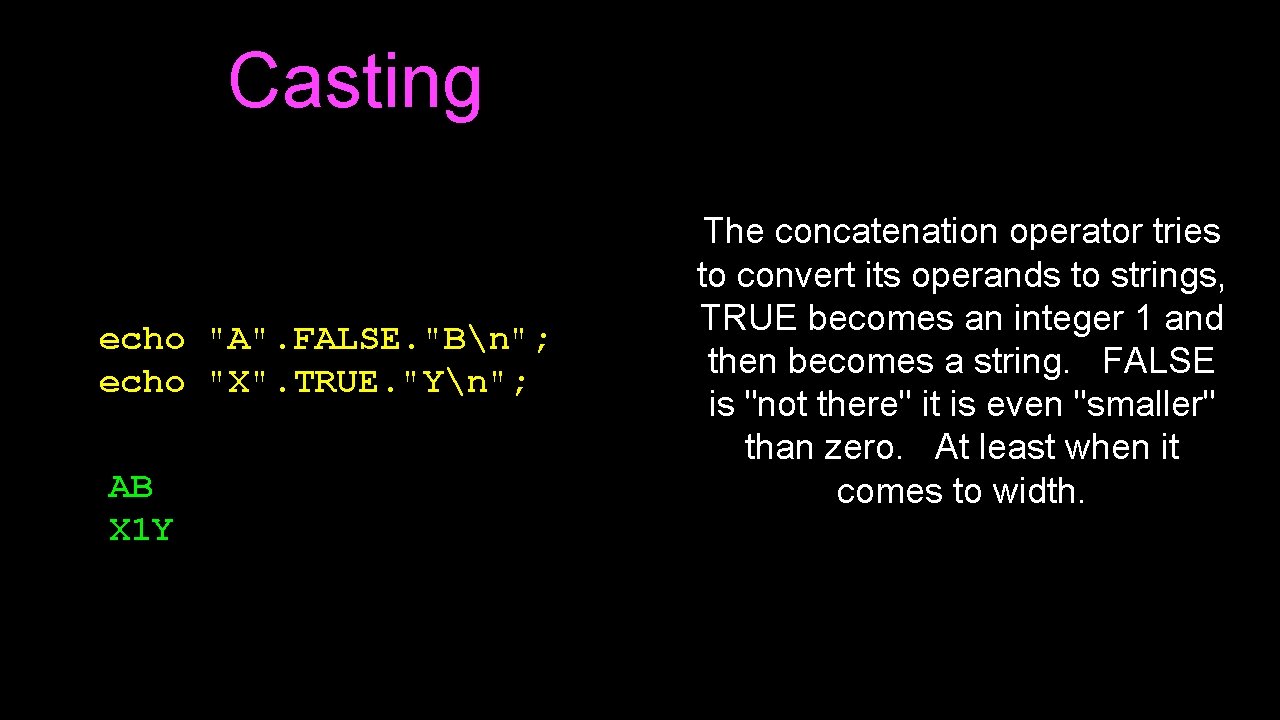
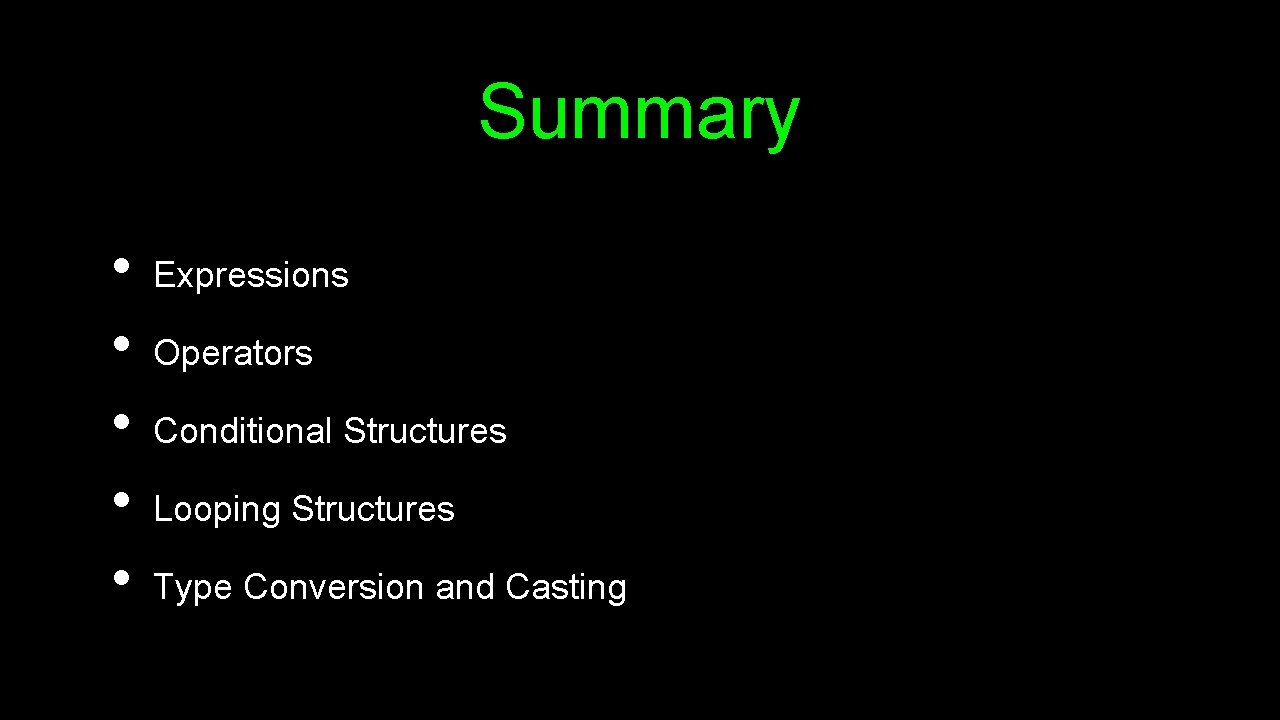
- Slides: 34
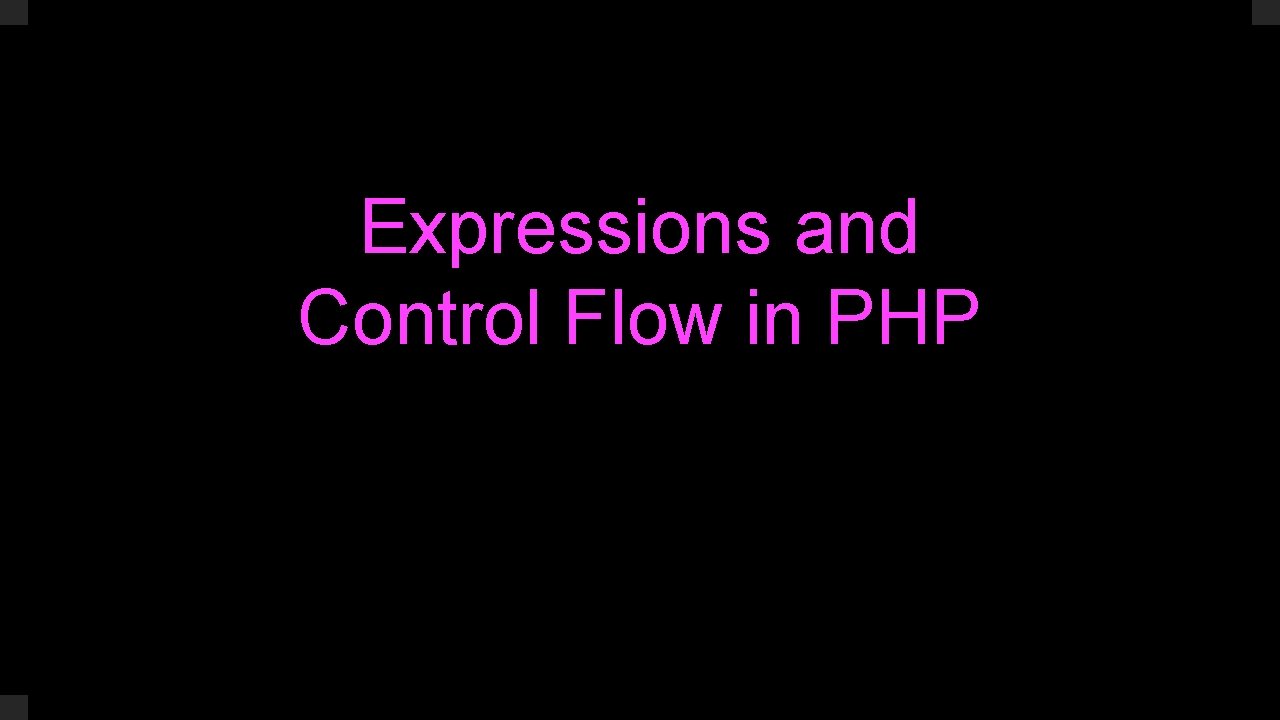
Expressions and Control Flow in PHP
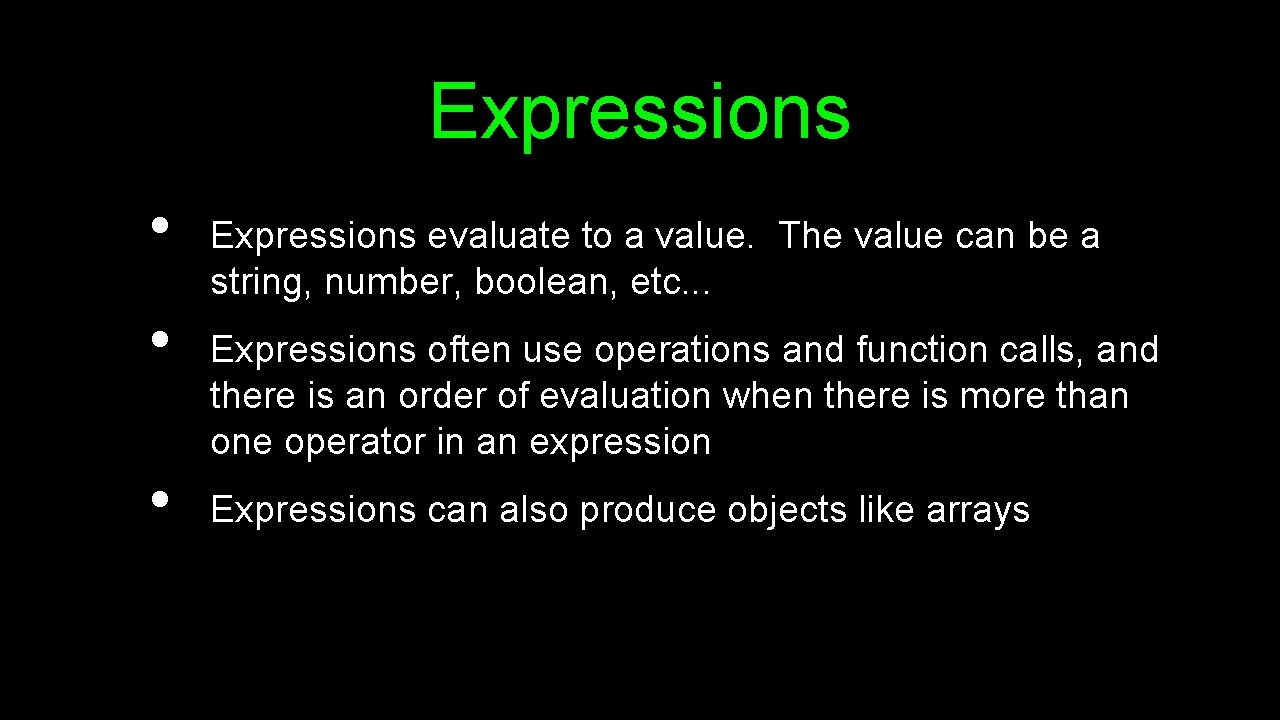
Expressions • • • Expressions evaluate to a value. The value can be a string, number, boolean, etc. . . Expressions often use operations and function calls, and there is an order of evaluation when there is more than one operator in an expression Expressions can also produce objects like arrays
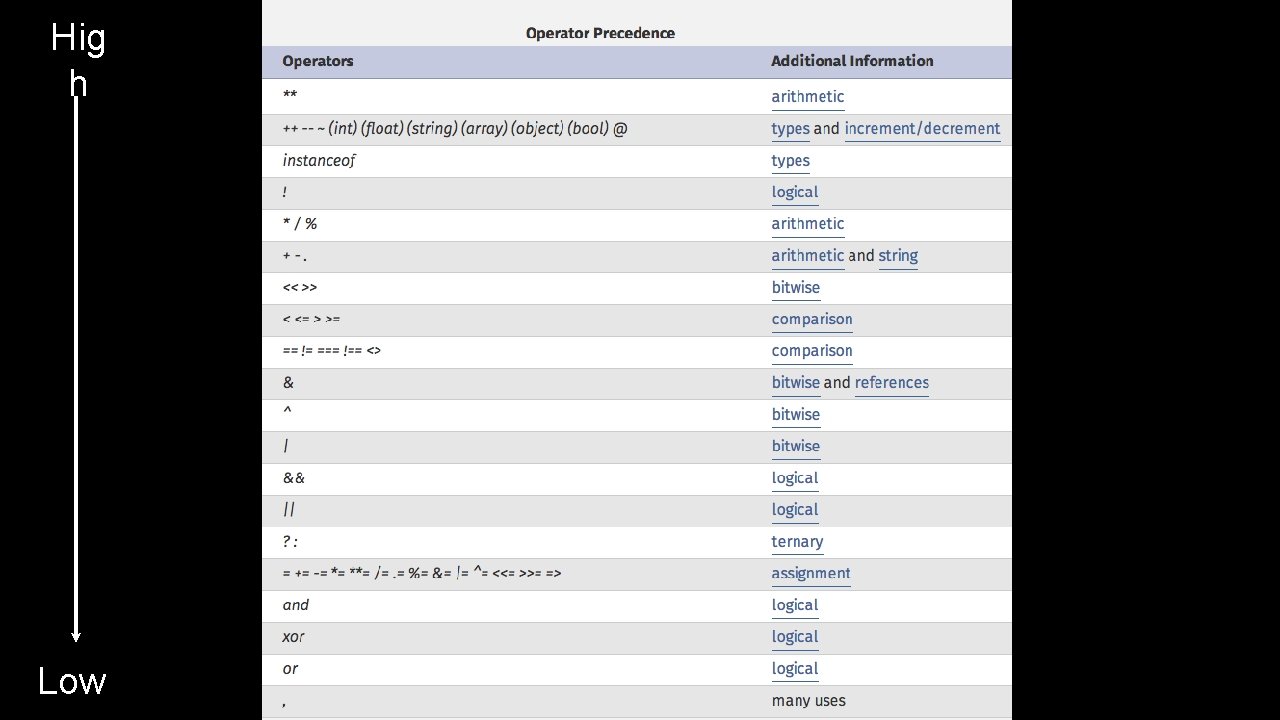
Hig h Low
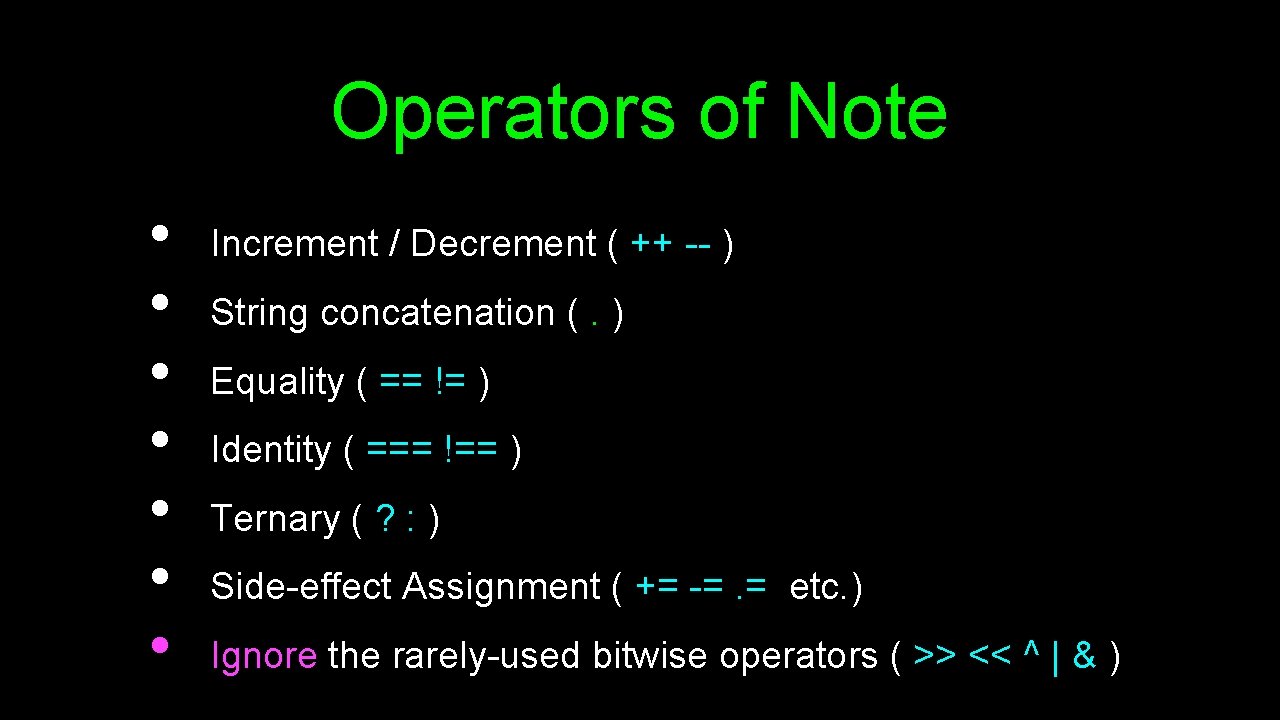
Operators of Note • • Increment / Decrement ( ++ -- ) String concatenation (. ) Equality ( == != ) Identity ( === !== ) Ternary ( ? : ) Side-effect Assignment ( += -=. = etc. ) Ignore the rarely-used bitwise operators ( >> << ^ | & )
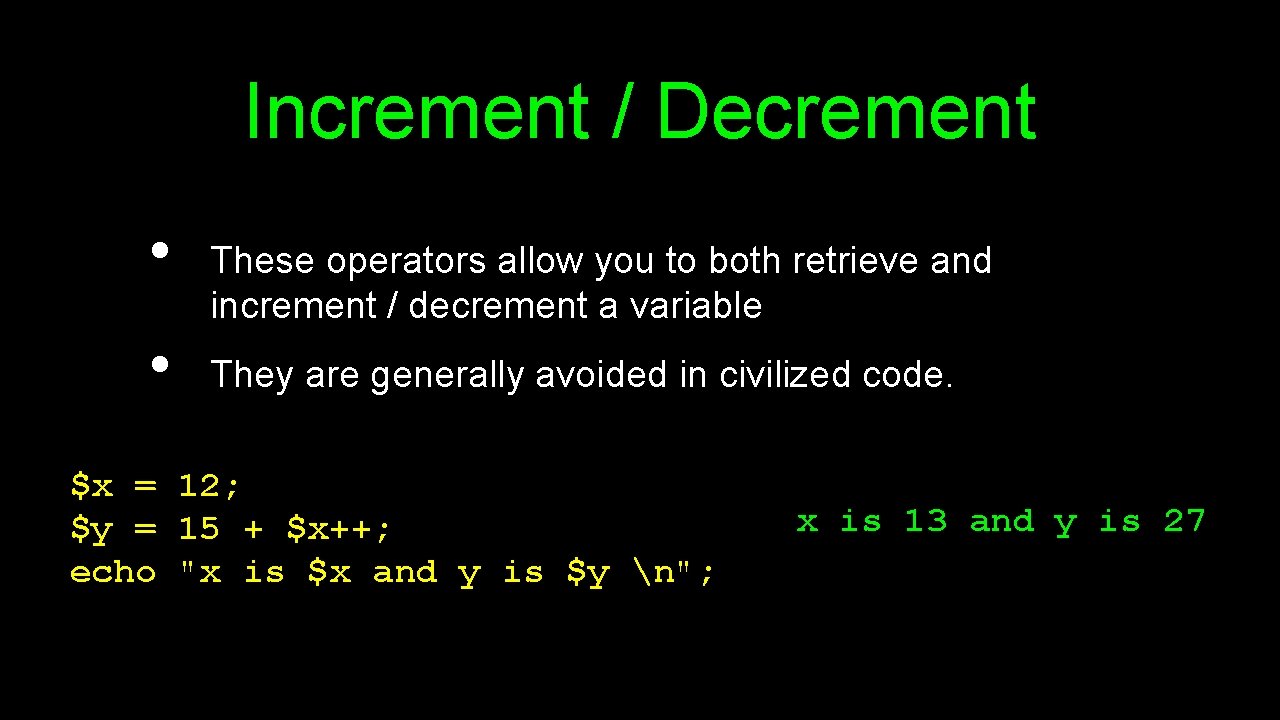
Increment / Decrement • • These operators allow you to both retrieve and increment / decrement a variable They are generally avoided in civilized code. $x = 12; $y = 15 + $x++; echo "x is $x and y is $y n"; x is 13 and y is 27
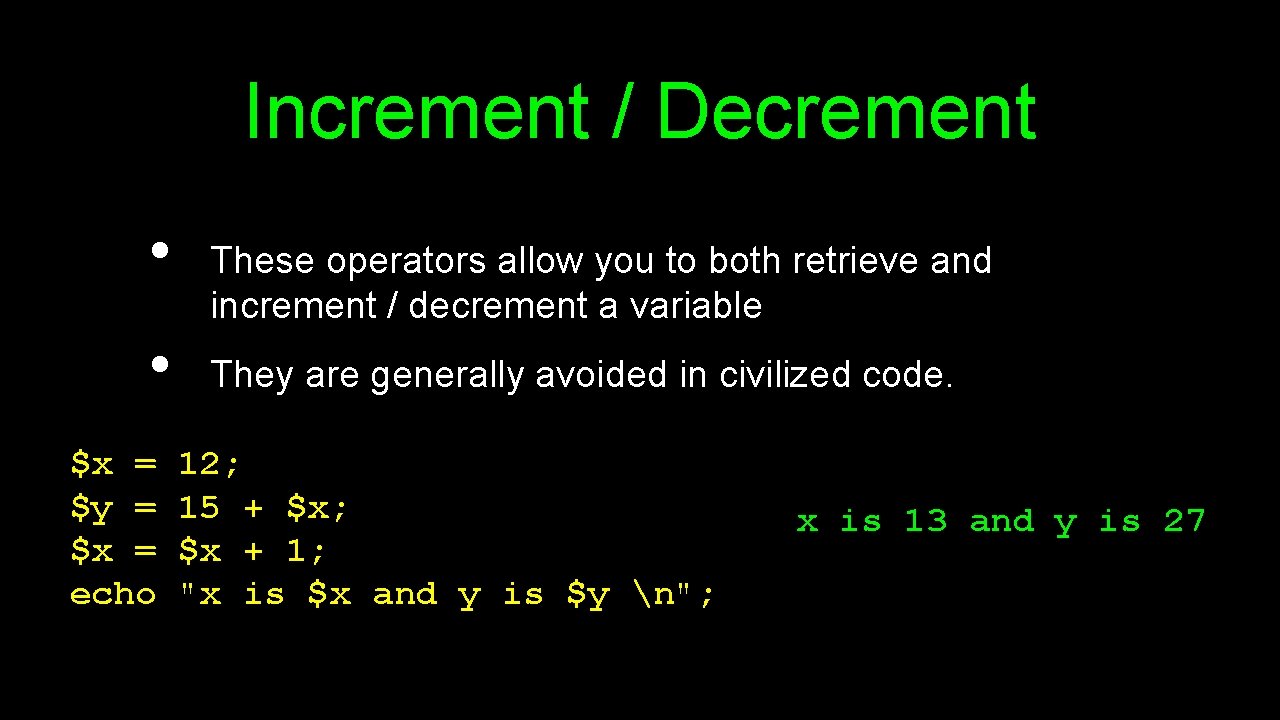
Increment / Decrement • • $x = $y = $x = echo These operators allow you to both retrieve and increment / decrement a variable They are generally avoided in civilized code. 12; 15 + $x; $x + 1; "x is $x and y is $y n"; x is 13 and y is 27
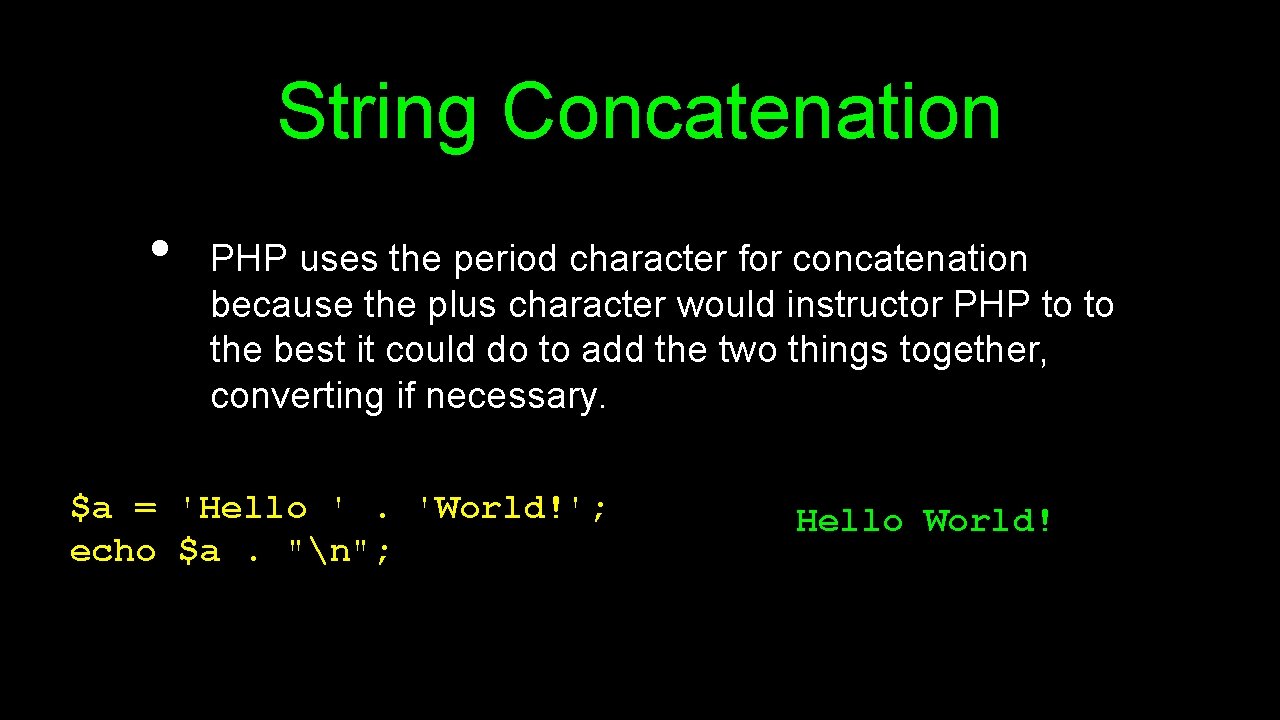
String Concatenation • PHP uses the period character for concatenation because the plus character would instructor PHP to to the best it could do to add the two things together, converting if necessary. $a = 'Hello '. 'World!'; echo $a. "n"; Hello World!
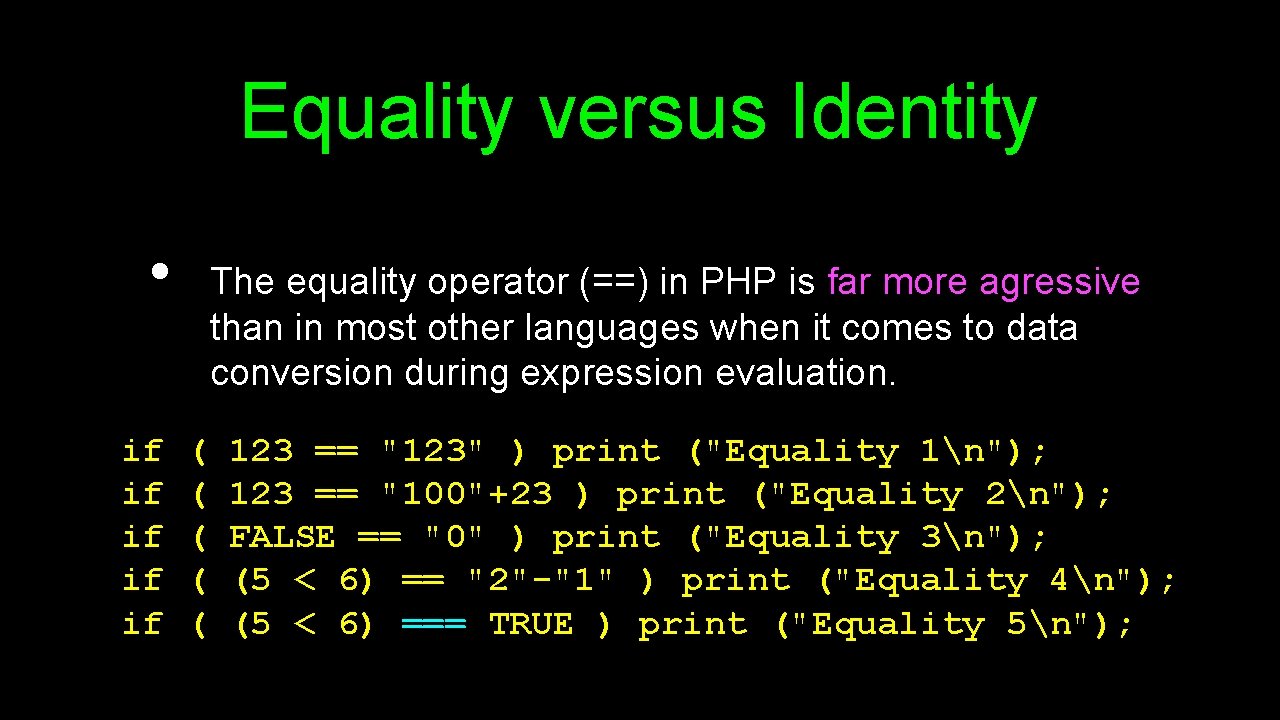
Equality versus Identity • if if if The equality operator (==) in PHP is far more agressive than in most other languages when it comes to data conversion during expression evaluation. ( ( ( 123 == "123" ) print ("Equality 1n"); 123 == "100"+23 ) print ("Equality 2n"); FALSE == "0" ) print ("Equality 3n"); (5 < 6) == "2"-"1" ) print ("Equality 4n"); (5 < 6) === TRUE ) print ("Equality 5n");
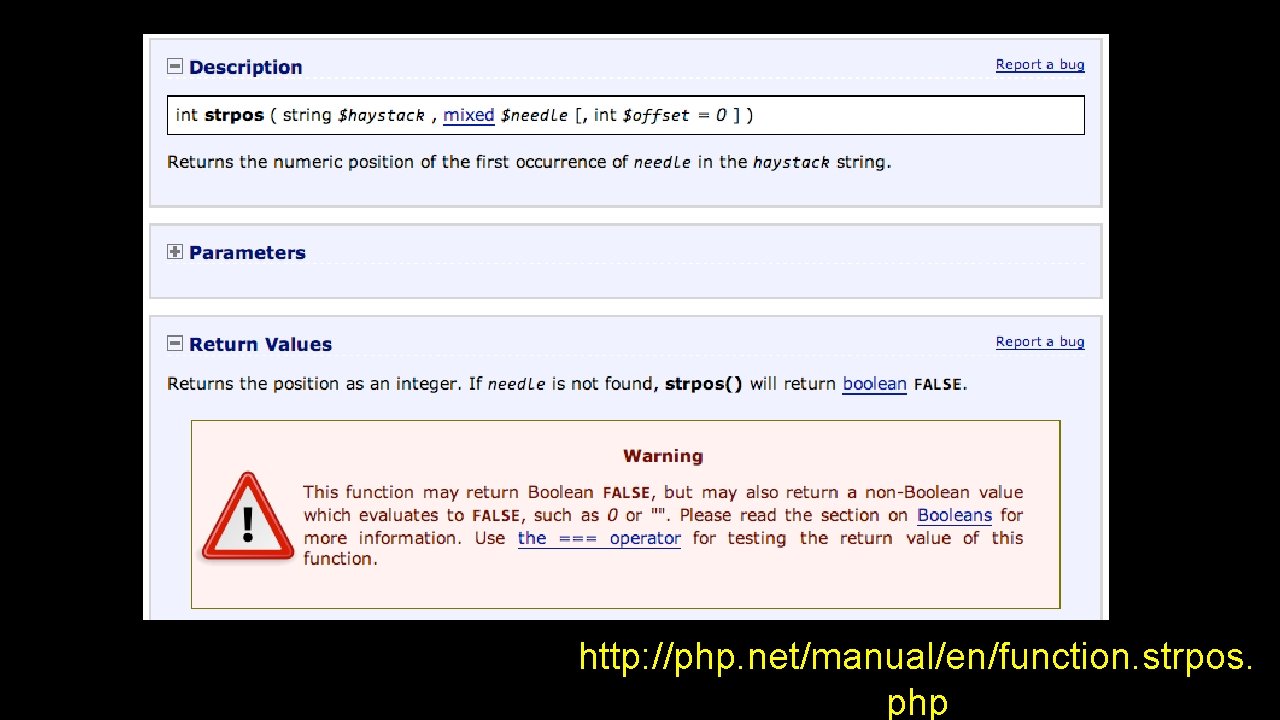
http: //php. net/manual/en/function. strpos. php
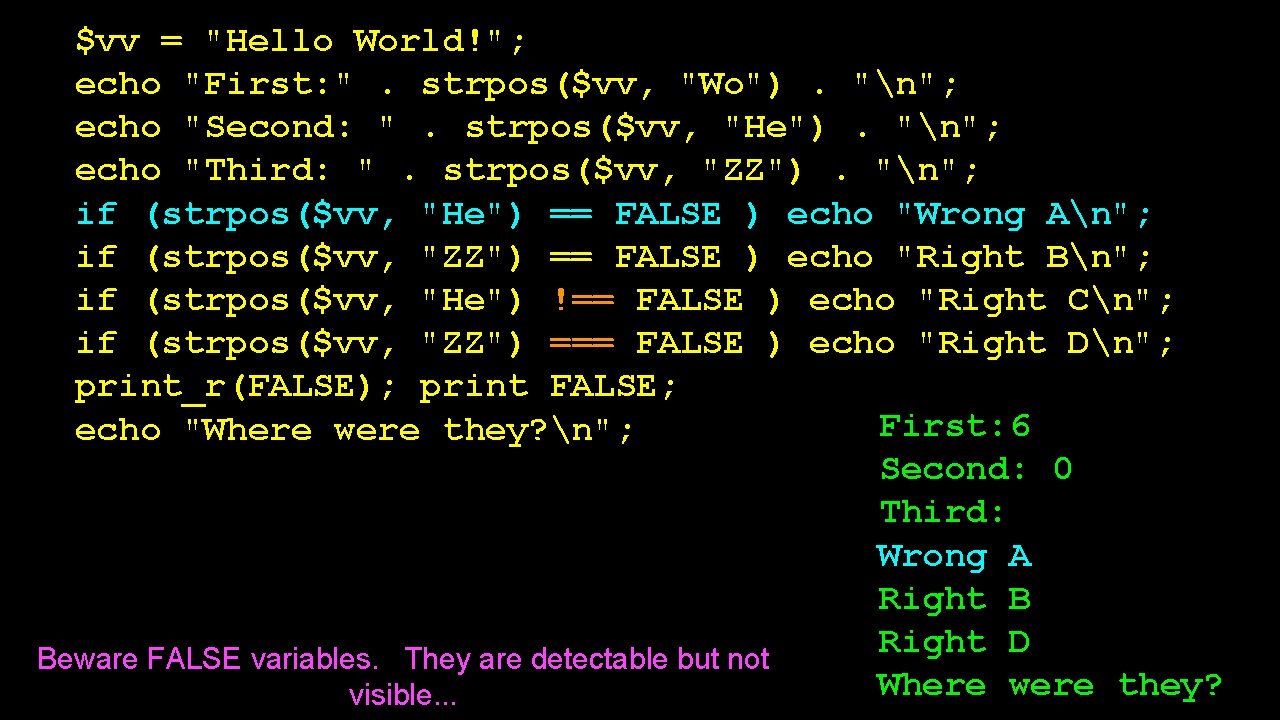
$vv = "Hello World!"; echo "First: ". strpos($vv, "Wo"). "n"; echo "Second: ". strpos($vv, "He"). "n"; echo "Third: ". strpos($vv, "ZZ"). "n"; if (strpos($vv, "He") == FALSE ) echo "Wrong An"; if (strpos($vv, "ZZ") == FALSE ) echo "Right Bn"; if (strpos($vv, "He") !== FALSE ) echo "Right Cn"; if (strpos($vv, "ZZ") === FALSE ) echo "Right Dn"; print_r(FALSE); print FALSE; First: 6 echo "Where were they? n"; Second: 0 Third: Wrong A Right B Right D Beware FALSE variables. They are detectable but not Where were they? visible. . .
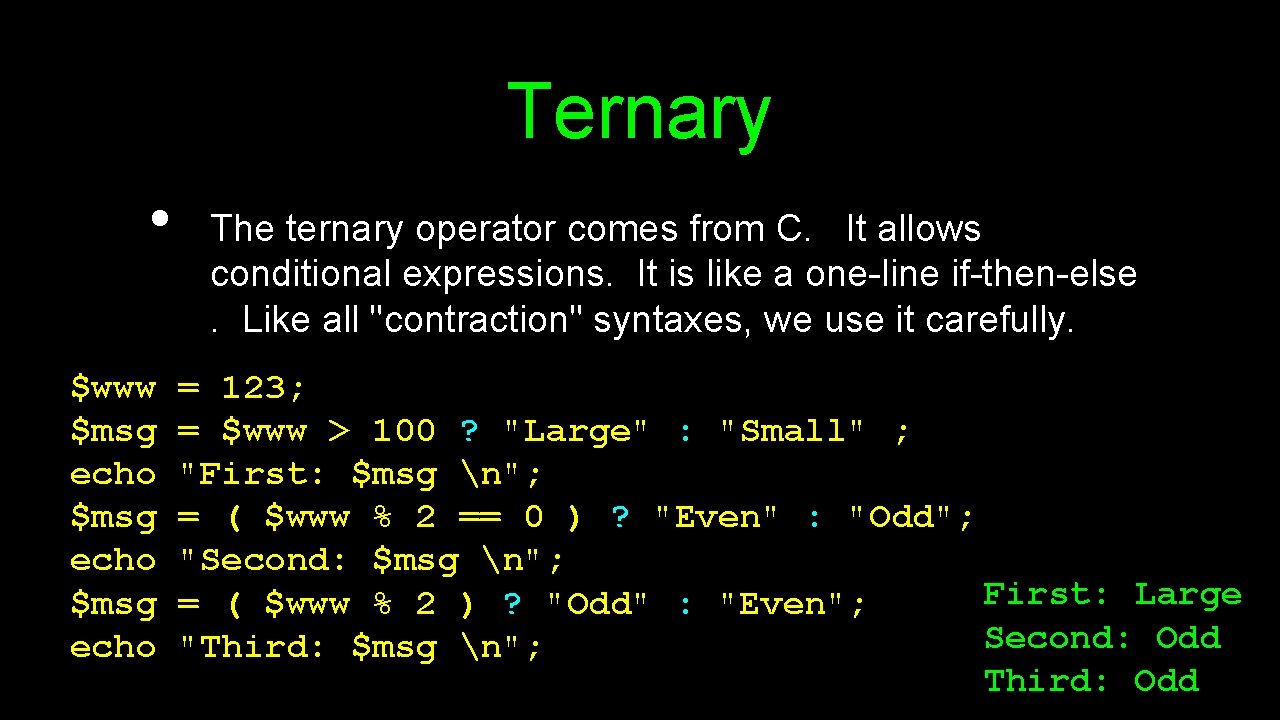
Ternary • $www $msg echo The ternary operator comes from C. It allows conditional expressions. It is like a one-line if-then-else. Like all "contraction" syntaxes, we use it carefully. = 123; = $www > 100 ? "Large" : "Small" ; "First: $msg n"; = ( $www % 2 == 0 ) ? "Even" : "Odd"; "Second: $msg n"; First: Large = ( $www % 2 ) ? "Odd" : "Even"; Second: Odd "Third: $msg n"; Third: Odd
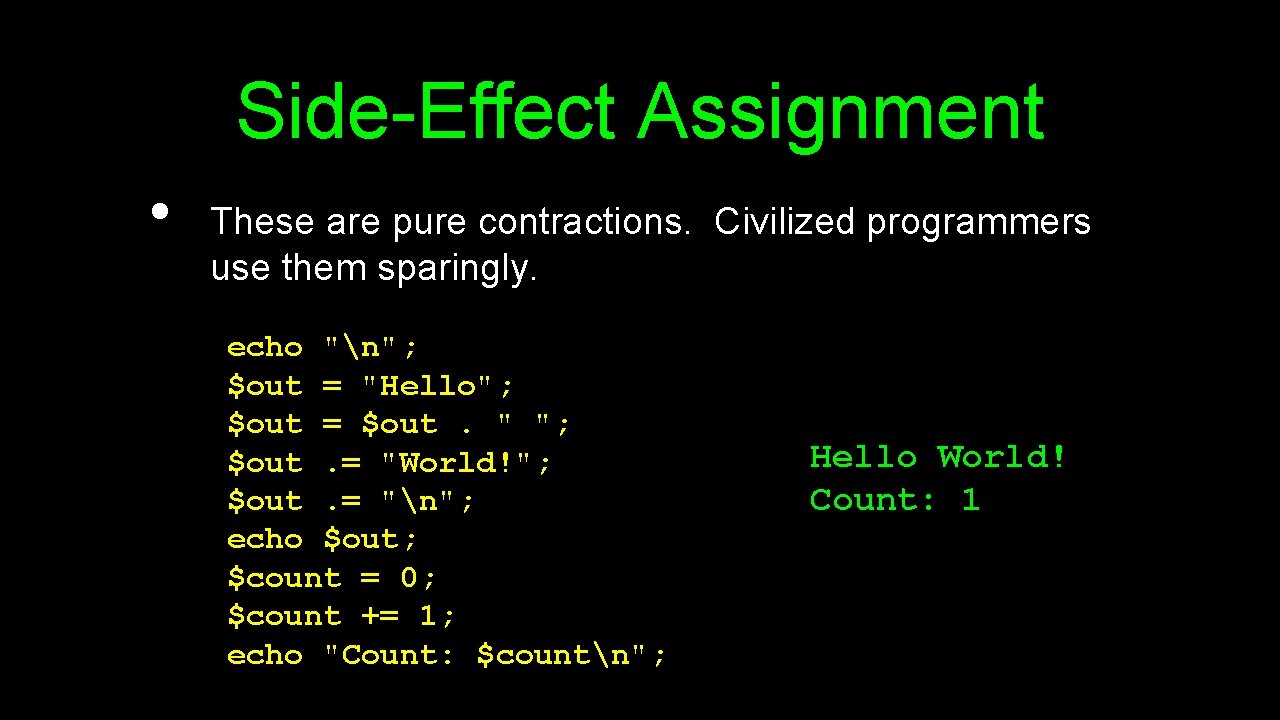
Side-Effect Assignment • These are pure contractions. Civilized programmers use them sparingly. echo "n"; $out = "Hello"; $out = $out. " "; $out. = "World!"; $out. = "n"; echo $out; $count = 0; $count += 1; echo "Count: $countn"; Hello World! Count: 1
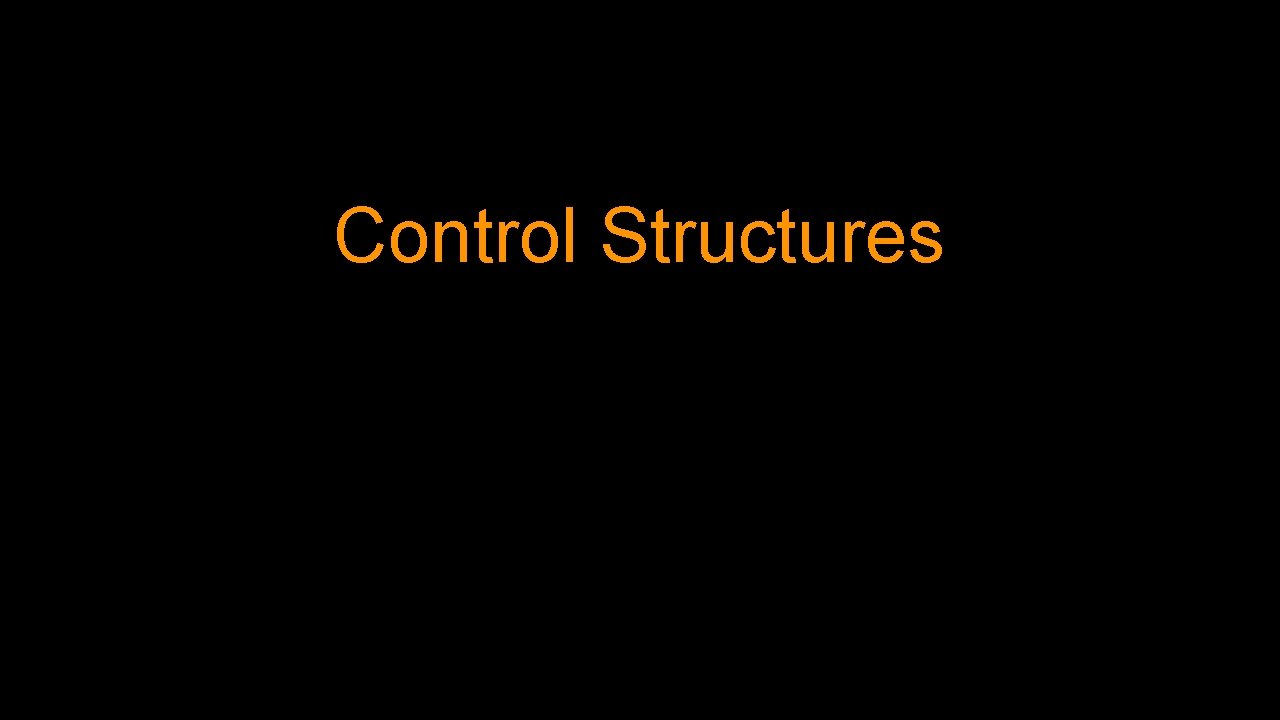
Control Structures
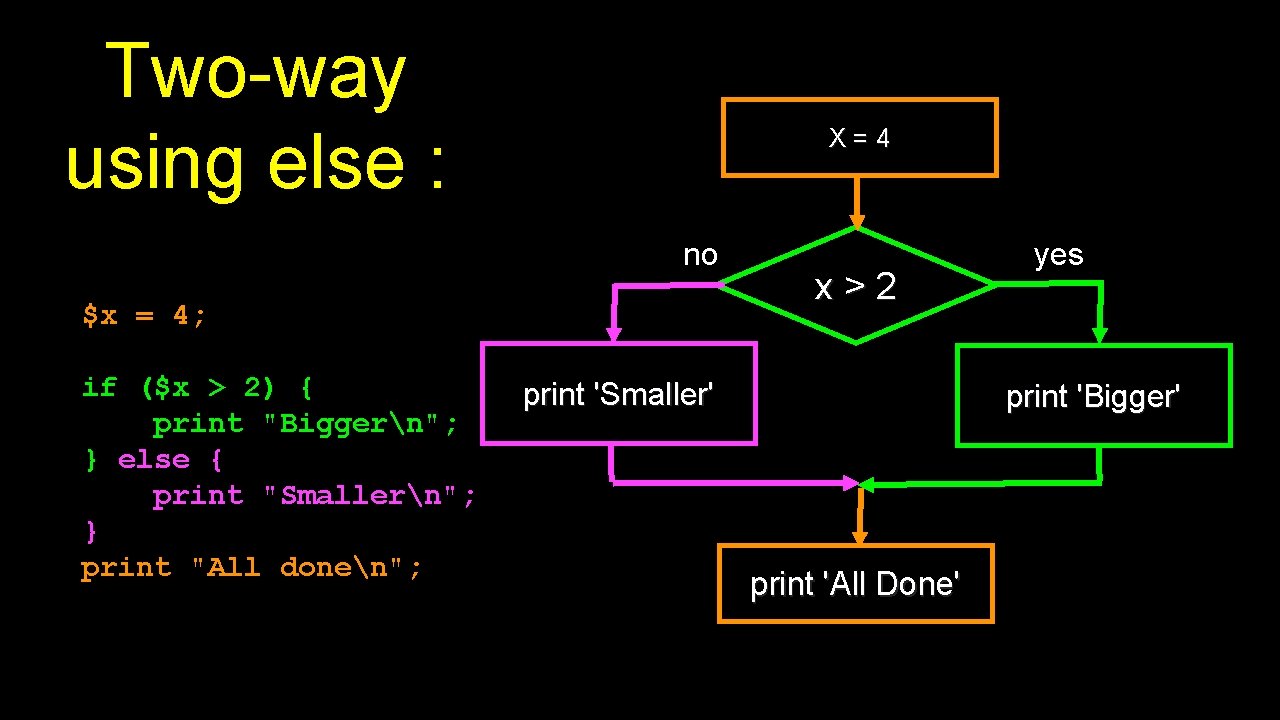
Two-way using else : X=4 no $x = 4; if ($x > 2) { print "Biggern"; } else { print "Smallern"; } print "All donen"; x>2 print 'Smaller' yes print 'Bigger' print 'All Done'
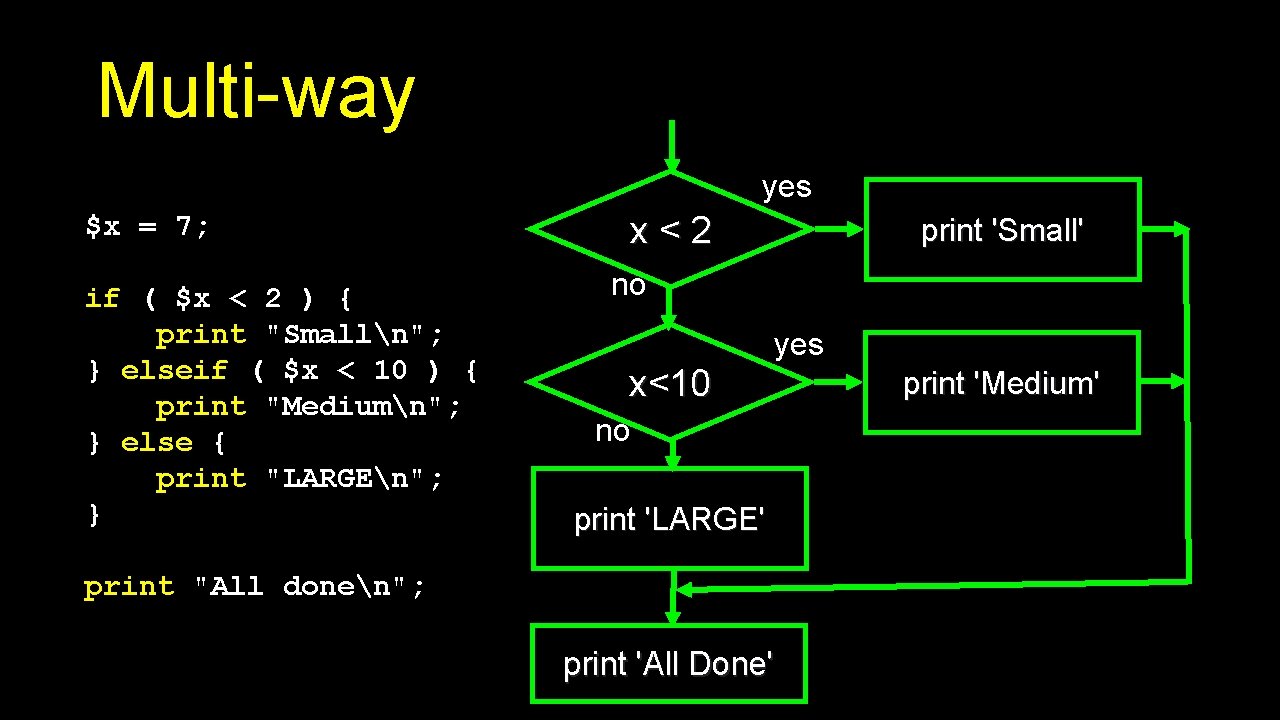
Multi-way yes $x = 7; if ( $x < 2 ) { print "Smalln"; } elseif ( $x < 10 ) { print "Mediumn"; } else { print "LARGEn"; } x<2 print 'Small' no yes x<10 no print 'LARGE' print "All donen"; print 'All Done' print 'Medium'
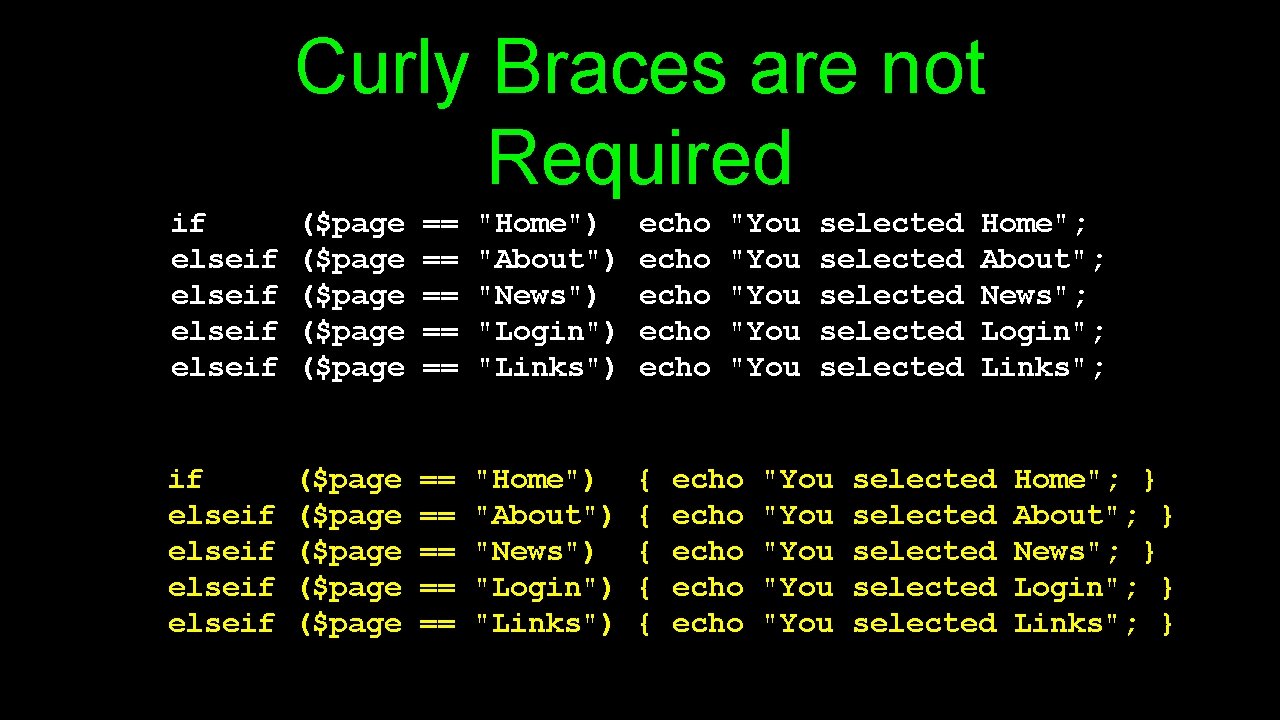
Curly Braces are not Required if elseif ($page ($page == == == "Home") "About") "News") "Login") "Links") echo echo if elseif ($page ($page == == == "Home") "About") "News") "Login") "Links") { { { "You "You echo echo selected selected "You "You Home"; About"; News"; Login"; Links"; selected selected Home"; } About"; } News"; } Login"; } Links"; }
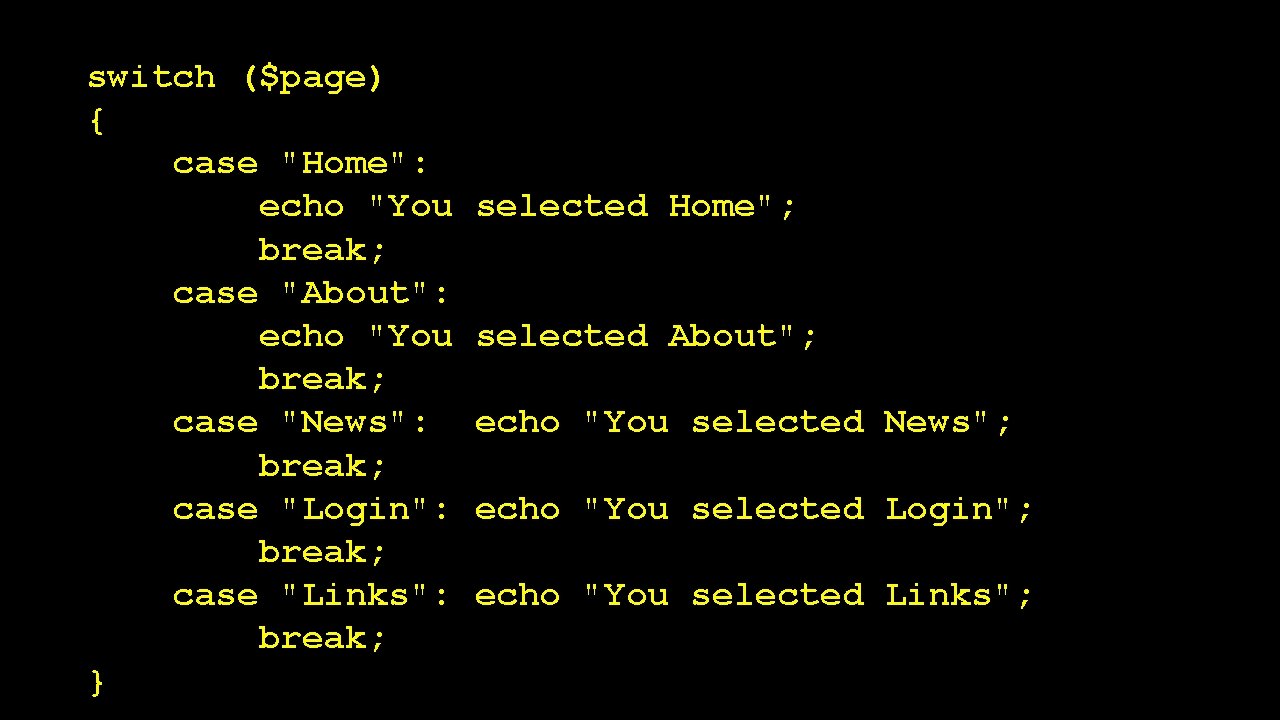
switch ($page) { case "Home": echo "You break; case "About": echo "You break; case "News": break; case "Login": break; case "Links": break; } selected Home"; selected About"; echo "You selected News"; echo "You selected Login"; echo "You selected Links";
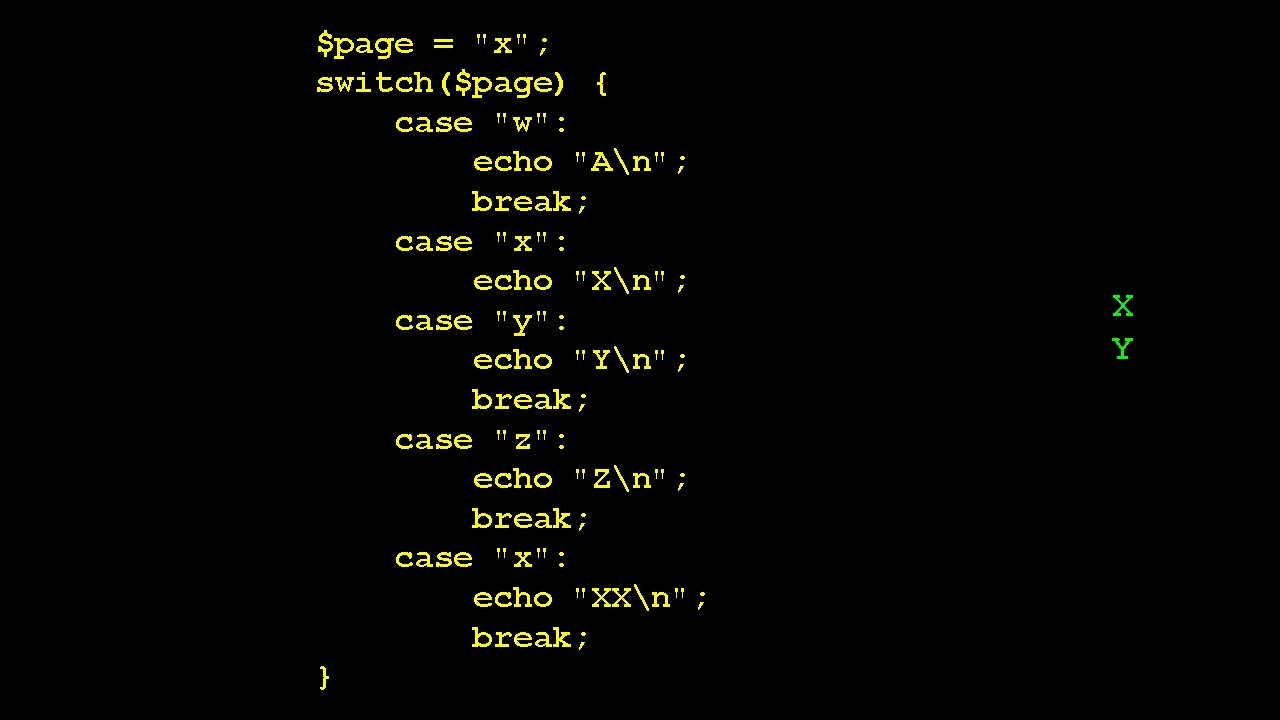
$page = "x"; switch($page) { case "w": echo "An"; break; case "x": echo "Xn"; case "y": echo "Yn"; break; case "z": echo "Zn"; break; case "x": echo "XXn"; break; } X Y
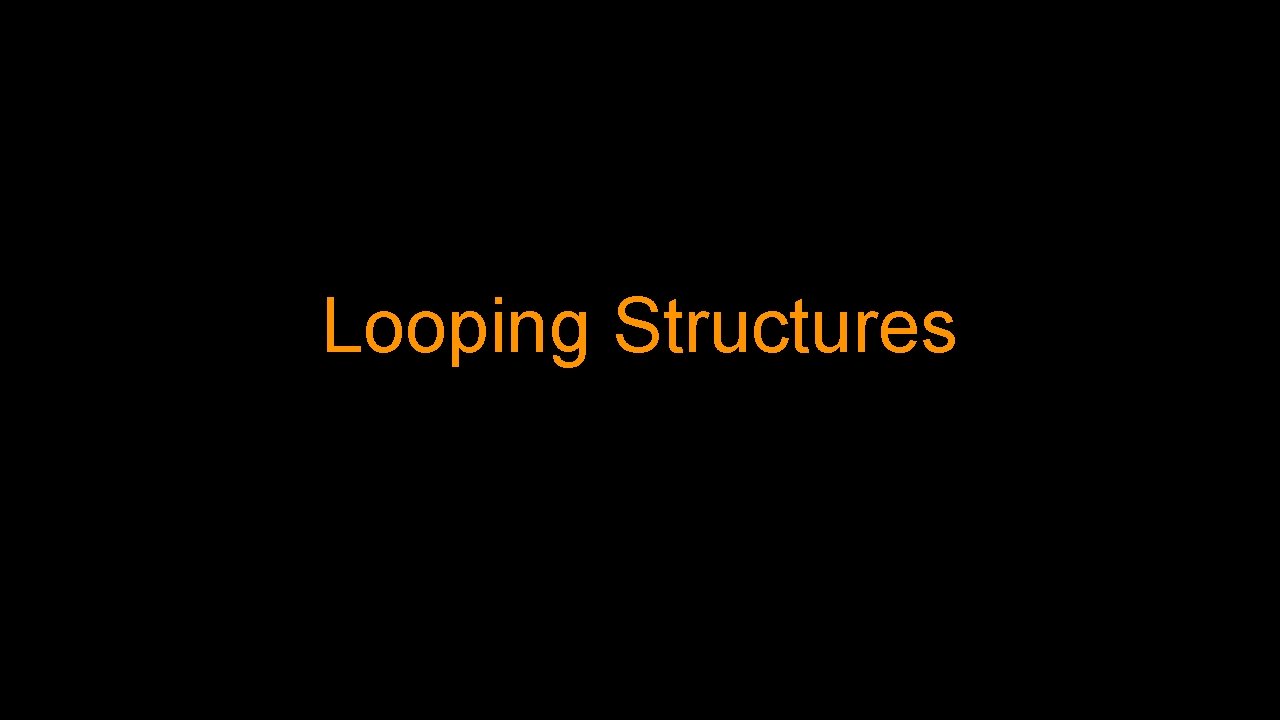
Looping Structures
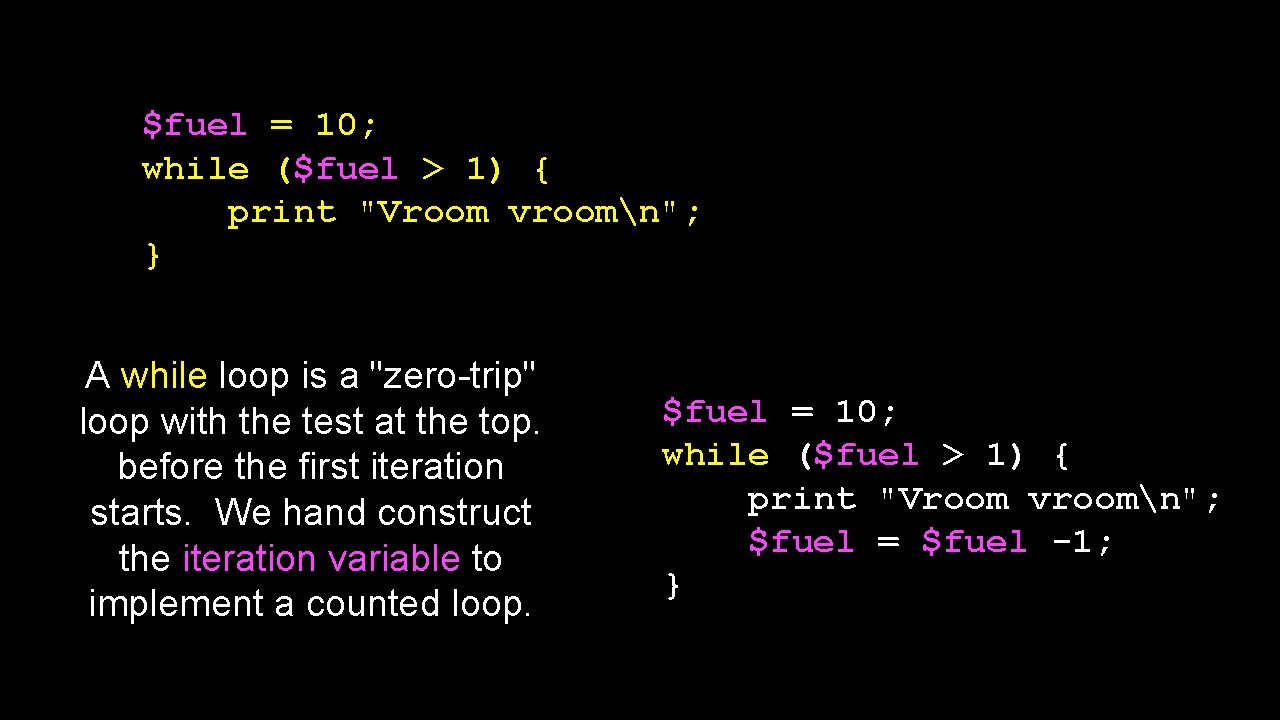
$fuel = 10; while ($fuel > 1) { print "Vroom vroomn"; } A while loop is a "zero-trip" loop with the test at the top. before the first iteration starts. We hand construct the iteration variable to implement a counted loop. $fuel = 10; while ($fuel > 1) { print "Vroom vroomn"; $fuel = $fuel -1; }
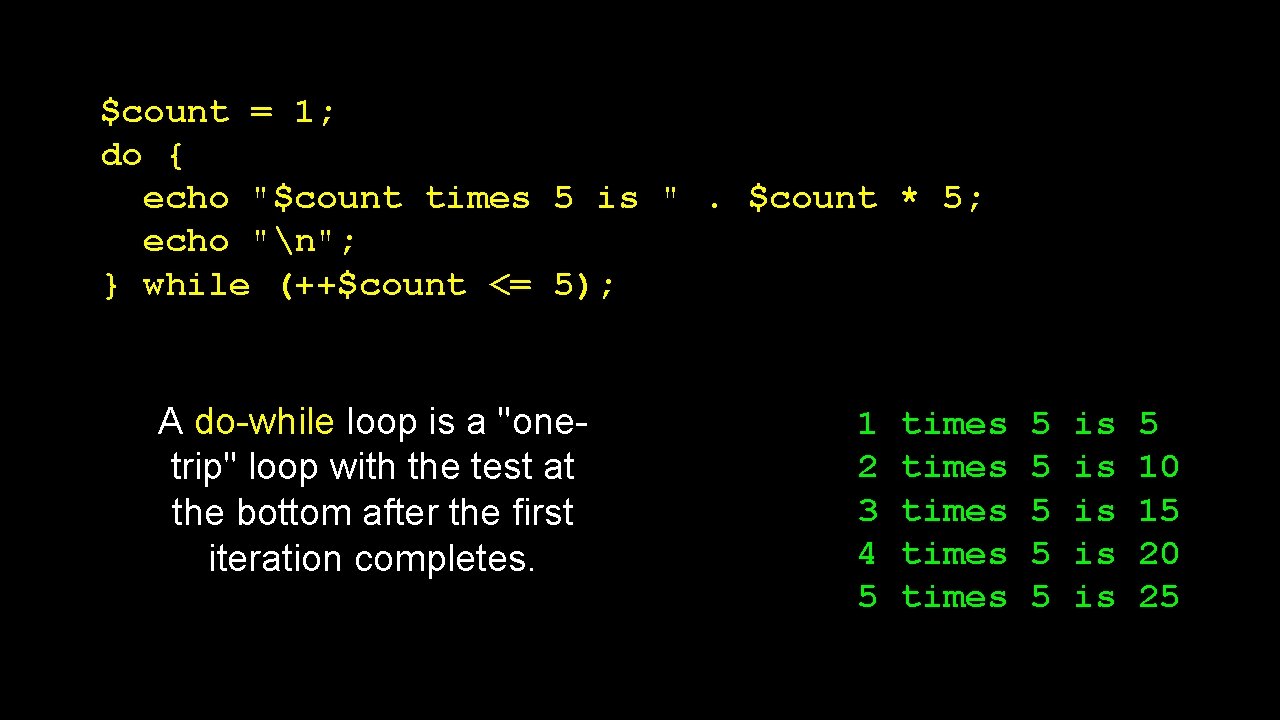
$count = 1; do { echo "$count times 5 is ". $count * 5; echo "n"; } while (++$count <= 5); A do-while loop is a "onetrip" loop with the test at the bottom after the first iteration completes. 1 2 3 4 5 times times 5 5 5 is is is 5 10 15 20 25
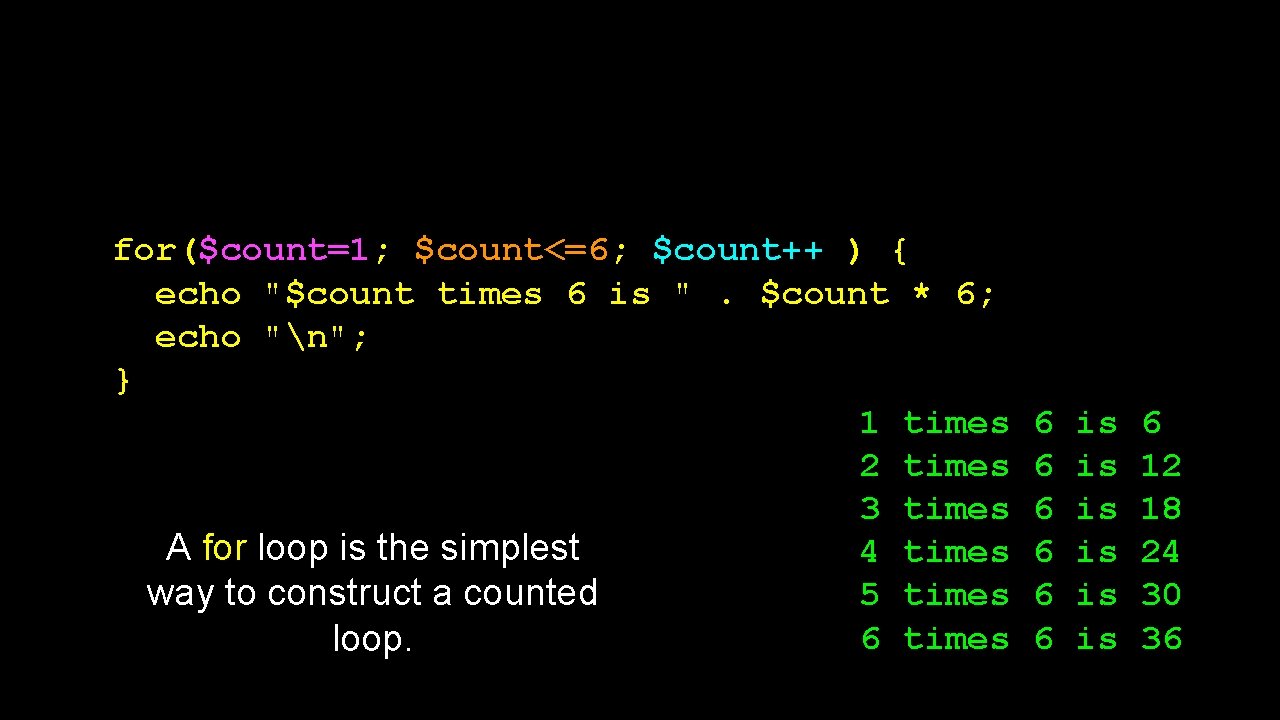
for($count=1; $count<=6; $count++ ) { echo "$count times 6 is ". $count * 6; echo "n"; } 1 times 2 times 3 times A for loop is the simplest 4 times way to construct a counted 5 times 6 times loop. 6 6 6 is is is 6 12 18 24 30 36
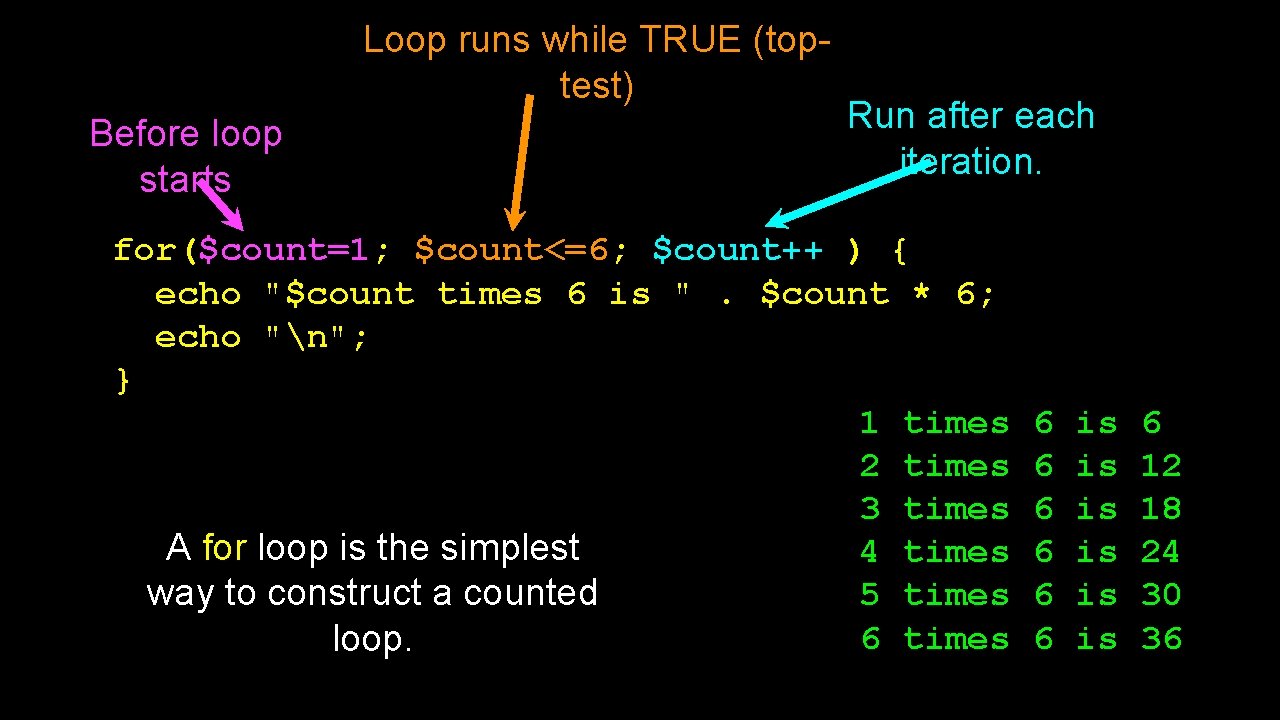
Loop runs while TRUE (toptest) Before loop starts Run after each iteration. for($count=1; $count<=6; $count++ ) { echo "$count times 6 is ". $count * 6; echo "n"; } 1 times 2 times 3 times A for loop is the simplest 4 times way to construct a counted 5 times 6 times loop. 6 6 6 is is is 6 12 18 24 30 36
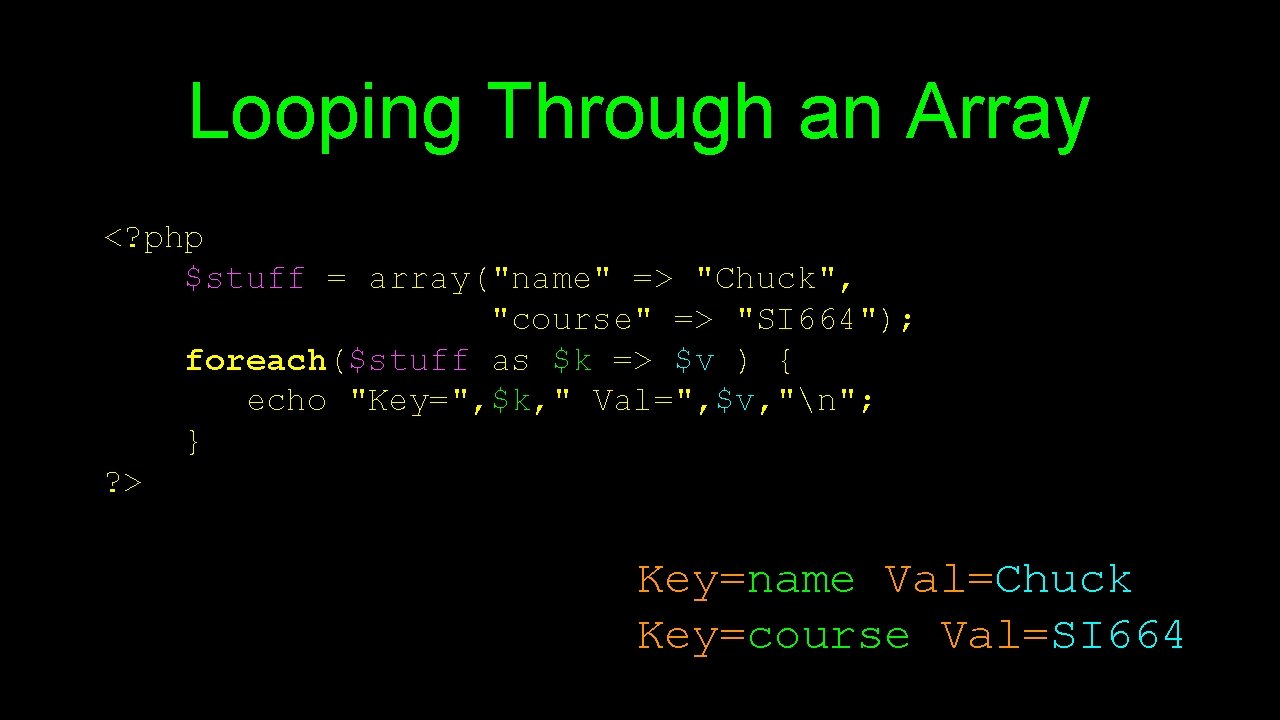
Looping Through an Array <? php $stuff = array("name" => "Chuck", "course" => "SI 664"); foreach($stuff as $k => $v ) { echo "Key=", $k, " Val=", $v, "n"; } ? > Key=name Val=Chuck Key=course Val=SI 664
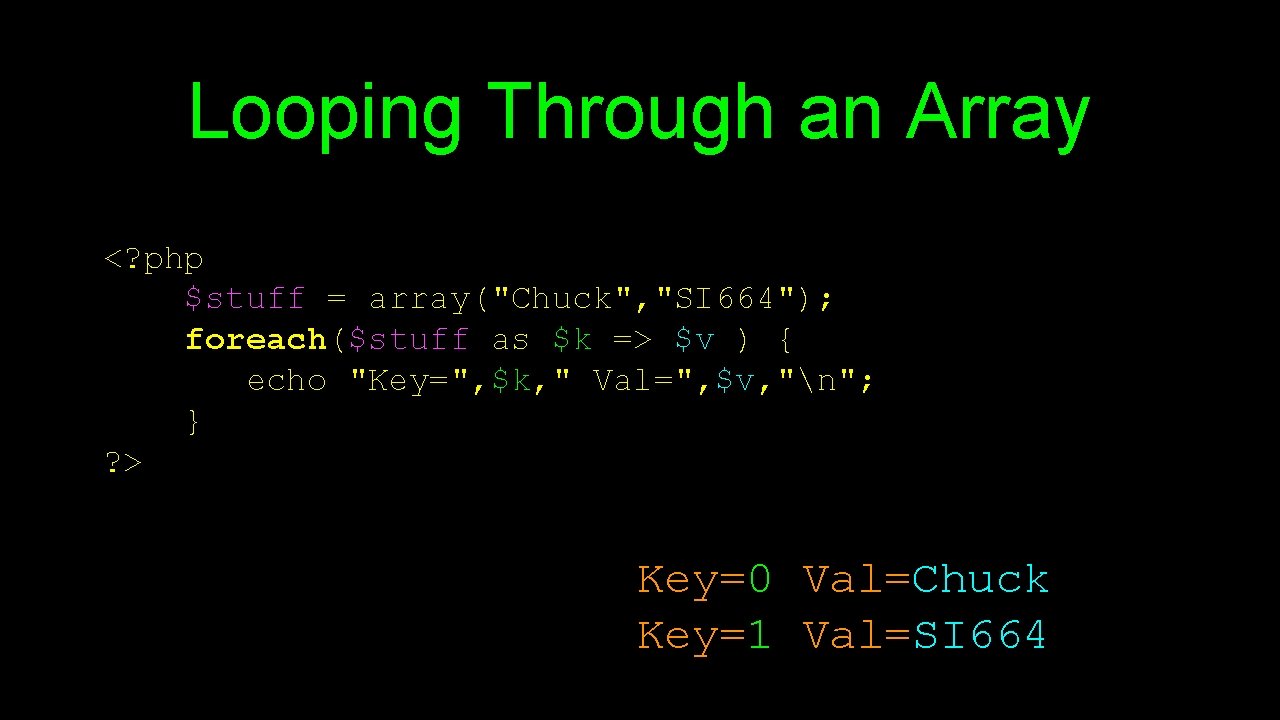
Looping Through an Array <? php $stuff = array("Chuck", "SI 664"); foreach($stuff as $k => $v ) { echo "Key=", $k, " Val=", $v, "n"; } ? > Key=0 Val=Chuck Key=1 Val=SI 664
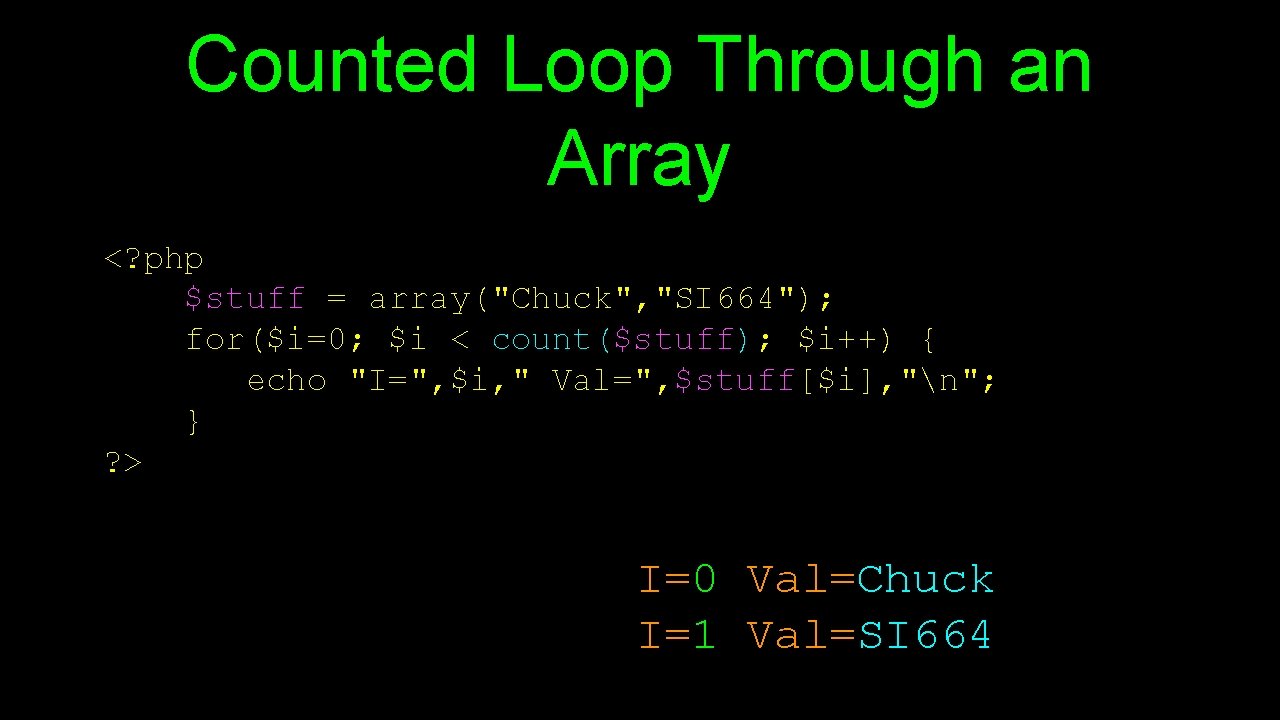
Counted Loop Through an Array <? php $stuff = array("Chuck", "SI 664"); for($i=0; $i < count($stuff); $i++) { echo "I=", $i, " Val=", $stuff[$i], "n"; } ? > I=0 Val=Chuck I=1 Val=SI 664
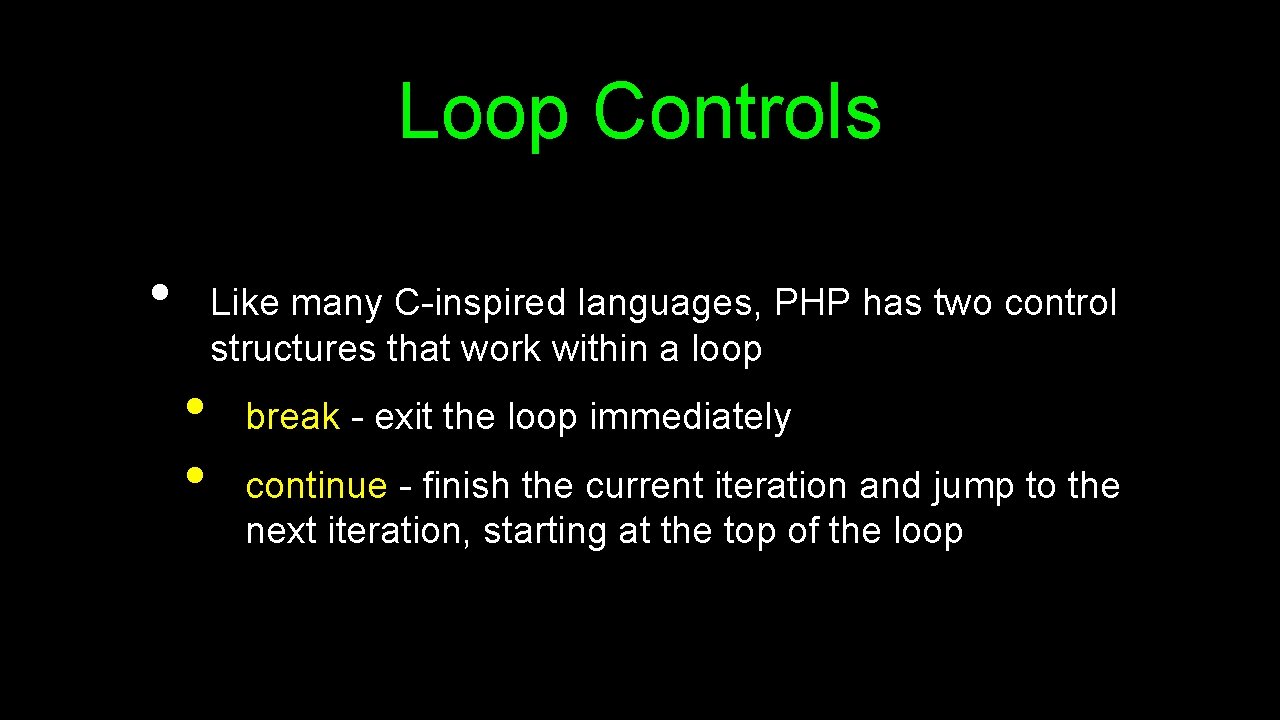
Loop Controls • Like many C-inspired languages, PHP has two control structures that work within a loop • • break - exit the loop immediately continue - finish the current iteration and jump to the next iteration, starting at the top of the loop
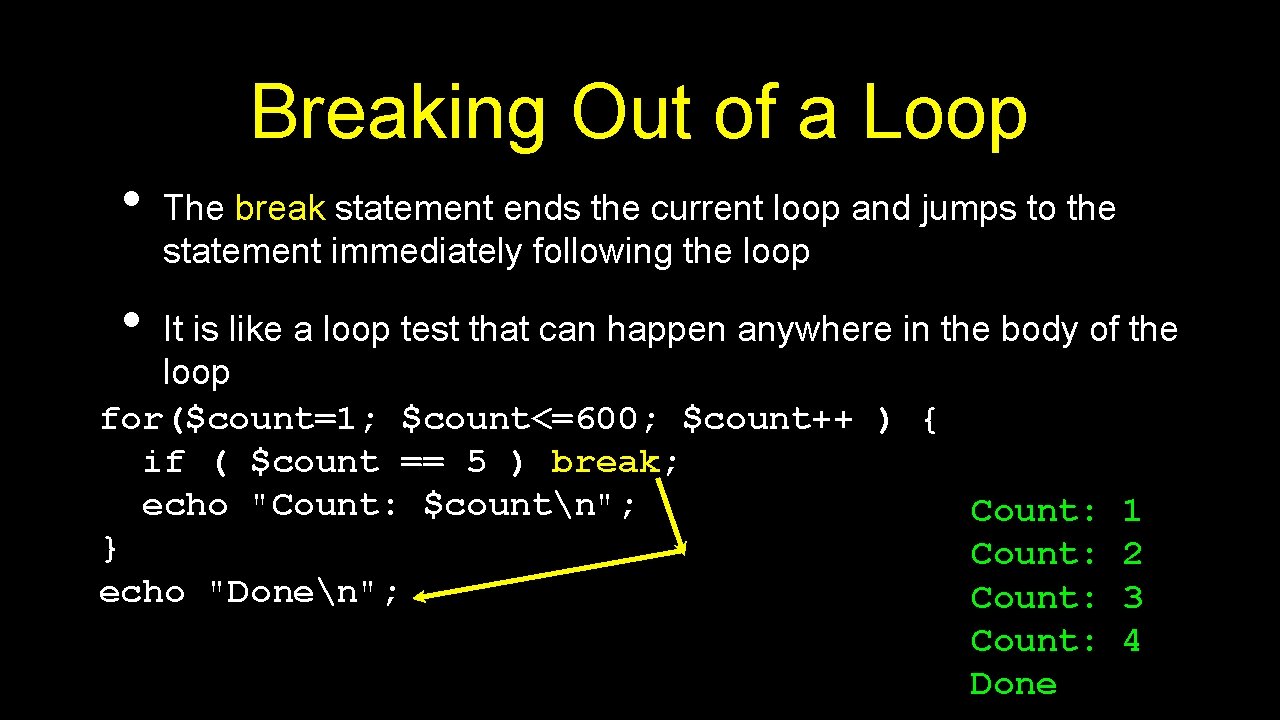
Breaking Out of a Loop • • The break statement ends the current loop and jumps to the statement immediately following the loop It is like a loop test that can happen anywhere in the body of the loop for($count=1; $count<=600; $count++ ) { if ( $count == 5 ) break; echo "Count: $countn"; Count: 1 } Count: 2 echo "Donen"; Count: 3 Count: 4 Done
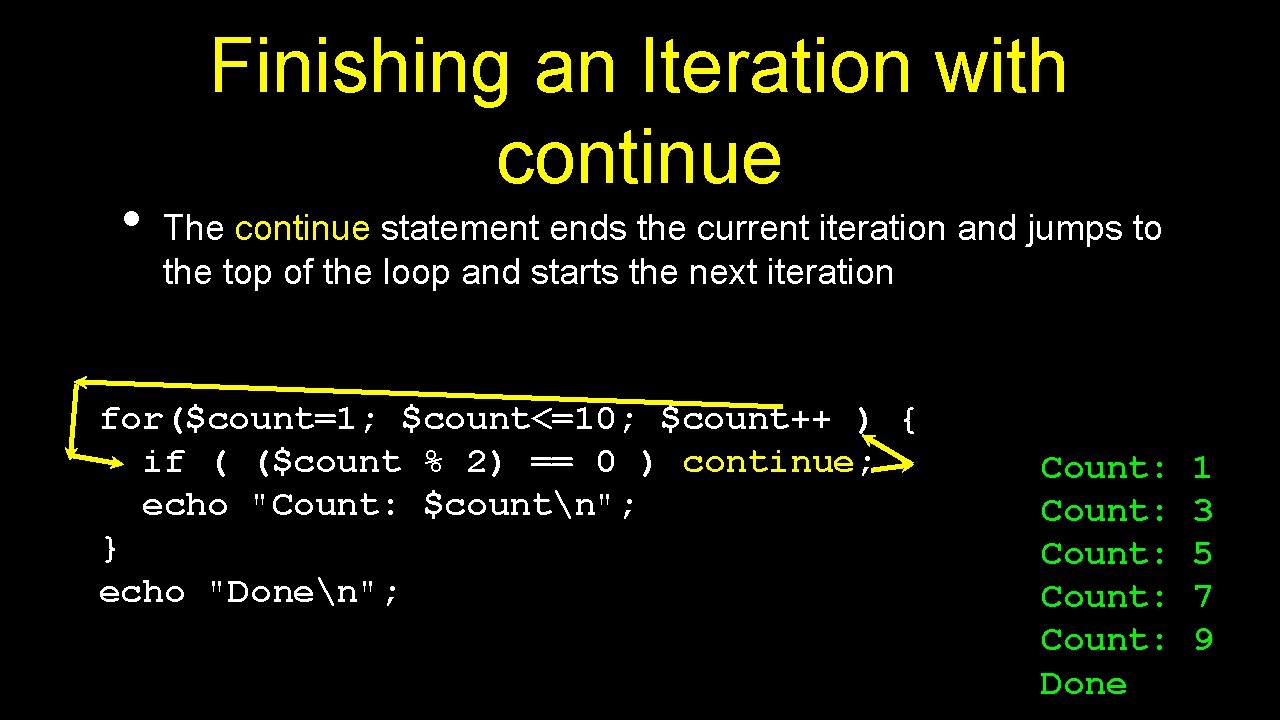
• Finishing an Iteration with continue The continue statement ends the current iteration and jumps to the top of the loop and starts the next iteration for($count=1; $count<=10; $count++ ) { if ( ($count % 2) == 0 ) continue; echo "Count: $countn"; } echo "Donen"; Count: Count: Done 1 3 5 7 9
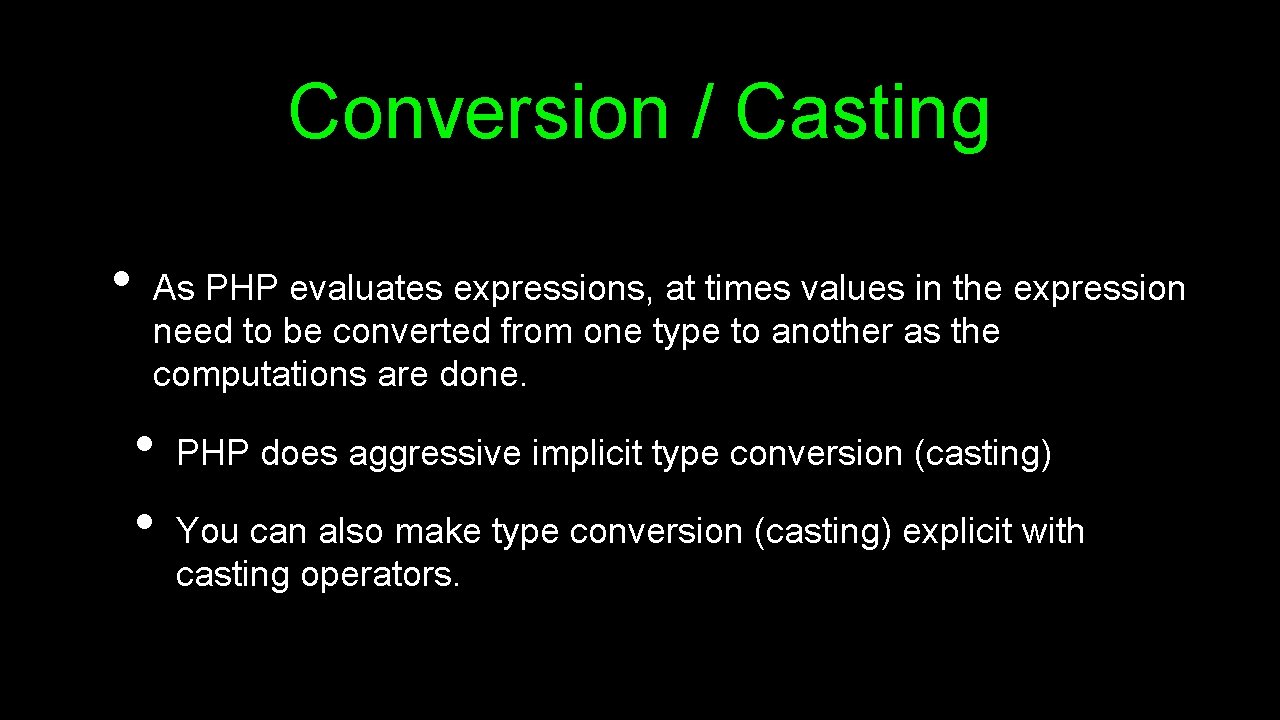
Conversion / Casting • As PHP evaluates expressions, at times values in the expression need to be converted from one type to another as the computations are done. • • PHP does aggressive implicit type conversion (casting) You can also make type conversion (casting) explicit with casting operators.
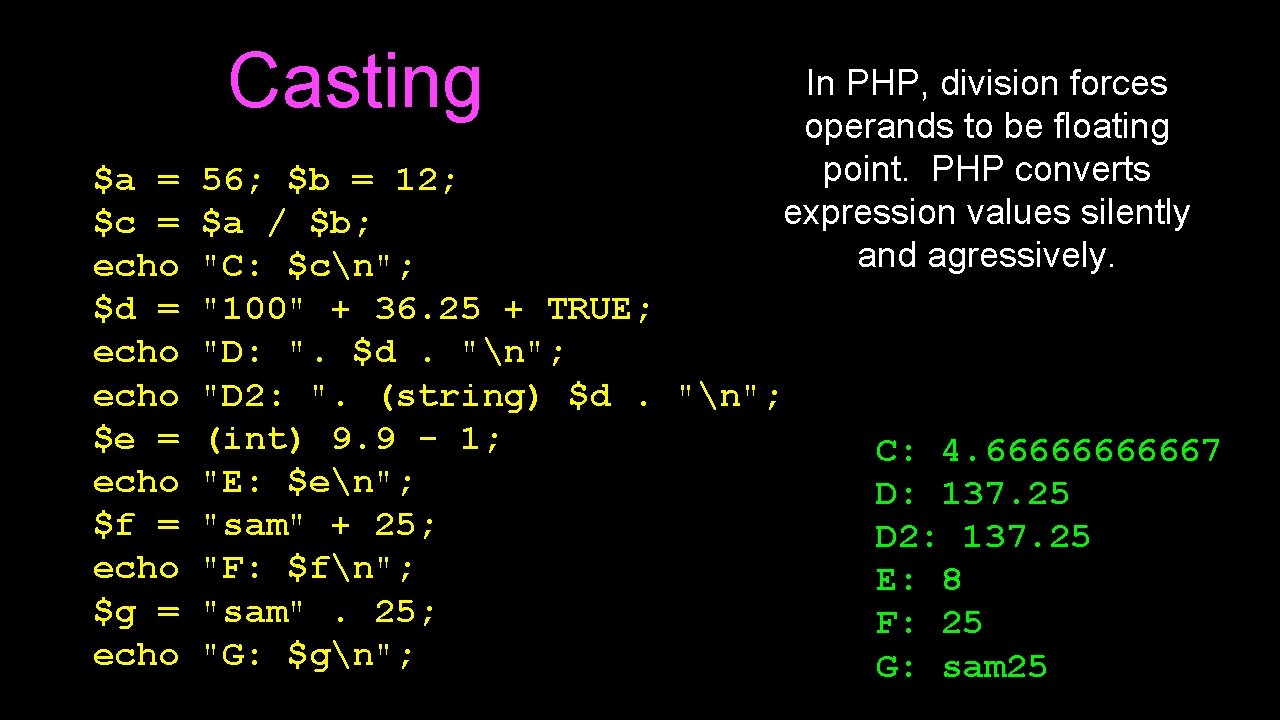
Casting $a = $c = echo $d = echo $e = echo $f = echo $g = echo In PHP, division forces operands to be floating point. PHP converts expression values silently and agressively. 56; $b = 12; $a / $b; "C: $cn"; "100" + 36. 25 + TRUE; "D: ". $d. "n"; "D 2: ". (string) $d. "n"; (int) 9. 9 - 1; "E: $en"; "sam" + 25; "F: $fn"; "sam". 25; "G: $gn"; C: 4. 666667 D: 137. 25 D 2: 137. 25 E: 8 F: 25 G: sam 25
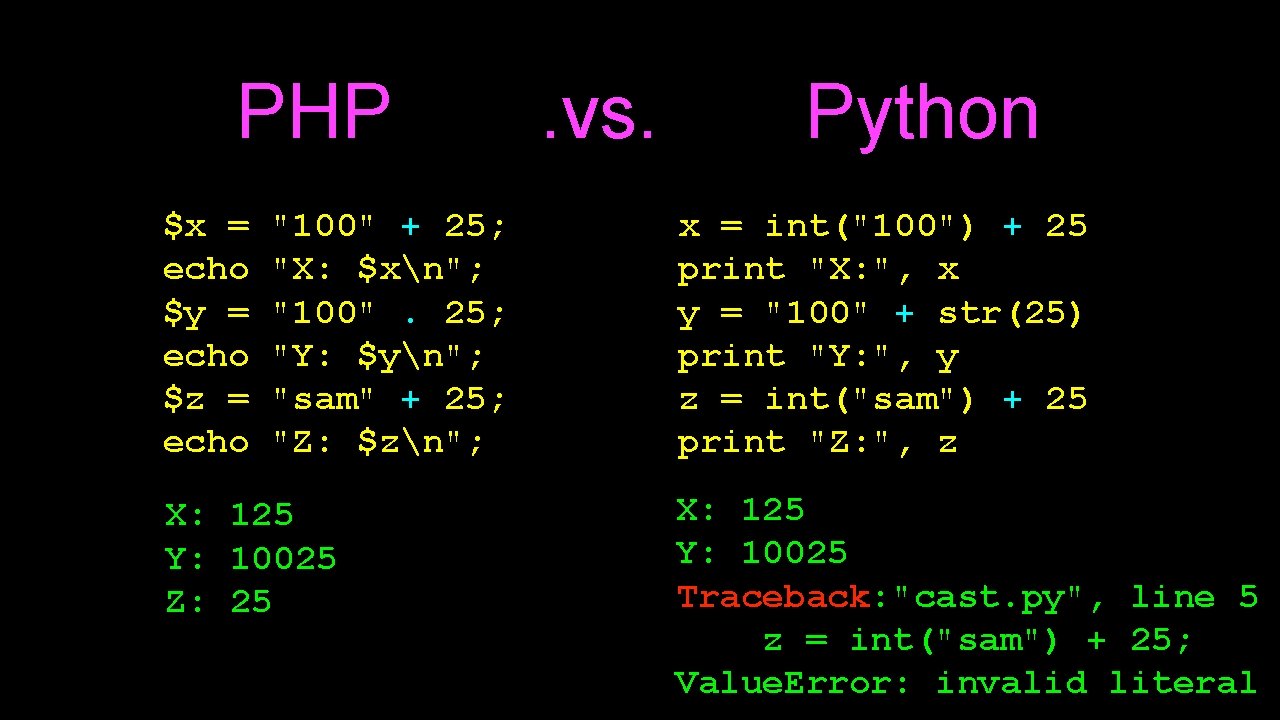
PHP $x = echo $y = echo $z = echo "100" + 25; "X: $xn"; "100". 25; "Y: $yn"; "sam" + 25; "Z: $zn"; X: 125 Y: 10025 Z: 25 . vs. Python x = int("100") + 25 print "X: ", x y = "100" + str(25) print "Y: ", y z = int("sam") + 25 print "Z: ", z X: 125 Y: 10025 Traceback: "cast. py", line 5 z = int("sam") + 25; Value. Error: invalid literal
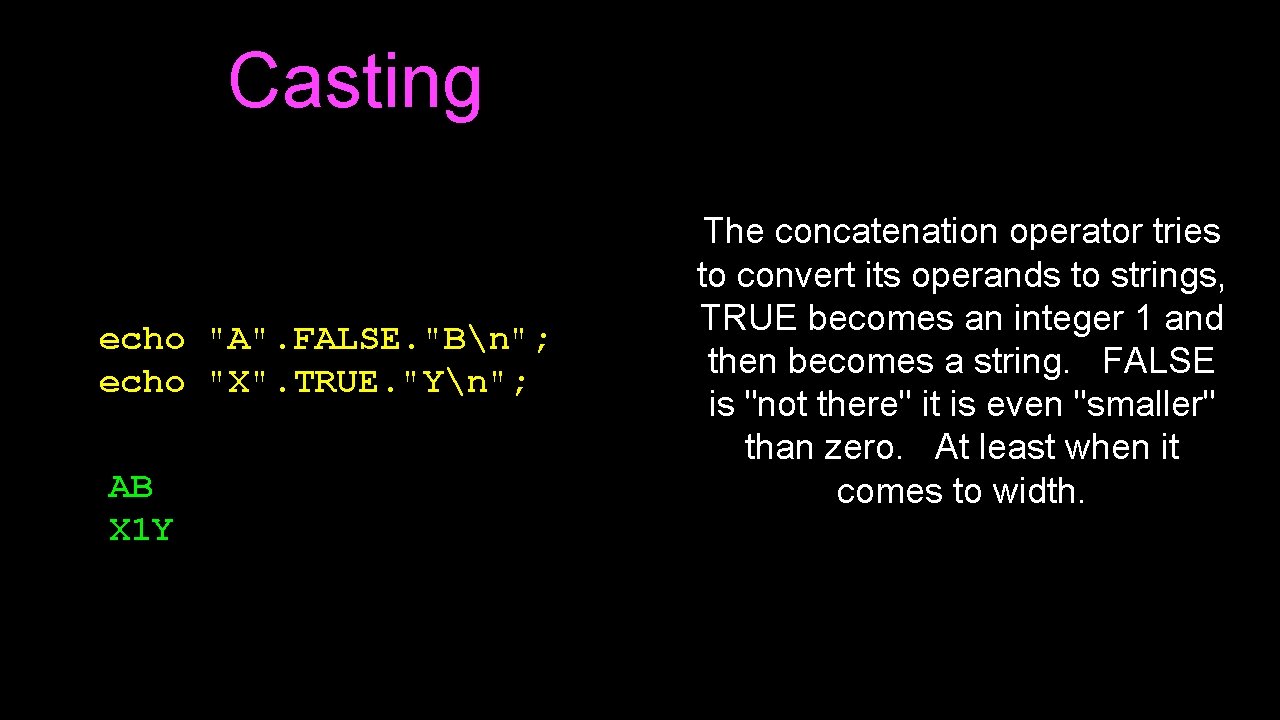
Casting echo "A". FALSE. "Bn"; echo "X". TRUE. "Yn"; AB X 1 Y The concatenation operator tries to convert its operands to strings, TRUE becomes an integer 1 and then becomes a string. FALSE is "not there" it is even "smaller" than zero. At least when it comes to width.
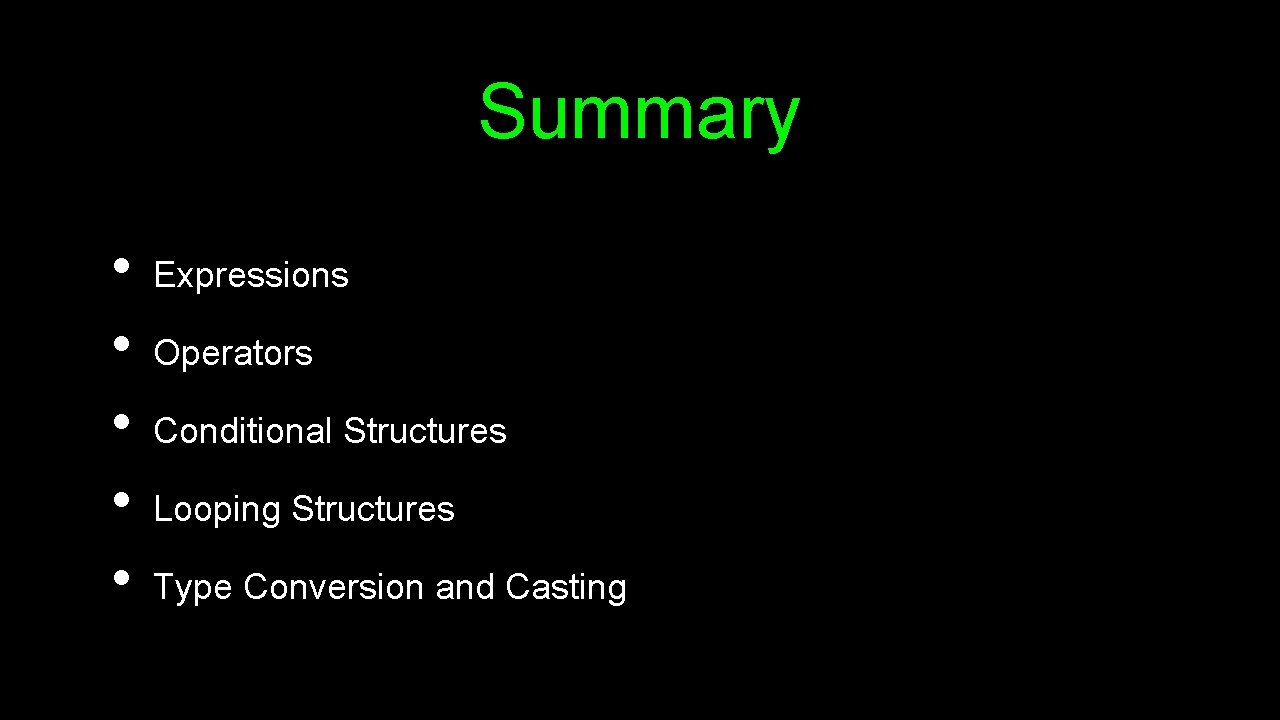
Summary • • • Expressions Operators Conditional Structures Looping Structures Type Conversion and Casting