Fundamental of Programming C Lecturer Omid Jafarinezhad Lecture
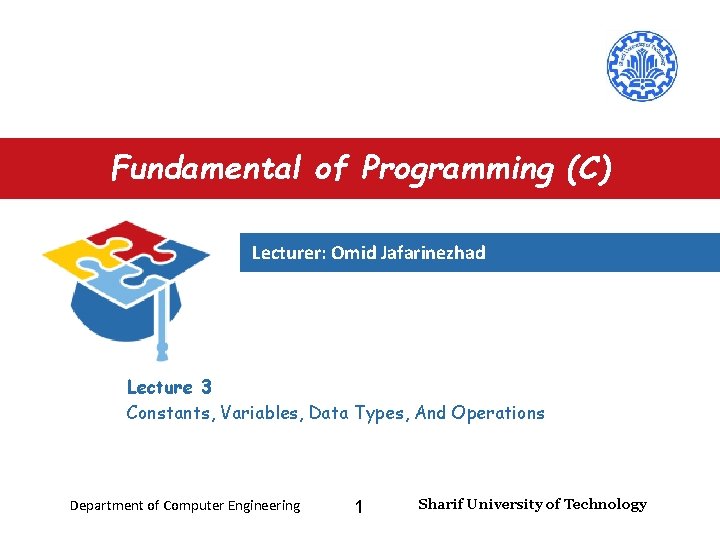
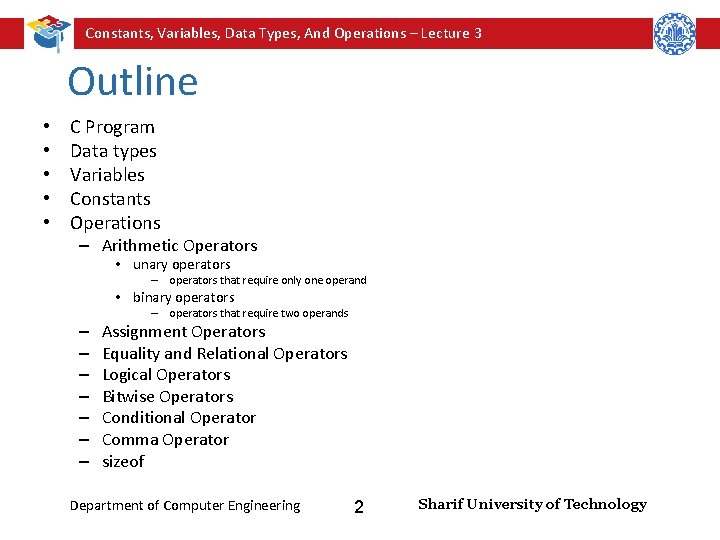
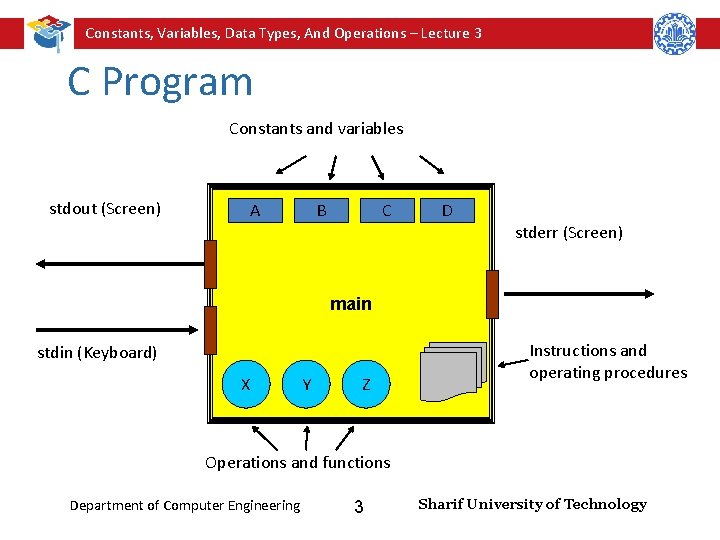
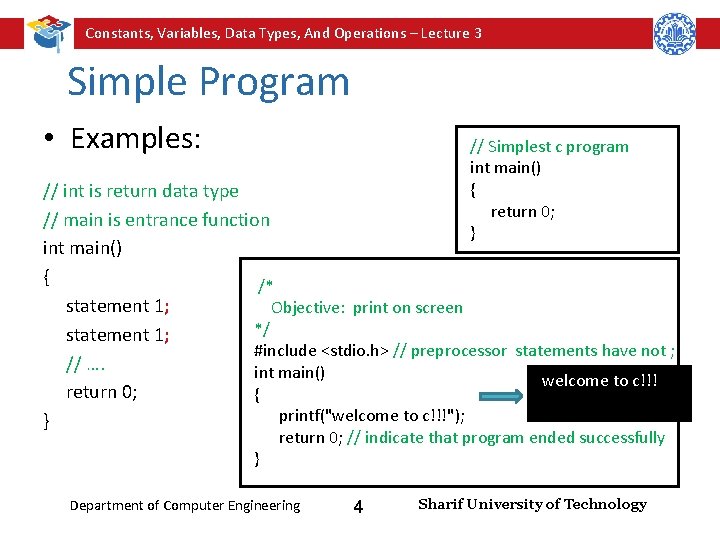
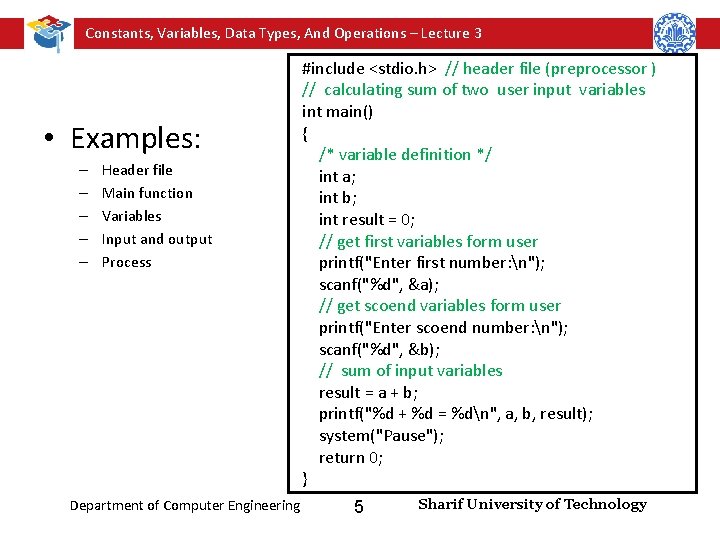
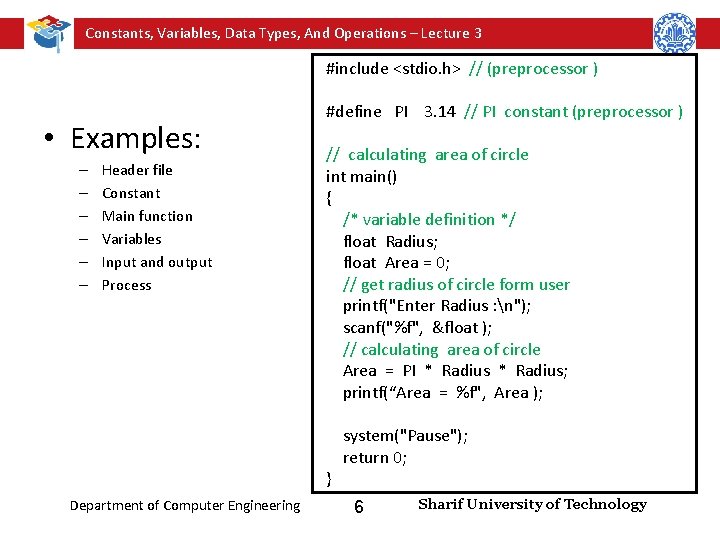
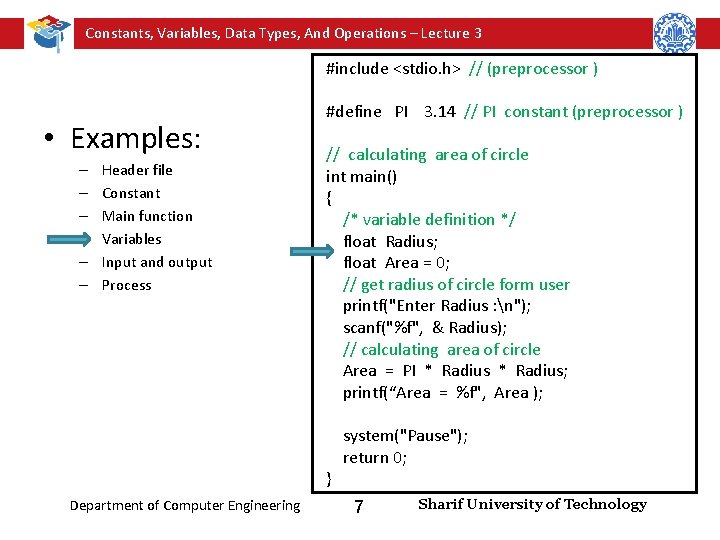
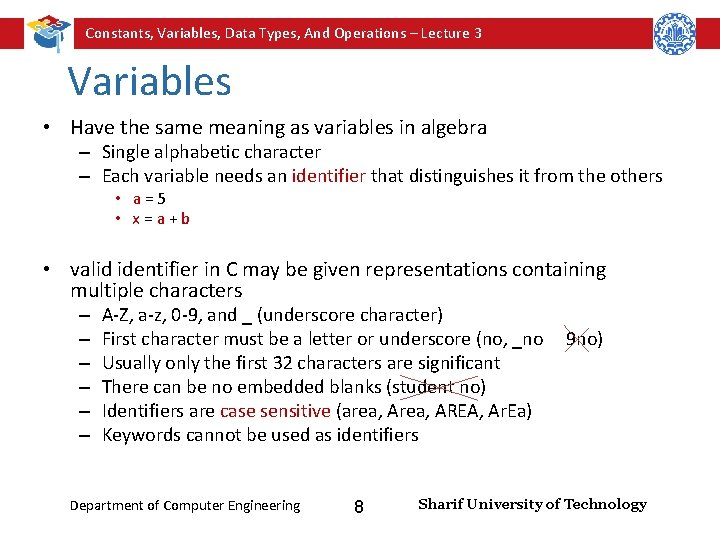
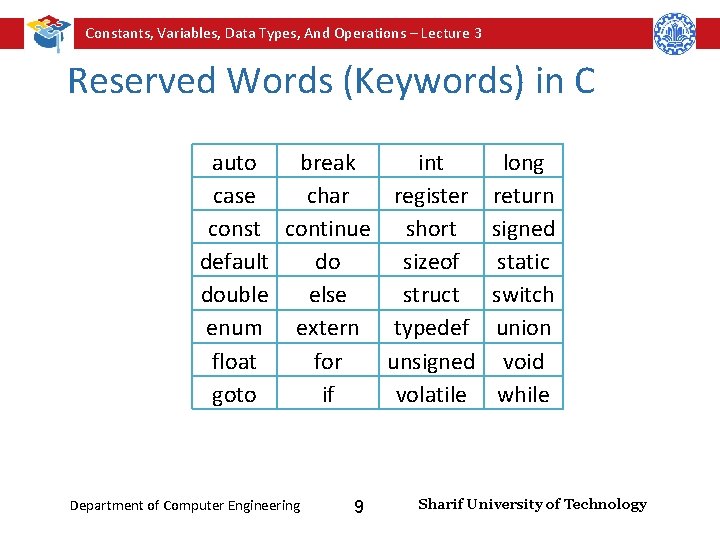
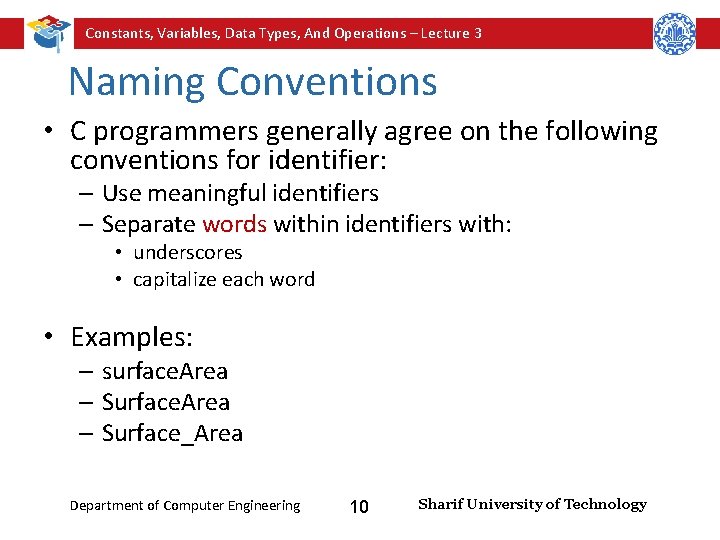
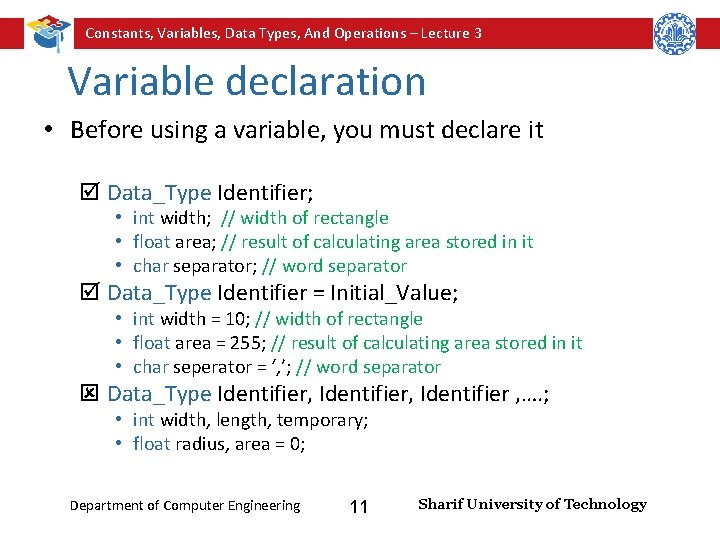
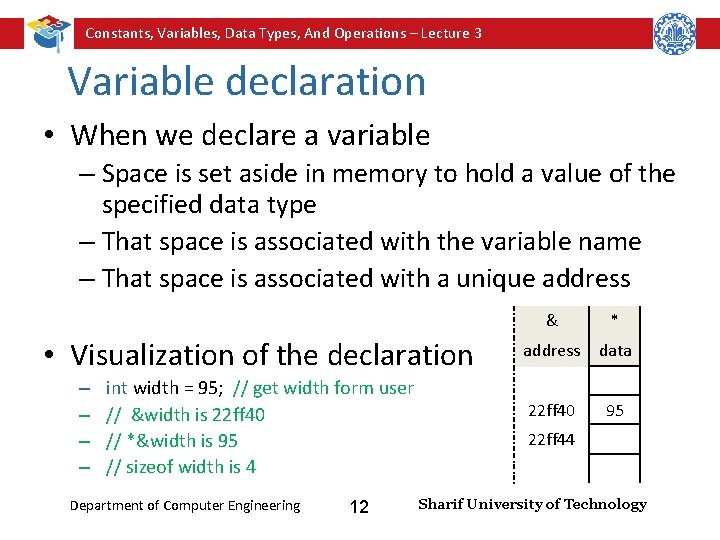
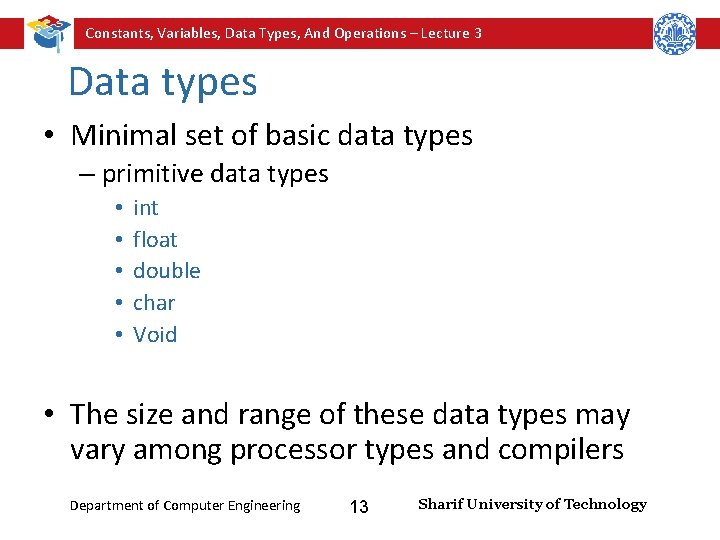
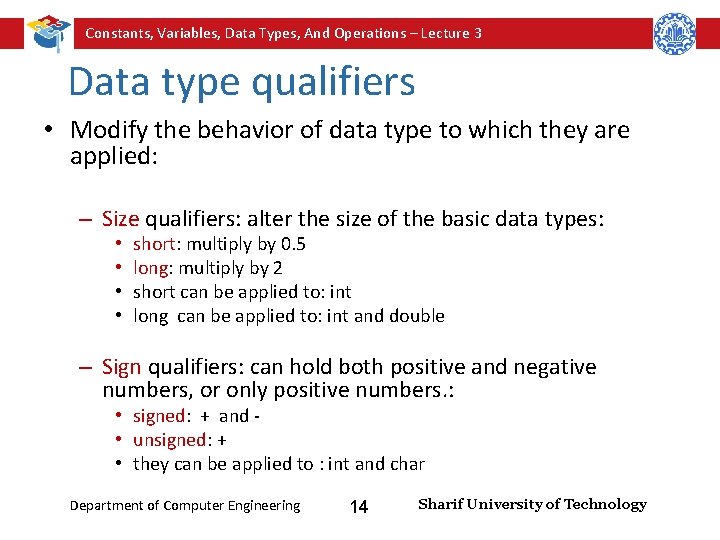
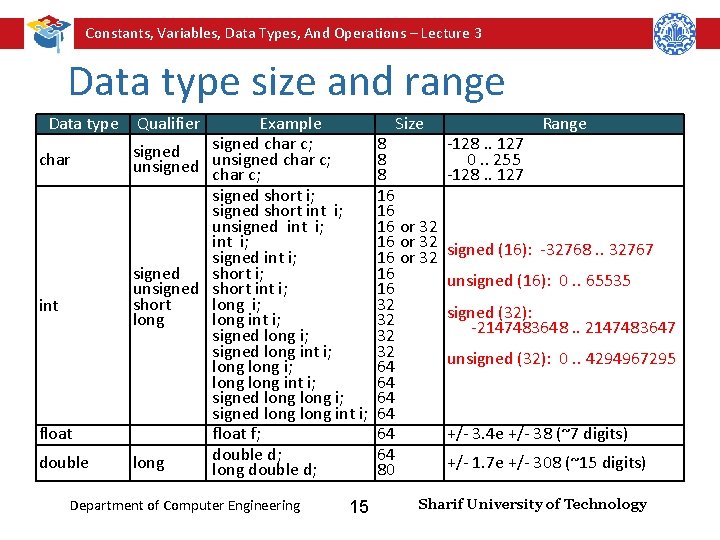
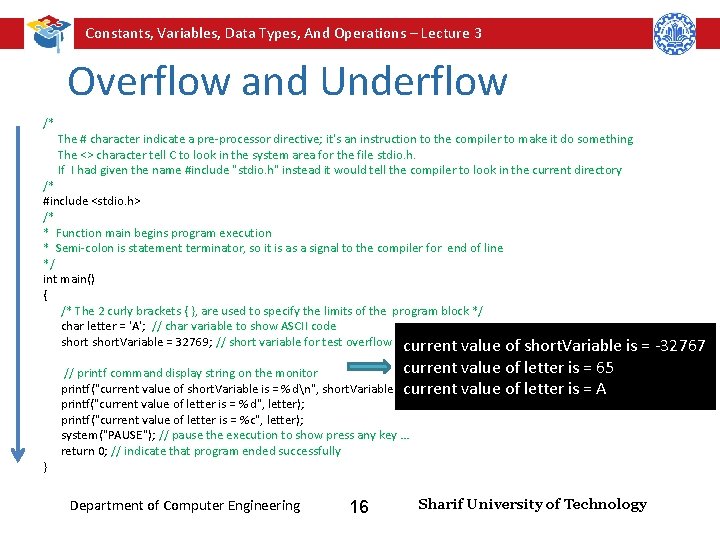
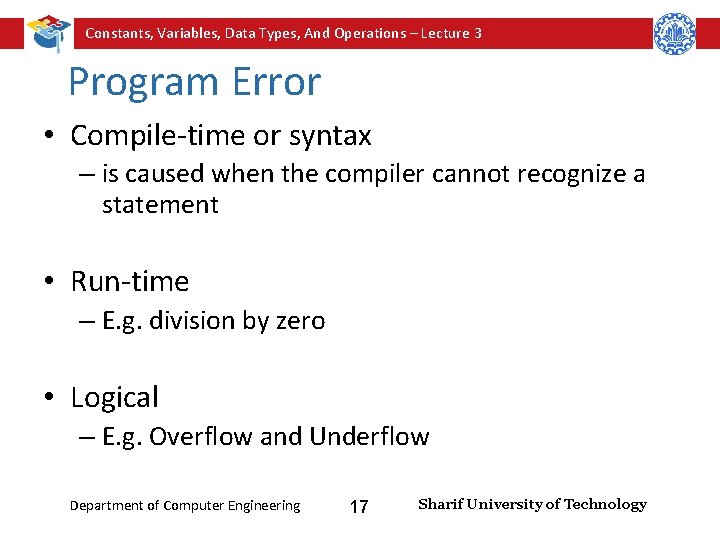
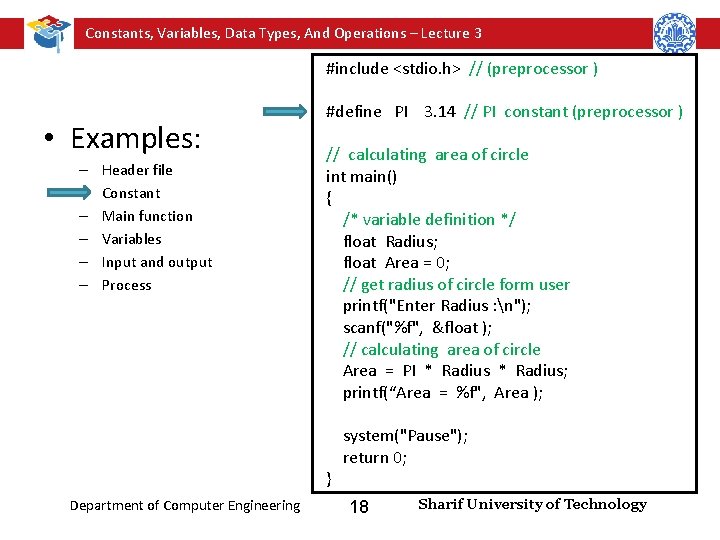
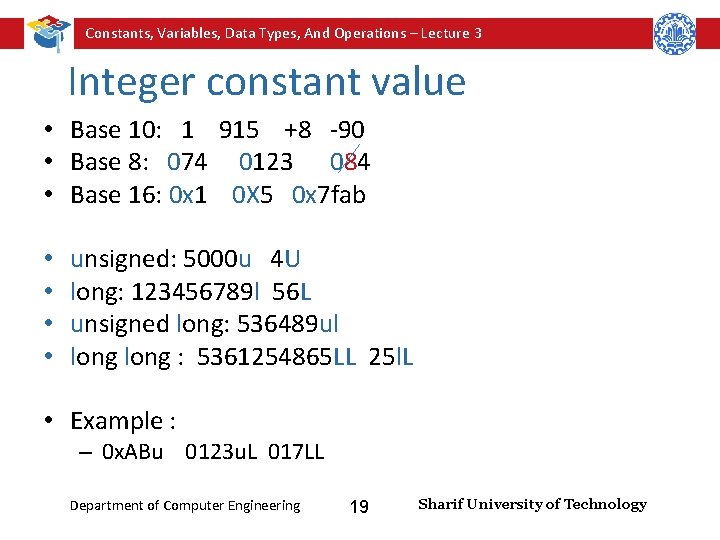
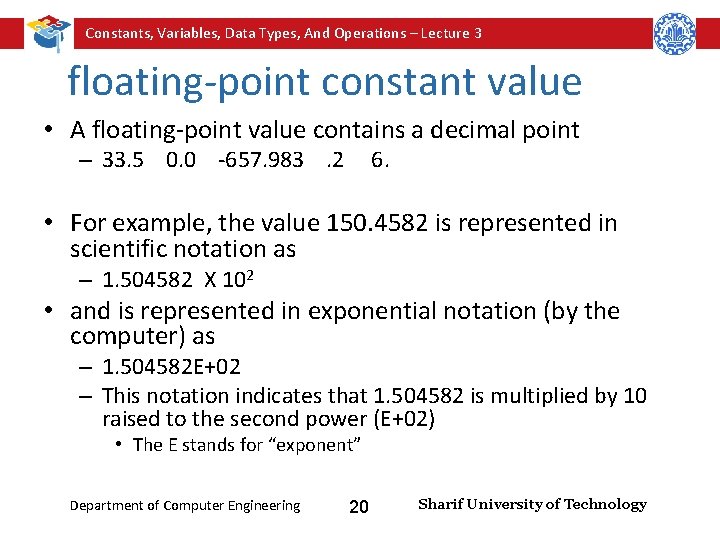
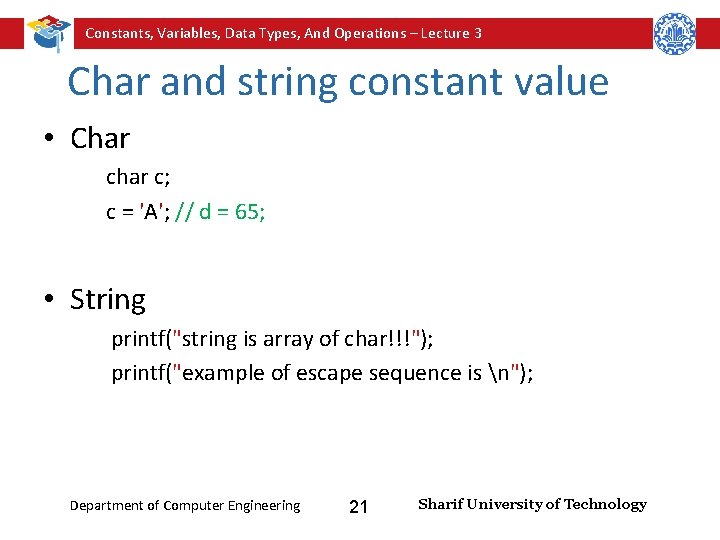
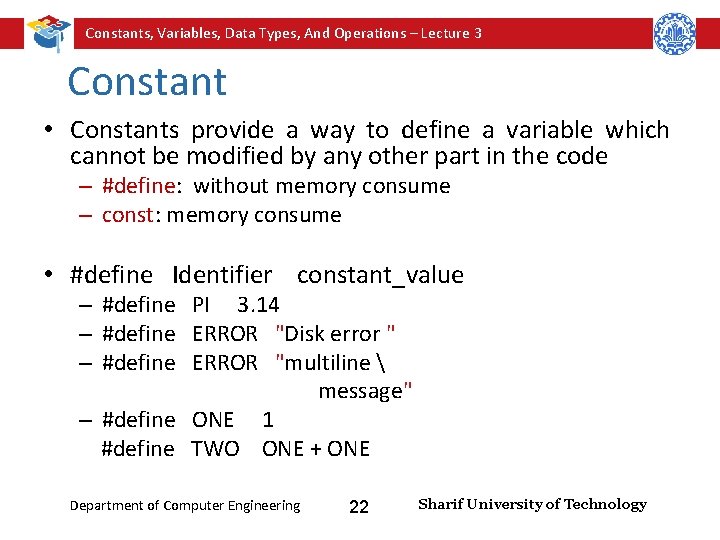
![Constants, Variables, Data Types, And Operations – Lecture 3 Constant • const [Data_Type] Identifier Constants, Variables, Data Types, And Operations – Lecture 3 Constant • const [Data_Type] Identifier](https://slidetodoc.com/presentation_image_h2/604401fbd50d99da71f7f8c2f3a179fd/image-23.jpg)
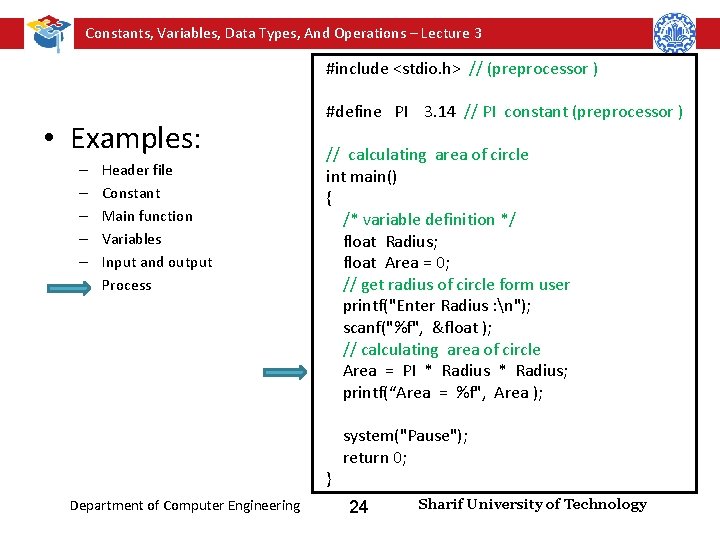
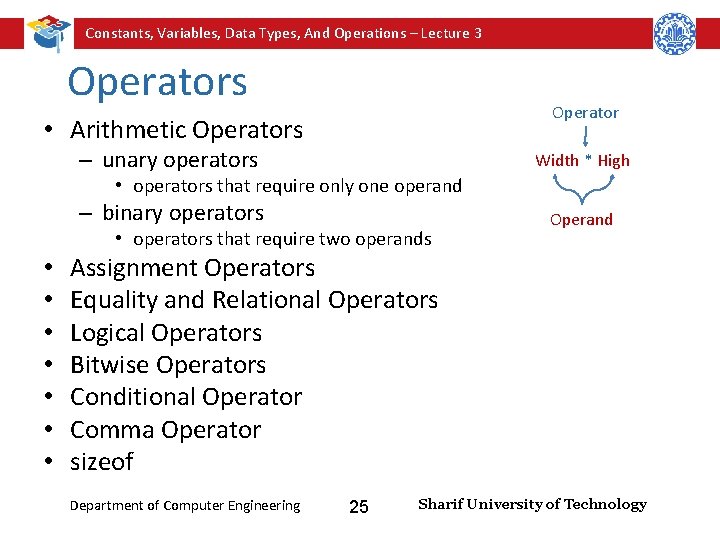
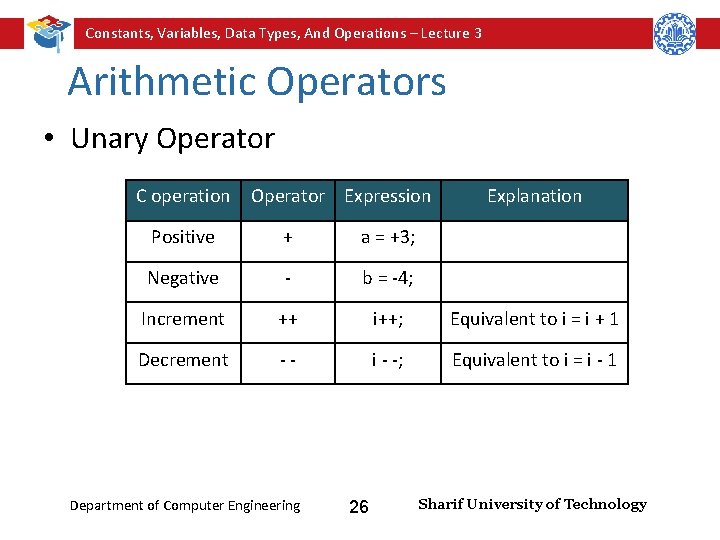
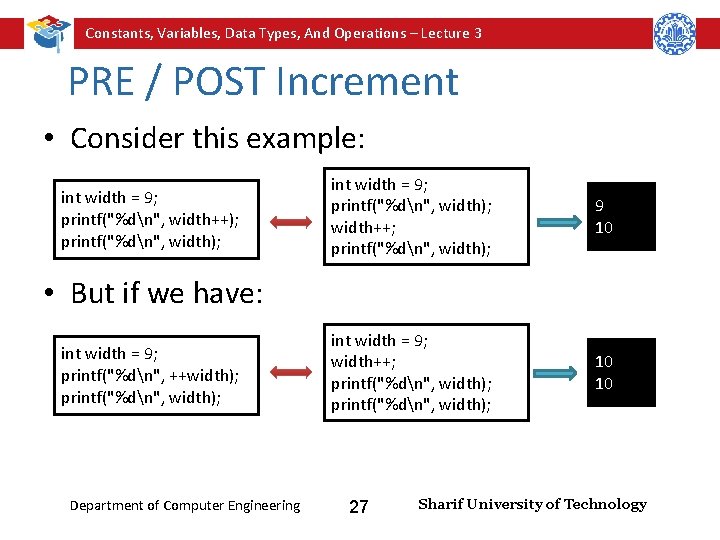
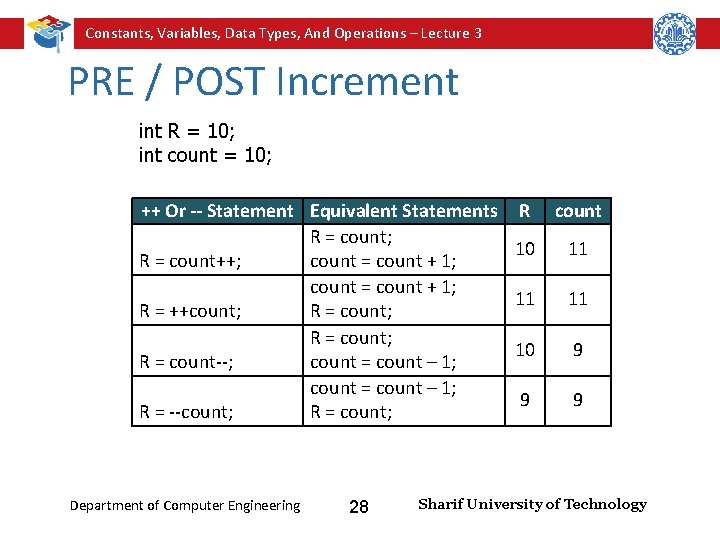
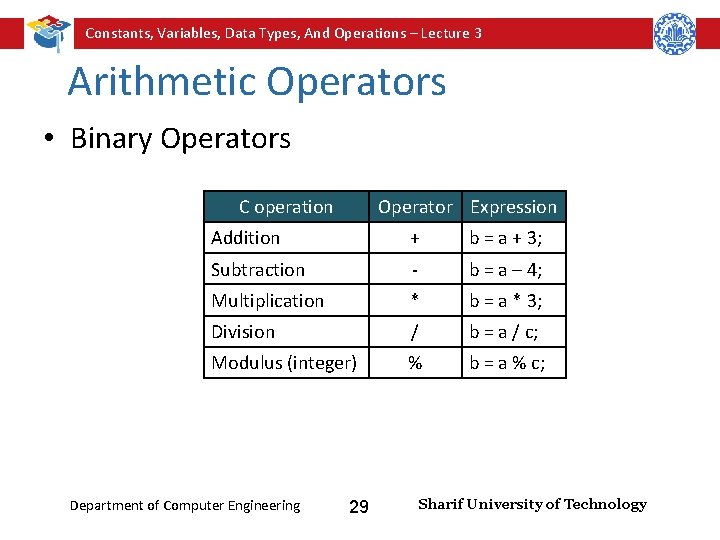
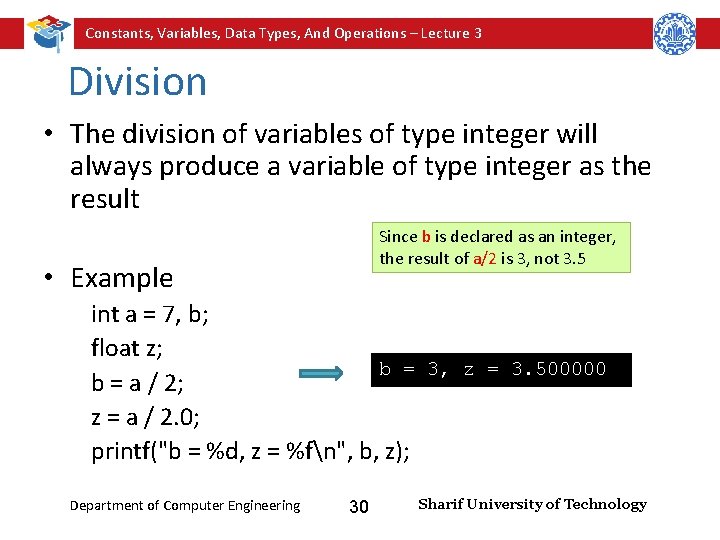
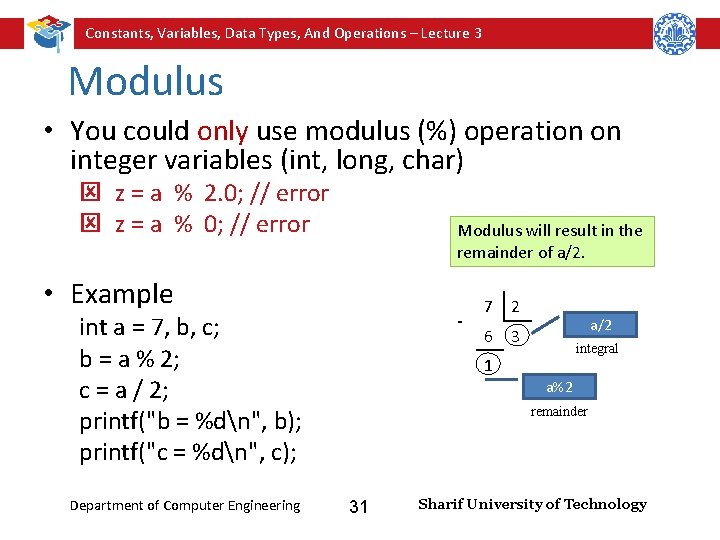
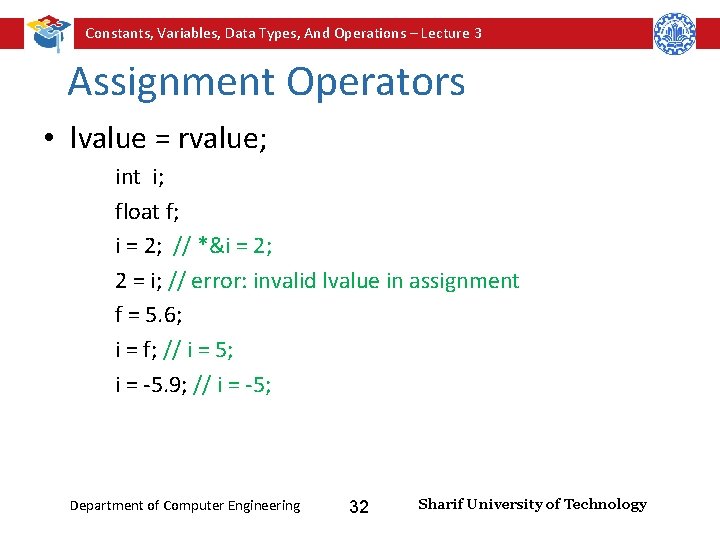
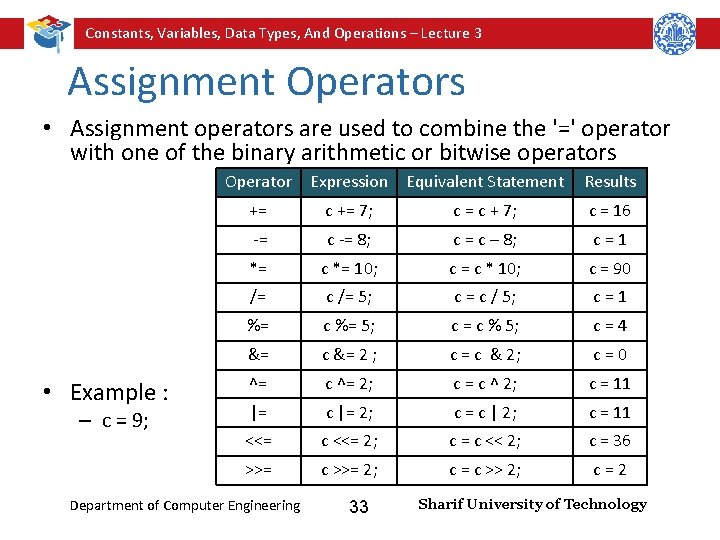
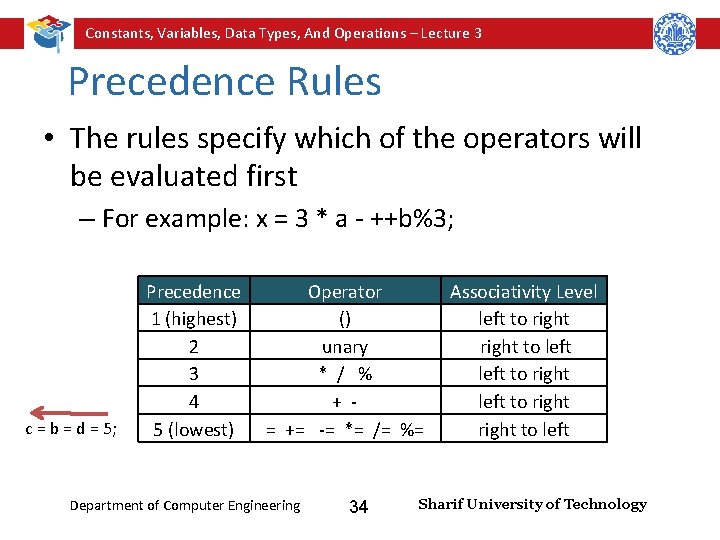
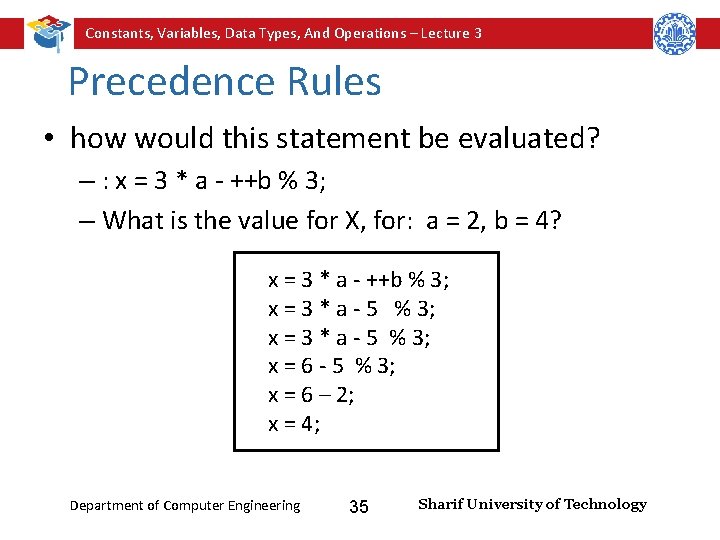
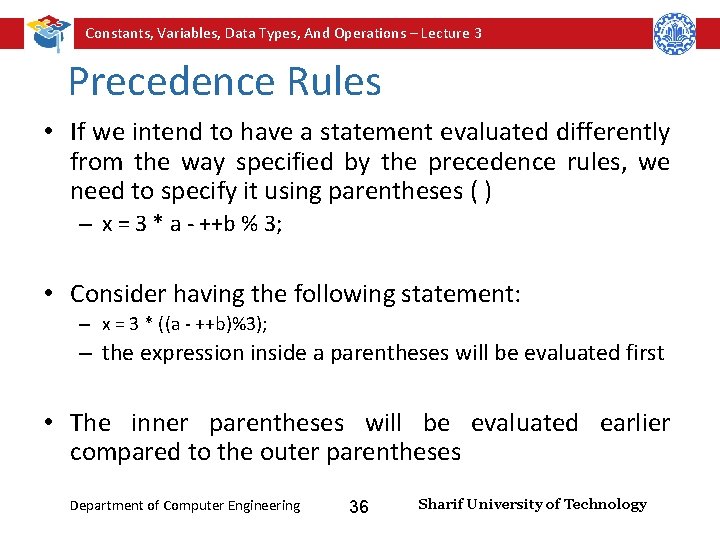
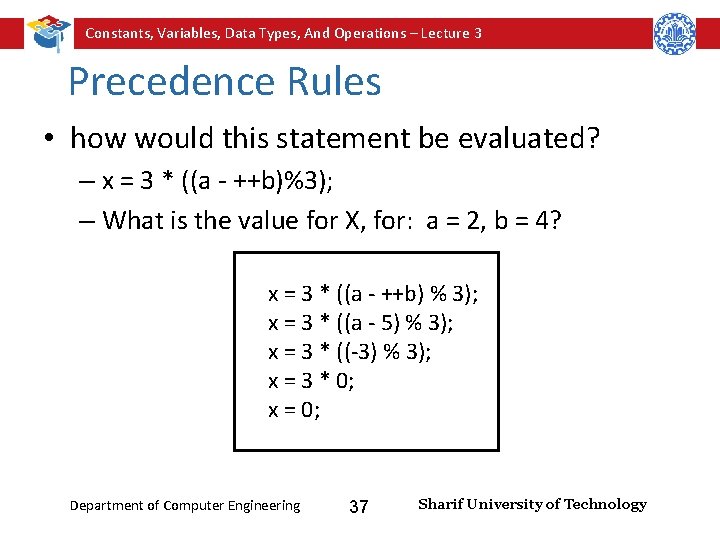
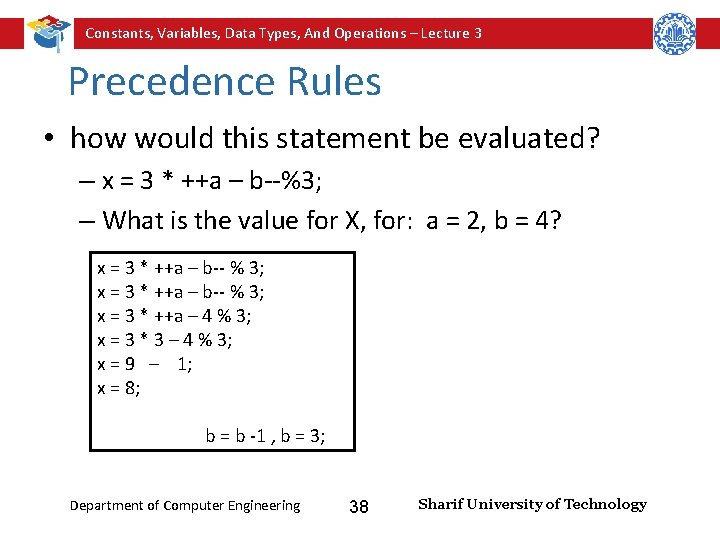
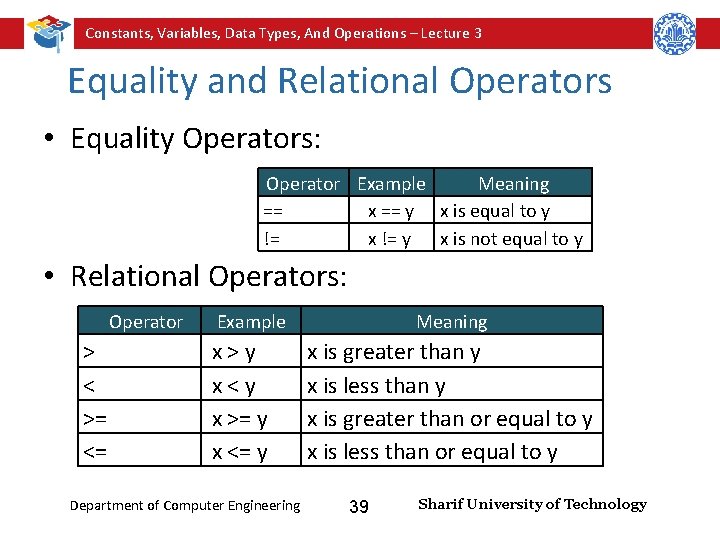
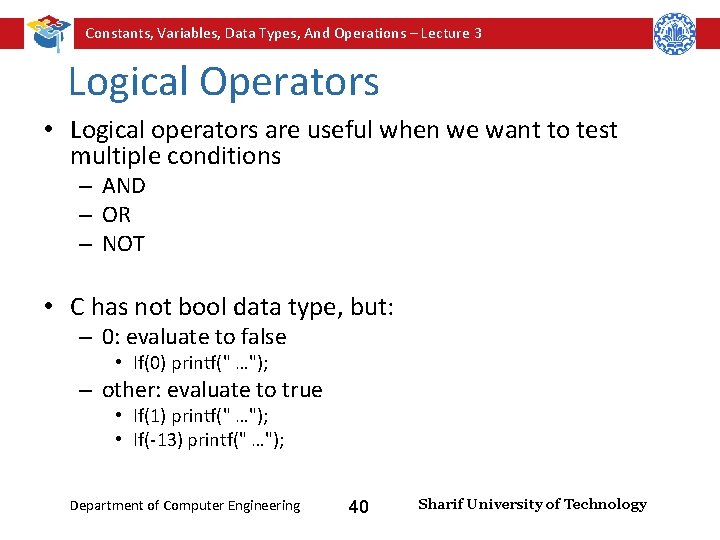
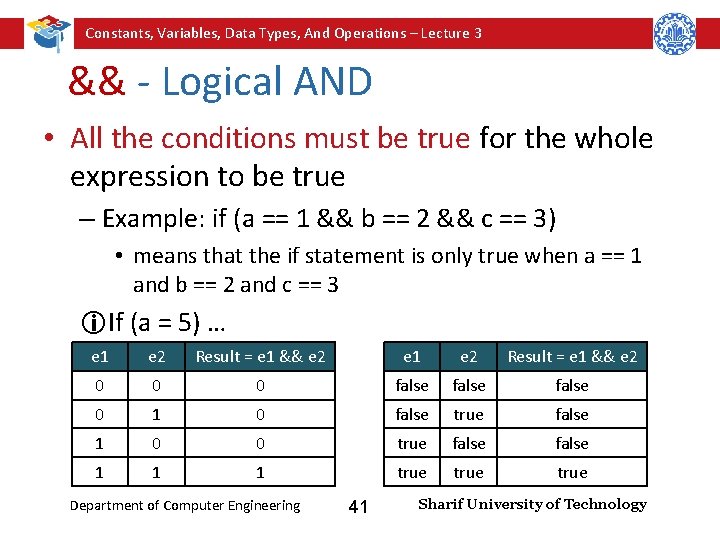
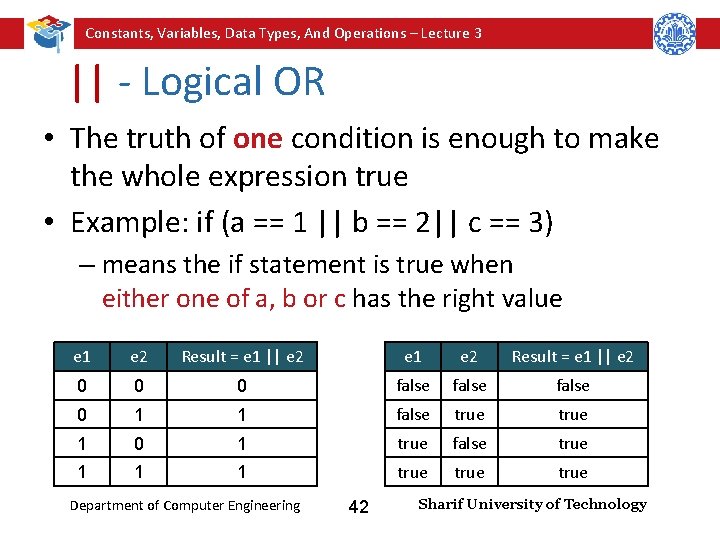
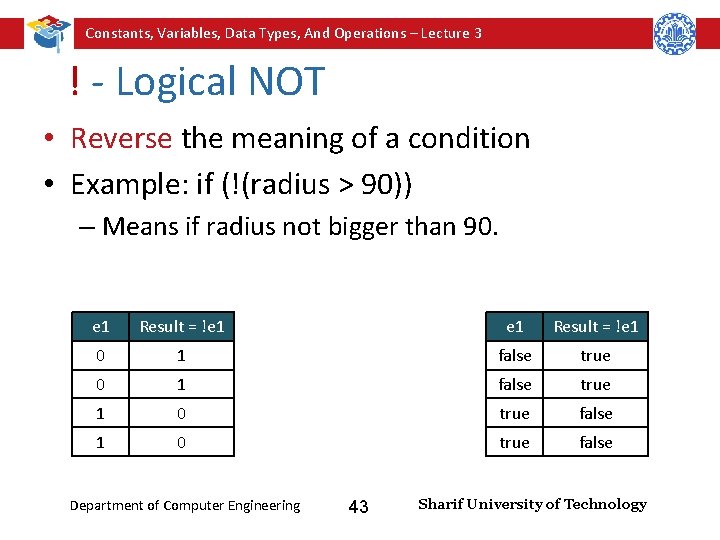
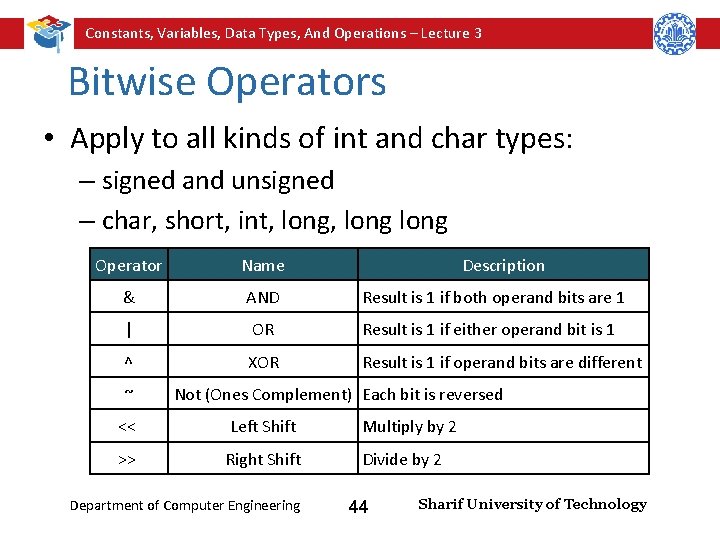
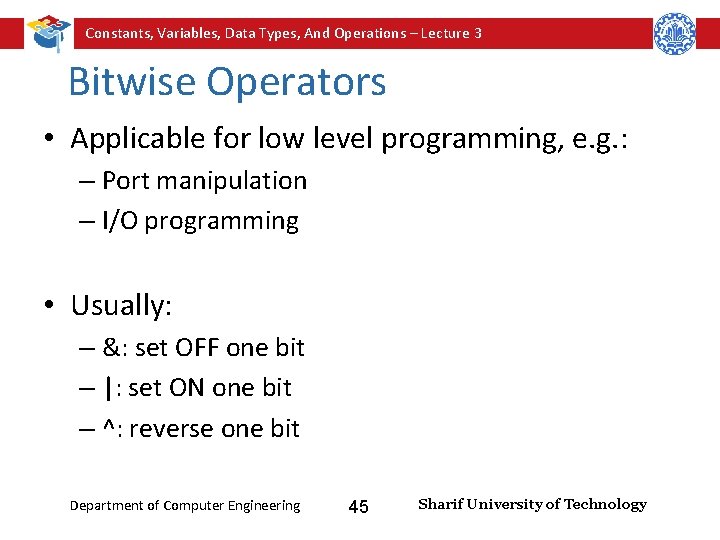
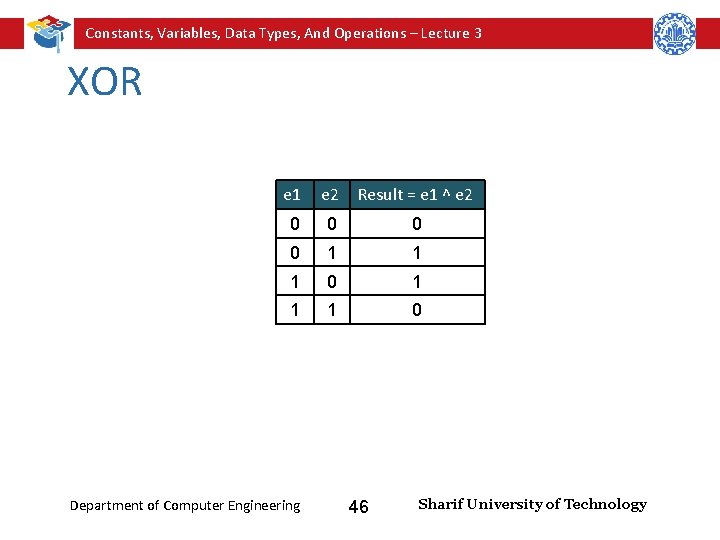
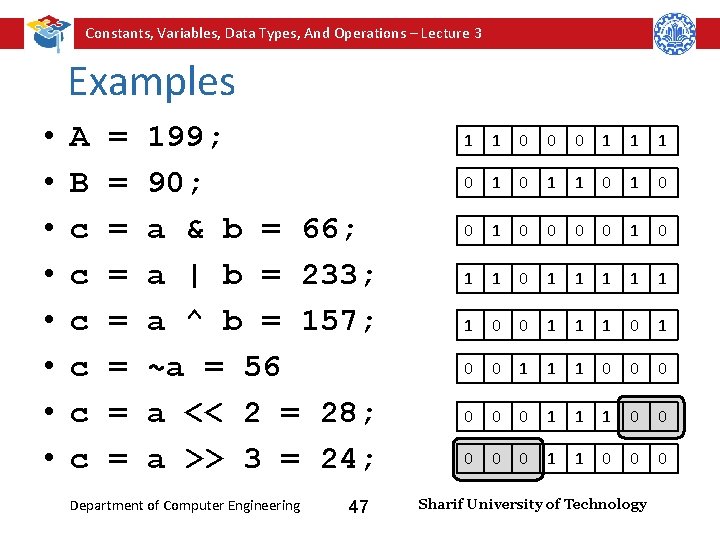
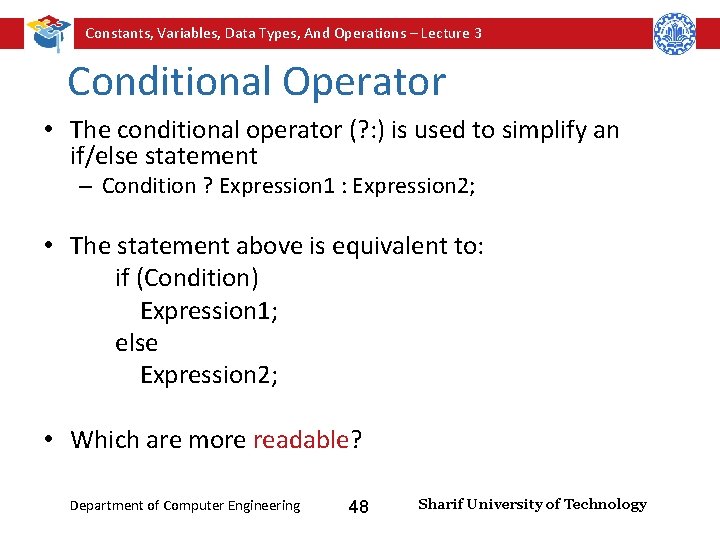
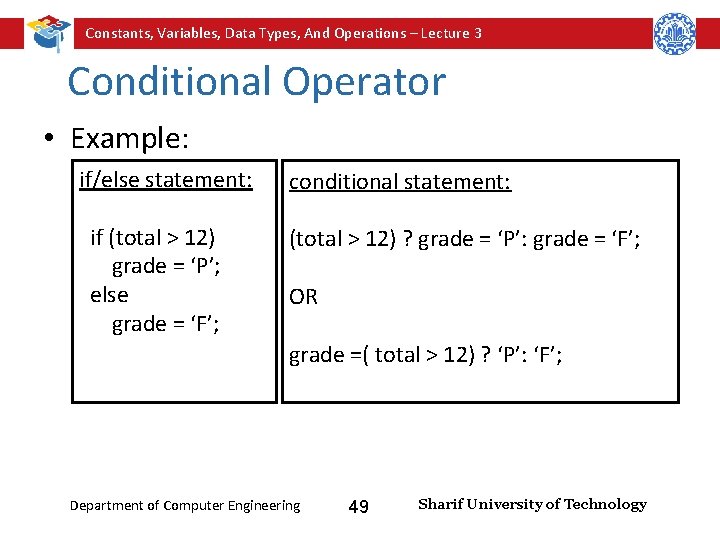
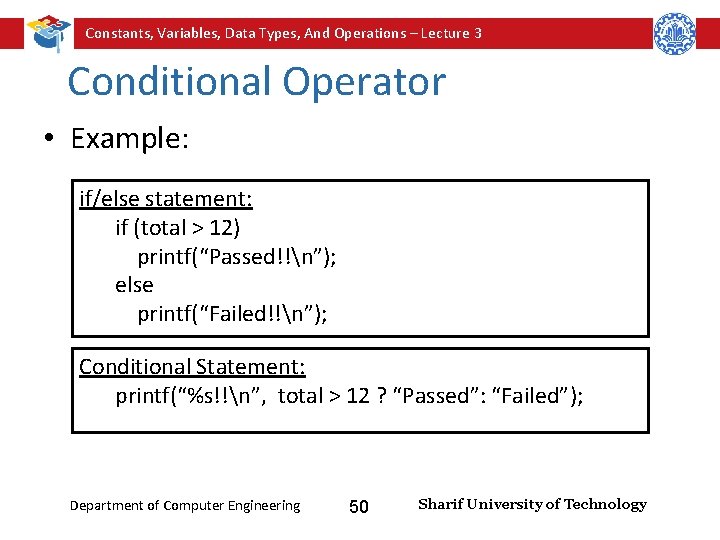
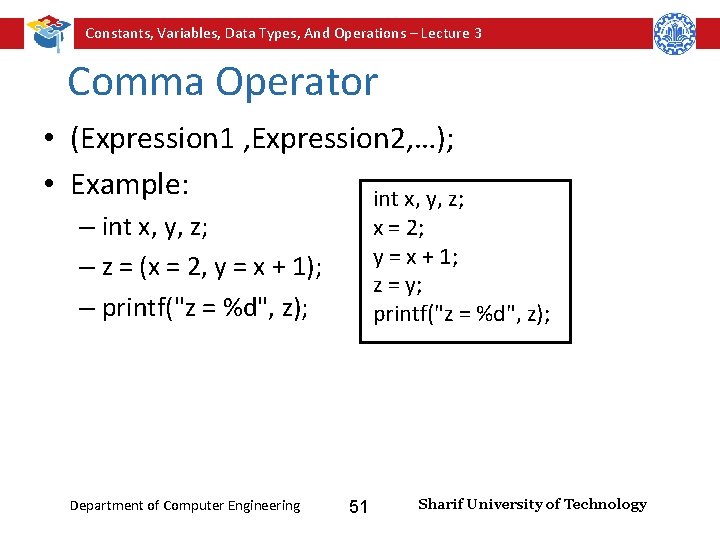
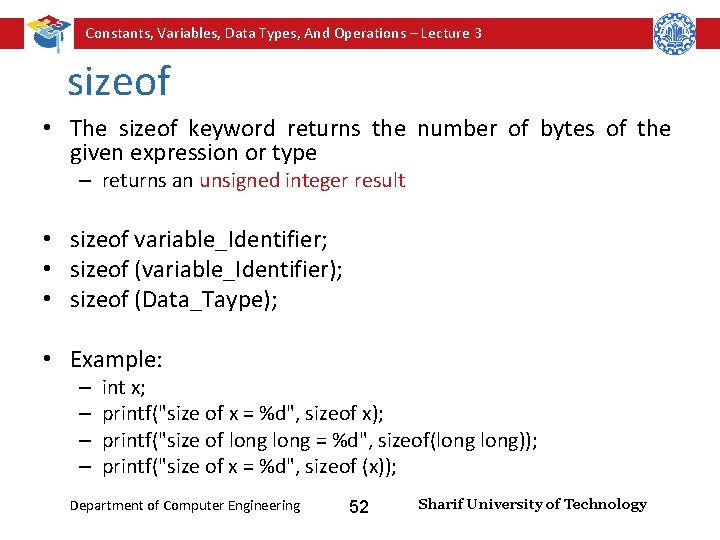
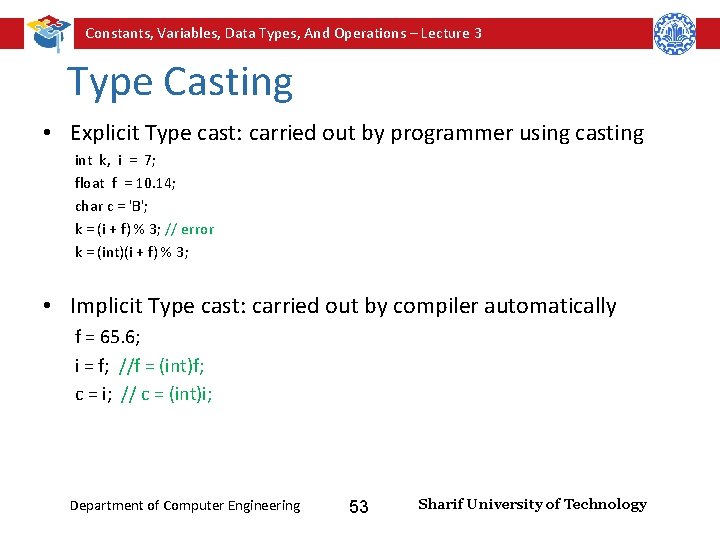
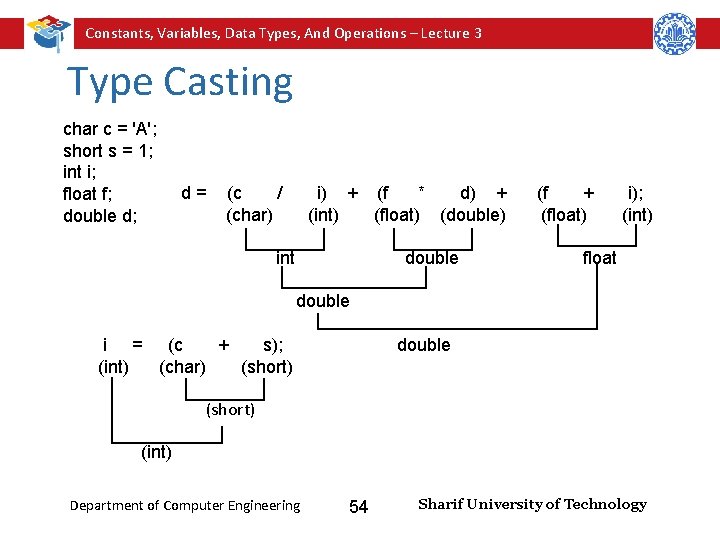
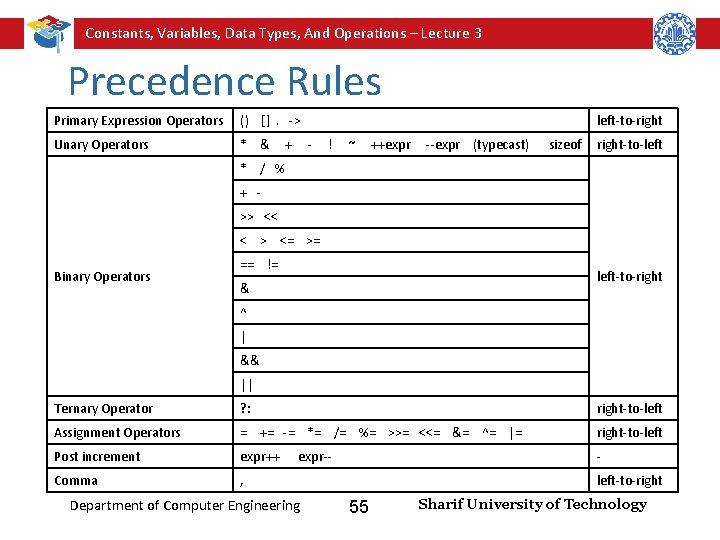
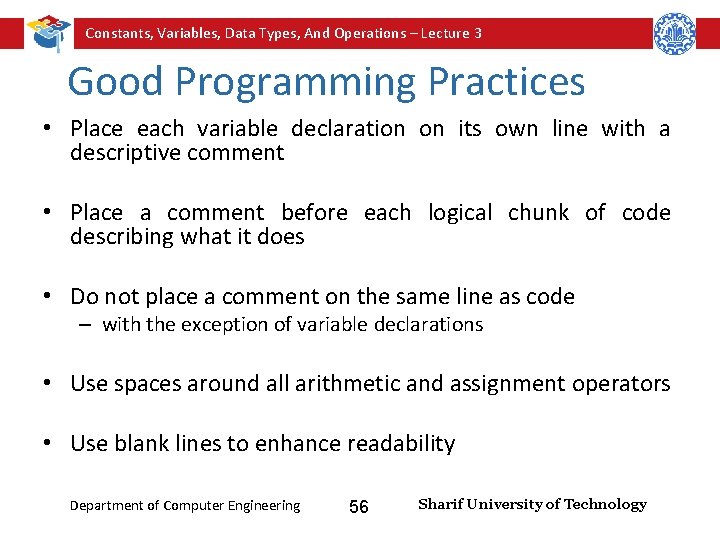
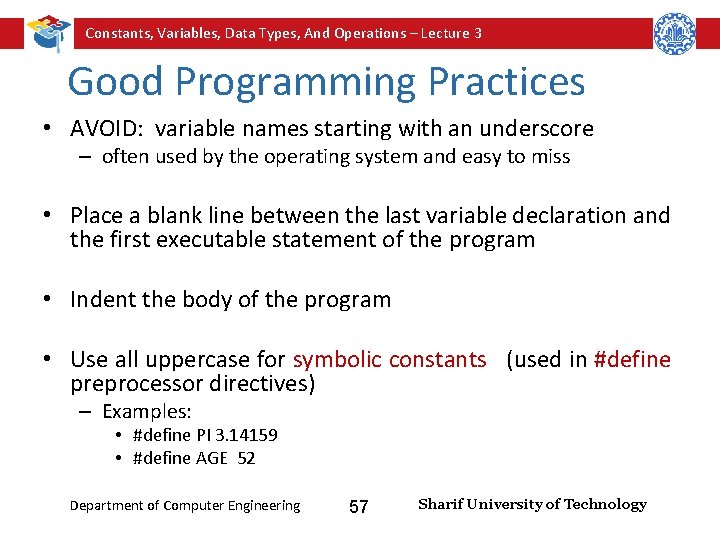
- Slides: 57
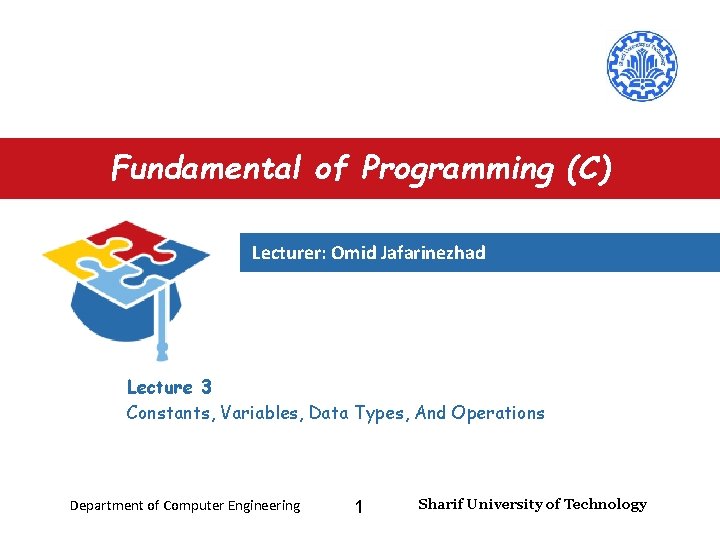
Fundamental of Programming (C) Lecturer: Omid Jafarinezhad Lecture 3 Constants, Variables, Data Types, And Operations Department of Computer Engineering 1 Sharif University of Technology
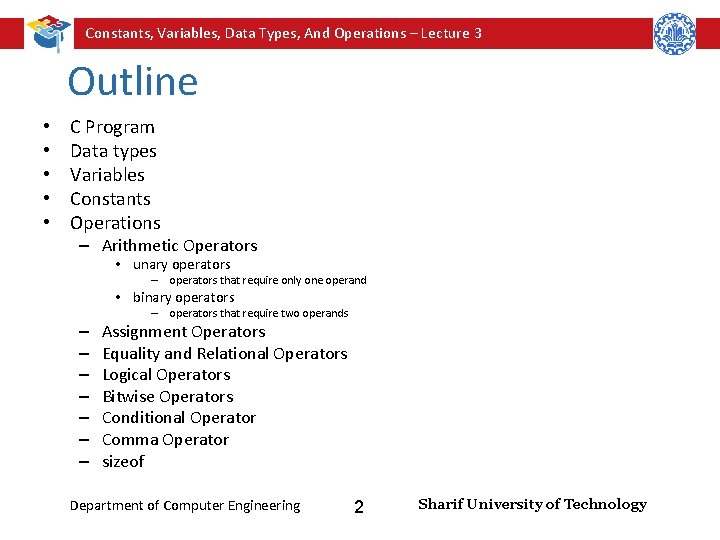
Constants, Variables, Data Types, And Operations – Lecture 3 Outline • • • C Program Data types Variables Constants Operations – Arithmetic Operators • unary operators – operators that require only one operand • binary operators – operators that require two operands – – – – Assignment Operators Equality and Relational Operators Logical Operators Bitwise Operators Conditional Operator Comma Operator sizeof Department of Computer Engineering 2 Sharif University of Technology
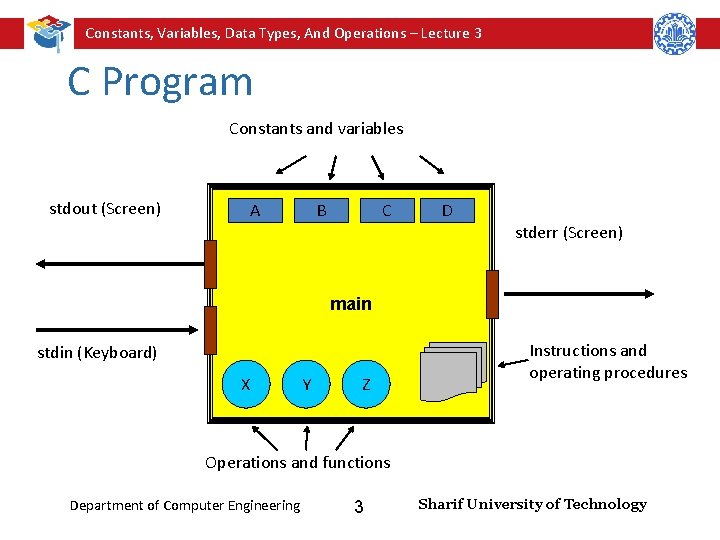
Constants, Variables, Data Types, And Operations – Lecture 3 C Program Constants and variables stdout (Screen) A B C D stderr (Screen) main stdin (Keyboard) X Y Z Instructions and operating procedures Operations and functions Department of Computer Engineering 3 Sharif University of Technology
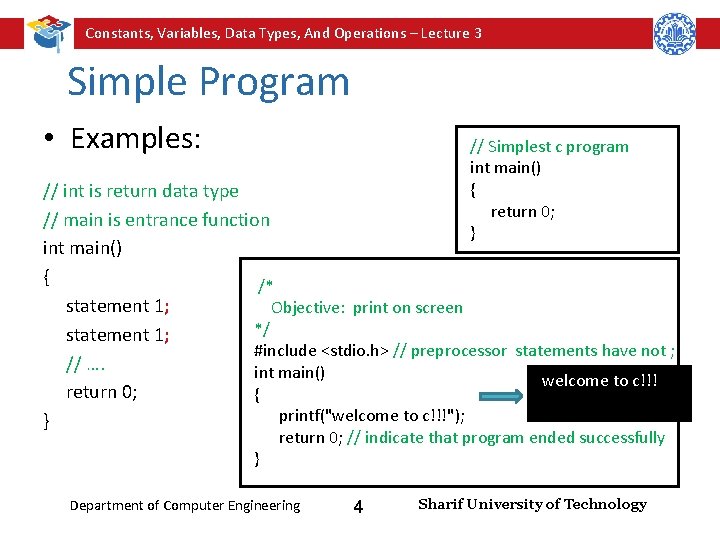
Constants, Variables, Data Types, And Operations – Lecture 3 Simple Program • Examples: // Simplest c program int main() { return 0; } // int is return data type // main is entrance function int main() { /* statement 1; Objective: print on screen */ statement 1; #include <stdio. h> // preprocessor statements have not ; // …. int main() welcome to c!!! return 0; { printf("welcome to c!!!"); } } return 0; // indicate that program ended successfully Department of Computer Engineering 4 Sharif University of Technology
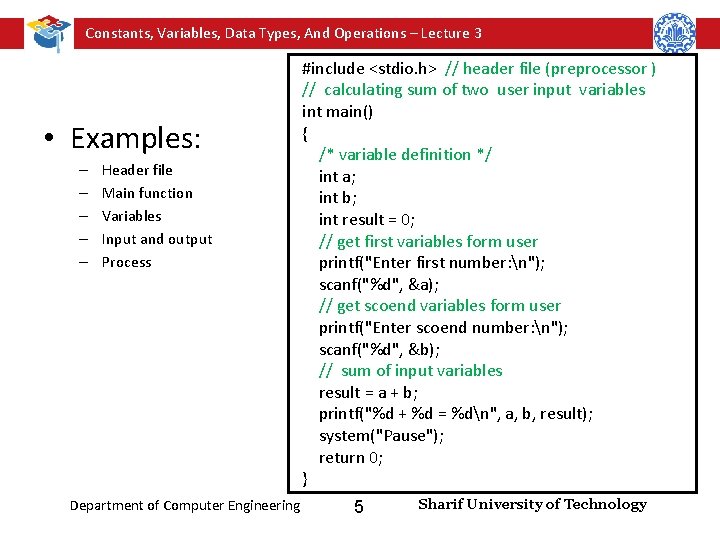
Constants, Variables, Data Types, And Operations – Lecture 3 • Examples: – – – Header file Main function Variables Input and output Process Department of Computer Engineering #include <stdio. h> // header file (preprocessor ) // calculating sum of two user input variables int main() { /* variable definition */ int a; int b; int result = 0; // get first variables form user printf("Enter first number: n"); scanf("%d", &a); // get scoend variables form user printf("Enter scoend number: n"); scanf("%d", &b); // sum of input variables result = a + b; printf("%d + %d = %dn", a, b, result); system("Pause"); return 0; } 5 Sharif University of Technology
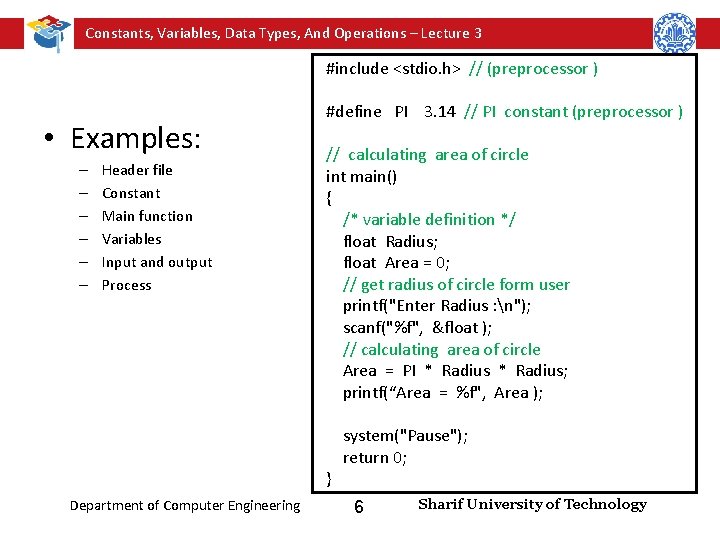
Constants, Variables, Data Types, And Operations – Lecture 3 #include <stdio. h> // (preprocessor ) • Examples: – – – Header file Constant Main function Variables Input and output Process #define PI 3. 14 // PI constant (preprocessor ) // calculating area of circle int main() { /* variable definition */ float Radius; float Area = 0; // get radius of circle form user printf("Enter Radius : n"); scanf("%f", &float ); // calculating area of circle Area = PI * Radius; printf(“Area = %f", Area ); } Department of Computer Engineering system("Pause"); return 0; 6 Sharif University of Technology
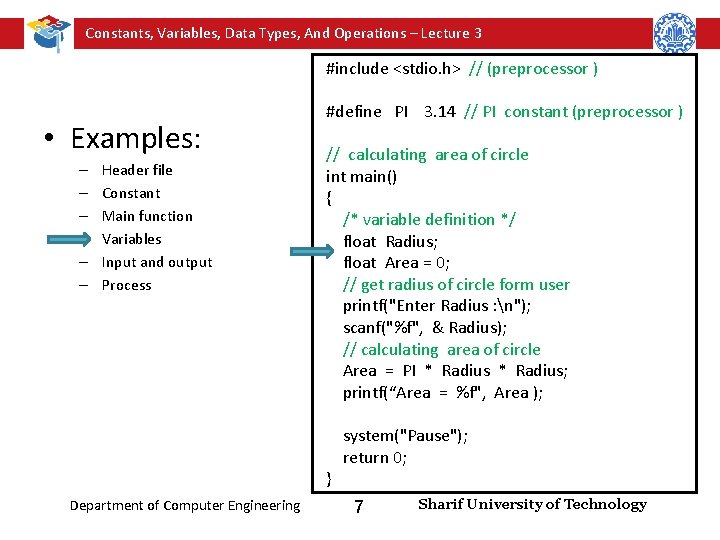
Constants, Variables, Data Types, And Operations – Lecture 3 #include <stdio. h> // (preprocessor ) • Examples: – – – Header file Constant Main function Variables Input and output Process #define PI 3. 14 // PI constant (preprocessor ) // calculating area of circle int main() { /* variable definition */ float Radius; float Area = 0; // get radius of circle form user printf("Enter Radius : n"); scanf("%f", & Radius); // calculating area of circle Area = PI * Radius; printf(“Area = %f", Area ); } Department of Computer Engineering system("Pause"); return 0; 7 Sharif University of Technology
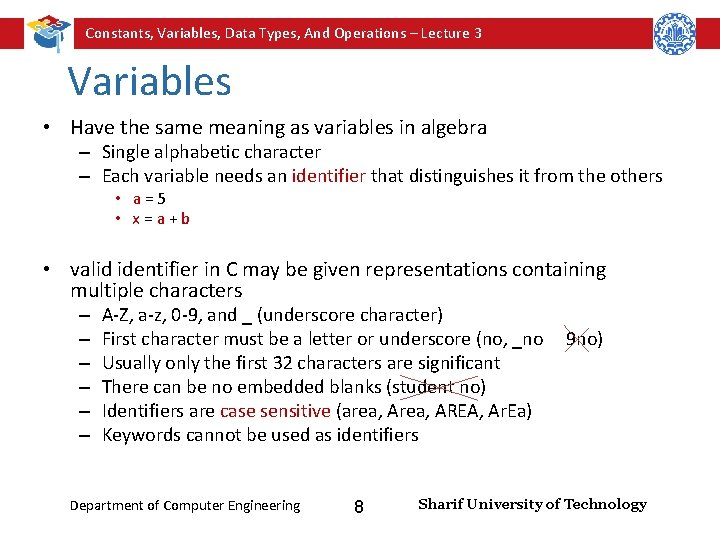
Constants, Variables, Data Types, And Operations – Lecture 3 Variables • Have the same meaning as variables in algebra – Single alphabetic character – Each variable needs an identifier that distinguishes it from the others • a=5 • x=a+b • valid identifier in C may be given representations containing multiple characters – – – A-Z, a-z, 0 -9, and _ (underscore character) First character must be a letter or underscore (no, _no Usually only the first 32 characters are significant There can be no embedded blanks (student no) Identifiers are case sensitive (area, AREA, Ar. Ea) Keywords cannot be used as identifiers Department of Computer Engineering 8 9 no) Sharif University of Technology
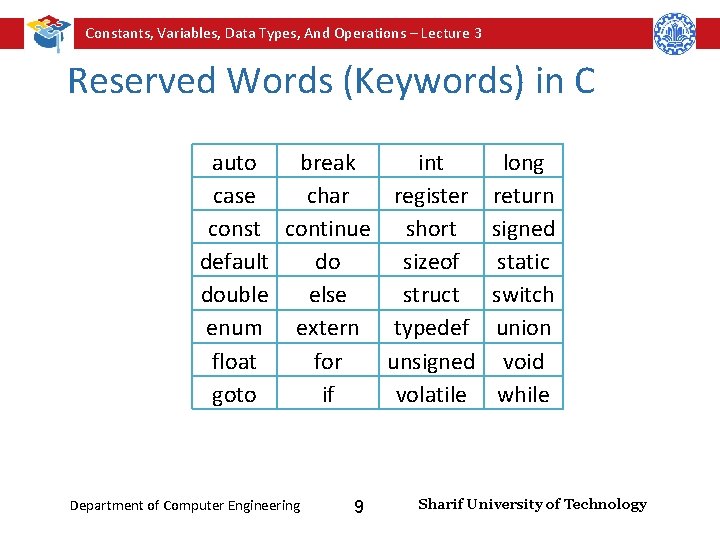
Constants, Variables, Data Types, And Operations – Lecture 3 Reserved Words (Keywords) in C auto break int case char register const continue short default do sizeof double else struct enum extern typedef float for unsigned goto if volatile Department of Computer Engineering 9 long return signed static switch union void while Sharif University of Technology
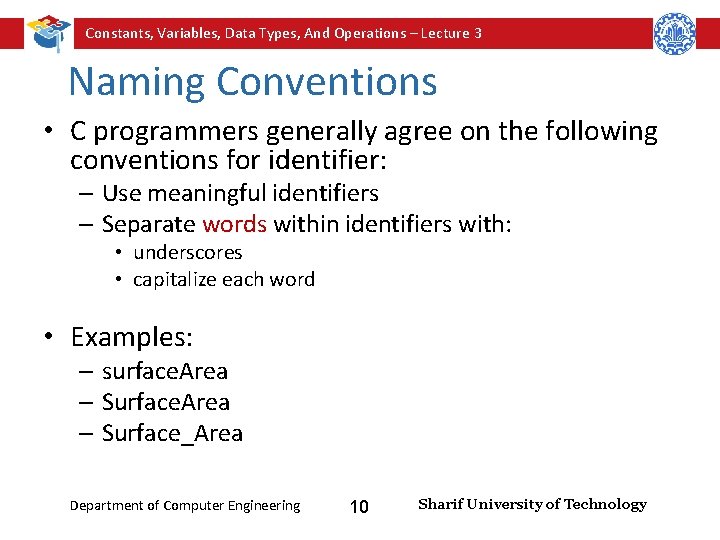
Constants, Variables, Data Types, And Operations – Lecture 3 Naming Conventions • C programmers generally agree on the following conventions for identifier: – Use meaningful identifiers – Separate words within identifiers with: • underscores • capitalize each word • Examples: – surface. Area – Surface_Area Department of Computer Engineering 10 Sharif University of Technology
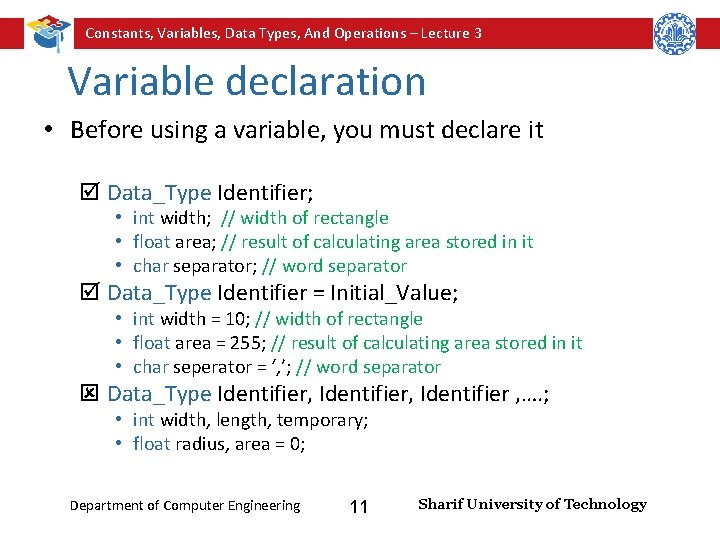
Constants, Variables, Data Types, And Operations – Lecture 3 Variable declaration • Before using a variable, you must declare it þ Data_Type Identifier; • int width; // width of rectangle • float area; // result of calculating area stored in it • char separator; // word separator þ Data_Type Identifier = Initial_Value; • int width = 10; // width of rectangle • float area = 255; // result of calculating area stored in it • char seperator = ‘, ’; // word separator Data_Type Identifier, Identifier , …. ; • int width, length, temporary; • float radius, area = 0; Department of Computer Engineering 11 Sharif University of Technology
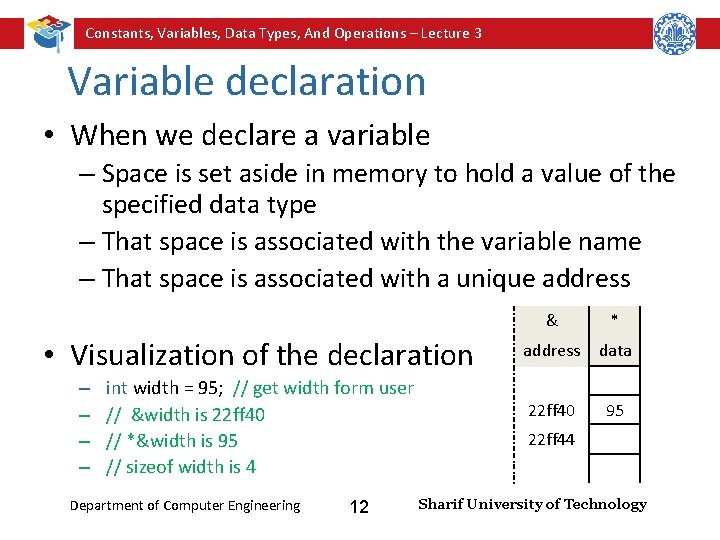
Constants, Variables, Data Types, And Operations – Lecture 3 Variable declaration • When we declare a variable – Space is set aside in memory to hold a value of the specified data type – That space is associated with the variable name – That space is associated with a unique address • Visualization of the declaration – – int width = 95; // get width form user // &width is 22 ff 40 // *&width is 95 // sizeof width is 4 Department of Computer Engineering 12 & * address data 22 ff 40 95 22 ff 44 Sharif University of Technology
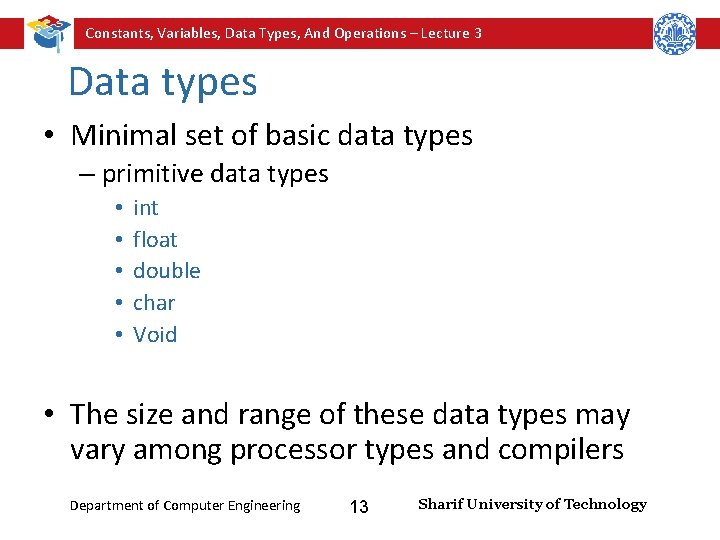
Constants, Variables, Data Types, And Operations – Lecture 3 Data types • Minimal set of basic data types – primitive data types • • • int float double char Void • The size and range of these data types may vary among processor types and compilers Department of Computer Engineering 13 Sharif University of Technology
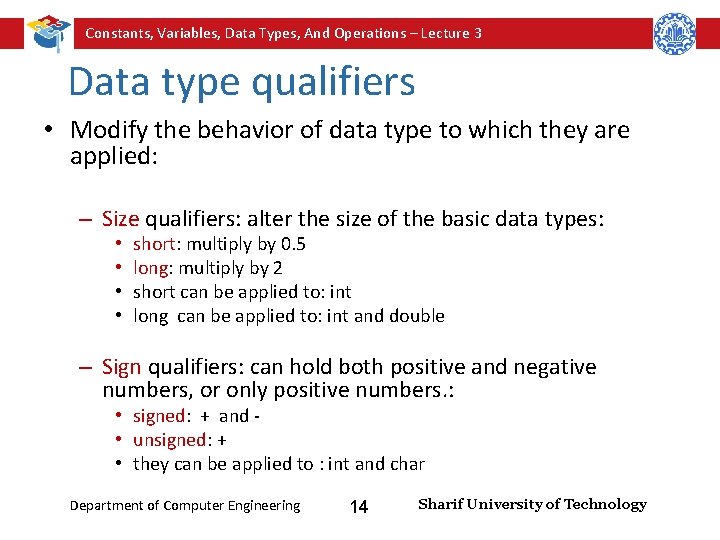
Constants, Variables, Data Types, And Operations – Lecture 3 Data type qualifiers • Modify the behavior of data type to which they are applied: – Size qualifiers: alter the size of the basic data types: • • short: multiply by 0. 5 long: multiply by 2 short can be applied to: int long can be applied to: int and double – Sign qualifiers: can hold both positive and negative numbers, or only positive numbers. : • signed: + and • unsigned: + • they can be applied to : int and char Department of Computer Engineering 14 Sharif University of Technology
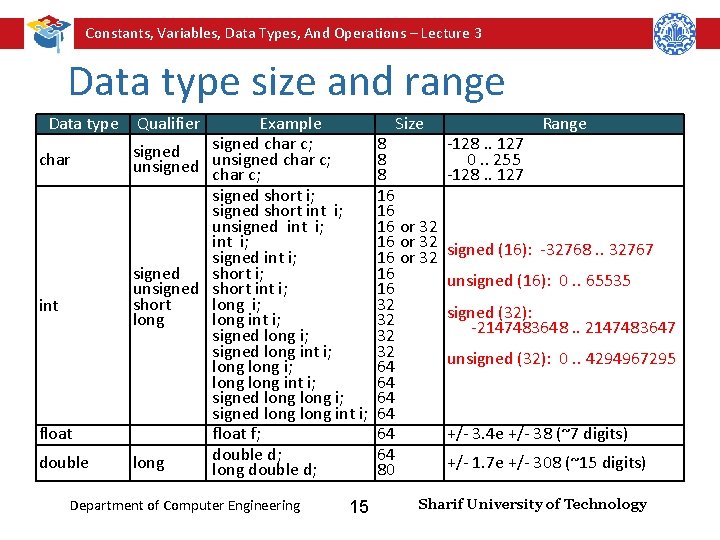
Constants, Variables, Data Types, And Operations – Lecture 3 Data type size and range Data type Qualifier char int float double Example signed char c; unsigned char c; signed short int i; unsigned int i; short i; signed unsigned short int i; long i; short long int i; long signed long i; signed long int i; long int i; signed long int i; float f; double d; long double d; Department of Computer Engineering 15 Size 8 8 8 16 16 16 or 32 16 16 32 32 64 64 64 80 -128. . 127 0. . 255 -128. . 127 Range signed (16): -32768. . 32767 unsigned (16): 0. . 65535 signed (32): -2147483648. . 2147483647 unsigned (32): 0. . 4294967295 +/- 3. 4 e +/- 38 (~7 digits) +/- 1. 7 e +/- 308 (~15 digits) Sharif University of Technology
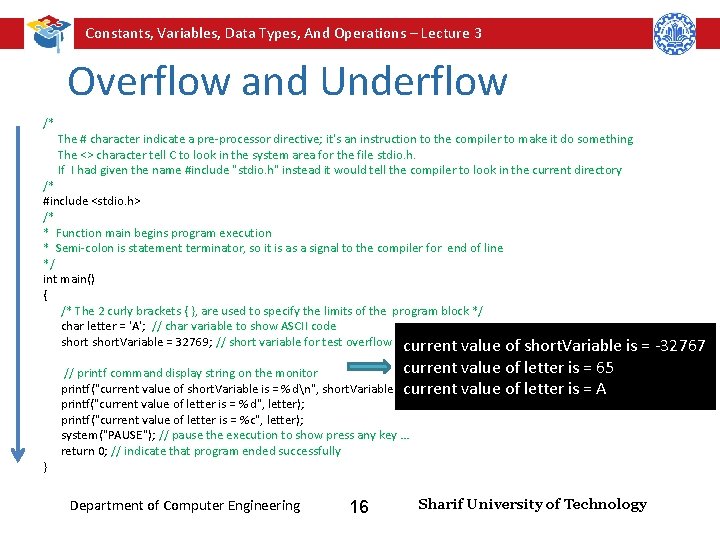
Constants, Variables, Data Types, And Operations – Lecture 3 Overflow and Underflow /* The # character indicate a pre-processor directive; it's an instruction to the compiler to make it do something The <> character tell C to look in the system area for the file stdio. h. If I had given the name #include "stdio. h" instead it would tell the compiler to look in the current directory /* #include <stdio. h> /* * Function main begins program execution * Semi-colon is statement terminator, so it is as a signal to the compiler for end of line */ int main() { /* The 2 curly brackets { }, are used to specify the limits of the program block */ char letter = 'A'; // char variable to show ASCII code short. Variable = 32769; // short variable for test overflow current value of short. Variable is = -32767 current value of letter is = 65 // printf command display string on the monitor printf("current value of short. Variable is = %dn", short. Variable); current value of letter is = A } printf("current value of letter is = % d", letter); printf("current value of letter is = %c", letter); system("PAUSE"); // pause the execution to show press any key … return 0; // indicate that program ended successfully Department of Computer Engineering 16 Sharif University of Technology
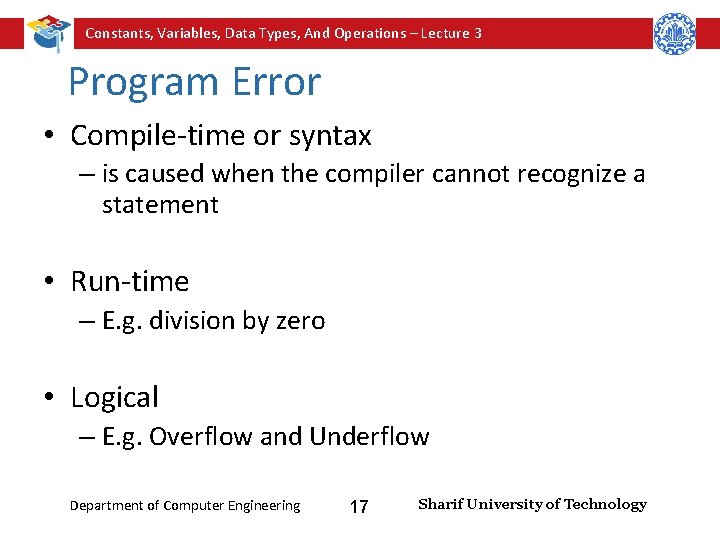
Constants, Variables, Data Types, And Operations – Lecture 3 Program Error • Compile-time or syntax – is caused when the compiler cannot recognize a statement • Run-time – E. g. division by zero • Logical – E. g. Overflow and Underflow Department of Computer Engineering 17 Sharif University of Technology
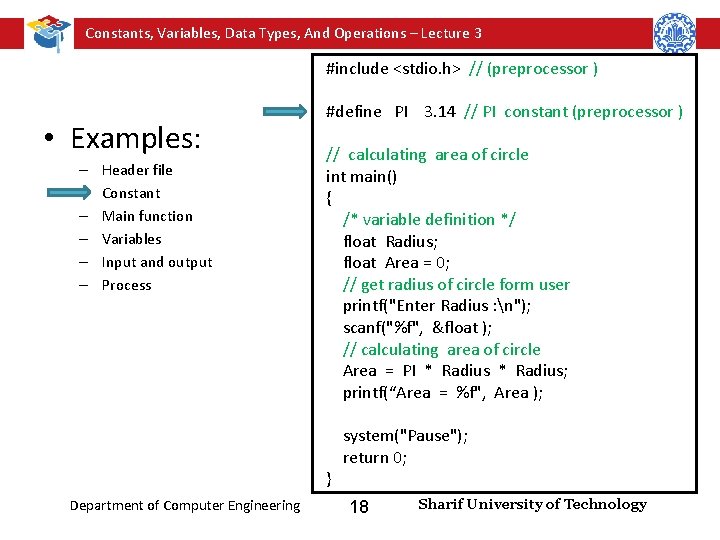
Constants, Variables, Data Types, And Operations – Lecture 3 #include <stdio. h> // (preprocessor ) • Examples: – – – Header file Constant Main function Variables Input and output Process #define PI 3. 14 // PI constant (preprocessor ) // calculating area of circle int main() { /* variable definition */ float Radius; float Area = 0; // get radius of circle form user printf("Enter Radius : n"); scanf("%f", &float ); // calculating area of circle Area = PI * Radius; printf(“Area = %f", Area ); } Department of Computer Engineering system("Pause"); return 0; 18 Sharif University of Technology
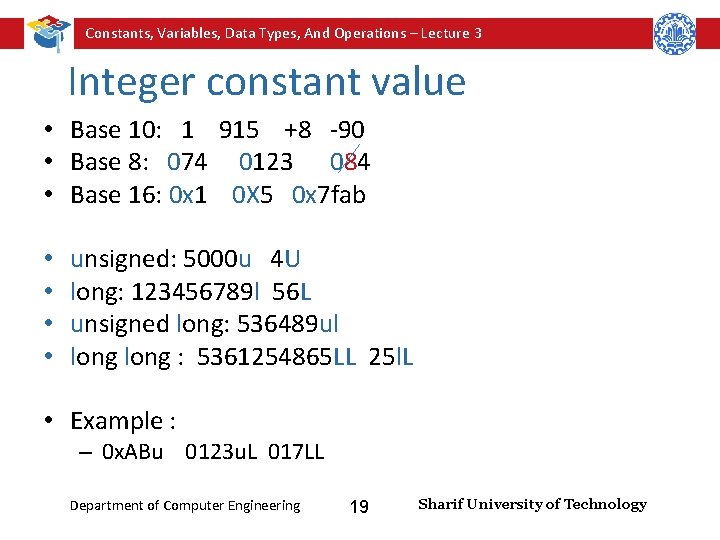
Constants, Variables, Data Types, And Operations – Lecture 3 Integer constant value • Base 10: 1 915 +8 -90 • Base 8: 074 0123 084 • Base 16: 0 x 1 0 X 5 0 x 7 fab • • unsigned: 5000 u 4 U long: 123456789 l 56 L unsigned long: 536489 ul long : 5361254865 LL 25 l. L • Example : – 0 x. ABu 0123 u. L 017 LL Department of Computer Engineering 19 Sharif University of Technology
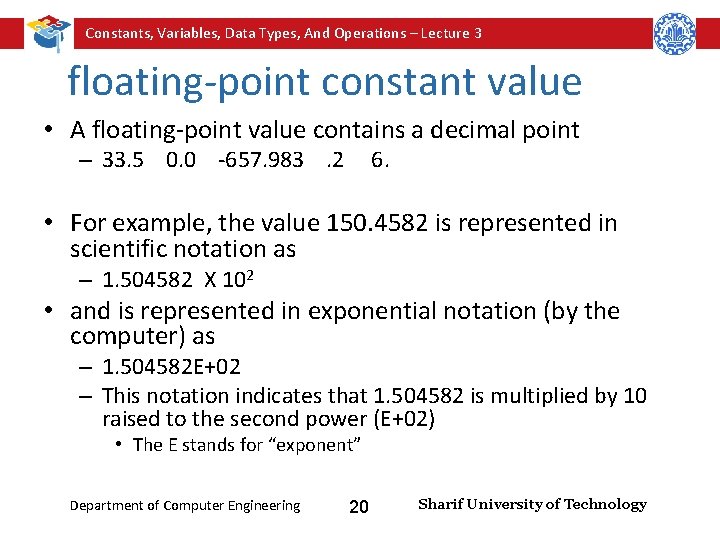
Constants, Variables, Data Types, And Operations – Lecture 3 floating-point constant value • A floating-point value contains a decimal point – 33. 5 0. 0 -657. 983. 2 6. • For example, the value 150. 4582 is represented in scientific notation as – 1. 504582 X 102 • and is represented in exponential notation (by the computer) as – 1. 504582 E+02 – This notation indicates that 1. 504582 is multiplied by 10 raised to the second power (E+02) • The E stands for “exponent” Department of Computer Engineering 20 Sharif University of Technology
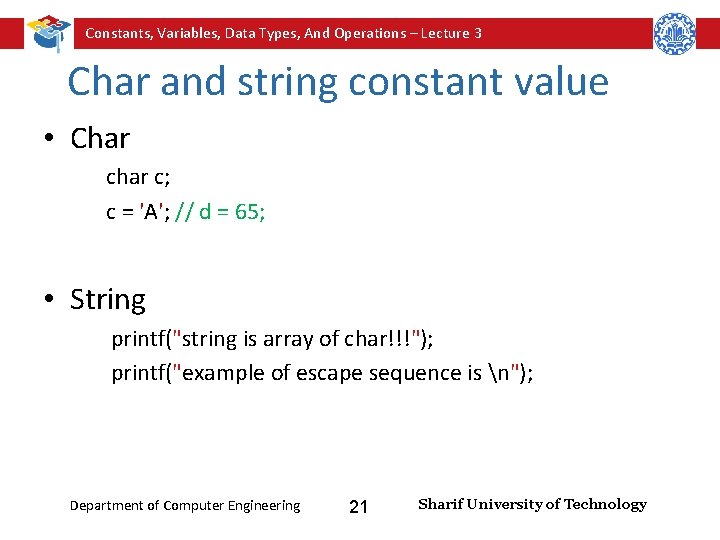
Constants, Variables, Data Types, And Operations – Lecture 3 Char and string constant value • Char c; c = 'A'; // d = 65; • String printf("string is array of char!!!"); printf("example of escape sequence is n"); Department of Computer Engineering 21 Sharif University of Technology
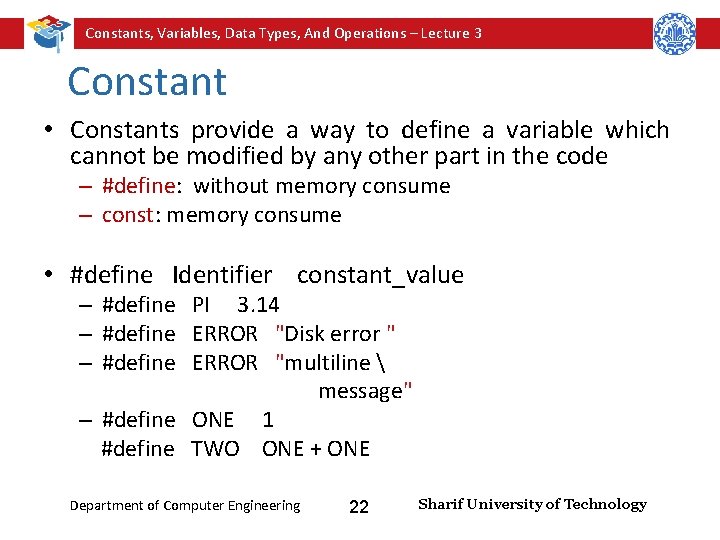
Constants, Variables, Data Types, And Operations – Lecture 3 Constant • Constants provide a way to define a variable which cannot be modified by any other part in the code – #define: without memory consume – const: memory consume • #define Identifier constant_value – #define PI 3. 14 – #define ERROR "Disk error " – #define ERROR "multiline message" – #define ONE 1 #define TWO ONE + ONE Department of Computer Engineering 22 Sharif University of Technology
![Constants Variables Data Types And Operations Lecture 3 Constant const DataType Identifier Constants, Variables, Data Types, And Operations – Lecture 3 Constant • const [Data_Type] Identifier](https://slidetodoc.com/presentation_image_h2/604401fbd50d99da71f7f8c2f3a179fd/image-23.jpg)
Constants, Variables, Data Types, And Operations – Lecture 3 Constant • const [Data_Type] Identifier = constant_value; – const p = 3; // const int p = 3; – const p; p = 3. 14; // compile error – const p = 3. 14; // p = 3 because default is int – const float p = 3. 14; Department of Computer Engineering 23 Sharif University of Technology
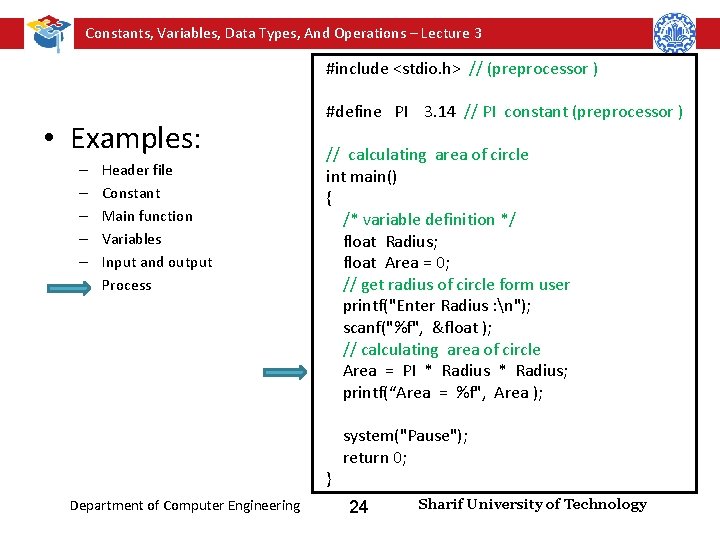
Constants, Variables, Data Types, And Operations – Lecture 3 #include <stdio. h> // (preprocessor ) • Examples: – – – Header file Constant Main function Variables Input and output Process #define PI 3. 14 // PI constant (preprocessor ) // calculating area of circle int main() { /* variable definition */ float Radius; float Area = 0; // get radius of circle form user printf("Enter Radius : n"); scanf("%f", &float ); // calculating area of circle Area = PI * Radius; printf(“Area = %f", Area ); } Department of Computer Engineering system("Pause"); return 0; 24 Sharif University of Technology
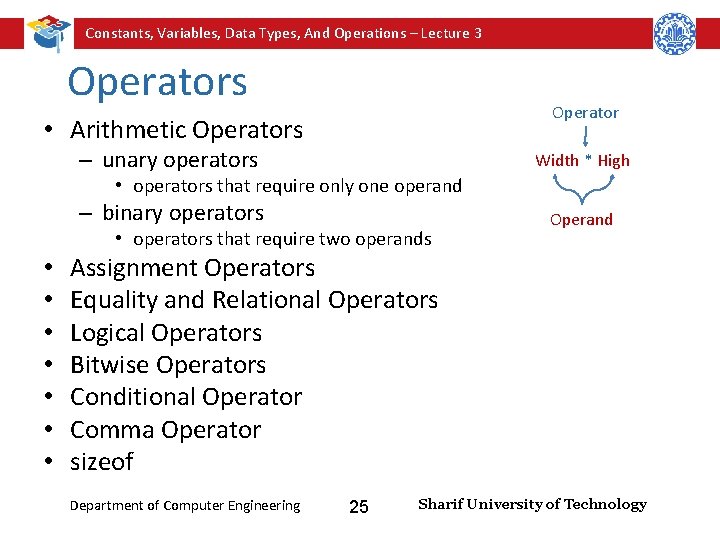
Constants, Variables, Data Types, And Operations – Lecture 3 Operators Operator • Arithmetic Operators – unary operators Width * High – binary operators Operand • operators that require only one operand • operators that require two operands • • Assignment Operators Equality and Relational Operators Logical Operators Bitwise Operators Conditional Operator Comma Operator sizeof Department of Computer Engineering 25 Sharif University of Technology
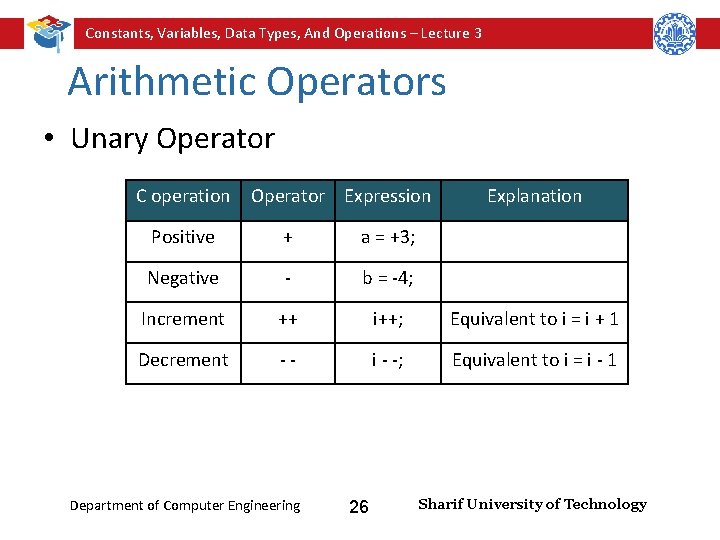
Constants, Variables, Data Types, And Operations – Lecture 3 Arithmetic Operators • Unary Operator C operation Operator Expression Explanation Positive + a = +3; Negative - b = -4; Increment ++ i++; Equivalent to i = i + 1 Decrement -- i - -; Equivalent to i = i - 1 Department of Computer Engineering 26 Sharif University of Technology
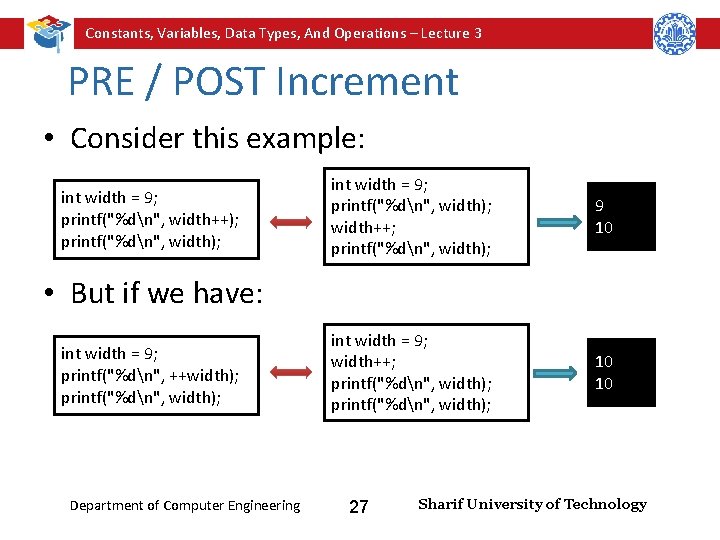
Constants, Variables, Data Types, And Operations – Lecture 3 PRE / POST Increment • Consider this example: int width = 9; printf("%dn", width++); printf("%dn", width); int width = 9; printf("%dn", width); width++; printf("%dn", width); 9 10 int width = 9; width++; printf("%dn", width); 10 10 • But if we have: int width = 9; printf("%dn", ++width); printf("%dn", width); Department of Computer Engineering 27 Sharif University of Technology
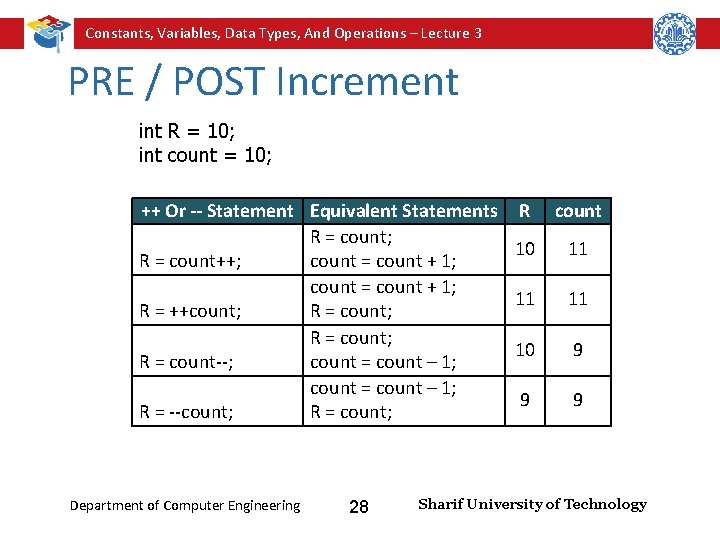
Constants, Variables, Data Types, And Operations – Lecture 3 PRE / POST Increment int R = 10; int count = 10; ++ Or -- Statement Equivalent Statements R count R = count; 10 11 R = count++; count = count + 1; 11 11 R = ++count; R = count; 10 9 R = count--; count = count – 1; 9 9 R = --count; R = count; Department of Computer Engineering 28 Sharif University of Technology
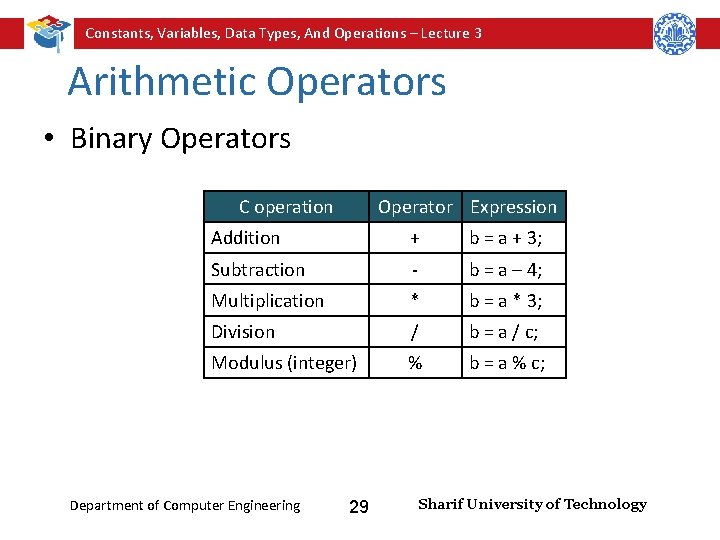
Constants, Variables, Data Types, And Operations – Lecture 3 Arithmetic Operators • Binary Operators C operation Operator Expression Addition + b = a + 3; Subtraction - b = a – 4; Multiplication * b = a * 3; Division / b = a / c; Modulus (integer) % b = a % c; Department of Computer Engineering 29 Sharif University of Technology
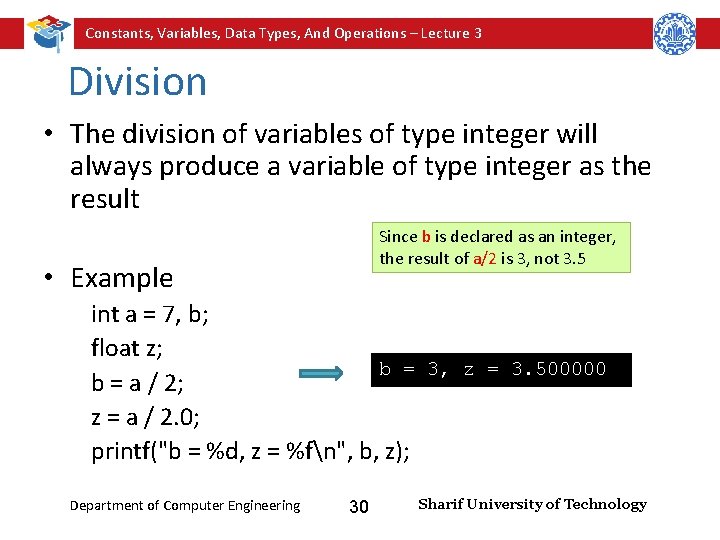
Constants, Variables, Data Types, And Operations – Lecture 3 Division • The division of variables of type integer will always produce a variable of type integer as the result Since b is declared as an integer, the result of a/2 is 3, not 3. 5 • Example int a = 7, b; float z; b = a / 2; z = a / 2. 0; printf("b = %d, z = %fn", b, z); Department of Computer Engineering 30 3, z = 3. 500000 Sharif University of Technology
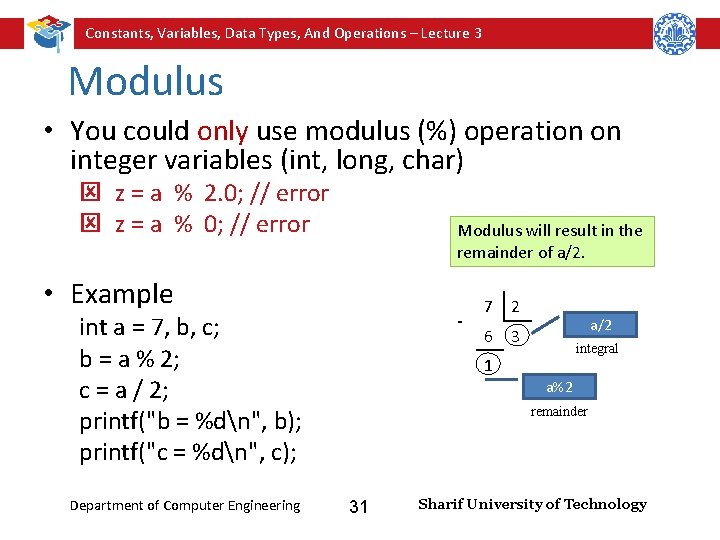
Constants, Variables, Data Types, And Operations – Lecture 3 Modulus • You could only use modulus (%) operation on integer variables (int, long, char) z = a % 2. 0; // error z = a % 0; // error Modulus will result in the remainder of a/2. • Example - int a = 7, b, c; b = a % 2; c = a / 2; printf("b = %dn", b); printf("c = %dn", c); Department of Computer Engineering 7 2 6 3 a/2 integral 1 a%2 remainder 31 Sharif University of Technology
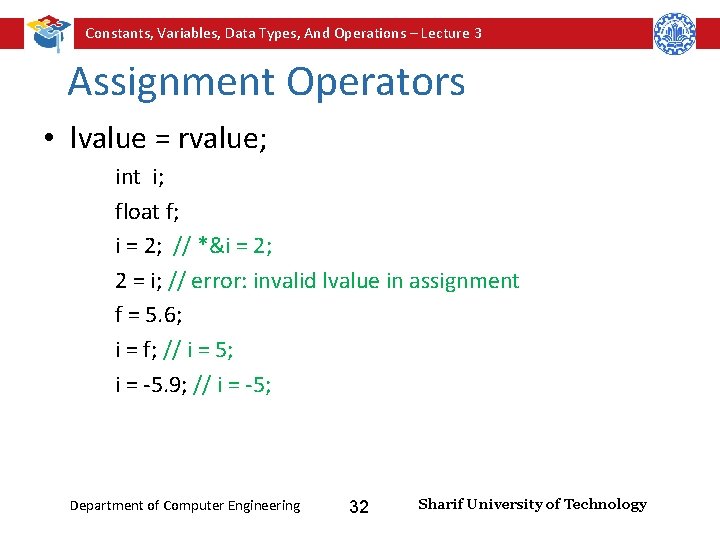
Constants, Variables, Data Types, And Operations – Lecture 3 Assignment Operators • lvalue = rvalue; int i; float f; i = 2; // *&i = 2; 2 = i; // error: invalid lvalue in assignment f = 5. 6; i = f; // i = 5; i = -5. 9; // i = -5; Department of Computer Engineering 32 Sharif University of Technology
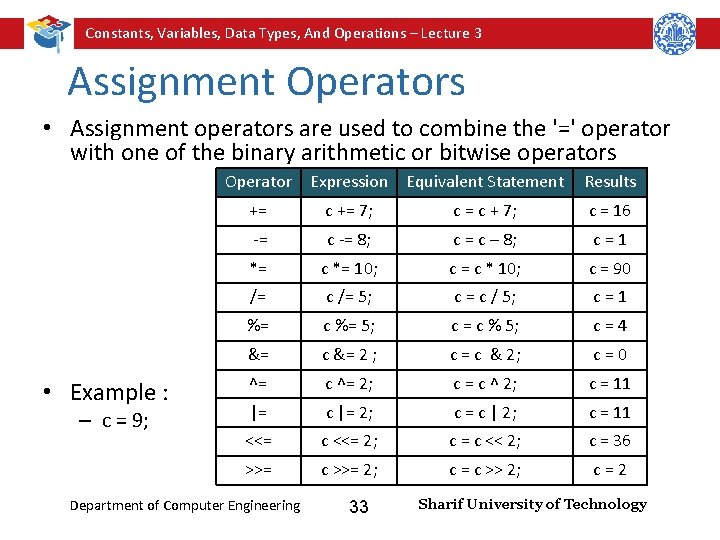
Constants, Variables, Data Types, And Operations – Lecture 3 Assignment Operators • Assignment operators are used to combine the '=' operator with one of the binary arithmetic or bitwise operators Operator • Example : – c = 9; Expression Equivalent Statement Results += c += 7; c = c + 7; c = 16 -= c -= 8; c = c – 8; c=1 *= c *= 10; c = c * 10; c = 90 /= c /= 5; c = c / 5; c=1 %= c %= 5; c = c % 5; c=4 &= c &= 2 ; c = c & 2; c=0 ^= c ^= 2; c = c ^ 2; c = 11 |= c |= 2; c = c | 2; c = 11 <<= c <<= 2; c = c << 2; c = 36 >>= c >>= 2; c = c >> 2; c=2 Department of Computer Engineering 33 Sharif University of Technology
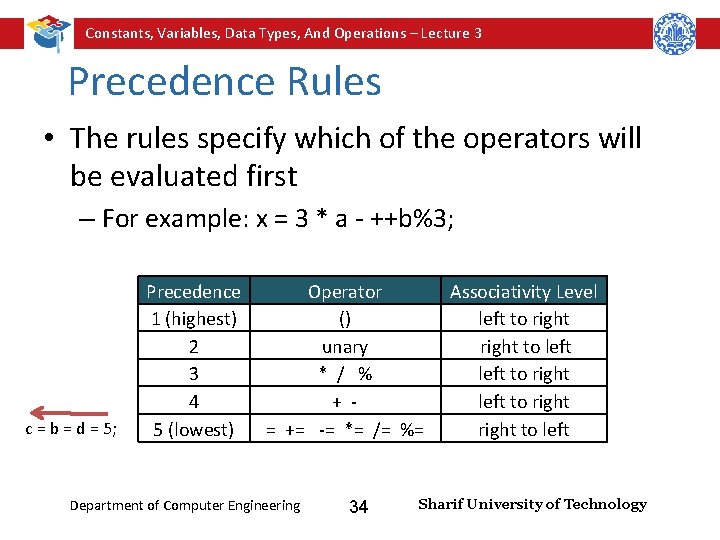
Constants, Variables, Data Types, And Operations – Lecture 3 Precedence Rules • The rules specify which of the operators will be evaluated first – For example: x = 3 * a - ++b%3; c = b = d = 5; Precedence 1 (highest) 2 3 4 5 (lowest) Operator () unary * / % + = += -= *= /= %= Department of Computer Engineering 34 Associativity Level left to right to left to right to left Sharif University of Technology
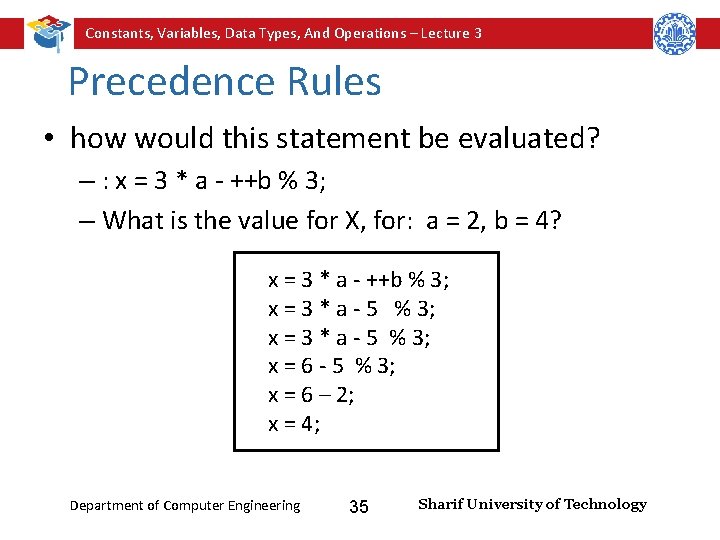
Constants, Variables, Data Types, And Operations – Lecture 3 Precedence Rules • how would this statement be evaluated? – : x = 3 * a - ++b % 3; – What is the value for X, for: a = 2, b = 4? x = 3 * a - ++b % 3; x = 3 * a - 5 % 3; x = 6 – 2; x = 4; Department of Computer Engineering 35 Sharif University of Technology
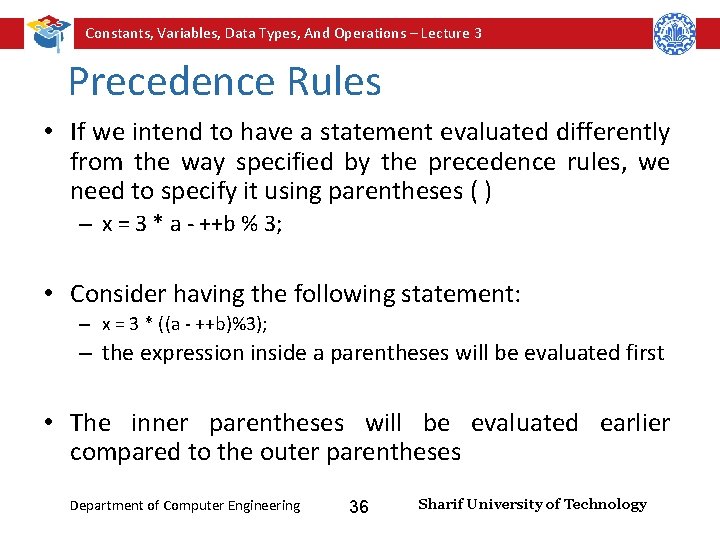
Constants, Variables, Data Types, And Operations – Lecture 3 Precedence Rules • If we intend to have a statement evaluated differently from the way specified by the precedence rules, we need to specify it using parentheses ( ) – x = 3 * a - ++b % 3; • Consider having the following statement: – x = 3 * ((a - ++b)%3); – the expression inside a parentheses will be evaluated first • The inner parentheses will be evaluated earlier compared to the outer parentheses Department of Computer Engineering 36 Sharif University of Technology
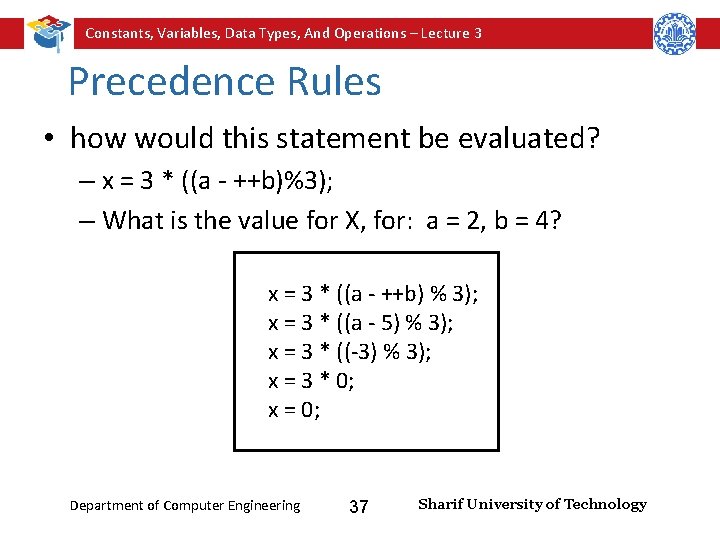
Constants, Variables, Data Types, And Operations – Lecture 3 Precedence Rules • how would this statement be evaluated? – x = 3 * ((a - ++b)%3); – What is the value for X, for: a = 2, b = 4? x = 3 * ((a - ++b) % 3); x = 3 * ((a - 5) % 3); x = 3 * ((-3) % 3); x = 3 * 0; x = 0; Department of Computer Engineering 37 Sharif University of Technology
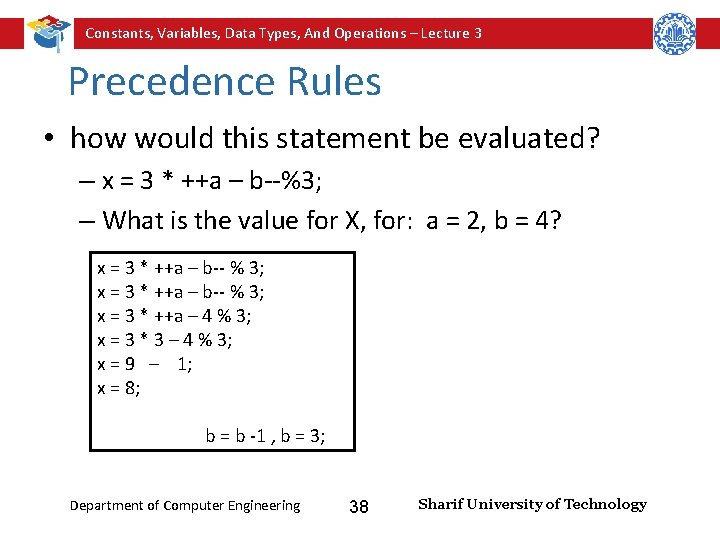
Constants, Variables, Data Types, And Operations – Lecture 3 Precedence Rules • how would this statement be evaluated? – x = 3 * ++a – b--%3; – What is the value for X, for: a = 2, b = 4? x = 3 * ++a – b-- % 3; x = 3 * ++a – 4 % 3; x = 3 * 3 – 4 % 3; x = 9 – 1; x = 8; b = b -1 , b = 3; Department of Computer Engineering 38 Sharif University of Technology
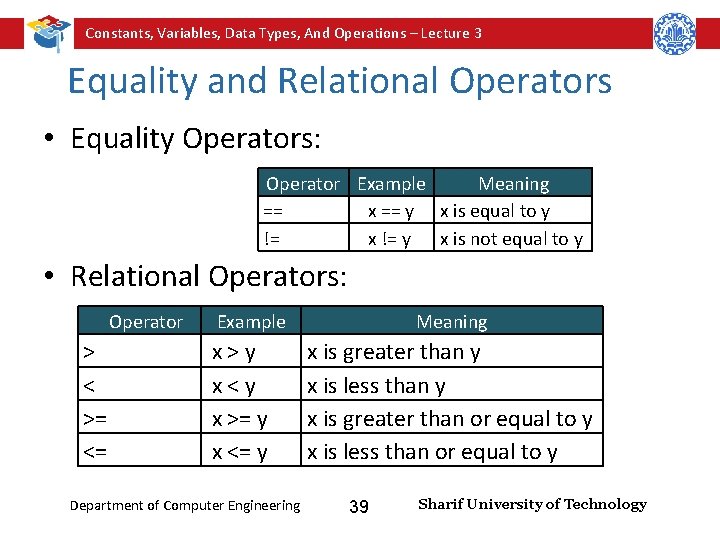
Constants, Variables, Data Types, And Operations – Lecture 3 Equality and Relational Operators • Equality Operators: Operator Example Meaning == x == y x is equal to y != x != y x is not equal to y • Relational Operators: Operator > < >= <= Example x>y x<y x >= y x <= y Department of Computer Engineering Meaning x is greater than y x is less than y x is greater than or equal to y x is less than or equal to y 39 Sharif University of Technology
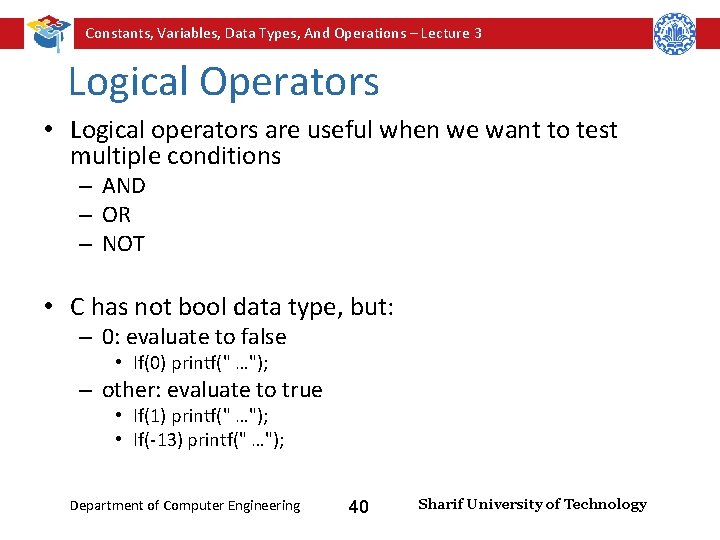
Constants, Variables, Data Types, And Operations – Lecture 3 Logical Operators • Logical operators are useful when we want to test multiple conditions – AND – OR – NOT • C has not bool data type, but: – 0: evaluate to false • If(0) printf(" …"); – other: evaluate to true • If(1) printf(" …"); • If(-13) printf(" …"); Department of Computer Engineering 40 Sharif University of Technology
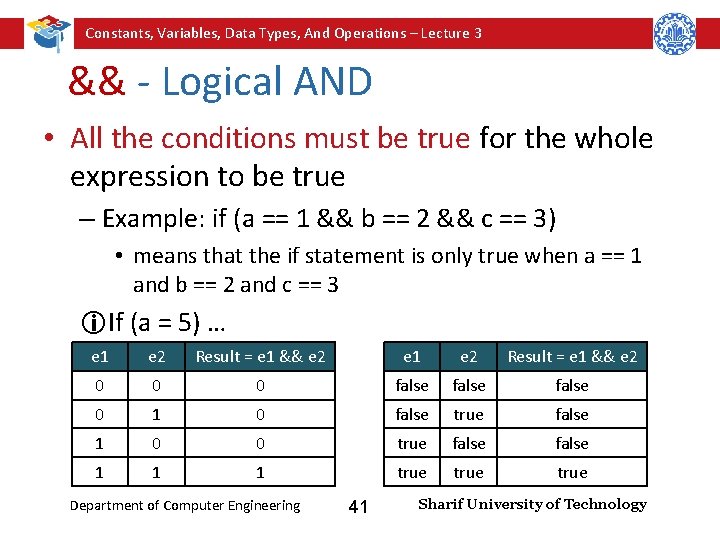
Constants, Variables, Data Types, And Operations – Lecture 3 && - Logical AND • All the conditions must be true for the whole expression to be true – Example: if (a == 1 && b == 2 && c == 3) • means that the if statement is only true when a == 1 and b == 2 and c == 3 If (a = 5) … e 1 e 2 Result = e 1 && e 2 0 0 0 false 0 1 0 false true false 1 0 0 true false 1 1 1 true Department of Computer Engineering 41 Sharif University of Technology
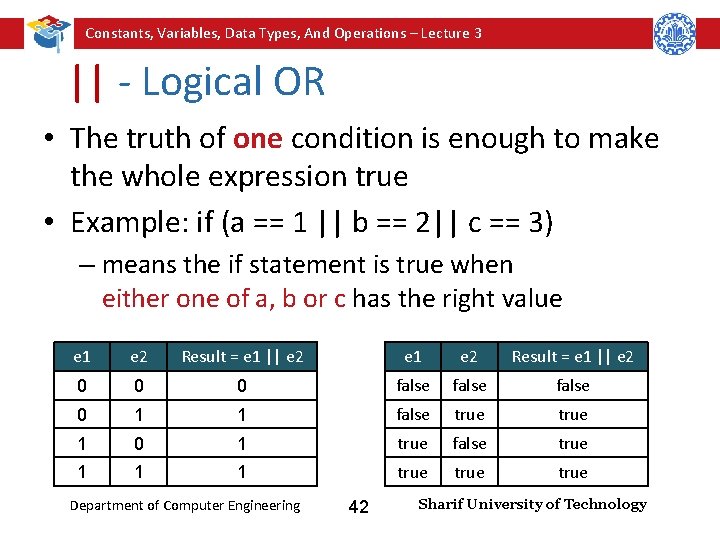
Constants, Variables, Data Types, And Operations – Lecture 3 || - Logical OR • The truth of one condition is enough to make the whole expression true • Example: if (a == 1 || b == 2|| c == 3) – means the if statement is true when either one of a, b or c has the right value e 1 e 2 Result = e 1 || e 2 0 0 0 false 0 1 1 false true 1 0 1 true false true 1 1 1 true Department of Computer Engineering 42 Sharif University of Technology
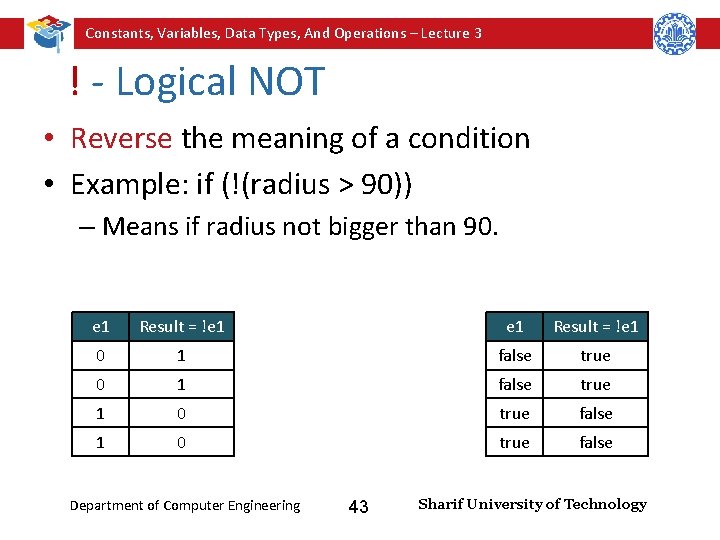
Constants, Variables, Data Types, And Operations – Lecture 3 ! - Logical NOT • Reverse the meaning of a condition • Example: if (!(radius > 90)) – Means if radius not bigger than 90. e 1 Result = !e 1 0 1 false true 1 0 true false Department of Computer Engineering 43 Sharif University of Technology
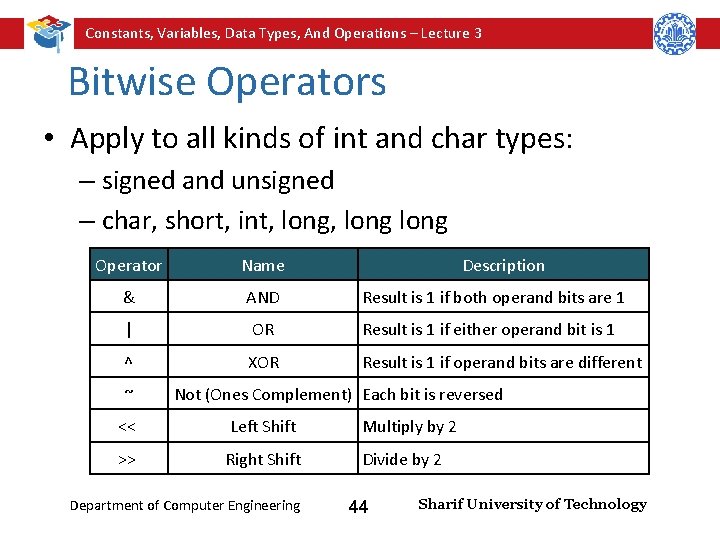
Constants, Variables, Data Types, And Operations – Lecture 3 Bitwise Operators • Apply to all kinds of int and char types: – signed and unsigned – char, short, int, long Operator Name & AND Result is 1 if both operand bits are 1 | OR Result is 1 if either operand bit is 1 ^ XOR Result is 1 if operand bits are different ~ Description Not (Ones Complement) Each bit is reversed << Left Shift Multiply by 2 >> Right Shift Divide by 2 Department of Computer Engineering 44 Sharif University of Technology
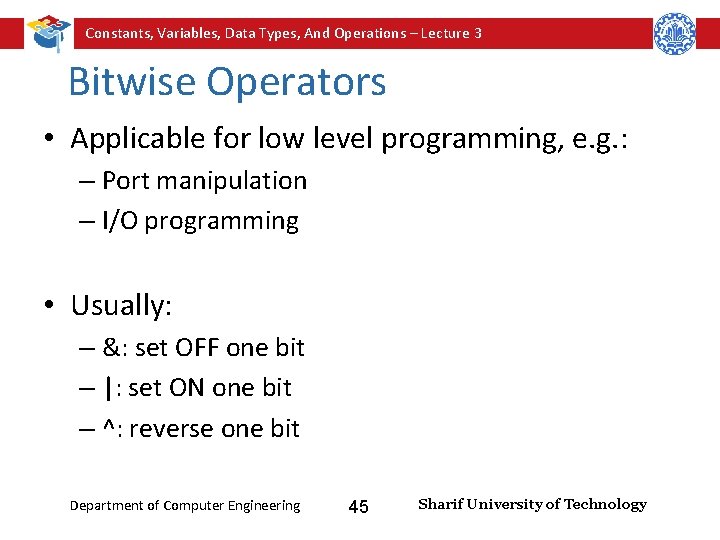
Constants, Variables, Data Types, And Operations – Lecture 3 Bitwise Operators • Applicable for low level programming, e. g. : – Port manipulation – I/O programming • Usually: – &: set OFF one bit – |: set ON one bit – ^: reverse one bit Department of Computer Engineering 45 Sharif University of Technology
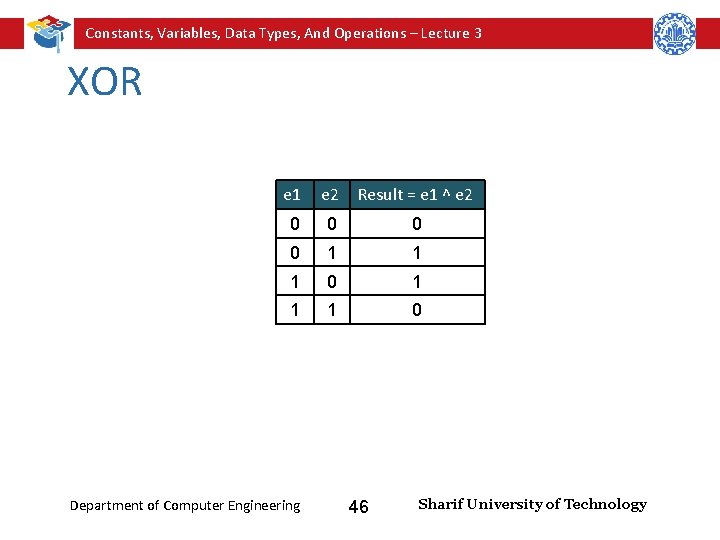
Constants, Variables, Data Types, And Operations – Lecture 3 XOR e 1 e 2 Result = e 1 ^ e 2 0 0 1 1 1 0 Department of Computer Engineering 46 Sharif University of Technology
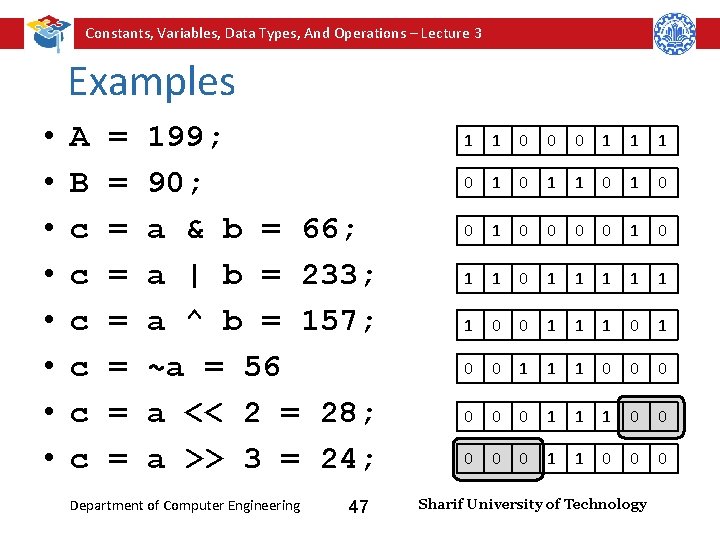
Constants, Variables, Data Types, And Operations – Lecture 3 Examples • • A B c c c = = = = 199; 90; a & b = 66; a | b = 233; a ^ b = 157; ~a = 56 a << 2 = 28; a >> 3 = 24; Department of Computer Engineering 47 1 1 0 0 0 1 1 1 0 1 0 0 1 0 1 1 1 1 0 0 0 0 1 1 1 0 0 0 Sharif University of Technology
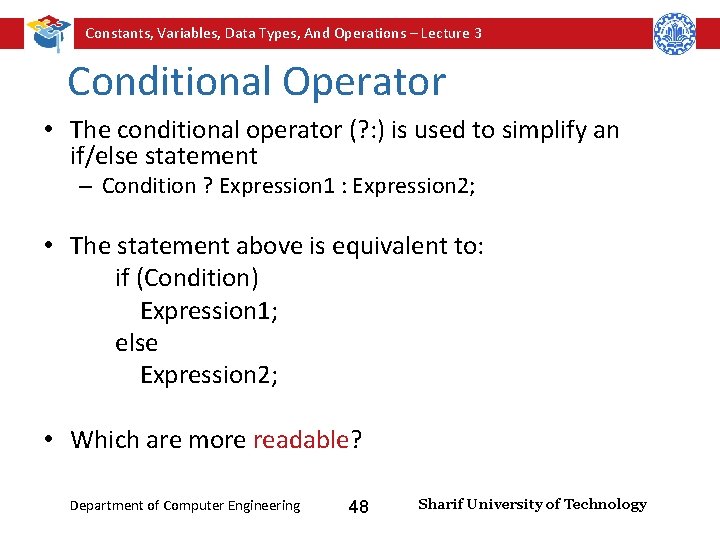
Constants, Variables, Data Types, And Operations – Lecture 3 Conditional Operator • The conditional operator (? : ) is used to simplify an if/else statement – Condition ? Expression 1 : Expression 2; • The statement above is equivalent to: if (Condition) Expression 1; else Expression 2; • Which are more readable? Department of Computer Engineering 48 Sharif University of Technology
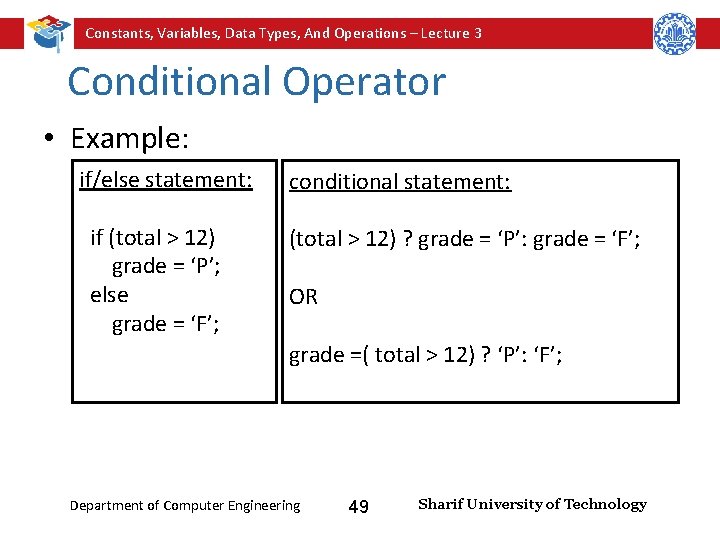
Constants, Variables, Data Types, And Operations – Lecture 3 Conditional Operator • Example: if/else statement: if (total > 12) grade = ‘P’; else grade = ‘F’; conditional statement: (total > 12) ? grade = ‘P’: grade = ‘F’; OR grade =( total > 12) ? ‘P’: ‘F’; Department of Computer Engineering 49 Sharif University of Technology
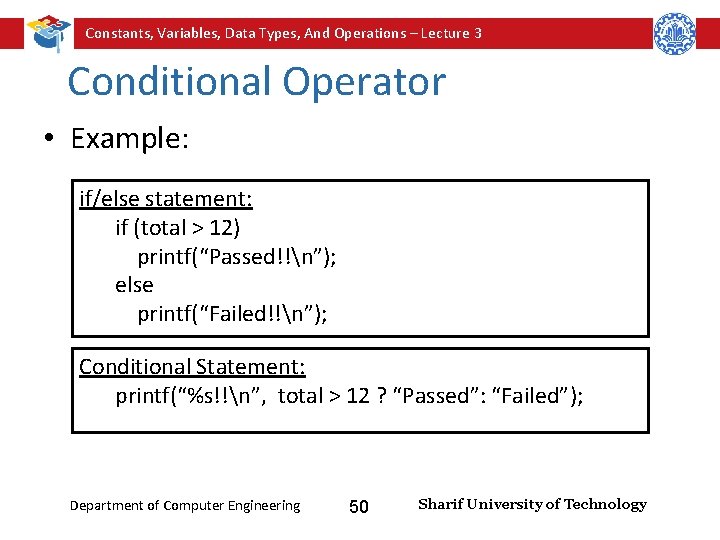
Constants, Variables, Data Types, And Operations – Lecture 3 Conditional Operator • Example: if/else statement: if (total > 12) printf(“Passed!!n”); else printf(“Failed!!n”); Conditional Statement: printf(“%s!!n”, total > 12 ? “Passed”: “Failed”); Department of Computer Engineering 50 Sharif University of Technology
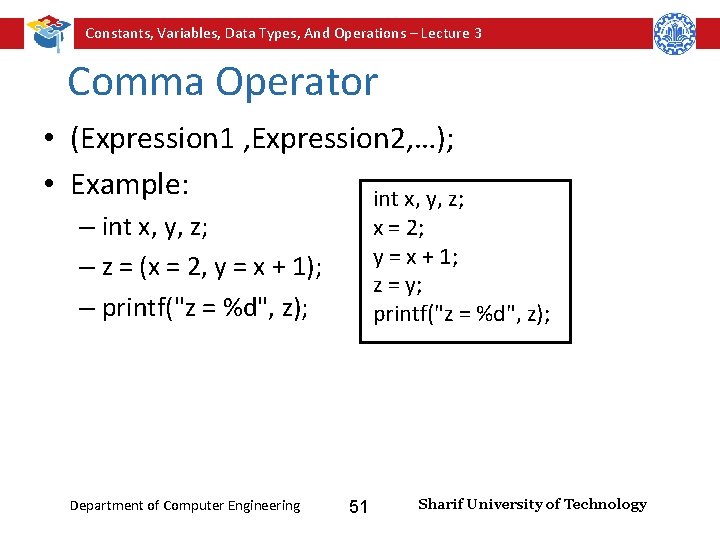
Constants, Variables, Data Types, And Operations – Lecture 3 Comma Operator • (Expression 1 , Expression 2, …); • Example: int x, y, z; – z = (x = 2, y = x + 1); – printf("z = %d", z); Department of Computer Engineering x = 2; y = x + 1; z = y; printf("z = %d", z); 51 Sharif University of Technology
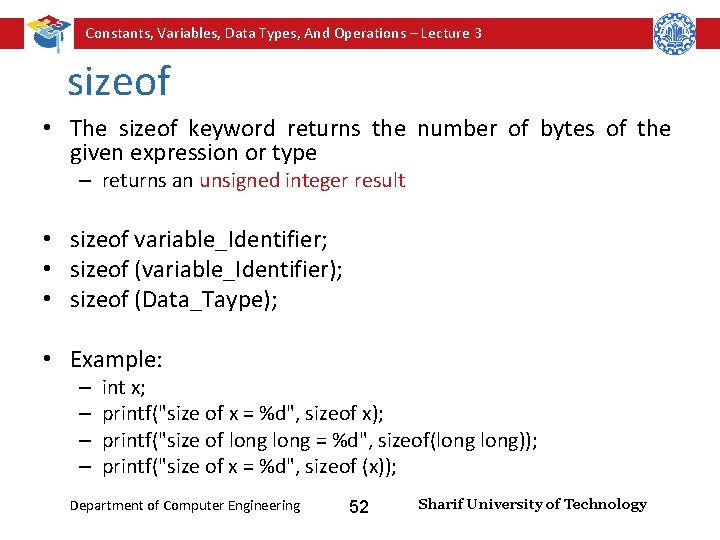
Constants, Variables, Data Types, And Operations – Lecture 3 sizeof • The sizeof keyword returns the number of bytes of the given expression or type – returns an unsigned integer result • sizeof variable_Identifier; • sizeof (variable_Identifier); • sizeof (Data_Taype); • Example: – – int x; printf("size of x = %d", sizeof x); printf("size of long = %d", sizeof(long)); printf("size of x = %d", sizeof (x)); Department of Computer Engineering 52 Sharif University of Technology
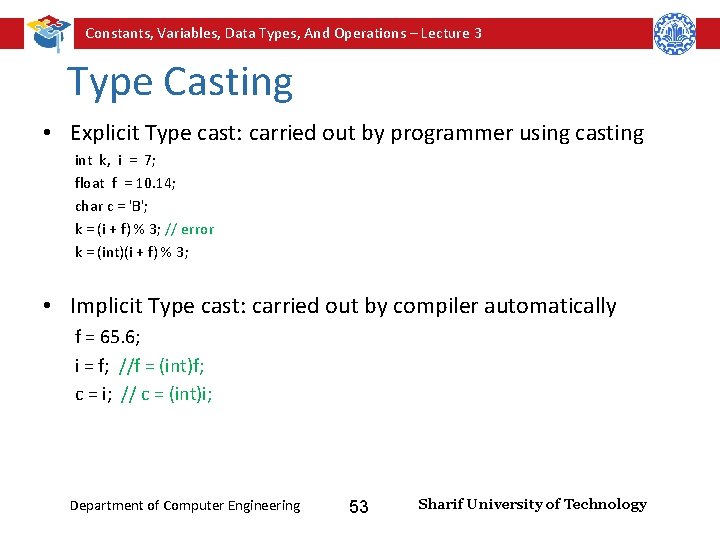
Constants, Variables, Data Types, And Operations – Lecture 3 Type Casting • Explicit Type cast: carried out by programmer using casting int k, i = 7; float f = 10. 14; char c = 'B'; k = (i + f) % 3; // error k = (int)(i + f) % 3; • Implicit Type cast: carried out by compiler automatically f = 65. 6; i = f; //f = (int)f; c = i; // c = (int)i; Department of Computer Engineering 53 Sharif University of Technology
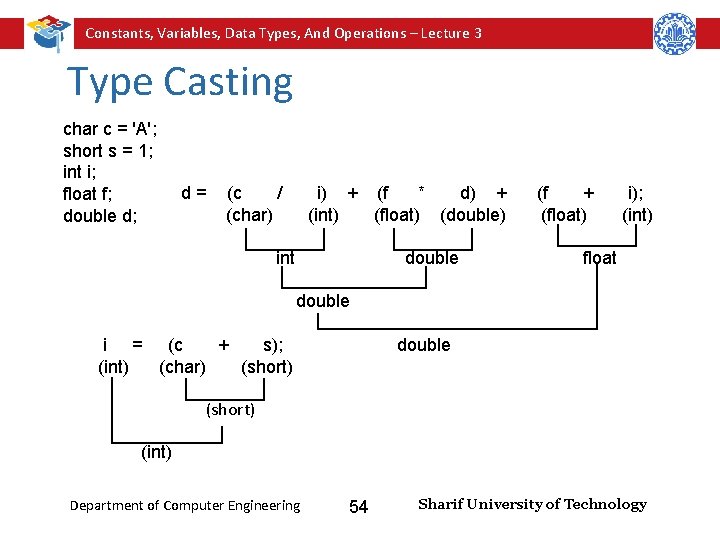
Constants, Variables, Data Types, And Operations – Lecture 3 Type Casting char c = 'A'; short s = 1; int i; float f; double d; d= (c / (char) i) + (f * d) + (int) (float) (double) int double (f + (float) i); (int) float double i = (c + s); (int) (char) (short) (int) Department of Computer Engineering 54 Sharif University of Technology
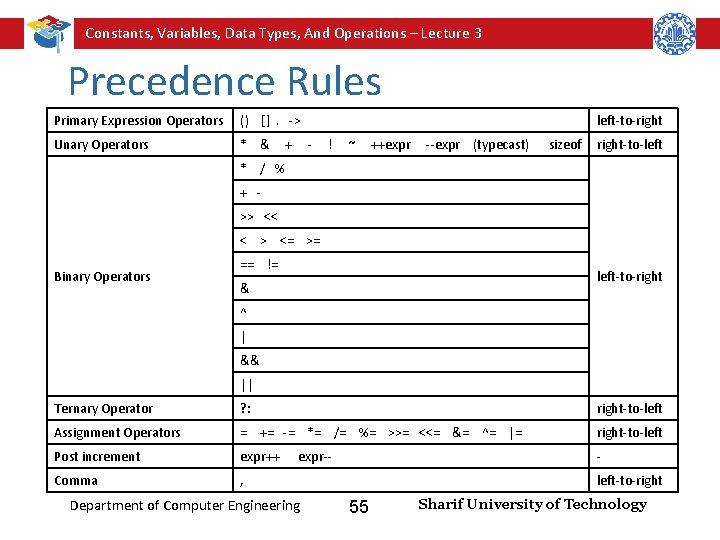
Constants, Variables, Data Types, And Operations – Lecture 3 Precedence Rules Primary Expression Operators () []. -> Unary Operators * & + left-to-right - ! ~ ++expr --expr (typecast) sizeof right-to-left * / % + >> << < > <= >= Binary Operators == != left-to-right & ^ | && || Ternary Operator ? : right-to-left Assignment Operators = += -= *= /= %= >>= <<= &= ^= |= right-to-left Post increment expr++ - Comma , expr-- Department of Computer Engineering left-to-right 55 Sharif University of Technology
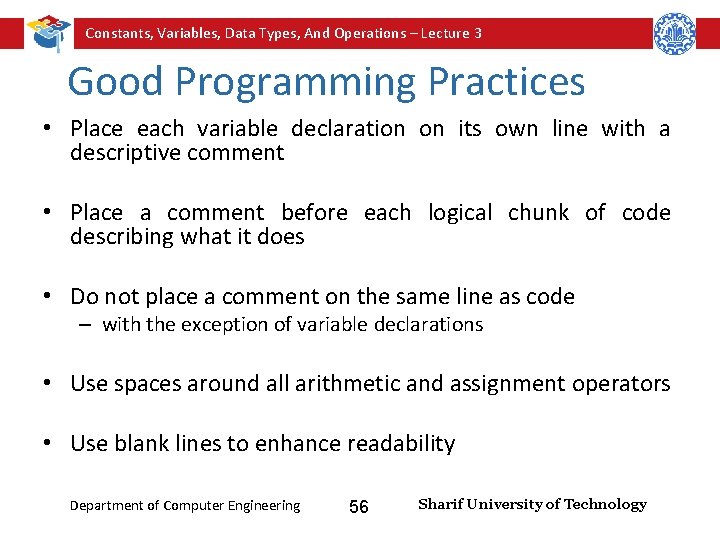
Constants, Variables, Data Types, And Operations – Lecture 3 Good Programming Practices • Place each variable declaration on its own line with a descriptive comment • Place a comment before each logical chunk of code describing what it does • Do not place a comment on the same line as code – with the exception of variable declarations • Use spaces around all arithmetic and assignment operators • Use blank lines to enhance readability Department of Computer Engineering 56 Sharif University of Technology
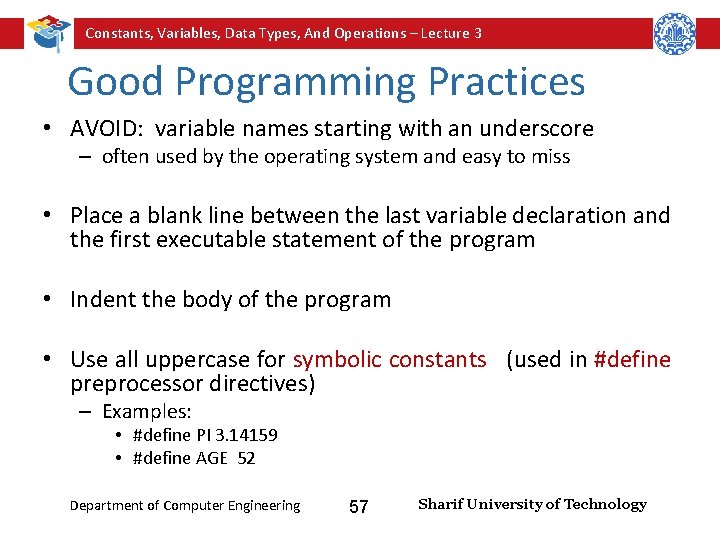
Constants, Variables, Data Types, And Operations – Lecture 3 Good Programming Practices • AVOID: variable names starting with an underscore – often used by the operating system and easy to miss • Place a blank line between the last variable declaration and the first executable statement of the program • Indent the body of the program • Use all uppercase for symbolic constants (used in #define preprocessor directives) – Examples: • #define PI 3. 14159 • #define AGE 52 Department of Computer Engineering 57 Sharif University of Technology
Omid jafarinezhad
Omid jafarinezhad
Lecturer's name
01:640:244 lecture notes - lecture 15: plat, idah, farad
Lecturer name
Spe distinguished lecturer
Cfa lecturer handbook
Designation lecturer
Pearson lecturer resources
How do you greet your teacher in the afternoon
Lecturer asad ali
Designation of lecturer
Lector vs lecturer
140000/120
Photography lecturer
Gcwak
Physician associate lecturer
Lecturer in charge
Lecturer in charge
Omid ahangar
Omid asadi artist
Martin ordonez
Omid fatahi valilai
Omid fatahi valilai
Omid mirmotahari
Omid parhizkar
Omid mirmotahari
Omid kashefi
Omid aghazadeh
Omid askari
C programming lecture
Integer programming vs linear programming
Perbedaan linear programming dan integer programming
Definisi linear
Greedy vs dynamic
System programming definition
Lecture sound systems
Bjt equations
Floor of femoral triangle
Othello lecture
Mass spectrometry lecture
The parsec lecture tutorial answers
Matlab lecture notes
Sangam period
Lecture title
Lecture records transaction in
Project cost management lecture notes
Drawing lecture
Introduction vs abstract
Financial engineering notes
Flame hardening mild steel
At the end of the lecture
Ophthalmology lecture
Crisis communications lecture
Business research methods lecture notes ppt
Vertical
Proportioning system anesthesia machine
Double lecture