Control Structures I Selection Control Structures The programs
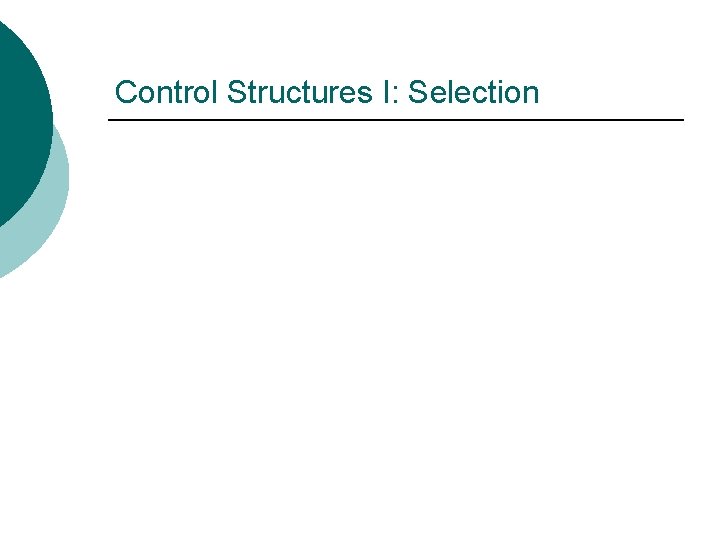
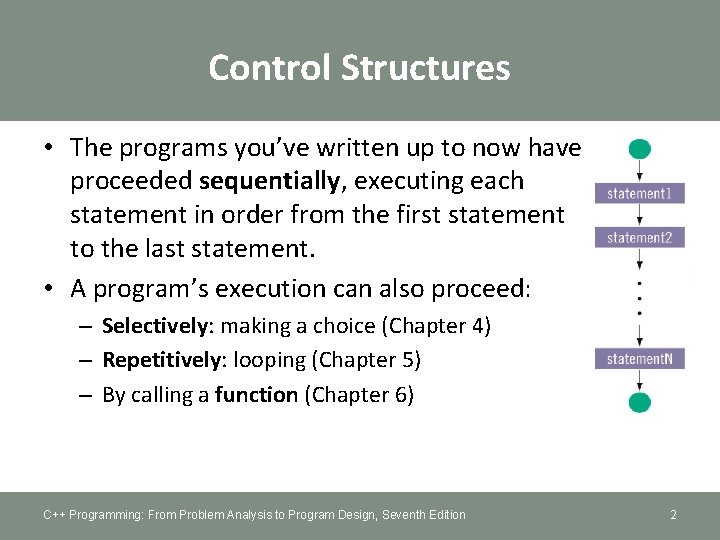
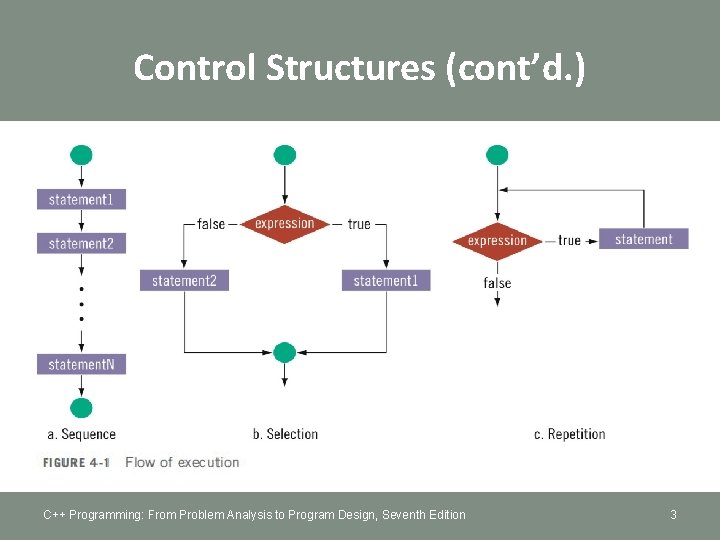
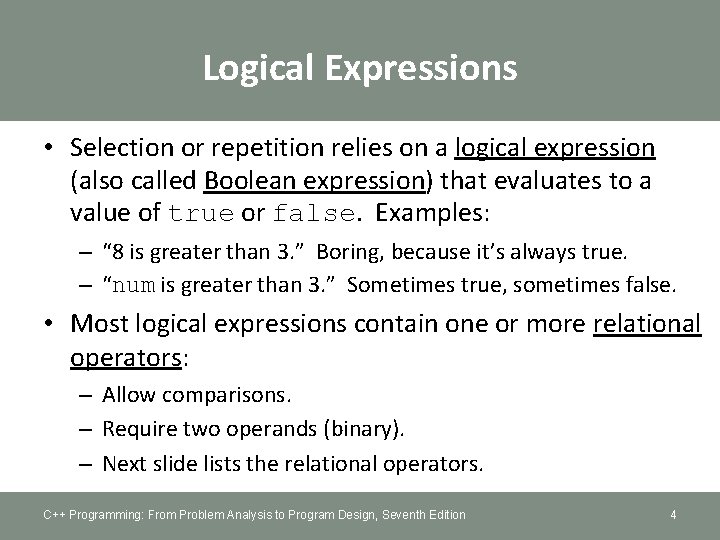
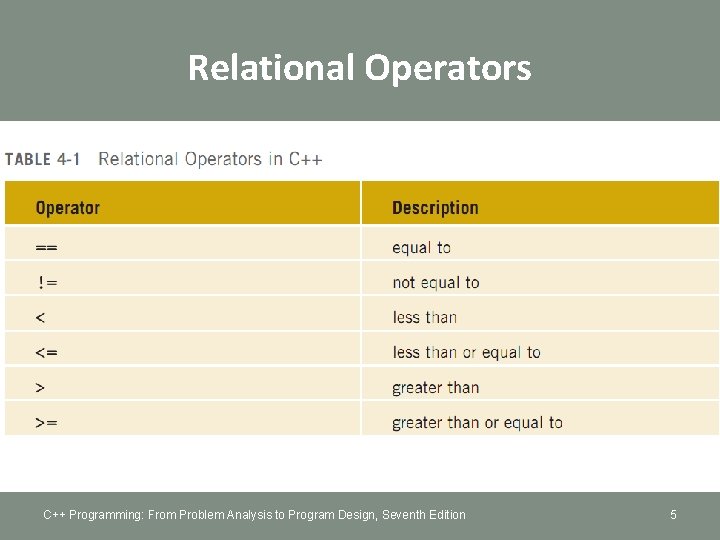
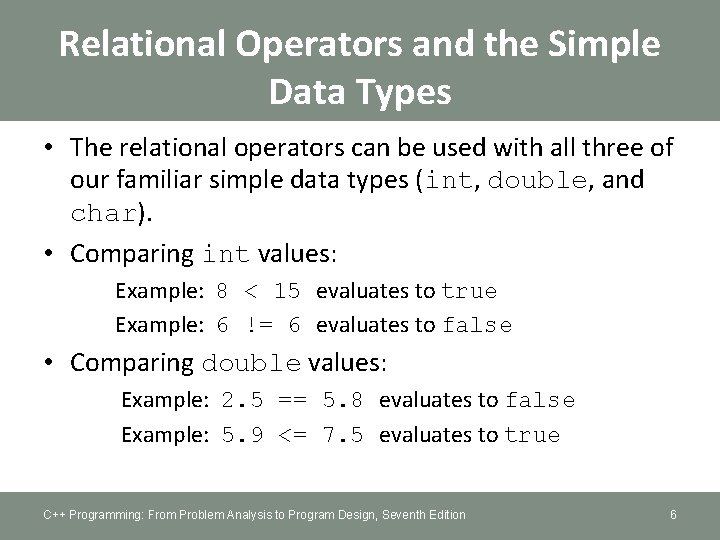
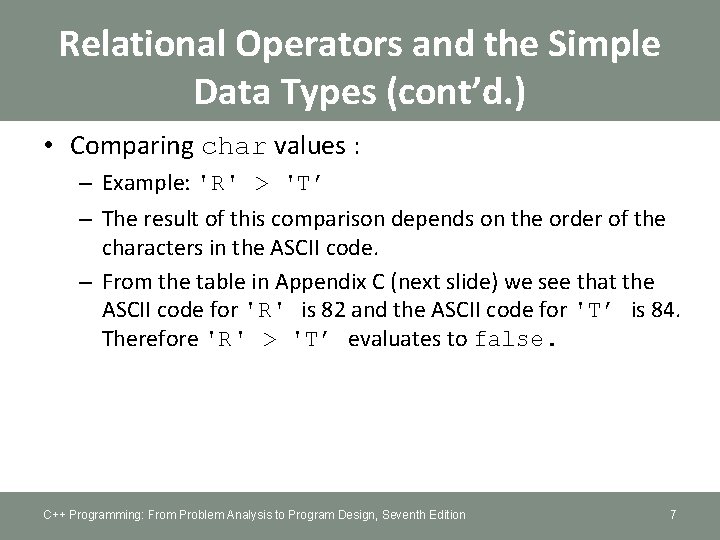
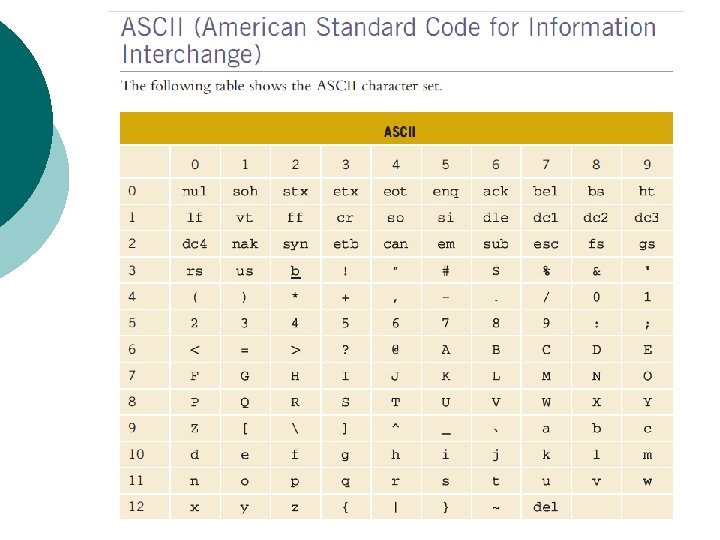
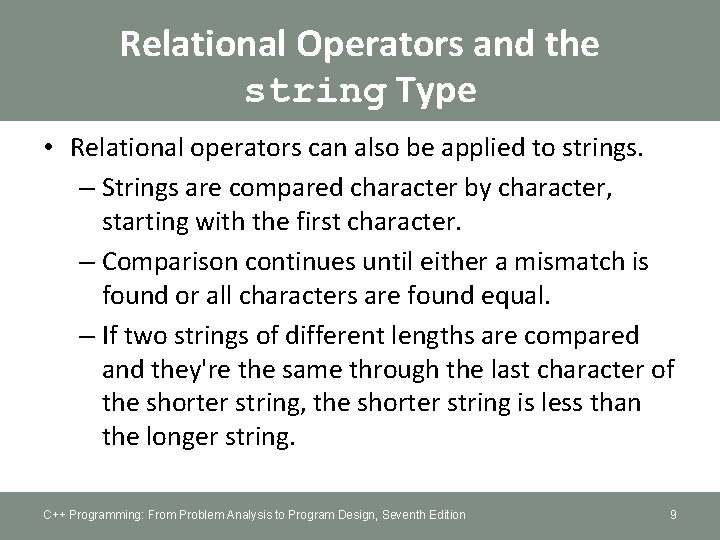
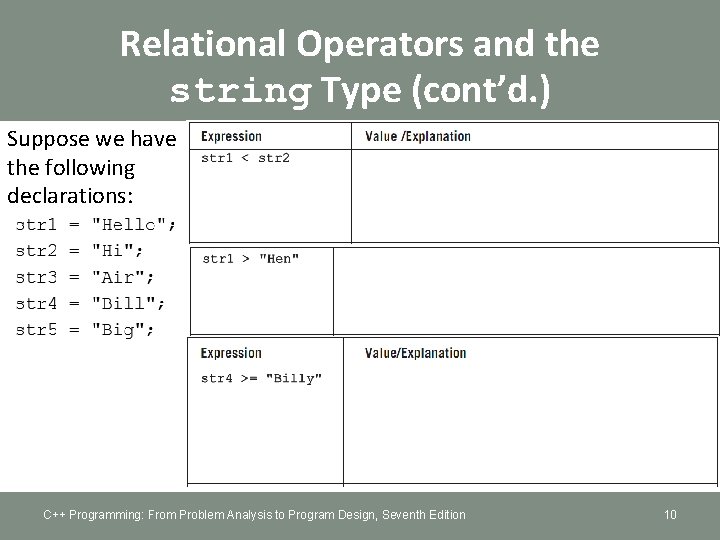
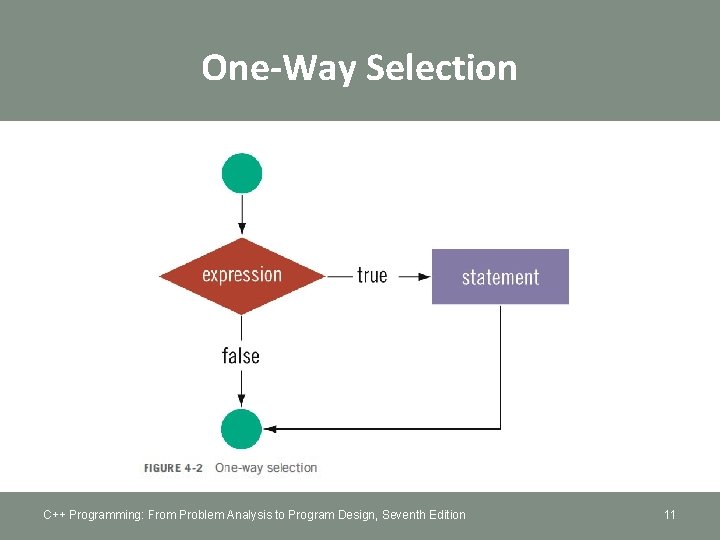
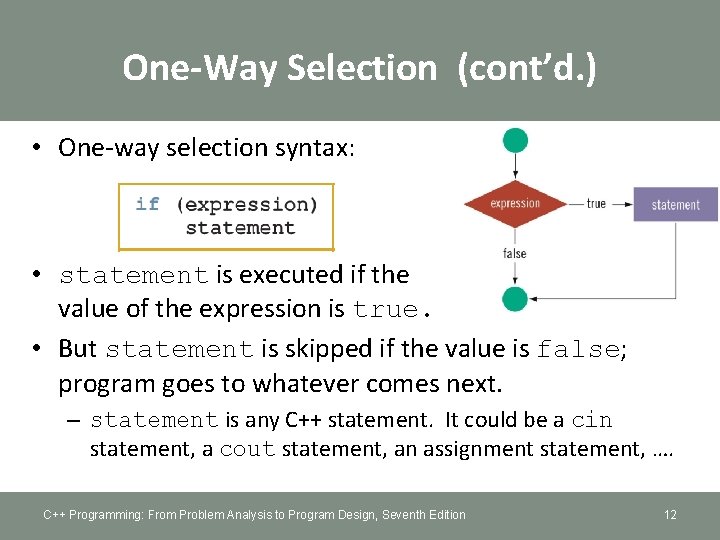
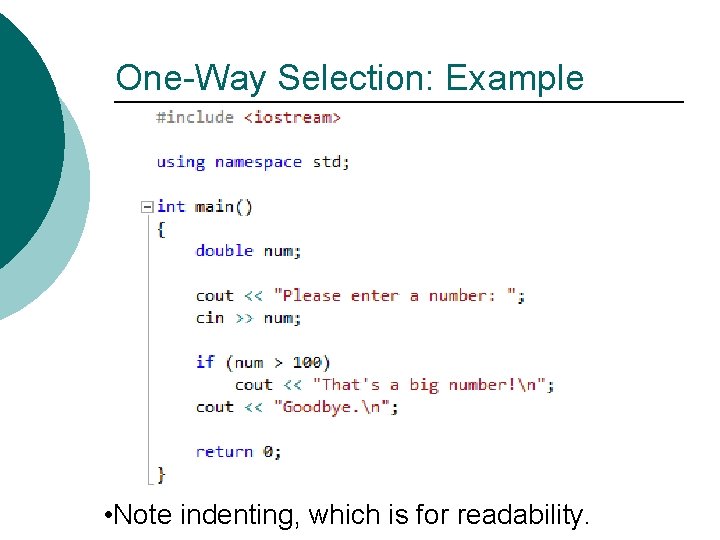
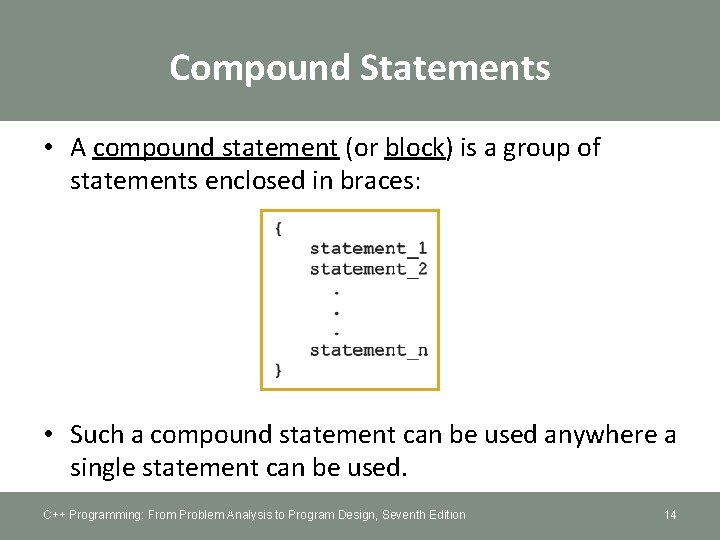
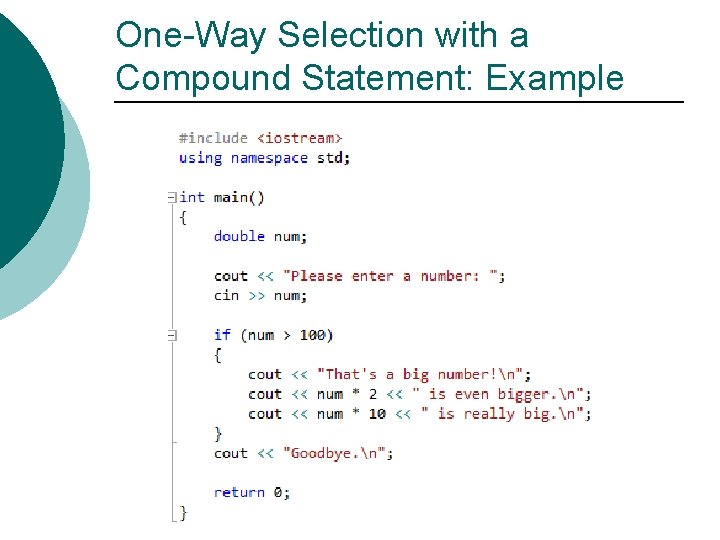
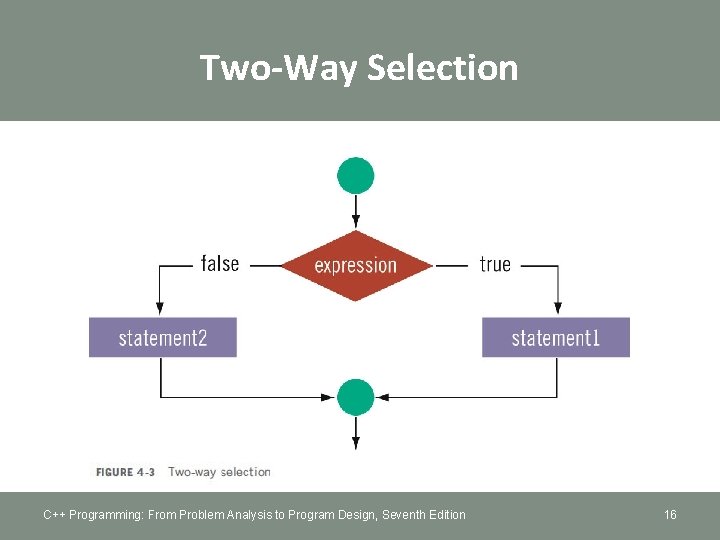
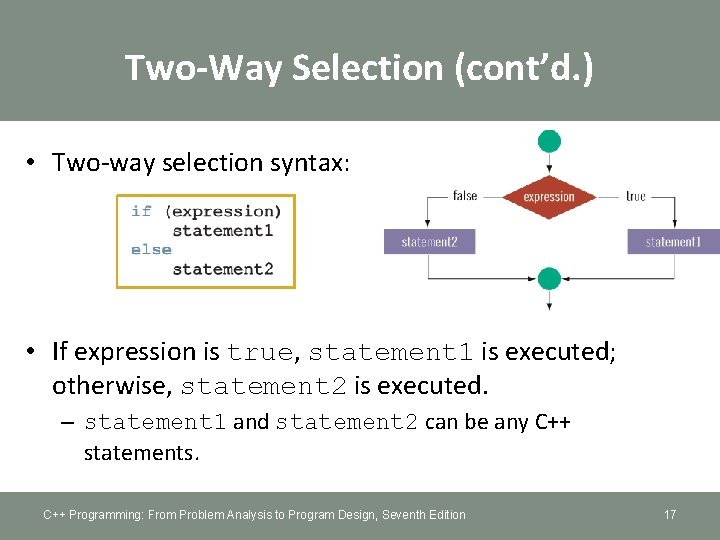
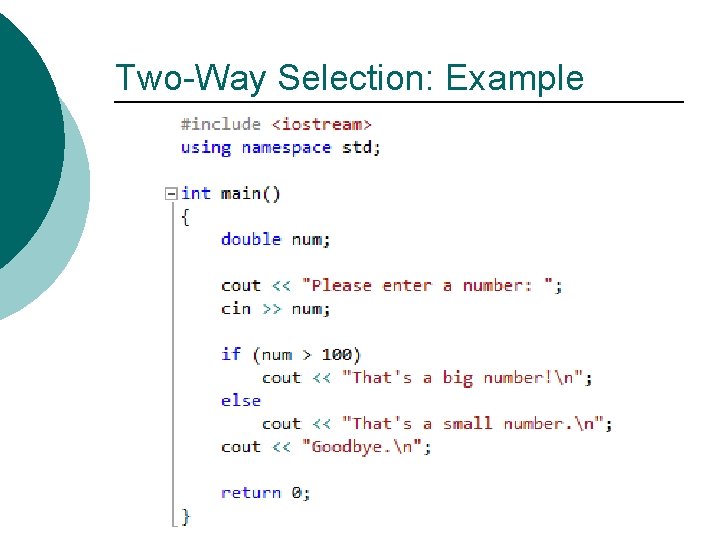
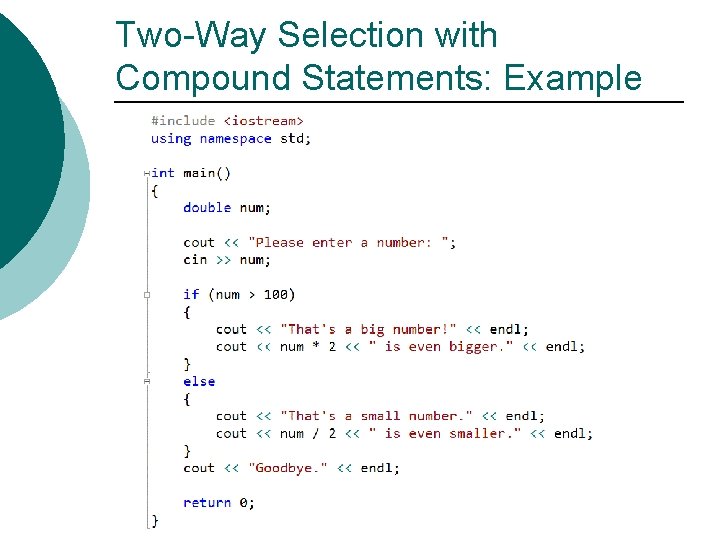
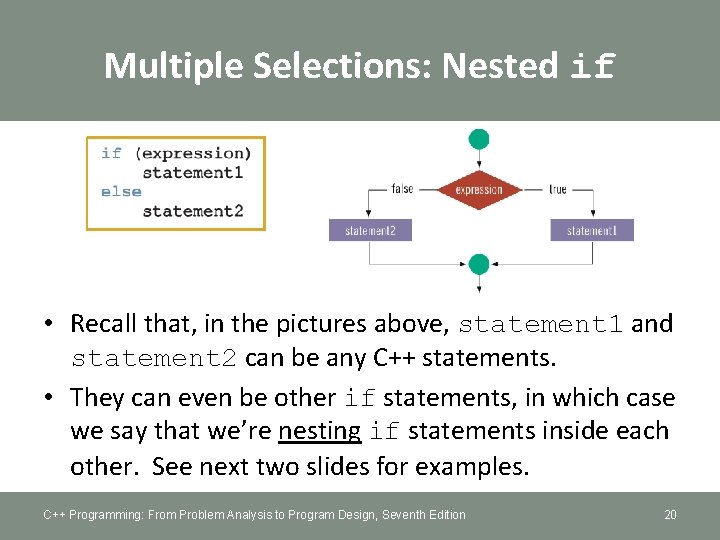
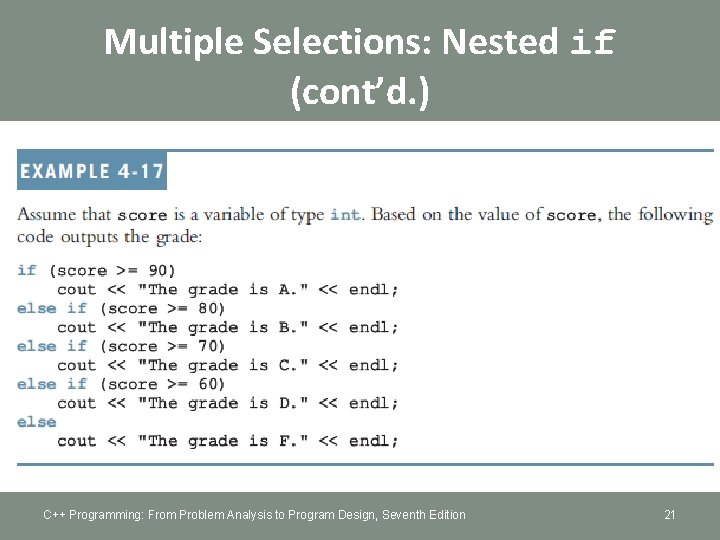
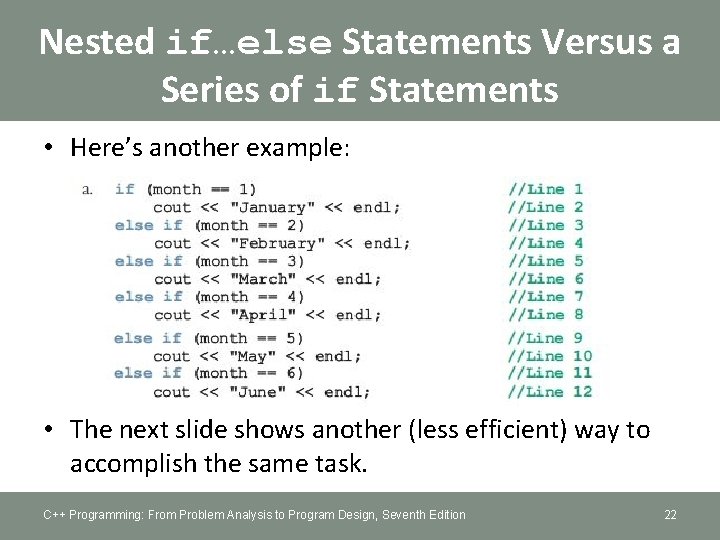
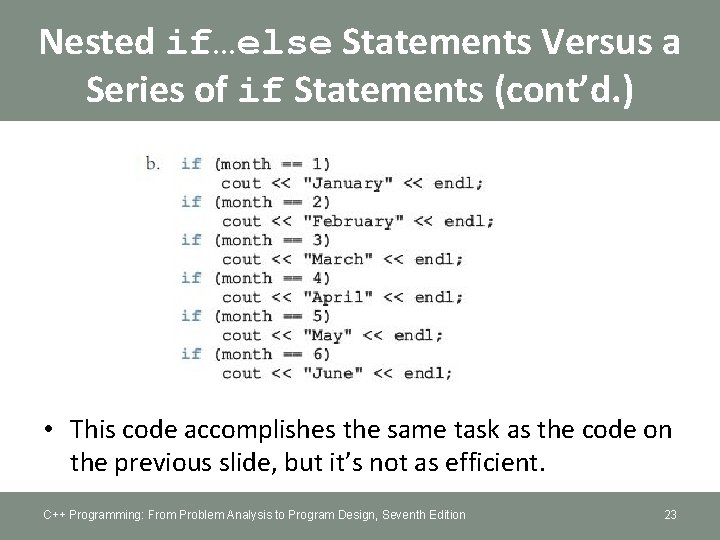
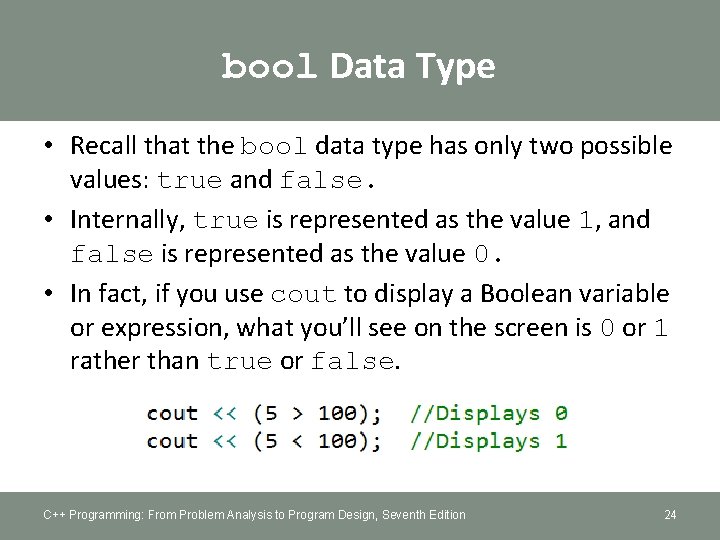
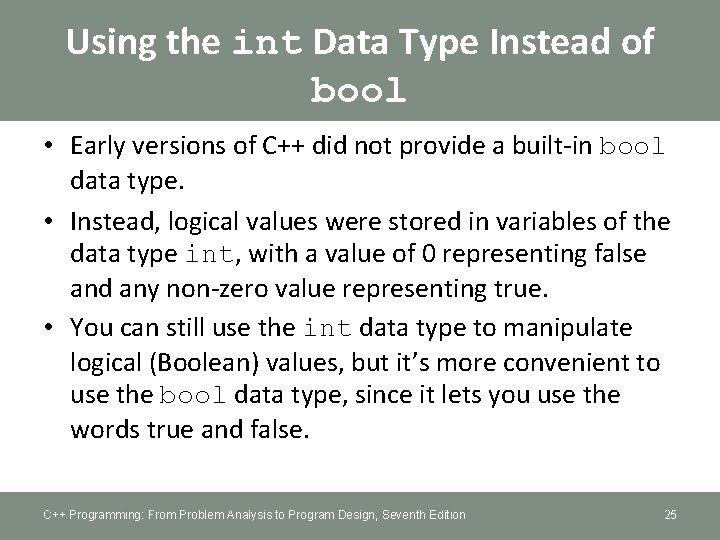
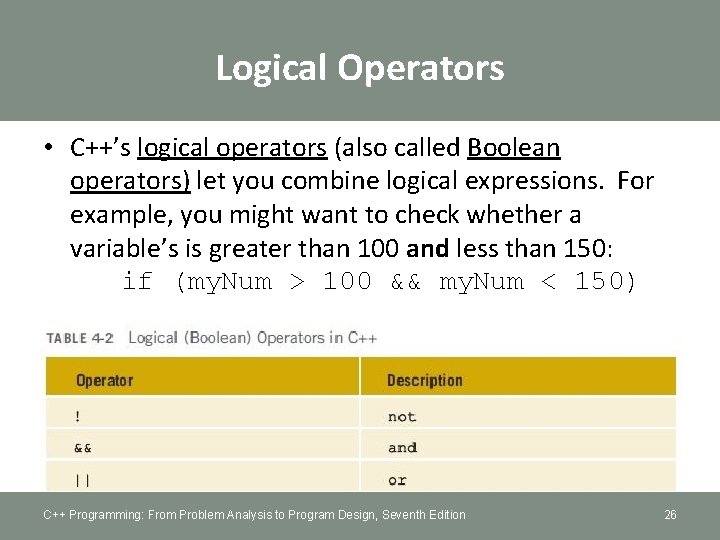
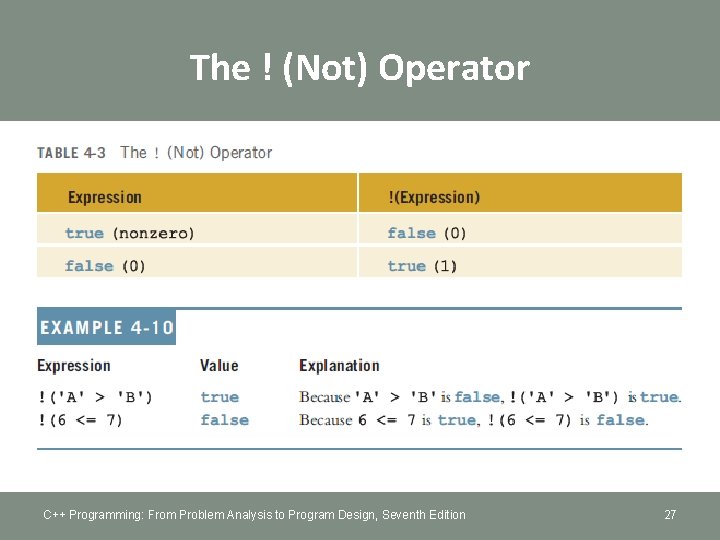
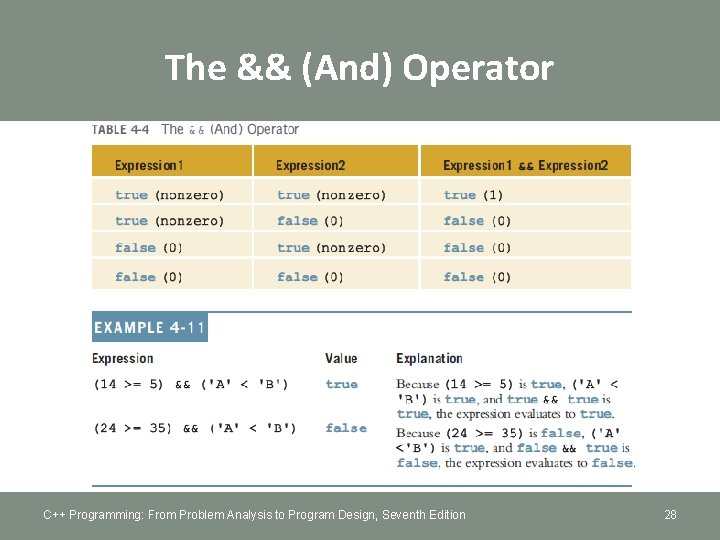
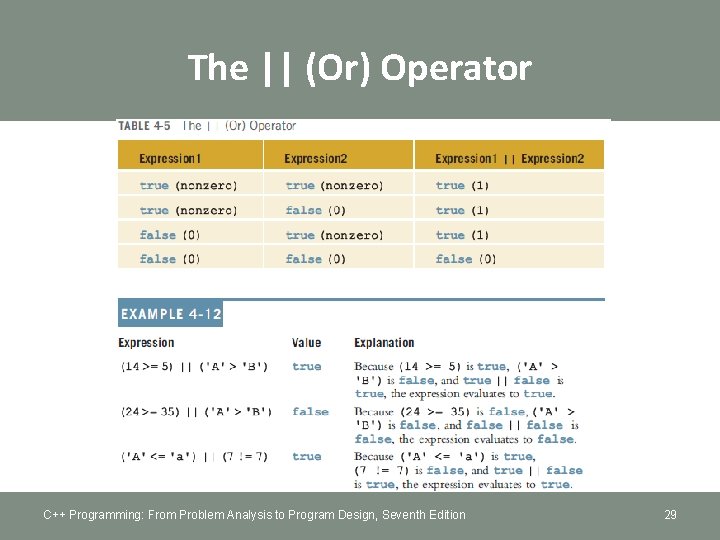
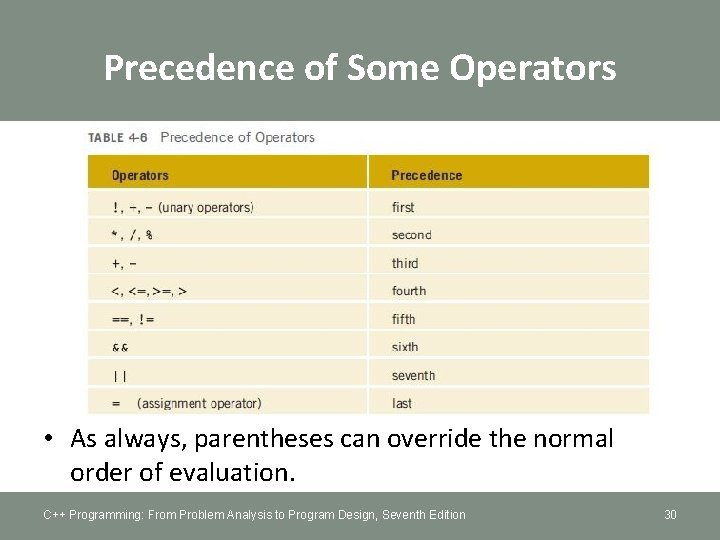
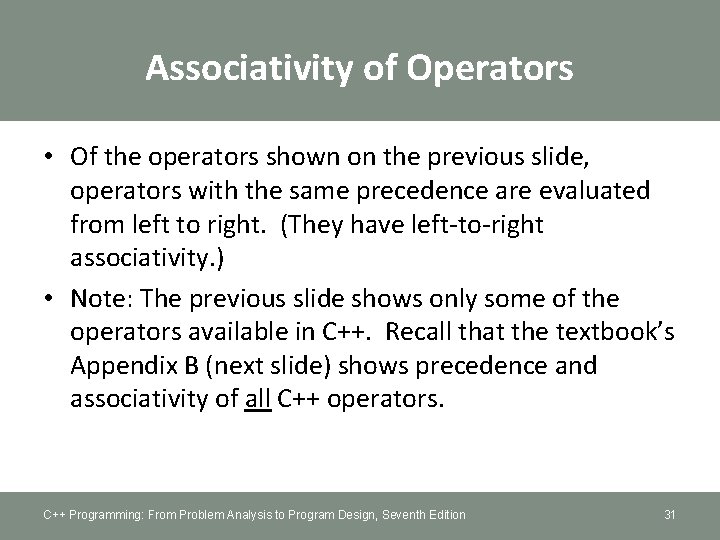
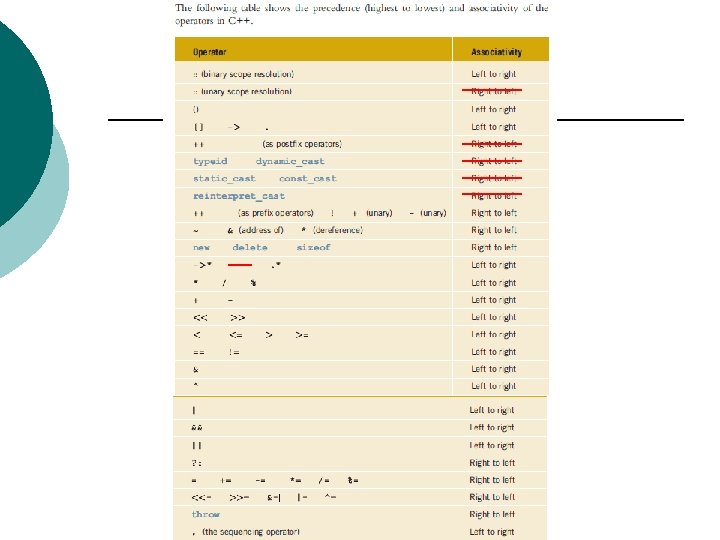
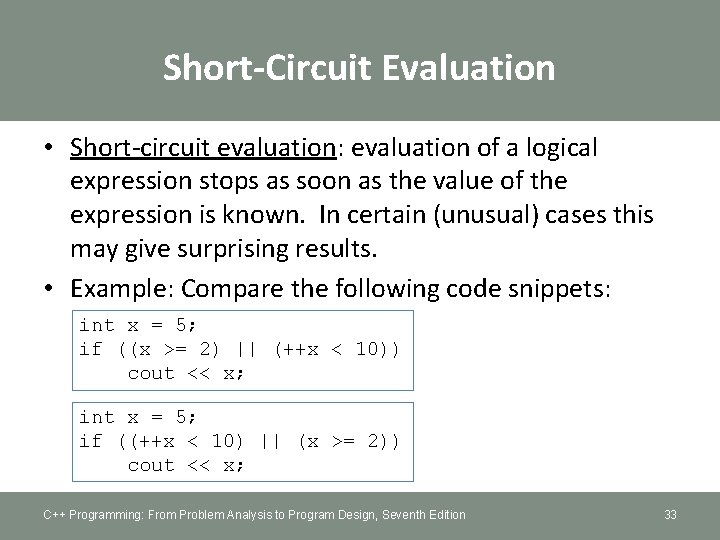
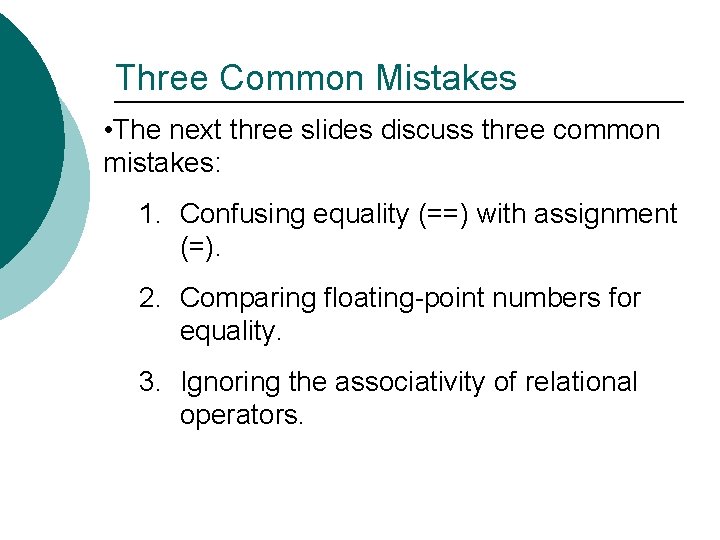
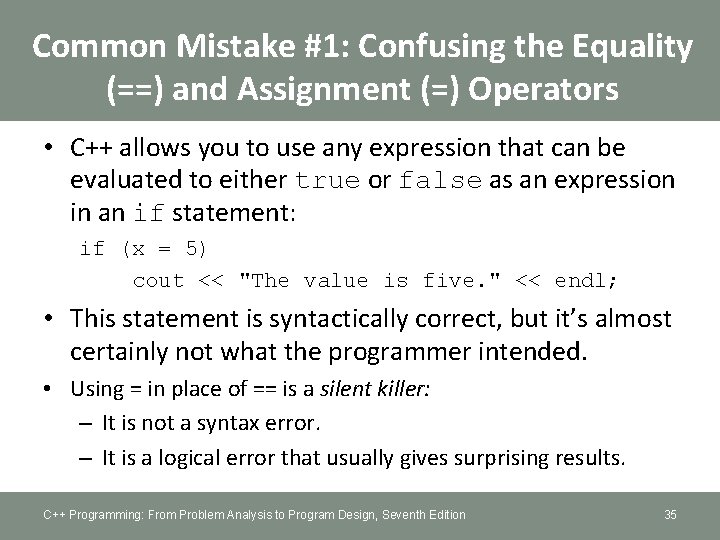
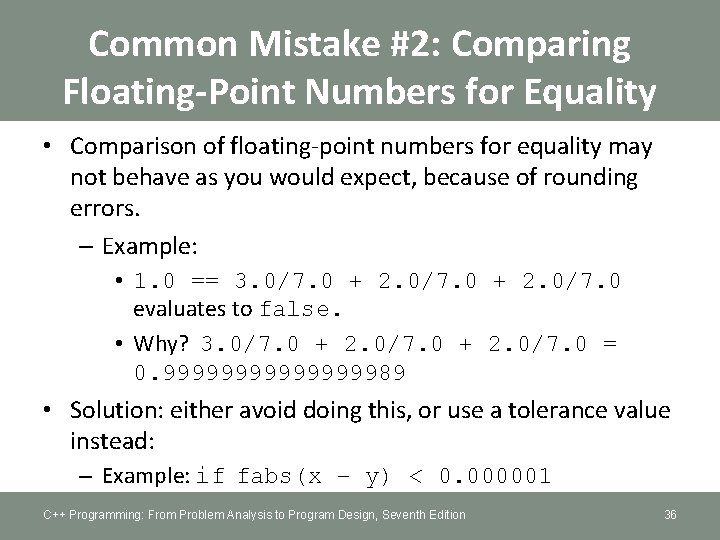
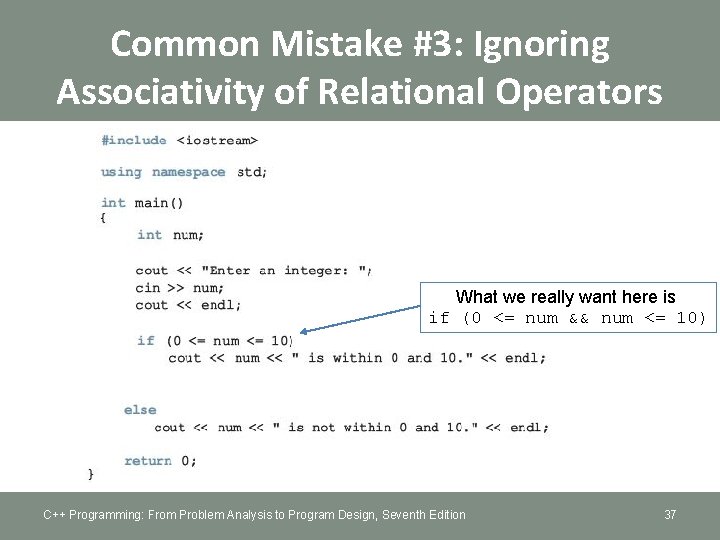
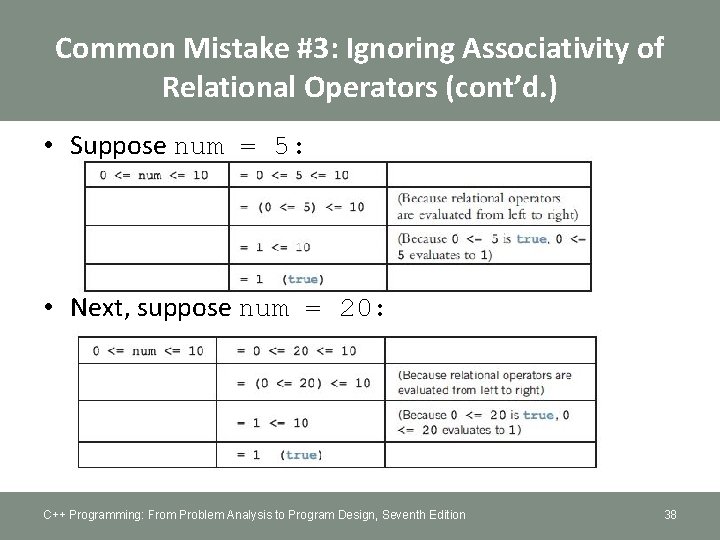
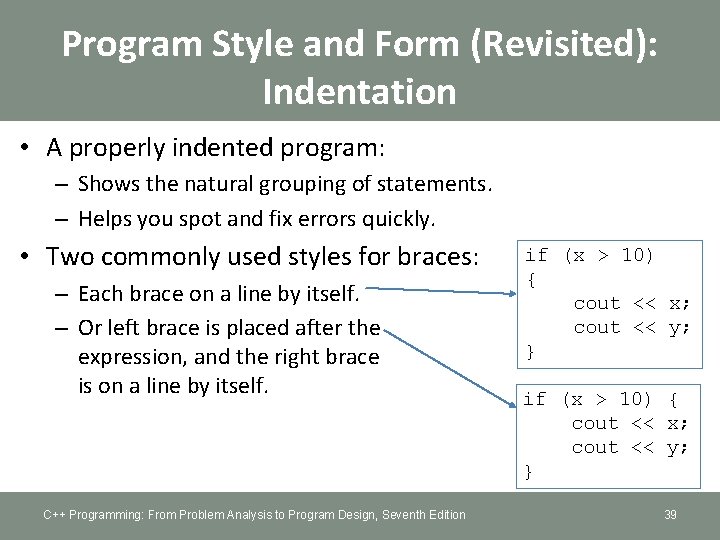
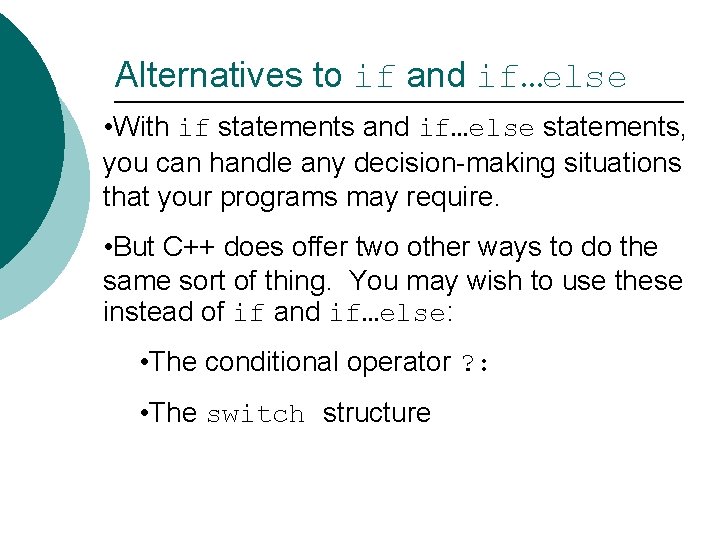
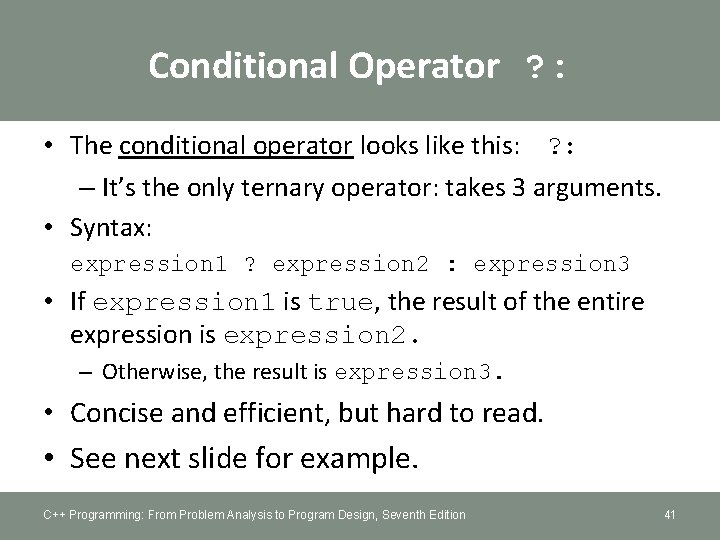
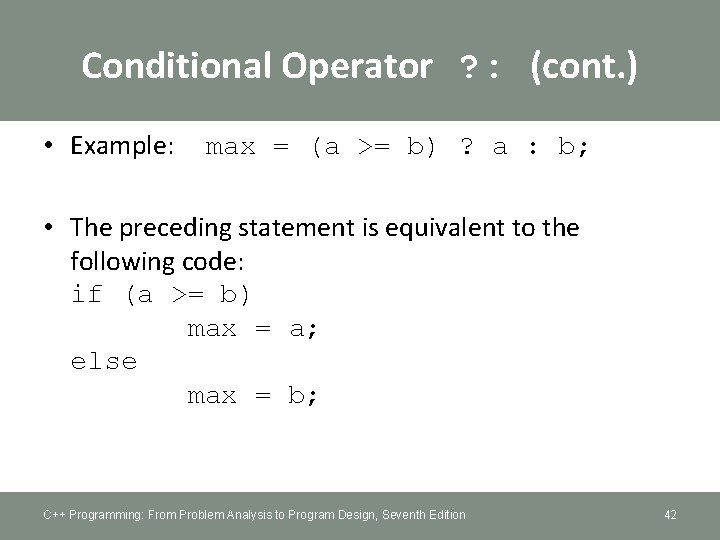
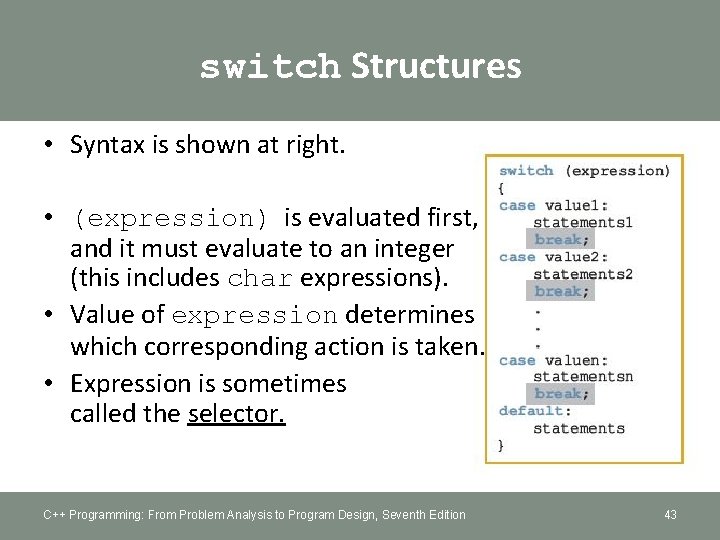
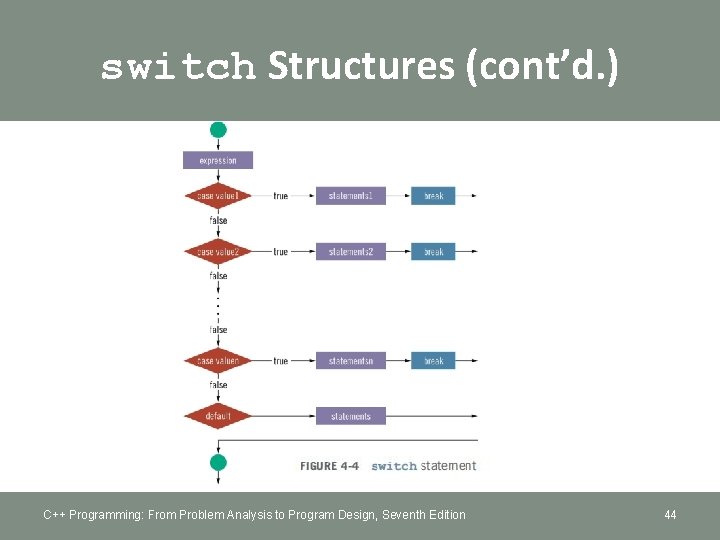
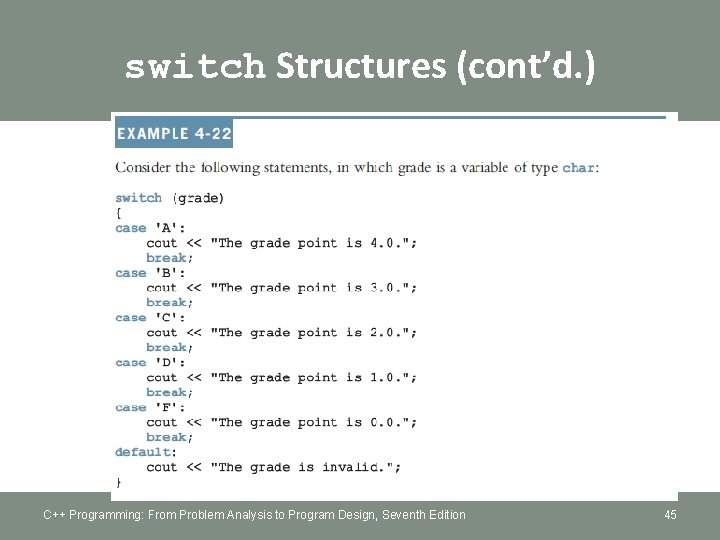
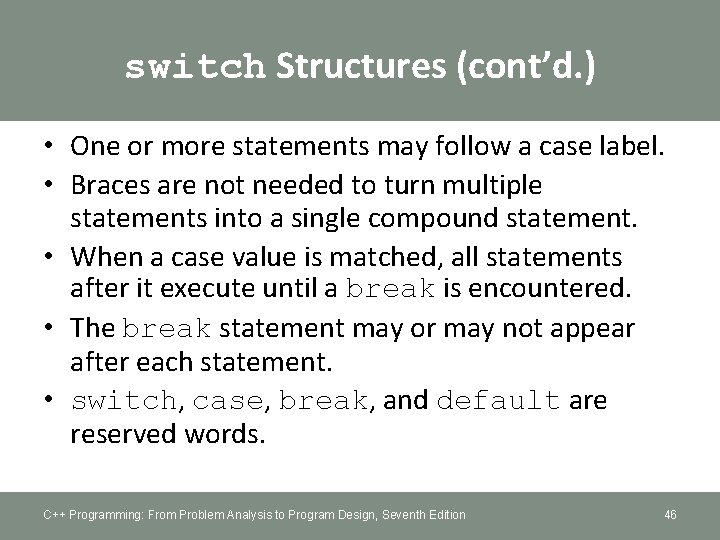
- Slides: 46
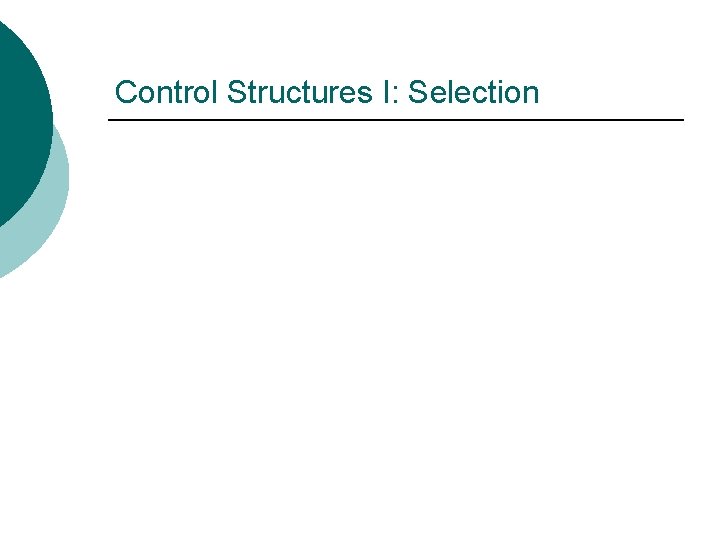
Control Structures I: Selection
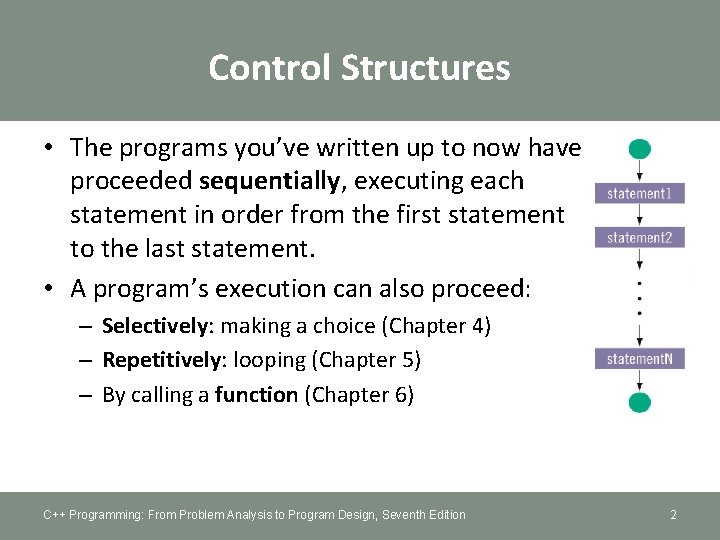
Control Structures • The programs you’ve written up to now have proceeded sequentially, executing each statement in order from the first statement to the last statement. • A program’s execution can also proceed: – Selectively: making a choice (Chapter 4) – Repetitively: looping (Chapter 5) – By calling a function (Chapter 6) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 2
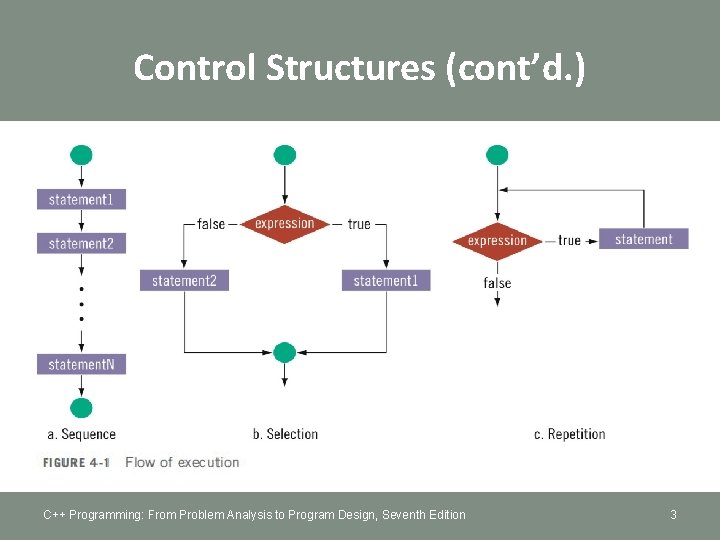
Control Structures (cont’d. ) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 3
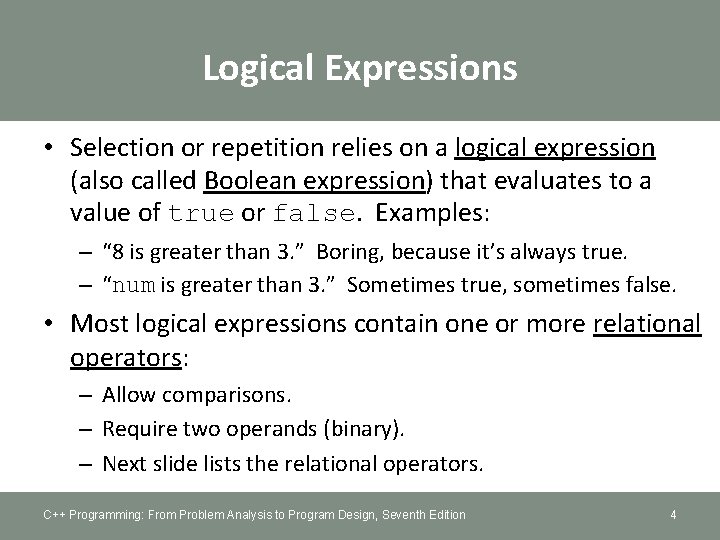
Logical Expressions • Selection or repetition relies on a logical expression (also called Boolean expression) that evaluates to a value of true or false. Examples: – “ 8 is greater than 3. ” Boring, because it’s always true. – “num is greater than 3. ” Sometimes true, sometimes false. • Most logical expressions contain one or more relational operators: – Allow comparisons. – Require two operands (binary). – Next slide lists the relational operators. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 4
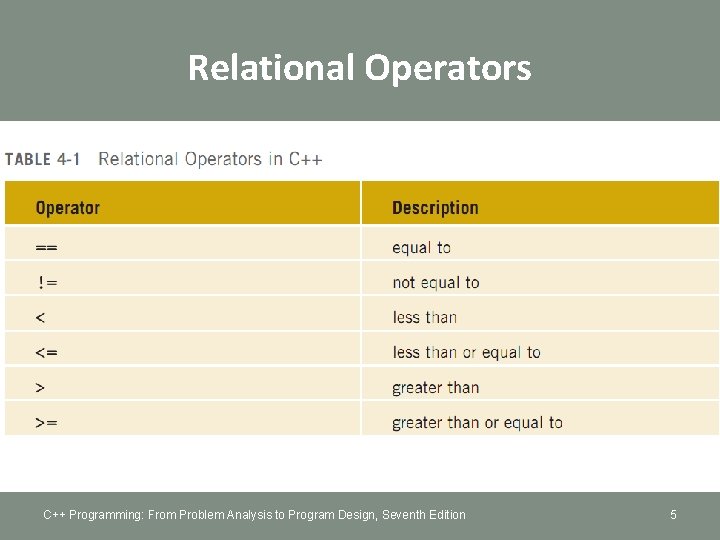
Relational Operators C++ Programming: From Problem Analysis to Program Design, Seventh Edition 5
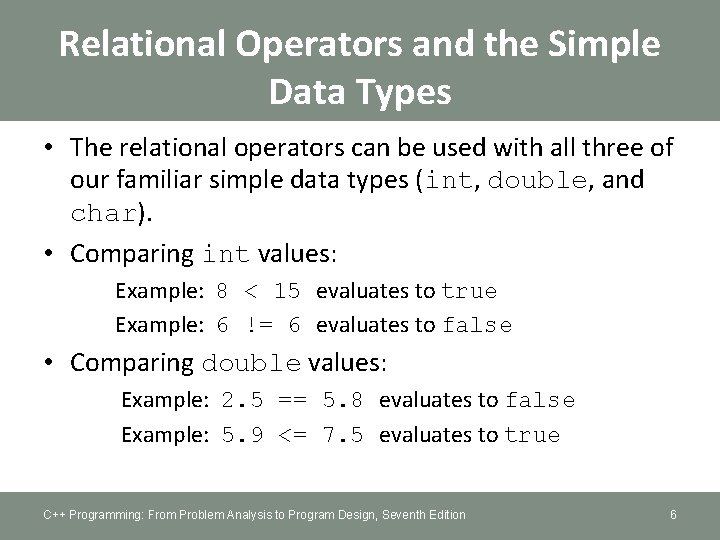
Relational Operators and the Simple Data Types • The relational operators can be used with all three of our familiar simple data types (int, double, and char). • Comparing int values: Example: 8 < 15 evaluates to true Example: 6 != 6 evaluates to false • Comparing double values: Example: 2. 5 == 5. 8 evaluates to false Example: 5. 9 <= 7. 5 evaluates to true C++ Programming: From Problem Analysis to Program Design, Seventh Edition 6
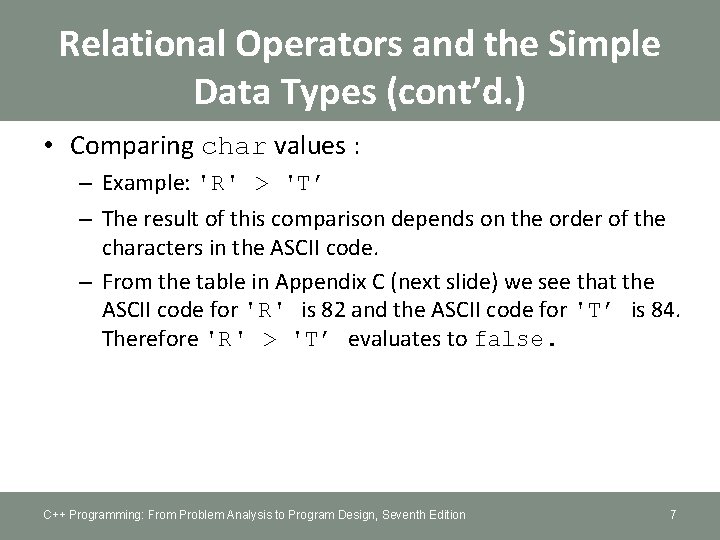
Relational Operators and the Simple Data Types (cont’d. ) • Comparing char values : – Example: 'R' > 'T’ – The result of this comparison depends on the order of the characters in the ASCII code. – From the table in Appendix C (next slide) we see that the ASCII code for 'R' is 82 and the ASCII code for 'T’ is 84. Therefore 'R' > 'T’ evaluates to false. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 7
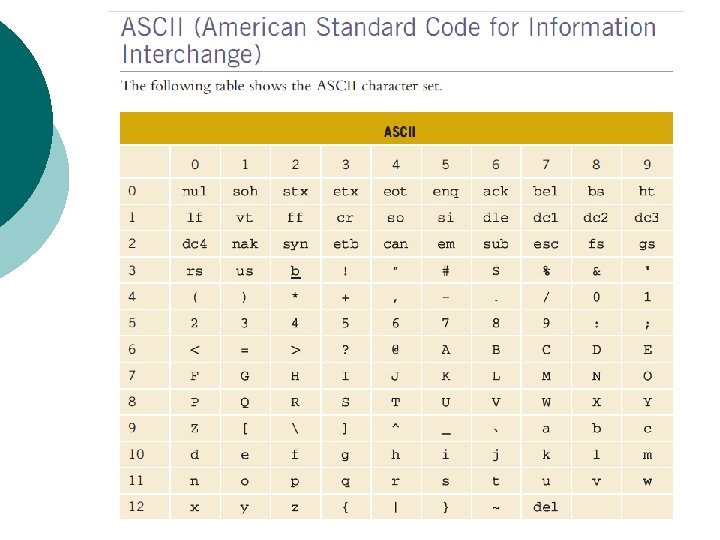
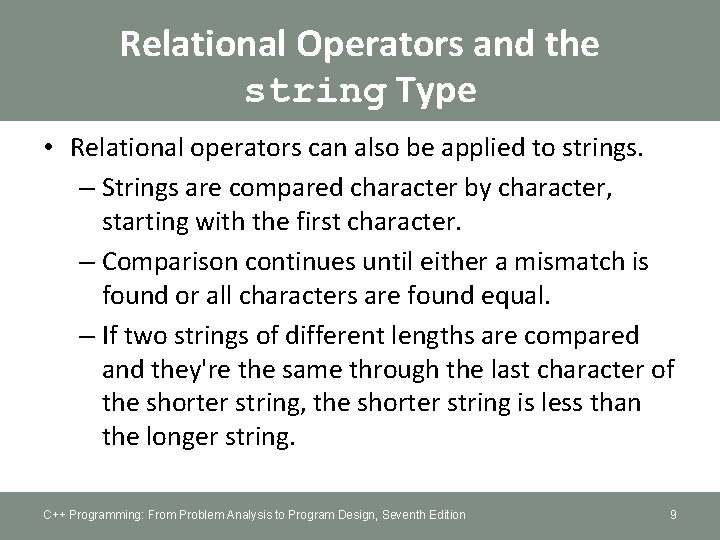
Relational Operators and the string Type • Relational operators can also be applied to strings. – Strings are compared character by character, starting with the first character. – Comparison continues until either a mismatch is found or all characters are found equal. – If two strings of different lengths are compared and they're the same through the last character of the shorter string, the shorter string is less than the longer string. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 9
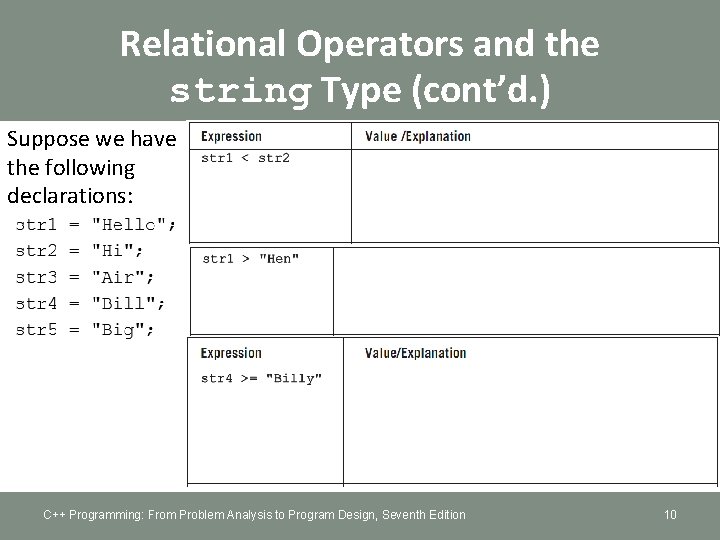
Relational Operators and the string Type (cont’d. ) Suppose we have the following declarations: C++ Programming: From Problem Analysis to Program Design, Seventh Edition 10
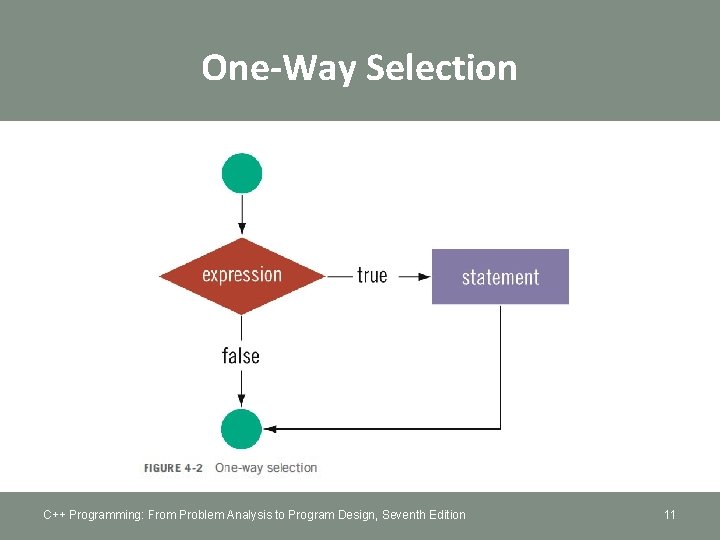
One-Way Selection C++ Programming: From Problem Analysis to Program Design, Seventh Edition 11
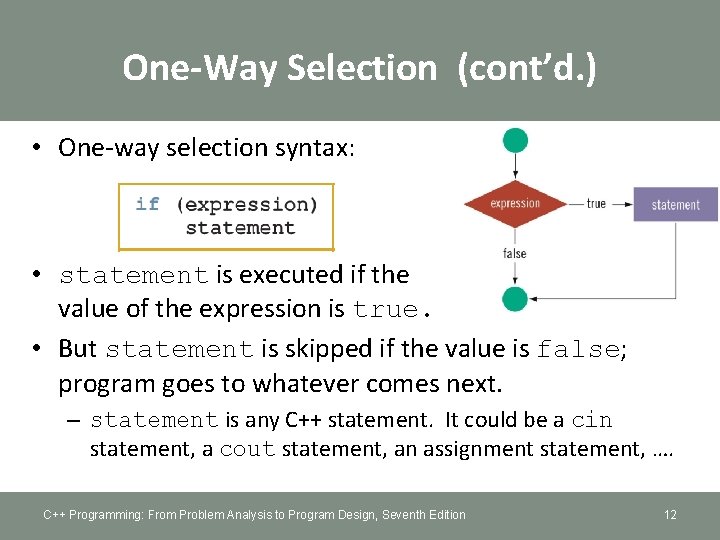
One-Way Selection (cont’d. ) • One-way selection syntax: • statement is executed if the value of the expression is true. • But statement is skipped if the value is false; program goes to whatever comes next. – statement is any C++ statement. It could be a cin statement, a cout statement, an assignment statement, …. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 12
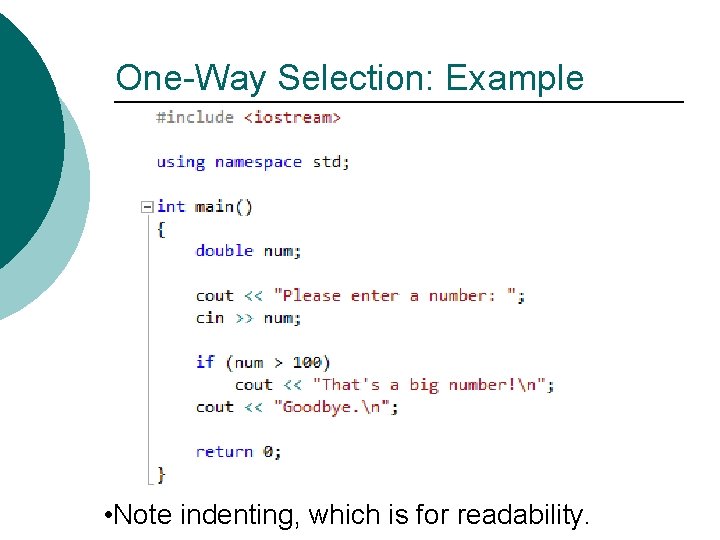
One-Way Selection: Example • Note indenting, which is for readability.
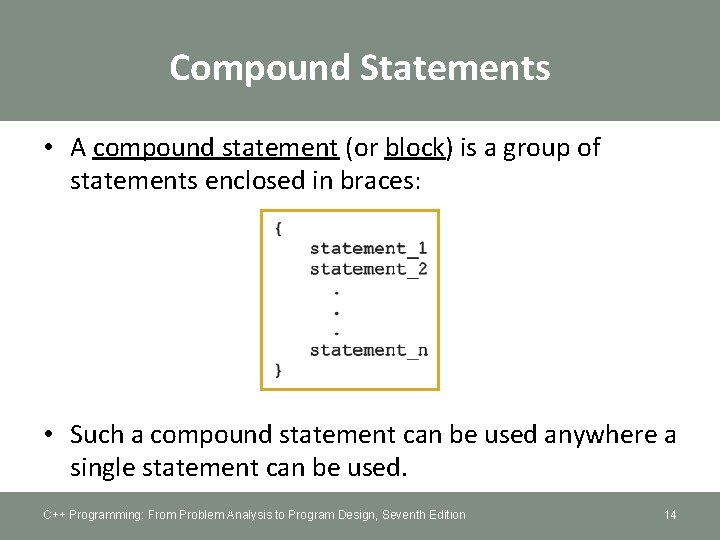
Compound Statements • A compound statement (or block) is a group of statements enclosed in braces: • Such a compound statement can be used anywhere a single statement can be used. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 14
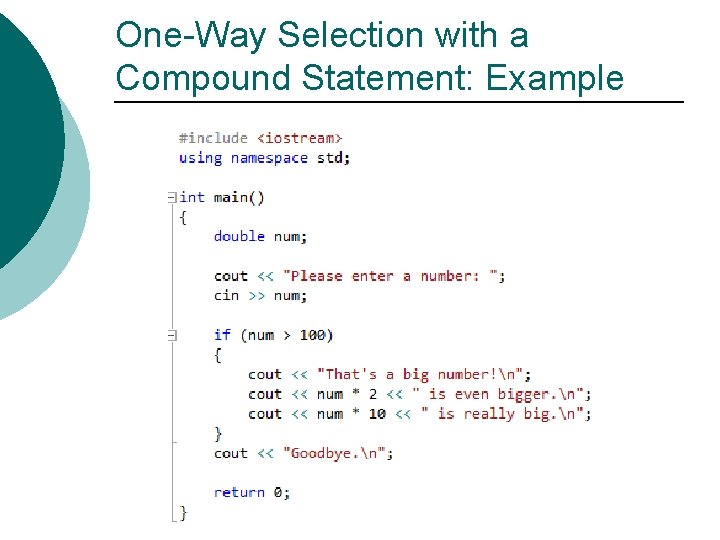
One-Way Selection with a Compound Statement: Example
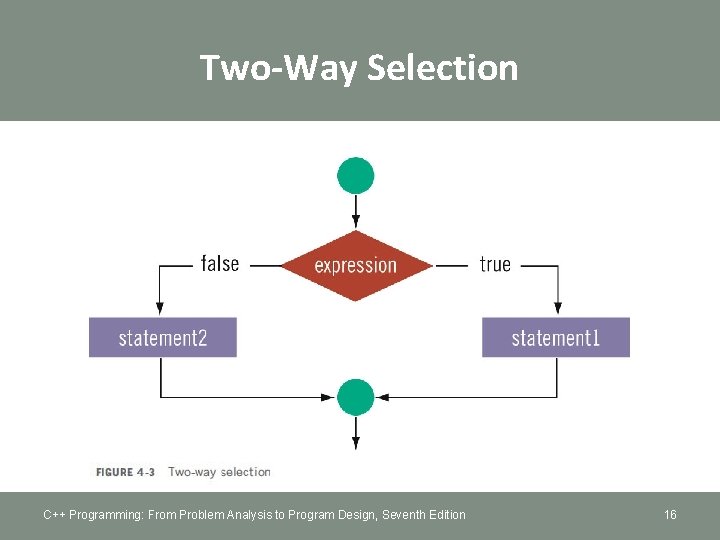
Two-Way Selection C++ Programming: From Problem Analysis to Program Design, Seventh Edition 16
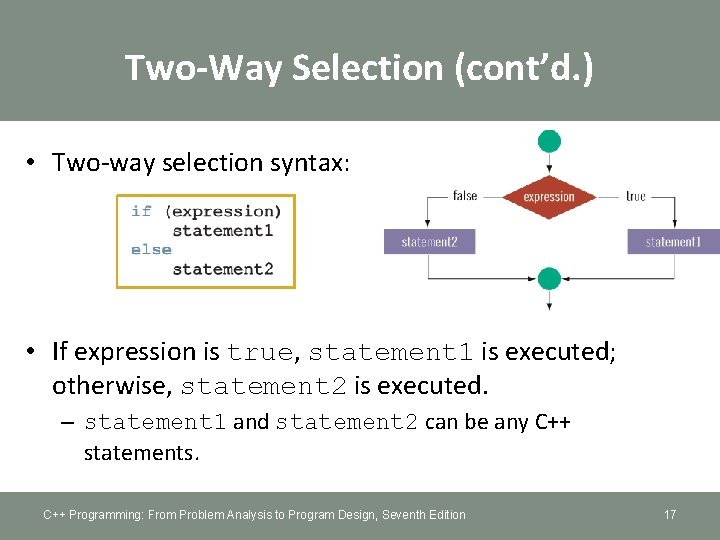
Two-Way Selection (cont’d. ) • Two-way selection syntax: • If expression is true, statement 1 is executed; otherwise, statement 2 is executed. – statement 1 and statement 2 can be any C++ statements. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 17
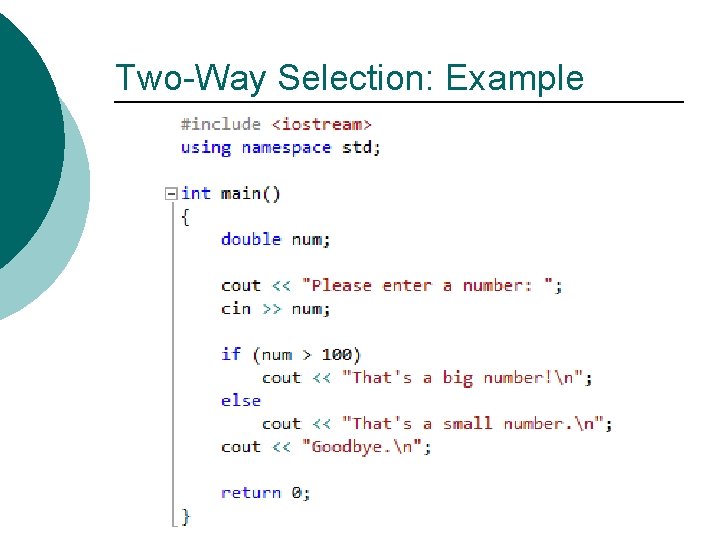
Two-Way Selection: Example
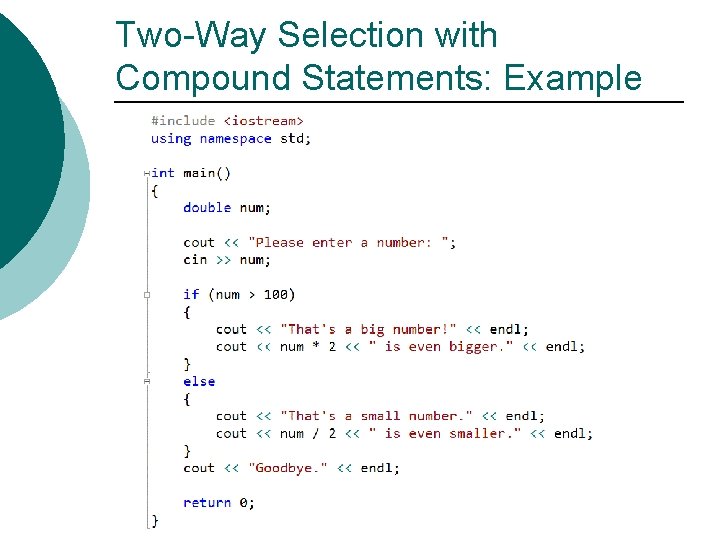
Two-Way Selection with Compound Statements: Example
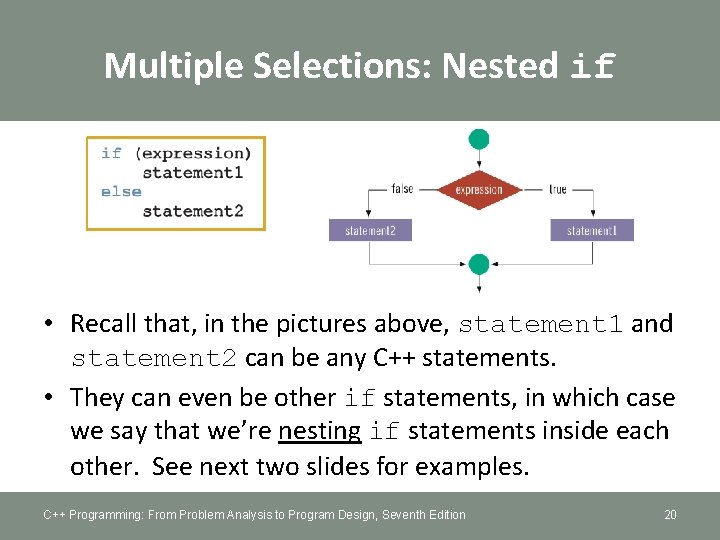
Multiple Selections: Nested if • Recall that, in the pictures above, statement 1 and statement 2 can be any C++ statements. • They can even be other if statements, in which case we say that we’re nesting if statements inside each other. See next two slides for examples. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 20
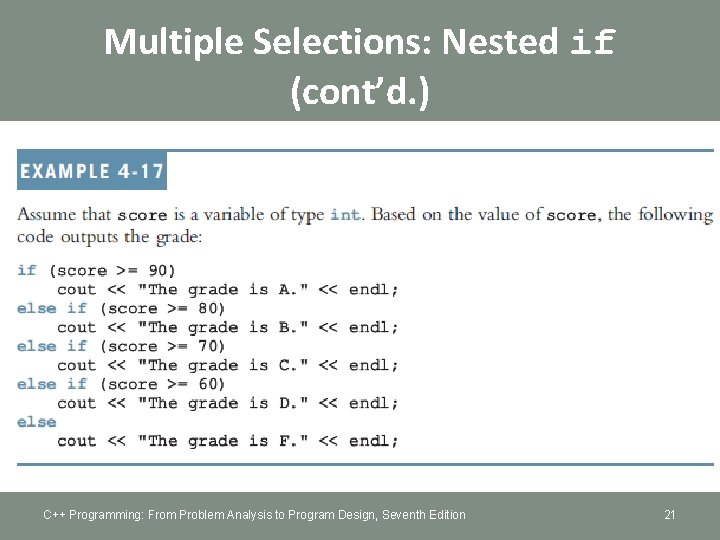
Multiple Selections: Nested if (cont’d. ) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 21
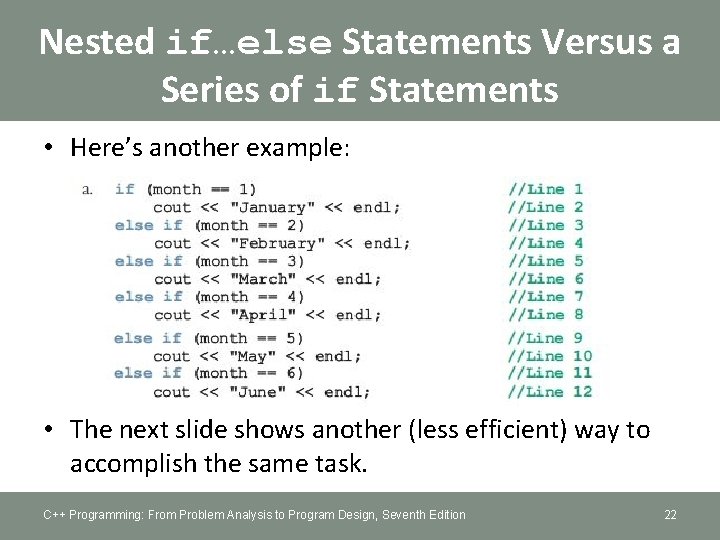
Nested if…else Statements Versus a Series of if Statements • Here’s another example: • The next slide shows another (less efficient) way to accomplish the same task. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 22
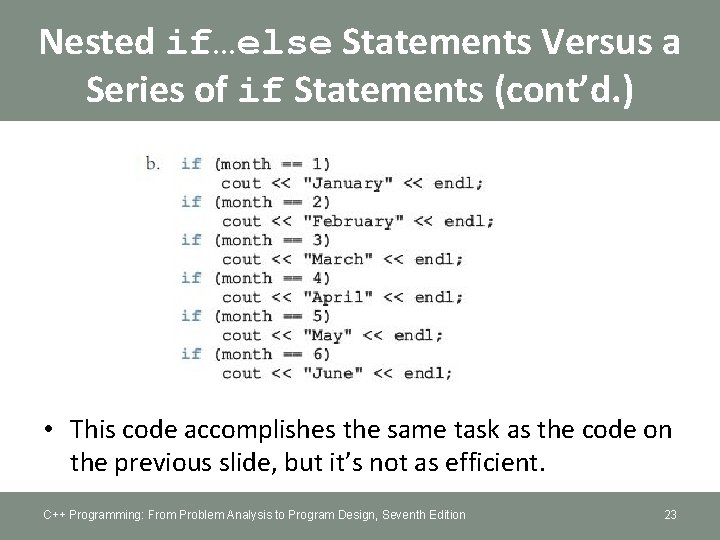
Nested if…else Statements Versus a Series of if Statements (cont’d. ) • This code accomplishes the same task as the code on the previous slide, but it’s not as efficient. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 23
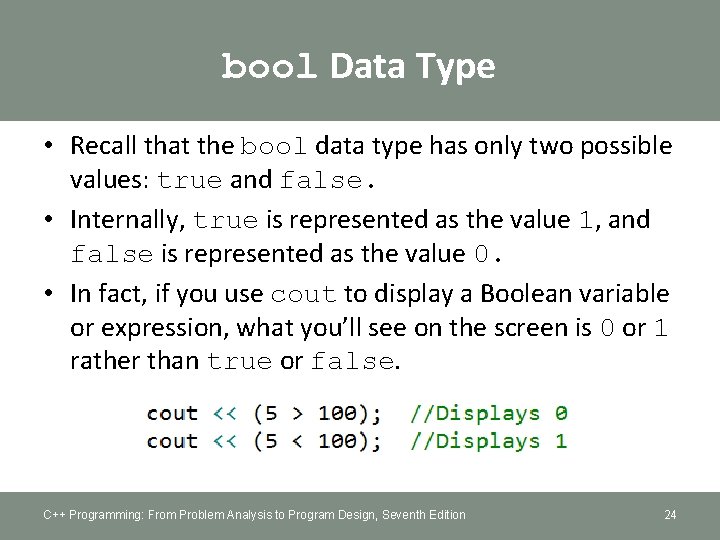
bool Data Type • Recall that the bool data type has only two possible values: true and false. • Internally, true is represented as the value 1, and false is represented as the value 0. • In fact, if you use cout to display a Boolean variable or expression, what you’ll see on the screen is 0 or 1 rather than true or false. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 24
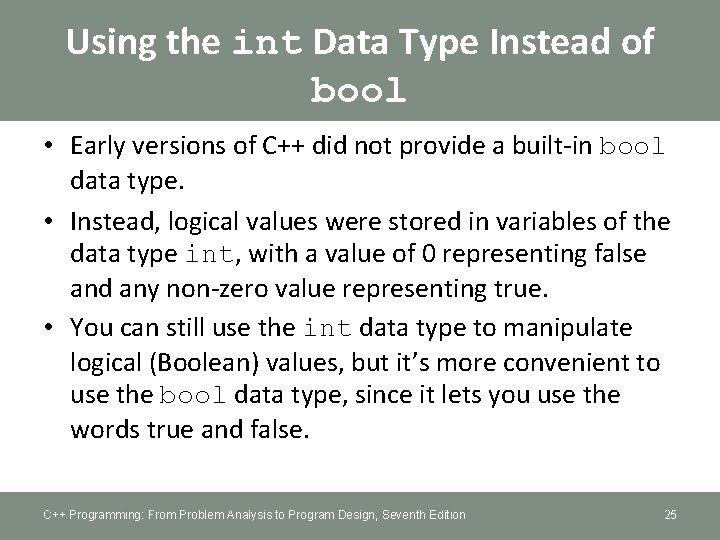
Using the int Data Type Instead of bool • Early versions of C++ did not provide a built-in bool data type. • Instead, logical values were stored in variables of the data type int, with a value of 0 representing false and any non-zero value representing true. • You can still use the int data type to manipulate logical (Boolean) values, but it’s more convenient to use the bool data type, since it lets you use the words true and false. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 25
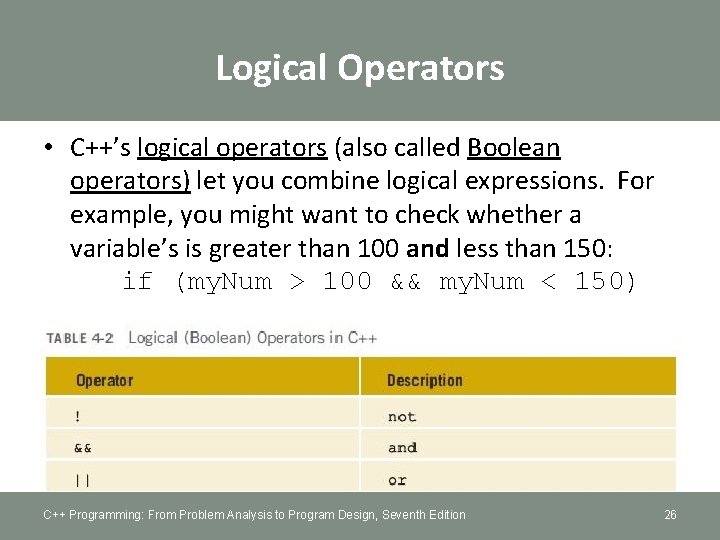
Logical Operators • C++’s logical operators (also called Boolean operators) let you combine logical expressions. For example, you might want to check whether a variable’s is greater than 100 and less than 150: if (my. Num > 100 && my. Num < 150) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 26
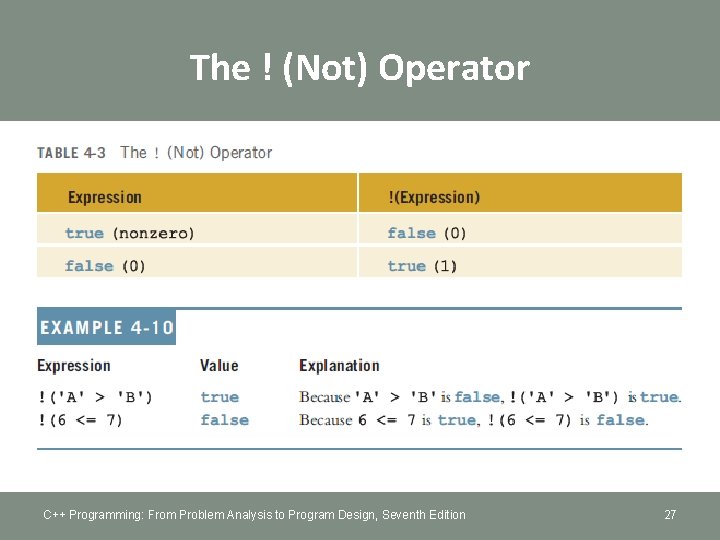
The ! (Not) Operator C++ Programming: From Problem Analysis to Program Design, Seventh Edition 27
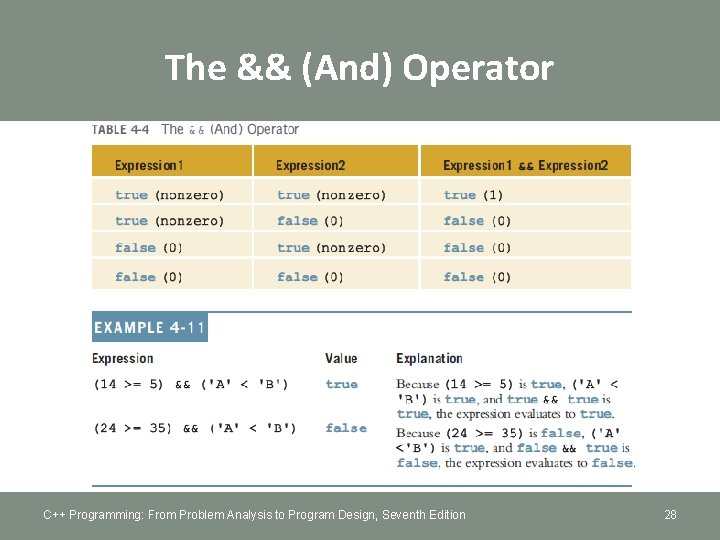
The && (And) Operator C++ Programming: From Problem Analysis to Program Design, Seventh Edition 28
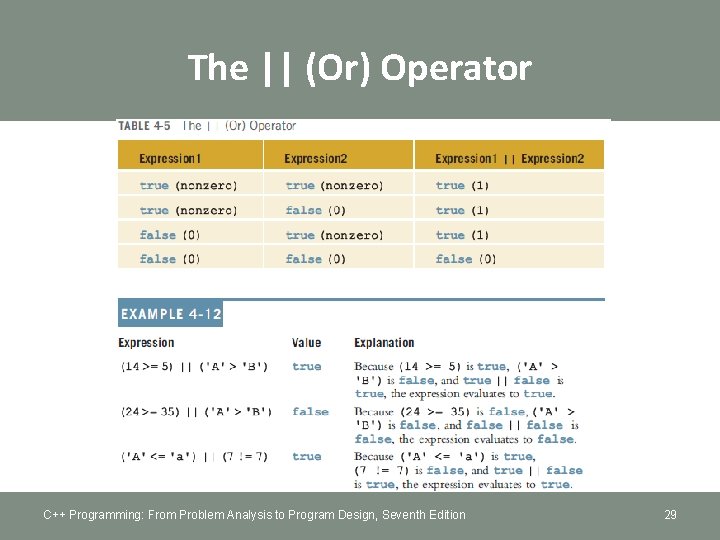
The || (Or) Operator C++ Programming: From Problem Analysis to Program Design, Seventh Edition 29
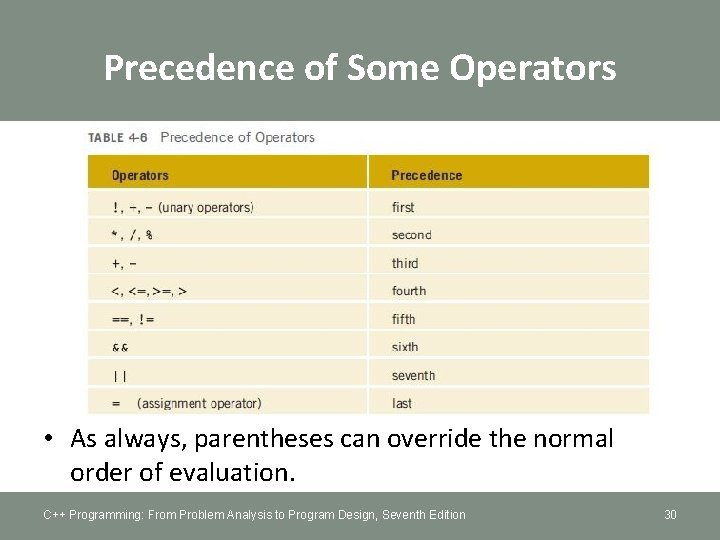
Precedence of Some Operators • As always, parentheses can override the normal order of evaluation. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 30
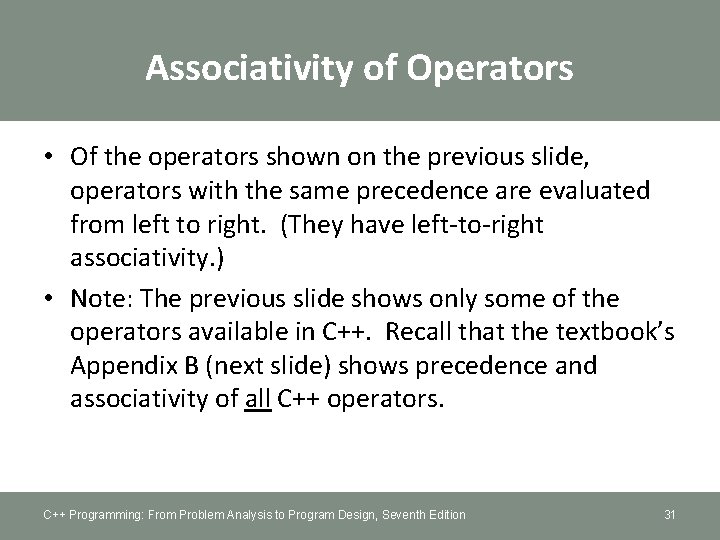
Associativity of Operators • Of the operators shown on the previous slide, operators with the same precedence are evaluated from left to right. (They have left-to-right associativity. ) • Note: The previous slide shows only some of the operators available in C++. Recall that the textbook’s Appendix B (next slide) shows precedence and associativity of all C++ operators. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 31
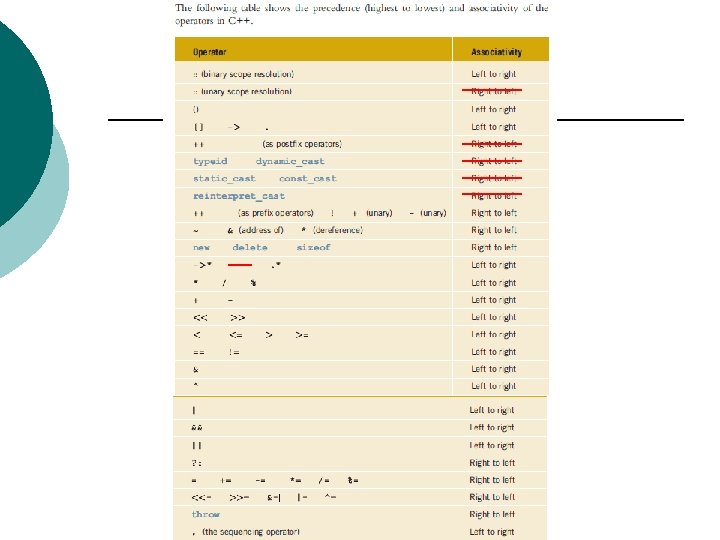
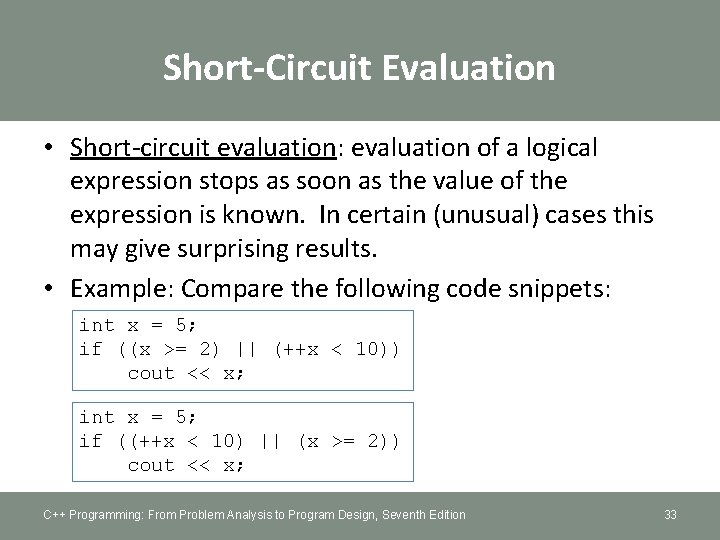
Short-Circuit Evaluation • Short-circuit evaluation: evaluation of a logical expression stops as soon as the value of the expression is known. In certain (unusual) cases this may give surprising results. • Example: Compare the following code snippets: int x = 5; if ((x >= 2) || (++x < 10)) cout << x; int x = 5; if ((++x < 10) || (x >= 2)) cout << x; C++ Programming: From Problem Analysis to Program Design, Seventh Edition 33
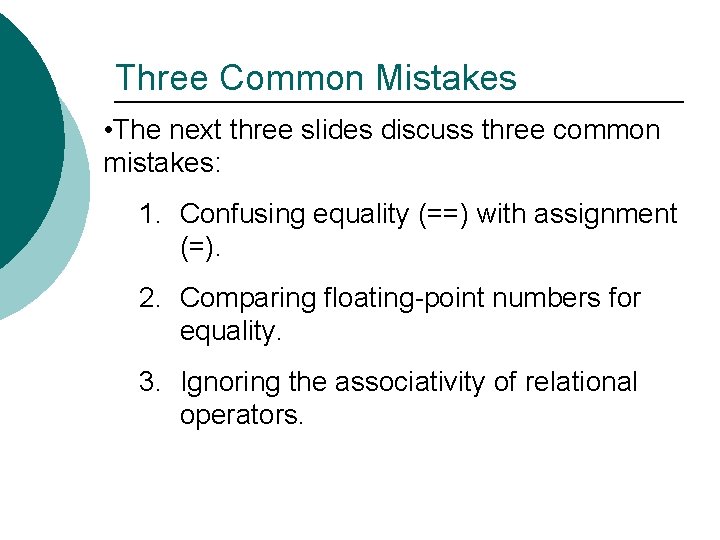
Three Common Mistakes • The next three slides discuss three common mistakes: 1. Confusing equality (==) with assignment (=). 2. Comparing floating-point numbers for equality. 3. Ignoring the associativity of relational operators.
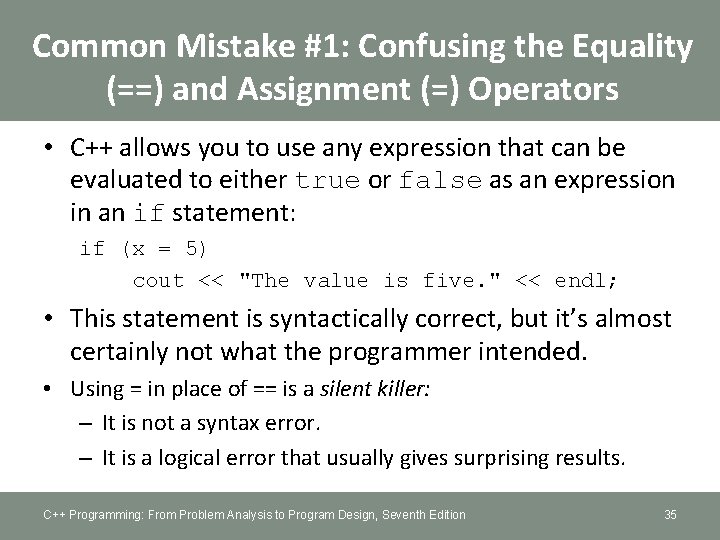
Common Mistake #1: Confusing the Equality (==) and Assignment (=) Operators • C++ allows you to use any expression that can be evaluated to either true or false as an expression in an if statement: if (x = 5) cout << "The value is five. " << endl; • This statement is syntactically correct, but it’s almost certainly not what the programmer intended. • Using = in place of == is a silent killer: – It is not a syntax error. – It is a logical error that usually gives surprising results. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 35
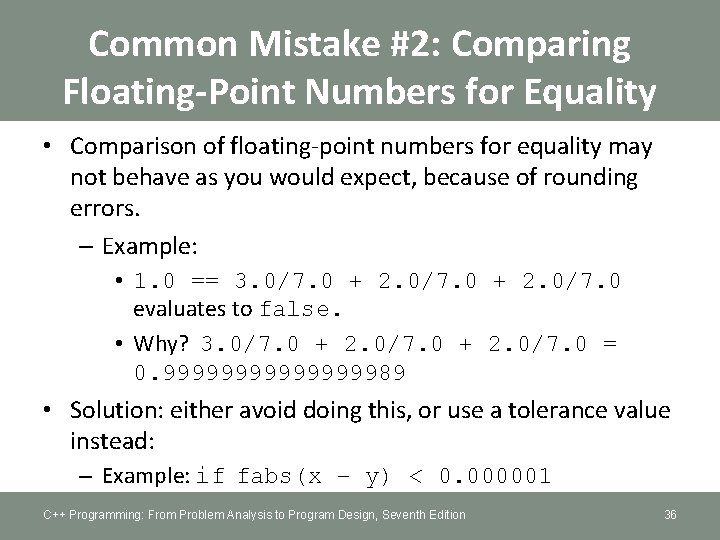
Common Mistake #2: Comparing Floating-Point Numbers for Equality • Comparison of floating-point numbers for equality may not behave as you would expect, because of rounding errors. – Example: • 1. 0 == 3. 0/7. 0 + 2. 0/7. 0 evaluates to false. • Why? 3. 0/7. 0 + 2. 0/7. 0 = 0. 9999999989 • Solution: either avoid doing this, or use a tolerance value instead: – Example: if fabs(x – y) < 0. 000001 C++ Programming: From Problem Analysis to Program Design, Seventh Edition 36
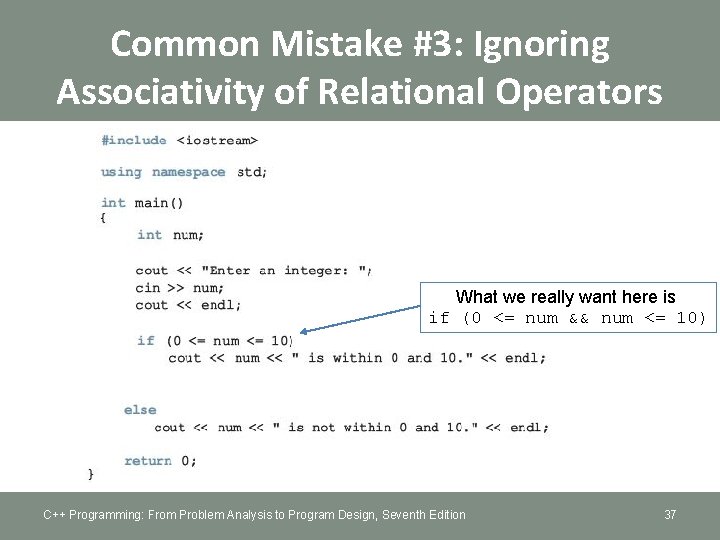
Common Mistake #3: Ignoring Associativity of Relational Operators What we really want here is if (0 <= num && num <= 10) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 37
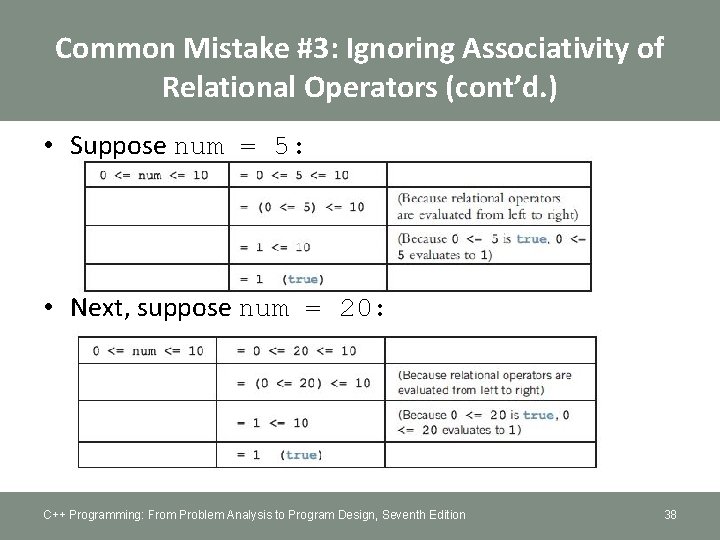
Common Mistake #3: Ignoring Associativity of Relational Operators (cont’d. ) • Suppose num = 5: • Next, suppose num = 20: C++ Programming: From Problem Analysis to Program Design, Seventh Edition 38
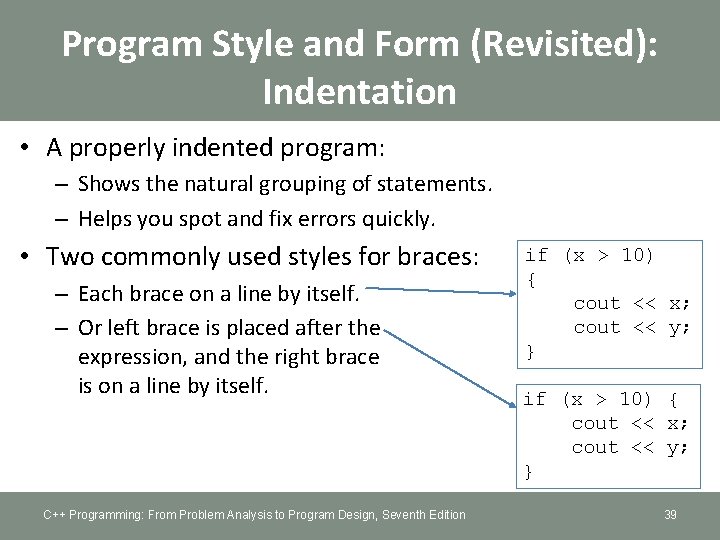
Program Style and Form (Revisited): Indentation • A properly indented program: – Shows the natural grouping of statements. – Helps you spot and fix errors quickly. • Two commonly used styles for braces: – Each brace on a line by itself. – Or left brace is placed after the expression, and the right brace is on a line by itself. C++ Programming: From Problem Analysis to Program Design, Seventh Edition if (x > 10) { cout << x; cout << y; } 39
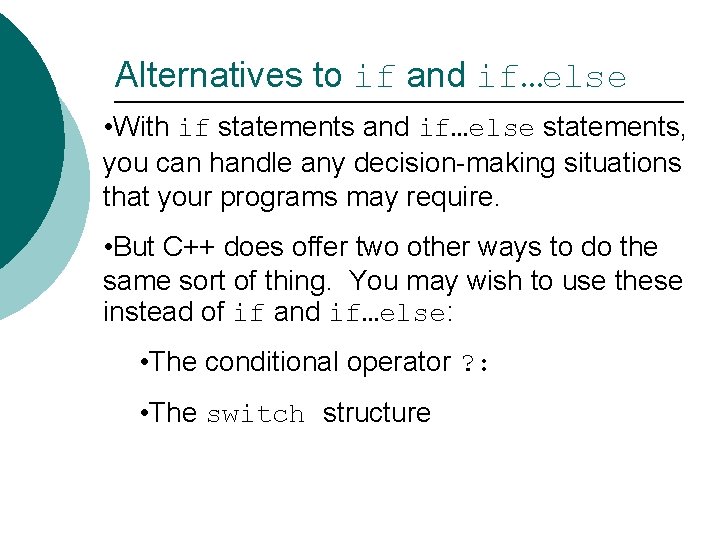
Alternatives to if and if…else • With if statements and if…else statements, you can handle any decision-making situations that your programs may require. • But C++ does offer two other ways to do the same sort of thing. You may wish to use these instead of if and if…else: • The conditional operator ? : • The switch structure
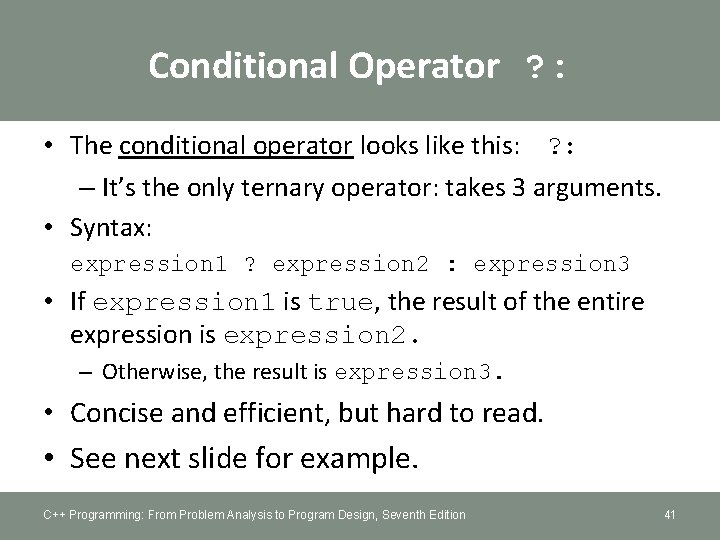
Conditional Operator ? : • The conditional operator looks like this: ? : – It’s the only ternary operator: takes 3 arguments. • Syntax: expression 1 ? expression 2 : expression 3 • If expression 1 is true, the result of the entire expression is expression 2. – Otherwise, the result is expression 3. • Concise and efficient, but hard to read. • See next slide for example. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 41
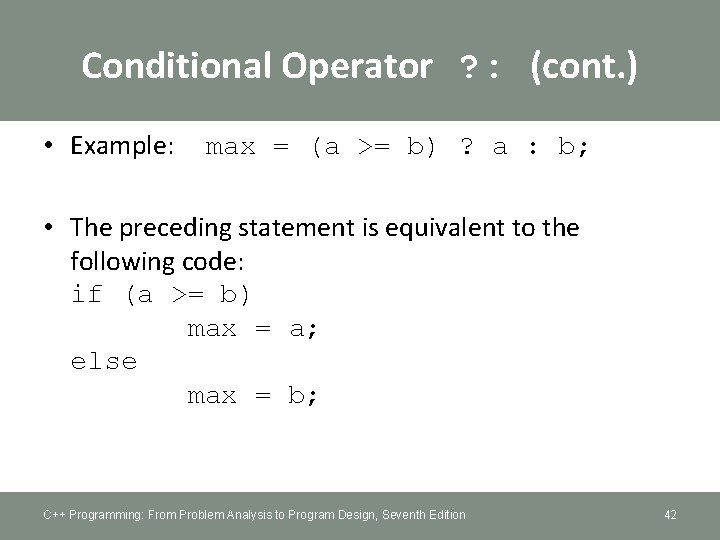
Conditional Operator ? : (cont. ) • Example: max = (a >= b) ? a : b; • The preceding statement is equivalent to the following code: if (a >= b) max = a; else max = b; C++ Programming: From Problem Analysis to Program Design, Seventh Edition 42
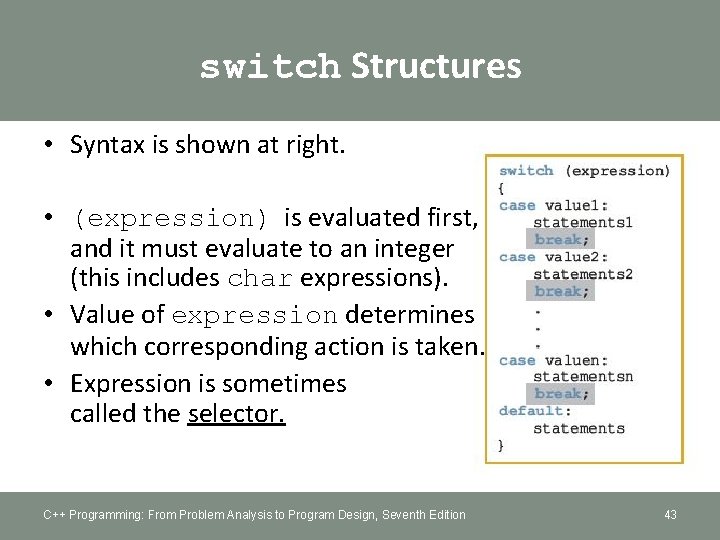
switch Structures • Syntax is shown at right. • (expression) is evaluated first, and it must evaluate to an integer (this includes char expressions). • Value of expression determines which corresponding action is taken. • Expression is sometimes called the selector. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 43
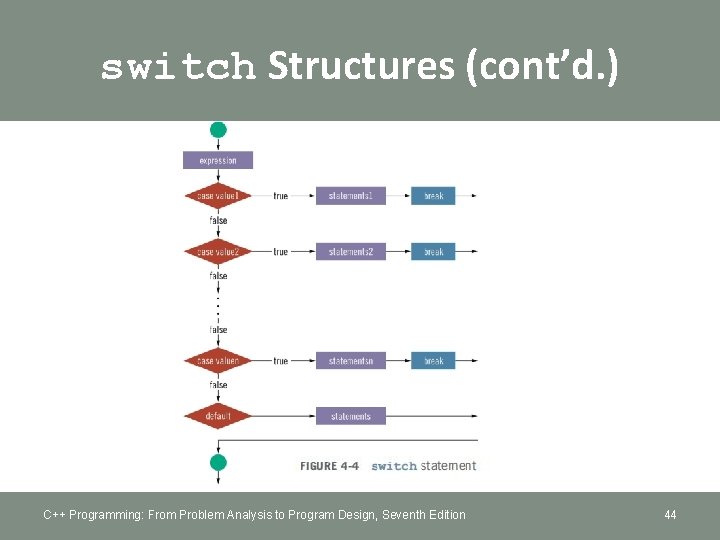
switch Structures (cont’d. ) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 44
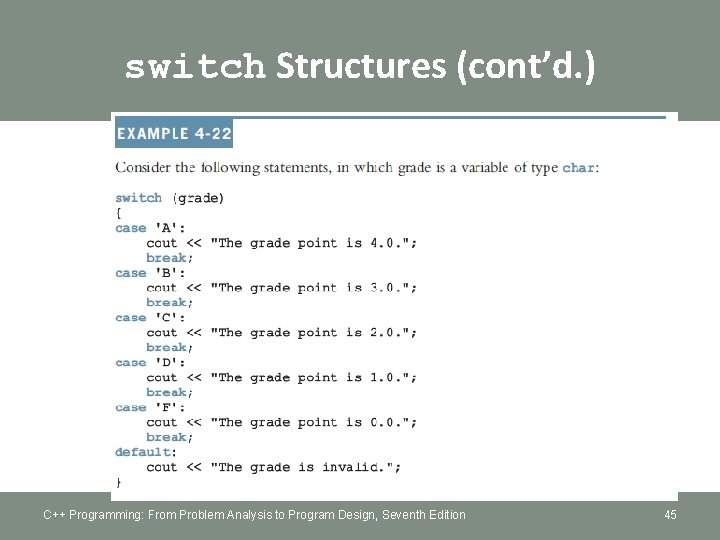
switch Structures (cont’d. ) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 45
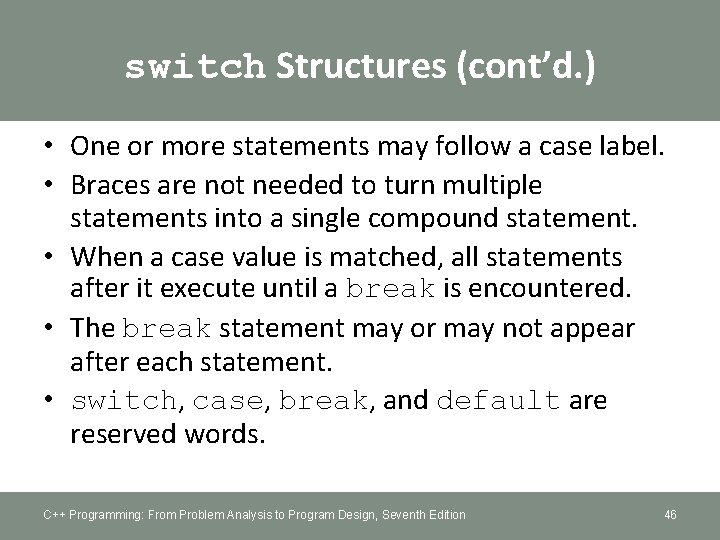
switch Structures (cont’d. ) • One or more statements may follow a case label. • Braces are not needed to turn multiple statements into a single compound statement. • When a case value is matched, all statements after it execute until a break is encountered. • The break statement may or may not appear after each statement. • switch, case, break, and default are reserved words. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 46
Collection of programs written to service other programs.
Algorithms + data structures = programs
Balancing selection vs stabilizing selection
Similarities
K selection r selection
Natural selection vs artificial selection
Artificial selection vs natural selection
Directional selection
Clumped dispersion
Natural selection vs artificial selection
Two way selection and multiway selection in c
Multiway selection in c
Procedure of pure line selection
Logical functions matlab
Selection structures
Homology
Hình ảnh bộ gõ cơ thể búng tay
Ng-html
Bổ thể
Tỉ lệ cơ thể trẻ em
Chó sói
Glasgow thang điểm
Hát lên người ơi alleluia
Môn thể thao bắt đầu bằng chữ f
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công của trọng lực
Trời xanh đây là của chúng ta thể thơ
Cách giải mật thư tọa độ
Làm thế nào để 102-1=99
độ dài liên kết
Các châu lục và đại dương trên thế giới
Thơ thất ngôn tứ tuyệt đường luật
Quá trình desamine hóa có thể tạo ra
Một số thể thơ truyền thống
Cái miệng xinh xinh thế chỉ nói điều hay thôi
Vẽ hình chiếu vuông góc của vật thể sau
Biện pháp chống mỏi cơ
đặc điểm cơ thể của người tối cổ
Ví dụ giọng cùng tên
Vẽ hình chiếu đứng bằng cạnh của vật thể
Vẽ hình chiếu vuông góc của vật thể sau
Thẻ vin
đại từ thay thế
điện thế nghỉ
Tư thế ngồi viết
Diễn thế sinh thái là