Adding psuedocode pre instantiate a date with todays
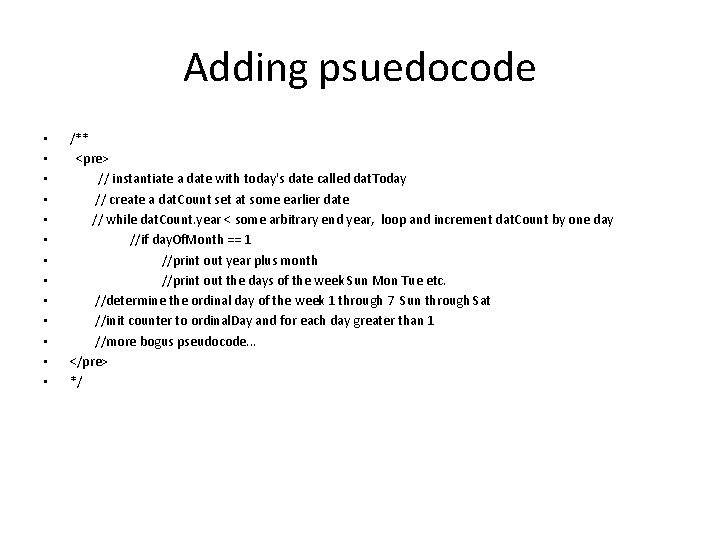
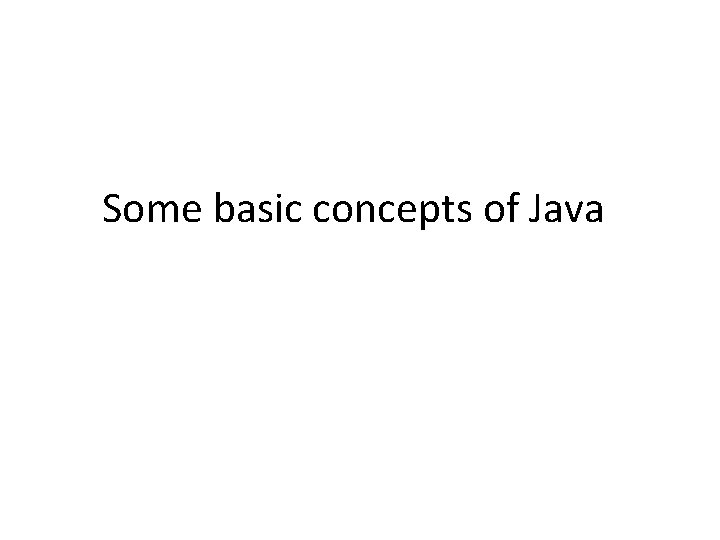
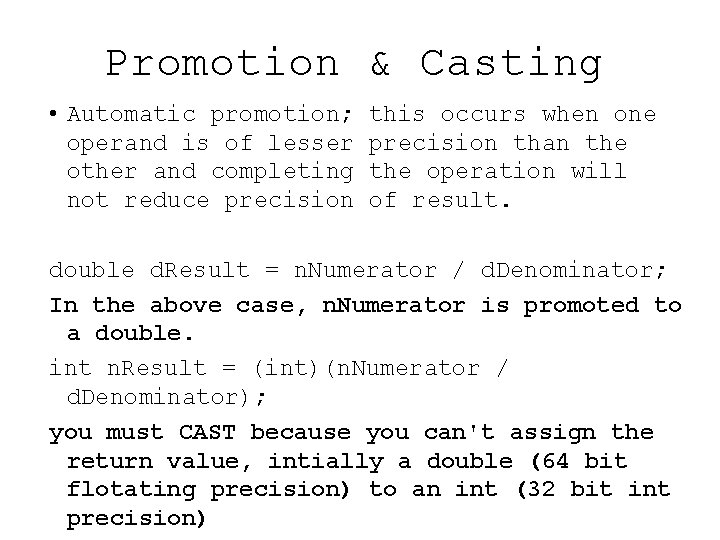
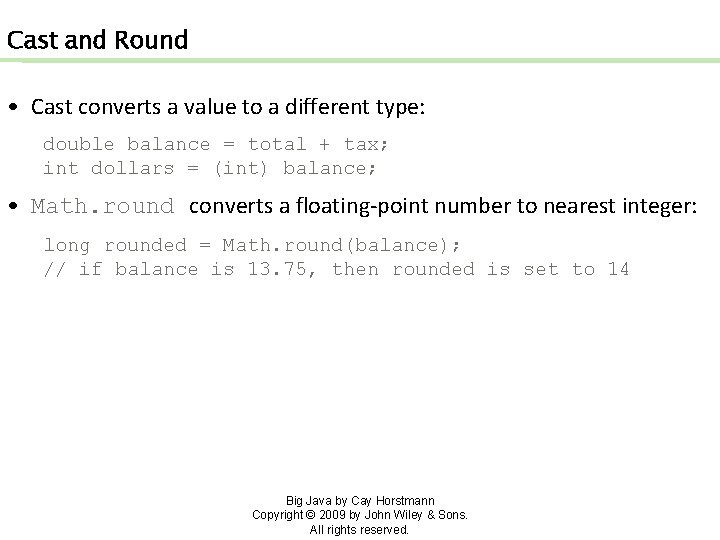
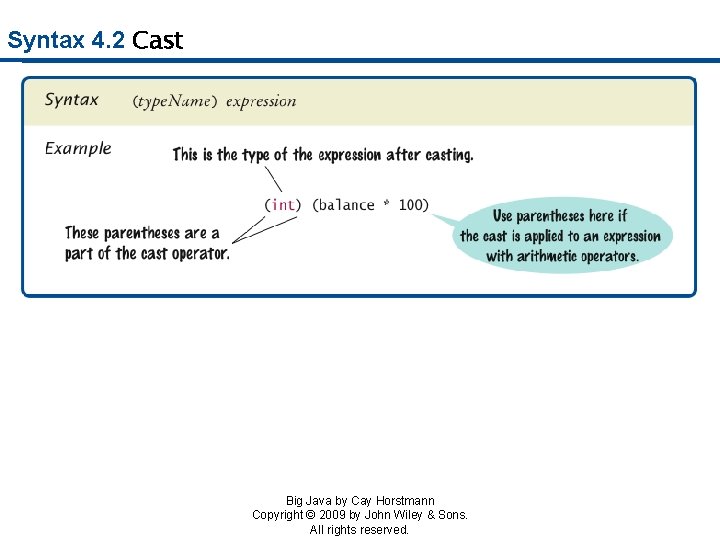
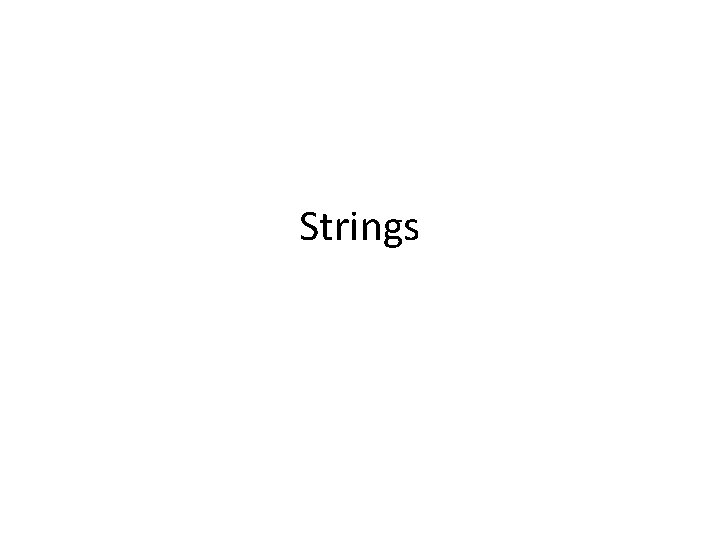
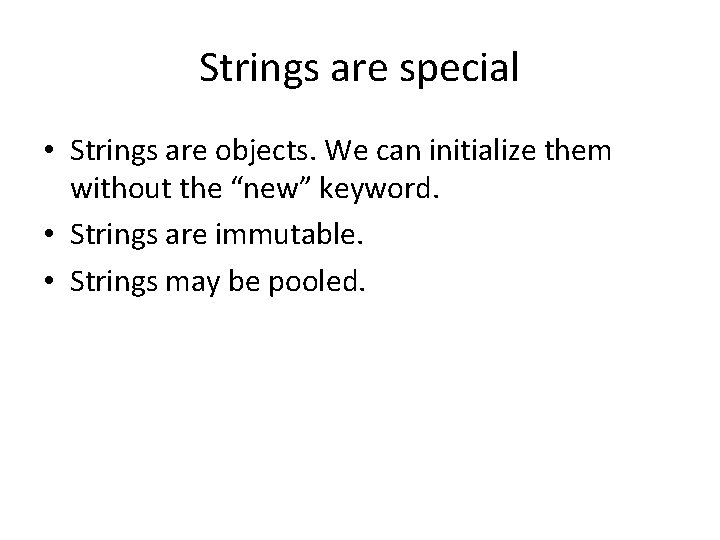
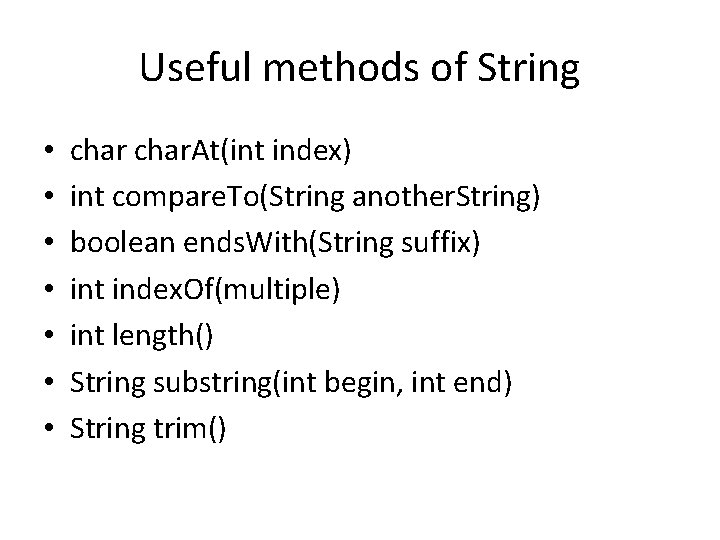
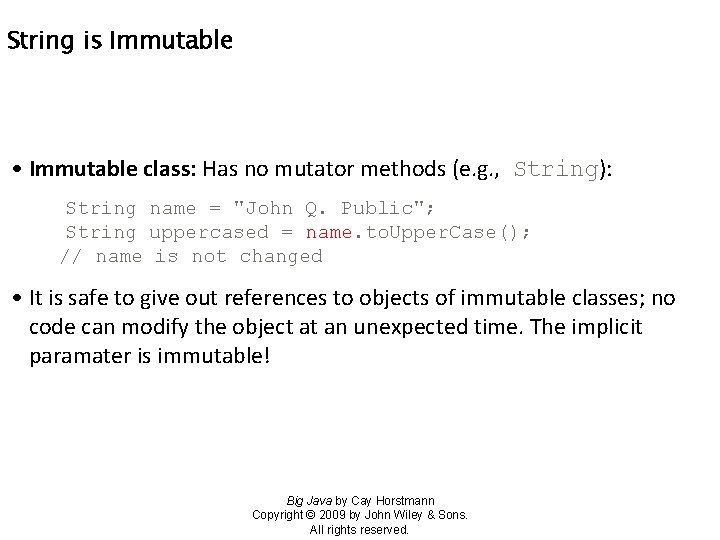
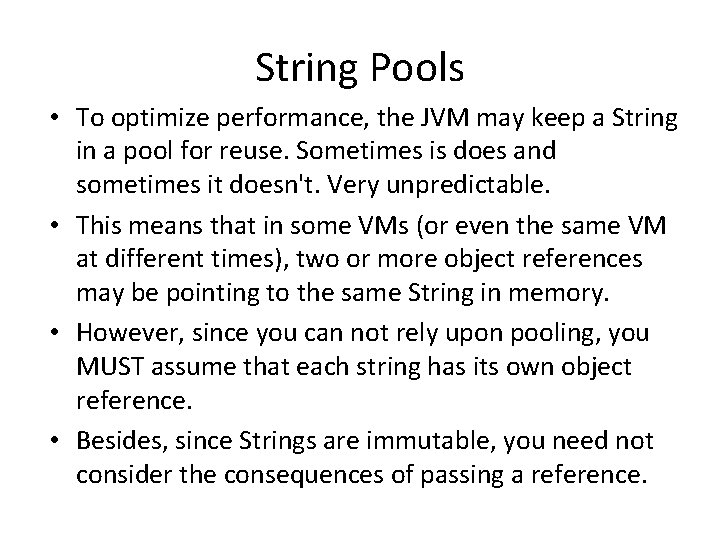
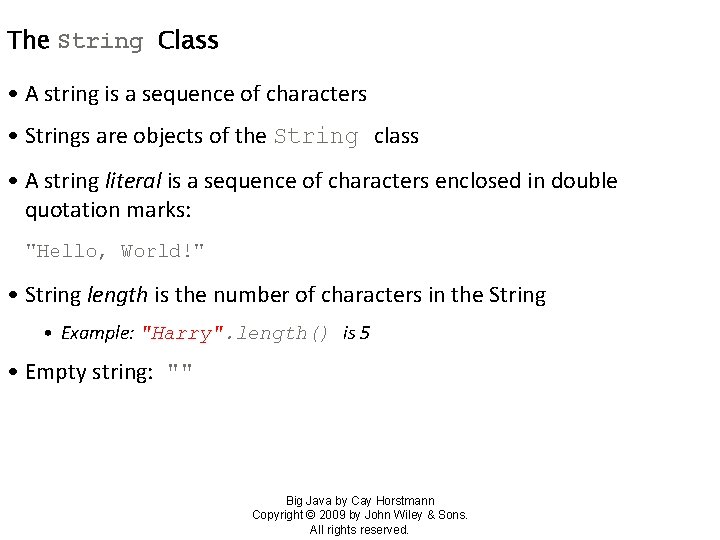
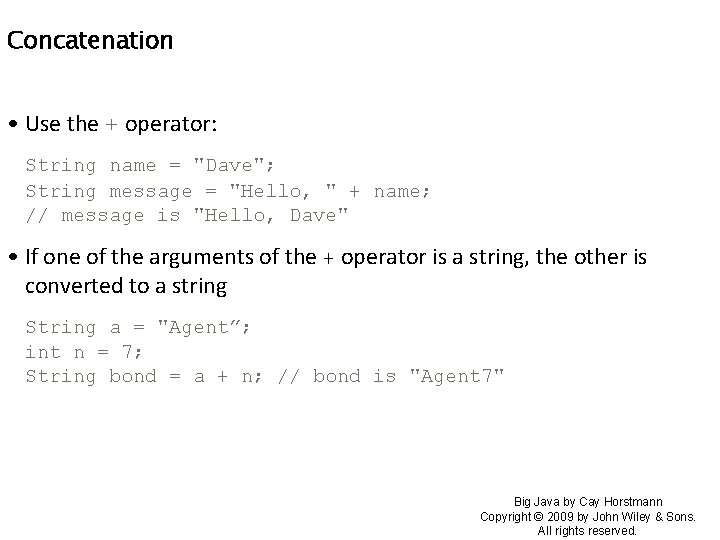
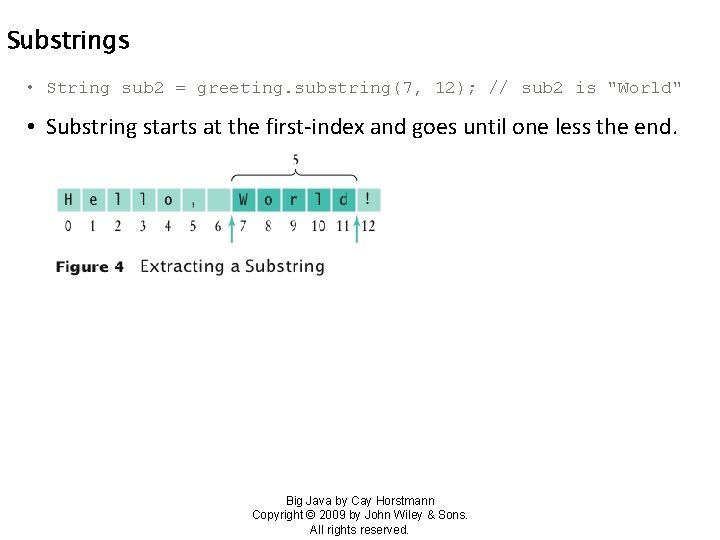
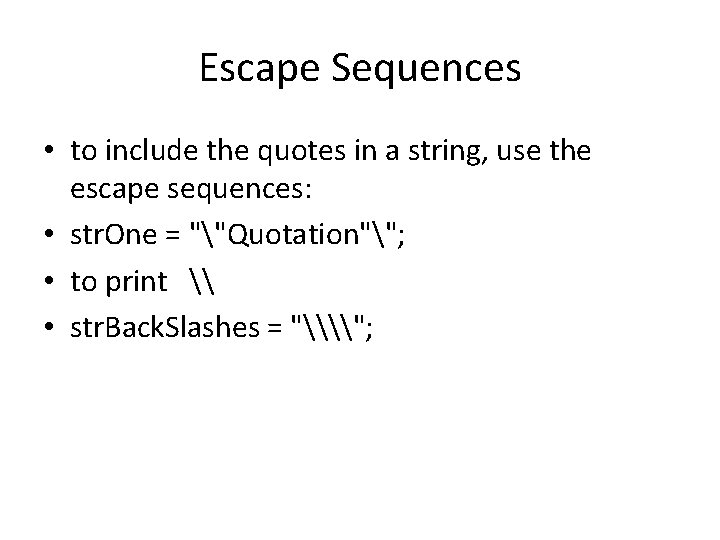
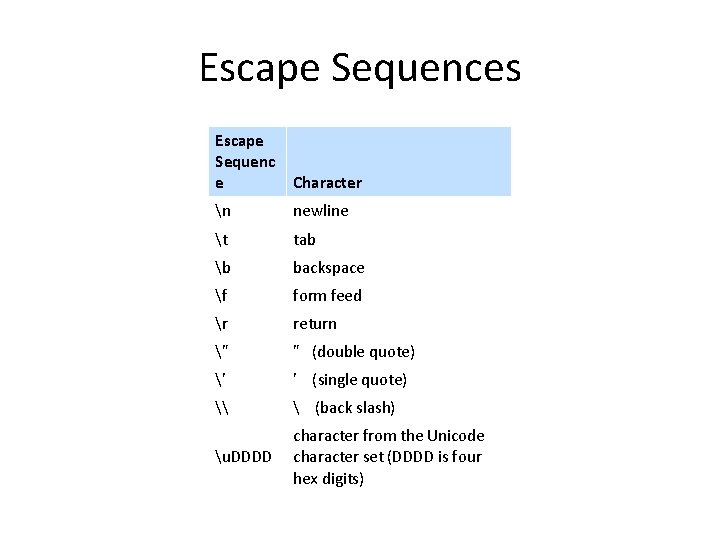
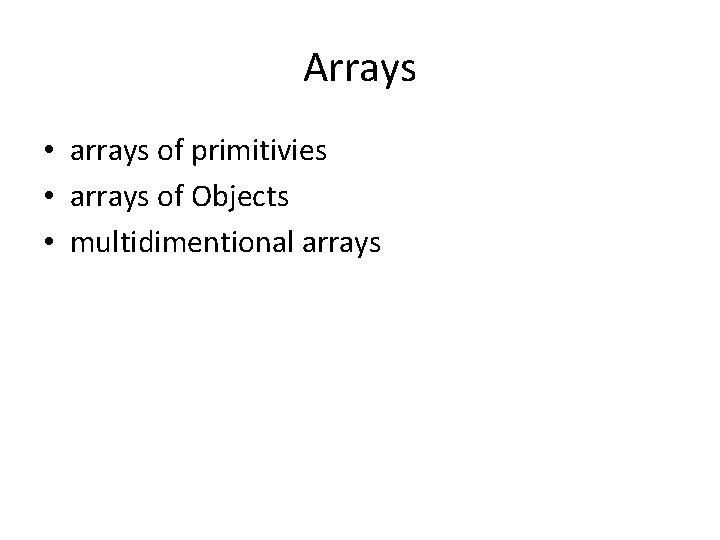
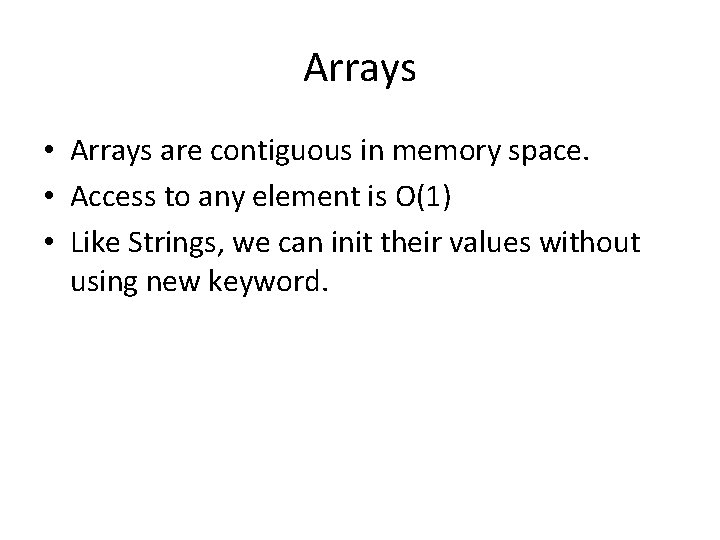
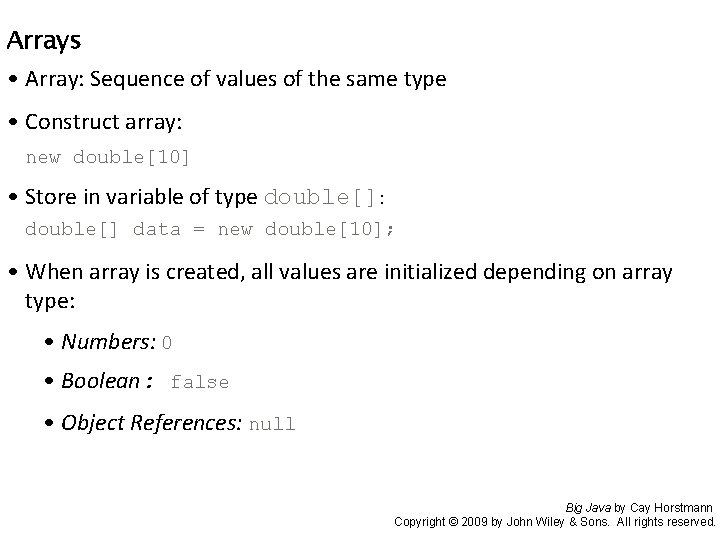
![Arrays: declare and init • Array: Sequence of values of the same type double[] Arrays: declare and init • Array: Sequence of values of the same type double[]](https://slidetodoc.com/presentation_image_h2/520214fe588c7300271032fb2c5a400c/image-19.jpg)
![Arrays Use [] to access an element: double[] values = new double[10]; values[2] = Arrays Use [] to access an element: double[] values = new double[10]; values[2] =](https://slidetodoc.com/presentation_image_h2/520214fe588c7300271032fb2c5a400c/image-20.jpg)
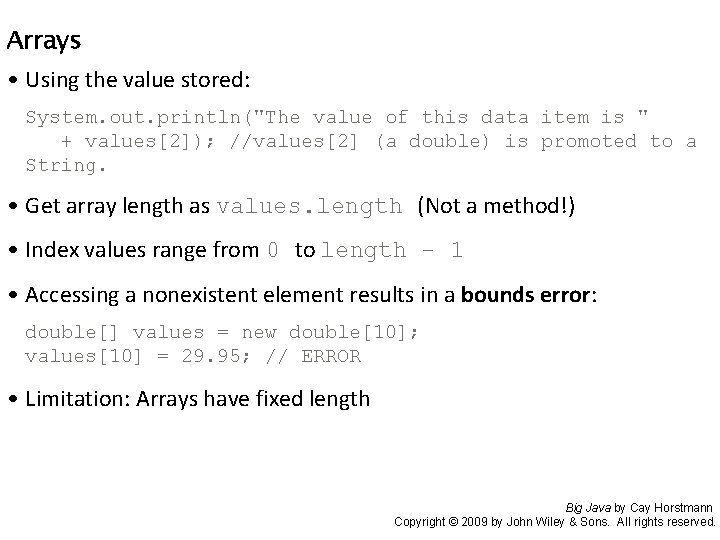
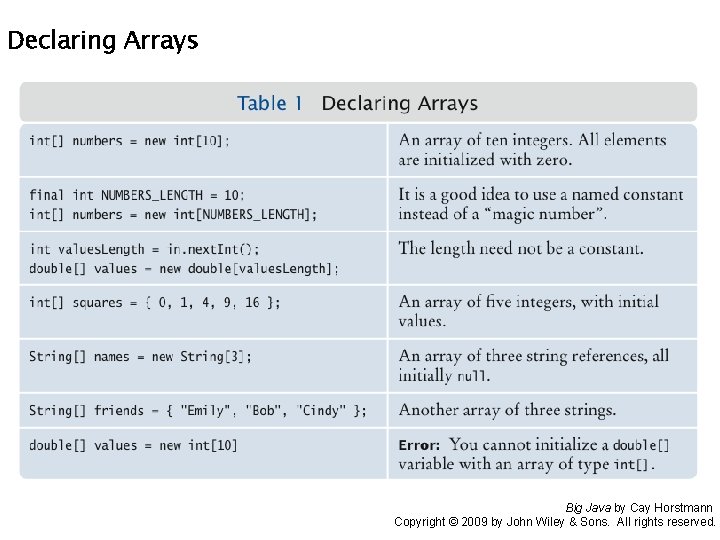
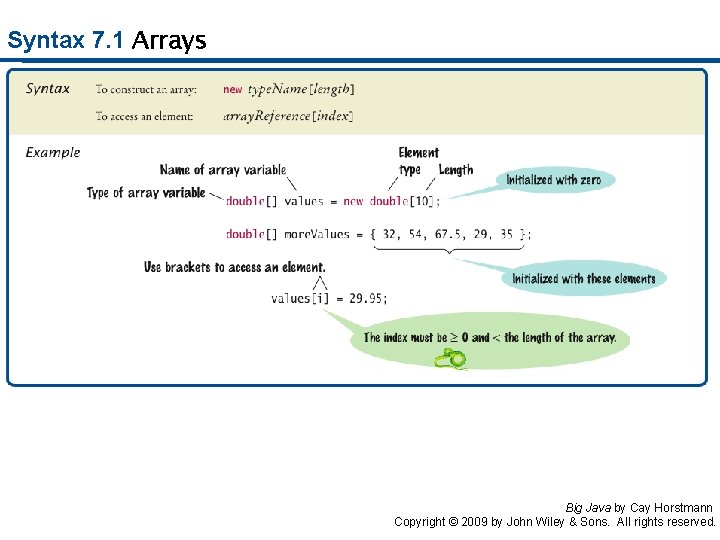
![Make Parallel Arrays into Arrays of Objects // Don't do this int[] account. Numbers; Make Parallel Arrays into Arrays of Objects // Don't do this int[] account. Numbers;](https://slidetodoc.com/presentation_image_h2/520214fe588c7300271032fb2c5a400c/image-24.jpg)
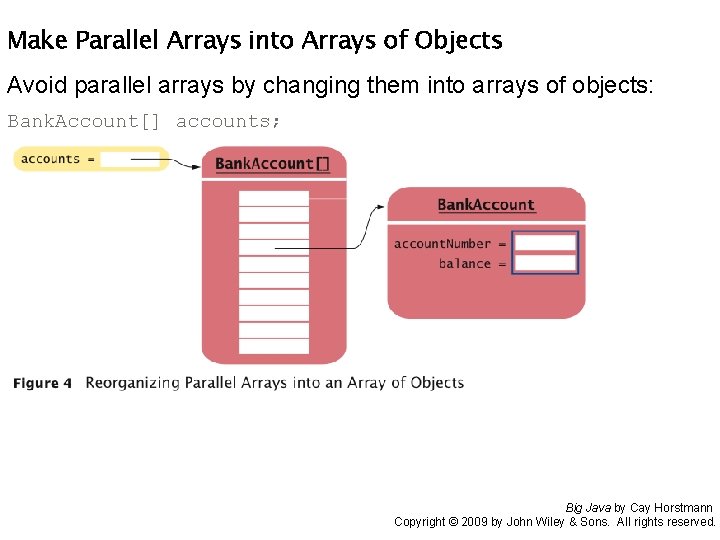
![multidimensional arrays • int[][] n. Numbers = new int[3][4]; • boolean[][] b. Exams = multidimensional arrays • int[][] n. Numbers = new int[3][4]; • boolean[][] b. Exams =](https://slidetodoc.com/presentation_image_h2/520214fe588c7300271032fb2c5a400c/image-26.jpg)
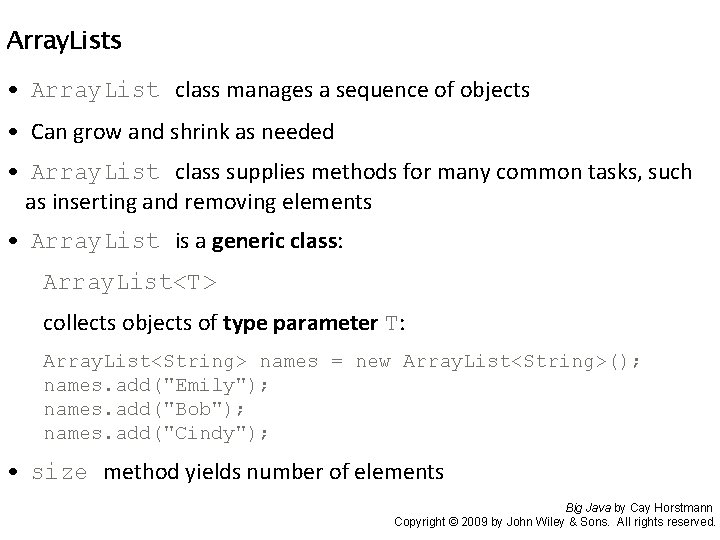
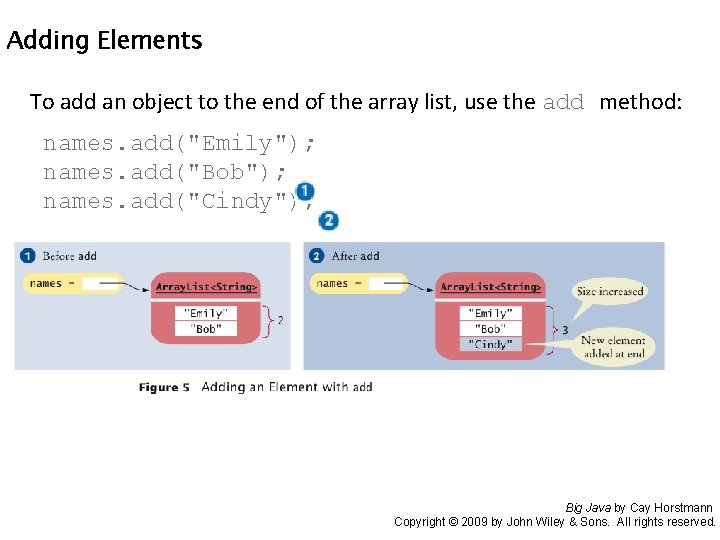
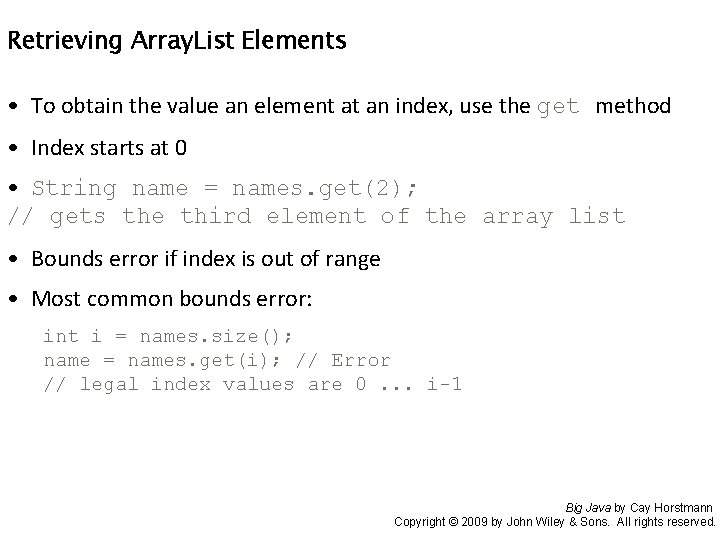
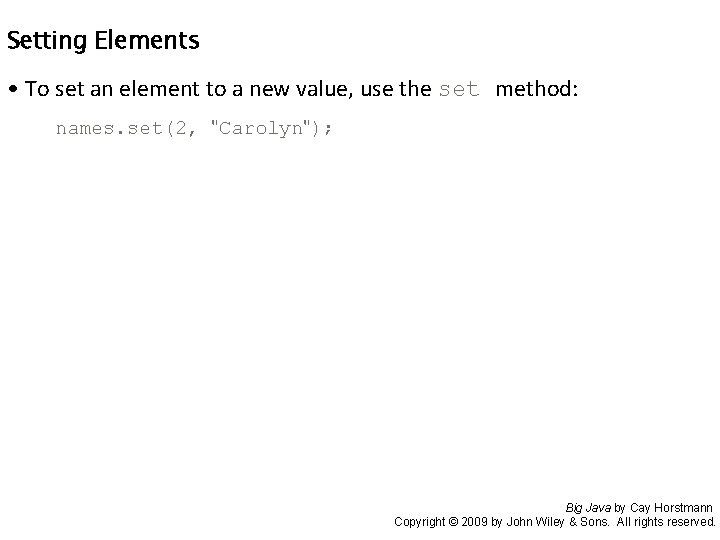
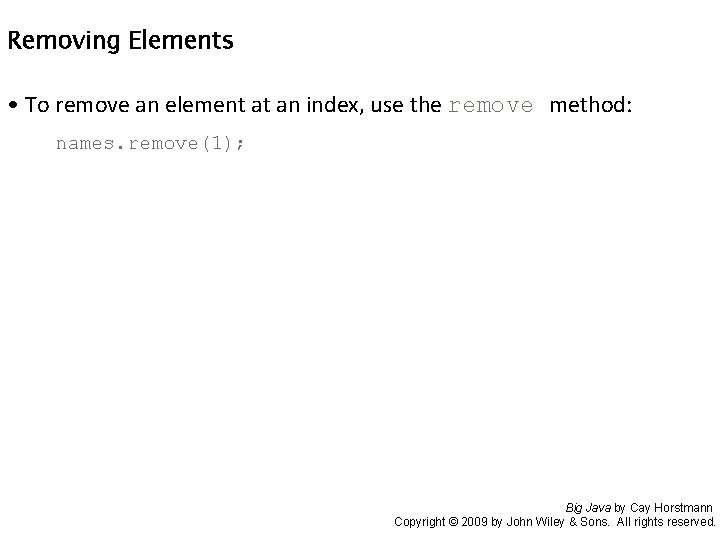
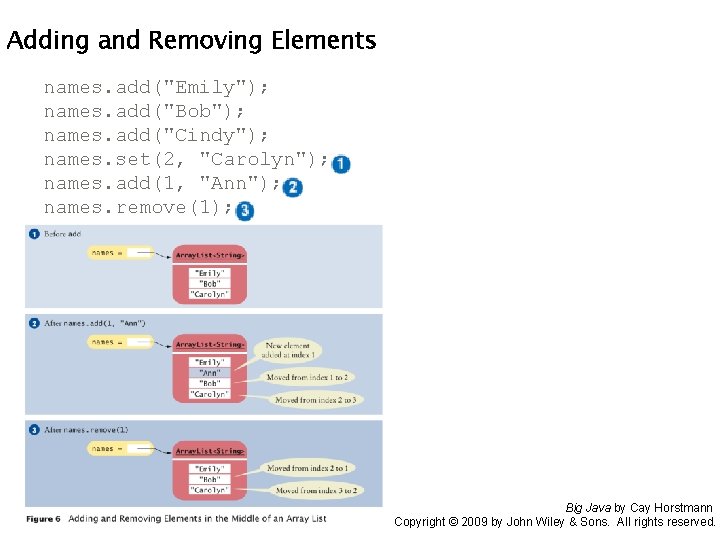
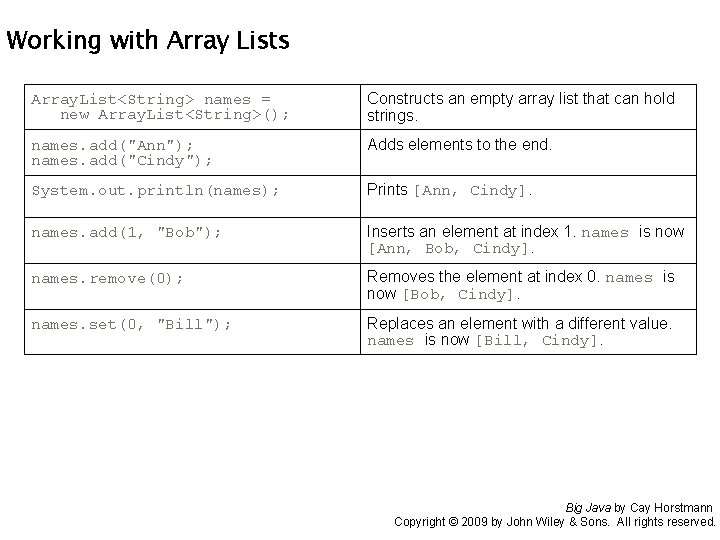
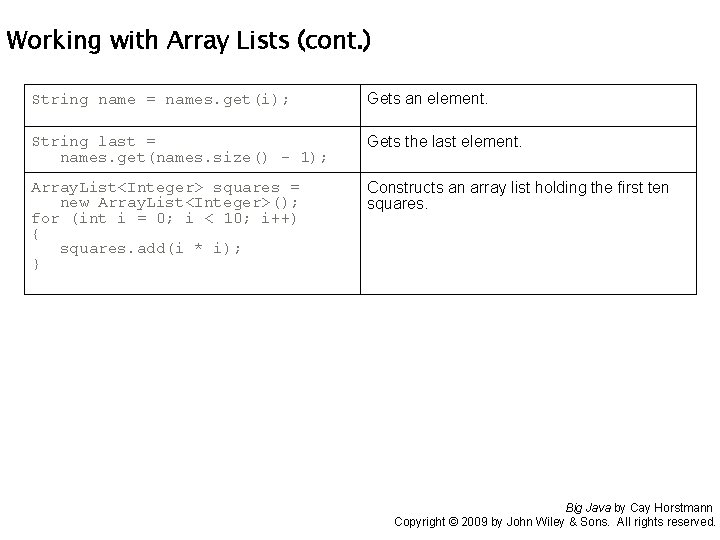
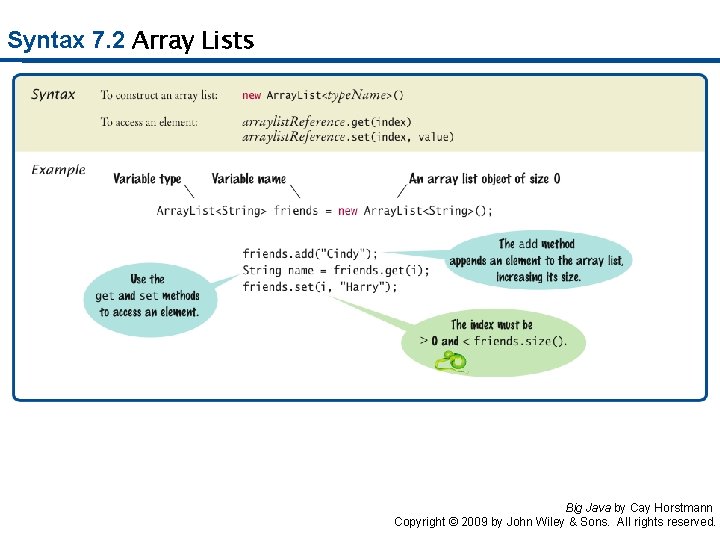
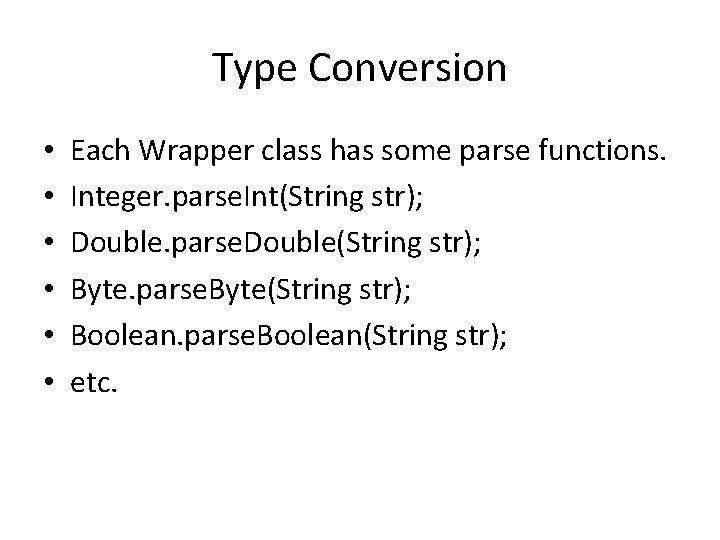
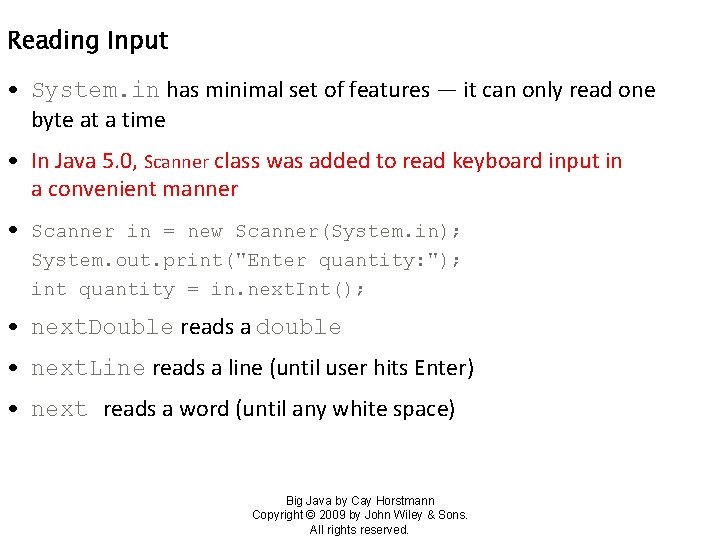
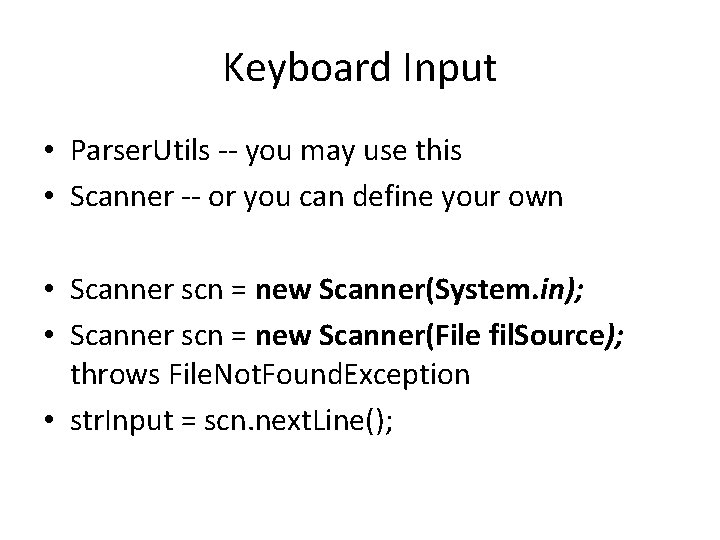
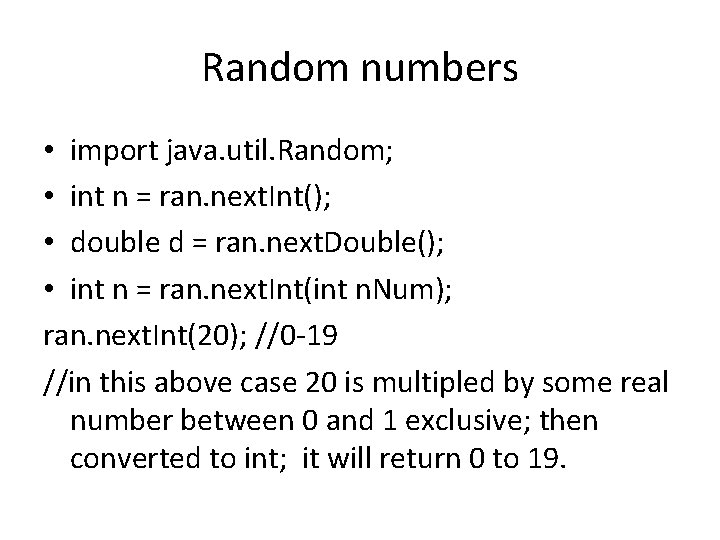
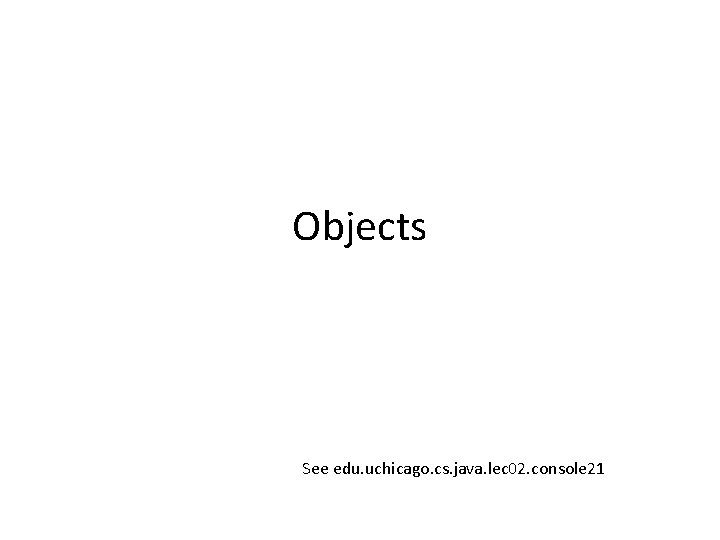
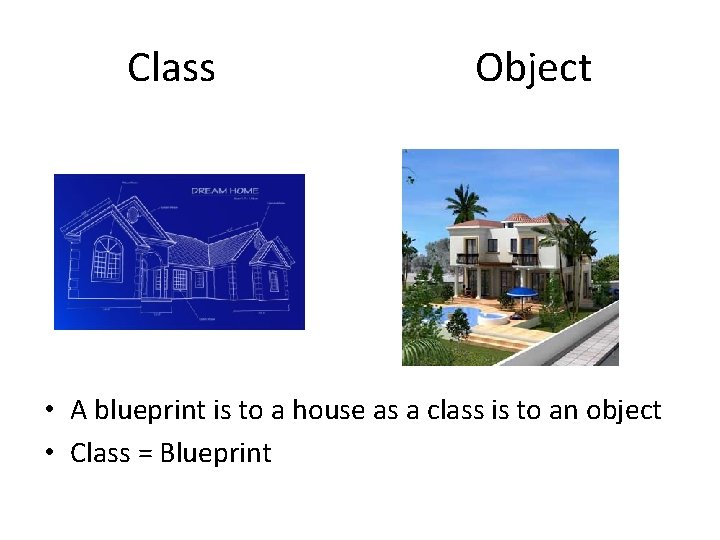
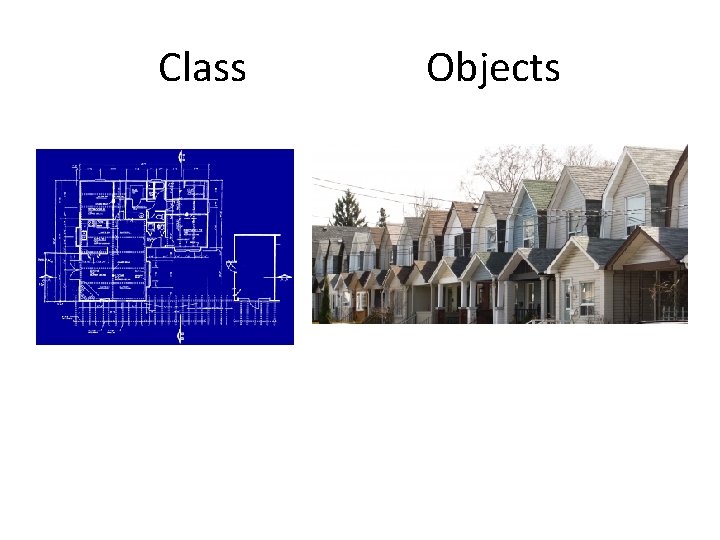
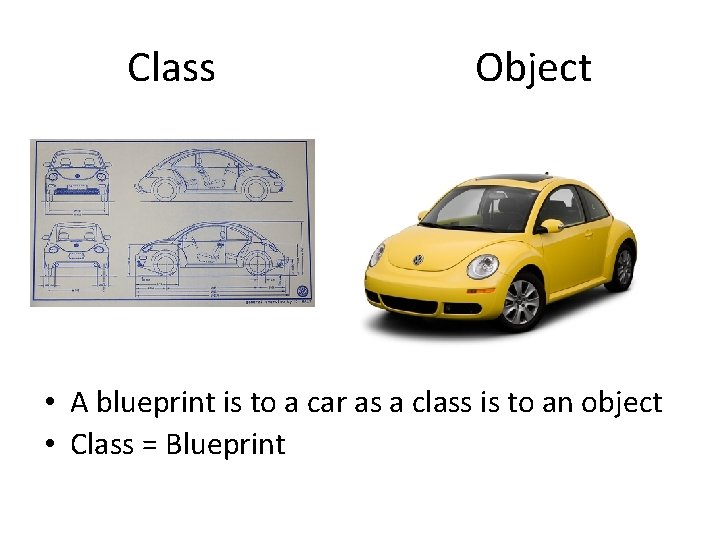
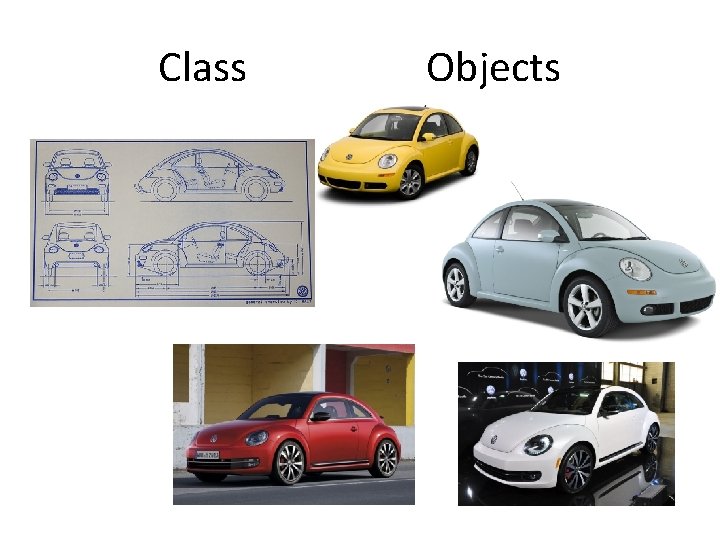
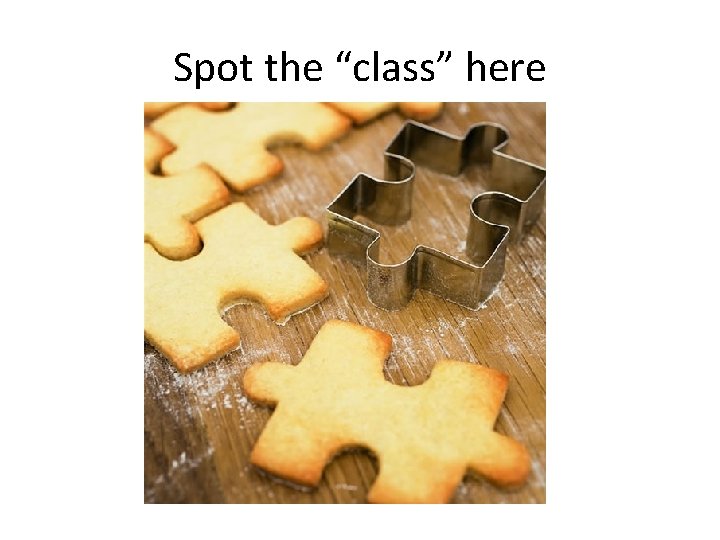
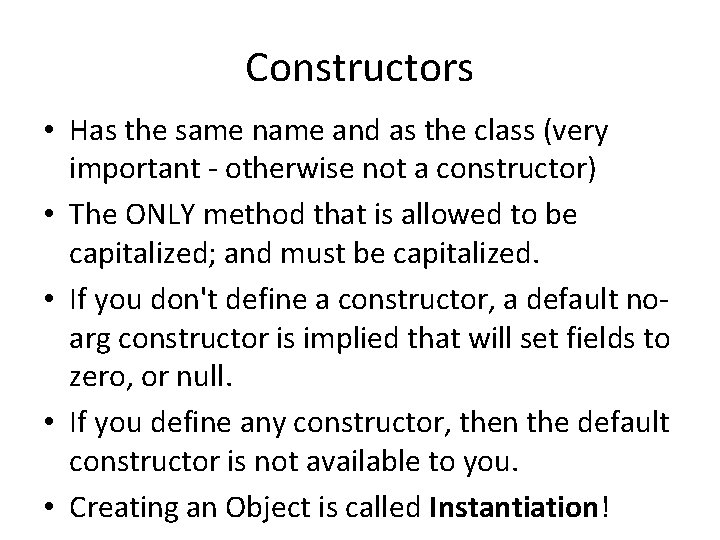
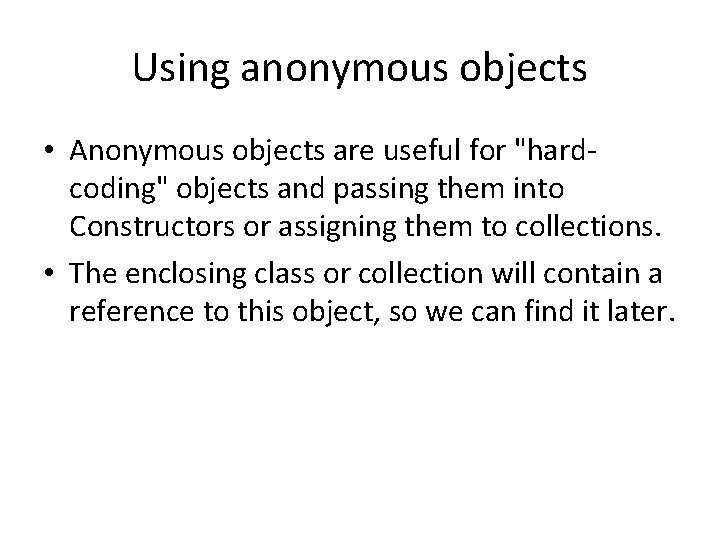
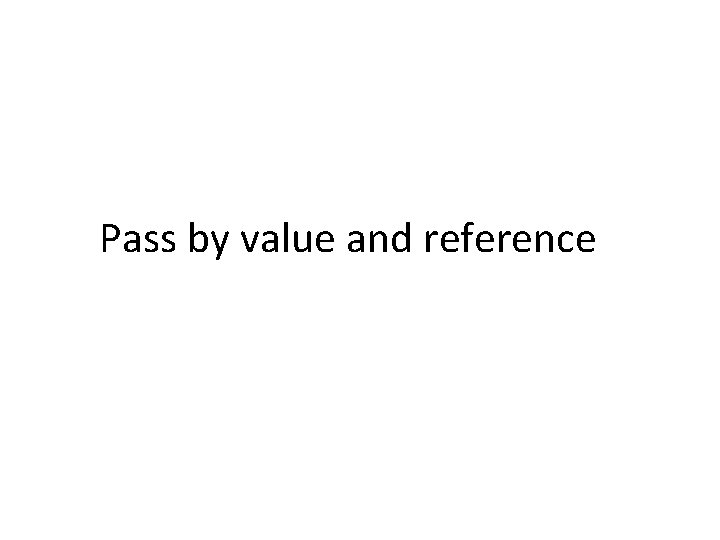
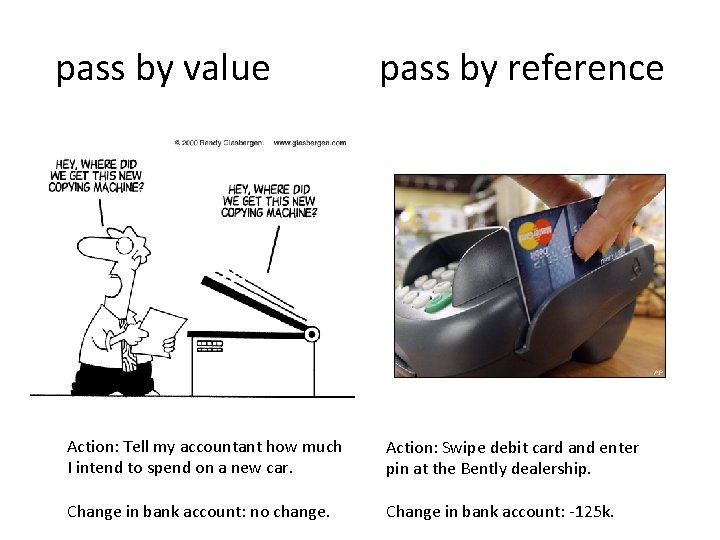
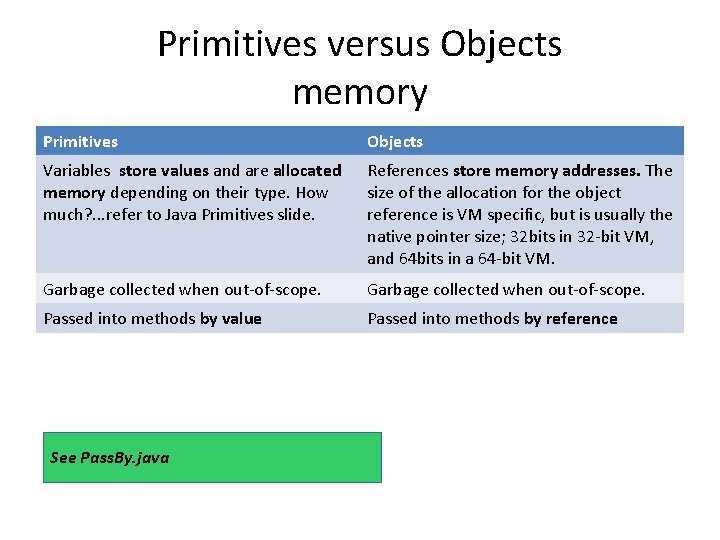
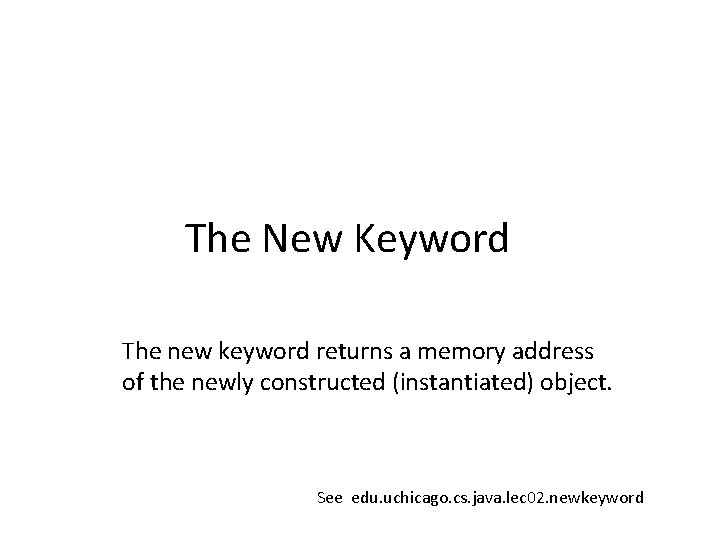
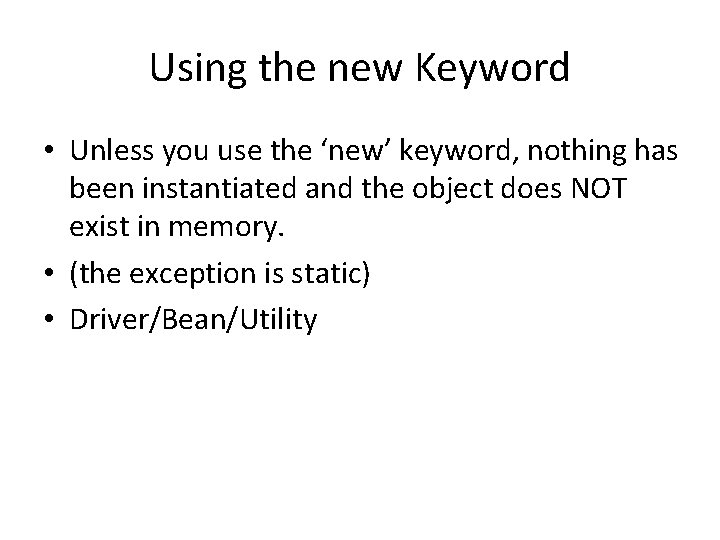
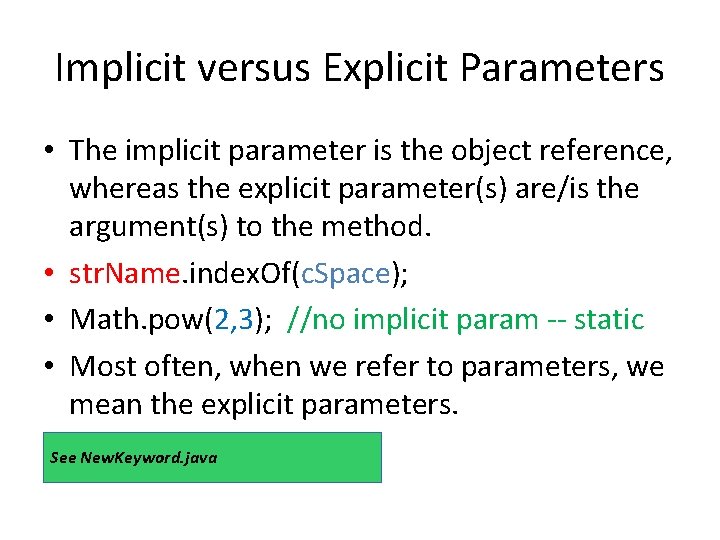
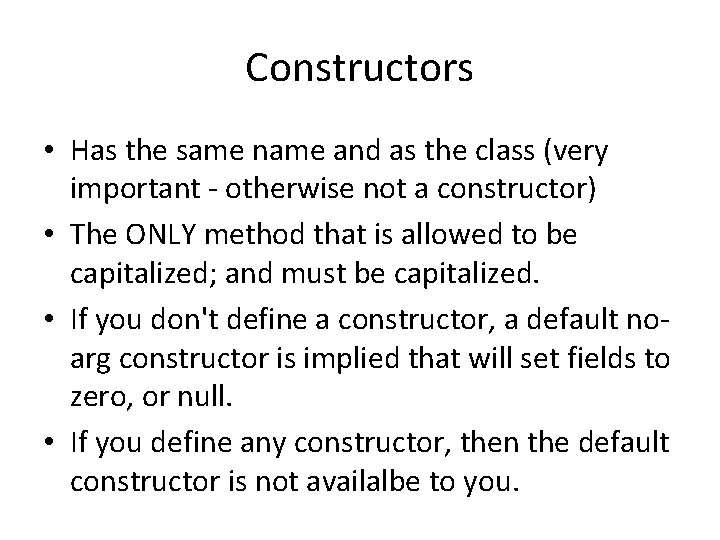
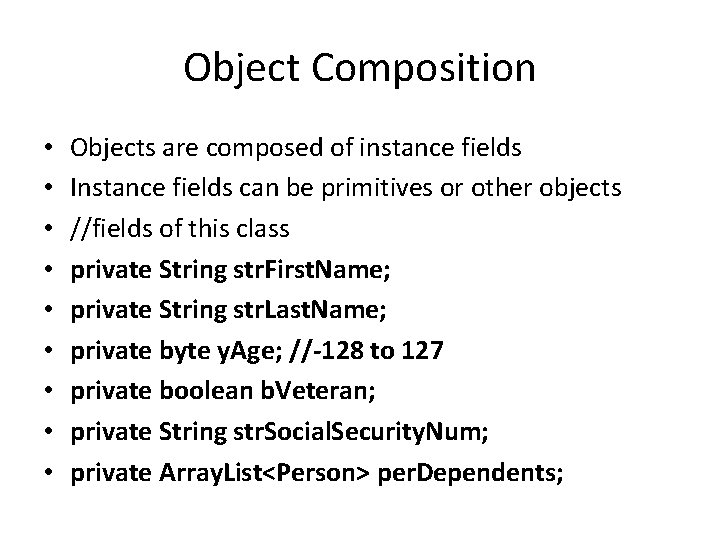
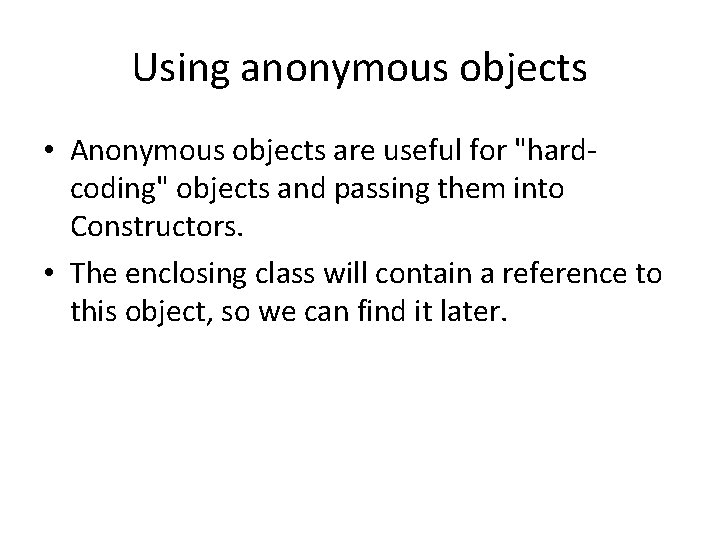
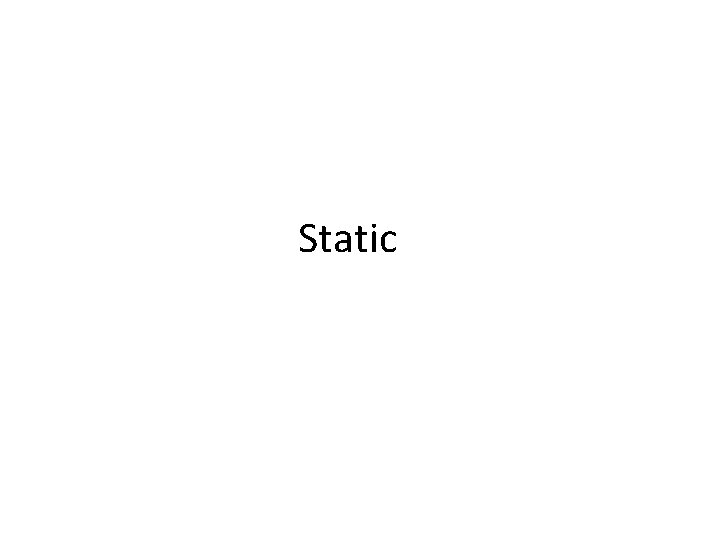
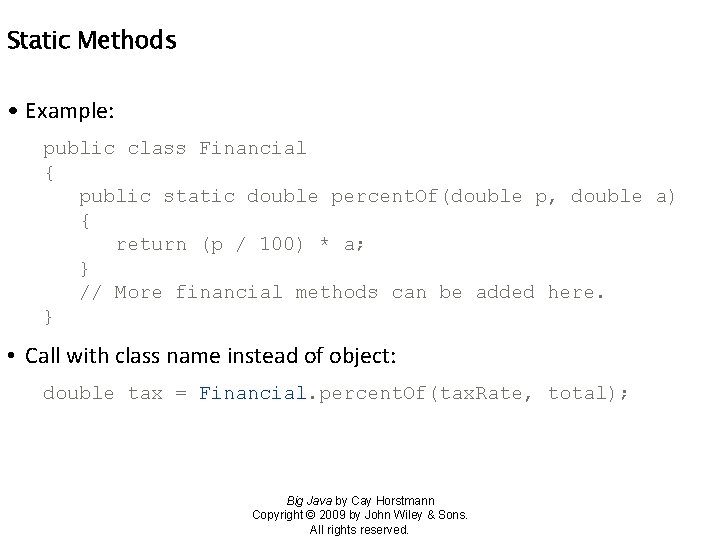
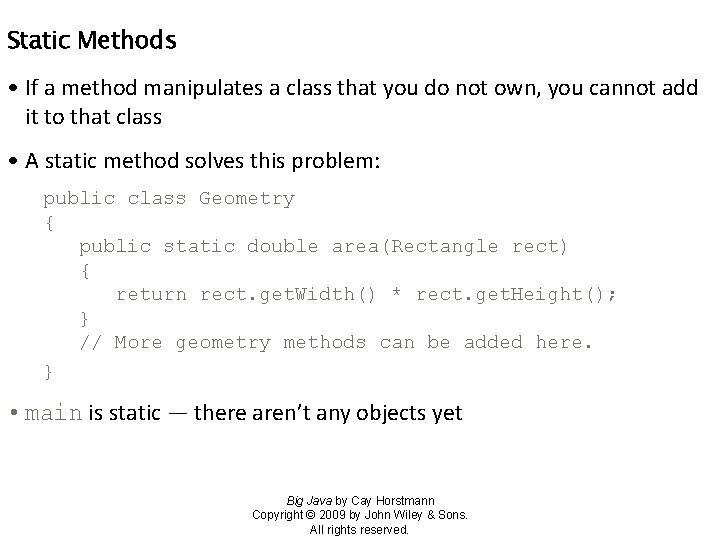
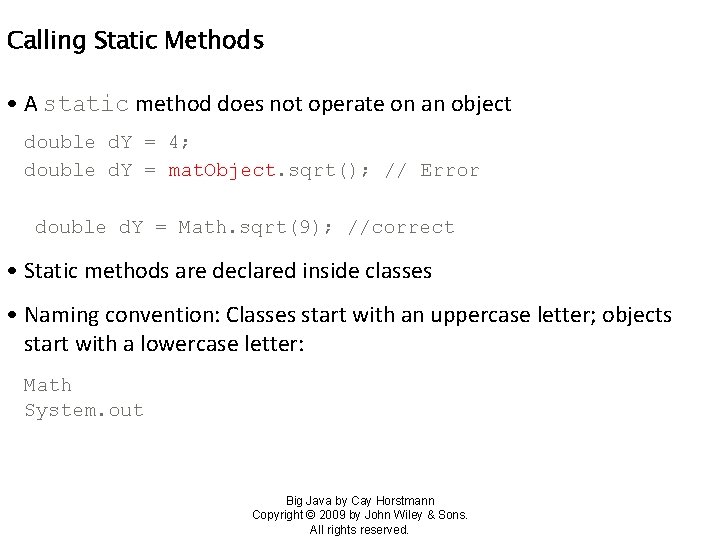
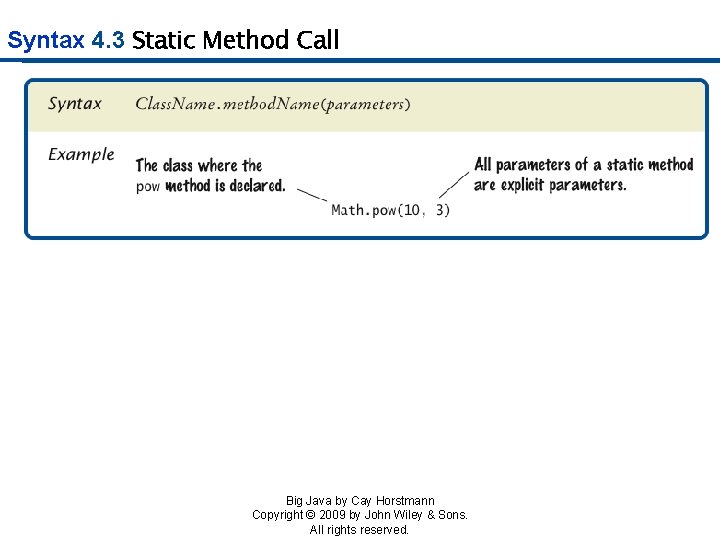
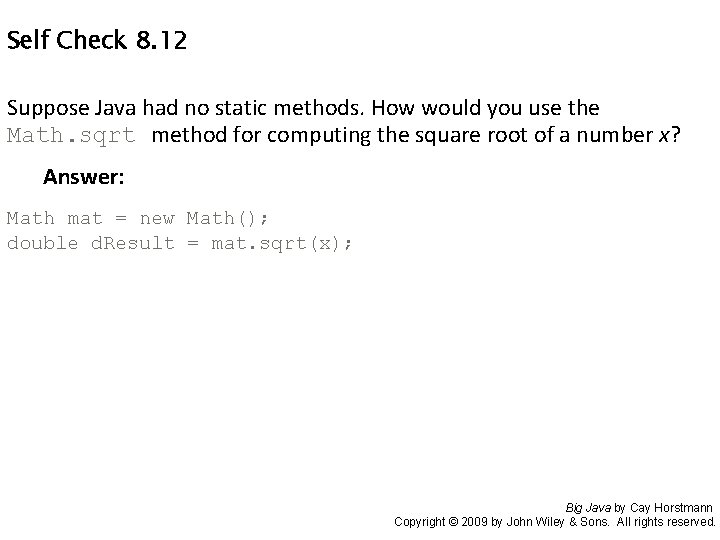
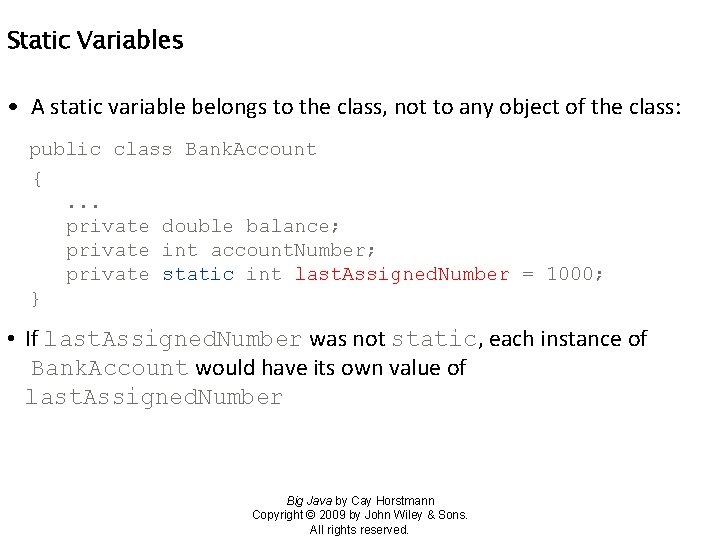
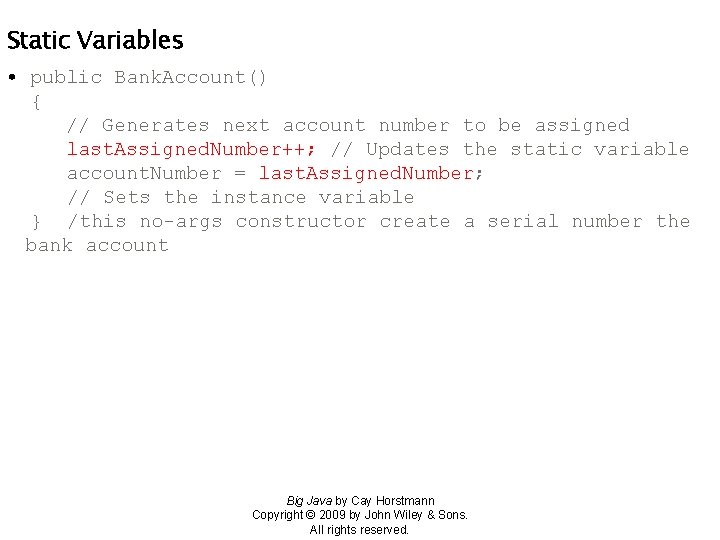
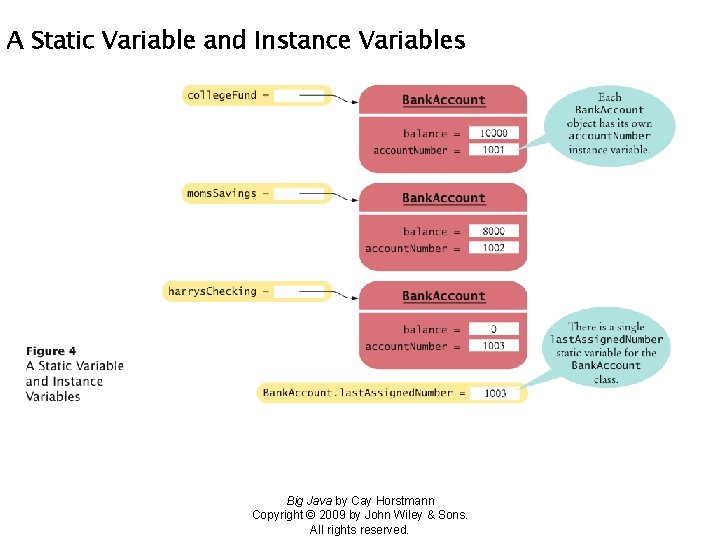
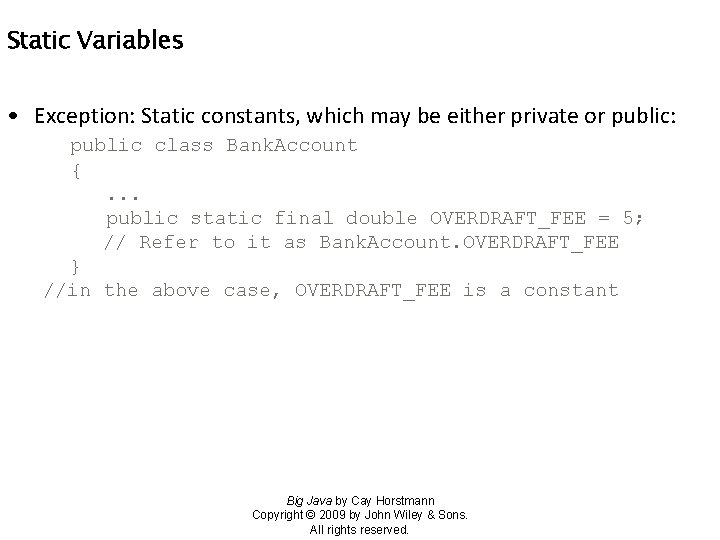
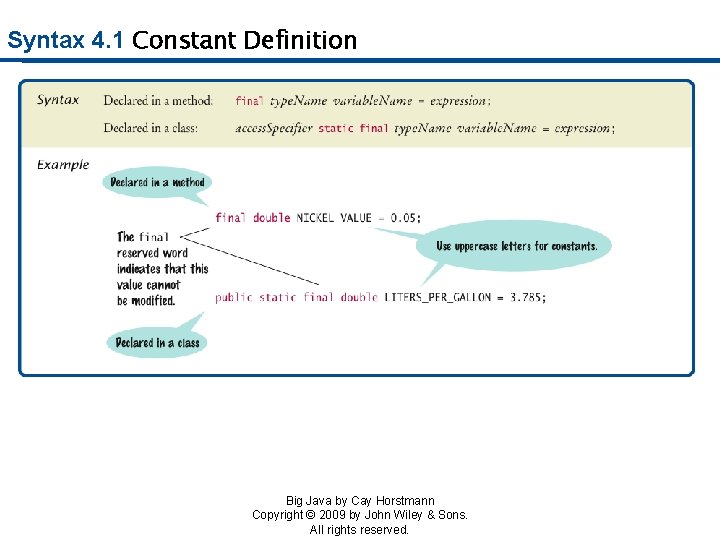
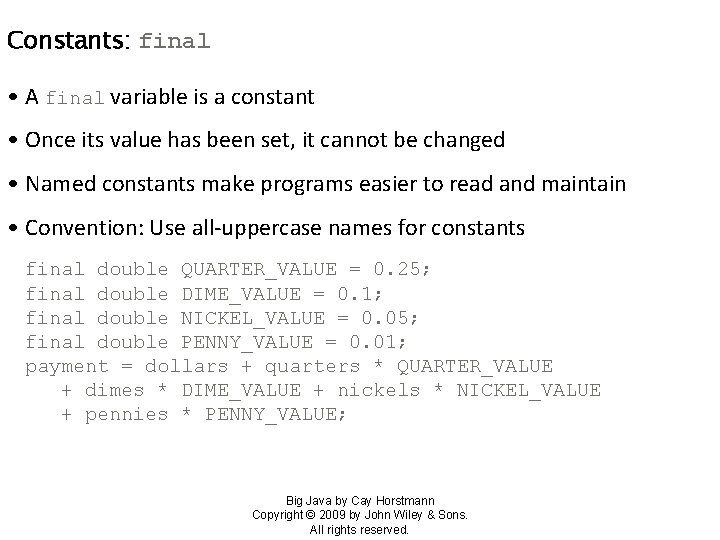
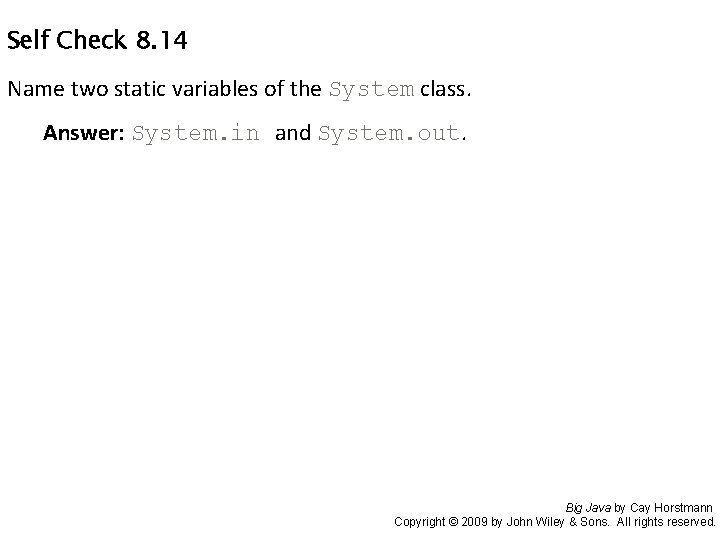
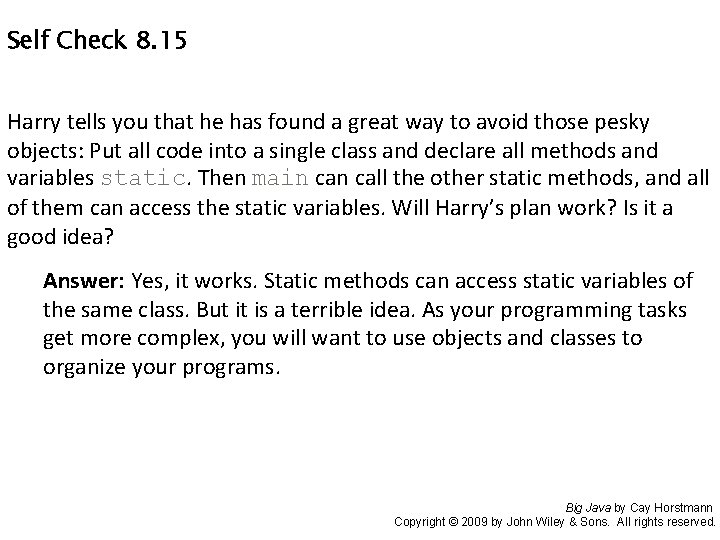
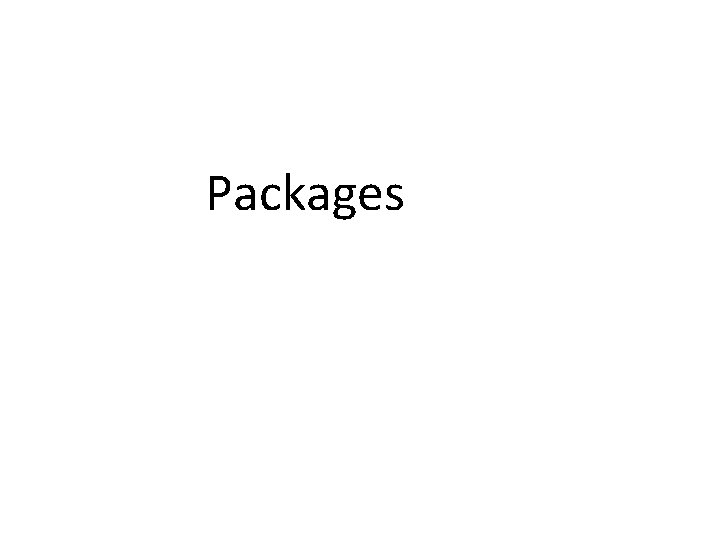
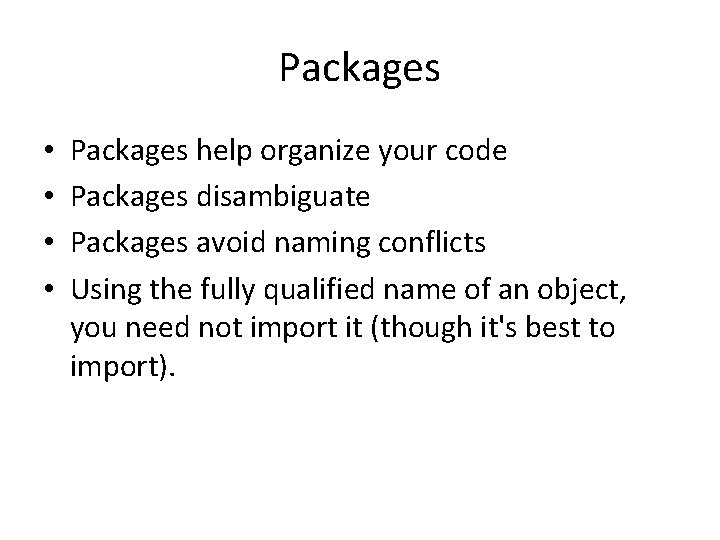
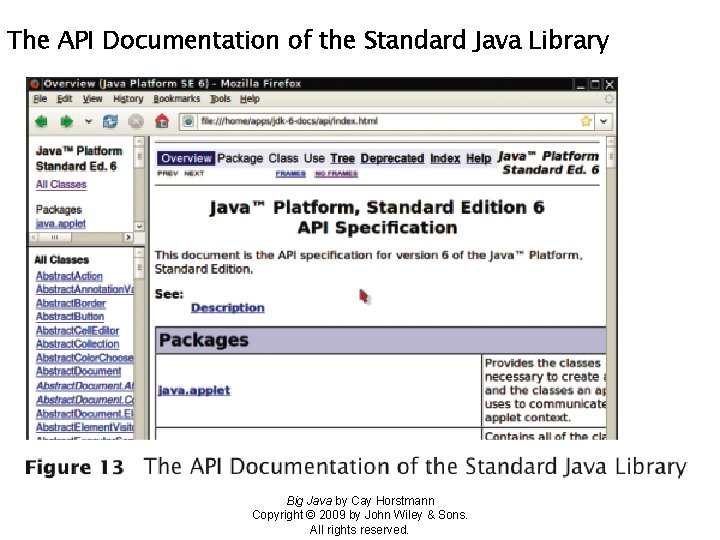
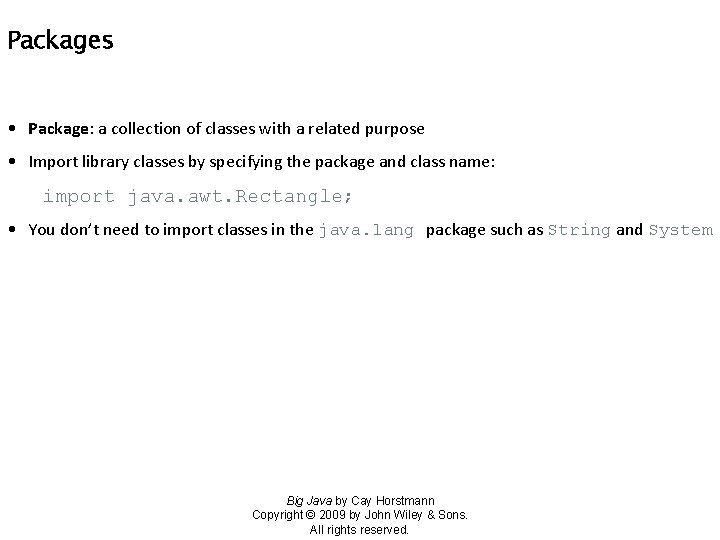
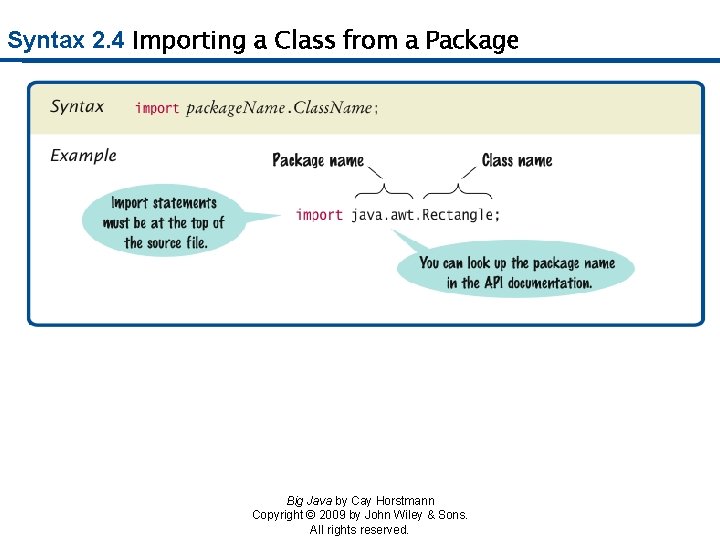
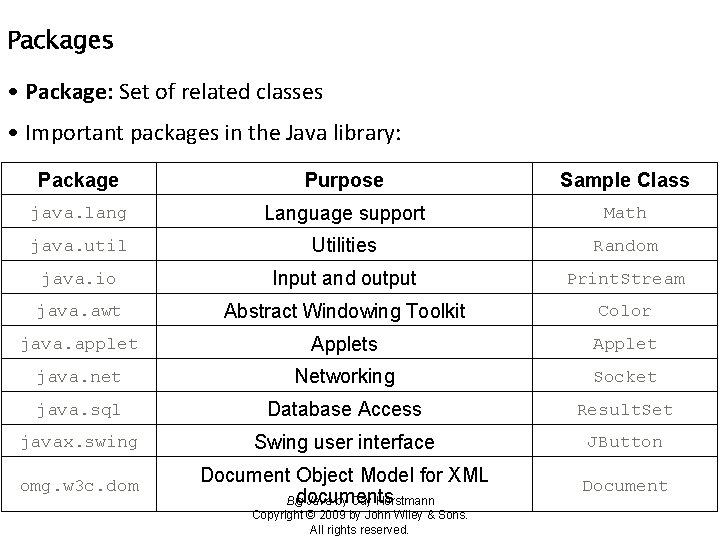
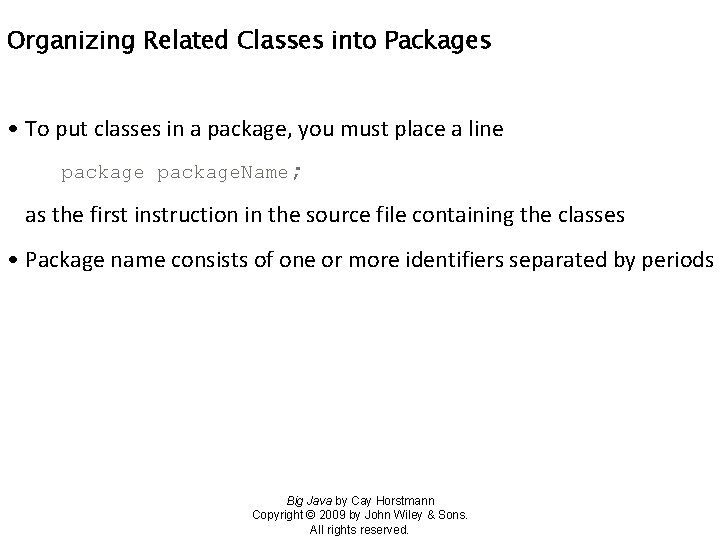
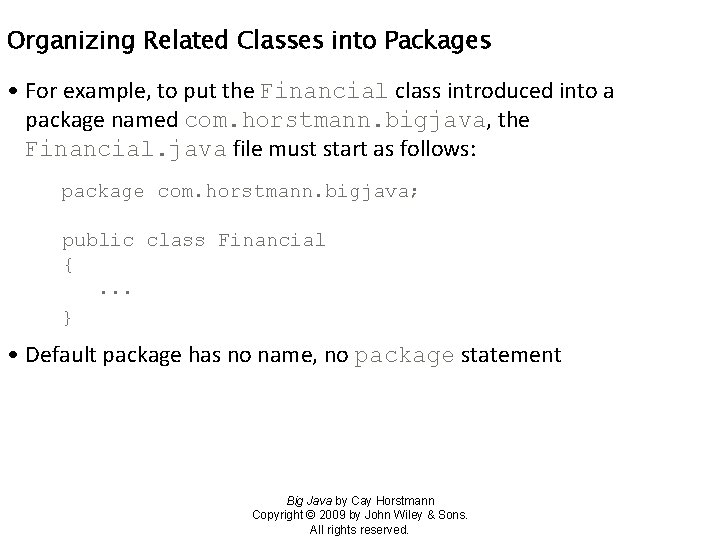
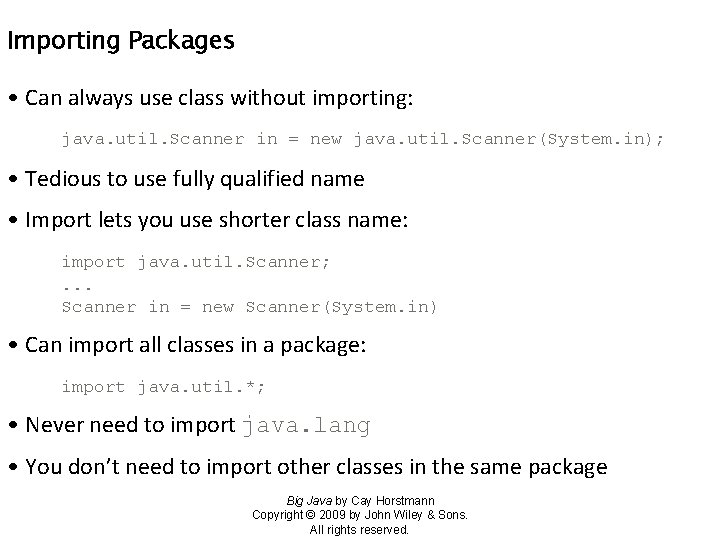
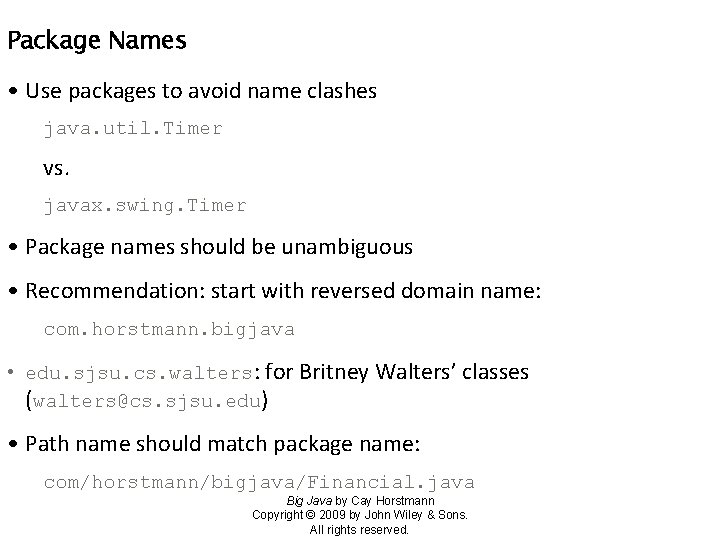
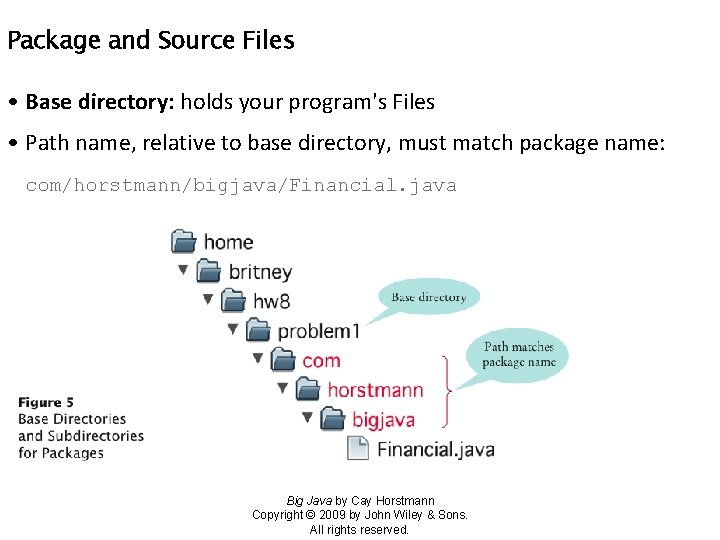
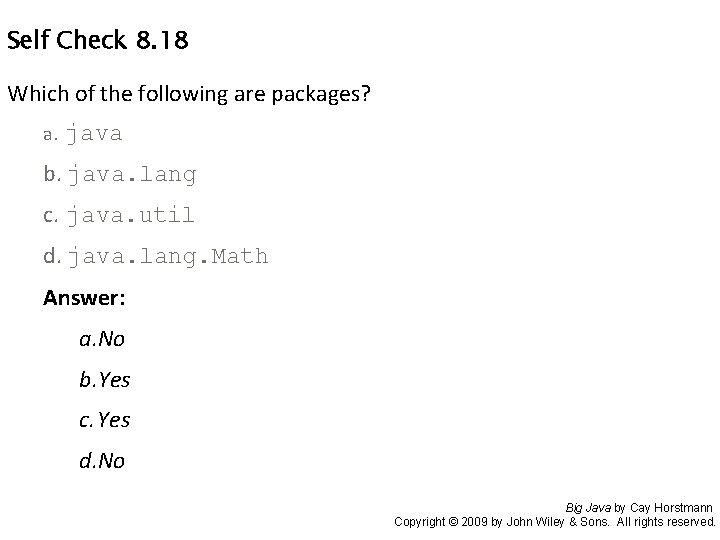
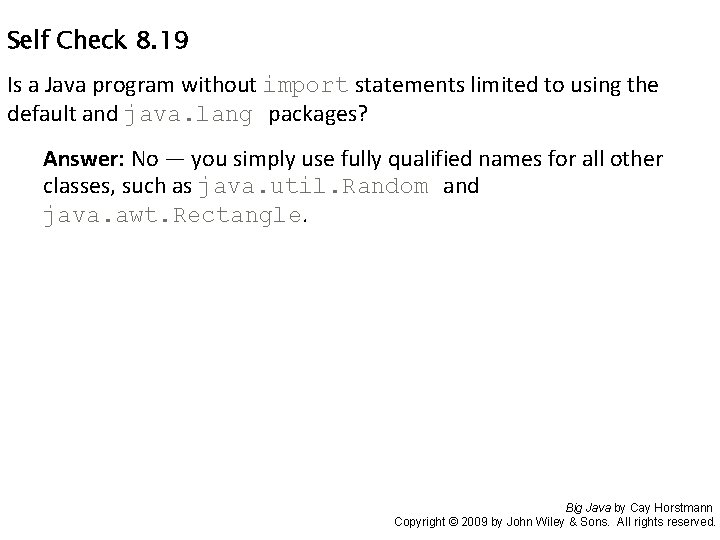
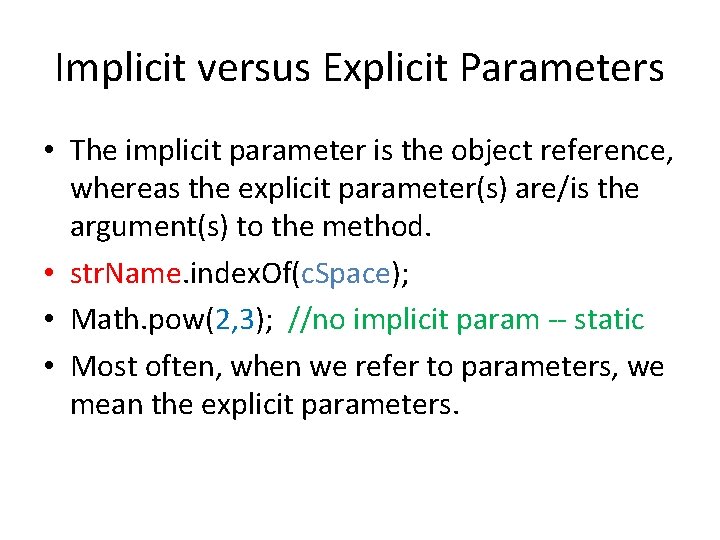
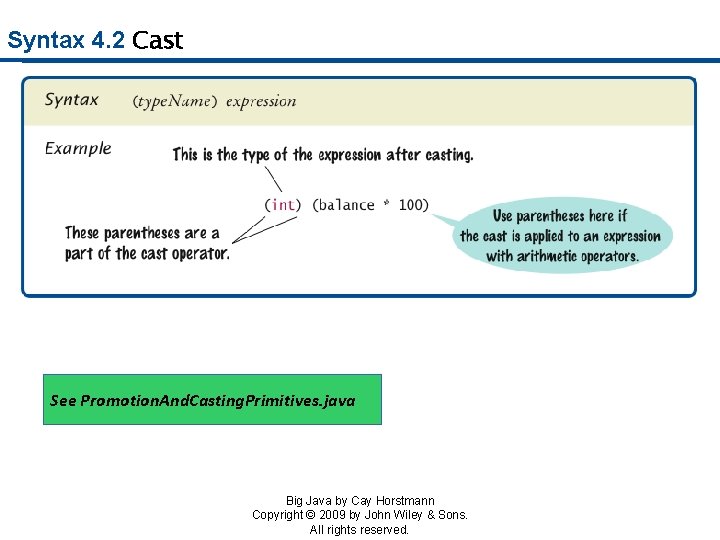
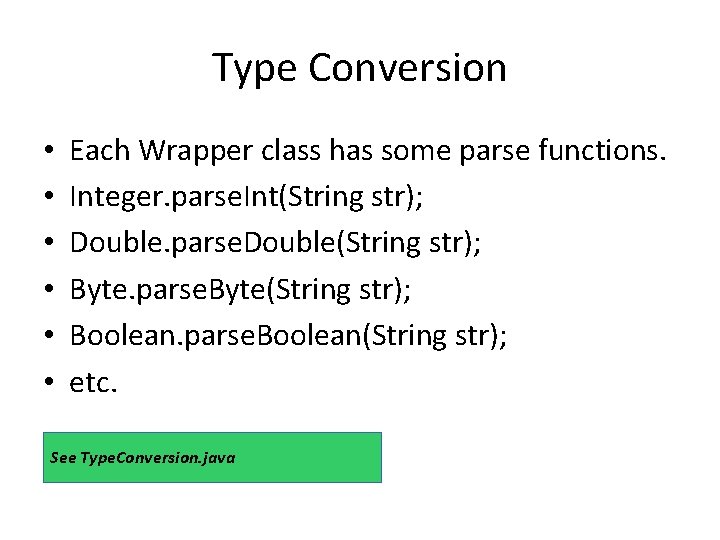
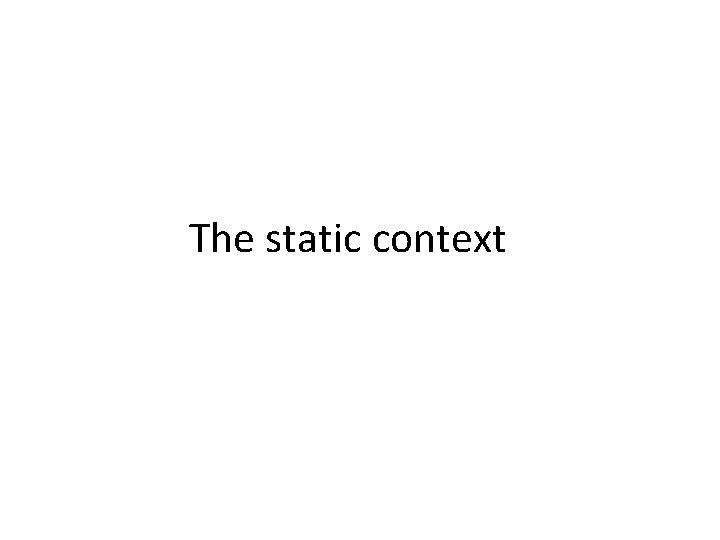
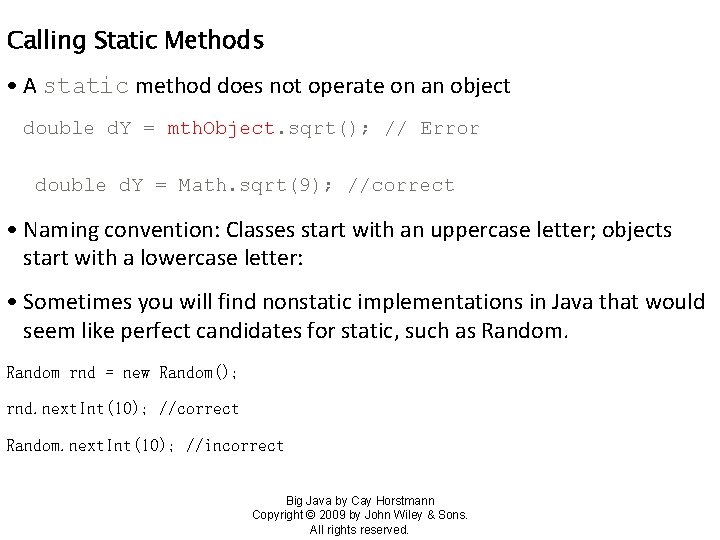
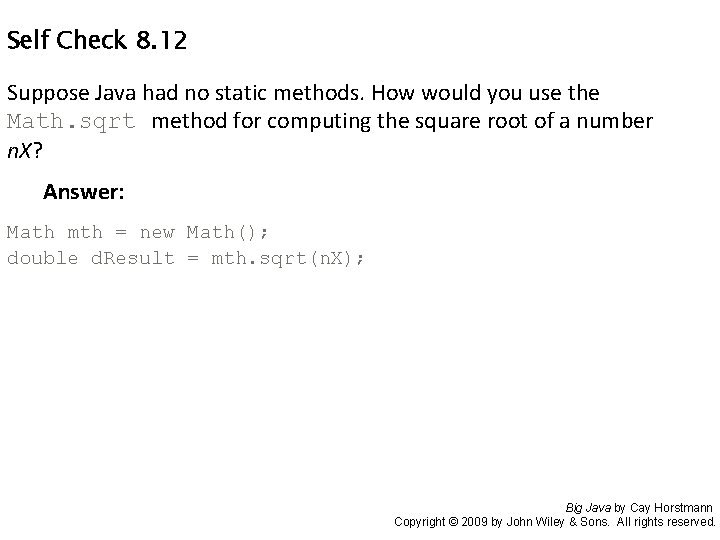
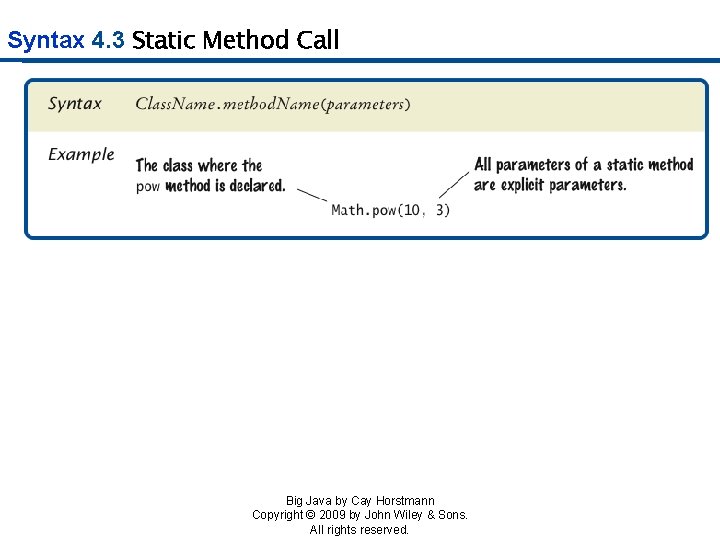
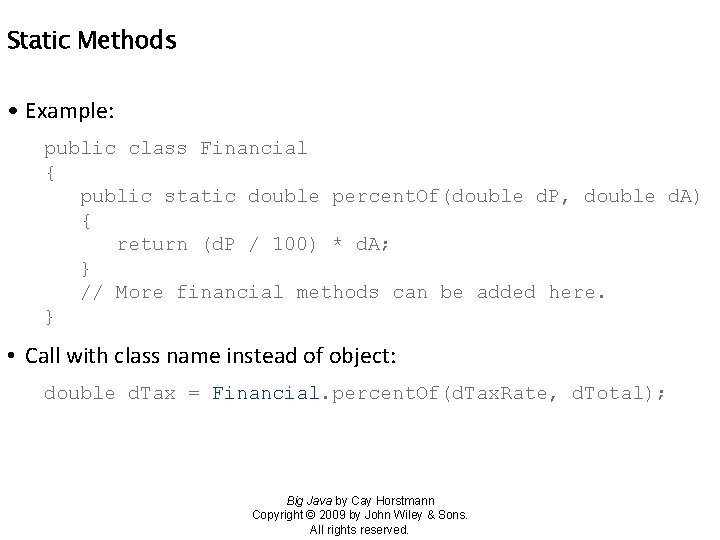
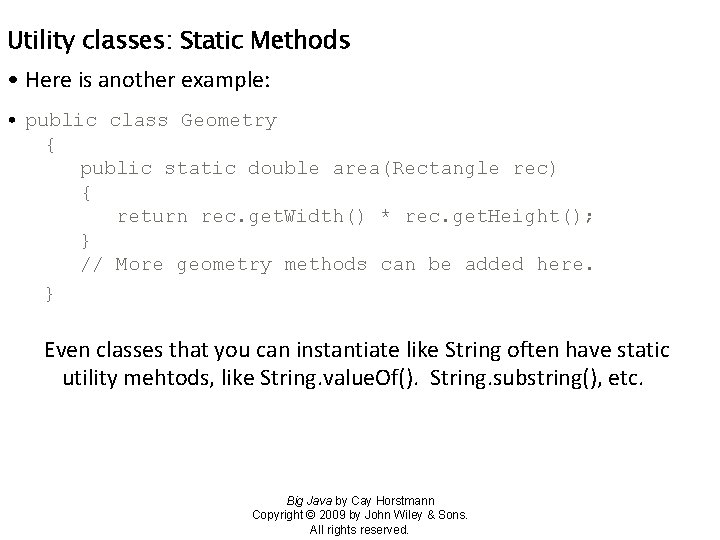
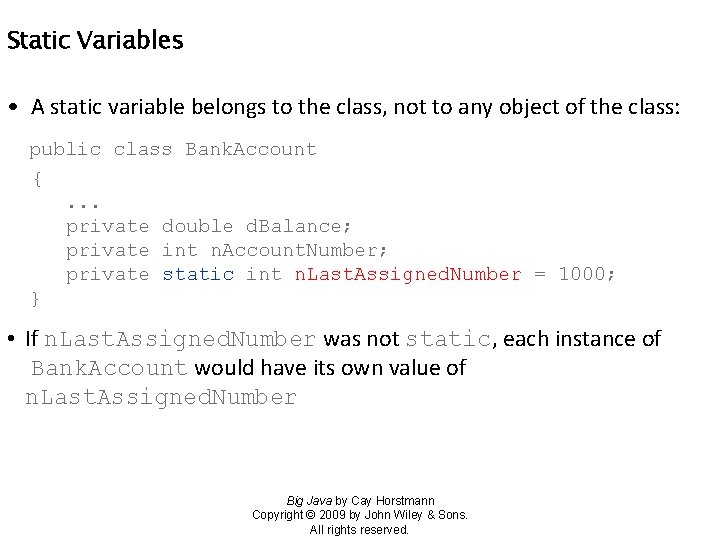
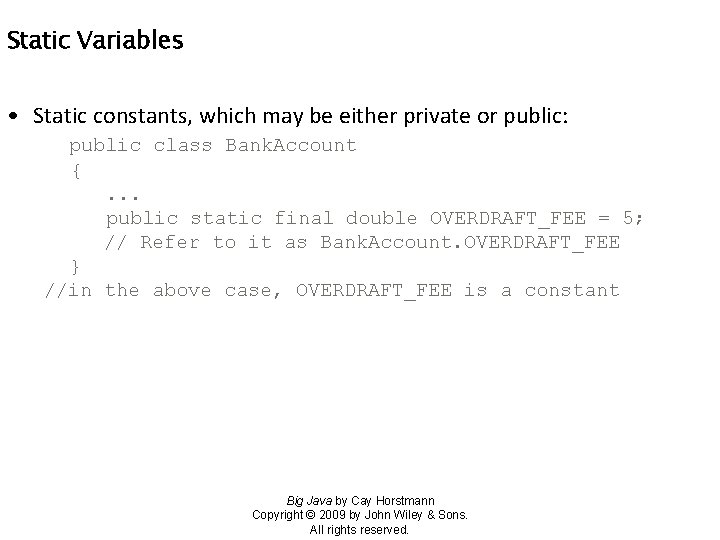
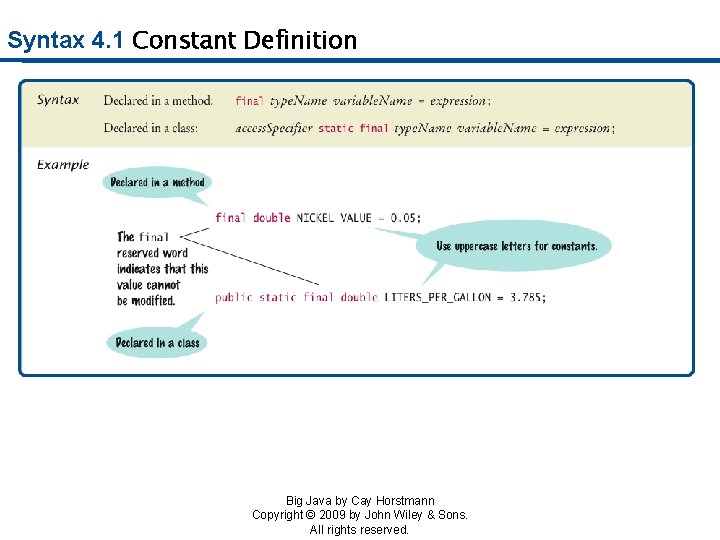
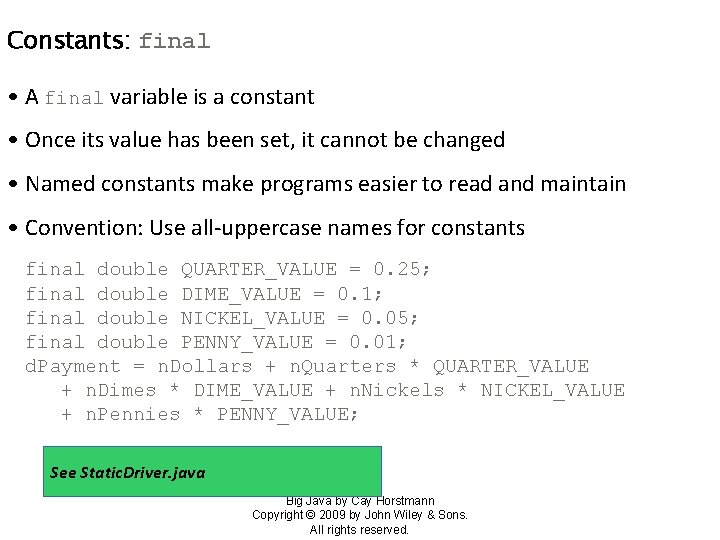
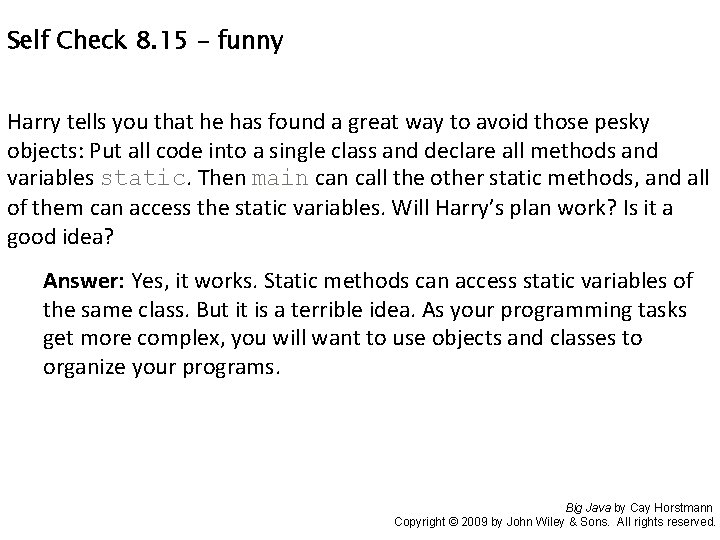
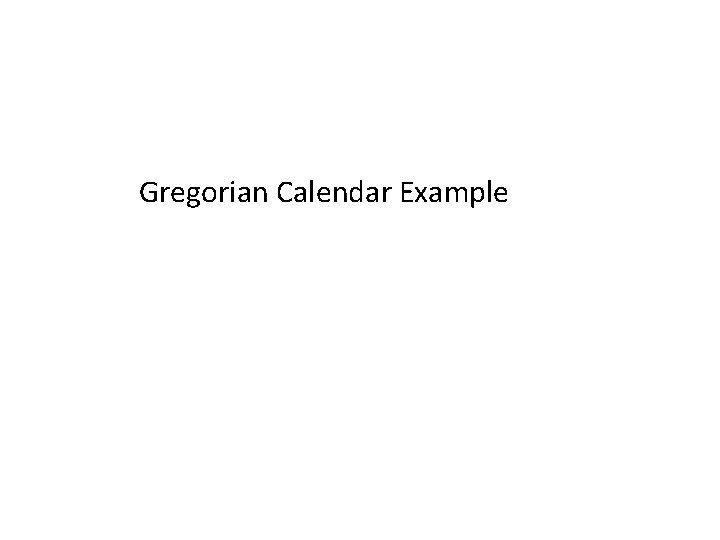
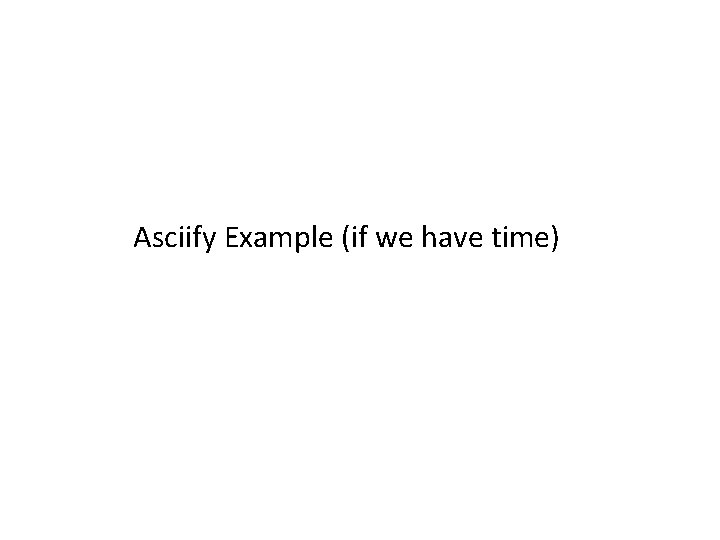
- Slides: 99
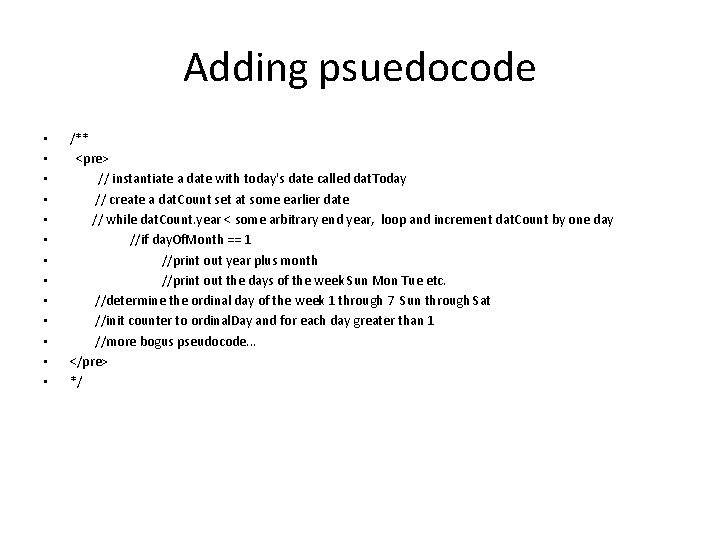
Adding psuedocode • • • • /** <pre> // instantiate a date with today's date called dat. Today // create a dat. Count set at some earlier date // while dat. Count. year < some arbitrary end year, loop and increment dat. Count by one day //if day. Of. Month == 1 //print out year plus month //print out the days of the week Sun Mon Tue etc. //determine the ordinal day of the week 1 through 7 Sun through Sat //init counter to ordinal. Day and for each day greater than 1 //more bogus pseudocode. . . </pre> */
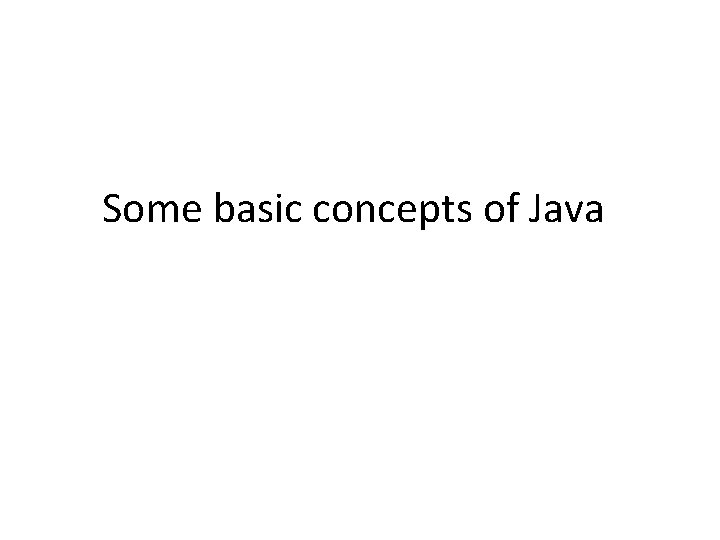
Some basic concepts of Java
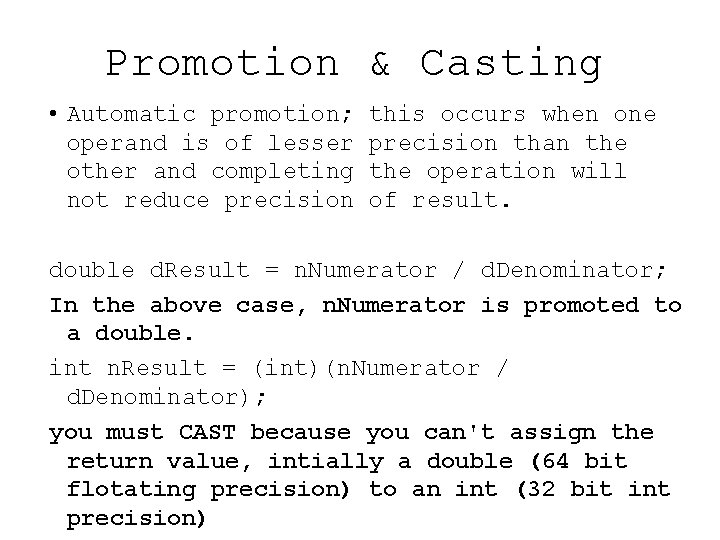
Promotion & Casting • Automatic promotion; operand is of lesser other and completing not reduce precision this occurs when one precision than the operation will of result. double d. Result = n. Numerator / d. Denominator; In the above case, n. Numerator is promoted to a double. int n. Result = (int)(n. Numerator / d. Denominator); you must CAST because you can't assign the return value, intially a double (64 bit flotating precision) to an int (32 bit int precision)
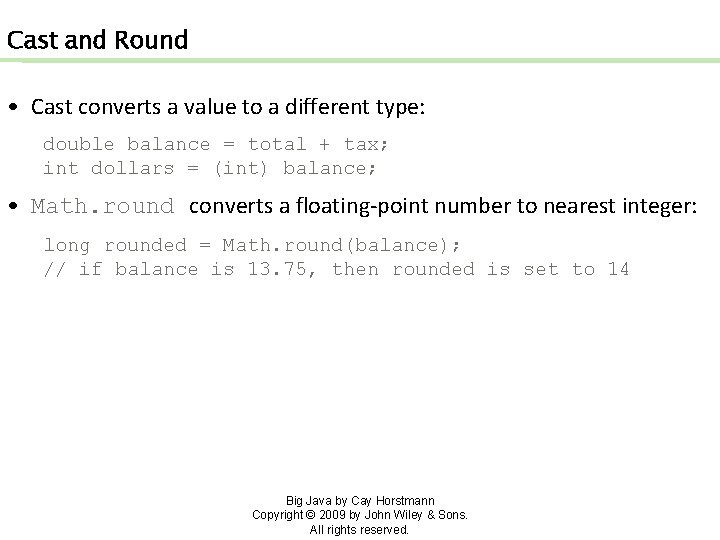
Cast and Round • Cast converts a value to a different type: double balance = total + tax; int dollars = (int) balance; • Math. round converts a floating-point number to nearest integer: long rounded = Math. round(balance); // if balance is 13. 75, then rounded is set to 14 Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
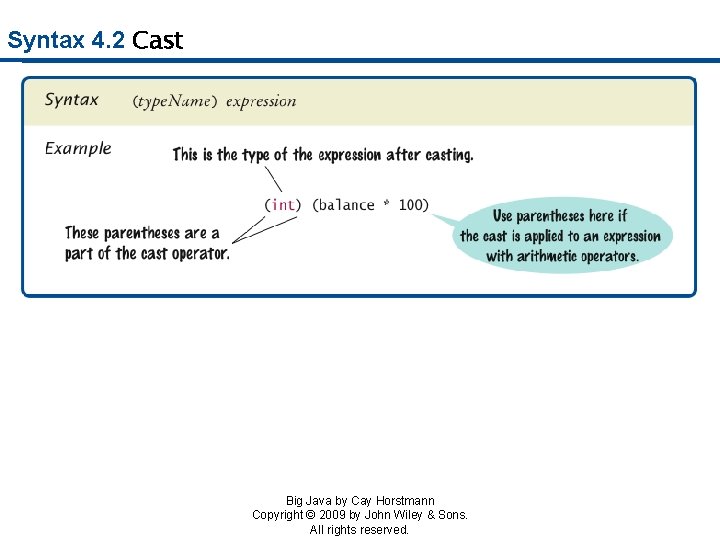
Syntax 4. 2 Cast Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
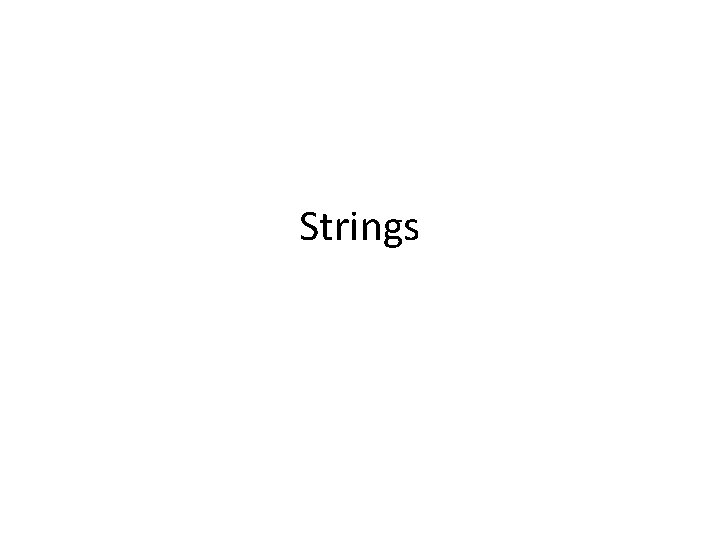
Strings
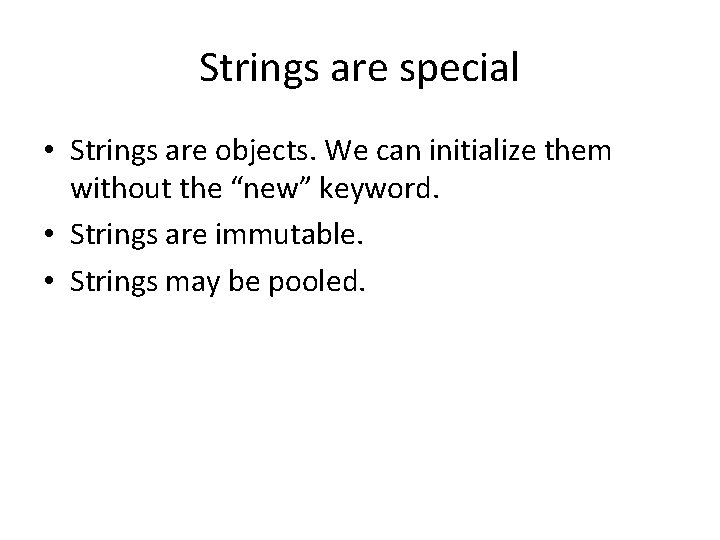
Strings are special • Strings are objects. We can initialize them without the “new” keyword. • Strings are immutable. • Strings may be pooled.
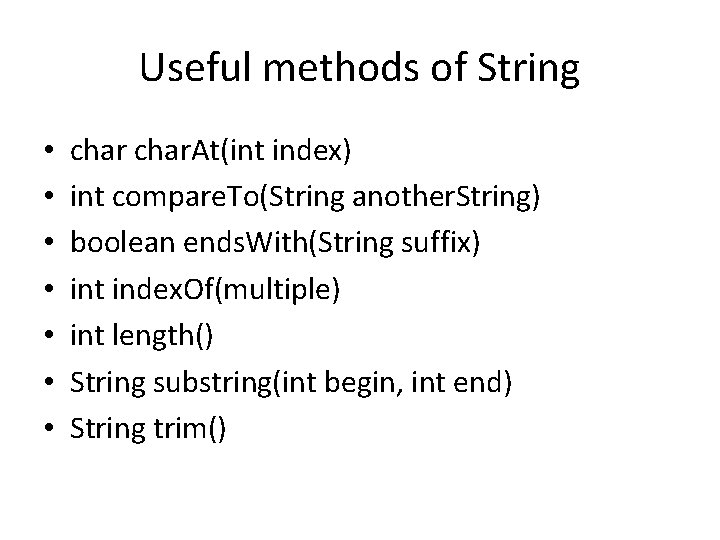
Useful methods of String • • char. At(int index) int compare. To(String another. String) boolean ends. With(String suffix) int index. Of(multiple) int length() String substring(int begin, int end) String trim()
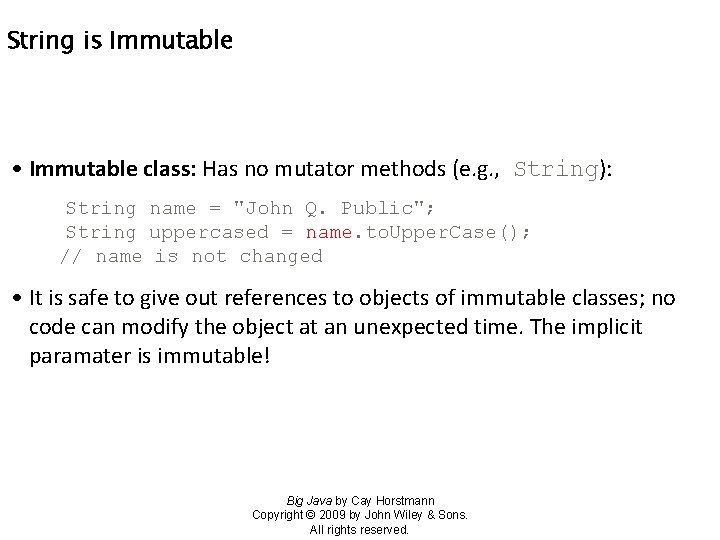
String is Immutable • Immutable class: Has no mutator methods (e. g. , String): String name = "John Q. Public"; String uppercased = name. to. Upper. Case(); // name is not changed • It is safe to give out references to objects of immutable classes; no code can modify the object at an unexpected time. The implicit paramater is immutable! Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
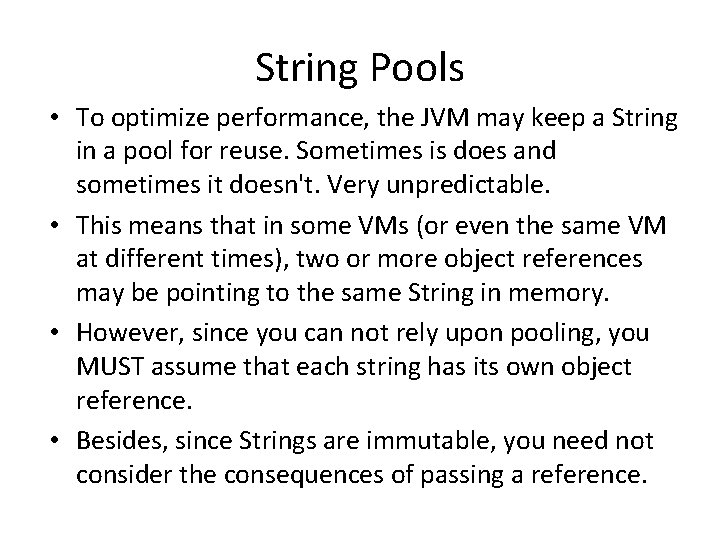
String Pools • To optimize performance, the JVM may keep a String in a pool for reuse. Sometimes is does and sometimes it doesn't. Very unpredictable. • This means that in some VMs (or even the same VM at different times), two or more object references may be pointing to the same String in memory. • However, since you can not rely upon pooling, you MUST assume that each string has its own object reference. • Besides, since Strings are immutable, you need not consider the consequences of passing a reference.
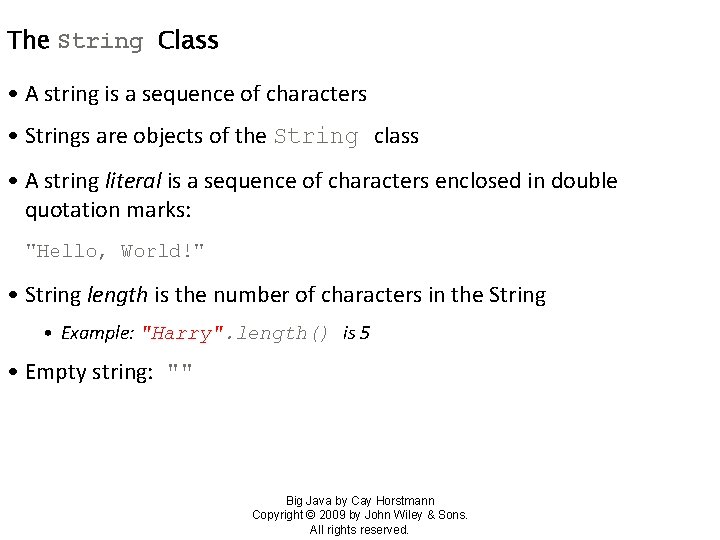
The String Class • A string is a sequence of characters • Strings are objects of the String class • A string literal is a sequence of characters enclosed in double quotation marks: "Hello, World!" • String length is the number of characters in the String • Example: "Harry". length() is 5 • Empty string: "" Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
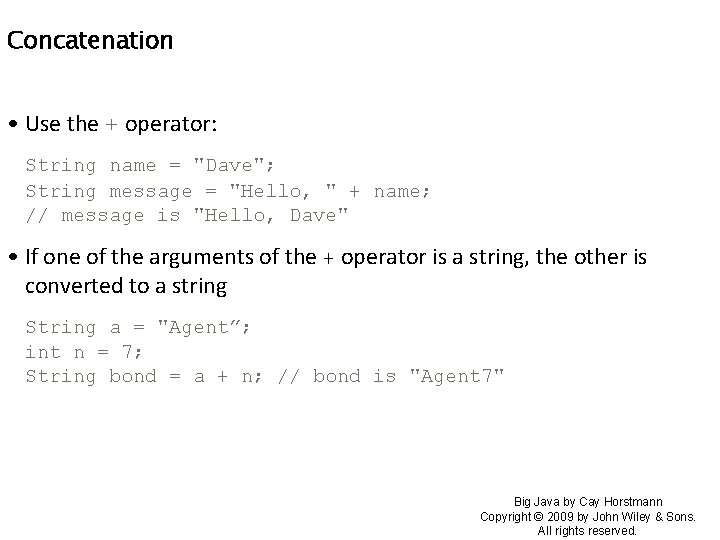
Concatenation • Use the + operator: String name = "Dave"; String message = "Hello, " + name; // message is "Hello, Dave" • If one of the arguments of the + operator is a string, the other is converted to a string String a = "Agent”; int n = 7; String bond = a + n; // bond is "Agent 7" Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
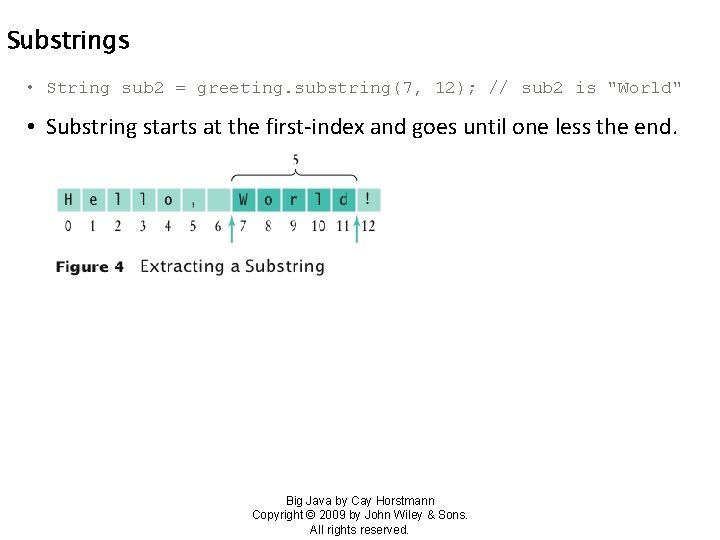
Substrings • String sub 2 = greeting. substring(7, 12); // sub 2 is "World" • Substring starts at the first-index and goes until one less the end. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
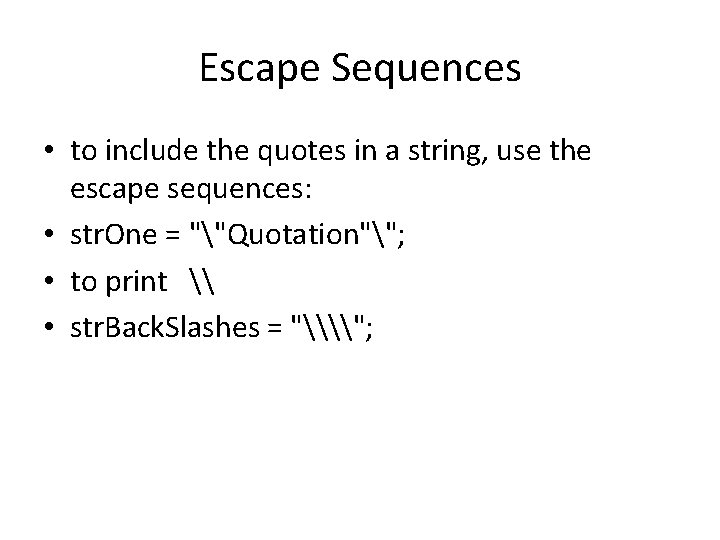
Escape Sequences • to include the quotes in a string, use the escape sequences: • str. One = ""Quotation""; • to print \ • str. Back. Slashes = "\\";
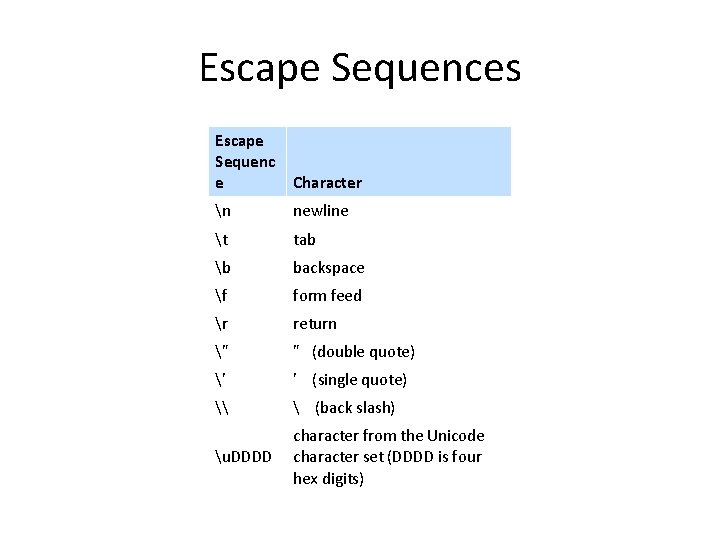
Escape Sequences Escape Sequenc e Character n newline t tab b backspace f form feed r return " " (double quote) ' ' (single quote) \ (back slash) u. DDDD character from the Unicode character set (DDDD is four hex digits)
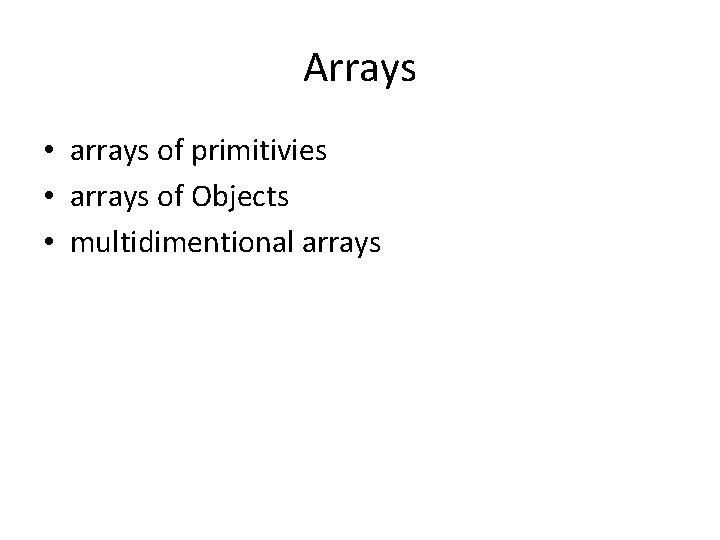
Arrays • arrays of primitivies • arrays of Objects • multidimentional arrays
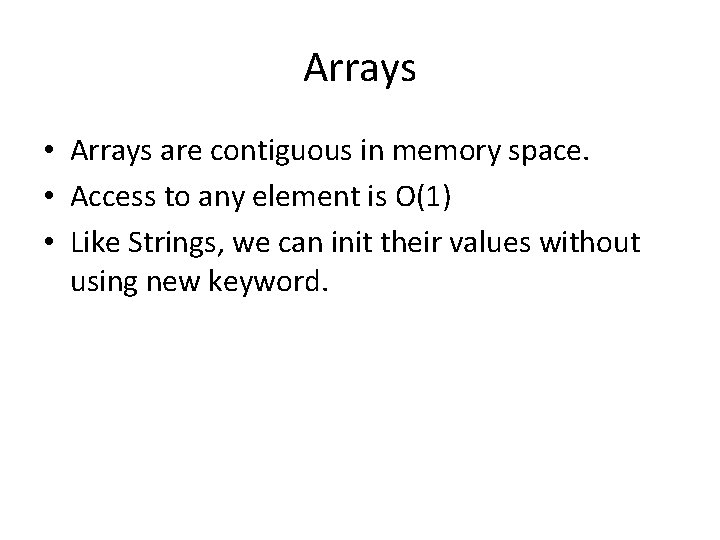
Arrays • Arrays are contiguous in memory space. • Access to any element is O(1) • Like Strings, we can init their values without using new keyword.
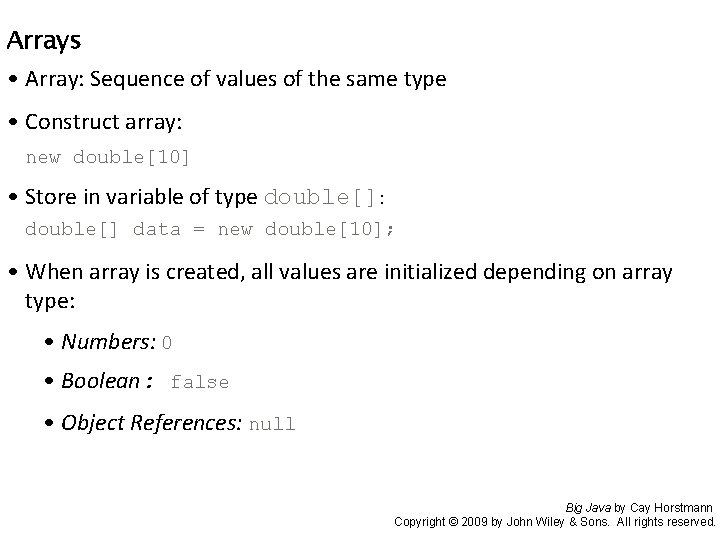
Arrays • Array: Sequence of values of the same type • Construct array: new double[10] • Store in variable of type double[]: double[] data = new double[10]; • When array is created, all values are initialized depending on array type: • Numbers: 0 • Boolean: false • Object References: null Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
![Arrays declare and init Array Sequence of values of the same type double Arrays: declare and init • Array: Sequence of values of the same type double[]](https://slidetodoc.com/presentation_image_h2/520214fe588c7300271032fb2c5a400c/image-19.jpg)
Arrays: declare and init • Array: Sequence of values of the same type double[] d. Values = {2. 5, 8. 1, 3. 79, 0, 6. 0}; The above declares and intializes an array of double[] String[] str. Names = {"Harry", "Lary", "Mary", "Perry"}; The above declares and intializes an array of String[]
![Arrays Use to access an element double values new double10 values2 Arrays Use [] to access an element: double[] values = new double[10]; values[2] =](https://slidetodoc.com/presentation_image_h2/520214fe588c7300271032fb2c5a400c/image-20.jpg)
Arrays Use [] to access an element: double[] values = new double[10]; values[2] = 29. 95; Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
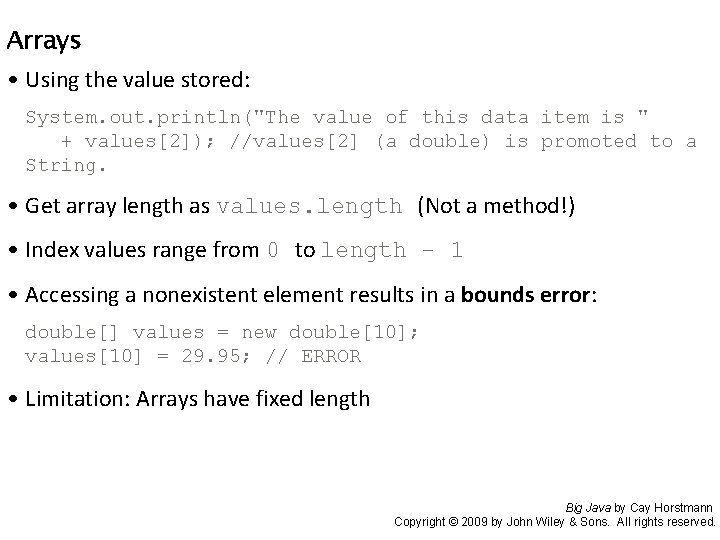
Arrays • Using the value stored: System. out. println("The value of this data item is " + values[2]); //values[2] (a double) is promoted to a String. • Get array length as values. length (Not a method!) • Index values range from 0 to length - 1 • Accessing a nonexistent element results in a bounds error: double[] values = new double[10]; values[10] = 29. 95; // ERROR • Limitation: Arrays have fixed length Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
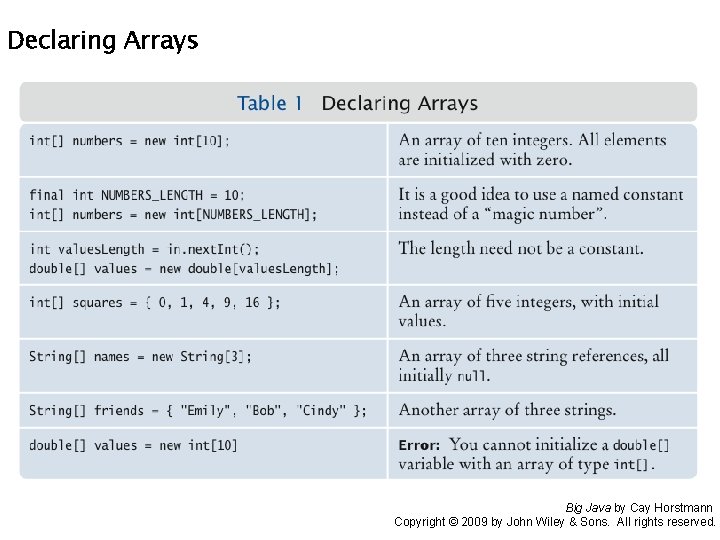
Declaring Arrays Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
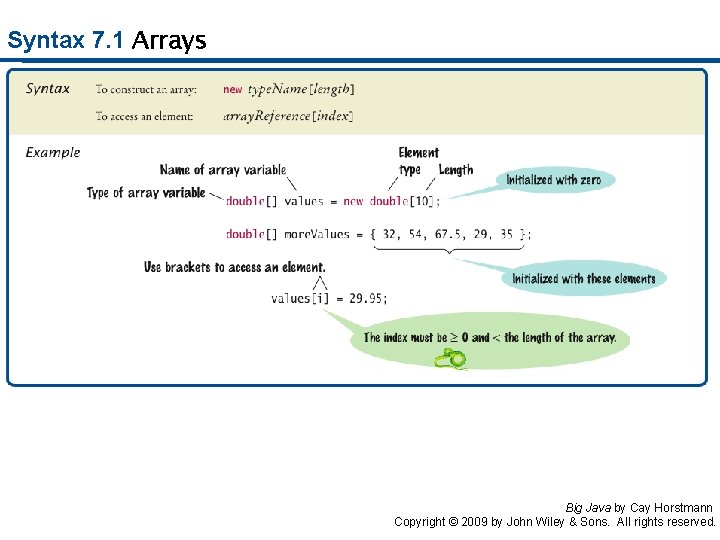
Syntax 7. 1 Arrays Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
![Make Parallel Arrays into Arrays of Objects Dont do this int account Numbers Make Parallel Arrays into Arrays of Objects // Don't do this int[] account. Numbers;](https://slidetodoc.com/presentation_image_h2/520214fe588c7300271032fb2c5a400c/image-24.jpg)
Make Parallel Arrays into Arrays of Objects // Don't do this int[] account. Numbers; double[] balances; Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
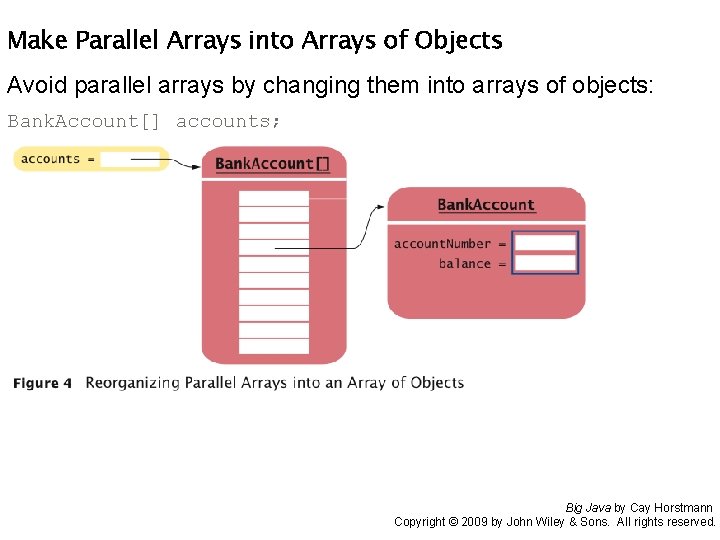
Make Parallel Arrays into Arrays of Objects Avoid parallel arrays by changing them into arrays of objects: Bank. Account[] accounts; Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
![multidimensional arrays int n Numbers new int34 boolean b Exams multidimensional arrays • int[][] n. Numbers = new int[3][4]; • boolean[][] b. Exams =](https://slidetodoc.com/presentation_image_h2/520214fe588c7300271032fb2c5a400c/image-26.jpg)
multidimensional arrays • int[][] n. Numbers = new int[3][4]; • boolean[][] b. Exams = { new boolean[6], new boolean[9], new boolean[3], new boolean[8] }; //ragged array
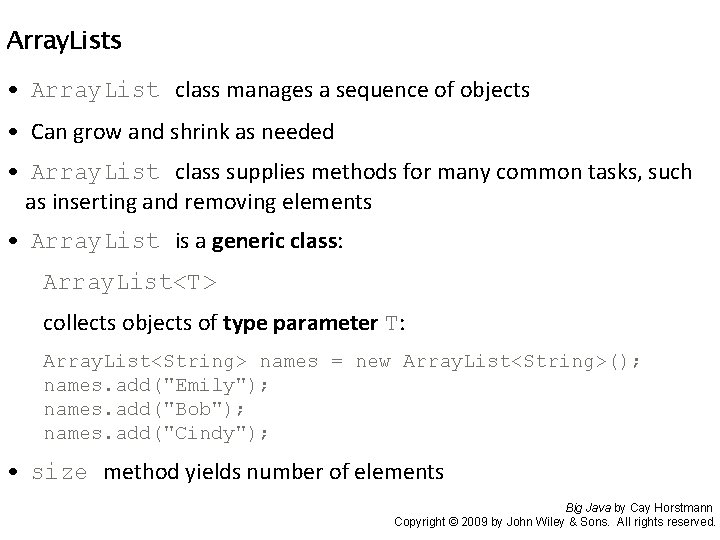
Array. Lists • Array. List class manages a sequence of objects • Can grow and shrink as needed • Array. List class supplies methods for many common tasks, such as inserting and removing elements • Array. List is a generic class: Array. List<T> collects objects of type parameter T: Array. List<String> names = new Array. List<String>(); names. add("Emily"); names. add("Bob"); names. add("Cindy"); • size method yields number of elements Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
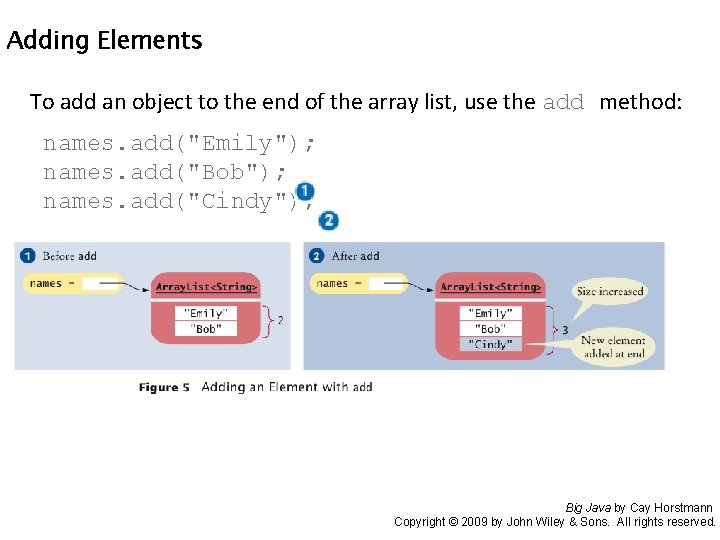
Adding Elements To add an object to the end of the array list, use the add method: names. add("Emily"); names. add("Bob"); names. add("Cindy"); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
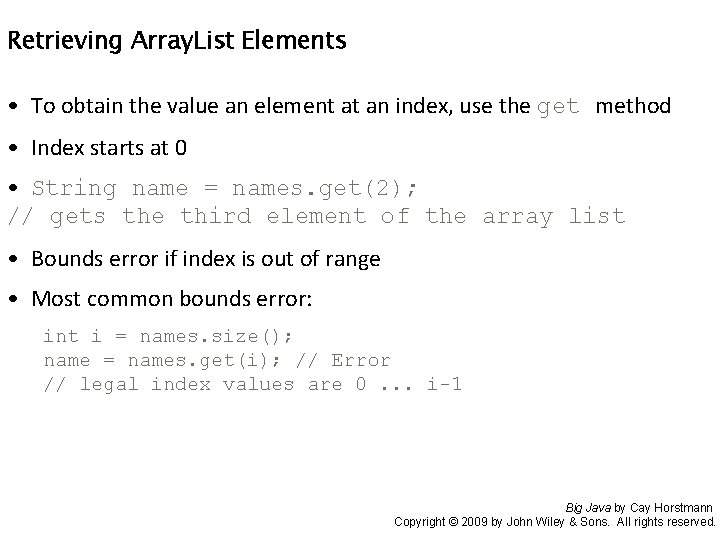
Retrieving Array. List Elements • To obtain the value an element at an index, use the get method • Index starts at 0 • String name = names. get(2); // gets the third element of the array list • Bounds error if index is out of range • Most common bounds error: int i = names. size(); name = names. get(i); // Error // legal index values are 0. . . i-1 Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
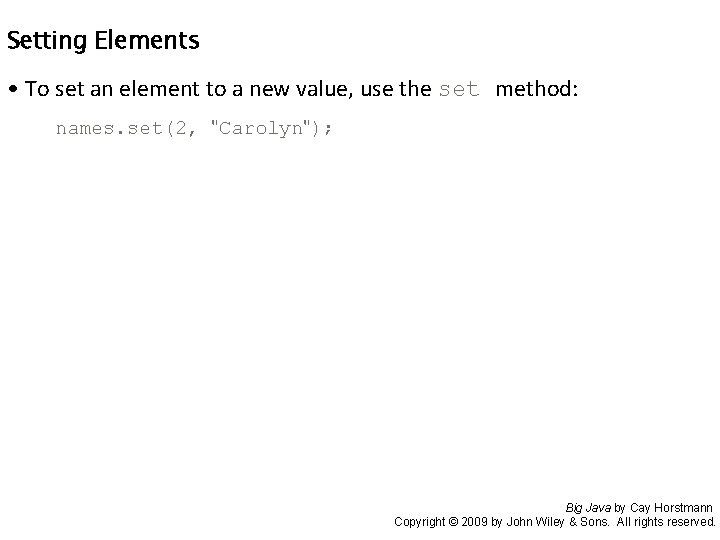
Setting Elements • To set an element to a new value, use the set method: names. set(2, "Carolyn"); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
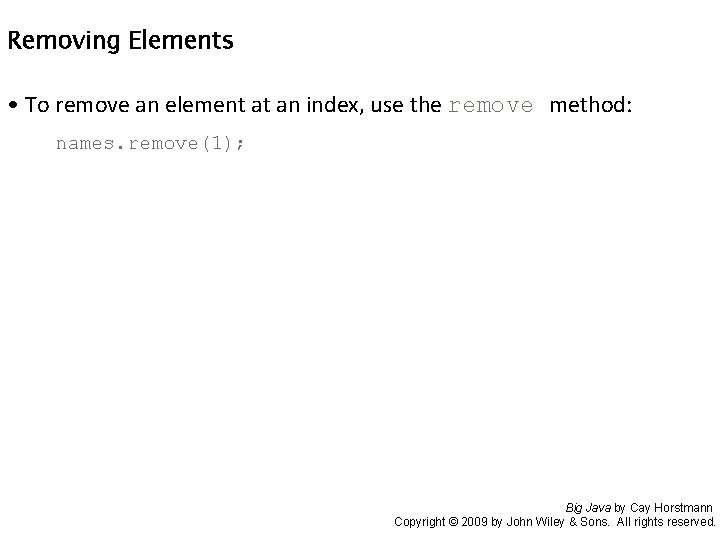
Removing Elements • To remove an element at an index, use the remove method: names. remove(1); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
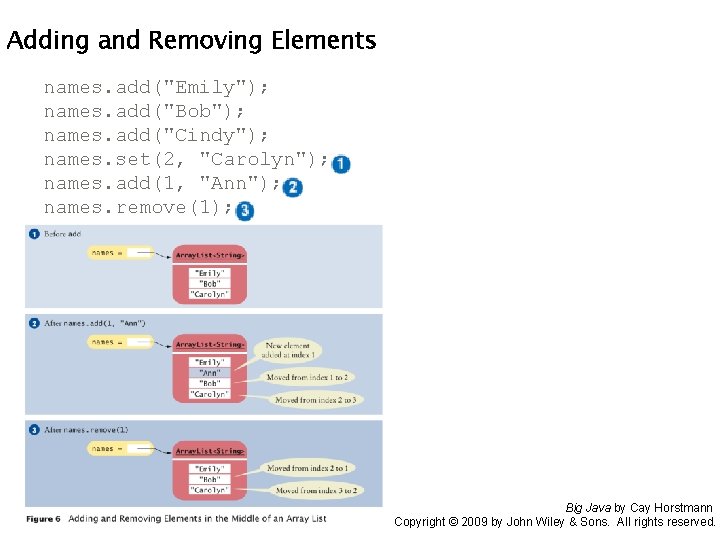
Adding and Removing Elements names. add("Emily"); names. add("Bob"); names. add("Cindy"); names. set(2, "Carolyn"); names. add(1, "Ann"); names. remove(1); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
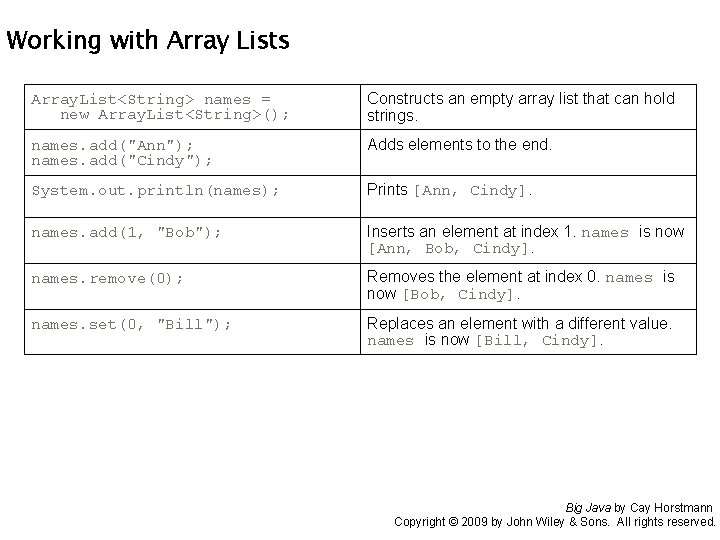
Working with Array Lists Array. List<String> names = new Array. List<String>(); Constructs an empty array list that can hold strings. names. add("Ann"); names. add("Cindy"); Adds elements to the end. System. out. println(names); Prints [Ann, Cindy]. names. add(1, "Bob"); Inserts an element at index 1. names is now [Ann, Bob, Cindy]. names. remove(0); Removes the element at index 0. names is now [Bob, Cindy]. names. set(0, "Bill"); Replaces an element with a different value. names is now [Bill, Cindy]. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
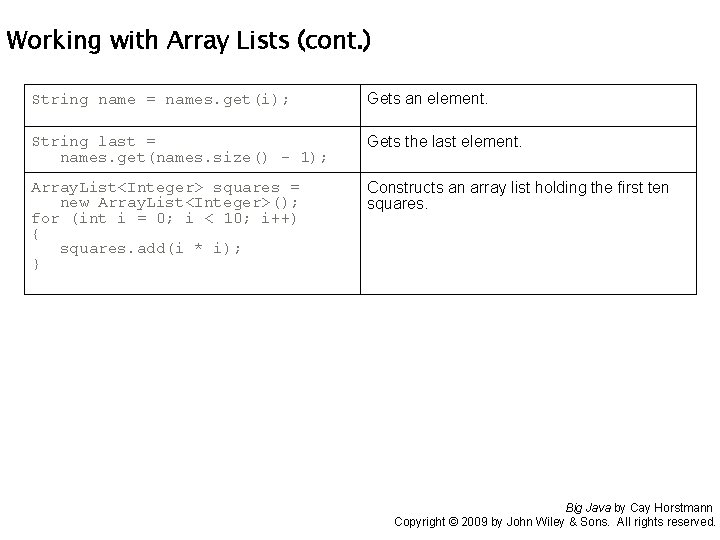
Working with Array Lists (cont. ) String name = names. get(i); Gets an element. String last = names. get(names. size() - 1); Gets the last element. Array. List<Integer> squares = new Array. List<Integer>(); for (int i = 0; i < 10; i++) { squares. add(i * i); } Constructs an array list holding the first ten squares. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
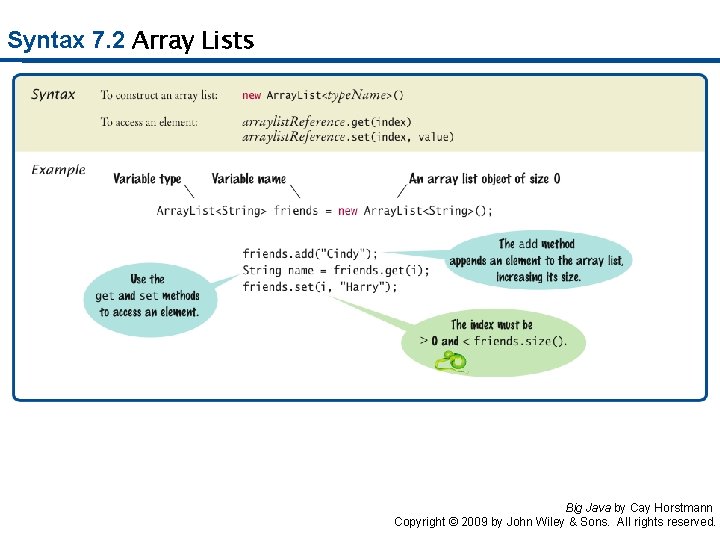
Syntax 7. 2 Array Lists Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
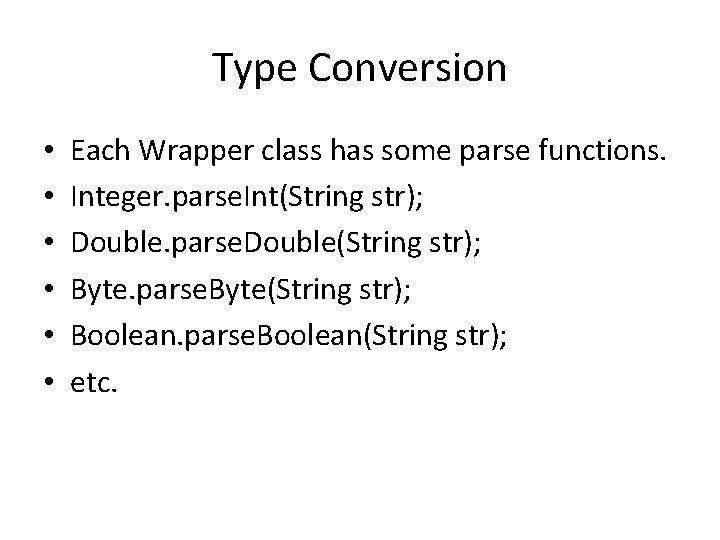
Type Conversion • • • Each Wrapper class has some parse functions. Integer. parse. Int(String str); Double. parse. Double(String str); Byte. parse. Byte(String str); Boolean. parse. Boolean(String str); etc.
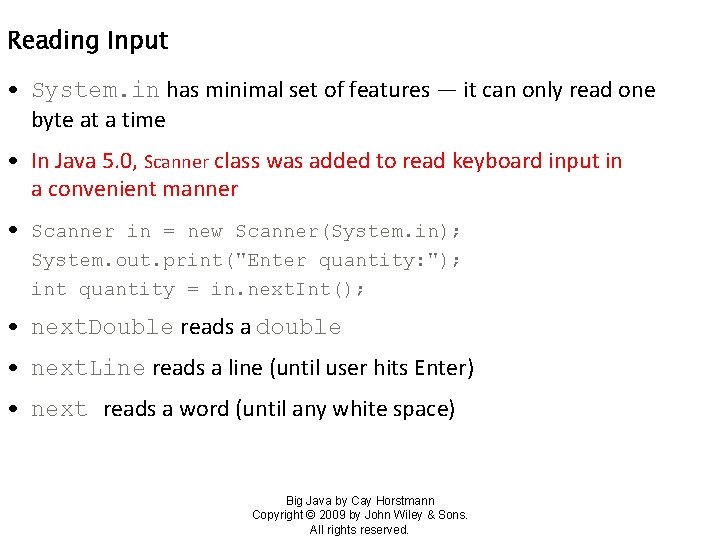
Reading Input • System. in has minimal set of features — it can only read one byte at a time • In Java 5. 0, Scanner class was added to read keyboard input in a convenient manner • Scanner in = new Scanner(System. in); System. out. print("Enter quantity: "); int quantity = in. next. Int(); • next. Double reads a double • next. Line reads a line (until user hits Enter) • next reads a word (until any white space) Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
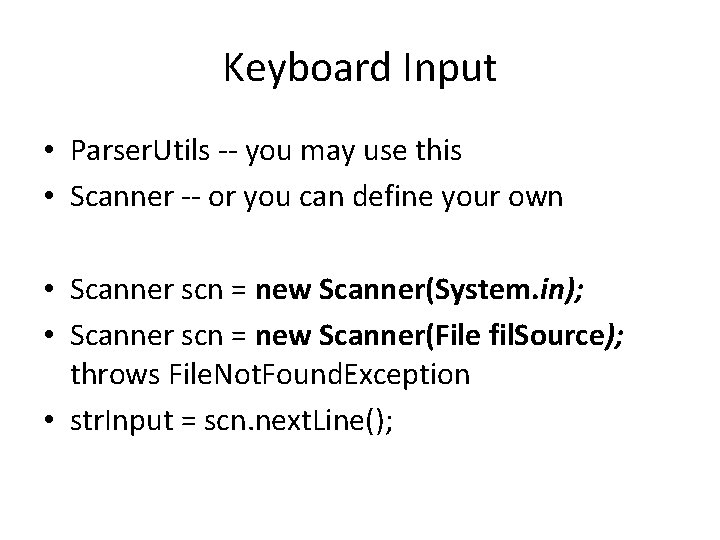
Keyboard Input • Parser. Utils -- you may use this • Scanner -- or you can define your own • Scanner scn = new Scanner(System. in); • Scanner scn = new Scanner(File fil. Source); throws File. Not. Found. Exception • str. Input = scn. next. Line();
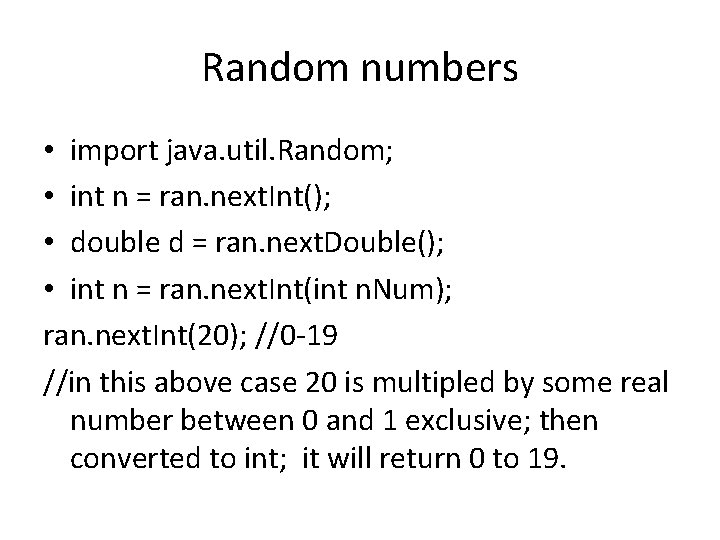
Random numbers • import java. util. Random; • int n = ran. next. Int(); • double d = ran. next. Double(); • int n = ran. next. Int(int n. Num); ran. next. Int(20); //0 -19 //in this above case 20 is multipled by some real number between 0 and 1 exclusive; then converted to int; it will return 0 to 19.
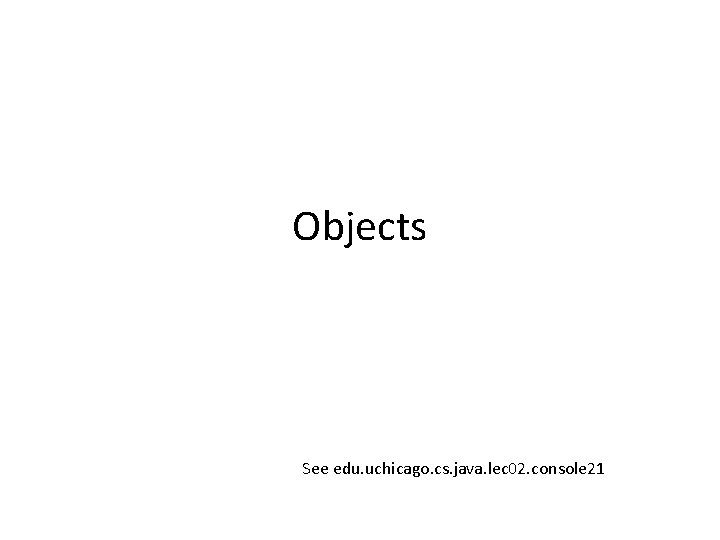
Objects See edu. uchicago. cs. java. lec 02. console 21
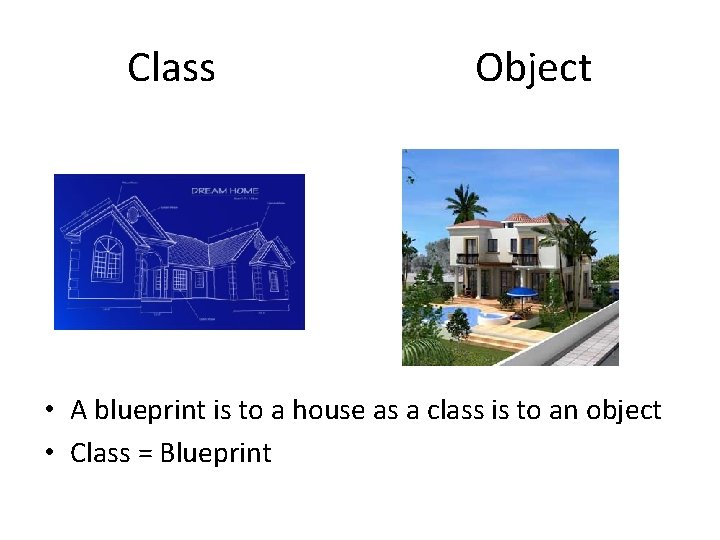
Class Object • A blueprint is to a house as a class is to an object • Class = Blueprint
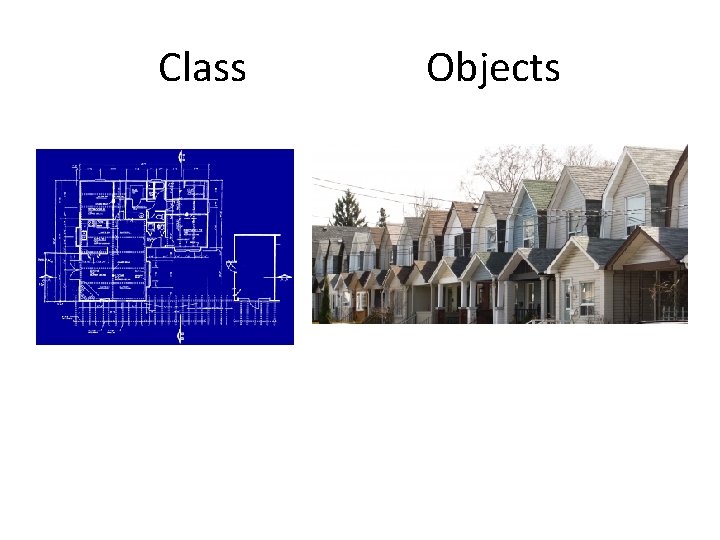
Class Objects
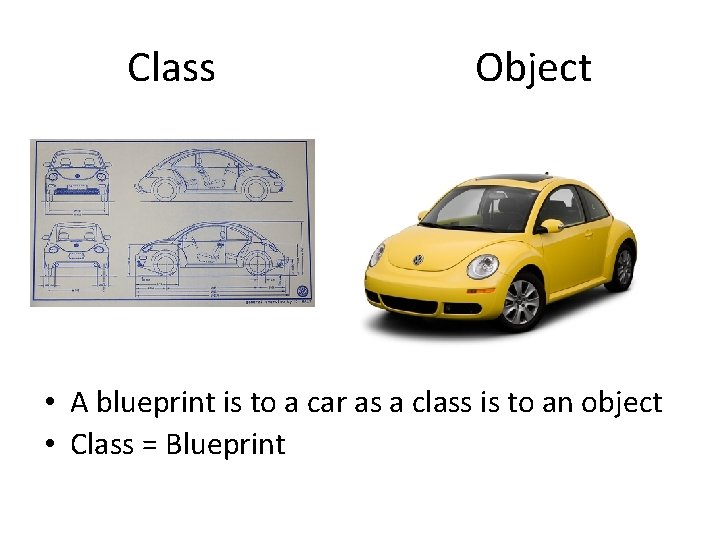
Class Object • A blueprint is to a car as a class is to an object • Class = Blueprint
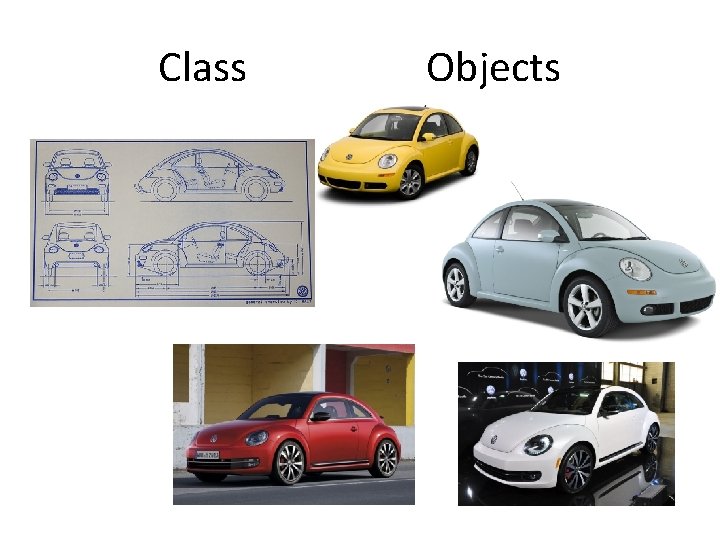
Class Objects
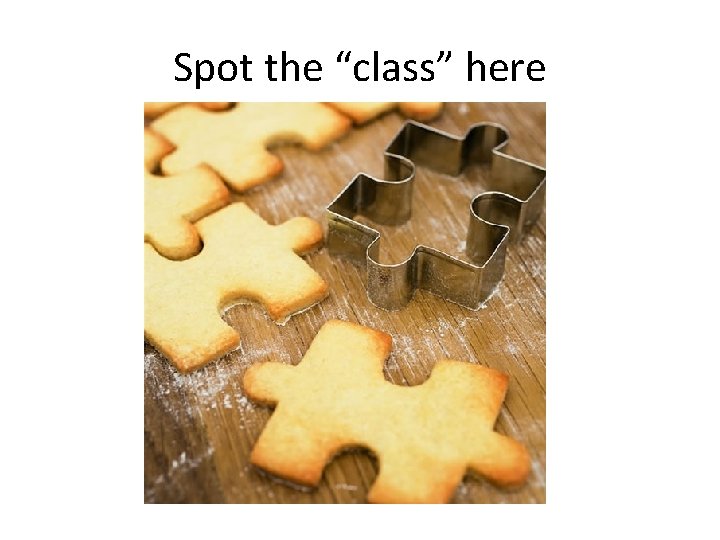
Spot the “class” here
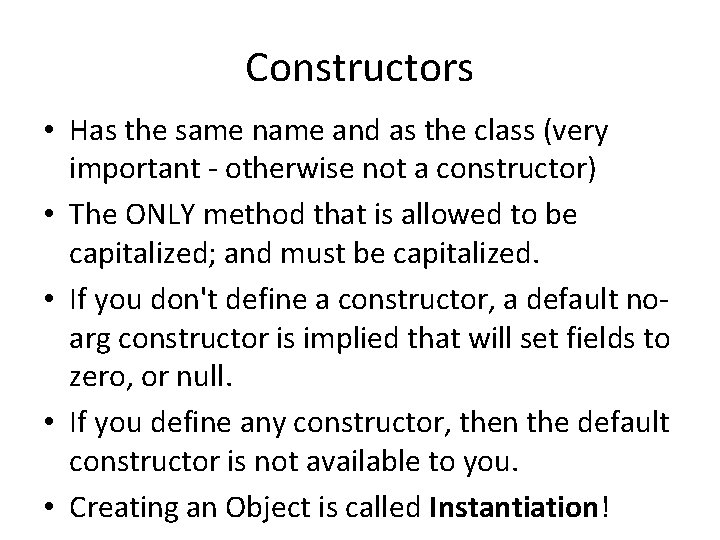
Constructors • Has the same name and as the class (very important - otherwise not a constructor) • The ONLY method that is allowed to be capitalized; and must be capitalized. • If you don't define a constructor, a default noarg constructor is implied that will set fields to zero, or null. • If you define any constructor, then the default constructor is not available to you. • Creating an Object is called Instantiation!
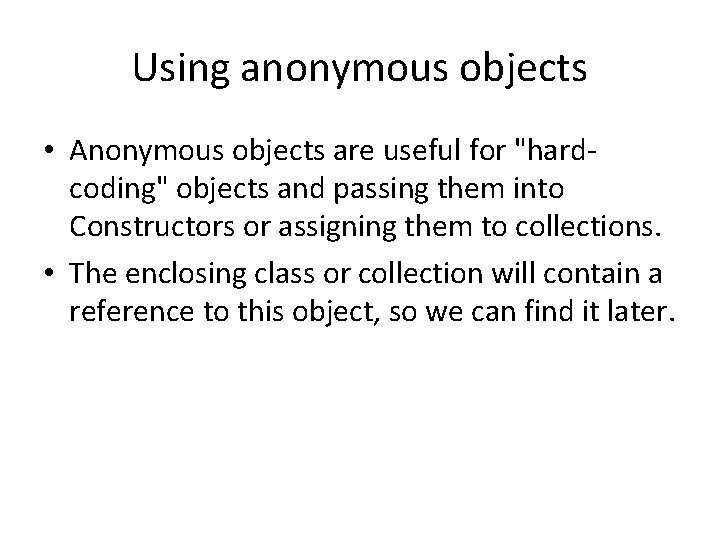
Using anonymous objects • Anonymous objects are useful for "hardcoding" objects and passing them into Constructors or assigning them to collections. • The enclosing class or collection will contain a reference to this object, so we can find it later.
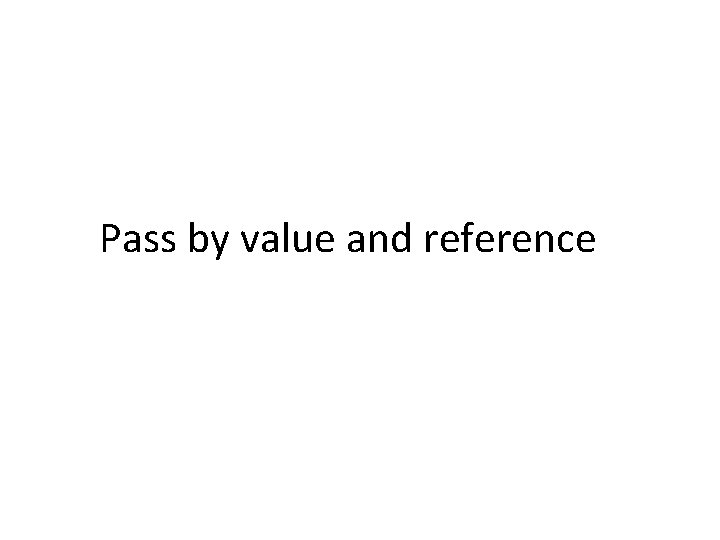
Pass by value and reference
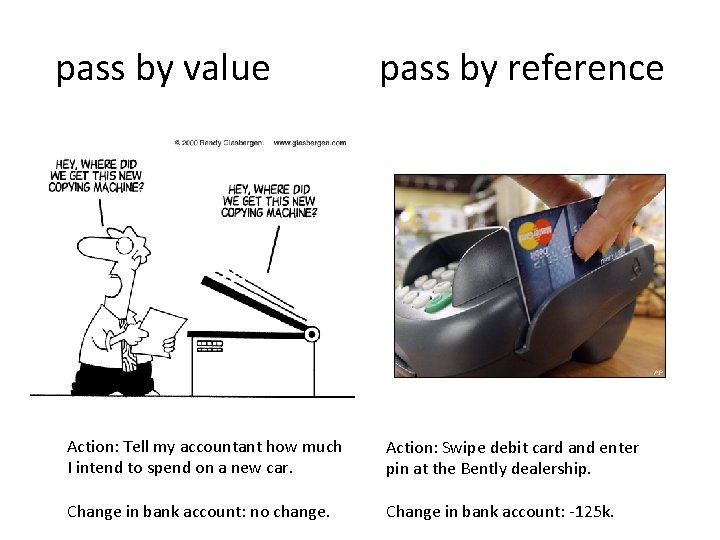
pass by value pass by reference Action: Tell my accountant how much I intend to spend on a new car. Action: Swipe debit card and enter pin at the Bently dealership. Change in bank account: no change. Change in bank account: -125 k.
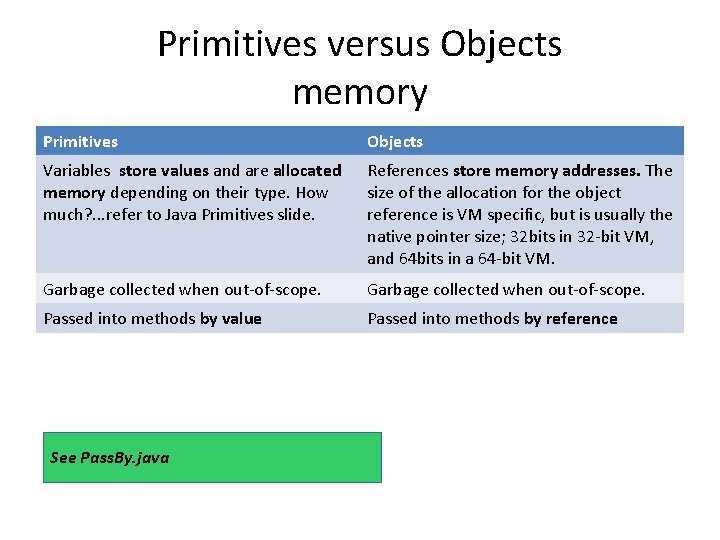
Primitives versus Objects memory Primitives Objects Variables store values and are allocated memory depending on their type. How much? . . . refer to Java Primitives slide. References store memory addresses. The size of the allocation for the object reference is VM specific, but is usually the native pointer size; 32 bits in 32 -bit VM, and 64 bits in a 64 -bit VM. Garbage collected when out-of-scope. Passed into methods by value Passed into methods by reference See Pass. By. java
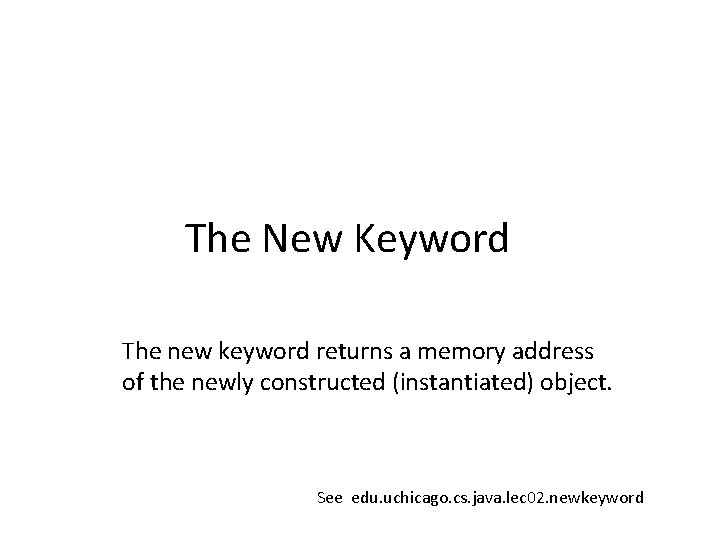
The New Keyword The new keyword returns a memory address of the newly constructed (instantiated) object. See edu. uchicago. cs. java. lec 02. newkeyword
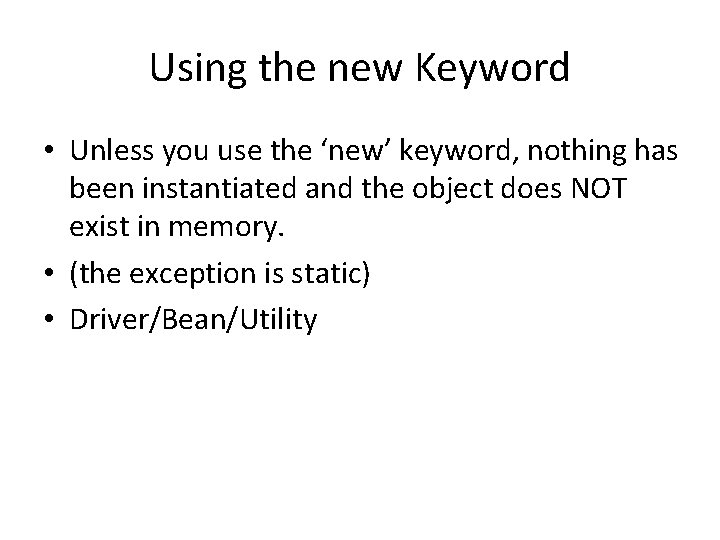
Using the new Keyword • Unless you use the ‘new’ keyword, nothing has been instantiated and the object does NOT exist in memory. • (the exception is static) • Driver/Bean/Utility
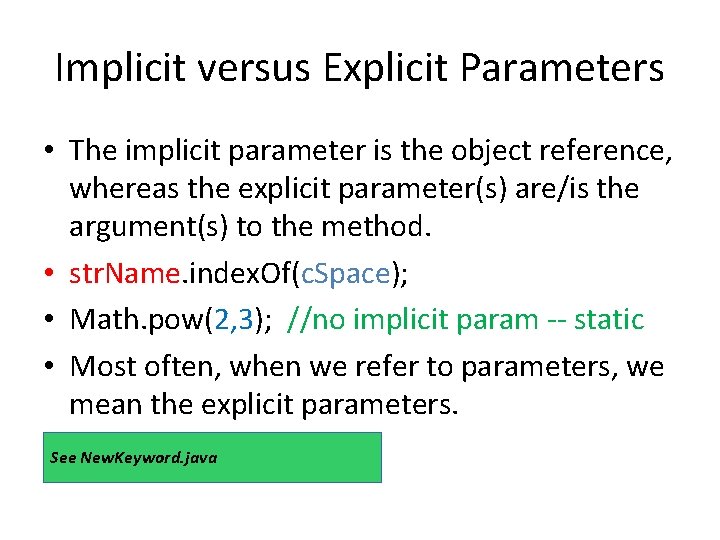
Implicit versus Explicit Parameters • The implicit parameter is the object reference, whereas the explicit parameter(s) are/is the argument(s) to the method. • str. Name. index. Of(c. Space); • Math. pow(2, 3); //no implicit param -- static • Most often, when we refer to parameters, we mean the explicit parameters. See New. Keyword. java
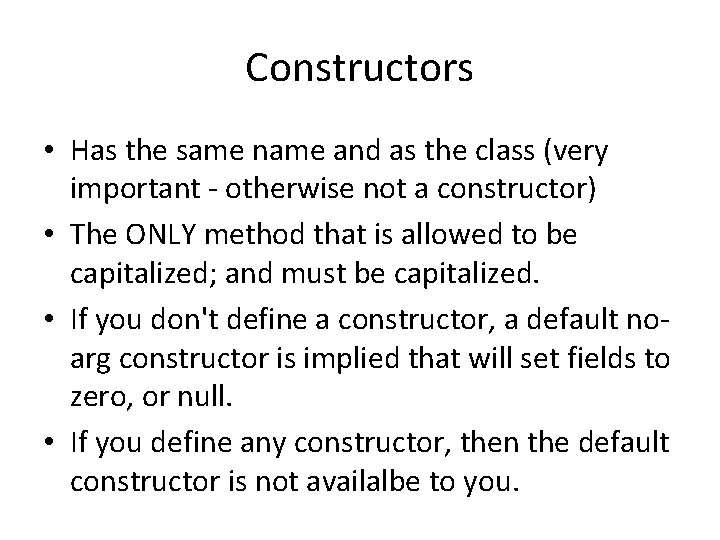
Constructors • Has the same name and as the class (very important - otherwise not a constructor) • The ONLY method that is allowed to be capitalized; and must be capitalized. • If you don't define a constructor, a default noarg constructor is implied that will set fields to zero, or null. • If you define any constructor, then the default constructor is not availalbe to you.
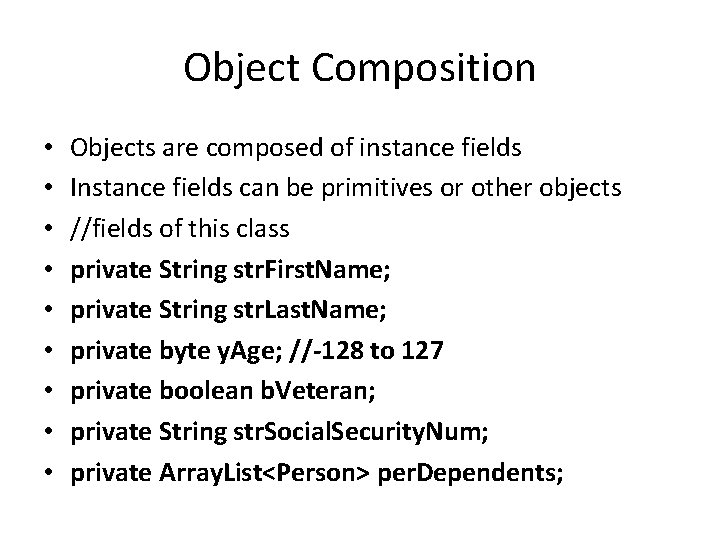
Object Composition • • • Objects are composed of instance fields Instance fields can be primitives or other objects //fields of this class private String str. First. Name; private String str. Last. Name; private byte y. Age; //-128 to 127 private boolean b. Veteran; private String str. Social. Security. Num; private Array. List<Person> per. Dependents;
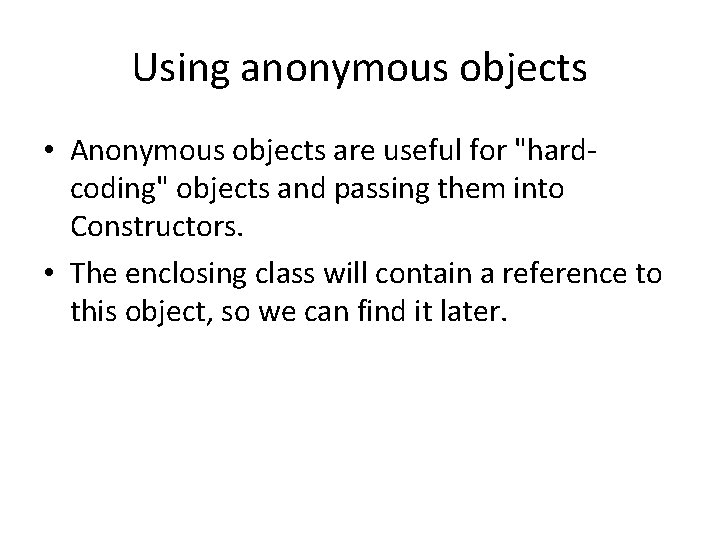
Using anonymous objects • Anonymous objects are useful for "hardcoding" objects and passing them into Constructors. • The enclosing class will contain a reference to this object, so we can find it later.
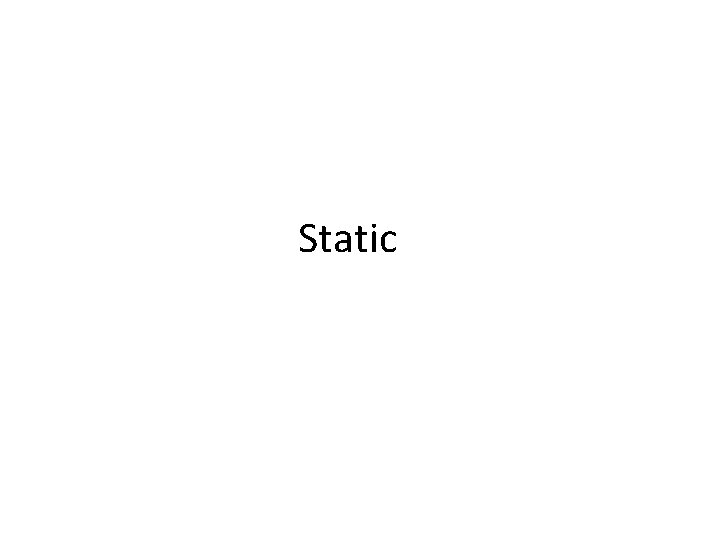
Static
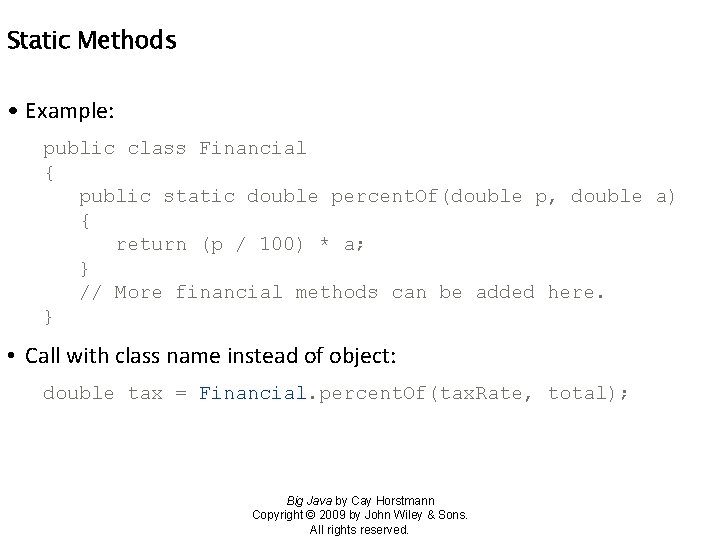
Static Methods • Example: public class Financial { public static double percent. Of(double p, double a) { return (p / 100) * a; } // More financial methods can be added here. } • Call with class name instead of object: double tax = Financial. percent. Of(tax. Rate, total); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
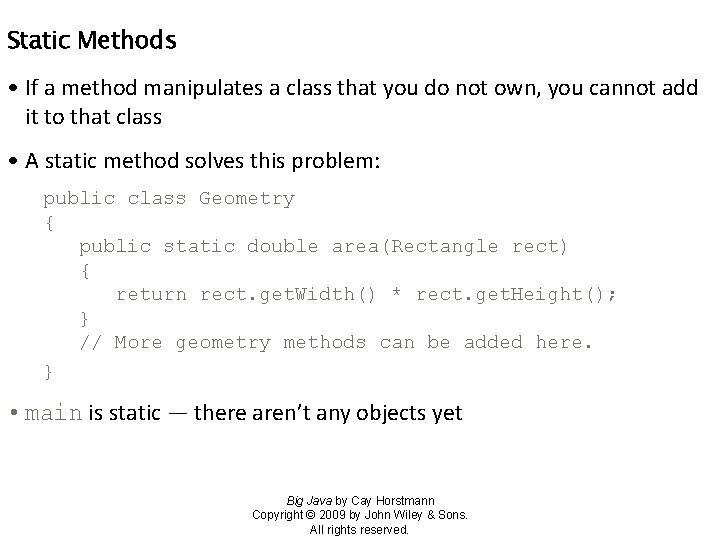
Static Methods • If a method manipulates a class that you do not own, you cannot add it to that class • A static method solves this problem: public class Geometry { public static double area(Rectangle rect) { return rect. get. Width() * rect. get. Height(); } // More geometry methods can be added here. } • main is static — there aren’t any objects yet Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
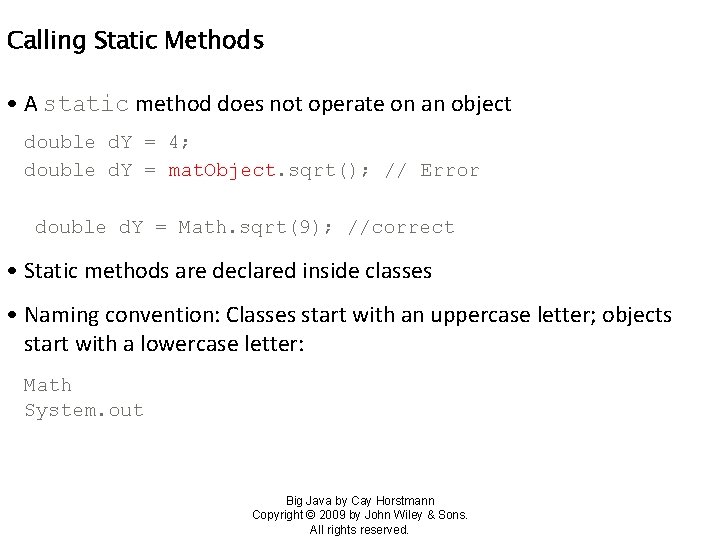
Calling Static Methods • A static method does not operate on an object double d. Y = 4; double d. Y = mat. Object. sqrt(); // Error double d. Y = Math. sqrt(9); //correct • Static methods are declared inside classes • Naming convention: Classes start with an uppercase letter; objects start with a lowercase letter: Math System. out Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
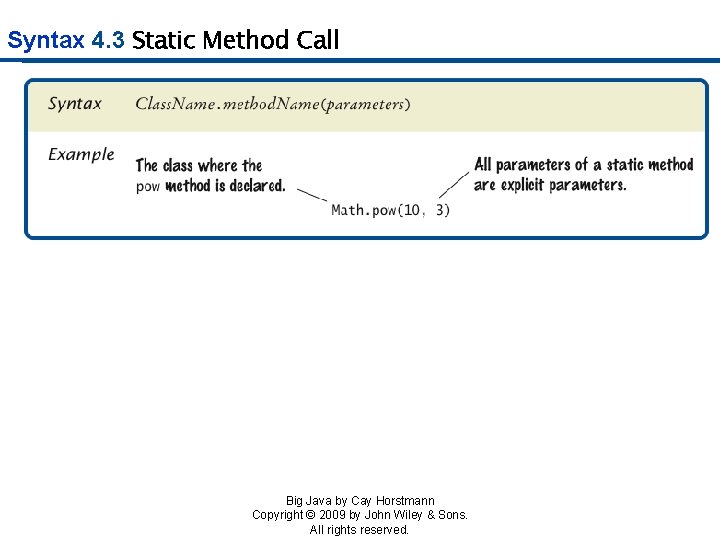
Syntax 4. 3 Static Method Call Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
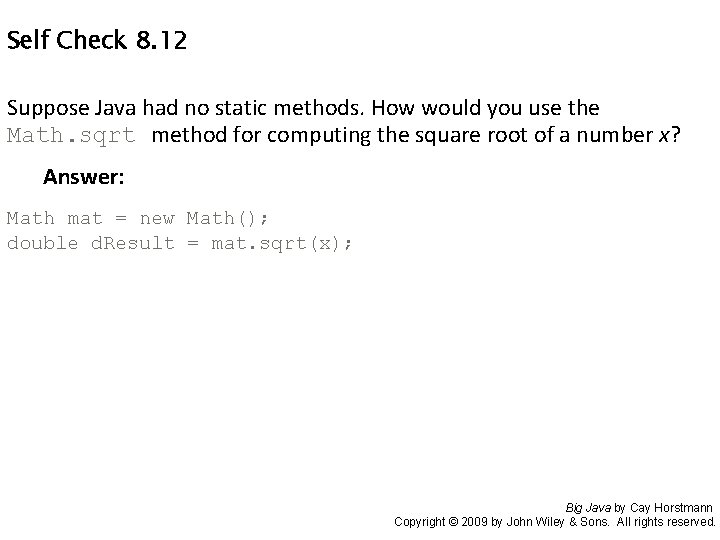
Self Check 8. 12 Suppose Java had no static methods. How would you use the Math. sqrt method for computing the square root of a number x? Answer: Math mat = new Math(); double d. Result = mat. sqrt(x); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
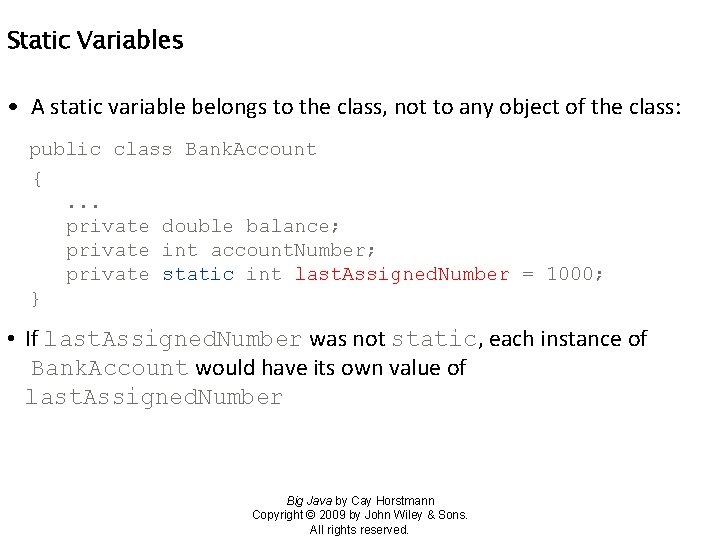
Static Variables • A static variable belongs to the class, not to any object of the class: public class Bank. Account {. . . private double balance; private int account. Number; private static int last. Assigned. Number = 1000; } • If last. Assigned. Number was not static, each instance of Bank. Account would have its own value of last. Assigned. Number Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
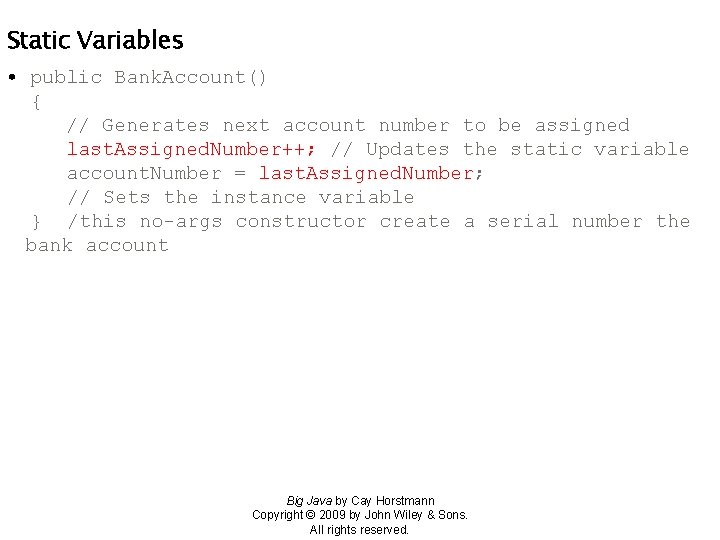
Static Variables • public Bank. Account() { // Generates next account number to be assigned last. Assigned. Number++; // Updates the static variable account. Number = last. Assigned. Number; // Sets the instance variable } /this no-args constructor create a serial number the bank account Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
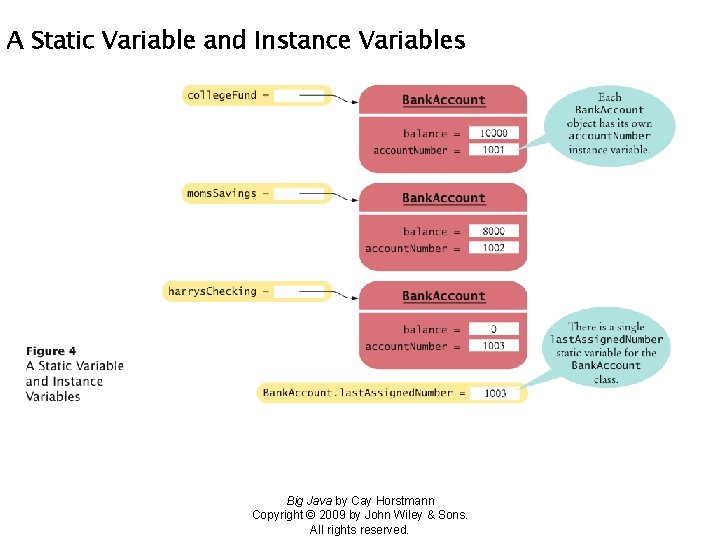
A Static Variable and Instance Variables Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
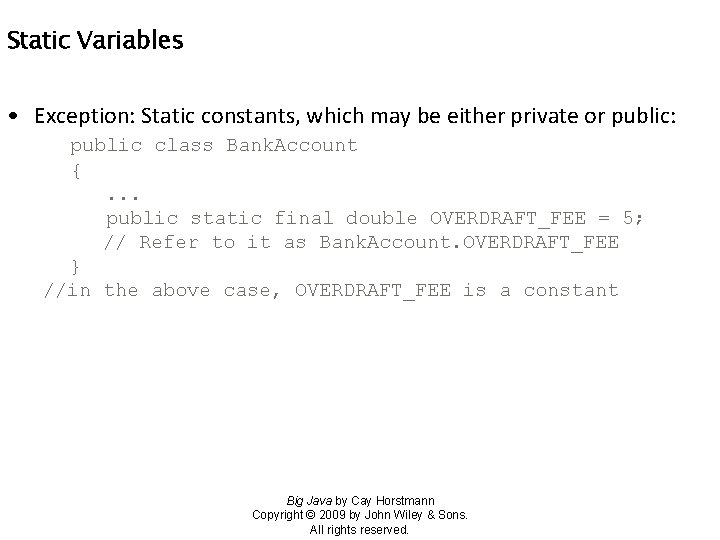
Static Variables • Exception: Static constants, which may be either private or public: public class Bank. Account {. . . public static final double OVERDRAFT_FEE = 5; // Refer to it as Bank. Account. OVERDRAFT_FEE } //in the above case, OVERDRAFT_FEE is a constant Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
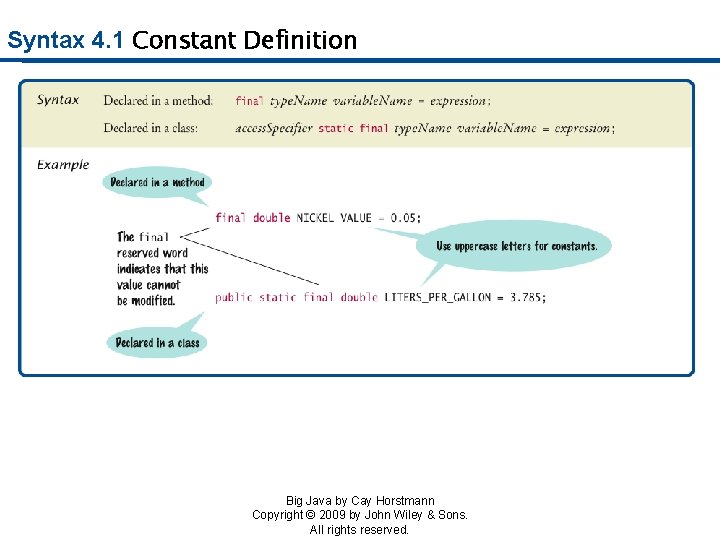
Syntax 4. 1 Constant Definition Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
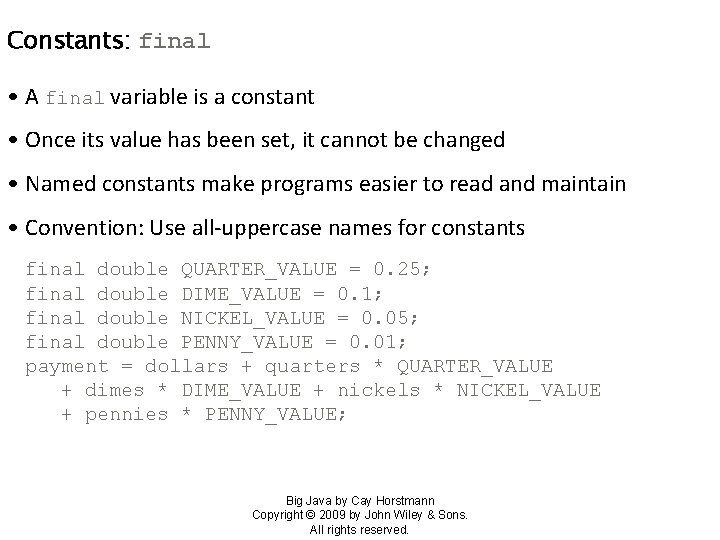
Constants: final • A final variable is a constant • Once its value has been set, it cannot be changed • Named constants make programs easier to read and maintain • Convention: Use all-uppercase names for constants final double QUARTER_VALUE = 0. 25; final double DIME_VALUE = 0. 1; final double NICKEL_VALUE = 0. 05; final double PENNY_VALUE = 0. 01; payment = dollars + quarters * QUARTER_VALUE + dimes * DIME_VALUE + nickels * NICKEL_VALUE + pennies * PENNY_VALUE; Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
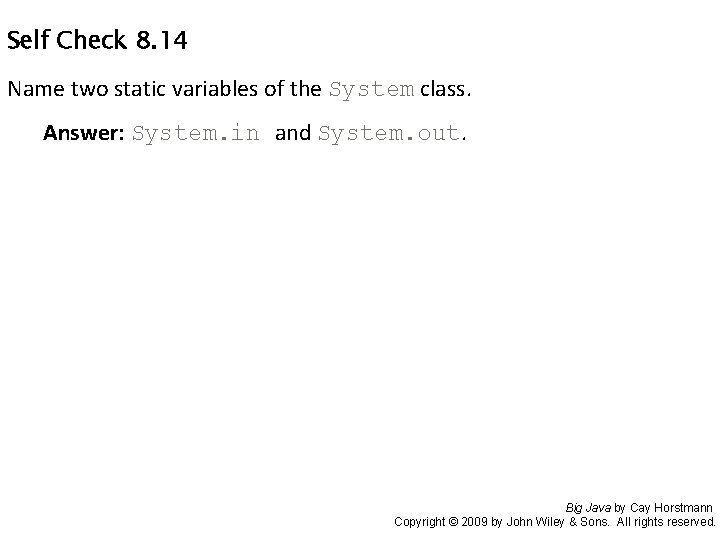
Self Check 8. 14 Name two static variables of the System class. Answer: System. in and System. out. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
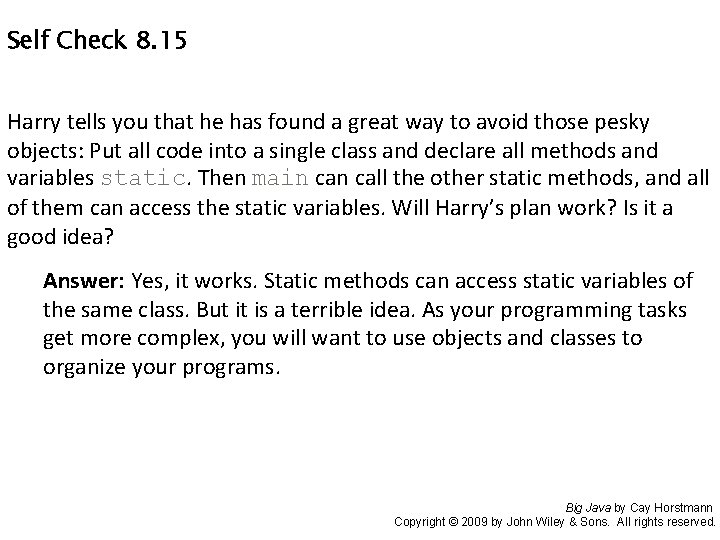
Self Check 8. 15 Harry tells you that he has found a great way to avoid those pesky objects: Put all code into a single class and declare all methods and variables static. Then main call the other static methods, and all of them can access the static variables. Will Harry’s plan work? Is it a good idea? Answer: Yes, it works. Static methods can access static variables of the same class. But it is a terrible idea. As your programming tasks get more complex, you will want to use objects and classes to organize your programs. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
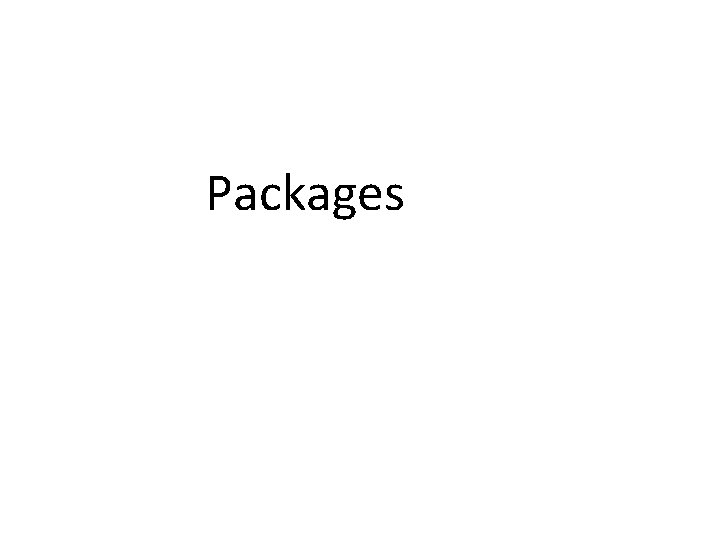
Packages
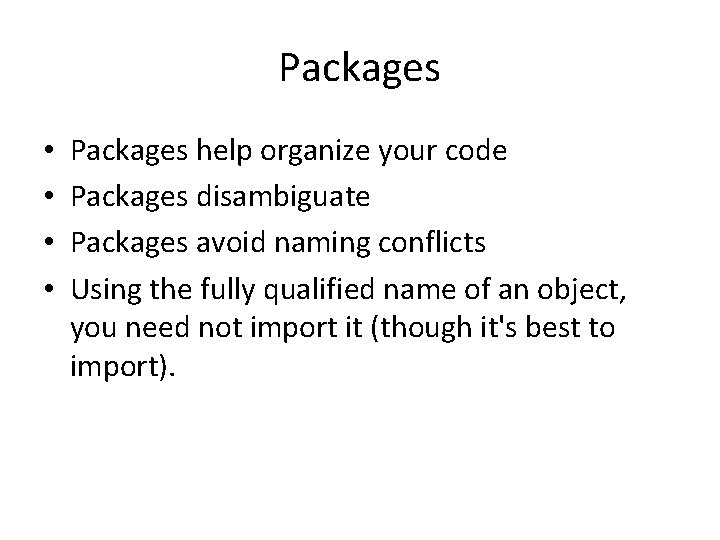
Packages • • Packages help organize your code Packages disambiguate Packages avoid naming conflicts Using the fully qualified name of an object, you need not import it (though it's best to import).
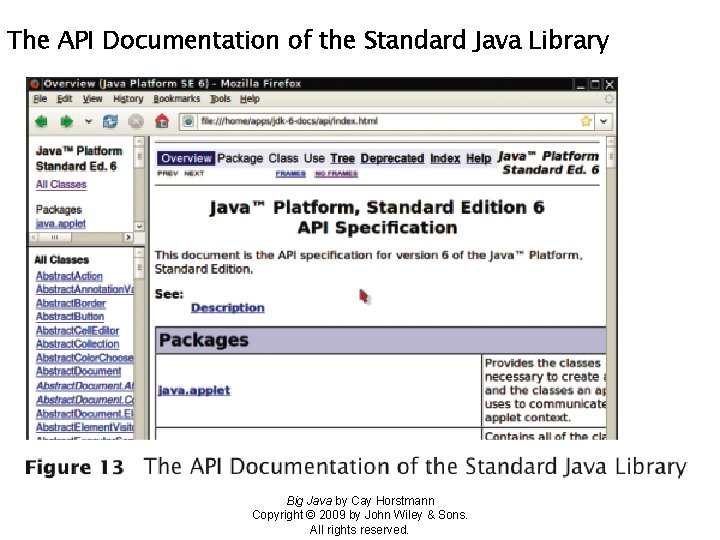
The API Documentation of the Standard Java Library Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
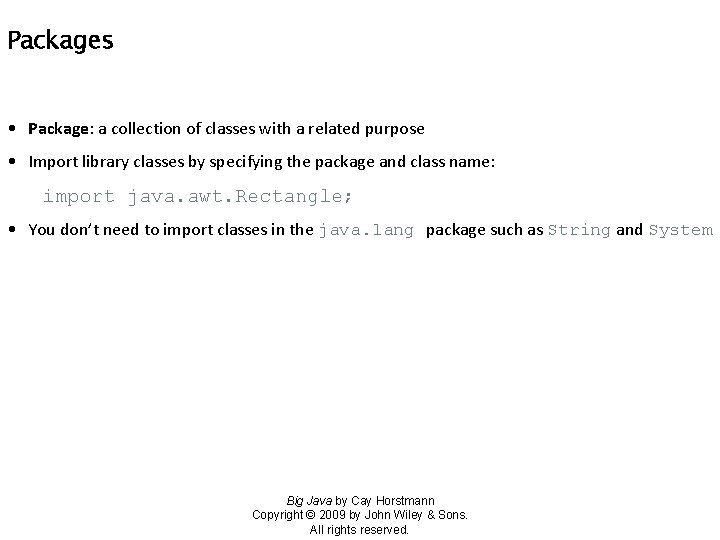
Packages • Package: a collection of classes with a related purpose • Import library classes by specifying the package and class name: import java. awt. Rectangle; • You don’t need to import classes in the java. lang package such as String and System Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
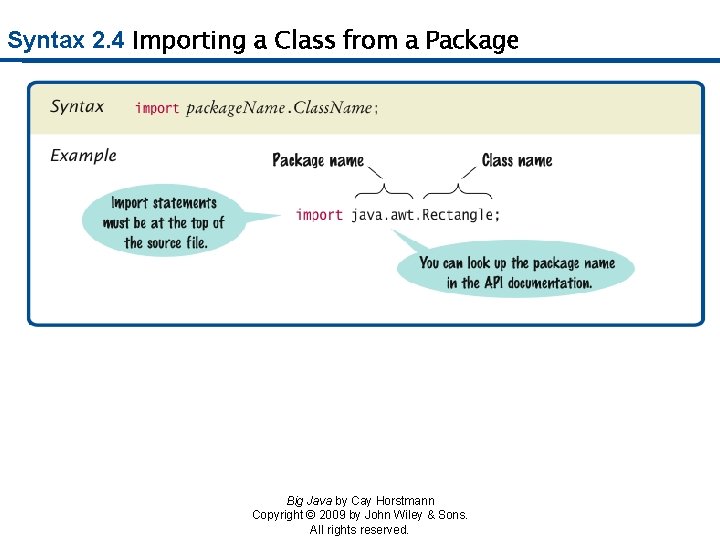
Syntax 2. 4 Importing a Class from a Package Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
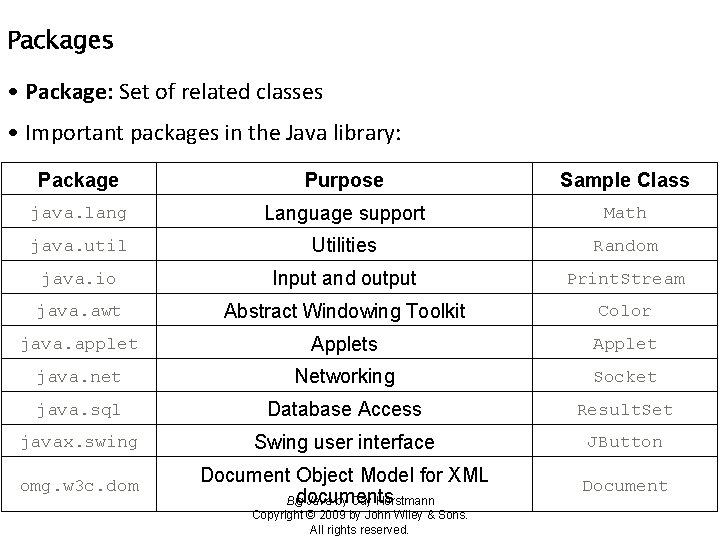
Packages • Package: Set of related classes • Important packages in the Java library: Package Purpose Sample Class java. lang Language support Math java. util Utilities Random java. io Input and output Print. Stream java. awt Abstract Windowing Toolkit Color java. applet Applets Applet java. net Networking Socket java. sql Database Access Result. Set javax. swing Swing user interface JButton omg. w 3 c. dom Document Object Model for XML documents Big Java by Cay Horstmann Document Copyright © 2009 by John Wiley & Sons. All rights reserved.
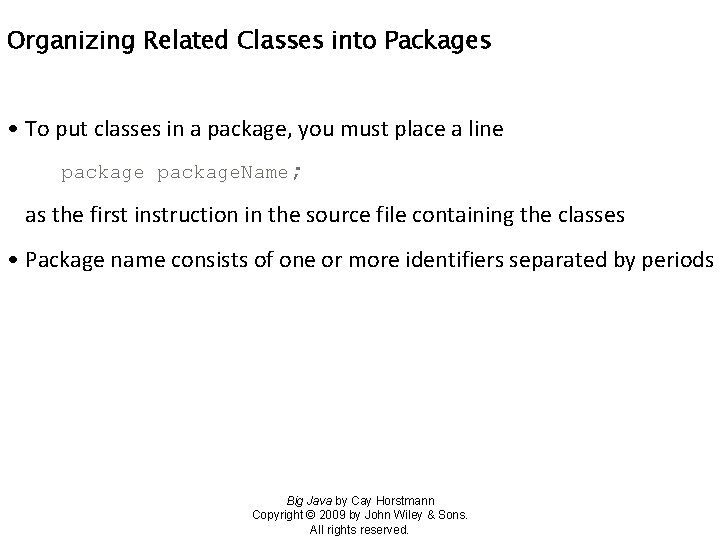
Organizing Related Classes into Packages • To put classes in a package, you must place a line package. Name; as the first instruction in the source file containing the classes • Package name consists of one or more identifiers separated by periods Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
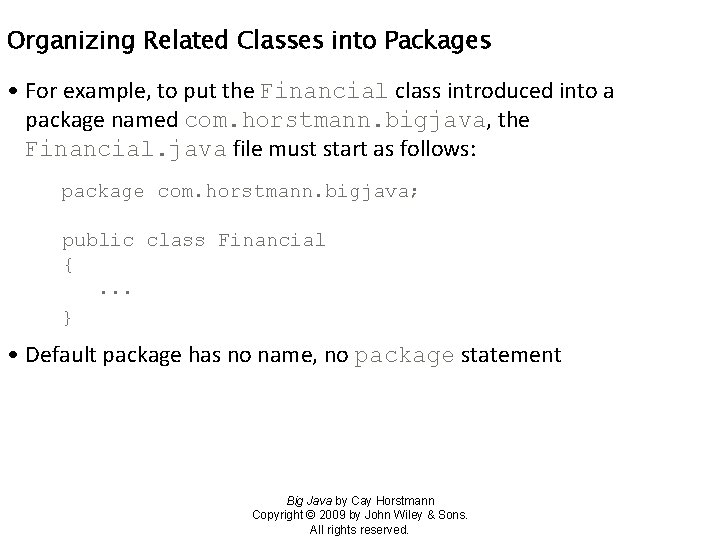
Organizing Related Classes into Packages • For example, to put the Financial class introduced into a package named com. horstmann. bigjava, the Financial. java file must start as follows: package com. horstmann. bigjava; public class Financial {. . . } • Default package has no name, no package statement Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
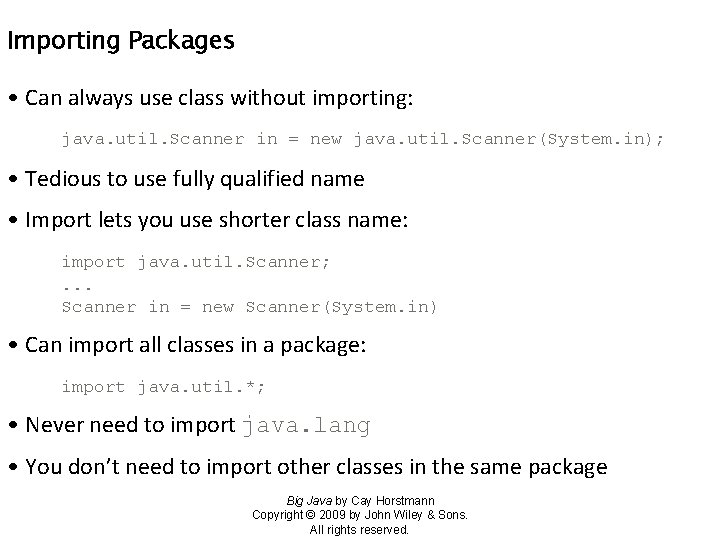
Importing Packages • Can always use class without importing: java. util. Scanner in = new java. util. Scanner(System. in); • Tedious to use fully qualified name • Import lets you use shorter class name: import java. util. Scanner; . . . Scanner in = new Scanner(System. in) • Can import all classes in a package: import java. util. *; • Never need to import java. lang • You don’t need to import other classes in the same package Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
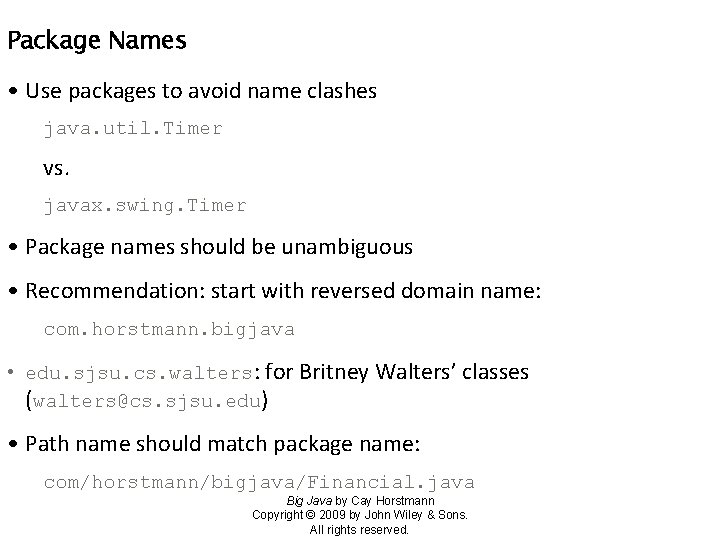
Package Names • Use packages to avoid name clashes java. util. Timer vs. javax. swing. Timer • Package names should be unambiguous • Recommendation: start with reversed domain name: com. horstmann. bigjava • edu. sjsu. cs. walters: for Britney Walters’ classes (walters@cs. sjsu. edu) • Path name should match package name: com/horstmann/bigjava/Financial. java Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
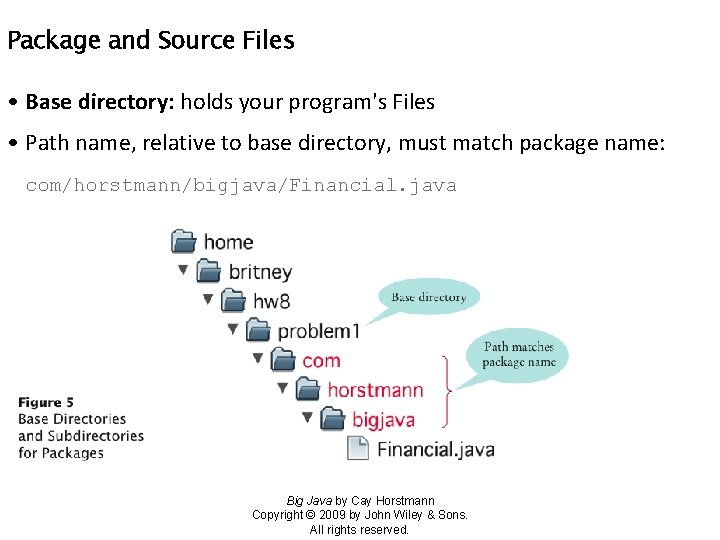
Package and Source Files • Base directory: holds your program's Files • Path name, relative to base directory, must match package name: com/horstmann/bigjava/Financial. java Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
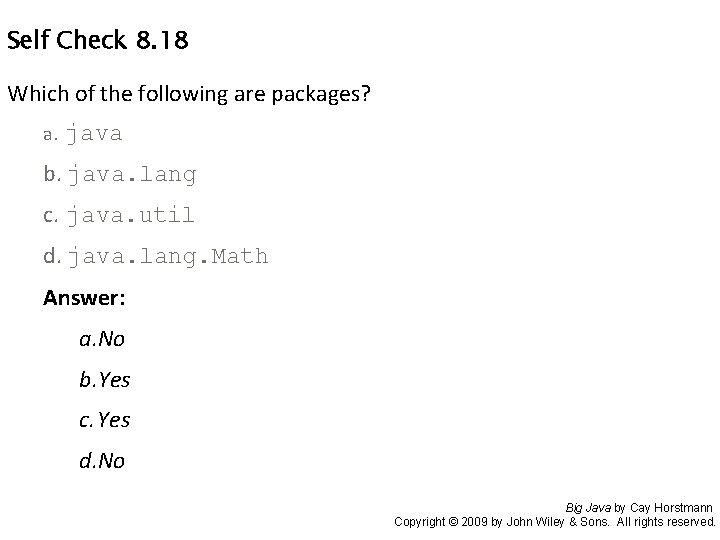
Self Check 8. 18 Which of the following are packages? a. java b. java. lang c. java. util d. java. lang. Math Answer: a. No b. Yes c. Yes d. No Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
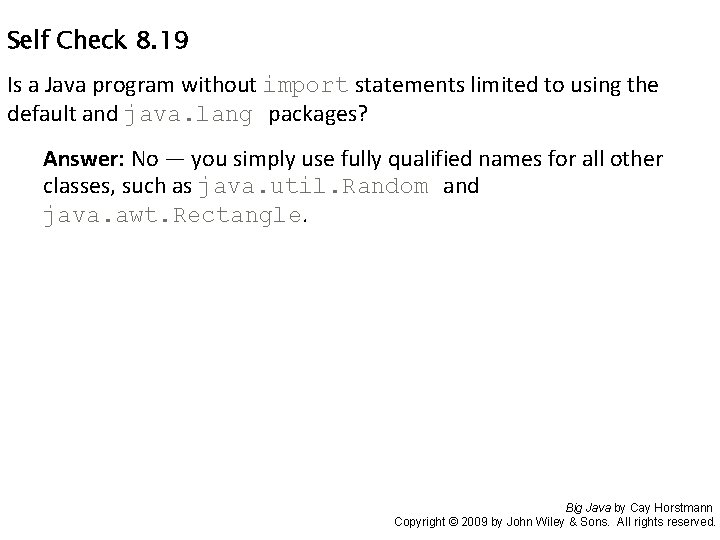
Self Check 8. 19 Is a Java program without import statements limited to using the default and java. lang packages? Answer: No — you simply use fully qualified names for all other classes, such as java. util. Random and java. awt. Rectangle. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
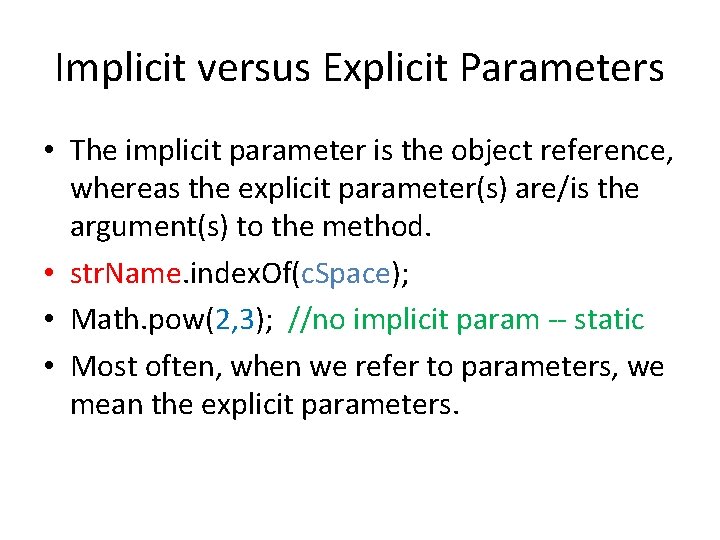
Implicit versus Explicit Parameters • The implicit parameter is the object reference, whereas the explicit parameter(s) are/is the argument(s) to the method. • str. Name. index. Of(c. Space); • Math. pow(2, 3); //no implicit param -- static • Most often, when we refer to parameters, we mean the explicit parameters.
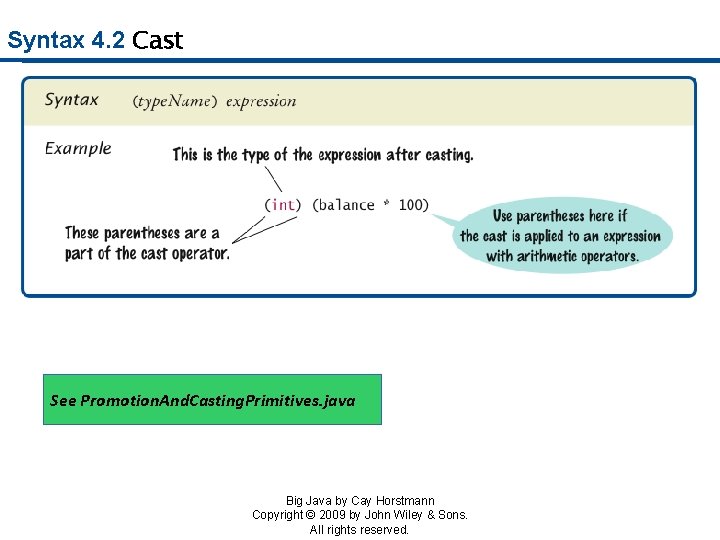
Syntax 4. 2 Cast See Promotion. And. Casting. Primitives. java Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
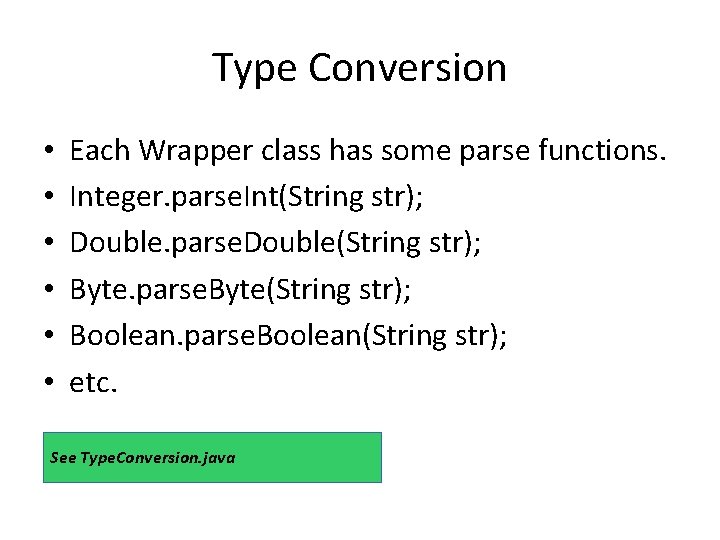
Type Conversion • • • Each Wrapper class has some parse functions. Integer. parse. Int(String str); Double. parse. Double(String str); Byte. parse. Byte(String str); Boolean. parse. Boolean(String str); etc. See Type. Conversion. java
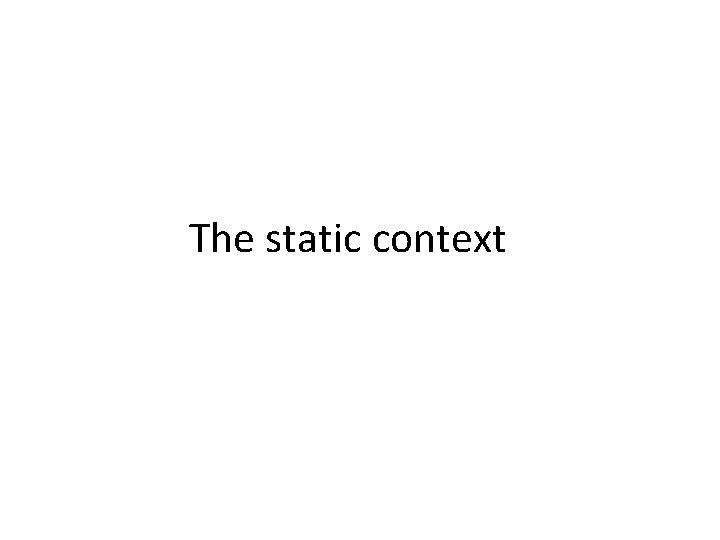
The static context
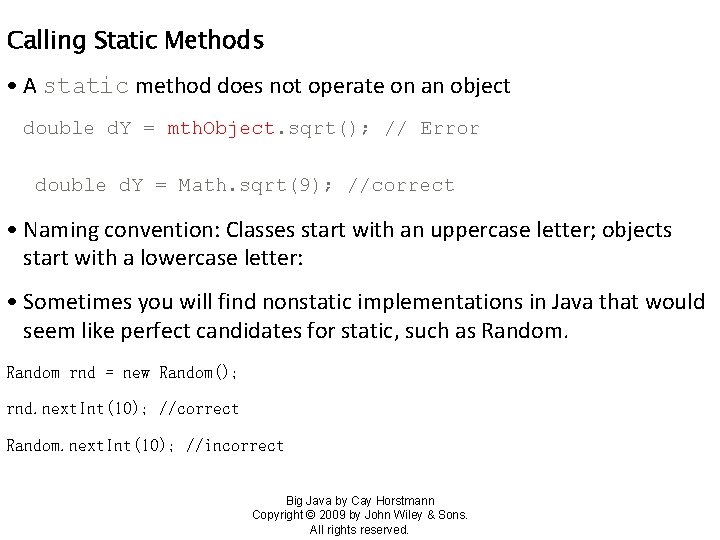
Calling Static Methods • A static method does not operate on an object double d. Y = mth. Object. sqrt(); // Error double d. Y = Math. sqrt(9); //correct • Naming convention: Classes start with an uppercase letter; objects start with a lowercase letter: • Sometimes you will find nonstatic implementations in Java that would seem like perfect candidates for static, such as Random rnd = new Random(); rnd. next. Int(10); //correct Random. next. Int(10); //incorrect Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
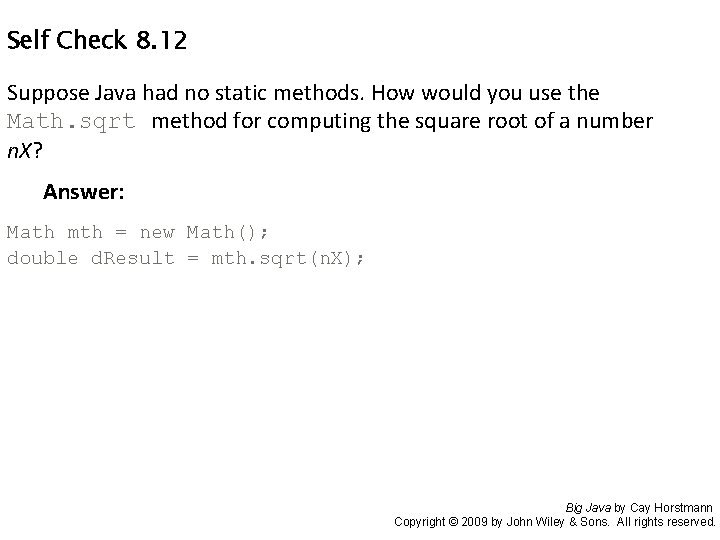
Self Check 8. 12 Suppose Java had no static methods. How would you use the Math. sqrt method for computing the square root of a number n. X? Answer: Math mth = new Math(); double d. Result = mth. sqrt(n. X); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
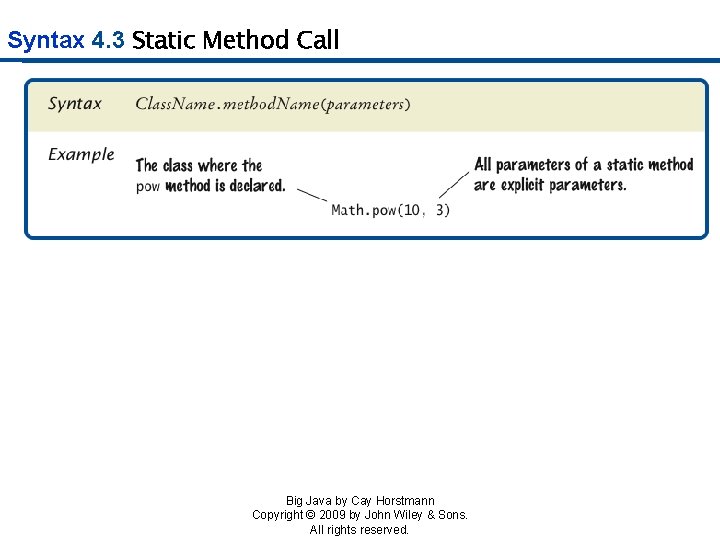
Syntax 4. 3 Static Method Call Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
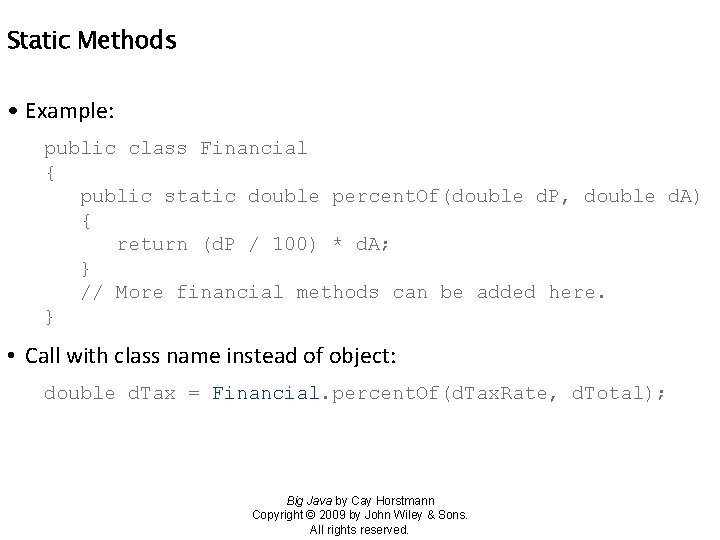
Static Methods • Example: public class Financial { public static double percent. Of(double d. P, double d. A) { return (d. P / 100) * d. A; } // More financial methods can be added here. } • Call with class name instead of object: double d. Tax = Financial. percent. Of(d. Tax. Rate, d. Total); Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
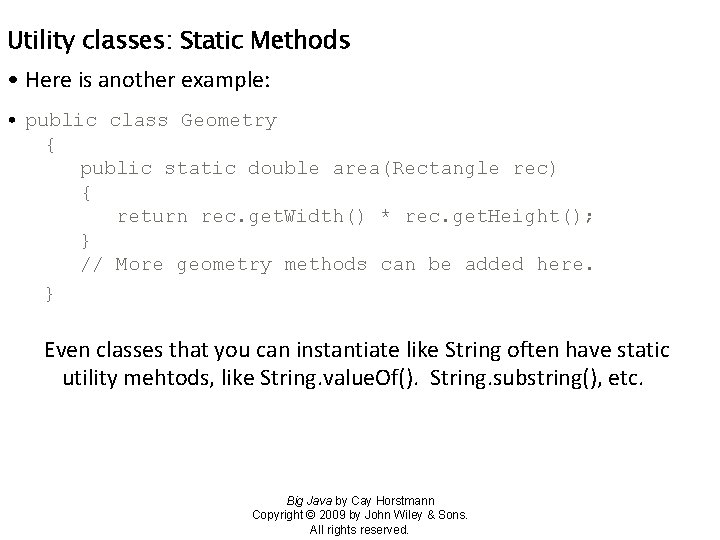
Utility classes: Static Methods • Here is another example: • public class Geometry { public static double area(Rectangle rec) { return rec. get. Width() * rec. get. Height(); } // More geometry methods can be added here. } Even classes that you can instantiate like String often have static utility mehtods, like String. value. Of(). String. substring(), etc. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
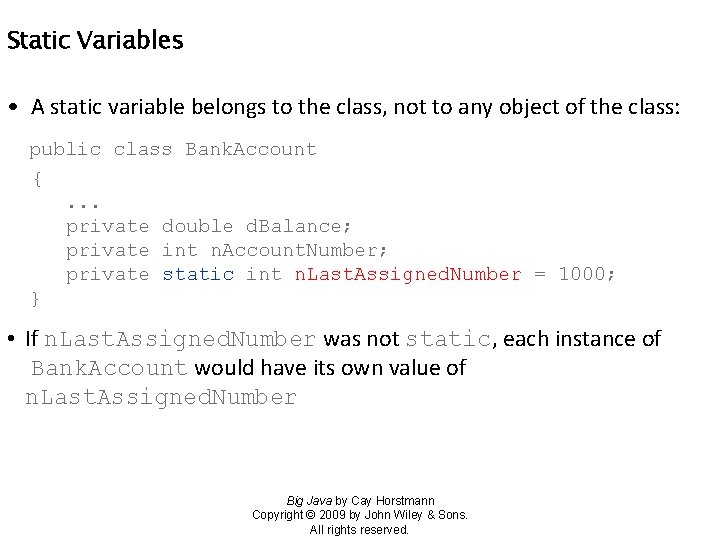
Static Variables • A static variable belongs to the class, not to any object of the class: public class Bank. Account {. . . private double d. Balance; private int n. Account. Number; private static int n. Last. Assigned. Number = 1000; } • If n. Last. Assigned. Number was not static, each instance of Bank. Account would have its own value of n. Last. Assigned. Number Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
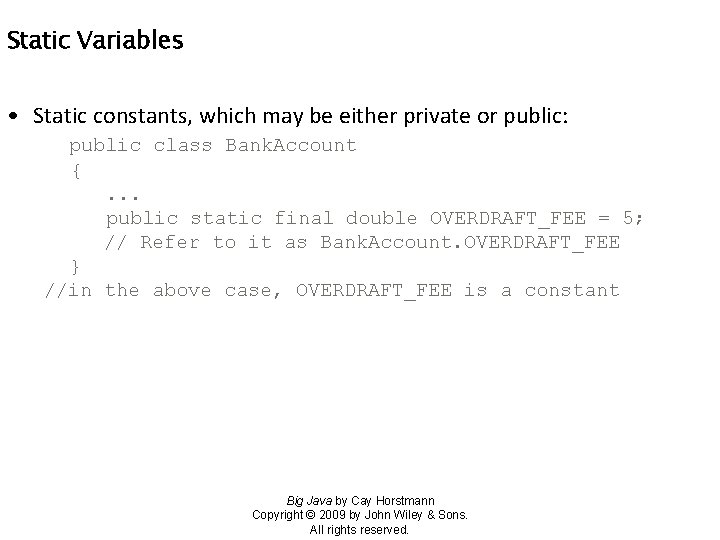
Static Variables • Static constants, which may be either private or public: public class Bank. Account {. . . public static final double OVERDRAFT_FEE = 5; // Refer to it as Bank. Account. OVERDRAFT_FEE } //in the above case, OVERDRAFT_FEE is a constant Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
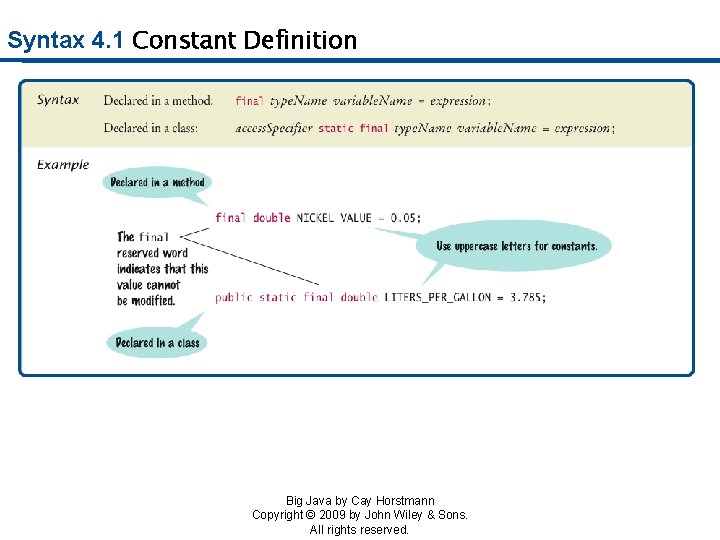
Syntax 4. 1 Constant Definition Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
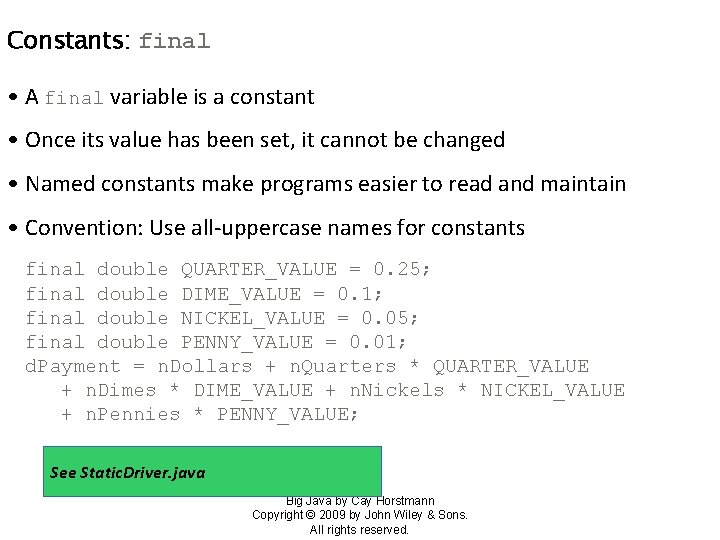
Constants: final • A final variable is a constant • Once its value has been set, it cannot be changed • Named constants make programs easier to read and maintain • Convention: Use all-uppercase names for constants final double QUARTER_VALUE = 0. 25; final double DIME_VALUE = 0. 1; final double NICKEL_VALUE = 0. 05; final double PENNY_VALUE = 0. 01; d. Payment = n. Dollars + n. Quarters * QUARTER_VALUE + n. Dimes * DIME_VALUE + n. Nickels * NICKEL_VALUE + n. Pennies * PENNY_VALUE; See Static. Driver. java Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
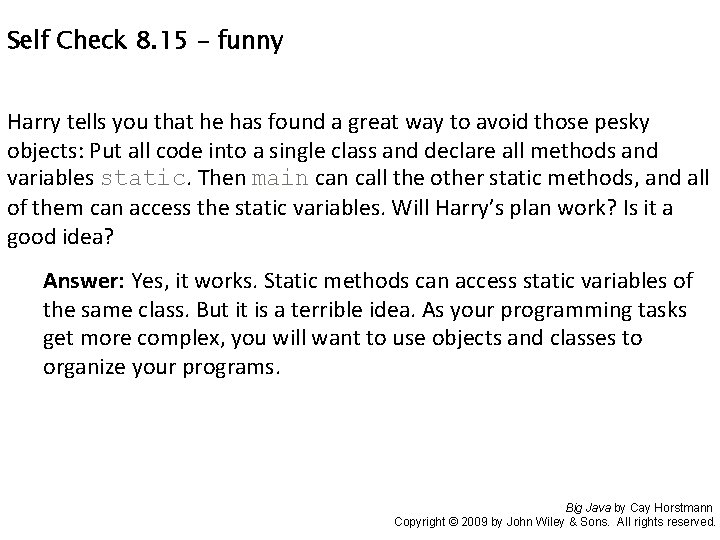
Self Check 8. 15 - funny Harry tells you that he has found a great way to avoid those pesky objects: Put all code into a single class and declare all methods and variables static. Then main call the other static methods, and all of them can access the static variables. Will Harry’s plan work? Is it a good idea? Answer: Yes, it works. Static methods can access static variables of the same class. But it is a terrible idea. As your programming tasks get more complex, you will want to use objects and classes to organize your programs. Big Java by Cay Horstmann Copyright © 2009 by John Wiley & Sons. All rights reserved.
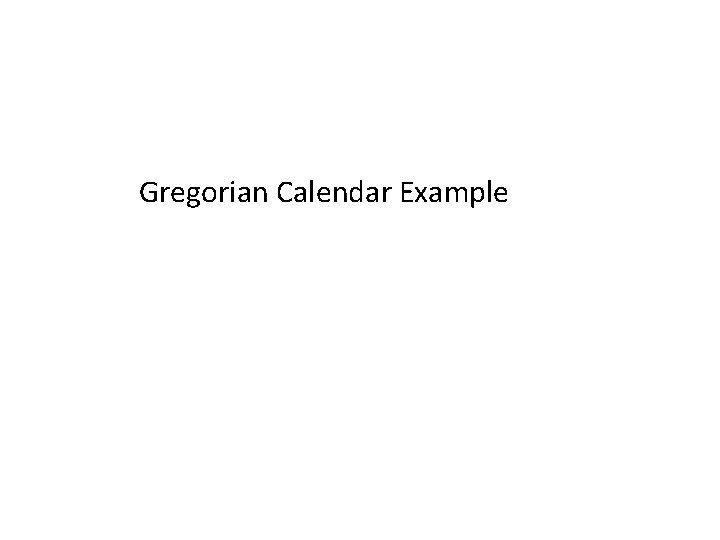
Gregorian Calendar Example
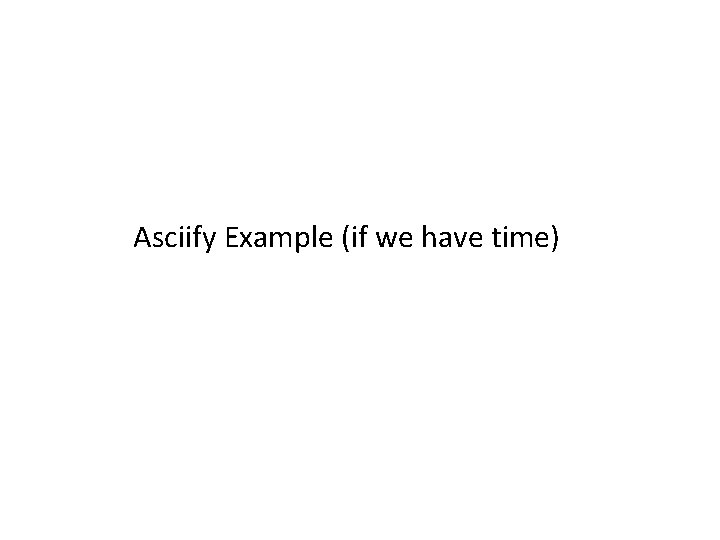
Asciify Example (if we have time)
Cover page in mla
Date frui
Dividend declaration date
Record date for dividend
Znacznik pre /pre jest stosowany w celu wyświetlenia
No thats not it
Whats todays temperature
Todays objective
Todays wordlw
Objective on resume example
Todays objective
Walsall rugby
Objective of cyberbullying
Today's public relations departments:
Stuttering jeopardy
Todays jeopardy
Todays weather hull
Todays worldld
Digestive system of ruminants
Todays objective
Todays jeopardy
Today meeting or today's meeting
Todays agenda
Chapter 13 marketing in today's world
Planetary positions today
Todays generations
Todays health
Todays final jeopardy
Geographic regions final jeopardy
Todays software
Todays objective
Whats thermal energy
Todays objective
Todays whether
Todays lab
Rprefer
Todays price of asda shares
Todays plan
Todays jeopardy
Today's classes
Todays final jeopardy answer
How to identify simile
Todays concept
Todays science
Todays objective
Todays globl
Safe online talk
Todays vision
Welcome remarks for sabbath school program
Organelle that modifies packages and transports
Todays class
Welcome to today's class
Todays sabbath lesson
Good morning campers today's challenge is simple
Todays with apostrophe
Standing handcuffing techniques
Adding and subtracting real numbers
Differentiation strategy
Adding rational expressions examples
Adding hexadecimal numbers
Adding and subtracting inequalities
Unit 1: the real numbers
Adding pronouns to email signature
Multiplying polynomials jeopardy
Adding integers notes
Adding vectors geometrically
Adding er
How to add and subtract rational expressions
How do significant figures work
Adding integers examples
Adding monomial
Adding this dimension will overconstrain the sketch
Intro to polynomials
What's 8 + 5
6-1 adding and subtracting polynomials
Adding water
Adding scientific notation
Adding integers song
Adding subtracting and multiplying radical expressions
When the sign are the same and keep the sign
Why is adding menthol to cigarettes dangerous?
Adding ment
What is addition
Adding fractions notes
Integers warm up
Practice 9-5 adding and subtracting rational expressions
Adding value to agricultural products
Adding polynomials vertically
How to add subtract and multiply polynomials
Add and subtract complex numbers
Adding and subtracting fractions review
Is 3/10 a rational number
Simplifying rational expressions
You are adding vectors of length 20 and 40 units
Adding and subtracting inequalities
3x 5 is a monomial
Adding and subtracting negative numbers calculator
Improper fraction to mixed number quiz
Multiplying exponents with same base
654 to 1 significant figure