www hndit com Data Structures and Algorithms IT
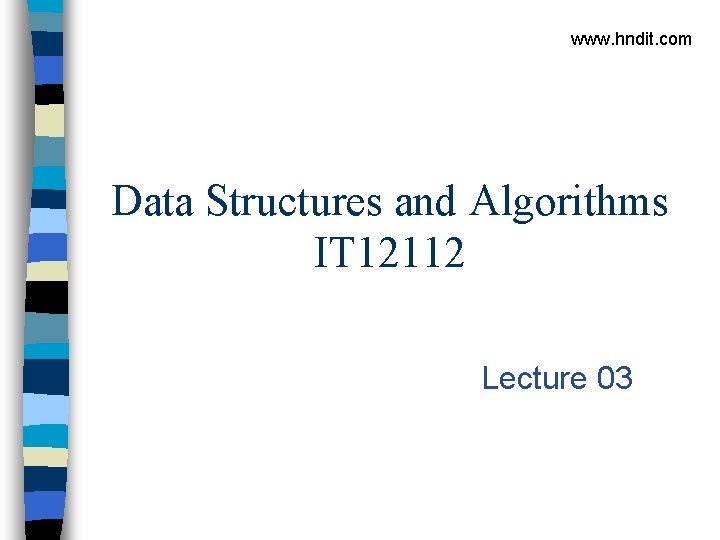
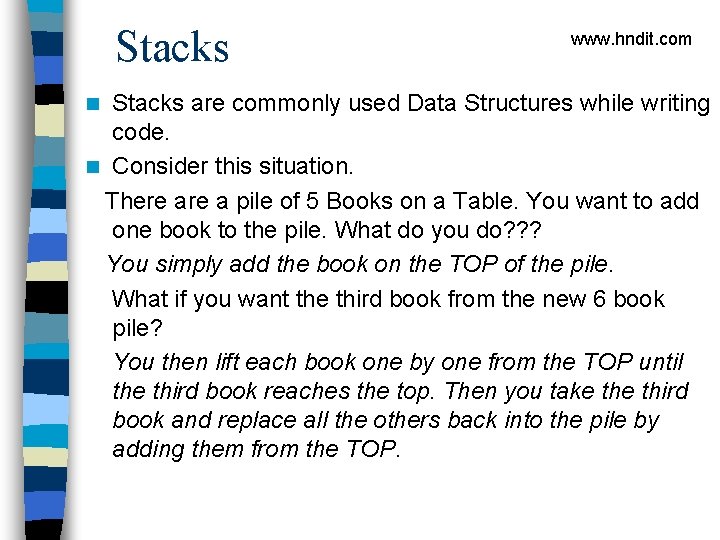
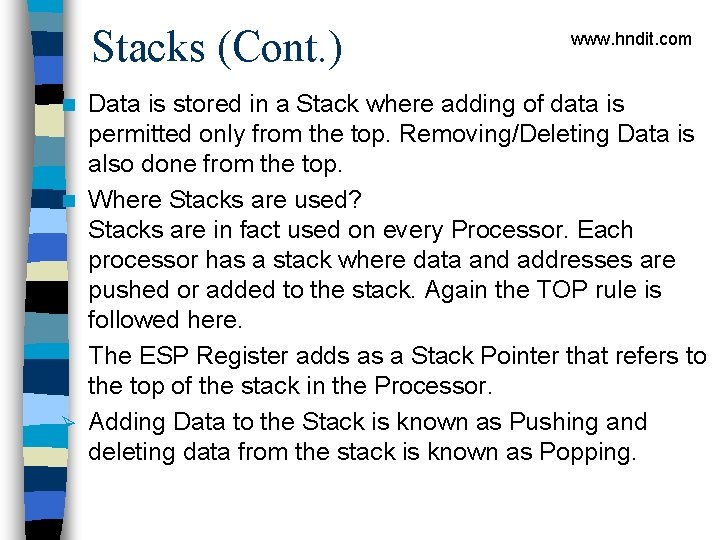
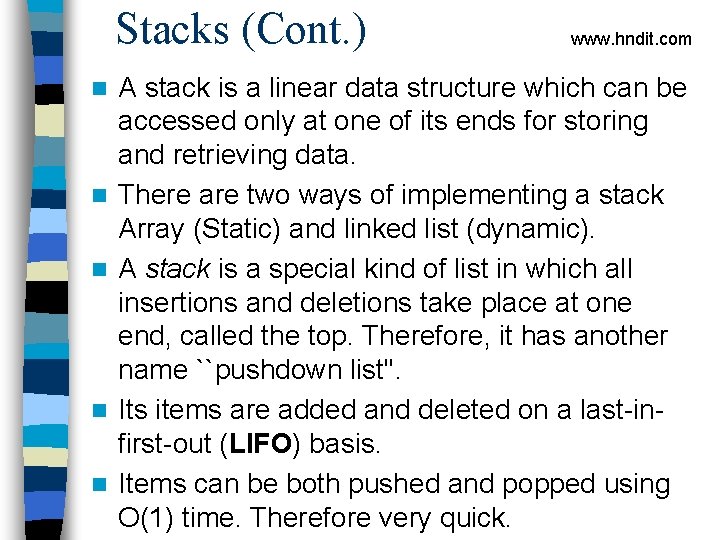
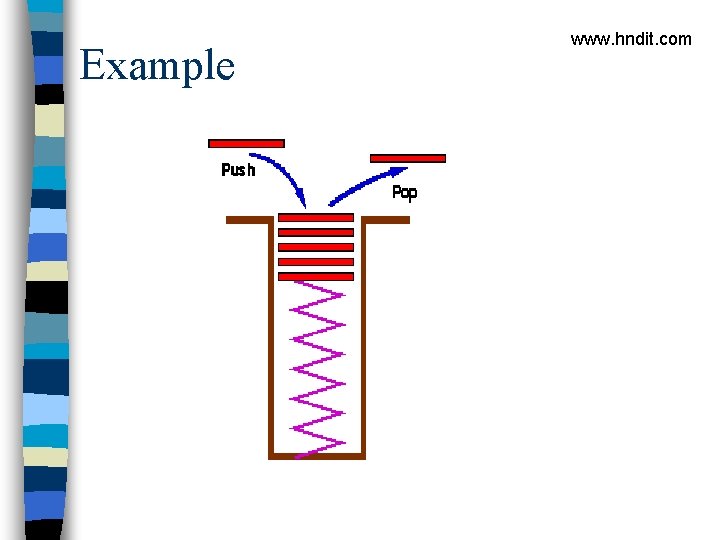
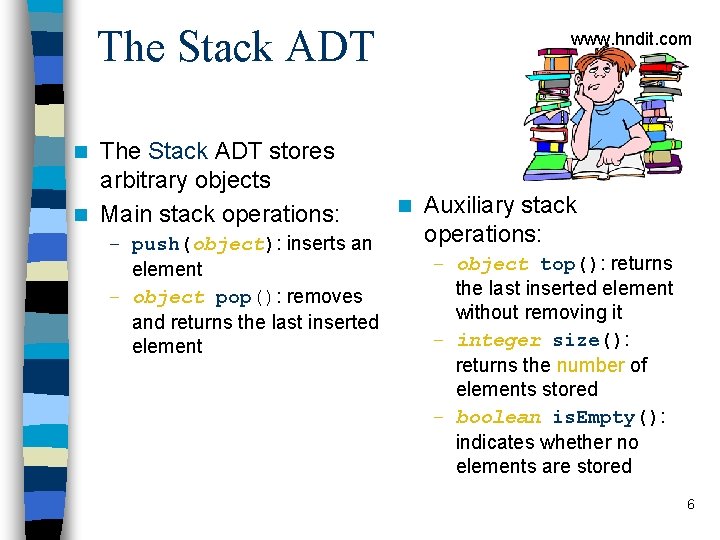
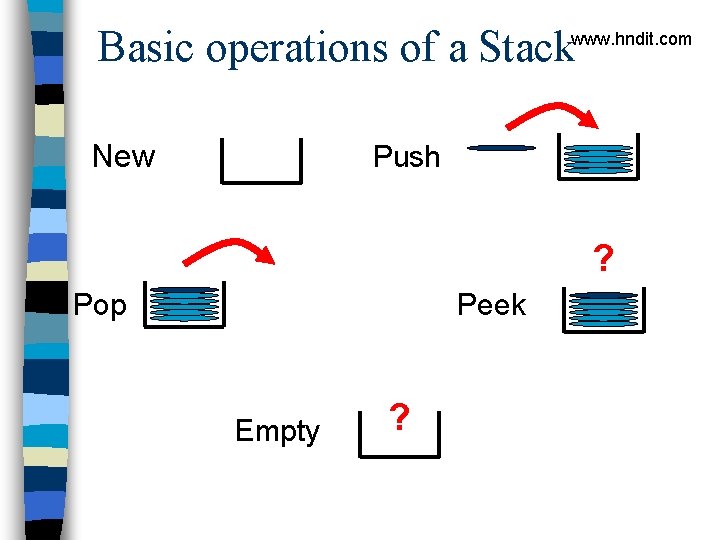
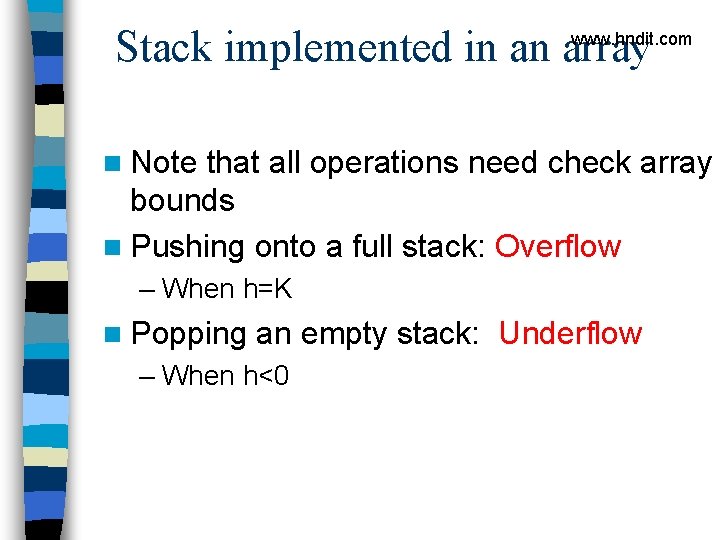
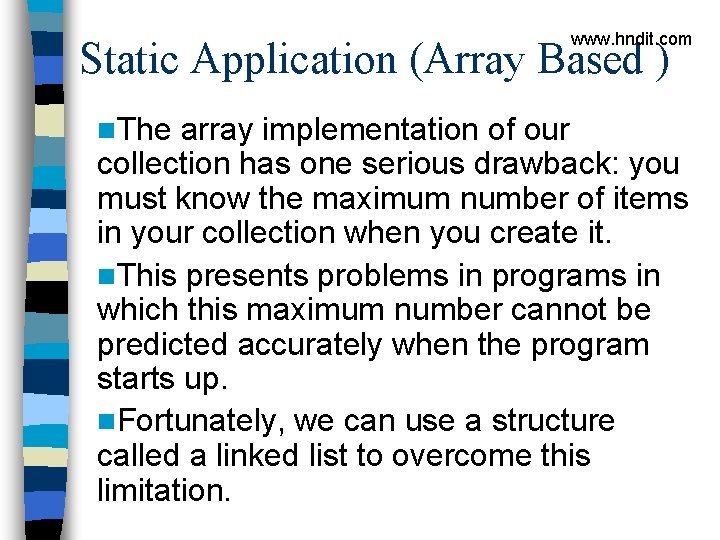
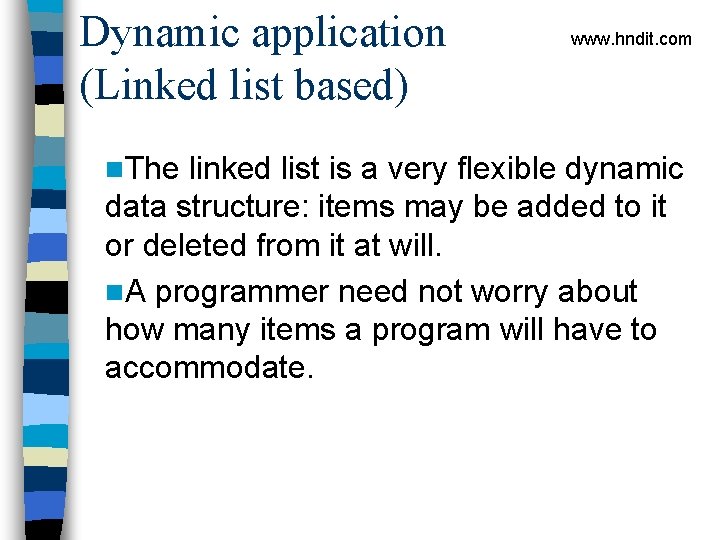
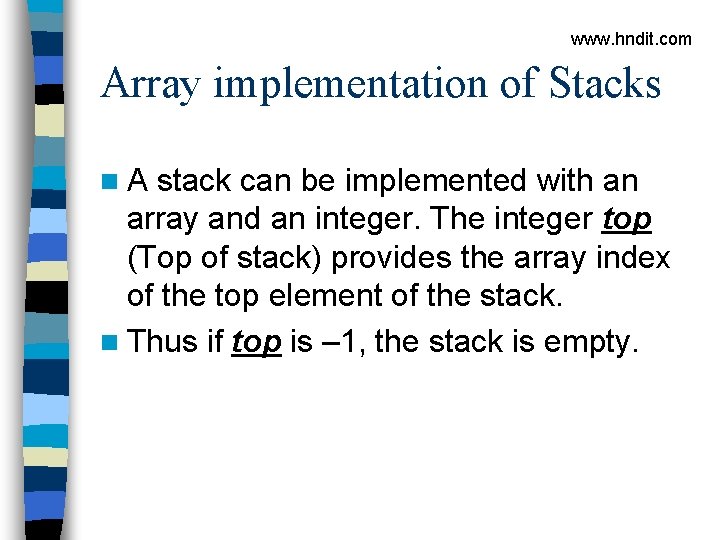
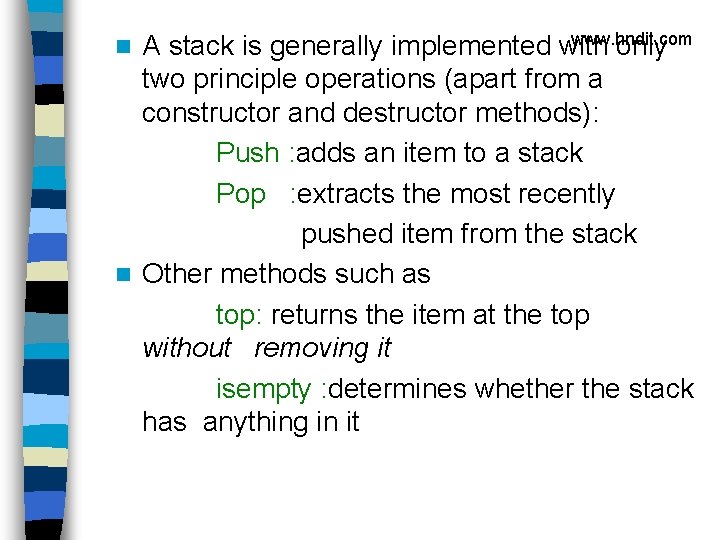
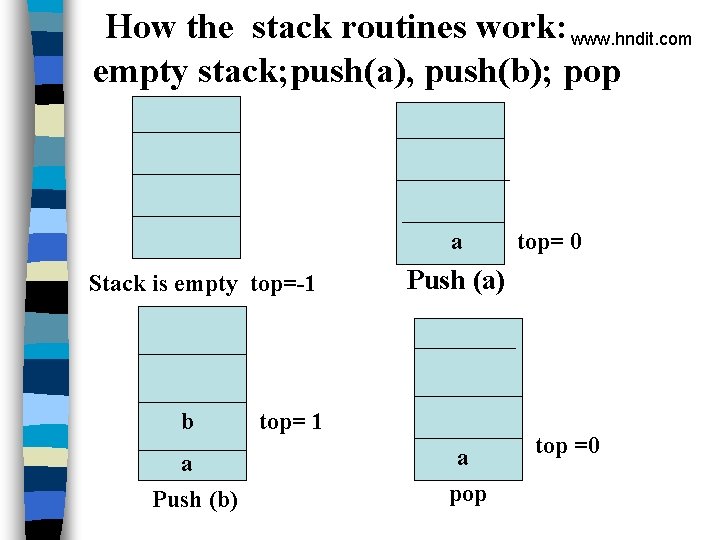
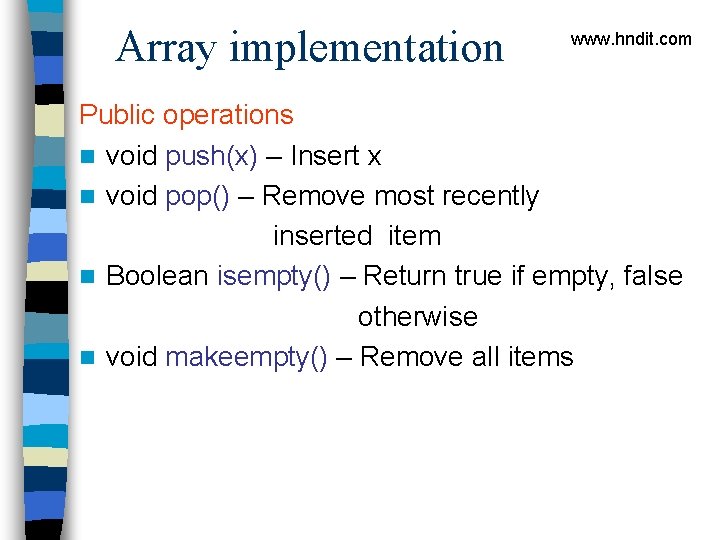
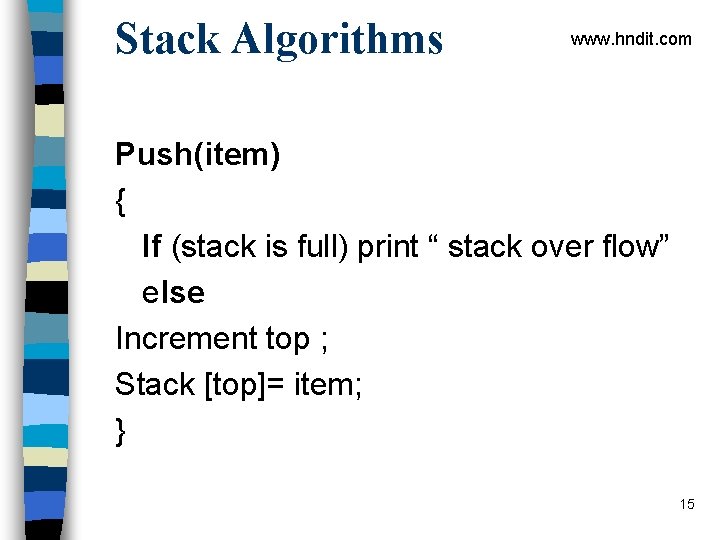
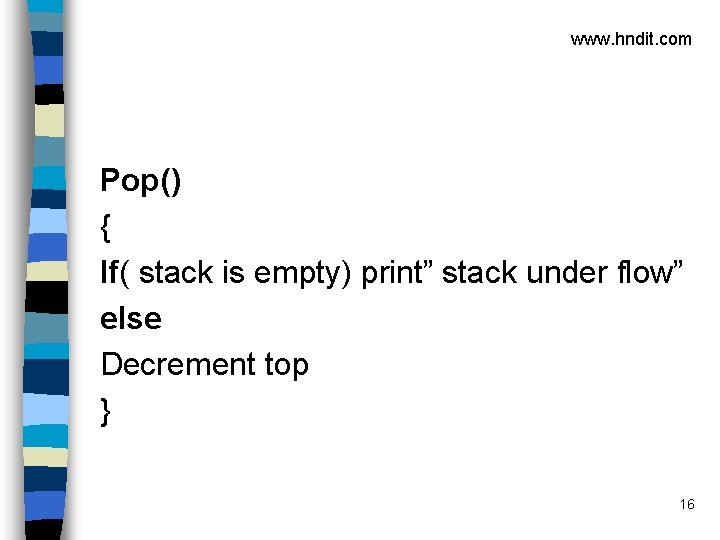
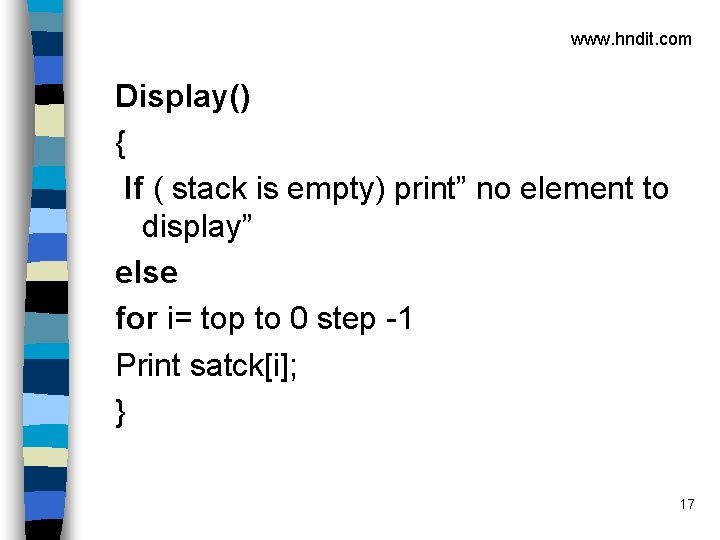
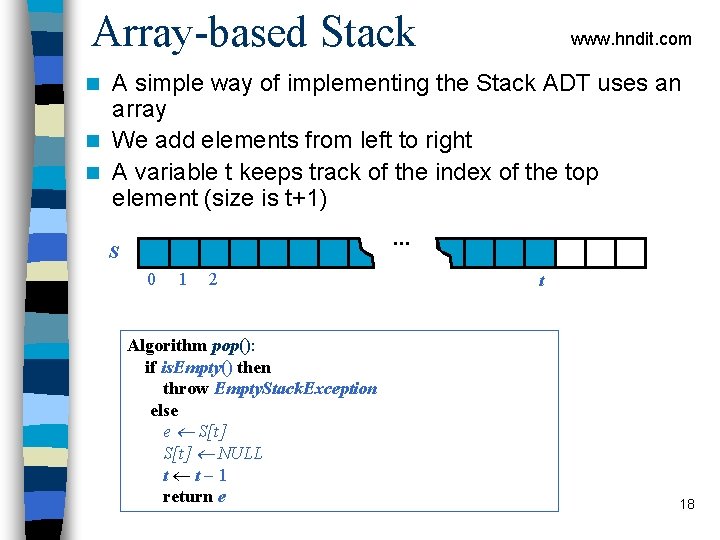
![Algorithm push(o): if Size()=N then throw Full. Stack. Exception else t t+1 S[t] o Algorithm push(o): if Size()=N then throw Full. Stack. Exception else t t+1 S[t] o](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-19.jpg)
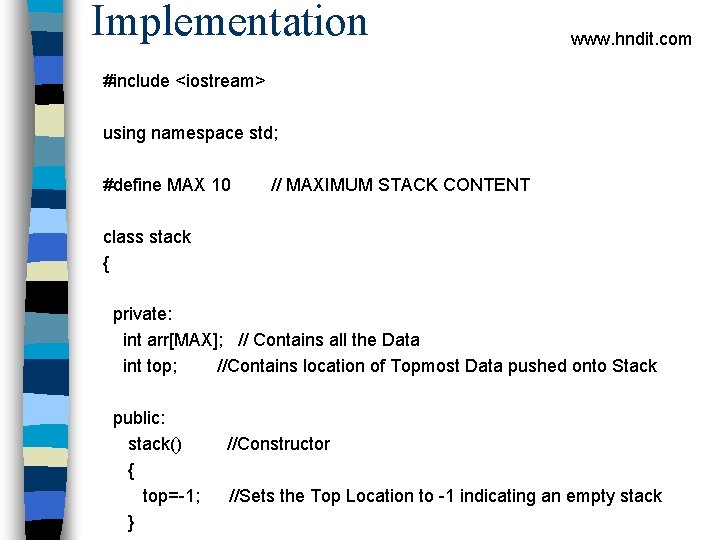
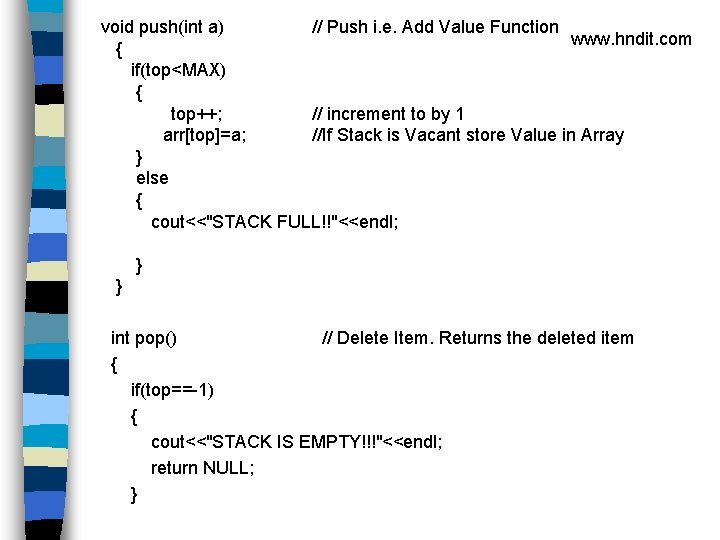
![else { www. hndit. com int data=arr[top]; arr[top]=NULL; top--; return data; } } }; else { www. hndit. com int data=arr[top]; arr[top]=NULL; top--; return data; } } };](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-22.jpg)
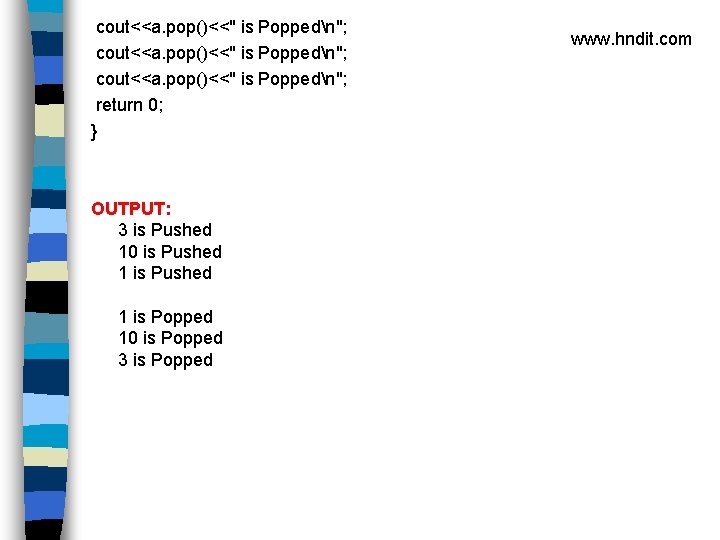
![Implementation #include<iostream. h> #include<conio. h> #include<stdlib. h> class stack { int stk[5]; int top; Implementation #include<iostream. h> #include<conio. h> #include<stdlib. h> class stack { int stk[5]; int top;](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-24.jpg)
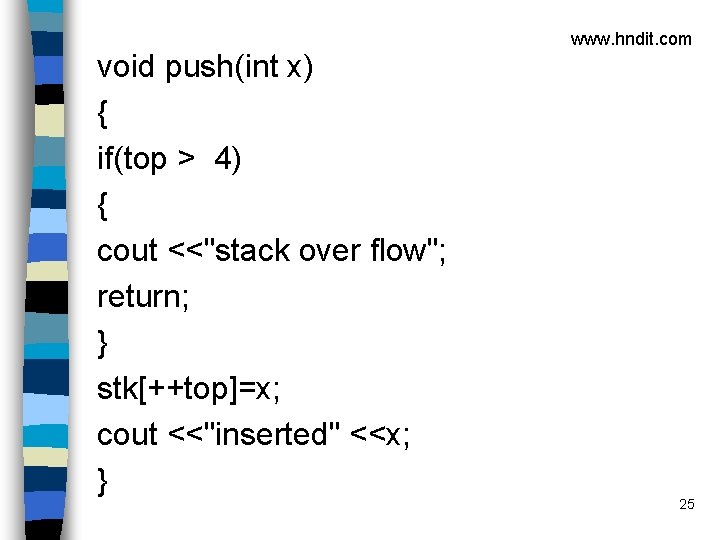
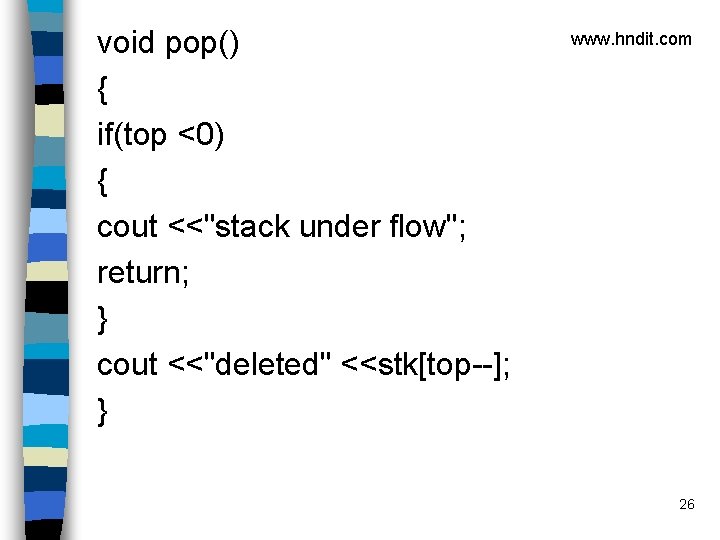
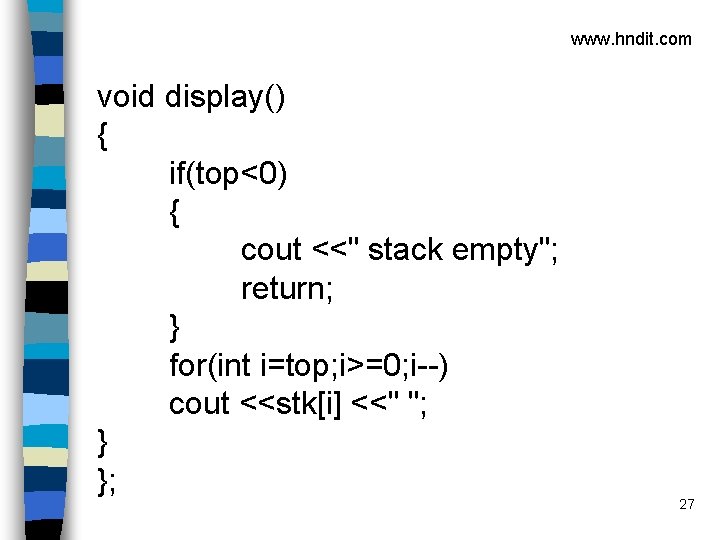
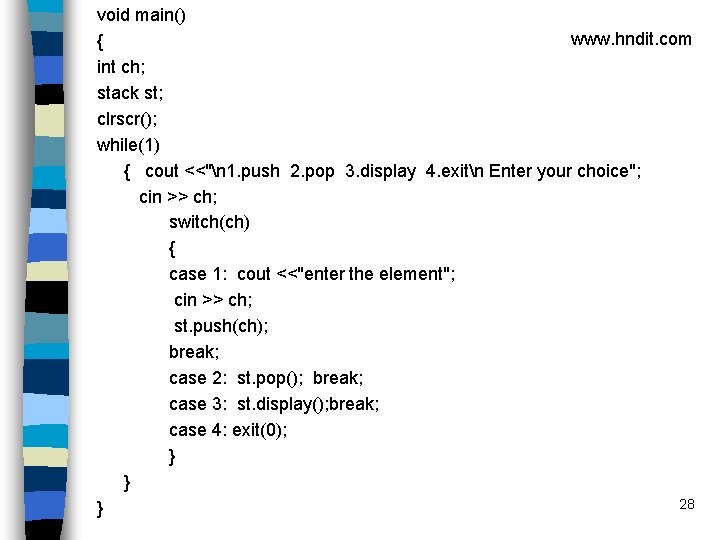
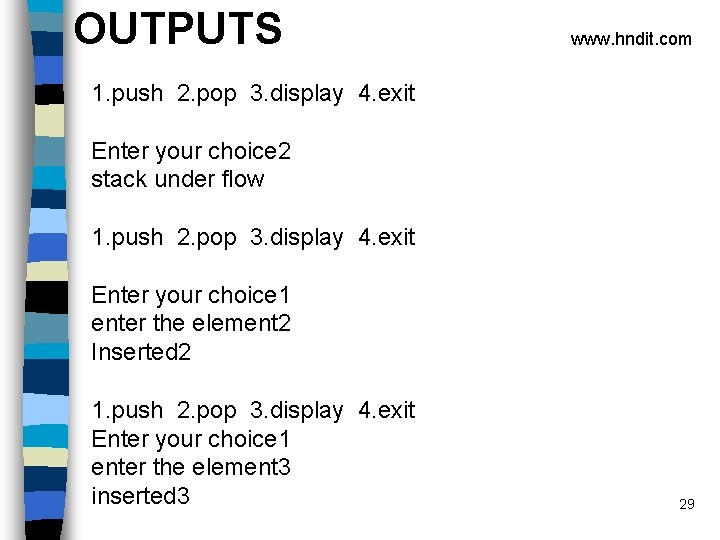
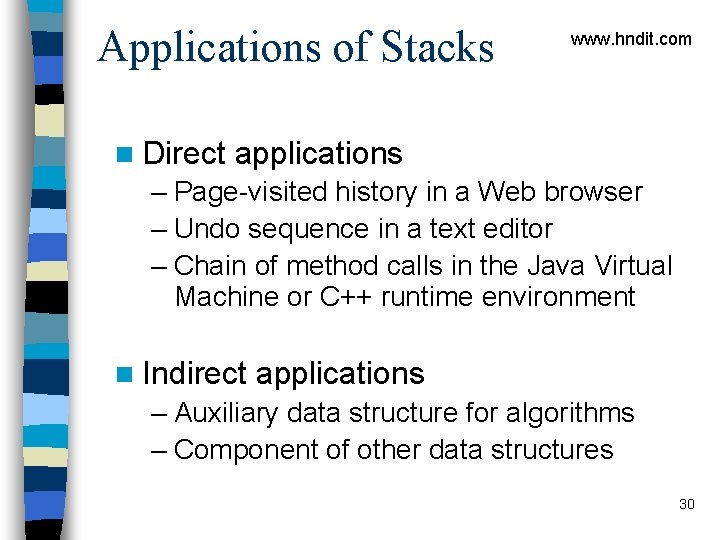
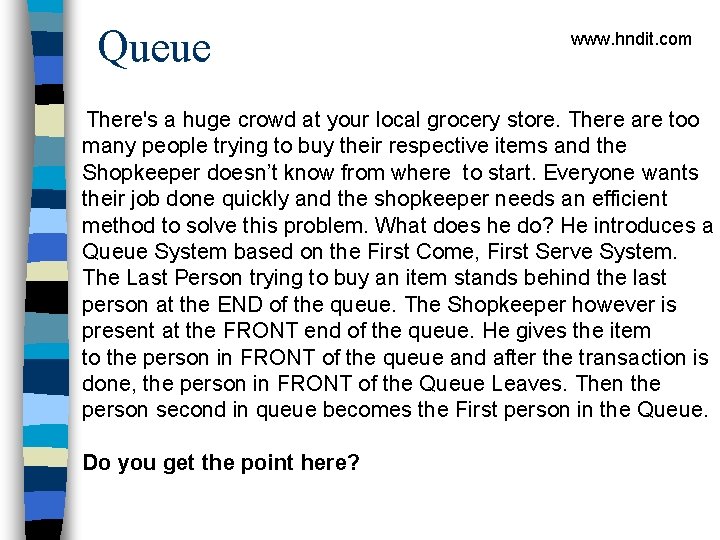
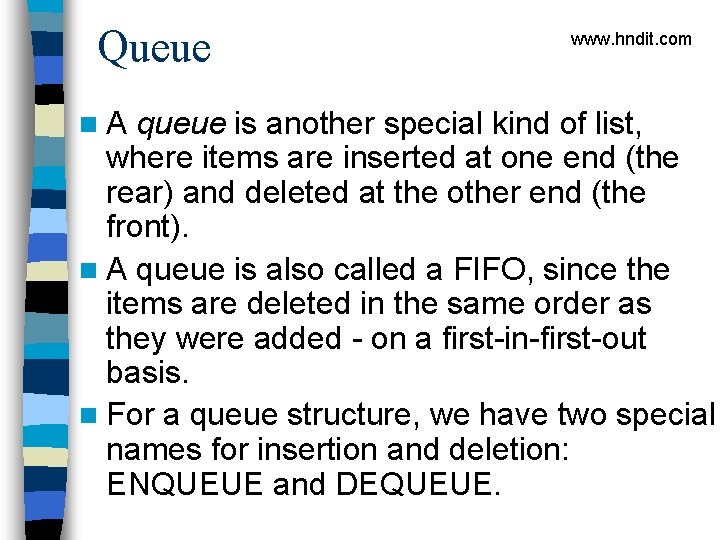
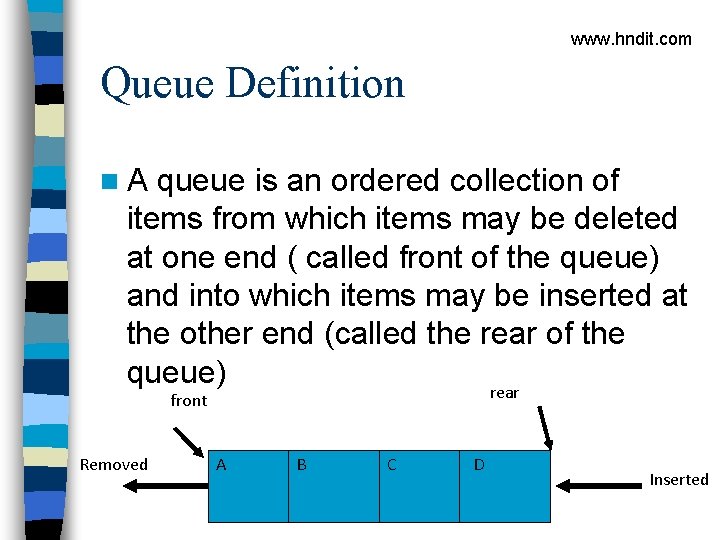
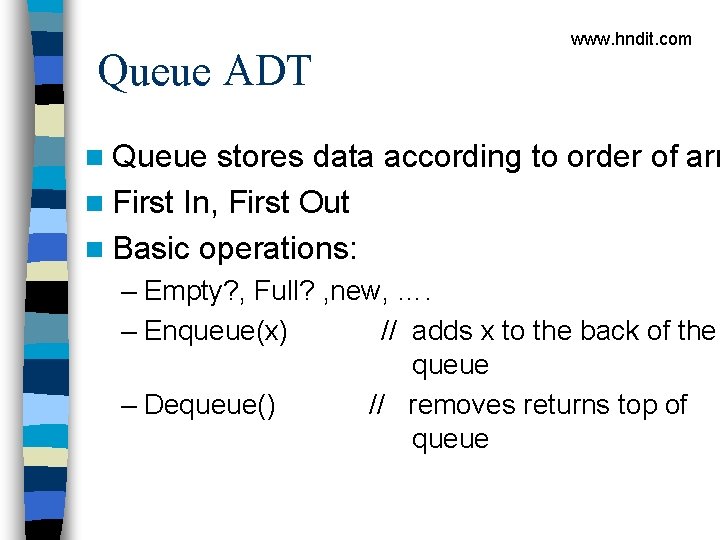
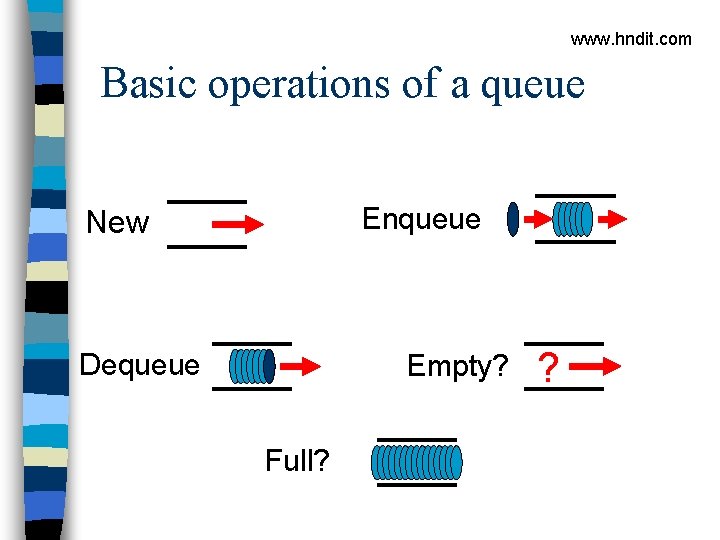
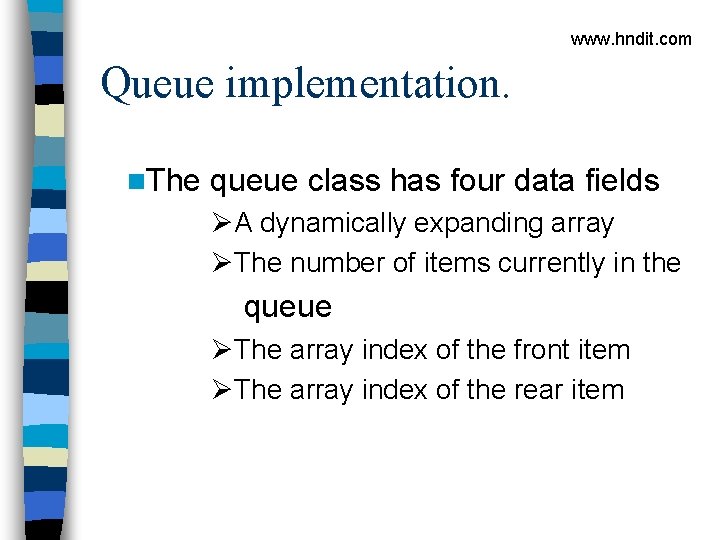
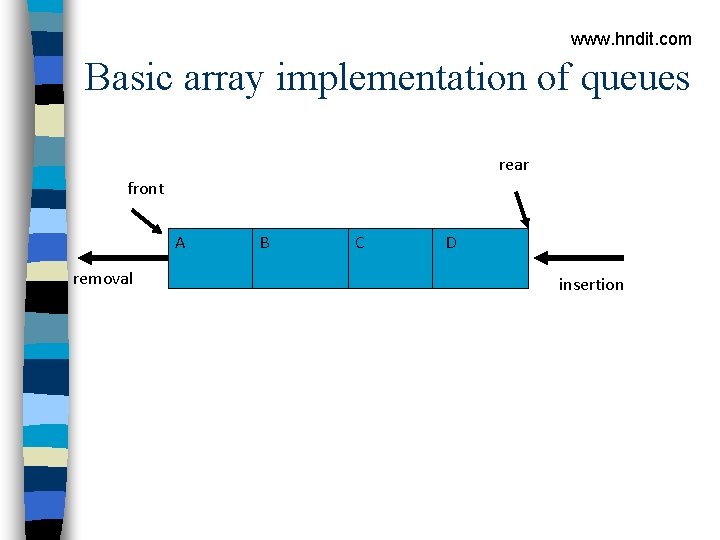
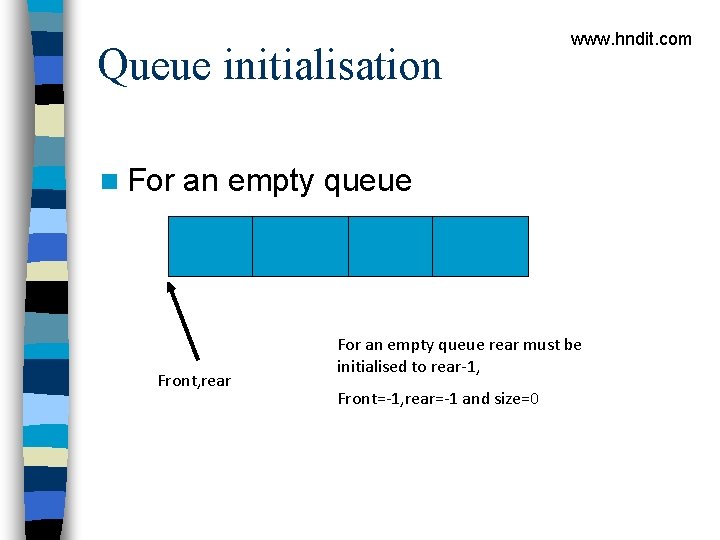
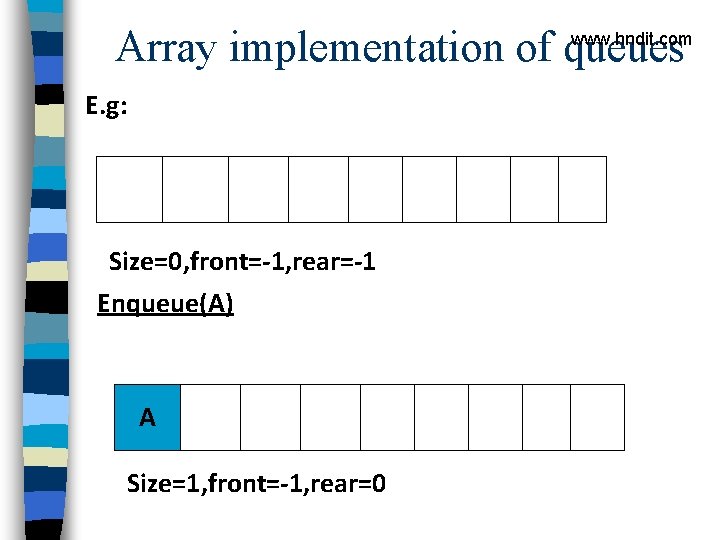
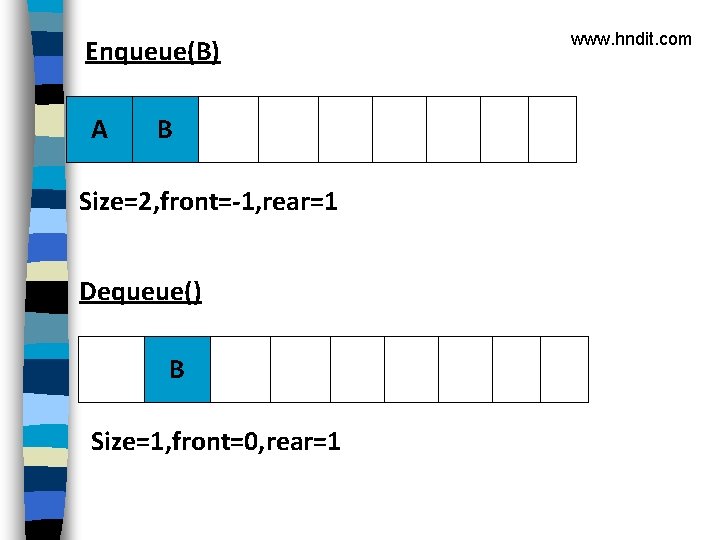
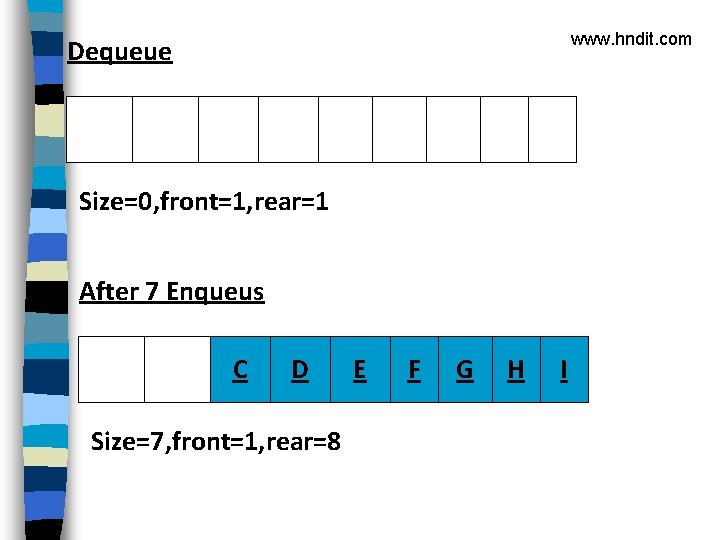
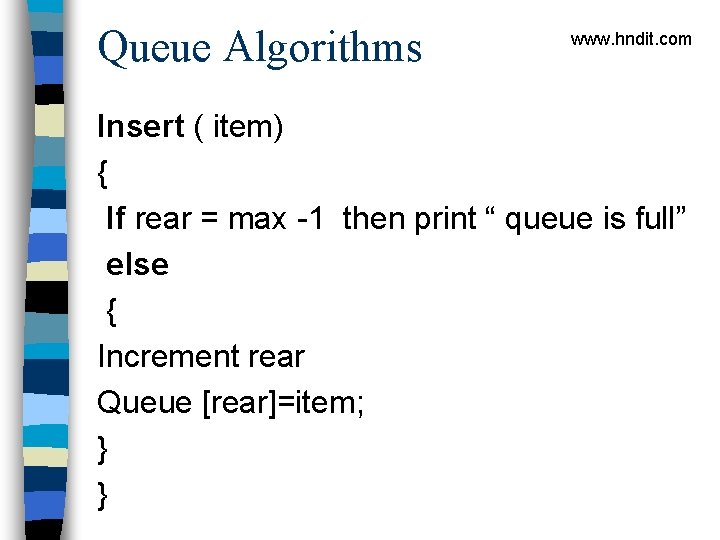
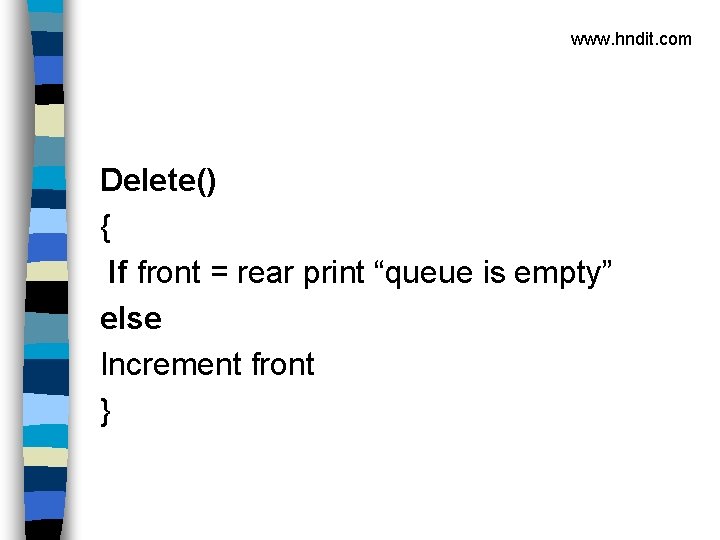
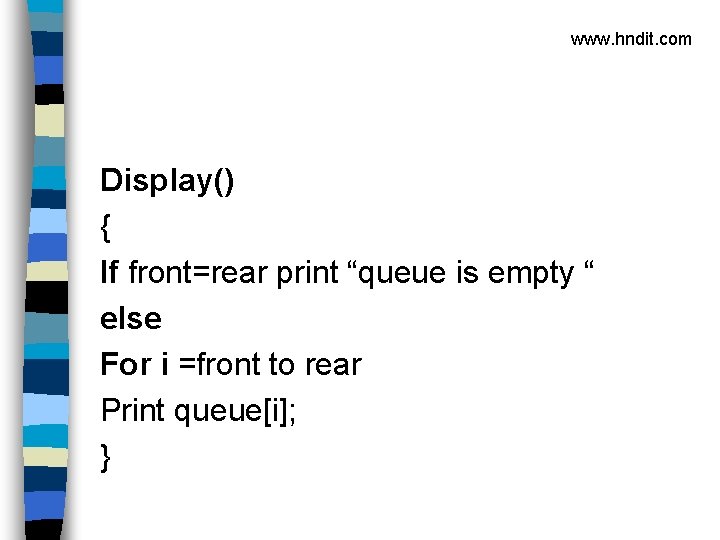
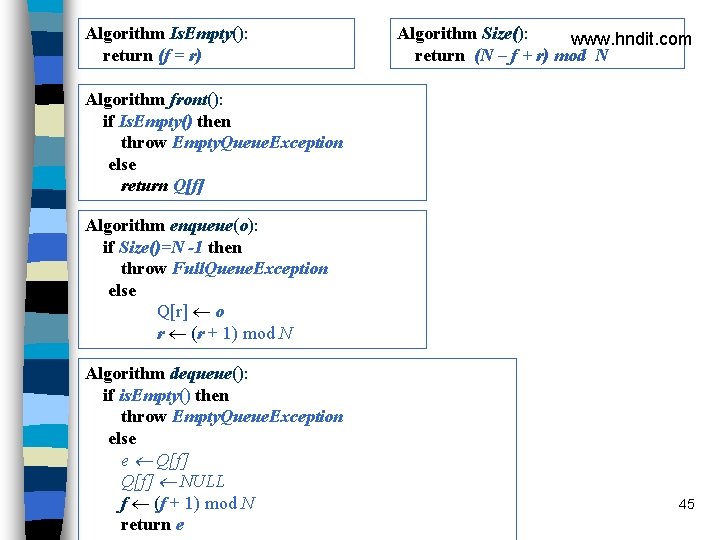
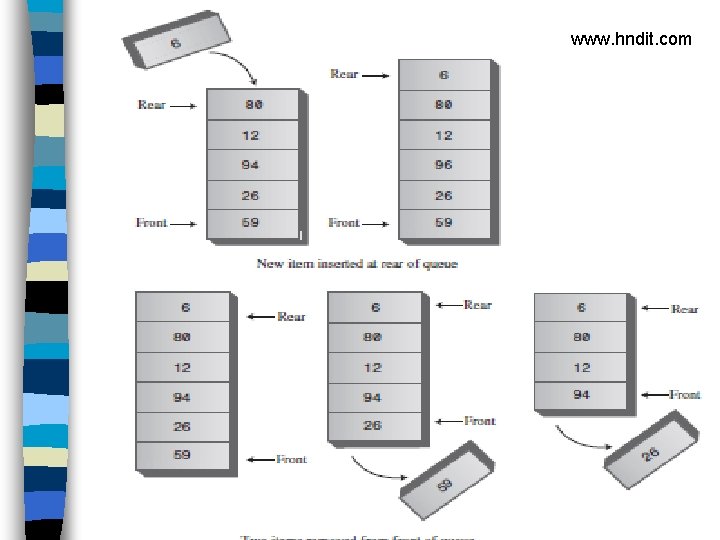
![Queue Implementation #include<iostream. h> #include<conio. h> #include<stdlib. h> class queue { int queue[5]; int Queue Implementation #include<iostream. h> #include<conio. h> #include<stdlib. h> class queue { int queue[5]; int](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-47.jpg)
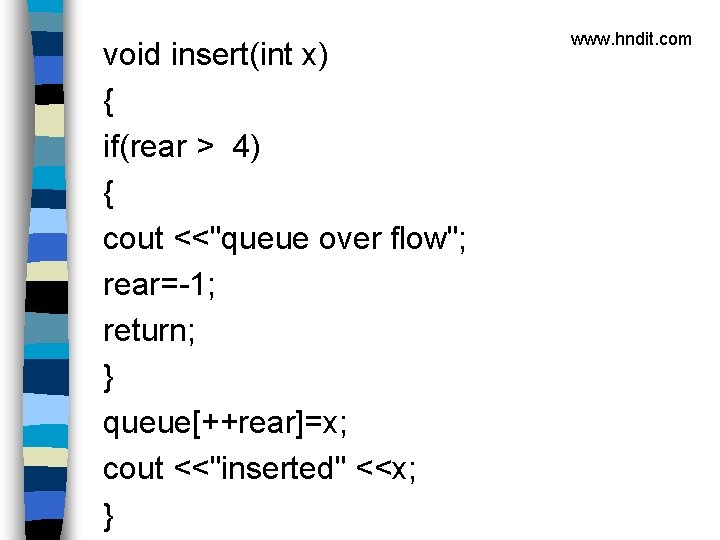
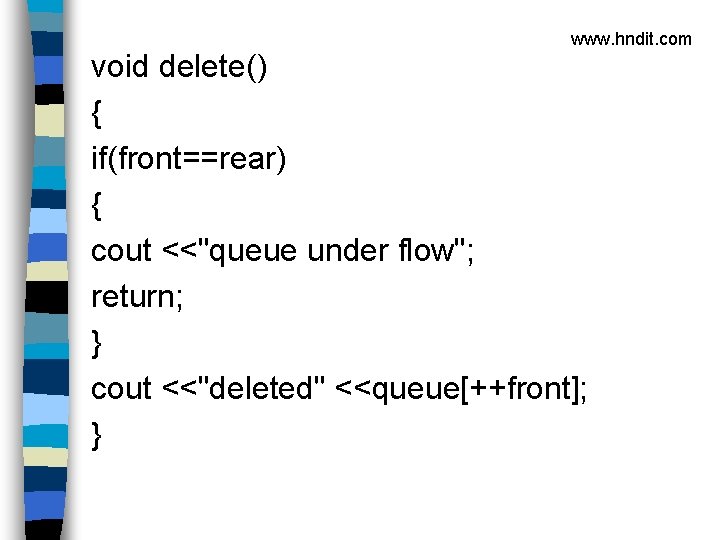
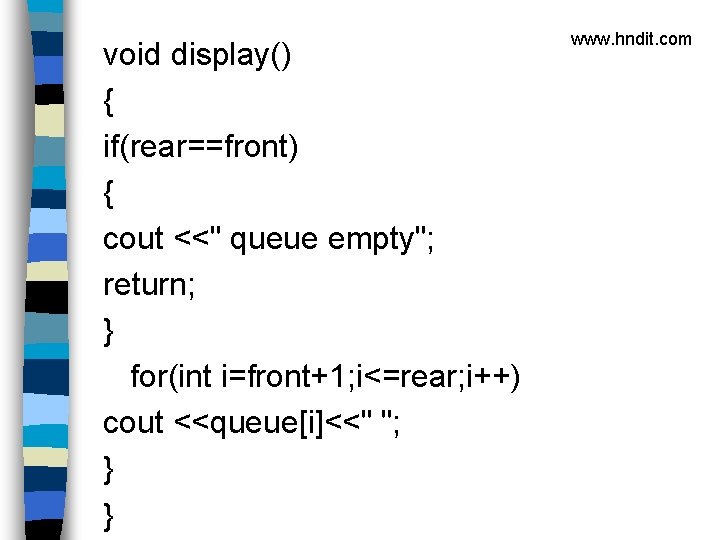
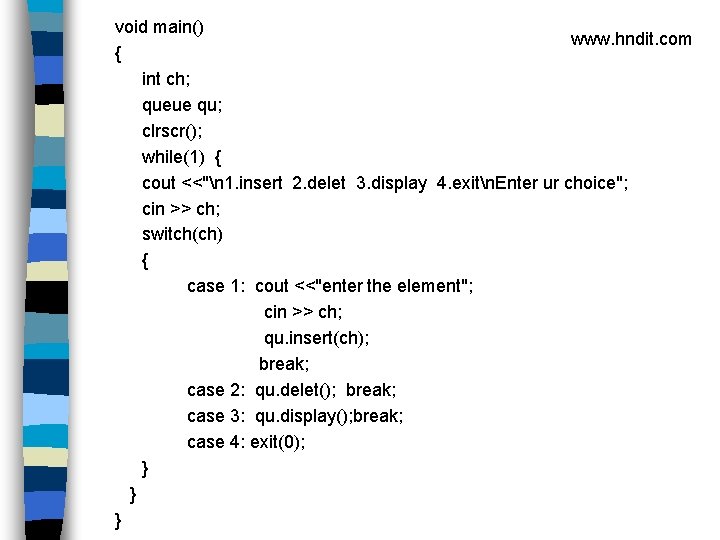
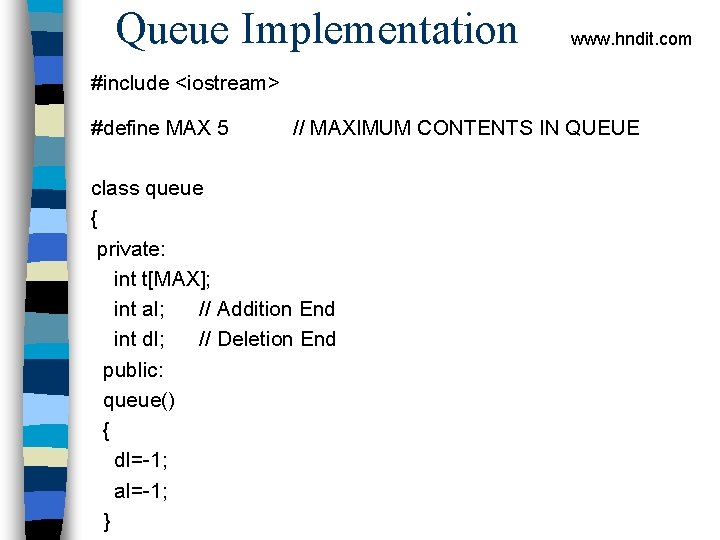
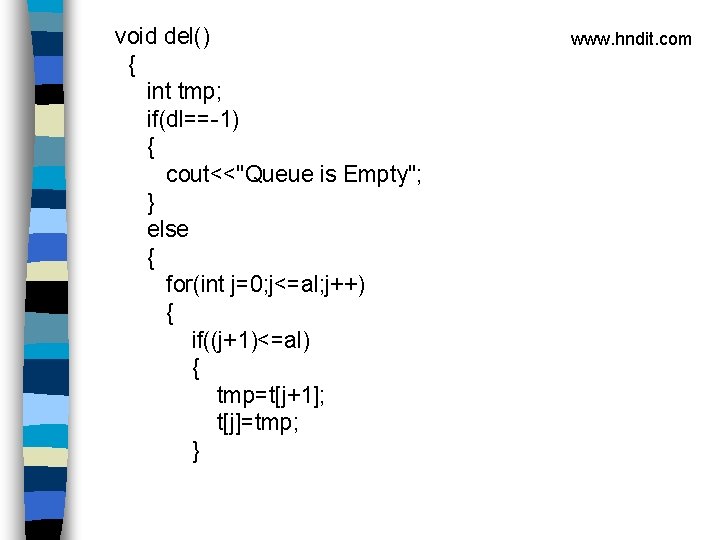
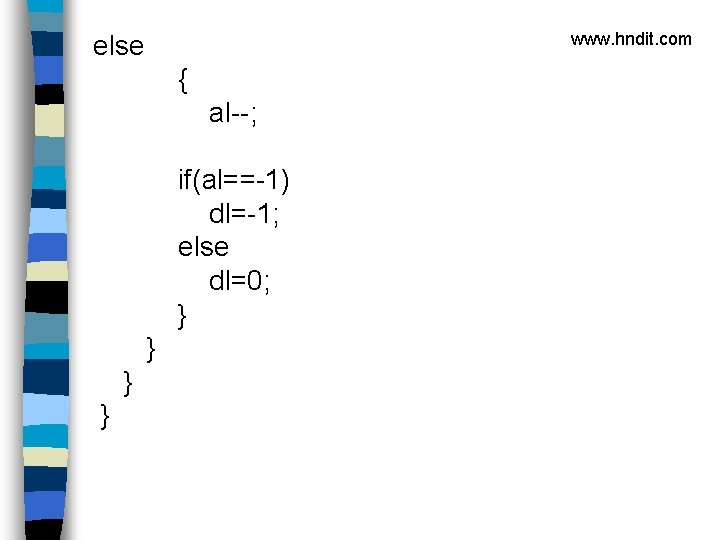
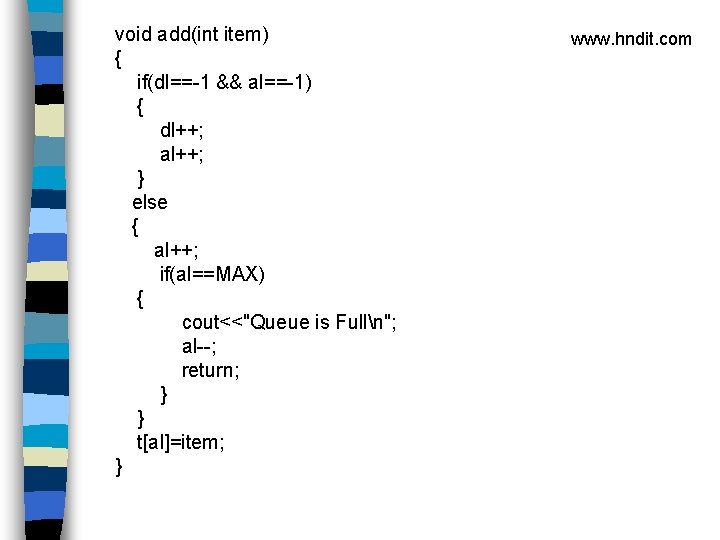
![void display() { if(dl!=-1) { for(int iter=0 ; iter<=al ; iter++) cout<<t[iter]<<" "; } void display() { if(dl!=-1) { for(int iter=0 ; iter<=al ; iter++) cout<<t[iter]<<" "; }](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-56.jpg)
![int main() { queue a; int data[5]={32, 23, 45, 99, 24}; www. hndit. com int main() { queue a; int data[5]={32, 23, 45, 99, 24}; www. hndit. com](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-57.jpg)
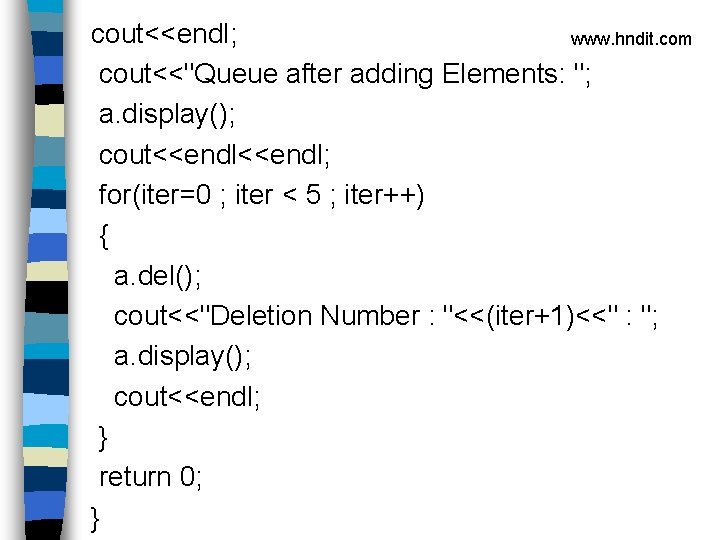
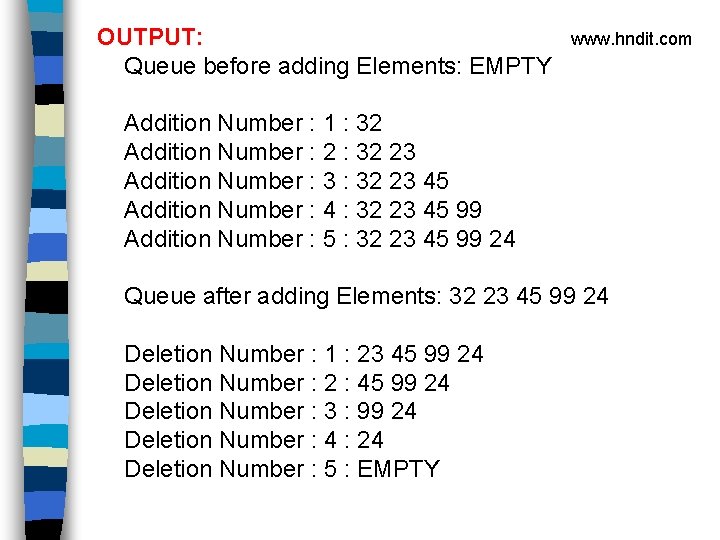
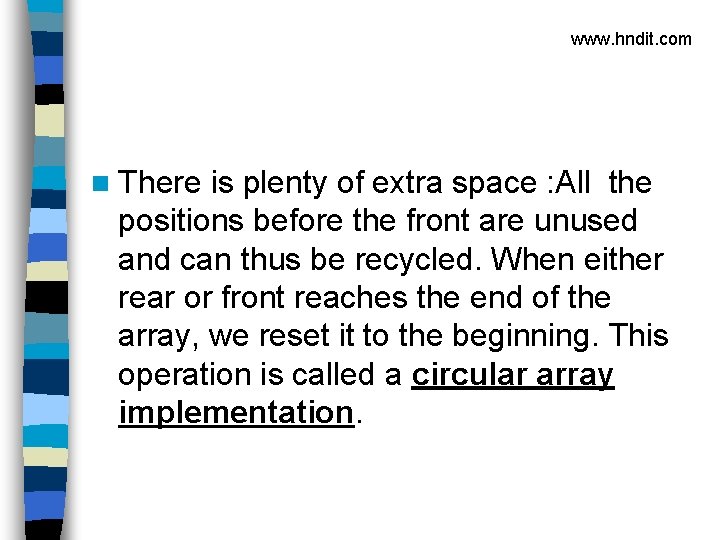
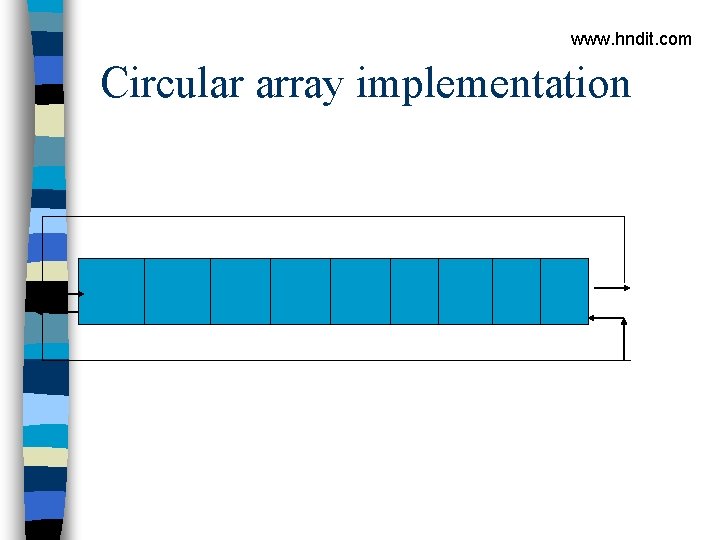
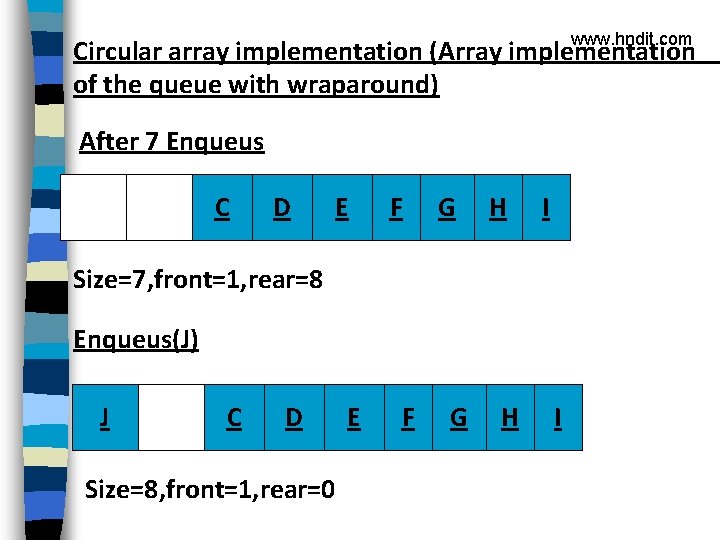
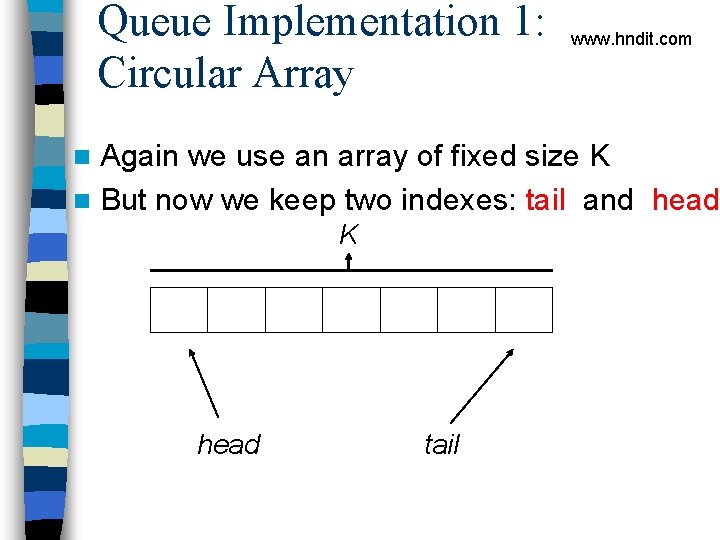
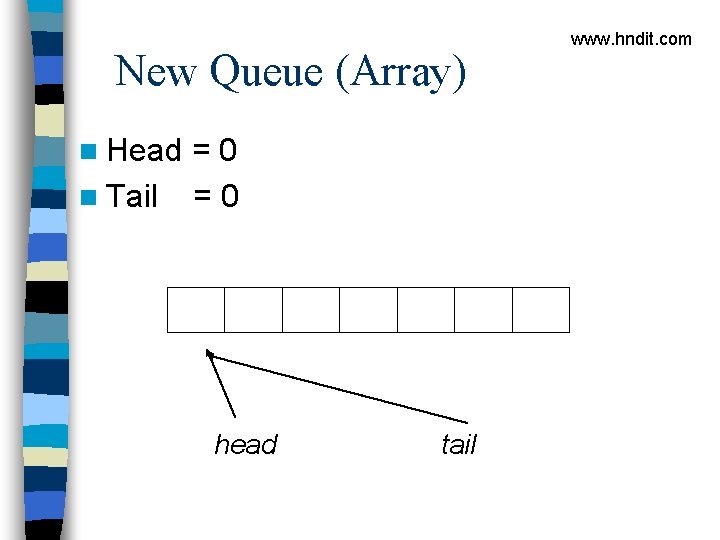
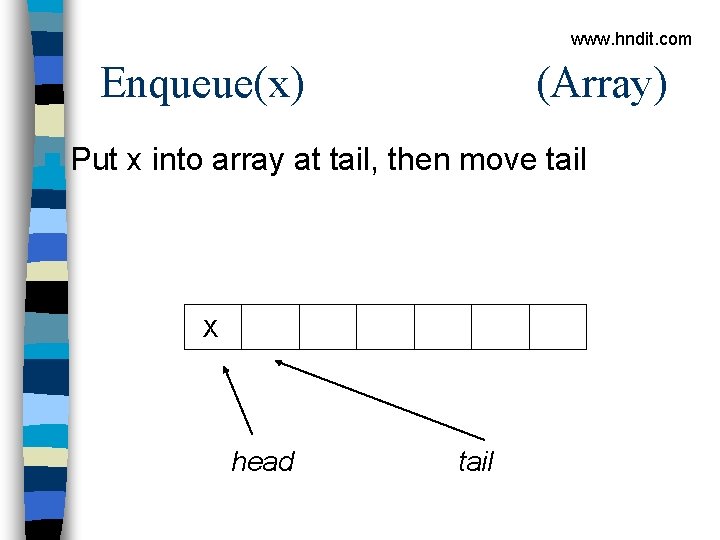
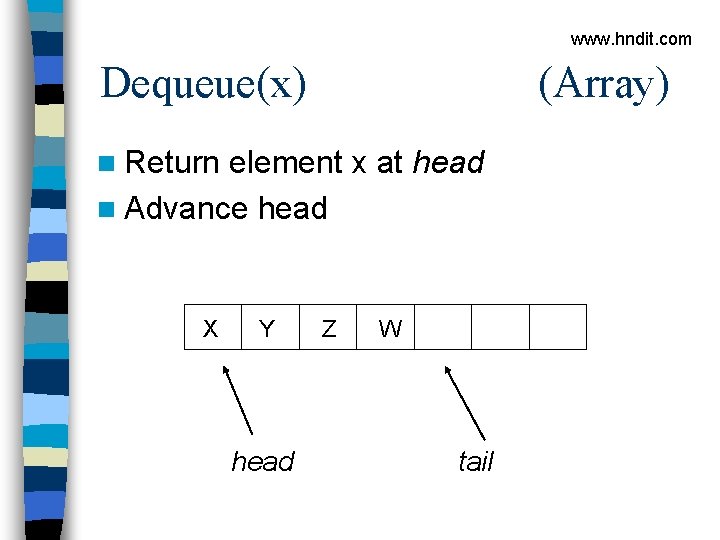
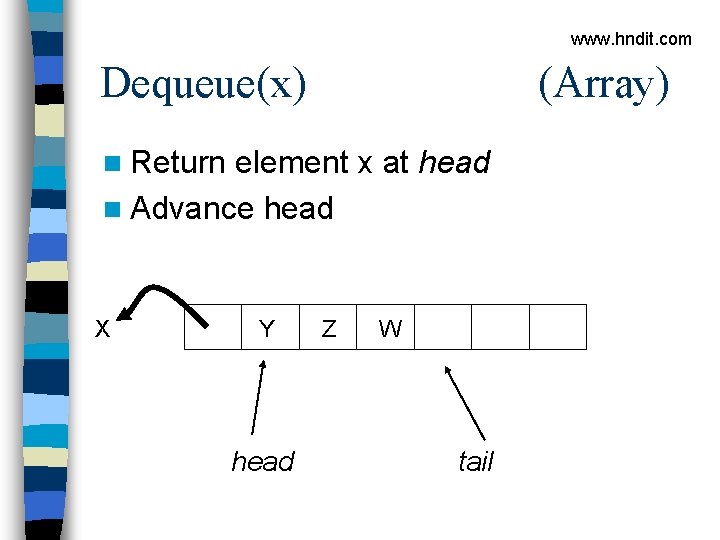
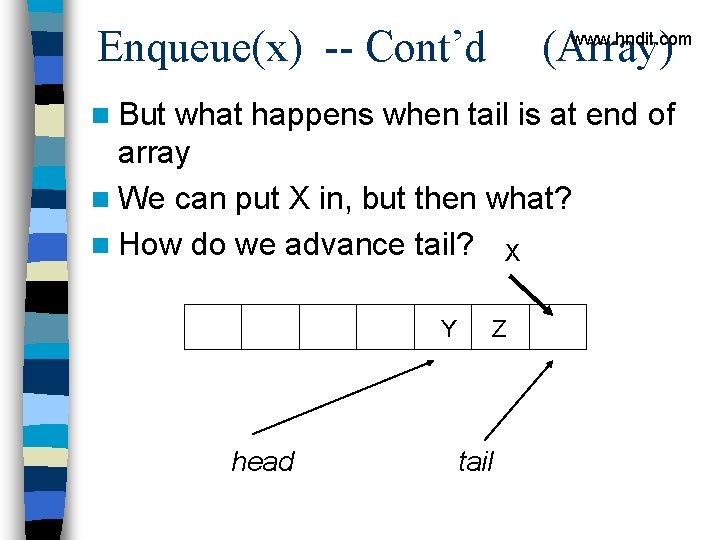
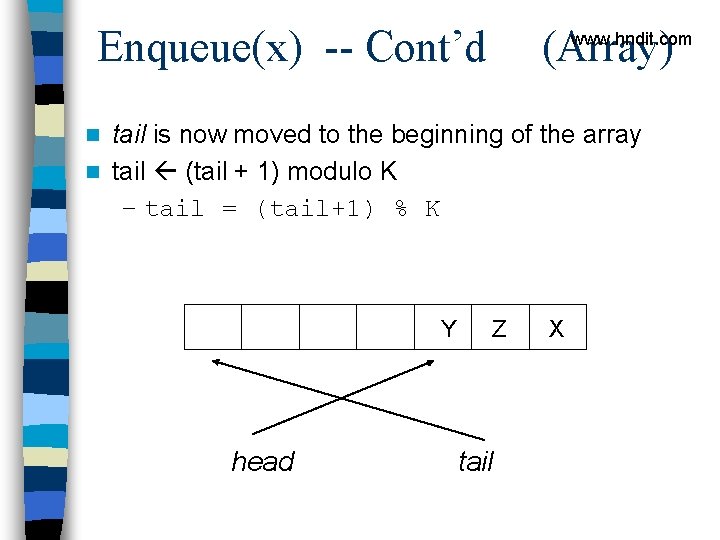
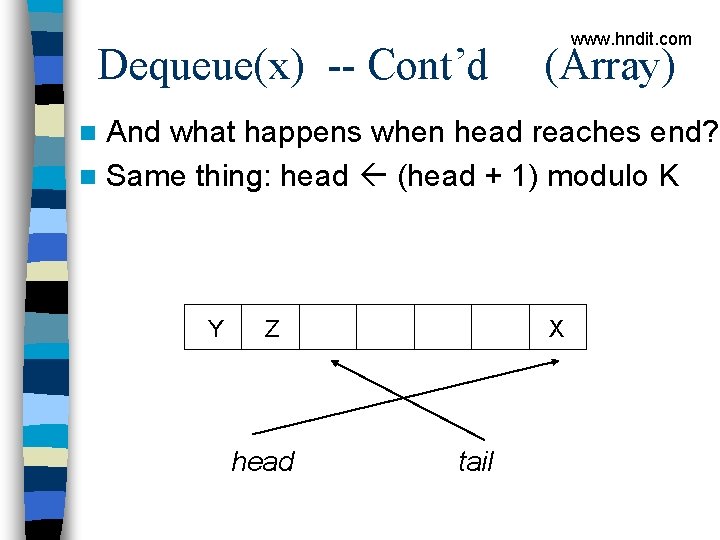
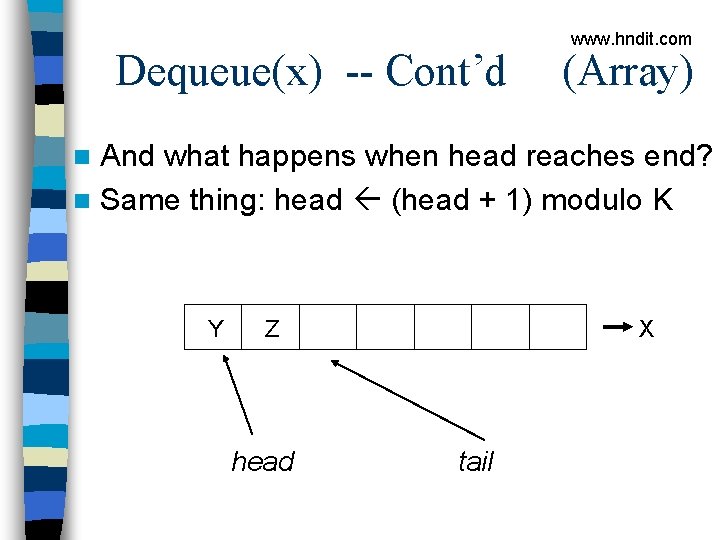
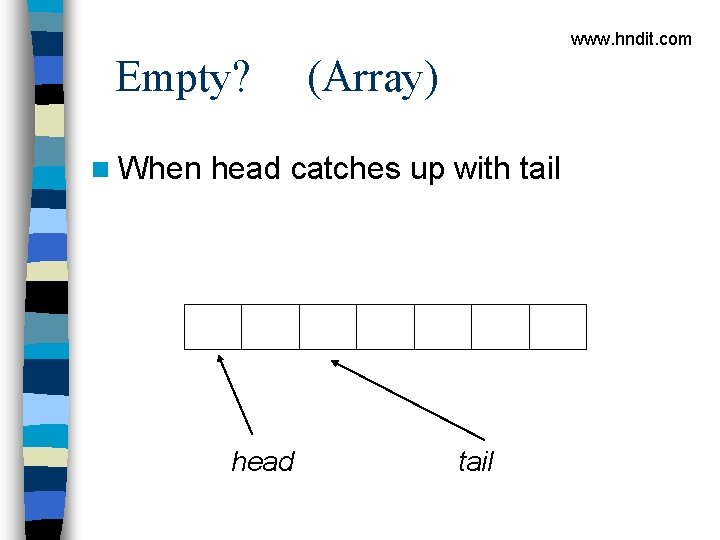
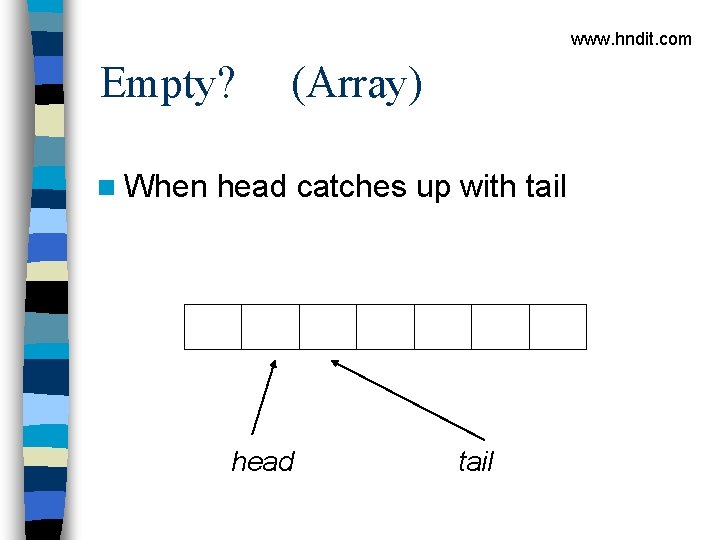
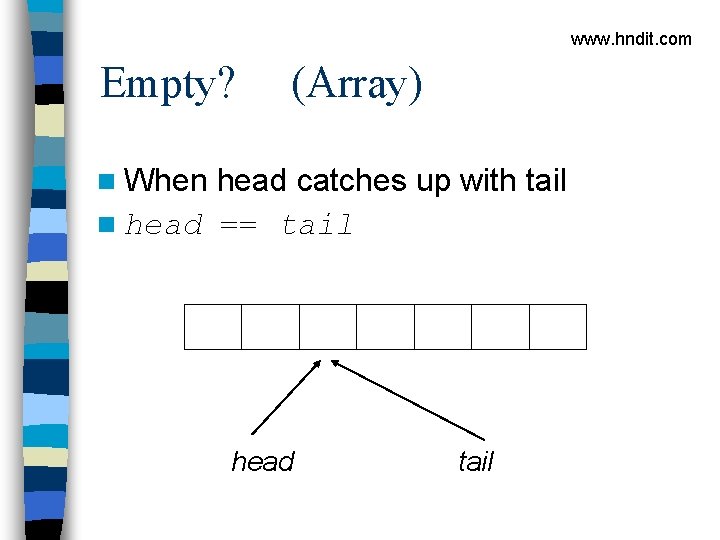
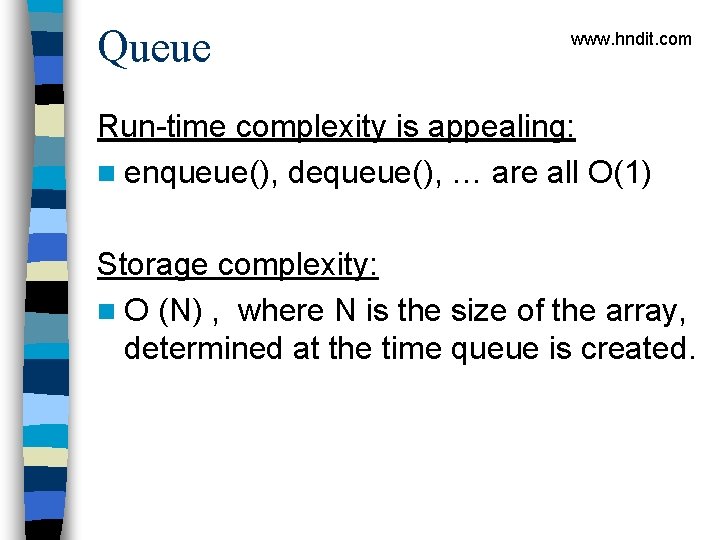
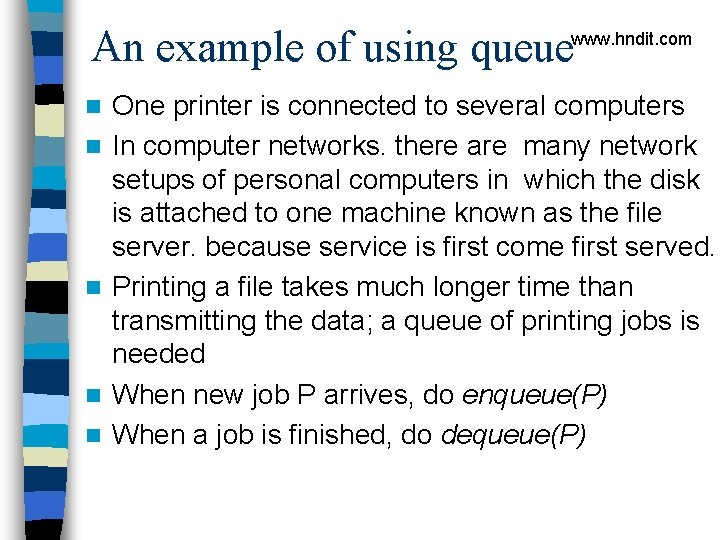
- Slides: 76
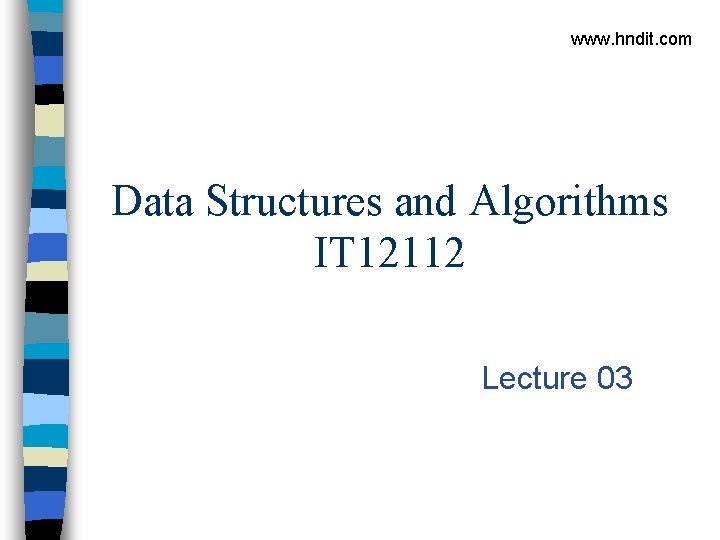
www. hndit. com Data Structures and Algorithms IT 12112 Lecture 03
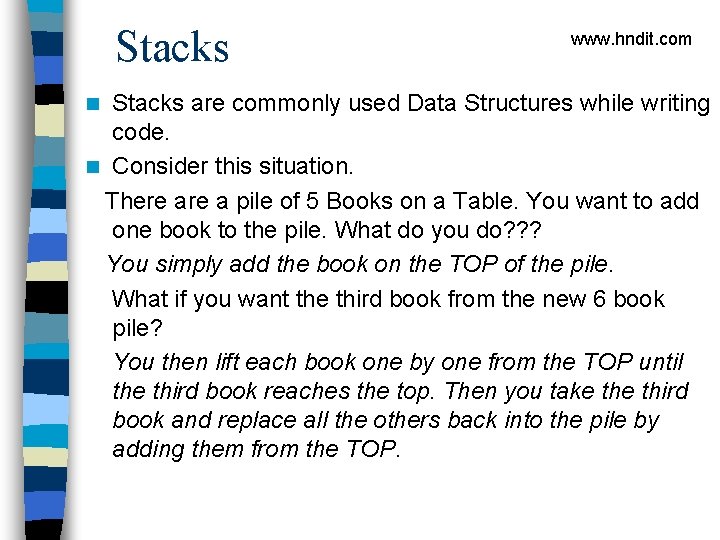
Stacks www. hndit. com Stacks are commonly used Data Structures while writing code. n Consider this situation. There a pile of 5 Books on a Table. You want to add one book to the pile. What do you do? ? ? You simply add the book on the TOP of the pile. What if you want the third book from the new 6 book pile? You then lift each book one by one from the TOP until the third book reaches the top. Then you take third book and replace all the others back into the pile by adding them from the TOP. n
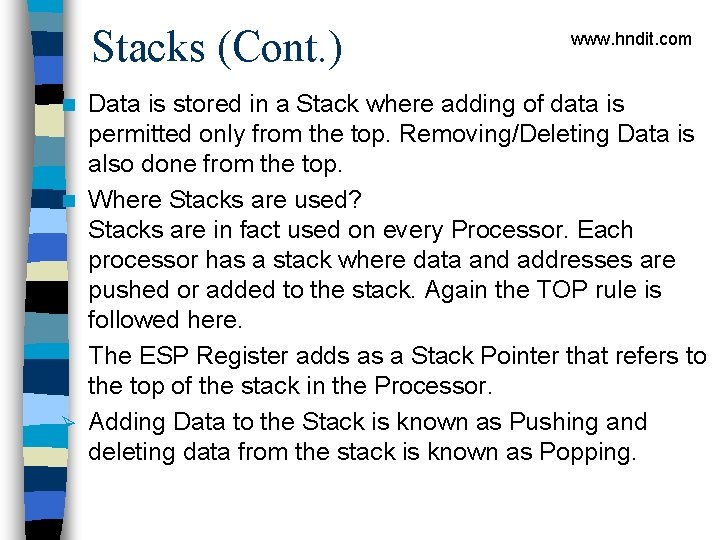
Stacks (Cont. ) www. hndit. com Data is stored in a Stack where adding of data is permitted only from the top. Removing/Deleting Data is also done from the top. n Where Stacks are used? Stacks are in fact used on every Processor. Each processor has a stack where data and addresses are pushed or added to the stack. Again the TOP rule is followed here. The ESP Register adds as a Stack Pointer that refers to the top of the stack in the Processor. Ø Adding Data to the Stack is known as Pushing and deleting data from the stack is known as Popping. n
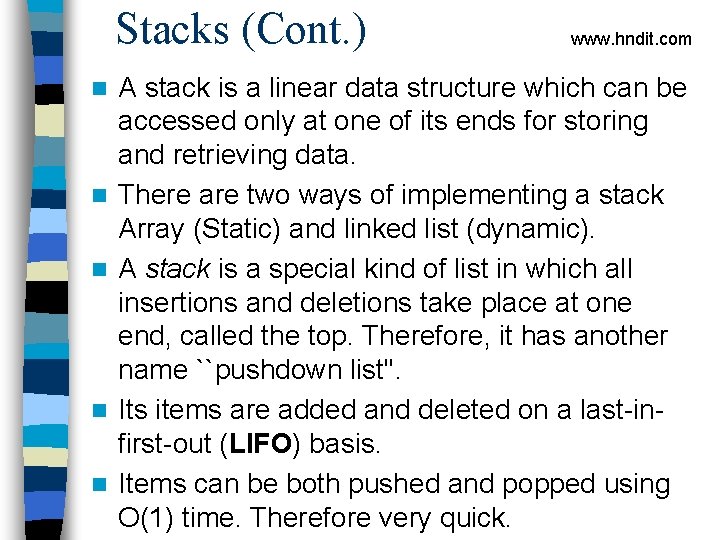
Stacks (Cont. ) n n n www. hndit. com A stack is a linear data structure which can be accessed only at one of its ends for storing and retrieving data. There are two ways of implementing a stack Array (Static) and linked list (dynamic). A stack is a special kind of list in which all insertions and deletions take place at one end, called the top. Therefore, it has another name ``pushdown list''. Its items are added and deleted on a last-infirst-out (LIFO) basis. Items can be both pushed and popped using O(1) time. Therefore very quick.
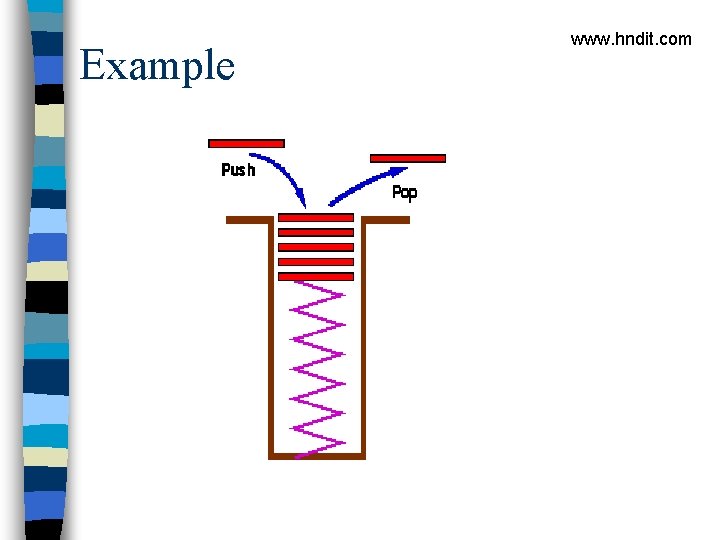
Example www. hndit. com
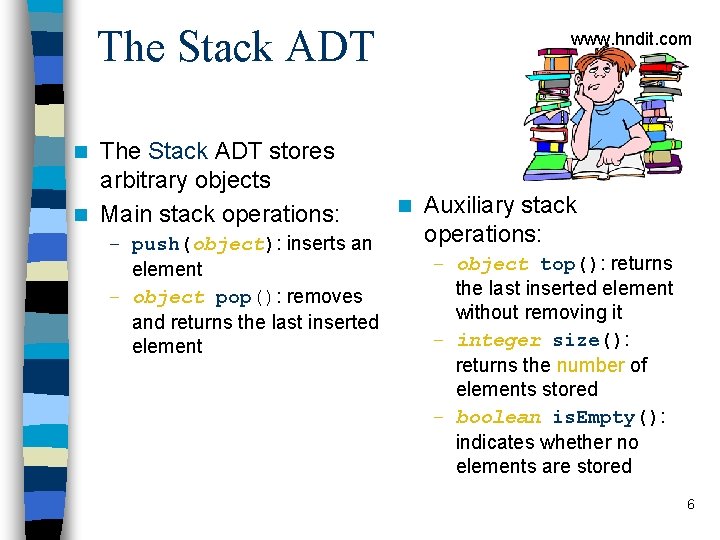
The Stack ADT stores arbitrary objects n Main stack operations: www. hndit. com n – push(object): inserts an element – object pop(): removes and returns the last inserted element n Auxiliary stack operations: – object top(): returns the last inserted element without removing it – integer size(): returns the number of elements stored – boolean is. Empty(): indicates whether no elements are stored 6
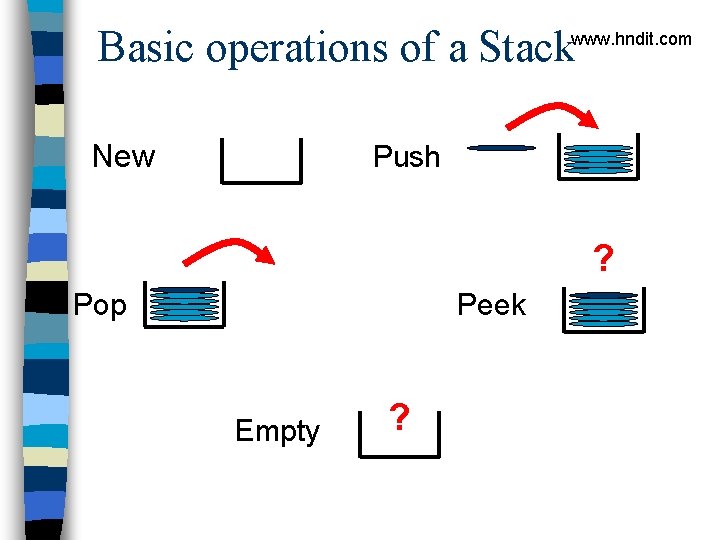
Basic operations of a Stack www. hndit. com New Push ? Pop Peek Empty ?
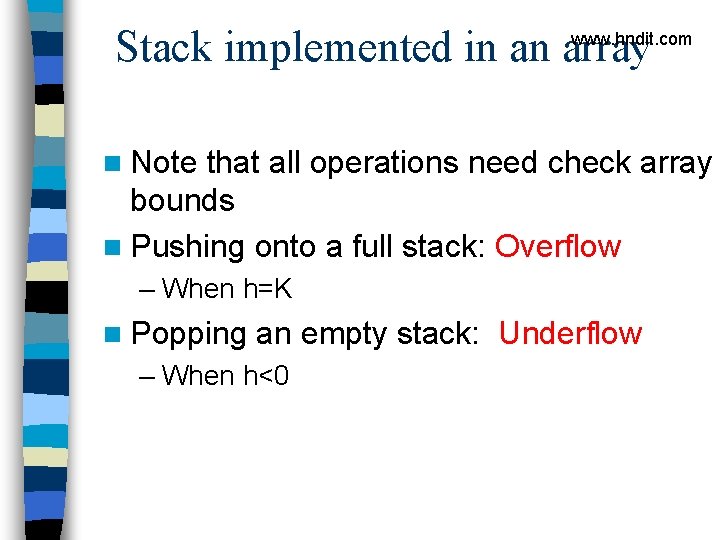
Stack implemented in an array www. hndit. com n Note that all operations need check array bounds n Pushing onto a full stack: Overflow – When h=K n Popping an empty stack: Underflow – When h<0
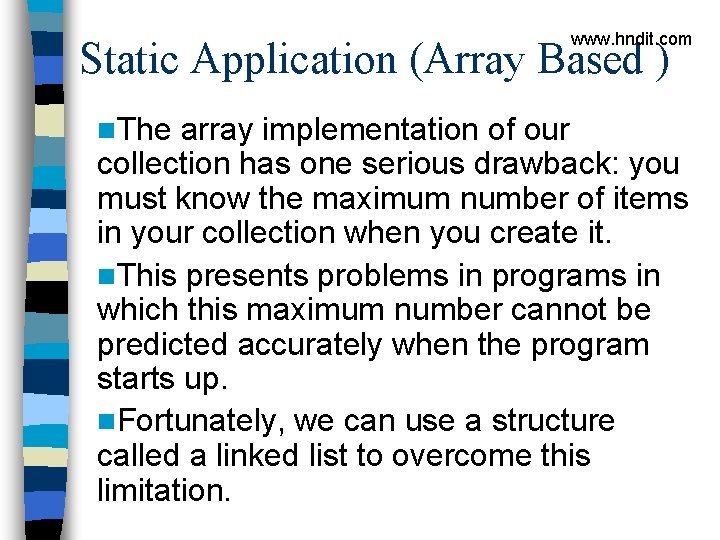
www. hndit. com Static Application (Array Based ) n. The array implementation of our collection has one serious drawback: you must know the maximum number of items in your collection when you create it. n. This presents problems in programs in which this maximum number cannot be predicted accurately when the program starts up. n. Fortunately, we can use a structure called a linked list to overcome this limitation.
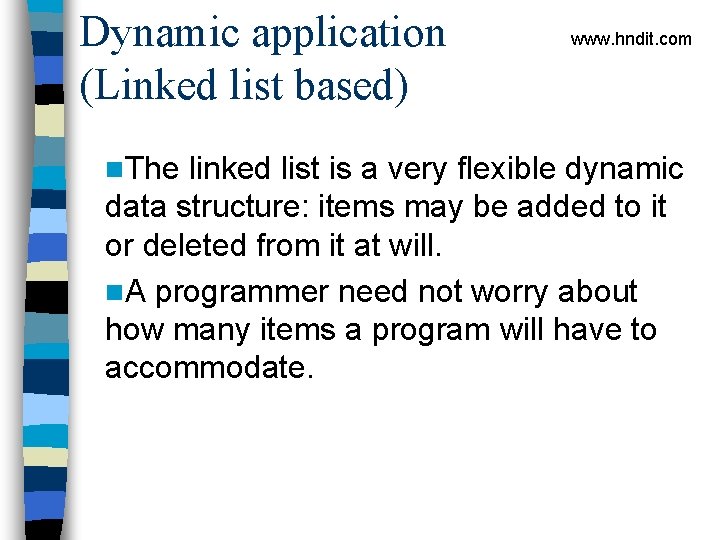
Dynamic application (Linked list based) n. The www. hndit. com linked list is a very flexible dynamic data structure: items may be added to it or deleted from it at will. n. A programmer need not worry about how many items a program will have to accommodate.
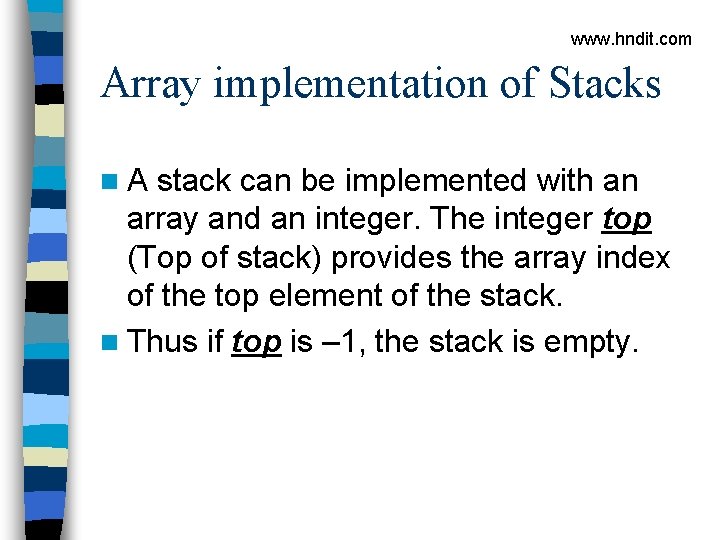
www. hndit. com Array implementation of Stacks n. A stack can be implemented with an array and an integer. The integer top (Top of stack) provides the array index of the top element of the stack. n Thus if top is – 1, the stack is empty.
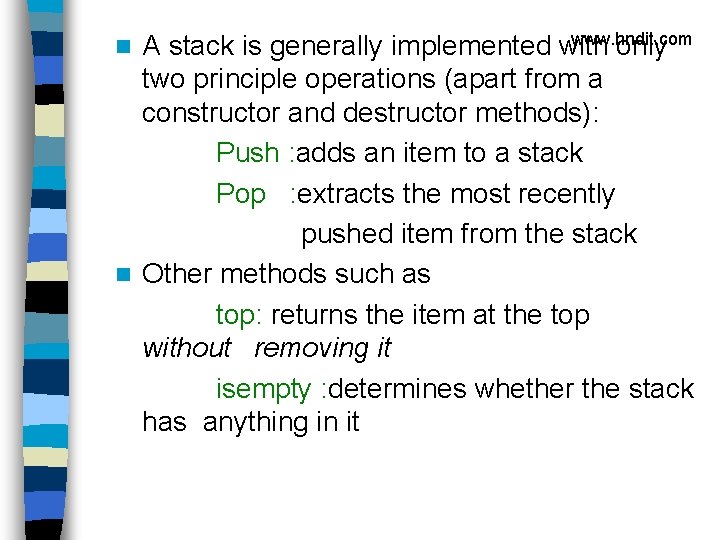
www. hndit. com A stack is generally implemented with only two principle operations (apart from a constructor and destructor methods): Push : adds an item to a stack Pop : extracts the most recently pushed item from the stack n Other methods such as top: returns the item at the top without removing it isempty : determines whether the stack has anything in it n
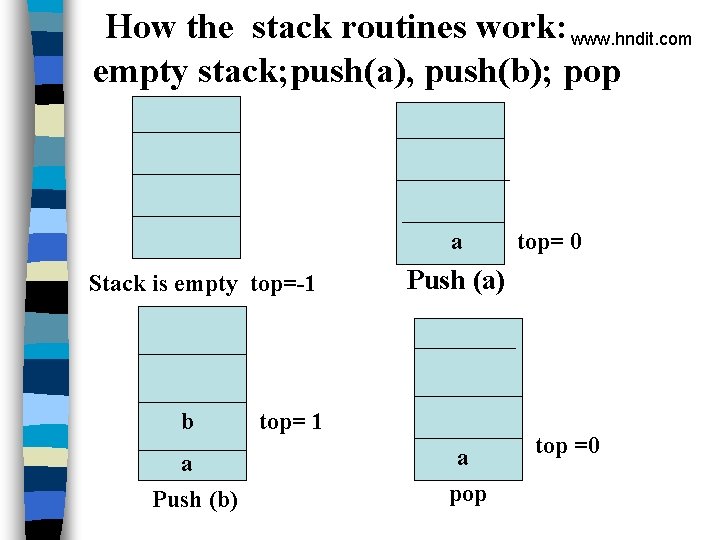
How the stack routines work: www. hndit. com empty stack; push(a), push(b); pop a Stack is empty top=-1 b a Push (b) top= 0 Push (a) top= 1 a pop top =0
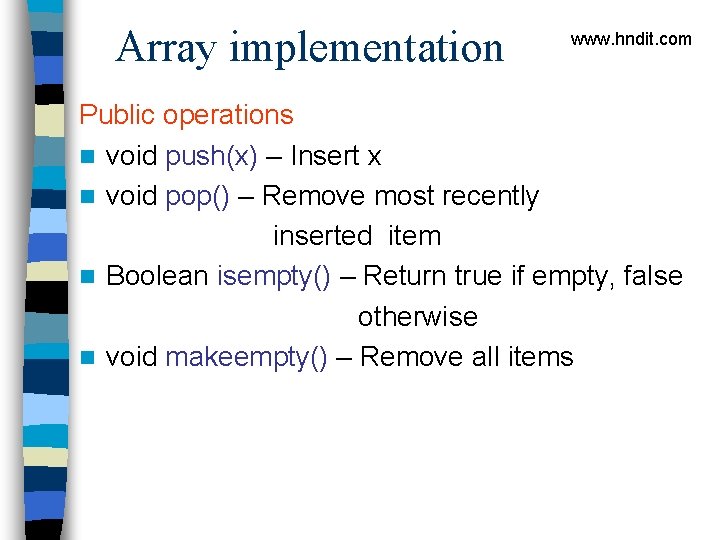
Array implementation www. hndit. com Public operations n void push(x) – Insert x n void pop() – Remove most recently inserted item n Boolean isempty() – Return true if empty, false otherwise n void makeempty() – Remove all items
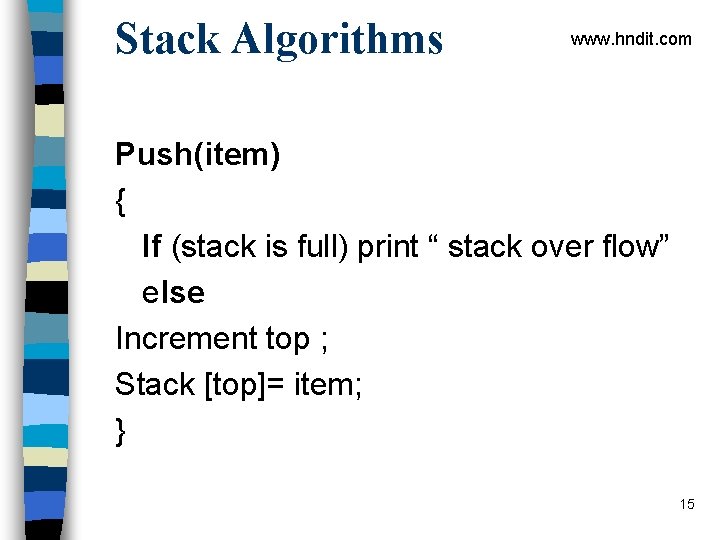
Stack Algorithms www. hndit. com Push(item) { If (stack is full) print “ stack over flow” else Increment top ; Stack [top]= item; } 15
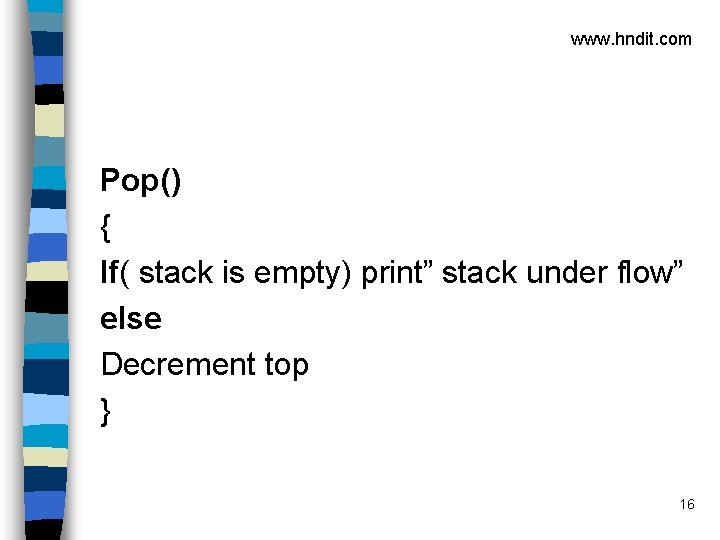
www. hndit. com Pop() { If( stack is empty) print” stack under flow” else Decrement top } 16
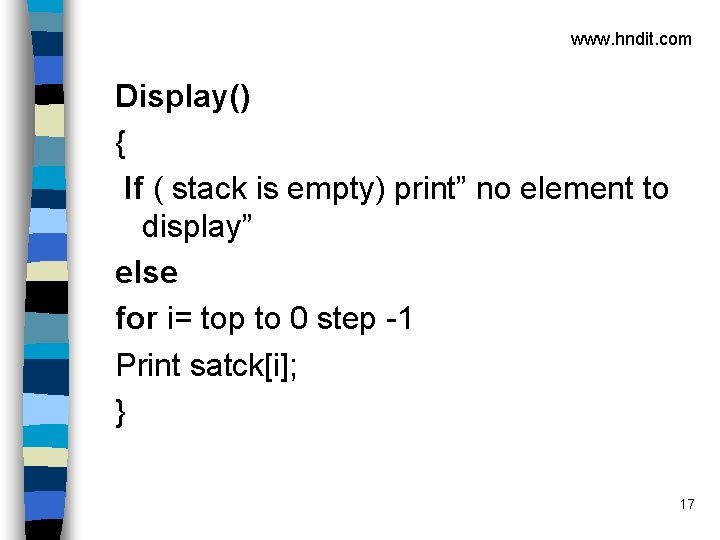
www. hndit. com Display() { If ( stack is empty) print” no element to display” else for i= top to 0 step -1 Print satck[i]; } 17
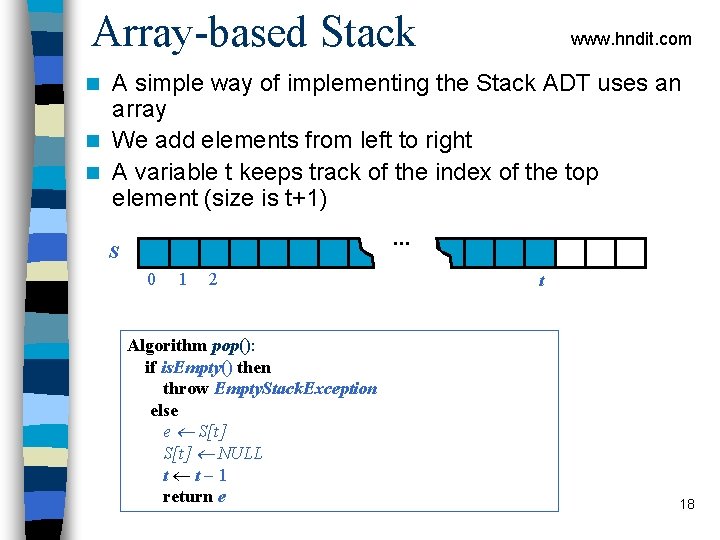
Array-based Stack www. hndit. com A simple way of implementing the Stack ADT uses an array n We add elements from left to right n A variable t keeps track of the index of the top element (size is t+1) n … S 0 1 2 Algorithm pop(): if is. Empty() then throw Empty. Stack. Exception else e S[t] NULL t t 1 return e t 18
![Algorithm pusho if SizeN then throw Full Stack Exception else t t1 St o Algorithm push(o): if Size()=N then throw Full. Stack. Exception else t t+1 S[t] o](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-19.jpg)
Algorithm push(o): if Size()=N then throw Full. Stack. Exception else t t+1 S[t] o www. hndit. com Algorithm top(): if Is. Empty() then throw Empty. Stack. Exception else return S[t] Algorithm Is. Empty(): return (t<0) Algorithm Size(): return t+1 19
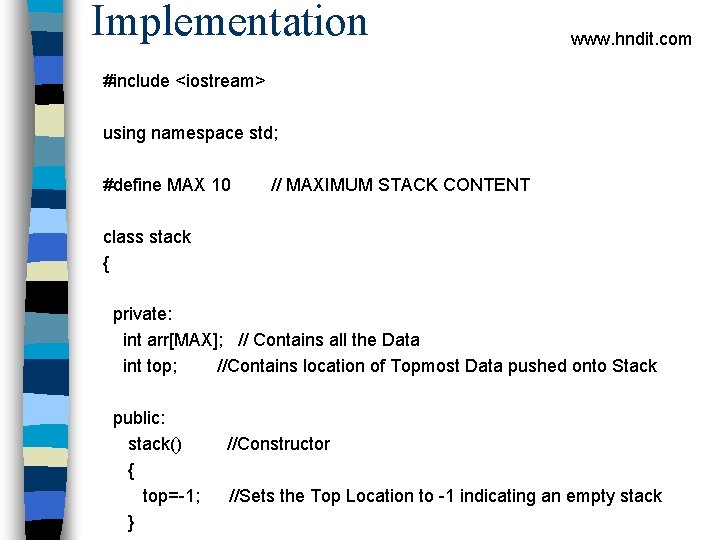
Implementation www. hndit. com #include <iostream> using namespace std; #define MAX 10 // MAXIMUM STACK CONTENT class stack { private: int arr[MAX]; // Contains all the Data int top; //Contains location of Topmost Data pushed onto Stack public: stack() { top=-1; } //Constructor //Sets the Top Location to -1 indicating an empty stack
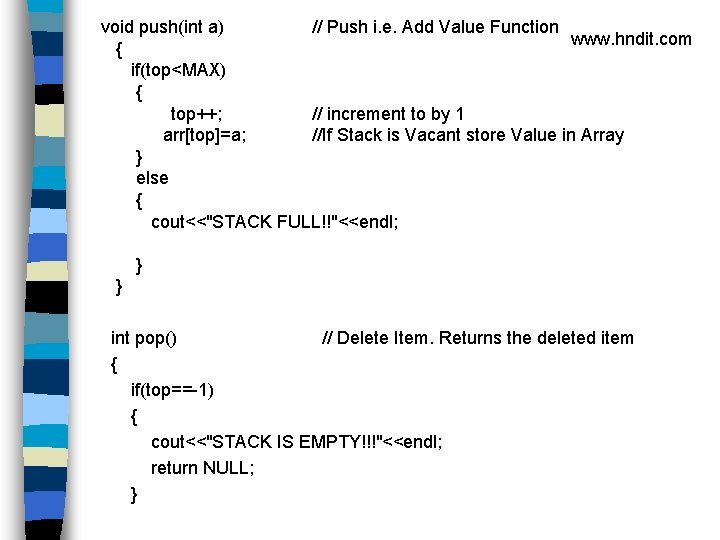
void push(int a) // Push i. e. Add Value Function www. hndit. com { if(top<MAX) { top++; // increment to by 1 arr[top]=a; //If Stack is Vacant store Value in Array } else { cout<<"STACK FULL!!"<<endl; } } int pop() // Delete Item. Returns the deleted item { if(top==-1) { cout<<"STACK IS EMPTY!!!"<<endl; return NULL; }
![else www hndit com int dataarrtop arrtopNULL top return data else { www. hndit. com int data=arr[top]; arr[top]=NULL; top--; return data; } } };](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-22.jpg)
else { www. hndit. com int data=arr[top]; arr[top]=NULL; top--; return data; } } }; int main() { stack a; a. push(3); cout<<"3 is Pushedn"; a. push(10); cout<<"10 is Pushedn"; a. push(1); cout<<"1 is Pushednn"; //Set Topmost Value in data //Set Original Location to NULL // Decrement top by 1 // Return deleted item
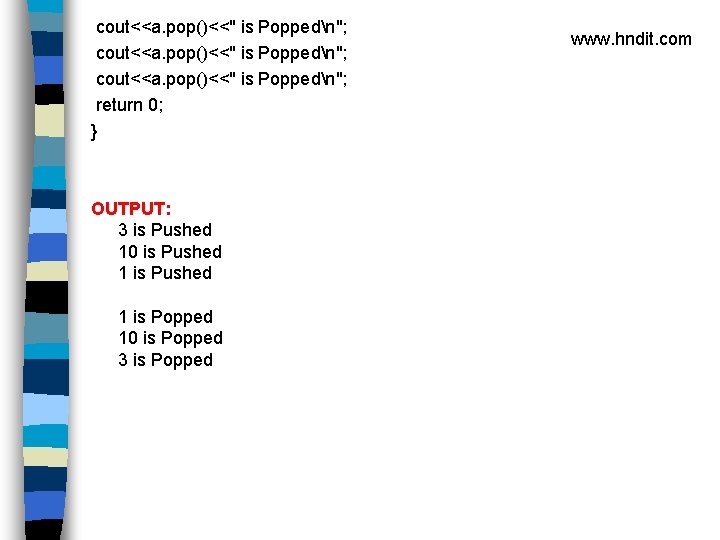
cout<<a. pop()<<" is Poppedn"; return 0; } OUTPUT: 3 is Pushed 10 is Pushed 1 is Popped 10 is Popped 3 is Popped www. hndit. com
![Implementation includeiostream h includeconio h includestdlib h class stack int stk5 int top Implementation #include<iostream. h> #include<conio. h> #include<stdlib. h> class stack { int stk[5]; int top;](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-24.jpg)
Implementation #include<iostream. h> #include<conio. h> #include<stdlib. h> class stack { int stk[5]; int top; public: stack() { top=-1; } www. hndit. com 24
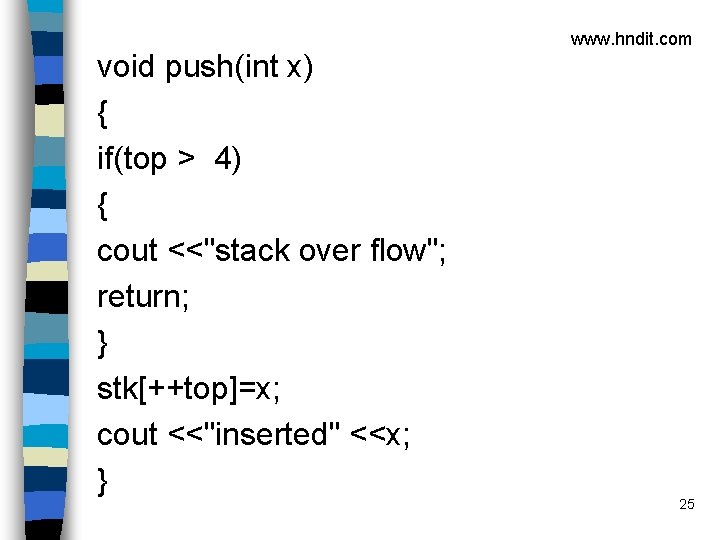
void push(int x) { if(top > 4) { cout <<"stack over flow"; return; } stk[++top]=x; cout <<"inserted" <<x; } www. hndit. com 25
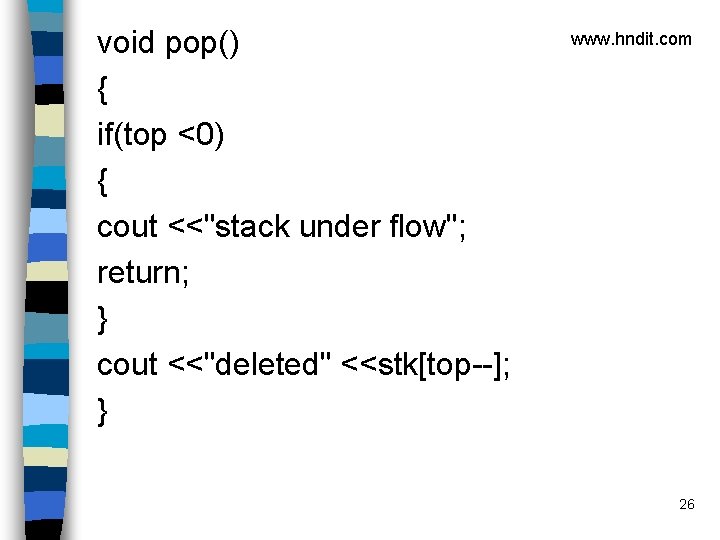
void pop() { if(top <0) { cout <<"stack under flow"; return; } cout <<"deleted" <<stk[top--]; } www. hndit. com 26
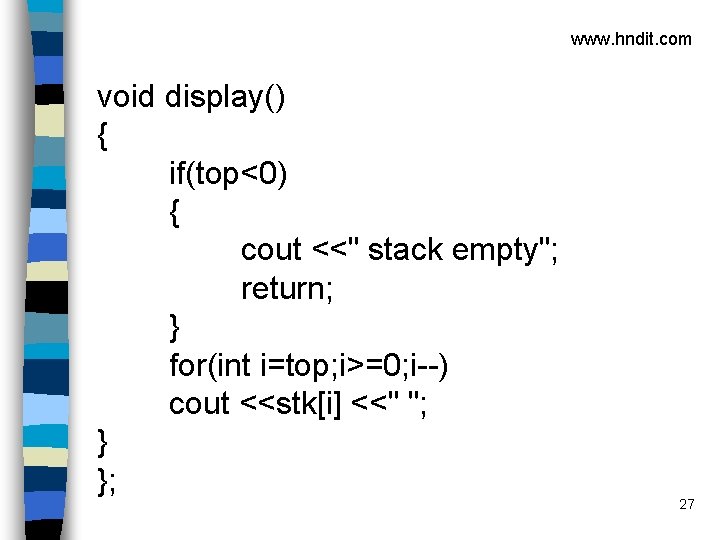
www. hndit. com void display() { if(top<0) { cout <<" stack empty"; return; } for(int i=top; i>=0; i--) cout <<stk[i] <<" "; } }; 27
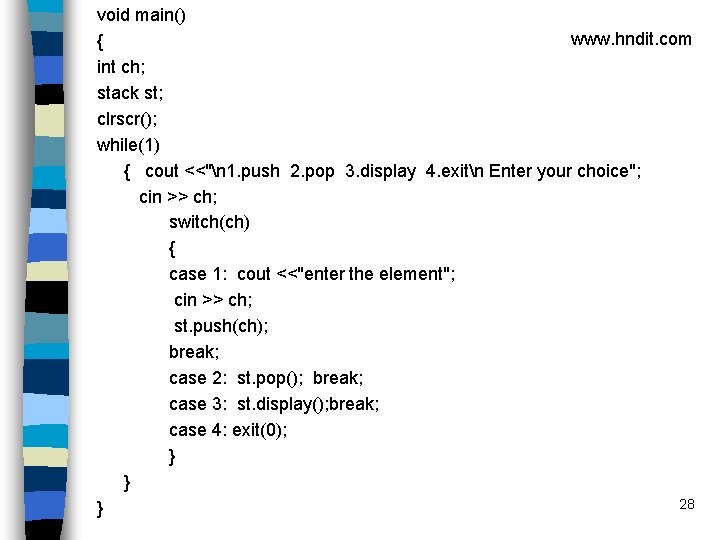
void main() www. hndit. com { int ch; stack st; clrscr(); while(1) { cout <<"n 1. push 2. pop 3. display 4. exitn Enter your choice"; cin >> ch; switch(ch) { case 1: cout <<"enter the element"; cin >> ch; st. push(ch); break; case 2: st. pop(); break; case 3: st. display(); break; case 4: exit(0); } } 28 }
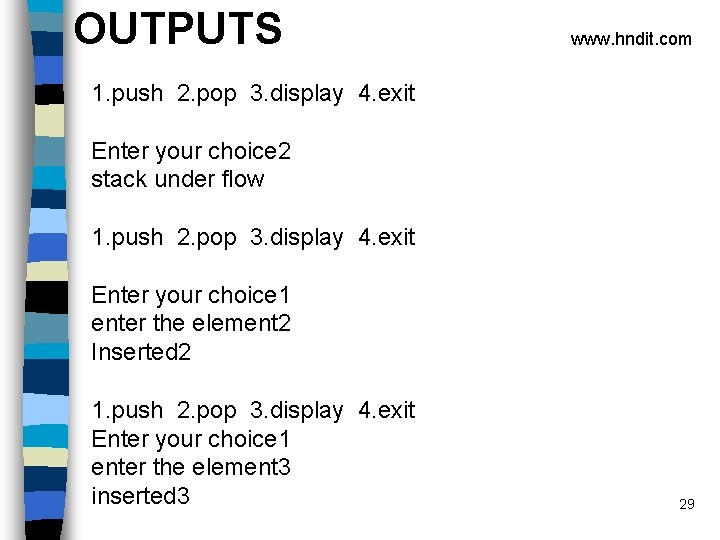
OUTPUTS www. hndit. com 1. push 2. pop 3. display 4. exit Enter your choice 2 stack under flow 1. push 2. pop 3. display 4. exit Enter your choice 1 enter the element 2 Inserted 2 1. push 2. pop 3. display 4. exit Enter your choice 1 enter the element 3 inserted 3 29
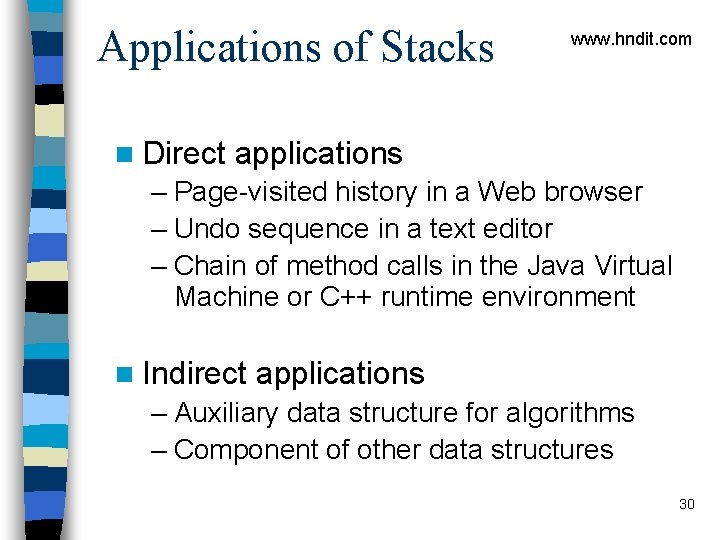
Applications of Stacks n Direct www. hndit. com applications – Page-visited history in a Web browser – Undo sequence in a text editor – Chain of method calls in the Java Virtual Machine or C++ runtime environment n Indirect applications – Auxiliary data structure for algorithms – Component of other data structures 30
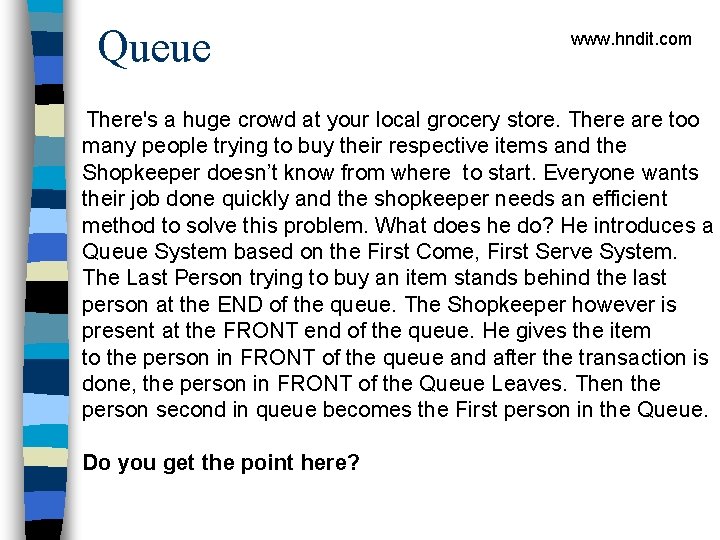
Queue www. hndit. com There's a huge crowd at your local grocery store. There are too many people trying to buy their respective items and the Shopkeeper doesn’t know from where to start. Everyone wants their job done quickly and the shopkeeper needs an efficient method to solve this problem. What does he do? He introduces a Queue System based on the First Come, First Serve System. The Last Person trying to buy an item stands behind the last person at the END of the queue. The Shopkeeper however is present at the FRONT end of the queue. He gives the item to the person in FRONT of the queue and after the transaction is done, the person in FRONT of the Queue Leaves. Then the person second in queue becomes the First person in the Queue. Do you get the point here?
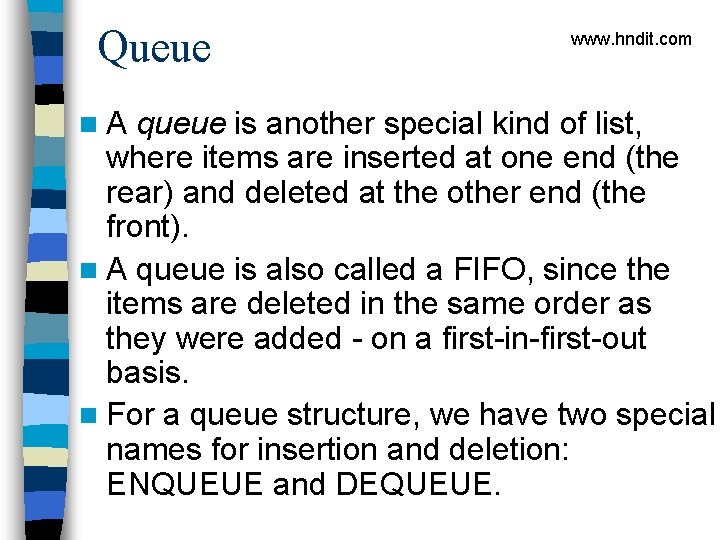
Queue n. A www. hndit. com queue is another special kind of list, where items are inserted at one end (the rear) and deleted at the other end (the front). n A queue is also called a FIFO, since the items are deleted in the same order as they were added - on a first-in-first-out basis. n For a queue structure, we have two special names for insertion and deletion: ENQUEUE and DEQUEUE.
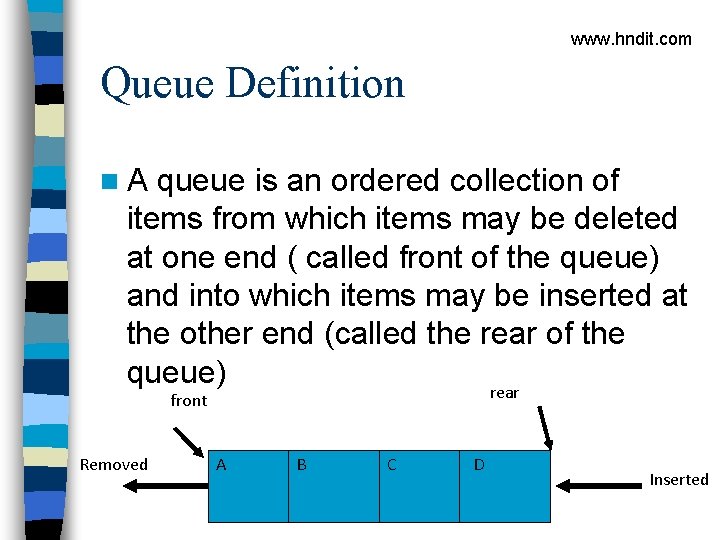
www. hndit. com Queue Definition n. A queue is an ordered collection of items from which items may be deleted at one end ( called front of the queue) and into which items may be inserted at the other end (called the rear of the queue) rear front Removed A B C D Inserted
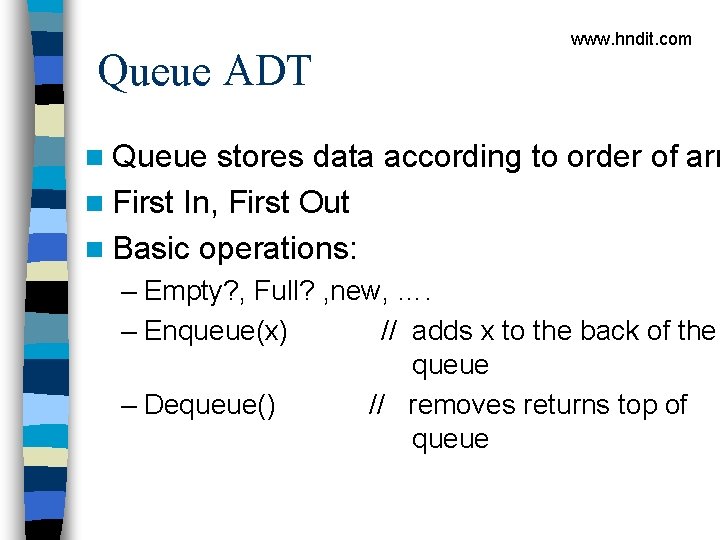
Queue ADT www. hndit. com n Queue stores data according to order of arr n First In, First Out n Basic operations: – Empty? , Full? , new, …. – Enqueue(x) // adds x to the back of the queue – Dequeue() // removes returns top of queue
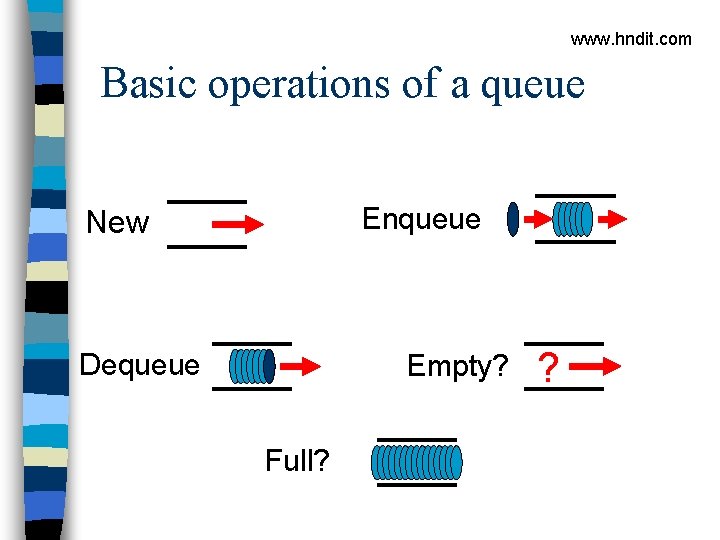
www. hndit. com Basic operations of a queue Enqueue New Dequeue Empty? Full? ?
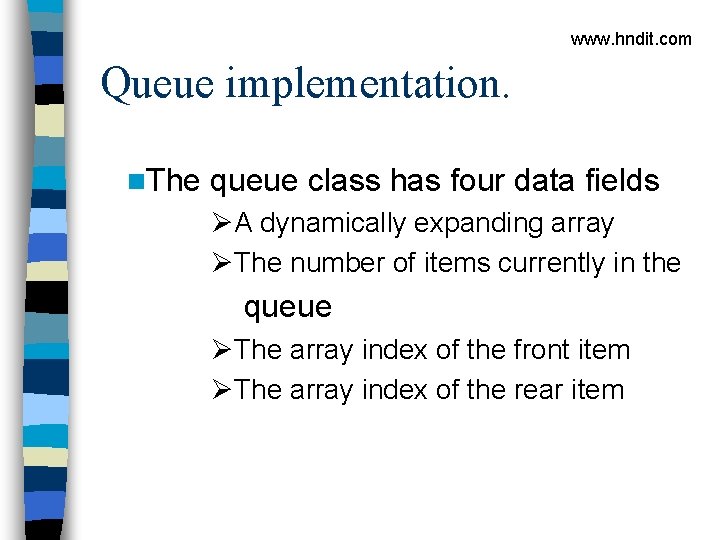
www. hndit. com Queue implementation. n. The queue class has four data fields ØA dynamically expanding array ØThe number of items currently in the queue ØThe array index of the front item ØThe array index of the rear item
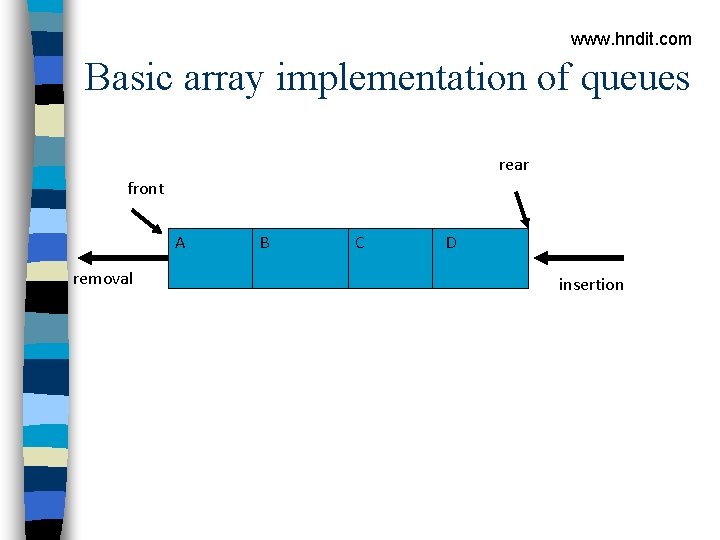
www. hndit. com Basic array implementation of queues rear front A removal B C D insertion
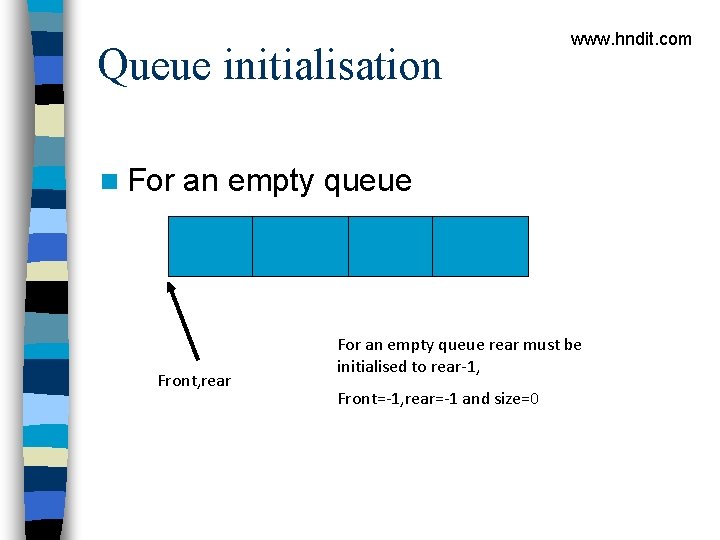
Queue initialisation n For www. hndit. com an empty queue Front, rear For an empty queue rear must be initialised to rear-1, Front=-1, rear=-1 and size=0
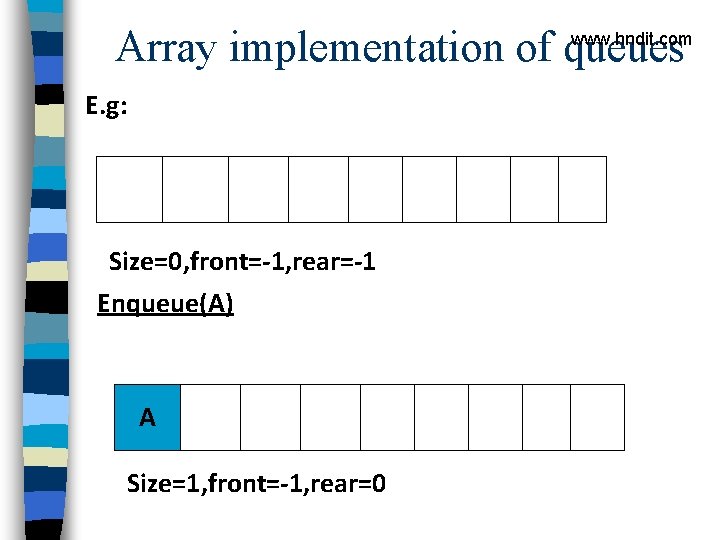
Array implementation of queues www. hndit. com E. g: Size=0, front=-1, rear=-1 Enqueue(A) A Size=1, front=-1, rear=0
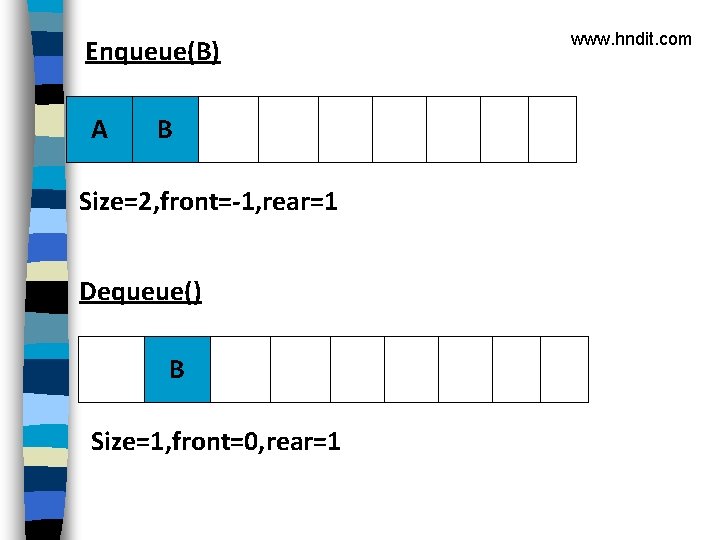
Enqueue(B) A B Size=2, front=-1, rear=1 Dequeue() B Size=1, front=0, rear=1 www. hndit. com
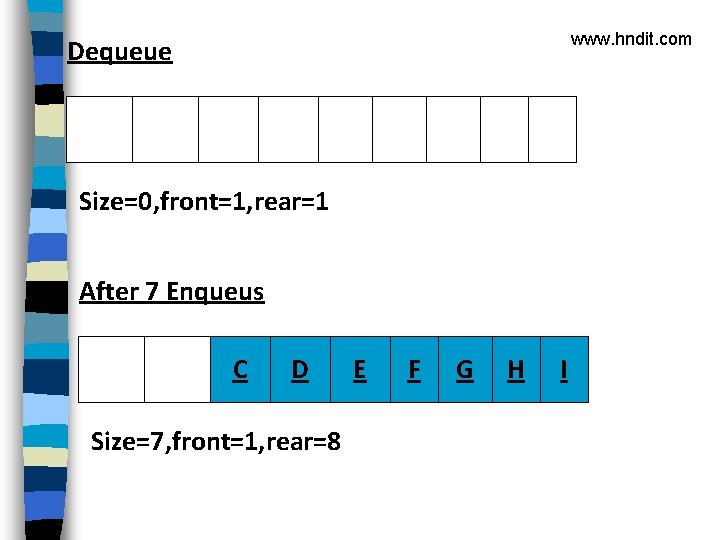
www. hndit. com Dequeue Size=0, front=1, rear=1 After 7 Enqueus C D Size=7, front=1, rear=8 E F G H I
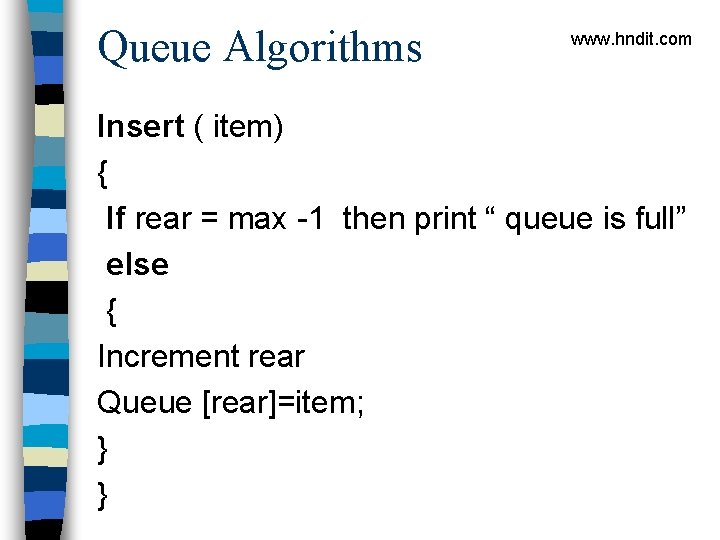
Queue Algorithms www. hndit. com Insert ( item) { If rear = max -1 then print “ queue is full” else { Increment rear Queue [rear]=item; } }
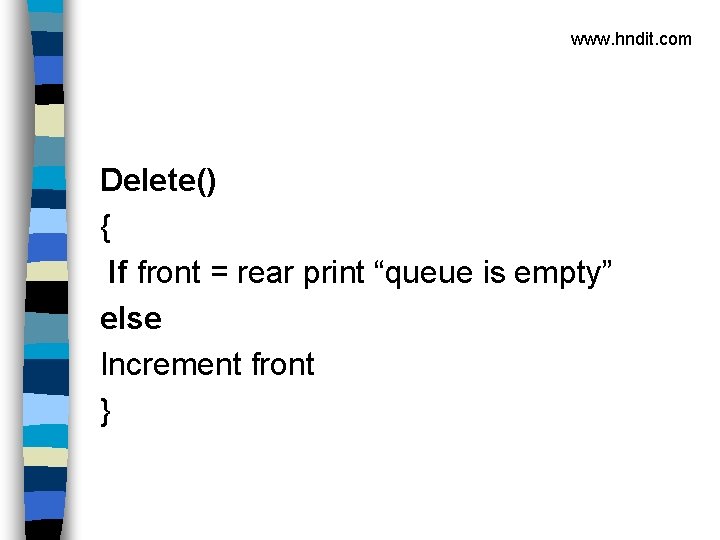
www. hndit. com Delete() { If front = rear print “queue is empty” else Increment front }
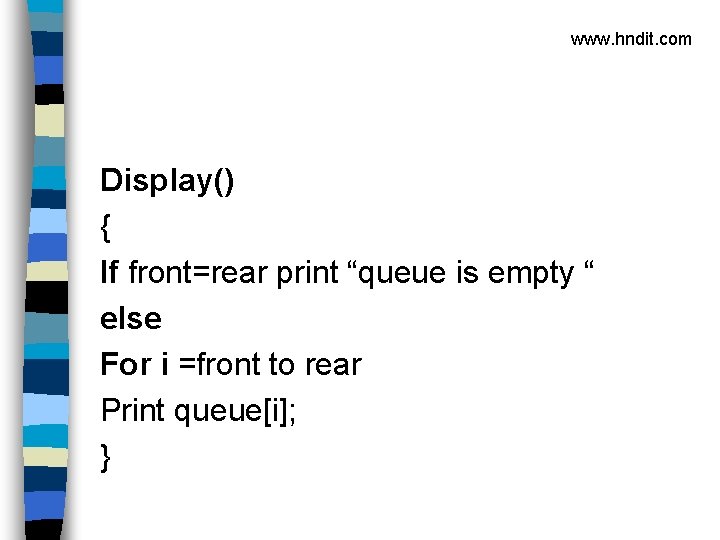
www. hndit. com Display() { If front=rear print “queue is empty “ else For i =front to rear Print queue[i]; }
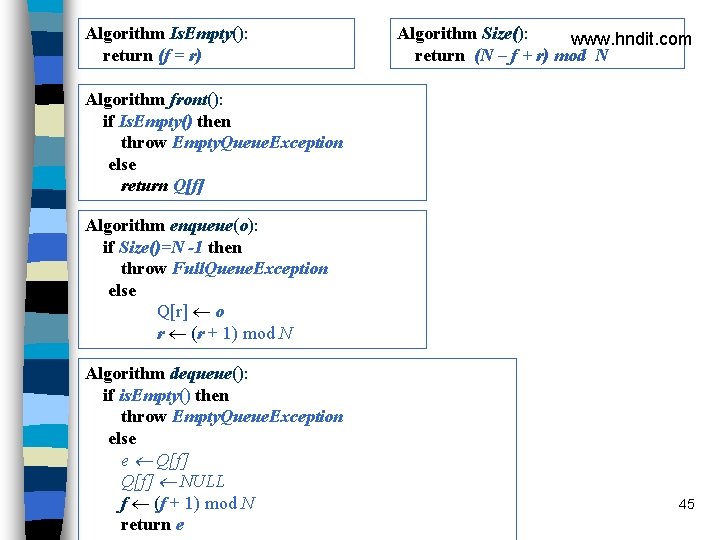
Algorithm Is. Empty(): return (f = r) Algorithm Size(): www. hndit. com return (N – f + r) mod N Algorithm front(): if Is. Empty() then throw Empty. Queue. Exception else return Q[f] Algorithm enqueue(o): if Size()=N -1 then throw Full. Queue. Exception else Q[r] o r (r + 1) mod N Algorithm dequeue(): if is. Empty() then throw Empty. Queue. Exception else e Q[f] NULL f (f + 1) mod N return e 45
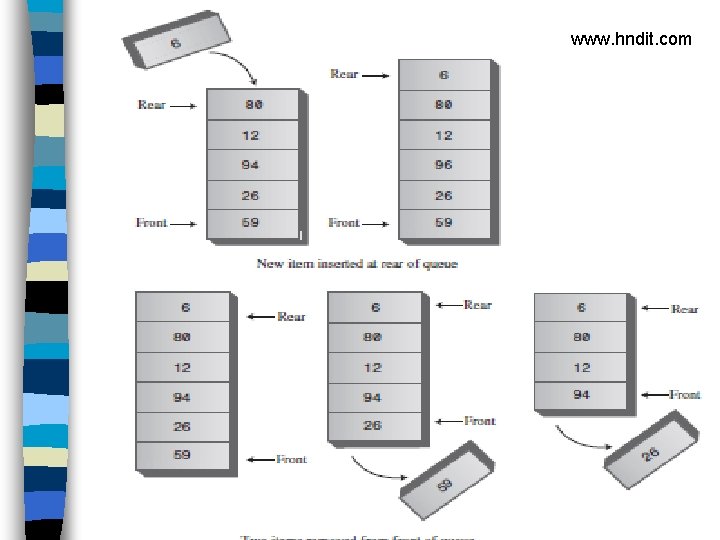
www. hndit. com
![Queue Implementation includeiostream h includeconio h includestdlib h class queue int queue5 int Queue Implementation #include<iostream. h> #include<conio. h> #include<stdlib. h> class queue { int queue[5]; int](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-47.jpg)
Queue Implementation #include<iostream. h> #include<conio. h> #include<stdlib. h> class queue { int queue[5]; int rear, front; public: queue() {rear=-1; front=-1; } www. hndit. com
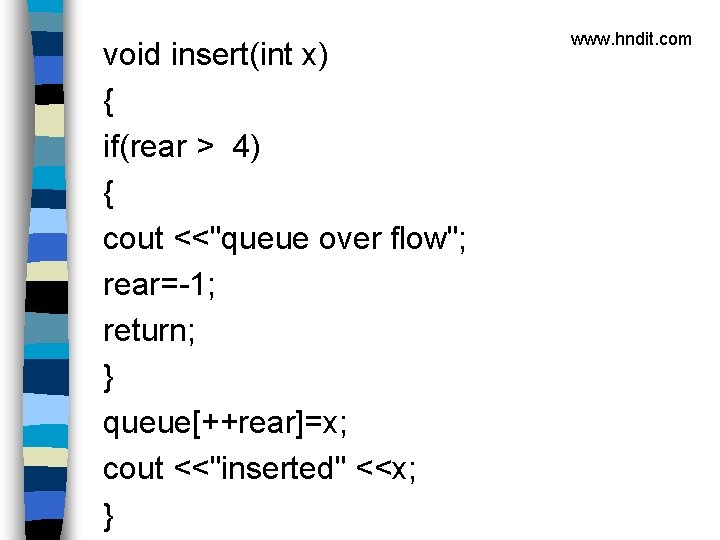
void insert(int x) { if(rear > 4) { cout <<"queue over flow"; rear=-1; return; } queue[++rear]=x; cout <<"inserted" <<x; } www. hndit. com
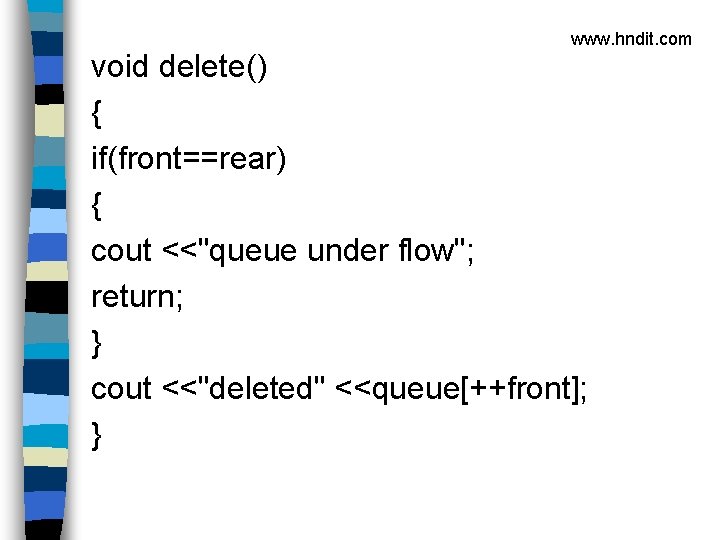
www. hndit. com void delete() { if(front==rear) { cout <<"queue under flow"; return; } cout <<"deleted" <<queue[++front]; }
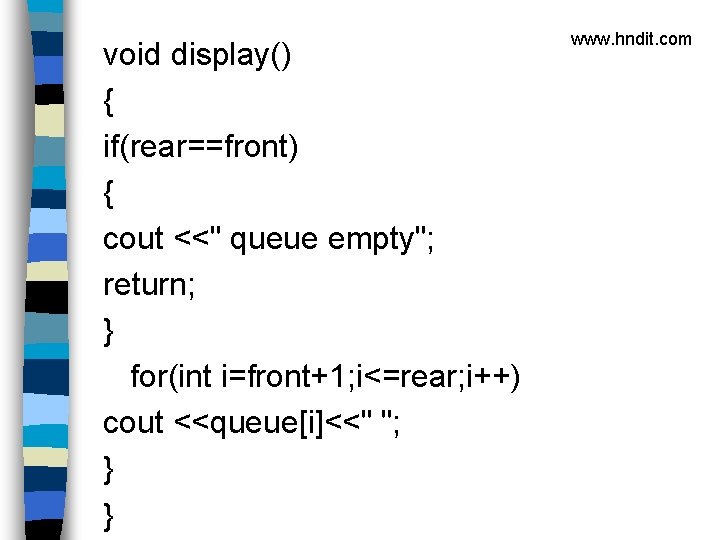
void display() { if(rear==front) { cout <<" queue empty"; return; } for(int i=front+1; i<=rear; i++) cout <<queue[i]<<" "; } } www. hndit. com
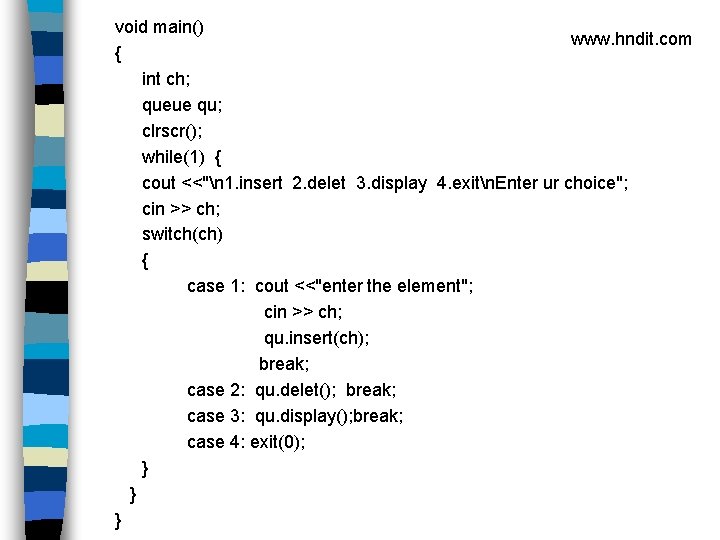
void main() www. hndit. com { int ch; queue qu; clrscr(); while(1) { cout <<"n 1. insert 2. delet 3. display 4. exitn. Enter ur choice"; cin >> ch; switch(ch) { case 1: cout <<"enter the element"; cin >> ch; qu. insert(ch); break; case 2: qu. delet(); break; case 3: qu. display(); break; case 4: exit(0); } } }
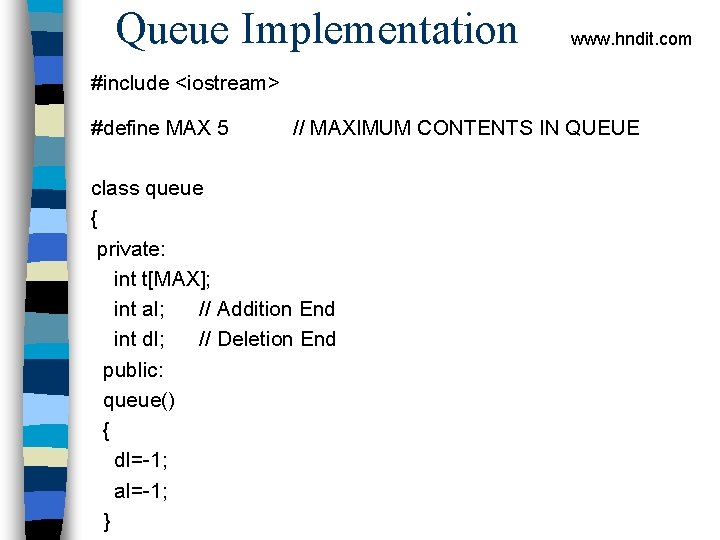
Queue Implementation www. hndit. com #include <iostream> #define MAX 5 // MAXIMUM CONTENTS IN QUEUE class queue { private: int t[MAX]; int al; // Addition End int dl; // Deletion End public: queue() { dl=-1; al=-1; }
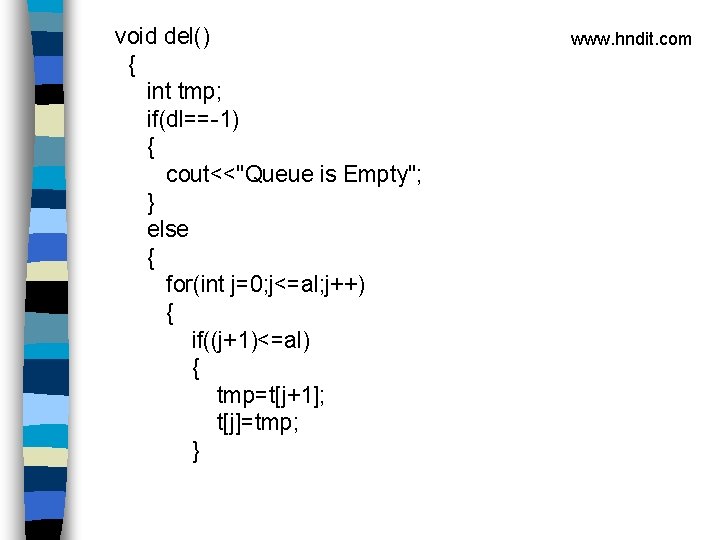
void del() { int tmp; if(dl==-1) { cout<<"Queue is Empty"; } else { for(int j=0; j<=al; j++) { if((j+1)<=al) { tmp=t[j+1]; t[j]=tmp; } www. hndit. com
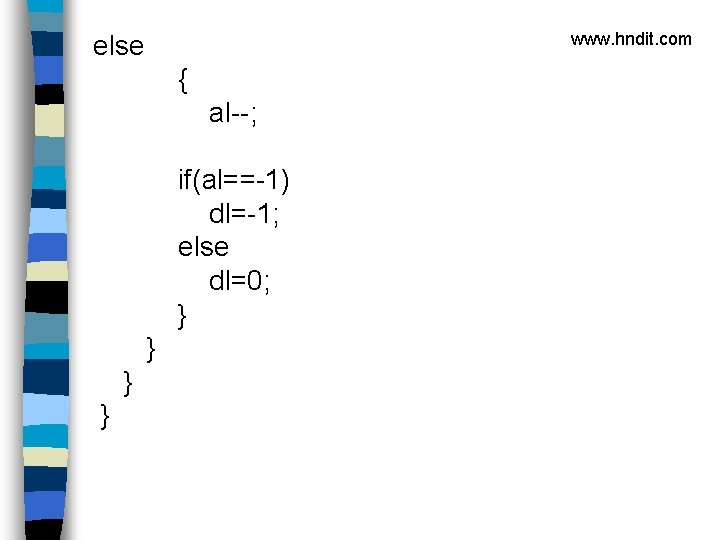
www. hndit. com else { al--; if(al==-1) dl=-1; else dl=0; } }
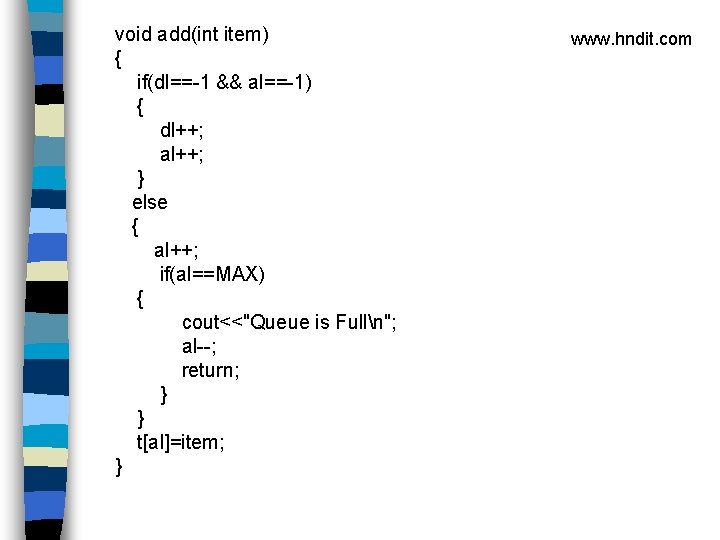
void add(int item) { if(dl==-1 && al==-1) { dl++; al++; } else { al++; if(al==MAX) { cout<<"Queue is Fulln"; al--; return; } } t[al]=item; } www. hndit. com
![void display ifdl1 forint iter0 iteral iter couttiter void display() { if(dl!=-1) { for(int iter=0 ; iter<=al ; iter++) cout<<t[iter]<<" "; }](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-56.jpg)
void display() { if(dl!=-1) { for(int iter=0 ; iter<=al ; iter++) cout<<t[iter]<<" "; } else cout<<"EMPTY"; } }; www. hndit. com
![int main queue a int data532 23 45 99 24 www hndit com int main() { queue a; int data[5]={32, 23, 45, 99, 24}; www. hndit. com](https://slidetodoc.com/presentation_image_h2/e4c1f821d2ab35d373075fd5a6518d51/image-57.jpg)
int main() { queue a; int data[5]={32, 23, 45, 99, 24}; www. hndit. com cout<<"Queue before adding Elements: "; a. display(); cout<<endl; for(int iter = 0 ; iter < 5 ; iter++) { a. add(data[iter]); cout<<"Addition Number : "<<(iter+1)<<" : "; a. display(); cout<<endl; }
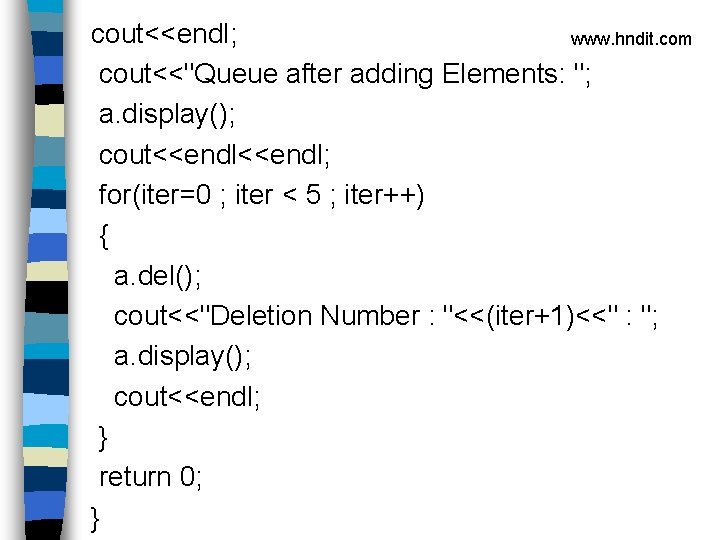
cout<<endl; www. hndit. com cout<<"Queue after adding Elements: "; a. display(); cout<<endl; for(iter=0 ; iter < 5 ; iter++) { a. del(); cout<<"Deletion Number : "<<(iter+1)<<" : "; a. display(); cout<<endl; } return 0; }
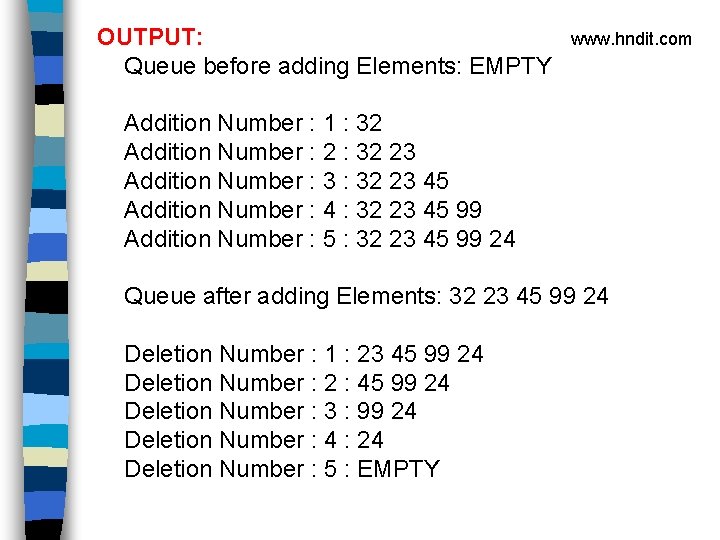
OUTPUT: Queue before adding Elements: EMPTY www. hndit. com Addition Number : 1 : 32 Addition Number : 2 : 32 23 Addition Number : 32 23 45 Addition Number : 4 : 32 23 45 99 Addition Number : 5 : 32 23 45 99 24 Queue after adding Elements: 32 23 45 99 24 Deletion Number : 1 : 23 45 99 24 Deletion Number : 2 : 45 99 24 Deletion Number : 3 : 99 24 Deletion Number : 4 : 24 Deletion Number : 5 : EMPTY
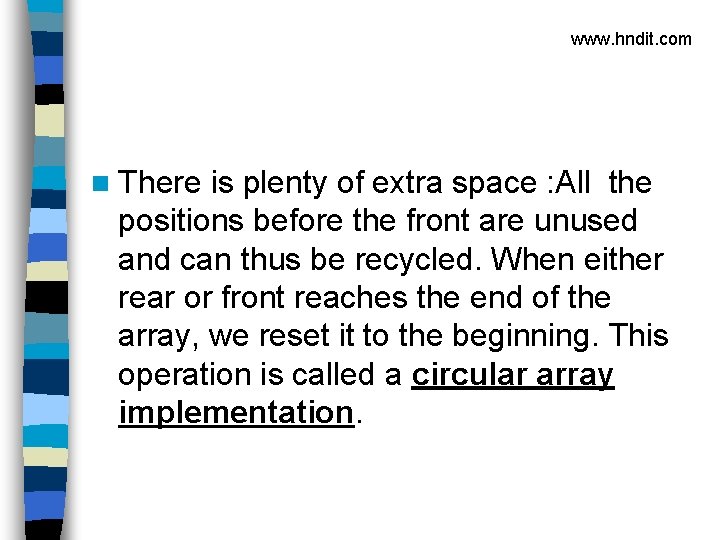
www. hndit. com n There is plenty of extra space : All the positions before the front are unused and can thus be recycled. When either rear or front reaches the end of the array, we reset it to the beginning. This operation is called a circular array implementation.
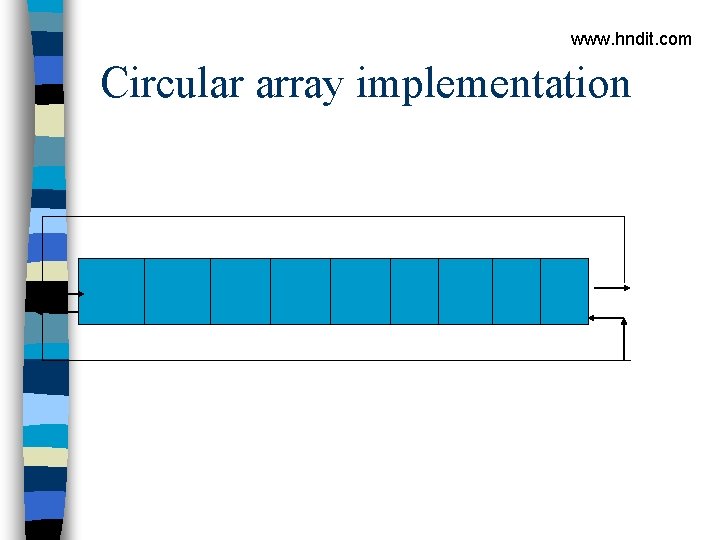
www. hndit. com Circular array implementation
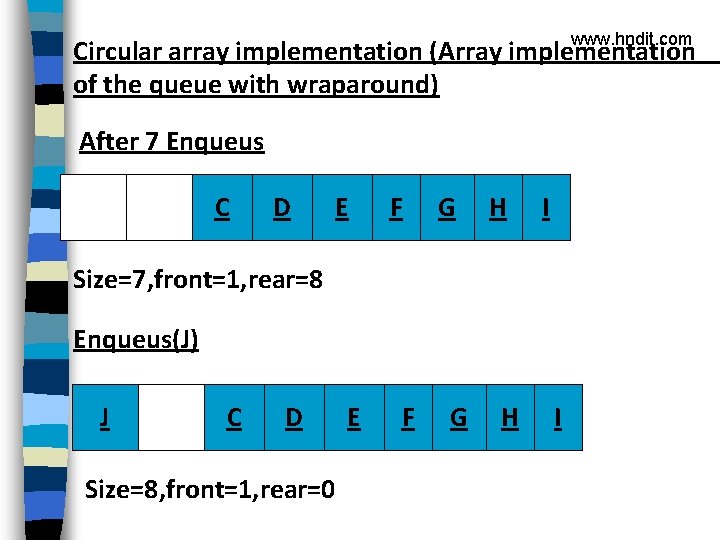
www. hndit. com Circular array implementation (Array implementation of the queue with wraparound) After 7 Enqueus C D E F G H I Size=7, front=1, rear=8 Enqueus(J) J C D Size=8, front=1, rear=0 E F G H I
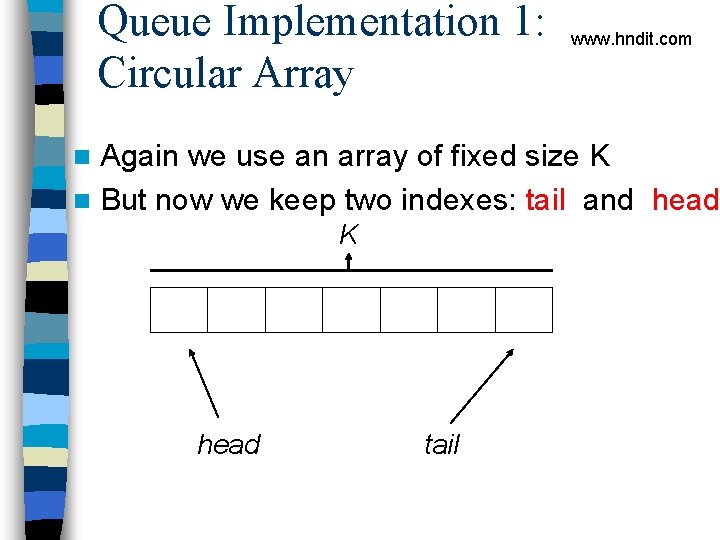
Queue Implementation 1: Circular Array www. hndit. com Again we use an array of fixed size K n But now we keep two indexes: tail and head n K head tail
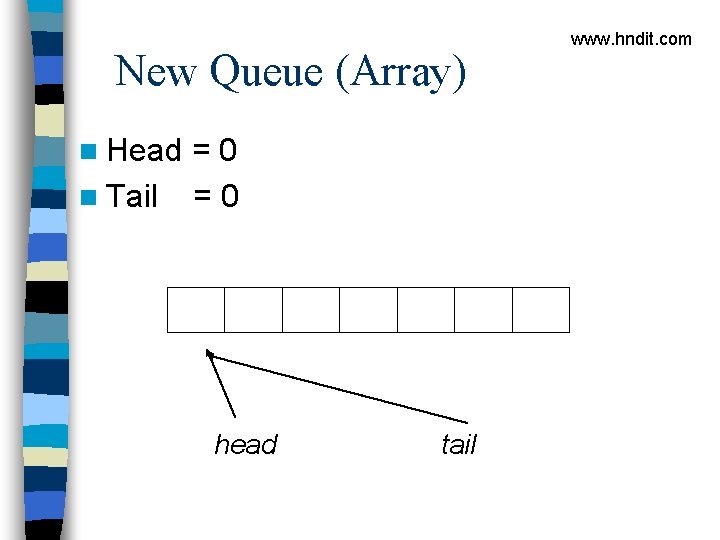
New Queue (Array) n Head n Tail =0 =0 head tail www. hndit. com
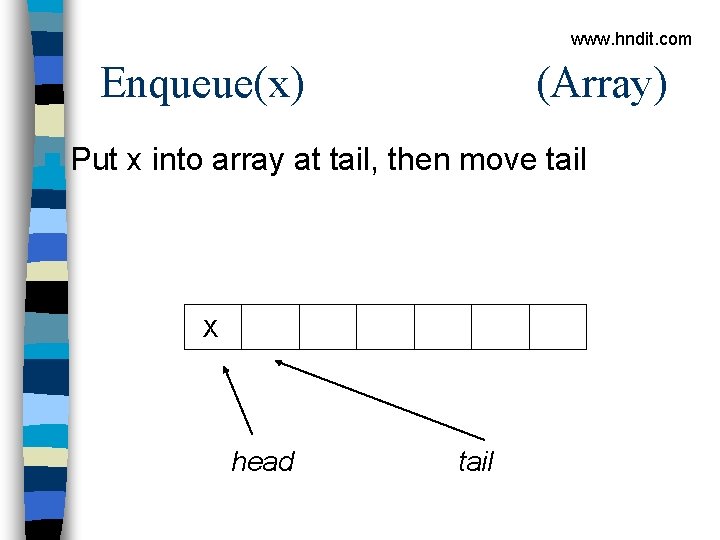
www. hndit. com Enqueue(x) n Put (Array) x into array at tail, then move tail X head tail
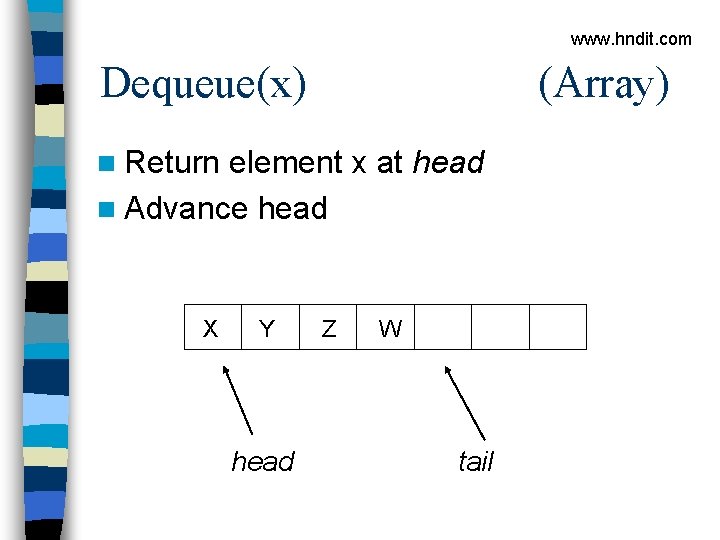
www. hndit. com Dequeue(x) (Array) n Return element x at head n Advance head X Y head Z W tail
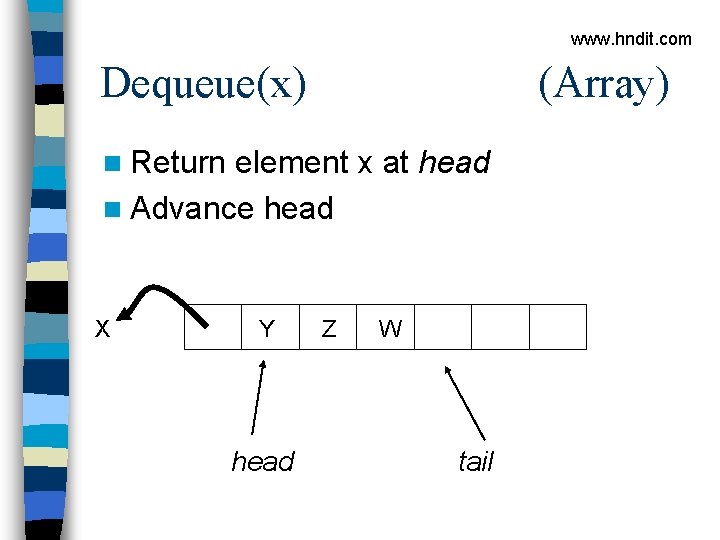
www. hndit. com Dequeue(x) (Array) n Return element x at head n Advance head X Y head Z W tail
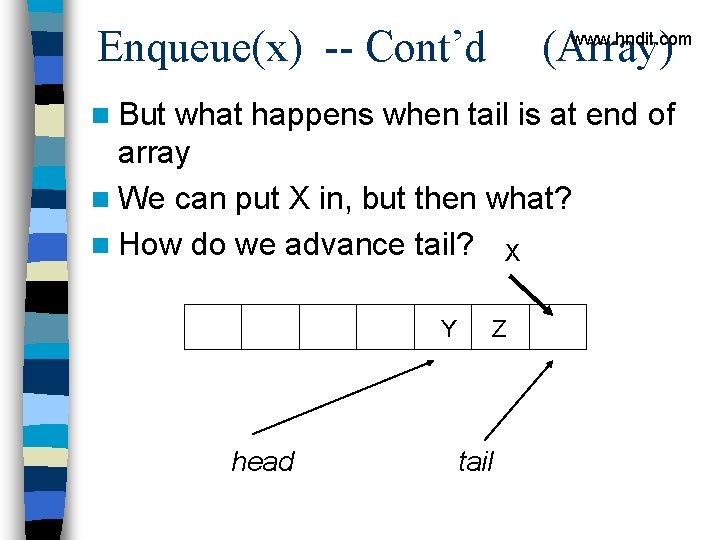
Enqueue(x) -- Cont’d (Array) www. hndit. com n But what happens when tail is at end of array n We can put X in, but then what? n How do we advance tail? X Y head Z tail
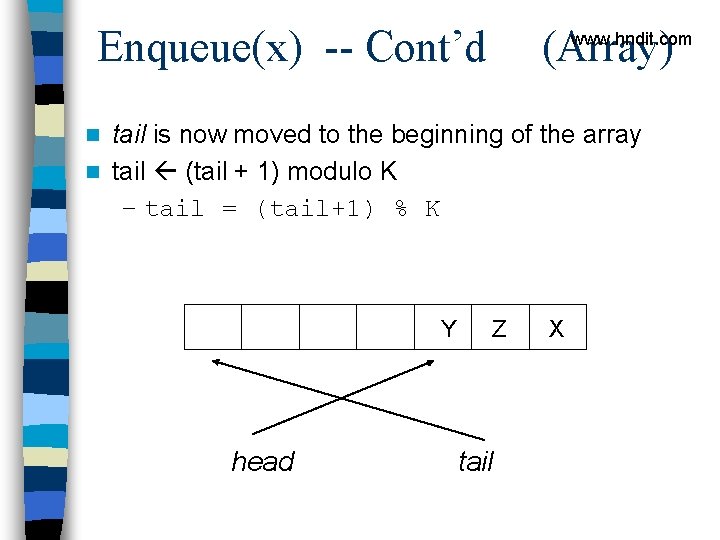
Enqueue(x) -- Cont’d (Array) www. hndit. com tail is now moved to the beginning of the array n tail (tail + 1) modulo K – tail = (tail+1) % K n Y head Z tail X
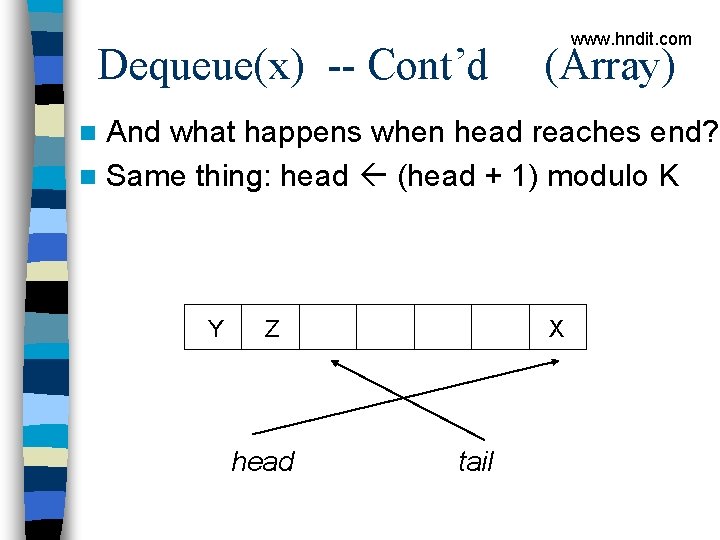
Dequeue(x) -- Cont’d www. hndit. com (Array) And what happens when head reaches end? n Same thing: head (head + 1) modulo K n Y Z head X tail
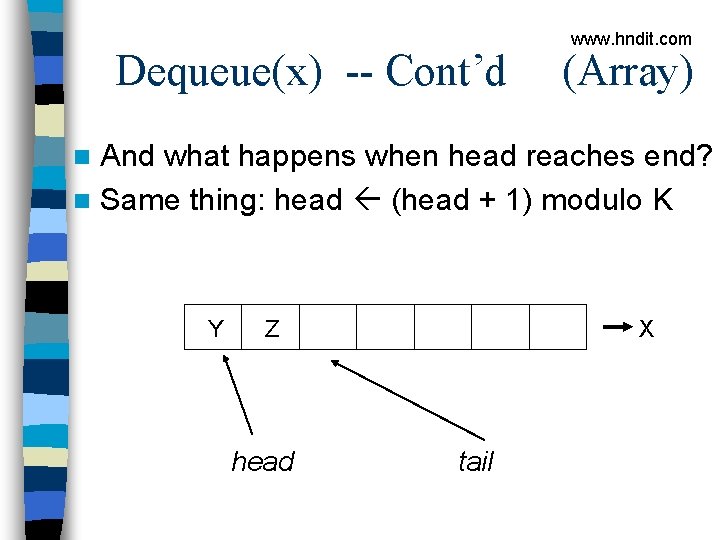
Dequeue(x) -- Cont’d www. hndit. com (Array) And what happens when head reaches end? n Same thing: head (head + 1) modulo K n Y Z head X tail
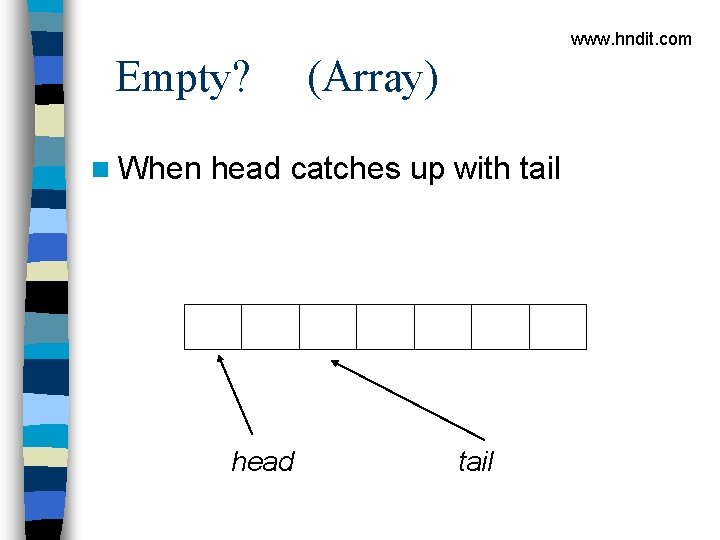
www. hndit. com Empty? n When (Array) head catches up with tail head tail
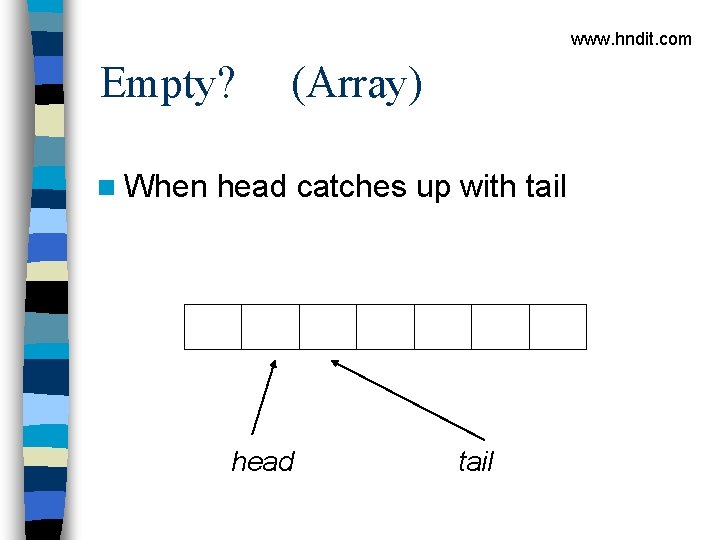
www. hndit. com Empty? n When (Array) head catches up with tail head tail
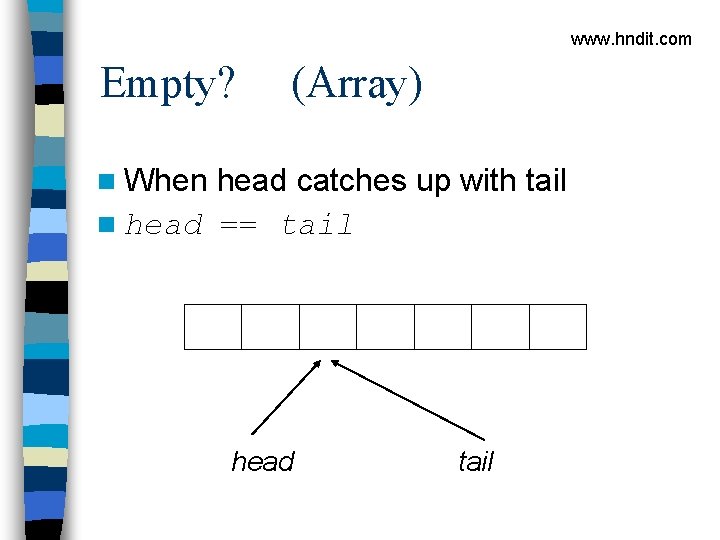
www. hndit. com Empty? (Array) n When head catches up with tail n head == tail head tail
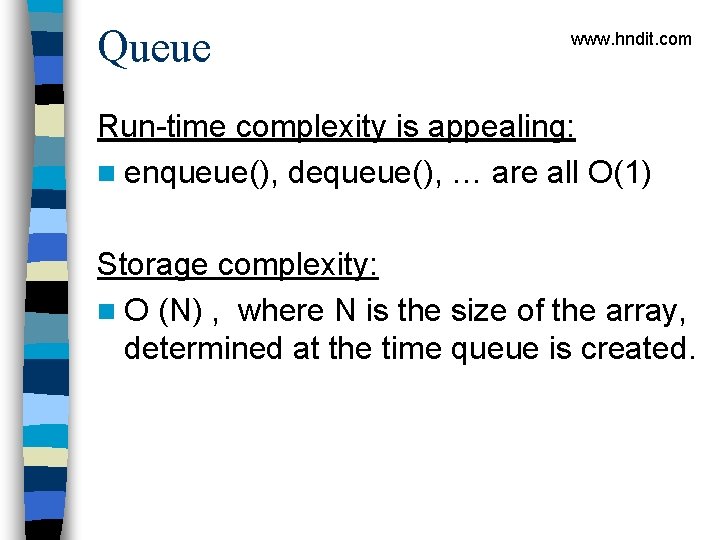
Queue www. hndit. com Run-time complexity is appealing: n enqueue(), dequeue(), … are all O(1) Storage complexity: n O (N) , where N is the size of the array, determined at the time queue is created.
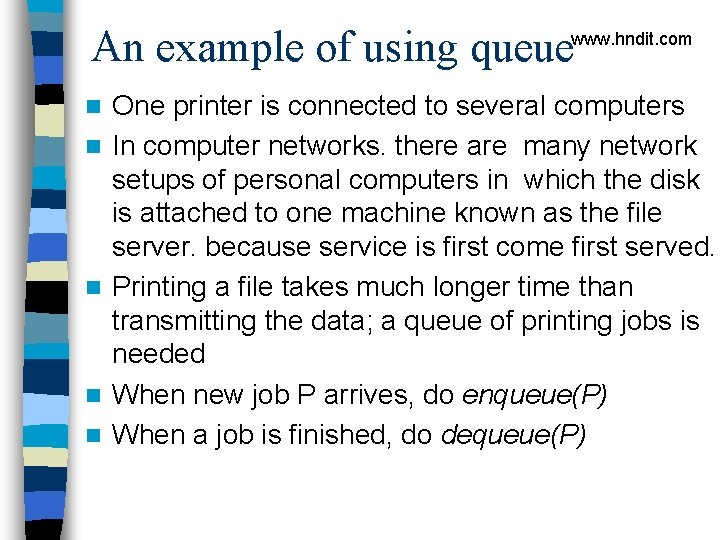
An example of using queue www. hndit. com n n n One printer is connected to several computers In computer networks. there are many network setups of personal computers in which the disk is attached to one machine known as the file server. because service is first come first served. Printing a file takes much longer time than transmitting the data; a queue of printing jobs is needed When new job P arrives, do enqueue(P) When a job is finished, do dequeue(P)
Bài thơ mẹ đi làm từ sáng sớm
Cơm
Ajit diwan iit bombay
Cos 423 princeton
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Data structures and algorithms iit bombay
Data structures and algorithms
Data structures and algorithms
Waterloo data structures and algorithms
Signature file structure in information retrieval system
Data structures and algorithms
Algorithms + data structures = programs
Hndit syllabus pdf
Hndit syllabus
Hndit.com
Hndit.com
Hndit
Data structure
Hndit
Hndit
Hndit
Hndit application 2020
Hndit
Homology
Muthukrishnan data stream algorithms
One pass macro processor
Assembler data structures
Data structures and abstractions with java
Adts, data structures, and problem solving with c++
Data structures and algorithm
Persistent and ephemeral data structures
Computational thinking algorithms and programming
1001 design
Association analysis: basic concepts and algorithms
Fftooo
Algorithms for select and join operations
Algorithms and flowcharts
Undecidable problems and unreasonable time algorithms.
Cluster analysis: basic concepts and algorithms
Randomized algorithms and probabilistic analysis
Introduction of design and analysis of algorithms
Algorithms for query processing and optimization
Synchronization algorithms and concurrent programming
Parallel and distributed algorithms
Lerp
Cluster analysis basic concepts and algorithms
Cluster analysis basic concepts and algorithms
Aries recovery algorithm
Dsp algorithms and architecture notes
Parametric and non parametric algorithms
Exercise 24
Binary search in design and analysis of algorithms
Introduction to the design and analysis of algorithms
Undecidable problems and unreasonable time algorithms
Design and analysis of algorithms
Design and analysis of algorithms
Cluster analysis basic concepts and algorithms
Comp 482
Btechsmartclass data structures
Types of data structures in r
Oblivious data structures
Linux kernel data structures
Introduction to data structures
Introduction to data structures
Esoteric data structures
Geometric data structures
Hadoop io
Advanced data structures in java
Samantacomputer
Persistent vs ephemeral data structures
Php data structures
What is data structure in gis
Dynamic data structure in java
Recurrence data structures
Data structures in c ppt