Iteration Chapter 6 Fall 2005 CS 101 Aaron
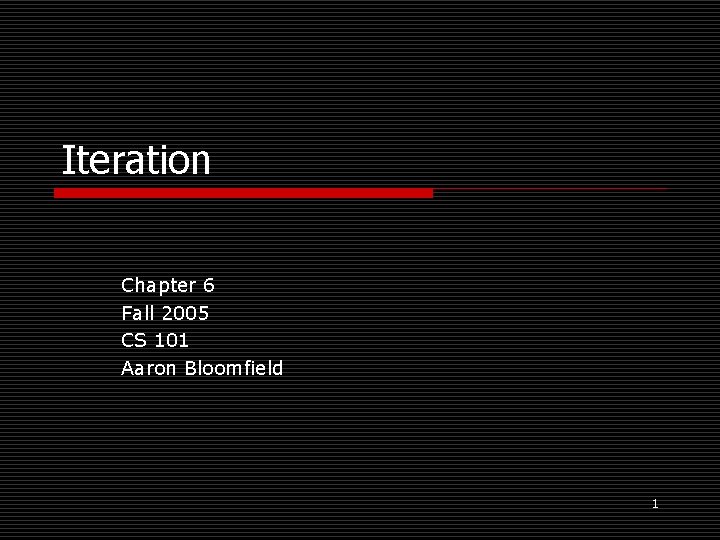
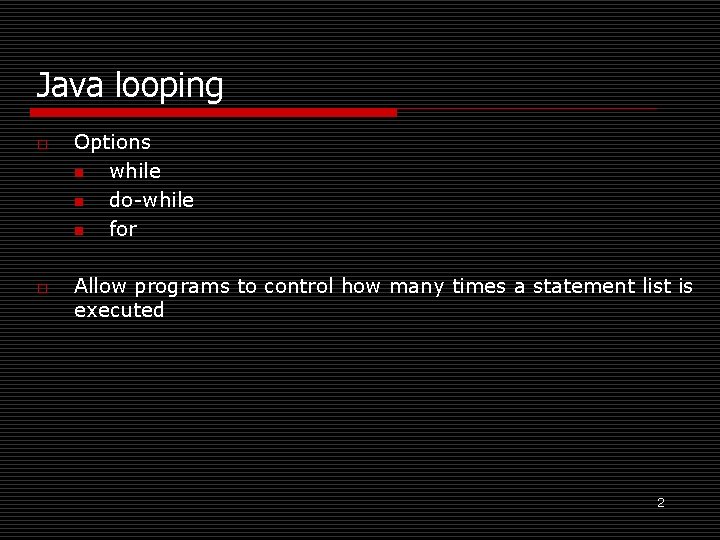
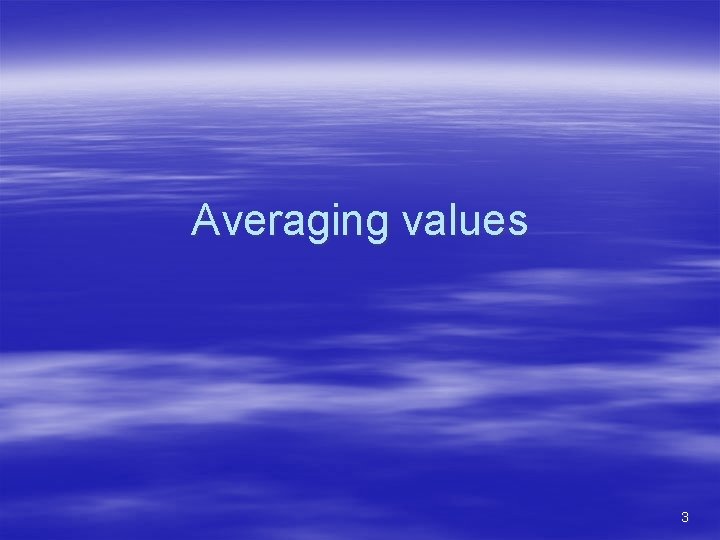
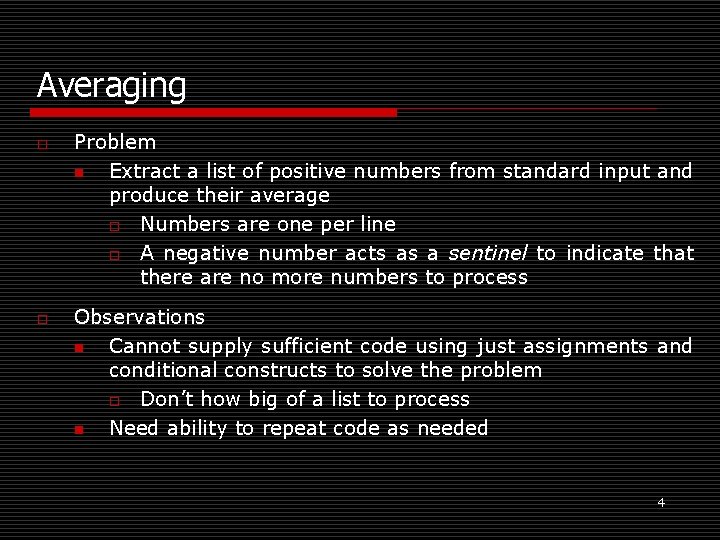
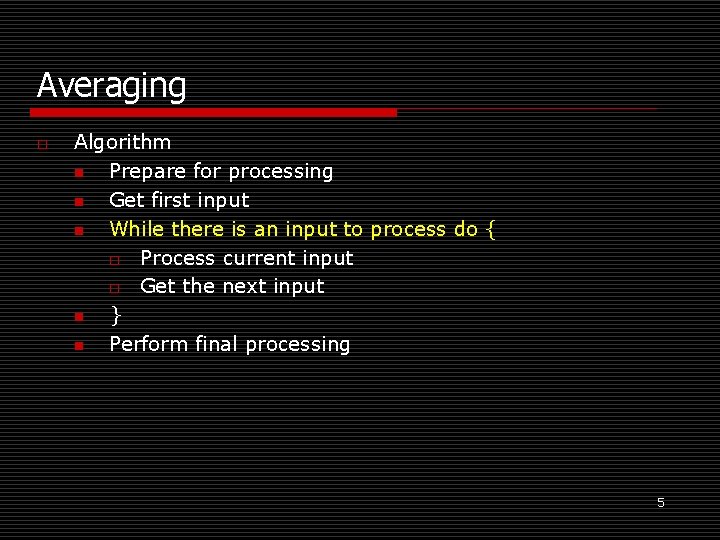
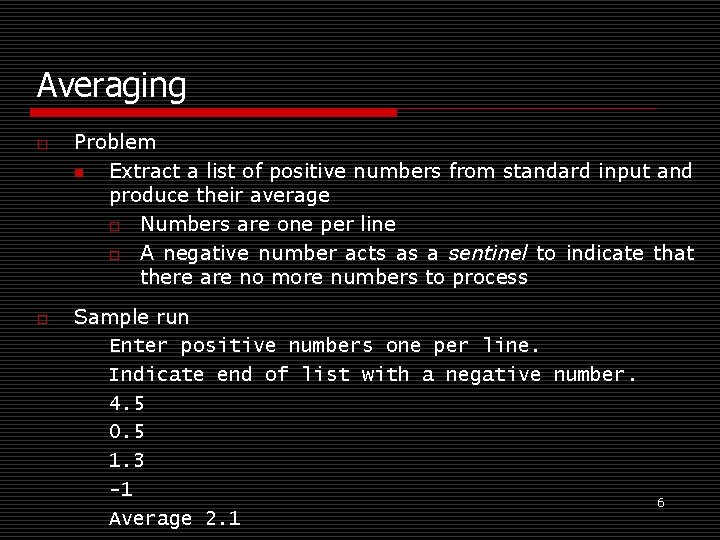
![public class Number. Average { // main(): application entry point public static void main(String[] public class Number. Average { // main(): application entry point public static void main(String[]](https://slidetodoc.com/presentation_image/f31e04bae69c2a009fc956421a67c2b8/image-7.jpg)
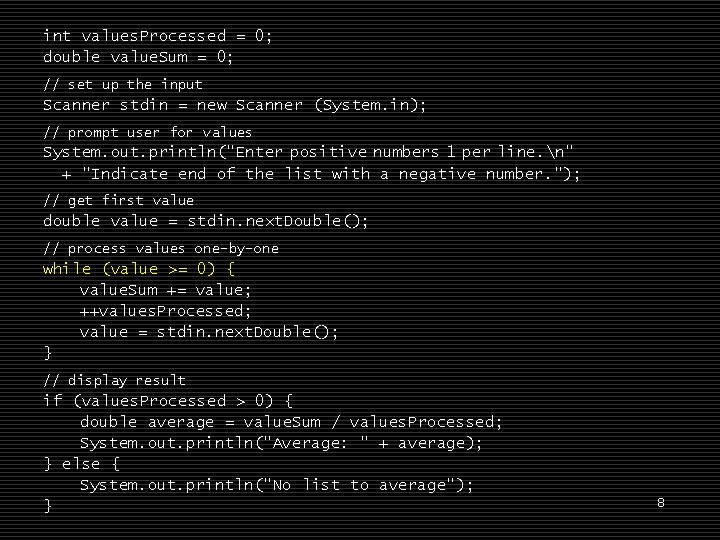
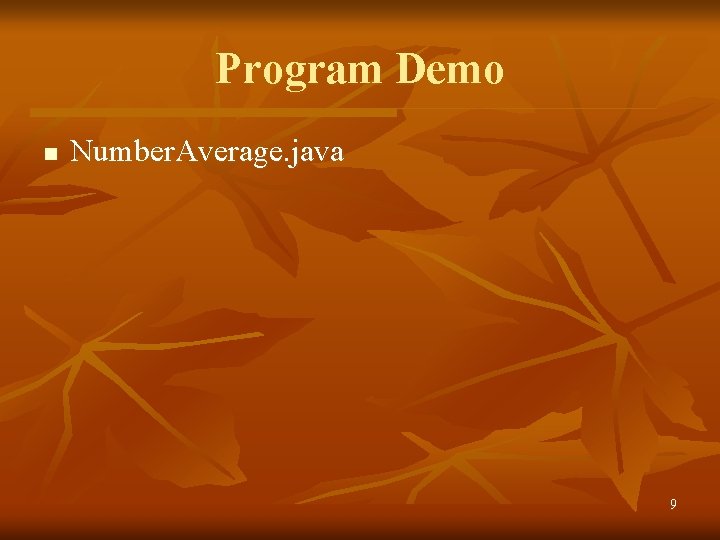
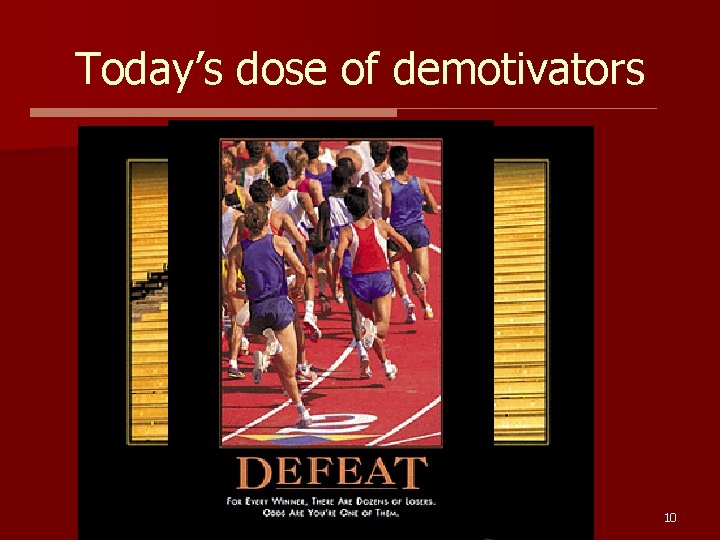
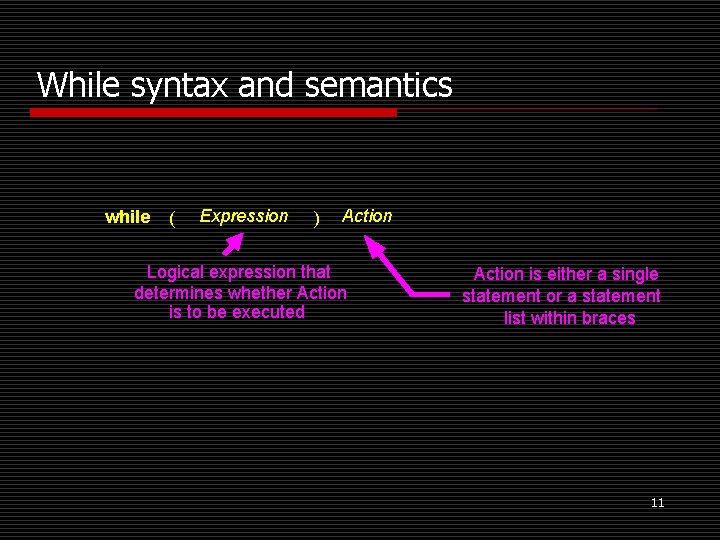
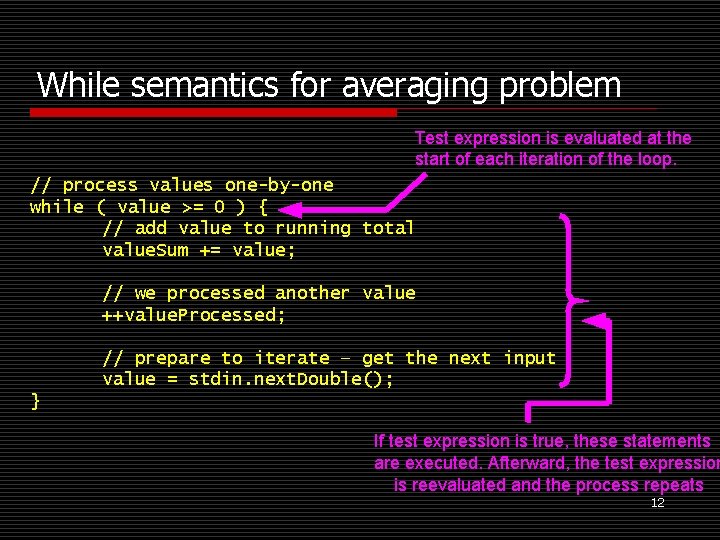
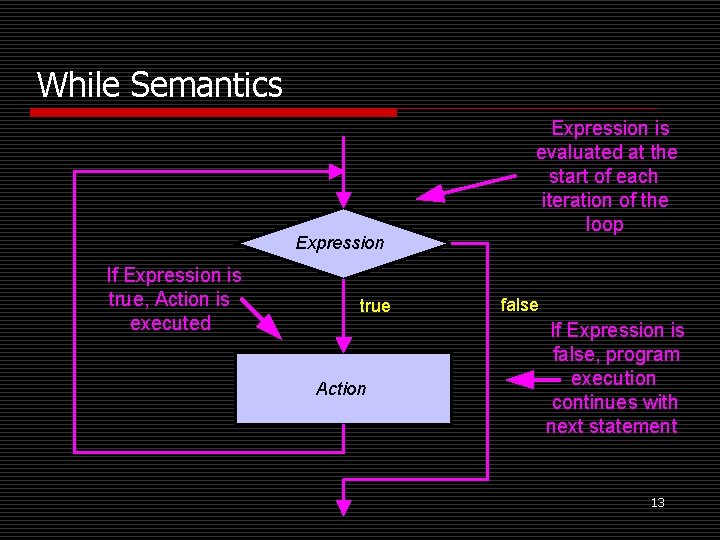
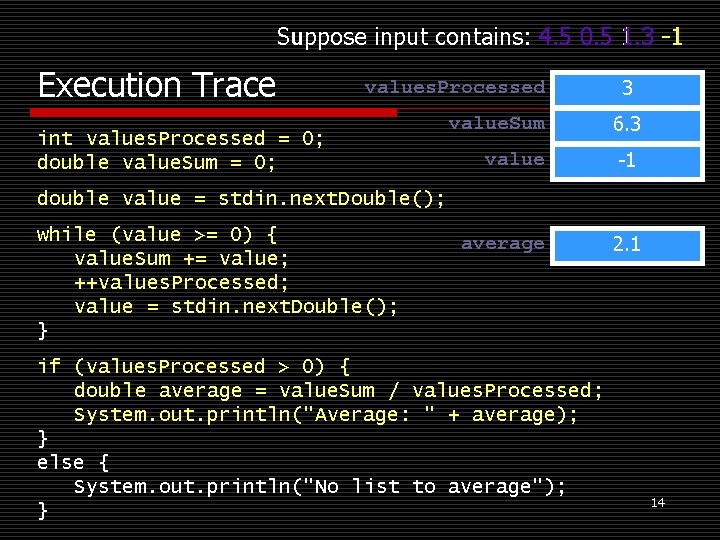
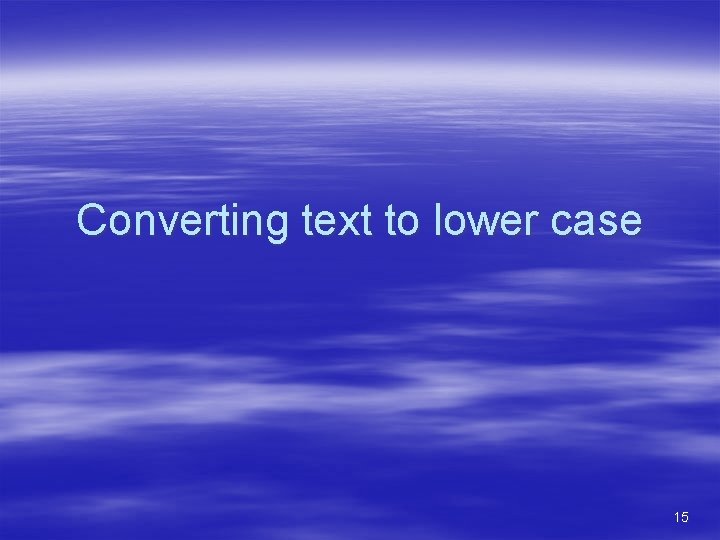
![Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin = Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin =](https://slidetodoc.com/presentation_image/f31e04bae69c2a009fc956421a67c2b8/image-16.jpg)
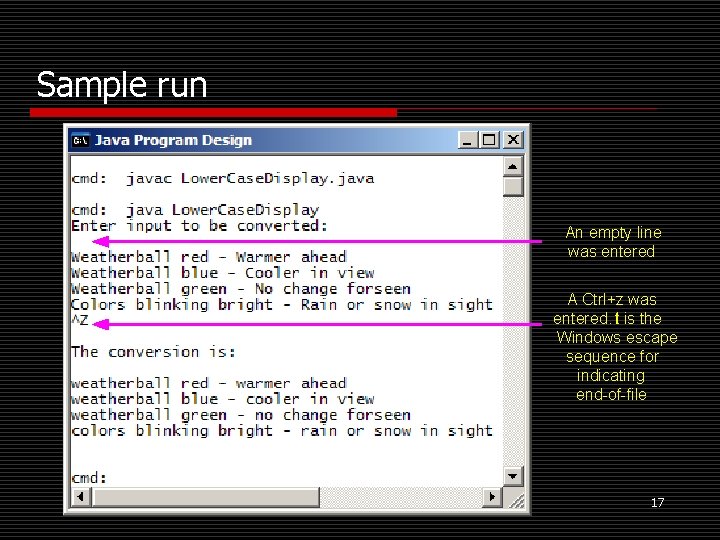
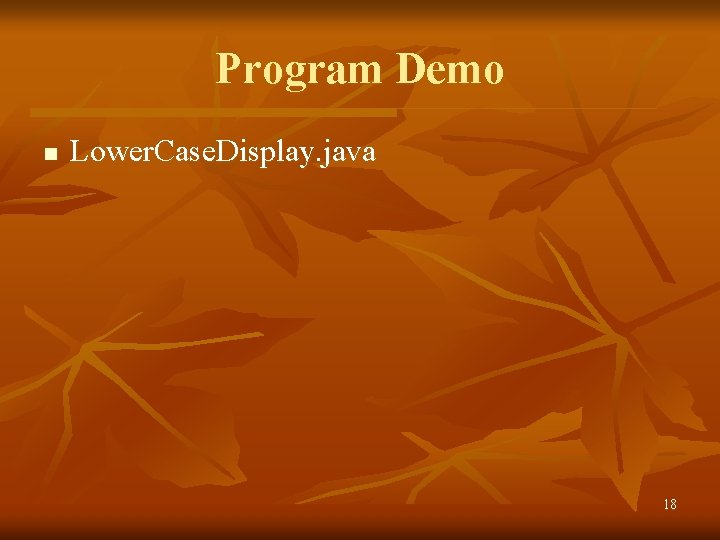
![Program trace public static void main(String[] args) { Scanner stdin = new Scanner (System. Program trace public static void main(String[] args) { Scanner stdin = new Scanner (System.](https://slidetodoc.com/presentation_image/f31e04bae69c2a009fc956421a67c2b8/image-19.jpg)
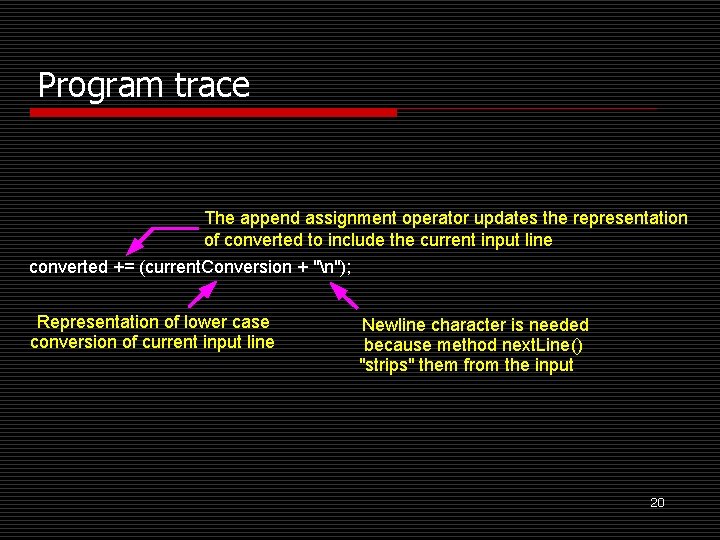
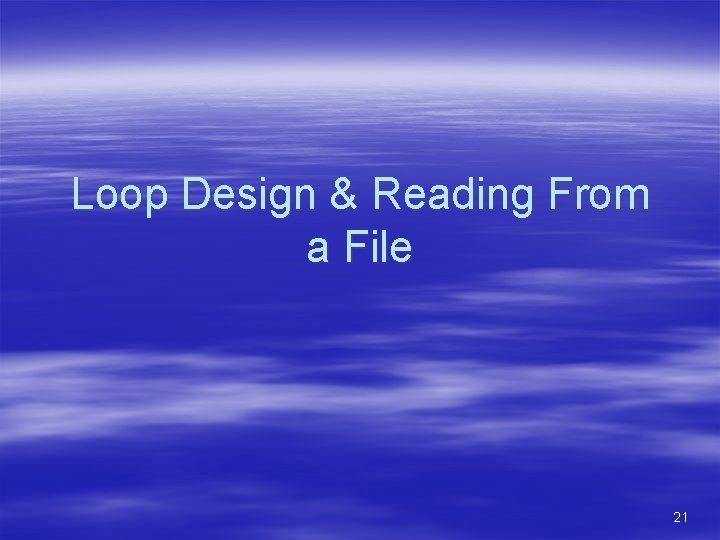
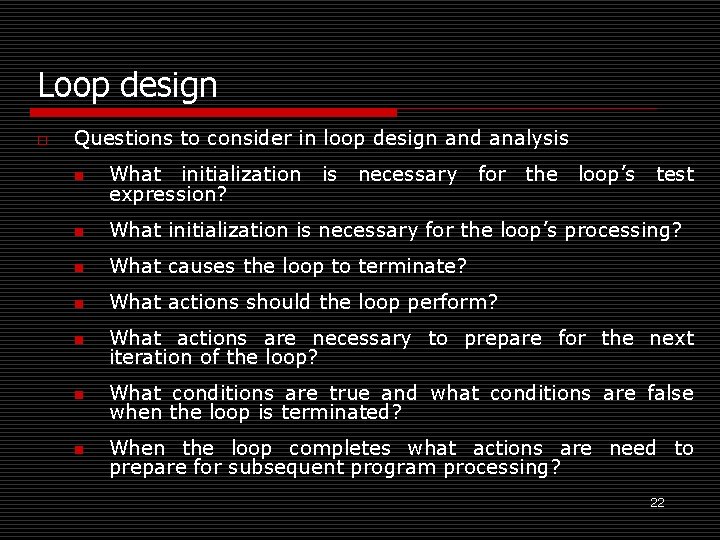
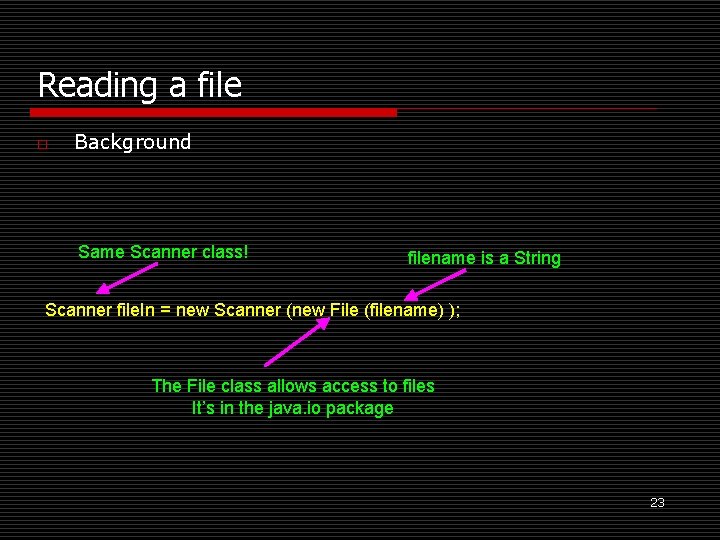
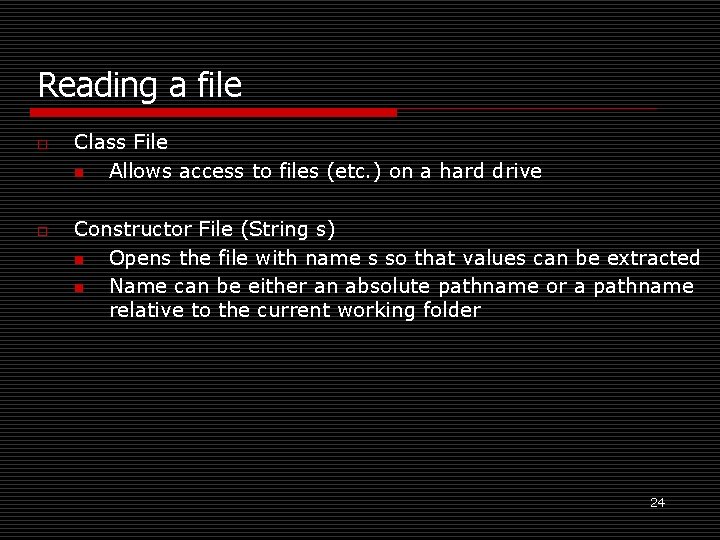
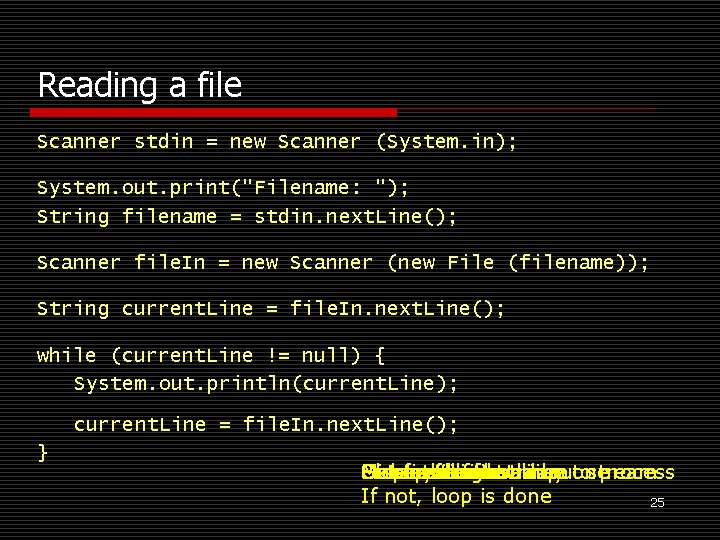
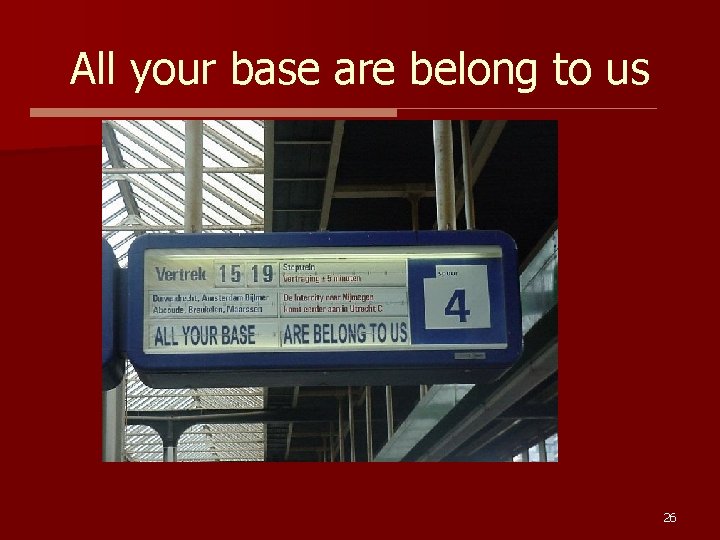
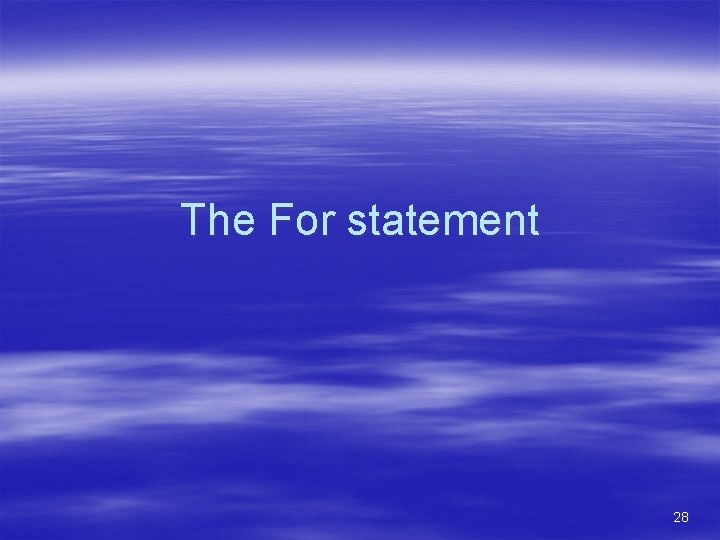
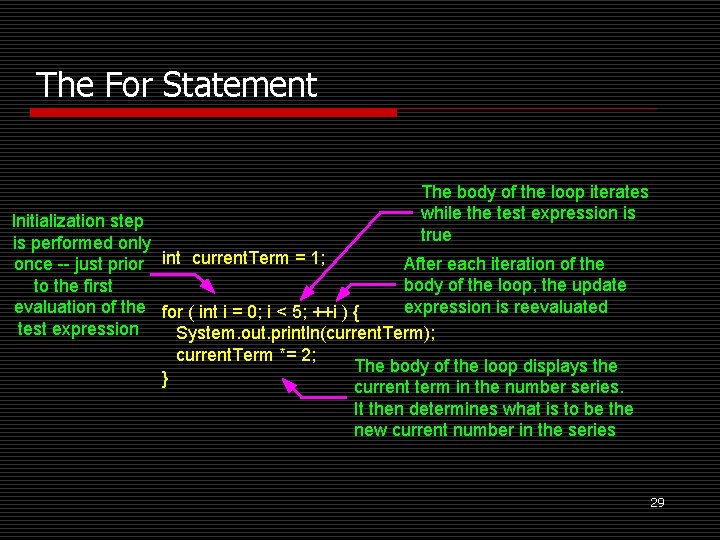
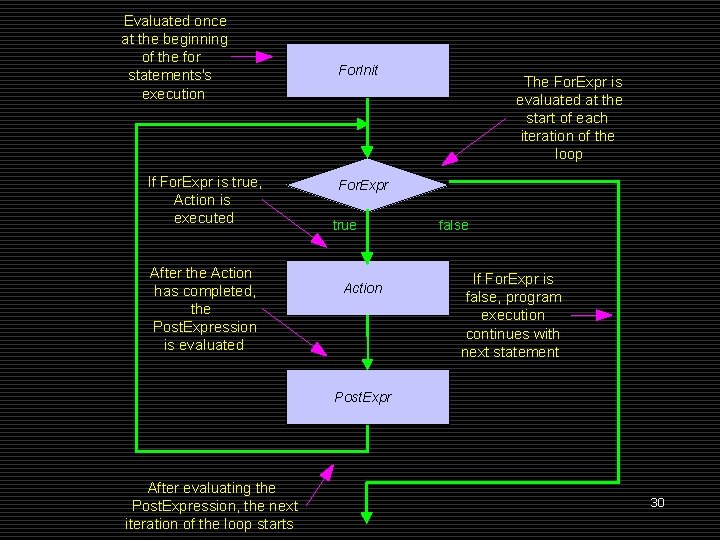
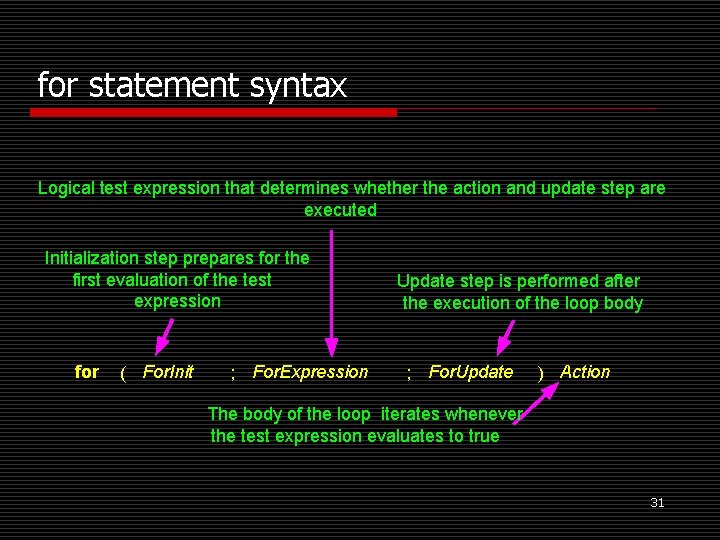
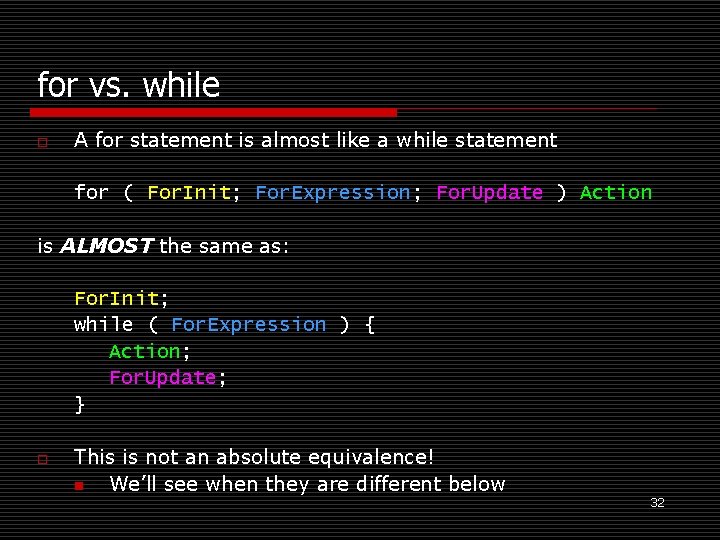
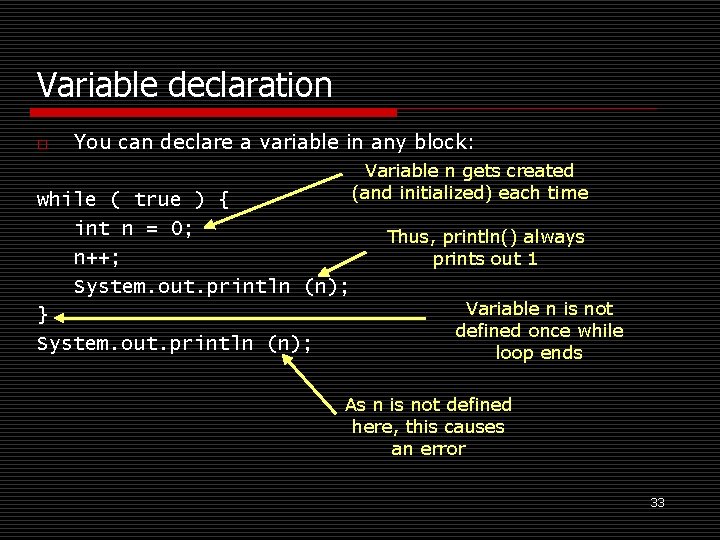
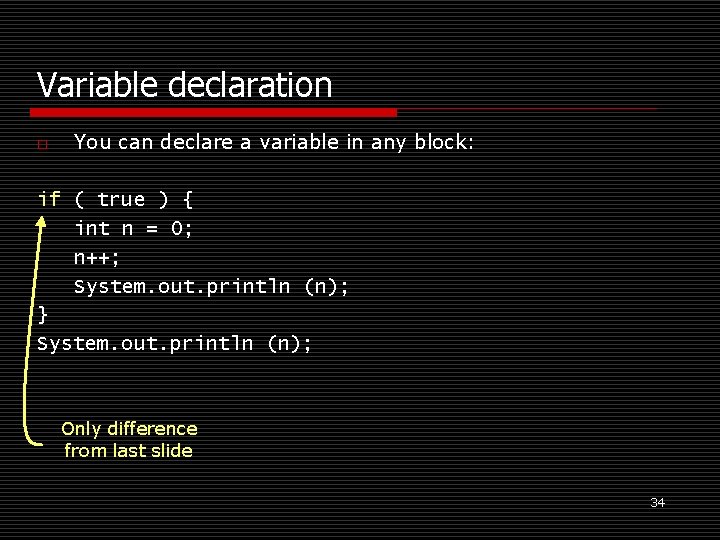
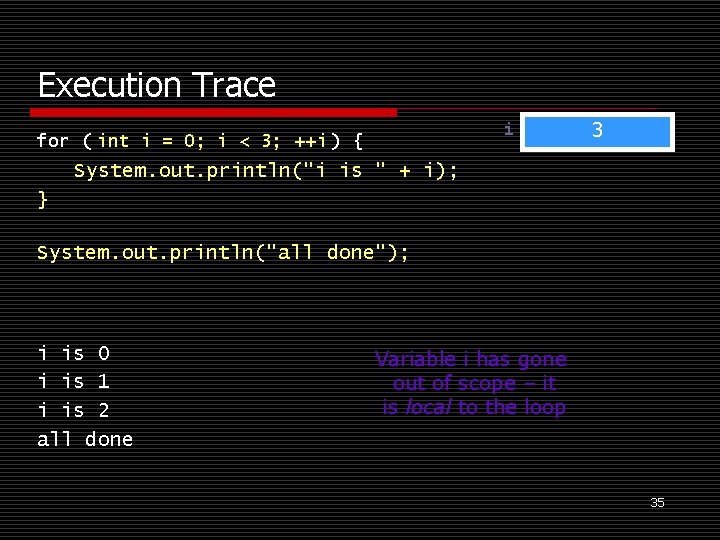
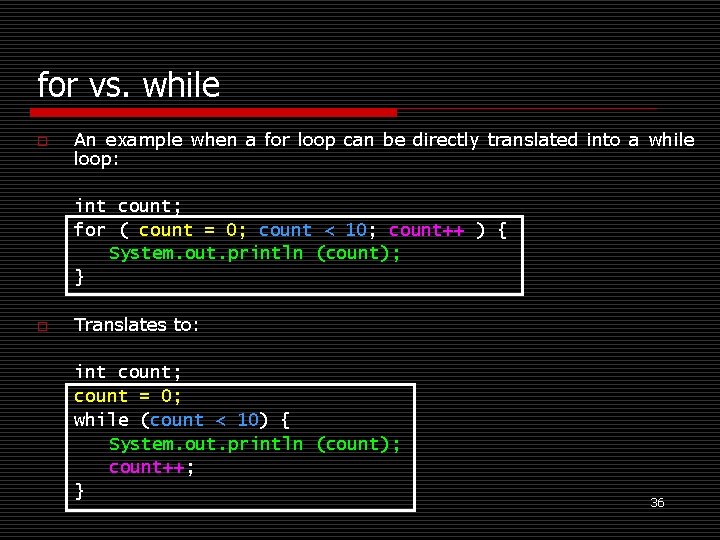
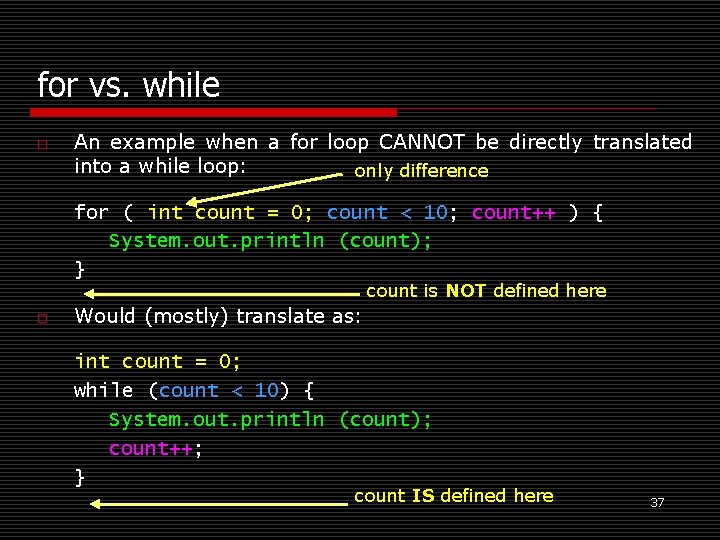
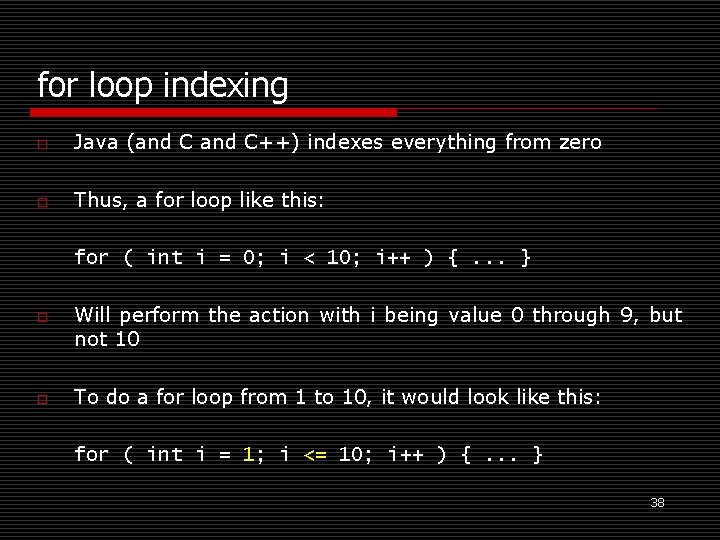
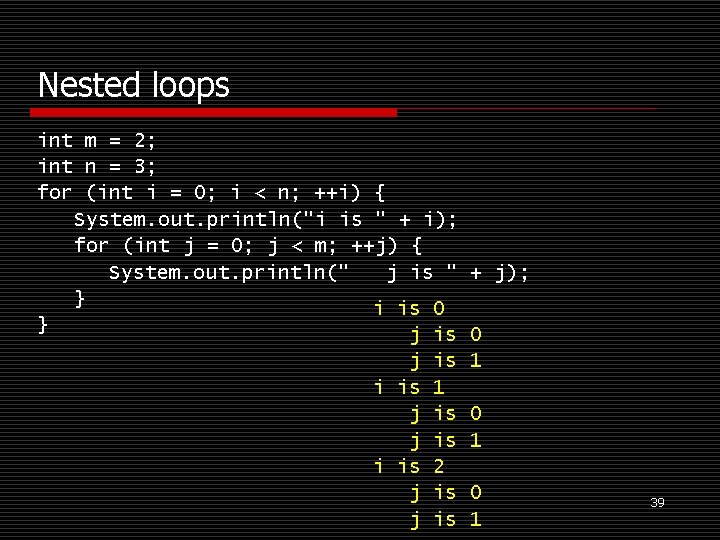
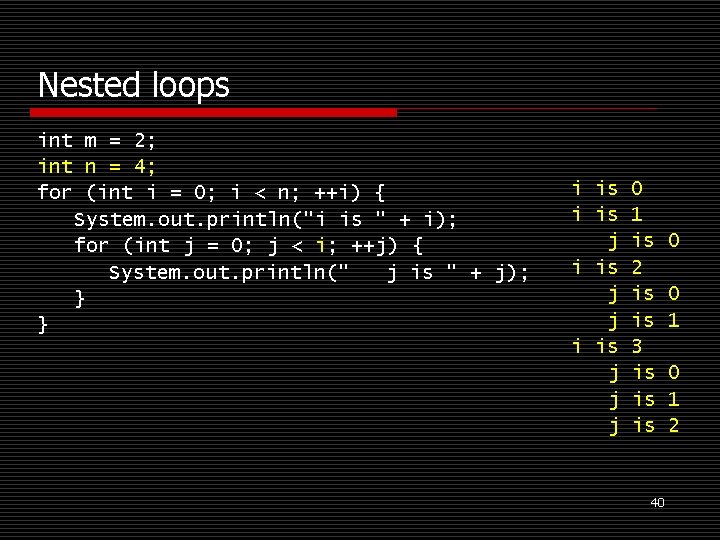
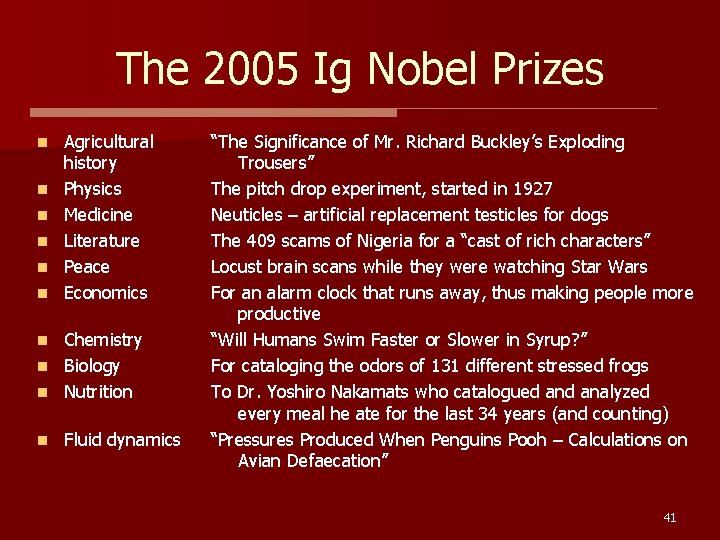
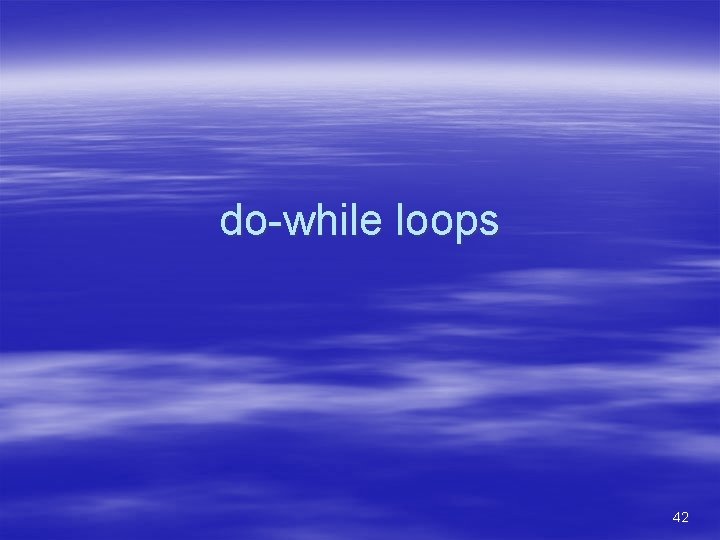
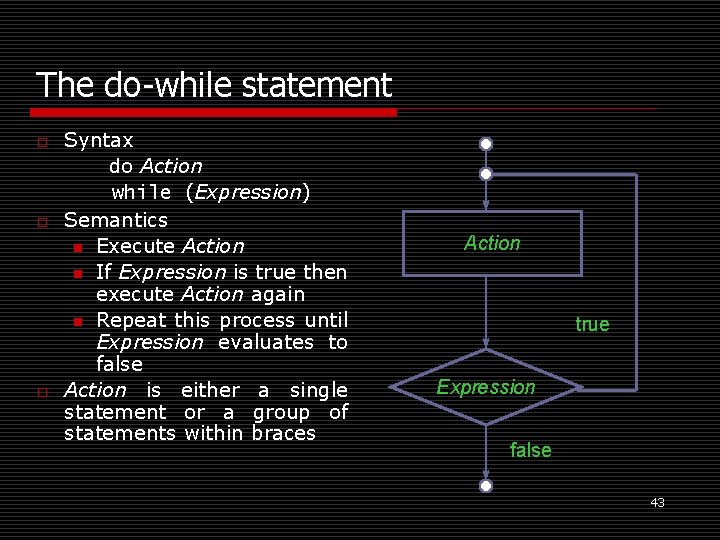
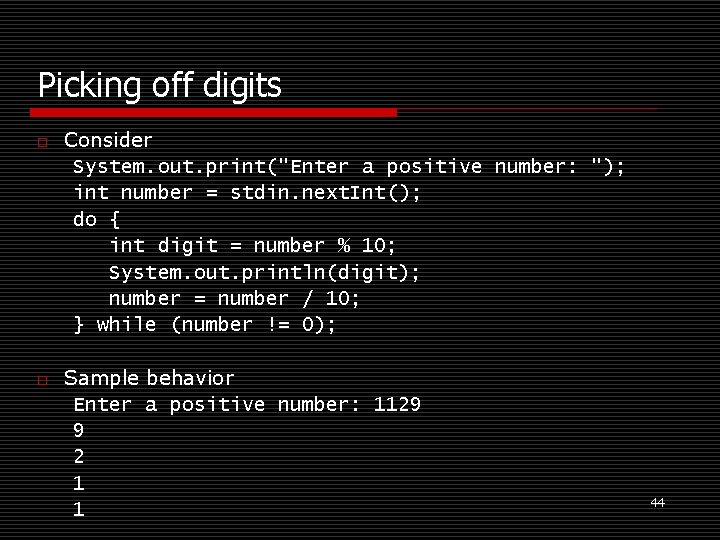
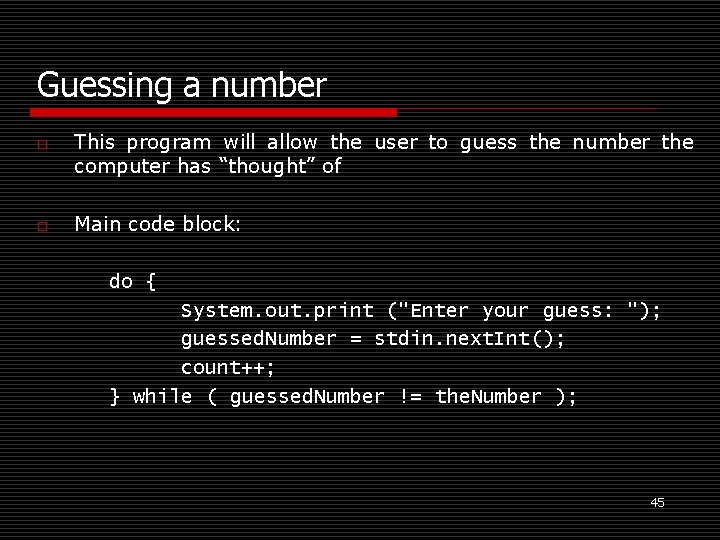
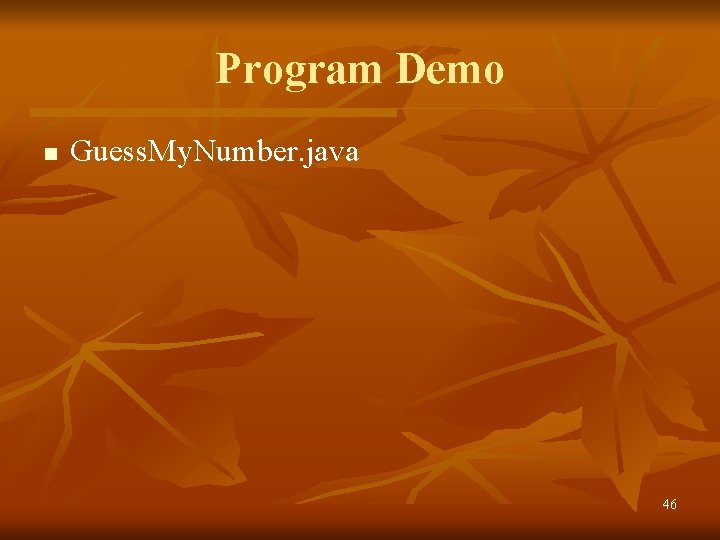
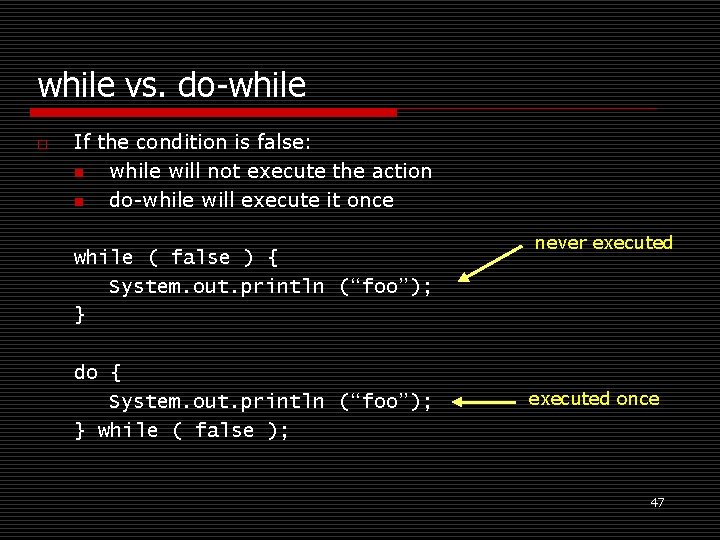
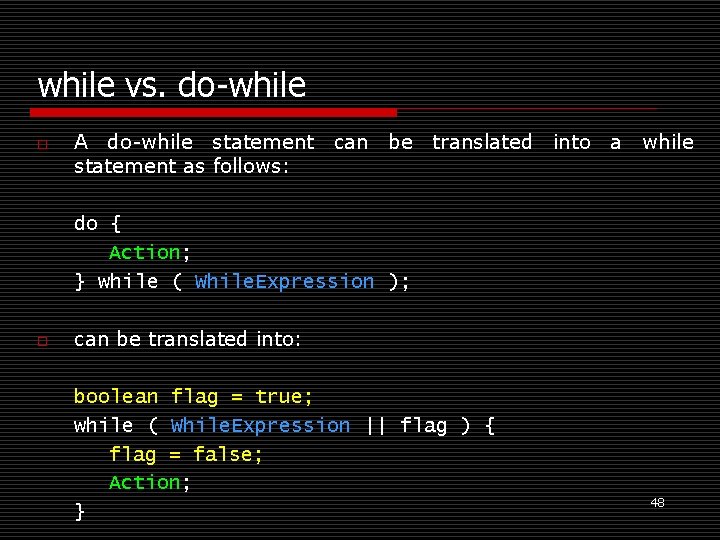
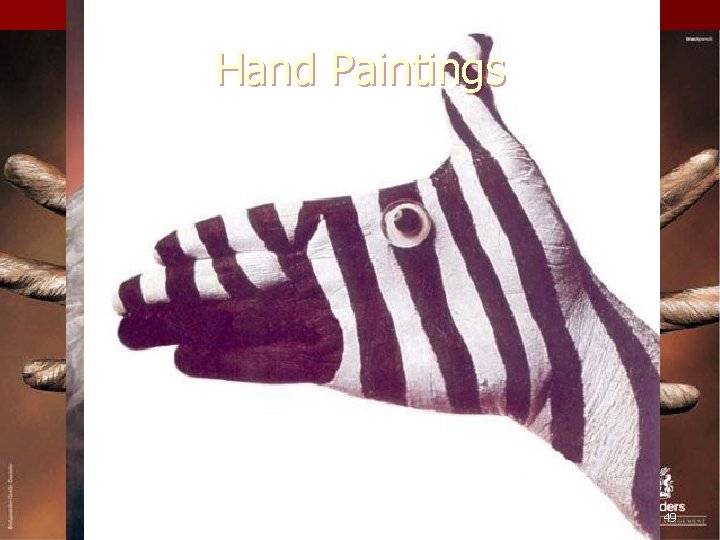
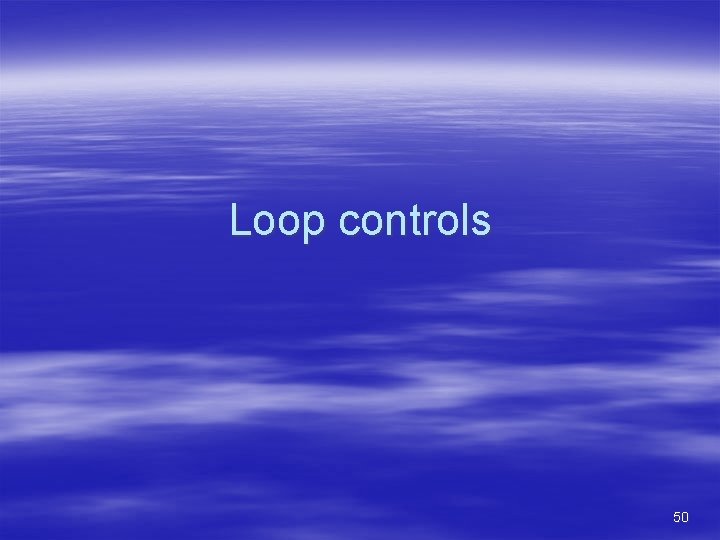
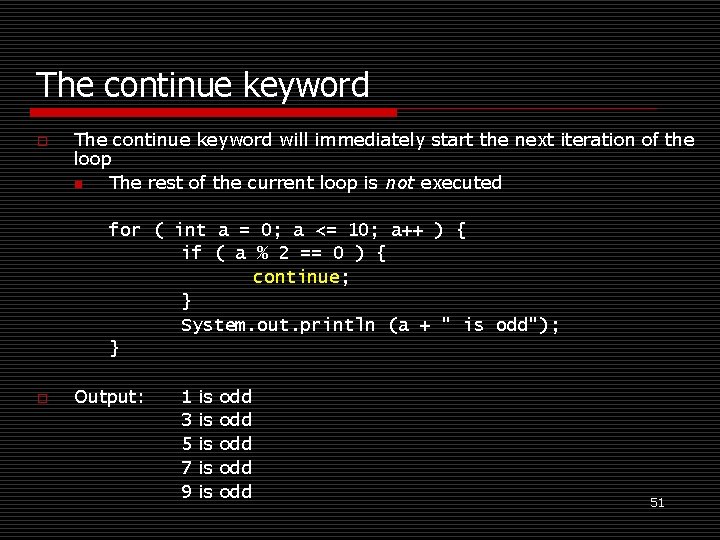
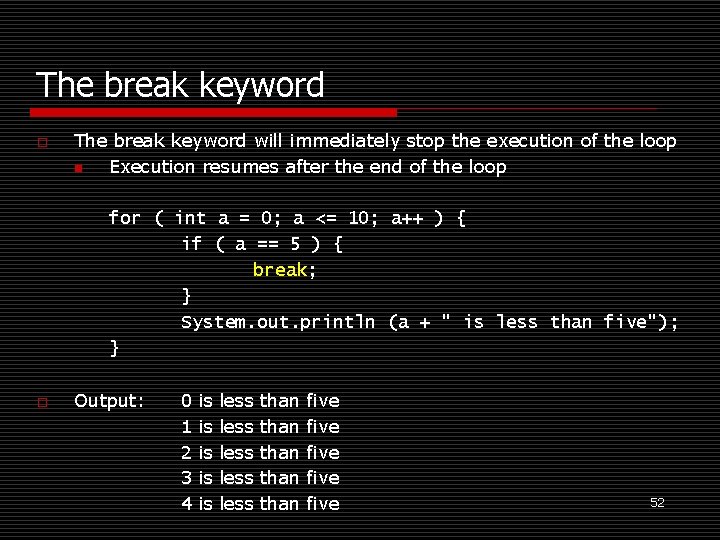
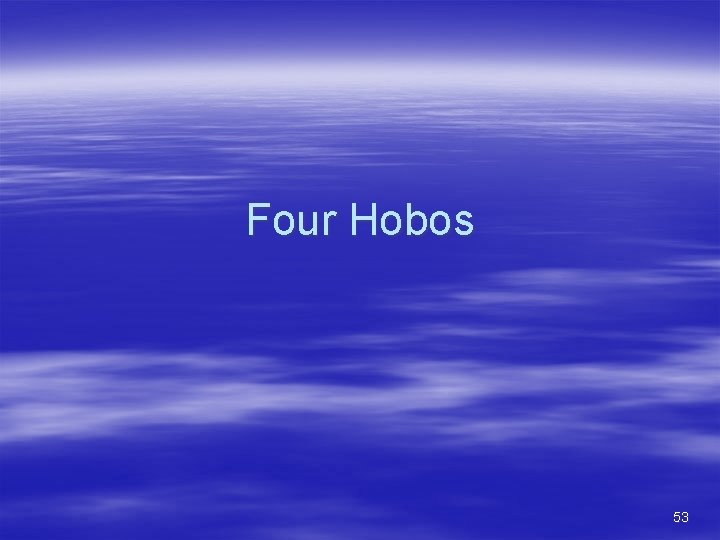
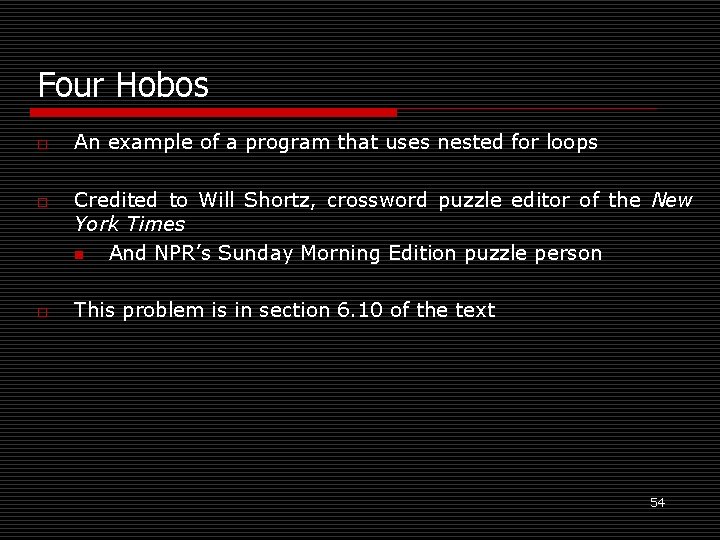
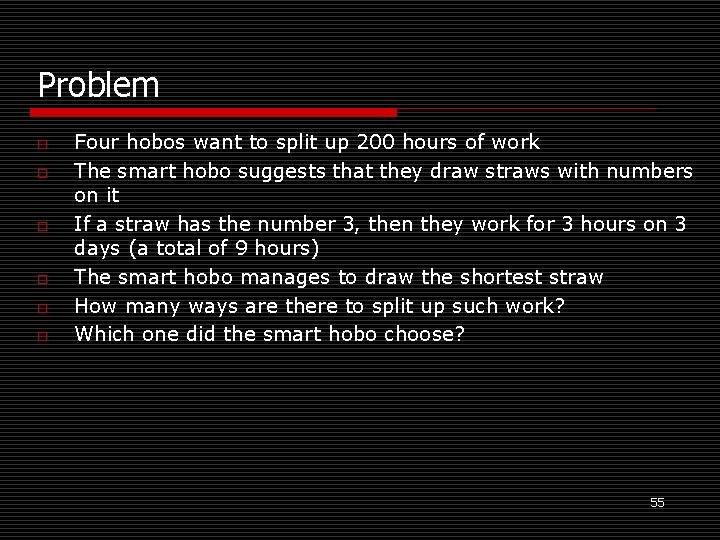
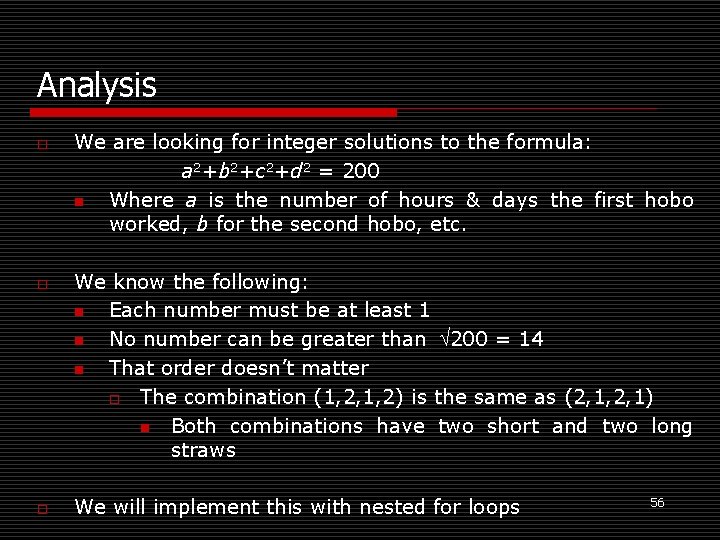
![Implementation public class Four. Hobos { public static void main (String[] args) { for Implementation public class Four. Hobos { public static void main (String[] args) { for](https://slidetodoc.com/presentation_image/f31e04bae69c2a009fc956421a67c2b8/image-56.jpg)
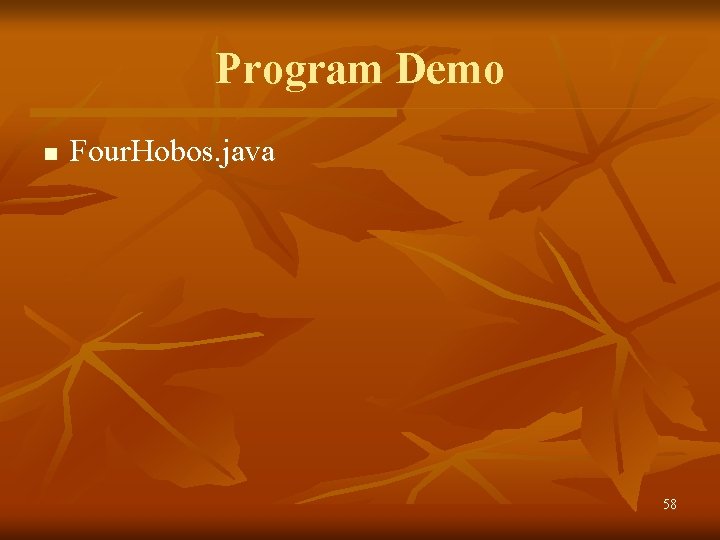
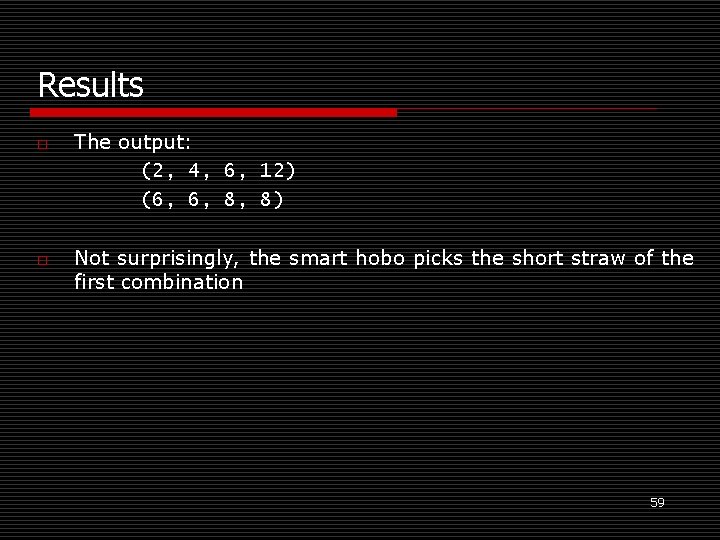
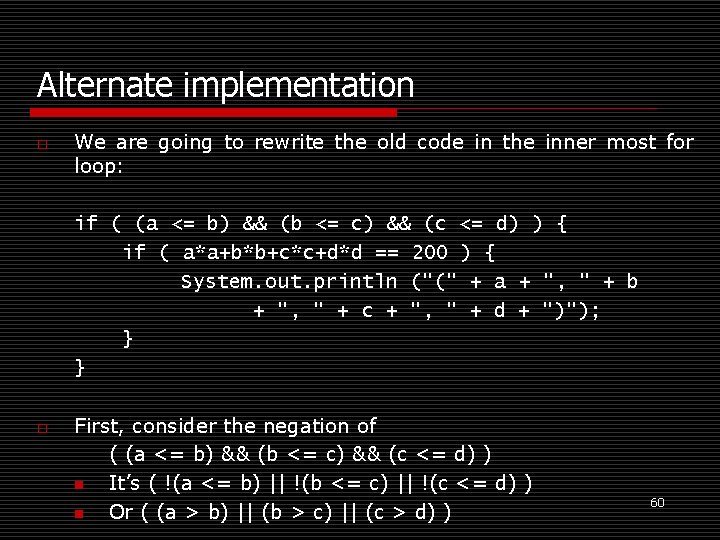
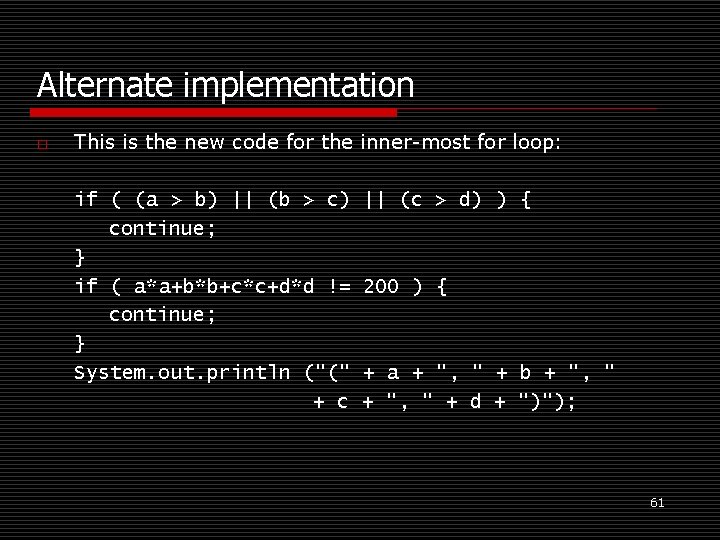
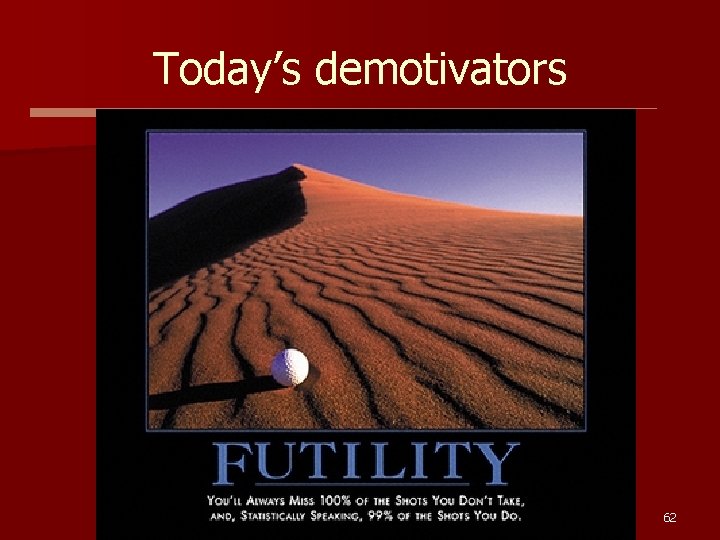
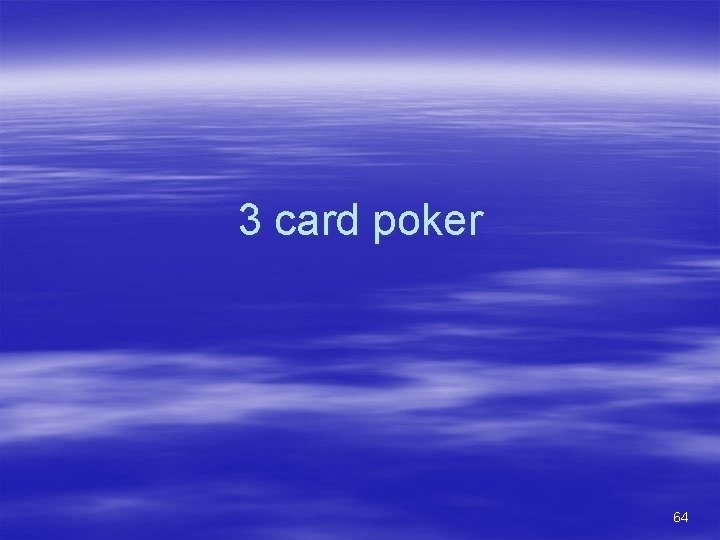
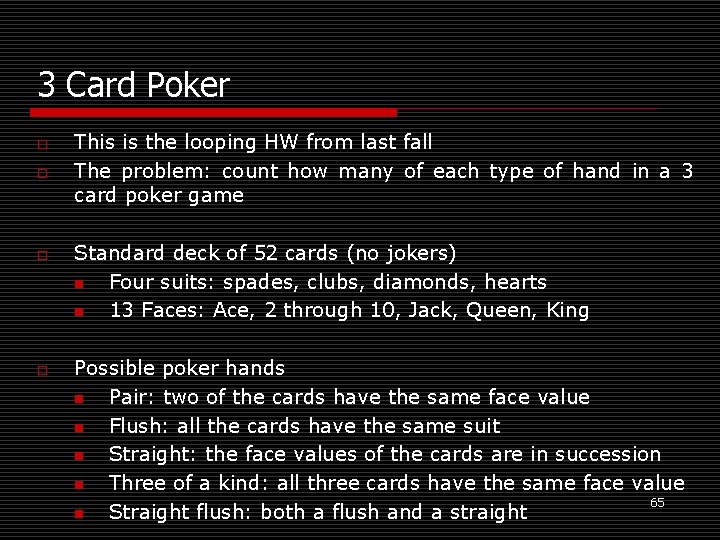
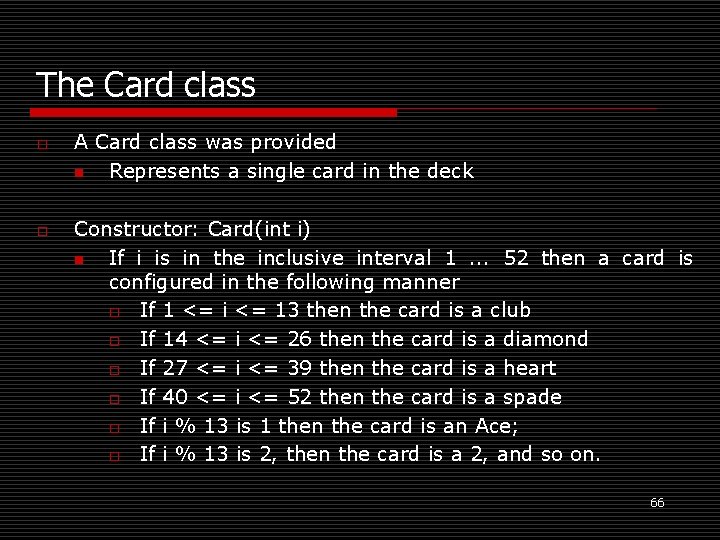
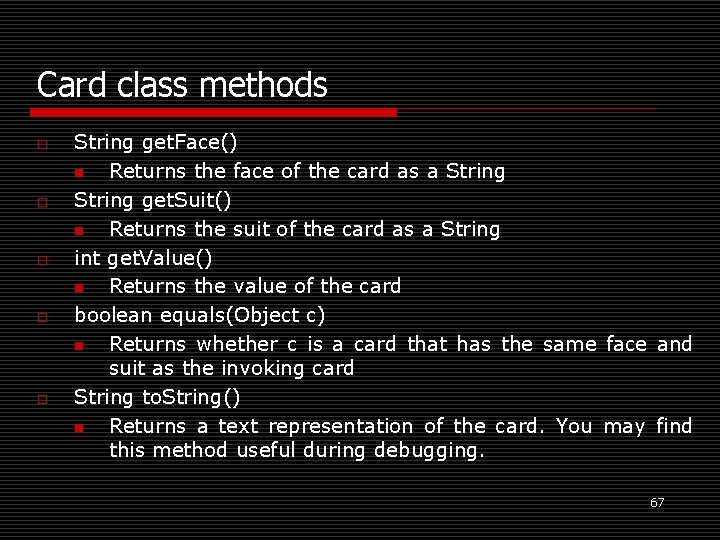
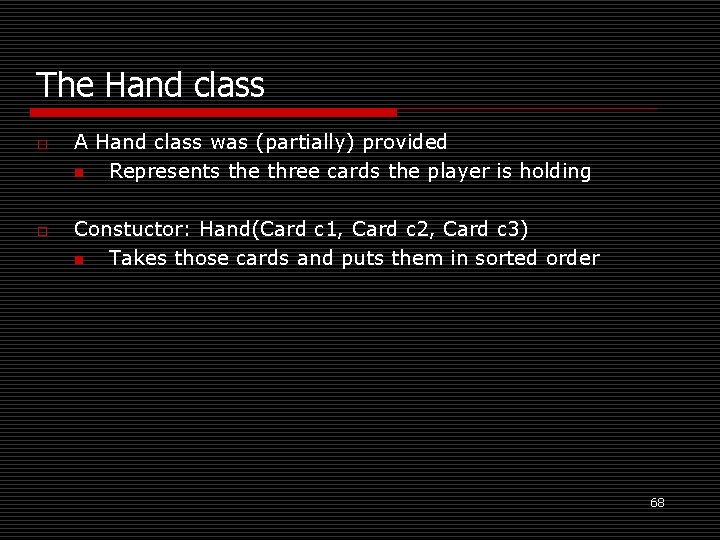
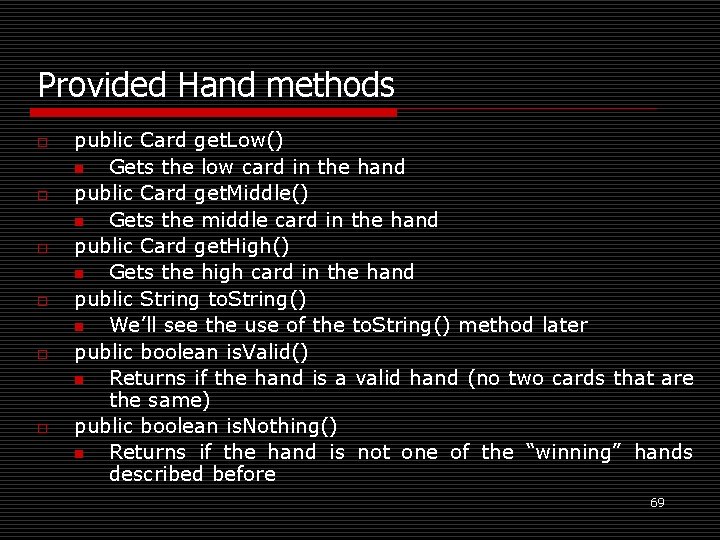
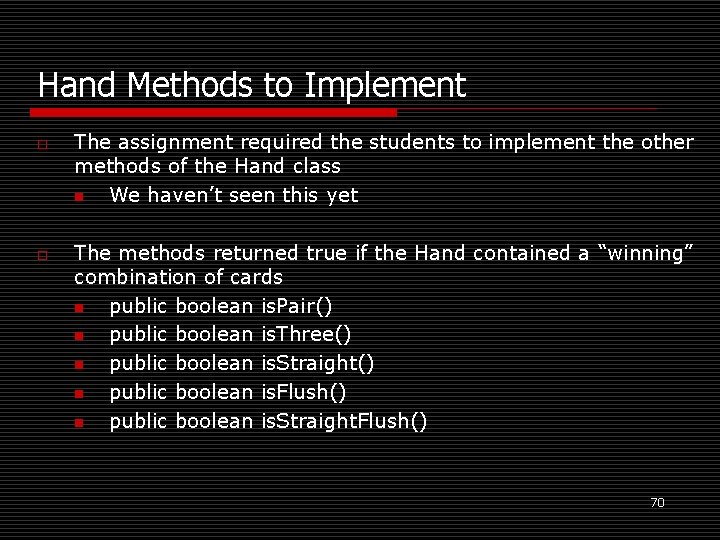
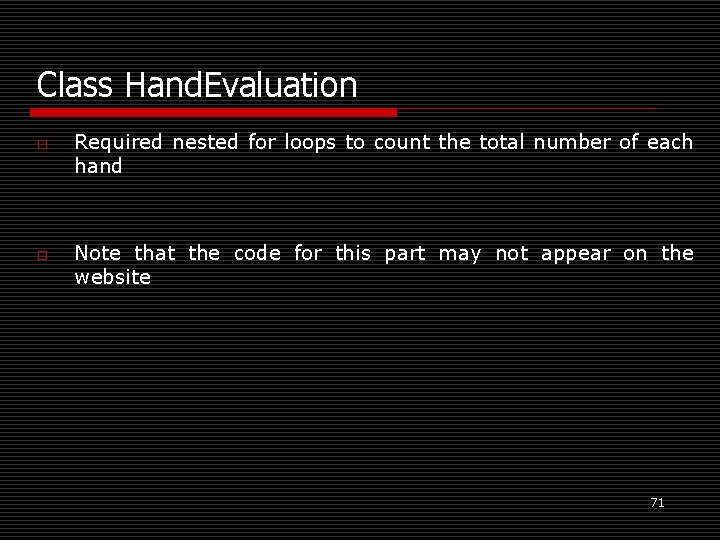
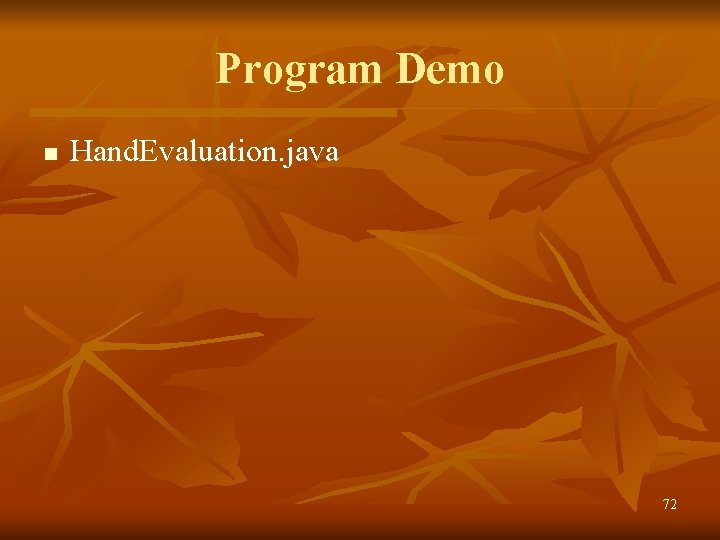
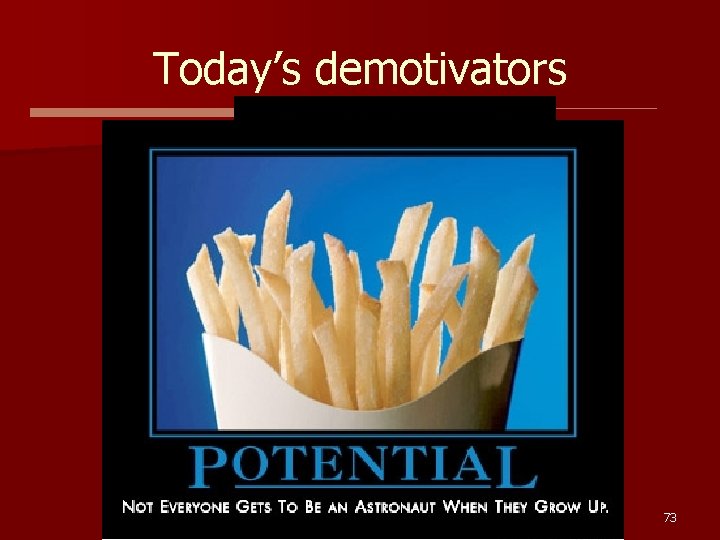
- Slides: 71
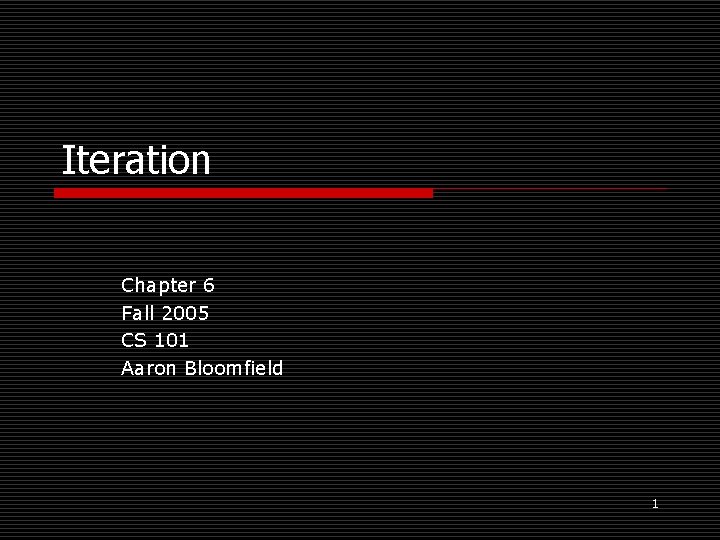
Iteration Chapter 6 Fall 2005 CS 101 Aaron Bloomfield 1
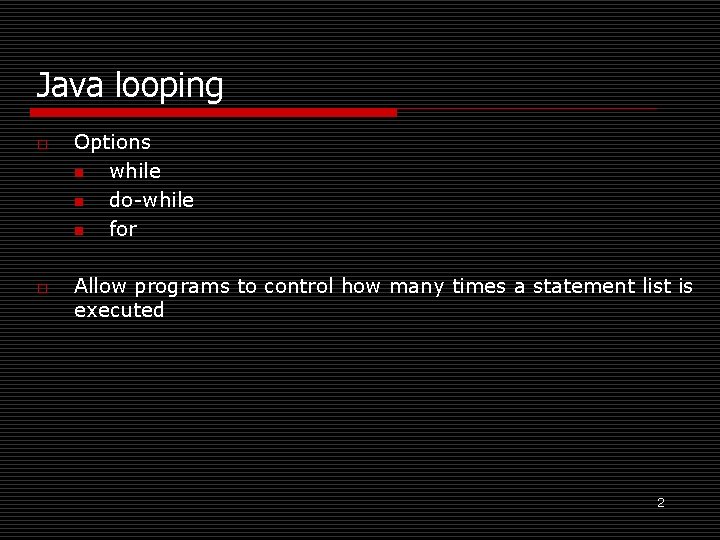
Java looping o o Options n while n do-while n for Allow programs to control how many times a statement list is executed 2
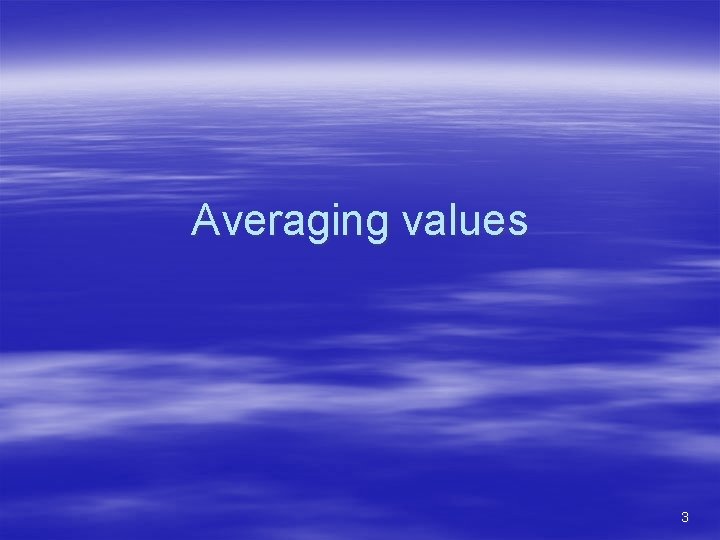
Averaging values 3
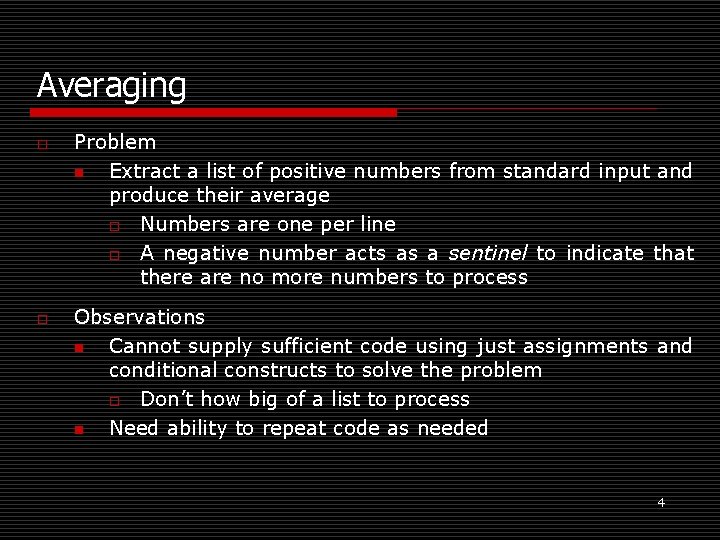
Averaging o o Problem n Extract a list of positive numbers from standard input and produce their average o Numbers are one per line o A negative number acts as a sentinel to indicate that there are no more numbers to process Observations n Cannot supply sufficient code using just assignments and conditional constructs to solve the problem o Don’t how big of a list to process n Need ability to repeat code as needed 4
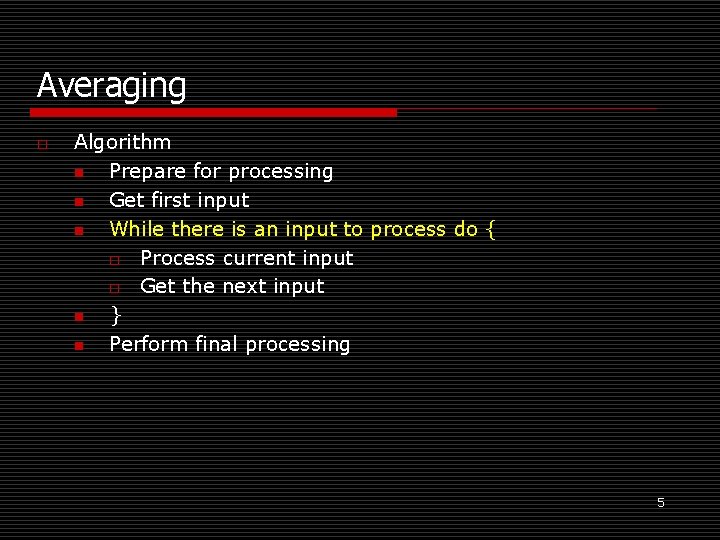
Averaging o Algorithm n Prepare for processing n Get first input n While there is an input to process do { o Process current input o Get the next input n } n Perform final processing 5
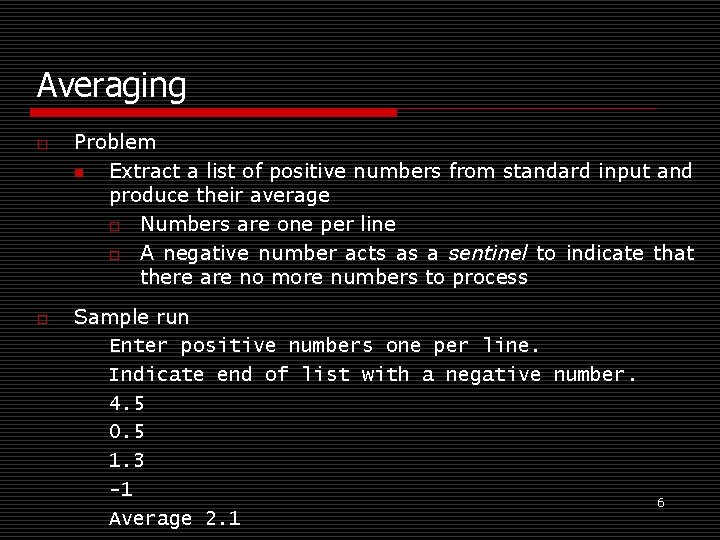
Averaging o o Problem n Extract a list of positive numbers from standard input and produce their average o Numbers are one per line o A negative number acts as a sentinel to indicate that there are no more numbers to process Sample run Enter positive numbers one per line. Indicate end of list with a negative number. 4. 5 0. 5 1. 3 -1 Average 2. 1 6
![public class Number Average main application entry point public static void mainString public class Number. Average { // main(): application entry point public static void main(String[]](https://slidetodoc.com/presentation_image/f31e04bae69c2a009fc956421a67c2b8/image-7.jpg)
public class Number. Average { // main(): application entry point public static void main(String[] args) { // set up the input // prompt user for values // get first value // process values one-by-one while (value >= 0) { // add value to running total // processed another value // prepare next iteration - get next value } // display result if (values. Processed > 0) // compute and display average else // indicate no average to display } } 7
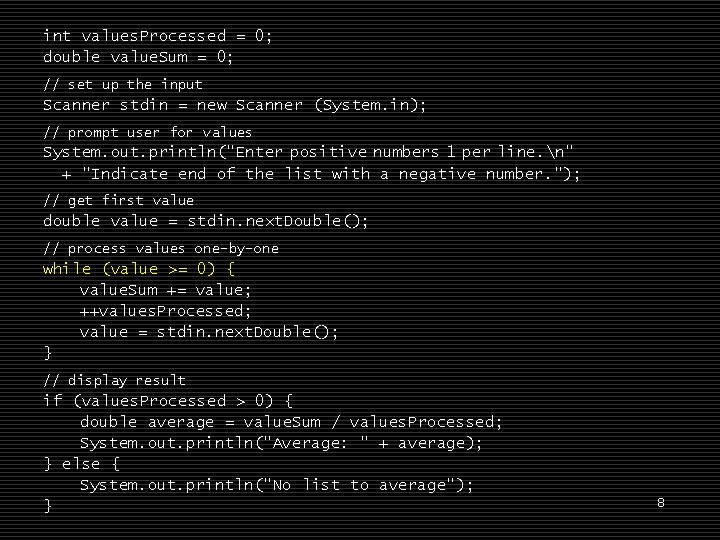
int values. Processed = 0; double value. Sum = 0; // set up the input Scanner stdin = new Scanner (System. in); // prompt user for values System. out. println("Enter positive numbers 1 per line. n" + "Indicate end of the list with a negative number. "); // get first value double value = stdin. next. Double(); // process values one-by-one while (value >= 0) { value. Sum += value; ++values. Processed; value = stdin. next. Double(); } // display result if (values. Processed > 0) { double average = value. Sum / values. Processed; System. out. println("Average: " + average); } else { System. out. println("No list to average"); } 8
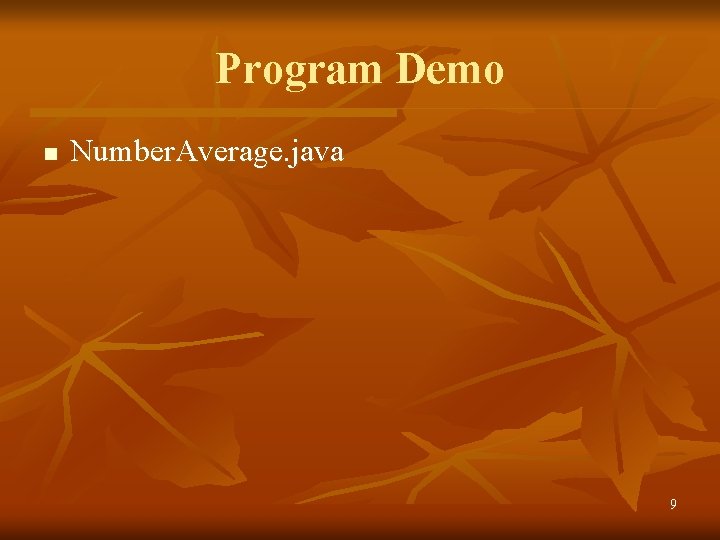
Program Demo n Number. Average. java 9
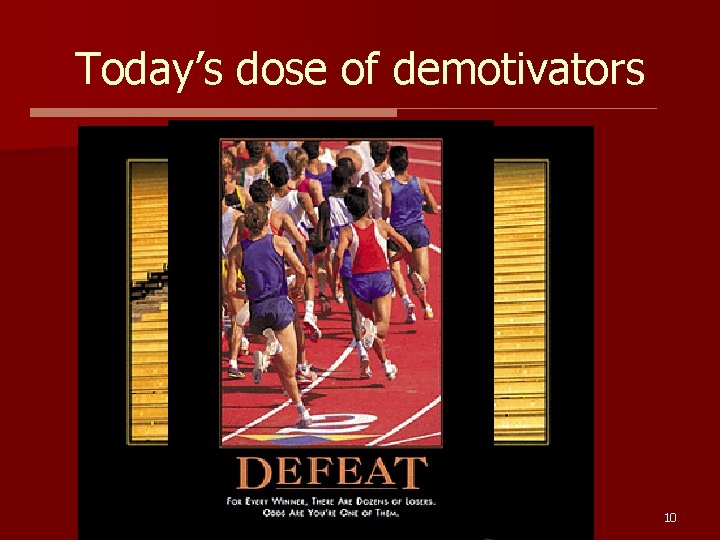
Today’s dose of demotivators 10
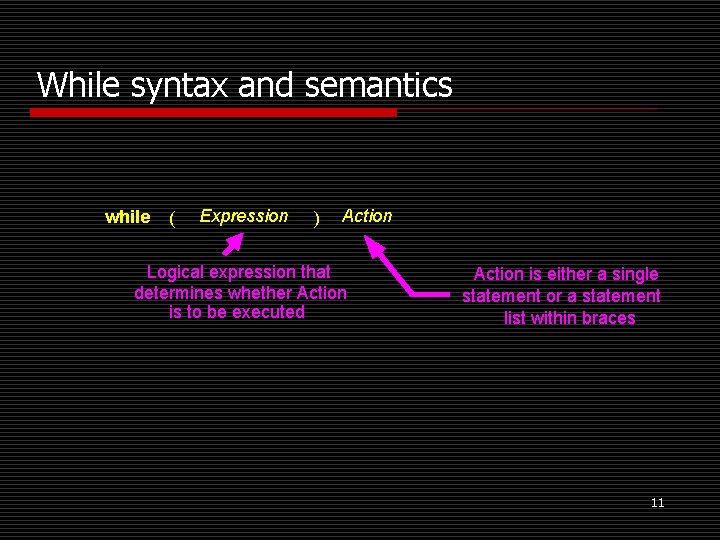
While syntax and semantics while ( Expression ) Action Logical expression that determines whether Action is to be executed Action is either a single statement or a statement list within braces 11
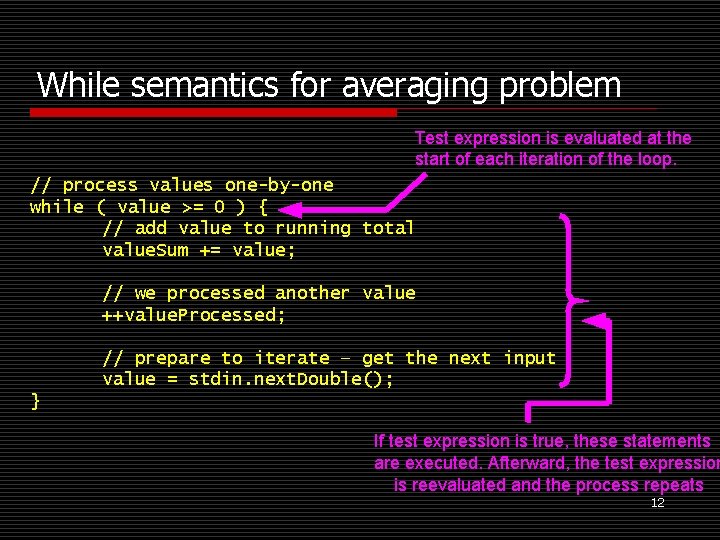
While semantics for averaging problem Test expression is evaluated at the start of each iteration of the loop. // process values one-by-one while ( value >= 0 ) { // add value to running total value. Sum += value; // we processed another value ++value. Processed; // prepare to iterate – get the next input value = stdin. next. Double(); } If test expression is true, these statements are executed. Afterward, the test expression is reevaluated and the process repeats 12
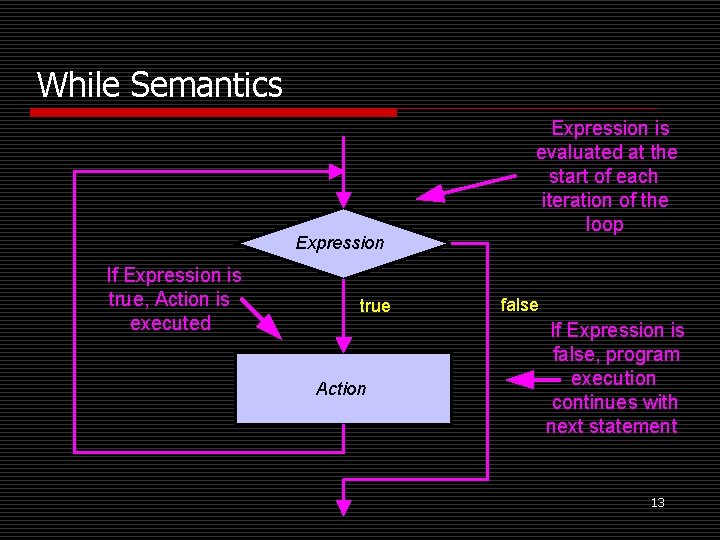
While Semantics Expression If Expression is true, Action is executed true Action Expression is evaluated at the start of each iteration of the loop false If Expression is false, program execution continues with next statement 13
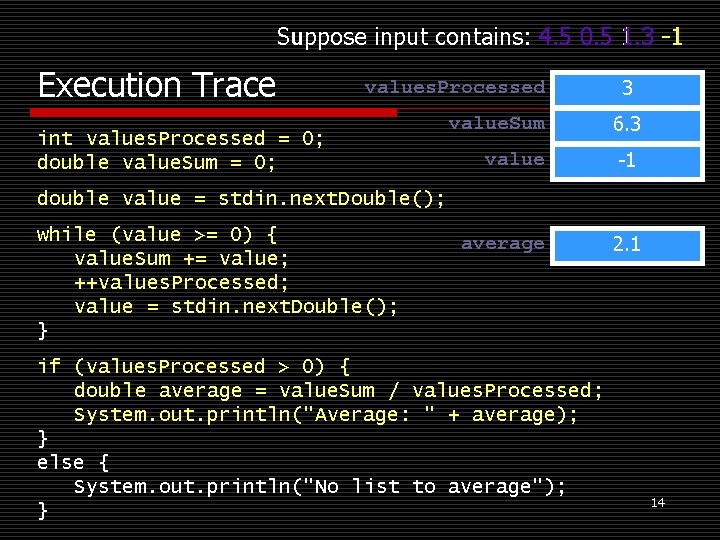
Suppose input contains: 4. 5 0. 5 1. 3 -1 Execution Trace values. Processed int values. Processed = 0; double value. Sum = 0; 0 1 2 3 value. Sum 4. 5 5. 0 6. 3 0 value 4. 5 0. 5 1. 3 -1 average 2. 1 double value = stdin. next. Double(); while (value >= 0) { value. Sum += value; ++values. Processed; value = stdin. next. Double(); } if (values. Processed > 0) { double average = value. Sum / values. Processed; System. out. println("Average: " + average); } else { System. out. println("No list to average"); } 14
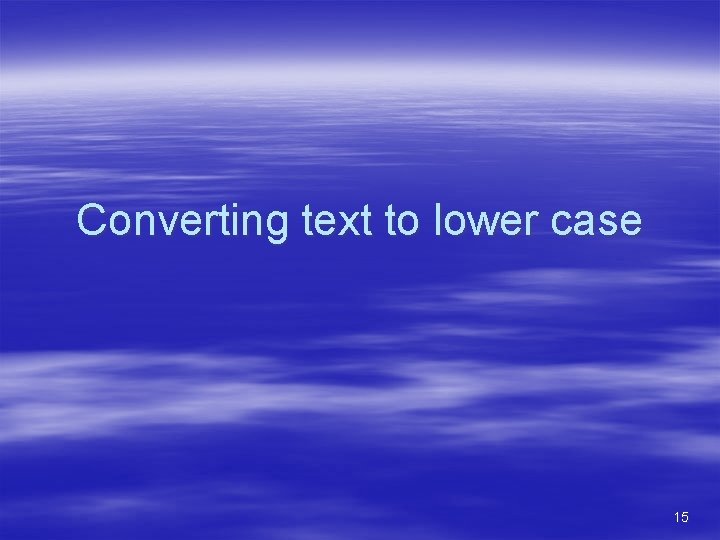
Converting text to lower case 15
![Converting text to strictly lowercase public static void mainString args Scanner stdin Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin =](https://slidetodoc.com/presentation_image/f31e04bae69c2a009fc956421a67c2b8/image-16.jpg)
Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin = new Scanner (System. in); System. out. println("Enter input to be converted: "); String converted = ""; while (stdin. has. Next()) { String current. Line = stdin. next. Line(); String current. Conversion = current. Line. to. Lower. Case(); converted += (current. Conversion + "n"); } System. out. println("n. Conversion is: n" + converted); } 16
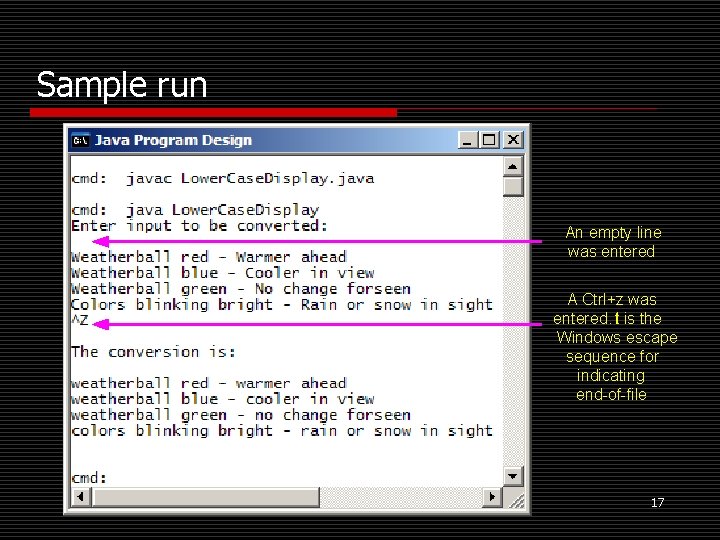
Sample run An empty line was entered A Ctrl+z was entered. t. I is the Windows escape sequence for indicating end-of-file 17
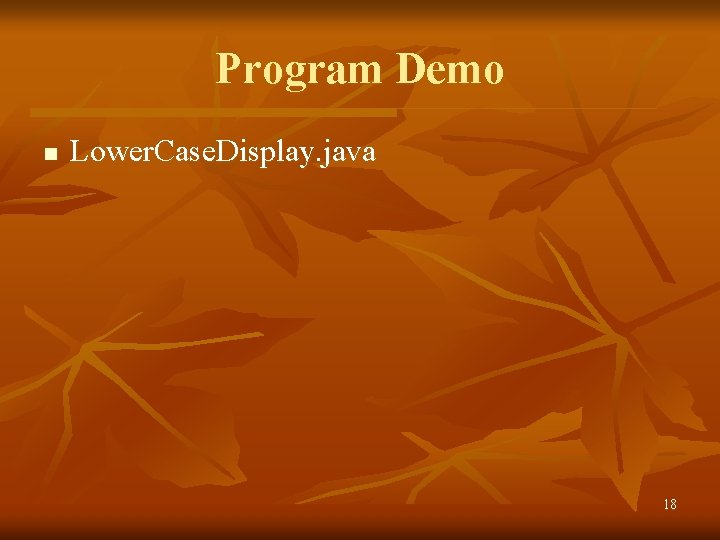
Program Demo n Lower. Case. Display. java 18
![Program trace public static void mainString args Scanner stdin new Scanner System Program trace public static void main(String[] args) { Scanner stdin = new Scanner (System.](https://slidetodoc.com/presentation_image/f31e04bae69c2a009fc956421a67c2b8/image-19.jpg)
Program trace public static void main(String[] args) { Scanner stdin = new Scanner (System. in); System. out. println("Enter input to be converted: "); String converted = ""; while (stdin. has. Next()) { String current. Line = stdin. next. Line(); String current. Conversion = current. Line. to. Lower. Case(); converted += (current. Conversion + "n"); } System. out. println("n. Conversion is: n" + converted); 19
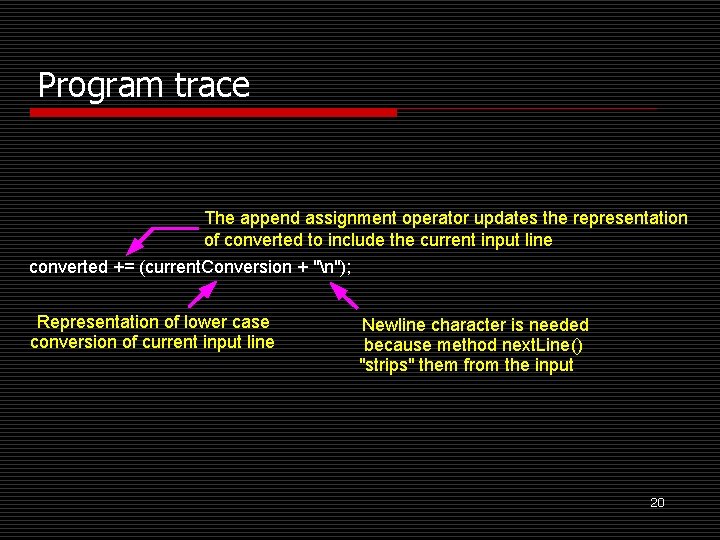
Program trace The append assignment operator updates the representation of converted to include the current input line converted += (current. Conversion + "n"); Representation of lower case conversion of current input line Newline character is needed because method next. Line() "strips" them from the input 20
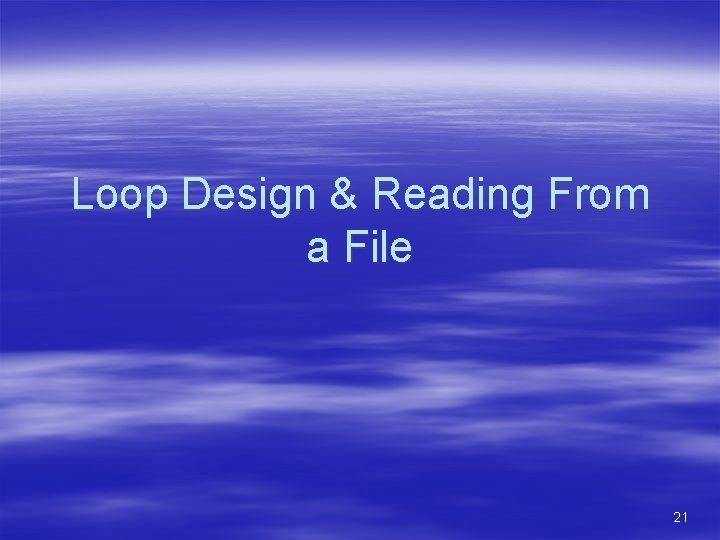
Loop Design & Reading From a File 21
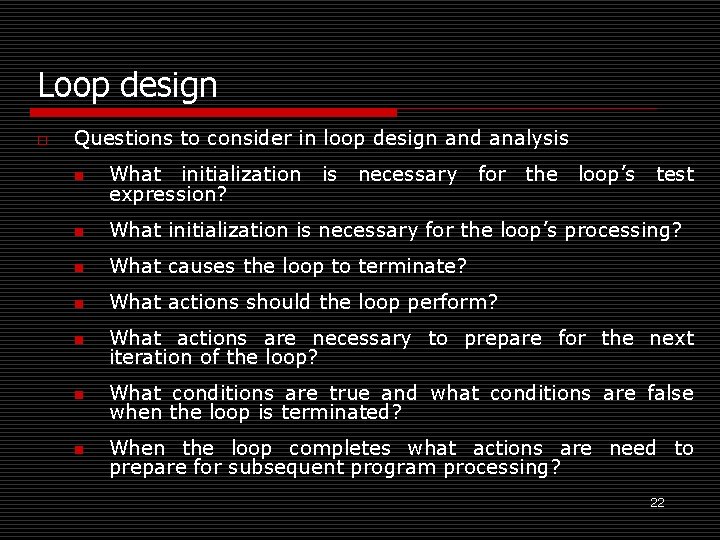
Loop design o Questions to consider in loop design and analysis n What initialization expression? is necessary for the loop’s test n What initialization is necessary for the loop’s processing? n What causes the loop to terminate? n What actions should the loop perform? n What actions are necessary to prepare for the next iteration of the loop? n What conditions are true and what conditions are false when the loop is terminated? n When the loop completes what actions are need to prepare for subsequent program processing? 22
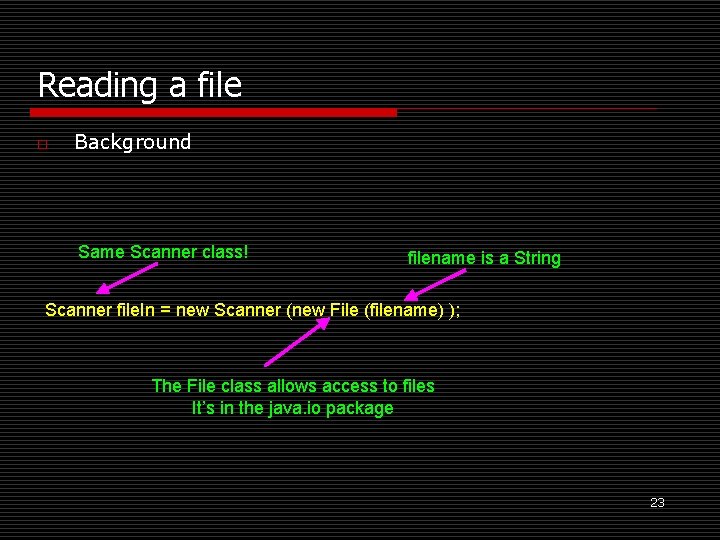
Reading a file o Background Same Scanner class! filename is a String Scanner file. In = new Scanner (new File (filename) ); The File class allows access to files It’s in the java. io package 23
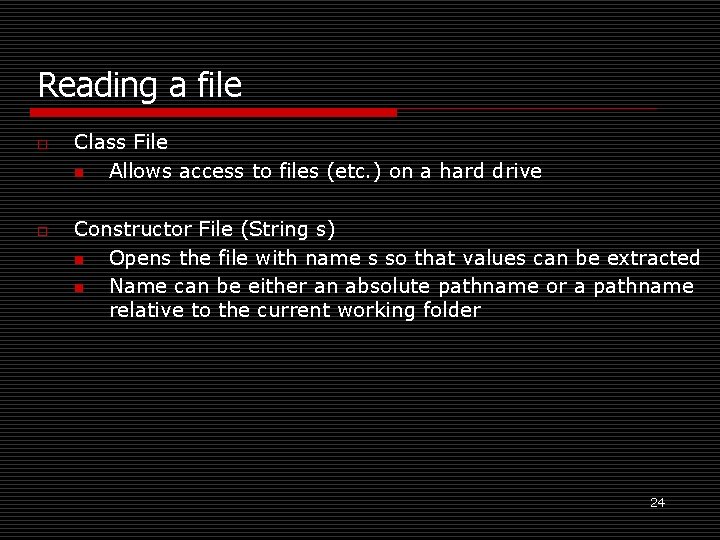
Reading a file o o Class File n Allows access to files (etc. ) on a hard drive Constructor File (String s) n Opens the file with name s so that values can be extracted n Name can be either an absolute pathname or a pathname relative to the current working folder 24
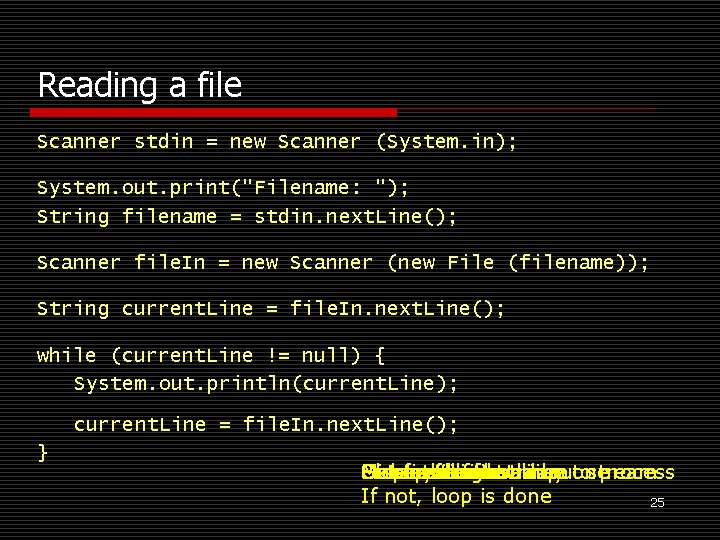
Reading a file Scanner stdin = new Scanner (System. in); System. out. print("Filename: "); String filename = stdin. next. Line(); Scanner file. In = new Scanner (new File (filename)); String current. Line = file. In. next. Line(); while (current. Line != null) { System. out. println(current. Line); current. Line = file. In. next. Line(); } Close Determine Set Process Display Get Make up first next sure the standard file current lines line file stream got fileone stream name aline input line by one tostream process If not, loop is done 25
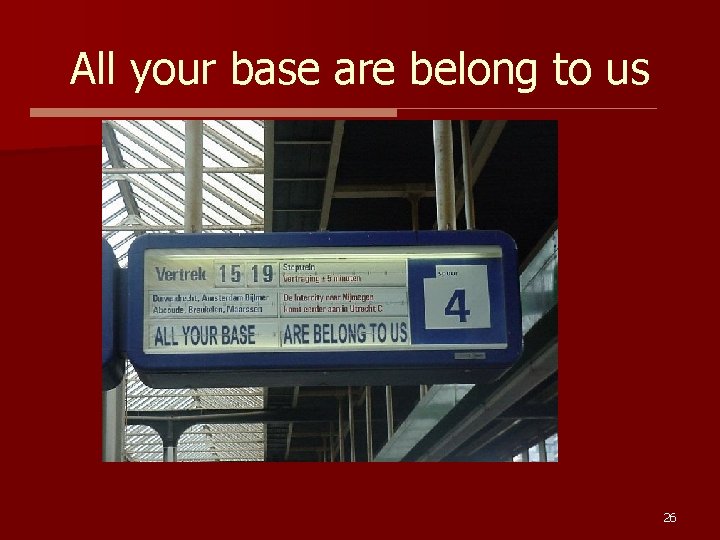
All your base are belong to us 26
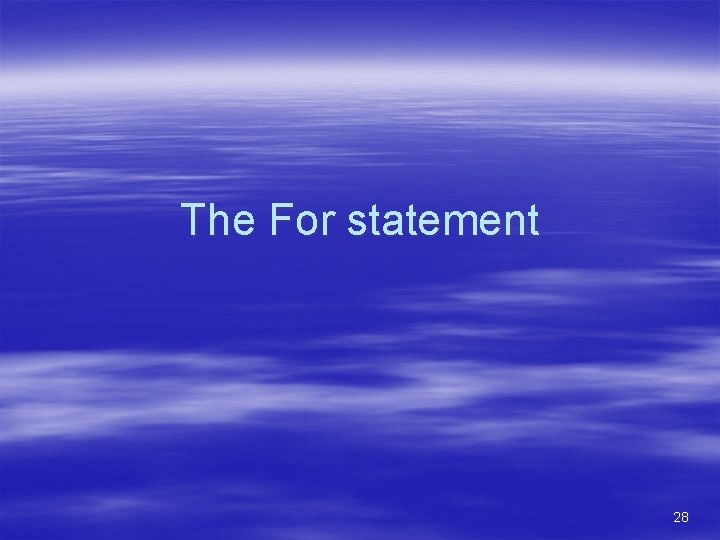
The For statement 28
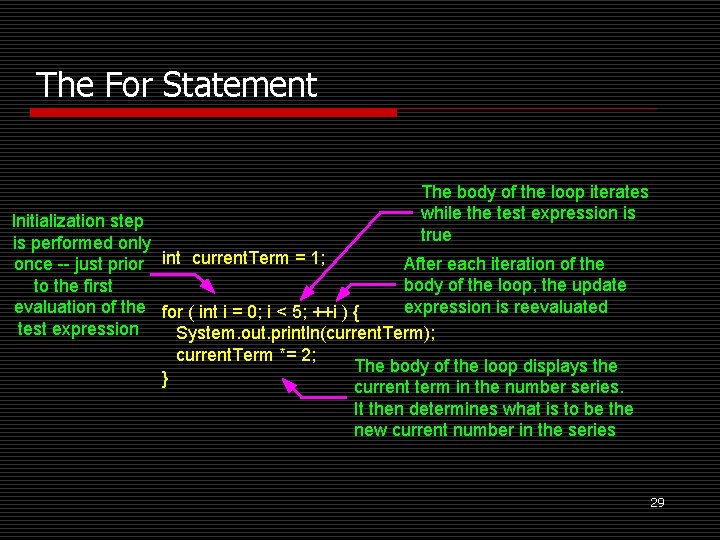
The For Statement The body of the loop iterates while the test expression is true Initialization step is performed only After each iteration of the once -- just prior int current. Term = 1; body of the loop, the update to the first expression is reevaluated evaluation of the for ( int i = 0; i < 5; ++i ) { test expression System. out. println(current. Term); current. Term *= 2; The body of the loop displays the } current term in the number series. It then determines what is to be the new current number in the series 29
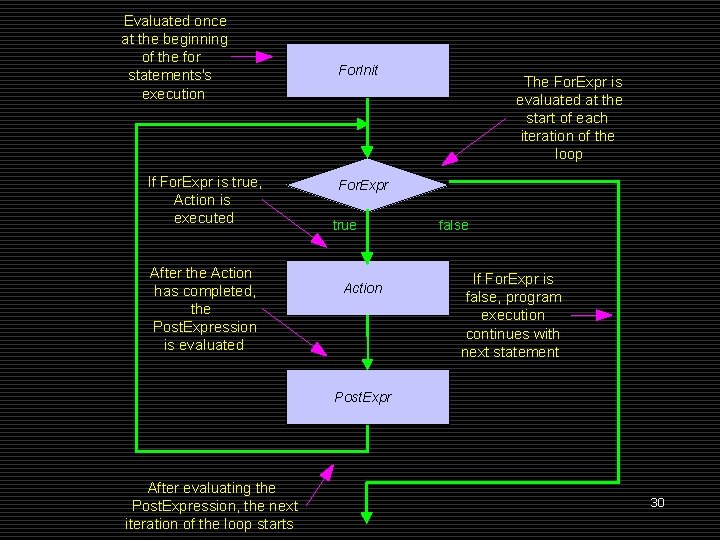
Evaluated once at the beginning of the for statements's execution If For. Expr is true, Action is executed After the Action has completed, the Post. Expression is evaluated For. Init The For. Expr is evaluated at the start of each iteration of the loop For. Expr true Action false If For. Expr is false, program execution continues with next statement Post. Expr After evaluating the Post. Expression, the next iteration of the loop starts 30
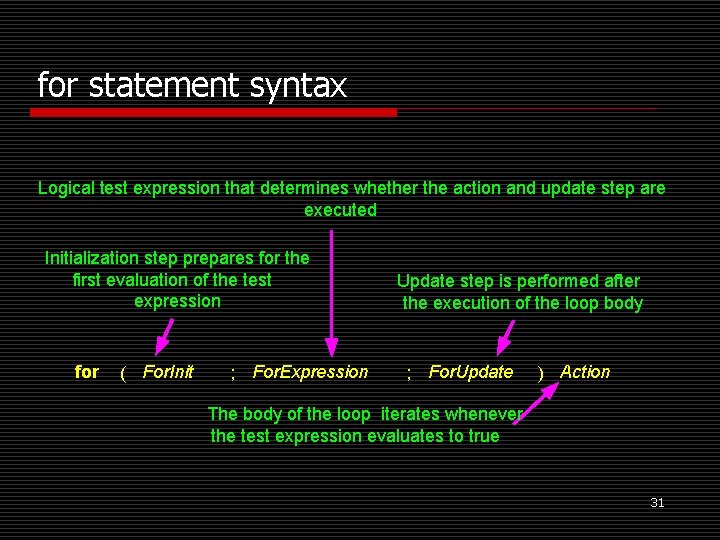
for statement syntax Logical test expression that determines whether the action and update step are executed Initialization step prepares for the first evaluation of the test expression for ( For. Init ; For. Expression Update step is performed after the execution of the loop body ; For. Update ) Action The body of the loop iterates whenever the test expression evaluates to true 31
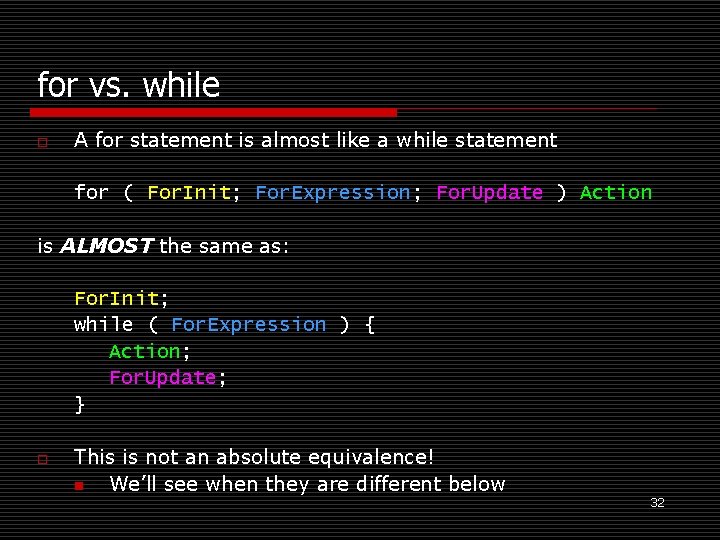
for vs. while o A for statement is almost like a while statement for ( For. Init; For. Expression; For. Update ) Action is ALMOST the same as: For. Init; while ( For. Expression ) { Action; For. Update; } o This is not an absolute equivalence! n We’ll see when they are different below 32
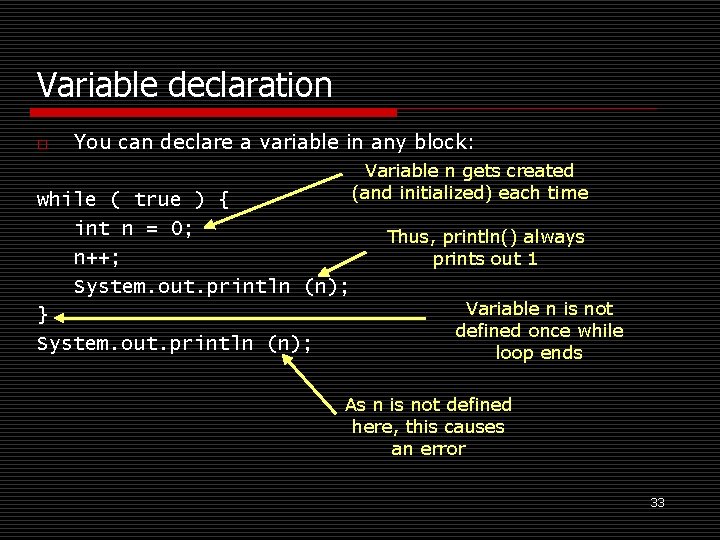
Variable declaration o You can declare a variable in any block: while ( true ) { int n = 0; n++; System. out. println (n); } System. out. println (n); Variable n gets created (and initialized) each time Thus, println() always prints out 1 Variable n is not defined once while loop ends As n is not defined here, this causes an error 33
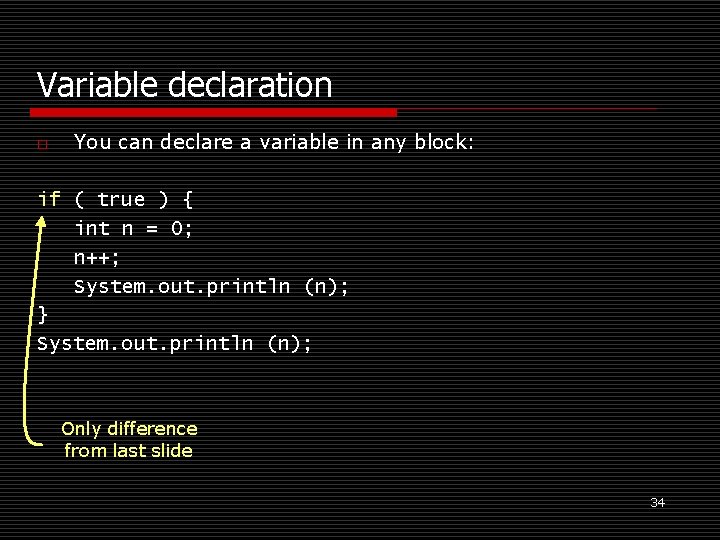
Variable declaration o You can declare a variable in any block: if ( true ) { int n = 0; n++; System. out. println (n); } System. out. println (n); Only difference from last slide 34
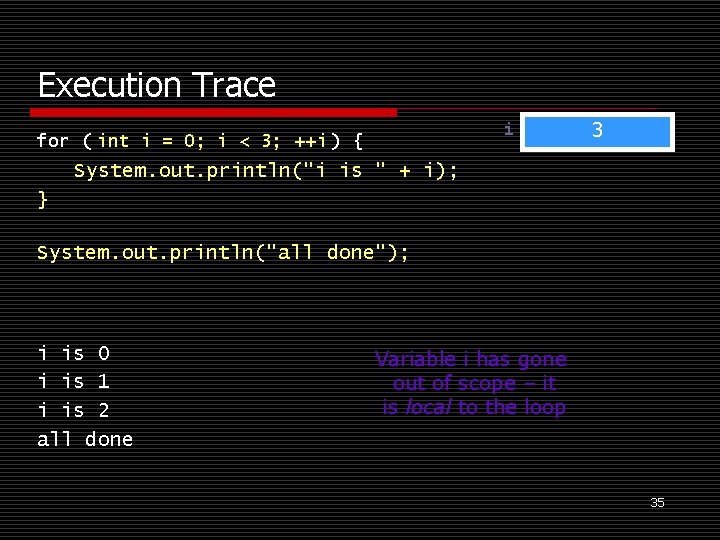
Execution Trace i for ( int i = 0; i < 3; ++i ) { 0 3 2 1 System. out. println("i is " + i); } System. out. println("all done"); i is 0 i is 1 i is 2 all done Variable i has gone out of scope – it is local to the loop 35
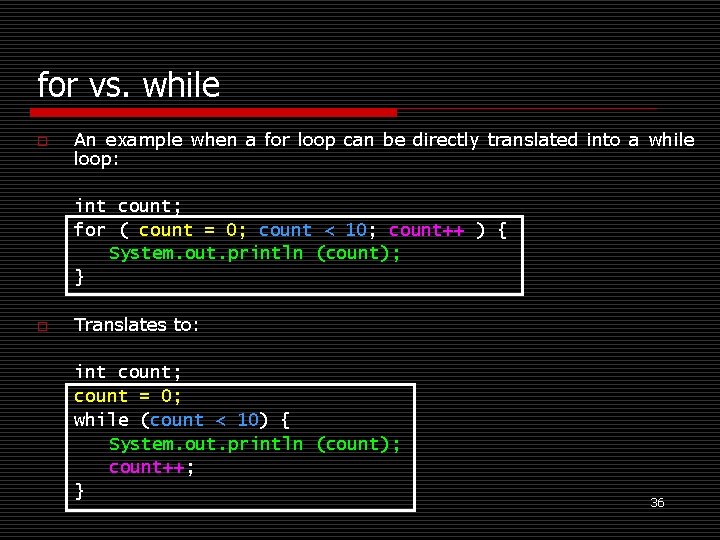
for vs. while o An example when a for loop can be directly translated into a while loop: int count; for ( count = 0; count < 10; count++ ) { System. out. println (count); } o Translates to: int count; count = 0; while (count < 10) { System. out. println (count); count++; } 36
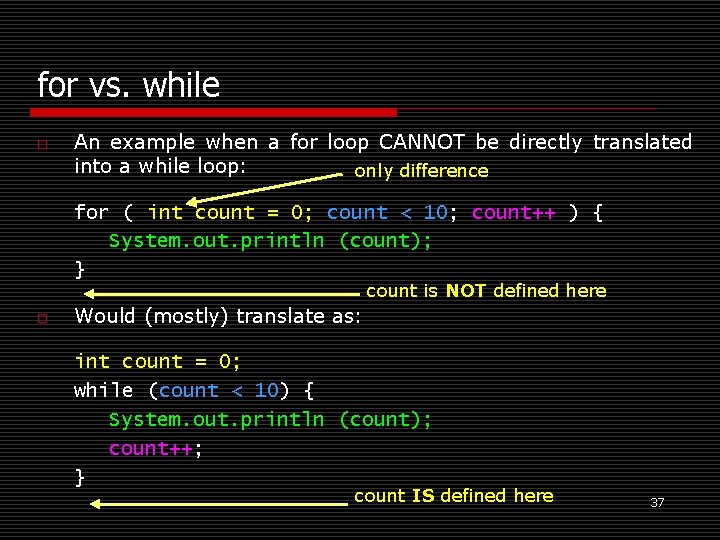
for vs. while o An example when a for loop CANNOT be directly translated into a while loop: only difference for ( int count = 0; count < 10; count++ ) { System. out. println (count); } count is NOT defined here o Would (mostly) translate as: int count = 0; while (count < 10) { System. out. println (count); count++; } count IS defined here 37
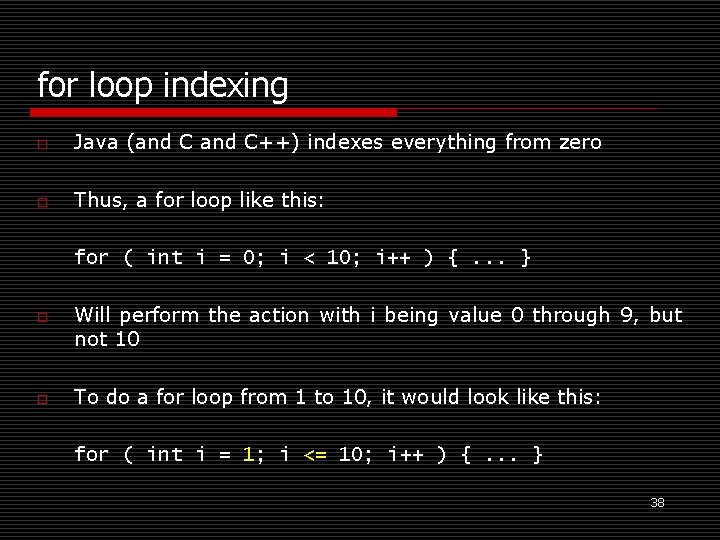
for loop indexing o Java (and C++) indexes everything from zero o Thus, a for loop like this: for ( int i = 0; i < 10; i++ ) {. . . } o o Will perform the action with i being value 0 through 9, but not 10 To do a for loop from 1 to 10, it would look like this: for ( int i = 1; i <= 10; i++ ) {. . . } 38
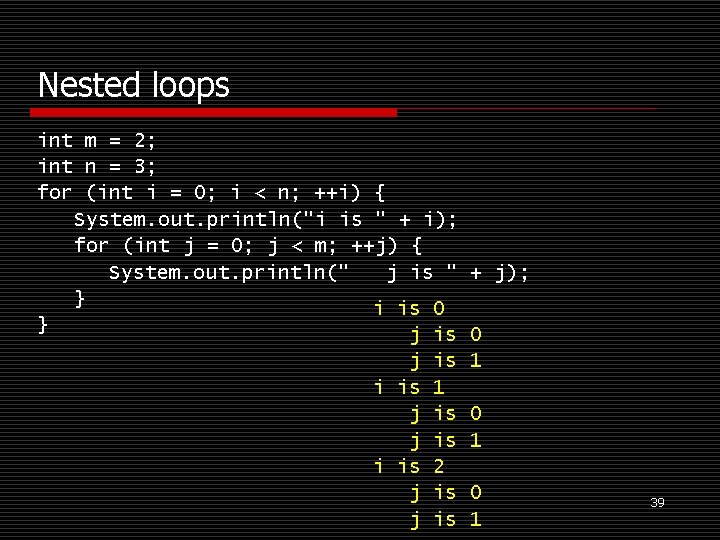
Nested loops int m = 2; int n = 3; for (int i = 0; i < n; ++i) { System. out. println("i is " + i); for (int j = 0; j < m; ++j) { System. out. println(" j is " + j); } i is 0 } j is 0 j is 1 i is 1 j is 0 j is 1 i is 2 j is 0 j is 1 39
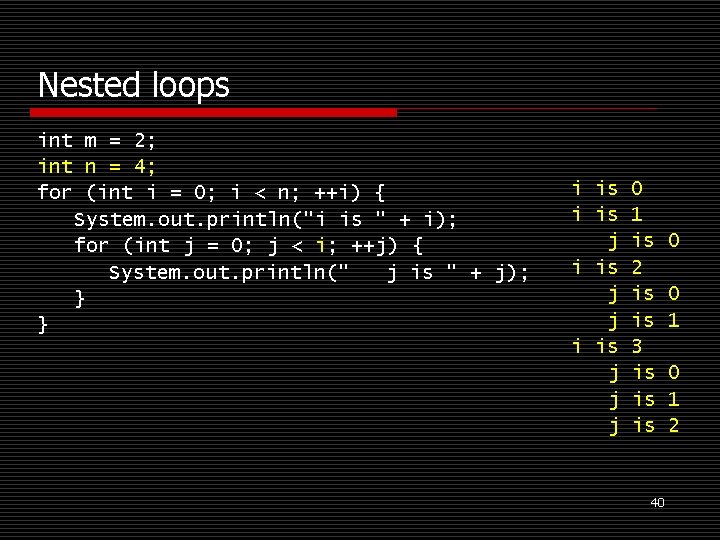
Nested loops int m = 2; int n = 4; for (int i = 0; i < n; ++i) { System. out. println("i is " + i); for (int j = 0; j < i; ++j) { System. out. println(" j is " + j); } } i is 0 i is 1 j is i is 2 j is i is 3 j is 40 0 0 1 2
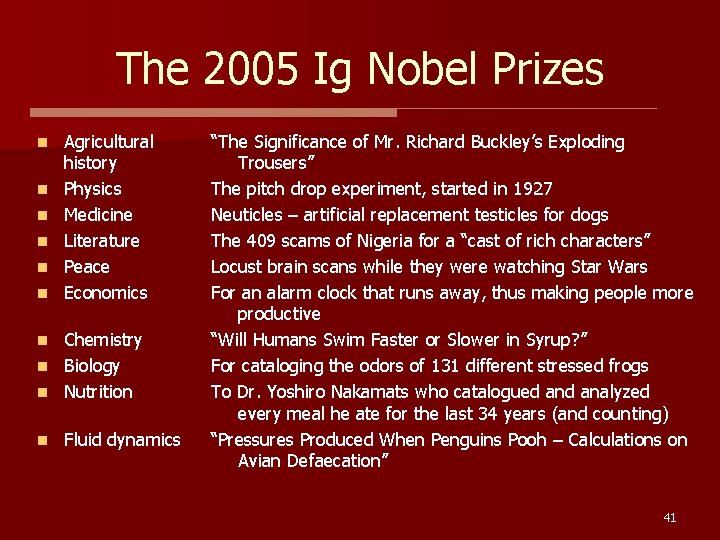
The 2005 Ig Nobel Prizes n n n Agricultural history Physics Medicine Literature Peace Economics Chemistry n Biology n Nutrition n n Fluid dynamics “The Significance of Mr. Richard Buckley’s Exploding Trousers” The pitch drop experiment, started in 1927 Neuticles – artificial replacement testicles for dogs The 409 scams of Nigeria for a “cast of rich characters” Locust brain scans while they were watching Star Wars For an alarm clock that runs away, thus making people more productive “Will Humans Swim Faster or Slower in Syrup? ” For cataloging the odors of 131 different stressed frogs To Dr. Yoshiro Nakamats who catalogued analyzed every meal he ate for the last 34 years (and counting) “Pressures Produced When Penguins Pooh – Calculations on Avian Defaecation” 41
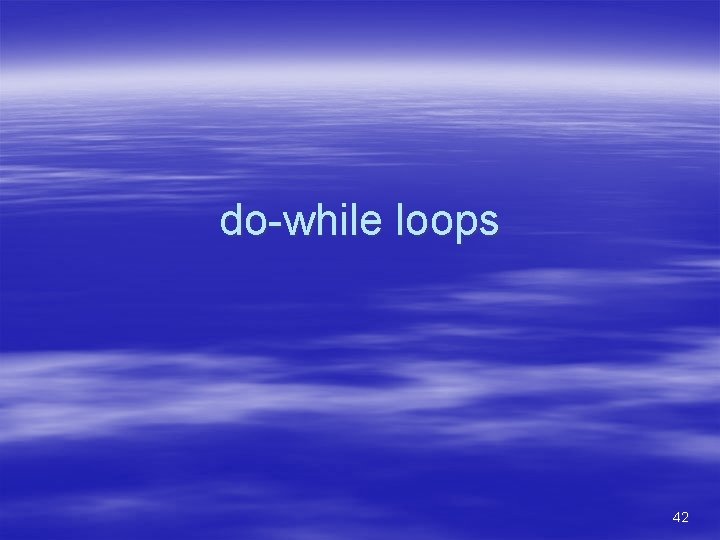
do-while loops 42
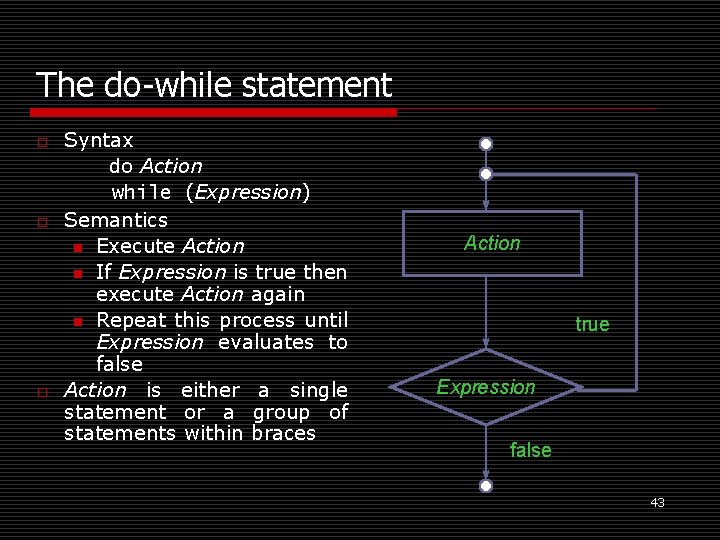
The do-while statement o o o Syntax do Action while (Expression) Semantics n Execute Action n If Expression is true then execute Action again n Repeat this process until Expression evaluates to false Action is either a single statement or a group of statements within braces Action true Expression false 43
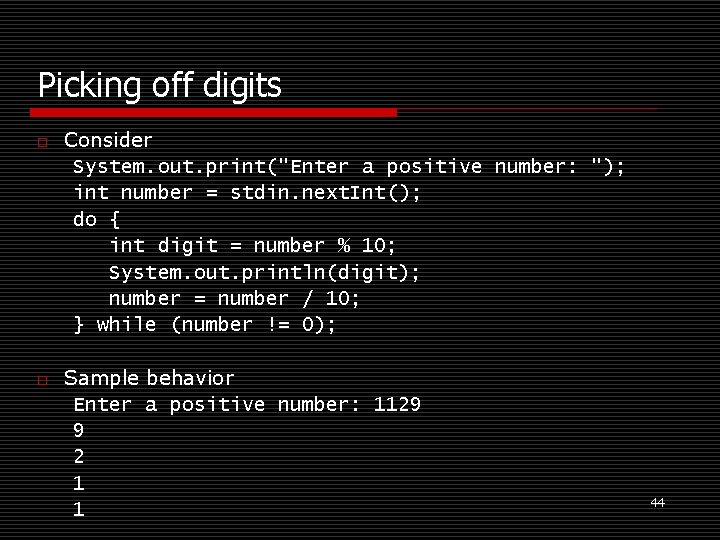
Picking off digits o o Consider System. out. print("Enter a positive number: "); int number = stdin. next. Int(); do { int digit = number % 10; System. out. println(digit); number = number / 10; } while (number != 0); Sample behavior Enter a positive number: 1129 9 2 1 1 44
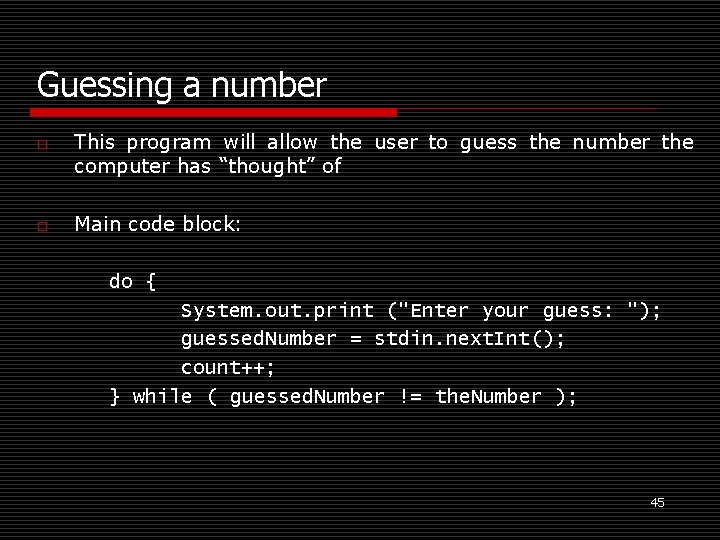
Guessing a number o o This program will allow the user to guess the number the computer has “thought” of Main code block: do { System. out. print ("Enter your guess: "); guessed. Number = stdin. next. Int(); count++; } while ( guessed. Number != the. Number ); 45
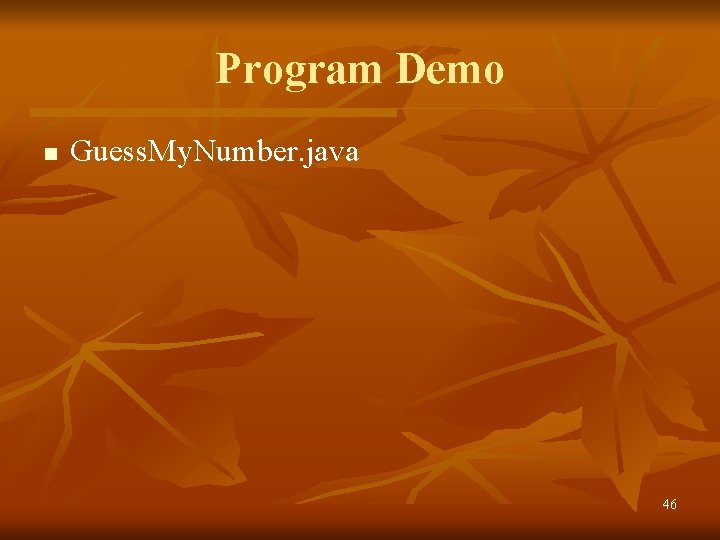
Program Demo n Guess. My. Number. java 46
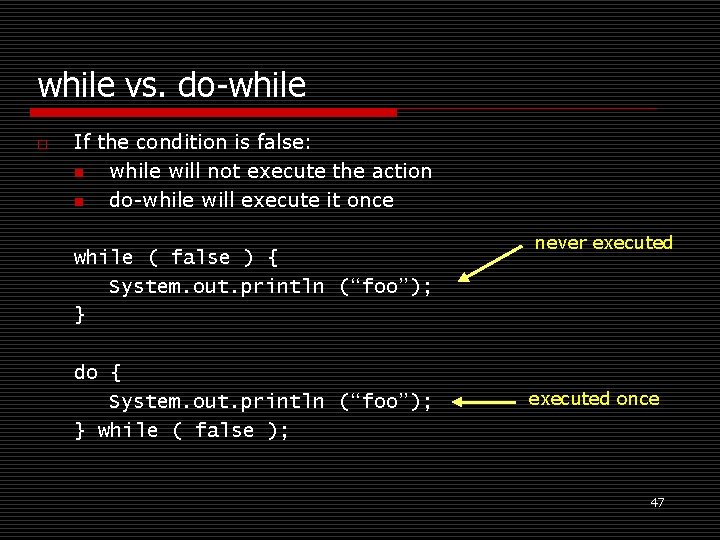
while vs. do-while o If the condition is false: n while will not execute the action n do-while will execute it once while ( false ) { System. out. println (“foo”); } do { System. out. println (“foo”); } while ( false ); never executed once 47
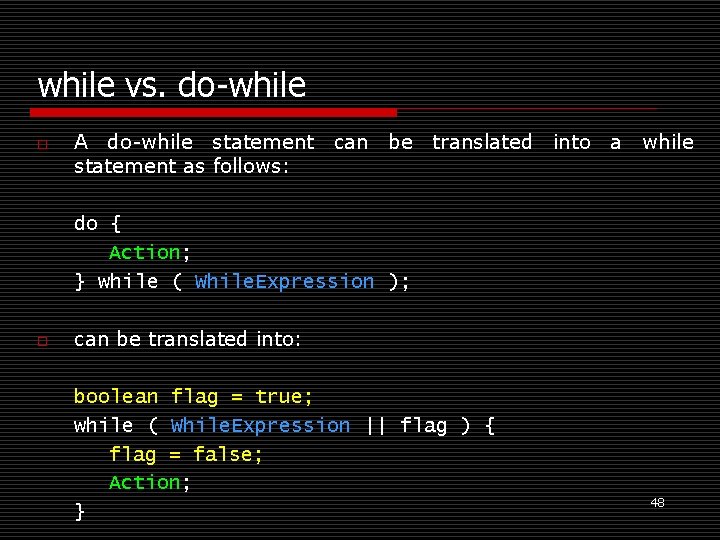
while vs. do-while o A do-while statement can be translated into a while statement as follows: do { Action; } while ( While. Expression ); o can be translated into: boolean flag = true; while ( While. Expression || flag ) { flag = false; Action; } 48
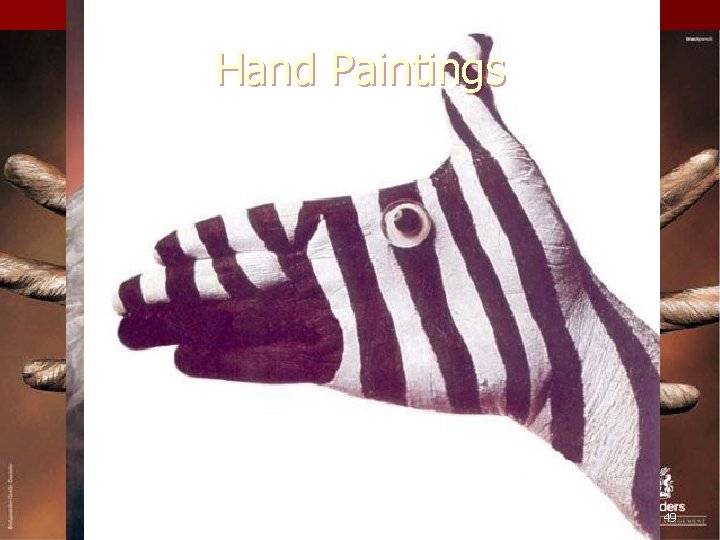
Hand Paintings 49
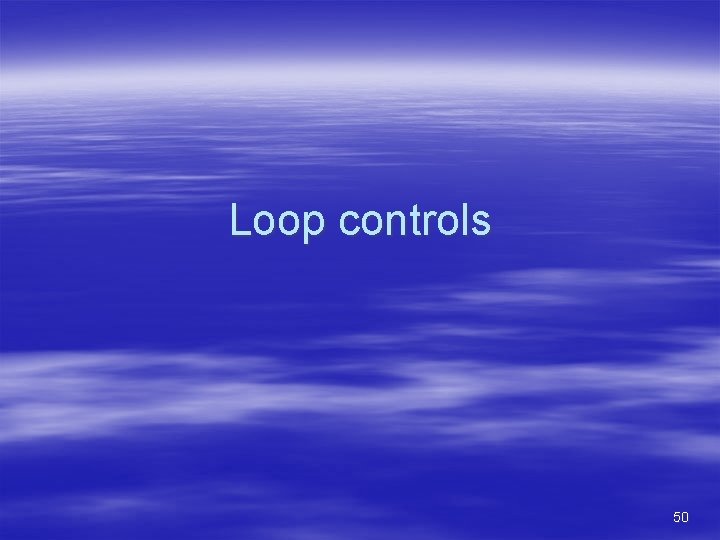
Loop controls 50
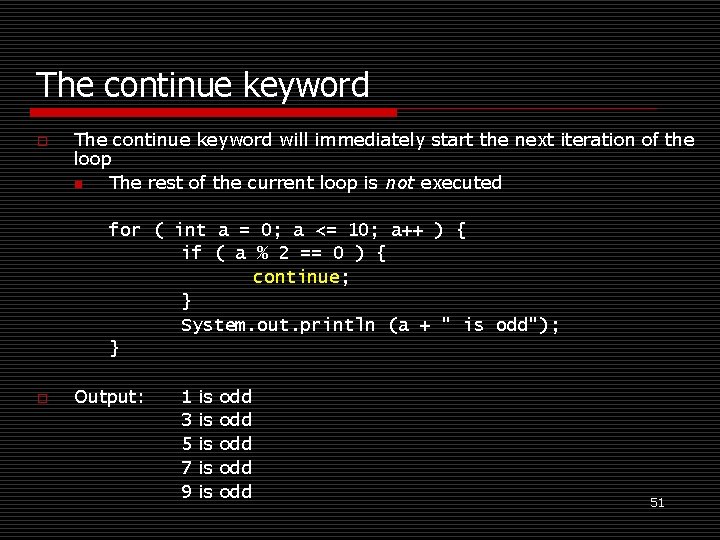
The continue keyword o The continue keyword will immediately start the next iteration of the loop n The rest of the current loop is not executed for ( int a = 0; a <= 10; a++ ) { if ( a % 2 == 0 ) { continue; } System. out. println (a + " is odd"); } o Output: 1 3 5 7 9 is is is odd odd odd 51
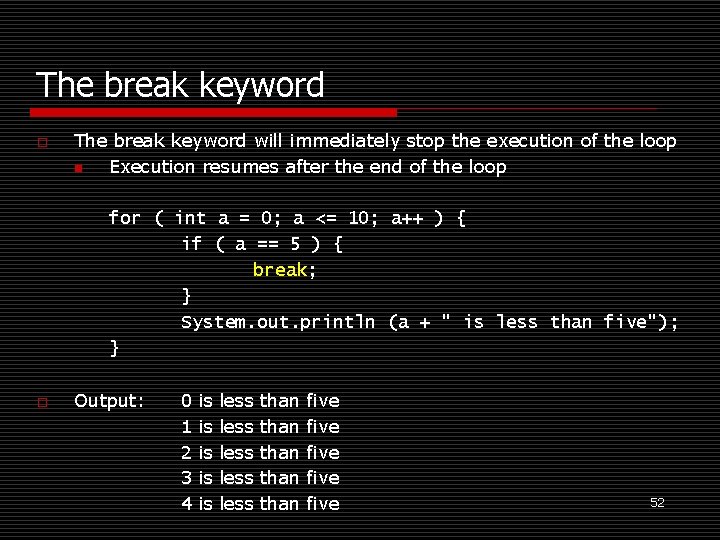
The break keyword o The break keyword will immediately stop the execution of the loop n Execution resumes after the end of the loop for ( int a = 0; a <= 10; a++ ) { if ( a == 5 ) { break; } System. out. println (a + " is less than five"); } o Output: 0 1 2 3 4 is is is less less than than five five 52
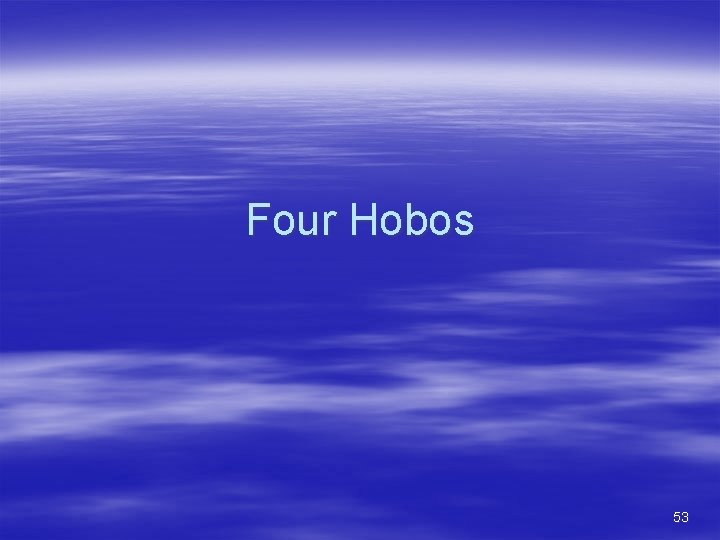
Four Hobos 53
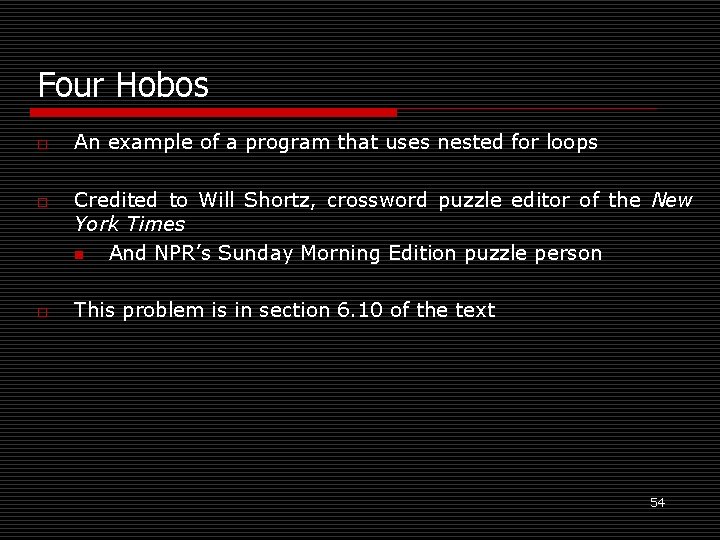
Four Hobos o o o An example of a program that uses nested for loops Credited to Will Shortz, crossword puzzle editor of the New York Times n And NPR’s Sunday Morning Edition puzzle person This problem is in section 6. 10 of the text 54
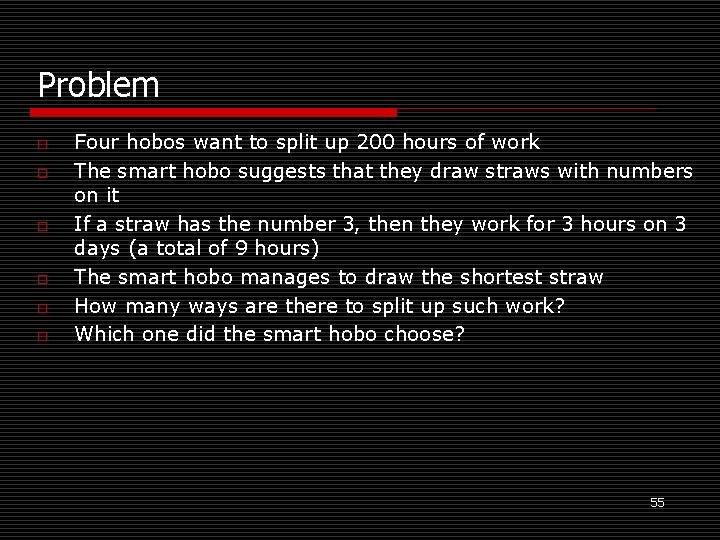
Problem o o o Four hobos want to split up 200 hours of work The smart hobo suggests that they draw straws with numbers on it If a straw has the number 3, then they work for 3 hours on 3 days (a total of 9 hours) The smart hobo manages to draw the shortest straw How many ways are there to split up such work? Which one did the smart hobo choose? 55
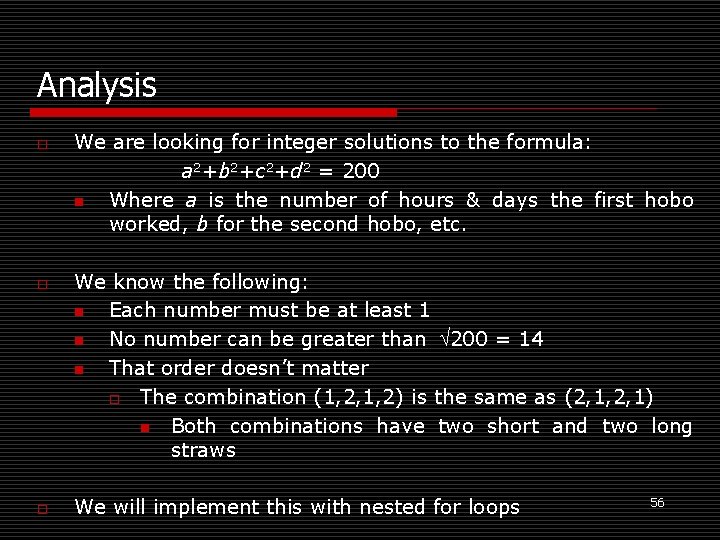
Analysis o o o We are looking for integer solutions to the formula: a 2+b 2+c 2+d 2 = 200 n Where a is the number of hours & days the first hobo worked, b for the second hobo, etc. We know the following: n Each number must be at least 1 n No number can be greater than 200 = 14 n That order doesn’t matter o The combination (1, 2, 1, 2) is the same as (2, 1, 2, 1) n Both combinations have two short and two long straws We will implement this with nested for loops 56
![Implementation public class Four Hobos public static void main String args for Implementation public class Four. Hobos { public static void main (String[] args) { for](https://slidetodoc.com/presentation_image/f31e04bae69c2a009fc956421a67c2b8/image-56.jpg)
Implementation public class Four. Hobos { public static void main (String[] args) { for ( int a = 1; a <= 14; a++ ) { for ( int b = 1; b <= 14; b++ ) { for ( int c = 1; c <= 14; c++ ) { for ( int d = 1; d <= 14; d++ ) { if ( (a <= b) && (b <= c) && (c <= d) ) { if ( a*a+b*b+c*c+d*d == 200 ) { System. out. println ("(" + a + ", " + b + ", " + c + ", " + d + ")"); } } } } 57
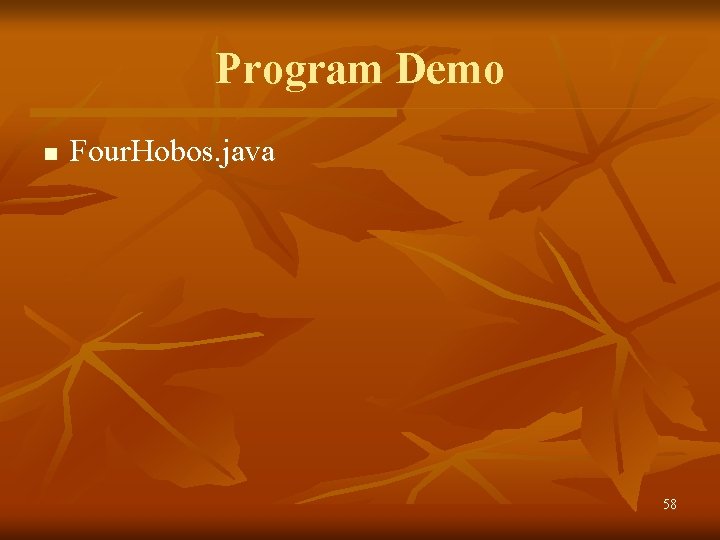
Program Demo n Four. Hobos. java 58
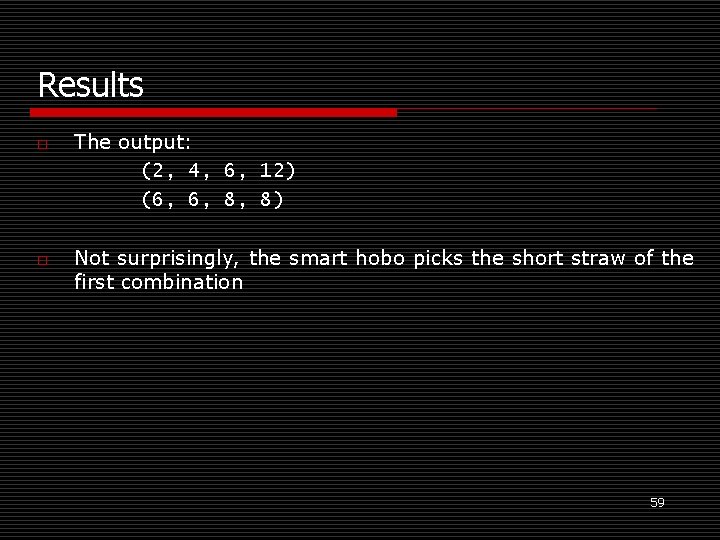
Results o o The output: (2, 4, 6, 12) (6, 6, 8, 8) Not surprisingly, the smart hobo picks the short straw of the first combination 59
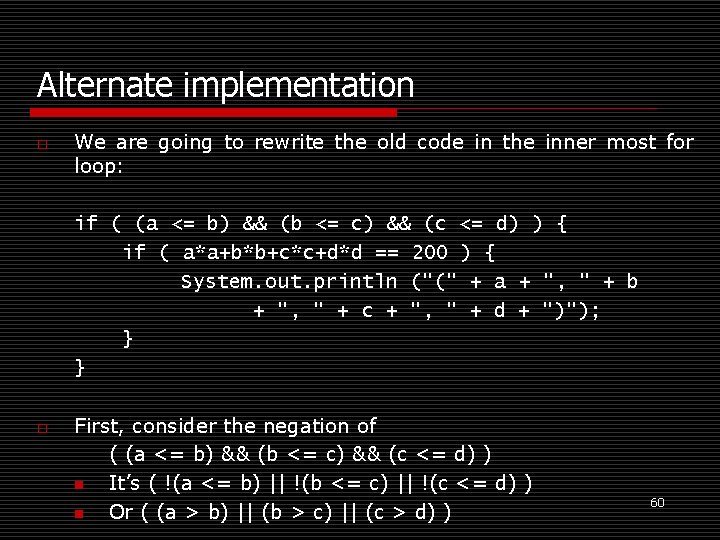
Alternate implementation o We are going to rewrite the old code in the inner most for loop: if ( (a <= b) && (b <= c) && (c <= d) ) { if ( a*a+b*b+c*c+d*d == 200 ) { System. out. println ("(" + a + ", " + b + ", " + c + ", " + d + ")"); } } o First, consider the negation of ( (a <= b) && (b <= c) && (c <= d) ) n It’s ( !(a <= b) || !(b <= c) || !(c <= d) ) n Or ( (a > b) || (b > c) || (c > d) ) 60
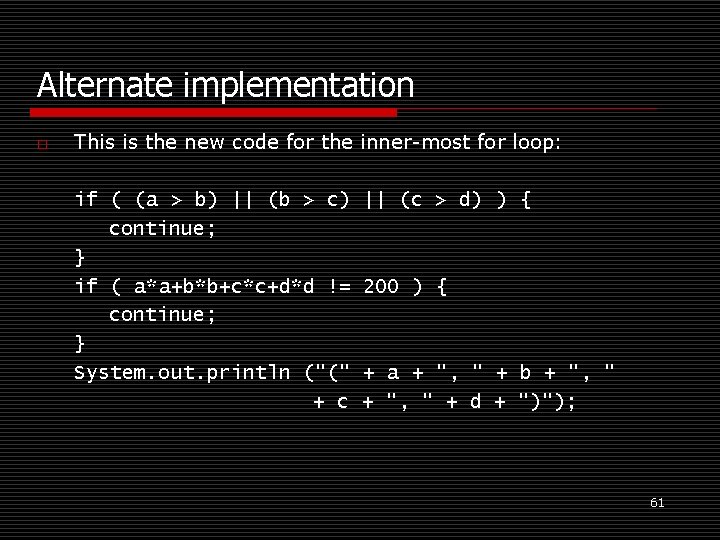
Alternate implementation o This is the new code for the inner-most for loop: if ( (a > b) || (b > c) continue; } if ( a*a+b*b+c*c+d*d != continue; } System. out. println ("(" + c || (c > d) ) { 200 ) { + a + ", " + b + ", " + d + ")"); 61
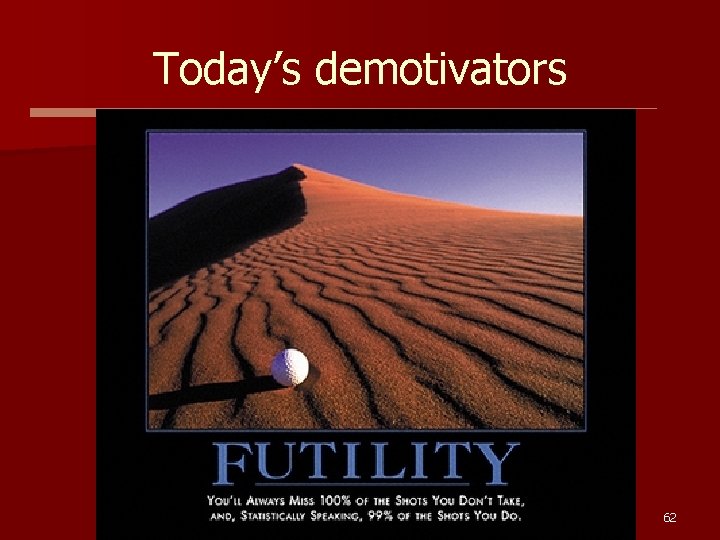
Today’s demotivators 62
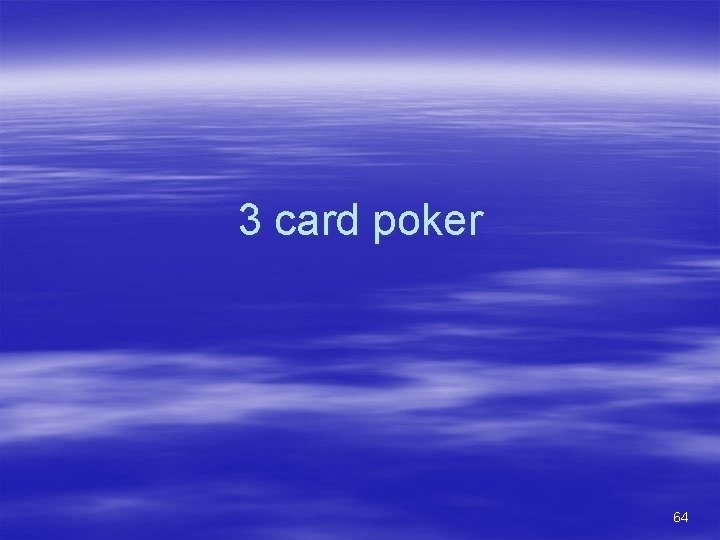
3 card poker 64
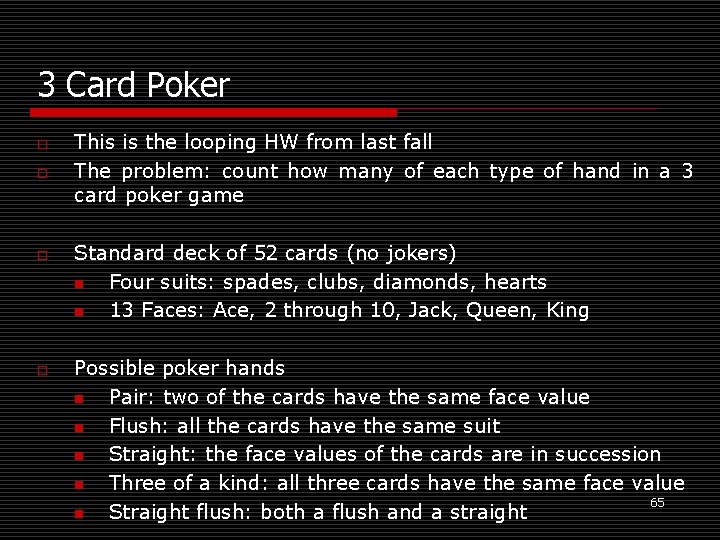
3 Card Poker o o This is the looping HW from last fall The problem: count how many of each type of hand in a 3 card poker game Standard deck of 52 cards (no jokers) n Four suits: spades, clubs, diamonds, hearts n 13 Faces: Ace, 2 through 10, Jack, Queen, King Possible poker hands n Pair: two of the cards have the same face value n Flush: all the cards have the same suit n Straight: the face values of the cards are in succession n Three of a kind: all three cards have the same face value 65 n Straight flush: both a flush and a straight
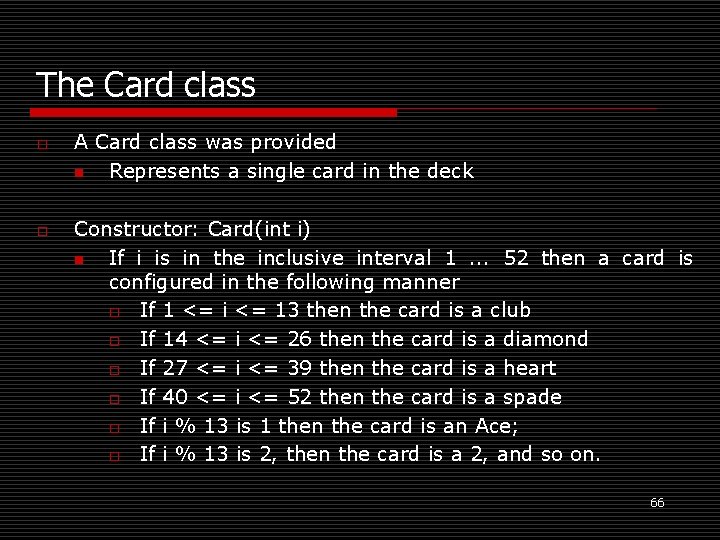
The Card class o o A Card class was provided n Represents a single card in the deck Constructor: Card(int i) n If i is in the inclusive interval 1. . . 52 then a card is configured in the following manner o If 1 <= i <= 13 then the card is a club o If 14 <= i <= 26 then the card is a diamond o If 27 <= i <= 39 then the card is a heart o If 40 <= i <= 52 then the card is a spade o If i % 13 is 1 then the card is an Ace; o If i % 13 is 2, then the card is a 2, and so on. 66
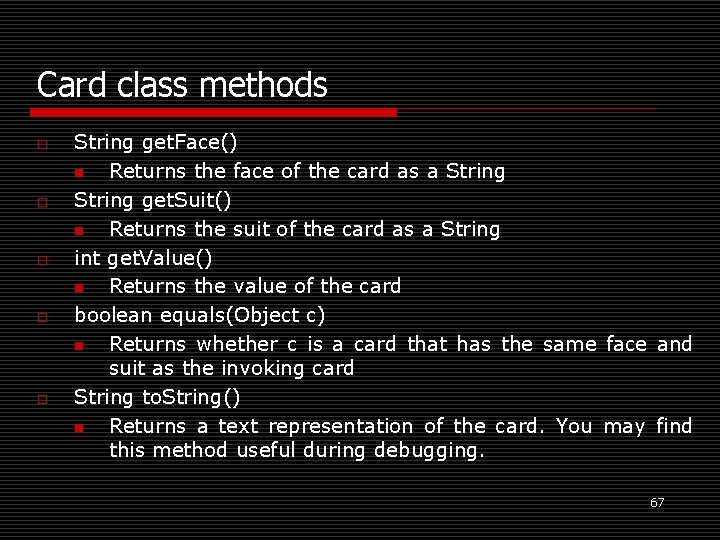
Card class methods o o o String get. Face() n Returns the face of the card as a String get. Suit() n Returns the suit of the card as a String int get. Value() n Returns the value of the card boolean equals(Object c) n Returns whether c is a card that has the same face and suit as the invoking card String to. String() n Returns a text representation of the card. You may find this method useful during debugging. 67
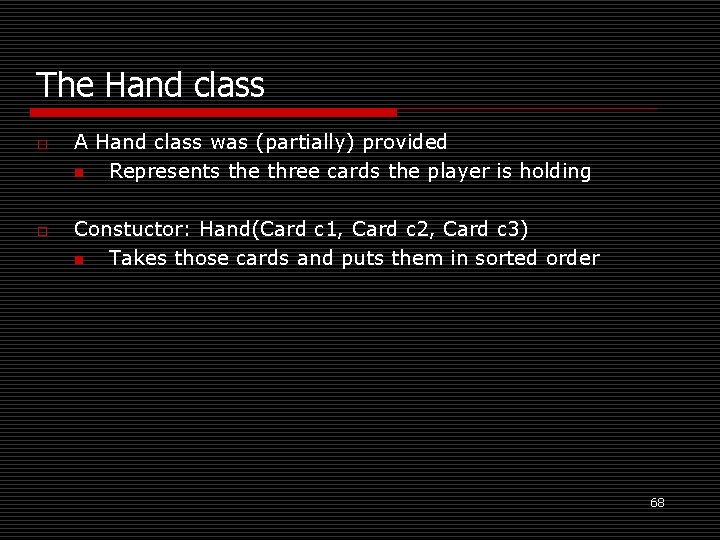
The Hand class o o A Hand class was (partially) provided n Represents the three cards the player is holding Constuctor: Hand(Card c 1, Card c 2, Card c 3) n Takes those cards and puts them in sorted order 68
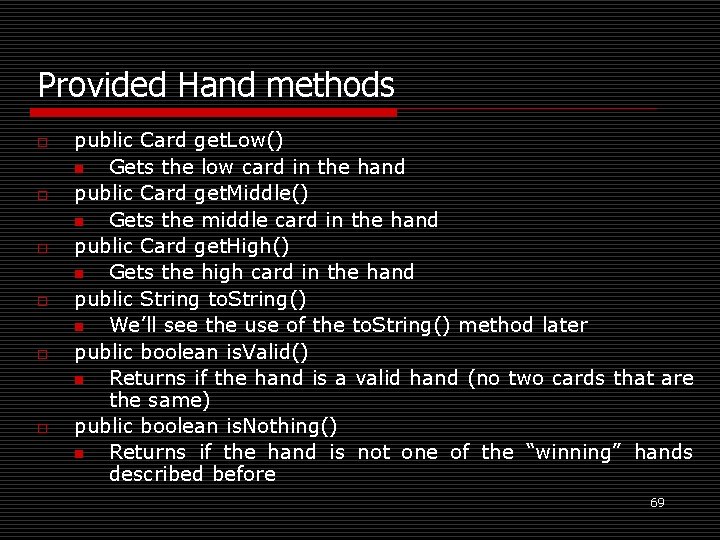
Provided Hand methods o o o public Card get. Low() n Gets the low card in the hand public Card get. Middle() n Gets the middle card in the hand public Card get. High() n Gets the high card in the hand public String to. String() n We’ll see the use of the to. String() method later public boolean is. Valid() n Returns if the hand is a valid hand (no two cards that are the same) public boolean is. Nothing() n Returns if the hand is not one of the “winning” hands described before 69
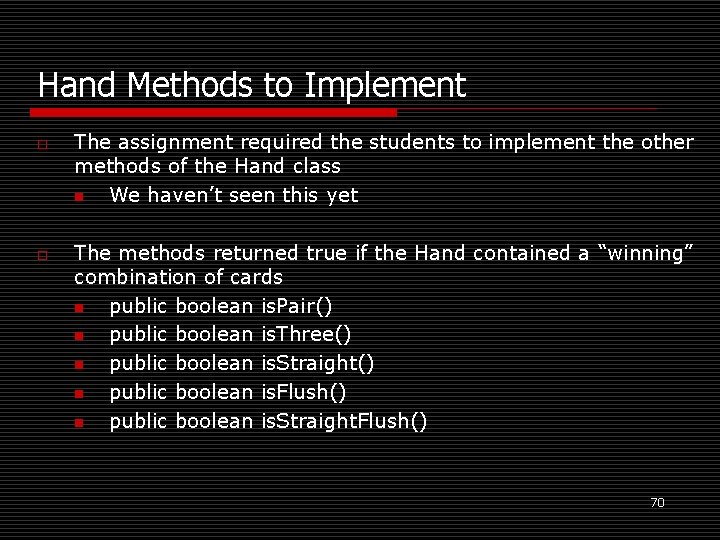
Hand Methods to Implement o o The assignment required the students to implement the other methods of the Hand class n We haven’t seen this yet The methods returned true if the Hand contained a “winning” combination of cards n public boolean is. Pair() n public boolean is. Three() n public boolean is. Straight() n public boolean is. Flush() n public boolean is. Straight. Flush() 70
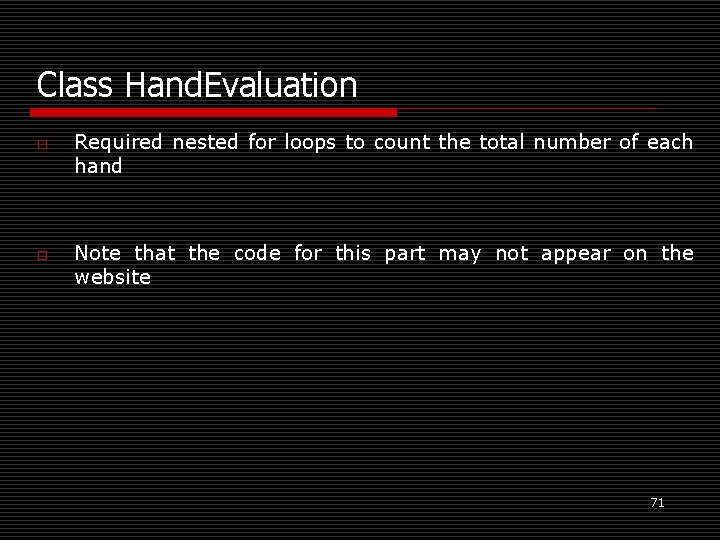
Class Hand. Evaluation o o Required nested for loops to count the total number of each hand Note that the code for this part may not appear on the website 71
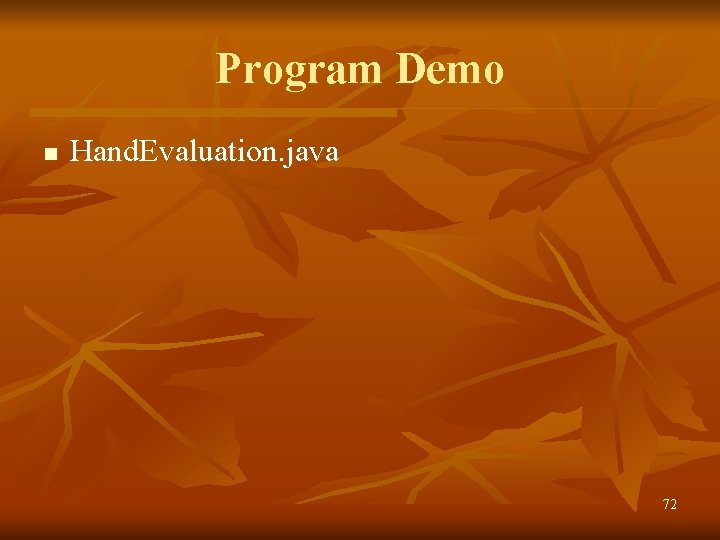
Program Demo n Hand. Evaluation. java 72
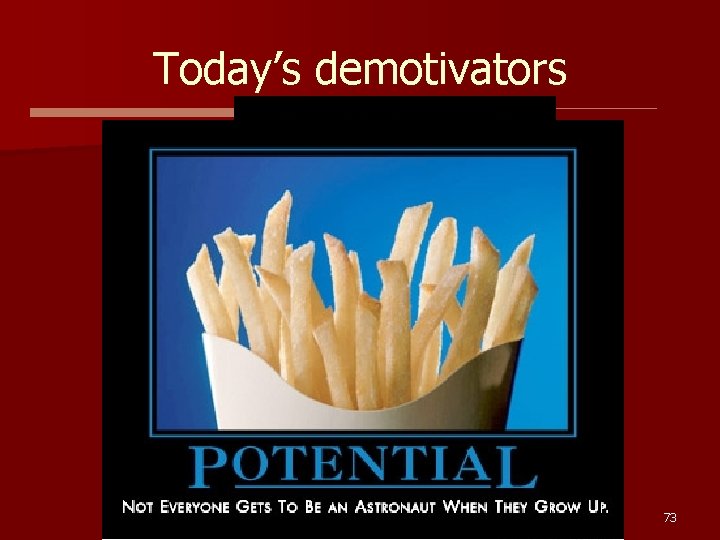
Today’s demotivators 73
Condition for convergence of newton raphson method
4115
Solving recurrence relations by iteration
Recursion vs iteration
What is sequencing selection and iteration
Value iteration
Value iteration
Orthogonal iteration
Value iteration algorithm
Value iteration algorithm
What is the purpose of an iteration recap
What is iteration in mathematics
What is iteration in computer science
Recursion
Iteration definition computer science
What is the purpose of iteration goals?
Birge-vieta method uses formula of
Iteration variable
Final iteration definition
Replace recursion with iteration
Value iteration
Value iteration
Alpenbeta
What is embarrassingly parallel
Iteration abstraction
Sequential conditional and iterative
Get sequence get another sequence pseudocode
Jurassic park chaos theory
Bbc iteration
Iteration demo
Lambda iteration
Iteration statements in java
What is meant by an iteration goal
Arnoldi iteration example
Lambda iteration
Lambda iteration
Iteration control structures
Iteration control structures
Iteration control structures
Modern process transitions in spm
Iteration.n5 - how many panels
Value iteration
Iteration
Iteration
Iteration
Which statement defines the purpose of iteration planning?
метод зейделя
Iteration demo
Value iteration
Sequence selection iteration
Iteration structure
Fixed point iteration method
Tail recursion vs iteration
Ncut
Jacobi method
Math 175
Iteration.n5
Py4e chapter 5
Policy iteration
Scratch iteration
Fitted q iteration
Iteration variable
Aaron’s priestly garments
Aaron collington troy ny
Aaron mulder
Paula squitieri
Aaron's robe in exodus
Aaron beck
Aaron egelman
Aaron prager
Why did god choose moses
Harold cohen aaron