Iteration Statements Iteration Statements Loop structures allows a
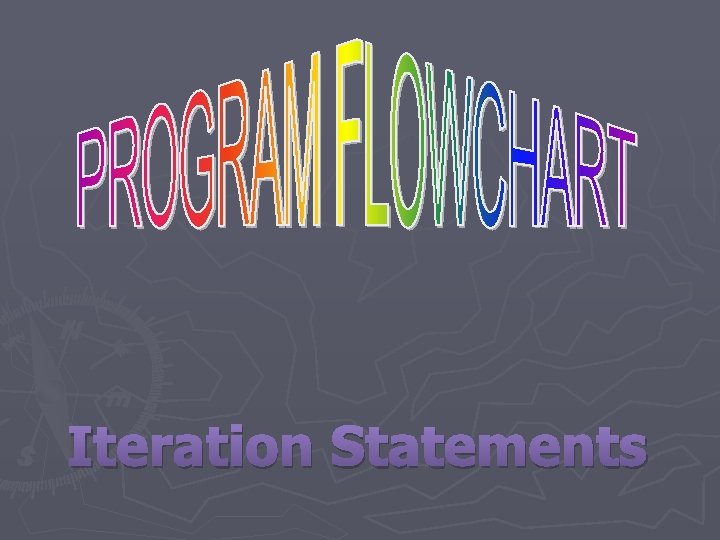
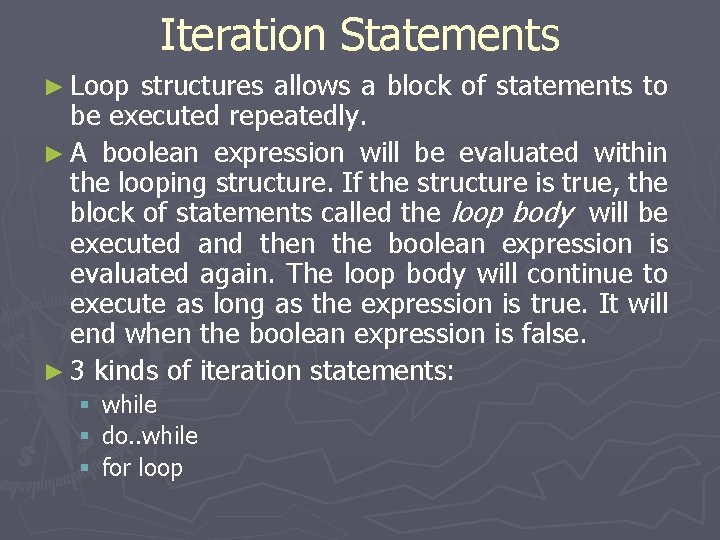
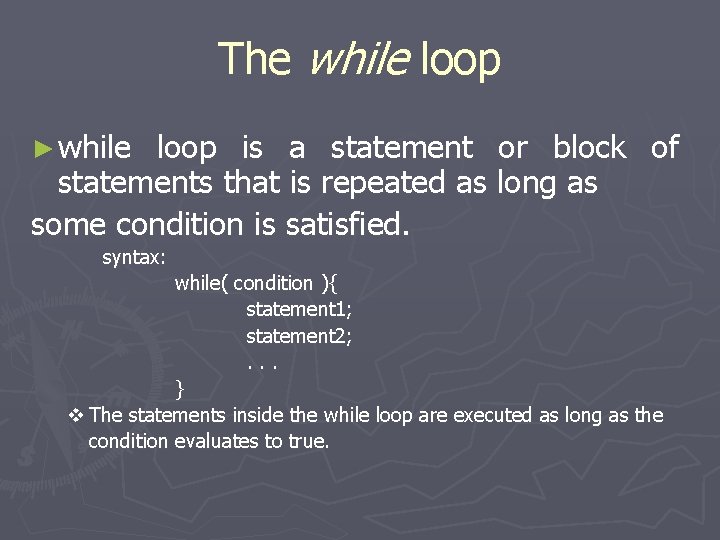
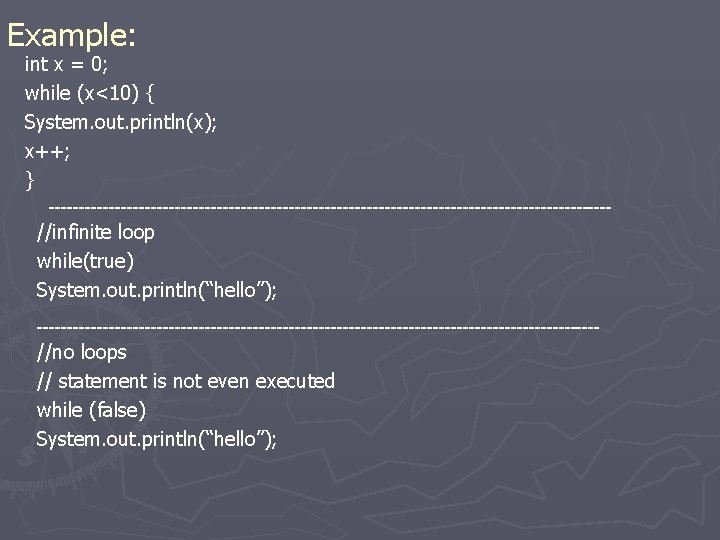
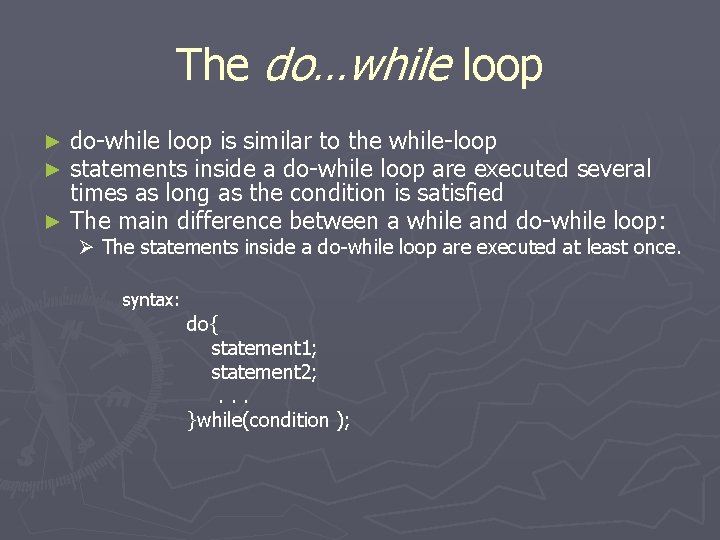
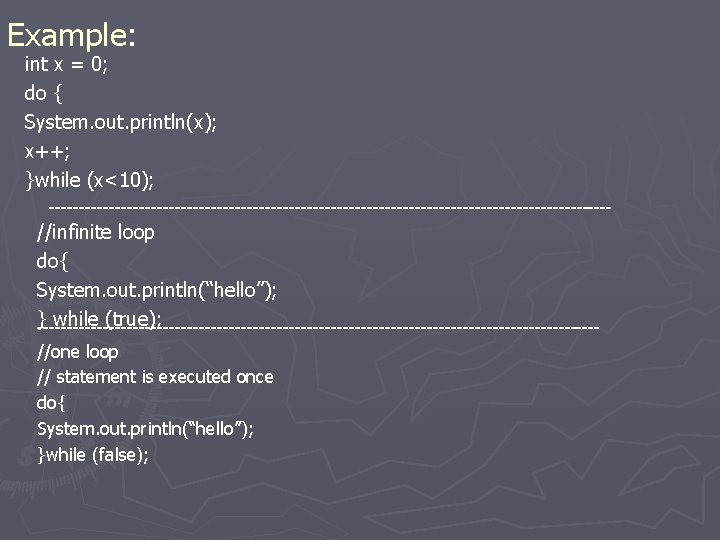
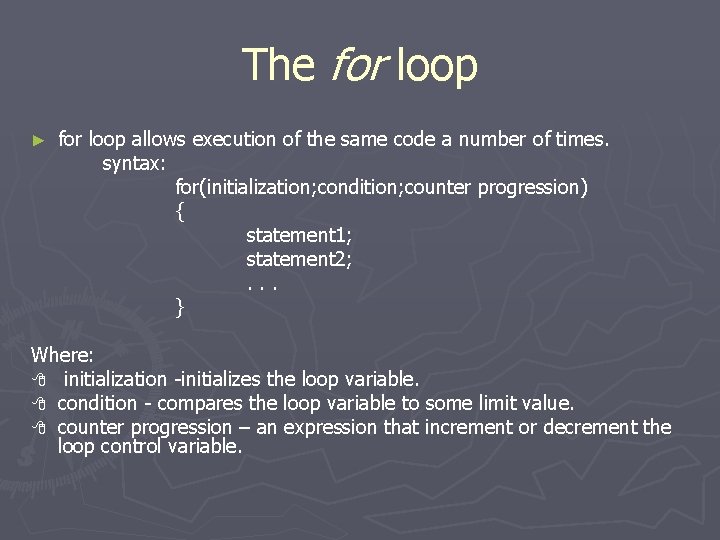
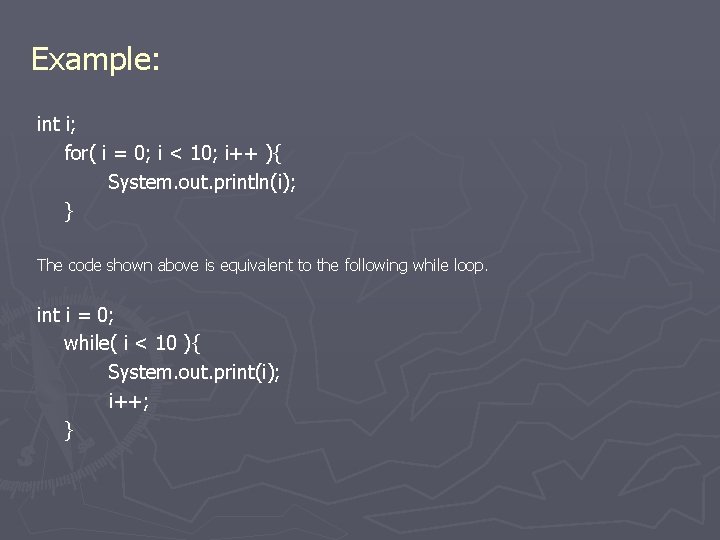
- Slides: 8
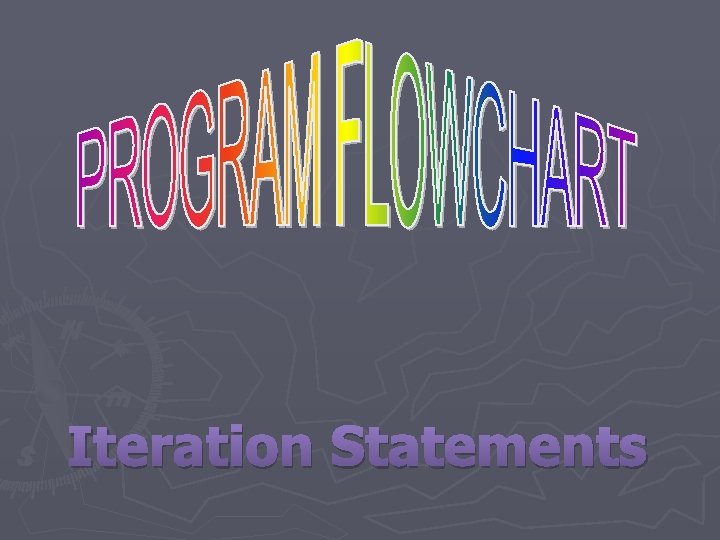
Iteration Statements
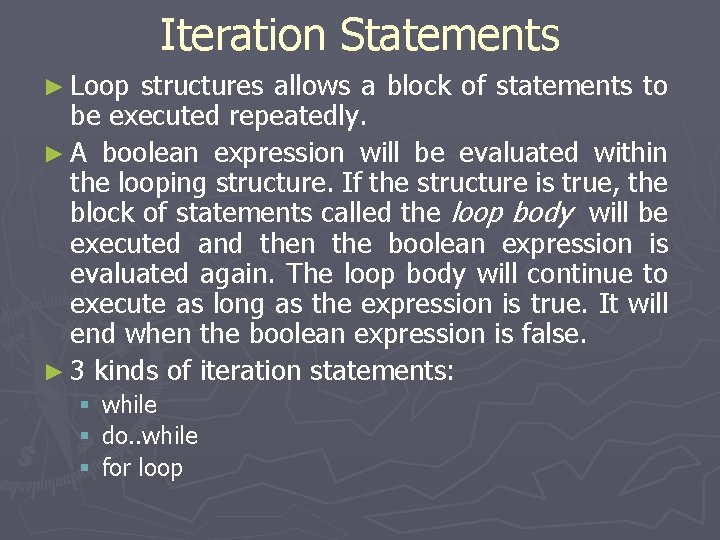
Iteration Statements ► Loop structures allows a block of statements to be executed repeatedly. ► A boolean expression will be evaluated within the looping structure. If the structure is true, the block of statements called the loop body will be executed and then the boolean expression is evaluated again. The loop body will continue to execute as long as the expression is true. It will end when the boolean expression is false. ► 3 kinds of iteration statements: § while § do. . while § for loop
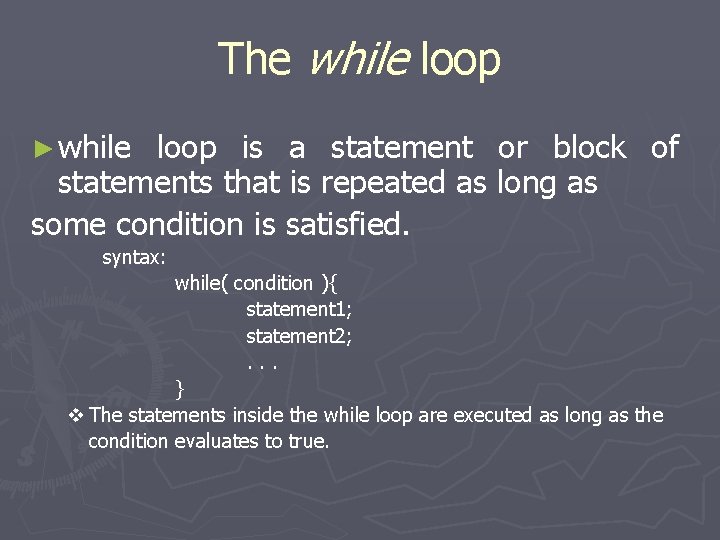
The while loop ► while loop is a statement or block of statements that is repeated as long as some condition is satisfied. syntax: while( condition ){ statement 1; statement 2; . . . } v The statements inside the while loop are executed as long as the condition evaluates to true.
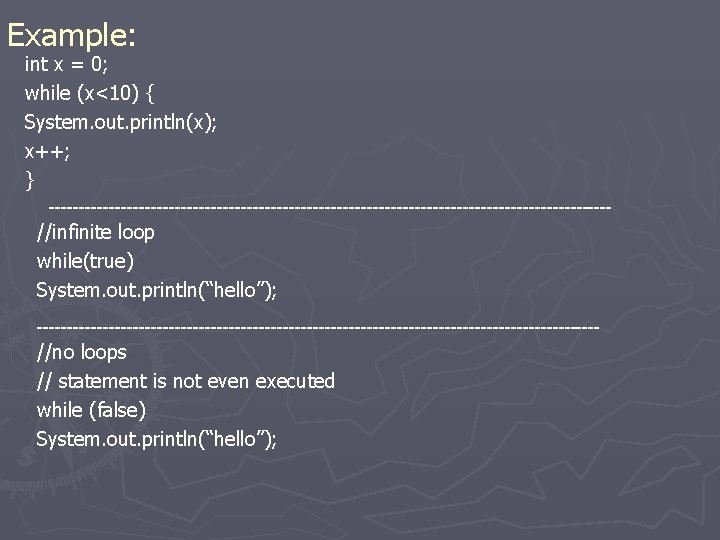
Example: int x = 0; while (x<10) { System. out. println(x); x++; } ----------------------------------------------- //infinite loop while(true) System. out. println(“hello”); ----------------------------------------------- //no loops // statement is not even executed while (false) System. out. println(“hello”);
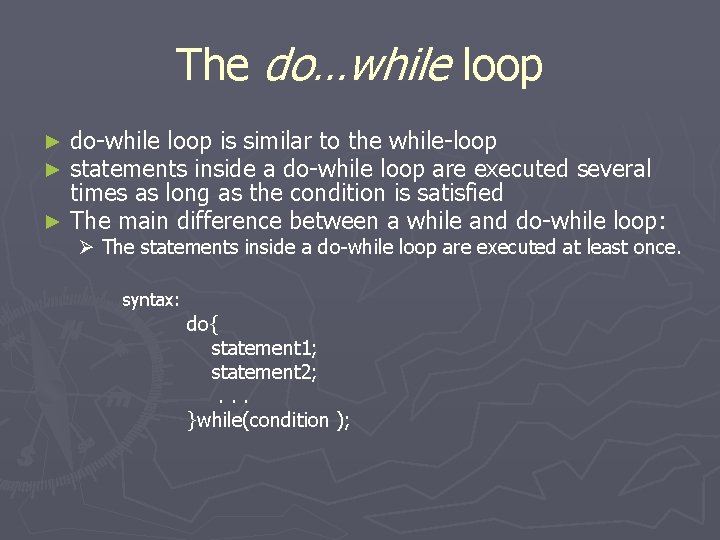
The do…while loop do-while loop is similar to the while-loop statements inside a do-while loop are executed several times as long as the condition is satisfied ► The main difference between a while and do-while loop: ► ► Ø The statements inside a do-while loop are executed at least once. syntax: do{ statement 1; statement 2; . . . }while(condition );
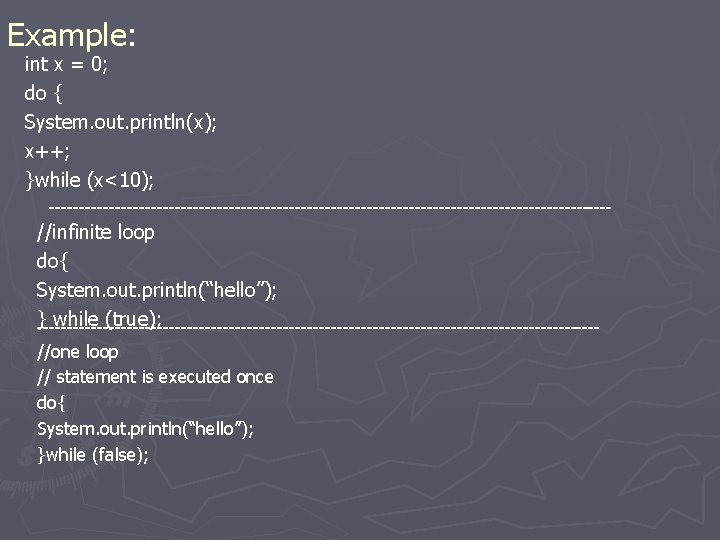
Example: int x = 0; do { System. out. println(x); x++; }while (x<10); ----------------------------------------------- //infinite loop do{ System. out. println(“hello”); }-----------------------------------------------while (true); //one loop // statement is executed once do{ System. out. println(“hello”); }while (false);
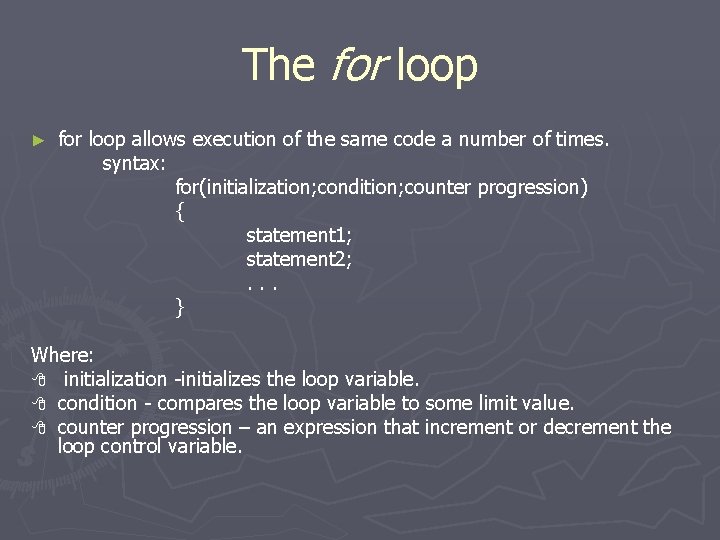
The for loop ► for loop allows execution of the same code a number of times. syntax: for(initialization; condition; counter progression) { statement 1; statement 2; . . . } Where: 8 initialization -initializes the loop variable. 8 condition - compares the loop variable to some limit value. 8 counter progression – an expression that increment or decrement the loop control variable.
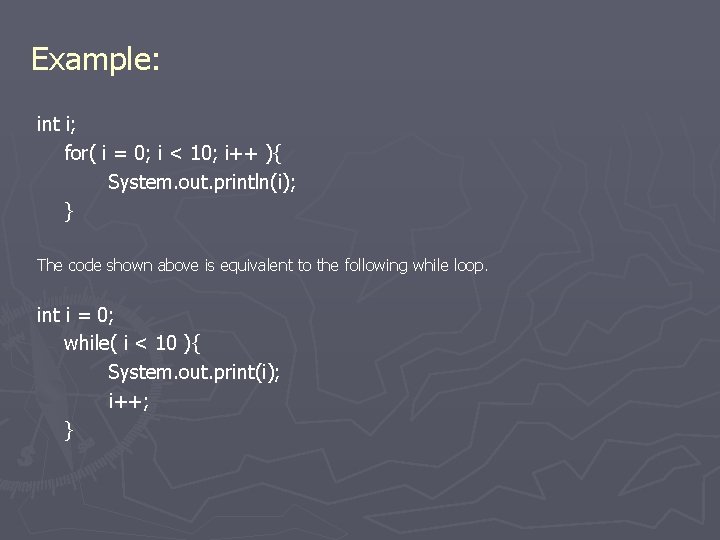
Example: int i; for( i = 0; i < 10; i++ ){ System. out. println(i); } The code shown above is equivalent to the following while loop. int i = 0; while( i < 10 ){ System. out. print(i); i++; }