Decisions decisions Chapter 5 Spring 2006 CS 101
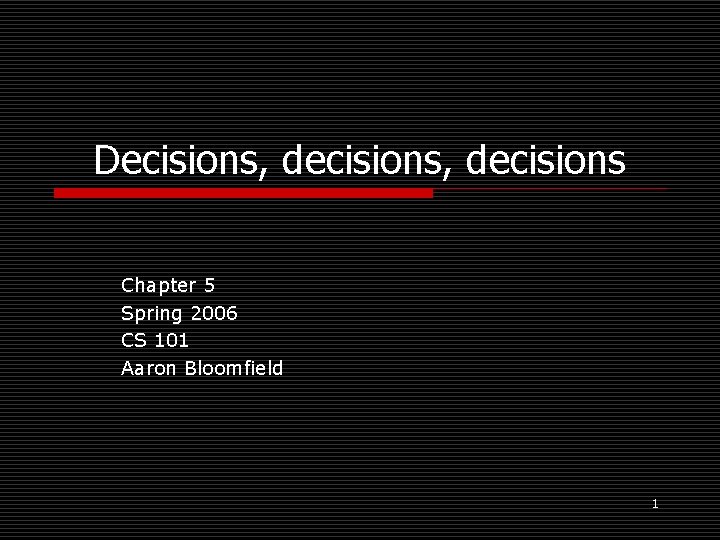
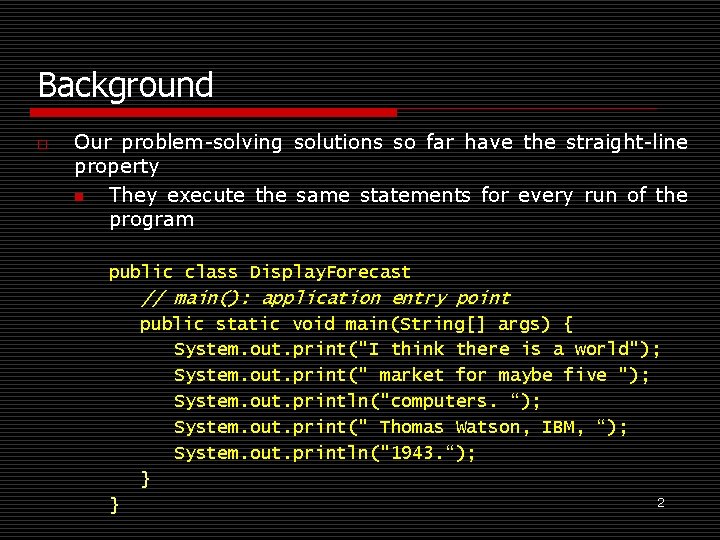
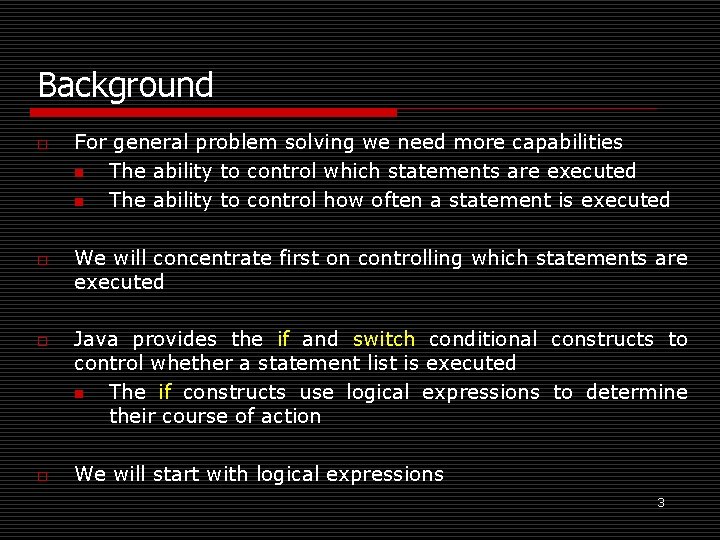
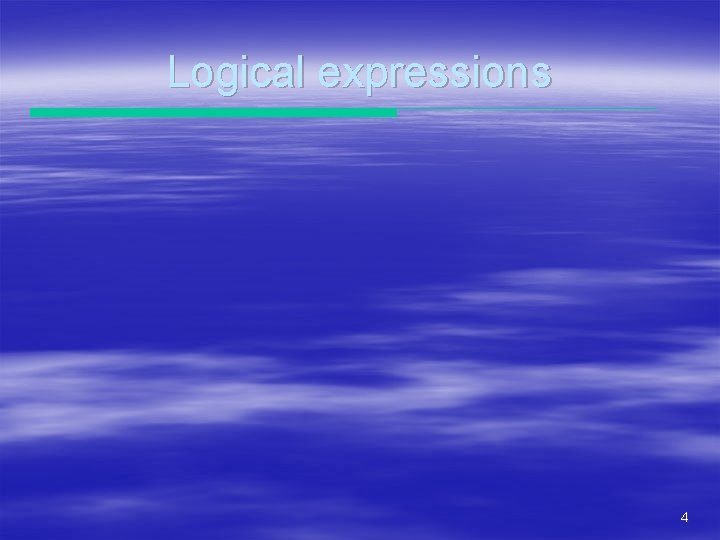
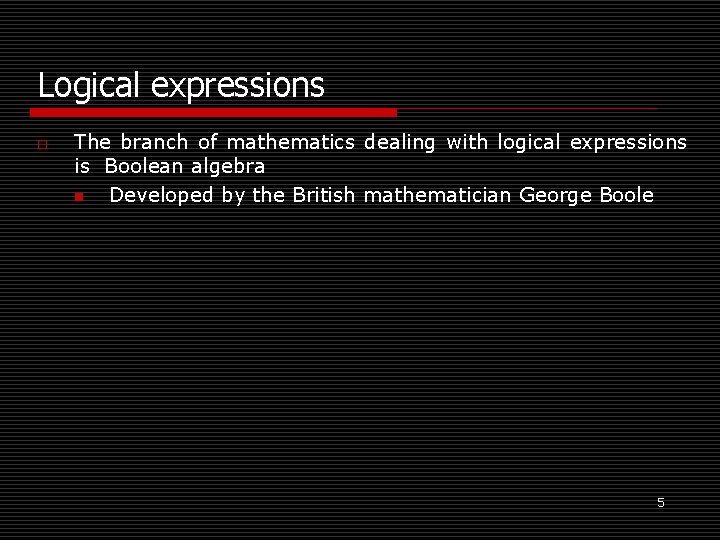
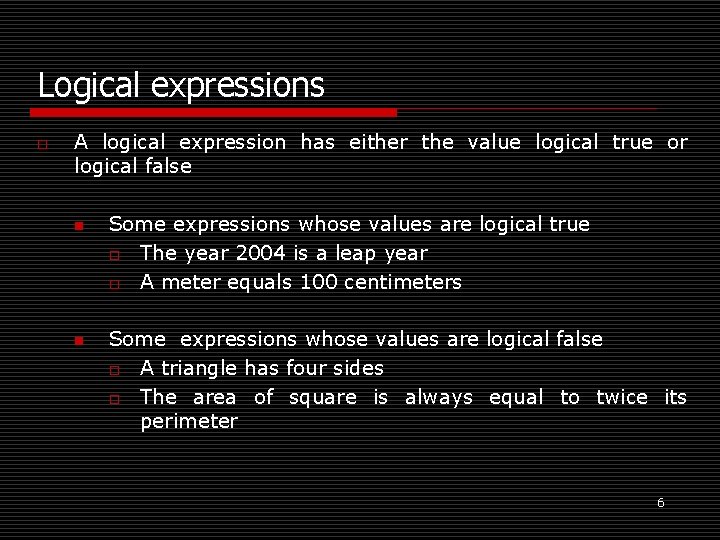
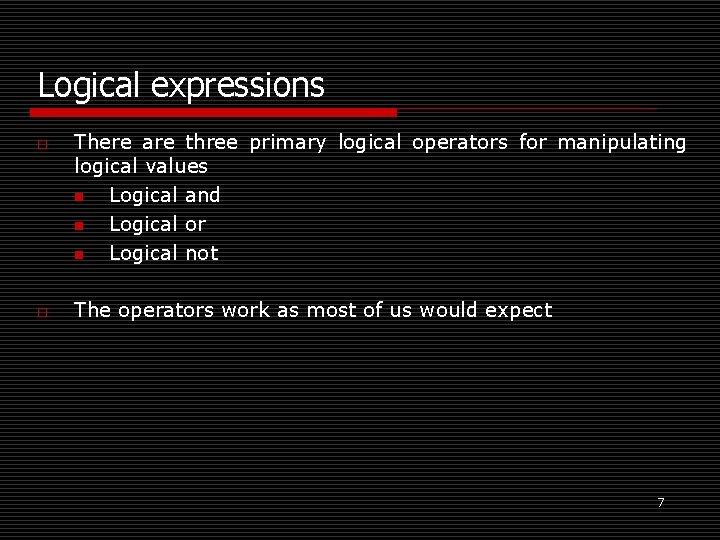
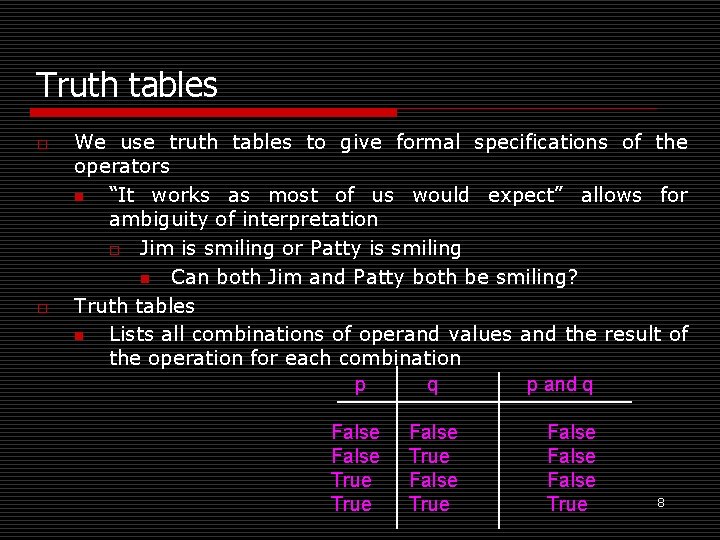
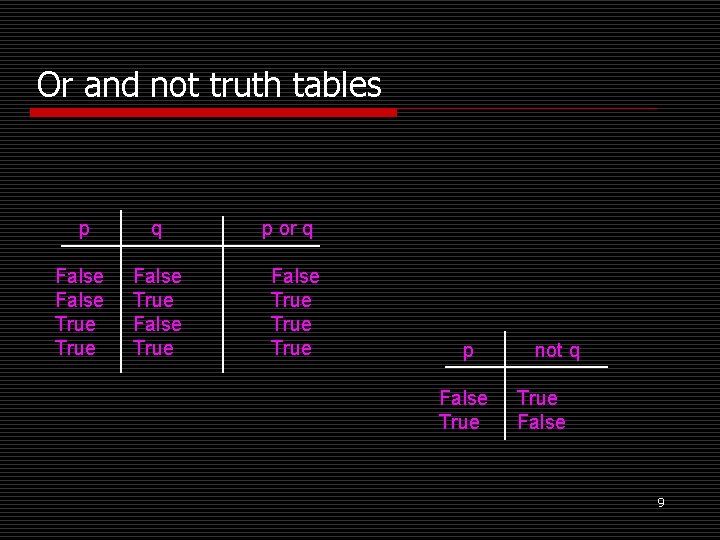
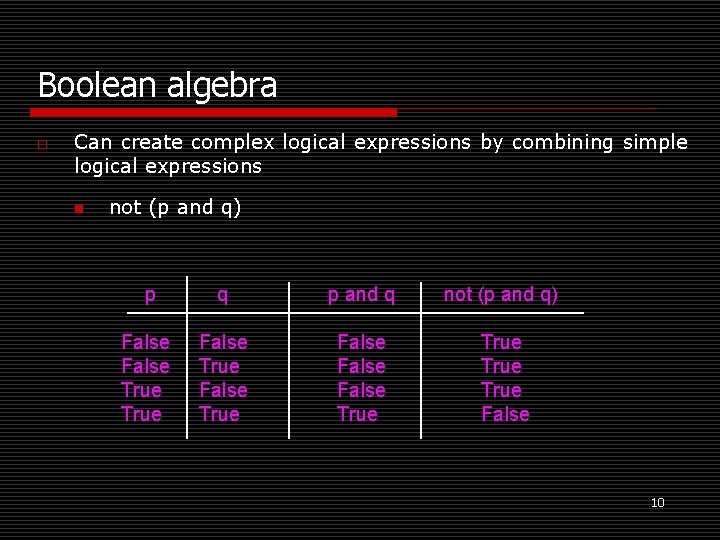
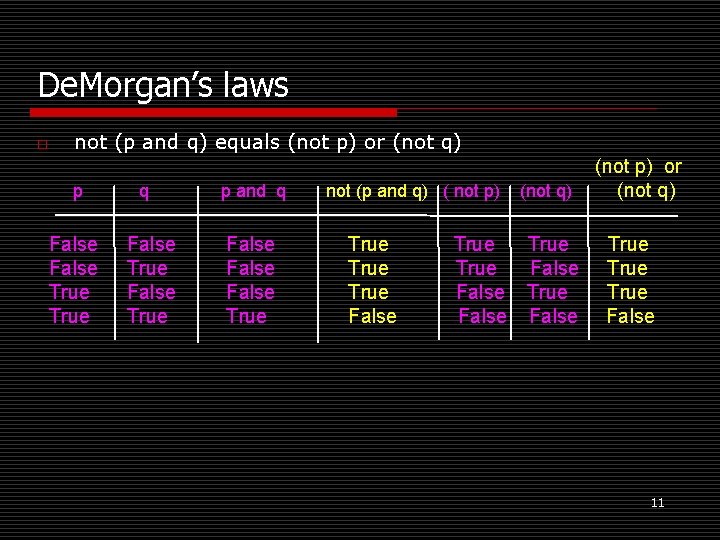
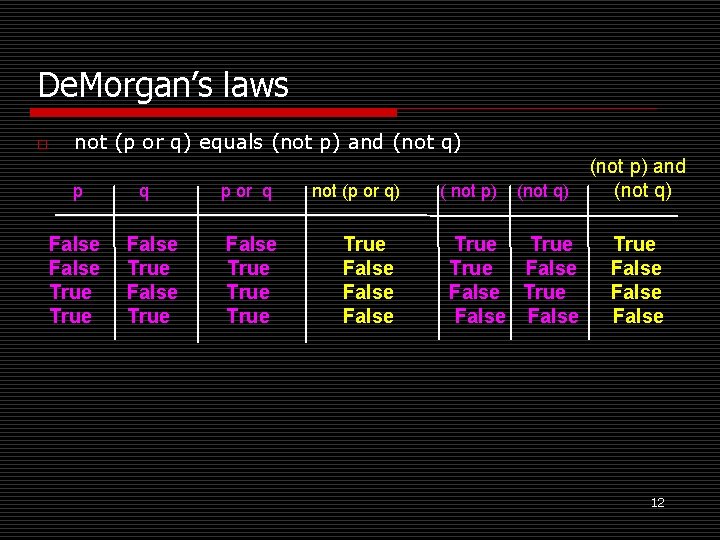
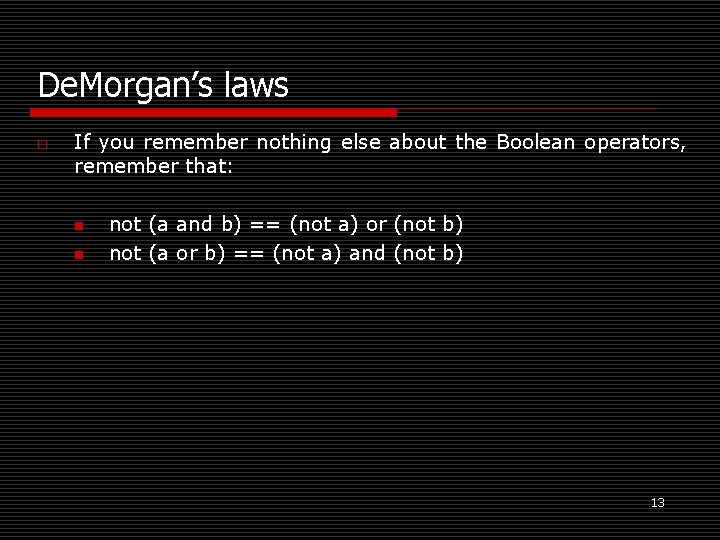
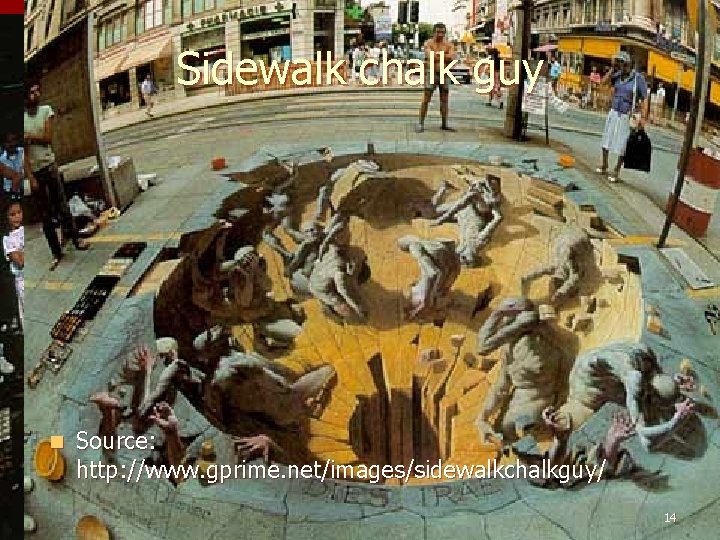
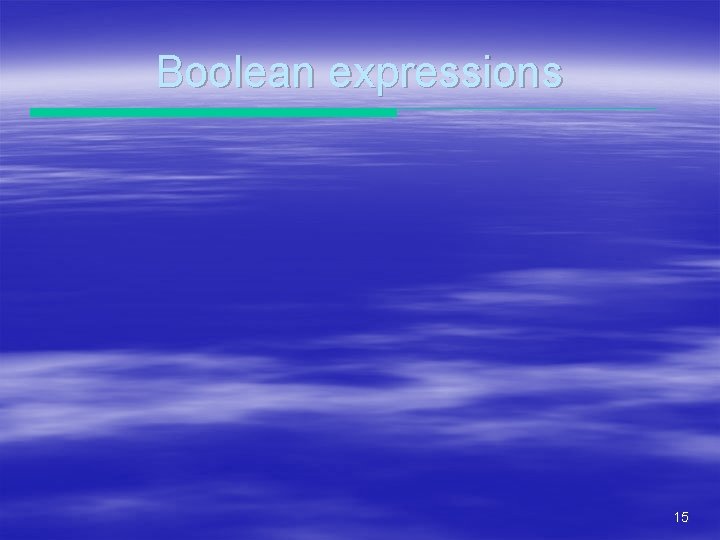
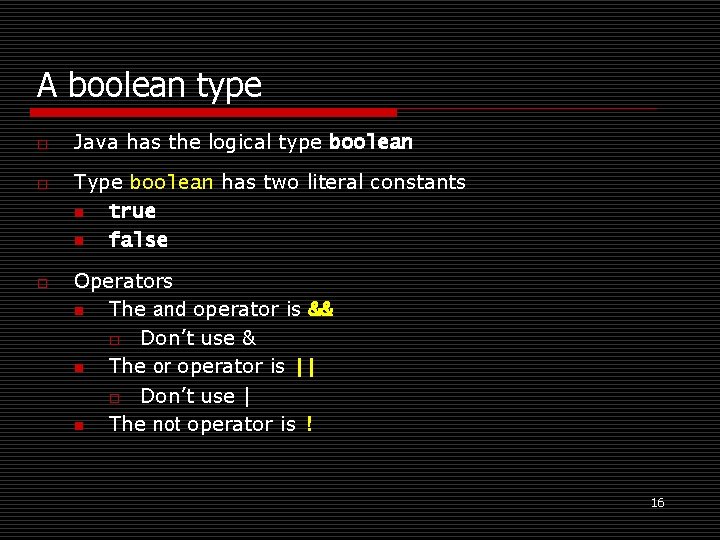
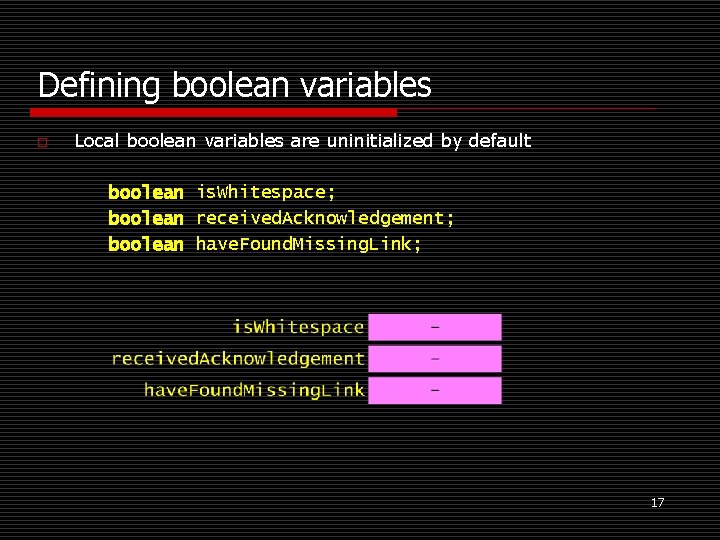
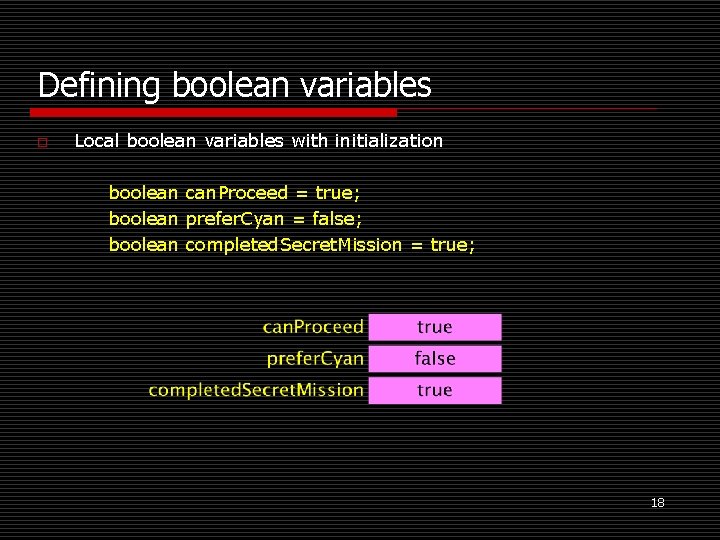
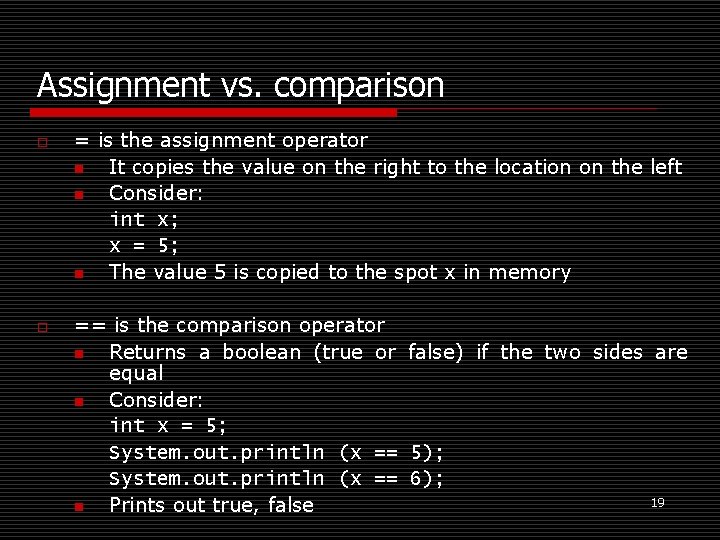
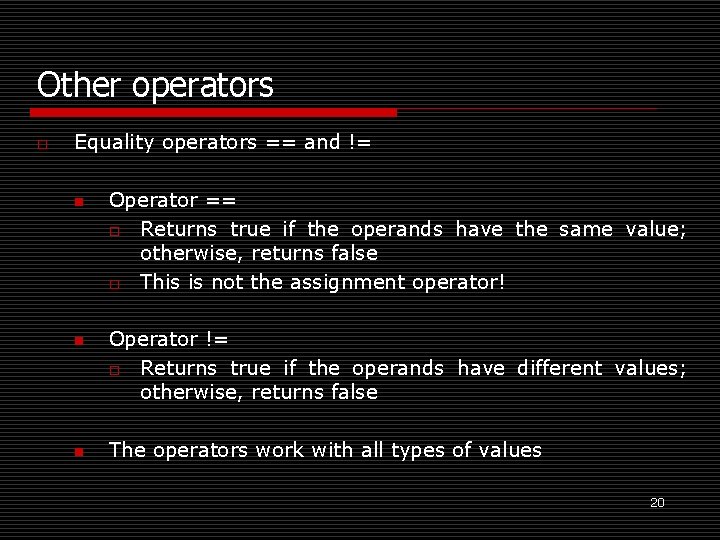
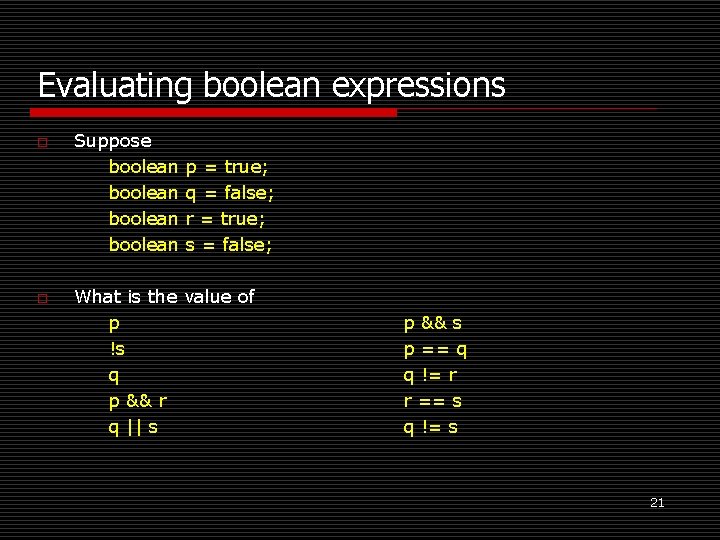
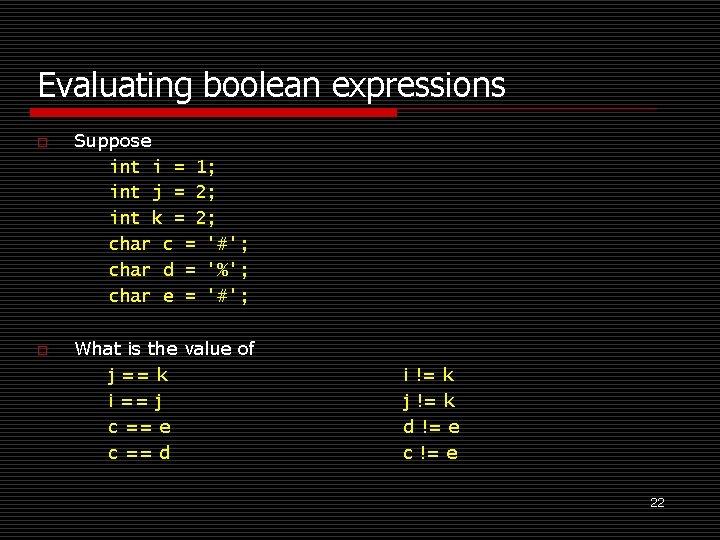
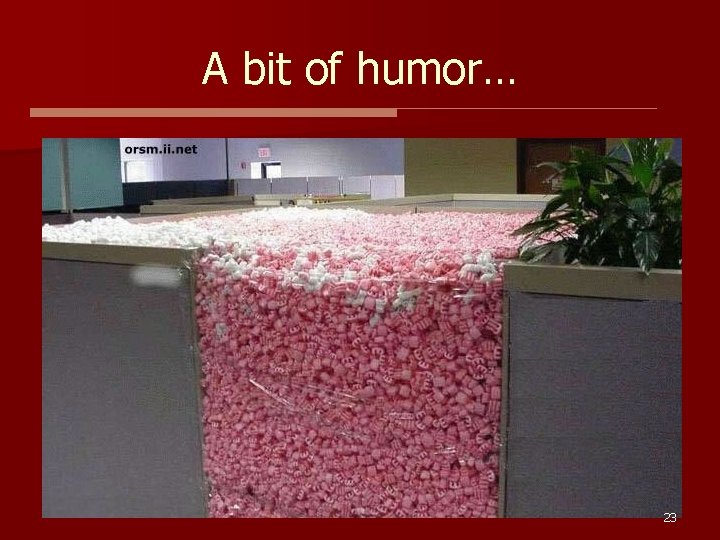
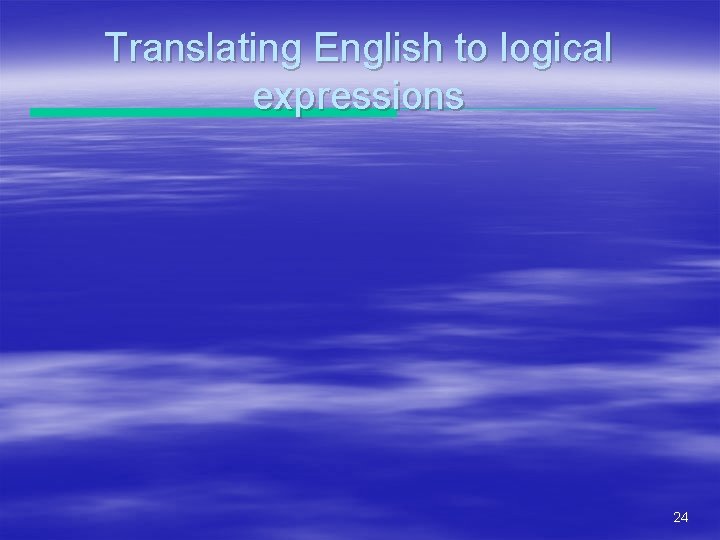
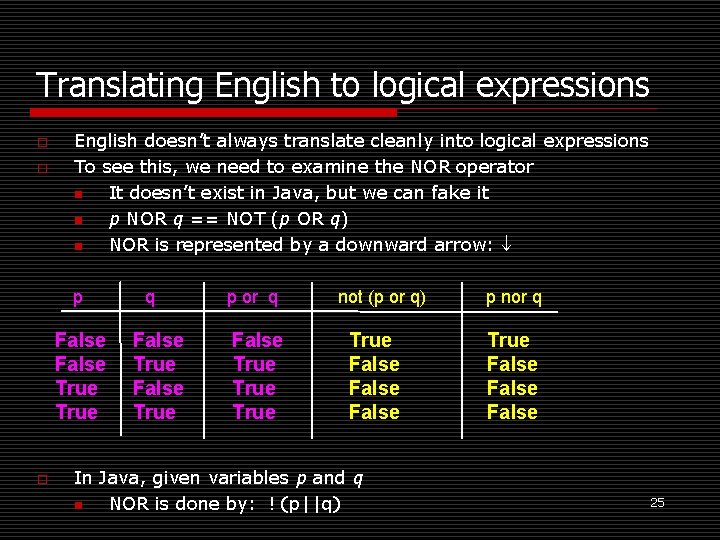
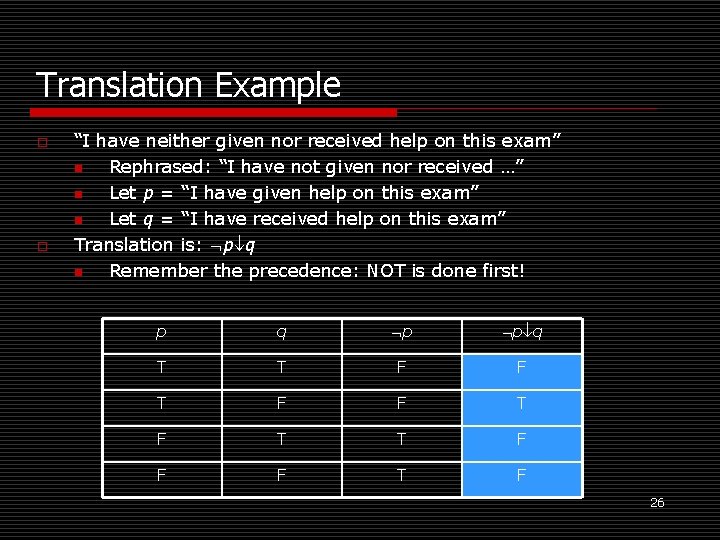
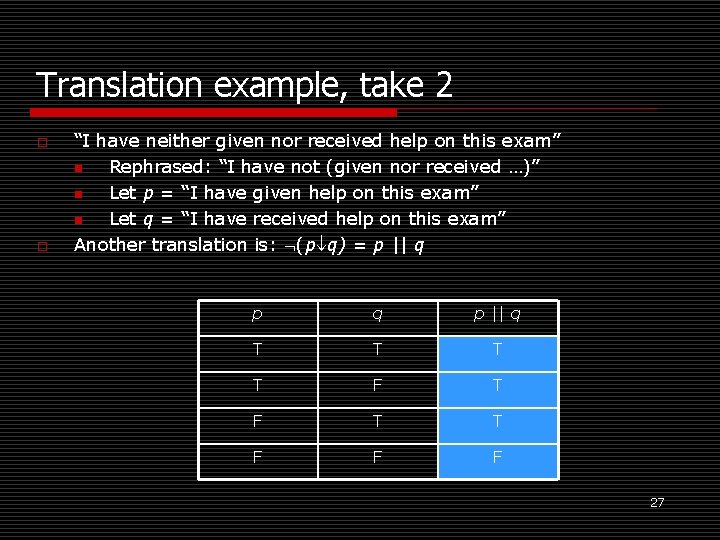
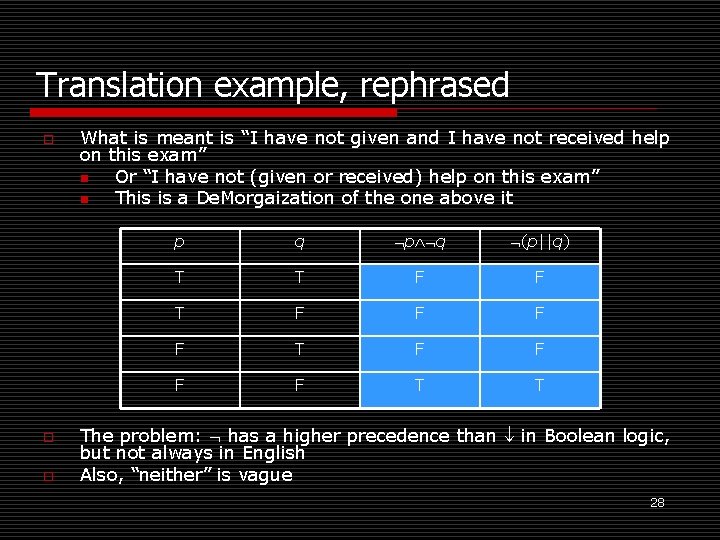
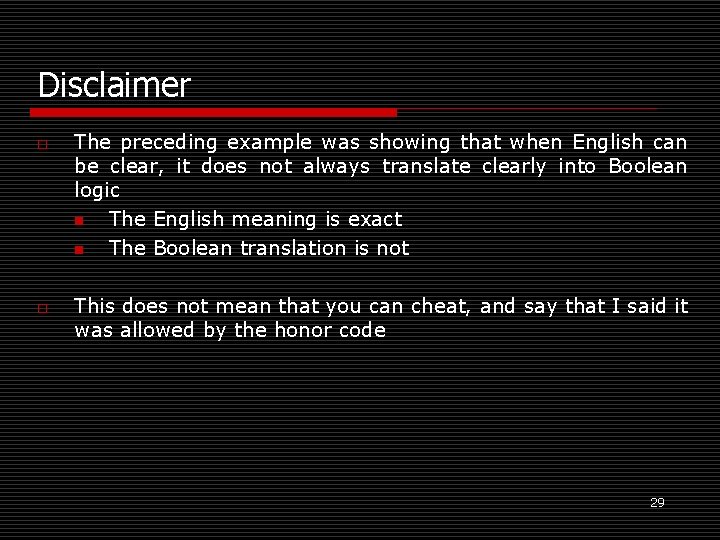
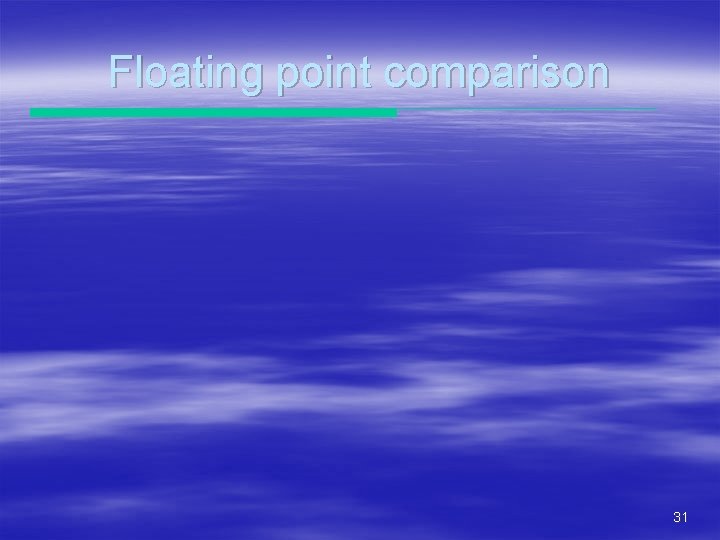
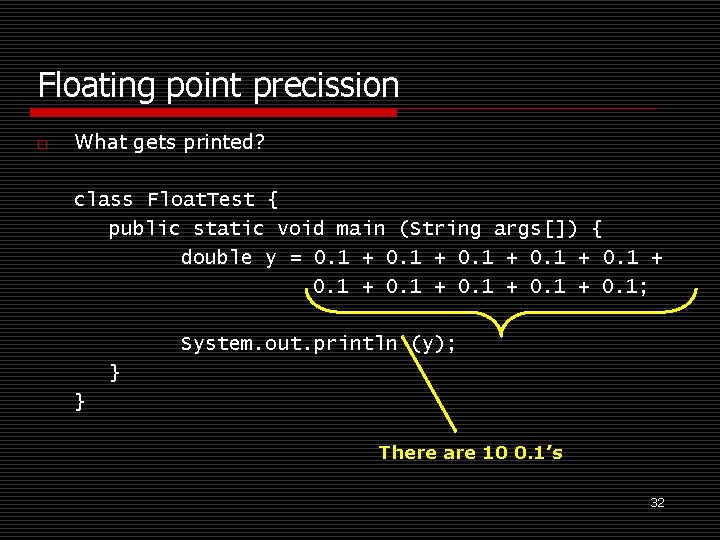
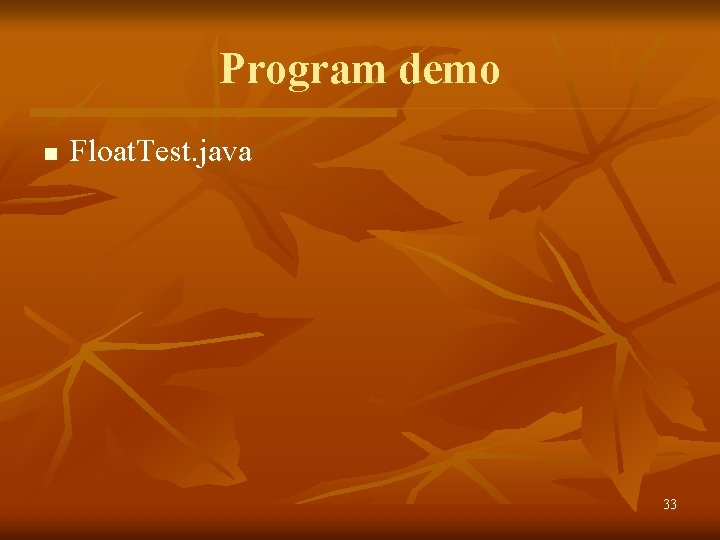
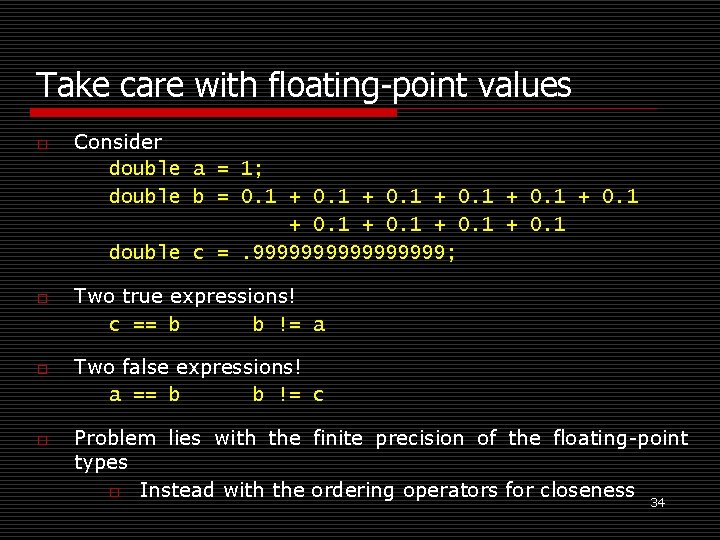
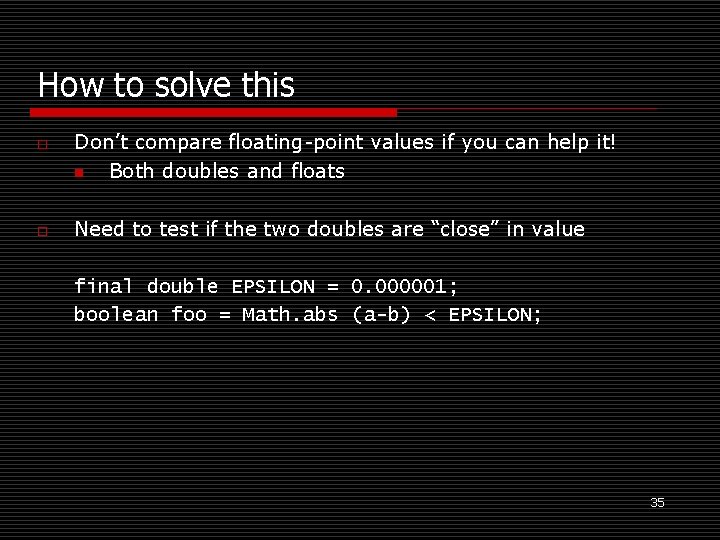
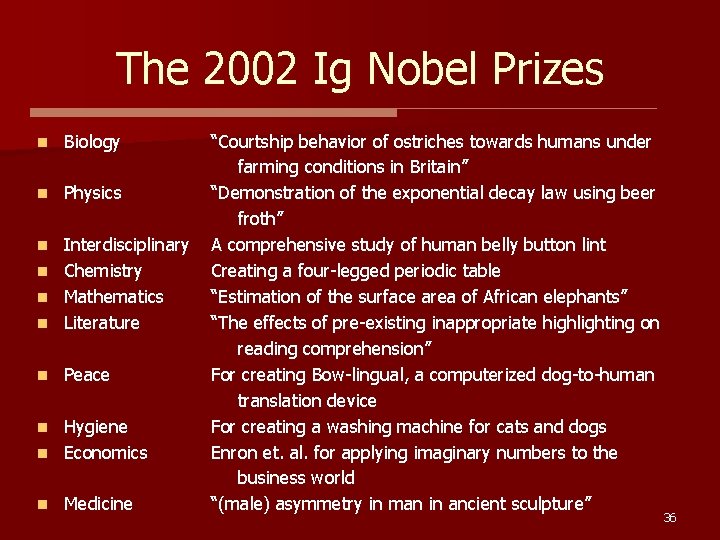
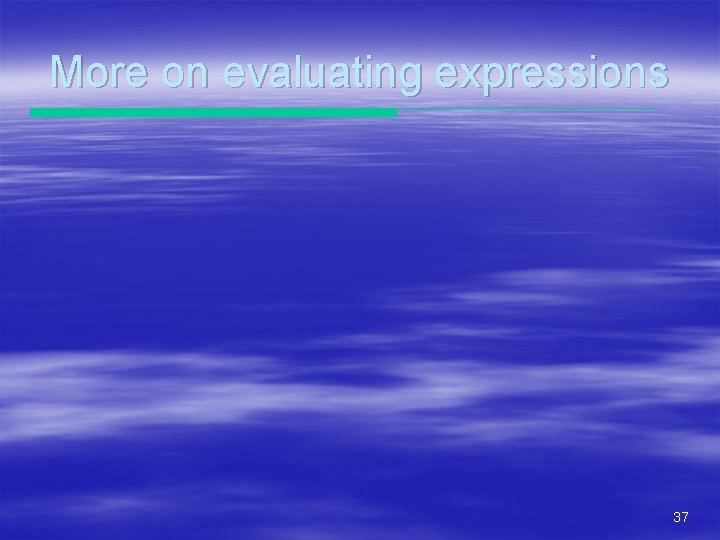
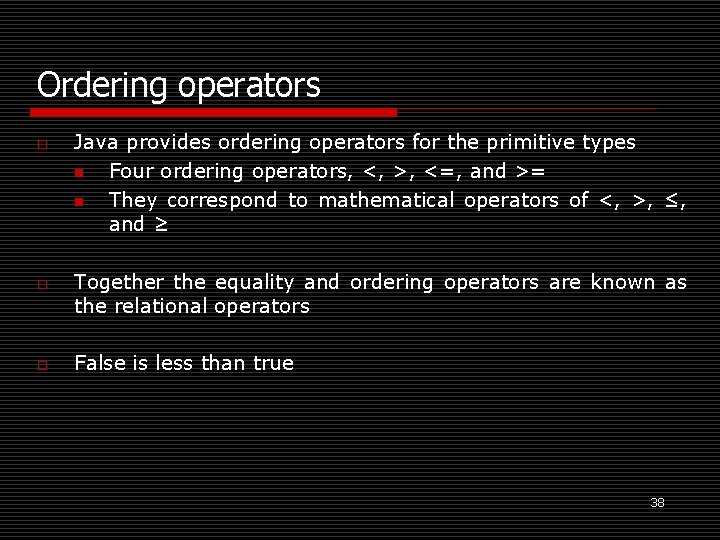
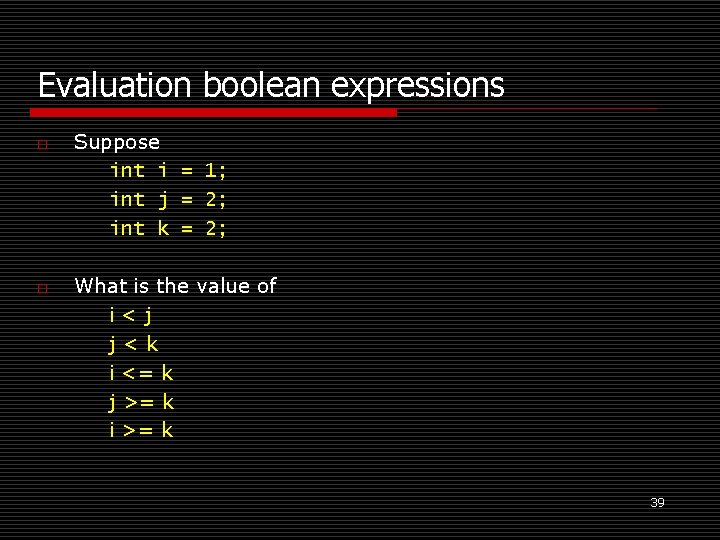
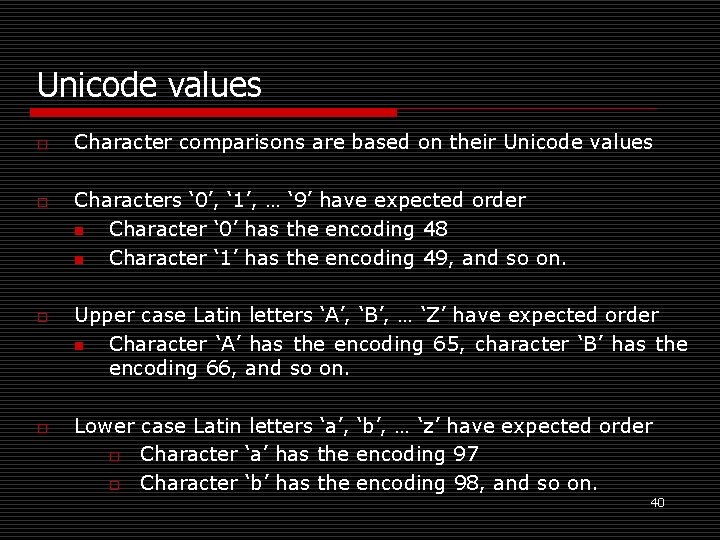
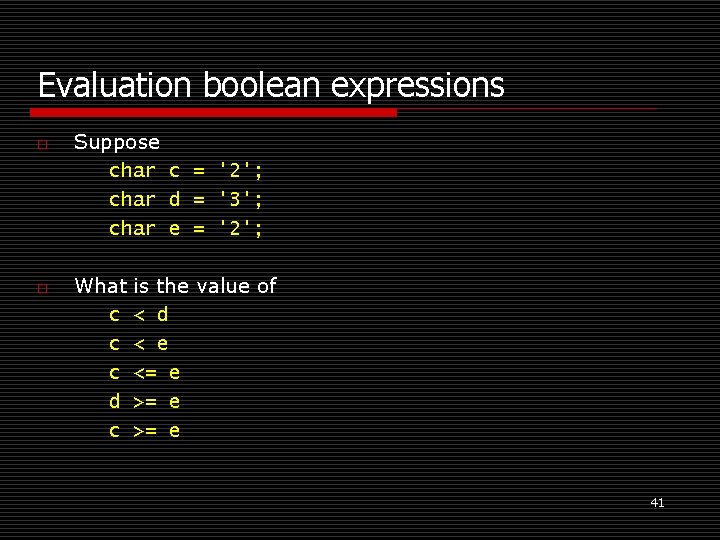
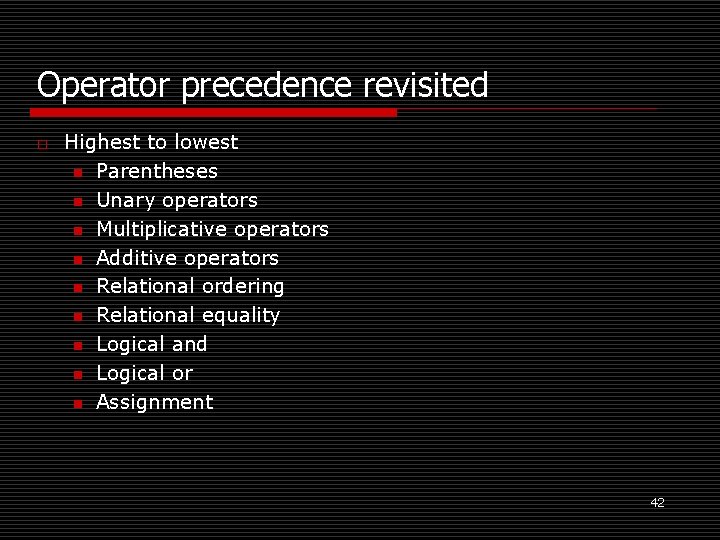
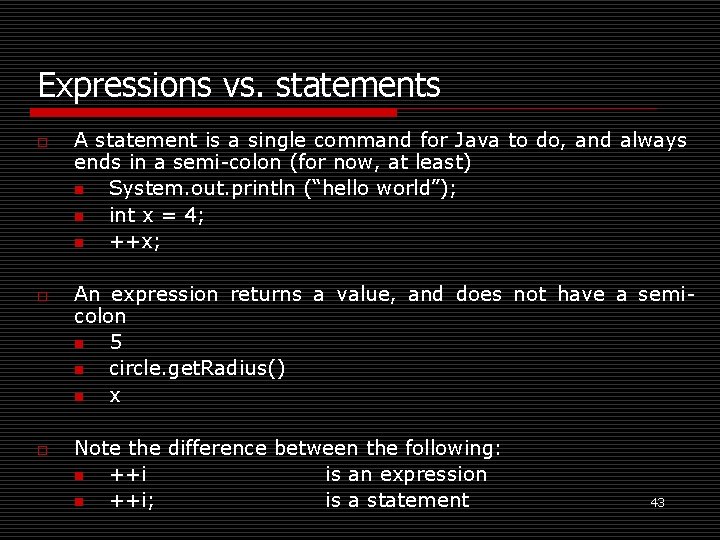
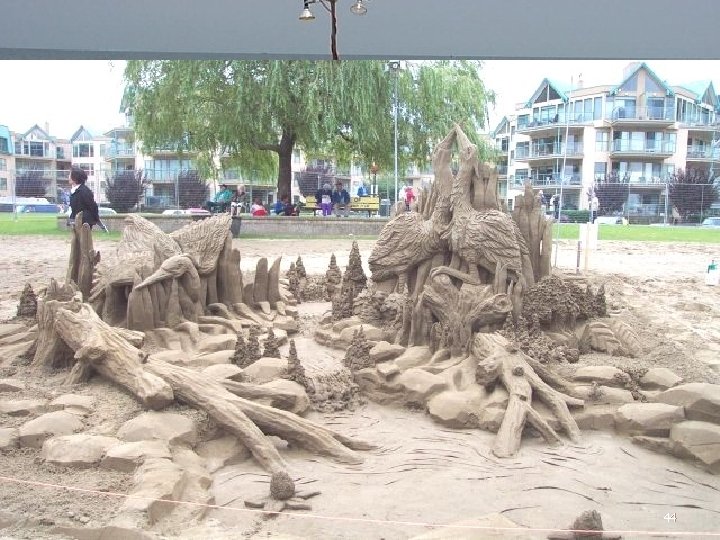
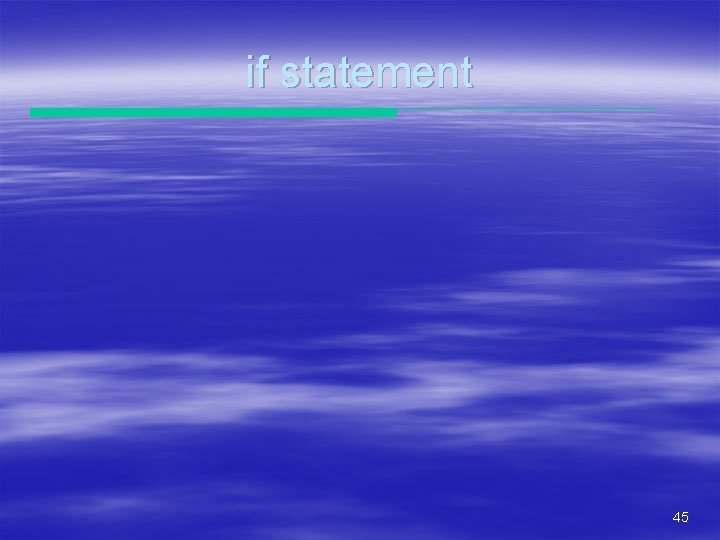
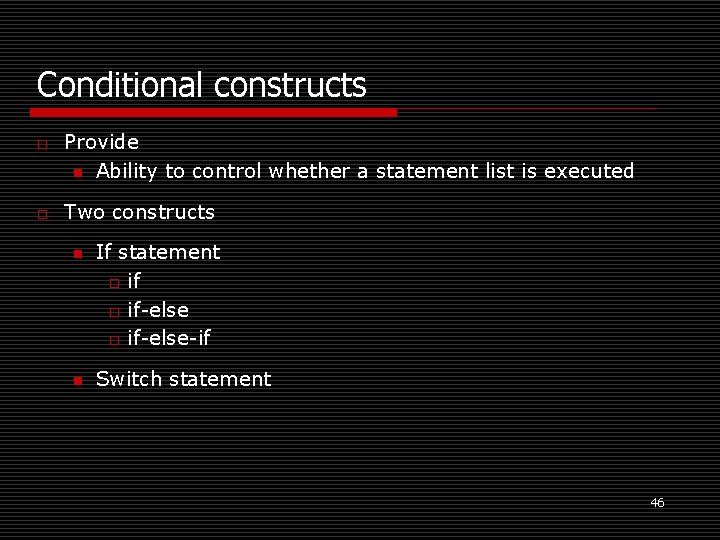
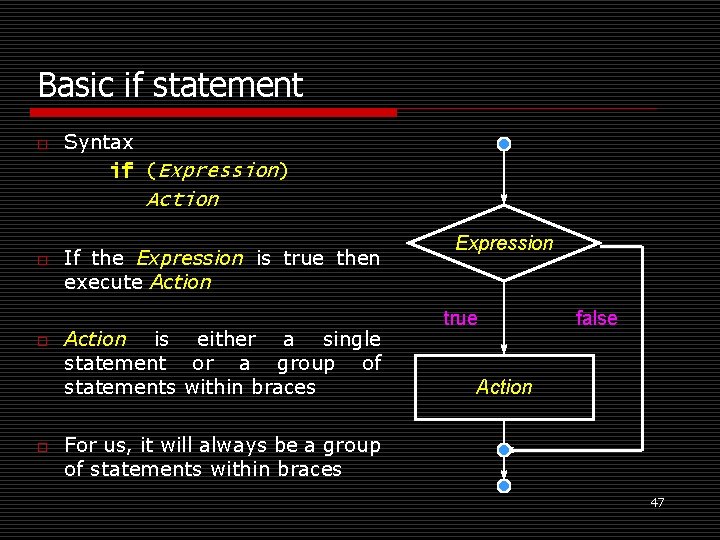
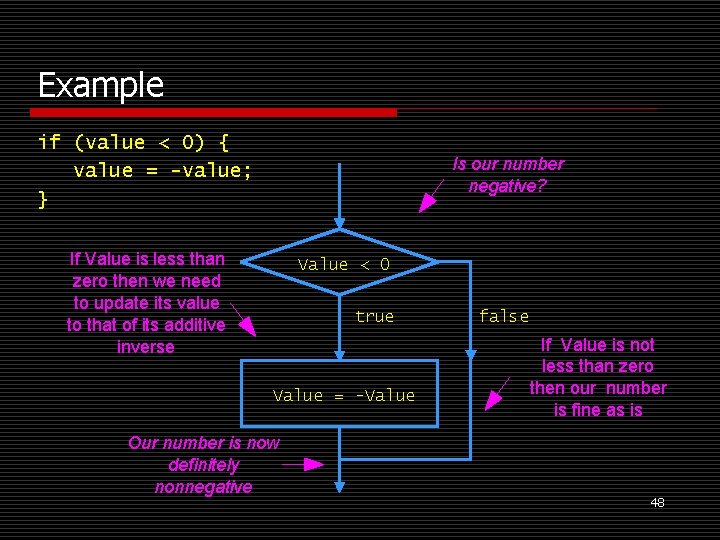
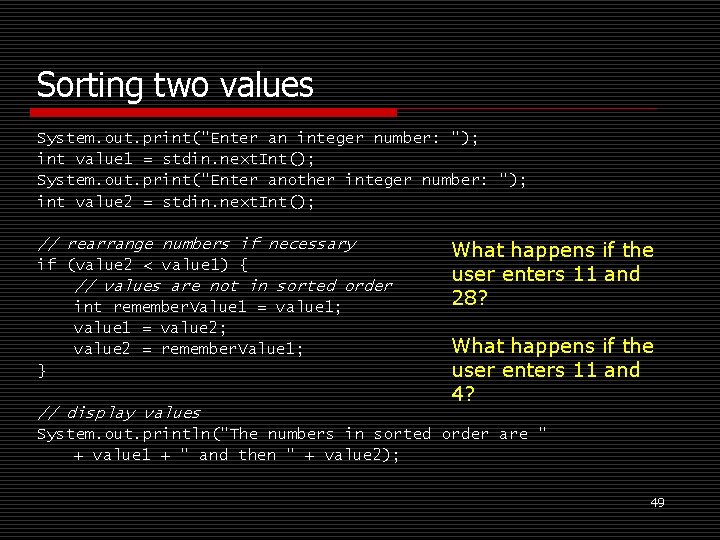
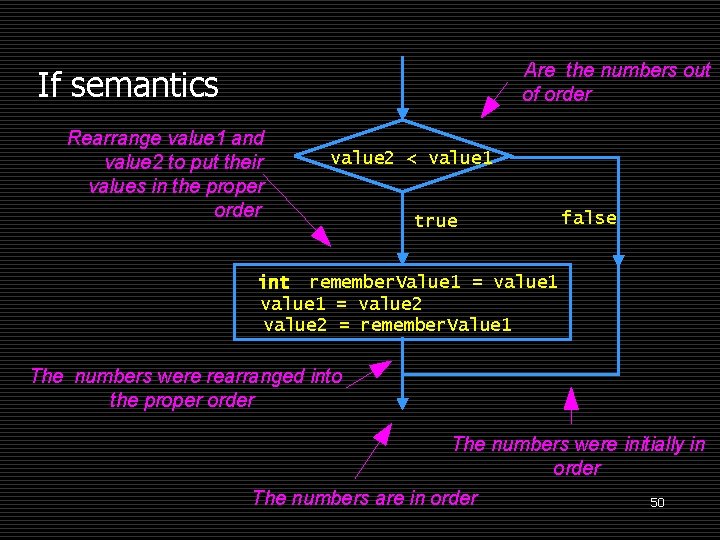
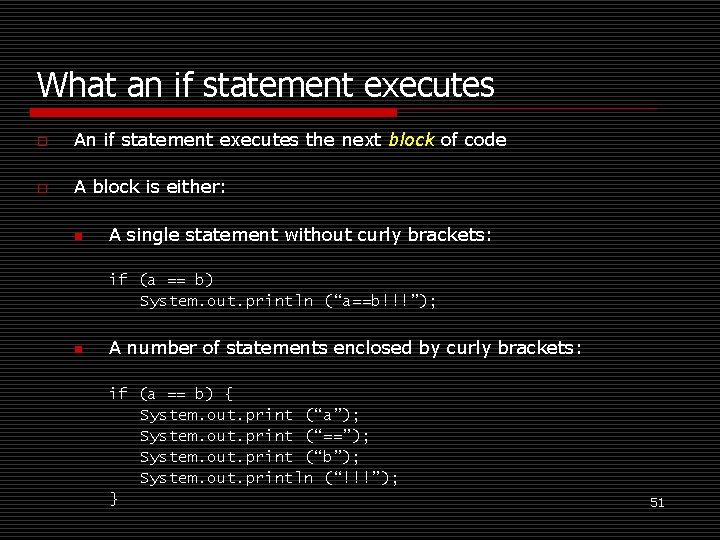
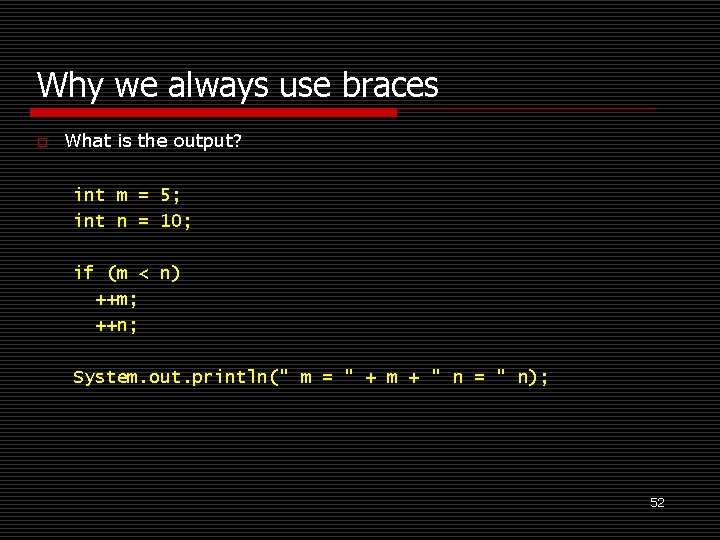
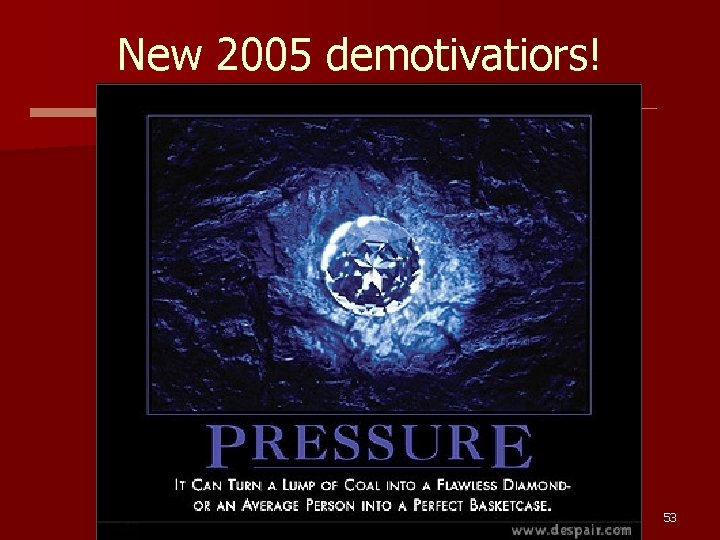
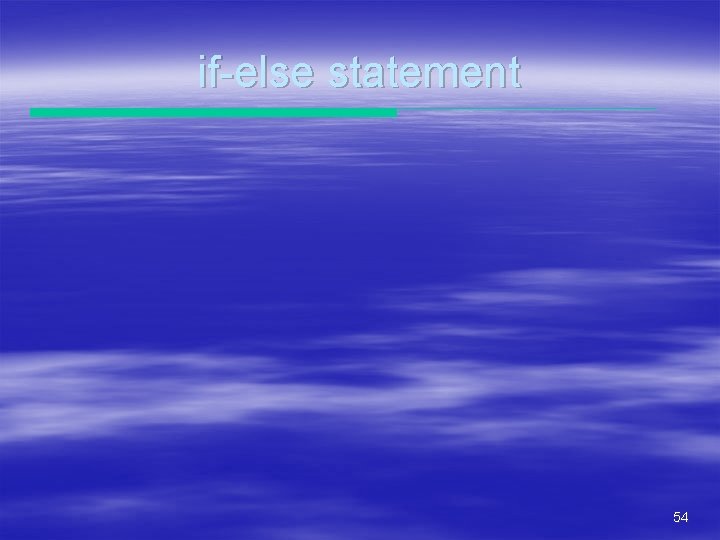
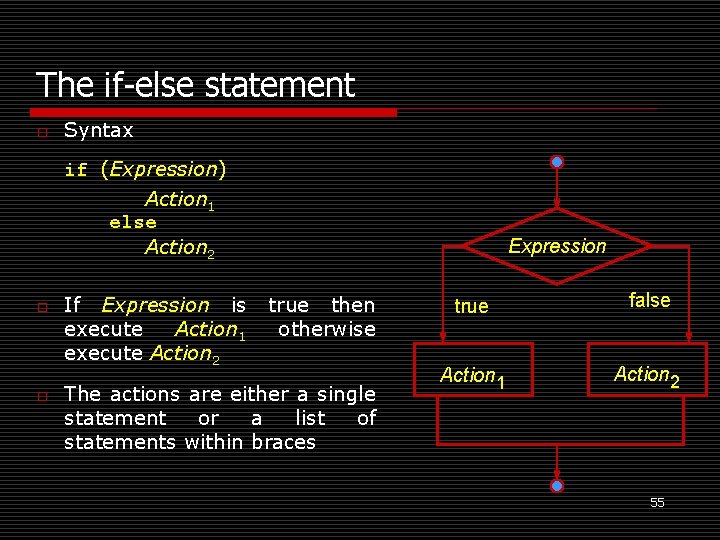
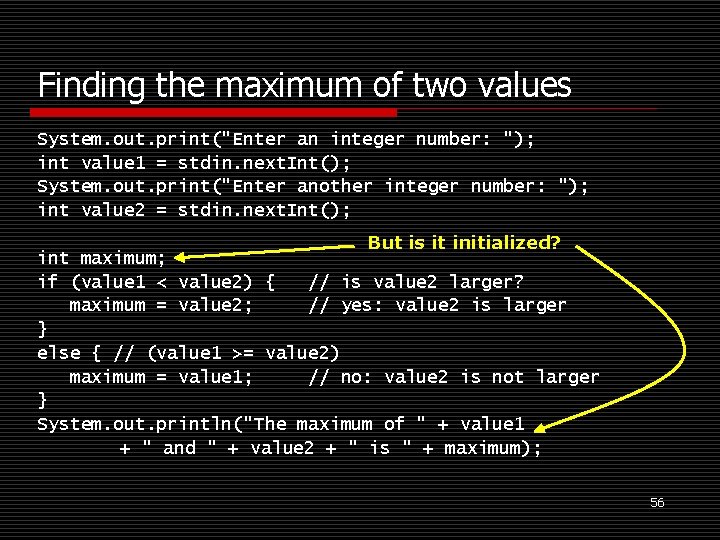
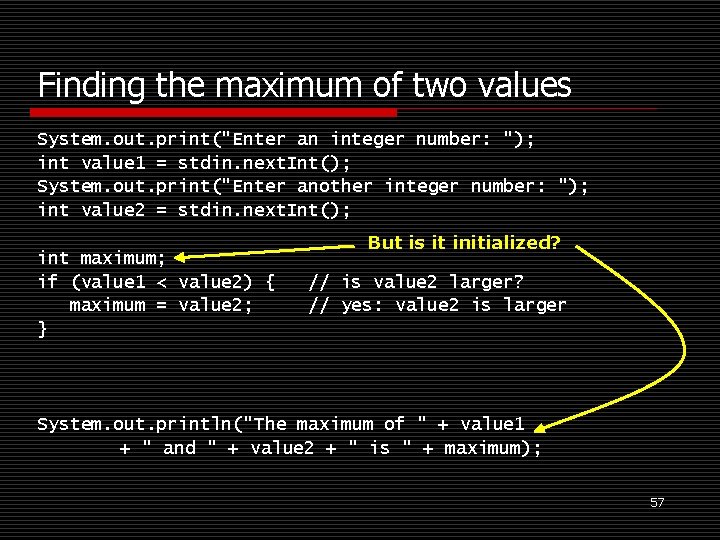
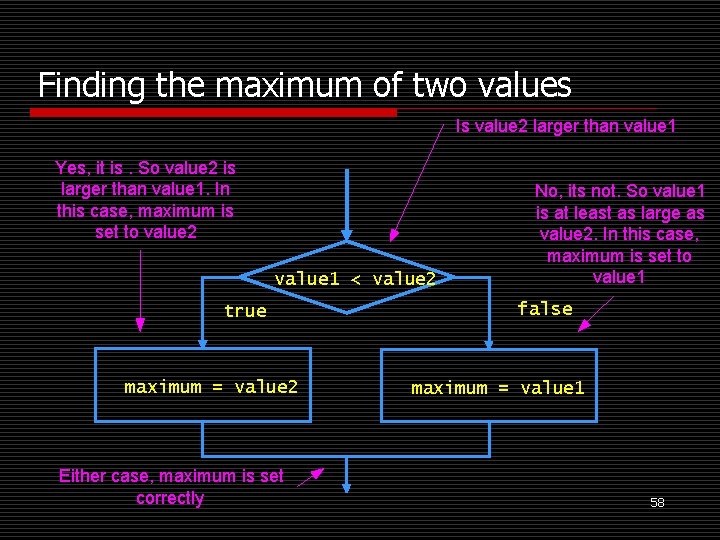
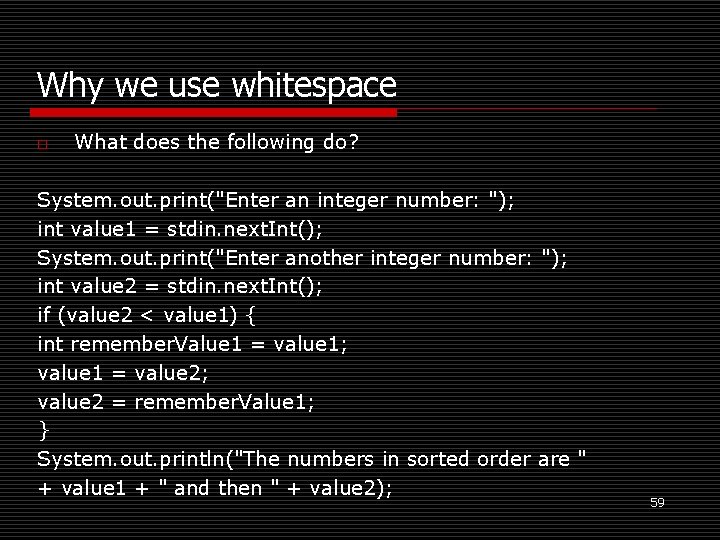
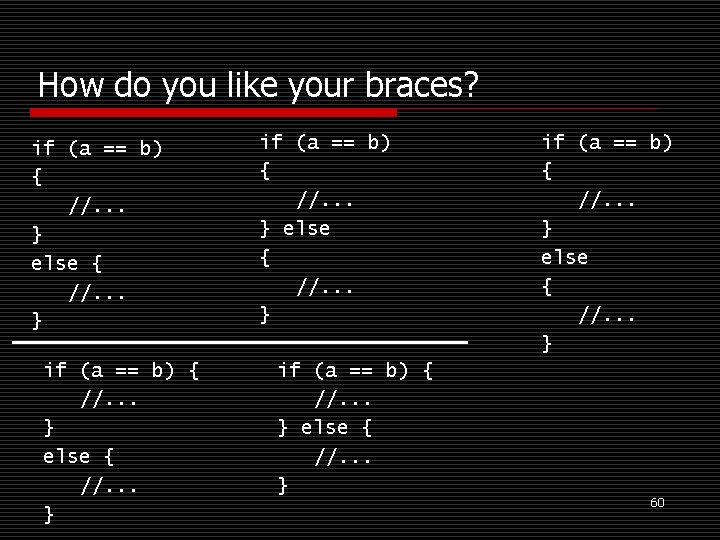
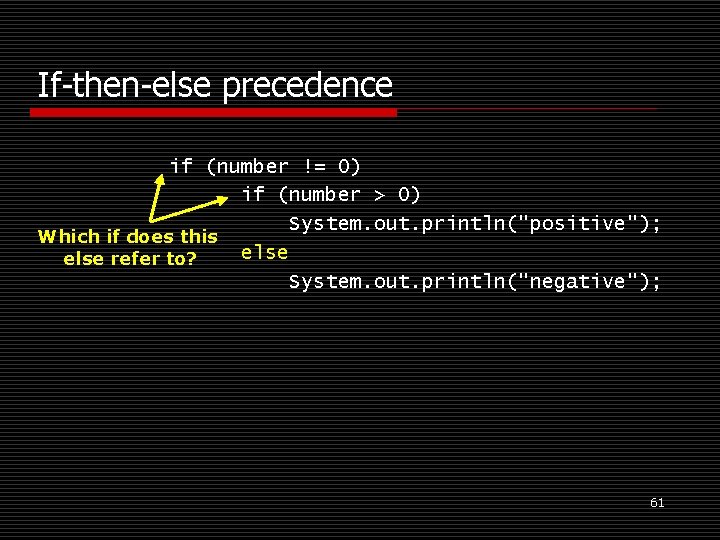
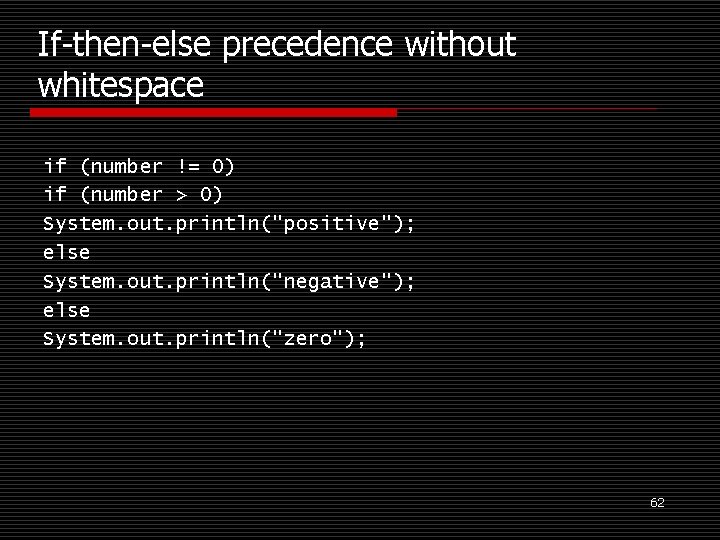
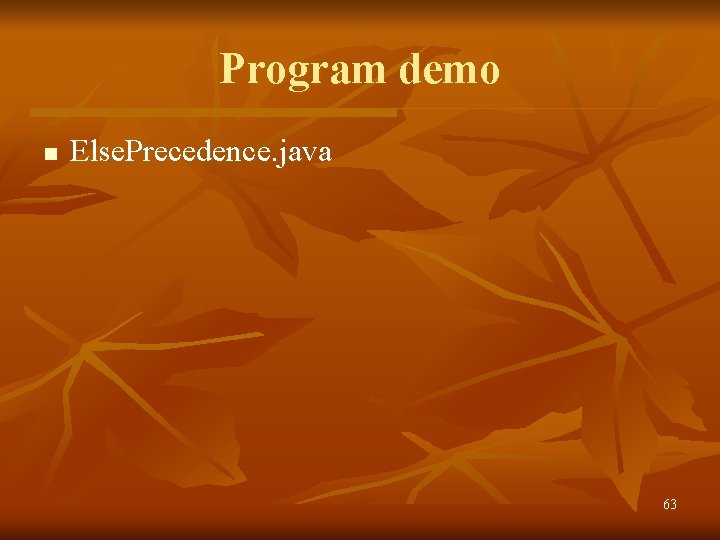
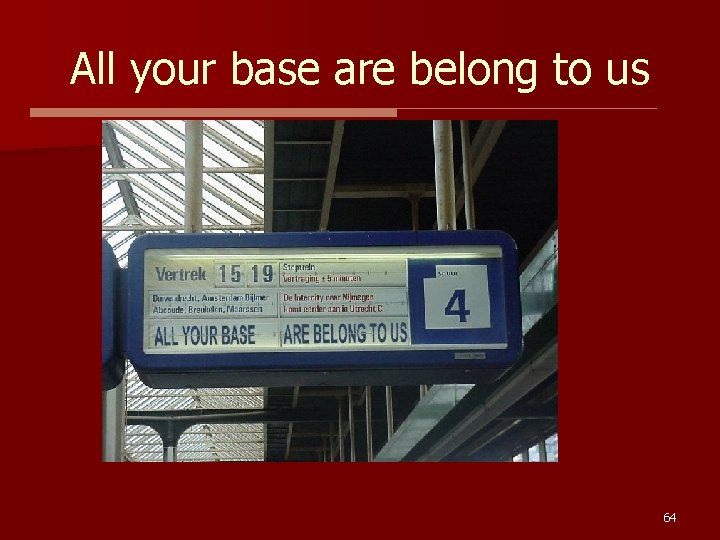
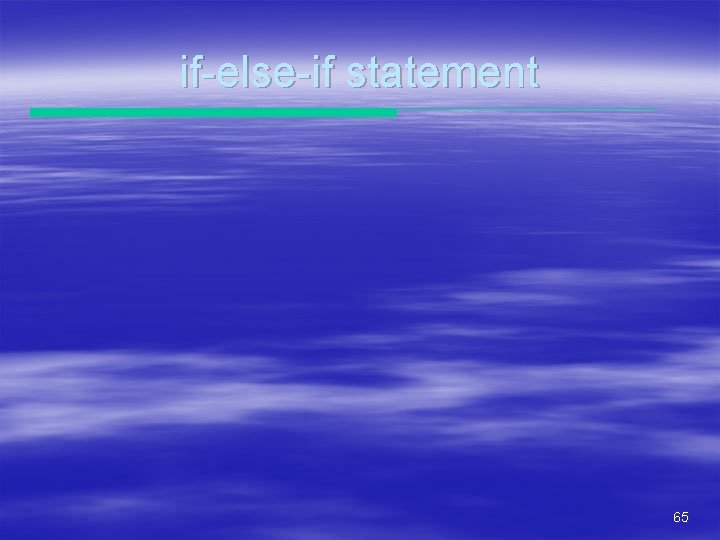
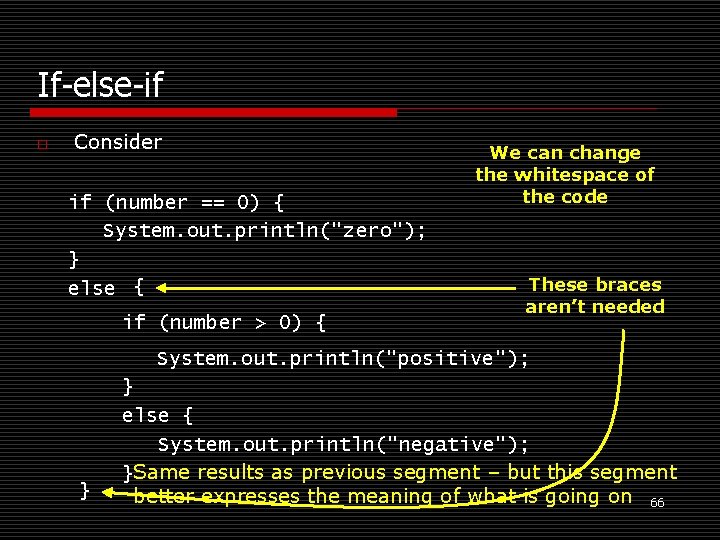
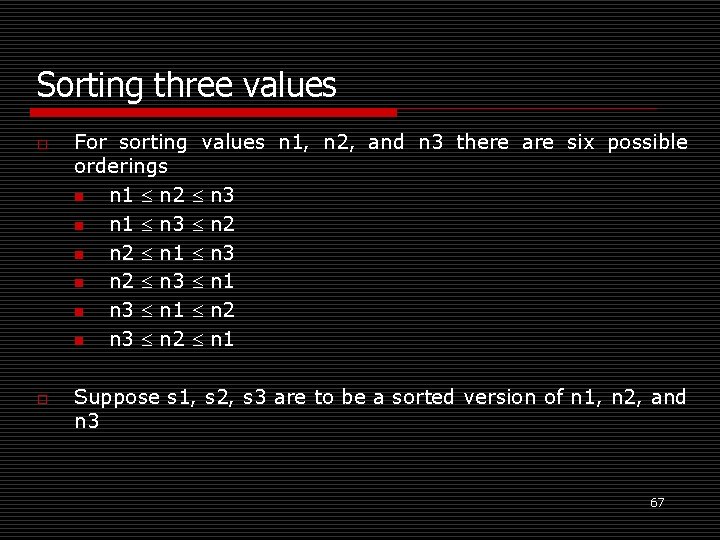
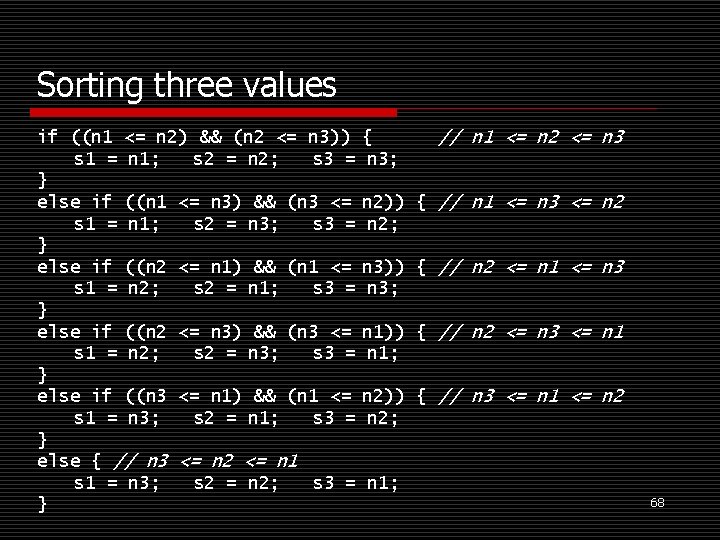
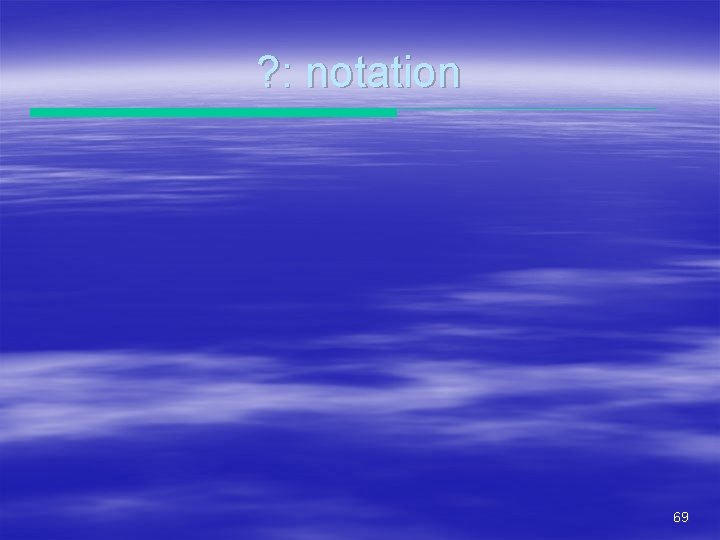
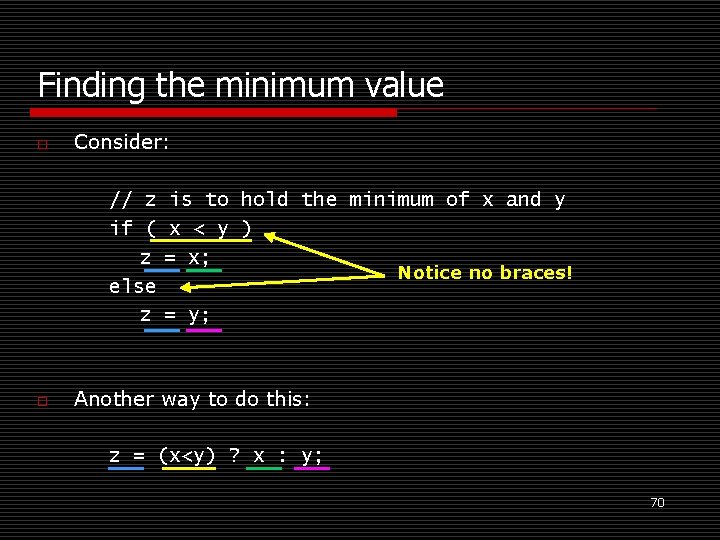
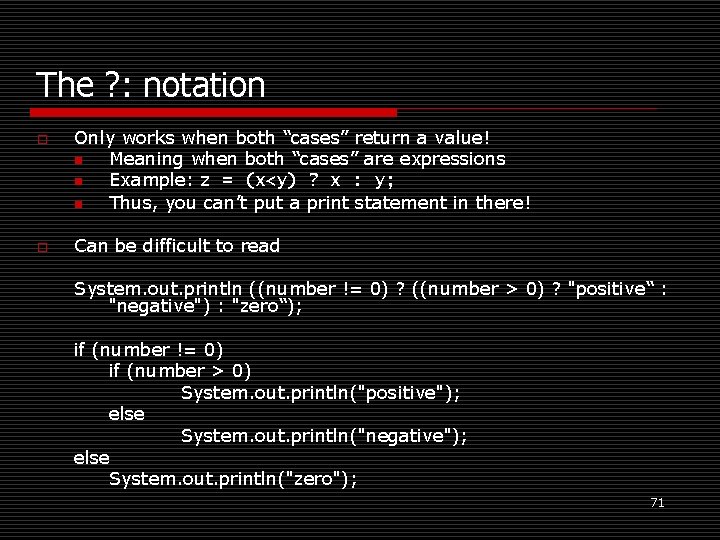
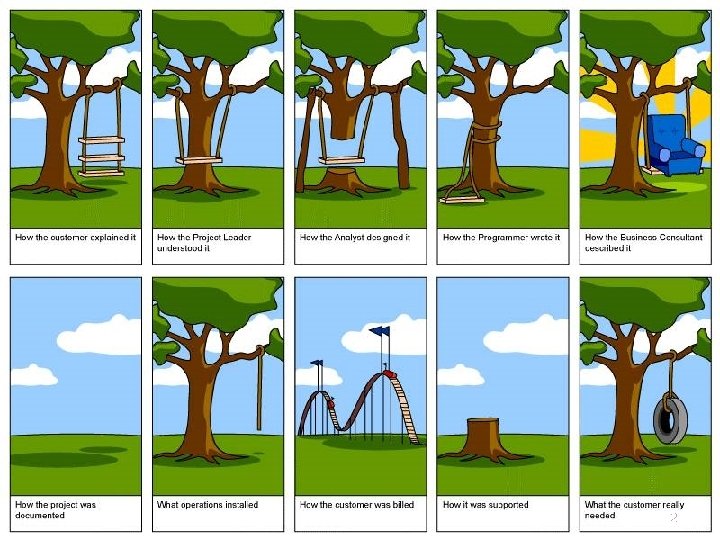
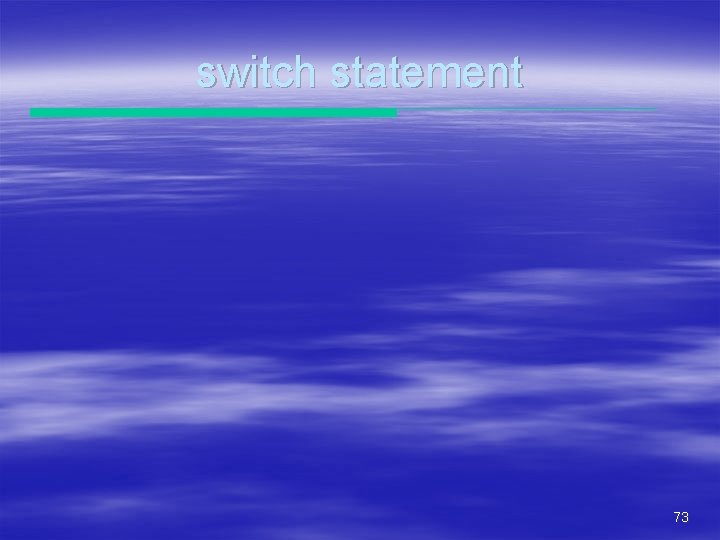
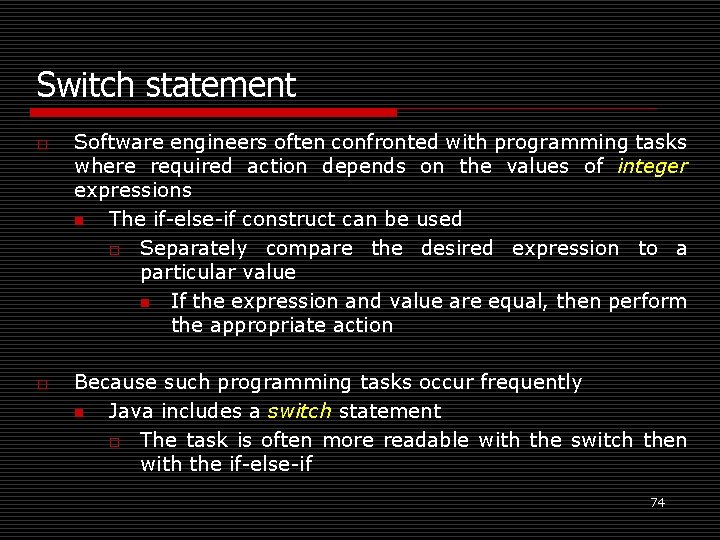
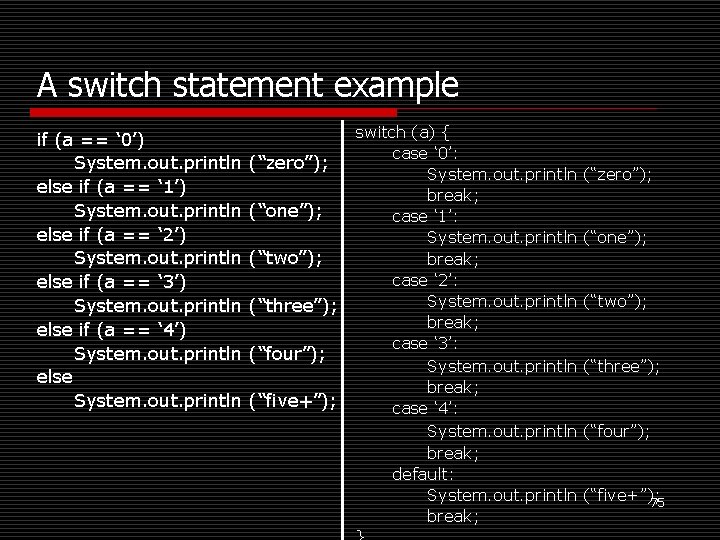
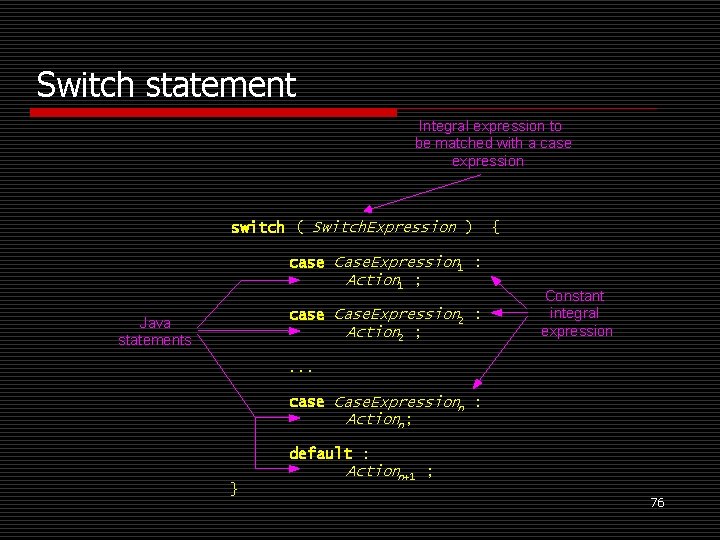
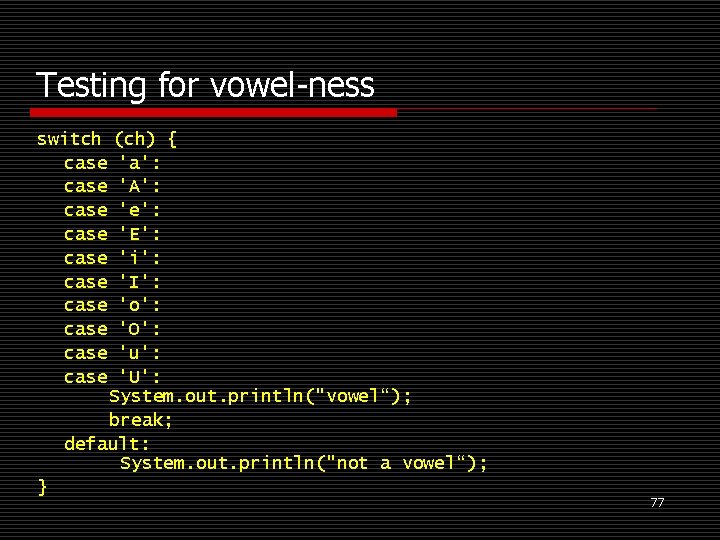
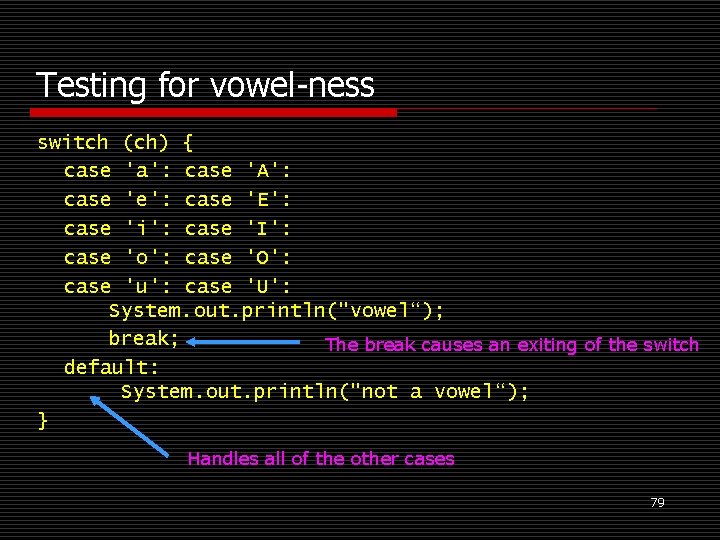
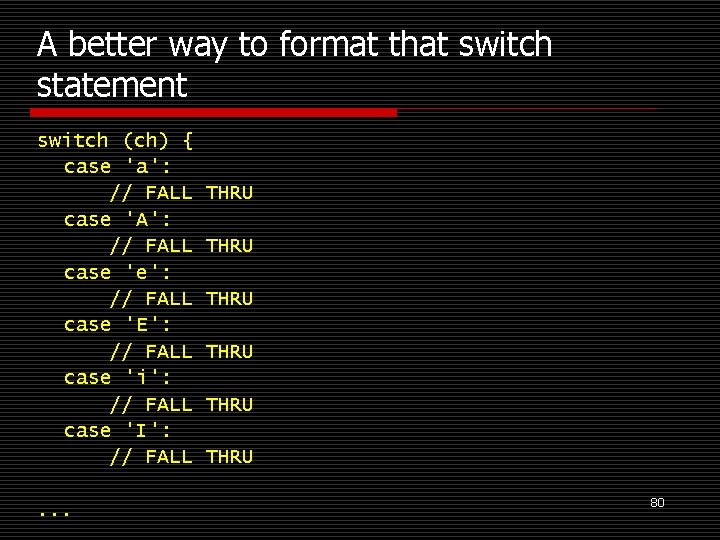
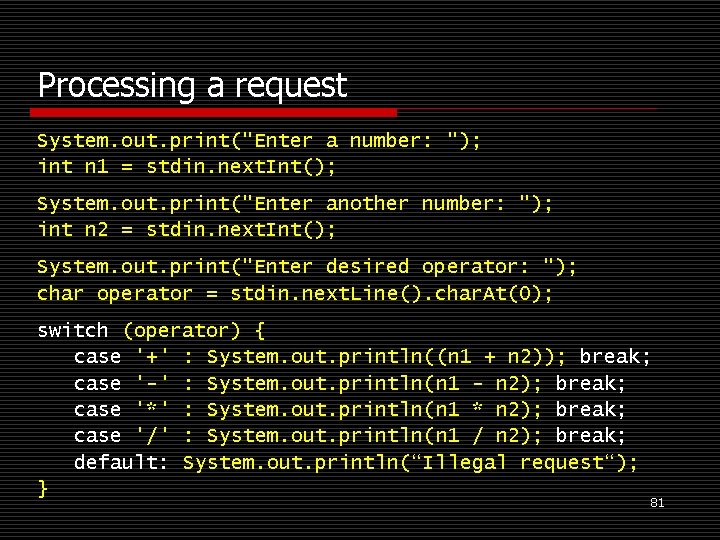
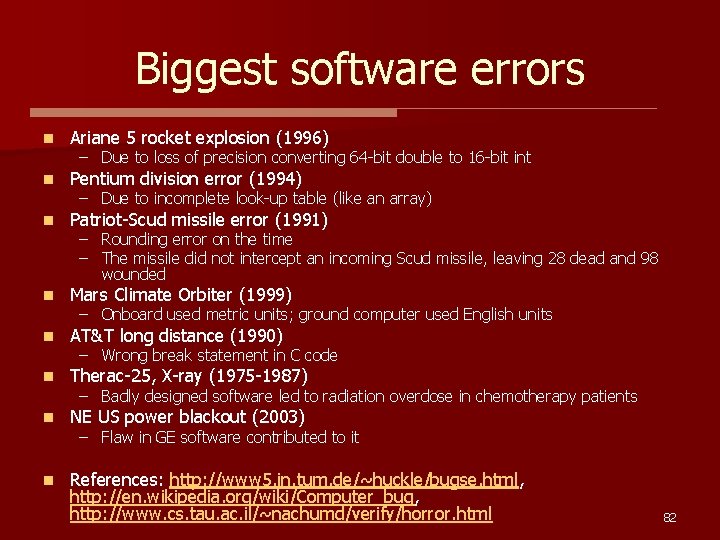
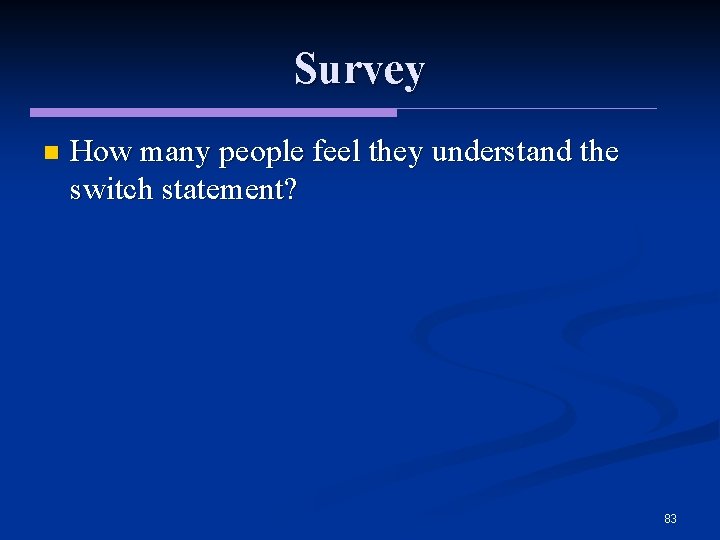
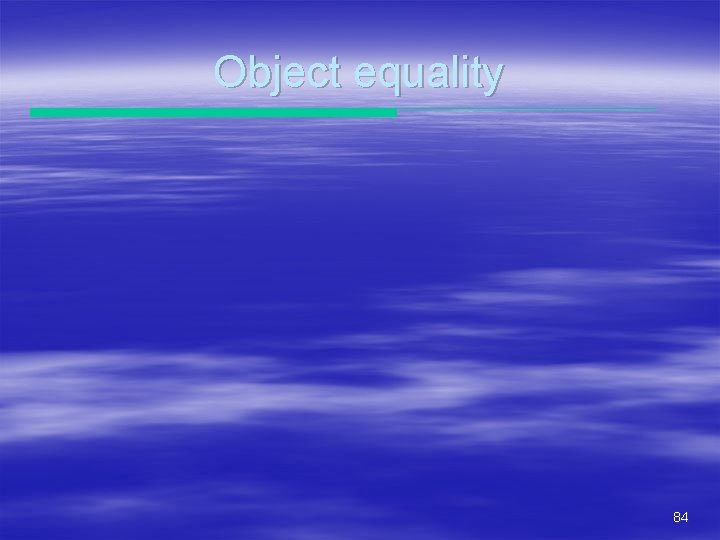
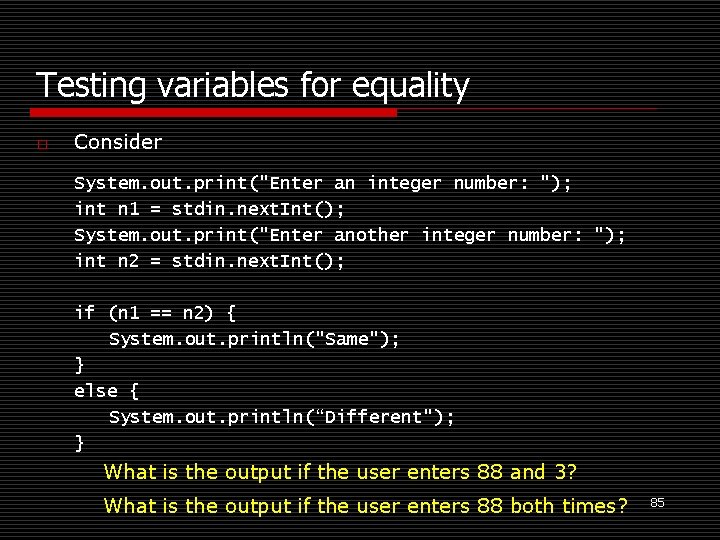
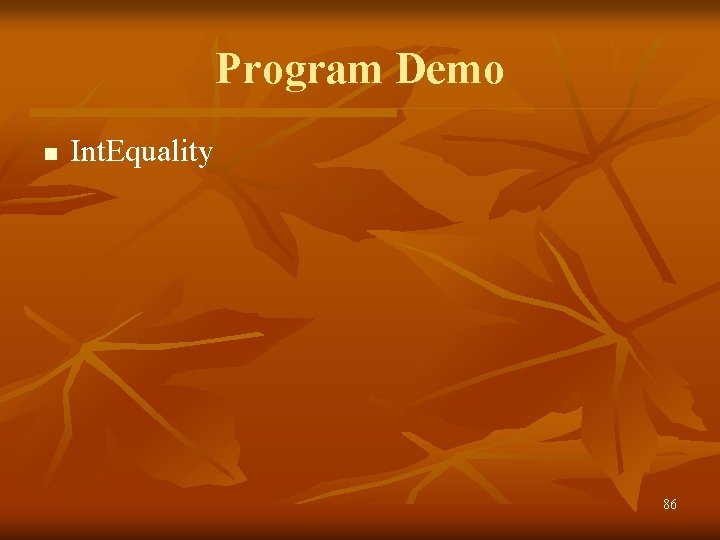
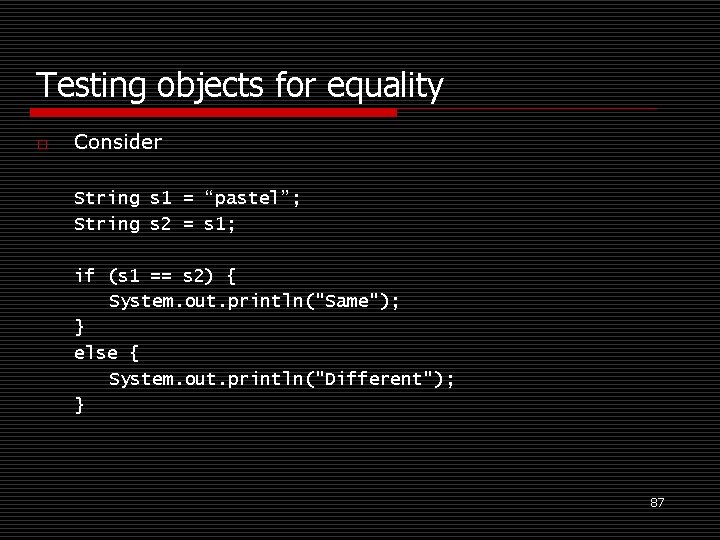
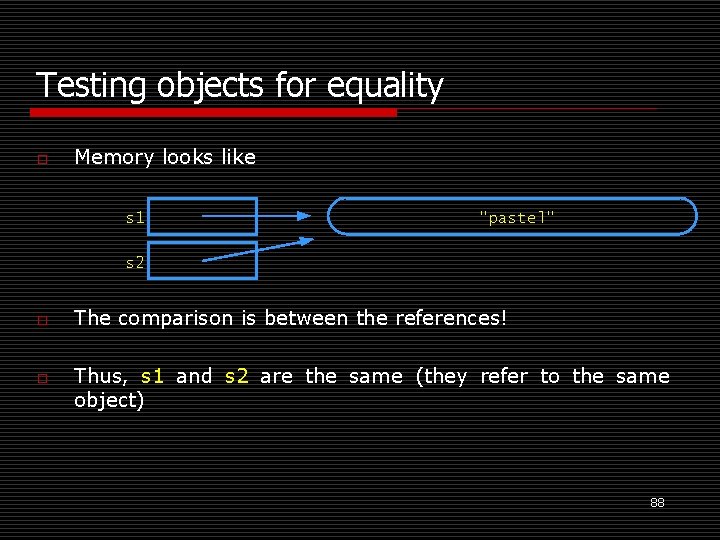
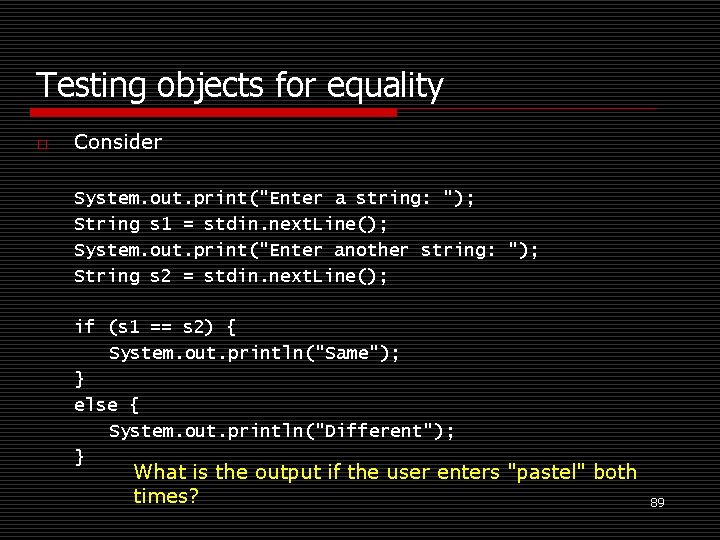
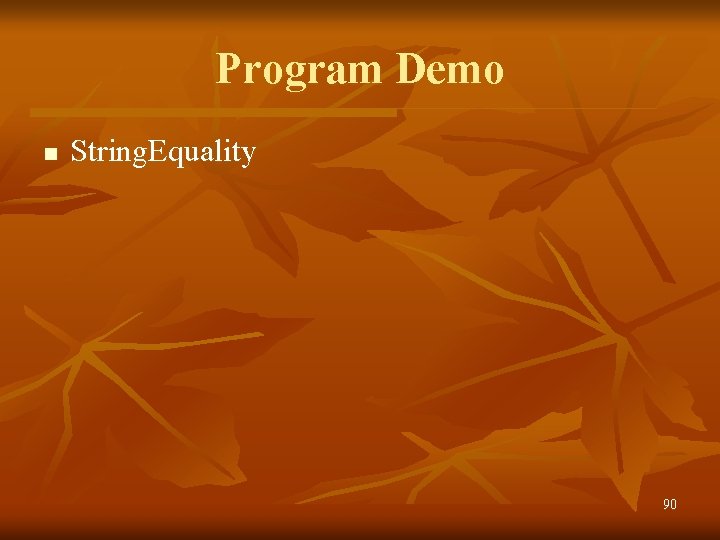
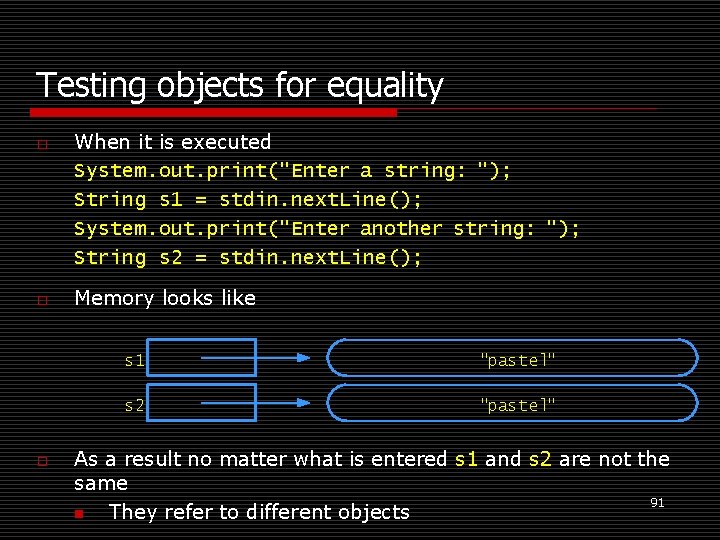
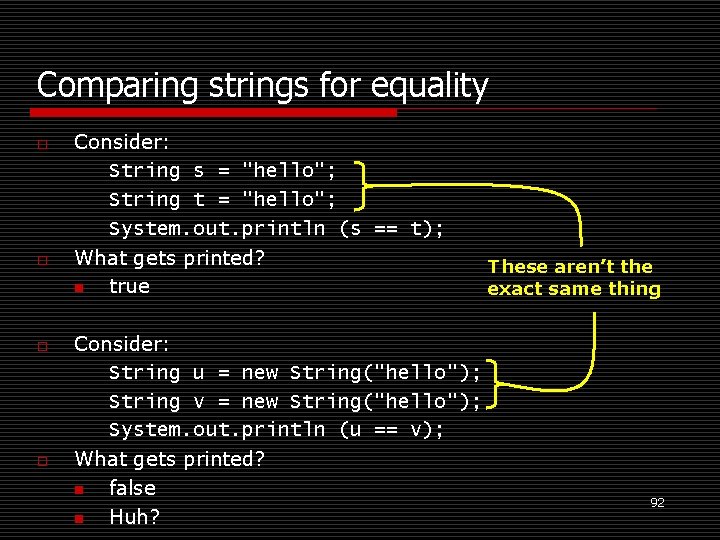
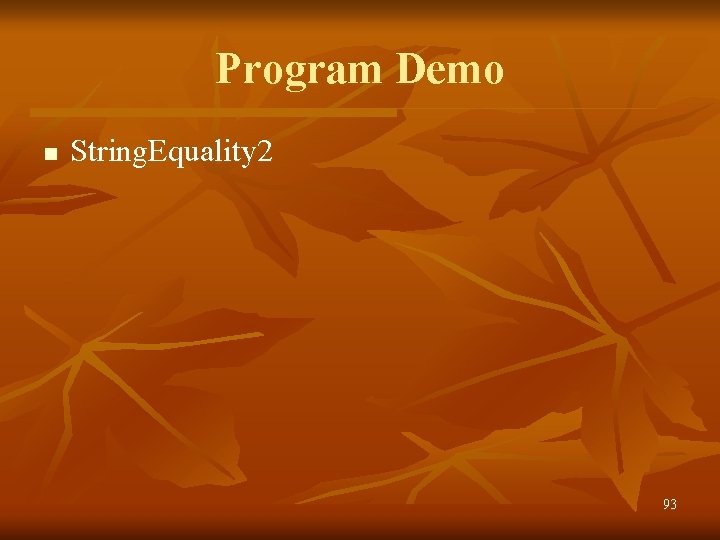
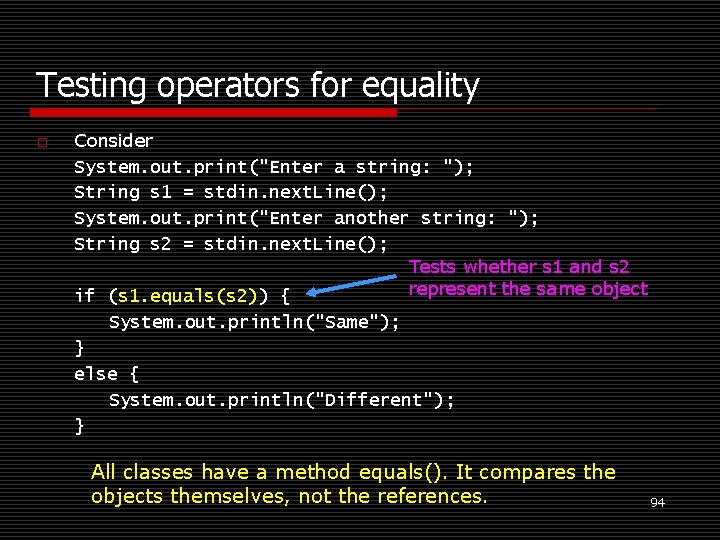
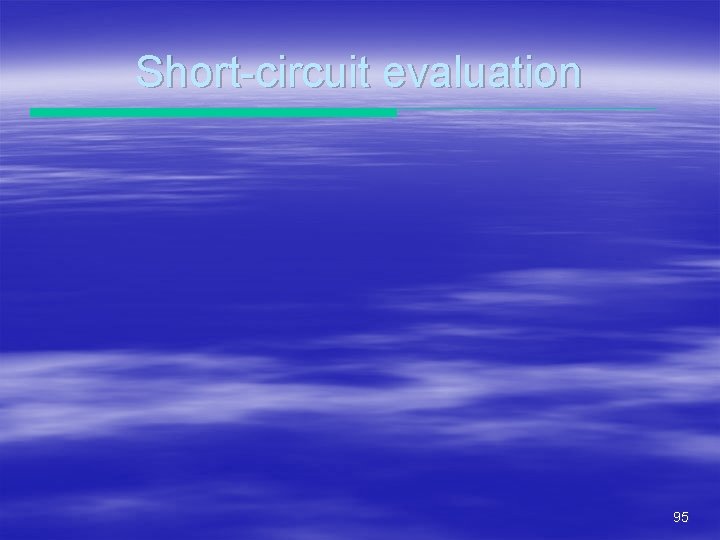
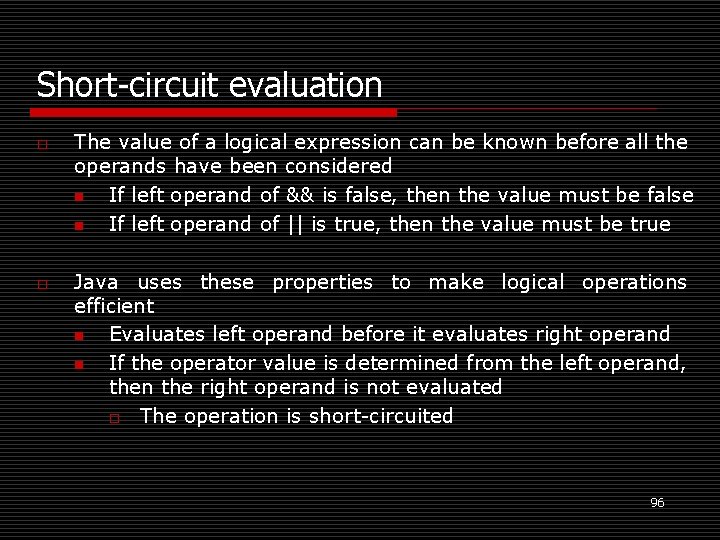
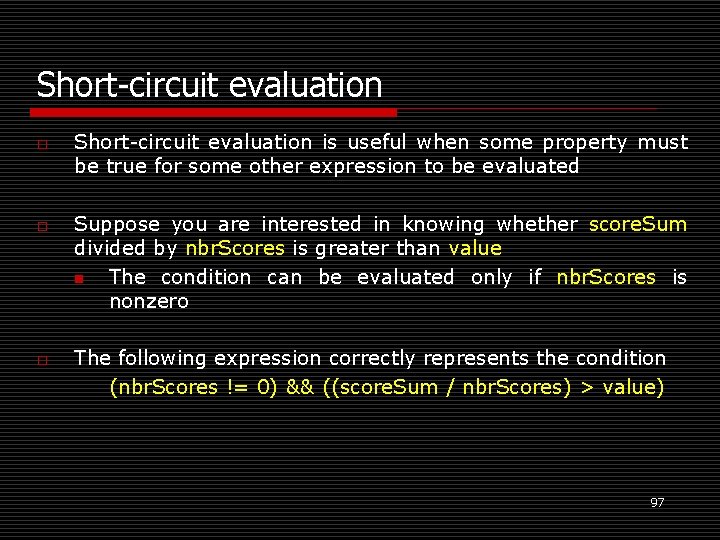
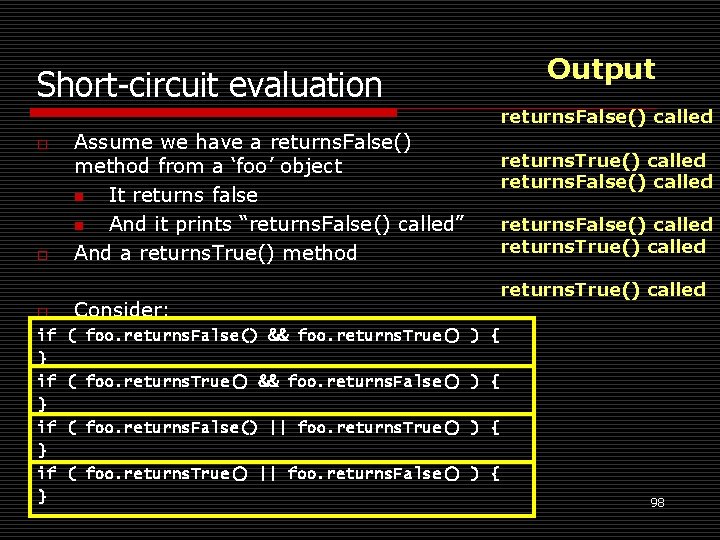
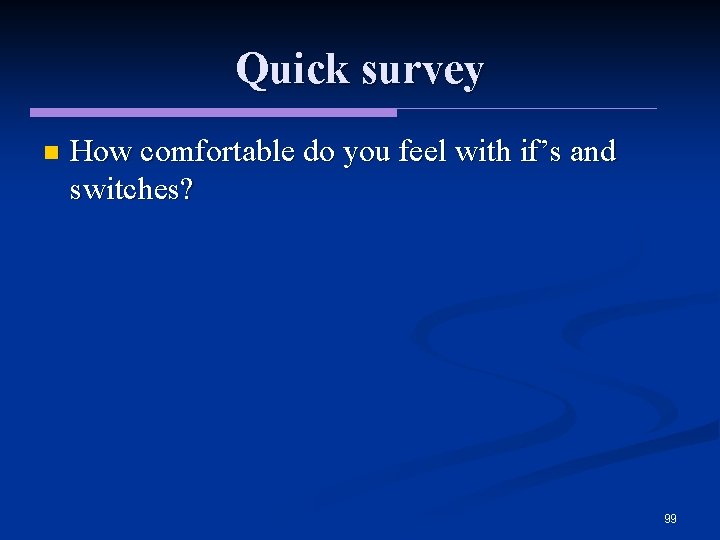
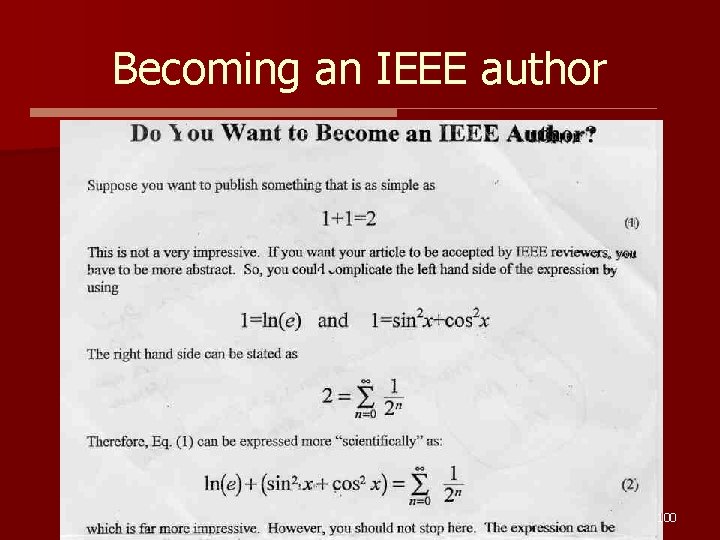
- Slides: 98
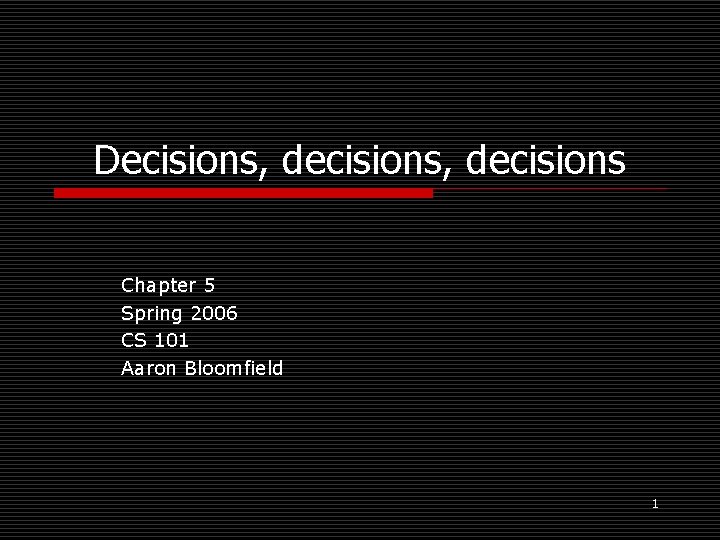
Decisions, decisions Chapter 5 Spring 2006 CS 101 Aaron Bloomfield 1
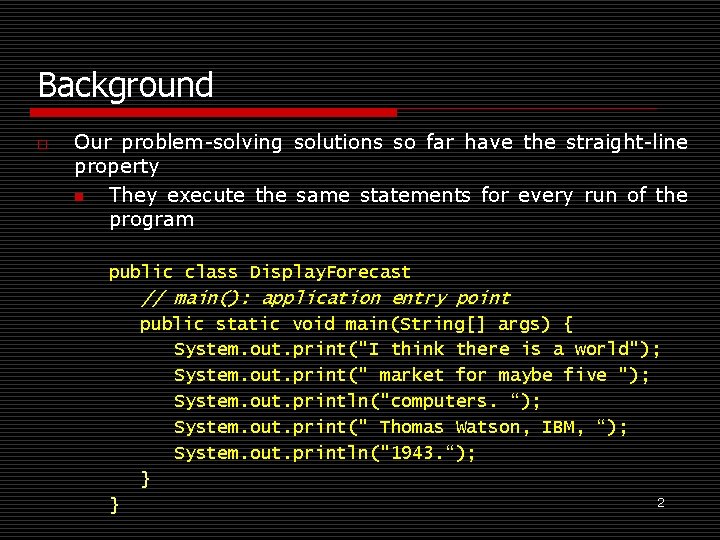
Background o Our problem-solving solutions so far have the straight-line property n They execute the same statements for every run of the program public class Display. Forecast // main(): application entry point public static void main(String[] args) { System. out. print("I think there is a world"); System. out. print(" market for maybe five "); System. out. println("computers. “); System. out. print(" Thomas Watson, IBM, “); System. out. println("1943. “); } } 2
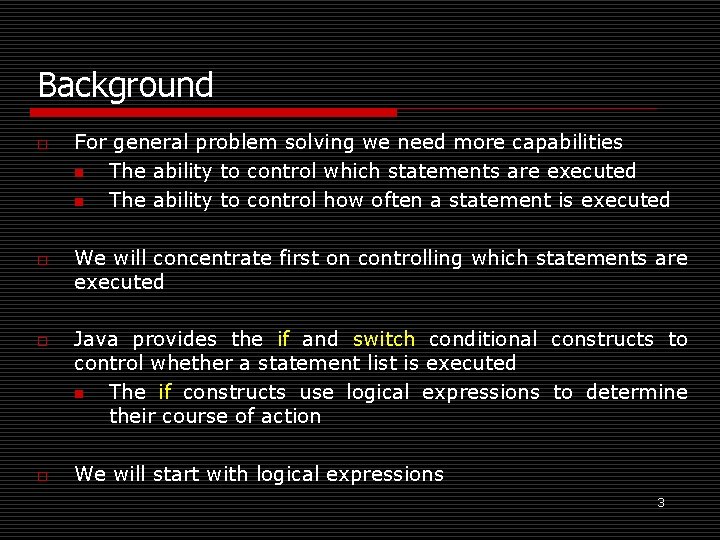
Background o o For general problem solving we need more capabilities n The ability to control which statements are executed n The ability to control how often a statement is executed We will concentrate first on controlling which statements are executed Java provides the if and switch conditional constructs to control whether a statement list is executed n The if constructs use logical expressions to determine their course of action We will start with logical expressions 3
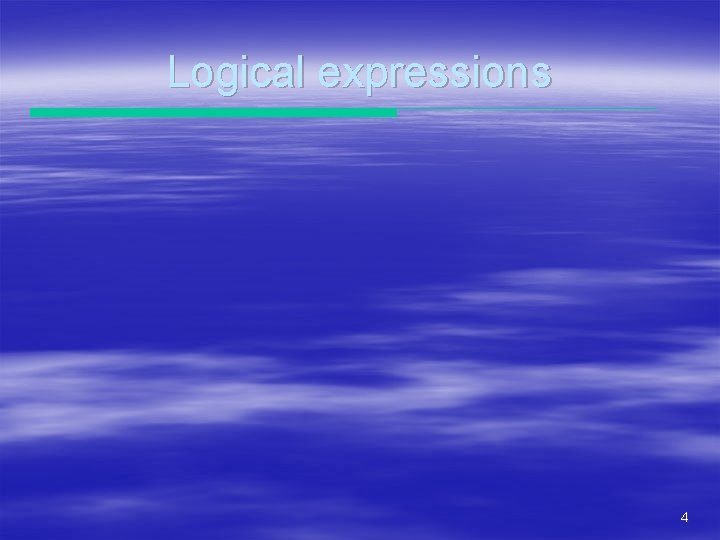
Logical expressions 4
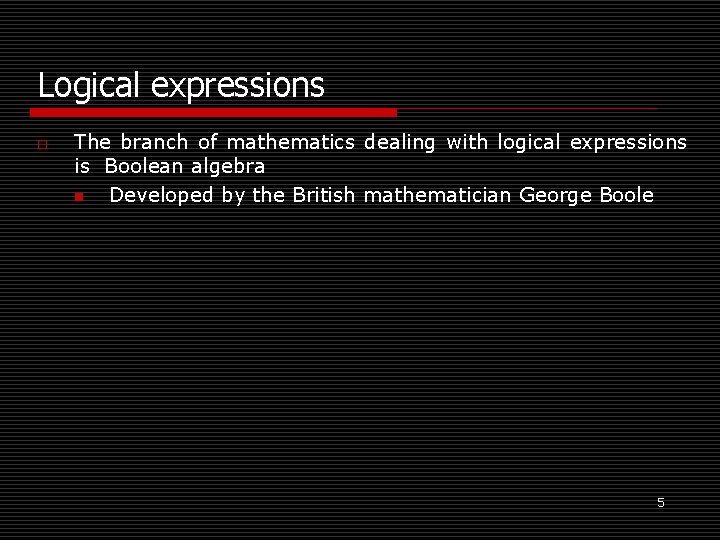
Logical expressions o The branch of mathematics dealing with logical expressions is Boolean algebra n Developed by the British mathematician George Boole 5
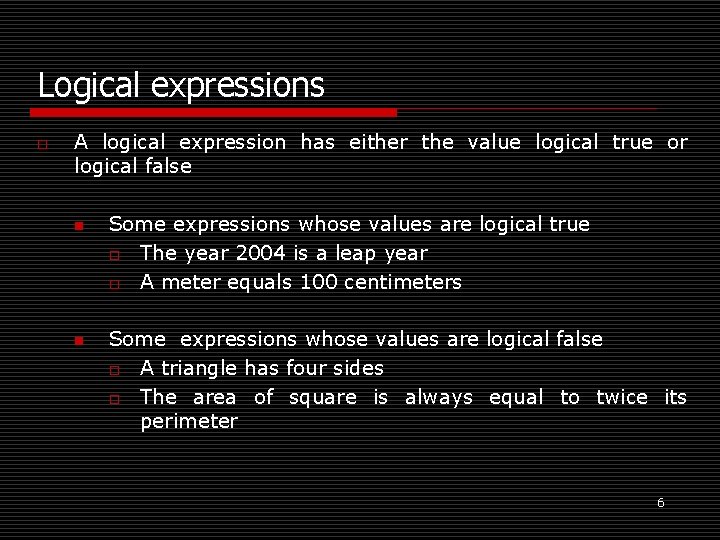
Logical expressions o A logical expression has either the value logical true or logical false n n Some expressions whose values are logical true o The year 2004 is a leap year o A meter equals 100 centimeters Some expressions whose values are logical false o A triangle has four sides o The area of square is always equal to twice its perimeter 6
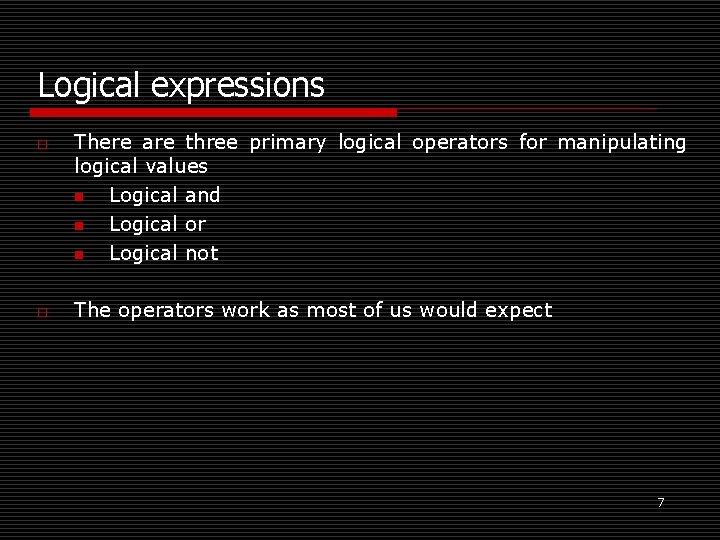
Logical expressions o o There are three primary logical operators for manipulating logical values n Logical and n Logical or n Logical not The operators work as most of us would expect 7
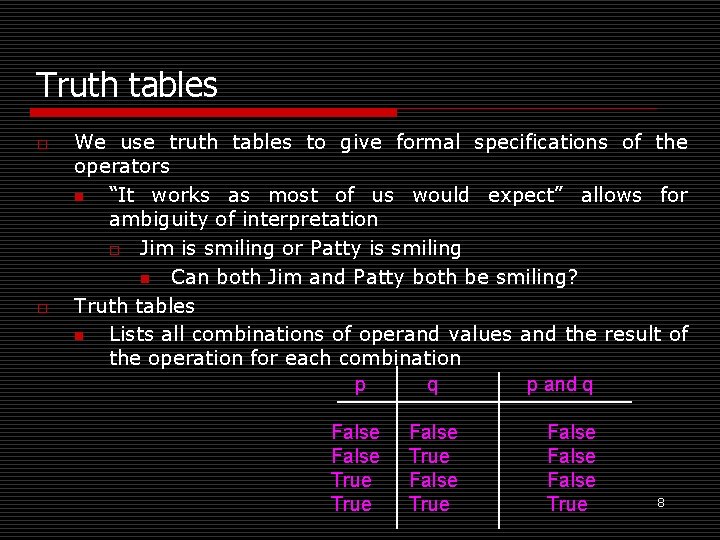
Truth tables o o We use truth tables to give formal specifications of the operators n “It works as most of us would expect” allows for ambiguity of interpretation o Jim is smiling or Patty is smiling n Can both Jim and Patty both be smiling? Truth tables n Lists all combinations of operand values and the result of the operation for each combination p q p and q False True False True 8
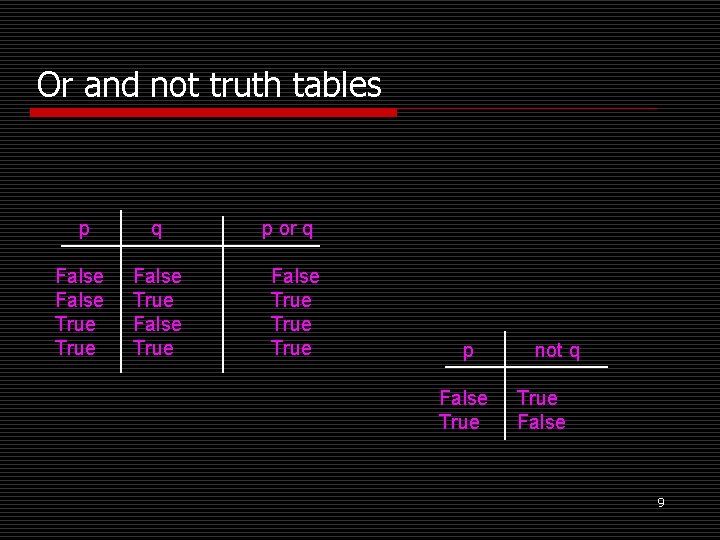
Or and not truth tables p q False True p or q False True p False True not q True False 9
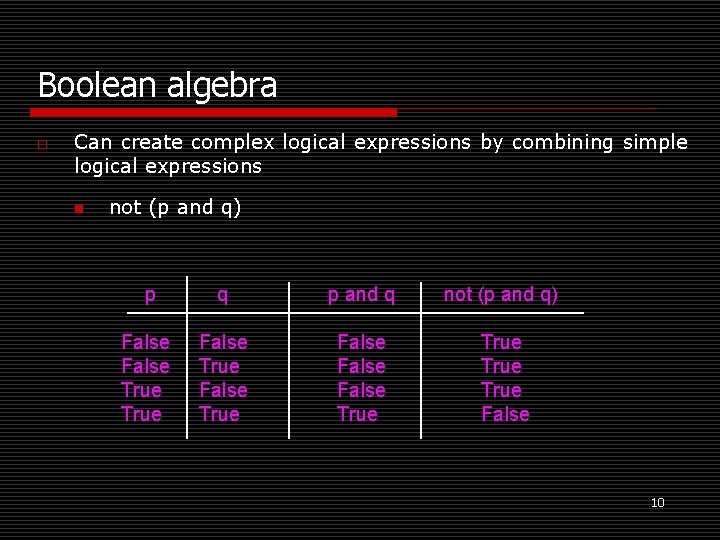
Boolean algebra o Can create complex logical expressions by combining simple logical expressions n not (p and q) p q p and q not (p and q) False True False True False 10
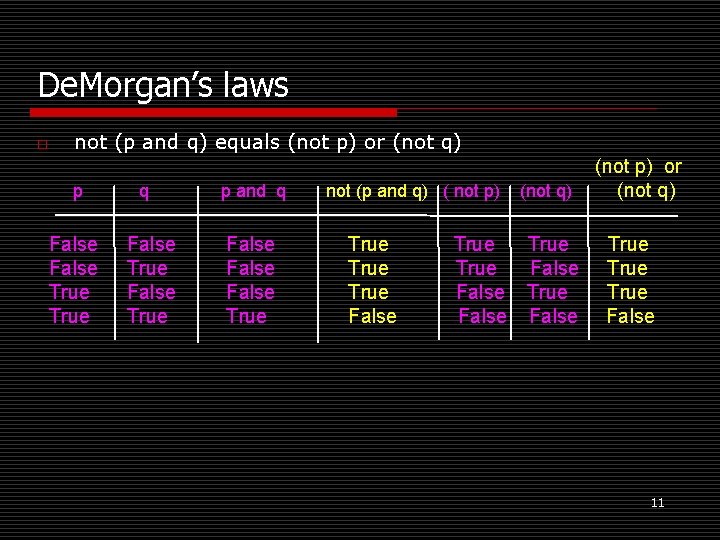
De. Morgan’s laws o not (p and q) equals (not p) or (not q) p False True q False True p and q False True not (p and q) ( not p) True False (not q) True False (not p) or (not q) True False 11
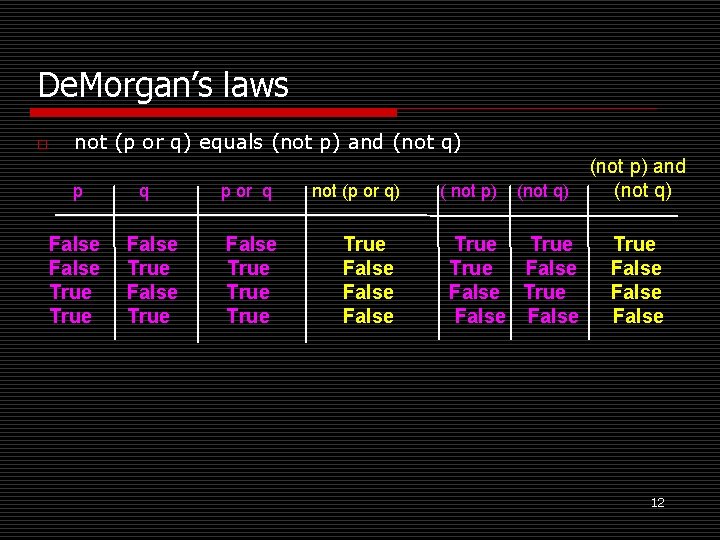
De. Morgan’s laws o not (p or q) equals (not p) and (not q) p False True q False True p or q False True not (p or q) True False ( not p) (not q) True False (not p) and (not q) True False 12
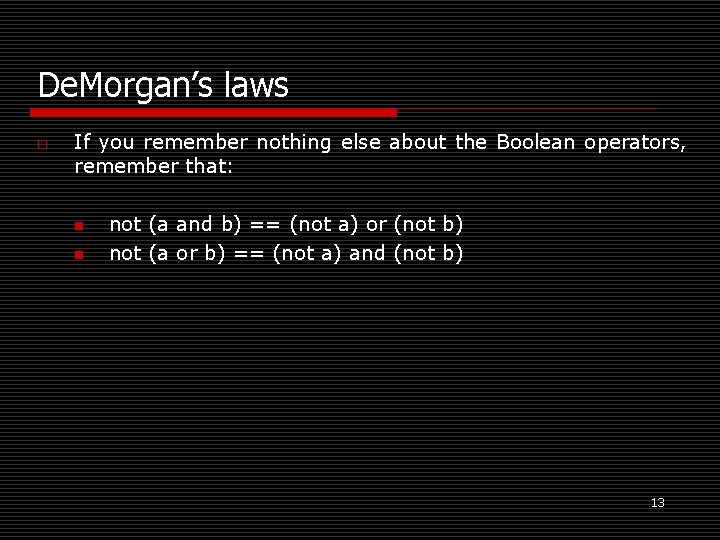
De. Morgan’s laws o If you remember nothing else about the Boolean operators, remember that: n n not (a and b) == (not a) or (not b) not (a or b) == (not a) and (not b) 13
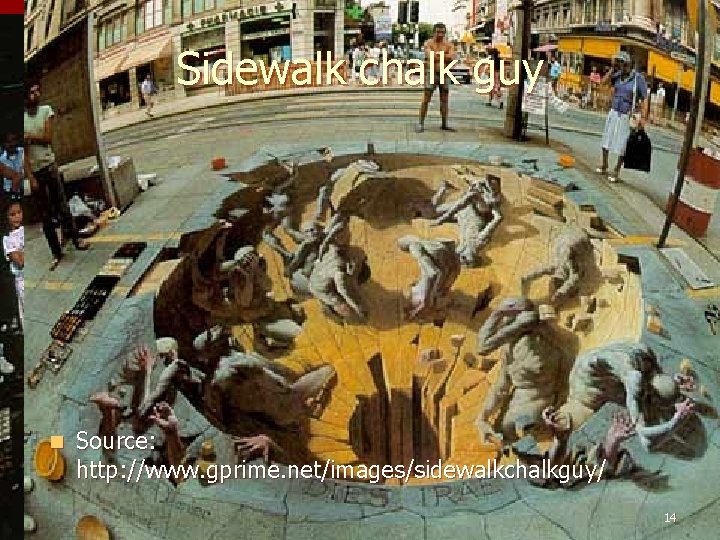
Sidewalk chalk guy n Source: http: //www. gprime. net/images/sidewalkchalkguy/ 14
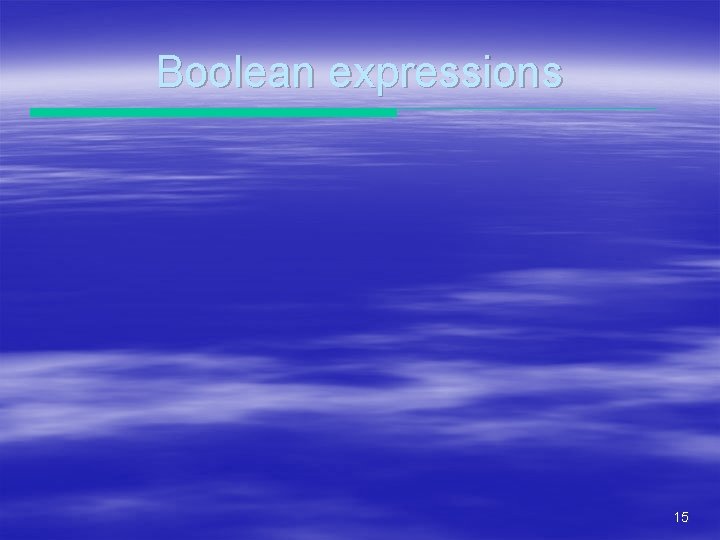
Boolean expressions 15
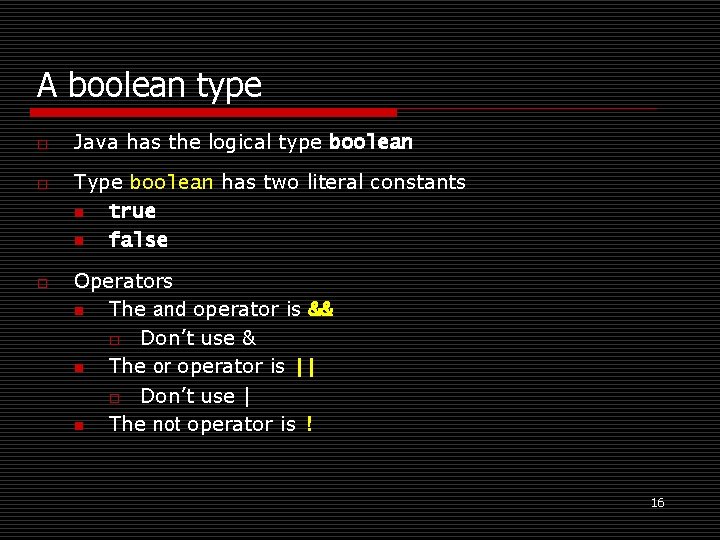
A boolean type o o o Java has the logical type boolean Type boolean has two literal constants n true n false Operators n The and operator is && o Don’t use & n The or operator is || o Don’t use | n The not operator is ! 16
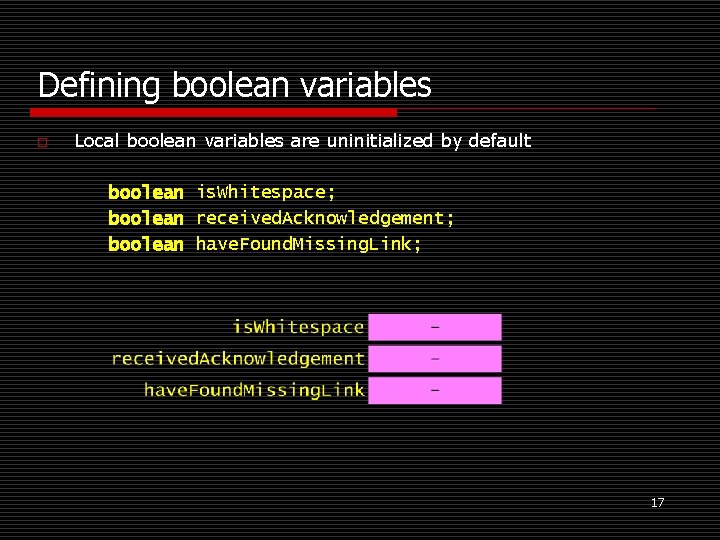
Defining boolean variables o Local boolean variables are uninitialized by default boolean is. Whitespace; boolean received. Acknowledgement; boolean have. Found. Missing. Link; 17
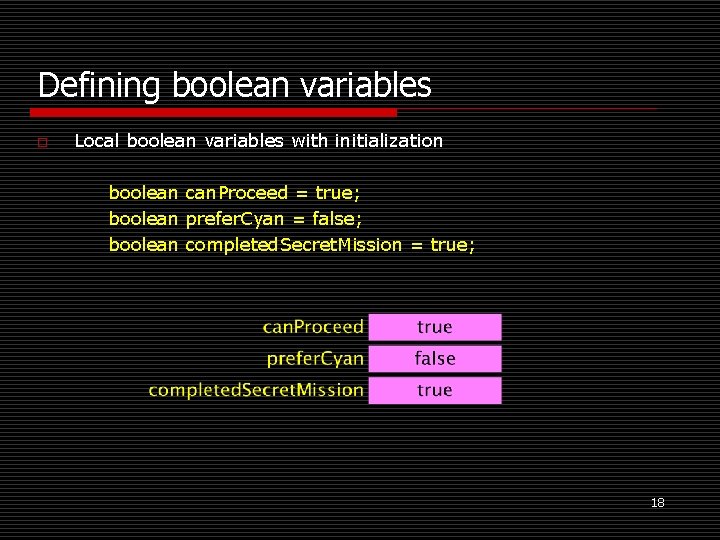
Defining boolean variables o Local boolean variables with initialization boolean can. Proceed = true; boolean prefer. Cyan = false; boolean completed. Secret. Mission = true; 18
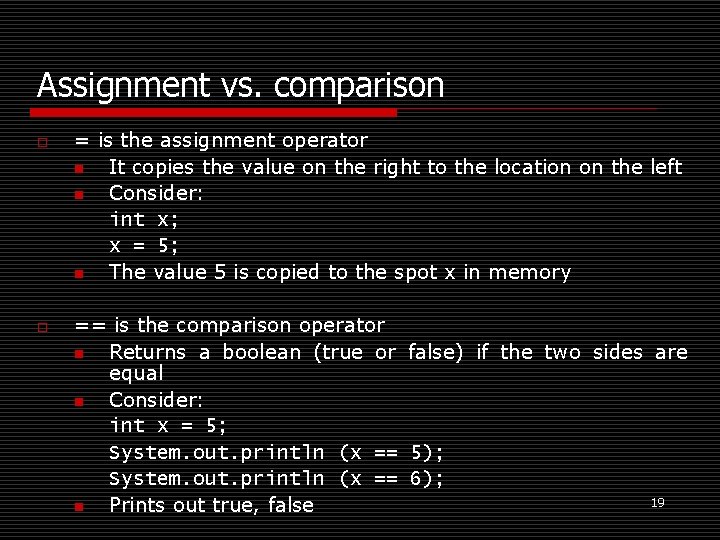
Assignment vs. comparison o o = is the assignment operator n It copies the value on the right to the location on the left n Consider: int x; x = 5; n The value 5 is copied to the spot x in memory == is the comparison operator n Returns a boolean (true or false) if the two sides are equal n Consider: int x = 5; System. out. println (x == 5); System. out. println (x == 6); 19 n Prints out true, false
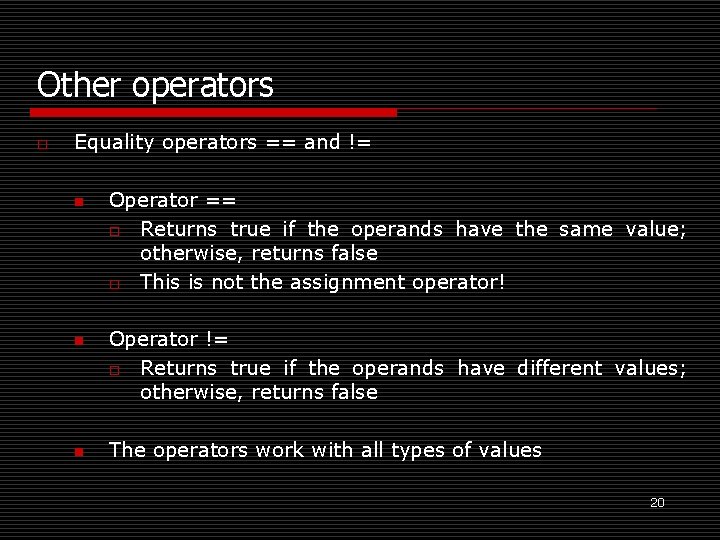
Other operators o Equality operators == and != n n n Operator == o Returns true if the operands have the same value; otherwise, returns false o This is not the assignment operator! Operator != o Returns true if the operands have different values; otherwise, returns false The operators work with all types of values 20
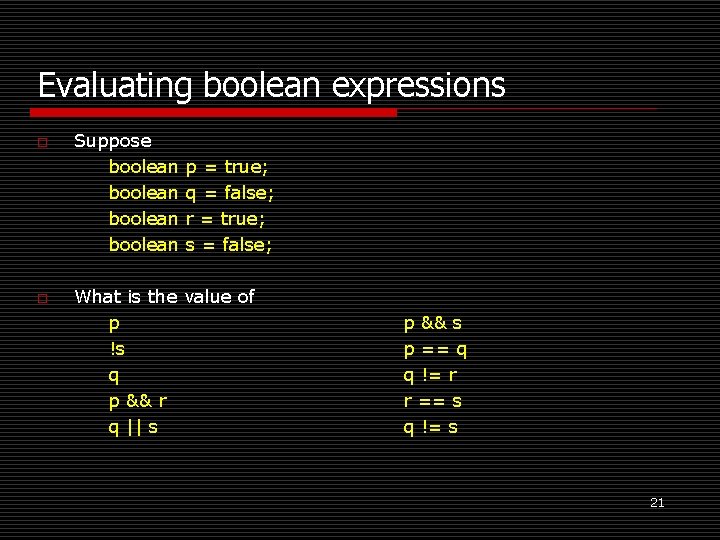
Evaluating boolean expressions o o Suppose boolean p = true; q = false; r = true; s = false; What is the value of p !s q p && r q || s p && s p == q q != r r == s q != s 21
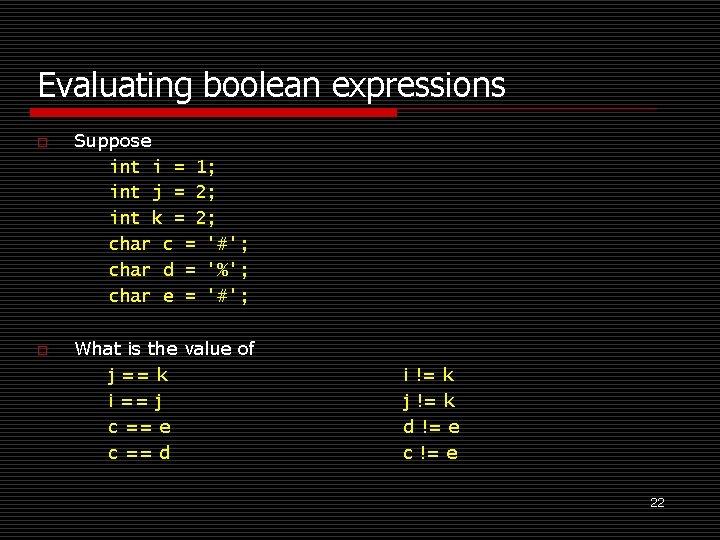
Evaluating boolean expressions o o Suppose int i = 1; int j = 2; int k = 2; char c = '#'; char d = '%'; char e = '#'; What is the value of j == k i == j c == e c == d i != k j != k d != e c != e 22
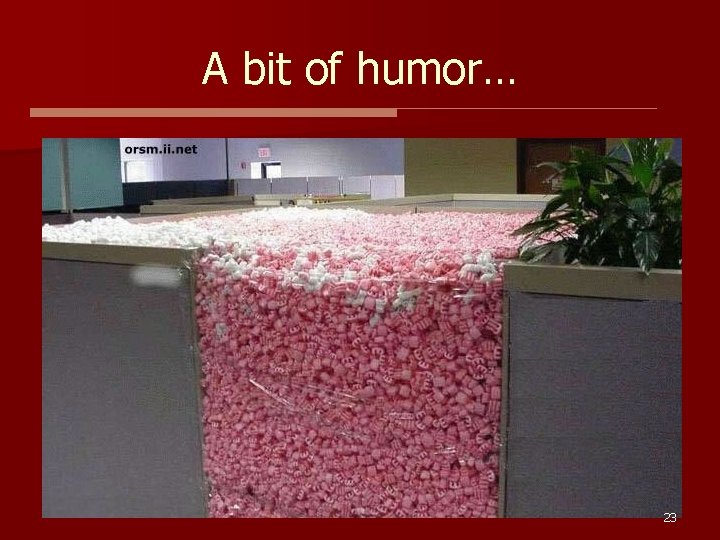
A bit of humor… 23
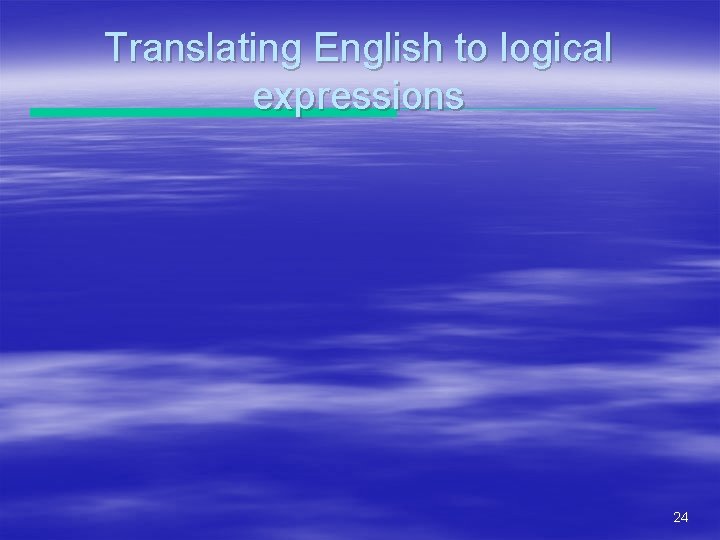
Translating English to logical expressions 24
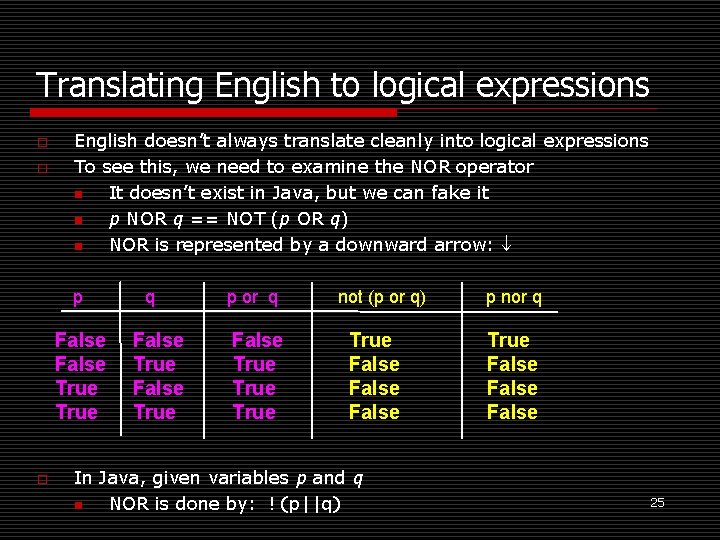
Translating English to logical expressions o o English doesn’t always translate cleanly into logical expressions To see this, we need to examine the NOR operator n It doesn’t exist in Java, but we can fake it n p NOR q == NOT (p OR q) n NOR is represented by a downward arrow: p False True o q False True p or q False True not (p or q) True False In Java, given variables p and q n NOR is done by: !(p||q) p nor q True False 25
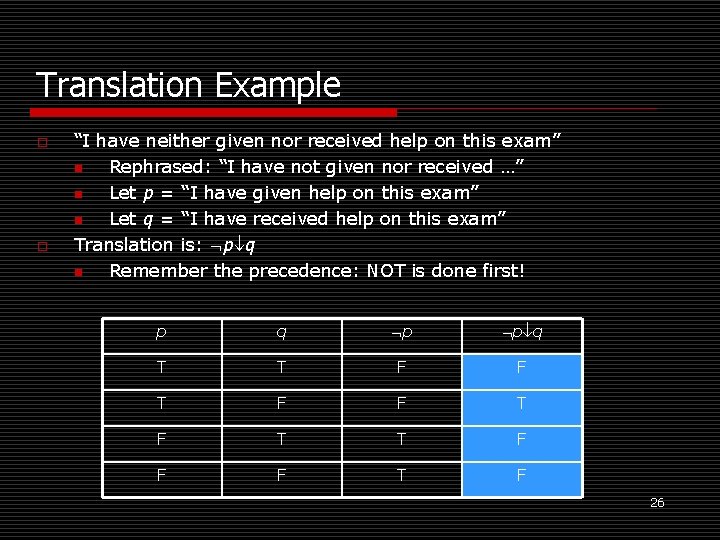
Translation Example o o “I have neither given nor received help on this exam” n Rephrased: “I have not given nor received …” n Let p = “I have given help on this exam” n Let q = “I have received help on this exam” Translation is: p q n Remember the precedence: NOT is done first! p q p p q T T F F T F T T F F F T F 26
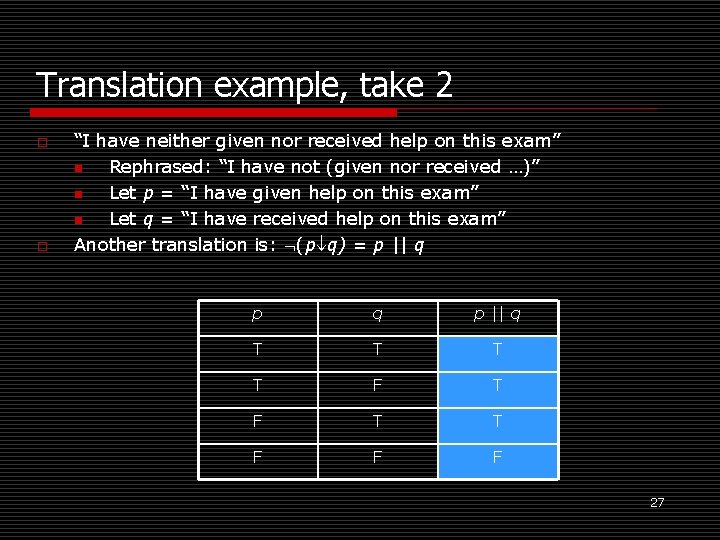
Translation example, take 2 o o “I have neither given nor received help on this exam” n Rephrased: “I have not (given nor received …)” n Let p = “I have given help on this exam” n Let q = “I have received help on this exam” Another translation is: (p q) = p || q T T F F F 27
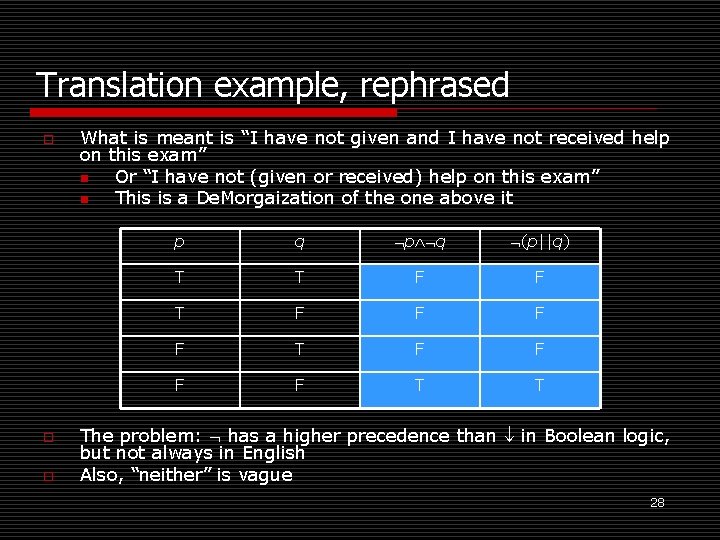
Translation example, rephrased o o o What is meant is “I have not given and I have not received help on this exam” n Or “I have not (given or received) help on this exam” n This is a De. Morgaization of the one above it p q (p||q) T T F F F F T T The problem: has a higher precedence than in Boolean logic, but not always in English Also, “neither” is vague 28
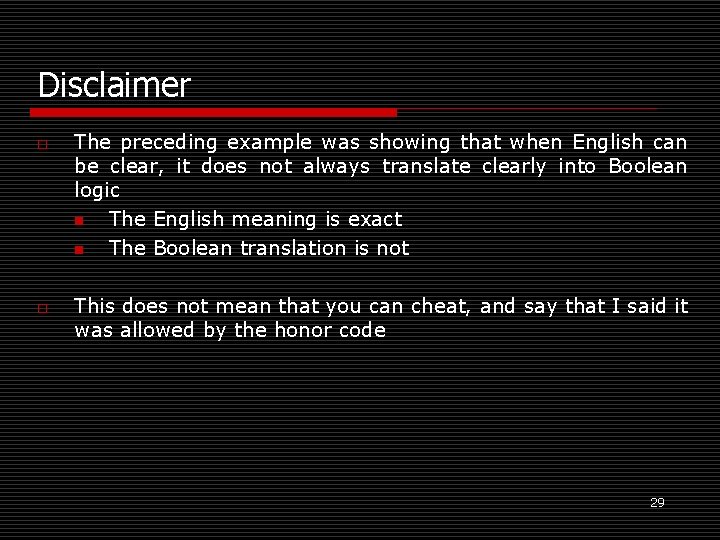
Disclaimer o o The preceding example was showing that when English can be clear, it does not always translate clearly into Boolean logic n The English meaning is exact n The Boolean translation is not This does not mean that you can cheat, and say that I said it was allowed by the honor code 29
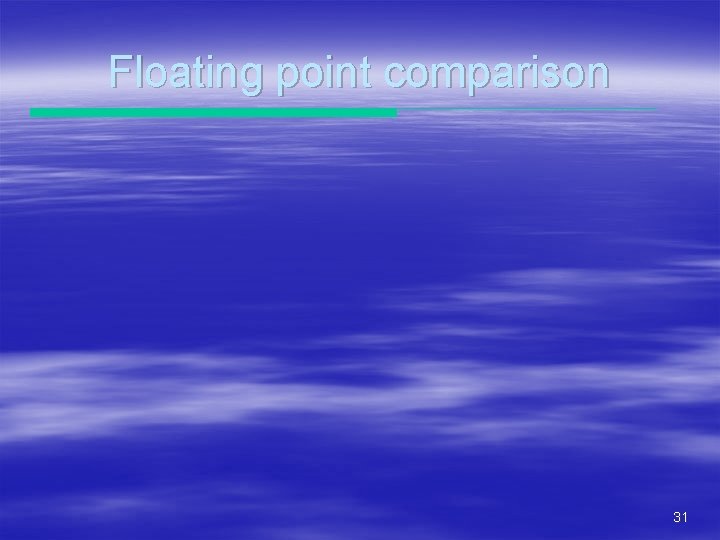
Floating point comparison 31
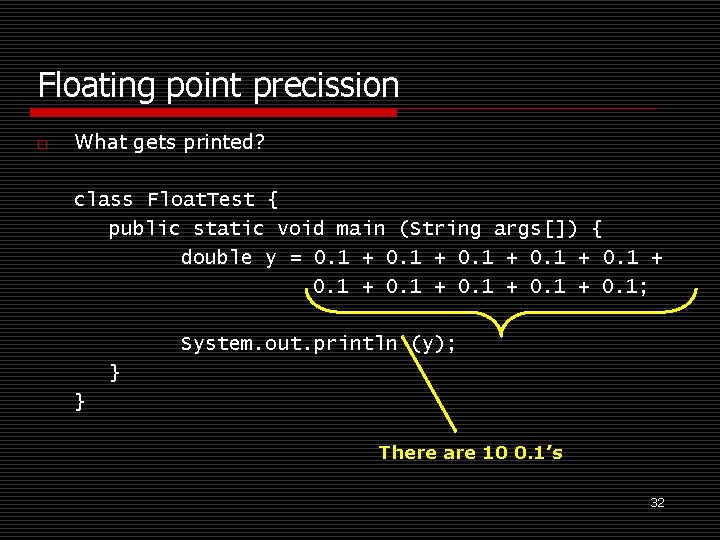
Floating point precission o What gets printed? class Float. Test { public static void main (String args[]) { double y = 0. 1 + 0. 1; System. out. println (y); } } There are 10 0. 1’s 32
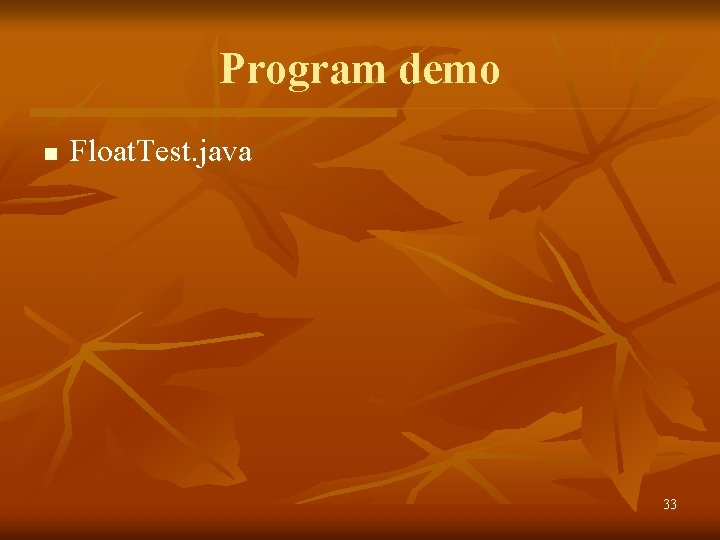
Program demo n Float. Test. java 33
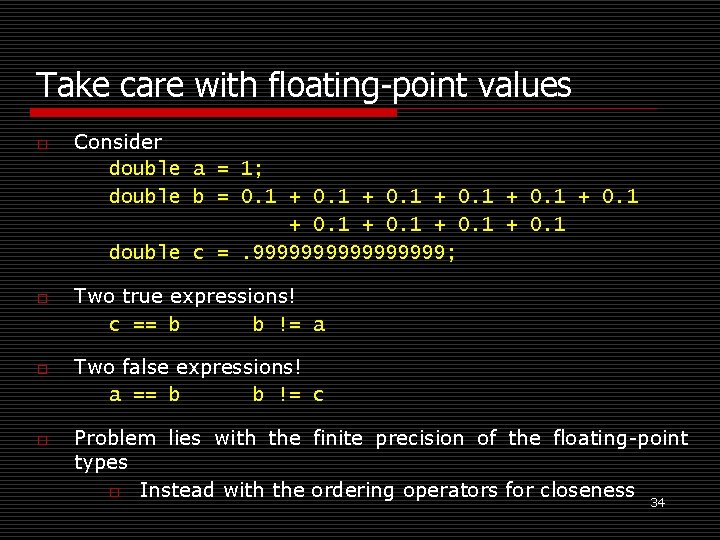
Take care with floating-point values o o Consider double a = 1; double b = 0. 1 + 0. 1 double c =. 99999999; Two true expressions! c == b b != a Two false expressions! a == b b != c Problem lies with the finite precision of the floating-point types o Instead with the ordering operators for closeness 34
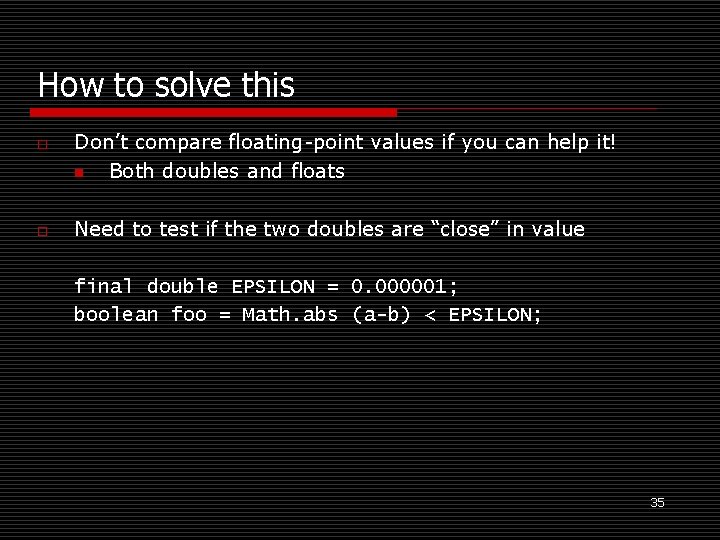
How to solve this o o Don’t compare floating-point values if you can help it! n Both doubles and floats Need to test if the two doubles are “close” in value final double EPSILON = 0. 000001; boolean foo = Math. abs (a-b) < EPSILON; 35
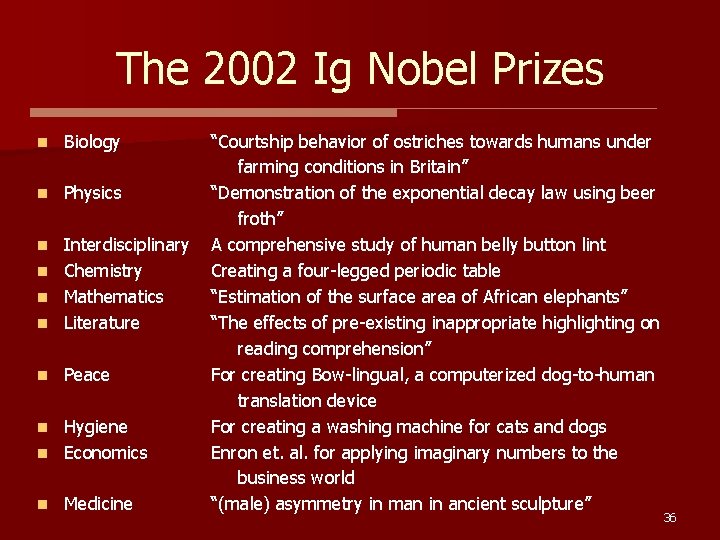
The 2002 Ig Nobel Prizes n Biology n Physics Interdisciplinary n Chemistry n Mathematics n Literature n n Peace Hygiene n Economics n n Medicine “Courtship behavior of ostriches towards humans under farming conditions in Britain” “Demonstration of the exponential decay law using beer froth” A comprehensive study of human belly button lint Creating a four-legged periodic table “Estimation of the surface area of African elephants” “The effects of pre-existing inappropriate highlighting on reading comprehension” For creating Bow-lingual, a computerized dog-to-human translation device For creating a washing machine for cats and dogs Enron et. al. for applying imaginary numbers to the business world “(male) asymmetry in man in ancient sculpture” 36
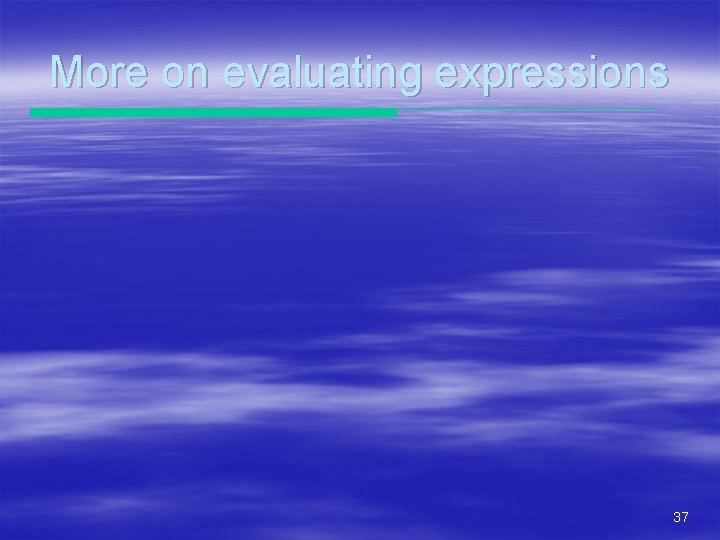
More on evaluating expressions 37
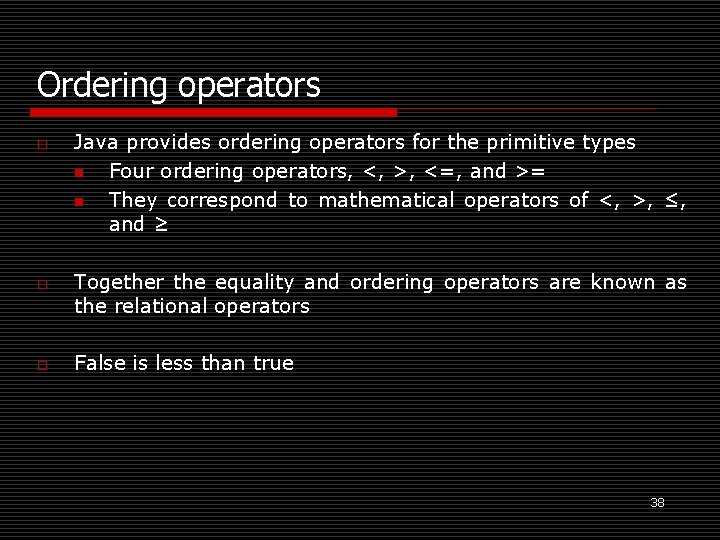
Ordering operators o o o Java provides ordering operators for the primitive types n Four ordering operators, <, >, <=, and >= n They correspond to mathematical operators of <, >, ≤, and ≥ Together the equality and ordering operators are known as the relational operators False is less than true 38
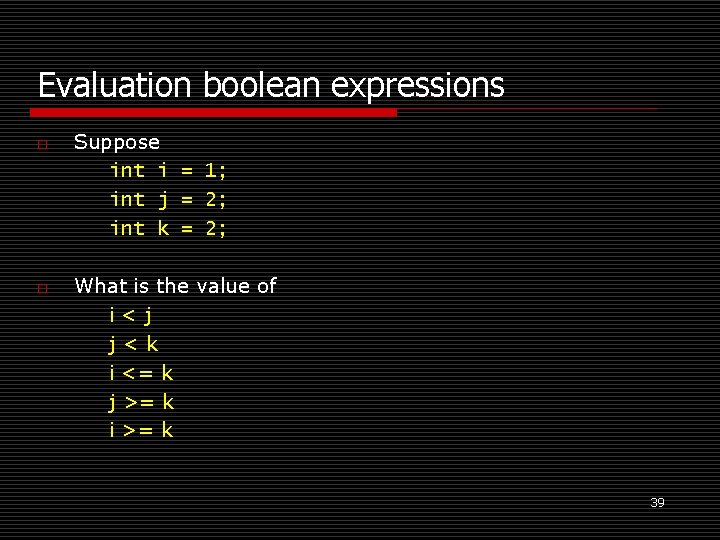
Evaluation boolean expressions o o Suppose int i = 1; int j = 2; int k = 2; What is the value of i<j j<k i <= k j >= k i >= k 39
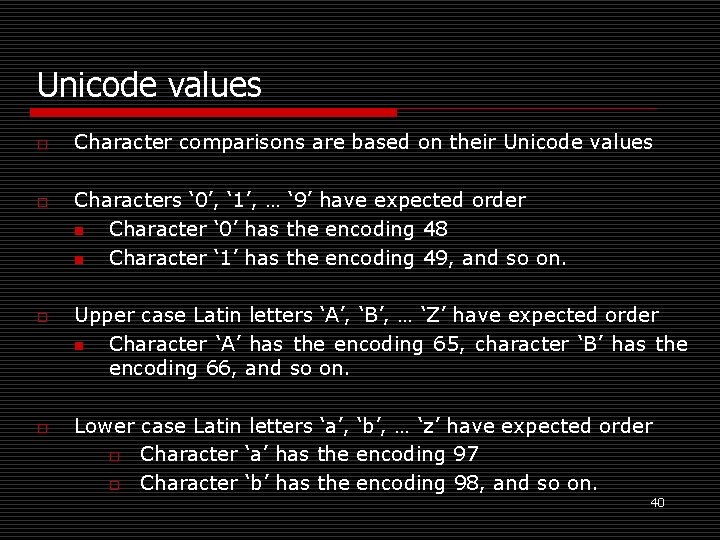
Unicode values o o Character comparisons are based on their Unicode values Characters ‘ 0’, ‘ 1’, … ‘ 9’ have expected order n Character ‘ 0’ has the encoding 48 n Character ‘ 1’ has the encoding 49, and so on. Upper case Latin letters ‘A’, ‘B’, … ‘Z’ have expected order n Character ‘A’ has the encoding 65, character ‘B’ has the encoding 66, and so on. Lower case Latin letters ‘a’, ‘b’, … ‘z’ have expected order o Character ‘a’ has the encoding 97 o Character ‘b’ has the encoding 98, and so on. 40
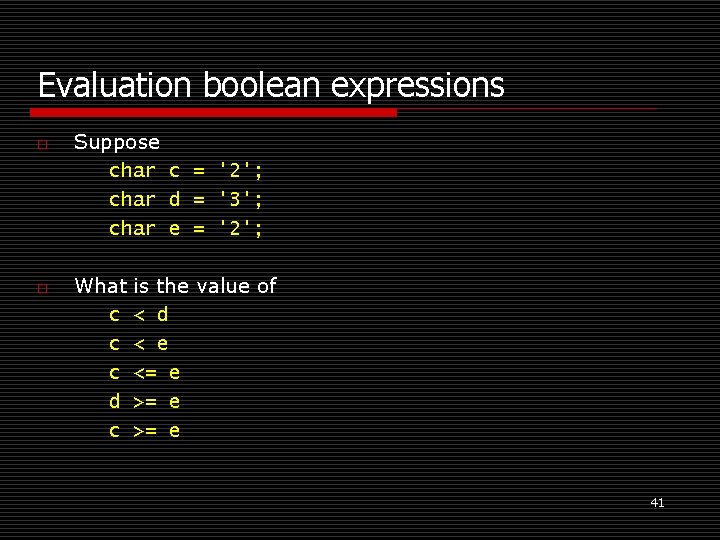
Evaluation boolean expressions o o Suppose char c = '2'; char d = '3'; char e = '2'; What c c c d c is the value of < d < e <= e >= e 41
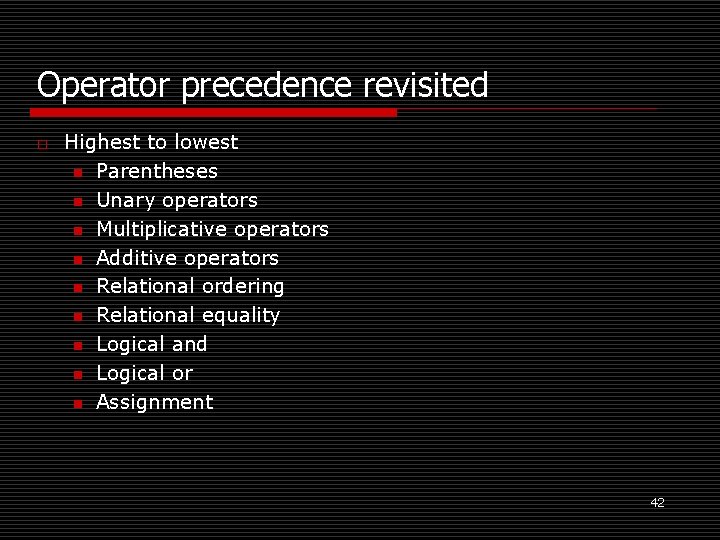
Operator precedence revisited o Highest to lowest n Parentheses n Unary operators n Multiplicative operators n Additive operators n Relational ordering n Relational equality n Logical and n Logical or n Assignment 42
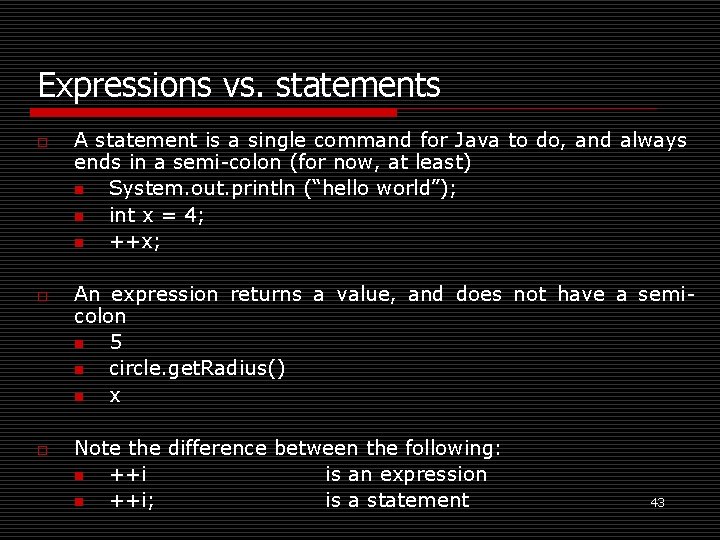
Expressions vs. statements o o o A statement is a single command for Java to do, and always ends in a semi-colon (for now, at least) n System. out. println (“hello world”); n int x = 4; n ++x; An expression returns a value, and does not have a semicolon n 5 n circle. get. Radius() n x Note the difference between the following: n ++i is an expression n ++i; is a statement 43
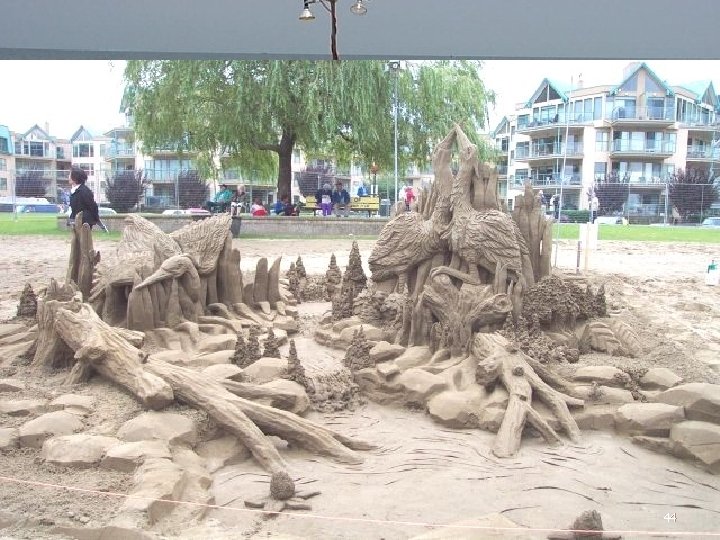
Sand Castles 44
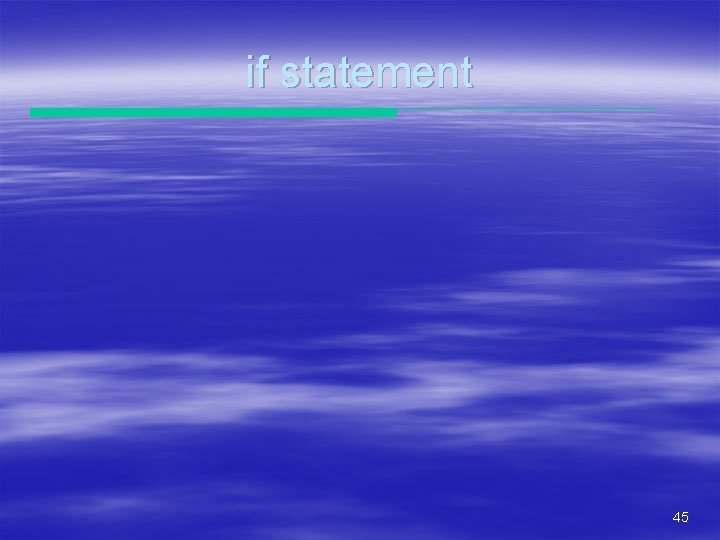
if statement 45
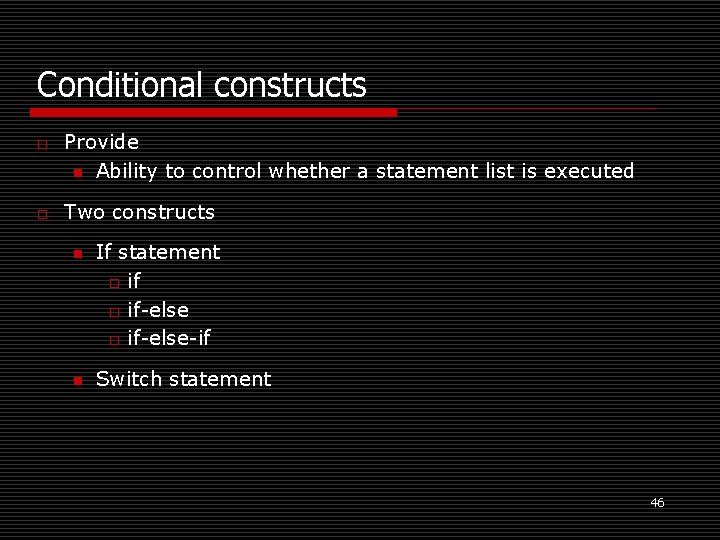
Conditional constructs o o Provide n Ability to control whether a statement list is executed Two constructs n n If statement o if-else-if Switch statement 46
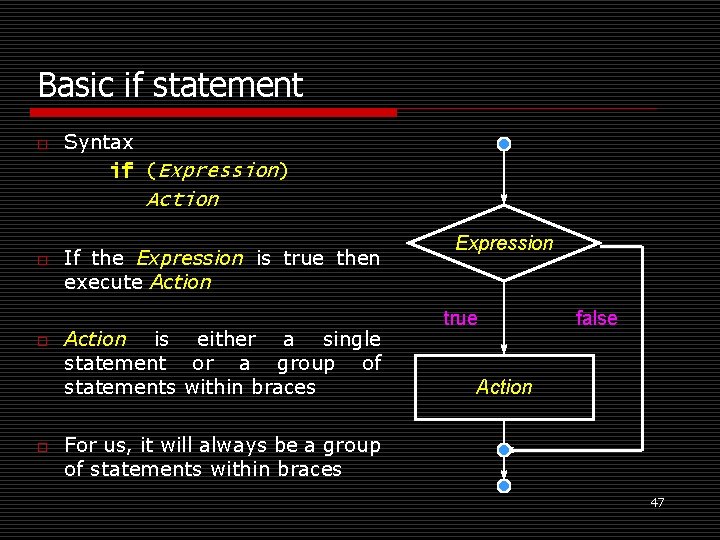
Basic if statement o Syntax if (Expression) Action o o o If the Expression is true then execute Action is either a single statement or a group of statements within braces Expression true false Action For us, it will always be a group of statements within braces 47
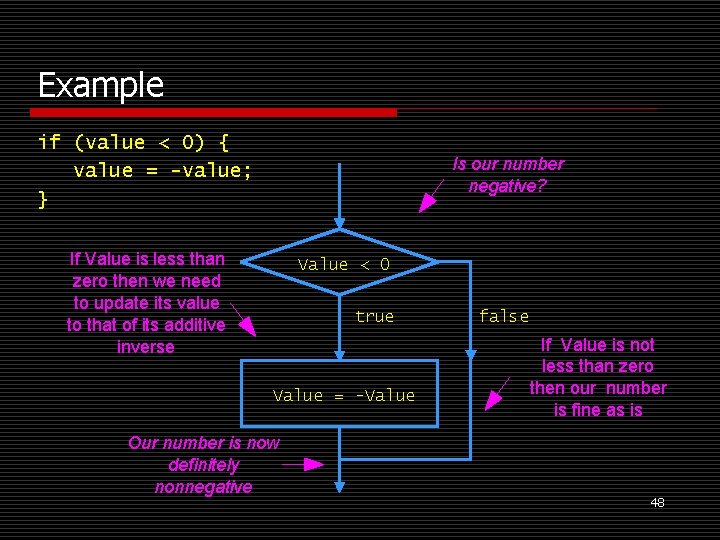
Example if (value < 0) { value = -value; } Is our number negative? If Value is less than zero then we need to update its value to that of its additive inverse Value < 0 true Value = -Value Our number is now definitely nonnegative false If Value is not less than zero then our number is fine as is 48
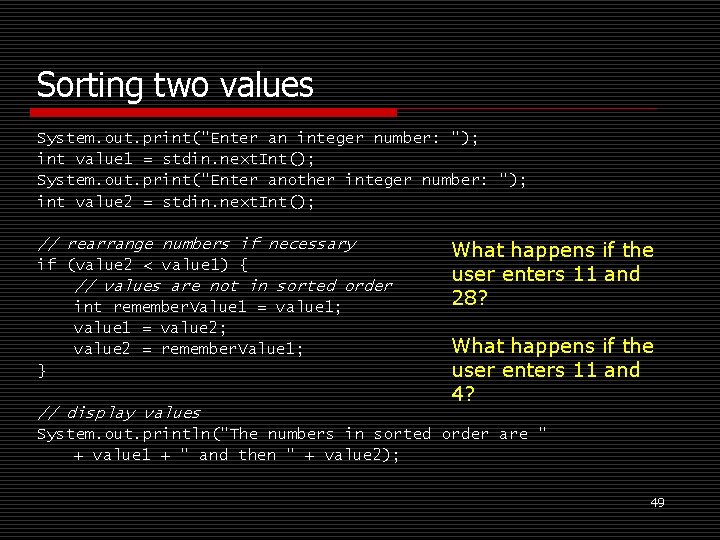
Sorting two values System. out. print("Enter an integer number: "); int value 1 = stdin. next. Int(); System. out. print("Enter another integer number: "); int value 2 = stdin. next. Int(); // rearrange numbers if necessary if (value 2 < value 1) { // values are not in sorted order int remember. Value 1 = value 1; value 1 = value 2; value 2 = remember. Value 1; } // display values What happens if the user enters 11 and 28? What happens if the user enters 11 and 4? System. out. println("The numbers in sorted order are " + value 1 + " and then " + value 2); 49
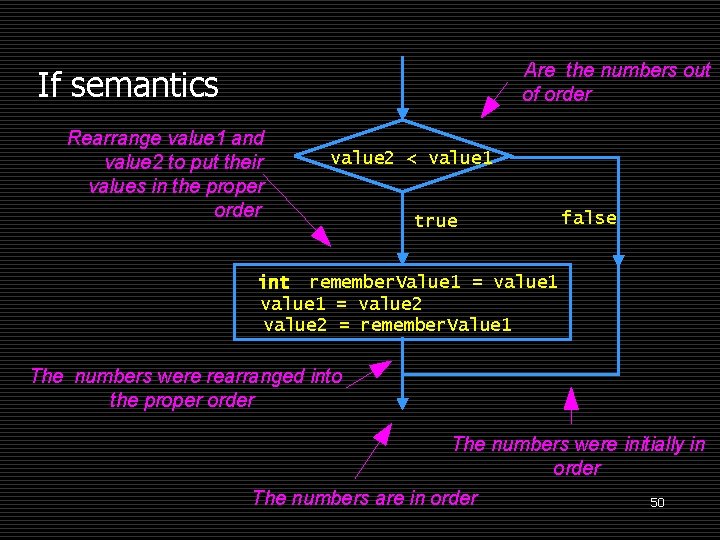
Are the numbers out of order If semantics Rearrange value 1 and value 2 to put their values in the proper order value 2 < value 1 true false int remember. Value 1 = value 2 = remember. Value 1 The numbers were rearranged into the proper order The numbers were initially in order The numbers are in order 50
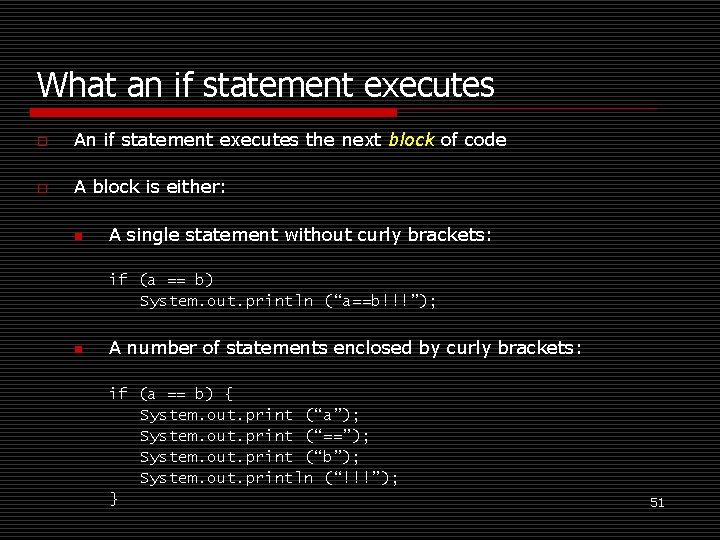
What an if statement executes o An if statement executes the next block of code o A block is either: n A single statement without curly brackets: if (a == b) System. out. println (“a==b!!!”); n A number of statements enclosed by curly brackets: if (a == b) { System. out. print (“a”); System. out. print (“==”); System. out. print (“b”); System. out. println (“!!!”); } 51
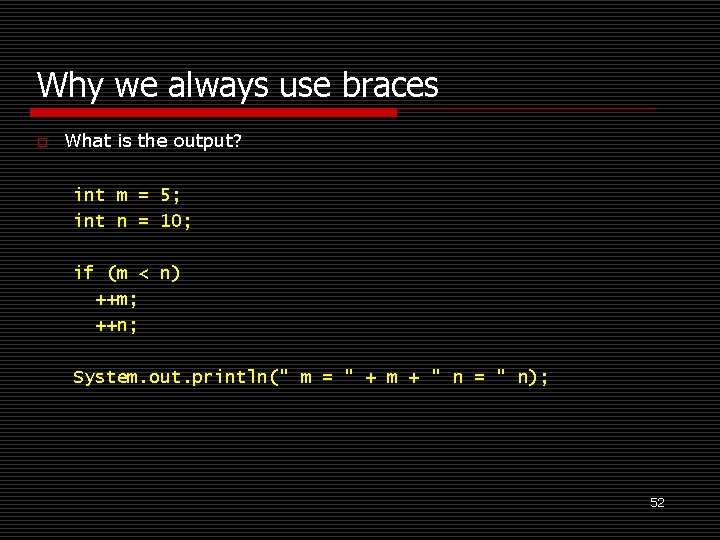
Why we always use braces o What is the output? int m = 5; int n = 10; if (m < n) ++m; ++n; System. out. println(" m = " + m + " n = " n); 52
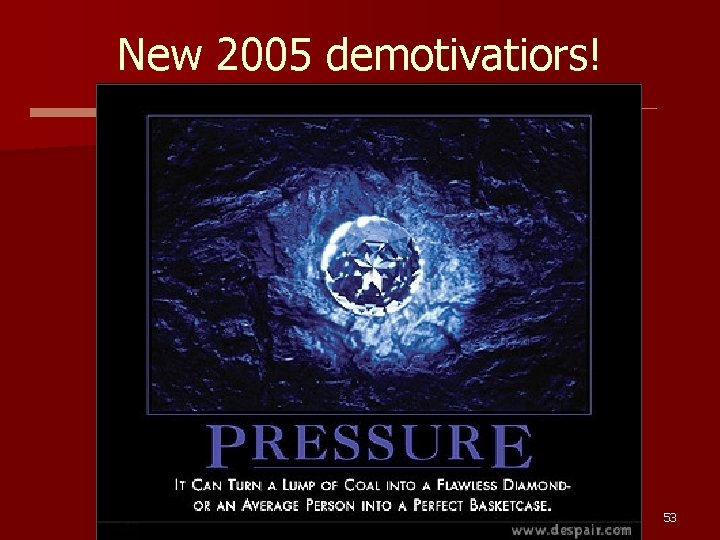
New 2005 demotivatiors! 53
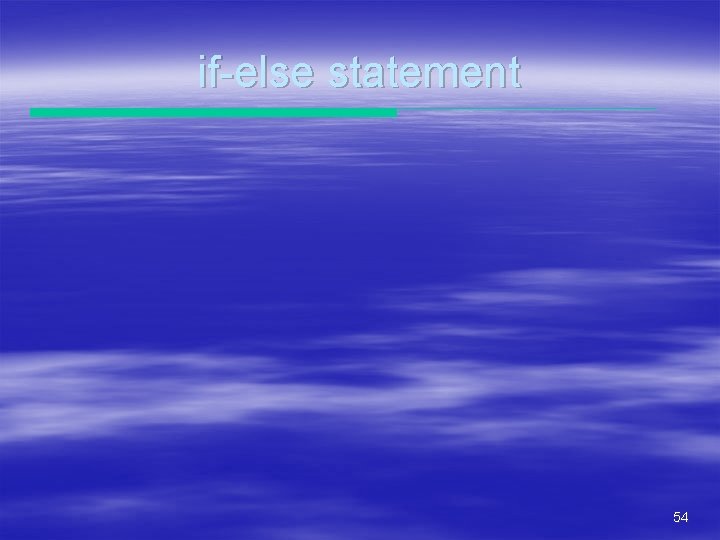
if-else statement 54
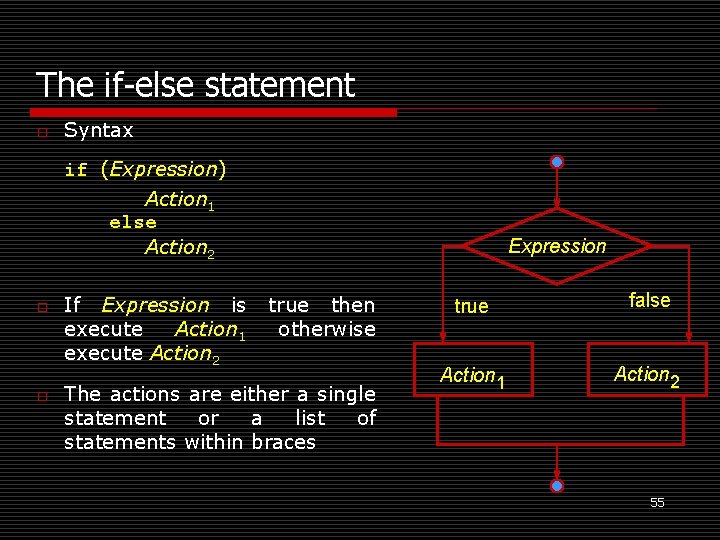
The if-else statement o Syntax if (Expression) Action 1 else Action 2 o o If Expression is execute Action 1 execute Action 2 Expression true then otherwise The actions are either a single statement or a list of statements within braces true false Action 1 Action 2 55
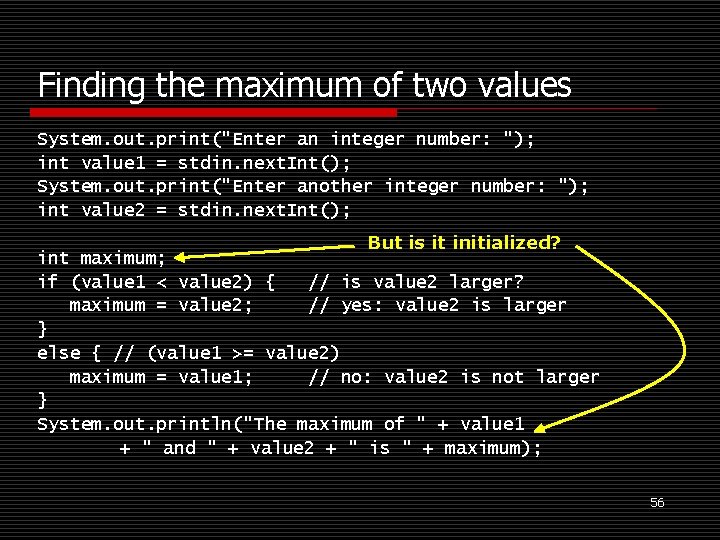
Finding the maximum of two values System. out. print("Enter an integer number: "); int value 1 = stdin. next. Int(); System. out. print("Enter another integer number: "); int value 2 = stdin. next. Int(); But is it initialized? int maximum; if (value 1 < value 2) { // is value 2 larger? maximum = value 2; // yes: value 2 is larger } else { // (value 1 >= value 2) maximum = value 1; // no: value 2 is not larger } System. out. println("The maximum of " + value 1 + " and " + value 2 + " is " + maximum); 56
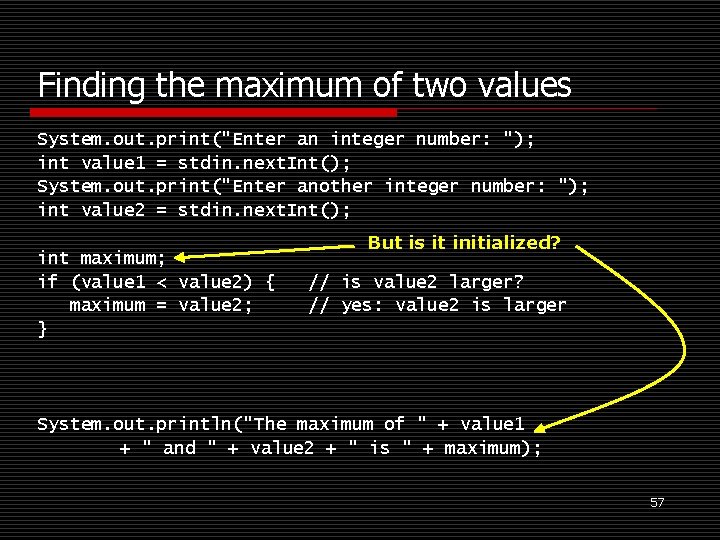
Finding the maximum of two values System. out. print("Enter an integer number: "); int value 1 = stdin. next. Int(); System. out. print("Enter another integer number: "); int value 2 = stdin. next. Int(); int maximum; if (value 1 < value 2) { maximum = value 2; } But is it initialized? // is value 2 larger? // yes: value 2 is larger System. out. println("The maximum of " + value 1 + " and " + value 2 + " is " + maximum); 57
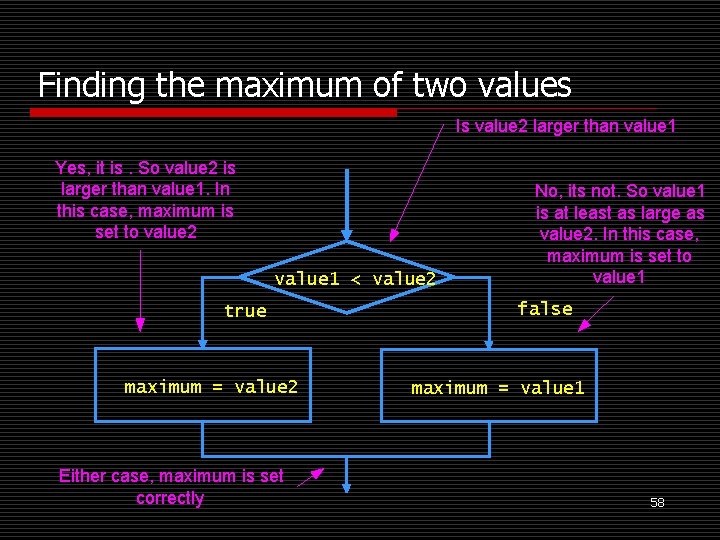
Finding the maximum of two values Is value 2 larger than value 1 Yes, it is. So value 2 is larger than value 1. In this case, maximum is set to value 2 value 1 < value 2 true maximum = value 2 Either case, maximum is set correctly No, its not. So value 1 is at least as large as value 2. In this case, maximum is set to value 1 false maximum = value 1 58
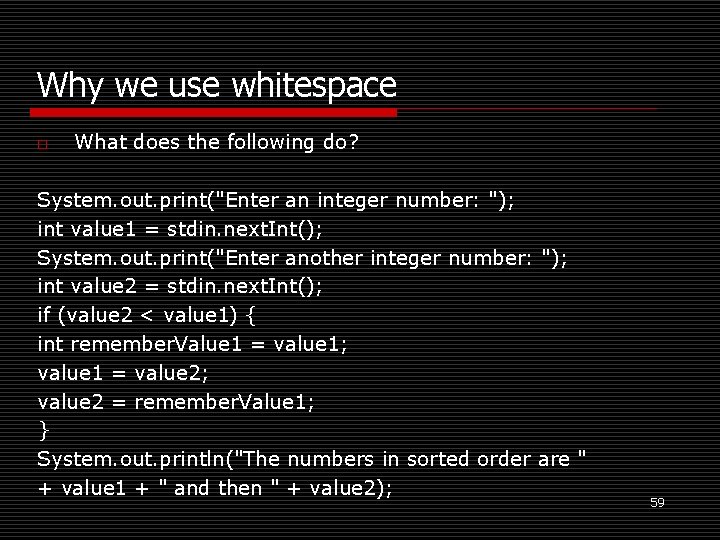
Why we use whitespace o What does the following do? System. out. print("Enter an integer number: "); int value 1 = stdin. next. Int(); System. out. print("Enter another integer number: "); int value 2 = stdin. next. Int(); if (value 2 < value 1) { int remember. Value 1 = value 1; value 1 = value 2; value 2 = remember. Value 1; } System. out. println("The numbers in sorted order are " + value 1 + " and then " + value 2); 59
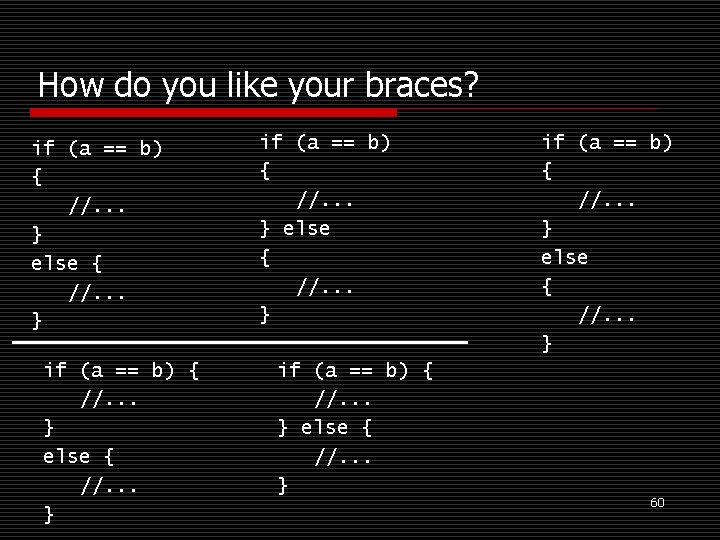
How do you like your braces? if (a == b) { //. . . } else { //. . . } 60
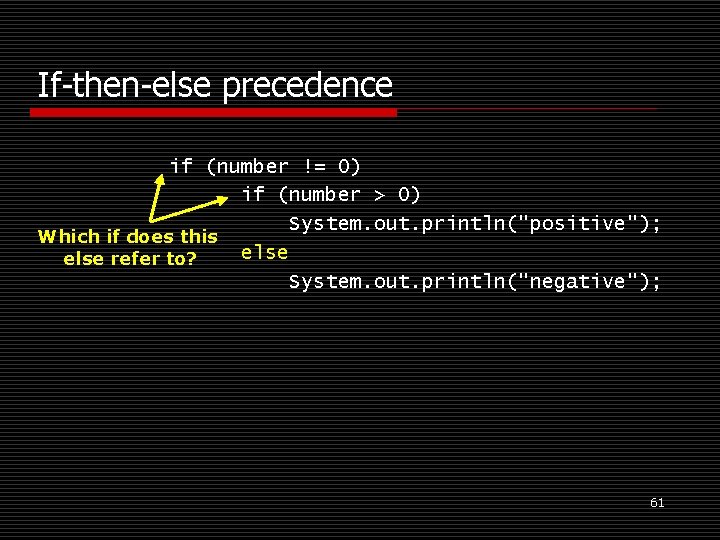
If-then-else precedence if (number != 0) if (number > 0) System. out. println("positive"); Which if does this else refer to? System. out. println("negative"); 61
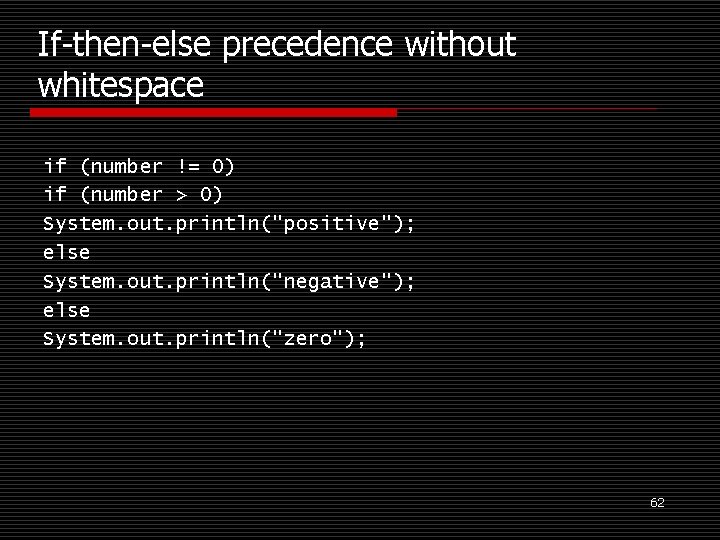
If-then-else precedence without whitespace if (number != 0) if (number > 0) System. out. println("positive"); else System. out. println("negative"); else System. out. println("zero"); 62
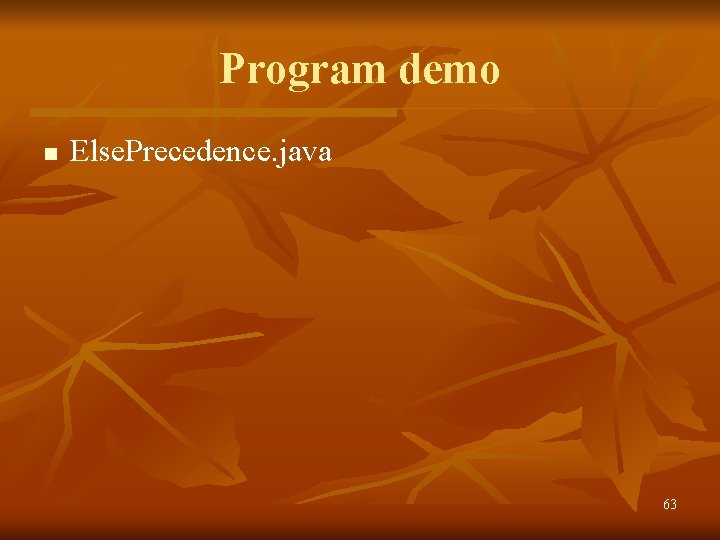
Program demo n Else. Precedence. java 63
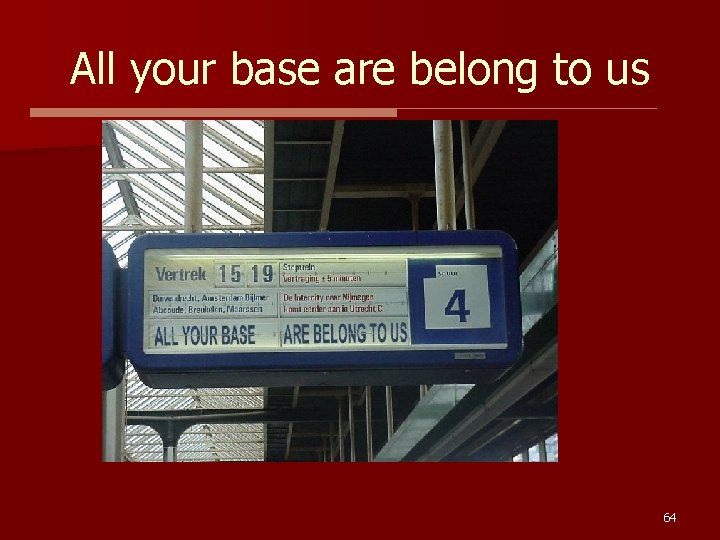
All your base are belong to us 64
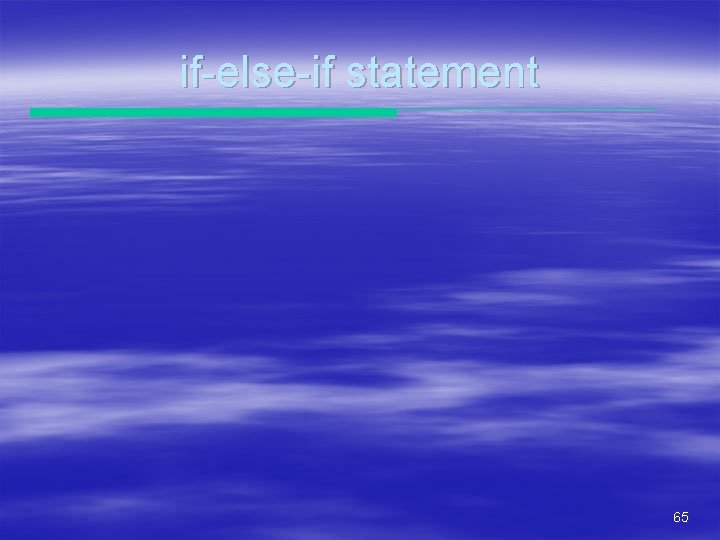
if-else-if statement 65
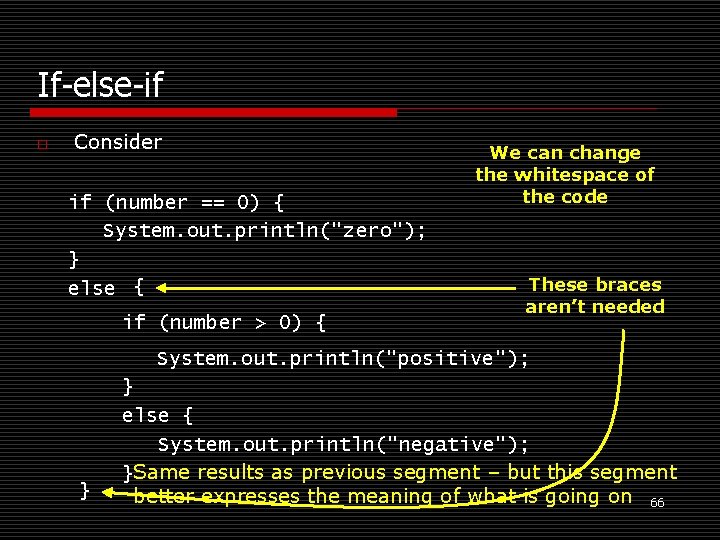
If-else-if o Consider if (number == 0) { System. out. println("zero"); } else { if (number > 0) { We can change the whitespace of the code These braces aren’t needed System. out. println("positive"); } } else { System. out. println("negative"); }Same results as previous segment – but this segment better expresses the meaning of what is going on 66
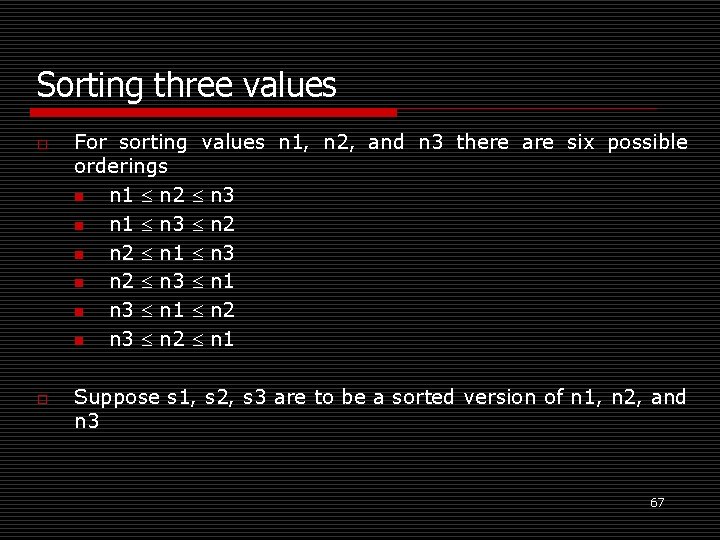
Sorting three values o o For sorting values n 1, n 2, and n 3 there are six possible orderings n n 1 £ n 2 £ n 3 n n 1 £ n 3 £ n 2 n n 2 £ n 1 £ n 3 n n 2 £ n 3 £ n 1 n n 3 £ n 1 £ n 2 n n 3 £ n 2 £ n 1 Suppose s 1, s 2, s 3 are to be a sorted version of n 1, n 2, and n 3 67
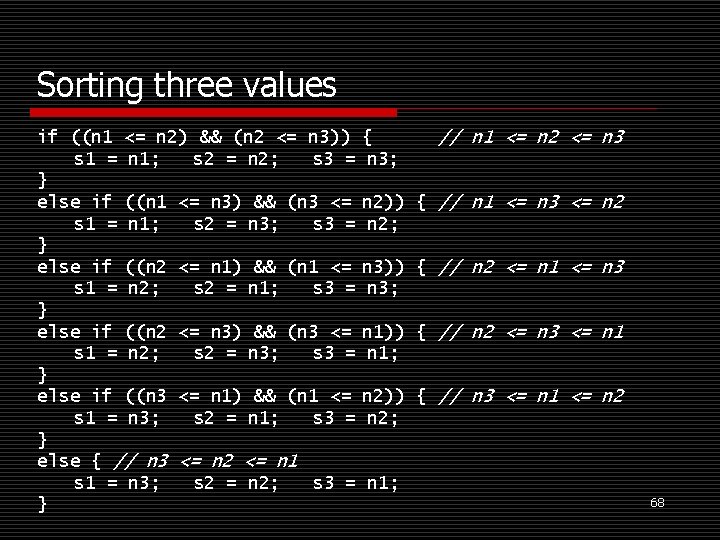
Sorting three values if ((n 1 <= n 2) && (n 2 <= n 3)) s 1 = n 1; s 2 = n 2; s 3 = } else if ((n 1 <= n 3) && (n 3 <= s 1 = n 1; s 2 = n 3; s 3 = } else if ((n 2 <= n 1) && (n 1 <= s 1 = n 2; s 2 = n 1; s 3 = } else if ((n 2 <= n 3) && (n 3 <= s 1 = n 2; s 2 = n 3; s 3 = } else if ((n 3 <= n 1) && (n 1 <= s 1 = n 3; s 2 = n 1; s 3 = } else { // n 3 <= n 2 <= n 1 s 1 = n 3; s 2 = n 2; s 3 = } { n 3; // n 1 <= n 2 <= n 3 n 2)) { // n 1 <= n 3 <= n 2; n 3)) { // n 2 <= n 1 <= n 3; n 1)) { // n 2 <= n 3 <= n 1; n 2)) { // n 3 <= n 1 <= n 2; n 1; 68
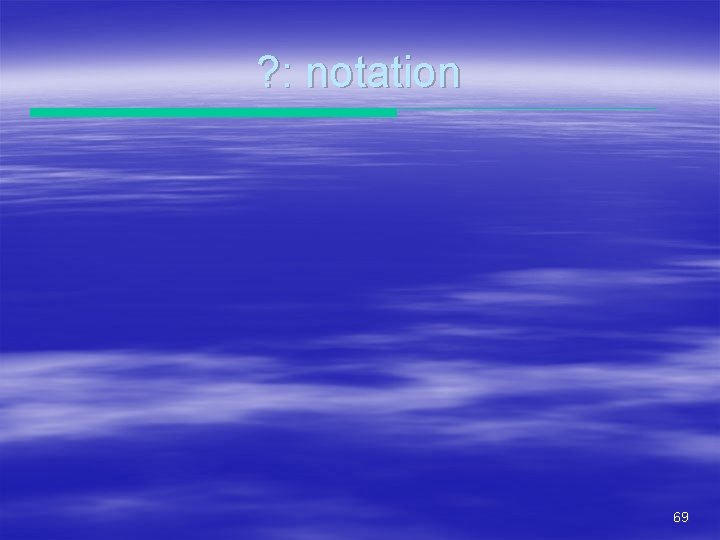
? : notation 69
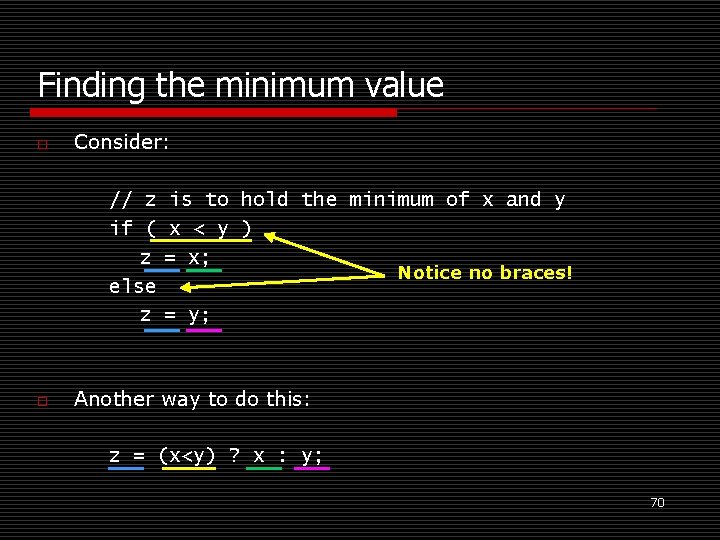
Finding the minimum value o Consider: // z is to hold the minimum of x and y if ( x < y ) z = x; Notice no braces! else z = y; o Another way to do this: z = (x<y) ? x : y; 70
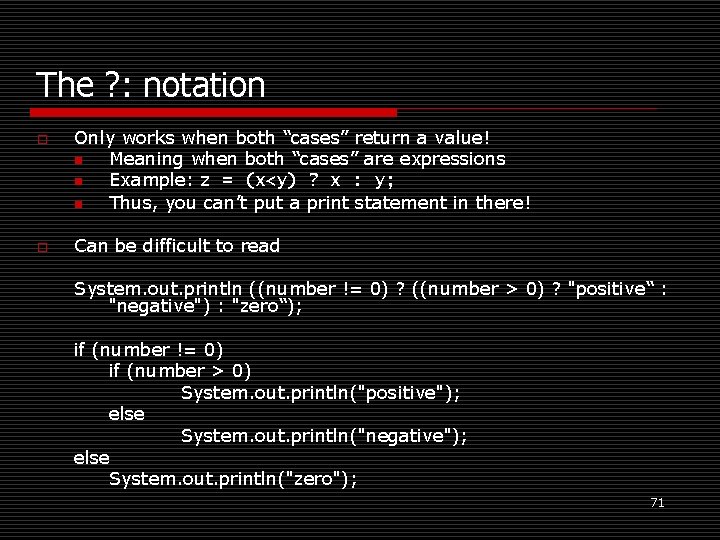
The ? : notation o o Only works when both “cases” return a value! n Meaning when both “cases” are expressions n Example: z = (x<y) ? x : y; n Thus, you can’t put a print statement in there! Can be difficult to read System. out. println ((number != 0) ? ((number > 0) ? "positive“ : "negative") : "zero“); if (number != 0) if (number > 0) System. out. println("positive"); else System. out. println("negative"); else System. out. println("zero"); 71
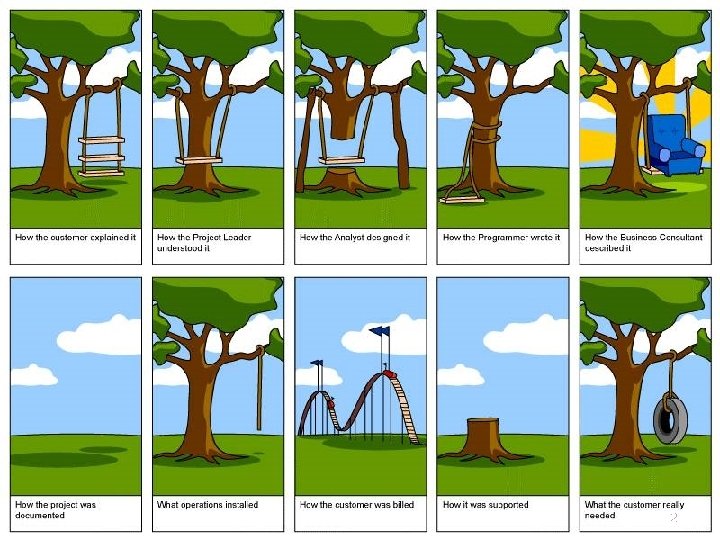
A bit of humor… 72
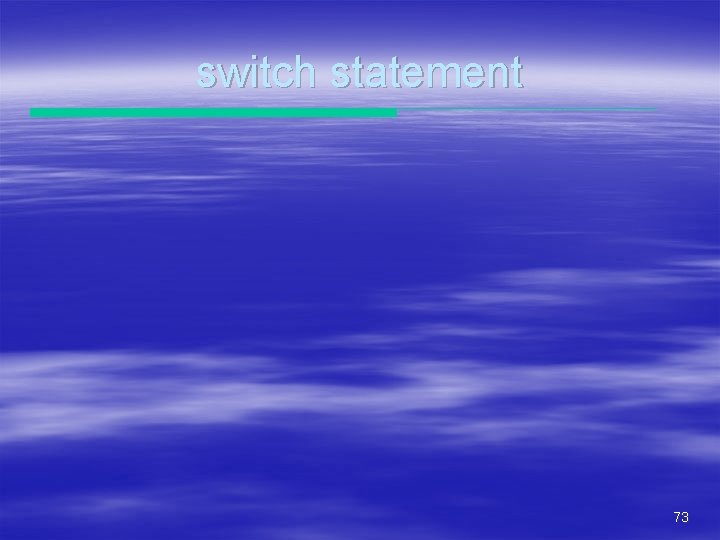
switch statement 73
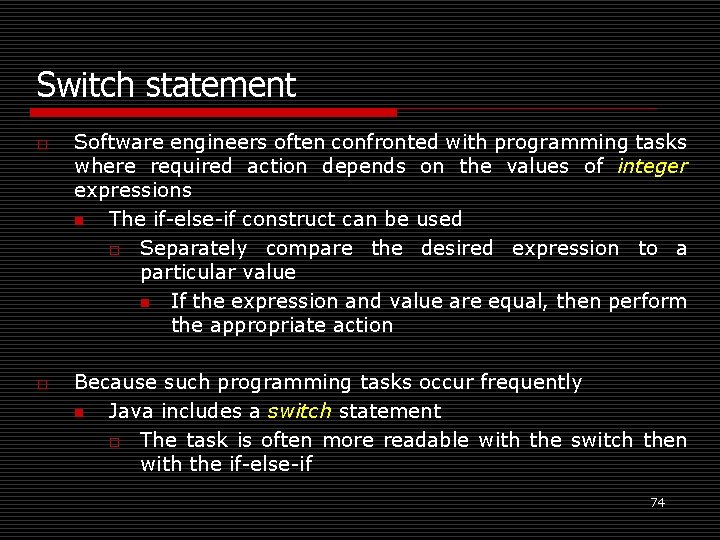
Switch statement o o Software engineers often confronted with programming tasks where required action depends on the values of integer expressions n The if-else-if construct can be used o Separately compare the desired expression to a particular value n If the expression and value are equal, then perform the appropriate action Because such programming tasks occur frequently n Java includes a switch statement o The task is often more readable with the switch then with the if-else-if 74
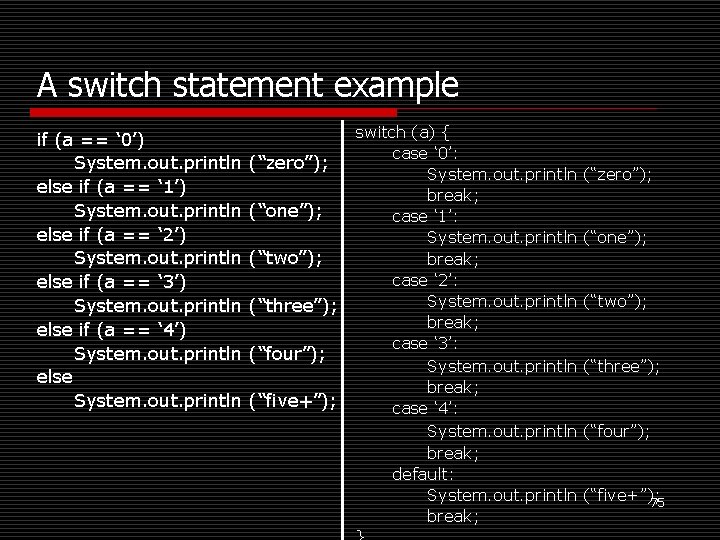
A switch statement example if (a == ‘ 0’) System. out. println else if (a == ‘ 1’) System. out. println else if (a == ‘ 2’) System. out. println else if (a == ‘ 3’) System. out. println else if (a == ‘ 4’) System. out. println else System. out. println (“zero”); (“one”); (“two”); (“three”); (“four”); (“five+”); switch (a) { case ‘ 0’: System. out. println break; case ‘ 1’: System. out. println break; case ‘ 2’: System. out. println break; case ‘ 3’: System. out. println break; case ‘ 4’: System. out. println break; default: System. out. println break; (“zero”); (“one”); (“two”); (“three”); (“four”); (“five+”); 75
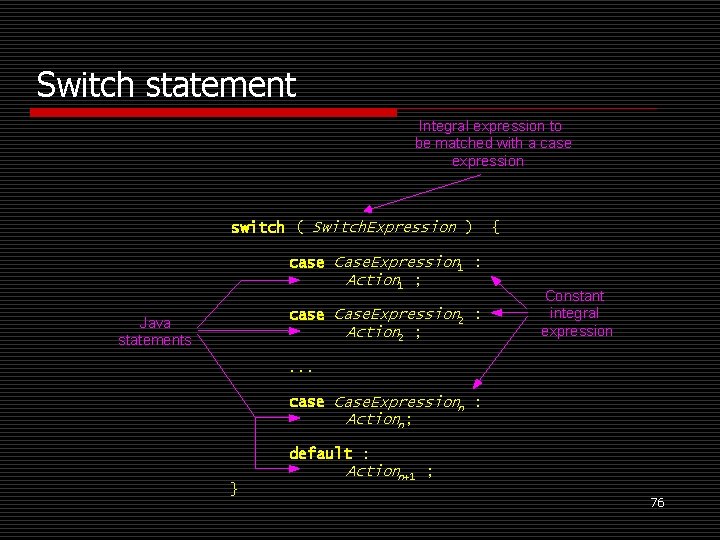
Switch statement Integral expression to be matched with a case expression switch ( Switch. Expression ) case Case. Expression 1 : Action 1 ; case Case. Expression 2 : Action 2 ; Java statements { Constant integral expression . . . case Case. Expressionn : Actionn ; default : } Actionn+1 ; 76
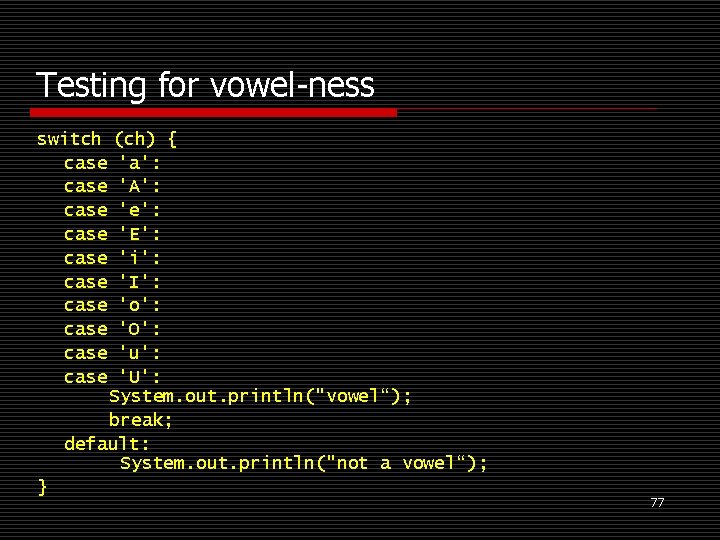
Testing for vowel-ness switch case case case (ch) { 'a': 'A': 'e': 'E': 'i': 'I': 'o': 'O': 'u': 'U': System. out. println("vowel“); break; default: System. out. println("not a vowel“); } 77
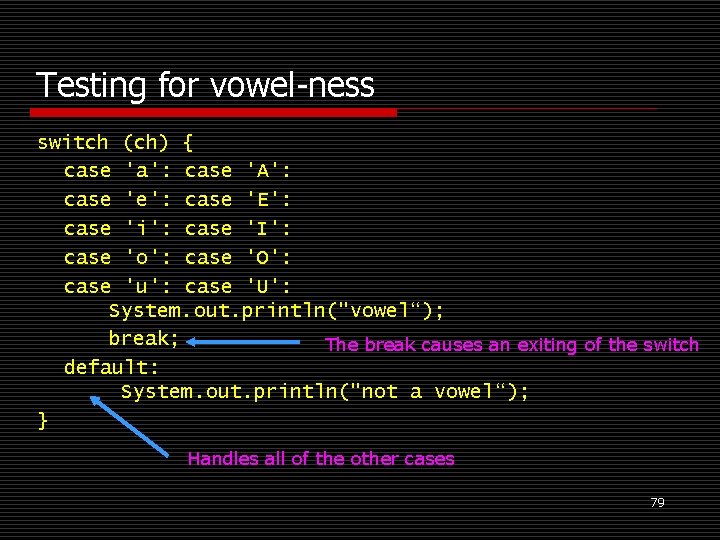
Testing for vowel-ness switch (ch) { case 'a': case 'A': case 'e': case 'E': case 'i': case 'I': case 'o': case 'O': case 'u': case 'U': System. out. println("vowel“); break; The break causes an exiting of the switch default: System. out. println("not a vowel“); } Handles all of the other cases 79
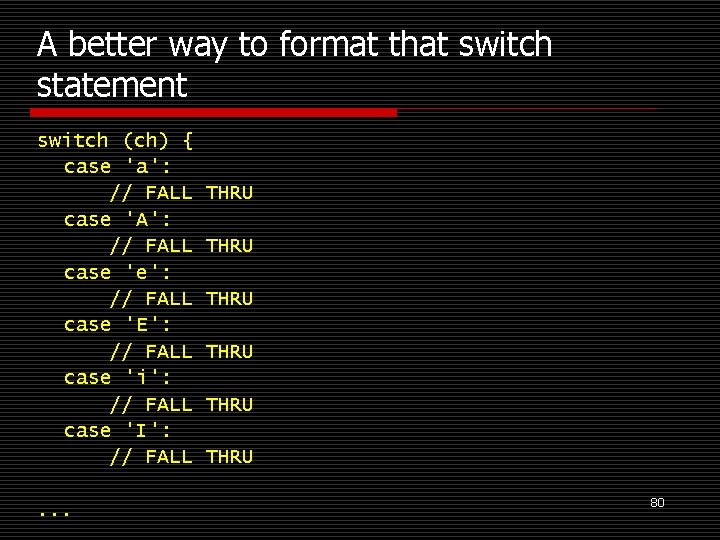
A better way to format that switch statement switch (ch) { case 'a': // FALL case 'A': // FALL case 'e': // FALL case 'E': // FALL case 'i': // FALL case 'I': // FALL. . . THRU THRU 80
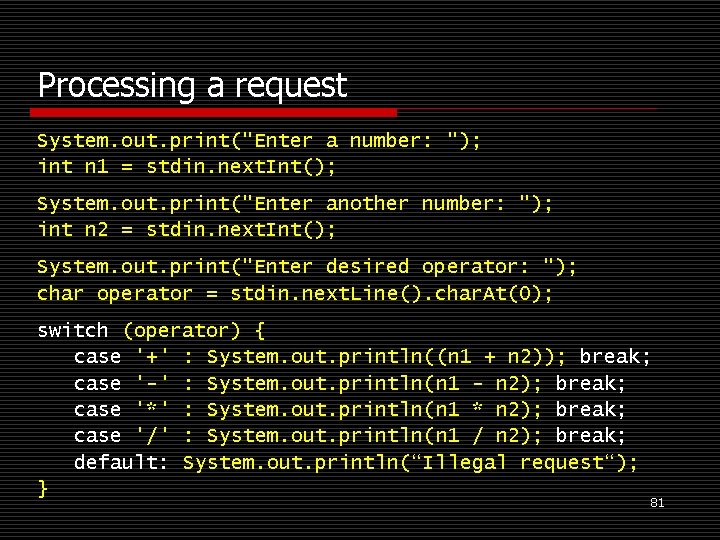
Processing a request System. out. print("Enter a number: "); int n 1 = stdin. next. Int(); System. out. print("Enter another number: "); int n 2 = stdin. next. Int(); System. out. print("Enter desired operator: "); char operator = stdin. next. Line(). char. At(0); switch (operator) { case '+' : System. out. println((n 1 + n 2)); break; case '-' : System. out. println(n 1 - n 2); break; case '*' : System. out. println(n 1 * n 2); break; case '/' : System. out. println(n 1 / n 2); break; default: System. out. println(“Illegal request“); } 81
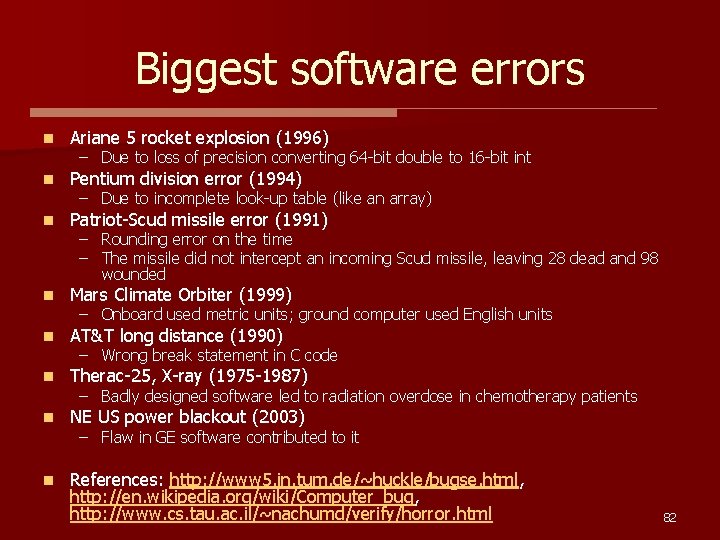
Biggest software errors n Ariane 5 rocket explosion (1996) n Pentium division error (1994) n Patriot-Scud missile error (1991) n Mars Climate Orbiter (1999) n AT&T long distance (1990) n Therac-25, X-ray (1975 -1987) n NE US power blackout (2003) n References: http: //www 5. in. tum. de/~huckle/bugse. html, http: //en. wikipedia. org/wiki/Computer_bug, http: //www. cs. tau. ac. il/~nachumd/verify/horror. html – Due to loss of precision converting 64 -bit double to 16 -bit int – Due to incomplete look-up table (like an array) – Rounding error on the time – The missile did not intercept an incoming Scud missile, leaving 28 dead and 98 wounded – Onboard used metric units; ground computer used English units – Wrong break statement in C code – Badly designed software led to radiation overdose in chemotherapy patients – Flaw in GE software contributed to it 82
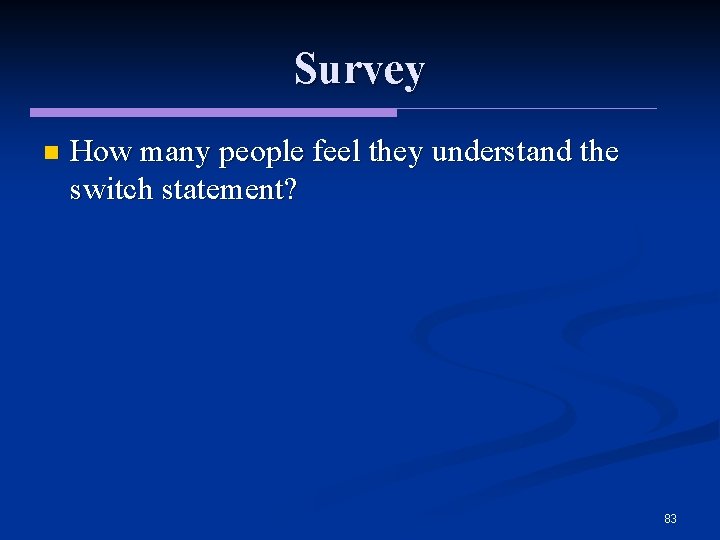
Survey n How many people feel they understand the switch statement? 83
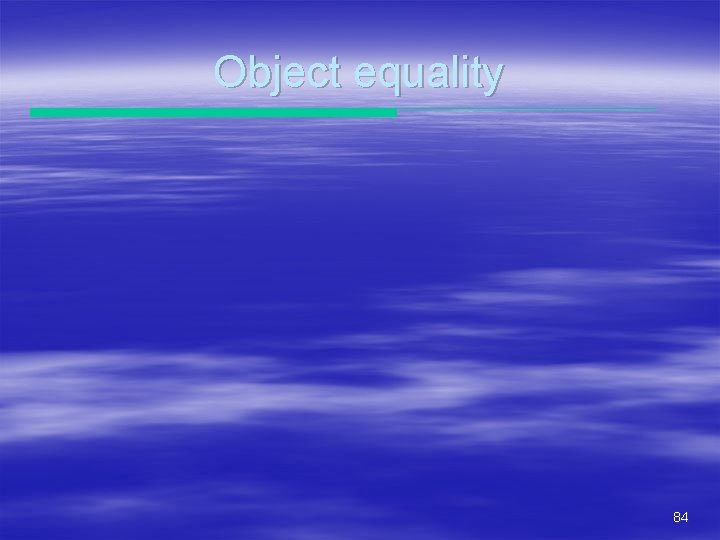
Object equality 84
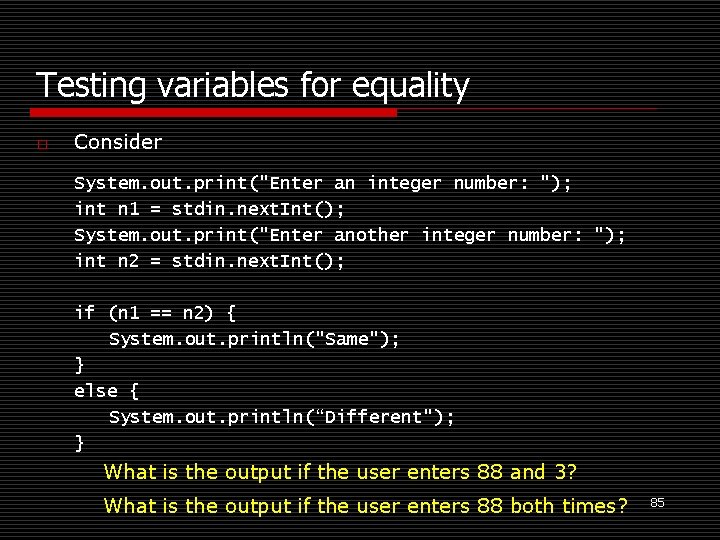
Testing variables for equality o Consider System. out. print("Enter an integer number: "); int n 1 = stdin. next. Int(); System. out. print("Enter another integer number: "); int n 2 = stdin. next. Int(); if (n 1 == n 2) { System. out. println("Same"); } else { System. out. println(“Different"); } What is the output if the user enters 88 and 3? What is the output if the user enters 88 both times? 85
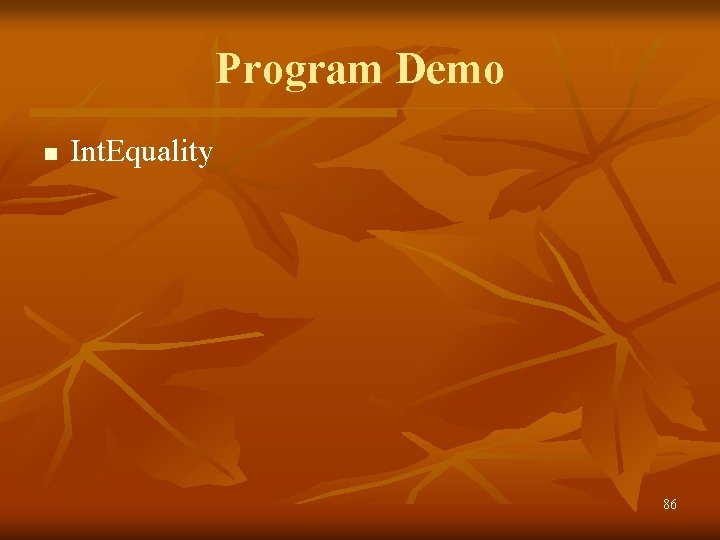
Program Demo n Int. Equality 86
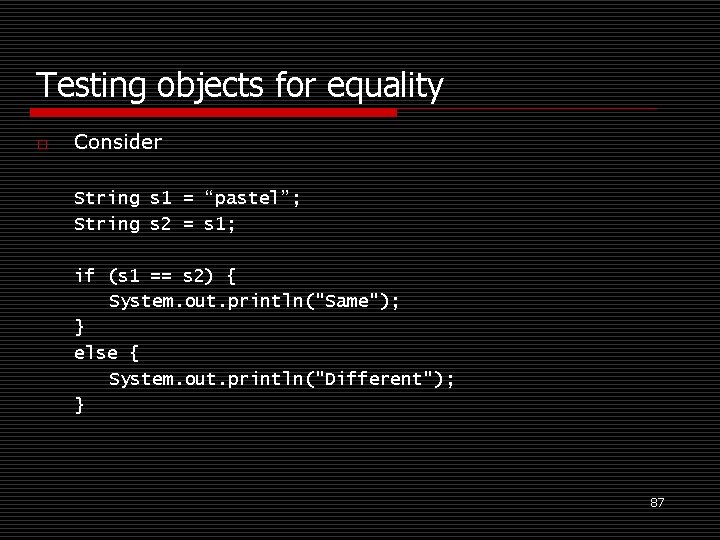
Testing objects for equality o Consider String s 1 = “pastel”; String s 2 = s 1; if (s 1 == s 2) { System. out. println("Same"); } else { System. out. println("Different"); } 87
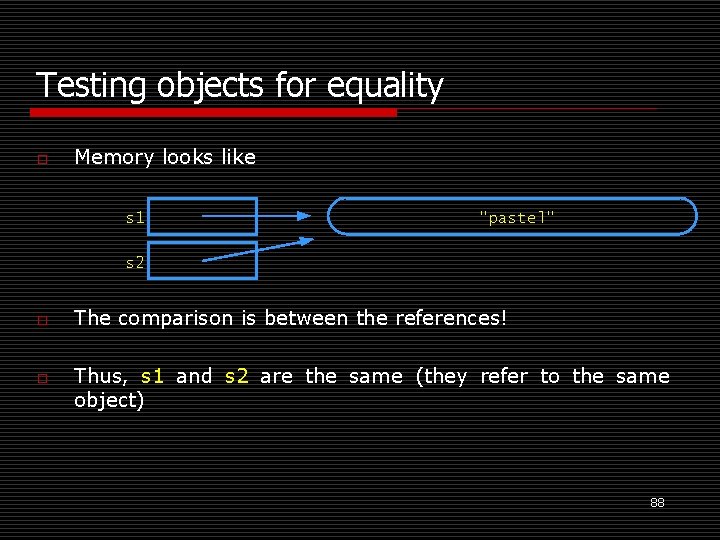
Testing objects for equality o Memory looks like s 1 "pastel" s 2 o o The comparison is between the references! Thus, s 1 and s 2 are the same (they refer to the same object) 88
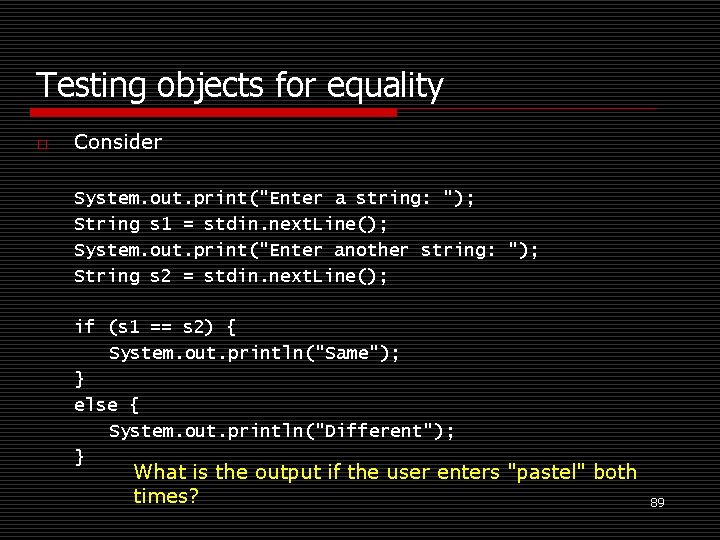
Testing objects for equality o Consider System. out. print("Enter a string: "); String s 1 = stdin. next. Line(); System. out. print("Enter another string: "); String s 2 = stdin. next. Line(); if (s 1 == s 2) { System. out. println("Same"); } else { System. out. println("Different"); } What is the output if the user enters "pastel" both times? 89
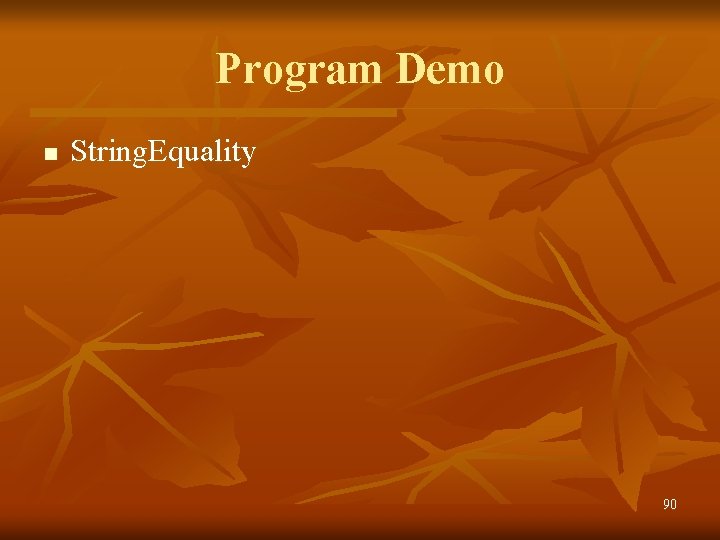
Program Demo n String. Equality 90
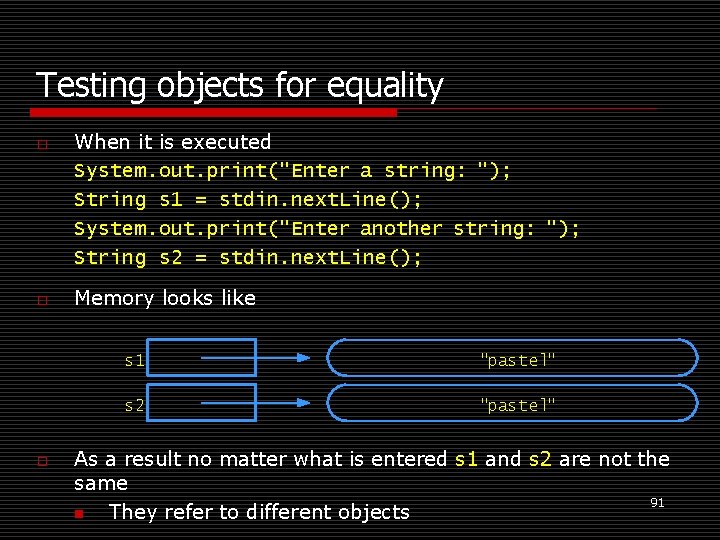
Testing objects for equality o o o When it is executed System. out. print("Enter a string: "); String s 1 = stdin. next. Line(); System. out. print("Enter another string: "); String s 2 = stdin. next. Line(); Memory looks like s 1 "pastel" s 2 "pastel" As a result no matter what is entered s 1 and s 2 are not the same 91 n They refer to different objects
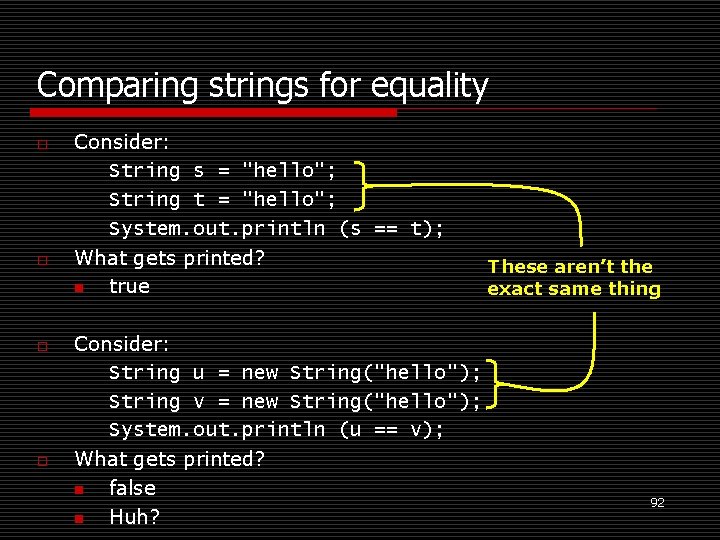
Comparing strings for equality o o Consider: String s = "hello"; String t = "hello"; System. out. println (s == t); What gets printed? n true Consider: String u = new String("hello"); String v = new String("hello"); System. out. println (u == v); What gets printed? n false n Huh? These aren’t the exact same thing 92
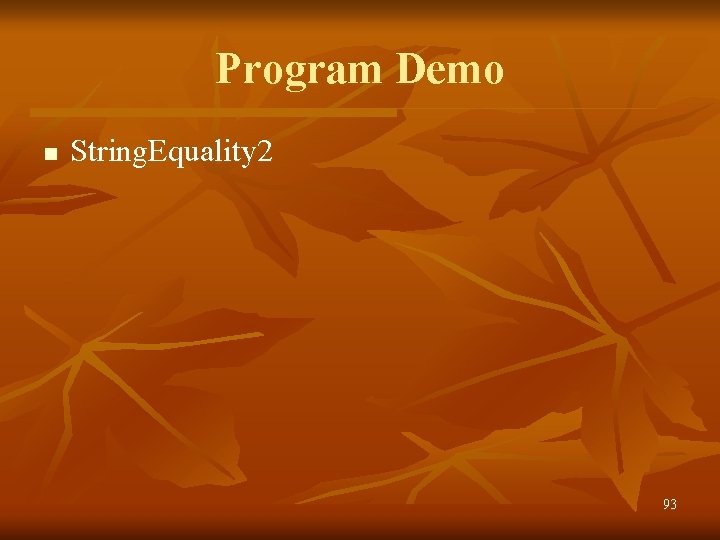
Program Demo n String. Equality 2 93
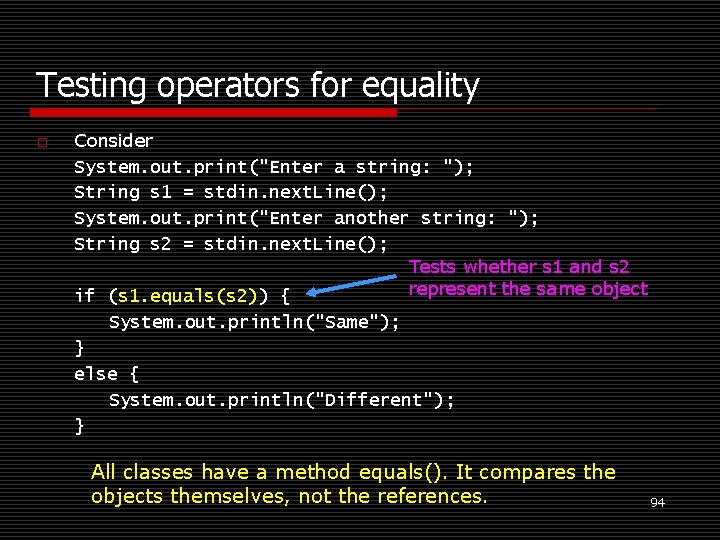
Testing operators for equality o Consider System. out. print("Enter a string: "); String s 1 = stdin. next. Line(); System. out. print("Enter another string: "); String s 2 = stdin. next. Line(); Tests whether s 1 and s 2 represent the same object if (s 1. equals(s 2)) { System. out. println("Same"); } else { System. out. println("Different"); } All classes have a method equals(). It compares the objects themselves, not the references. 94
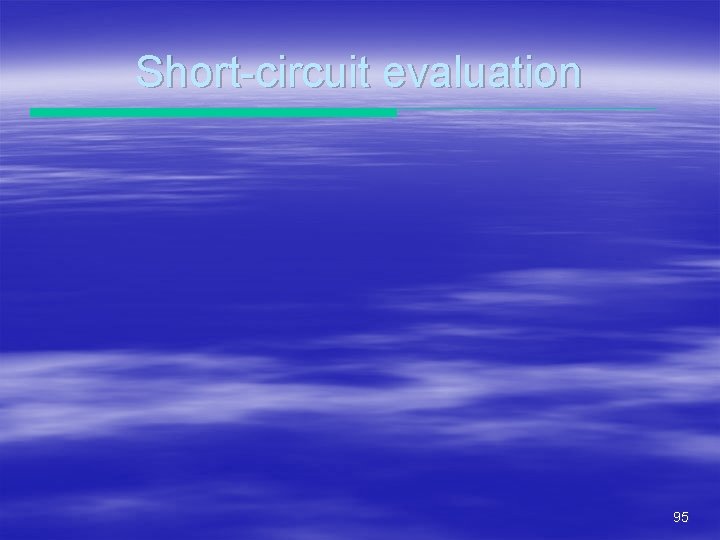
Short-circuit evaluation 95
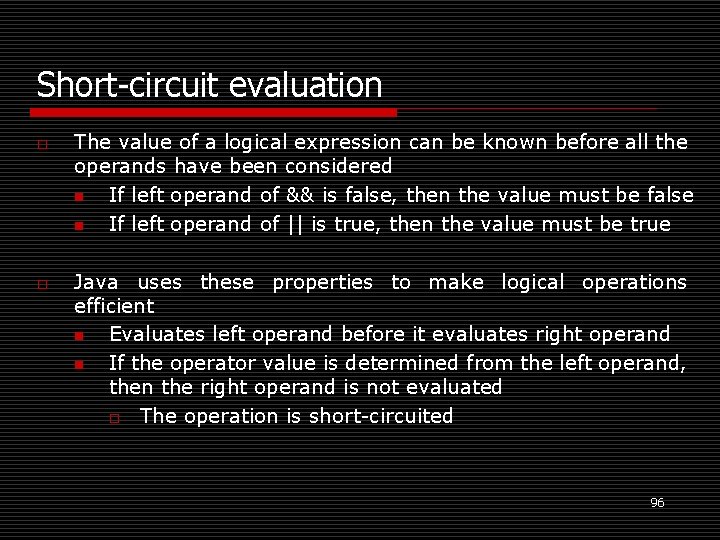
Short-circuit evaluation o o The value of a logical expression can be known before all the operands have been considered n If left operand of && is false, then the value must be false n If left operand of || is true, then the value must be true Java uses these properties to make logical operations efficient n Evaluates left operand before it evaluates right operand n If the operator value is determined from the left operand, then the right operand is not evaluated o The operation is short-circuited 96
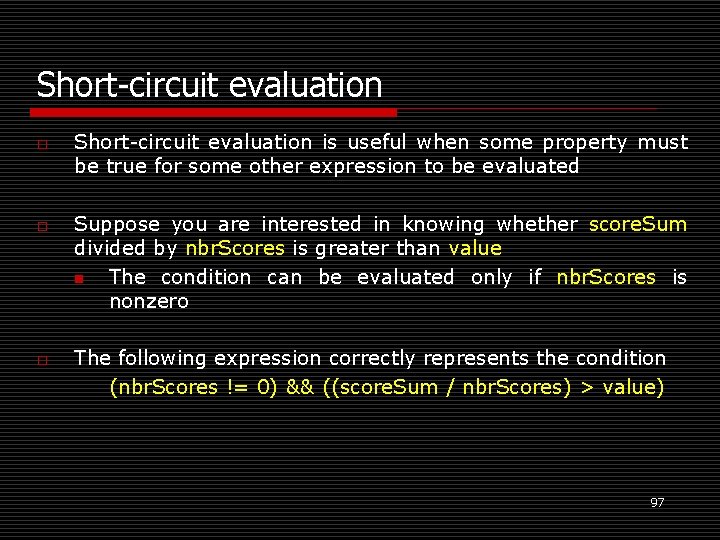
Short-circuit evaluation o o o Short-circuit evaluation is useful when some property must be true for some other expression to be evaluated Suppose you are interested in knowing whether score. Sum divided by nbr. Scores is greater than value n The condition can be evaluated only if nbr. Scores is nonzero The following expression correctly represents the condition (nbr. Scores != 0) && ((score. Sum / nbr. Scores) > value) 97
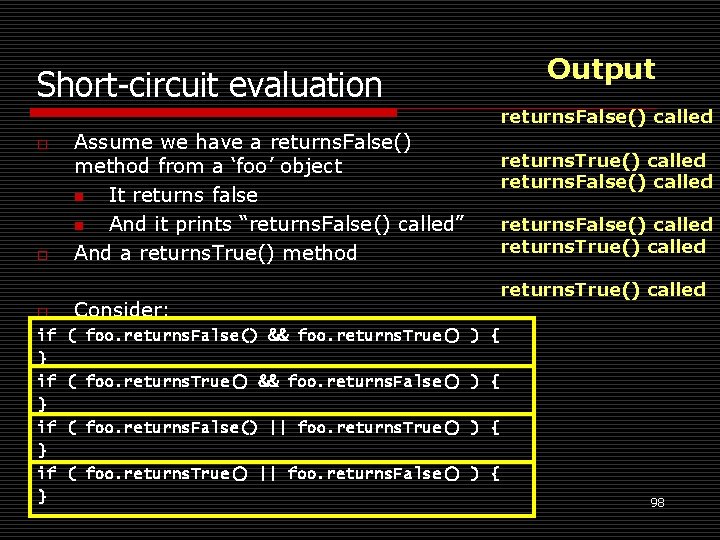
Short-circuit evaluation Output returns. False() called o o o if } Assume we have a returns. False() method from a ‘foo’ object n It returns false n And it prints “returns. False() called” And a returns. True() method Consider: returns. True() called returns. False() called returns. True() called ( foo. returns. False() && foo. returns. True() ) { ( foo. returns. True() && foo. returns. False() ) { ( foo. returns. False() || foo. returns. True() ) { ( foo. returns. True() || foo. returns. False() ) { 98
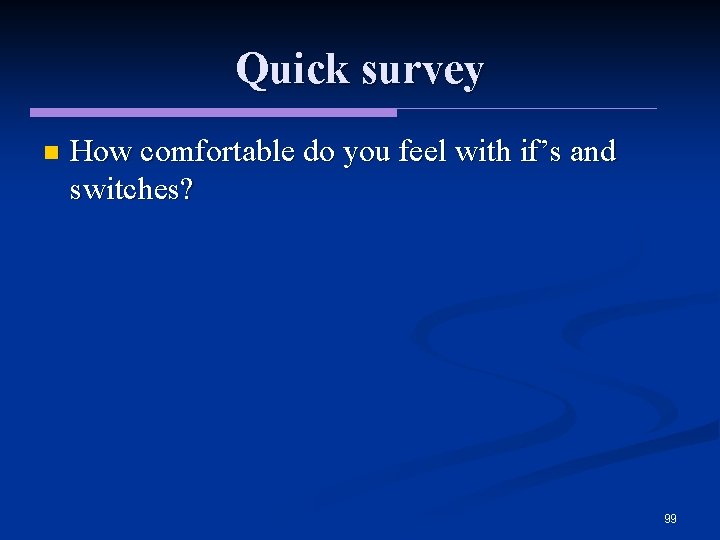
Quick survey n How comfortable do you feel with if’s and switches? 99
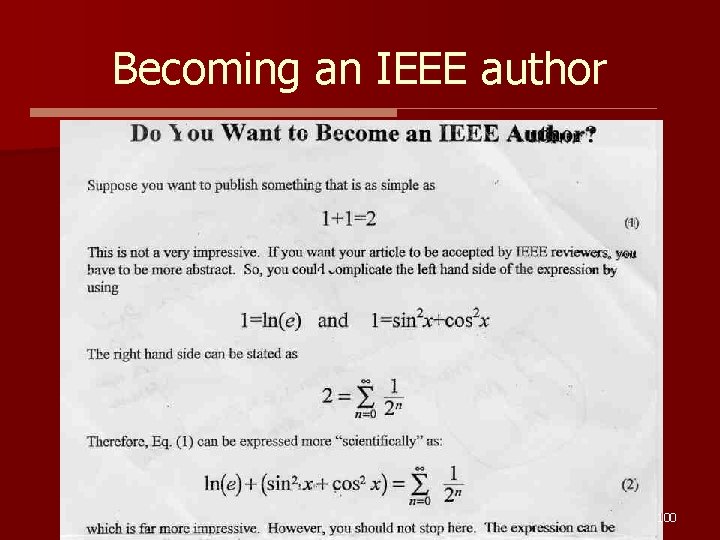
Becoming an IEEE author 100
Good decision making poster
Four seasons korean movie
Spring summer fall winter months
Screening decisions and preference decisions
Blue spring from a distance chapter 1
Chapter 4 financial decisions and planning
Chapter 4 financial decisions and planning
An organized method for making good buying decisions.
An organized method for making good buying decisions
Chapter 10 personal loans and purchasing decisions
Chapter 1 economic decisions and systems answer key
Depreciation tax shield formula
Chapter 4 financial decisions and planning
Chapter 4 financial decisions and planning
Chapter 4 financial decisions and planning
Chapter 10 making capital investment decisions
Chapter 3 budgeting
Chemistry 101 chapter 1
A real friend 2006
Sentencia c-355 de 2006
Pengiktirafan ukm 2006
Monarch awards 2006
Syawal 2006
T. trimpe 2006 http //sciencespot.net/ answer key
Sexenio 2000 a 2006
Sentencia c-355 de 2006.
Pengiktirafan ukm 2006
Rdc 214 de 2006
Rose report 2006
Perbedaan kurikulum 1994 dengan kurikulum 2004
Monarch awards 2006
2006-1971
Maturita 2006
Imagenes del codigo de infancia y adolescencia
Jana 2006
Ramadan 2006
Giec 2006
Apa hakikat fungsi dan tujuan pkn di sd
T trimpe 2006 http //sciencespot.net/ answer key
Ss-en 12097:2006
Jost 2006
Pam contract 2006
Lee 2006
Guinsaugon landslide 2006 case study
Het panel
2 mars 2006
Rabt 2006
Luthans 2006
April 2006 calendar
Bacterial structure
Lee 2006
Rg 2141/2006
Contoh surat penghapusan piutang tak tertagih
Law society of tasmania v richardson [2003] tassc 9
Xxxxxx me
Use colored pencils to circle the common atoms
El paso flood 2006
Deped school calendar 2005-2006
Boardworks ltd 2006
Boardworks ltd 2006
Boardworks ltd 2006
Boardworks ltd 2006
The moon on 1/7/2006
What is unfair competition
Unfair commercial practices directive
Astronomy picture of the day march 29 2006
Giddens 2006
Giddens 2006
02/06/2006 lunaf
Http://sciencespot.net
T. trimpe 2006 http //sciencespot.net/
T. trimpe 2003 http //sciencespot.net/
T. trimpe 2006 http //sciencespot.net/
Sciencespot fingerprint challenge
T. trimpe 2006 http //sciencespot.net/
T.trimpe 2006 http sciencespot.net
Okada
R a 9344
2006 kids
31 desember 2006
Rs 234-2006
Nasa october 26 2006
October 29 2006 nasa
2006
Vandelay art. seinfeld the show about nothing. penguin 1997
Maturita 2006
Maturita 2006
Lei 11 346
Jane eyre characterization
Ibraop projeto básico
T. trimpe 2006 http //sciencespot.net/
Ego pharma birthday 10th february 2006
Pelan induk pembangunan pendidikan 2006 hingga 2010
Xxxx2006
76-a/2006
The moon in 5/8/2006
Picture nasa took on feb 14 2006
There was nothing leon the driver could do
Caltrans standard specifications 2015