Lecture 6 What is the difference in pictures
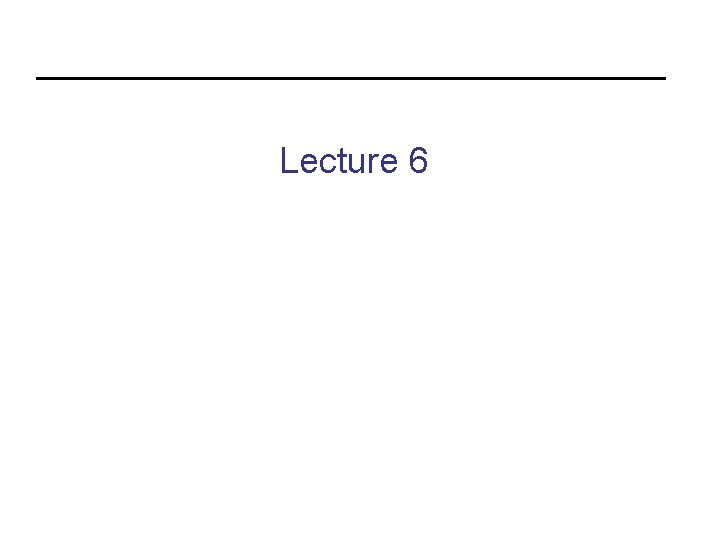
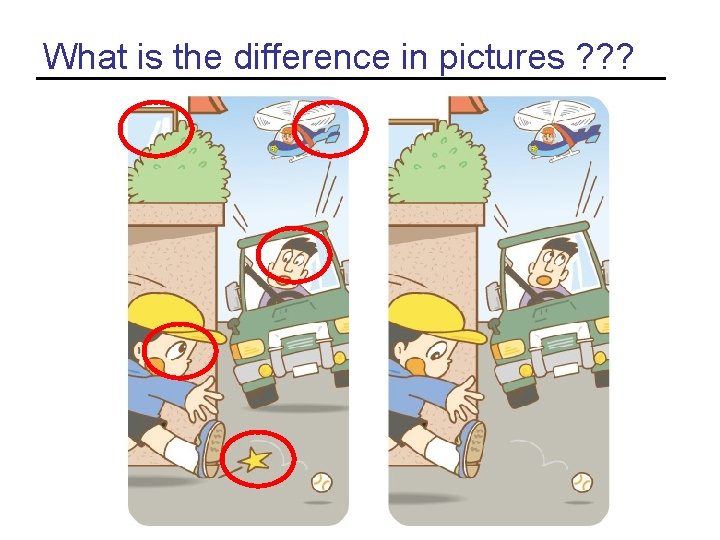
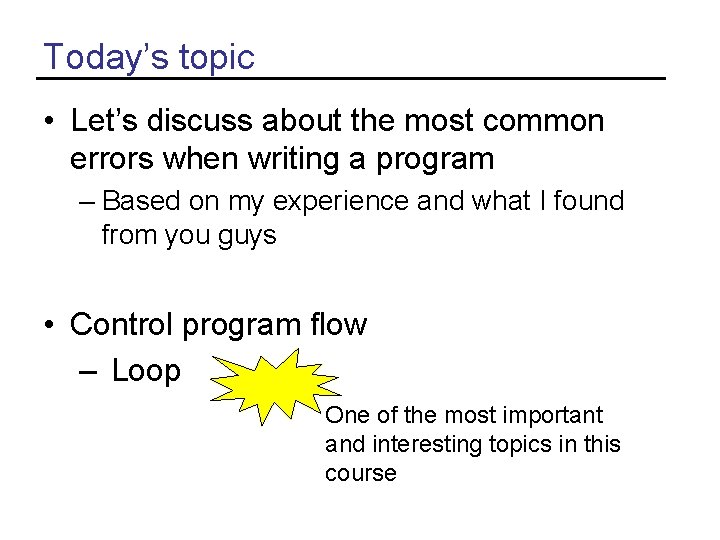
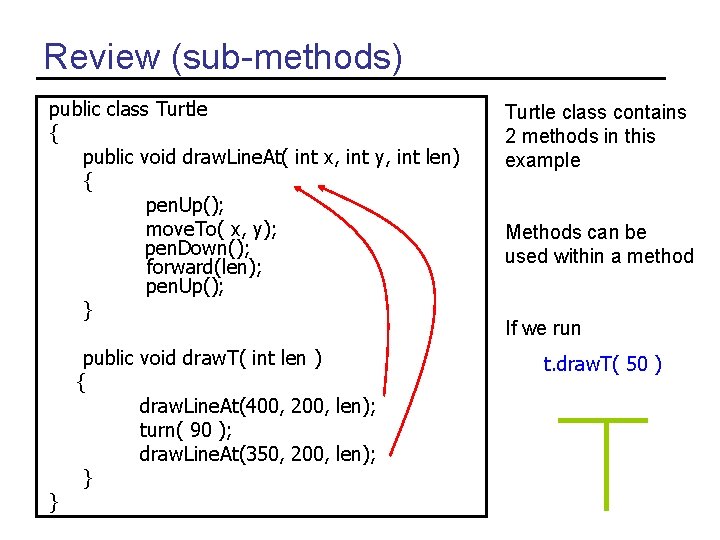
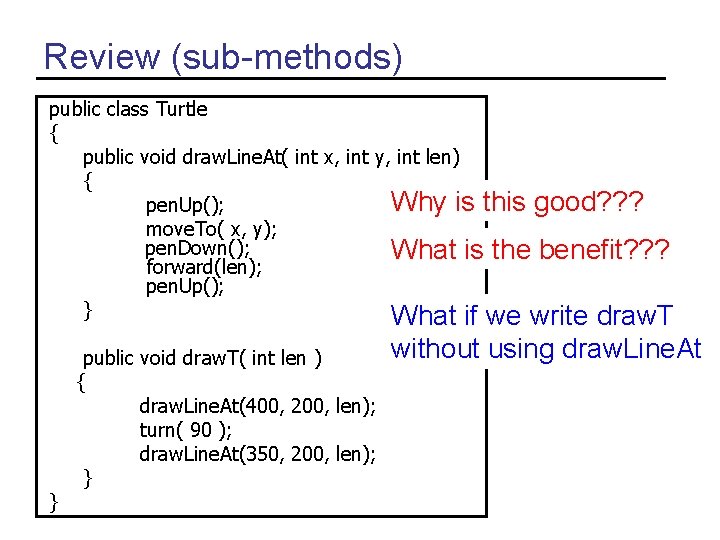
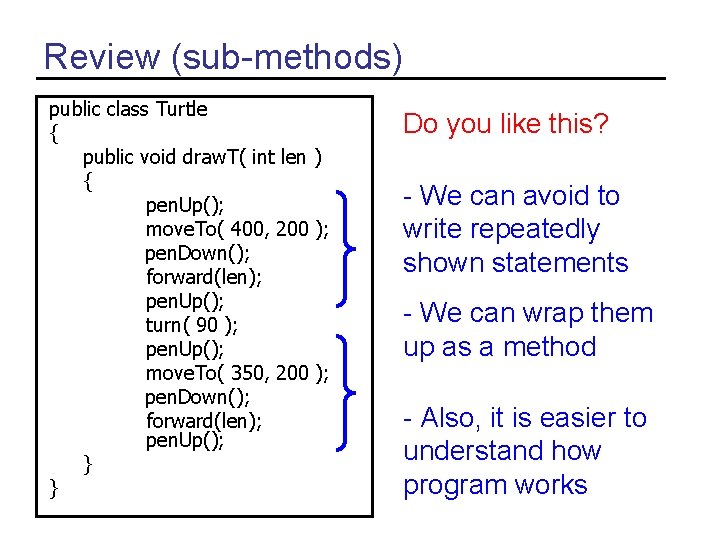
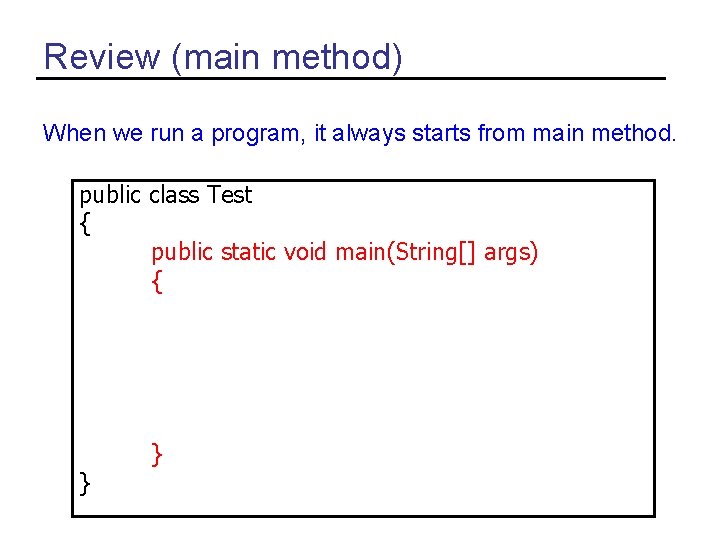
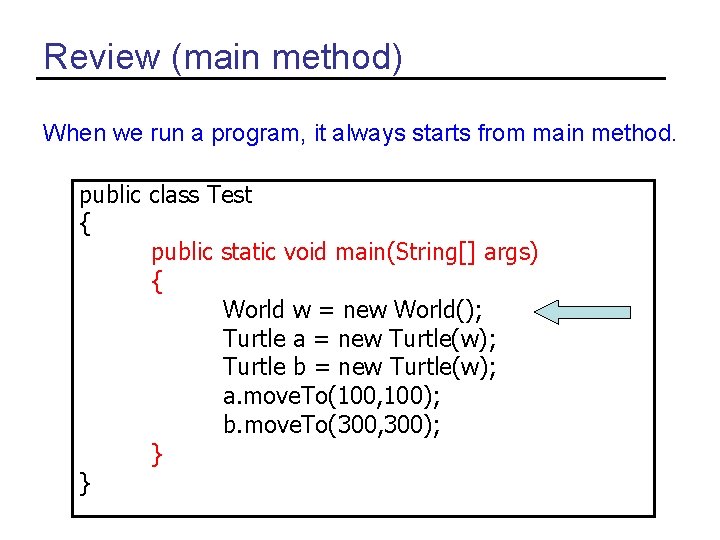
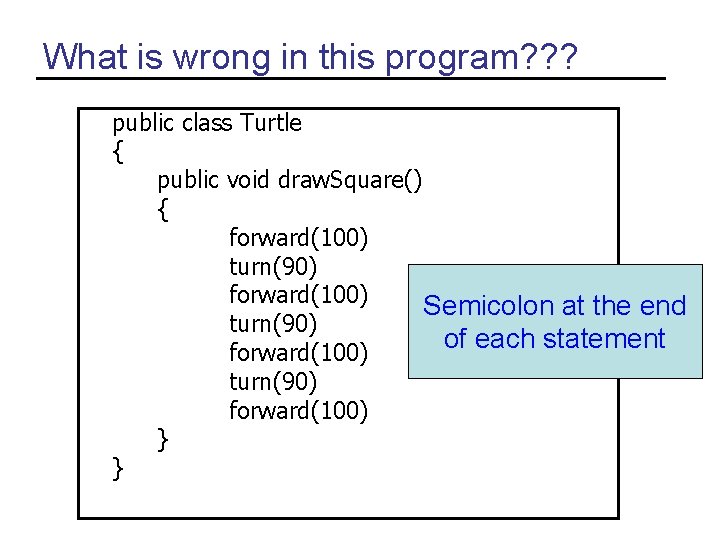
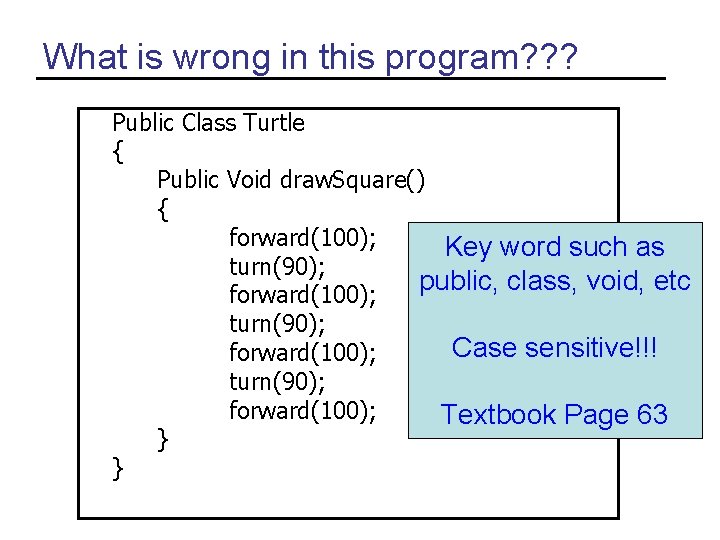
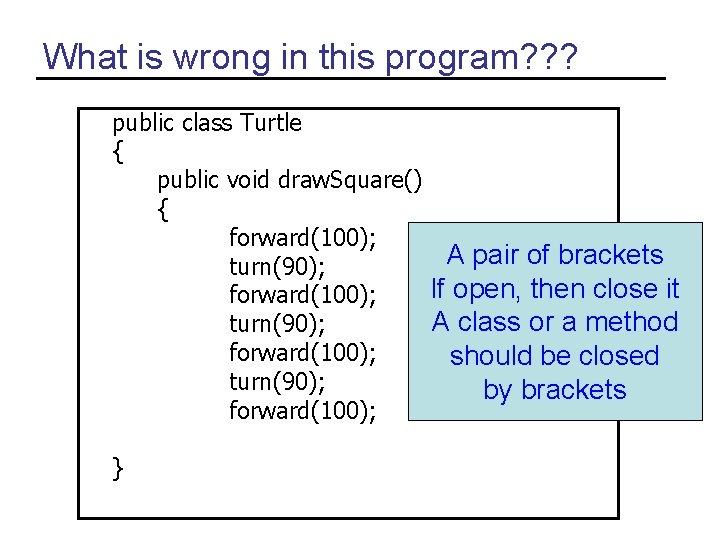
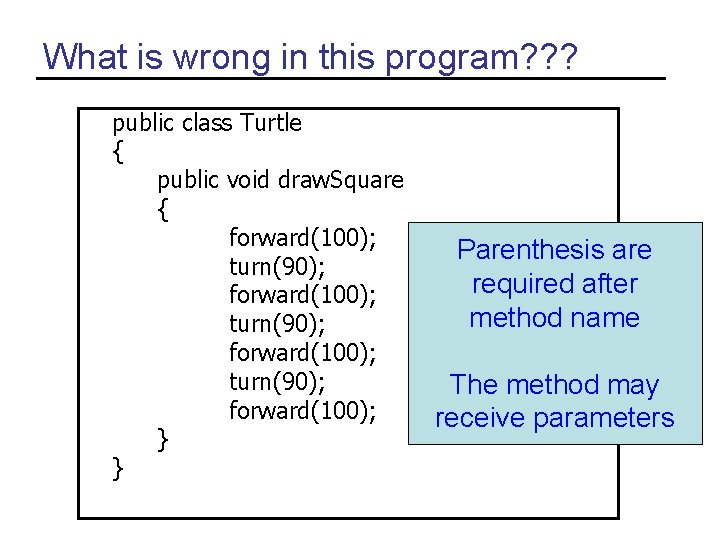
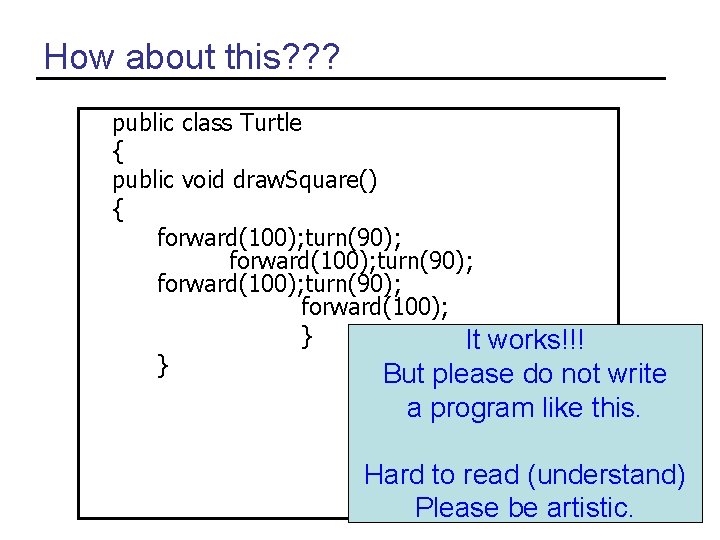
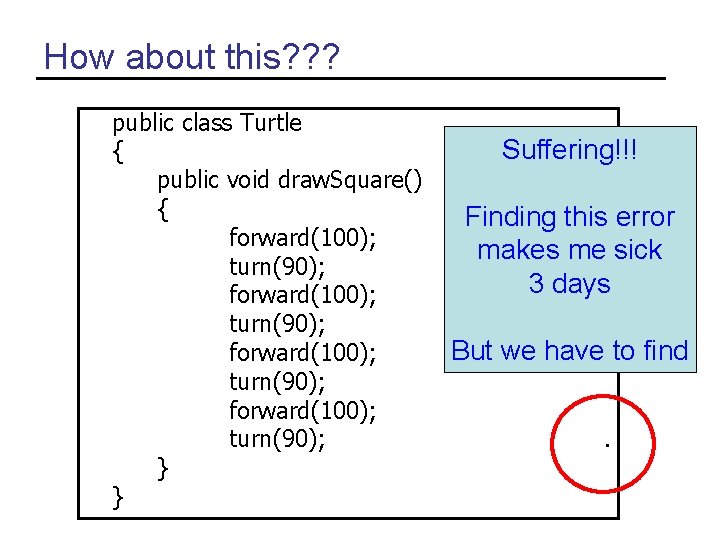
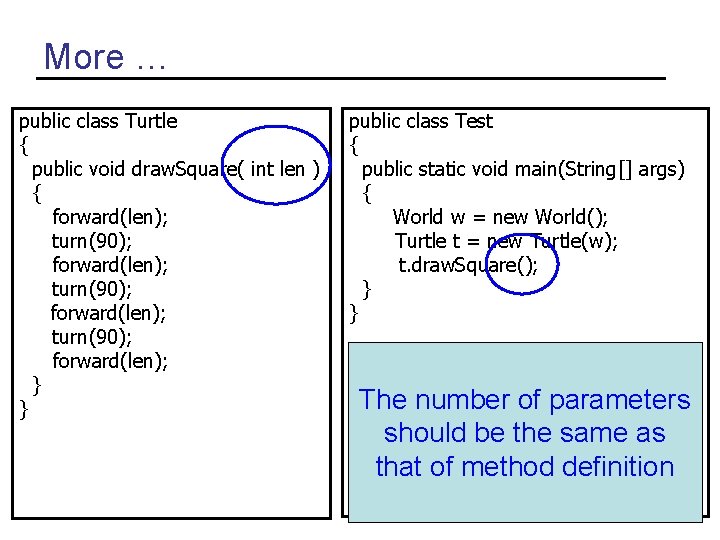
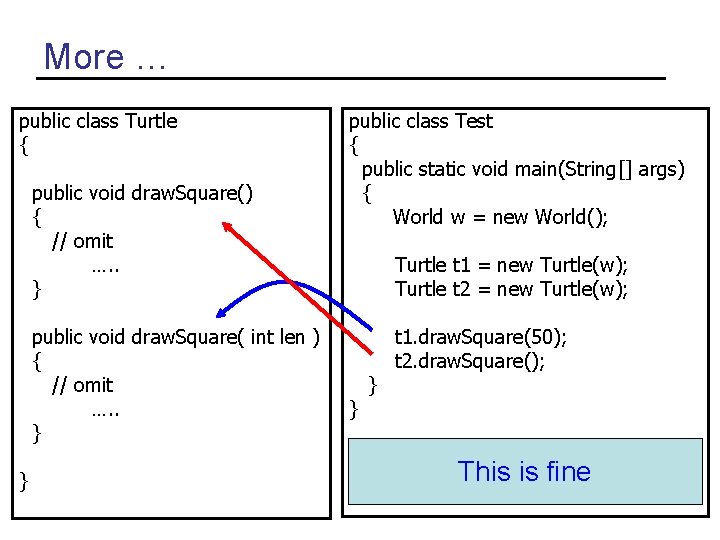
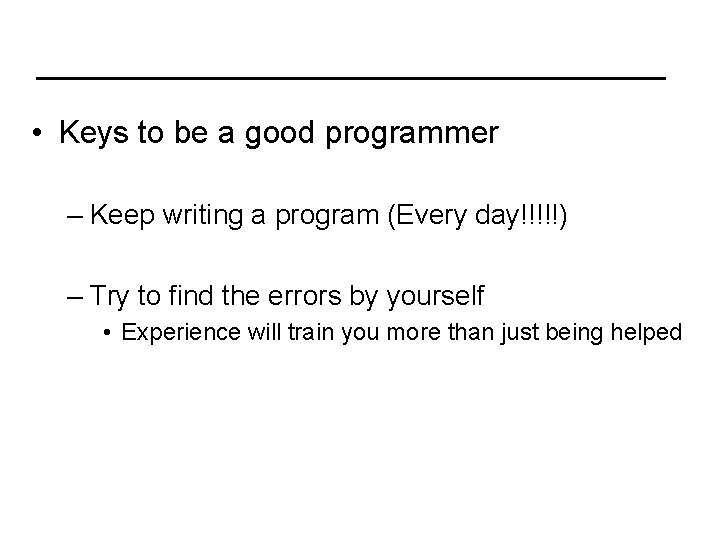
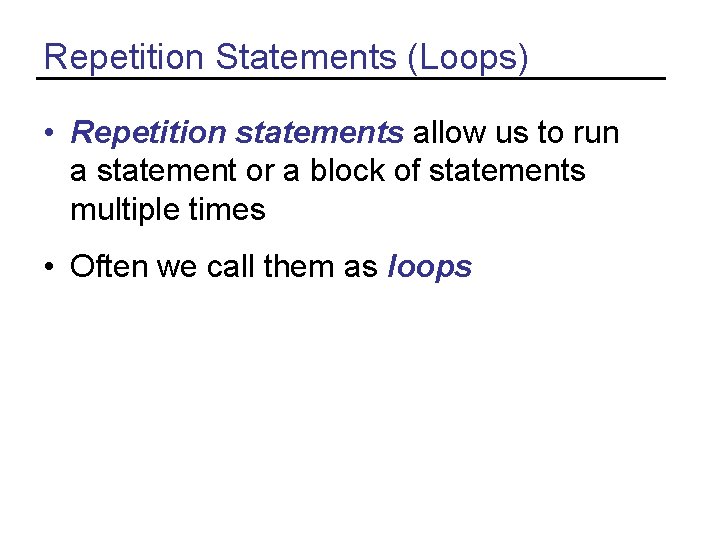
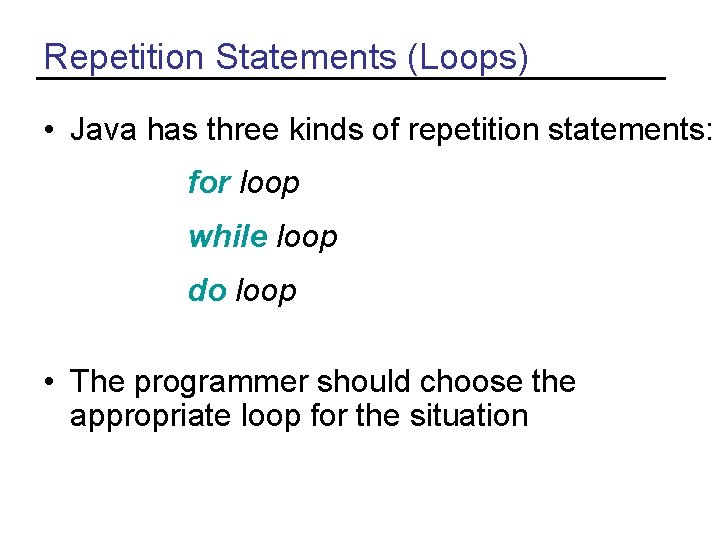
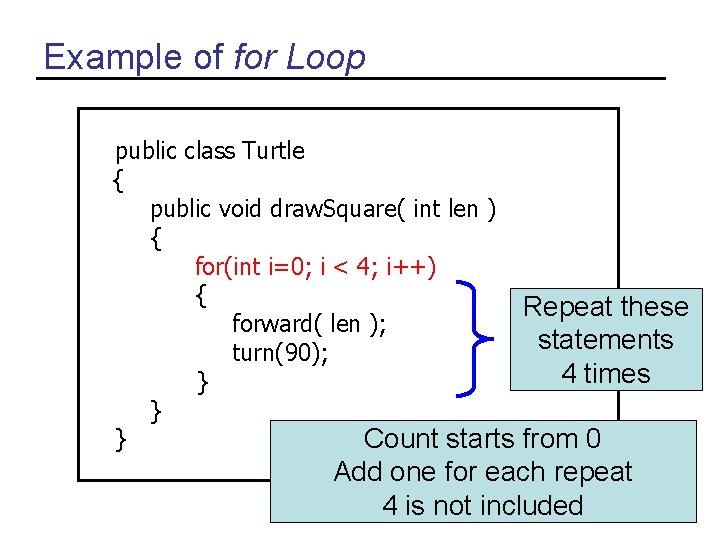
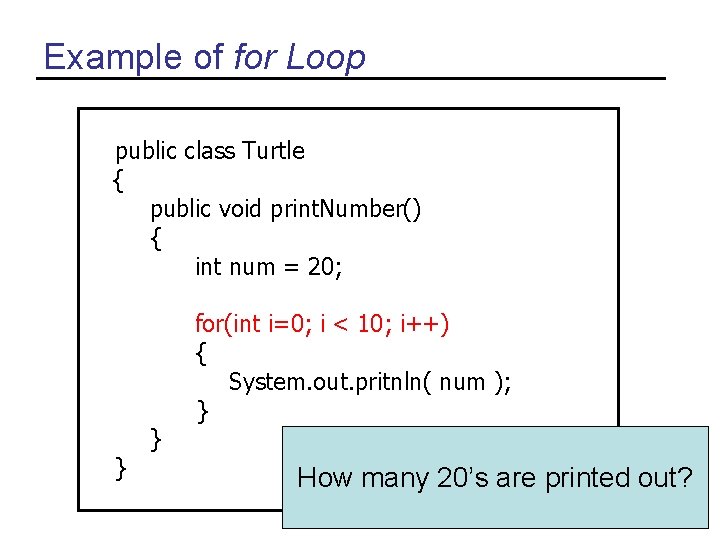
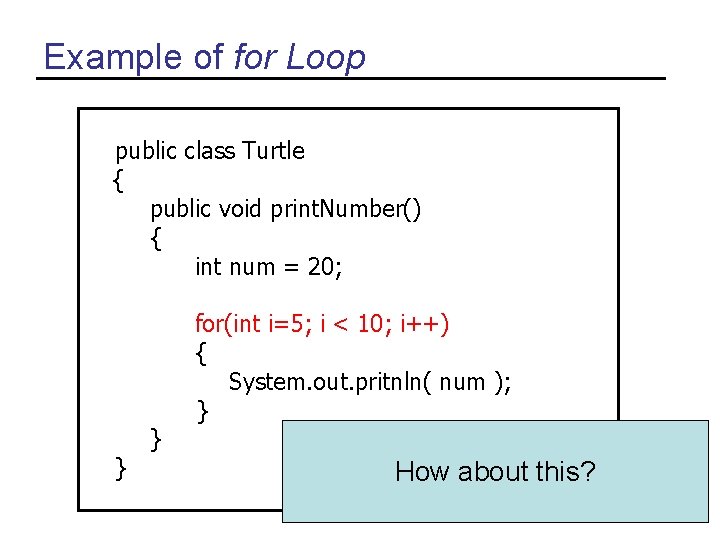
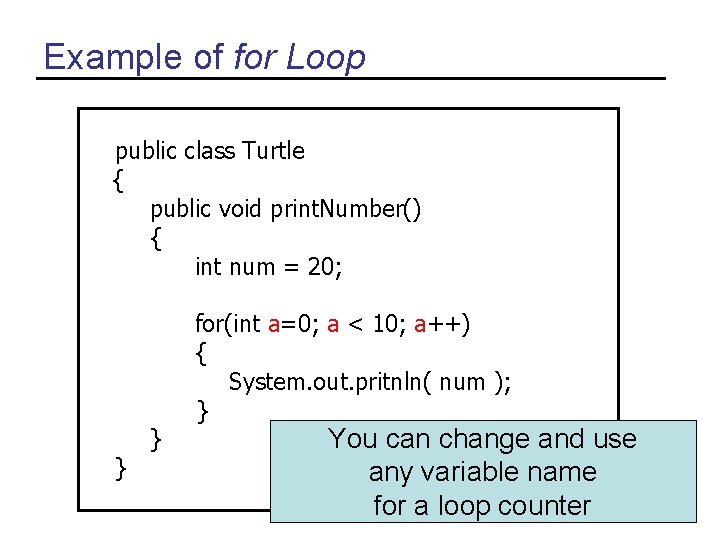
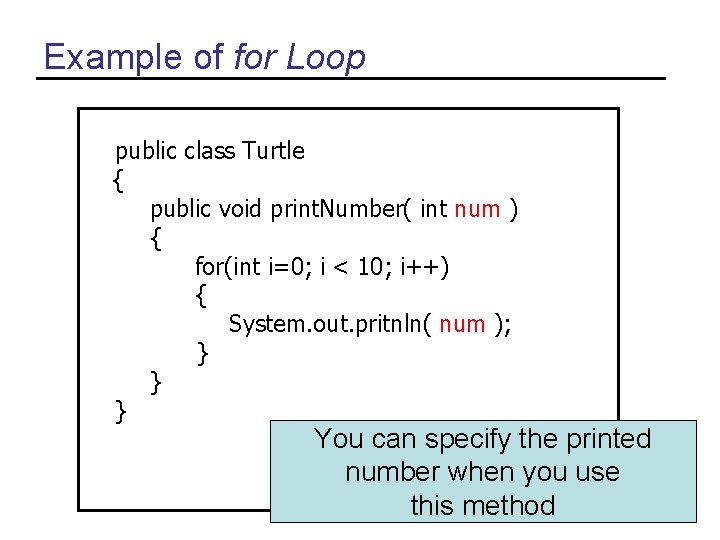
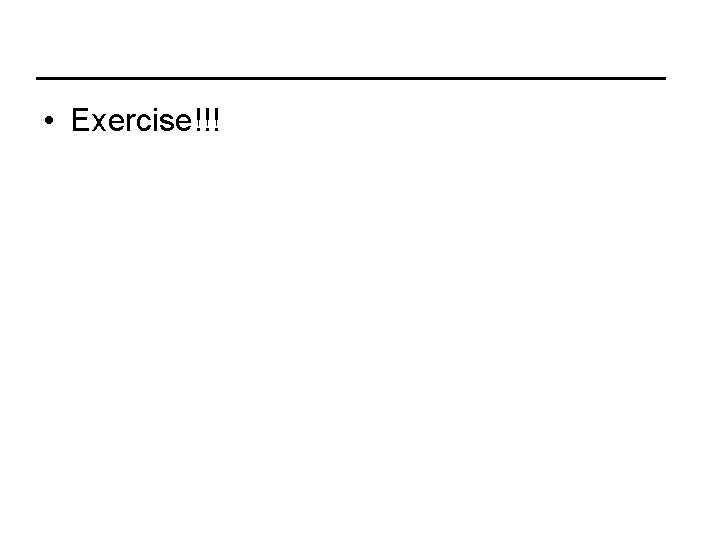
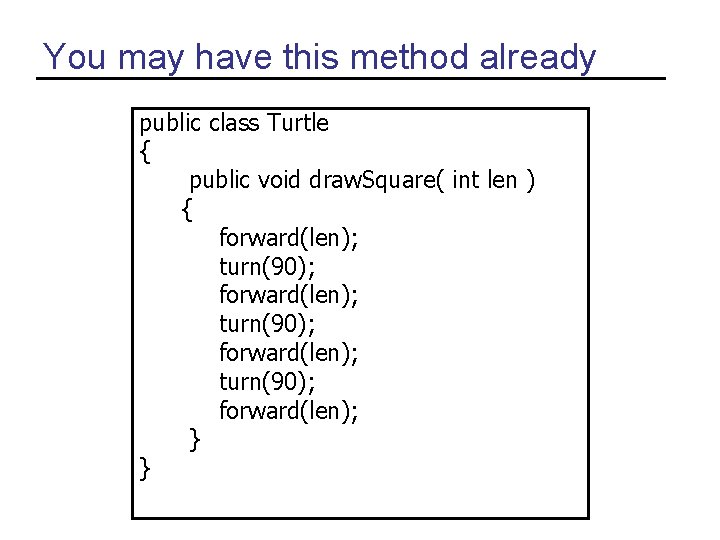
![Exercise 1 public class Test { public static void main(String[] args) { World w Exercise 1 public class Test { public static void main(String[] args) { World w](https://slidetodoc.com/presentation_image_h/9eeabf7a46f3bcf13f8e040eb3c1aaaa/image-27.jpg)
![Exercise 2 public class Test { public static void main(String[] args) { World w Exercise 2 public class Test { public static void main(String[] args) { World w](https://slidetodoc.com/presentation_image_h/9eeabf7a46f3bcf13f8e040eb3c1aaaa/image-28.jpg)
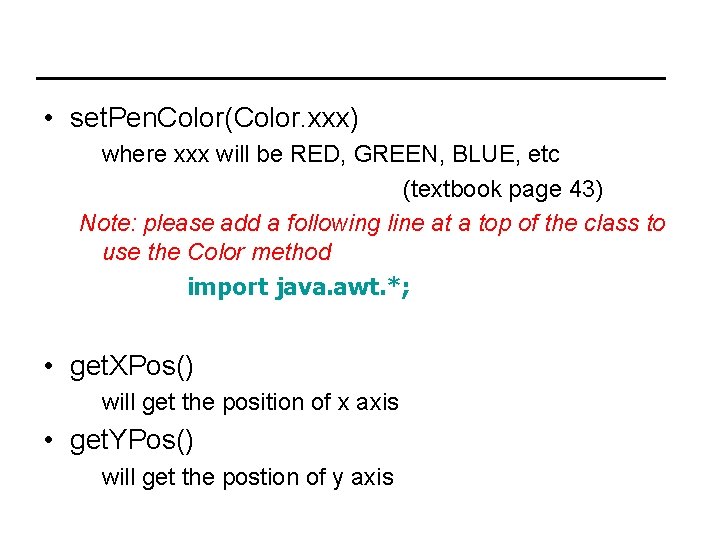
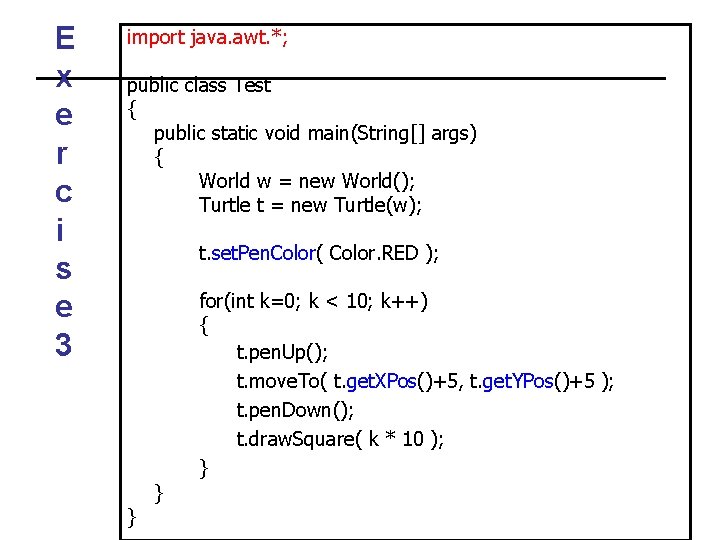
![Go back to Exercise 1 public class Test { public static void main(String[] args) Go back to Exercise 1 public class Test { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/9eeabf7a46f3bcf13f8e040eb3c1aaaa/image-31.jpg)
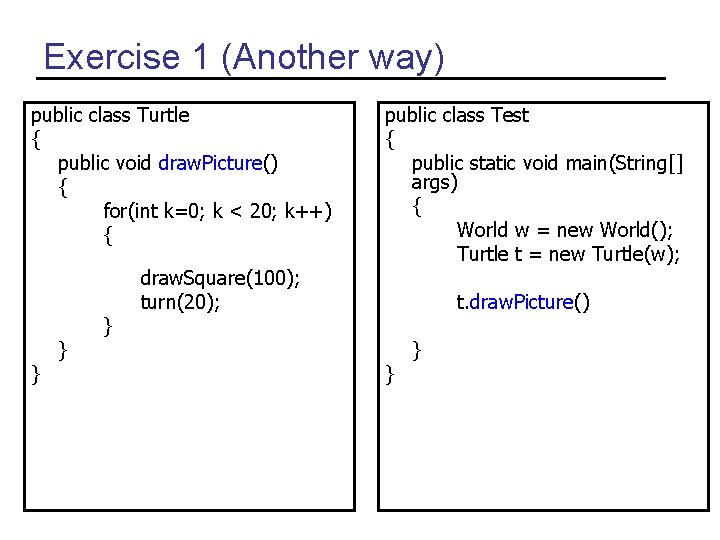
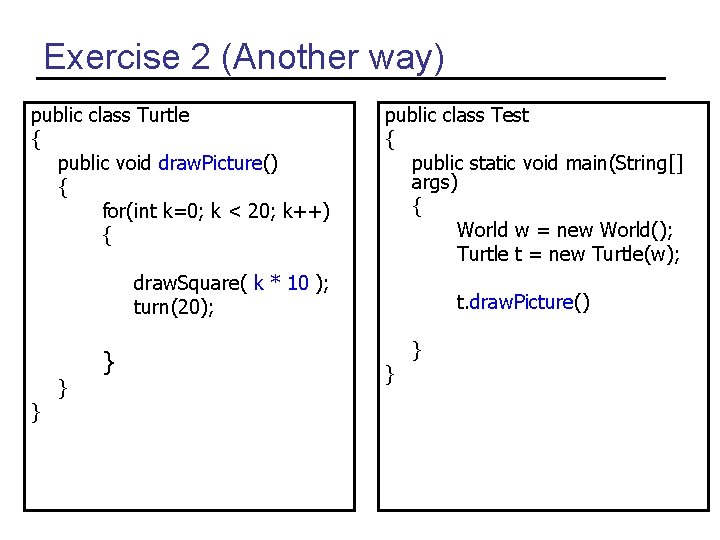
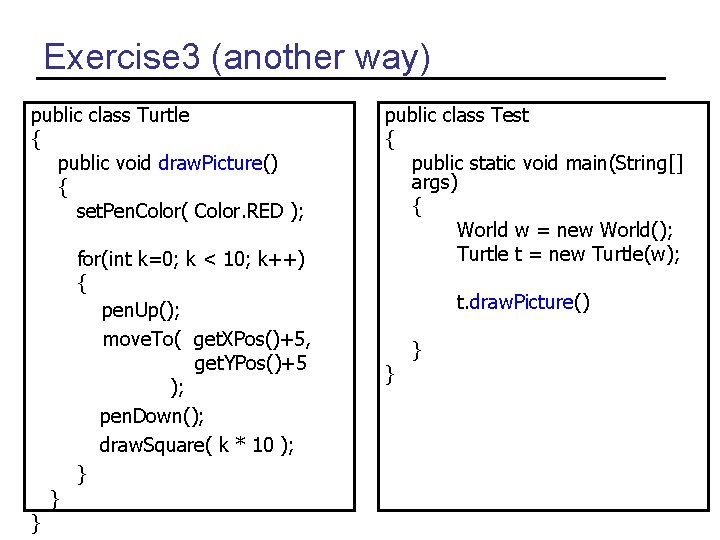
- Slides: 34
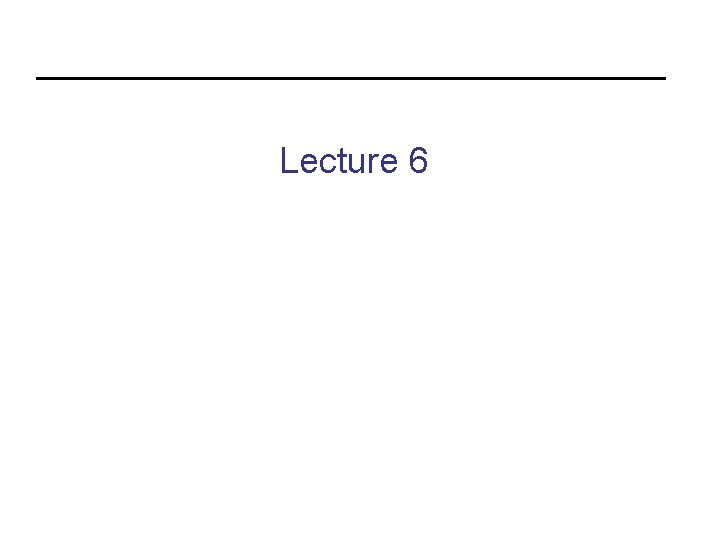
Lecture 6
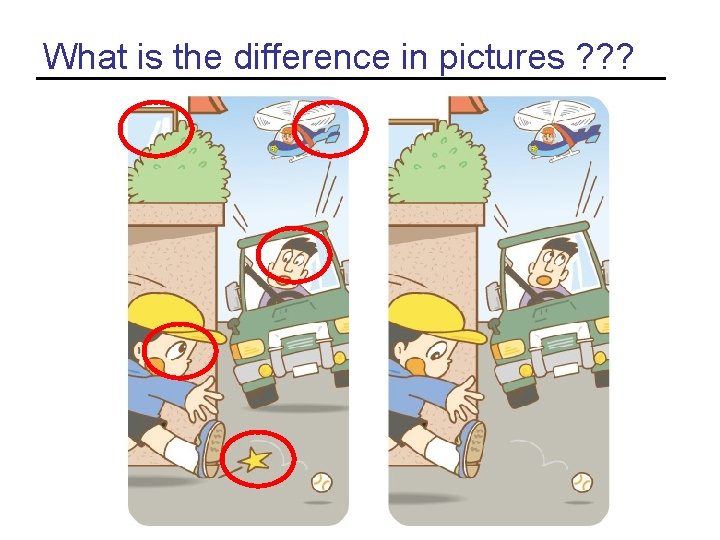
What is the difference in pictures ? ? ?
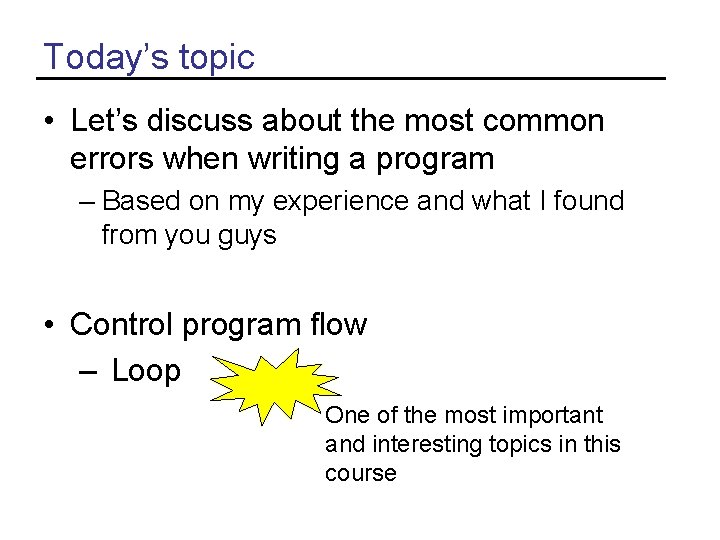
Today’s topic • Let’s discuss about the most common errors when writing a program – Based on my experience and what I found from you guys • Control program flow – Loop One of the most important and interesting topics in this course
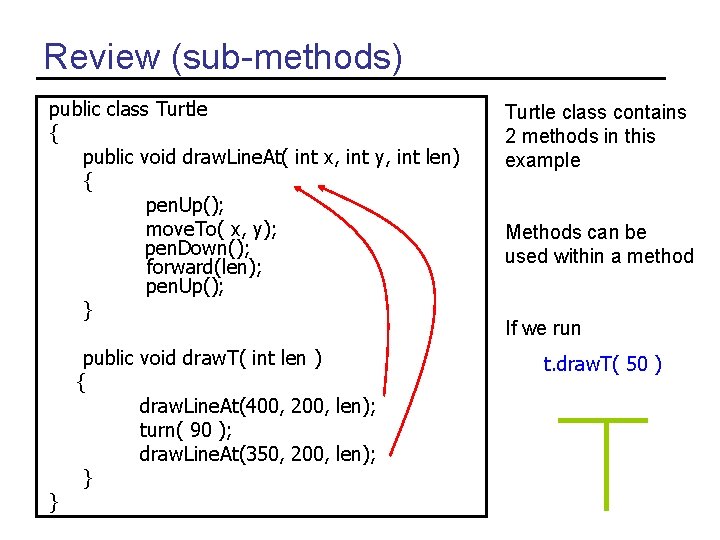
Review (sub-methods) public class Turtle { public void draw. Line. At( int x, int y, int len) { pen. Up(); move. To( x, y); pen. Down(); forward(len); pen. Up(); } } public void draw. T( int len ) { draw. Line. At(400, 200, len); turn( 90 ); draw. Line. At(350, 200, len); } Turtle class contains 2 methods in this example Methods can be used within a method If we run t. draw. T( 50 )
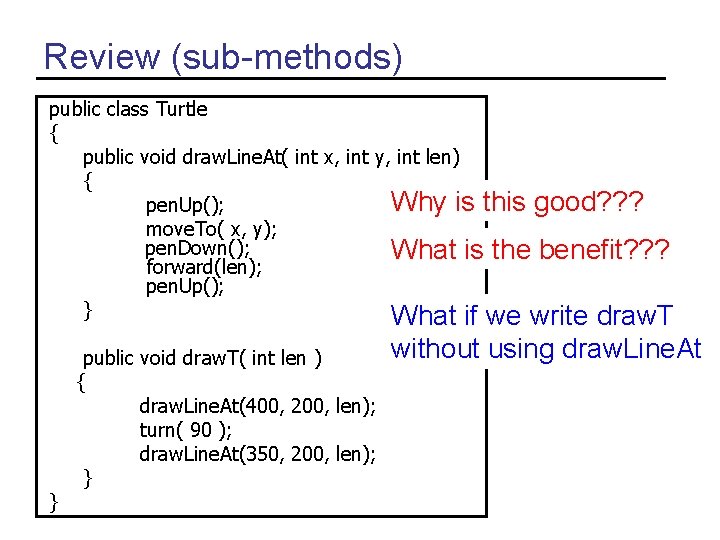
Review (sub-methods) public class Turtle { public void draw. Line. At( int x, int y, int len) { Why is this good? ? ? pen. Up(); move. To( x, y); pen. Down(); What is the benefit? ? ? forward(len); pen. Up(); } What if we write draw. T } public void draw. T( int len ) { draw. Line. At(400, 200, len); turn( 90 ); draw. Line. At(350, 200, len); } without using draw. Line. At
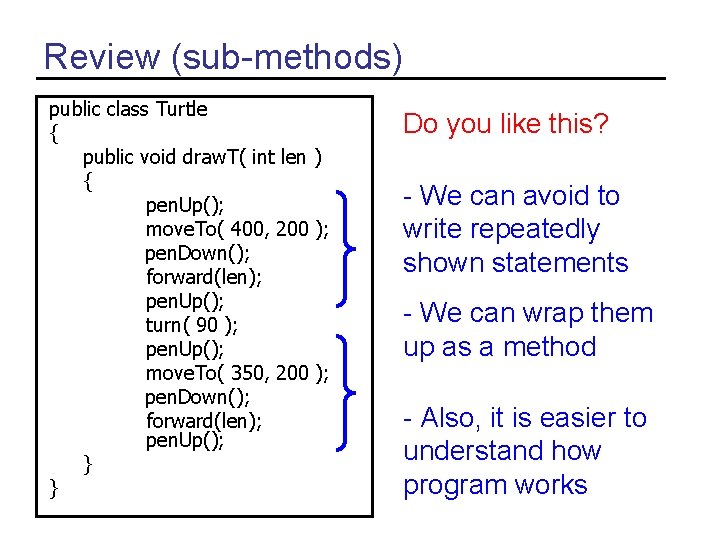
Review (sub-methods) public class Turtle { public void draw. T( int len ) { pen. Up(); move. To( 400, 200 ); pen. Down(); forward(len); pen. Up(); turn( 90 ); pen. Up(); move. To( 350, 200 ); pen. Down(); forward(len); pen. Up(); } } Do you like this? - We can avoid to write repeatedly shown statements - We can wrap them up as a method - Also, it is easier to understand how program works
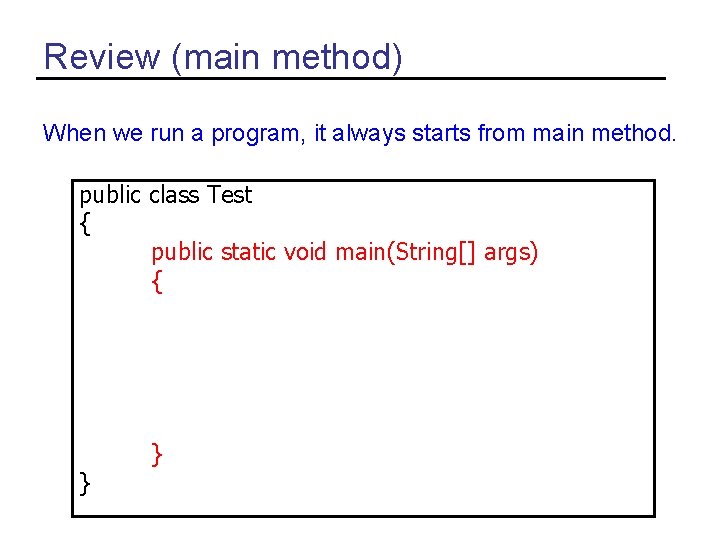
Review (main method) When we run a program, it always starts from main method. public class Test { public static void main(String[] args) { } }
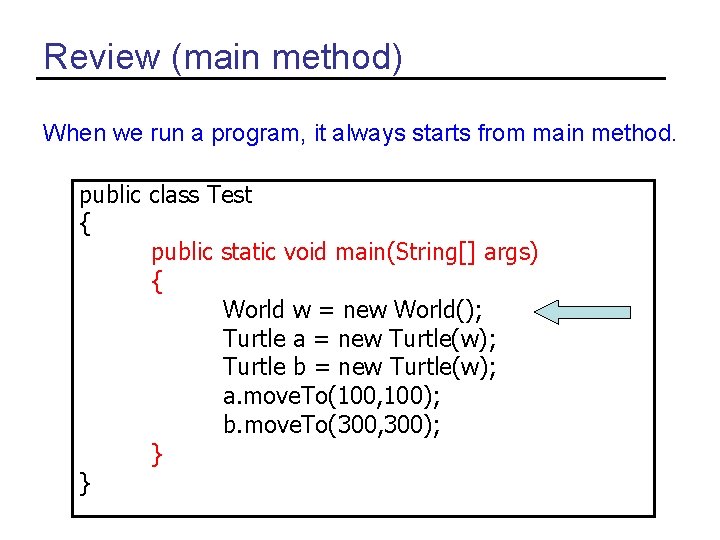
Review (main method) When we run a program, it always starts from main method. public class Test { public static void main(String[] args) { World w = new World(); Turtle a = new Turtle(w); Turtle b = new Turtle(w); a. move. To(100, 100); b. move. To(300, 300); } }
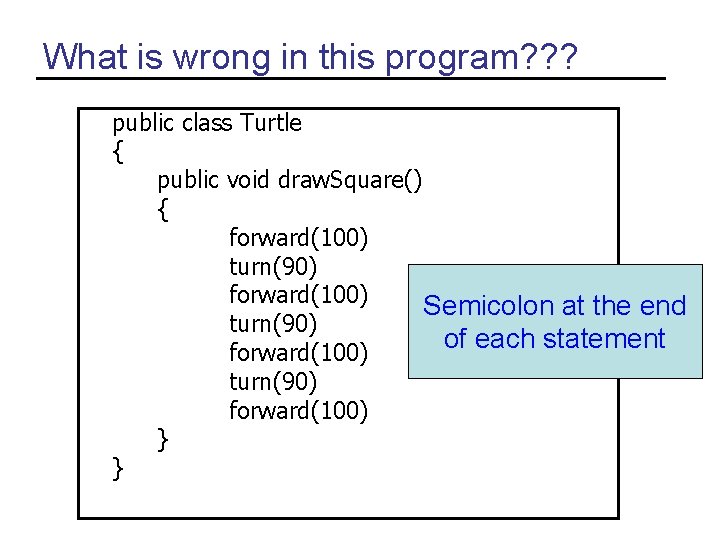
What is wrong in this program? ? ? public class Turtle { public void draw. Square() { forward(100) turn(90) forward(100) Semicolon at the end turn(90) of each statement forward(100) turn(90) forward(100) } }
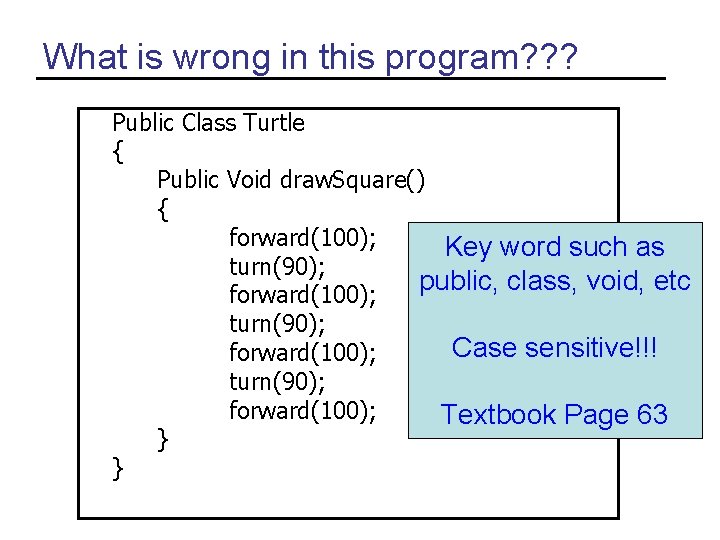
What is wrong in this program? ? ? Public Class Turtle { Public Void draw. Square() { forward(100); Key word such as turn(90); public, class, void, etc forward(100); turn(90); Case sensitive!!! forward(100); turn(90); forward(100); Textbook Page 63 } }
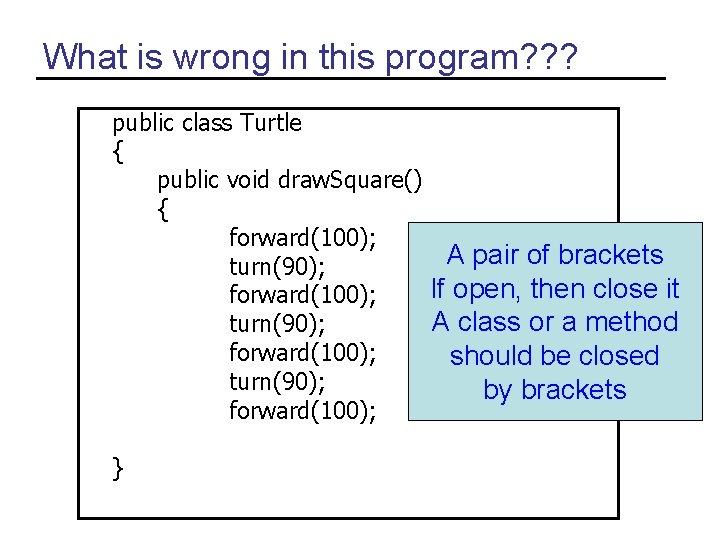
What is wrong in this program? ? ? public class Turtle { public void draw. Square() { forward(100); A pair of brackets turn(90); If open, then close it forward(100); A class or a method turn(90); forward(100); should be closed turn(90); by brackets forward(100); }
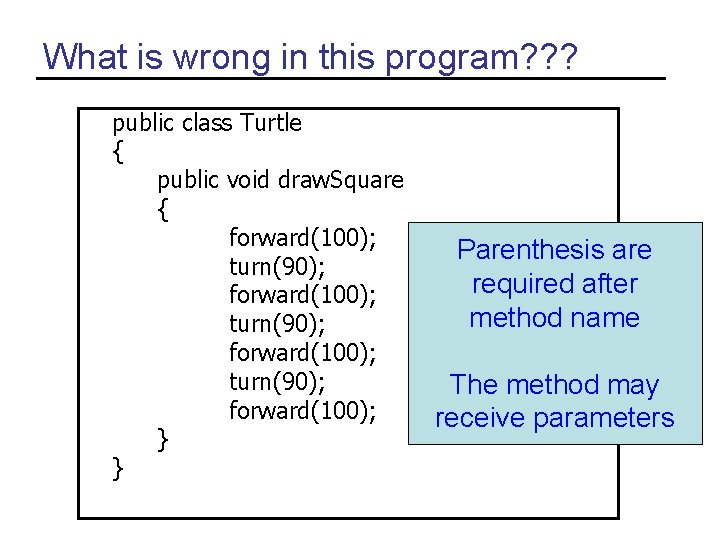
What is wrong in this program? ? ? public class Turtle { public void draw. Square { forward(100); turn(90); forward(100); } } Parenthesis are required after method name The method may receive parameters
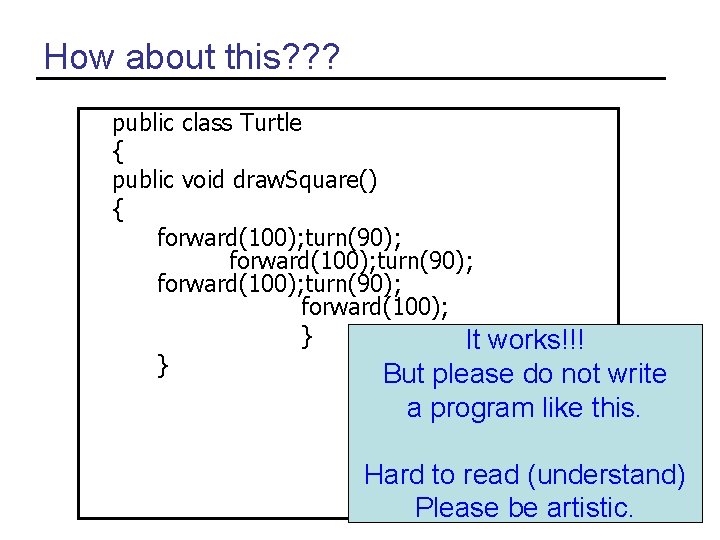
How about this? ? ? public class Turtle { public void draw. Square() { forward(100); turn(90); forward(100); } It works!!! } But please do not write a program like this. Hard to read (understand) Please be artistic.
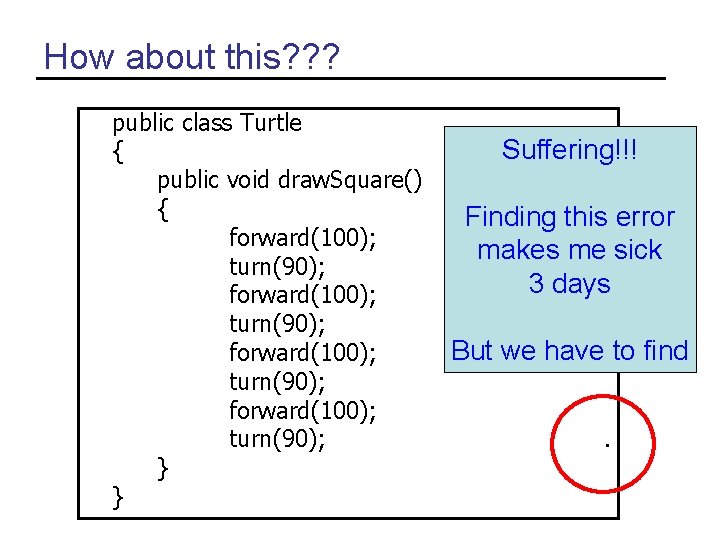
How about this? ? ? public class Turtle { public void draw. Square() { forward(100); turn(90); } } Suffering!!! Finding this error makes me sick 3 days But we have to find.
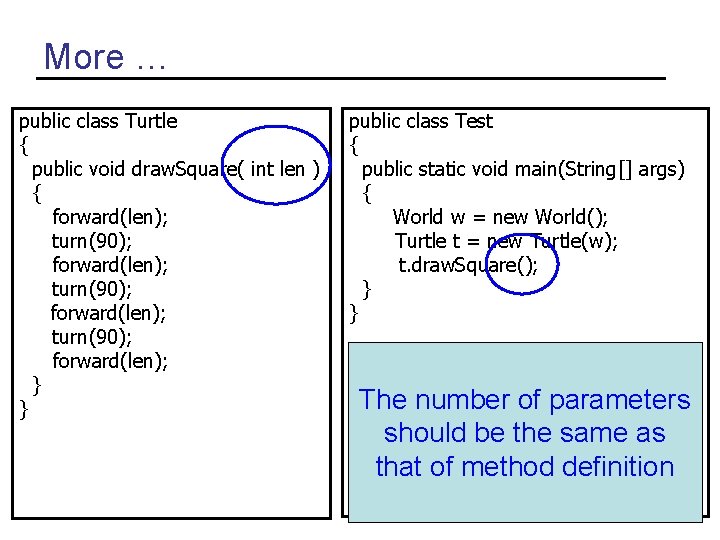
More … public class Turtle { public void draw. Square( int len ) { forward(len); turn(90); forward(len); } } public class Test { public static void main(String[] args) { World w = new World(); Turtle t = new Turtle(w); t. draw. Square(); } } The number of parameters should be the same as that of method definition
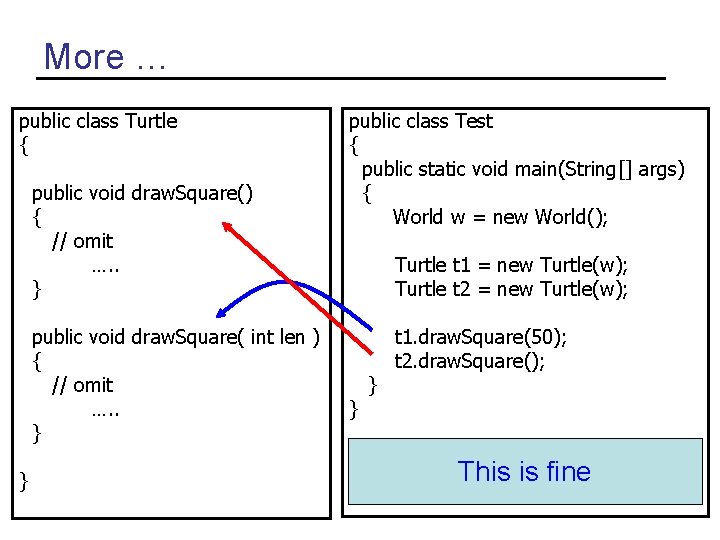
More … public class Turtle { public void draw. Square() { // omit …. . } public void draw. Square( int len ) { // omit …. . } } public class Test { public static void main(String[] args) { World w = new World(); Turtle t 1 = new Turtle(w); Turtle t 2 = new Turtle(w); } } t 1. draw. Square(50); t 2. draw. Square(); This is fine
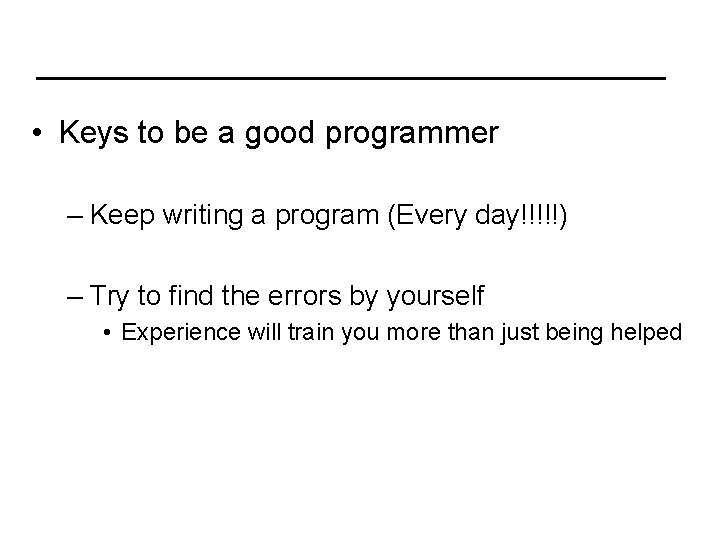
• Keys to be a good programmer – Keep writing a program (Every day!!!!!) – Try to find the errors by yourself • Experience will train you more than just being helped
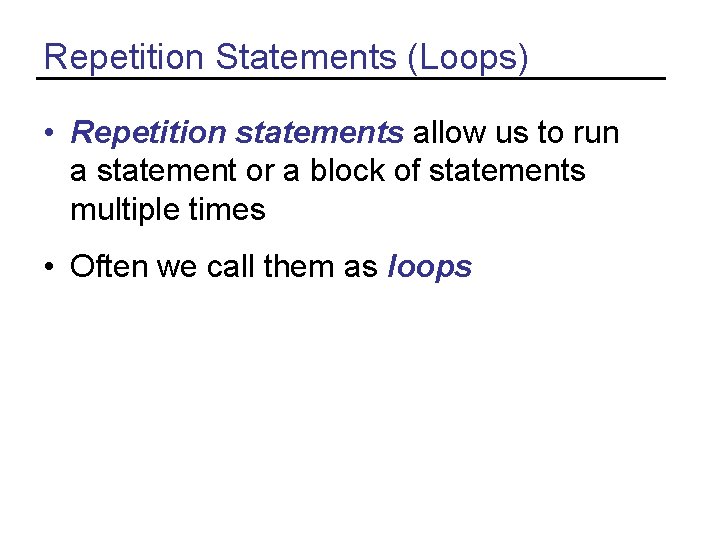
Repetition Statements (Loops) • Repetition statements allow us to run a statement or a block of statements multiple times • Often we call them as loops
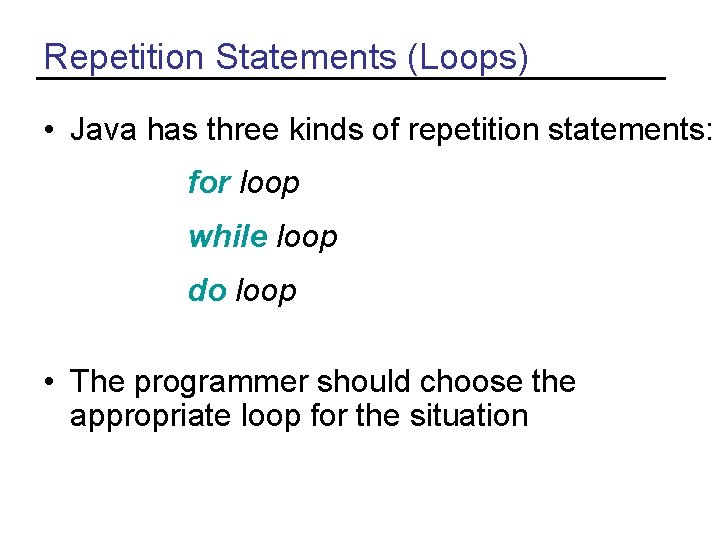
Repetition Statements (Loops) • Java has three kinds of repetition statements: for loop while loop do loop • The programmer should choose the appropriate loop for the situation
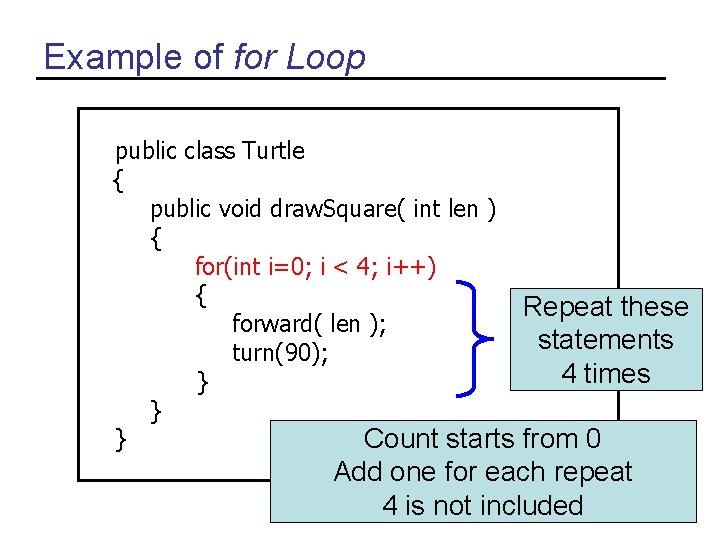
Example of for Loop public class Turtle { public void draw. Square( int len ) { for(int i=0; i < 4; i++) { Repeat these forward( len ); statements turn(90); 4 times } } } Count starts from 0 Add one for each repeat 4 is not included
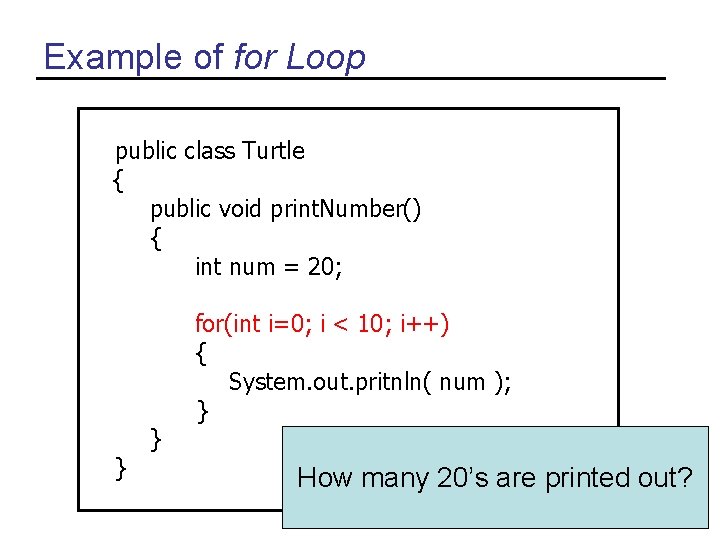
Example of for Loop public class Turtle { public void print. Number() { int num = 20; } } for(int i=0; i < 10; i++) { System. out. pritnln( num ); } How many 20’s are printed out?
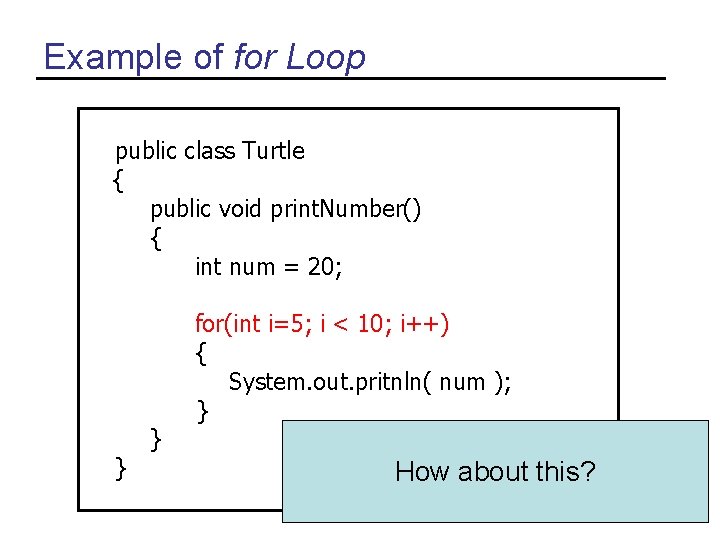
Example of for Loop public class Turtle { public void print. Number() { int num = 20; } } for(int i=5; i < 10; i++) { System. out. pritnln( num ); } How about this?
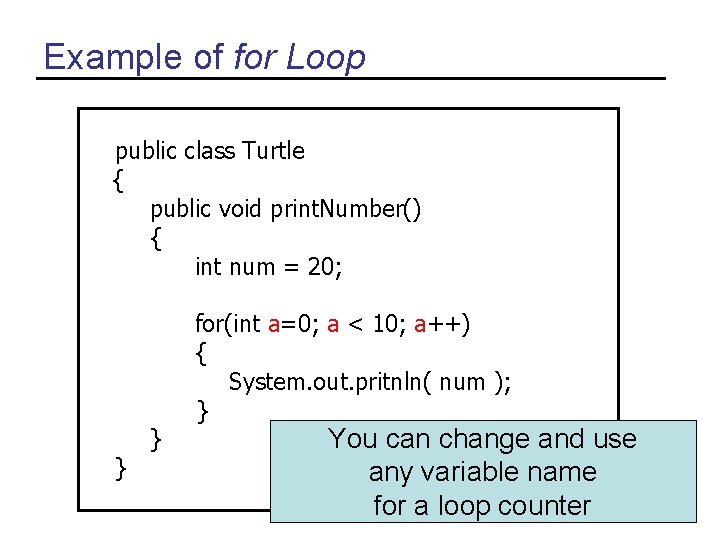
Example of for Loop public class Turtle { public void print. Number() { int num = 20; } } for(int a=0; a < 10; a++) { System. out. pritnln( num ); } You can change and use any variable name for a loop counter
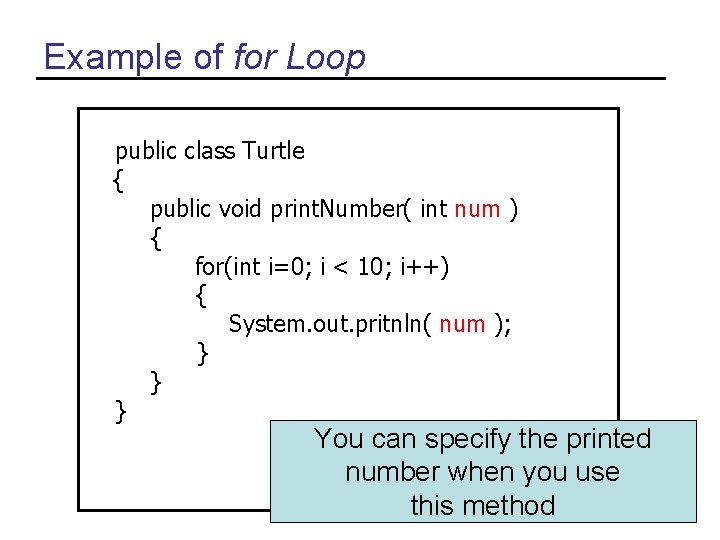
Example of for Loop public class Turtle { public void print. Number( int num ) { for(int i=0; i < 10; i++) { System. out. pritnln( num ); } } } You can specify the printed number when you use this method
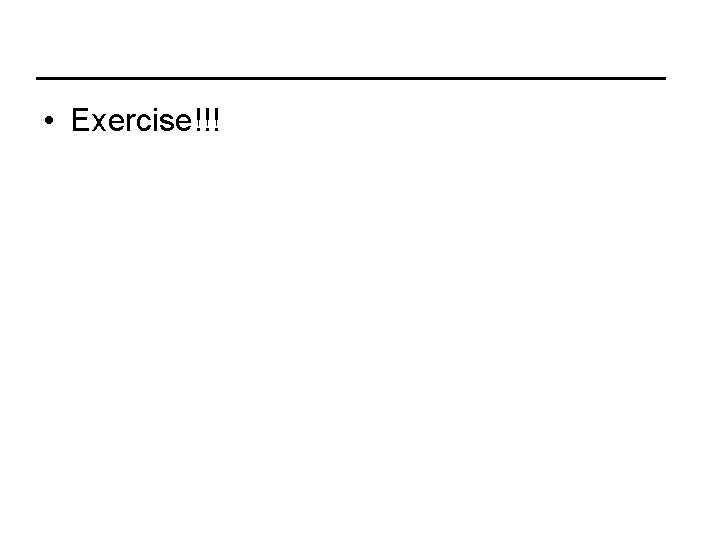
• Exercise!!!
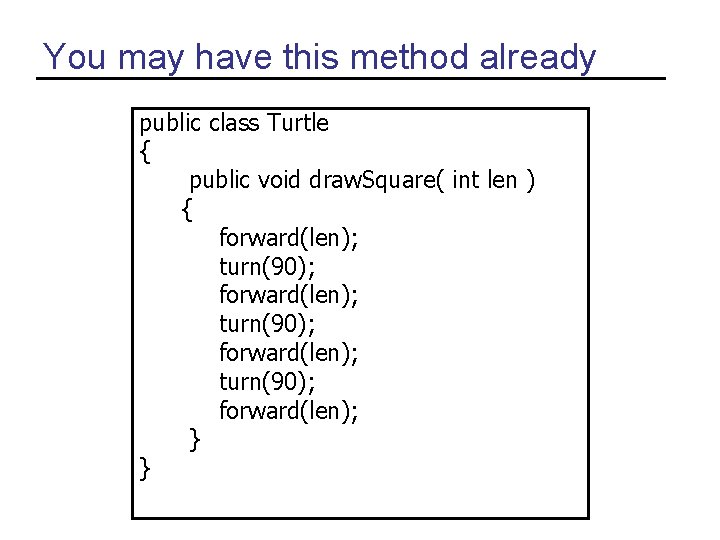
You may have this method already public class Turtle { public void draw. Square( int len ) { forward(len); turn(90); forward(len); } }
![Exercise 1 public class Test public static void mainString args World w Exercise 1 public class Test { public static void main(String[] args) { World w](https://slidetodoc.com/presentation_image_h/9eeabf7a46f3bcf13f8e040eb3c1aaaa/image-27.jpg)
Exercise 1 public class Test { public static void main(String[] args) { World w = new World(); Turtle t = new Turtle(w); } } for(int k=0; k < 20; k++) { t. draw. Square(100); t. turn(20); }
![Exercise 2 public class Test public static void mainString args World w Exercise 2 public class Test { public static void main(String[] args) { World w](https://slidetodoc.com/presentation_image_h/9eeabf7a46f3bcf13f8e040eb3c1aaaa/image-28.jpg)
Exercise 2 public class Test { public static void main(String[] args) { World w = new World(); Turtle t = new Turtle(w); } } for(int k=0; k < 20; k++) { t. draw. Square( k * 10 ); t. turn(20); }
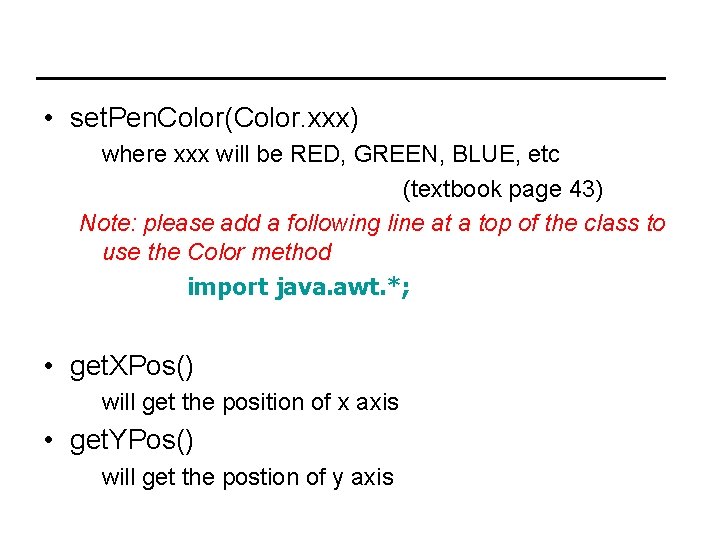
• set. Pen. Color(Color. xxx) where xxx will be RED, GREEN, BLUE, etc (textbook page 43) Note: please add a following line at a top of the class to use the Color method import java. awt. *; • get. XPos() will get the position of x axis • get. YPos() will get the postion of y axis
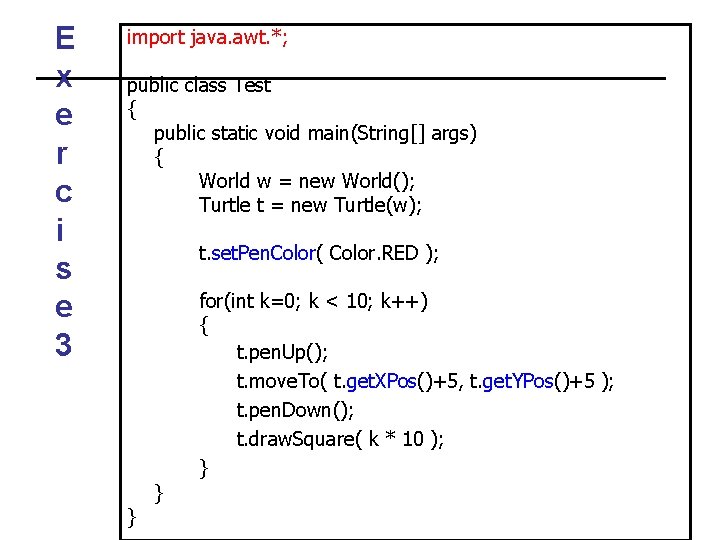
E x e r c i s e 3 import java. awt. *; public class Test { public static void main(String[] args) { World w = new World(); Turtle t = new Turtle(w); t. set. Pen. Color( Color. RED ); for(int k=0; k < 10; k++) { t. pen. Up(); t. move. To( t. get. XPos()+5, t. get. YPos()+5 ); t. pen. Down(); t. draw. Square( k * 10 ); } } }
![Go back to Exercise 1 public class Test public static void mainString args Go back to Exercise 1 public class Test { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h/9eeabf7a46f3bcf13f8e040eb3c1aaaa/image-31.jpg)
Go back to Exercise 1 public class Test { public static void main(String[] args) { World w = new World(); Turtle t = new Turtle(w); } } for(int k=0; k < 20; k++) { t. draw. Square(100); t. turn(20); } Can be written as a method
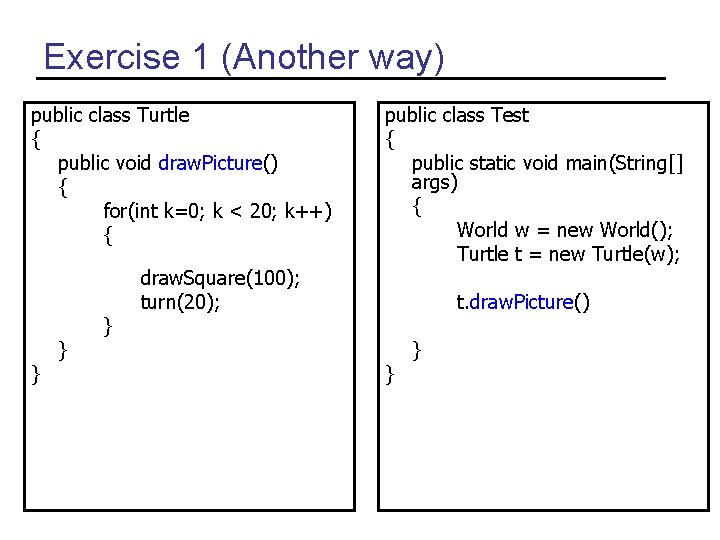
Exercise 1 (Another way) public class Turtle { public void draw. Picture() { for(int k=0; k < 20; k++) { } } } draw. Square(100); turn(20); public class Test { public static void main(String[] args) { World w = new World(); Turtle t = new Turtle(w); t. draw. Picture() } }
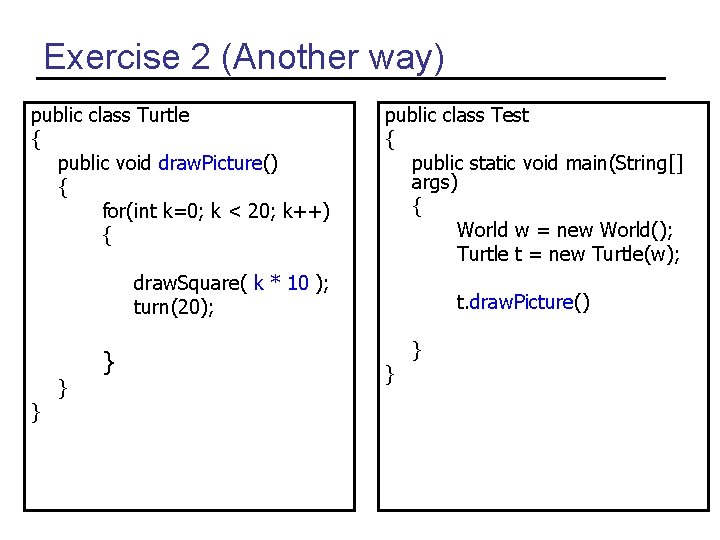
Exercise 2 (Another way) public class Turtle { public void draw. Picture() { for(int k=0; k < 20; k++) { public class Test { public static void main(String[] args) { World w = new World(); Turtle t = new Turtle(w); draw. Square( k * 10 ); turn(20); } } } t. draw. Picture() } }
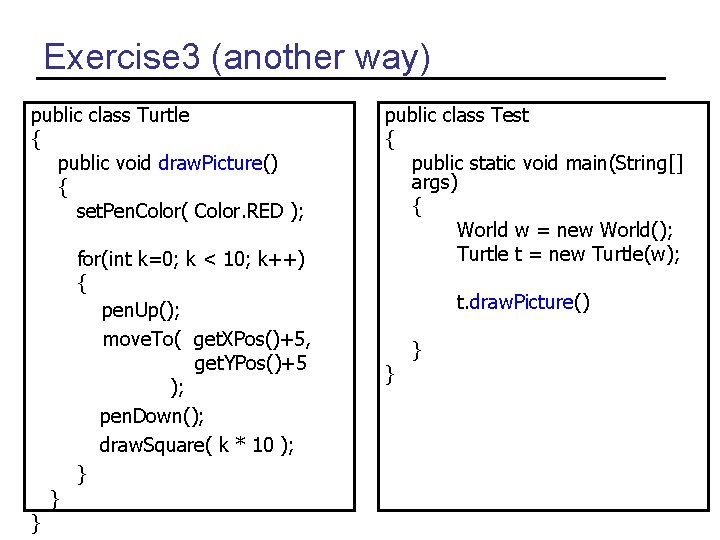
Exercise 3 (another way) public class Turtle { public void draw. Picture() { set. Pen. Color( Color. RED ); for(int k=0; k < 10; k++) { pen. Up(); move. To( get. XPos()+5, get. YPos()+5 ); pen. Down(); draw. Square( k * 10 ); } } } public class Test { public static void main(String[] args) { World w = new World(); Turtle t = new Turtle(w); t. draw. Picture() } }
01:640:244 lecture notes - lecture 15: plat, idah, farad
Columbia pictures and universal pictures
Colombia paramount
Hát kết hợp bộ gõ cơ thể
Frameset trong html5
Bổ thể
Tỉ lệ cơ thể trẻ em
Voi kéo gỗ như thế nào
Chụp tư thế worms-breton
Bài hát chúa yêu trần thế alleluia
Môn thể thao bắt đầu bằng từ đua
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công thức tiính động năng
Trời xanh đây là của chúng ta thể thơ
Cách giải mật thư tọa độ
101012 bằng
Phản ứng thế ankan
Các châu lục và đại dương trên thế giới
Thơ thất ngôn tứ tuyệt đường luật
Quá trình desamine hóa có thể tạo ra
Một số thể thơ truyền thống
Cái miệng bé xinh thế chỉ nói điều hay thôi
Vẽ hình chiếu vuông góc của vật thể sau
Thế nào là sự mỏi cơ
đặc điểm cơ thể của người tối cổ
Ví dụ về giọng cùng tên
Vẽ hình chiếu đứng bằng cạnh của vật thể
Tia chieu sa te
Thẻ vin
đại từ thay thế
điện thế nghỉ
Tư thế ngồi viết
Diễn thế sinh thái là
Dạng đột biến một nhiễm là