CS 367 Introduction to Data Structures Lecture 2
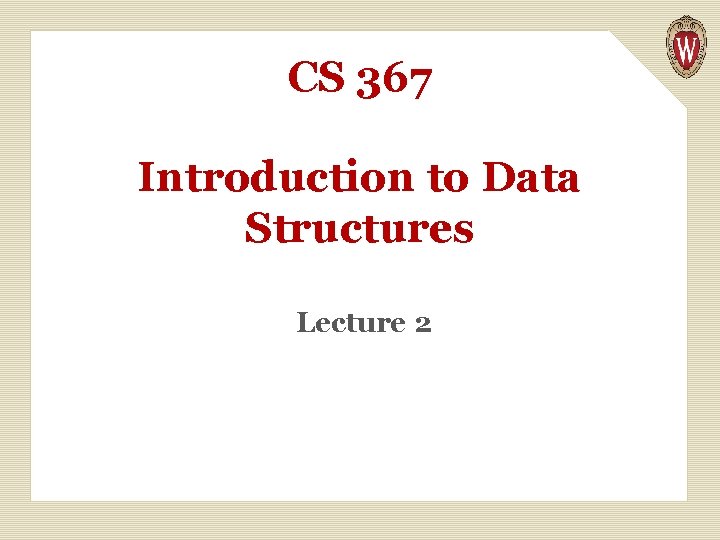
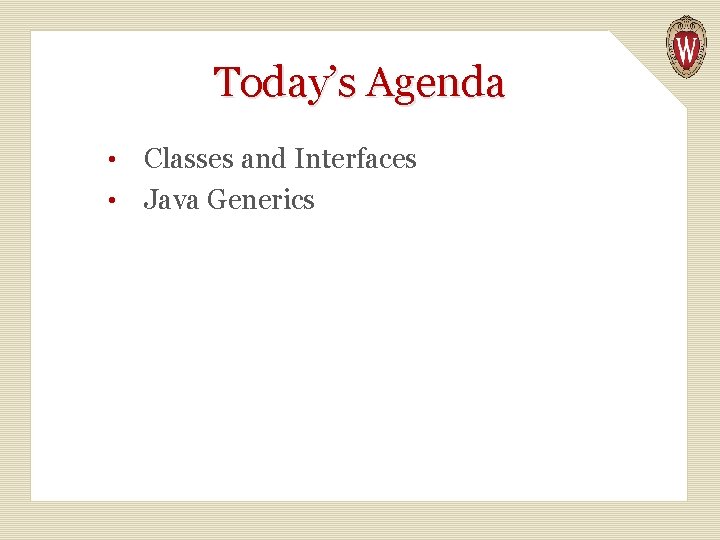
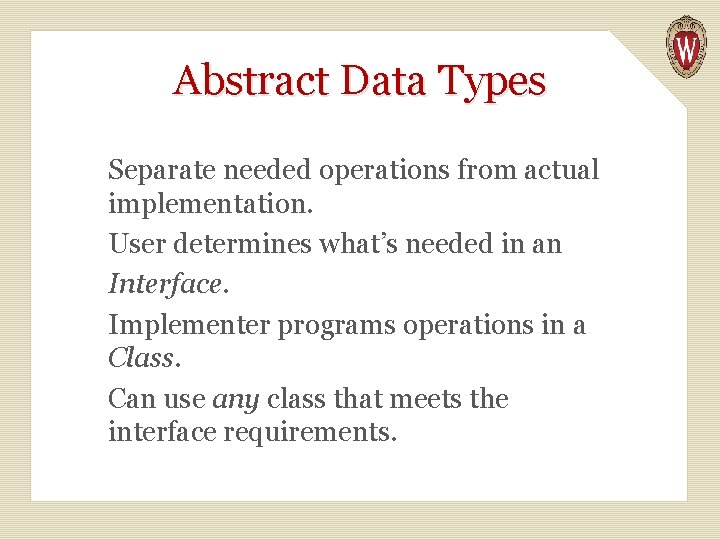
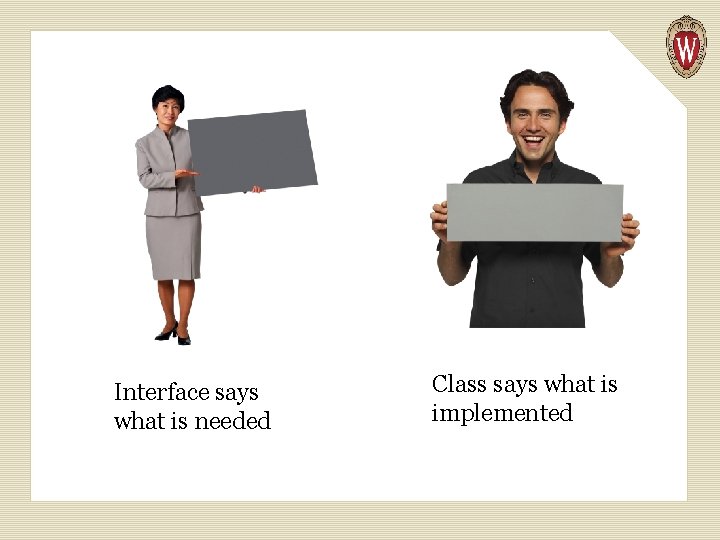
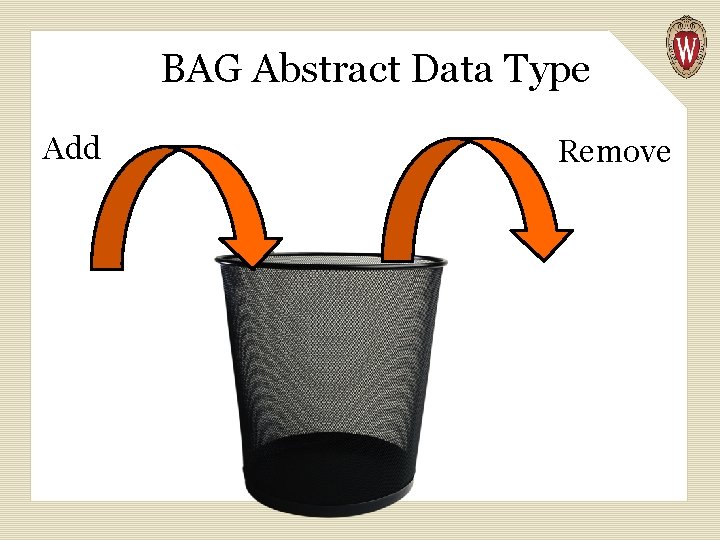
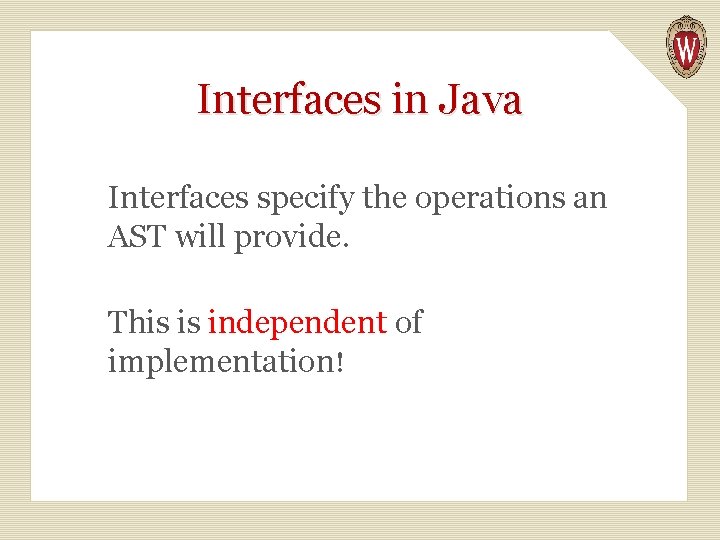
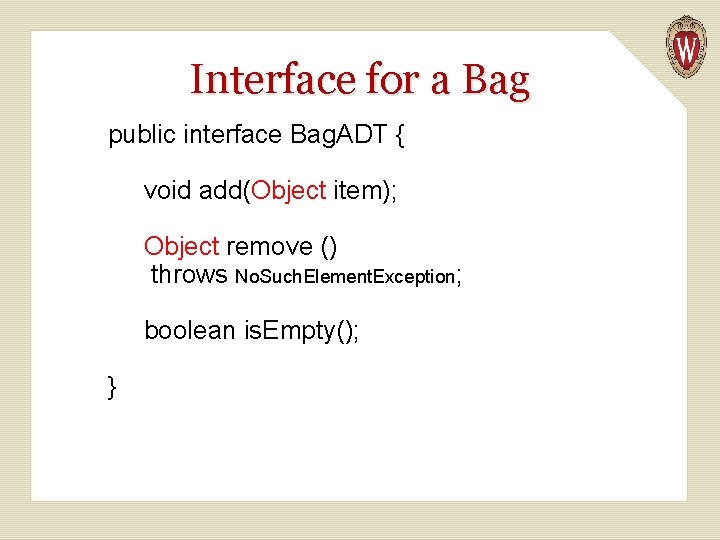
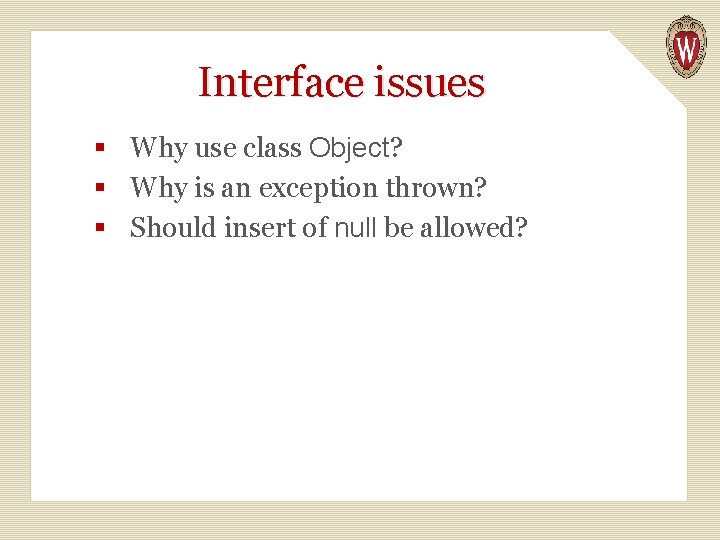
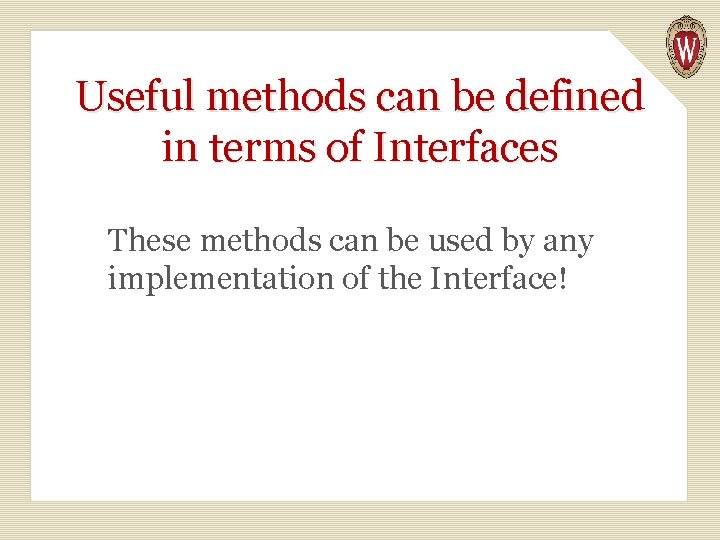
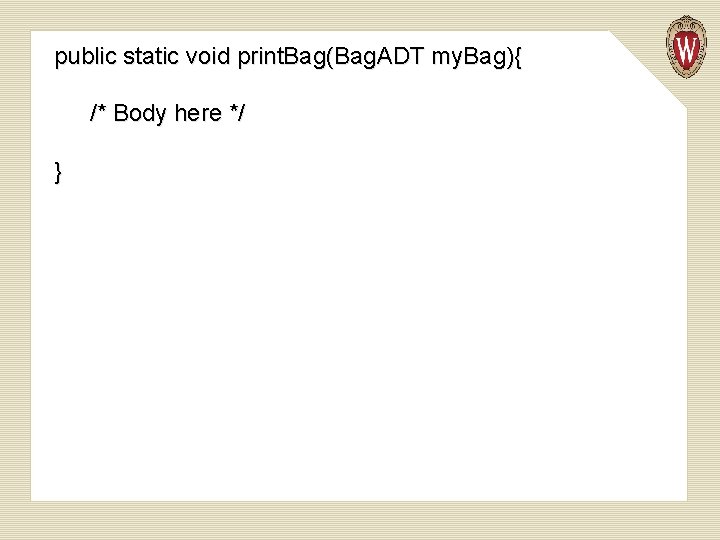
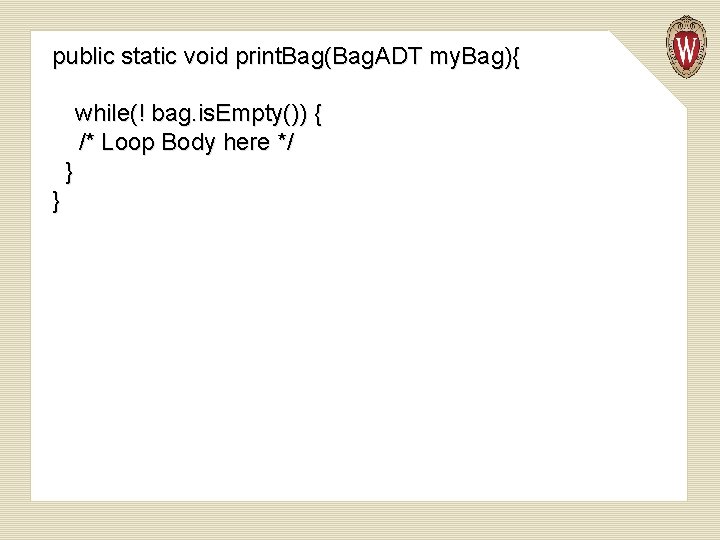
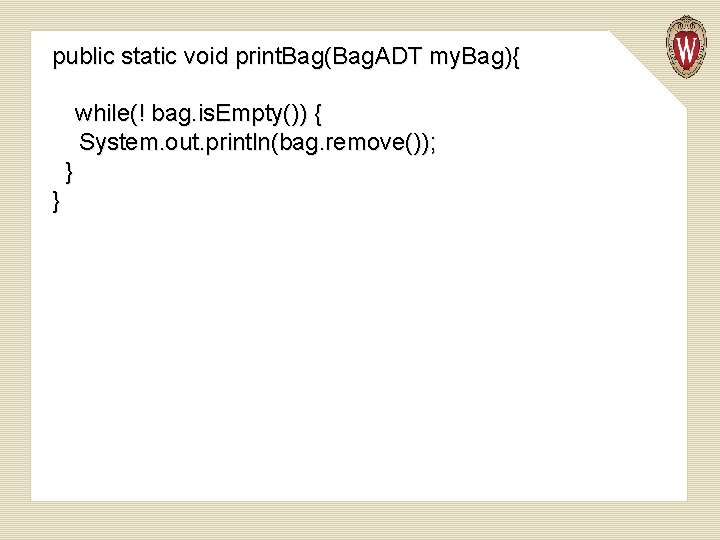
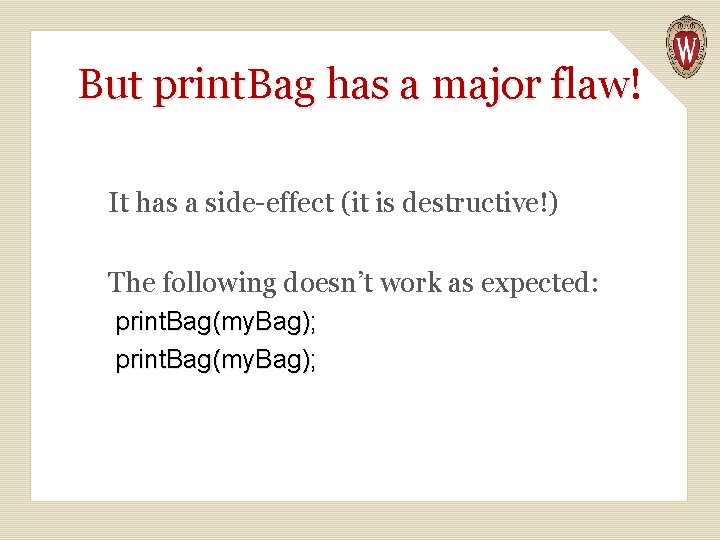
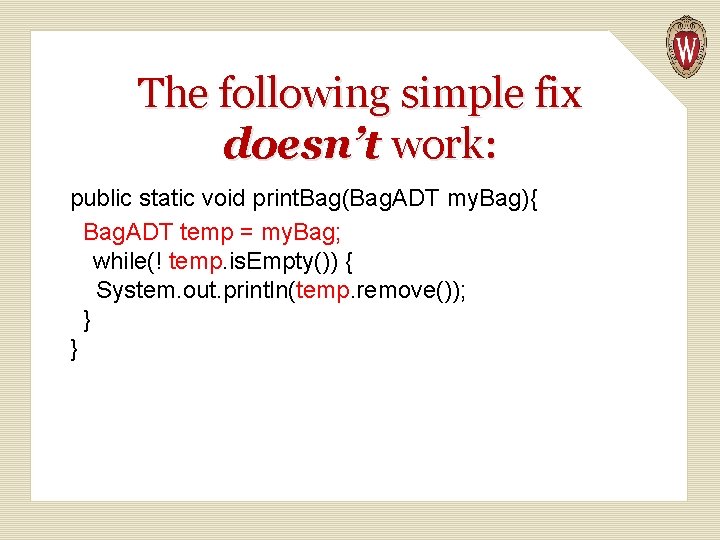
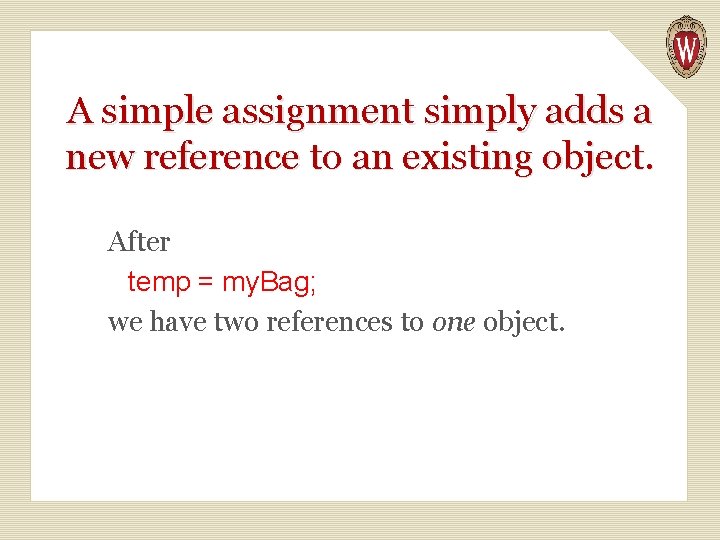
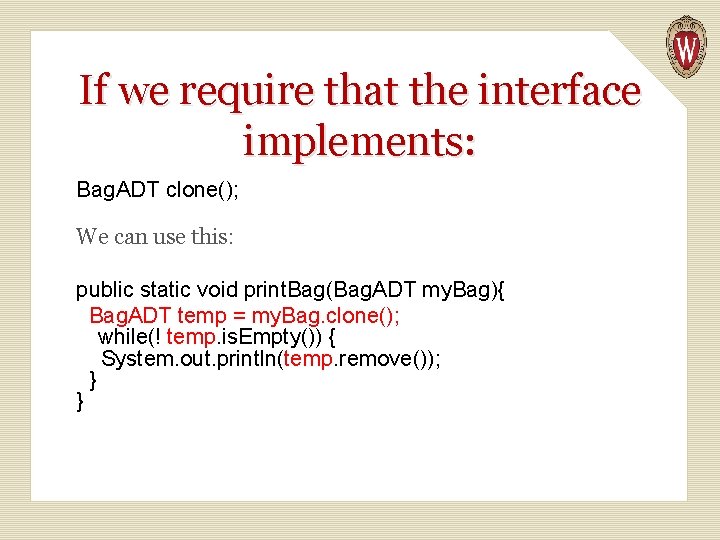
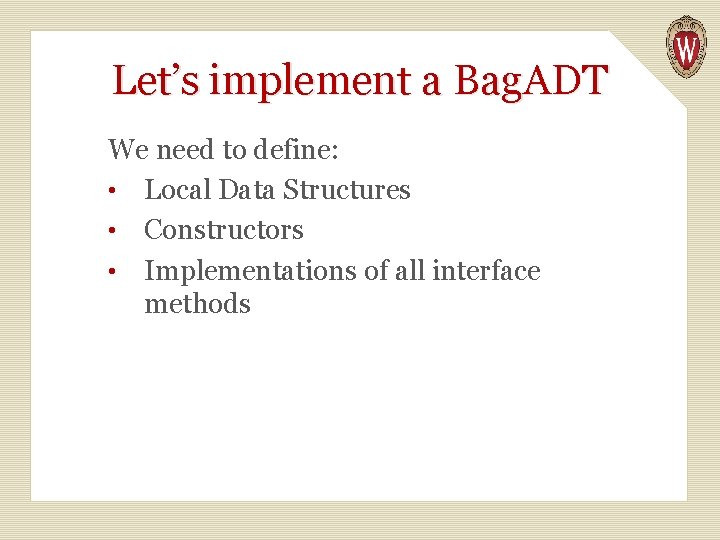
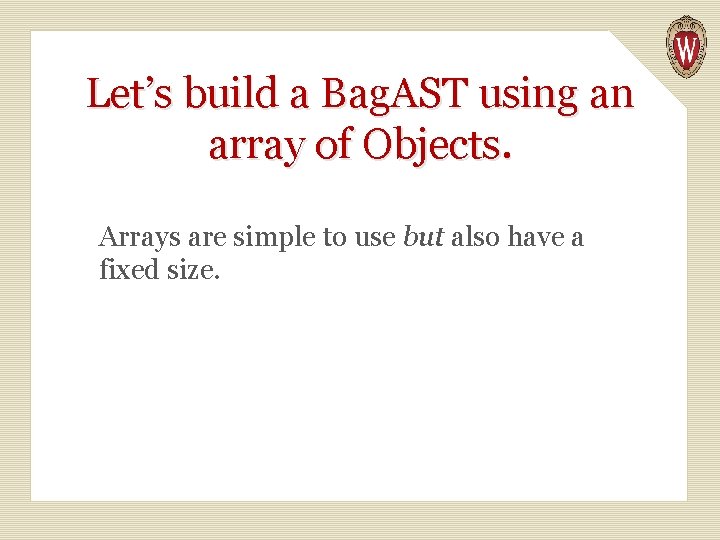
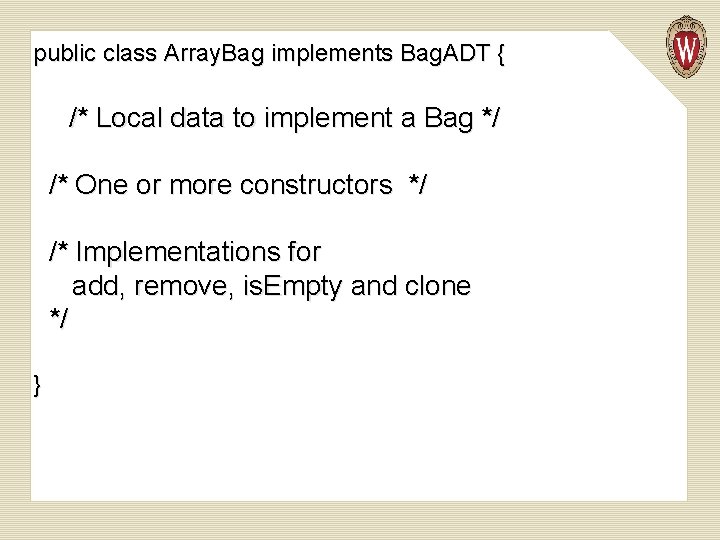
![public class Array. Bag implements Bag. ADT { private Object[] items; private int item. public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-20.jpg)
![public class Array. Bag implements Bag. ADT { private Object[] items; private int item. public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-21.jpg)
![public class Array. Bag implements Bag. ADT { private Object[] items; private int item. public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-22.jpg)
![public class Array. Bag implements Bag. ADT { private Object[] items; private int item. public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-23.jpg)
![public class Array. Bag implements Bag. ADT { private Object[] items; private int item. public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-24.jpg)
![public class Array. Bag implements Bag. ADT { private Object[] items; private int item. public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-25.jpg)
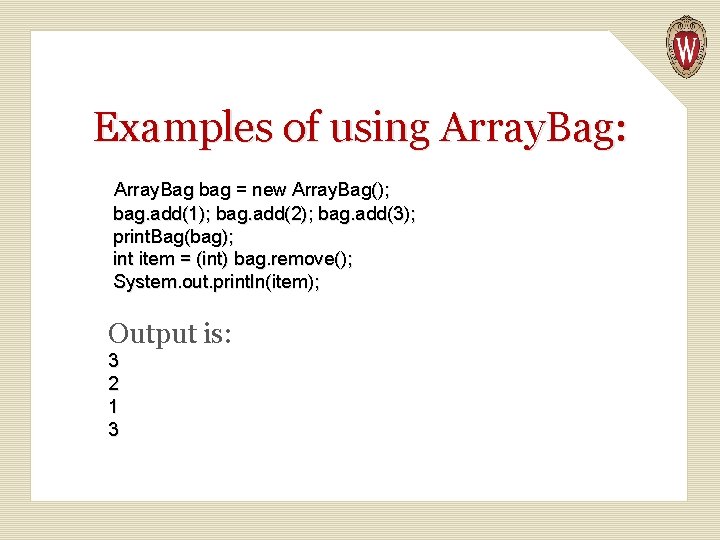
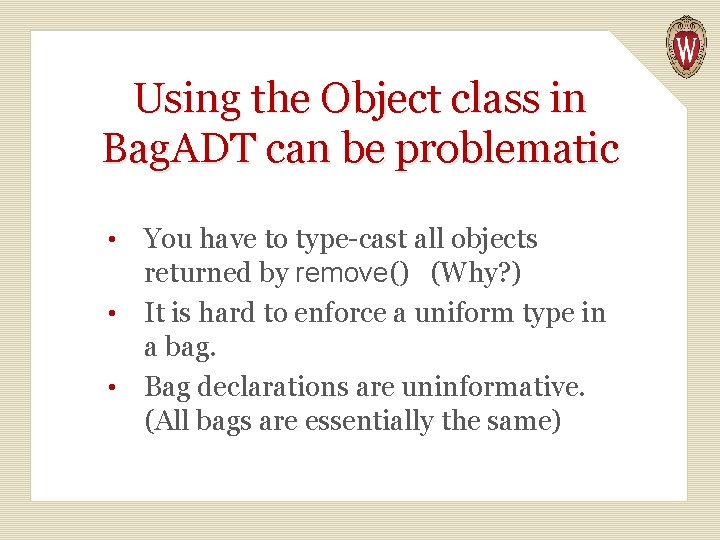
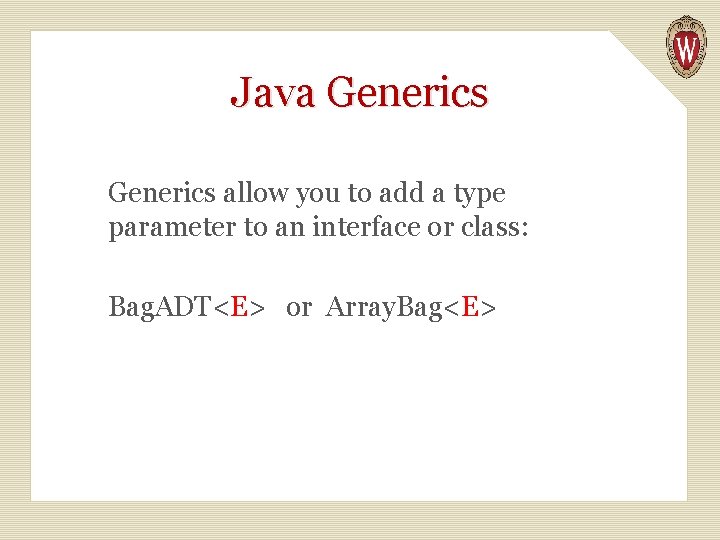
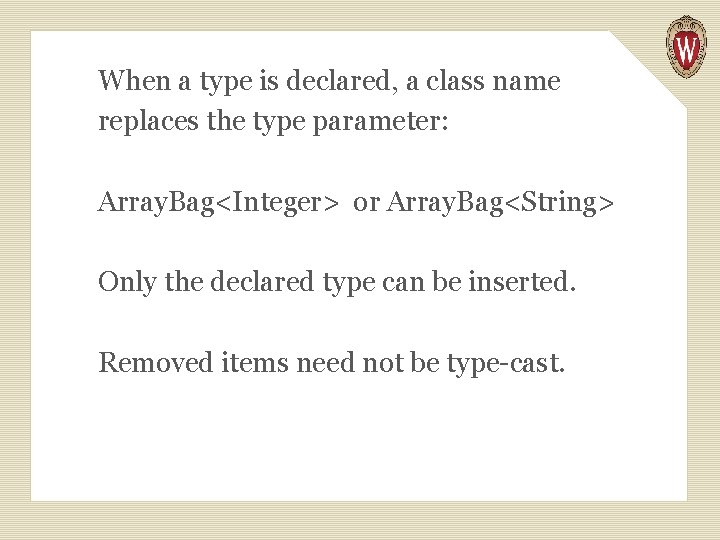
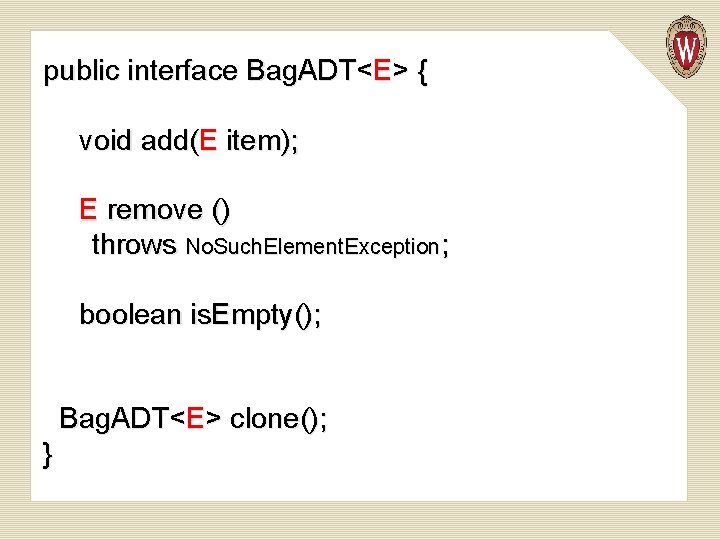
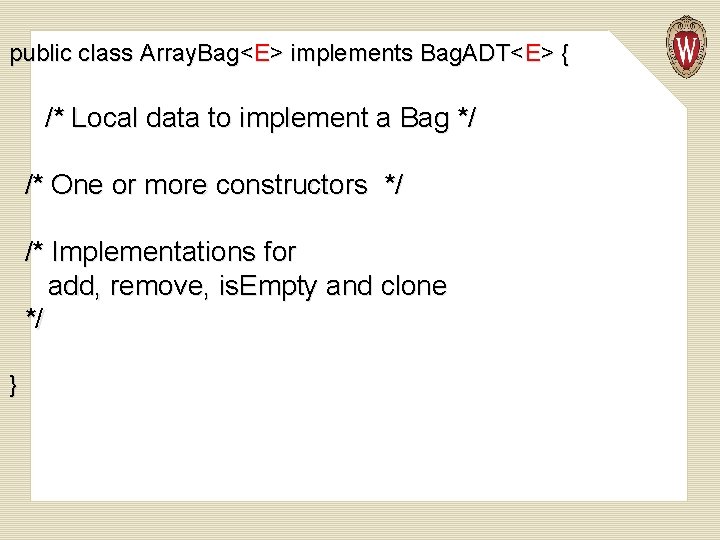
![public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-32.jpg)
![public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-33.jpg)
![public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-34.jpg)
![public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-35.jpg)
![public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-36.jpg)
![public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-37.jpg)
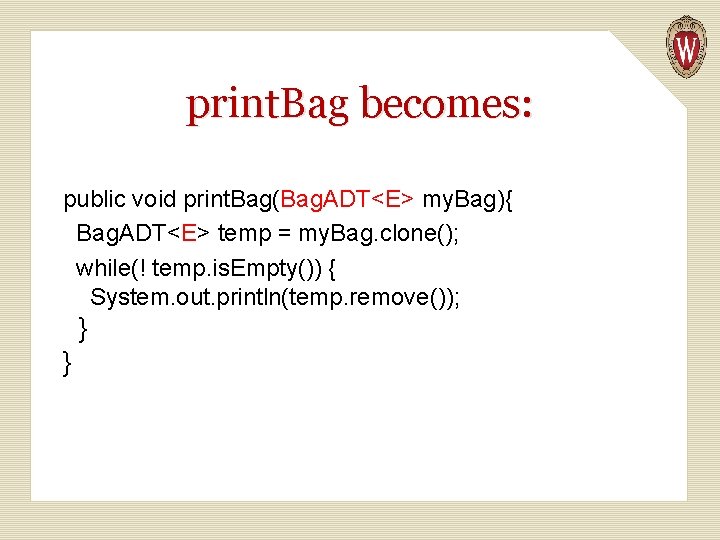
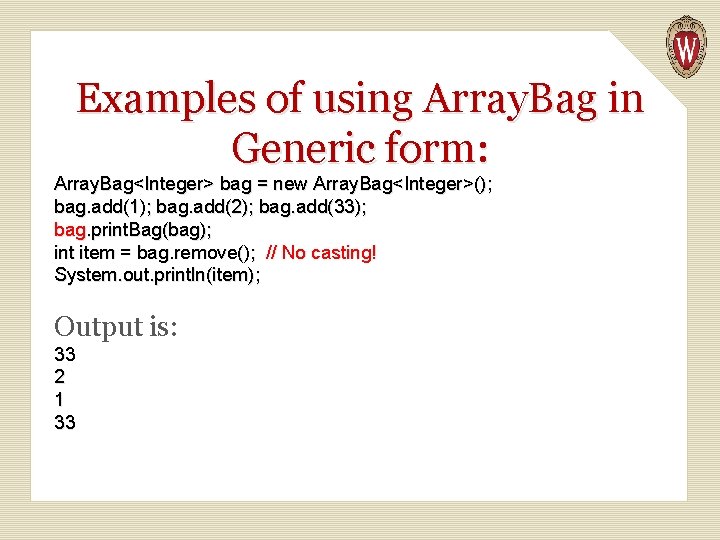
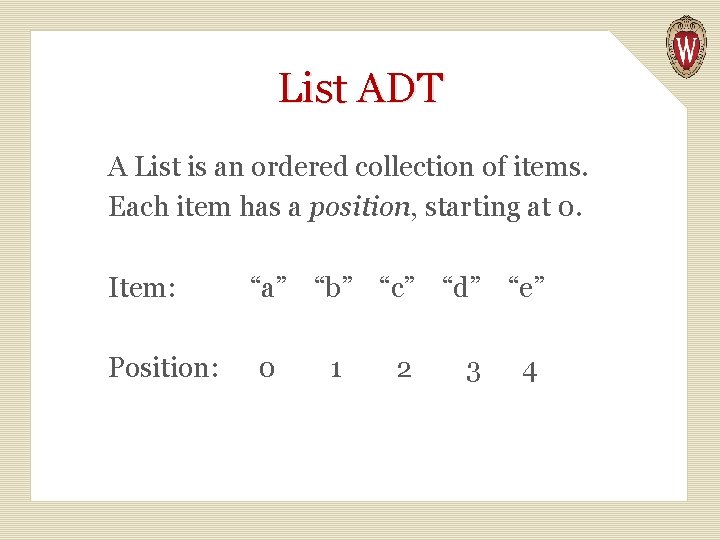
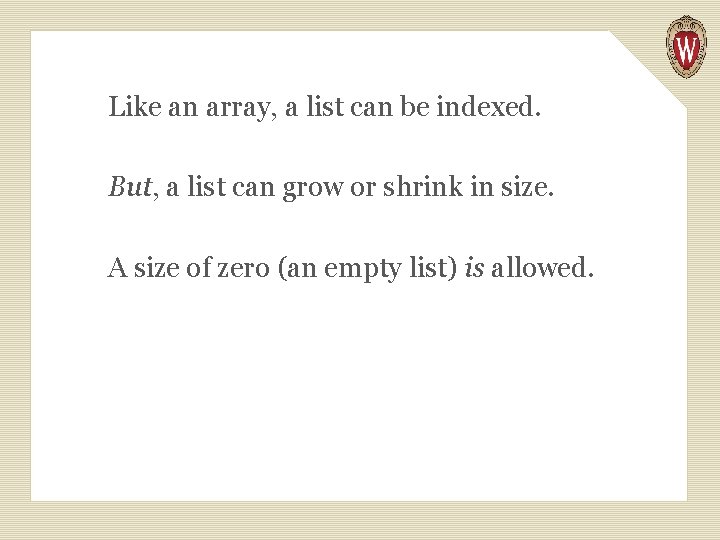
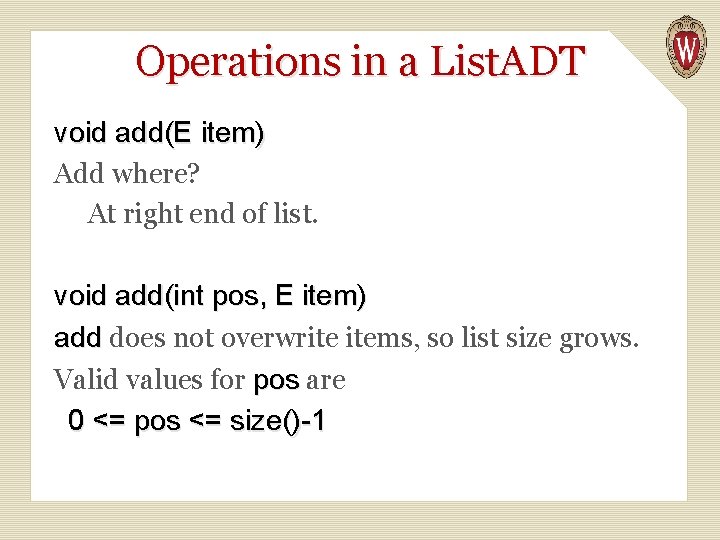
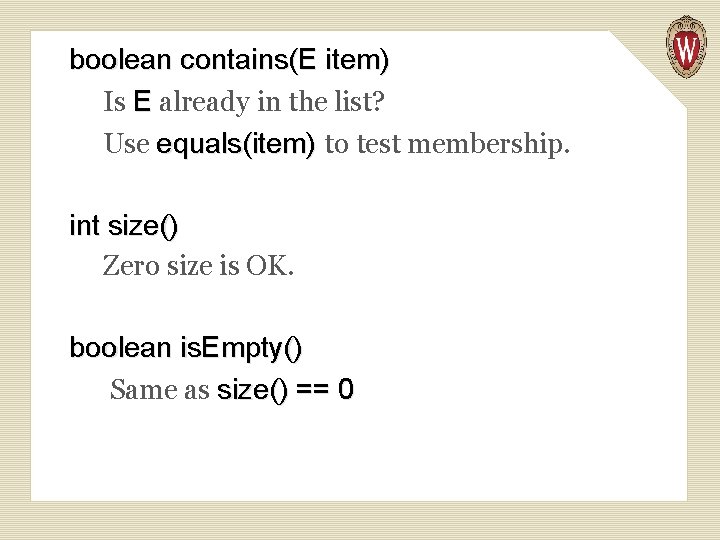
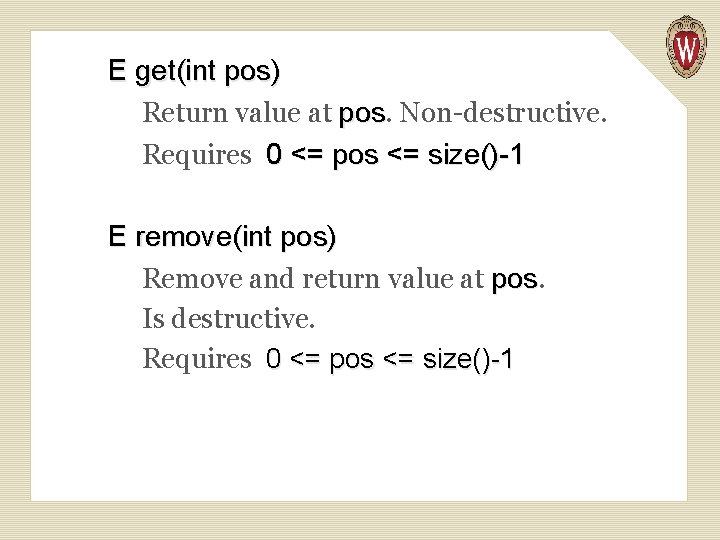
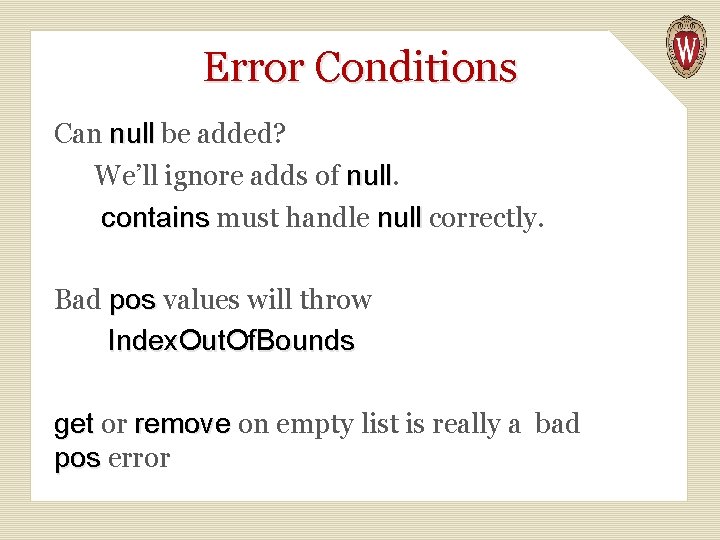
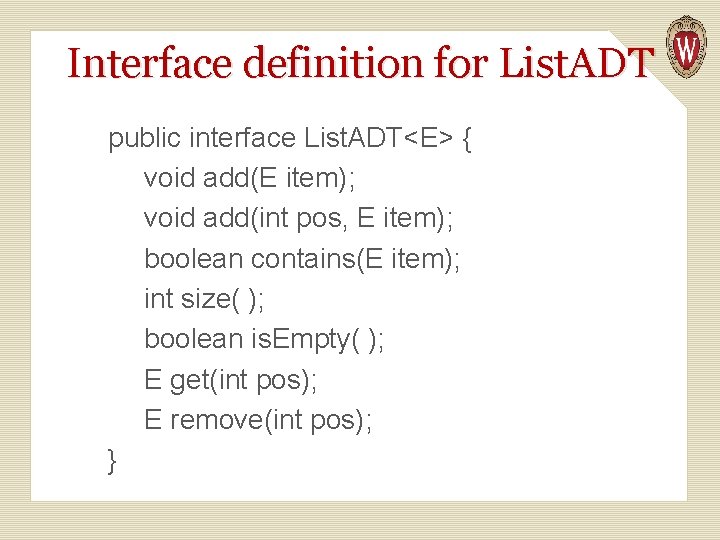
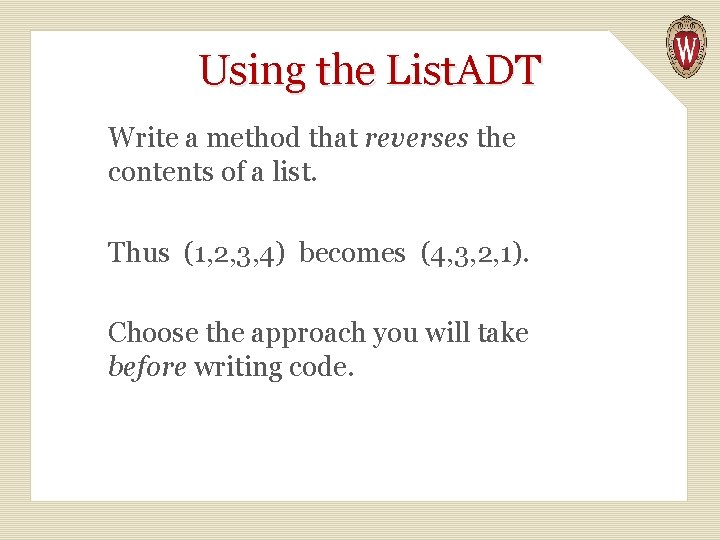
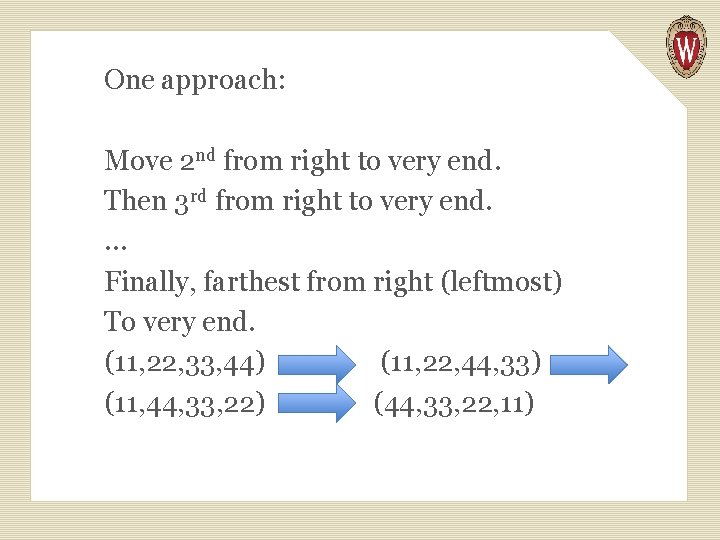
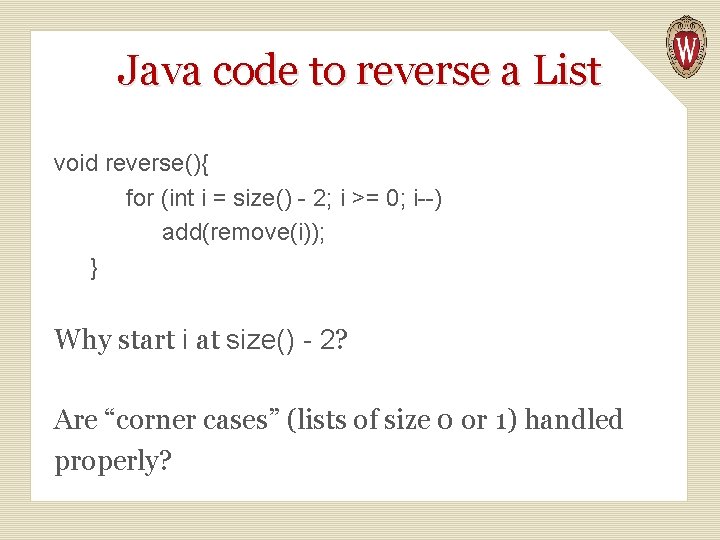
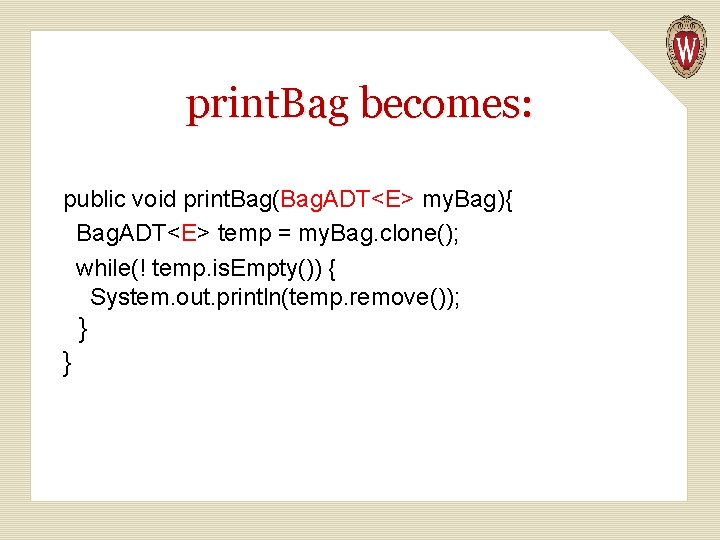
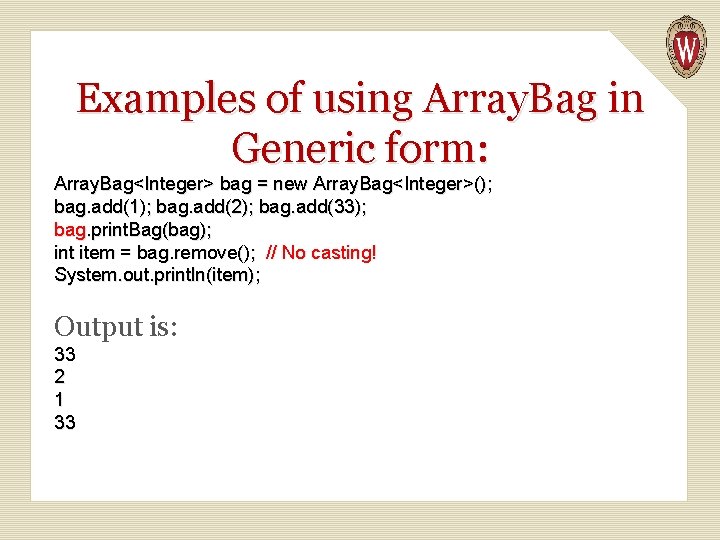
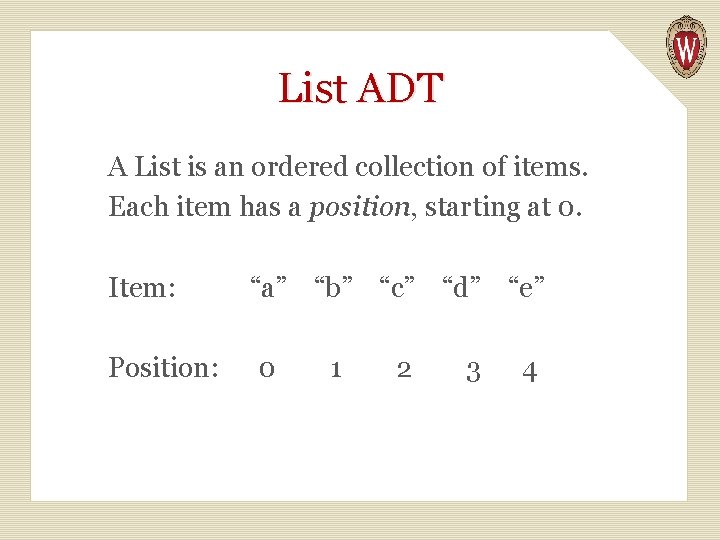
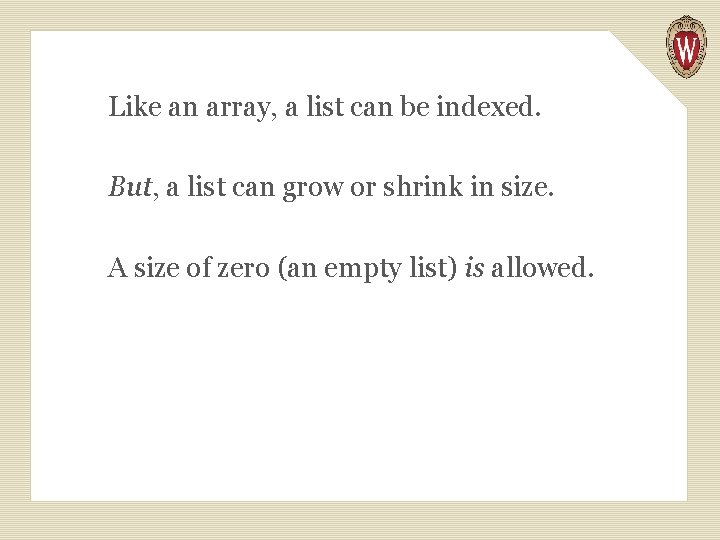
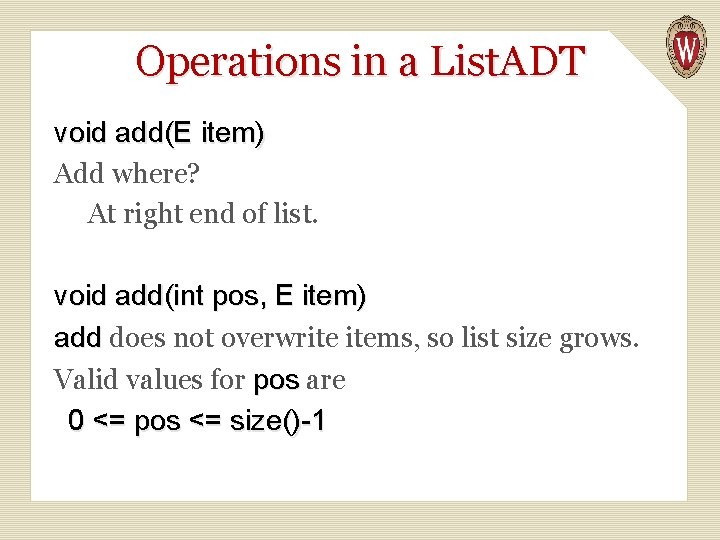
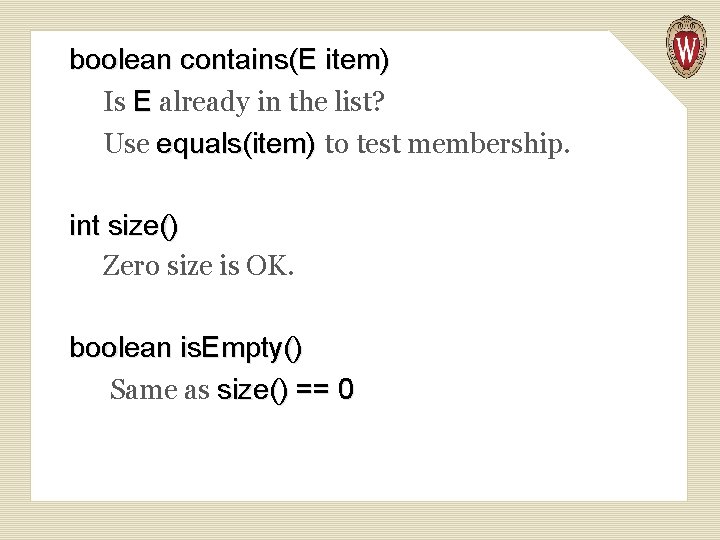
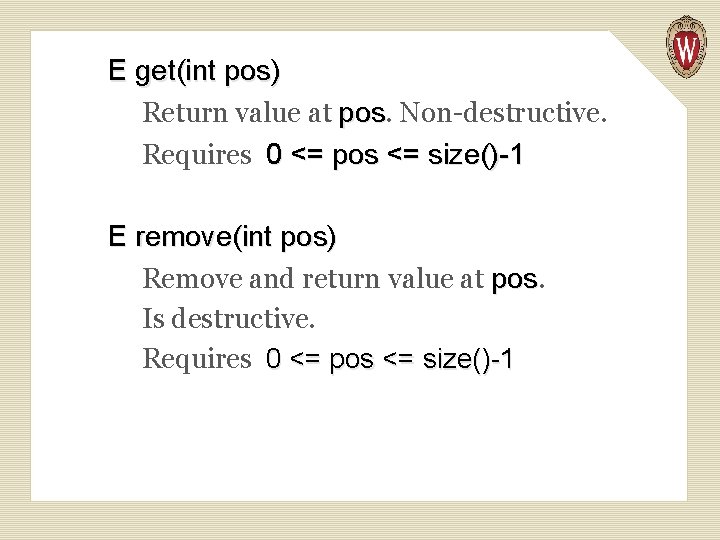
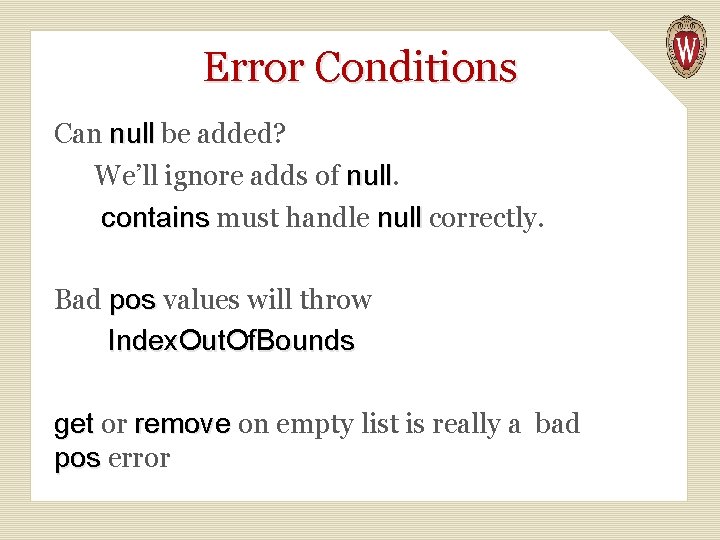
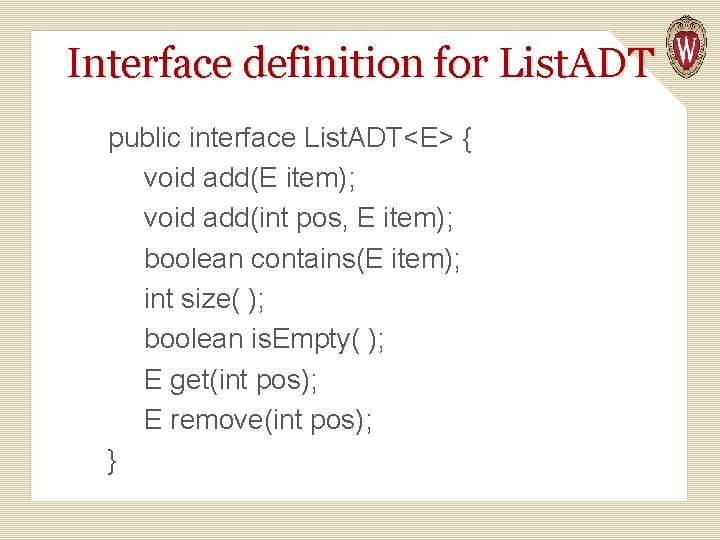
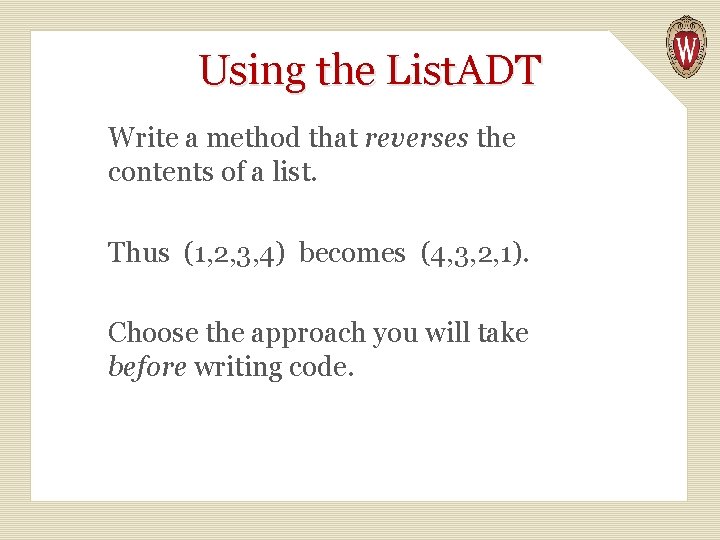
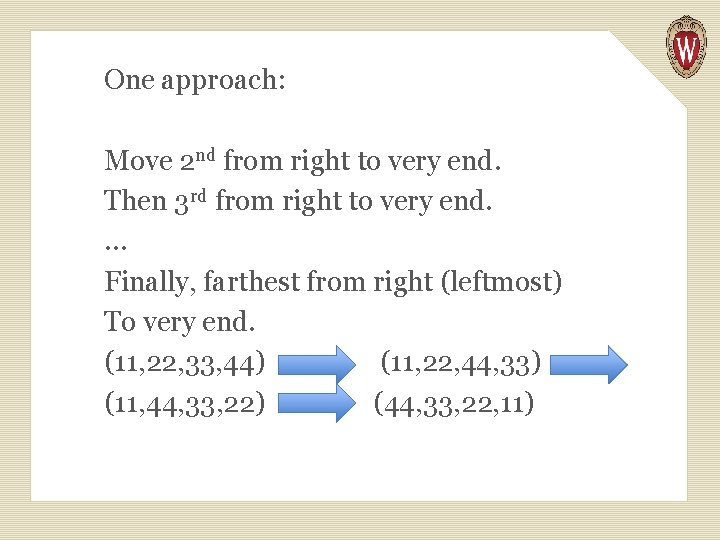
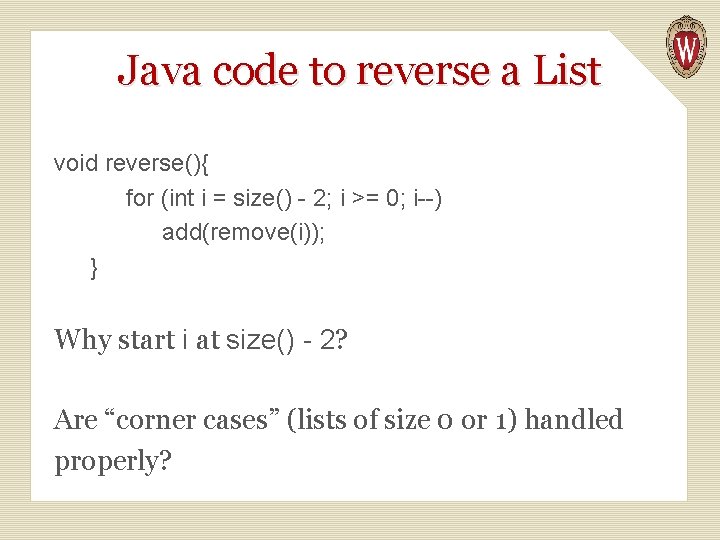
- Slides: 61
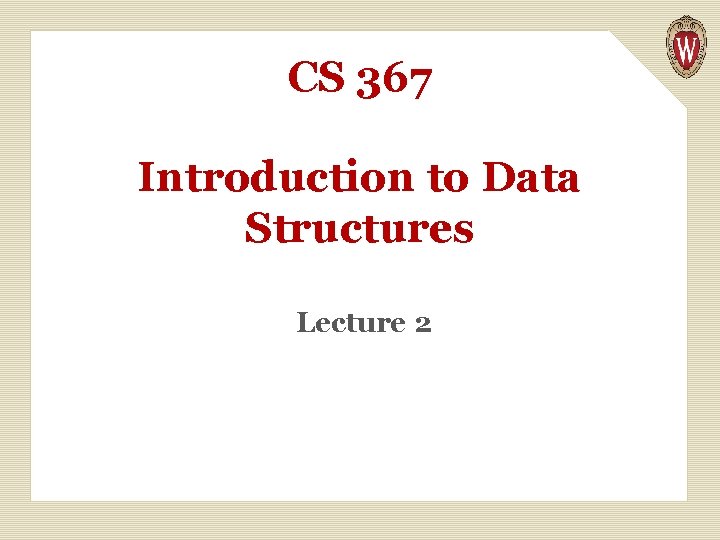
CS 367 Introduction to Data Structures Lecture 2
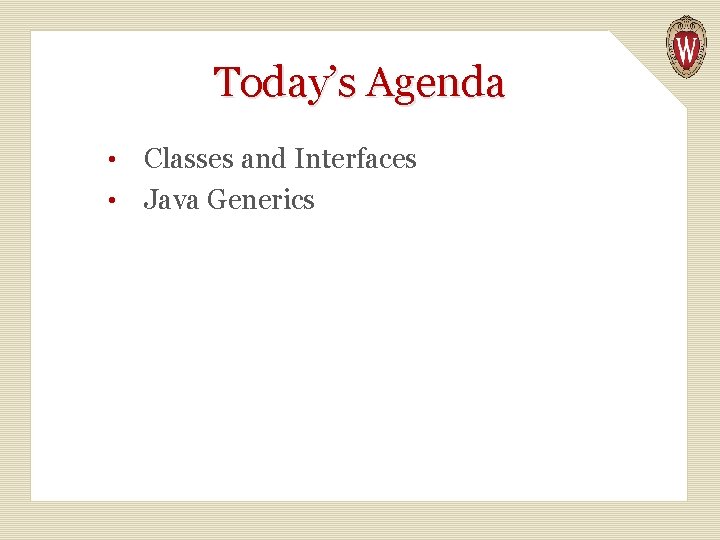
Today’s Agenda Classes and Interfaces • Java Generics •
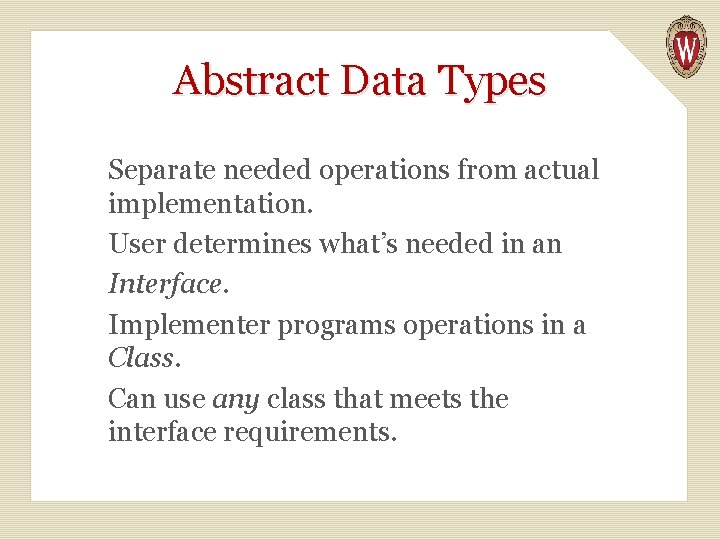
Abstract Data Types Separate needed operations from actual implementation. User determines what’s needed in an Interface. Implementer programs operations in a Class. Can use any class that meets the interface requirements.
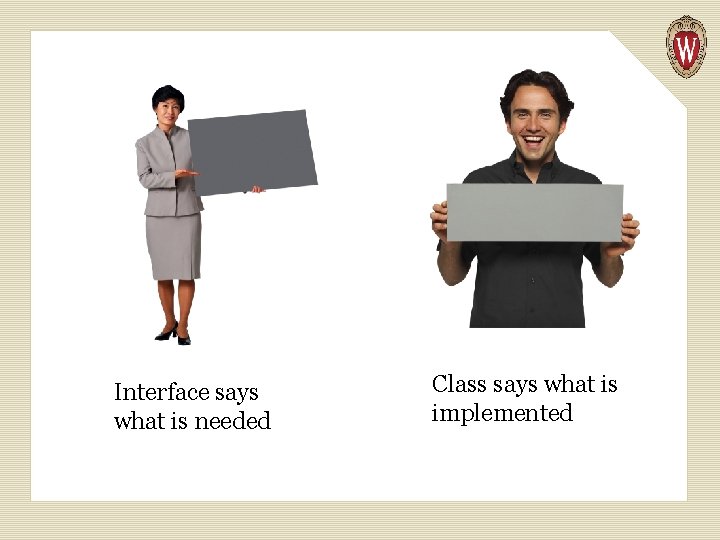
Interface says what is needed Class says what is implemented
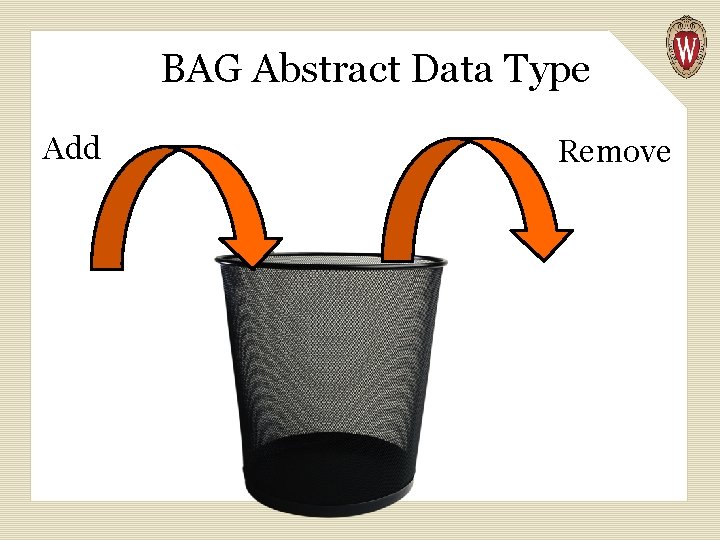
BAG Abstract Data Type Add Remove
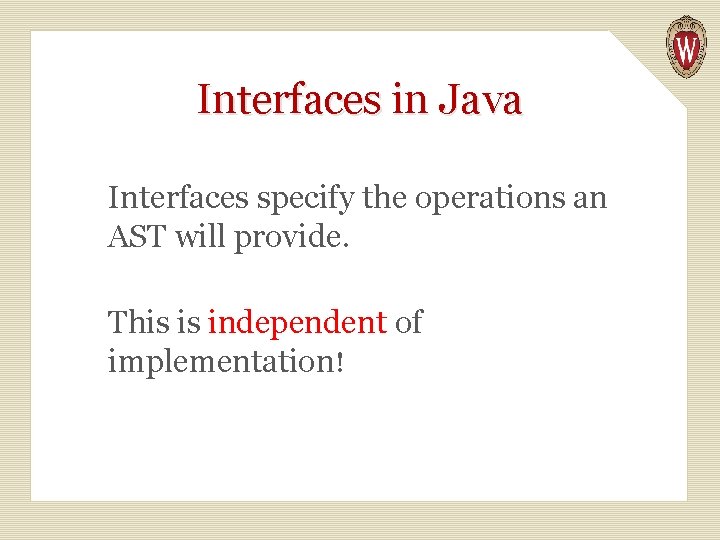
Interfaces in Java Interfaces specify the operations an AST will provide. This is independent of implementation!
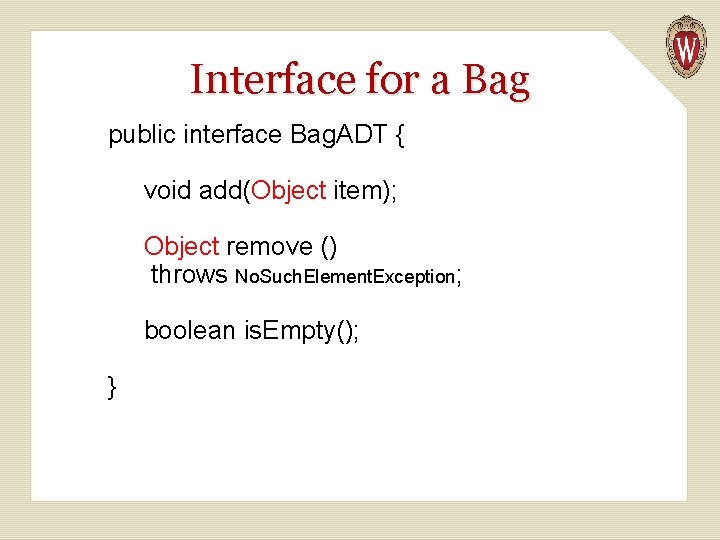
Interface for a Bag public interface Bag. ADT { void add(Object item); Object remove () throws No. Such. Element. Exception; boolean is. Empty(); }
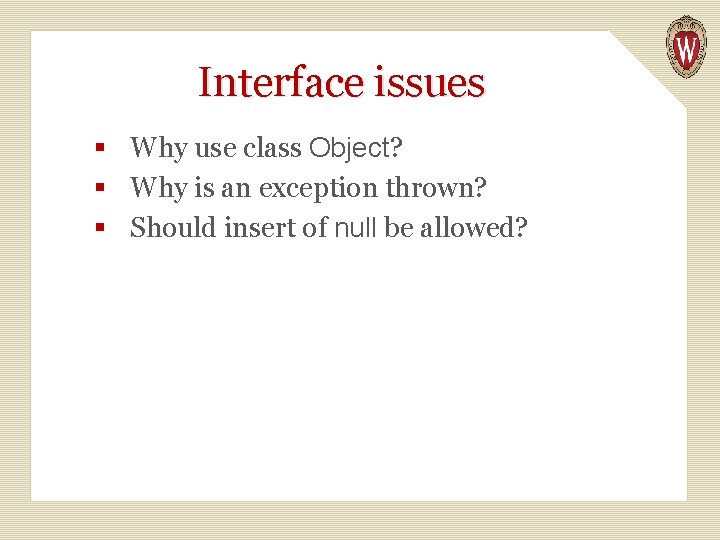
Interface issues § Why use class Object? § Why is an exception thrown? § Should insert of null be allowed?
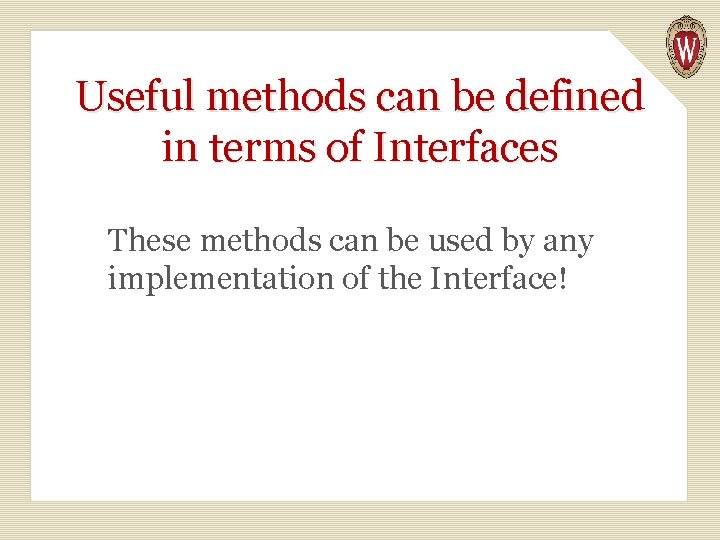
Useful methods can be defined in terms of Interfaces These methods can be used by any implementation of the Interface!
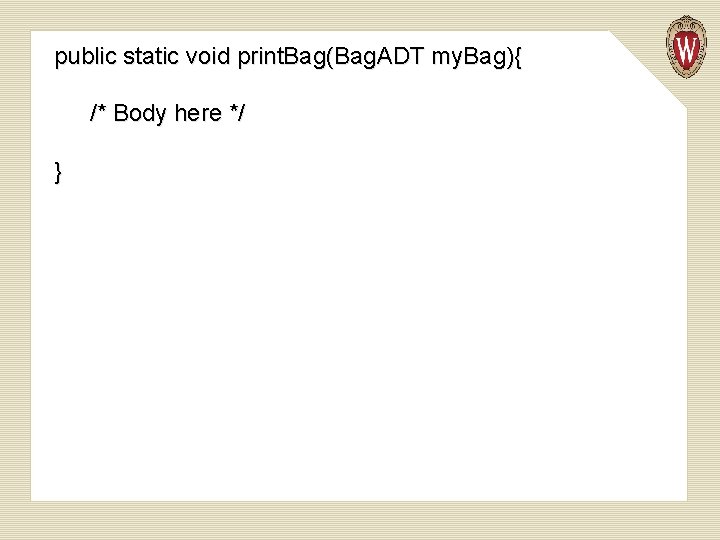
public static void print. Bag(Bag. ADT my. Bag){ /* Body here */ }
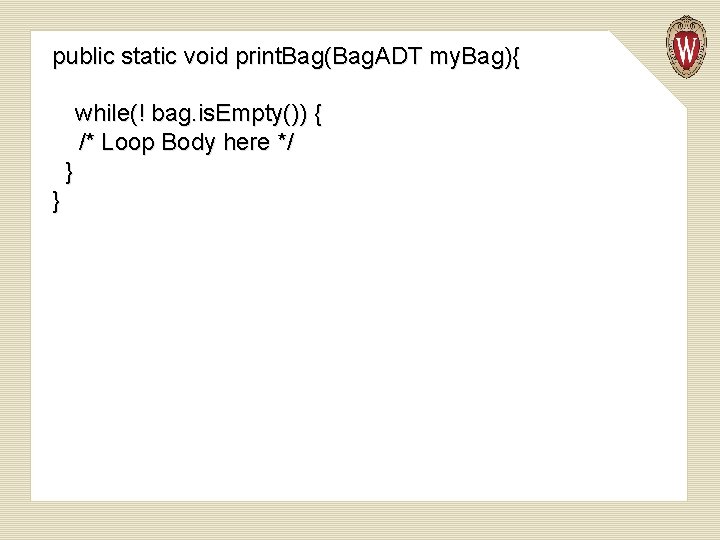
public static void print. Bag(Bag. ADT my. Bag){ while(! bag. is. Empty()) { /* Loop Body here */ } }
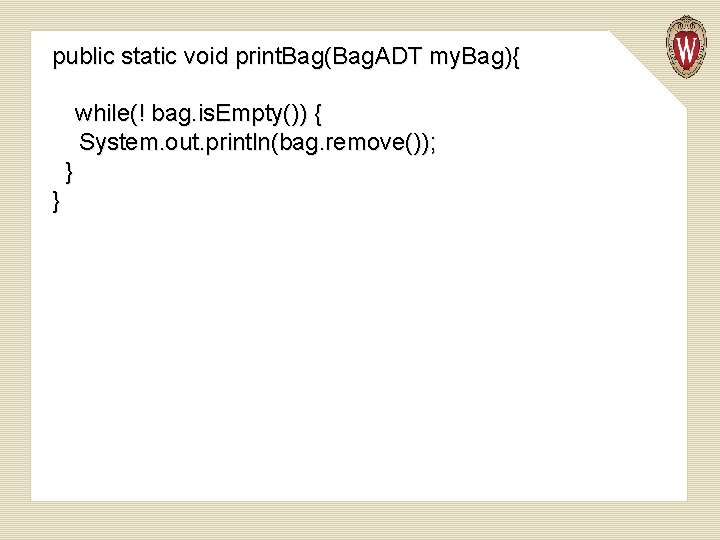
public static void print. Bag(Bag. ADT my. Bag){ while(! bag. is. Empty()) { System. out. println(bag. remove()); } }
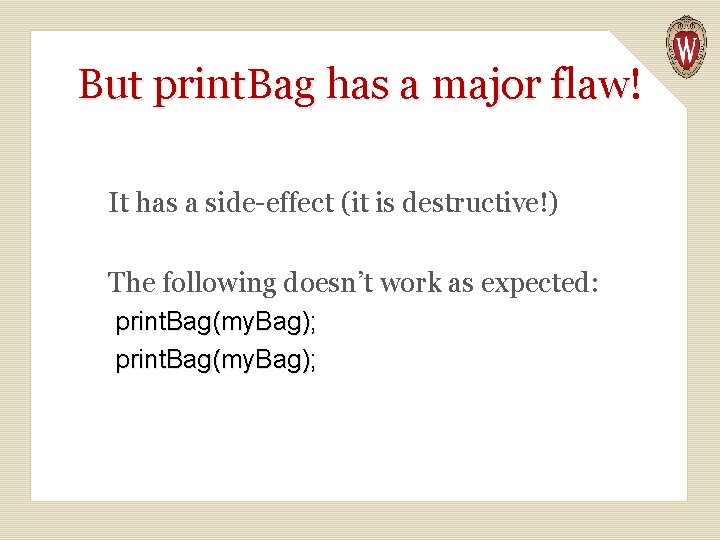
But print. Bag has a major flaw! It has a side-effect (it is destructive!) The following doesn’t work as expected: print. Bag(my. Bag);
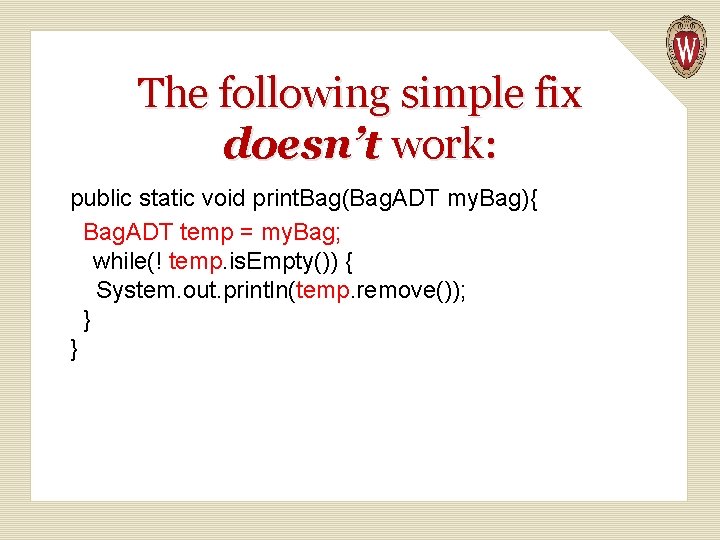
The following simple fix doesn’t work: public static void print. Bag(Bag. ADT my. Bag){ Bag. ADT temp = my. Bag; while(! temp. is. Empty()) { System. out. println(temp. remove()); } }
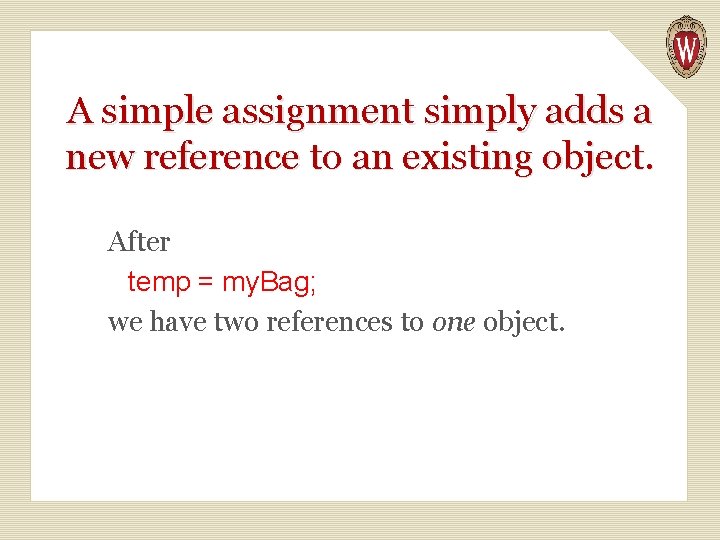
A simple assignment simply adds a new reference to an existing object. After temp = my. Bag; we have two references to one object.
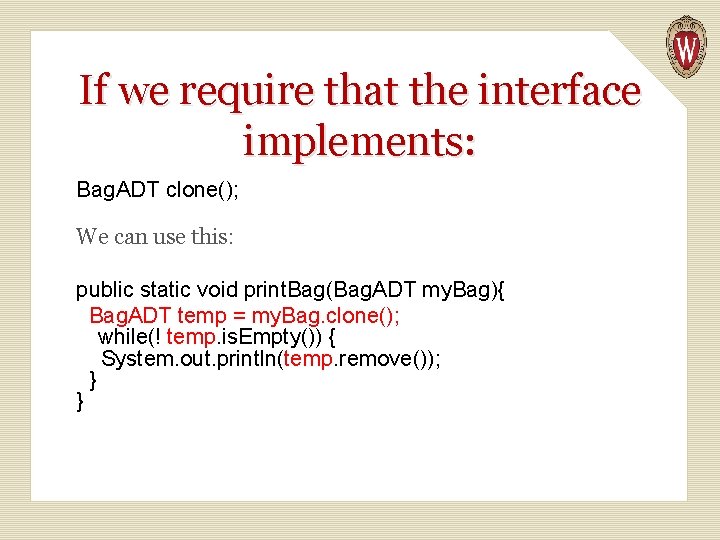
If we require that the interface implements: Bag. ADT clone(); We can use this: public static void print. Bag(Bag. ADT my. Bag){ Bag. ADT temp = my. Bag. clone(); while(! temp. is. Empty()) { System. out. println(temp. remove()); } }
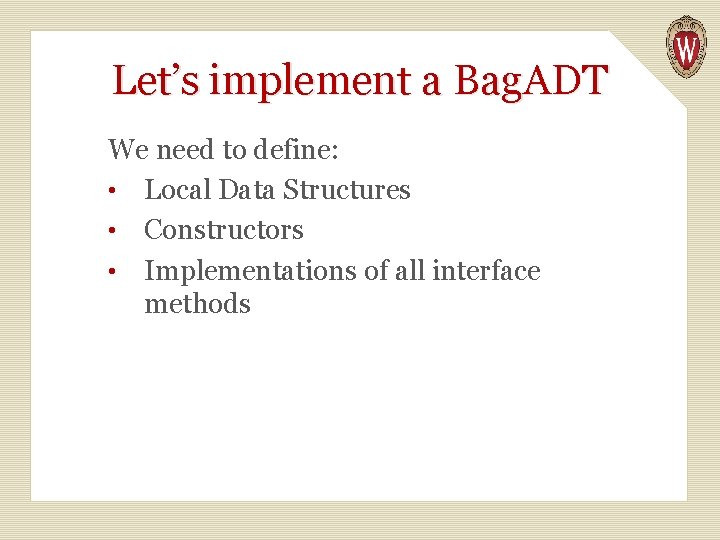
Let’s implement a Bag. ADT We need to define: • Local Data Structures • Constructors • Implementations of all interface methods
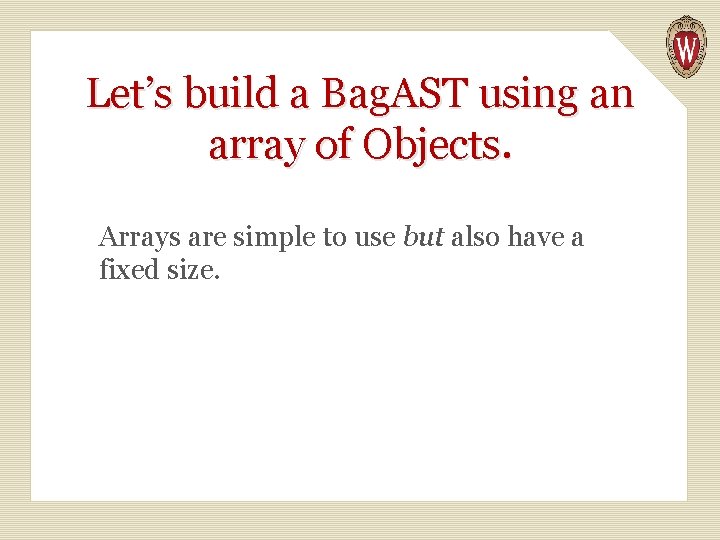
Let’s build a Bag. AST using an array of Objects. Arrays are simple to use but also have a fixed size.
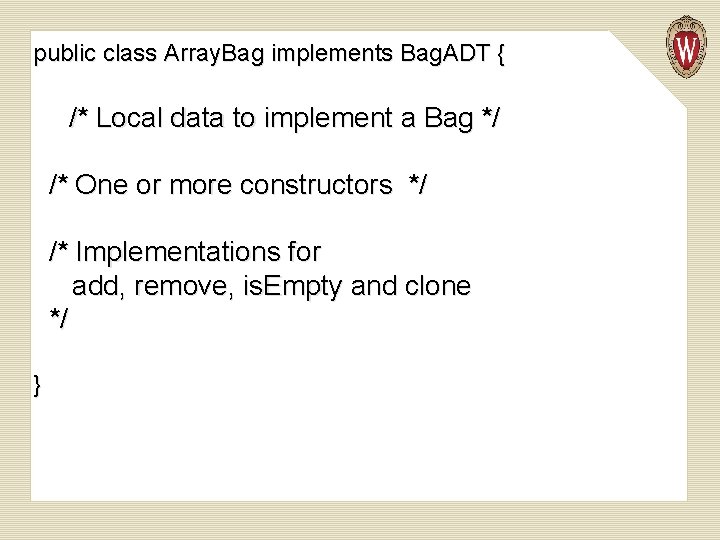
public class Array. Bag implements Bag. ADT { /* Local data to implement a Bag */ /* One or more constructors */ /* Implementations for add, remove, is. Empty and clone */ }
![public class Array Bag implements Bag ADT private Object items private int item public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-20.jpg)
public class Array. Bag implements Bag. ADT { private Object[] items; private int item. Count; private final int INIT_SIZE; /* One or more constructors */ /* Implementations for add, remove, is. Empty and clone */ }
![public class Array Bag implements Bag ADT private Object items private int item public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-21.jpg)
public class Array. Bag implements Bag. ADT { private Object[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { item. Count = 0; INIT_SIZE = 100; items = new Object[INIT_SIZE]; } /* Implementations for add, remove, is. Empty and clone */ }
![public class Array Bag implements Bag ADT private Object items private int item public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-22.jpg)
public class Array. Bag implements Bag. ADT { private Object[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { … } public boolean is. Empty() { return (item. Count == 0); } }
![public class Array Bag implements Bag ADT private Object items private int item public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-23.jpg)
public class Array. Bag implements Bag. ADT { private Object[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { … } public boolean is. Empty() { … } public void add(Object item) { if (item == null) throw new Null. Pointer. Exception(); if (item. Count >= INIT_SIZE) throw new Error(); items[item. Count] = item; item. Count++; }}
![public class Array Bag implements Bag ADT private Object items private int item public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-24.jpg)
public class Array. Bag implements Bag. ADT { private Object[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { … } public boolean is. Empty() { … } public void add(Object item) {…} public Object remove() throws No. Such. Element. Exception { if (item. Count == 0) throw new No. Such. Element. Exception(); else { item. Count--; return items[item. Count]; } }
![public class Array Bag implements Bag ADT private Object items private int item public class Array. Bag implements Bag. ADT { private Object[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-25.jpg)
public class Array. Bag implements Bag. ADT { private Object[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { … } public boolean is. Empty() { … } public void add(Object item) {…} public Object remove() throws No. Such. Element. Exception {…} public Array. Bag clone() { Array. Bag copy = new Array. Bag(); copy. item. Count = item. Count; copy. items = items. clone(); return copy; } }
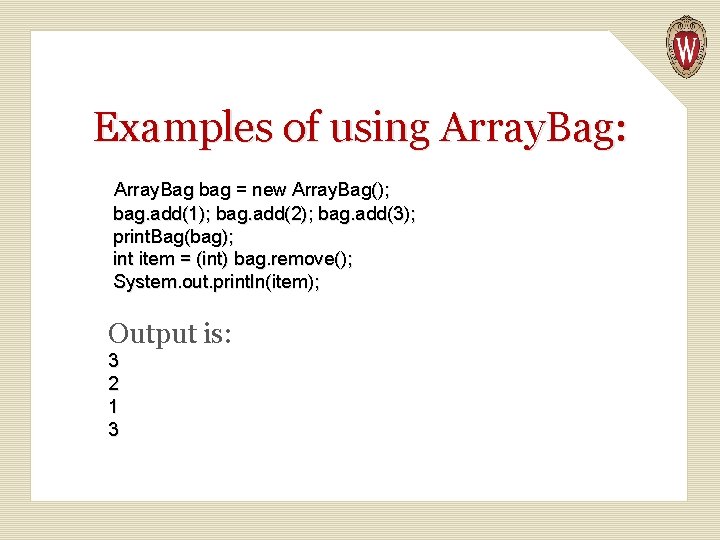
Examples of using Array. Bag: Array. Bag bag = new Array. Bag(); bag. add(1); bag. add(2); bag. add(3); print. Bag(bag); int item = (int) bag. remove(); System. out. println(item); Output is: 3 2 1 3
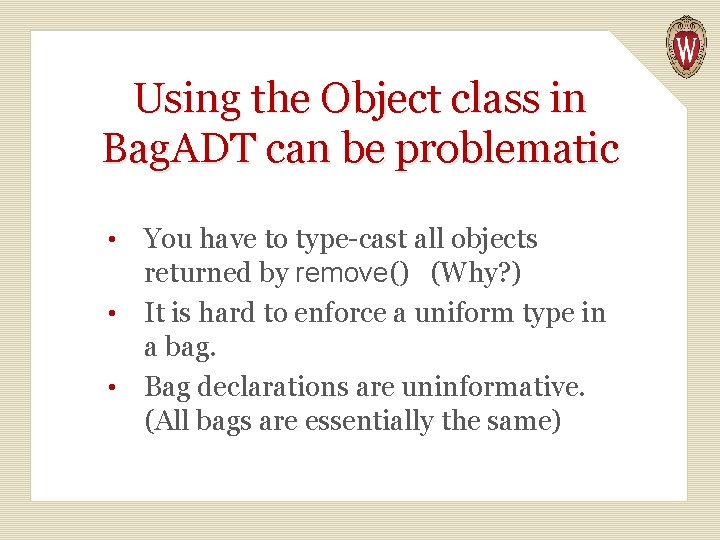
Using the Object class in Bag. ADT can be problematic You have to type-cast all objects returned by remove() (Why? ) • It is hard to enforce a uniform type in a bag. • Bag declarations are uninformative. (All bags are essentially the same) •
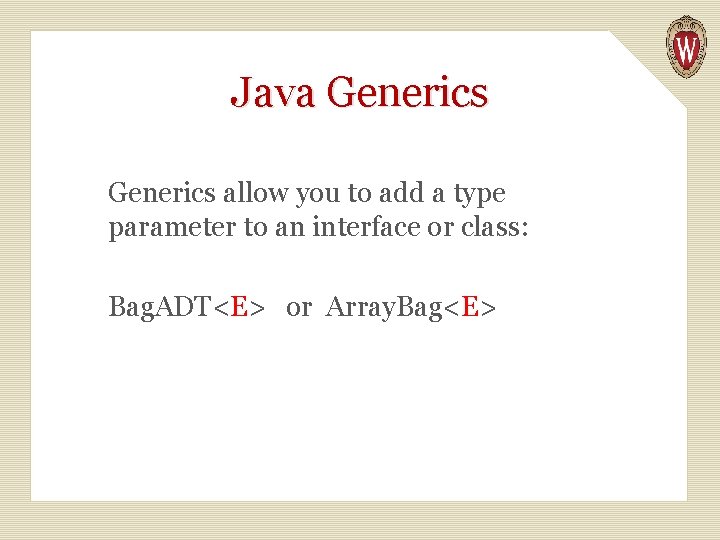
Java Generics allow you to add a type parameter to an interface or class: Bag. ADT<E> or Array. Bag<E>
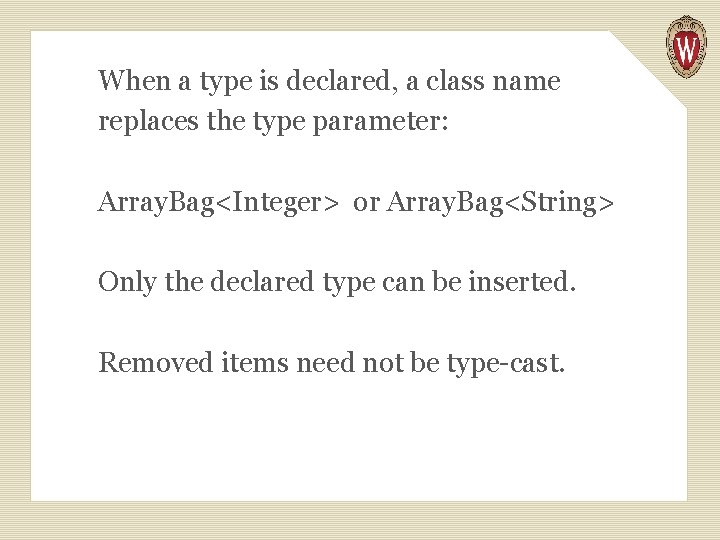
When a type is declared, a class name replaces the type parameter: Array. Bag<Integer> or Array. Bag<String> Only the declared type can be inserted. Removed items need not be type-cast.
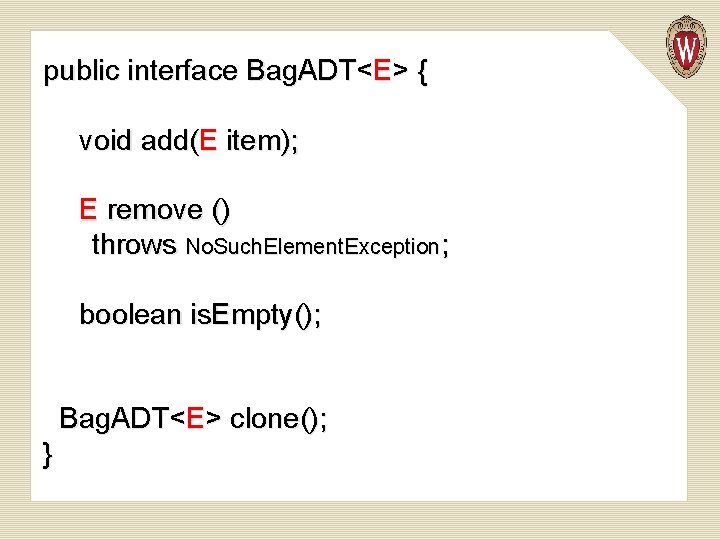
public interface Bag. ADT<E> { void add(E item); E remove () throws No. Such. Element. Exception; boolean is. Empty(); Bag. ADT<E> clone(); }
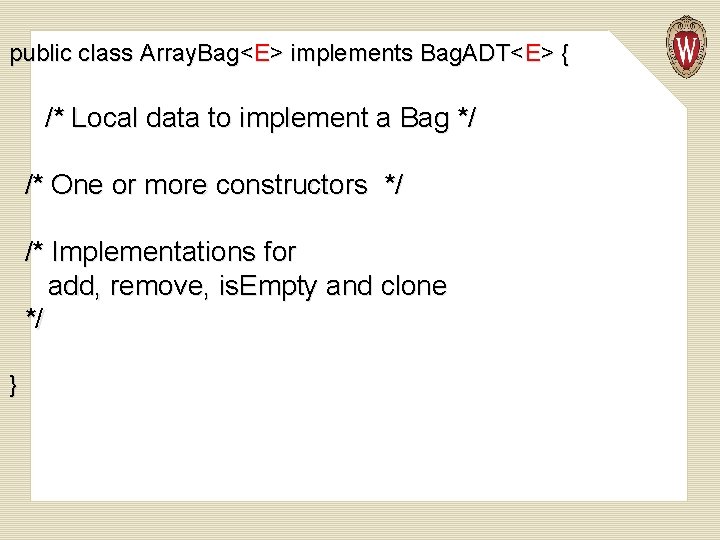
public class Array. Bag<E> implements Bag. ADT<E> { /* Local data to implement a Bag */ /* One or more constructors */ /* Implementations for add, remove, is. Empty and clone */ }
![public class Array BagE implements Bag ADTE private E items private int item public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-32.jpg)
public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. Count; private final int INIT_SIZE; /* One or more constructors */ /* Implementations for add, remove, is. Empty and clone */ }
![public class Array BagE implements Bag ADTE private E items private int item public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-33.jpg)
public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { item. Count = 0; INIT_SIZE = 100; // Kludge alert! items = (E[]) new Object[INIT_SIZE]; } /* Implementations for add, remove, is. Empty and clone */ }
![public class Array BagE implements Bag ADTE private E items private int item public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-34.jpg)
public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { … } public boolean is. Empty() { return (item. Count == 0); } }
![public class Array BagE implements Bag ADTE private E items private int item public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-35.jpg)
public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { … } public boolean is. Empty() { … } public void add(E item) { if (item == null) throw new Null. Pointer. Exception(); if (item. Count >= INIT_SIZE) throw new Error(); items[item. Count] = item; item. Count++; }}
![public class Array BagE implements Bag ADTE private E items private int item public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-36.jpg)
public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { … } public boolean is. Empty() { … } public void add(Object item) {…} public E remove() throws No. Such. Element. Exception { if (item. Count == 0) throw new No. Such. Element. Exception(); else { item. Count--; return items[item. Count]; } }
![public class Array BagE implements Bag ADTE private E items private int item public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item.](https://slidetodoc.com/presentation_image_h/e77e02c50d444c7d1da2839765faf3f7/image-37.jpg)
public class Array. Bag<E> implements Bag. ADT<E> { private E[] items; private int item. Count; private final int INIT_SIZE; public Array. Bag() { … } public boolean is. Empty() { … } public void add(Object item) {…} public Object remove() throws No. Such. Element. Exception {…} public Array. Bag<E> clone() { Array. Bag<E> copy = new Array. Bag<E>(); copy. item. Count = item. Count; copy. items = items. clone(); return copy; } }
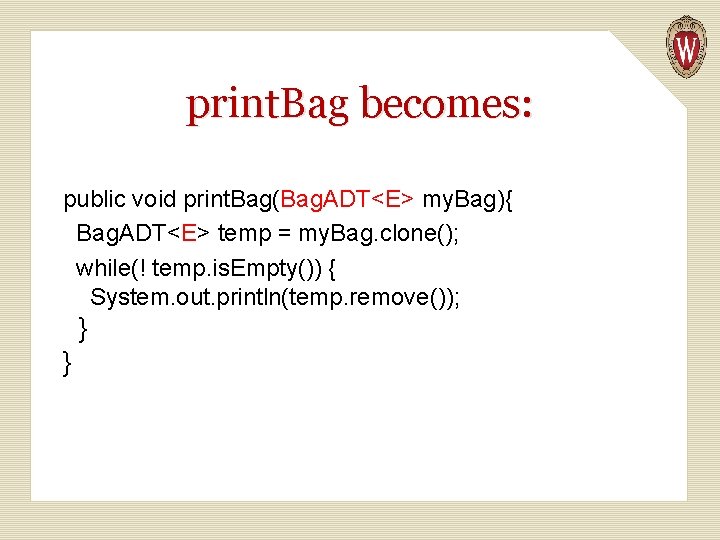
print. Bag becomes: public void print. Bag(Bag. ADT<E> my. Bag){ Bag. ADT<E> temp = my. Bag. clone(); while(! temp. is. Empty()) { System. out. println(temp. remove()); } }
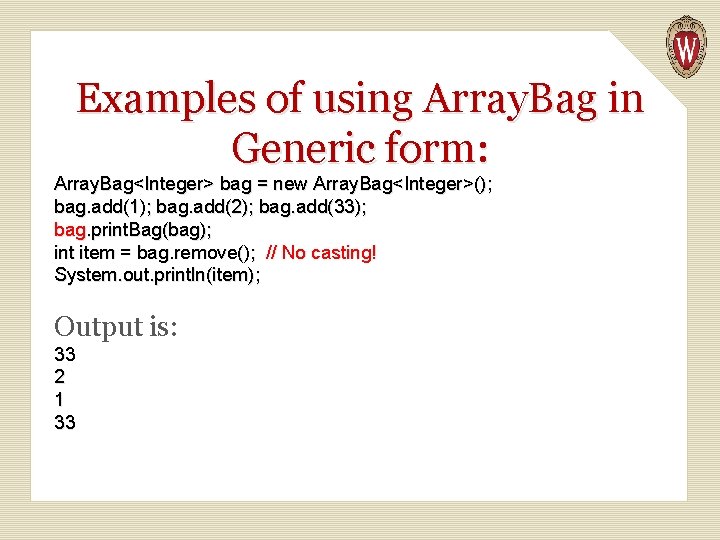
Examples of using Array. Bag in Generic form: Array. Bag<Integer> bag = new Array. Bag<Integer>(); bag. add(1); bag. add(2); bag. add(33); bag. print. Bag(bag); int item = bag. remove(); // No casting! System. out. println(item); Output is: 33 2 1 33
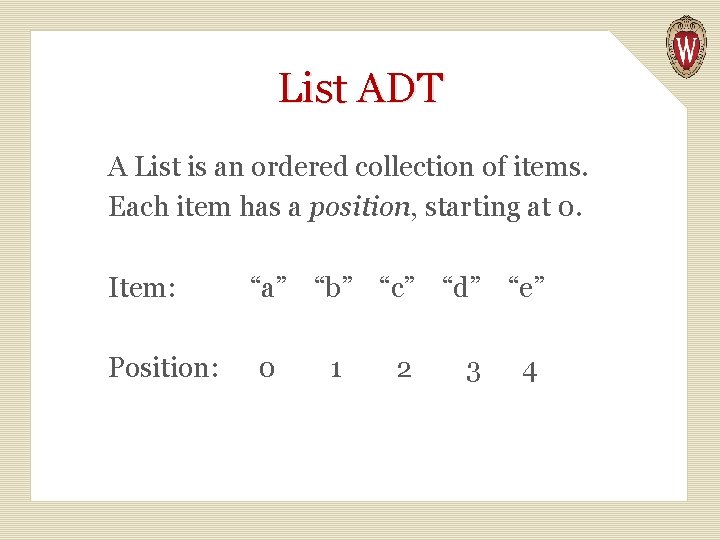
List ADT A List is an ordered collection of items. Each item has a position, starting at 0. Item: Position: “a” “b” “c” “d” “e” 0 1 2 3 4
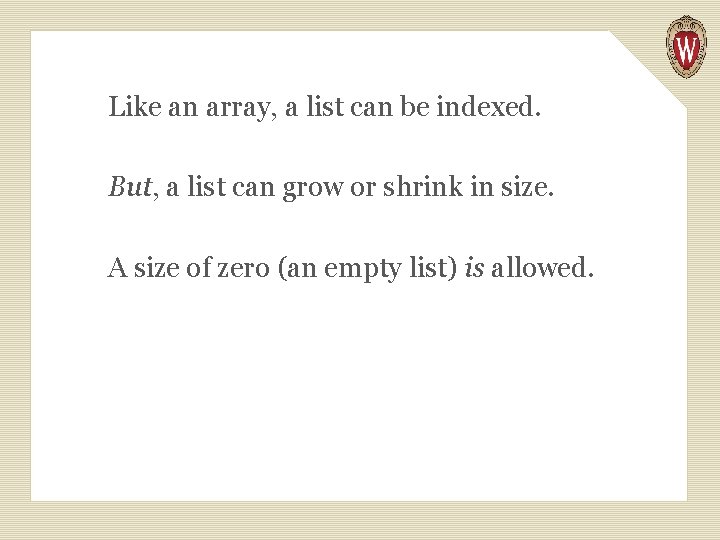
Like an array, a list can be indexed. But, a list can grow or shrink in size. A size of zero (an empty list) is allowed.
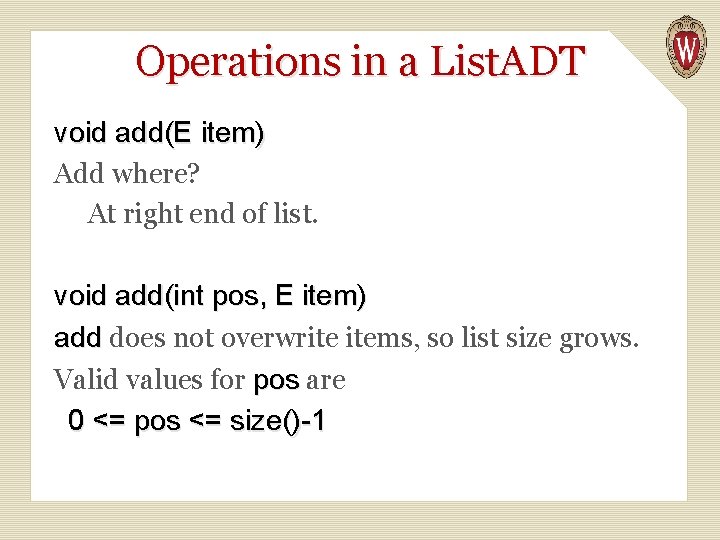
Operations in a List. ADT void add(E item) Add where? At right end of list. void add(int pos, E item) add does not overwrite items, so list size grows. Valid values for pos are 0 <= pos <= size()-1
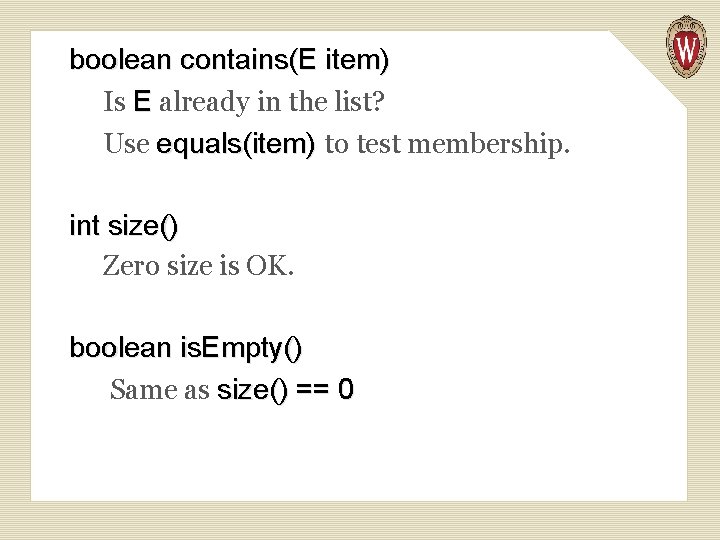
boolean contains(E item) Is E already in the list? Use equals(item) to test membership. int size() Zero size is OK. boolean is. Empty() Same as size() == 0
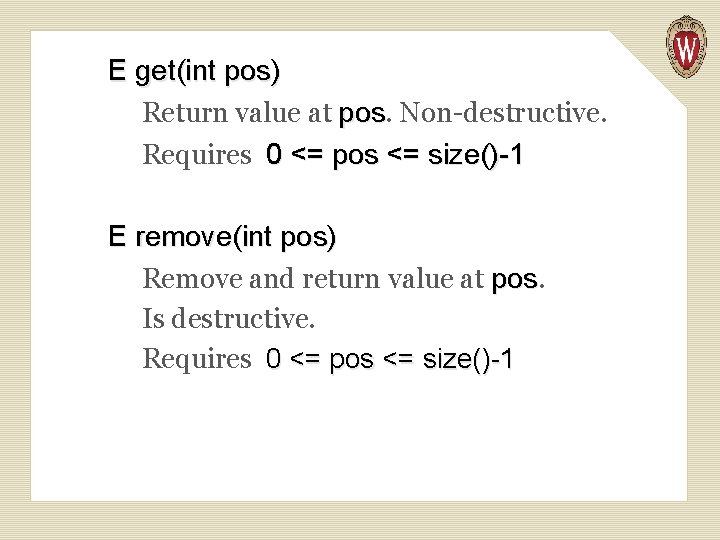
E get(int pos) Return value at pos. Non-destructive. Requires 0 <= pos <= size()-1 E remove(int pos) Remove and return value at pos. Is destructive. Requires 0 <= pos <= size()-1
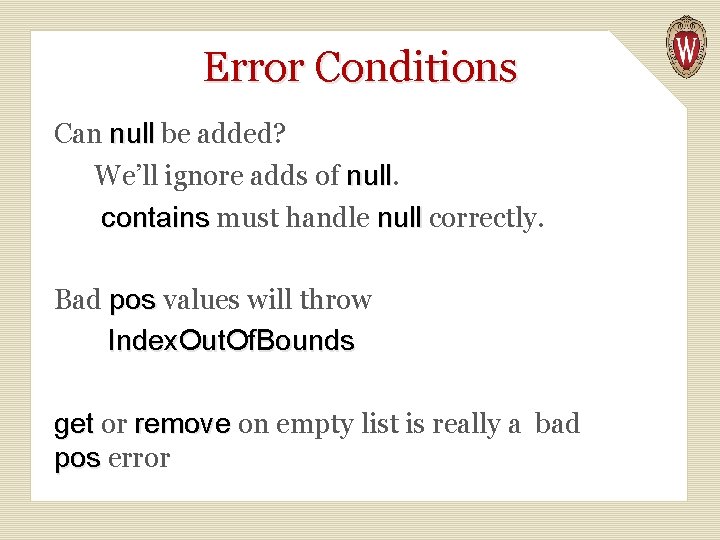
Error Conditions Can null be added? We’ll ignore adds of null. contains must handle null correctly. Bad pos values will throw Index. Out. Of. Bounds get or remove on empty list is really a bad pos error
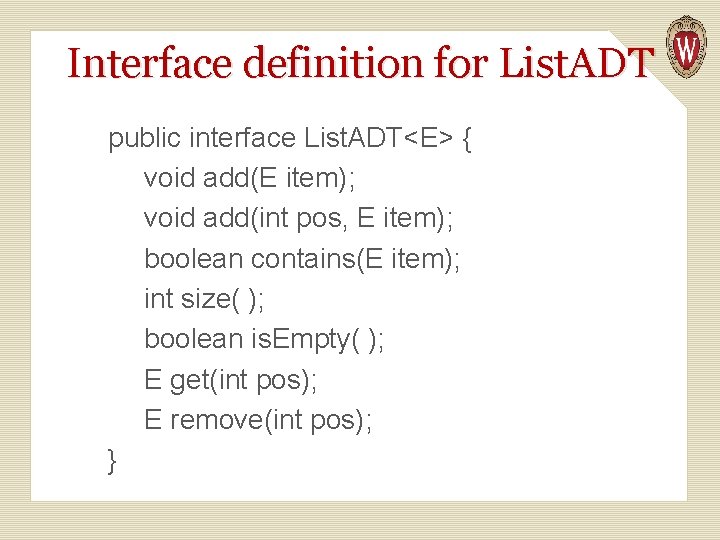
Interface definition for List. ADT public interface List. ADT<E> { void add(E item); void add(int pos, E item); boolean contains(E item); int size( ); boolean is. Empty( ); E get(int pos); E remove(int pos); }
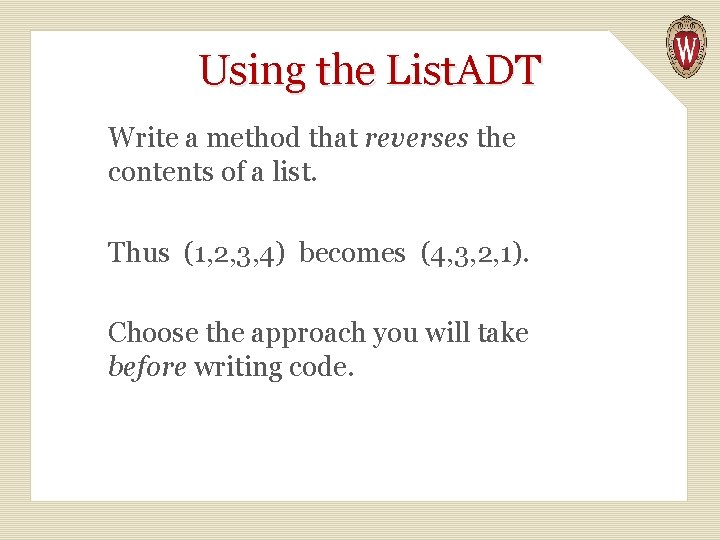
Using the List. ADT Write a method that reverses the contents of a list. Thus (1, 2, 3, 4) becomes (4, 3, 2, 1). Choose the approach you will take before writing code.
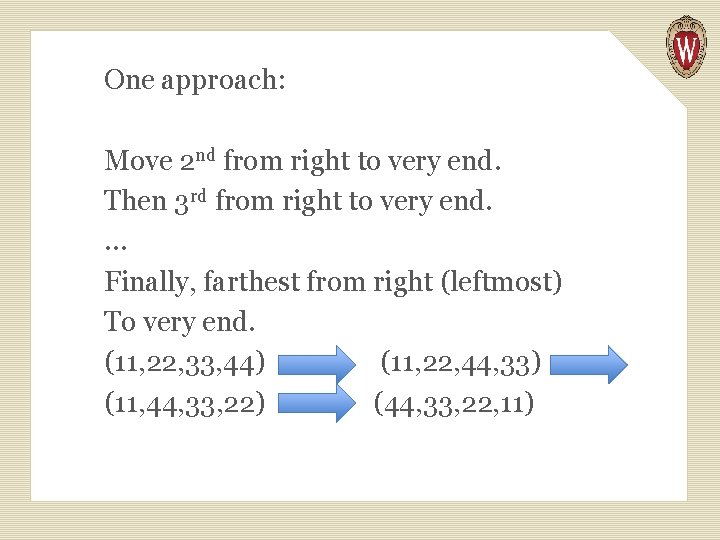
One approach: Move 2 nd from right to very end. Then 3 rd from right to very end. … Finally, farthest from right (leftmost) To very end. (11, 22, 33, 44) (11, 22, 44, 33) (11, 44, 33, 22) (44, 33, 22, 11)
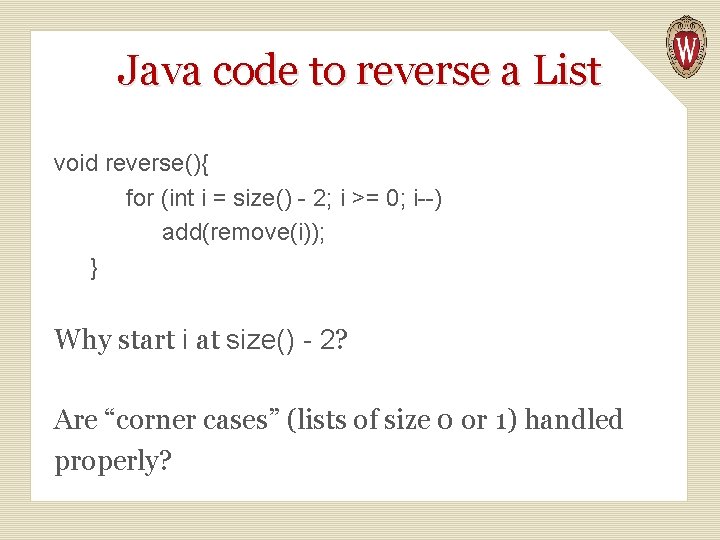
Java code to reverse a List void reverse(){ for (int i = size() - 2; i >= 0; i--) add(remove(i)); } Why start i at size() - 2? Are “corner cases” (lists of size 0 or 1) handled properly?
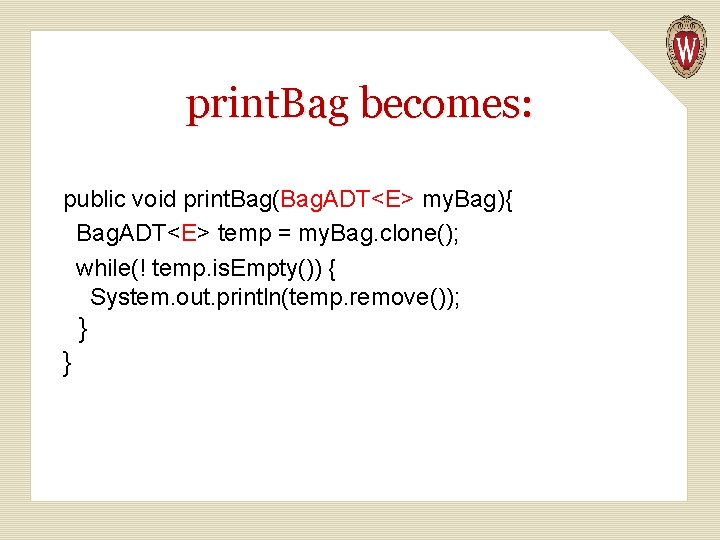
print. Bag becomes: public void print. Bag(Bag. ADT<E> my. Bag){ Bag. ADT<E> temp = my. Bag. clone(); while(! temp. is. Empty()) { System. out. println(temp. remove()); } }
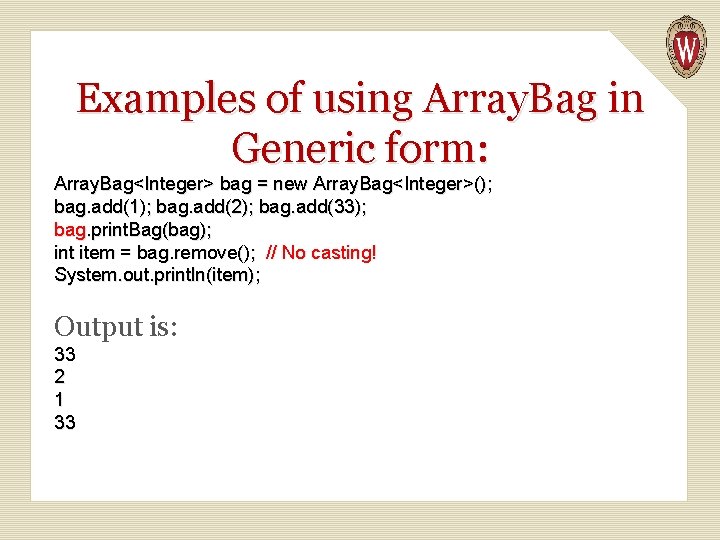
Examples of using Array. Bag in Generic form: Array. Bag<Integer> bag = new Array. Bag<Integer>(); bag. add(1); bag. add(2); bag. add(33); bag. print. Bag(bag); int item = bag. remove(); // No casting! System. out. println(item); Output is: 33 2 1 33
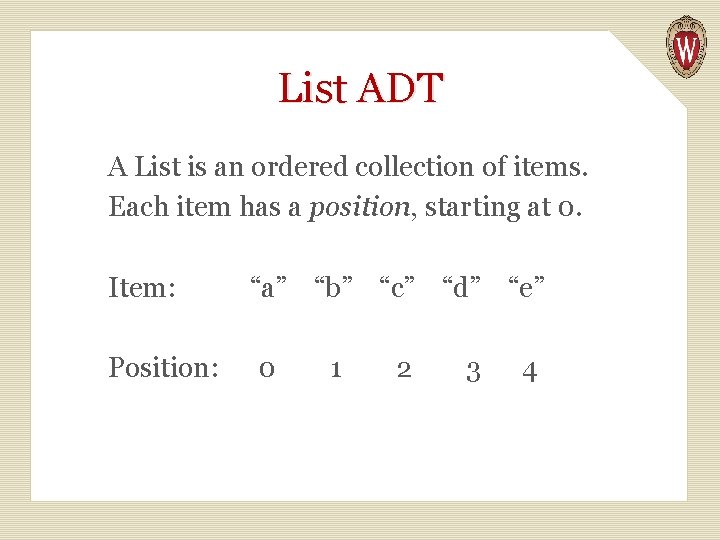
List ADT A List is an ordered collection of items. Each item has a position, starting at 0. Item: Position: “a” “b” “c” “d” “e” 0 1 2 3 4
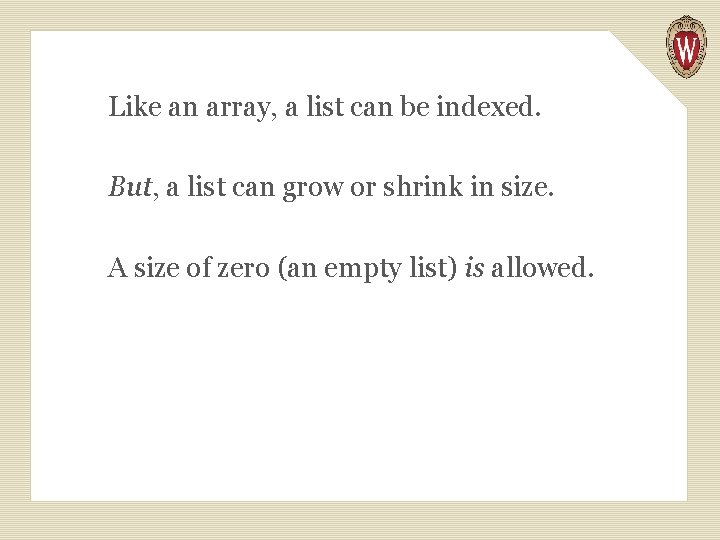
Like an array, a list can be indexed. But, a list can grow or shrink in size. A size of zero (an empty list) is allowed.
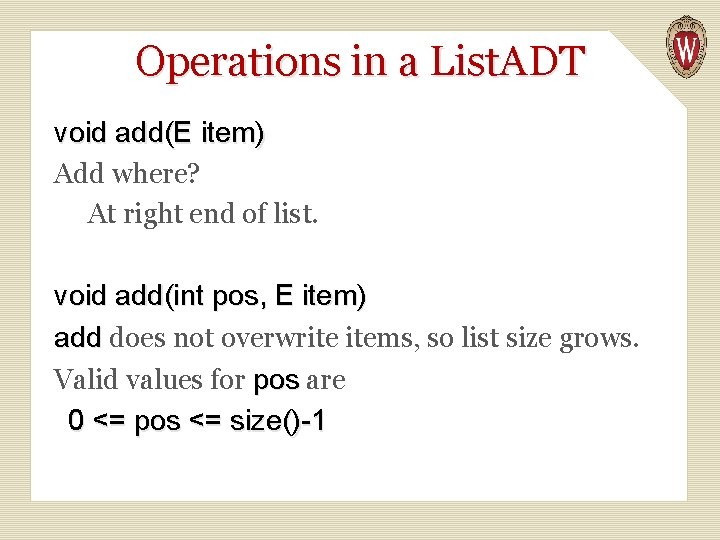
Operations in a List. ADT void add(E item) Add where? At right end of list. void add(int pos, E item) add does not overwrite items, so list size grows. Valid values for pos are 0 <= pos <= size()-1
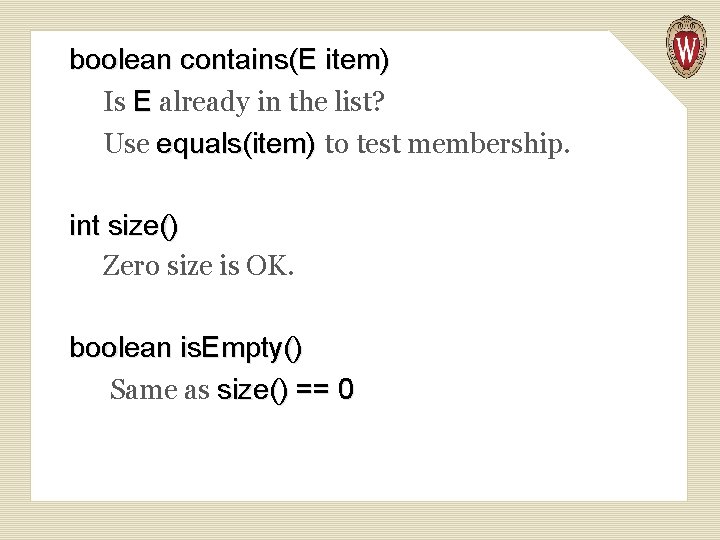
boolean contains(E item) Is E already in the list? Use equals(item) to test membership. int size() Zero size is OK. boolean is. Empty() Same as size() == 0
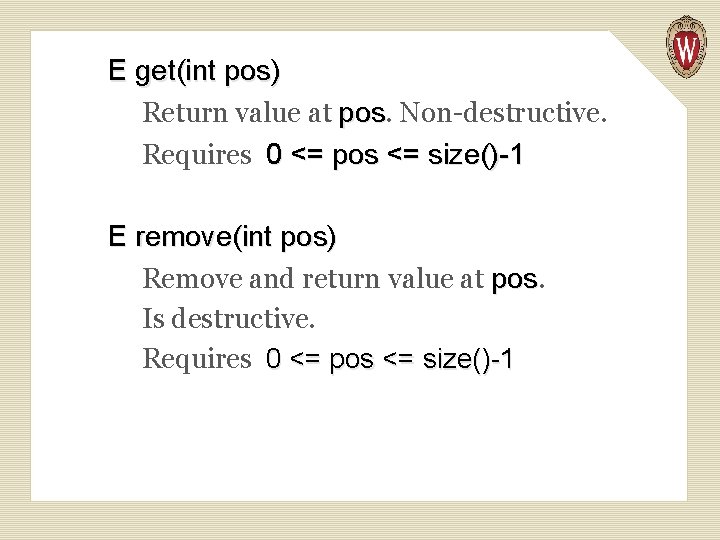
E get(int pos) Return value at pos. Non-destructive. Requires 0 <= pos <= size()-1 E remove(int pos) Remove and return value at pos. Is destructive. Requires 0 <= pos <= size()-1
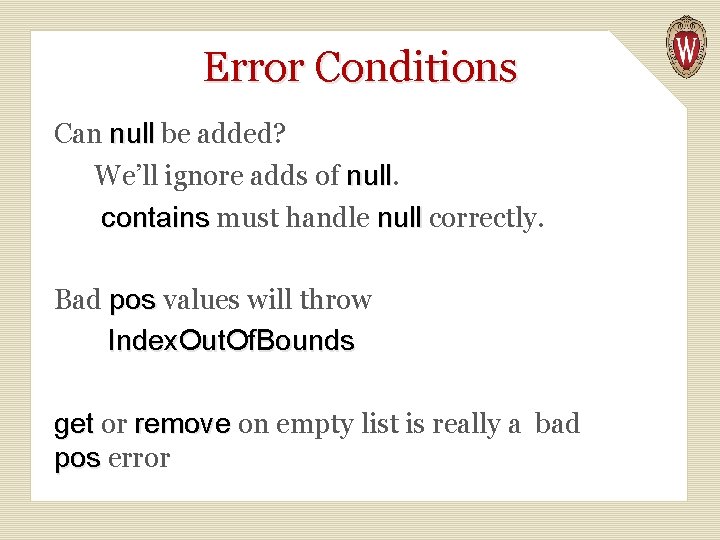
Error Conditions Can null be added? We’ll ignore adds of null. contains must handle null correctly. Bad pos values will throw Index. Out. Of. Bounds get or remove on empty list is really a bad pos error
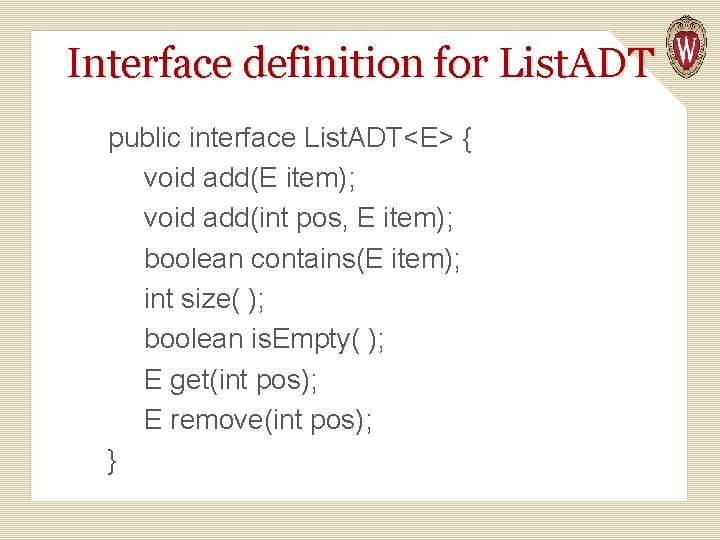
Interface definition for List. ADT public interface List. ADT<E> { void add(E item); void add(int pos, E item); boolean contains(E item); int size( ); boolean is. Empty( ); E get(int pos); E remove(int pos); }
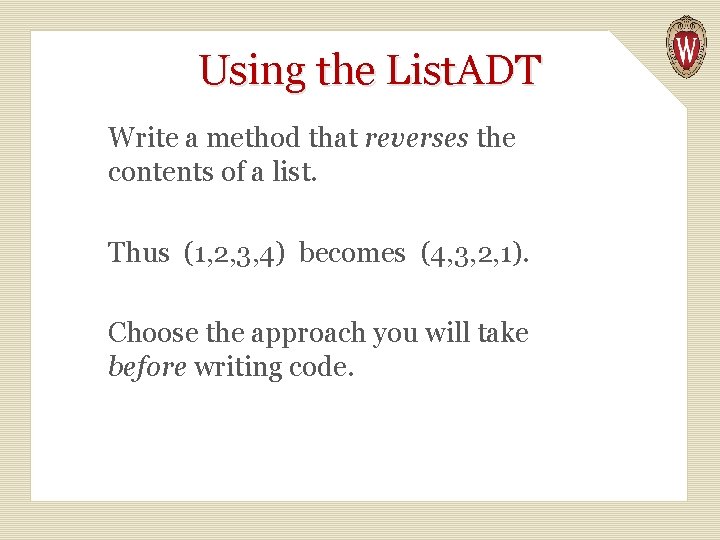
Using the List. ADT Write a method that reverses the contents of a list. Thus (1, 2, 3, 4) becomes (4, 3, 2, 1). Choose the approach you will take before writing code.
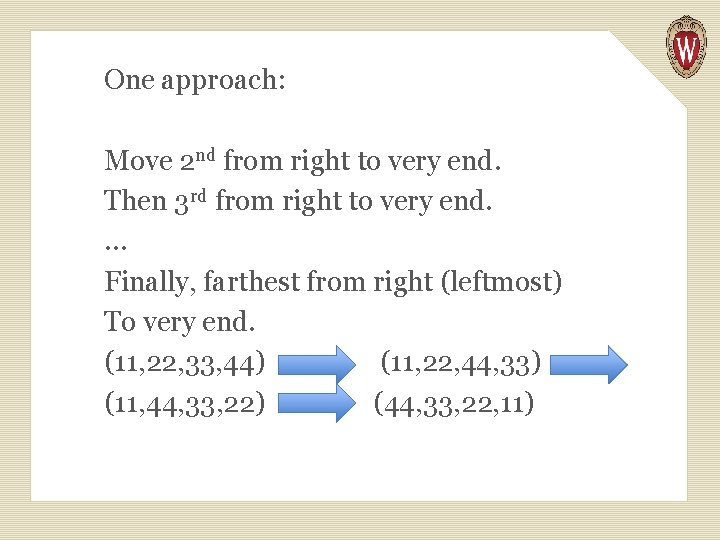
One approach: Move 2 nd from right to very end. Then 3 rd from right to very end. … Finally, farthest from right (leftmost) To very end. (11, 22, 33, 44) (11, 22, 44, 33) (11, 44, 33, 22) (44, 33, 22, 11)
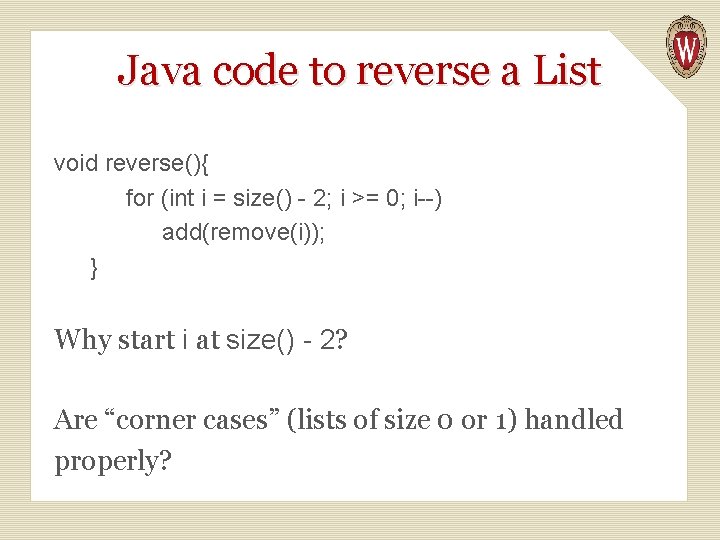
Java code to reverse a List void reverse(){ for (int i = size() - 2; i >= 0; i--) add(remove(i)); } Why start i at size() - 2? Are “corner cases” (lists of size 0 or 1) handled properly?
Whitely v chappel (1868)
Disadvantage of literal rule
Adler v george (1964)
Cmput 367
R v allen (1872) lr 1 ccr 367
Cs 367
631 to the nearest 10
Direct instruction strategies
Cs 367
01:640:244 lecture notes - lecture 15: plat, idah, farad
Introduction to data structures
Introduction to data structures
Examples of homologous
Introduction to biochemistry lecture notes
Introduction to psychology lecture
Introduction to algorithms lecture notes
Exploratory data analysis lecture notes
Bayesian classification in data mining lecture notes
Data mining lecture notes
Data visualization lecture
Data mining lecture notes
Data mining lecture notes
Introduction to data warehouse
Btechsmartclass data structures
Data structures in r
Oblivious data structures
Kernel data structures
Ajit diwan
Esoteric data structures
Geometric data structures
Cos 423
Data structures and algorithms tutorial
Writable wrappers for java primitives
Conditional macro expansion in system software
Advanced data structures in java
Assembler algorithm and data structures
Debasis samanta data structure
Persistent vs ephemeral data structures
Php data structures
What is data structure in gis
Information retrieval data structures and algorithms
Java dynamic data structures
Recurrence data structures
Data structures in c ppt
Data structures for parallel computing
Data structures and abstractions with java
Data structures for language processing
Data structures and algorithms bits pilani
Cos 423
Disjoint set adt
Stacks in data structures
Fundamentals of data structures in c
Data structures in prolog
Unit 1 introduction
Polynomial addition in data structure using linked list
Adts, data structures, and problem solving with c++
Dynamic data structures
Data structures and algorithms iit bombay
Hybrid data structures
Graphical user interface in data structures
Data structures and algorithm
Java data structures