Sorting Part I CS 367 Introduction to Data
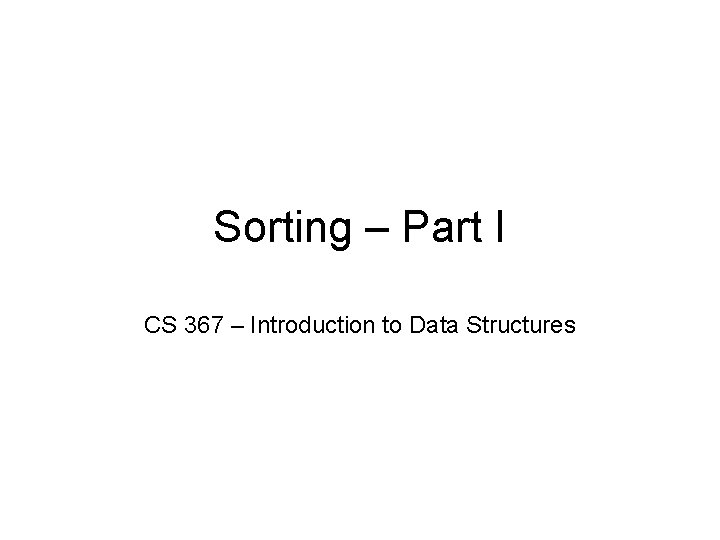
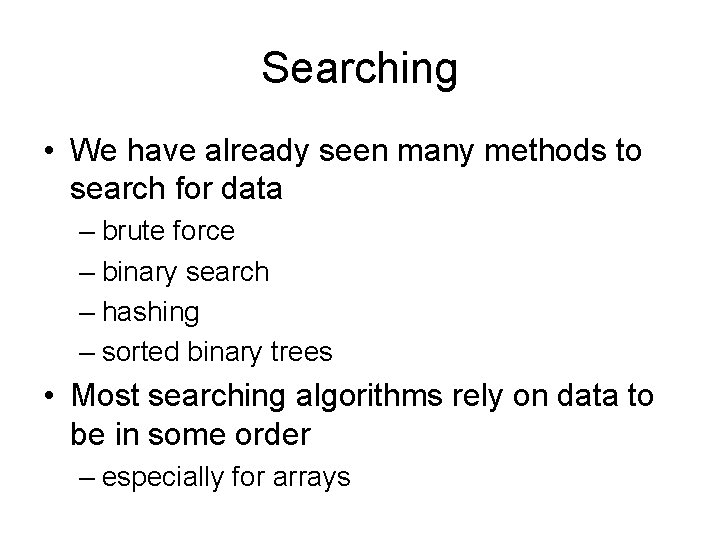
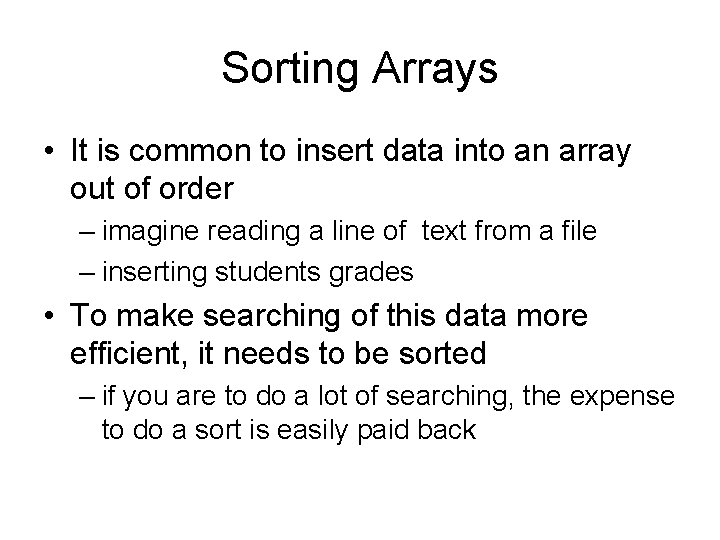
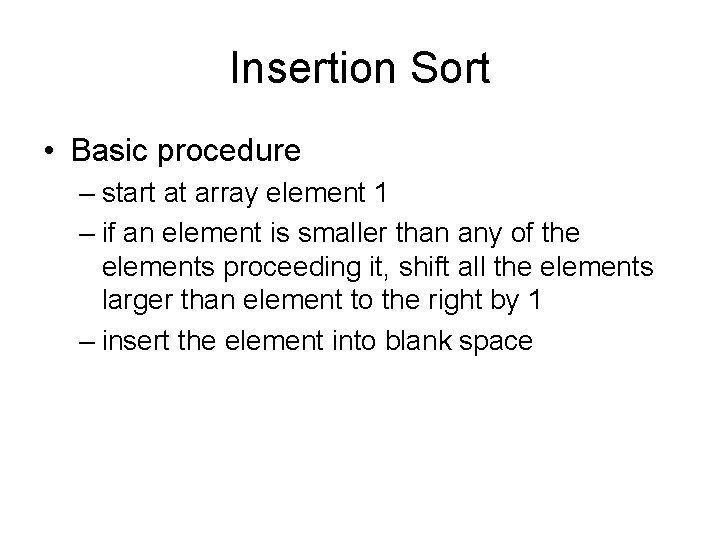
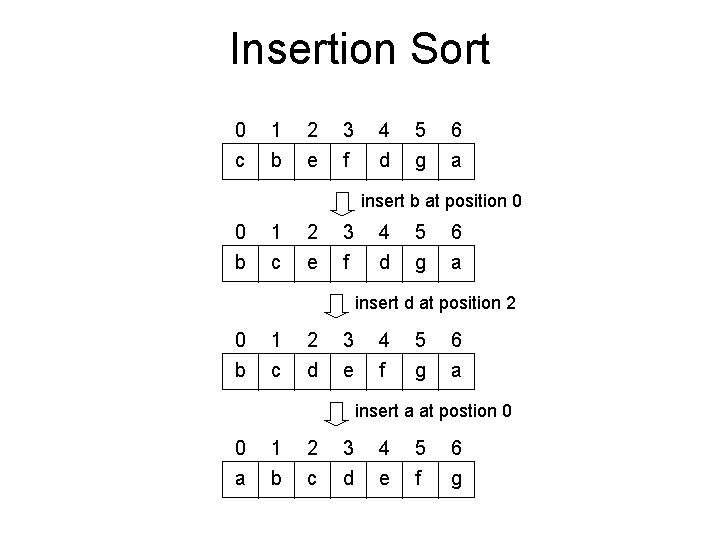
![Insertion Sort • Code public void insertion. Sort(Object[ ] data) { Comparable tmp; int Insertion Sort • Code public void insertion. Sort(Object[ ] data) { Comparable tmp; int](https://slidetodoc.com/presentation_image_h2/2e7100ed4f91e1c03932f855bd6a8d68/image-6.jpg)
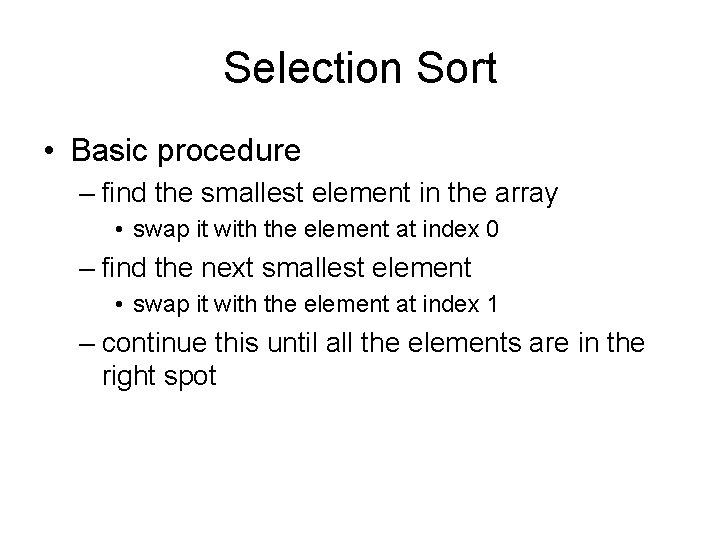
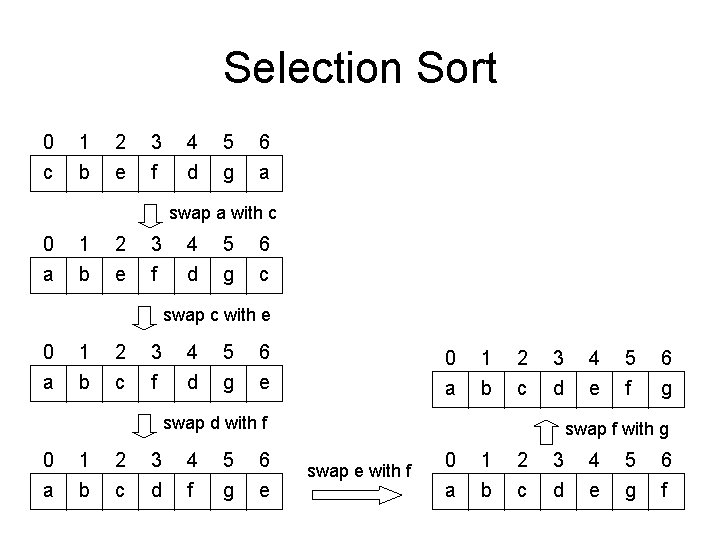
![Selection Sort • Code public void selection. Sort(Object[ ] data) { int i, k, Selection Sort • Code public void selection. Sort(Object[ ] data) { int i, k,](https://slidetodoc.com/presentation_image_h2/2e7100ed4f91e1c03932f855bd6a8d68/image-9.jpg)
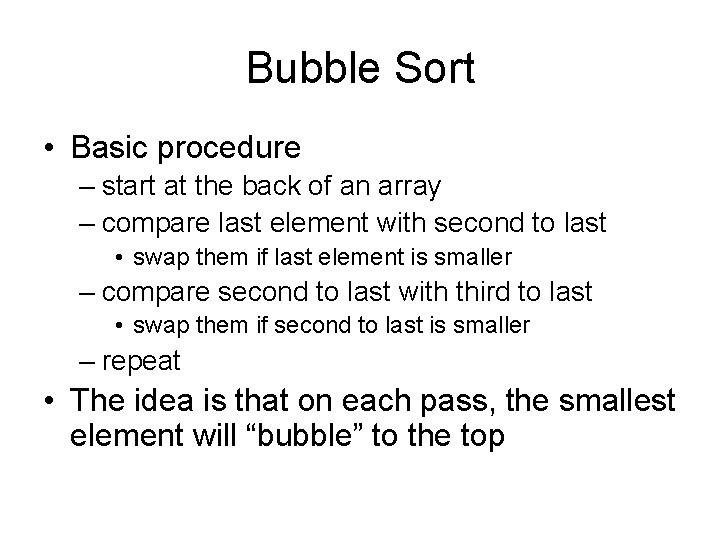
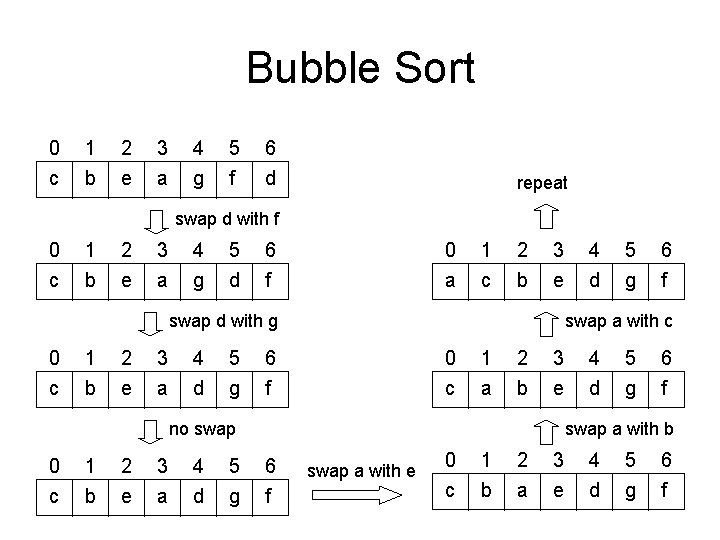
![Bubble Sort • Code public void bubble. Sort(Object[ ] data) { int i, k; Bubble Sort • Code public void bubble. Sort(Object[ ] data) { int i, k;](https://slidetodoc.com/presentation_image_h2/2e7100ed4f91e1c03932f855bd6a8d68/image-12.jpg)
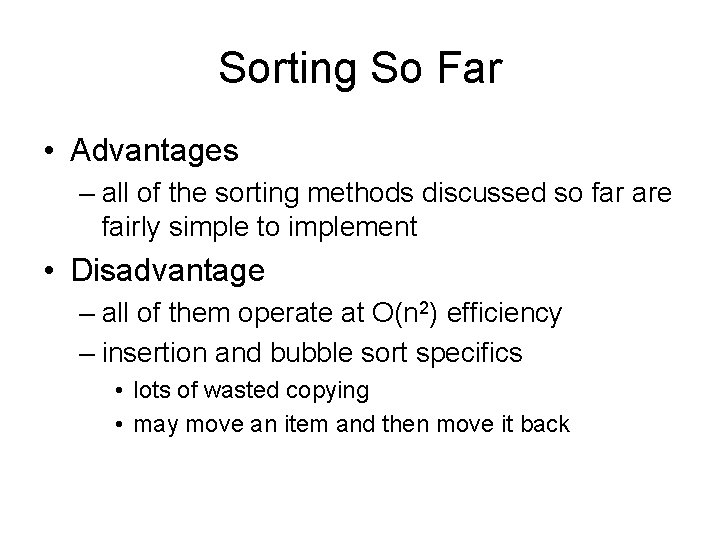
- Slides: 13
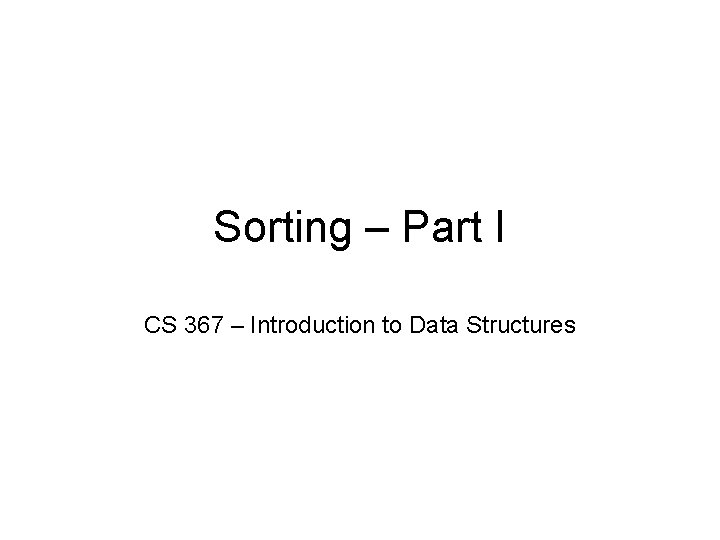
Sorting – Part I CS 367 – Introduction to Data Structures
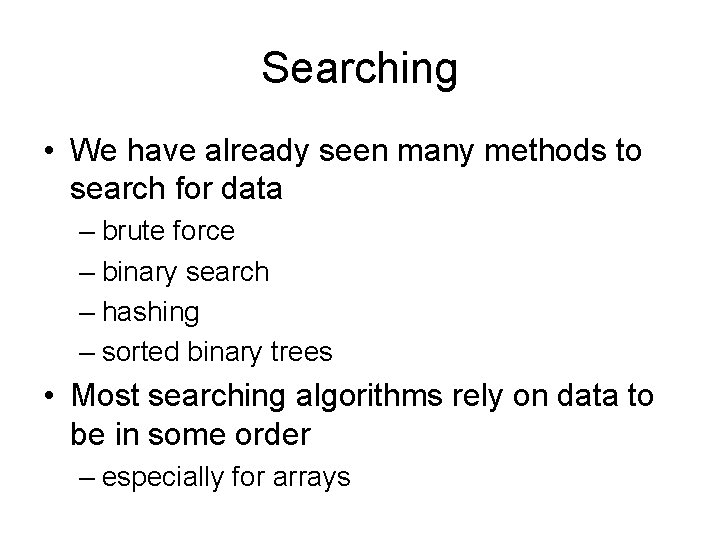
Searching • We have already seen many methods to search for data – brute force – binary search – hashing – sorted binary trees • Most searching algorithms rely on data to be in some order – especially for arrays
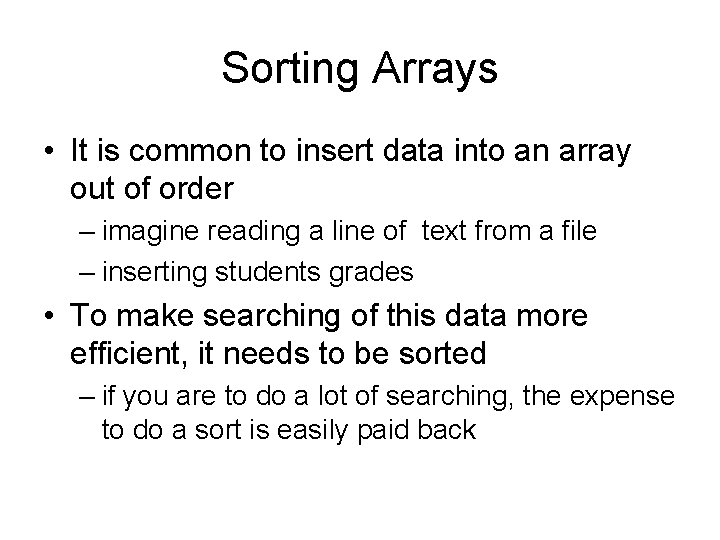
Sorting Arrays • It is common to insert data into an array out of order – imagine reading a line of text from a file – inserting students grades • To make searching of this data more efficient, it needs to be sorted – if you are to do a lot of searching, the expense to do a sort is easily paid back
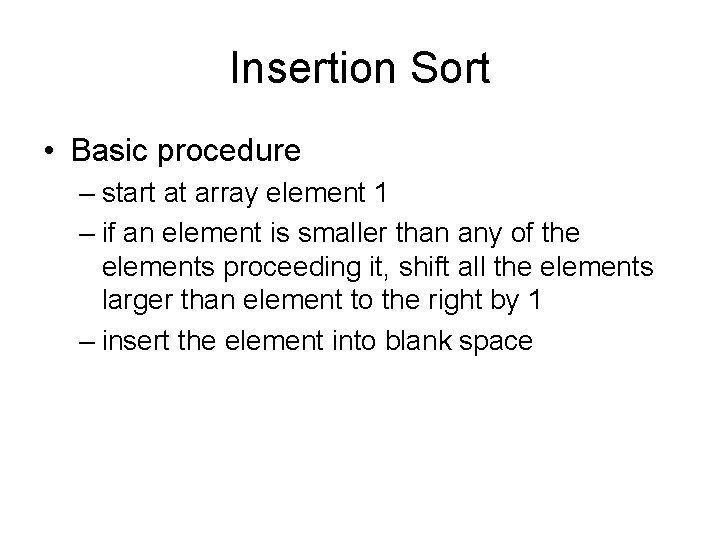
Insertion Sort • Basic procedure – start at array element 1 – if an element is smaller than any of the elements proceeding it, shift all the elements larger than element to the right by 1 – insert the element into blank space
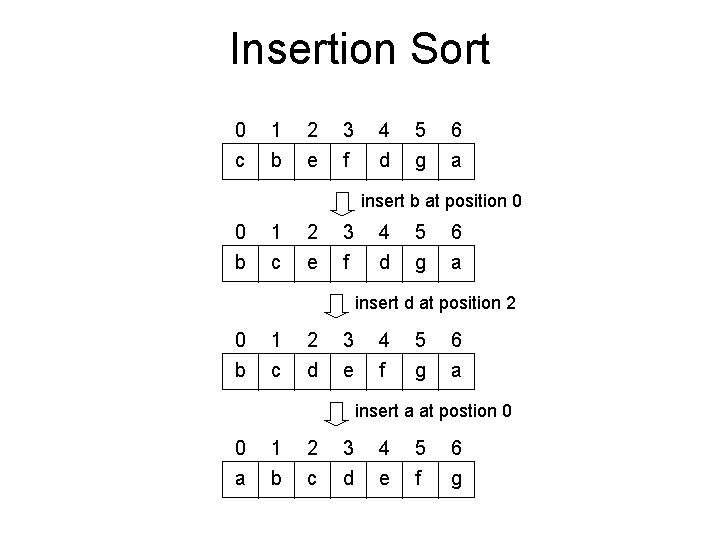
Insertion Sort 0 1 2 3 4 5 6 c b e f d g a insert b at position 0 0 b 1 c 2 e 3 f 4 d 5 g 6 a insert d at position 2 0 b 1 c 2 d 3 e 4 f 5 g 6 a insert a at postion 0 0 a 1 b 2 c 3 d 4 e 5 f 6 g
![Insertion Sort Code public void insertion SortObject data Comparable tmp int Insertion Sort • Code public void insertion. Sort(Object[ ] data) { Comparable tmp; int](https://slidetodoc.com/presentation_image_h2/2e7100ed4f91e1c03932f855bd6a8d68/image-6.jpg)
Insertion Sort • Code public void insertion. Sort(Object[ ] data) { Comparable tmp; int i, k; for(i = 1; i < data. length; i++) { tmp = Object[i]; for(k = i; (k>0) && tmp. compare. To(data[k-1]) < 0; k--) data[k] = data[k-1]; data[i] = tmp; } }
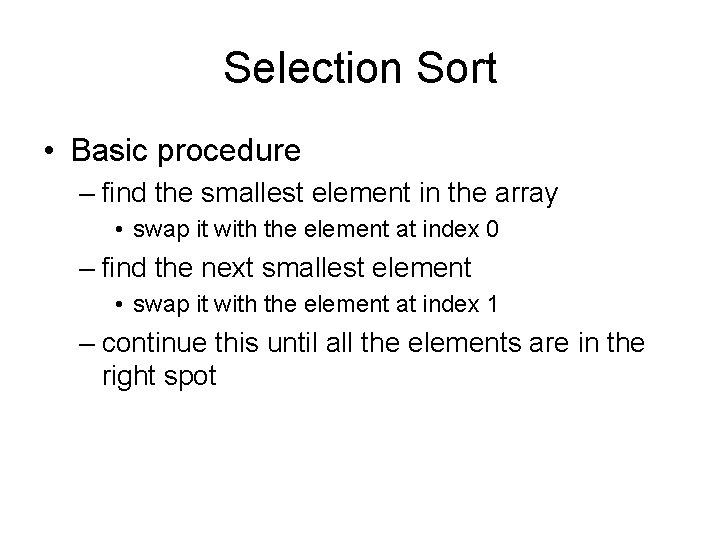
Selection Sort • Basic procedure – find the smallest element in the array • swap it with the element at index 0 – find the next smallest element • swap it with the element at index 1 – continue this until all the elements are in the right spot
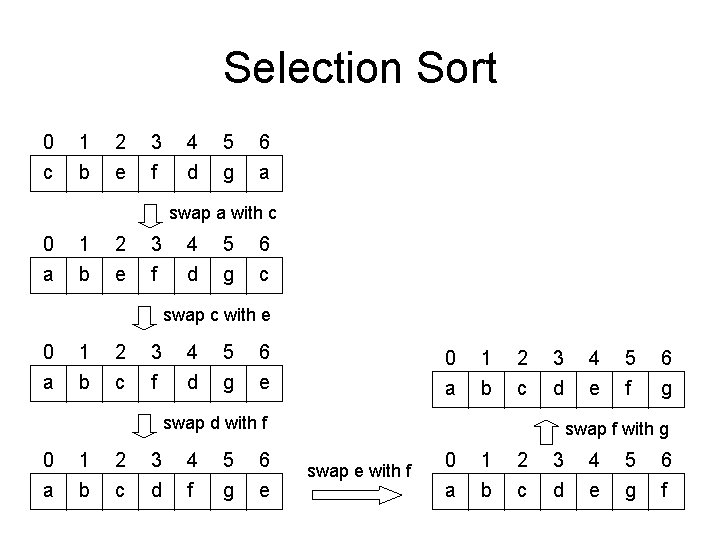
Selection Sort 0 c 1 b 2 e 3 f 4 d 5 g 6 a swap a with c 0 a 1 b 2 e 3 f 4 d 5 g 6 c swap c with e 0 a 1 b 2 c 3 f 4 d 5 g 6 e 0 a 1 b 2 c 3 d swap d with f 0 a 1 b 2 c 3 d 4 f 5 g 6 e 4 e 5 f 6 g swap f with g swap e with f 0 a 1 b 2 c 3 d 4 e 5 g 6 f
![Selection Sort Code public void selection SortObject data int i k Selection Sort • Code public void selection. Sort(Object[ ] data) { int i, k,](https://slidetodoc.com/presentation_image_h2/2e7100ed4f91e1c03932f855bd6a8d68/image-9.jpg)
Selection Sort • Code public void selection. Sort(Object[ ] data) { int i, k, least; for(i=0; i<data. length-1; i++) { least = i; for(k = i+1; k<data. length; k++) { if(((Comparable)data[k]). compare. To(data[least]) < 0) least = k; } swap(i, least); } }
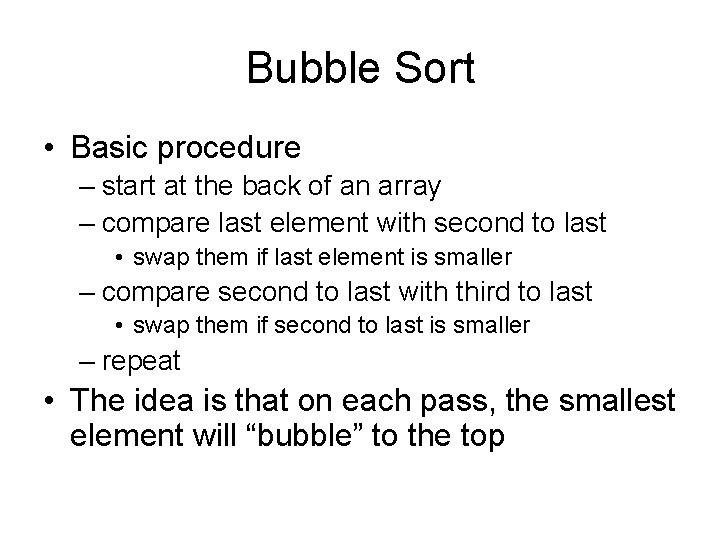
Bubble Sort • Basic procedure – start at the back of an array – compare last element with second to last • swap them if last element is smaller – compare second to last with third to last • swap them if second to last is smaller – repeat • The idea is that on each pass, the smallest element will “bubble” to the top
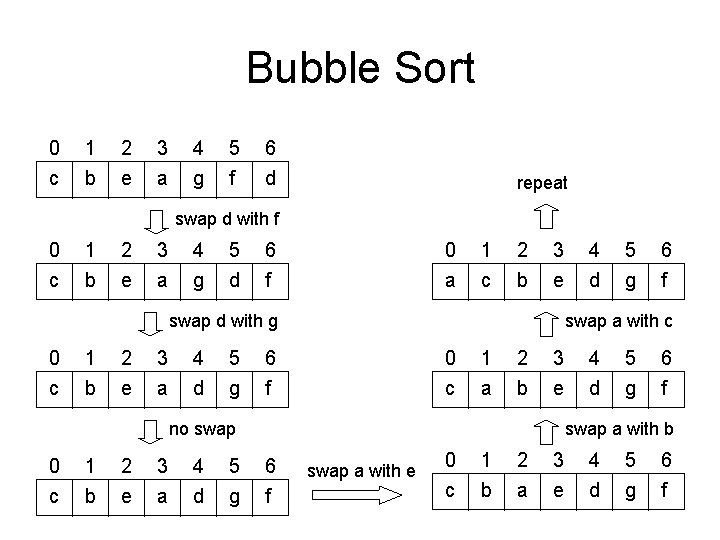
Bubble Sort 0 c 1 b 2 e 3 a 4 g 5 f 6 d repeat swap d with f 0 c 1 b 2 e 3 a 4 g 5 d 6 f 0 a 1 c 2 b 3 e swap d with g 0 c 1 b 2 e 3 a 4 d 5 g 5 g 6 f swap a with c 6 f 0 c 1 a 2 b 3 e no swap 0 c 4 d 5 g 6 f swap a with b 6 f swap a with e 0 c 1 b 2 a 3 e 4 d 5 g 6 f
![Bubble Sort Code public void bubble SortObject data int i k Bubble Sort • Code public void bubble. Sort(Object[ ] data) { int i, k;](https://slidetodoc.com/presentation_image_h2/2e7100ed4f91e1c03932f855bd6a8d68/image-12.jpg)
Bubble Sort • Code public void bubble. Sort(Object[ ] data) { int i, k; for(i = 0; i<data. length – 1; i++) { for(k = data. length – 1; k > i; k- - ) if(((Comparable)data[k]). compare. To(data[k-1]) < 0) swap(k, k-1); } }
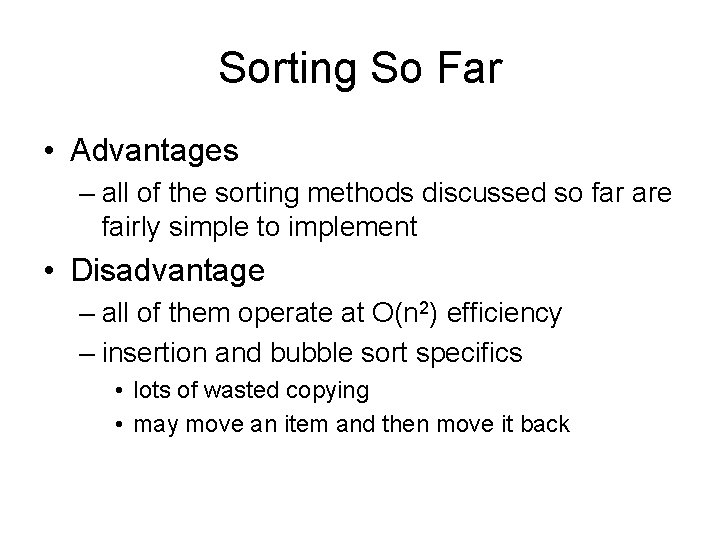
Sorting So Far • Advantages – all of the sorting methods discussed so far are fairly simple to implement • Disadvantage – all of them operate at O(n 2) efficiency – insertion and bubble sort specifics • lots of wasted copying • may move an item and then move it back
Internal vs external sorting
Poder judicial
Advantages of mischief rule
R v allen (1872)
Cmput 367
R v allen (1872) lr 1 ccr 367
Cs367
What is 248 rounded to the nearest hundred
Direct vs indirect instruction
Cs 367
Introduction to sorting
Introduction to sorting algorithms
Tujuan dari sorting adalah
Restricting and sorting data in oracle