CHAPTER 2 Advanced flow control and data aggregates
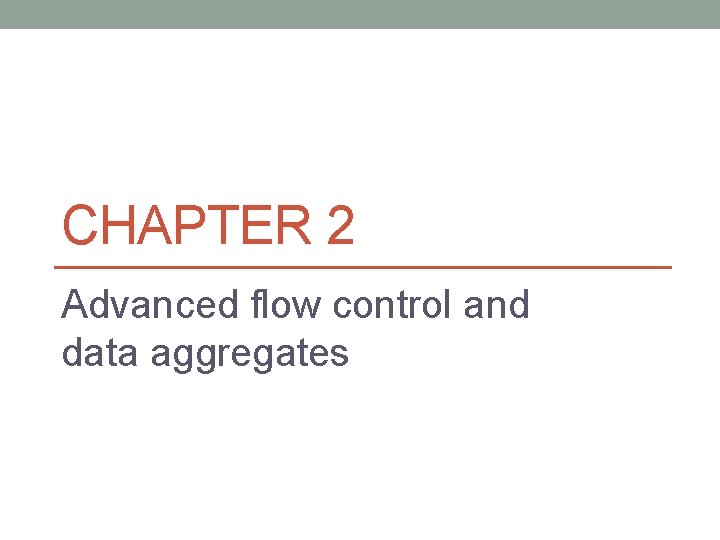
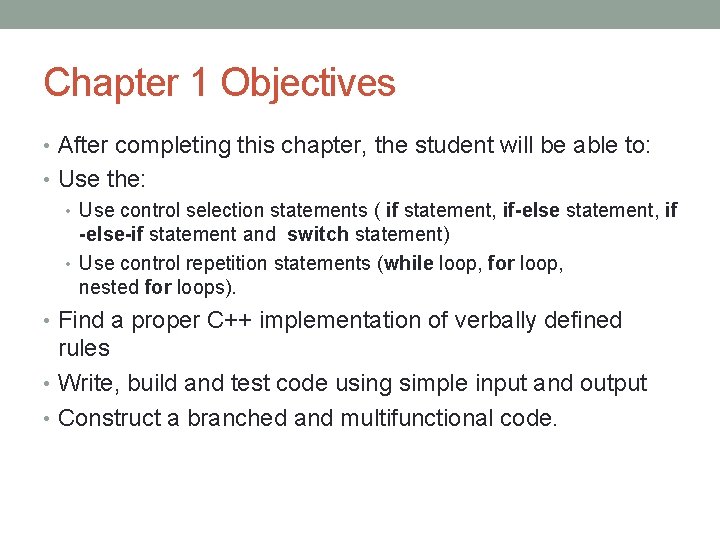
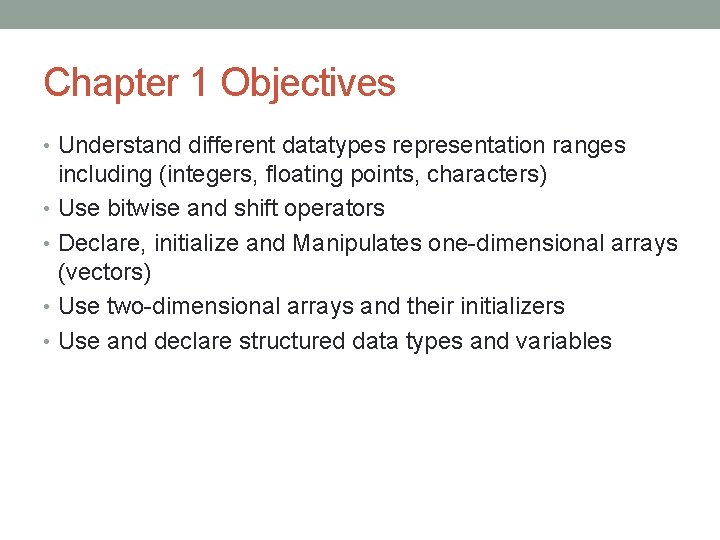
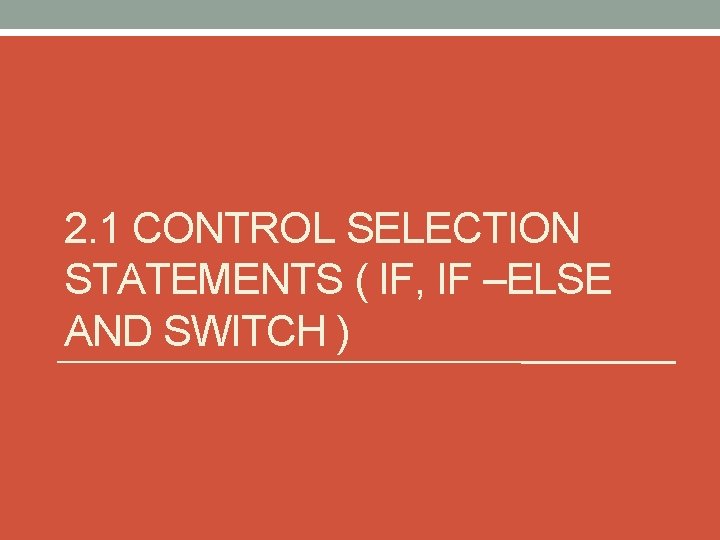
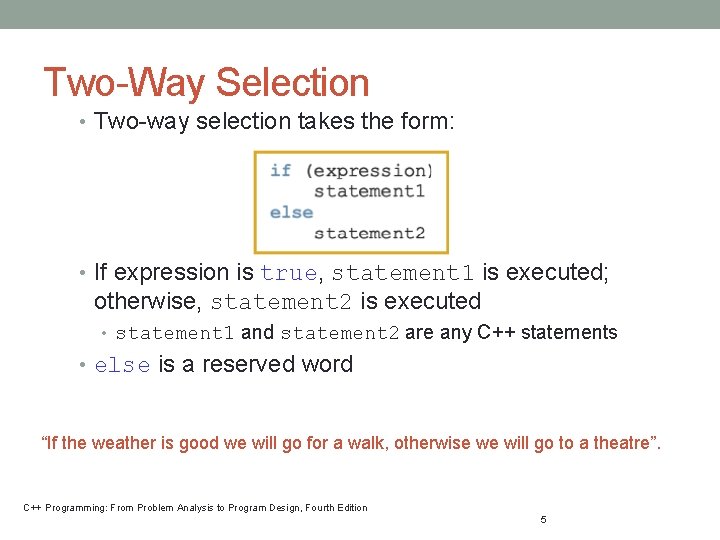
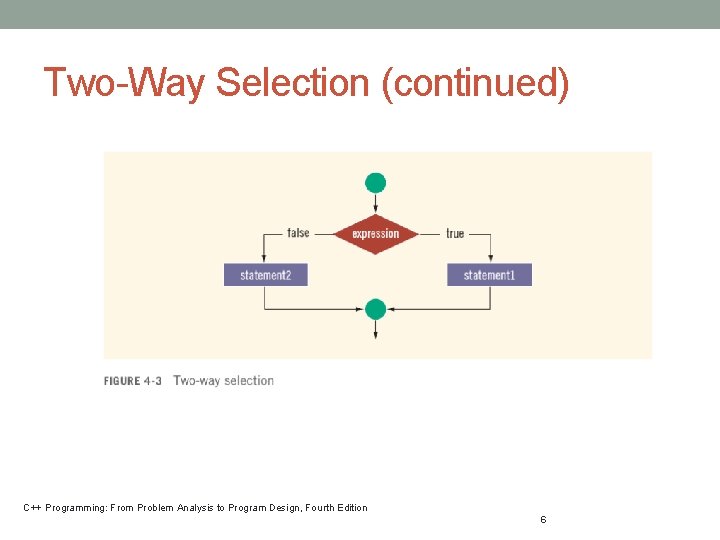
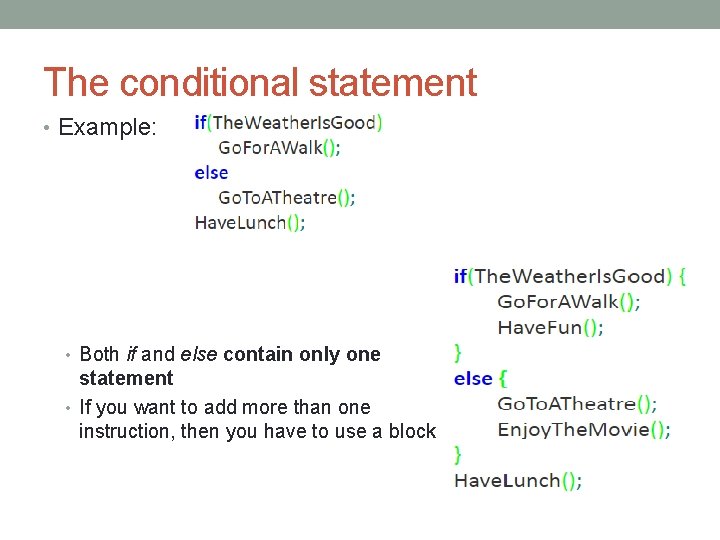
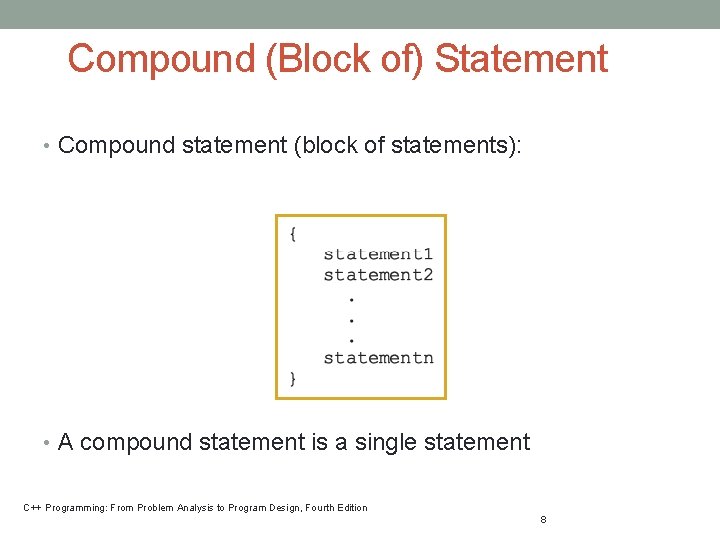
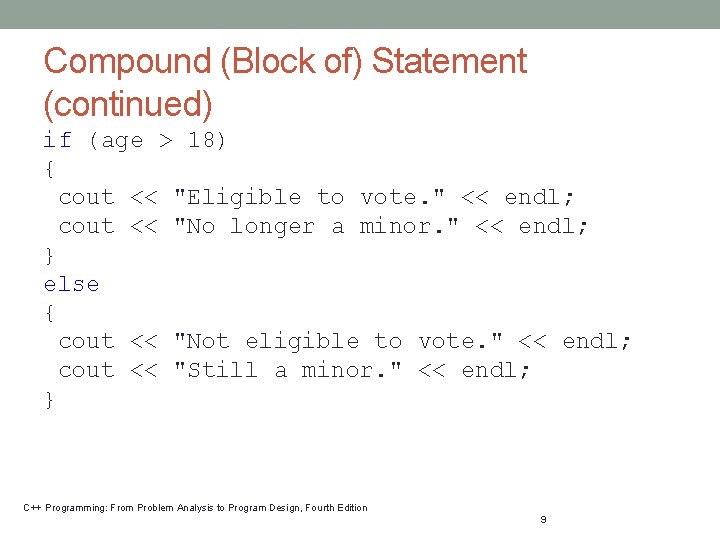
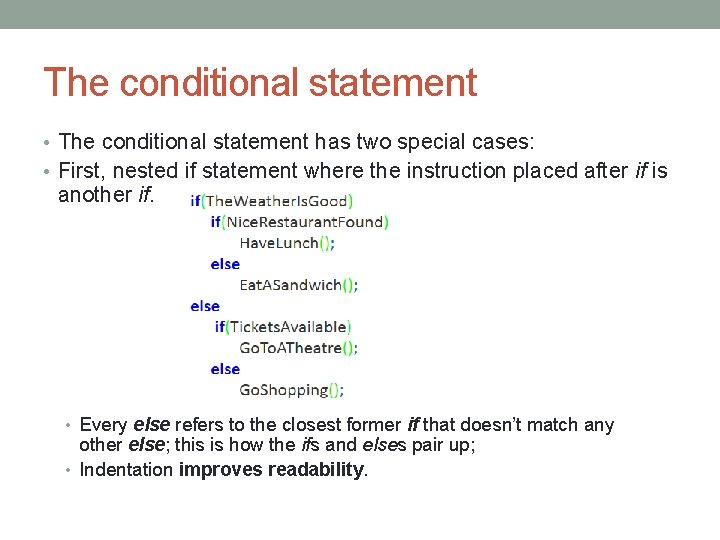
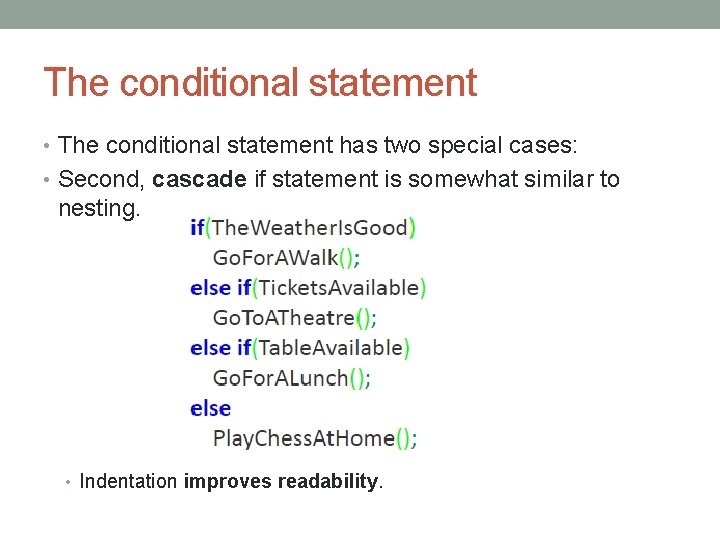
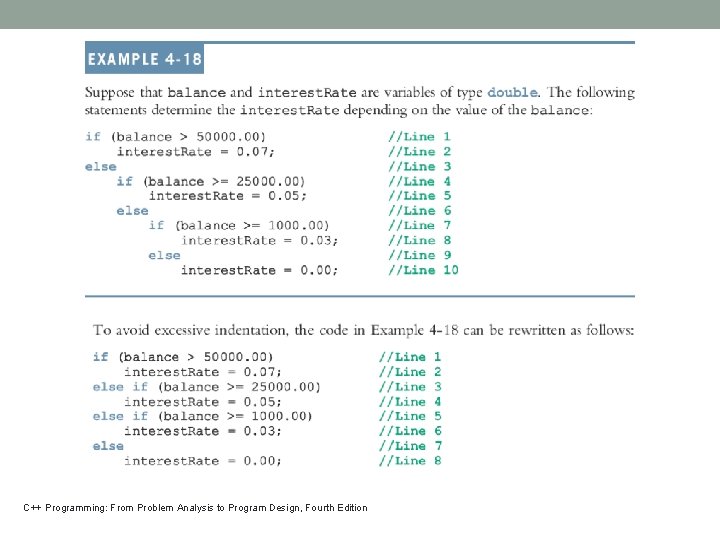
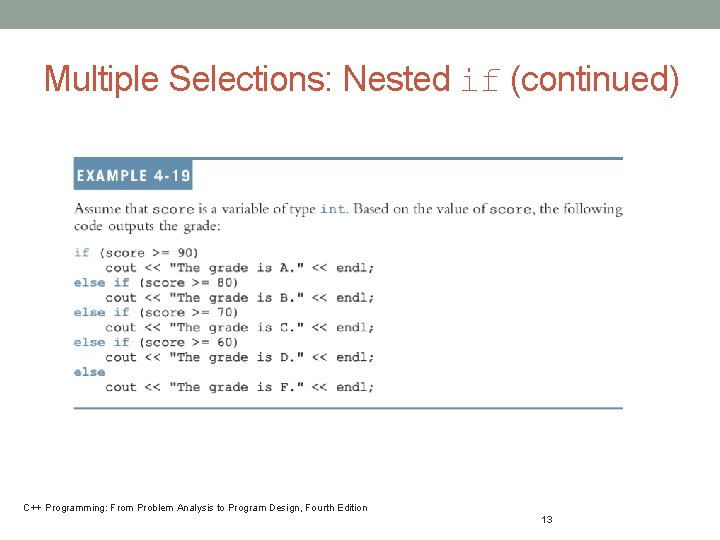
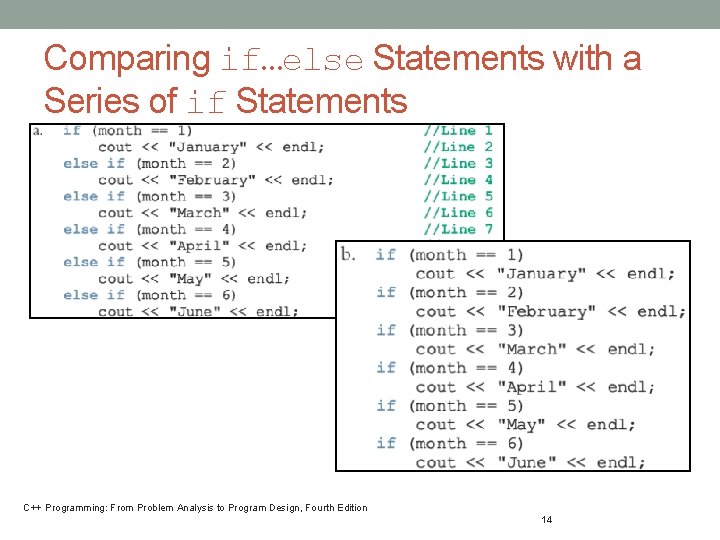
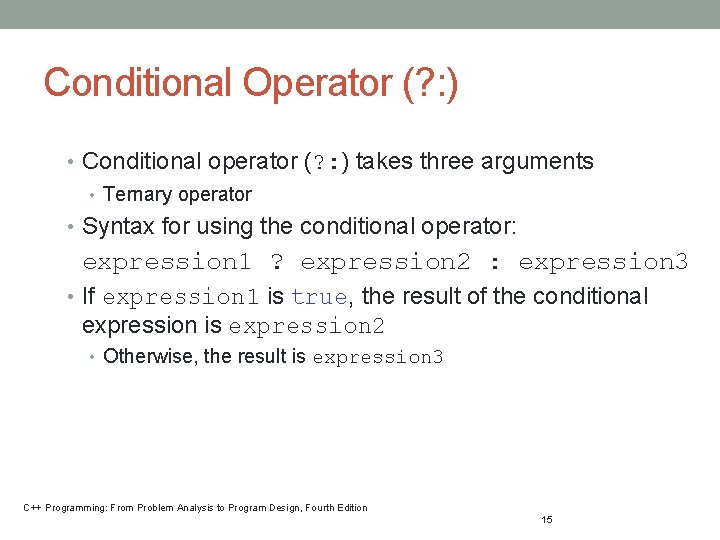
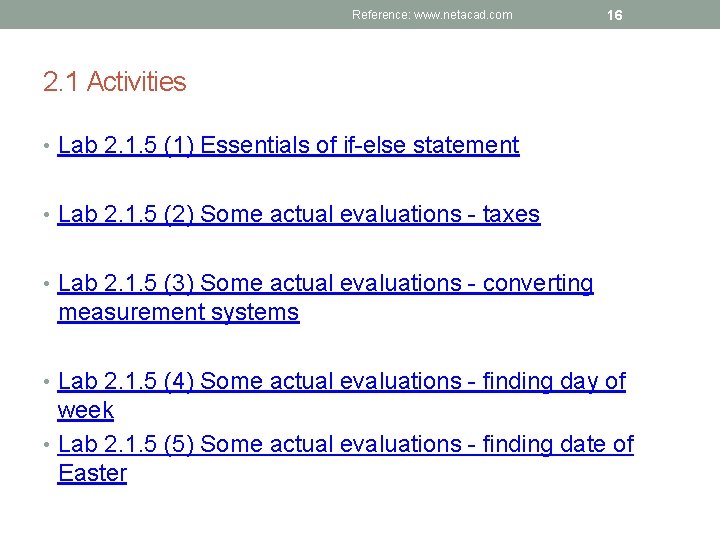
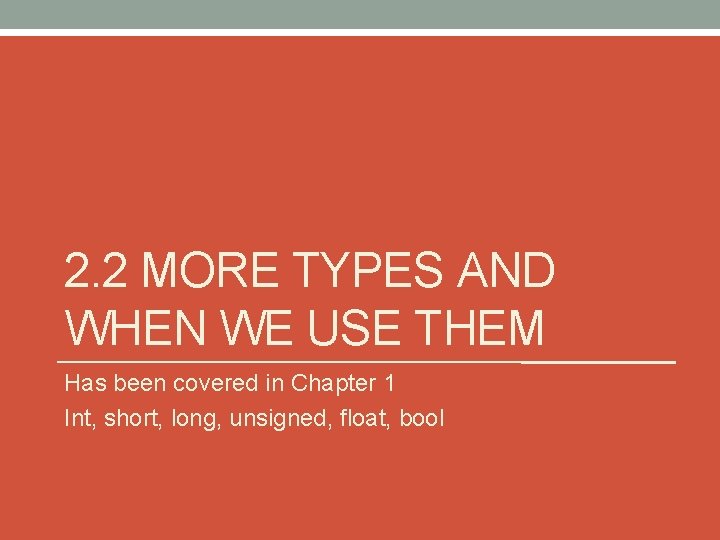
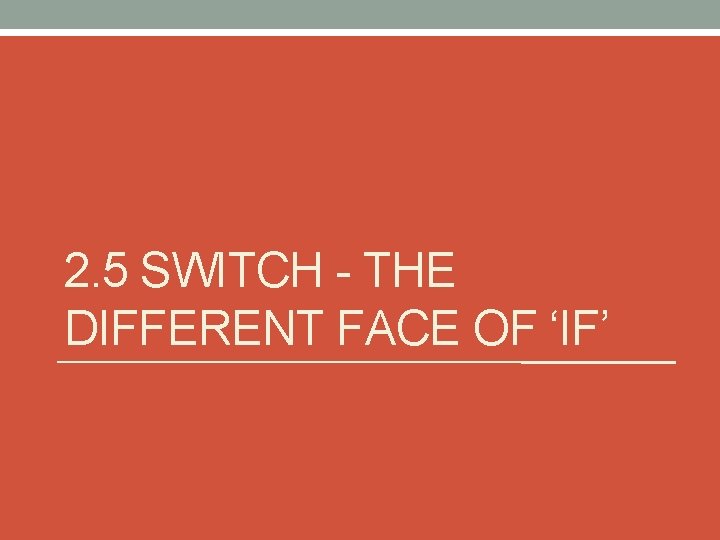
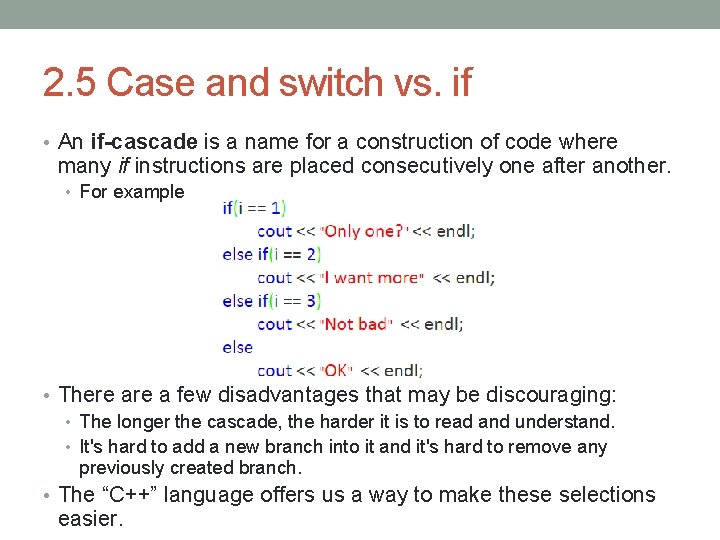
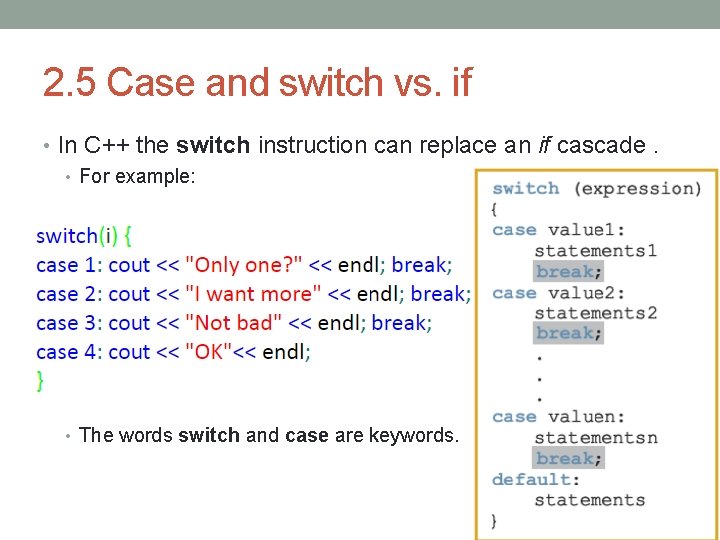
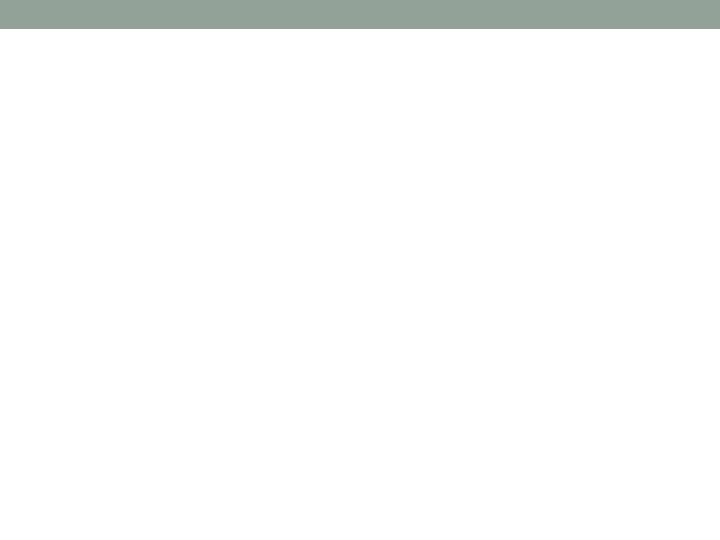
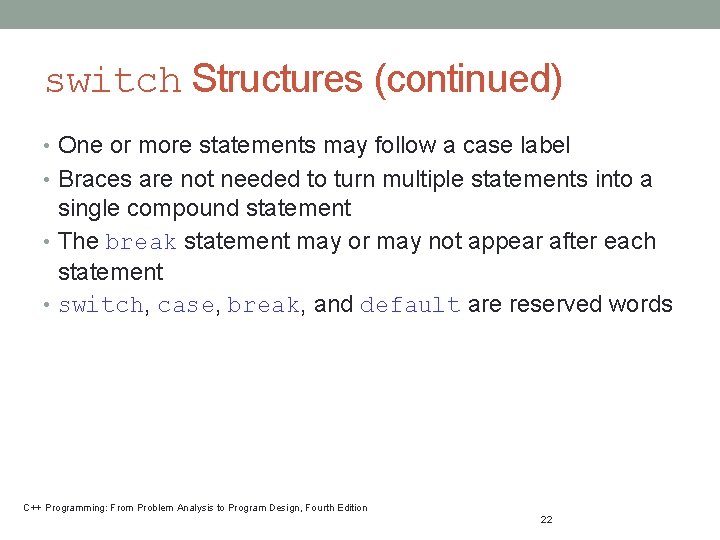
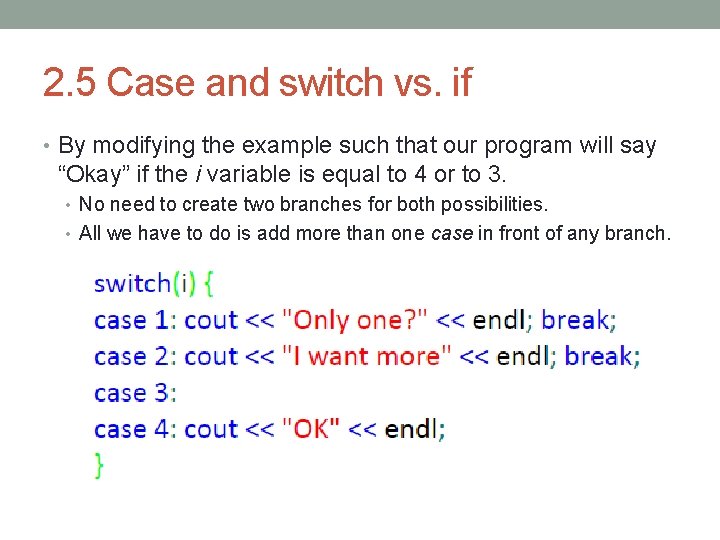
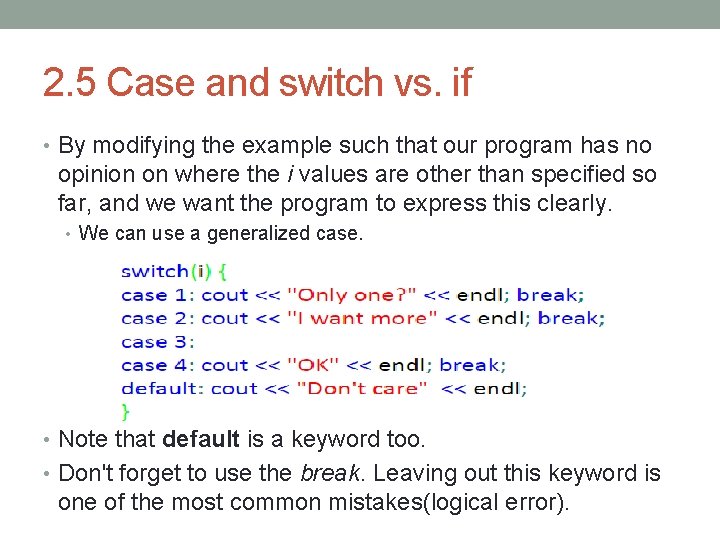
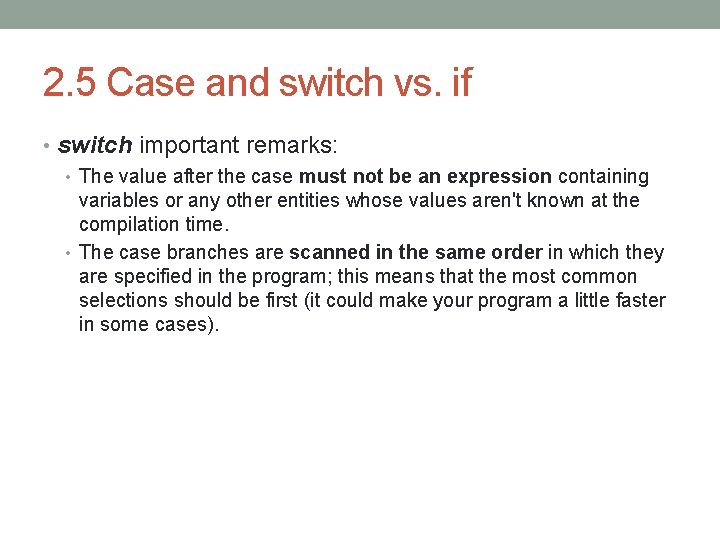
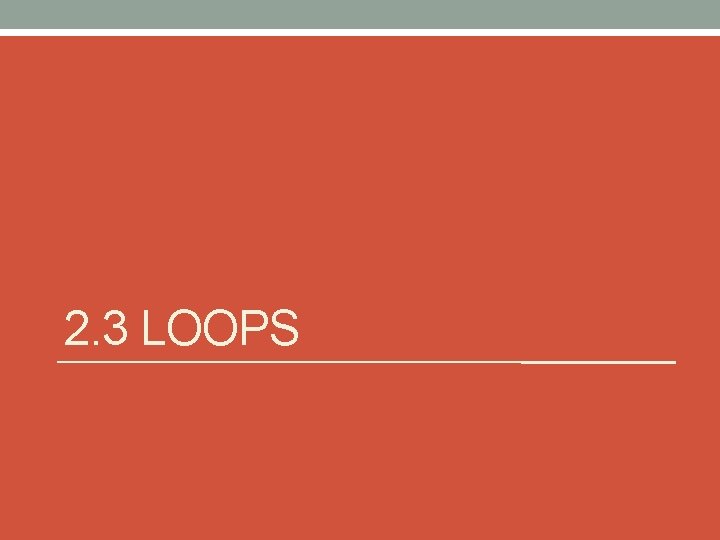
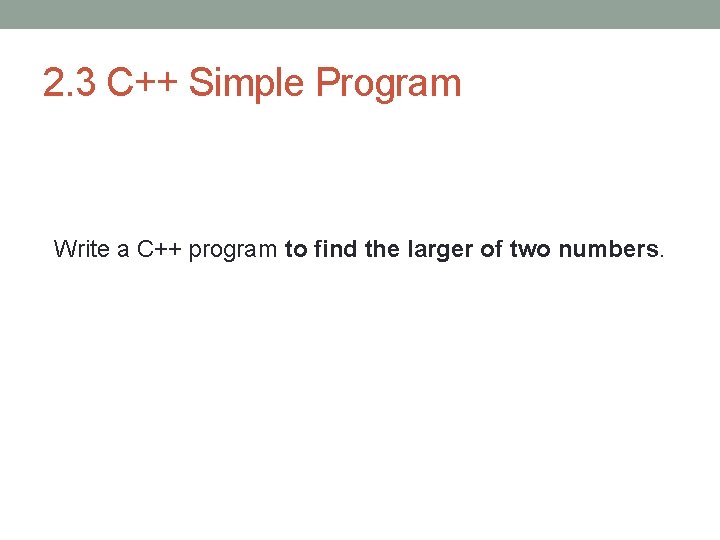
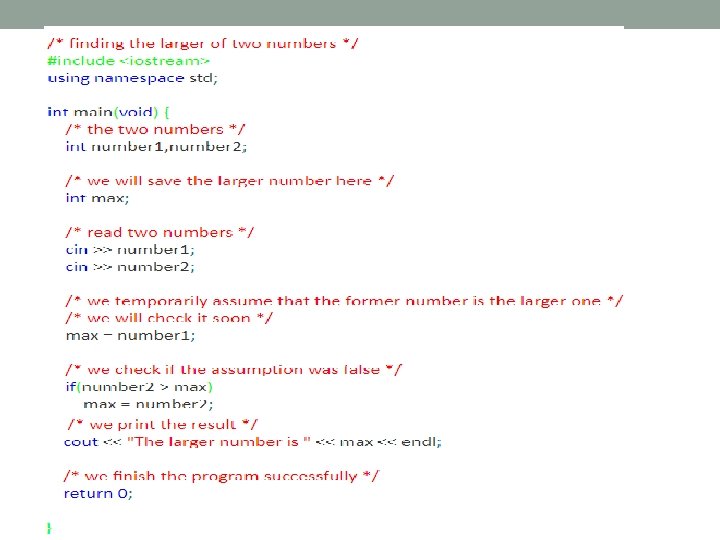
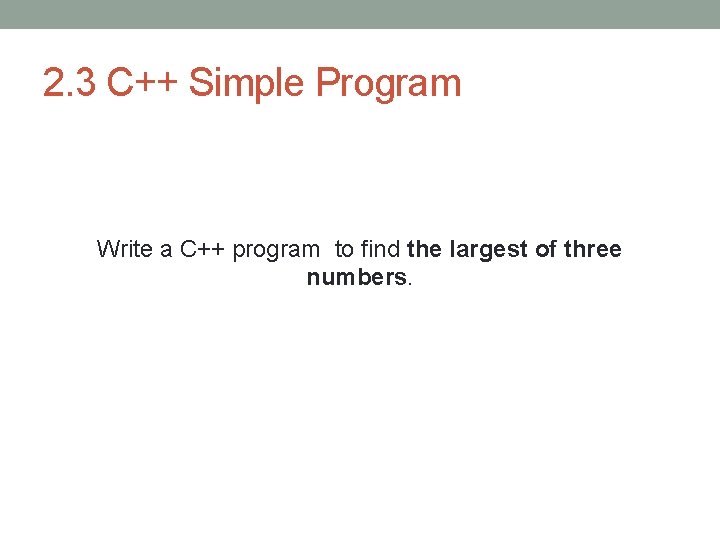
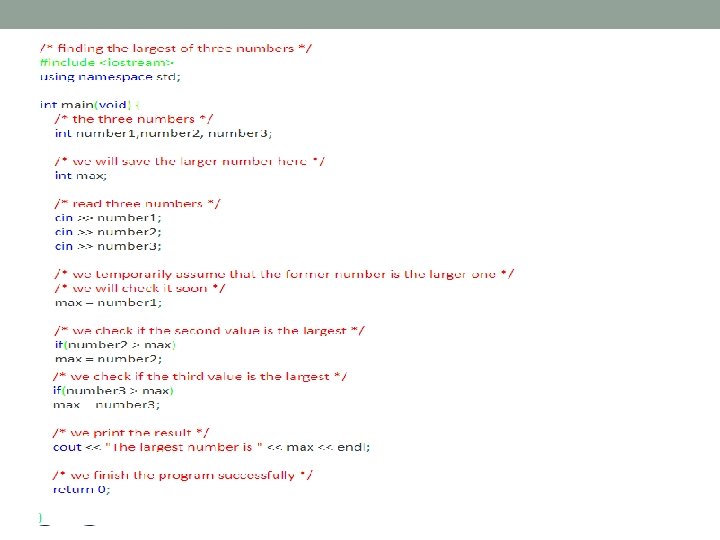
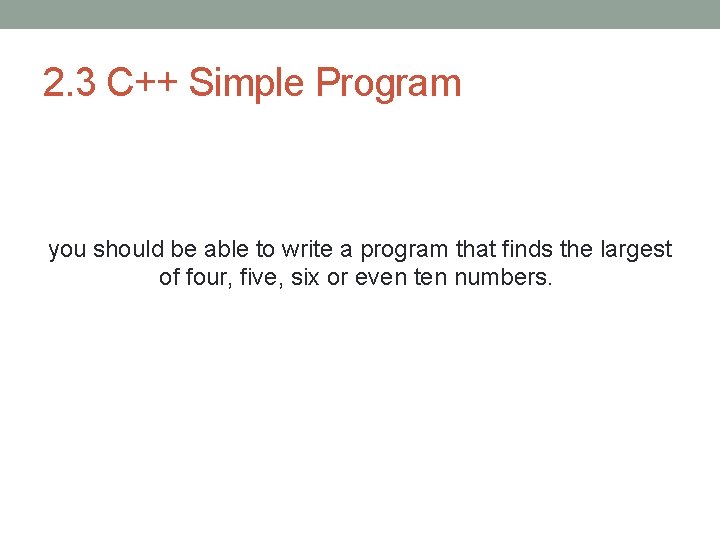
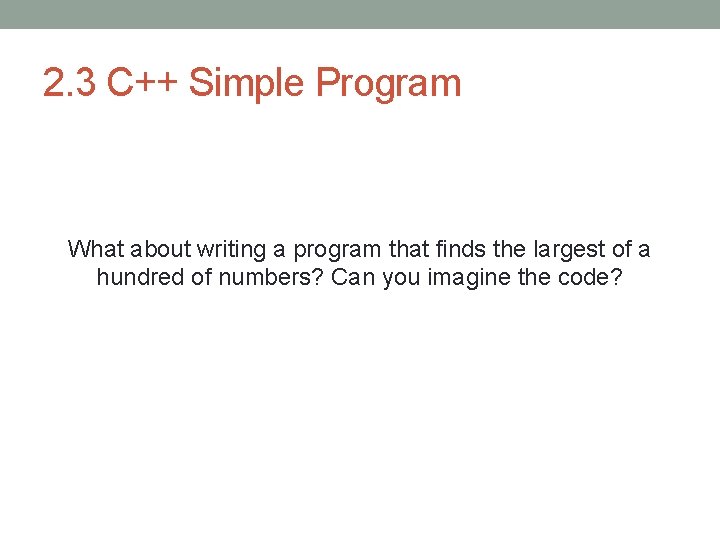
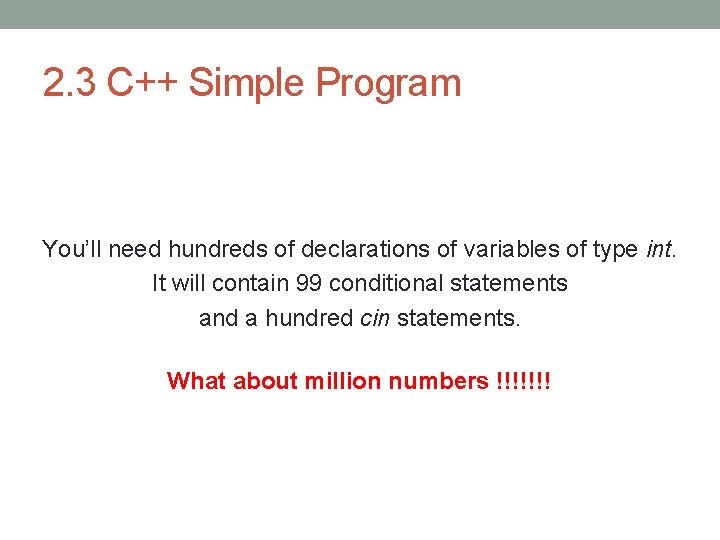
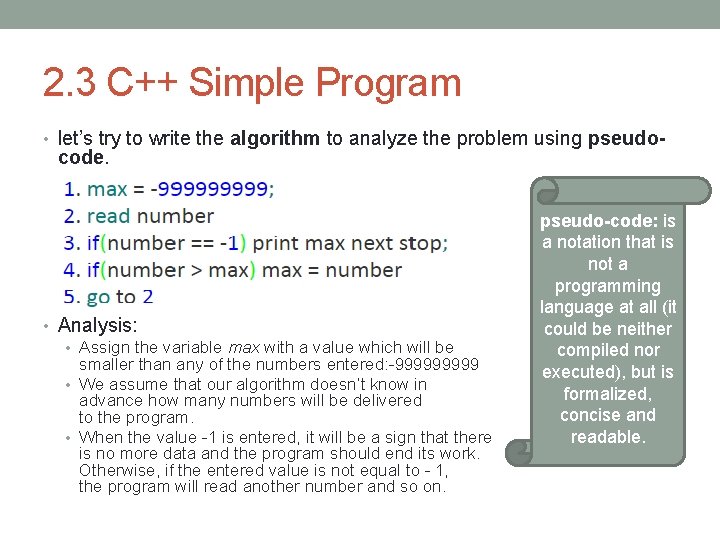
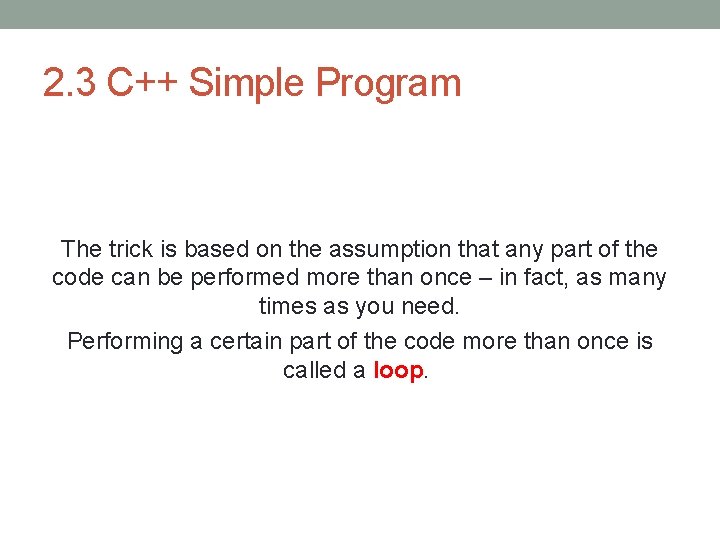
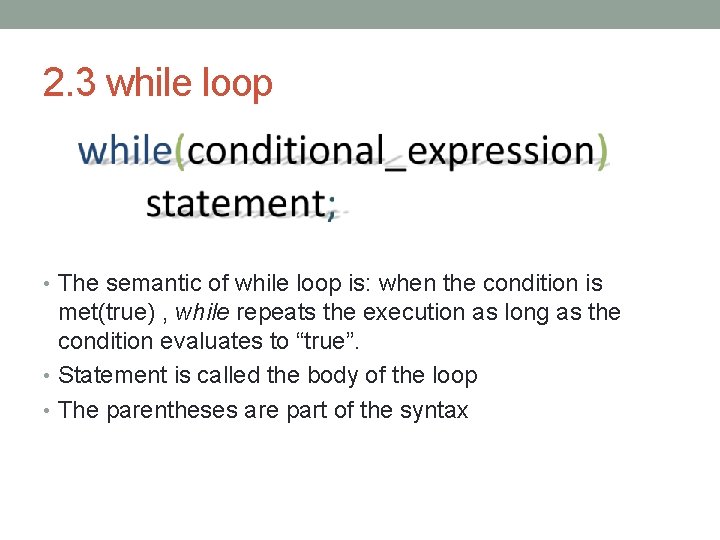
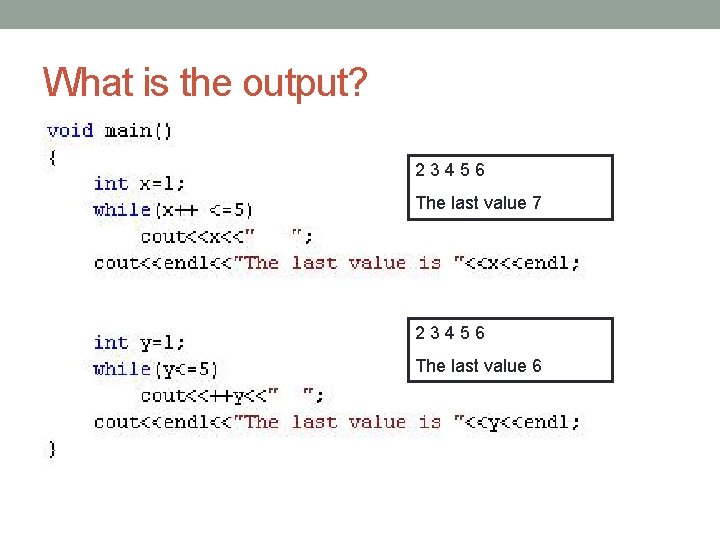
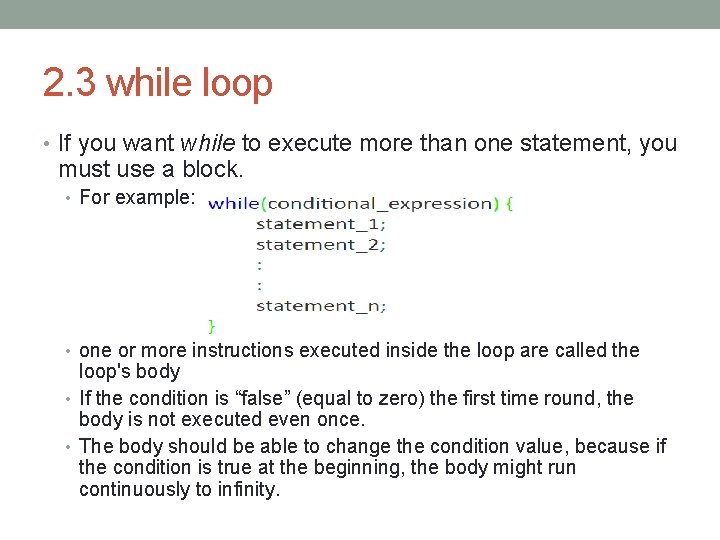
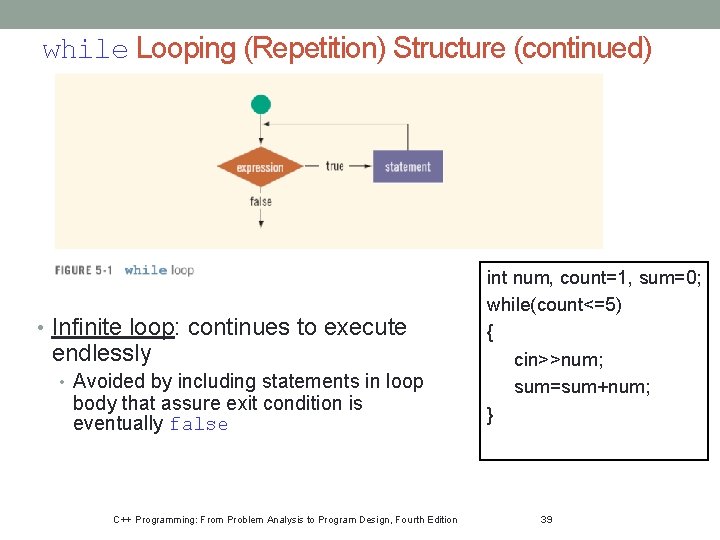
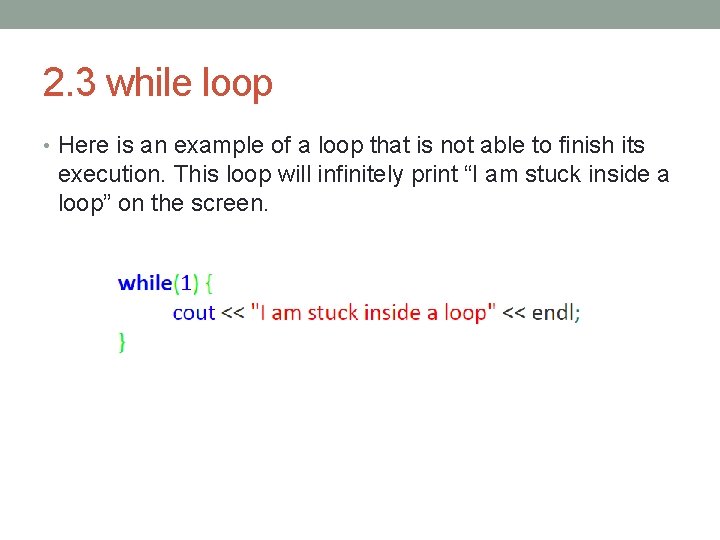
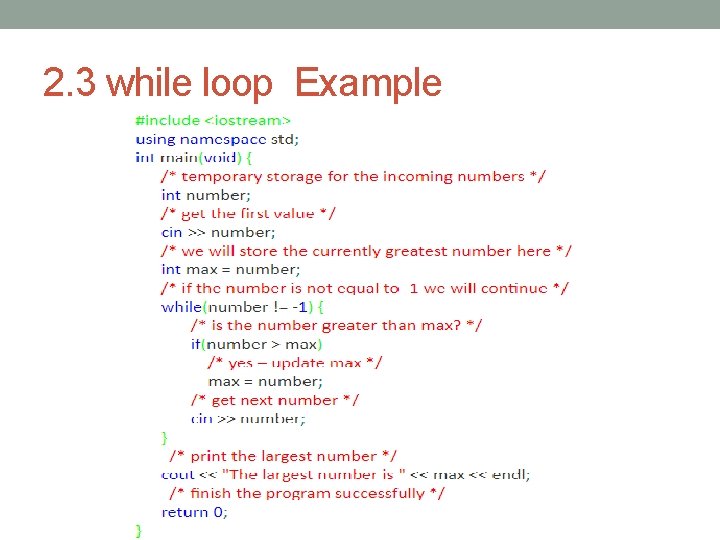
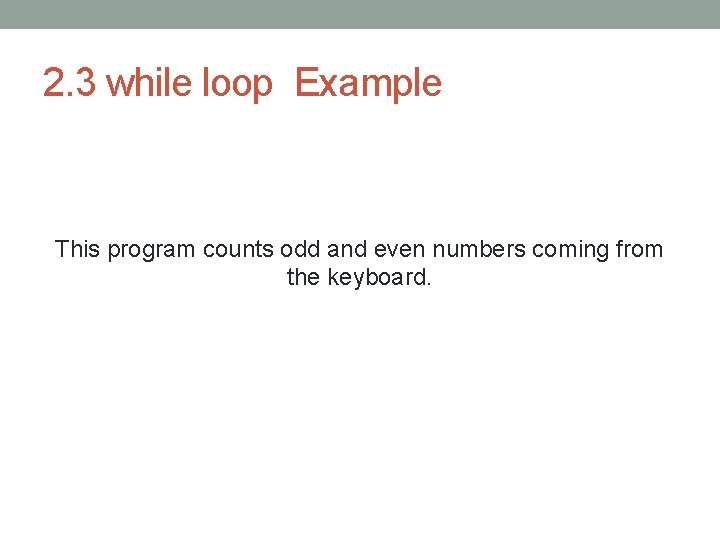
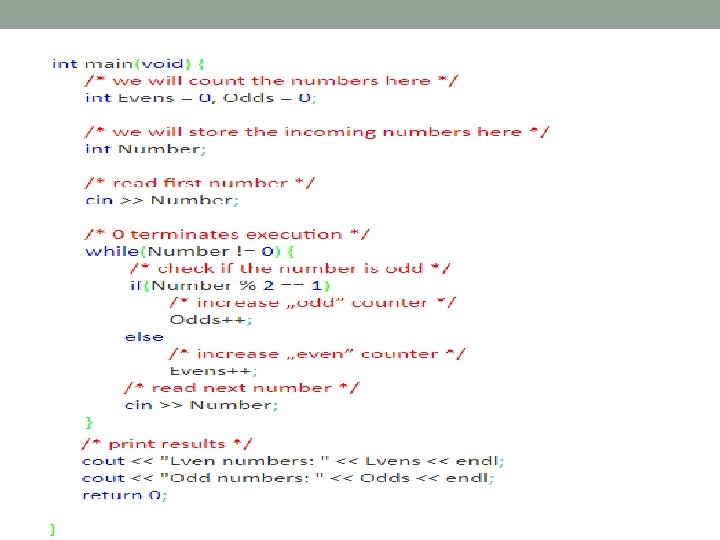
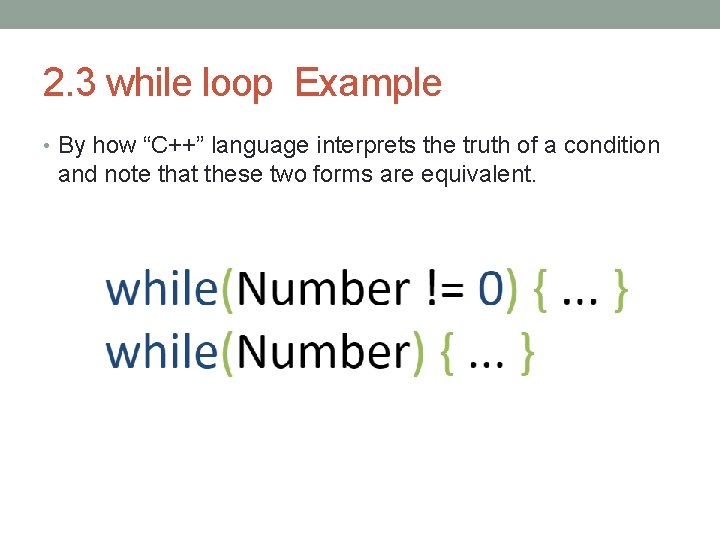
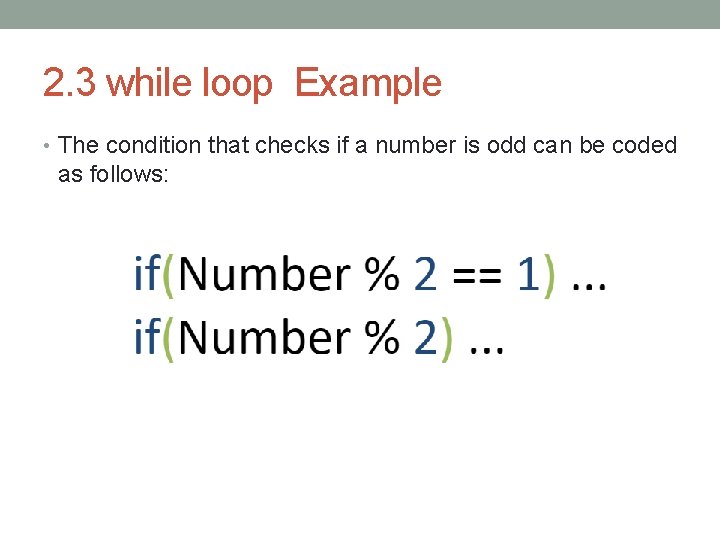
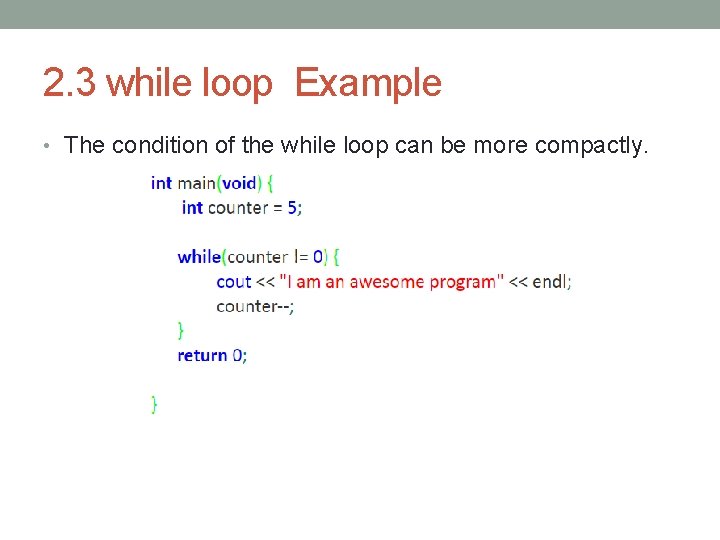
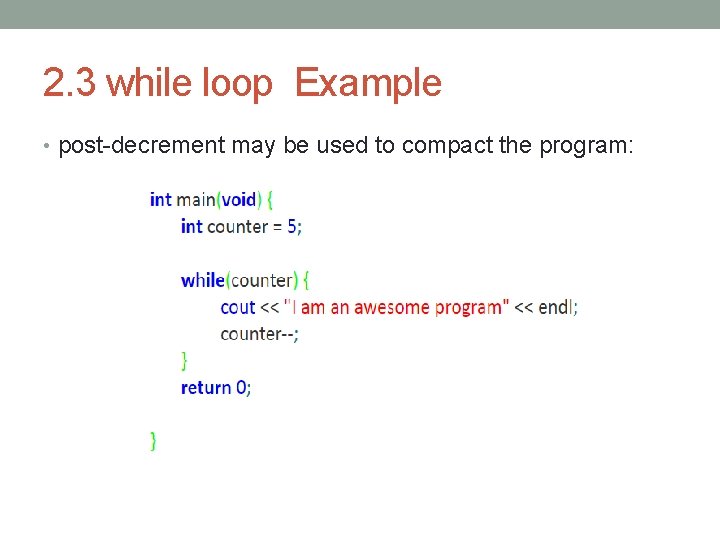
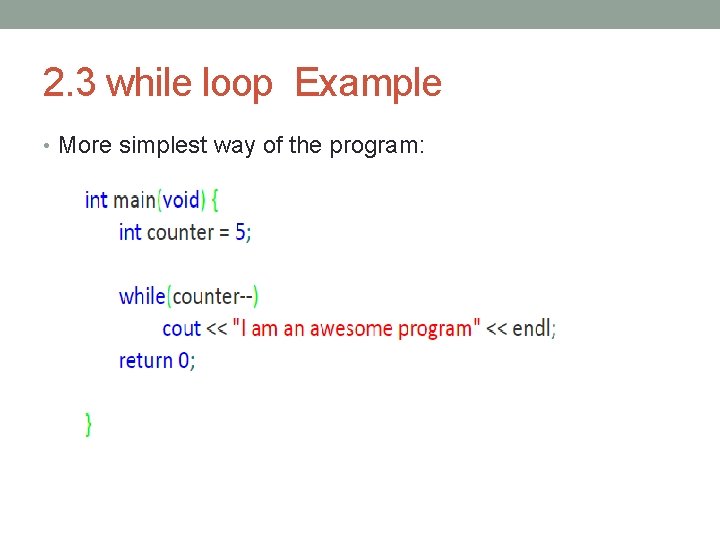
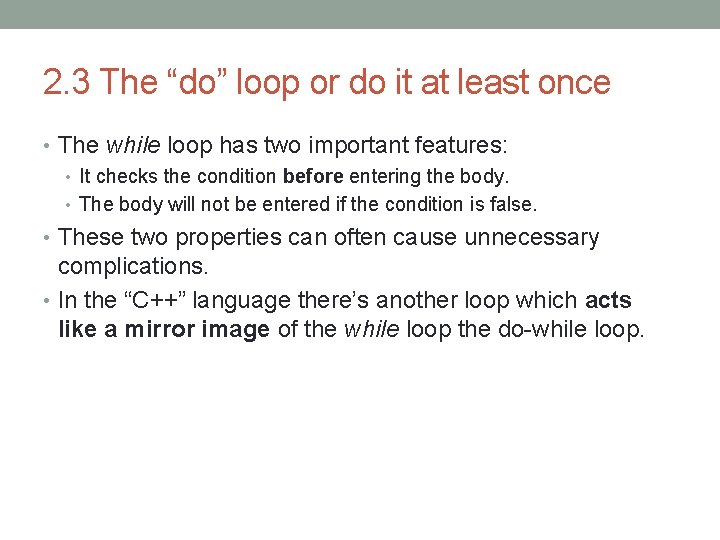
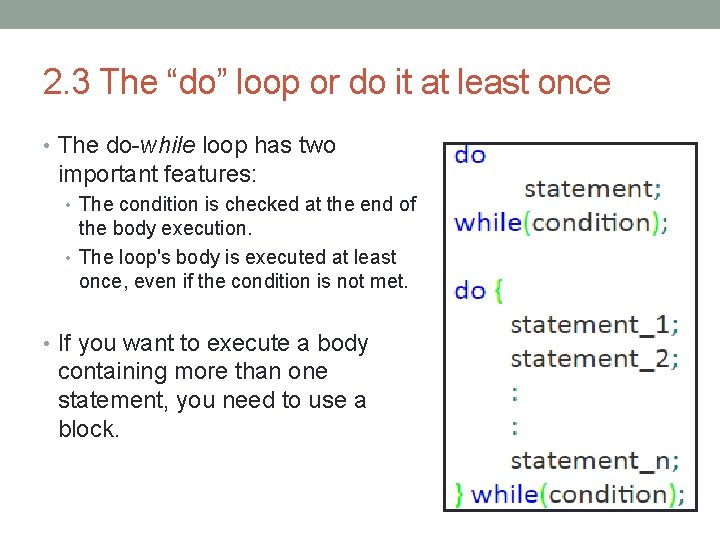
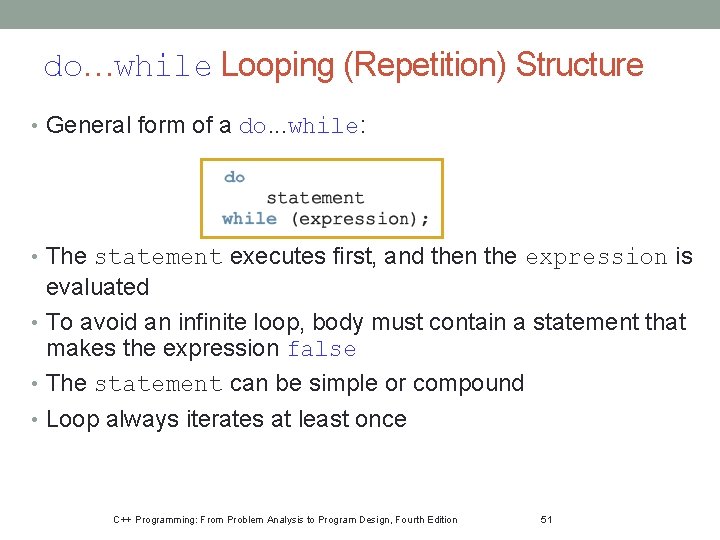
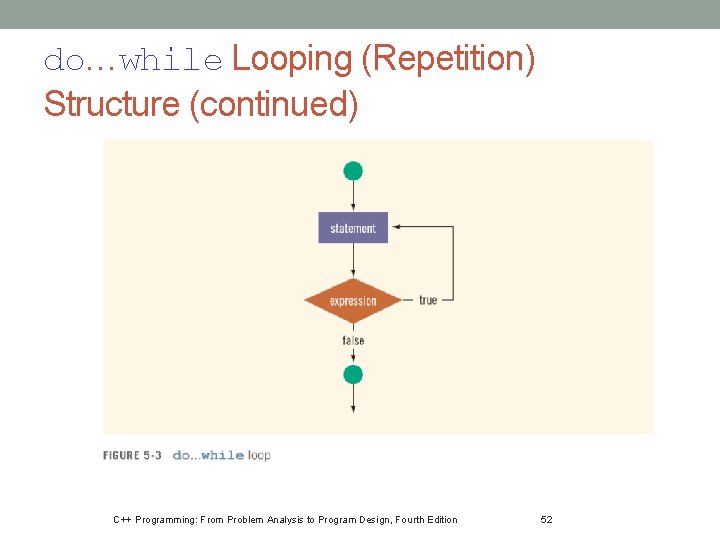
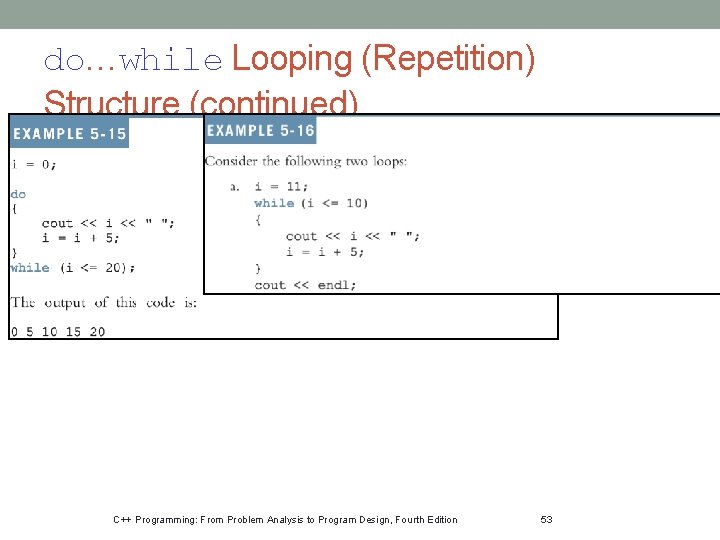
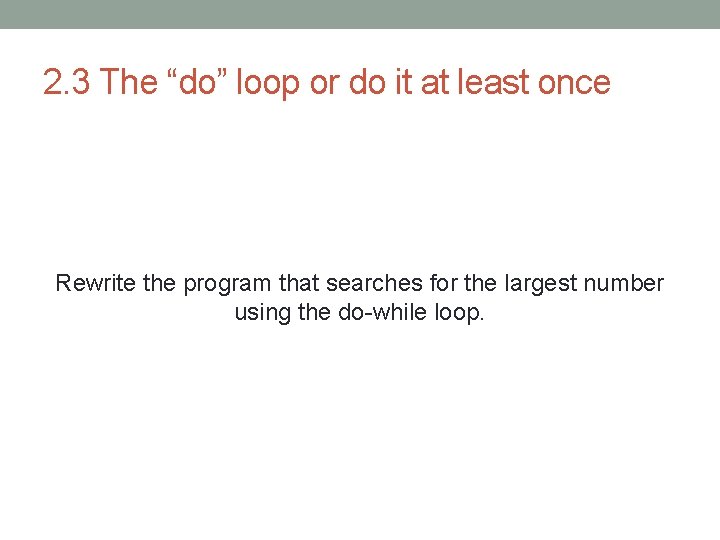
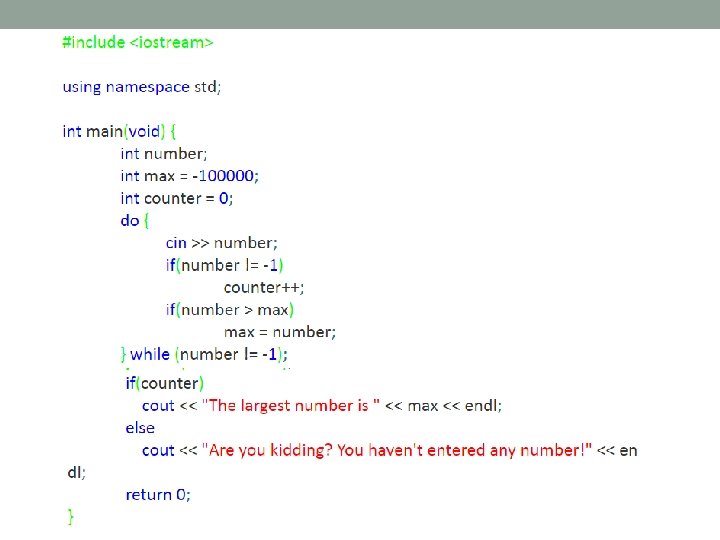
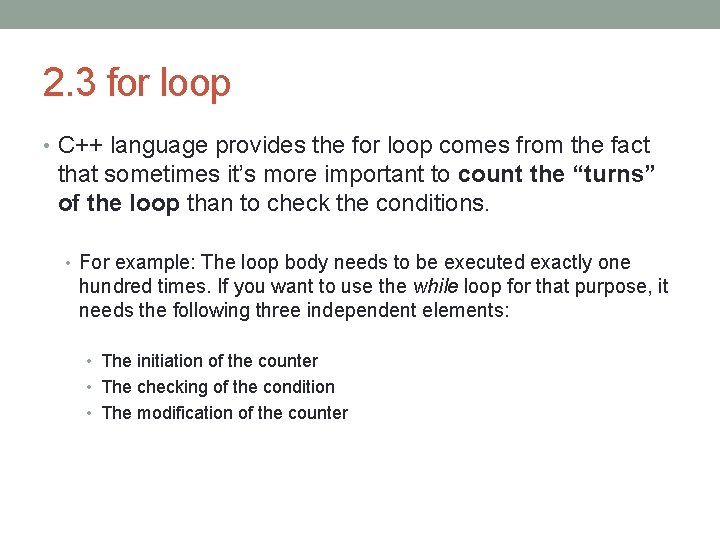
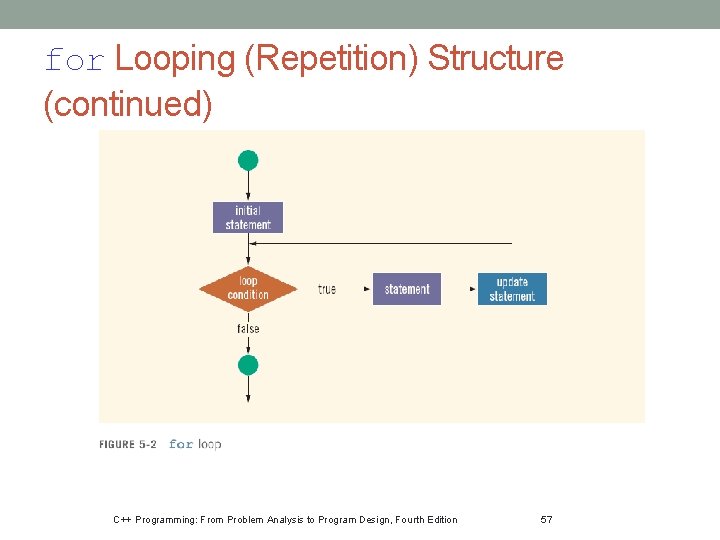
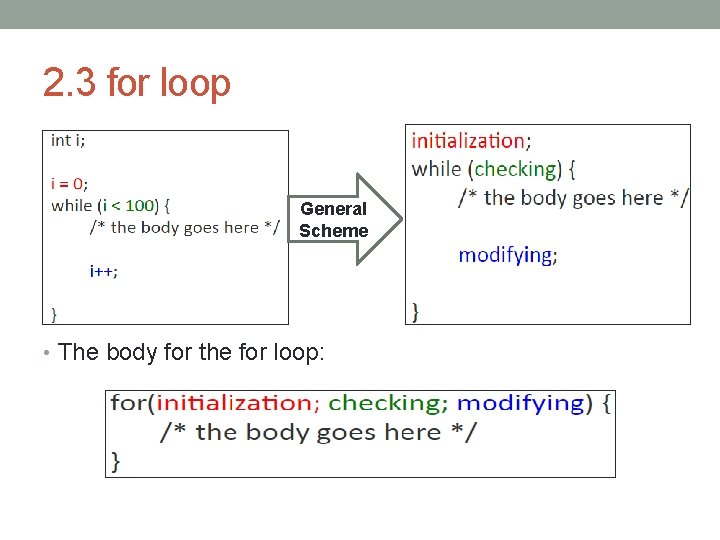
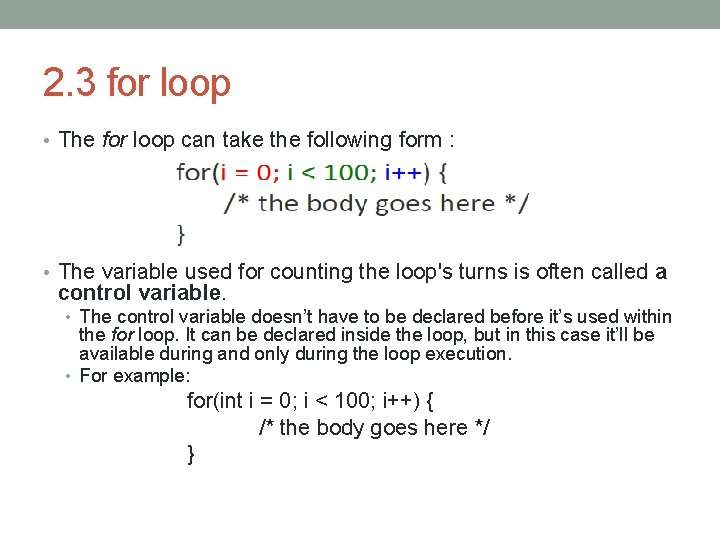
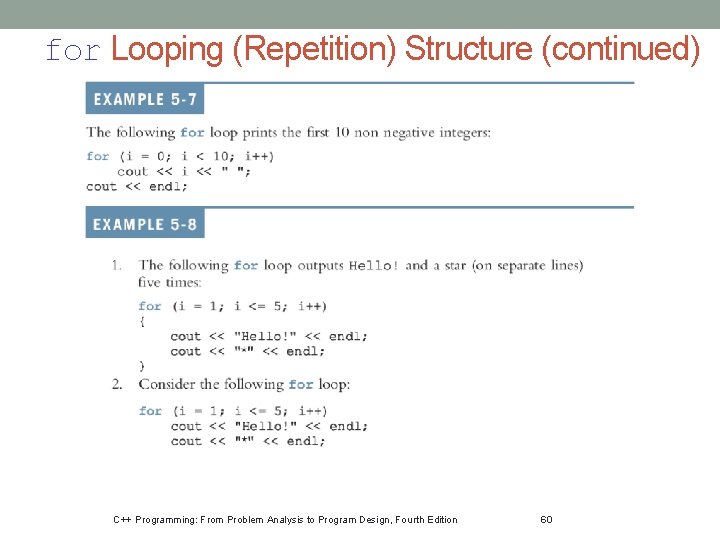
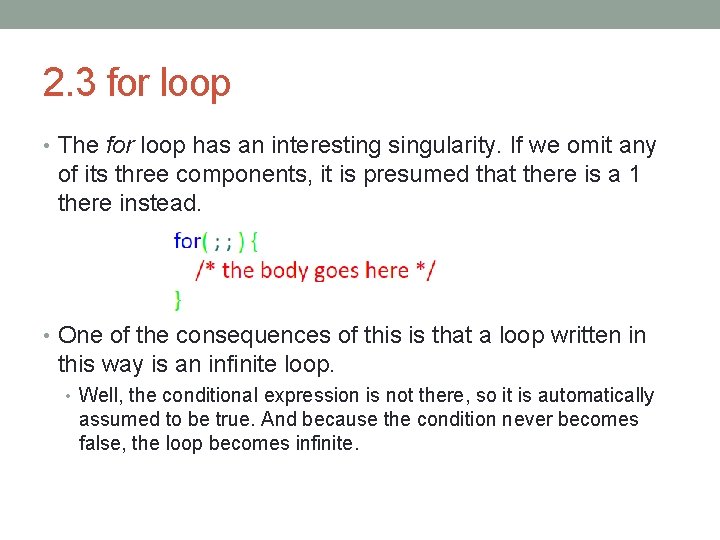
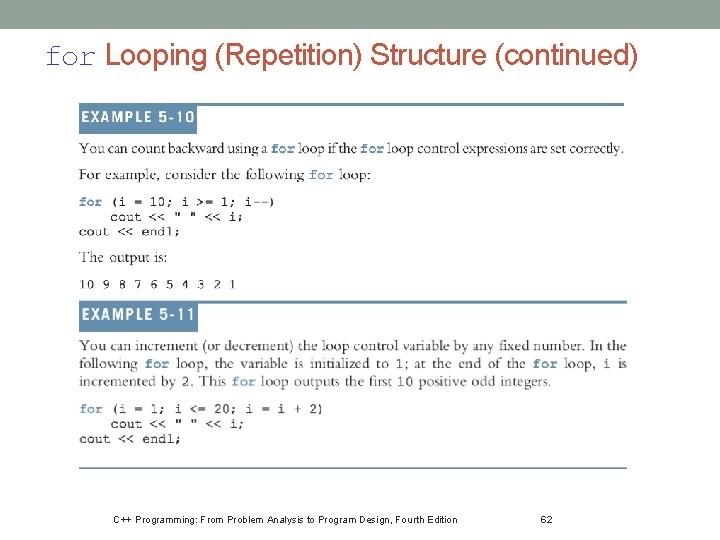
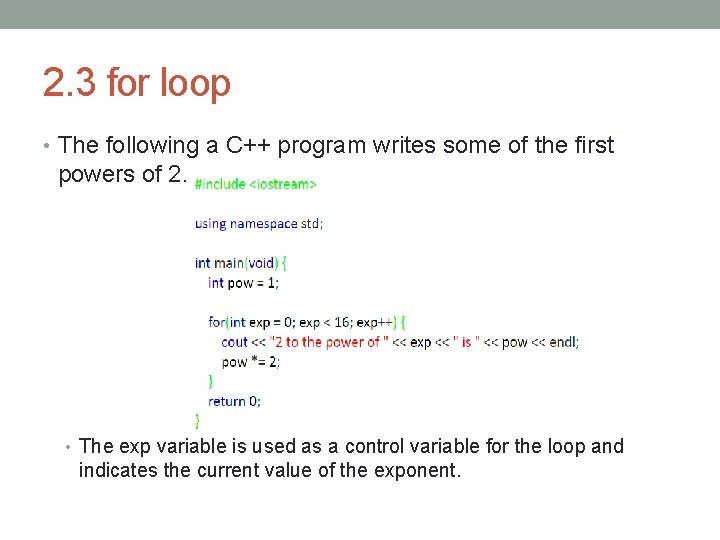
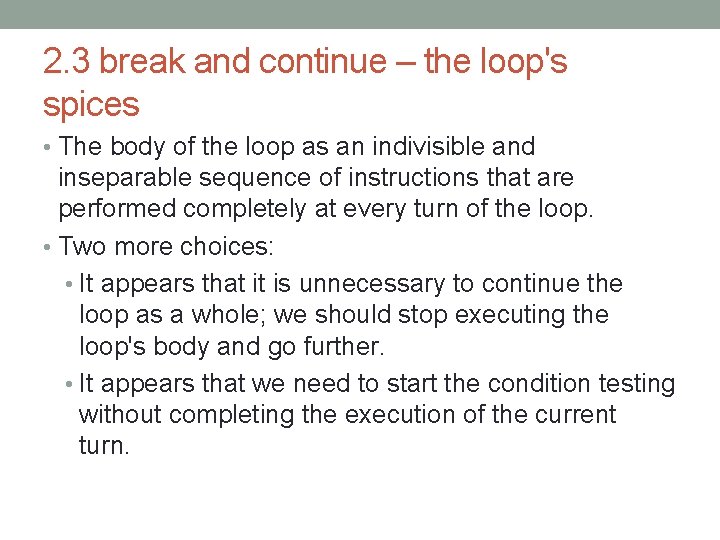
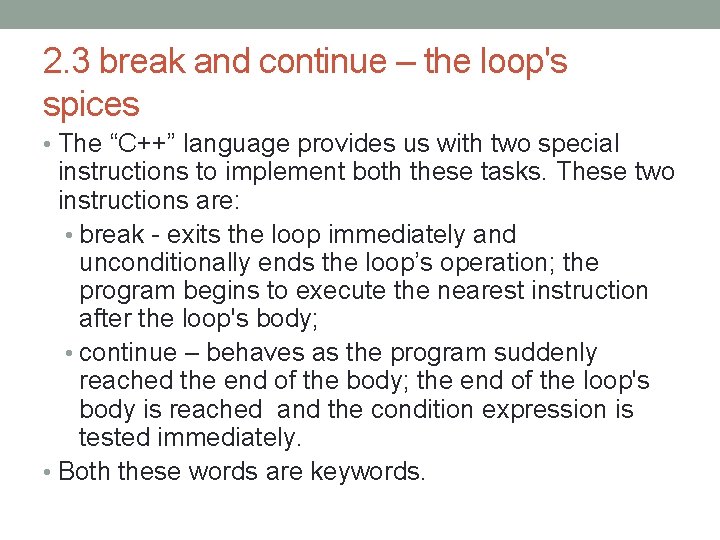
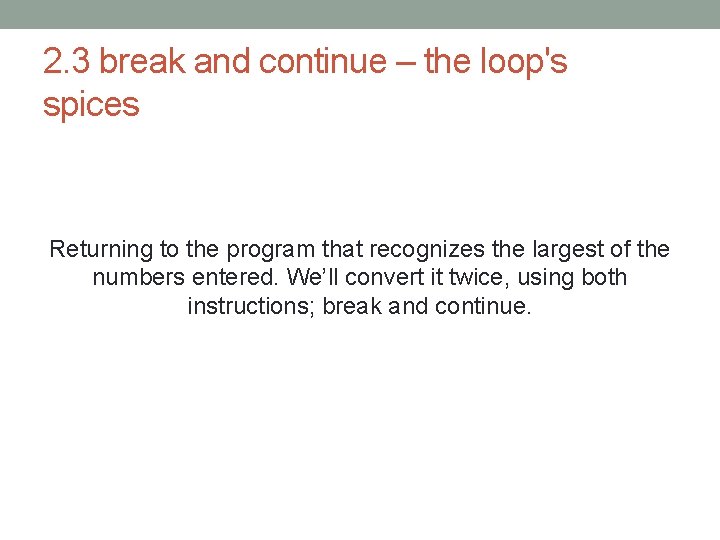
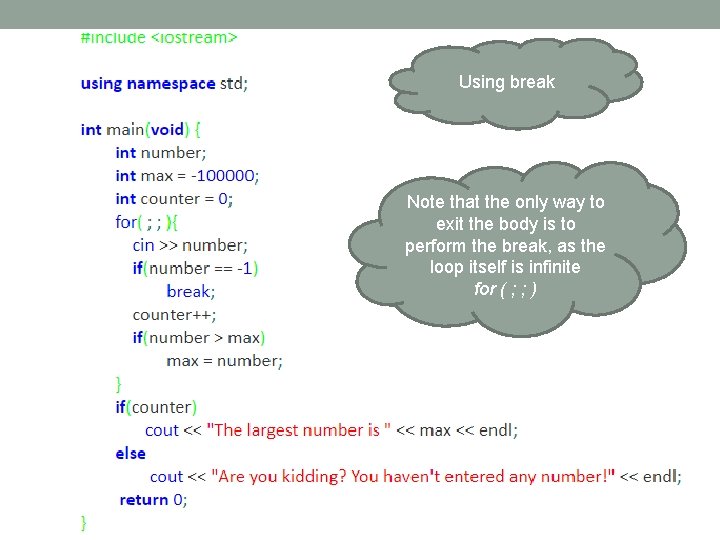
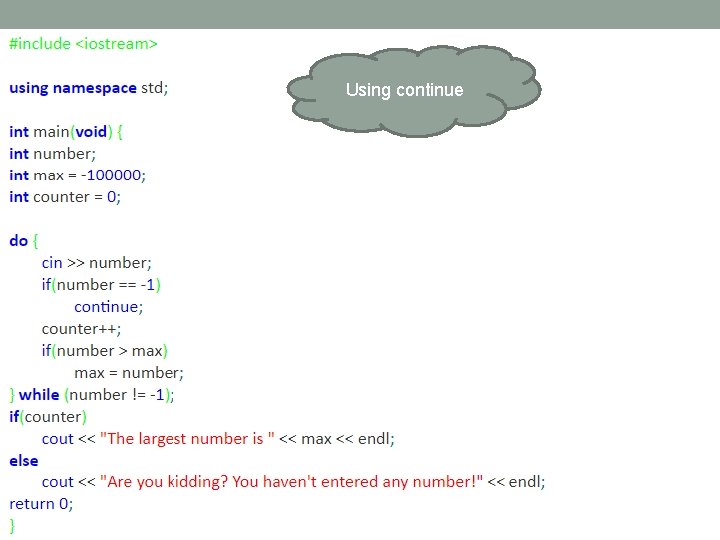
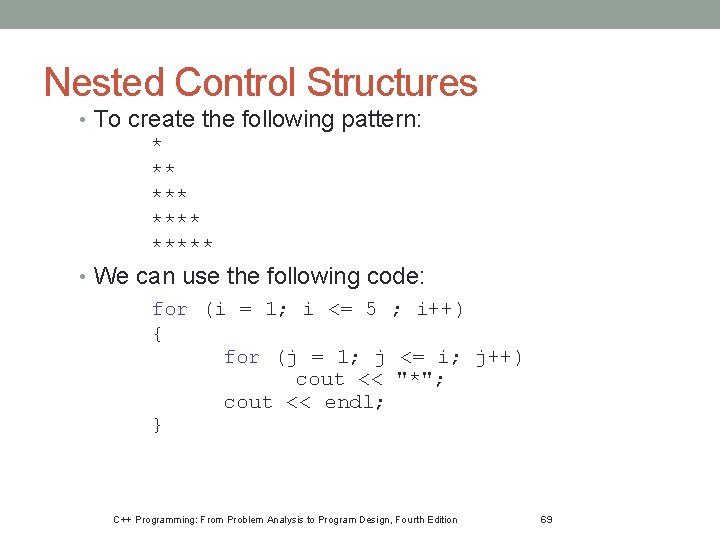
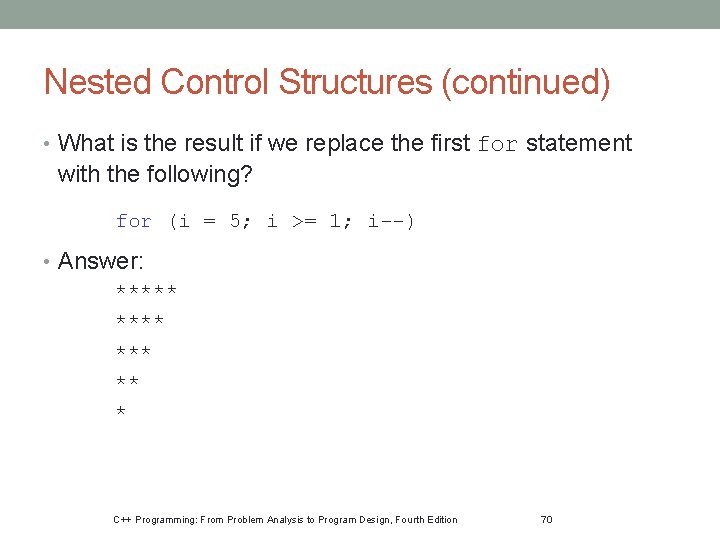
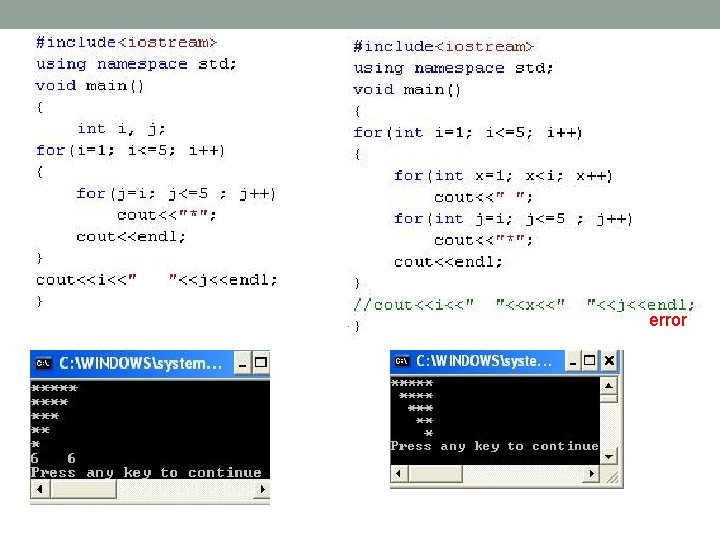
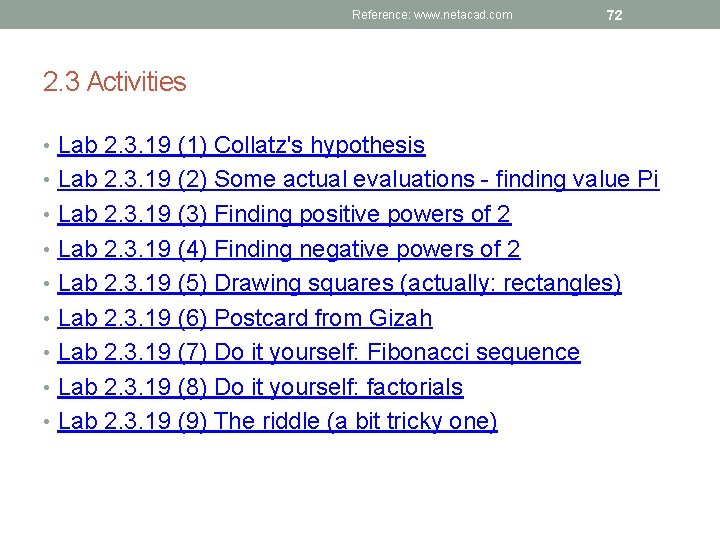
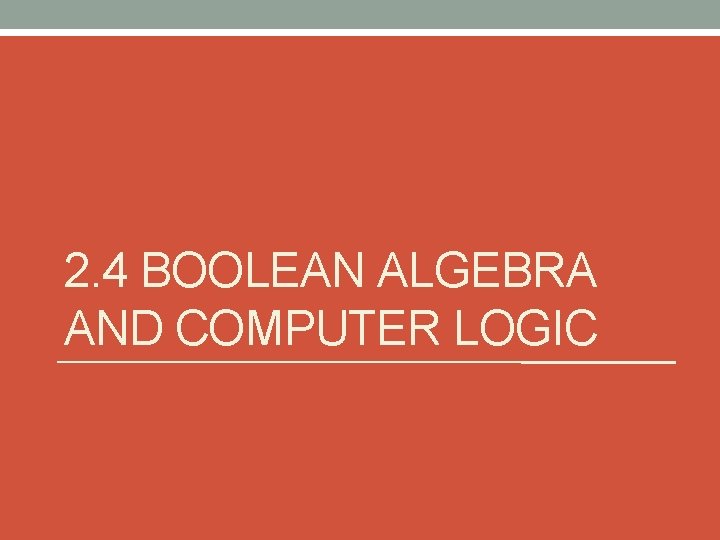
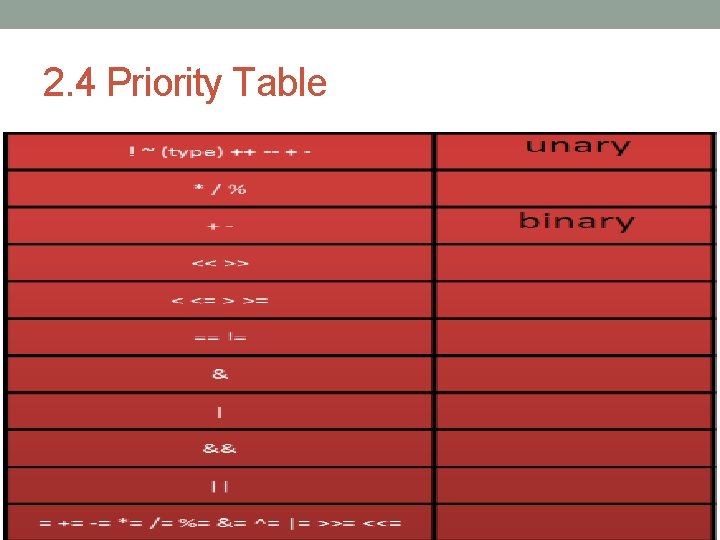
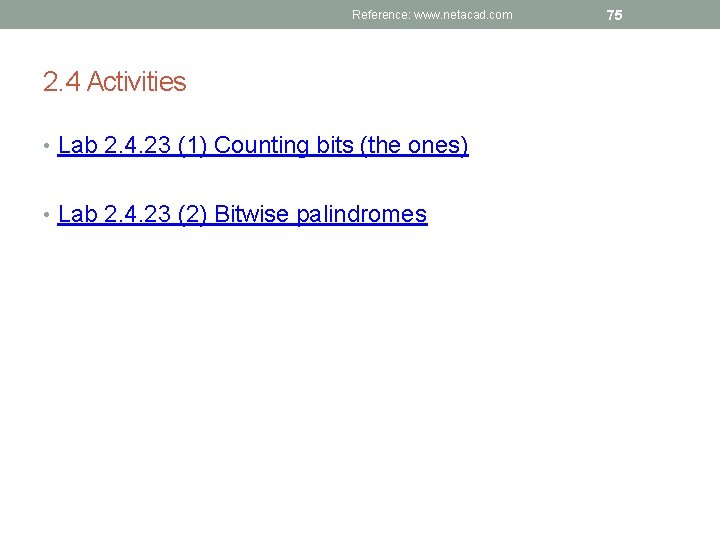
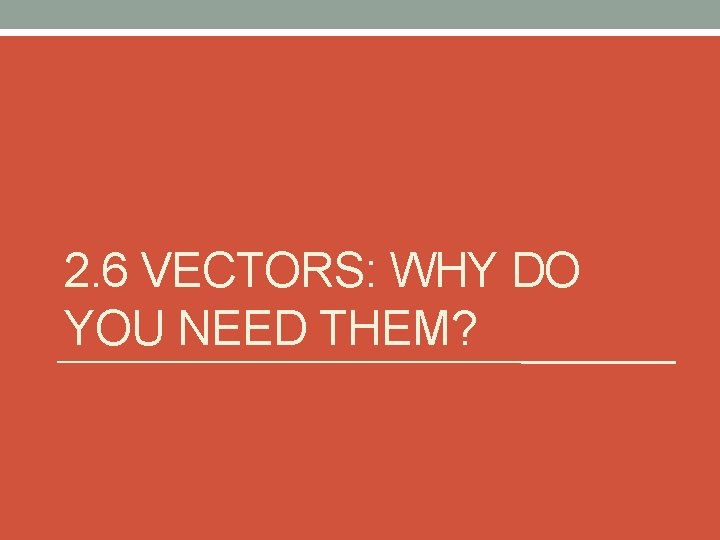
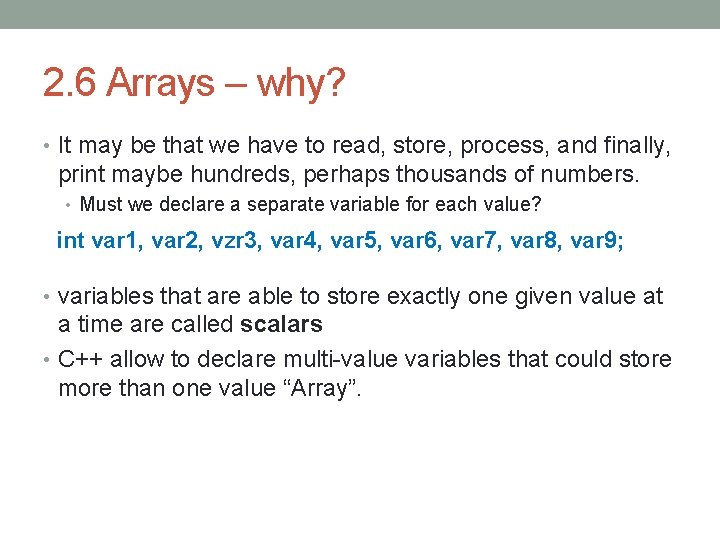
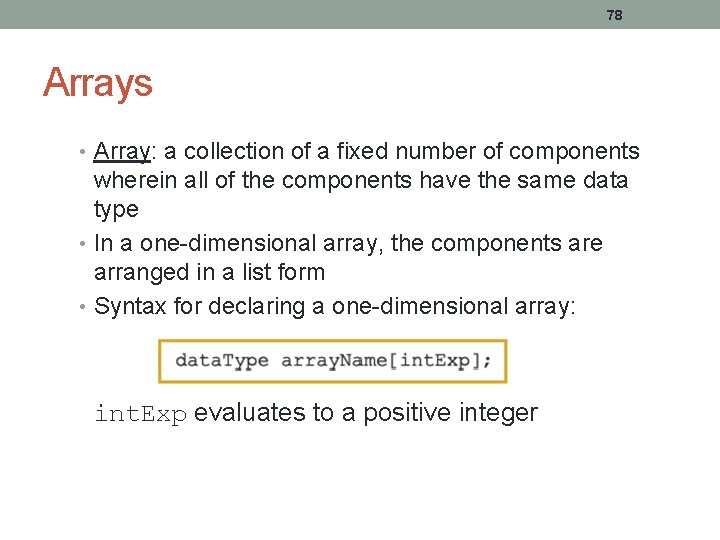
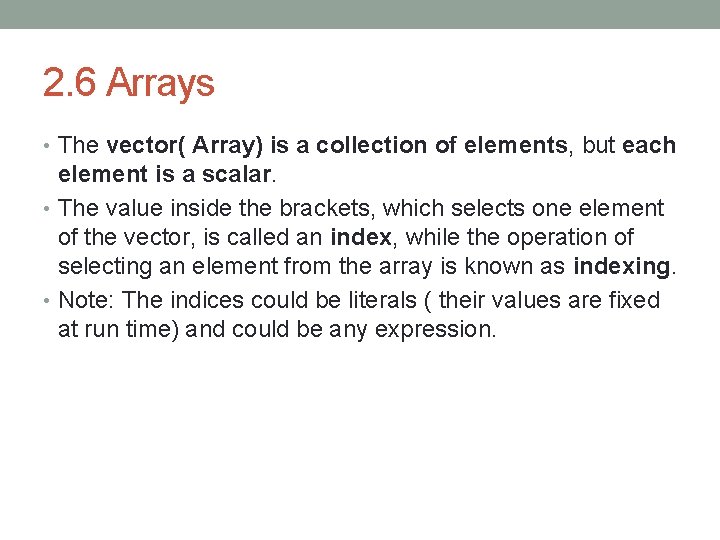
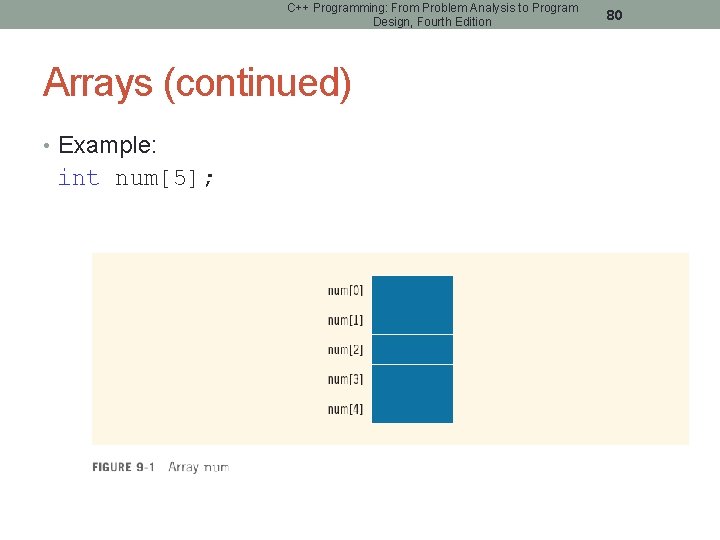
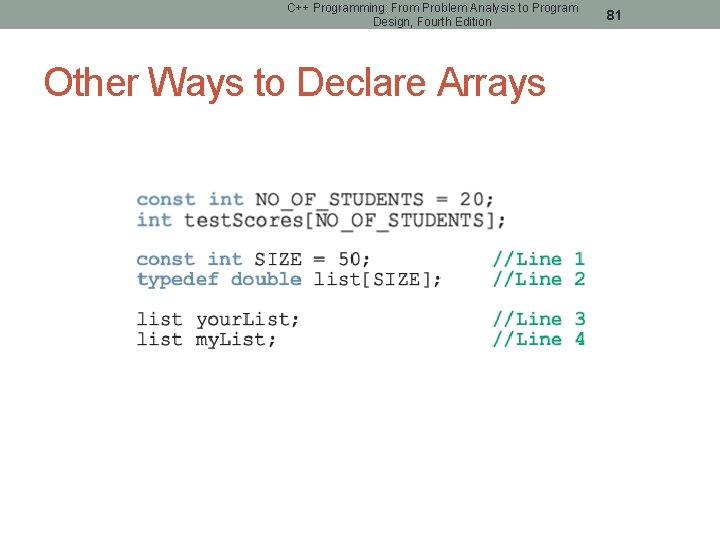
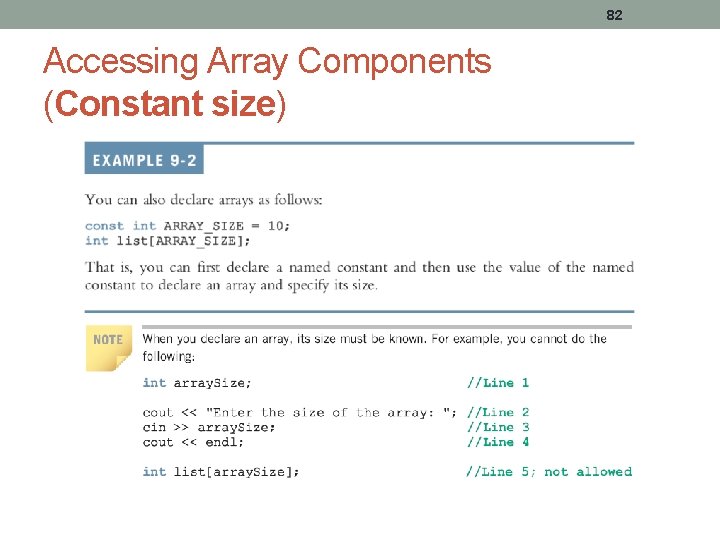
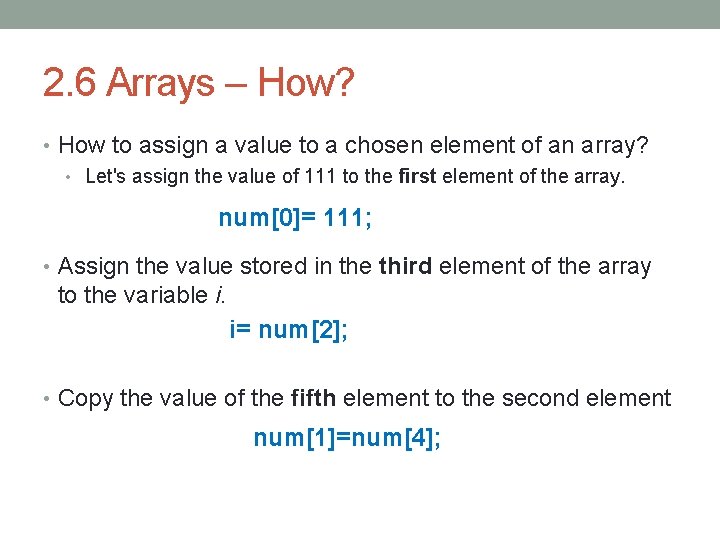
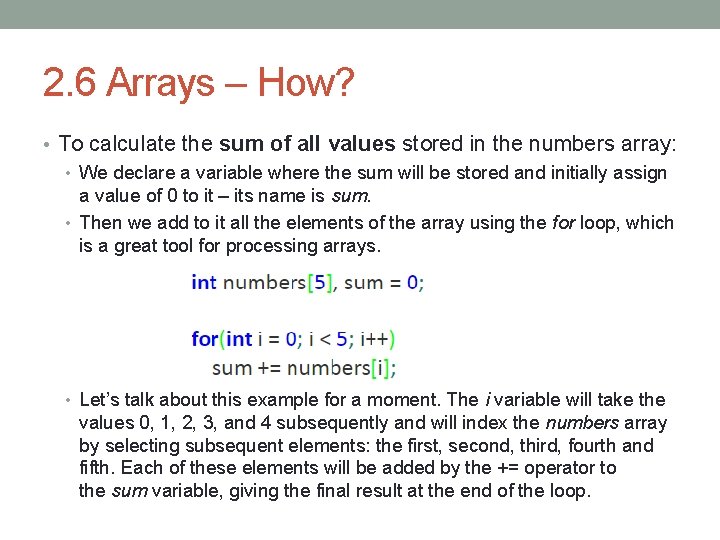
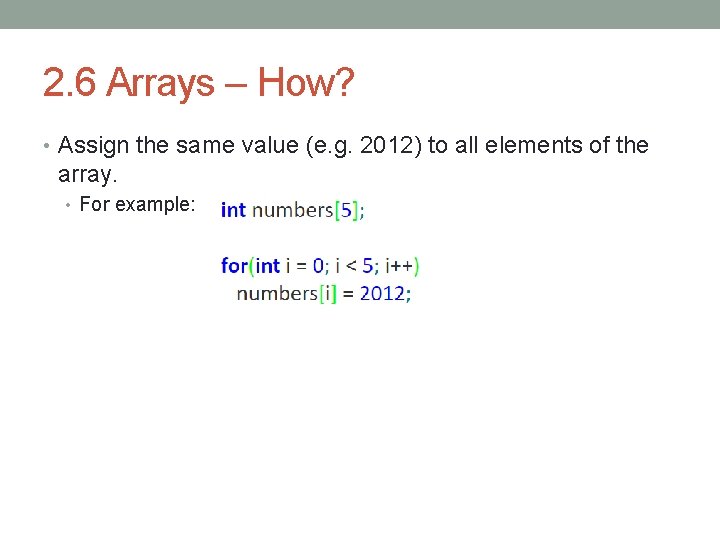
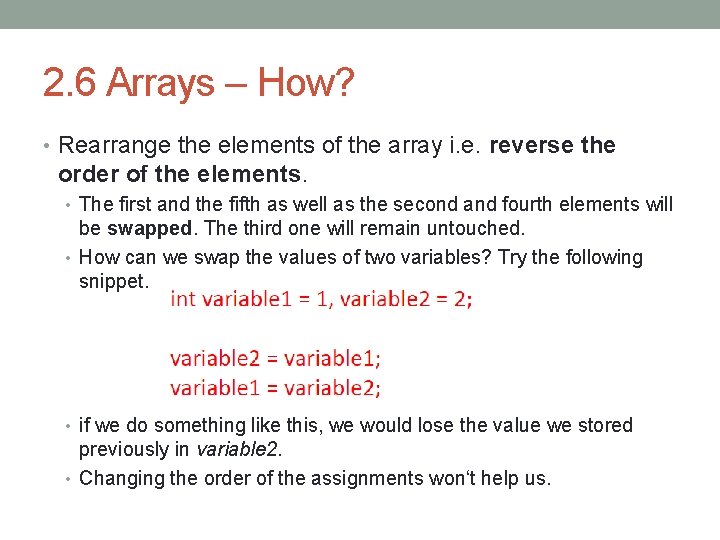
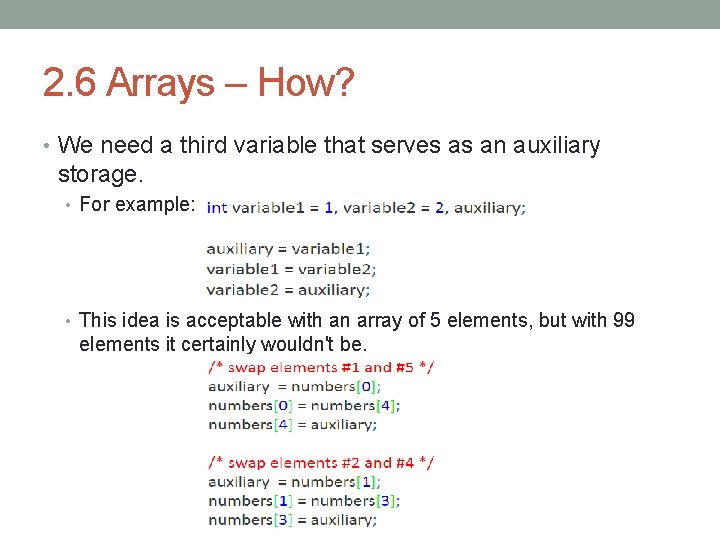
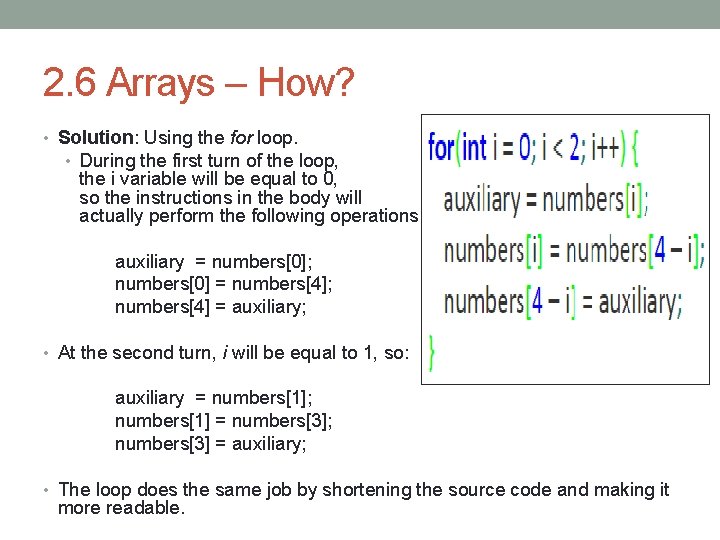
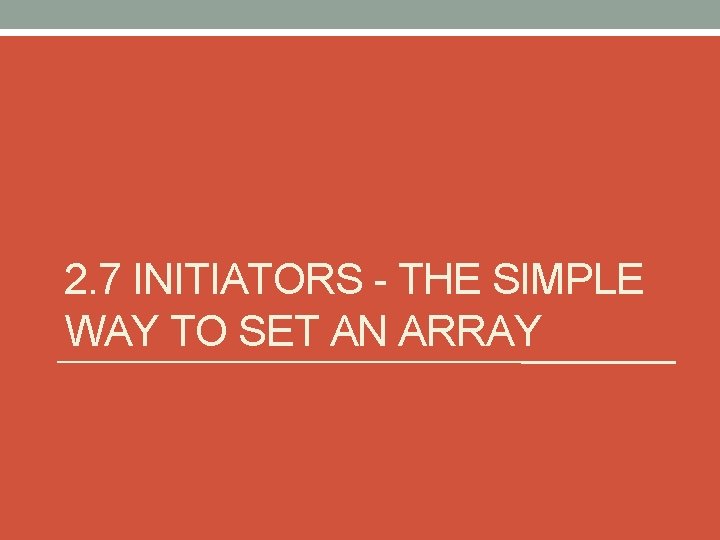
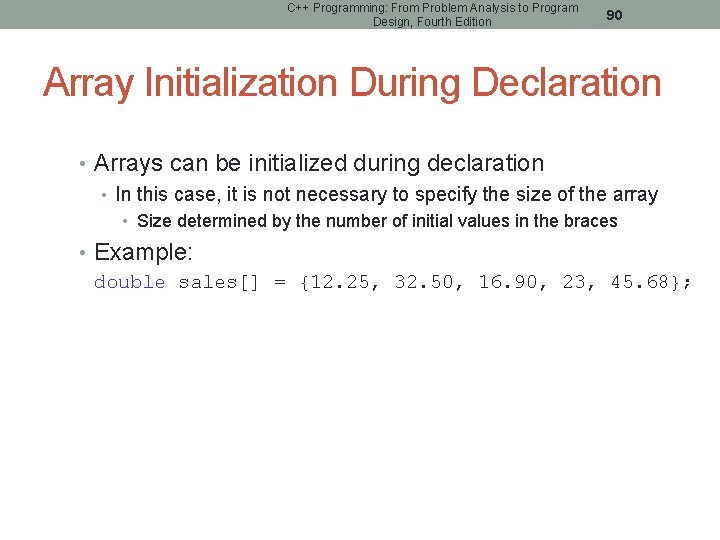
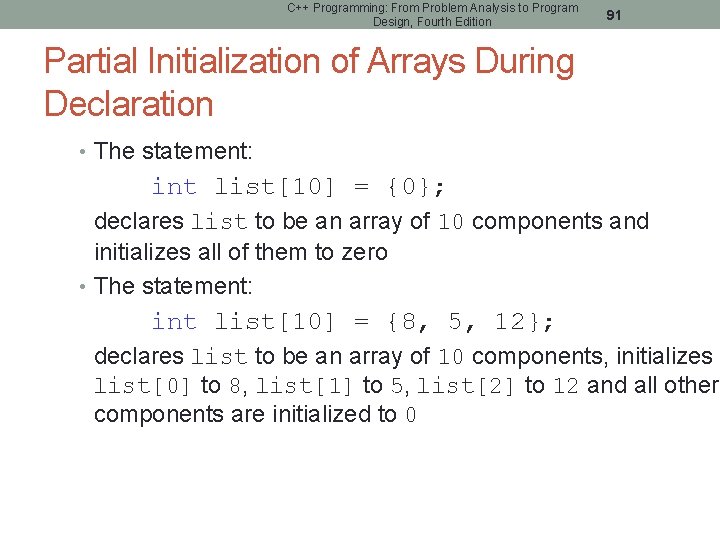
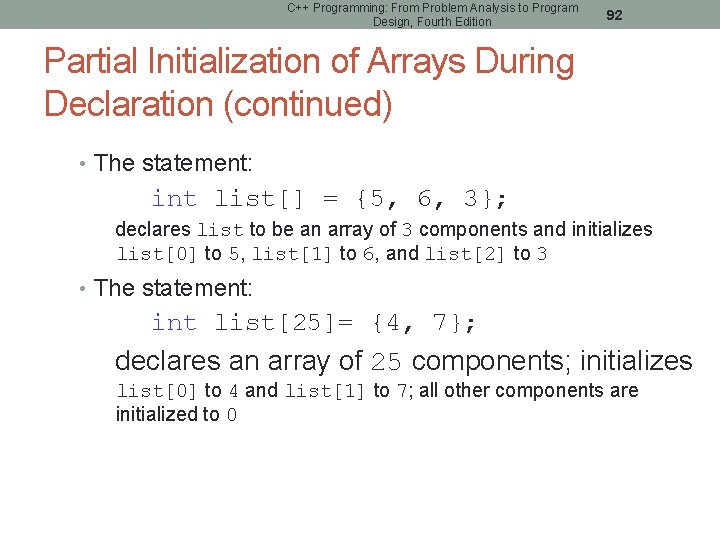
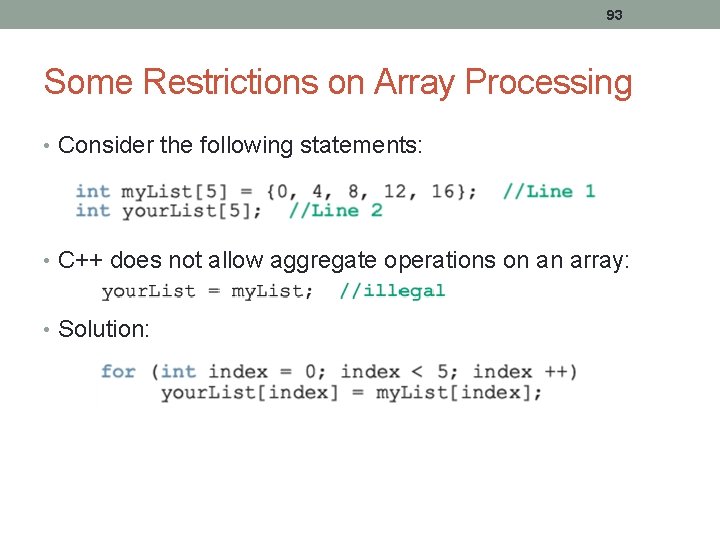
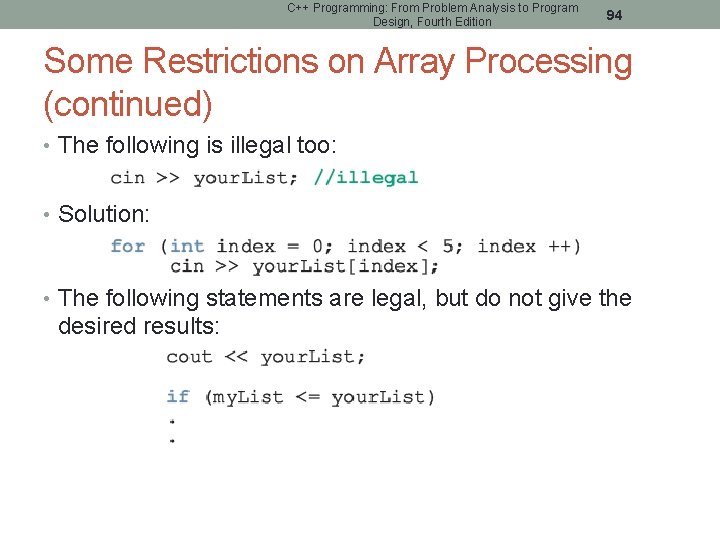
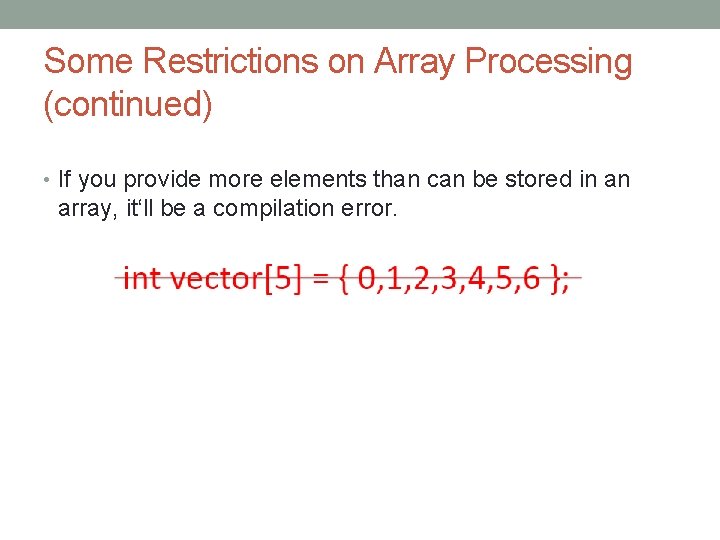
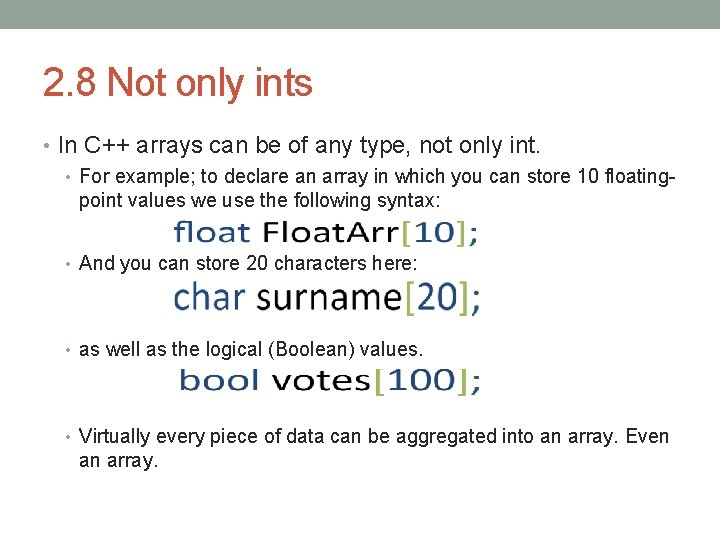
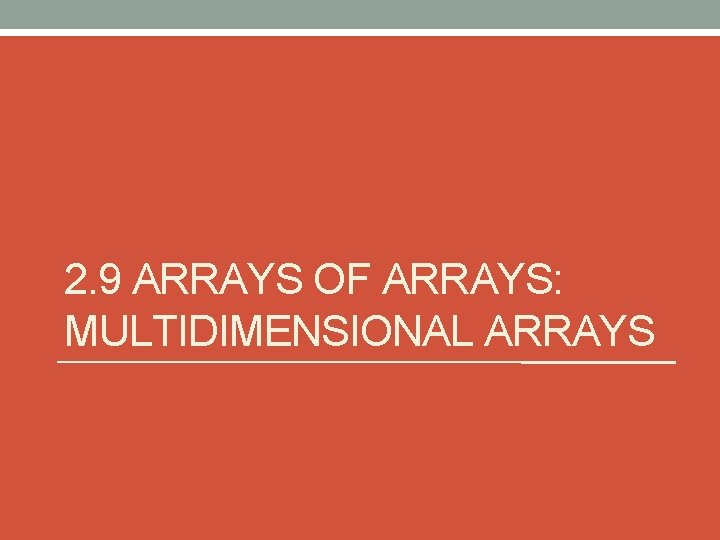
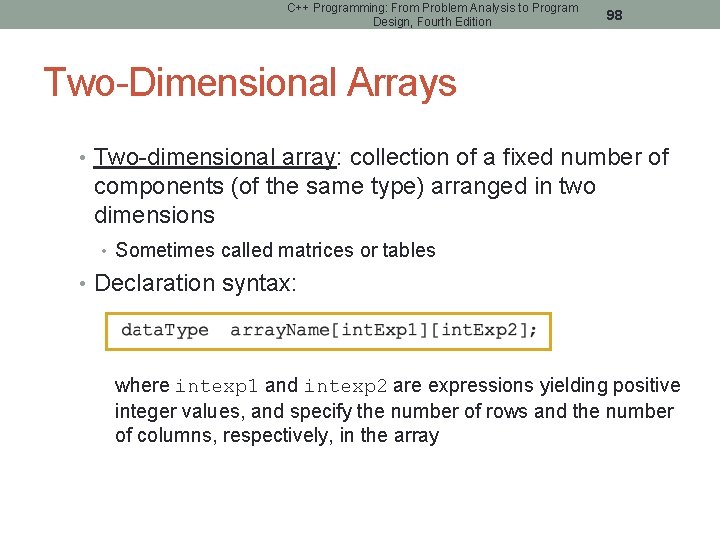
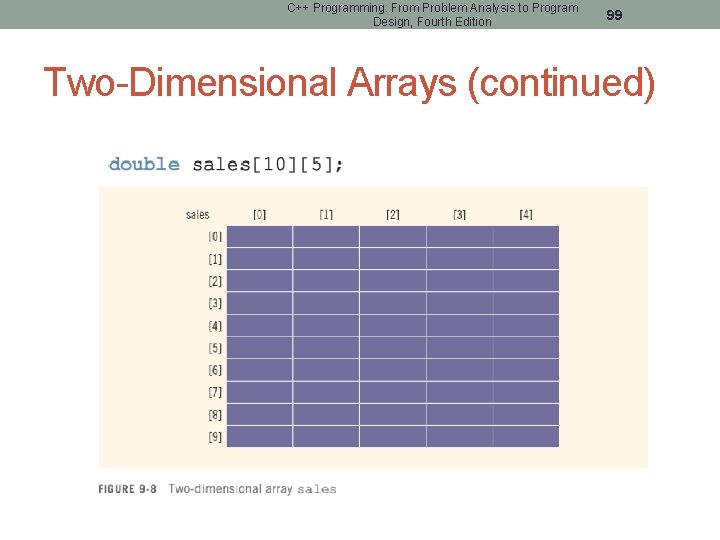
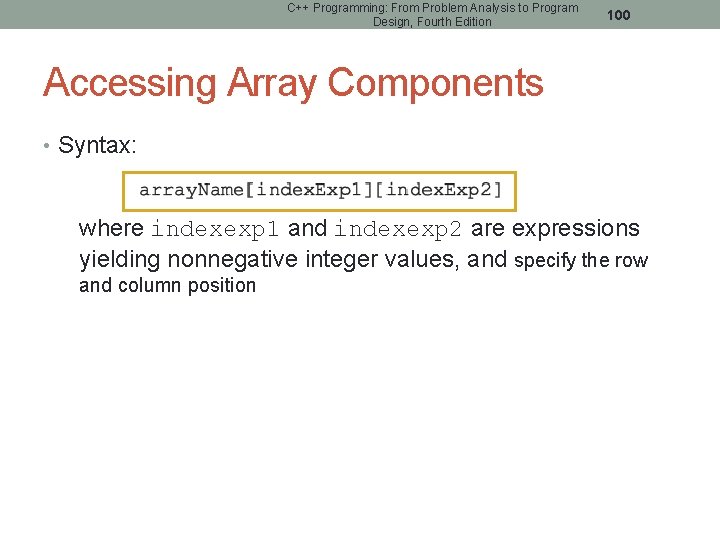
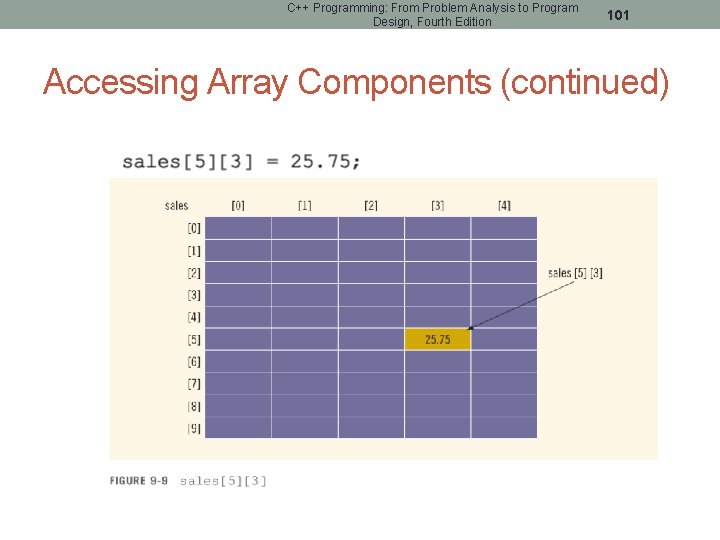
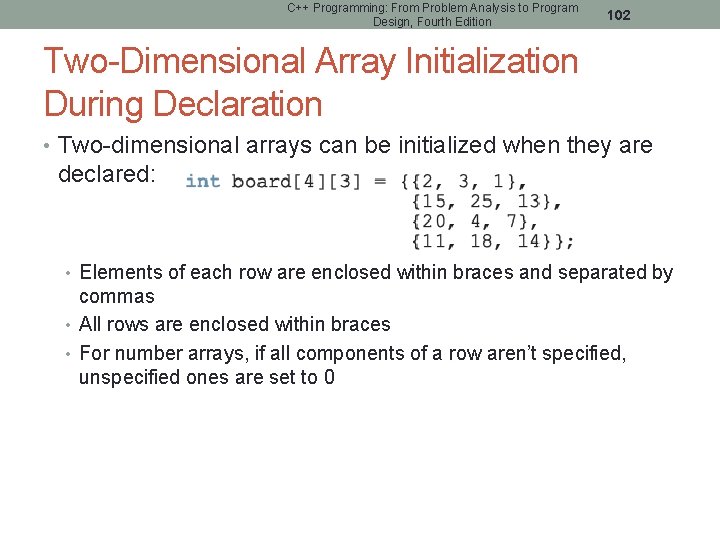
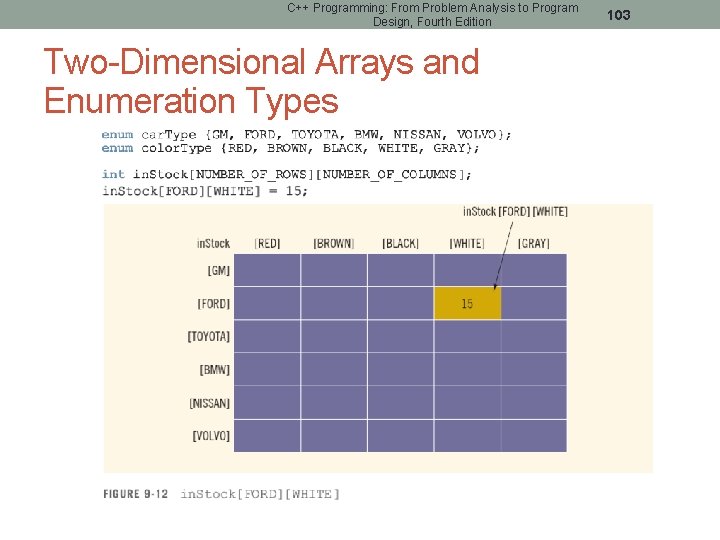
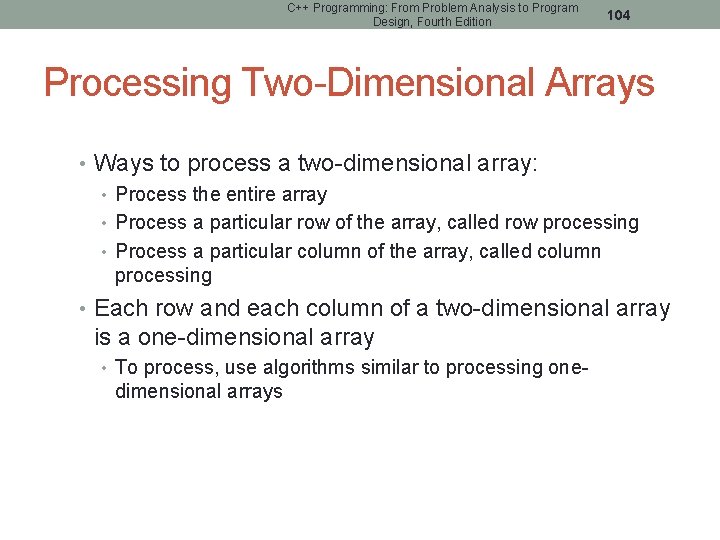
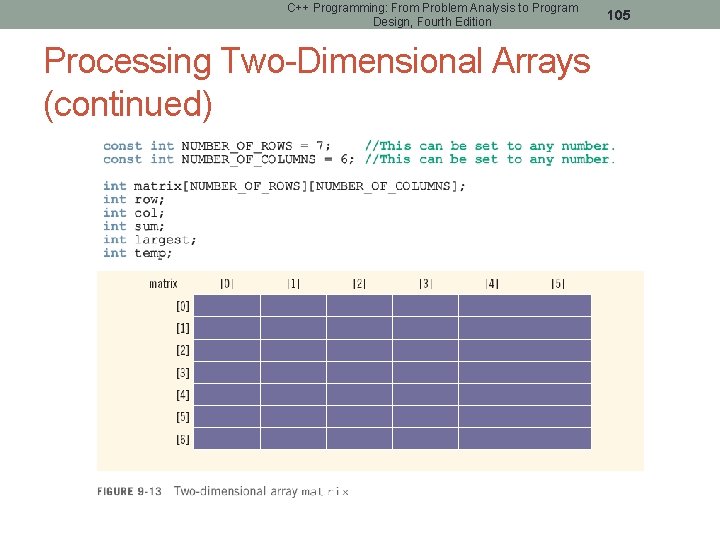
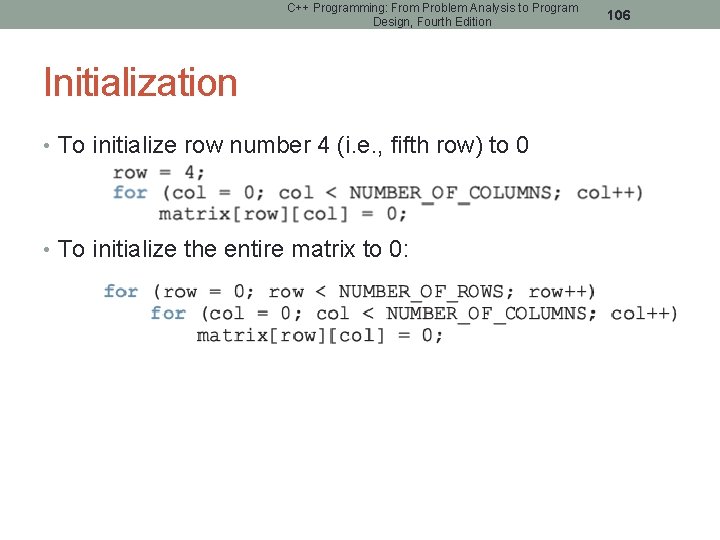
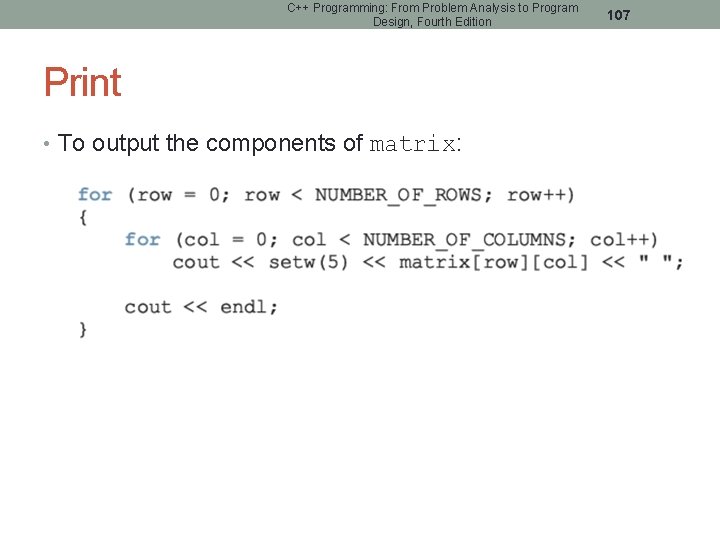
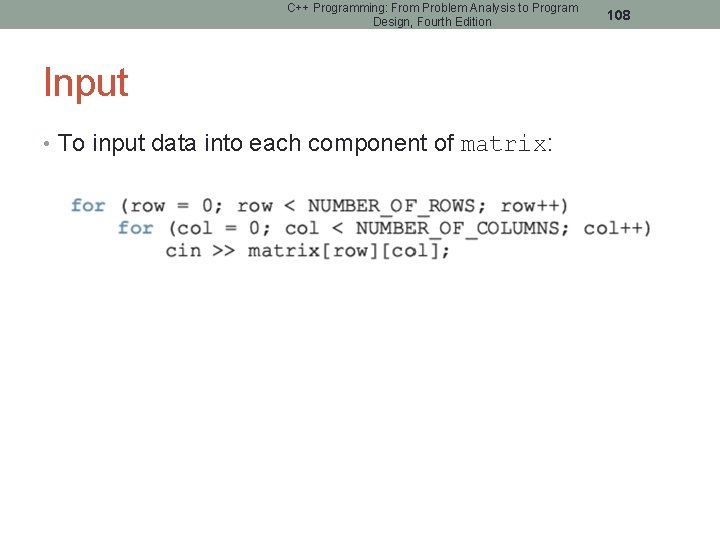
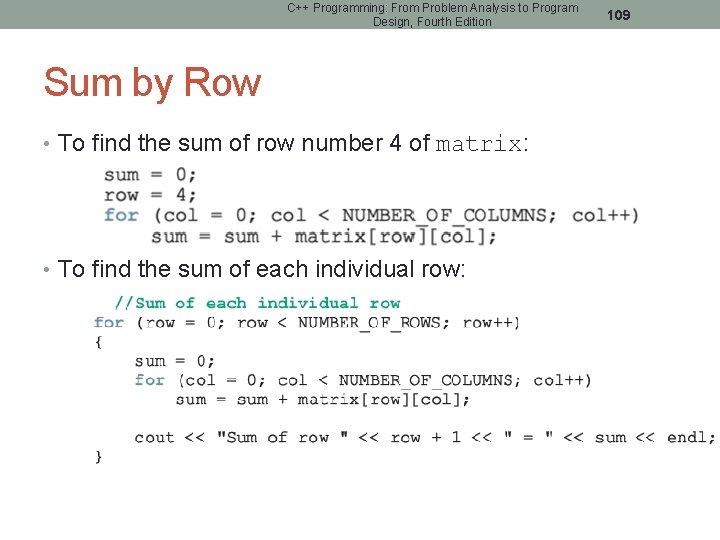
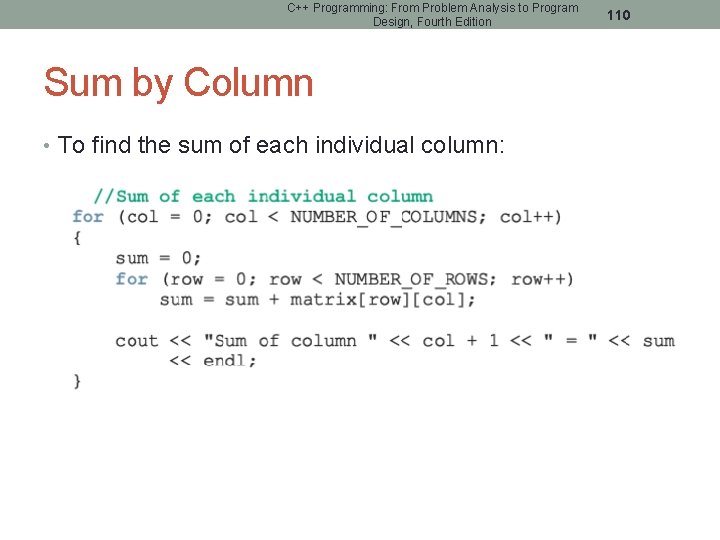
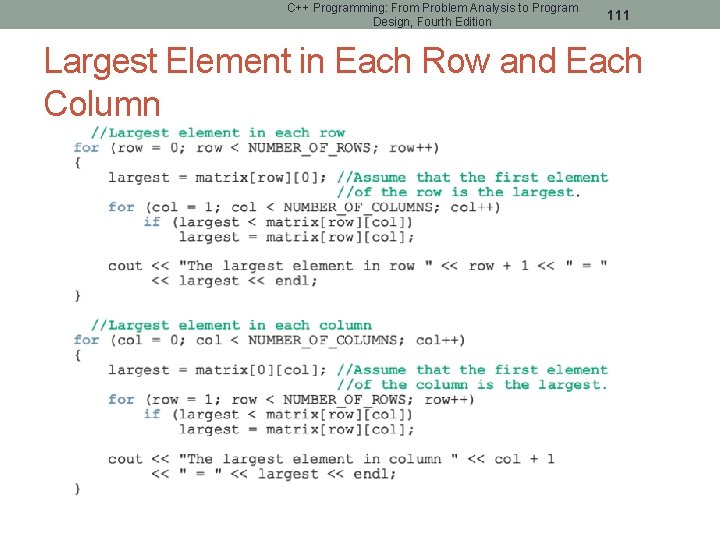
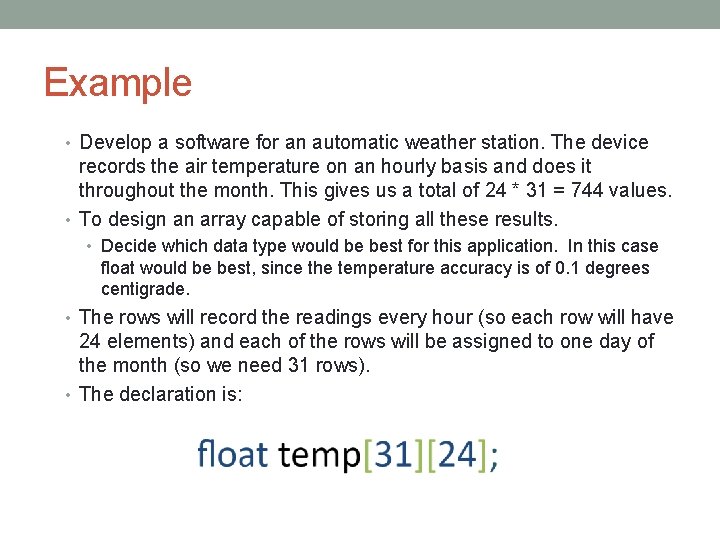
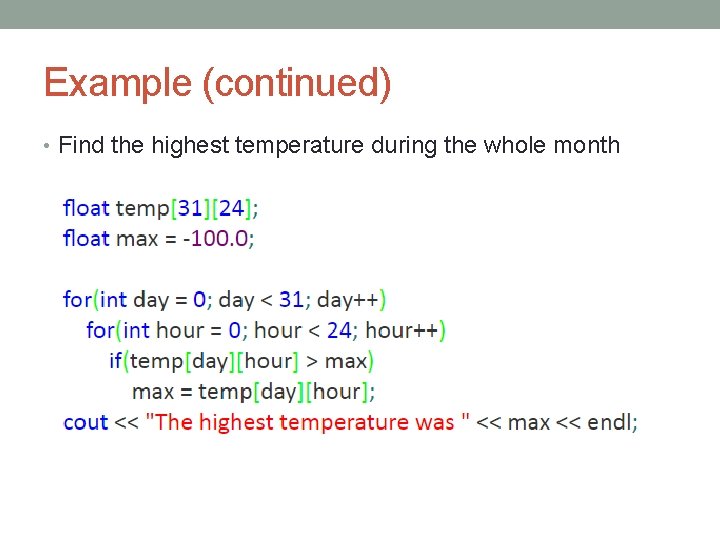
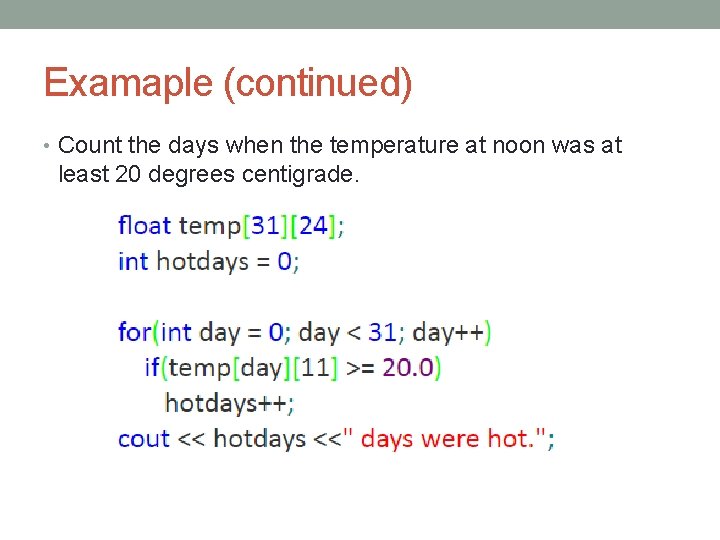
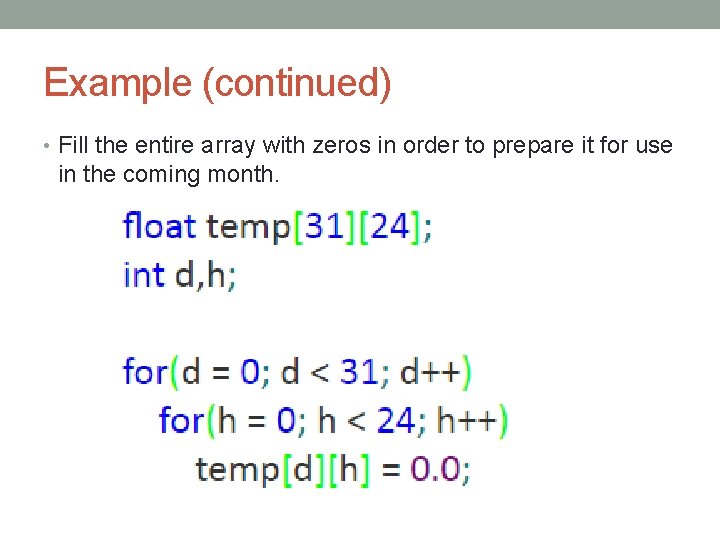
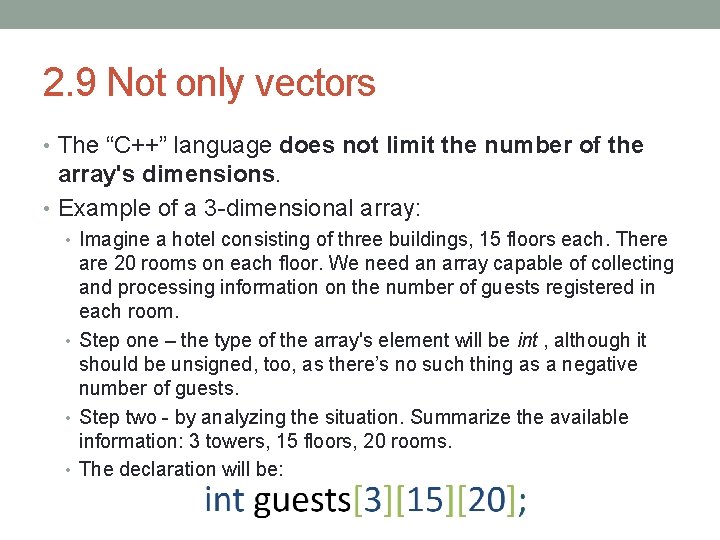
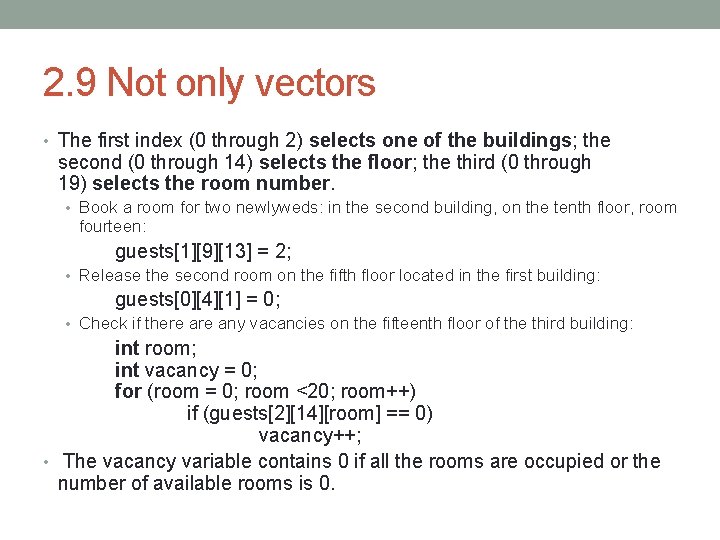
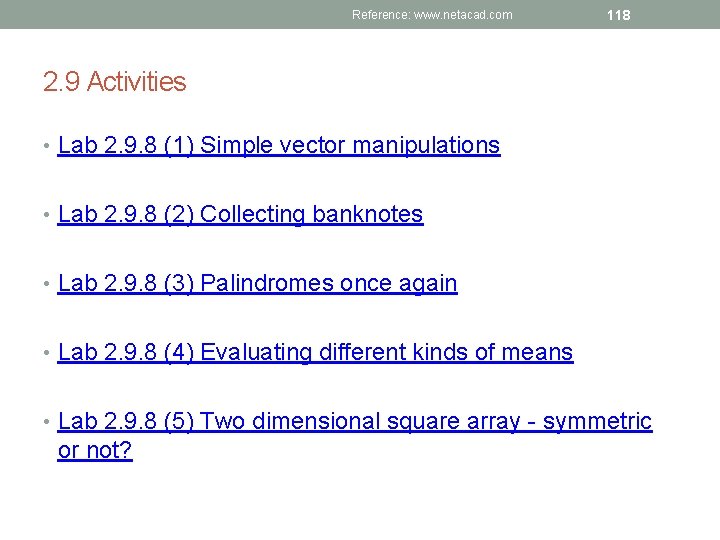
- Slides: 118
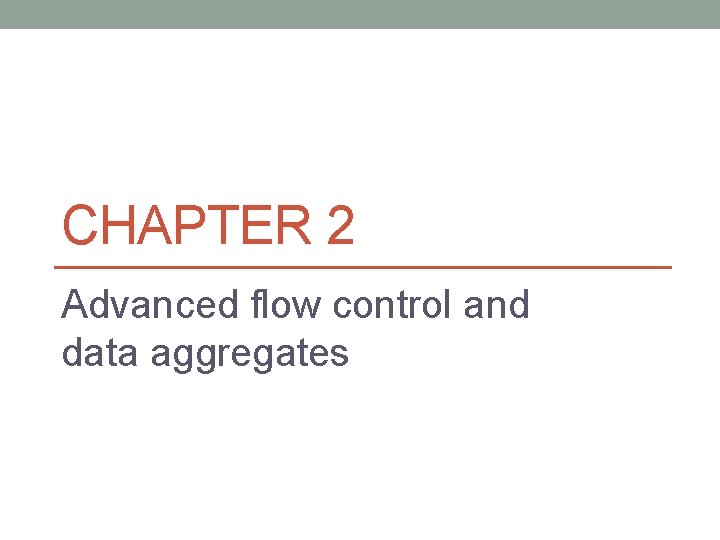
CHAPTER 2 Advanced flow control and data aggregates
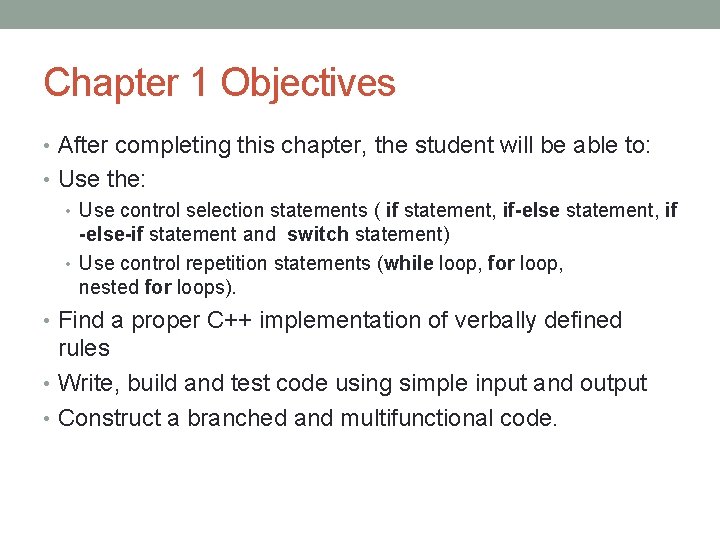
Chapter 1 Objectives • After completing this chapter, the student will be able to: • Use the: • Use control selection statements ( if statement, if-else statement, if -else-if statement and switch statement) • Use control repetition statements (while loop, for loop, nested for loops). • Find a proper C++ implementation of verbally defined rules • Write, build and test code using simple input and output • Construct a branched and multifunctional code.
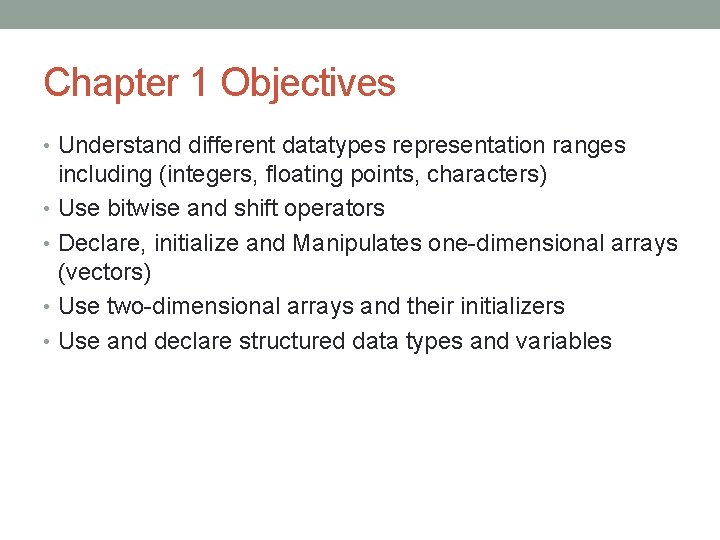
Chapter 1 Objectives • Understand different datatypes representation ranges including (integers, floating points, characters) • Use bitwise and shift operators • Declare, initialize and Manipulates one-dimensional arrays (vectors) • Use two-dimensional arrays and their initializers • Use and declare structured data types and variables
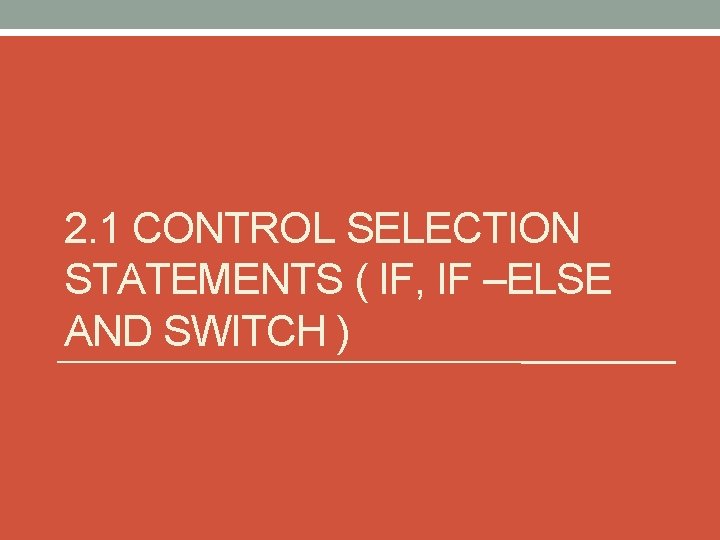
2. 1 CONTROL SELECTION STATEMENTS ( IF, IF –ELSE AND SWITCH )
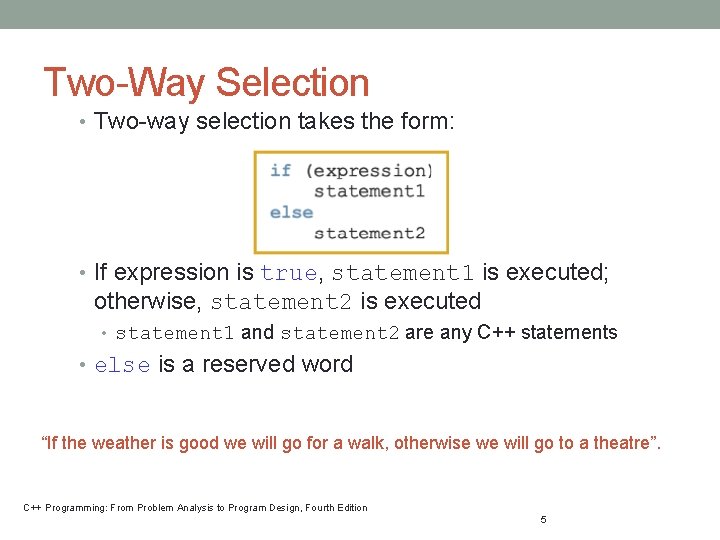
Two-Way Selection • Two-way selection takes the form: • If expression is true, statement 1 is executed; otherwise, statement 2 is executed • statement 1 and statement 2 are any C++ statements • else is a reserved word “If the weather is good we will go for a walk, otherwise we will go to a theatre”. C++ Programming: From Problem Analysis to Program Design, Fourth Edition 5
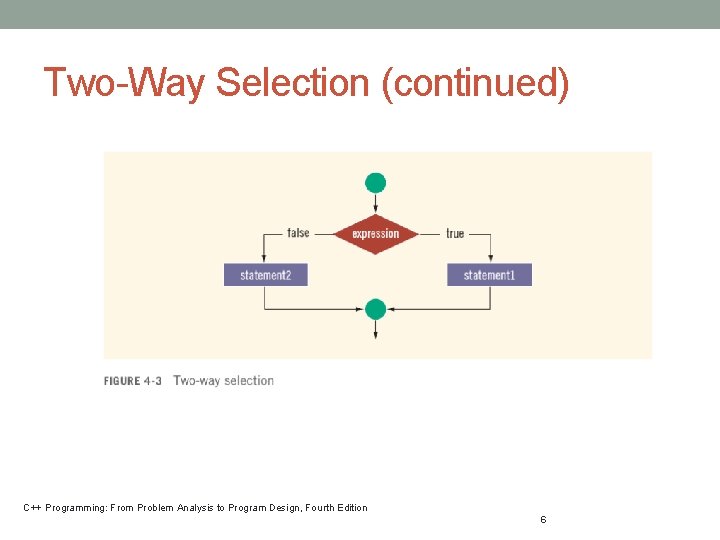
Two-Way Selection (continued) C++ Programming: From Problem Analysis to Program Design, Fourth Edition 6
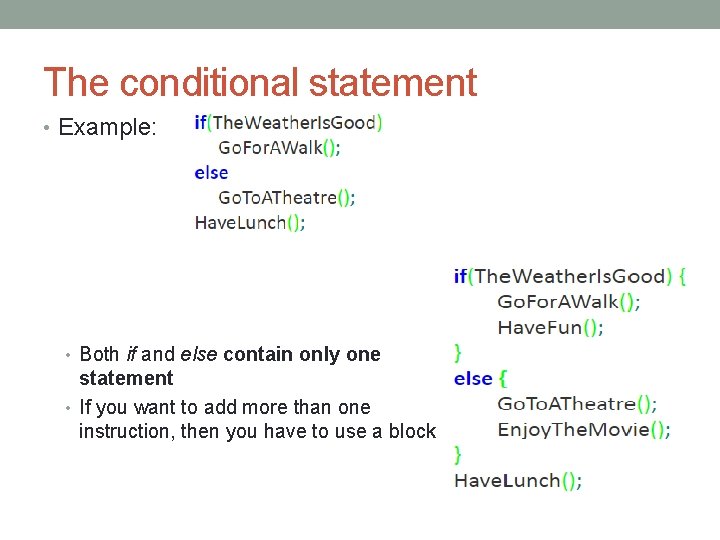
The conditional statement • Example: • Both if and else contain only one statement • If you want to add more than one instruction, then you have to use a block
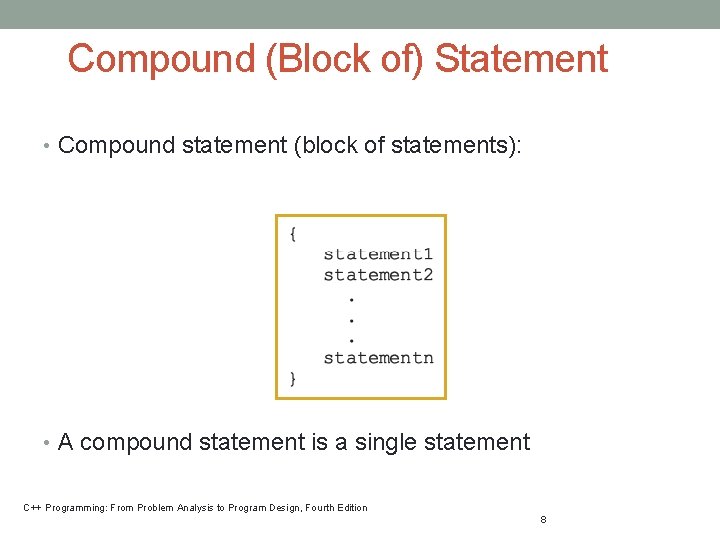
Compound (Block of) Statement • Compound statement (block of statements): • A compound statement is a single statement C++ Programming: From Problem Analysis to Program Design, Fourth Edition 8
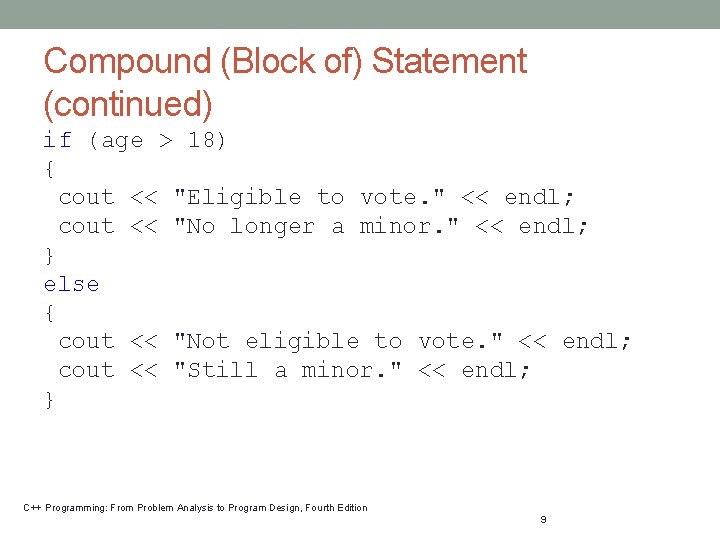
Compound (Block of) Statement (continued) if (age > 18) { cout << "Eligible to vote. " << endl; cout << "No longer a minor. " << endl; } else { cout << "Not eligible to vote. " << endl; cout << "Still a minor. " << endl; } C++ Programming: From Problem Analysis to Program Design, Fourth Edition 9
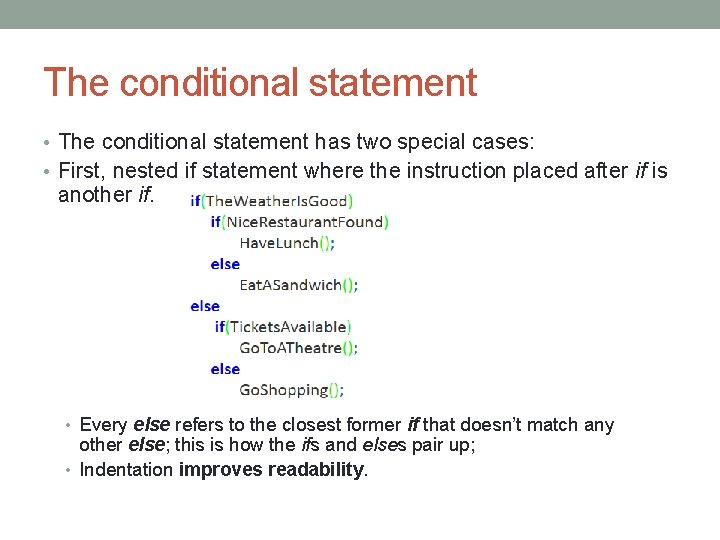
The conditional statement • The conditional statement has two special cases: • First, nested if statement where the instruction placed after if is another if. • Every else refers to the closest former if that doesn’t match any other else; this is how the ifs and elses pair up; • Indentation improves readability.
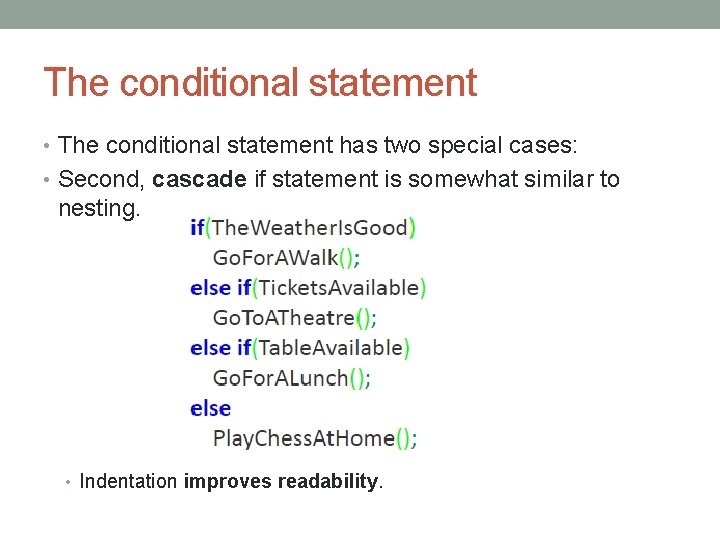
The conditional statement • The conditional statement has two special cases: • Second, cascade if statement is somewhat similar to nesting. • Indentation improves readability.
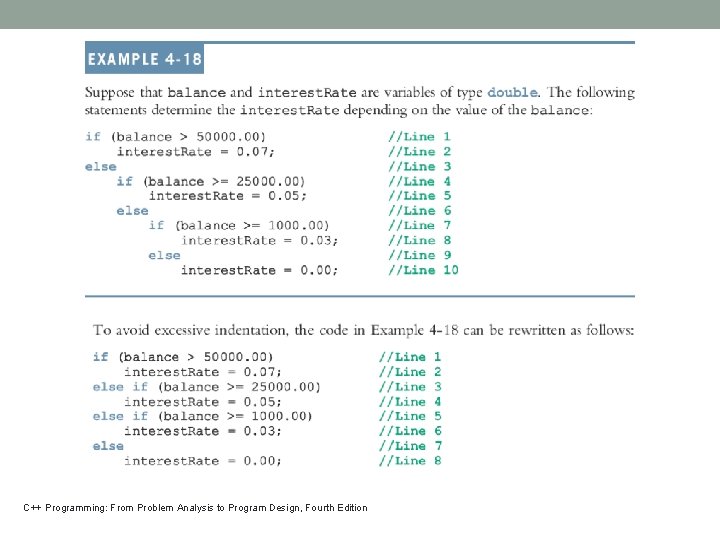
C++ Programming: From Problem Analysis to Program Design, Fourth Edition
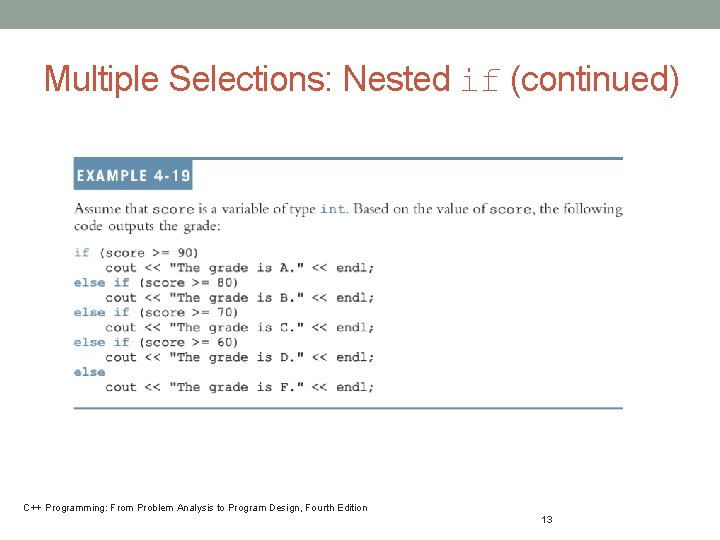
Multiple Selections: Nested if (continued) C++ Programming: From Problem Analysis to Program Design, Fourth Edition 13
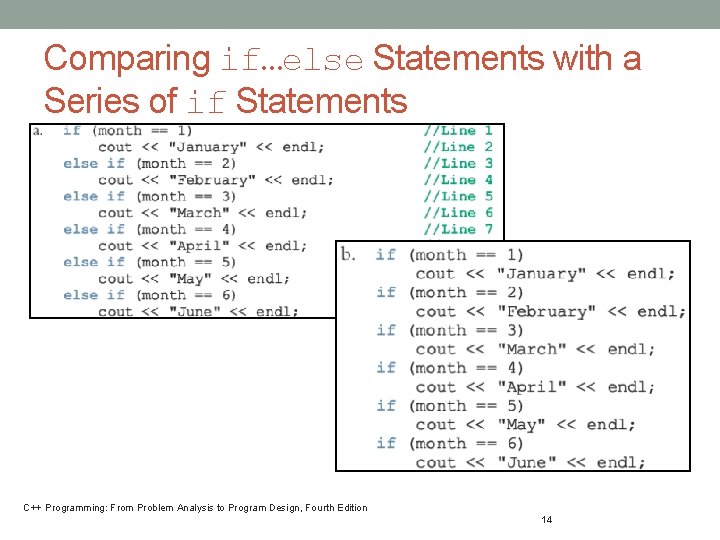
Comparing if…else Statements with a Series of if Statements C++ Programming: From Problem Analysis to Program Design, Fourth Edition 14
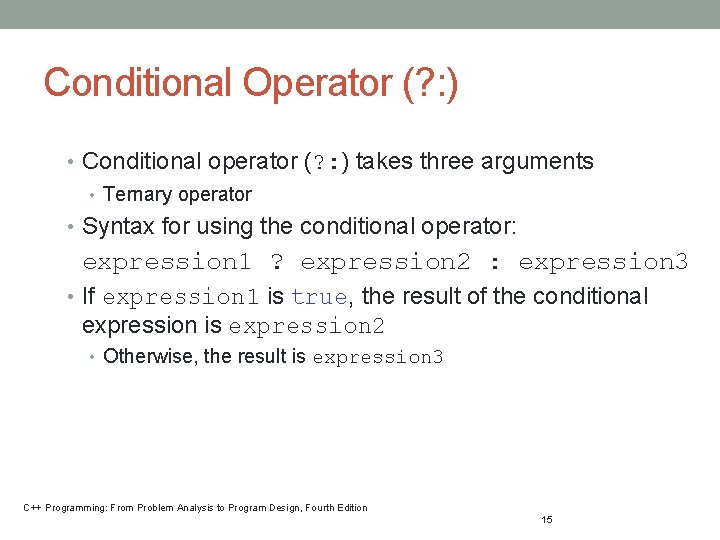
Conditional Operator (? : ) • Conditional operator (? : ) takes three arguments • Ternary operator • Syntax for using the conditional operator: expression 1 ? expression 2 : expression 3 • If expression 1 is true, the result of the conditional expression is expression 2 • Otherwise, the result is expression 3 C++ Programming: From Problem Analysis to Program Design, Fourth Edition 15
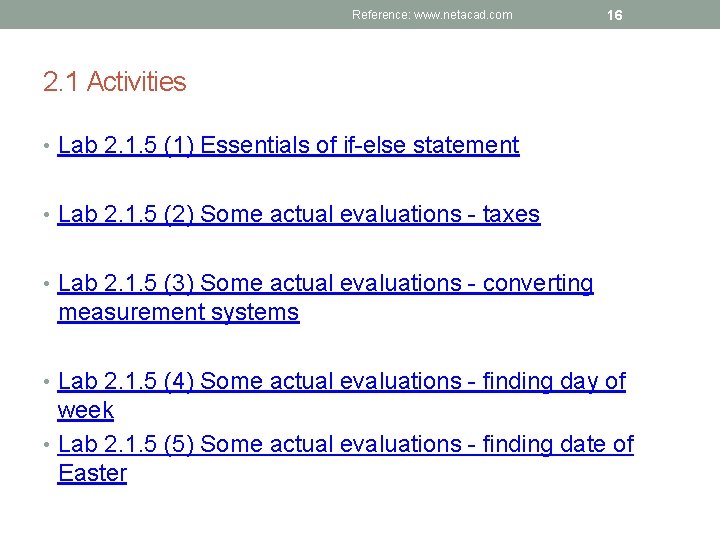
Reference: www. netacad. com 16 2. 1 Activities • Lab 2. 1. 5 (1) Essentials of if-else statement • Lab 2. 1. 5 (2) Some actual evaluations - taxes • Lab 2. 1. 5 (3) Some actual evaluations - converting measurement systems • Lab 2. 1. 5 (4) Some actual evaluations - finding day of week • Lab 2. 1. 5 (5) Some actual evaluations - finding date of Easter
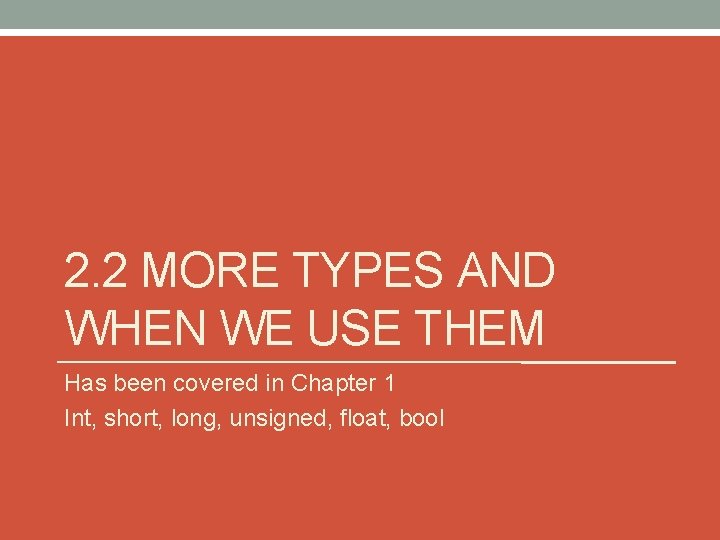
2. 2 MORE TYPES AND WHEN WE USE THEM Has been covered in Chapter 1 Int, short, long, unsigned, float, bool
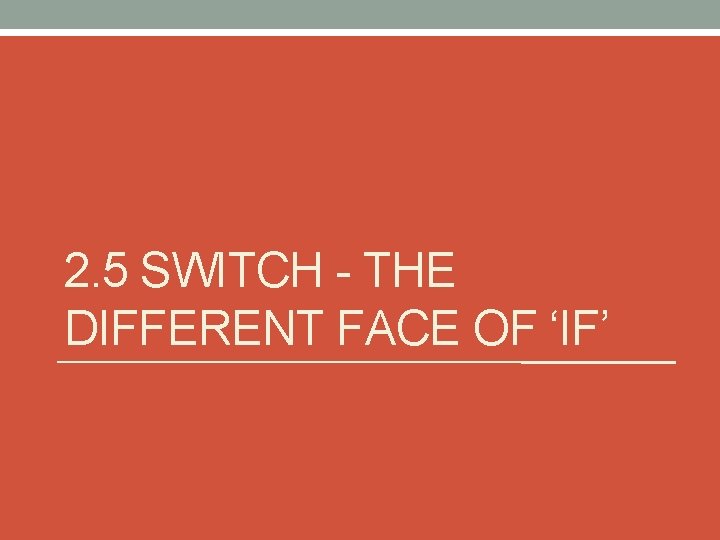
2. 5 SWITCH - THE DIFFERENT FACE OF ‘IF’
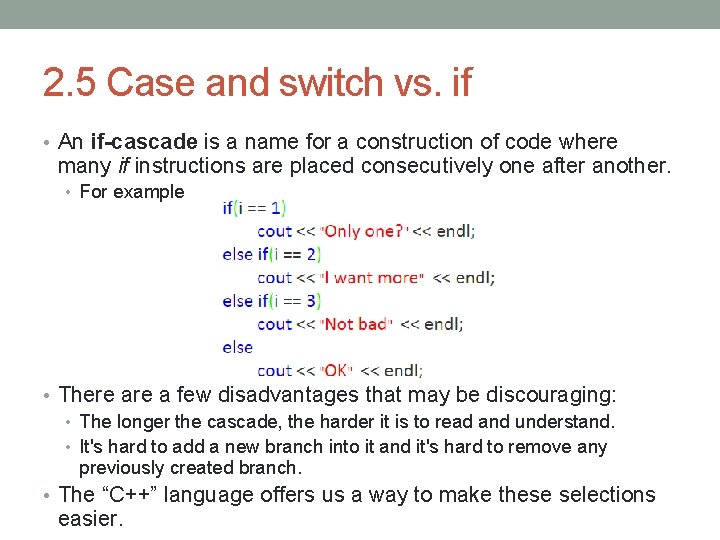
2. 5 Case and switch vs. if • An if-cascade is a name for a construction of code where many if instructions are placed consecutively one after another. • For example • There a few disadvantages that may be discouraging: • The longer the cascade, the harder it is to read and understand. • It's hard to add a new branch into it and it's hard to remove any previously created branch. • The “C++” language offers us a way to make these selections easier.
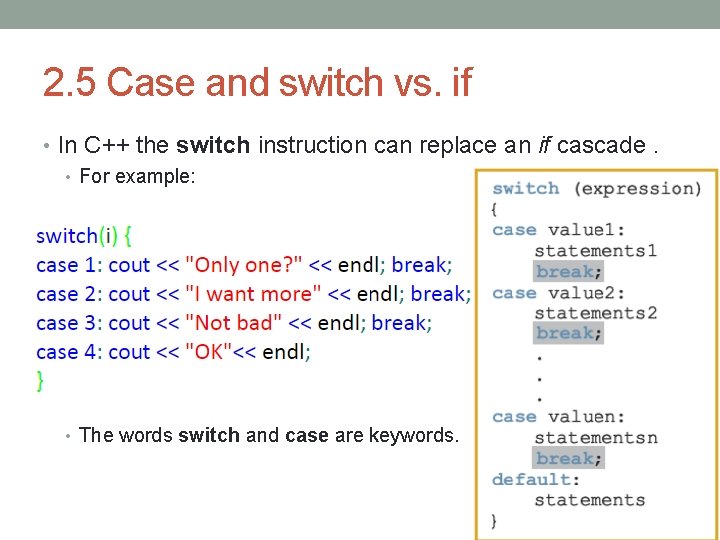
2. 5 Case and switch vs. if • In C++ the switch instruction can replace an if cascade. • For example: • The words switch and case are keywords.
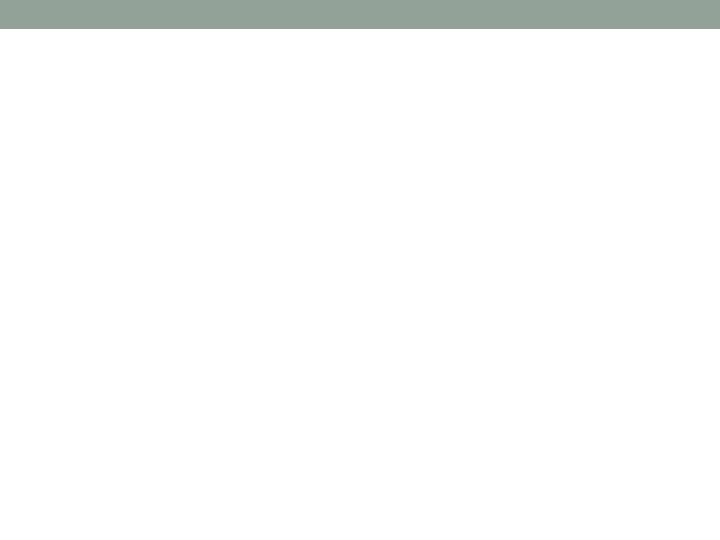
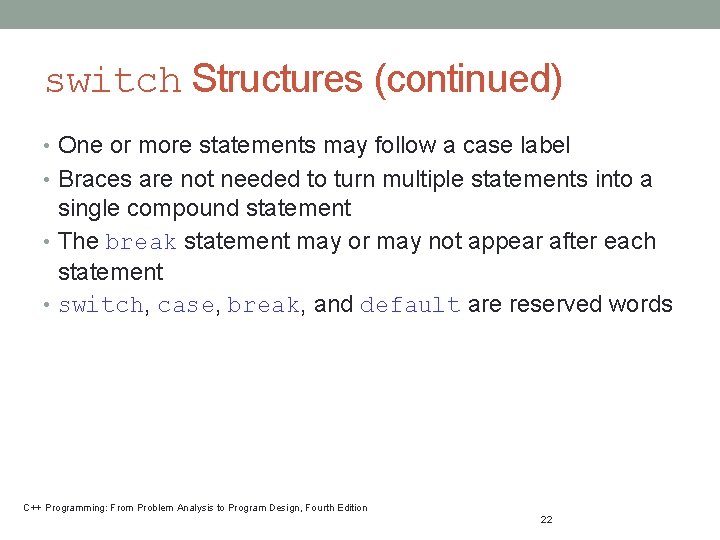
switch Structures (continued) • One or more statements may follow a case label • Braces are not needed to turn multiple statements into a single compound statement • The break statement may or may not appear after each statement • switch, case, break, and default are reserved words C++ Programming: From Problem Analysis to Program Design, Fourth Edition 22
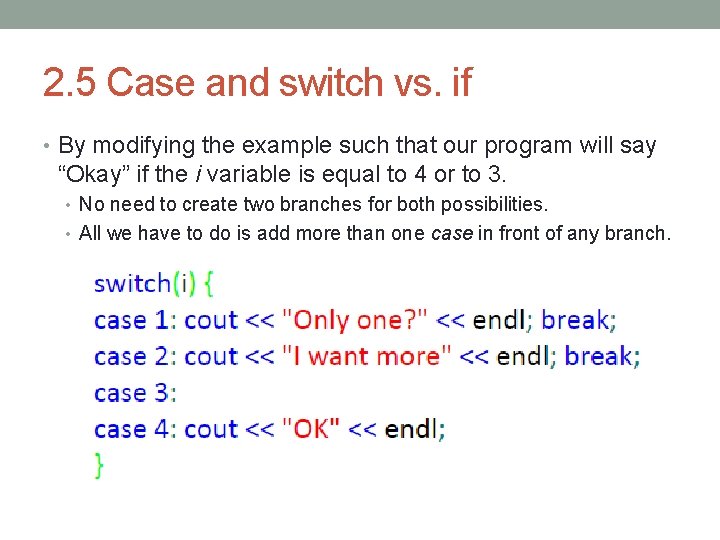
2. 5 Case and switch vs. if • By modifying the example such that our program will say “Okay” if the i variable is equal to 4 or to 3. • No need to create two branches for both possibilities. • All we have to do is add more than one case in front of any branch.
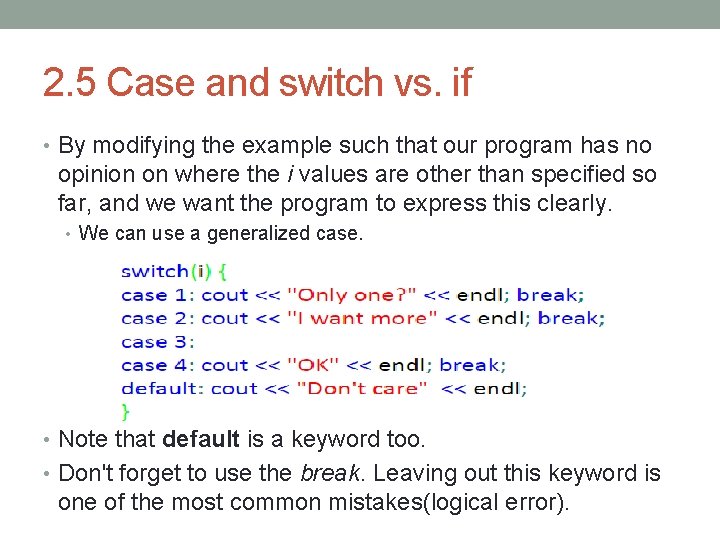
2. 5 Case and switch vs. if • By modifying the example such that our program has no opinion on where the i values are other than specified so far, and we want the program to express this clearly. • We can use a generalized case. • Note that default is a keyword too. • Don't forget to use the break. Leaving out this keyword is one of the most common mistakes(logical error).
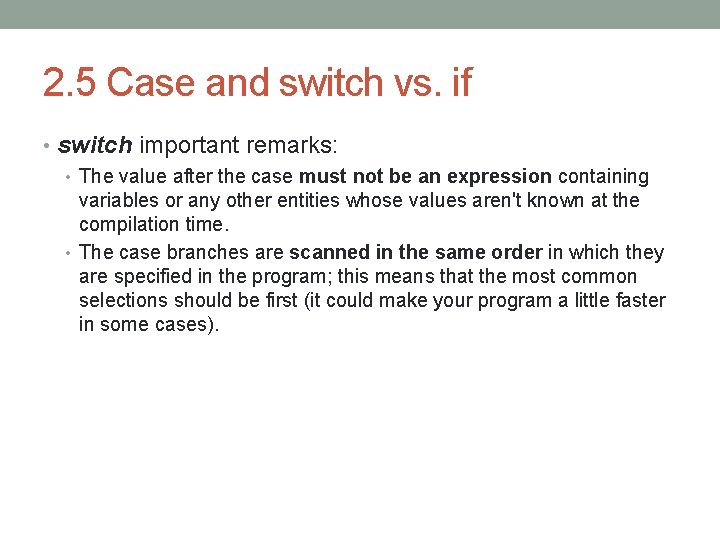
2. 5 Case and switch vs. if • switch important remarks: • The value after the case must not be an expression containing variables or any other entities whose values aren't known at the compilation time. • The case branches are scanned in the same order in which they are specified in the program; this means that the most common selections should be first (it could make your program a little faster in some cases).
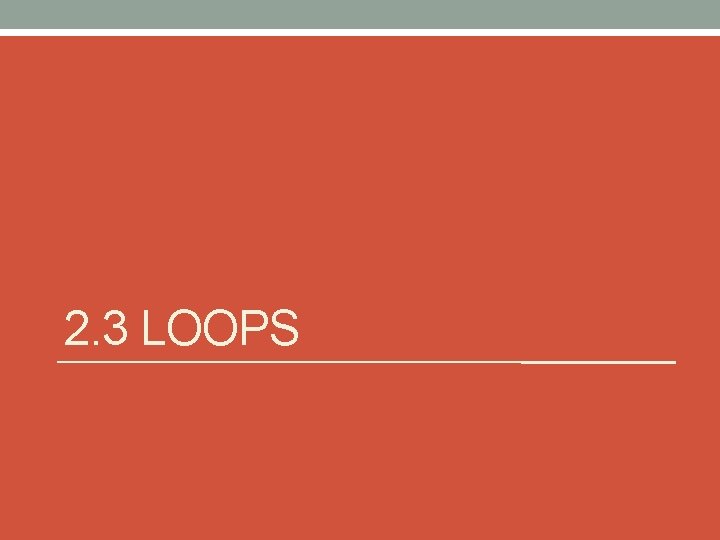
2. 3 LOOPS
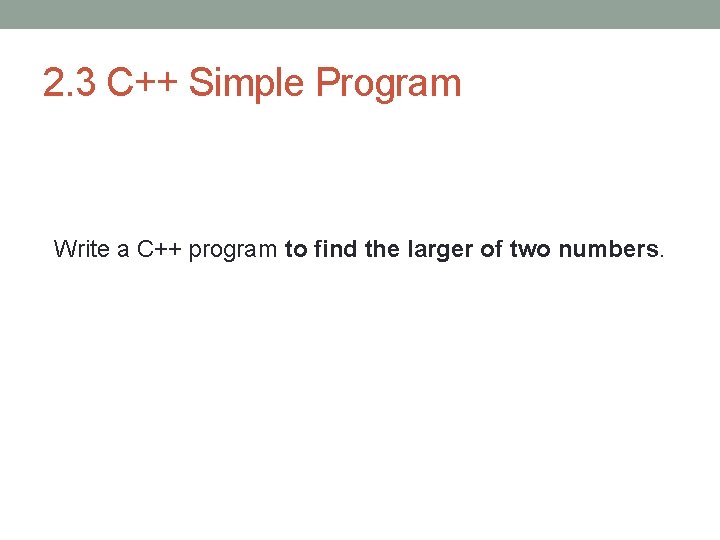
2. 3 C++ Simple Program Write a C++ program to find the larger of two numbers.
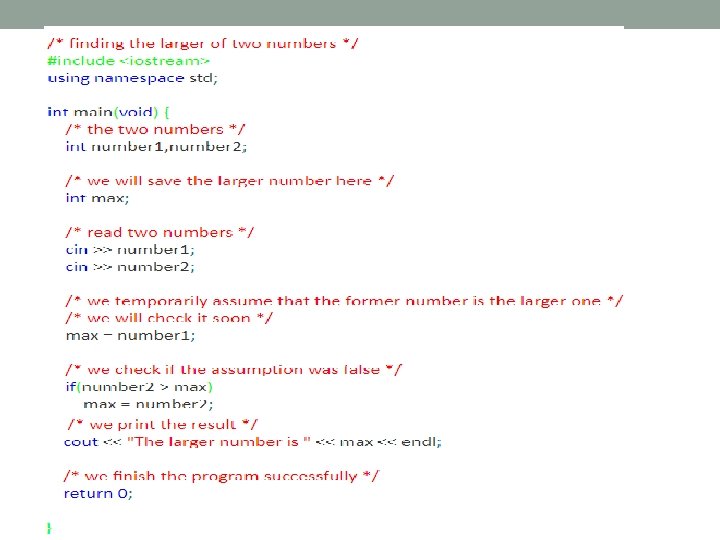
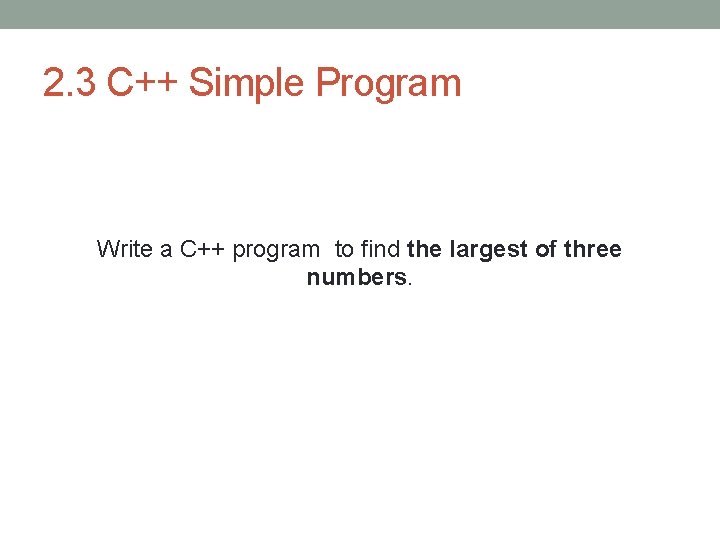
2. 3 C++ Simple Program Write a C++ program to find the largest of three numbers.
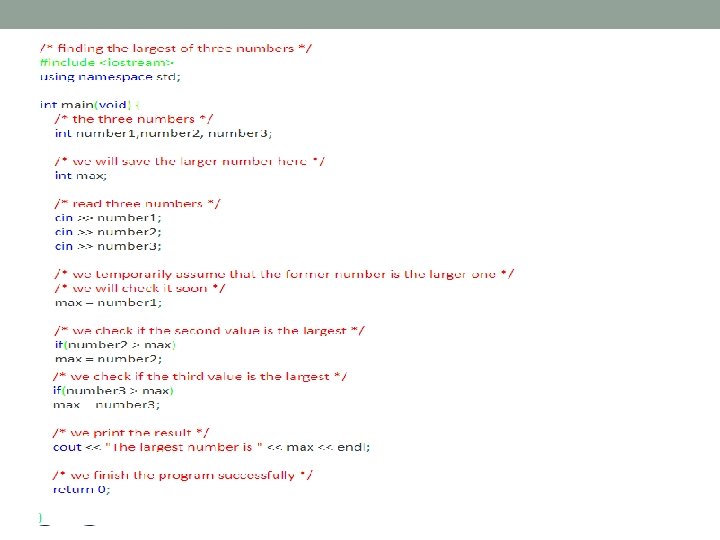
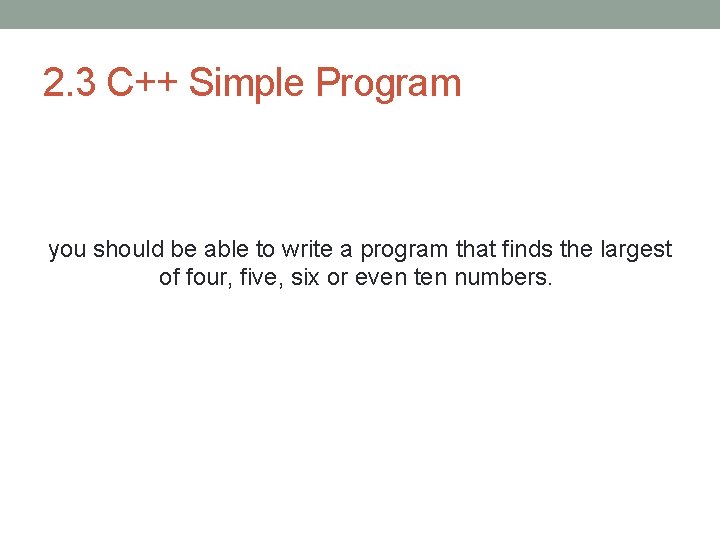
2. 3 C++ Simple Program you should be able to write a program that finds the largest of four, five, six or even ten numbers.
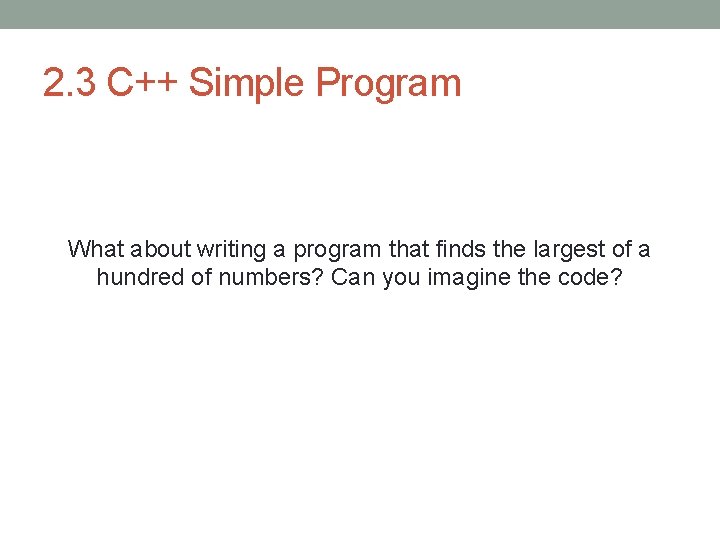
2. 3 C++ Simple Program What about writing a program that finds the largest of a hundred of numbers? Can you imagine the code?
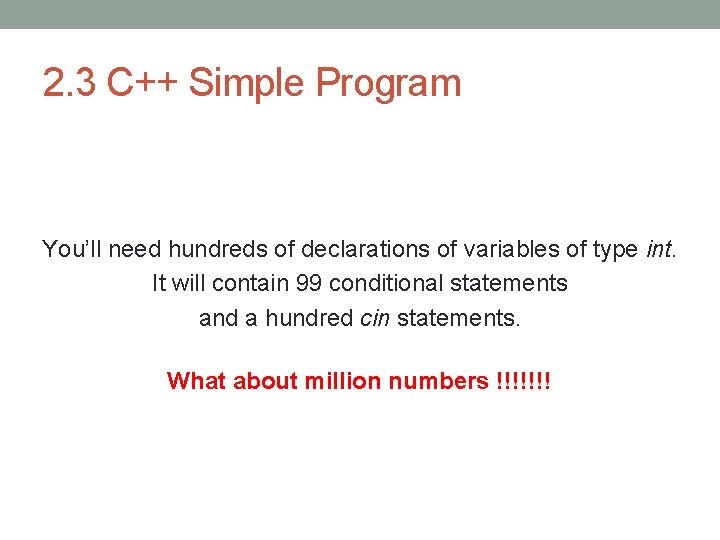
2. 3 C++ Simple Program You’ll need hundreds of declarations of variables of type int. It will contain 99 conditional statements and a hundred cin statements. What about million numbers !!!!!!!
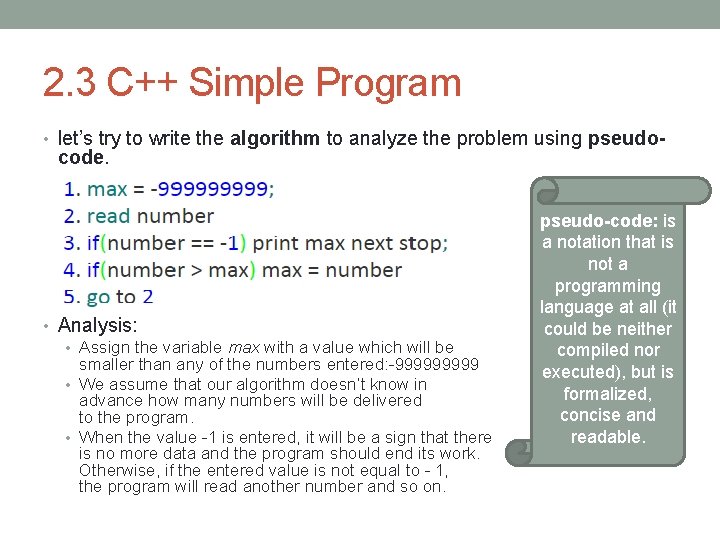
2. 3 C++ Simple Program • let’s try to write the algorithm to analyze the problem using pseudo- code. • Analysis: • Assign the variable max with a value which will be smaller than any of the numbers entered: -99999 • We assume that our algorithm doesn’t know in advance how many numbers will be delivered to the program. • When the value -1 is entered, it will be a sign that there is no more data and the program should end its work. Otherwise, if the entered value is not equal to - 1, the program will read another number and so on. pseudo-code: is a notation that is not a programming language at all (it could be neither compiled nor executed), but is formalized, concise and readable.
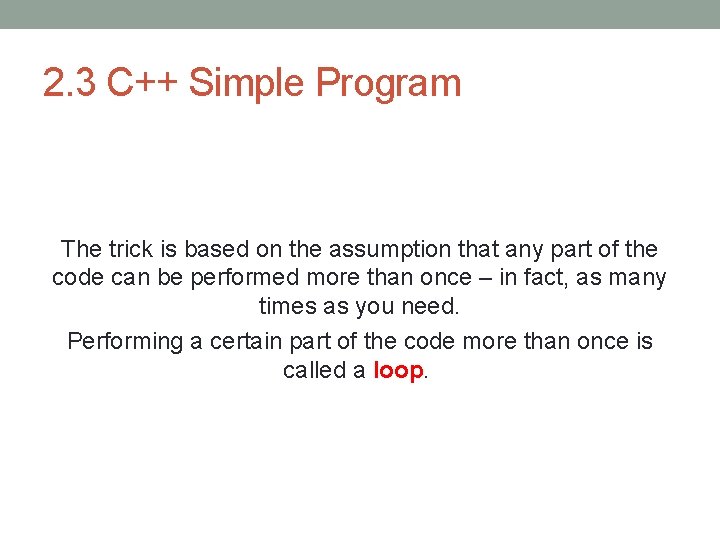
2. 3 C++ Simple Program The trick is based on the assumption that any part of the code can be performed more than once – in fact, as many times as you need. Performing a certain part of the code more than once is called a loop.
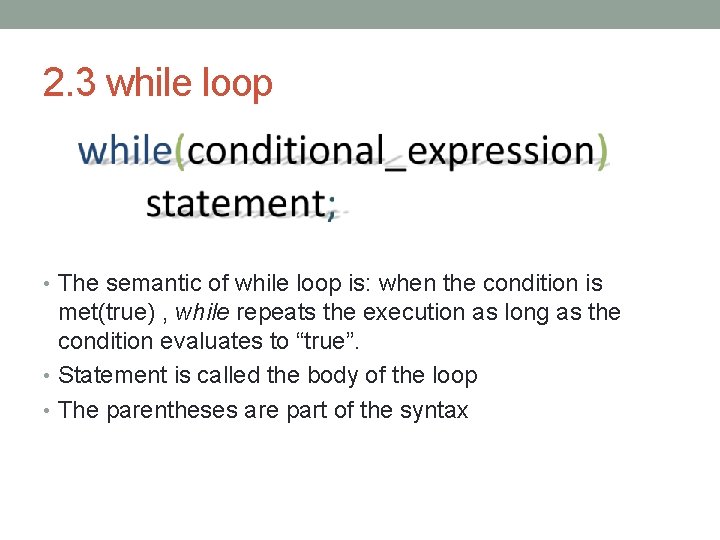
2. 3 while loop • The semantic of while loop is: when the condition is met(true) , while repeats the execution as long as the condition evaluates to “true”. • Statement is called the body of the loop • The parentheses are part of the syntax
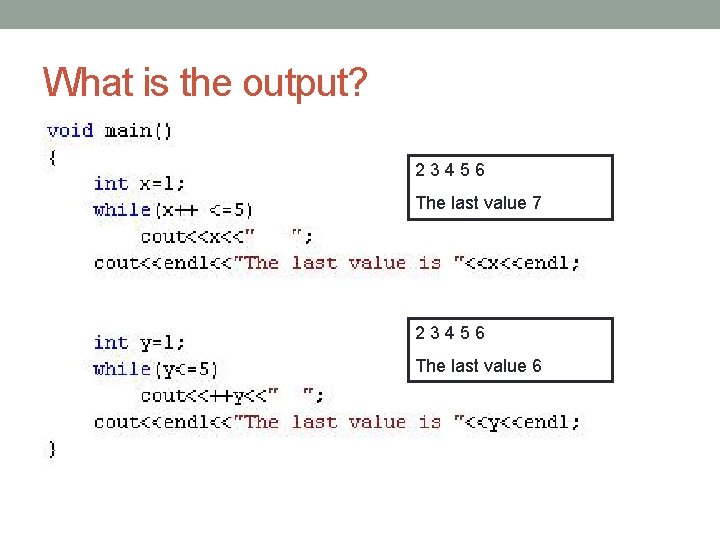
What is the output? 23456 The last value 7 23456 The last value 6
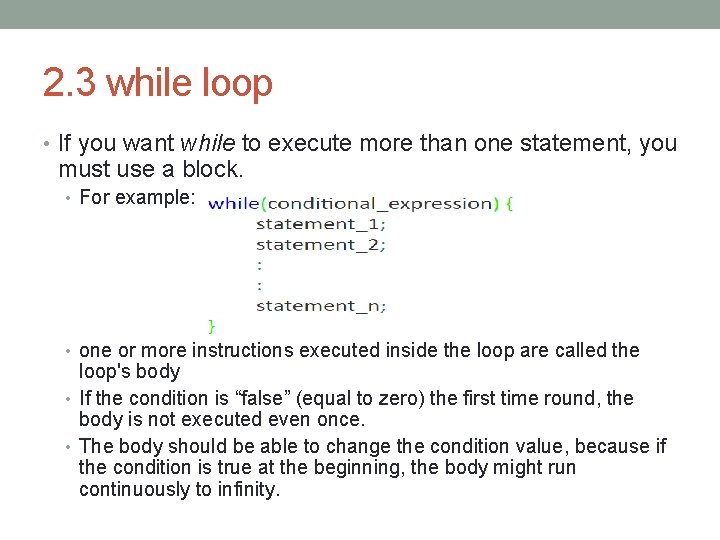
2. 3 while loop • If you want while to execute more than one statement, you must use a block. • For example: • one or more instructions executed inside the loop are called the loop's body • If the condition is “false” (equal to zero) the first time round, the body is not executed even once. • The body should be able to change the condition value, because if the condition is true at the beginning, the body might run continuously to infinity.
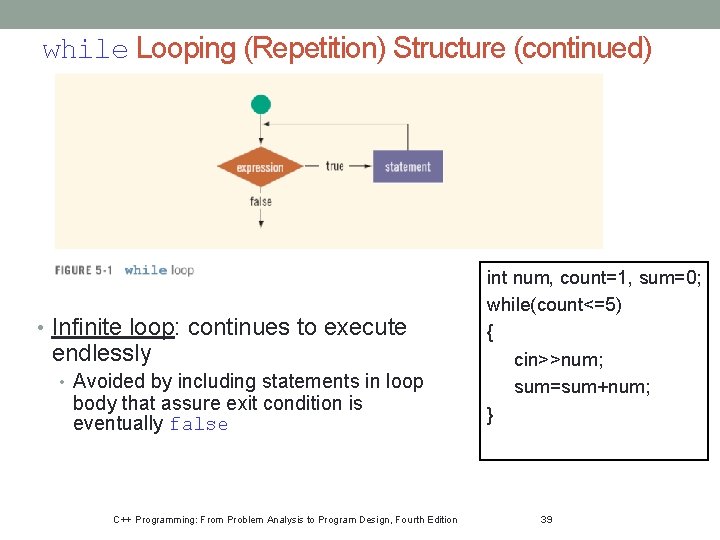
while Looping (Repetition) Structure (continued) • Infinite loop: continues to execute endlessly • Avoided by including statements in loop body that assure exit condition is eventually false C++ Programming: From Problem Analysis to Program Design, Fourth Edition int num, count=1, sum=0; while(count<=5) { cin>>num; sum=sum+num; } 39
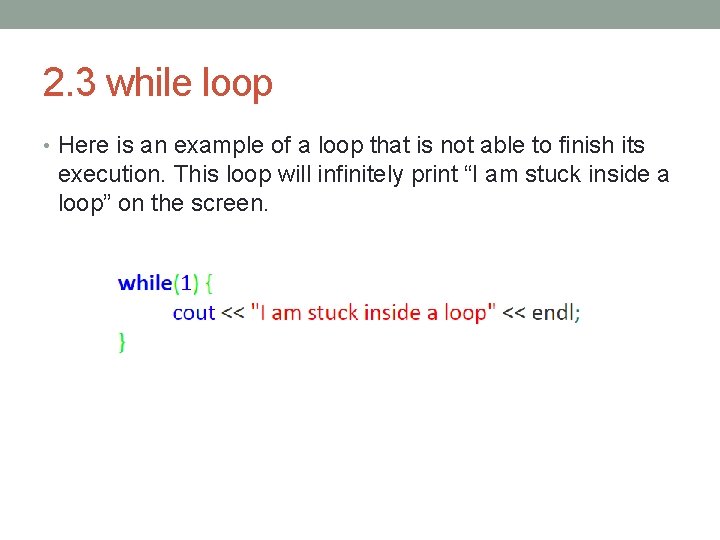
2. 3 while loop • Here is an example of a loop that is not able to finish its execution. This loop will infinitely print “I am stuck inside a loop” on the screen.
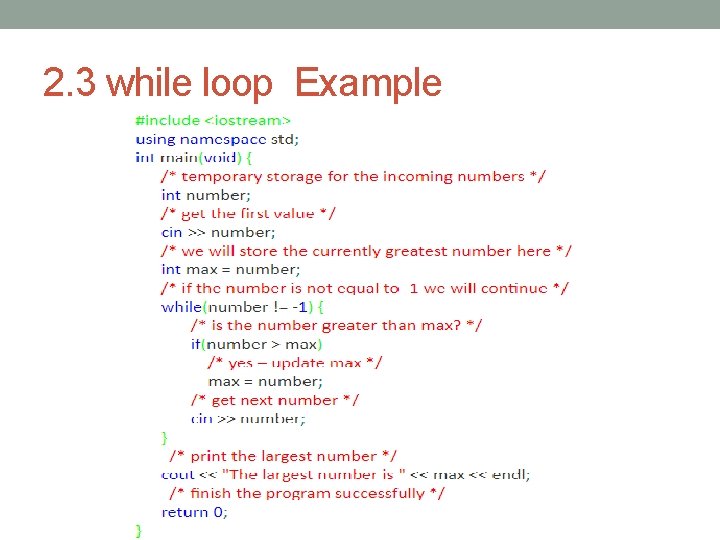
2. 3 while loop Example
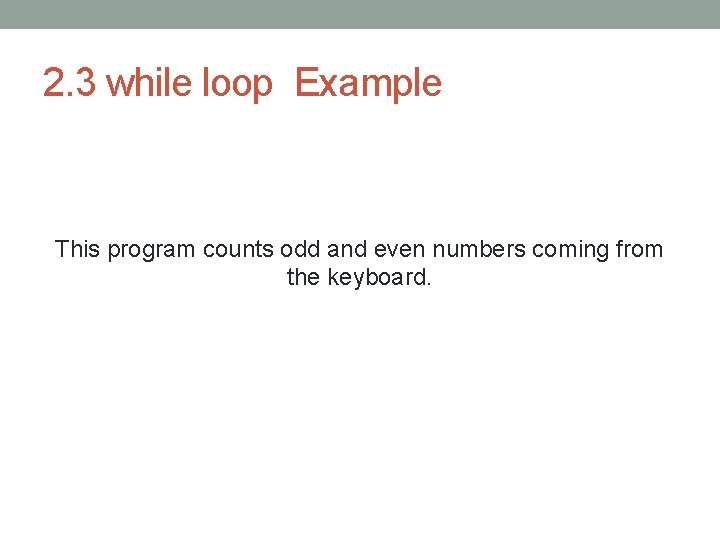
2. 3 while loop Example This program counts odd and even numbers coming from the keyboard.
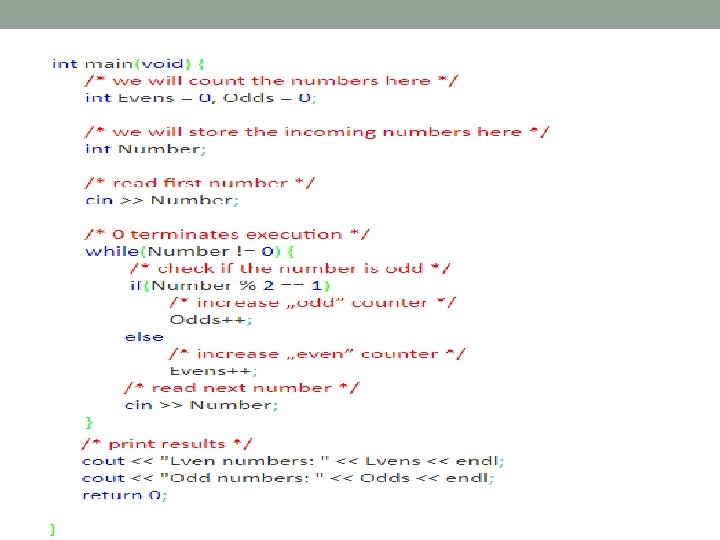
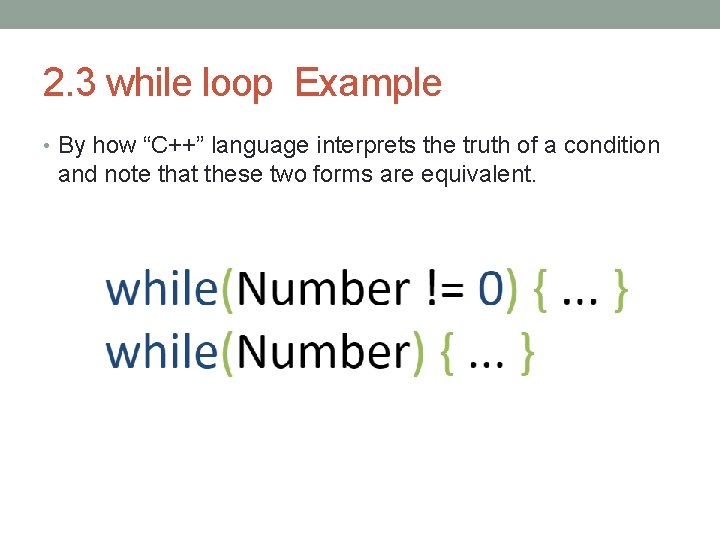
2. 3 while loop Example • By how “C++” language interprets the truth of a condition and note that these two forms are equivalent.
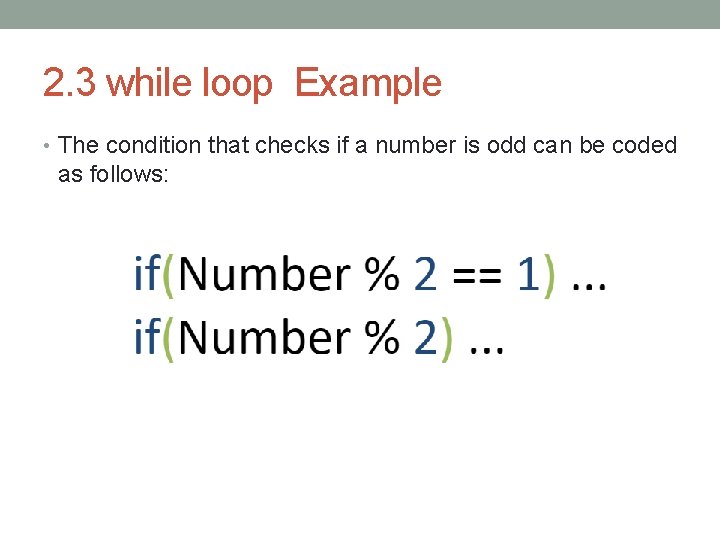
2. 3 while loop Example • The condition that checks if a number is odd can be coded as follows:
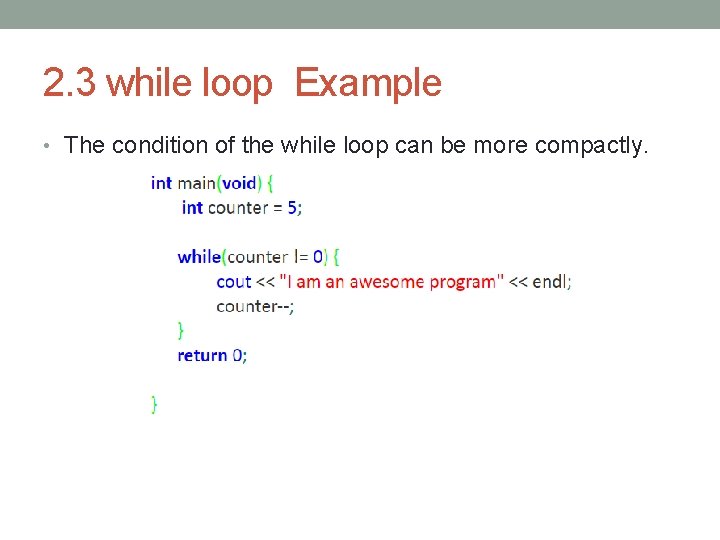
2. 3 while loop Example • The condition of the while loop can be more compactly.
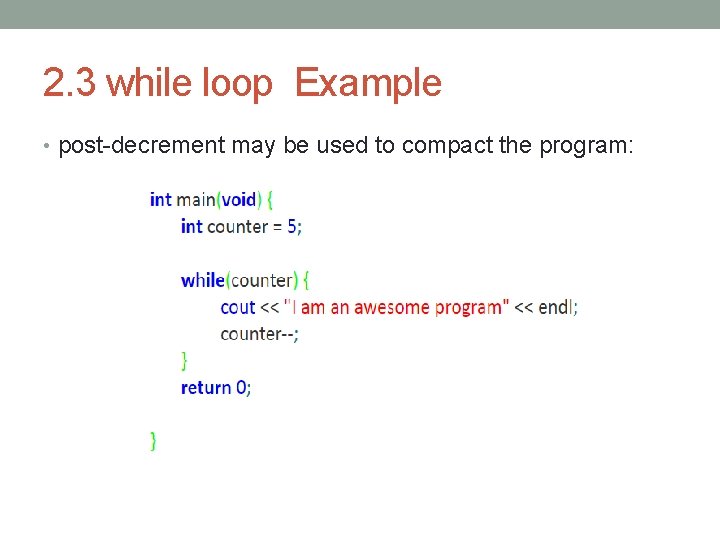
2. 3 while loop Example • post-decrement may be used to compact the program:
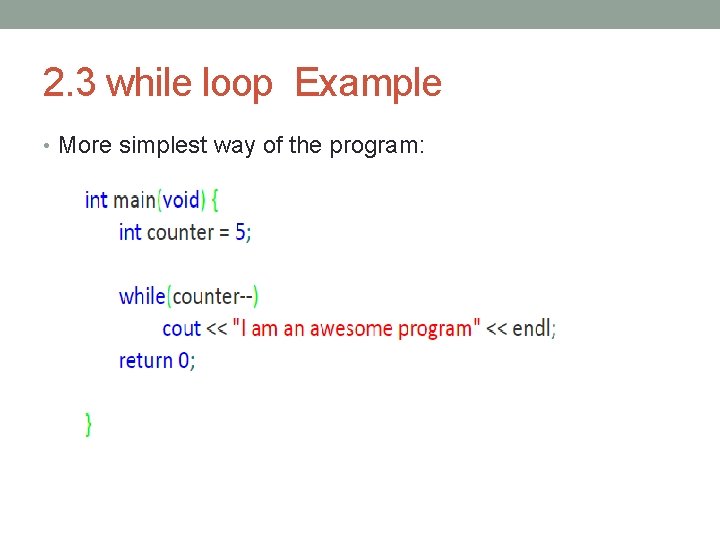
2. 3 while loop Example • More simplest way of the program:
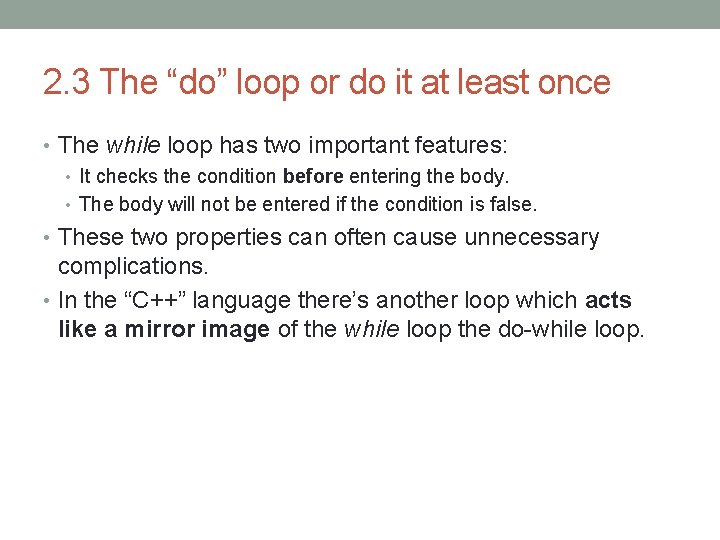
2. 3 The “do” loop or do it at least once • The while loop has two important features: • It checks the condition before entering the body. • The body will not be entered if the condition is false. • These two properties can often cause unnecessary complications. • In the “C++” language there’s another loop which acts like a mirror image of the while loop the do-while loop.
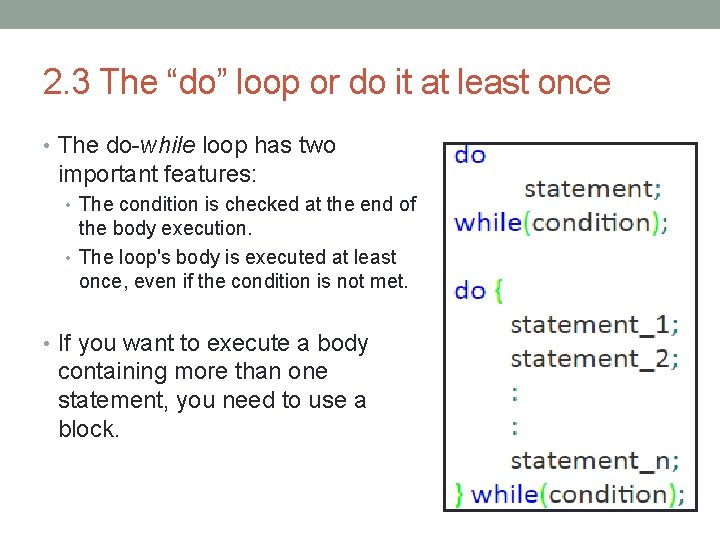
2. 3 The “do” loop or do it at least once • The do-while loop has two important features: • The condition is checked at the end of the body execution. • The loop's body is executed at least once, even if the condition is not met. • If you want to execute a body containing more than one statement, you need to use a block.
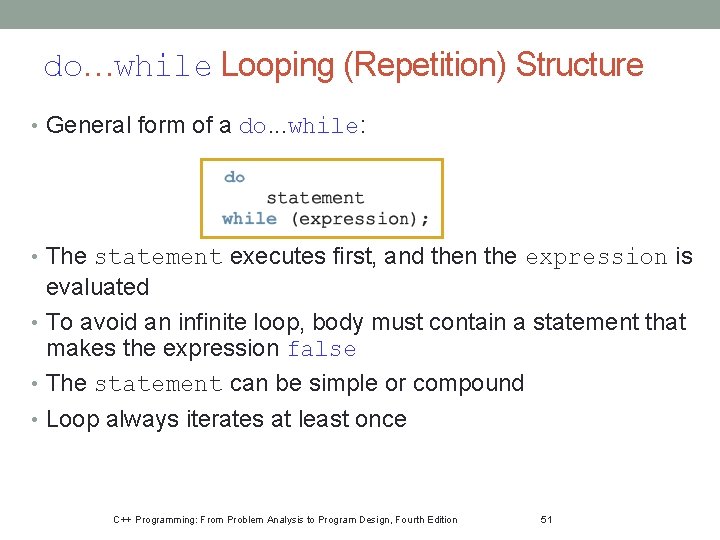
do…while Looping (Repetition) Structure • General form of a do. . . while: • The statement executes first, and then the expression is evaluated • To avoid an infinite loop, body must contain a statement that makes the expression false • The statement can be simple or compound • Loop always iterates at least once C++ Programming: From Problem Analysis to Program Design, Fourth Edition 51
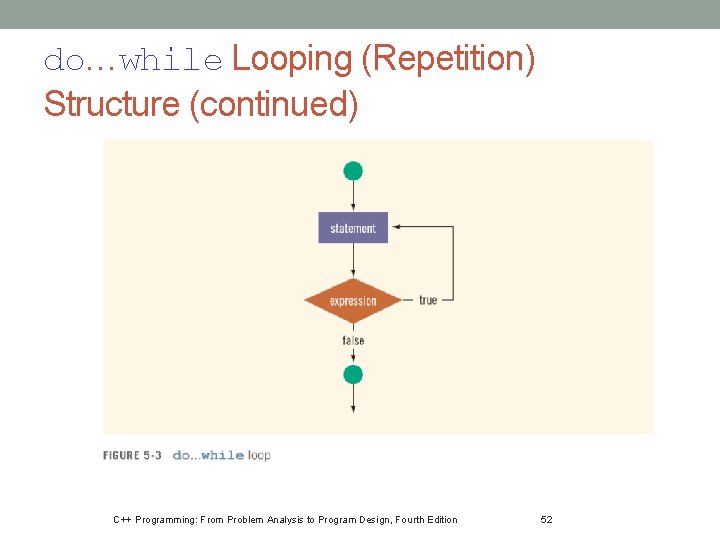
do…while Looping (Repetition) Structure (continued) C++ Programming: From Problem Analysis to Program Design, Fourth Edition 52
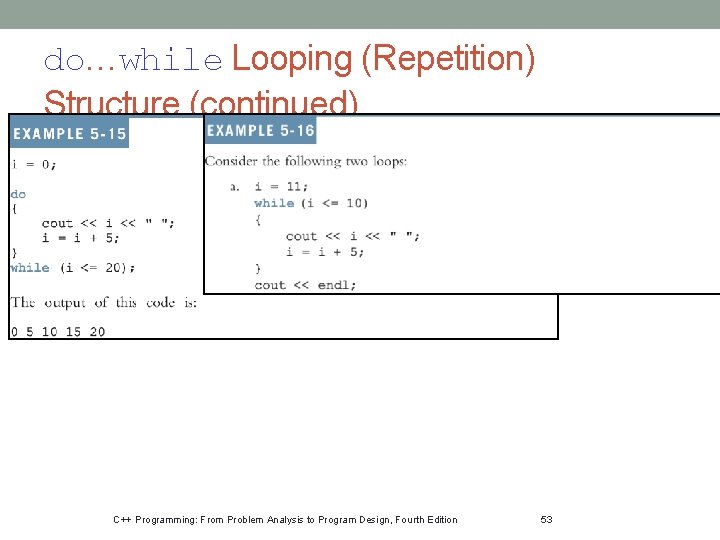
do…while Looping (Repetition) Structure (continued) C++ Programming: From Problem Analysis to Program Design, Fourth Edition 53
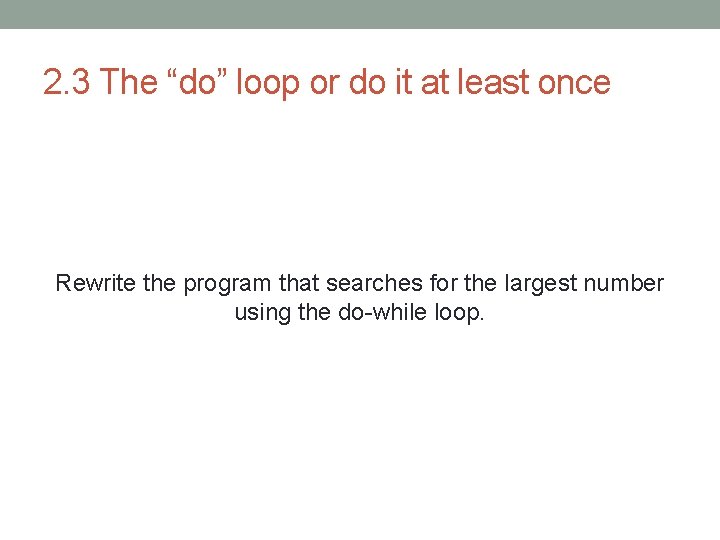
2. 3 The “do” loop or do it at least once Rewrite the program that searches for the largest number using the do-while loop.
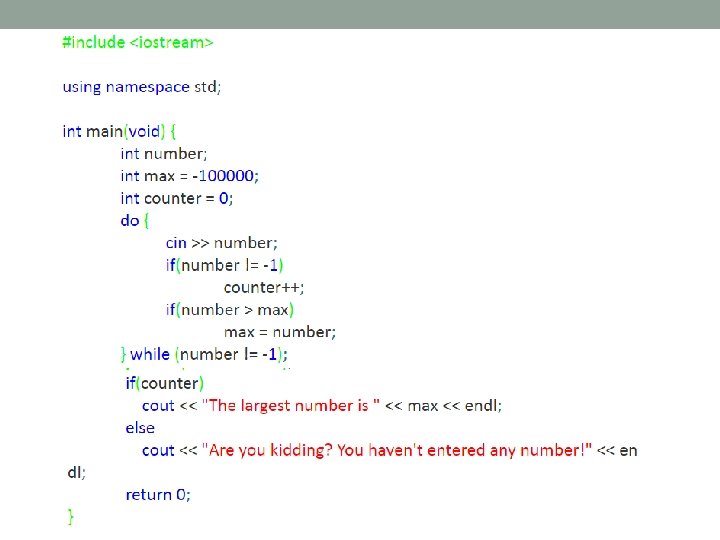
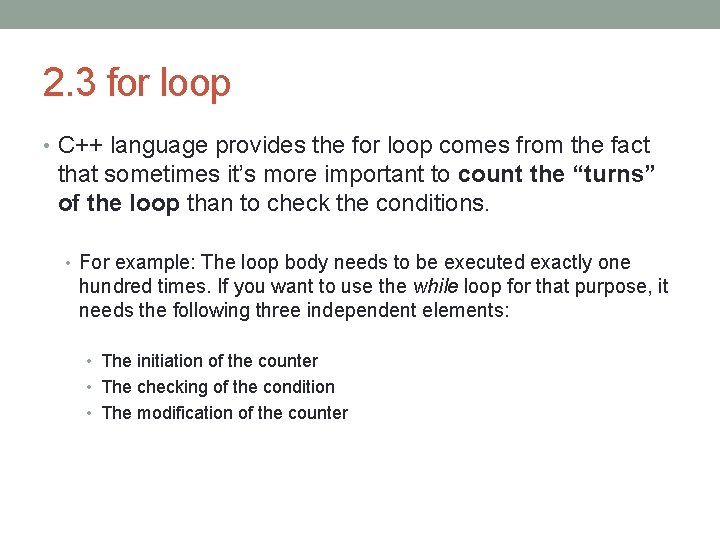
2. 3 for loop • C++ language provides the for loop comes from the fact that sometimes it’s more important to count the “turns” of the loop than to check the conditions. • For example: The loop body needs to be executed exactly one hundred times. If you want to use the while loop for that purpose, it needs the following three independent elements: • The initiation of the counter • The checking of the condition • The modification of the counter
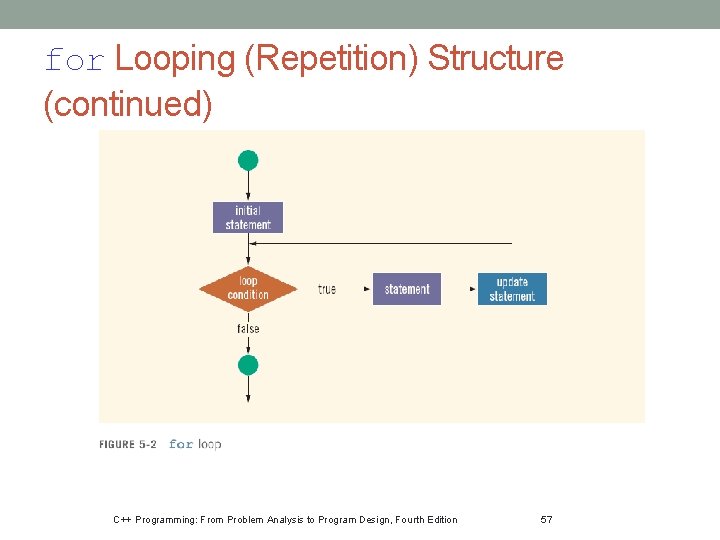
for Looping (Repetition) Structure (continued) C++ Programming: From Problem Analysis to Program Design, Fourth Edition 57
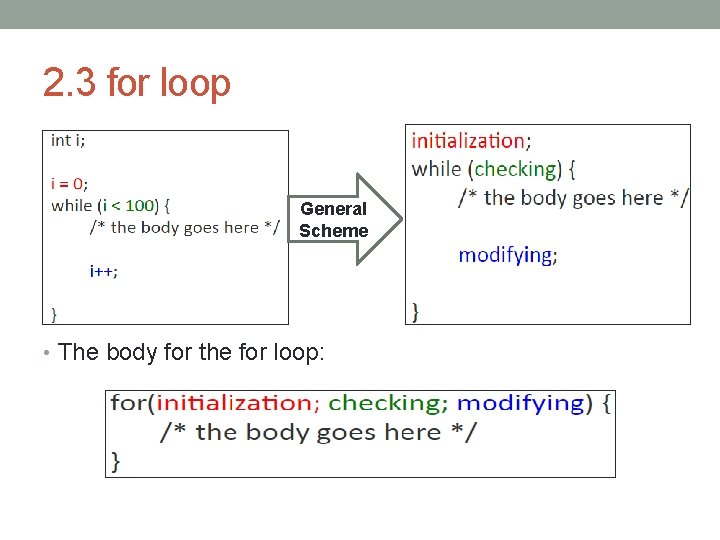
2. 3 for loop General Scheme • The body for the for loop:
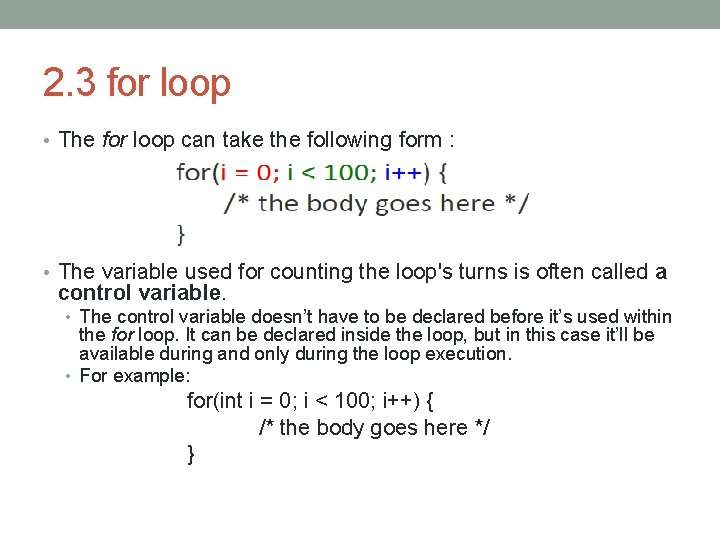
2. 3 for loop • The for loop can take the following form : • The variable used for counting the loop's turns is often called a control variable. • The control variable doesn’t have to be declared before it’s used within the for loop. It can be declared inside the loop, but in this case it’ll be available during and only during the loop execution. • For example: for(int i = 0; i < 100; i++) { /* the body goes here */ }
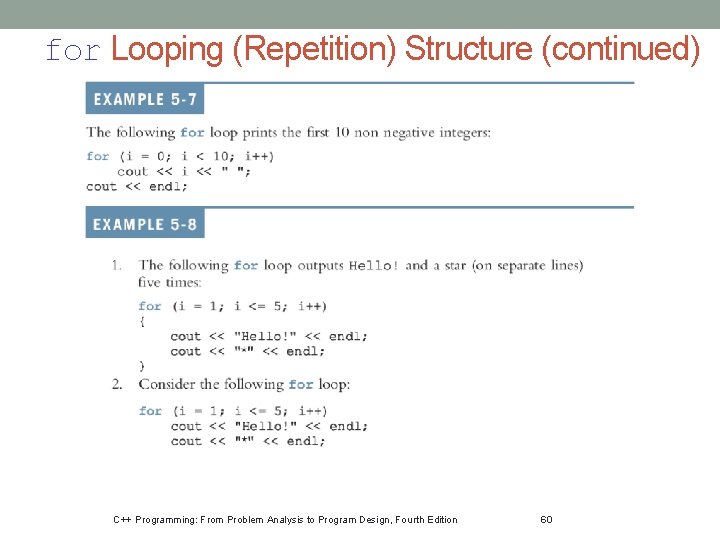
for Looping (Repetition) Structure (continued) C++ Programming: From Problem Analysis to Program Design, Fourth Edition 60
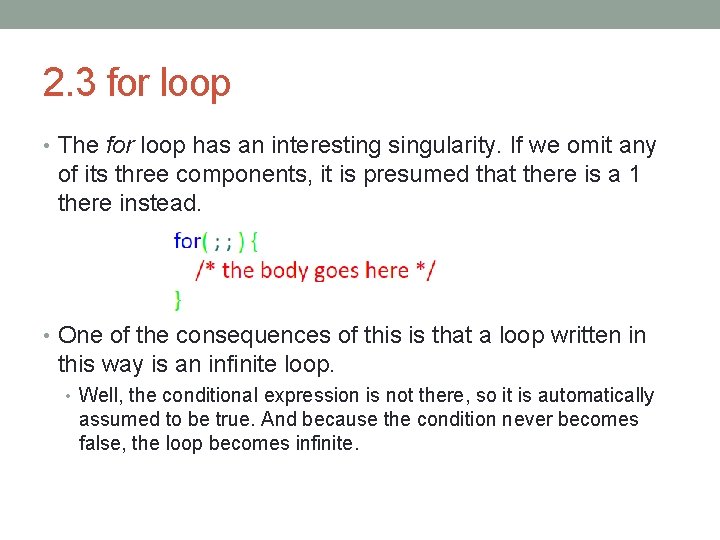
2. 3 for loop • The for loop has an interesting singularity. If we omit any of its three components, it is presumed that there is a 1 there instead. • One of the consequences of this is that a loop written in this way is an infinite loop. • Well, the conditional expression is not there, so it is automatically assumed to be true. And because the condition never becomes false, the loop becomes infinite.
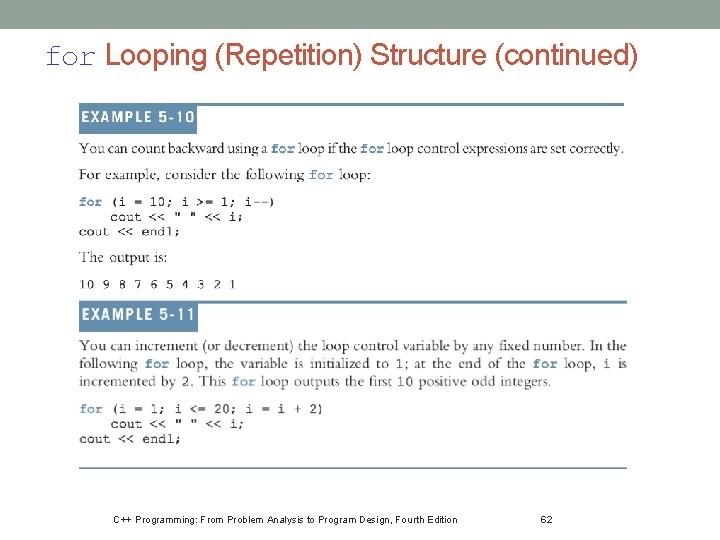
for Looping (Repetition) Structure (continued) C++ Programming: From Problem Analysis to Program Design, Fourth Edition 62
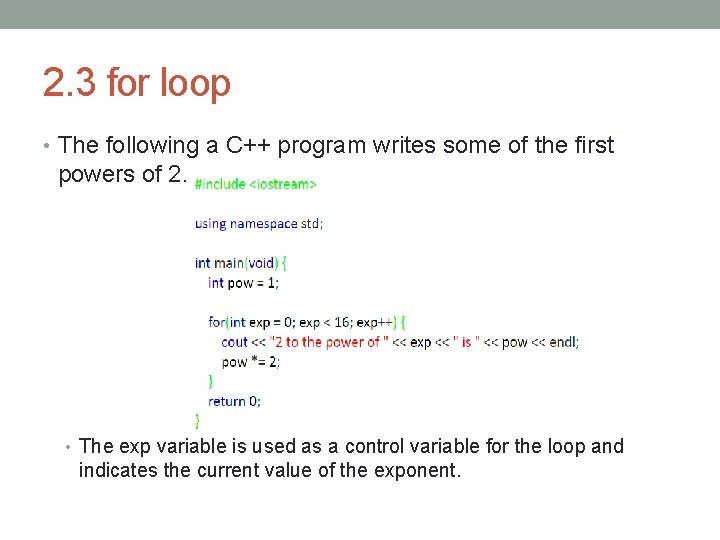
2. 3 for loop • The following a C++ program writes some of the first powers of 2. • The exp variable is used as a control variable for the loop and indicates the current value of the exponent.
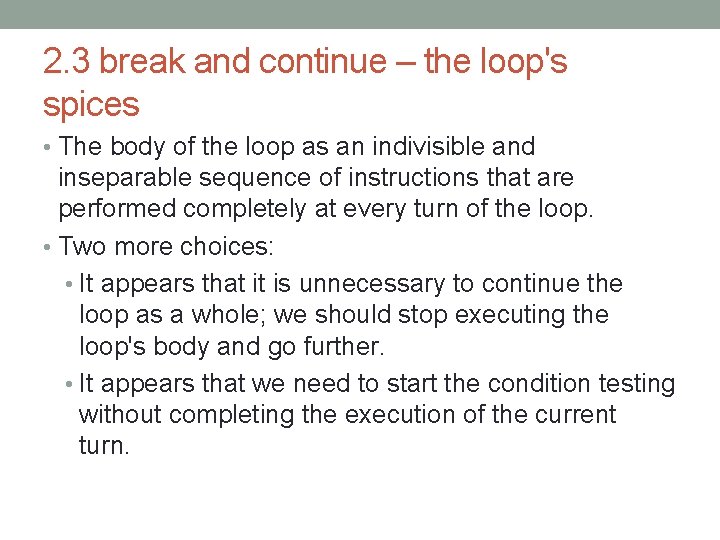
2. 3 break and continue – the loop's spices • The body of the loop as an indivisible and inseparable sequence of instructions that are performed completely at every turn of the loop. • Two more choices: • It appears that it is unnecessary to continue the loop as a whole; we should stop executing the loop's body and go further. • It appears that we need to start the condition testing without completing the execution of the current turn.
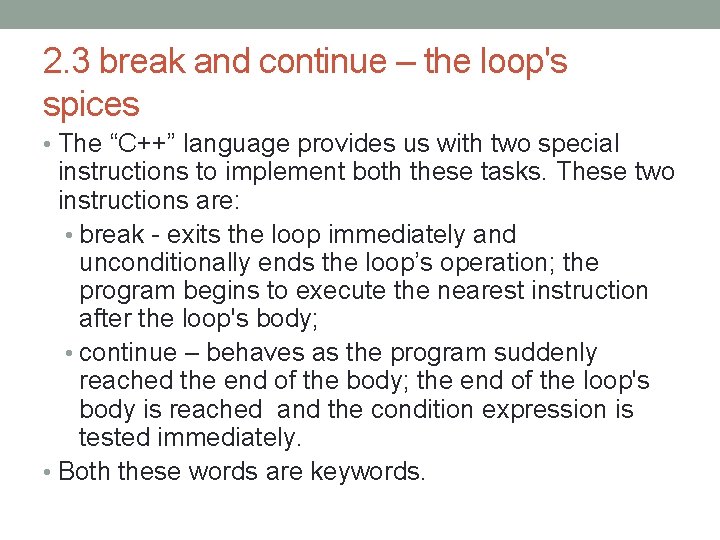
2. 3 break and continue – the loop's spices • The “C++” language provides us with two special instructions to implement both these tasks. These two instructions are: • break - exits the loop immediately and unconditionally ends the loop’s operation; the program begins to execute the nearest instruction after the loop's body; • continue – behaves as the program suddenly reached the end of the body; the end of the loop's body is reached and the condition expression is tested immediately. • Both these words are keywords.
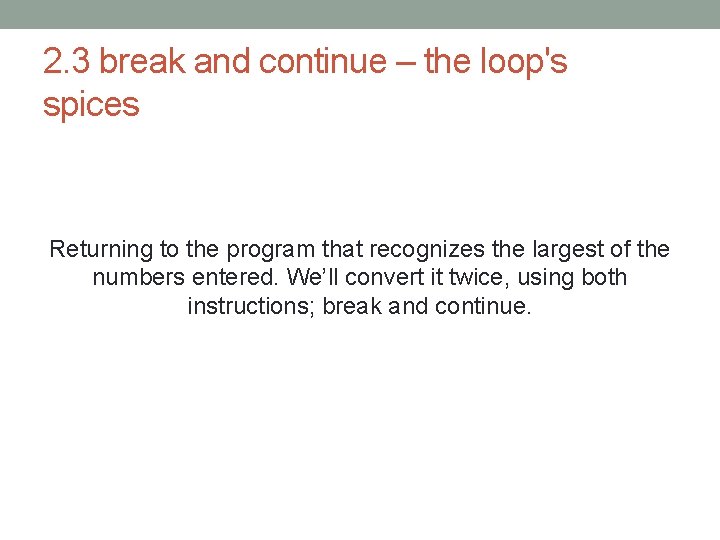
2. 3 break and continue – the loop's spices Returning to the program that recognizes the largest of the numbers entered. We’ll convert it twice, using both instructions; break and continue.
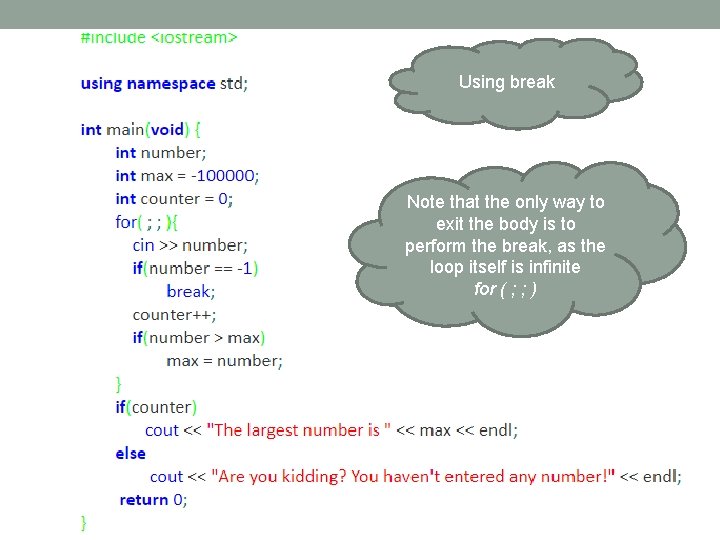
Using break Note that the only way to exit the body is to perform the break, as the loop itself is infinite for ( ; ; )
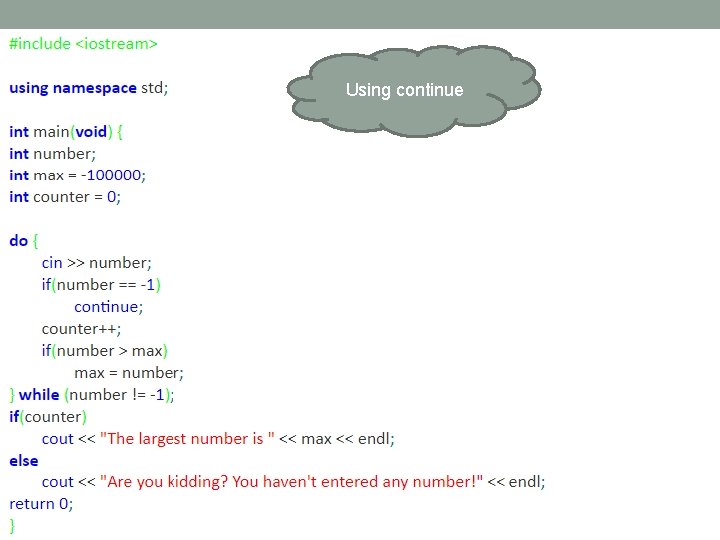
Using continue
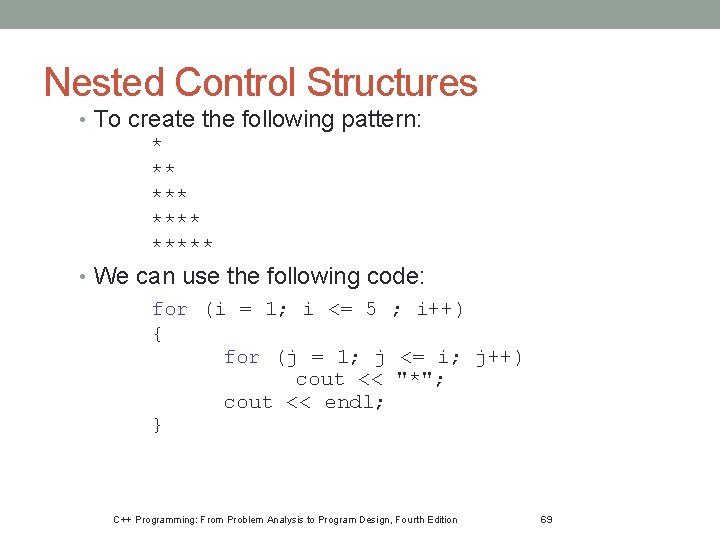
Nested Control Structures • To create the following pattern: * ** ***** • We can use the following code: for (i = 1; i <= 5 ; i++) { for (j = 1; j <= i; j++) cout << "*"; cout << endl; } C++ Programming: From Problem Analysis to Program Design, Fourth Edition 69
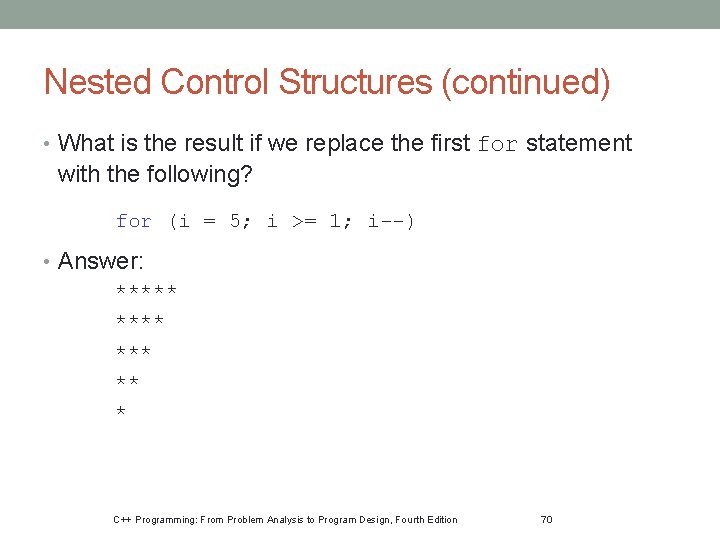
Nested Control Structures (continued) • What is the result if we replace the first for statement with the following? for (i = 5; i >= 1; i--) • Answer: ***** *** ** * C++ Programming: From Problem Analysis to Program Design, Fourth Edition 70
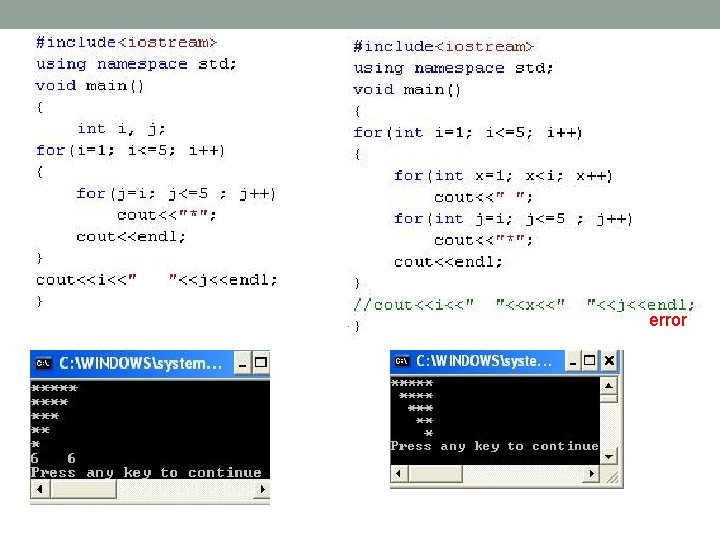
error Updated by: Malak Abdullah
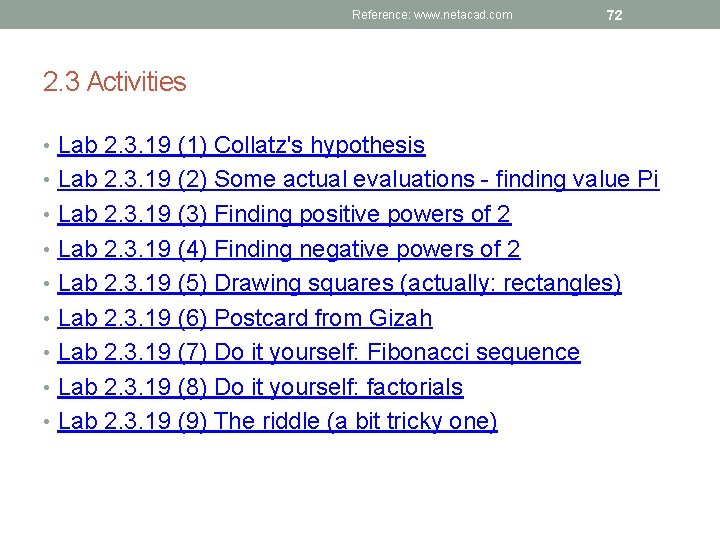
Reference: www. netacad. com 72 2. 3 Activities • Lab 2. 3. 19 (1) Collatz's hypothesis • Lab 2. 3. 19 (2) Some actual evaluations - finding value Pi • Lab 2. 3. 19 (3) Finding positive powers of 2 • Lab 2. 3. 19 (4) Finding negative powers of 2 • Lab 2. 3. 19 (5) Drawing squares (actually: rectangles) • Lab 2. 3. 19 (6) Postcard from Gizah • Lab 2. 3. 19 (7) Do it yourself: Fibonacci sequence • Lab 2. 3. 19 (8) Do it yourself: factorials • Lab 2. 3. 19 (9) The riddle (a bit tricky one)
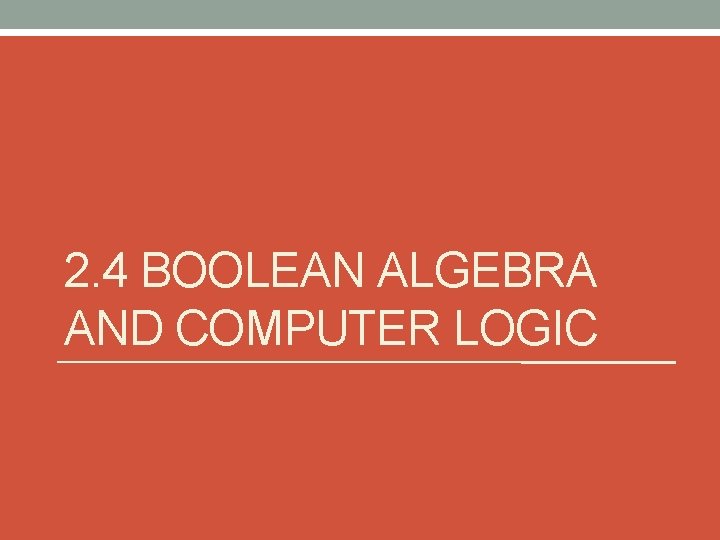
2. 4 BOOLEAN ALGEBRA AND COMPUTER LOGIC
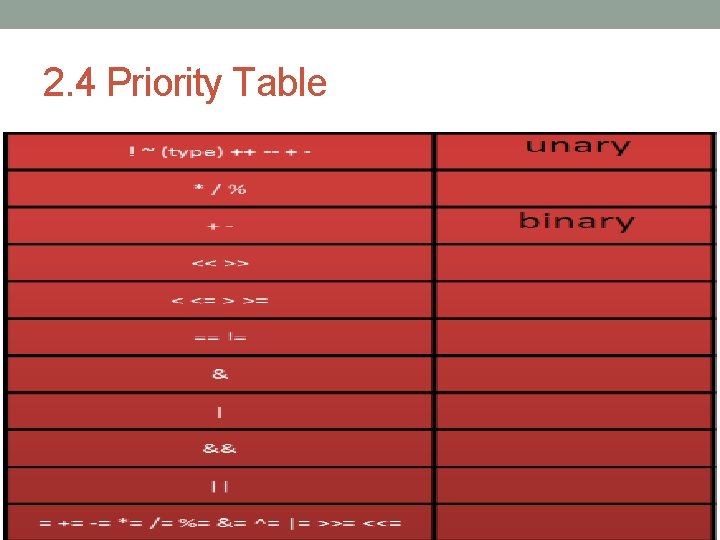
2. 4 Priority Table
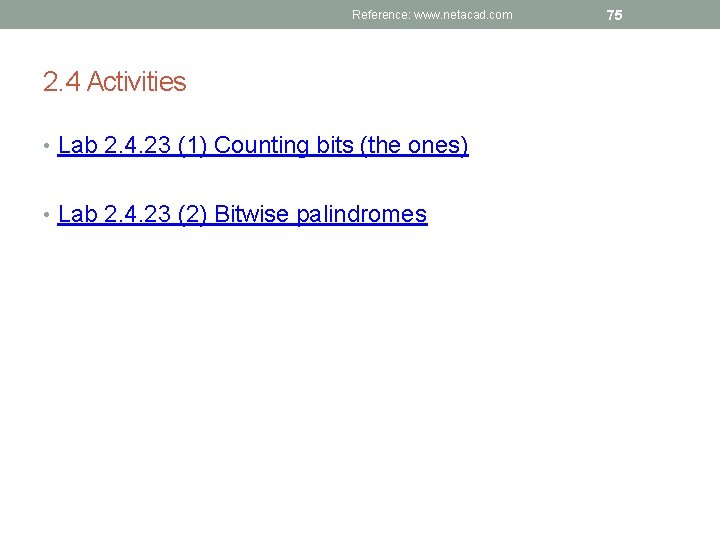
Reference: www. netacad. com 2. 4 Activities • Lab 2. 4. 23 (1) Counting bits (the ones) • Lab 2. 4. 23 (2) Bitwise palindromes 75
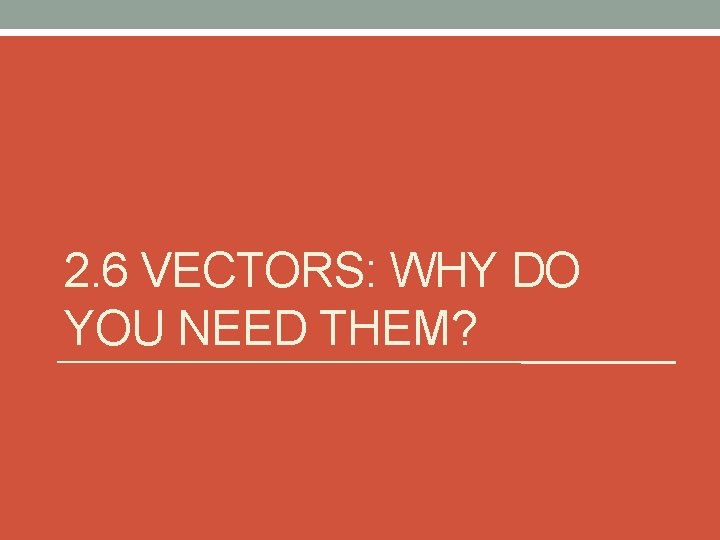
2. 6 VECTORS: WHY DO YOU NEED THEM?
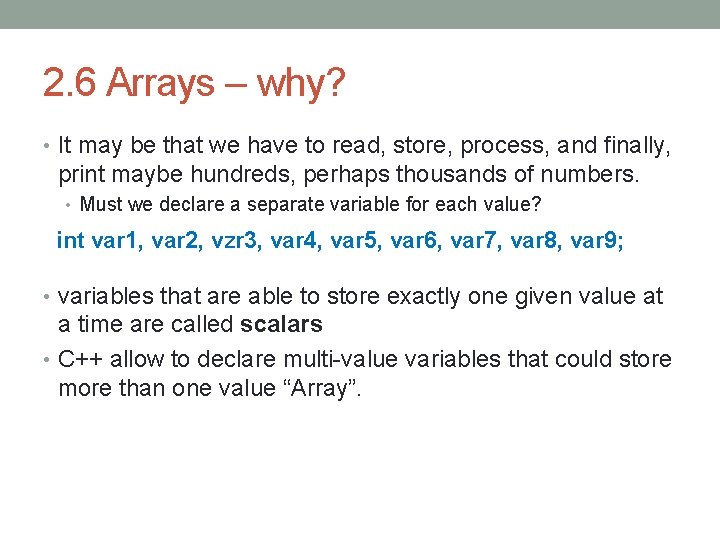
2. 6 Arrays – why? • It may be that we have to read, store, process, and finally, print maybe hundreds, perhaps thousands of numbers. • Must we declare a separate variable for each value? int var 1, var 2, vzr 3, var 4, var 5, var 6, var 7, var 8, var 9; • variables that are able to store exactly one given value at a time are called scalars • C++ allow to declare multi-value variables that could store more than one value “Array”.
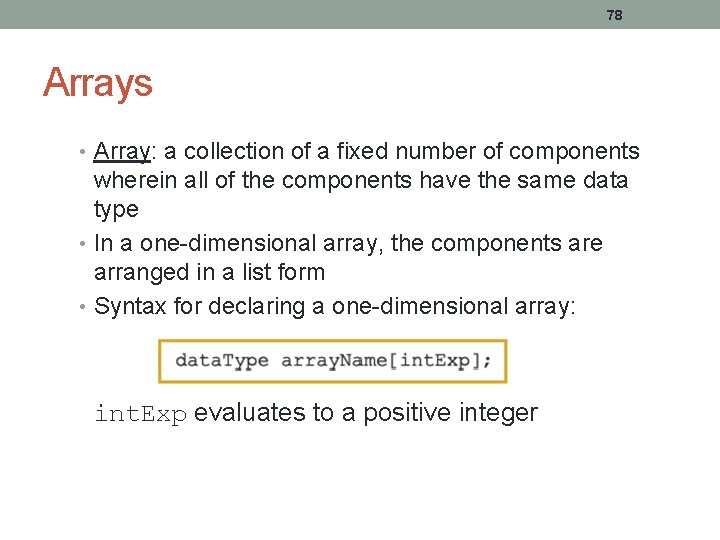
78 Arrays • Array: a collection of a fixed number of components wherein all of the components have the same data type • In a one-dimensional array, the components are arranged in a list form • Syntax for declaring a one-dimensional array: int. Exp evaluates to a positive integer
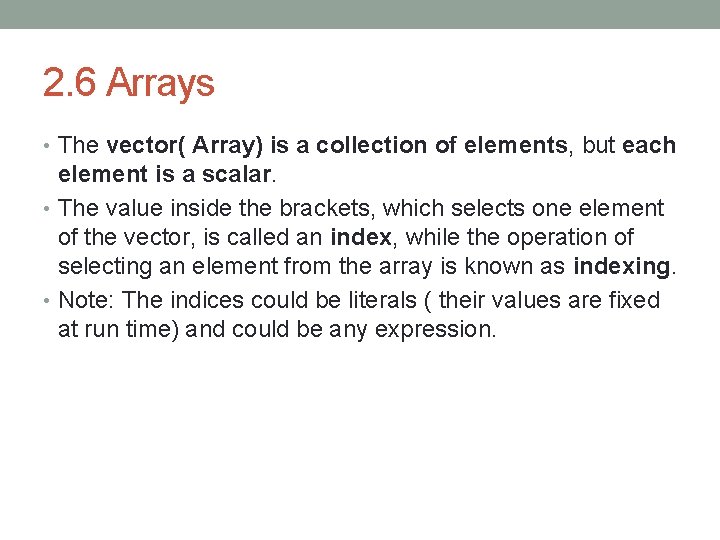
2. 6 Arrays • The vector( Array) is a collection of elements, but each element is a scalar. • The value inside the brackets, which selects one element of the vector, is called an index, while the operation of selecting an element from the array is known as indexing. • Note: The indices could be literals ( their values are fixed at run time) and could be any expression.
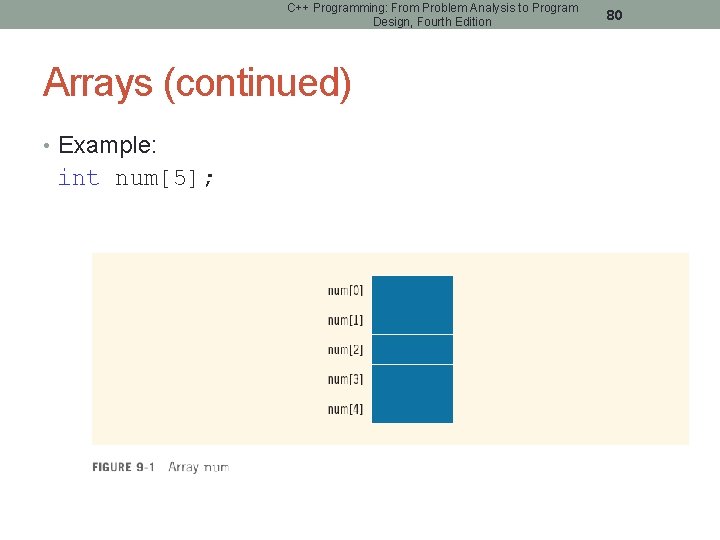
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Arrays (continued) • Example: int num[5]; 80
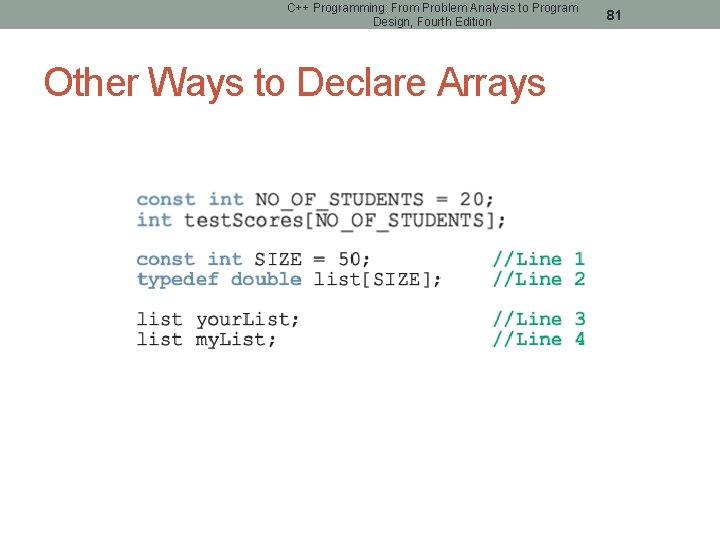
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Other Ways to Declare Arrays 81
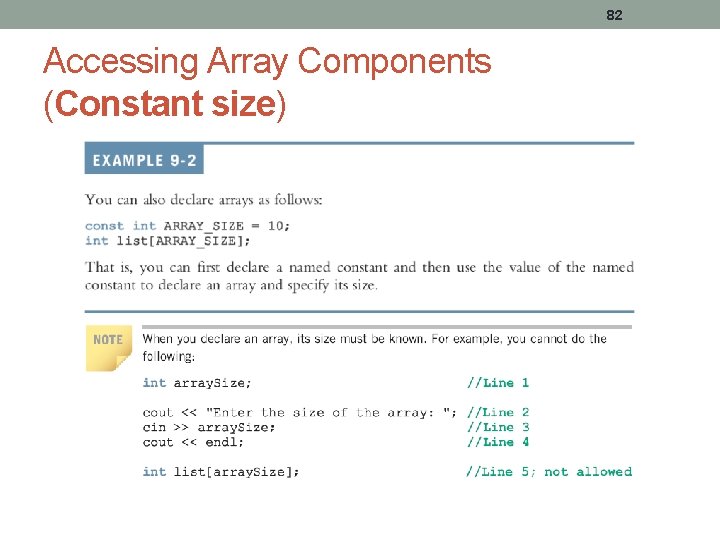
82 Accessing Array Components (Constant size)
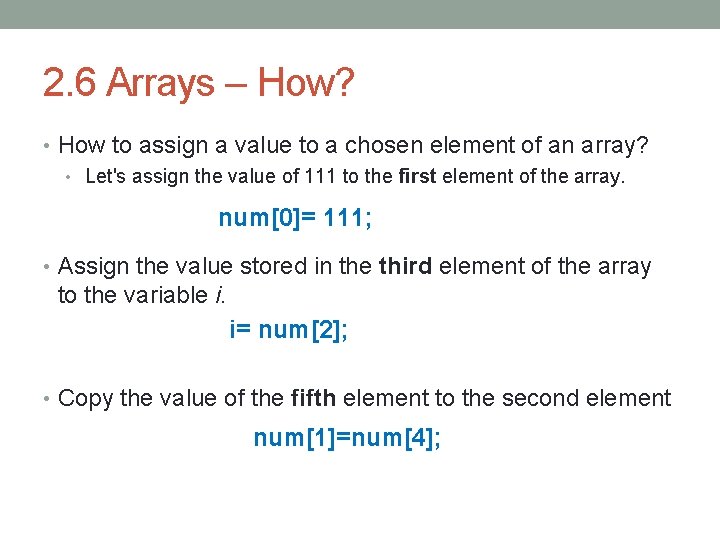
2. 6 Arrays – How? • How to assign a value to a chosen element of an array? • Let's assign the value of 111 to the first element of the array. num[0]= 111; • Assign the value stored in the third element of the array to the variable i. i= num[2]; • Copy the value of the fifth element to the second element num[1]=num[4];
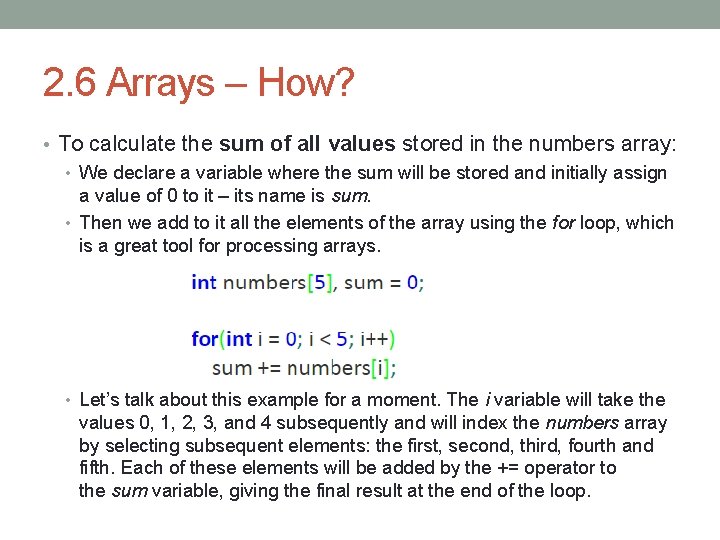
2. 6 Arrays – How? • To calculate the sum of all values stored in the numbers array: • We declare a variable where the sum will be stored and initially assign a value of 0 to it – its name is sum. • Then we add to it all the elements of the array using the for loop, which is a great tool for processing arrays. • Let’s talk about this example for a moment. The i variable will take the values 0, 1, 2, 3, and 4 subsequently and will index the numbers array by selecting subsequent elements: the first, second, third, fourth and fifth. Each of these elements will be added by the += operator to the sum variable, giving the final result at the end of the loop.
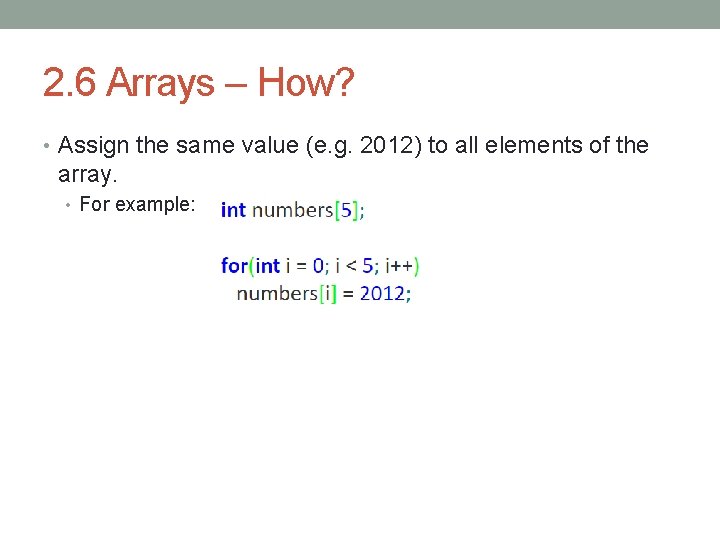
2. 6 Arrays – How? • Assign the same value (e. g. 2012) to all elements of the array. • For example:
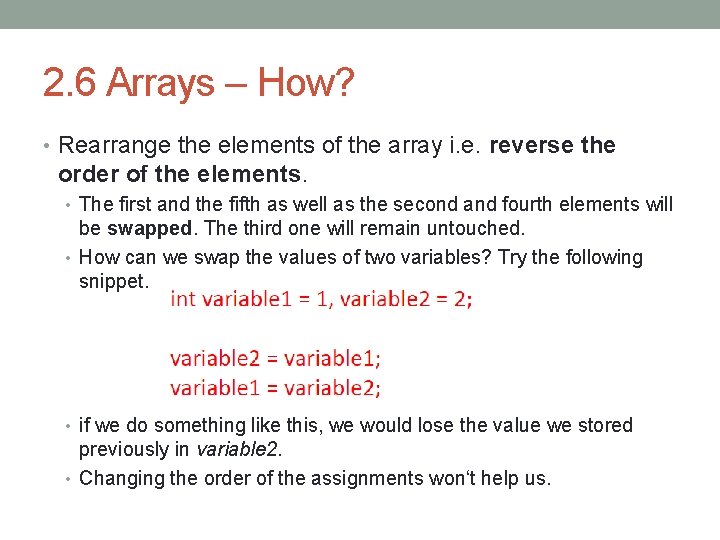
2. 6 Arrays – How? • Rearrange the elements of the array i. e. reverse the order of the elements. • The first and the fifth as well as the second and fourth elements will be swapped. The third one will remain untouched. • How can we swap the values of two variables? Try the following snippet. • if we do something like this, we would lose the value we stored previously in variable 2. • Changing the order of the assignments won‘t help us.
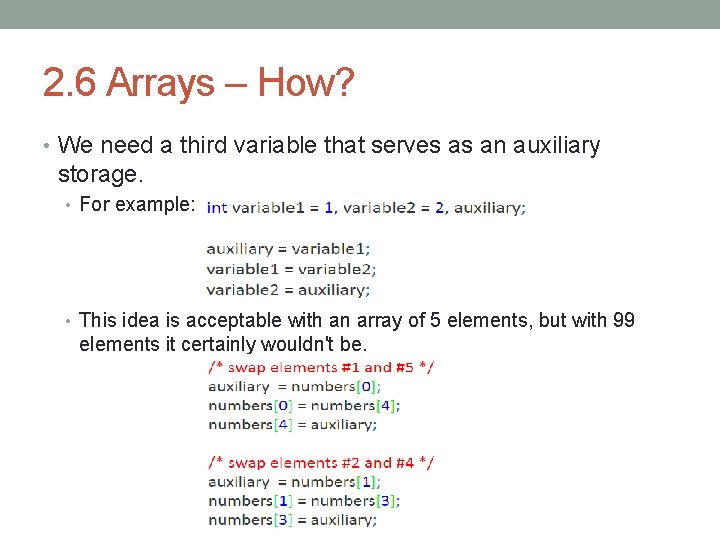
2. 6 Arrays – How? • We need a third variable that serves as an auxiliary storage. • For example: • This idea is acceptable with an array of 5 elements, but with 99 elements it certainly wouldn't be.
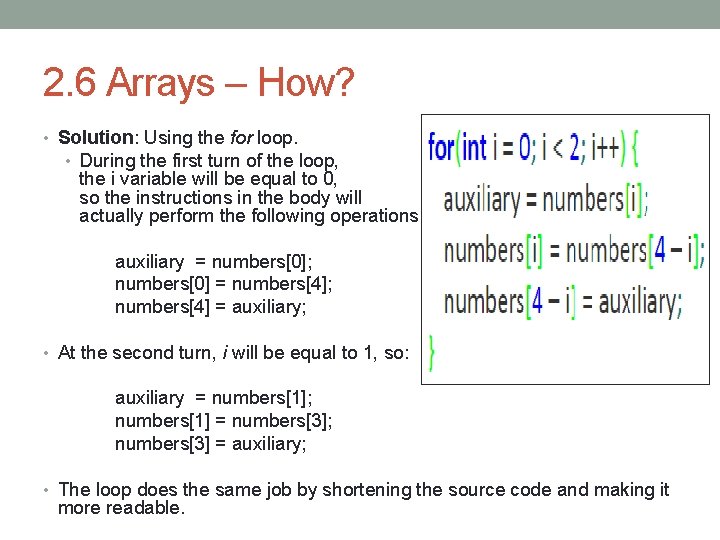
2. 6 Arrays – How? • Solution: Using the for loop. • During the first turn of the loop, the i variable will be equal to 0, so the instructions in the body will actually perform the following operations: auxiliary = numbers[0]; numbers[0] = numbers[4]; numbers[4] = auxiliary; • At the second turn, i will be equal to 1, so: auxiliary = numbers[1]; numbers[1] = numbers[3]; numbers[3] = auxiliary; • The loop does the same job by shortening the source code and making it more readable.
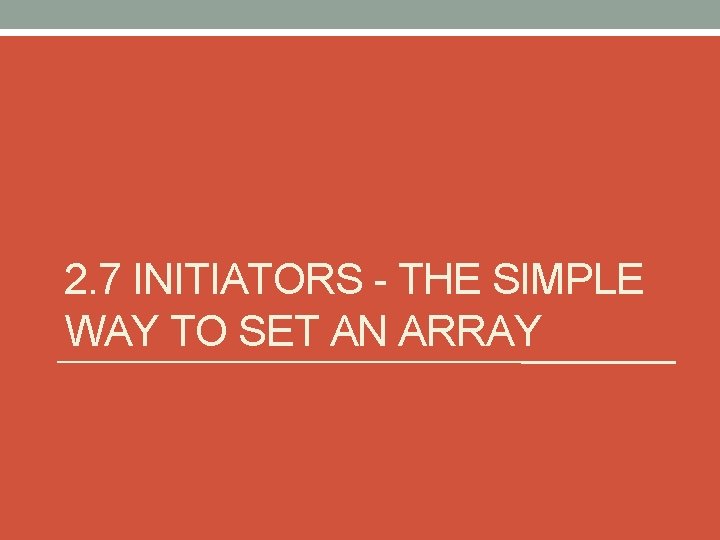
2. 7 INITIATORS - THE SIMPLE WAY TO SET AN ARRAY
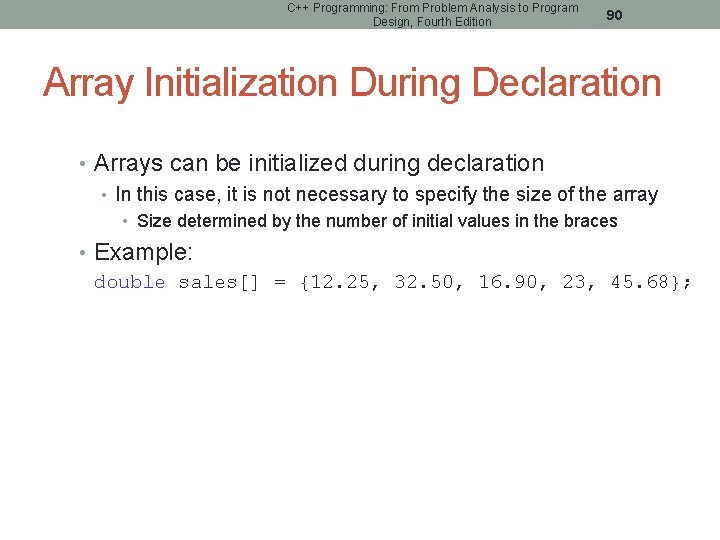
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 90 Array Initialization During Declaration • Arrays can be initialized during declaration • In this case, it is not necessary to specify the size of the array • Size determined by the number of initial values in the braces • Example: double sales[] = {12. 25, 32. 50, 16. 90, 23, 45. 68};
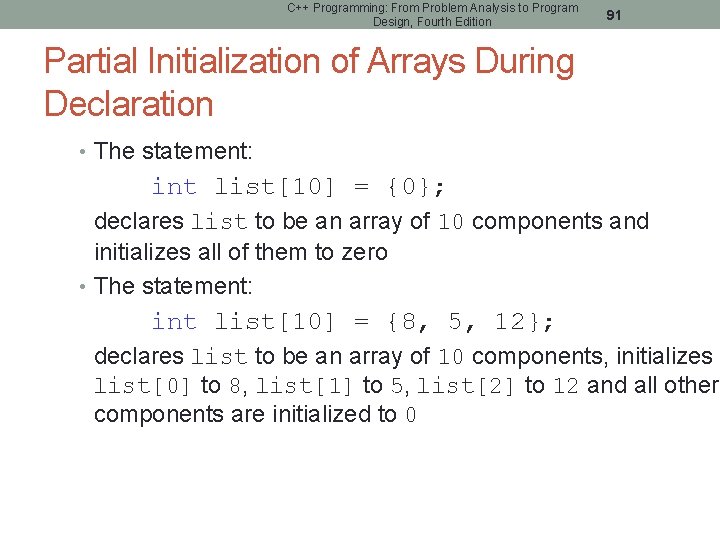
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 91 Partial Initialization of Arrays During Declaration • The statement: int list[10] = {0}; declares list to be an array of 10 components and initializes all of them to zero • The statement: int list[10] = {8, 5, 12}; declares list to be an array of 10 components, initializes list[0] to 8, list[1] to 5, list[2] to 12 and all other components are initialized to 0
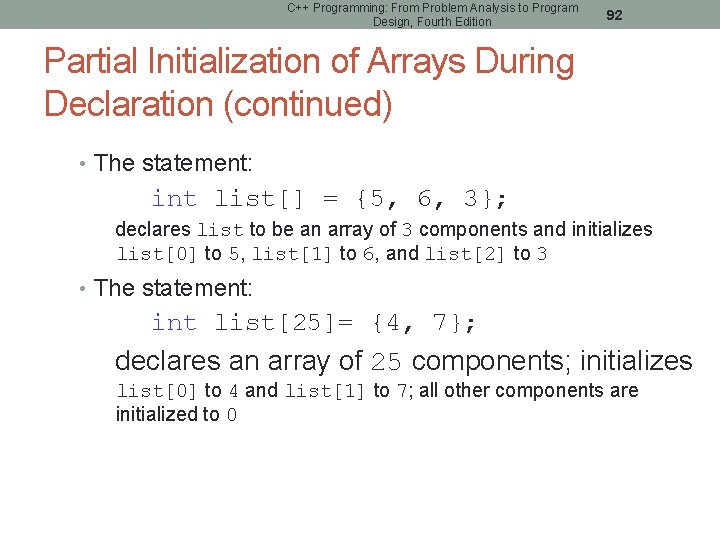
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 92 Partial Initialization of Arrays During Declaration (continued) • The statement: int list[] = {5, 6, 3}; declares list to be an array of 3 components and initializes list[0] to 5, list[1] to 6, and list[2] to 3 • The statement: int list[25]= {4, 7}; declares an array of 25 components; initializes list[0] to 4 and list[1] to 7; all other components are initialized to 0
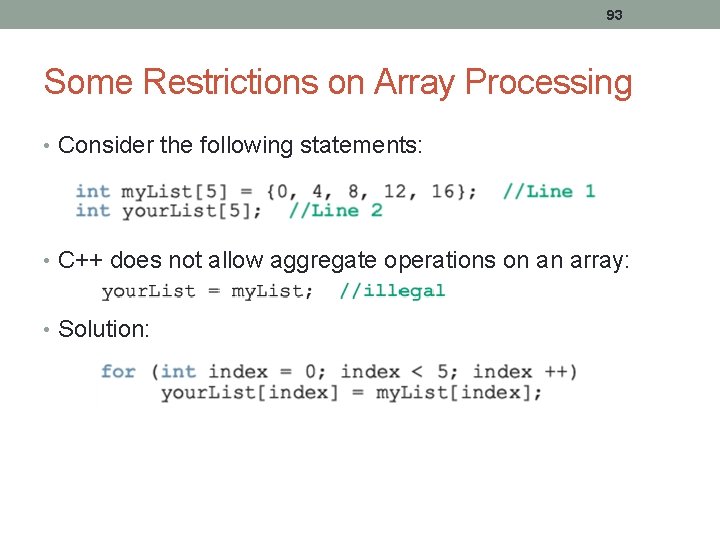
93 Some Restrictions on Array Processing • Consider the following statements: • C++ does not allow aggregate operations on an array: • Solution:
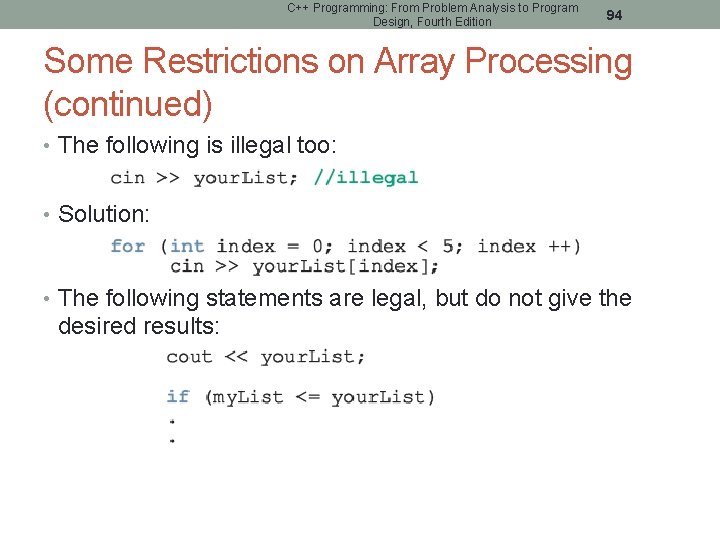
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 94 Some Restrictions on Array Processing (continued) • The following is illegal too: • Solution: • The following statements are legal, but do not give the desired results:
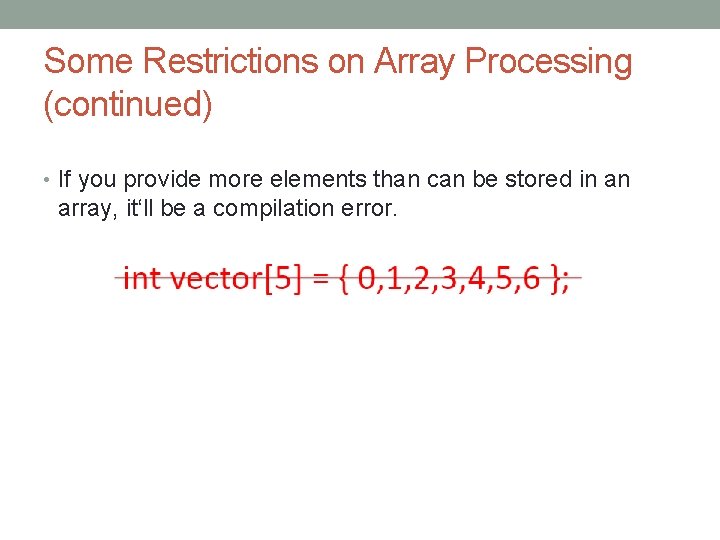
Some Restrictions on Array Processing (continued) • If you provide more elements than can be stored in an array, it‘ll be a compilation error.
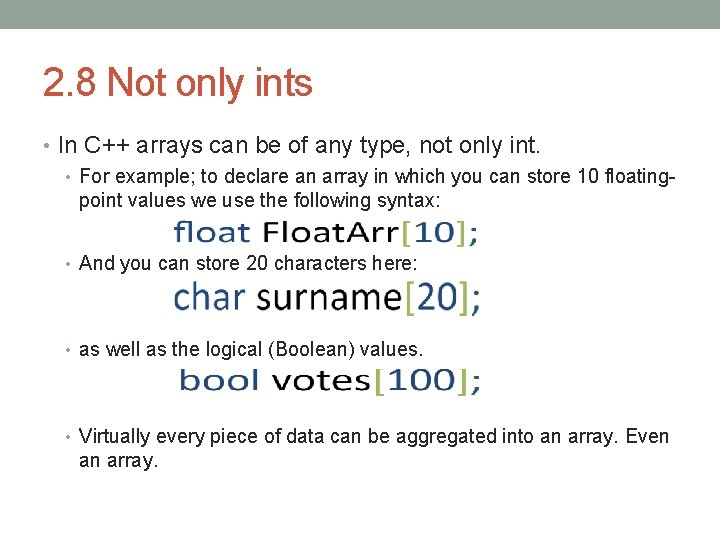
2. 8 Not only ints • In C++ arrays can be of any type, not only int. • For example; to declare an array in which you can store 10 floatingpoint values we use the following syntax: • And you can store 20 characters here: • as well as the logical (Boolean) values. • Virtually every piece of data can be aggregated into an array. Even an array.
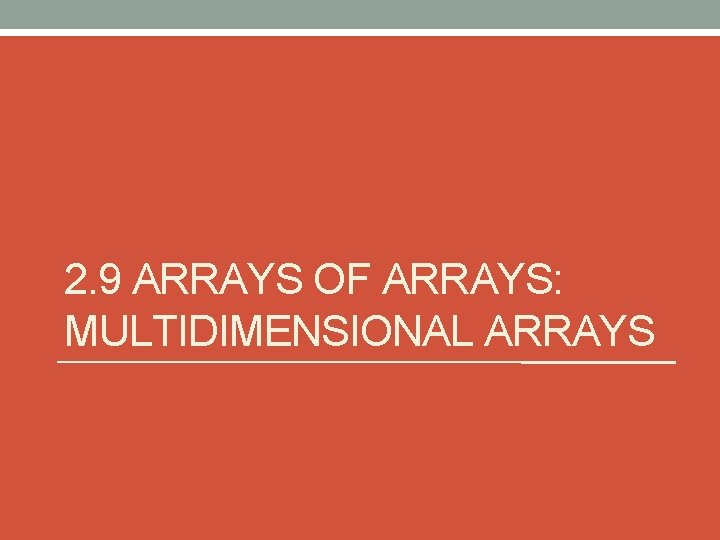
2. 9 ARRAYS OF ARRAYS: MULTIDIMENSIONAL ARRAYS
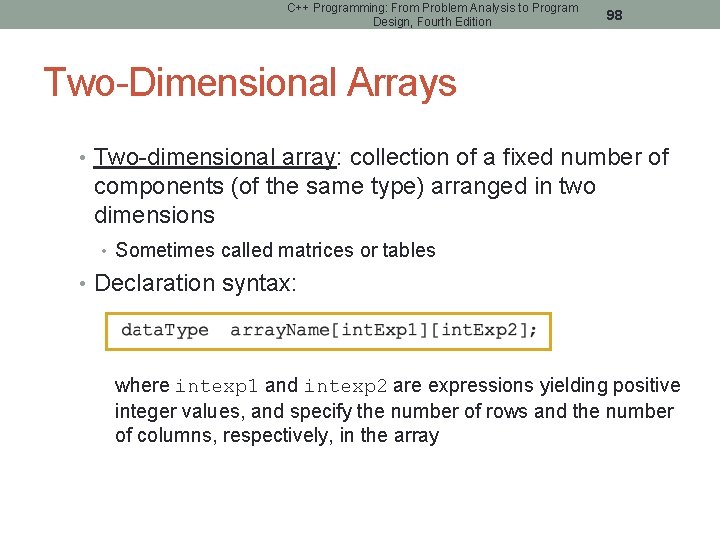
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 98 Two-Dimensional Arrays • Two-dimensional array: collection of a fixed number of components (of the same type) arranged in two dimensions • Sometimes called matrices or tables • Declaration syntax: where intexp 1 and intexp 2 are expressions yielding positive integer values, and specify the number of rows and the number of columns, respectively, in the array
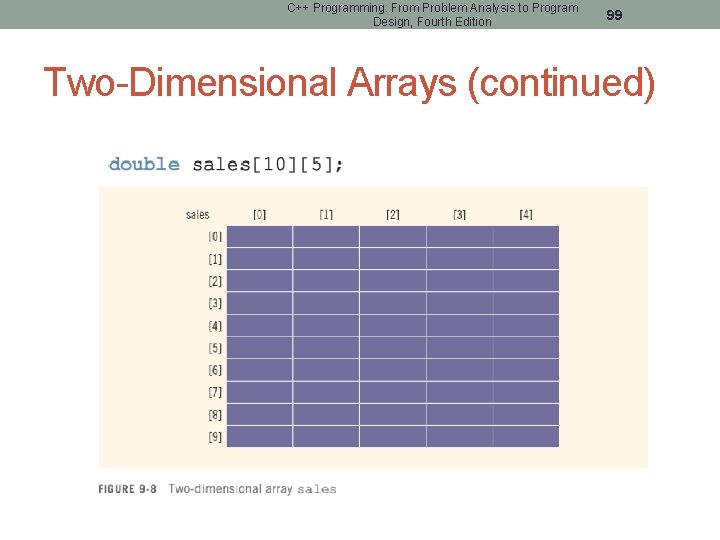
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 99 Two-Dimensional Arrays (continued)
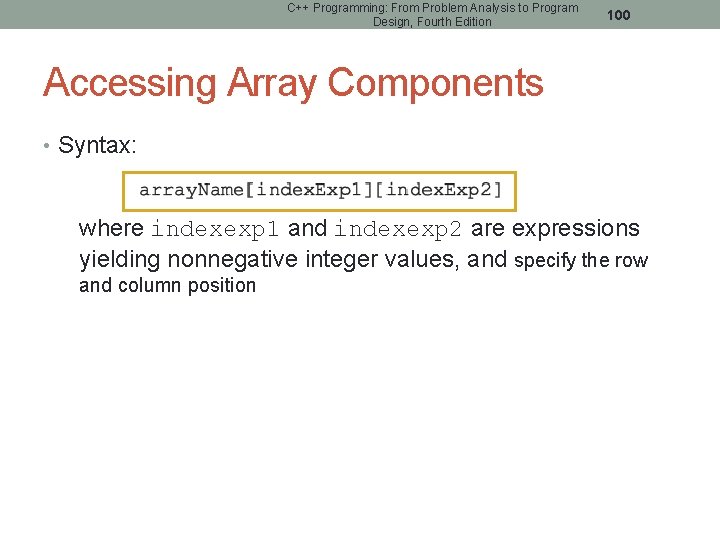
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 100 Accessing Array Components • Syntax: where indexexp 1 and indexexp 2 are expressions yielding nonnegative integer values, and specify the row and column position
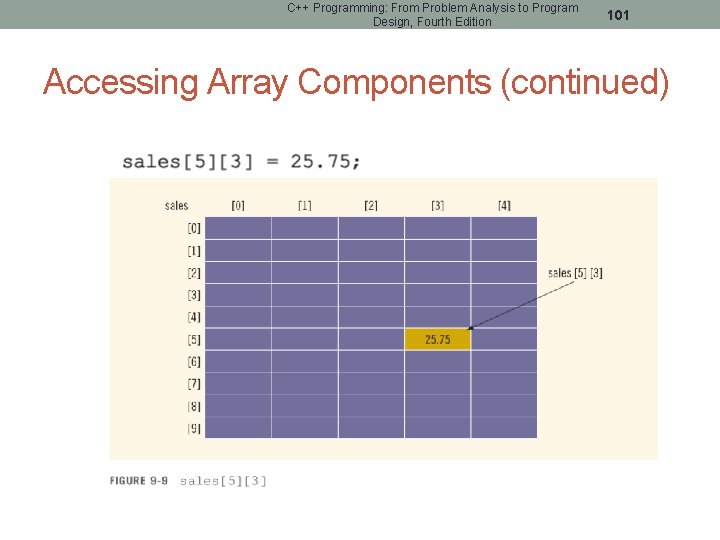
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 101 Accessing Array Components (continued)
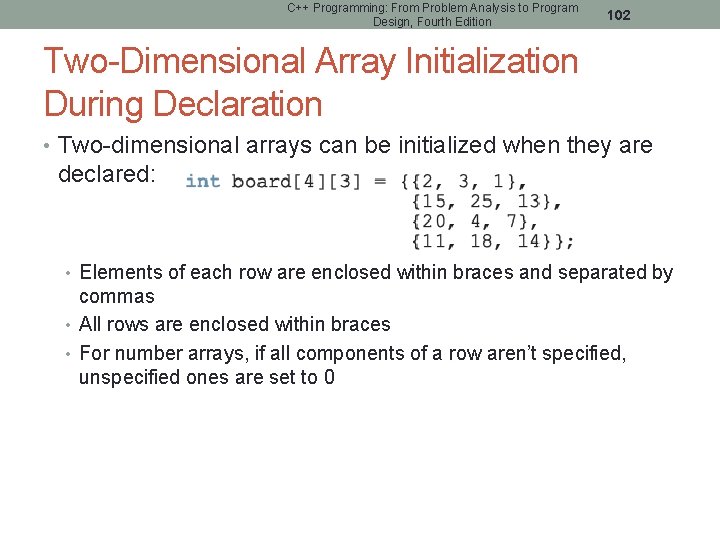
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 102 Two-Dimensional Array Initialization During Declaration • Two-dimensional arrays can be initialized when they are declared: • Elements of each row are enclosed within braces and separated by commas • All rows are enclosed within braces • For number arrays, if all components of a row aren’t specified, unspecified ones are set to 0
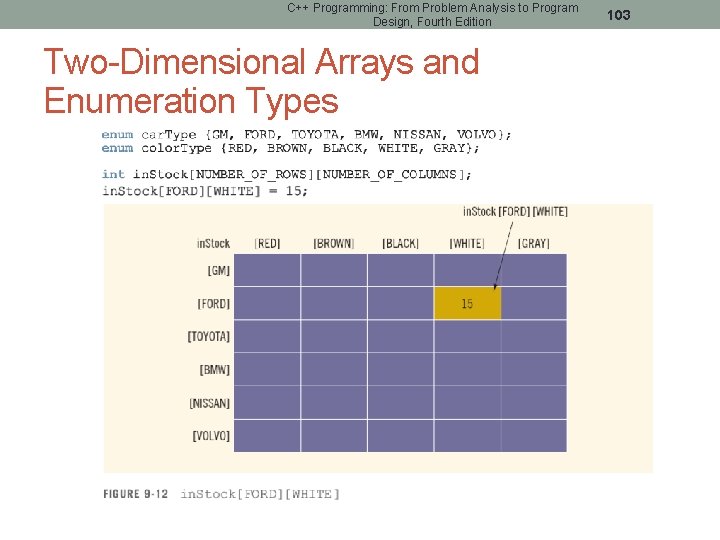
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Two-Dimensional Arrays and Enumeration Types 103
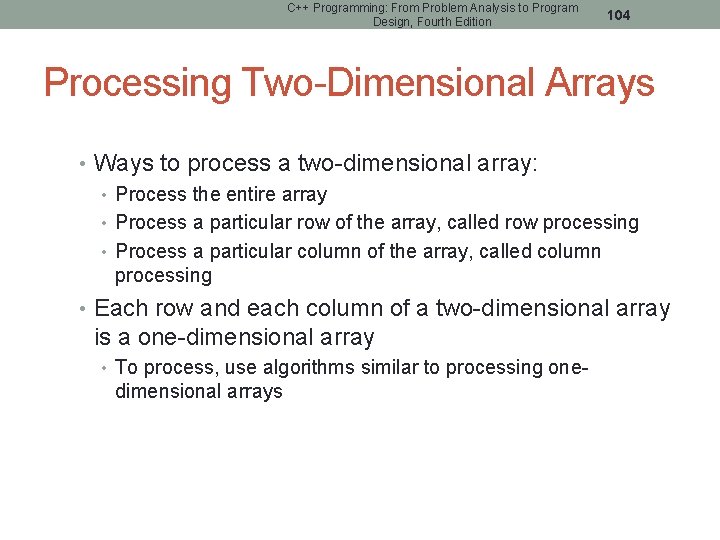
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 104 Processing Two-Dimensional Arrays • Ways to process a two-dimensional array: • Process the entire array • Process a particular row of the array, called row processing • Process a particular column of the array, called column processing • Each row and each column of a two-dimensional array is a one-dimensional array • To process, use algorithms similar to processing one- dimensional arrays
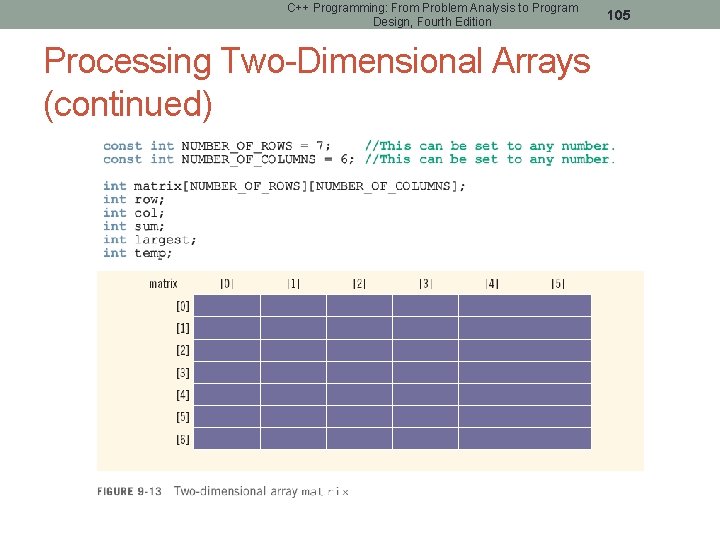
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Processing Two-Dimensional Arrays (continued) 105
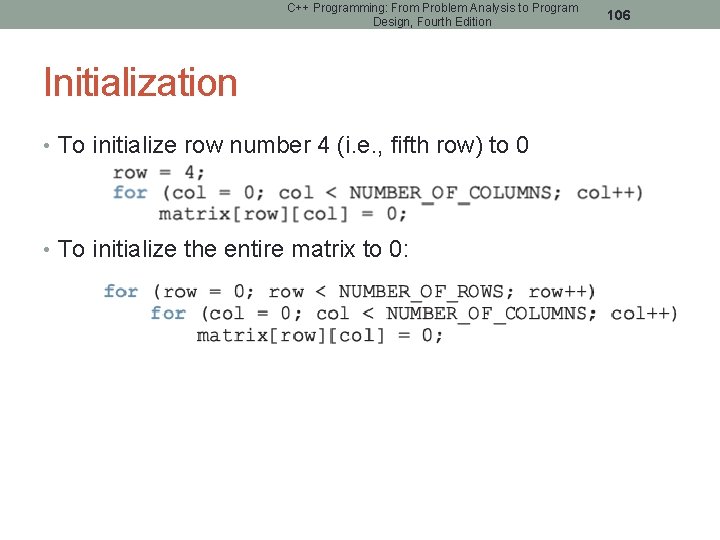
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Initialization • To initialize row number 4 (i. e. , fifth row) to 0 • To initialize the entire matrix to 0: 106
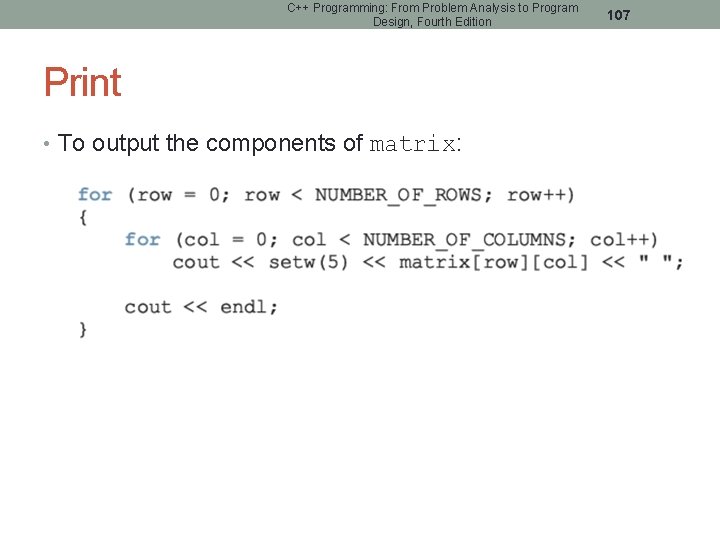
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Print • To output the components of matrix: 107
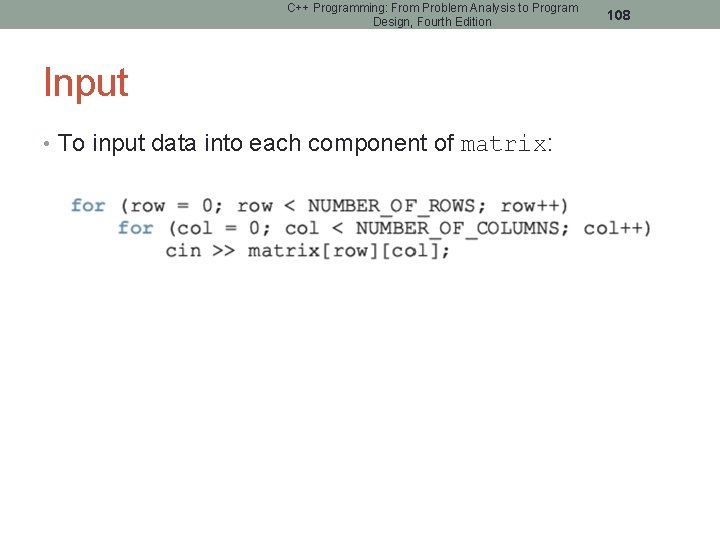
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Input • To input data into each component of matrix: 108
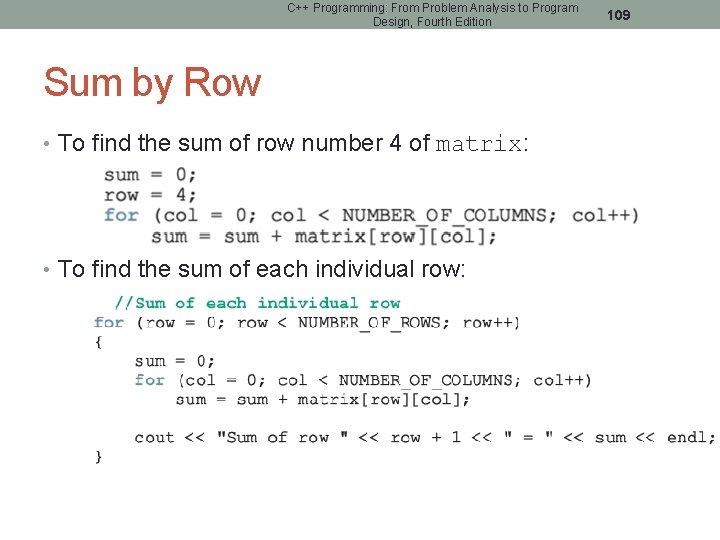
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Sum by Row • To find the sum of row number 4 of matrix: • To find the sum of each individual row: 109
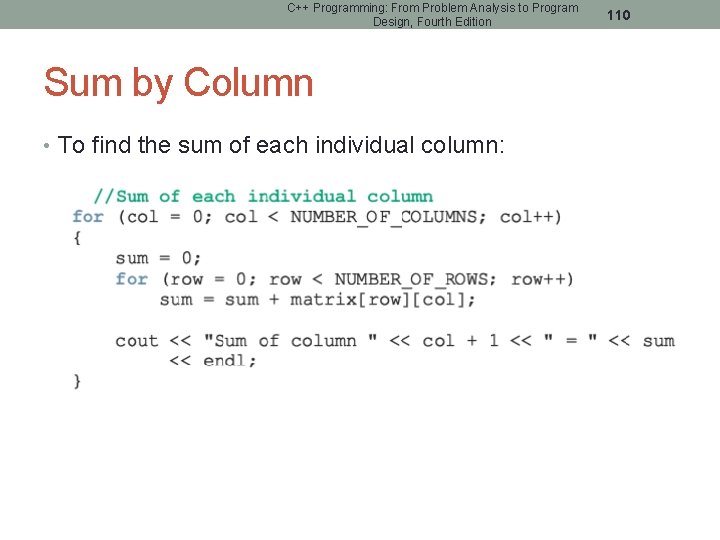
C++ Programming: From Problem Analysis to Program Design, Fourth Edition Sum by Column • To find the sum of each individual column: 110
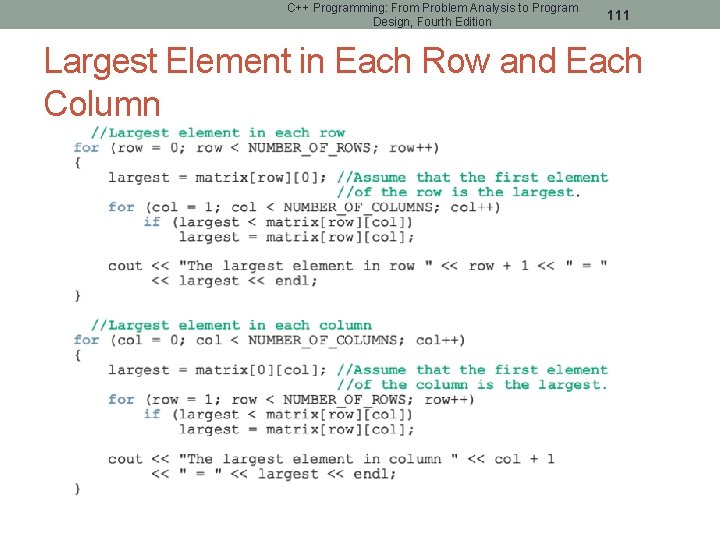
C++ Programming: From Problem Analysis to Program Design, Fourth Edition 111 Largest Element in Each Row and Each Column
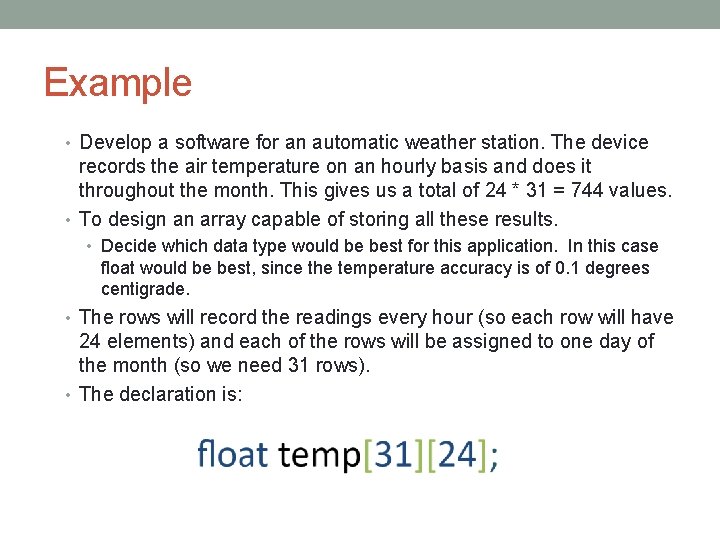
Example • Develop a software for an automatic weather station. The device records the air temperature on an hourly basis and does it throughout the month. This gives us a total of 24 * 31 = 744 values. • To design an array capable of storing all these results. • Decide which data type would be best for this application. In this case float would be best, since the temperature accuracy is of 0. 1 degrees centigrade. • The rows will record the readings every hour (so each row will have 24 elements) and each of the rows will be assigned to one day of the month (so we need 31 rows). • The declaration is:
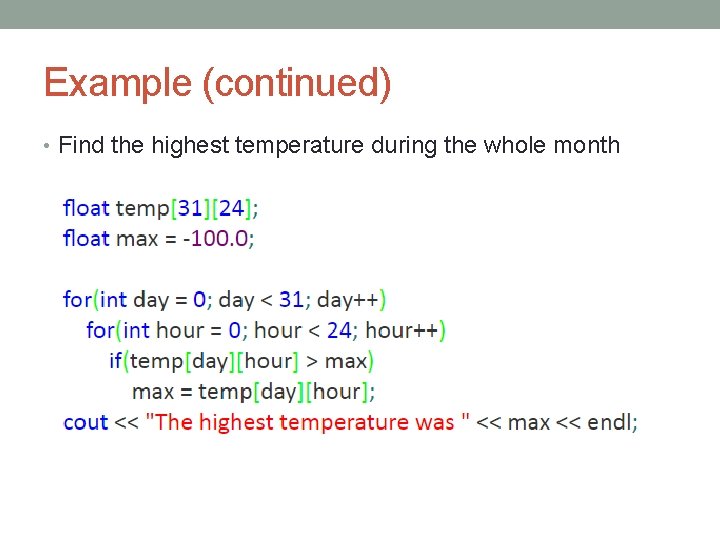
Example (continued) • Find the highest temperature during the whole month
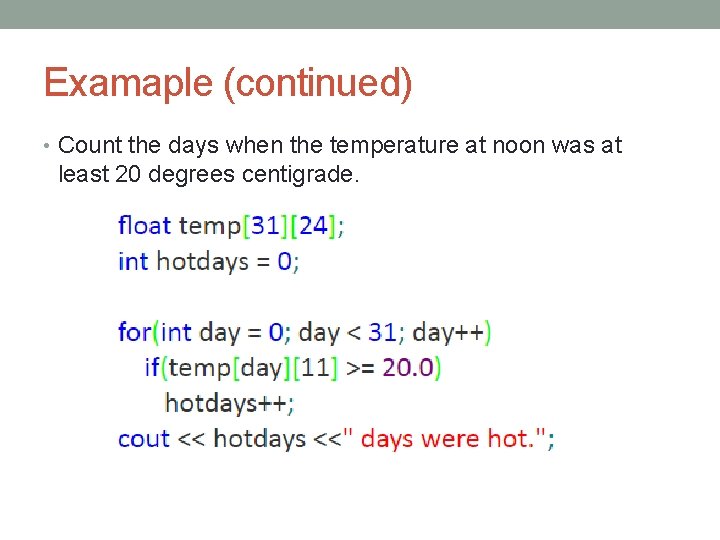
Examaple (continued) • Count the days when the temperature at noon was at least 20 degrees centigrade.
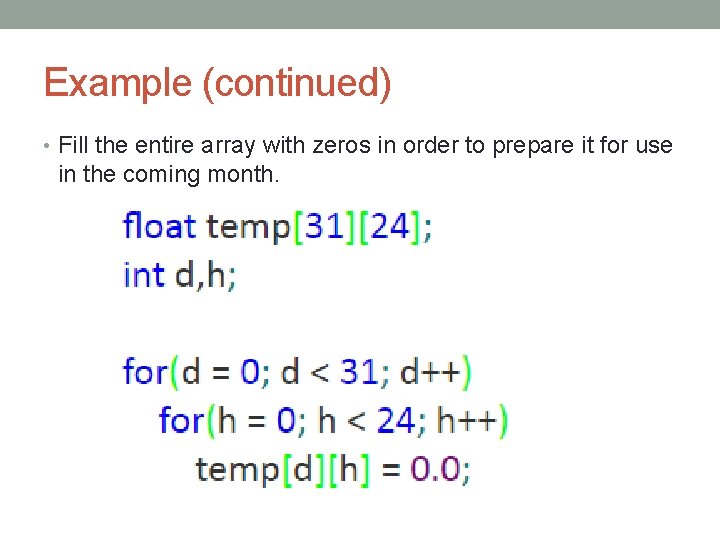
Example (continued) • Fill the entire array with zeros in order to prepare it for use in the coming month.
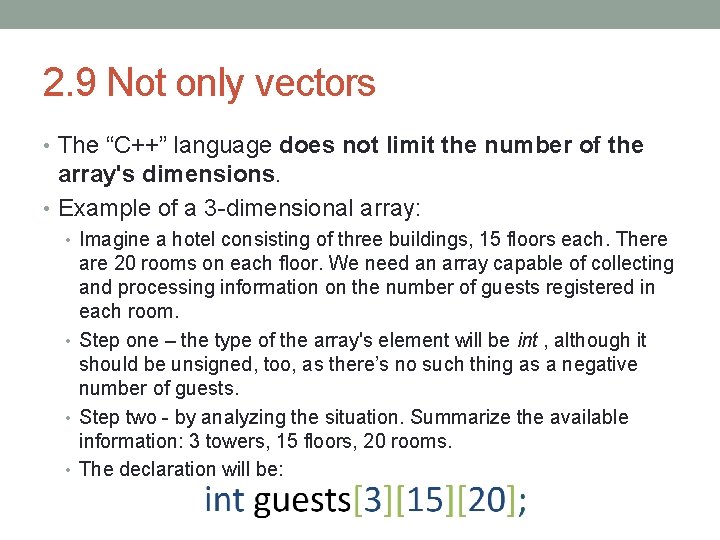
2. 9 Not only vectors • The “C++” language does not limit the number of the array's dimensions. • Example of a 3 -dimensional array: • Imagine a hotel consisting of three buildings, 15 floors each. There are 20 rooms on each floor. We need an array capable of collecting and processing information on the number of guests registered in each room. • Step one – the type of the array's element will be int , although it should be unsigned, too, as there’s no such thing as a negative number of guests. • Step two - by analyzing the situation. Summarize the available information: 3 towers, 15 floors, 20 rooms. • The declaration will be:
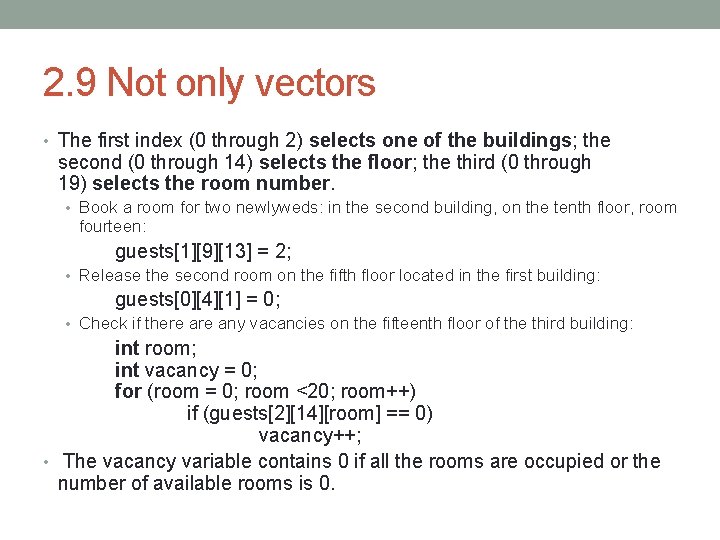
2. 9 Not only vectors • The first index (0 through 2) selects one of the buildings; the second (0 through 14) selects the floor; the third (0 through 19) selects the room number. • Book a room for two newlyweds: in the second building, on the tenth floor, room fourteen: guests[1][9][13] = 2; • Release the second room on the fifth floor located in the first building: guests[0][4][1] = 0; • Check if there any vacancies on the fifteenth floor of the third building: int room; int vacancy = 0; for (room = 0; room <20; room++) if (guests[2][14][room] == 0) vacancy++; • The vacancy variable contains 0 if all the rooms are occupied or the number of available rooms is 0.
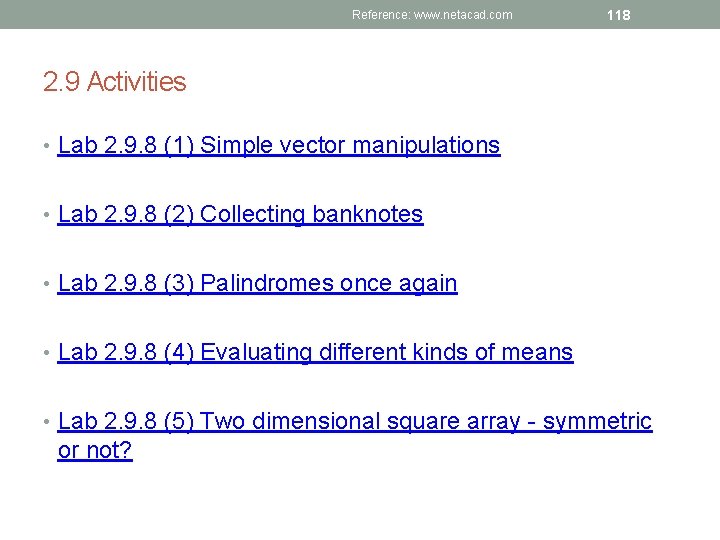
Reference: www. netacad. com 118 2. 9 Activities • Lab 2. 9. 8 (1) Simple vector manipulations • Lab 2. 9. 8 (2) Collecting banknotes • Lab 2. 9. 8 (3) Palindromes once again • Lab 2. 9. 8 (4) Evaluating different kinds of means • Lab 2. 9. 8 (5) Two dimensional square array - symmetric or not?
Control flow and data flow computers
Data flow vs control flow
Rocks are aggregates of minerals
Uce first grade aggregates
Scalar replacement of aggregates
Blending aggregates
Grading of aggregates ppt
Tests on aggregates ppt
Gordon aggregates
F’c concrete compressive strength
Ibas aggregates
Flow and error control
Transaction flow graph
Stock control e flow control
Flow control layer
Unrestricted simplex protocol program in c
Data link control
Data link control
Big data flow diagram
Data dictionary notation
Hijacking attacks
Advanced vehicle control
Dfd to structure chart
Transform flow and transaction flow
Example of rotational and irrotational flow
Internal and external flow
Laminar vs turbulent flow
Cash flow and cost control
Program flow of control without and with interrupts
Oxygen flow rate chart
Cylinder oxygen
Non rebreather mask nursing considerations
Internal vs external flow
Energy naturally flows from warmer matter to cooler matter.
Oikos meaning
Cheese making flow chart
Azure sql advanced threat protection
Advanced data structures in java
Advanced data modeling
Advanced field artillery tactical data system
Advanced data structures in python
Advanced data processing
Advanced higher physics notes
Advanced data visualization techniques
Dairy plant management
Lac operon positive control
Orifice valve symbol
Udp flow control
If else in 8086
Exceptional control flow
Repl capture/apply: flow control
Material flow control
Control flow graph for bubble sort
Dcv symbol
Unity flow chart
Define database security
Trim valves
Lactic acid vasodilation
Example of control hijacking
Control flow graph
Linearly independent paths in control flow graph
Deceleration valve symbol
Supply air throttling
Supply air throttling in pneumatic system
Flow control functions in php
Branching instructions in 8086
Www.mbaknol.com
Control flow graph python
Flow control frame format
Control refers to
Tcp flow control
Tcp flow control
Inbound flow control
In sctp only data chunks consume
Interrupts execution flow
Flow control instructions
Empty statement in sequential block
Control-flow graph
Assembly language
Control flow graph for bubble sort
Flow control
Control structure flow chart
Single acting pneumatic cylinder diagram
What are flow control techniques
Microsoft flow concurrency control
Ghidra control flow graph
Control structure flow chart
Sequential control flow instructions
What is flow control in computer network
Contoh flow control
Flow control
Tcp
Flow control
Tcp flow control diagram
Flow control windows
Tcp sliding window
Windows flow control
Sliding window in tcp
Flow control
Sequential control structure
Rxjava backpressure
Answer
Fc flow control
Go back n
Tcp congestion control
Unrestricted simplex protocol
Data link control protocols in computer networks
Which protocol has neither flow nor error control
Control flow constructs
Useless control flow
Qc control integrator
Chapter 6 advanced shielded metal arc welding
Pseudocde
Advanced evolution chapter 4
Advanced evolution chapter 8
Advanced accounting chapter 1
Data flow diagram in system analysis and design
Balancing of dfd
Data flow and mutation testing