Functions and Data Aggregates Advanced Programming Functions Named
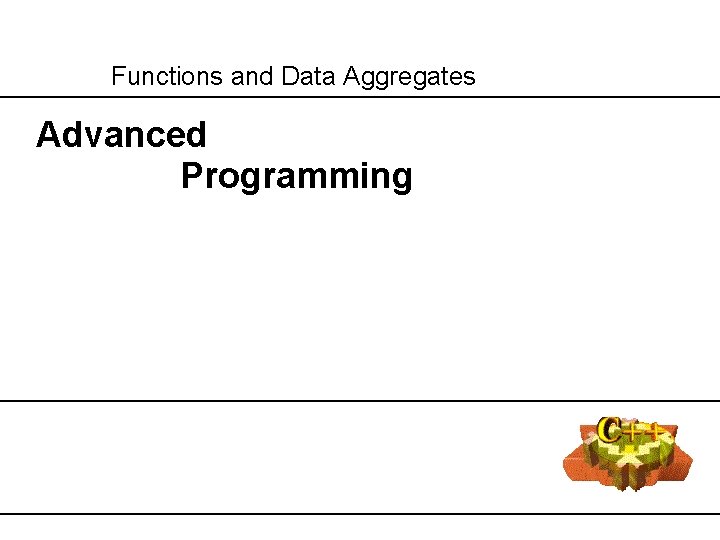
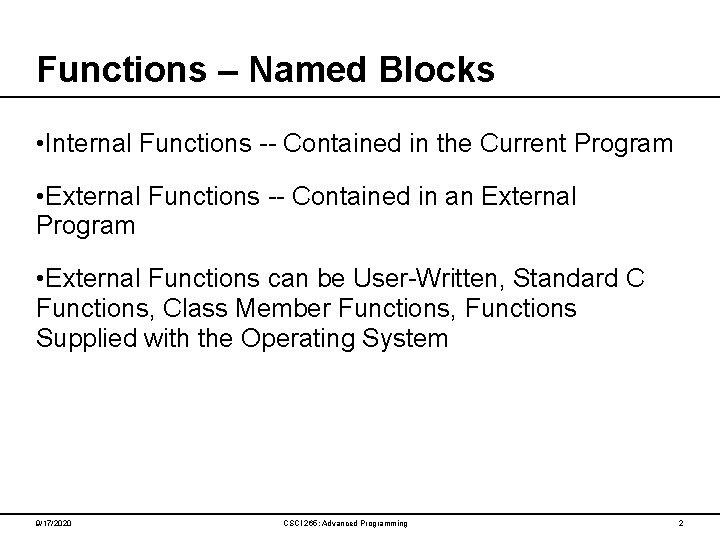
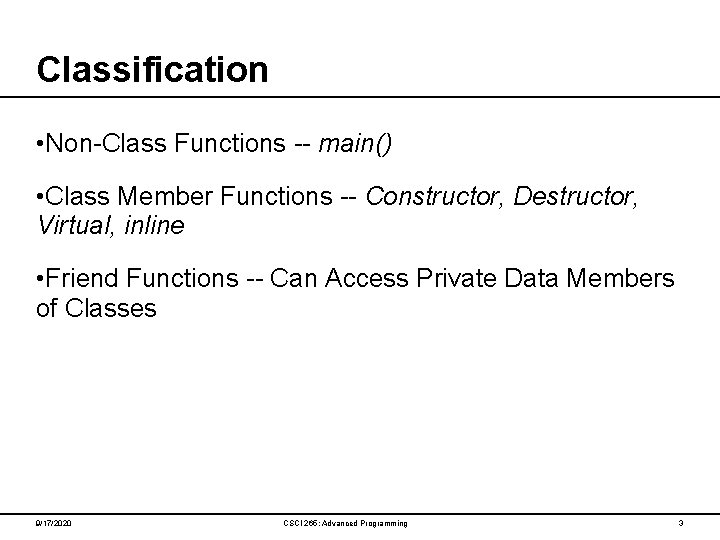
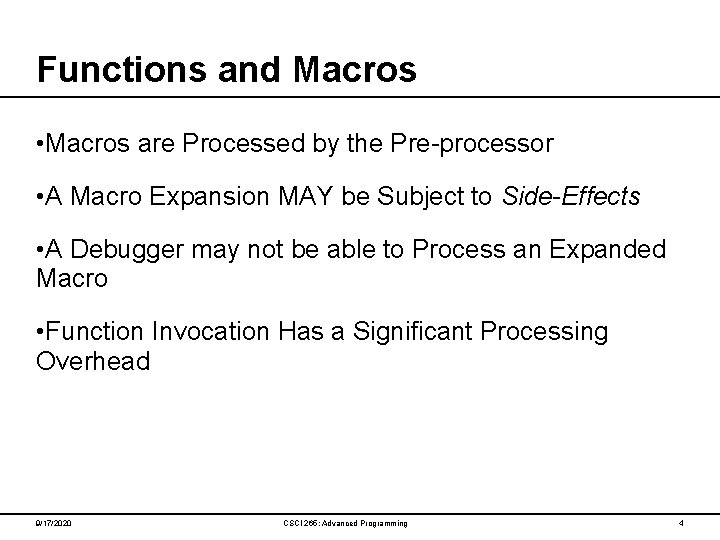
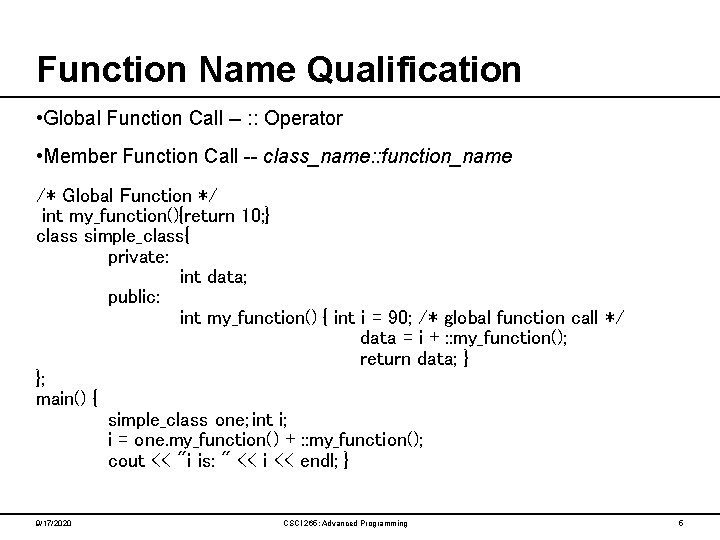
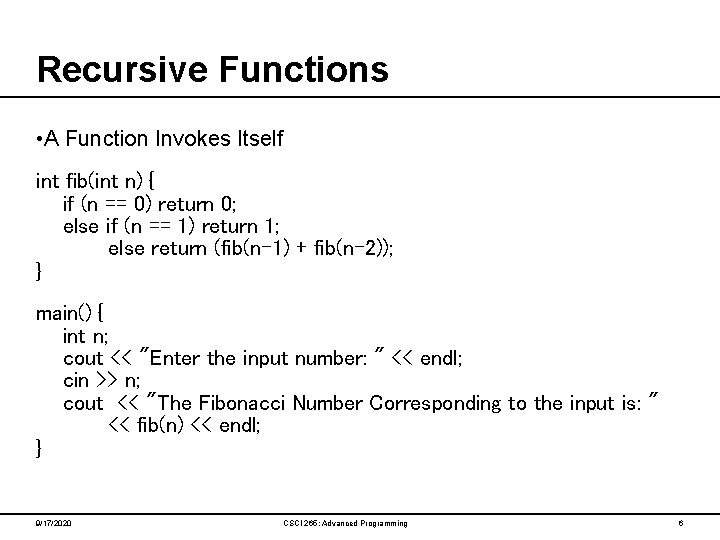
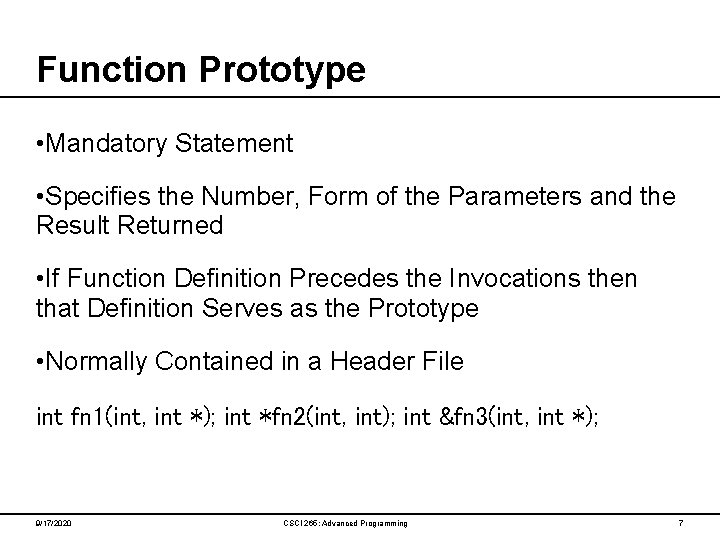
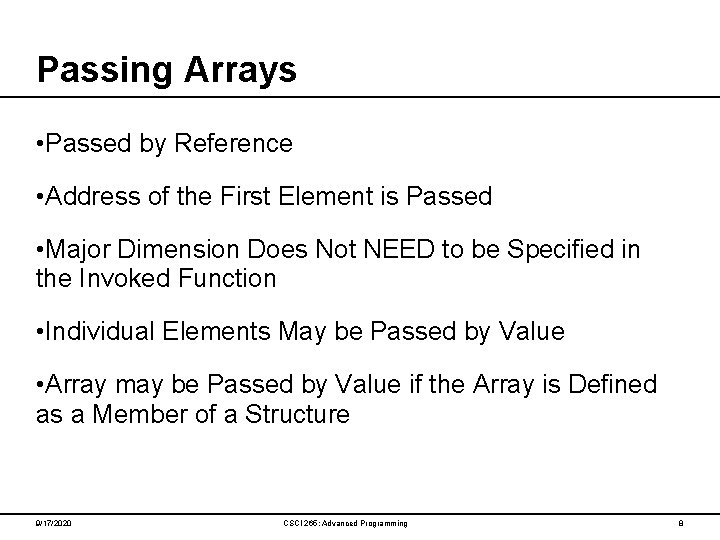
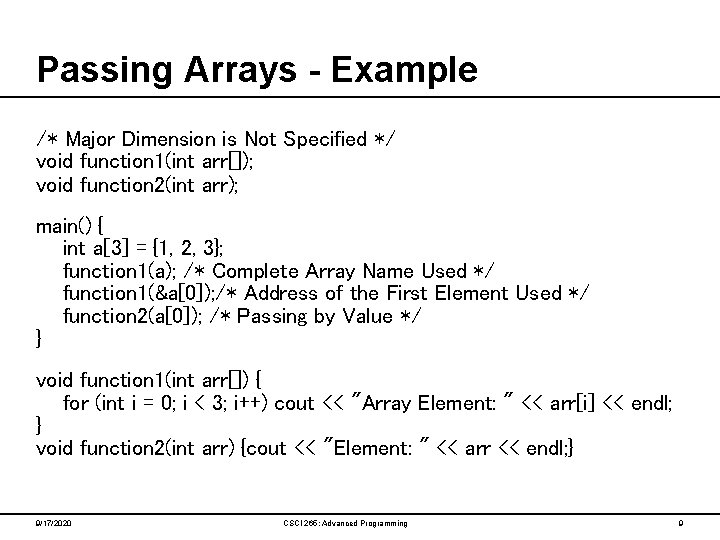
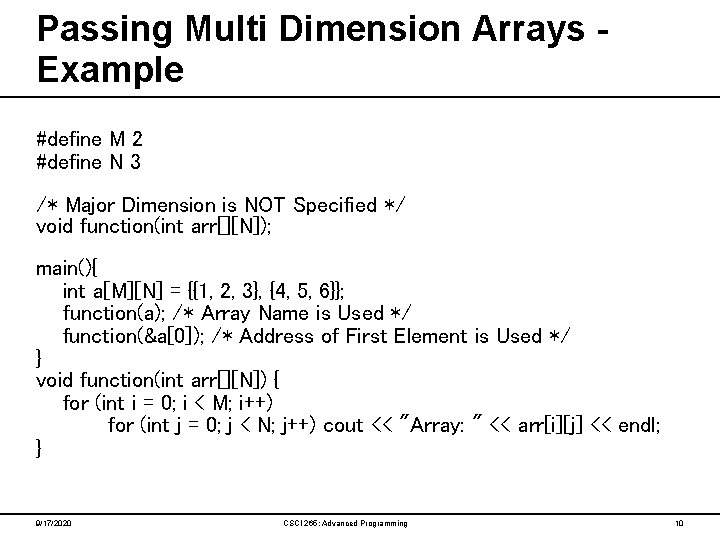
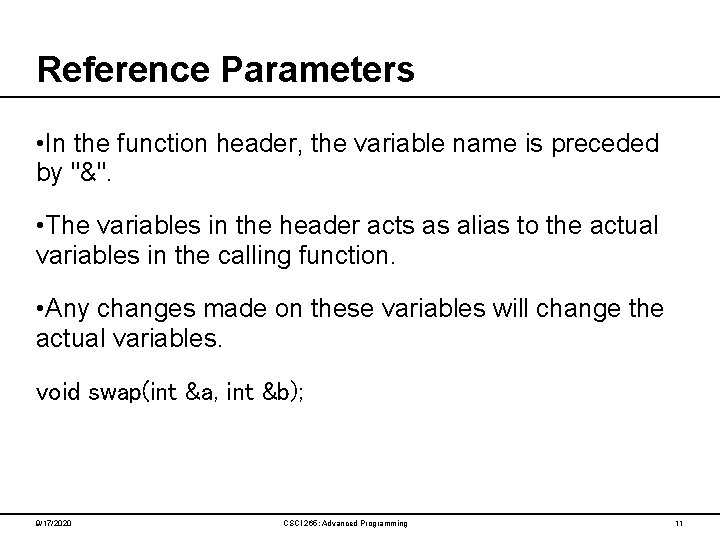
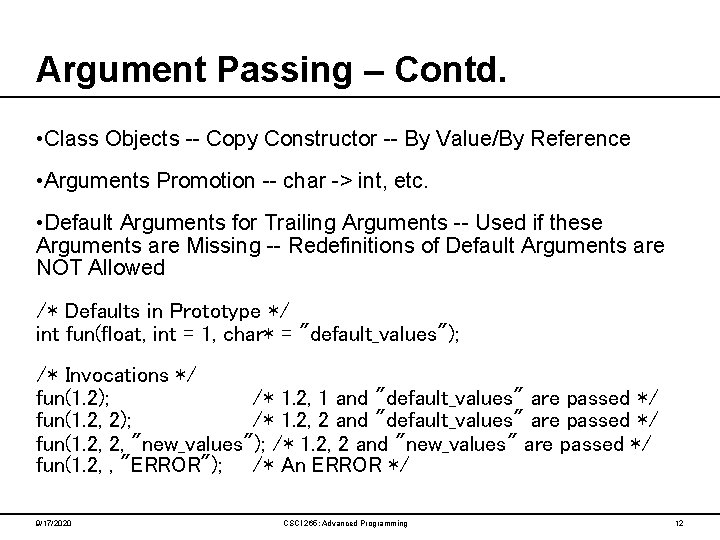
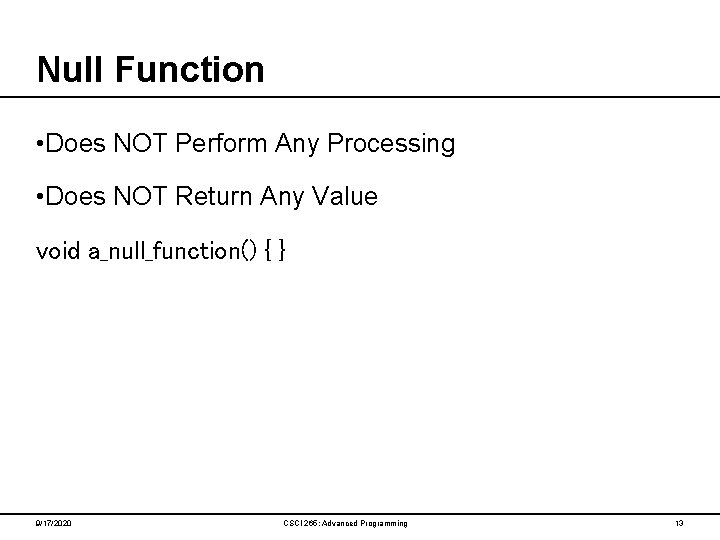
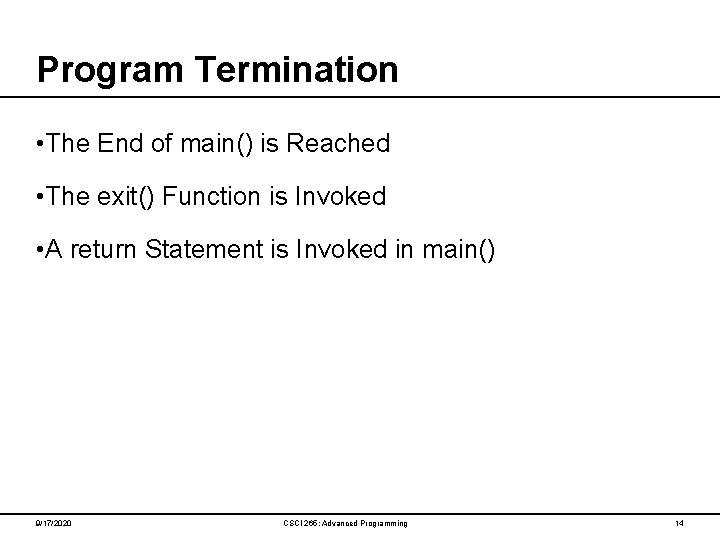
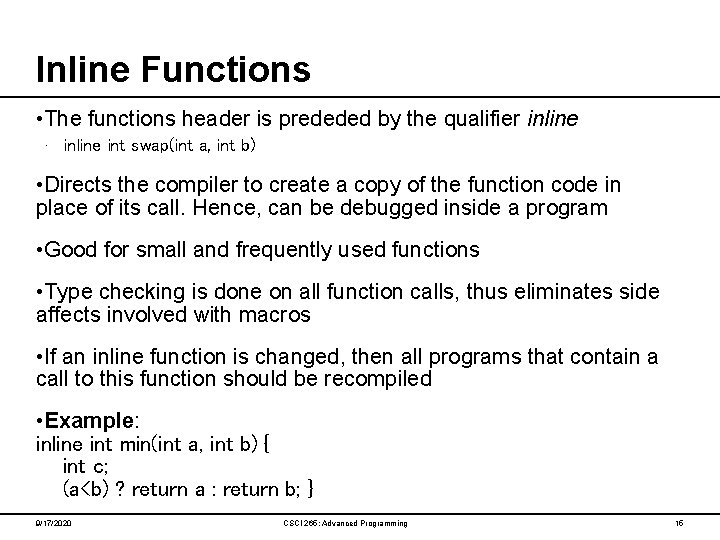
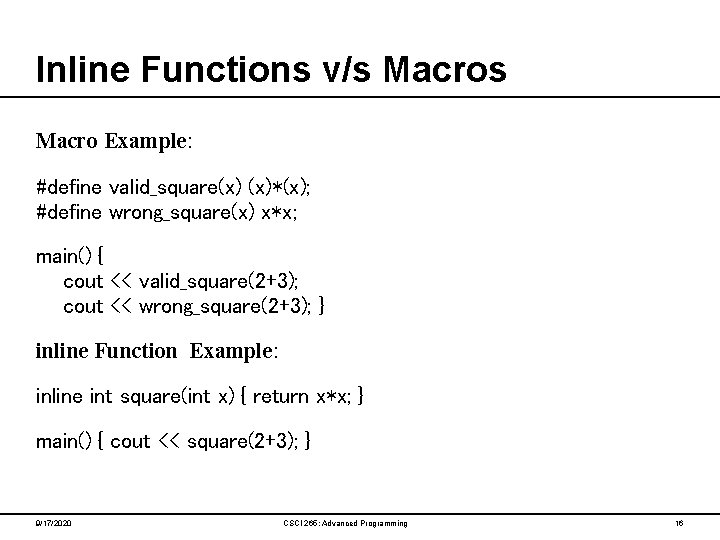
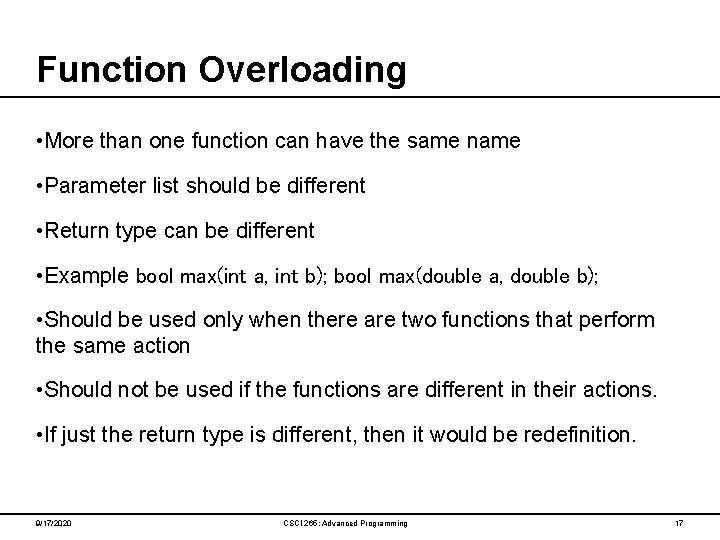
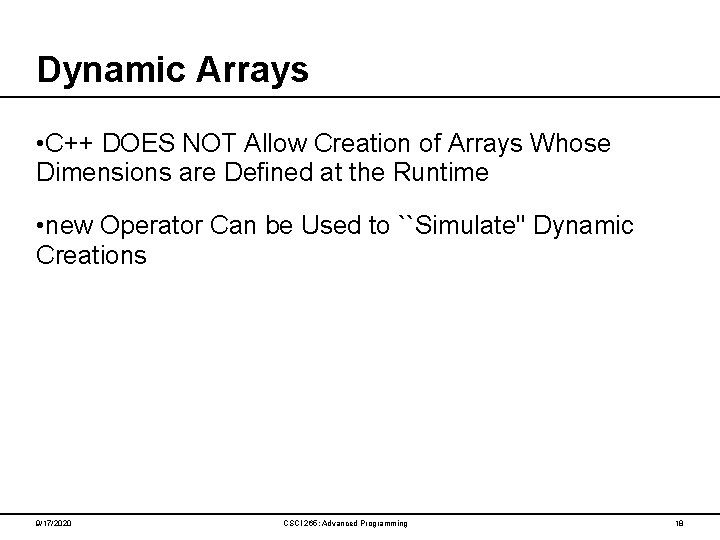
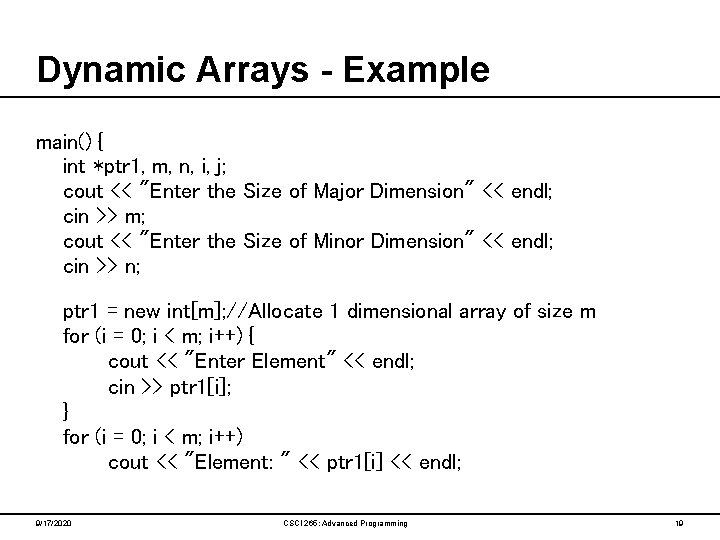
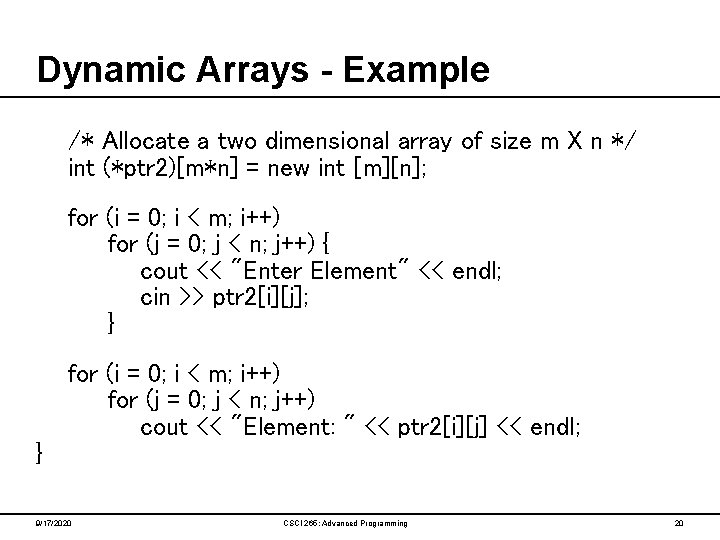
![Multi-Dimension Array Addressing main() { int a[2][2] = {1, 2, 3, 4}; int i, Multi-Dimension Array Addressing main() { int a[2][2] = {1, 2, 3, 4}; int i,](https://slidetodoc.com/presentation_image/509648c25e0d0b489049b3adb5b76309/image-21.jpg)
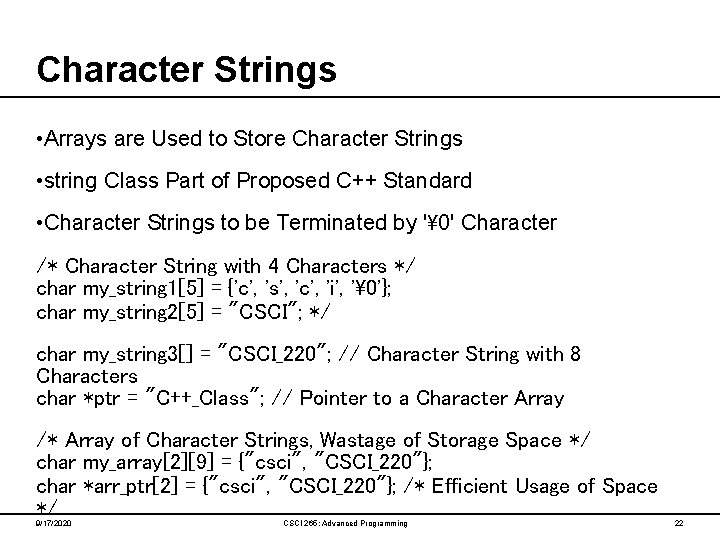
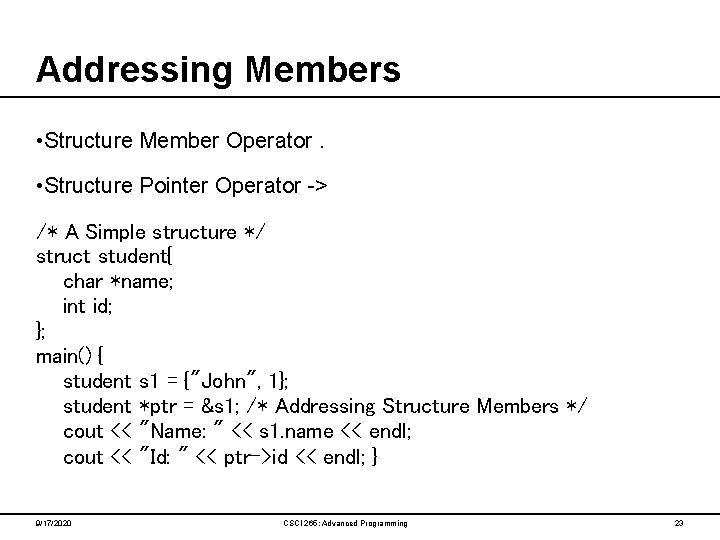
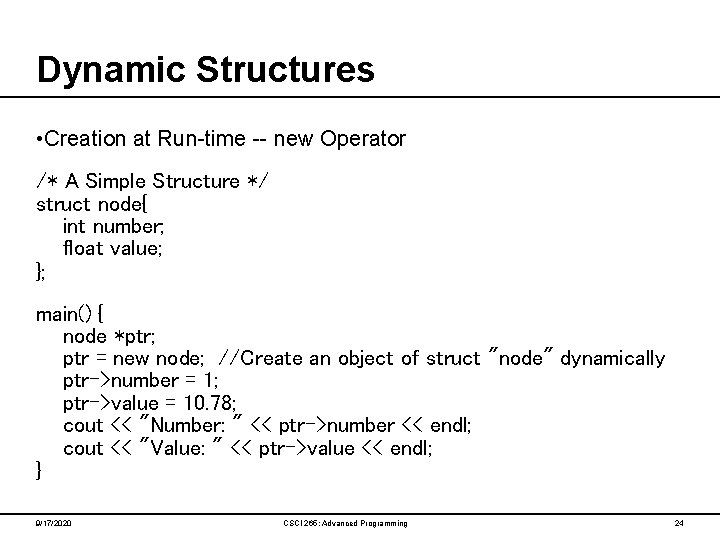
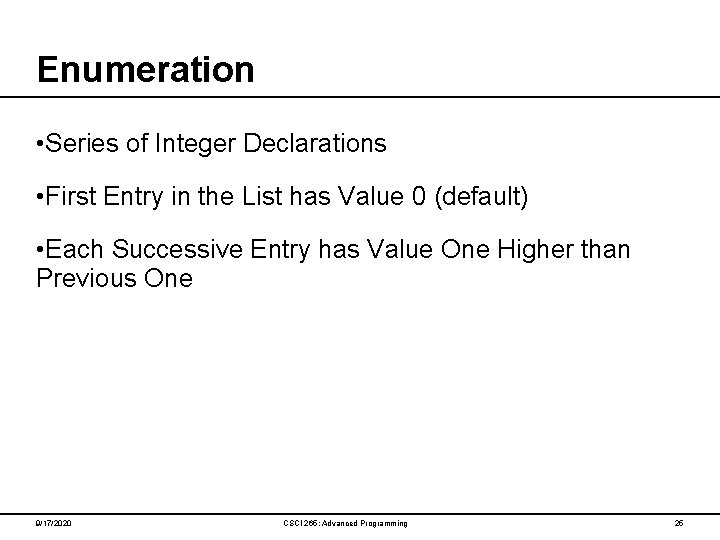
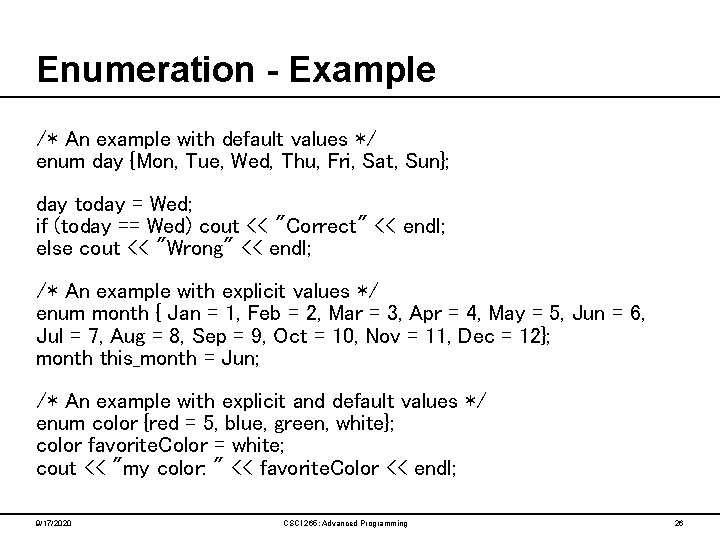
- Slides: 26
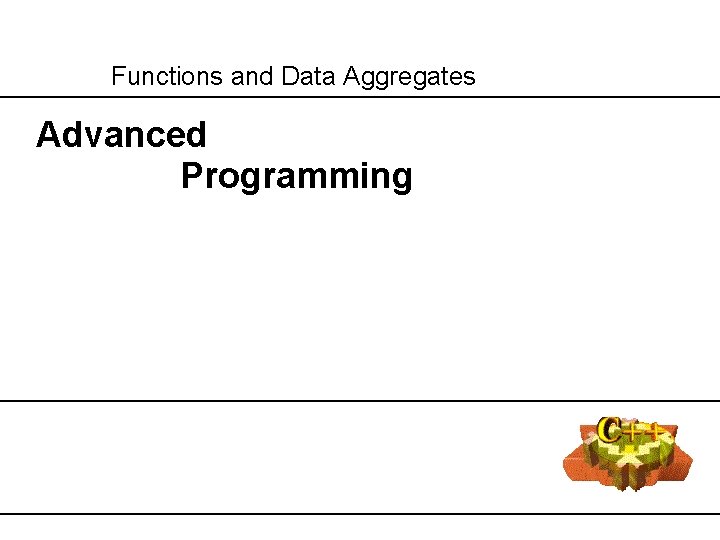
Functions and Data Aggregates Advanced Programming
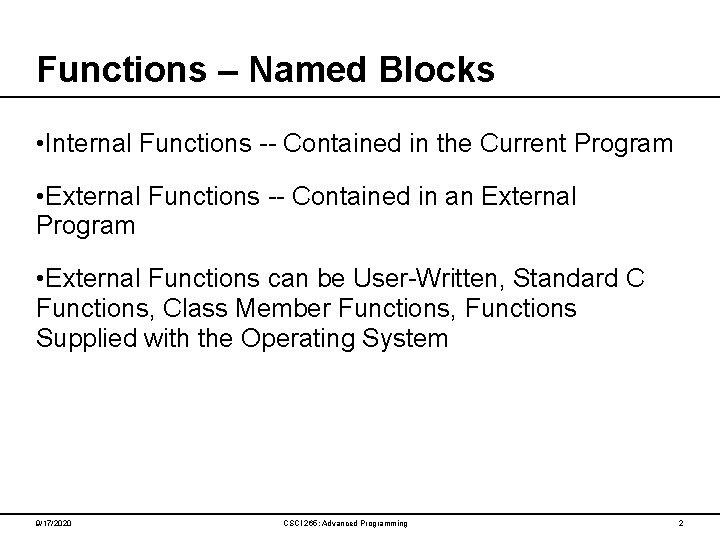
Functions – Named Blocks • Internal Functions -- Contained in the Current Program • External Functions -- Contained in an External Program • External Functions can be User-Written, Standard C Functions, Class Member Functions, Functions Supplied with the Operating System 9/17/2020 CSCI 265: Advanced Programming 2
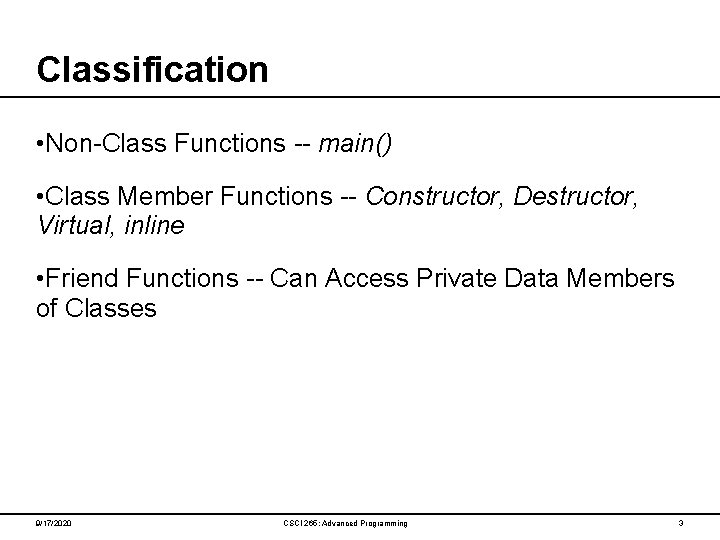
Classification • Non-Class Functions -- main() • Class Member Functions -- Constructor, Destructor, Virtual, inline • Friend Functions -- Can Access Private Data Members of Classes 9/17/2020 CSCI 265: Advanced Programming 3
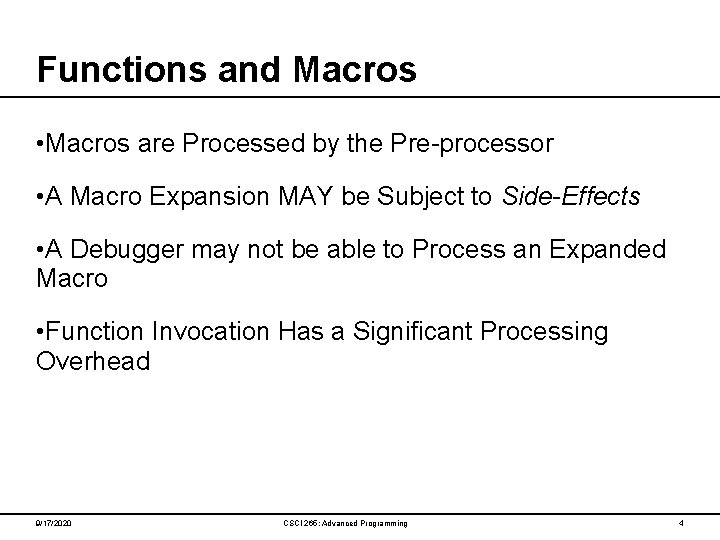
Functions and Macros • Macros are Processed by the Pre-processor • A Macro Expansion MAY be Subject to Side-Effects • A Debugger may not be able to Process an Expanded Macro • Function Invocation Has a Significant Processing Overhead 9/17/2020 CSCI 265: Advanced Programming 4
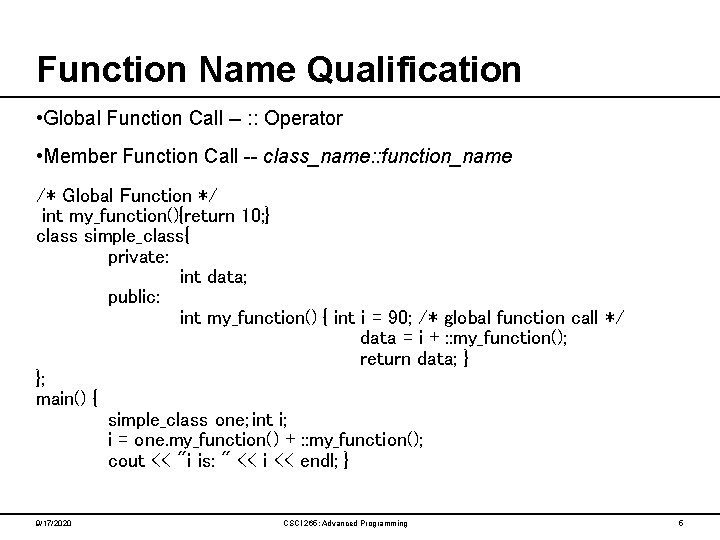
Function Name Qualification • Global Function Call -- : : Operator • Member Function Call -- class_name: : function_name /* Global Function */ int my_function(){return 10; } class simple_class{ private: int data; public: int my_function() { int i = 90; /* global function call */ data = i + : : my_function(); return data; } }; main() { simple_class one; int i; i = one. my_function() + : : my_function(); cout << "i is: " << i << endl; } 9/17/2020 CSCI 265: Advanced Programming 5
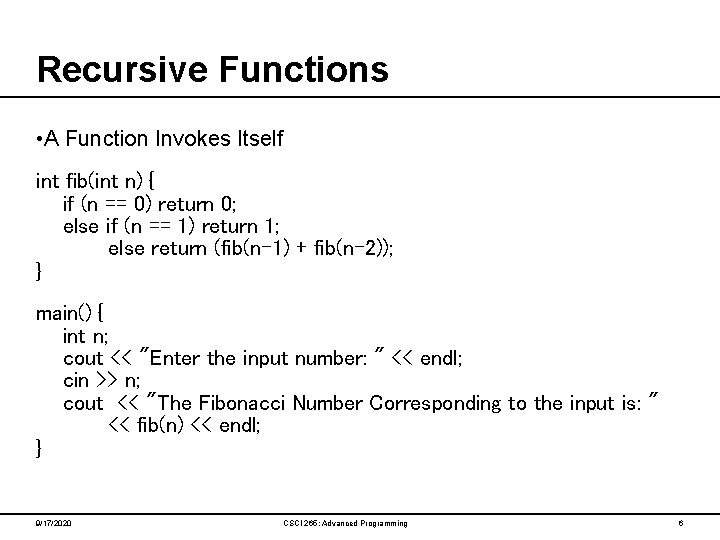
Recursive Functions • A Function Invokes Itself int fib(int n) { if (n == 0) return 0; else if (n == 1) return 1; else return (fib(n-1) + fib(n-2)); } main() { int n; cout << "Enter the input number: " << endl; cin >> n; cout << "The Fibonacci Number Corresponding to the input is: " << fib(n) << endl; } 9/17/2020 CSCI 265: Advanced Programming 6
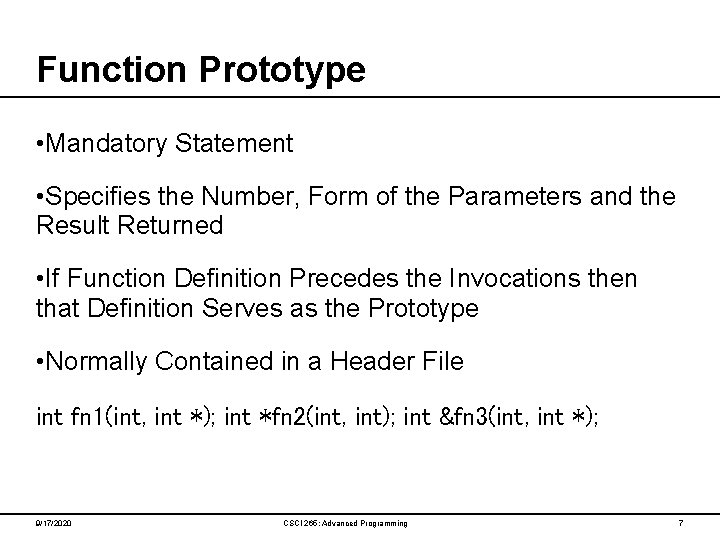
Function Prototype • Mandatory Statement • Specifies the Number, Form of the Parameters and the Result Returned • If Function Definition Precedes the Invocations then that Definition Serves as the Prototype • Normally Contained in a Header File int fn 1(int, int *); int *fn 2(int, int); int &fn 3(int, int *); 9/17/2020 CSCI 265: Advanced Programming 7
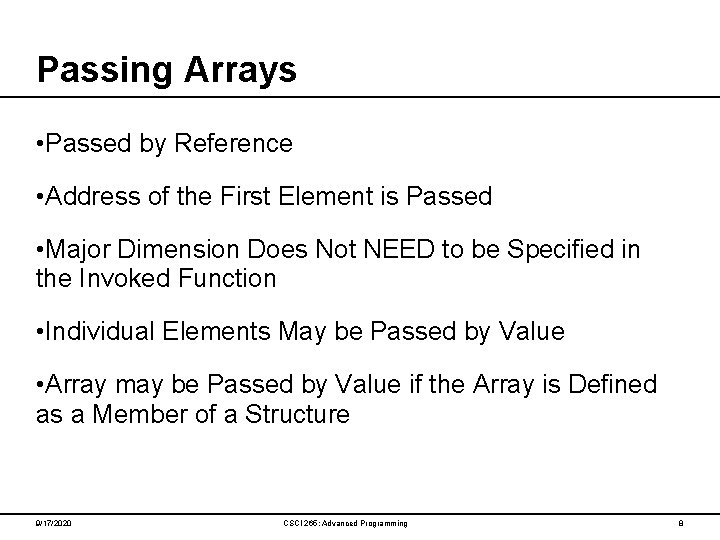
Passing Arrays • Passed by Reference • Address of the First Element is Passed • Major Dimension Does Not NEED to be Specified in the Invoked Function • Individual Elements May be Passed by Value • Array may be Passed by Value if the Array is Defined as a Member of a Structure 9/17/2020 CSCI 265: Advanced Programming 8
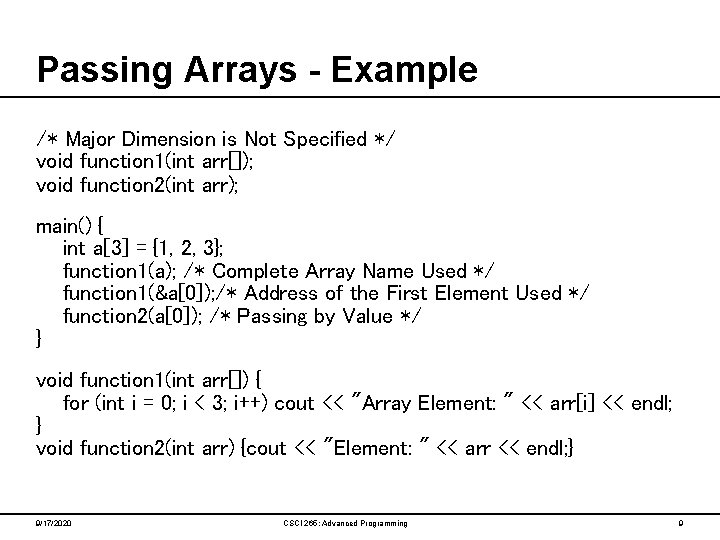
Passing Arrays - Example /* Major Dimension is Not Specified */ void function 1(int arr[]); void function 2(int arr); main() { int a[3] = {1, 2, 3}; function 1(a); /* Complete Array Name Used */ function 1(&a[0]); /* Address of the First Element Used */ function 2(a[0]); /* Passing by Value */ } void function 1(int arr[]) { for (int i = 0; i < 3; i++) cout << "Array Element: " << arr[i] << endl; } void function 2(int arr) {cout << "Element: " << arr << endl; } 9/17/2020 CSCI 265: Advanced Programming 9
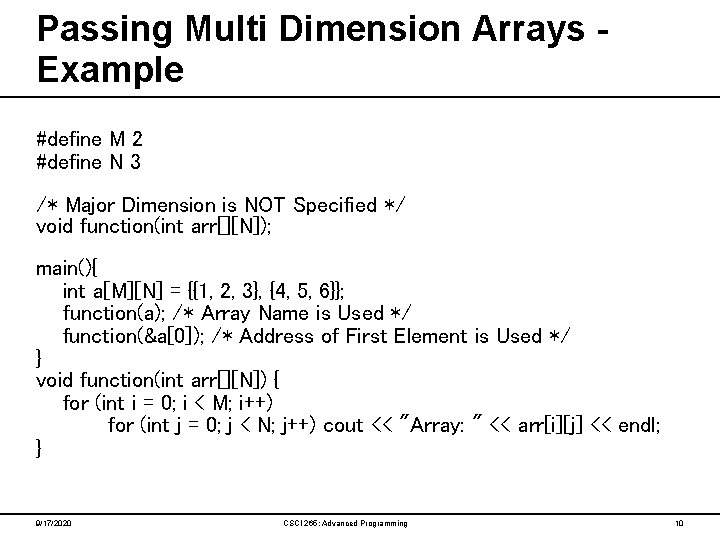
Passing Multi Dimension Arrays Example #define M 2 #define N 3 /* Major Dimension is NOT Specified */ void function(int arr[][N]); main(){ int a[M][N] = {{1, 2, 3}, {4, 5, 6}}; function(a); /* Array Name is Used */ function(&a[0]); /* Address of First Element is Used */ } void function(int arr[][N]) { for (int i = 0; i < M; i++) for (int j = 0; j < N; j++) cout << "Array: " << arr[i][j] << endl; } 9/17/2020 CSCI 265: Advanced Programming 10
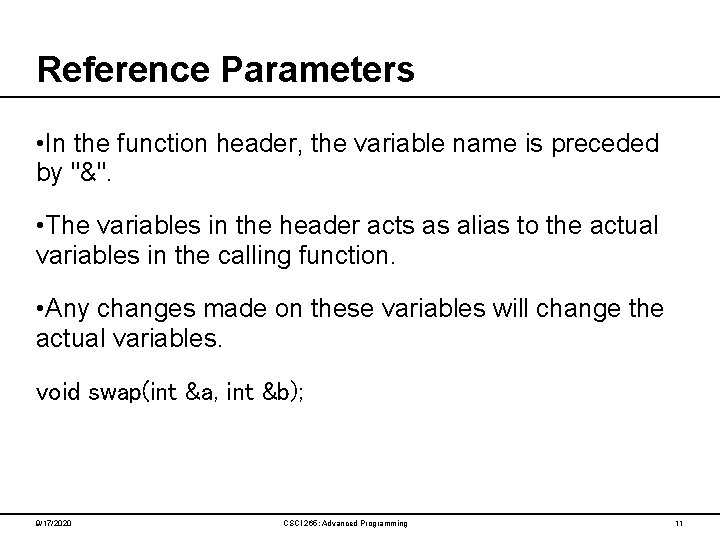
Reference Parameters • In the function header, the variable name is preceded by "&". • The variables in the header acts as alias to the actual variables in the calling function. • Any changes made on these variables will change the actual variables. void swap(int &a, int &b); 9/17/2020 CSCI 265: Advanced Programming 11
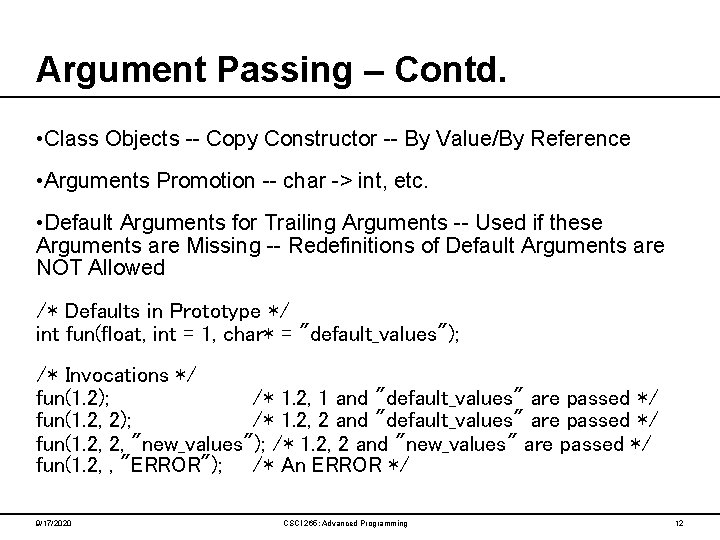
Argument Passing – Contd. • Class Objects -- Copy Constructor -- By Value/By Reference • Arguments Promotion -- char -> int, etc. • Default Arguments for Trailing Arguments -- Used if these Arguments are Missing -- Redefinitions of Default Arguments are NOT Allowed /* Defaults in Prototype */ int fun(float, int = 1, char* = "default_values"); /* Invocations */ fun(1. 2); /* 1. 2, 1 and "default_values" are passed */ fun(1. 2, 2); /* 1. 2, 2 and "default_values" are passed */ fun(1. 2, 2, "new_values"); /* 1. 2, 2 and "new_values" are passed */ fun(1. 2, , "ERROR"); /* An ERROR */ 9/17/2020 CSCI 265: Advanced Programming 12
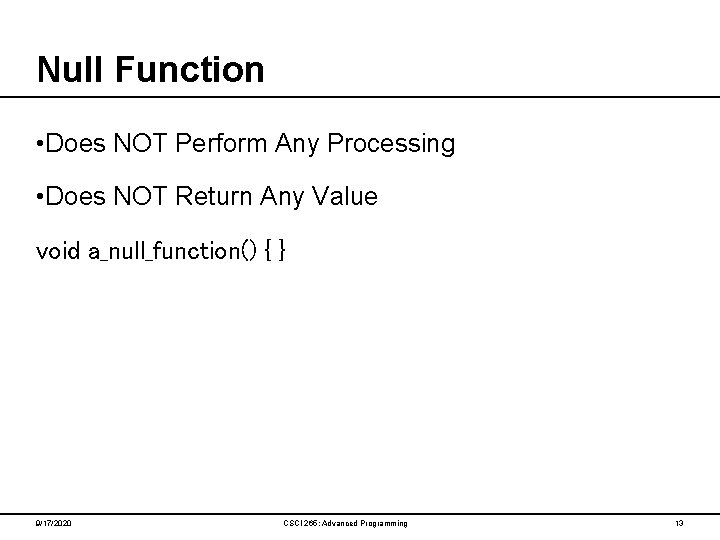
Null Function • Does NOT Perform Any Processing • Does NOT Return Any Value void a_null_function() { } 9/17/2020 CSCI 265: Advanced Programming 13
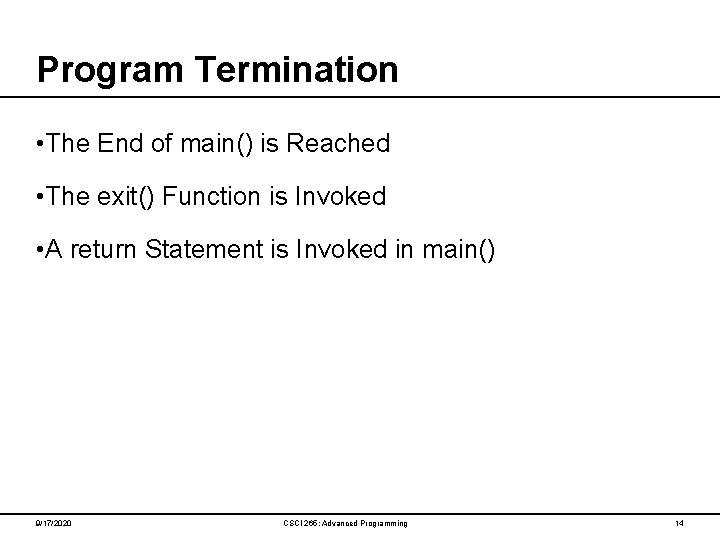
Program Termination • The End of main() is Reached • The exit() Function is Invoked • A return Statement is Invoked in main() 9/17/2020 CSCI 265: Advanced Programming 14
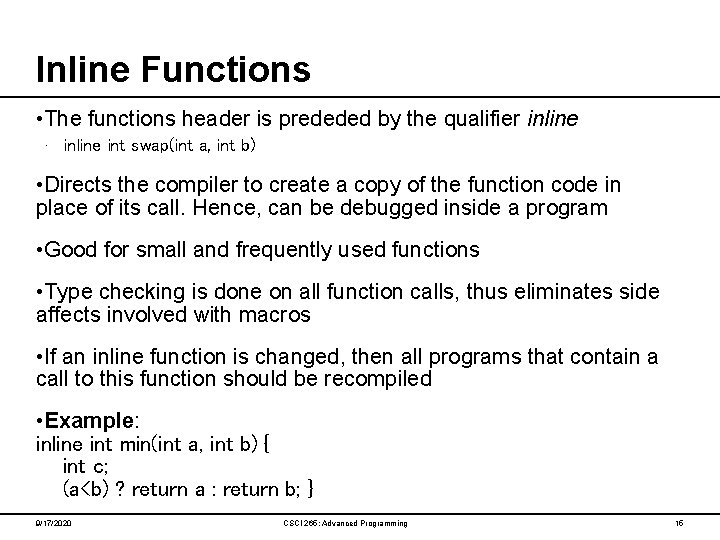
Inline Functions • The functions header is prededed by the qualifier inline • inline int swap(int a, int b) • Directs the compiler to create a copy of the function code in place of its call. Hence, can be debugged inside a program • Good for small and frequently used functions • Type checking is done on all function calls, thus eliminates side affects involved with macros • If an inline function is changed, then all programs that contain a call to this function should be recompiled • Example: inline int min(int a, int b) { int c; (a<b) ? return a : return b; } 9/17/2020 CSCI 265: Advanced Programming 15
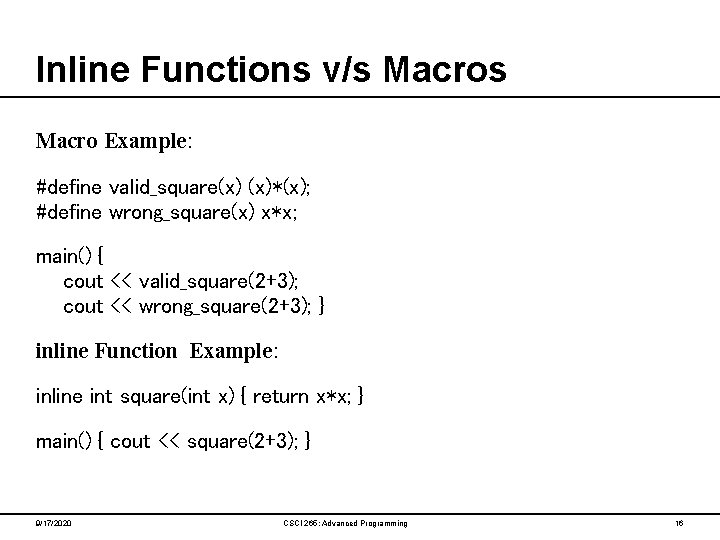
Inline Functions v/s Macro Example: #define valid_square(x) (x)*(x); #define wrong_square(x) x*x; main() { cout << valid_square(2+3); cout << wrong_square(2+3); } inline Function Example: inline int square(int x) { return x*x; } main() { cout << square(2+3); } 9/17/2020 CSCI 265: Advanced Programming 16
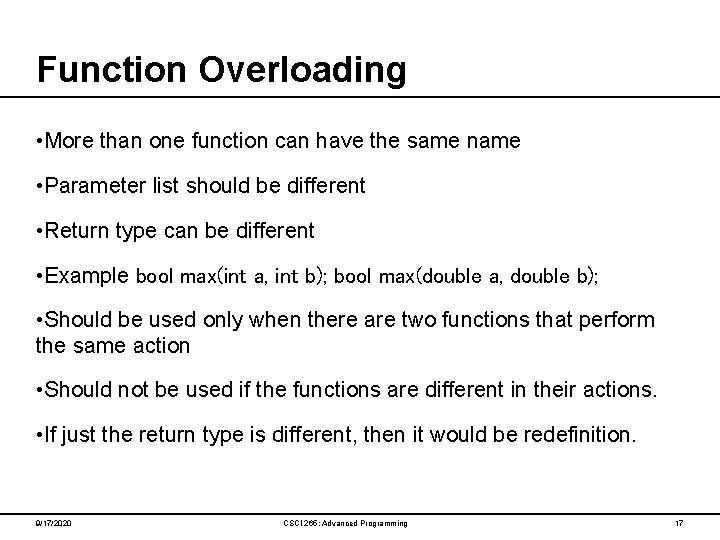
Function Overloading • More than one function can have the same name • Parameter list should be different • Return type can be different • Example bool max(int a, int b); bool max(double a, double b); • Should be used only when there are two functions that perform the same action • Should not be used if the functions are different in their actions. • If just the return type is different, then it would be redefinition. 9/17/2020 CSCI 265: Advanced Programming 17
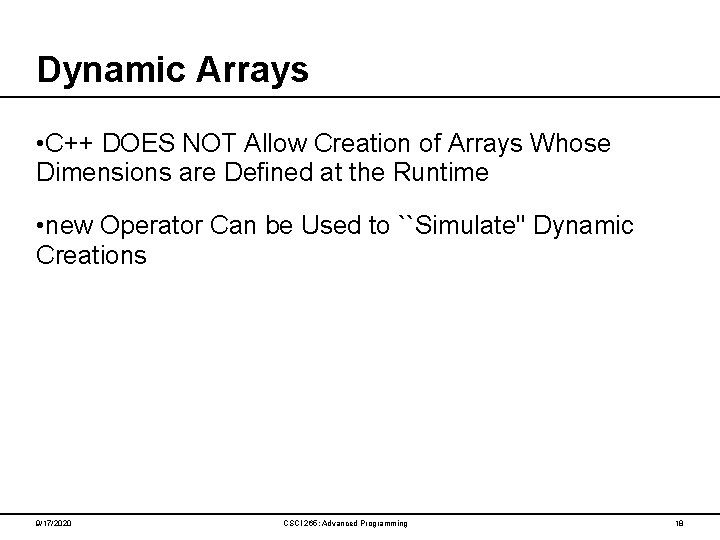
Dynamic Arrays • C++ DOES NOT Allow Creation of Arrays Whose Dimensions are Defined at the Runtime • new Operator Can be Used to ``Simulate'' Dynamic Creations 9/17/2020 CSCI 265: Advanced Programming 18
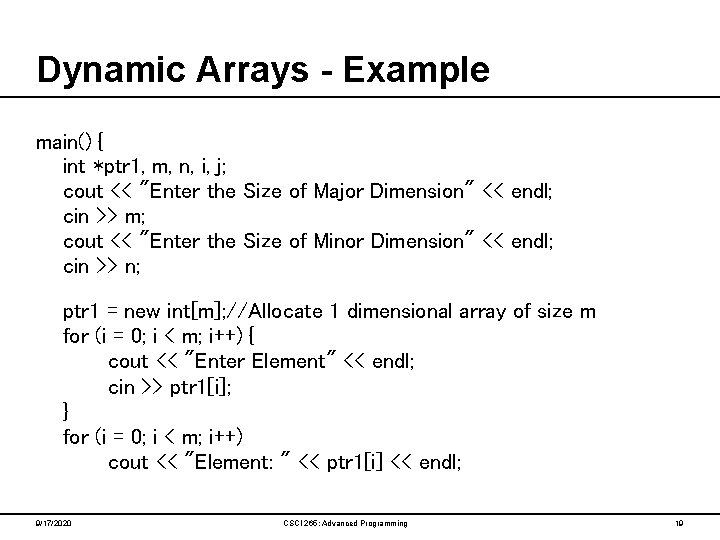
Dynamic Arrays - Example main() { int *ptr 1, m, n, i, j; cout << "Enter the Size of Major Dimension" << endl; cin >> m; cout << "Enter the Size of Minor Dimension" << endl; cin >> n; ptr 1 = new int[m]; //Allocate 1 dimensional array of size m for (i = 0; i < m; i++) { cout << "Enter Element" << endl; cin >> ptr 1[i]; } for (i = 0; i < m; i++) cout << "Element: " << ptr 1[i] << endl; 9/17/2020 CSCI 265: Advanced Programming 19
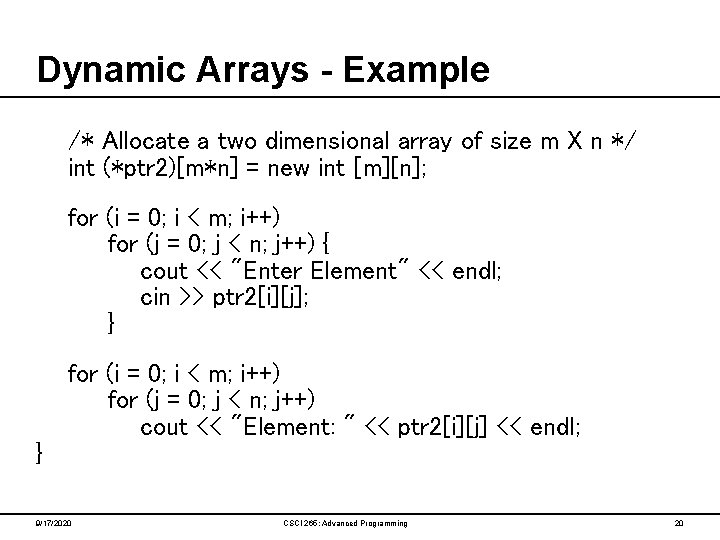
Dynamic Arrays - Example /* Allocate a two dimensional array of size m X n */ int (*ptr 2)[m*n] = new int [m][n]; for (i = 0; i < m; i++) for (j = 0; j < n; j++) { cout << "Enter Element" << endl; cin >> ptr 2[i][j]; } for (i = 0; i < m; i++) for (j = 0; j < n; j++) cout << "Element: " << ptr 2[i][j] << endl; } 9/17/2020 CSCI 265: Advanced Programming 20
![MultiDimension Array Addressing main int a22 1 2 3 4 int i Multi-Dimension Array Addressing main() { int a[2][2] = {1, 2, 3, 4}; int i,](https://slidetodoc.com/presentation_image/509648c25e0d0b489049b3adb5b76309/image-21.jpg)
Multi-Dimension Array Addressing main() { int a[2][2] = {1, 2, 3, 4}; int i, j, *ptr 1; int (*ptr 2) [2]; for (i = 0; i < 2; i++) // Prints out 1, 2, 3, 4 for (j = 0; j < 2; j ++) cout << "Array: " << a[i][j] << endl; ptr 1 = a[0]; // ptr 1 is pointing to the first row/element for (i = 0; i < 2; i++, ptr 1++) //Prints out 1, 2 cout << "Array: " << *ptr 1 << endl; ptr 2 = &a[0]; // Prints out 1, 3 for (i = 0; i < 2; i++, ptr 2++) cout << "Array: " << **ptr 2 << endl; } 9/17/2020 CSCI 265: Advanced Programming 21
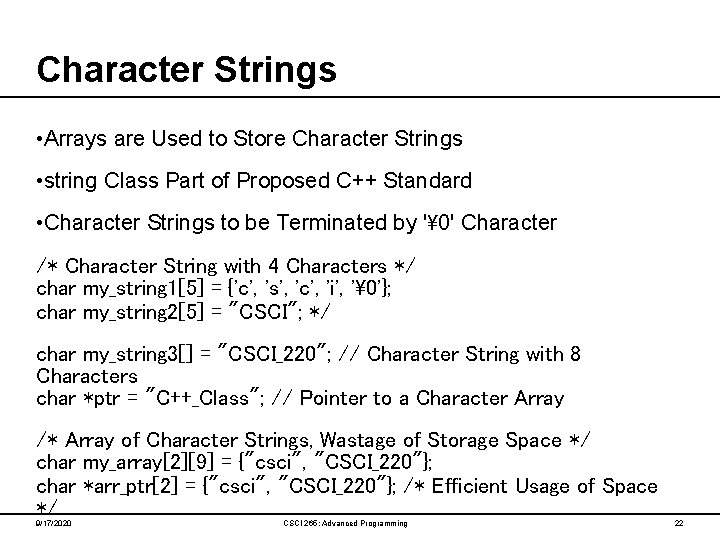
Character Strings • Arrays are Used to Store Character Strings • string Class Part of Proposed C++ Standard • Character Strings to be Terminated by '