Recall that char str 8 str is the
![Recall that. . . char str [ 8 ]; str is the base address Recall that. . . char str [ 8 ]; str is the base address](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-1.jpg)
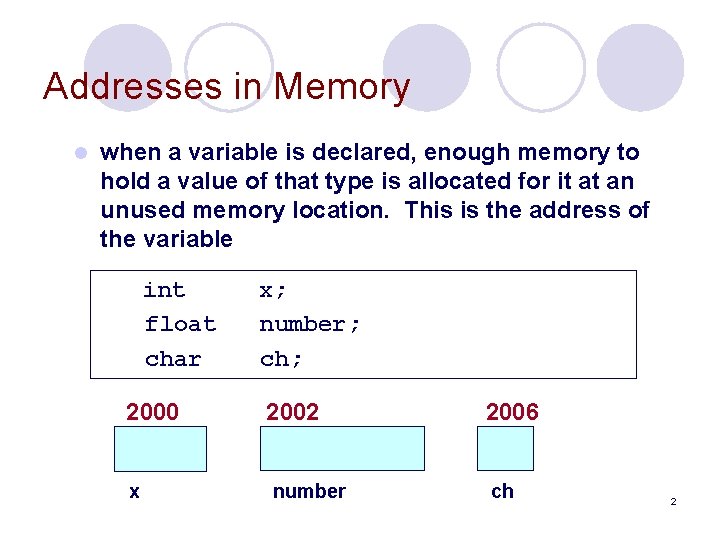
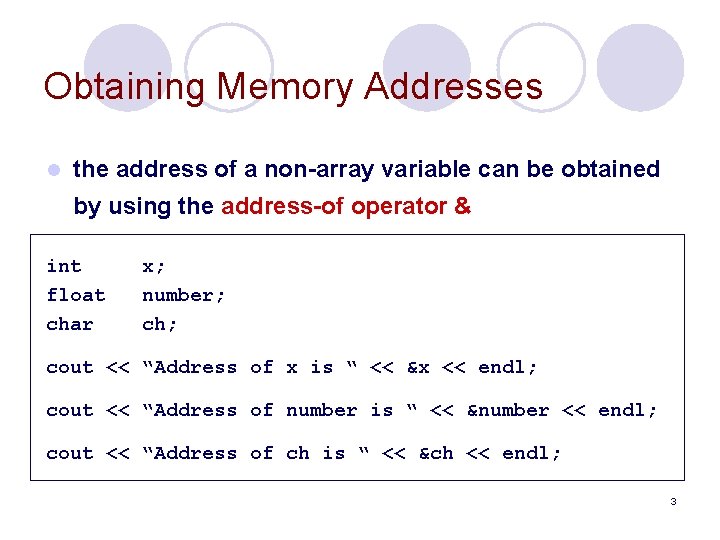
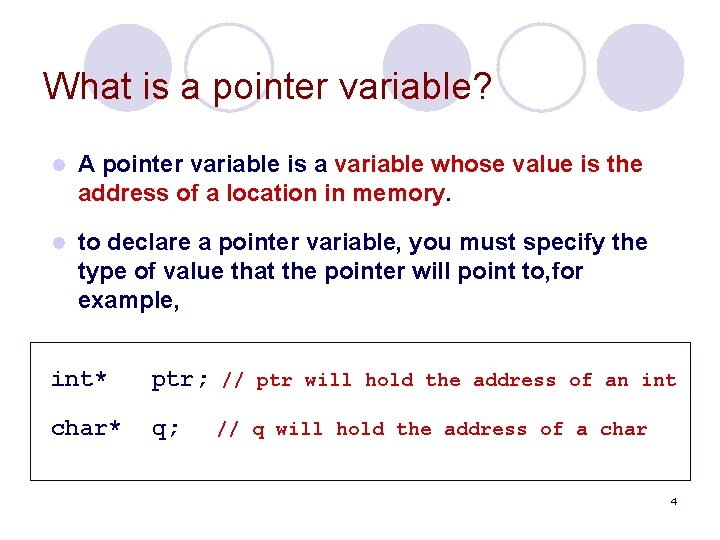
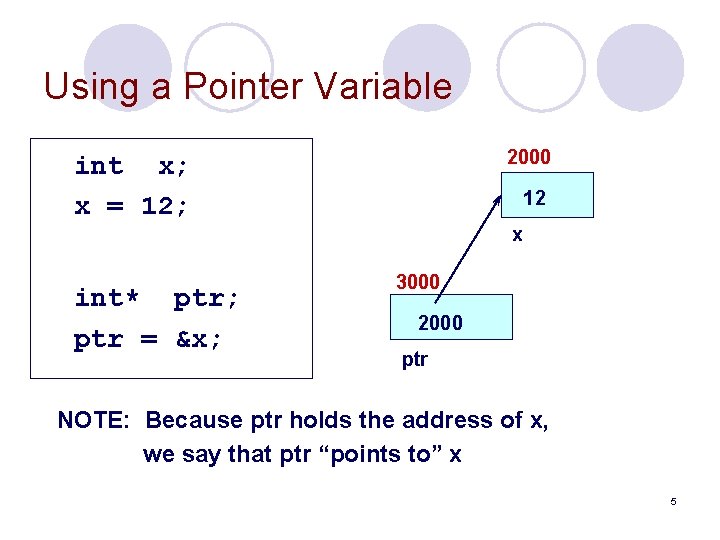
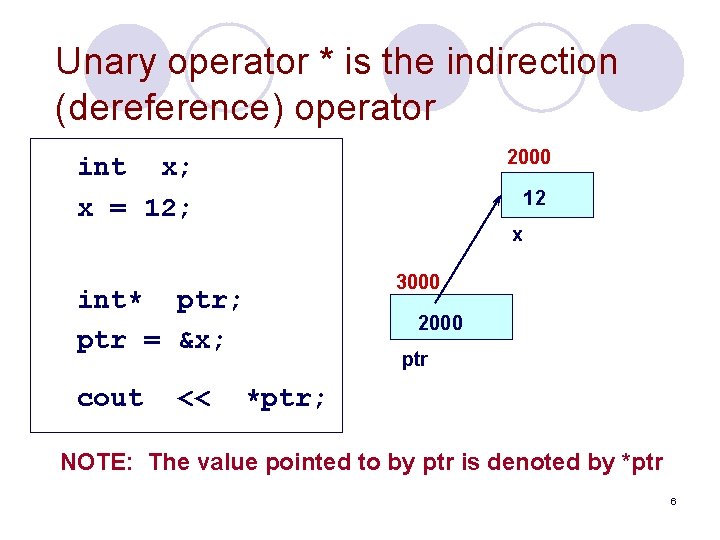
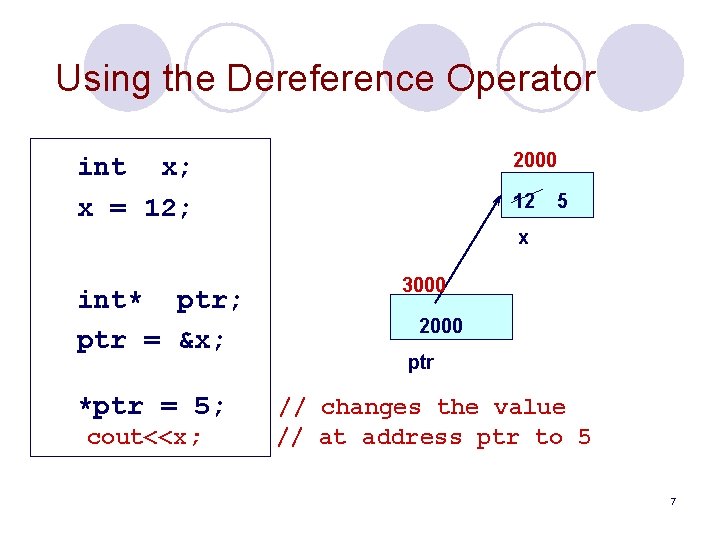
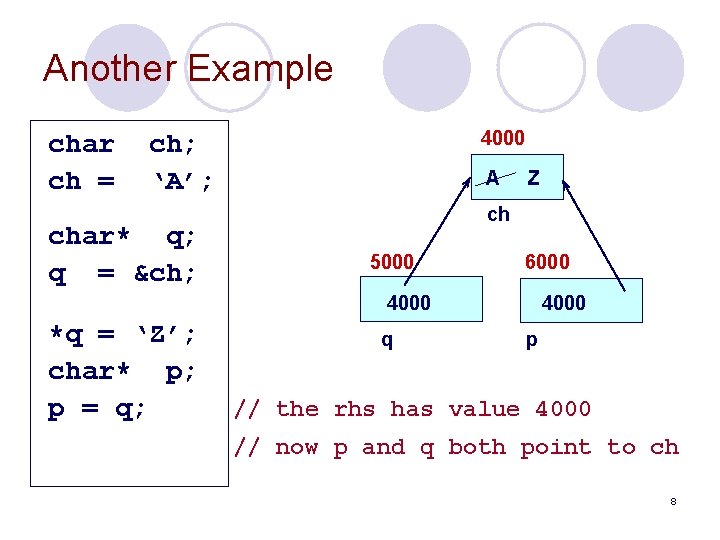
![Using a Pointer to Access the Elements of a String char msg[ ] = Using a Pointer to Access the Elements of a String char msg[ ] =](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-9.jpg)
![int String. Length ( /* in */ const char str[ ] ) // - int String. Length ( /* in */ const char str[ ] ) // -](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-10.jpg)
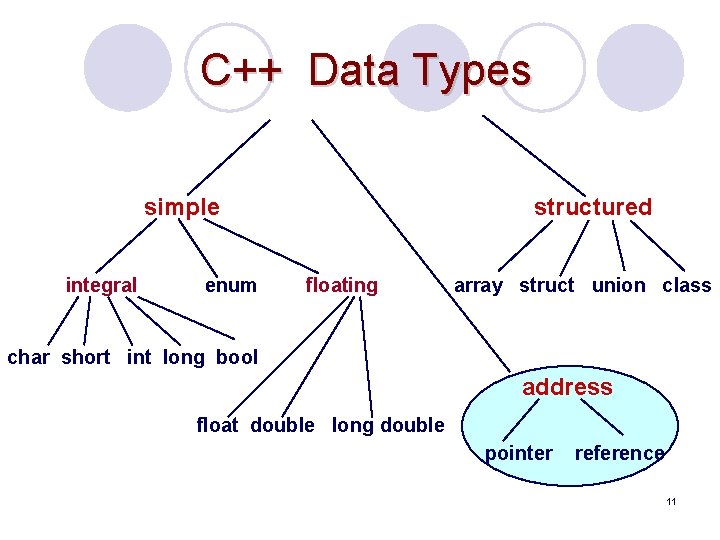
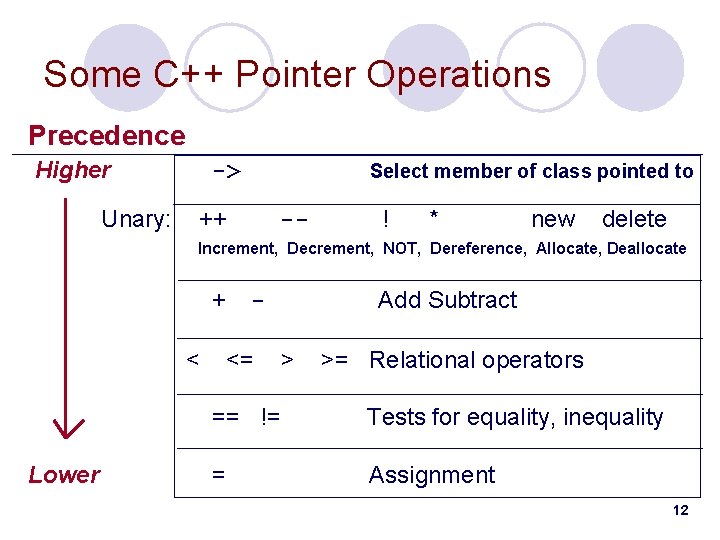
![Operator new Syntax new Data. Type [Int. Expression] If memory is available, in an Operator new Syntax new Data. Type [Int. Expression] If memory is available, in an](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-13.jpg)
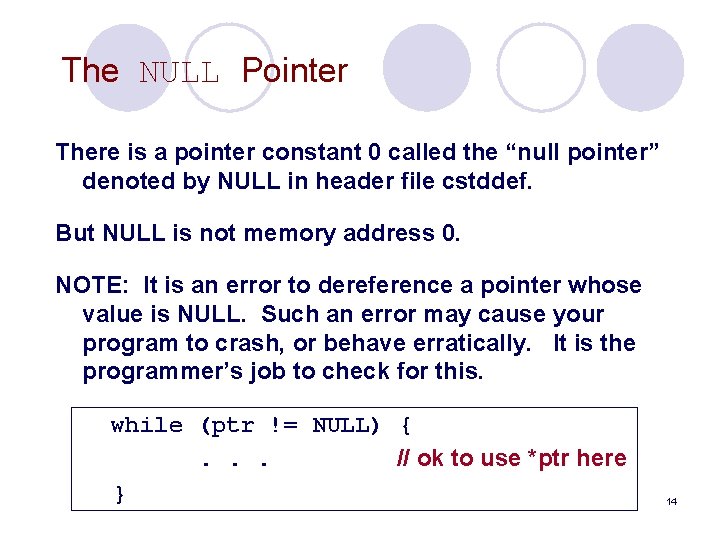
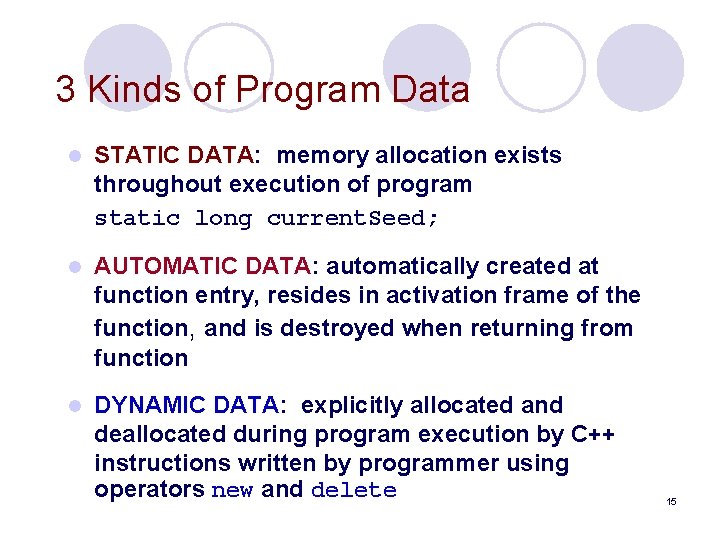
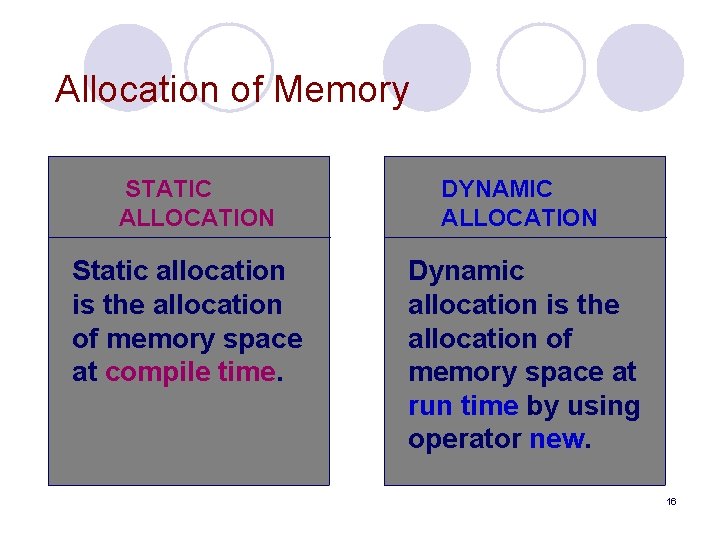
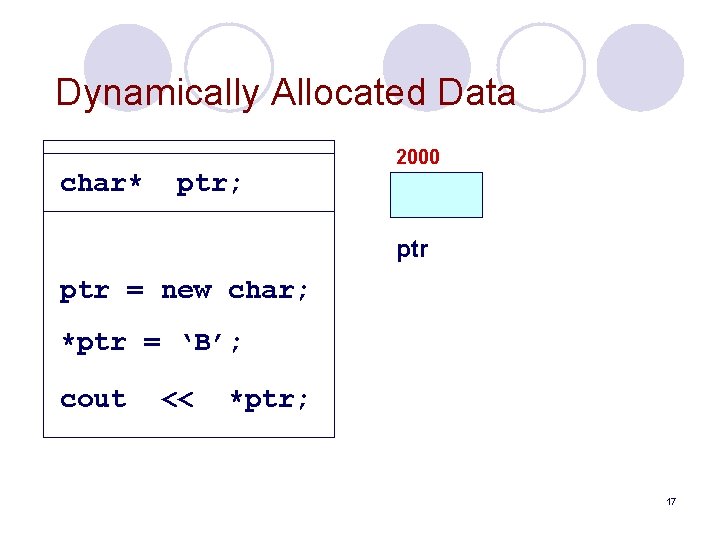
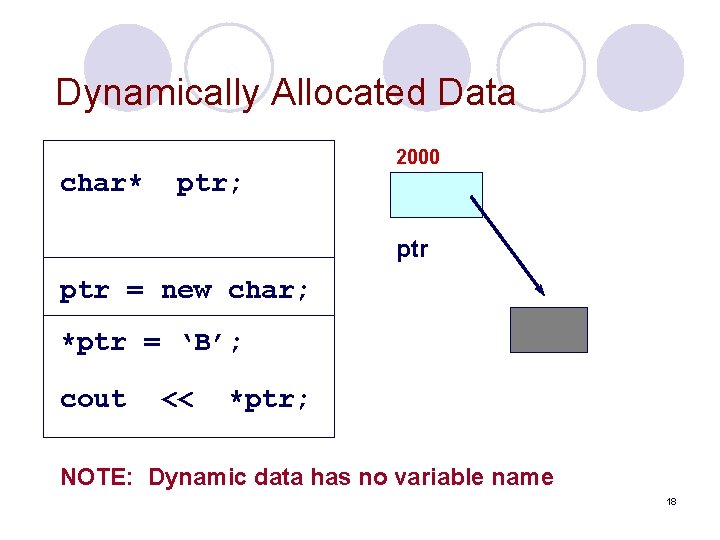
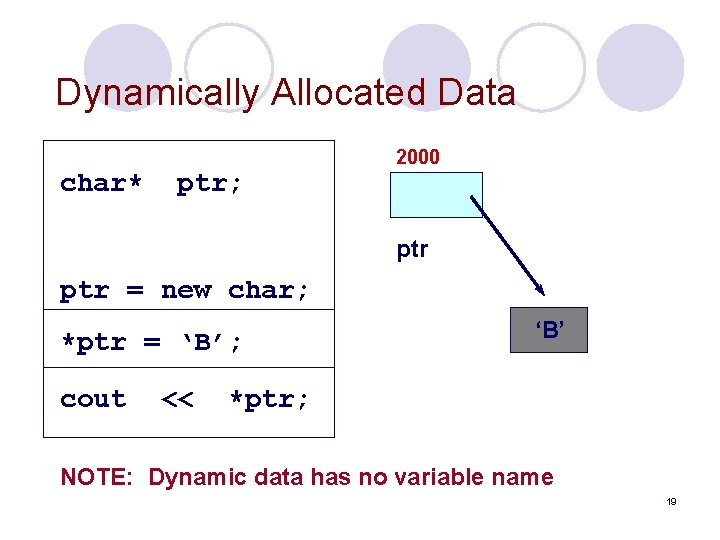
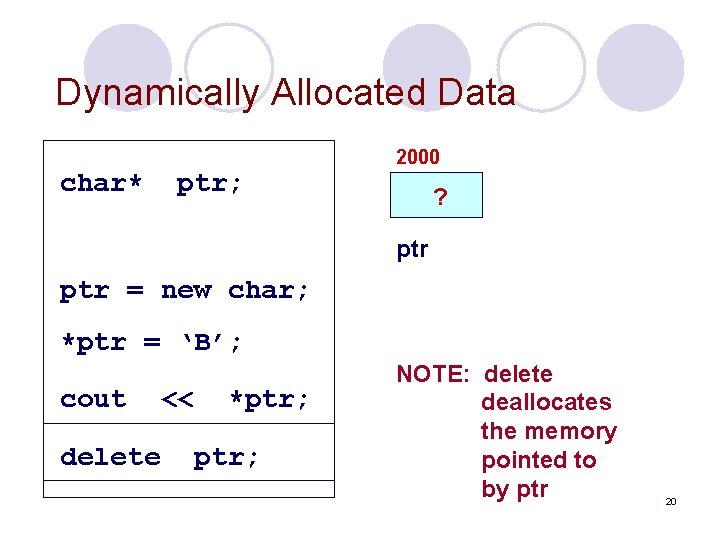
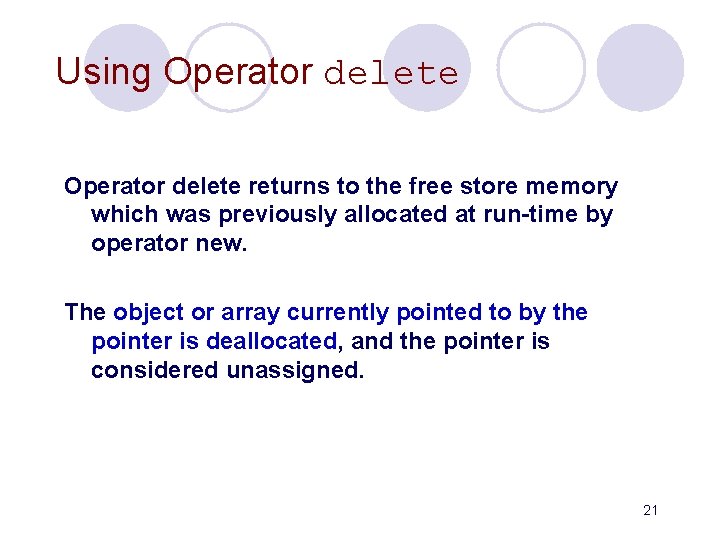
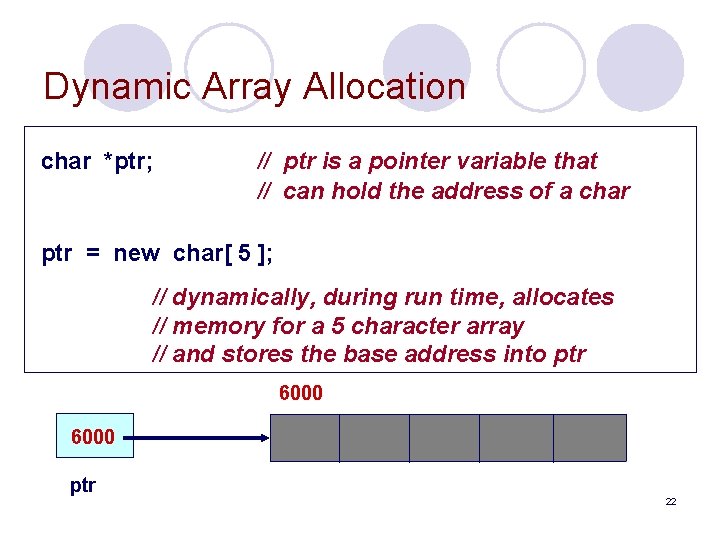
![Dynamic Array Allocation char *ptr ; ptr = new char[ 5 ]; strcpy( ptr, Dynamic Array Allocation char *ptr ; ptr = new char[ 5 ]; strcpy( ptr,](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-23.jpg)
![Operator delete Syntax delete Pointer delete [ ] Pointer If the value of the Operator delete Syntax delete Pointer delete [ ] Pointer If the value of the](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-24.jpg)
![Dynamic Array Deallocation char *ptr ; ptr = new char[ 5 ]; strcpy( ptr, Dynamic Array Deallocation char *ptr ; ptr = new char[ 5 ]; strcpy( ptr,](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-25.jpg)
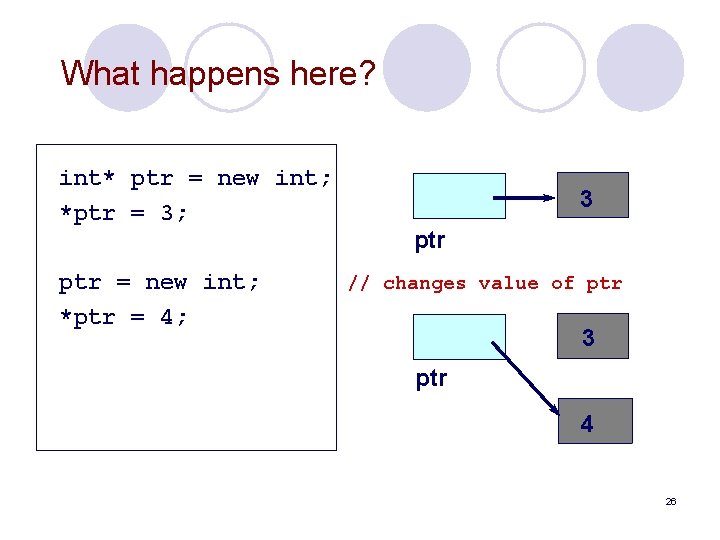
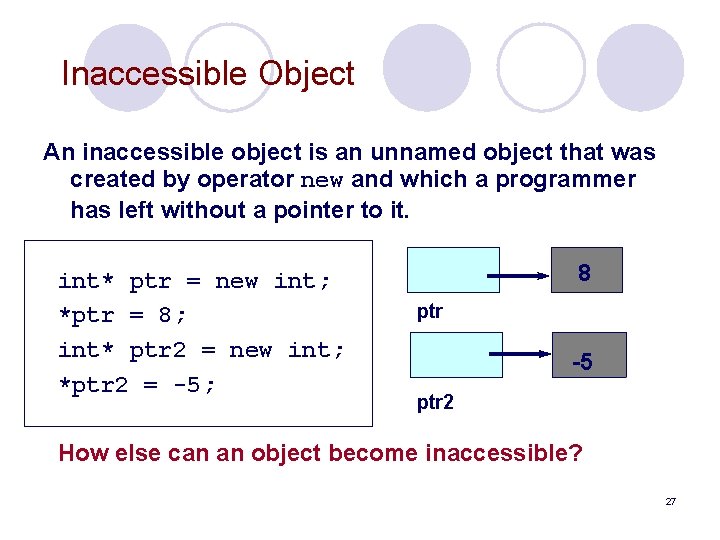
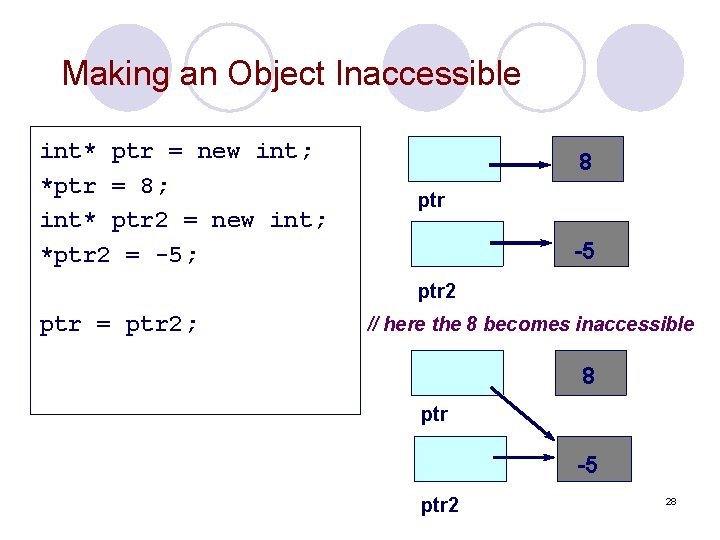
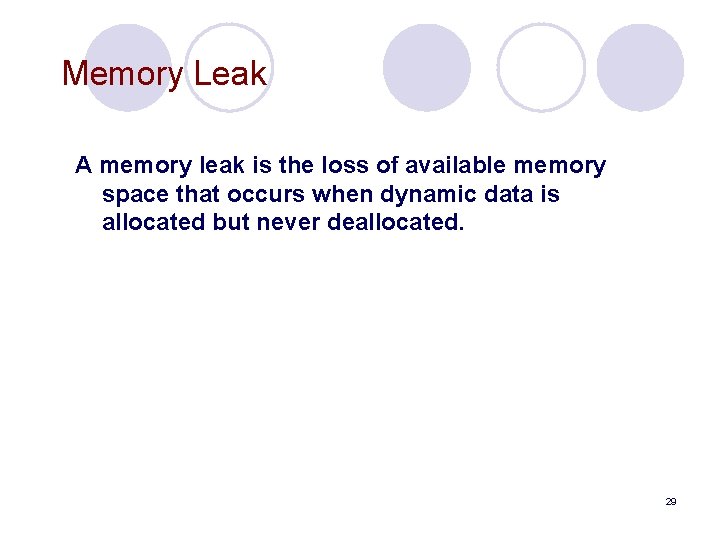
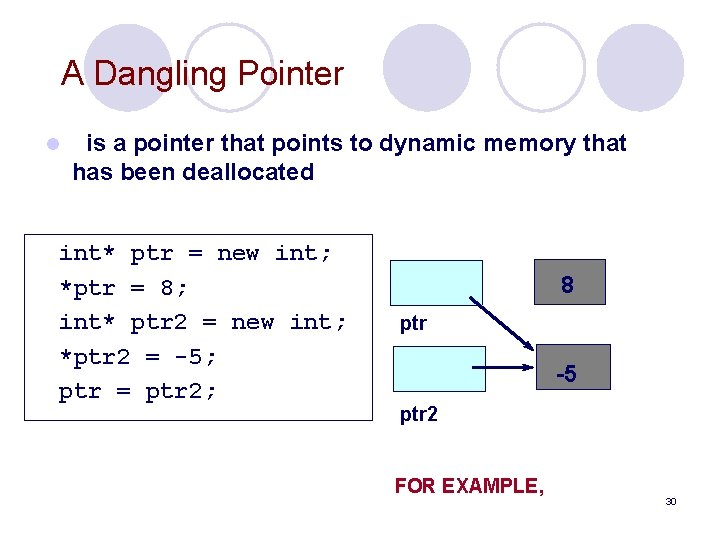
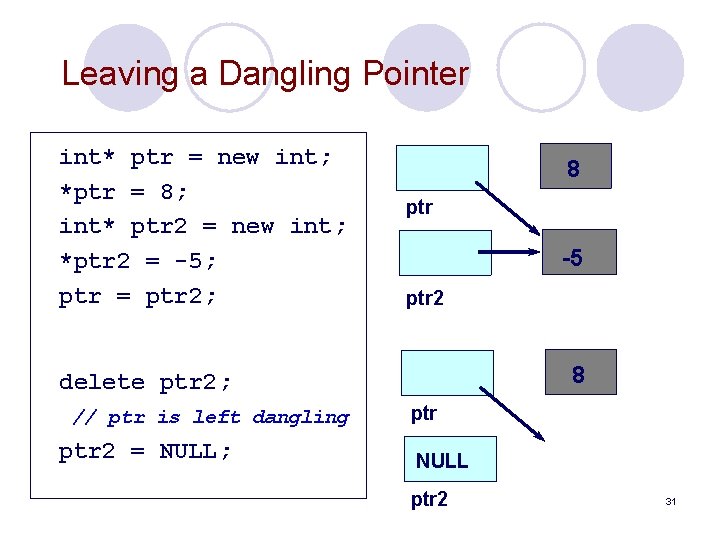
- Slides: 31
![Recall that char str 8 str is the base address Recall that. . . char str [ 8 ]; str is the base address](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-1.jpg)
Recall that. . . char str [ 8 ]; str is the base address of the array. We say str is a pointer because its value is an address. It is a pointer constant because the value of str itself cannot be changed by assignment. It “points” to the memory location of a char. 6000 ‘H’ ‘e’ ‘l’ ‘o’ ‘ ’ str [0] [1] [2] [3] [4] [5] [6] [7] 1
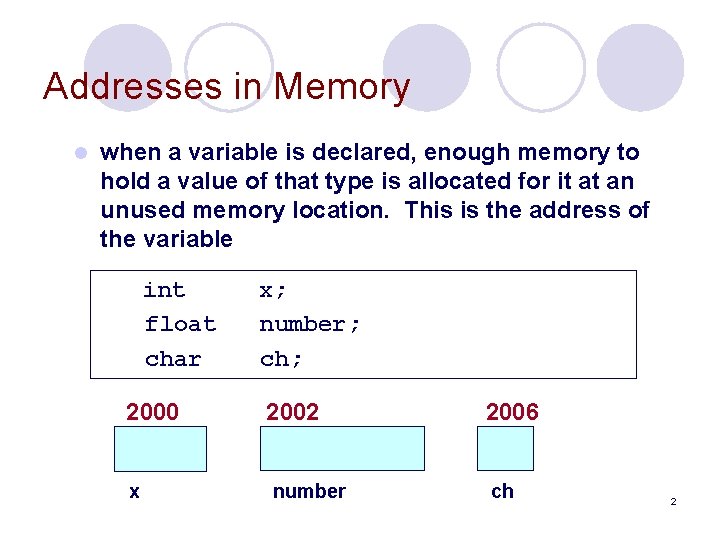
Addresses in Memory l when a variable is declared, enough memory to hold a value of that type is allocated for it at an unused memory location. This is the address of the variable int float char 2000 x x; number; ch; 2002 number 2006 ch 2
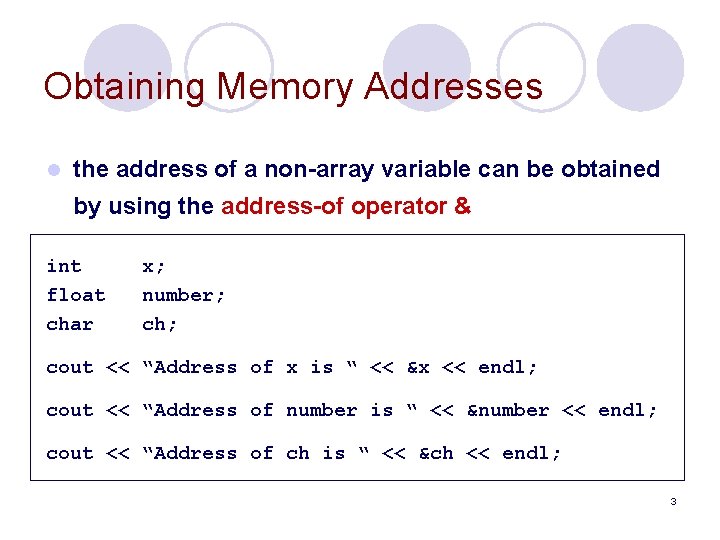
Obtaining Memory Addresses l the address of a non-array variable can be obtained by using the address-of operator & int float char x; number; ch; cout << “Address of x is “ << &x << endl; cout << “Address of number is “ << &number << endl; cout << “Address of ch is “ << &ch << endl; 3
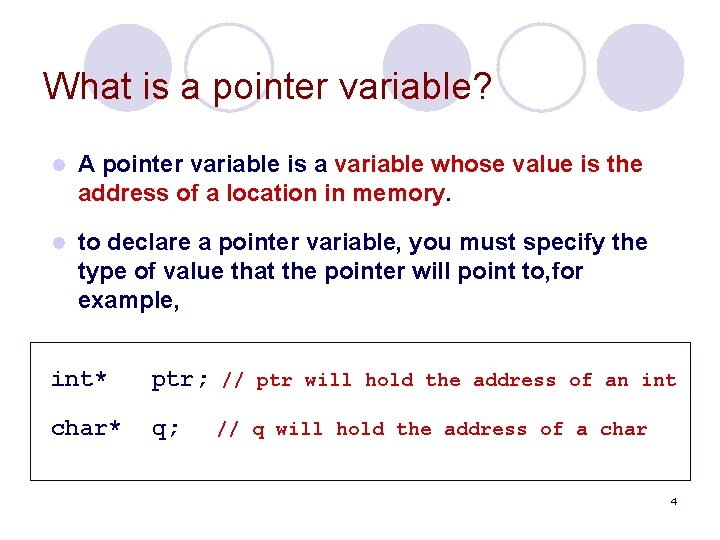
What is a pointer variable? l A pointer variable is a variable whose value is the address of a location in memory. l to declare a pointer variable, you must specify the type of value that the pointer will point to, for example, int* ptr; // ptr will hold the address of an int char* q; // q will hold the address of a char 4
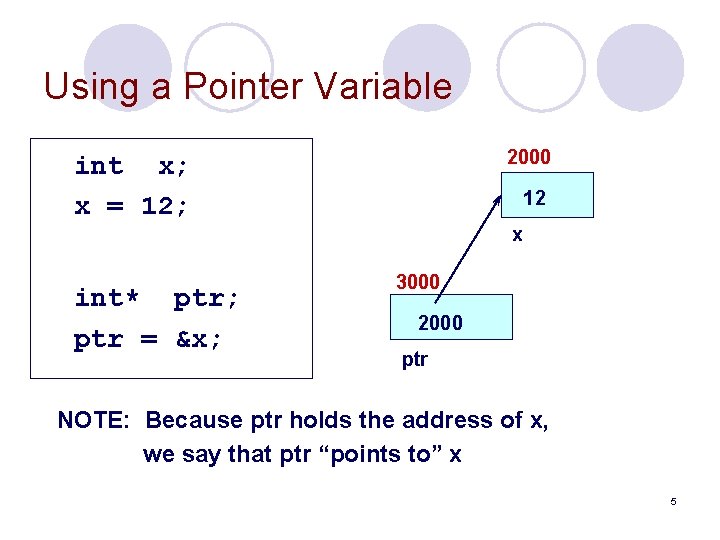
Using a Pointer Variable 2000 int x; x = 12; 12 x int* ptr; ptr = &x; 3000 2000 ptr NOTE: Because ptr holds the address of x, we say that ptr “points to” x 5
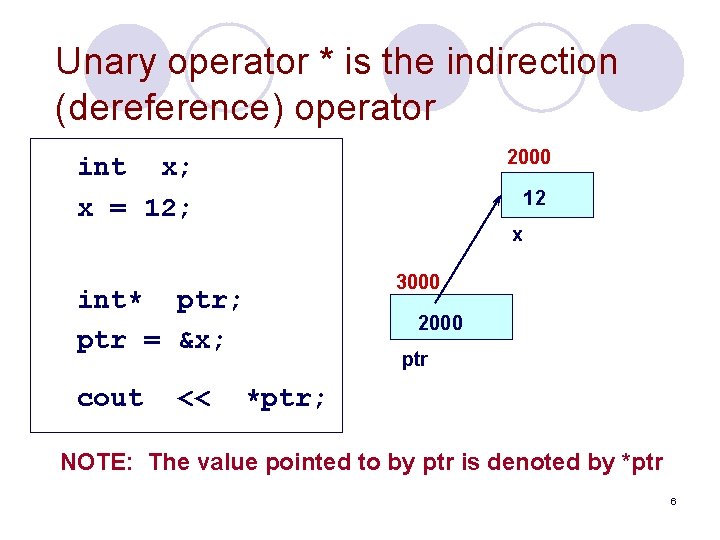
Unary operator * is the indirection (dereference) operator 2000 int x; x = 12; 12 x 3000 int* ptr; ptr = &x; cout << 2000 ptr *ptr; NOTE: The value pointed to by ptr is denoted by *ptr 6
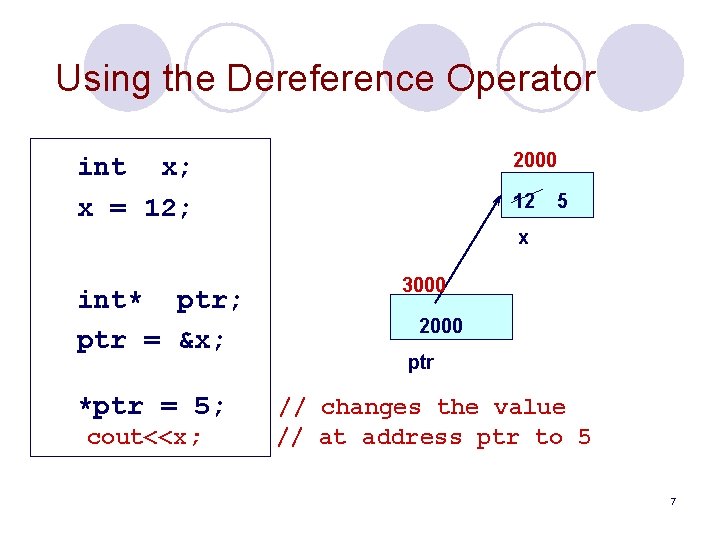
Using the Dereference Operator 2000 int x; x = 12; 12 5 x int* ptr; ptr = &x; *ptr = 5; cout<<x; 3000 2000 ptr // changes the value // at address ptr to 5 7
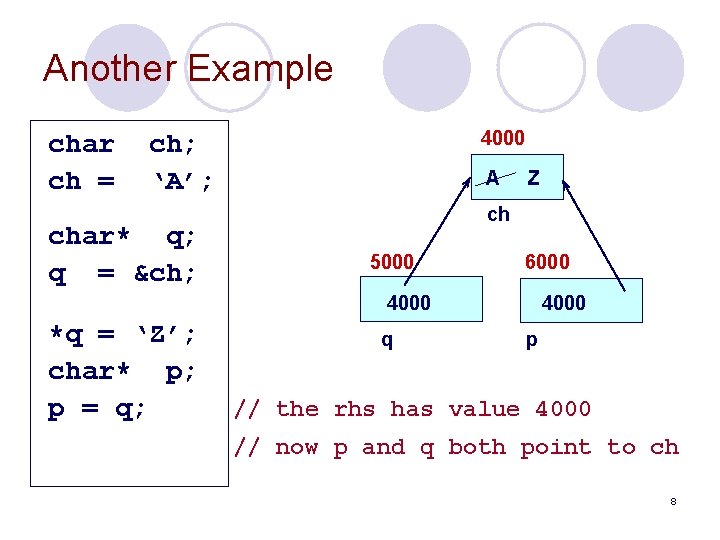
Another Example char ch = 4000 ch; ‘A’; char* q; q = &ch; A Z ch 5000 6000 4000 *q = ‘Z’; char* p; p = q; q 4000 p // the rhs has value 4000 // now p and q both point to ch 8
![Using a Pointer to Access the Elements of a String char msg Using a Pointer to Access the Elements of a String char msg[ ] =](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-9.jpg)
Using a Pointer to Access the Elements of a String char msg[ ] = “Hello”; char* ptr; 3000 ‘M’ ‘a’ ‘H’ ‘e’ ‘l’ ‘o’ ‘ ’ 3001 3000 ptr = msg; // recall that msg == &msg[ 0 ] *ptr = ‘M’ ; ptr++; *ptr = ‘a’; // increments the address in ptr 9
![int String Length in const char str int String. Length ( /* in */ const char str[ ] ) // -](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-10.jpg)
int String. Length ( /* in */ const char str[ ] ) // - - - - - - - - - - - // Precondition: str is a null-terminated string // Postcondition: FCTVAL == length of str (not counting ‘ ’) // - - - - - - - - - - - { char* p ; int count = 0; p = str; while ( *p != ‘ ’ ) { count++ ; p++ ; // increments the address p by sizeof char } return count; } 10 10
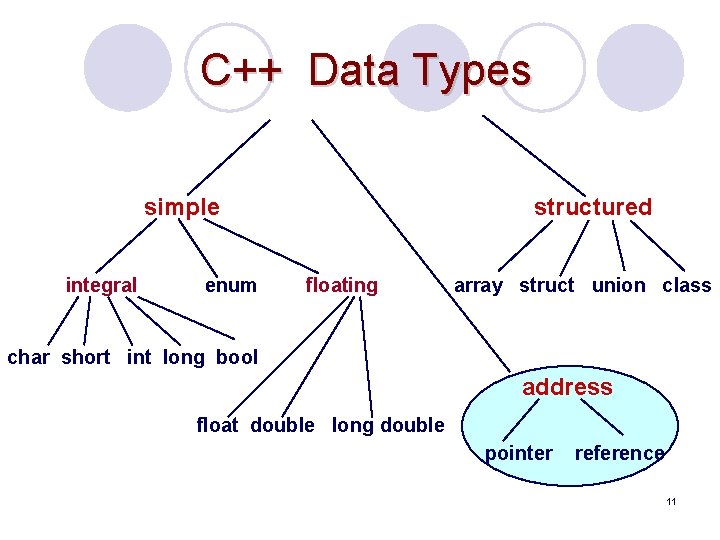
C++ Data Types simple integral enum structured floating array struct union class char short int long bool address float double long double pointer reference 11
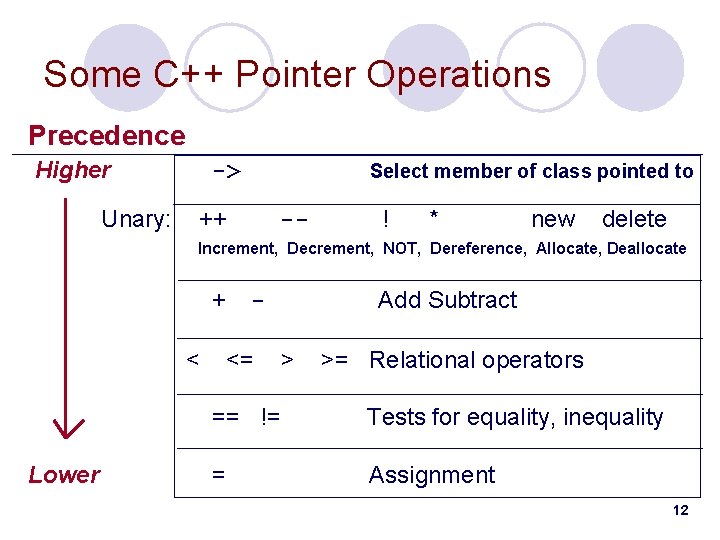
Some C++ Pointer Operations Precedence Higher Unary: -> Select member of class pointed to ++ -- ! * new delete Increment, Decrement, NOT, Dereference, Allocate, Deallocate + < Lower Add Subtract <= > >= Relational operators == != Tests for equality, inequality = Assignment 12
![Operator new Syntax new Data Type Int Expression If memory is available in an Operator new Syntax new Data. Type [Int. Expression] If memory is available, in an](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-13.jpg)
Operator new Syntax new Data. Type [Int. Expression] If memory is available, in an area called the heap (or free store) new allocates the requested object or array, and returns a pointer to (address of ) the memory allocated. Otherwise, program terminates with error message. The dynamically allocated object exists until the delete operator destroys it. 13
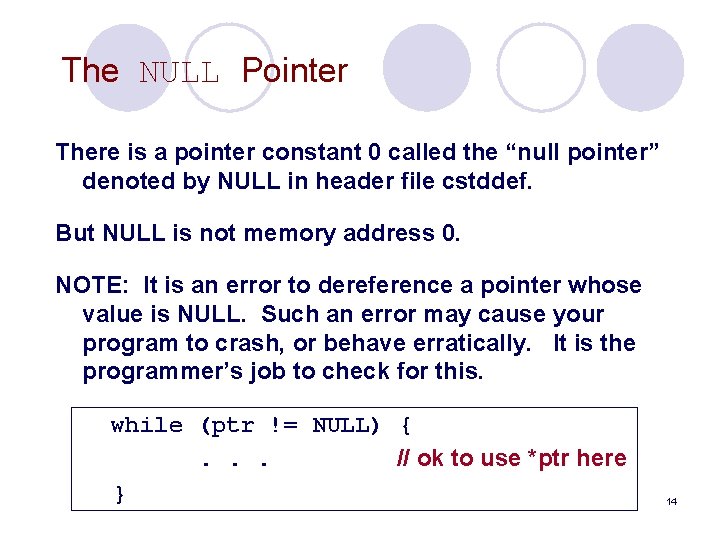
The NULL Pointer There is a pointer constant 0 called the “null pointer” denoted by NULL in header file cstddef. But NULL is not memory address 0. NOTE: It is an error to dereference a pointer whose value is NULL. Such an error may cause your program to crash, or behave erratically. It is the programmer’s job to check for this. while (ptr != NULL) {. . . // ok to use *ptr here } 14
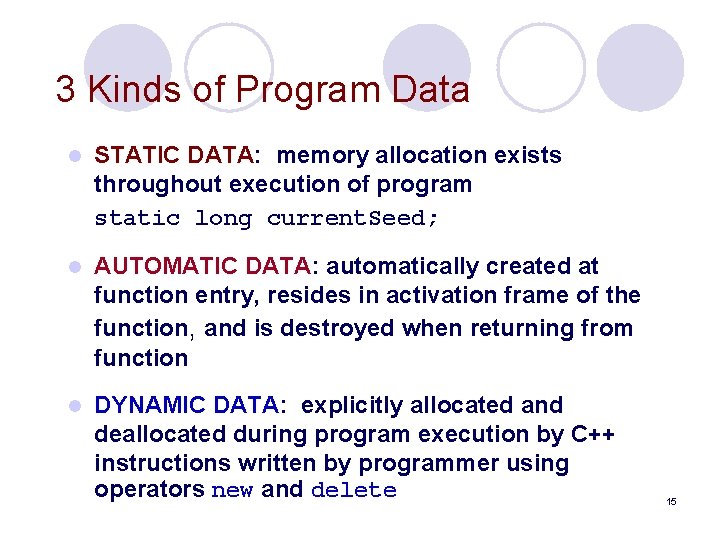
3 Kinds of Program Data l STATIC DATA: memory allocation exists throughout execution of program static long current. Seed; l AUTOMATIC DATA: automatically created at function entry, resides in activation frame of the function, and is destroyed when returning from function l DYNAMIC DATA: explicitly allocated and deallocated during program execution by C++ instructions written by programmer using operators new and delete 15
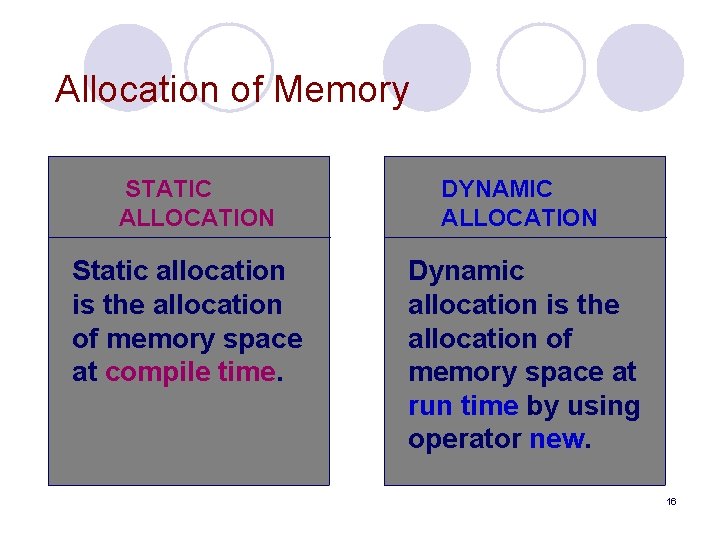
Allocation of Memory STATIC ALLOCATION Static allocation is the allocation of memory space at compile time. DYNAMIC ALLOCATION Dynamic allocation is the allocation of memory space at run time by using operator new. 16
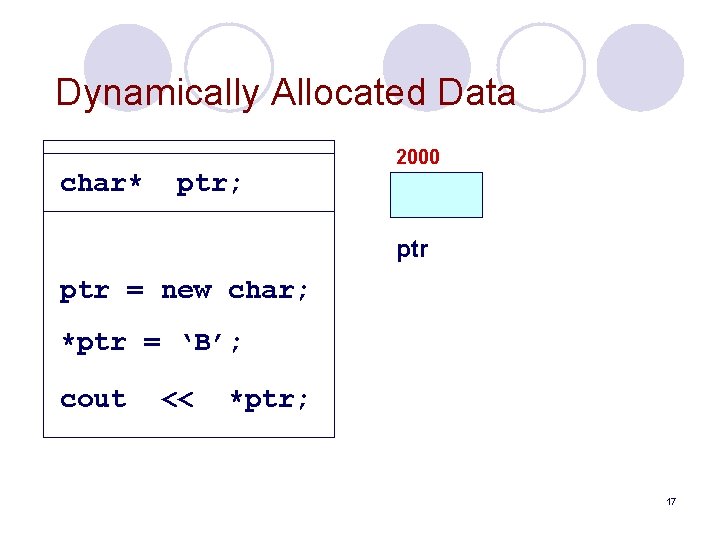
Dynamically Allocated Data char* ptr; 2000 ptr = new char; *ptr = ‘B’; cout << *ptr; 17
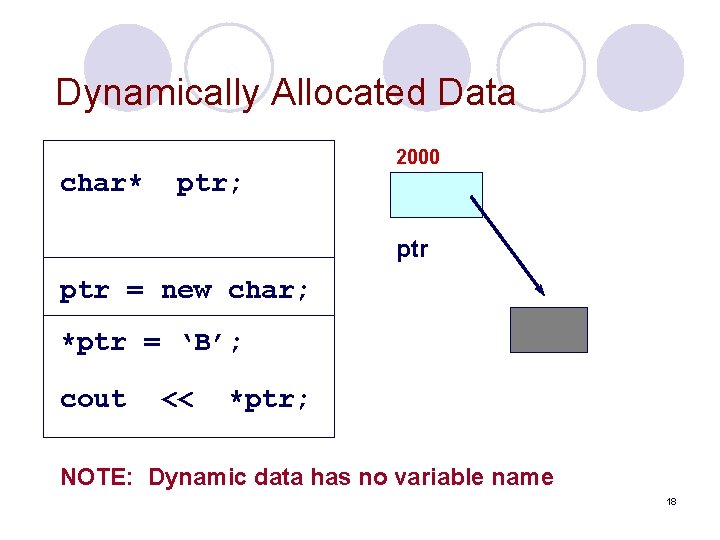
Dynamically Allocated Data char* ptr; 2000 ptr = new char; *ptr = ‘B’; cout << *ptr; NOTE: Dynamic data has no variable name 18
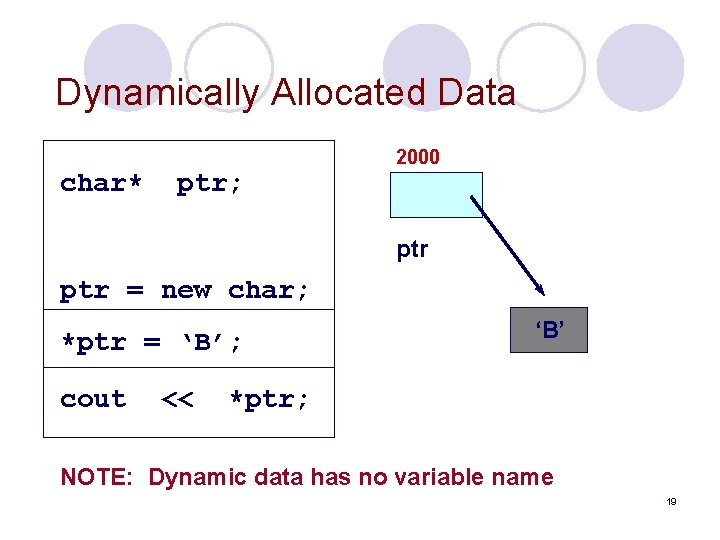
Dynamically Allocated Data char* ptr; 2000 ptr = new char; *ptr = ‘B’; cout << ‘B’ *ptr; NOTE: Dynamic data has no variable name 19
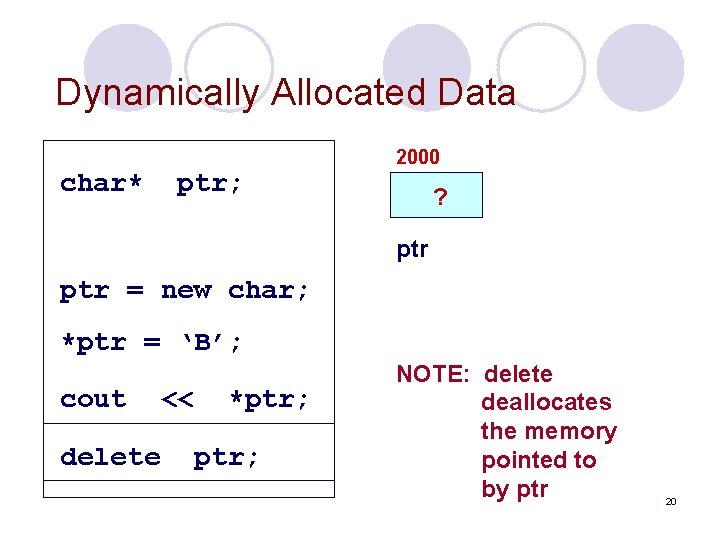
Dynamically Allocated Data char* ptr; 2000 ? ptr = new char; *ptr = ‘B’; cout delete << *ptr; NOTE: delete deallocates the memory pointed to by ptr 20
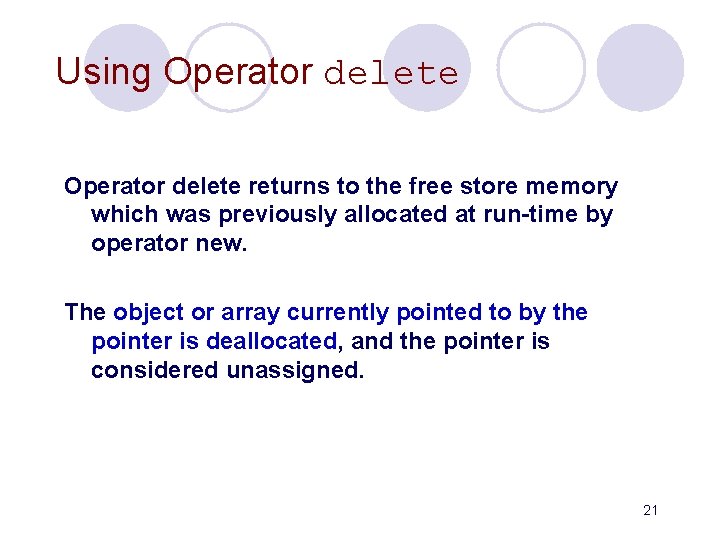
Using Operator delete returns to the free store memory which was previously allocated at run-time by operator new. The object or array currently pointed to by the pointer is deallocated, and the pointer is considered unassigned. 21
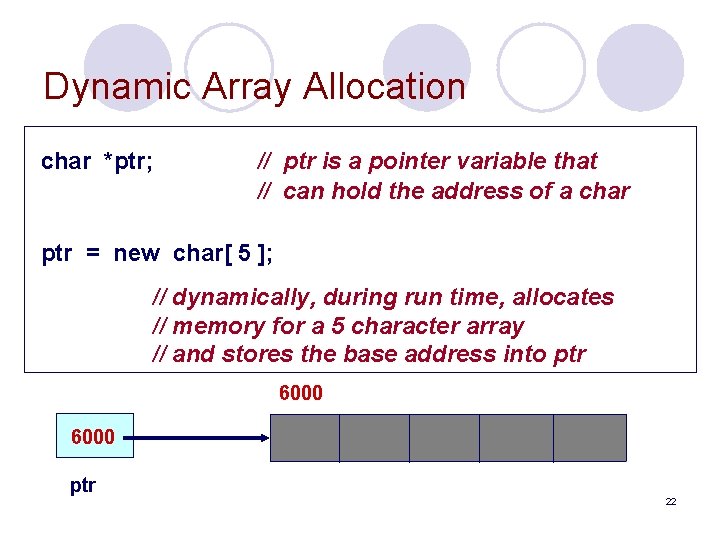
Dynamic Array Allocation char *ptr; // ptr is a pointer variable that // can hold the address of a char ptr = new char[ 5 ]; // dynamically, during run time, allocates // memory for a 5 character array // and stores the base address into ptr 6000 ptr 22
![Dynamic Array Allocation char ptr ptr new char 5 strcpy ptr Dynamic Array Allocation char *ptr ; ptr = new char[ 5 ]; strcpy( ptr,](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-23.jpg)
Dynamic Array Allocation char *ptr ; ptr = new char[ 5 ]; strcpy( ptr, “Bye” ); ptr[ 1 ] = ‘u’; // a pointer can be subscripted cout << ptr[ 2] ; 6000 ‘B’ ‘u’ ‘y’ ‘e’ ‘ ’ ptr 23
![Operator delete Syntax delete Pointer delete Pointer If the value of the Operator delete Syntax delete Pointer delete [ ] Pointer If the value of the](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-24.jpg)
Operator delete Syntax delete Pointer delete [ ] Pointer If the value of the pointer is 0 there is no effect. Otherwise, the object or array currently pointed to by Pointer is deallocated, and the value of Pointer is undefined. The memory is returned to the free store. Square brackets are used with delete to deallocate a dynamically allocated array. 24
![Dynamic Array Deallocation char ptr ptr new char 5 strcpy ptr Dynamic Array Deallocation char *ptr ; ptr = new char[ 5 ]; strcpy( ptr,](https://slidetodoc.com/presentation_image/0663b0850b43aab740293ab2a5905989/image-25.jpg)
Dynamic Array Deallocation char *ptr ; ptr = new char[ 5 ]; strcpy( ptr, “Bye” ); ptr[ 1 ] = ‘u’; delete ptr; // deallocates array pointed to by ptr // ptr itself is not deallocated // the value of ptr is undefined. ? ptr 25
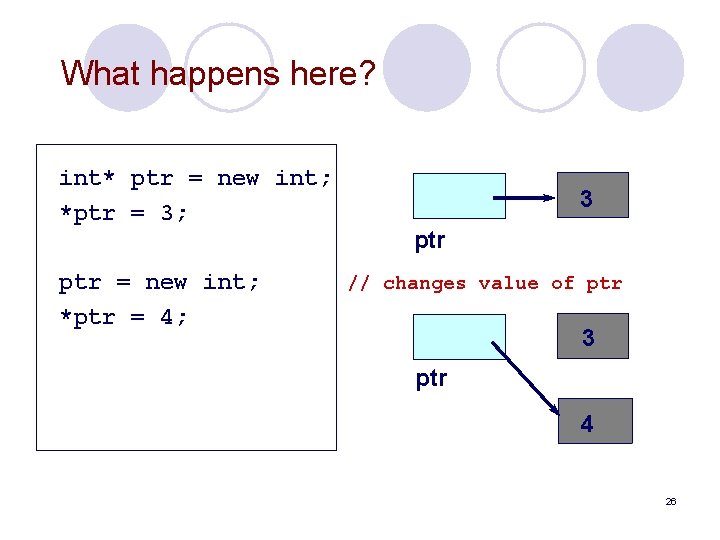
What happens here? int* ptr = new int; *ptr = 3; ptr = new int; *ptr = 4; 3 ptr // changes value of ptr 3 ptr 4 26
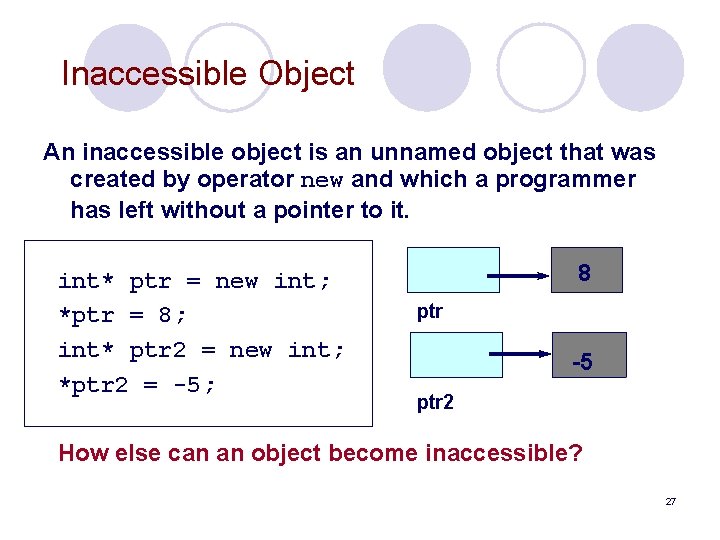
Inaccessible Object An inaccessible object is an unnamed object that was created by operator new and which a programmer has left without a pointer to it. int* ptr = new int; *ptr = 8; int* ptr 2 = new int; *ptr 2 = -5; 8 ptr -5 ptr 2 How else can an object become inaccessible? 27
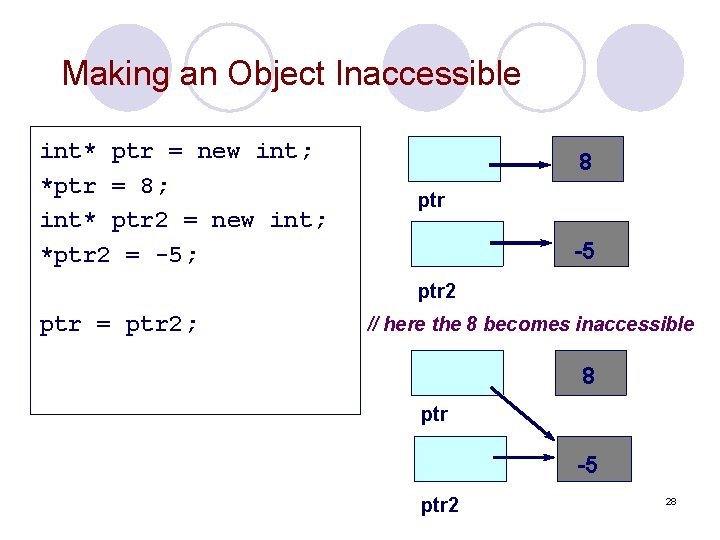
Making an Object Inaccessible int* ptr = new int; *ptr = 8; int* ptr 2 = new int; *ptr 2 = -5; 8 ptr -5 ptr 2 ptr = ptr 2; // here the 8 becomes inaccessible 8 ptr -5 ptr 2 28
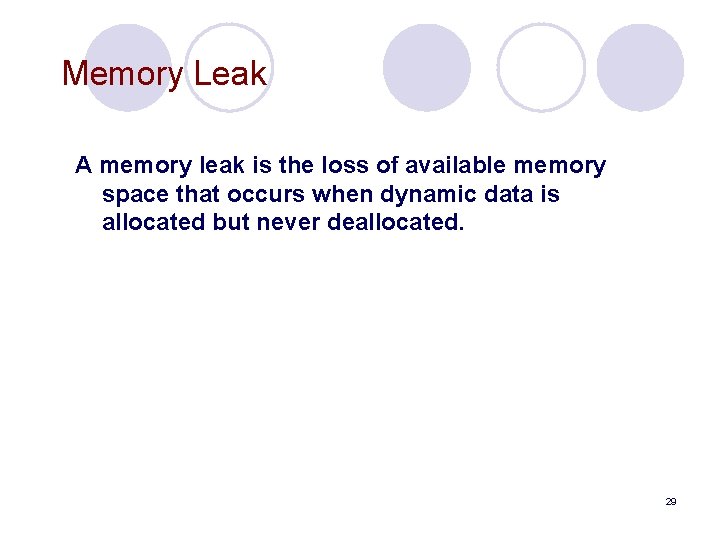
Memory Leak A memory leak is the loss of available memory space that occurs when dynamic data is allocated but never deallocated. 29
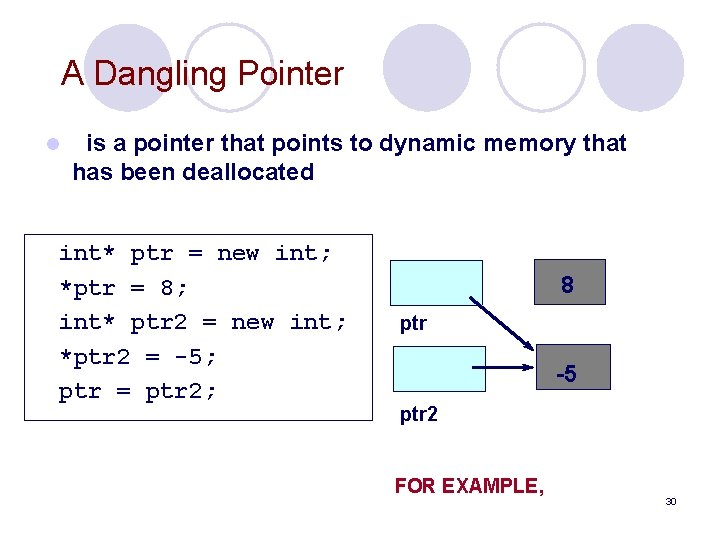
A Dangling Pointer l is a pointer that points to dynamic memory that has been deallocated int* ptr = new int; *ptr = 8; int* ptr 2 = new int; *ptr 2 = -5; ptr = ptr 2; 8 ptr -5 ptr 2 FOR EXAMPLE, 30
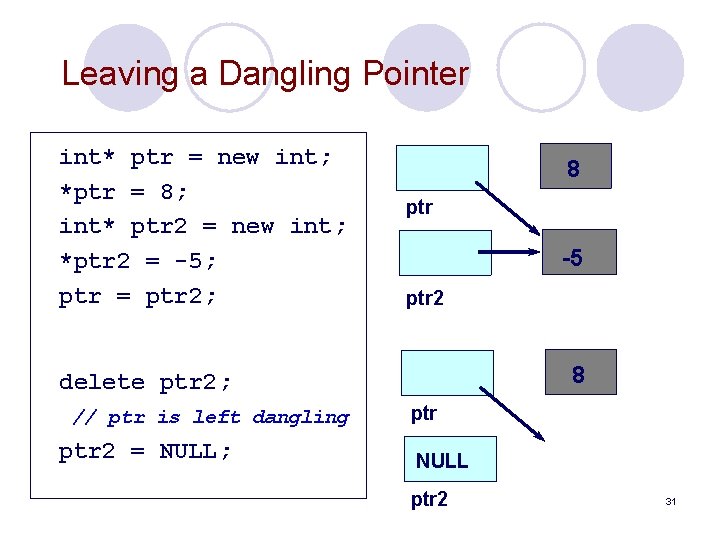
Leaving a Dangling Pointer int* ptr = new int; *ptr = 8; int* ptr 2 = new int; *ptr 2 = -5; ptr = ptr 2; 8 ptr -5 ptr 2 8 delete ptr 2; // ptr is left dangling ptr 2 = NULL; ptr NULL ptr 2 31
Str
Char str
Char res
Const int size=18; string *tbl2 = new string[size];
Auto break case char
Thơ thất ngôn tứ tuyệt đường luật
Tôn thất thuyết là ai
Phân độ lown
Chiến lược kinh doanh quốc tế của walmart
Gây tê cơ vuông thắt lưng
Block nhĩ thất độ 1
Tìm độ lớn thật của tam giác abc
Sau thất bại ở hồ điển triệt
Thơ thất ngôn tứ tuyệt đường luật
Hãy nói thật ít để làm được nhiều
Công của trọng lực
Tỉ lệ cơ thể trẻ em
Thiếu nhi thế giới liên hoan
Tia chieu sa te
Phản ứng thế ankan
Một số thể thơ truyền thống
Môn thể thao bắt đầu bằng từ đua
Hình ảnh bộ gõ cơ thể búng tay
Hệ hô hấp
Tư thế ngồi viết
Số nguyên tố là
đặc điểm cơ thể của người tối cổ
Trời xanh đây là của chúng ta thể thơ
Gấu đi như thế nào
ưu thế lai là gì
Thẻ vin
Cái miệng nó xinh thế chỉ nói điều hay thôi