The character data type char Character type char
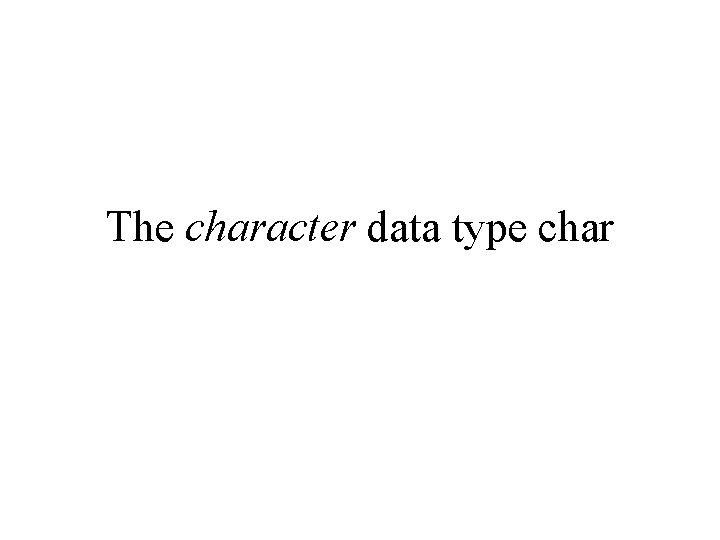
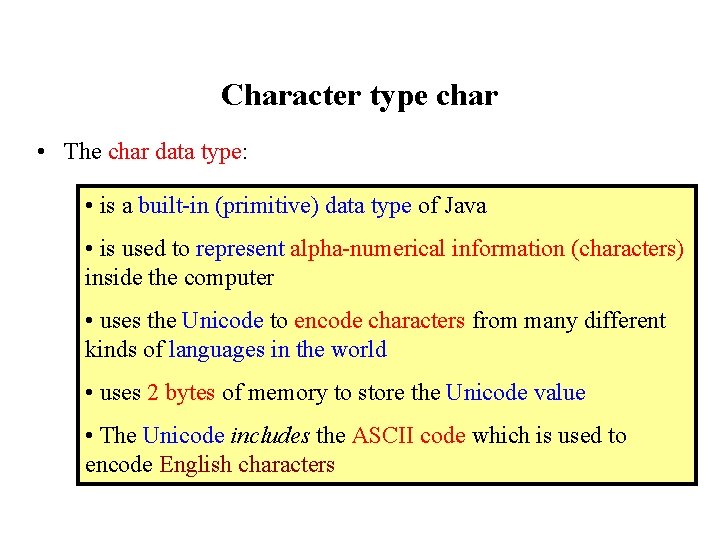
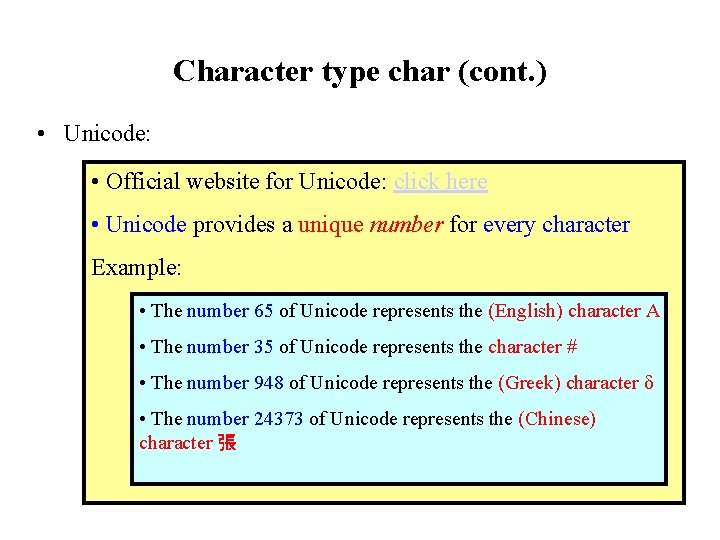
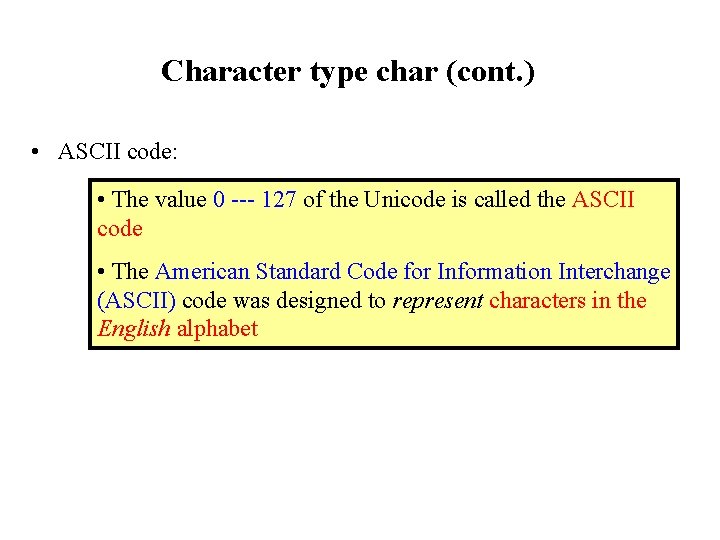
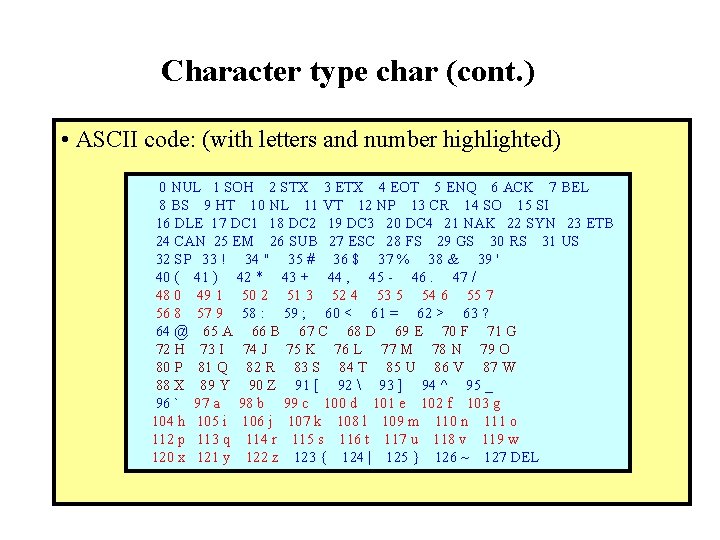
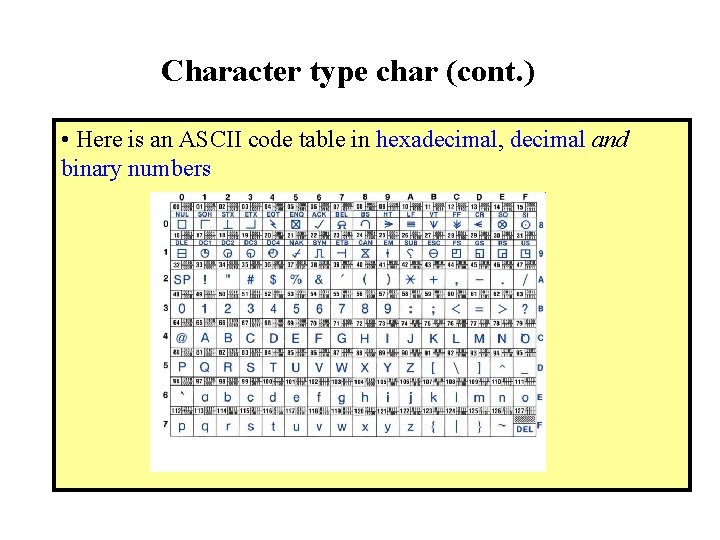
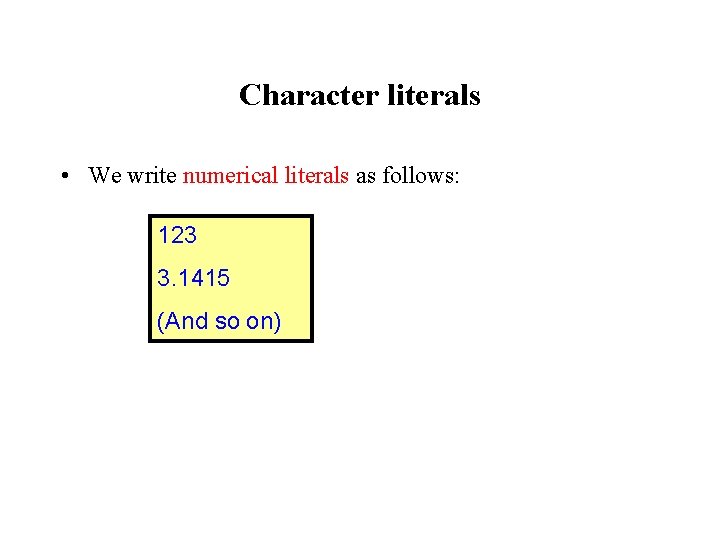
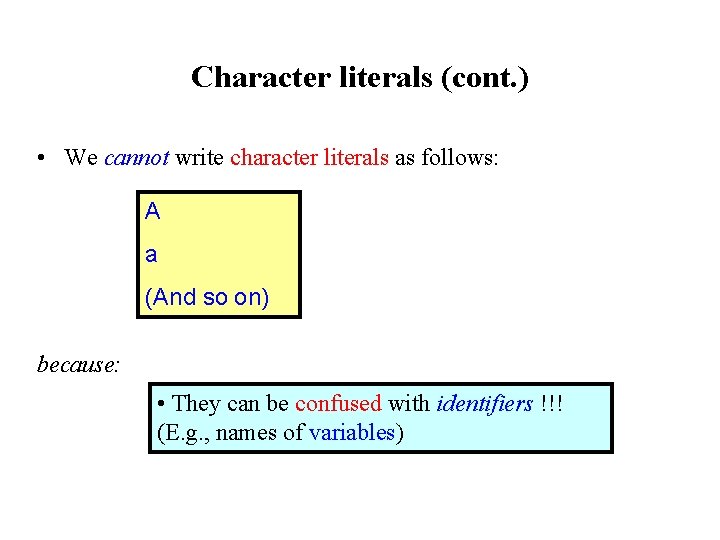
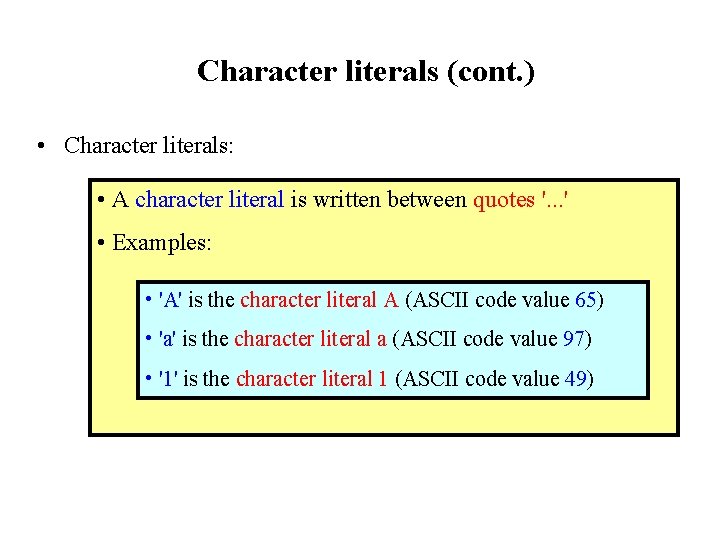
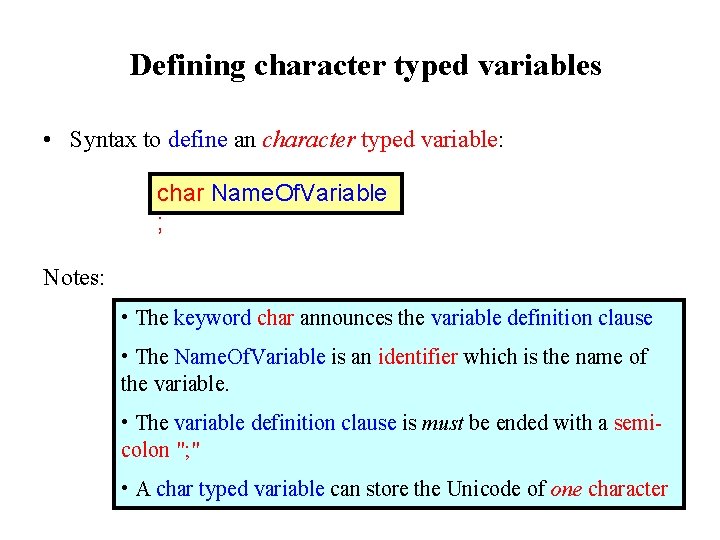
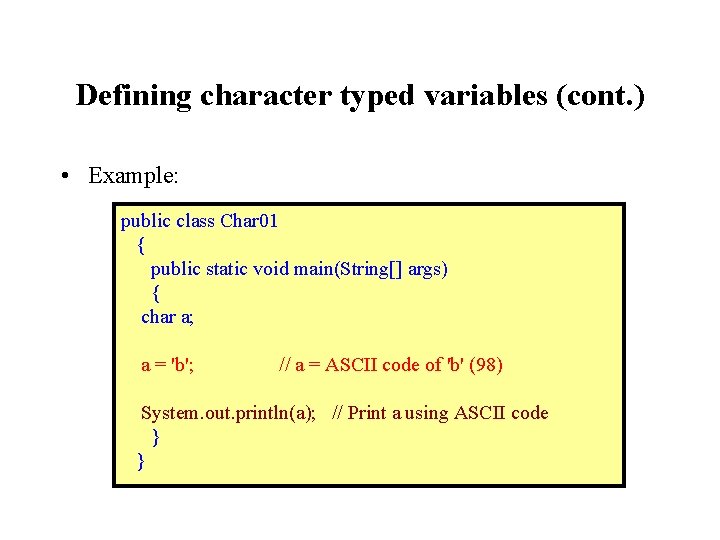
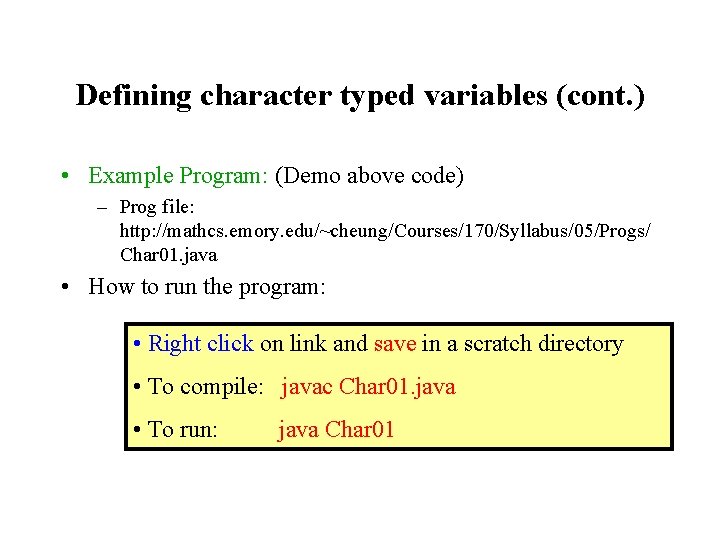
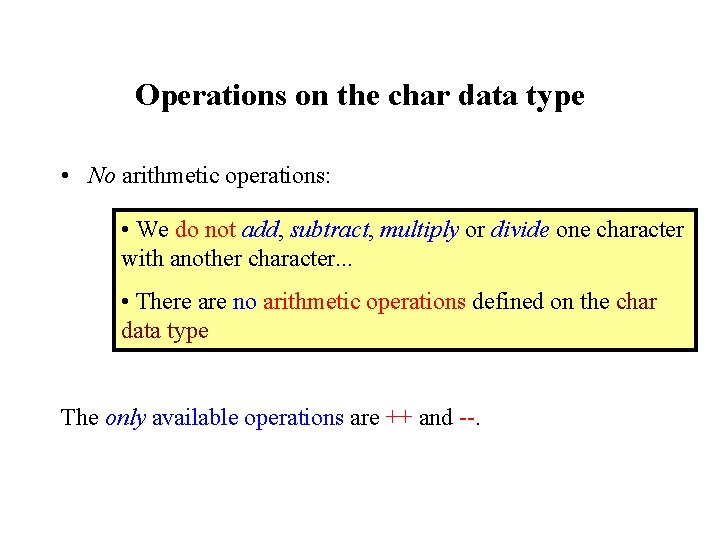
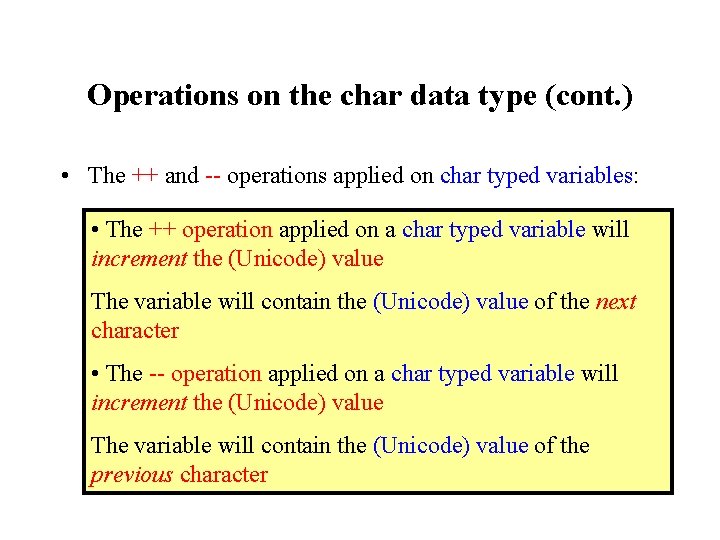
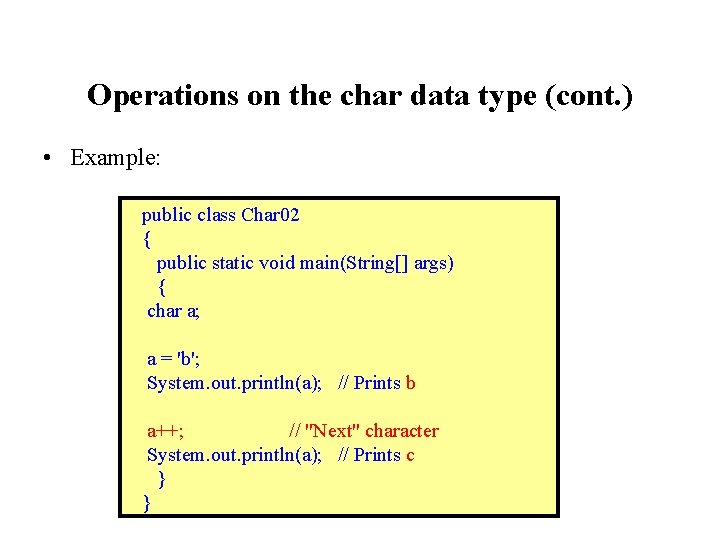
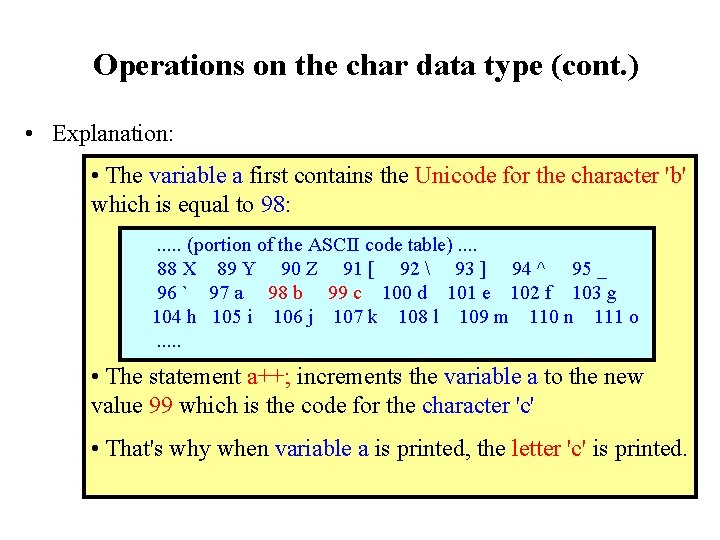
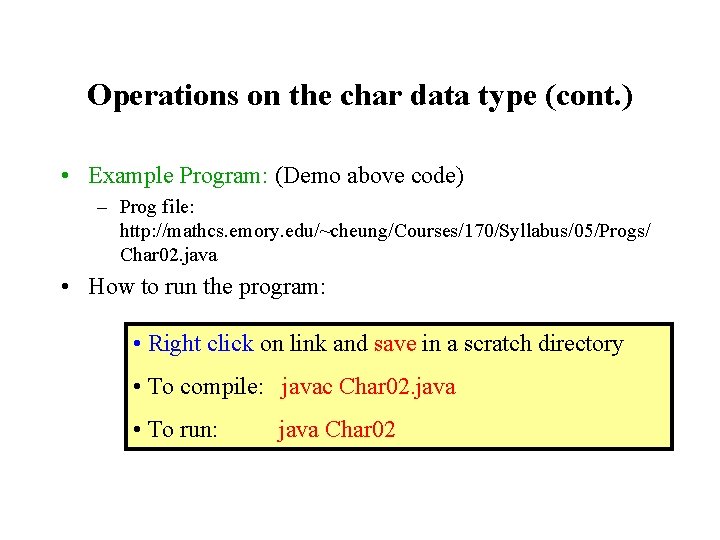
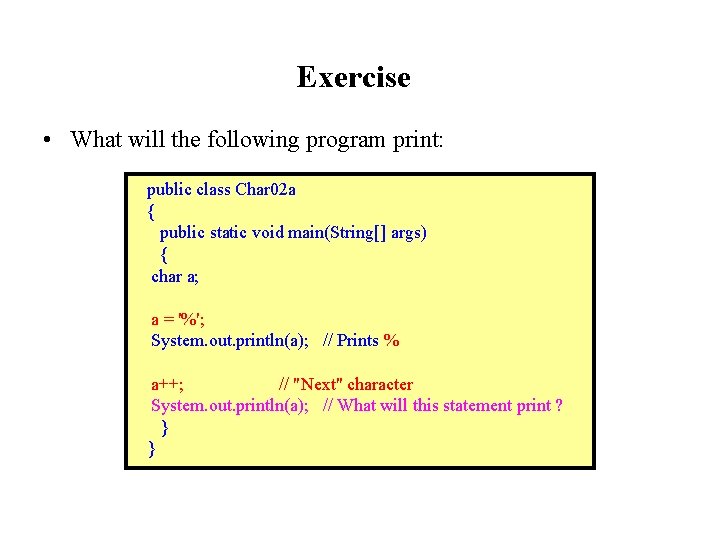
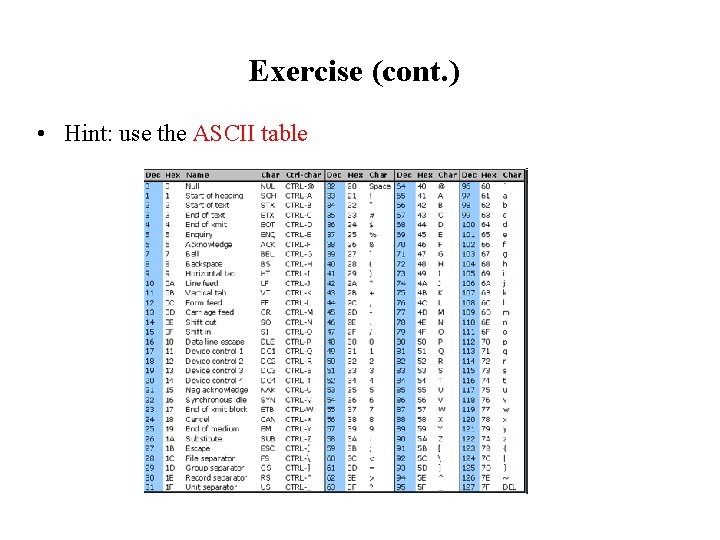
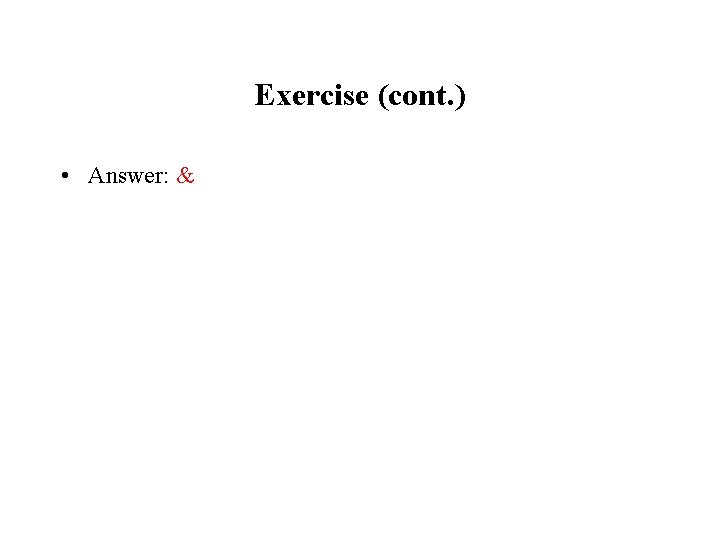
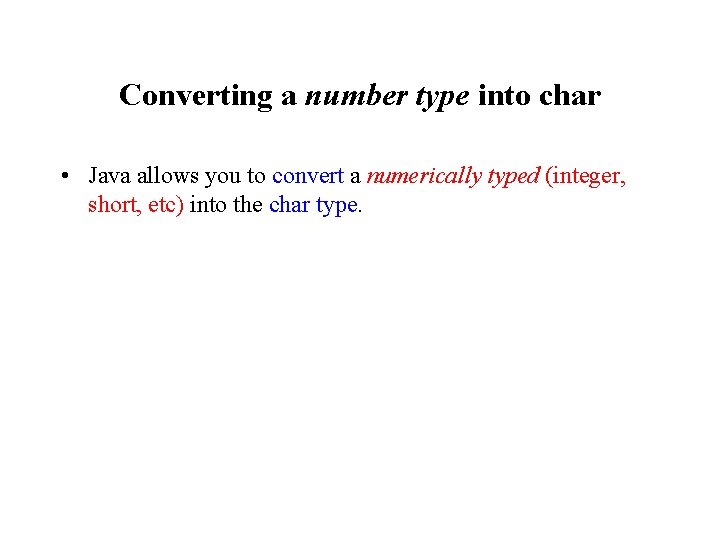
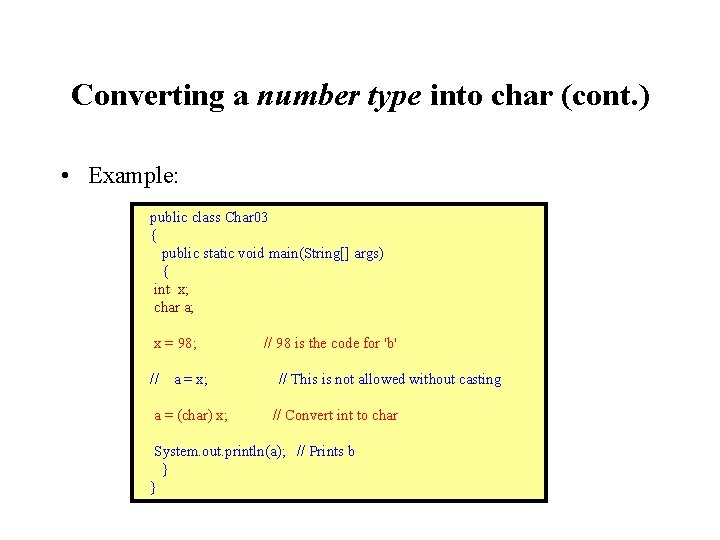
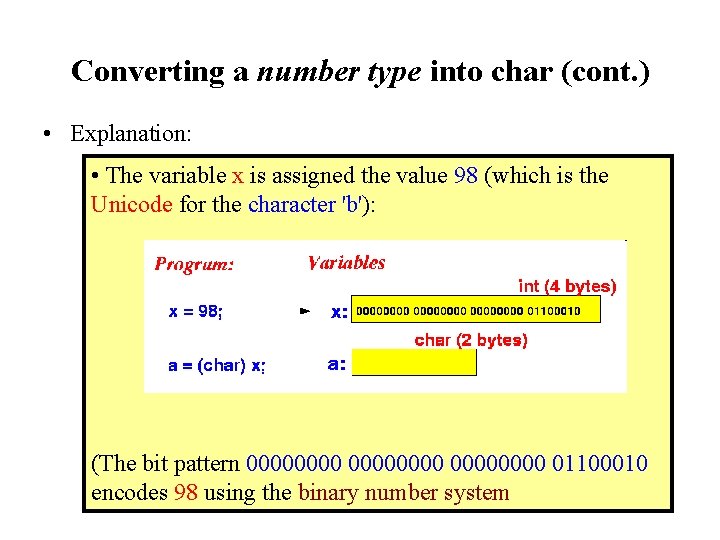
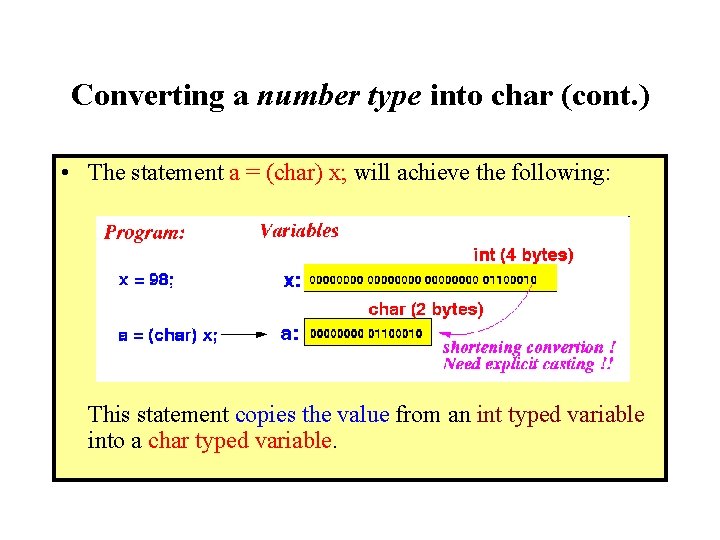
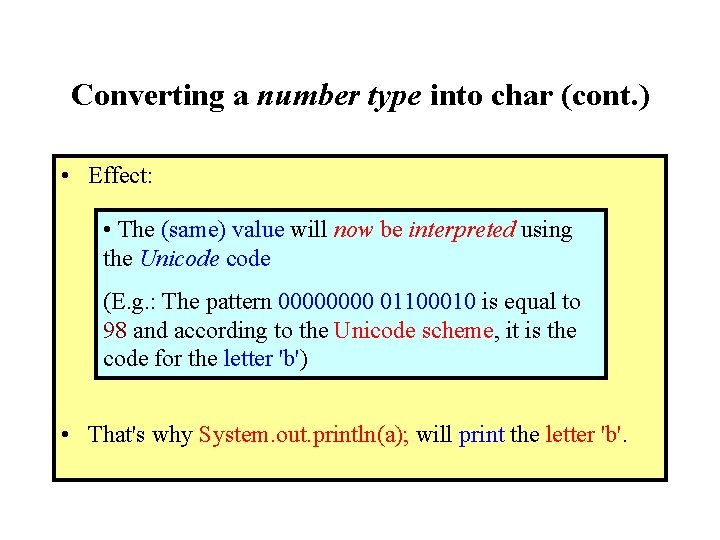
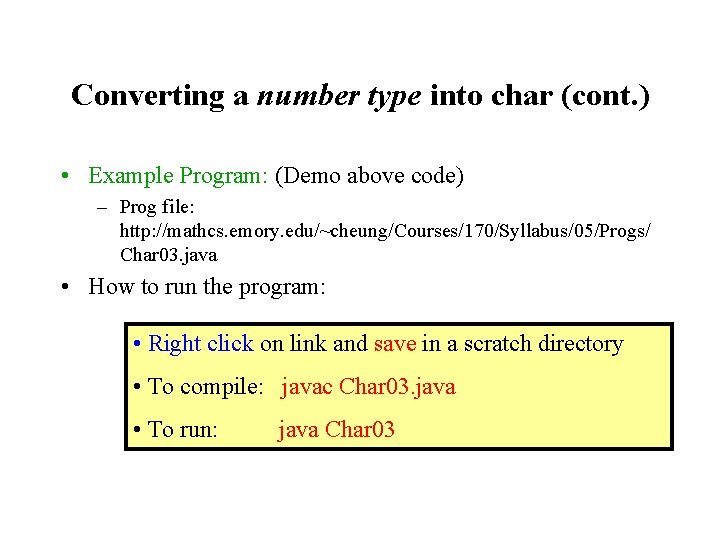
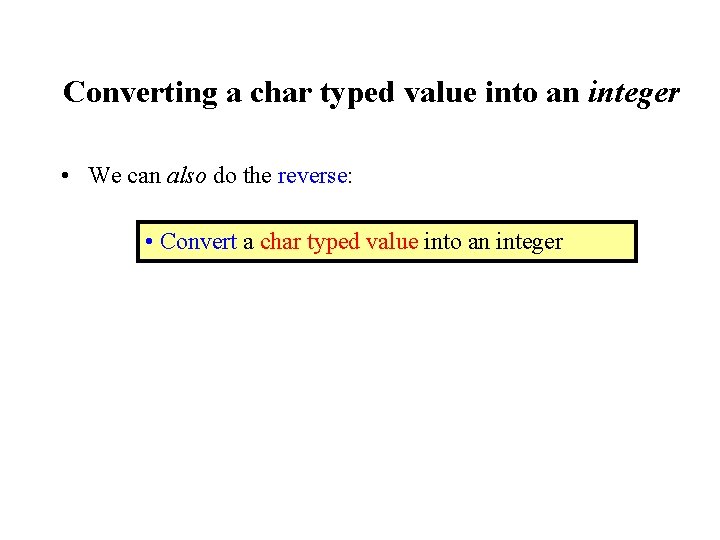
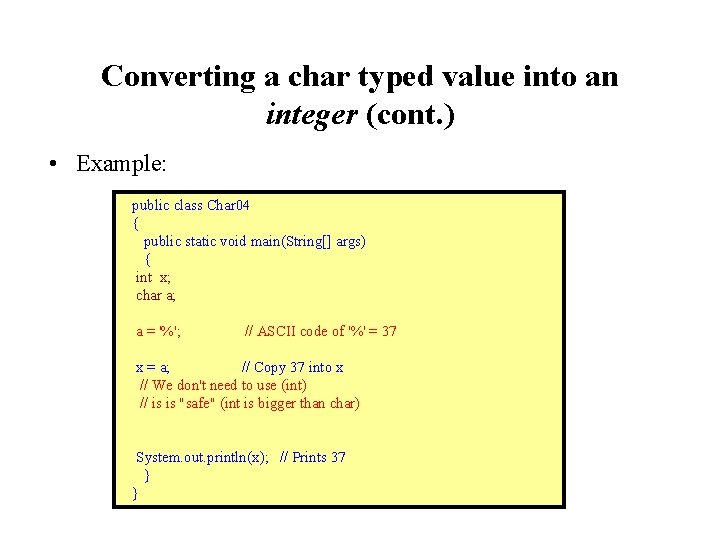
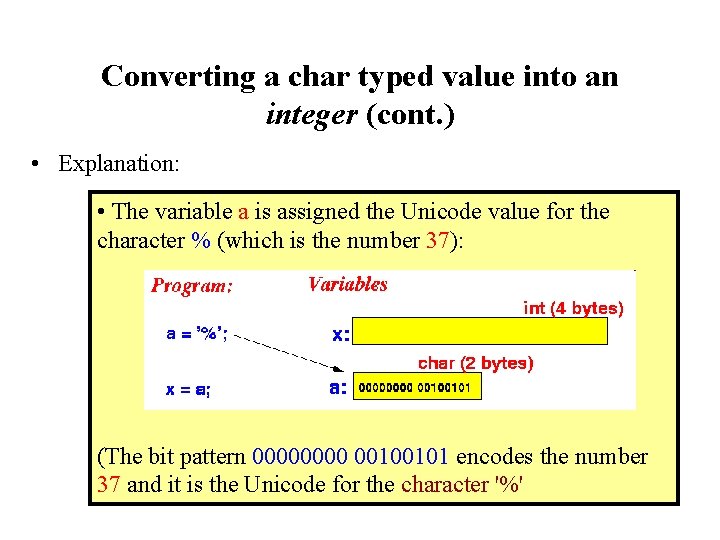
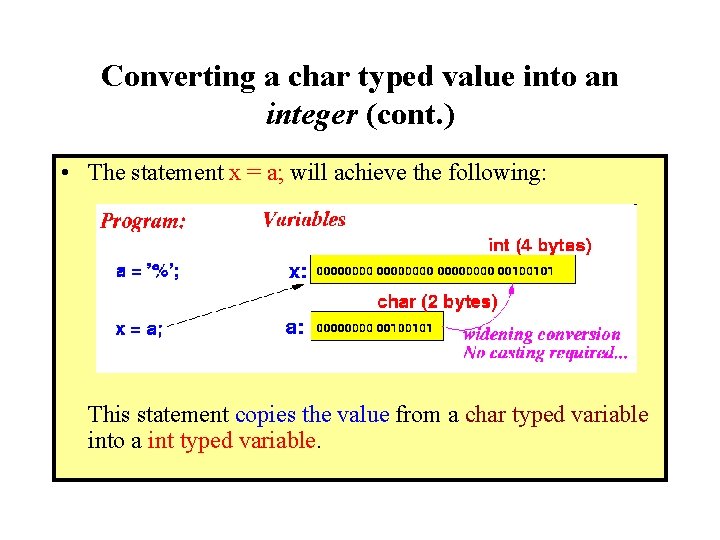
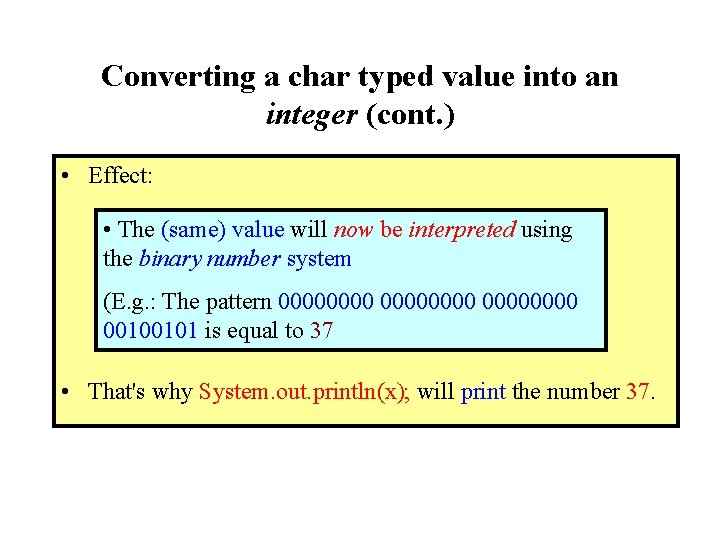
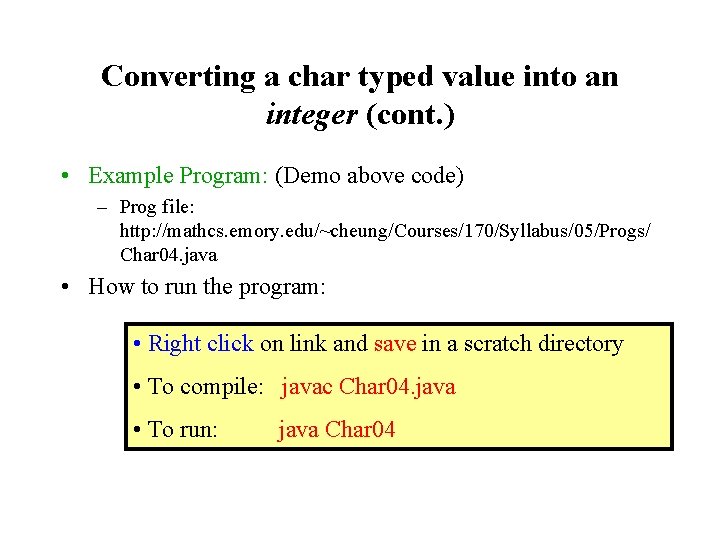
- Slides: 32
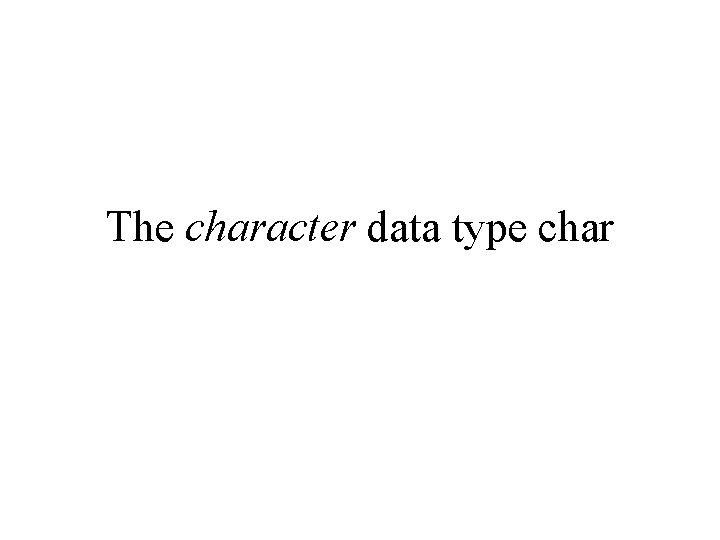
The character data type char
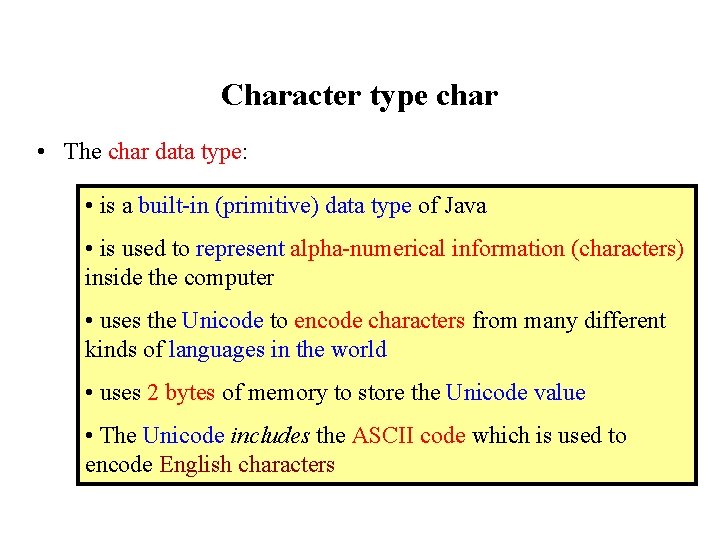
Character type char • The char data type: • is a built-in (primitive) data type of Java • is used to represent alpha-numerical information (characters) inside the computer • uses the Unicode to encode characters from many different kinds of languages in the world • uses 2 bytes of memory to store the Unicode value • The Unicode includes the ASCII code which is used to encode English characters
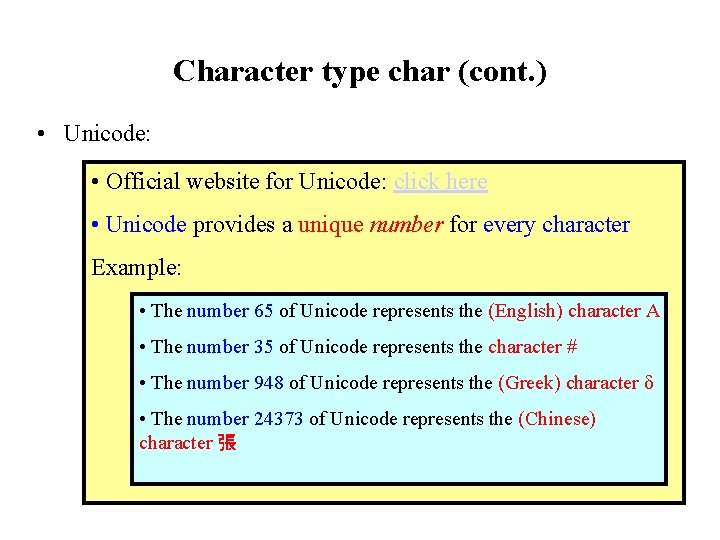
Character type char (cont. ) • Unicode: • Official website for Unicode: click here • Unicode provides a unique number for every character Example: • The number 65 of Unicode represents the (English) character A • The number 35 of Unicode represents the character # • The number 948 of Unicode represents the (Greek) character δ • The number 24373 of Unicode represents the (Chinese) character 張
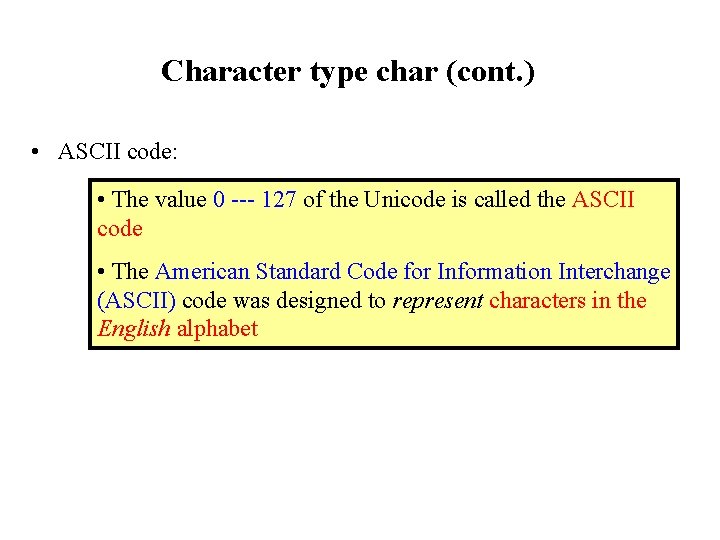
Character type char (cont. ) • ASCII code: • The value 0 --- 127 of the Unicode is called the ASCII code • The American Standard Code for Information Interchange (ASCII) code was designed to represent characters in the English alphabet
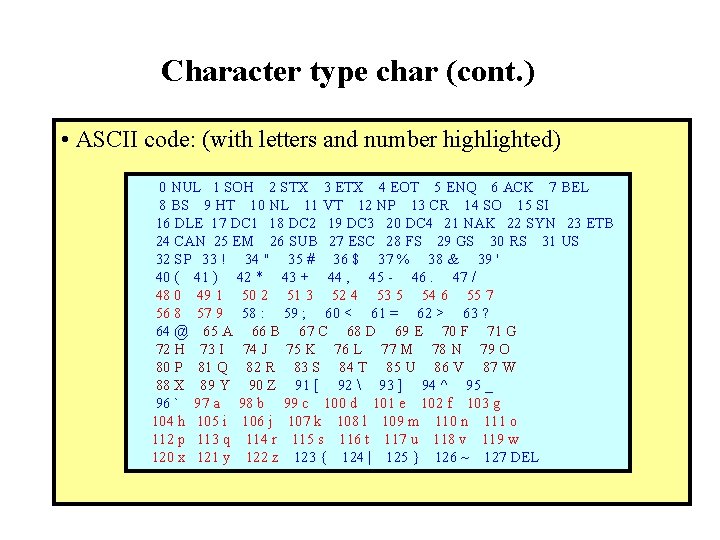
Character type char (cont. ) • ASCII code: (with letters and number highlighted) 0 NUL 1 SOH 2 STX 3 ETX 4 EOT 5 ENQ 6 ACK 7 BEL 8 BS 9 HT 10 NL 11 VT 12 NP 13 CR 14 SO 15 SI 16 DLE 17 DC 1 18 DC 2 19 DC 3 20 DC 4 21 NAK 22 SYN 23 ETB 24 CAN 25 EM 26 SUB 27 ESC 28 FS 29 GS 30 RS 31 US 32 SP 33 ! 34 " 35 # 36 $ 37 % 38 & 39 ' 40 ( 41 ) 42 * 43 + 44 , 45 - 46. 47 / 48 0 49 1 50 2 51 3 52 4 53 5 54 6 55 7 56 8 57 9 58 : 59 ; 60 < 61 = 62 > 63 ? 64 @ 65 A 66 B 67 C 68 D 69 E 70 F 71 G 72 H 73 I 74 J 75 K 76 L 77 M 78 N 79 O 80 P 81 Q 82 R 83 S 84 T 85 U 86 V 87 W 88 X 89 Y 90 Z 91 [ 92 93 ] 94 ^ 95 _ 96 ` 97 a 98 b 99 c 100 d 101 e 102 f 103 g 104 h 105 i 106 j 107 k 108 l 109 m 110 n 111 o 112 p 113 q 114 r 115 s 116 t 117 u 118 v 119 w 120 x 121 y 122 z 123 { 124 | 125 } 126 ~ 127 DEL
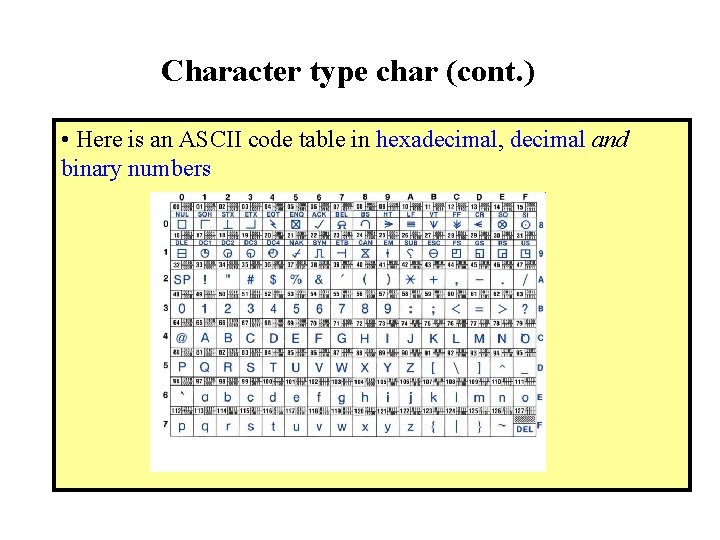
Character type char (cont. ) • Here is an ASCII code table in hexadecimal, decimal and binary numbers
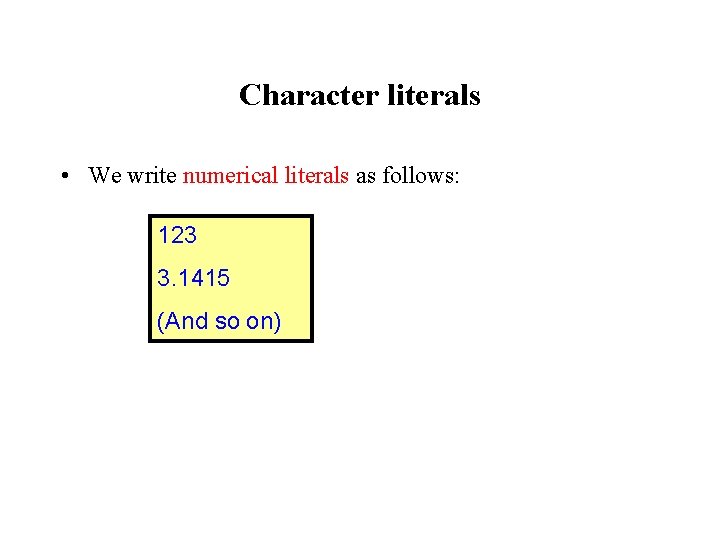
Character literals • We write numerical literals as follows: 123 3. 1415 (And so on)
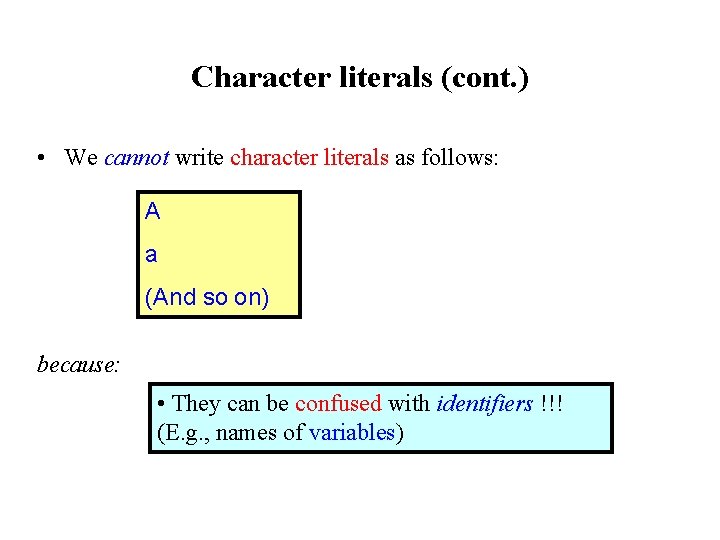
Character literals (cont. ) • We cannot write character literals as follows: A a (And so on) because: • They can be confused with identifiers !!! (E. g. , names of variables)
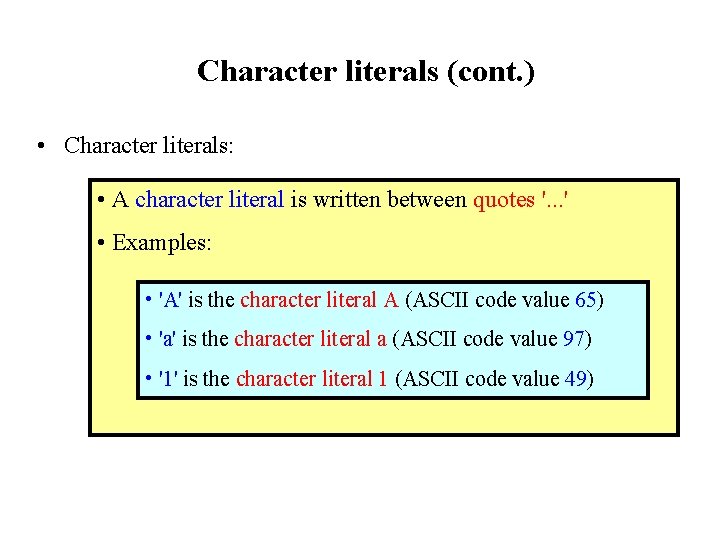
Character literals (cont. ) • Character literals: • A character literal is written between quotes '. . . ' • Examples: • 'A' is the character literal A (ASCII code value 65) • 'a' is the character literal a (ASCII code value 97) • '1' is the character literal 1 (ASCII code value 49)
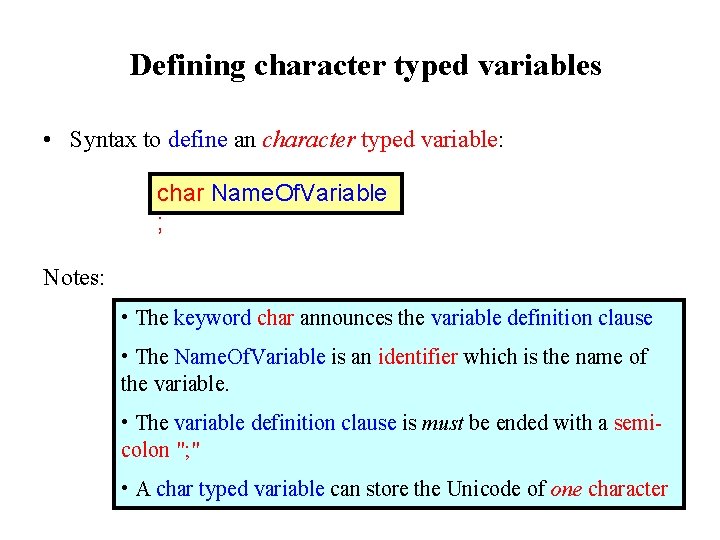
Defining character typed variables • Syntax to define an character typed variable: char Name. Of. Variable ; Notes: • The keyword char announces the variable definition clause • The Name. Of. Variable is an identifier which is the name of the variable. • The variable definition clause is must be ended with a semicolon "; " • A char typed variable can store the Unicode of one character
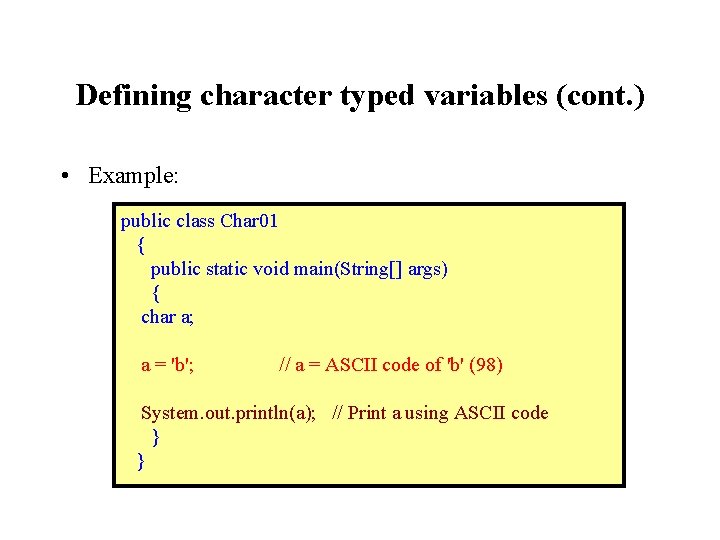
Defining character typed variables (cont. ) • Example: public class Char 01 { public static void main(String[] args) { char a; a = 'b'; // a = ASCII code of 'b' (98) System. out. println(a); // Print a using ASCII code } }
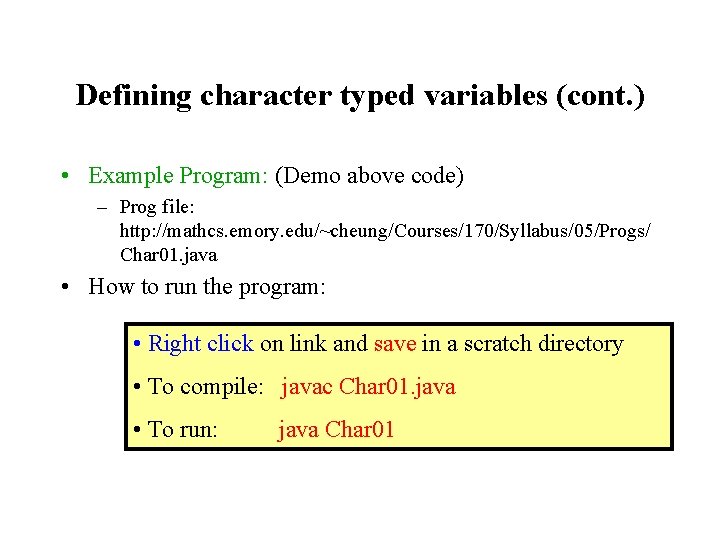
Defining character typed variables (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/05/Progs/ Char 01. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Char 01. java • To run: java Char 01
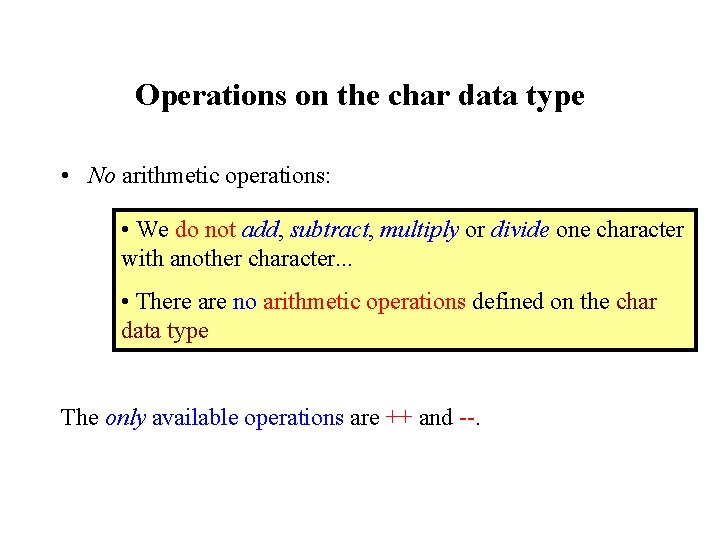
Operations on the char data type • No arithmetic operations: • We do not add, subtract, multiply or divide one character with another character. . . • There are no arithmetic operations defined on the char data type The only available operations are ++ and --.
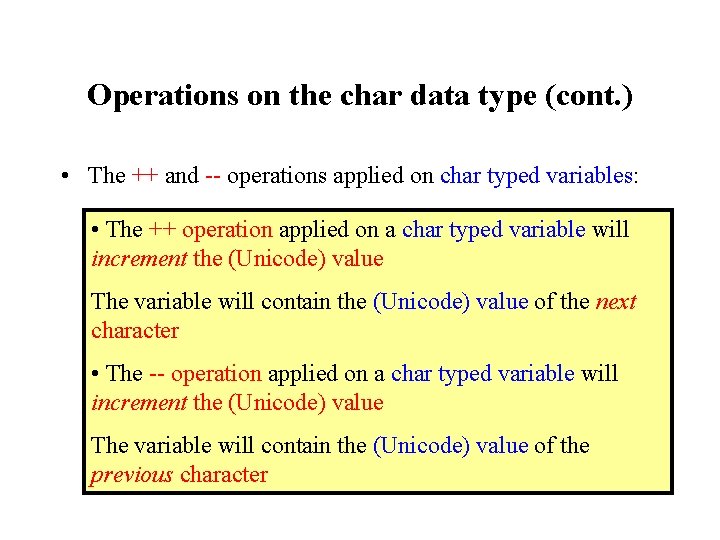
Operations on the char data type (cont. ) • The ++ and -- operations applied on char typed variables: • The ++ operation applied on a char typed variable will increment the (Unicode) value The variable will contain the (Unicode) value of the next character • The -- operation applied on a char typed variable will increment the (Unicode) value The variable will contain the (Unicode) value of the previous character
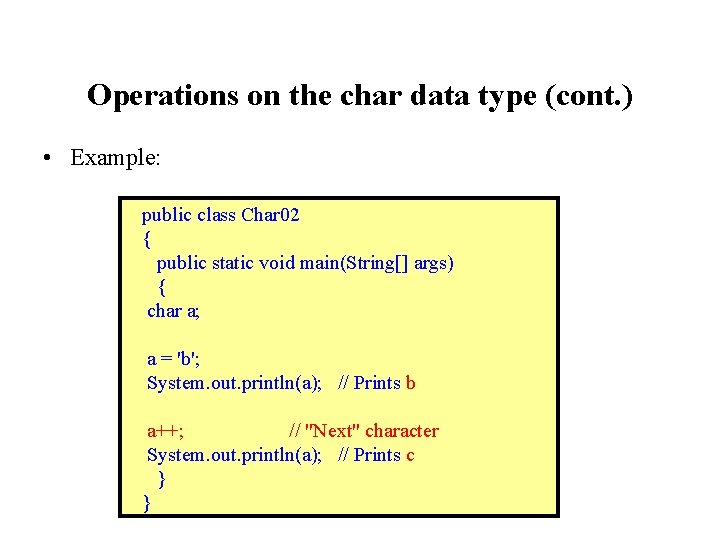
Operations on the char data type (cont. ) • Example: public class Char 02 { public static void main(String[] args) { char a; a = 'b'; System. out. println(a); // Prints b a++; // "Next" character System. out. println(a); // Prints c } }
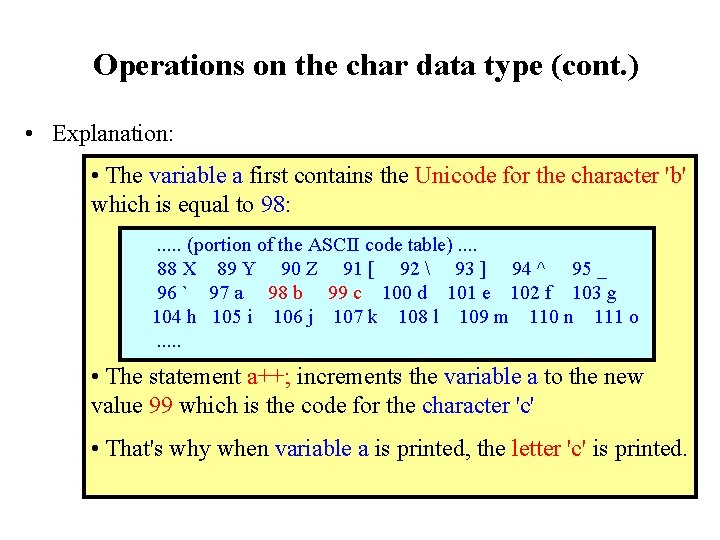
Operations on the char data type (cont. ) • Explanation: • The variable a first contains the Unicode for the character 'b' which is equal to 98: . . . (portion of the ASCII code table). . 88 X 89 Y 90 Z 91 [ 92 93 ] 94 ^ 95 _ 96 ` 97 a 98 b 99 c 100 d 101 e 102 f 103 g 104 h 105 i 106 j 107 k 108 l 109 m 110 n 111 o . . . • The statement a++; increments the variable a to the new value 99 which is the code for the character 'c' • That's why when variable a is printed, the letter 'c' is printed.
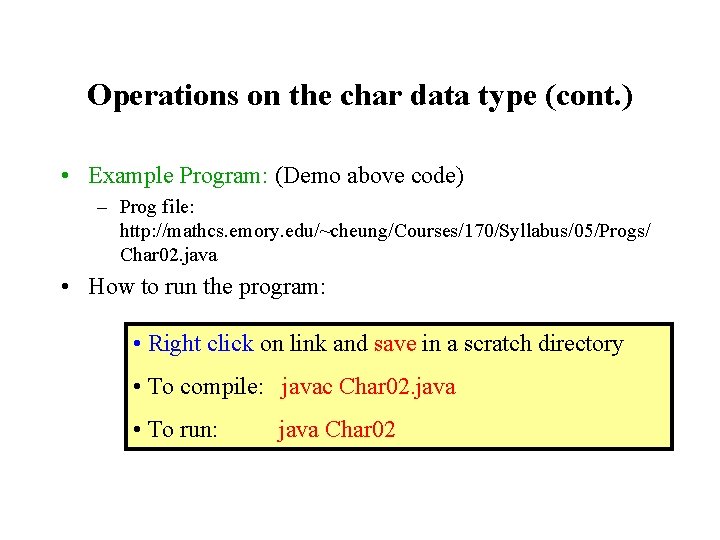
Operations on the char data type (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/05/Progs/ Char 02. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Char 02. java • To run: java Char 02
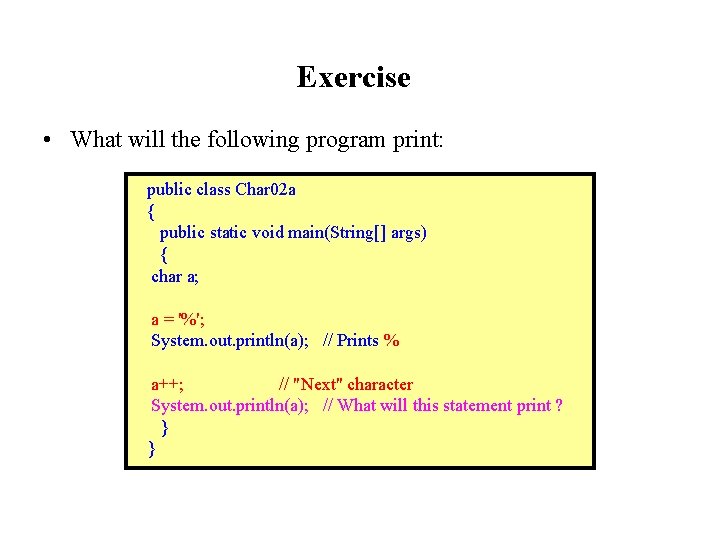
Exercise • What will the following program print: public class Char 02 a { public static void main(String[] args) { char a; a = '%'; System. out. println(a); // Prints % a++; // "Next" character System. out. println(a); // What will this statement print ? } }
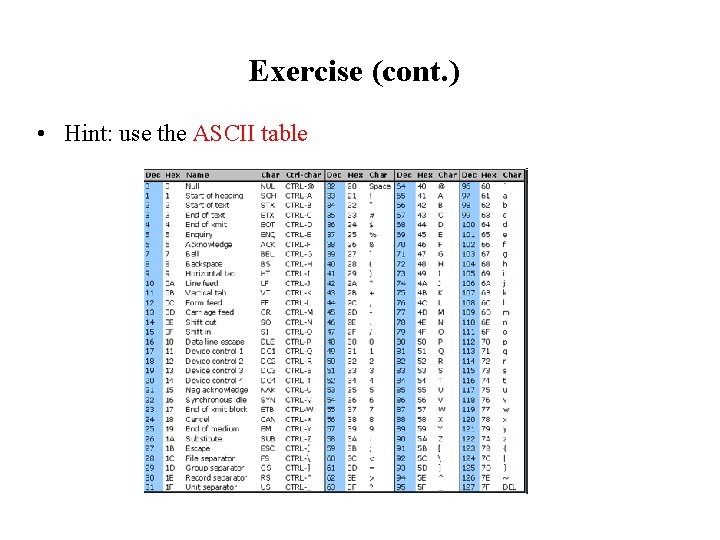
Exercise (cont. ) • Hint: use the ASCII table
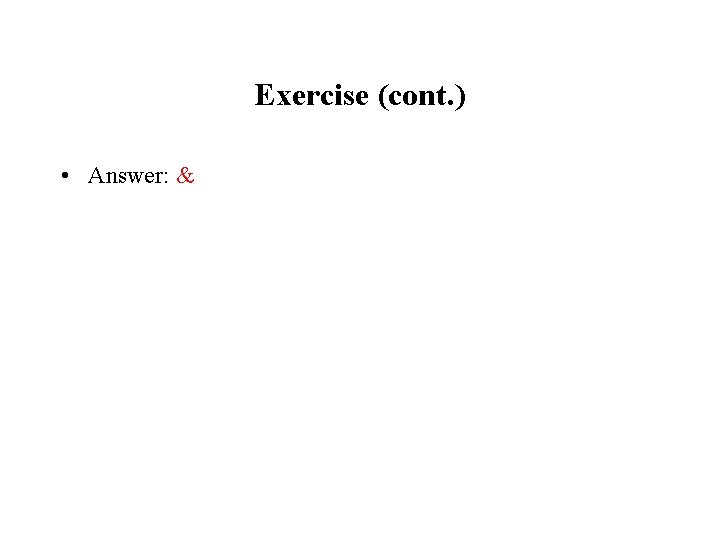
Exercise (cont. ) • Answer: &
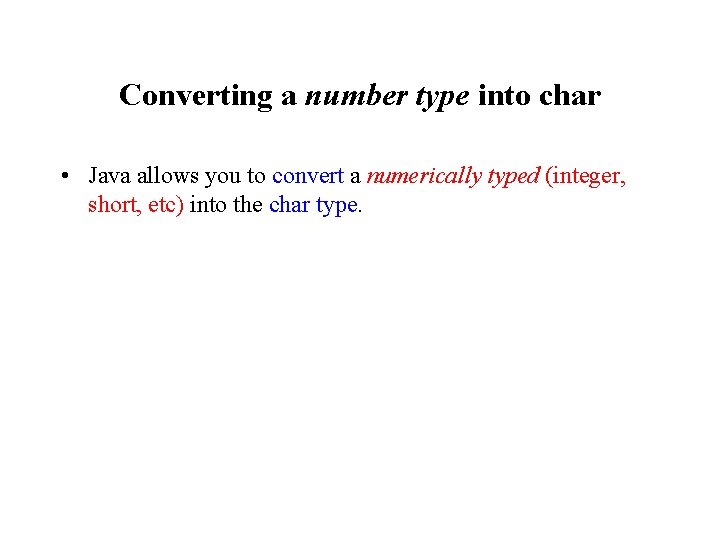
Converting a number type into char • Java allows you to convert a numerically typed (integer, short, etc) into the char type.
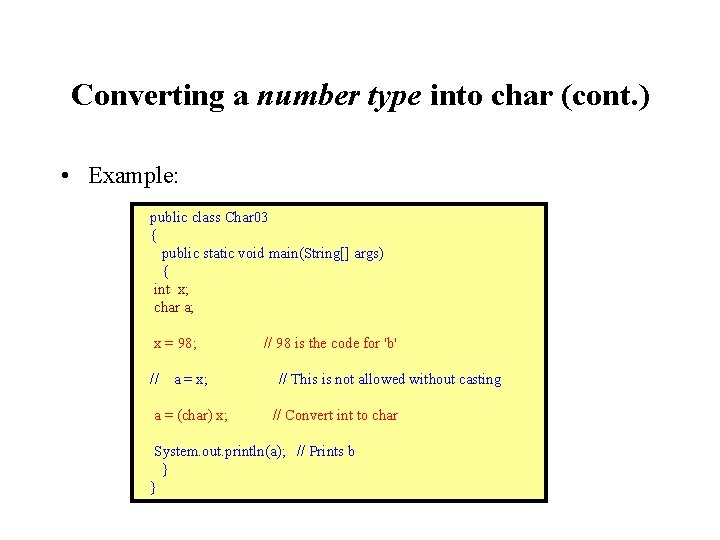
Converting a number type into char (cont. ) • Example: public class Char 03 { public static void main(String[] args) { int x; char a; x = 98; // 98 is the code for 'b' // a = x; // This is not allowed without casting a = (char) x; // Convert int to char System. out. println(a); // Prints b } }
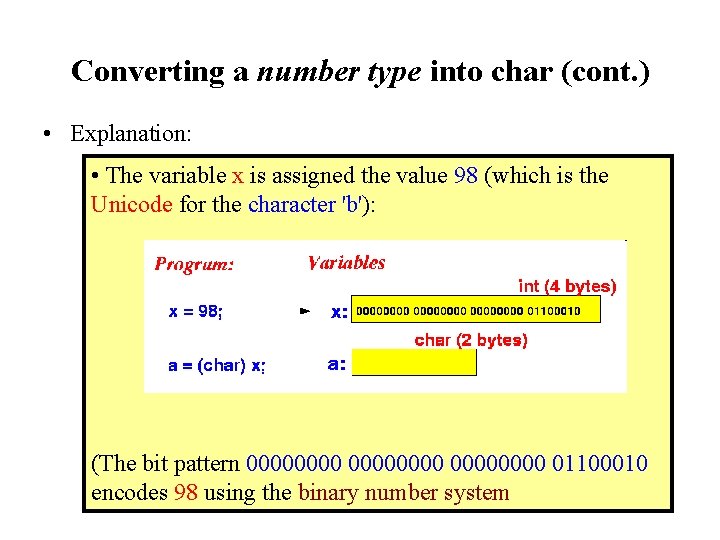
Converting a number type into char (cont. ) • Explanation: • The variable x is assigned the value 98 (which is the Unicode for the character 'b'): (The bit pattern 00000000 01100010 encodes 98 using the binary number system
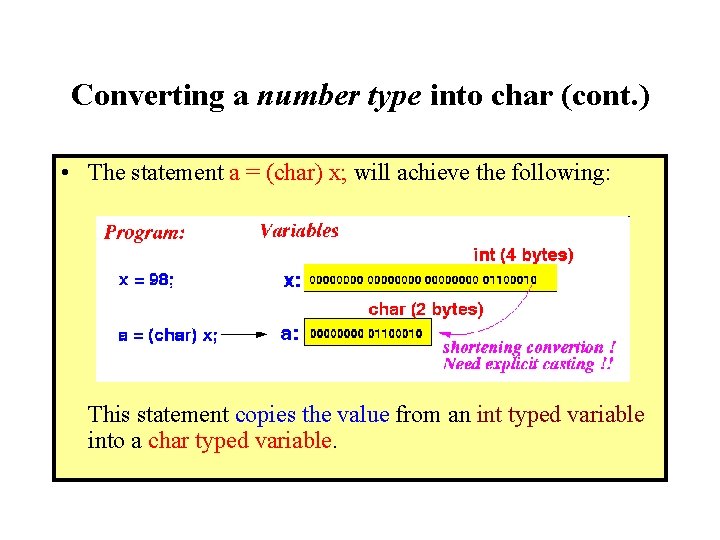
Converting a number type into char (cont. ) • The statement a = (char) x; will achieve the following: This statement copies the value from an int typed variable into a char typed variable.
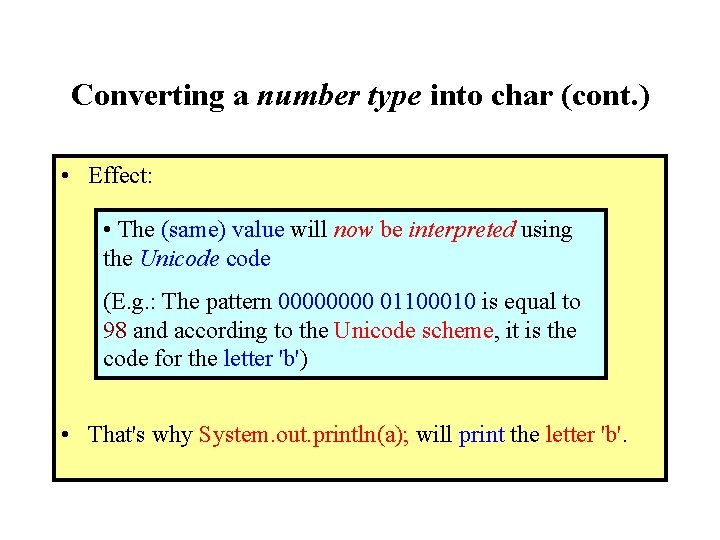
Converting a number type into char (cont. ) • Effect: • The (same) value will now be interpreted using the Unicode (E. g. : The pattern 0000 01100010 is equal to 98 and according to the Unicode scheme, it is the code for the letter 'b') • That's why System. out. println(a); will print the letter 'b'.
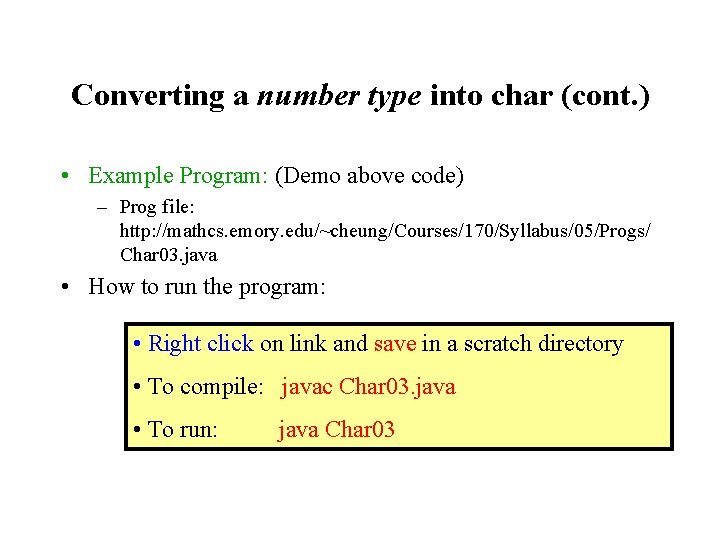
Converting a number type into char (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/05/Progs/ Char 03. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Char 03. java • To run: java Char 03
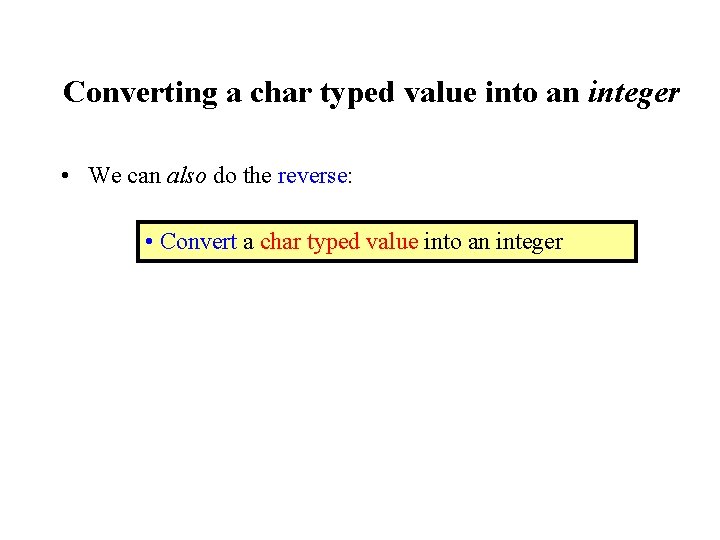
Converting a char typed value into an integer • We can also do the reverse: • Convert a char typed value into an integer
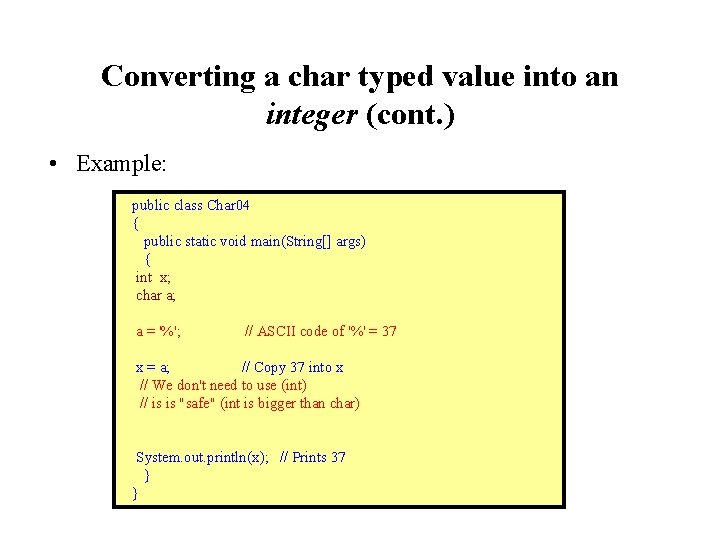
Converting a char typed value into an integer (cont. ) • Example: public class Char 04 { public static void main(String[] args) { int x; char a; a = '%'; // ASCII code of '%' = 37 x = a; // Copy 37 into x // We don't need to use (int) // is is "safe" (int is bigger than char) System. out. println(x); // Prints 37 } }
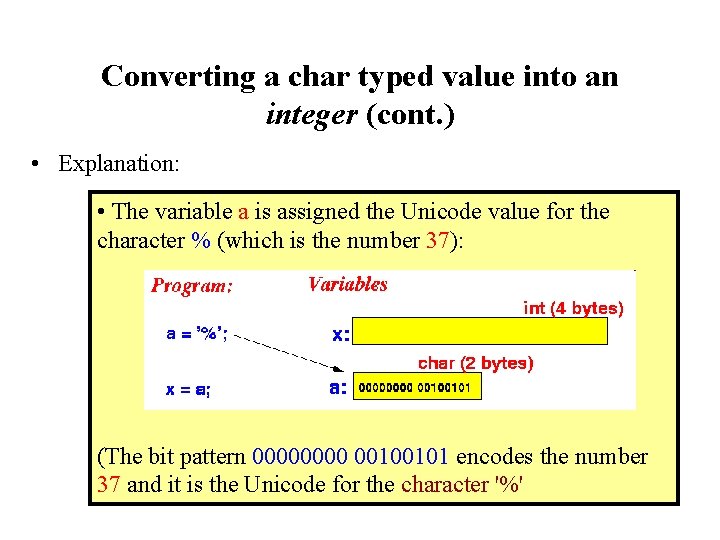
Converting a char typed value into an integer (cont. ) • Explanation: • The variable a is assigned the Unicode value for the character % (which is the number 37): (The bit pattern 0000 00100101 encodes the number 37 and it is the Unicode for the character '%'
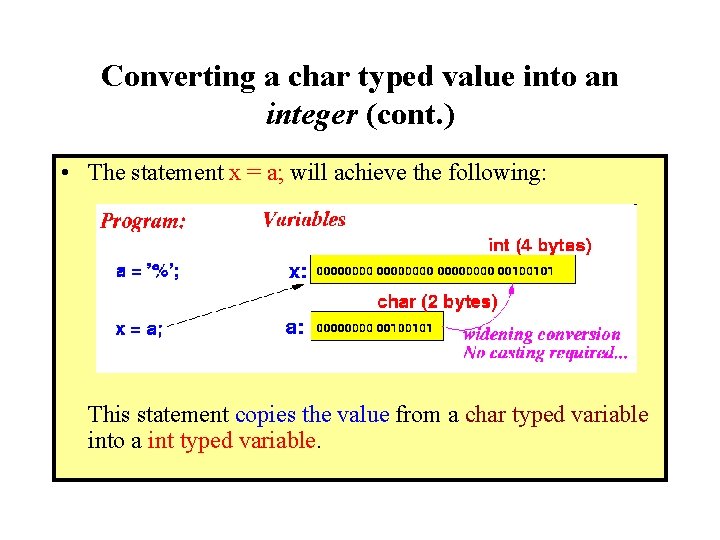
Converting a char typed value into an integer (cont. ) • The statement x = a; will achieve the following: This statement copies the value from a char typed variable into a int typed variable.
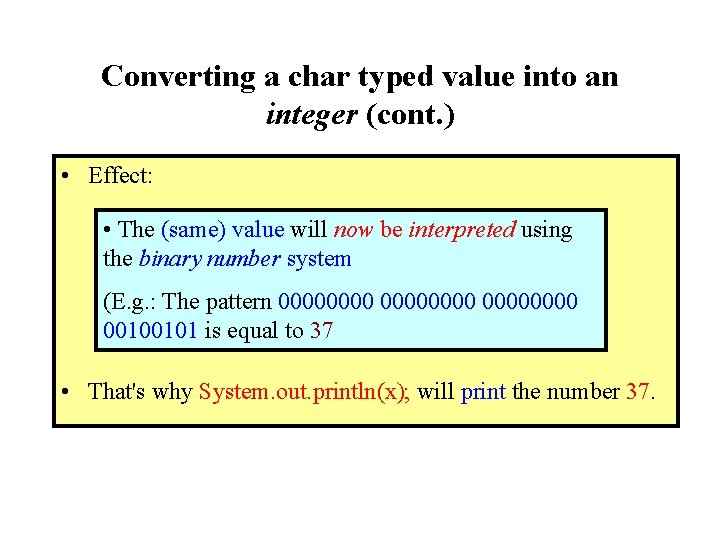
Converting a char typed value into an integer (cont. ) • Effect: • The (same) value will now be interpreted using the binary number system (E. g. : The pattern 00000000 00100101 is equal to 37 • That's why System. out. println(x); will print the number 37.
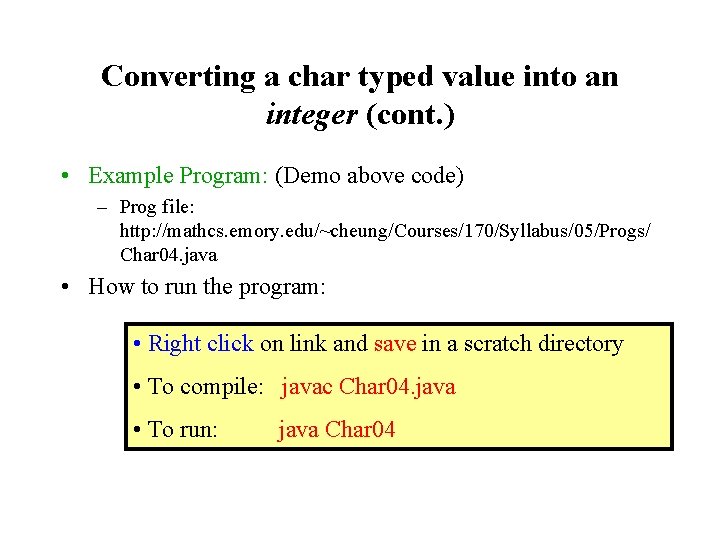
Converting a char typed value into an integer (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/05/Progs/ Char 04. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Char 04. java • To run: java Char 04