Sequential Logic in Verilog Taken From Digital Design
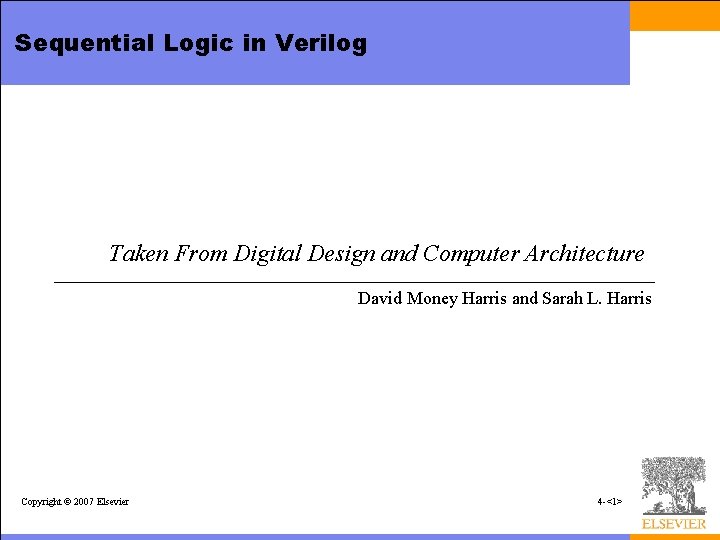
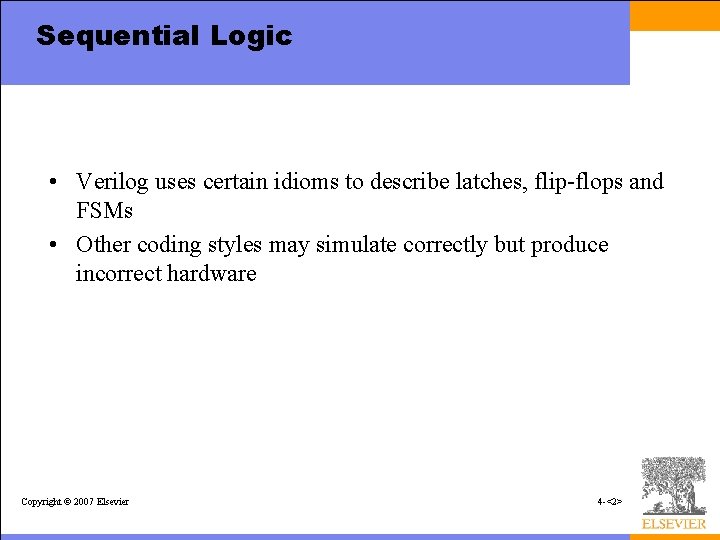
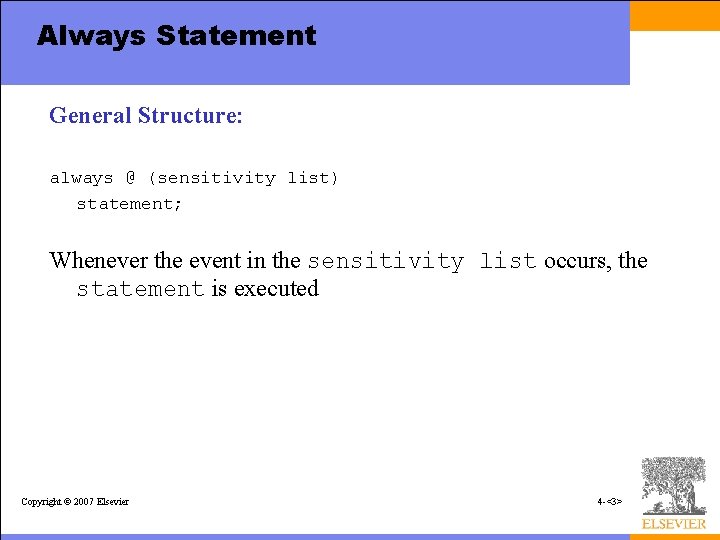
![D Flip-Flop module flop(input clk, input [3: 0] d, output reg [3: 0] q); D Flip-Flop module flop(input clk, input [3: 0] d, output reg [3: 0] q);](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-4.jpg)
![Resettable D Flip-Flop module flopr(input clk, input reset, input [3: 0] d, output reg Resettable D Flip-Flop module flopr(input clk, input reset, input [3: 0] d, output reg](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-5.jpg)
![Resettable D Flip-Flop module flopr(input clk, input reset, input [3: 0] d, output reg Resettable D Flip-Flop module flopr(input clk, input reset, input [3: 0] d, output reg](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-6.jpg)
![D Flip-Flop with Enable module flopren(input clk, input reset, input en, input [3: 0] D Flip-Flop with Enable module flopren(input clk, input reset, input en, input [3: 0]](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-7.jpg)
![Latch module latch(input clk, input [3: 0] d, output reg [3: 0] q); always Latch module latch(input clk, input [3: 0] d, output reg [3: 0] q); always](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-8.jpg)
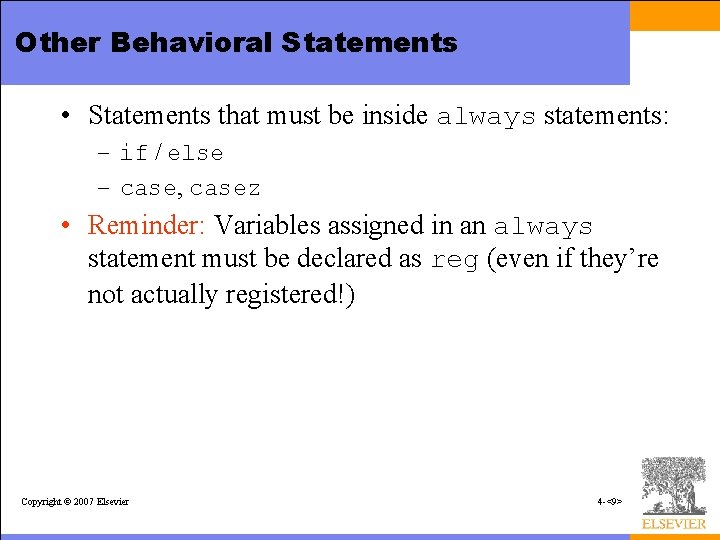
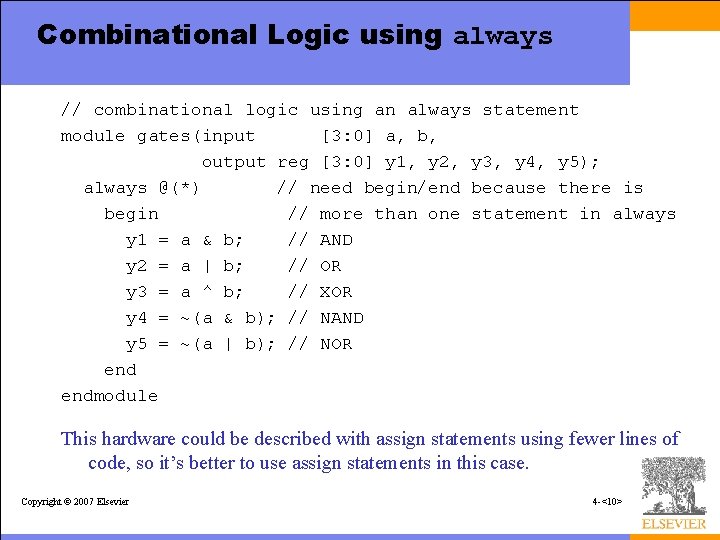
![Combinational Logic using assign (from Lecture 4) module gates(input [3: 0] a, b, output Combinational Logic using assign (from Lecture 4) module gates(input [3: 0] a, b, output](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-11.jpg)
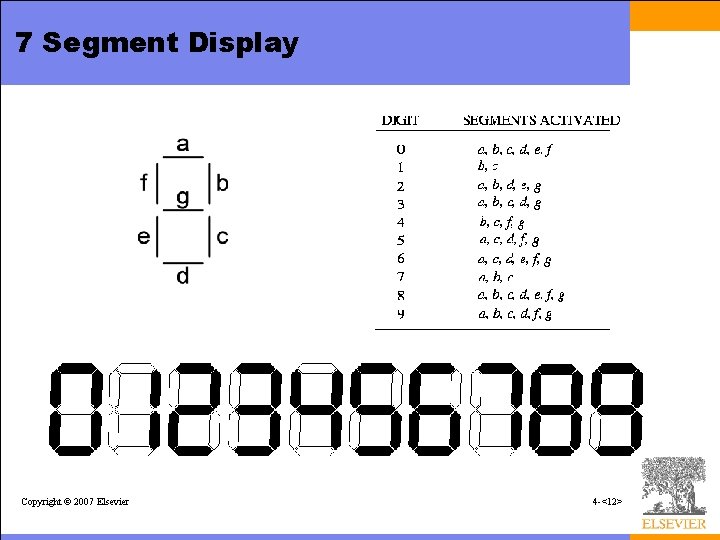
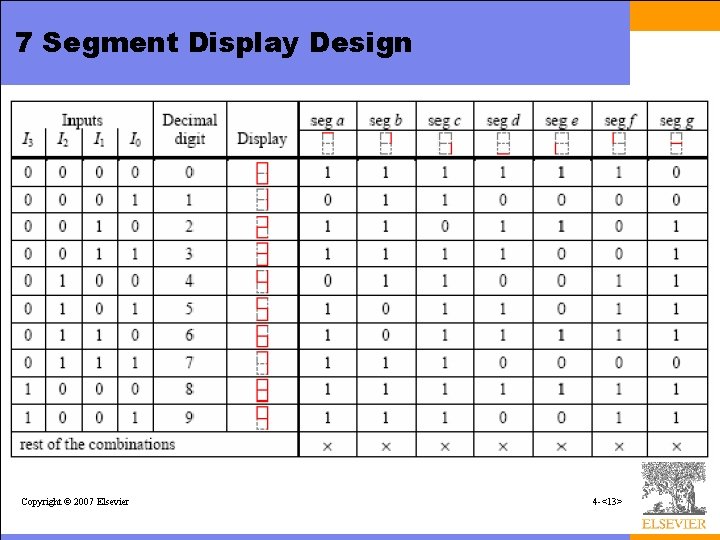
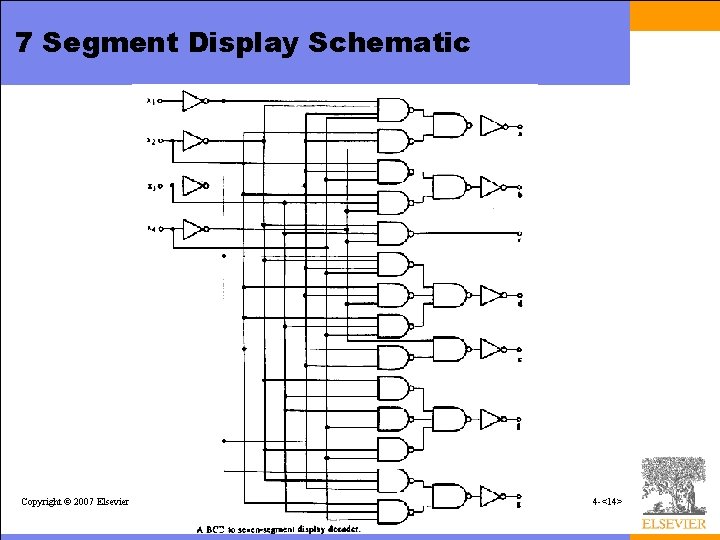
![Combinational Logic using case module sevenseg(input [3: 0] data, output reg [6: 0] segments); Combinational Logic using case module sevenseg(input [3: 0] data, output reg [6: 0] segments);](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-15.jpg)
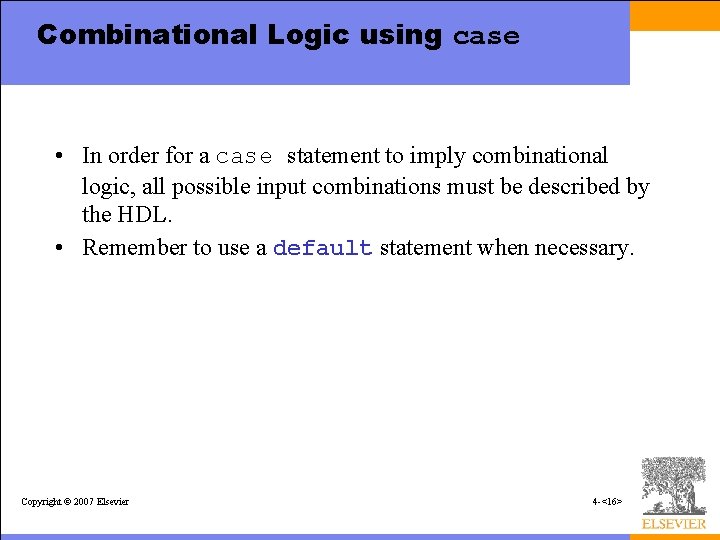
![Combinational Logic using casez module priority_casez(input [3: 0] a, output reg [3: 0] y); Combinational Logic using casez module priority_casez(input [3: 0] a, output reg [3: 0] y);](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-17.jpg)
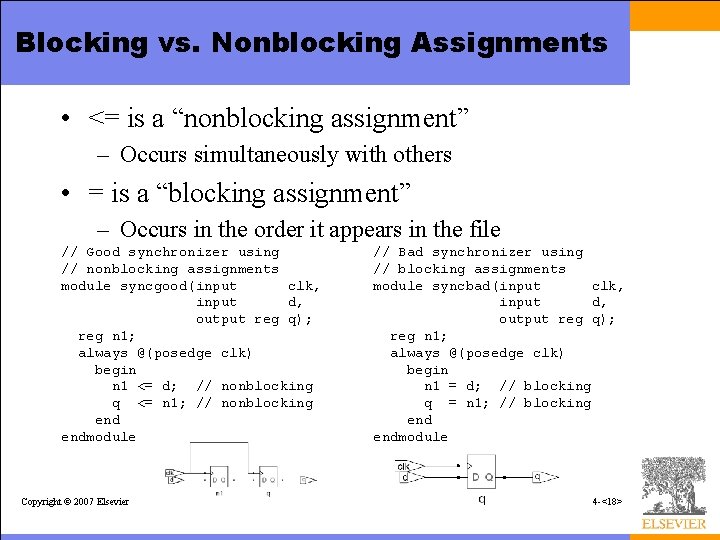
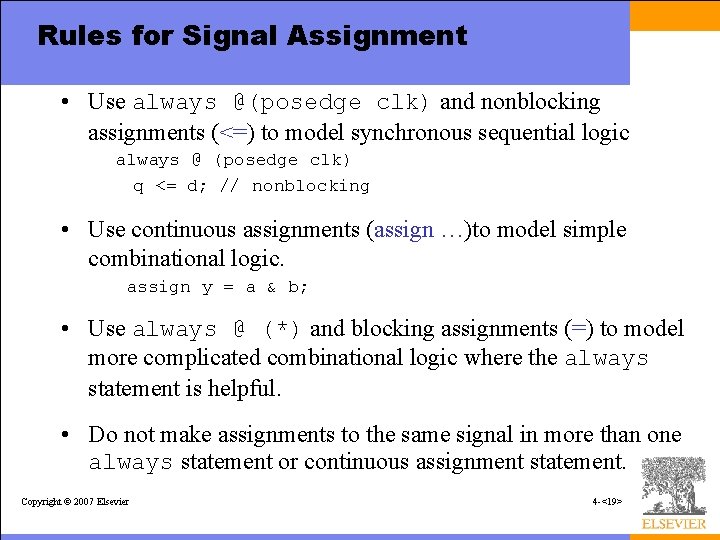
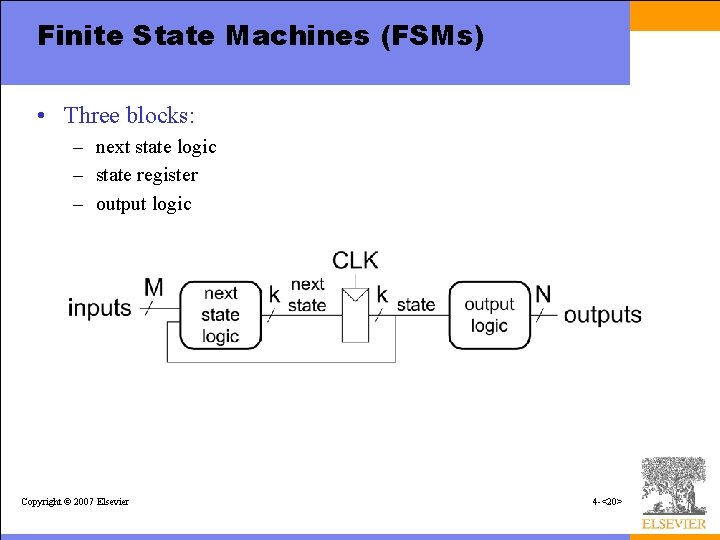
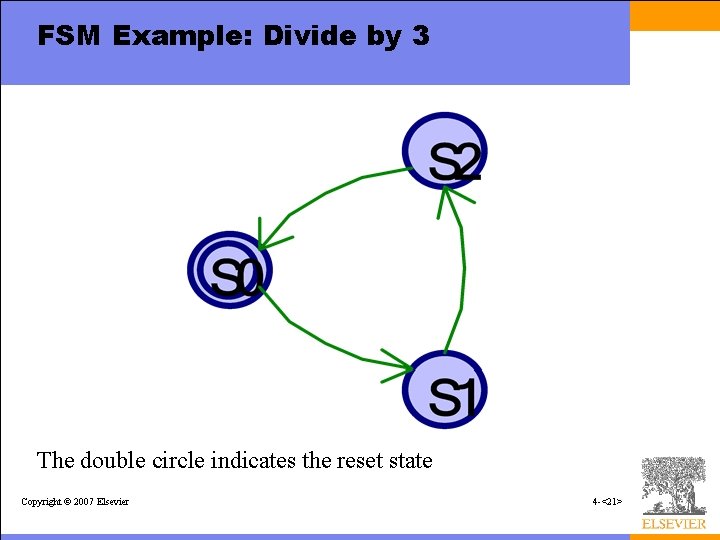
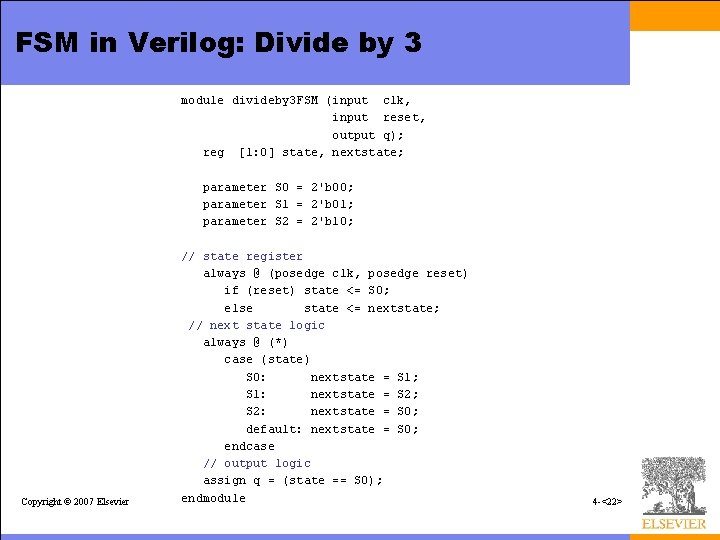
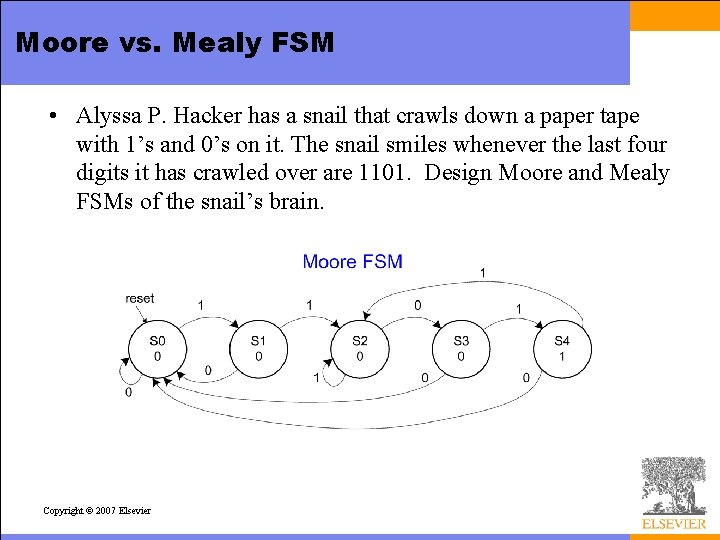
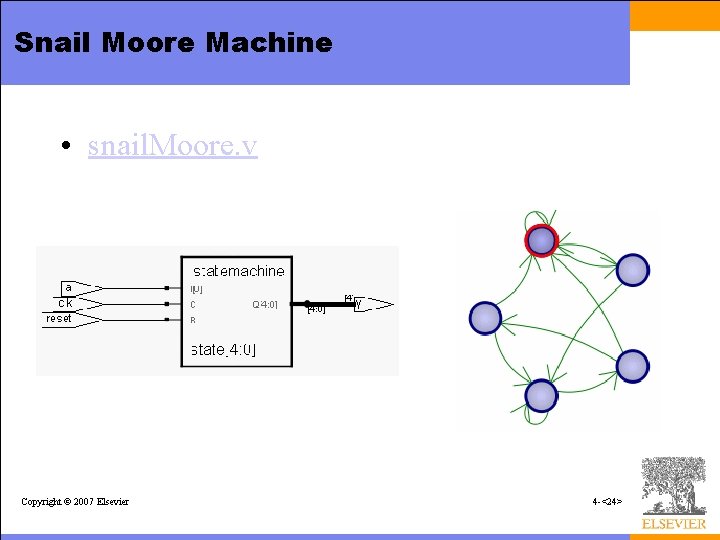
- Slides: 24
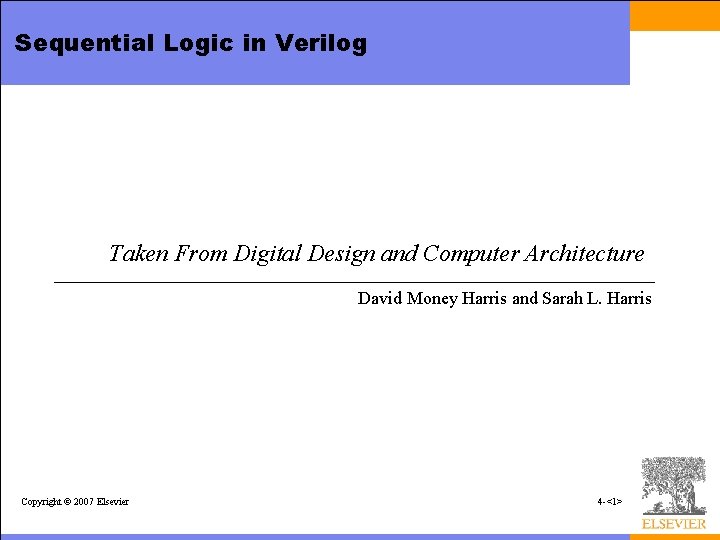
Sequential Logic in Verilog Taken From Digital Design and Computer Architecture David Money Harris and Sarah L. Harris Copyright © 2007 Elsevier 4 -<1>
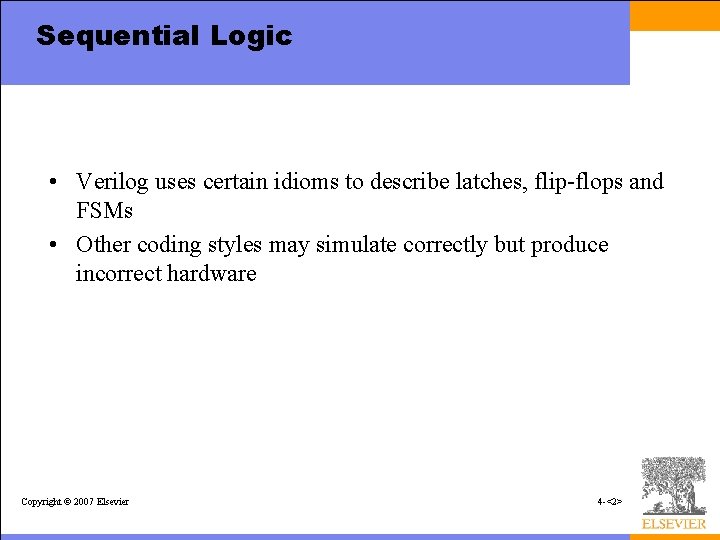
Sequential Logic • Verilog uses certain idioms to describe latches, flip-flops and FSMs • Other coding styles may simulate correctly but produce incorrect hardware Copyright © 2007 Elsevier 4 -<2>
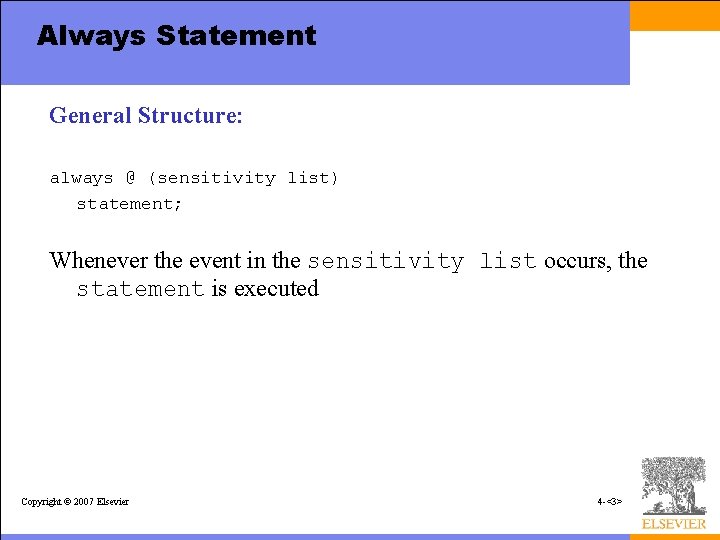
Always Statement General Structure: always @ (sensitivity list) statement; Whenever the event in the sensitivity list occurs, the statement is executed Copyright © 2007 Elsevier 4 -<3>
![D FlipFlop module flopinput clk input 3 0 d output reg 3 0 q D Flip-Flop module flop(input clk, input [3: 0] d, output reg [3: 0] q);](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-4.jpg)
D Flip-Flop module flop(input clk, input [3: 0] d, output reg [3: 0] q); always @ (posedge clk) q <= d; // pronounced “q gets d” endmodule Any signal assigned in an always statement must be declared reg. In this case q is declared as reg Beware: A variable declared reg is not necessarily a registered output. We will show examples of this later. Copyright © 2007 Elsevier 4 -<4>
![Resettable D FlipFlop module floprinput clk input reset input 3 0 d output reg Resettable D Flip-Flop module flopr(input clk, input reset, input [3: 0] d, output reg](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-5.jpg)
Resettable D Flip-Flop module flopr(input clk, input reset, input [3: 0] d, output reg [3: 0] q); // synchronous reset always @ (posedge clk) if (reset) q <= 4'b 0; else q <= d; endmodule Copyright © 2007 Elsevier 4 -<5>
![Resettable D FlipFlop module floprinput clk input reset input 3 0 d output reg Resettable D Flip-Flop module flopr(input clk, input reset, input [3: 0] d, output reg](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-6.jpg)
Resettable D Flip-Flop module flopr(input clk, input reset, input [3: 0] d, output reg [3: 0] q); // asynchronous reset always @ (posedge clk, posedge reset) if (reset) q <= 4'b 0; else q <= d; endmodule Copyright © 2007 Elsevier 4 -<6>
![D FlipFlop with Enable module flopreninput clk input reset input en input 3 0 D Flip-Flop with Enable module flopren(input clk, input reset, input en, input [3: 0]](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-7.jpg)
D Flip-Flop with Enable module flopren(input clk, input reset, input en, input [3: 0] d, output reg [3: 0] q); // asynchronous reset and enable always @ (posedge clk, posedge reset) if (reset) q <= 4'b 0; else if (en) q <= d; endmodule Copyright © 2007 Elsevier 4 -<7>
![Latch module latchinput clk input 3 0 d output reg 3 0 q always Latch module latch(input clk, input [3: 0] d, output reg [3: 0] q); always](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-8.jpg)
Latch module latch(input clk, input [3: 0] d, output reg [3: 0] q); always @ (clk, d) if (clk) q <= d; endmodule Warning: We won’t use latches in this course, but you might write code that inadvertently implies a latch. So if your synthesized hardware has latches in it, this indicates an error. Copyright © 2007 Elsevier 4 -<8>
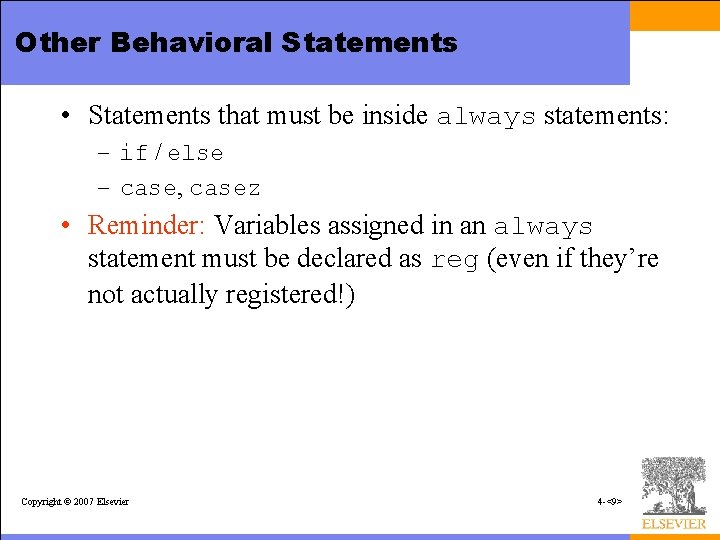
Other Behavioral Statements • Statements that must be inside always statements: – if / else – case, casez • Reminder: Variables assigned in an always statement must be declared as reg (even if they’re not actually registered!) Copyright © 2007 Elsevier 4 -<9>
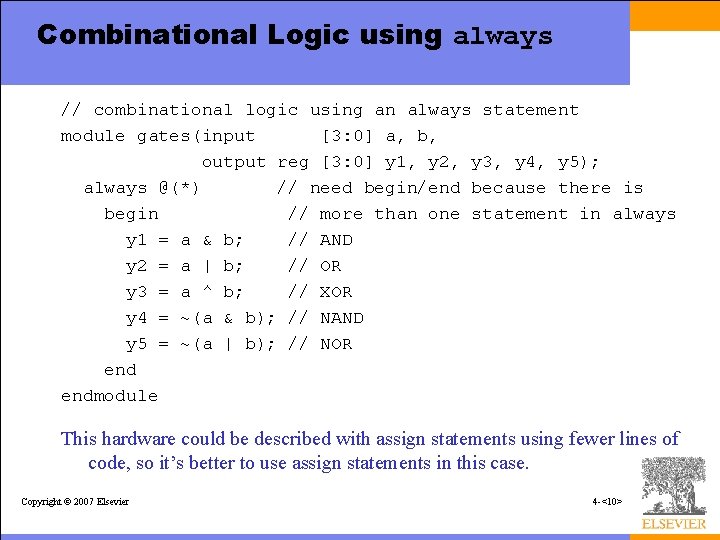
Combinational Logic using always // combinational logic using an always statement module gates(input [3: 0] a, b, output reg [3: 0] y 1, y 2, y 3, y 4, y 5); always @(*) // need begin/end because there is begin // more than one statement in always y 1 = a & b; // AND y 2 = a | b; // OR y 3 = a ^ b; // XOR y 4 = ~(a & b); // NAND y 5 = ~(a | b); // NOR endmodule This hardware could be described with assign statements using fewer lines of code, so it’s better to use assign statements in this case. Copyright © 2007 Elsevier 4 -<10>
![Combinational Logic using assign from Lecture 4 module gatesinput 3 0 a b output Combinational Logic using assign (from Lecture 4) module gates(input [3: 0] a, b, output](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-11.jpg)
Combinational Logic using assign (from Lecture 4) module gates(input [3: 0] a, b, output [3: 0] y 1, y 2, y 3, y 4, y 5); /* Five different two-input logic gates acting on 4 bit busses */ assign y 1 = a & b; // AND assign y 2 = a | b; // OR assign y 3 = a ^ b; // XOR assign y 4 = ~(a & b); // NAND assign y 5 = ~(a | b); // NOR endmodule Copyright © 2007 Elsevier 4 -<11>
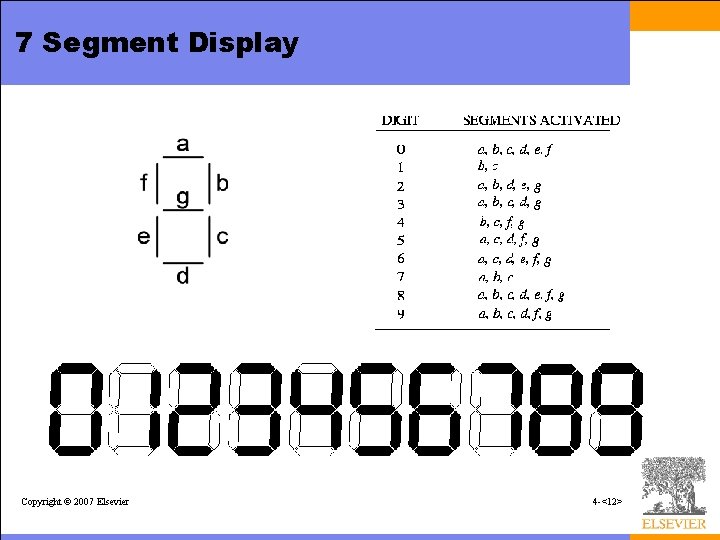
7 Segment Display Copyright © 2007 Elsevier 4 -<12>
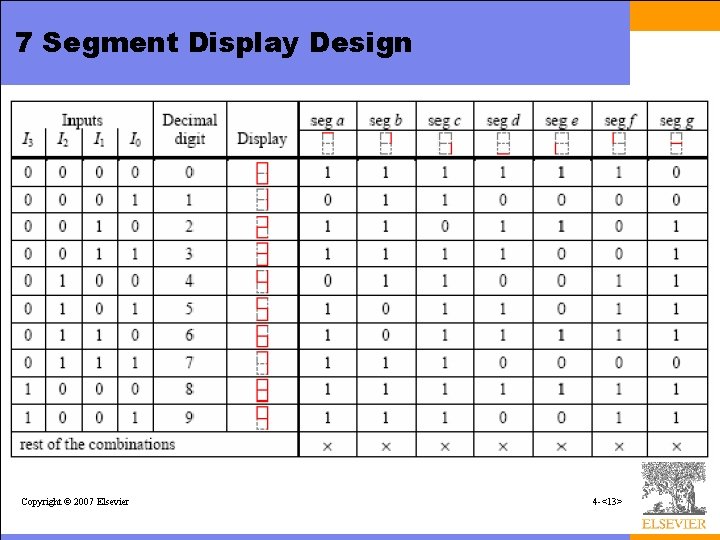
7 Segment Display Design Copyright © 2007 Elsevier 4 -<13>
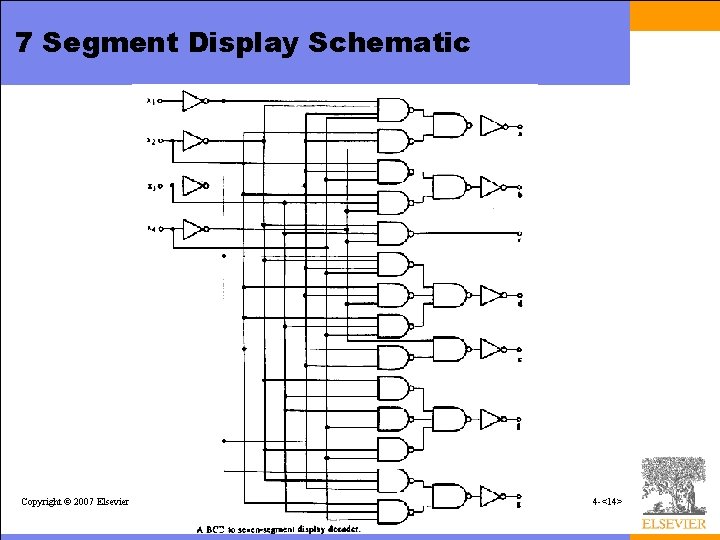
7 Segment Display Schematic Copyright © 2007 Elsevier 4 -<14>
![Combinational Logic using case module sevenseginput 3 0 data output reg 6 0 segments Combinational Logic using case module sevenseg(input [3: 0] data, output reg [6: 0] segments);](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-15.jpg)
Combinational Logic using case module sevenseg(input [3: 0] data, output reg [6: 0] segments); always @(*) case (data) // abc_defg 0: segments = 7'b 111_1110; 1: segments = 7'b 011_0000; 2: segments = 7'b 110_1101; 3: segments = 7'b 111_1001; 4: segments = 7'b 011_0011; 5: segments = 7'b 101_1011; 6: segments = 7'b 101_1111; 7: segments = 7'b 111_0000; 8: segments = 7'b 111_1111; 9: segments = 7'b 111_1011; default: segments = 7'b 000_0000; // required endcase endmodule Copyright © 2007 Elsevier 4 -<15>
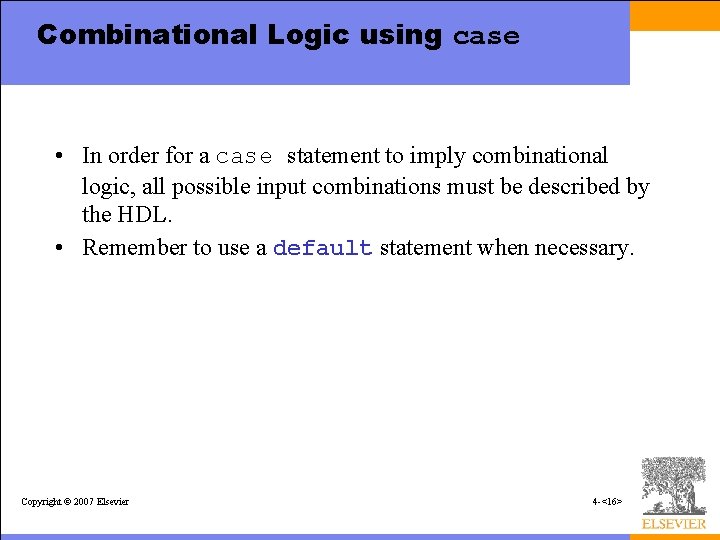
Combinational Logic using case • In order for a case statement to imply combinational logic, all possible input combinations must be described by the HDL. • Remember to use a default statement when necessary. Copyright © 2007 Elsevier 4 -<16>
![Combinational Logic using casez module prioritycasezinput 3 0 a output reg 3 0 y Combinational Logic using casez module priority_casez(input [3: 0] a, output reg [3: 0] y);](https://slidetodoc.com/presentation_image_h/0db644abda50016f38e355e819054a0d/image-17.jpg)
Combinational Logic using casez module priority_casez(input [3: 0] a, output reg [3: 0] y); always @(*) casez(a) 4'b 1? ? ? : 4'b 01? ? : 4'b 001? : 4'b 0001: default: endcase y y y = = = 4'b 1000; 4'b 0100; 4'b 0010; 4'b 0001; 4'b 0000; // ? = don’t care endmodule Copyright © 2007 Elsevier 4 -<17>
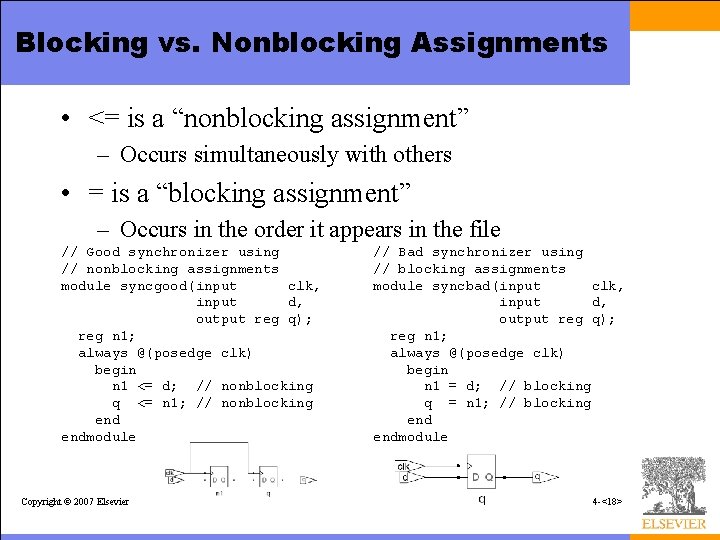
Blocking vs. Nonblocking Assignments • <= is a “nonblocking assignment” – Occurs simultaneously with others • = is a “blocking assignment” – Occurs in the order it appears in the file // Good synchronizer using // nonblocking assignments module syncgood(input clk, input d, output reg q); reg n 1; always @(posedge clk) begin n 1 <= d; // nonblocking q <= n 1; // nonblocking endmodule Copyright © 2007 Elsevier // Bad synchronizer using // blocking assignments module syncbad(input clk, input d, output reg q); reg n 1; always @(posedge clk) begin n 1 = d; // blocking q = n 1; // blocking endmodule 4 -<18>
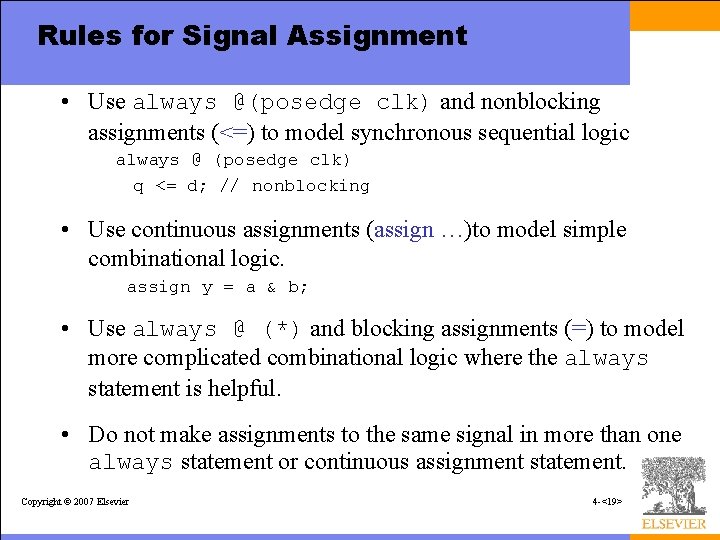
Rules for Signal Assignment • Use always @(posedge clk) and nonblocking assignments (<=) to model synchronous sequential logic always @ (posedge clk) q <= d; // nonblocking • Use continuous assignments (assign …)to model simple combinational logic. assign y = a & b; • Use always @ (*) and blocking assignments (=) to model more complicated combinational logic where the always statement is helpful. • Do not make assignments to the same signal in more than one always statement or continuous assignment statement. Copyright © 2007 Elsevier 4 -<19>
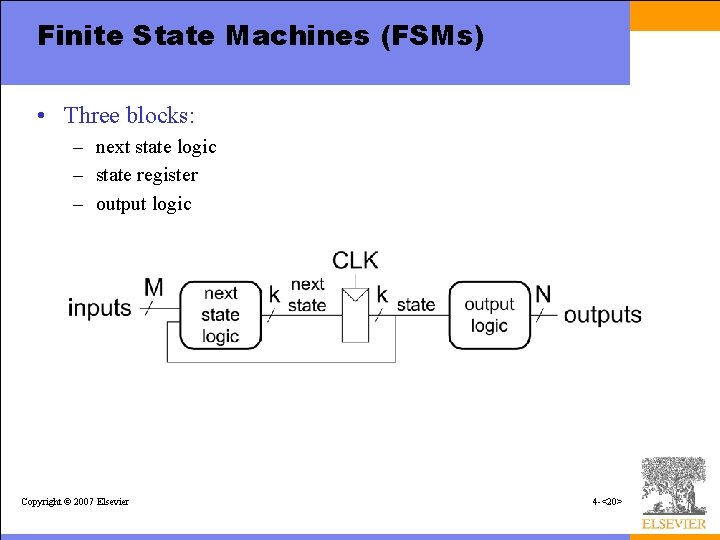
Finite State Machines (FSMs) • Three blocks: – next state logic – state register – output logic Copyright © 2007 Elsevier 4 -<20>
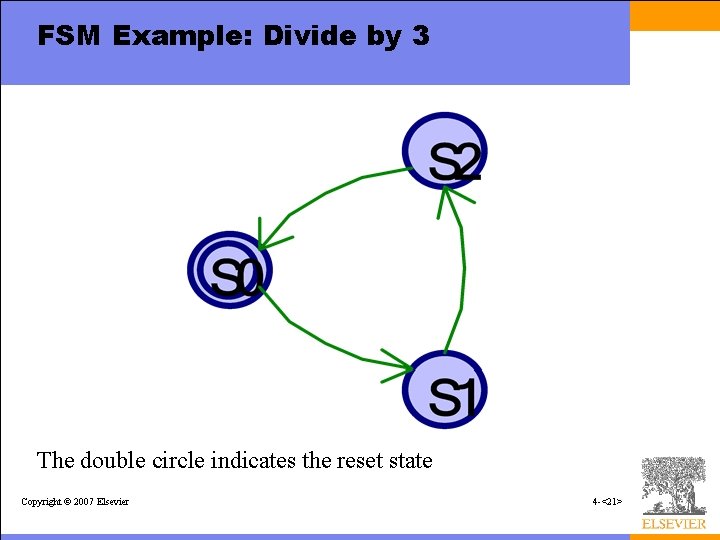
FSM Example: Divide by 3 The double circle indicates the reset state Copyright © 2007 Elsevier 4 -<21>
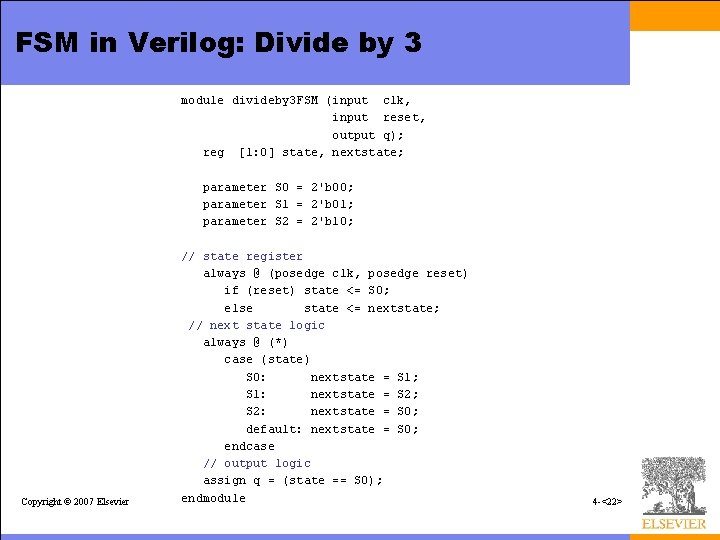
FSM in Verilog: Divide by 3 module divideby 3 FSM (input clk, input reset, output q); reg [1: 0] state, nextstate; parameter S 0 = 2'b 00; parameter S 1 = 2'b 01; parameter S 2 = 2'b 10; Copyright © 2007 Elsevier // state register always @ (posedge clk, posedge reset) if (reset) state <= S 0; else state <= nextstate; // next state logic always @ (*) case (state) S 0: nextstate = S 1; S 1: nextstate = S 2; S 2: nextstate = S 0; default: nextstate = S 0; endcase // output logic assign q = (state == S 0); endmodule 4 -<22>
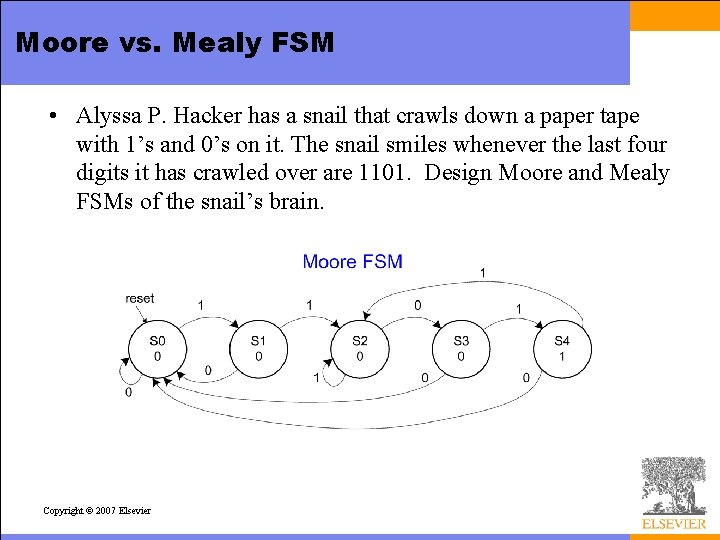
Moore vs. Mealy FSM • Alyssa P. Hacker has a snail that crawls down a paper tape with 1’s and 0’s on it. The snail smiles whenever the last four digits it has crawled over are 1101. Design Moore and Mealy FSMs of the snail’s brain. Copyright © 2007 Elsevier
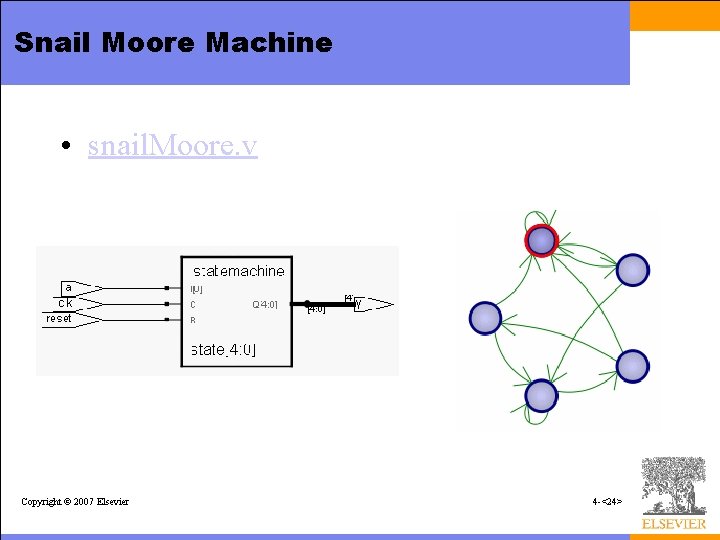
Snail Moore Machine • snail. Moore. v Copyright © 2007 Elsevier 4 -<24>
Combinational vs sequential logic
Combinational logic sequential logic 차이
Is it x y or y x
Combinational logic sequential logic 차이
Combinational logic sequential logic
Digital system design using verilog
Verilog hdl a guide to digital design and synthesis
Advanced digital design with the verilog hdl
Verilog hdl: a guide to digital design and synthesis
Verilog
Sequential file in vb
Verilog combinational logic
Digital logic design tutorial
Digital logic design number system
Digital logic design practice problems
Digital logic design lectures
Digital logic design
What is a nonserual sequential logic in project
What is a nonserual sequential logic in project
Spld in digital electronics
S r q logic
First order logic vs propositional logic
First order logic vs propositional logic
First order logic vs propositional logic
Tw