Python FirstClass Functions Copyright Software Carpentry 2010 This
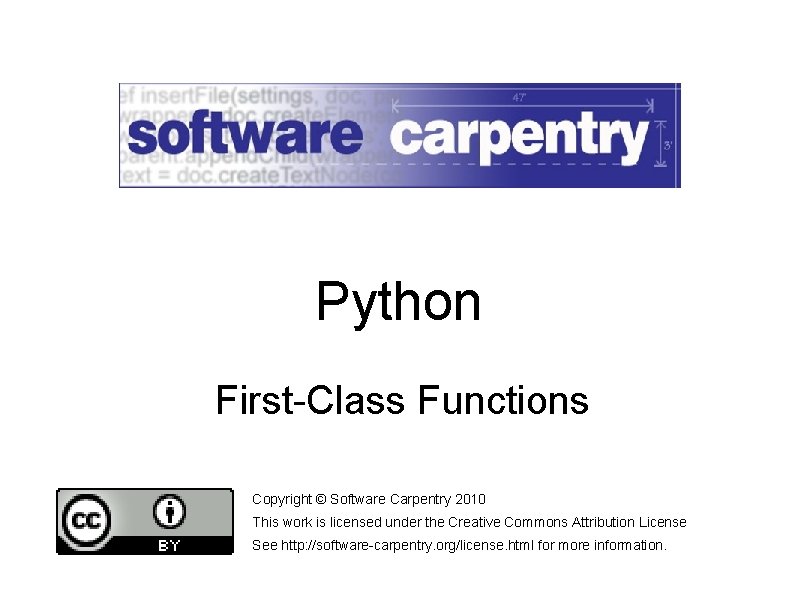
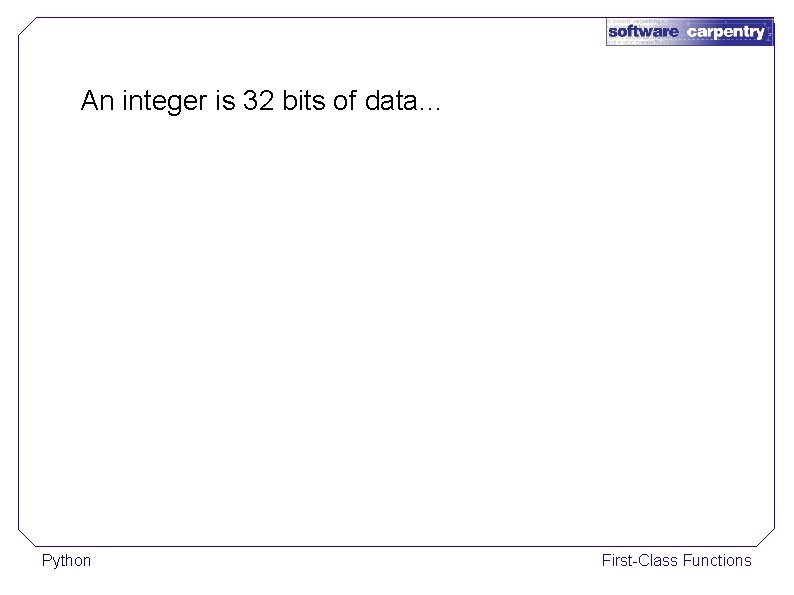
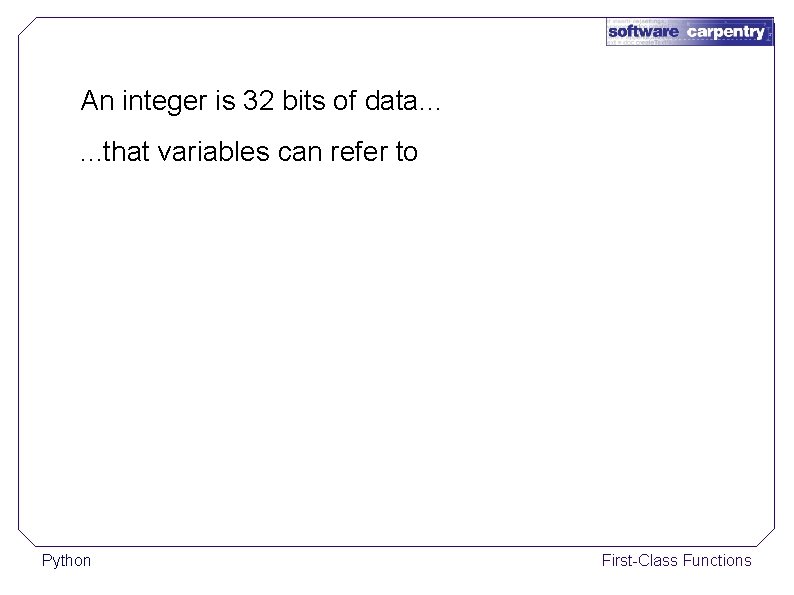
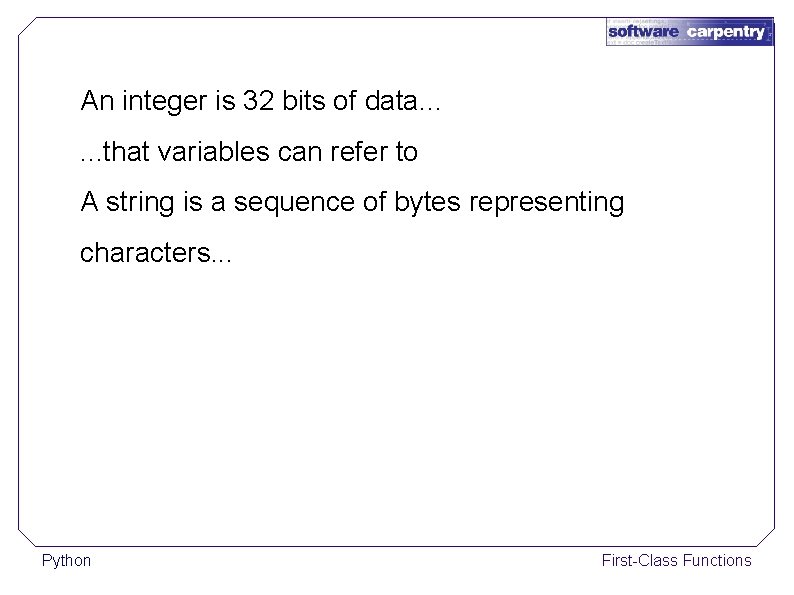
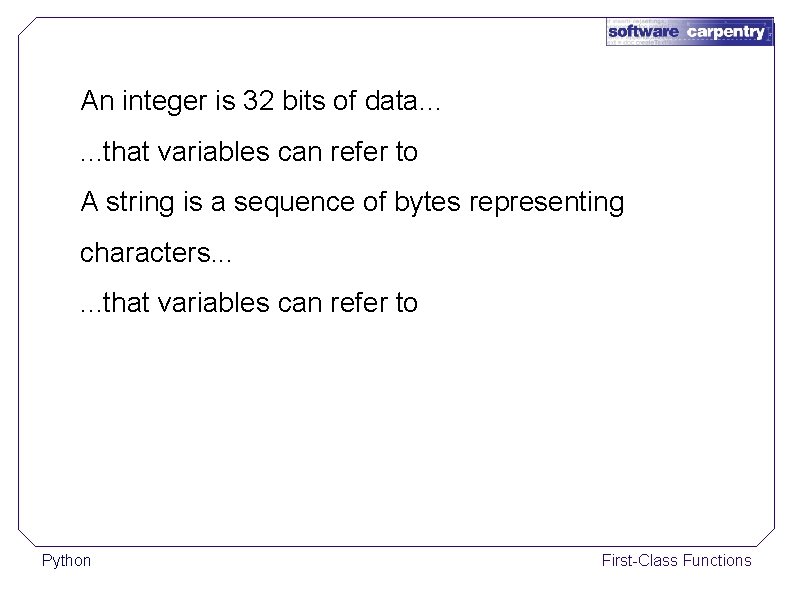
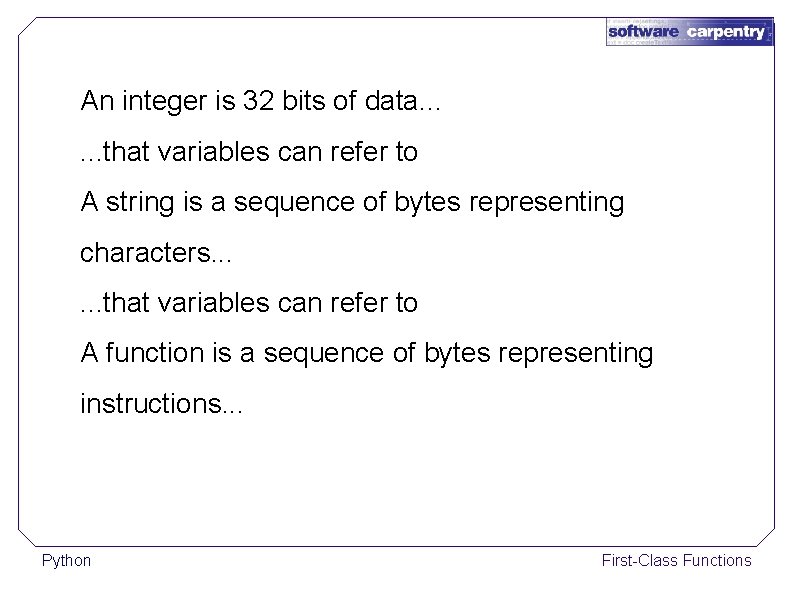
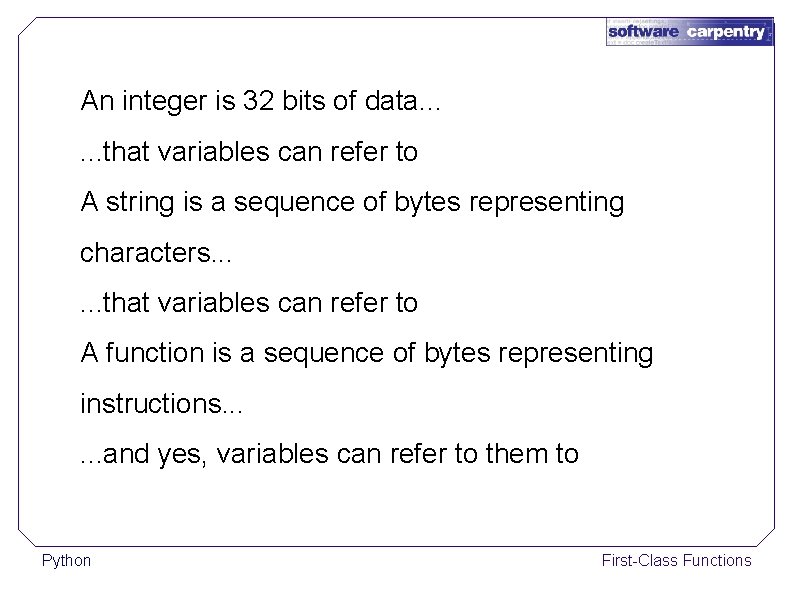
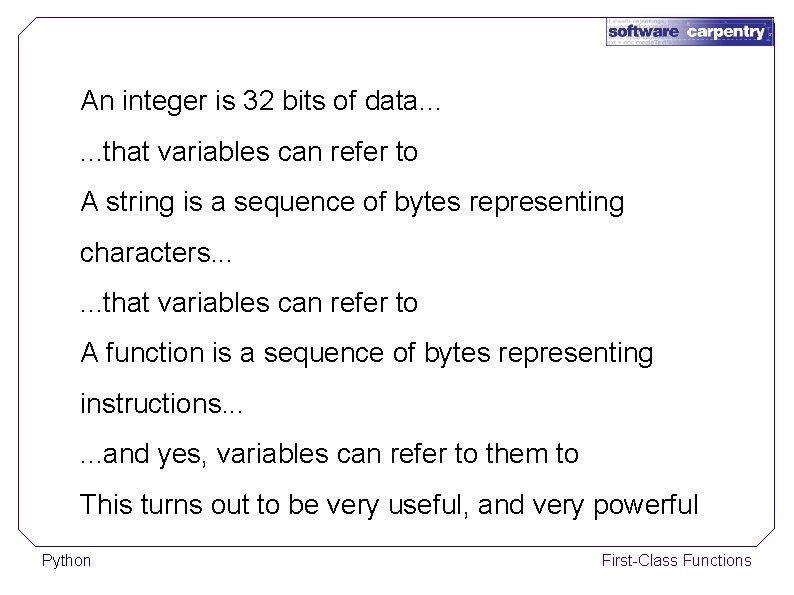
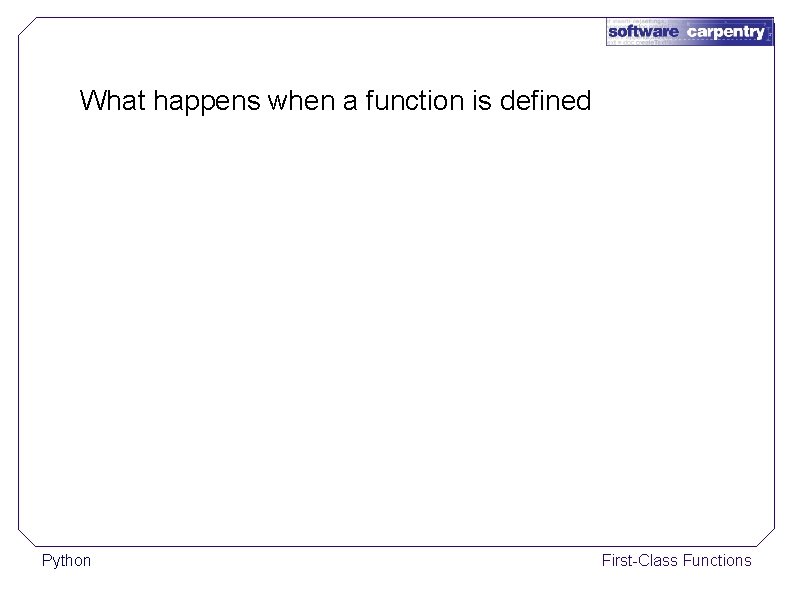
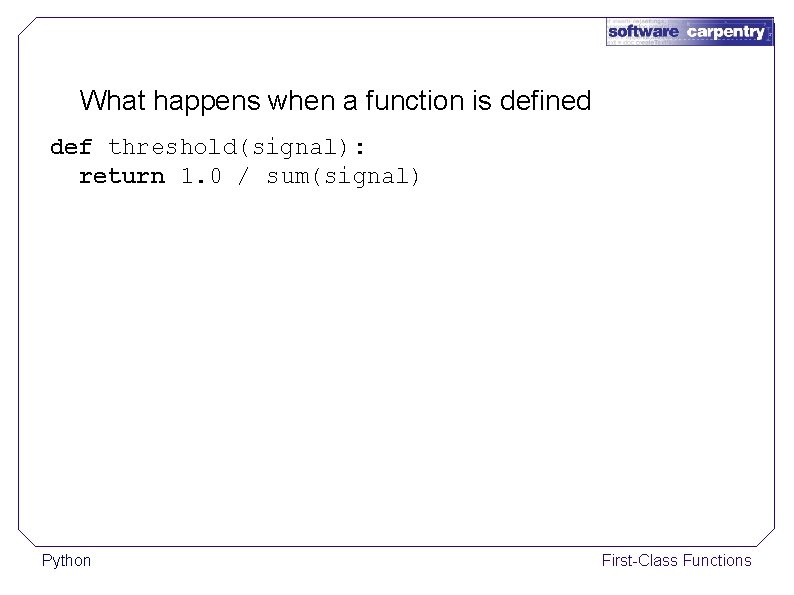
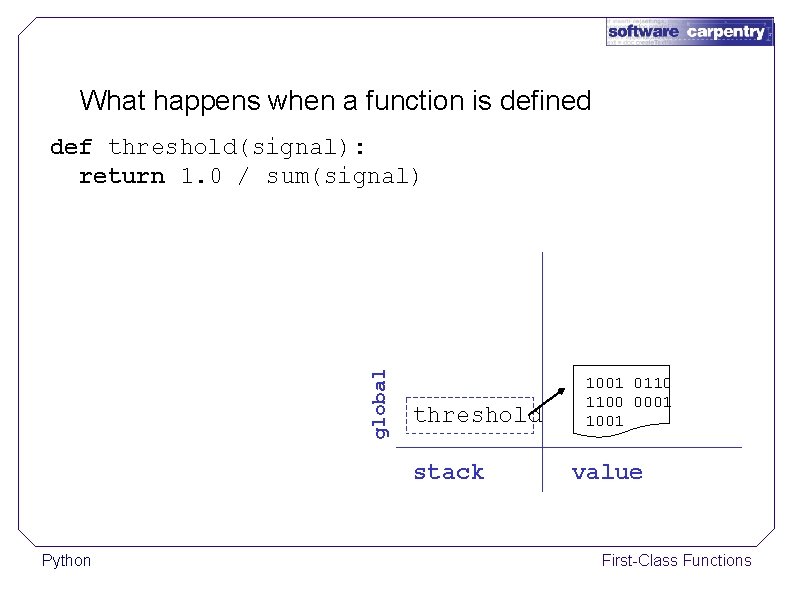
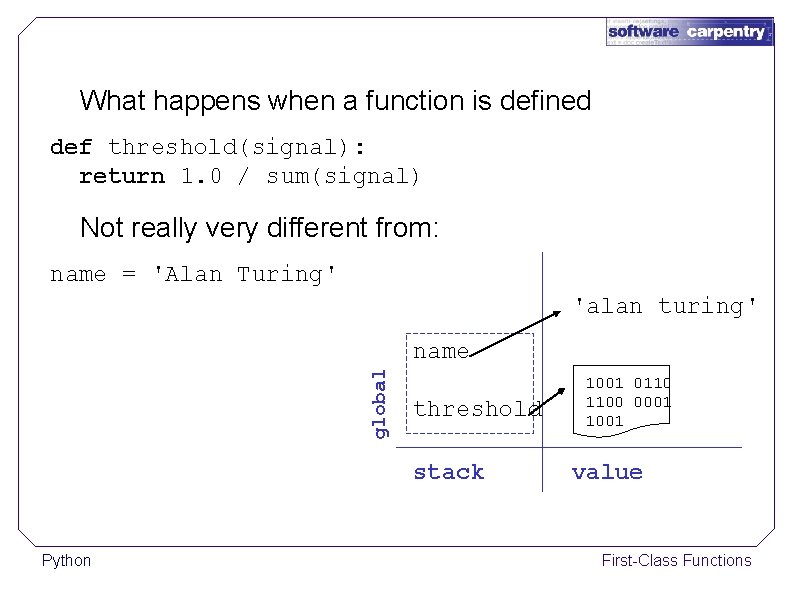
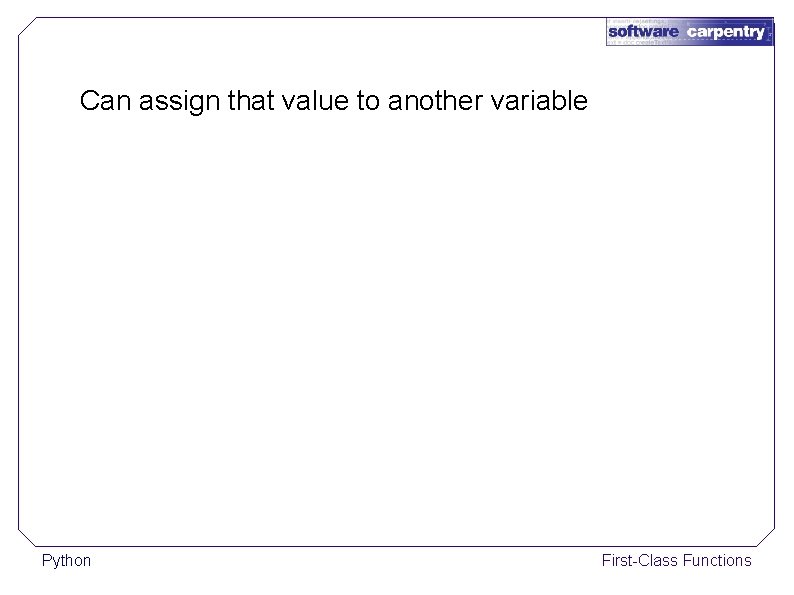
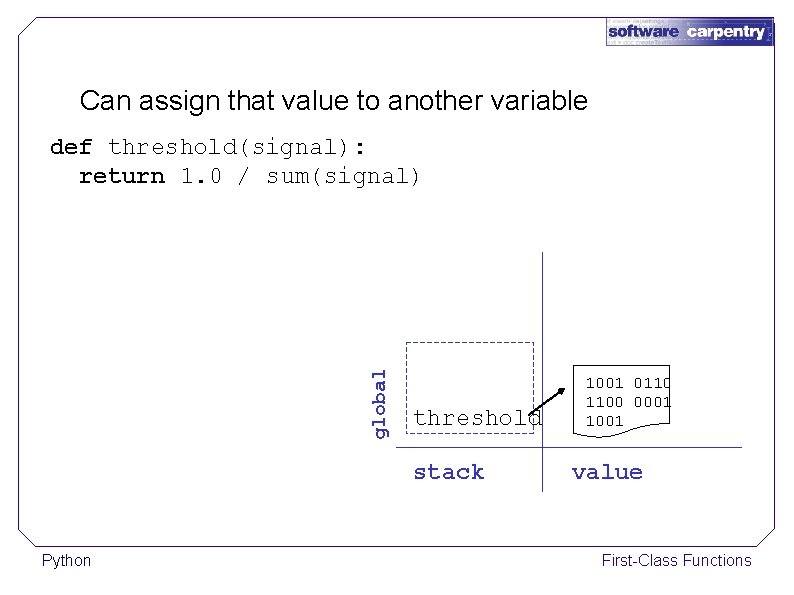
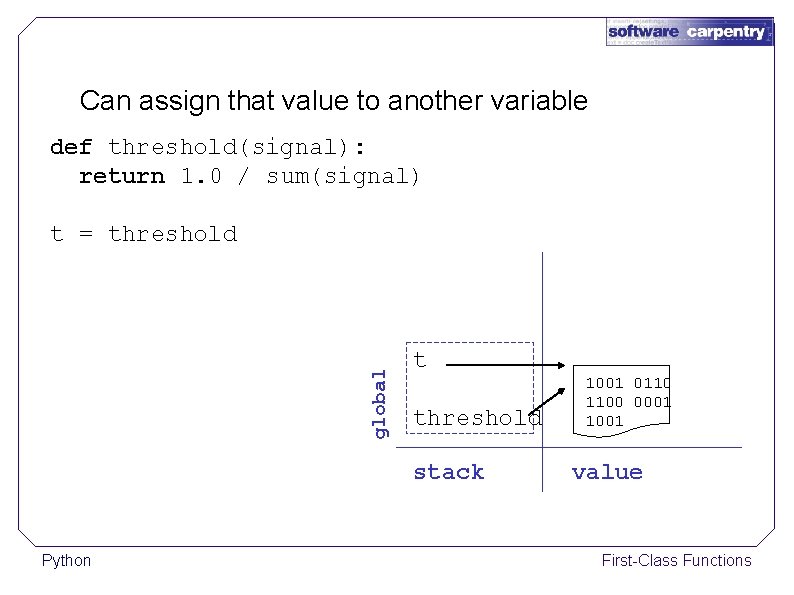
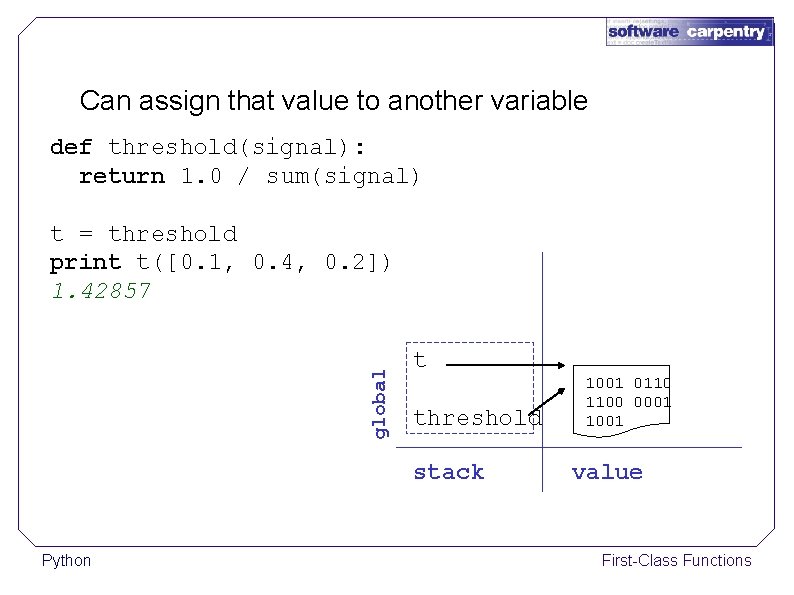
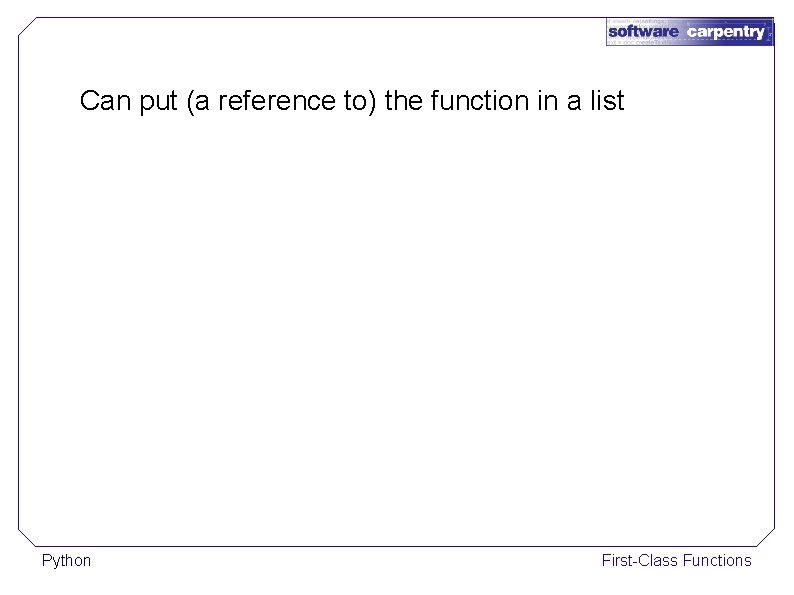
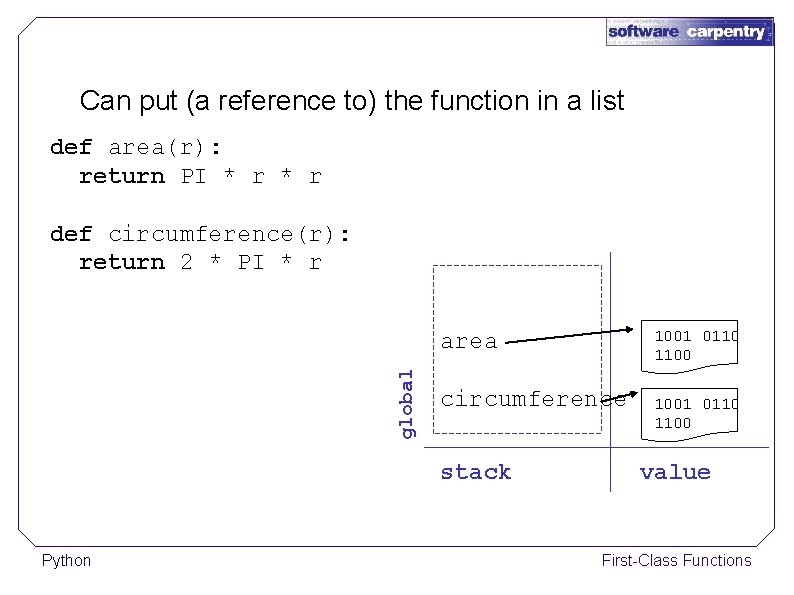
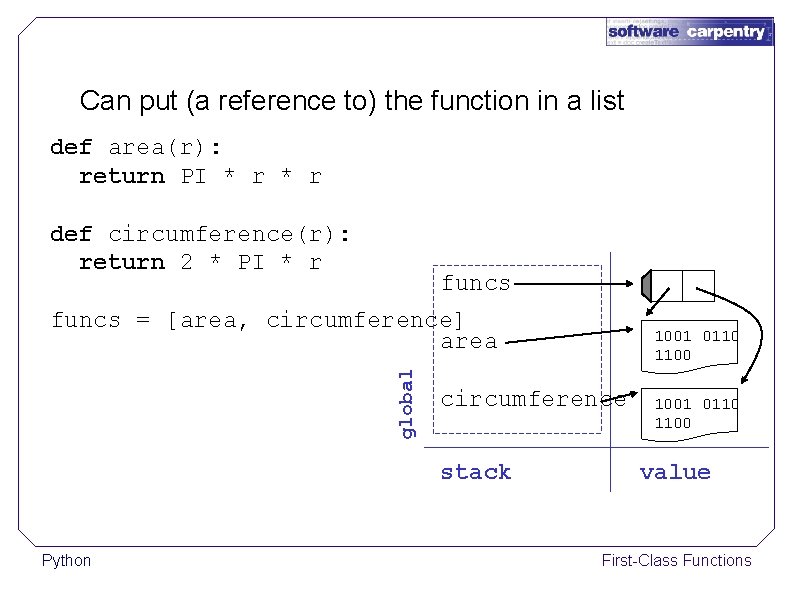
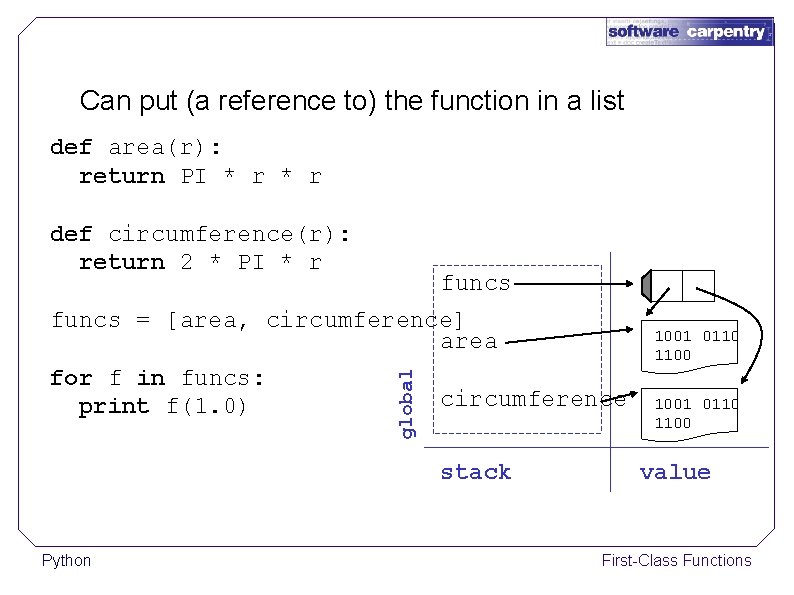
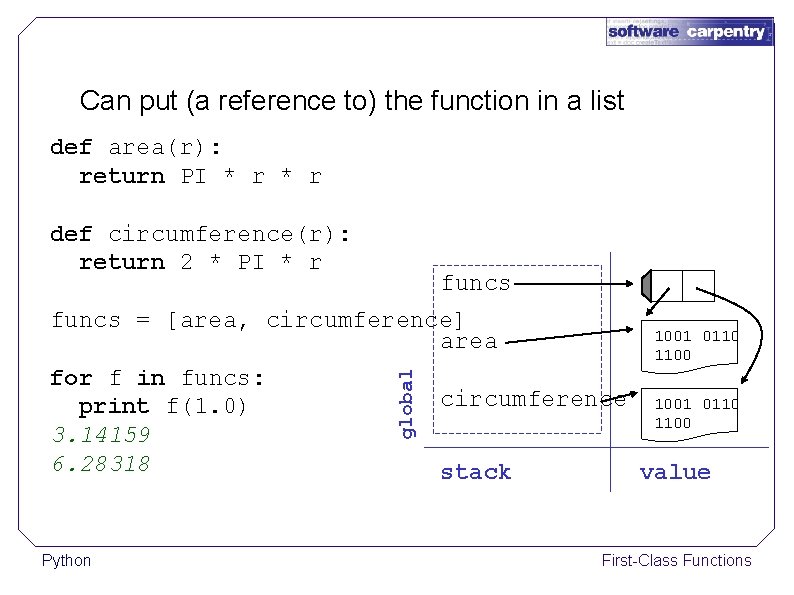
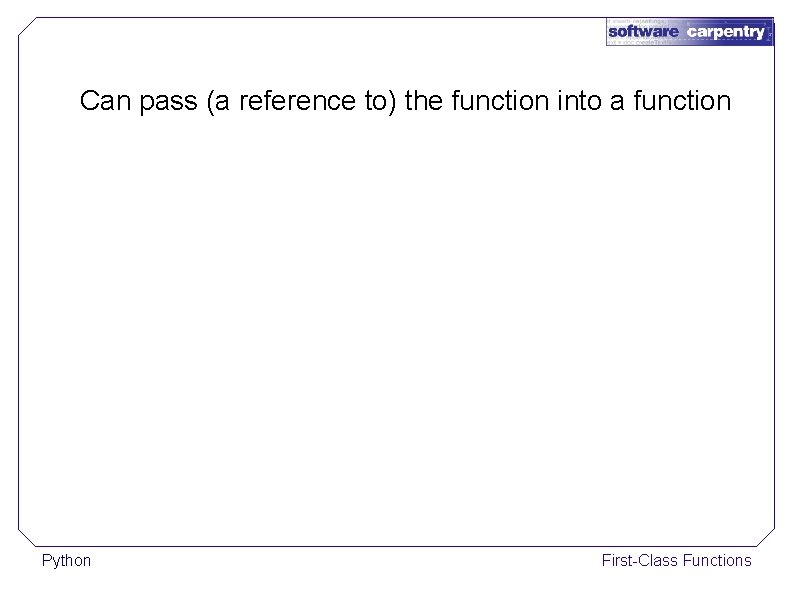
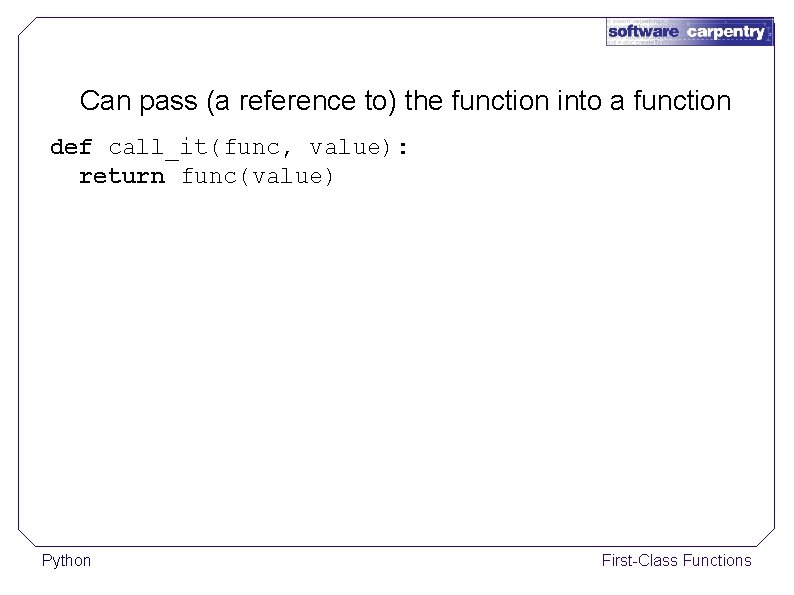
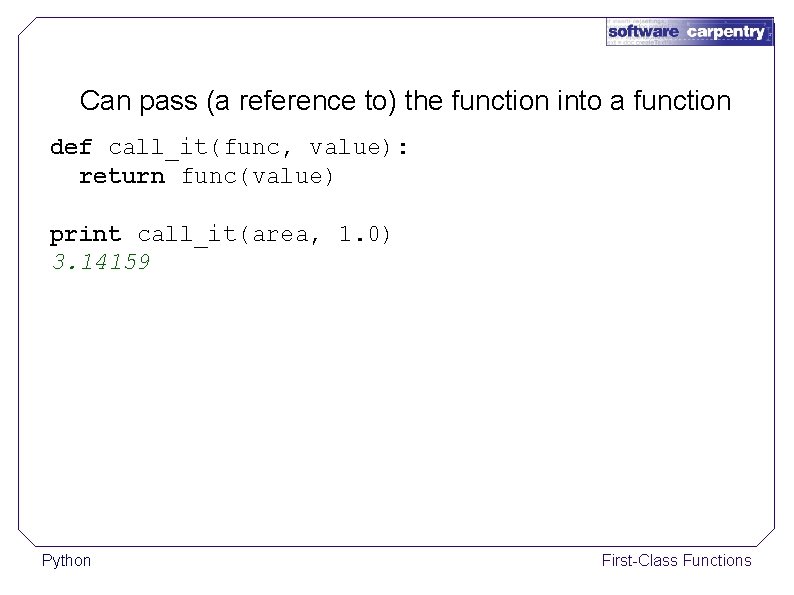
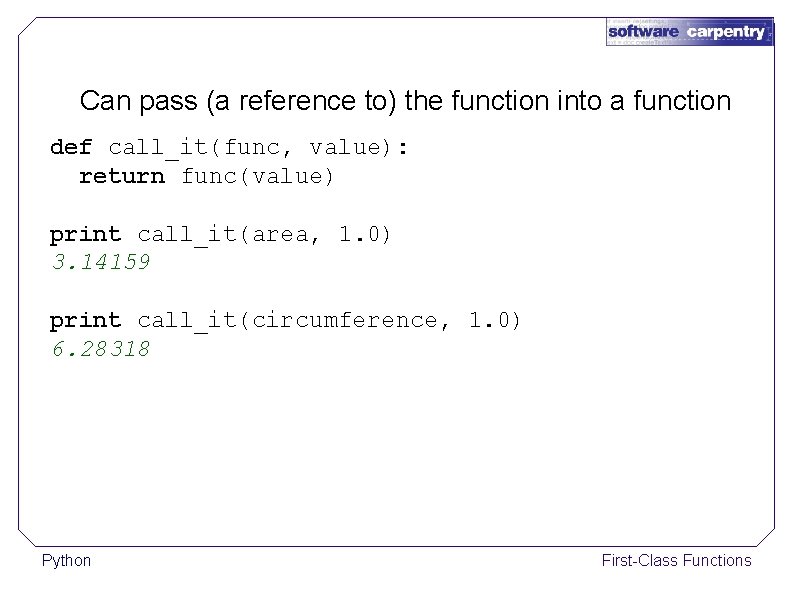
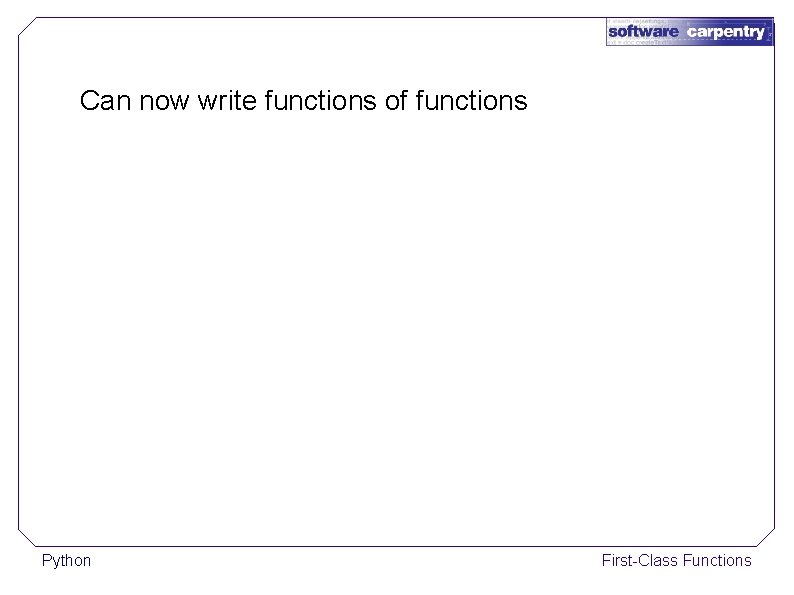
![Can now write functions of functions def do_all(func, values): result = [] for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-27.jpg)
![Can now write functions of functions def do_all(func, values): result = [] for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-28.jpg)
![Can now write functions of functions def do_all(func, values): result = [] for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-29.jpg)
![Can now write functions of functions def do_all(func, values): result = [] for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-30.jpg)
![Can now write functions of functions def do_all(func, values): result = [] for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-31.jpg)
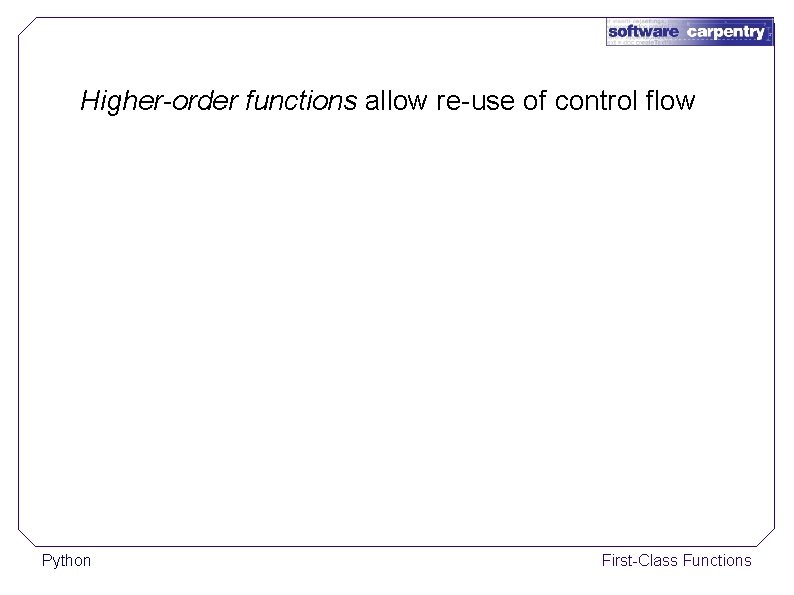
![Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-33.jpg)
![Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-34.jpg)
![Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-35.jpg)
![Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-36.jpg)
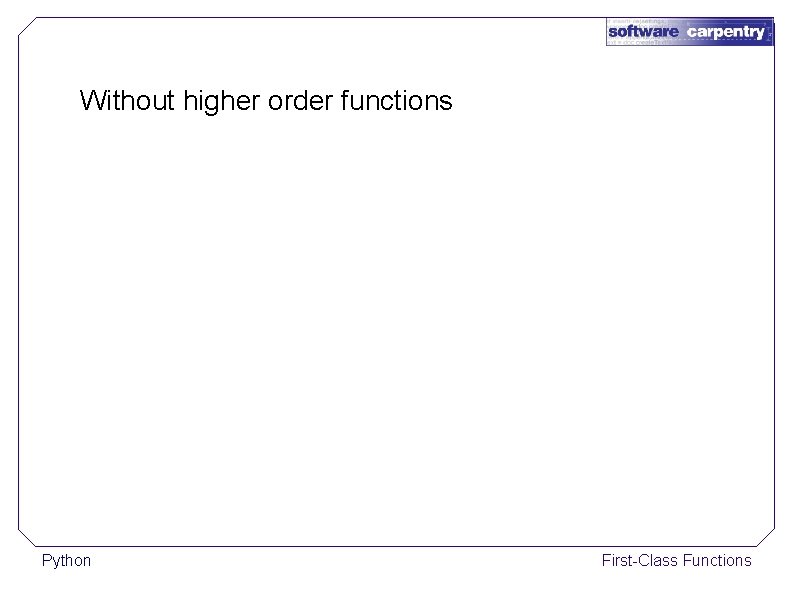
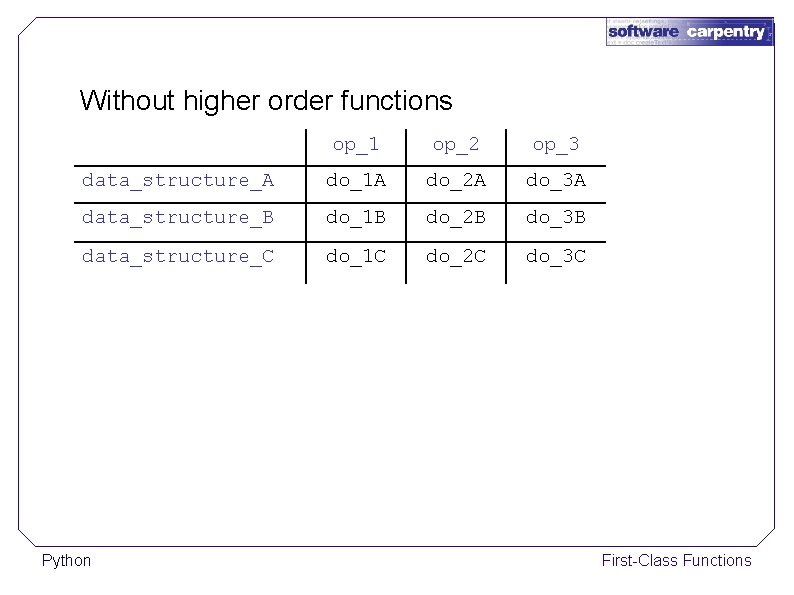
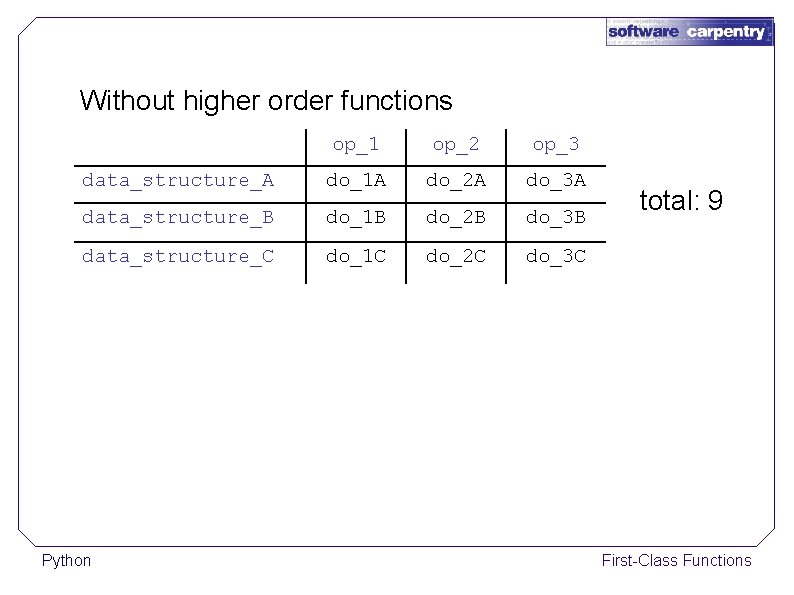
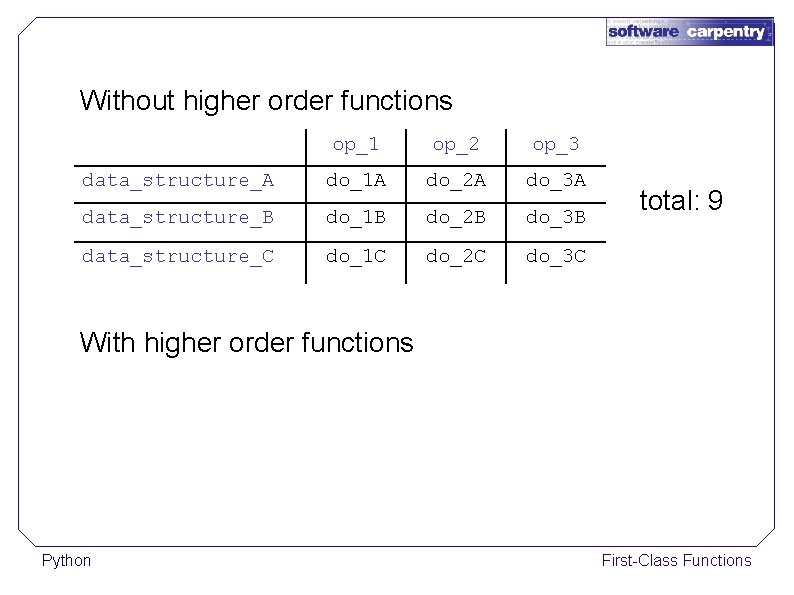
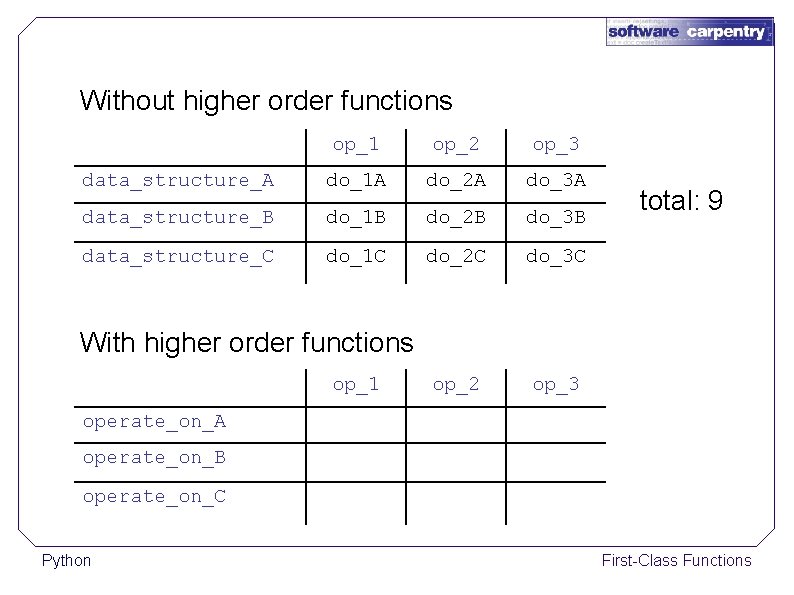
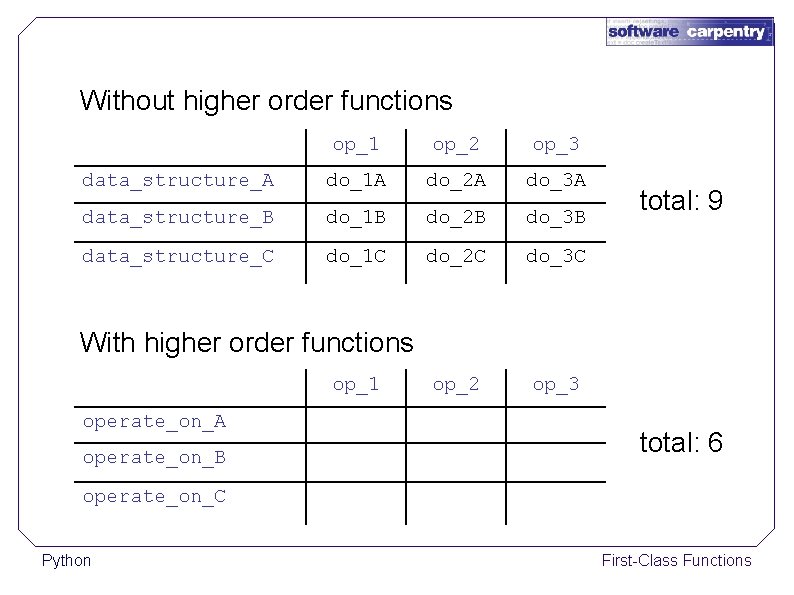
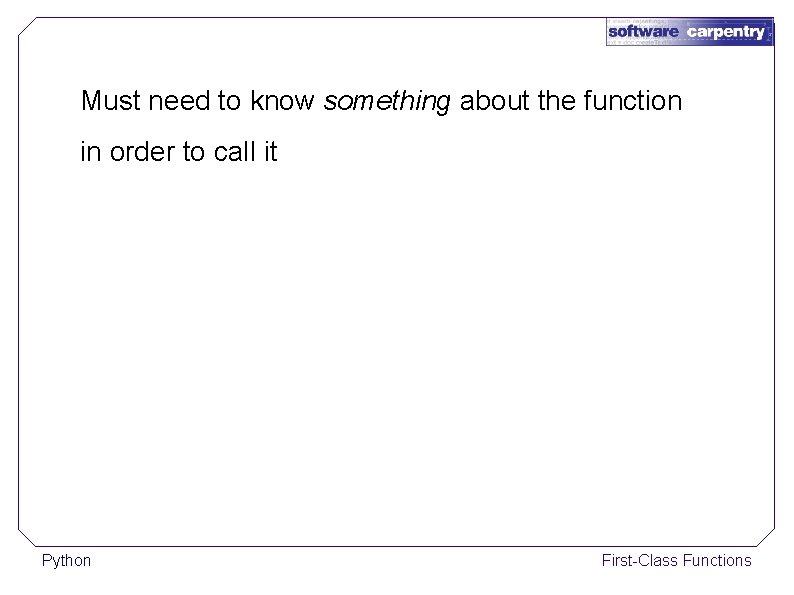
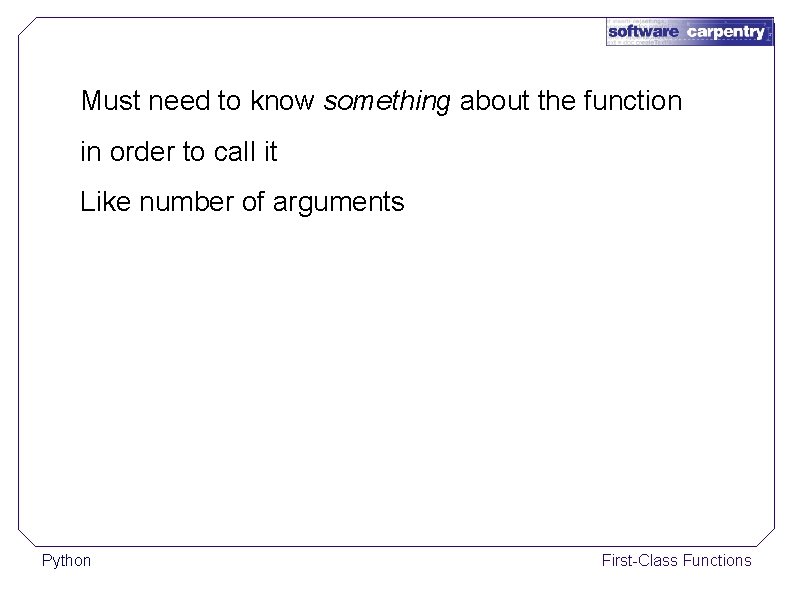
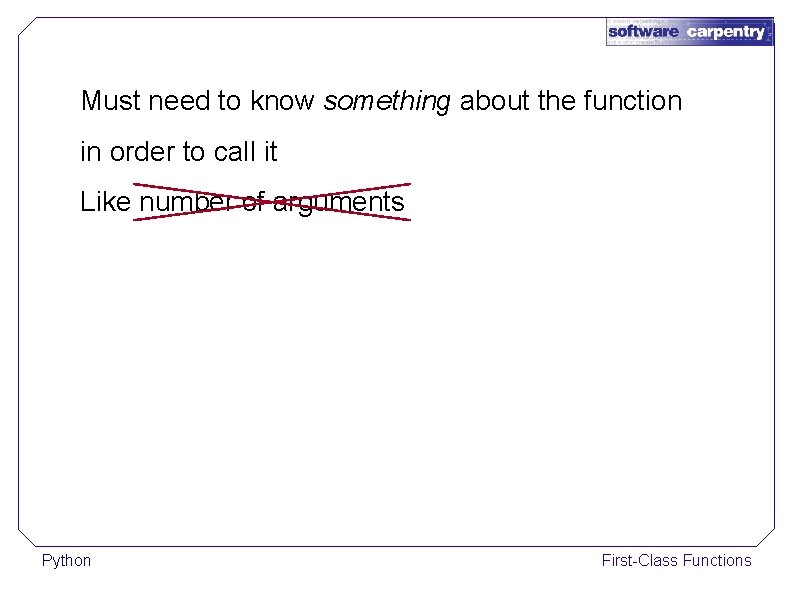
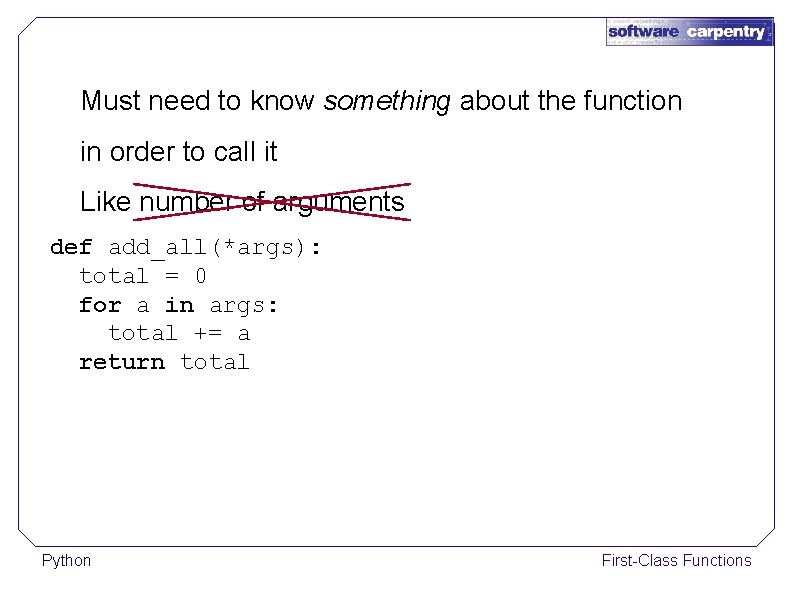
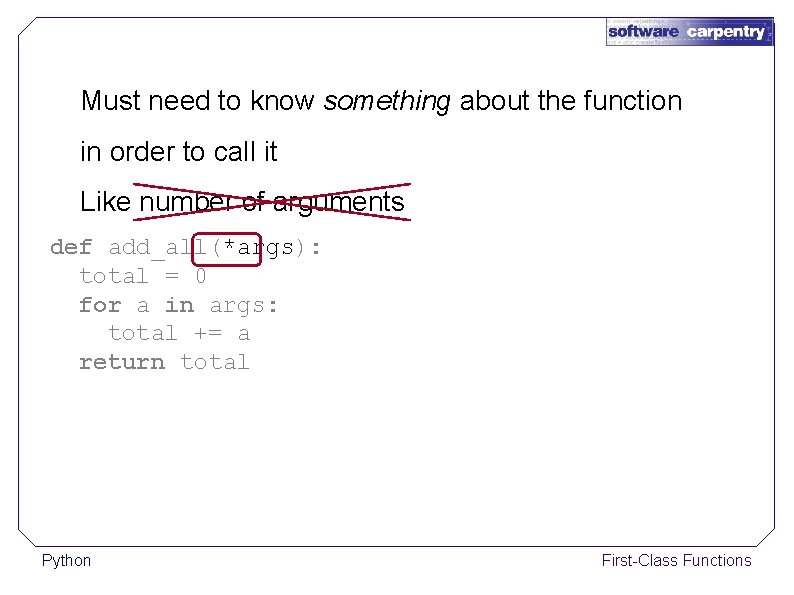
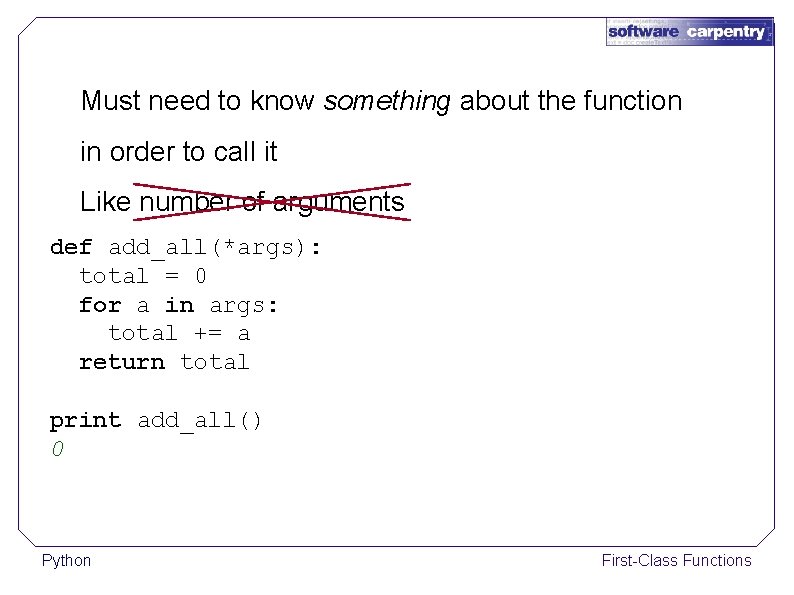
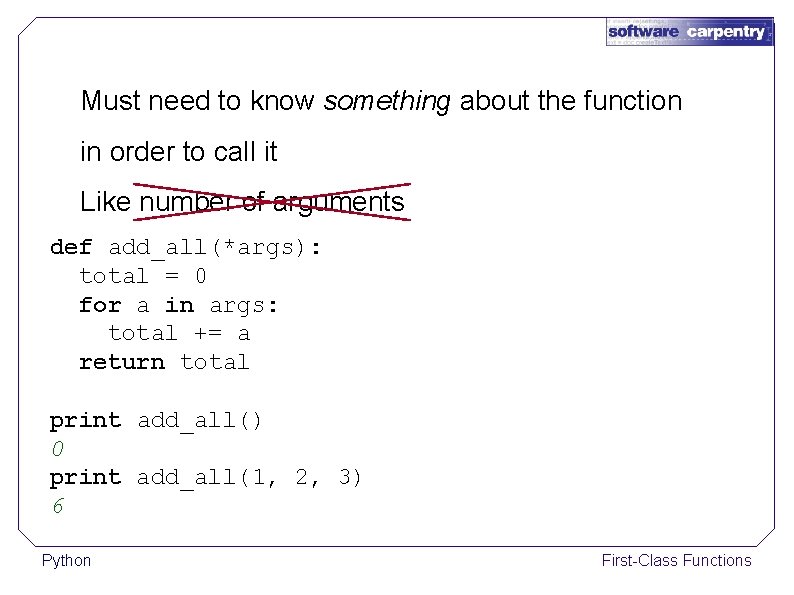
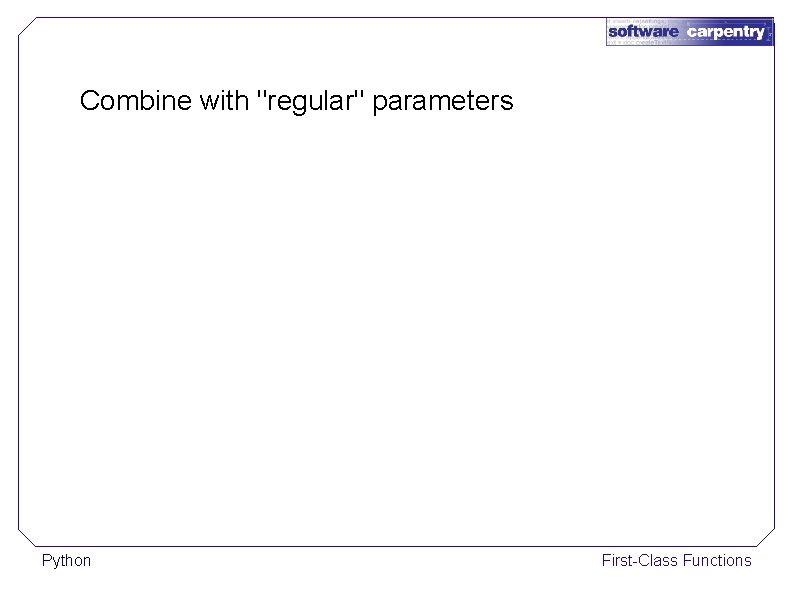
![Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-51.jpg)
![Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-52.jpg)
![Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-53.jpg)
![Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-54.jpg)
![Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-55.jpg)
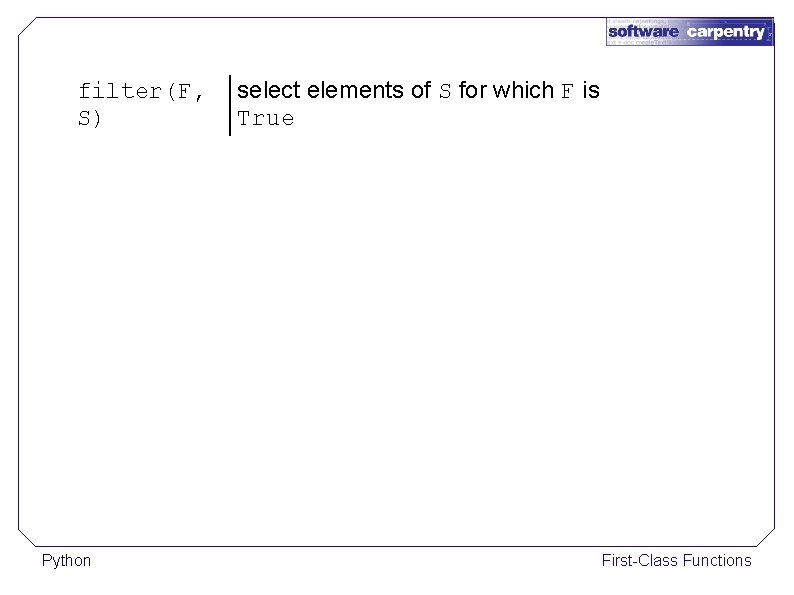
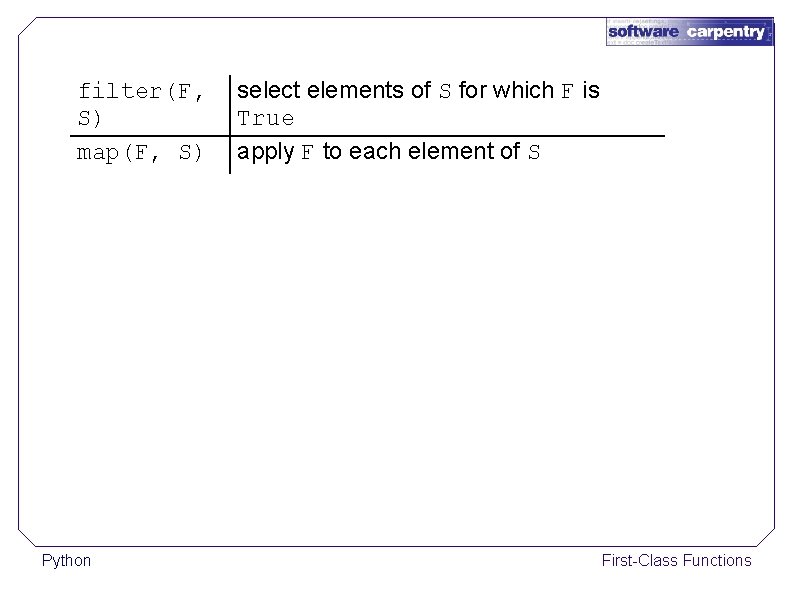
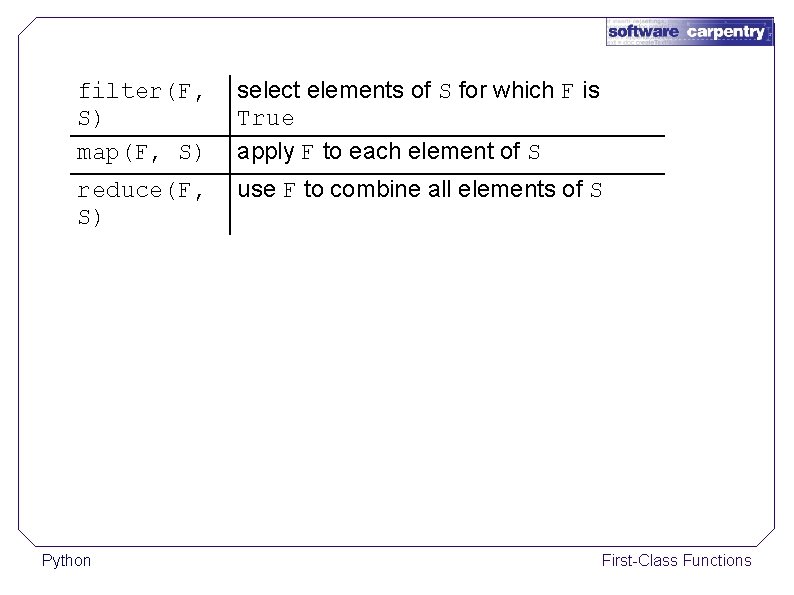
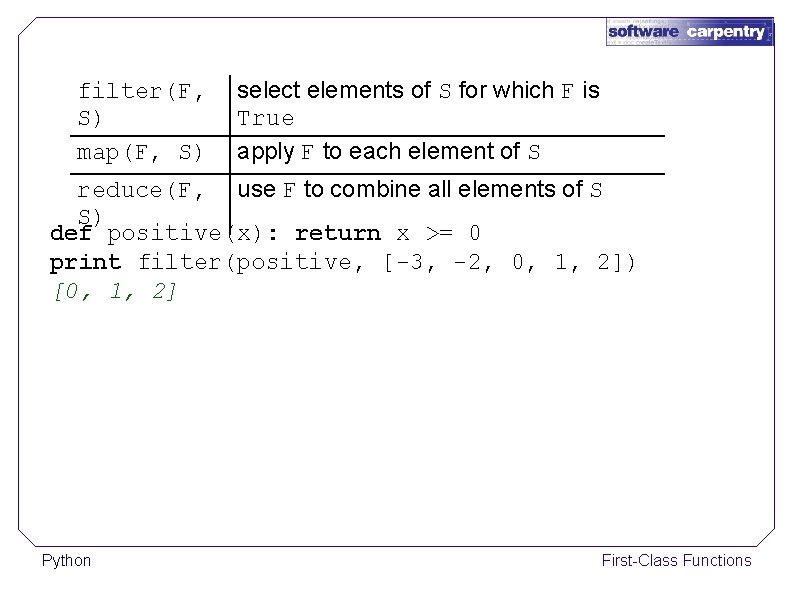
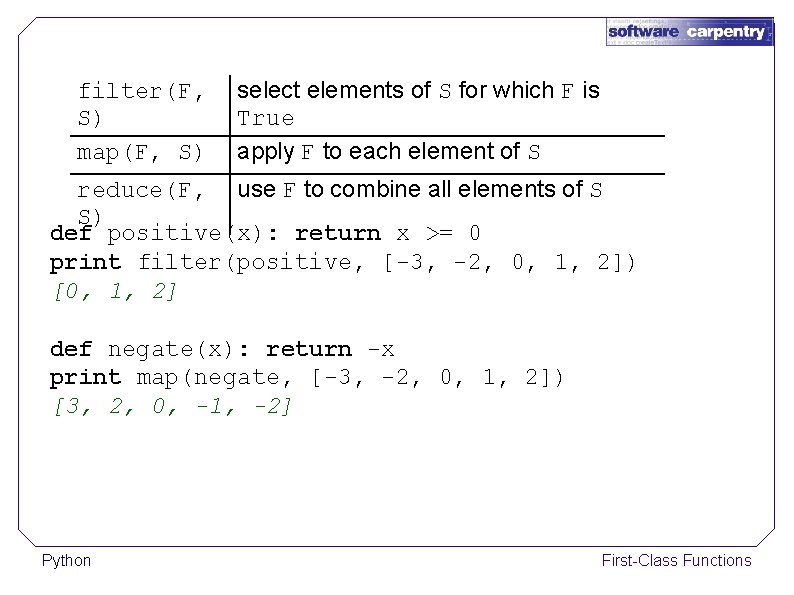
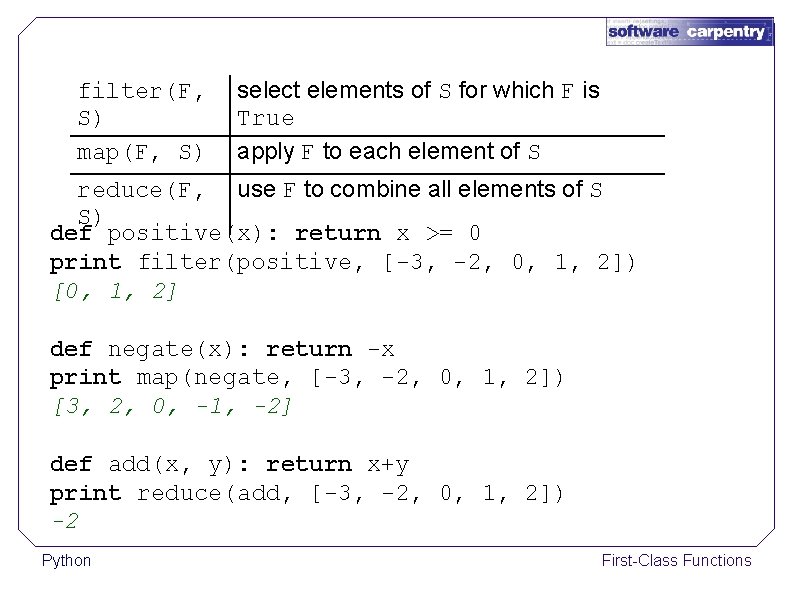
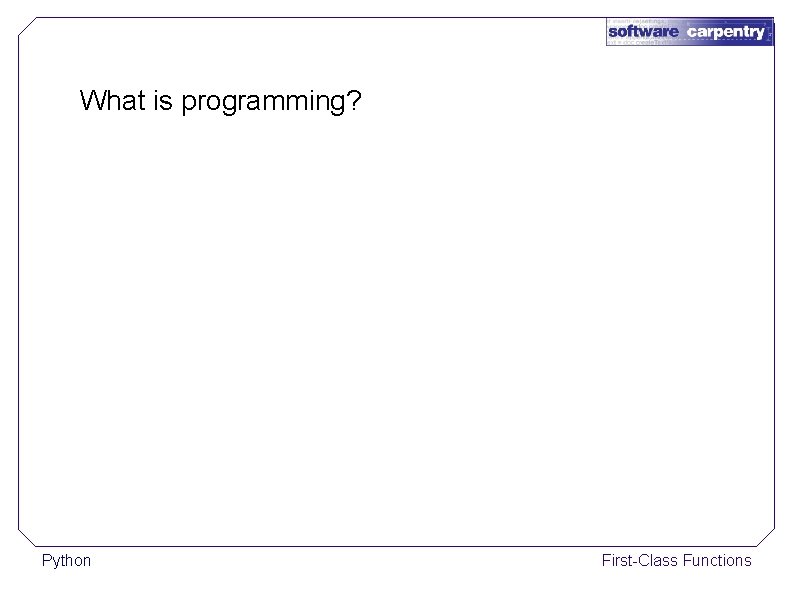
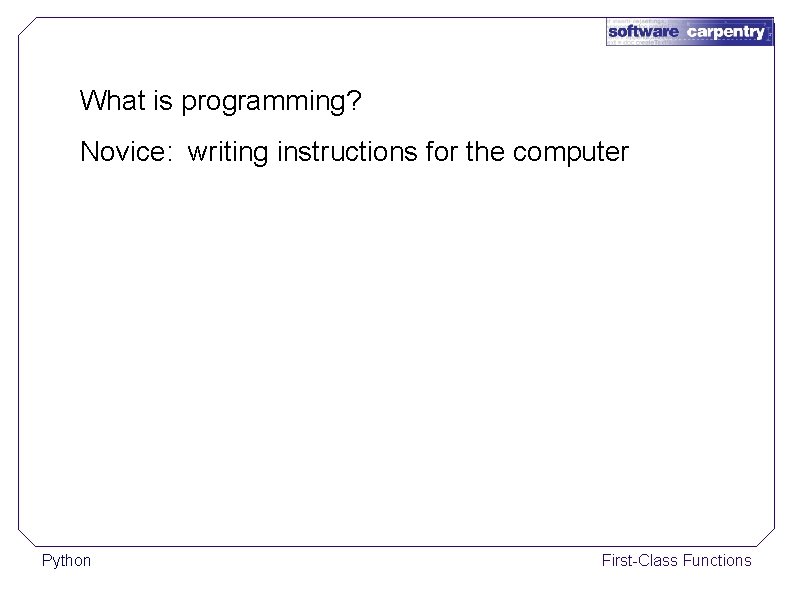
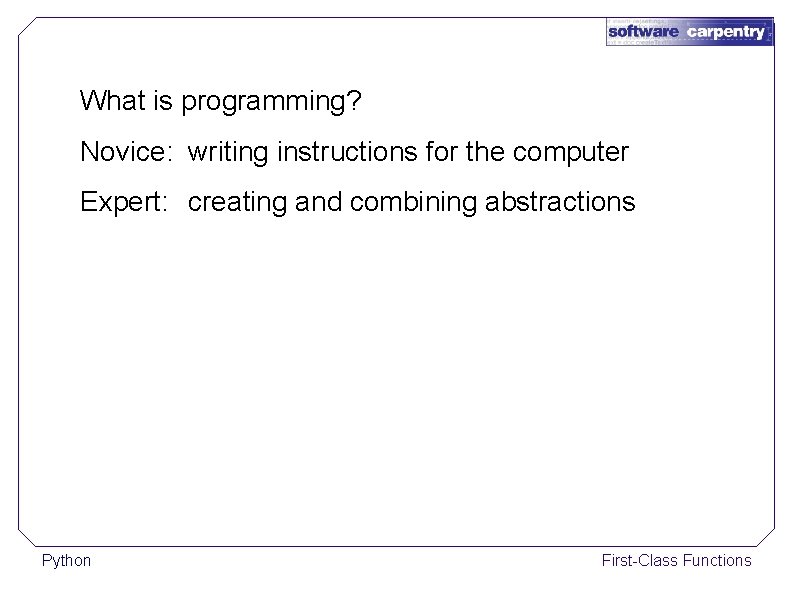
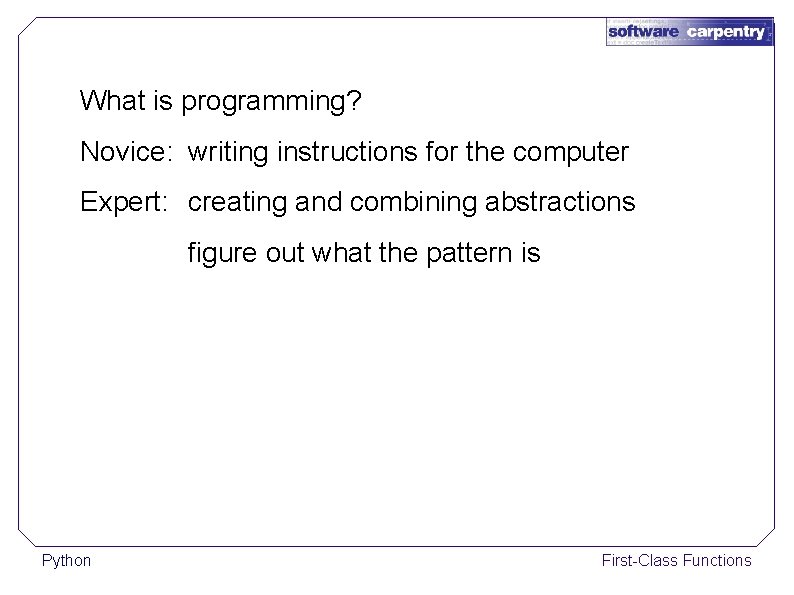
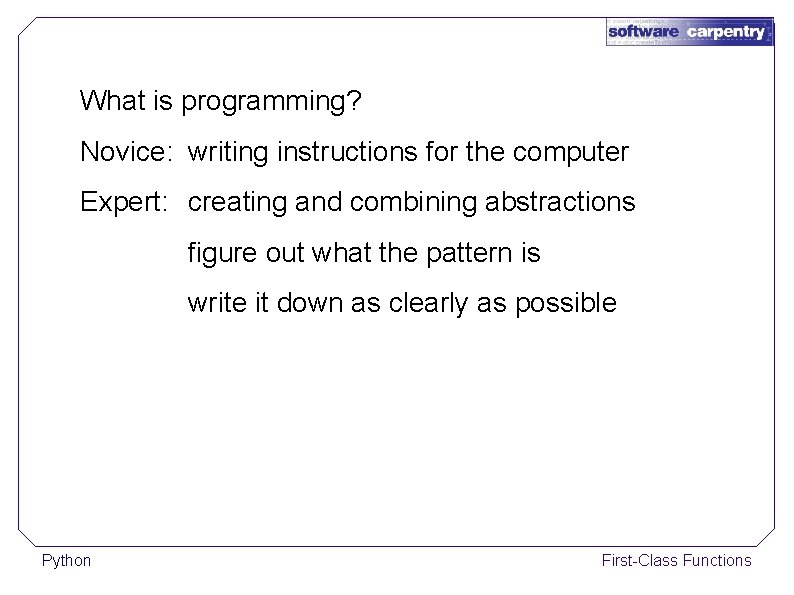
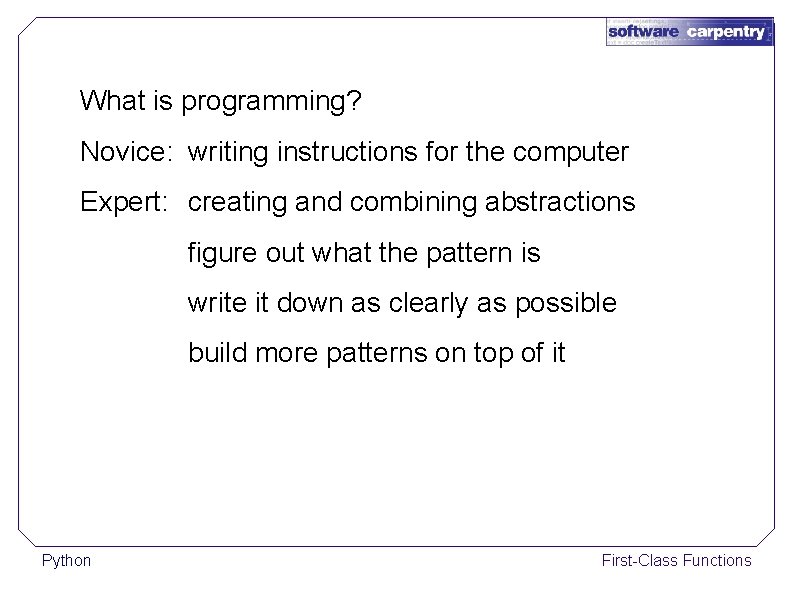
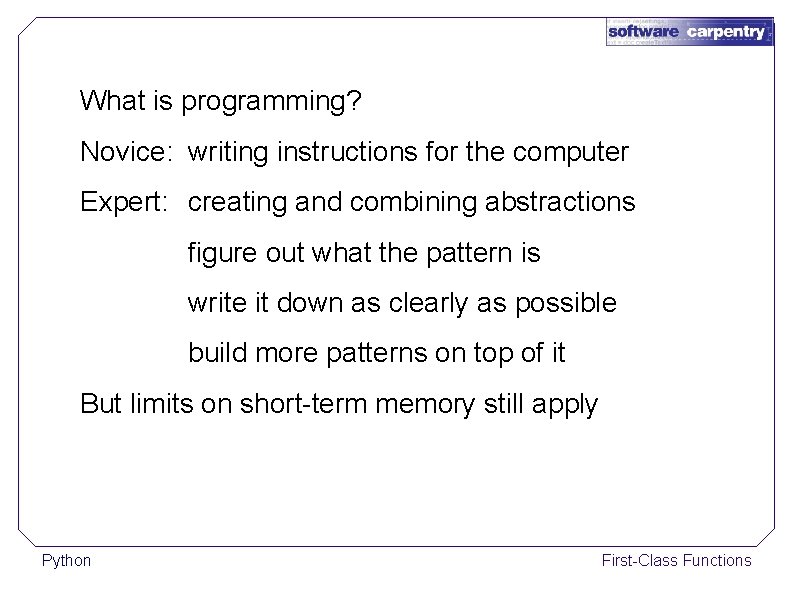
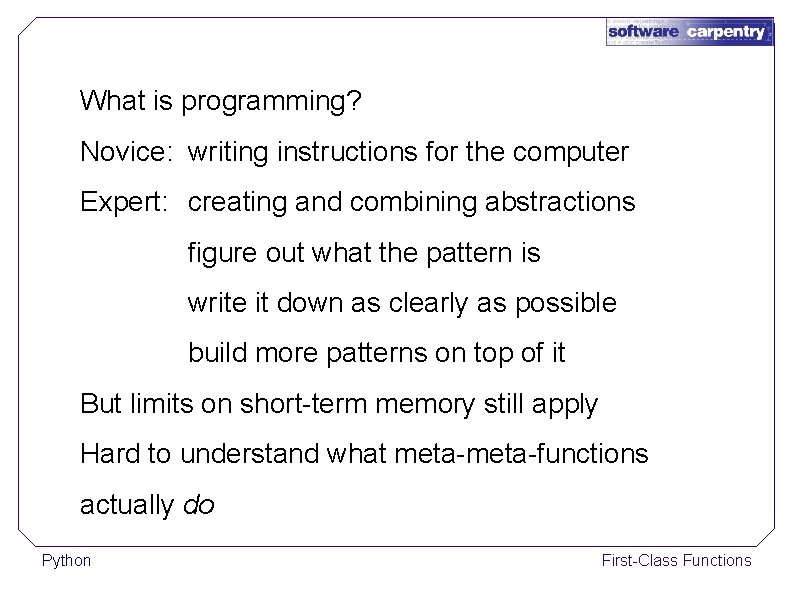
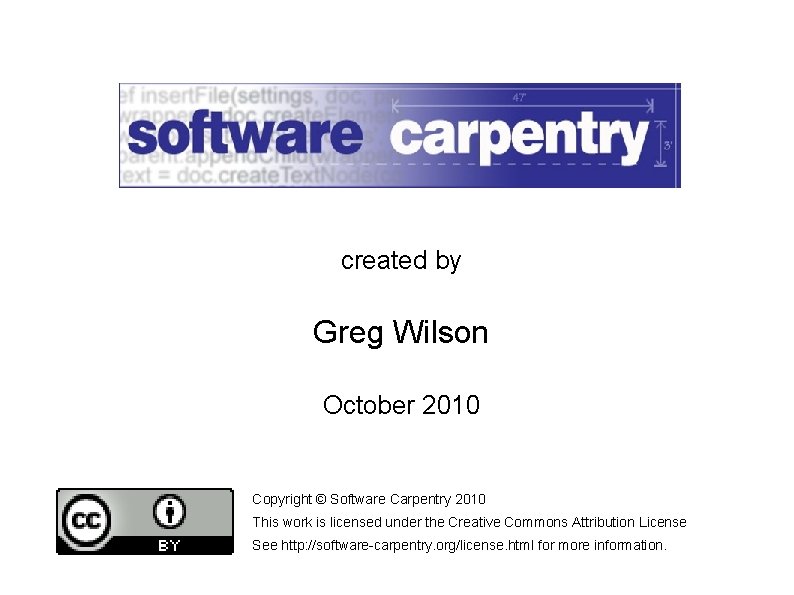
- Slides: 70
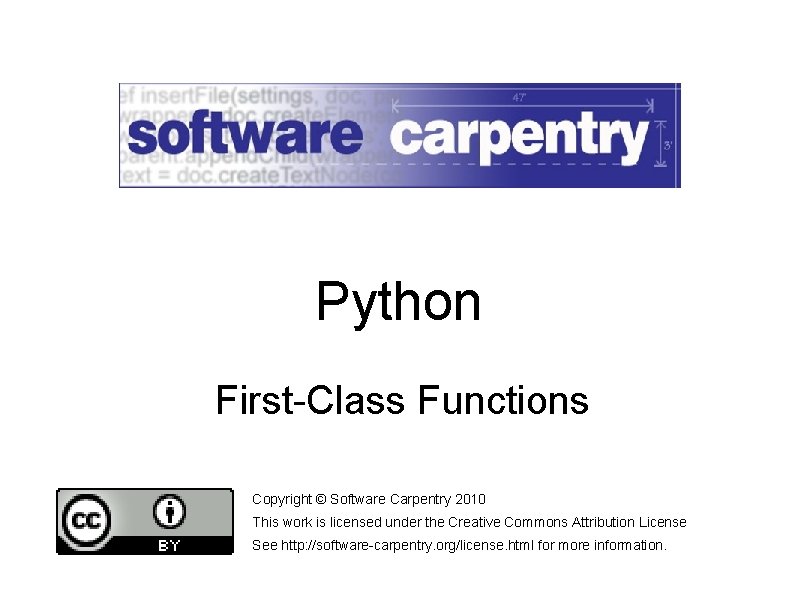
Python First-Class Functions Copyright © Software Carpentry 2010 This work is licensed under the Creative Commons Attribution License See http: //software-carpentry. org/license. html for more information.
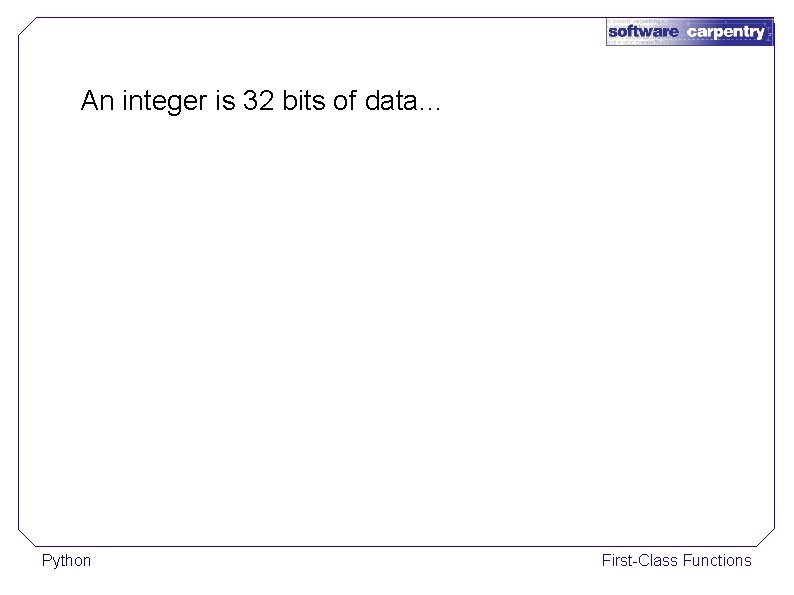
An integer is 32 bits of data. . . Python First-Class Functions
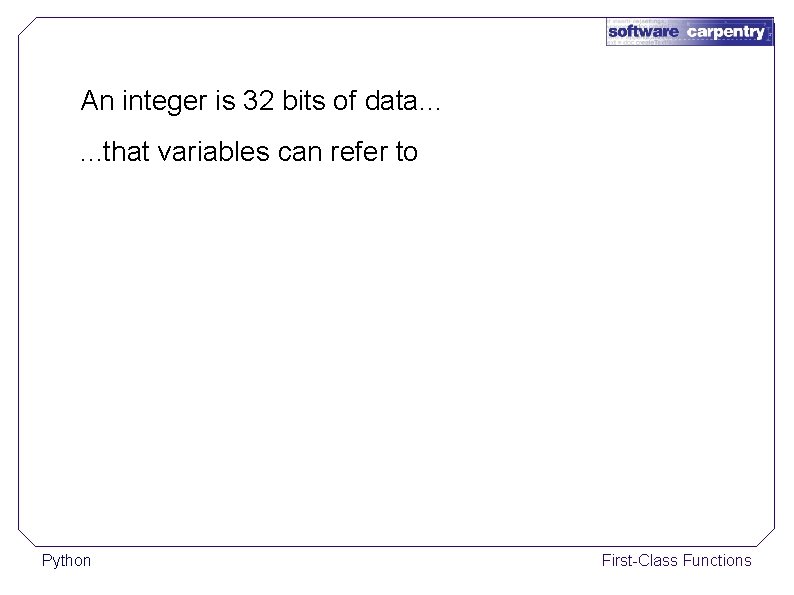
An integer is 32 bits of data. . . that variables can refer to Python First-Class Functions
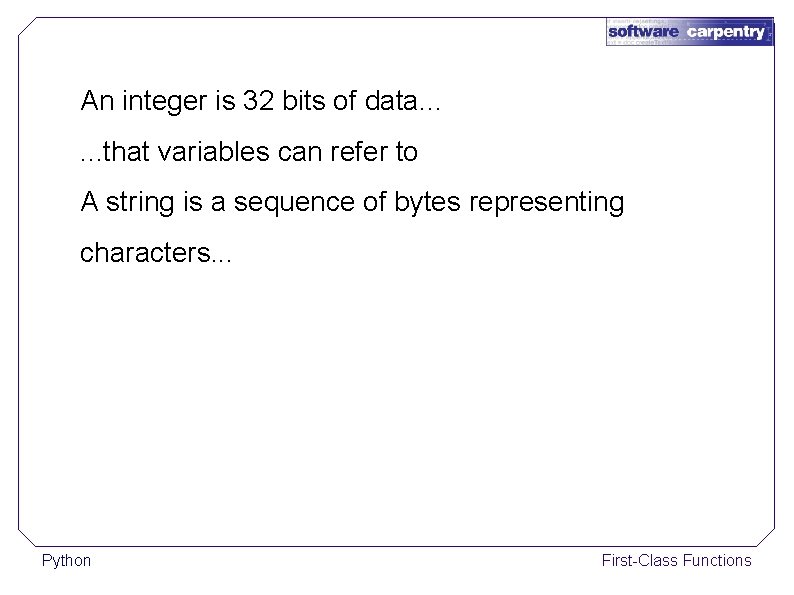
An integer is 32 bits of data. . . that variables can refer to A string is a sequence of bytes representing characters. . . Python First-Class Functions
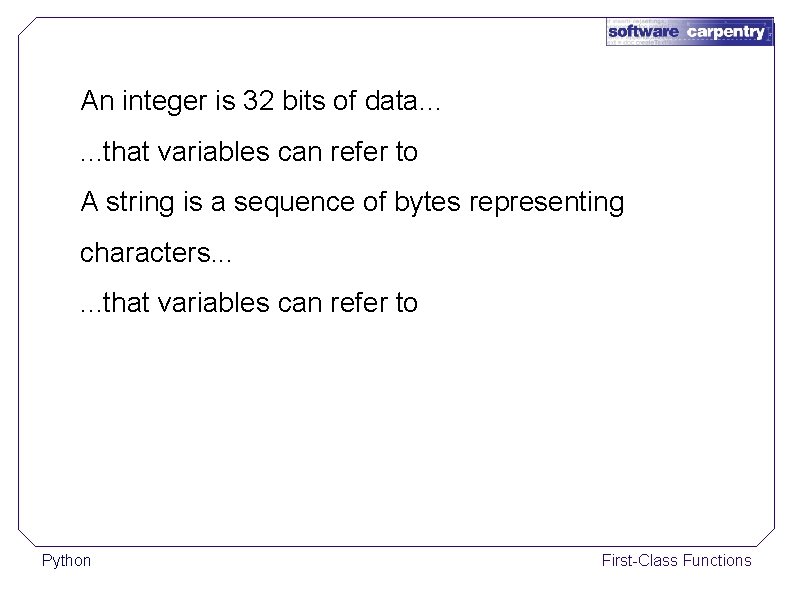
An integer is 32 bits of data. . . that variables can refer to A string is a sequence of bytes representing characters. . . that variables can refer to Python First-Class Functions
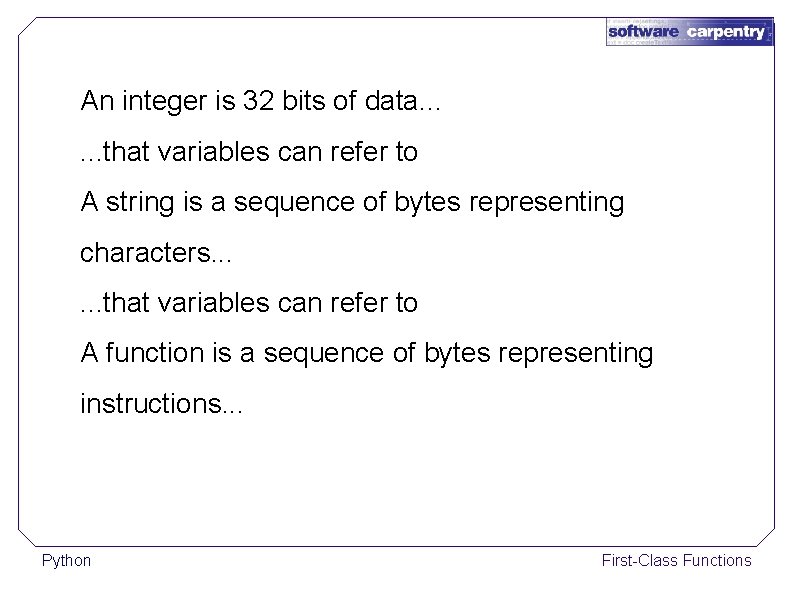
An integer is 32 bits of data. . . that variables can refer to A string is a sequence of bytes representing characters. . . that variables can refer to A function is a sequence of bytes representing instructions. . . Python First-Class Functions
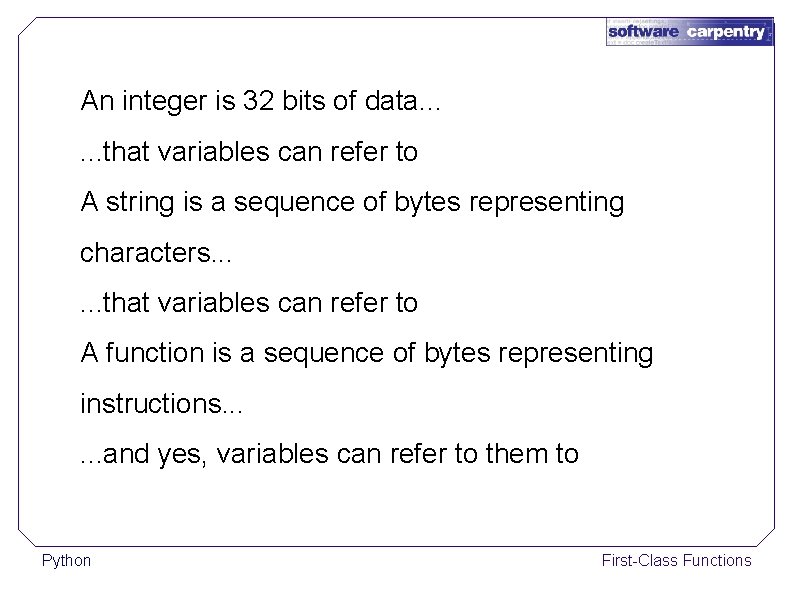
An integer is 32 bits of data. . . that variables can refer to A string is a sequence of bytes representing characters. . . that variables can refer to A function is a sequence of bytes representing instructions. . . and yes, variables can refer to them to Python First-Class Functions
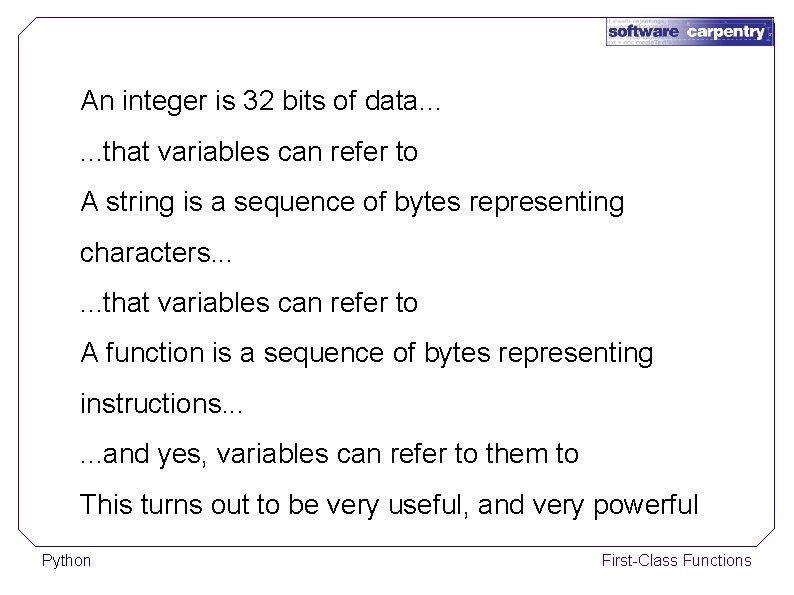
An integer is 32 bits of data. . . that variables can refer to A string is a sequence of bytes representing characters. . . that variables can refer to A function is a sequence of bytes representing instructions. . . and yes, variables can refer to them to This turns out to be very useful, and very powerful Python First-Class Functions
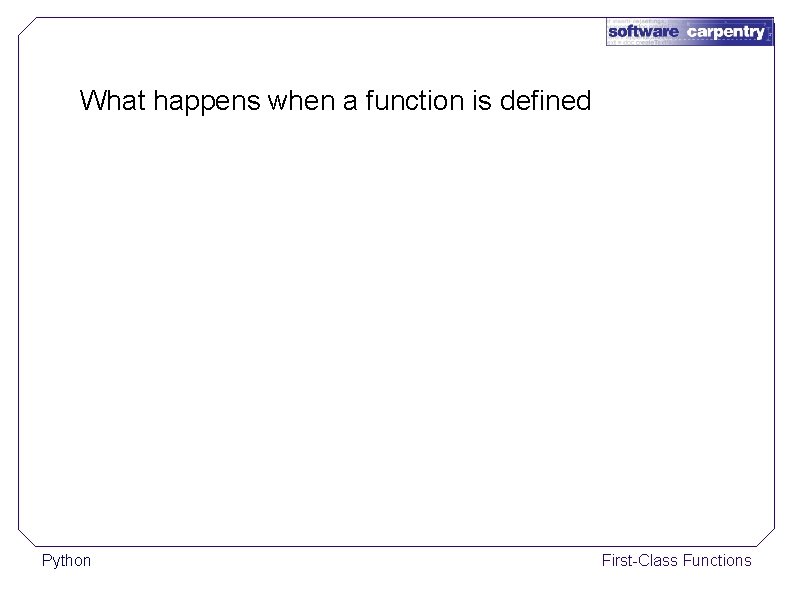
What happens when a function is defined Python First-Class Functions
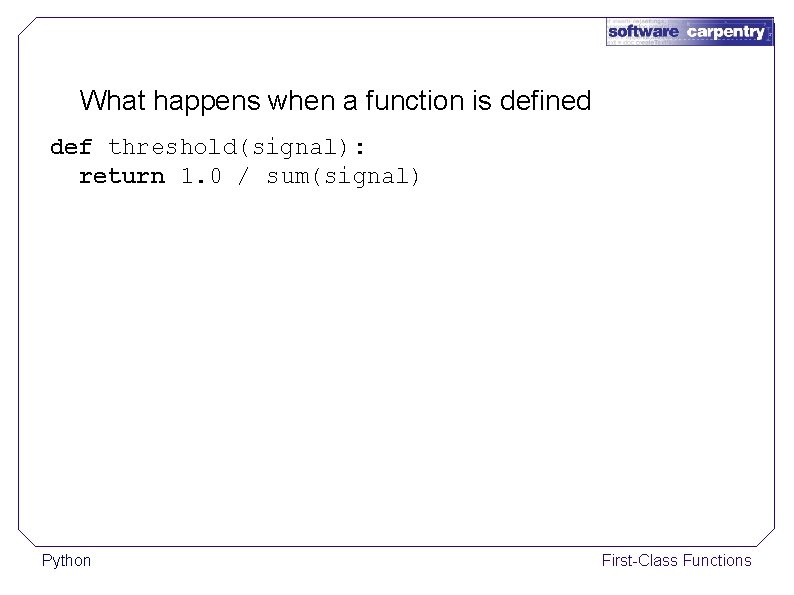
What happens when a function is defined def threshold(signal): return 1. 0 / sum(signal) Python First-Class Functions
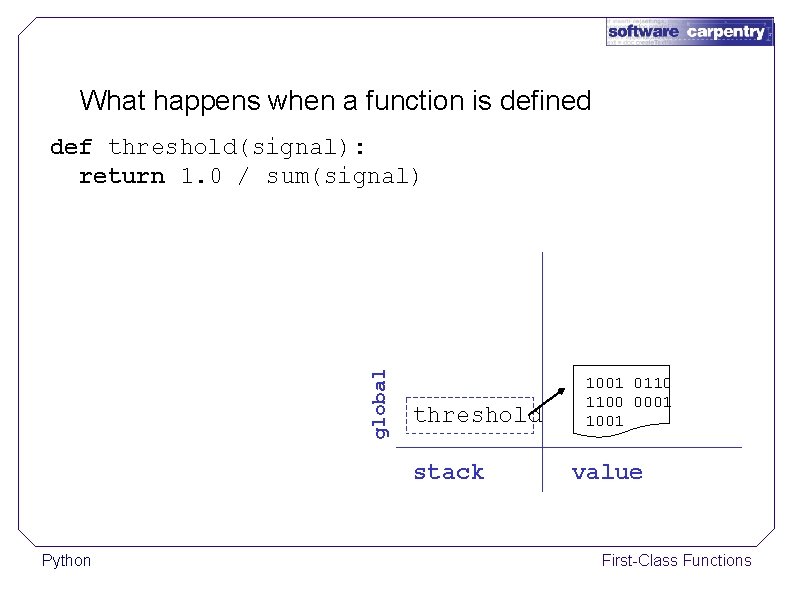
What happens when a function is defined global def threshold(signal): return 1. 0 / sum(signal) threshold stack Python 1001 0110 1100 0001 1001 value First-Class Functions
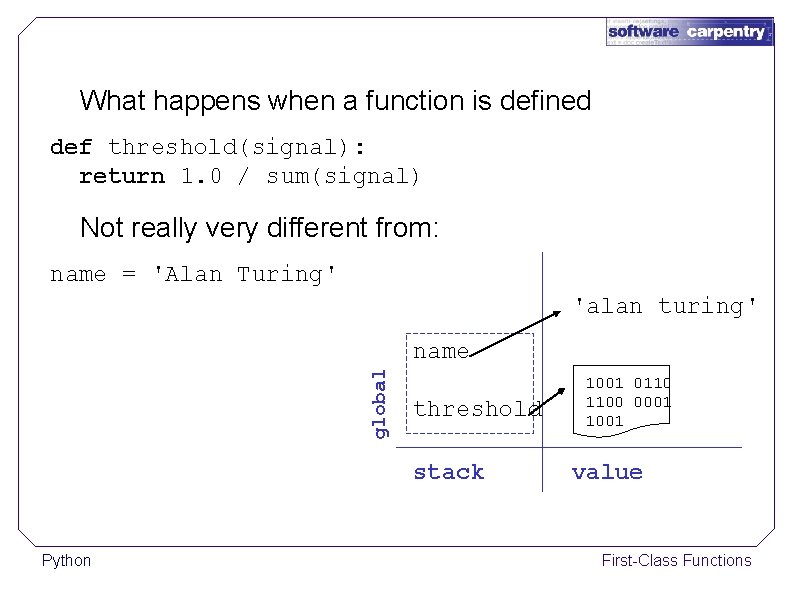
What happens when a function is defined def threshold(signal): return 1. 0 / sum(signal) Not really very different from: name = 'Alan Turing' 'alan turing' global name threshold stack Python 1001 0110 1100 0001 1001 value First-Class Functions
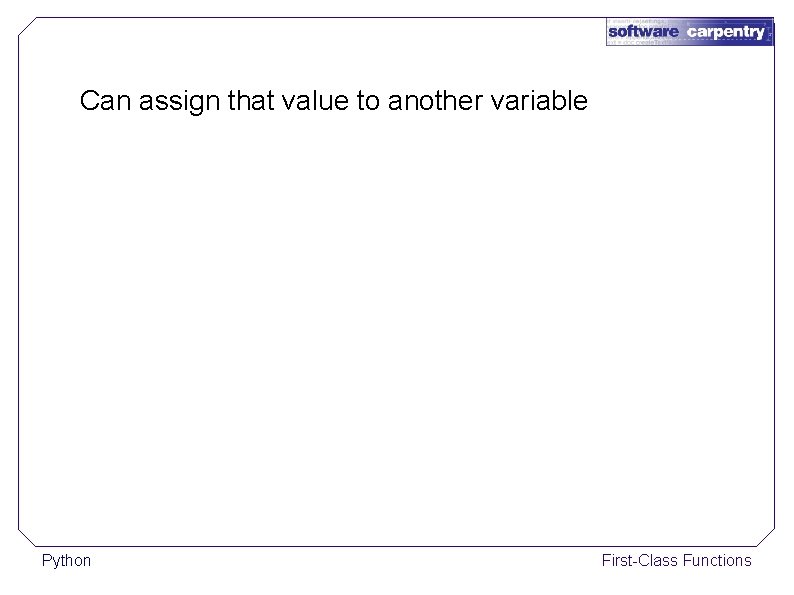
Can assign that value to another variable Python First-Class Functions
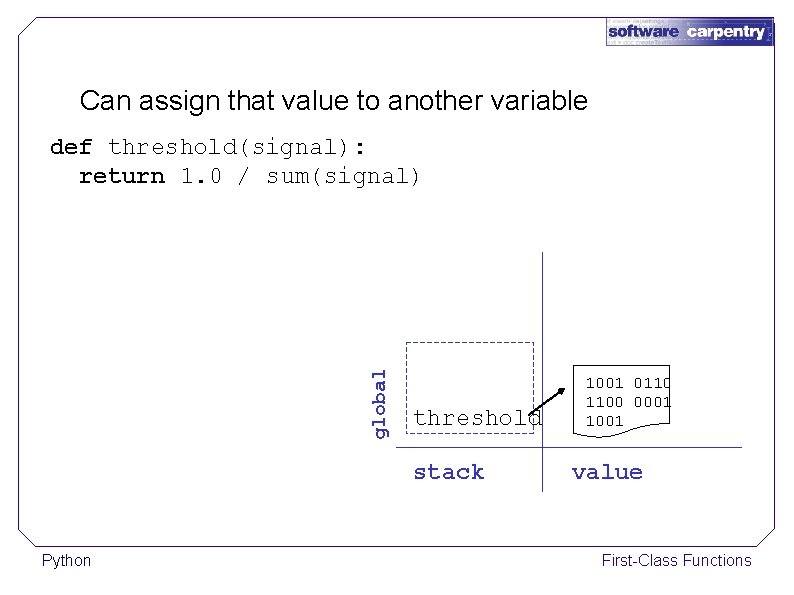
Can assign that value to another variable global def threshold(signal): return 1. 0 / sum(signal) threshold stack Python 1001 0110 1100 0001 1001 value First-Class Functions
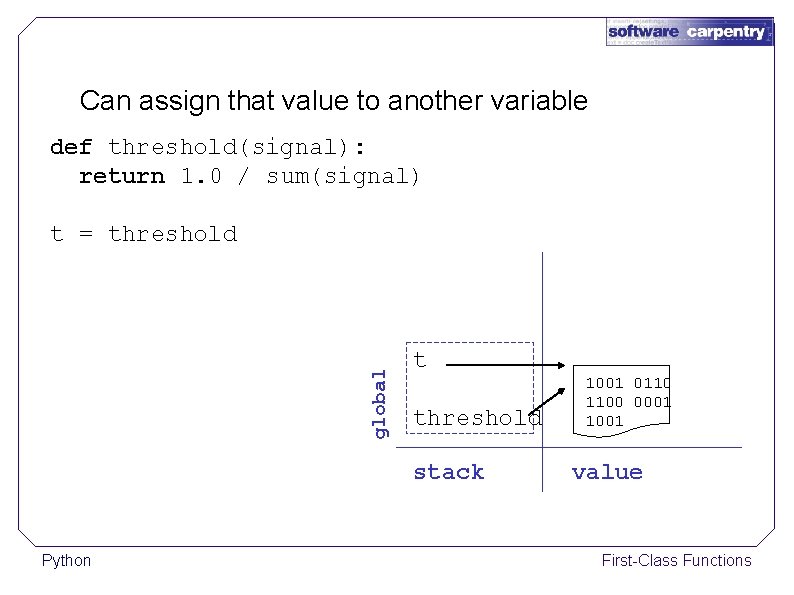
Can assign that value to another variable def threshold(signal): return 1. 0 / sum(signal) global t = threshold t threshold stack Python 1001 0110 1100 0001 1001 value First-Class Functions
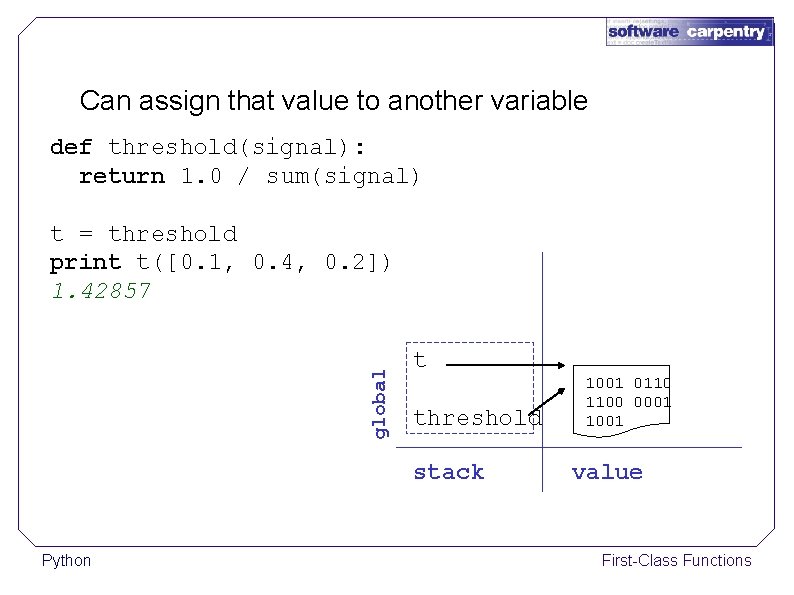
Can assign that value to another variable def threshold(signal): return 1. 0 / sum(signal) global t = threshold print t([0. 1, 0. 4, 0. 2]) 1. 42857 t threshold stack Python 1001 0110 1100 0001 1001 value First-Class Functions
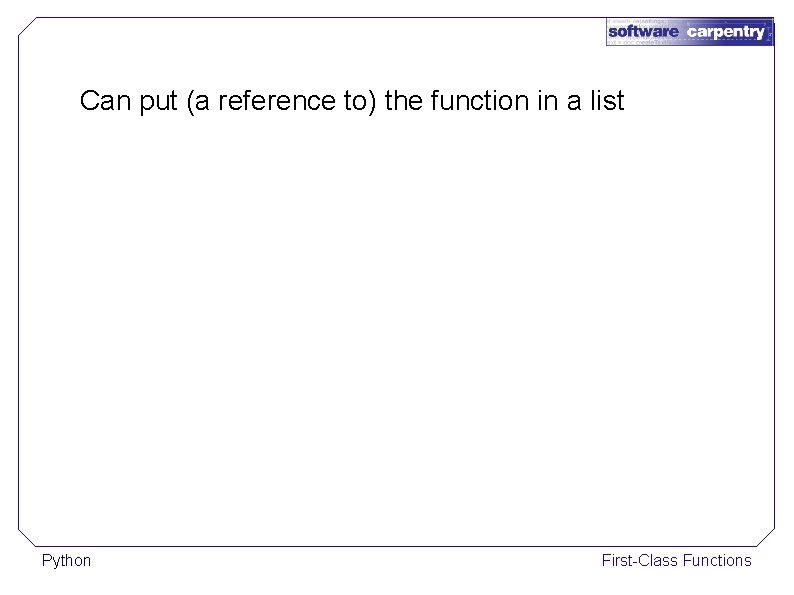
Can put (a reference to) the function in a list Python First-Class Functions
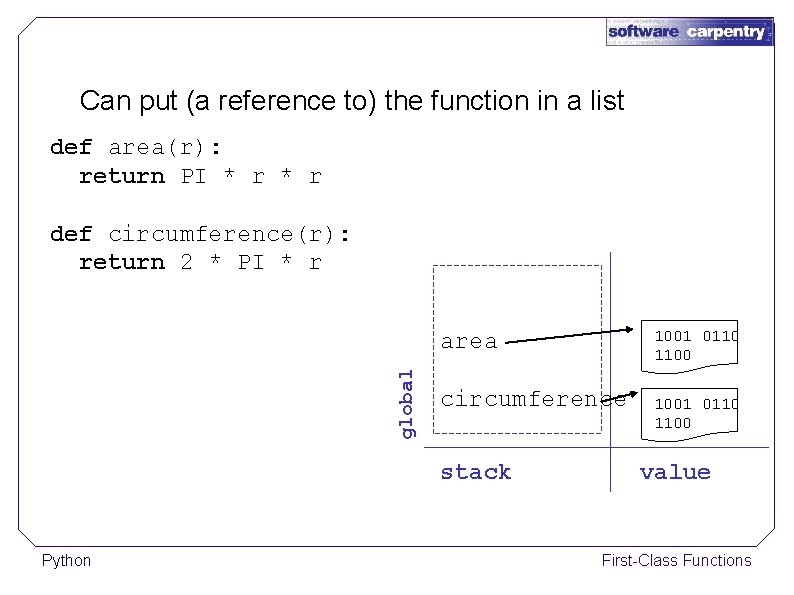
Can put (a reference to) the function in a list def area(r): return PI * r def circumference(r): return 2 * PI * r 1001 0110 1100 global area circumference stack Python 1001 0110 1100 value First-Class Functions
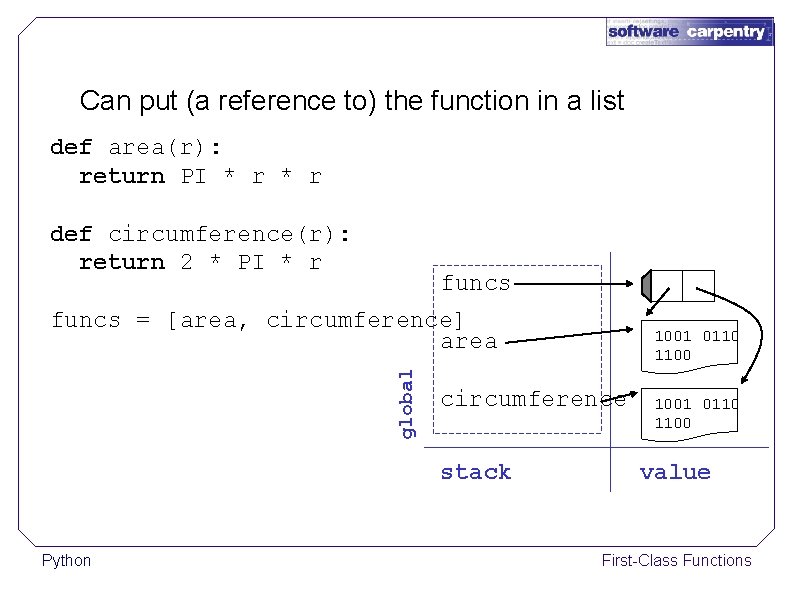
Can put (a reference to) the function in a list def area(r): return PI * r def circumference(r): return 2 * PI * r funcs global funcs = [area, circumference] area circumference stack Python 1001 0110 1100 value First-Class Functions
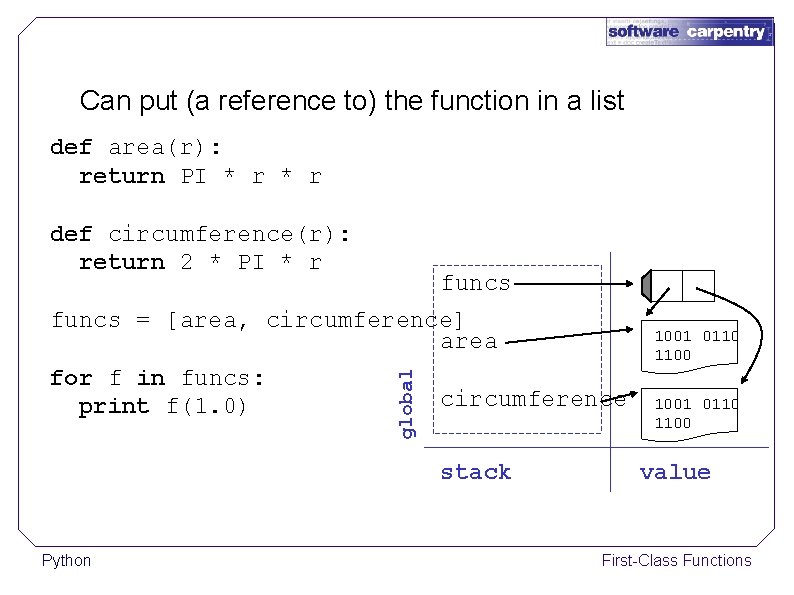
Can put (a reference to) the function in a list def area(r): return PI * r def circumference(r): return 2 * PI * r funcs for f in funcs: print f(1. 0) global funcs = [area, circumference] area circumference stack Python 1001 0110 1100 value First-Class Functions
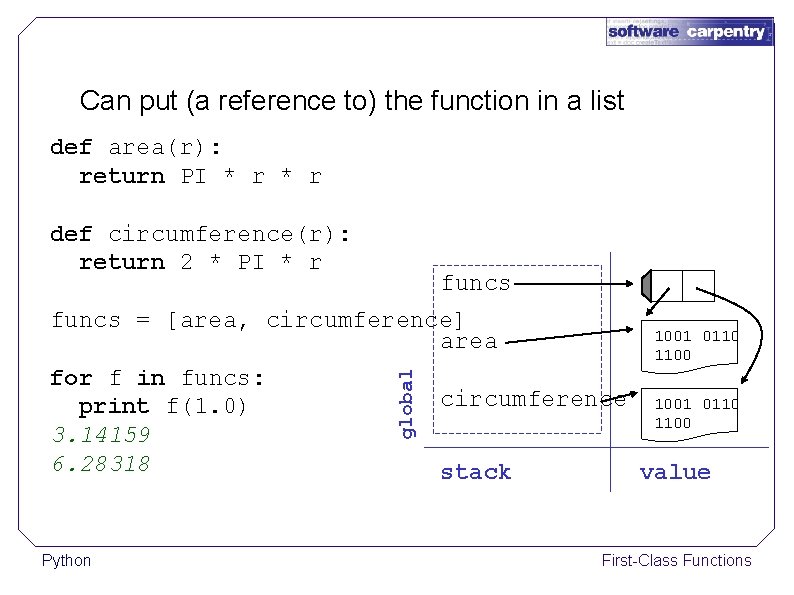
Can put (a reference to) the function in a list def area(r): return PI * r def circumference(r): return 2 * PI * r funcs for f in funcs: print f(1. 0) 3. 14159 6. 28318 Python global funcs = [area, circumference] area 1001 0110 1100 circumference stack 1001 0110 1100 value First-Class Functions
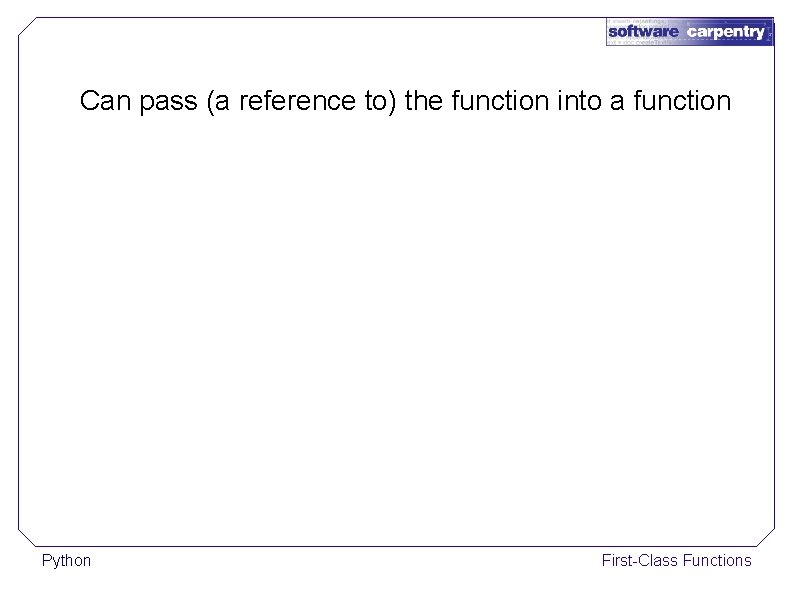
Can pass (a reference to) the function into a function Python First-Class Functions
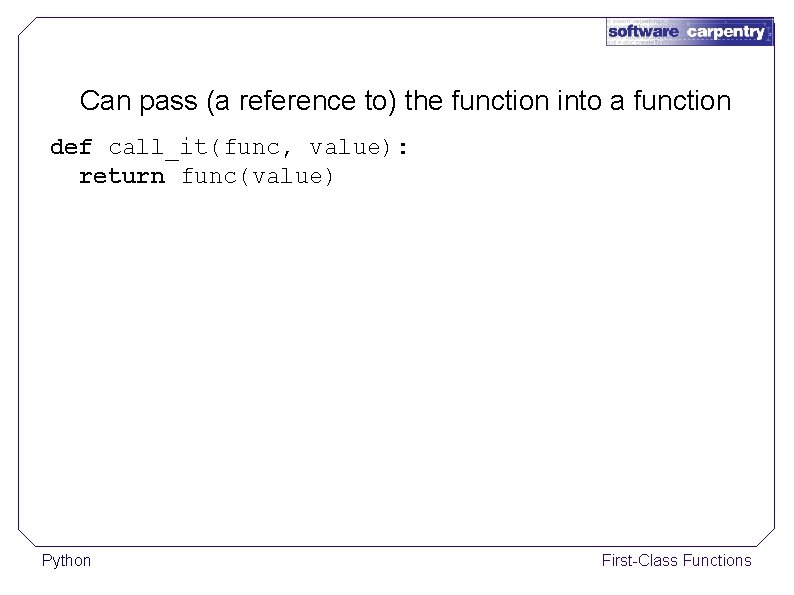
Can pass (a reference to) the function into a function def call_it(func, value): return func(value) Python First-Class Functions
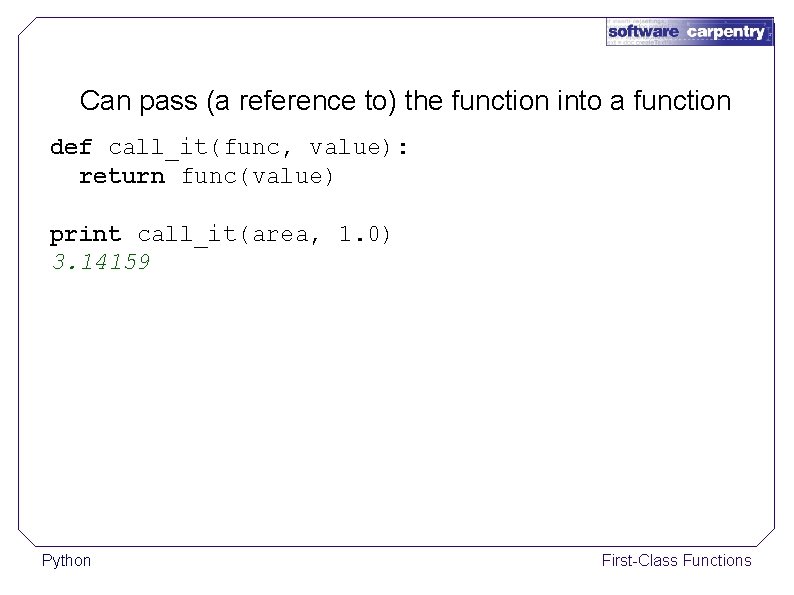
Can pass (a reference to) the function into a function def call_it(func, value): return func(value) print call_it(area, 1. 0) 3. 14159 Python First-Class Functions
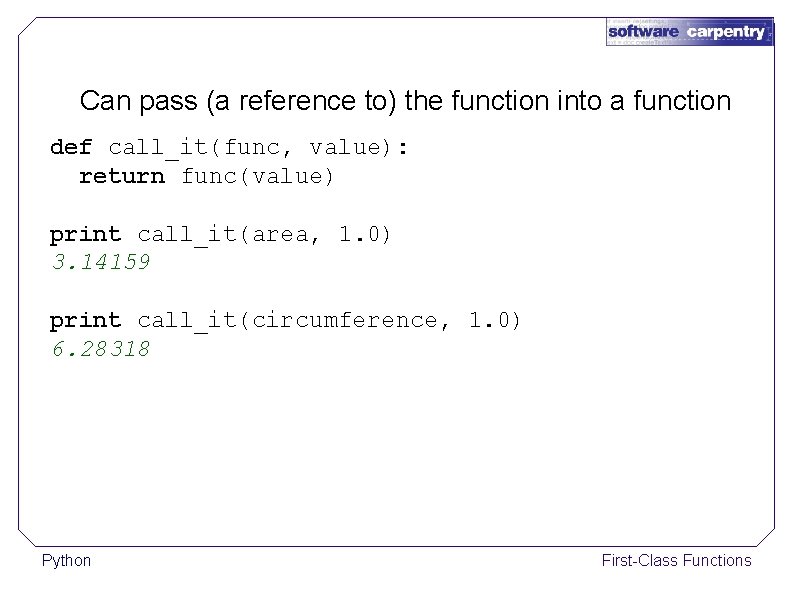
Can pass (a reference to) the function into a function def call_it(func, value): return func(value) print call_it(area, 1. 0) 3. 14159 print call_it(circumference, 1. 0) 6. 28318 Python First-Class Functions
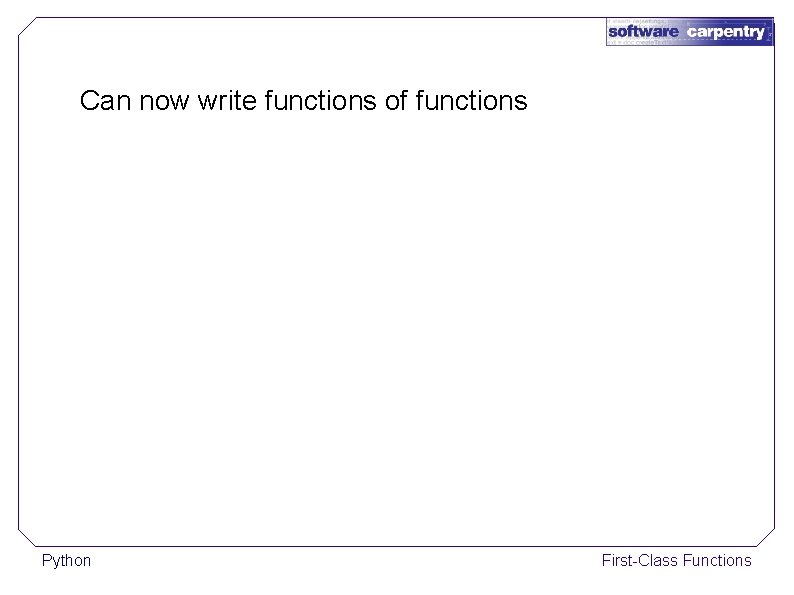
Can now write functions of functions Python First-Class Functions
![Can now write functions of functions def doallfunc values result for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-27.jpg)
Can now write functions of functions def do_all(func, values): result = [] for v in values: temp = func(v) result. append(temp) return result Python First-Class Functions
![Can now write functions of functions def doallfunc values result for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-28.jpg)
Can now write functions of functions def do_all(func, values): result = [] for v in values: temp = func(v) result. append(temp) return result print do_all(area, [1. 0, 2. 0, 3. 0] Python First-Class Functions
![Can now write functions of functions def doallfunc values result for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-29.jpg)
Can now write functions of functions def do_all(func, values): result = [] for v in values: temp = func(v) result. append(temp) return result print do_all(area, [1. 0, 2. 0, 3. 0] [3. 14159, 12. 56636, 28. 27431] Python First-Class Functions
![Can now write functions of functions def doallfunc values result for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-30.jpg)
Can now write functions of functions def do_all(func, values): result = [] for v in values: temp = func(v) result. append(temp) return result print do_all(area, [1. 0, 2. 0, 3. 0] [3. 14159, 12. 56636, 28. 27431] def slim(text): return text[1: -1] Python First-Class Functions
![Can now write functions of functions def doallfunc values result for v Can now write functions of functions def do_all(func, values): result = [] for v](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-31.jpg)
Can now write functions of functions def do_all(func, values): result = [] for v in values: temp = func(v) result. append(temp) return result print do_all(area, [1. 0, 2. 0, 3. 0] [3. 14159, 12. 56636, 28. 27431] def slim(text): return text[1: -1] print do_all(slim, ['abc', 'defgh' b efg Python First-Class Functions
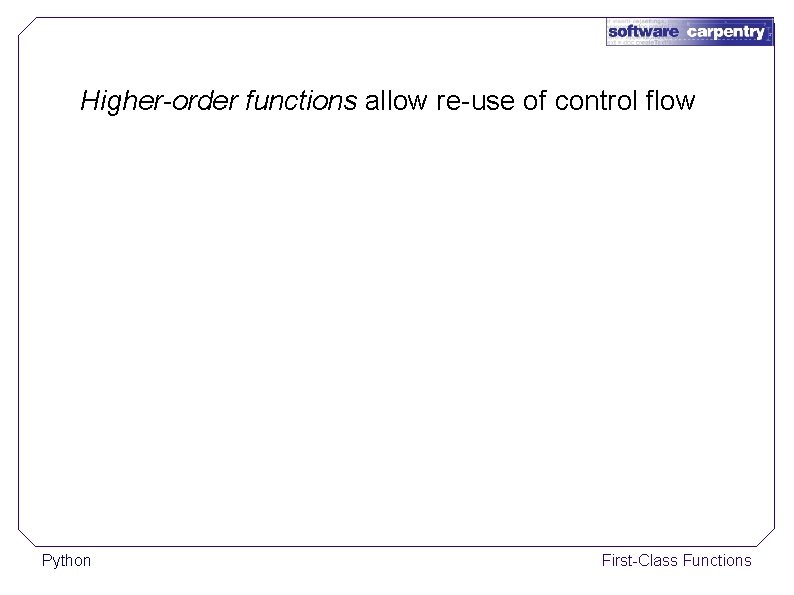
Higher-order functions allow re-use of control flow Python First-Class Functions
![Higherorder functions allow reuse of control flow def combinevaluesfunc values current values0 for Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-33.jpg)
Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current Python First-Class Functions
![Higherorder functions allow reuse of control flow def combinevaluesfunc values current values0 for Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-34.jpg)
Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current def add(x, y): return x + y def mul(x, y): return x * y Python First-Class Functions
![Higherorder functions allow reuse of control flow def combinevaluesfunc values current values0 for Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-35.jpg)
Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current def add(x, y): return x + y def mul(x, y): return x * y print combine_values(add, [1, 3, 5]) 9 Python First-Class Functions
![Higherorder functions allow reuse of control flow def combinevaluesfunc values current values0 for Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-36.jpg)
Higher-order functions allow re-use of control flow def combine_values(func, values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current def add(x, y): return x + y def mul(x, y): return x * y print combine_values(add, [1, 3, 5]) 9 print combine_values(mul, [1, 3, 5]) 15 Python First-Class Functions
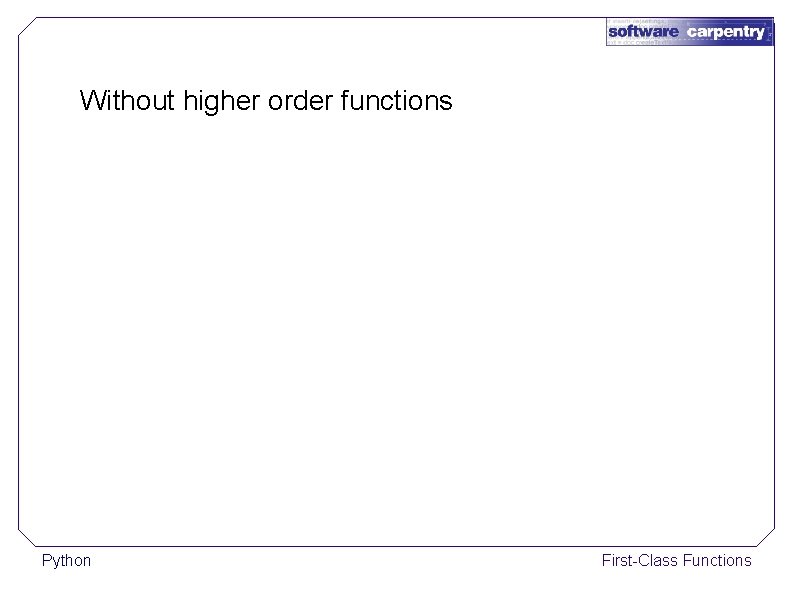
Without higher order functions Python First-Class Functions
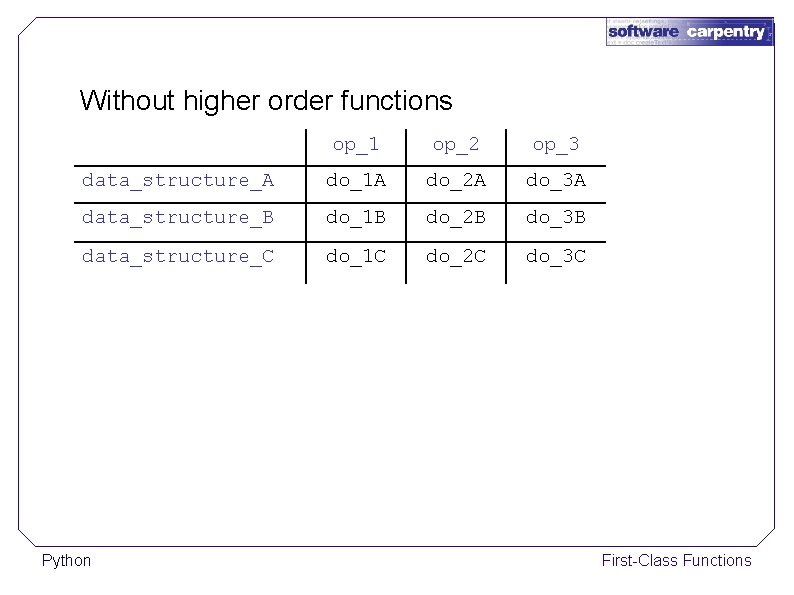
Without higher order functions op_1 op_2 op_3 data_structure_A do_1 A do_2 A do_3 A data_structure_B do_1 B do_2 B do_3 B data_structure_C do_1 C do_2 C do_3 C Python First-Class Functions
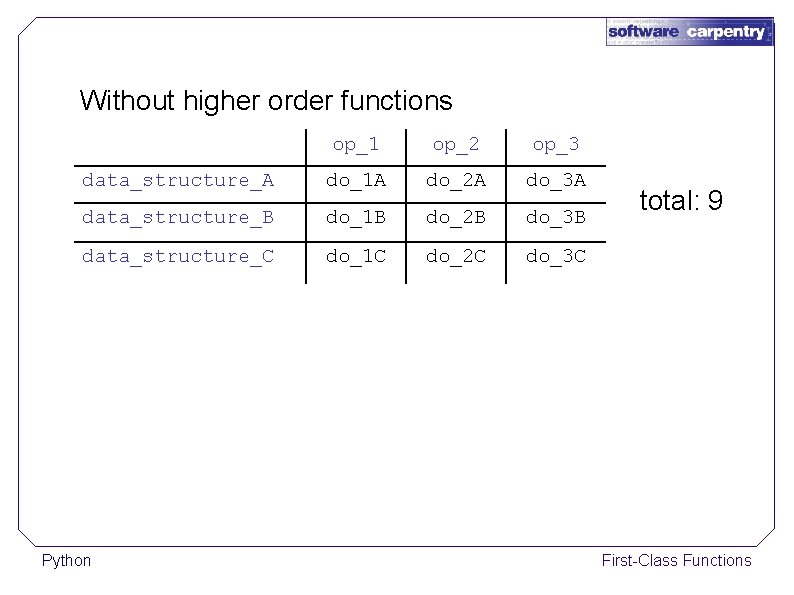
Without higher order functions op_1 op_2 op_3 data_structure_A do_1 A do_2 A do_3 A data_structure_B do_1 B do_2 B do_3 B data_structure_C do_1 C do_2 C do_3 C Python total: 9 First-Class Functions
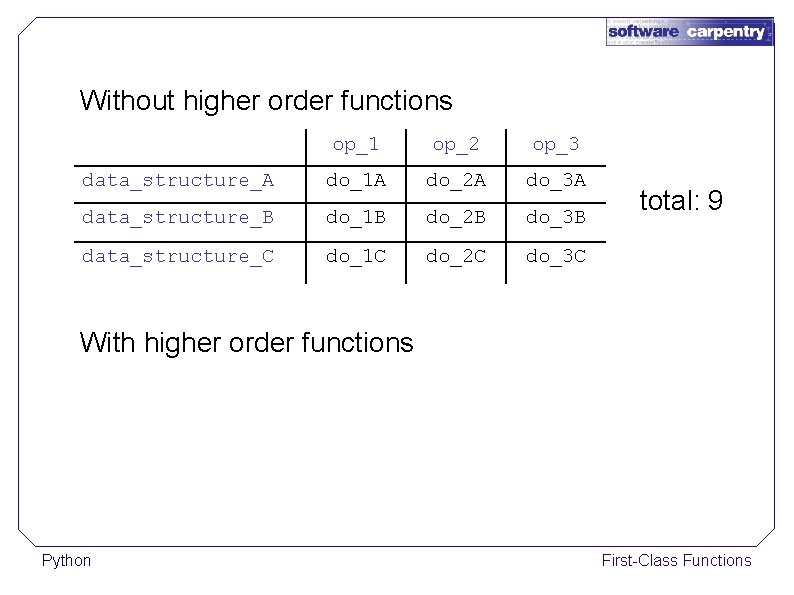
Without higher order functions op_1 op_2 op_3 data_structure_A do_1 A do_2 A do_3 A data_structure_B do_1 B do_2 B do_3 B data_structure_C do_1 C do_2 C do_3 C total: 9 With higher order functions Python First-Class Functions
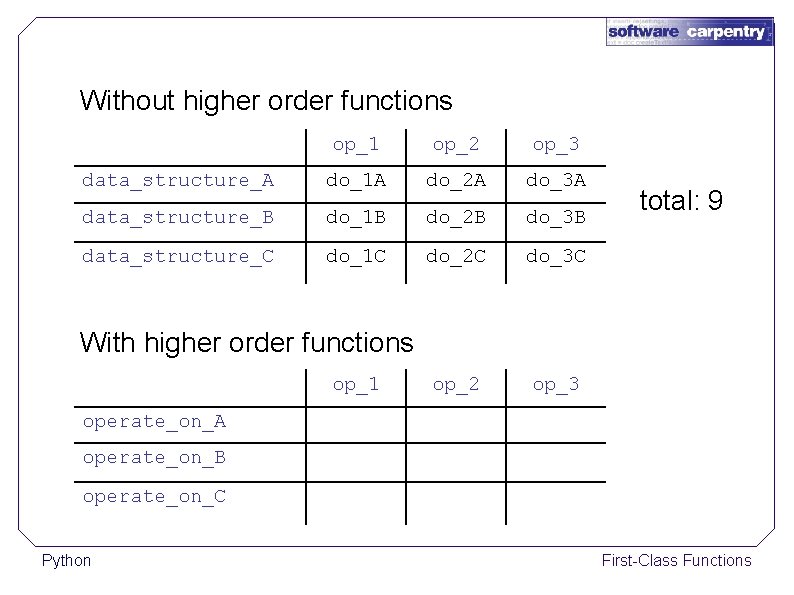
Without higher order functions op_1 op_2 op_3 data_structure_A do_1 A do_2 A do_3 A data_structure_B do_1 B do_2 B do_3 B data_structure_C do_1 C do_2 C do_3 C op_2 op_3 total: 9 With higher order functions op_1 operate_on_A operate_on_B operate_on_C Python First-Class Functions
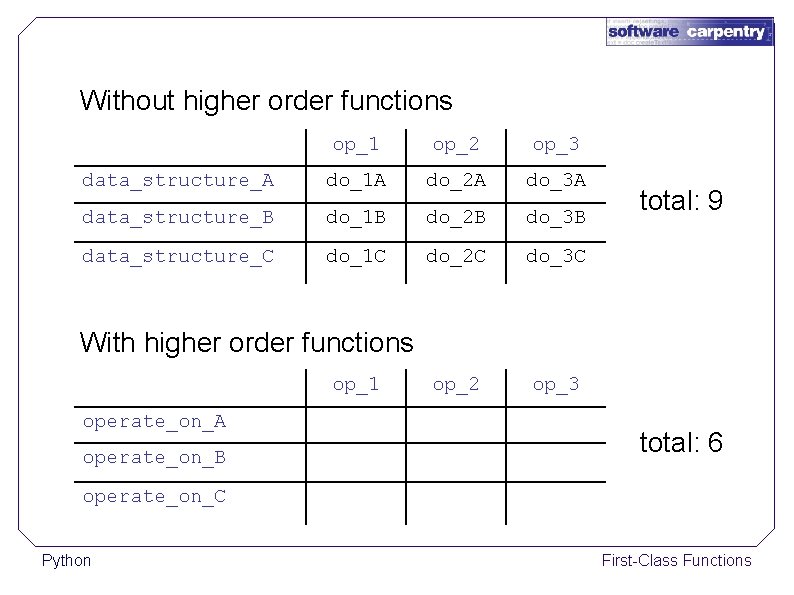
Without higher order functions op_1 op_2 op_3 data_structure_A do_1 A do_2 A do_3 A data_structure_B do_1 B do_2 B do_3 B data_structure_C do_1 C do_2 C do_3 C op_2 op_3 total: 9 With higher order functions op_1 operate_on_A operate_on_B total: 6 operate_on_C Python First-Class Functions
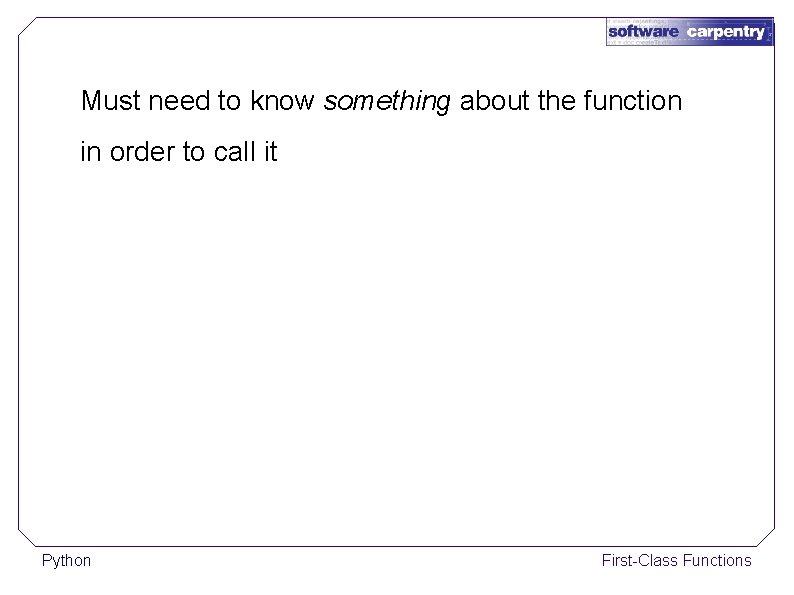
Must need to know something about the function in order to call it Python First-Class Functions
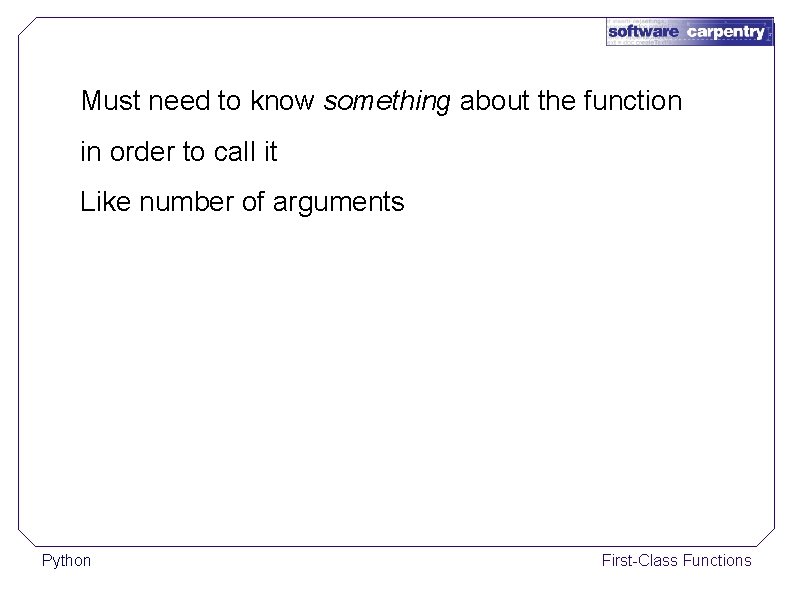
Must need to know something about the function in order to call it Like number of arguments Python First-Class Functions
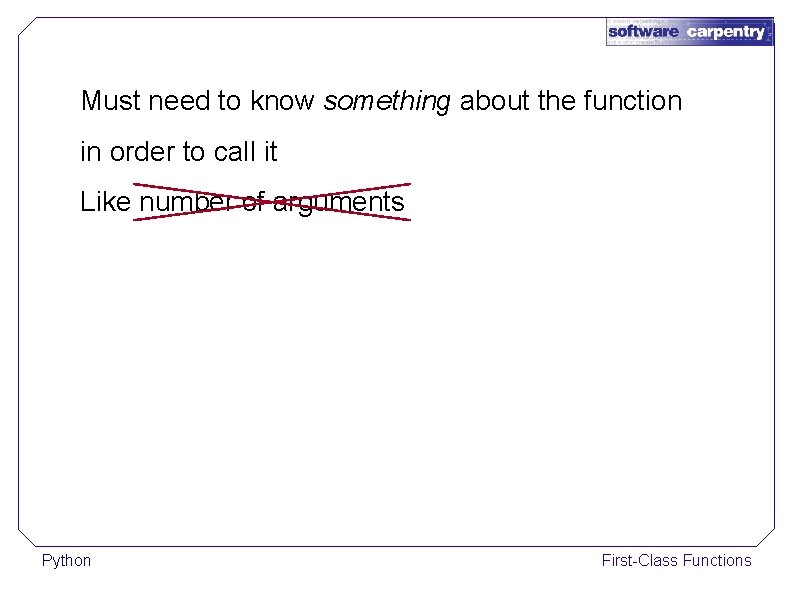
Must need to know something about the function in order to call it Like number of arguments Python First-Class Functions
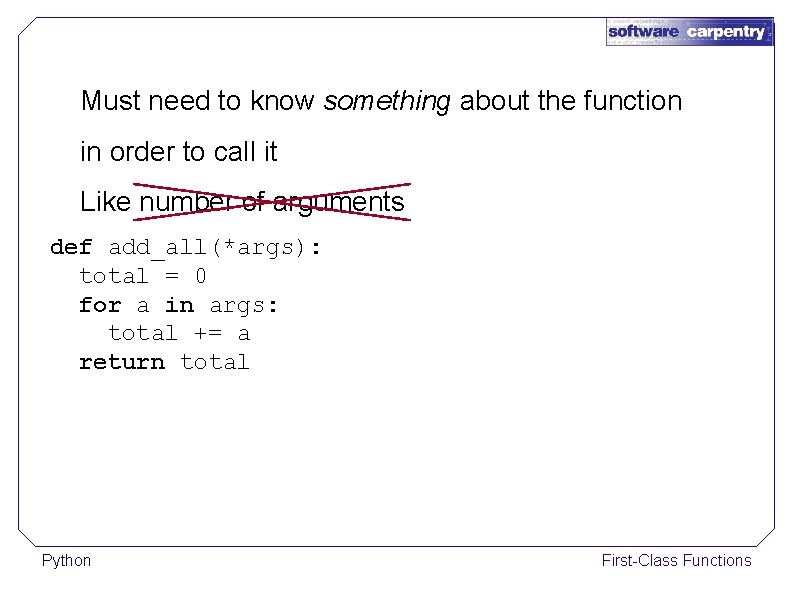
Must need to know something about the function in order to call it Like number of arguments def add_all(*args): total = 0 for a in args: total += a return total Python First-Class Functions
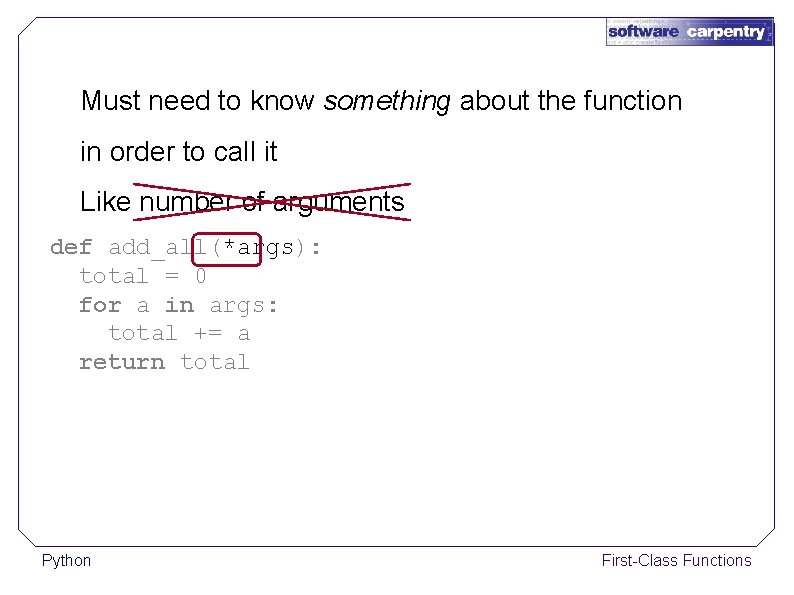
Must need to know something about the function in order to call it Like number of arguments def add_all(*args): total = 0 for a in args: total += a return total Python First-Class Functions
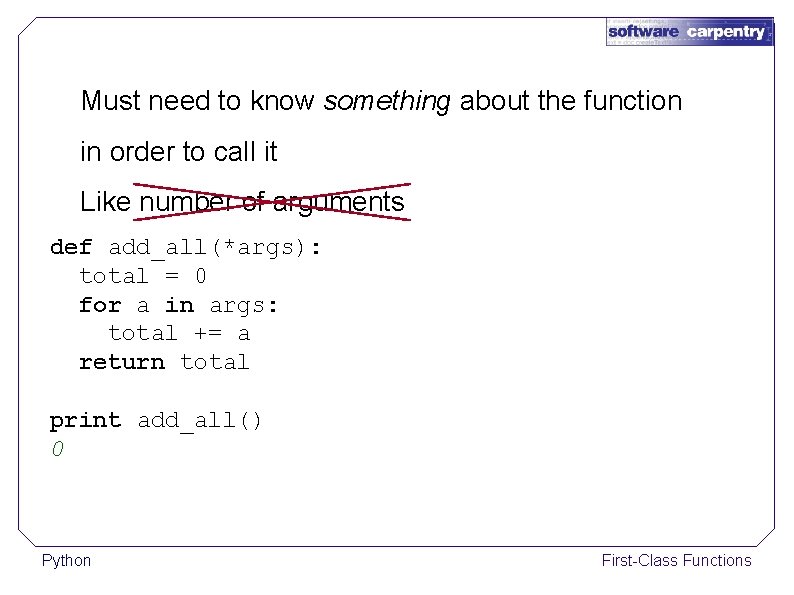
Must need to know something about the function in order to call it Like number of arguments def add_all(*args): total = 0 for a in args: total += a return total print add_all() 0 Python First-Class Functions
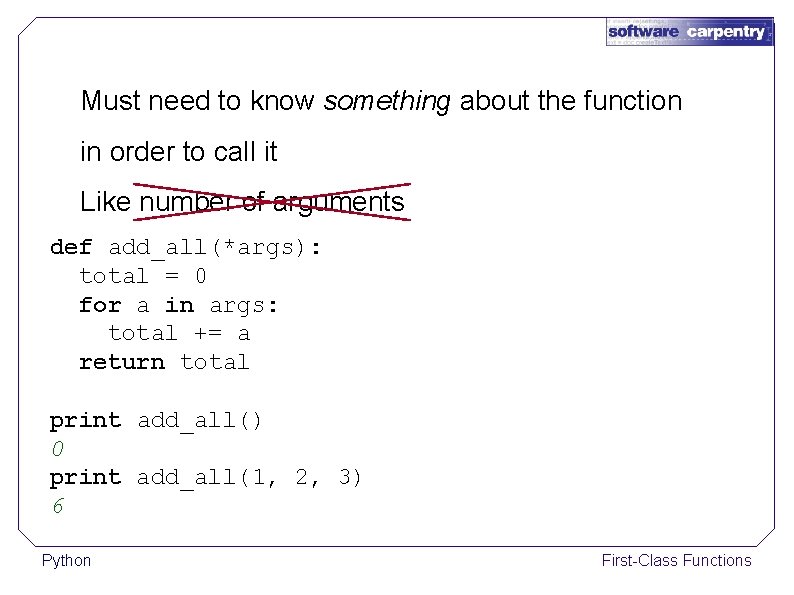
Must need to know something about the function in order to call it Like number of arguments def add_all(*args): total = 0 for a in args: total += a return total print add_all() 0 print add_all(1, 2, 3) 6 Python First-Class Functions
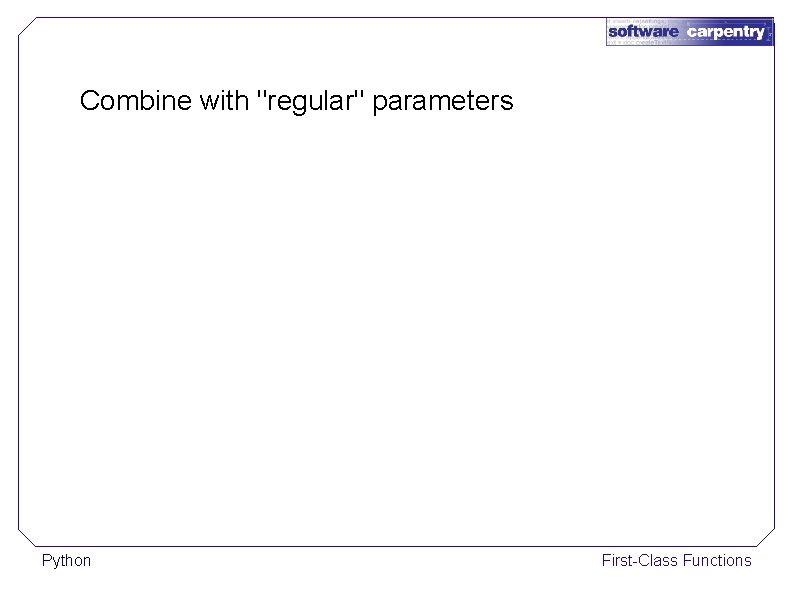
Combine with "regular" parameters Python First-Class Functions
![Combine with regular parameters def combinevaluesfunc values current values0 for i in range1 Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-51.jpg)
Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current Python First-Class Functions
![Combine with regular parameters def combinevaluesfunc values current values0 for i in range1 Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-52.jpg)
Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current Python First-Class Functions
![Combine with regular parameters def combinevaluesfunc values current values0 for i in range1 Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-53.jpg)
Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current print combine_values(add, 1, 3, 5) 9 Python First-Class Functions
![Combine with regular parameters def combinevaluesfunc values current values0 for i in range1 Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-54.jpg)
Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current print combine_values(add, 1, 3, 5) 9 What does combine_values(add) do? Python First-Class Functions
![Combine with regular parameters def combinevaluesfunc values current values0 for i in range1 Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1,](https://slidetodoc.com/presentation_image_h/030c1008e30c211ef7ddbeb24c17597e/image-55.jpg)
Combine with "regular" parameters def combine_values(func, *values): current = values[0] for i in range(1, len(values)): current = func(current, v) return current print combine_values(add, 1, 3, 5) 9 What does combine_values(add) do? What should it do? Python First-Class Functions
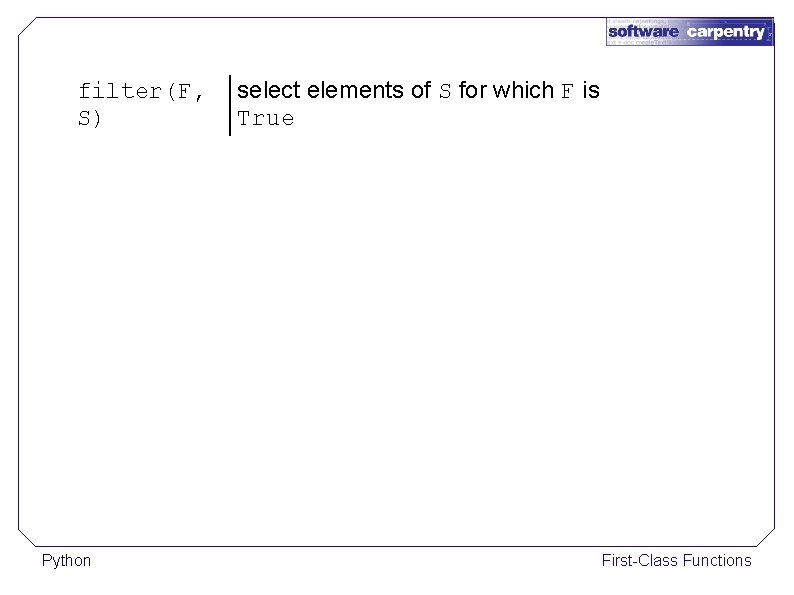
filter(F, S) Python select elements of S for which F is True First-Class Functions
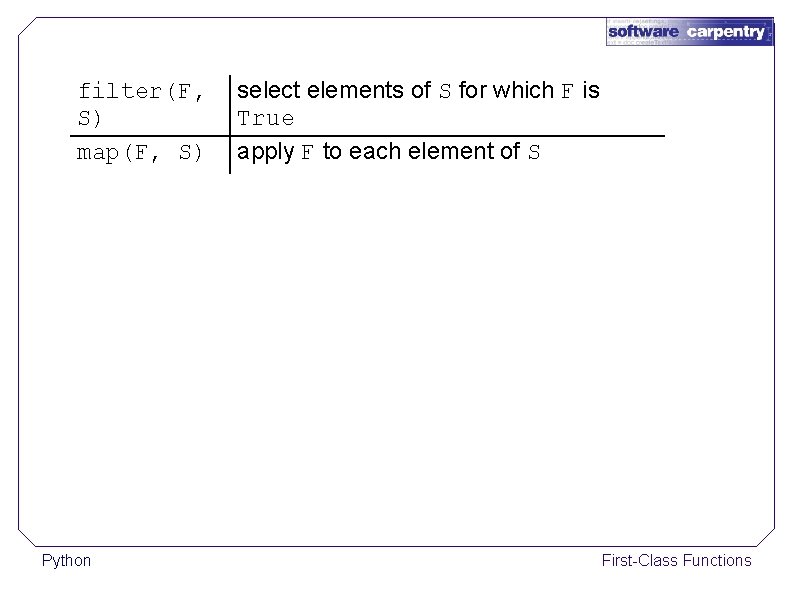
filter(F, S) map(F, S) Python select elements of S for which F is True apply F to each element of S First-Class Functions
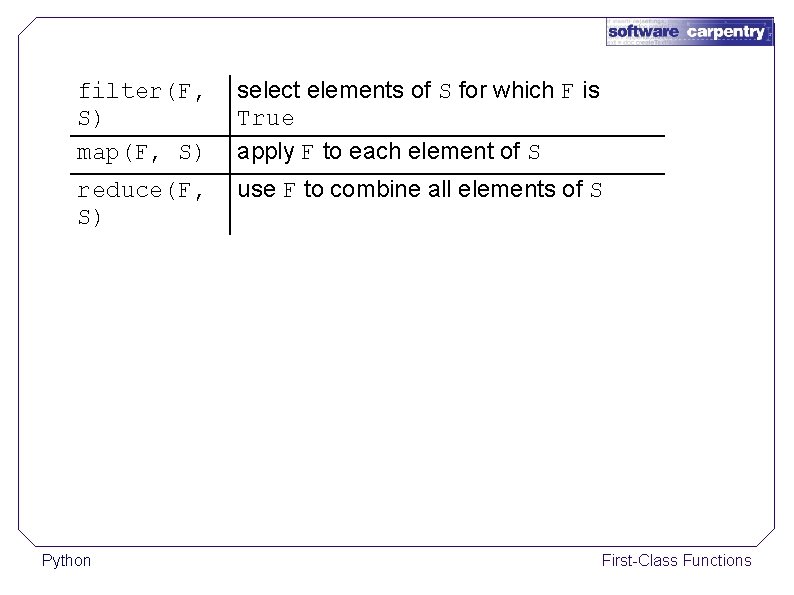
filter(F, S) map(F, S) select elements of S for which F is True apply F to each element of S reduce(F, S) use F to combine all elements of S Python First-Class Functions
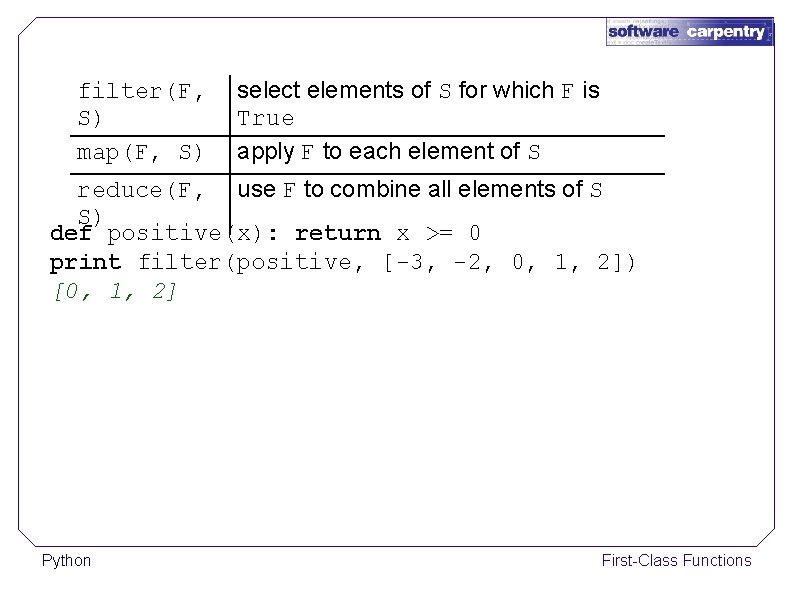
filter(F, S) map(F, S) select elements of S for which F is True apply F to each element of S reduce(F, use F to combine all elements of S S) def positive(x): return x >= 0 print filter(positive, [-3, -2, 0, 1, 2]) [0, 1, 2] Python First-Class Functions
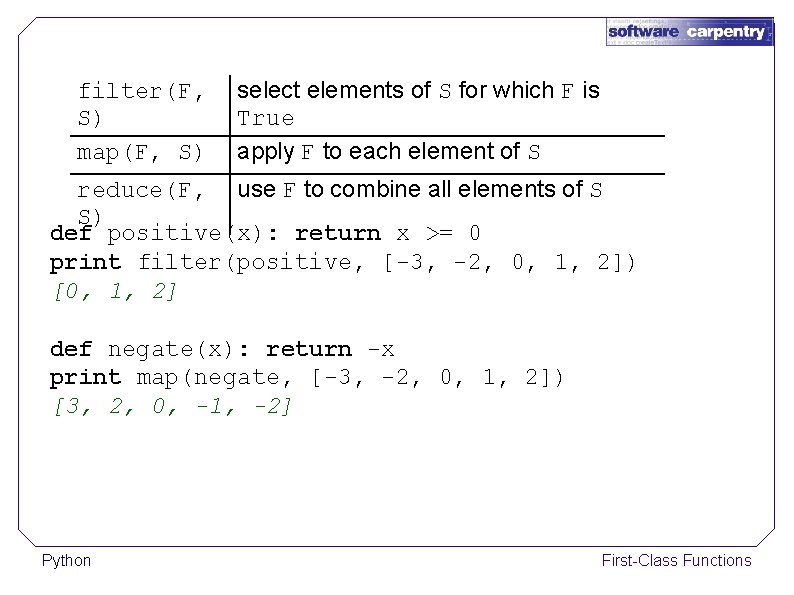
filter(F, S) map(F, S) select elements of S for which F is True apply F to each element of S reduce(F, use F to combine all elements of S S) def positive(x): return x >= 0 print filter(positive, [-3, -2, 0, 1, 2]) [0, 1, 2] def negate(x): return -x print map(negate, [-3, -2, 0, 1, 2]) [3, 2, 0, -1, -2] Python First-Class Functions
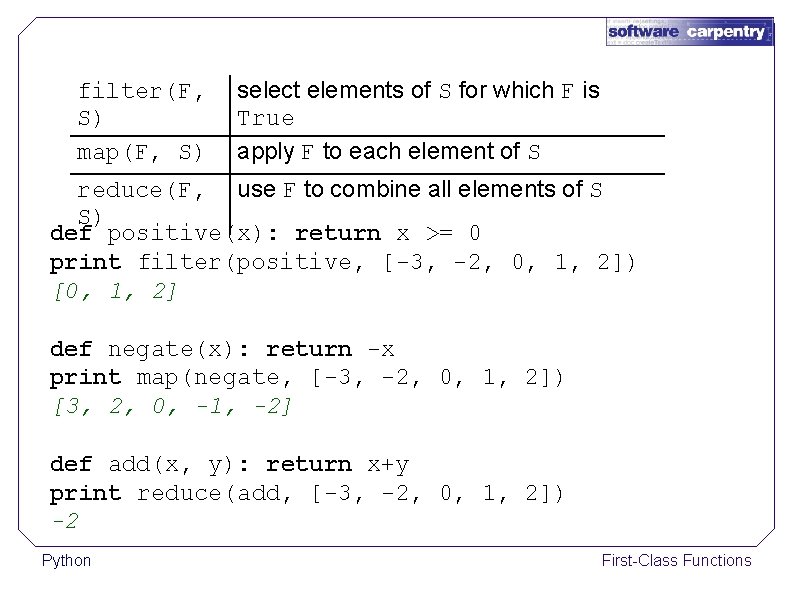
filter(F, S) map(F, S) select elements of S for which F is True apply F to each element of S reduce(F, use F to combine all elements of S S) def positive(x): return x >= 0 print filter(positive, [-3, -2, 0, 1, 2]) [0, 1, 2] def negate(x): return -x print map(negate, [-3, -2, 0, 1, 2]) [3, 2, 0, -1, -2] def add(x, y): return x+y print reduce(add, [-3, -2, 0, 1, 2]) -2 Python First-Class Functions
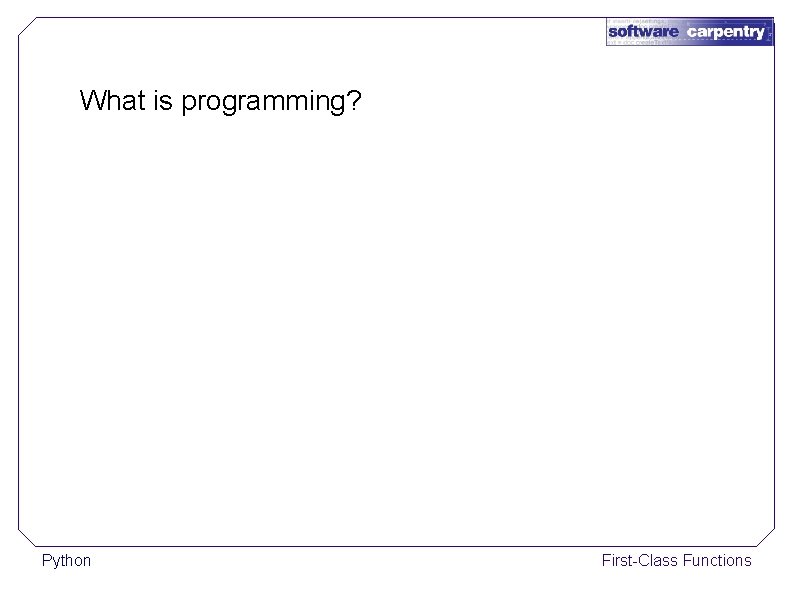
What is programming? Python First-Class Functions
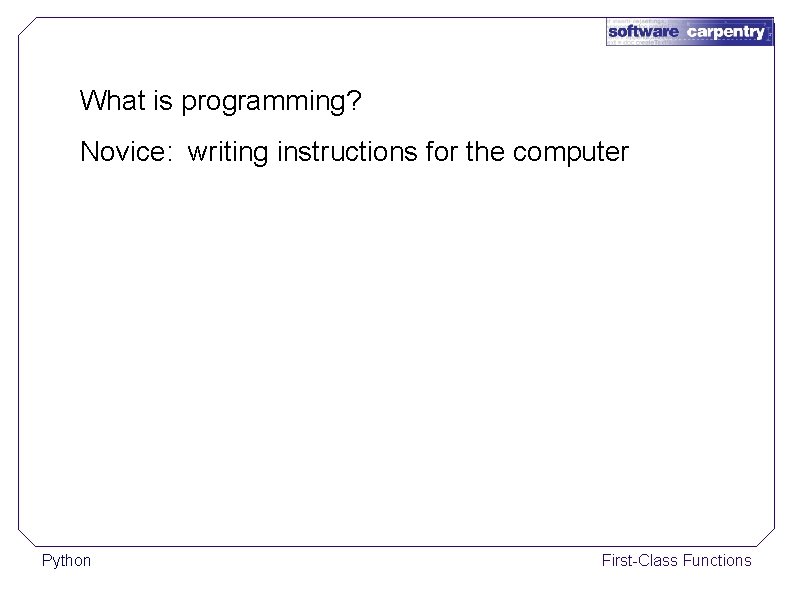
What is programming? Novice: writing instructions for the computer Python First-Class Functions
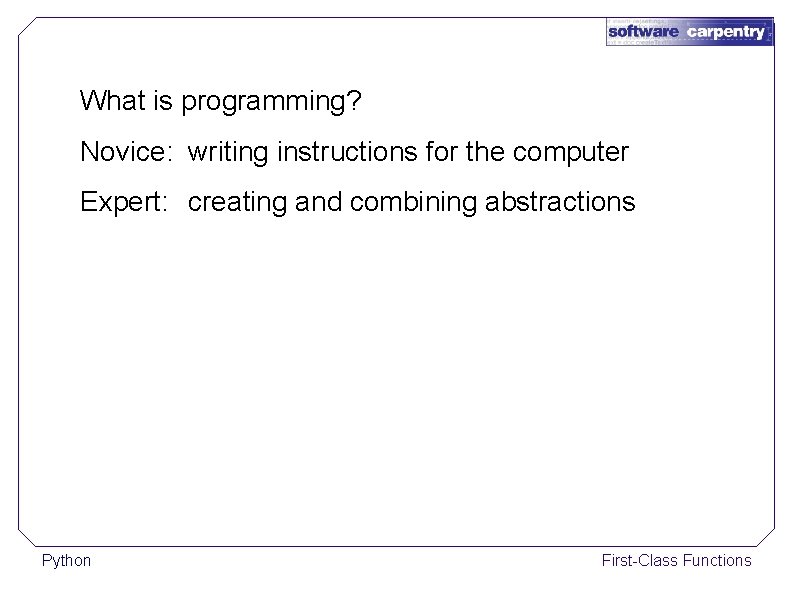
What is programming? Novice: writing instructions for the computer Expert: creating and combining abstractions Python First-Class Functions
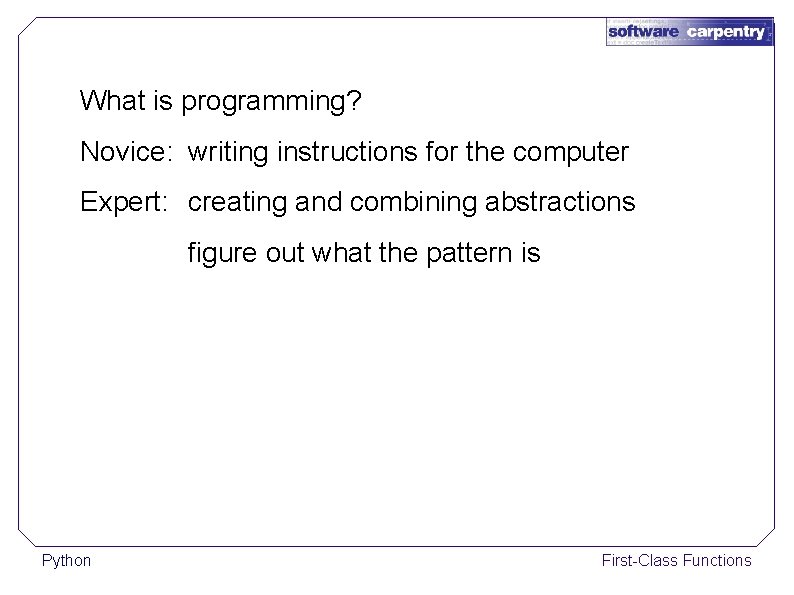
What is programming? Novice: writing instructions for the computer Expert: creating and combining abstractions figure out what the pattern is Python First-Class Functions
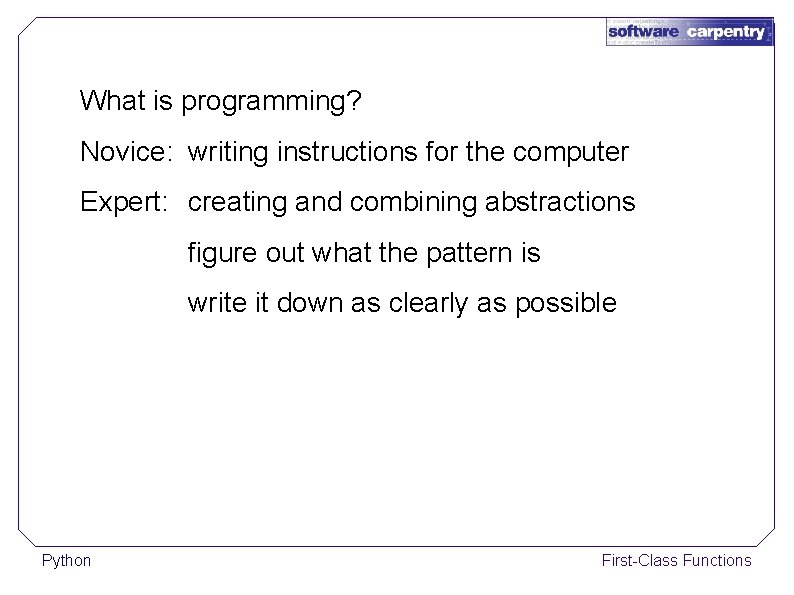
What is programming? Novice: writing instructions for the computer Expert: creating and combining abstractions figure out what the pattern is write it down as clearly as possible Python First-Class Functions
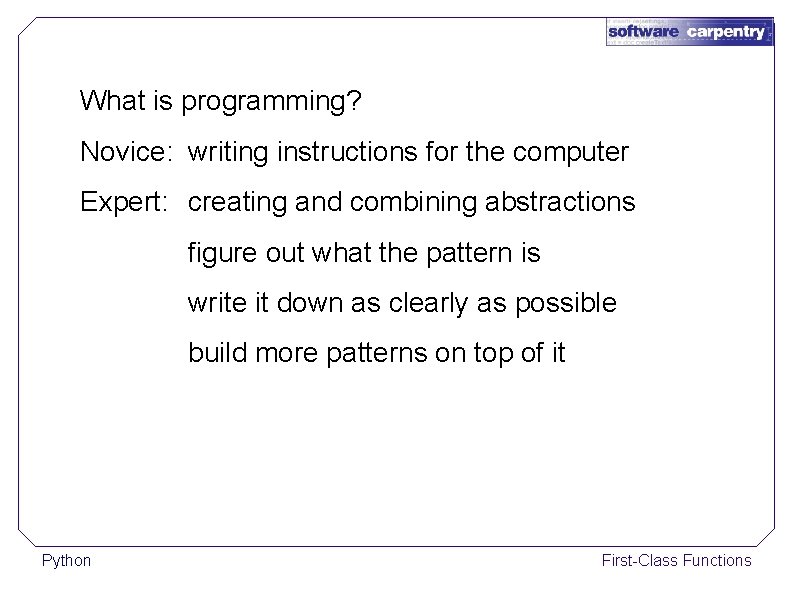
What is programming? Novice: writing instructions for the computer Expert: creating and combining abstractions figure out what the pattern is write it down as clearly as possible build more patterns on top of it Python First-Class Functions
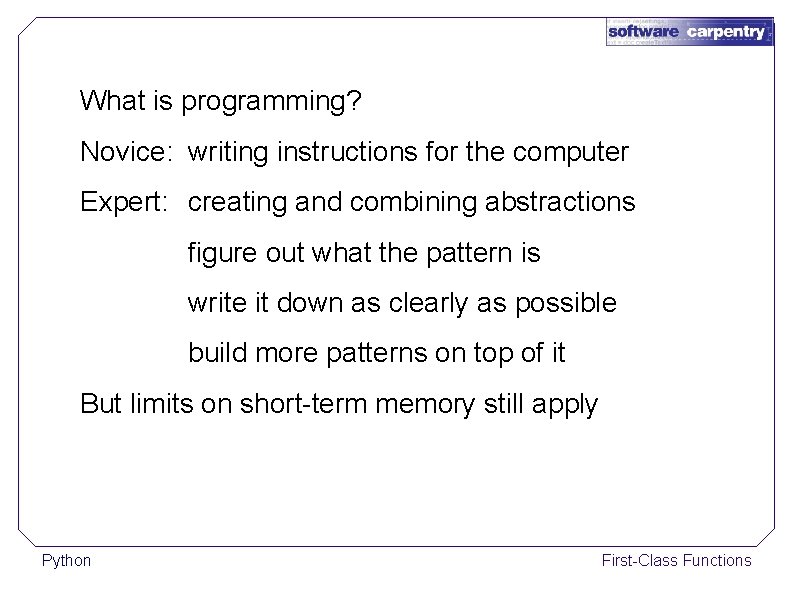
What is programming? Novice: writing instructions for the computer Expert: creating and combining abstractions figure out what the pattern is write it down as clearly as possible build more patterns on top of it But limits on short-term memory still apply Python First-Class Functions
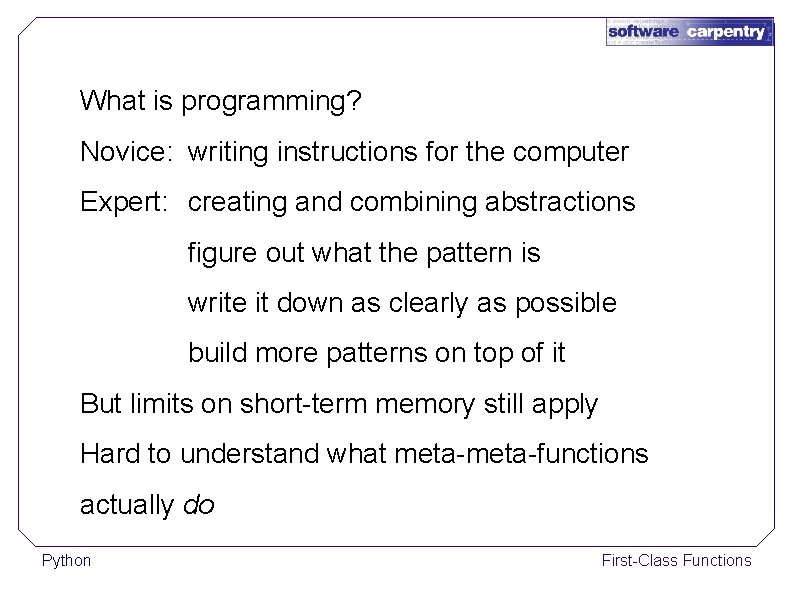
What is programming? Novice: writing instructions for the computer Expert: creating and combining abstractions figure out what the pattern is write it down as clearly as possible build more patterns on top of it But limits on short-term memory still apply Hard to understand what meta-functions actually do Python First-Class Functions
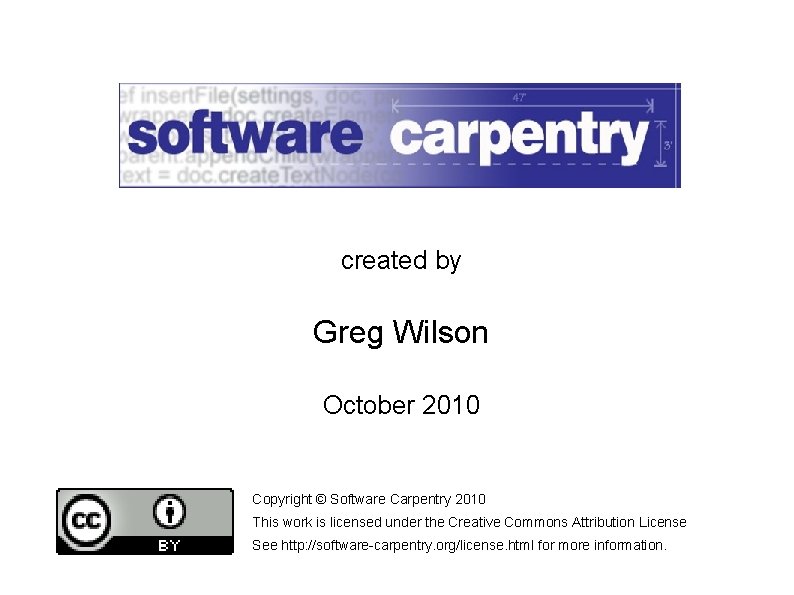
created by Greg Wilson October 2010 Copyright © Software Carpentry 2010 This work is licensed under the Creative Commons Attribution License See http: //software-carpentry. org/license. html for more information.
Gmail
2010 pearson education inc
Copyright 2010
Variazioni finanziarie attive e passive
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010
Copyright 2010
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
Copyright 2010 pearson education inc
2010 pearson education inc
Copyright 2010 pearson education inc
Composition copyright example
Copyright 2010 pearson education inc
Method vs function python
Python lists functions
Pure functions and modifiers in python
Pure functions and modifiers in python
Up until this point
Fruitful function python
Fruitful functions
Columbus custom carpentry
Classification of tools used to assemble wood
Dowel widening joint
A carpentry hammer with a slightly rounded
Big carpentry
Holding tools classification
Wood joints
Python event manager
Absolute value as a piecewise function
How to solve evaluating functions
Evaluating functions and operations on functions
Functions of compiler
System software functions
Types of utility programs
Software maintenance in software engineering ppt
What is software implementation in software engineering
Improve software economics
Software engineering vs computer science
Metrics computer science
Skills and applications chapter 3
Generic and customized software
Difference between student software and industrial software
Software crisis of 1960s
Examples of product metrics
Is an os system software or application software
Eic software reviews
Real time software design in software engineering
Design principles in software engineering
Multimedia software uses
Electronic copyright office login
Tmg copyright
Copyright houghton mifflin company
The hunger games copyright
Copyright is debit or credit in trial balance
Copyright 2015 all rights reserved
Population growth factors
It fw
Copyright 2008
Trademark and trade secret
Proper netiquette to avoid copyright
Copyright
Encyclopedia britannica copyright
Copyright
A right handed operator generally begins seated at
Ntuition
Copyright disclaimer
Copyright quiz for students
Copyright notice on powerpoint slides
Berne convention